code
stringlengths 501
5.19M
| package
stringlengths 2
81
| path
stringlengths 9
304
| filename
stringlengths 4
145
|
---|---|---|---|
.. include:: /defs.txt
#################################
Parsing and displaying quantities
#################################
.. code-block:: python
>>> from zero.format import Quantity
|Zero| contains a powerful quantity parser and formatter, allowing you to specify and display
component values, frequencies and other values in a natural way without resorting to mathematical
notation or special formatting.
Parsing quantities
------------------
The quantity parser accepts SI units and prefixes:
.. code-block:: python
>>> Quantity("1.23 kHz")
1230.0
>>> Quantity("1e-3 mF")
1e-06
Mathematical notation and SI prefixes can be combined arbitrarily, and spaces are ignored.
Displaying quantities
---------------------
A quantity's unit travels with its value. You can print a quantity with its value by calling for its
string representation:
.. code-block:: python
>>> str(Quantity("45.678MHz"))
'45.678 MHz'
An appropriate prefix is chosen even if the quantity is instantiated with another prefix:
.. code-block:: python
>>> str(Quantity("45678 kHz"))
'45.678 MHz'
More control can be gained over the display of the quantity by calling the :meth:`~.format.Quantity.format`
method. This allows units to be hidden, scale factors to be removed, and for a custom precision to
be specified.
Mathematical operations with quantities
---------------------------------------
For all intents and purposes, quantities behave like :class:`floats <float>`. That means you can
multiply, divide, add and subtract them from other quantities or scalars, and use them with standard
Python code.
Units are not carried over to the results of mathematical operations; the results of operations
involving quantities are always :class:`floats <float>`. Unit propagation is beyond the scope of
|Zero|.
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/format/index.rst | index.rst |
.. include:: /defs.txt
Introduction
============
Circuit analyses with |Zero| revolve around building a :ref:`circuit <circuit/index:Circuits>` from
:ref:`components <components/index:Components>` and applying an :ref:`analysis <analyses/index:Analyses>`.
The output from an analysis is a :ref:`solution <solution/index:Solutions>`, with which the results
can be plotted or saved.
|Zero| can be used either as a Python library or using its :ref:`command line interface <cli/index:Command line interface>`.
|Zero| also somewhat understands LISO input and output files (see :ref:`liso/index:LISO Compatibility`).
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/introduction/index.rst | index.rst |
Analyses
========
.. code-block:: python
>>> from zero.analysis import AcSignalAnalysis, AcNoiseAnalysis
The circuit can be solved in order to compute responses or noise in a single run. If both responses
*and* noise are required, then these must be obtained in separate calls.
Responses between the circuit input and an output (or outputs) can be computed with
:class:`.AcSignalAnalysis`. Noise from components and nodes in the circuit at a particular node can
be calculated with :class:`.AcNoiseAnalysis`.
.. toctree::
:maxdepth: 2
ac/index
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/analyses/index.rst | index.rst |
.. include:: /defs.txt
AC analyses
===========
The available AC analyses are performed assuming the circuit to be linear time invariant (LTI),
meaning that the parameters of the components within the circuit, and the circuit itself, cannot
change over time. This is usually a reasonable assumption to make for circuits containing passive
components such as resistors, capacitors and inductors, and op-amps far from saturation, and where
only the frequency response or noise spectral density is required to be computed.
The linearity property implies the principle of superposition, such that if you double the circuit's
input voltage, the current through each component will also double. This restriction implies
that components with a non-linear relationship between current and voltage cannot be simulated.
Components such as diodes and transistors fall within this category, but it also means that certain
component properties such as the output swing limit of op-amps cannot be simulated. In certain
circumstances it is possible to approximate a linear relationship between a component's voltage and
current around the operating point, allowing the component to be simulated in the LTI regime, and
this property is exploited in the case of :class:`op-amps <.OpAmp>`.
The small-signal AC response of a circuit input to any of its components or nodes can be computed
with the :ref:`AC small signal analysis <analyses/ac/signal:Small AC signal analysis>`.
The noise spectral density at a node arising from components and nodes elsewhere in the circuit can
be computed using the :ref:`AC small signal noise analysis <analyses/ac/noise:Small AC noise analysis>`.
.. toctree::
:maxdepth: 2
signal
noise
Implementation
##############
|Zero| uses a `modified nodal analysis <https://en.wikipedia.org/wiki/Modified_nodal_analysis>`_ to
compute circuit voltages and currents. Standard nodal analysis only allows voltages to be determined
across components by using `Kirchoff's current law <https://en.wikipedia.org/wiki/Kirchhoff%27s_circuit_laws#Kirchhoff's_current_law_(KCL)https://en.wikipedia.org/wiki/Kirchhoff%27s_circuit_laws>`_
(which states that the sum of all currents flowing into or out of each node equals zero). A series
of equations representing the inverse impedance of each component are built into a so-called
`admittance` matrix, which is then solved to find the voltage drop across each component. This works
well for circuits containing only passive components, but not those containing voltage sources.
Ideal voltage sources considered in |Zero| have by definition no internal resistance (in other
words, they can supply infinitely high current), and this makes the matrix representation of
such a circuit singular due to the row corresponding to the voltage source effectively
stating that ``0 = 1``! To handle voltage sources as well as other voltage-producing components like
op-amps, |Zero| extends the equations derived from Kirchoff's current law to include columns
representing voltage drops between nodes. The circuit can then be solved for a number of unknown
voltages and currents.
Consider the following voltage divider, defined in :ref:`LISO syntax <liso/input:LISO input file parsing>`:
.. code-block:: text
r r1 1k n1 n2
r r2 2k n2 gnd
freq log 1 100 101
uinput n1 0
uoutput n2
The following circuit matrix is generated for this circuit (using
``zero liso /path/to/script.fil --print-matrix``):
.. code-block:: text
╒═══════╤═════════╤═════════╤════════════╤═════════╤═════════╤═══════╕
│ │ i[r1] │ i[r2] │ i[input] │ V[n2] │ V[n1] │ RHS │
╞═══════╪═════════╪═════════╪════════════╪═════════╪═════════╪═══════╡
│ r1 │ 1.00e3 │ --- │ --- │ 1 │ -1 │ --- │
├───────┼─────────┼─────────┼────────────┼─────────┼─────────┼───────┤
│ r2 │ --- │ 2.00e3 │ --- │ -1 │ --- │ --- │
├───────┼─────────┼─────────┼────────────┼─────────┼─────────┼───────┤
│ input │ --- │ --- │ --- │ --- │ 1 │ 1 │
├───────┼─────────┼─────────┼────────────┼─────────┼─────────┼───────┤
│ n2 │ 1 │ -1 │ --- │ --- │ --- │ --- │
├───────┼─────────┼─────────┼────────────┼─────────┼─────────┼───────┤
│ n1 │ -1 │ --- │ 1 │ --- │ --- │ --- │
╘═══════╧═════════╧═════════╧════════════╧═════════╧═════════╧═══════╛
The entries containing ``---`` represent zero in sparse matrix form. The equations this matrix
represents look like this (using ``zero liso /path/to/script.fil --print-equations``):
.. code-block:: text
1.00 × 10 ^ 3 × I[r1] + V[n2] - V[n1] = 0
2.00 × 10 ^ 3 × I[r2] - V[n2] = 0
V[n1] = 1
I[r1] - I[r2] = 0
- I[r1] + I[input] = 0
In the matrix, this equation is arranged to equal ``0``
on the right hand side; however, we can rearrange the equation for ``r1`` to read: "voltage drop
across ``r1`` equals the voltage difference between nodes ``n2`` and ``n1``". The equation for
``r2`` is similar, but since one of its nodes is attached to ground (which has ``0`` potential), the
voltage difference between its nodes is simply equal to the potential at ``n2``.
.. note::
In |Zero|, the circuit is solved under the assumption that the circuit's ground is ``0``, which
allows the use of standard linear analysis techniques. One side-effect of this approach is that
circuits cannot contain floating loops, i.e. loops without a defined ground connection. This
is usually not important, but has an effect on e.g. transformer circuits with intermediate
or isolated loops. If reasonable for the application, consider supplying a weak connection to
ground using e.g. a large valued resistor.
This property does *not* affect the ability to include floating voltage sources in circuits,
as long as these are contained in grounded loops.
In the row corresponding to the voltage input, there is no voltage drop across the source (as per
its definition as an ideal source), and its right hand side equals ``1``. This means that the
circuit is solved with the constraint that the voltage between ``n1`` and ground must always be
``1``. The solver can adjust all of the other non-zero matrix elements in the left hand side until
this condition is met within some level of tolerance.
Available analyses
~~~~~~~~~~~~~~~~~~
Signals
.......
The solution ``x`` to the matrix equation ``Ax = b``, where ``A`` is the circuit matrix above and
``b`` is the right hand side vector, gives the current through each component and voltage at each
node.
Noise
.....
Noise analysis requires an essentially identical approach to building the circuit matrix, except
that the matrix is transposed and the right hand side is given a ``1`` in the row corresponding to
the chosen noise output node instead of the input. This results in the solution ``x`` in the matrix
equation ``Ax = b`` instead providing what amounts to the reverse responses between the component
and nodes in the circuit and the chosen noise output node. These reverse responses are as a last
step multiplied by the noise at each component and node to infer the noise at the noise output node.
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/analyses/ac/index.rst | index.rst |
.. currentmodule:: zero.analysis.ac.signal
Small AC signal analysis
========================
The small AC signal analysis calculates the signal at all :class:`nodes <.Node>` and
:class:`components <.Component>` within a circuit due to either a voltage or a current applied to
the circuit's input. The input is unity, meaning that the resulting signals represent the
:ref:`responses <data/index:Responses>` from the input to each node or component. The analysis
assumes that the input is small enough not to influence the operating point and gain of the circuit.
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/analyses/ac/signal.rst | signal.rst |
.. include:: /defs.txt
.. currentmodule:: zero.analysis.ac.noise
Small AC noise analysis
=======================
The small signal AC noise analysis calculates the :ref:`noise spectral densities <data/index:Noise
spectral densities>` at a particular :class:`.Node` or :class:`.Component` within a circuit due to
noise sources within the circuit, assuming that the noise is small enough not to influence the
operating point and gain of the circuit.
Generating noise sums
---------------------
Incoherent noise sums can be created as part of the analysis and added to the :class:`.Solution`.
This is governed by the ``incoherent_sum`` parameter of :meth:`~.AcNoiseAnalysis.calculate`.
Setting ``incoherent_sum`` to ``True`` results in the incoherent sum of all noise in the circuit at
the specified noise sink being calculated and added as a single function to the solution.
Alternatively, ``incoherent_sum`` can be specified as a :class:`dict` containing legend labels as
keys and sequences of noise spectra as values. The noise spectra can either be
:class:`.NoiseDensity` objects or :ref:`noise specifier strings <solution/index:Specifying noise
sources and sinks>` as supported by :meth:`.Solution.get_noise`. The values may alternatively be the
strings "all", "allop" or "allr" to compute noise from all components, all op-amps and all
resistors, respectively.
Sums are plotted in shades of grey determined by the plotting configuration's
``sum_greyscale_cycle_start``, ``sum_greyscale_cycle_stop`` and ``sum_greyscale_cycle_count``
values.
Examples
~~~~~~~~
Add a total incoherent sum to the solution:
.. code-block:: python
solution = analysis.calculate(frequencies=frequencies, input_type="voltage", node="n1",
sink="nout", incoherent_sum=True)
Add an incoherent sum of all resistor noise:
.. code-block:: python
solution = analysis.calculate(frequencies=frequencies, input_type="voltage", node="n1",
sink="nout", incoherent_sum={"resistors": "allr"})
Add incoherent sums of all resistor and op-amp noise:
.. code-block:: python
# Shorthand syntax.
solution = analysis.calculate(frequencies=frequencies, input_type="voltage", node="n1",
sink="nout", incoherent_sum={"resistors": "allr",
"op-amps": "allop"})
# Alternatively specify components directly using noise specifiers.
solution = analysis.calculate(frequencies=frequencies, input_type="voltage", node="n1",
sink="nout", incoherent_sum={"sum": ["R(r1)", "V(op1)"]})
Referring noise to the input
----------------------------
It is often desirable to refer the noise at a node or component to the input. This is particularly
useful when modelling readout circuits (e.g. for photodetectors), where the input referred noise
shows the smallest equivalent signal spectral density that can be detected above the noise.
Noise analyses can refer noise at a node or component to the input by setting the ``input_refer``
flag to ``True`` in :meth:`~.AcNoiseAnalysis.calculate`, which makes |Zero| apply a response
function (from the noise sink to the input) to the noise computed at the noise sink. The resulting
noise has its ``sink`` property changed to the input. If ``input_type`` was set to ``voltage``, this
is the input node; whereas if ``input_type`` was set to ``current``, this is the input component.
.. note::
The input referring response function is obtained by performing a separate :ref:`signal analysis
<analyses/ac/signal:Small AC signal analysis>` with the same circuit as the noise analysis. The
response from the input to the sink is then extracted and inverted to give the response from the
sink to the input. The noise at the sink in the noise analysis is then multiplied by this input
referring response function.
| zero | /zero-0.9.1.tar.gz/zero-0.9.1/docs/analyses/ac/noise.rst | noise.rst |
import os, time, numpy as np
from cryptography.fernet import Fernet
import matplotlib.pyplot as plt
vBin = ['544', '432', '252', '541', '441', '414', '555', '513', '322', '242',
'152', '132', '342', '455', '253', '512', '531', '155', '352', '241',
'532', '112', '344', '234', '412', '511', '141', '353', '521', '323',
'153', '142', '453', '535', '345', '452', '121', '543', '552', '214',
'231', '553', '534', '114', '522', '135', '424', '154', '354', '111',
'244', '113', '331', '444', '215', '134', '355', '434', '133', '212',
'251', '523', '451', '334', '144', '514', '411', '341', '324', '545',
'124', '413', '255', '533', '145', '315', '313', '123', '213', '515',
'211', '311', '122', '554', '314', '222', '332', '243', '321', '232',
'542', '454', '223', '425', '351', '131', '443', '423', '125', '254',
'335', '245', '221', '235', '325', '415', '435', '442', '225', '343',
'233', '312', '525', '551', '431', '224', '433', '445', '151', '143',
'333', '115', '422', '421', '524', '100','001', '010']
vChar = ['\x00', '\x01', '\x02', '\x03', '\x04', '\x05', '\x06', '\x07',
'\x08', '\t', '\n', '\x0b', '\x0c', '\r', '\x0e', '\x0f', '\x10',
'\x11', '\x12', '\x13', '\x14', '\x15', '\x16', '\x17', '\x18',
'\x19', '\x1a', '\x1b', '\x1c', '\x1d', '\x1e', '\x1f', ' ', '!',
'"', '#', '$', '%', '&', "'", '(', ')', '*', '+', ',', '-', '.',
'/', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', ':', ';',
'<', '=', '>', '?', '@', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H',
'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U',
'V', 'W', 'X', 'Y', 'Z', '[', '\\', ']', '^', '_', '`', 'a', 'b',
'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o',
'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', '{', '|',
'}', '~', '\x80']
keyPath = ''
#Voltize the Encrypted Value
def toVolt(encrypted):
en = ''
try :
encrypted = encrypted.decode()
except : pass
for i in encrypted:
for j in range(len(vChar)):
if i == vChar[j]:
en += vBin[j]
return en
#Unvoltize the Voltized Value and return the Encrypted value
def toUnvolt(volt):
de = ''
volt = [(volt[i:i+3]) for i in range(0, len(volt), 3)]
for i in volt:
for j in range(len(vBin)):
if i == vBin[j]:
de += vChar[j]
return de
#Convert String to Binary including Special CHaracters
def toBinary(word):
try:
binary = ''.join([format(ord(i),'08b') for i in word])
return binary
except:
print("toBinary Error : Check the Input")
#Convert Binary to String including Special CHaracters
def toString(binary,n):
try:
binary = [(binary[i:i+n]) for i in range(0, len(binary), n)]
# word = ''.join([chr(i) for i in binary])
return binary
except:
print("toString Error : Check the Input")
#Encrypt String to Bytes and save the Key in Key.txt
def toEncrypt(value):
try:
Key = Fernet.generate_key()
with open('Key.txt', 'w') as f:
f.write(Key.decode())
except:
with open('Key.txt', 'rb') as f:
key = f.read()
Key = key.encode()
fernet = Fernet(Key)
encrypted = fernet.encrypt(value.encode())
return(encrypted)
#To Decrypt Bytes to String and uses the Key in Key.txt
def toDecrypt(encrypted):
#Check for Key File Exists
try:
with open('Key.txt', 'r') as f:
key = f.read()
Key = key.encode()
fernet = Fernet(Key)
try :
encrypted = encrypted.encode()
except : pass
decrypted = fernet.decrypt(encrypted)
return(decrypted)
#Print Error if the Key File doesn't Exists
except:
print("toDecrypt Error : Key File is Missing")
#Returns the Words with Count for a File in Location
def countFile(fileLocation):
#Check for File Exists
if os.path.exists(fileLocation):
ind_word = []
count = []
with open(fileLocation, 'r') as f:
message = f.read()
message = message.lower()
message = message.split()
[ind_word.append(i) for i in message if not i in ind_word]
ind_word.sort()
[count.append(message.count(i)) for i in ind_word]
#Returns the Individual_Words, Counts respectively
return ind_word, count
#Print Error if the File doesn't Exists
else:
print("countFile Error : Check File Location")
#Returns the Words with Count for Multiple in Location
def countFolder(folderLocation):
#Check for Folder Exists
try:
name = []
word = []
count = []
files = os.scandir(folderLocation)
files = [f.name for f in files if f.is_file()]
for i in range(len(files)):
w, c = countFile(folderLocation + files[i])
name.append(files[i])
word.append(w)
count.append(c)
#Returns the File_Name, Words, Counts respectively
return name, word, count
#Print Error if the Folder doesn't Exists
except:
print("countFile Error : Check Folder Location")
#Retrns and Print the Summary of the File
def summaryFile(fileLocation):
wBin = []
summ = ''
try:
#Call the countFile function
w, c = countFile(fileLocation)
#sMax for the Maximum Word display width
sMax = len(max(w, key=len)) + 4
#Print the Summary of All Files
summ += ("\nSummary : \n{x:<{x_w}}{y:<{y_w}}{z}".format(
x=' Word', x_w = sMax, y = "Count", y_w = 3,z=' Binary'))
for l in range(len(w)):
bin = toBinary(w[l])
summ += ("\n{x:<{x_w}}""{y:<{y_w}}{z}".format(x = w[l], x_w= sMax,
y = " " + str(c[l]), y_w = 5,z = " " + str(bin)))
wBin.append(str(bin))
summ += ("\n-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-_-")
print( summ )
#Returns the Words, Counts, Binary Values respectively
return w, c, wBin, summ
except :
print("summaryFile Error : Check File Location")
#Retrns and Print the Summary of the All Files in the Folder
def summaryFolder(folderLocation):
wBin = []
word = []
count = []
summ = ''
try:
#Get the Names of the All Files
n, _, _ = countFolder(folderLocation)
#Print the Summary of All Files
for i in n:
summ += ("\nFile : " + i)
print("\nFile : " + i)
w, c, b, s = summaryFile(folderLocation + i)
wBin.append(b)
word.append(w)
count.append(c)
summ += s
#Returns the File_Name, Words, Counts, Binary Values respectively
return n, word, count, wBin, summ
except:
print("summaryFolder Error : Check Folder Location")
#Returns the Both X and Y Axis Values for the Graph
def graph(volt):
x_axis = []
y_axis = []
for i in range(len(volt)):
x_axis.append(i)
y_axis.append(volt[i])
return x_axis, y_axis
#To Set the Path of the Key.txt
def setKeyPath(path):
global keyPath
keyPath = path
#To Convert Binary to Bipolar
def toBipolar(binary):
bp = []
for i in binary:
if i == '0' :
bp.append('-1')
else:
bp.append('1')
return bp
#To form Square and Sine Wave and returns Coordinates
def squareSine(word, graph):
xQ, yQ = squareWave(word, 0)
xS, yS = sineWave(word, 0)
#Plot the Graph if Graph is HIGH
while graph:
yQ = yQ[1:]+yQ[1:2]
yS = yS[10:]+yS[:10]
plt.plot(xS, yS, color='b')
plt.step(xQ, yQ, color='r', where='post')
plt.axhline(0.5, color='g')
plt.xlabel('Time')
plt.ylabel('Analog & Digital Values')
plt.title('Square & Sine Wave')
plt.show()
time.sleep(0.5)
return xQ, yQ, xS, yS
#To form Sine Wave and returns Coordinates
def sineWave(word, graph):
binary = toBinary(word)
xNew = []
yNew = []
xT = []
yT = []
direction = -1
#Loop to find the Zero Crossing Points on X-Axis
s = int(not(binary[0]))
for i in range(len(binary)):
if s!=binary[i]:
s=binary[i]
xT.append(i)
yT.append(binary[i])
xT.append(len(binary)-1)
yT.append(binary[-1])
#Loop to find the Sine Wave Coordinates
for i in range(len(xT)-1):
d = (xT[i+1] - xT[i]) #Difference btw Two Zero Crossing Points - X
for j in range(d*10):
xNew.append(xT[i]+(j/10))
if s:
yNew.append((direction*np.sin(22/7*j/10/d)+1.0)/2.0)
else:
yNew.append((direction*np.sin(22/7*j/10/d)+1.0)/2.0)
if direction == 1 :
direction = -1
else:
direction = 1
#Plot the Graph if Graph is HIGH
while graph:
yNew = yNew[1:]+yNew[1:2]
plt.plot(xNew, yNew)
plt.axhline(0.5, color='g')
plt.xlabel('Time')
plt.ylabel('Analog Representation')
plt.title('Sine Wave')
plt.show()
time.sleep(0.05)
return xNew, yNew
#To plot the Square Wave Graph with Word
def squareWave(word, graph):
binary = toBinary(word)
y = m = [int(i) for i in binary]
x = n = [i for i in range(len(y))]
#Plot the Graph if Graph is HIGH
while graph:
tmp = y[0]
for i in range(len(y)-1):
y[i] = y[i+1]
y[-1] = tmp
plt.step(x, y, where='post')
plt.axhline(0.5, color='g')
plt.xlabel('Time')
plt.ylabel('Digital Representation')
plt.title('Square Wave')
plt.show()
time.sleep(0.5)
return n, m | zeroBYT | /zeroBYT-0.2-py3-none-any.whl/zeroBYT.py | zeroBYT.py |
zero_buffer
===========
.. image:: https://travis-ci.org/alex/zero_buffer.png?branch=master
:target: https://travis-ci.org/alex/zero_buffer
``zero_buffer`` is a high-performance, zero-copy, implementation of a
byte-buffer for Python.
`Documentation`_ is available on ReadTheDocs.
.. code-block:: python
from zero_buffer import Buffer
# Create a buffer which has space for 8192 bytes.
b = Buffer.allocate(8192)
with open(path, "rb") as f:
# Read up to 8192 bytes from the file into the buffer
b.read_from(f.fileno())
# Create a read-only view of the buffer, this performs no copying.
view = b.view()
# Split the view on colons, this returns a generator which yields sub-views
# of the view.
for part in view.split(b":"):
print(part)
``zero_buffer`` works on Python 2.6, 2.7, 3.2, 3.3, and PyPy.
.. _`Documentation`: https://zero-buffer.readthedocs.org/en/latest/
| zero_buffer | /zero_buffer-0.5.1.tar.gz/zero_buffer-0.5.1/README.rst | README.rst |
import os
import six
from six.moves import xrange
import cffi
_ffi = cffi.FFI()
_ffi.cdef("""
ssize_t read(int, void *, size_t);
ssize_t write(int, const void *, size_t);
int memcmp(const void *, const void *, size_t);
void *memchr(const void *, int, size_t);
void *Zero_memrchr(const void *, int, size_t);
void *memcpy(void *, const void *, size_t);
""")
_lib = _ffi.verify("""
#include <string.h>
#include <sys/types.h>
#include <sys/uio.h>
#include <unistd.h>
#ifdef __GNU_SOURCE
#define Zero_memrchr memrchr
#else
void *Zero_memrchr(const void *s, int c, size_t n) {
const unsigned char *cp;
if (n != 0) {
cp = (unsigned char *)s + n;
do {
if (*(--cp) == (unsigned char)c) {
return (void *)cp;
}
} while (--n != 0);
}
return NULL;
}
#endif
""", extra_compile_args=["-D_GNU_SOURCE"])
BLOOM_WIDTH = _ffi.sizeof("long") * 8
class BufferFull(Exception):
pass
class Buffer(object):
def __init__(self, data, writepos):
self._data = data
self._writepos = writepos
@classmethod
def allocate(cls, size):
return cls(_ffi.new("uint8_t[]", size), 0)
def __repr__(self):
return "Buffer(data=%r, capacity=%d, free=%d)" % (
[self._data[i] for i in xrange(self.writepos)],
self.capacity, self.free
)
@property
def writepos(self):
return self._writepos
@property
def capacity(self):
return len(self._data)
@property
def free(self):
return self.capacity - self.writepos
def read_from(self, fd):
if not self.free:
raise BufferFull
res = _lib.read(fd, self._data + self.writepos, self.free)
if res == -1:
raise OSError(_ffi.errno, os.strerror(_ffi.errno))
elif res == 0:
raise EOFError
self._writepos += res
return res
def add_bytes(self, b):
if not self.free:
raise BufferFull
bytes_written = min(len(b), self.free)
for i in xrange(bytes_written):
self._data[self.writepos] = six.indexbytes(b, i)
self._writepos += 1
return bytes_written
def view(self, start=0, stop=None):
if stop is None:
stop = self.writepos
if stop < start:
raise ValueError("stop is less than start")
if not (0 <= start <= self.writepos):
raise ValueError(
"The start is either negative or after the writepos"
)
if stop > self.writepos:
raise ValueError("stop is after the writepos")
return BufferView(self, self._data, start, stop)
class BufferView(object):
def __init__(self, buf, data, start, stop):
self._keepalive = buf
self._data = data + start
self._length = stop - start
def __bytes__(self):
return _ffi.buffer(self._data, self._length)[:]
if six.PY2:
__str__ = __bytes__
def __repr__(self):
return "BufferView(data=%r)" % (
[self._data[i] for i in xrange(len(self))]
)
def __len__(self):
return self._length
def __eq__(self, other):
if len(self) != len(other):
return False
if isinstance(other, BufferView):
return _lib.memcmp(self._data, other._data, len(self)) == 0
elif isinstance(other, bytes):
for i in xrange(len(self)):
if self[i] != six.indexbytes(other, i):
return False
return True
else:
return NotImplemented
def __ne__(self, other):
return not (self == other)
def __contains__(self, data):
return self.find(data) != -1
def __getitem__(self, idx):
if isinstance(idx, slice):
start, stop, step = idx.indices(len(self))
if step != 1:
raise ValueError("Can't slice with non-1 step.")
if start > stop:
raise ValueError("Can't slice backwards.")
return BufferView(self._keepalive, self._data, start, stop)
else:
if idx < 0:
idx += len(self)
if not (0 <= idx < len(self)):
raise IndexError(idx)
return self._data[idx]
def __add__(self, other):
if isinstance(other, BufferView):
collator = BufferCollator()
collator.append(self)
collator.append(other)
return collator.collapse()
else:
return NotImplemented
def find(self, needle, start=0, stop=None):
stop = stop or len(self)
if start < 0:
start = 0
if stop > len(self):
stop = len(self)
if stop - start < 0:
return -1
if len(needle) == 0:
return start
elif len(needle) == 1:
res = _lib.memchr(self._data + start, ord(needle), stop - start)
if res == _ffi.NULL:
return -1
else:
return _ffi.cast("uint8_t *", res) - self._data
else:
mask, skip = self._make_find_mask(needle)
return self._multi_char_find(needle, start, stop, mask, skip)
def index(self, needle, start=0, stop=None):
idx = self.find(needle, start, stop)
if idx == -1:
raise ValueError("substring not found")
return idx
def rfind(self, needle, start=0, stop=None):
stop = stop or len(self)
if start < 0:
start = 0
if stop > len(self):
stop = len(self)
if stop - start < 0:
return -1
if len(needle) == 0:
return start
elif len(needle) == 1:
res = _lib.Zero_memrchr(
self._data + start, ord(needle), stop - start
)
if res == _ffi.NULL:
return -1
else:
return _ffi.cast("uint8_t *", res) - self._data
else:
mask, skip = self._make_rfind_mask(needle)
return self._multi_char_rfind(needle, start, stop, mask, skip)
def rindex(self, needle, start=0, stop=None):
idx = self.rfind(needle, start, stop)
if idx == -1:
raise ValueError("substring not found")
return idx
def split(self, by, maxsplit=-1):
if len(by) == 0:
raise ValueError("empty separator")
elif len(by) == 1:
return self._split_char(by, maxsplit)
else:
return self._split_multi_char(by, maxsplit)
def _split_char(self, by, maxsplit):
start = 0
while maxsplit != 0:
next = self.find(by, start)
if next == -1:
break
yield self[start:next]
start = next + 1
maxsplit -= 1
yield self[start:]
def _split_multi_char(self, by, maxsplit):
start = 0
mask, skip = self._make_find_mask(by)
while maxsplit != 0:
next = self._multi_char_find(by, start, len(self), mask, skip)
if next < 0:
break
yield self[start:next]
start = next + len(by)
maxsplit -= 1
yield self[start:]
def _bloom_add(self, mask, c):
return mask | (1 << (c & (BLOOM_WIDTH - 1)))
def _bloom(self, mask, c):
return mask & (1 << (c & (BLOOM_WIDTH - 1)))
def _make_find_mask(self, needle):
mlast = len(needle) - 1
mask = 0
skip = mlast - 1
for i in xrange(mlast):
mask = self._bloom_add(mask, six.indexbytes(needle, i))
if needle[i] == needle[mlast]:
skip = mlast - i - 1
mask = self._bloom_add(mask, six.indexbytes(needle, mlast))
return mask, skip
def _multi_char_find(self, needle, start, stop, mask, skip):
i = start - 1
w = (stop - start) - len(needle)
while i + 1 <= start + w:
i += 1
if self._data[i + len(needle) - 1] == six.indexbytes(needle, -1):
for j in xrange(len(needle) - 1):
if self._data[i + j] != six.indexbytes(needle, j):
break
else:
return i
if (
i + len(needle) < len(self) and
not self._bloom(mask, self._data[i + len(needle)])
):
i += len(needle)
else:
i += skip
else:
if (
i + len(needle) < len(self) and
not self._bloom(mask, self._data[i + len(needle)])
):
i += len(needle)
return -1
def _make_rfind_mask(self, needle):
mask = self._bloom_add(0, six.indexbytes(needle, 0))
skip = len(needle) - 1
for i in xrange(len(needle) - 1, 0, -1):
mask = self._bloom_add(mask, six.indexbytes(needle, i))
if needle[i] == needle[0]:
skip = i - 1
return mask, skip
def _multi_char_rfind(self, needle, start, stop, mask, skip):
i = start + (stop - start - len(needle)) + 1
while i - 1 >= start:
i -= 1
if self[i] == six.indexbytes(needle, 0):
for j in xrange(len(needle) - 1, 0, -1):
if self[i + j] != six.indexbytes(needle, j):
break
else:
return i
if i - 1 >= 0 and not self._bloom(mask, self[i - 1]):
i -= len(needle)
else:
i -= skip
else:
if i - 1 >= 0 and not self._bloom(mask, self[i - 1]):
i -= len(needle)
return -1
def splitlines(self, keepends=False):
i = 0
j = 0
while j < len(self):
while (
i < len(self) and
self[i] != ord(b"\n") and self[i] != ord(b"\r")
):
i += 1
eol = i
if i < len(self):
if (
self[i] == ord(b"\r") and
i + 1 < len(self) and self[i + 1] == ord(b"\n")
):
i += 2
else:
i += 1
if keepends:
eol = i
yield self[j:eol]
j = i
def isspace(self):
if not self:
return False
for ch in self:
if ch != 32 and not (9 <= ch <= 13):
return False
return True
def isdigit(self):
if not self:
return False
for ch in self:
if not (ord("0") <= ch <= ord("9")):
return False
return True
def isalpha(self):
if not self:
return False
for ch in self:
if not (65 <= ch <= 90 or 97 <= ch <= 122):
return False
return True
def _strip_none(self, left, right):
lpos = 0
rpos = len(self)
if left:
while lpos < rpos and chr(self[lpos]).isspace():
lpos += 1
if right:
while rpos > lpos and chr(self[rpos - 1]).isspace():
rpos -= 1
return self[lpos:rpos]
def _strip_chars(self, chars, left, right):
lpos = 0
rpos = len(self)
if left:
while lpos < rpos and six.int2byte(self[lpos]) in chars:
lpos += 1
if right:
while rpos > lpos and six.int2byte(self[rpos - 1]) in chars:
rpos -= 1
return self[lpos:rpos]
def strip(self, chars=None):
if chars is None:
return self._strip_none(left=True, right=True)
else:
return self._strip_chars(chars, left=True, right=True)
def lstrip(self, chars=None):
if chars is None:
return self._strip_none(left=True, right=False)
else:
return self._strip_chars(chars, left=True, right=False)
def rstrip(self, chars=None):
if chars is None:
return self._strip_none(left=False, right=True)
else:
return self._strip_chars(chars, left=False, right=True)
def write_to(self, fd):
res = _lib.write(fd, self._data, self._length)
if res == -1:
raise OSError(_ffi.errno, os.strerror(_ffi.errno))
return res
class BufferCollator(object):
def __init__(self):
self._views = []
self._total_length = 0
def __len__(self):
return self._total_length
def append(self, view):
if self._views:
last_view = self._views[-1]
if (
last_view._keepalive is view._keepalive and
last_view._data + len(last_view) == view._data
):
self._views[-1] = BufferView(
last_view._keepalive,
last_view._data,
0,
len(last_view) + len(view)
)
else:
self._views.append(view)
else:
self._views.append(view)
self._total_length += len(view)
def collapse(self):
if len(self._views) == 1:
result = self._views[0]
else:
data = _ffi.new("uint8_t[]", self._total_length)
pos = 0
for view in self._views:
_lib.memcpy(data + pos, view._data, len(view))
pos += len(view)
result = Buffer(data, self._total_length).view()
del self._views[:]
self._total_length = 0
return result | zero_buffer | /zero_buffer-0.5.1.tar.gz/zero_buffer-0.5.1/zero_buffer.py | zero_buffer.py |
Welcome to Zero Buffer
======================
``zero_buffer`` is a high-performance, zero-copy, implementation of a
byte-buffer for Python.
.. code-block:: python
from zero_buffer import Buffer
# Create a buffer which has space for 8192 bytes.
b = Buffer.allocate(8192)
with open(path, "rb") as f:
# Read up to 8192 bytes from the file into the buffer
b.read_from(f.fileno())
# Create a read-only view of the buffer, this performs no copying.
view = b.view()
# Split the view on colons, this returns a generator which yields sub-views
# of the view.
for part in view.split(b":"):
print(part)
``zero_buffer`` works on Python 2.6, 2.7, 3.2, 3.3, and PyPy.
Install it with ``pip``:
.. code-block:: console
$ pip install zero_buffer
Contents
--------
.. toctree::
:maxdepth: 2
api-reference
| zero_buffer | /zero_buffer-0.5.1.tar.gz/zero_buffer-0.5.1/docs/index.rst | index.rst |
API Reference
=============
.. currentmodule:: zero_buffer
.. class:: Buffer
A buffer is a fixed-size, append only, contigious region of memory. Once
data is in it, that data cannot be mutated, however data can be read into
the buffer with multiple calls.
.. classmethod:: allocate(size)
:param int size: Number of bytes.
:return Buffer: The new buffer.
Allocates a new buffer of ``size`` bytes.
.. attribute:: capacity
Returns the size of the underlying buffer. This is the same as what it
was allocated with.
.. attribute:: writepos
Returns the current, internal writing position, this increases on calls
to :meth:`read_from` and :meth:`add_bytes`.
.. attribute:: free
Returns the remaining space in the :class:`Buffer`.
.. method:: read_from(fd)
:param int fd: A file descriptor.
:return int: Number of bytes read.
:raises OSError: on an error reading from the file descriptor.
:raises EOFError: when the read position of the file is at the end.
:raises BufferFull: when the buffer has no remaining space when called
Reads from the file descriptor into the :class:`Buffer`. Note that the
number of bytes copied may be less than the number of bytes in the
file.
.. method:: add_bytes(b)
:param bytes b: Bytes to copy into the buffer.
:return int: Number of bytes copied into the buffer.
:raises BufferFull: when the buffer has no remaining space when called
Copies the bytes into the :class:`Buffer`. Note that the number of
bytes copied may be less than ``len(b)`` if there isn't space in the
:class:`Buffer`.
.. method:: view(start=0, stop=None)
:param int start: The byte-offset from the beggining of the buffer.
:param int stop: The byte-offset from start.
:return BufferView:
:raises ValueError: If the stop is before the start, if the start is
negative or after the writepos, or if the stop is
after the writepos.
Returns a view of the buffer's data. This does not perform any copying.
.. class:: BufferView
A buffer view is an immutable, fixed-size, view over a contigious region of
memory. It exposes much of the same API as :class:`bytes`, except most
methods return BufferViews and do not make copies of the data. A buffer
view is either a view into a :class:`Buffer` or into another
:class:`BufferView`.
.. method:: __bytes__()
Returns a copy of the contents of the view as a :class:`bytes`.
.. method:: __len__()
Returns the length of the view.
.. method:: __eq__(other)
Checks whether the contents of the view are equal to ``other``, which
can be either a :class:`bytes` or a :class:`BufferView`.
.. method:: __contains__(needle)
Returns whether or not the ``needle`` exists in the view as a
contigious series of bytes.
.. method:: __getitem__(idx)
If ``idx`` is a :class:`slice`, returns a :class:`BufferView` over that
data, it does not perform a copy. If ``idx`` is an integer, it returns
the ordinal value of the byte at that index.
Unlike other containers in Python, this does not support slices with
steps (``view[::2]``).
.. method:: __add__(other)
:param BufferView other:
Returns a :class:`BufferView` over the concatenated contents. If
``other`` is contigious with ``self`` in memory, no copying is
performed, otherwise both views are copied into a new one.
.. method:: find(needle, start=0, stop=None)
The same as :meth:`bytes.find`.
.. method:: index(needle, start=0, stop=None)
The same as :meth:`bytes.index`.
.. method:: rfind(needle, start=0, stop=None)
The same as :meth:`bytes.rfind`.
.. method:: rindex(needle, start=0, stop=None)
The same as :meth:`bytes.rindex`.
.. method:: split(by, maxsplit=-1)
Similar to :meth:`bytes.split`, except it returns an iterator (not a
:class:`list`) over the results, and each result is a
:class:`BufferView` (not a :class:`bytes`).
.. method:: splitlines(keepends=False)
Similar to :meth:`bytes.splitlines`, except it returns an iterator (not
a :class:`list`) over the results, and each result is a
:class:`BufferView` (not a :class:`bytes`).
.. method:: isspace()
The same as :meth:`bytes.isspace`.
.. method:: isdigit()
The same as :meth:`bytes.isdigit`.
.. method:: isalpha()
The same as :meth:`bytes.isalpha`.
.. method:: strip(chars=None)
The same as :meth:`bytes.strip` except it returns a :class:`BufferView`
(and not a :class:`bytes`).
.. method:: lstrip(chars=None)
The same as :meth:`bytes.lstrip` except it returns a
:class:`BufferView` (and not a :class:`bytes`).
.. method:: rstrip(chars=None)
The same as :meth:`bytes.rstrip` except it returns a
:class:`BufferView` (and not a :class:`bytes`).
.. method:: write_to(fd)
:param int fd: A file descriptor.
:return int: Number of bytes written.
:raises OSError: on an error writing to the file descriptor.
Writes the contents of the buffer to a file descriptor. Note that the
number of bytes written may be less than the number of bytes in the
buffer view.
.. class:: BufferCollator
A buffer collator is a collection of :class:`BufferView` objects which can
be collapsed into a single :class:`BufferView`.
.. method:: __len__()
Returns the sum of the lengths of the views inside the collator.
.. method:: append(view)
:param BufferView view:
Adds the contents of a view to the collator.
.. method:: collapse()
Collapses the contents of the collator into a single
:class:`BufferView`. Also resets the internal state of the collator, so
if you call it twice successively, the second call will return an empty
:class:`BufferView`.
| zero_buffer | /zero_buffer-0.5.1.tar.gz/zero_buffer-0.5.1/docs/api-reference.rst | api-reference.rst |
============
zeroae's cli
============
.. image:: https://img.shields.io/github/workflow/status/zeroae/zeroae-cli/pypa-conda?label=pypa-conda&logo=github&style=flat-square
:target: https://github.com/zeroae/zeroae-cli/actions?query=workflow%3Apypa-conda
.. image:: https://img.shields.io/conda/v/zeroae/zeroae-cli?logo=anaconda&style=flat-square
:target: https://anaconda.org/zeroae/zeroae-cli
.. image:: https://img.shields.io/codecov/c/gh/zeroae/zeroae-cli?logo=codecov&style=flat-square
:target: https://codecov.io/gh/zeroae/zeroae-cli
.. image:: https://img.shields.io/codacy/grade/d0799708f30942368739c3c54d4f2b92?logo=codacy&style=flat-square
:target: https://www.codacy.com/app/zeroae/zeroae-cli
:alt: Codacy Badge
.. image:: https://img.shields.io/badge/code--style-black-black?style=flat-square
:target: https://github.com/psf/black
.. image:: https://img.shields.io/pypi/v/zeroae-cli?logo=pypi&style=flat-square
:target: https://pypi.python.org/pypi/zeroae-cli
.. image:: https://readthedocs.org/projects/zeroae-cli/badge/?version=latest&style=flat-square
:target: https://zeroae-cli.readthedocs.io/en/latest/?badge=latest
:alt: Documentation Status
ZeroAE's CLI
* Free software: MIT
* Documentation: https://zeroae-cli.readthedocs.io.
Features
--------
* TODO
-------
This package was created with ght-render_ and the `sodre/ght-pypackage`_ project template.
.. _ght-render: https://github.com/sodre/action-ght-render
.. _`sodre/ght-pypackage`: https://github.com/sodre/ght-pypackage
| zeroae-cli | /zeroae-cli-0.0.5.tar.gz/zeroae-cli-0.0.5/README.rst | README.rst |
.. highlight:: shell
============
Contributing
============
Contributions are welcome, and they are greatly appreciated! Every little bit
helps, and credit will always be given.
You can contribute in many ways:
Types of Contributions
----------------------
Report Bugs
~~~~~~~~~~~
Report bugs at https://github.com/zeroae/zeroae-cli/issues.
If you are reporting a bug, please include:
* Your operating system name and version.
* Any details about your local setup that might be helpful in troubleshooting.
* Detailed steps to reproduce the bug.
Fix Bugs
~~~~~~~~
Look through the GitHub issues for bugs. Anything tagged with "bug" and "help
wanted" is open to whoever wants to implement it.
Implement Features
~~~~~~~~~~~~~~~~~~
Look through the GitHub issues for features. Anything tagged with "enhancement"
and "help wanted" is open to whoever wants to implement it.
Write Documentation
~~~~~~~~~~~~~~~~~~~
zeroae's cli could always use more documentation, whether as part of the
official zeroae's cli docs, in docstrings, or even on the web in blog posts,
articles, and such.
Submit Feedback
~~~~~~~~~~~~~~~
The best way to send feedback is to file an issue at https://github.com/zeroae/zeroae-cli/issues.
If you are proposing a feature:
* Explain in detail how it would work.
* Keep the scope as narrow as possible, to make it easier to implement.
* Remember that this is a volunteer-driven project, and that contributions
are welcome :)
Get Started!
------------
Ready to contribute? Here's how to set up `zeroae-cli` for local development.
1. Fork the `zeroae-cli` repo on GitHub.
2. Clone your fork locally::
$ git clone [email protected]:your_name_here/zeroae-cli.git
3. Install your local copy into a conda environment. Assuming you have conda installed, this is how you set up your fork for local development::
$ cd zeroae-cli/
$ make init
$ conda activate zeroae-cli-dev
$ pip install -e . --no-deps
4. Create a branch for local development, use the ``f-``, ``i-`` or ``chore-`` prefixes to auto-label your PR::
$ git checkout -b (f|i|chore)-name-of-your-contribution
Now you can make your changes locally.
5. When you're done making changes, check that your changes pass black, flake8 and coverage
tests::
$ make format lint test
All the dependencies should already be included in your conda environment.
6. Commit your changes and push your branch to GitHub::
$ git add .
$ git commit -m "Your detailed description of your changes."
$ git push origin (f|i|chore)-name-of-your-contribution
7. Submit a pull request through the GitHub website.
Pull Request Guidelines
-----------------------
Before you submit a pull request, check that it meets these guidelines:
1. The pull request should include tests.
2. If the pull request adds functionality, the docs should be updated. Put
your new functionality into a function with a docstring, and add the
feature to the list in README.rst.
3. The pull request should pass all our GitHub tests.
Tips
----
To run a subset of tests::
$ pytest tests.test_cli
Deploying
---------
A reminder for the maintainers on how to deploy.
Make sure all your changes are committed (including an entry in HISTORY.rst).
Then run::
$ bump2version patch # possible: major / minor / patch
$ git push
$ git push --tags
Travis will then deploy to PyPI if tests pass.
| zeroae-cli | /zeroae-cli-0.0.5.tar.gz/zeroae-cli-0.0.5/CONTRIBUTING.rst | CONTRIBUTING.rst |
.. highlight:: shell
============
Installation
============
Stable release
--------------
To install zeroae's cli, run this command in your terminal:
.. code-block:: console
$ pip install zeroae-cli
This is the preferred method to install zeroae's cli, as it will always install the most recent stable release.
If you don't have `pip`_ installed, this `Python installation guide`_ can guide
you through the process.
.. _pip: https://pip.pypa.io
.. _Python installation guide: http://docs.python-guide.org/en/latest/starting/installation/
From sources
------------
The sources for zeroae's cli can be downloaded from the `Github repo`_.
You can either clone the public repository:
.. code-block:: console
$ git clone git://github.com/sodre/zeroae-cli
Or download the `tarball`_:
.. code-block:: console
$ curl -OJL https://github.com/sodre/zeroae-cli/tarball/master
Once you have a copy of the source, you can install it with:
.. code-block:: console
$ python setup.py install
.. _Github repo: https://github.com/zeroae/zeroae-cli
.. _tarball: https://github.com/zeroae/zeroae-cli/tarball/master
| zeroae-cli | /zeroae-cli-0.0.5.tar.gz/zeroae-cli-0.0.5/docs/installation.rst | installation.rst |
set -euf
GHT_CONF=.github/ght.yaml
render()
{
in=$1
out=$2
ght_conf=${3:-$GHT_CONF}
jinja2 \
--format yaml\
-e jinja2_time.TimeExtension \
-o $out \
$in $ght_conf
}
converged()
{
diff -q $1 $2 > /dev/null
}
tree_converged()
{
templ=$1;
rendered=$2;
# List all the types under the repo, ignoring the .git dir
find . -path ./.git -prune -o ${@:3} | grep -v '^\./\.git' | sort > ${templ}
render $templ $rendered
converged $templ $rendered
}
render_configuration()
{
set -e
ght_temp=$(mktemp)
declare -i i=1
until converged $GHT_CONF $ght_temp; do
echo "Rendering $GHT_CONF: Pass $i"
stop_rendering_lines=false
mv $GHT_CONF $ght_temp
while IFS= read line; do
in=$(mktemp)
printf "%s\n" "$line" > $in
if [ "$stop_rendering_lines" == false ]; then
out=$(mktemp)
render $in $out $ght_temp
cat < $out >> $GHT_CONF
if ! converged $in $out; then
stop_rendering_lines=true
fi
else
cat < $in >> $GHT_CONF
fi
done < $ght_temp
let i++
done
#git commit -m "ght: configuration rendered" $GHT_CONF
}
render_tree_structure()
{
for filter in "-type d" "-type f"; do
ght_temp=$(mktemp)
until tree_converged $ght_temp ${ght_temp}.rendered $filter ; do
paste -- $ght_temp $ght_temp.rendered |
while IFS=$'\t' read -r d1 d2; do
if [ $d1 != $d2 ]; then
git mv $d1 $d2 || true
fi
done
done
done
#git commit -m 'ght: template structure rendered'
}
render_tree_content()
{
find . -path ./.git -prune -o -type f |
grep -E -v './.git$|./.github' |
sort |
while IFS=$'\n' read -r in; do
out=$(mktemp)
echo "rendering ${in}"
render $in $out
cp -a ${out} ${in}
git add ${in}
done
#git commit -m 'ght: content rendered'
}
render_tree_ght_content()
{
find . -path ./.github -prune -o -type f -name '*.ght' |
grep -E -v './.github$' |
sort |
while IFS=$'\n' read -r in; do
out=${in/.ght/}
echo "rendering ${in}"
render $in $out
git rm ${in}
git add ${out}
done
#git commit -m 'ght: *.j2 content rendered and renamed'
}
remove_workflows()
{
git rm -f .github/workflows/ght-init.yml
git rm -f .github/workflows/ght-render-repo.yml
}
# This is so we can test things locally without screwing up the master branch
# tmp=$(basename $(mktemp -t ght-XXXX))
# git checkout -b $tmp
cmd=${1//-/_}
if [ ! -z "$cmd" ]; then
shift 1
$cmd "$@"
exit
fi | zeroae-cli | /zeroae-cli-0.0.5.tar.gz/zeroae-cli-0.0.5/.github/bin/ght-render.sh | ght-render.sh |
=============================
ZeroAE's GitHub App Framework
=============================
.. image:: https://img.shields.io/github/workflow/status/zeroae/zeroae-goblet/pypa-conda?label=pypa-conda&logo=github&style=flat-square
:target: https://github.com/zeroae/zeroae-goblet/actions?query=workflow%3Apypa-conda
.. image:: https://img.shields.io/conda/v/zeroae/zeroae-goblet?logo=anaconda&style=flat-square
:target: https://anaconda.org/zeroae/zeroae-goblet
.. image:: https://img.shields.io/codecov/c/gh/zeroae/zeroae-goblet?logo=codecov&style=flat-square
:target: https://codecov.io/gh/zeroae/zeroae-goblet
.. image:: https://img.shields.io/codacy/grade/2b7e8dd25f3a40e08a98d5b094181c4b?logo=codacy&style=flat-square
:target: https://www.codacy.com/app/zeroae/zeroae-goblet
:alt: Codacy Badge
.. image:: https://img.shields.io/badge/code--style-black-black?style=flat-square
:target: https://github.com/psf/black
.. image:: https://img.shields.io/pypi/v/zeroae-goblet?logo=pypi&style=flat-square
:target: https://pypi.python.org/pypi/zeroae-goblet
.. image:: https://readthedocs.org/projects/zeroae-goblet/badge/?version=latest&style=flat-square
:target: https://zeroae-goblet.readthedocs.io/en/latest/?badge=latest
:alt: Documentation Status
A Chalice_ Blueprint for creating `GitHub Apps`_ inspired by Probot_
* Free software: Apache-2.0
* Documentation: https://zeroae-goblet.readthedocs.io.
Features
--------
* TODO
-------
This package was created with ght-render_ and the `sodre/ght-pypackage`_ project template.
.. _Chalice: https://github.com/aws/chalice
.. _`GitHub Apps`: https://developer.github.com/apps
.. _Probot: https://probot.github.io
.. _ght-render: https://github.com/sodre/action-ght-render
.. _`sodre/ght-pypackage`: https://github.com/sodre/ght-pypackage
| zeroae-goblet | /zeroae-goblet-0.0.1.tar.gz/zeroae-goblet-0.0.1/README.rst | README.rst |
.. highlight:: shell
============
Contributing
============
Contributions are welcome, and they are greatly appreciated! Every little bit
helps, and credit will always be given.
You can contribute in many ways:
Types of Contributions
----------------------
Report Bugs
~~~~~~~~~~~
Report bugs at https://github.com/zeroae/zeroae-goblet/issues.
If you are reporting a bug, please include:
* Your operating system name and version.
* Any details about your local setup that might be helpful in troubleshooting.
* Detailed steps to reproduce the bug.
Fix Bugs
~~~~~~~~
Look through the GitHub issues for bugs. Anything tagged with "bug" and "help
wanted" is open to whoever wants to implement it.
Implement Features
~~~~~~~~~~~~~~~~~~
Look through the GitHub issues for features. Anything tagged with "enhancement"
and "help wanted" is open to whoever wants to implement it.
Write Documentation
~~~~~~~~~~~~~~~~~~~
ZeroAE's GitHub App framework could always use more documentation, whether as part of the
official ZeroAE's GitHub App framework docs, in docstrings, or even on the web in blog posts,
articles, and such.
Submit Feedback
~~~~~~~~~~~~~~~
The best way to send feedback is to file an issue at https://github.com/zeroae/zeroae-goblet/issues.
If you are proposing a feature:
* Explain in detail how it would work.
* Keep the scope as narrow as possible, to make it easier to implement.
* Remember that this is a volunteer-driven project, and that contributions
are welcome :)
Get Started!
------------
Ready to contribute? Here's how to set up `zeroae-goblet` for local development.
1. Fork the `zeroae-goblet` repo on GitHub.
2. Clone your fork locally::
$ git clone [email protected]:your_name_here/zeroae-goblet.git
3. Install your local copy into a conda environment. Assuming you have conda installed, this is how you set up your fork for local development::
$ cd zeroae-goblet/
$ make init
$ conda activate zeroae-goblet-dev
$ pip install -e . --no-deps
4. Create a branch for local development, use the ``f-``, ``i-`` or ``chore-`` prefixes to auto-label your PR::
$ git checkout -b (f|i|chore)-name-of-your-contribution
Now you can make your changes locally.
5. When you're done making changes, check that your changes pass black, flake8 and coverage
tests::
$ make format lint test
All the dependencies should already be included in your conda environment.
6. Commit your changes and push your branch to GitHub::
$ git add .
$ git commit -m "Your detailed description of your changes."
$ git push origin (f|i|chore)-name-of-your-contribution
7. Submit a pull request through the GitHub website.
Pull Request Guidelines
-----------------------
Before you submit a pull request, check that it meets these guidelines:
1. The pull request should include tests.
2. If the pull request adds functionality, the docs should be updated. Put
your new functionality into a function with a docstring, and add the
feature to the list in README.rst.
3. The pull request should pass all our GitHub tests.
Tips
----
To run a subset of tests::
$ pytest tests.test_goblet
Deploying
---------
A reminder for the maintainers on how to deploy.
Make sure all your changes are committed (including an entry in HISTORY.rst).
Then run::
$ bump2version patch # possible: major / minor / patch
$ git push
$ git push --tags
Travis will then deploy to PyPI if tests pass. | zeroae-goblet | /zeroae-goblet-0.0.1.tar.gz/zeroae-goblet-0.0.1/CONTRIBUTING.rst | CONTRIBUTING.rst |
.. highlight:: shell
============
Installation
============
Stable release
--------------
To install ZeroAE's GitHub App framework, run this command in your terminal:
.. code-block:: console
$ pip install zeroae-goblet
This is the preferred method to install ZeroAE's GitHub App framework, as it will always install the most recent stable release.
If you don't have `pip`_ installed, this `Python installation guide`_ can guide
you through the process.
.. _pip: https://pip.pypa.io
.. _Python installation guide: http://docs.python-guide.org/en/latest/starting/installation/
From sources
------------
The sources for ZeroAE's GitHub App framework can be downloaded from the `Github repo`_.
You can either clone the public repository:
.. code-block:: console
$ git clone git://github.com/sodre/zeroae-goblet
Or download the `tarball`_:
.. code-block:: console
$ curl -OJL https://github.com/sodre/zeroae-goblet/tarball/master
Once you have a copy of the source, you can install it with:
.. code-block:: console
$ python setup.py install
.. _Github repo: https://github.com/zeroae/zeroae-goblet
.. _tarball: https://github.com/zeroae/zeroae-goblet/tarball/master | zeroae-goblet | /zeroae-goblet-0.0.1.tar.gz/zeroae-goblet-0.0.1/docs/installation.rst | installation.rst |
import os
from typing import Dict
from urllib.parse import urlparse
from dotenv import set_key
from environs import Env
from marshmallow.validate import OneOf, ContainsOnly
from octokit_routes import specifications
# GitHub API Config
GHE_HOST = None
GHE_PROTO = None
GHE_API_URL = None
GHE_API_SPEC = None
# GitHub Application Manifest Config
APP_NAME = None
APP_DESCRIPTION = None
APP_ORGANIZATION = None
APP_PUBLIC = None
APP_DEFAULT_EVENTS = None
APP_DEFAULT_PERMISSIONS = None
# GitHub Application Registration Config
APP_ID = None
APP_CLIENT_ID = None
APP_CLIENT_SECRET = None
APP_PEM = None
APP_WEBHOOK_SECRET = None
# Other Options
WEBHOOK_PROXY_URL = None
def configure(env: Env):
_load_ghe_options(env)
_load_app_options(env)
_load_app_registration_options(env)
_load_other_options(env)
def _load_other_options(env: Env):
global WEBHOOK_PROXY_URL
WEBHOOK_PROXY_URL = env.url("WEBHOOK_PROXY_URL", None)
def _load_ghe_options(env: Env):
global GHE_HOST, GHE_PROTO, GHE_API_URL, GHE_API_SPEC
with env.prefixed("GHE_"):
GHE_API_SPEC = env.str(
"API_SPEC",
"api.github.com",
validate=OneOf(
specifications.keys(), error="GHE_API_SPEC must be one of: {choices}"
),
)
if GHE_API_SPEC == "api.github.com":
GHE_PROTO = "https"
GHE_HOST = "github.com"
GHE_API_URL = urlparse("https://api.github.com")
else:
GHE_PROTO = env.str(
"PROTO",
"https",
validate=OneOf(
["http", "https"], error="GHE_PROTO must be one of: {choices}"
),
)
GHE_HOST = env.str("HOST")
GHE_API_URL = env.url("GHE_API_URL", f"{GHE_PROTO}://{GHE_HOST}/api/v3")
def _load_app_options(env: Env):
from octokit.utils import get_json_data as octokit_get_json_data
global APP_NAME, APP_DESCRIPTION, APP_ORGANIZATION, APP_PUBLIC
global APP_DEFAULT_EVENTS, APP_DEFAULT_PERMISSIONS
valid_events = octokit_get_json_data("events.json")
with env.prefixed("APP_"):
APP_NAME = env.str("NAME", "gh-app")
APP_DESCRIPTION = env.str("DESCRIPTION", "")
APP_ORGANIZATION = env.str("ORGANIZATION", None)
APP_PUBLIC = env.bool("PUBLIC", True)
with env.prefixed("DEFAULT_"):
APP_DEFAULT_EVENTS = env.list(
"EVENTS", "push", validate=ContainsOnly(valid_events)
)
APP_DEFAULT_PERMISSIONS = env.dict("PERMISSIONS", "metadata=read")
def _load_app_registration_options(env: Env):
"""
TODO: decrypt these with Credstash, SecretsManager, or ParameterStore.
ref: https://github.com/aws/chalice/issues/859#issuecomment-547676237
ref: https://environ-config.readthedocs.io/en/stable/tutorial.html
ref: https://bit.ly/why-you-shouldnt-use-env-variables-for-secret-data
:param env:
:return:
"""
global APP_ID, APP_CLIENT_ID, APP_CLIENT_SECRET, APP_PEM, APP_WEBHOOK_SECRET
with env.prefixed("APP_"):
APP_ID = env.str("ID", None)
APP_CLIENT_ID = env.str("CLIENT_ID", None)
APP_CLIENT_SECRET = env.str("CLIENT_SECRET", None)
APP_PEM = env.str("PEM", None)
APP_WEBHOOK_SECRET = env.str("WEBHOOK_SECRET", None)
def save_app_registration(registration: Dict):
# TODO: encrypt these in Credstash, SecretsManager, or ParameterStore
# dotenv backing store (create if not exists)
if not os.path.exists(".env"):
f = open(".env", "w")
f.close()
keys = ["id", "client_id", "client_secret", "html_url", "pem", "webhook_secret"]
for key in keys:
set_key(".env", f"APP_{key.upper()}", str(registration[key]))
env = Env()
env.read_env(".env")
_load_app_registration_options(env) | zeroae-goblet | /zeroae-goblet-0.0.1.tar.gz/zeroae-goblet-0.0.1/zeroae/goblet/config.py | config.py |
from http import HTTPStatus
from urllib.parse import urljoin
from chalice import Blueprint, Response
from chalice.app import Request, ForbiddenError
from octokit import webhook
from pubsub import pub
from pubsub.utils import ExcPublisher
from zeroae.goblet.utils import (
create_app_manifest,
infer_app_url,
get_create_app_url,
get_configured_octokit,
)
from .. import config
from ..views import render_setup_html
class GitHubAppBlueprint(Blueprint):
def __init__(self, import_name):
"""Creates the Chalice GitHubApp Blueprint."""
super().__init__(import_name)
pub.setListenerExcHandler(ExcPublisher(pub.getDefaultTopicMgr()))
@staticmethod
def on_gh_event(topic):
"""
:param topic: The GitHub webhook topic
:return: decorator
"""
def decorator(f):
l, _ = pub.subscribe(f, "gh" if topic == "*" else f"gh.{topic}")
f.listener = l
return f
return decorator
bp: GitHubAppBlueprint = GitHubAppBlueprint(__name__)
@bp.route("/")
def register():
"""
Note: HTTP Post Redirect, 307 code
ref: bit.ly/why-doesnt-http-have-post-redirect
:return:
"""
# Get GitHub's create_app_url, for user/owner application
create_app_url = get_create_app_url()
request: Request = bp.current_request
app_url = infer_app_url(request.headers, request.context["path"])
app_manifest = create_app_manifest(app_url)
body = render_setup_html(app_manifest, create_app_url)
return Response(
body=body, status_code=HTTPStatus.OK, headers={"Content-Type": "text/html"}
)
@bp.route("/callback")
def register_callback():
"""
Finish the GitHub application registration flow.
Converts code for clientId, clientSecret, webhook secret, and App PEM
Saves result in the configuration backend
:return:
"""
query_params = bp.current_request.query_params
query_params = query_params if query_params else dict()
code = query_params.get("code", None)
if code is None:
return Response(
body='{"error": "The GitHub application-manifest code is missing."}',
status_code=HTTPStatus.EXPECTATION_FAILED,
headers={"Content-Type": "text/html"},
)
o = get_configured_octokit()
o = o.apps.create_from_manifest(code=code)
config.save_app_registration(o.json)
return Response(
body="",
status_code=HTTPStatus.SEE_OTHER,
headers={"Location": urljoin(f'{o.json["html_url"]}/', "installations/new")},
)
@bp.route("/events", methods=["POST"])
def events():
if not webhook.verify(
bp.current_request.headers,
bp.current_request.raw_body.decode("utf-8"),
config.APP_WEBHOOK_SECRET,
events=["*"],
):
raise ForbiddenError(
f"Error validating the event: {bp.current_request.to_dict()}"
)
r: Request = bp.current_request
event_topic = r.headers["x-github-event"]
pub.sendMessage(f"gh.{event_topic}", payload=r.json_body)
return {} | zeroae-goblet | /zeroae-goblet-0.0.1.tar.gz/zeroae-goblet-0.0.1/zeroae/goblet/chalice/blueprint.py | blueprint.py |
<p align="center">
<img height="300px" src="https://ananto30.github.io/i/1200xCL_TP.png" />
</p>
<p align="center">
<em>Zero is a simple Python framework (RPC like) to build fast and high performance microservices or distributed servers</em>
</p>
<p align="center">
<a href="https://codecov.io/gh/Ananto30/zero" target="_blank">
<img src="https://codecov.io/gh/Ananto30/zero/branch/main/graph/badge.svg?token=k0aA0G6NLs" />
</a>
<a href="https://pypi.org/project/zeroapi/" target="_blank">
<img src="https://img.shields.io/pypi/v/zeroapi" />
</a>
<br>
<a href="https://app.codacy.com/gh/Ananto30/zero/dashboard?utm_source=gh&utm_medium=referral&utm_content=&utm_campaign=Badge_grade">
<img src="https://app.codacy.com/project/badge/Grade/f6d4db49974b470f95999565f7901595"/>
</a>
<a href="https://codeclimate.com/github/Ananto30/zero/maintainability" target="_blank">
<img src="https://api.codeclimate.com/v1/badges/4f2fd83bee97326699bc/maintainability" />
</a>
</p>
<hr>
**Features**:
* Zero provides **faster communication** (see [benchmarks](https://github.com/Ananto30/zero#benchmarks-)) between the microservices using [zeromq](https://zeromq.org/) under the hood.
* Zero uses messages for communication and traditional **client-server** or **request-reply** pattern is supported.
* Support for both **async** and **sync**.
* The base server (ZeroServer) **utilizes all cpu cores**.
* **Code generation**! See [example](https://github.com/Ananto30/zero#code-generation-) 👇
**Philosophy** behind Zero:
* **Zero learning curve**: The learning curve is tends to zero. Just add functions and spin up a server, literally that's it! The framework hides the complexity of messaging pattern that enables faster communication.
* **ZeroMQ**: An awesome messaging library enables the power of Zero.
Let's get started!
# Getting started 🚀
*Ensure Python 3.8+*
pip install zeroapi
**For Windows**, [tornado](https://pypi.org/project/tornado/) needs to be installed separately (for async operations). It's not included with `zeroapi` because for linux and mac-os, tornado is not needed as they have their own event loops.
* Create a `server.py`
```python
from zero import ZeroServer
app = ZeroServer(port=5559)
@app.register_rpc
def echo(msg: str) -> str:
return msg
@app.register_rpc
async def hello_world() -> str:
return "hello world"
if __name__ == "__main__":
app.run()
```
* The **RPC functions only support one argument** (`msg`) for now.
* Also note that server **RPC functions are type hinted**. Type hint is **must** in Zero server. Supported types can be found [here](/zero/utils/type_util.py#L11).
* Run the server
```shell
python -m server
```
* Call the rpc methods
```python
from zero import ZeroClient
zero_client = ZeroClient("localhost", 5559)
def echo():
resp = zero_client.call("echo", "Hi there!")
print(resp)
def hello():
resp = zero_client.call("hello_world", None)
print(resp)
if __name__ == "__main__":
echo()
hello()
```
* Or using async client -
```python
import asyncio
from zero import AsyncZeroClient
zero_client = AsyncZeroClient("localhost", 5559)
async def echo():
resp = await zero_client.call("echo", "Hi there!")
print(resp)
async def hello():
resp = await zero_client.call("hello_world", None)
print(resp)
if __name__ == "__main__":
loop = asyncio.get_event_loop()
loop.run_until_complete(echo())
loop.run_until_complete(hello())
```
# Serialization 📦
## Default serializer
[Msgspec](https://jcristharif.com/msgspec/) is the default serializer. So `msgspec.Struct` (for high performance) or `dataclass` or any [supported types](https://jcristharif.com/msgspec/supported-types.html) can be used easily to pass complex arguments, i.e.
```python
from dataclasses import dataclass
from msgspec import Struct
from zero import ZeroServer
app = ZeroServer()
class Person(Struct):
name: str
age: int
dob: datetime
@dataclass
class Order:
id: int
amount: float
created_at: datetime
@app.register_rpc
def save_person(person: Person) -> None:
# save person to db
...
@app.register_rpc
def save_order(order: Order) -> None:
# save order to db
...
```
## Return type
The return type of the RPC function can be any of the [supported types](https://jcristharif.com/msgspec/supported-types.html). If `return_type` is set in the client `call` method, then the return type will be converted to that type.
```python
@dataclass
class Order:
id: int
amount: float
created_at: datetime
def get_order(id: str) -> Order:
return zero_client.call("get_order", id, return_type=Order)
```
# Code Generation 🤖
Easy to use code generation tool is also provided!
After running the server, like above, call the server to get the client code. This makes it easy to know what functions are available in the local or remote server.
Using `zero.generate_client` generate client code for even remote servers using the `--host` and `--port` options.
```shell
python -m zero.generate_client --host localhost --port 5559 --overwrite-dir ./my_client
```
It will generate client like this -
```python
import typing # remove this if not needed
from typing import List, Dict, Union, Optional, Tuple # remove this if not needed
from zero import ZeroClient
zero_client = ZeroClient("localhost", 5559)
class RpcClient:
def __init__(self, zero_client: ZeroClient):
self._zero_client = zero_client
def echo(self, msg: str) -> str:
return self._zero_client.call("echo", msg)
def hello_world(self, msg: str) -> str:
return self._zero_client.call("hello_world", msg)
```
Use the client -
```python
from my_client import RpcClient, zero_client
client = RpcClient(zero_client)
if __name__ == "__main__":
client.echo("Hi there!")
client.hello_world(None)
```
Currently, the code generation tool supports only `ZeroClient` and not `AsyncZeroClient`.
*WIP - Generate models from server code.*
# Important notes 📝
* `ZeroServer` should always be run under `if __name__ == "__main__":`, as it uses multiprocessing.
* The methods which are under `register_rpc()` in `ZeroServer` should have **type hinting**, like `def echo(msg: str) -> str:`
# Let's do some benchmarking! 🏎
Zero is all about inter service communication. In most real life scenarios, we need to call another microservice.
So we will be testing a gateway calling another server for some data. Check the [benchmark/dockerize](https://github.com/Ananto30/zero/tree/main/benchmarks/dockerize) folder for details.
There are two endpoints in every tests,
* `/hello`: Just call for a hello world response 😅
* `/order`: Save a Order object in redis
Compare the results! 👇
# Benchmarks 🏆
11th Gen Intel® Core™ i7-11800H @ 2.30GHz, 8 cores, 16 threads, 16GB RAM (Docker in Ubuntu 22.04.2 LTS)
*(Sorted alphabetically)*
Framework | "hello world" (req/s) | 99% latency (ms) | redis save (req/s) | 99% latency (ms)
----------- | --------------------- | ---------------- | ------------------ | ----------------
aiohttp | 14391.38 | 10.96 | 9470.74 | 12.94
aiozmq | 15121.86 | 9.42 | 5904.84 | 21.57
fastApi | 9590.96 | 18.31 | 6669.81 | 24.41
sanic | 18790.49 | 8.69 | 12259.29 | 13.52
zero(sync) | 24805.61 | 4.57 | 16498.83 | 7.80
zero(async) | 22716.84 | 5.61 | 17446.19 | 7.24
# Roadmap 🗺
* [x] Make msgspec as default serializer
* [ ] Add support for async server (currently the sync server runs async functions in the eventloop, which is blocking)
* [ ] Add pub/sub support
# Contribution
Contributors are welcomed 🙏
**Please leave a star ⭐ if you like Zero!**
[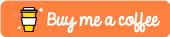](https://www.buymeacoffee.com/ananto30)
| zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/README.md | README.md |
import logging
import os
import sys
import zmq
import zmq.asyncio
class _AsyncLogger: # pragma: no cover
"""
*We don't have any support for async logger now.*
The idea is to have a push pull based logger to reduce io time in main process.
Logger will serve the methods like info, warn, error, exception
and push to the desired tcp or ipc.
The problem is, as the server runs in several processes,
the logger makes each instance in each process.
If we run two servers, we cannot connect to the same ipc or tcp.
So our logger's tcp or ipc should be dynamic.
But as we got several instances of the logger,
we cannot centralize a place from where logger can get the tcp or ipc.
The end user have to provide the tcp or ipc themselves.
"""
port = 12345
ipc = "zerologger"
def __init__(self):
# pass
self.init_push_logger()
def init_push_logger(self):
ctx = zmq.asyncio.Context()
self.log_pusher = ctx.socket(zmq.PUSH)
if os.name == "posix":
self.log_pusher.connect(f"ipc://{_AsyncLogger.ipc}")
else:
self.log_pusher.connect(f"tcp://127.0.0.1:{_AsyncLogger.port}")
def log(self, msg):
self.log_pusher.send_string(str(msg))
@classmethod
def start_log_poller(cls, ipc, port):
_AsyncLogger.port = port
_AsyncLogger.ipc = ipc
ctx = zmq.Context()
log_listener = ctx.socket(zmq.PULL)
if os.name == "posix":
log_listener.bind(f"ipc://{_AsyncLogger.ipc}")
logging.info("Async logger starting at ipc://%s", _AsyncLogger.ipc)
else:
log_listener.bind(f"tcp://127.0.0.1:{_AsyncLogger.port}")
logging.info(
"Async logger starting at tcp://127.0.0.1:%s", _AsyncLogger.port
)
try:
while True:
log = log_listener.recv_string()
logging.info(log)
except KeyboardInterrupt:
print("Caught KeyboardInterrupt, terminating async logger")
except Exception as exc:
print(exc)
finally:
log_listener.close()
sys.exit() | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/logger.py | logger.py |
import asyncio
import logging
import sys
from multiprocessing import Process
from typing import Callable, Dict
import msgspec
import zmq
import zmq.asyncio
class ZeroSubscriber: # pragma: no cover
def __init__(self, host: str = "127.0.0.1", port: int = 5558):
self._host = host
self._port = port
self._ctx = zmq.Context.instance()
self._topic_map: Dict[str, Callable] = {}
def register_listener(self, topic: str, func: Callable):
if not isinstance(func, Callable): # type: ignore
raise ValueError(f"topic should listen to function not {type(func)}")
self._topic_map[topic] = func
return func
def run(self):
processes = []
try:
processes = [
Process(
target=Listener.spawn_listener_worker,
args=(topic, func),
)
for topic, func in self._topic_map.items()
]
for prcs in processes:
prcs.start()
self._create_zmq_device()
except KeyboardInterrupt:
print("Caught KeyboardInterrupt, terminating workers")
for prcs in processes:
prcs.terminate()
else:
print("Normal termination")
for prcs in processes:
prcs.close()
for prcs in processes:
prcs.join()
def _create_zmq_device(self):
gateway: zmq.Socket = None
backend: zmq.Socket = None
try:
gateway = self._ctx.socket(zmq.SUB)
gateway.bind(f"tcp://*:{self._port}")
gateway.setsockopt_string(zmq.SUBSCRIBE, "")
logging.info("Starting server at %d", self._port)
backend = self._ctx.socket(zmq.PUB)
if sys.platform == "posix":
backend.bind("ipc://backendworker")
else:
backend.bind("tcp://127.0.0.1:6667")
zmq.device(zmq.FORWARDER, gateway, backend)
except Exception as exc: # pylint: disable=broad-except
logging.error(exc)
logging.error("bringing down zmq device")
finally:
gateway.close()
backend.close()
self._ctx.term()
class Listener: # pragma: no cover
@classmethod
def spawn_listener_worker(cls, topic, func):
worker = cls(topic, func)
asyncio.run(worker._create_worker())
def __init__(self, topic, func):
self._topic = topic
self._func = func
async def _create_worker(self):
ctx = zmq.asyncio.Context()
socket = ctx.socket(zmq.SUB)
if sys.platform == "posix":
socket.connect("ipc://backendworker")
else:
socket.connect("tcp://127.0.0.1:6667")
socket.setsockopt_string(zmq.SUBSCRIBE, self._topic)
logging.info("Starting listener for: %s", self._topic)
while True:
topic, msg = await socket.recv_multipart()
if topic.decode() != self._topic:
continue
try:
await self._func(msgspec.msgpack.decode(msg))
except Exception as exc: # pylint: disable=broad-except
logging.error(exc) | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/pubsub/subscriber.py | subscriber.py |
import asyncio
import logging
from typing import Any, Dict, Optional, Tuple, Type, TypeVar, Union
from zero import config
from zero.encoder import Encoder, get_encoder
from zero.error import MethodNotFoundException, RemoteException, TimeoutException
from zero.utils import util
from zero.zero_mq import AsyncZeroMQClient, ZeroMQClient, get_async_client, get_client
from zero.zero_mq.helpers import zpipe_async
T = TypeVar("T")
class ZeroClient:
def __init__(
self,
host: str,
port: int,
default_timeout: int = 2000,
encoder: Optional[Encoder] = None,
):
"""
ZeroClient provides the client interface for calling the ZeroServer.
Zero use tcp protocol for communication.
So a connection needs to be established to make a call.
The connection creation is done lazily.
So the first call will take some time to establish the connection.
If the connection is dropped the client might timeout.
But in the next call the connection will be re-established.
Parameters
----------
host: str
Host of the ZeroServer.
port: int
Port of the ZeroServer.
default_timeout: int
Default timeout for the ZeroClient for all calls.
Default is 2000 ms.
encoder: Optional[Encoder]
Encoder to encode/decode messages from/to client.
Default is msgspec.
If any other encoder is used, the server should use the same encoder.
Implement custom encoder by inheriting from `zero.encoder.Encoder`.
"""
self._address = f"tcp://{host}:{port}"
self._default_timeout = default_timeout
self._encoder = encoder or get_encoder(config.ENCODER)
self.zmqc: ZeroMQClient = None # type: ignore
def call(
self,
rpc_func_name: str,
msg: Union[int, float, str, dict, list, tuple, None],
timeout: Optional[int] = None,
return_type: Optional[Type[T]] = None,
) -> T:
"""
Call the rpc function resides on the ZeroServer.
Parameters
----------
rpc_func_name: str
Function name should be string.
This funtion should reside on the ZeroServer to get a successful response.
msg: Union[int, float, str, dict, list, tuple, None]
The only argument of the rpc function.
This should be of the same type as the rpc function's argument.
timeout: Optional[int]
Timeout for the call. In milliseconds.
Default is 2000 milliseconds.
return_type: Optional[Type[T]]
The return type of the rpc function.
If return_type is set, the response will be parsed to the return_type.
Returns
-------
Any
The return value of the rpc function.
Raises
------
TimeoutException
If the call times out or the connection is dropped.
MethodNotFoundException
If the rpc function is not found on the ZeroServer.
ConnectionException
If zeromq connection is not established.
Or zeromq cannot send the message to the server.
Or zeromq cannot receive the response from the server.
Mainly represents zmq.error.Again exception.
"""
self._ensure_connected()
_timeout = self._default_timeout if timeout is None else timeout
def _poll_data():
if not self.zmqc.poll(_timeout):
raise TimeoutException(
f"Timeout while sending message at {self._address}"
)
resp_id, resp_data = (
self._encoder.decode(self.zmqc.recv())
if return_type is None
else self._encoder.decode_type(
self.zmqc.recv(), Tuple[str, return_type]
)
)
return resp_id, resp_data
req_id = util.unique_id()
frames = [req_id, rpc_func_name, "" if msg is None else msg]
self.zmqc.send(self._encoder.encode(frames))
resp_id, resp_data = None, None
# as the client is synchronous, we know that the response will be available any next poll
# we try to get the response until timeout because a previous call might be timed out
# and the response is still in the socket,
# so we poll until we get the response for this call
while resp_id != req_id:
resp_id, resp_data = _poll_data()
check_response(resp_data)
return resp_data # type: ignore
def close(self):
if self.zmqc is not None:
self.zmqc.close()
self.zmqc = None
def _ensure_connected(self):
if self.zmqc is not None:
return
self._init()
self._try_connect()
def _init(self):
self.zmqc = get_client(config.ZEROMQ_PATTERN, self._default_timeout)
self.zmqc.connect(self._address)
def _try_connect(self):
frames = [util.unique_id(), "connect", ""]
self.zmqc.send(self._encoder.encode(frames))
self._encoder.decode(self.zmqc.recv())
logging.info("Connected to server at %s", self._address)
class AsyncZeroClient:
def __init__(
self,
host: str,
port: int,
default_timeout: int = 2000,
encoder: Optional[Encoder] = None,
):
"""
AsyncZeroClient provides the asynchronous client interface for calling the ZeroServer.
Python's async/await can be used to make the calls.
Naturally async client is faster.
Zero use tcp protocol for communication.
So a connection needs to be established to make a call.
The connection creation is done lazily.
So the first call will take some time to establish the connection.
If the connection is dropped the client might timeout.
But in the next call the connection will be re-established.
Parameters
----------
host: str
Host of the ZeroServer.
port: int
Port of the ZeroServer.
default_timeout: int
Default timeout for the AsyncZeroClient for all calls.
Default is 2000 ms.
encoder: Optional[Encoder]
Encoder to encode/decode messages from/to client.
Default is msgspec.
If any other encoder is used, the server should use the same encoder.
Implement custom encoder by inheriting from `zero.encoder.Encoder`.
"""
self._address = f"tcp://{host}:{port}"
self._default_timeout = default_timeout
self._encoder = encoder or get_encoder(config.ENCODER)
self._resp_map: Dict[str, Any] = {}
self.zmqc: AsyncZeroMQClient = None # type: ignore
self.peer1 = self.peer2 = None
async def call(
self,
rpc_func_name: str,
msg: Union[int, float, str, dict, list, tuple, None],
timeout: Optional[int] = None,
return_type: Optional[Type[T]] = None,
) -> T:
"""
Call the rpc function resides on the ZeroServer.
Parameters
----------
rpc_func_name: str
Function name should be string.
This funtion should reside on the ZeroServer to get a successful response.
msg: Union[int, float, str, dict, list, tuple, None]
The only argument of the rpc function.
This should be of the same type as the rpc function's argument.
timeout: Optional[int]
Timeout for the call. In milliseconds.
Default is 2000 milliseconds.
return_type: Optional[Type[T]]
The return type of the rpc function.
If return_type is set, the response will be parsed to the return_type.
Returns
-------
T
The return value of the rpc function.
If return_type is set, the response will be parsed to the return_type.
Raises
------
TimeoutException
If the call times out or the connection is dropped.
MethodNotFoundException
If the rpc function is not found on the ZeroServer.
ConnectionException
If zeromq connection is not established.
Or zeromq cannot send the message to the server.
Or zeromq cannot receive the response from the server.
Mainly represents zmq.error.Again exception.
"""
await self._ensure_connected()
_timeout = self._default_timeout if timeout is None else timeout
expire_at = util.current_time_us() + (_timeout * 1000)
async def _poll_data():
# TODO async has issue with poller, after 3-4 calls, it returns empty
# if not await self.zmq_client.poll(_timeout):
# raise TimeoutException(f"Timeout while sending message at {self._address}")
resp = await self.zmqc.recv()
resp_id, resp_data = (
self._encoder.decode(resp)
if return_type is None
else self._encoder.decode_type(resp, Tuple[str, return_type])
)
self._resp_map[resp_id] = resp_data
# TODO try to use pipe instead of sleep
# await self.peer1.send(b"")
req_id = util.unique_id()
frames = [req_id, rpc_func_name, "" if msg is None else msg]
await self.zmqc.send(self._encoder.encode(frames))
# every request poll the data, so whenever a response comes, it will be stored in __resps
# dont need to poll again in the while loop
await _poll_data()
while req_id not in self._resp_map and util.current_time_us() <= expire_at:
# TODO the problem with the pipe is that we can miss some response
# when we come to this line
# await self.peer2.recv()
await asyncio.sleep(1e-6)
if util.current_time_us() > expire_at:
raise TimeoutException(
f"Timeout while waiting for response at {self._address}"
)
resp_data = self._resp_map.pop(req_id)
check_response(resp_data)
return resp_data
def close(self):
if self.zmqc is not None:
self.zmqc.close()
self.zmqc = None
self._resp_map = {}
async def _ensure_connected(self):
if self.zmqc is not None:
return
self._init()
await self._try_connect()
def _init(self):
self.zmqc = get_async_client(config.ZEROMQ_PATTERN, self._default_timeout)
self.zmqc.connect(self._address)
self._resp_map: Dict[str, Any] = {}
self.peer1, self.peer2 = zpipe_async(self.zmqc.context, 10000)
# TODO try to use pipe instead of sleep
# asyncio.create_task(self._poll_data())
async def _try_connect(self):
frames = [util.unique_id(), "connect", ""]
await self.zmqc.send(self._encoder.encode(frames))
self._encoder.decode(await self.zmqc.recv())
logging.info("Connected to server at %s", self._address)
async def _poll_data(self): # pragma: no cover
while True:
try:
if not await self.zmqc.poll(self._default_timeout):
continue
frames = await self.zmqc.recv()
resp_id, data = self._encoder.decode(frames)
self._resp_map[resp_id] = data
await self.peer1.send(b"")
except Exception as exc: # pylint: disable=broad-except
logging.error("Error while polling data: %s", exc)
def check_response(resp_data):
if isinstance(resp_data, dict):
if exc := resp_data.get("__zerror__function_not_found"):
raise MethodNotFoundException(exc)
if exc := resp_data.get("__zerror__server_exception"):
raise RemoteException(exc) | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/client_server/client.py | client.py |
import asyncio
import inspect
import logging
import time
from typing import Optional
from zero import config
from zero.codegen.codegen import CodeGen
from zero.encoder.protocols import Encoder
from zero.zero_mq.factory import get_worker
class _Worker:
def __init__(
self,
rpc_router: dict,
device_comm_channel: str,
encoder: Encoder,
rpc_input_type_map: dict,
rpc_return_type_map: dict,
):
self._rpc_router = rpc_router
self._device_comm_channel = device_comm_channel
self._encoder = encoder
self._rpc_input_type_map = rpc_input_type_map
self._rpc_return_type_map = rpc_return_type_map
self._loop = asyncio.new_event_loop() or asyncio.get_event_loop()
self.codegen = CodeGen(
self._rpc_router,
self._rpc_input_type_map,
self._rpc_return_type_map,
)
def start_dealer_worker(self, worker_id):
def process_message(data: bytes) -> Optional[bytes]:
try:
decoded = self._encoder.decode(data)
req_id, func_name, msg = decoded
response = self.handle_msg(func_name, msg)
return self._encoder.encode([req_id, response])
except Exception as inner_exc: # pylint: disable=broad-except
logging.exception(inner_exc)
# TODO what to return
return None
worker = get_worker(config.ZEROMQ_PATTERN, worker_id)
try:
worker.listen(self._device_comm_channel, process_message)
except KeyboardInterrupt:
logging.warning(
"Caught KeyboardInterrupt, terminating worker %d", worker_id
)
except Exception as exc: # pylint: disable=broad-except
logging.exception(exc)
finally:
logging.warning("Closing worker %d", worker_id)
worker.close()
def handle_msg(self, rpc, msg):
if rpc == "get_rpc_contract":
return self.generate_rpc_contract(msg)
if rpc == "connect":
return "connected"
if rpc not in self._rpc_router:
logging.error("Function `%s` not found!", rpc)
return {"__zerror__function_not_found": f"Function `{rpc}` not found!"}
func = self._rpc_router[rpc]
ret = None
try:
# TODO: is this a bottleneck
if inspect.iscoroutinefunction(func):
# this is blocking
ret = self._loop.run_until_complete(func(msg) if msg else func())
else:
ret = func(msg) if msg else func()
except Exception as exc: # pylint: disable=broad-except
logging.exception(exc)
ret = {"__zerror__server_exception": repr(exc)}
return ret
def generate_rpc_contract(self, msg):
try:
return self.codegen.generate_code(msg[0], msg[1])
except Exception as exc: # pylint: disable=broad-except
logging.exception(exc)
return {"__zerror__failed_to_generate_client_code": str(exc)}
@classmethod
def spawn_worker(
cls,
rpc_router: dict,
device_comm_channel: str,
encoder: Encoder,
rpc_input_type_map: dict,
rpc_return_type_map: dict,
worker_id: int,
) -> None:
"""
Spawn a worker process.
A class method is used because the worker process is spawned using multiprocessing.Process.
The class method is used to avoid pickling the class instance (which can lead to errors).
"""
# give some time for the broker to start
time.sleep(0.2)
worker = _Worker(
rpc_router,
device_comm_channel,
encoder,
rpc_input_type_map,
rpc_return_type_map,
)
worker.start_dealer_worker(worker_id) | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/client_server/worker.py | worker.py |
import logging
import os
import signal
import sys
from functools import partial
from multiprocessing.pool import Pool
from typing import Callable, Dict, Optional
import zmq.utils.win32
from zero import config
from zero.encoder import Encoder, get_encoder
from zero.utils import type_util, util
from zero.zero_mq import ZeroMQBroker, get_broker
from .worker import _Worker
# import uvloop
class ZeroServer:
def __init__(
self,
host: str = "0.0.0.0",
port: int = 5559,
encoder: Optional[Encoder] = None,
):
"""
ZeroServer registers and exposes rpc functions that can be called from a ZeroClient.
By default ZeroServer uses all of the cores for best performance possible.
A "zmq proxy" load balances the requests and runs on the main thread.
Parameters
----------
host: str
Host of the ZeroServer.
port: int
Port of the ZeroServer.
encoder: Optional[Encoder]
Encoder to encode/decode messages from/to client.
Default is msgspec.
If any other encoder is used, the client should use the same encoder.
Implement custom encoder by inheriting from `zero.encoder.Encoder`.
"""
self._broker: ZeroMQBroker = None # type: ignore
self._device_comm_channel: str = None # type: ignore
self._pool: Pool = None # type: ignore
self._device_ipc: str = None # type: ignore
self._host = host
self._port = port
self._address = f"tcp://{self._host}:{self._port}"
# to encode/decode messages from/to client
self._encoder = encoder or get_encoder(config.ENCODER)
# Stores rpc functions against their names
self._rpc_router: Dict[str, Callable] = {}
# Stores rpc functions `msg` types
self._rpc_input_type_map: Dict[str, Optional[type]] = {}
self._rpc_return_type_map: Dict[str, Optional[type]] = {}
def register_rpc(self, func: Callable):
"""
Register a function available for clients.
Function should have a single argument.
Argument and return should have a type hint.
If the function got exception, client will get None as return value.
Parameters
----------
func: Callable
RPC function.
"""
self._verify_function_name(func)
type_util.verify_function_args(func)
type_util.verify_function_input_type(func)
type_util.verify_function_return(func)
type_util.verify_function_return_type(func)
self._rpc_input_type_map[func.__name__] = type_util.get_function_input_class(
func
)
self._rpc_return_type_map[func.__name__] = type_util.get_function_return_class(
func
)
self._rpc_router[func.__name__] = func
return func
def run(self, workers: int = os.cpu_count() or 1):
"""
Run the ZeroServer. This is a blocking operation.
By default it uses all the cores available.
Ensure to run the server inside
`if __name__ == "__main__":`
As the server runs on multiple processes.
It starts a zmq proxy on the main process and spawns workers on the background.
It uses a pool of processes to spawn workers. Each worker is a zmq router.
A proxy device is used to load balance the requests.
Parameters
----------
workers: int
Number of workers to spawn.
Each worker is a zmq router and runs on a separate process.
"""
self._broker = get_broker(config.ZEROMQ_PATTERN)
# for device-worker communication
self._device_comm_channel = self._get_comm_channel()
spawn_worker = partial(
_Worker.spawn_worker,
self._rpc_router,
self._device_comm_channel,
self._encoder,
self._rpc_input_type_map,
self._rpc_return_type_map,
)
try:
self._start_server(workers, spawn_worker)
except KeyboardInterrupt:
logging.warning("Caught KeyboardInterrupt, terminating server")
except Exception as exc: # pylint: disable=broad-except
logging.exception(exc)
finally:
self._terminate_server()
def _start_server(self, workers: int, spawn_worker: Callable):
self._pool = Pool(workers)
# process termination signals
util.register_signal_term(self._sig_handler)
# TODO: by default we start the workers with processes,
# but we need support to run only router, without workers
self._pool.map_async(spawn_worker, list(range(1, workers + 1)))
# blocking
with zmq.utils.win32.allow_interrupt(self._terminate_server):
self._broker.listen(self._address, self._device_comm_channel)
def _get_comm_channel(self) -> str:
if os.name == "posix":
ipc_id = util.unique_id()
self._device_ipc = f"{ipc_id}.ipc"
return f"ipc://{ipc_id}.ipc"
# device port is used for non-posix env
return f"tcp://127.0.0.1:{util.get_next_available_port(6666)}"
def _verify_function_name(self, func):
if not isinstance(func, Callable):
raise ValueError(f"register function; not {type(func)}")
if func.__name__ in self._rpc_router:
raise ValueError(
f"cannot have two RPC function same name: `{func.__name__}`"
)
if func.__name__ in config.RESERVED_FUNCTIONS:
raise ValueError(
f"{func.__name__} is a reserved function; cannot have `{func.__name__}` "
"as a RPC function"
)
def _sig_handler(self, signum, frame): # pylint: disable=unused-argument
logging.warning("%s signal called", signal.Signals(signum).name)
self._terminate_server()
def _terminate_server(self):
logging.warning("Terminating server at %d", self._port)
if self._broker is not None:
self._broker.close()
self._terminate_pool()
self._remove_ipc()
sys.exit(0)
@util.log_error
def _remove_ipc(self):
if (
os.name == "posix"
and self._device_ipc is not None
and os.path.exists(self._device_ipc)
):
os.remove(self._device_ipc)
@util.log_error
def _terminate_pool(self):
self._pool.terminate()
self._pool.close()
self._pool.join() | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/client_server/server.py | server.py |
import asyncio
import sys
from typing import Optional
import zmq
import zmq.asyncio
from zero.error import ConnectionException, TimeoutException
class ZeroMQClient:
def __init__(self, default_timeout):
self._address = None
self._default_timeout = default_timeout
self._context = zmq.Context.instance()
self.socket: zmq.Socket = self._context.socket(zmq.DEALER)
self.socket.setsockopt(zmq.LINGER, 0) # dont buffer messages
self.socket.setsockopt(zmq.RCVTIMEO, default_timeout)
self.socket.setsockopt(zmq.SNDTIMEO, default_timeout)
self.poller = zmq.Poller()
self.poller.register(self.socket, zmq.POLLIN)
@property
def context(self):
return self._context
def connect(self, address: str) -> None:
self._address = address
self.socket.connect(address)
def close(self) -> None:
self.socket.close()
def send(self, message: bytes) -> None:
try:
self.socket.send(message, zmq.DONTWAIT)
except zmq.error.Again as exc:
raise ConnectionException(
f"Connection error for send at {self._address}"
) from exc
def poll(self, timeout: int) -> bool:
socks = dict(self.poller.poll(timeout))
return self.socket in socks
def recv(self) -> bytes:
try:
return self.socket.recv()
except zmq.error.Again as exc:
raise ConnectionException(
f"Connection error for recv at {self._address}"
) from exc
def request(self, message: bytes, timeout: Optional[int] = None) -> bytes:
try:
self.send(message)
if self.poll(timeout or self._default_timeout):
return self.recv()
raise TimeoutException(f"Timeout waiting for response from {self._address}")
except zmq.error.Again as exc:
raise ConnectionException(
f"Connection error for request at {self._address}"
) from exc
class AsyncZeroMQClient:
def __init__(self, default_timeout: int = 2000):
if sys.platform == "win32":
# windows need special event loop policy to work with zmq
asyncio.set_event_loop_policy(asyncio.WindowsSelectorEventLoopPolicy())
self._address: str = None # type: ignore
self._default_timeout = default_timeout
self._context = zmq.asyncio.Context.instance()
self.socket: zmq.asyncio.Socket = self._context.socket(zmq.DEALER)
self.socket.setsockopt(zmq.LINGER, 0) # dont buffer messages
self.socket.setsockopt(zmq.RCVTIMEO, default_timeout)
self.socket.setsockopt(zmq.SNDTIMEO, default_timeout)
self.poller = zmq.asyncio.Poller()
self.poller.register(self.socket, zmq.POLLIN)
@property
def context(self):
return self._context
def connect(self, address: str) -> None:
self._address = address
self.socket.connect(address)
def close(self) -> None:
self.socket.close()
async def send(self, message: bytes) -> None:
try:
await self.socket.send(message, zmq.DONTWAIT)
except zmq.error.Again as exc:
raise ConnectionException(
f"Connection error for send at {self._address}"
) from exc
async def poll(self, timeout: int) -> bool:
socks = dict(await self.poller.poll(timeout))
return self.socket in socks
async def recv(self) -> bytes:
try:
return await self.socket.recv() # type: ignore
except zmq.error.Again as exc:
raise ConnectionException(
f"Connection error for recv at {self._address}"
) from exc
async def request(self, message: bytes, timeout: Optional[int] = None) -> bytes:
try:
await self.send(message)
# TODO async has issue with poller, after 3-4 calls, it returns empty
# await self.poll(timeout or self._default_timeout)
return await self.recv()
except zmq.error.Again as exc:
raise ConnectionException(
f"Conection error for request at {self._address}"
) from exc | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/zero_mq/queue_device/client.py | client.py |
import logging
import signal
import socket
import sys
import time
import uuid
from typing import Callable
def get_next_available_port(port: int) -> int:
"""
Get the next available port.
Parameters
----------
port: int
Port to start with.
Returns
-------
int
Next available port.
"""
def is_port_available() -> bool:
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
sock.bind(("localhost", port))
return True
except socket.error:
return False
finally:
sock.close()
while not is_port_available():
port += 1
return port
def unique_id() -> str:
"""
Generate a unique id.
UUID without dashes.
Returns
-------
str
Unique id.
"""
return str(uuid.uuid4()).replace("-", "")
def current_time_us() -> int:
"""
Get current time in microseconds.
Returns
-------
int
Current time in microseconds.
"""
return int(time.time() * 1e6)
def register_signal_term(sigterm_handler: Callable):
"""
Register the signal term handler.
Parameters
----------
signal_term_handler: typing.Callable
Signal term handler.
"""
# this is important to catch KeyboardInterrupt
original_sigint_handler = signal.signal(signal.SIGINT, signal.SIG_IGN)
signal.signal(signal.SIGTERM, sigterm_handler)
signal.signal(signal.SIGINT, original_sigint_handler)
if sys.platform == "win32":
signal.signal(signal.SIGBREAK, sigterm_handler)
else:
signal.signal(signal.SIGQUIT, sigterm_handler)
signal.signal(signal.SIGHUP, sigterm_handler)
def log_error(func):
"""
Decorator to log errors.
"""
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except Exception as exc: # pylint: disable=broad-except
logging.exception(exc)
return None
return wrapper | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/utils/util.py | util.py |
import dataclasses
import datetime
import decimal
import enum
import typing
import uuid
from typing import Callable, Optional, get_origin, get_type_hints
import msgspec
builtin_types: typing.List = [
None,
bool,
int,
float,
str,
bytes,
bytearray,
tuple,
typing.Tuple,
list,
typing.List,
dict,
typing.Dict,
set,
typing.Set,
frozenset,
typing.FrozenSet,
]
std_lib_types: typing.List = [
datetime.datetime,
datetime.date,
datetime.time,
uuid.UUID,
decimal.Decimal,
enum.Enum,
enum.IntEnum,
dataclasses.dataclass,
]
typing_types: typing.List = [
typing.Any,
typing.Union,
typing.Optional,
]
msgspec_types: typing.List = [
msgspec.Struct,
msgspec.Raw,
]
allowed_types = builtin_types + std_lib_types + typing_types
def verify_function_args(func: Callable) -> None:
arg_count = func.__code__.co_argcount
if arg_count < 1:
return
if arg_count > 1:
raise ValueError(
f"`{func.__name__}` has more than 1 args; "
"RPC functions can have only one arg - msg, or no arg"
)
arg_name = func.__code__.co_varnames[0]
func_arg_type = get_type_hints(func)
if arg_name not in func_arg_type:
raise TypeError(
f"`{func.__name__}` has no type hinting; RPC functions must have type hints"
)
def verify_function_return(func: Callable) -> None:
return_count = func.__code__.co_argcount
if return_count > 1:
raise ValueError(
f"`{func.__name__}` has more than 1 return values; "
"RPC functions can have only one return value"
)
types = get_type_hints(func)
if not types.get("return"):
raise TypeError(
f"`{func.__name__}` has no return type hinting; RPC functions must have type hints"
)
def get_function_input_class(func: Callable) -> Optional[type]:
arg_count = func.__code__.co_argcount
if arg_count == 0:
return None
if arg_count == 1:
arg_name = func.__code__.co_varnames[0]
func_arg_type = get_type_hints(func)
return func_arg_type[arg_name]
return None
def get_function_return_class(func: Callable):
types = get_type_hints(func)
return types.get("return")
def verify_function_input_type(func: Callable):
input_type = get_function_input_class(func)
if input_type in allowed_types:
return
origin_type = get_origin(input_type)
if origin_type is not None and origin_type in allowed_types:
return
for mtype in msgspec_types:
if input_type is not None and issubclass(input_type, mtype):
return
raise TypeError(
f"{func.__name__} has type {input_type} which is not allowed; "
"allowed types are: \n" + "\n".join([str(t) for t in allowed_types])
)
def verify_function_return_type(func: Callable):
return_type = get_function_return_class(func)
if return_type in allowed_types:
return
origin_type = get_origin(return_type)
if origin_type is not None and origin_type in allowed_types:
return
for t in msgspec_types:
if issubclass(return_type, t):
return
raise TypeError(
f"{func.__name__} has return type {return_type} which is not allowed; "
"allowed types are: \n" + "\n".join([str(t) for t in allowed_types])
)
def verify_allowed_type(msg, rpc_method: Optional[str] = None):
if not isinstance(msg, tuple(allowed_types)):
method_name = f"for method `{rpc_method}`" if rpc_method else ""
raise TypeError(
f"{msg} is not allowed {method_name}; allowed types are: \n"
+ "\n".join([str(t) for t in allowed_types])
)
def verify_incoming_rpc_call_input_type(
msg, rpc_method: str, rpc_input_type_map: dict
): # pragma: no cover
input_type = rpc_input_type_map[rpc_method]
if input_type is None:
return
if input_type in builtin_types:
if input_type != type(msg):
raise TypeError(
f"{msg} is not allowed for method `{rpc_method}`; allowed type: {input_type}"
)
origin_type = get_origin(input_type)
if origin_type in builtin_types:
if origin_type != type(msg):
raise TypeError(
f"{msg} is not allowed for method `{rpc_method}`; allowed type: {input_type}"
) | zeroapi | /zeroapi-0.4.1.tar.gz/zeroapi-0.4.1/zero/utils/type_util.py | type_util.py |
0bin
====
0bin is a client side encrypted pastebin that can run without a database.
* Try it: `0bin.net <http://0bin.net>`_
* `Report a bug <https://github.com/sametmax/0bin/issues>`_
0bin allows anybody to host a pastebin while welcoming any type of content to
be pasted in it. The idea is that one can (probably...) not be legally entitled
to `moderate the pastebin content`_ as they have no way to decrypt it.
It's an Python implementation of the
`zerobin project`_ under the `WTF licence`_. It's easy to
install even if you know nothing about Python.
For now tested with IE9, and the last opera, safari, chrome and FF.
There is a `good doc <http://readthedocs.org/docs/0bin/en/latest/>`_,
but in short::
pip install zerobin
zerobin
0bin runs on Python 2.7 and Python 3.4.
How it works
=============
When creating the paste:
- the browser generates a random key;
- the pasted content is encrypted with this key using AES256;
- the encrypted pasted content is sent to the server;
- the browser receives the paste URL and adds the key in the URL hash (#).
When reading the paste:
- the browser makes the GET request to the paste URL;
- because the key is in the hash, the key is not part of the request;
- browser gets the encrypted content end decrypts it using the key;
- the pasted decrypted content is displayed and sourcecode is highlighted.
Key points:
- because the key is in the hash, the key is never sent to the server;
- therefore it won't appear in the server logs;
- all operations, including code coloration, happen on the client-side;
- the server is no more than a fancy recipient for the encrypted data.
Other features
======================
- automatic code coloration (no need to specify);
- pastebin expiration: 1 day, 1 month or never;
- burn after reading: the paste is destroyed after the first reading;
- clone paste: you can't edit a paste, but you can duplicate any of them;
- code upload: if a file is too big, you can upload it instead of using copy/paste;
- copy paste to clipboard in a click;
- get paste short URL in a click;
- own previous pastes history;
- visual hash of a paste to easily tell it apart from others in a list;
- optional command-line tool to encrypt and paste data from shell or scripts.
Technologies used
==================
- Python_
- `The Bottle Python Web microframework`_
- SJCL_ (js crypto tools)
- jQuery_
- Bootstrap_, the Twitter HTML5/CSS3 framework
- VizHash.js_ to create visual hashes from pastes
- Cherrypy_ (server only)
- `node.js`_ (for optional command-line tool only)
Known issues
============
- 0bin uses several HTML5/CSS3 features that are not widely supported. In that case we handle the degradation as gracefully as we can.
- The "copy to clipboard" feature is buggy under linux. It's flash, so we won't fix it. Better wait for the HTML5 clipboard API to be implemented in major browsers.
- The pasted content size limit check is not accurate. It's just a safety net, so we think it's ok.
- Some url shorteners and other services storing URLs break the encryption key. We will sanitize the URL as much as we can, but there is a limit to what we can do.
What does 0bin not implement?
=================================
* Request throttling. It would be inefficient to do it at the app level, and web servers have robust implementations for it.
* Hash collision prevention: the ratio "probability it happens/consequence seriousness" `is not worth it`_
* Comments: it was initially planed. But comes with a lot of issues so we chose to focus on lower hanging fruits.
.. _moderate the pastebin content: http://www.zdnet.com/blog/security/pastebin-to-hunt-for-hacker-pastes-anonymous-cries-censorship/11336
.. _zerobin project: https://github.com/sebsauvage/ZeroBin/
.. _Python: https://en.wikipedia.org/wiki/Python_(programming_language)
.. _The Bottle Python Web microframework: http://bottlepy.org/
.. _SJCL: http://crypto.stanford.edu/sjcl/
.. _jQuery: http://jquery.com/
.. _Bootstrap: http://twitter.github.com/bootstrap/
.. _VizHash.js: https://github.com/sametmax/VizHash.js
.. _Cherrypy: http://www.cherrypy.org/
.. _node.js: http://nodejs.org/
.. _is not worth it: http://stackoverflow.com/questions/201705/how-many-random-elements-before-md5-produces-collisions
.. _WTF licence: http://en.wikipedia.org/wiki/WTFPL
Contributing
=============
Please fork the project, clone your repository and add the original repo as an upstream remote to keep yours in sync.
For small fixes (typo and such), you can work on master.
For features, you should create a dedicated branch.
In any case, if you modify Javascript or CSS files, you shall run compress.sh afterward to provide the minified files. It requires your to have yui-compressor installed (apt-get install yui-compressor on the debian family).
We don't require you to rebase/merge, ordinary merging is alright.
Once it's ready, just request a PR.
| zerobin | /zerobin-0.5.tar.gz/zerobin-0.5/README.rst | README.rst |
from datetime import datetime
from . import ZBValidateStatus, ZBValidateSubStatus
from ._zb_response import ZBResponse
class ZBValidateResponse(ZBResponse):
"""This is the response for the GET /validate request."""
address: str = None
"""The email address you are validating."""
status: ZBValidateStatus = None
"""one of [valid, invalid, catch-all, unknown, spamtrap, abuse, do_not_mail]"""
sub_status: ZBValidateSubStatus = None
"""one of [antispam_system, greylisted, mail_server_temporary_error, forcible_disconnect,
mail_server_did_not_respond, timeout_exceeded, failed_smtp_connection, mailbox_quota_exceeded,
exception_occurred, possible_trap, role_based, global_suppression, mailbox_not_found, no_dns_entries,
failed_syntax_check, possible_typo, unroutable_ip_address, leading_period_removed, does_not_accept_mail,
alias_address, role_based_catch_all, disposable, toxic, alternate, mx_forward, blocked, allowed]"""
account: str = None
"""The portion of the email address before the "@" symbol."""
domain: str = None
"""The portion of the email address after the "@" symbol."""
did_you_mean: str = None
"""Suggestive Fix for an email typo"""
domain_age_days: str = None
"""Age of the email domain in days or [null]."""
free_email: bool = False
"""[true/false] If the email comes from a free provider."""
mx_found: bool = False
"""[true/false] Does the domain have an MX record."""
mx_record: str = None
""" The preferred MX record of the domain"""
smtp_provider: str = None
"""The SMTP Provider of the email or [null] [BETA]."""
firstname: str = None
"""The first name of the owner of the email when available or [null]."""
lastname: str = None
"""The last name of the owner of the email when available or [null]."""
gender: str = None
"""The gender of the owner of the email when available or [null]."""
city: str = None
"""The city of the IP passed in or [null]"""
region: str = None
"""The region/state of the IP passed in or [null]"""
zipcode: str = None
"""The zipcode of the IP passed in or [null]"""
country: str = None
"""The country of the IP passed in or [null]"""
processed_at: datetime = None
"""The UTC time the email was validated."""
def __init__(self, data):
super().__init__(data)
self.status = None if self.status is None else ZBValidateStatus(self.status)
self.sub_status = None if self.sub_status is None else ZBValidateSubStatus(self.sub_status)
if self.processed_at is not None:
self.processed_at = datetime.strptime(self.processed_at, "%Y-%m-%d %H:%M:%S.%f") | zerobounceindiasdk | /zerobounceindiasdk-1.0.0-py3-none-any.whl/zerobouncesdk/zb_validate_response.py | zb_validate_response.py |
from datetime import date
import os
from typing import List
import requests
from . import (
ZBApiException,
ZBClientException,
ZBGetCreditsResponse,
ZBGetApiUsageResponse,
ZBGetActivityResponse,
ZBValidateResponse,
ZBValidateBatchElement,
ZBValidateBatchResponse,
ZBSendFileResponse,
ZBFileStatusResponse,
ZBGetFileResponse,
ZBDeleteFileResponse,
)
class ZeroBounce:
"""The ZeroBounce main class. All the requests are implemented here."""
BASE_URL = "https://api.zerobounce.in/v2"
BULK_BASE_URL = "https://bulkapi.zerobounce.in/v2"
SCORING_BASE_URL = "https://bulkapi.zerobounce.in/v2/scoring"
def __init__(self, api_key: str):
if not api_key.strip():
raise ZBClientException("Empty parameter: api_key")
self._api_key = api_key
def _request(self, url, response_class, params=None):
if not params:
params = {}
params["api_key"] = self._api_key
response = requests.get(url, params=params)
try:
json_response = response.json()
except ValueError as e:
raise ZBApiException from e
error = json_response.pop("error", None)
if error:
raise ZBApiException(error)
return response_class(json_response)
def get_credits(self):
"""Tells you how many credits you have left on your account.
It's simple, fast and easy to use.
Raises
------
ZBApiException
Returns
-------
response: ZBGetCreditsResponse
Returns a ZBGetCreditsResponse object if the request was successful
"""
return self._request(
f"{self.BASE_URL}/getcredits",
ZBGetCreditsResponse
)
def get_api_usage(self, start_date: date, end_date: date):
"""Returns the API usage between the given dates.
Parameters
----------
start_date: date
The start date of when you want to view API usage
end_date: date
The end date of when you want to view API usage
Raises
------
ZBApiException
Returns
-------
response: ZBGetApiUsageResponse
Returns a ZBGetApiUsageResponse object if the request was successful
"""
return self._request(
f"{self.BASE_URL}/getapiusage",
ZBGetApiUsageResponse,
params={
"start_date": start_date.strftime("%Y-%m-%d"),
"end_date": end_date.strftime("%Y-%m-%d"),
}
)
def get_activity(self, email: str):
"""Allows you to gather insights into your subscribers' overall email engagement
Parameters
----------
email: str
The email whose activity you want to check
Raises
------
ZBApiException
Returns
-------
response: ZBGetActivityResponse
Returns a ZBGetActivityResponse object if the request was successful
"""
return self._request(
f"{self.BASE_URL}/activity",
ZBGetActivityResponse,
params={"email": email}
)
def validate(self, email: str, ip_address: str = None):
"""Validates the given email address.
Parameters
----------
email: str
The email address you want to validate
ip_address: str or None
The IP Address the email signed up from (Can be blank)
Raises
------
ZBApiException
Returns
-------
response: ZBValidateResponse
Returns a ZBValidateResponse object if the request was successful
"""
return self._request(
f"{self.BASE_URL}/validate",
ZBValidateResponse,
params={
"email": email,
"ip_address": ip_address,
}
)
def validate_batch(self, email_batch: List[ZBValidateBatchElement]):
"""Allows you to send us batches up to 100 emails at a time.
Parameters
----------
email_batch: List[ZBValidateBatchElement]
Array of ZBValidateBatchElement
Raises
------
ZBApiException
ZBClientException
Returns
-------
response: ZBValidateBatchResponse
Returns a ZBValidateBatchResponse object if the request was successful
"""
if not email_batch:
raise ZBClientException("Empty parameter: email_batch")
response = requests.post(
f"{self.BULK_BASE_URL}/validatebatch",
json={
"api_key": self._api_key,
"email_batch": [
batch_element.to_json() for batch_element in email_batch
],
}
)
json_response = response.json()
try:
return ZBValidateBatchResponse(json_response)
except KeyError:
error = list(json_response.values())[0]
raise ZBApiException(error)
def _send_file(
self,
scoring: bool,
file_path: str,
email_address_column: int,
data: dict,
):
data.update({
"api_key": self._api_key,
"email_address_column": email_address_column,
})
with open(file_path, "rb") as file:
response = requests.post(
f"{self.SCORING_BASE_URL if scoring else self.BULK_BASE_URL}/sendfile",
data=data,
files={
"file": (os.path.basename(file_path), file, "text/csv")
},
)
try:
json_response = response.json()
except ValueError as e:
raise ZBApiException from e
return ZBSendFileResponse(json_response)
def send_file(
self,
file_path: str,
email_address_column: int,
return_url: str = None,
first_name_column: int = None,
last_name_column: int = None,
gender_column: int = None,
ip_address_column: int = None,
has_header_row: bool = False,
remove_duplicate: bool = True,
):
"""Allows user to send a file for bulk email validation
Parameters
----------
file_path: str
The path of the csv or txt file to be submitted.
email_address_column: int
The column index of the email address in the file. Index starts from 1.
return_url: str or None
The URL will be used to call back when the validation is completed.
first_name_column: int or None
The column index of the first name column.
last_name_column: int or None
The column index of the last name column.
gender_column: int or None
The column index of the gender column.
ip_address_column: int or None
The IP Address the email signed up from.
has_header_row: bool
If the first row from the submitted file is a header row.
remove_duplicate: bool
If you want the system to remove duplicate emails.
Raises
------
ZBApiException
Returns
-------
response: ZBSendFileResponse
Returns a ZBSendFileResponse object if the request was successful
"""
data = {}
if return_url is not None:
data["return_url"] = return_url
if first_name_column is not None:
data["first_name_column"] = first_name_column
if last_name_column is not None:
data["last_name_column"] = last_name_column
if gender_column is not None:
data["gender_column"] = gender_column
if ip_address_column is not None:
data["ip_address_column"] = ip_address_column
if has_header_row is not None:
data["has_header_row"] = has_header_row
if remove_duplicate is not None:
data["remove_duplicate"] = remove_duplicate
return self._send_file(False, file_path, email_address_column, data)
def scoring_send_file(
self,
file_path: str,
email_address_column: int,
return_url: str = None,
has_header_row: bool = False,
remove_duplicate: bool = True,
):
"""Allows user to send a file for bulk email scoring
Parameters
----------
file_path: str
The path of the csv or txt file to be submitted.
email_address_column: int
The column index of the email address in the file. Index starts from 1.
return_url: str or None
The URL will be used to call back when the validation is completed.
has_header_row: bool
If the first row from the submitted file is a header row.
remove_duplicate: bool
If you want the system to remove duplicate emails.
Raises
------
ZBApiException
Returns
-------
response: ZBSendFileResponse
Returns a ZBSendFileResponse object if the request was successful
"""
data = {}
if return_url is not None:
data["return_url"] = return_url
if has_header_row is not None:
data["has_header_row"] = has_header_row
if remove_duplicate is not None:
data["remove_duplicate"] = remove_duplicate
return self._send_file(True, file_path, email_address_column, data)
def _file_status(self, scoring: bool, file_id: str):
if not file_id.strip():
raise ZBClientException("Empty parameter: file_id")
return self._request(
f"{self.SCORING_BASE_URL if scoring else self.BULK_BASE_URL}/filestatus",
ZBFileStatusResponse,
params={"file_id": file_id}
)
def file_status(self, file_id: str):
"""Returns the file processing status for the file that has been submitted
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
Raises
------
ZBClientException
Returns
-------
response: ZBSendFileResponse
Returns a ZBSendFileResponse object if the request was successful
"""
return self._file_status(False, file_id)
def scoring_file_status(self, file_id: str):
"""Returns the file processing status for the file that has been submitted
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
Raises
------
ZBClientException
Returns
-------
response: ZBSendFileResponse
Returns a ZBSendFileResponse object if the request was successful
"""
return self._file_status(True, file_id)
def _get_file(self, scoring: bool, file_id: str, download_path: str):
if not file_id.strip():
raise ZBClientException("Empty parameter: file_id")
response = requests.get(
f"{self.SCORING_BASE_URL if scoring else self.BULK_BASE_URL}/getfile",
params={
"api_key": self._api_key,
"file_id": file_id,
}
)
if response.headers['Content-Type'] == "application/json":
json_response = response.json()
return ZBGetFileResponse(json_response)
dirname = os.path.dirname(download_path)
if dirname:
os.makedirs(dirname, exist_ok=True)
with open(download_path, "wb") as f:
f.write(response.content)
return ZBGetFileResponse({"local_file_path": download_path})
def get_file(self, file_id: str, download_path: str):
"""Allows you to get the validation results for the file you submitted
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
download_path: str
The local path where the file will be downloaded.
Raises
------
ZBClientException
Returns
-------
response: ZBGetFileResponse
Returns a ZBGetFileResponse object if the request was successful
"""
return self._get_file(False, file_id, download_path)
def scoring_get_file(self, file_id: str, download_path: str):
"""Allows you to get the validation results for the file you submitted
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
download_path: str
The local path where the file will be downloaded.
Raises
------
ZBClientException
Returns
-------
response: ZBGetFileResponse
Returns a ZBGetFileResponse object if the request was successful
"""
return self._get_file(True, file_id, download_path)
def _delete_file(self, scoring: bool, file_id: str):
if not file_id.strip():
raise ZBClientException("Empty parameter: file_id")
return self._request(
f"{self.SCORING_BASE_URL if scoring else self.BULK_BASE_URL}/deletefile",
ZBDeleteFileResponse,
params={"file_id": file_id}
)
def delete_file(self, file_id: str):
"""Deletes the file that you submitted
Please note that the file can be deleted only when its status is Complete
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
Raises
------
ZBApiException
ZBClientException
Returns
-------
response: ZBDeleteFileResponse
Returns a ZBDeleteFileResponse object if the request was successful
"""
return self._delete_file(False, file_id)
def scoring_delete_file(self, file_id: str):
"""Deletes the file that you submitted
Please note that the file can be deleted only when its status is Complete
Parameters
----------
file_id: str
The returned file ID when calling sendfile API.
Raises
------
ZBApiException
ZBClientException
Returns
-------
response: ZBDeleteFileResponse
Returns a ZBDeleteFileResponse object if the request was successful
"""
return self._delete_file(True, file_id) | zerobounceindiasdk | /zerobounceindiasdk-1.0.0-py3-none-any.whl/zerobouncesdk/zerobouncesdk.py | zerobouncesdk.py |
from datetime import date, datetime
from ._zb_response import ZBResponse
class ZBGetApiUsageResponse(ZBResponse):
"""This is the response for the GET /apiusage request."""
total: int = 0
"""Total number of times the API has been called"""
status_valid: int = 0
"""Total valid email addresses returned by the API"""
status_invalid: int = 0
"""Total invalid email addresses returned by the API"""
status_catch_all: int = 0
"""Total catch-all email addresses returned by the API"""
status_do_not_mail: int = 0
"""Total do not mail email addresses returned by the API"""
status_spamtrap: int = 0
"""Total spamtrap email addresses returned by the API"""
status_unknown: int = 0
"""Total unknown email addresses returned by the API"""
sub_status_toxic: int = 0
"""Total number of times the API has a sub status of toxic"""
sub_status_disposable: int = 0
"""Total number of times the API has a sub status of disposable"""
sub_status_role_based: int = 0
"""Total number of times the API has a sub status of role_based"""
sub_status_possible_trap: int = 0
"""Total number of times the API has a sub status of possible_trap"""
sub_status_global_suppression: int = 0
"""Total number of times the API has a sub status of global_suppression"""
sub_status_timeout_exceeded: int = 0
"""Total number of times the API has a sub status of timeout_exceeded"""
sub_status_mail_server_temporary_error: int = 0
"""Total number of times the API has a sub status of mail_server_temporary_error"""
sub_status_mail_server_did_not_respond: int = 0
"""Total number of times the API has a sub status of mail_server_did_not_respond"""
sub_status_greylisted: int = 0
"""Total number of times the API has a sub status of greylisted"""
sub_status_antispam_system: int = 0
"""Total number of times the API has a sub status of antispam_system"""
sub_status_does_not_accept_mail: int = 0
"""Total number of times the API has a sub status of does_not_accept_mail"""
sub_status_exception_occurred: int = 0
"""Total number of times the API has a sub status of exception_occurred"""
sub_status_failed_syntax_check: int = 0
"""Total number of times the API has a sub status of failed_syntax_check"""
sub_status_mailbox_not_found: int = 0
"""Total number of times the API has a sub status of mailbox_not_found"""
sub_status_unroutable_ip_address: int = 0
"""Total number of times the API has a sub status of unroutable_ip_address"""
sub_status_possible_typo: int = 0
"""Total number of times the API has a sub status of possible_typo"""
sub_status_no_dns_entries: int = 0
"""Total number of times the API has a sub status of no_dns_entries"""
sub_status_role_based_catch_all: int = 0
"""Total number of times the API has a sub status of role_based_catch_all"""
sub_status_mailbox_quota_exceeded: int = 0
"""Total number of times the API has a sub status of mailbox_quota_exceeded"""
sub_status_forcible_disconnect: int = 0
"""Total number of times the API has a sub status of forcible_disconnect"""
sub_status_failed_smtp_connection: int = 0
"""Total number of times the API has a sub status of failed_smtp_connection"""
sub_status_mx_forward: int = 0
"""Total number of times the API has a sub status of mx_forward"""
sub_status_alternate: int = 0
"""Total number of times the API has a sub status of alternate"""
sub_status_allowed: int = 0
"""Total number of times the API has a sub status of allowed"""
sub_status_blocked: int = 0
"""Total number of times the API has a sub status of blocked"""
start_date: date = None
"""Start date of query"""
end_date: date = None
"""End date of query"""
def __init__(self, data):
super().__init__(data)
if self.start_date is not None:
self.start_date = datetime.strptime(self.start_date, "%m/%d/%Y").date()
if self.end_date is not None:
self.end_date = datetime.strptime(self.end_date, "%m/%d/%Y").date() | zerobounceindiasdk | /zerobounceindiasdk-1.0.0-py3-none-any.whl/zerobouncesdk/zb_get_api_usage_response.py | zb_get_api_usage_response.py |
======
2.0.9
======
|Maturity| |license gpl|
Overview
========
Zeroincombenze® continuous testing framework for python and bash programs
-------------------------------------------------------------------------
This library can run unit test of target package software.
Supported languages are *python* (through z0testlib.py) and *bash* (through z0testrc)
*zerobug* supports test automation, aggregation of tests into collections
and independence of the tests from the reporting framework.
The *zerobug* module provides all code that make it easy to support testing
both for python programs both for bash scripts.
*zerobug* shows execution test with a message like "n/tot message"
where *n* is current unit test and *tot* is the total unit test to execute,
that is a sort of advancing test progress.
You can use z0bug_odoo that is the odoo integration to test Odoo modules.
*zerobug* is built on follow concepts:
* test main - it is a main program to executes all test runners
* test runner - it is a program to executes one or more test suites
* test suite - it is a collection of test cases
* test case - it is a smallest unit test
The main file is the command **zerobug** of this package; it searches for test runner files
named `[id_]test_` where 'id' is the shor name of testing package.
Test suite is a collection of test case named `test_[0-9]+` inside the runner file,
executed in sorted order.
Every suit can contains one or more test case, the smallest unit test;
every unit test terminates with success or with failure.
Because **zerobug** can show total number of unit test to execute, it runs tests
in 2 passes. In the first pass it counts the number of test, in second pass executes really
it. This behavior can be overridden by -0 switch.
|
Features
--------
* Test execution log
* Autodiscovery test modules and functions
* Python 2.7+ and 3.5+
* coverage integration
* travis integration
|
Usage
=====
::
usage: zerobug [-h] [-B] [-C] [-e] [-J] [-k] [-l file] [-N] [-n] [-O]
[-p file_list] [-q] [-r number] [-s number] [-V] [-v] [-x] [-X]
[-z number] [-0] [-1] [-3]
Regression test on z0bug_odoo
optional arguments:
-h, --help show this help message and exit
-B, --debug trace msgs in zerobug.tracehis
-C, --no-coverage run tests without coverage
-e, --echo enable echoing even if not interactive tty
-J load travisrc
-k, --keep keep current logfile
-l file, --logname file
set logfile name
-N, --new create new logfile
-n, --dry-run count and display # unit tests
-O load odoorc
-p file_list, --search-pattern file_list
test file pattern
-q, --quiet run tests without output (quiet mode)
-r number, --restart number
set to counted tests, 1st one next to this
-s number, --start number
deprecated
-V, --version show program's version number and exit
-v, --verbose verbose mode
-x, --qsanity like -X but run silently
-X, --esanity execute test library sanity check and exit
-z number, --end number
display total # tests when execute them
-0, --no-count no count # unit tests
-1, --coverage run tests for coverage (obsolete)
-3, --python3 use python3
Code example
------------
*zerobug* makes avaiable following functions to test:
|
`Z0BUG.setup(ctx)` (python)
`Z0BUG_setup` (bash)
Setup for test. It is called before all tests.
|
`Z0BUG.teardown(ctx)` (python)
`Z0BUG_teardown` (bash)
Setup for test. It is called after all tests.
|
`Z0BUG.build_os_tree(ctx, list_of_paths)` (python)
`Z0BUG_build_os_tree list_of_paths` (bash)
Build a full os tree from supplied list.
If python, list of paths is a list of strings.
If bash, list is one string of paths separated by spaces.
Function reads list of paths and then create all directories.
If directory is an absolute path, it is created with the supplied path.
If directory is a relative path, the directory is created under "tests/res" directory.
Warning!
To check is made is parent dir does not exit. Please, supply path from parent
to children, if you want to build a nested tree.
::
# (python)
from zerobug import Z0BUG
class RegressionTest():
def __init__(self, Z0BUG):
self.Z0BUG = Z0BUG
def test_01(self, ctx):
os_tree = ['10.0',
'10.0/addons',
'10.0/odoo',]
root = self.Z0BUG.build_os_tree(ctx, os_tree)
::
# (bash)
Z0BUG_setup() {
Z0BUG_build_os_tree "10.0 10.0/addons 10.0/odoo"
}
|
`Z0BUG.remove_os_tree(ctx, list_of_paths)` (python)
`Z0BUG_remove_os_tree list_of_paths` (bash)
Remove a full os tree created by `build_os_tree`
If python, list of paths is a list of strings.
If bash, list is a string of paths separated by spaces.
Function reads list of paths and remove all directories.
If directory is an absolute path, the supplied path is dropped.
If directory is a relative path, the directory is dropped from tests/res directory.
Warning!
This function remove directory and all sub-directories without any control.
::
# (python)
from zerobug import Z0BUG
class RegressionTest():
def __init__(self, Z0BUG):
self.Z0BUG = Z0BUG
def test_01(self, ctx):
os_tree = ['10.0',
'10.0/addons',
'10.0/odoo',]
root = self.Z0BUG.remove_os_tree(ctx, os_tree)
|
`Z0BUG.build_odoo_env(ctx, version)` (python)
Like build_os_tree but create a specific odoo os tree.
::
# (python)
from zerobug import Z0BUG
from zerobug import Z0testOdoo
class RegressionTest():
def __init__(self, Z0BUG):
self.Z0BUG = Z0BUG
def test_01(self, ctx):
root = Z0testOdoo.build_odoo_env(ctx, '10.0')
|
`Z0BUG.git_clone(remote, reponame, branch, odoo_path, force=None)` (python)
Execute git clone of `remote:reponame:branch` into local directory `odoo_path`.
In local travis emulation, if repository uses local repository, if exists.
Return odoo root directory
::
# (python)
from zerobug import Z0BUG
from zerobug import Z0testOdoo
from zerobug import Z0BUG
class RegressionTest():
def __init__(self, Z0BUG):
self.Z0BUG = Z0BUG
def test_01(self, ctx):
remote = 'OCA'
reponame = 'OCB'
branch = '10.0'
odoo_path = '/opt/odoo/10.0'
Z0testOdoo.git_clone(remote, reponame, branch, odoo_path)
Unit test can run in package directory or in ./tests directory of package.
Every test can inquire internal context.
::
this_fqn parent caller full qualified name (i.e. /opt/odoo/z0bug.pyc)
this parent name, w/o extension (i.e. z0bug)
ctr test counter [both bash and python tests]
dry_run dry-run (do nothing) [opt_dry_run in bash test] "-n"
esanity True if required sanity check with echo "-X"
max_test # of tests to execute [both bash and python tests] "-z"
min_test # of test executed before this one "-r"
on_error behavior after error, 'continue' or 'raise' (default)
opt_echo True if echo test result onto std output "-e"
opt_new new log file [both bash and python tests] "-N"
opt_noctr do not count # tests [both bash and python tests] "-0"
opt_verbose show messages during execution "-v"
logfn real trace log file name from switch "-l"
qsanity True if required sanity check w/o echo "-x"
run4cover Run tests for coverage (use coverage run rather python) "-C"
python3 Execute test in python3 "-3"
run_daemon True if execution w/o tty as stdio
run_on_top Top test (not parent)
run_tty Opposite of run_daemon
tlog default tracelog file name
_run_autotest True if running auto-test
_parser cmd line parser
_opt_obj parser obj, to acquire optional switches
WLOGCMD override opt_echo; may be None, 'echo', 'echo-1', 'echo-0'
Z0 this library object
Environment read:
DEV_ENVIRONMENT Name of package; if set test is under travis emulator control
COVERAGE_PROCESS_START
Name of coverage conf file; if set test is running for coverage
|
|
Getting started
===============
Installation
------------
Zeroincombenze tools require:
* Linux Centos 7/8 or Debian 9/10 or Ubuntu 18/20/22
* python 2.7+, some tools require python 3.6+
* bash 5.0+
Stable version via Python Package
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
::
pip install
|
Current version via Git
~~~~~~~~~~~~~~~~~~~~~~~
::
cd $HOME
git clone https://github.com/zeroincombenze/tools.git
cd ./tools
./install_tools.sh -p
source $HOME/devel/activate_tools
Upgrade
-------
Stable version via Python Package
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
::
pip install -U
|
Current version via Git
~~~~~~~~~~~~~~~~~~~~~~~
::
cd $HOME
./install_tools.sh -U
source $HOME/devel/activate_tools
History
-------
2.0.9 (2023-07-12)
~~~~~~~~~~~~~~~~~~
* [IMP] zerobug implementation with unittest
* [FIX] z0testlib.py: build_odoo_env, odoo-bin / openerp-server are executable
* [FIX] z0testlib.py: minor fixes
2.0.7 (2023-05-14)
~~~~~~~~~~~~~~~~~~
* [IMP] travis_run_pypi_tests: new switch -p PATTERN
2.0.6 (2023-05-08)
~~~~~~~~~~~~~~~~~~
* [IMP] Now all_tests is ignored
* [IMP] Build Odoo environment for Odoo 16.0
2.0.5 (2023-03-24)
~~~~~~~~~~~~~~~~~~
* [FIX] travis_install_env: ensure list_requirements is executable
* [IMP] flake8 configuration
* [IMP] coveralls and codecov are not more dependencies
* [IMP] Test for Odoo 16.0
2.0.4 (2022-12-08)
~~~~~~~~~~~~~~~~~~
* [FIX] run_pypi_test: best recognition of python version
* [FIX] build_cmd: best recognition of python version
* [FIX] travis_install_env: ensure coverage version
* [IMP] odoo environment to test more precise
2.0.3 (2022-11-08)
~~~~~~~~~~~~~~~~~~
* [IMP] npm management
2.0.2.1 (2022-10-31)
~~~~~~~~~~~~~~~~~~~~
* [FIX] Odoo 11.0+
* [FIX] Ensure coverage 5.0+
2.0.2 (2022-10-20)
~~~~~~~~~~~~~~~~~~
* [IMP] Stable version
2.0.1.1 (2022-10-12)
~~~~~~~~~~~~~~~~~~~~
* [IMP] minor improvements
2.0.1 (2022-10-12)
~~~~~~~~~~~~~~~~~~
* [IMP] stable version
2.0.0.2 (2022-10-05)
~~~~~~~~~~~~~~~~~~~~
* [IMP] travis_install_env: python2 tests
2.0.0.1 (2022-09-06)
~~~~~~~~~~~~~~~~~~~~
* [FIX] travis_install_env: minor fixes
* [IMP] z0testlib: show coverage result
|
|
Credits
=======
Copyright
---------
SHS-AV s.r.l. <https://www.shs-av.com/>
Contributors
------------
* Antonio Maria Vigliotti <[email protected]>
|
This module is part of project.
Last Update / Ultimo aggiornamento:
.. |Maturity| image:: https://img.shields.io/badge/maturity-Beta-yellow.png
:target: https://odoo-community.org/page/development-status
:alt:
.. |license gpl| image:: https://img.shields.io/badge/licence-AGPL--3-blue.svg
:target: http://www.gnu.org/licenses/agpl-3.0-standalone.html
:alt: License: AGPL-3
.. |license opl| image:: https://img.shields.io/badge/licence-OPL-7379c3.svg
:target: https://www.odoo.com/documentation/user/9.0/legal/licenses/licenses.html
:alt: License: OPL
.. |Tech Doc| image:: https://www.zeroincombenze.it/wp-content/uploads/ci-ct/prd/button-docs-2.svg
:target: https://wiki.zeroincombenze.org/en/Odoo/2.0/dev
:alt: Technical Documentation
.. |Help| image:: https://www.zeroincombenze.it/wp-content/uploads/ci-ct/prd/button-help-2.svg
:target: https://wiki.zeroincombenze.org/it/Odoo/2.0/man
:alt: Technical Documentation
.. |Try Me| image:: https://www.zeroincombenze.it/wp-content/uploads/ci-ct/prd/button-try-it-2.svg
:target: https://erp2.zeroincombenze.it
:alt: Try Me
.. |Zeroincombenze| image:: https://avatars0.githubusercontent.com/u/6972555?s=460&v=4
:target: https://www.zeroincombenze.it/
:alt: Zeroincombenze
.. |en| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/flags/en_US.png
:target: https://www.facebook.com/Zeroincombenze-Software-gestionale-online-249494305219415/
.. |it| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/flags/it_IT.png
:target: https://www.facebook.com/Zeroincombenze-Software-gestionale-online-249494305219415/
.. |check| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/check.png
.. |no_check| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/no_check.png
.. |menu| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/menu.png
.. |right_do| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/right_do.png
.. |exclamation| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/exclamation.png
.. |warning| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/warning.png
.. |same| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/same.png
.. |late| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/late.png
.. |halt| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/halt.png
.. |info| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/awesome/info.png
.. |xml_schema| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/certificates/iso/icons/xml-schema.png
:target: https://github.com/zeroincombenze/grymb/blob/master/certificates/iso/scope/xml-schema.md
.. |DesktopTelematico| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/certificates/ade/icons/DesktopTelematico.png
:target: https://github.com/zeroincombenze/grymb/blob/master/certificates/ade/scope/Desktoptelematico.md
.. |FatturaPA| image:: https://raw.githubusercontent.com/zeroincombenze/grymb/master/certificates/ade/icons/fatturapa.png
:target: https://github.com/zeroincombenze/grymb/blob/master/certificates/ade/scope/fatturapa.md
.. |chat_with_us| image:: https://www.shs-av.com/wp-content/chat_with_us.gif
:target: https://t.me/Assitenza_clienti_powERP
| zerobug | /zerobug-2.0.9.tar.gz/zerobug-2.0.9/README.rst | README.rst |
import numpy as np
#import faiss
import ngtpy
from numpy import genfromtxt
from anytree import Node, RenderTree
import operator
import os.path
import time
class DataPoint():
def __init__ (self, id):
self.point_id = id
self.class_id = 0 # 0 not clustered but -1 means a noise
self.refer_to = []
self.referred_by = []
self.reference = []
self.visited = False
self.homogeneity = 0
class DENMUNE():
def __init__ (self, data, k_nearest=0, max_knn=200, dp_dis=[]):
self.data = data
self.dp_count = self.data.shape[0]
self.dp_dim = self.data.shape[1]
self.k_nearest = k_nearest
self.max_knn =max_knn
self.dp_dis = dp_dis
self.DataPoints = []
self.ClassPoints = {}
self.KernelPoints = []
self.init_DataPoints()
self.kd_NGT()
self.load_DataPoints() # load_DataPoints must come after kd_NGT()
self.compute_Links()
#self.semi_init_DataPoints #I think it is usuful with csharp and cnune only
self.find_Noise()
self.sort_DataPoints()
self.prepare_Clusters()
self.attach_Points()
self.print_Clusters()
self.save_Dis()
return None # __init__ should return Nine
def save_Dis(self):
return self.dp_dis
def save_Data(self):
return self.data
def kd_NGT(self):
if len(self.dp_dis) == 0:
ngtpy.create(b"tmp", self.dp_dim)
index = ngtpy.Index(b"tmp")
index.batch_insert(self.data)
index.save()
k= self.max_knn
start = time.time()
self.dp_dis = []
for i in range(self.dp_count):
query = self.data[i]
result = index.search(query, k+1) [1:] #we skip first distance from a point to itself
self.dp_dis.append(result)
end = time.time()
#print ('Calc proximity tooks: ', end-start)
self.data = None
def getValue(self, dic, what, who, other=False):
if what == 'max' and who == 'key' and other==False:
val = max(dic.items(), key=operator.itemgetter(0))[0] #max key
elif what == 'max' and who == 'key' and other==True:
val = max(dic.items(), key=operator.itemgetter(0))[1] #max key==>Value
elif what == 'max' and who == 'value' and other==True:
val = max(dic.items(), key=operator.itemgetter(1))[0] #max value==>key
elif what == 'max' and who == 'value'and other == False:
val = max(dic.items(), key=operator.itemgetter(1))[1] #max value
return val
def init_DataPoints(self):
self.DataPoints = []
self.KernelPoints = []
for i in range (self.dp_count):
dp = DataPoint(i)
#no need since datapoint is initialised with these values
"""
dp.refer_to = []
dp.referred_by = []
dp.reference = []
dp.class_id = 0
dp.visited = False
dp.homogeneity = 0.0
"""
self.DataPoints.append(dp)
return 0
def semi_init_DataPoints(self):
for dp in self.DataPoints:
dp.visited= False
dp.class_id = 0
dp.homogeneity = 0
return 0
def find_Noise(self):
self.ClassPoints[-1] = Node(-1, parent=None)
self.ClassPoints[0] = Node(0, parent=None)
for i in range (self.dp_count):
dp = self.DataPoints[i]
if len(dp.reference) == 0:
dp.class_id = -1
self.ClassPoints[i] = self.ClassPoints[-1] # Node(-1, parent=None) # this it is a noise
else : # at least one point
dp.class_id = 0 #this is allready set initally
self.ClassPoints[i] = self.ClassPoints[0] #Node(0, parent=None) # this it is a non-clustered point
# where -1 is noise and 0 is non-clustered
return 0
def sort_DataPoints(self):
for dp in self.DataPoints:
if len(dp.reference) != 0:
self.KernelPoints.append([dp.point_id, dp.homogeneity])
self.KernelPoints = self.sort_Tuple(self.KernelPoints, reverse=True)
return 0
def compute_Links(self):
start = time.time()
for i in range (self.dp_count) :
for pos in self.DataPoints[i].refer_to:
for pos2 in self.DataPoints[i].referred_by:
if pos[0] == pos2[0]:
self.DataPoints[i].reference.append(pos)
break
for i in range (self.dp_count) :
self.DataPoints[i].referred_by = self.sort_Tuple(self.DataPoints[i].referred_by, reverse=False)
self.DataPoints[i].reference = self.sort_Tuple(self.DataPoints[i].reference, reverse=False)
homogeneity = (100 * len(self.DataPoints[i].referred_by)) + len(self.DataPoints[i].reference)
self.DataPoints[i].homogeneity = homogeneity
end = time.time()
#print ('Compute links tools:', end-start)
return 0
def sort_Tuple(self, li, reverse=False):
# reverse = None (Sorts in Ascending order)
# key is set to sort using second element of
# sublist lambda has been used
li.sort(key = lambda x: x[1], reverse=reverse)
return li
def load_DataPoints(self):
#initialize datapoints to its default values
self.init_DataPoints()
for i in range (self.dp_count) :
result = self.dp_dis[i]
for k, o in enumerate(result):
if k >= self.k_nearest:
break
#if k != 0:
_dis = round(o[1], 6)
_point = o[0]
self.DataPoints[i].refer_to.append([_point, _dis])
self.DataPoints[_point].referred_by.append([i, _dis])
#print (i, k, _dis, _point)
return 0
def prepare_Clusters(self):
start = time.time()
class_id = 0
for dp_kern in self.KernelPoints:
dp_core = self.DataPoints[dp_kern[0]]
#remember no strong points & weak points in Tirann
#all points with at least one refernce are considered (ignore noises)
if len(dp_core.reference) > 0 and len(dp_core.referred_by) >= len(dp_core.refer_to):
class_id += 1
dp_core.visited = True
dp_core.class_id = class_id
self.ClassPoints[class_id] = Node(class_id, parent=None)
max_class = -1
weight_map = {}
#Class_Points[class_id] = new TreeCls::Node(class_id)
for pos2 in dp_core.reference :
# if DataPoints[*pos2].visited && visited was tested not to affect on results, so you can ommit it
if self.DataPoints[pos2[0]].class_id > 0 and len(self.DataPoints[pos2[0]].referred_by) >= len(self.DataPoints[pos2[0]].refer_to):
# this condition is a must, as some points may be visited but not classified yet
# maa we may neglect is noise as long as it is in our refernce points
_cls = self.DataPoints[pos2[0]].class_id
_class_id = self.ClassPoints[_cls].root.name
#_class_id = _cls
if _class_id not in weight_map.keys():
weight_map[_class_id] = 1
else:
weight_map[_class_id] += 1
elif self.DataPoints[pos2[0]].visited == False:
self.DataPoints[pos2[0]].visited = True # this point is visited but not classified yet
while len(weight_map) > 0 :
#weight_no = self.getValue(dic=weight_map, what='max', who='value') # no need to it in DenMune
max_class = self.getValue(dic=weight_map, what='max', who='value', other=True)
if max_class != -1 and max_class != class_id:
self.ClassPoints[max_class].parent = self.ClassPoints[class_id]
del weight_map[max_class]
for i in range (self.dp_count):
clsid = self.DataPoints[i].class_id
clsroot = self.ClassPoints[clsid].root.name
self.DataPoints[i].class_id = clsroot
end = time.time()
#print ('prepare Clusters took:', end-start)
return 0
def attach_Points(self):
start = time.time()
olditr = 0
newitr = -1
while olditr != newitr:
newitr = olditr
olditr = 0
for pos in self.KernelPoints:
if self.DataPoints[pos[0]].class_id == 0 :
self.DataPoints[pos[0]].class_id = self.attach_StrongPoint(pos[0])
olditr += 1
olditr = 0
newitr = -1
while olditr != newitr:
newitr = olditr
olditr = 0
for pos in self.KernelPoints:
if self.DataPoints[pos[0]].class_id == 0:
self.DataPoints[pos[0]].class_id = self.attach_WeakPoint(pos[0])
olditr += 1
end = time.time()
#print ('Attach points tooks:', end-start)
def attach_StrongPoint(self, point_id):
weight_map = {}
max_class = 0 # max_class in attach point = 0 , thus if a point faild to merge with any cluster, it has one more time
#to merge in attach weak point
dp_core = self.DataPoints[point_id]
if len(dp_core.reference) != 0:
dp_core.visited = True
for pos2 in dp_core.reference:
if self.DataPoints[pos2[0]].visited == True and len(self.DataPoints[pos2[0]].referred_by) >= len(self.DataPoints[pos2[0]].refer_to):
clsid = self.DataPoints[pos2[0]].class_id
clsroot = self.ClassPoints[clsid].root.name
self.DataPoints[pos2[0]].class_id = clsroot
if clsroot not in weight_map.keys():
weight_map[clsroot] = 1
else:
weight_map[clsroot] += 1
if len (weight_map) != 0:
weight_map = dict(sorted(weight_map.items()))
max_class = self.getValue(dic=weight_map, what='max', who='value', other=True)
return max_class # this will return get_Root(max_class) as we computed earlier _class_id = get_Root(_cls)
def attach_WeakPoint(self, point_id):
weight_map = {}
max_class = -1 # max_class in attach weak point = -1 , thus if a point faild to merge with any cluster it is a noise
dp_core = self.DataPoints[point_id]
if len(dp_core.reference) != 0:
dp_core.visited = True
for pos2 in dp_core.reference:
if self.DataPoints[pos2[0]].visited == True :
clsid = self.DataPoints[pos2[0]].class_id
clsroot = self.ClassPoints[clsid].root.name
self.DataPoints[pos2[0]].class_id = clsroot
if clsroot not in weight_map.keys():
weight_map[clsroot] = 1
else:
weight_map[clsroot] += 1
if len (weight_map) != 0:
weight_map = dict(sorted(weight_map.items()))
max_class = self.getValue(dic=weight_map, what='max', who='value', other=True)
return max_class # this will return get_Root(max_class) as we computed earlier _class_id = get_Root(_cls)
def print_Clusters(self):
solution_file = 'solution.txt'
#solution_file = alg_name + "/" + data_type + "/solution.txt"
if os.path.isfile(solution_file):
os.remove(solution_file)
pred_list = []
for dp in self.DataPoints:
pred_list.append(dp.class_id)
with open(solution_file, 'w') as f:
f.writelines("%s\n" % pred for pred in pred_list)
return 0 | zerobytes | /zerobytes-0.0.0.0.tar.gz/zerobytes-0.0.0.0/src/denmune/denmune.py | denmune.py |
from sklearn.metrics import confusion_matrix
import os.path
from sklearn import metrics
import numpy as np
from numpy import genfromtxt
import matplotlib.pyplot as plt
import seaborn as sns
import sklearn.cluster as cluster
import time
from sklearn.manifold import TSNE
sns.set_context('poster')
sns.set_color_codes()
plot_kwds = {'alpha' : 0.99, 's' : 80, 'linewidths':0}
class Functions():
def backline(self):
print('\r', end='')
def calc_Metrics(self, labels_true, labels_pred):
# Score the clustering
from sklearn.metrics.cluster import adjusted_mutual_info_score #2010
from sklearn.metrics.cluster import adjusted_rand_score # 1985
from sklearn.metrics import f1_score
from sklearn.metrics import accuracy_score
from sklearn.metrics import precision_score
from sklearn.metrics import recall_score
#from sklearn.metrics import davies_bouldin_score
# #1975 - 2001 ## no ground truth ##Values closer to zero indicate a better partition.
from sklearn.metrics import pairwise_distances # for calinski_harabasz_score
## also known as the Variance Ratio Criterion - can be used to evaluate the model,
## where a higher Calinski-Harabasz score relates to a model with better defined clusters.
from sklearn import metrics # for homogeneity, completeness, fowlkes
## homogeneity: each cluster contains only members of a single class.
## completeness: all members of a given class are assigned to the same cluster.
#v-measure the harmonic mean of homogeneity and completeness called V-measure 2007
acc = metrics.accuracy_score(labels_true, labels_pred, normalize=False)
#mi = metrics.mutual_info_score(labels_true, labels_pred)
#print("mutual_info_score: %f." % mi)
nmi = metrics.normalized_mutual_info_score(labels_true, labels_pred, average_method='arithmetic')
#print("normalized_mutual_info_score: %f." % nmi)
ami = adjusted_mutual_info_score(labels_true, labels_pred, average_method='arithmetic')
#print("Adjusted_mutual_info_score: %f." % adj_nmi)
homogeneity = metrics.homogeneity_score(labels_true, labels_pred)
#print("homogeneity_score: %f." % homogeneity_score)
completeness = metrics.completeness_score(labels_true, labels_pred)
#print("completeness_score: %f." % completeness_score)
f1_weight = metrics.f1_score(labels_true, labels_pred, average='weighted')
#f1_micro = metrics.f1_score(labels_true, labels_pred, average='micro')
#f1_macro = metrics.f1_score(labels_true, labels_pred, average='macro')
#print("f1_score: %f." % f1_score)
ari = adjusted_rand_score(labels_true, labels_pred)
#print("adjusted_rand_score: %f." % adj_rand)
f1 = f1_weight
val = ['0', acc, f1, nmi, ami, ari, homogeneity, completeness, '0' ]
return val
def plot_clusters(self, data, labels, alg_name, dp_name, show=False):
palette = sns.color_palette('deep', np.unique(labels).max() + 1) #deep, dark, bright, muted, pastel, colorblind
colors = [palette[x] if x >= 0 else (0.0, 0.0, 0.0) for x in labels]
plt.scatter(data.T[0], data.T[1], c=colors, **plot_kwds)
frame = plt.gca()
frame.axes.get_xaxis().set_visible(False)
frame.axes.get_yaxis().set_visible(False)
plt.savefig('results/' + alg_name +'/images/' + alg_name + '_' + dp_name + '.png')
if show == True:
plt.show()
plt.clf() # this is a must to clear figures if you plot continously
return 0
def generate_tsne(self, f_name, k):
filename = f_name
filename2 = 'data/' + filename + '-2d'
file_to_save = filename2 + ".txt"
data = genfromtxt('data/' + filename + '.txt' , delimiter='\t')
dim_two = TSNE(n_components=k).fit_transform(data)
mystr = ""
data_len = len (dim_two)
for i in range(data_len):
for n in range(k):
mystr += str(round(dim_two[i][n],6))
if (n < k-1):mystr += '\t'
if (n == k-1): mystr += '\n'
file_to_save = filename2 + ".txt"
text_file = open(file_to_save, "w")
text_file.write(mystr)
text_file.close()
return file_to_save
def match_Labels(self, labels_pred, labels_true):
list_pred = labels_pred.tolist()
pred_set = set(list_pred)
index = []
x = 1
old_item = labels_true[0]
old_x = 0
for item in labels_true:
if item != old_item:
count = x - old_x
index.append([old_x, old_item, count])
old_item = item
old_x = x
x+= 1
ln = len(labels_true)
count = x - old_x
index.append([old_x, old_item, count])
index[0][2] = index[0][2] -1
index.sort(key=lambda x: x[2], reverse=True)
lebeled = []
for n in range (len(index)):
newval = index[n][1]
max_class = max(set(list_pred), key = list_pred[index[n][0]:index[n][0]+index[n][2]-1].count)
if max_class not in lebeled:
list_pred = [newval if x==max_class else x for x in list_pred]
lebeled.append(newval)
list_pred = np.array(list_pred)
list_pred = list_pred.astype(np.int64)
return list_pred | zerobytes | /zerobytes-0.0.0.0.tar.gz/zerobytes-0.0.0.0/src/denmune/functions.py | functions.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Metrics_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module IceMX
_M_IceMX = Ice.openModule('IceMX')
# Start of module IceMX
__name__ = 'IceMX'
if 'TopicMetrics' not in _M_IceMX.__dict__:
_M_IceMX.TopicMetrics = Ice.createTempClass()
class TopicMetrics(_M_IceMX.Metrics):
"""
Provides information on IceStorm topics.
Members:
published -- Number of events published on the topic by publishers.
forwarded -- Number of events forwarded on the topic by IceStorm topic links.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, published=0, forwarded=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.published = published
self.forwarded = forwarded
def ice_id(self):
return '::IceMX::TopicMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::TopicMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_TopicMetrics)
__repr__ = __str__
_M_IceMX._t_TopicMetrics = IcePy.defineValue('::IceMX::TopicMetrics', TopicMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('published', (), IcePy._t_long, False, 0),
('forwarded', (), IcePy._t_long, False, 0)
))
TopicMetrics._ice_type = _M_IceMX._t_TopicMetrics
_M_IceMX.TopicMetrics = TopicMetrics
del TopicMetrics
if 'SubscriberMetrics' not in _M_IceMX.__dict__:
_M_IceMX.SubscriberMetrics = Ice.createTempClass()
class SubscriberMetrics(_M_IceMX.Metrics):
"""
Provides information on IceStorm subscribers.
Members:
queued -- Number of queued events.
outstanding -- Number of outstanding events.
delivered -- Number of forwarded events.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, queued=0, outstanding=0, delivered=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.queued = queued
self.outstanding = outstanding
self.delivered = delivered
def ice_id(self):
return '::IceMX::SubscriberMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::SubscriberMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_SubscriberMetrics)
__repr__ = __str__
_M_IceMX._t_SubscriberMetrics = IcePy.defineValue('::IceMX::SubscriberMetrics', SubscriberMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('queued', (), IcePy._t_int, False, 0),
('outstanding', (), IcePy._t_int, False, 0),
('delivered', (), IcePy._t_long, False, 0)
))
SubscriberMetrics._ice_type = _M_IceMX._t_SubscriberMetrics
_M_IceMX.SubscriberMetrics = SubscriberMetrics
del SubscriberMetrics
# End of module IceMX
Ice.sliceChecksums["::IceMX::SubscriberMetrics"] = "ab5eddbb2d0449f94b4808b6cf92552"
Ice.sliceChecksums["::IceMX::TopicMetrics"] = "afc516f773371c41f4f612d9e9629c" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceStorm/Metrics_ice.py | Metrics_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.SliceChecksumDict_ice
import IceStorm.Metrics_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module IceMX
_M_IceMX = Ice.openModule('IceMX')
# Start of module IceStorm
_M_IceStorm = Ice.openModule('IceStorm')
__name__ = 'IceStorm'
_M_IceStorm.__doc__ = """
A messaging service with support for federation. In contrast to
most other messaging or event services, IceStorm supports typed
events, meaning that broadcasting a message over a federation is as
easy as invoking a method on an interface.
"""
if 'Topic' not in _M_IceStorm.__dict__:
_M_IceStorm._t_TopicDisp = IcePy.declareClass('::IceStorm::Topic')
_M_IceStorm._t_TopicPrx = IcePy.declareProxy('::IceStorm::Topic')
if 'LinkInfo' not in _M_IceStorm.__dict__:
_M_IceStorm.LinkInfo = Ice.createTempClass()
class LinkInfo(object):
"""
Information on the topic links.
Members:
theTopic -- The linked topic.
name -- The name of the linked topic.
cost -- The cost of traversing this link.
"""
def __init__(self, theTopic=None, name='', cost=0):
self.theTopic = theTopic
self.name = name
self.cost = cost
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceStorm.LinkInfo):
return NotImplemented
else:
if self.theTopic != other.theTopic:
return False
if self.name != other.name:
return False
if self.cost != other.cost:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceStorm._t_LinkInfo)
__repr__ = __str__
_M_IceStorm._t_LinkInfo = IcePy.defineStruct('::IceStorm::LinkInfo', LinkInfo, (), (
('theTopic', (), _M_IceStorm._t_TopicPrx),
('name', (), IcePy._t_string),
('cost', (), IcePy._t_int)
))
_M_IceStorm.LinkInfo = LinkInfo
del LinkInfo
if '_t_LinkInfoSeq' not in _M_IceStorm.__dict__:
_M_IceStorm._t_LinkInfoSeq = IcePy.defineSequence('::IceStorm::LinkInfoSeq', (), _M_IceStorm._t_LinkInfo)
if '_t_QoS' not in _M_IceStorm.__dict__:
_M_IceStorm._t_QoS = IcePy.defineDictionary('::IceStorm::QoS', (), IcePy._t_string, IcePy._t_string)
if 'LinkExists' not in _M_IceStorm.__dict__:
_M_IceStorm.LinkExists = Ice.createTempClass()
class LinkExists(Ice.UserException):
"""
This exception indicates that an attempt was made to create a link
that already exists.
Members:
name -- The name of the linked topic.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::LinkExists'
_M_IceStorm._t_LinkExists = IcePy.defineException('::IceStorm::LinkExists', LinkExists, (), False, None, (('name', (), IcePy._t_string, False, 0),))
LinkExists._ice_type = _M_IceStorm._t_LinkExists
_M_IceStorm.LinkExists = LinkExists
del LinkExists
if 'NoSuchLink' not in _M_IceStorm.__dict__:
_M_IceStorm.NoSuchLink = Ice.createTempClass()
class NoSuchLink(Ice.UserException):
"""
This exception indicates that an attempt was made to remove a
link that does not exist.
Members:
name -- The name of the link that does not exist.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::NoSuchLink'
_M_IceStorm._t_NoSuchLink = IcePy.defineException('::IceStorm::NoSuchLink', NoSuchLink, (), False, None, (('name', (), IcePy._t_string, False, 0),))
NoSuchLink._ice_type = _M_IceStorm._t_NoSuchLink
_M_IceStorm.NoSuchLink = NoSuchLink
del NoSuchLink
if 'AlreadySubscribed' not in _M_IceStorm.__dict__:
_M_IceStorm.AlreadySubscribed = Ice.createTempClass()
class AlreadySubscribed(Ice.UserException):
"""
This exception indicates that an attempt was made to subscribe
a proxy for which a subscription already exists.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::AlreadySubscribed'
_M_IceStorm._t_AlreadySubscribed = IcePy.defineException('::IceStorm::AlreadySubscribed', AlreadySubscribed, (), False, None, ())
AlreadySubscribed._ice_type = _M_IceStorm._t_AlreadySubscribed
_M_IceStorm.AlreadySubscribed = AlreadySubscribed
del AlreadySubscribed
if 'InvalidSubscriber' not in _M_IceStorm.__dict__:
_M_IceStorm.InvalidSubscriber = Ice.createTempClass()
class InvalidSubscriber(Ice.UserException):
"""
This exception indicates that an attempt was made to subscribe
a proxy that is null.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::InvalidSubscriber'
_M_IceStorm._t_InvalidSubscriber = IcePy.defineException('::IceStorm::InvalidSubscriber', InvalidSubscriber, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
InvalidSubscriber._ice_type = _M_IceStorm._t_InvalidSubscriber
_M_IceStorm.InvalidSubscriber = InvalidSubscriber
del InvalidSubscriber
if 'BadQoS' not in _M_IceStorm.__dict__:
_M_IceStorm.BadQoS = Ice.createTempClass()
class BadQoS(Ice.UserException):
"""
This exception indicates that a subscription failed due to an
invalid QoS.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::BadQoS'
_M_IceStorm._t_BadQoS = IcePy.defineException('::IceStorm::BadQoS', BadQoS, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
BadQoS._ice_type = _M_IceStorm._t_BadQoS
_M_IceStorm.BadQoS = BadQoS
del BadQoS
_M_IceStorm._t_Topic = IcePy.defineValue('::IceStorm::Topic', Ice.Value, -1, (), False, True, None, ())
if 'TopicPrx' not in _M_IceStorm.__dict__:
_M_IceStorm.TopicPrx = Ice.createTempClass()
class TopicPrx(Ice.ObjectPrx):
"""
Get the name of this topic.
Arguments:
context -- The request context for the invocation.
Returns: The name of the topic.
"""
def getName(self, context=None):
return _M_IceStorm.Topic._op_getName.invoke(self, ((), context))
"""
Get the name of this topic.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNameAsync(self, context=None):
return _M_IceStorm.Topic._op_getName.invokeAsync(self, ((), context))
"""
Get the name of this topic.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getName(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_getName.begin(self, ((), _response, _ex, _sent, context))
"""
Get the name of this topic.
Arguments:
Returns: The name of the topic.
"""
def end_getName(self, _r):
return _M_IceStorm.Topic._op_getName.end(self, _r)
"""
Get a proxy to a publisher object for this topic. To publish
data to a topic, the publisher calls getPublisher and then
casts to the topic type. An unchecked cast must be used on this
proxy. If a replicated IceStorm deployment is used this call
may return a replicated proxy.
Arguments:
context -- The request context for the invocation.
Returns: A proxy to publish data on this topic.
"""
def getPublisher(self, context=None):
return _M_IceStorm.Topic._op_getPublisher.invoke(self, ((), context))
"""
Get a proxy to a publisher object for this topic. To publish
data to a topic, the publisher calls getPublisher and then
casts to the topic type. An unchecked cast must be used on this
proxy. If a replicated IceStorm deployment is used this call
may return a replicated proxy.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getPublisherAsync(self, context=None):
return _M_IceStorm.Topic._op_getPublisher.invokeAsync(self, ((), context))
"""
Get a proxy to a publisher object for this topic. To publish
data to a topic, the publisher calls getPublisher and then
casts to the topic type. An unchecked cast must be used on this
proxy. If a replicated IceStorm deployment is used this call
may return a replicated proxy.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getPublisher(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_getPublisher.begin(self, ((), _response, _ex, _sent, context))
"""
Get a proxy to a publisher object for this topic. To publish
data to a topic, the publisher calls getPublisher and then
casts to the topic type. An unchecked cast must be used on this
proxy. If a replicated IceStorm deployment is used this call
may return a replicated proxy.
Arguments:
Returns: A proxy to publish data on this topic.
"""
def end_getPublisher(self, _r):
return _M_IceStorm.Topic._op_getPublisher.end(self, _r)
"""
Get a non-replicated proxy to a publisher object for this
topic. To publish data to a topic, the publisher calls
getPublisher and then casts to the topic type. An unchecked
cast must be used on this proxy.
Arguments:
context -- The request context for the invocation.
Returns: A proxy to publish data on this topic.
"""
def getNonReplicatedPublisher(self, context=None):
return _M_IceStorm.Topic._op_getNonReplicatedPublisher.invoke(self, ((), context))
"""
Get a non-replicated proxy to a publisher object for this
topic. To publish data to a topic, the publisher calls
getPublisher and then casts to the topic type. An unchecked
cast must be used on this proxy.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNonReplicatedPublisherAsync(self, context=None):
return _M_IceStorm.Topic._op_getNonReplicatedPublisher.invokeAsync(self, ((), context))
"""
Get a non-replicated proxy to a publisher object for this
topic. To publish data to a topic, the publisher calls
getPublisher and then casts to the topic type. An unchecked
cast must be used on this proxy.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNonReplicatedPublisher(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_getNonReplicatedPublisher.begin(self, ((), _response, _ex, _sent, context))
"""
Get a non-replicated proxy to a publisher object for this
topic. To publish data to a topic, the publisher calls
getPublisher and then casts to the topic type. An unchecked
cast must be used on this proxy.
Arguments:
Returns: A proxy to publish data on this topic.
"""
def end_getNonReplicatedPublisher(self, _r):
return _M_IceStorm.Topic._op_getNonReplicatedPublisher.end(self, _r)
"""
Subscribe with the given qos to this topic. A
per-subscriber publisher object is returned.
Arguments:
theQoS -- The quality of service parameters for this subscription.
subscriber -- The subscriber's proxy.
context -- The request context for the invocation.
Returns: The per-subscriber publisher object.
Throws:
AlreadySubscribed -- Raised if the subscriber object is already subscribed.
BadQoS -- Raised if the requested quality of service is unavailable or invalid.
InvalidSubscriber -- Raised if the subscriber object is null.
"""
def subscribeAndGetPublisher(self, theQoS, subscriber, context=None):
return _M_IceStorm.Topic._op_subscribeAndGetPublisher.invoke(self, ((theQoS, subscriber), context))
"""
Subscribe with the given qos to this topic. A
per-subscriber publisher object is returned.
Arguments:
theQoS -- The quality of service parameters for this subscription.
subscriber -- The subscriber's proxy.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def subscribeAndGetPublisherAsync(self, theQoS, subscriber, context=None):
return _M_IceStorm.Topic._op_subscribeAndGetPublisher.invokeAsync(self, ((theQoS, subscriber), context))
"""
Subscribe with the given qos to this topic. A
per-subscriber publisher object is returned.
Arguments:
theQoS -- The quality of service parameters for this subscription.
subscriber -- The subscriber's proxy.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_subscribeAndGetPublisher(self, theQoS, subscriber, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_subscribeAndGetPublisher.begin(self, ((theQoS, subscriber), _response, _ex, _sent, context))
"""
Subscribe with the given qos to this topic. A
per-subscriber publisher object is returned.
Arguments:
theQoS -- The quality of service parameters for this subscription.
subscriber -- The subscriber's proxy.
Returns: The per-subscriber publisher object.
Throws:
AlreadySubscribed -- Raised if the subscriber object is already subscribed.
BadQoS -- Raised if the requested quality of service is unavailable or invalid.
InvalidSubscriber -- Raised if the subscriber object is null.
"""
def end_subscribeAndGetPublisher(self, _r):
return _M_IceStorm.Topic._op_subscribeAndGetPublisher.end(self, _r)
"""
Unsubscribe the given subscriber.
Arguments:
subscriber -- The proxy of an existing subscriber.
context -- The request context for the invocation.
"""
def unsubscribe(self, subscriber, context=None):
return _M_IceStorm.Topic._op_unsubscribe.invoke(self, ((subscriber, ), context))
"""
Unsubscribe the given subscriber.
Arguments:
subscriber -- The proxy of an existing subscriber.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def unsubscribeAsync(self, subscriber, context=None):
return _M_IceStorm.Topic._op_unsubscribe.invokeAsync(self, ((subscriber, ), context))
"""
Unsubscribe the given subscriber.
Arguments:
subscriber -- The proxy of an existing subscriber.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_unsubscribe(self, subscriber, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_unsubscribe.begin(self, ((subscriber, ), _response, _ex, _sent, context))
"""
Unsubscribe the given subscriber.
Arguments:
subscriber -- The proxy of an existing subscriber.
"""
def end_unsubscribe(self, _r):
return _M_IceStorm.Topic._op_unsubscribe.end(self, _r)
"""
Create a link to the given topic. All events originating
on this topic will also be sent to linkTo.
Arguments:
linkTo -- The topic to link to.
cost -- The cost to the linked topic.
context -- The request context for the invocation.
Throws:
LinkExists -- Raised if a link to the same topic already exists.
"""
def link(self, linkTo, cost, context=None):
return _M_IceStorm.Topic._op_link.invoke(self, ((linkTo, cost), context))
"""
Create a link to the given topic. All events originating
on this topic will also be sent to linkTo.
Arguments:
linkTo -- The topic to link to.
cost -- The cost to the linked topic.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def linkAsync(self, linkTo, cost, context=None):
return _M_IceStorm.Topic._op_link.invokeAsync(self, ((linkTo, cost), context))
"""
Create a link to the given topic. All events originating
on this topic will also be sent to linkTo.
Arguments:
linkTo -- The topic to link to.
cost -- The cost to the linked topic.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_link(self, linkTo, cost, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_link.begin(self, ((linkTo, cost), _response, _ex, _sent, context))
"""
Create a link to the given topic. All events originating
on this topic will also be sent to linkTo.
Arguments:
linkTo -- The topic to link to.
cost -- The cost to the linked topic.
Throws:
LinkExists -- Raised if a link to the same topic already exists.
"""
def end_link(self, _r):
return _M_IceStorm.Topic._op_link.end(self, _r)
"""
Destroy the link from this topic to the given topic linkTo.
Arguments:
linkTo -- The topic to destroy the link to.
context -- The request context for the invocation.
Throws:
NoSuchLink -- Raised if a link to the topic does not exist.
"""
def unlink(self, linkTo, context=None):
return _M_IceStorm.Topic._op_unlink.invoke(self, ((linkTo, ), context))
"""
Destroy the link from this topic to the given topic linkTo.
Arguments:
linkTo -- The topic to destroy the link to.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def unlinkAsync(self, linkTo, context=None):
return _M_IceStorm.Topic._op_unlink.invokeAsync(self, ((linkTo, ), context))
"""
Destroy the link from this topic to the given topic linkTo.
Arguments:
linkTo -- The topic to destroy the link to.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_unlink(self, linkTo, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_unlink.begin(self, ((linkTo, ), _response, _ex, _sent, context))
"""
Destroy the link from this topic to the given topic linkTo.
Arguments:
linkTo -- The topic to destroy the link to.
Throws:
NoSuchLink -- Raised if a link to the topic does not exist.
"""
def end_unlink(self, _r):
return _M_IceStorm.Topic._op_unlink.end(self, _r)
"""
Retrieve information on the current links.
Arguments:
context -- The request context for the invocation.
Returns: A sequence of LinkInfo objects.
"""
def getLinkInfoSeq(self, context=None):
return _M_IceStorm.Topic._op_getLinkInfoSeq.invoke(self, ((), context))
"""
Retrieve information on the current links.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLinkInfoSeqAsync(self, context=None):
return _M_IceStorm.Topic._op_getLinkInfoSeq.invokeAsync(self, ((), context))
"""
Retrieve information on the current links.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLinkInfoSeq(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_getLinkInfoSeq.begin(self, ((), _response, _ex, _sent, context))
"""
Retrieve information on the current links.
Arguments:
Returns: A sequence of LinkInfo objects.
"""
def end_getLinkInfoSeq(self, _r):
return _M_IceStorm.Topic._op_getLinkInfoSeq.end(self, _r)
"""
Retrieve the list of subscribers for this topic.
Arguments:
context -- The request context for the invocation.
Returns: The sequence of Ice identities for the subscriber objects.
"""
def getSubscribers(self, context=None):
return _M_IceStorm.Topic._op_getSubscribers.invoke(self, ((), context))
"""
Retrieve the list of subscribers for this topic.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSubscribersAsync(self, context=None):
return _M_IceStorm.Topic._op_getSubscribers.invokeAsync(self, ((), context))
"""
Retrieve the list of subscribers for this topic.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSubscribers(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_getSubscribers.begin(self, ((), _response, _ex, _sent, context))
"""
Retrieve the list of subscribers for this topic.
Arguments:
Returns: The sequence of Ice identities for the subscriber objects.
"""
def end_getSubscribers(self, _r):
return _M_IceStorm.Topic._op_getSubscribers.end(self, _r)
"""
Destroy the topic.
Arguments:
context -- The request context for the invocation.
"""
def destroy(self, context=None):
return _M_IceStorm.Topic._op_destroy.invoke(self, ((), context))
"""
Destroy the topic.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def destroyAsync(self, context=None):
return _M_IceStorm.Topic._op_destroy.invokeAsync(self, ((), context))
"""
Destroy the topic.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_destroy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Topic._op_destroy.begin(self, ((), _response, _ex, _sent, context))
"""
Destroy the topic.
Arguments:
"""
def end_destroy(self, _r):
return _M_IceStorm.Topic._op_destroy.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceStorm.TopicPrx.ice_checkedCast(proxy, '::IceStorm::Topic', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceStorm.TopicPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceStorm::Topic'
_M_IceStorm._t_TopicPrx = IcePy.defineProxy('::IceStorm::Topic', TopicPrx)
_M_IceStorm.TopicPrx = TopicPrx
del TopicPrx
_M_IceStorm.Topic = Ice.createTempClass()
class Topic(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceStorm::Topic')
def ice_id(self, current=None):
return '::IceStorm::Topic'
@staticmethod
def ice_staticId():
return '::IceStorm::Topic'
def getName(self, current=None):
"""
Get the name of this topic.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getName' not implemented")
def getPublisher(self, current=None):
"""
Get a proxy to a publisher object for this topic. To publish
data to a topic, the publisher calls getPublisher and then
casts to the topic type. An unchecked cast must be used on this
proxy. If a replicated IceStorm deployment is used this call
may return a replicated proxy.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getPublisher' not implemented")
def getNonReplicatedPublisher(self, current=None):
"""
Get a non-replicated proxy to a publisher object for this
topic. To publish data to a topic, the publisher calls
getPublisher and then casts to the topic type. An unchecked
cast must be used on this proxy.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getNonReplicatedPublisher' not implemented")
def subscribeAndGetPublisher(self, theQoS, subscriber, current=None):
"""
Subscribe with the given qos to this topic. A
per-subscriber publisher object is returned.
Arguments:
theQoS -- The quality of service parameters for this subscription.
subscriber -- The subscriber's proxy.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AlreadySubscribed -- Raised if the subscriber object is already subscribed.
BadQoS -- Raised if the requested quality of service is unavailable or invalid.
InvalidSubscriber -- Raised if the subscriber object is null.
"""
raise NotImplementedError("servant method 'subscribeAndGetPublisher' not implemented")
def unsubscribe(self, subscriber, current=None):
"""
Unsubscribe the given subscriber.
Arguments:
subscriber -- The proxy of an existing subscriber.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'unsubscribe' not implemented")
def link(self, linkTo, cost, current=None):
"""
Create a link to the given topic. All events originating
on this topic will also be sent to linkTo.
Arguments:
linkTo -- The topic to link to.
cost -- The cost to the linked topic.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
LinkExists -- Raised if a link to the same topic already exists.
"""
raise NotImplementedError("servant method 'link' not implemented")
def unlink(self, linkTo, current=None):
"""
Destroy the link from this topic to the given topic linkTo.
Arguments:
linkTo -- The topic to destroy the link to.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NoSuchLink -- Raised if a link to the topic does not exist.
"""
raise NotImplementedError("servant method 'unlink' not implemented")
def getLinkInfoSeq(self, current=None):
"""
Retrieve information on the current links.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getLinkInfoSeq' not implemented")
def getSubscribers(self, current=None):
"""
Retrieve the list of subscribers for this topic.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSubscribers' not implemented")
def destroy(self, current=None):
"""
Destroy the topic.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceStorm._t_TopicDisp)
__repr__ = __str__
_M_IceStorm._t_TopicDisp = IcePy.defineClass('::IceStorm::Topic', Topic, (), None, ())
Topic._ice_type = _M_IceStorm._t_TopicDisp
Topic._op_getName = IcePy.Operation('getName', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_string, False, 0), ())
Topic._op_getPublisher = IcePy.Operation('getPublisher', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Topic._op_getNonReplicatedPublisher = IcePy.Operation('getNonReplicatedPublisher', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Topic._op_subscribeAndGetPublisher = IcePy.Operation('subscribeAndGetPublisher', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceStorm._t_QoS, False, 0), ((), IcePy._t_ObjectPrx, False, 0)), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceStorm._t_AlreadySubscribed, _M_IceStorm._t_InvalidSubscriber, _M_IceStorm._t_BadQoS))
Topic._op_unsubscribe = IcePy.Operation('unsubscribe', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_ObjectPrx, False, 0),), (), None, ())
Topic._op_link = IcePy.Operation('link', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceStorm._t_TopicPrx, False, 0), ((), IcePy._t_int, False, 0)), (), None, (_M_IceStorm._t_LinkExists,))
Topic._op_unlink = IcePy.Operation('unlink', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceStorm._t_TopicPrx, False, 0),), (), None, (_M_IceStorm._t_NoSuchLink,))
Topic._op_getLinkInfoSeq = IcePy.Operation('getLinkInfoSeq', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_IceStorm._t_LinkInfoSeq, False, 0), ())
Topic._op_getSubscribers = IcePy.Operation('getSubscribers', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Ice._t_IdentitySeq, False, 0), ())
Topic._op_destroy = IcePy.Operation('destroy', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
_M_IceStorm.Topic = Topic
del Topic
if '_t_TopicDict' not in _M_IceStorm.__dict__:
_M_IceStorm._t_TopicDict = IcePy.defineDictionary('::IceStorm::TopicDict', (), IcePy._t_string, _M_IceStorm._t_TopicPrx)
if 'TopicExists' not in _M_IceStorm.__dict__:
_M_IceStorm.TopicExists = Ice.createTempClass()
class TopicExists(Ice.UserException):
"""
This exception indicates that an attempt was made to create a topic
that already exists.
Members:
name -- The name of the topic that already exists.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::TopicExists'
_M_IceStorm._t_TopicExists = IcePy.defineException('::IceStorm::TopicExists', TopicExists, (), False, None, (('name', (), IcePy._t_string, False, 0),))
TopicExists._ice_type = _M_IceStorm._t_TopicExists
_M_IceStorm.TopicExists = TopicExists
del TopicExists
if 'NoSuchTopic' not in _M_IceStorm.__dict__:
_M_IceStorm.NoSuchTopic = Ice.createTempClass()
class NoSuchTopic(Ice.UserException):
"""
This exception indicates that an attempt was made to retrieve a
topic that does not exist.
Members:
name -- The name of the topic that does not exist.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceStorm::NoSuchTopic'
_M_IceStorm._t_NoSuchTopic = IcePy.defineException('::IceStorm::NoSuchTopic', NoSuchTopic, (), False, None, (('name', (), IcePy._t_string, False, 0),))
NoSuchTopic._ice_type = _M_IceStorm._t_NoSuchTopic
_M_IceStorm.NoSuchTopic = NoSuchTopic
del NoSuchTopic
_M_IceStorm._t_TopicManager = IcePy.defineValue('::IceStorm::TopicManager', Ice.Value, -1, (), False, True, None, ())
if 'TopicManagerPrx' not in _M_IceStorm.__dict__:
_M_IceStorm.TopicManagerPrx = Ice.createTempClass()
class TopicManagerPrx(Ice.ObjectPrx):
"""
Create a new topic. The topic name must be unique.
Arguments:
name -- The name of the topic.
context -- The request context for the invocation.
Returns: A proxy to the topic instance.
Throws:
TopicExists -- Raised if a topic with the same name already exists.
"""
def create(self, name, context=None):
return _M_IceStorm.TopicManager._op_create.invoke(self, ((name, ), context))
"""
Create a new topic. The topic name must be unique.
Arguments:
name -- The name of the topic.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createAsync(self, name, context=None):
return _M_IceStorm.TopicManager._op_create.invokeAsync(self, ((name, ), context))
"""
Create a new topic. The topic name must be unique.
Arguments:
name -- The name of the topic.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_create(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.TopicManager._op_create.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Create a new topic. The topic name must be unique.
Arguments:
name -- The name of the topic.
Returns: A proxy to the topic instance.
Throws:
TopicExists -- Raised if a topic with the same name already exists.
"""
def end_create(self, _r):
return _M_IceStorm.TopicManager._op_create.end(self, _r)
"""
Retrieve a topic by name.
Arguments:
name -- The name of the topic.
context -- The request context for the invocation.
Returns: A proxy to the topic instance.
Throws:
NoSuchTopic -- Raised if the topic does not exist.
"""
def retrieve(self, name, context=None):
return _M_IceStorm.TopicManager._op_retrieve.invoke(self, ((name, ), context))
"""
Retrieve a topic by name.
Arguments:
name -- The name of the topic.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def retrieveAsync(self, name, context=None):
return _M_IceStorm.TopicManager._op_retrieve.invokeAsync(self, ((name, ), context))
"""
Retrieve a topic by name.
Arguments:
name -- The name of the topic.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_retrieve(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.TopicManager._op_retrieve.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Retrieve a topic by name.
Arguments:
name -- The name of the topic.
Returns: A proxy to the topic instance.
Throws:
NoSuchTopic -- Raised if the topic does not exist.
"""
def end_retrieve(self, _r):
return _M_IceStorm.TopicManager._op_retrieve.end(self, _r)
"""
Retrieve all topics managed by this topic manager.
Arguments:
context -- The request context for the invocation.
Returns: A dictionary of string, topic proxy pairs.
"""
def retrieveAll(self, context=None):
return _M_IceStorm.TopicManager._op_retrieveAll.invoke(self, ((), context))
"""
Retrieve all topics managed by this topic manager.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def retrieveAllAsync(self, context=None):
return _M_IceStorm.TopicManager._op_retrieveAll.invokeAsync(self, ((), context))
"""
Retrieve all topics managed by this topic manager.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_retrieveAll(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.TopicManager._op_retrieveAll.begin(self, ((), _response, _ex, _sent, context))
"""
Retrieve all topics managed by this topic manager.
Arguments:
Returns: A dictionary of string, topic proxy pairs.
"""
def end_retrieveAll(self, _r):
return _M_IceStorm.TopicManager._op_retrieveAll.end(self, _r)
"""
Returns the checksums for the IceStorm Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def getSliceChecksums(self, context=None):
return _M_IceStorm.TopicManager._op_getSliceChecksums.invoke(self, ((), context))
"""
Returns the checksums for the IceStorm Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSliceChecksumsAsync(self, context=None):
return _M_IceStorm.TopicManager._op_getSliceChecksums.invokeAsync(self, ((), context))
"""
Returns the checksums for the IceStorm Slice definitions.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSliceChecksums(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.TopicManager._op_getSliceChecksums.begin(self, ((), _response, _ex, _sent, context))
"""
Returns the checksums for the IceStorm Slice definitions.
Arguments:
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def end_getSliceChecksums(self, _r):
return _M_IceStorm.TopicManager._op_getSliceChecksums.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceStorm.TopicManagerPrx.ice_checkedCast(proxy, '::IceStorm::TopicManager', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceStorm.TopicManagerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceStorm::TopicManager'
_M_IceStorm._t_TopicManagerPrx = IcePy.defineProxy('::IceStorm::TopicManager', TopicManagerPrx)
_M_IceStorm.TopicManagerPrx = TopicManagerPrx
del TopicManagerPrx
_M_IceStorm.TopicManager = Ice.createTempClass()
class TopicManager(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceStorm::TopicManager')
def ice_id(self, current=None):
return '::IceStorm::TopicManager'
@staticmethod
def ice_staticId():
return '::IceStorm::TopicManager'
def create(self, name, current=None):
"""
Create a new topic. The topic name must be unique.
Arguments:
name -- The name of the topic.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
TopicExists -- Raised if a topic with the same name already exists.
"""
raise NotImplementedError("servant method 'create' not implemented")
def retrieve(self, name, current=None):
"""
Retrieve a topic by name.
Arguments:
name -- The name of the topic.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NoSuchTopic -- Raised if the topic does not exist.
"""
raise NotImplementedError("servant method 'retrieve' not implemented")
def retrieveAll(self, current=None):
"""
Retrieve all topics managed by this topic manager.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'retrieveAll' not implemented")
def getSliceChecksums(self, current=None):
"""
Returns the checksums for the IceStorm Slice definitions.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSliceChecksums' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceStorm._t_TopicManagerDisp)
__repr__ = __str__
_M_IceStorm._t_TopicManagerDisp = IcePy.defineClass('::IceStorm::TopicManager', TopicManager, (), None, ())
TopicManager._ice_type = _M_IceStorm._t_TopicManagerDisp
TopicManager._op_create = IcePy.Operation('create', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceStorm._t_TopicPrx, False, 0), (_M_IceStorm._t_TopicExists,))
TopicManager._op_retrieve = IcePy.Operation('retrieve', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceStorm._t_TopicPrx, False, 0), (_M_IceStorm._t_NoSuchTopic,))
TopicManager._op_retrieveAll = IcePy.Operation('retrieveAll', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_IceStorm._t_TopicDict, False, 0), ())
TopicManager._op_getSliceChecksums = IcePy.Operation('getSliceChecksums', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_SliceChecksumDict, False, 0), ())
_M_IceStorm.TopicManager = TopicManager
del TopicManager
_M_IceStorm._t_Finder = IcePy.defineValue('::IceStorm::Finder', Ice.Value, -1, (), False, True, None, ())
if 'FinderPrx' not in _M_IceStorm.__dict__:
_M_IceStorm.FinderPrx = Ice.createTempClass()
class FinderPrx(Ice.ObjectPrx):
"""
Get the topic manager proxy. The proxy might point to several
replicas.
Arguments:
context -- The request context for the invocation.
Returns: The topic manager proxy.
"""
def getTopicManager(self, context=None):
return _M_IceStorm.Finder._op_getTopicManager.invoke(self, ((), context))
"""
Get the topic manager proxy. The proxy might point to several
replicas.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getTopicManagerAsync(self, context=None):
return _M_IceStorm.Finder._op_getTopicManager.invokeAsync(self, ((), context))
"""
Get the topic manager proxy. The proxy might point to several
replicas.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getTopicManager(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceStorm.Finder._op_getTopicManager.begin(self, ((), _response, _ex, _sent, context))
"""
Get the topic manager proxy. The proxy might point to several
replicas.
Arguments:
Returns: The topic manager proxy.
"""
def end_getTopicManager(self, _r):
return _M_IceStorm.Finder._op_getTopicManager.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceStorm.FinderPrx.ice_checkedCast(proxy, '::IceStorm::Finder', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceStorm.FinderPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceStorm::Finder'
_M_IceStorm._t_FinderPrx = IcePy.defineProxy('::IceStorm::Finder', FinderPrx)
_M_IceStorm.FinderPrx = FinderPrx
del FinderPrx
_M_IceStorm.Finder = Ice.createTempClass()
class Finder(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceStorm::Finder')
def ice_id(self, current=None):
return '::IceStorm::Finder'
@staticmethod
def ice_staticId():
return '::IceStorm::Finder'
def getTopicManager(self, current=None):
"""
Get the topic manager proxy. The proxy might point to several
replicas.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getTopicManager' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceStorm._t_FinderDisp)
__repr__ = __str__
_M_IceStorm._t_FinderDisp = IcePy.defineClass('::IceStorm::Finder', Finder, (), None, ())
Finder._ice_type = _M_IceStorm._t_FinderDisp
Finder._op_getTopicManager = IcePy.Operation('getTopicManager', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_IceStorm._t_TopicManagerPrx, False, 0), ())
_M_IceStorm.Finder = Finder
del Finder
# End of module IceStorm
Ice.sliceChecksums["::IceStorm::AlreadySubscribed"] = "5a82e77b38f02f3118c536f9446a889e"
Ice.sliceChecksums["::IceStorm::BadQoS"] = "44f2de592dd62e3f7f4ffdf043692d"
Ice.sliceChecksums["::IceStorm::Finder"] = "74a058c59ac11fe9a98347ab96533e0"
Ice.sliceChecksums["::IceStorm::InvalidSubscriber"] = "79b17123acba6189e77285da82a862d3"
Ice.sliceChecksums["::IceStorm::LinkExists"] = "e11768febd56a8813729ce69be6c4c2"
Ice.sliceChecksums["::IceStorm::LinkInfo"] = "d0e073e5e0925ec95656f71d572e2e13"
Ice.sliceChecksums["::IceStorm::LinkInfoSeq"] = "a8921e43838692bbe6ca63f3dcf9b6"
Ice.sliceChecksums["::IceStorm::NoSuchLink"] = "fd8f652776796bffca2df1a3baf455a3"
Ice.sliceChecksums["::IceStorm::NoSuchTopic"] = "7a9479a5c39cdd32335d722bbc971176"
Ice.sliceChecksums["::IceStorm::QoS"] = "3e27cb32bc95cca7b013efbf5c254b35"
Ice.sliceChecksums["::IceStorm::Topic"] = "7058388fe7d6c582c2506237904eb7c9"
Ice.sliceChecksums["::IceStorm::TopicDict"] = "fff078a98be068c52d9e1d7d8f6df2a"
Ice.sliceChecksums["::IceStorm::TopicExists"] = "38e6913833539b8d616d114d4e7b28d"
Ice.sliceChecksums["::IceStorm::TopicManager"] = "ffc1baf19222891f8b432be6551fed5" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceStorm/IceStorm_ice.py | IceStorm_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Glacier2.Session_ice
import IceGrid.Exception_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Included module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
# Start of module IceGrid
__name__ = 'IceGrid'
_M_IceGrid._t_Session = IcePy.defineValue('::IceGrid::Session', Ice.Value, -1, (), False, True, None, ())
if 'SessionPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.SessionPrx = Ice.createTempClass()
class SessionPrx(_M_Glacier2.SessionPrx):
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
context -- The request context for the invocation.
"""
def keepAlive(self, context=None):
return _M_IceGrid.Session._op_keepAlive.invoke(self, ((), context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def keepAliveAsync(self, context=None):
return _M_IceGrid.Session._op_keepAlive.invokeAsync(self, ((), context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_keepAlive(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Session._op_keepAlive.begin(self, ((), _response, _ex, _sent, context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
"""
def end_keepAlive(self, _r):
return _M_IceGrid.Session._op_keepAlive.end(self, _r)
"""
Allocate an object. Depending on the allocation timeout, this
operation might hang until the object is available or until the
timeout is reached.
Arguments:
id -- The identity of the object to allocate.
context -- The request context for the invocation.
Returns: The proxy of the allocated object.
Throws:
AllocationException -- Raised if the object can't be allocated.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
def allocateObjectById(self, id, context=None):
return _M_IceGrid.Session._op_allocateObjectById.invoke(self, ((id, ), context))
"""
Allocate an object. Depending on the allocation timeout, this
operation might hang until the object is available or until the
timeout is reached.
Arguments:
id -- The identity of the object to allocate.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def allocateObjectByIdAsync(self, id, context=None):
return _M_IceGrid.Session._op_allocateObjectById.invokeAsync(self, ((id, ), context))
"""
Allocate an object. Depending on the allocation timeout, this
operation might hang until the object is available or until the
timeout is reached.
Arguments:
id -- The identity of the object to allocate.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_allocateObjectById(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Session._op_allocateObjectById.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Allocate an object. Depending on the allocation timeout, this
operation might hang until the object is available or until the
timeout is reached.
Arguments:
id -- The identity of the object to allocate.
Returns: The proxy of the allocated object.
Throws:
AllocationException -- Raised if the object can't be allocated.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
def end_allocateObjectById(self, _r):
return _M_IceGrid.Session._op_allocateObjectById.end(self, _r)
"""
Allocate an object with the given type. Depending on the
allocation timeout, this operation can block until an object
becomes available or until the timeout is reached.
Arguments:
type -- The type of the object.
context -- The request context for the invocation.
Returns: The proxy of the allocated object.
Throws:
AllocationException -- Raised if the object could not be allocated.
"""
def allocateObjectByType(self, type, context=None):
return _M_IceGrid.Session._op_allocateObjectByType.invoke(self, ((type, ), context))
"""
Allocate an object with the given type. Depending on the
allocation timeout, this operation can block until an object
becomes available or until the timeout is reached.
Arguments:
type -- The type of the object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def allocateObjectByTypeAsync(self, type, context=None):
return _M_IceGrid.Session._op_allocateObjectByType.invokeAsync(self, ((type, ), context))
"""
Allocate an object with the given type. Depending on the
allocation timeout, this operation can block until an object
becomes available or until the timeout is reached.
Arguments:
type -- The type of the object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_allocateObjectByType(self, type, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Session._op_allocateObjectByType.begin(self, ((type, ), _response, _ex, _sent, context))
"""
Allocate an object with the given type. Depending on the
allocation timeout, this operation can block until an object
becomes available or until the timeout is reached.
Arguments:
type -- The type of the object.
Returns: The proxy of the allocated object.
Throws:
AllocationException -- Raised if the object could not be allocated.
"""
def end_allocateObjectByType(self, _r):
return _M_IceGrid.Session._op_allocateObjectByType.end(self, _r)
"""
Release an object that was allocated using allocateObjectById or
allocateObjectByType.
Arguments:
id -- The identity of the object to release.
context -- The request context for the invocation.
Throws:
AllocationException -- Raised if the given object can't be released. This might happen if the object isn't allocatable or isn't allocated by the session.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
def releaseObject(self, id, context=None):
return _M_IceGrid.Session._op_releaseObject.invoke(self, ((id, ), context))
"""
Release an object that was allocated using allocateObjectById or
allocateObjectByType.
Arguments:
id -- The identity of the object to release.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def releaseObjectAsync(self, id, context=None):
return _M_IceGrid.Session._op_releaseObject.invokeAsync(self, ((id, ), context))
"""
Release an object that was allocated using allocateObjectById or
allocateObjectByType.
Arguments:
id -- The identity of the object to release.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_releaseObject(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Session._op_releaseObject.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Release an object that was allocated using allocateObjectById or
allocateObjectByType.
Arguments:
id -- The identity of the object to release.
Throws:
AllocationException -- Raised if the given object can't be released. This might happen if the object isn't allocatable or isn't allocated by the session.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
def end_releaseObject(self, _r):
return _M_IceGrid.Session._op_releaseObject.end(self, _r)
"""
Set the allocation timeout. If no objects are available for an
allocation request, a call to allocateObjectById or
allocateObjectByType will block for the duration of this
timeout.
Arguments:
timeout -- The timeout in milliseconds.
context -- The request context for the invocation.
"""
def setAllocationTimeout(self, timeout, context=None):
return _M_IceGrid.Session._op_setAllocationTimeout.invoke(self, ((timeout, ), context))
"""
Set the allocation timeout. If no objects are available for an
allocation request, a call to allocateObjectById or
allocateObjectByType will block for the duration of this
timeout.
Arguments:
timeout -- The timeout in milliseconds.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setAllocationTimeoutAsync(self, timeout, context=None):
return _M_IceGrid.Session._op_setAllocationTimeout.invokeAsync(self, ((timeout, ), context))
"""
Set the allocation timeout. If no objects are available for an
allocation request, a call to allocateObjectById or
allocateObjectByType will block for the duration of this
timeout.
Arguments:
timeout -- The timeout in milliseconds.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setAllocationTimeout(self, timeout, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Session._op_setAllocationTimeout.begin(self, ((timeout, ), _response, _ex, _sent, context))
"""
Set the allocation timeout. If no objects are available for an
allocation request, a call to allocateObjectById or
allocateObjectByType will block for the duration of this
timeout.
Arguments:
timeout -- The timeout in milliseconds.
"""
def end_setAllocationTimeout(self, _r):
return _M_IceGrid.Session._op_setAllocationTimeout.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.SessionPrx.ice_checkedCast(proxy, '::IceGrid::Session', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.SessionPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::Session'
_M_IceGrid._t_SessionPrx = IcePy.defineProxy('::IceGrid::Session', SessionPrx)
_M_IceGrid.SessionPrx = SessionPrx
del SessionPrx
_M_IceGrid.Session = Ice.createTempClass()
class Session(_M_Glacier2.Session):
def ice_ids(self, current=None):
return ('::Glacier2::Session', '::Ice::Object', '::IceGrid::Session')
def ice_id(self, current=None):
return '::IceGrid::Session'
@staticmethod
def ice_staticId():
return '::IceGrid::Session'
def keepAlive(self, current=None):
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'keepAlive' not implemented")
def allocateObjectById(self, id, current=None):
"""
Allocate an object. Depending on the allocation timeout, this
operation might hang until the object is available or until the
timeout is reached.
Arguments:
id -- The identity of the object to allocate.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AllocationException -- Raised if the object can't be allocated.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
raise NotImplementedError("servant method 'allocateObjectById' not implemented")
def allocateObjectByType(self, type, current=None):
"""
Allocate an object with the given type. Depending on the
allocation timeout, this operation can block until an object
becomes available or until the timeout is reached.
Arguments:
type -- The type of the object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AllocationException -- Raised if the object could not be allocated.
"""
raise NotImplementedError("servant method 'allocateObjectByType' not implemented")
def releaseObject(self, id, current=None):
"""
Release an object that was allocated using allocateObjectById or
allocateObjectByType.
Arguments:
id -- The identity of the object to release.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AllocationException -- Raised if the given object can't be released. This might happen if the object isn't allocatable or isn't allocated by the session.
ObjectNotRegisteredException -- Raised if the object with the given identity is not registered with the registry.
"""
raise NotImplementedError("servant method 'releaseObject' not implemented")
def setAllocationTimeout(self, timeout, current=None):
"""
Set the allocation timeout. If no objects are available for an
allocation request, a call to allocateObjectById or
allocateObjectByType will block for the duration of this
timeout.
Arguments:
timeout -- The timeout in milliseconds.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'setAllocationTimeout' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_SessionDisp)
__repr__ = __str__
_M_IceGrid._t_SessionDisp = IcePy.defineClass('::IceGrid::Session', Session, (), None, (_M_Glacier2._t_SessionDisp,))
Session._ice_type = _M_IceGrid._t_SessionDisp
Session._op_keepAlive = IcePy.Operation('keepAlive', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), None, ())
Session._op_allocateObjectById = IcePy.Operation('allocateObjectById', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), _M_Ice._t_Identity, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceGrid._t_ObjectNotRegisteredException, _M_IceGrid._t_AllocationException))
Session._op_allocateObjectByType = IcePy.Operation('allocateObjectByType', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceGrid._t_AllocationException,))
Session._op_releaseObject = IcePy.Operation('releaseObject', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_Identity, False, 0),), (), None, (_M_IceGrid._t_ObjectNotRegisteredException, _M_IceGrid._t_AllocationException))
Session._op_setAllocationTimeout = IcePy.Operation('setAllocationTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_int, False, 0),), (), None, ())
_M_IceGrid.Session = Session
del Session
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::Session"] = "cf4206d0a8aff6c1b0f2c437f34c5d" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/Session_ice.py | Session_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import IceGrid.Exception_ice
import IceGrid.Session_ice
import IceGrid.Admin_ice
import Ice.Locator_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Start of module IceGrid
__name__ = 'IceGrid'
if 'LoadSample' not in _M_IceGrid.__dict__:
_M_IceGrid.LoadSample = Ice.createTempClass()
class LoadSample(Ice.EnumBase):
"""
Determines which load sampling interval to use.
Enumerators:
LoadSample1 -- Sample every minute.
LoadSample5 -- Sample every five minutes.
LoadSample15 -- Sample every fifteen minutes.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
LoadSample.LoadSample1 = LoadSample("LoadSample1", 0)
LoadSample.LoadSample5 = LoadSample("LoadSample5", 1)
LoadSample.LoadSample15 = LoadSample("LoadSample15", 2)
LoadSample._enumerators = { 0:LoadSample.LoadSample1, 1:LoadSample.LoadSample5, 2:LoadSample.LoadSample15 }
_M_IceGrid._t_LoadSample = IcePy.defineEnum('::IceGrid::LoadSample', LoadSample, (), LoadSample._enumerators)
_M_IceGrid.LoadSample = LoadSample
del LoadSample
_M_IceGrid._t_Query = IcePy.defineValue('::IceGrid::Query', Ice.Value, -1, (), False, True, None, ())
if 'QueryPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.QueryPrx = Ice.createTempClass()
class QueryPrx(Ice.ObjectPrx):
"""
Find a well-known object by identity.
Arguments:
id -- The identity.
context -- The request context for the invocation.
Returns: The proxy or null if no such object has been found.
"""
def findObjectById(self, id, context=None):
return _M_IceGrid.Query._op_findObjectById.invoke(self, ((id, ), context))
"""
Find a well-known object by identity.
Arguments:
id -- The identity.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findObjectByIdAsync(self, id, context=None):
return _M_IceGrid.Query._op_findObjectById.invokeAsync(self, ((id, ), context))
"""
Find a well-known object by identity.
Arguments:
id -- The identity.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findObjectById(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Query._op_findObjectById.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Find a well-known object by identity.
Arguments:
id -- The identity.
Returns: The proxy or null if no such object has been found.
"""
def end_findObjectById(self, _r):
return _M_IceGrid.Query._op_findObjectById.end(self, _r)
"""
Find a well-known object by type. If there are several objects
registered for the given type, the object is randomly
selected.
Arguments:
type -- The object type.
context -- The request context for the invocation.
Returns: The proxy or null, if no such object has been found.
"""
def findObjectByType(self, type, context=None):
return _M_IceGrid.Query._op_findObjectByType.invoke(self, ((type, ), context))
"""
Find a well-known object by type. If there are several objects
registered for the given type, the object is randomly
selected.
Arguments:
type -- The object type.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findObjectByTypeAsync(self, type, context=None):
return _M_IceGrid.Query._op_findObjectByType.invokeAsync(self, ((type, ), context))
"""
Find a well-known object by type. If there are several objects
registered for the given type, the object is randomly
selected.
Arguments:
type -- The object type.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findObjectByType(self, type, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Query._op_findObjectByType.begin(self, ((type, ), _response, _ex, _sent, context))
"""
Find a well-known object by type. If there are several objects
registered for the given type, the object is randomly
selected.
Arguments:
type -- The object type.
Returns: The proxy or null, if no such object has been found.
"""
def end_findObjectByType(self, _r):
return _M_IceGrid.Query._op_findObjectByType.end(self, _r)
"""
Find a well-known object by type on the least-loaded node. If
the registry does not know which node hosts the object
(for example, because the object was registered with a direct proxy), the
registry assumes the object is hosted on a node that has a load
average of 1.0.
Arguments:
type -- The object type.
sample -- The sampling interval.
context -- The request context for the invocation.
Returns: The proxy or null, if no such object has been found.
"""
def findObjectByTypeOnLeastLoadedNode(self, type, sample, context=None):
return _M_IceGrid.Query._op_findObjectByTypeOnLeastLoadedNode.invoke(self, ((type, sample), context))
"""
Find a well-known object by type on the least-loaded node. If
the registry does not know which node hosts the object
(for example, because the object was registered with a direct proxy), the
registry assumes the object is hosted on a node that has a load
average of 1.0.
Arguments:
type -- The object type.
sample -- The sampling interval.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findObjectByTypeOnLeastLoadedNodeAsync(self, type, sample, context=None):
return _M_IceGrid.Query._op_findObjectByTypeOnLeastLoadedNode.invokeAsync(self, ((type, sample), context))
"""
Find a well-known object by type on the least-loaded node. If
the registry does not know which node hosts the object
(for example, because the object was registered with a direct proxy), the
registry assumes the object is hosted on a node that has a load
average of 1.0.
Arguments:
type -- The object type.
sample -- The sampling interval.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findObjectByTypeOnLeastLoadedNode(self, type, sample, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Query._op_findObjectByTypeOnLeastLoadedNode.begin(self, ((type, sample), _response, _ex, _sent, context))
"""
Find a well-known object by type on the least-loaded node. If
the registry does not know which node hosts the object
(for example, because the object was registered with a direct proxy), the
registry assumes the object is hosted on a node that has a load
average of 1.0.
Arguments:
type -- The object type.
sample -- The sampling interval.
Returns: The proxy or null, if no such object has been found.
"""
def end_findObjectByTypeOnLeastLoadedNode(self, _r):
return _M_IceGrid.Query._op_findObjectByTypeOnLeastLoadedNode.end(self, _r)
"""
Find all the well-known objects with the given type.
Arguments:
type -- The object type.
context -- The request context for the invocation.
Returns: The proxies or an empty sequence, if no such objects have been found.
"""
def findAllObjectsByType(self, type, context=None):
return _M_IceGrid.Query._op_findAllObjectsByType.invoke(self, ((type, ), context))
"""
Find all the well-known objects with the given type.
Arguments:
type -- The object type.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findAllObjectsByTypeAsync(self, type, context=None):
return _M_IceGrid.Query._op_findAllObjectsByType.invokeAsync(self, ((type, ), context))
"""
Find all the well-known objects with the given type.
Arguments:
type -- The object type.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findAllObjectsByType(self, type, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Query._op_findAllObjectsByType.begin(self, ((type, ), _response, _ex, _sent, context))
"""
Find all the well-known objects with the given type.
Arguments:
type -- The object type.
Returns: The proxies or an empty sequence, if no such objects have been found.
"""
def end_findAllObjectsByType(self, _r):
return _M_IceGrid.Query._op_findAllObjectsByType.end(self, _r)
"""
Find all the object replicas associated with the given
proxy. If the given proxy is not an indirect proxy from a
replica group, an empty sequence is returned.
Arguments:
proxy -- The object proxy.
context -- The request context for the invocation.
Returns: The proxies of each object replica or an empty sequence, if the given proxy is not from a replica group.
"""
def findAllReplicas(self, proxy, context=None):
return _M_IceGrid.Query._op_findAllReplicas.invoke(self, ((proxy, ), context))
"""
Find all the object replicas associated with the given
proxy. If the given proxy is not an indirect proxy from a
replica group, an empty sequence is returned.
Arguments:
proxy -- The object proxy.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findAllReplicasAsync(self, proxy, context=None):
return _M_IceGrid.Query._op_findAllReplicas.invokeAsync(self, ((proxy, ), context))
"""
Find all the object replicas associated with the given
proxy. If the given proxy is not an indirect proxy from a
replica group, an empty sequence is returned.
Arguments:
proxy -- The object proxy.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findAllReplicas(self, proxy, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Query._op_findAllReplicas.begin(self, ((proxy, ), _response, _ex, _sent, context))
"""
Find all the object replicas associated with the given
proxy. If the given proxy is not an indirect proxy from a
replica group, an empty sequence is returned.
Arguments:
proxy -- The object proxy.
Returns: The proxies of each object replica or an empty sequence, if the given proxy is not from a replica group.
"""
def end_findAllReplicas(self, _r):
return _M_IceGrid.Query._op_findAllReplicas.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.QueryPrx.ice_checkedCast(proxy, '::IceGrid::Query', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.QueryPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::Query'
_M_IceGrid._t_QueryPrx = IcePy.defineProxy('::IceGrid::Query', QueryPrx)
_M_IceGrid.QueryPrx = QueryPrx
del QueryPrx
_M_IceGrid.Query = Ice.createTempClass()
class Query(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::Query')
def ice_id(self, current=None):
return '::IceGrid::Query'
@staticmethod
def ice_staticId():
return '::IceGrid::Query'
def findObjectById(self, id, current=None):
"""
Find a well-known object by identity.
Arguments:
id -- The identity.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'findObjectById' not implemented")
def findObjectByType(self, type, current=None):
"""
Find a well-known object by type. If there are several objects
registered for the given type, the object is randomly
selected.
Arguments:
type -- The object type.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'findObjectByType' not implemented")
def findObjectByTypeOnLeastLoadedNode(self, type, sample, current=None):
"""
Find a well-known object by type on the least-loaded node. If
the registry does not know which node hosts the object
(for example, because the object was registered with a direct proxy), the
registry assumes the object is hosted on a node that has a load
average of 1.0.
Arguments:
type -- The object type.
sample -- The sampling interval.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'findObjectByTypeOnLeastLoadedNode' not implemented")
def findAllObjectsByType(self, type, current=None):
"""
Find all the well-known objects with the given type.
Arguments:
type -- The object type.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'findAllObjectsByType' not implemented")
def findAllReplicas(self, proxy, current=None):
"""
Find all the object replicas associated with the given
proxy. If the given proxy is not an indirect proxy from a
replica group, an empty sequence is returned.
Arguments:
proxy -- The object proxy.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'findAllReplicas' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_QueryDisp)
__repr__ = __str__
_M_IceGrid._t_QueryDisp = IcePy.defineClass('::IceGrid::Query', Query, (), None, ())
Query._ice_type = _M_IceGrid._t_QueryDisp
Query._op_findObjectById = IcePy.Operation('findObjectById', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), _M_Ice._t_Identity, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Query._op_findObjectByType = IcePy.Operation('findObjectByType', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Query._op_findObjectByTypeOnLeastLoadedNode = IcePy.Operation('findObjectByTypeOnLeastLoadedNode', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0), ((), _M_IceGrid._t_LoadSample, False, 0)), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Query._op_findAllObjectsByType = IcePy.Operation('findAllObjectsByType', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_Ice._t_ObjectProxySeq, False, 0), ())
Query._op_findAllReplicas = IcePy.Operation('findAllReplicas', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_ObjectPrx, False, 0),), (), ((), _M_Ice._t_ObjectProxySeq, False, 0), ())
_M_IceGrid.Query = Query
del Query
_M_IceGrid._t_Registry = IcePy.defineValue('::IceGrid::Registry', Ice.Value, -1, (), False, True, None, ())
if 'RegistryPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryPrx = Ice.createTempClass()
class RegistryPrx(Ice.ObjectPrx):
"""
Create a client session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def createSession(self, userId, password, context=None):
return _M_IceGrid.Registry._op_createSession.invoke(self, ((userId, password), context))
"""
Create a client session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createSessionAsync(self, userId, password, context=None):
return _M_IceGrid.Registry._op_createSession.invokeAsync(self, ((userId, password), context))
"""
Create a client session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createSession(self, userId, password, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_createSession.begin(self, ((userId, password), _response, _ex, _sent, context))
"""
Create a client session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def end_createSession(self, _r):
return _M_IceGrid.Registry._op_createSession.end(self, _r)
"""
Create an administrative session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def createAdminSession(self, userId, password, context=None):
return _M_IceGrid.Registry._op_createAdminSession.invoke(self, ((userId, password), context))
"""
Create an administrative session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createAdminSessionAsync(self, userId, password, context=None):
return _M_IceGrid.Registry._op_createAdminSession.invokeAsync(self, ((userId, password), context))
"""
Create an administrative session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createAdminSession(self, userId, password, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_createAdminSession.begin(self, ((userId, password), _response, _ex, _sent, context))
"""
Create an administrative session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def end_createAdminSession(self, _r):
return _M_IceGrid.Registry._op_createAdminSession.end(self, _r)
"""
Create a client session from a secure connection.
Arguments:
context -- The request context for the invocation.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def createSessionFromSecureConnection(self, context=None):
return _M_IceGrid.Registry._op_createSessionFromSecureConnection.invoke(self, ((), context))
"""
Create a client session from a secure connection.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createSessionFromSecureConnectionAsync(self, context=None):
return _M_IceGrid.Registry._op_createSessionFromSecureConnection.invokeAsync(self, ((), context))
"""
Create a client session from a secure connection.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createSessionFromSecureConnection(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_createSessionFromSecureConnection.begin(self, ((), _response, _ex, _sent, context))
"""
Create a client session from a secure connection.
Arguments:
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def end_createSessionFromSecureConnection(self, _r):
return _M_IceGrid.Registry._op_createSessionFromSecureConnection.end(self, _r)
"""
Create an administrative session from a secure connection.
Arguments:
context -- The request context for the invocation.
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def createAdminSessionFromSecureConnection(self, context=None):
return _M_IceGrid.Registry._op_createAdminSessionFromSecureConnection.invoke(self, ((), context))
"""
Create an administrative session from a secure connection.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createAdminSessionFromSecureConnectionAsync(self, context=None):
return _M_IceGrid.Registry._op_createAdminSessionFromSecureConnection.invokeAsync(self, ((), context))
"""
Create an administrative session from a secure connection.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createAdminSessionFromSecureConnection(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_createAdminSessionFromSecureConnection.begin(self, ((), _response, _ex, _sent, context))
"""
Create an administrative session from a secure connection.
Arguments:
Returns: A proxy for the newly created session.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def end_createAdminSessionFromSecureConnection(self, _r):
return _M_IceGrid.Registry._op_createAdminSessionFromSecureConnection.end(self, _r)
"""
Get the session timeout. If a client or administrative client
doesn't call the session keepAlive method in the time interval
defined by this timeout, IceGrid might reap the session.
Arguments:
context -- The request context for the invocation.
Returns: The timeout (in seconds).
"""
def getSessionTimeout(self, context=None):
return _M_IceGrid.Registry._op_getSessionTimeout.invoke(self, ((), context))
"""
Get the session timeout. If a client or administrative client
doesn't call the session keepAlive method in the time interval
defined by this timeout, IceGrid might reap the session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSessionTimeoutAsync(self, context=None):
return _M_IceGrid.Registry._op_getSessionTimeout.invokeAsync(self, ((), context))
"""
Get the session timeout. If a client or administrative client
doesn't call the session keepAlive method in the time interval
defined by this timeout, IceGrid might reap the session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSessionTimeout(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_getSessionTimeout.begin(self, ((), _response, _ex, _sent, context))
"""
Get the session timeout. If a client or administrative client
doesn't call the session keepAlive method in the time interval
defined by this timeout, IceGrid might reap the session.
Arguments:
Returns: The timeout (in seconds).
"""
def end_getSessionTimeout(self, _r):
return _M_IceGrid.Registry._op_getSessionTimeout.end(self, _r)
"""
Get the value of the ACM timeout. Clients supporting ACM
connection heartbeats can enable them instead of explicitly
sending keep alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
context -- The request context for the invocation.
Returns: The timeout (in seconds).
"""
def getACMTimeout(self, context=None):
return _M_IceGrid.Registry._op_getACMTimeout.invoke(self, ((), context))
"""
Get the value of the ACM timeout. Clients supporting ACM
connection heartbeats can enable them instead of explicitly
sending keep alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getACMTimeoutAsync(self, context=None):
return _M_IceGrid.Registry._op_getACMTimeout.invokeAsync(self, ((), context))
"""
Get the value of the ACM timeout. Clients supporting ACM
connection heartbeats can enable them instead of explicitly
sending keep alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getACMTimeout(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Registry._op_getACMTimeout.begin(self, ((), _response, _ex, _sent, context))
"""
Get the value of the ACM timeout. Clients supporting ACM
connection heartbeats can enable them instead of explicitly
sending keep alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
Returns: The timeout (in seconds).
"""
def end_getACMTimeout(self, _r):
return _M_IceGrid.Registry._op_getACMTimeout.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.RegistryPrx.ice_checkedCast(proxy, '::IceGrid::Registry', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.RegistryPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::Registry'
_M_IceGrid._t_RegistryPrx = IcePy.defineProxy('::IceGrid::Registry', RegistryPrx)
_M_IceGrid.RegistryPrx = RegistryPrx
del RegistryPrx
_M_IceGrid.Registry = Ice.createTempClass()
class Registry(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::Registry')
def ice_id(self, current=None):
return '::IceGrid::Registry'
@staticmethod
def ice_staticId():
return '::IceGrid::Registry'
def createSession(self, userId, password, current=None):
"""
Create a client session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createSession' not implemented")
def createAdminSession(self, userId, password, current=None):
"""
Create an administrative session.
Arguments:
userId -- The user id.
password -- The password for the given user id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createAdminSession' not implemented")
def createSessionFromSecureConnection(self, current=None):
"""
Create a client session from a secure connection.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createSessionFromSecureConnection' not implemented")
def createAdminSessionFromSecureConnection(self, current=None):
"""
Create an administrative session from a secure connection.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createAdminSessionFromSecureConnection' not implemented")
def getSessionTimeout(self, current=None):
"""
Get the session timeout. If a client or administrative client
doesn't call the session keepAlive method in the time interval
defined by this timeout, IceGrid might reap the session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSessionTimeout' not implemented")
def getACMTimeout(self, current=None):
"""
Get the value of the ACM timeout. Clients supporting ACM
connection heartbeats can enable them instead of explicitly
sending keep alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getACMTimeout' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RegistryDisp)
__repr__ = __str__
_M_IceGrid._t_RegistryDisp = IcePy.defineClass('::IceGrid::Registry', Registry, (), None, ())
Registry._ice_type = _M_IceGrid._t_RegistryDisp
Registry._op_createSession = IcePy.Operation('createSession', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), ((), _M_IceGrid._t_SessionPrx, False, 0), (_M_IceGrid._t_PermissionDeniedException,))
Registry._op_createAdminSession = IcePy.Operation('createAdminSession', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), ((), _M_IceGrid._t_AdminSessionPrx, False, 0), (_M_IceGrid._t_PermissionDeniedException,))
Registry._op_createSessionFromSecureConnection = IcePy.Operation('createSessionFromSecureConnection', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_IceGrid._t_SessionPrx, False, 0), (_M_IceGrid._t_PermissionDeniedException,))
Registry._op_createAdminSessionFromSecureConnection = IcePy.Operation('createAdminSessionFromSecureConnection', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_IceGrid._t_AdminSessionPrx, False, 0), (_M_IceGrid._t_PermissionDeniedException,))
Registry._op_getSessionTimeout = IcePy.Operation('getSessionTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_int, False, 0), ())
Registry._op_getACMTimeout = IcePy.Operation('getACMTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_int, False, 0), ())
_M_IceGrid.Registry = Registry
del Registry
_M_IceGrid._t_Locator = IcePy.defineValue('::IceGrid::Locator', Ice.Value, -1, (), False, True, None, ())
if 'LocatorPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.LocatorPrx = Ice.createTempClass()
class LocatorPrx(_M_Ice.LocatorPrx):
"""
Get the proxy of the registry object hosted by this IceGrid
registry.
Arguments:
context -- The request context for the invocation.
Returns: The proxy of the registry object.
"""
def getLocalRegistry(self, context=None):
return _M_IceGrid.Locator._op_getLocalRegistry.invoke(self, ((), context))
"""
Get the proxy of the registry object hosted by this IceGrid
registry.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLocalRegistryAsync(self, context=None):
return _M_IceGrid.Locator._op_getLocalRegistry.invokeAsync(self, ((), context))
"""
Get the proxy of the registry object hosted by this IceGrid
registry.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLocalRegistry(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Locator._op_getLocalRegistry.begin(self, ((), _response, _ex, _sent, context))
"""
Get the proxy of the registry object hosted by this IceGrid
registry.
Arguments:
Returns: The proxy of the registry object.
"""
def end_getLocalRegistry(self, _r):
return _M_IceGrid.Locator._op_getLocalRegistry.end(self, _r)
"""
Get the proxy of the query object hosted by this IceGrid
registry.
Arguments:
context -- The request context for the invocation.
Returns: The proxy of the query object.
"""
def getLocalQuery(self, context=None):
return _M_IceGrid.Locator._op_getLocalQuery.invoke(self, ((), context))
"""
Get the proxy of the query object hosted by this IceGrid
registry.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLocalQueryAsync(self, context=None):
return _M_IceGrid.Locator._op_getLocalQuery.invokeAsync(self, ((), context))
"""
Get the proxy of the query object hosted by this IceGrid
registry.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLocalQuery(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Locator._op_getLocalQuery.begin(self, ((), _response, _ex, _sent, context))
"""
Get the proxy of the query object hosted by this IceGrid
registry.
Arguments:
Returns: The proxy of the query object.
"""
def end_getLocalQuery(self, _r):
return _M_IceGrid.Locator._op_getLocalQuery.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.LocatorPrx.ice_checkedCast(proxy, '::IceGrid::Locator', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.LocatorPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::Locator'
_M_IceGrid._t_LocatorPrx = IcePy.defineProxy('::IceGrid::Locator', LocatorPrx)
_M_IceGrid.LocatorPrx = LocatorPrx
del LocatorPrx
_M_IceGrid.Locator = Ice.createTempClass()
class Locator(_M_Ice.Locator):
def ice_ids(self, current=None):
return ('::Ice::Locator', '::Ice::Object', '::IceGrid::Locator')
def ice_id(self, current=None):
return '::IceGrid::Locator'
@staticmethod
def ice_staticId():
return '::IceGrid::Locator'
def getLocalRegistry(self, current=None):
"""
Get the proxy of the registry object hosted by this IceGrid
registry.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getLocalRegistry' not implemented")
def getLocalQuery(self, current=None):
"""
Get the proxy of the query object hosted by this IceGrid
registry.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getLocalQuery' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_LocatorDisp)
__repr__ = __str__
_M_IceGrid._t_LocatorDisp = IcePy.defineClass('::IceGrid::Locator', Locator, (), None, (_M_Ice._t_LocatorDisp,))
Locator._ice_type = _M_IceGrid._t_LocatorDisp
Locator._op_getLocalRegistry = IcePy.Operation('getLocalRegistry', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), _M_IceGrid._t_RegistryPrx, False, 0), ())
Locator._op_getLocalQuery = IcePy.Operation('getLocalQuery', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), _M_IceGrid._t_QueryPrx, False, 0), ())
_M_IceGrid.Locator = Locator
del Locator
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::LoadSample"] = "ec48c06fa099138a5fbbce121a9a290"
Ice.sliceChecksums["::IceGrid::Locator"] = "816e9d7a3cb39b8c80fe342e7f18ae"
Ice.sliceChecksums["::IceGrid::Query"] = "39dbe5f62c19aa42c2e0fbaf220b4f1"
Ice.sliceChecksums["::IceGrid::Registry"] = "8298cc0aba1a722d75eb79034fbb076" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/Registry_ice.py | Registry_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
__name__ = 'IceGrid'
if 'ApplicationNotExistException' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationNotExistException = Ice.createTempClass()
class ApplicationNotExistException(Ice.UserException):
"""
This exception is raised if an application does not exist.
Members:
name -- The name of the application.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ApplicationNotExistException'
_M_IceGrid._t_ApplicationNotExistException = IcePy.defineException('::IceGrid::ApplicationNotExistException', ApplicationNotExistException, (), False, None, (('name', (), IcePy._t_string, False, 0),))
ApplicationNotExistException._ice_type = _M_IceGrid._t_ApplicationNotExistException
_M_IceGrid.ApplicationNotExistException = ApplicationNotExistException
del ApplicationNotExistException
if 'ServerNotExistException' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerNotExistException = Ice.createTempClass()
class ServerNotExistException(Ice.UserException):
"""
This exception is raised if a server does not exist.
Members:
id -- The identifier of the server.
"""
def __init__(self, id=''):
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ServerNotExistException'
_M_IceGrid._t_ServerNotExistException = IcePy.defineException('::IceGrid::ServerNotExistException', ServerNotExistException, (), False, None, (('id', (), IcePy._t_string, False, 0),))
ServerNotExistException._ice_type = _M_IceGrid._t_ServerNotExistException
_M_IceGrid.ServerNotExistException = ServerNotExistException
del ServerNotExistException
if 'ServerStartException' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerStartException = Ice.createTempClass()
class ServerStartException(Ice.UserException):
"""
This exception is raised if a server failed to start.
Members:
id -- The identifier of the server.
reason -- The reason for the failure.
"""
def __init__(self, id='', reason=''):
self.id = id
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ServerStartException'
_M_IceGrid._t_ServerStartException = IcePy.defineException('::IceGrid::ServerStartException', ServerStartException, (), False, None, (
('id', (), IcePy._t_string, False, 0),
('reason', (), IcePy._t_string, False, 0)
))
ServerStartException._ice_type = _M_IceGrid._t_ServerStartException
_M_IceGrid.ServerStartException = ServerStartException
del ServerStartException
if 'ServerStopException' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerStopException = Ice.createTempClass()
class ServerStopException(Ice.UserException):
"""
This exception is raised if a server failed to stop.
Members:
id -- The identifier of the server.
reason -- The reason for the failure.
"""
def __init__(self, id='', reason=''):
self.id = id
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ServerStopException'
_M_IceGrid._t_ServerStopException = IcePy.defineException('::IceGrid::ServerStopException', ServerStopException, (), False, None, (
('id', (), IcePy._t_string, False, 0),
('reason', (), IcePy._t_string, False, 0)
))
ServerStopException._ice_type = _M_IceGrid._t_ServerStopException
_M_IceGrid.ServerStopException = ServerStopException
del ServerStopException
if 'AdapterNotExistException' not in _M_IceGrid.__dict__:
_M_IceGrid.AdapterNotExistException = Ice.createTempClass()
class AdapterNotExistException(Ice.UserException):
"""
This exception is raised if an adapter does not exist.
Members:
id -- The id of the object adapter.
"""
def __init__(self, id=''):
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::AdapterNotExistException'
_M_IceGrid._t_AdapterNotExistException = IcePy.defineException('::IceGrid::AdapterNotExistException', AdapterNotExistException, (), False, None, (('id', (), IcePy._t_string, False, 0),))
AdapterNotExistException._ice_type = _M_IceGrid._t_AdapterNotExistException
_M_IceGrid.AdapterNotExistException = AdapterNotExistException
del AdapterNotExistException
if 'ObjectExistsException' not in _M_IceGrid.__dict__:
_M_IceGrid.ObjectExistsException = Ice.createTempClass()
class ObjectExistsException(Ice.UserException):
"""
This exception is raised if an object already exists.
Members:
id -- The identity of the object.
"""
def __init__(self, id=Ice._struct_marker):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ObjectExistsException'
_M_IceGrid._t_ObjectExistsException = IcePy.defineException('::IceGrid::ObjectExistsException', ObjectExistsException, (), False, None, (('id', (), _M_Ice._t_Identity, False, 0),))
ObjectExistsException._ice_type = _M_IceGrid._t_ObjectExistsException
_M_IceGrid.ObjectExistsException = ObjectExistsException
del ObjectExistsException
if 'ObjectNotRegisteredException' not in _M_IceGrid.__dict__:
_M_IceGrid.ObjectNotRegisteredException = Ice.createTempClass()
class ObjectNotRegisteredException(Ice.UserException):
"""
This exception is raised if an object is not registered.
Members:
id -- The identity of the object.
"""
def __init__(self, id=Ice._struct_marker):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ObjectNotRegisteredException'
_M_IceGrid._t_ObjectNotRegisteredException = IcePy.defineException('::IceGrid::ObjectNotRegisteredException', ObjectNotRegisteredException, (), False, None, (('id', (), _M_Ice._t_Identity, False, 0),))
ObjectNotRegisteredException._ice_type = _M_IceGrid._t_ObjectNotRegisteredException
_M_IceGrid.ObjectNotRegisteredException = ObjectNotRegisteredException
del ObjectNotRegisteredException
if 'NodeNotExistException' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeNotExistException = Ice.createTempClass()
class NodeNotExistException(Ice.UserException):
"""
This exception is raised if a node does not exist.
Members:
name -- The node name.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::NodeNotExistException'
_M_IceGrid._t_NodeNotExistException = IcePy.defineException('::IceGrid::NodeNotExistException', NodeNotExistException, (), False, None, (('name', (), IcePy._t_string, False, 0),))
NodeNotExistException._ice_type = _M_IceGrid._t_NodeNotExistException
_M_IceGrid.NodeNotExistException = NodeNotExistException
del NodeNotExistException
if 'RegistryNotExistException' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryNotExistException = Ice.createTempClass()
class RegistryNotExistException(Ice.UserException):
"""
This exception is raised if a registry does not exist.
Members:
name -- The registry name.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::RegistryNotExistException'
_M_IceGrid._t_RegistryNotExistException = IcePy.defineException('::IceGrid::RegistryNotExistException', RegistryNotExistException, (), False, None, (('name', (), IcePy._t_string, False, 0),))
RegistryNotExistException._ice_type = _M_IceGrid._t_RegistryNotExistException
_M_IceGrid.RegistryNotExistException = RegistryNotExistException
del RegistryNotExistException
if 'DeploymentException' not in _M_IceGrid.__dict__:
_M_IceGrid.DeploymentException = Ice.createTempClass()
class DeploymentException(Ice.UserException):
"""
An exception for deployment errors.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::DeploymentException'
_M_IceGrid._t_DeploymentException = IcePy.defineException('::IceGrid::DeploymentException', DeploymentException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
DeploymentException._ice_type = _M_IceGrid._t_DeploymentException
_M_IceGrid.DeploymentException = DeploymentException
del DeploymentException
if 'NodeUnreachableException' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeUnreachableException = Ice.createTempClass()
class NodeUnreachableException(Ice.UserException):
"""
This exception is raised if a node could not be reached.
Members:
name -- The name of the node that is not reachable.
reason -- The reason why the node couldn't be reached.
"""
def __init__(self, name='', reason=''):
self.name = name
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::NodeUnreachableException'
_M_IceGrid._t_NodeUnreachableException = IcePy.defineException('::IceGrid::NodeUnreachableException', NodeUnreachableException, (), False, None, (
('name', (), IcePy._t_string, False, 0),
('reason', (), IcePy._t_string, False, 0)
))
NodeUnreachableException._ice_type = _M_IceGrid._t_NodeUnreachableException
_M_IceGrid.NodeUnreachableException = NodeUnreachableException
del NodeUnreachableException
if 'ServerUnreachableException' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerUnreachableException = Ice.createTempClass()
class ServerUnreachableException(Ice.UserException):
"""
This exception is raised if a server could not be reached.
Members:
name -- The id of the server that is not reachable.
reason -- The reason why the server couldn't be reached.
"""
def __init__(self, name='', reason=''):
self.name = name
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ServerUnreachableException'
_M_IceGrid._t_ServerUnreachableException = IcePy.defineException('::IceGrid::ServerUnreachableException', ServerUnreachableException, (), False, None, (
('name', (), IcePy._t_string, False, 0),
('reason', (), IcePy._t_string, False, 0)
))
ServerUnreachableException._ice_type = _M_IceGrid._t_ServerUnreachableException
_M_IceGrid.ServerUnreachableException = ServerUnreachableException
del ServerUnreachableException
if 'RegistryUnreachableException' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryUnreachableException = Ice.createTempClass()
class RegistryUnreachableException(Ice.UserException):
"""
This exception is raised if a registry could not be reached.
Members:
name -- The name of the registry that is not reachable.
reason -- The reason why the registry couldn't be reached.
"""
def __init__(self, name='', reason=''):
self.name = name
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::RegistryUnreachableException'
_M_IceGrid._t_RegistryUnreachableException = IcePy.defineException('::IceGrid::RegistryUnreachableException', RegistryUnreachableException, (), False, None, (
('name', (), IcePy._t_string, False, 0),
('reason', (), IcePy._t_string, False, 0)
))
RegistryUnreachableException._ice_type = _M_IceGrid._t_RegistryUnreachableException
_M_IceGrid.RegistryUnreachableException = RegistryUnreachableException
del RegistryUnreachableException
if 'BadSignalException' not in _M_IceGrid.__dict__:
_M_IceGrid.BadSignalException = Ice.createTempClass()
class BadSignalException(Ice.UserException):
"""
This exception is raised if an unknown signal was sent to
to a server.
Members:
reason -- The details of the unknown signal.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::BadSignalException'
_M_IceGrid._t_BadSignalException = IcePy.defineException('::IceGrid::BadSignalException', BadSignalException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
BadSignalException._ice_type = _M_IceGrid._t_BadSignalException
_M_IceGrid.BadSignalException = BadSignalException
del BadSignalException
if 'PatchException' not in _M_IceGrid.__dict__:
_M_IceGrid.PatchException = Ice.createTempClass()
class PatchException(Ice.UserException):
"""
This exception is raised if a patch failed.
Members:
reasons -- The reasons why the patch failed.
"""
def __init__(self, reasons=None):
self.reasons = reasons
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::PatchException'
_M_IceGrid._t_PatchException = IcePy.defineException('::IceGrid::PatchException', PatchException, (), False, None, (('reasons', (), _M_Ice._t_StringSeq, False, 0),))
PatchException._ice_type = _M_IceGrid._t_PatchException
_M_IceGrid.PatchException = PatchException
del PatchException
if 'AccessDeniedException' not in _M_IceGrid.__dict__:
_M_IceGrid.AccessDeniedException = Ice.createTempClass()
class AccessDeniedException(Ice.UserException):
"""
This exception is raised if a registry lock wasn't
acquired or is already held by a session.
Members:
lockUserId -- The id of the user holding the lock (if any).
"""
def __init__(self, lockUserId=''):
self.lockUserId = lockUserId
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::AccessDeniedException'
_M_IceGrid._t_AccessDeniedException = IcePy.defineException('::IceGrid::AccessDeniedException', AccessDeniedException, (), False, None, (('lockUserId', (), IcePy._t_string, False, 0),))
AccessDeniedException._ice_type = _M_IceGrid._t_AccessDeniedException
_M_IceGrid.AccessDeniedException = AccessDeniedException
del AccessDeniedException
if 'AllocationException' not in _M_IceGrid.__dict__:
_M_IceGrid.AllocationException = Ice.createTempClass()
class AllocationException(Ice.UserException):
"""
This exception is raised if the allocation of an object failed.
Members:
reason -- The reason why the object couldn't be allocated.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::AllocationException'
_M_IceGrid._t_AllocationException = IcePy.defineException('::IceGrid::AllocationException', AllocationException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
AllocationException._ice_type = _M_IceGrid._t_AllocationException
_M_IceGrid.AllocationException = AllocationException
del AllocationException
if 'AllocationTimeoutException' not in _M_IceGrid.__dict__:
_M_IceGrid.AllocationTimeoutException = Ice.createTempClass()
class AllocationTimeoutException(_M_IceGrid.AllocationException):
"""
This exception is raised if the request to allocate an object times
out.
"""
def __init__(self, reason=''):
_M_IceGrid.AllocationException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::AllocationTimeoutException'
_M_IceGrid._t_AllocationTimeoutException = IcePy.defineException('::IceGrid::AllocationTimeoutException', AllocationTimeoutException, (), False, _M_IceGrid._t_AllocationException, ())
AllocationTimeoutException._ice_type = _M_IceGrid._t_AllocationTimeoutException
_M_IceGrid.AllocationTimeoutException = AllocationTimeoutException
del AllocationTimeoutException
if 'PermissionDeniedException' not in _M_IceGrid.__dict__:
_M_IceGrid.PermissionDeniedException = Ice.createTempClass()
class PermissionDeniedException(Ice.UserException):
"""
This exception is raised if a client is denied the ability to create
a session with IceGrid.
Members:
reason -- The reason why permission was denied.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::PermissionDeniedException'
_M_IceGrid._t_PermissionDeniedException = IcePy.defineException('::IceGrid::PermissionDeniedException', PermissionDeniedException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
PermissionDeniedException._ice_type = _M_IceGrid._t_PermissionDeniedException
_M_IceGrid.PermissionDeniedException = PermissionDeniedException
del PermissionDeniedException
if 'ObserverAlreadyRegisteredException' not in _M_IceGrid.__dict__:
_M_IceGrid.ObserverAlreadyRegisteredException = Ice.createTempClass()
class ObserverAlreadyRegisteredException(Ice.UserException):
"""
This exception is raised if an observer is already registered with
the registry.
Members:
id -- The identity of the observer.
"""
def __init__(self, id=Ice._struct_marker):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ObserverAlreadyRegisteredException'
_M_IceGrid._t_ObserverAlreadyRegisteredException = IcePy.defineException('::IceGrid::ObserverAlreadyRegisteredException', ObserverAlreadyRegisteredException, (), False, None, (('id', (), _M_Ice._t_Identity, False, 0),))
ObserverAlreadyRegisteredException._ice_type = _M_IceGrid._t_ObserverAlreadyRegisteredException
_M_IceGrid.ObserverAlreadyRegisteredException = ObserverAlreadyRegisteredException
del ObserverAlreadyRegisteredException
if 'FileNotAvailableException' not in _M_IceGrid.__dict__:
_M_IceGrid.FileNotAvailableException = Ice.createTempClass()
class FileNotAvailableException(Ice.UserException):
"""
This exception is raised if a file is not available.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::FileNotAvailableException'
_M_IceGrid._t_FileNotAvailableException = IcePy.defineException('::IceGrid::FileNotAvailableException', FileNotAvailableException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
FileNotAvailableException._ice_type = _M_IceGrid._t_FileNotAvailableException
_M_IceGrid.FileNotAvailableException = FileNotAvailableException
del FileNotAvailableException
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::AccessDeniedException"] = "e39e5ad60577c1e7b52e190e1d906b"
Ice.sliceChecksums["::IceGrid::AdapterNotExistException"] = "cee552cb69227f723030cd78b0cccc97"
Ice.sliceChecksums["::IceGrid::AllocationException"] = "ea85a8e5e5f281709bf6aa88d742"
Ice.sliceChecksums["::IceGrid::AllocationTimeoutException"] = "6695f5713499ac6de0626277e167f553"
Ice.sliceChecksums["::IceGrid::ApplicationNotExistException"] = "93fdaabe25dcf75485ffd4972223610"
Ice.sliceChecksums["::IceGrid::BadSignalException"] = "13e67e2d3f46a84aa73fd56d5812caf1"
Ice.sliceChecksums["::IceGrid::DeploymentException"] = "e316fdba8e93ef72d58bd61bbfe29e4"
Ice.sliceChecksums["::IceGrid::FileNotAvailableException"] = "a3e88ae3be93ecd4c82797ad26d6076"
Ice.sliceChecksums["::IceGrid::NodeNotExistException"] = "f07ddace1aa3cb1bbed37c3fbf862dff"
Ice.sliceChecksums["::IceGrid::NodeUnreachableException"] = "8f894a5022704f4dde30bb2a3ea326f9"
Ice.sliceChecksums["::IceGrid::ObjectExistsException"] = "833f69d3ebc872974a9f096352d2ddb"
Ice.sliceChecksums["::IceGrid::ObjectNotRegisteredException"] = "cb181c92b4dfb6e6b97f4ca806899e7"
Ice.sliceChecksums["::IceGrid::ObserverAlreadyRegisteredException"] = "e1267578f9666e2bda9952d7106fd12c"
Ice.sliceChecksums["::IceGrid::PatchException"] = "c28994d76c834b99b94cf4535a13d3"
Ice.sliceChecksums["::IceGrid::PermissionDeniedException"] = "27def8d4569ab203b629b9162d530ba"
Ice.sliceChecksums["::IceGrid::RegistryNotExistException"] = "9e1c1b717e9c5ef72886f16dbfce56f"
Ice.sliceChecksums["::IceGrid::RegistryUnreachableException"] = "514020cac28c588ae487a628e227699"
Ice.sliceChecksums["::IceGrid::ServerNotExistException"] = "6df151f3ce87bd522ed095f7ad97a941"
Ice.sliceChecksums["::IceGrid::ServerStartException"] = "ce92acafa218dd1d1e8aafab20d1"
Ice.sliceChecksums["::IceGrid::ServerStopException"] = "edb57abb5393b8b31b41f3a8e5bd111"
Ice.sliceChecksums["::IceGrid::ServerUnreachableException"] = "f3233583ef7ad8eac2f961aedafdd64" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/Exception_ice.py | Exception_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
__name__ = 'IceGrid'
if '_t_StringStringDict' not in _M_IceGrid.__dict__:
_M_IceGrid._t_StringStringDict = IcePy.defineDictionary('::IceGrid::StringStringDict', (), IcePy._t_string, IcePy._t_string)
if 'PropertyDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.PropertyDescriptor = Ice.createTempClass()
class PropertyDescriptor(object):
"""
Property descriptor.
Members:
name -- The name of the property.
value -- The value of the property.
"""
def __init__(self, name='', value=''):
self.name = name
self.value = value
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.value)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.PropertyDescriptor):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.value is None or other.value is None:
if self.value != other.value:
return (-1 if self.value is None else 1)
else:
if self.value < other.value:
return -1
elif self.value > other.value:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_PropertyDescriptor)
__repr__ = __str__
_M_IceGrid._t_PropertyDescriptor = IcePy.defineStruct('::IceGrid::PropertyDescriptor', PropertyDescriptor, (), (
('name', (), IcePy._t_string),
('value', (), IcePy._t_string)
))
_M_IceGrid.PropertyDescriptor = PropertyDescriptor
del PropertyDescriptor
if '_t_PropertyDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_PropertyDescriptorSeq = IcePy.defineSequence('::IceGrid::PropertyDescriptorSeq', (), _M_IceGrid._t_PropertyDescriptor)
if 'PropertySetDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.PropertySetDescriptor = Ice.createTempClass()
class PropertySetDescriptor(object):
"""
A property set descriptor.
Members:
references -- References to named property sets.
properties -- The property set properties.
"""
def __init__(self, references=None, properties=None):
self.references = references
self.properties = properties
def __hash__(self):
_h = 0
if self.references:
for _i0 in self.references:
_h = 5 * _h + Ice.getHash(_i0)
if self.properties:
for _i1 in self.properties:
_h = 5 * _h + Ice.getHash(_i1)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.PropertySetDescriptor):
return NotImplemented
else:
if self.references is None or other.references is None:
if self.references != other.references:
return (-1 if self.references is None else 1)
else:
if self.references < other.references:
return -1
elif self.references > other.references:
return 1
if self.properties is None or other.properties is None:
if self.properties != other.properties:
return (-1 if self.properties is None else 1)
else:
if self.properties < other.properties:
return -1
elif self.properties > other.properties:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_PropertySetDescriptor)
__repr__ = __str__
_M_IceGrid._t_PropertySetDescriptor = IcePy.defineStruct('::IceGrid::PropertySetDescriptor', PropertySetDescriptor, (), (
('references', (), _M_Ice._t_StringSeq),
('properties', (), _M_IceGrid._t_PropertyDescriptorSeq)
))
_M_IceGrid.PropertySetDescriptor = PropertySetDescriptor
del PropertySetDescriptor
if '_t_PropertySetDescriptorDict' not in _M_IceGrid.__dict__:
_M_IceGrid._t_PropertySetDescriptorDict = IcePy.defineDictionary('::IceGrid::PropertySetDescriptorDict', (), IcePy._t_string, _M_IceGrid._t_PropertySetDescriptor)
if 'ObjectDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ObjectDescriptor = Ice.createTempClass()
class ObjectDescriptor(object):
"""
An Ice object descriptor.
Members:
id -- The identity of the object.
type -- The object type.
proxyOptions -- Proxy options to use with the proxy created for this Ice object. If empty,
the proxy will be created with the proxy options specified on the object
adapter or replica group.
"""
def __init__(self, id=Ice._struct_marker, type='', proxyOptions=''):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
self.type = type
self.proxyOptions = proxyOptions
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.id)
_h = 5 * _h + Ice.getHash(self.type)
_h = 5 * _h + Ice.getHash(self.proxyOptions)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.ObjectDescriptor):
return NotImplemented
else:
if self.id is None or other.id is None:
if self.id != other.id:
return (-1 if self.id is None else 1)
else:
if self.id < other.id:
return -1
elif self.id > other.id:
return 1
if self.type is None or other.type is None:
if self.type != other.type:
return (-1 if self.type is None else 1)
else:
if self.type < other.type:
return -1
elif self.type > other.type:
return 1
if self.proxyOptions is None or other.proxyOptions is None:
if self.proxyOptions != other.proxyOptions:
return (-1 if self.proxyOptions is None else 1)
else:
if self.proxyOptions < other.proxyOptions:
return -1
elif self.proxyOptions > other.proxyOptions:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ObjectDescriptor)
__repr__ = __str__
_M_IceGrid._t_ObjectDescriptor = IcePy.defineStruct('::IceGrid::ObjectDescriptor', ObjectDescriptor, (), (
('id', (), _M_Ice._t_Identity),
('type', (), IcePy._t_string),
('proxyOptions', (), IcePy._t_string)
))
_M_IceGrid.ObjectDescriptor = ObjectDescriptor
del ObjectDescriptor
if '_t_ObjectDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ObjectDescriptorSeq = IcePy.defineSequence('::IceGrid::ObjectDescriptorSeq', (), _M_IceGrid._t_ObjectDescriptor)
if 'AdapterDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.AdapterDescriptor = Ice.createTempClass()
class AdapterDescriptor(object):
"""
An Ice object adapter descriptor.
Members:
name -- The object adapter name.
description -- The description of this object adapter.
id -- The object adapter id.
replicaGroupId -- The replica id of this adapter.
priority -- The adapter priority. This is eventually used when the adapter
is member of a replica group to sort the adapter endpoints by
priority.
registerProcess -- Flag to specify if the object adapter will register a process object.
serverLifetime -- If true the lifetime of this object adapter is the same of the
server lifetime. This information is used by the IceGrid node
to figure out the server state: the server is active only if
all its "server lifetime" adapters are active.
objects -- The well-known object descriptors associated with this object adapter.
allocatables -- The allocatable object descriptors associated with this object adapter.
"""
def __init__(self, name='', description='', id='', replicaGroupId='', priority='', registerProcess=False, serverLifetime=False, objects=None, allocatables=None):
self.name = name
self.description = description
self.id = id
self.replicaGroupId = replicaGroupId
self.priority = priority
self.registerProcess = registerProcess
self.serverLifetime = serverLifetime
self.objects = objects
self.allocatables = allocatables
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.description)
_h = 5 * _h + Ice.getHash(self.id)
_h = 5 * _h + Ice.getHash(self.replicaGroupId)
_h = 5 * _h + Ice.getHash(self.priority)
_h = 5 * _h + Ice.getHash(self.registerProcess)
_h = 5 * _h + Ice.getHash(self.serverLifetime)
if self.objects:
for _i0 in self.objects:
_h = 5 * _h + Ice.getHash(_i0)
if self.allocatables:
for _i1 in self.allocatables:
_h = 5 * _h + Ice.getHash(_i1)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.AdapterDescriptor):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.description is None or other.description is None:
if self.description != other.description:
return (-1 if self.description is None else 1)
else:
if self.description < other.description:
return -1
elif self.description > other.description:
return 1
if self.id is None or other.id is None:
if self.id != other.id:
return (-1 if self.id is None else 1)
else:
if self.id < other.id:
return -1
elif self.id > other.id:
return 1
if self.replicaGroupId is None or other.replicaGroupId is None:
if self.replicaGroupId != other.replicaGroupId:
return (-1 if self.replicaGroupId is None else 1)
else:
if self.replicaGroupId < other.replicaGroupId:
return -1
elif self.replicaGroupId > other.replicaGroupId:
return 1
if self.priority is None or other.priority is None:
if self.priority != other.priority:
return (-1 if self.priority is None else 1)
else:
if self.priority < other.priority:
return -1
elif self.priority > other.priority:
return 1
if self.registerProcess is None or other.registerProcess is None:
if self.registerProcess != other.registerProcess:
return (-1 if self.registerProcess is None else 1)
else:
if self.registerProcess < other.registerProcess:
return -1
elif self.registerProcess > other.registerProcess:
return 1
if self.serverLifetime is None or other.serverLifetime is None:
if self.serverLifetime != other.serverLifetime:
return (-1 if self.serverLifetime is None else 1)
else:
if self.serverLifetime < other.serverLifetime:
return -1
elif self.serverLifetime > other.serverLifetime:
return 1
if self.objects is None or other.objects is None:
if self.objects != other.objects:
return (-1 if self.objects is None else 1)
else:
if self.objects < other.objects:
return -1
elif self.objects > other.objects:
return 1
if self.allocatables is None or other.allocatables is None:
if self.allocatables != other.allocatables:
return (-1 if self.allocatables is None else 1)
else:
if self.allocatables < other.allocatables:
return -1
elif self.allocatables > other.allocatables:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdapterDescriptor)
__repr__ = __str__
_M_IceGrid._t_AdapterDescriptor = IcePy.defineStruct('::IceGrid::AdapterDescriptor', AdapterDescriptor, (), (
('name', (), IcePy._t_string),
('description', (), IcePy._t_string),
('id', (), IcePy._t_string),
('replicaGroupId', (), IcePy._t_string),
('priority', (), IcePy._t_string),
('registerProcess', (), IcePy._t_bool),
('serverLifetime', (), IcePy._t_bool),
('objects', (), _M_IceGrid._t_ObjectDescriptorSeq),
('allocatables', (), _M_IceGrid._t_ObjectDescriptorSeq)
))
_M_IceGrid.AdapterDescriptor = AdapterDescriptor
del AdapterDescriptor
if '_t_AdapterDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_AdapterDescriptorSeq = IcePy.defineSequence('::IceGrid::AdapterDescriptorSeq', (), _M_IceGrid._t_AdapterDescriptor)
if 'DbEnvDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.DbEnvDescriptor = Ice.createTempClass()
class DbEnvDescriptor(object):
"""
A Freeze database environment descriptor.
Members:
name -- The name of the database environment.
description -- The description of this database environment.
dbHome -- The home of the database environment (i.e., the directory where
the database files will be stored). If empty, the node will
provide a default database directory, otherwise the directory
must exist.
properties -- The configuration properties of the database environment.
"""
def __init__(self, name='', description='', dbHome='', properties=None):
self.name = name
self.description = description
self.dbHome = dbHome
self.properties = properties
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.description)
_h = 5 * _h + Ice.getHash(self.dbHome)
if self.properties:
for _i0 in self.properties:
_h = 5 * _h + Ice.getHash(_i0)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.DbEnvDescriptor):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.description is None or other.description is None:
if self.description != other.description:
return (-1 if self.description is None else 1)
else:
if self.description < other.description:
return -1
elif self.description > other.description:
return 1
if self.dbHome is None or other.dbHome is None:
if self.dbHome != other.dbHome:
return (-1 if self.dbHome is None else 1)
else:
if self.dbHome < other.dbHome:
return -1
elif self.dbHome > other.dbHome:
return 1
if self.properties is None or other.properties is None:
if self.properties != other.properties:
return (-1 if self.properties is None else 1)
else:
if self.properties < other.properties:
return -1
elif self.properties > other.properties:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_DbEnvDescriptor)
__repr__ = __str__
_M_IceGrid._t_DbEnvDescriptor = IcePy.defineStruct('::IceGrid::DbEnvDescriptor', DbEnvDescriptor, (), (
('name', (), IcePy._t_string),
('description', (), IcePy._t_string),
('dbHome', (), IcePy._t_string),
('properties', (), _M_IceGrid._t_PropertyDescriptorSeq)
))
_M_IceGrid.DbEnvDescriptor = DbEnvDescriptor
del DbEnvDescriptor
if '_t_DbEnvDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_DbEnvDescriptorSeq = IcePy.defineSequence('::IceGrid::DbEnvDescriptorSeq', (), _M_IceGrid._t_DbEnvDescriptor)
if 'CommunicatorDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.CommunicatorDescriptor = Ice.createTempClass()
class CommunicatorDescriptor(Ice.Value):
"""
A communicator descriptor.
Members:
adapters -- The object adapters.
propertySet -- The property set.
dbEnvs -- The database environments.
logs -- The path of each log file.
description -- A description of this descriptor.
"""
def __init__(self, adapters=None, propertySet=Ice._struct_marker, dbEnvs=None, logs=None, description=''):
self.adapters = adapters
if propertySet is Ice._struct_marker:
self.propertySet = _M_IceGrid.PropertySetDescriptor()
else:
self.propertySet = propertySet
self.dbEnvs = dbEnvs
self.logs = logs
self.description = description
def ice_id(self):
return '::IceGrid::CommunicatorDescriptor'
@staticmethod
def ice_staticId():
return '::IceGrid::CommunicatorDescriptor'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_CommunicatorDescriptor)
__repr__ = __str__
_M_IceGrid._t_CommunicatorDescriptor = IcePy.defineValue('::IceGrid::CommunicatorDescriptor', CommunicatorDescriptor, -1, (), False, False, None, (
('adapters', (), _M_IceGrid._t_AdapterDescriptorSeq, False, 0),
('propertySet', (), _M_IceGrid._t_PropertySetDescriptor, False, 0),
('dbEnvs', (), _M_IceGrid._t_DbEnvDescriptorSeq, False, 0),
('logs', (), _M_Ice._t_StringSeq, False, 0),
('description', (), IcePy._t_string, False, 0)
))
CommunicatorDescriptor._ice_type = _M_IceGrid._t_CommunicatorDescriptor
_M_IceGrid.CommunicatorDescriptor = CommunicatorDescriptor
del CommunicatorDescriptor
if 'DistributionDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.DistributionDescriptor = Ice.createTempClass()
class DistributionDescriptor(object):
"""
A distribution descriptor defines an IcePatch2 server and the
directories to retrieve from the patch server.
Members:
icepatch -- The proxy of the IcePatch2 server.
directories -- The source directories.
"""
def __init__(self, icepatch='', directories=None):
self.icepatch = icepatch
self.directories = directories
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.icepatch)
if self.directories:
for _i0 in self.directories:
_h = 5 * _h + Ice.getHash(_i0)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.DistributionDescriptor):
return NotImplemented
else:
if self.icepatch is None or other.icepatch is None:
if self.icepatch != other.icepatch:
return (-1 if self.icepatch is None else 1)
else:
if self.icepatch < other.icepatch:
return -1
elif self.icepatch > other.icepatch:
return 1
if self.directories is None or other.directories is None:
if self.directories != other.directories:
return (-1 if self.directories is None else 1)
else:
if self.directories < other.directories:
return -1
elif self.directories > other.directories:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_DistributionDescriptor)
__repr__ = __str__
_M_IceGrid._t_DistributionDescriptor = IcePy.defineStruct('::IceGrid::DistributionDescriptor', DistributionDescriptor, (), (
('icepatch', (), IcePy._t_string),
('directories', (), _M_Ice._t_StringSeq)
))
_M_IceGrid.DistributionDescriptor = DistributionDescriptor
del DistributionDescriptor
if 'ServerDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerDescriptor = Ice.createTempClass()
class ServerDescriptor(_M_IceGrid.CommunicatorDescriptor):
"""
An Ice server descriptor.
Members:
id -- The server id.
exe -- The path of the server executable.
iceVersion -- The Ice version used by this server. This is only required if
backward compatibility with servers using old Ice versions is
needed (otherwise the registry will assume the server is using
the same Ice version).
For example "3.1.1", "3.2", "3.3.0".
pwd -- The path to the server working directory.
options -- The command line options to pass to the server executable.
envs -- The server environment variables.
activation -- The server activation mode (possible values are "on-demand" or
"manual").
activationTimeout -- The activation timeout (an integer value representing the
number of seconds to wait for activation).
deactivationTimeout -- The deactivation timeout (an integer value representing the
number of seconds to wait for deactivation).
applicationDistrib -- Specifies if the server depends on the application
distribution.
distrib -- The distribution descriptor.
allocatable -- Specifies if the server is allocatable.
user -- The user account used to run the server.
"""
def __init__(self, adapters=None, propertySet=Ice._struct_marker, dbEnvs=None, logs=None, description='', id='', exe='', iceVersion='', pwd='', options=None, envs=None, activation='', activationTimeout='', deactivationTimeout='', applicationDistrib=False, distrib=Ice._struct_marker, allocatable=False, user=''):
_M_IceGrid.CommunicatorDescriptor.__init__(self, adapters, propertySet, dbEnvs, logs, description)
self.id = id
self.exe = exe
self.iceVersion = iceVersion
self.pwd = pwd
self.options = options
self.envs = envs
self.activation = activation
self.activationTimeout = activationTimeout
self.deactivationTimeout = deactivationTimeout
self.applicationDistrib = applicationDistrib
if distrib is Ice._struct_marker:
self.distrib = _M_IceGrid.DistributionDescriptor()
else:
self.distrib = distrib
self.allocatable = allocatable
self.user = user
def ice_id(self):
return '::IceGrid::ServerDescriptor'
@staticmethod
def ice_staticId():
return '::IceGrid::ServerDescriptor'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServerDescriptor)
__repr__ = __str__
_M_IceGrid._t_ServerDescriptor = IcePy.defineValue('::IceGrid::ServerDescriptor', ServerDescriptor, -1, (), False, False, _M_IceGrid._t_CommunicatorDescriptor, (
('id', (), IcePy._t_string, False, 0),
('exe', (), IcePy._t_string, False, 0),
('iceVersion', (), IcePy._t_string, False, 0),
('pwd', (), IcePy._t_string, False, 0),
('options', (), _M_Ice._t_StringSeq, False, 0),
('envs', (), _M_Ice._t_StringSeq, False, 0),
('activation', (), IcePy._t_string, False, 0),
('activationTimeout', (), IcePy._t_string, False, 0),
('deactivationTimeout', (), IcePy._t_string, False, 0),
('applicationDistrib', (), IcePy._t_bool, False, 0),
('distrib', (), _M_IceGrid._t_DistributionDescriptor, False, 0),
('allocatable', (), IcePy._t_bool, False, 0),
('user', (), IcePy._t_string, False, 0)
))
ServerDescriptor._ice_type = _M_IceGrid._t_ServerDescriptor
_M_IceGrid.ServerDescriptor = ServerDescriptor
del ServerDescriptor
if '_t_ServerDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ServerDescriptorSeq = IcePy.defineSequence('::IceGrid::ServerDescriptorSeq', (), _M_IceGrid._t_ServerDescriptor)
if 'ServiceDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ServiceDescriptor = Ice.createTempClass()
class ServiceDescriptor(_M_IceGrid.CommunicatorDescriptor):
"""
An IceBox service descriptor.
Members:
name -- The service name.
entry -- The entry point of the IceBox service.
"""
def __init__(self, adapters=None, propertySet=Ice._struct_marker, dbEnvs=None, logs=None, description='', name='', entry=''):
_M_IceGrid.CommunicatorDescriptor.__init__(self, adapters, propertySet, dbEnvs, logs, description)
self.name = name
self.entry = entry
def ice_id(self):
return '::IceGrid::ServiceDescriptor'
@staticmethod
def ice_staticId():
return '::IceGrid::ServiceDescriptor'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServiceDescriptor)
__repr__ = __str__
_M_IceGrid._t_ServiceDescriptor = IcePy.defineValue('::IceGrid::ServiceDescriptor', ServiceDescriptor, -1, (), False, False, _M_IceGrid._t_CommunicatorDescriptor, (
('name', (), IcePy._t_string, False, 0),
('entry', (), IcePy._t_string, False, 0)
))
ServiceDescriptor._ice_type = _M_IceGrid._t_ServiceDescriptor
_M_IceGrid.ServiceDescriptor = ServiceDescriptor
del ServiceDescriptor
if '_t_ServiceDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ServiceDescriptorSeq = IcePy.defineSequence('::IceGrid::ServiceDescriptorSeq', (), _M_IceGrid._t_ServiceDescriptor)
if 'ServerInstanceDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerInstanceDescriptor = Ice.createTempClass()
class ServerInstanceDescriptor(object):
"""
A server template instance descriptor.
Members:
template -- The template used by this instance.
parameterValues -- The template parameter values.
propertySet -- The property set.
servicePropertySets -- The services property sets. It's only valid to set these
property sets if the template is an IceBox server template.
"""
def __init__(self, template='', parameterValues=None, propertySet=Ice._struct_marker, servicePropertySets=None):
self.template = template
self.parameterValues = parameterValues
if propertySet is Ice._struct_marker:
self.propertySet = _M_IceGrid.PropertySetDescriptor()
else:
self.propertySet = propertySet
self.servicePropertySets = servicePropertySets
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ServerInstanceDescriptor):
return NotImplemented
else:
if self.template != other.template:
return False
if self.parameterValues != other.parameterValues:
return False
if self.propertySet != other.propertySet:
return False
if self.servicePropertySets != other.servicePropertySets:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServerInstanceDescriptor)
__repr__ = __str__
_M_IceGrid._t_ServerInstanceDescriptor = IcePy.defineStruct('::IceGrid::ServerInstanceDescriptor', ServerInstanceDescriptor, (), (
('template', (), IcePy._t_string),
('parameterValues', (), _M_IceGrid._t_StringStringDict),
('propertySet', (), _M_IceGrid._t_PropertySetDescriptor),
('servicePropertySets', (), _M_IceGrid._t_PropertySetDescriptorDict)
))
_M_IceGrid.ServerInstanceDescriptor = ServerInstanceDescriptor
del ServerInstanceDescriptor
if '_t_ServerInstanceDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ServerInstanceDescriptorSeq = IcePy.defineSequence('::IceGrid::ServerInstanceDescriptorSeq', (), _M_IceGrid._t_ServerInstanceDescriptor)
if 'TemplateDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.TemplateDescriptor = Ice.createTempClass()
class TemplateDescriptor(object):
"""
A template descriptor for server or service templates.
Members:
descriptor -- The template.
parameters -- The parameters required to instantiate the template.
parameterDefaults -- The parameters default values.
"""
def __init__(self, descriptor=None, parameters=None, parameterDefaults=None):
self.descriptor = descriptor
self.parameters = parameters
self.parameterDefaults = parameterDefaults
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.TemplateDescriptor):
return NotImplemented
else:
if self.descriptor != other.descriptor:
return False
if self.parameters != other.parameters:
return False
if self.parameterDefaults != other.parameterDefaults:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_TemplateDescriptor)
__repr__ = __str__
_M_IceGrid._t_TemplateDescriptor = IcePy.defineStruct('::IceGrid::TemplateDescriptor', TemplateDescriptor, (), (
('descriptor', (), _M_IceGrid._t_CommunicatorDescriptor),
('parameters', (), _M_Ice._t_StringSeq),
('parameterDefaults', (), _M_IceGrid._t_StringStringDict)
))
_M_IceGrid.TemplateDescriptor = TemplateDescriptor
del TemplateDescriptor
if '_t_TemplateDescriptorDict' not in _M_IceGrid.__dict__:
_M_IceGrid._t_TemplateDescriptorDict = IcePy.defineDictionary('::IceGrid::TemplateDescriptorDict', (), IcePy._t_string, _M_IceGrid._t_TemplateDescriptor)
if 'ServiceInstanceDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ServiceInstanceDescriptor = Ice.createTempClass()
class ServiceInstanceDescriptor(object):
"""
A service template instance descriptor.
Members:
template -- The template used by this instance.
parameterValues -- The template parameter values.
descriptor -- The service definition if the instance isn't a template
instance (i.e.: if the template attribute is empty).
propertySet -- The property set.
"""
def __init__(self, template='', parameterValues=None, descriptor=None, propertySet=Ice._struct_marker):
self.template = template
self.parameterValues = parameterValues
self.descriptor = descriptor
if propertySet is Ice._struct_marker:
self.propertySet = _M_IceGrid.PropertySetDescriptor()
else:
self.propertySet = propertySet
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ServiceInstanceDescriptor):
return NotImplemented
else:
if self.template != other.template:
return False
if self.parameterValues != other.parameterValues:
return False
if self.descriptor != other.descriptor:
return False
if self.propertySet != other.propertySet:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServiceInstanceDescriptor)
__repr__ = __str__
_M_IceGrid._t_ServiceInstanceDescriptor = IcePy.defineStruct('::IceGrid::ServiceInstanceDescriptor', ServiceInstanceDescriptor, (), (
('template', (), IcePy._t_string),
('parameterValues', (), _M_IceGrid._t_StringStringDict),
('descriptor', (), _M_IceGrid._t_ServiceDescriptor),
('propertySet', (), _M_IceGrid._t_PropertySetDescriptor)
))
_M_IceGrid.ServiceInstanceDescriptor = ServiceInstanceDescriptor
del ServiceInstanceDescriptor
if '_t_ServiceInstanceDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ServiceInstanceDescriptorSeq = IcePy.defineSequence('::IceGrid::ServiceInstanceDescriptorSeq', (), _M_IceGrid._t_ServiceInstanceDescriptor)
if 'IceBoxDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.IceBoxDescriptor = Ice.createTempClass()
class IceBoxDescriptor(_M_IceGrid.ServerDescriptor):
"""
An IceBox server descriptor.
Members:
services -- The service instances.
"""
def __init__(self, adapters=None, propertySet=Ice._struct_marker, dbEnvs=None, logs=None, description='', id='', exe='', iceVersion='', pwd='', options=None, envs=None, activation='', activationTimeout='', deactivationTimeout='', applicationDistrib=False, distrib=Ice._struct_marker, allocatable=False, user='', services=None):
_M_IceGrid.ServerDescriptor.__init__(self, adapters, propertySet, dbEnvs, logs, description, id, exe, iceVersion, pwd, options, envs, activation, activationTimeout, deactivationTimeout, applicationDistrib, distrib, allocatable, user)
self.services = services
def ice_id(self):
return '::IceGrid::IceBoxDescriptor'
@staticmethod
def ice_staticId():
return '::IceGrid::IceBoxDescriptor'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_IceBoxDescriptor)
__repr__ = __str__
_M_IceGrid._t_IceBoxDescriptor = IcePy.declareValue('::IceGrid::IceBoxDescriptor')
_M_IceGrid._t_IceBoxDescriptor = IcePy.defineValue('::IceGrid::IceBoxDescriptor', IceBoxDescriptor, -1, (), False, False, _M_IceGrid._t_ServerDescriptor, (('services', (), _M_IceGrid._t_ServiceInstanceDescriptorSeq, False, 0),))
IceBoxDescriptor._ice_type = _M_IceGrid._t_IceBoxDescriptor
_M_IceGrid.IceBoxDescriptor = IceBoxDescriptor
del IceBoxDescriptor
if 'NodeDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeDescriptor = Ice.createTempClass()
class NodeDescriptor(object):
"""
A node descriptor.
Members:
variables -- The variables defined for the node.
serverInstances -- The server instances.
servers -- Servers (which are not template instances).
loadFactor -- Load factor of the node.
description -- The description of this node.
propertySets -- Property set descriptors.
"""
def __init__(self, variables=None, serverInstances=None, servers=None, loadFactor='', description='', propertySets=None):
self.variables = variables
self.serverInstances = serverInstances
self.servers = servers
self.loadFactor = loadFactor
self.description = description
self.propertySets = propertySets
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.NodeDescriptor):
return NotImplemented
else:
if self.variables != other.variables:
return False
if self.serverInstances != other.serverInstances:
return False
if self.servers != other.servers:
return False
if self.loadFactor != other.loadFactor:
return False
if self.description != other.description:
return False
if self.propertySets != other.propertySets:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_NodeDescriptor)
__repr__ = __str__
_M_IceGrid._t_NodeDescriptor = IcePy.defineStruct('::IceGrid::NodeDescriptor', NodeDescriptor, (), (
('variables', (), _M_IceGrid._t_StringStringDict),
('serverInstances', (), _M_IceGrid._t_ServerInstanceDescriptorSeq),
('servers', (), _M_IceGrid._t_ServerDescriptorSeq),
('loadFactor', (), IcePy._t_string),
('description', (), IcePy._t_string),
('propertySets', (), _M_IceGrid._t_PropertySetDescriptorDict)
))
_M_IceGrid.NodeDescriptor = NodeDescriptor
del NodeDescriptor
if '_t_NodeDescriptorDict' not in _M_IceGrid.__dict__:
_M_IceGrid._t_NodeDescriptorDict = IcePy.defineDictionary('::IceGrid::NodeDescriptorDict', (), IcePy._t_string, _M_IceGrid._t_NodeDescriptor)
if 'LoadBalancingPolicy' not in _M_IceGrid.__dict__:
_M_IceGrid.LoadBalancingPolicy = Ice.createTempClass()
class LoadBalancingPolicy(Ice.Value):
"""
A base class for load balancing policies.
Members:
nReplicas -- The number of replicas that will be used to gather the
endpoints of a replica group.
"""
def __init__(self, nReplicas=''):
self.nReplicas = nReplicas
def ice_id(self):
return '::IceGrid::LoadBalancingPolicy'
@staticmethod
def ice_staticId():
return '::IceGrid::LoadBalancingPolicy'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_LoadBalancingPolicy)
__repr__ = __str__
_M_IceGrid._t_LoadBalancingPolicy = IcePy.defineValue('::IceGrid::LoadBalancingPolicy', LoadBalancingPolicy, -1, (), False, False, None, (('nReplicas', (), IcePy._t_string, False, 0),))
LoadBalancingPolicy._ice_type = _M_IceGrid._t_LoadBalancingPolicy
_M_IceGrid.LoadBalancingPolicy = LoadBalancingPolicy
del LoadBalancingPolicy
if 'RandomLoadBalancingPolicy' not in _M_IceGrid.__dict__:
_M_IceGrid.RandomLoadBalancingPolicy = Ice.createTempClass()
class RandomLoadBalancingPolicy(_M_IceGrid.LoadBalancingPolicy):
"""
Random load balancing policy.
"""
def __init__(self, nReplicas=''):
_M_IceGrid.LoadBalancingPolicy.__init__(self, nReplicas)
def ice_id(self):
return '::IceGrid::RandomLoadBalancingPolicy'
@staticmethod
def ice_staticId():
return '::IceGrid::RandomLoadBalancingPolicy'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RandomLoadBalancingPolicy)
__repr__ = __str__
_M_IceGrid._t_RandomLoadBalancingPolicy = IcePy.defineValue('::IceGrid::RandomLoadBalancingPolicy', RandomLoadBalancingPolicy, -1, (), False, False, _M_IceGrid._t_LoadBalancingPolicy, ())
RandomLoadBalancingPolicy._ice_type = _M_IceGrid._t_RandomLoadBalancingPolicy
_M_IceGrid.RandomLoadBalancingPolicy = RandomLoadBalancingPolicy
del RandomLoadBalancingPolicy
if 'OrderedLoadBalancingPolicy' not in _M_IceGrid.__dict__:
_M_IceGrid.OrderedLoadBalancingPolicy = Ice.createTempClass()
class OrderedLoadBalancingPolicy(_M_IceGrid.LoadBalancingPolicy):
"""
Ordered load balancing policy.
"""
def __init__(self, nReplicas=''):
_M_IceGrid.LoadBalancingPolicy.__init__(self, nReplicas)
def ice_id(self):
return '::IceGrid::OrderedLoadBalancingPolicy'
@staticmethod
def ice_staticId():
return '::IceGrid::OrderedLoadBalancingPolicy'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_OrderedLoadBalancingPolicy)
__repr__ = __str__
_M_IceGrid._t_OrderedLoadBalancingPolicy = IcePy.defineValue('::IceGrid::OrderedLoadBalancingPolicy', OrderedLoadBalancingPolicy, -1, (), False, False, _M_IceGrid._t_LoadBalancingPolicy, ())
OrderedLoadBalancingPolicy._ice_type = _M_IceGrid._t_OrderedLoadBalancingPolicy
_M_IceGrid.OrderedLoadBalancingPolicy = OrderedLoadBalancingPolicy
del OrderedLoadBalancingPolicy
if 'RoundRobinLoadBalancingPolicy' not in _M_IceGrid.__dict__:
_M_IceGrid.RoundRobinLoadBalancingPolicy = Ice.createTempClass()
class RoundRobinLoadBalancingPolicy(_M_IceGrid.LoadBalancingPolicy):
"""
Round robin load balancing policy.
"""
def __init__(self, nReplicas=''):
_M_IceGrid.LoadBalancingPolicy.__init__(self, nReplicas)
def ice_id(self):
return '::IceGrid::RoundRobinLoadBalancingPolicy'
@staticmethod
def ice_staticId():
return '::IceGrid::RoundRobinLoadBalancingPolicy'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RoundRobinLoadBalancingPolicy)
__repr__ = __str__
_M_IceGrid._t_RoundRobinLoadBalancingPolicy = IcePy.defineValue('::IceGrid::RoundRobinLoadBalancingPolicy', RoundRobinLoadBalancingPolicy, -1, (), False, False, _M_IceGrid._t_LoadBalancingPolicy, ())
RoundRobinLoadBalancingPolicy._ice_type = _M_IceGrid._t_RoundRobinLoadBalancingPolicy
_M_IceGrid.RoundRobinLoadBalancingPolicy = RoundRobinLoadBalancingPolicy
del RoundRobinLoadBalancingPolicy
if 'AdaptiveLoadBalancingPolicy' not in _M_IceGrid.__dict__:
_M_IceGrid.AdaptiveLoadBalancingPolicy = Ice.createTempClass()
class AdaptiveLoadBalancingPolicy(_M_IceGrid.LoadBalancingPolicy):
"""
Adaptive load balancing policy.
Members:
loadSample -- The load sample to use for the load balancing. The allowed
values for this attribute are "1", "5" and "15", representing
respectively the load average over the past minute, the past 5
minutes and the past 15 minutes.
"""
def __init__(self, nReplicas='', loadSample=''):
_M_IceGrid.LoadBalancingPolicy.__init__(self, nReplicas)
self.loadSample = loadSample
def ice_id(self):
return '::IceGrid::AdaptiveLoadBalancingPolicy'
@staticmethod
def ice_staticId():
return '::IceGrid::AdaptiveLoadBalancingPolicy'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdaptiveLoadBalancingPolicy)
__repr__ = __str__
_M_IceGrid._t_AdaptiveLoadBalancingPolicy = IcePy.defineValue('::IceGrid::AdaptiveLoadBalancingPolicy', AdaptiveLoadBalancingPolicy, -1, (), False, False, _M_IceGrid._t_LoadBalancingPolicy, (('loadSample', (), IcePy._t_string, False, 0),))
AdaptiveLoadBalancingPolicy._ice_type = _M_IceGrid._t_AdaptiveLoadBalancingPolicy
_M_IceGrid.AdaptiveLoadBalancingPolicy = AdaptiveLoadBalancingPolicy
del AdaptiveLoadBalancingPolicy
if 'ReplicaGroupDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ReplicaGroupDescriptor = Ice.createTempClass()
class ReplicaGroupDescriptor(object):
"""
A replica group descriptor.
Members:
id -- The id of the replica group.
loadBalancing -- The load balancing policy.
proxyOptions -- Default options for proxies created for the replica group.
objects -- The object descriptors associated with this object adapter.
description -- The description of this replica group.
filter -- The filter to use for this replica group.
"""
def __init__(self, id='', loadBalancing=None, proxyOptions='', objects=None, description='', filter=''):
self.id = id
self.loadBalancing = loadBalancing
self.proxyOptions = proxyOptions
self.objects = objects
self.description = description
self.filter = filter
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ReplicaGroupDescriptor):
return NotImplemented
else:
if self.id != other.id:
return False
if self.loadBalancing != other.loadBalancing:
return False
if self.proxyOptions != other.proxyOptions:
return False
if self.objects != other.objects:
return False
if self.description != other.description:
return False
if self.filter != other.filter:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ReplicaGroupDescriptor)
__repr__ = __str__
_M_IceGrid._t_ReplicaGroupDescriptor = IcePy.defineStruct('::IceGrid::ReplicaGroupDescriptor', ReplicaGroupDescriptor, (), (
('id', (), IcePy._t_string),
('loadBalancing', (), _M_IceGrid._t_LoadBalancingPolicy),
('proxyOptions', (), IcePy._t_string),
('objects', (), _M_IceGrid._t_ObjectDescriptorSeq),
('description', (), IcePy._t_string),
('filter', (), IcePy._t_string)
))
_M_IceGrid.ReplicaGroupDescriptor = ReplicaGroupDescriptor
del ReplicaGroupDescriptor
if '_t_ReplicaGroupDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ReplicaGroupDescriptorSeq = IcePy.defineSequence('::IceGrid::ReplicaGroupDescriptorSeq', (), _M_IceGrid._t_ReplicaGroupDescriptor)
if 'ApplicationDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationDescriptor = Ice.createTempClass()
class ApplicationDescriptor(object):
"""
An application descriptor.
Members:
name -- The application name.
variables -- The variables defined in the application descriptor.
replicaGroups -- The replica groups.
serverTemplates -- The server templates.
serviceTemplates -- The service templates.
nodes -- The application nodes.
distrib -- The application distribution.
description -- The description of this application.
propertySets -- Property set descriptors.
"""
def __init__(self, name='', variables=None, replicaGroups=None, serverTemplates=None, serviceTemplates=None, nodes=None, distrib=Ice._struct_marker, description='', propertySets=None):
self.name = name
self.variables = variables
self.replicaGroups = replicaGroups
self.serverTemplates = serverTemplates
self.serviceTemplates = serviceTemplates
self.nodes = nodes
if distrib is Ice._struct_marker:
self.distrib = _M_IceGrid.DistributionDescriptor()
else:
self.distrib = distrib
self.description = description
self.propertySets = propertySets
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ApplicationDescriptor):
return NotImplemented
else:
if self.name != other.name:
return False
if self.variables != other.variables:
return False
if self.replicaGroups != other.replicaGroups:
return False
if self.serverTemplates != other.serverTemplates:
return False
if self.serviceTemplates != other.serviceTemplates:
return False
if self.nodes != other.nodes:
return False
if self.distrib != other.distrib:
return False
if self.description != other.description:
return False
if self.propertySets != other.propertySets:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ApplicationDescriptor)
__repr__ = __str__
_M_IceGrid._t_ApplicationDescriptor = IcePy.defineStruct('::IceGrid::ApplicationDescriptor', ApplicationDescriptor, (), (
('name', (), IcePy._t_string),
('variables', (), _M_IceGrid._t_StringStringDict),
('replicaGroups', (), _M_IceGrid._t_ReplicaGroupDescriptorSeq),
('serverTemplates', (), _M_IceGrid._t_TemplateDescriptorDict),
('serviceTemplates', (), _M_IceGrid._t_TemplateDescriptorDict),
('nodes', (), _M_IceGrid._t_NodeDescriptorDict),
('distrib', (), _M_IceGrid._t_DistributionDescriptor),
('description', (), IcePy._t_string),
('propertySets', (), _M_IceGrid._t_PropertySetDescriptorDict)
))
_M_IceGrid.ApplicationDescriptor = ApplicationDescriptor
del ApplicationDescriptor
if '_t_ApplicationDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ApplicationDescriptorSeq = IcePy.defineSequence('::IceGrid::ApplicationDescriptorSeq', (), _M_IceGrid._t_ApplicationDescriptor)
if 'BoxedString' not in _M_IceGrid.__dict__:
_M_IceGrid.BoxedString = Ice.createTempClass()
class BoxedString(Ice.Value):
"""
A "boxed" string.
Members:
value -- The value of the boxed string.
"""
def __init__(self, value=''):
self.value = value
def ice_id(self):
return '::IceGrid::BoxedString'
@staticmethod
def ice_staticId():
return '::IceGrid::BoxedString'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_BoxedString)
__repr__ = __str__
_M_IceGrid._t_BoxedString = IcePy.defineValue('::IceGrid::BoxedString', BoxedString, -1, (), False, False, None, (('value', (), IcePy._t_string, False, 0),))
BoxedString._ice_type = _M_IceGrid._t_BoxedString
_M_IceGrid.BoxedString = BoxedString
del BoxedString
if 'NodeUpdateDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeUpdateDescriptor = Ice.createTempClass()
class NodeUpdateDescriptor(object):
"""
A node update descriptor to describe the updates to apply to a
node of a deployed application.
Members:
name -- The name of the node to update.
description -- The updated description (or null if the description wasn't
updated.)
variables -- The variables to update.
removeVariables -- The variables to remove.
propertySets -- The property sets to update.
removePropertySets -- The property sets to remove.
serverInstances -- The server instances to update.
servers -- The servers which are not template instances to update.
removeServers -- The ids of the servers to remove.
loadFactor -- The updated load factor of the node (or null if the load factor
was not updated).
"""
def __init__(self, name='', description=None, variables=None, removeVariables=None, propertySets=None, removePropertySets=None, serverInstances=None, servers=None, removeServers=None, loadFactor=None):
self.name = name
self.description = description
self.variables = variables
self.removeVariables = removeVariables
self.propertySets = propertySets
self.removePropertySets = removePropertySets
self.serverInstances = serverInstances
self.servers = servers
self.removeServers = removeServers
self.loadFactor = loadFactor
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.NodeUpdateDescriptor):
return NotImplemented
else:
if self.name != other.name:
return False
if self.description != other.description:
return False
if self.variables != other.variables:
return False
if self.removeVariables != other.removeVariables:
return False
if self.propertySets != other.propertySets:
return False
if self.removePropertySets != other.removePropertySets:
return False
if self.serverInstances != other.serverInstances:
return False
if self.servers != other.servers:
return False
if self.removeServers != other.removeServers:
return False
if self.loadFactor != other.loadFactor:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_NodeUpdateDescriptor)
__repr__ = __str__
_M_IceGrid._t_NodeUpdateDescriptor = IcePy.defineStruct('::IceGrid::NodeUpdateDescriptor', NodeUpdateDescriptor, (), (
('name', (), IcePy._t_string),
('description', (), _M_IceGrid._t_BoxedString),
('variables', (), _M_IceGrid._t_StringStringDict),
('removeVariables', (), _M_Ice._t_StringSeq),
('propertySets', (), _M_IceGrid._t_PropertySetDescriptorDict),
('removePropertySets', (), _M_Ice._t_StringSeq),
('serverInstances', (), _M_IceGrid._t_ServerInstanceDescriptorSeq),
('servers', (), _M_IceGrid._t_ServerDescriptorSeq),
('removeServers', (), _M_Ice._t_StringSeq),
('loadFactor', (), _M_IceGrid._t_BoxedString)
))
_M_IceGrid.NodeUpdateDescriptor = NodeUpdateDescriptor
del NodeUpdateDescriptor
if '_t_NodeUpdateDescriptorSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_NodeUpdateDescriptorSeq = IcePy.defineSequence('::IceGrid::NodeUpdateDescriptorSeq', (), _M_IceGrid._t_NodeUpdateDescriptor)
if 'BoxedDistributionDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.BoxedDistributionDescriptor = Ice.createTempClass()
class BoxedDistributionDescriptor(Ice.Value):
"""
A "boxed" distribution descriptor.
Members:
value -- The value of the boxed distribution descriptor.
"""
def __init__(self, value=Ice._struct_marker):
if value is Ice._struct_marker:
self.value = _M_IceGrid.DistributionDescriptor()
else:
self.value = value
def ice_id(self):
return '::IceGrid::BoxedDistributionDescriptor'
@staticmethod
def ice_staticId():
return '::IceGrid::BoxedDistributionDescriptor'
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_BoxedDistributionDescriptor)
__repr__ = __str__
_M_IceGrid._t_BoxedDistributionDescriptor = IcePy.defineValue('::IceGrid::BoxedDistributionDescriptor', BoxedDistributionDescriptor, -1, (), False, False, None, (('value', (), _M_IceGrid._t_DistributionDescriptor, False, 0),))
BoxedDistributionDescriptor._ice_type = _M_IceGrid._t_BoxedDistributionDescriptor
_M_IceGrid.BoxedDistributionDescriptor = BoxedDistributionDescriptor
del BoxedDistributionDescriptor
if 'ApplicationUpdateDescriptor' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationUpdateDescriptor = Ice.createTempClass()
class ApplicationUpdateDescriptor(object):
"""
An application update descriptor to describe the updates to apply
to a deployed application.
Members:
name -- The name of the application to update.
description -- The updated description (or null if the description wasn't
updated.)
distrib -- The updated distribution application descriptor.
variables -- The variables to update.
removeVariables -- The variables to remove.
propertySets -- The property sets to update.
removePropertySets -- The property sets to remove.
replicaGroups -- The replica groups to update.
removeReplicaGroups -- The replica groups to remove.
serverTemplates -- The server templates to update.
removeServerTemplates -- The ids of the server template to remove.
serviceTemplates -- The service templates to update.
removeServiceTemplates -- The ids of the service template to remove.
nodes -- The application nodes to update.
removeNodes -- The nodes to remove.
"""
def __init__(self, name='', description=None, distrib=None, variables=None, removeVariables=None, propertySets=None, removePropertySets=None, replicaGroups=None, removeReplicaGroups=None, serverTemplates=None, removeServerTemplates=None, serviceTemplates=None, removeServiceTemplates=None, nodes=None, removeNodes=None):
self.name = name
self.description = description
self.distrib = distrib
self.variables = variables
self.removeVariables = removeVariables
self.propertySets = propertySets
self.removePropertySets = removePropertySets
self.replicaGroups = replicaGroups
self.removeReplicaGroups = removeReplicaGroups
self.serverTemplates = serverTemplates
self.removeServerTemplates = removeServerTemplates
self.serviceTemplates = serviceTemplates
self.removeServiceTemplates = removeServiceTemplates
self.nodes = nodes
self.removeNodes = removeNodes
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ApplicationUpdateDescriptor):
return NotImplemented
else:
if self.name != other.name:
return False
if self.description != other.description:
return False
if self.distrib != other.distrib:
return False
if self.variables != other.variables:
return False
if self.removeVariables != other.removeVariables:
return False
if self.propertySets != other.propertySets:
return False
if self.removePropertySets != other.removePropertySets:
return False
if self.replicaGroups != other.replicaGroups:
return False
if self.removeReplicaGroups != other.removeReplicaGroups:
return False
if self.serverTemplates != other.serverTemplates:
return False
if self.removeServerTemplates != other.removeServerTemplates:
return False
if self.serviceTemplates != other.serviceTemplates:
return False
if self.removeServiceTemplates != other.removeServiceTemplates:
return False
if self.nodes != other.nodes:
return False
if self.removeNodes != other.removeNodes:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ApplicationUpdateDescriptor)
__repr__ = __str__
_M_IceGrid._t_ApplicationUpdateDescriptor = IcePy.defineStruct('::IceGrid::ApplicationUpdateDescriptor', ApplicationUpdateDescriptor, (), (
('name', (), IcePy._t_string),
('description', (), _M_IceGrid._t_BoxedString),
('distrib', (), _M_IceGrid._t_BoxedDistributionDescriptor),
('variables', (), _M_IceGrid._t_StringStringDict),
('removeVariables', (), _M_Ice._t_StringSeq),
('propertySets', (), _M_IceGrid._t_PropertySetDescriptorDict),
('removePropertySets', (), _M_Ice._t_StringSeq),
('replicaGroups', (), _M_IceGrid._t_ReplicaGroupDescriptorSeq),
('removeReplicaGroups', (), _M_Ice._t_StringSeq),
('serverTemplates', (), _M_IceGrid._t_TemplateDescriptorDict),
('removeServerTemplates', (), _M_Ice._t_StringSeq),
('serviceTemplates', (), _M_IceGrid._t_TemplateDescriptorDict),
('removeServiceTemplates', (), _M_Ice._t_StringSeq),
('nodes', (), _M_IceGrid._t_NodeUpdateDescriptorSeq),
('removeNodes', (), _M_Ice._t_StringSeq)
))
_M_IceGrid.ApplicationUpdateDescriptor = ApplicationUpdateDescriptor
del ApplicationUpdateDescriptor
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::AdapterDescriptor"] = "4ae12581eab9d8ecba56534d28960f0"
Ice.sliceChecksums["::IceGrid::AdapterDescriptorSeq"] = "61bb9118038552b5e80bf14cf41719c"
Ice.sliceChecksums["::IceGrid::AdaptiveLoadBalancingPolicy"] = "eae551a45bf88ecdfdcbd169e3502816"
Ice.sliceChecksums["::IceGrid::ApplicationDescriptor"] = "fc17fb9c4c7fc8f17ad10bc5da634a0"
Ice.sliceChecksums["::IceGrid::ApplicationDescriptorSeq"] = "b56d6d3091e8c0199e924bbdc074"
Ice.sliceChecksums["::IceGrid::ApplicationUpdateDescriptor"] = "9aef62072a0ecc3ee4be33bc46e0da"
Ice.sliceChecksums["::IceGrid::BoxedDistributionDescriptor"] = "bab8796f5dc33ebe6955d4bb3219c5e9"
Ice.sliceChecksums["::IceGrid::BoxedString"] = "f6bfc069c5150c34e14331c921218d7"
Ice.sliceChecksums["::IceGrid::CommunicatorDescriptor"] = "b7cdae49f8df0d1d93afb857875ec15"
Ice.sliceChecksums["::IceGrid::DbEnvDescriptor"] = "19c130dac4bf7fa2f82375a85e5f421"
Ice.sliceChecksums["::IceGrid::DbEnvDescriptorSeq"] = "d0e45f67b942541727ae69d6cda2fdd8"
Ice.sliceChecksums["::IceGrid::DistributionDescriptor"] = "109eee8e2dc57e518243806796d756"
Ice.sliceChecksums["::IceGrid::IceBoxDescriptor"] = "814eec3d42ab727f75f7b183e1b02c38"
Ice.sliceChecksums["::IceGrid::LoadBalancingPolicy"] = "dfbd5166bbdcac620f2d7f5de185afe"
Ice.sliceChecksums["::IceGrid::NodeDescriptor"] = "be38d2d0b946fea6266f7a97d493d4"
Ice.sliceChecksums["::IceGrid::NodeDescriptorDict"] = "600e78031867992f2fbd18719cb494"
Ice.sliceChecksums["::IceGrid::NodeUpdateDescriptor"] = "d1c0a29ce34753b44e54285c49c9780"
Ice.sliceChecksums["::IceGrid::NodeUpdateDescriptorSeq"] = "3416e1746e2acedfb8192d9d83d9dc3"
Ice.sliceChecksums["::IceGrid::ObjectDescriptor"] = "913039a22b7b5fc0fd156ce764a4237d"
Ice.sliceChecksums["::IceGrid::ObjectDescriptorSeq"] = "57236a6ef224f825849907a344412bb"
Ice.sliceChecksums["::IceGrid::OrderedLoadBalancingPolicy"] = "bef5dacddeeae0e0b58945adaea2121"
Ice.sliceChecksums["::IceGrid::PropertyDescriptor"] = "8b2145a8b1c5c8ffc9eac6a13e731798"
Ice.sliceChecksums["::IceGrid::PropertyDescriptorSeq"] = "5f4143ef7e2c87b63136a3177b7a2830"
Ice.sliceChecksums["::IceGrid::PropertySetDescriptor"] = "d07a6de61ed833b349d869bacb7d857"
Ice.sliceChecksums["::IceGrid::PropertySetDescriptorDict"] = "30fc60d722ab4ba7affa70387730322f"
Ice.sliceChecksums["::IceGrid::RandomLoadBalancingPolicy"] = "b52a26591c76fe2d6d134d954568c1a"
Ice.sliceChecksums["::IceGrid::ReplicaGroupDescriptor"] = "dadc1d584d51fe9a16ea846b2796717"
Ice.sliceChecksums["::IceGrid::ReplicaGroupDescriptorSeq"] = "5a3d3e7b4dc5f21b74f7adb5a6b24ccc"
Ice.sliceChecksums["::IceGrid::RoundRobinLoadBalancingPolicy"] = "d9c7e987c732d89b7aa79621a788fcb4"
Ice.sliceChecksums["::IceGrid::ServerDescriptor"] = "45903227dd1968cedd1811b9d71bc374"
Ice.sliceChecksums["::IceGrid::ServerDescriptorSeq"] = "1bf128cadf1974b22258f66617a1ed"
Ice.sliceChecksums["::IceGrid::ServerInstanceDescriptor"] = "56938d38e0189cdbd57d16e5a6bbc0fb"
Ice.sliceChecksums["::IceGrid::ServerInstanceDescriptorSeq"] = "2a8ae55ccef7917d96691c0a84778dd"
Ice.sliceChecksums["::IceGrid::ServiceDescriptor"] = "7c2496565248aa7d9732565ee5fe7c"
Ice.sliceChecksums["::IceGrid::ServiceDescriptorSeq"] = "cc519ed2b7f626b896cdc062823166"
Ice.sliceChecksums["::IceGrid::ServiceInstanceDescriptor"] = "8581f0afc39ae7daab937244b28c1394"
Ice.sliceChecksums["::IceGrid::ServiceInstanceDescriptorSeq"] = "eb22cd2a50e79f648d803c4b54755"
Ice.sliceChecksums["::IceGrid::StringStringDict"] = "87cdc9524ba3964efc9091e5b3346f29"
Ice.sliceChecksums["::IceGrid::TemplateDescriptor"] = "d1229192d114f32db747493becd5765"
Ice.sliceChecksums["::IceGrid::TemplateDescriptorDict"] = "7b9427f03e8ce3b67decd2cc35baa1" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/Descriptor_ice.py | Descriptor_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
__name__ = 'IceGrid'
if 'UserAccountNotFoundException' not in _M_IceGrid.__dict__:
_M_IceGrid.UserAccountNotFoundException = Ice.createTempClass()
class UserAccountNotFoundException(Ice.UserException):
"""
This exception is raised if a user account for a given session
identifier can't be found.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::UserAccountNotFoundException'
_M_IceGrid._t_UserAccountNotFoundException = IcePy.defineException('::IceGrid::UserAccountNotFoundException', UserAccountNotFoundException, (), False, None, ())
UserAccountNotFoundException._ice_type = _M_IceGrid._t_UserAccountNotFoundException
_M_IceGrid.UserAccountNotFoundException = UserAccountNotFoundException
del UserAccountNotFoundException
_M_IceGrid._t_UserAccountMapper = IcePy.defineValue('::IceGrid::UserAccountMapper', Ice.Value, -1, (), False, True, None, ())
if 'UserAccountMapperPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.UserAccountMapperPrx = Ice.createTempClass()
class UserAccountMapperPrx(Ice.ObjectPrx):
"""
Get the name of the user account for the given user. This is
used by IceGrid nodes to figure out the user account to use
to run servers.
Arguments:
user -- The value of the server descriptor's user attribute. If this attribute is not defined, and the server's activation mode is session, the default value of user is the session identifier.
context -- The request context for the invocation.
Returns: The user account name.
Throws:
UserAccountNotFoundException -- Raised if no user account is found for the given user.
"""
def getUserAccount(self, user, context=None):
return _M_IceGrid.UserAccountMapper._op_getUserAccount.invoke(self, ((user, ), context))
"""
Get the name of the user account for the given user. This is
used by IceGrid nodes to figure out the user account to use
to run servers.
Arguments:
user -- The value of the server descriptor's user attribute. If this attribute is not defined, and the server's activation mode is session, the default value of user is the session identifier.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getUserAccountAsync(self, user, context=None):
return _M_IceGrid.UserAccountMapper._op_getUserAccount.invokeAsync(self, ((user, ), context))
"""
Get the name of the user account for the given user. This is
used by IceGrid nodes to figure out the user account to use
to run servers.
Arguments:
user -- The value of the server descriptor's user attribute. If this attribute is not defined, and the server's activation mode is session, the default value of user is the session identifier.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getUserAccount(self, user, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.UserAccountMapper._op_getUserAccount.begin(self, ((user, ), _response, _ex, _sent, context))
"""
Get the name of the user account for the given user. This is
used by IceGrid nodes to figure out the user account to use
to run servers.
Arguments:
user -- The value of the server descriptor's user attribute. If this attribute is not defined, and the server's activation mode is session, the default value of user is the session identifier.
Returns: The user account name.
Throws:
UserAccountNotFoundException -- Raised if no user account is found for the given user.
"""
def end_getUserAccount(self, _r):
return _M_IceGrid.UserAccountMapper._op_getUserAccount.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.UserAccountMapperPrx.ice_checkedCast(proxy, '::IceGrid::UserAccountMapper', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.UserAccountMapperPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::UserAccountMapper'
_M_IceGrid._t_UserAccountMapperPrx = IcePy.defineProxy('::IceGrid::UserAccountMapper', UserAccountMapperPrx)
_M_IceGrid.UserAccountMapperPrx = UserAccountMapperPrx
del UserAccountMapperPrx
_M_IceGrid.UserAccountMapper = Ice.createTempClass()
class UserAccountMapper(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::UserAccountMapper')
def ice_id(self, current=None):
return '::IceGrid::UserAccountMapper'
@staticmethod
def ice_staticId():
return '::IceGrid::UserAccountMapper'
def getUserAccount(self, user, current=None):
"""
Get the name of the user account for the given user. This is
used by IceGrid nodes to figure out the user account to use
to run servers.
Arguments:
user -- The value of the server descriptor's user attribute. If this attribute is not defined, and the server's activation mode is session, the default value of user is the session identifier.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UserAccountNotFoundException -- Raised if no user account is found for the given user.
"""
raise NotImplementedError("servant method 'getUserAccount' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_UserAccountMapperDisp)
__repr__ = __str__
_M_IceGrid._t_UserAccountMapperDisp = IcePy.defineClass('::IceGrid::UserAccountMapper', UserAccountMapper, (), None, ())
UserAccountMapper._ice_type = _M_IceGrid._t_UserAccountMapperDisp
UserAccountMapper._op_getUserAccount = IcePy.Operation('getUserAccount', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_string, False, 0), (_M_IceGrid._t_UserAccountNotFoundException,))
_M_IceGrid.UserAccountMapper = UserAccountMapper
del UserAccountMapper
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::UserAccountMapper"] = "779fd561878e199444e04cdebaf9ffd4"
Ice.sliceChecksums["::IceGrid::UserAccountNotFoundException"] = "fe2dc4d87f21b9b2cf6f1339d1666281" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/UserAccountMapper_ice.py | UserAccountMapper_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import IceGrid.Admin_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Included module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
# Start of module IceGrid
__name__ = 'IceGrid'
if 'ParseException' not in _M_IceGrid.__dict__:
_M_IceGrid.ParseException = Ice.createTempClass()
class ParseException(Ice.UserException):
"""
This exception is raised if an error occurs during parsing.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceGrid::ParseException'
_M_IceGrid._t_ParseException = IcePy.defineException('::IceGrid::ParseException', ParseException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
ParseException._ice_type = _M_IceGrid._t_ParseException
_M_IceGrid.ParseException = ParseException
del ParseException
_M_IceGrid._t_FileParser = IcePy.defineValue('::IceGrid::FileParser', Ice.Value, -1, (), False, True, None, ())
if 'FileParserPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.FileParserPrx = Ice.createTempClass()
class FileParserPrx(Ice.ObjectPrx):
"""
Parse a file.
Arguments:
xmlFile -- Full pathname to the file.
adminProxy -- An Admin proxy, used only to retrieve default templates when needed. May be null.
context -- The request context for the invocation.
Returns: The application descriptor.
Throws:
ParseException -- Raised if an error occurred during parsing.
"""
def parse(self, xmlFile, adminProxy, context=None):
return _M_IceGrid.FileParser._op_parse.invoke(self, ((xmlFile, adminProxy), context))
"""
Parse a file.
Arguments:
xmlFile -- Full pathname to the file.
adminProxy -- An Admin proxy, used only to retrieve default templates when needed. May be null.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def parseAsync(self, xmlFile, adminProxy, context=None):
return _M_IceGrid.FileParser._op_parse.invokeAsync(self, ((xmlFile, adminProxy), context))
"""
Parse a file.
Arguments:
xmlFile -- Full pathname to the file.
adminProxy -- An Admin proxy, used only to retrieve default templates when needed. May be null.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_parse(self, xmlFile, adminProxy, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.FileParser._op_parse.begin(self, ((xmlFile, adminProxy), _response, _ex, _sent, context))
"""
Parse a file.
Arguments:
xmlFile -- Full pathname to the file.
adminProxy -- An Admin proxy, used only to retrieve default templates when needed. May be null.
Returns: The application descriptor.
Throws:
ParseException -- Raised if an error occurred during parsing.
"""
def end_parse(self, _r):
return _M_IceGrid.FileParser._op_parse.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.FileParserPrx.ice_checkedCast(proxy, '::IceGrid::FileParser', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.FileParserPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::FileParser'
_M_IceGrid._t_FileParserPrx = IcePy.defineProxy('::IceGrid::FileParser', FileParserPrx)
_M_IceGrid.FileParserPrx = FileParserPrx
del FileParserPrx
_M_IceGrid.FileParser = Ice.createTempClass()
class FileParser(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::FileParser')
def ice_id(self, current=None):
return '::IceGrid::FileParser'
@staticmethod
def ice_staticId():
return '::IceGrid::FileParser'
def parse(self, xmlFile, adminProxy, current=None):
"""
Parse a file.
Arguments:
xmlFile -- Full pathname to the file.
adminProxy -- An Admin proxy, used only to retrieve default templates when needed. May be null.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ParseException -- Raised if an error occurred during parsing.
"""
raise NotImplementedError("servant method 'parse' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_FileParserDisp)
__repr__ = __str__
_M_IceGrid._t_FileParserDisp = IcePy.defineClass('::IceGrid::FileParser', FileParser, (), None, ())
FileParser._ice_type = _M_IceGrid._t_FileParserDisp
FileParser._op_parse = IcePy.Operation('parse', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0), ((), _M_IceGrid._t_AdminPrx, False, 0)), (), ((), _M_IceGrid._t_ApplicationDescriptor, False, 0), (_M_IceGrid._t_ParseException,))
_M_IceGrid.FileParser = FileParser
del FileParser
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::FileParser"] = "b847ccf3e3db7cbba649ec7cc464faf"
Ice.sliceChecksums["::IceGrid::ParseException"] = "dec9aacba8b3ba76afc5de1cc3489598" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/FileParser_ice.py | FileParser_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
import Ice.Current_ice
import IceGrid.Admin_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Included module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
# Start of module IceGrid
__name__ = 'IceGrid'
if 'ReplicaGroupFilter' not in _M_IceGrid.__dict__:
_M_IceGrid.ReplicaGroupFilter = Ice.createTempClass()
class ReplicaGroupFilter(object):
"""
The ReplicaGroupFilter is used by IceGrid to filter adapters
returned to the client when it resolves a filtered replica group.
IceGrid provides the list of available adapters. The implementation
of this method can use the provided context and connection to
filter and return the filtered set of adapters.
"""
def __init__(self):
if Ice.getType(self) == _M_IceGrid.ReplicaGroupFilter:
raise RuntimeError('IceGrid.ReplicaGroupFilter is an abstract class')
def filter(self, replicaGroupId, adapterIds, con, ctx):
"""
Filter the the given set of adapters.
Arguments:
replicaGroupId -- The replica group ID.
adapterIds -- The adpater IDs to filter.
con -- The connection from the Ice client which is resolving the replica group endpoints.
ctx -- The context from the Ice client which is resolving the replica group endpoints.
Returns: The filtered adapter IDs.
"""
raise NotImplementedError("method 'filter' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ReplicaGroupFilter)
__repr__ = __str__
_M_IceGrid._t_ReplicaGroupFilter = IcePy.defineValue('::IceGrid::ReplicaGroupFilter', ReplicaGroupFilter, -1, (), False, True, None, ())
ReplicaGroupFilter._ice_type = _M_IceGrid._t_ReplicaGroupFilter
_M_IceGrid.ReplicaGroupFilter = ReplicaGroupFilter
del ReplicaGroupFilter
if 'TypeFilter' not in _M_IceGrid.__dict__:
_M_IceGrid.TypeFilter = Ice.createTempClass()
class TypeFilter(object):
"""
The TypeFilter is used by IceGrid to filter well-known proxies
returned to the client when it searches a well-known object by
type.
IceGrid provides the list of available proxies. The implementation
of this method can use the provided context and connection to
filter and return the filtered set of proxies.
"""
def __init__(self):
if Ice.getType(self) == _M_IceGrid.TypeFilter:
raise RuntimeError('IceGrid.TypeFilter is an abstract class')
def filter(self, type, proxies, con, ctx):
"""
Filter the the given set of proxies.
Arguments:
type -- The type.
proxies -- The proxies to filter.
con -- The connection from the Ice client which is looking up well-known objects by type.
ctx -- The context from the Ice client which is looking up well-known objects by type.
Returns: The filtered proxies.
"""
raise NotImplementedError("method 'filter' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_TypeFilter)
__repr__ = __str__
_M_IceGrid._t_TypeFilter = IcePy.defineValue('::IceGrid::TypeFilter', TypeFilter, -1, (), False, True, None, ())
TypeFilter._ice_type = _M_IceGrid._t_TypeFilter
_M_IceGrid.TypeFilter = TypeFilter
del TypeFilter
if 'RegistryPluginFacade' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryPluginFacade = Ice.createTempClass()
class RegistryPluginFacade(object):
"""
The RegistryPluginFacade is implemented by IceGrid and can be used
by plugins and filter implementations to retrieve information from
IceGrid about the well-known objects or adapters. It's also used to
register/unregister replica group and type filters.
"""
def __init__(self):
if Ice.getType(self) == _M_IceGrid.RegistryPluginFacade:
raise RuntimeError('IceGrid.RegistryPluginFacade is an abstract class')
def getApplicationInfo(self, name):
"""
Get an application descriptor.
Arguments:
name -- The application name.
Returns: The application descriptor.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
"""
raise NotImplementedError("method 'getApplicationInfo' not implemented")
def getServerInfo(self, id):
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
Returns: The server information.
Throws:
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("method 'getServerInfo' not implemented")
def getAdapterServer(self, adapterId):
"""
Get the ID of the server to which the given adapter belongs.
Arguments:
adapterId -- The adapter ID.
Returns: The server ID or the empty string if the given identifier is not associated to an object adapter defined with an application descriptor.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
"""
raise NotImplementedError("method 'getAdapterServer' not implemented")
def getAdapterApplication(self, adapterId):
"""
Get the name of the application to which the given adapter belongs.
Arguments:
adapterId -- The adapter ID.
Returns: The application name or the empty string if the given identifier is not associated to a replica group or object adapter defined with an application descriptor.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
"""
raise NotImplementedError("method 'getAdapterApplication' not implemented")
def getAdapterNode(self, adapterId):
"""
Get the name of the node to which the given adapter belongs.
Arguments:
adapterId -- The adapter ID.
Returns: The node name or the empty string if the given identifier is not associated to an object adapter defined with an application descriptor.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
"""
raise NotImplementedError("method 'getAdapterNode' not implemented")
def getAdapterInfo(self, id):
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
Returns: A sequence of adapter information structures. If the given id refers to an adapter, this sequence will contain only one element. If the given id refers to a replica group, the sequence will contain the adapter information of each member of the replica group.
Throws:
AdapterNotExistException -- Raised if the adapter or replica group doesn't exist.
"""
raise NotImplementedError("method 'getAdapterInfo' not implemented")
def getObjectInfo(self, id):
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
Returns: The object info.
Throws:
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
raise NotImplementedError("method 'getObjectInfo' not implemented")
def getNodeInfo(self, name):
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
Returns: The node information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("method 'getNodeInfo' not implemented")
def getNodeLoad(self, name):
"""
Get the load averages of the node.
Arguments:
name -- The node name.
Returns: The node load information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("method 'getNodeLoad' not implemented")
def getPropertyForAdapter(self, adapterId, name):
"""
Get the property value for the given property and adapter. The
property is looked up in the server or service descriptor where
the adapter is defined.
Arguments:
adapterId -- The adapter ID
name -- The name of the property.
Returns: The property value.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
"""
raise NotImplementedError("method 'getPropertyForAdapter' not implemented")
def addReplicaGroupFilter(self, id, filter):
"""
Add a replica group filter.
Arguments:
id -- The identifier of the filter. This identifier must match the value of the "filter" attribute specified in the replica group descriptor. To filter dynamically registered replica groups, you should use the empty filter id.
filter -- The filter implementation.
"""
raise NotImplementedError("method 'addReplicaGroupFilter' not implemented")
def removeReplicaGroupFilter(self, id, filter):
"""
Remove a replica group filter.
Arguments:
id -- The identifier of the filter.
filter -- The filter implementation.
Returns: True of the filter was removed, false otherwise.
"""
raise NotImplementedError("method 'removeReplicaGroupFilter' not implemented")
def addTypeFilter(self, type, filter):
"""
Add a type filter.
Arguments:
type -- The type to register this filter with.
filter -- The filter implementation.
"""
raise NotImplementedError("method 'addTypeFilter' not implemented")
def removeTypeFilter(self, type, filter):
"""
Remove a type filter.
Arguments:
type -- The type to register this filter with.
filter -- The filter implementation.
Returns: True of the filter was removed, false otherwise.
"""
raise NotImplementedError("method 'removeTypeFilter' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RegistryPluginFacade)
__repr__ = __str__
_M_IceGrid._t_RegistryPluginFacade = IcePy.defineValue('::IceGrid::RegistryPluginFacade', RegistryPluginFacade, -1, (), False, True, None, ())
RegistryPluginFacade._ice_type = _M_IceGrid._t_RegistryPluginFacade
_M_IceGrid.RegistryPluginFacade = RegistryPluginFacade
del RegistryPluginFacade
# End of module IceGrid | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/PluginFacade_ice.py | PluginFacade_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.BuiltinSequences_ice
import Ice.Properties_ice
import Ice.SliceChecksumDict_ice
import Glacier2.Session_ice
import IceGrid.Exception_ice
import IceGrid.Descriptor_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Included module IceGrid
_M_IceGrid = Ice.openModule('IceGrid')
# Start of module IceGrid
__name__ = 'IceGrid'
if 'ServerState' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerState = Ice.createTempClass()
class ServerState(Ice.EnumBase):
"""
An enumeration representing the state of the server.
Enumerators:
Inactive -- The server is not running.
Activating -- The server is being activated and will change to the active
state when the registered server object adapters are activated
or to the activation timed out state if the activation timeout
expires.
ActivationTimedOut -- The activation timed out state indicates that the server
activation timed out.
Active -- The server is running.
Deactivating -- The server is being deactivated.
Destroying -- The server is being destroyed.
Destroyed -- The server is destroyed.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ServerState.Inactive = ServerState("Inactive", 0)
ServerState.Activating = ServerState("Activating", 1)
ServerState.ActivationTimedOut = ServerState("ActivationTimedOut", 2)
ServerState.Active = ServerState("Active", 3)
ServerState.Deactivating = ServerState("Deactivating", 4)
ServerState.Destroying = ServerState("Destroying", 5)
ServerState.Destroyed = ServerState("Destroyed", 6)
ServerState._enumerators = { 0:ServerState.Inactive, 1:ServerState.Activating, 2:ServerState.ActivationTimedOut, 3:ServerState.Active, 4:ServerState.Deactivating, 5:ServerState.Destroying, 6:ServerState.Destroyed }
_M_IceGrid._t_ServerState = IcePy.defineEnum('::IceGrid::ServerState', ServerState, (), ServerState._enumerators)
_M_IceGrid.ServerState = ServerState
del ServerState
if '_t_StringObjectProxyDict' not in _M_IceGrid.__dict__:
_M_IceGrid._t_StringObjectProxyDict = IcePy.defineDictionary('::IceGrid::StringObjectProxyDict', (), IcePy._t_string, IcePy._t_ObjectPrx)
if 'ObjectInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.ObjectInfo = Ice.createTempClass()
class ObjectInfo(object):
"""
Information about an Ice object.
Members:
proxy -- The proxy of the object.
type -- The type of the object.
"""
def __init__(self, proxy=None, type=''):
self.proxy = proxy
self.type = type
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ObjectInfo):
return NotImplemented
else:
if self.proxy != other.proxy:
return False
if self.type != other.type:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ObjectInfo)
__repr__ = __str__
_M_IceGrid._t_ObjectInfo = IcePy.defineStruct('::IceGrid::ObjectInfo', ObjectInfo, (), (
('proxy', (), IcePy._t_ObjectPrx),
('type', (), IcePy._t_string)
))
_M_IceGrid.ObjectInfo = ObjectInfo
del ObjectInfo
if '_t_ObjectInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ObjectInfoSeq = IcePy.defineSequence('::IceGrid::ObjectInfoSeq', (), _M_IceGrid._t_ObjectInfo)
if 'AdapterInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.AdapterInfo = Ice.createTempClass()
class AdapterInfo(object):
"""
Information about an adapter registered with the IceGrid registry.
Members:
id -- The id of the adapter.
proxy -- A dummy direct proxy that contains the adapter endpoints.
replicaGroupId -- The replica group id of the object adapter, or empty if the
adapter doesn't belong to a replica group.
"""
def __init__(self, id='', proxy=None, replicaGroupId=''):
self.id = id
self.proxy = proxy
self.replicaGroupId = replicaGroupId
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.AdapterInfo):
return NotImplemented
else:
if self.id != other.id:
return False
if self.proxy != other.proxy:
return False
if self.replicaGroupId != other.replicaGroupId:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdapterInfo)
__repr__ = __str__
_M_IceGrid._t_AdapterInfo = IcePy.defineStruct('::IceGrid::AdapterInfo', AdapterInfo, (), (
('id', (), IcePy._t_string),
('proxy', (), IcePy._t_ObjectPrx),
('replicaGroupId', (), IcePy._t_string)
))
_M_IceGrid.AdapterInfo = AdapterInfo
del AdapterInfo
if '_t_AdapterInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_AdapterInfoSeq = IcePy.defineSequence('::IceGrid::AdapterInfoSeq', (), _M_IceGrid._t_AdapterInfo)
if 'ServerInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerInfo = Ice.createTempClass()
class ServerInfo(object):
"""
Information about a server managed by an IceGrid node.
Members:
application -- The server application.
uuid -- The application uuid.
revision -- The application revision.
node -- The server node.
descriptor -- The server descriptor.
sessionId -- The id of the session which allocated the server.
"""
def __init__(self, application='', uuid='', revision=0, node='', descriptor=None, sessionId=''):
self.application = application
self.uuid = uuid
self.revision = revision
self.node = node
self.descriptor = descriptor
self.sessionId = sessionId
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ServerInfo):
return NotImplemented
else:
if self.application != other.application:
return False
if self.uuid != other.uuid:
return False
if self.revision != other.revision:
return False
if self.node != other.node:
return False
if self.descriptor != other.descriptor:
return False
if self.sessionId != other.sessionId:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServerInfo)
__repr__ = __str__
_M_IceGrid._t_ServerInfo = IcePy.defineStruct('::IceGrid::ServerInfo', ServerInfo, (), (
('application', (), IcePy._t_string),
('uuid', (), IcePy._t_string),
('revision', (), IcePy._t_int),
('node', (), IcePy._t_string),
('descriptor', (), _M_IceGrid._t_ServerDescriptor),
('sessionId', (), IcePy._t_string)
))
_M_IceGrid.ServerInfo = ServerInfo
del ServerInfo
if 'NodeInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeInfo = Ice.createTempClass()
class NodeInfo(object):
"""
Information about an IceGrid node.
Members:
name -- The name of the node.
os -- The operating system name.
hostname -- The network name of the host running this node (as defined in
uname()).
release -- The operation system release level (as defined in uname()).
version -- The operation system version (as defined in uname()).
machine -- The machine hardware type (as defined in uname()).
nProcessors -- The number of processor threads on the node.
For example, nProcessors is 8 on a computer with a single quad-core
processor and two HT threads per core.
dataDir -- The path to the node data directory.
"""
def __init__(self, name='', os='', hostname='', release='', version='', machine='', nProcessors=0, dataDir=''):
self.name = name
self.os = os
self.hostname = hostname
self.release = release
self.version = version
self.machine = machine
self.nProcessors = nProcessors
self.dataDir = dataDir
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.os)
_h = 5 * _h + Ice.getHash(self.hostname)
_h = 5 * _h + Ice.getHash(self.release)
_h = 5 * _h + Ice.getHash(self.version)
_h = 5 * _h + Ice.getHash(self.machine)
_h = 5 * _h + Ice.getHash(self.nProcessors)
_h = 5 * _h + Ice.getHash(self.dataDir)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.NodeInfo):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.os is None or other.os is None:
if self.os != other.os:
return (-1 if self.os is None else 1)
else:
if self.os < other.os:
return -1
elif self.os > other.os:
return 1
if self.hostname is None or other.hostname is None:
if self.hostname != other.hostname:
return (-1 if self.hostname is None else 1)
else:
if self.hostname < other.hostname:
return -1
elif self.hostname > other.hostname:
return 1
if self.release is None or other.release is None:
if self.release != other.release:
return (-1 if self.release is None else 1)
else:
if self.release < other.release:
return -1
elif self.release > other.release:
return 1
if self.version is None or other.version is None:
if self.version != other.version:
return (-1 if self.version is None else 1)
else:
if self.version < other.version:
return -1
elif self.version > other.version:
return 1
if self.machine is None or other.machine is None:
if self.machine != other.machine:
return (-1 if self.machine is None else 1)
else:
if self.machine < other.machine:
return -1
elif self.machine > other.machine:
return 1
if self.nProcessors is None or other.nProcessors is None:
if self.nProcessors != other.nProcessors:
return (-1 if self.nProcessors is None else 1)
else:
if self.nProcessors < other.nProcessors:
return -1
elif self.nProcessors > other.nProcessors:
return 1
if self.dataDir is None or other.dataDir is None:
if self.dataDir != other.dataDir:
return (-1 if self.dataDir is None else 1)
else:
if self.dataDir < other.dataDir:
return -1
elif self.dataDir > other.dataDir:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_NodeInfo)
__repr__ = __str__
_M_IceGrid._t_NodeInfo = IcePy.defineStruct('::IceGrid::NodeInfo', NodeInfo, (), (
('name', (), IcePy._t_string),
('os', (), IcePy._t_string),
('hostname', (), IcePy._t_string),
('release', (), IcePy._t_string),
('version', (), IcePy._t_string),
('machine', (), IcePy._t_string),
('nProcessors', (), IcePy._t_int),
('dataDir', (), IcePy._t_string)
))
_M_IceGrid.NodeInfo = NodeInfo
del NodeInfo
if 'RegistryInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryInfo = Ice.createTempClass()
class RegistryInfo(object):
"""
Information about an IceGrid registry replica.
Members:
name -- The name of the registry.
hostname -- The network name of the host running this registry (as defined in
uname()).
"""
def __init__(self, name='', hostname=''):
self.name = name
self.hostname = hostname
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.hostname)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.RegistryInfo):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.hostname is None or other.hostname is None:
if self.hostname != other.hostname:
return (-1 if self.hostname is None else 1)
else:
if self.hostname < other.hostname:
return -1
elif self.hostname > other.hostname:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RegistryInfo)
__repr__ = __str__
_M_IceGrid._t_RegistryInfo = IcePy.defineStruct('::IceGrid::RegistryInfo', RegistryInfo, (), (
('name', (), IcePy._t_string),
('hostname', (), IcePy._t_string)
))
_M_IceGrid.RegistryInfo = RegistryInfo
del RegistryInfo
if '_t_RegistryInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_RegistryInfoSeq = IcePy.defineSequence('::IceGrid::RegistryInfoSeq', (), _M_IceGrid._t_RegistryInfo)
if 'LoadInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.LoadInfo = Ice.createTempClass()
class LoadInfo(object):
"""
Information about the load of a node.
Members:
avg1 -- The load average over the past minute.
avg5 -- The load average over the past 5 minutes.
avg15 -- The load average over the past 15 minutes.
"""
def __init__(self, avg1=0.0, avg5=0.0, avg15=0.0):
self.avg1 = avg1
self.avg5 = avg5
self.avg15 = avg15
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.LoadInfo):
return NotImplemented
else:
if self.avg1 != other.avg1:
return False
if self.avg5 != other.avg5:
return False
if self.avg15 != other.avg15:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_LoadInfo)
__repr__ = __str__
_M_IceGrid._t_LoadInfo = IcePy.defineStruct('::IceGrid::LoadInfo', LoadInfo, (), (
('avg1', (), IcePy._t_float),
('avg5', (), IcePy._t_float),
('avg15', (), IcePy._t_float)
))
_M_IceGrid.LoadInfo = LoadInfo
del LoadInfo
if 'ApplicationInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationInfo = Ice.createTempClass()
class ApplicationInfo(object):
"""
Information about an IceGrid application.
Members:
uuid -- Unique application identifier.
createTime -- The creation time.
createUser -- The user who created the application.
updateTime -- The update time.
updateUser -- The user who updated the application.
revision -- The application revision number.
descriptor -- The application descriptor
"""
def __init__(self, uuid='', createTime=0, createUser='', updateTime=0, updateUser='', revision=0, descriptor=Ice._struct_marker):
self.uuid = uuid
self.createTime = createTime
self.createUser = createUser
self.updateTime = updateTime
self.updateUser = updateUser
self.revision = revision
if descriptor is Ice._struct_marker:
self.descriptor = _M_IceGrid.ApplicationDescriptor()
else:
self.descriptor = descriptor
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ApplicationInfo):
return NotImplemented
else:
if self.uuid != other.uuid:
return False
if self.createTime != other.createTime:
return False
if self.createUser != other.createUser:
return False
if self.updateTime != other.updateTime:
return False
if self.updateUser != other.updateUser:
return False
if self.revision != other.revision:
return False
if self.descriptor != other.descriptor:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ApplicationInfo)
__repr__ = __str__
_M_IceGrid._t_ApplicationInfo = IcePy.defineStruct('::IceGrid::ApplicationInfo', ApplicationInfo, (), (
('uuid', (), IcePy._t_string),
('createTime', (), IcePy._t_long),
('createUser', (), IcePy._t_string),
('updateTime', (), IcePy._t_long),
('updateUser', (), IcePy._t_string),
('revision', (), IcePy._t_int),
('descriptor', (), _M_IceGrid._t_ApplicationDescriptor)
))
_M_IceGrid.ApplicationInfo = ApplicationInfo
del ApplicationInfo
if '_t_ApplicationInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ApplicationInfoSeq = IcePy.defineSequence('::IceGrid::ApplicationInfoSeq', (), _M_IceGrid._t_ApplicationInfo)
if 'ApplicationUpdateInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationUpdateInfo = Ice.createTempClass()
class ApplicationUpdateInfo(object):
"""
Information about updates to an IceGrid application.
Members:
updateTime -- The update time.
updateUser -- The user who updated the application.
revision -- The application revision number.
descriptor -- The update descriptor.
"""
def __init__(self, updateTime=0, updateUser='', revision=0, descriptor=Ice._struct_marker):
self.updateTime = updateTime
self.updateUser = updateUser
self.revision = revision
if descriptor is Ice._struct_marker:
self.descriptor = _M_IceGrid.ApplicationUpdateDescriptor()
else:
self.descriptor = descriptor
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.ApplicationUpdateInfo):
return NotImplemented
else:
if self.updateTime != other.updateTime:
return False
if self.updateUser != other.updateUser:
return False
if self.revision != other.revision:
return False
if self.descriptor != other.descriptor:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ApplicationUpdateInfo)
__repr__ = __str__
_M_IceGrid._t_ApplicationUpdateInfo = IcePy.defineStruct('::IceGrid::ApplicationUpdateInfo', ApplicationUpdateInfo, (), (
('updateTime', (), IcePy._t_long),
('updateUser', (), IcePy._t_string),
('revision', (), IcePy._t_int),
('descriptor', (), _M_IceGrid._t_ApplicationUpdateDescriptor)
))
_M_IceGrid.ApplicationUpdateInfo = ApplicationUpdateInfo
del ApplicationUpdateInfo
_M_IceGrid._t_Admin = IcePy.defineValue('::IceGrid::Admin', Ice.Value, -1, (), False, True, None, ())
if 'AdminPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.AdminPrx = Ice.createTempClass()
class AdminPrx(Ice.ObjectPrx):
"""
Add an application to IceGrid.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
DeploymentException -- Raised if application deployment failed.
"""
def addApplication(self, descriptor, context=None):
return _M_IceGrid.Admin._op_addApplication.invoke(self, ((descriptor, ), context))
"""
Add an application to IceGrid.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addApplicationAsync(self, descriptor, context=None):
return _M_IceGrid.Admin._op_addApplication.invokeAsync(self, ((descriptor, ), context))
"""
Add an application to IceGrid.
Arguments:
descriptor -- The application descriptor.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_addApplication(self, descriptor, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_addApplication.begin(self, ((descriptor, ), _response, _ex, _sent, context))
"""
Add an application to IceGrid.
Arguments:
descriptor -- The application descriptor.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
DeploymentException -- Raised if application deployment failed.
"""
def end_addApplication(self, _r):
return _M_IceGrid.Admin._op_addApplication.end(self, _r)
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def syncApplication(self, descriptor, context=None):
return _M_IceGrid.Admin._op_syncApplication.invoke(self, ((descriptor, ), context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def syncApplicationAsync(self, descriptor, context=None):
return _M_IceGrid.Admin._op_syncApplication.invokeAsync(self, ((descriptor, ), context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor.
Arguments:
descriptor -- The application descriptor.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_syncApplication(self, descriptor, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_syncApplication.begin(self, ((descriptor, ), _response, _ex, _sent, context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor.
Arguments:
descriptor -- The application descriptor.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_syncApplication(self, _r):
return _M_IceGrid.Admin._op_syncApplication.end(self, _r)
"""
Update a deployed application with the given update application
descriptor.
Arguments:
descriptor -- The update descriptor.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def updateApplication(self, descriptor, context=None):
return _M_IceGrid.Admin._op_updateApplication.invoke(self, ((descriptor, ), context))
"""
Update a deployed application with the given update application
descriptor.
Arguments:
descriptor -- The update descriptor.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def updateApplicationAsync(self, descriptor, context=None):
return _M_IceGrid.Admin._op_updateApplication.invokeAsync(self, ((descriptor, ), context))
"""
Update a deployed application with the given update application
descriptor.
Arguments:
descriptor -- The update descriptor.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_updateApplication(self, descriptor, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_updateApplication.begin(self, ((descriptor, ), _response, _ex, _sent, context))
"""
Update a deployed application with the given update application
descriptor.
Arguments:
descriptor -- The update descriptor.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_updateApplication(self, _r):
return _M_IceGrid.Admin._op_updateApplication.end(self, _r)
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor only if no server restarts are
necessary for the update of the application. If some servers
need to be restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def syncApplicationWithoutRestart(self, descriptor, context=None):
return _M_IceGrid.Admin._op_syncApplicationWithoutRestart.invoke(self, ((descriptor, ), context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor only if no server restarts are
necessary for the update of the application. If some servers
need to be restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The application descriptor.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def syncApplicationWithoutRestartAsync(self, descriptor, context=None):
return _M_IceGrid.Admin._op_syncApplicationWithoutRestart.invokeAsync(self, ((descriptor, ), context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor only if no server restarts are
necessary for the update of the application. If some servers
need to be restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The application descriptor.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_syncApplicationWithoutRestart(self, descriptor, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_syncApplicationWithoutRestart.begin(self, ((descriptor, ), _response, _ex, _sent, context))
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor only if no server restarts are
necessary for the update of the application. If some servers
need to be restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The application descriptor.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_syncApplicationWithoutRestart(self, _r):
return _M_IceGrid.Admin._op_syncApplicationWithoutRestart.end(self, _r)
"""
Update a deployed application with the given update application
descriptor only if no server restarts are necessary for the
update of the application. If some servers need to be
restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The update descriptor.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def updateApplicationWithoutRestart(self, descriptor, context=None):
return _M_IceGrid.Admin._op_updateApplicationWithoutRestart.invoke(self, ((descriptor, ), context))
"""
Update a deployed application with the given update application
descriptor only if no server restarts are necessary for the
update of the application. If some servers need to be
restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The update descriptor.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def updateApplicationWithoutRestartAsync(self, descriptor, context=None):
return _M_IceGrid.Admin._op_updateApplicationWithoutRestart.invokeAsync(self, ((descriptor, ), context))
"""
Update a deployed application with the given update application
descriptor only if no server restarts are necessary for the
update of the application. If some servers need to be
restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The update descriptor.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_updateApplicationWithoutRestart(self, descriptor, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_updateApplicationWithoutRestart.begin(self, ((descriptor, ), _response, _ex, _sent, context))
"""
Update a deployed application with the given update application
descriptor only if no server restarts are necessary for the
update of the application. If some servers need to be
restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The update descriptor.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_updateApplicationWithoutRestart(self, _r):
return _M_IceGrid.Admin._op_updateApplicationWithoutRestart.end(self, _r)
"""
Remove an application from IceGrid.
Arguments:
name -- The application name.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def removeApplication(self, name, context=None):
return _M_IceGrid.Admin._op_removeApplication.invoke(self, ((name, ), context))
"""
Remove an application from IceGrid.
Arguments:
name -- The application name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def removeApplicationAsync(self, name, context=None):
return _M_IceGrid.Admin._op_removeApplication.invokeAsync(self, ((name, ), context))
"""
Remove an application from IceGrid.
Arguments:
name -- The application name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_removeApplication(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_removeApplication.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Remove an application from IceGrid.
Arguments:
name -- The application name.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_removeApplication(self, _r):
return _M_IceGrid.Admin._op_removeApplication.end(self, _r)
"""
Instantiate a server template from an application on the given
node.
Arguments:
application -- The application name.
node -- The name of the node where the server will be deployed.
desc -- The descriptor of the server instance to deploy.
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if server instantiation failed.
"""
def instantiateServer(self, application, node, desc, context=None):
return _M_IceGrid.Admin._op_instantiateServer.invoke(self, ((application, node, desc), context))
"""
Instantiate a server template from an application on the given
node.
Arguments:
application -- The application name.
node -- The name of the node where the server will be deployed.
desc -- The descriptor of the server instance to deploy.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def instantiateServerAsync(self, application, node, desc, context=None):
return _M_IceGrid.Admin._op_instantiateServer.invokeAsync(self, ((application, node, desc), context))
"""
Instantiate a server template from an application on the given
node.
Arguments:
application -- The application name.
node -- The name of the node where the server will be deployed.
desc -- The descriptor of the server instance to deploy.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_instantiateServer(self, application, node, desc, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_instantiateServer.begin(self, ((application, node, desc), _response, _ex, _sent, context))
"""
Instantiate a server template from an application on the given
node.
Arguments:
application -- The application name.
node -- The name of the node where the server will be deployed.
desc -- The descriptor of the server instance to deploy.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if server instantiation failed.
"""
def end_instantiateServer(self, _r):
return _M_IceGrid.Admin._op_instantiateServer.end(self, _r)
"""
Patch the given application data.
Arguments:
name -- The application name.
shutdown -- If true, the servers depending on the data to patch will be shut down if necessary.
context -- The request context for the invocation.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
PatchException -- Raised if the patch failed.
"""
def patchApplication(self, name, shutdown, context=None):
return _M_IceGrid.Admin._op_patchApplication.invoke(self, ((name, shutdown), context))
"""
Patch the given application data.
Arguments:
name -- The application name.
shutdown -- If true, the servers depending on the data to patch will be shut down if necessary.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def patchApplicationAsync(self, name, shutdown, context=None):
return _M_IceGrid.Admin._op_patchApplication.invokeAsync(self, ((name, shutdown), context))
"""
Patch the given application data.
Arguments:
name -- The application name.
shutdown -- If true, the servers depending on the data to patch will be shut down if necessary.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_patchApplication(self, name, shutdown, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_patchApplication.begin(self, ((name, shutdown), _response, _ex, _sent, context))
"""
Patch the given application data.
Arguments:
name -- The application name.
shutdown -- If true, the servers depending on the data to patch will be shut down if necessary.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
PatchException -- Raised if the patch failed.
"""
def end_patchApplication(self, _r):
return _M_IceGrid.Admin._op_patchApplication.end(self, _r)
"""
Get an application descriptor.
Arguments:
name -- The application name.
context -- The request context for the invocation.
Returns: The application descriptor.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
"""
def getApplicationInfo(self, name, context=None):
return _M_IceGrid.Admin._op_getApplicationInfo.invoke(self, ((name, ), context))
"""
Get an application descriptor.
Arguments:
name -- The application name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getApplicationInfoAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getApplicationInfo.invokeAsync(self, ((name, ), context))
"""
Get an application descriptor.
Arguments:
name -- The application name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getApplicationInfo(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getApplicationInfo.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get an application descriptor.
Arguments:
name -- The application name.
Returns: The application descriptor.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
"""
def end_getApplicationInfo(self, _r):
return _M_IceGrid.Admin._op_getApplicationInfo.end(self, _r)
"""
Get the default application descriptor.
Arguments:
context -- The request context for the invocation.
Returns: The default application descriptor.
Throws:
DeploymentException -- Raised if the default application descriptor can't be accessed or is invalid.
"""
def getDefaultApplicationDescriptor(self, context=None):
return _M_IceGrid.Admin._op_getDefaultApplicationDescriptor.invoke(self, ((), context))
"""
Get the default application descriptor.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getDefaultApplicationDescriptorAsync(self, context=None):
return _M_IceGrid.Admin._op_getDefaultApplicationDescriptor.invokeAsync(self, ((), context))
"""
Get the default application descriptor.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getDefaultApplicationDescriptor(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getDefaultApplicationDescriptor.begin(self, ((), _response, _ex, _sent, context))
"""
Get the default application descriptor.
Arguments:
Returns: The default application descriptor.
Throws:
DeploymentException -- Raised if the default application descriptor can't be accessed or is invalid.
"""
def end_getDefaultApplicationDescriptor(self, _r):
return _M_IceGrid.Admin._op_getDefaultApplicationDescriptor.end(self, _r)
"""
Get all the IceGrid applications currently registered.
Arguments:
context -- The request context for the invocation.
Returns: The application names.
"""
def getAllApplicationNames(self, context=None):
return _M_IceGrid.Admin._op_getAllApplicationNames.invoke(self, ((), context))
"""
Get all the IceGrid applications currently registered.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllApplicationNamesAsync(self, context=None):
return _M_IceGrid.Admin._op_getAllApplicationNames.invokeAsync(self, ((), context))
"""
Get all the IceGrid applications currently registered.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllApplicationNames(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllApplicationNames.begin(self, ((), _response, _ex, _sent, context))
"""
Get all the IceGrid applications currently registered.
Arguments:
Returns: The application names.
"""
def end_getAllApplicationNames(self, _r):
return _M_IceGrid.Admin._op_getAllApplicationNames.end(self, _r)
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: The server information.
Throws:
ServerNotExistException -- Raised if the server doesn't exist.
"""
def getServerInfo(self, id, context=None):
return _M_IceGrid.Admin._op_getServerInfo.invoke(self, ((id, ), context))
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerInfoAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getServerInfo.invokeAsync(self, ((id, ), context))
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerInfo(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getServerInfo.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
Returns: The server information.
Throws:
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_getServerInfo(self, _r):
return _M_IceGrid.Admin._op_getServerInfo.end(self, _r)
"""
Get a server's state.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: The server state.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def getServerState(self, id, context=None):
return _M_IceGrid.Admin._op_getServerState.invoke(self, ((id, ), context))
"""
Get a server's state.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerStateAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getServerState.invokeAsync(self, ((id, ), context))
"""
Get a server's state.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerState(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getServerState.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get a server's state.
Arguments:
id -- The server id.
Returns: The server state.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_getServerState(self, _r):
return _M_IceGrid.Admin._op_getServerState.end(self, _r)
"""
Get a server's system process id. The process id is operating
system dependent.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: The server's process id.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def getServerPid(self, id, context=None):
return _M_IceGrid.Admin._op_getServerPid.invoke(self, ((id, ), context))
"""
Get a server's system process id. The process id is operating
system dependent.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerPidAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getServerPid.invokeAsync(self, ((id, ), context))
"""
Get a server's system process id. The process id is operating
system dependent.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerPid(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getServerPid.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get a server's system process id. The process id is operating
system dependent.
Arguments:
id -- The server id.
Returns: The server's process id.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_getServerPid(self, _r):
return _M_IceGrid.Admin._op_getServerPid.end(self, _r)
"""
Get the category for server admin objects. You can manufacture a server admin
proxy from the admin proxy by changing its identity: use the server ID as name
and the returned category as category.
Arguments:
context -- The request context for the invocation.
Returns: The category for server admin objects.
"""
def getServerAdminCategory(self, context=None):
return _M_IceGrid.Admin._op_getServerAdminCategory.invoke(self, ((), context))
"""
Get the category for server admin objects. You can manufacture a server admin
proxy from the admin proxy by changing its identity: use the server ID as name
and the returned category as category.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerAdminCategoryAsync(self, context=None):
return _M_IceGrid.Admin._op_getServerAdminCategory.invokeAsync(self, ((), context))
"""
Get the category for server admin objects. You can manufacture a server admin
proxy from the admin proxy by changing its identity: use the server ID as name
and the returned category as category.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerAdminCategory(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getServerAdminCategory.begin(self, ((), _response, _ex, _sent, context))
"""
Get the category for server admin objects. You can manufacture a server admin
proxy from the admin proxy by changing its identity: use the server ID as name
and the returned category as category.
Arguments:
Returns: The category for server admin objects.
"""
def end_getServerAdminCategory(self, _r):
return _M_IceGrid.Admin._op_getServerAdminCategory.end(self, _r)
"""
Get a proxy to the server's admin object.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A proxy to the server's admin object
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def getServerAdmin(self, id, context=None):
return _M_IceGrid.Admin._op_getServerAdmin.invoke(self, ((id, ), context))
"""
Get a proxy to the server's admin object.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerAdminAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getServerAdmin.invokeAsync(self, ((id, ), context))
"""
Get a proxy to the server's admin object.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerAdmin(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getServerAdmin.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get a proxy to the server's admin object.
Arguments:
id -- The server id.
Returns: A proxy to the server's admin object
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_getServerAdmin(self, _r):
return _M_IceGrid.Admin._op_getServerAdmin.end(self, _r)
"""
Enable or disable a server. A disabled server can't be started
on demand or administratively. The enable state of the server
is not persistent: if the node is shut down and restarted, the
server will be enabled by default.
Arguments:
id -- The server id.
enabled -- True to enable the server, false to disable it.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def enableServer(self, id, enabled, context=None):
return _M_IceGrid.Admin._op_enableServer.invoke(self, ((id, enabled), context))
"""
Enable or disable a server. A disabled server can't be started
on demand or administratively. The enable state of the server
is not persistent: if the node is shut down and restarted, the
server will be enabled by default.
Arguments:
id -- The server id.
enabled -- True to enable the server, false to disable it.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def enableServerAsync(self, id, enabled, context=None):
return _M_IceGrid.Admin._op_enableServer.invokeAsync(self, ((id, enabled), context))
"""
Enable or disable a server. A disabled server can't be started
on demand or administratively. The enable state of the server
is not persistent: if the node is shut down and restarted, the
server will be enabled by default.
Arguments:
id -- The server id.
enabled -- True to enable the server, false to disable it.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_enableServer(self, id, enabled, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_enableServer.begin(self, ((id, enabled), _response, _ex, _sent, context))
"""
Enable or disable a server. A disabled server can't be started
on demand or administratively. The enable state of the server
is not persistent: if the node is shut down and restarted, the
server will be enabled by default.
Arguments:
id -- The server id.
enabled -- True to enable the server, false to disable it.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_enableServer(self, _r):
return _M_IceGrid.Admin._op_enableServer.end(self, _r)
"""
Check if the server is enabled or disabled.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: True if the server is enabled.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def isServerEnabled(self, id, context=None):
return _M_IceGrid.Admin._op_isServerEnabled.invoke(self, ((id, ), context))
"""
Check if the server is enabled or disabled.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def isServerEnabledAsync(self, id, context=None):
return _M_IceGrid.Admin._op_isServerEnabled.invokeAsync(self, ((id, ), context))
"""
Check if the server is enabled or disabled.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_isServerEnabled(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_isServerEnabled.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Check if the server is enabled or disabled.
Arguments:
id -- The server id.
Returns: True if the server is enabled.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_isServerEnabled(self, _r):
return _M_IceGrid.Admin._op_isServerEnabled.end(self, _r)
"""
Start a server and wait for its activation.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStartException -- Raised if the server couldn't be started.
"""
def startServer(self, id, context=None):
return _M_IceGrid.Admin._op_startServer.invoke(self, ((id, ), context))
"""
Start a server and wait for its activation.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def startServerAsync(self, id, context=None):
return _M_IceGrid.Admin._op_startServer.invokeAsync(self, ((id, ), context))
"""
Start a server and wait for its activation.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_startServer(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_startServer.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Start a server and wait for its activation.
Arguments:
id -- The server id.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStartException -- Raised if the server couldn't be started.
"""
def end_startServer(self, _r):
return _M_IceGrid.Admin._op_startServer.end(self, _r)
"""
Stop a server.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStopException -- Raised if the server couldn't be stopped.
"""
def stopServer(self, id, context=None):
return _M_IceGrid.Admin._op_stopServer.invoke(self, ((id, ), context))
"""
Stop a server.
Arguments:
id -- The server id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def stopServerAsync(self, id, context=None):
return _M_IceGrid.Admin._op_stopServer.invokeAsync(self, ((id, ), context))
"""
Stop a server.
Arguments:
id -- The server id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_stopServer(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_stopServer.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Stop a server.
Arguments:
id -- The server id.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStopException -- Raised if the server couldn't be stopped.
"""
def end_stopServer(self, _r):
return _M_IceGrid.Admin._op_stopServer.end(self, _r)
"""
Patch a server.
Arguments:
id -- The server id.
shutdown -- If true, servers depending on the data to patch will be shut down if necessary.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
PatchException -- Raised if the patch failed.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def patchServer(self, id, shutdown, context=None):
return _M_IceGrid.Admin._op_patchServer.invoke(self, ((id, shutdown), context))
"""
Patch a server.
Arguments:
id -- The server id.
shutdown -- If true, servers depending on the data to patch will be shut down if necessary.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def patchServerAsync(self, id, shutdown, context=None):
return _M_IceGrid.Admin._op_patchServer.invokeAsync(self, ((id, shutdown), context))
"""
Patch a server.
Arguments:
id -- The server id.
shutdown -- If true, servers depending on the data to patch will be shut down if necessary.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_patchServer(self, id, shutdown, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_patchServer.begin(self, ((id, shutdown), _response, _ex, _sent, context))
"""
Patch a server.
Arguments:
id -- The server id.
shutdown -- If true, servers depending on the data to patch will be shut down if necessary.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
PatchException -- Raised if the patch failed.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_patchServer(self, _r):
return _M_IceGrid.Admin._op_patchServer.end(self, _r)
"""
Send signal to a server.
Arguments:
id -- The server id.
signal -- The signal, for example SIGTERM or 15.
context -- The request context for the invocation.
Throws:
BadSignalException -- Raised if the signal is not recognized by the target server.
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def sendSignal(self, id, signal, context=None):
return _M_IceGrid.Admin._op_sendSignal.invoke(self, ((id, signal), context))
"""
Send signal to a server.
Arguments:
id -- The server id.
signal -- The signal, for example SIGTERM or 15.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def sendSignalAsync(self, id, signal, context=None):
return _M_IceGrid.Admin._op_sendSignal.invokeAsync(self, ((id, signal), context))
"""
Send signal to a server.
Arguments:
id -- The server id.
signal -- The signal, for example SIGTERM or 15.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_sendSignal(self, id, signal, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_sendSignal.begin(self, ((id, signal), _response, _ex, _sent, context))
"""
Send signal to a server.
Arguments:
id -- The server id.
signal -- The signal, for example SIGTERM or 15.
Throws:
BadSignalException -- Raised if the signal is not recognized by the target server.
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_sendSignal(self, _r):
return _M_IceGrid.Admin._op_sendSignal.end(self, _r)
"""
Get all the server ids registered with IceGrid.
Arguments:
context -- The request context for the invocation.
Returns: The server ids.
"""
def getAllServerIds(self, context=None):
return _M_IceGrid.Admin._op_getAllServerIds.invoke(self, ((), context))
"""
Get all the server ids registered with IceGrid.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllServerIdsAsync(self, context=None):
return _M_IceGrid.Admin._op_getAllServerIds.invokeAsync(self, ((), context))
"""
Get all the server ids registered with IceGrid.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllServerIds(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllServerIds.begin(self, ((), _response, _ex, _sent, context))
"""
Get all the server ids registered with IceGrid.
Arguments:
Returns: The server ids.
"""
def end_getAllServerIds(self, _r):
return _M_IceGrid.Admin._op_getAllServerIds.end(self, _r)
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Returns: A sequence of adapter information structures. If the given id refers to an adapter, this sequence will contain only one element. If the given id refers to a replica group, the sequence will contain the adapter information of each member of the replica group.
Throws:
AdapterNotExistException -- Raised if the adapter or replica group doesn't exist.
"""
def getAdapterInfo(self, id, context=None):
return _M_IceGrid.Admin._op_getAdapterInfo.invoke(self, ((id, ), context))
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAdapterInfoAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getAdapterInfo.invokeAsync(self, ((id, ), context))
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAdapterInfo(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAdapterInfo.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
Returns: A sequence of adapter information structures. If the given id refers to an adapter, this sequence will contain only one element. If the given id refers to a replica group, the sequence will contain the adapter information of each member of the replica group.
Throws:
AdapterNotExistException -- Raised if the adapter or replica group doesn't exist.
"""
def end_getAdapterInfo(self, _r):
return _M_IceGrid.Admin._op_getAdapterInfo.end(self, _r)
"""
Remove the adapter with the given id.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def removeAdapter(self, id, context=None):
return _M_IceGrid.Admin._op_removeAdapter.invoke(self, ((id, ), context))
"""
Remove the adapter with the given id.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def removeAdapterAsync(self, id, context=None):
return _M_IceGrid.Admin._op_removeAdapter.invokeAsync(self, ((id, ), context))
"""
Remove the adapter with the given id.
Arguments:
id -- The adapter id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_removeAdapter(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_removeAdapter.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Remove the adapter with the given id.
Arguments:
id -- The adapter id.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
def end_removeAdapter(self, _r):
return _M_IceGrid.Admin._op_removeAdapter.end(self, _r)
"""
Get all the adapter ids registered with IceGrid.
Arguments:
context -- The request context for the invocation.
Returns: The adapter ids.
"""
def getAllAdapterIds(self, context=None):
return _M_IceGrid.Admin._op_getAllAdapterIds.invoke(self, ((), context))
"""
Get all the adapter ids registered with IceGrid.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllAdapterIdsAsync(self, context=None):
return _M_IceGrid.Admin._op_getAllAdapterIds.invokeAsync(self, ((), context))
"""
Get all the adapter ids registered with IceGrid.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllAdapterIds(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllAdapterIds.begin(self, ((), _response, _ex, _sent, context))
"""
Get all the adapter ids registered with IceGrid.
Arguments:
Returns: The adapter ids.
"""
def end_getAllAdapterIds(self, _r):
return _M_IceGrid.Admin._op_getAllAdapterIds.end(self, _r)
"""
Add an object to the object registry. IceGrid will get the
object type by calling ice_id on the given proxy. The object
must be reachable.
Arguments:
obj -- The object to be added to the registry.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the object can't be added. This might be raised if the invocation on the proxy to get the object type failed.
ObjectExistsException -- Raised if the object is already registered.
"""
def addObject(self, obj, context=None):
return _M_IceGrid.Admin._op_addObject.invoke(self, ((obj, ), context))
"""
Add an object to the object registry. IceGrid will get the
object type by calling ice_id on the given proxy. The object
must be reachable.
Arguments:
obj -- The object to be added to the registry.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addObjectAsync(self, obj, context=None):
return _M_IceGrid.Admin._op_addObject.invokeAsync(self, ((obj, ), context))
"""
Add an object to the object registry. IceGrid will get the
object type by calling ice_id on the given proxy. The object
must be reachable.
Arguments:
obj -- The object to be added to the registry.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_addObject(self, obj, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_addObject.begin(self, ((obj, ), _response, _ex, _sent, context))
"""
Add an object to the object registry. IceGrid will get the
object type by calling ice_id on the given proxy. The object
must be reachable.
Arguments:
obj -- The object to be added to the registry.
Throws:
DeploymentException -- Raised if the object can't be added. This might be raised if the invocation on the proxy to get the object type failed.
ObjectExistsException -- Raised if the object is already registered.
"""
def end_addObject(self, _r):
return _M_IceGrid.Admin._op_addObject.end(self, _r)
"""
Update an object in the object registry. Only objects added
with this interface can be updated with this operation. Objects
added with deployment descriptors should be updated with the
deployment mechanism.
Arguments:
obj -- The object to be updated to the registry.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the object can't be updated. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def updateObject(self, obj, context=None):
return _M_IceGrid.Admin._op_updateObject.invoke(self, ((obj, ), context))
"""
Update an object in the object registry. Only objects added
with this interface can be updated with this operation. Objects
added with deployment descriptors should be updated with the
deployment mechanism.
Arguments:
obj -- The object to be updated to the registry.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def updateObjectAsync(self, obj, context=None):
return _M_IceGrid.Admin._op_updateObject.invokeAsync(self, ((obj, ), context))
"""
Update an object in the object registry. Only objects added
with this interface can be updated with this operation. Objects
added with deployment descriptors should be updated with the
deployment mechanism.
Arguments:
obj -- The object to be updated to the registry.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_updateObject(self, obj, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_updateObject.begin(self, ((obj, ), _response, _ex, _sent, context))
"""
Update an object in the object registry. Only objects added
with this interface can be updated with this operation. Objects
added with deployment descriptors should be updated with the
deployment mechanism.
Arguments:
obj -- The object to be updated to the registry.
Throws:
DeploymentException -- Raised if the object can't be updated. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def end_updateObject(self, _r):
return _M_IceGrid.Admin._op_updateObject.end(self, _r)
"""
Add an object to the object registry and explicitly specify
its type.
Arguments:
obj -- The object to be added to the registry.
type -- The object type.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if application deployment failed.
ObjectExistsException -- Raised if the object is already registered.
"""
def addObjectWithType(self, obj, type, context=None):
return _M_IceGrid.Admin._op_addObjectWithType.invoke(self, ((obj, type), context))
"""
Add an object to the object registry and explicitly specify
its type.
Arguments:
obj -- The object to be added to the registry.
type -- The object type.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addObjectWithTypeAsync(self, obj, type, context=None):
return _M_IceGrid.Admin._op_addObjectWithType.invokeAsync(self, ((obj, type), context))
"""
Add an object to the object registry and explicitly specify
its type.
Arguments:
obj -- The object to be added to the registry.
type -- The object type.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_addObjectWithType(self, obj, type, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_addObjectWithType.begin(self, ((obj, type), _response, _ex, _sent, context))
"""
Add an object to the object registry and explicitly specify
its type.
Arguments:
obj -- The object to be added to the registry.
type -- The object type.
Throws:
DeploymentException -- Raised if application deployment failed.
ObjectExistsException -- Raised if the object is already registered.
"""
def end_addObjectWithType(self, _r):
return _M_IceGrid.Admin._op_addObjectWithType.end(self, _r)
"""
Remove an object from the object registry. Only objects added
with this interface can be removed with this operation. Objects
added with deployment descriptors should be removed with the
deployment mechanism.
Arguments:
id -- The identity of the object to be removed from the registry.
context -- The request context for the invocation.
Throws:
DeploymentException -- Raised if the object can't be removed. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def removeObject(self, id, context=None):
return _M_IceGrid.Admin._op_removeObject.invoke(self, ((id, ), context))
"""
Remove an object from the object registry. Only objects added
with this interface can be removed with this operation. Objects
added with deployment descriptors should be removed with the
deployment mechanism.
Arguments:
id -- The identity of the object to be removed from the registry.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def removeObjectAsync(self, id, context=None):
return _M_IceGrid.Admin._op_removeObject.invokeAsync(self, ((id, ), context))
"""
Remove an object from the object registry. Only objects added
with this interface can be removed with this operation. Objects
added with deployment descriptors should be removed with the
deployment mechanism.
Arguments:
id -- The identity of the object to be removed from the registry.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_removeObject(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_removeObject.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Remove an object from the object registry. Only objects added
with this interface can be removed with this operation. Objects
added with deployment descriptors should be removed with the
deployment mechanism.
Arguments:
id -- The identity of the object to be removed from the registry.
Throws:
DeploymentException -- Raised if the object can't be removed. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def end_removeObject(self, _r):
return _M_IceGrid.Admin._op_removeObject.end(self, _r)
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
context -- The request context for the invocation.
Returns: The object info.
Throws:
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def getObjectInfo(self, id, context=None):
return _M_IceGrid.Admin._op_getObjectInfo.invoke(self, ((id, ), context))
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getObjectInfoAsync(self, id, context=None):
return _M_IceGrid.Admin._op_getObjectInfo.invokeAsync(self, ((id, ), context))
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getObjectInfo(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getObjectInfo.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
Returns: The object info.
Throws:
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
def end_getObjectInfo(self, _r):
return _M_IceGrid.Admin._op_getObjectInfo.end(self, _r)
"""
Get the object info of all the registered objects with the
given type.
Arguments:
type -- The type of the object.
context -- The request context for the invocation.
Returns: The object infos.
"""
def getObjectInfosByType(self, type, context=None):
return _M_IceGrid.Admin._op_getObjectInfosByType.invoke(self, ((type, ), context))
"""
Get the object info of all the registered objects with the
given type.
Arguments:
type -- The type of the object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getObjectInfosByTypeAsync(self, type, context=None):
return _M_IceGrid.Admin._op_getObjectInfosByType.invokeAsync(self, ((type, ), context))
"""
Get the object info of all the registered objects with the
given type.
Arguments:
type -- The type of the object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getObjectInfosByType(self, type, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getObjectInfosByType.begin(self, ((type, ), _response, _ex, _sent, context))
"""
Get the object info of all the registered objects with the
given type.
Arguments:
type -- The type of the object.
Returns: The object infos.
"""
def end_getObjectInfosByType(self, _r):
return _M_IceGrid.Admin._op_getObjectInfosByType.end(self, _r)
"""
Get the object info of all the registered objects whose stringified
identities match the given expression.
Arguments:
expr -- The expression to match against the stringified identities of registered objects. The expression may contain a trailing wildcard (*) character.
context -- The request context for the invocation.
Returns: All the object infos with a stringified identity matching the given expression.
"""
def getAllObjectInfos(self, expr, context=None):
return _M_IceGrid.Admin._op_getAllObjectInfos.invoke(self, ((expr, ), context))
"""
Get the object info of all the registered objects whose stringified
identities match the given expression.
Arguments:
expr -- The expression to match against the stringified identities of registered objects. The expression may contain a trailing wildcard (*) character.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllObjectInfosAsync(self, expr, context=None):
return _M_IceGrid.Admin._op_getAllObjectInfos.invokeAsync(self, ((expr, ), context))
"""
Get the object info of all the registered objects whose stringified
identities match the given expression.
Arguments:
expr -- The expression to match against the stringified identities of registered objects. The expression may contain a trailing wildcard (*) character.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllObjectInfos(self, expr, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllObjectInfos.begin(self, ((expr, ), _response, _ex, _sent, context))
"""
Get the object info of all the registered objects whose stringified
identities match the given expression.
Arguments:
expr -- The expression to match against the stringified identities of registered objects. The expression may contain a trailing wildcard (*) character.
Returns: All the object infos with a stringified identity matching the given expression.
"""
def end_getAllObjectInfos(self, _r):
return _M_IceGrid.Admin._op_getAllObjectInfos.end(self, _r)
"""
Ping an IceGrid node to see if it is active.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: true if the node ping succeeded, false otherwise.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
"""
def pingNode(self, name, context=None):
return _M_IceGrid.Admin._op_pingNode.invoke(self, ((name, ), context))
"""
Ping an IceGrid node to see if it is active.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def pingNodeAsync(self, name, context=None):
return _M_IceGrid.Admin._op_pingNode.invokeAsync(self, ((name, ), context))
"""
Ping an IceGrid node to see if it is active.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_pingNode(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_pingNode.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Ping an IceGrid node to see if it is active.
Arguments:
name -- The node name.
Returns: true if the node ping succeeded, false otherwise.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
"""
def end_pingNode(self, _r):
return _M_IceGrid.Admin._op_pingNode.end(self, _r)
"""
Get the load averages of the node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: The node load information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def getNodeLoad(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeLoad.invoke(self, ((name, ), context))
"""
Get the load averages of the node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNodeLoadAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeLoad.invokeAsync(self, ((name, ), context))
"""
Get the load averages of the node.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNodeLoad(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getNodeLoad.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get the load averages of the node.
Arguments:
name -- The node name.
Returns: The node load information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_getNodeLoad(self, _r):
return _M_IceGrid.Admin._op_getNodeLoad.end(self, _r)
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: The node information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def getNodeInfo(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeInfo.invoke(self, ((name, ), context))
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNodeInfoAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeInfo.invokeAsync(self, ((name, ), context))
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNodeInfo(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getNodeInfo.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
Returns: The node information.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_getNodeInfo(self, _r):
return _M_IceGrid.Admin._op_getNodeInfo.end(self, _r)
"""
Get a proxy to the IceGrid node's admin object.
Arguments:
name -- The IceGrid node name
context -- The request context for the invocation.
Returns: A proxy to the IceGrid node's admin object
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def getNodeAdmin(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeAdmin.invoke(self, ((name, ), context))
"""
Get a proxy to the IceGrid node's admin object.
Arguments:
name -- The IceGrid node name
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNodeAdminAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeAdmin.invokeAsync(self, ((name, ), context))
"""
Get a proxy to the IceGrid node's admin object.
Arguments:
name -- The IceGrid node name
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNodeAdmin(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getNodeAdmin.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get a proxy to the IceGrid node's admin object.
Arguments:
name -- The IceGrid node name
Returns: A proxy to the IceGrid node's admin object
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_getNodeAdmin(self, _r):
return _M_IceGrid.Admin._op_getNodeAdmin.end(self, _r)
"""
Get the number of physical processor sockets for the machine
running the node with the given name.
Note that this method will return 1 on operating systems where
this can't be automatically determined and where the
IceGrid.Node.ProcessorSocketCount property for the node is not
set.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: The number of processor sockets or 1 if the number of sockets can't determined.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def getNodeProcessorSocketCount(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeProcessorSocketCount.invoke(self, ((name, ), context))
"""
Get the number of physical processor sockets for the machine
running the node with the given name.
Note that this method will return 1 on operating systems where
this can't be automatically determined and where the
IceGrid.Node.ProcessorSocketCount property for the node is not
set.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNodeProcessorSocketCountAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeProcessorSocketCount.invokeAsync(self, ((name, ), context))
"""
Get the number of physical processor sockets for the machine
running the node with the given name.
Note that this method will return 1 on operating systems where
this can't be automatically determined and where the
IceGrid.Node.ProcessorSocketCount property for the node is not
set.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNodeProcessorSocketCount(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getNodeProcessorSocketCount.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get the number of physical processor sockets for the machine
running the node with the given name.
Note that this method will return 1 on operating systems where
this can't be automatically determined and where the
IceGrid.Node.ProcessorSocketCount property for the node is not
set.
Arguments:
name -- The node name.
Returns: The number of processor sockets or 1 if the number of sockets can't determined.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_getNodeProcessorSocketCount(self, _r):
return _M_IceGrid.Admin._op_getNodeProcessorSocketCount.end(self, _r)
"""
Shutdown an IceGrid node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def shutdownNode(self, name, context=None):
return _M_IceGrid.Admin._op_shutdownNode.invoke(self, ((name, ), context))
"""
Shutdown an IceGrid node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def shutdownNodeAsync(self, name, context=None):
return _M_IceGrid.Admin._op_shutdownNode.invokeAsync(self, ((name, ), context))
"""
Shutdown an IceGrid node.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_shutdownNode(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_shutdownNode.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Shutdown an IceGrid node.
Arguments:
name -- The node name.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_shutdownNode(self, _r):
return _M_IceGrid.Admin._op_shutdownNode.end(self, _r)
"""
Get the hostname of this node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: The node hostname.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def getNodeHostname(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeHostname.invoke(self, ((name, ), context))
"""
Get the hostname of this node.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getNodeHostnameAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getNodeHostname.invokeAsync(self, ((name, ), context))
"""
Get the hostname of this node.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getNodeHostname(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getNodeHostname.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get the hostname of this node.
Arguments:
name -- The node name.
Returns: The node hostname.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_getNodeHostname(self, _r):
return _M_IceGrid.Admin._op_getNodeHostname.end(self, _r)
"""
Get all the IceGrid nodes currently registered.
Arguments:
context -- The request context for the invocation.
Returns: The node names.
"""
def getAllNodeNames(self, context=None):
return _M_IceGrid.Admin._op_getAllNodeNames.invoke(self, ((), context))
"""
Get all the IceGrid nodes currently registered.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllNodeNamesAsync(self, context=None):
return _M_IceGrid.Admin._op_getAllNodeNames.invokeAsync(self, ((), context))
"""
Get all the IceGrid nodes currently registered.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllNodeNames(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllNodeNames.begin(self, ((), _response, _ex, _sent, context))
"""
Get all the IceGrid nodes currently registered.
Arguments:
Returns: The node names.
"""
def end_getAllNodeNames(self, _r):
return _M_IceGrid.Admin._op_getAllNodeNames.end(self, _r)
"""
Ping an IceGrid registry to see if it is active.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Returns: true if the registry ping succeeded, false otherwise.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
def pingRegistry(self, name, context=None):
return _M_IceGrid.Admin._op_pingRegistry.invoke(self, ((name, ), context))
"""
Ping an IceGrid registry to see if it is active.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def pingRegistryAsync(self, name, context=None):
return _M_IceGrid.Admin._op_pingRegistry.invokeAsync(self, ((name, ), context))
"""
Ping an IceGrid registry to see if it is active.
Arguments:
name -- The registry name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_pingRegistry(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_pingRegistry.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Ping an IceGrid registry to see if it is active.
Arguments:
name -- The registry name.
Returns: true if the registry ping succeeded, false otherwise.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
def end_pingRegistry(self, _r):
return _M_IceGrid.Admin._op_pingRegistry.end(self, _r)
"""
Get the registry information for the registry with the given name.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Returns: The registry information.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def getRegistryInfo(self, name, context=None):
return _M_IceGrid.Admin._op_getRegistryInfo.invoke(self, ((name, ), context))
"""
Get the registry information for the registry with the given name.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getRegistryInfoAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getRegistryInfo.invokeAsync(self, ((name, ), context))
"""
Get the registry information for the registry with the given name.
Arguments:
name -- The registry name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getRegistryInfo(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getRegistryInfo.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get the registry information for the registry with the given name.
Arguments:
name -- The registry name.
Returns: The registry information.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def end_getRegistryInfo(self, _r):
return _M_IceGrid.Admin._op_getRegistryInfo.end(self, _r)
"""
Get a proxy to the IceGrid registry's admin object.
Arguments:
name -- The registry name
context -- The request context for the invocation.
Returns: A proxy to the IceGrid registry's admin object
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
def getRegistryAdmin(self, name, context=None):
return _M_IceGrid.Admin._op_getRegistryAdmin.invoke(self, ((name, ), context))
"""
Get a proxy to the IceGrid registry's admin object.
Arguments:
name -- The registry name
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getRegistryAdminAsync(self, name, context=None):
return _M_IceGrid.Admin._op_getRegistryAdmin.invokeAsync(self, ((name, ), context))
"""
Get a proxy to the IceGrid registry's admin object.
Arguments:
name -- The registry name
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getRegistryAdmin(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getRegistryAdmin.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Get a proxy to the IceGrid registry's admin object.
Arguments:
name -- The registry name
Returns: A proxy to the IceGrid registry's admin object
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
def end_getRegistryAdmin(self, _r):
return _M_IceGrid.Admin._op_getRegistryAdmin.end(self, _r)
"""
Shutdown an IceGrid registry.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def shutdownRegistry(self, name, context=None):
return _M_IceGrid.Admin._op_shutdownRegistry.invoke(self, ((name, ), context))
"""
Shutdown an IceGrid registry.
Arguments:
name -- The registry name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def shutdownRegistryAsync(self, name, context=None):
return _M_IceGrid.Admin._op_shutdownRegistry.invokeAsync(self, ((name, ), context))
"""
Shutdown an IceGrid registry.
Arguments:
name -- The registry name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_shutdownRegistry(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_shutdownRegistry.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Shutdown an IceGrid registry.
Arguments:
name -- The registry name.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def end_shutdownRegistry(self, _r):
return _M_IceGrid.Admin._op_shutdownRegistry.end(self, _r)
"""
Get all the IceGrid registries currently registered.
Arguments:
context -- The request context for the invocation.
Returns: The registry names.
"""
def getAllRegistryNames(self, context=None):
return _M_IceGrid.Admin._op_getAllRegistryNames.invoke(self, ((), context))
"""
Get all the IceGrid registries currently registered.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAllRegistryNamesAsync(self, context=None):
return _M_IceGrid.Admin._op_getAllRegistryNames.invokeAsync(self, ((), context))
"""
Get all the IceGrid registries currently registered.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAllRegistryNames(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getAllRegistryNames.begin(self, ((), _response, _ex, _sent, context))
"""
Get all the IceGrid registries currently registered.
Arguments:
Returns: The registry names.
"""
def end_getAllRegistryNames(self, _r):
return _M_IceGrid.Admin._op_getAllRegistryNames.end(self, _r)
"""
Shut down the IceGrid registry.
Arguments:
context -- The request context for the invocation.
"""
def shutdown(self, context=None):
return _M_IceGrid.Admin._op_shutdown.invoke(self, ((), context))
"""
Shut down the IceGrid registry.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def shutdownAsync(self, context=None):
return _M_IceGrid.Admin._op_shutdown.invokeAsync(self, ((), context))
"""
Shut down the IceGrid registry.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_shutdown(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_shutdown.begin(self, ((), _response, _ex, _sent, context))
"""
Shut down the IceGrid registry.
Arguments:
"""
def end_shutdown(self, _r):
return _M_IceGrid.Admin._op_shutdown.end(self, _r)
"""
Returns the checksums for the IceGrid Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def getSliceChecksums(self, context=None):
return _M_IceGrid.Admin._op_getSliceChecksums.invoke(self, ((), context))
"""
Returns the checksums for the IceGrid Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSliceChecksumsAsync(self, context=None):
return _M_IceGrid.Admin._op_getSliceChecksums.invokeAsync(self, ((), context))
"""
Returns the checksums for the IceGrid Slice definitions.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSliceChecksums(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.Admin._op_getSliceChecksums.begin(self, ((), _response, _ex, _sent, context))
"""
Returns the checksums for the IceGrid Slice definitions.
Arguments:
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def end_getSliceChecksums(self, _r):
return _M_IceGrid.Admin._op_getSliceChecksums.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.AdminPrx.ice_checkedCast(proxy, '::IceGrid::Admin', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.AdminPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::Admin'
_M_IceGrid._t_AdminPrx = IcePy.defineProxy('::IceGrid::Admin', AdminPrx)
_M_IceGrid.AdminPrx = AdminPrx
del AdminPrx
_M_IceGrid.Admin = Ice.createTempClass()
class Admin(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::Admin')
def ice_id(self, current=None):
return '::IceGrid::Admin'
@staticmethod
def ice_staticId():
return '::IceGrid::Admin'
def addApplication(self, descriptor, current=None):
"""
Add an application to IceGrid.
Arguments:
descriptor -- The application descriptor.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'addApplication' not implemented")
def syncApplication(self, descriptor, current=None):
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor.
Arguments:
descriptor -- The application descriptor.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'syncApplication' not implemented")
def updateApplication(self, descriptor, current=None):
"""
Update a deployed application with the given update application
descriptor.
Arguments:
descriptor -- The update descriptor.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'updateApplication' not implemented")
def syncApplicationWithoutRestart(self, descriptor, current=None):
"""
Synchronize a deployed application with the given application
descriptor. This operation will replace the current descriptor
with this new descriptor only if no server restarts are
necessary for the update of the application. If some servers
need to be restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The application descriptor.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'syncApplicationWithoutRestart' not implemented")
def updateApplicationWithoutRestart(self, descriptor, current=None):
"""
Update a deployed application with the given update application
descriptor only if no server restarts are necessary for the
update of the application. If some servers need to be
restarted, the synchronization is rejected with a
DeploymentException.
Arguments:
descriptor -- The update descriptor.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'updateApplicationWithoutRestart' not implemented")
def removeApplication(self, name, current=None):
"""
Remove an application from IceGrid.
Arguments:
name -- The application name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'removeApplication' not implemented")
def instantiateServer(self, application, node, desc, current=None):
"""
Instantiate a server template from an application on the given
node.
Arguments:
application -- The application name.
node -- The name of the node where the server will be deployed.
desc -- The descriptor of the server instance to deploy.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock or if another session is holding the lock.
ApplicationNotExistException -- Raised if the application doesn't exist.
DeploymentException -- Raised if server instantiation failed.
"""
raise NotImplementedError("servant method 'instantiateServer' not implemented")
def patchApplication(self, name, shutdown, current=None):
"""
Patch the given application data.
Arguments:
name -- The application name.
shutdown -- If true, the servers depending on the data to patch will be shut down if necessary.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
PatchException -- Raised if the patch failed.
"""
raise NotImplementedError("servant method 'patchApplication' not implemented")
def getApplicationInfo(self, name, current=None):
"""
Get an application descriptor.
Arguments:
name -- The application name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ApplicationNotExistException -- Raised if the application doesn't exist.
"""
raise NotImplementedError("servant method 'getApplicationInfo' not implemented")
def getDefaultApplicationDescriptor(self, current=None):
"""
Get the default application descriptor.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the default application descriptor can't be accessed or is invalid.
"""
raise NotImplementedError("servant method 'getDefaultApplicationDescriptor' not implemented")
def getAllApplicationNames(self, current=None):
"""
Get all the IceGrid applications currently registered.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllApplicationNames' not implemented")
def getServerInfo(self, id, current=None):
"""
Get the server information for the server with the given id.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'getServerInfo' not implemented")
def getServerState(self, id, current=None):
"""
Get a server's state.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'getServerState' not implemented")
def getServerPid(self, id, current=None):
"""
Get a server's system process id. The process id is operating
system dependent.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'getServerPid' not implemented")
def getServerAdminCategory(self, current=None):
"""
Get the category for server admin objects. You can manufacture a server admin
proxy from the admin proxy by changing its identity: use the server ID as name
and the returned category as category.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getServerAdminCategory' not implemented")
def getServerAdmin(self, id, current=None):
"""
Get a proxy to the server's admin object.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'getServerAdmin' not implemented")
def enableServer(self, id, enabled, current=None):
"""
Enable or disable a server. A disabled server can't be started
on demand or administratively. The enable state of the server
is not persistent: if the node is shut down and restarted, the
server will be enabled by default.
Arguments:
id -- The server id.
enabled -- True to enable the server, false to disable it.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'enableServer' not implemented")
def isServerEnabled(self, id, current=None):
"""
Check if the server is enabled or disabled.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'isServerEnabled' not implemented")
def startServer(self, id, current=None):
"""
Start a server and wait for its activation.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStartException -- Raised if the server couldn't be started.
"""
raise NotImplementedError("servant method 'startServer' not implemented")
def stopServer(self, id, current=None):
"""
Stop a server.
Arguments:
id -- The server id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
ServerStopException -- Raised if the server couldn't be stopped.
"""
raise NotImplementedError("servant method 'stopServer' not implemented")
def patchServer(self, id, shutdown, current=None):
"""
Patch a server.
Arguments:
id -- The server id.
shutdown -- If true, servers depending on the data to patch will be shut down if necessary.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
PatchException -- Raised if the patch failed.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'patchServer' not implemented")
def sendSignal(self, id, signal, current=None):
"""
Send signal to a server.
Arguments:
id -- The server id.
signal -- The signal, for example SIGTERM or 15.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
BadSignalException -- Raised if the signal is not recognized by the target server.
DeploymentException -- Raised if the server couldn't be deployed on the node.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'sendSignal' not implemented")
def getAllServerIds(self, current=None):
"""
Get all the server ids registered with IceGrid.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllServerIds' not implemented")
def getAdapterInfo(self, id, current=None):
"""
Get the adapter information for the replica group or adapter
with the given id.
Arguments:
id -- The adapter id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AdapterNotExistException -- Raised if the adapter or replica group doesn't exist.
"""
raise NotImplementedError("servant method 'getAdapterInfo' not implemented")
def removeAdapter(self, id, current=None):
"""
Remove the adapter with the given id.
Arguments:
id -- The adapter id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AdapterNotExistException -- Raised if the adapter doesn't exist.
DeploymentException -- Raised if application deployment failed.
"""
raise NotImplementedError("servant method 'removeAdapter' not implemented")
def getAllAdapterIds(self, current=None):
"""
Get all the adapter ids registered with IceGrid.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllAdapterIds' not implemented")
def addObject(self, obj, current=None):
"""
Add an object to the object registry. IceGrid will get the
object type by calling ice_id on the given proxy. The object
must be reachable.
Arguments:
obj -- The object to be added to the registry.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the object can't be added. This might be raised if the invocation on the proxy to get the object type failed.
ObjectExistsException -- Raised if the object is already registered.
"""
raise NotImplementedError("servant method 'addObject' not implemented")
def updateObject(self, obj, current=None):
"""
Update an object in the object registry. Only objects added
with this interface can be updated with this operation. Objects
added with deployment descriptors should be updated with the
deployment mechanism.
Arguments:
obj -- The object to be updated to the registry.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the object can't be updated. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
raise NotImplementedError("servant method 'updateObject' not implemented")
def addObjectWithType(self, obj, type, current=None):
"""
Add an object to the object registry and explicitly specify
its type.
Arguments:
obj -- The object to be added to the registry.
type -- The object type.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if application deployment failed.
ObjectExistsException -- Raised if the object is already registered.
"""
raise NotImplementedError("servant method 'addObjectWithType' not implemented")
def removeObject(self, id, current=None):
"""
Remove an object from the object registry. Only objects added
with this interface can be removed with this operation. Objects
added with deployment descriptors should be removed with the
deployment mechanism.
Arguments:
id -- The identity of the object to be removed from the registry.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the object can't be removed. This might happen if the object was added with a deployment descriptor.
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
raise NotImplementedError("servant method 'removeObject' not implemented")
def getObjectInfo(self, id, current=None):
"""
Get the object info for the object with the given identity.
Arguments:
id -- The identity of the object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ObjectNotRegisteredException -- Raised if the object isn't registered with the registry.
"""
raise NotImplementedError("servant method 'getObjectInfo' not implemented")
def getObjectInfosByType(self, type, current=None):
"""
Get the object info of all the registered objects with the
given type.
Arguments:
type -- The type of the object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getObjectInfosByType' not implemented")
def getAllObjectInfos(self, expr, current=None):
"""
Get the object info of all the registered objects whose stringified
identities match the given expression.
Arguments:
expr -- The expression to match against the stringified identities of registered objects. The expression may contain a trailing wildcard (*) character.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllObjectInfos' not implemented")
def pingNode(self, name, current=None):
"""
Ping an IceGrid node to see if it is active.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
"""
raise NotImplementedError("servant method 'pingNode' not implemented")
def getNodeLoad(self, name, current=None):
"""
Get the load averages of the node.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'getNodeLoad' not implemented")
def getNodeInfo(self, name, current=None):
"""
Get the node information for the node with the given name.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'getNodeInfo' not implemented")
def getNodeAdmin(self, name, current=None):
"""
Get a proxy to the IceGrid node's admin object.
Arguments:
name -- The IceGrid node name
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'getNodeAdmin' not implemented")
def getNodeProcessorSocketCount(self, name, current=None):
"""
Get the number of physical processor sockets for the machine
running the node with the given name.
Note that this method will return 1 on operating systems where
this can't be automatically determined and where the
IceGrid.Node.ProcessorSocketCount property for the node is not
set.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'getNodeProcessorSocketCount' not implemented")
def shutdownNode(self, name, current=None):
"""
Shutdown an IceGrid node.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'shutdownNode' not implemented")
def getNodeHostname(self, name, current=None):
"""
Get the hostname of this node.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'getNodeHostname' not implemented")
def getAllNodeNames(self, current=None):
"""
Get all the IceGrid nodes currently registered.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllNodeNames' not implemented")
def pingRegistry(self, name, current=None):
"""
Ping an IceGrid registry to see if it is active.
Arguments:
name -- The registry name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
raise NotImplementedError("servant method 'pingRegistry' not implemented")
def getRegistryInfo(self, name, current=None):
"""
Get the registry information for the registry with the given name.
Arguments:
name -- The registry name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
raise NotImplementedError("servant method 'getRegistryInfo' not implemented")
def getRegistryAdmin(self, name, current=None):
"""
Get a proxy to the IceGrid registry's admin object.
Arguments:
name -- The registry name
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
"""
raise NotImplementedError("servant method 'getRegistryAdmin' not implemented")
def shutdownRegistry(self, name, current=None):
"""
Shutdown an IceGrid registry.
Arguments:
name -- The registry name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
raise NotImplementedError("servant method 'shutdownRegistry' not implemented")
def getAllRegistryNames(self, current=None):
"""
Get all the IceGrid registries currently registered.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAllRegistryNames' not implemented")
def shutdown(self, current=None):
"""
Shut down the IceGrid registry.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'shutdown' not implemented")
def getSliceChecksums(self, current=None):
"""
Returns the checksums for the IceGrid Slice definitions.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSliceChecksums' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdminDisp)
__repr__ = __str__
_M_IceGrid._t_AdminDisp = IcePy.defineClass('::IceGrid::Admin', Admin, (), None, ())
Admin._ice_type = _M_IceGrid._t_AdminDisp
Admin._op_addApplication = IcePy.Operation('addApplication', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ApplicationDescriptor, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException))
Admin._op_syncApplication = IcePy.Operation('syncApplication', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ApplicationDescriptor, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_ApplicationNotExistException))
Admin._op_updateApplication = IcePy.Operation('updateApplication', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ApplicationUpdateDescriptor, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_ApplicationNotExistException))
Admin._op_syncApplicationWithoutRestart = IcePy.Operation('syncApplicationWithoutRestart', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ApplicationDescriptor, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_ApplicationNotExistException))
Admin._op_updateApplicationWithoutRestart = IcePy.Operation('updateApplicationWithoutRestart', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ApplicationUpdateDescriptor, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_ApplicationNotExistException))
Admin._op_removeApplication = IcePy.Operation('removeApplication', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_ApplicationNotExistException))
Admin._op_instantiateServer = IcePy.Operation('instantiateServer', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0), ((), _M_IceGrid._t_ServerInstanceDescriptor, False, 0)), (), None, (_M_IceGrid._t_AccessDeniedException, _M_IceGrid._t_ApplicationNotExistException, _M_IceGrid._t_DeploymentException))
Admin._op_patchApplication = IcePy.Operation('patchApplication', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_bool, False, 0)), (), None, (_M_IceGrid._t_ApplicationNotExistException, _M_IceGrid._t_PatchException))
Admin._op_getApplicationInfo = IcePy.Operation('getApplicationInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_ApplicationInfo, False, 0), (_M_IceGrid._t_ApplicationNotExistException,))
Admin._op_getDefaultApplicationDescriptor = IcePy.Operation('getDefaultApplicationDescriptor', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_IceGrid._t_ApplicationDescriptor, False, 0), (_M_IceGrid._t_DeploymentException,))
Admin._op_getAllApplicationNames = IcePy.Operation('getAllApplicationNames', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
Admin._op_getServerInfo = IcePy.Operation('getServerInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_ServerInfo, False, 0), (_M_IceGrid._t_ServerNotExistException,))
Admin._op_getServerState = IcePy.Operation('getServerState', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_ServerState, False, 0), (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_getServerPid = IcePy.Operation('getServerPid', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_int, False, 0), (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_getServerAdminCategory = IcePy.Operation('getServerAdminCategory', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), IcePy._t_string, False, 0), ())
Admin._op_getServerAdmin = IcePy.Operation('getServerAdmin', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_enableServer = IcePy.Operation('enableServer', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_bool, False, 0)), (), None, (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_isServerEnabled = IcePy.Operation('isServerEnabled', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_bool, False, 0), (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_startServer = IcePy.Operation('startServer', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_ServerStartException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_stopServer = IcePy.Operation('stopServer', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_ServerStopException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
Admin._op_patchServer = IcePy.Operation('patchServer', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_bool, False, 0)), (), None, (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_PatchException))
Admin._op_sendSignal = IcePy.Operation('sendSignal', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), None, (_M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException, _M_IceGrid._t_BadSignalException))
Admin._op_getAllServerIds = IcePy.Operation('getAllServerIds', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
Admin._op_getAdapterInfo = IcePy.Operation('getAdapterInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_AdapterInfoSeq, False, 0), (_M_IceGrid._t_AdapterNotExistException,))
Admin._op_removeAdapter = IcePy.Operation('removeAdapter', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_AdapterNotExistException, _M_IceGrid._t_DeploymentException))
Admin._op_getAllAdapterIds = IcePy.Operation('getAllAdapterIds', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
Admin._op_addObject = IcePy.Operation('addObject', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_ObjectPrx, False, 0),), (), None, (_M_IceGrid._t_ObjectExistsException, _M_IceGrid._t_DeploymentException))
Admin._op_updateObject = IcePy.Operation('updateObject', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_ObjectPrx, False, 0),), (), None, (_M_IceGrid._t_ObjectNotRegisteredException, _M_IceGrid._t_DeploymentException))
Admin._op_addObjectWithType = IcePy.Operation('addObjectWithType', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_ObjectPrx, False, 0), ((), IcePy._t_string, False, 0)), (), None, (_M_IceGrid._t_ObjectExistsException, _M_IceGrid._t_DeploymentException))
Admin._op_removeObject = IcePy.Operation('removeObject', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_Identity, False, 0),), (), None, (_M_IceGrid._t_ObjectNotRegisteredException, _M_IceGrid._t_DeploymentException))
Admin._op_getObjectInfo = IcePy.Operation('getObjectInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), _M_Ice._t_Identity, False, 0),), (), ((), _M_IceGrid._t_ObjectInfo, False, 0), (_M_IceGrid._t_ObjectNotRegisteredException,))
Admin._op_getObjectInfosByType = IcePy.Operation('getObjectInfosByType', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_ObjectInfoSeq, False, 0), ())
Admin._op_getAllObjectInfos = IcePy.Operation('getAllObjectInfos', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_ObjectInfoSeq, False, 0), ())
Admin._op_pingNode = IcePy.Operation('pingNode', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_bool, False, 0), (_M_IceGrid._t_NodeNotExistException,))
Admin._op_getNodeLoad = IcePy.Operation('getNodeLoad', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_LoadInfo, False, 0), (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_getNodeInfo = IcePy.Operation('getNodeInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_NodeInfo, False, 0), (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_getNodeAdmin = IcePy.Operation('getNodeAdmin', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_getNodeProcessorSocketCount = IcePy.Operation('getNodeProcessorSocketCount', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_int, False, 0), (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_shutdownNode = IcePy.Operation('shutdownNode', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_getNodeHostname = IcePy.Operation('getNodeHostname', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_string, False, 0), (_M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
Admin._op_getAllNodeNames = IcePy.Operation('getAllNodeNames', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
Admin._op_pingRegistry = IcePy.Operation('pingRegistry', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_bool, False, 0), (_M_IceGrid._t_RegistryNotExistException,))
Admin._op_getRegistryInfo = IcePy.Operation('getRegistryInfo', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_IceGrid._t_RegistryInfo, False, 0), (_M_IceGrid._t_RegistryNotExistException, _M_IceGrid._t_RegistryUnreachableException))
Admin._op_getRegistryAdmin = IcePy.Operation('getRegistryAdmin', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_IceGrid._t_RegistryNotExistException,))
Admin._op_shutdownRegistry = IcePy.Operation('shutdownRegistry', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceGrid._t_RegistryNotExistException, _M_IceGrid._t_RegistryUnreachableException))
Admin._op_getAllRegistryNames = IcePy.Operation('getAllRegistryNames', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
Admin._op_shutdown = IcePy.Operation('shutdown', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
Admin._op_getSliceChecksums = IcePy.Operation('getSliceChecksums', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_SliceChecksumDict, False, 0), ())
_M_IceGrid.Admin = Admin
del Admin
_M_IceGrid._t_FileIterator = IcePy.defineValue('::IceGrid::FileIterator', Ice.Value, -1, (), False, True, None, ())
if 'FileIteratorPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.FileIteratorPrx = Ice.createTempClass()
class FileIteratorPrx(Ice.ObjectPrx):
"""
Read lines from the log file.
Arguments:
size -- Specifies the maximum number of bytes to be received. The server will ensure that the returned message doesn't exceed the given size.
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- True if EOF is encountered.
lines -- The lines read from the file. If there was nothing to read from the file since the last call to read, an empty sequence is returned. The last line of the sequence is always incomplete (and therefore no '\n' should be added when writing the last line to the to the output device).
Throws:
FileNotAvailableException -- Raised if there was a problem to read lines from the file.
"""
def read(self, size, context=None):
return _M_IceGrid.FileIterator._op_read.invoke(self, ((size, ), context))
"""
Read lines from the log file.
Arguments:
size -- Specifies the maximum number of bytes to be received. The server will ensure that the returned message doesn't exceed the given size.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def readAsync(self, size, context=None):
return _M_IceGrid.FileIterator._op_read.invokeAsync(self, ((size, ), context))
"""
Read lines from the log file.
Arguments:
size -- Specifies the maximum number of bytes to be received. The server will ensure that the returned message doesn't exceed the given size.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_read(self, size, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.FileIterator._op_read.begin(self, ((size, ), _response, _ex, _sent, context))
"""
Read lines from the log file.
Arguments:
size -- Specifies the maximum number of bytes to be received. The server will ensure that the returned message doesn't exceed the given size.
Returns a tuple containing the following:
_retval -- True if EOF is encountered.
lines -- The lines read from the file. If there was nothing to read from the file since the last call to read, an empty sequence is returned. The last line of the sequence is always incomplete (and therefore no '\n' should be added when writing the last line to the to the output device).
Throws:
FileNotAvailableException -- Raised if there was a problem to read lines from the file.
"""
def end_read(self, _r):
return _M_IceGrid.FileIterator._op_read.end(self, _r)
"""
Destroy the iterator.
Arguments:
context -- The request context for the invocation.
"""
def destroy(self, context=None):
return _M_IceGrid.FileIterator._op_destroy.invoke(self, ((), context))
"""
Destroy the iterator.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def destroyAsync(self, context=None):
return _M_IceGrid.FileIterator._op_destroy.invokeAsync(self, ((), context))
"""
Destroy the iterator.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_destroy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.FileIterator._op_destroy.begin(self, ((), _response, _ex, _sent, context))
"""
Destroy the iterator.
Arguments:
"""
def end_destroy(self, _r):
return _M_IceGrid.FileIterator._op_destroy.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.FileIteratorPrx.ice_checkedCast(proxy, '::IceGrid::FileIterator', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.FileIteratorPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::FileIterator'
_M_IceGrid._t_FileIteratorPrx = IcePy.defineProxy('::IceGrid::FileIterator', FileIteratorPrx)
_M_IceGrid.FileIteratorPrx = FileIteratorPrx
del FileIteratorPrx
_M_IceGrid.FileIterator = Ice.createTempClass()
class FileIterator(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::FileIterator')
def ice_id(self, current=None):
return '::IceGrid::FileIterator'
@staticmethod
def ice_staticId():
return '::IceGrid::FileIterator'
def read(self, size, current=None):
"""
Read lines from the log file.
Arguments:
size -- Specifies the maximum number of bytes to be received. The server will ensure that the returned message doesn't exceed the given size.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileNotAvailableException -- Raised if there was a problem to read lines from the file.
"""
raise NotImplementedError("servant method 'read' not implemented")
def destroy(self, current=None):
"""
Destroy the iterator.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_FileIteratorDisp)
__repr__ = __str__
_M_IceGrid._t_FileIteratorDisp = IcePy.defineClass('::IceGrid::FileIterator', FileIterator, (), None, ())
FileIterator._ice_type = _M_IceGrid._t_FileIteratorDisp
FileIterator._op_read = IcePy.Operation('read', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_int, False, 0),), (((), _M_Ice._t_StringSeq, False, 0),), ((), IcePy._t_bool, False, 0), (_M_IceGrid._t_FileNotAvailableException,))
FileIterator._op_destroy = IcePy.Operation('destroy', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
_M_IceGrid.FileIterator = FileIterator
del FileIterator
if 'ServerDynamicInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.ServerDynamicInfo = Ice.createTempClass()
class ServerDynamicInfo(object):
"""
Dynamic information about the state of a server.
Members:
id -- The id of the server.
state -- The state of the server.
pid -- The process id of the server.
enabled -- Indicates whether the server is enabled.
"""
def __init__(self, id='', state=_M_IceGrid.ServerState.Inactive, pid=0, enabled=False):
self.id = id
self.state = state
self.pid = pid
self.enabled = enabled
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.id)
_h = 5 * _h + Ice.getHash(self.state)
_h = 5 * _h + Ice.getHash(self.pid)
_h = 5 * _h + Ice.getHash(self.enabled)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IceGrid.ServerDynamicInfo):
return NotImplemented
else:
if self.id is None or other.id is None:
if self.id != other.id:
return (-1 if self.id is None else 1)
else:
if self.id < other.id:
return -1
elif self.id > other.id:
return 1
if self.state is None or other.state is None:
if self.state != other.state:
return (-1 if self.state is None else 1)
else:
if self.state < other.state:
return -1
elif self.state > other.state:
return 1
if self.pid is None or other.pid is None:
if self.pid != other.pid:
return (-1 if self.pid is None else 1)
else:
if self.pid < other.pid:
return -1
elif self.pid > other.pid:
return 1
if self.enabled is None or other.enabled is None:
if self.enabled != other.enabled:
return (-1 if self.enabled is None else 1)
else:
if self.enabled < other.enabled:
return -1
elif self.enabled > other.enabled:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ServerDynamicInfo)
__repr__ = __str__
_M_IceGrid._t_ServerDynamicInfo = IcePy.defineStruct('::IceGrid::ServerDynamicInfo', ServerDynamicInfo, (), (
('id', (), IcePy._t_string),
('state', (), _M_IceGrid._t_ServerState),
('pid', (), IcePy._t_int),
('enabled', (), IcePy._t_bool)
))
_M_IceGrid.ServerDynamicInfo = ServerDynamicInfo
del ServerDynamicInfo
if '_t_ServerDynamicInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_ServerDynamicInfoSeq = IcePy.defineSequence('::IceGrid::ServerDynamicInfoSeq', (), _M_IceGrid._t_ServerDynamicInfo)
if 'AdapterDynamicInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.AdapterDynamicInfo = Ice.createTempClass()
class AdapterDynamicInfo(object):
"""
Dynamic information about the state of an adapter.
Members:
id -- The id of the adapter.
proxy -- The direct proxy containing the adapter endpoints.
"""
def __init__(self, id='', proxy=None):
self.id = id
self.proxy = proxy
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.AdapterDynamicInfo):
return NotImplemented
else:
if self.id != other.id:
return False
if self.proxy != other.proxy:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdapterDynamicInfo)
__repr__ = __str__
_M_IceGrid._t_AdapterDynamicInfo = IcePy.defineStruct('::IceGrid::AdapterDynamicInfo', AdapterDynamicInfo, (), (
('id', (), IcePy._t_string),
('proxy', (), IcePy._t_ObjectPrx)
))
_M_IceGrid.AdapterDynamicInfo = AdapterDynamicInfo
del AdapterDynamicInfo
if '_t_AdapterDynamicInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_AdapterDynamicInfoSeq = IcePy.defineSequence('::IceGrid::AdapterDynamicInfoSeq', (), _M_IceGrid._t_AdapterDynamicInfo)
if 'NodeDynamicInfo' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeDynamicInfo = Ice.createTempClass()
class NodeDynamicInfo(object):
"""
Dynamic information about the state of a node.
Members:
info -- Some static information about the node.
servers -- The dynamic information of the servers deployed on this node.
adapters -- The dynamic information of the adapters deployed on this node.
"""
def __init__(self, info=Ice._struct_marker, servers=None, adapters=None):
if info is Ice._struct_marker:
self.info = _M_IceGrid.NodeInfo()
else:
self.info = info
self.servers = servers
self.adapters = adapters
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceGrid.NodeDynamicInfo):
return NotImplemented
else:
if self.info != other.info:
return False
if self.servers != other.servers:
return False
if self.adapters != other.adapters:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_NodeDynamicInfo)
__repr__ = __str__
_M_IceGrid._t_NodeDynamicInfo = IcePy.defineStruct('::IceGrid::NodeDynamicInfo', NodeDynamicInfo, (), (
('info', (), _M_IceGrid._t_NodeInfo),
('servers', (), _M_IceGrid._t_ServerDynamicInfoSeq),
('adapters', (), _M_IceGrid._t_AdapterDynamicInfoSeq)
))
_M_IceGrid.NodeDynamicInfo = NodeDynamicInfo
del NodeDynamicInfo
_M_IceGrid._t_RegistryObserver = IcePy.defineValue('::IceGrid::RegistryObserver', Ice.Value, -1, (), False, True, None, ())
if 'RegistryObserverPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.RegistryObserverPrx = Ice.createTempClass()
class RegistryObserverPrx(Ice.ObjectPrx):
"""
The registryInit operation is called after registration of
an observer to indicate the state of the registries.
Arguments:
registries -- The current state of the registries.
context -- The request context for the invocation.
"""
def registryInit(self, registries, context=None):
return _M_IceGrid.RegistryObserver._op_registryInit.invoke(self, ((registries, ), context))
"""
The registryInit operation is called after registration of
an observer to indicate the state of the registries.
Arguments:
registries -- The current state of the registries.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def registryInitAsync(self, registries, context=None):
return _M_IceGrid.RegistryObserver._op_registryInit.invokeAsync(self, ((registries, ), context))
"""
The registryInit operation is called after registration of
an observer to indicate the state of the registries.
Arguments:
registries -- The current state of the registries.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_registryInit(self, registries, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.RegistryObserver._op_registryInit.begin(self, ((registries, ), _response, _ex, _sent, context))
"""
The registryInit operation is called after registration of
an observer to indicate the state of the registries.
Arguments:
registries -- The current state of the registries.
"""
def end_registryInit(self, _r):
return _M_IceGrid.RegistryObserver._op_registryInit.end(self, _r)
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
context -- The request context for the invocation.
"""
def registryUp(self, node, context=None):
return _M_IceGrid.RegistryObserver._op_registryUp.invoke(self, ((node, ), context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def registryUpAsync(self, node, context=None):
return _M_IceGrid.RegistryObserver._op_registryUp.invokeAsync(self, ((node, ), context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_registryUp(self, node, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.RegistryObserver._op_registryUp.begin(self, ((node, ), _response, _ex, _sent, context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
"""
def end_registryUp(self, _r):
return _M_IceGrid.RegistryObserver._op_registryUp.end(self, _r)
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
context -- The request context for the invocation.
"""
def registryDown(self, name, context=None):
return _M_IceGrid.RegistryObserver._op_registryDown.invoke(self, ((name, ), context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def registryDownAsync(self, name, context=None):
return _M_IceGrid.RegistryObserver._op_registryDown.invokeAsync(self, ((name, ), context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_registryDown(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.RegistryObserver._op_registryDown.begin(self, ((name, ), _response, _ex, _sent, context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
"""
def end_registryDown(self, _r):
return _M_IceGrid.RegistryObserver._op_registryDown.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.RegistryObserverPrx.ice_checkedCast(proxy, '::IceGrid::RegistryObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.RegistryObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::RegistryObserver'
_M_IceGrid._t_RegistryObserverPrx = IcePy.defineProxy('::IceGrid::RegistryObserver', RegistryObserverPrx)
_M_IceGrid.RegistryObserverPrx = RegistryObserverPrx
del RegistryObserverPrx
_M_IceGrid.RegistryObserver = Ice.createTempClass()
class RegistryObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::RegistryObserver')
def ice_id(self, current=None):
return '::IceGrid::RegistryObserver'
@staticmethod
def ice_staticId():
return '::IceGrid::RegistryObserver'
def registryInit(self, registries, current=None):
"""
The registryInit operation is called after registration of
an observer to indicate the state of the registries.
Arguments:
registries -- The current state of the registries.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'registryInit' not implemented")
def registryUp(self, node, current=None):
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'registryUp' not implemented")
def registryDown(self, name, current=None):
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'registryDown' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_RegistryObserverDisp)
__repr__ = __str__
_M_IceGrid._t_RegistryObserverDisp = IcePy.defineClass('::IceGrid::RegistryObserver', RegistryObserver, (), None, ())
RegistryObserver._ice_type = _M_IceGrid._t_RegistryObserverDisp
RegistryObserver._op_registryInit = IcePy.Operation('registryInit', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_RegistryInfoSeq, False, 0),), (), None, ())
RegistryObserver._op_registryUp = IcePy.Operation('registryUp', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_RegistryInfo, False, 0),), (), None, ())
RegistryObserver._op_registryDown = IcePy.Operation('registryDown', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, ())
_M_IceGrid.RegistryObserver = RegistryObserver
del RegistryObserver
if '_t_NodeDynamicInfoSeq' not in _M_IceGrid.__dict__:
_M_IceGrid._t_NodeDynamicInfoSeq = IcePy.defineSequence('::IceGrid::NodeDynamicInfoSeq', (), _M_IceGrid._t_NodeDynamicInfo)
_M_IceGrid._t_NodeObserver = IcePy.defineValue('::IceGrid::NodeObserver', Ice.Value, -1, (), False, True, None, ())
if 'NodeObserverPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.NodeObserverPrx = Ice.createTempClass()
class NodeObserverPrx(Ice.ObjectPrx):
"""
The nodeInit operation indicates the current state
of nodes. It is called after the registration of an observer.
Arguments:
nodes -- The current state of the nodes.
context -- The request context for the invocation.
"""
def nodeInit(self, nodes, context=None):
return _M_IceGrid.NodeObserver._op_nodeInit.invoke(self, ((nodes, ), context))
"""
The nodeInit operation indicates the current state
of nodes. It is called after the registration of an observer.
Arguments:
nodes -- The current state of the nodes.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def nodeInitAsync(self, nodes, context=None):
return _M_IceGrid.NodeObserver._op_nodeInit.invokeAsync(self, ((nodes, ), context))
"""
The nodeInit operation indicates the current state
of nodes. It is called after the registration of an observer.
Arguments:
nodes -- The current state of the nodes.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_nodeInit(self, nodes, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.NodeObserver._op_nodeInit.begin(self, ((nodes, ), _response, _ex, _sent, context))
"""
The nodeInit operation indicates the current state
of nodes. It is called after the registration of an observer.
Arguments:
nodes -- The current state of the nodes.
"""
def end_nodeInit(self, _r):
return _M_IceGrid.NodeObserver._op_nodeInit.end(self, _r)
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
context -- The request context for the invocation.
"""
def nodeUp(self, node, context=None):
return _M_IceGrid.NodeObserver._op_nodeUp.invoke(self, ((node, ), context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def nodeUpAsync(self, node, context=None):
return _M_IceGrid.NodeObserver._op_nodeUp.invokeAsync(self, ((node, ), context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_nodeUp(self, node, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.NodeObserver._op_nodeUp.begin(self, ((node, ), _response, _ex, _sent, context))
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
"""
def end_nodeUp(self, _r):
return _M_IceGrid.NodeObserver._op_nodeUp.end(self, _r)
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
context -- The request context for the invocation.
"""
def nodeDown(self, name, context=None):
return _M_IceGrid.NodeObserver._op_nodeDown.invoke(self, ((name, ), context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def nodeDownAsync(self, name, context=None):
return _M_IceGrid.NodeObserver._op_nodeDown.invokeAsync(self, ((name, ), context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_nodeDown(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.NodeObserver._op_nodeDown.begin(self, ((name, ), _response, _ex, _sent, context))
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
"""
def end_nodeDown(self, _r):
return _M_IceGrid.NodeObserver._op_nodeDown.end(self, _r)
"""
The updateServer operation is called to notify an observer that
the state of a server changed.
Arguments:
node -- The node hosting the server.
updatedInfo -- The new server state.
context -- The request context for the invocation.
"""
def updateServer(self, node, updatedInfo, context=None):
return _M_IceGrid.NodeObserver._op_updateServer.invoke(self, ((node, updatedInfo), context))
"""
The updateServer operation is called to notify an observer that
the state of a server changed.
Arguments:
node -- The node hosting the server.
updatedInfo -- The new server state.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def updateServerAsync(self, node, updatedInfo, context=None):
return _M_IceGrid.NodeObserver._op_updateServer.invokeAsync(self, ((node, updatedInfo), context))
"""
The updateServer operation is called to notify an observer that
the state of a server changed.
Arguments:
node -- The node hosting the server.
updatedInfo -- The new server state.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_updateServer(self, node, updatedInfo, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.NodeObserver._op_updateServer.begin(self, ((node, updatedInfo), _response, _ex, _sent, context))
"""
The updateServer operation is called to notify an observer that
the state of a server changed.
Arguments:
node -- The node hosting the server.
updatedInfo -- The new server state.
"""
def end_updateServer(self, _r):
return _M_IceGrid.NodeObserver._op_updateServer.end(self, _r)
"""
The updateAdapter operation is called to notify an observer that
the state of an adapter changed.
Arguments:
node -- The node hosting the adapter.
updatedInfo -- The new adapter state.
context -- The request context for the invocation.
"""
def updateAdapter(self, node, updatedInfo, context=None):
return _M_IceGrid.NodeObserver._op_updateAdapter.invoke(self, ((node, updatedInfo), context))
"""
The updateAdapter operation is called to notify an observer that
the state of an adapter changed.
Arguments:
node -- The node hosting the adapter.
updatedInfo -- The new adapter state.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def updateAdapterAsync(self, node, updatedInfo, context=None):
return _M_IceGrid.NodeObserver._op_updateAdapter.invokeAsync(self, ((node, updatedInfo), context))
"""
The updateAdapter operation is called to notify an observer that
the state of an adapter changed.
Arguments:
node -- The node hosting the adapter.
updatedInfo -- The new adapter state.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_updateAdapter(self, node, updatedInfo, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.NodeObserver._op_updateAdapter.begin(self, ((node, updatedInfo), _response, _ex, _sent, context))
"""
The updateAdapter operation is called to notify an observer that
the state of an adapter changed.
Arguments:
node -- The node hosting the adapter.
updatedInfo -- The new adapter state.
"""
def end_updateAdapter(self, _r):
return _M_IceGrid.NodeObserver._op_updateAdapter.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.NodeObserverPrx.ice_checkedCast(proxy, '::IceGrid::NodeObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.NodeObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::NodeObserver'
_M_IceGrid._t_NodeObserverPrx = IcePy.defineProxy('::IceGrid::NodeObserver', NodeObserverPrx)
_M_IceGrid.NodeObserverPrx = NodeObserverPrx
del NodeObserverPrx
_M_IceGrid.NodeObserver = Ice.createTempClass()
class NodeObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::NodeObserver')
def ice_id(self, current=None):
return '::IceGrid::NodeObserver'
@staticmethod
def ice_staticId():
return '::IceGrid::NodeObserver'
def nodeInit(self, nodes, current=None):
"""
The nodeInit operation indicates the current state
of nodes. It is called after the registration of an observer.
Arguments:
nodes -- The current state of the nodes.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'nodeInit' not implemented")
def nodeUp(self, node, current=None):
"""
The nodeUp operation is called to notify an observer that a node
came up.
Arguments:
node -- The node state.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'nodeUp' not implemented")
def nodeDown(self, name, current=None):
"""
The nodeDown operation is called to notify an observer that a node
went down.
Arguments:
name -- The node name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'nodeDown' not implemented")
def updateServer(self, node, updatedInfo, current=None):
"""
The updateServer operation is called to notify an observer that
the state of a server changed.
Arguments:
node -- The node hosting the server.
updatedInfo -- The new server state.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'updateServer' not implemented")
def updateAdapter(self, node, updatedInfo, current=None):
"""
The updateAdapter operation is called to notify an observer that
the state of an adapter changed.
Arguments:
node -- The node hosting the adapter.
updatedInfo -- The new adapter state.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'updateAdapter' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_NodeObserverDisp)
__repr__ = __str__
_M_IceGrid._t_NodeObserverDisp = IcePy.defineClass('::IceGrid::NodeObserver', NodeObserver, (), None, ())
NodeObserver._ice_type = _M_IceGrid._t_NodeObserverDisp
NodeObserver._op_nodeInit = IcePy.Operation('nodeInit', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_NodeDynamicInfoSeq, False, 0),), (), None, ())
NodeObserver._op_nodeUp = IcePy.Operation('nodeUp', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_NodeDynamicInfo, False, 0),), (), None, ())
NodeObserver._op_nodeDown = IcePy.Operation('nodeDown', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, ())
NodeObserver._op_updateServer = IcePy.Operation('updateServer', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), _M_IceGrid._t_ServerDynamicInfo, False, 0)), (), None, ())
NodeObserver._op_updateAdapter = IcePy.Operation('updateAdapter', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), _M_IceGrid._t_AdapterDynamicInfo, False, 0)), (), None, ())
_M_IceGrid.NodeObserver = NodeObserver
del NodeObserver
_M_IceGrid._t_ApplicationObserver = IcePy.defineValue('::IceGrid::ApplicationObserver', Ice.Value, -1, (), False, True, None, ())
if 'ApplicationObserverPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.ApplicationObserverPrx = Ice.createTempClass()
class ApplicationObserverPrx(Ice.ObjectPrx):
"""
applicationInit is called after the registration
of an observer to indicate the state of the registry.
Arguments:
serial -- The current serial number of the registry database. This serial number allows observers to make sure that their internal state is synchronized with the registry.
applications -- The applications currently registered with the registry.
context -- The request context for the invocation.
"""
def applicationInit(self, serial, applications, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationInit.invoke(self, ((serial, applications), context))
"""
applicationInit is called after the registration
of an observer to indicate the state of the registry.
Arguments:
serial -- The current serial number of the registry database. This serial number allows observers to make sure that their internal state is synchronized with the registry.
applications -- The applications currently registered with the registry.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def applicationInitAsync(self, serial, applications, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationInit.invokeAsync(self, ((serial, applications), context))
"""
applicationInit is called after the registration
of an observer to indicate the state of the registry.
Arguments:
serial -- The current serial number of the registry database. This serial number allows observers to make sure that their internal state is synchronized with the registry.
applications -- The applications currently registered with the registry.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_applicationInit(self, serial, applications, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationInit.begin(self, ((serial, applications), _response, _ex, _sent, context))
"""
applicationInit is called after the registration
of an observer to indicate the state of the registry.
Arguments:
serial -- The current serial number of the registry database. This serial number allows observers to make sure that their internal state is synchronized with the registry.
applications -- The applications currently registered with the registry.
"""
def end_applicationInit(self, _r):
return _M_IceGrid.ApplicationObserver._op_applicationInit.end(self, _r)
"""
The applicationAdded operation is called to notify an observer
that an application was added.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the new application.
context -- The request context for the invocation.
"""
def applicationAdded(self, serial, desc, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationAdded.invoke(self, ((serial, desc), context))
"""
The applicationAdded operation is called to notify an observer
that an application was added.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the new application.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def applicationAddedAsync(self, serial, desc, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationAdded.invokeAsync(self, ((serial, desc), context))
"""
The applicationAdded operation is called to notify an observer
that an application was added.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the new application.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_applicationAdded(self, serial, desc, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationAdded.begin(self, ((serial, desc), _response, _ex, _sent, context))
"""
The applicationAdded operation is called to notify an observer
that an application was added.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the new application.
"""
def end_applicationAdded(self, _r):
return _M_IceGrid.ApplicationObserver._op_applicationAdded.end(self, _r)
"""
The applicationRemoved operation is called to notify an observer
that an application was removed.
Arguments:
serial -- The new serial number of the registry database.
name -- The name of the application that was removed.
context -- The request context for the invocation.
"""
def applicationRemoved(self, serial, name, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationRemoved.invoke(self, ((serial, name), context))
"""
The applicationRemoved operation is called to notify an observer
that an application was removed.
Arguments:
serial -- The new serial number of the registry database.
name -- The name of the application that was removed.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def applicationRemovedAsync(self, serial, name, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationRemoved.invokeAsync(self, ((serial, name), context))
"""
The applicationRemoved operation is called to notify an observer
that an application was removed.
Arguments:
serial -- The new serial number of the registry database.
name -- The name of the application that was removed.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_applicationRemoved(self, serial, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationRemoved.begin(self, ((serial, name), _response, _ex, _sent, context))
"""
The applicationRemoved operation is called to notify an observer
that an application was removed.
Arguments:
serial -- The new serial number of the registry database.
name -- The name of the application that was removed.
"""
def end_applicationRemoved(self, _r):
return _M_IceGrid.ApplicationObserver._op_applicationRemoved.end(self, _r)
"""
The applicationUpdated operation is called to notify an observer
that an application was updated.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the update.
context -- The request context for the invocation.
"""
def applicationUpdated(self, serial, desc, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationUpdated.invoke(self, ((serial, desc), context))
"""
The applicationUpdated operation is called to notify an observer
that an application was updated.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the update.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def applicationUpdatedAsync(self, serial, desc, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationUpdated.invokeAsync(self, ((serial, desc), context))
"""
The applicationUpdated operation is called to notify an observer
that an application was updated.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the update.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_applicationUpdated(self, serial, desc, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ApplicationObserver._op_applicationUpdated.begin(self, ((serial, desc), _response, _ex, _sent, context))
"""
The applicationUpdated operation is called to notify an observer
that an application was updated.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the update.
"""
def end_applicationUpdated(self, _r):
return _M_IceGrid.ApplicationObserver._op_applicationUpdated.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.ApplicationObserverPrx.ice_checkedCast(proxy, '::IceGrid::ApplicationObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.ApplicationObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::ApplicationObserver'
_M_IceGrid._t_ApplicationObserverPrx = IcePy.defineProxy('::IceGrid::ApplicationObserver', ApplicationObserverPrx)
_M_IceGrid.ApplicationObserverPrx = ApplicationObserverPrx
del ApplicationObserverPrx
_M_IceGrid.ApplicationObserver = Ice.createTempClass()
class ApplicationObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::ApplicationObserver')
def ice_id(self, current=None):
return '::IceGrid::ApplicationObserver'
@staticmethod
def ice_staticId():
return '::IceGrid::ApplicationObserver'
def applicationInit(self, serial, applications, current=None):
"""
applicationInit is called after the registration
of an observer to indicate the state of the registry.
Arguments:
serial -- The current serial number of the registry database. This serial number allows observers to make sure that their internal state is synchronized with the registry.
applications -- The applications currently registered with the registry.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'applicationInit' not implemented")
def applicationAdded(self, serial, desc, current=None):
"""
The applicationAdded operation is called to notify an observer
that an application was added.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the new application.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'applicationAdded' not implemented")
def applicationRemoved(self, serial, name, current=None):
"""
The applicationRemoved operation is called to notify an observer
that an application was removed.
Arguments:
serial -- The new serial number of the registry database.
name -- The name of the application that was removed.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'applicationRemoved' not implemented")
def applicationUpdated(self, serial, desc, current=None):
"""
The applicationUpdated operation is called to notify an observer
that an application was updated.
Arguments:
serial -- The new serial number of the registry database.
desc -- The descriptor of the update.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'applicationUpdated' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ApplicationObserverDisp)
__repr__ = __str__
_M_IceGrid._t_ApplicationObserverDisp = IcePy.defineClass('::IceGrid::ApplicationObserver', ApplicationObserver, (), None, ())
ApplicationObserver._ice_type = _M_IceGrid._t_ApplicationObserverDisp
ApplicationObserver._op_applicationInit = IcePy.Operation('applicationInit', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_int, False, 0), ((), _M_IceGrid._t_ApplicationInfoSeq, False, 0)), (), None, ())
ApplicationObserver._op_applicationAdded = IcePy.Operation('applicationAdded', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_int, False, 0), ((), _M_IceGrid._t_ApplicationInfo, False, 0)), (), None, ())
ApplicationObserver._op_applicationRemoved = IcePy.Operation('applicationRemoved', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_int, False, 0), ((), IcePy._t_string, False, 0)), (), None, ())
ApplicationObserver._op_applicationUpdated = IcePy.Operation('applicationUpdated', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_int, False, 0), ((), _M_IceGrid._t_ApplicationUpdateInfo, False, 0)), (), None, ())
_M_IceGrid.ApplicationObserver = ApplicationObserver
del ApplicationObserver
_M_IceGrid._t_AdapterObserver = IcePy.defineValue('::IceGrid::AdapterObserver', Ice.Value, -1, (), False, True, None, ())
if 'AdapterObserverPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.AdapterObserverPrx = Ice.createTempClass()
class AdapterObserverPrx(Ice.ObjectPrx):
"""
adapterInit is called after registration of
an observer to indicate the state of the registry.
Arguments:
adpts -- The adapters that were dynamically registered with the registry (not through the deployment mechanism).
context -- The request context for the invocation.
"""
def adapterInit(self, adpts, context=None):
return _M_IceGrid.AdapterObserver._op_adapterInit.invoke(self, ((adpts, ), context))
"""
adapterInit is called after registration of
an observer to indicate the state of the registry.
Arguments:
adpts -- The adapters that were dynamically registered with the registry (not through the deployment mechanism).
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def adapterInitAsync(self, adpts, context=None):
return _M_IceGrid.AdapterObserver._op_adapterInit.invokeAsync(self, ((adpts, ), context))
"""
adapterInit is called after registration of
an observer to indicate the state of the registry.
Arguments:
adpts -- The adapters that were dynamically registered with the registry (not through the deployment mechanism).
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_adapterInit(self, adpts, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdapterObserver._op_adapterInit.begin(self, ((adpts, ), _response, _ex, _sent, context))
"""
adapterInit is called after registration of
an observer to indicate the state of the registry.
Arguments:
adpts -- The adapters that were dynamically registered with the registry (not through the deployment mechanism).
"""
def end_adapterInit(self, _r):
return _M_IceGrid.AdapterObserver._op_adapterInit.end(self, _r)
"""
The adapterAdded operation is called to notify an observer when
a dynamically-registered adapter was added.
Arguments:
info -- The details of the new adapter.
context -- The request context for the invocation.
"""
def adapterAdded(self, info, context=None):
return _M_IceGrid.AdapterObserver._op_adapterAdded.invoke(self, ((info, ), context))
"""
The adapterAdded operation is called to notify an observer when
a dynamically-registered adapter was added.
Arguments:
info -- The details of the new adapter.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def adapterAddedAsync(self, info, context=None):
return _M_IceGrid.AdapterObserver._op_adapterAdded.invokeAsync(self, ((info, ), context))
"""
The adapterAdded operation is called to notify an observer when
a dynamically-registered adapter was added.
Arguments:
info -- The details of the new adapter.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_adapterAdded(self, info, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdapterObserver._op_adapterAdded.begin(self, ((info, ), _response, _ex, _sent, context))
"""
The adapterAdded operation is called to notify an observer when
a dynamically-registered adapter was added.
Arguments:
info -- The details of the new adapter.
"""
def end_adapterAdded(self, _r):
return _M_IceGrid.AdapterObserver._op_adapterAdded.end(self, _r)
"""
The adapterUpdated operation is called to notify an observer when
a dynamically-registered adapter was updated.
Arguments:
info -- The details of the updated adapter.
context -- The request context for the invocation.
"""
def adapterUpdated(self, info, context=None):
return _M_IceGrid.AdapterObserver._op_adapterUpdated.invoke(self, ((info, ), context))
"""
The adapterUpdated operation is called to notify an observer when
a dynamically-registered adapter was updated.
Arguments:
info -- The details of the updated adapter.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def adapterUpdatedAsync(self, info, context=None):
return _M_IceGrid.AdapterObserver._op_adapterUpdated.invokeAsync(self, ((info, ), context))
"""
The adapterUpdated operation is called to notify an observer when
a dynamically-registered adapter was updated.
Arguments:
info -- The details of the updated adapter.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_adapterUpdated(self, info, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdapterObserver._op_adapterUpdated.begin(self, ((info, ), _response, _ex, _sent, context))
"""
The adapterUpdated operation is called to notify an observer when
a dynamically-registered adapter was updated.
Arguments:
info -- The details of the updated adapter.
"""
def end_adapterUpdated(self, _r):
return _M_IceGrid.AdapterObserver._op_adapterUpdated.end(self, _r)
"""
The adapterRemoved operation is called to notify an observer when
a dynamically-registered adapter was removed.
Arguments:
id -- The ID of the removed adapter.
context -- The request context for the invocation.
"""
def adapterRemoved(self, id, context=None):
return _M_IceGrid.AdapterObserver._op_adapterRemoved.invoke(self, ((id, ), context))
"""
The adapterRemoved operation is called to notify an observer when
a dynamically-registered adapter was removed.
Arguments:
id -- The ID of the removed adapter.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def adapterRemovedAsync(self, id, context=None):
return _M_IceGrid.AdapterObserver._op_adapterRemoved.invokeAsync(self, ((id, ), context))
"""
The adapterRemoved operation is called to notify an observer when
a dynamically-registered adapter was removed.
Arguments:
id -- The ID of the removed adapter.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_adapterRemoved(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdapterObserver._op_adapterRemoved.begin(self, ((id, ), _response, _ex, _sent, context))
"""
The adapterRemoved operation is called to notify an observer when
a dynamically-registered adapter was removed.
Arguments:
id -- The ID of the removed adapter.
"""
def end_adapterRemoved(self, _r):
return _M_IceGrid.AdapterObserver._op_adapterRemoved.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.AdapterObserverPrx.ice_checkedCast(proxy, '::IceGrid::AdapterObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.AdapterObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::AdapterObserver'
_M_IceGrid._t_AdapterObserverPrx = IcePy.defineProxy('::IceGrid::AdapterObserver', AdapterObserverPrx)
_M_IceGrid.AdapterObserverPrx = AdapterObserverPrx
del AdapterObserverPrx
_M_IceGrid.AdapterObserver = Ice.createTempClass()
class AdapterObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::AdapterObserver')
def ice_id(self, current=None):
return '::IceGrid::AdapterObserver'
@staticmethod
def ice_staticId():
return '::IceGrid::AdapterObserver'
def adapterInit(self, adpts, current=None):
"""
adapterInit is called after registration of
an observer to indicate the state of the registry.
Arguments:
adpts -- The adapters that were dynamically registered with the registry (not through the deployment mechanism).
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'adapterInit' not implemented")
def adapterAdded(self, info, current=None):
"""
The adapterAdded operation is called to notify an observer when
a dynamically-registered adapter was added.
Arguments:
info -- The details of the new adapter.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'adapterAdded' not implemented")
def adapterUpdated(self, info, current=None):
"""
The adapterUpdated operation is called to notify an observer when
a dynamically-registered adapter was updated.
Arguments:
info -- The details of the updated adapter.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'adapterUpdated' not implemented")
def adapterRemoved(self, id, current=None):
"""
The adapterRemoved operation is called to notify an observer when
a dynamically-registered adapter was removed.
Arguments:
id -- The ID of the removed adapter.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'adapterRemoved' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdapterObserverDisp)
__repr__ = __str__
_M_IceGrid._t_AdapterObserverDisp = IcePy.defineClass('::IceGrid::AdapterObserver', AdapterObserver, (), None, ())
AdapterObserver._ice_type = _M_IceGrid._t_AdapterObserverDisp
AdapterObserver._op_adapterInit = IcePy.Operation('adapterInit', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_AdapterInfoSeq, False, 0),), (), None, ())
AdapterObserver._op_adapterAdded = IcePy.Operation('adapterAdded', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_AdapterInfo, False, 0),), (), None, ())
AdapterObserver._op_adapterUpdated = IcePy.Operation('adapterUpdated', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_AdapterInfo, False, 0),), (), None, ())
AdapterObserver._op_adapterRemoved = IcePy.Operation('adapterRemoved', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, ())
_M_IceGrid.AdapterObserver = AdapterObserver
del AdapterObserver
_M_IceGrid._t_ObjectObserver = IcePy.defineValue('::IceGrid::ObjectObserver', Ice.Value, -1, (), False, True, None, ())
if 'ObjectObserverPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.ObjectObserverPrx = Ice.createTempClass()
class ObjectObserverPrx(Ice.ObjectPrx):
"""
objectInit is called after the registration of
an observer to indicate the state of the registry.
Arguments:
objects -- The objects registered with the Admin interface (not through the deployment mechanism).
context -- The request context for the invocation.
"""
def objectInit(self, objects, context=None):
return _M_IceGrid.ObjectObserver._op_objectInit.invoke(self, ((objects, ), context))
"""
objectInit is called after the registration of
an observer to indicate the state of the registry.
Arguments:
objects -- The objects registered with the Admin interface (not through the deployment mechanism).
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def objectInitAsync(self, objects, context=None):
return _M_IceGrid.ObjectObserver._op_objectInit.invokeAsync(self, ((objects, ), context))
"""
objectInit is called after the registration of
an observer to indicate the state of the registry.
Arguments:
objects -- The objects registered with the Admin interface (not through the deployment mechanism).
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_objectInit(self, objects, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ObjectObserver._op_objectInit.begin(self, ((objects, ), _response, _ex, _sent, context))
"""
objectInit is called after the registration of
an observer to indicate the state of the registry.
Arguments:
objects -- The objects registered with the Admin interface (not through the deployment mechanism).
"""
def end_objectInit(self, _r):
return _M_IceGrid.ObjectObserver._op_objectInit.end(self, _r)
"""
The objectAdded operation is called to notify an observer when an
object was added to the Admin interface.
Arguments:
info -- The details of the added object.
context -- The request context for the invocation.
"""
def objectAdded(self, info, context=None):
return _M_IceGrid.ObjectObserver._op_objectAdded.invoke(self, ((info, ), context))
"""
The objectAdded operation is called to notify an observer when an
object was added to the Admin interface.
Arguments:
info -- The details of the added object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def objectAddedAsync(self, info, context=None):
return _M_IceGrid.ObjectObserver._op_objectAdded.invokeAsync(self, ((info, ), context))
"""
The objectAdded operation is called to notify an observer when an
object was added to the Admin interface.
Arguments:
info -- The details of the added object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_objectAdded(self, info, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ObjectObserver._op_objectAdded.begin(self, ((info, ), _response, _ex, _sent, context))
"""
The objectAdded operation is called to notify an observer when an
object was added to the Admin interface.
Arguments:
info -- The details of the added object.
"""
def end_objectAdded(self, _r):
return _M_IceGrid.ObjectObserver._op_objectAdded.end(self, _r)
"""
objectUpdated is called to notify an observer when
an object registered with the Admin interface was updated.
Arguments:
info -- The details of the updated object.
context -- The request context for the invocation.
"""
def objectUpdated(self, info, context=None):
return _M_IceGrid.ObjectObserver._op_objectUpdated.invoke(self, ((info, ), context))
"""
objectUpdated is called to notify an observer when
an object registered with the Admin interface was updated.
Arguments:
info -- The details of the updated object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def objectUpdatedAsync(self, info, context=None):
return _M_IceGrid.ObjectObserver._op_objectUpdated.invokeAsync(self, ((info, ), context))
"""
objectUpdated is called to notify an observer when
an object registered with the Admin interface was updated.
Arguments:
info -- The details of the updated object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_objectUpdated(self, info, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ObjectObserver._op_objectUpdated.begin(self, ((info, ), _response, _ex, _sent, context))
"""
objectUpdated is called to notify an observer when
an object registered with the Admin interface was updated.
Arguments:
info -- The details of the updated object.
"""
def end_objectUpdated(self, _r):
return _M_IceGrid.ObjectObserver._op_objectUpdated.end(self, _r)
"""
objectRemoved is called to notify an observer when
an object registered with the Admin interface was removed.
Arguments:
id -- The identity of the removed object.
context -- The request context for the invocation.
"""
def objectRemoved(self, id, context=None):
return _M_IceGrid.ObjectObserver._op_objectRemoved.invoke(self, ((id, ), context))
"""
objectRemoved is called to notify an observer when
an object registered with the Admin interface was removed.
Arguments:
id -- The identity of the removed object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def objectRemovedAsync(self, id, context=None):
return _M_IceGrid.ObjectObserver._op_objectRemoved.invokeAsync(self, ((id, ), context))
"""
objectRemoved is called to notify an observer when
an object registered with the Admin interface was removed.
Arguments:
id -- The identity of the removed object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_objectRemoved(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.ObjectObserver._op_objectRemoved.begin(self, ((id, ), _response, _ex, _sent, context))
"""
objectRemoved is called to notify an observer when
an object registered with the Admin interface was removed.
Arguments:
id -- The identity of the removed object.
"""
def end_objectRemoved(self, _r):
return _M_IceGrid.ObjectObserver._op_objectRemoved.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.ObjectObserverPrx.ice_checkedCast(proxy, '::IceGrid::ObjectObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.ObjectObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::ObjectObserver'
_M_IceGrid._t_ObjectObserverPrx = IcePy.defineProxy('::IceGrid::ObjectObserver', ObjectObserverPrx)
_M_IceGrid.ObjectObserverPrx = ObjectObserverPrx
del ObjectObserverPrx
_M_IceGrid.ObjectObserver = Ice.createTempClass()
class ObjectObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceGrid::ObjectObserver')
def ice_id(self, current=None):
return '::IceGrid::ObjectObserver'
@staticmethod
def ice_staticId():
return '::IceGrid::ObjectObserver'
def objectInit(self, objects, current=None):
"""
objectInit is called after the registration of
an observer to indicate the state of the registry.
Arguments:
objects -- The objects registered with the Admin interface (not through the deployment mechanism).
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'objectInit' not implemented")
def objectAdded(self, info, current=None):
"""
The objectAdded operation is called to notify an observer when an
object was added to the Admin interface.
Arguments:
info -- The details of the added object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'objectAdded' not implemented")
def objectUpdated(self, info, current=None):
"""
objectUpdated is called to notify an observer when
an object registered with the Admin interface was updated.
Arguments:
info -- The details of the updated object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'objectUpdated' not implemented")
def objectRemoved(self, id, current=None):
"""
objectRemoved is called to notify an observer when
an object registered with the Admin interface was removed.
Arguments:
id -- The identity of the removed object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'objectRemoved' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_ObjectObserverDisp)
__repr__ = __str__
_M_IceGrid._t_ObjectObserverDisp = IcePy.defineClass('::IceGrid::ObjectObserver', ObjectObserver, (), None, ())
ObjectObserver._ice_type = _M_IceGrid._t_ObjectObserverDisp
ObjectObserver._op_objectInit = IcePy.Operation('objectInit', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ObjectInfoSeq, False, 0),), (), None, ())
ObjectObserver._op_objectAdded = IcePy.Operation('objectAdded', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ObjectInfo, False, 0),), (), None, ())
ObjectObserver._op_objectUpdated = IcePy.Operation('objectUpdated', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceGrid._t_ObjectInfo, False, 0),), (), None, ())
ObjectObserver._op_objectRemoved = IcePy.Operation('objectRemoved', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_Identity, False, 0),), (), None, ())
_M_IceGrid.ObjectObserver = ObjectObserver
del ObjectObserver
_M_IceGrid._t_AdminSession = IcePy.defineValue('::IceGrid::AdminSession', Ice.Value, -1, (), False, True, None, ())
if 'AdminSessionPrx' not in _M_IceGrid.__dict__:
_M_IceGrid.AdminSessionPrx = Ice.createTempClass()
class AdminSessionPrx(_M_Glacier2.SessionPrx):
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
context -- The request context for the invocation.
"""
def keepAlive(self, context=None):
return _M_IceGrid.AdminSession._op_keepAlive.invoke(self, ((), context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def keepAliveAsync(self, context=None):
return _M_IceGrid.AdminSession._op_keepAlive.invokeAsync(self, ((), context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_keepAlive(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_keepAlive.begin(self, ((), _response, _ex, _sent, context))
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
"""
def end_keepAlive(self, _r):
return _M_IceGrid.AdminSession._op_keepAlive.end(self, _r)
"""
Get the admin interface. The admin object returned by this
operation can only be accessed by the session.
Arguments:
context -- The request context for the invocation.
Returns: The admin interface proxy.
"""
def getAdmin(self, context=None):
return _M_IceGrid.AdminSession._op_getAdmin.invoke(self, ((), context))
"""
Get the admin interface. The admin object returned by this
operation can only be accessed by the session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAdminAsync(self, context=None):
return _M_IceGrid.AdminSession._op_getAdmin.invokeAsync(self, ((), context))
"""
Get the admin interface. The admin object returned by this
operation can only be accessed by the session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAdmin(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_getAdmin.begin(self, ((), _response, _ex, _sent, context))
"""
Get the admin interface. The admin object returned by this
operation can only be accessed by the session.
Arguments:
Returns: The admin interface proxy.
"""
def end_getAdmin(self, _r):
return _M_IceGrid.AdminSession._op_getAdmin.end(self, _r)
"""
Get a "template" proxy for admin callback objects.
An Admin client uses this proxy to set the category of its callback
objects, and the published endpoints of the object adapter hosting
the admin callback objects.
Arguments:
context -- The request context for the invocation.
Returns: A template proxy. The returned proxy is null when the Admin session was established using Glacier2.
"""
def getAdminCallbackTemplate(self, context=None):
return _M_IceGrid.AdminSession._op_getAdminCallbackTemplate.invoke(self, ((), context))
"""
Get a "template" proxy for admin callback objects.
An Admin client uses this proxy to set the category of its callback
objects, and the published endpoints of the object adapter hosting
the admin callback objects.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAdminCallbackTemplateAsync(self, context=None):
return _M_IceGrid.AdminSession._op_getAdminCallbackTemplate.invokeAsync(self, ((), context))
"""
Get a "template" proxy for admin callback objects.
An Admin client uses this proxy to set the category of its callback
objects, and the published endpoints of the object adapter hosting
the admin callback objects.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getAdminCallbackTemplate(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_getAdminCallbackTemplate.begin(self, ((), _response, _ex, _sent, context))
"""
Get a "template" proxy for admin callback objects.
An Admin client uses this proxy to set the category of its callback
objects, and the published endpoints of the object adapter hosting
the admin callback objects.
Arguments:
Returns: A template proxy. The returned proxy is null when the Admin session was established using Glacier2.
"""
def end_getAdminCallbackTemplate(self, _r):
return _M_IceGrid.AdminSession._op_getAdminCallbackTemplate.end(self, _r)
"""
Set the observer proxies that receive
notifications when the state of the registry
or nodes changes.
Arguments:
registryObs -- The registry observer.
nodeObs -- The node observer.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
context -- The request context for the invocation.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
def setObservers(self, registryObs, nodeObs, appObs, adptObs, objObs, context=None):
return _M_IceGrid.AdminSession._op_setObservers.invoke(self, ((registryObs, nodeObs, appObs, adptObs, objObs), context))
"""
Set the observer proxies that receive
notifications when the state of the registry
or nodes changes.
Arguments:
registryObs -- The registry observer.
nodeObs -- The node observer.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setObserversAsync(self, registryObs, nodeObs, appObs, adptObs, objObs, context=None):
return _M_IceGrid.AdminSession._op_setObservers.invokeAsync(self, ((registryObs, nodeObs, appObs, adptObs, objObs), context))
"""
Set the observer proxies that receive
notifications when the state of the registry
or nodes changes.
Arguments:
registryObs -- The registry observer.
nodeObs -- The node observer.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setObservers(self, registryObs, nodeObs, appObs, adptObs, objObs, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_setObservers.begin(self, ((registryObs, nodeObs, appObs, adptObs, objObs), _response, _ex, _sent, context))
"""
Set the observer proxies that receive
notifications when the state of the registry
or nodes changes.
Arguments:
registryObs -- The registry observer.
nodeObs -- The node observer.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
def end_setObservers(self, _r):
return _M_IceGrid.AdminSession._op_setObservers.end(self, _r)
"""
Set the observer identities that receive
notifications the state of the registry
or nodes changes. This operation should be used by clients that
are using a bidirectional connection to communicate with the
session.
Arguments:
registryObs -- The registry observer identity.
nodeObs -- The node observer identity.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
context -- The request context for the invocation.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
def setObserversByIdentity(self, registryObs, nodeObs, appObs, adptObs, objObs, context=None):
return _M_IceGrid.AdminSession._op_setObserversByIdentity.invoke(self, ((registryObs, nodeObs, appObs, adptObs, objObs), context))
"""
Set the observer identities that receive
notifications the state of the registry
or nodes changes. This operation should be used by clients that
are using a bidirectional connection to communicate with the
session.
Arguments:
registryObs -- The registry observer identity.
nodeObs -- The node observer identity.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setObserversByIdentityAsync(self, registryObs, nodeObs, appObs, adptObs, objObs, context=None):
return _M_IceGrid.AdminSession._op_setObserversByIdentity.invokeAsync(self, ((registryObs, nodeObs, appObs, adptObs, objObs), context))
"""
Set the observer identities that receive
notifications the state of the registry
or nodes changes. This operation should be used by clients that
are using a bidirectional connection to communicate with the
session.
Arguments:
registryObs -- The registry observer identity.
nodeObs -- The node observer identity.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setObserversByIdentity(self, registryObs, nodeObs, appObs, adptObs, objObs, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_setObserversByIdentity.begin(self, ((registryObs, nodeObs, appObs, adptObs, objObs), _response, _ex, _sent, context))
"""
Set the observer identities that receive
notifications the state of the registry
or nodes changes. This operation should be used by clients that
are using a bidirectional connection to communicate with the
session.
Arguments:
registryObs -- The registry observer identity.
nodeObs -- The node observer identity.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
def end_setObserversByIdentity(self, _r):
return _M_IceGrid.AdminSession._op_setObserversByIdentity.end(self, _r)
"""
Acquires an exclusive lock to start updating the registry applications.
Arguments:
context -- The request context for the invocation.
Returns: The current serial.
Throws:
AccessDeniedException -- Raised if the exclusive lock can't be acquired. This might happen if the lock is currently acquired by another session.
"""
def startUpdate(self, context=None):
return _M_IceGrid.AdminSession._op_startUpdate.invoke(self, ((), context))
"""
Acquires an exclusive lock to start updating the registry applications.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def startUpdateAsync(self, context=None):
return _M_IceGrid.AdminSession._op_startUpdate.invokeAsync(self, ((), context))
"""
Acquires an exclusive lock to start updating the registry applications.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_startUpdate(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_startUpdate.begin(self, ((), _response, _ex, _sent, context))
"""
Acquires an exclusive lock to start updating the registry applications.
Arguments:
Returns: The current serial.
Throws:
AccessDeniedException -- Raised if the exclusive lock can't be acquired. This might happen if the lock is currently acquired by another session.
"""
def end_startUpdate(self, _r):
return _M_IceGrid.AdminSession._op_startUpdate.end(self, _r)
"""
Finish updating the registry and release the exclusive lock.
Arguments:
context -- The request context for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock.
"""
def finishUpdate(self, context=None):
return _M_IceGrid.AdminSession._op_finishUpdate.invoke(self, ((), context))
"""
Finish updating the registry and release the exclusive lock.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def finishUpdateAsync(self, context=None):
return _M_IceGrid.AdminSession._op_finishUpdate.invokeAsync(self, ((), context))
"""
Finish updating the registry and release the exclusive lock.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_finishUpdate(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_finishUpdate.begin(self, ((), _response, _ex, _sent, context))
"""
Finish updating the registry and release the exclusive lock.
Arguments:
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock.
"""
def end_finishUpdate(self, _r):
return _M_IceGrid.AdminSession._op_finishUpdate.end(self, _r)
"""
Get the name of the registry replica hosting this session.
Arguments:
context -- The request context for the invocation.
Returns: The replica name of the registry.
"""
def getReplicaName(self, context=None):
return _M_IceGrid.AdminSession._op_getReplicaName.invoke(self, ((), context))
"""
Get the name of the registry replica hosting this session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getReplicaNameAsync(self, context=None):
return _M_IceGrid.AdminSession._op_getReplicaName.invokeAsync(self, ((), context))
"""
Get the name of the registry replica hosting this session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getReplicaName(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_getReplicaName.begin(self, ((), _response, _ex, _sent, context))
"""
Get the name of the registry replica hosting this session.
Arguments:
Returns: The replica name of the registry.
"""
def end_getReplicaName(self, _r):
return _M_IceGrid.AdminSession._op_getReplicaName.end(self, _r)
"""
Open the given server log file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
path -- The path of the log file. A log file can be opened only if it's declared in the server or service deployment descriptor.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def openServerLog(self, id, path, count, context=None):
return _M_IceGrid.AdminSession._op_openServerLog.invoke(self, ((id, path, count), context))
"""
Open the given server log file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
path -- The path of the log file. A log file can be opened only if it's declared in the server or service deployment descriptor.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openServerLogAsync(self, id, path, count, context=None):
return _M_IceGrid.AdminSession._op_openServerLog.invokeAsync(self, ((id, path, count), context))
"""
Open the given server log file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
path -- The path of the log file. A log file can be opened only if it's declared in the server or service deployment descriptor.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openServerLog(self, id, path, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openServerLog.begin(self, ((id, path, count), _response, _ex, _sent, context))
"""
Open the given server log file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
path -- The path of the log file. A log file can be opened only if it's declared in the server or service deployment descriptor.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_openServerLog(self, _r):
return _M_IceGrid.AdminSession._op_openServerLog.end(self, _r)
"""
Open the given server stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def openServerStdErr(self, id, count, context=None):
return _M_IceGrid.AdminSession._op_openServerStdErr.invoke(self, ((id, count), context))
"""
Open the given server stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openServerStdErrAsync(self, id, count, context=None):
return _M_IceGrid.AdminSession._op_openServerStdErr.invokeAsync(self, ((id, count), context))
"""
Open the given server stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openServerStdErr(self, id, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openServerStdErr.begin(self, ((id, count), _response, _ex, _sent, context))
"""
Open the given server stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_openServerStdErr(self, _r):
return _M_IceGrid.AdminSession._op_openServerStdErr.end(self, _r)
"""
Open the given server stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def openServerStdOut(self, id, count, context=None):
return _M_IceGrid.AdminSession._op_openServerStdOut.invoke(self, ((id, count), context))
"""
Open the given server stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openServerStdOutAsync(self, id, count, context=None):
return _M_IceGrid.AdminSession._op_openServerStdOut.invokeAsync(self, ((id, count), context))
"""
Open the given server stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openServerStdOut(self, id, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openServerStdOut.begin(self, ((id, count), _response, _ex, _sent, context))
"""
Open the given server stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
def end_openServerStdOut(self, _r):
return _M_IceGrid.AdminSession._op_openServerStdOut.end(self, _r)
"""
Open the given node stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def openNodeStdErr(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdErr.invoke(self, ((name, count), context))
"""
Open the given node stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openNodeStdErrAsync(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdErr.invokeAsync(self, ((name, count), context))
"""
Open the given node stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openNodeStdErr(self, name, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdErr.begin(self, ((name, count), _response, _ex, _sent, context))
"""
Open the given node stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_openNodeStdErr(self, _r):
return _M_IceGrid.AdminSession._op_openNodeStdErr.end(self, _r)
"""
Open the given node stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def openNodeStdOut(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdOut.invoke(self, ((name, count), context))
"""
Open the given node stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openNodeStdOutAsync(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdOut.invokeAsync(self, ((name, count), context))
"""
Open the given node stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openNodeStdOut(self, name, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openNodeStdOut.begin(self, ((name, count), _response, _ex, _sent, context))
"""
Open the given node stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
def end_openNodeStdOut(self, _r):
return _M_IceGrid.AdminSession._op_openNodeStdOut.end(self, _r)
"""
Open the given registry stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def openRegistryStdErr(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdErr.invoke(self, ((name, count), context))
"""
Open the given registry stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openRegistryStdErrAsync(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdErr.invokeAsync(self, ((name, count), context))
"""
Open the given registry stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openRegistryStdErr(self, name, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdErr.begin(self, ((name, count), _response, _ex, _sent, context))
"""
Open the given registry stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def end_openRegistryStdErr(self, _r):
return _M_IceGrid.AdminSession._op_openRegistryStdErr.end(self, _r)
"""
Open the given registry stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def openRegistryStdOut(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdOut.invoke(self, ((name, count), context))
"""
Open the given registry stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def openRegistryStdOutAsync(self, name, count, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdOut.invokeAsync(self, ((name, count), context))
"""
Open the given registry stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_openRegistryStdOut(self, name, count, _response=None, _ex=None, _sent=None, context=None):
return _M_IceGrid.AdminSession._op_openRegistryStdOut.begin(self, ((name, count), _response, _ex, _sent, context))
"""
Open the given registry stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
Returns: An iterator to read the file.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
def end_openRegistryStdOut(self, _r):
return _M_IceGrid.AdminSession._op_openRegistryStdOut.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceGrid.AdminSessionPrx.ice_checkedCast(proxy, '::IceGrid::AdminSession', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceGrid.AdminSessionPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceGrid::AdminSession'
_M_IceGrid._t_AdminSessionPrx = IcePy.defineProxy('::IceGrid::AdminSession', AdminSessionPrx)
_M_IceGrid.AdminSessionPrx = AdminSessionPrx
del AdminSessionPrx
_M_IceGrid.AdminSession = Ice.createTempClass()
class AdminSession(_M_Glacier2.Session):
def ice_ids(self, current=None):
return ('::Glacier2::Session', '::Ice::Object', '::IceGrid::AdminSession')
def ice_id(self, current=None):
return '::IceGrid::AdminSession'
@staticmethod
def ice_staticId():
return '::IceGrid::AdminSession'
def keepAlive(self, current=None):
"""
Keep the session alive. Clients should call this operation
regularly to prevent the server from reaping the session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'keepAlive' not implemented")
def getAdmin(self, current=None):
"""
Get the admin interface. The admin object returned by this
operation can only be accessed by the session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAdmin' not implemented")
def getAdminCallbackTemplate(self, current=None):
"""
Get a "template" proxy for admin callback objects.
An Admin client uses this proxy to set the category of its callback
objects, and the published endpoints of the object adapter hosting
the admin callback objects.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getAdminCallbackTemplate' not implemented")
def setObservers(self, registryObs, nodeObs, appObs, adptObs, objObs, current=None):
"""
Set the observer proxies that receive
notifications when the state of the registry
or nodes changes.
Arguments:
registryObs -- The registry observer.
nodeObs -- The node observer.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
raise NotImplementedError("servant method 'setObservers' not implemented")
def setObserversByIdentity(self, registryObs, nodeObs, appObs, adptObs, objObs, current=None):
"""
Set the observer identities that receive
notifications the state of the registry
or nodes changes. This operation should be used by clients that
are using a bidirectional connection to communicate with the
session.
Arguments:
registryObs -- The registry observer identity.
nodeObs -- The node observer identity.
appObs -- The application observer.
adptObs -- The adapter observer.
objObs -- The object observer.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ObserverAlreadyRegisteredException -- Raised if an observer is already registered with this registry.
"""
raise NotImplementedError("servant method 'setObserversByIdentity' not implemented")
def startUpdate(self, current=None):
"""
Acquires an exclusive lock to start updating the registry applications.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the exclusive lock can't be acquired. This might happen if the lock is currently acquired by another session.
"""
raise NotImplementedError("servant method 'startUpdate' not implemented")
def finishUpdate(self, current=None):
"""
Finish updating the registry and release the exclusive lock.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AccessDeniedException -- Raised if the session doesn't hold the exclusive lock.
"""
raise NotImplementedError("servant method 'finishUpdate' not implemented")
def getReplicaName(self, current=None):
"""
Get the name of the registry replica hosting this session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getReplicaName' not implemented")
def openServerLog(self, id, path, count, current=None):
"""
Open the given server log file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
path -- The path of the log file. A log file can be opened only if it's declared in the server or service deployment descriptor.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'openServerLog' not implemented")
def openServerStdErr(self, id, count, current=None):
"""
Open the given server stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'openServerStdErr' not implemented")
def openServerStdOut(self, id, count, current=None):
"""
Open the given server stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
id -- The server id.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
DeploymentException -- Raised if the server couldn't be deployed on the node.
FileNotAvailableException -- Raised if the file can't be read.
NodeUnreachableException -- Raised if the node could not be reached.
ServerNotExistException -- Raised if the server doesn't exist.
"""
raise NotImplementedError("servant method 'openServerStdOut' not implemented")
def openNodeStdErr(self, name, count, current=None):
"""
Open the given node stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'openNodeStdErr' not implemented")
def openNodeStdOut(self, name, count, current=None):
"""
Open the given node stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The node name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
NodeNotExistException -- Raised if the node doesn't exist.
NodeUnreachableException -- Raised if the node could not be reached.
"""
raise NotImplementedError("servant method 'openNodeStdOut' not implemented")
def openRegistryStdErr(self, name, count, current=None):
"""
Open the given registry stderr file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
raise NotImplementedError("servant method 'openRegistryStdErr' not implemented")
def openRegistryStdOut(self, name, count, current=None):
"""
Open the given registry stdout file for reading. The file can be
read with the returned file iterator.
Arguments:
name -- The registry name.
count -- Specifies where to start reading the file. If negative, the file is read from the begining. If 0 or positive, the file is read from the last count lines.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileNotAvailableException -- Raised if the file can't be read.
RegistryNotExistException -- Raised if the registry doesn't exist.
RegistryUnreachableException -- Raised if the registry could not be reached.
"""
raise NotImplementedError("servant method 'openRegistryStdOut' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceGrid._t_AdminSessionDisp)
__repr__ = __str__
_M_IceGrid._t_AdminSessionDisp = IcePy.defineClass('::IceGrid::AdminSession', AdminSession, (), None, (_M_Glacier2._t_SessionDisp,))
AdminSession._ice_type = _M_IceGrid._t_AdminSessionDisp
AdminSession._op_keepAlive = IcePy.Operation('keepAlive', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), None, ())
AdminSession._op_getAdmin = IcePy.Operation('getAdmin', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_IceGrid._t_AdminPrx, False, 0), ())
AdminSession._op_getAdminCallbackTemplate = IcePy.Operation('getAdminCallbackTemplate', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), IcePy._t_ObjectPrx, False, 0), ())
AdminSession._op_setObservers = IcePy.Operation('setObservers', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_IceGrid._t_RegistryObserverPrx, False, 0), ((), _M_IceGrid._t_NodeObserverPrx, False, 0), ((), _M_IceGrid._t_ApplicationObserverPrx, False, 0), ((), _M_IceGrid._t_AdapterObserverPrx, False, 0), ((), _M_IceGrid._t_ObjectObserverPrx, False, 0)), (), None, (_M_IceGrid._t_ObserverAlreadyRegisteredException,))
AdminSession._op_setObserversByIdentity = IcePy.Operation('setObserversByIdentity', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_Identity, False, 0), ((), _M_Ice._t_Identity, False, 0), ((), _M_Ice._t_Identity, False, 0), ((), _M_Ice._t_Identity, False, 0), ((), _M_Ice._t_Identity, False, 0)), (), None, (_M_IceGrid._t_ObserverAlreadyRegisteredException,))
AdminSession._op_startUpdate = IcePy.Operation('startUpdate', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), IcePy._t_int, False, 0), (_M_IceGrid._t_AccessDeniedException,))
AdminSession._op_finishUpdate = IcePy.Operation('finishUpdate', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, (_M_IceGrid._t_AccessDeniedException,))
AdminSession._op_getReplicaName = IcePy.Operation('getReplicaName', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), IcePy._t_string, False, 0), ())
AdminSession._op_openServerLog = IcePy.Operation('openServerLog', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
AdminSession._op_openServerStdErr = IcePy.Operation('openServerStdErr', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
AdminSession._op_openServerStdOut = IcePy.Operation('openServerStdOut', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_ServerNotExistException, _M_IceGrid._t_NodeUnreachableException, _M_IceGrid._t_DeploymentException))
AdminSession._op_openNodeStdErr = IcePy.Operation('openNodeStdErr', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
AdminSession._op_openNodeStdOut = IcePy.Operation('openNodeStdOut', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_NodeNotExistException, _M_IceGrid._t_NodeUnreachableException))
AdminSession._op_openRegistryStdErr = IcePy.Operation('openRegistryStdErr', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_RegistryNotExistException, _M_IceGrid._t_RegistryUnreachableException))
AdminSession._op_openRegistryStdOut = IcePy.Operation('openRegistryStdOut', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_IceGrid._t_FileIteratorPrx, False, 0), (_M_IceGrid._t_FileNotAvailableException, _M_IceGrid._t_RegistryNotExistException, _M_IceGrid._t_RegistryUnreachableException))
_M_IceGrid.AdminSession = AdminSession
del AdminSession
# End of module IceGrid
Ice.sliceChecksums["::IceGrid::AdapterDynamicInfo"] = "b371e9a58f115e6ebfbcda735fee57f7"
Ice.sliceChecksums["::IceGrid::AdapterDynamicInfoSeq"] = "54465843167a2f93fa96d13b7f41ea32"
Ice.sliceChecksums["::IceGrid::AdapterInfo"] = "a22de437e0d82d91cca7d476992b2a43"
Ice.sliceChecksums["::IceGrid::AdapterInfoSeq"] = "9fdbbb3c2d938b4e5f3bf5a21f234147"
Ice.sliceChecksums["::IceGrid::AdapterObserver"] = "7f4ed59e236da9d6c35ad7e6ad9cb0"
Ice.sliceChecksums["::IceGrid::Admin"] = "35cb721cd375fb13818304b7c87217a"
Ice.sliceChecksums["::IceGrid::AdminSession"] = "ca6f21e8ff4210158f382cdbc66c2566"
Ice.sliceChecksums["::IceGrid::ApplicationInfo"] = "44ab5928481a1441216f93965f9e6c5"
Ice.sliceChecksums["::IceGrid::ApplicationInfoSeq"] = "dc7429d6b923c3e66eea573eccc1598"
Ice.sliceChecksums["::IceGrid::ApplicationObserver"] = "2862cdcba54714282f68b13a8fb4ae"
Ice.sliceChecksums["::IceGrid::ApplicationUpdateInfo"] = "c21c8cfe85e332fd9ad194e611bc6b7f"
Ice.sliceChecksums["::IceGrid::FileIterator"] = "54341a38932f89d199f28ffc4712c7"
Ice.sliceChecksums["::IceGrid::LoadInfo"] = "c28c339f5af52a46ac64c33864ae6"
Ice.sliceChecksums["::IceGrid::NodeDynamicInfo"] = "3ad52341f32973212d26a9a6dda08b"
Ice.sliceChecksums["::IceGrid::NodeDynamicInfoSeq"] = "f61633c5e3992f718dba78b7f165c2"
Ice.sliceChecksums["::IceGrid::NodeInfo"] = "f348b389deb653ac28b2b991e23d63b9"
Ice.sliceChecksums["::IceGrid::NodeObserver"] = "e06c1ad6807d2876de9e818855a65738"
Ice.sliceChecksums["::IceGrid::ObjectInfo"] = "6c8a382c348df5cbda50e58d87189e33"
Ice.sliceChecksums["::IceGrid::ObjectInfoSeq"] = "1491c01cb93b575c602baed26ed0f989"
Ice.sliceChecksums["::IceGrid::ObjectObserver"] = "5364683a872f127e46cc5e215d98c3c"
Ice.sliceChecksums["::IceGrid::RegistryInfo"] = "60e64fc1e37ce59ecbeed4a0e276ba"
Ice.sliceChecksums["::IceGrid::RegistryInfoSeq"] = "fabb868b9f2164f68bc9eb68240c8a6"
Ice.sliceChecksums["::IceGrid::RegistryObserver"] = "fd83b1558e7c77f2d322b25449518"
Ice.sliceChecksums["::IceGrid::ServerDynamicInfo"] = "fd4b9177ca54ae4688b51fa51d6870"
Ice.sliceChecksums["::IceGrid::ServerDynamicInfoSeq"] = "e3fda58997d5cd946e78cae739174cb"
Ice.sliceChecksums["::IceGrid::ServerInfo"] = "7f99dc872345b2c3c741c8b4c23440da"
Ice.sliceChecksums["::IceGrid::ServerState"] = "21e8ecba86a4678f3b783de286583093"
Ice.sliceChecksums["::IceGrid::StringObjectProxyDict"] = "978c325e58cebefb212e5ebde28acdc" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceGrid/Admin_ice.py | Admin_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.ObjectAdapterF_ice
import Ice.Current_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'ServantLocator' not in _M_Ice.__dict__:
_M_Ice.ServantLocator = Ice.createTempClass()
class ServantLocator(object):
"""
A servant locator is called by an object adapter to
locate a servant that is not found in its active servant map.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.ServantLocator:
raise RuntimeError('Ice.ServantLocator is an abstract class')
def locate(self, curr):
"""
Called before a request is dispatched if a
servant cannot be found in the object adapter's active servant
map. Note that the object adapter does not automatically insert
the returned servant into its active servant map. This must be
done by the servant locator implementation, if this is desired.
locate can throw any user exception. If it does, that exception
is marshaled back to the client. If the Slice definition for the
corresponding operation includes that user exception, the client
receives that user exception; otherwise, the client receives
UnknownUserException.
If locate throws any exception, the Ice run time does not
call finished.
If you call locate from your own code, you
must also call finished when you have finished using the
servant, provided that locate returned a non-null servant;
otherwise, you will get undefined behavior if you use
servant locators such as the Freeze Evictor.
Arguments:
curr -- Information about the current operation for which a servant is required.
Returns a tuple containing the following:
_retval -- The located servant, or null if no suitable servant has been found.
cookie -- A "cookie" that will be passed to finished.
Throws:
UserException -- The implementation can raise a UserException and the run time will marshal it as the result of the invocation.
"""
raise NotImplementedError("method 'locate' not implemented")
def finished(self, curr, servant, cookie):
"""
Called by the object adapter after a request has been
made. This operation is only called if locate was called
prior to the request and returned a non-null servant. This
operation can be used for cleanup purposes after a request.
finished can throw any user exception. If it does, that exception
is marshaled back to the client. If the Slice definition for the
corresponding operation includes that user exception, the client
receives that user exception; otherwise, the client receives
UnknownUserException.
If both the operation and finished throw an exception, the
exception thrown by finished is marshaled back to the client.
Arguments:
curr -- Information about the current operation call for which a servant was located by locate.
servant -- The servant that was returned by locate.
cookie -- The cookie that was returned by locate.
Throws:
UserException -- The implementation can raise a UserException and the run time will marshal it as the result of the invocation.
"""
raise NotImplementedError("method 'finished' not implemented")
def deactivate(self, category):
"""
Called when the object adapter in which this servant locator is
installed is destroyed.
Arguments:
category -- Indicates for which category the servant locator is being deactivated.
"""
raise NotImplementedError("method 'deactivate' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ServantLocator)
__repr__ = __str__
_M_Ice._t_ServantLocator = IcePy.defineValue('::Ice::ServantLocator', ServantLocator, -1, (), False, True, None, ())
ServantLocator._ice_type = _M_Ice._t_ServantLocator
_M_Ice.ServantLocator = ServantLocator
del ServantLocator
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/ServantLocator_ice.py | ServantLocator_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module IceMX
_M_IceMX = Ice.openModule('IceMX')
__name__ = 'IceMX'
_M_IceMX.__doc__ = """
The Ice Management eXtension facility. It provides the
IceMX#MetricsAdmin interface for management clients to
retrieve metrics from Ice applications.
"""
if '_t_StringIntDict' not in _M_IceMX.__dict__:
_M_IceMX._t_StringIntDict = IcePy.defineDictionary('::IceMX::StringIntDict', (), IcePy._t_string, IcePy._t_int)
if 'Metrics' not in _M_IceMX.__dict__:
_M_IceMX.Metrics = Ice.createTempClass()
class Metrics(Ice.Value):
"""
The base class for metrics. A metrics object represents a
collection of measurements associated to a given a system.
Members:
id -- The metrics identifier.
total -- The total number of objects observed by this metrics. This includes
the number of currently observed objects and the number of objects
observed in the past.
current -- The number of objects currently observed by this metrics.
totalLifetime -- The sum of the lifetime of each observed objects. This does not
include the lifetime of objects which are currently observed,
only the objects observed in the past.
failures -- The number of failures observed.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0):
self.id = id
self.total = total
self.current = current
self.totalLifetime = totalLifetime
self.failures = failures
def ice_id(self):
return '::IceMX::Metrics'
@staticmethod
def ice_staticId():
return '::IceMX::Metrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_Metrics)
__repr__ = __str__
_M_IceMX._t_Metrics = IcePy.defineValue('::IceMX::Metrics', Metrics, -1, (), False, False, None, (
('id', (), IcePy._t_string, False, 0),
('total', (), IcePy._t_long, False, 0),
('current', (), IcePy._t_int, False, 0),
('totalLifetime', (), IcePy._t_long, False, 0),
('failures', (), IcePy._t_int, False, 0)
))
Metrics._ice_type = _M_IceMX._t_Metrics
_M_IceMX.Metrics = Metrics
del Metrics
if 'MetricsFailures' not in _M_IceMX.__dict__:
_M_IceMX.MetricsFailures = Ice.createTempClass()
class MetricsFailures(object):
"""
A structure to keep track of failures associated with a given
metrics.
Members:
id -- The identifier of the metrics object associated to the
failures.
failures -- The failures observed for this metrics.
"""
def __init__(self, id='', failures=None):
self.id = id
self.failures = failures
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_IceMX.MetricsFailures):
return NotImplemented
else:
if self.id != other.id:
return False
if self.failures != other.failures:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_MetricsFailures)
__repr__ = __str__
_M_IceMX._t_MetricsFailures = IcePy.defineStruct('::IceMX::MetricsFailures', MetricsFailures, (), (
('id', (), IcePy._t_string),
('failures', (), _M_IceMX._t_StringIntDict)
))
_M_IceMX.MetricsFailures = MetricsFailures
del MetricsFailures
if '_t_MetricsFailuresSeq' not in _M_IceMX.__dict__:
_M_IceMX._t_MetricsFailuresSeq = IcePy.defineSequence('::IceMX::MetricsFailuresSeq', (), _M_IceMX._t_MetricsFailures)
if '_t_MetricsMap' not in _M_IceMX.__dict__:
_M_IceMX._t_MetricsMap = IcePy.defineSequence('::IceMX::MetricsMap', (), _M_IceMX._t_Metrics)
if '_t_MetricsView' not in _M_IceMX.__dict__:
_M_IceMX._t_MetricsView = IcePy.defineDictionary('::IceMX::MetricsView', (), IcePy._t_string, _M_IceMX._t_MetricsMap)
if 'UnknownMetricsView' not in _M_IceMX.__dict__:
_M_IceMX.UnknownMetricsView = Ice.createTempClass()
class UnknownMetricsView(Ice.UserException):
"""
Raised if a metrics view cannot be found.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceMX::UnknownMetricsView'
_M_IceMX._t_UnknownMetricsView = IcePy.defineException('::IceMX::UnknownMetricsView', UnknownMetricsView, (), False, None, ())
UnknownMetricsView._ice_type = _M_IceMX._t_UnknownMetricsView
_M_IceMX.UnknownMetricsView = UnknownMetricsView
del UnknownMetricsView
_M_IceMX._t_MetricsAdmin = IcePy.defineValue('::IceMX::MetricsAdmin', Ice.Value, -1, (), False, True, None, ())
if 'MetricsAdminPrx' not in _M_IceMX.__dict__:
_M_IceMX.MetricsAdminPrx = Ice.createTempClass()
class MetricsAdminPrx(Ice.ObjectPrx):
"""
Get the names of enabled and disabled metrics.
Arguments:
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- The name of the enabled views.
disabledViews -- The names of the disabled views.
"""
def getMetricsViewNames(self, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsViewNames.invoke(self, ((), context))
"""
Get the names of enabled and disabled metrics.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getMetricsViewNamesAsync(self, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsViewNames.invokeAsync(self, ((), context))
"""
Get the names of enabled and disabled metrics.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getMetricsViewNames(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsViewNames.begin(self, ((), _response, _ex, _sent, context))
"""
Get the names of enabled and disabled metrics.
Arguments:
Returns a tuple containing the following:
_retval -- The name of the enabled views.
disabledViews -- The names of the disabled views.
"""
def end_getMetricsViewNames(self, _r):
return _M_IceMX.MetricsAdmin._op_getMetricsViewNames.end(self, _r)
"""
Enables a metrics view.
Arguments:
name -- The metrics view name.
context -- The request context for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def enableMetricsView(self, name, context=None):
return _M_IceMX.MetricsAdmin._op_enableMetricsView.invoke(self, ((name, ), context))
"""
Enables a metrics view.
Arguments:
name -- The metrics view name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def enableMetricsViewAsync(self, name, context=None):
return _M_IceMX.MetricsAdmin._op_enableMetricsView.invokeAsync(self, ((name, ), context))
"""
Enables a metrics view.
Arguments:
name -- The metrics view name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_enableMetricsView(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_enableMetricsView.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Enables a metrics view.
Arguments:
name -- The metrics view name.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def end_enableMetricsView(self, _r):
return _M_IceMX.MetricsAdmin._op_enableMetricsView.end(self, _r)
"""
Disable a metrics view.
Arguments:
name -- The metrics view name.
context -- The request context for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def disableMetricsView(self, name, context=None):
return _M_IceMX.MetricsAdmin._op_disableMetricsView.invoke(self, ((name, ), context))
"""
Disable a metrics view.
Arguments:
name -- The metrics view name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def disableMetricsViewAsync(self, name, context=None):
return _M_IceMX.MetricsAdmin._op_disableMetricsView.invokeAsync(self, ((name, ), context))
"""
Disable a metrics view.
Arguments:
name -- The metrics view name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_disableMetricsView(self, name, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_disableMetricsView.begin(self, ((name, ), _response, _ex, _sent, context))
"""
Disable a metrics view.
Arguments:
name -- The metrics view name.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def end_disableMetricsView(self, _r):
return _M_IceMX.MetricsAdmin._op_disableMetricsView.end(self, _r)
"""
Get the metrics objects for the given metrics view. This
returns a dictionnary of metric maps for each metrics class
configured with the view. The timestamp allows the client to
compute averages which are not dependent of the invocation
latency for this operation.
Arguments:
view -- The name of the metrics view.
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- The metrics view data.
timestamp -- The local time of the process when the metrics object were retrieved.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def getMetricsView(self, view, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsView.invoke(self, ((view, ), context))
"""
Get the metrics objects for the given metrics view. This
returns a dictionnary of metric maps for each metrics class
configured with the view. The timestamp allows the client to
compute averages which are not dependent of the invocation
latency for this operation.
Arguments:
view -- The name of the metrics view.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getMetricsViewAsync(self, view, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsView.invokeAsync(self, ((view, ), context))
"""
Get the metrics objects for the given metrics view. This
returns a dictionnary of metric maps for each metrics class
configured with the view. The timestamp allows the client to
compute averages which are not dependent of the invocation
latency for this operation.
Arguments:
view -- The name of the metrics view.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getMetricsView(self, view, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsView.begin(self, ((view, ), _response, _ex, _sent, context))
"""
Get the metrics objects for the given metrics view. This
returns a dictionnary of metric maps for each metrics class
configured with the view. The timestamp allows the client to
compute averages which are not dependent of the invocation
latency for this operation.
Arguments:
view -- The name of the metrics view.
Returns a tuple containing the following:
_retval -- The metrics view data.
timestamp -- The local time of the process when the metrics object were retrieved.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def end_getMetricsView(self, _r):
return _M_IceMX.MetricsAdmin._op_getMetricsView.end(self, _r)
"""
Get the metrics failures associated with the given view and map.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
context -- The request context for the invocation.
Returns: The metrics failures associated with the map.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def getMapMetricsFailures(self, view, map, context=None):
return _M_IceMX.MetricsAdmin._op_getMapMetricsFailures.invoke(self, ((view, map), context))
"""
Get the metrics failures associated with the given view and map.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getMapMetricsFailuresAsync(self, view, map, context=None):
return _M_IceMX.MetricsAdmin._op_getMapMetricsFailures.invokeAsync(self, ((view, map), context))
"""
Get the metrics failures associated with the given view and map.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getMapMetricsFailures(self, view, map, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_getMapMetricsFailures.begin(self, ((view, map), _response, _ex, _sent, context))
"""
Get the metrics failures associated with the given view and map.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
Returns: The metrics failures associated with the map.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def end_getMapMetricsFailures(self, _r):
return _M_IceMX.MetricsAdmin._op_getMapMetricsFailures.end(self, _r)
"""
Get the metrics failure associated for the given metrics.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
id -- The ID of the metrics.
context -- The request context for the invocation.
Returns: The metrics failures associated with the metrics.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def getMetricsFailures(self, view, map, id, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsFailures.invoke(self, ((view, map, id), context))
"""
Get the metrics failure associated for the given metrics.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
id -- The ID of the metrics.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getMetricsFailuresAsync(self, view, map, id, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsFailures.invokeAsync(self, ((view, map, id), context))
"""
Get the metrics failure associated for the given metrics.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
id -- The ID of the metrics.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getMetricsFailures(self, view, map, id, _response=None, _ex=None, _sent=None, context=None):
return _M_IceMX.MetricsAdmin._op_getMetricsFailures.begin(self, ((view, map, id), _response, _ex, _sent, context))
"""
Get the metrics failure associated for the given metrics.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
id -- The ID of the metrics.
Returns: The metrics failures associated with the metrics.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
def end_getMetricsFailures(self, _r):
return _M_IceMX.MetricsAdmin._op_getMetricsFailures.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceMX.MetricsAdminPrx.ice_checkedCast(proxy, '::IceMX::MetricsAdmin', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceMX.MetricsAdminPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceMX::MetricsAdmin'
_M_IceMX._t_MetricsAdminPrx = IcePy.defineProxy('::IceMX::MetricsAdmin', MetricsAdminPrx)
_M_IceMX.MetricsAdminPrx = MetricsAdminPrx
del MetricsAdminPrx
_M_IceMX.MetricsAdmin = Ice.createTempClass()
class MetricsAdmin(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceMX::MetricsAdmin')
def ice_id(self, current=None):
return '::IceMX::MetricsAdmin'
@staticmethod
def ice_staticId():
return '::IceMX::MetricsAdmin'
def getMetricsViewNames(self, current=None):
"""
Get the names of enabled and disabled metrics.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getMetricsViewNames' not implemented")
def enableMetricsView(self, name, current=None):
"""
Enables a metrics view.
Arguments:
name -- The metrics view name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
raise NotImplementedError("servant method 'enableMetricsView' not implemented")
def disableMetricsView(self, name, current=None):
"""
Disable a metrics view.
Arguments:
name -- The metrics view name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
raise NotImplementedError("servant method 'disableMetricsView' not implemented")
def getMetricsView(self, view, current=None):
"""
Get the metrics objects for the given metrics view. This
returns a dictionnary of metric maps for each metrics class
configured with the view. The timestamp allows the client to
compute averages which are not dependent of the invocation
latency for this operation.
Arguments:
view -- The name of the metrics view.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
raise NotImplementedError("servant method 'getMetricsView' not implemented")
def getMapMetricsFailures(self, view, map, current=None):
"""
Get the metrics failures associated with the given view and map.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
raise NotImplementedError("servant method 'getMapMetricsFailures' not implemented")
def getMetricsFailures(self, view, map, id, current=None):
"""
Get the metrics failure associated for the given metrics.
Arguments:
view -- The name of the metrics view.
map -- The name of the metrics map.
id -- The ID of the metrics.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
UnknownMetricsView -- Raised if the metrics view cannot be found.
"""
raise NotImplementedError("servant method 'getMetricsFailures' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_MetricsAdminDisp)
__repr__ = __str__
_M_IceMX._t_MetricsAdminDisp = IcePy.defineClass('::IceMX::MetricsAdmin', MetricsAdmin, (), None, ())
MetricsAdmin._ice_type = _M_IceMX._t_MetricsAdminDisp
MetricsAdmin._op_getMetricsViewNames = IcePy.Operation('getMetricsViewNames', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (), (((), _M_Ice._t_StringSeq, False, 0),), ((), _M_Ice._t_StringSeq, False, 0), ())
MetricsAdmin._op_enableMetricsView = IcePy.Operation('enableMetricsView', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceMX._t_UnknownMetricsView,))
MetricsAdmin._op_disableMetricsView = IcePy.Operation('disableMetricsView', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceMX._t_UnknownMetricsView,))
MetricsAdmin._op_getMetricsView = IcePy.Operation('getMetricsView', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0),), (((), IcePy._t_long, False, 0),), ((), _M_IceMX._t_MetricsView, False, 0), (_M_IceMX._t_UnknownMetricsView,))
MetricsAdmin._op_getMapMetricsFailures = IcePy.Operation('getMapMetricsFailures', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), ((), _M_IceMX._t_MetricsFailuresSeq, False, 0), (_M_IceMX._t_UnknownMetricsView,))
MetricsAdmin._op_getMetricsFailures = IcePy.Operation('getMetricsFailures', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), ((), _M_IceMX._t_MetricsFailures, False, 0), (_M_IceMX._t_UnknownMetricsView,))
_M_IceMX.MetricsAdmin = MetricsAdmin
del MetricsAdmin
if 'ThreadMetrics' not in _M_IceMX.__dict__:
_M_IceMX.ThreadMetrics = Ice.createTempClass()
class ThreadMetrics(_M_IceMX.Metrics):
"""
Provides information on the number of threads currently in use and
their activity.
Members:
inUseForIO -- The number of threads which are currently performing socket
read or writes.
inUseForUser -- The number of threads which are currently calling user code
(servant dispatch, AMI callbacks, etc).
inUseForOther -- The number of threads which are currently performing other
activities. These are all other that are not counted with
inUseForUser or inUseForIO, such as DNS
lookups, garbage collection).
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, inUseForIO=0, inUseForUser=0, inUseForOther=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.inUseForIO = inUseForIO
self.inUseForUser = inUseForUser
self.inUseForOther = inUseForOther
def ice_id(self):
return '::IceMX::ThreadMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::ThreadMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_ThreadMetrics)
__repr__ = __str__
_M_IceMX._t_ThreadMetrics = IcePy.defineValue('::IceMX::ThreadMetrics', ThreadMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('inUseForIO', (), IcePy._t_int, False, 0),
('inUseForUser', (), IcePy._t_int, False, 0),
('inUseForOther', (), IcePy._t_int, False, 0)
))
ThreadMetrics._ice_type = _M_IceMX._t_ThreadMetrics
_M_IceMX.ThreadMetrics = ThreadMetrics
del ThreadMetrics
if 'DispatchMetrics' not in _M_IceMX.__dict__:
_M_IceMX.DispatchMetrics = Ice.createTempClass()
class DispatchMetrics(_M_IceMX.Metrics):
"""
Provides information on servant dispatch.
Members:
userException -- The number of dispatch that failed with a user exception.
size -- The size of the dispatch. This corresponds to the size of the
marshalled input parameters.
replySize -- The size of the dispatch reply. This corresponds to the size of
the marshalled output and return parameters.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, userException=0, size=0, replySize=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.userException = userException
self.size = size
self.replySize = replySize
def ice_id(self):
return '::IceMX::DispatchMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::DispatchMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_DispatchMetrics)
__repr__ = __str__
_M_IceMX._t_DispatchMetrics = IcePy.defineValue('::IceMX::DispatchMetrics', DispatchMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('userException', (), IcePy._t_int, False, 0),
('size', (), IcePy._t_long, False, 0),
('replySize', (), IcePy._t_long, False, 0)
))
DispatchMetrics._ice_type = _M_IceMX._t_DispatchMetrics
_M_IceMX.DispatchMetrics = DispatchMetrics
del DispatchMetrics
if 'ChildInvocationMetrics' not in _M_IceMX.__dict__:
_M_IceMX.ChildInvocationMetrics = Ice.createTempClass()
class ChildInvocationMetrics(_M_IceMX.Metrics):
"""
Provides information on child invocations. A child invocation is
either remote (sent over an Ice connection) or collocated. An
invocation can have multiple child invocation if it is
retried. Child invocation metrics are embedded within
InvocationMetrics.
Members:
size -- The size of the invocation. This corresponds to the size of the
marshalled input parameters.
replySize -- The size of the invocation reply. This corresponds to the size
of the marshalled output and return parameters.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, size=0, replySize=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.size = size
self.replySize = replySize
def ice_id(self):
return '::IceMX::ChildInvocationMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::ChildInvocationMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_ChildInvocationMetrics)
__repr__ = __str__
_M_IceMX._t_ChildInvocationMetrics = IcePy.defineValue('::IceMX::ChildInvocationMetrics', ChildInvocationMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('size', (), IcePy._t_long, False, 0),
('replySize', (), IcePy._t_long, False, 0)
))
ChildInvocationMetrics._ice_type = _M_IceMX._t_ChildInvocationMetrics
_M_IceMX.ChildInvocationMetrics = ChildInvocationMetrics
del ChildInvocationMetrics
if 'CollocatedMetrics' not in _M_IceMX.__dict__:
_M_IceMX.CollocatedMetrics = Ice.createTempClass()
class CollocatedMetrics(_M_IceMX.ChildInvocationMetrics):
"""
Provides information on invocations that are collocated. Collocated
metrics are embedded within InvocationMetrics.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, size=0, replySize=0):
_M_IceMX.ChildInvocationMetrics.__init__(self, id, total, current, totalLifetime, failures, size, replySize)
def ice_id(self):
return '::IceMX::CollocatedMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::CollocatedMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_CollocatedMetrics)
__repr__ = __str__
_M_IceMX._t_CollocatedMetrics = IcePy.defineValue('::IceMX::CollocatedMetrics', CollocatedMetrics, -1, (), False, False, _M_IceMX._t_ChildInvocationMetrics, ())
CollocatedMetrics._ice_type = _M_IceMX._t_CollocatedMetrics
_M_IceMX.CollocatedMetrics = CollocatedMetrics
del CollocatedMetrics
if 'RemoteMetrics' not in _M_IceMX.__dict__:
_M_IceMX.RemoteMetrics = Ice.createTempClass()
class RemoteMetrics(_M_IceMX.ChildInvocationMetrics):
"""
Provides information on invocations that are specifically sent over
Ice connections. Remote metrics are embedded within InvocationMetrics.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, size=0, replySize=0):
_M_IceMX.ChildInvocationMetrics.__init__(self, id, total, current, totalLifetime, failures, size, replySize)
def ice_id(self):
return '::IceMX::RemoteMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::RemoteMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_RemoteMetrics)
__repr__ = __str__
_M_IceMX._t_RemoteMetrics = IcePy.defineValue('::IceMX::RemoteMetrics', RemoteMetrics, -1, (), False, False, _M_IceMX._t_ChildInvocationMetrics, ())
RemoteMetrics._ice_type = _M_IceMX._t_RemoteMetrics
_M_IceMX.RemoteMetrics = RemoteMetrics
del RemoteMetrics
if 'InvocationMetrics' not in _M_IceMX.__dict__:
_M_IceMX.InvocationMetrics = Ice.createTempClass()
class InvocationMetrics(_M_IceMX.Metrics):
"""
Provide measurements for proxy invocations. Proxy invocations can
either be sent over the wire or be collocated.
Members:
retry -- The number of retries for the invocation(s).
userException -- The number of invocations that failed with a user exception.
remotes -- The remote invocation metrics map.
collocated -- The collocated invocation metrics map.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, retry=0, userException=0, remotes=None, collocated=None):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.retry = retry
self.userException = userException
self.remotes = remotes
self.collocated = collocated
def ice_id(self):
return '::IceMX::InvocationMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::InvocationMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_InvocationMetrics)
__repr__ = __str__
_M_IceMX._t_InvocationMetrics = IcePy.declareValue('::IceMX::InvocationMetrics')
_M_IceMX._t_InvocationMetrics = IcePy.defineValue('::IceMX::InvocationMetrics', InvocationMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('retry', (), IcePy._t_int, False, 0),
('userException', (), IcePy._t_int, False, 0),
('remotes', (), _M_IceMX._t_MetricsMap, False, 0),
('collocated', (), _M_IceMX._t_MetricsMap, False, 0)
))
InvocationMetrics._ice_type = _M_IceMX._t_InvocationMetrics
_M_IceMX.InvocationMetrics = InvocationMetrics
del InvocationMetrics
if 'ConnectionMetrics' not in _M_IceMX.__dict__:
_M_IceMX.ConnectionMetrics = Ice.createTempClass()
class ConnectionMetrics(_M_IceMX.Metrics):
"""
Provides information on the data sent and received over Ice
connections.
Members:
receivedBytes -- The number of bytes received by the connection.
sentBytes -- The number of bytes sent by the connection.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, receivedBytes=0, sentBytes=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.receivedBytes = receivedBytes
self.sentBytes = sentBytes
def ice_id(self):
return '::IceMX::ConnectionMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::ConnectionMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_ConnectionMetrics)
__repr__ = __str__
_M_IceMX._t_ConnectionMetrics = IcePy.defineValue('::IceMX::ConnectionMetrics', ConnectionMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('receivedBytes', (), IcePy._t_long, False, 0),
('sentBytes', (), IcePy._t_long, False, 0)
))
ConnectionMetrics._ice_type = _M_IceMX._t_ConnectionMetrics
_M_IceMX.ConnectionMetrics = ConnectionMetrics
del ConnectionMetrics
# End of module IceMX
Ice.sliceChecksums["::IceMX::ChildInvocationMetrics"] = "e8d7639c4944abd2b46e1676712e3914"
Ice.sliceChecksums["::IceMX::CollocatedMetrics"] = "3249804089f8e0882d12276c8e6d4165"
Ice.sliceChecksums["::IceMX::ConnectionMetrics"] = "ff705e359a88ff9bc35ab28283c8e29"
Ice.sliceChecksums["::IceMX::DispatchMetrics"] = "e583ad5ced38253d9ff59929af294ba"
Ice.sliceChecksums["::IceMX::InvocationMetrics"] = "64216b477afc9431249a60ae5913f8"
Ice.sliceChecksums["::IceMX::Metrics"] = "94c8036c48f8749deb5f33a364ecee"
Ice.sliceChecksums["::IceMX::MetricsAdmin"] = "88697f8371c057b7177760281b33a5"
Ice.sliceChecksums["::IceMX::MetricsFailures"] = "19ecb6e915befa597421fa4c92a1ac"
Ice.sliceChecksums["::IceMX::MetricsFailuresSeq"] = "a62163545a1a5a54ade5d7d826aac8bd"
Ice.sliceChecksums["::IceMX::MetricsMap"] = "22667dd9415a83de99a17cf19f63975"
Ice.sliceChecksums["::IceMX::MetricsView"] = "c8c150b17e594dea2e3c7c2d4738b6"
Ice.sliceChecksums["::IceMX::RemoteMetrics"] = "1dda65bb856a2c2fcaf32ea2b40682f"
Ice.sliceChecksums["::IceMX::StringIntDict"] = "e6c7aa764386f6528aa38c89cbff5dd4"
Ice.sliceChecksums["::IceMX::ThreadMetrics"] = "54ca6eb235a9769ade47e8ae200ff18"
Ice.sliceChecksums["::IceMX::UnknownMetricsView"] = "18de0c2a8812deb6facfd521d84ba6" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Metrics_ice.py | Metrics_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.Version_ice
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'InitializationException' not in _M_Ice.__dict__:
_M_Ice.InitializationException = Ice.createTempClass()
class InitializationException(Ice.LocalException):
"""
This exception is raised when a failure occurs during initialization.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::InitializationException'
_M_Ice._t_InitializationException = IcePy.defineException('::Ice::InitializationException', InitializationException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
InitializationException._ice_type = _M_Ice._t_InitializationException
_M_Ice.InitializationException = InitializationException
del InitializationException
if 'PluginInitializationException' not in _M_Ice.__dict__:
_M_Ice.PluginInitializationException = Ice.createTempClass()
class PluginInitializationException(Ice.LocalException):
"""
This exception indicates that a failure occurred while initializing
a plug-in.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::PluginInitializationException'
_M_Ice._t_PluginInitializationException = IcePy.defineException('::Ice::PluginInitializationException', PluginInitializationException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
PluginInitializationException._ice_type = _M_Ice._t_PluginInitializationException
_M_Ice.PluginInitializationException = PluginInitializationException
del PluginInitializationException
if 'CollocationOptimizationException' not in _M_Ice.__dict__:
_M_Ice.CollocationOptimizationException = Ice.createTempClass()
class CollocationOptimizationException(Ice.LocalException):
"""
This exception is raised if a feature is requested that is not
supported with collocation optimization.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CollocationOptimizationException'
_M_Ice._t_CollocationOptimizationException = IcePy.defineException('::Ice::CollocationOptimizationException', CollocationOptimizationException, (), False, None, ())
CollocationOptimizationException._ice_type = _M_Ice._t_CollocationOptimizationException
_M_Ice.CollocationOptimizationException = CollocationOptimizationException
del CollocationOptimizationException
if 'AlreadyRegisteredException' not in _M_Ice.__dict__:
_M_Ice.AlreadyRegisteredException = Ice.createTempClass()
class AlreadyRegisteredException(Ice.LocalException):
"""
An attempt was made to register something more than once with
the Ice run time.
This exception is raised if an attempt is made to register a
servant, servant locator, facet, value factory, plug-in, object
adapter, object, or user exception factory more than once for the
same ID.
Members:
kindOfObject -- The kind of object that could not be removed: "servant", "facet",
"object", "default servant", "servant locator", "value factory", "plugin",
"object adapter", "object adapter with router", "replica group".
id -- The ID (or name) of the object that is registered already.
"""
def __init__(self, kindOfObject='', id=''):
self.kindOfObject = kindOfObject
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::AlreadyRegisteredException'
_M_Ice._t_AlreadyRegisteredException = IcePy.defineException('::Ice::AlreadyRegisteredException', AlreadyRegisteredException, (), False, None, (
('kindOfObject', (), IcePy._t_string, False, 0),
('id', (), IcePy._t_string, False, 0)
))
AlreadyRegisteredException._ice_type = _M_Ice._t_AlreadyRegisteredException
_M_Ice.AlreadyRegisteredException = AlreadyRegisteredException
del AlreadyRegisteredException
if 'NotRegisteredException' not in _M_Ice.__dict__:
_M_Ice.NotRegisteredException = Ice.createTempClass()
class NotRegisteredException(Ice.LocalException):
"""
An attempt was made to find or deregister something that is not
registered with the Ice run time or Ice locator.
This exception is raised if an attempt is made to remove a servant,
servant locator, facet, value factory, plug-in, object adapter,
object, or user exception factory that is not currently registered.
It's also raised if the Ice locator can't find an object or object
adapter when resolving an indirect proxy or when an object adapter
is activated.
Members:
kindOfObject -- The kind of object that could not be removed: "servant", "facet",
"object", "default servant", "servant locator", "value factory", "plugin",
"object adapter", "object adapter with router", "replica group".
id -- The ID (or name) of the object that could not be removed.
"""
def __init__(self, kindOfObject='', id=''):
self.kindOfObject = kindOfObject
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::NotRegisteredException'
_M_Ice._t_NotRegisteredException = IcePy.defineException('::Ice::NotRegisteredException', NotRegisteredException, (), False, None, (
('kindOfObject', (), IcePy._t_string, False, 0),
('id', (), IcePy._t_string, False, 0)
))
NotRegisteredException._ice_type = _M_Ice._t_NotRegisteredException
_M_Ice.NotRegisteredException = NotRegisteredException
del NotRegisteredException
if 'TwowayOnlyException' not in _M_Ice.__dict__:
_M_Ice.TwowayOnlyException = Ice.createTempClass()
class TwowayOnlyException(Ice.LocalException):
"""
The operation can only be invoked with a twoway request.
This exception is raised if an attempt is made to invoke an
operation with ice_oneway, ice_batchOneway, ice_datagram,
or ice_batchDatagram and the operation has a return value,
out-parameters, or an exception specification.
Members:
operation -- The name of the operation that was invoked.
"""
def __init__(self, operation=''):
self.operation = operation
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::TwowayOnlyException'
_M_Ice._t_TwowayOnlyException = IcePy.defineException('::Ice::TwowayOnlyException', TwowayOnlyException, (), False, None, (('operation', (), IcePy._t_string, False, 0),))
TwowayOnlyException._ice_type = _M_Ice._t_TwowayOnlyException
_M_Ice.TwowayOnlyException = TwowayOnlyException
del TwowayOnlyException
if 'CloneNotImplementedException' not in _M_Ice.__dict__:
_M_Ice.CloneNotImplementedException = Ice.createTempClass()
class CloneNotImplementedException(Ice.LocalException):
"""
An attempt was made to clone a class that does not support
cloning.
This exception is raised if ice_clone is called on
a class that is derived from an abstract Slice class (that is,
a class containing operations), and the derived class does not
provide an implementation of the ice_clone operation (C++ only).
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CloneNotImplementedException'
_M_Ice._t_CloneNotImplementedException = IcePy.defineException('::Ice::CloneNotImplementedException', CloneNotImplementedException, (), False, None, ())
CloneNotImplementedException._ice_type = _M_Ice._t_CloneNotImplementedException
_M_Ice.CloneNotImplementedException = CloneNotImplementedException
del CloneNotImplementedException
if 'UnknownException' not in _M_Ice.__dict__:
_M_Ice.UnknownException = Ice.createTempClass()
class UnknownException(Ice.LocalException):
"""
This exception is raised if an operation call on a server raises an
unknown exception. For example, for C++, this exception is raised
if the server throws a C++ exception that is not directly or
indirectly derived from Ice::LocalException or
Ice::UserException.
Members:
unknown -- This field is set to the textual representation of the unknown
exception if available.
"""
def __init__(self, unknown=''):
self.unknown = unknown
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownException'
_M_Ice._t_UnknownException = IcePy.defineException('::Ice::UnknownException', UnknownException, (), False, None, (('unknown', (), IcePy._t_string, False, 0),))
UnknownException._ice_type = _M_Ice._t_UnknownException
_M_Ice.UnknownException = UnknownException
del UnknownException
if 'UnknownLocalException' not in _M_Ice.__dict__:
_M_Ice.UnknownLocalException = Ice.createTempClass()
class UnknownLocalException(_M_Ice.UnknownException):
"""
This exception is raised if an operation call on a server raises a
local exception. Because local exceptions are not transmitted by
the Ice protocol, the client receives all local exceptions raised
by the server as UnknownLocalException. The only exception to this
rule are all exceptions derived from RequestFailedException,
which are transmitted by the Ice protocol even though they are
declared local.
"""
def __init__(self, unknown=''):
_M_Ice.UnknownException.__init__(self, unknown)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownLocalException'
_M_Ice._t_UnknownLocalException = IcePy.defineException('::Ice::UnknownLocalException', UnknownLocalException, (), False, _M_Ice._t_UnknownException, ())
UnknownLocalException._ice_type = _M_Ice._t_UnknownLocalException
_M_Ice.UnknownLocalException = UnknownLocalException
del UnknownLocalException
if 'UnknownUserException' not in _M_Ice.__dict__:
_M_Ice.UnknownUserException = Ice.createTempClass()
class UnknownUserException(_M_Ice.UnknownException):
"""
An operation raised an incorrect user exception.
This exception is raised if an operation raises a
user exception that is not declared in the exception's
throws clause. Such undeclared exceptions are
not transmitted from the server to the client by the Ice
protocol, but instead the client just gets an
UnknownUserException. This is necessary in order to not violate
the contract established by an operation's signature: Only local
exceptions and user exceptions declared in the
throws clause can be raised.
"""
def __init__(self, unknown=''):
_M_Ice.UnknownException.__init__(self, unknown)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownUserException'
_M_Ice._t_UnknownUserException = IcePy.defineException('::Ice::UnknownUserException', UnknownUserException, (), False, _M_Ice._t_UnknownException, ())
UnknownUserException._ice_type = _M_Ice._t_UnknownUserException
_M_Ice.UnknownUserException = UnknownUserException
del UnknownUserException
if 'VersionMismatchException' not in _M_Ice.__dict__:
_M_Ice.VersionMismatchException = Ice.createTempClass()
class VersionMismatchException(Ice.LocalException):
"""
This exception is raised if the Ice library version does not match
the version in the Ice header files.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::VersionMismatchException'
_M_Ice._t_VersionMismatchException = IcePy.defineException('::Ice::VersionMismatchException', VersionMismatchException, (), False, None, ())
VersionMismatchException._ice_type = _M_Ice._t_VersionMismatchException
_M_Ice.VersionMismatchException = VersionMismatchException
del VersionMismatchException
if 'CommunicatorDestroyedException' not in _M_Ice.__dict__:
_M_Ice.CommunicatorDestroyedException = Ice.createTempClass()
class CommunicatorDestroyedException(Ice.LocalException):
"""
This exception is raised if the Communicator has been destroyed.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CommunicatorDestroyedException'
_M_Ice._t_CommunicatorDestroyedException = IcePy.defineException('::Ice::CommunicatorDestroyedException', CommunicatorDestroyedException, (), False, None, ())
CommunicatorDestroyedException._ice_type = _M_Ice._t_CommunicatorDestroyedException
_M_Ice.CommunicatorDestroyedException = CommunicatorDestroyedException
del CommunicatorDestroyedException
if 'ObjectAdapterDeactivatedException' not in _M_Ice.__dict__:
_M_Ice.ObjectAdapterDeactivatedException = Ice.createTempClass()
class ObjectAdapterDeactivatedException(Ice.LocalException):
"""
This exception is raised if an attempt is made to use a deactivated
ObjectAdapter.
Members:
name -- Name of the adapter.
"""
def __init__(self, name=''):
self.name = name
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ObjectAdapterDeactivatedException'
_M_Ice._t_ObjectAdapterDeactivatedException = IcePy.defineException('::Ice::ObjectAdapterDeactivatedException', ObjectAdapterDeactivatedException, (), False, None, (('name', (), IcePy._t_string, False, 0),))
ObjectAdapterDeactivatedException._ice_type = _M_Ice._t_ObjectAdapterDeactivatedException
_M_Ice.ObjectAdapterDeactivatedException = ObjectAdapterDeactivatedException
del ObjectAdapterDeactivatedException
if 'ObjectAdapterIdInUseException' not in _M_Ice.__dict__:
_M_Ice.ObjectAdapterIdInUseException = Ice.createTempClass()
class ObjectAdapterIdInUseException(Ice.LocalException):
"""
This exception is raised if an ObjectAdapter cannot be activated.
This happens if the Locator detects another active ObjectAdapter with
the same adapter id.
Members:
id -- Adapter ID.
"""
def __init__(self, id=''):
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ObjectAdapterIdInUseException'
_M_Ice._t_ObjectAdapterIdInUseException = IcePy.defineException('::Ice::ObjectAdapterIdInUseException', ObjectAdapterIdInUseException, (), False, None, (('id', (), IcePy._t_string, False, 0),))
ObjectAdapterIdInUseException._ice_type = _M_Ice._t_ObjectAdapterIdInUseException
_M_Ice.ObjectAdapterIdInUseException = ObjectAdapterIdInUseException
del ObjectAdapterIdInUseException
if 'NoEndpointException' not in _M_Ice.__dict__:
_M_Ice.NoEndpointException = Ice.createTempClass()
class NoEndpointException(Ice.LocalException):
"""
This exception is raised if no suitable endpoint is available.
Members:
proxy -- The stringified proxy for which no suitable endpoint is
available.
"""
def __init__(self, proxy=''):
self.proxy = proxy
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::NoEndpointException'
_M_Ice._t_NoEndpointException = IcePy.defineException('::Ice::NoEndpointException', NoEndpointException, (), False, None, (('proxy', (), IcePy._t_string, False, 0),))
NoEndpointException._ice_type = _M_Ice._t_NoEndpointException
_M_Ice.NoEndpointException = NoEndpointException
del NoEndpointException
if 'EndpointParseException' not in _M_Ice.__dict__:
_M_Ice.EndpointParseException = Ice.createTempClass()
class EndpointParseException(Ice.LocalException):
"""
This exception is raised if there was an error while parsing an
endpoint.
Members:
str -- Describes the failure and includes the string that could not be parsed.
"""
def __init__(self, str=''):
self.str = str
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::EndpointParseException'
_M_Ice._t_EndpointParseException = IcePy.defineException('::Ice::EndpointParseException', EndpointParseException, (), False, None, (('str', (), IcePy._t_string, False, 0),))
EndpointParseException._ice_type = _M_Ice._t_EndpointParseException
_M_Ice.EndpointParseException = EndpointParseException
del EndpointParseException
if 'EndpointSelectionTypeParseException' not in _M_Ice.__dict__:
_M_Ice.EndpointSelectionTypeParseException = Ice.createTempClass()
class EndpointSelectionTypeParseException(Ice.LocalException):
"""
This exception is raised if there was an error while parsing an
endpoint selection type.
Members:
str -- Describes the failure and includes the string that could not be parsed.
"""
def __init__(self, str=''):
self.str = str
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::EndpointSelectionTypeParseException'
_M_Ice._t_EndpointSelectionTypeParseException = IcePy.defineException('::Ice::EndpointSelectionTypeParseException', EndpointSelectionTypeParseException, (), False, None, (('str', (), IcePy._t_string, False, 0),))
EndpointSelectionTypeParseException._ice_type = _M_Ice._t_EndpointSelectionTypeParseException
_M_Ice.EndpointSelectionTypeParseException = EndpointSelectionTypeParseException
del EndpointSelectionTypeParseException
if 'VersionParseException' not in _M_Ice.__dict__:
_M_Ice.VersionParseException = Ice.createTempClass()
class VersionParseException(Ice.LocalException):
"""
This exception is raised if there was an error while parsing a
version.
Members:
str -- Describes the failure and includes the string that could not be parsed.
"""
def __init__(self, str=''):
self.str = str
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::VersionParseException'
_M_Ice._t_VersionParseException = IcePy.defineException('::Ice::VersionParseException', VersionParseException, (), False, None, (('str', (), IcePy._t_string, False, 0),))
VersionParseException._ice_type = _M_Ice._t_VersionParseException
_M_Ice.VersionParseException = VersionParseException
del VersionParseException
if 'IdentityParseException' not in _M_Ice.__dict__:
_M_Ice.IdentityParseException = Ice.createTempClass()
class IdentityParseException(Ice.LocalException):
"""
This exception is raised if there was an error while parsing a
stringified identity.
Members:
str -- Describes the failure and includes the string that could not be parsed.
"""
def __init__(self, str=''):
self.str = str
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::IdentityParseException'
_M_Ice._t_IdentityParseException = IcePy.defineException('::Ice::IdentityParseException', IdentityParseException, (), False, None, (('str', (), IcePy._t_string, False, 0),))
IdentityParseException._ice_type = _M_Ice._t_IdentityParseException
_M_Ice.IdentityParseException = IdentityParseException
del IdentityParseException
if 'ProxyParseException' not in _M_Ice.__dict__:
_M_Ice.ProxyParseException = Ice.createTempClass()
class ProxyParseException(Ice.LocalException):
"""
This exception is raised if there was an error while parsing a
stringified proxy.
Members:
str -- Describes the failure and includes the string that could not be parsed.
"""
def __init__(self, str=''):
self.str = str
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ProxyParseException'
_M_Ice._t_ProxyParseException = IcePy.defineException('::Ice::ProxyParseException', ProxyParseException, (), False, None, (('str', (), IcePy._t_string, False, 0),))
ProxyParseException._ice_type = _M_Ice._t_ProxyParseException
_M_Ice.ProxyParseException = ProxyParseException
del ProxyParseException
if 'IllegalIdentityException' not in _M_Ice.__dict__:
_M_Ice.IllegalIdentityException = Ice.createTempClass()
class IllegalIdentityException(Ice.LocalException):
"""
This exception is raised if an illegal identity is encountered.
Members:
id -- The illegal identity.
"""
def __init__(self, id=Ice._struct_marker):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::IllegalIdentityException'
_M_Ice._t_IllegalIdentityException = IcePy.defineException('::Ice::IllegalIdentityException', IllegalIdentityException, (), False, None, (('id', (), _M_Ice._t_Identity, False, 0),))
IllegalIdentityException._ice_type = _M_Ice._t_IllegalIdentityException
_M_Ice.IllegalIdentityException = IllegalIdentityException
del IllegalIdentityException
if 'IllegalServantException' not in _M_Ice.__dict__:
_M_Ice.IllegalServantException = Ice.createTempClass()
class IllegalServantException(Ice.LocalException):
"""
This exception is raised to reject an illegal servant (typically
a null servant)
Members:
reason -- Describes why this servant is illegal.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::IllegalServantException'
_M_Ice._t_IllegalServantException = IcePy.defineException('::Ice::IllegalServantException', IllegalServantException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
IllegalServantException._ice_type = _M_Ice._t_IllegalServantException
_M_Ice.IllegalServantException = IllegalServantException
del IllegalServantException
if 'RequestFailedException' not in _M_Ice.__dict__:
_M_Ice.RequestFailedException = Ice.createTempClass()
class RequestFailedException(Ice.LocalException):
"""
This exception is raised if a request failed. This exception, and
all exceptions derived from RequestFailedException, are
transmitted by the Ice protocol, even though they are declared
local.
Members:
id -- The identity of the Ice Object to which the request was sent.
facet -- The facet to which the request was sent.
operation -- The operation name of the request.
"""
def __init__(self, id=Ice._struct_marker, facet='', operation=''):
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
self.facet = facet
self.operation = operation
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::RequestFailedException'
_M_Ice._t_RequestFailedException = IcePy.defineException('::Ice::RequestFailedException', RequestFailedException, (), False, None, (
('id', (), _M_Ice._t_Identity, False, 0),
('facet', (), IcePy._t_string, False, 0),
('operation', (), IcePy._t_string, False, 0)
))
RequestFailedException._ice_type = _M_Ice._t_RequestFailedException
_M_Ice.RequestFailedException = RequestFailedException
del RequestFailedException
if 'ObjectNotExistException' not in _M_Ice.__dict__:
_M_Ice.ObjectNotExistException = Ice.createTempClass()
class ObjectNotExistException(_M_Ice.RequestFailedException):
"""
This exception is raised if an object does not exist on the server,
that is, if no facets with the given identity exist.
"""
def __init__(self, id=Ice._struct_marker, facet='', operation=''):
_M_Ice.RequestFailedException.__init__(self, id, facet, operation)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ObjectNotExistException'
_M_Ice._t_ObjectNotExistException = IcePy.defineException('::Ice::ObjectNotExistException', ObjectNotExistException, (), False, _M_Ice._t_RequestFailedException, ())
ObjectNotExistException._ice_type = _M_Ice._t_ObjectNotExistException
_M_Ice.ObjectNotExistException = ObjectNotExistException
del ObjectNotExistException
if 'FacetNotExistException' not in _M_Ice.__dict__:
_M_Ice.FacetNotExistException = Ice.createTempClass()
class FacetNotExistException(_M_Ice.RequestFailedException):
"""
This exception is raised if no facet with the given name exists,
but at least one facet with the given identity exists.
"""
def __init__(self, id=Ice._struct_marker, facet='', operation=''):
_M_Ice.RequestFailedException.__init__(self, id, facet, operation)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::FacetNotExistException'
_M_Ice._t_FacetNotExistException = IcePy.defineException('::Ice::FacetNotExistException', FacetNotExistException, (), False, _M_Ice._t_RequestFailedException, ())
FacetNotExistException._ice_type = _M_Ice._t_FacetNotExistException
_M_Ice.FacetNotExistException = FacetNotExistException
del FacetNotExistException
if 'OperationNotExistException' not in _M_Ice.__dict__:
_M_Ice.OperationNotExistException = Ice.createTempClass()
class OperationNotExistException(_M_Ice.RequestFailedException):
"""
This exception is raised if an operation for a given object does
not exist on the server. Typically this is caused by either the
client or the server using an outdated Slice specification.
"""
def __init__(self, id=Ice._struct_marker, facet='', operation=''):
_M_Ice.RequestFailedException.__init__(self, id, facet, operation)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::OperationNotExistException'
_M_Ice._t_OperationNotExistException = IcePy.defineException('::Ice::OperationNotExistException', OperationNotExistException, (), False, _M_Ice._t_RequestFailedException, ())
OperationNotExistException._ice_type = _M_Ice._t_OperationNotExistException
_M_Ice.OperationNotExistException = OperationNotExistException
del OperationNotExistException
if 'SyscallException' not in _M_Ice.__dict__:
_M_Ice.SyscallException = Ice.createTempClass()
class SyscallException(Ice.LocalException):
"""
This exception is raised if a system error occurred in the server
or client process. There are many possible causes for such a system
exception. For details on the cause, SyscallException#error
should be inspected.
Members:
error -- The error number describing the system exception. For C++ and
Unix, this is equivalent to errno. For C++
and Windows, this is the value returned by
GetLastError() or
WSAGetLastError().
"""
def __init__(self, error=0):
self.error = error
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::SyscallException'
_M_Ice._t_SyscallException = IcePy.defineException('::Ice::SyscallException', SyscallException, (), False, None, (('error', (), IcePy._t_int, False, 0),))
SyscallException._ice_type = _M_Ice._t_SyscallException
_M_Ice.SyscallException = SyscallException
del SyscallException
if 'SocketException' not in _M_Ice.__dict__:
_M_Ice.SocketException = Ice.createTempClass()
class SocketException(_M_Ice.SyscallException):
"""
This exception indicates socket errors.
"""
def __init__(self, error=0):
_M_Ice.SyscallException.__init__(self, error)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::SocketException'
_M_Ice._t_SocketException = IcePy.defineException('::Ice::SocketException', SocketException, (), False, _M_Ice._t_SyscallException, ())
SocketException._ice_type = _M_Ice._t_SocketException
_M_Ice.SocketException = SocketException
del SocketException
if 'CFNetworkException' not in _M_Ice.__dict__:
_M_Ice.CFNetworkException = Ice.createTempClass()
class CFNetworkException(_M_Ice.SocketException):
"""
This exception indicates CFNetwork errors.
Members:
domain -- The domain of the error.
"""
def __init__(self, error=0, domain=''):
_M_Ice.SocketException.__init__(self, error)
self.domain = domain
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CFNetworkException'
_M_Ice._t_CFNetworkException = IcePy.defineException('::Ice::CFNetworkException', CFNetworkException, (), False, _M_Ice._t_SocketException, (('domain', (), IcePy._t_string, False, 0),))
CFNetworkException._ice_type = _M_Ice._t_CFNetworkException
_M_Ice.CFNetworkException = CFNetworkException
del CFNetworkException
if 'FileException' not in _M_Ice.__dict__:
_M_Ice.FileException = Ice.createTempClass()
class FileException(_M_Ice.SyscallException):
"""
This exception indicates file errors.
Members:
path -- The path of the file responsible for the error.
"""
def __init__(self, error=0, path=''):
_M_Ice.SyscallException.__init__(self, error)
self.path = path
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::FileException'
_M_Ice._t_FileException = IcePy.defineException('::Ice::FileException', FileException, (), False, _M_Ice._t_SyscallException, (('path', (), IcePy._t_string, False, 0),))
FileException._ice_type = _M_Ice._t_FileException
_M_Ice.FileException = FileException
del FileException
if 'ConnectFailedException' not in _M_Ice.__dict__:
_M_Ice.ConnectFailedException = Ice.createTempClass()
class ConnectFailedException(_M_Ice.SocketException):
"""
This exception indicates connection failures.
"""
def __init__(self, error=0):
_M_Ice.SocketException.__init__(self, error)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectFailedException'
_M_Ice._t_ConnectFailedException = IcePy.defineException('::Ice::ConnectFailedException', ConnectFailedException, (), False, _M_Ice._t_SocketException, ())
ConnectFailedException._ice_type = _M_Ice._t_ConnectFailedException
_M_Ice.ConnectFailedException = ConnectFailedException
del ConnectFailedException
if 'ConnectionRefusedException' not in _M_Ice.__dict__:
_M_Ice.ConnectionRefusedException = Ice.createTempClass()
class ConnectionRefusedException(_M_Ice.ConnectFailedException):
"""
This exception indicates a connection failure for which
the server host actively refuses a connection.
"""
def __init__(self, error=0):
_M_Ice.ConnectFailedException.__init__(self, error)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectionRefusedException'
_M_Ice._t_ConnectionRefusedException = IcePy.defineException('::Ice::ConnectionRefusedException', ConnectionRefusedException, (), False, _M_Ice._t_ConnectFailedException, ())
ConnectionRefusedException._ice_type = _M_Ice._t_ConnectionRefusedException
_M_Ice.ConnectionRefusedException = ConnectionRefusedException
del ConnectionRefusedException
if 'ConnectionLostException' not in _M_Ice.__dict__:
_M_Ice.ConnectionLostException = Ice.createTempClass()
class ConnectionLostException(_M_Ice.SocketException):
"""
This exception indicates a lost connection.
"""
def __init__(self, error=0):
_M_Ice.SocketException.__init__(self, error)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectionLostException'
_M_Ice._t_ConnectionLostException = IcePy.defineException('::Ice::ConnectionLostException', ConnectionLostException, (), False, _M_Ice._t_SocketException, ())
ConnectionLostException._ice_type = _M_Ice._t_ConnectionLostException
_M_Ice.ConnectionLostException = ConnectionLostException
del ConnectionLostException
if 'DNSException' not in _M_Ice.__dict__:
_M_Ice.DNSException = Ice.createTempClass()
class DNSException(Ice.LocalException):
"""
This exception indicates a DNS problem. For details on the cause,
DNSException#error should be inspected.
Members:
error -- The error number describing the DNS problem. For C++ and Unix,
this is equivalent to h_errno. For C++ and
Windows, this is the value returned by
WSAGetLastError().
host -- The host name that could not be resolved.
"""
def __init__(self, error=0, host=''):
self.error = error
self.host = host
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::DNSException'
_M_Ice._t_DNSException = IcePy.defineException('::Ice::DNSException', DNSException, (), False, None, (
('error', (), IcePy._t_int, False, 0),
('host', (), IcePy._t_string, False, 0)
))
DNSException._ice_type = _M_Ice._t_DNSException
_M_Ice.DNSException = DNSException
del DNSException
if 'OperationInterruptedException' not in _M_Ice.__dict__:
_M_Ice.OperationInterruptedException = Ice.createTempClass()
class OperationInterruptedException(Ice.LocalException):
"""
This exception indicates a request was interrupted.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::OperationInterruptedException'
_M_Ice._t_OperationInterruptedException = IcePy.defineException('::Ice::OperationInterruptedException', OperationInterruptedException, (), False, None, ())
OperationInterruptedException._ice_type = _M_Ice._t_OperationInterruptedException
_M_Ice.OperationInterruptedException = OperationInterruptedException
del OperationInterruptedException
if 'TimeoutException' not in _M_Ice.__dict__:
_M_Ice.TimeoutException = Ice.createTempClass()
class TimeoutException(Ice.LocalException):
"""
This exception indicates a timeout condition.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::TimeoutException'
_M_Ice._t_TimeoutException = IcePy.defineException('::Ice::TimeoutException', TimeoutException, (), False, None, ())
TimeoutException._ice_type = _M_Ice._t_TimeoutException
_M_Ice.TimeoutException = TimeoutException
del TimeoutException
if 'ConnectTimeoutException' not in _M_Ice.__dict__:
_M_Ice.ConnectTimeoutException = Ice.createTempClass()
class ConnectTimeoutException(_M_Ice.TimeoutException):
"""
This exception indicates a connection establishment timeout condition.
"""
def __init__(self):
_M_Ice.TimeoutException.__init__(self)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectTimeoutException'
_M_Ice._t_ConnectTimeoutException = IcePy.defineException('::Ice::ConnectTimeoutException', ConnectTimeoutException, (), False, _M_Ice._t_TimeoutException, ())
ConnectTimeoutException._ice_type = _M_Ice._t_ConnectTimeoutException
_M_Ice.ConnectTimeoutException = ConnectTimeoutException
del ConnectTimeoutException
if 'CloseTimeoutException' not in _M_Ice.__dict__:
_M_Ice.CloseTimeoutException = Ice.createTempClass()
class CloseTimeoutException(_M_Ice.TimeoutException):
"""
This exception indicates a connection closure timeout condition.
"""
def __init__(self):
_M_Ice.TimeoutException.__init__(self)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CloseTimeoutException'
_M_Ice._t_CloseTimeoutException = IcePy.defineException('::Ice::CloseTimeoutException', CloseTimeoutException, (), False, _M_Ice._t_TimeoutException, ())
CloseTimeoutException._ice_type = _M_Ice._t_CloseTimeoutException
_M_Ice.CloseTimeoutException = CloseTimeoutException
del CloseTimeoutException
if 'ConnectionTimeoutException' not in _M_Ice.__dict__:
_M_Ice.ConnectionTimeoutException = Ice.createTempClass()
class ConnectionTimeoutException(_M_Ice.TimeoutException):
"""
This exception indicates that a connection has been shut down because it has been
idle for some time.
"""
def __init__(self):
_M_Ice.TimeoutException.__init__(self)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectionTimeoutException'
_M_Ice._t_ConnectionTimeoutException = IcePy.defineException('::Ice::ConnectionTimeoutException', ConnectionTimeoutException, (), False, _M_Ice._t_TimeoutException, ())
ConnectionTimeoutException._ice_type = _M_Ice._t_ConnectionTimeoutException
_M_Ice.ConnectionTimeoutException = ConnectionTimeoutException
del ConnectionTimeoutException
if 'InvocationTimeoutException' not in _M_Ice.__dict__:
_M_Ice.InvocationTimeoutException = Ice.createTempClass()
class InvocationTimeoutException(_M_Ice.TimeoutException):
"""
This exception indicates that an invocation failed because it timed
out.
"""
def __init__(self):
_M_Ice.TimeoutException.__init__(self)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::InvocationTimeoutException'
_M_Ice._t_InvocationTimeoutException = IcePy.defineException('::Ice::InvocationTimeoutException', InvocationTimeoutException, (), False, _M_Ice._t_TimeoutException, ())
InvocationTimeoutException._ice_type = _M_Ice._t_InvocationTimeoutException
_M_Ice.InvocationTimeoutException = InvocationTimeoutException
del InvocationTimeoutException
if 'InvocationCanceledException' not in _M_Ice.__dict__:
_M_Ice.InvocationCanceledException = Ice.createTempClass()
class InvocationCanceledException(Ice.LocalException):
"""
This exception indicates that an asynchronous invocation failed
because it was canceled explicitly by the user.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::InvocationCanceledException'
_M_Ice._t_InvocationCanceledException = IcePy.defineException('::Ice::InvocationCanceledException', InvocationCanceledException, (), False, None, ())
InvocationCanceledException._ice_type = _M_Ice._t_InvocationCanceledException
_M_Ice.InvocationCanceledException = InvocationCanceledException
del InvocationCanceledException
if 'ProtocolException' not in _M_Ice.__dict__:
_M_Ice.ProtocolException = Ice.createTempClass()
class ProtocolException(Ice.LocalException):
"""
A generic exception base for all kinds of protocol error
conditions.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ProtocolException'
_M_Ice._t_ProtocolException = IcePy.defineException('::Ice::ProtocolException', ProtocolException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
ProtocolException._ice_type = _M_Ice._t_ProtocolException
_M_Ice.ProtocolException = ProtocolException
del ProtocolException
if 'BadMagicException' not in _M_Ice.__dict__:
_M_Ice.BadMagicException = Ice.createTempClass()
class BadMagicException(_M_Ice.ProtocolException):
"""
This exception indicates that a message did not start with the expected
magic number ('I', 'c', 'e', 'P').
Members:
badMagic -- A sequence containing the first four bytes of the incorrect message.
"""
def __init__(self, reason='', badMagic=None):
_M_Ice.ProtocolException.__init__(self, reason)
self.badMagic = badMagic
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::BadMagicException'
_M_Ice._t_BadMagicException = IcePy.defineException('::Ice::BadMagicException', BadMagicException, (), False, _M_Ice._t_ProtocolException, (('badMagic', (), _M_Ice._t_ByteSeq, False, 0),))
BadMagicException._ice_type = _M_Ice._t_BadMagicException
_M_Ice.BadMagicException = BadMagicException
del BadMagicException
if 'UnsupportedProtocolException' not in _M_Ice.__dict__:
_M_Ice.UnsupportedProtocolException = Ice.createTempClass()
class UnsupportedProtocolException(_M_Ice.ProtocolException):
"""
This exception indicates an unsupported protocol version.
Members:
bad -- The version of the unsupported protocol.
supported -- The version of the protocol that is supported.
"""
def __init__(self, reason='', bad=Ice._struct_marker, supported=Ice._struct_marker):
_M_Ice.ProtocolException.__init__(self, reason)
if bad is Ice._struct_marker:
self.bad = _M_Ice.ProtocolVersion()
else:
self.bad = bad
if supported is Ice._struct_marker:
self.supported = _M_Ice.ProtocolVersion()
else:
self.supported = supported
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnsupportedProtocolException'
_M_Ice._t_UnsupportedProtocolException = IcePy.defineException('::Ice::UnsupportedProtocolException', UnsupportedProtocolException, (), False, _M_Ice._t_ProtocolException, (
('bad', (), _M_Ice._t_ProtocolVersion, False, 0),
('supported', (), _M_Ice._t_ProtocolVersion, False, 0)
))
UnsupportedProtocolException._ice_type = _M_Ice._t_UnsupportedProtocolException
_M_Ice.UnsupportedProtocolException = UnsupportedProtocolException
del UnsupportedProtocolException
if 'UnsupportedEncodingException' not in _M_Ice.__dict__:
_M_Ice.UnsupportedEncodingException = Ice.createTempClass()
class UnsupportedEncodingException(_M_Ice.ProtocolException):
"""
This exception indicates an unsupported data encoding version.
Members:
bad -- The version of the unsupported encoding.
supported -- The version of the encoding that is supported.
"""
def __init__(self, reason='', bad=Ice._struct_marker, supported=Ice._struct_marker):
_M_Ice.ProtocolException.__init__(self, reason)
if bad is Ice._struct_marker:
self.bad = _M_Ice.EncodingVersion()
else:
self.bad = bad
if supported is Ice._struct_marker:
self.supported = _M_Ice.EncodingVersion()
else:
self.supported = supported
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnsupportedEncodingException'
_M_Ice._t_UnsupportedEncodingException = IcePy.defineException('::Ice::UnsupportedEncodingException', UnsupportedEncodingException, (), False, _M_Ice._t_ProtocolException, (
('bad', (), _M_Ice._t_EncodingVersion, False, 0),
('supported', (), _M_Ice._t_EncodingVersion, False, 0)
))
UnsupportedEncodingException._ice_type = _M_Ice._t_UnsupportedEncodingException
_M_Ice.UnsupportedEncodingException = UnsupportedEncodingException
del UnsupportedEncodingException
if 'UnknownMessageException' not in _M_Ice.__dict__:
_M_Ice.UnknownMessageException = Ice.createTempClass()
class UnknownMessageException(_M_Ice.ProtocolException):
"""
This exception indicates that an unknown protocol message has been received.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownMessageException'
_M_Ice._t_UnknownMessageException = IcePy.defineException('::Ice::UnknownMessageException', UnknownMessageException, (), False, _M_Ice._t_ProtocolException, ())
UnknownMessageException._ice_type = _M_Ice._t_UnknownMessageException
_M_Ice.UnknownMessageException = UnknownMessageException
del UnknownMessageException
if 'ConnectionNotValidatedException' not in _M_Ice.__dict__:
_M_Ice.ConnectionNotValidatedException = Ice.createTempClass()
class ConnectionNotValidatedException(_M_Ice.ProtocolException):
"""
This exception is raised if a message is received over a connection
that is not yet validated.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectionNotValidatedException'
_M_Ice._t_ConnectionNotValidatedException = IcePy.defineException('::Ice::ConnectionNotValidatedException', ConnectionNotValidatedException, (), False, _M_Ice._t_ProtocolException, ())
ConnectionNotValidatedException._ice_type = _M_Ice._t_ConnectionNotValidatedException
_M_Ice.ConnectionNotValidatedException = ConnectionNotValidatedException
del ConnectionNotValidatedException
if 'UnknownRequestIdException' not in _M_Ice.__dict__:
_M_Ice.UnknownRequestIdException = Ice.createTempClass()
class UnknownRequestIdException(_M_Ice.ProtocolException):
"""
This exception indicates that a response for an unknown request ID has been
received.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownRequestIdException'
_M_Ice._t_UnknownRequestIdException = IcePy.defineException('::Ice::UnknownRequestIdException', UnknownRequestIdException, (), False, _M_Ice._t_ProtocolException, ())
UnknownRequestIdException._ice_type = _M_Ice._t_UnknownRequestIdException
_M_Ice.UnknownRequestIdException = UnknownRequestIdException
del UnknownRequestIdException
if 'UnknownReplyStatusException' not in _M_Ice.__dict__:
_M_Ice.UnknownReplyStatusException = Ice.createTempClass()
class UnknownReplyStatusException(_M_Ice.ProtocolException):
"""
This exception indicates that an unknown reply status has been received.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnknownReplyStatusException'
_M_Ice._t_UnknownReplyStatusException = IcePy.defineException('::Ice::UnknownReplyStatusException', UnknownReplyStatusException, (), False, _M_Ice._t_ProtocolException, ())
UnknownReplyStatusException._ice_type = _M_Ice._t_UnknownReplyStatusException
_M_Ice.UnknownReplyStatusException = UnknownReplyStatusException
del UnknownReplyStatusException
if 'CloseConnectionException' not in _M_Ice.__dict__:
_M_Ice.CloseConnectionException = Ice.createTempClass()
class CloseConnectionException(_M_Ice.ProtocolException):
"""
This exception indicates that the connection has been gracefully shut down by the
server. The operation call that caused this exception has not been
executed by the server. In most cases you will not get this
exception, because the client will automatically retry the
operation call in case the server shut down the connection. However,
if upon retry the server shuts down the connection again, and the
retry limit has been reached, then this exception is propagated to
the application code.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CloseConnectionException'
_M_Ice._t_CloseConnectionException = IcePy.defineException('::Ice::CloseConnectionException', CloseConnectionException, (), False, _M_Ice._t_ProtocolException, ())
CloseConnectionException._ice_type = _M_Ice._t_CloseConnectionException
_M_Ice.CloseConnectionException = CloseConnectionException
del CloseConnectionException
if 'ConnectionManuallyClosedException' not in _M_Ice.__dict__:
_M_Ice.ConnectionManuallyClosedException = Ice.createTempClass()
class ConnectionManuallyClosedException(Ice.LocalException):
"""
This exception is raised by an operation call if the application
closes the connection locally using Connection#close.
Members:
graceful -- True if the connection was closed gracefully, false otherwise.
"""
def __init__(self, graceful=False):
self.graceful = graceful
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ConnectionManuallyClosedException'
_M_Ice._t_ConnectionManuallyClosedException = IcePy.defineException('::Ice::ConnectionManuallyClosedException', ConnectionManuallyClosedException, (), False, None, (('graceful', (), IcePy._t_bool, False, 0),))
ConnectionManuallyClosedException._ice_type = _M_Ice._t_ConnectionManuallyClosedException
_M_Ice.ConnectionManuallyClosedException = ConnectionManuallyClosedException
del ConnectionManuallyClosedException
if 'IllegalMessageSizeException' not in _M_Ice.__dict__:
_M_Ice.IllegalMessageSizeException = Ice.createTempClass()
class IllegalMessageSizeException(_M_Ice.ProtocolException):
"""
This exception indicates that a message size is less
than the minimum required size.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::IllegalMessageSizeException'
_M_Ice._t_IllegalMessageSizeException = IcePy.defineException('::Ice::IllegalMessageSizeException', IllegalMessageSizeException, (), False, _M_Ice._t_ProtocolException, ())
IllegalMessageSizeException._ice_type = _M_Ice._t_IllegalMessageSizeException
_M_Ice.IllegalMessageSizeException = IllegalMessageSizeException
del IllegalMessageSizeException
if 'CompressionException' not in _M_Ice.__dict__:
_M_Ice.CompressionException = Ice.createTempClass()
class CompressionException(_M_Ice.ProtocolException):
"""
This exception indicates a problem with compressing or uncompressing data.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::CompressionException'
_M_Ice._t_CompressionException = IcePy.defineException('::Ice::CompressionException', CompressionException, (), False, _M_Ice._t_ProtocolException, ())
CompressionException._ice_type = _M_Ice._t_CompressionException
_M_Ice.CompressionException = CompressionException
del CompressionException
if 'DatagramLimitException' not in _M_Ice.__dict__:
_M_Ice.DatagramLimitException = Ice.createTempClass()
class DatagramLimitException(_M_Ice.ProtocolException):
"""
A datagram exceeds the configured size.
This exception is raised if a datagram exceeds the configured send or receive buffer
size, or exceeds the maximum payload size of a UDP packet (65507 bytes).
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::DatagramLimitException'
_M_Ice._t_DatagramLimitException = IcePy.defineException('::Ice::DatagramLimitException', DatagramLimitException, (), False, _M_Ice._t_ProtocolException, ())
DatagramLimitException._ice_type = _M_Ice._t_DatagramLimitException
_M_Ice.DatagramLimitException = DatagramLimitException
del DatagramLimitException
if 'MarshalException' not in _M_Ice.__dict__:
_M_Ice.MarshalException = Ice.createTempClass()
class MarshalException(_M_Ice.ProtocolException):
"""
This exception is raised for errors during marshaling or unmarshaling data.
"""
def __init__(self, reason=''):
_M_Ice.ProtocolException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::MarshalException'
_M_Ice._t_MarshalException = IcePy.defineException('::Ice::MarshalException', MarshalException, (), False, _M_Ice._t_ProtocolException, ())
MarshalException._ice_type = _M_Ice._t_MarshalException
_M_Ice.MarshalException = MarshalException
del MarshalException
if 'ProxyUnmarshalException' not in _M_Ice.__dict__:
_M_Ice.ProxyUnmarshalException = Ice.createTempClass()
class ProxyUnmarshalException(_M_Ice.MarshalException):
"""
This exception is raised if inconsistent data is received while unmarshaling a proxy.
"""
def __init__(self, reason=''):
_M_Ice.MarshalException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ProxyUnmarshalException'
_M_Ice._t_ProxyUnmarshalException = IcePy.defineException('::Ice::ProxyUnmarshalException', ProxyUnmarshalException, (), False, _M_Ice._t_MarshalException, ())
ProxyUnmarshalException._ice_type = _M_Ice._t_ProxyUnmarshalException
_M_Ice.ProxyUnmarshalException = ProxyUnmarshalException
del ProxyUnmarshalException
if 'UnmarshalOutOfBoundsException' not in _M_Ice.__dict__:
_M_Ice.UnmarshalOutOfBoundsException = Ice.createTempClass()
class UnmarshalOutOfBoundsException(_M_Ice.MarshalException):
"""
This exception is raised if an out-of-bounds condition occurs during unmarshaling.
"""
def __init__(self, reason=''):
_M_Ice.MarshalException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnmarshalOutOfBoundsException'
_M_Ice._t_UnmarshalOutOfBoundsException = IcePy.defineException('::Ice::UnmarshalOutOfBoundsException', UnmarshalOutOfBoundsException, (), False, _M_Ice._t_MarshalException, ())
UnmarshalOutOfBoundsException._ice_type = _M_Ice._t_UnmarshalOutOfBoundsException
_M_Ice.UnmarshalOutOfBoundsException = UnmarshalOutOfBoundsException
del UnmarshalOutOfBoundsException
if 'NoValueFactoryException' not in _M_Ice.__dict__:
_M_Ice.NoValueFactoryException = Ice.createTempClass()
class NoValueFactoryException(_M_Ice.MarshalException):
"""
This exception is raised if no suitable value factory was found during
unmarshaling of a Slice class instance.
Members:
type -- The Slice type ID of the class instance for which no
no factory could be found.
"""
def __init__(self, reason='', type=''):
_M_Ice.MarshalException.__init__(self, reason)
self.type = type
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::NoValueFactoryException'
_M_Ice._t_NoValueFactoryException = IcePy.defineException('::Ice::NoValueFactoryException', NoValueFactoryException, (), False, _M_Ice._t_MarshalException, (('type', (), IcePy._t_string, False, 0),))
NoValueFactoryException._ice_type = _M_Ice._t_NoValueFactoryException
_M_Ice.NoValueFactoryException = NoValueFactoryException
del NoValueFactoryException
if 'UnexpectedObjectException' not in _M_Ice.__dict__:
_M_Ice.UnexpectedObjectException = Ice.createTempClass()
class UnexpectedObjectException(_M_Ice.MarshalException):
"""
This exception is raised if the type of an unmarshaled Slice class instance does
not match its expected type.
This can happen if client and server are compiled with mismatched Slice
definitions or if a class of the wrong type is passed as a parameter
or return value using dynamic invocation. This exception can also be
raised if IceStorm is used to send Slice class instances and
an operation is subscribed to the wrong topic.
Members:
type -- The Slice type ID of the class instance that was unmarshaled.
expectedType -- The Slice type ID that was expected by the receiving operation.
"""
def __init__(self, reason='', type='', expectedType=''):
_M_Ice.MarshalException.__init__(self, reason)
self.type = type
self.expectedType = expectedType
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::UnexpectedObjectException'
_M_Ice._t_UnexpectedObjectException = IcePy.defineException('::Ice::UnexpectedObjectException', UnexpectedObjectException, (), False, _M_Ice._t_MarshalException, (
('type', (), IcePy._t_string, False, 0),
('expectedType', (), IcePy._t_string, False, 0)
))
UnexpectedObjectException._ice_type = _M_Ice._t_UnexpectedObjectException
_M_Ice.UnexpectedObjectException = UnexpectedObjectException
del UnexpectedObjectException
if 'MemoryLimitException' not in _M_Ice.__dict__:
_M_Ice.MemoryLimitException = Ice.createTempClass()
class MemoryLimitException(_M_Ice.MarshalException):
"""
This exception is raised when Ice receives a request or reply
message whose size exceeds the limit specified by the
Ice.MessageSizeMax property.
"""
def __init__(self, reason=''):
_M_Ice.MarshalException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::MemoryLimitException'
_M_Ice._t_MemoryLimitException = IcePy.defineException('::Ice::MemoryLimitException', MemoryLimitException, (), False, _M_Ice._t_MarshalException, ())
MemoryLimitException._ice_type = _M_Ice._t_MemoryLimitException
_M_Ice.MemoryLimitException = MemoryLimitException
del MemoryLimitException
if 'StringConversionException' not in _M_Ice.__dict__:
_M_Ice.StringConversionException = Ice.createTempClass()
class StringConversionException(_M_Ice.MarshalException):
"""
This exception is raised when a string conversion to or from UTF-8
fails during marshaling or unmarshaling.
"""
def __init__(self, reason=''):
_M_Ice.MarshalException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::StringConversionException'
_M_Ice._t_StringConversionException = IcePy.defineException('::Ice::StringConversionException', StringConversionException, (), False, _M_Ice._t_MarshalException, ())
StringConversionException._ice_type = _M_Ice._t_StringConversionException
_M_Ice.StringConversionException = StringConversionException
del StringConversionException
if 'EncapsulationException' not in _M_Ice.__dict__:
_M_Ice.EncapsulationException = Ice.createTempClass()
class EncapsulationException(_M_Ice.MarshalException):
"""
This exception indicates a malformed data encapsulation.
"""
def __init__(self, reason=''):
_M_Ice.MarshalException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::EncapsulationException'
_M_Ice._t_EncapsulationException = IcePy.defineException('::Ice::EncapsulationException', EncapsulationException, (), False, _M_Ice._t_MarshalException, ())
EncapsulationException._ice_type = _M_Ice._t_EncapsulationException
_M_Ice.EncapsulationException = EncapsulationException
del EncapsulationException
if 'FeatureNotSupportedException' not in _M_Ice.__dict__:
_M_Ice.FeatureNotSupportedException = Ice.createTempClass()
class FeatureNotSupportedException(Ice.LocalException):
"""
This exception is raised if an unsupported feature is used. The
unsupported feature string contains the name of the unsupported
feature
Members:
unsupportedFeature -- The name of the unsupported feature.
"""
def __init__(self, unsupportedFeature=''):
self.unsupportedFeature = unsupportedFeature
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::FeatureNotSupportedException'
_M_Ice._t_FeatureNotSupportedException = IcePy.defineException('::Ice::FeatureNotSupportedException', FeatureNotSupportedException, (), False, None, (('unsupportedFeature', (), IcePy._t_string, False, 0),))
FeatureNotSupportedException._ice_type = _M_Ice._t_FeatureNotSupportedException
_M_Ice.FeatureNotSupportedException = FeatureNotSupportedException
del FeatureNotSupportedException
if 'SecurityException' not in _M_Ice.__dict__:
_M_Ice.SecurityException = Ice.createTempClass()
class SecurityException(Ice.LocalException):
"""
This exception indicates a failure in a security subsystem,
such as the IceSSL plug-in.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::SecurityException'
_M_Ice._t_SecurityException = IcePy.defineException('::Ice::SecurityException', SecurityException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
SecurityException._ice_type = _M_Ice._t_SecurityException
_M_Ice.SecurityException = SecurityException
del SecurityException
if 'FixedProxyException' not in _M_Ice.__dict__:
_M_Ice.FixedProxyException = Ice.createTempClass()
class FixedProxyException(Ice.LocalException):
"""
This exception indicates that an attempt has been made to
change the connection properties of a fixed proxy.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::FixedProxyException'
_M_Ice._t_FixedProxyException = IcePy.defineException('::Ice::FixedProxyException', FixedProxyException, (), False, None, ())
FixedProxyException._ice_type = _M_Ice._t_FixedProxyException
_M_Ice.FixedProxyException = FixedProxyException
del FixedProxyException
if 'ResponseSentException' not in _M_Ice.__dict__:
_M_Ice.ResponseSentException = Ice.createTempClass()
class ResponseSentException(Ice.LocalException):
"""
Indicates that the response to a request has already been sent;
re-dispatching such a request is not possible.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ResponseSentException'
_M_Ice._t_ResponseSentException = IcePy.defineException('::Ice::ResponseSentException', ResponseSentException, (), False, None, ())
ResponseSentException._ice_type = _M_Ice._t_ResponseSentException
_M_Ice.ResponseSentException = ResponseSentException
del ResponseSentException
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/LocalException_ice.py | LocalException_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.ObjectAdapterF_ice
import Ice.ConnectionF_ice
import Ice.Identity_ice
import Ice.Version_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if '_t_Context' not in _M_Ice.__dict__:
_M_Ice._t_Context = IcePy.defineDictionary('::Ice::Context', (), IcePy._t_string, IcePy._t_string)
if 'OperationMode' not in _M_Ice.__dict__:
_M_Ice.OperationMode = Ice.createTempClass()
class OperationMode(Ice.EnumBase):
"""
Determines the retry behavior an invocation in case of a (potentially) recoverable error.
Enumerators:
Normal -- Ordinary operations have Normal mode. These operations
modify object state; invoking such an operation twice in a row
has different semantics than invoking it once. The Ice run time
guarantees that it will not violate at-most-once semantics for
Normal operations.
Nonmutating -- Operations that use the Slice nonmutating keyword must not
modify object state. For C++, nonmutating operations generate
const member functions in the skeleton. In addition, the Ice
run time will attempt to transparently recover from certain
run-time errors by re-issuing a failed request and propagate
the failure to the application only if the second attempt
fails.
Nonmutating is deprecated; Use the
idempotent keyword instead. For C++, to retain the mapping
of nonmutating operations to C++ const
member functions, use the \["cpp:const"] metadata
directive.
Idempotent -- Operations that use the Slice idempotent keyword can modify
object state, but invoking an operation twice in a row must
result in the same object state as invoking it once. For
example, x = 1 is an idempotent statement,
whereas x += 1 is not. For idempotent
operations, the Ice run-time uses the same retry behavior
as for nonmutating operations in case of a potentially
recoverable error.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
OperationMode.Normal = OperationMode("Normal", 0)
OperationMode.Nonmutating = OperationMode("Nonmutating", 1)
OperationMode.Idempotent = OperationMode("Idempotent", 2)
OperationMode._enumerators = { 0:OperationMode.Normal, 1:OperationMode.Nonmutating, 2:OperationMode.Idempotent }
_M_Ice._t_OperationMode = IcePy.defineEnum('::Ice::OperationMode', OperationMode, (), OperationMode._enumerators)
_M_Ice.OperationMode = OperationMode
del OperationMode
if 'Current' not in _M_Ice.__dict__:
_M_Ice.Current = Ice.createTempClass()
class Current(object):
"""
Information about the current method invocation for servers. Each
operation on the server has a Current as its implicit final
parameter. Current is mostly used for Ice services. Most
applications ignore this parameter.
Members:
adapter -- The object adapter.
con -- Information about the connection over which the current method
invocation was received. If the invocation is direct due to
collocation optimization, this value is set to null.
id -- The Ice object identity.
facet -- The facet.
operation -- The operation name.
mode -- The mode of the operation.
ctx -- The request context, as received from the client.
requestId -- The request id unless oneway (0).
encoding -- The encoding version used to encode the input and output parameters.
"""
def __init__(self, adapter=None, con=None, id=Ice._struct_marker, facet='', operation='', mode=_M_Ice.OperationMode.Normal, ctx=None, requestId=0, encoding=Ice._struct_marker):
self.adapter = adapter
self.con = con
if id is Ice._struct_marker:
self.id = _M_Ice.Identity()
else:
self.id = id
self.facet = facet
self.operation = operation
self.mode = mode
self.ctx = ctx
self.requestId = requestId
if encoding is Ice._struct_marker:
self.encoding = _M_Ice.EncodingVersion()
else:
self.encoding = encoding
def __eq__(self, other):
if other is None:
return False
elif not isinstance(other, _M_Ice.Current):
return NotImplemented
else:
if self.adapter != other.adapter:
return False
if self.con != other.con:
return False
if self.id != other.id:
return False
if self.facet != other.facet:
return False
if self.operation != other.operation:
return False
if self.mode != other.mode:
return False
if self.ctx != other.ctx:
return False
if self.requestId != other.requestId:
return False
if self.encoding != other.encoding:
return False
return True
def __ne__(self, other):
return not self.__eq__(other)
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Current)
__repr__ = __str__
_M_Ice._t_Current = IcePy.defineStruct('::Ice::Current', Current, (), (
('adapter', (), _M_Ice._t_ObjectAdapter),
('con', (), _M_Ice._t_Connection),
('id', (), _M_Ice._t_Identity),
('facet', (), IcePy._t_string),
('operation', (), IcePy._t_string),
('mode', (), _M_Ice._t_OperationMode),
('ctx', (), _M_Ice._t_Context),
('requestId', (), IcePy._t_int),
('encoding', (), _M_Ice._t_EncodingVersion)
))
_M_Ice.Current = Current
del Current
# End of module Ice
Ice.sliceChecksums["::Ice::Context"] = "e6cb8aba8a3ca160eab3597c6fbbeba"
Ice.sliceChecksums["::Ice::OperationMode"] = "56db1e0dd464f97828282bdb11d8955" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Current_ice.py | Current_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.LocalException_ice
import Ice.Current_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'ImplicitContext' not in _M_Ice.__dict__:
_M_Ice.ImplicitContext = Ice.createTempClass()
class ImplicitContext(object):
"""
An interface to associate implict contexts with communicators.
When you make a remote invocation without an explicit context parameter,
Ice uses the per-proxy context (if any) combined with the ImplicitContext
associated with the communicator.
Ice provides several implementations of ImplicitContext. The implementation
used depends on the value of the Ice.ImplicitContext property.
None (default)
No implicit context at all.
PerThread
The implementation maintains a context per thread.
Shared
The implementation maintains a single context shared by all threads.
ImplicitContext also provides a number of operations to create, update or retrieve
an entry in the underlying context without first retrieving a copy of the entire
context. These operations correspond to a subset of the java.util.Map methods,
with java.lang.Object replaced by string and null replaced by the empty-string.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.ImplicitContext:
raise RuntimeError('Ice.ImplicitContext is an abstract class')
def getContext(self):
"""
Get a copy of the underlying context.
Returns: A copy of the underlying context.
"""
raise NotImplementedError("method 'getContext' not implemented")
def setContext(self, newContext):
"""
Set the underlying context.
Arguments:
newContext -- The new context.
"""
raise NotImplementedError("method 'setContext' not implemented")
def containsKey(self, key):
"""
Check if this key has an associated value in the underlying context.
Arguments:
key -- The key.
Returns: True if the key has an associated value, False otherwise.
"""
raise NotImplementedError("method 'containsKey' not implemented")
def get(self, key):
"""
Get the value associated with the given key in the underlying context.
Returns an empty string if no value is associated with the key.
containsKey allows you to distinguish between an empty-string value and
no value at all.
Arguments:
key -- The key.
Returns: The value associated with the key.
"""
raise NotImplementedError("method 'get' not implemented")
def put(self, key, value):
"""
Create or update a key/value entry in the underlying context.
Arguments:
key -- The key.
value -- The value.
Returns: The previous value associated with the key, if any.
"""
raise NotImplementedError("method 'put' not implemented")
def remove(self, key):
"""
Remove the entry for the given key in the underlying context.
Arguments:
key -- The key.
Returns: The value associated with the key, if any.
"""
raise NotImplementedError("method 'remove' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ImplicitContext)
__repr__ = __str__
_M_Ice._t_ImplicitContext = IcePy.defineValue('::Ice::ImplicitContext', ImplicitContext, -1, (), False, True, None, ())
ImplicitContext._ice_type = _M_Ice._t_ImplicitContext
_M_Ice.ImplicitContext = ImplicitContext
del ImplicitContext
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/ImplicitContext_ice.py | ImplicitContext_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Version_ice
import Ice.BuiltinSequences_ice
import Ice.EndpointF_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
_M_Ice.TCPEndpointType = 1
_M_Ice.SSLEndpointType = 2
_M_Ice.UDPEndpointType = 3
_M_Ice.WSEndpointType = 4
_M_Ice.WSSEndpointType = 5
_M_Ice.BTEndpointType = 6
_M_Ice.BTSEndpointType = 7
_M_Ice.iAPEndpointType = 8
_M_Ice.iAPSEndpointType = 9
if 'EndpointInfo' not in _M_Ice.__dict__:
_M_Ice.EndpointInfo = Ice.createTempClass()
class EndpointInfo(object):
"""
Base class providing access to the endpoint details.
Members:
underlying -- The information of the underyling endpoint of null if there's
no underlying endpoint.
timeout -- The timeout for the endpoint in milliseconds. 0 means
non-blocking, -1 means no timeout.
compress -- Specifies whether or not compression should be used if
available when using this endpoint.
"""
def __init__(self, underlying=None, timeout=0, compress=False):
if Ice.getType(self) == _M_Ice.EndpointInfo:
raise RuntimeError('Ice.EndpointInfo is an abstract class')
self.underlying = underlying
self.timeout = timeout
self.compress = compress
def type(self):
"""
Returns the type of the endpoint.
Returns: The endpoint type.
"""
raise NotImplementedError("method 'type' not implemented")
def datagram(self):
"""
Returns true if this endpoint is a datagram endpoint.
Returns: True for a datagram endpoint.
"""
raise NotImplementedError("method 'datagram' not implemented")
def secure(self):
"""
Returns true if this endpoint is a secure endpoint.
Returns: True for a secure endpoint.
"""
raise NotImplementedError("method 'secure' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_EndpointInfo)
__repr__ = __str__
_M_Ice._t_EndpointInfo = IcePy.declareValue('::Ice::EndpointInfo')
_M_Ice._t_EndpointInfo = IcePy.defineValue('::Ice::EndpointInfo', EndpointInfo, -1, (), False, False, None, (
('underlying', (), _M_Ice._t_EndpointInfo, False, 0),
('timeout', (), IcePy._t_int, False, 0),
('compress', (), IcePy._t_bool, False, 0)
))
EndpointInfo._ice_type = _M_Ice._t_EndpointInfo
_M_Ice.EndpointInfo = EndpointInfo
del EndpointInfo
if 'Endpoint' not in _M_Ice.__dict__:
_M_Ice.Endpoint = Ice.createTempClass()
class Endpoint(object):
"""
The user-level interface to an endpoint.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Endpoint:
raise RuntimeError('Ice.Endpoint is an abstract class')
def toString(self):
"""
Return a string representation of the endpoint.
Returns: The string representation of the endpoint.
"""
raise NotImplementedError("method 'toString' not implemented")
def getInfo(self):
"""
Returns the endpoint information.
Returns: The endpoint information class.
"""
raise NotImplementedError("method 'getInfo' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Endpoint)
__repr__ = __str__
_M_Ice._t_Endpoint = IcePy.defineValue('::Ice::Endpoint', Endpoint, -1, (), False, True, None, ())
Endpoint._ice_type = _M_Ice._t_Endpoint
_M_Ice.Endpoint = Endpoint
del Endpoint
if 'IPEndpointInfo' not in _M_Ice.__dict__:
_M_Ice.IPEndpointInfo = Ice.createTempClass()
class IPEndpointInfo(_M_Ice.EndpointInfo):
"""
Provides access to the address details of a IP endpoint.
Members:
host -- The host or address configured with the endpoint.
port -- The port number.
sourceAddress -- The source IP address.
"""
def __init__(self, underlying=None, timeout=0, compress=False, host='', port=0, sourceAddress=''):
if Ice.getType(self) == _M_Ice.IPEndpointInfo:
raise RuntimeError('Ice.IPEndpointInfo is an abstract class')
_M_Ice.EndpointInfo.__init__(self, underlying, timeout, compress)
self.host = host
self.port = port
self.sourceAddress = sourceAddress
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_IPEndpointInfo)
__repr__ = __str__
_M_Ice._t_IPEndpointInfo = IcePy.declareValue('::Ice::IPEndpointInfo')
_M_Ice._t_IPEndpointInfo = IcePy.defineValue('::Ice::IPEndpointInfo', IPEndpointInfo, -1, (), False, False, _M_Ice._t_EndpointInfo, (
('host', (), IcePy._t_string, False, 0),
('port', (), IcePy._t_int, False, 0),
('sourceAddress', (), IcePy._t_string, False, 0)
))
IPEndpointInfo._ice_type = _M_Ice._t_IPEndpointInfo
_M_Ice.IPEndpointInfo = IPEndpointInfo
del IPEndpointInfo
if 'TCPEndpointInfo' not in _M_Ice.__dict__:
_M_Ice.TCPEndpointInfo = Ice.createTempClass()
class TCPEndpointInfo(_M_Ice.IPEndpointInfo):
"""
Provides access to a TCP endpoint information.
"""
def __init__(self, underlying=None, timeout=0, compress=False, host='', port=0, sourceAddress=''):
if Ice.getType(self) == _M_Ice.TCPEndpointInfo:
raise RuntimeError('Ice.TCPEndpointInfo is an abstract class')
_M_Ice.IPEndpointInfo.__init__(self, underlying, timeout, compress, host, port, sourceAddress)
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_TCPEndpointInfo)
__repr__ = __str__
_M_Ice._t_TCPEndpointInfo = IcePy.declareValue('::Ice::TCPEndpointInfo')
_M_Ice._t_TCPEndpointInfo = IcePy.defineValue('::Ice::TCPEndpointInfo', TCPEndpointInfo, -1, (), False, False, _M_Ice._t_IPEndpointInfo, ())
TCPEndpointInfo._ice_type = _M_Ice._t_TCPEndpointInfo
_M_Ice.TCPEndpointInfo = TCPEndpointInfo
del TCPEndpointInfo
if 'UDPEndpointInfo' not in _M_Ice.__dict__:
_M_Ice.UDPEndpointInfo = Ice.createTempClass()
class UDPEndpointInfo(_M_Ice.IPEndpointInfo):
"""
Provides access to an UDP endpoint information.
Members:
mcastInterface -- The multicast interface.
mcastTtl -- The multicast time-to-live (or hops).
"""
def __init__(self, underlying=None, timeout=0, compress=False, host='', port=0, sourceAddress='', mcastInterface='', mcastTtl=0):
if Ice.getType(self) == _M_Ice.UDPEndpointInfo:
raise RuntimeError('Ice.UDPEndpointInfo is an abstract class')
_M_Ice.IPEndpointInfo.__init__(self, underlying, timeout, compress, host, port, sourceAddress)
self.mcastInterface = mcastInterface
self.mcastTtl = mcastTtl
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_UDPEndpointInfo)
__repr__ = __str__
_M_Ice._t_UDPEndpointInfo = IcePy.declareValue('::Ice::UDPEndpointInfo')
_M_Ice._t_UDPEndpointInfo = IcePy.defineValue('::Ice::UDPEndpointInfo', UDPEndpointInfo, -1, (), False, False, _M_Ice._t_IPEndpointInfo, (
('mcastInterface', (), IcePy._t_string, False, 0),
('mcastTtl', (), IcePy._t_int, False, 0)
))
UDPEndpointInfo._ice_type = _M_Ice._t_UDPEndpointInfo
_M_Ice.UDPEndpointInfo = UDPEndpointInfo
del UDPEndpointInfo
if 'WSEndpointInfo' not in _M_Ice.__dict__:
_M_Ice.WSEndpointInfo = Ice.createTempClass()
class WSEndpointInfo(_M_Ice.EndpointInfo):
"""
Provides access to a WebSocket endpoint information.
Members:
resource -- The URI configured with the endpoint.
"""
def __init__(self, underlying=None, timeout=0, compress=False, resource=''):
if Ice.getType(self) == _M_Ice.WSEndpointInfo:
raise RuntimeError('Ice.WSEndpointInfo is an abstract class')
_M_Ice.EndpointInfo.__init__(self, underlying, timeout, compress)
self.resource = resource
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_WSEndpointInfo)
__repr__ = __str__
_M_Ice._t_WSEndpointInfo = IcePy.declareValue('::Ice::WSEndpointInfo')
_M_Ice._t_WSEndpointInfo = IcePy.defineValue('::Ice::WSEndpointInfo', WSEndpointInfo, -1, (), False, False, _M_Ice._t_EndpointInfo, (('resource', (), IcePy._t_string, False, 0),))
WSEndpointInfo._ice_type = _M_Ice._t_WSEndpointInfo
_M_Ice.WSEndpointInfo = WSEndpointInfo
del WSEndpointInfo
if 'OpaqueEndpointInfo' not in _M_Ice.__dict__:
_M_Ice.OpaqueEndpointInfo = Ice.createTempClass()
class OpaqueEndpointInfo(_M_Ice.EndpointInfo):
"""
Provides access to the details of an opaque endpoint.
Members:
rawEncoding -- The encoding version of the opaque endpoint (to decode or
encode the rawBytes).
rawBytes -- The raw encoding of the opaque endpoint.
"""
def __init__(self, underlying=None, timeout=0, compress=False, rawEncoding=Ice._struct_marker, rawBytes=None):
if Ice.getType(self) == _M_Ice.OpaqueEndpointInfo:
raise RuntimeError('Ice.OpaqueEndpointInfo is an abstract class')
_M_Ice.EndpointInfo.__init__(self, underlying, timeout, compress)
if rawEncoding is Ice._struct_marker:
self.rawEncoding = _M_Ice.EncodingVersion()
else:
self.rawEncoding = rawEncoding
self.rawBytes = rawBytes
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_OpaqueEndpointInfo)
__repr__ = __str__
_M_Ice._t_OpaqueEndpointInfo = IcePy.declareValue('::Ice::OpaqueEndpointInfo')
_M_Ice._t_OpaqueEndpointInfo = IcePy.defineValue('::Ice::OpaqueEndpointInfo', OpaqueEndpointInfo, -1, (), False, False, _M_Ice._t_EndpointInfo, (
('rawEncoding', (), _M_Ice._t_EncodingVersion, False, 0),
('rawBytes', (), _M_Ice._t_ByteSeq, False, 0)
))
OpaqueEndpointInfo._ice_type = _M_Ice._t_OpaqueEndpointInfo
_M_Ice.OpaqueEndpointInfo = OpaqueEndpointInfo
del OpaqueEndpointInfo
# End of module Ice
Ice.sliceChecksums["::Ice::BTEndpointType"] = "bf2c14e5c7ae9b47c4306e35b744efbd"
Ice.sliceChecksums["::Ice::BTSEndpointType"] = "91c6c150f7d5678cdc541a82852a8f1"
Ice.sliceChecksums["::Ice::SSLEndpointType"] = "846ad753a0862cc0c55a4ff278181b15"
Ice.sliceChecksums["::Ice::TCPEndpointType"] = "19d61f4b55a285927bb1648f7a943c29"
Ice.sliceChecksums["::Ice::UDPEndpointType"] = "20cb65b86f212e8c67dfa0314854eaf1"
Ice.sliceChecksums["::Ice::WSEndpointType"] = "9d96d5f9ecade1e2a84f6187d644b92d"
Ice.sliceChecksums["::Ice::WSSEndpointType"] = "e4c22485e7afe76aadc2921852b6182b"
Ice.sliceChecksums["::Ice::iAPEndpointType"] = "c170d414154fdef5ae94dedb8ec07bf"
Ice.sliceChecksums["::Ice::iAPSEndpointType"] = "87796981d5b8ab436f975e663c75d4a" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Endpoint_ice.py | Endpoint_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.EndpointF_ice
import Ice.ConnectionF_ice
import Ice.Current_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
# Start of module Ice.Instrumentation
_M_Ice.Instrumentation = Ice.openModule('Ice.Instrumentation')
__name__ = 'Ice.Instrumentation'
_M_Ice.Instrumentation.__doc__ = """
The Instrumentation local interfaces enable observing a number of
Ice core internal components (threads, connections, etc).
"""
if 'Observer' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.Observer = Ice.createTempClass()
class Observer(object):
"""
The object observer interface used by instrumented objects to
notify the observer of their existence.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.Observer:
raise RuntimeError('Ice.Instrumentation.Observer is an abstract class')
def attach(self):
"""
This method is called when the instrumented object is created
or when the observer is attached to an existing object.
"""
raise NotImplementedError("method 'attach' not implemented")
def detach(self):
"""
This method is called when the instrumented object is destroyed
and as a result the observer detached from the object.
"""
raise NotImplementedError("method 'detach' not implemented")
def failed(self, exceptionName):
"""
Notification of a failure.
Arguments:
exceptionName -- The name of the exception.
"""
raise NotImplementedError("method 'failed' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_Observer)
__repr__ = __str__
_M_Ice.Instrumentation._t_Observer = IcePy.defineValue('::Ice::Instrumentation::Observer', Observer, -1, (), False, True, None, ())
Observer._ice_type = _M_Ice.Instrumentation._t_Observer
_M_Ice.Instrumentation.Observer = Observer
del Observer
if 'ThreadState' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ThreadState = Ice.createTempClass()
class ThreadState(Ice.EnumBase):
"""
The thread state enumeration keeps track of the different possible
states of Ice threads.
Enumerators:
ThreadStateIdle -- The thread is idle.
ThreadStateInUseForIO -- The thread is in use performing reads or writes for Ice
connections. This state is only for threads from an Ice thread
pool.
ThreadStateInUseForUser -- The thread is calling user code (servant implementation, AMI
callbacks). This state is only for threads from an Ice thread
pool.
ThreadStateInUseForOther -- The thread is performing other internal activities (DNS
lookups, timer callbacks, etc).
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ThreadState.ThreadStateIdle = ThreadState("ThreadStateIdle", 0)
ThreadState.ThreadStateInUseForIO = ThreadState("ThreadStateInUseForIO", 1)
ThreadState.ThreadStateInUseForUser = ThreadState("ThreadStateInUseForUser", 2)
ThreadState.ThreadStateInUseForOther = ThreadState("ThreadStateInUseForOther", 3)
ThreadState._enumerators = { 0:ThreadState.ThreadStateIdle, 1:ThreadState.ThreadStateInUseForIO, 2:ThreadState.ThreadStateInUseForUser, 3:ThreadState.ThreadStateInUseForOther }
_M_Ice.Instrumentation._t_ThreadState = IcePy.defineEnum('::Ice::Instrumentation::ThreadState', ThreadState, (), ThreadState._enumerators)
_M_Ice.Instrumentation.ThreadState = ThreadState
del ThreadState
if 'ThreadObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ThreadObserver = Ice.createTempClass()
class ThreadObserver(_M_Ice.Instrumentation.Observer):
"""
The thread observer interface to instrument Ice threads. This can
be threads from the Ice thread pool or utility threads used by the
Ice core.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.ThreadObserver:
raise RuntimeError('Ice.Instrumentation.ThreadObserver is an abstract class')
def stateChanged(self, oldState, newState):
"""
Notification of thread state change.
Arguments:
oldState -- The previous thread state.
newState -- The new thread state.
"""
raise NotImplementedError("method 'stateChanged' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_ThreadObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_ThreadObserver = IcePy.defineValue('::Ice::Instrumentation::ThreadObserver', ThreadObserver, -1, (), False, True, None, ())
ThreadObserver._ice_type = _M_Ice.Instrumentation._t_ThreadObserver
_M_Ice.Instrumentation.ThreadObserver = ThreadObserver
del ThreadObserver
if 'ConnectionState' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ConnectionState = Ice.createTempClass()
class ConnectionState(Ice.EnumBase):
"""
The state of an Ice connection.
Enumerators:
ConnectionStateValidating -- The connection is being validated.
ConnectionStateHolding -- The connection is holding the reception of new messages.
ConnectionStateActive -- The connection is active and can send and receive messages.
ConnectionStateClosing -- The connection is being gracefully shutdown and waits for the
peer to close its end of the connection.
ConnectionStateClosed -- The connection is closed and waits for potential dispatch to be
finished before being destroyed and detached from the observer.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ConnectionState.ConnectionStateValidating = ConnectionState("ConnectionStateValidating", 0)
ConnectionState.ConnectionStateHolding = ConnectionState("ConnectionStateHolding", 1)
ConnectionState.ConnectionStateActive = ConnectionState("ConnectionStateActive", 2)
ConnectionState.ConnectionStateClosing = ConnectionState("ConnectionStateClosing", 3)
ConnectionState.ConnectionStateClosed = ConnectionState("ConnectionStateClosed", 4)
ConnectionState._enumerators = { 0:ConnectionState.ConnectionStateValidating, 1:ConnectionState.ConnectionStateHolding, 2:ConnectionState.ConnectionStateActive, 3:ConnectionState.ConnectionStateClosing, 4:ConnectionState.ConnectionStateClosed }
_M_Ice.Instrumentation._t_ConnectionState = IcePy.defineEnum('::Ice::Instrumentation::ConnectionState', ConnectionState, (), ConnectionState._enumerators)
_M_Ice.Instrumentation.ConnectionState = ConnectionState
del ConnectionState
if 'ConnectionObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ConnectionObserver = Ice.createTempClass()
class ConnectionObserver(_M_Ice.Instrumentation.Observer):
"""
The connection observer interface to instrument Ice connections.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.ConnectionObserver:
raise RuntimeError('Ice.Instrumentation.ConnectionObserver is an abstract class')
def sentBytes(self, num):
"""
Notification of sent bytes over the connection.
Arguments:
num -- The number of bytes sent.
"""
raise NotImplementedError("method 'sentBytes' not implemented")
def receivedBytes(self, num):
"""
Notification of received bytes over the connection.
Arguments:
num -- The number of bytes received.
"""
raise NotImplementedError("method 'receivedBytes' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_ConnectionObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_ConnectionObserver = IcePy.defineValue('::Ice::Instrumentation::ConnectionObserver', ConnectionObserver, -1, (), False, True, None, ())
ConnectionObserver._ice_type = _M_Ice.Instrumentation._t_ConnectionObserver
_M_Ice.Instrumentation.ConnectionObserver = ConnectionObserver
del ConnectionObserver
if 'DispatchObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.DispatchObserver = Ice.createTempClass()
class DispatchObserver(_M_Ice.Instrumentation.Observer):
"""
The dispatch observer to instrument servant dispatch.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.DispatchObserver:
raise RuntimeError('Ice.Instrumentation.DispatchObserver is an abstract class')
def userException(self):
"""
Notification of a user exception.
"""
raise NotImplementedError("method 'userException' not implemented")
def reply(self, size):
"""
Reply notification.
Arguments:
size -- The size of the reply.
"""
raise NotImplementedError("method 'reply' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_DispatchObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_DispatchObserver = IcePy.defineValue('::Ice::Instrumentation::DispatchObserver', DispatchObserver, -1, (), False, True, None, ())
DispatchObserver._ice_type = _M_Ice.Instrumentation._t_DispatchObserver
_M_Ice.Instrumentation.DispatchObserver = DispatchObserver
del DispatchObserver
if 'ChildInvocationObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ChildInvocationObserver = Ice.createTempClass()
class ChildInvocationObserver(_M_Ice.Instrumentation.Observer):
"""
The child invocation observer to instrument remote or collocated
invocations.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.ChildInvocationObserver:
raise RuntimeError('Ice.Instrumentation.ChildInvocationObserver is an abstract class')
def reply(self, size):
"""
Reply notification.
Arguments:
size -- The size of the reply.
"""
raise NotImplementedError("method 'reply' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_ChildInvocationObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_ChildInvocationObserver = IcePy.defineValue('::Ice::Instrumentation::ChildInvocationObserver', ChildInvocationObserver, -1, (), False, True, None, ())
ChildInvocationObserver._ice_type = _M_Ice.Instrumentation._t_ChildInvocationObserver
_M_Ice.Instrumentation.ChildInvocationObserver = ChildInvocationObserver
del ChildInvocationObserver
if 'RemoteObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.RemoteObserver = Ice.createTempClass()
class RemoteObserver(_M_Ice.Instrumentation.ChildInvocationObserver):
"""
The remote observer to instrument invocations that are sent over
the wire.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.RemoteObserver:
raise RuntimeError('Ice.Instrumentation.RemoteObserver is an abstract class')
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_RemoteObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_RemoteObserver = IcePy.defineValue('::Ice::Instrumentation::RemoteObserver', RemoteObserver, -1, (), False, True, None, ())
RemoteObserver._ice_type = _M_Ice.Instrumentation._t_RemoteObserver
_M_Ice.Instrumentation.RemoteObserver = RemoteObserver
del RemoteObserver
if 'CollocatedObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.CollocatedObserver = Ice.createTempClass()
class CollocatedObserver(_M_Ice.Instrumentation.ChildInvocationObserver):
"""
The collocated observer to instrument invocations that are
collocated.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.CollocatedObserver:
raise RuntimeError('Ice.Instrumentation.CollocatedObserver is an abstract class')
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_CollocatedObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_CollocatedObserver = IcePy.defineValue('::Ice::Instrumentation::CollocatedObserver', CollocatedObserver, -1, (), False, True, None, ())
CollocatedObserver._ice_type = _M_Ice.Instrumentation._t_CollocatedObserver
_M_Ice.Instrumentation.CollocatedObserver = CollocatedObserver
del CollocatedObserver
if 'InvocationObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.InvocationObserver = Ice.createTempClass()
class InvocationObserver(_M_Ice.Instrumentation.Observer):
"""
The invocation observer to instrument invocations on proxies. A
proxy invocation can either result in a collocated or remote
invocation. If it results in a remote invocation, a sub-observer is
requested for the remote invocation.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.InvocationObserver:
raise RuntimeError('Ice.Instrumentation.InvocationObserver is an abstract class')
def retried(self):
"""
Notification of the invocation being retried.
"""
raise NotImplementedError("method 'retried' not implemented")
def userException(self):
"""
Notification of a user exception.
"""
raise NotImplementedError("method 'userException' not implemented")
def getRemoteObserver(self, con, endpt, requestId, size):
"""
Get a remote observer for this invocation.
Arguments:
con -- The connection information.
endpt -- The connection endpoint.
requestId -- The ID of the invocation.
size -- The size of the invocation.
Returns: The observer to instrument the remote invocation.
"""
raise NotImplementedError("method 'getRemoteObserver' not implemented")
def getCollocatedObserver(self, adapter, requestId, size):
"""
Get a collocated observer for this invocation.
Arguments:
adapter -- The object adapter hosting the collocated Ice object.
requestId -- The ID of the invocation.
size -- The size of the invocation.
Returns: The observer to instrument the collocated invocation.
"""
raise NotImplementedError("method 'getCollocatedObserver' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_InvocationObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_InvocationObserver = IcePy.defineValue('::Ice::Instrumentation::InvocationObserver', InvocationObserver, -1, (), False, True, None, ())
InvocationObserver._ice_type = _M_Ice.Instrumentation._t_InvocationObserver
_M_Ice.Instrumentation.InvocationObserver = InvocationObserver
del InvocationObserver
if 'ObserverUpdater' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.ObserverUpdater = Ice.createTempClass()
class ObserverUpdater(object):
"""
The observer updater interface. This interface is implemented by
the Ice run-time and an instance of this interface is provided by
the Ice communicator on initialization to the
CommunicatorObserver object set with the communicator
initialization data. The Ice communicator calls
CommunicatorObserver#setObserverUpdater to provide the observer
updater.
This interface can be used by add-ins implementing the
CommunicatorObserver interface to update the observers of
connections and threads.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.ObserverUpdater:
raise RuntimeError('Ice.Instrumentation.ObserverUpdater is an abstract class')
def updateConnectionObservers(self):
"""
Update connection observers associated with each of the Ice
connection from the communicator and its object adapters.
When called, this method goes through all the connections and
for each connection CommunicatorObserver#getConnectionObserver
is called. The implementation of getConnectionObserver has the
possibility to return an updated observer if necessary.
"""
raise NotImplementedError("method 'updateConnectionObservers' not implemented")
def updateThreadObservers(self):
"""
Update thread observers associated with each of the Ice thread
from the communicator and its object adapters.
When called, this method goes through all the threads and for
each thread CommunicatorObserver#getThreadObserver is
called. The implementation of getThreadObserver has the
possibility to return an updated observer if necessary.
"""
raise NotImplementedError("method 'updateThreadObservers' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_ObserverUpdater)
__repr__ = __str__
_M_Ice.Instrumentation._t_ObserverUpdater = IcePy.defineValue('::Ice::Instrumentation::ObserverUpdater', ObserverUpdater, -1, (), False, True, None, ())
ObserverUpdater._ice_type = _M_Ice.Instrumentation._t_ObserverUpdater
_M_Ice.Instrumentation.ObserverUpdater = ObserverUpdater
del ObserverUpdater
if 'CommunicatorObserver' not in _M_Ice.Instrumentation.__dict__:
_M_Ice.Instrumentation.CommunicatorObserver = Ice.createTempClass()
class CommunicatorObserver(object):
"""
The communicator observer interface used by the Ice run-time to
obtain and update observers for its observable objects. This
interface should be implemented by add-ins that wish to observe Ice
objects in order to collect statistics. An instance of this
interface can be provided to the Ice run-time through the Ice
communicator initialization data.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Instrumentation.CommunicatorObserver:
raise RuntimeError('Ice.Instrumentation.CommunicatorObserver is an abstract class')
def getConnectionEstablishmentObserver(self, endpt, connector):
"""
This method should return an observer for the given endpoint
information and connector. The Ice run-time calls this method
for each connection establishment attempt.
Arguments:
endpt -- The endpoint.
connector -- The description of the connector. For IP transports, this is typically the IP address to connect to.
Returns: The observer to instrument the connection establishment.
"""
raise NotImplementedError("method 'getConnectionEstablishmentObserver' not implemented")
def getEndpointLookupObserver(self, endpt):
"""
This method should return an observer for the given endpoint
information. The Ice run-time calls this method to resolve an
endpoint and obtain the list of connectors.
For IP endpoints, this typically involves doing a DNS lookup to
obtain the IP addresses associated with the DNS name.
Arguments:
endpt -- The endpoint.
Returns: The observer to instrument the endpoint lookup.
"""
raise NotImplementedError("method 'getEndpointLookupObserver' not implemented")
def getConnectionObserver(self, c, e, s, o):
"""
This method should return a connection observer for the given
connection. The Ice run-time calls this method for each new
connection and for all the Ice communicator connections when
ObserverUpdater#updateConnectionObservers is called.
Arguments:
c -- The connection information.
e -- The connection endpoint.
s -- The state of the connection.
o -- The old connection observer if one is already set or a null reference otherwise.
Returns: The connection observer to instrument the connection.
"""
raise NotImplementedError("method 'getConnectionObserver' not implemented")
def getThreadObserver(self, parent, id, s, o):
"""
This method should return a thread observer for the given
thread. The Ice run-time calls this method for each new thread
and for all the Ice communicator threads when
ObserverUpdater#updateThreadObservers is called.
Arguments:
parent -- The parent of the thread.
id -- The ID of the thread to observe.
s -- The state of the thread.
o -- The old thread observer if one is already set or a null reference otherwise.
Returns: The thread observer to instrument the thread.
"""
raise NotImplementedError("method 'getThreadObserver' not implemented")
def getInvocationObserver(self, prx, operation, ctx):
"""
This method should return an invocation observer for the given
invocation. The Ice run-time calls this method for each new
invocation on a proxy.
Arguments:
prx -- The proxy used for the invocation.
operation -- The name of the invocation.
ctx -- The context specified by the user.
Returns: The invocation observer to instrument the invocation.
"""
raise NotImplementedError("method 'getInvocationObserver' not implemented")
def getDispatchObserver(self, c, size):
"""
This method should return a dispatch observer for the given
dispatch. The Ice run-time calls this method each time it
receives an incoming invocation to be dispatched for an Ice
object.
Arguments:
c -- The current object as provided to the Ice servant dispatching the invocation.
size -- The size of the dispatch.
Returns: The dispatch observer to instrument the dispatch.
"""
raise NotImplementedError("method 'getDispatchObserver' not implemented")
def setObserverUpdater(self, updater):
"""
The Ice run-time calls this method when the communicator is
initialized. The add-in implementing this interface can use
this object to get the Ice run-time to re-obtain observers for
observed objects.
Arguments:
updater -- The observer updater object.
"""
raise NotImplementedError("method 'setObserverUpdater' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice.Instrumentation._t_CommunicatorObserver)
__repr__ = __str__
_M_Ice.Instrumentation._t_CommunicatorObserver = IcePy.defineValue('::Ice::Instrumentation::CommunicatorObserver', CommunicatorObserver, -1, (), False, True, None, ())
CommunicatorObserver._ice_type = _M_Ice.Instrumentation._t_CommunicatorObserver
_M_Ice.Instrumentation.CommunicatorObserver = CommunicatorObserver
del CommunicatorObserver
# End of module Ice.Instrumentation
__name__ = 'Ice'
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Instrumentation_ice.py | Instrumentation_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if '_t_PropertyDict' not in _M_Ice.__dict__:
_M_Ice._t_PropertyDict = IcePy.defineDictionary('::Ice::PropertyDict', (), IcePy._t_string, IcePy._t_string)
_M_Ice._t_PropertiesAdmin = IcePy.defineValue('::Ice::PropertiesAdmin', Ice.Value, -1, (), False, True, None, ())
if 'PropertiesAdminPrx' not in _M_Ice.__dict__:
_M_Ice.PropertiesAdminPrx = Ice.createTempClass()
class PropertiesAdminPrx(Ice.ObjectPrx):
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
context -- The request context for the invocation.
Returns: The property value.
"""
def getProperty(self, key, context=None):
return _M_Ice.PropertiesAdmin._op_getProperty.invoke(self, ((key, ), context))
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getPropertyAsync(self, key, context=None):
return _M_Ice.PropertiesAdmin._op_getProperty.invokeAsync(self, ((key, ), context))
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getProperty(self, key, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.PropertiesAdmin._op_getProperty.begin(self, ((key, ), _response, _ex, _sent, context))
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
Returns: The property value.
"""
def end_getProperty(self, _r):
return _M_Ice.PropertiesAdmin._op_getProperty.end(self, _r)
"""
Get all properties whose keys begin with prefix. If
prefix is an empty string then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
context -- The request context for the invocation.
Returns: The matching property set.
"""
def getPropertiesForPrefix(self, prefix, context=None):
return _M_Ice.PropertiesAdmin._op_getPropertiesForPrefix.invoke(self, ((prefix, ), context))
"""
Get all properties whose keys begin with prefix. If
prefix is an empty string then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getPropertiesForPrefixAsync(self, prefix, context=None):
return _M_Ice.PropertiesAdmin._op_getPropertiesForPrefix.invokeAsync(self, ((prefix, ), context))
"""
Get all properties whose keys begin with prefix. If
prefix is an empty string then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getPropertiesForPrefix(self, prefix, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.PropertiesAdmin._op_getPropertiesForPrefix.begin(self, ((prefix, ), _response, _ex, _sent, context))
"""
Get all properties whose keys begin with prefix. If
prefix is an empty string then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
Returns: The matching property set.
"""
def end_getPropertiesForPrefix(self, _r):
return _M_Ice.PropertiesAdmin._op_getPropertiesForPrefix.end(self, _r)
"""
Update the communicator's properties with the given property set.
Arguments:
newProperties -- Properties to be added, changed, or removed. If an entry in newProperties matches the name of an existing property, that property's value is replaced with the new value. If the new value is an empty string, the property is removed. Any existing properties that are not modified or removed by the entries in newProperties are retained with their original values.
context -- The request context for the invocation.
"""
def setProperties(self, newProperties, context=None):
return _M_Ice.PropertiesAdmin._op_setProperties.invoke(self, ((newProperties, ), context))
"""
Update the communicator's properties with the given property set.
Arguments:
newProperties -- Properties to be added, changed, or removed. If an entry in newProperties matches the name of an existing property, that property's value is replaced with the new value. If the new value is an empty string, the property is removed. Any existing properties that are not modified or removed by the entries in newProperties are retained with their original values.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setPropertiesAsync(self, newProperties, context=None):
return _M_Ice.PropertiesAdmin._op_setProperties.invokeAsync(self, ((newProperties, ), context))
"""
Update the communicator's properties with the given property set.
Arguments:
newProperties -- Properties to be added, changed, or removed. If an entry in newProperties matches the name of an existing property, that property's value is replaced with the new value. If the new value is an empty string, the property is removed. Any existing properties that are not modified or removed by the entries in newProperties are retained with their original values.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setProperties(self, newProperties, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.PropertiesAdmin._op_setProperties.begin(self, ((newProperties, ), _response, _ex, _sent, context))
"""
Update the communicator's properties with the given property set.
Arguments:
newProperties -- Properties to be added, changed, or removed. If an entry in newProperties matches the name of an existing property, that property's value is replaced with the new value. If the new value is an empty string, the property is removed. Any existing properties that are not modified or removed by the entries in newProperties are retained with their original values.
"""
def end_setProperties(self, _r):
return _M_Ice.PropertiesAdmin._op_setProperties.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.PropertiesAdminPrx.ice_checkedCast(proxy, '::Ice::PropertiesAdmin', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.PropertiesAdminPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::PropertiesAdmin'
_M_Ice._t_PropertiesAdminPrx = IcePy.defineProxy('::Ice::PropertiesAdmin', PropertiesAdminPrx)
_M_Ice.PropertiesAdminPrx = PropertiesAdminPrx
del PropertiesAdminPrx
_M_Ice.PropertiesAdmin = Ice.createTempClass()
class PropertiesAdmin(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::Ice::PropertiesAdmin')
def ice_id(self, current=None):
return '::Ice::PropertiesAdmin'
@staticmethod
def ice_staticId():
return '::Ice::PropertiesAdmin'
def getProperty(self, key, current=None):
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getProperty' not implemented")
def getPropertiesForPrefix(self, prefix, current=None):
"""
Get all properties whose keys begin with prefix. If
prefix is an empty string then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getPropertiesForPrefix' not implemented")
def setProperties(self, newProperties, current=None):
"""
Update the communicator's properties with the given property set.
Arguments:
newProperties -- Properties to be added, changed, or removed. If an entry in newProperties matches the name of an existing property, that property's value is replaced with the new value. If the new value is an empty string, the property is removed. Any existing properties that are not modified or removed by the entries in newProperties are retained with their original values.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'setProperties' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_PropertiesAdminDisp)
__repr__ = __str__
_M_Ice._t_PropertiesAdminDisp = IcePy.defineClass('::Ice::PropertiesAdmin', PropertiesAdmin, (), None, ())
PropertiesAdmin._ice_type = _M_Ice._t_PropertiesAdminDisp
PropertiesAdmin._op_getProperty = IcePy.Operation('getProperty', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_string, False, 0), ())
PropertiesAdmin._op_getPropertiesForPrefix = IcePy.Operation('getPropertiesForPrefix', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), _M_Ice._t_PropertyDict, False, 0), ())
PropertiesAdmin._op_setProperties = IcePy.Operation('setProperties', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_PropertyDict, False, 0),), (), None, ())
_M_Ice.PropertiesAdmin = PropertiesAdmin
del PropertiesAdmin
# End of module Ice
Ice.sliceChecksums["::Ice::PropertiesAdmin"] = "2fdc55e4b6d63dcc2fa612b79b57c6e"
Ice.sliceChecksums["::Ice::PropertyDict"] = "28c9538d4ffc29a24c3cf15fff4f329" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/PropertiesAdmin_ice.py | PropertiesAdmin_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.LoggerF_ice
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'Plugin' not in _M_Ice.__dict__:
_M_Ice.Plugin = Ice.createTempClass()
class Plugin(object):
"""
A communicator plug-in. A plug-in generally adds a feature to a
communicator, such as support for a protocol.
The communicator loads its plug-ins in two stages: the first stage
creates the plug-ins, and the second stage invokes Plugin#initialize on
each one.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Plugin:
raise RuntimeError('Ice.Plugin is an abstract class')
def initialize(self):
"""
Perform any necessary initialization steps.
"""
raise NotImplementedError("method 'initialize' not implemented")
def destroy(self):
"""
Called when the communicator is being destroyed.
"""
raise NotImplementedError("method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Plugin)
__repr__ = __str__
_M_Ice._t_Plugin = IcePy.defineValue('::Ice::Plugin', Plugin, -1, (), False, True, None, ())
Plugin._ice_type = _M_Ice._t_Plugin
_M_Ice.Plugin = Plugin
del Plugin
if 'PluginManager' not in _M_Ice.__dict__:
_M_Ice.PluginManager = Ice.createTempClass()
class PluginManager(object):
"""
Each communicator has a plug-in manager to administer the set of
plug-ins.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.PluginManager:
raise RuntimeError('Ice.PluginManager is an abstract class')
def initializePlugins(self):
"""
Initialize the configured plug-ins. The communicator automatically initializes
the plug-ins by default, but an application may need to interact directly with
a plug-in prior to initialization. In this case, the application must set
Ice.InitPlugins=0 and then invoke initializePlugins
manually. The plug-ins are initialized in the order in which they are loaded.
If a plug-in raises an exception during initialization, the communicator
invokes destroy on the plug-ins that have already been initialized.
Throws:
InitializationException -- Raised if the plug-ins have already been initialized.
"""
raise NotImplementedError("method 'initializePlugins' not implemented")
def getPlugins(self):
"""
Get a list of plugins installed.
Returns: The names of the plugins installed.
"""
raise NotImplementedError("method 'getPlugins' not implemented")
def getPlugin(self, name):
"""
Obtain a plug-in by name.
Arguments:
name -- The plug-in's name.
Returns: The plug-in.
Throws:
NotRegisteredException -- Raised if no plug-in is found with the given name.
"""
raise NotImplementedError("method 'getPlugin' not implemented")
def addPlugin(self, name, pi):
"""
Install a new plug-in.
Arguments:
name -- The plug-in's name.
pi -- The plug-in.
Throws:
AlreadyRegisteredException -- Raised if a plug-in already exists with the given name.
"""
raise NotImplementedError("method 'addPlugin' not implemented")
def destroy(self):
"""
Called when the communicator is being destroyed.
"""
raise NotImplementedError("method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_PluginManager)
__repr__ = __str__
_M_Ice._t_PluginManager = IcePy.defineValue('::Ice::PluginManager', PluginManager, -1, (), False, True, None, ())
PluginManager._ice_type = _M_Ice._t_PluginManager
_M_Ice.PluginManager = PluginManager
del PluginManager
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Plugin_ice.py | Plugin_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Identity_ice
import Ice.Process_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'AdapterNotFoundException' not in _M_Ice.__dict__:
_M_Ice.AdapterNotFoundException = Ice.createTempClass()
class AdapterNotFoundException(Ice.UserException):
"""
This exception is raised if an adapter cannot be found.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::AdapterNotFoundException'
_M_Ice._t_AdapterNotFoundException = IcePy.defineException('::Ice::AdapterNotFoundException', AdapterNotFoundException, (), False, None, ())
AdapterNotFoundException._ice_type = _M_Ice._t_AdapterNotFoundException
_M_Ice.AdapterNotFoundException = AdapterNotFoundException
del AdapterNotFoundException
if 'InvalidReplicaGroupIdException' not in _M_Ice.__dict__:
_M_Ice.InvalidReplicaGroupIdException = Ice.createTempClass()
class InvalidReplicaGroupIdException(Ice.UserException):
"""
This exception is raised if the replica group provided by the
server is invalid.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::InvalidReplicaGroupIdException'
_M_Ice._t_InvalidReplicaGroupIdException = IcePy.defineException('::Ice::InvalidReplicaGroupIdException', InvalidReplicaGroupIdException, (), False, None, ())
InvalidReplicaGroupIdException._ice_type = _M_Ice._t_InvalidReplicaGroupIdException
_M_Ice.InvalidReplicaGroupIdException = InvalidReplicaGroupIdException
del InvalidReplicaGroupIdException
if 'AdapterAlreadyActiveException' not in _M_Ice.__dict__:
_M_Ice.AdapterAlreadyActiveException = Ice.createTempClass()
class AdapterAlreadyActiveException(Ice.UserException):
"""
This exception is raised if a server tries to set endpoints for
an adapter that is already active.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::AdapterAlreadyActiveException'
_M_Ice._t_AdapterAlreadyActiveException = IcePy.defineException('::Ice::AdapterAlreadyActiveException', AdapterAlreadyActiveException, (), False, None, ())
AdapterAlreadyActiveException._ice_type = _M_Ice._t_AdapterAlreadyActiveException
_M_Ice.AdapterAlreadyActiveException = AdapterAlreadyActiveException
del AdapterAlreadyActiveException
if 'ObjectNotFoundException' not in _M_Ice.__dict__:
_M_Ice.ObjectNotFoundException = Ice.createTempClass()
class ObjectNotFoundException(Ice.UserException):
"""
This exception is raised if an object cannot be found.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ObjectNotFoundException'
_M_Ice._t_ObjectNotFoundException = IcePy.defineException('::Ice::ObjectNotFoundException', ObjectNotFoundException, (), False, None, ())
ObjectNotFoundException._ice_type = _M_Ice._t_ObjectNotFoundException
_M_Ice.ObjectNotFoundException = ObjectNotFoundException
del ObjectNotFoundException
if 'ServerNotFoundException' not in _M_Ice.__dict__:
_M_Ice.ServerNotFoundException = Ice.createTempClass()
class ServerNotFoundException(Ice.UserException):
"""
This exception is raised if a server cannot be found.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::ServerNotFoundException'
_M_Ice._t_ServerNotFoundException = IcePy.defineException('::Ice::ServerNotFoundException', ServerNotFoundException, (), False, None, ())
ServerNotFoundException._ice_type = _M_Ice._t_ServerNotFoundException
_M_Ice.ServerNotFoundException = ServerNotFoundException
del ServerNotFoundException
if 'LocatorRegistry' not in _M_Ice.__dict__:
_M_Ice._t_LocatorRegistryDisp = IcePy.declareClass('::Ice::LocatorRegistry')
_M_Ice._t_LocatorRegistryPrx = IcePy.declareProxy('::Ice::LocatorRegistry')
_M_Ice._t_Locator = IcePy.defineValue('::Ice::Locator', Ice.Value, -1, (), False, True, None, ())
if 'LocatorPrx' not in _M_Ice.__dict__:
_M_Ice.LocatorPrx = Ice.createTempClass()
class LocatorPrx(Ice.ObjectPrx):
"""
Find an object by identity and return a proxy that contains
the adapter ID or endpoints which can be used to access the
object.
Arguments:
id -- The identity.
context -- The request context for the invocation.
Returns: The proxy, or null if the object is not active.
Throws:
ObjectNotFoundException -- Raised if the object cannot be found.
"""
def findObjectById(self, id, context=None):
return _M_Ice.Locator._op_findObjectById.invoke(self, ((id, ), context))
"""
Find an object by identity and return a proxy that contains
the adapter ID or endpoints which can be used to access the
object.
Arguments:
id -- The identity.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findObjectByIdAsync(self, id, context=None):
return _M_Ice.Locator._op_findObjectById.invokeAsync(self, ((id, ), context))
"""
Find an object by identity and return a proxy that contains
the adapter ID or endpoints which can be used to access the
object.
Arguments:
id -- The identity.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findObjectById(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Locator._op_findObjectById.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Find an object by identity and return a proxy that contains
the adapter ID or endpoints which can be used to access the
object.
Arguments:
id -- The identity.
Returns: The proxy, or null if the object is not active.
Throws:
ObjectNotFoundException -- Raised if the object cannot be found.
"""
def end_findObjectById(self, _r):
return _M_Ice.Locator._op_findObjectById.end(self, _r)
"""
Find an adapter by id and return a proxy that contains
its endpoints.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Returns: The adapter proxy, or null if the adapter is not active.
Throws:
AdapterNotFoundException -- Raised if the adapter cannot be found.
"""
def findAdapterById(self, id, context=None):
return _M_Ice.Locator._op_findAdapterById.invoke(self, ((id, ), context))
"""
Find an adapter by id and return a proxy that contains
its endpoints.
Arguments:
id -- The adapter id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def findAdapterByIdAsync(self, id, context=None):
return _M_Ice.Locator._op_findAdapterById.invokeAsync(self, ((id, ), context))
"""
Find an adapter by id and return a proxy that contains
its endpoints.
Arguments:
id -- The adapter id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_findAdapterById(self, id, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Locator._op_findAdapterById.begin(self, ((id, ), _response, _ex, _sent, context))
"""
Find an adapter by id and return a proxy that contains
its endpoints.
Arguments:
id -- The adapter id.
Returns: The adapter proxy, or null if the adapter is not active.
Throws:
AdapterNotFoundException -- Raised if the adapter cannot be found.
"""
def end_findAdapterById(self, _r):
return _M_Ice.Locator._op_findAdapterById.end(self, _r)
"""
Get the locator registry.
Arguments:
context -- The request context for the invocation.
Returns: The locator registry.
"""
def getRegistry(self, context=None):
return _M_Ice.Locator._op_getRegistry.invoke(self, ((), context))
"""
Get the locator registry.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getRegistryAsync(self, context=None):
return _M_Ice.Locator._op_getRegistry.invokeAsync(self, ((), context))
"""
Get the locator registry.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getRegistry(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Locator._op_getRegistry.begin(self, ((), _response, _ex, _sent, context))
"""
Get the locator registry.
Arguments:
Returns: The locator registry.
"""
def end_getRegistry(self, _r):
return _M_Ice.Locator._op_getRegistry.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.LocatorPrx.ice_checkedCast(proxy, '::Ice::Locator', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.LocatorPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::Locator'
_M_Ice._t_LocatorPrx = IcePy.defineProxy('::Ice::Locator', LocatorPrx)
_M_Ice.LocatorPrx = LocatorPrx
del LocatorPrx
_M_Ice.Locator = Ice.createTempClass()
class Locator(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Locator', '::Ice::Object')
def ice_id(self, current=None):
return '::Ice::Locator'
@staticmethod
def ice_staticId():
return '::Ice::Locator'
def findObjectById(self, id, current=None):
"""
Find an object by identity and return a proxy that contains
the adapter ID or endpoints which can be used to access the
object.
Arguments:
id -- The identity.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ObjectNotFoundException -- Raised if the object cannot be found.
"""
raise NotImplementedError("servant method 'findObjectById' not implemented")
def findAdapterById(self, id, current=None):
"""
Find an adapter by id and return a proxy that contains
its endpoints.
Arguments:
id -- The adapter id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AdapterNotFoundException -- Raised if the adapter cannot be found.
"""
raise NotImplementedError("servant method 'findAdapterById' not implemented")
def getRegistry(self, current=None):
"""
Get the locator registry.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getRegistry' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_LocatorDisp)
__repr__ = __str__
_M_Ice._t_LocatorDisp = IcePy.defineClass('::Ice::Locator', Locator, (), None, ())
Locator._ice_type = _M_Ice._t_LocatorDisp
Locator._op_findObjectById = IcePy.Operation('findObjectById', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, True, None, (), (((), _M_Ice._t_Identity, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_Ice._t_ObjectNotFoundException,))
Locator._op_findAdapterById = IcePy.Operation('findAdapterById', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, True, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_ObjectPrx, False, 0), (_M_Ice._t_AdapterNotFoundException,))
Locator._op_getRegistry = IcePy.Operation('getRegistry', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_LocatorRegistryPrx, False, 0), ())
_M_Ice.Locator = Locator
del Locator
_M_Ice._t_LocatorRegistry = IcePy.defineValue('::Ice::LocatorRegistry', Ice.Value, -1, (), False, True, None, ())
if 'LocatorRegistryPrx' not in _M_Ice.__dict__:
_M_Ice.LocatorRegistryPrx = Ice.createTempClass()
class LocatorRegistryPrx(Ice.ObjectPrx):
"""
Set the adapter endpoints with the locator registry.
Arguments:
id -- The adapter id.
proxy -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
context -- The request context for the invocation.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
"""
def setAdapterDirectProxy(self, id, proxy, context=None):
return _M_Ice.LocatorRegistry._op_setAdapterDirectProxy.invoke(self, ((id, proxy), context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
id -- The adapter id.
proxy -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setAdapterDirectProxyAsync(self, id, proxy, context=None):
return _M_Ice.LocatorRegistry._op_setAdapterDirectProxy.invokeAsync(self, ((id, proxy), context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
id -- The adapter id.
proxy -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setAdapterDirectProxy(self, id, proxy, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LocatorRegistry._op_setAdapterDirectProxy.begin(self, ((id, proxy), _response, _ex, _sent, context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
id -- The adapter id.
proxy -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
"""
def end_setAdapterDirectProxy(self, _r):
return _M_Ice.LocatorRegistry._op_setAdapterDirectProxy.end(self, _r)
"""
Set the adapter endpoints with the locator registry.
Arguments:
adapterId -- The adapter id.
replicaGroupId -- The replica group id.
p -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
context -- The request context for the invocation.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
InvalidReplicaGroupIdException -- Raised if the given replica group doesn't match the one registered with the locator registry for this object adapter.
"""
def setReplicatedAdapterDirectProxy(self, adapterId, replicaGroupId, p, context=None):
return _M_Ice.LocatorRegistry._op_setReplicatedAdapterDirectProxy.invoke(self, ((adapterId, replicaGroupId, p), context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
adapterId -- The adapter id.
replicaGroupId -- The replica group id.
p -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setReplicatedAdapterDirectProxyAsync(self, adapterId, replicaGroupId, p, context=None):
return _M_Ice.LocatorRegistry._op_setReplicatedAdapterDirectProxy.invokeAsync(self, ((adapterId, replicaGroupId, p), context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
adapterId -- The adapter id.
replicaGroupId -- The replica group id.
p -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setReplicatedAdapterDirectProxy(self, adapterId, replicaGroupId, p, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LocatorRegistry._op_setReplicatedAdapterDirectProxy.begin(self, ((adapterId, replicaGroupId, p), _response, _ex, _sent, context))
"""
Set the adapter endpoints with the locator registry.
Arguments:
adapterId -- The adapter id.
replicaGroupId -- The replica group id.
p -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
InvalidReplicaGroupIdException -- Raised if the given replica group doesn't match the one registered with the locator registry for this object adapter.
"""
def end_setReplicatedAdapterDirectProxy(self, _r):
return _M_Ice.LocatorRegistry._op_setReplicatedAdapterDirectProxy.end(self, _r)
"""
Set the process proxy for a server.
Arguments:
id -- The server id.
proxy -- The process proxy.
context -- The request context for the invocation.
Throws:
ServerNotFoundException -- Raised if the server cannot be found.
"""
def setServerProcessProxy(self, id, proxy, context=None):
return _M_Ice.LocatorRegistry._op_setServerProcessProxy.invoke(self, ((id, proxy), context))
"""
Set the process proxy for a server.
Arguments:
id -- The server id.
proxy -- The process proxy.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def setServerProcessProxyAsync(self, id, proxy, context=None):
return _M_Ice.LocatorRegistry._op_setServerProcessProxy.invokeAsync(self, ((id, proxy), context))
"""
Set the process proxy for a server.
Arguments:
id -- The server id.
proxy -- The process proxy.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_setServerProcessProxy(self, id, proxy, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LocatorRegistry._op_setServerProcessProxy.begin(self, ((id, proxy), _response, _ex, _sent, context))
"""
Set the process proxy for a server.
Arguments:
id -- The server id.
proxy -- The process proxy.
Throws:
ServerNotFoundException -- Raised if the server cannot be found.
"""
def end_setServerProcessProxy(self, _r):
return _M_Ice.LocatorRegistry._op_setServerProcessProxy.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.LocatorRegistryPrx.ice_checkedCast(proxy, '::Ice::LocatorRegistry', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.LocatorRegistryPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::LocatorRegistry'
_M_Ice._t_LocatorRegistryPrx = IcePy.defineProxy('::Ice::LocatorRegistry', LocatorRegistryPrx)
_M_Ice.LocatorRegistryPrx = LocatorRegistryPrx
del LocatorRegistryPrx
_M_Ice.LocatorRegistry = Ice.createTempClass()
class LocatorRegistry(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::LocatorRegistry', '::Ice::Object')
def ice_id(self, current=None):
return '::Ice::LocatorRegistry'
@staticmethod
def ice_staticId():
return '::Ice::LocatorRegistry'
def setAdapterDirectProxy(self, id, proxy, current=None):
"""
Set the adapter endpoints with the locator registry.
Arguments:
id -- The adapter id.
proxy -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
"""
raise NotImplementedError("servant method 'setAdapterDirectProxy' not implemented")
def setReplicatedAdapterDirectProxy(self, adapterId, replicaGroupId, p, current=None):
"""
Set the adapter endpoints with the locator registry.
Arguments:
adapterId -- The adapter id.
replicaGroupId -- The replica group id.
p -- The adapter proxy (a dummy direct proxy created by the adapter). The direct proxy contains the adapter endpoints.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AdapterAlreadyActiveException -- Raised if an adapter with the same id is already active.
AdapterNotFoundException -- Raised if the adapter cannot be found, or if the locator only allows registered adapters to set their active proxy and the adapter is not registered with the locator.
InvalidReplicaGroupIdException -- Raised if the given replica group doesn't match the one registered with the locator registry for this object adapter.
"""
raise NotImplementedError("servant method 'setReplicatedAdapterDirectProxy' not implemented")
def setServerProcessProxy(self, id, proxy, current=None):
"""
Set the process proxy for a server.
Arguments:
id -- The server id.
proxy -- The process proxy.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
ServerNotFoundException -- Raised if the server cannot be found.
"""
raise NotImplementedError("servant method 'setServerProcessProxy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_LocatorRegistryDisp)
__repr__ = __str__
_M_Ice._t_LocatorRegistryDisp = IcePy.defineClass('::Ice::LocatorRegistry', LocatorRegistry, (), None, ())
LocatorRegistry._ice_type = _M_Ice._t_LocatorRegistryDisp
LocatorRegistry._op_setAdapterDirectProxy = IcePy.Operation('setAdapterDirectProxy', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_ObjectPrx, False, 0)), (), None, (_M_Ice._t_AdapterNotFoundException, _M_Ice._t_AdapterAlreadyActiveException))
LocatorRegistry._op_setReplicatedAdapterDirectProxy = IcePy.Operation('setReplicatedAdapterDirectProxy', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0), ((), IcePy._t_ObjectPrx, False, 0)), (), None, (_M_Ice._t_AdapterNotFoundException, _M_Ice._t_AdapterAlreadyActiveException, _M_Ice._t_InvalidReplicaGroupIdException))
LocatorRegistry._op_setServerProcessProxy = IcePy.Operation('setServerProcessProxy', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, True, None, (), (((), IcePy._t_string, False, 0), ((), _M_Ice._t_ProcessPrx, False, 0)), (), None, (_M_Ice._t_ServerNotFoundException,))
_M_Ice.LocatorRegistry = LocatorRegistry
del LocatorRegistry
_M_Ice._t_LocatorFinder = IcePy.defineValue('::Ice::LocatorFinder', Ice.Value, -1, (), False, True, None, ())
if 'LocatorFinderPrx' not in _M_Ice.__dict__:
_M_Ice.LocatorFinderPrx = Ice.createTempClass()
class LocatorFinderPrx(Ice.ObjectPrx):
"""
Get the locator proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
context -- The request context for the invocation.
Returns: The locator proxy.
"""
def getLocator(self, context=None):
return _M_Ice.LocatorFinder._op_getLocator.invoke(self, ((), context))
"""
Get the locator proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLocatorAsync(self, context=None):
return _M_Ice.LocatorFinder._op_getLocator.invokeAsync(self, ((), context))
"""
Get the locator proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLocator(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LocatorFinder._op_getLocator.begin(self, ((), _response, _ex, _sent, context))
"""
Get the locator proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
Returns: The locator proxy.
"""
def end_getLocator(self, _r):
return _M_Ice.LocatorFinder._op_getLocator.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.LocatorFinderPrx.ice_checkedCast(proxy, '::Ice::LocatorFinder', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.LocatorFinderPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::LocatorFinder'
_M_Ice._t_LocatorFinderPrx = IcePy.defineProxy('::Ice::LocatorFinder', LocatorFinderPrx)
_M_Ice.LocatorFinderPrx = LocatorFinderPrx
del LocatorFinderPrx
_M_Ice.LocatorFinder = Ice.createTempClass()
class LocatorFinder(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::LocatorFinder', '::Ice::Object')
def ice_id(self, current=None):
return '::Ice::LocatorFinder'
@staticmethod
def ice_staticId():
return '::Ice::LocatorFinder'
def getLocator(self, current=None):
"""
Get the locator proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getLocator' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_LocatorFinderDisp)
__repr__ = __str__
_M_Ice._t_LocatorFinderDisp = IcePy.defineClass('::Ice::LocatorFinder', LocatorFinder, (), None, ())
LocatorFinder._ice_type = _M_Ice._t_LocatorFinderDisp
LocatorFinder._op_getLocator = IcePy.Operation('getLocator', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Ice._t_LocatorPrx, False, 0), ())
_M_Ice.LocatorFinder = LocatorFinder
del LocatorFinder
# End of module Ice
Ice.sliceChecksums["::Ice::AdapterAlreadyActiveException"] = "bf5f24acc569fea5251d9f8532a83ab8"
Ice.sliceChecksums["::Ice::AdapterNotFoundException"] = "55f1a93f62d3937d94533ad2894ad9c"
Ice.sliceChecksums["::Ice::InvalidReplicaGroupIdException"] = "7876104b5711f2b49ae078686a03f"
Ice.sliceChecksums["::Ice::Locator"] = "5efd321bc74cc794fed432e3f5186d9"
Ice.sliceChecksums["::Ice::LocatorFinder"] = "19b7a40de7be4cae27f4f8d867bd682"
Ice.sliceChecksums["::Ice::LocatorRegistry"] = "e9f8ca2c8ce174f9214961e8596b7ed"
Ice.sliceChecksums["::Ice::ObjectNotFoundException"] = "23fe4ef042d6496b97c4e2313f6c4675"
Ice.sliceChecksums["::Ice::ServerNotFoundException"] = "bafa988368a55c1471e3c7be16baa74" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Locator_ice.py | Locator_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
if 'ValueFactory' not in _M_Ice.__dict__:
_M_Ice.ValueFactory = Ice.createTempClass()
class ValueFactory(object):
"""
A factory for values. Value factories are used in several
places, such as when Ice receives a class instance and
when Freeze restores a persistent value. Value factories
must be implemented by the application writer and registered
with the communicator.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.ValueFactory:
raise RuntimeError('Ice.ValueFactory is an abstract class')
def create(self, type):
"""
Create a new value for a given value type. The type is the
absolute Slice type id, i.e., the id relative to the
unnamed top-level Slice module. For example, the absolute
Slice type id for an interface Bar in the module
Foo is "::Foo::Bar".
Note that the leading "::" is required.
Arguments:
type -- The value type.
Returns: The value created for the given type, or nil if the factory is unable to create the value.
"""
raise NotImplementedError("method 'create' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ValueFactory)
__repr__ = __str__
_M_Ice._t_ValueFactory = IcePy.defineValue('::Ice::ValueFactory', ValueFactory, -1, (), False, True, None, ())
ValueFactory._ice_type = _M_Ice._t_ValueFactory
_M_Ice.ValueFactory = ValueFactory
del ValueFactory
if 'ValueFactoryManager' not in _M_Ice.__dict__:
_M_Ice.ValueFactoryManager = Ice.createTempClass()
class ValueFactoryManager(object):
"""
A value factory manager maintains a collection of value factories.
An application can supply a custom implementation during communicator
initialization, otherwise Ice provides a default implementation.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.ValueFactoryManager:
raise RuntimeError('Ice.ValueFactoryManager is an abstract class')
def add(self, factory, id):
"""
Add a value factory. Attempting to add a factory with an id for
which a factory is already registered throws AlreadyRegisteredException.
When unmarshaling an Ice value, the Ice run time reads the
most-derived type id off the wire and attempts to create an
instance of the type using a factory. If no instance is created,
either because no factory was found, or because all factories
returned nil, the behavior of the Ice run time depends on the
format with which the value was marshaled:
If the value uses the "sliced" format, Ice ascends the class
hierarchy until it finds a type that is recognized by a factory,
or it reaches the least-derived type. If no factory is found that
can create an instance, the run time throws NoValueFactoryException.
If the value uses the "compact" format, Ice immediately raises
NoValueFactoryException.
The following order is used to locate a factory for a type:
The Ice run-time looks for a factory registered
specifically for the type.
If no instance has been created, the Ice run-time looks
for the default factory, which is registered with an empty type id.
If no instance has been created by any of the preceding
steps, the Ice run-time looks for a factory that may have been
statically generated by the language mapping for non-abstract classes.
Arguments:
factory -- The factory to add.
id -- The type id for which the factory can create instances, or an empty string for the default factory.
"""
raise NotImplementedError("method 'add' not implemented")
def find(self, id):
"""
Find an value factory registered with this communicator.
Arguments:
id -- The type id for which the factory can create instances, or an empty string for the default factory.
Returns: The value factory, or null if no value factory was found for the given id.
"""
raise NotImplementedError("method 'find' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ValueFactoryManager)
__repr__ = __str__
_M_Ice._t_ValueFactoryManager = IcePy.defineValue('::Ice::ValueFactoryManager', ValueFactoryManager, -1, (), False, True, None, ())
ValueFactoryManager._ice_type = _M_Ice._t_ValueFactoryManager
_M_Ice.ValueFactoryManager = ValueFactoryManager
del ValueFactoryManager
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/ValueFactory_ice.py | ValueFactory_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
_M_Ice._t_Process = IcePy.defineValue('::Ice::Process', Ice.Value, -1, (), False, True, None, ())
if 'ProcessPrx' not in _M_Ice.__dict__:
_M_Ice.ProcessPrx = Ice.createTempClass()
class ProcessPrx(Ice.ObjectPrx):
"""
Initiate a graceful shut-down.
Arguments:
context -- The request context for the invocation.
"""
def shutdown(self, context=None):
return _M_Ice.Process._op_shutdown.invoke(self, ((), context))
"""
Initiate a graceful shut-down.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def shutdownAsync(self, context=None):
return _M_Ice.Process._op_shutdown.invokeAsync(self, ((), context))
"""
Initiate a graceful shut-down.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_shutdown(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Process._op_shutdown.begin(self, ((), _response, _ex, _sent, context))
"""
Initiate a graceful shut-down.
Arguments:
"""
def end_shutdown(self, _r):
return _M_Ice.Process._op_shutdown.end(self, _r)
"""
Write a message on the process' stdout or stderr.
Arguments:
message -- The message.
fd -- 1 for stdout, 2 for stderr.
context -- The request context for the invocation.
"""
def writeMessage(self, message, fd, context=None):
return _M_Ice.Process._op_writeMessage.invoke(self, ((message, fd), context))
"""
Write a message on the process' stdout or stderr.
Arguments:
message -- The message.
fd -- 1 for stdout, 2 for stderr.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def writeMessageAsync(self, message, fd, context=None):
return _M_Ice.Process._op_writeMessage.invokeAsync(self, ((message, fd), context))
"""
Write a message on the process' stdout or stderr.
Arguments:
message -- The message.
fd -- 1 for stdout, 2 for stderr.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_writeMessage(self, message, fd, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Process._op_writeMessage.begin(self, ((message, fd), _response, _ex, _sent, context))
"""
Write a message on the process' stdout or stderr.
Arguments:
message -- The message.
fd -- 1 for stdout, 2 for stderr.
"""
def end_writeMessage(self, _r):
return _M_Ice.Process._op_writeMessage.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.ProcessPrx.ice_checkedCast(proxy, '::Ice::Process', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.ProcessPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::Process'
_M_Ice._t_ProcessPrx = IcePy.defineProxy('::Ice::Process', ProcessPrx)
_M_Ice.ProcessPrx = ProcessPrx
del ProcessPrx
_M_Ice.Process = Ice.createTempClass()
class Process(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::Ice::Process')
def ice_id(self, current=None):
return '::Ice::Process'
@staticmethod
def ice_staticId():
return '::Ice::Process'
def shutdown(self, current=None):
"""
Initiate a graceful shut-down.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'shutdown' not implemented")
def writeMessage(self, message, fd, current=None):
"""
Write a message on the process' stdout or stderr.
Arguments:
message -- The message.
fd -- 1 for stdout, 2 for stderr.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'writeMessage' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ProcessDisp)
__repr__ = __str__
_M_Ice._t_ProcessDisp = IcePy.defineClass('::Ice::Process', Process, (), None, ())
Process._ice_type = _M_Ice._t_ProcessDisp
Process._op_shutdown = IcePy.Operation('shutdown', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
Process._op_writeMessage = IcePy.Operation('writeMessage', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0)), (), None, ())
_M_Ice.Process = Process
del Process
# End of module Ice
Ice.sliceChecksums["::Ice::Process"] = "e3a9673d486a5bf031844cac6f9d287e" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Process_ice.py | Process_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
if '_t_BoolSeq' not in _M_Ice.__dict__:
_M_Ice._t_BoolSeq = IcePy.defineSequence('::Ice::BoolSeq', (), IcePy._t_bool)
if '_t_ByteSeq' not in _M_Ice.__dict__:
_M_Ice._t_ByteSeq = IcePy.defineSequence('::Ice::ByteSeq', (), IcePy._t_byte)
if '_t_ShortSeq' not in _M_Ice.__dict__:
_M_Ice._t_ShortSeq = IcePy.defineSequence('::Ice::ShortSeq', (), IcePy._t_short)
if '_t_IntSeq' not in _M_Ice.__dict__:
_M_Ice._t_IntSeq = IcePy.defineSequence('::Ice::IntSeq', (), IcePy._t_int)
if '_t_LongSeq' not in _M_Ice.__dict__:
_M_Ice._t_LongSeq = IcePy.defineSequence('::Ice::LongSeq', (), IcePy._t_long)
if '_t_FloatSeq' not in _M_Ice.__dict__:
_M_Ice._t_FloatSeq = IcePy.defineSequence('::Ice::FloatSeq', (), IcePy._t_float)
if '_t_DoubleSeq' not in _M_Ice.__dict__:
_M_Ice._t_DoubleSeq = IcePy.defineSequence('::Ice::DoubleSeq', (), IcePy._t_double)
if '_t_StringSeq' not in _M_Ice.__dict__:
_M_Ice._t_StringSeq = IcePy.defineSequence('::Ice::StringSeq', (), IcePy._t_string)
if '_t_ObjectSeq' not in _M_Ice.__dict__:
_M_Ice._t_ObjectSeq = IcePy.defineSequence('::Ice::ObjectSeq', (), IcePy._t_Value)
if '_t_ObjectProxySeq' not in _M_Ice.__dict__:
_M_Ice._t_ObjectProxySeq = IcePy.defineSequence('::Ice::ObjectProxySeq', (), IcePy._t_ObjectPrx)
# End of module Ice
Ice.sliceChecksums["::Ice::BoolSeq"] = "321b1d4186eaf796937e82eed711fe7f"
Ice.sliceChecksums["::Ice::ByteSeq"] = "eae189e6d7b57b6f9628e78293d6d7"
Ice.sliceChecksums["::Ice::DoubleSeq"] = "7fb8b78b3ab4b2e358d27dc4d6f1e330"
Ice.sliceChecksums["::Ice::FloatSeq"] = "eb848491fad1518427fd58a7330958e"
Ice.sliceChecksums["::Ice::IntSeq"] = "826eab2e95b89b0476198b6a36991dc"
Ice.sliceChecksums["::Ice::LongSeq"] = "325a51044cdee10b14ecc3c1672a86"
Ice.sliceChecksums["::Ice::ObjectProxySeq"] = "45ccc241c2bbfebfbd031e733a5832"
Ice.sliceChecksums["::Ice::ObjectSeq"] = "a1e80bc6b87e9687455a4faa4c2f295"
Ice.sliceChecksums["::Ice::ShortSeq"] = "32aadcc785b88fc7198ae3d262ff3acd"
Ice.sliceChecksums["::Ice::StringSeq"] = "7986f19514e3b04fbe4af53c7446e563" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/BuiltinSequences_ice.py | BuiltinSequences_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.PropertiesAdmin_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'Properties' not in _M_Ice.__dict__:
_M_Ice.Properties = Ice.createTempClass()
class Properties(object):
"""
A property set used to configure Ice and Ice applications.
Properties are key/value pairs, with both keys and values
being strings. By convention, property keys should have the form
application-name\[.category\[.sub-category]].name.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Properties:
raise RuntimeError('Ice.Properties is an abstract class')
def getProperty(self, key):
"""
Get a property by key. If the property is not set, an empty
string is returned.
Arguments:
key -- The property key.
Returns: The property value.
"""
raise NotImplementedError("method 'getProperty' not implemented")
def getPropertyWithDefault(self, key, value):
"""
Get a property by key. If the property is not set, the
given default value is returned.
Arguments:
key -- The property key.
value -- The default value to use if the property does not exist.
Returns: The property value or the default value.
"""
raise NotImplementedError("method 'getPropertyWithDefault' not implemented")
def getPropertyAsInt(self, key):
"""
Get a property as an integer. If the property is not set, 0
is returned.
Arguments:
key -- The property key.
Returns: The property value interpreted as an integer.
"""
raise NotImplementedError("method 'getPropertyAsInt' not implemented")
def getPropertyAsIntWithDefault(self, key, value):
"""
Get a property as an integer. If the property is not set, the
given default value is returned.
Arguments:
key -- The property key.
value -- The default value to use if the property does not exist.
Returns: The property value interpreted as an integer, or the default value.
"""
raise NotImplementedError("method 'getPropertyAsIntWithDefault' not implemented")
def getPropertyAsList(self, key):
"""
Get a property as a list of strings. The strings must be
separated by whitespace or comma. If the property is not set,
an empty list is returned. The strings in the list can contain
whitespace and commas if they are enclosed in single or double
quotes. If quotes are mismatched, an empty list is returned.
Within single quotes or double quotes, you can escape the
quote in question with \, e.g. O'Reilly can be written as
O'Reilly, "O'Reilly" or 'O\'Reilly'.
Arguments:
key -- The property key.
Returns: The property value interpreted as a list of strings.
"""
raise NotImplementedError("method 'getPropertyAsList' not implemented")
def getPropertyAsListWithDefault(self, key, value):
"""
Get a property as a list of strings. The strings must be
separated by whitespace or comma. If the property is not set,
the default list is returned. The strings in the list can contain
whitespace and commas if they are enclosed in single or double
quotes. If quotes are mismatched, the default list is returned.
Within single quotes or double quotes, you can escape the
quote in question with \, e.g. O'Reilly can be written as
O'Reilly, "O'Reilly" or 'O\'Reilly'.
Arguments:
key -- The property key.
value -- The default value to use if the property is not set.
Returns: The property value interpreted as list of strings, or the default value.
"""
raise NotImplementedError("method 'getPropertyAsListWithDefault' not implemented")
def getPropertiesForPrefix(self, prefix):
"""
Get all properties whose keys begins with
prefix. If
prefix is an empty string,
then all properties are returned.
Arguments:
prefix -- The prefix to search for (empty string if none).
Returns: The matching property set.
"""
raise NotImplementedError("method 'getPropertiesForPrefix' not implemented")
def setProperty(self, key, value):
"""
Set a property. To unset a property, set it to
the empty string.
Arguments:
key -- The property key.
value -- The property value.
"""
raise NotImplementedError("method 'setProperty' not implemented")
def getCommandLineOptions(self):
"""
Get a sequence of command-line options that is equivalent to
this property set. Each element of the returned sequence is
a command-line option of the form
--key=value.
Returns: The command line options for this property set.
"""
raise NotImplementedError("method 'getCommandLineOptions' not implemented")
def parseCommandLineOptions(self, prefix, options):
"""
Convert a sequence of command-line options into properties.
All options that begin with
--prefix. are
converted into properties. If the prefix is empty, all options
that begin with -- are converted to properties.
Arguments:
prefix -- The property prefix, or an empty string to convert all options starting with --.
options -- The command-line options.
Returns: The command-line options that do not start with the specified prefix, in their original order.
"""
raise NotImplementedError("method 'parseCommandLineOptions' not implemented")
def parseIceCommandLineOptions(self, options):
"""
Convert a sequence of command-line options into properties.
All options that begin with one of the following prefixes
are converted into properties: --Ice, --IceBox, --IceGrid,
--IcePatch2, --IceSSL, --IceStorm, --Freeze, and --Glacier2.
Arguments:
options -- The command-line options.
Returns: The command-line options that do not start with one of the listed prefixes, in their original order.
"""
raise NotImplementedError("method 'parseIceCommandLineOptions' not implemented")
def load(self, file):
"""
Load properties from a file.
Arguments:
file -- The property file.
"""
raise NotImplementedError("method 'load' not implemented")
def clone(self):
"""
Create a copy of this property set.
Returns: A copy of this property set.
"""
raise NotImplementedError("method 'clone' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Properties)
__repr__ = __str__
_M_Ice._t_Properties = IcePy.defineValue('::Ice::Properties', Properties, -1, (), False, True, None, ())
Properties._ice_type = _M_Ice._t_Properties
_M_Ice.Properties = Properties
del Properties
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Properties_ice.py | Properties_ice.py |
import sys, string, os, threading, warnings, datetime, logging, time, inspect, traceback, types, array
#
# RTTI problems can occur in C++ code unless we modify Python's dlopen flags.
# Note that changing these flags might cause problems for other extensions
# loaded by the application (see bug 3660), so we restore the original settings
# after loading IcePy.
#
_dlopenflags = -1
try:
_dlopenflags = sys.getdlopenflags()
try:
import dl
sys.setdlopenflags(dl.RTLD_NOW|dl.RTLD_GLOBAL)
except ImportError:
#
# If the dl module is not available and we're running on a Linux
# platform, use the hard coded value of RTLD_NOW|RTLD_GLOBAL.
#
if sys.platform.startswith("linux"):
sys.setdlopenflags(258)
pass
except AttributeError:
#
# sys.getdlopenflags() is not supported (we're probably running on Windows).
#
pass
#
# Import the Python extension.
#
import IcePy
#
# Restore the dlopen flags.
#
if _dlopenflags >= 0:
sys.setdlopenflags(_dlopenflags)
#
# Give the extension an opportunity to clean up before a graceful exit.
#
import atexit
atexit.register(IcePy.cleanup)
#
# Add some symbols to the Ice module.
#
ObjectPrx = IcePy.ObjectPrx
stringVersion = IcePy.stringVersion
intVersion = IcePy.intVersion
currentProtocol = IcePy.currentProtocol
currentProtocolEncoding = IcePy.currentProtocolEncoding
currentEncoding = IcePy.currentEncoding
stringToProtocolVersion = IcePy.stringToProtocolVersion
protocolVersionToString = IcePy.protocolVersionToString
stringToEncodingVersion = IcePy.stringToEncodingVersion
encodingVersionToString = IcePy.encodingVersionToString
generateUUID = IcePy.generateUUID
loadSlice = IcePy.loadSlice
AsyncResult = IcePy.AsyncResult
Unset = IcePy.Unset
def Python35():
return sys.version_info[:2] >= (3, 5)
if Python35():
from Ice.Py3.IceFuture import FutureBase, wrap_future
else:
FutureBase = object
class Future(FutureBase):
def __init__(self):
self._result = None
self._exception = None
self._condition = threading.Condition()
self._doneCallbacks = []
self._state = Future.StateRunning
def cancel(self):
callbacks = []
with self._condition:
if self._state == Future.StateDone:
return False
if self._state == Future.StateCancelled:
return True
self._state = Future.StateCancelled
callbacks = self._doneCallbacks
self._doneCallbacks = []
self._condition.notify_all()
self._callCallbacks(callbacks)
return True
def cancelled(self):
with self._condition:
return self._state == Future.StateCancelled
def running(self):
with self._condition:
return self._state == Future.StateRunning
def done(self):
with self._condition:
return self._state in [Future.StateCancelled, Future.StateDone]
def add_done_callback(self, fn):
with self._condition:
if self._state == Future.StateRunning:
self._doneCallbacks.append(fn)
return
fn(self)
def result(self, timeout=None):
with self._condition:
if not self._wait(timeout, lambda: self._state == Future.StateRunning):
raise TimeoutException()
if self._state == Future.StateCancelled:
raise InvocationCanceledException()
elif self._exception:
raise self._exception
else:
return self._result
def exception(self, timeout=None):
with self._condition:
if not self._wait(timeout, lambda: self._state == Future.StateRunning):
raise TimeoutException()
if self._state == Future.StateCancelled:
raise InvocationCanceledException()
else:
return self._exception
def set_result(self, result):
callbacks = []
with self._condition:
if self._state != Future.StateRunning:
return
self._result = result
self._state = Future.StateDone
callbacks = self._doneCallbacks
self._doneCallbacks = []
self._condition.notify_all()
self._callCallbacks(callbacks)
def set_exception(self, ex):
callbacks = []
with self._condition:
if self._state != Future.StateRunning:
return
self._exception = ex
self._state = Future.StateDone
callbacks = self._doneCallbacks
self._doneCallbacks = []
self._condition.notify_all()
self._callCallbacks(callbacks)
@staticmethod
def completed(result):
f = Future()
f.set_result(result)
return f
def _wait(self, timeout, testFn=None):
# Must be called with _condition acquired
while testFn():
if timeout:
start = time.time()
self._condition.wait(timeout)
# Subtract the elapsed time so far from the timeout
timeout -= (time.time() - start)
if timeout <= 0:
return False
else:
self._condition.wait()
return True
def _callCallbacks(self, callbacks):
for callback in callbacks:
try:
callback(self)
except:
self._warn('done callback raised exception')
def _warn(self, msg):
logging.getLogger("Ice.Future").exception(msg)
StateRunning = 'running'
StateCancelled = 'cancelled'
StateDone = 'done'
class InvocationFuture(Future):
def __init__(self, operation, asyncResult):
Future.__init__(self)
assert(asyncResult)
self._operation = operation
self._asyncResult = asyncResult # May be None for a batch invocation.
self._sent = False
self._sentSynchronously = False
self._sentCallbacks = []
def cancel(self):
self._asyncResult.cancel()
return Future.cancel(self)
def add_done_callback_async(self, fn):
def callback():
try:
fn(self)
except:
self._warn('done callback raised exception')
with self._condition:
if self._state == Future.StateRunning:
self._doneCallbacks.append(fn)
return
self._asyncResult.callLater(callback)
def is_sent(self):
with self._condition:
return self._sent
def is_sent_synchronously(self):
with self._condition:
return self._sentSynchronously
def add_sent_callback(self, fn):
with self._condition:
if not self._sent:
self._sentCallbacks.append(fn)
return
fn(self, self._sentSynchronously)
def add_sent_callback_async(self, fn):
def callback():
try:
fn(self, self._sentSynchronously)
except:
self._warn('sent callback raised exception')
with self._condition:
if not self._sent:
self._sentCallbacks.append(fn)
return
self._asyncResult.callLater(callback)
def sent(self, timeout=None):
with self._condition:
if not self._wait(timeout, lambda: not self._sent):
raise TimeoutException()
if self._state == Future.StateCancelled:
raise InvocationCanceledException()
elif self._exception:
raise self._exception
else:
return self._sentSynchronously
def set_sent(self, sentSynchronously):
callbacks = []
with self._condition:
if self._sent:
return
self._sent = True
self._sentSynchronously = sentSynchronously
callbacks = self._sentCallbacks
self._sentCallbacks = []
self._condition.notify_all()
for callback in callbacks:
try:
callback(self, sentSynchronously)
except Exception:
self._warn('sent callback raised exception')
def operation(self):
return self._operation
def proxy(self):
return self._asyncResult.getProxy()
def connection(self):
return self._asyncResult.getConnection()
def communicator(self):
return self._asyncResult.getCommunicator()
def _warn(self, msg):
communicator = self.communicator()
if communicator:
if communicator.getProperties().getPropertyAsIntWithDefault("Ice.Warn.AMICallback", 1) > 0:
communicator.getLogger().warning("Ice.Future: " + msg + ":\n" + traceback.format_exc())
else:
logging.getLogger("Ice.Future").exception(msg)
#
# This value is used as the default value for struct types in the constructors
# of user-defined types. It allows us to determine whether the application has
# supplied a value. (See bug 3676)
#
_struct_marker = object()
#
# Core Ice types.
#
class Value(object):
def ice_id(self):
'''Obtains the type id corresponding to the most-derived Slice
interface supported by the target object.
Returns:
The type id.
'''
return '::Ice::Object'
@staticmethod
def ice_staticId():
'''Obtains the type id of this Slice class or interface.
Returns:
The type id.
'''
return '::Ice::Object'
#
# Do not define these here. They will be invoked if defined by a subclass.
#
#def ice_preMarshal(self):
# pass
#
#def ice_postUnmarshal(self):
# pass
def ice_getSlicedData(self):
'''Returns the sliced data if the value has a preserved-slice base class and has been sliced during
un-marshaling of the value, null is returned otherwise.
Returns:
The sliced data or null.
'''
return getattr(self, "_ice_slicedData", None);
class InterfaceByValue(Value):
def __init__(self, id):
self.id = id
def ice_id(self):
return self.id
class Object(object):
def ice_isA(self, id, current=None):
'''Determines whether the target object supports the interface denoted
by the given Slice type id.
Arguments:
id The Slice type id
Returns:
True if the target object supports the interface, or False otherwise.
'''
return id in self.ice_ids(current)
def ice_ping(self, current=None):
'''A reachability test for the target object.'''
pass
def ice_ids(self, current=None):
'''Obtains the type ids corresponding to the Slice interface
that are supported by the target object.
Returns:
A list of type ids.
'''
return [ self.ice_id(current) ]
def ice_id(self, current=None):
'''Obtains the type id corresponding to the most-derived Slice
interface supported by the target object.
Returns:
The type id.
'''
return '::Ice::Object'
@staticmethod
def ice_staticId():
'''Obtains the type id of this Slice class or interface.
Returns:
The type id.
'''
return '::Ice::Object'
def _iceDispatch(self, cb, method, args):
# Invoke the given servant method. Exceptions can propagate to the caller.
result = method(*args)
# Check for a future.
if isinstance(result, Future) or callable(getattr(result, "add_done_callback", None)):
def handler(future):
try:
cb.response(future.result())
except:
cb.exception(sys.exc_info()[1])
result.add_done_callback(handler)
elif Python35() and inspect.iscoroutine(result): # The iscoroutine() function was added in Python 3.5.
self._iceDispatchCoroutine(cb, result)
else:
cb.response(result)
def _iceDispatchCoroutine(self, cb, coro, value=None, exception=None):
try:
if exception:
result = coro.throw(exception)
else:
result = coro.send(value)
if result is None:
# The result can be None if the coroutine performs a bare yield (such as asyncio.sleep(0))
cb.response(None)
elif isinstance(result, Future) or callable(getattr(result, "add_done_callback", None)):
# If we've received a future from the coroutine setup a done callback to continue the dispatching
# when the future completes.
def handler(future):
try:
self._iceDispatchCoroutine(cb, coro, value=future.result())
except:
self._iceDispatchCoroutine(cb, coro, exception=sys.exc_info()[1])
result.add_done_callback(handler)
else:
raise RuntimeError('unexpected value of type ' + str(type(result)) + ' provided by coroutine')
except StopIteration as ex:
# StopIteration is raised when the coroutine completes.
cb.response(ex.value)
except:
cb.exception(sys.exc_info()[1])
class Blobject(Object):
'''Special-purpose servant base class that allows a subclass to
handle synchronous Ice invocations as "blobs" of bytes.'''
def ice_invoke(self, bytes, current):
'''Dispatch a synchronous Ice invocation. The operation's
arguments are encoded in the bytes argument. The return
value must be a tuple of two values: the first is a
boolean indicating whether the operation succeeded (True)
or raised a user exception (False), and the second is
the encoded form of the operation's results or the user
exception.
'''
pass
class BlobjectAsync(Object):
'''Special-purpose servant base class that allows a subclass to
handle asynchronous Ice invocations as "blobs" of bytes.'''
def ice_invoke(self, bytes, current):
'''Dispatch an asynchronous Ice invocation. The operation's
arguments are encoded in the bytes argument. The result must be
a tuple of two values: the first is a boolean indicating whether the
operation succeeded (True) or raised a user exception (False), and
the second is the encoded form of the operation's results or the user
exception. The subclass can either return the tuple directly (for
synchronous completion) or return a future that is eventually
completed with the tuple.
'''
pass
#
# Exceptions.
#
class Exception(Exception): # Derives from built-in base 'Exception' class.
'''The base class for all Ice exceptions.'''
def __str__(self):
return self.__class__.__name__
def ice_name(self):
'''Returns the type name of this exception.'''
return self.ice_id()[2:]
def ice_id(self):
'''Returns the type id of this exception.'''
return self._ice_id
class LocalException(Exception):
'''The base class for all Ice run-time exceptions.'''
def __init__(self, args=''):
self.args = args
class UserException(Exception):
'''The base class for all user-defined exceptions.'''
def ice_getSlicedData(self):
'''Returns the sliced data if the value has a preserved-slice base class and has been sliced during
un-marshaling of the value, null is returned otherwise.
Returns:
The sliced data or null.
'''
return getattr(self, "_ice_slicedData", None);
class EnumBase(object):
def __init__(self, _n, _v):
self._name = _n
self._value = _v
def __str__(self):
return self._name
__repr__ = __str__
def __hash__(self):
return self._value
def __lt__(self, other):
if isinstance(other, self.__class__):
return self._value < other._value
elif other == None:
return False
return NotImplemented
def __le__(self, other):
if isinstance(other, self.__class__):
return self._value <= other._value
elif other is None:
return False
return NotImplemented
def __eq__(self, other):
if isinstance(other, self.__class__):
return self._value == other._value
elif other == None:
return False
return NotImplemented
def __ne__(self, other):
if isinstance(other, self.__class__):
return self._value != other._value
elif other == None:
return False
return NotImplemented
def __gt__(self, other):
if isinstance(other, self.__class__):
return self._value > other._value;
elif other == None:
return False
return NotImplemented
def __ge__(self, other):
if isinstance(other, self.__class__):
return self._value >= other._value
elif other == None:
return False
return NotImplemented
def _getName(self):
return self._name
def _getValue(self):
return self._value
name = property(_getName)
value = property(_getValue)
class SlicedData(object):
#
# Members:
#
# slices - tuple of SliceInfo
#
pass
class SliceInfo(object):
#
# Members:
#
# typeId - string
# compactId - int
# bytes - string/bytes
# instances - tuple of Ice.Value
# hasOptionalMembers - boolean
# isLastSlice - boolean
pass
#
# Native PropertiesAdmin admin facet.
#
NativePropertiesAdmin = IcePy.NativePropertiesAdmin
class PropertiesAdminUpdateCallback(object):
'''Callback class to get notifications of property updates passed
through the Properties admin facet'''
def updated(self, props):
pass
class UnknownSlicedValue(Value):
#
# Members:
#
# unknownTypeId - string
def ice_id(self):
return self.unknownTypeId
def getSliceDir():
'''Convenience function for locating the directory containing the Slice files.'''
#
# Get the parent of the directory containing this file (__init__.py).
#
pyHome = os.path.join(os.path.dirname(__file__), "..")
#
# Detect setup.py installation in site-packages. The slice
# files live one level above this file.
#
dir = os.path.join(pyHome, "slice")
if os.path.isdir(dir):
return dir
#
# For an installation from a source distribution, a binary tarball, or a
# Windows installer, the "slice" directory is a sibling of the "python"
# directory.
#
dir = os.path.join(pyHome, "..", "slice")
if os.path.exists(dir):
return os.path.normpath(dir)
#
# In a source distribution, the "slice" directory is an extra level higher.
# directory.
#
dir = os.path.join(pyHome, "..", "..", "slice")
if os.path.exists(dir):
return os.path.normpath(dir)
if sys.platform[:5] == "linux":
#
# Check the default Linux location.
#
dir = os.path.join("/", "usr", "share", "ice", "slice")
if os.path.exists(dir):
return dir
elif sys.platform == "darwin":
#
# Check the default macOS homebrew location.
#
dir = os.path.join("/", "usr", "local", "share", "ice", "slice")
if os.path.exists(dir):
return dir
return None
#
# Utilities for use by generated code.
#
_pendingModules = {}
def openModule(name):
global _pendingModules
if name in sys.modules:
result = sys.modules[name]
elif name in _pendingModules:
result = _pendingModules[name]
else:
result = createModule(name)
return result
def createModule(name):
global _pendingModules
l = name.split(".")
curr = ''
mod = None
for s in l:
curr = curr + s
if curr in sys.modules:
mod = sys.modules[curr]
elif curr in _pendingModules:
mod = _pendingModules[curr]
else:
nmod = types.ModuleType(curr)
_pendingModules[curr] = nmod
mod = nmod
curr = curr + "."
return mod
def updateModule(name):
global _pendingModules
if name in _pendingModules:
pendingModule = _pendingModules[name]
mod = sys.modules[name]
mod.__dict__.update(pendingModule.__dict__)
del _pendingModules[name]
def updateModules():
global _pendingModules
for name in _pendingModules.keys():
if name in sys.modules:
sys.modules[name].__dict__.update(_pendingModules[name].__dict__)
else:
sys.modules[name] = _pendingModules[name]
_pendingModules = {}
def createTempClass():
class __temp: pass
return __temp
class FormatType(object):
def __init__(self, val):
assert(val >= 0 and val < 3)
self.value = val
FormatType.DefaultFormat = FormatType(0)
FormatType.CompactFormat = FormatType(1)
FormatType.SlicedFormat = FormatType(2)
#
# Forward declarations.
#
IcePy._t_Object = IcePy.declareClass('::Ice::Object')
IcePy._t_Value = IcePy.declareValue('::Ice::Object')
IcePy._t_ObjectPrx = IcePy.declareProxy('::Ice::Object')
IcePy._t_LocalObject = IcePy.declareValue('::Ice::LocalObject')
#
# Slice checksum dictionary.
#
sliceChecksums = {}
#
# Import generated Ice modules.
#
import Ice.BuiltinSequences_ice
import Ice.Current_ice
import Ice.Communicator_ice
import Ice.ImplicitContext_ice
import Ice.Endpoint_ice
import Ice.EndpointTypes_ice
import Ice.Identity_ice
import Ice.LocalException_ice
import Ice.Locator_ice
import Ice.Logger_ice
import Ice.ObjectAdapter_ice
import Ice.ObjectFactory_ice
import Ice.ValueFactory_ice
import Ice.Process_ice
import Ice.Properties_ice
import Ice.RemoteLogger_ice
import Ice.Router_ice
import Ice.ServantLocator_ice
import Ice.Connection_ice
import Ice.Version_ice
import Ice.Instrumentation_ice
import Ice.Metrics_ice
#
# Replace EndpointInfo with our implementation.
#
del EndpointInfo
EndpointInfo = IcePy.EndpointInfo
del IPEndpointInfo
IPEndpointInfo = IcePy.IPEndpointInfo
del TCPEndpointInfo
TCPEndpointInfo = IcePy.TCPEndpointInfo
del UDPEndpointInfo
UDPEndpointInfo = IcePy.UDPEndpointInfo
del WSEndpointInfo
WSEndpointInfo = IcePy.WSEndpointInfo
del OpaqueEndpointInfo
OpaqueEndpointInfo = IcePy.OpaqueEndpointInfo
SSLEndpointInfo = IcePy.SSLEndpointInfo
#
# Replace ConnectionInfo with our implementation.
#
del ConnectionInfo
ConnectionInfo = IcePy.ConnectionInfo
del IPConnectionInfo
IPConnectionInfo = IcePy.IPConnectionInfo
del TCPConnectionInfo
TCPConnectionInfo = IcePy.TCPConnectionInfo
del UDPConnectionInfo
UDPConnectionInfo = IcePy.UDPConnectionInfo
del WSConnectionInfo
WSConnectionInfo = IcePy.WSConnectionInfo
SSLConnectionInfo = IcePy.SSLConnectionInfo
class ThreadNotification(object):
'''Base class for thread notification callbacks. A subclass must
define the start and stop methods.'''
def __init__(self):
pass
def start():
'''Invoked in the context of a thread created by the Ice run time.'''
pass
def stop():
'''Invoked in the context of an Ice run-time thread that is about
to terminate.'''
pass
class BatchRequestInterceptor(object):
'''Base class for batch request interceptor. A subclass must
define the enqueue method.'''
def __init__(self):
pass
def enqueue(self, request, queueCount, queueSize):
'''Invoked when a request is batched.'''
pass
#
# Initialization data.
#
class InitializationData(object):
'''The attributes of this class are used to initialize a new
communicator instance. The supported attributes are as follows:
properties: An instance of Ice.Properties. You can use the
Ice.createProperties function to create a new property set.
logger: An instance of Ice.Logger.
threadStart: A callable that is invoked for each new Ice thread that is started.
threadStop: A callable that is invoked when an Ice thread is stopped.
dispatcher: A callable that is invoked when Ice needs to dispatch an activity. The callable
receives two arguments: a callable and an Ice.Connection object. The dispatcher must
eventually invoke the callable with no arguments.
batchRequestInterceptor: A callable that will be invoked when a batch request is queued.
The callable receives three arguments: a BatchRequest object, an integer representing
the number of requests in the queue, and an integer representing the number of bytes
consumed by the requests in the queue. The interceptor must eventually invoke the
enqueue method on the BatchRequest object.
valueFactoryManager: An object that implements ValueFactoryManager.
'''
def __init__(self):
self.properties = None
self.logger = None
self.threadHook = None # Deprecated.
self.threadStart = None
self.threadStop = None
self.dispatcher = None
self.batchRequestInterceptor = None
self.valueFactoryManager = None
#
# Communicator wrapper.
#
class CommunicatorI(Communicator):
def __init__(self, impl):
self._impl = impl
impl._setWrapper(self)
def __enter__(self):
return self
def __exit__(self, type, value, traceback):
self._impl.destroy()
def getImpl(self):
return self._impl
def destroy(self):
self._impl.destroy()
def shutdown(self):
self._impl.shutdown()
def waitForShutdown(self):
#
# If invoked by the main thread, waitForShutdown only blocks for
# the specified timeout in order to give us a chance to handle
# signals.
#
while not self._impl.waitForShutdown(500):
pass
def isShutdown(self):
return self._impl.isShutdown()
def stringToProxy(self, str):
return self._impl.stringToProxy(str)
def proxyToString(self, obj):
return self._impl.proxyToString(obj)
def propertyToProxy(self, str):
return self._impl.propertyToProxy(str)
def proxyToProperty(self, obj, str):
return self._impl.proxyToProperty(obj, str)
def stringToIdentity(self, str):
return self._impl.stringToIdentity(str)
def identityToString(self, ident):
return self._impl.identityToString(ident)
def createObjectAdapter(self, name):
adapter = self._impl.createObjectAdapter(name)
return ObjectAdapterI(adapter)
def createObjectAdapterWithEndpoints(self, name, endpoints):
adapter = self._impl.createObjectAdapterWithEndpoints(name, endpoints)
return ObjectAdapterI(adapter)
def createObjectAdapterWithRouter(self, name, router):
adapter = self._impl.createObjectAdapterWithRouter(name, router)
return ObjectAdapterI(adapter)
def addObjectFactory(self, factory, id):
# The extension implementation requires an extra argument that is a value factory
self._impl.addObjectFactory(factory, id, lambda s, factory=factory: factory.create(s))
def findObjectFactory(self, id):
return self._impl.findObjectFactory(id)
def getValueFactoryManager(self):
return self._impl.getValueFactoryManager()
def getImplicitContext(self):
context = self._impl.getImplicitContext()
if context == None:
return None;
else:
return ImplicitContextI(context)
def getProperties(self):
properties = self._impl.getProperties()
return PropertiesI(properties)
def getLogger(self):
logger = self._impl.getLogger()
if isinstance(logger, Logger):
return logger
else:
return LoggerI(logger)
def getStats(self):
raise RuntimeError("operation `getStats' not implemented")
def getDefaultRouter(self):
return self._impl.getDefaultRouter()
def setDefaultRouter(self, rtr):
self._impl.setDefaultRouter(rtr)
def getDefaultLocator(self):
return self._impl.getDefaultLocator()
def setDefaultLocator(self, loc):
self._impl.setDefaultLocator(loc)
def getPluginManager(self):
raise RuntimeError("operation `getPluginManager' not implemented")
def flushBatchRequests(self, compress):
self._impl.flushBatchRequests(compress)
def flushBatchRequestsAsync(self, compress):
return self._impl.flushBatchRequestsAsync(compress)
def begin_flushBatchRequests(self, compress, _ex=None, _sent=None):
return self._impl.begin_flushBatchRequests(compress, _ex, _sent)
def end_flushBatchRequests(self, r):
return self._impl.end_flushBatchRequests(r)
def createAdmin(self, adminAdapter, adminIdentity):
return self._impl.createAdmin(adminAdapter, adminIdentity)
def getAdmin(self):
return self._impl.getAdmin()
def addAdminFacet(self, servant, facet):
self._impl.addAdminFacet(servant, facet)
def findAdminFacet(self, facet):
return self._impl.findAdminFacet(facet)
def findAllAdminFacets(self):
return self._impl.findAllAdminFacets()
def removeAdminFacet(self, facet):
return self._impl.removeAdminFacet(facet)
#
# Ice.initialize()
#
def initialize(args=None, data=None):
'''Initializes a new communicator. The optional arguments represent
an argument list (such as sys.argv) and an instance of InitializationData.
You can invoke this function as follows:
Ice.initialize()
Ice.initialize(args)
Ice.initialize(data)
Ice.initialize(args, data)
If you supply an argument list, the function removes those arguments from
the list that were recognized by the Ice run time.
'''
communicator = IcePy.Communicator(args, data)
return CommunicatorI(communicator)
#
# Ice.identityToString
#
def identityToString(id, toStringMode=None):
return IcePy.identityToString(id, toStringMode)
#
# Ice.stringToIdentity
#
def stringToIdentity(str):
return IcePy.stringToIdentity(str)
#
# ObjectAdapter wrapper.
#
class ObjectAdapterI(ObjectAdapter):
def __init__(self, impl):
self._impl = impl
def getName(self):
return self._impl.getName()
def getCommunicator(self):
communicator = self._impl.getCommunicator()
return communicator._getWrapper()
def activate(self):
self._impl.activate()
def hold(self):
self._impl.hold()
def waitForHold(self):
#
# If invoked by the main thread, waitForHold only blocks for
# the specified timeout in order to give us a chance to handle
# signals.
#
while not self._impl.waitForHold(1000):
pass
def deactivate(self):
self._impl.deactivate()
def waitForDeactivate(self):
#
# If invoked by the main thread, waitForDeactivate only blocks for
# the specified timeout in order to give us a chance to handle
# signals.
#
while not self._impl.waitForDeactivate(1000):
pass
def isDeactivated(self):
self._impl.isDeactivated()
def destroy(self):
self._impl.destroy()
def add(self, servant, id):
return self._impl.add(servant, id)
def addFacet(self, servant, id, facet):
return self._impl.addFacet(servant, id, facet)
def addWithUUID(self, servant):
return self._impl.addWithUUID(servant)
def addFacetWithUUID(self, servant, facet):
return self._impl.addFacetWIthUUID(servant, facet)
def addDefaultServant(self, servant, category):
self._impl.addDefaultServant(servant, category)
def remove(self, id):
return self._impl.remove(id)
def removeFacet(self, id, facet):
return self._impl.removeFacet(id, facet)
def removeAllFacets(self, id):
return self._impl.removeAllFacets(id)
def removeDefaultServant(self, category):
return self._impl.removeDefaultServant(category)
def find(self, id):
return self._impl.find(id)
def findFacet(self, id, facet):
return self._impl.findFacet(id, facet)
def findAllFacets(self, id):
return self._impl.findAllFacets(id)
def findByProxy(self, proxy):
return self._impl.findByProxy(proxy)
def findDefaultServant(self, category):
return self._impl.findDefaultServant(category)
def addServantLocator(self, locator, category):
self._impl.addServantLocator(locator, category)
def removeServantLocator(self, category):
return self._impl.removeServantLocator(category)
def findServantLocator(self, category):
return self._impl.findServantLocator(category)
def createProxy(self, id):
return self._impl.createProxy(id)
def createDirectProxy(self, id):
return self._impl.createDirectProxy(id)
def createIndirectProxy(self, id):
return self._impl.createIndirectProxy(id)
def createReverseProxy(self, id):
return self._impl.createReverseProxy(id)
def setLocator(self, loc):
self._impl.setLocator(loc)
def getLocator(self):
return self._impl.getLocator()
def getEndpoints(self):
return self._impl.getEndpoints()
def refreshPublishedEndpoints(self):
self._impl.refreshPublishedEndpoints()
def getPublishedEndpoints(self):
return self._impl.getPublishedEndpoints()
def setPublishedEndpoints(self, newEndpoints):
self._impl.setPublishedEndpoints(newEndpoints)
#
# Logger wrapper.
#
class LoggerI(Logger):
def __init__(self, impl):
self._impl = impl
def _print(self, message):
return self._impl._print(message)
def trace(self, category, message):
return self._impl.trace(category, message)
def warning(self, message):
return self._impl.warning(message)
def error(self, message):
return self._impl.error(message)
def getPrefix(self):
return self._impl.getPrefix()
def cloneWithPrefix(self, prefix):
logger = self._impl.cloneWithPrefix(prefix)
return LoggerI(logger)
#
# Properties wrapper.
#
class PropertiesI(Properties):
def __init__(self, impl):
self._impl = impl
def getProperty(self, key):
return self._impl.getProperty(key)
def getPropertyWithDefault(self, key, value):
return self._impl.getPropertyWithDefault(key, value)
def getPropertyAsInt(self, key):
return self._impl.getPropertyAsInt(key)
def getPropertyAsIntWithDefault(self, key, value):
return self._impl.getPropertyAsIntWithDefault(key, value)
def getPropertyAsList(self, key):
return self._impl.getPropertyAsList(key)
def getPropertyAsListWithDefault(self, key, value):
return self._impl.getPropertyAsListWithDefault(key, value)
def getPropertiesForPrefix(self, prefix):
return self._impl.getPropertiesForPrefix(prefix)
def setProperty(self, key, value):
self._impl.setProperty(key, value)
def getCommandLineOptions(self):
return self._impl.getCommandLineOptions()
def parseCommandLineOptions(self, prefix, options):
return self._impl.parseCommandLineOptions(prefix, options)
def parseIceCommandLineOptions(self, options):
return self._impl.parseIceCommandLineOptions(options)
def load(self, file):
self._impl.load(file)
def clone(self):
properties = self._impl.clone()
return PropertiesI(properties)
def __iter__(self):
dict = self._impl.getPropertiesForPrefix('')
return iter(dict)
def __str__(self):
return str(self._impl)
#
# Ice.createProperties()
#
def createProperties(args=None, defaults=None):
'''Creates a new property set. The optional arguments represent
an argument list (such as sys.argv) and a property set that supplies
default values. You can invoke this function as follows:
Ice.createProperties()
Ice.createProperties(args)
Ice.createProperties(defaults)
Ice.createProperties(args, defaults)
If you supply an argument list, the function removes those arguments
from the list that were recognized by the Ice run time.
'''
properties = IcePy.createProperties(args, defaults)
return PropertiesI(properties)
#
# Ice.getProcessLogger()
# Ice.setProcessLogger()
#
def getProcessLogger():
'''Returns the default logger object.'''
logger = IcePy.getProcessLogger()
if isinstance(logger, Logger):
return logger
else:
return LoggerI(logger)
def setProcessLogger(logger):
'''Sets the default logger object.'''
IcePy.setProcessLogger(logger)
#
# ImplicitContext wrapper
#
class ImplicitContextI(ImplicitContext):
def __init__(self, impl):
self._impl = impl
def setContext(self, ctx):
self._impl.setContext(ctx)
def getContext(self):
return self._impl.getContext()
def containsKey(self, key):
return self._impl.containsKey(key)
def get(self, key):
return self._impl.get(key)
def put(self, key, value):
return self._impl.put(key, value)
def remove(self, key):
return self._impl.remove(key)
#
# Its not possible to block in a python signal handler since this
# blocks the main thread from doing further work. As such we queue the
# signal with a worker thread which then "dispatches" the signal to
# the registered callback object.
#
# Note the interface is the same as the C++ CtrlCHandler
# implementation, however, the implementation is different.
#
class CtrlCHandler(threading.Thread):
# Class variable referring to the one and only handler for use
# from the signal handling callback.
_self = None
def __init__(self):
threading.Thread.__init__(self)
if CtrlCHandler._self != None:
raise RuntimeError("Only a single instance of a CtrlCHandler can be instantiated.")
CtrlCHandler._self = self
# State variables. These are not class static variables.
self._condVar = threading.Condition()
self._queue = []
self._done = False
self._callback = None
#
# Setup and install signal handlers
#
if 'SIGHUP' in signal.__dict__:
signal.signal(signal.SIGHUP, CtrlCHandler.signalHandler)
if 'SIGBREAK' in signal.__dict__:
signal.signal(signal.SIGBREAK, CtrlCHandler.signalHandler)
signal.signal(signal.SIGINT, CtrlCHandler.signalHandler)
signal.signal(signal.SIGTERM, CtrlCHandler.signalHandler)
# Start the thread once everything else is done.
self.start()
# Dequeue and dispatch signals.
def run(self):
while True:
self._condVar.acquire()
while len(self._queue) == 0 and not self._done:
self._condVar.wait()
if self._done:
self._condVar.release()
break
sig, callback = self._queue.pop()
self._condVar.release()
if callback:
callback(sig)
# Destroy the object. Wait for the thread to terminate and cleanup
# the internal state.
def destroy(self):
self._condVar.acquire()
self._done = True
self._condVar.notify()
self._condVar.release()
# Wait for the thread to terminate
self.join()
#
# Cleanup any state set by the CtrlCHandler.
#
if 'SIGHUP' in signal.__dict__:
signal.signal(signal.SIGHUP, signal.SIG_DFL)
if 'SIGBREAK' in signal.__dict__:
signal.signal(signal.SIGBREAK, signal.SIG_DFL)
signal.signal(signal.SIGINT, signal.SIG_DFL)
signal.signal(signal.SIGTERM, signal.SIG_DFL)
CtrlCHandler._self = None
def setCallback(self, callback):
self._condVar.acquire()
self._callback = callback
self._condVar.release()
def getCallback(self):
self._condVar.acquire()
callback = self._callback
self._condVar.release()
return callback
# Private. Only called by the signal handling mechanism.
def signalHandler(self, sig, frame):
self._self._condVar.acquire()
#
# The signal AND the current callback are queued together.
#
self._self._queue.append([sig, self._self._callback])
self._self._condVar.notify()
self._self._condVar.release()
signalHandler = classmethod(signalHandler)
#
# Application logger.
#
class _ApplicationLoggerI(Logger):
def __init__(self, prefix):
if len(prefix) > 0:
self._prefix = prefix + ": "
else:
self._prefix = ""
self._outputMutex = threading.Lock()
def _print(self, message):
s = "[ " + str(datetime.datetime.now()) + " " + self._prefix
self._outputMutex.acquire()
sys.stderr.write(message + "\n")
self._outputMutex.release()
def trace(self, category, message):
s = "[ " + str(datetime.datetime.now()) + " " + self._prefix
if len(category) > 0:
s += category + ": "
s += message + " ]"
s = s.replace("\n", "\n ")
self._outputMutex.acquire()
sys.stderr.write(s + "\n")
self._outputMutex.release()
def warning(self, message):
self._outputMutex.acquire()
sys.stderr.write(str(datetime.datetime.now()) + " " + self._prefix + "warning: " + message + "\n")
self._outputMutex.release()
def error(self, message):
self._outputMutex.acquire()
sys.stderr.write(str(datetime.datetime.now()) + " " + self._prefix + "error: " + message + "\n")
self._outputMutex.release()
#
# Application class.
#
import signal
class Application(object):
'''Convenience class that initializes a communicator and reacts
gracefully to signals. An application must define a subclass
of this class and supply an implementation of the run method.
'''
def __init__(self, signalPolicy=0): # HandleSignals=0
'''The constructor accepts an optional argument indicating
whether to handle signals. The value should be either
Application.HandleSignals (the default) or
Application.NoSignalHandling.
'''
if type(self) == Application:
raise RuntimeError("Ice.Application is an abstract class")
Application._signalPolicy = signalPolicy
def main(self, args, configFile=None, initData=None):
'''The main entry point for the Application class. The arguments
are an argument list (such as sys.argv), the name of an Ice
configuration file (optional), and an instance of
InitializationData (optional). This method does not return
until after the completion of the run method. The return
value is an integer representing the exit status.
'''
if Application._communicator:
getProcessLogger().error(args[0] + ": only one instance of the Application class can be used")
return 1
#
# We parse the properties here to extract Ice.ProgramName.
#
if not initData:
initData = InitializationData()
if configFile:
try:
initData.properties = createProperties(None, initData.properties)
initData.properties.load(configFile)
except:
getProcessLogger().error(traceback.format_exc())
return 1
initData.properties = createProperties(args, initData.properties)
#
# If the process logger is the default logger, we replace it with a
# a logger which is using the program name for the prefix.
#
if isinstance(getProcessLogger(), LoggerI):
setProcessLogger(_ApplicationLoggerI(initData.properties.getProperty("Ice.ProgramName")))
#
# Install our handler for the signals we are interested in. We assume main()
# is called from the main thread.
#
if Application._signalPolicy == Application.HandleSignals:
Application._ctrlCHandler = CtrlCHandler()
try:
Application._interrupted = False
Application._appName = initData.properties.getPropertyWithDefault("Ice.ProgramName", args[0])
Application._application = self
#
# Used by _destroyOnInterruptCallback and _shutdownOnInterruptCallback.
#
Application._nohup = initData.properties.getPropertyAsInt("Ice.Nohup") > 0
#
# The default is to destroy when a signal is received.
#
if Application._signalPolicy == Application.HandleSignals:
Application.destroyOnInterrupt()
status = self.doMain(args, initData)
except:
getProcessLogger().error(traceback.format_exc())
status = 1
#
# Set _ctrlCHandler to 0 only once communicator.destroy() has
# completed.
#
if Application._signalPolicy == Application.HandleSignals:
Application._ctrlCHandler.destroy()
Application._ctrlCHandler = None
return status
def doMain(self, args, initData):
try:
Application._communicator = initialize(args, initData)
Application._destroyed = False
status = self.run(args)
except:
getProcessLogger().error(traceback.format_exc())
status = 1
#
# Don't want any new interrupt and at this point (post-run),
# it would not make sense to release a held signal to run
# shutdown or destroy.
#
if Application._signalPolicy == Application.HandleSignals:
Application.ignoreInterrupt()
Application._condVar.acquire()
while Application._callbackInProgress:
Application._condVar.wait()
if Application._destroyed:
Application._communicator = None
else:
Application._destroyed = True
#
# And _communicator != 0, meaning will be destroyed
# next, _destroyed = true also ensures that any
# remaining callback won't do anything
#
Application._application = None
Application._condVar.release()
if Application._communicator:
try:
Application._communicator.destroy()
except:
getProcessLogger().error(traceback.format_exc())
status = 1
Application._communicator = None
return status
def run(self, args):
'''This method must be overridden in a subclass. The base
class supplies an argument list from which all Ice arguments
have already been removed. The method returns an integer
exit status (0 is success, non-zero is failure).
'''
raise RuntimeError('run() not implemented')
def interruptCallback(self, sig):
'''Subclass hook to intercept an interrupt.'''
pass
def appName(self):
'''Returns the application name (the first element of
the argument list).'''
return self._appName
appName = classmethod(appName)
def communicator(self):
'''Returns the communicator that was initialized for
the application.'''
return self._communicator
communicator = classmethod(communicator)
def destroyOnInterrupt(self):
'''Configures the application to destroy its communicator
when interrupted by a signal.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() == self._holdInterruptCallback:
self._released = True
self._condVar.notify()
self._ctrlCHandler.setCallback(self._destroyOnInterruptCallback)
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
destroyOnInterrupt = classmethod(destroyOnInterrupt)
def shutdownOnInterrupt(self):
'''Configures the application to shutdown its communicator
when interrupted by a signal.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() == self._holdInterruptCallback:
self._released = True
self._condVar.notify()
self._ctrlCHandler.setCallback(self._shutdownOnInterruptCallback)
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
shutdownOnInterrupt = classmethod(shutdownOnInterrupt)
def ignoreInterrupt(self):
'''Configures the application to ignore signals.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() == self._holdInterruptCallback:
self._released = True
self._condVar.notify()
self._ctrlCHandler.setCallback(None)
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
ignoreInterrupt = classmethod(ignoreInterrupt)
def callbackOnInterrupt(self):
'''Configures the application to invoke interruptCallback
when interrupted by a signal.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() == self._holdInterruptCallback:
self._released = True
self._condVar.notify()
self._ctrlCHandler.setCallback(self._callbackOnInterruptCallback)
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
callbackOnInterrupt = classmethod(callbackOnInterrupt)
def holdInterrupt(self):
'''Configures the application to queue an interrupt for
later processing.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() != self._holdInterruptCallback:
self._previousCallback = self._ctrlCHandler.getCallback()
self._released = False
self._ctrlCHandler.setCallback(self._holdInterruptCallback)
# else, we were already holding signals
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
holdInterrupt = classmethod(holdInterrupt)
def releaseInterrupt(self):
'''Instructs the application to process any queued interrupt.'''
if Application._signalPolicy == Application.HandleSignals:
self._condVar.acquire()
if self._ctrlCHandler.getCallback() == self._holdInterruptCallback:
#
# Note that it's very possible no signal is held;
# in this case the callback is just replaced and
# setting _released to true and signalling _condVar
# do no harm.
#
self._released = True
self._ctrlCHandler.setCallback(self._previousCallback)
self._condVar.notify()
# Else nothing to release.
self._condVar.release()
else:
getProcessLogger().error(Application._appName + \
": warning: interrupt method called on Application configured to not handle interrupts.")
releaseInterrupt = classmethod(releaseInterrupt)
def interrupted(self):
'''Returns True if the application was interrupted by a
signal, or False otherwise.'''
self._condVar.acquire()
result = self._interrupted
self._condVar.release()
return result
interrupted = classmethod(interrupted)
def _holdInterruptCallback(self, sig):
self._condVar.acquire()
while not self._released:
self._condVar.wait()
if self._destroyed:
#
# Being destroyed by main thread
#
self._condVar.release()
return
callback = self._ctrlCHandler.getCallback()
self._condVar.release()
if callback:
callback(sig)
_holdInterruptCallback = classmethod(_holdInterruptCallback)
def _destroyOnInterruptCallback(self, sig):
self._condVar.acquire()
if self._destroyed or self._nohup and sig == signal.SIGHUP:
#
# Being destroyed by main thread, or nohup.
#
self._condVar.release()
return
self._callbackInProcess = True
self._interrupted = True
self._destroyed = True
self._condVar.release()
try:
self._communicator.destroy()
except:
getProcessLogger().error(self._appName + " (while destroying in response to signal " + str(sig) + "):" + \
traceback.format_exc())
self._condVar.acquire()
self._callbackInProcess = False
self._condVar.notify()
self._condVar.release()
_destroyOnInterruptCallback = classmethod(_destroyOnInterruptCallback)
def _shutdownOnInterruptCallback(self, sig):
self._condVar.acquire()
if self._destroyed or self._nohup and sig == signal.SIGHUP:
#
# Being destroyed by main thread, or nohup.
#
self._condVar.release()
return
self._callbackInProcess = True
self._interrupted = True
self._condVar.release()
try:
self._communicator.shutdown()
except:
getProcessLogger().error(self._appName + " (while shutting down in response to signal " + str(sig) + \
"):" + traceback.format_exc())
self._condVar.acquire()
self._callbackInProcess = False
self._condVar.notify()
self._condVar.release()
_shutdownOnInterruptCallback = classmethod(_shutdownOnInterruptCallback)
def _callbackOnInterruptCallback(self, sig):
self._condVar.acquire()
if self._destroyed:
#
# Being destroyed by main thread.
#
self._condVar.release()
return
# For SIGHUP the user callback is always called. It can decide
# what to do.
self._callbackInProcess = True
self._interrupted = True
self._condVar.release()
try:
self._application.interruptCallback(sig)
except:
getProcessLogger().error(self._appName + " (while interrupting in response to signal " + str(sig) + \
"):" + traceback.format_exc())
self._condVar.acquire()
self._callbackInProcess = False
self._condVar.notify()
self._condVar.release()
_callbackOnInterruptCallback = classmethod(_callbackOnInterruptCallback)
HandleSignals = 0
NoSignalHandling = 1
_appName = None
_communicator = None
_application = None
_ctrlCHandler = None
_previousCallback = None
_interrupted = False
_released = False
_destroyed = False
_callbackInProgress = False
_condVar = threading.Condition()
_signalPolicy = HandleSignals
#
# Define Ice::Object and Ice::ObjectPrx.
#
IcePy._t_Object = IcePy.defineClass('::Ice::Object', Object, (), None, ())
IcePy._t_Value = IcePy.defineValue('::Ice::Object', Value, -1, (), False, False, None, ())
IcePy._t_ObjectPrx = IcePy.defineProxy('::Ice::Object', ObjectPrx)
Object._ice_type = IcePy._t_Object
Object._op_ice_isA = IcePy.Operation('ice_isA', OperationMode.Idempotent, OperationMode.Nonmutating, False, None, (), (((), IcePy._t_string, False, 0),), (), ((), IcePy._t_bool, False, 0), ())
Object._op_ice_ping = IcePy.Operation('ice_ping', OperationMode.Idempotent, OperationMode.Nonmutating, False, None, (), (), (), None, ())
Object._op_ice_ids = IcePy.Operation('ice_ids', OperationMode.Idempotent, OperationMode.Nonmutating, False, None, (), (), (), ((), _t_StringSeq, False, 0), ())
Object._op_ice_id = IcePy.Operation('ice_id', OperationMode.Idempotent, OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_string, False, 0), ())
IcePy._t_LocalObject = IcePy.defineValue('::Ice::LocalObject', object, -1, (), False, False, None, ())
IcePy._t_UnknownSlicedValue = IcePy.defineValue('::Ice::UnknownSlicedValue', UnknownSlicedValue, -1, (), True, False, None, ())
UnknownSlicedValue._ice_type = IcePy._t_UnknownSlicedValue
#
# Annotate some exceptions.
#
def SyscallException__str__(self):
return "Ice.SyscallException:\n" + os.strerror(self.error)
SyscallException.__str__ = SyscallException__str__
del SyscallException__str__
def SocketException__str__(self):
return "Ice.SocketException:\n" + os.strerror(self.error)
SocketException.__str__ = SocketException__str__
del SocketException__str__
def ConnectFailedException__str__(self):
return "Ice.ConnectFailedException:\n" + os.strerror(self.error)
ConnectFailedException.__str__ = ConnectFailedException__str__
del ConnectFailedException__str__
def ConnectionRefusedException__str__(self):
return "Ice.ConnectionRefusedException:\n" + os.strerror(self.error)
ConnectionRefusedException.__str__ = ConnectionRefusedException__str__
del ConnectionRefusedException__str__
def ConnectionLostException__str__(self):
if self.error == 0:
return "Ice.ConnectionLostException:\nrecv() returned zero"
else:
return "Ice.ConnectionLostException:\n" + os.strerror(self.error)
ConnectionLostException.__str__ = ConnectionLostException__str__
del ConnectionLostException__str__
#
# Proxy comparison functions.
#
def proxyIdentityEqual(lhs, rhs):
'''Determines whether the identities of two proxies are equal.'''
return proxyIdentityCompare(lhs, rhs) == 0
def proxyIdentityCompare(lhs, rhs):
'''Compares the identities of two proxies.'''
if (lhs and not isinstance(lhs, ObjectPrx)) or (rhs and not isinstance(rhs, ObjectPrx)):
raise ValueError('argument is not a proxy')
if not lhs and not rhs:
return 0
elif not lhs and rhs:
return -1
elif lhs and not rhs:
return 1
else:
lid = lhs.ice_getIdentity()
rid = rhs.ice_getIdentity()
return (lid > rid) - (lid < rid)
def proxyIdentityAndFacetEqual(lhs, rhs):
'''Determines whether the identities and facets of two
proxies are equal.'''
return proxyIdentityAndFacetCompare(lhs, rhs) == 0
def proxyIdentityAndFacetCompare(lhs, rhs):
'''Compares the identities and facets of two proxies.'''
if (lhs and not isinstance(lhs, ObjectPrx)) or (rhs and not isinstance(rhs, ObjectPrx)):
raise ValueError('argument is not a proxy')
if not lhs and not rhs:
return 0
elif not lhs and rhs:
return -1
elif lhs and not rhs:
return 1
elif lhs.ice_getIdentity() != rhs.ice_getIdentity():
lid = lhs.ice_getIdentity()
rid = rhs.ice_getIdentity()
return (lid > rid) - (lid < rid)
else:
lf = lhs.ice_getFacet()
rf = rhs.ice_getFacet()
return (lf > rf) - (lf < rf)
#
# Used by generated code. Defining these in the Ice module means the generated code
# can avoid the need to qualify the type() and hash() functions with their module
# names. Since the functions are in the __builtin__ module (for Python 2.x) and the
# builtins module (for Python 3.x), it's easier to define them here.
#
def getType(o):
return type(o)
#
# Used by generated code. Defining this in the Ice module means the generated code
# can avoid the need to qualify the hash() function with its module name. Since
# the function is in the __builtin__ module (for Python 2.x) and the builtins
# module (for Python 3.x), it's easier to define it here.
#
def getHash(o):
return hash(o)
Protocol_1_0 = ProtocolVersion(1, 0)
Encoding_1_0 = EncodingVersion(1, 0)
Encoding_1_1 = EncodingVersion(1, 1)
BuiltinBool = 0
BuiltinByte = 1
BuiltinShort = 2
BuiltinInt = 3
BuiltinLong = 4
BuiltinFloat = 5
BuiltinDouble = 6
BuiltinTypes = [BuiltinBool, BuiltinByte, BuiltinShort, BuiltinInt, BuiltinLong, BuiltinFloat, BuiltinDouble]
BuiltinArrayTypes = ["b", "b", "h", "i", "q", "f", "d"]
#
# The array "q" type specifier is new in Python 3.3
#
if sys.version_info[:2] >= (3, 3):
def createArray(view, t, copy):
if t not in BuiltinTypes:
raise ValueError("`{0}' is not an array builtin type".format(t))
a = array.array(BuiltinArrayTypes[t])
a.frombytes(view)
return a
#
# The array.frombytes method is new in Python 3.2
#
elif sys.version_info[:2] >= (3, 2):
def createArray(view, t, copy):
if t not in BuiltinTypes:
raise ValueError("`{0}' is not an array builtin type".format(t))
elif t == BuiltinLong:
raise ValueError("array.array 'q' specifier is only supported with Python >= 3.3")
a = array.array(BuiltinArrayTypes[t])
a.frombytes(view)
return a
else:
def createArray(view, t, copy):
if t not in BuiltinTypes:
raise ValueError("`{0}' is not an array builtin type".format(t))
elif t == BuiltinLong:
raise ValueError("array.array 'q' specifier is only supported with Python >= 3.3")
a = array.array(BuiltinArrayTypes[t])
a.fromstring(str(view.tobytes()))
return a
try:
import numpy
BuiltinNumpyTypes = [numpy.bool_, numpy.int8, numpy.int16, numpy.int32, numpy.int64, numpy.float32, numpy.float64]
#
# With Python2.7 we cannot initialize numpy array from memoryview
# See: https://github.com/numpy/numpy/issues/5935
#
if sys.version_info[0] >= 3:
def createNumPyArray(view, t, copy):
if t not in BuiltinTypes:
raise ValueError("`{0}' is not an array builtin type".format(t))
return numpy.frombuffer(view.tobytes() if copy else view, BuiltinNumpyTypes[t])
else:
def createNumPyArray(view, t, copy):
if t not in BuiltinTypes:
raise ValueError("`{0}' is not an array builtin type".format(t))
return numpy.frombuffer(view.tobytes(), BuiltinNumpyTypes[t])
except ImportError:
pass | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/__init__.py | __init__.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'LogMessageType' not in _M_Ice.__dict__:
_M_Ice.LogMessageType = Ice.createTempClass()
class LogMessageType(Ice.EnumBase):
"""
An enumeration representing the different types of log messages.
Enumerators:
PrintMessage -- The Logger received a print message.
TraceMessage -- The Logger received a trace message.
WarningMessage -- The Logger received a warning message.
ErrorMessage -- The Logger received an error message.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
LogMessageType.PrintMessage = LogMessageType("PrintMessage", 0)
LogMessageType.TraceMessage = LogMessageType("TraceMessage", 1)
LogMessageType.WarningMessage = LogMessageType("WarningMessage", 2)
LogMessageType.ErrorMessage = LogMessageType("ErrorMessage", 3)
LogMessageType._enumerators = { 0:LogMessageType.PrintMessage, 1:LogMessageType.TraceMessage, 2:LogMessageType.WarningMessage, 3:LogMessageType.ErrorMessage }
_M_Ice._t_LogMessageType = IcePy.defineEnum('::Ice::LogMessageType', LogMessageType, (), LogMessageType._enumerators)
_M_Ice.LogMessageType = LogMessageType
del LogMessageType
if '_t_LogMessageTypeSeq' not in _M_Ice.__dict__:
_M_Ice._t_LogMessageTypeSeq = IcePy.defineSequence('::Ice::LogMessageTypeSeq', (), _M_Ice._t_LogMessageType)
if 'LogMessage' not in _M_Ice.__dict__:
_M_Ice.LogMessage = Ice.createTempClass()
class LogMessage(object):
"""
A complete log message.
Members:
type -- The type of message sent to the Logger.
timestamp -- The date and time when the Logger received this message, expressed
as the number of microseconds since the Unix Epoch (00:00:00 UTC on 1 January 1970)
traceCategory -- For a message of type trace, the trace category of this log message;
otherwise, the empty string.
message -- The log message itself.
"""
def __init__(self, type=_M_Ice.LogMessageType.PrintMessage, timestamp=0, traceCategory='', message=''):
self.type = type
self.timestamp = timestamp
self.traceCategory = traceCategory
self.message = message
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.type)
_h = 5 * _h + Ice.getHash(self.timestamp)
_h = 5 * _h + Ice.getHash(self.traceCategory)
_h = 5 * _h + Ice.getHash(self.message)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Ice.LogMessage):
return NotImplemented
else:
if self.type is None or other.type is None:
if self.type != other.type:
return (-1 if self.type is None else 1)
else:
if self.type < other.type:
return -1
elif self.type > other.type:
return 1
if self.timestamp is None or other.timestamp is None:
if self.timestamp != other.timestamp:
return (-1 if self.timestamp is None else 1)
else:
if self.timestamp < other.timestamp:
return -1
elif self.timestamp > other.timestamp:
return 1
if self.traceCategory is None or other.traceCategory is None:
if self.traceCategory != other.traceCategory:
return (-1 if self.traceCategory is None else 1)
else:
if self.traceCategory < other.traceCategory:
return -1
elif self.traceCategory > other.traceCategory:
return 1
if self.message is None or other.message is None:
if self.message != other.message:
return (-1 if self.message is None else 1)
else:
if self.message < other.message:
return -1
elif self.message > other.message:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_LogMessage)
__repr__ = __str__
_M_Ice._t_LogMessage = IcePy.defineStruct('::Ice::LogMessage', LogMessage, (), (
('type', (), _M_Ice._t_LogMessageType),
('timestamp', (), IcePy._t_long),
('traceCategory', (), IcePy._t_string),
('message', (), IcePy._t_string)
))
_M_Ice.LogMessage = LogMessage
del LogMessage
if '_t_LogMessageSeq' not in _M_Ice.__dict__:
_M_Ice._t_LogMessageSeq = IcePy.defineSequence('::Ice::LogMessageSeq', (), _M_Ice._t_LogMessage)
_M_Ice._t_RemoteLogger = IcePy.defineValue('::Ice::RemoteLogger', Ice.Value, -1, (), False, True, None, ())
if 'RemoteLoggerPrx' not in _M_Ice.__dict__:
_M_Ice.RemoteLoggerPrx = Ice.createTempClass()
class RemoteLoggerPrx(Ice.ObjectPrx):
"""
init is called by attachRemoteLogger when a RemoteLogger proxy is attached.
Arguments:
prefix -- The prefix of the associated local Logger.
logMessages -- Old log messages generated before "now".
context -- The request context for the invocation.
"""
def init(self, prefix, logMessages, context=None):
return _M_Ice.RemoteLogger._op_init.invoke(self, ((prefix, logMessages), context))
"""
init is called by attachRemoteLogger when a RemoteLogger proxy is attached.
Arguments:
prefix -- The prefix of the associated local Logger.
logMessages -- Old log messages generated before "now".
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def initAsync(self, prefix, logMessages, context=None):
return _M_Ice.RemoteLogger._op_init.invokeAsync(self, ((prefix, logMessages), context))
"""
init is called by attachRemoteLogger when a RemoteLogger proxy is attached.
Arguments:
prefix -- The prefix of the associated local Logger.
logMessages -- Old log messages generated before "now".
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_init(self, prefix, logMessages, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.RemoteLogger._op_init.begin(self, ((prefix, logMessages), _response, _ex, _sent, context))
"""
init is called by attachRemoteLogger when a RemoteLogger proxy is attached.
Arguments:
prefix -- The prefix of the associated local Logger.
logMessages -- Old log messages generated before "now".
"""
def end_init(self, _r):
return _M_Ice.RemoteLogger._op_init.end(self, _r)
"""
Log a LogMessage. Note that log may be called by LoggerAdmin before init.
Arguments:
message -- The message to log.
context -- The request context for the invocation.
"""
def log(self, message, context=None):
return _M_Ice.RemoteLogger._op_log.invoke(self, ((message, ), context))
"""
Log a LogMessage. Note that log may be called by LoggerAdmin before init.
Arguments:
message -- The message to log.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def logAsync(self, message, context=None):
return _M_Ice.RemoteLogger._op_log.invokeAsync(self, ((message, ), context))
"""
Log a LogMessage. Note that log may be called by LoggerAdmin before init.
Arguments:
message -- The message to log.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_log(self, message, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.RemoteLogger._op_log.begin(self, ((message, ), _response, _ex, _sent, context))
"""
Log a LogMessage. Note that log may be called by LoggerAdmin before init.
Arguments:
message -- The message to log.
"""
def end_log(self, _r):
return _M_Ice.RemoteLogger._op_log.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.RemoteLoggerPrx.ice_checkedCast(proxy, '::Ice::RemoteLogger', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.RemoteLoggerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::RemoteLogger'
_M_Ice._t_RemoteLoggerPrx = IcePy.defineProxy('::Ice::RemoteLogger', RemoteLoggerPrx)
_M_Ice.RemoteLoggerPrx = RemoteLoggerPrx
del RemoteLoggerPrx
_M_Ice.RemoteLogger = Ice.createTempClass()
class RemoteLogger(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::Ice::RemoteLogger')
def ice_id(self, current=None):
return '::Ice::RemoteLogger'
@staticmethod
def ice_staticId():
return '::Ice::RemoteLogger'
def init(self, prefix, logMessages, current=None):
"""
init is called by attachRemoteLogger when a RemoteLogger proxy is attached.
Arguments:
prefix -- The prefix of the associated local Logger.
logMessages -- Old log messages generated before "now".
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'init' not implemented")
def log(self, message, current=None):
"""
Log a LogMessage. Note that log may be called by LoggerAdmin before init.
Arguments:
message -- The message to log.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'log' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_RemoteLoggerDisp)
__repr__ = __str__
_M_Ice._t_RemoteLoggerDisp = IcePy.defineClass('::Ice::RemoteLogger', RemoteLogger, (), None, ())
RemoteLogger._ice_type = _M_Ice._t_RemoteLoggerDisp
RemoteLogger._op_init = IcePy.Operation('init', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0), ((), _M_Ice._t_LogMessageSeq, False, 0)), (), None, ())
RemoteLogger._op_log = IcePy.Operation('log', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_LogMessage, False, 0),), (), None, ())
_M_Ice.RemoteLogger = RemoteLogger
del RemoteLogger
if 'RemoteLoggerAlreadyAttachedException' not in _M_Ice.__dict__:
_M_Ice.RemoteLoggerAlreadyAttachedException = Ice.createTempClass()
class RemoteLoggerAlreadyAttachedException(Ice.UserException):
"""
Thrown when the provided RemoteLogger was previously attached to a LoggerAdmin.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Ice::RemoteLoggerAlreadyAttachedException'
_M_Ice._t_RemoteLoggerAlreadyAttachedException = IcePy.defineException('::Ice::RemoteLoggerAlreadyAttachedException', RemoteLoggerAlreadyAttachedException, (), False, None, ())
RemoteLoggerAlreadyAttachedException._ice_type = _M_Ice._t_RemoteLoggerAlreadyAttachedException
_M_Ice.RemoteLoggerAlreadyAttachedException = RemoteLoggerAlreadyAttachedException
del RemoteLoggerAlreadyAttachedException
_M_Ice._t_LoggerAdmin = IcePy.defineValue('::Ice::LoggerAdmin', Ice.Value, -1, (), False, True, None, ())
if 'LoggerAdminPrx' not in _M_Ice.__dict__:
_M_Ice.LoggerAdminPrx = Ice.createTempClass()
class LoggerAdminPrx(Ice.ObjectPrx):
"""
Attaches a RemoteLogger object to the local logger.
attachRemoteLogger calls init on the provided RemoteLogger proxy.
Arguments:
prx -- A proxy to the remote logger.
messageTypes -- The list of message types that the remote logger wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that the remote logger wishes to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be provided to init. A negative value requests all messages available.
context -- The request context for the invocation.
Throws:
RemoteLoggerAlreadyAttachedException -- Raised if this remote logger is already attached to this admin object.
"""
def attachRemoteLogger(self, prx, messageTypes, traceCategories, messageMax, context=None):
return _M_Ice.LoggerAdmin._op_attachRemoteLogger.invoke(self, ((prx, messageTypes, traceCategories, messageMax), context))
"""
Attaches a RemoteLogger object to the local logger.
attachRemoteLogger calls init on the provided RemoteLogger proxy.
Arguments:
prx -- A proxy to the remote logger.
messageTypes -- The list of message types that the remote logger wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that the remote logger wishes to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be provided to init. A negative value requests all messages available.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def attachRemoteLoggerAsync(self, prx, messageTypes, traceCategories, messageMax, context=None):
return _M_Ice.LoggerAdmin._op_attachRemoteLogger.invokeAsync(self, ((prx, messageTypes, traceCategories, messageMax), context))
"""
Attaches a RemoteLogger object to the local logger.
attachRemoteLogger calls init on the provided RemoteLogger proxy.
Arguments:
prx -- A proxy to the remote logger.
messageTypes -- The list of message types that the remote logger wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that the remote logger wishes to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be provided to init. A negative value requests all messages available.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_attachRemoteLogger(self, prx, messageTypes, traceCategories, messageMax, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LoggerAdmin._op_attachRemoteLogger.begin(self, ((prx, messageTypes, traceCategories, messageMax), _response, _ex, _sent, context))
"""
Attaches a RemoteLogger object to the local logger.
attachRemoteLogger calls init on the provided RemoteLogger proxy.
Arguments:
prx -- A proxy to the remote logger.
messageTypes -- The list of message types that the remote logger wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that the remote logger wishes to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be provided to init. A negative value requests all messages available.
Throws:
RemoteLoggerAlreadyAttachedException -- Raised if this remote logger is already attached to this admin object.
"""
def end_attachRemoteLogger(self, _r):
return _M_Ice.LoggerAdmin._op_attachRemoteLogger.end(self, _r)
"""
Detaches a RemoteLogger object from the local logger.
Arguments:
prx -- A proxy to the remote logger.
context -- The request context for the invocation.
Returns: True if the provided remote logger proxy was detached, and false otherwise.
"""
def detachRemoteLogger(self, prx, context=None):
return _M_Ice.LoggerAdmin._op_detachRemoteLogger.invoke(self, ((prx, ), context))
"""
Detaches a RemoteLogger object from the local logger.
Arguments:
prx -- A proxy to the remote logger.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def detachRemoteLoggerAsync(self, prx, context=None):
return _M_Ice.LoggerAdmin._op_detachRemoteLogger.invokeAsync(self, ((prx, ), context))
"""
Detaches a RemoteLogger object from the local logger.
Arguments:
prx -- A proxy to the remote logger.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_detachRemoteLogger(self, prx, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LoggerAdmin._op_detachRemoteLogger.begin(self, ((prx, ), _response, _ex, _sent, context))
"""
Detaches a RemoteLogger object from the local logger.
Arguments:
prx -- A proxy to the remote logger.
Returns: True if the provided remote logger proxy was detached, and false otherwise.
"""
def end_detachRemoteLogger(self, _r):
return _M_Ice.LoggerAdmin._op_detachRemoteLogger.end(self, _r)
"""
Retrieves log messages recently logged.
Arguments:
messageTypes -- The list of message types that the caller wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that caller wish to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be returned. A negative value requests all messages available.
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- The Log messages.
prefix -- The prefix of the associated local logger.
"""
def getLog(self, messageTypes, traceCategories, messageMax, context=None):
return _M_Ice.LoggerAdmin._op_getLog.invoke(self, ((messageTypes, traceCategories, messageMax), context))
"""
Retrieves log messages recently logged.
Arguments:
messageTypes -- The list of message types that the caller wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that caller wish to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be returned. A negative value requests all messages available.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLogAsync(self, messageTypes, traceCategories, messageMax, context=None):
return _M_Ice.LoggerAdmin._op_getLog.invokeAsync(self, ((messageTypes, traceCategories, messageMax), context))
"""
Retrieves log messages recently logged.
Arguments:
messageTypes -- The list of message types that the caller wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that caller wish to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be returned. A negative value requests all messages available.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLog(self, messageTypes, traceCategories, messageMax, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.LoggerAdmin._op_getLog.begin(self, ((messageTypes, traceCategories, messageMax), _response, _ex, _sent, context))
"""
Retrieves log messages recently logged.
Arguments:
messageTypes -- The list of message types that the caller wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that caller wish to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be returned. A negative value requests all messages available.
Returns a tuple containing the following:
_retval -- The Log messages.
prefix -- The prefix of the associated local logger.
"""
def end_getLog(self, _r):
return _M_Ice.LoggerAdmin._op_getLog.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.LoggerAdminPrx.ice_checkedCast(proxy, '::Ice::LoggerAdmin', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.LoggerAdminPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::LoggerAdmin'
_M_Ice._t_LoggerAdminPrx = IcePy.defineProxy('::Ice::LoggerAdmin', LoggerAdminPrx)
_M_Ice.LoggerAdminPrx = LoggerAdminPrx
del LoggerAdminPrx
_M_Ice.LoggerAdmin = Ice.createTempClass()
class LoggerAdmin(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::LoggerAdmin', '::Ice::Object')
def ice_id(self, current=None):
return '::Ice::LoggerAdmin'
@staticmethod
def ice_staticId():
return '::Ice::LoggerAdmin'
def attachRemoteLogger(self, prx, messageTypes, traceCategories, messageMax, current=None):
"""
Attaches a RemoteLogger object to the local logger.
attachRemoteLogger calls init on the provided RemoteLogger proxy.
Arguments:
prx -- A proxy to the remote logger.
messageTypes -- The list of message types that the remote logger wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that the remote logger wishes to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be provided to init. A negative value requests all messages available.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
RemoteLoggerAlreadyAttachedException -- Raised if this remote logger is already attached to this admin object.
"""
raise NotImplementedError("servant method 'attachRemoteLogger' not implemented")
def detachRemoteLogger(self, prx, current=None):
"""
Detaches a RemoteLogger object from the local logger.
Arguments:
prx -- A proxy to the remote logger.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'detachRemoteLogger' not implemented")
def getLog(self, messageTypes, traceCategories, messageMax, current=None):
"""
Retrieves log messages recently logged.
Arguments:
messageTypes -- The list of message types that the caller wishes to receive. An empty list means no filtering (send all message types).
traceCategories -- The categories of traces that caller wish to receive. This parameter is ignored if messageTypes is not empty and does not include trace. An empty list means no filtering (send all trace categories).
messageMax -- The maximum number of log messages (of all types) to be returned. A negative value requests all messages available.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getLog' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_LoggerAdminDisp)
__repr__ = __str__
_M_Ice._t_LoggerAdminDisp = IcePy.defineClass('::Ice::LoggerAdmin', LoggerAdmin, (), None, ())
LoggerAdmin._ice_type = _M_Ice._t_LoggerAdminDisp
LoggerAdmin._op_attachRemoteLogger = IcePy.Operation('attachRemoteLogger', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_RemoteLoggerPrx, False, 0), ((), _M_Ice._t_LogMessageTypeSeq, False, 0), ((), _M_Ice._t_StringSeq, False, 0), ((), IcePy._t_int, False, 0)), (), None, (_M_Ice._t_RemoteLoggerAlreadyAttachedException,))
LoggerAdmin._op_detachRemoteLogger = IcePy.Operation('detachRemoteLogger', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_RemoteLoggerPrx, False, 0),), (), ((), IcePy._t_bool, False, 0), ())
LoggerAdmin._op_getLog = IcePy.Operation('getLog', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_LogMessageTypeSeq, False, 0), ((), _M_Ice._t_StringSeq, False, 0), ((), IcePy._t_int, False, 0)), (((), IcePy._t_string, False, 0),), ((), _M_Ice._t_LogMessageSeq, False, 0), ())
_M_Ice.LoggerAdmin = LoggerAdmin
del LoggerAdmin
# End of module Ice
Ice.sliceChecksums["::Ice::LogMessage"] = "b3e905f1b794f505c1f817935be760"
Ice.sliceChecksums["::Ice::LogMessageSeq"] = "26c45475ae04c87944249ec14ebe9c"
Ice.sliceChecksums["::Ice::LogMessageType"] = "938bafca5970dc253c1e82a4c8ee234b"
Ice.sliceChecksums["::Ice::LogMessageTypeSeq"] = "cb11159511adf1a74eb2901796137d7"
Ice.sliceChecksums["::Ice::LoggerAdmin"] = "cba78eeef4c84241e3677c18bbccfb0"
Ice.sliceChecksums["::Ice::RemoteLogger"] = "8c4657be5994cd2ccf54ae795b97273"
Ice.sliceChecksums["::Ice::RemoteLoggerAlreadyAttachedException"] = "c0cffbaeb5ca2d0e1d4a0f328a701b" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/RemoteLogger_ice.py | RemoteLogger_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
if 'ProtocolVersion' not in _M_Ice.__dict__:
_M_Ice.ProtocolVersion = Ice.createTempClass()
class ProtocolVersion(object):
"""
A version structure for the protocol version.
"""
def __init__(self, major=0, minor=0):
self.major = major
self.minor = minor
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.major)
_h = 5 * _h + Ice.getHash(self.minor)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Ice.ProtocolVersion):
return NotImplemented
else:
if self.major is None or other.major is None:
if self.major != other.major:
return (-1 if self.major is None else 1)
else:
if self.major < other.major:
return -1
elif self.major > other.major:
return 1
if self.minor is None or other.minor is None:
if self.minor != other.minor:
return (-1 if self.minor is None else 1)
else:
if self.minor < other.minor:
return -1
elif self.minor > other.minor:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ProtocolVersion)
__repr__ = __str__
_M_Ice._t_ProtocolVersion = IcePy.defineStruct('::Ice::ProtocolVersion', ProtocolVersion, (), (
('major', (), IcePy._t_byte),
('minor', (), IcePy._t_byte)
))
_M_Ice.ProtocolVersion = ProtocolVersion
del ProtocolVersion
if 'EncodingVersion' not in _M_Ice.__dict__:
_M_Ice.EncodingVersion = Ice.createTempClass()
class EncodingVersion(object):
"""
A version structure for the encoding version.
"""
def __init__(self, major=0, minor=0):
self.major = major
self.minor = minor
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.major)
_h = 5 * _h + Ice.getHash(self.minor)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Ice.EncodingVersion):
return NotImplemented
else:
if self.major is None or other.major is None:
if self.major != other.major:
return (-1 if self.major is None else 1)
else:
if self.major < other.major:
return -1
elif self.major > other.major:
return 1
if self.minor is None or other.minor is None:
if self.minor != other.minor:
return (-1 if self.minor is None else 1)
else:
if self.minor < other.minor:
return -1
elif self.minor > other.minor:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_EncodingVersion)
__repr__ = __str__
_M_Ice._t_EncodingVersion = IcePy.defineStruct('::Ice::EncodingVersion', EncodingVersion, (), (
('major', (), IcePy._t_byte),
('minor', (), IcePy._t_byte)
))
_M_Ice.EncodingVersion = EncodingVersion
del EncodingVersion
# End of module Ice
Ice.sliceChecksums["::Ice::EncodingVersion"] = "68abf5e483fdca188a95d11537ee5c8"
Ice.sliceChecksums["::Ice::ProtocolVersion"] = "59bbb2793242c1ad2cb72e920ff65b0" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Version_ice.py | Version_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
_M_Ice._t_Router = IcePy.defineValue('::Ice::Router', Ice.Value, -1, (), False, True, None, ())
if 'RouterPrx' not in _M_Ice.__dict__:
_M_Ice.RouterPrx = Ice.createTempClass()
class RouterPrx(Ice.ObjectPrx):
"""
Get the router's client proxy, i.e., the proxy to use for
forwarding requests from the client to the router.
If a null proxy is returned, the client will forward requests
to the router's endpoints.
Arguments:
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- The router's client proxy.
hasRoutingTable -- Indicates whether or not the router supports a routing table. If it is supported, the Ice runtime will call addProxies to populate the routing table. This out parameter is only supported starting with Ice 3.7. The Ice runtime assumes the router has a routing table if the optional is not set.
"""
def getClientProxy(self, context=None):
return _M_Ice.Router._op_getClientProxy.invoke(self, ((), context))
"""
Get the router's client proxy, i.e., the proxy to use for
forwarding requests from the client to the router.
If a null proxy is returned, the client will forward requests
to the router's endpoints.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getClientProxyAsync(self, context=None):
return _M_Ice.Router._op_getClientProxy.invokeAsync(self, ((), context))
"""
Get the router's client proxy, i.e., the proxy to use for
forwarding requests from the client to the router.
If a null proxy is returned, the client will forward requests
to the router's endpoints.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getClientProxy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Router._op_getClientProxy.begin(self, ((), _response, _ex, _sent, context))
"""
Get the router's client proxy, i.e., the proxy to use for
forwarding requests from the client to the router.
If a null proxy is returned, the client will forward requests
to the router's endpoints.
Arguments:
Returns a tuple containing the following:
_retval -- The router's client proxy.
hasRoutingTable -- Indicates whether or not the router supports a routing table. If it is supported, the Ice runtime will call addProxies to populate the routing table. This out parameter is only supported starting with Ice 3.7. The Ice runtime assumes the router has a routing table if the optional is not set.
"""
def end_getClientProxy(self, _r):
return _M_Ice.Router._op_getClientProxy.end(self, _r)
"""
Get the router's server proxy, i.e., the proxy to use for
forwarding requests from the server to the router.
Arguments:
context -- The request context for the invocation.
Returns: The router's server proxy.
"""
def getServerProxy(self, context=None):
return _M_Ice.Router._op_getServerProxy.invoke(self, ((), context))
"""
Get the router's server proxy, i.e., the proxy to use for
forwarding requests from the server to the router.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getServerProxyAsync(self, context=None):
return _M_Ice.Router._op_getServerProxy.invokeAsync(self, ((), context))
"""
Get the router's server proxy, i.e., the proxy to use for
forwarding requests from the server to the router.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getServerProxy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Router._op_getServerProxy.begin(self, ((), _response, _ex, _sent, context))
"""
Get the router's server proxy, i.e., the proxy to use for
forwarding requests from the server to the router.
Arguments:
Returns: The router's server proxy.
"""
def end_getServerProxy(self, _r):
return _M_Ice.Router._op_getServerProxy.end(self, _r)
"""
Add new proxy information to the router's routing table.
Arguments:
proxies -- The proxies to add.
context -- The request context for the invocation.
Returns: Proxies discarded by the router.
"""
def addProxies(self, proxies, context=None):
return _M_Ice.Router._op_addProxies.invoke(self, ((proxies, ), context))
"""
Add new proxy information to the router's routing table.
Arguments:
proxies -- The proxies to add.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addProxiesAsync(self, proxies, context=None):
return _M_Ice.Router._op_addProxies.invokeAsync(self, ((proxies, ), context))
"""
Add new proxy information to the router's routing table.
Arguments:
proxies -- The proxies to add.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_addProxies(self, proxies, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.Router._op_addProxies.begin(self, ((proxies, ), _response, _ex, _sent, context))
"""
Add new proxy information to the router's routing table.
Arguments:
proxies -- The proxies to add.
Returns: Proxies discarded by the router.
"""
def end_addProxies(self, _r):
return _M_Ice.Router._op_addProxies.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.RouterPrx.ice_checkedCast(proxy, '::Ice::Router', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.RouterPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::Router'
_M_Ice._t_RouterPrx = IcePy.defineProxy('::Ice::Router', RouterPrx)
_M_Ice.RouterPrx = RouterPrx
del RouterPrx
_M_Ice.Router = Ice.createTempClass()
class Router(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::Ice::Router')
def ice_id(self, current=None):
return '::Ice::Router'
@staticmethod
def ice_staticId():
return '::Ice::Router'
def getClientProxy(self, current=None):
"""
Get the router's client proxy, i.e., the proxy to use for
forwarding requests from the client to the router.
If a null proxy is returned, the client will forward requests
to the router's endpoints.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getClientProxy' not implemented")
def getServerProxy(self, current=None):
"""
Get the router's server proxy, i.e., the proxy to use for
forwarding requests from the server to the router.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getServerProxy' not implemented")
def addProxies(self, proxies, current=None):
"""
Add new proxy information to the router's routing table.
Arguments:
proxies -- The proxies to add.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'addProxies' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_RouterDisp)
__repr__ = __str__
_M_Ice._t_RouterDisp = IcePy.defineClass('::Ice::Router', Router, (), None, ())
Router._ice_type = _M_Ice._t_RouterDisp
Router._op_getClientProxy = IcePy.Operation('getClientProxy', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (((), IcePy._t_bool, True, 1),), ((), IcePy._t_ObjectPrx, False, 0), ())
Router._op_getServerProxy = IcePy.Operation('getServerProxy', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_ObjectPrx, False, 0), ())
Router._op_addProxies = IcePy.Operation('addProxies', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_ObjectProxySeq, False, 0),), (), ((), _M_Ice._t_ObjectProxySeq, False, 0), ())
_M_Ice.Router = Router
del Router
_M_Ice._t_RouterFinder = IcePy.defineValue('::Ice::RouterFinder', Ice.Value, -1, (), False, True, None, ())
if 'RouterFinderPrx' not in _M_Ice.__dict__:
_M_Ice.RouterFinderPrx = Ice.createTempClass()
class RouterFinderPrx(Ice.ObjectPrx):
"""
Get the router proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
context -- The request context for the invocation.
Returns: The router proxy.
"""
def getRouter(self, context=None):
return _M_Ice.RouterFinder._op_getRouter.invoke(self, ((), context))
"""
Get the router proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getRouterAsync(self, context=None):
return _M_Ice.RouterFinder._op_getRouter.invokeAsync(self, ((), context))
"""
Get the router proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getRouter(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Ice.RouterFinder._op_getRouter.begin(self, ((), _response, _ex, _sent, context))
"""
Get the router proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
Returns: The router proxy.
"""
def end_getRouter(self, _r):
return _M_Ice.RouterFinder._op_getRouter.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Ice.RouterFinderPrx.ice_checkedCast(proxy, '::Ice::RouterFinder', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Ice.RouterFinderPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Ice::RouterFinder'
_M_Ice._t_RouterFinderPrx = IcePy.defineProxy('::Ice::RouterFinder', RouterFinderPrx)
_M_Ice.RouterFinderPrx = RouterFinderPrx
del RouterFinderPrx
_M_Ice.RouterFinder = Ice.createTempClass()
class RouterFinder(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::Ice::RouterFinder')
def ice_id(self, current=None):
return '::Ice::RouterFinder'
@staticmethod
def ice_staticId():
return '::Ice::RouterFinder'
def getRouter(self, current=None):
"""
Get the router proxy implemented by the process hosting this
finder object. The proxy might point to several replicas.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getRouter' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_RouterFinderDisp)
__repr__ = __str__
_M_Ice._t_RouterFinderDisp = IcePy.defineClass('::Ice::RouterFinder', RouterFinder, (), None, ())
RouterFinder._ice_type = _M_Ice._t_RouterFinderDisp
RouterFinder._op_getRouter = IcePy.Operation('getRouter', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Ice._t_RouterPrx, False, 0), ())
_M_Ice.RouterFinder = RouterFinder
del RouterFinder
# End of module Ice
Ice.sliceChecksums["::Ice::Router"] = "5d699ae7ef13629643981f91ff236c55"
Ice.sliceChecksums["::Ice::RouterFinder"] = "94c0f14a95fc3b15b808186b4c3c512" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Router_ice.py | Router_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.ObjectAdapterF_ice
import Ice.Identity_ice
import Ice.Endpoint_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'CompressBatch' not in _M_Ice.__dict__:
_M_Ice.CompressBatch = Ice.createTempClass()
class CompressBatch(Ice.EnumBase):
"""
The batch compression option when flushing queued batch requests.
Enumerators:
Yes -- Compress the batch requests.
No -- Don't compress the batch requests.
BasedOnProxy -- Compress the batch requests if at least one request was
made on a compressed proxy.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
CompressBatch.Yes = CompressBatch("Yes", 0)
CompressBatch.No = CompressBatch("No", 1)
CompressBatch.BasedOnProxy = CompressBatch("BasedOnProxy", 2)
CompressBatch._enumerators = { 0:CompressBatch.Yes, 1:CompressBatch.No, 2:CompressBatch.BasedOnProxy }
_M_Ice._t_CompressBatch = IcePy.defineEnum('::Ice::CompressBatch', CompressBatch, (), CompressBatch._enumerators)
_M_Ice.CompressBatch = CompressBatch
del CompressBatch
if 'ConnectionInfo' not in _M_Ice.__dict__:
_M_Ice.ConnectionInfo = Ice.createTempClass()
class ConnectionInfo(object):
"""
Base class providing access to the connection details.
Members:
underlying -- The information of the underyling transport or null if there's
no underlying transport.
incoming -- Whether or not the connection is an incoming or outgoing
connection.
adapterName -- The name of the adapter associated with the connection.
connectionId -- The connection id.
"""
def __init__(self, underlying=None, incoming=False, adapterName='', connectionId=''):
self.underlying = underlying
self.incoming = incoming
self.adapterName = adapterName
self.connectionId = connectionId
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ConnectionInfo)
__repr__ = __str__
_M_Ice._t_ConnectionInfo = IcePy.declareValue('::Ice::ConnectionInfo')
_M_Ice._t_ConnectionInfo = IcePy.defineValue('::Ice::ConnectionInfo', ConnectionInfo, -1, (), False, False, None, (
('underlying', (), _M_Ice._t_ConnectionInfo, False, 0),
('incoming', (), IcePy._t_bool, False, 0),
('adapterName', (), IcePy._t_string, False, 0),
('connectionId', (), IcePy._t_string, False, 0)
))
ConnectionInfo._ice_type = _M_Ice._t_ConnectionInfo
_M_Ice.ConnectionInfo = ConnectionInfo
del ConnectionInfo
if 'Connection' not in _M_Ice.__dict__:
_M_Ice._t_Connection = IcePy.declareValue('::Ice::Connection')
if 'CloseCallback' not in _M_Ice.__dict__:
_M_Ice.CloseCallback = Ice.createTempClass()
class CloseCallback(object):
"""
An application can implement this interface to receive notifications when
a connection closes.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.CloseCallback:
raise RuntimeError('Ice.CloseCallback is an abstract class')
def closed(self, con):
"""
This method is called by the the connection when the connection
is closed. If the callback needs more information about the closure,
it can call Connection#throwException.
Arguments:
con -- The connection that closed.
"""
raise NotImplementedError("method 'closed' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_CloseCallback)
__repr__ = __str__
_M_Ice._t_CloseCallback = IcePy.defineValue('::Ice::CloseCallback', CloseCallback, -1, (), False, True, None, ())
CloseCallback._ice_type = _M_Ice._t_CloseCallback
_M_Ice.CloseCallback = CloseCallback
del CloseCallback
if 'HeartbeatCallback' not in _M_Ice.__dict__:
_M_Ice.HeartbeatCallback = Ice.createTempClass()
class HeartbeatCallback(object):
"""
An application can implement this interface to receive notifications when
a connection receives a heartbeat message.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.HeartbeatCallback:
raise RuntimeError('Ice.HeartbeatCallback is an abstract class')
def heartbeat(self, con):
"""
This method is called by the the connection when a heartbeat is
received from the peer.
Arguments:
con -- The connection on which a heartbeat was received.
"""
raise NotImplementedError("method 'heartbeat' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_HeartbeatCallback)
__repr__ = __str__
_M_Ice._t_HeartbeatCallback = IcePy.defineValue('::Ice::HeartbeatCallback', HeartbeatCallback, -1, (), False, True, None, ())
HeartbeatCallback._ice_type = _M_Ice._t_HeartbeatCallback
_M_Ice.HeartbeatCallback = HeartbeatCallback
del HeartbeatCallback
if 'ACMClose' not in _M_Ice.__dict__:
_M_Ice.ACMClose = Ice.createTempClass()
class ACMClose(Ice.EnumBase):
"""
Specifies the close semantics for Active Connection Management.
Enumerators:
CloseOff -- Disables automatic connection closure.
CloseOnIdle -- Gracefully closes a connection that has been idle for the configured timeout period.
CloseOnInvocation -- Forcefully closes a connection that has been idle for the configured timeout period,
but only if the connection has pending invocations.
CloseOnInvocationAndIdle -- Combines the behaviors of CloseOnIdle and CloseOnInvocation.
CloseOnIdleForceful -- Forcefully closes a connection that has been idle for the configured timeout period,
regardless of whether the connection has pending invocations or dispatch.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ACMClose.CloseOff = ACMClose("CloseOff", 0)
ACMClose.CloseOnIdle = ACMClose("CloseOnIdle", 1)
ACMClose.CloseOnInvocation = ACMClose("CloseOnInvocation", 2)
ACMClose.CloseOnInvocationAndIdle = ACMClose("CloseOnInvocationAndIdle", 3)
ACMClose.CloseOnIdleForceful = ACMClose("CloseOnIdleForceful", 4)
ACMClose._enumerators = { 0:ACMClose.CloseOff, 1:ACMClose.CloseOnIdle, 2:ACMClose.CloseOnInvocation, 3:ACMClose.CloseOnInvocationAndIdle, 4:ACMClose.CloseOnIdleForceful }
_M_Ice._t_ACMClose = IcePy.defineEnum('::Ice::ACMClose', ACMClose, (), ACMClose._enumerators)
_M_Ice.ACMClose = ACMClose
del ACMClose
if 'ACMHeartbeat' not in _M_Ice.__dict__:
_M_Ice.ACMHeartbeat = Ice.createTempClass()
class ACMHeartbeat(Ice.EnumBase):
"""
Specifies the heartbeat semantics for Active Connection Management.
Enumerators:
HeartbeatOff -- Disables heartbeats.
HeartbeatOnDispatch -- Send a heartbeat at regular intervals if the connection is idle and only if there are pending dispatch.
HeartbeatOnIdle -- Send a heartbeat at regular intervals when the connection is idle.
HeartbeatAlways -- Send a heartbeat at regular intervals until the connection is closed.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ACMHeartbeat.HeartbeatOff = ACMHeartbeat("HeartbeatOff", 0)
ACMHeartbeat.HeartbeatOnDispatch = ACMHeartbeat("HeartbeatOnDispatch", 1)
ACMHeartbeat.HeartbeatOnIdle = ACMHeartbeat("HeartbeatOnIdle", 2)
ACMHeartbeat.HeartbeatAlways = ACMHeartbeat("HeartbeatAlways", 3)
ACMHeartbeat._enumerators = { 0:ACMHeartbeat.HeartbeatOff, 1:ACMHeartbeat.HeartbeatOnDispatch, 2:ACMHeartbeat.HeartbeatOnIdle, 3:ACMHeartbeat.HeartbeatAlways }
_M_Ice._t_ACMHeartbeat = IcePy.defineEnum('::Ice::ACMHeartbeat', ACMHeartbeat, (), ACMHeartbeat._enumerators)
_M_Ice.ACMHeartbeat = ACMHeartbeat
del ACMHeartbeat
if 'ACM' not in _M_Ice.__dict__:
_M_Ice.ACM = Ice.createTempClass()
class ACM(object):
"""
A collection of Active Connection Management configuration settings.
Members:
timeout -- A timeout value in seconds.
close -- The close semantics.
heartbeat -- The heartbeat semantics.
"""
def __init__(self, timeout=0, close=_M_Ice.ACMClose.CloseOff, heartbeat=_M_Ice.ACMHeartbeat.HeartbeatOff):
self.timeout = timeout
self.close = close
self.heartbeat = heartbeat
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.timeout)
_h = 5 * _h + Ice.getHash(self.close)
_h = 5 * _h + Ice.getHash(self.heartbeat)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Ice.ACM):
return NotImplemented
else:
if self.timeout is None or other.timeout is None:
if self.timeout != other.timeout:
return (-1 if self.timeout is None else 1)
else:
if self.timeout < other.timeout:
return -1
elif self.timeout > other.timeout:
return 1
if self.close is None or other.close is None:
if self.close != other.close:
return (-1 if self.close is None else 1)
else:
if self.close < other.close:
return -1
elif self.close > other.close:
return 1
if self.heartbeat is None or other.heartbeat is None:
if self.heartbeat != other.heartbeat:
return (-1 if self.heartbeat is None else 1)
else:
if self.heartbeat < other.heartbeat:
return -1
elif self.heartbeat > other.heartbeat:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ACM)
__repr__ = __str__
_M_Ice._t_ACM = IcePy.defineStruct('::Ice::ACM', ACM, (), (
('timeout', (), IcePy._t_int),
('close', (), _M_Ice._t_ACMClose),
('heartbeat', (), _M_Ice._t_ACMHeartbeat)
))
_M_Ice.ACM = ACM
del ACM
if 'ConnectionClose' not in _M_Ice.__dict__:
_M_Ice.ConnectionClose = Ice.createTempClass()
class ConnectionClose(Ice.EnumBase):
"""
Determines the behavior when manually closing a connection.
Enumerators:
Forcefully -- Close the connection immediately without sending a close connection protocol message to the peer
and waiting for the peer to acknowledge it.
Gracefully -- Close the connection by notifying the peer but do not wait for pending outgoing invocations to complete.
On the server side, the connection will not be closed until all incoming invocations have completed.
GracefullyWithWait -- Wait for all pending invocations to complete before closing the connection.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ConnectionClose.Forcefully = ConnectionClose("Forcefully", 0)
ConnectionClose.Gracefully = ConnectionClose("Gracefully", 1)
ConnectionClose.GracefullyWithWait = ConnectionClose("GracefullyWithWait", 2)
ConnectionClose._enumerators = { 0:ConnectionClose.Forcefully, 1:ConnectionClose.Gracefully, 2:ConnectionClose.GracefullyWithWait }
_M_Ice._t_ConnectionClose = IcePy.defineEnum('::Ice::ConnectionClose', ConnectionClose, (), ConnectionClose._enumerators)
_M_Ice.ConnectionClose = ConnectionClose
del ConnectionClose
if 'Connection' not in _M_Ice.__dict__:
_M_Ice.Connection = Ice.createTempClass()
class Connection(object):
"""
The user-level interface to a connection.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Connection:
raise RuntimeError('Ice.Connection is an abstract class')
def close(self, mode):
"""
Manually close the connection using the specified closure mode.
Arguments:
mode -- Determines how the connection will be closed.
"""
raise NotImplementedError("method 'close' not implemented")
def createProxy(self, id):
"""
Create a special proxy that always uses this connection. This
can be used for callbacks from a server to a client if the
server cannot directly establish a connection to the client,
for example because of firewalls. In this case, the server
would create a proxy using an already established connection
from the client.
Arguments:
id -- The identity for which a proxy is to be created.
Returns: A proxy that matches the given identity and uses this connection.
"""
raise NotImplementedError("method 'createProxy' not implemented")
def setAdapter(self, adapter):
"""
Explicitly set an object adapter that dispatches requests that
are received over this connection. A client can invoke an
operation on a server using a proxy, and then set an object
adapter for the outgoing connection that is used by the proxy
in order to receive callbacks. This is useful if the server
cannot establish a connection back to the client, for example
because of firewalls.
Arguments:
adapter -- The object adapter that should be used by this connection to dispatch requests. The object adapter must be activated. When the object adapter is deactivated, it is automatically removed from the connection. Attempts to use a deactivated object adapter raise ObjectAdapterDeactivatedException
"""
raise NotImplementedError("method 'setAdapter' not implemented")
def getAdapter(self):
"""
Get the object adapter that dispatches requests for this
connection.
Returns: The object adapter that dispatches requests for the connection, or null if no adapter is set.
"""
raise NotImplementedError("method 'getAdapter' not implemented")
def getEndpoint(self):
"""
Get the endpoint from which the connection was created.
Returns: The endpoint from which the connection was created.
"""
raise NotImplementedError("method 'getEndpoint' not implemented")
def flushBatchRequests(self, compress):
"""
Flush any pending batch requests for this connection.
This means all batch requests invoked on fixed proxies
associated with the connection.
Arguments:
compress -- Specifies whether or not the queued batch requests should be compressed before being sent over the wire.
"""
raise NotImplementedError("method 'flushBatchRequests' not implemented")
def setCloseCallback(self, callback):
"""
Set a close callback on the connection. The callback is called by the
connection when it's closed. The callback is called from the
Ice thread pool associated with the connection. If the callback needs
more information about the closure, it can call Connection#throwException.
Arguments:
callback -- The close callback object.
"""
raise NotImplementedError("method 'setCloseCallback' not implemented")
def setHeartbeatCallback(self, callback):
"""
Set a heartbeat callback on the connection. The callback is called by the
connection when a heartbeat is received. The callback is called
from the Ice thread pool associated with the connection.
Arguments:
callback -- The heartbeat callback object.
"""
raise NotImplementedError("method 'setHeartbeatCallback' not implemented")
def heartbeat(self):
"""
Send a heartbeat message.
"""
raise NotImplementedError("method 'heartbeat' not implemented")
def setACM(self, timeout, close, heartbeat):
"""
Set the active connection management parameters.
Arguments:
timeout -- The timeout value in seconds, must be >= 0.
close -- The close condition
heartbeat -- The hertbeat condition
"""
raise NotImplementedError("method 'setACM' not implemented")
def getACM(self):
"""
Get the ACM parameters.
Returns: The ACM parameters.
"""
raise NotImplementedError("method 'getACM' not implemented")
def type(self):
"""
Return the connection type. This corresponds to the endpoint
type, i.e., "tcp", "udp", etc.
Returns: The type of the connection.
"""
raise NotImplementedError("method 'type' not implemented")
def timeout(self):
"""
Get the timeout for the connection.
Returns: The connection's timeout.
"""
raise NotImplementedError("method 'timeout' not implemented")
def toString(self):
"""
Return a description of the connection as human readable text,
suitable for logging or error messages.
Returns: The description of the connection as human readable text.
"""
raise NotImplementedError("method 'toString' not implemented")
def getInfo(self):
"""
Returns the connection information.
Returns: The connection information.
"""
raise NotImplementedError("method 'getInfo' not implemented")
def setBufferSize(self, rcvSize, sndSize):
"""
Set the connection buffer receive/send size.
Arguments:
rcvSize -- The connection receive buffer size.
sndSize -- The connection send buffer size.
"""
raise NotImplementedError("method 'setBufferSize' not implemented")
def throwException(self):
"""
Throw an exception indicating the reason for connection closure. For example,
CloseConnectionException is raised if the connection was closed gracefully,
whereas ConnectionManuallyClosedException is raised if the connection was
manually closed by the application. This operation does nothing if the connection is
not yet closed.
"""
raise NotImplementedError("method 'throwException' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Connection)
__repr__ = __str__
_M_Ice._t_Connection = IcePy.defineValue('::Ice::Connection', Connection, -1, (), False, True, None, ())
Connection._ice_type = _M_Ice._t_Connection
_M_Ice.Connection = Connection
del Connection
if 'IPConnectionInfo' not in _M_Ice.__dict__:
_M_Ice.IPConnectionInfo = Ice.createTempClass()
class IPConnectionInfo(_M_Ice.ConnectionInfo):
"""
Provides access to the connection details of an IP connection
Members:
localAddress -- The local address.
localPort -- The local port.
remoteAddress -- The remote address.
remotePort -- The remote port.
"""
def __init__(self, underlying=None, incoming=False, adapterName='', connectionId='', localAddress="", localPort=-1, remoteAddress="", remotePort=-1):
_M_Ice.ConnectionInfo.__init__(self, underlying, incoming, adapterName, connectionId)
self.localAddress = localAddress
self.localPort = localPort
self.remoteAddress = remoteAddress
self.remotePort = remotePort
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_IPConnectionInfo)
__repr__ = __str__
_M_Ice._t_IPConnectionInfo = IcePy.declareValue('::Ice::IPConnectionInfo')
_M_Ice._t_IPConnectionInfo = IcePy.defineValue('::Ice::IPConnectionInfo', IPConnectionInfo, -1, (), False, False, _M_Ice._t_ConnectionInfo, (
('localAddress', (), IcePy._t_string, False, 0),
('localPort', (), IcePy._t_int, False, 0),
('remoteAddress', (), IcePy._t_string, False, 0),
('remotePort', (), IcePy._t_int, False, 0)
))
IPConnectionInfo._ice_type = _M_Ice._t_IPConnectionInfo
_M_Ice.IPConnectionInfo = IPConnectionInfo
del IPConnectionInfo
if 'TCPConnectionInfo' not in _M_Ice.__dict__:
_M_Ice.TCPConnectionInfo = Ice.createTempClass()
class TCPConnectionInfo(_M_Ice.IPConnectionInfo):
"""
Provides access to the connection details of a TCP connection
Members:
rcvSize -- The connection buffer receive size.
sndSize -- The connection buffer send size.
"""
def __init__(self, underlying=None, incoming=False, adapterName='', connectionId='', localAddress="", localPort=-1, remoteAddress="", remotePort=-1, rcvSize=0, sndSize=0):
_M_Ice.IPConnectionInfo.__init__(self, underlying, incoming, adapterName, connectionId, localAddress, localPort, remoteAddress, remotePort)
self.rcvSize = rcvSize
self.sndSize = sndSize
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_TCPConnectionInfo)
__repr__ = __str__
_M_Ice._t_TCPConnectionInfo = IcePy.declareValue('::Ice::TCPConnectionInfo')
_M_Ice._t_TCPConnectionInfo = IcePy.defineValue('::Ice::TCPConnectionInfo', TCPConnectionInfo, -1, (), False, False, _M_Ice._t_IPConnectionInfo, (
('rcvSize', (), IcePy._t_int, False, 0),
('sndSize', (), IcePy._t_int, False, 0)
))
TCPConnectionInfo._ice_type = _M_Ice._t_TCPConnectionInfo
_M_Ice.TCPConnectionInfo = TCPConnectionInfo
del TCPConnectionInfo
if 'UDPConnectionInfo' not in _M_Ice.__dict__:
_M_Ice.UDPConnectionInfo = Ice.createTempClass()
class UDPConnectionInfo(_M_Ice.IPConnectionInfo):
"""
Provides access to the connection details of a UDP connection
Members:
mcastAddress -- The multicast address.
mcastPort -- The multicast port.
rcvSize -- The connection buffer receive size.
sndSize -- The connection buffer send size.
"""
def __init__(self, underlying=None, incoming=False, adapterName='', connectionId='', localAddress="", localPort=-1, remoteAddress="", remotePort=-1, mcastAddress='', mcastPort=-1, rcvSize=0, sndSize=0):
_M_Ice.IPConnectionInfo.__init__(self, underlying, incoming, adapterName, connectionId, localAddress, localPort, remoteAddress, remotePort)
self.mcastAddress = mcastAddress
self.mcastPort = mcastPort
self.rcvSize = rcvSize
self.sndSize = sndSize
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_UDPConnectionInfo)
__repr__ = __str__
_M_Ice._t_UDPConnectionInfo = IcePy.declareValue('::Ice::UDPConnectionInfo')
_M_Ice._t_UDPConnectionInfo = IcePy.defineValue('::Ice::UDPConnectionInfo', UDPConnectionInfo, -1, (), False, False, _M_Ice._t_IPConnectionInfo, (
('mcastAddress', (), IcePy._t_string, False, 0),
('mcastPort', (), IcePy._t_int, False, 0),
('rcvSize', (), IcePy._t_int, False, 0),
('sndSize', (), IcePy._t_int, False, 0)
))
UDPConnectionInfo._ice_type = _M_Ice._t_UDPConnectionInfo
_M_Ice.UDPConnectionInfo = UDPConnectionInfo
del UDPConnectionInfo
if '_t_HeaderDict' not in _M_Ice.__dict__:
_M_Ice._t_HeaderDict = IcePy.defineDictionary('::Ice::HeaderDict', (), IcePy._t_string, IcePy._t_string)
if 'WSConnectionInfo' not in _M_Ice.__dict__:
_M_Ice.WSConnectionInfo = Ice.createTempClass()
class WSConnectionInfo(_M_Ice.ConnectionInfo):
"""
Provides access to the connection details of a WebSocket connection
Members:
headers -- The headers from the HTTP upgrade request.
"""
def __init__(self, underlying=None, incoming=False, adapterName='', connectionId='', headers=None):
_M_Ice.ConnectionInfo.__init__(self, underlying, incoming, adapterName, connectionId)
self.headers = headers
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_WSConnectionInfo)
__repr__ = __str__
_M_Ice._t_WSConnectionInfo = IcePy.declareValue('::Ice::WSConnectionInfo')
_M_Ice._t_WSConnectionInfo = IcePy.defineValue('::Ice::WSConnectionInfo', WSConnectionInfo, -1, (), False, False, _M_Ice._t_ConnectionInfo, (('headers', (), _M_Ice._t_HeaderDict, False, 0),))
WSConnectionInfo._ice_type = _M_Ice._t_WSConnectionInfo
_M_Ice.WSConnectionInfo = WSConnectionInfo
del WSConnectionInfo
# End of module Ice
Ice.sliceChecksums["::Ice::HeaderDict"] = "9998817e127863d3f3d6e31fe248bbb" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Connection_ice.py | Connection_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
if 'Identity' not in _M_Ice.__dict__:
_M_Ice.Identity = Ice.createTempClass()
class Identity(object):
"""
The identity of an Ice object. In a proxy, an empty Identity#name denotes a nil
proxy. An identity with an empty Identity#name and a non-empty Identity#category
is illegal. You cannot add a servant with an empty name to the Active Servant Map.
Members:
name -- The name of the Ice object.
category -- The Ice object category.
"""
def __init__(self, name='', category=''):
self.name = name
self.category = category
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.name)
_h = 5 * _h + Ice.getHash(self.category)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Ice.Identity):
return NotImplemented
else:
if self.name is None or other.name is None:
if self.name != other.name:
return (-1 if self.name is None else 1)
else:
if self.name < other.name:
return -1
elif self.name > other.name:
return 1
if self.category is None or other.category is None:
if self.category != other.category:
return (-1 if self.category is None else 1)
else:
if self.category < other.category:
return -1
elif self.category > other.category:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Identity)
__repr__ = __str__
_M_Ice._t_Identity = IcePy.defineStruct('::Ice::Identity', Identity, (), (
('name', (), IcePy._t_string),
('category', (), IcePy._t_string)
))
_M_Ice.Identity = Identity
del Identity
if '_t_ObjectDict' not in _M_Ice.__dict__:
_M_Ice._t_ObjectDict = IcePy.defineDictionary('::Ice::ObjectDict', (), _M_Ice._t_Identity, IcePy._t_Value)
if '_t_IdentitySeq' not in _M_Ice.__dict__:
_M_Ice._t_IdentitySeq = IcePy.defineSequence('::Ice::IdentitySeq', (), _M_Ice._t_Identity)
# End of module Ice
Ice.sliceChecksums["::Ice::Identity"] = "a0c37867a69924a9fcc4bdf078f06da"
Ice.sliceChecksums["::Ice::IdentitySeq"] = "90afa299fd9e7d7a17268cc22b5ca42" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Identity_ice.py | Identity_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
# Start of module Ice
_M_Ice = Ice.openModule('Ice')
__name__ = 'Ice'
if 'Logger' not in _M_Ice.__dict__:
_M_Ice.Logger = Ice.createTempClass()
class Logger(object):
"""
The Ice message logger. Applications can provide their own logger
by implementing this interface and installing it in a communicator.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Logger:
raise RuntimeError('Ice.Logger is an abstract class')
def _print(self, message):
"""
Print a message. The message is printed literally, without
any decorations such as executable name or time stamp.
Arguments:
message -- The message to log.
"""
raise NotImplementedError("method '_print' not implemented")
def trace(self, category, message):
"""
Log a trace message.
Arguments:
category -- The trace category.
message -- The trace message to log.
"""
raise NotImplementedError("method 'trace' not implemented")
def warning(self, message):
"""
Log a warning message.
Arguments:
message -- The warning message to log.
"""
raise NotImplementedError("method 'warning' not implemented")
def error(self, message):
"""
Log an error message.
Arguments:
message -- The error message to log.
"""
raise NotImplementedError("method 'error' not implemented")
def getPrefix(self):
"""
Returns this logger's prefix.
Returns: The prefix.
"""
raise NotImplementedError("method 'getPrefix' not implemented")
def cloneWithPrefix(self, prefix):
"""
Returns a clone of the logger with a new prefix.
Arguments:
prefix -- The new prefix for the logger.
Returns: A logger instance.
"""
raise NotImplementedError("method 'cloneWithPrefix' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Logger)
__repr__ = __str__
_M_Ice._t_Logger = IcePy.defineValue('::Ice::Logger', Logger, -1, (), False, True, None, ())
Logger._ice_type = _M_Ice._t_Logger
_M_Ice.Logger = Logger
del Logger
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Logger_ice.py | Logger_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.LoggerF_ice
import Ice.InstrumentationF_ice
import Ice.ObjectAdapterF_ice
import Ice.ObjectFactory_ice
import Ice.ValueFactory_ice
import Ice.Router_ice
import Ice.Locator_ice
import Ice.PluginF_ice
import Ice.ImplicitContextF_ice
import Ice.Current_ice
import Ice.Properties_ice
import Ice.FacetMap_ice
import Ice.Connection_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Ice.Instrumentation
_M_Ice.Instrumentation = Ice.openModule('Ice.Instrumentation')
# Start of module Ice
__name__ = 'Ice'
_M_Ice.__doc__ = """
The Ice core library. Among many other features, the Ice core
library manages all the communication tasks using an efficient
protocol (including protocol compression and support for both TCP
and UDP), provides a thread pool for multi-threaded servers, and
additional functionality that supports high scalability.
"""
if 'Communicator' not in _M_Ice.__dict__:
_M_Ice.Communicator = Ice.createTempClass()
class Communicator(object):
"""
The central object in Ice. One or more communicators can be
instantiated for an Ice application. Communicator instantiation
is language-specific, and not specified in Slice code.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.Communicator:
raise RuntimeError('Ice.Communicator is an abstract class')
def destroy(self):
"""
Destroy the communicator. This operation calls shutdown
implicitly. Calling destroy cleans up memory, and shuts down
this communicator's client functionality and destroys all object
adapters. Subsequent calls to destroy are ignored.
"""
raise NotImplementedError("method 'destroy' not implemented")
def shutdown(self):
"""
Shuts down this communicator's server functionality, which
includes the deactivation of all object adapters. Attempts to use a
deactivated object adapter raise ObjectAdapterDeactivatedException.
Subsequent calls to shutdown are ignored.
After shutdown returns, no new requests are processed. However, requests
that have been started before shutdown was called might still be active.
You can use waitForShutdown to wait for the completion of all
requests.
"""
raise NotImplementedError("method 'shutdown' not implemented")
def waitForShutdown(self):
"""
Wait until the application has called shutdown (or destroy).
On the server side, this operation blocks the calling thread
until all currently-executing operations have completed.
On the client side, the operation simply blocks until another
thread has called shutdown or destroy.
A typical use of this operation is to call it from the main thread,
which then waits until some other thread calls shutdown.
After shut-down is complete, the main thread returns and can do some
cleanup work before it finally calls destroy to shut down
the client functionality, and then exits the application.
"""
raise NotImplementedError("method 'waitForShutdown' not implemented")
def isShutdown(self):
"""
Check whether communicator has been shut down.
Returns: True if the communicator has been shut down; false otherwise.
"""
raise NotImplementedError("method 'isShutdown' not implemented")
def stringToProxy(self, str):
"""
Convert a stringified proxy into a proxy. For example,
MyCategory/MyObject:tcp -h some_host -p
10000 creates a proxy that refers to the Ice object
having an identity with a name "MyObject" and a category
"MyCategory", with the server running on host "some_host", port
10000. If the stringified proxy does not parse correctly, the
operation throws one of ProxyParseException, EndpointParseException,
or IdentityParseException. Refer to the Ice manual for a detailed
description of the syntax supported by stringified proxies.
Arguments:
str -- The stringified proxy to convert into a proxy.
Returns: The proxy, or nil if str is an empty string.
"""
raise NotImplementedError("method 'stringToProxy' not implemented")
def proxyToString(self, obj):
"""
Convert a proxy into a string.
Arguments:
obj -- The proxy to convert into a stringified proxy.
Returns: The stringified proxy, or an empty string if obj is nil.
"""
raise NotImplementedError("method 'proxyToString' not implemented")
def propertyToProxy(self, property):
"""
Convert a set of proxy properties into a proxy. The "base"
name supplied in the property argument refers to a
property containing a stringified proxy, such as
MyProxy=id:tcp -h localhost -p 10000. Additional
properties configure local settings for the proxy, such as
MyProxy.PreferSecure=1. The "Properties"
appendix in the Ice manual describes each of the supported
proxy properties.
Arguments:
property -- The base property name.
Returns: The proxy.
"""
raise NotImplementedError("method 'propertyToProxy' not implemented")
def proxyToProperty(self, proxy, property):
"""
Convert a proxy to a set of proxy properties.
Arguments:
proxy -- The proxy.
property -- The base property name.
Returns: The property set.
"""
raise NotImplementedError("method 'proxyToProperty' not implemented")
def stringToIdentity(self, str):
"""
Convert a string into an identity. If the string does not parse
correctly, the operation throws IdentityParseException.
Arguments:
str -- The string to convert into an identity.
Returns: The identity.
"""
raise NotImplementedError("method 'stringToIdentity' not implemented")
def identityToString(self, ident):
"""
Convert an identity into a string.
Arguments:
ident -- The identity to convert into a string.
Returns: The "stringified" identity.
"""
raise NotImplementedError("method 'identityToString' not implemented")
def createObjectAdapter(self, name):
"""
Create a new object adapter. The endpoints for the object
adapter are taken from the property name.Endpoints.
It is legal to create an object adapter with the empty string as
its name. Such an object adapter is accessible via bidirectional
connections or by collocated invocations that originate from the
same communicator as is used by the adapter.
Attempts to create a named object adapter for which no configuration
can be found raise InitializationException.
Arguments:
name -- The object adapter name.
Returns: The new object adapter.
"""
raise NotImplementedError("method 'createObjectAdapter' not implemented")
def createObjectAdapterWithEndpoints(self, name, endpoints):
"""
Create a new object adapter with endpoints. This operation sets
the property name.Endpoints, and then calls
createObjectAdapter. It is provided as a convenience
function.
Calling this operation with an empty name will result in a
UUID being generated for the name.
Arguments:
name -- The object adapter name.
endpoints -- The endpoints for the object adapter.
Returns: The new object adapter.
"""
raise NotImplementedError("method 'createObjectAdapterWithEndpoints' not implemented")
def createObjectAdapterWithRouter(self, name, rtr):
"""
Create a new object adapter with a router. This operation
creates a routed object adapter.
Calling this operation with an empty name will result in a
UUID being generated for the name.
Arguments:
name -- The object adapter name.
rtr -- The router.
Returns: The new object adapter.
"""
raise NotImplementedError("method 'createObjectAdapterWithRouter' not implemented")
def addObjectFactory(self, factory, id):
"""
Add an object factory to this communicator. Installing a
factory with an id for which a factory is already registered
throws AlreadyRegisteredException.
When unmarshaling an Ice object, the Ice run time reads the
most-derived type id off the wire and attempts to create an
instance of the type using a factory. If no instance is created,
either because no factory was found, or because all factories
returned nil, the behavior of the Ice run time depends on the
format with which the object was marshaled:
If the object uses the "sliced" format, Ice ascends the class
hierarchy until it finds a type that is recognized by a factory,
or it reaches the least-derived type. If no factory is found that
can create an instance, the run time throws NoValueFactoryException.
If the object uses the "compact" format, Ice immediately raises
NoValueFactoryException.
The following order is used to locate a factory for a type:
The Ice run-time looks for a factory registered
specifically for the type.
If no instance has been created, the Ice run-time looks
for the default factory, which is registered with an empty type id.
If no instance has been created by any of the preceding
steps, the Ice run-time looks for a factory that may have been
statically generated by the language mapping for non-abstract classes.
Arguments:
factory -- The factory to add.
id -- The type id for which the factory can create instances, or an empty string for the default factory.
"""
raise NotImplementedError("method 'addObjectFactory' not implemented")
def findObjectFactory(self, id):
"""
Find an object factory registered with this communicator.
Arguments:
id -- The type id for which the factory can create instances, or an empty string for the default factory.
Returns: The object factory, or null if no object factory was found for the given id.
"""
raise NotImplementedError("method 'findObjectFactory' not implemented")
def getImplicitContext(self):
"""
Get the implicit context associated with this communicator.
Returns: The implicit context associated with this communicator; returns null when the property Ice.ImplicitContext is not set or is set to None.
"""
raise NotImplementedError("method 'getImplicitContext' not implemented")
def getProperties(self):
"""
Get the properties for this communicator.
Returns: This communicator's properties.
"""
raise NotImplementedError("method 'getProperties' not implemented")
def getLogger(self):
"""
Get the logger for this communicator.
Returns: This communicator's logger.
"""
raise NotImplementedError("method 'getLogger' not implemented")
def getObserver(self):
"""
Get the observer resolver object for this communicator.
Returns: This communicator's observer resolver object.
"""
raise NotImplementedError("method 'getObserver' not implemented")
def getDefaultRouter(self):
"""
Get the default router this communicator.
Returns: The default router for this communicator.
"""
raise NotImplementedError("method 'getDefaultRouter' not implemented")
def setDefaultRouter(self, rtr):
"""
Set a default router for this communicator. All newly
created proxies will use this default router. To disable the
default router, null can be used. Note that this
operation has no effect on existing proxies.
You can also set a router for an individual proxy
by calling the operation ice_router on the proxy.
Arguments:
rtr -- The default router to use for this communicator.
"""
raise NotImplementedError("method 'setDefaultRouter' not implemented")
def getDefaultLocator(self):
"""
Get the default locator this communicator.
Returns: The default locator for this communicator.
"""
raise NotImplementedError("method 'getDefaultLocator' not implemented")
def setDefaultLocator(self, loc):
"""
Set a default Ice locator for this communicator. All newly
created proxy and object adapters will use this default
locator. To disable the default locator, null can be used.
Note that this operation has no effect on existing proxies or
object adapters.
You can also set a locator for an individual proxy by calling the
operation ice_locator on the proxy, or for an object adapter
by calling ObjectAdapter#setLocator on the object adapter.
Arguments:
loc -- The default locator to use for this communicator.
"""
raise NotImplementedError("method 'setDefaultLocator' not implemented")
def getPluginManager(self):
"""
Get the plug-in manager for this communicator.
Returns: This communicator's plug-in manager.
"""
raise NotImplementedError("method 'getPluginManager' not implemented")
def getValueFactoryManager(self):
"""
Get the value factory manager for this communicator.
Returns: This communicator's value factory manager.
"""
raise NotImplementedError("method 'getValueFactoryManager' not implemented")
def flushBatchRequests(self, compress):
"""
Flush any pending batch requests for this communicator.
This means all batch requests invoked on fixed proxies
for all connections associated with the communicator.
Any errors that occur while flushing a connection are ignored.
Arguments:
compress -- Specifies whether or not the queued batch requests should be compressed before being sent over the wire.
"""
raise NotImplementedError("method 'flushBatchRequests' not implemented")
def createAdmin(self, adminAdapter, adminId):
"""
Add the Admin object with all its facets to the provided object adapter.
If Ice.Admin.ServerId is set and the provided object adapter has a Locator,
createAdmin registers the Admin's Process facet with the Locator's LocatorRegistry.
createAdmin must only be called once; subsequent calls raise InitializationException.
Arguments:
adminAdapter -- The object adapter used to host the Admin object; if null and Ice.Admin.Endpoints is set, create, activate and use the Ice.Admin object adapter.
adminId -- The identity of the Admin object.
Returns: A proxy to the main ("") facet of the Admin object. Never returns a null proxy.
"""
raise NotImplementedError("method 'createAdmin' not implemented")
def getAdmin(self):
"""
Get a proxy to the main facet of the Admin object.
getAdmin also creates the Admin object and creates and activates the Ice.Admin object
adapter to host this Admin object if Ice.Admin.Enpoints is set. The identity of the Admin
object created by getAdmin is {value of Ice.Admin.InstanceName}/admin, or {UUID}/admin
when Ice.Admin.InstanceName is not set.
If Ice.Admin.DelayCreation is 0 or not set, getAdmin is called by the communicator
initialization, after initialization of all plugins.
Returns: A proxy to the main ("") facet of the Admin object, or a null proxy if no Admin object is configured.
"""
raise NotImplementedError("method 'getAdmin' not implemented")
def addAdminFacet(self, servant, facet):
"""
Add a new facet to the Admin object.
Adding a servant with a facet that is already registered
throws AlreadyRegisteredException.
Arguments:
servant -- The servant that implements the new Admin facet.
facet -- The name of the new Admin facet.
"""
raise NotImplementedError("method 'addAdminFacet' not implemented")
def removeAdminFacet(self, facet):
"""
Remove the following facet to the Admin object.
Removing a facet that was not previously registered throws
NotRegisteredException.
Arguments:
facet -- The name of the Admin facet.
Returns: The servant associated with this Admin facet.
"""
raise NotImplementedError("method 'removeAdminFacet' not implemented")
def findAdminFacet(self, facet):
"""
Returns a facet of the Admin object.
Arguments:
facet -- The name of the Admin facet.
Returns: The servant associated with this Admin facet, or null if no facet is registered with the given name.
"""
raise NotImplementedError("method 'findAdminFacet' not implemented")
def findAllAdminFacets(self):
"""
Returns a map of all facets of the Admin object.
Returns: A collection containing all the facet names and servants of the Admin object.
"""
raise NotImplementedError("method 'findAllAdminFacets' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_Communicator)
__repr__ = __str__
_M_Ice._t_Communicator = IcePy.defineValue('::Ice::Communicator', Communicator, -1, (), False, True, None, ())
Communicator._ice_type = _M_Ice._t_Communicator
_M_Ice.Communicator = Communicator
del Communicator
if 'ToStringMode' not in _M_Ice.__dict__:
_M_Ice.ToStringMode = Ice.createTempClass()
class ToStringMode(Ice.EnumBase):
"""
The output mode for xxxToString method such as identityToString and proxyToString.
The actual encoding format for the string is the same for all modes: you
don't need to specify an encoding format or mode when reading such a string.
Enumerators:
Unicode -- Characters with ordinal values greater than 127 are kept as-is in the resulting string.
Non-printable ASCII characters with ordinal values 127 and below are encoded as \\t, \\n (etc.)
or \\unnnn.
ASCII -- Characters with ordinal values greater than 127 are encoded as universal character names in
the resulting string: \\unnnn for BMP characters and \\Unnnnnnnn for non-BMP characters.
Non-printable ASCII characters with ordinal values 127 and below are encoded as \\t, \\n (etc.)
or \\unnnn.
Compat -- Characters with ordinal values greater than 127 are encoded as a sequence of UTF-8 bytes using
octal escapes. Characters with ordinal values 127 and below are encoded as \\t, \\n (etc.) or
an octal escape. Use this mode to generate strings compatible with Ice 3.6 and earlier.
"""
def __init__(self, _n, _v):
Ice.EnumBase.__init__(self, _n, _v)
def valueOf(self, _n):
if _n in self._enumerators:
return self._enumerators[_n]
return None
valueOf = classmethod(valueOf)
ToStringMode.Unicode = ToStringMode("Unicode", 0)
ToStringMode.ASCII = ToStringMode("ASCII", 1)
ToStringMode.Compat = ToStringMode("Compat", 2)
ToStringMode._enumerators = { 0:ToStringMode.Unicode, 1:ToStringMode.ASCII, 2:ToStringMode.Compat }
_M_Ice._t_ToStringMode = IcePy.defineEnum('::Ice::ToStringMode', ToStringMode, (), ToStringMode._enumerators)
_M_Ice.ToStringMode = ToStringMode
del ToStringMode
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/Communicator_ice.py | Communicator_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.CommunicatorF_ice
import Ice.ServantLocatorF_ice
import Ice.Locator_ice
import Ice.FacetMap_ice
import Ice.Endpoint_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Ice
__name__ = 'Ice'
if 'ObjectAdapter' not in _M_Ice.__dict__:
_M_Ice.ObjectAdapter = Ice.createTempClass()
class ObjectAdapter(object):
"""
The object adapter provides an up-call interface from the Ice
run time to the implementation of Ice objects.
The object adapter is responsible for receiving requests
from endpoints, and for mapping between servants, identities, and
proxies.
"""
def __init__(self):
if Ice.getType(self) == _M_Ice.ObjectAdapter:
raise RuntimeError('Ice.ObjectAdapter is an abstract class')
def getName(self):
"""
Get the name of this object adapter.
Returns: This object adapter's name.
"""
raise NotImplementedError("method 'getName' not implemented")
def getCommunicator(self):
"""
Get the communicator this object adapter belongs to.
Returns: This object adapter's communicator.
"""
raise NotImplementedError("method 'getCommunicator' not implemented")
def activate(self):
"""
Activate all endpoints that belong to this object adapter.
After activation, the object adapter can dispatch requests
received through its endpoints.
"""
raise NotImplementedError("method 'activate' not implemented")
def hold(self):
"""
Temporarily hold receiving and dispatching requests. The object
adapter can be reactivated with the activate operation.
Holding is not immediate, i.e., after hold
returns, the object adapter might still be active for some
time. You can use waitForHold to wait until holding is
complete.
"""
raise NotImplementedError("method 'hold' not implemented")
def waitForHold(self):
"""
Wait until the object adapter holds requests. Calling hold
initiates holding of requests, and waitForHold only returns
when holding of requests has been completed.
"""
raise NotImplementedError("method 'waitForHold' not implemented")
def deactivate(self):
"""
Deactivate all endpoints that belong to this object adapter.
After deactivation, the object adapter stops receiving
requests through its endpoints. Object adapters that have been
deactivated must not be reactivated again, and cannot be used
otherwise. Attempts to use a deactivated object adapter raise
ObjectAdapterDeactivatedException however, attempts to
deactivate an already deactivated object adapter are
ignored and do nothing. Once deactivated, it is possible to
destroy the adapter to clean up resources and then create and
activate a new adapter with the same name.
After deactivate returns, no new requests
are processed by the object adapter. However, requests that
have been started before deactivate was called might
still be active. You can use waitForDeactivate to wait
for the completion of all requests for this object adapter.
"""
raise NotImplementedError("method 'deactivate' not implemented")
def waitForDeactivate(self):
"""
Wait until the object adapter has deactivated. Calling
deactivate initiates object adapter deactivation, and
waitForDeactivate only returns when deactivation has
been completed.
"""
raise NotImplementedError("method 'waitForDeactivate' not implemented")
def isDeactivated(self):
"""
Check whether object adapter has been deactivated.
Returns: Whether adapter has been deactivated.
"""
raise NotImplementedError("method 'isDeactivated' not implemented")
def destroy(self):
"""
Destroys the object adapter and cleans up all resources held by
the object adapter. If the object adapter has not yet been
deactivated, destroy implicitly initiates the deactivation
and waits for it to finish. Subsequent calls to destroy are
ignored. Once destroy has returned, it is possible to create
another object adapter with the same name.
"""
raise NotImplementedError("method 'destroy' not implemented")
def add(self, servant, id):
"""
Add a servant to this object adapter's Active Servant Map. Note
that one servant can implement several Ice objects by registering
the servant with multiple identities. Adding a servant with an
identity that is in the map already throws AlreadyRegisteredException.
Arguments:
servant -- The servant to add.
id -- The identity of the Ice object that is implemented by the servant.
Returns: A proxy that matches the given identity and this object adapter.
"""
raise NotImplementedError("method 'add' not implemented")
def addFacet(self, servant, id, facet):
"""
Like add, but with a facet. Calling add(servant, id)
is equivalent to calling addFacet with an empty facet.
Arguments:
servant -- The servant to add.
id -- The identity of the Ice object that is implemented by the servant.
facet -- The facet. An empty facet means the default facet.
Returns: A proxy that matches the given identity, facet, and this object adapter.
"""
raise NotImplementedError("method 'addFacet' not implemented")
def addWithUUID(self, servant):
"""
Add a servant to this object adapter's Active Servant Map,
using an automatically generated UUID as its identity. Note that
the generated UUID identity can be accessed using the proxy's
ice_getIdentity operation.
Arguments:
servant -- The servant to add.
Returns: A proxy that matches the generated UUID identity and this object adapter.
"""
raise NotImplementedError("method 'addWithUUID' not implemented")
def addFacetWithUUID(self, servant, facet):
"""
Like addWithUUID, but with a facet. Calling
addWithUUID(servant) is equivalent to calling
addFacetWithUUID with an empty facet.
Arguments:
servant -- The servant to add.
facet -- The facet. An empty facet means the default facet.
Returns: A proxy that matches the generated UUID identity, facet, and this object adapter.
"""
raise NotImplementedError("method 'addFacetWithUUID' not implemented")
def addDefaultServant(self, servant, category):
"""
Add a default servant to handle requests for a specific
category. Adding a default servant for a category for
which a default servant is already registered throws
AlreadyRegisteredException. To dispatch operation
calls on servants, the object adapter tries to find a servant
for a given Ice object identity and facet in the following
order:
The object adapter tries to find a servant for the identity
and facet in the Active Servant Map.
If no servant has been found in the Active Servant Map, the
object adapter tries to find a default servant for the category
component of the identity.
If no servant has been found by any of the preceding steps,
the object adapter tries to find a default servant for an empty
category, regardless of the category contained in the identity.
If no servant has been found by any of the preceding steps,
the object adapter gives up and the caller receives
ObjectNotExistException or FacetNotExistException.
Arguments:
servant -- The default servant.
category -- The category for which the default servant is registered. An empty category means it will handle all categories.
"""
raise NotImplementedError("method 'addDefaultServant' not implemented")
def remove(self, id):
"""
Remove a servant (that is, the default facet) from the object
adapter's Active Servant Map.
Arguments:
id -- The identity of the Ice object that is implemented by the servant. If the servant implements multiple Ice objects, remove has to be called for all those Ice objects. Removing an identity that is not in the map throws NotRegisteredException.
Returns: The removed servant.
"""
raise NotImplementedError("method 'remove' not implemented")
def removeFacet(self, id, facet):
"""
Like remove, but with a facet. Calling remove(id)
is equivalent to calling removeFacet with an empty facet.
Arguments:
id -- The identity of the Ice object that is implemented by the servant.
facet -- The facet. An empty facet means the default facet.
Returns: The removed servant.
"""
raise NotImplementedError("method 'removeFacet' not implemented")
def removeAllFacets(self, id):
"""
Remove all facets with the given identity from the Active
Servant Map. The operation completely removes the Ice object,
including its default facet. Removing an identity that
is not in the map throws NotRegisteredException.
Arguments:
id -- The identity of the Ice object to be removed.
Returns: A collection containing all the facet names and servants of the removed Ice object.
"""
raise NotImplementedError("method 'removeAllFacets' not implemented")
def removeDefaultServant(self, category):
"""
Remove the default servant for a specific category. Attempting
to remove a default servant for a category that is not
registered throws NotRegisteredException.
Arguments:
category -- The category of the default servant to remove.
Returns: The default servant.
"""
raise NotImplementedError("method 'removeDefaultServant' not implemented")
def find(self, id):
"""
Look up a servant in this object adapter's Active Servant Map
by the identity of the Ice object it implements.
This operation only tries to look up a servant in
the Active Servant Map. It does not attempt to find a servant
by using any installed ServantLocator.
Arguments:
id -- The identity of the Ice object for which the servant should be returned.
Returns: The servant that implements the Ice object with the given identity, or null if no such servant has been found.
"""
raise NotImplementedError("method 'find' not implemented")
def findFacet(self, id, facet):
"""
Like find, but with a facet. Calling find(id)
is equivalent to calling findFacet with an empty
facet.
Arguments:
id -- The identity of the Ice object for which the servant should be returned.
facet -- The facet. An empty facet means the default facet.
Returns: The servant that implements the Ice object with the given identity and facet, or null if no such servant has been found.
"""
raise NotImplementedError("method 'findFacet' not implemented")
def findAllFacets(self, id):
"""
Find all facets with the given identity in the Active Servant
Map.
Arguments:
id -- The identity of the Ice object for which the facets should be returned.
Returns: A collection containing all the facet names and servants that have been found, or an empty map if there is no facet for the given identity.
"""
raise NotImplementedError("method 'findAllFacets' not implemented")
def findByProxy(self, proxy):
"""
Look up a servant in this object adapter's Active Servant Map,
given a proxy.
This operation only tries to lookup a servant in
the Active Servant Map. It does not attempt to find a servant
by using any installed ServantLocator.
Arguments:
proxy -- The proxy for which the servant should be returned.
Returns: The servant that matches the proxy, or null if no such servant has been found.
"""
raise NotImplementedError("method 'findByProxy' not implemented")
def addServantLocator(self, locator, category):
"""
Add a Servant Locator to this object adapter. Adding a servant
locator for a category for which a servant locator is already
registered throws AlreadyRegisteredException. To dispatch
operation calls on servants, the object adapter tries to find a
servant for a given Ice object identity and facet in the
following order:
The object adapter tries to find a servant for the identity
and facet in the Active Servant Map.
If no servant has been found in the Active Servant Map,
the object adapter tries to find a servant locator for the
category component of the identity. If a locator is found, the
object adapter tries to find a servant using this locator.
If no servant has been found by any of the preceding steps,
the object adapter tries to find a locator for an empty category,
regardless of the category contained in the identity. If a
locator is found, the object adapter tries to find a servant
using this locator.
If no servant has been found by any of the preceding steps,
the object adapter gives up and the caller receives
ObjectNotExistException or FacetNotExistException.
Only one locator for the empty category can be
installed.
Arguments:
locator -- The locator to add.
category -- The category for which the Servant Locator can locate servants, or an empty string if the Servant Locator does not belong to any specific category.
"""
raise NotImplementedError("method 'addServantLocator' not implemented")
def removeServantLocator(self, category):
"""
Remove a Servant Locator from this object adapter.
Arguments:
category -- The category for which the Servant Locator can locate servants, or an empty string if the Servant Locator does not belong to any specific category.
Returns: The Servant Locator, or throws NotRegisteredException if no Servant Locator was found for the given category.
"""
raise NotImplementedError("method 'removeServantLocator' not implemented")
def findServantLocator(self, category):
"""
Find a Servant Locator installed with this object adapter.
Arguments:
category -- The category for which the Servant Locator can locate servants, or an empty string if the Servant Locator does not belong to any specific category.
Returns: The Servant Locator, or null if no Servant Locator was found for the given category.
"""
raise NotImplementedError("method 'findServantLocator' not implemented")
def findDefaultServant(self, category):
"""
Find the default servant for a specific category.
Arguments:
category -- The category of the default servant to find.
Returns: The default servant or null if no default servant was registered for the category.
"""
raise NotImplementedError("method 'findDefaultServant' not implemented")
def createProxy(self, id):
"""
Create a proxy for the object with the given identity. If this
object adapter is configured with an adapter id, the return
value is an indirect proxy that refers to the adapter id. If
a replica group id is also defined, the return value is an
indirect proxy that refers to the replica group id. Otherwise,
if no adapter id is defined, the return value is a direct
proxy containing this object adapter's published endpoints.
Arguments:
id -- The object's identity.
Returns: A proxy for the object with the given identity.
"""
raise NotImplementedError("method 'createProxy' not implemented")
def createDirectProxy(self, id):
"""
Create a direct proxy for the object with the given identity.
The returned proxy contains this object adapter's published
endpoints.
Arguments:
id -- The object's identity.
Returns: A proxy for the object with the given identity.
"""
raise NotImplementedError("method 'createDirectProxy' not implemented")
def createIndirectProxy(self, id):
"""
Create an indirect proxy for the object with the given identity.
If this object adapter is configured with an adapter id, the
return value refers to the adapter id. Otherwise, the return
value contains only the object identity.
Arguments:
id -- The object's identity.
Returns: A proxy for the object with the given identity.
"""
raise NotImplementedError("method 'createIndirectProxy' not implemented")
def setLocator(self, loc):
"""
Set an Ice locator for this object adapter. By doing so, the
object adapter will register itself with the locator registry
when it is activated for the first time. Furthermore, the proxies
created by this object adapter will contain the adapter identifier
instead of its endpoints. The adapter identifier must be configured
using the AdapterId property.
Arguments:
loc -- The locator used by this object adapter.
"""
raise NotImplementedError("method 'setLocator' not implemented")
def getLocator(self):
"""
Get the Ice locator used by this object adapter.
Returns: The locator used by this object adapter, or null if no locator is used by this object adapter.
"""
raise NotImplementedError("method 'getLocator' not implemented")
def getEndpoints(self):
"""
Get the set of endpoints configured with this object adapter.
Returns: The set of endpoints.
"""
raise NotImplementedError("method 'getEndpoints' not implemented")
def refreshPublishedEndpoints(self):
"""
Refresh the set of published endpoints. The run time re-reads
the PublishedEndpoints property if it is set and re-reads the
list of local interfaces if the adapter is configured to listen
on all endpoints. This operation is useful to refresh the endpoint
information that is published in the proxies that are created by
an object adapter if the network interfaces used by a host changes.
"""
raise NotImplementedError("method 'refreshPublishedEndpoints' not implemented")
def getPublishedEndpoints(self):
"""
Get the set of endpoints that proxies created by this object
adapter will contain.
Returns: The set of published endpoints.
"""
raise NotImplementedError("method 'getPublishedEndpoints' not implemented")
def setPublishedEndpoints(self, newEndpoints):
"""
Set of the endpoints that proxies created by this object
adapter will contain.
Arguments:
newEndpoints -- The new set of endpoints that the object adapter will embed in proxies.
"""
raise NotImplementedError("method 'setPublishedEndpoints' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Ice._t_ObjectAdapter)
__repr__ = __str__
_M_Ice._t_ObjectAdapter = IcePy.defineValue('::Ice::ObjectAdapter', ObjectAdapter, -1, (), False, True, None, ())
ObjectAdapter._ice_type = _M_Ice._t_ObjectAdapter
_M_Ice.ObjectAdapter = ObjectAdapter
del ObjectAdapter
# End of module Ice | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Ice/ObjectAdapter_ice.py | ObjectAdapter_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module IcePatch2
_M_IcePatch2 = Ice.openModule('IcePatch2')
__name__ = 'IcePatch2'
if 'FileInfo' not in _M_IcePatch2.__dict__:
_M_IcePatch2.FileInfo = Ice.createTempClass()
class FileInfo(object):
"""
Basic information about a single file.
Members:
path -- The pathname.
checksum -- The SHA-1 checksum of the file.
size -- The size of the compressed file in number of bytes.
executable -- The executable flag.
"""
def __init__(self, path='', checksum=None, size=0, executable=False):
self.path = path
self.checksum = checksum
self.size = size
self.executable = executable
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.path)
if self.checksum:
for _i0 in self.checksum:
_h = 5 * _h + Ice.getHash(_i0)
_h = 5 * _h + Ice.getHash(self.size)
_h = 5 * _h + Ice.getHash(self.executable)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IcePatch2.FileInfo):
return NotImplemented
else:
if self.path is None or other.path is None:
if self.path != other.path:
return (-1 if self.path is None else 1)
else:
if self.path < other.path:
return -1
elif self.path > other.path:
return 1
if self.checksum is None or other.checksum is None:
if self.checksum != other.checksum:
return (-1 if self.checksum is None else 1)
else:
if self.checksum < other.checksum:
return -1
elif self.checksum > other.checksum:
return 1
if self.size is None or other.size is None:
if self.size != other.size:
return (-1 if self.size is None else 1)
else:
if self.size < other.size:
return -1
elif self.size > other.size:
return 1
if self.executable is None or other.executable is None:
if self.executable != other.executable:
return (-1 if self.executable is None else 1)
else:
if self.executable < other.executable:
return -1
elif self.executable > other.executable:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IcePatch2._t_FileInfo)
__repr__ = __str__
_M_IcePatch2._t_FileInfo = IcePy.defineStruct('::IcePatch2::FileInfo', FileInfo, (), (
('path', (), IcePy._t_string),
('checksum', (), _M_Ice._t_ByteSeq),
('size', (), IcePy._t_int),
('executable', (), IcePy._t_bool)
))
_M_IcePatch2.FileInfo = FileInfo
del FileInfo
if '_t_FileInfoSeq' not in _M_IcePatch2.__dict__:
_M_IcePatch2._t_FileInfoSeq = IcePy.defineSequence('::IcePatch2::FileInfoSeq', (), _M_IcePatch2._t_FileInfo)
if 'LargeFileInfo' not in _M_IcePatch2.__dict__:
_M_IcePatch2.LargeFileInfo = Ice.createTempClass()
class LargeFileInfo(object):
"""
Basic information about a single file.
Members:
path -- The pathname.
checksum -- The SHA-1 checksum of the file.
size -- The size of the compressed file in number of bytes.
executable -- The executable flag.
"""
def __init__(self, path='', checksum=None, size=0, executable=False):
self.path = path
self.checksum = checksum
self.size = size
self.executable = executable
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.path)
if self.checksum:
for _i0 in self.checksum:
_h = 5 * _h + Ice.getHash(_i0)
_h = 5 * _h + Ice.getHash(self.size)
_h = 5 * _h + Ice.getHash(self.executable)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_IcePatch2.LargeFileInfo):
return NotImplemented
else:
if self.path is None or other.path is None:
if self.path != other.path:
return (-1 if self.path is None else 1)
else:
if self.path < other.path:
return -1
elif self.path > other.path:
return 1
if self.checksum is None or other.checksum is None:
if self.checksum != other.checksum:
return (-1 if self.checksum is None else 1)
else:
if self.checksum < other.checksum:
return -1
elif self.checksum > other.checksum:
return 1
if self.size is None or other.size is None:
if self.size != other.size:
return (-1 if self.size is None else 1)
else:
if self.size < other.size:
return -1
elif self.size > other.size:
return 1
if self.executable is None or other.executable is None:
if self.executable != other.executable:
return (-1 if self.executable is None else 1)
else:
if self.executable < other.executable:
return -1
elif self.executable > other.executable:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_IcePatch2._t_LargeFileInfo)
__repr__ = __str__
_M_IcePatch2._t_LargeFileInfo = IcePy.defineStruct('::IcePatch2::LargeFileInfo', LargeFileInfo, (), (
('path', (), IcePy._t_string),
('checksum', (), _M_Ice._t_ByteSeq),
('size', (), IcePy._t_long),
('executable', (), IcePy._t_bool)
))
_M_IcePatch2.LargeFileInfo = LargeFileInfo
del LargeFileInfo
if '_t_LargeFileInfoSeq' not in _M_IcePatch2.__dict__:
_M_IcePatch2._t_LargeFileInfoSeq = IcePy.defineSequence('::IcePatch2::LargeFileInfoSeq', (), _M_IcePatch2._t_LargeFileInfo)
# End of module IcePatch2
Ice.sliceChecksums["::IcePatch2::FileInfo"] = "4c71622889c19c7d3b5ef8210245"
Ice.sliceChecksums["::IcePatch2::FileInfoSeq"] = "892945a7a7bfb532f6148c4be9889bd"
Ice.sliceChecksums["::IcePatch2::LargeFileInfo"] = "c711b1cd93bfe8cab428a3201e0f291"
Ice.sliceChecksums["::IcePatch2::LargeFileInfoSeq"] = "96e1aec0272b42f09289701462bb5921" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IcePatch2/FileInfo_ice.py | FileInfo_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import IcePatch2.FileInfo_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module IcePatch2
_M_IcePatch2 = Ice.openModule('IcePatch2')
# Start of module IcePatch2
__name__ = 'IcePatch2'
_M_IcePatch2.__doc__ = """
IcePatch can be used to update file hierarchies in a simple and
efficient manner. Checksums ensure file integrity, and data is
compressed before downloading.
"""
if '_t_ByteSeqSeq' not in _M_IcePatch2.__dict__:
_M_IcePatch2._t_ByteSeqSeq = IcePy.defineSequence('::IcePatch2::ByteSeqSeq', (), _M_Ice._t_ByteSeq)
if 'PartitionOutOfRangeException' not in _M_IcePatch2.__dict__:
_M_IcePatch2.PartitionOutOfRangeException = Ice.createTempClass()
class PartitionOutOfRangeException(Ice.UserException):
"""
A partition argument was not in the range 0-255.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IcePatch2::PartitionOutOfRangeException'
_M_IcePatch2._t_PartitionOutOfRangeException = IcePy.defineException('::IcePatch2::PartitionOutOfRangeException', PartitionOutOfRangeException, (), False, None, ())
PartitionOutOfRangeException._ice_type = _M_IcePatch2._t_PartitionOutOfRangeException
_M_IcePatch2.PartitionOutOfRangeException = PartitionOutOfRangeException
del PartitionOutOfRangeException
if 'FileAccessException' not in _M_IcePatch2.__dict__:
_M_IcePatch2.FileAccessException = Ice.createTempClass()
class FileAccessException(Ice.UserException):
"""
This exception is raised if a file's contents cannot be read.
Members:
reason -- An explanation of the reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IcePatch2::FileAccessException'
_M_IcePatch2._t_FileAccessException = IcePy.defineException('::IcePatch2::FileAccessException', FileAccessException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
FileAccessException._ice_type = _M_IcePatch2._t_FileAccessException
_M_IcePatch2.FileAccessException = FileAccessException
del FileAccessException
if 'FileSizeRangeException' not in _M_IcePatch2.__dict__:
_M_IcePatch2.FileSizeRangeException = Ice.createTempClass()
class FileSizeRangeException(_M_IcePatch2.FileAccessException):
"""
This exception is raised if an operation tries to use a file whose size is
larger than 2.1 GB. Use the "large" versions of the operations instead.
"""
def __init__(self, reason=''):
_M_IcePatch2.FileAccessException.__init__(self, reason)
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IcePatch2::FileSizeRangeException'
_M_IcePatch2._t_FileSizeRangeException = IcePy.defineException('::IcePatch2::FileSizeRangeException', FileSizeRangeException, (), False, _M_IcePatch2._t_FileAccessException, ())
FileSizeRangeException._ice_type = _M_IcePatch2._t_FileSizeRangeException
_M_IcePatch2.FileSizeRangeException = FileSizeRangeException
del FileSizeRangeException
_M_IcePatch2._t_FileServer = IcePy.defineValue('::IcePatch2::FileServer', Ice.Value, -1, (), False, True, None, ())
if 'FileServerPrx' not in _M_IcePatch2.__dict__:
_M_IcePatch2.FileServerPrx = Ice.createTempClass()
class FileServerPrx(Ice.ObjectPrx):
"""
Return file information for the specified partition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
partition -- The partition number in the range 0-255.
context -- The request context for the invocation.
Returns: A sequence containing information about the files in the specified partition.
Throws:
FileSizeRangeException -- If a file is larger than 2.1GB.
PartitionOutOfRangeException -- If the partition number is out of range.
"""
def getFileInfoSeq(self, partition, context=None):
return _M_IcePatch2.FileServer._op_getFileInfoSeq.invoke(self, ((partition, ), context))
"""
Return file information for the specified partition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
partition -- The partition number in the range 0-255.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getFileInfoSeqAsync(self, partition, context=None):
return _M_IcePatch2.FileServer._op_getFileInfoSeq.invokeAsync(self, ((partition, ), context))
"""
Return file information for the specified partition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
partition -- The partition number in the range 0-255.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getFileInfoSeq(self, partition, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getFileInfoSeq.begin(self, ((partition, ), _response, _ex, _sent, context))
"""
Return file information for the specified partition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
partition -- The partition number in the range 0-255.
Returns: A sequence containing information about the files in the specified partition.
Throws:
FileSizeRangeException -- If a file is larger than 2.1GB.
PartitionOutOfRangeException -- If the partition number is out of range.
"""
def end_getFileInfoSeq(self, _r):
return _M_IcePatch2.FileServer._op_getFileInfoSeq.end(self, _r)
"""
Returns file information for the specified partition.
Arguments:
partition -- The partition number in the range 0-255.
context -- The request context for the invocation.
Returns: A sequence containing information about the files in the specified partition.
Throws:
PartitionOutOfRangeException -- If the partition number is out of range.
"""
def getLargeFileInfoSeq(self, partition, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileInfoSeq.invoke(self, ((partition, ), context))
"""
Returns file information for the specified partition.
Arguments:
partition -- The partition number in the range 0-255.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLargeFileInfoSeqAsync(self, partition, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileInfoSeq.invokeAsync(self, ((partition, ), context))
"""
Returns file information for the specified partition.
Arguments:
partition -- The partition number in the range 0-255.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLargeFileInfoSeq(self, partition, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileInfoSeq.begin(self, ((partition, ), _response, _ex, _sent, context))
"""
Returns file information for the specified partition.
Arguments:
partition -- The partition number in the range 0-255.
Returns: A sequence containing information about the files in the specified partition.
Throws:
PartitionOutOfRangeException -- If the partition number is out of range.
"""
def end_getLargeFileInfoSeq(self, _r):
return _M_IcePatch2.FileServer._op_getLargeFileInfoSeq.end(self, _r)
"""
Return the checksums for all partitions.
Arguments:
context -- The request context for the invocation.
Returns: A sequence containing 256 checksums. Partitions with a checksum that differs from the previous checksum for the same partition contain updated files. Partitions with a checksum that is identical to the previous checksum do not contain updated files.
"""
def getChecksumSeq(self, context=None):
return _M_IcePatch2.FileServer._op_getChecksumSeq.invoke(self, ((), context))
"""
Return the checksums for all partitions.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getChecksumSeqAsync(self, context=None):
return _M_IcePatch2.FileServer._op_getChecksumSeq.invokeAsync(self, ((), context))
"""
Return the checksums for all partitions.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getChecksumSeq(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getChecksumSeq.begin(self, ((), _response, _ex, _sent, context))
"""
Return the checksums for all partitions.
Arguments:
Returns: A sequence containing 256 checksums. Partitions with a checksum that differs from the previous checksum for the same partition contain updated files. Partitions with a checksum that is identical to the previous checksum do not contain updated files.
"""
def end_getChecksumSeq(self, _r):
return _M_IcePatch2.FileServer._op_getChecksumSeq.end(self, _r)
"""
Return the master checksum for all partitions. If this checksum is the same
as for a previous run, the entire file set is up-to-date.
Arguments:
context -- The request context for the invocation.
Returns: The master checksum for the file set.
"""
def getChecksum(self, context=None):
return _M_IcePatch2.FileServer._op_getChecksum.invoke(self, ((), context))
"""
Return the master checksum for all partitions. If this checksum is the same
as for a previous run, the entire file set is up-to-date.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getChecksumAsync(self, context=None):
return _M_IcePatch2.FileServer._op_getChecksum.invokeAsync(self, ((), context))
"""
Return the master checksum for all partitions. If this checksum is the same
as for a previous run, the entire file set is up-to-date.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getChecksum(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getChecksum.begin(self, ((), _response, _ex, _sent, context))
"""
Return the master checksum for all partitions. If this checksum is the same
as for a previous run, the entire file set is up-to-date.
Arguments:
Returns: The master checksum for the file set.
"""
def end_getChecksum(self, _r):
return _M_IcePatch2.FileServer._op_getChecksum.end(self, _r)
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
context -- The request context for the invocation.
Returns: A sequence containing the compressed file contents.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
FileSizeRangeException -- If a file is larger than 2.1GB.
"""
def getFileCompressed(self, path, pos, num, context=None):
return _M_IcePatch2.FileServer._op_getFileCompressed.invoke(self, ((path, pos, num), context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getFileCompressedAsync(self, path, pos, num, context=None):
return _M_IcePatch2.FileServer._op_getFileCompressed.invokeAsync(self, ((path, pos, num), context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getFileCompressed(self, path, pos, num, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getFileCompressed.begin(self, ((path, pos, num), _response, _ex, _sent, context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
Returns: A sequence containing the compressed file contents.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
FileSizeRangeException -- If a file is larger than 2.1GB.
"""
def end_getFileCompressed(self, _r):
return _M_IcePatch2.FileServer._op_getFileCompressed.end(self, _r)
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
context -- The request context for the invocation.
Returns: A sequence containing the compressed file contents.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
"""
def getLargeFileCompressed(self, path, pos, num, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileCompressed.invoke(self, ((path, pos, num), context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getLargeFileCompressedAsync(self, path, pos, num, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileCompressed.invokeAsync(self, ((path, pos, num), context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getLargeFileCompressed(self, path, pos, num, _response=None, _ex=None, _sent=None, context=None):
return _M_IcePatch2.FileServer._op_getLargeFileCompressed.begin(self, ((path, pos, num), _response, _ex, _sent, context))
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
Returns: A sequence containing the compressed file contents.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
"""
def end_getLargeFileCompressed(self, _r):
return _M_IcePatch2.FileServer._op_getLargeFileCompressed.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IcePatch2.FileServerPrx.ice_checkedCast(proxy, '::IcePatch2::FileServer', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IcePatch2.FileServerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IcePatch2::FileServer'
_M_IcePatch2._t_FileServerPrx = IcePy.defineProxy('::IcePatch2::FileServer', FileServerPrx)
_M_IcePatch2.FileServerPrx = FileServerPrx
del FileServerPrx
_M_IcePatch2.FileServer = Ice.createTempClass()
class FileServer(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IcePatch2::FileServer')
def ice_id(self, current=None):
return '::IcePatch2::FileServer'
@staticmethod
def ice_staticId():
return '::IcePatch2::FileServer'
def getFileInfoSeq(self, partition, current=None):
"""
Return file information for the specified partition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
partition -- The partition number in the range 0-255.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileSizeRangeException -- If a file is larger than 2.1GB.
PartitionOutOfRangeException -- If the partition number is out of range.
"""
raise NotImplementedError("servant method 'getFileInfoSeq' not implemented")
def getLargeFileInfoSeq(self, partition, current=None):
"""
Returns file information for the specified partition.
Arguments:
partition -- The partition number in the range 0-255.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PartitionOutOfRangeException -- If the partition number is out of range.
"""
raise NotImplementedError("servant method 'getLargeFileInfoSeq' not implemented")
def getChecksumSeq(self, current=None):
"""
Return the checksums for all partitions.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getChecksumSeq' not implemented")
def getChecksum(self, current=None):
"""
Return the master checksum for all partitions. If this checksum is the same
as for a previous run, the entire file set is up-to-date.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getChecksum' not implemented")
def getFileCompressed(self, path, pos, num, current=None):
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
This operation is deprecated and only present for
compatibility with old Ice clients (older than version 3.6).
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
FileSizeRangeException -- If a file is larger than 2.1GB.
"""
raise NotImplementedError("servant method 'getFileCompressed' not implemented")
def getLargeFileCompressed(self, path, pos, num, current=None):
"""
Read the specified file. This operation may only return fewer bytes than requested
in case there was an end-of-file condition.
Arguments:
path -- The pathname (relative to the data directory) for the file to be read.
pos -- The file offset at which to begin reading.
num -- The number of bytes to be read.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
FileAccessException -- If an error occurred while trying to read the file.
"""
raise NotImplementedError("servant method 'getLargeFileCompressed' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IcePatch2._t_FileServerDisp)
__repr__ = __str__
_M_IcePatch2._t_FileServerDisp = IcePy.defineClass('::IcePatch2::FileServer', FileServer, (), None, ())
FileServer._ice_type = _M_IcePatch2._t_FileServerDisp
FileServer._op_getFileInfoSeq = IcePy.Operation('getFileInfoSeq', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_int, False, 0),), (), ((), _M_IcePatch2._t_FileInfoSeq, False, 0), (_M_IcePatch2._t_PartitionOutOfRangeException, _M_IcePatch2._t_FileSizeRangeException))
FileServer._op_getFileInfoSeq.deprecate("getFileInfoSeq() is deprecated, use getLargeFileInfoSeq() instead.")
FileServer._op_getLargeFileInfoSeq = IcePy.Operation('getLargeFileInfoSeq', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (((), IcePy._t_int, False, 0),), (), ((), _M_IcePatch2._t_LargeFileInfoSeq, False, 0), (_M_IcePatch2._t_PartitionOutOfRangeException,))
FileServer._op_getChecksumSeq = IcePy.Operation('getChecksumSeq', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_IcePatch2._t_ByteSeqSeq, False, 0), ())
FileServer._op_getChecksum = IcePy.Operation('getChecksum', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_ByteSeq, False, 0), ())
FileServer._op_getFileCompressed = IcePy.Operation('getFileCompressed', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_int, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_Ice._t_ByteSeq, False, 0), (_M_IcePatch2._t_FileAccessException, _M_IcePatch2._t_FileSizeRangeException))
FileServer._op_getFileCompressed.deprecate("getFileCompressed() is deprecated, use getLargeFileCompressed() instead.")
FileServer._op_getLargeFileCompressed = IcePy.Operation('getLargeFileCompressed', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, True, None, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_long, False, 0), ((), IcePy._t_int, False, 0)), (), ((), _M_Ice._t_ByteSeq, False, 0), (_M_IcePatch2._t_FileAccessException,))
_M_IcePatch2.FileServer = FileServer
del FileServer
# End of module IcePatch2
Ice.sliceChecksums["::IcePatch2::ByteSeqSeq"] = "4bef9684e41babda8aa55f759a854c"
Ice.sliceChecksums["::IcePatch2::FileAccessException"] = "e94ba15e1b6a3639c2358d2f384648"
Ice.sliceChecksums["::IcePatch2::FileServer"] = "4e2d29a4d5995edae6405e69b265943b"
Ice.sliceChecksums["::IcePatch2::FileSizeRangeException"] = "24ba4c71f6441c58b23a2058cd776c8"
Ice.sliceChecksums["::IcePatch2::PartitionOutOfRangeException"] = "edd324eb399a3f6fecc1a28c2296d8" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IcePatch2/FileServer_ice.py | FileServer_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Metrics_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module IceMX
_M_IceMX = Ice.openModule('IceMX')
# Start of module IceMX
__name__ = 'IceMX'
if 'SessionMetrics' not in _M_IceMX.__dict__:
_M_IceMX.SessionMetrics = Ice.createTempClass()
class SessionMetrics(_M_IceMX.Metrics):
"""
Provides information on Glacier2 sessions.
Members:
forwardedClient -- Number of client requests forwared.
forwardedServer -- Number of server requests forwared.
routingTableSize -- The size of the routing table.
queuedClient -- Number of client requests queued.
queuedServer -- Number of server requests queued.
overriddenClient -- Number of client requests overridden.
overriddenServer -- Number of server requests overridden.
"""
def __init__(self, id='', total=0, current=0, totalLifetime=0, failures=0, forwardedClient=0, forwardedServer=0, routingTableSize=0, queuedClient=0, queuedServer=0, overriddenClient=0, overriddenServer=0):
_M_IceMX.Metrics.__init__(self, id, total, current, totalLifetime, failures)
self.forwardedClient = forwardedClient
self.forwardedServer = forwardedServer
self.routingTableSize = routingTableSize
self.queuedClient = queuedClient
self.queuedServer = queuedServer
self.overriddenClient = overriddenClient
self.overriddenServer = overriddenServer
def ice_id(self):
return '::IceMX::SessionMetrics'
@staticmethod
def ice_staticId():
return '::IceMX::SessionMetrics'
def __str__(self):
return IcePy.stringify(self, _M_IceMX._t_SessionMetrics)
__repr__ = __str__
_M_IceMX._t_SessionMetrics = IcePy.defineValue('::IceMX::SessionMetrics', SessionMetrics, -1, (), False, False, _M_IceMX._t_Metrics, (
('forwardedClient', (), IcePy._t_int, False, 0),
('forwardedServer', (), IcePy._t_int, False, 0),
('routingTableSize', (), IcePy._t_int, False, 0),
('queuedClient', (), IcePy._t_int, False, 0),
('queuedServer', (), IcePy._t_int, False, 0),
('overriddenClient', (), IcePy._t_int, False, 0),
('overriddenServer', (), IcePy._t_int, False, 0)
))
SessionMetrics._ice_type = _M_IceMX._t_SessionMetrics
_M_IceMX.SessionMetrics = SessionMetrics
del SessionMetrics
# End of module IceMX
Ice.sliceChecksums["::IceMX::SessionMetrics"] = "221020be2c80301fb4dbb779e21b190" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/Metrics_ice.py | Metrics_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
import Ice.Identity_ice
import Glacier2.SSLInfo_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Start of module Glacier2
__name__ = 'Glacier2'
if 'CannotCreateSessionException' not in _M_Glacier2.__dict__:
_M_Glacier2.CannotCreateSessionException = Ice.createTempClass()
class CannotCreateSessionException(Ice.UserException):
"""
This exception is raised if an attempt to create a new session failed.
Members:
reason -- The reason why session creation has failed.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Glacier2::CannotCreateSessionException'
_M_Glacier2._t_CannotCreateSessionException = IcePy.defineException('::Glacier2::CannotCreateSessionException', CannotCreateSessionException, (), True, None, (('reason', (), IcePy._t_string, False, 0),))
CannotCreateSessionException._ice_type = _M_Glacier2._t_CannotCreateSessionException
_M_Glacier2.CannotCreateSessionException = CannotCreateSessionException
del CannotCreateSessionException
_M_Glacier2._t_Session = IcePy.defineValue('::Glacier2::Session', Ice.Value, -1, (), False, True, None, ())
if 'SessionPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.SessionPrx = Ice.createTempClass()
class SessionPrx(Ice.ObjectPrx):
"""
Destroy the session. This is called automatically when the router is destroyed.
Arguments:
context -- The request context for the invocation.
"""
def destroy(self, context=None):
return _M_Glacier2.Session._op_destroy.invoke(self, ((), context))
"""
Destroy the session. This is called automatically when the router is destroyed.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def destroyAsync(self, context=None):
return _M_Glacier2.Session._op_destroy.invokeAsync(self, ((), context))
"""
Destroy the session. This is called automatically when the router is destroyed.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_destroy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Session._op_destroy.begin(self, ((), _response, _ex, _sent, context))
"""
Destroy the session. This is called automatically when the router is destroyed.
Arguments:
"""
def end_destroy(self, _r):
return _M_Glacier2.Session._op_destroy.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.SessionPrx.ice_checkedCast(proxy, '::Glacier2::Session', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.SessionPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::Session'
_M_Glacier2._t_SessionPrx = IcePy.defineProxy('::Glacier2::Session', SessionPrx)
_M_Glacier2.SessionPrx = SessionPrx
del SessionPrx
_M_Glacier2.Session = Ice.createTempClass()
class Session(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::Session', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::Session'
@staticmethod
def ice_staticId():
return '::Glacier2::Session'
def destroy(self, current=None):
"""
Destroy the session. This is called automatically when the router is destroyed.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SessionDisp)
__repr__ = __str__
_M_Glacier2._t_SessionDisp = IcePy.defineClass('::Glacier2::Session', Session, (), None, ())
Session._ice_type = _M_Glacier2._t_SessionDisp
Session._op_destroy = IcePy.Operation('destroy', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
_M_Glacier2.Session = Session
del Session
_M_Glacier2._t_StringSet = IcePy.defineValue('::Glacier2::StringSet', Ice.Value, -1, (), False, True, None, ())
if 'StringSetPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.StringSetPrx = Ice.createTempClass()
class StringSetPrx(Ice.ObjectPrx):
"""
Add a sequence of strings to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of strings to be added.
context -- The request context for the invocation.
"""
def add(self, additions, context=None):
return _M_Glacier2.StringSet._op_add.invoke(self, ((additions, ), context))
"""
Add a sequence of strings to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of strings to be added.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addAsync(self, additions, context=None):
return _M_Glacier2.StringSet._op_add.invokeAsync(self, ((additions, ), context))
"""
Add a sequence of strings to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of strings to be added.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_add(self, additions, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.StringSet._op_add.begin(self, ((additions, ), _response, _ex, _sent, context))
"""
Add a sequence of strings to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of strings to be added.
"""
def end_add(self, _r):
return _M_Glacier2.StringSet._op_add.end(self, _r)
"""
Remove a sequence of strings from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of strings to be removed.
context -- The request context for the invocation.
"""
def remove(self, deletions, context=None):
return _M_Glacier2.StringSet._op_remove.invoke(self, ((deletions, ), context))
"""
Remove a sequence of strings from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of strings to be removed.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def removeAsync(self, deletions, context=None):
return _M_Glacier2.StringSet._op_remove.invokeAsync(self, ((deletions, ), context))
"""
Remove a sequence of strings from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of strings to be removed.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_remove(self, deletions, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.StringSet._op_remove.begin(self, ((deletions, ), _response, _ex, _sent, context))
"""
Remove a sequence of strings from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of strings to be removed.
"""
def end_remove(self, _r):
return _M_Glacier2.StringSet._op_remove.end(self, _r)
"""
Returns a sequence of strings describing the constraints in this
set.
Arguments:
context -- The request context for the invocation.
Returns: The sequence of strings for this set.
"""
def get(self, context=None):
return _M_Glacier2.StringSet._op_get.invoke(self, ((), context))
"""
Returns a sequence of strings describing the constraints in this
set.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAsync(self, context=None):
return _M_Glacier2.StringSet._op_get.invokeAsync(self, ((), context))
"""
Returns a sequence of strings describing the constraints in this
set.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_get(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.StringSet._op_get.begin(self, ((), _response, _ex, _sent, context))
"""
Returns a sequence of strings describing the constraints in this
set.
Arguments:
Returns: The sequence of strings for this set.
"""
def end_get(self, _r):
return _M_Glacier2.StringSet._op_get.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.StringSetPrx.ice_checkedCast(proxy, '::Glacier2::StringSet', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.StringSetPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::StringSet'
_M_Glacier2._t_StringSetPrx = IcePy.defineProxy('::Glacier2::StringSet', StringSetPrx)
_M_Glacier2.StringSetPrx = StringSetPrx
del StringSetPrx
_M_Glacier2.StringSet = Ice.createTempClass()
class StringSet(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::StringSet', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::StringSet'
@staticmethod
def ice_staticId():
return '::Glacier2::StringSet'
def add(self, additions, current=None):
"""
Add a sequence of strings to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of strings to be added.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'add' not implemented")
def remove(self, deletions, current=None):
"""
Remove a sequence of strings from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of strings to be removed.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'remove' not implemented")
def get(self, current=None):
"""
Returns a sequence of strings describing the constraints in this
set.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'get' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_StringSetDisp)
__repr__ = __str__
_M_Glacier2._t_StringSetDisp = IcePy.defineClass('::Glacier2::StringSet', StringSet, (), None, ())
StringSet._ice_type = _M_Glacier2._t_StringSetDisp
StringSet._op_add = IcePy.Operation('add', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_StringSeq, False, 0),), (), None, ())
StringSet._op_remove = IcePy.Operation('remove', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_StringSeq, False, 0),), (), None, ())
StringSet._op_get = IcePy.Operation('get', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), _M_Ice._t_StringSeq, False, 0), ())
_M_Glacier2.StringSet = StringSet
del StringSet
_M_Glacier2._t_IdentitySet = IcePy.defineValue('::Glacier2::IdentitySet', Ice.Value, -1, (), False, True, None, ())
if 'IdentitySetPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.IdentitySetPrx = Ice.createTempClass()
class IdentitySetPrx(Ice.ObjectPrx):
"""
Add a sequence of Ice identities to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of Ice identities to be added.
context -- The request context for the invocation.
"""
def add(self, additions, context=None):
return _M_Glacier2.IdentitySet._op_add.invoke(self, ((additions, ), context))
"""
Add a sequence of Ice identities to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of Ice identities to be added.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addAsync(self, additions, context=None):
return _M_Glacier2.IdentitySet._op_add.invokeAsync(self, ((additions, ), context))
"""
Add a sequence of Ice identities to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of Ice identities to be added.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_add(self, additions, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.IdentitySet._op_add.begin(self, ((additions, ), _response, _ex, _sent, context))
"""
Add a sequence of Ice identities to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of Ice identities to be added.
"""
def end_add(self, _r):
return _M_Glacier2.IdentitySet._op_add.end(self, _r)
"""
Remove a sequence of identities from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of Ice identities to be removed.
context -- The request context for the invocation.
"""
def remove(self, deletions, context=None):
return _M_Glacier2.IdentitySet._op_remove.invoke(self, ((deletions, ), context))
"""
Remove a sequence of identities from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of Ice identities to be removed.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def removeAsync(self, deletions, context=None):
return _M_Glacier2.IdentitySet._op_remove.invokeAsync(self, ((deletions, ), context))
"""
Remove a sequence of identities from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of Ice identities to be removed.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_remove(self, deletions, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.IdentitySet._op_remove.begin(self, ((deletions, ), _response, _ex, _sent, context))
"""
Remove a sequence of identities from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of Ice identities to be removed.
"""
def end_remove(self, _r):
return _M_Glacier2.IdentitySet._op_remove.end(self, _r)
"""
Returns a sequence of identities describing the constraints in this
set.
Arguments:
context -- The request context for the invocation.
Returns: The sequence of Ice identities for this set.
"""
def get(self, context=None):
return _M_Glacier2.IdentitySet._op_get.invoke(self, ((), context))
"""
Returns a sequence of identities describing the constraints in this
set.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getAsync(self, context=None):
return _M_Glacier2.IdentitySet._op_get.invokeAsync(self, ((), context))
"""
Returns a sequence of identities describing the constraints in this
set.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_get(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.IdentitySet._op_get.begin(self, ((), _response, _ex, _sent, context))
"""
Returns a sequence of identities describing the constraints in this
set.
Arguments:
Returns: The sequence of Ice identities for this set.
"""
def end_get(self, _r):
return _M_Glacier2.IdentitySet._op_get.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.IdentitySetPrx.ice_checkedCast(proxy, '::Glacier2::IdentitySet', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.IdentitySetPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::IdentitySet'
_M_Glacier2._t_IdentitySetPrx = IcePy.defineProxy('::Glacier2::IdentitySet', IdentitySetPrx)
_M_Glacier2.IdentitySetPrx = IdentitySetPrx
del IdentitySetPrx
_M_Glacier2.IdentitySet = Ice.createTempClass()
class IdentitySet(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::IdentitySet', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::IdentitySet'
@staticmethod
def ice_staticId():
return '::Glacier2::IdentitySet'
def add(self, additions, current=None):
"""
Add a sequence of Ice identities to this set of constraints. Order is
not preserved and duplicates are implicitly removed.
Arguments:
additions -- The sequence of Ice identities to be added.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'add' not implemented")
def remove(self, deletions, current=None):
"""
Remove a sequence of identities from this set of constraints. No
errors are returned if an entry is not found.
Arguments:
deletions -- The sequence of Ice identities to be removed.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'remove' not implemented")
def get(self, current=None):
"""
Returns a sequence of identities describing the constraints in this
set.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'get' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_IdentitySetDisp)
__repr__ = __str__
_M_Glacier2._t_IdentitySetDisp = IcePy.defineClass('::Glacier2::IdentitySet', IdentitySet, (), None, ())
IdentitySet._ice_type = _M_Glacier2._t_IdentitySetDisp
IdentitySet._op_add = IcePy.Operation('add', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_IdentitySeq, False, 0),), (), None, ())
IdentitySet._op_remove = IcePy.Operation('remove', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (((), _M_Ice._t_IdentitySeq, False, 0),), (), None, ())
IdentitySet._op_get = IcePy.Operation('get', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), _M_Ice._t_IdentitySeq, False, 0), ())
_M_Glacier2.IdentitySet = IdentitySet
del IdentitySet
_M_Glacier2._t_SessionControl = IcePy.defineValue('::Glacier2::SessionControl', Ice.Value, -1, (), False, True, None, ())
if 'SessionControlPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.SessionControlPrx = Ice.createTempClass()
class SessionControlPrx(Ice.ObjectPrx):
"""
Access the object that manages the allowable categories
for object identities for this session.
Arguments:
context -- The request context for the invocation.
Returns: A StringSet object.
"""
def categories(self, context=None):
return _M_Glacier2.SessionControl._op_categories.invoke(self, ((), context))
"""
Access the object that manages the allowable categories
for object identities for this session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def categoriesAsync(self, context=None):
return _M_Glacier2.SessionControl._op_categories.invokeAsync(self, ((), context))
"""
Access the object that manages the allowable categories
for object identities for this session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_categories(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionControl._op_categories.begin(self, ((), _response, _ex, _sent, context))
"""
Access the object that manages the allowable categories
for object identities for this session.
Arguments:
Returns: A StringSet object.
"""
def end_categories(self, _r):
return _M_Glacier2.SessionControl._op_categories.end(self, _r)
"""
Access the object that manages the allowable adapter identities
for objects for this session.
Arguments:
context -- The request context for the invocation.
Returns: A StringSet object.
"""
def adapterIds(self, context=None):
return _M_Glacier2.SessionControl._op_adapterIds.invoke(self, ((), context))
"""
Access the object that manages the allowable adapter identities
for objects for this session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def adapterIdsAsync(self, context=None):
return _M_Glacier2.SessionControl._op_adapterIds.invokeAsync(self, ((), context))
"""
Access the object that manages the allowable adapter identities
for objects for this session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_adapterIds(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionControl._op_adapterIds.begin(self, ((), _response, _ex, _sent, context))
"""
Access the object that manages the allowable adapter identities
for objects for this session.
Arguments:
Returns: A StringSet object.
"""
def end_adapterIds(self, _r):
return _M_Glacier2.SessionControl._op_adapterIds.end(self, _r)
"""
Access the object that manages the allowable object identities
for this session.
Arguments:
context -- The request context for the invocation.
Returns: An IdentitySet object.
"""
def identities(self, context=None):
return _M_Glacier2.SessionControl._op_identities.invoke(self, ((), context))
"""
Access the object that manages the allowable object identities
for this session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def identitiesAsync(self, context=None):
return _M_Glacier2.SessionControl._op_identities.invokeAsync(self, ((), context))
"""
Access the object that manages the allowable object identities
for this session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_identities(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionControl._op_identities.begin(self, ((), _response, _ex, _sent, context))
"""
Access the object that manages the allowable object identities
for this session.
Arguments:
Returns: An IdentitySet object.
"""
def end_identities(self, _r):
return _M_Glacier2.SessionControl._op_identities.end(self, _r)
"""
Get the session timeout.
Arguments:
context -- The request context for the invocation.
Returns: The timeout.
"""
def getSessionTimeout(self, context=None):
return _M_Glacier2.SessionControl._op_getSessionTimeout.invoke(self, ((), context))
"""
Get the session timeout.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSessionTimeoutAsync(self, context=None):
return _M_Glacier2.SessionControl._op_getSessionTimeout.invokeAsync(self, ((), context))
"""
Get the session timeout.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSessionTimeout(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionControl._op_getSessionTimeout.begin(self, ((), _response, _ex, _sent, context))
"""
Get the session timeout.
Arguments:
Returns: The timeout.
"""
def end_getSessionTimeout(self, _r):
return _M_Glacier2.SessionControl._op_getSessionTimeout.end(self, _r)
"""
Destroy the associated session.
Arguments:
context -- The request context for the invocation.
"""
def destroy(self, context=None):
return _M_Glacier2.SessionControl._op_destroy.invoke(self, ((), context))
"""
Destroy the associated session.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def destroyAsync(self, context=None):
return _M_Glacier2.SessionControl._op_destroy.invokeAsync(self, ((), context))
"""
Destroy the associated session.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_destroy(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionControl._op_destroy.begin(self, ((), _response, _ex, _sent, context))
"""
Destroy the associated session.
Arguments:
"""
def end_destroy(self, _r):
return _M_Glacier2.SessionControl._op_destroy.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.SessionControlPrx.ice_checkedCast(proxy, '::Glacier2::SessionControl', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.SessionControlPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::SessionControl'
_M_Glacier2._t_SessionControlPrx = IcePy.defineProxy('::Glacier2::SessionControl', SessionControlPrx)
_M_Glacier2.SessionControlPrx = SessionControlPrx
del SessionControlPrx
_M_Glacier2.SessionControl = Ice.createTempClass()
class SessionControl(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::SessionControl', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::SessionControl'
@staticmethod
def ice_staticId():
return '::Glacier2::SessionControl'
def categories(self, current=None):
"""
Access the object that manages the allowable categories
for object identities for this session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'categories' not implemented")
def adapterIds(self, current=None):
"""
Access the object that manages the allowable adapter identities
for objects for this session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'adapterIds' not implemented")
def identities(self, current=None):
"""
Access the object that manages the allowable object identities
for this session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'identities' not implemented")
def getSessionTimeout(self, current=None):
"""
Get the session timeout.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSessionTimeout' not implemented")
def destroy(self, current=None):
"""
Destroy the associated session.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'destroy' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SessionControlDisp)
__repr__ = __str__
_M_Glacier2._t_SessionControlDisp = IcePy.defineClass('::Glacier2::SessionControl', SessionControl, (), None, ())
SessionControl._ice_type = _M_Glacier2._t_SessionControlDisp
SessionControl._op_categories = IcePy.Operation('categories', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Glacier2._t_StringSetPrx, False, 0), ())
SessionControl._op_adapterIds = IcePy.Operation('adapterIds', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Glacier2._t_StringSetPrx, False, 0), ())
SessionControl._op_identities = IcePy.Operation('identities', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), ((), _M_Glacier2._t_IdentitySetPrx, False, 0), ())
SessionControl._op_getSessionTimeout = IcePy.Operation('getSessionTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Idempotent, False, None, (), (), (), ((), IcePy._t_int, False, 0), ())
SessionControl._op_destroy = IcePy.Operation('destroy', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
_M_Glacier2.SessionControl = SessionControl
del SessionControl
_M_Glacier2._t_SessionManager = IcePy.defineValue('::Glacier2::SessionManager', Ice.Value, -1, (), False, True, None, ())
if 'SessionManagerPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.SessionManagerPrx = Ice.createTempClass()
class SessionManagerPrx(Ice.ObjectPrx):
"""
Create a new session.
Arguments:
userId -- The user id for the session.
control -- A proxy to the session control object.
context -- The request context for the invocation.
Returns: A proxy to the newly created session.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
def create(self, userId, control, context=None):
return _M_Glacier2.SessionManager._op_create.invoke(self, ((userId, control), context))
"""
Create a new session.
Arguments:
userId -- The user id for the session.
control -- A proxy to the session control object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createAsync(self, userId, control, context=None):
return _M_Glacier2.SessionManager._op_create.invokeAsync(self, ((userId, control), context))
"""
Create a new session.
Arguments:
userId -- The user id for the session.
control -- A proxy to the session control object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_create(self, userId, control, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SessionManager._op_create.begin(self, ((userId, control), _response, _ex, _sent, context))
"""
Create a new session.
Arguments:
userId -- The user id for the session.
control -- A proxy to the session control object.
Returns: A proxy to the newly created session.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
def end_create(self, _r):
return _M_Glacier2.SessionManager._op_create.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.SessionManagerPrx.ice_checkedCast(proxy, '::Glacier2::SessionManager', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.SessionManagerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::SessionManager'
_M_Glacier2._t_SessionManagerPrx = IcePy.defineProxy('::Glacier2::SessionManager', SessionManagerPrx)
_M_Glacier2.SessionManagerPrx = SessionManagerPrx
del SessionManagerPrx
_M_Glacier2.SessionManager = Ice.createTempClass()
class SessionManager(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::SessionManager', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::SessionManager'
@staticmethod
def ice_staticId():
return '::Glacier2::SessionManager'
def create(self, userId, control, current=None):
"""
Create a new session.
Arguments:
userId -- The user id for the session.
control -- A proxy to the session control object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
raise NotImplementedError("servant method 'create' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SessionManagerDisp)
__repr__ = __str__
_M_Glacier2._t_SessionManagerDisp = IcePy.defineClass('::Glacier2::SessionManager', SessionManager, (), None, ())
SessionManager._ice_type = _M_Glacier2._t_SessionManagerDisp
SessionManager._op_create = IcePy.Operation('create', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0), ((), _M_Glacier2._t_SessionControlPrx, False, 0)), (), ((), _M_Glacier2._t_SessionPrx, False, 0), (_M_Glacier2._t_CannotCreateSessionException,))
_M_Glacier2.SessionManager = SessionManager
del SessionManager
_M_Glacier2._t_SSLSessionManager = IcePy.defineValue('::Glacier2::SSLSessionManager', Ice.Value, -1, (), False, True, None, ())
if 'SSLSessionManagerPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.SSLSessionManagerPrx = Ice.createTempClass()
class SSLSessionManagerPrx(Ice.ObjectPrx):
"""
Create a new session.
Arguments:
info -- The SSL info.
control -- A proxy to the session control object.
context -- The request context for the invocation.
Returns: A proxy to the newly created session.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
def create(self, info, control, context=None):
return _M_Glacier2.SSLSessionManager._op_create.invoke(self, ((info, control), context))
"""
Create a new session.
Arguments:
info -- The SSL info.
control -- A proxy to the session control object.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createAsync(self, info, control, context=None):
return _M_Glacier2.SSLSessionManager._op_create.invokeAsync(self, ((info, control), context))
"""
Create a new session.
Arguments:
info -- The SSL info.
control -- A proxy to the session control object.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_create(self, info, control, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SSLSessionManager._op_create.begin(self, ((info, control), _response, _ex, _sent, context))
"""
Create a new session.
Arguments:
info -- The SSL info.
control -- A proxy to the session control object.
Returns: A proxy to the newly created session.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
def end_create(self, _r):
return _M_Glacier2.SSLSessionManager._op_create.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.SSLSessionManagerPrx.ice_checkedCast(proxy, '::Glacier2::SSLSessionManager', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.SSLSessionManagerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::SSLSessionManager'
_M_Glacier2._t_SSLSessionManagerPrx = IcePy.defineProxy('::Glacier2::SSLSessionManager', SSLSessionManagerPrx)
_M_Glacier2.SSLSessionManagerPrx = SSLSessionManagerPrx
del SSLSessionManagerPrx
_M_Glacier2.SSLSessionManager = Ice.createTempClass()
class SSLSessionManager(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::SSLSessionManager', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::SSLSessionManager'
@staticmethod
def ice_staticId():
return '::Glacier2::SSLSessionManager'
def create(self, info, control, current=None):
"""
Create a new session.
Arguments:
info -- The SSL info.
control -- A proxy to the session control object.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
"""
raise NotImplementedError("servant method 'create' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SSLSessionManagerDisp)
__repr__ = __str__
_M_Glacier2._t_SSLSessionManagerDisp = IcePy.defineClass('::Glacier2::SSLSessionManager', SSLSessionManager, (), None, ())
SSLSessionManager._ice_type = _M_Glacier2._t_SSLSessionManagerDisp
SSLSessionManager._op_create = IcePy.Operation('create', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, Ice.FormatType.SlicedFormat, (), (((), _M_Glacier2._t_SSLInfo, False, 0), ((), _M_Glacier2._t_SessionControlPrx, False, 0)), (), ((), _M_Glacier2._t_SessionPrx, False, 0), (_M_Glacier2._t_CannotCreateSessionException,))
_M_Glacier2.SSLSessionManager = SSLSessionManager
del SSLSessionManager
# End of module Glacier2
Ice.sliceChecksums["::Glacier2::CannotCreateSessionException"] = "f3cf2057ea305ed04671164dfaeb6d95"
Ice.sliceChecksums["::Glacier2::IdentitySet"] = "622e43adfd1f535abaee1b089583847"
Ice.sliceChecksums["::Glacier2::SSLSessionManager"] = "4eb77cf437452f5296bf24dda4967d"
Ice.sliceChecksums["::Glacier2::Session"] = "8e47590dc18dd2a2e2e7749c941fc7"
Ice.sliceChecksums["::Glacier2::SessionControl"] = "83a11c547492ddc72db70659938222"
Ice.sliceChecksums["::Glacier2::SessionManager"] = "f3c67f2f29415754c0f1ccc1ab5558e"
Ice.sliceChecksums["::Glacier2::StringSet"] = "1b46953cdce5ef8b6fe92056adf3fda0" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/Session_ice.py | Session_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
__name__ = 'Glacier2'
if 'SSLInfo' not in _M_Glacier2.__dict__:
_M_Glacier2.SSLInfo = Ice.createTempClass()
class SSLInfo(object):
"""
Information taken from an SSL connection used for permissions
verification.
Members:
remoteHost -- The remote host.
remotePort -- The remote port.
localHost -- The router's host.
localPort -- The router's port.
cipher -- The negotiated cipher suite.
certs -- The certificate chain.
"""
def __init__(self, remoteHost='', remotePort=0, localHost='', localPort=0, cipher='', certs=None):
self.remoteHost = remoteHost
self.remotePort = remotePort
self.localHost = localHost
self.localPort = localPort
self.cipher = cipher
self.certs = certs
def __hash__(self):
_h = 0
_h = 5 * _h + Ice.getHash(self.remoteHost)
_h = 5 * _h + Ice.getHash(self.remotePort)
_h = 5 * _h + Ice.getHash(self.localHost)
_h = 5 * _h + Ice.getHash(self.localPort)
_h = 5 * _h + Ice.getHash(self.cipher)
if self.certs:
for _i0 in self.certs:
_h = 5 * _h + Ice.getHash(_i0)
return _h % 0x7fffffff
def __compare(self, other):
if other is None:
return 1
elif not isinstance(other, _M_Glacier2.SSLInfo):
return NotImplemented
else:
if self.remoteHost is None or other.remoteHost is None:
if self.remoteHost != other.remoteHost:
return (-1 if self.remoteHost is None else 1)
else:
if self.remoteHost < other.remoteHost:
return -1
elif self.remoteHost > other.remoteHost:
return 1
if self.remotePort is None or other.remotePort is None:
if self.remotePort != other.remotePort:
return (-1 if self.remotePort is None else 1)
else:
if self.remotePort < other.remotePort:
return -1
elif self.remotePort > other.remotePort:
return 1
if self.localHost is None or other.localHost is None:
if self.localHost != other.localHost:
return (-1 if self.localHost is None else 1)
else:
if self.localHost < other.localHost:
return -1
elif self.localHost > other.localHost:
return 1
if self.localPort is None or other.localPort is None:
if self.localPort != other.localPort:
return (-1 if self.localPort is None else 1)
else:
if self.localPort < other.localPort:
return -1
elif self.localPort > other.localPort:
return 1
if self.cipher is None or other.cipher is None:
if self.cipher != other.cipher:
return (-1 if self.cipher is None else 1)
else:
if self.cipher < other.cipher:
return -1
elif self.cipher > other.cipher:
return 1
if self.certs is None or other.certs is None:
if self.certs != other.certs:
return (-1 if self.certs is None else 1)
else:
if self.certs < other.certs:
return -1
elif self.certs > other.certs:
return 1
return 0
def __lt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r < 0
def __le__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r <= 0
def __gt__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r > 0
def __ge__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r >= 0
def __eq__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r == 0
def __ne__(self, other):
r = self.__compare(other)
if r is NotImplemented:
return r
else:
return r != 0
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SSLInfo)
__repr__ = __str__
_M_Glacier2._t_SSLInfo = IcePy.defineStruct('::Glacier2::SSLInfo', SSLInfo, (), (
('remoteHost', (), IcePy._t_string),
('remotePort', (), IcePy._t_int),
('localHost', (), IcePy._t_string),
('localPort', (), IcePy._t_int),
('cipher', (), IcePy._t_string),
('certs', (), _M_Ice._t_StringSeq)
))
_M_Glacier2.SSLInfo = SSLInfo
del SSLInfo
# End of module Glacier2
Ice.sliceChecksums["::Glacier2::SSLInfo"] = "ca63bc6d361a48471c4d16ea29818e5" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/SSLInfo_ice.py | SSLInfo_ice.py |
import threading, traceback, copy
#
# Import the Python extension.
#
import Ice
Ice.updateModule("Glacier2")
import Glacier2.Router_ice
import Glacier2.Session_ice
import Glacier2.PermissionsVerifier_ice
import Glacier2.SSLInfo_ice
import Glacier2.Metrics_ice
class SessionNotExistException(Exception):
def __init__(self):
pass
class RestartSessionException(Exception):
def __init__(self):
pass
class Application(Ice.Application):
def __init__(self, signalPolicy=0): # HandleSignals=0
'''The constructor accepts an optional argument indicating
whether to handle signals. The value should be either
Application.HandleSignals (the default) or
Application.NoSignalHandling.
'''
if type(self) == Application:
raise RuntimeError("Glacier2.Application is an abstract class")
Ice.Application.__init__(self, signalPolicy)
Application._adapter = None
Application._router = None
Application._session = None
Application._category = None
def run(self, args):
raise RuntimeError('run should not be called on Glacier2.Application - call runWithSession instead')
def createSession(self, args):
raise RuntimeError('createSession() not implemented')
def runWithSession(self, args):
raise RuntimeError('runWithSession() not implemented')
def sessionDestroyed(self):
pass
def restart(self):
raise RestartSessionException()
restart = classmethod(restart)
def router(self):
return Application._router
router = classmethod(router)
def session(self):
return Application._session
session = classmethod(session)
def categoryForClient(self):
if Application._router == None:
raise SessionNotExistException()
return Application._category
categoryForClient = classmethod(categoryForClient)
def createCallbackIdentity(self, name):
return Ice.Identity(name, self.categoryForClient())
createCallbackIdentity = classmethod(createCallbackIdentity)
def addWithUUID(self, servant):
return self.objectAdapter().add(servant, self.createCallbackIdentity(Ice.generateUUID()))
addWithUUID = classmethod(addWithUUID)
def objectAdapter(self):
if Application._router == None:
raise SessionNotExistException()
if Application._adapter == None:
Application._adapter = self.communicator().createObjectAdapterWithRouter("", Application._router)
Application._adapter.activate()
return Application._adapter
objectAdapter = classmethod(objectAdapter)
def doMainInternal(self, args, initData):
# Reset internal state variables from Ice.Application. The
# remainder are reset at the end of this method.
Ice.Application._callbackInProgress = False
Ice.Application._destroyed = False
Ice.Application._interrupted = False
restart = False
status = 0
sessionCreated = False
try:
Ice.Application._communicator = Ice.initialize(args, initData)
Application._router = RouterPrx.uncheckedCast(Ice.Application.communicator().getDefaultRouter())
if Application._router == None:
Ice.getProcessLogger().error("no glacier2 router configured")
status = 1
else:
#
# The default is to destroy when a signal is received.
#
if Ice.Application._signalPolicy == Ice.Application.HandleSignals:
Ice.Application.destroyOnInterrupt()
# If createSession throws, we're done.
try:
Application._session = self.createSession()
sessionCreated = True
except Ice.LocalException:
Ice.getProcessLogger().error(traceback.format_exc())
status = 1
if sessionCreated:
acmTimeout = 0
try:
acmTimeout = Application._router.getACMTimeout()
except(Ice.OperationNotExistException):
pass
if acmTimeout <= 0:
acmTimeout = Application._router.getSessionTimeout()
if acmTimeout > 0:
connection = Application._router.ice_getCachedConnection()
assert(connection)
connection.setACM(acmTimeout, Ice.Unset, Ice.ACMHeartbeat.HeartbeatAlways)
connection.setCloseCallback(lambda conn: self.sessionDestroyed())
Application._category = Application._router.getCategoryForClient()
status = self.runWithSession(args)
# We want to restart on those exceptions which indicate a
# break down in communications, but not those exceptions that
# indicate a programming logic error (ie: marshal, protocol
# failure, etc).
except(RestartSessionException):
restart = True
except(Ice.ConnectionRefusedException, Ice.ConnectionLostException, Ice.UnknownLocalException, \
Ice.RequestFailedException, Ice.TimeoutException):
Ice.getProcessLogger().error(traceback.format_exc())
restart = True
except:
Ice.getProcessLogger().error(traceback.format_exc())
status = 1
#
# Don't want any new interrupt and at this point (post-run),
# it would not make sense to release a held signal to run
# shutdown or destroy.
#
if Ice.Application._signalPolicy == Ice.Application.HandleSignals:
Ice.Application.ignoreInterrupt()
Ice.Application._condVar.acquire()
while Ice.Application._callbackInProgress:
Ice.Application._condVar.wait()
if Ice.Application._destroyed:
Ice.Application._communicator = None
else:
Ice.Application._destroyed = True
#
# And _communicator != None, meaning will be destroyed
# next, _destroyed = True also ensures that any
# remaining callback won't do anything
#
Ice.Application._condVar.release()
if sessionCreated and Application._router:
try:
Application._router.destroySession()
except (Ice.ConnectionLostException, SessionNotExistException):
pass
except:
Ice.getProcessLogger().error("unexpected exception when destroying the session " + \
traceback.format_exc())
Application._router = None
if Ice.Application._communicator:
try:
Ice.Application._communicator.destroy()
except:
getProcessLogger().error(traceback.format_exc())
status = 1
Ice.Application._communicator = None
# Reset internal state. We cannot reset the Application state
# here, since _destroyed must remain true until we re-run
# this method.
Application._adapter = None
Application._router = None
Application._session = None
sessionCreated = False
Application._category = None
return (restart, status)
def doMain(self, args, initData):
# Set the default properties for all Glacier2 applications.
initData.properties.setProperty("Ice.RetryIntervals", "-1")
restart = True
ret = 0
while restart:
# A copy of the initialization data and the string seq
# needs to be passed to doMainInternal, as these can be
# changed by the application.
id = copy.copy(initData)
if id.properties:
id.properties = id.properties.clone()
argsCopy = args[:]
(restart, ret) = self.doMainInternal(argsCopy, initData)
return ret | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/__init__.py | __init__.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Glacier2.SSLInfo_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Start of module Glacier2
__name__ = 'Glacier2'
if 'PermissionDeniedException' not in _M_Glacier2.__dict__:
_M_Glacier2.PermissionDeniedException = Ice.createTempClass()
class PermissionDeniedException(Ice.UserException):
"""
This exception is raised if a client is denied the ability to create
a session with the router.
Members:
reason -- The reason why permission was denied.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Glacier2::PermissionDeniedException'
_M_Glacier2._t_PermissionDeniedException = IcePy.defineException('::Glacier2::PermissionDeniedException', PermissionDeniedException, (), True, None, (('reason', (), IcePy._t_string, False, 0),))
PermissionDeniedException._ice_type = _M_Glacier2._t_PermissionDeniedException
_M_Glacier2.PermissionDeniedException = PermissionDeniedException
del PermissionDeniedException
_M_Glacier2._t_PermissionsVerifier = IcePy.defineValue('::Glacier2::PermissionsVerifier', Ice.Value, -1, (), False, True, None, ())
if 'PermissionsVerifierPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.PermissionsVerifierPrx = Ice.createTempClass()
class PermissionsVerifierPrx(Ice.ObjectPrx):
"""
Check whether a user has permission to access the router.
Arguments:
userId -- The user id for which to check permission.
password -- The user's password.
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- True if access is granted, or false otherwise.
reason -- The reason why access was denied.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
def checkPermissions(self, userId, password, context=None):
return _M_Glacier2.PermissionsVerifier._op_checkPermissions.invoke(self, ((userId, password), context))
"""
Check whether a user has permission to access the router.
Arguments:
userId -- The user id for which to check permission.
password -- The user's password.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def checkPermissionsAsync(self, userId, password, context=None):
return _M_Glacier2.PermissionsVerifier._op_checkPermissions.invokeAsync(self, ((userId, password), context))
"""
Check whether a user has permission to access the router.
Arguments:
userId -- The user id for which to check permission.
password -- The user's password.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_checkPermissions(self, userId, password, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.PermissionsVerifier._op_checkPermissions.begin(self, ((userId, password), _response, _ex, _sent, context))
"""
Check whether a user has permission to access the router.
Arguments:
userId -- The user id for which to check permission.
password -- The user's password.
Returns a tuple containing the following:
_retval -- True if access is granted, or false otherwise.
reason -- The reason why access was denied.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
def end_checkPermissions(self, _r):
return _M_Glacier2.PermissionsVerifier._op_checkPermissions.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.PermissionsVerifierPrx.ice_checkedCast(proxy, '::Glacier2::PermissionsVerifier', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.PermissionsVerifierPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::PermissionsVerifier'
_M_Glacier2._t_PermissionsVerifierPrx = IcePy.defineProxy('::Glacier2::PermissionsVerifier', PermissionsVerifierPrx)
_M_Glacier2.PermissionsVerifierPrx = PermissionsVerifierPrx
del PermissionsVerifierPrx
_M_Glacier2.PermissionsVerifier = Ice.createTempClass()
class PermissionsVerifier(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::PermissionsVerifier', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::PermissionsVerifier'
@staticmethod
def ice_staticId():
return '::Glacier2::PermissionsVerifier'
def checkPermissions(self, userId, password, current=None):
"""
Check whether a user has permission to access the router.
Arguments:
userId -- The user id for which to check permission.
password -- The user's password.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
raise NotImplementedError("servant method 'checkPermissions' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_PermissionsVerifierDisp)
__repr__ = __str__
_M_Glacier2._t_PermissionsVerifierDisp = IcePy.defineClass('::Glacier2::PermissionsVerifier', PermissionsVerifier, (), None, ())
PermissionsVerifier._ice_type = _M_Glacier2._t_PermissionsVerifierDisp
PermissionsVerifier._op_checkPermissions = IcePy.Operation('checkPermissions', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (((), IcePy._t_string, False, 0),), ((), IcePy._t_bool, False, 0), (_M_Glacier2._t_PermissionDeniedException,))
_M_Glacier2.PermissionsVerifier = PermissionsVerifier
del PermissionsVerifier
_M_Glacier2._t_SSLPermissionsVerifier = IcePy.defineValue('::Glacier2::SSLPermissionsVerifier', Ice.Value, -1, (), False, True, None, ())
if 'SSLPermissionsVerifierPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.SSLPermissionsVerifierPrx = Ice.createTempClass()
class SSLPermissionsVerifierPrx(Ice.ObjectPrx):
"""
Check whether a user has permission to access the router.
Arguments:
info -- The SSL information.
context -- The request context for the invocation.
Returns a tuple containing the following:
_retval -- True if access is granted, or false otherwise.
reason -- The reason why access was denied.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
def authorize(self, info, context=None):
return _M_Glacier2.SSLPermissionsVerifier._op_authorize.invoke(self, ((info, ), context))
"""
Check whether a user has permission to access the router.
Arguments:
info -- The SSL information.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def authorizeAsync(self, info, context=None):
return _M_Glacier2.SSLPermissionsVerifier._op_authorize.invokeAsync(self, ((info, ), context))
"""
Check whether a user has permission to access the router.
Arguments:
info -- The SSL information.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_authorize(self, info, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.SSLPermissionsVerifier._op_authorize.begin(self, ((info, ), _response, _ex, _sent, context))
"""
Check whether a user has permission to access the router.
Arguments:
info -- The SSL information.
Returns a tuple containing the following:
_retval -- True if access is granted, or false otherwise.
reason -- The reason why access was denied.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
def end_authorize(self, _r):
return _M_Glacier2.SSLPermissionsVerifier._op_authorize.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.SSLPermissionsVerifierPrx.ice_checkedCast(proxy, '::Glacier2::SSLPermissionsVerifier', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.SSLPermissionsVerifierPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::SSLPermissionsVerifier'
_M_Glacier2._t_SSLPermissionsVerifierPrx = IcePy.defineProxy('::Glacier2::SSLPermissionsVerifier', SSLPermissionsVerifierPrx)
_M_Glacier2.SSLPermissionsVerifierPrx = SSLPermissionsVerifierPrx
del SSLPermissionsVerifierPrx
_M_Glacier2.SSLPermissionsVerifier = Ice.createTempClass()
class SSLPermissionsVerifier(Ice.Object):
def ice_ids(self, current=None):
return ('::Glacier2::SSLPermissionsVerifier', '::Ice::Object')
def ice_id(self, current=None):
return '::Glacier2::SSLPermissionsVerifier'
@staticmethod
def ice_staticId():
return '::Glacier2::SSLPermissionsVerifier'
def authorize(self, info, current=None):
"""
Check whether a user has permission to access the router.
Arguments:
info -- The SSL information.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
PermissionDeniedException -- Raised if the user access is denied. This can be raised in place of returning false with a reason set in the reason out parameter.
"""
raise NotImplementedError("servant method 'authorize' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_SSLPermissionsVerifierDisp)
__repr__ = __str__
_M_Glacier2._t_SSLPermissionsVerifierDisp = IcePy.defineClass('::Glacier2::SSLPermissionsVerifier', SSLPermissionsVerifier, (), None, ())
SSLPermissionsVerifier._ice_type = _M_Glacier2._t_SSLPermissionsVerifierDisp
SSLPermissionsVerifier._op_authorize = IcePy.Operation('authorize', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, Ice.FormatType.SlicedFormat, (), (((), _M_Glacier2._t_SSLInfo, False, 0),), (((), IcePy._t_string, False, 0),), ((), IcePy._t_bool, False, 0), (_M_Glacier2._t_PermissionDeniedException,))
_M_Glacier2.SSLPermissionsVerifier = SSLPermissionsVerifier
del SSLPermissionsVerifier
# End of module Glacier2
Ice.sliceChecksums["::Glacier2::PermissionDeniedException"] = "27def8d4569ab203b629b9162d530ba"
Ice.sliceChecksums["::Glacier2::PermissionsVerifier"] = "fcf33ee75c6e0e651d33c88bbca148d"
Ice.sliceChecksums["::Glacier2::SSLPermissionsVerifier"] = "c9d5a5d4c26aae9cb2da81d56f9f9e1" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/PermissionsVerifier_ice.py | PermissionsVerifier_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.Router_ice
import Glacier2.Session_ice
import Glacier2.PermissionsVerifier_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Included module Glacier2
_M_Glacier2 = Ice.openModule('Glacier2')
# Start of module Glacier2
__name__ = 'Glacier2'
_M_Glacier2.__doc__ = """
Glacier2 is a firewall solution for Ice. Glacier2 authenticates
and filters client requests and allows callbacks to the client in a
secure fashion. In combination with IceSSL, Glacier2 provides a
security solution that is both non-intrusive and easy to configure.
"""
if 'SessionNotExistException' not in _M_Glacier2.__dict__:
_M_Glacier2.SessionNotExistException = Ice.createTempClass()
class SessionNotExistException(Ice.UserException):
"""
This exception is raised if a client tries to destroy a session
with a router, but no session exists for the client.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::Glacier2::SessionNotExistException'
_M_Glacier2._t_SessionNotExistException = IcePy.defineException('::Glacier2::SessionNotExistException', SessionNotExistException, (), False, None, ())
SessionNotExistException._ice_type = _M_Glacier2._t_SessionNotExistException
_M_Glacier2.SessionNotExistException = SessionNotExistException
del SessionNotExistException
_M_Glacier2._t_Router = IcePy.defineValue('::Glacier2::Router', Ice.Value, -1, (), False, True, None, ())
if 'RouterPrx' not in _M_Glacier2.__dict__:
_M_Glacier2.RouterPrx = Ice.createTempClass()
class RouterPrx(_M_Ice.RouterPrx):
"""
This category must be used in the identities of all of the client's
callback objects. This is necessary in order for the router to
forward callback requests to the intended client. If the Glacier2
server endpoints are not set, the returned category is an empty
string.
Arguments:
context -- The request context for the invocation.
Returns: The category.
"""
def getCategoryForClient(self, context=None):
return _M_Glacier2.Router._op_getCategoryForClient.invoke(self, ((), context))
"""
This category must be used in the identities of all of the client's
callback objects. This is necessary in order for the router to
forward callback requests to the intended client. If the Glacier2
server endpoints are not set, the returned category is an empty
string.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getCategoryForClientAsync(self, context=None):
return _M_Glacier2.Router._op_getCategoryForClient.invokeAsync(self, ((), context))
"""
This category must be used in the identities of all of the client's
callback objects. This is necessary in order for the router to
forward callback requests to the intended client. If the Glacier2
server endpoints are not set, the returned category is an empty
string.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getCategoryForClient(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_getCategoryForClient.begin(self, ((), _response, _ex, _sent, context))
"""
This category must be used in the identities of all of the client's
callback objects. This is necessary in order for the router to
forward callback requests to the intended client. If the Glacier2
server endpoints are not set, the returned category is an empty
string.
Arguments:
Returns: The category.
"""
def end_getCategoryForClient(self, _r):
return _M_Glacier2.Router._op_getCategoryForClient.end(self, _r)
"""
Create a per-client session with the router. If a
SessionManager has been installed, a proxy to a Session
object is returned to the client. Otherwise, null is returned
and only an internal session (i.e., not visible to the client)
is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
userId -- The user id for which to check the password.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A proxy for the newly created session, or null if no SessionManager has been installed.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def createSession(self, userId, password, context=None):
return _M_Glacier2.Router._op_createSession.invoke(self, ((userId, password), context))
"""
Create a per-client session with the router. If a
SessionManager has been installed, a proxy to a Session
object is returned to the client. Otherwise, null is returned
and only an internal session (i.e., not visible to the client)
is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
userId -- The user id for which to check the password.
password -- The password for the given user id.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createSessionAsync(self, userId, password, context=None):
return _M_Glacier2.Router._op_createSession.invokeAsync(self, ((userId, password), context))
"""
Create a per-client session with the router. If a
SessionManager has been installed, a proxy to a Session
object is returned to the client. Otherwise, null is returned
and only an internal session (i.e., not visible to the client)
is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
userId -- The user id for which to check the password.
password -- The password for the given user id.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createSession(self, userId, password, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_createSession.begin(self, ((userId, password), _response, _ex, _sent, context))
"""
Create a per-client session with the router. If a
SessionManager has been installed, a proxy to a Session
object is returned to the client. Otherwise, null is returned
and only an internal session (i.e., not visible to the client)
is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
userId -- The user id for which to check the password.
password -- The password for the given user id.
Returns: A proxy for the newly created session, or null if no SessionManager has been installed.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
def end_createSession(self, _r):
return _M_Glacier2.Router._op_createSession.end(self, _r)
"""
Create a per-client session with the router. The user is
authenticated through the SSL certificates that have been
associated with the connection. If a SessionManager has been
installed, a proxy to a Session object is returned to the
client. Otherwise, null is returned and only an internal
session (i.e., not visible to the client) is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
context -- The request context for the invocation.
Returns: A proxy for the newly created session, or null if no SessionManager has been installed.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the user cannot be authenticated or if the user is not allowed access.
"""
def createSessionFromSecureConnection(self, context=None):
return _M_Glacier2.Router._op_createSessionFromSecureConnection.invoke(self, ((), context))
"""
Create a per-client session with the router. The user is
authenticated through the SSL certificates that have been
associated with the connection. If a SessionManager has been
installed, a proxy to a Session object is returned to the
client. Otherwise, null is returned and only an internal
session (i.e., not visible to the client) is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def createSessionFromSecureConnectionAsync(self, context=None):
return _M_Glacier2.Router._op_createSessionFromSecureConnection.invokeAsync(self, ((), context))
"""
Create a per-client session with the router. The user is
authenticated through the SSL certificates that have been
associated with the connection. If a SessionManager has been
installed, a proxy to a Session object is returned to the
client. Otherwise, null is returned and only an internal
session (i.e., not visible to the client) is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_createSessionFromSecureConnection(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_createSessionFromSecureConnection.begin(self, ((), _response, _ex, _sent, context))
"""
Create a per-client session with the router. The user is
authenticated through the SSL certificates that have been
associated with the connection. If a SessionManager has been
installed, a proxy to a Session object is returned to the
client. Otherwise, null is returned and only an internal
session (i.e., not visible to the client) is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
Returns: A proxy for the newly created session, or null if no SessionManager has been installed.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the user cannot be authenticated or if the user is not allowed access.
"""
def end_createSessionFromSecureConnection(self, _r):
return _M_Glacier2.Router._op_createSessionFromSecureConnection.end(self, _r)
"""
Keep the calling client's session with this router alive.
Arguments:
context -- The request context for the invocation.
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
def refreshSession(self, context=None):
return _M_Glacier2.Router._op_refreshSession.invoke(self, ((), context))
"""
Keep the calling client's session with this router alive.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def refreshSessionAsync(self, context=None):
return _M_Glacier2.Router._op_refreshSession.invokeAsync(self, ((), context))
"""
Keep the calling client's session with this router alive.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_refreshSession(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_refreshSession.begin(self, ((), _response, _ex, _sent, context))
"""
Keep the calling client's session with this router alive.
Arguments:
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
def end_refreshSession(self, _r):
return _M_Glacier2.Router._op_refreshSession.end(self, _r)
"""
Destroy the calling client's session with this router.
Arguments:
context -- The request context for the invocation.
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
def destroySession(self, context=None):
return _M_Glacier2.Router._op_destroySession.invoke(self, ((), context))
"""
Destroy the calling client's session with this router.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def destroySessionAsync(self, context=None):
return _M_Glacier2.Router._op_destroySession.invokeAsync(self, ((), context))
"""
Destroy the calling client's session with this router.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_destroySession(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_destroySession.begin(self, ((), _response, _ex, _sent, context))
"""
Destroy the calling client's session with this router.
Arguments:
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
def end_destroySession(self, _r):
return _M_Glacier2.Router._op_destroySession.end(self, _r)
"""
Get the value of the session timeout. Sessions are destroyed
if they see no activity for this period of time.
Arguments:
context -- The request context for the invocation.
Returns: The timeout (in seconds).
"""
def getSessionTimeout(self, context=None):
return _M_Glacier2.Router._op_getSessionTimeout.invoke(self, ((), context))
"""
Get the value of the session timeout. Sessions are destroyed
if they see no activity for this period of time.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSessionTimeoutAsync(self, context=None):
return _M_Glacier2.Router._op_getSessionTimeout.invokeAsync(self, ((), context))
"""
Get the value of the session timeout. Sessions are destroyed
if they see no activity for this period of time.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSessionTimeout(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_getSessionTimeout.begin(self, ((), _response, _ex, _sent, context))
"""
Get the value of the session timeout. Sessions are destroyed
if they see no activity for this period of time.
Arguments:
Returns: The timeout (in seconds).
"""
def end_getSessionTimeout(self, _r):
return _M_Glacier2.Router._op_getSessionTimeout.end(self, _r)
"""
Get the value of the ACM timeout. Clients supporting connection
heartbeats can enable them instead of explicitly sending keep
alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
context -- The request context for the invocation.
Returns: The timeout (in seconds).
"""
def getACMTimeout(self, context=None):
return _M_Glacier2.Router._op_getACMTimeout.invoke(self, ((), context))
"""
Get the value of the ACM timeout. Clients supporting connection
heartbeats can enable them instead of explicitly sending keep
alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getACMTimeoutAsync(self, context=None):
return _M_Glacier2.Router._op_getACMTimeout.invokeAsync(self, ((), context))
"""
Get the value of the ACM timeout. Clients supporting connection
heartbeats can enable them instead of explicitly sending keep
alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getACMTimeout(self, _response=None, _ex=None, _sent=None, context=None):
return _M_Glacier2.Router._op_getACMTimeout.begin(self, ((), _response, _ex, _sent, context))
"""
Get the value of the ACM timeout. Clients supporting connection
heartbeats can enable them instead of explicitly sending keep
alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
Returns: The timeout (in seconds).
"""
def end_getACMTimeout(self, _r):
return _M_Glacier2.Router._op_getACMTimeout.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_Glacier2.RouterPrx.ice_checkedCast(proxy, '::Glacier2::Router', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_Glacier2.RouterPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::Glacier2::Router'
_M_Glacier2._t_RouterPrx = IcePy.defineProxy('::Glacier2::Router', RouterPrx)
_M_Glacier2.RouterPrx = RouterPrx
del RouterPrx
_M_Glacier2.Router = Ice.createTempClass()
class Router(_M_Ice.Router):
def ice_ids(self, current=None):
return ('::Glacier2::Router', '::Ice::Object', '::Ice::Router')
def ice_id(self, current=None):
return '::Glacier2::Router'
@staticmethod
def ice_staticId():
return '::Glacier2::Router'
def getCategoryForClient(self, current=None):
"""
This category must be used in the identities of all of the client's
callback objects. This is necessary in order for the router to
forward callback requests to the intended client. If the Glacier2
server endpoints are not set, the returned category is an empty
string.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getCategoryForClient' not implemented")
def createSession(self, userId, password, current=None):
"""
Create a per-client session with the router. If a
SessionManager has been installed, a proxy to a Session
object is returned to the client. Otherwise, null is returned
and only an internal session (i.e., not visible to the client)
is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
userId -- The user id for which to check the password.
password -- The password for the given user id.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the password for the given user id is not correct, or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createSession' not implemented")
def createSessionFromSecureConnection(self, current=None):
"""
Create a per-client session with the router. The user is
authenticated through the SSL certificates that have been
associated with the connection. If a SessionManager has been
installed, a proxy to a Session object is returned to the
client. Otherwise, null is returned and only an internal
session (i.e., not visible to the client) is created.
If a session proxy is returned, it must be configured to route
through the router that created it. This will happen automatically
if the router is configured as the client's default router at the
time the session proxy is created in the client process, otherwise
the client must configure the session proxy explicitly.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
CannotCreateSessionException -- Raised if the session cannot be created.
PermissionDeniedException -- Raised if the user cannot be authenticated or if the user is not allowed access.
"""
raise NotImplementedError("servant method 'createSessionFromSecureConnection' not implemented")
def refreshSession(self, current=None):
"""
Keep the calling client's session with this router alive.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
raise NotImplementedError("servant method 'refreshSession' not implemented")
def destroySession(self, current=None):
"""
Destroy the calling client's session with this router.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
SessionNotExistException -- Raised if no session exists for the calling client.
"""
raise NotImplementedError("servant method 'destroySession' not implemented")
def getSessionTimeout(self, current=None):
"""
Get the value of the session timeout. Sessions are destroyed
if they see no activity for this period of time.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSessionTimeout' not implemented")
def getACMTimeout(self, current=None):
"""
Get the value of the ACM timeout. Clients supporting connection
heartbeats can enable them instead of explicitly sending keep
alives requests.
NOTE: This method is only available since Ice 3.6.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getACMTimeout' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_Glacier2._t_RouterDisp)
__repr__ = __str__
_M_Glacier2._t_RouterDisp = IcePy.defineClass('::Glacier2::Router', Router, (), None, (_M_Ice._t_RouterDisp,))
Router._ice_type = _M_Glacier2._t_RouterDisp
Router._op_getCategoryForClient = IcePy.Operation('getCategoryForClient', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_string, False, 0), ())
Router._op_createSession = IcePy.Operation('createSession', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, Ice.FormatType.SlicedFormat, (), (((), IcePy._t_string, False, 0), ((), IcePy._t_string, False, 0)), (), ((), _M_Glacier2._t_SessionPrx, False, 0), (_M_Glacier2._t_PermissionDeniedException, _M_Glacier2._t_CannotCreateSessionException))
Router._op_createSessionFromSecureConnection = IcePy.Operation('createSessionFromSecureConnection', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, Ice.FormatType.SlicedFormat, (), (), (), ((), _M_Glacier2._t_SessionPrx, False, 0), (_M_Glacier2._t_PermissionDeniedException, _M_Glacier2._t_CannotCreateSessionException))
Router._op_refreshSession = IcePy.Operation('refreshSession', Ice.OperationMode.Normal, Ice.OperationMode.Normal, True, None, (), (), (), None, (_M_Glacier2._t_SessionNotExistException,))
Router._op_destroySession = IcePy.Operation('destroySession', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, (_M_Glacier2._t_SessionNotExistException,))
Router._op_getSessionTimeout = IcePy.Operation('getSessionTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_long, False, 0), ())
Router._op_getACMTimeout = IcePy.Operation('getACMTimeout', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), IcePy._t_int, False, 0), ())
_M_Glacier2.Router = Router
del Router
# End of module Glacier2
Ice.sliceChecksums["::Glacier2::Router"] = "dfe8817f11292a5582437c19a65b63"
Ice.sliceChecksums["::Glacier2::SessionNotExistException"] = "9b3392dc48a63f86d96c13662972328" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/Glacier2/Router_ice.py | Router_ice.py |
from sys import version_info as _version_info_
import Ice, IcePy
import Ice.BuiltinSequences_ice
import Ice.CommunicatorF_ice
import Ice.PropertiesF_ice
import Ice.SliceChecksumDict_ice
# Included module Ice
_M_Ice = Ice.openModule('Ice')
# Start of module IceBox
_M_IceBox = Ice.openModule('IceBox')
__name__ = 'IceBox'
_M_IceBox.__doc__ = """
IceBox is an application server specifically for Ice
applications. IceBox can easily run and administer Ice services
that are dynamically loaded as a DLL, shared library, or Java
class.
"""
if 'FailureException' not in _M_IceBox.__dict__:
_M_IceBox.FailureException = Ice.createTempClass()
class FailureException(Ice.LocalException):
"""
This exception is a general failure notification. It is thrown
for errors such as a service encountering an error during
initialization, or the service manager being unable
to load a service executable.
Members:
reason -- The reason for the failure.
"""
def __init__(self, reason=''):
self.reason = reason
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceBox::FailureException'
_M_IceBox._t_FailureException = IcePy.defineException('::IceBox::FailureException', FailureException, (), False, None, (('reason', (), IcePy._t_string, False, 0),))
FailureException._ice_type = _M_IceBox._t_FailureException
_M_IceBox.FailureException = FailureException
del FailureException
if 'AlreadyStartedException' not in _M_IceBox.__dict__:
_M_IceBox.AlreadyStartedException = Ice.createTempClass()
class AlreadyStartedException(Ice.UserException):
"""
This exception is thrown if an attempt is made to start an
already-started service.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceBox::AlreadyStartedException'
_M_IceBox._t_AlreadyStartedException = IcePy.defineException('::IceBox::AlreadyStartedException', AlreadyStartedException, (), False, None, ())
AlreadyStartedException._ice_type = _M_IceBox._t_AlreadyStartedException
_M_IceBox.AlreadyStartedException = AlreadyStartedException
del AlreadyStartedException
if 'AlreadyStoppedException' not in _M_IceBox.__dict__:
_M_IceBox.AlreadyStoppedException = Ice.createTempClass()
class AlreadyStoppedException(Ice.UserException):
"""
This exception is thrown if an attempt is made to stop an
already-stopped service.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceBox::AlreadyStoppedException'
_M_IceBox._t_AlreadyStoppedException = IcePy.defineException('::IceBox::AlreadyStoppedException', AlreadyStoppedException, (), False, None, ())
AlreadyStoppedException._ice_type = _M_IceBox._t_AlreadyStoppedException
_M_IceBox.AlreadyStoppedException = AlreadyStoppedException
del AlreadyStoppedException
if 'NoSuchServiceException' not in _M_IceBox.__dict__:
_M_IceBox.NoSuchServiceException = Ice.createTempClass()
class NoSuchServiceException(Ice.UserException):
"""
This exception is thrown if a service name does not refer
to an existing service.
"""
def __init__(self):
pass
def __str__(self):
return IcePy.stringifyException(self)
__repr__ = __str__
_ice_id = '::IceBox::NoSuchServiceException'
_M_IceBox._t_NoSuchServiceException = IcePy.defineException('::IceBox::NoSuchServiceException', NoSuchServiceException, (), False, None, ())
NoSuchServiceException._ice_type = _M_IceBox._t_NoSuchServiceException
_M_IceBox.NoSuchServiceException = NoSuchServiceException
del NoSuchServiceException
if 'Service' not in _M_IceBox.__dict__:
_M_IceBox.Service = Ice.createTempClass()
class Service(object):
"""
An application service managed by a ServiceManager.
"""
def __init__(self):
if Ice.getType(self) == _M_IceBox.Service:
raise RuntimeError('IceBox.Service is an abstract class')
def start(self, name, communicator, args):
"""
Start the service. The given communicator is created by the
ServiceManager for use by the service. This communicator may
also be used by other services, depending on the service
configuration.
The ServiceManager owns this communicator, and is
responsible for destroying it.
Arguments:
name -- The service's name, as determined by the configuration.
communicator -- A communicator for use by the service.
args -- The service arguments that were not converted into properties.
Throws:
FailureException -- Raised if start failed.
"""
raise NotImplementedError("method 'start' not implemented")
def stop(self):
"""
Stop the service.
"""
raise NotImplementedError("method 'stop' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceBox._t_Service)
__repr__ = __str__
_M_IceBox._t_Service = IcePy.defineValue('::IceBox::Service', Service, -1, (), False, True, None, ())
Service._ice_type = _M_IceBox._t_Service
_M_IceBox.Service = Service
del Service
_M_IceBox._t_ServiceObserver = IcePy.defineValue('::IceBox::ServiceObserver', Ice.Value, -1, (), False, True, None, ())
if 'ServiceObserverPrx' not in _M_IceBox.__dict__:
_M_IceBox.ServiceObserverPrx = Ice.createTempClass()
class ServiceObserverPrx(Ice.ObjectPrx):
"""
Receives the names of the services that were started.
Arguments:
services -- The names of the services.
context -- The request context for the invocation.
"""
def servicesStarted(self, services, context=None):
return _M_IceBox.ServiceObserver._op_servicesStarted.invoke(self, ((services, ), context))
"""
Receives the names of the services that were started.
Arguments:
services -- The names of the services.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def servicesStartedAsync(self, services, context=None):
return _M_IceBox.ServiceObserver._op_servicesStarted.invokeAsync(self, ((services, ), context))
"""
Receives the names of the services that were started.
Arguments:
services -- The names of the services.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_servicesStarted(self, services, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceObserver._op_servicesStarted.begin(self, ((services, ), _response, _ex, _sent, context))
"""
Receives the names of the services that were started.
Arguments:
services -- The names of the services.
"""
def end_servicesStarted(self, _r):
return _M_IceBox.ServiceObserver._op_servicesStarted.end(self, _r)
"""
Receives the names of the services that were stopped.
Arguments:
services -- The names of the services.
context -- The request context for the invocation.
"""
def servicesStopped(self, services, context=None):
return _M_IceBox.ServiceObserver._op_servicesStopped.invoke(self, ((services, ), context))
"""
Receives the names of the services that were stopped.
Arguments:
services -- The names of the services.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def servicesStoppedAsync(self, services, context=None):
return _M_IceBox.ServiceObserver._op_servicesStopped.invokeAsync(self, ((services, ), context))
"""
Receives the names of the services that were stopped.
Arguments:
services -- The names of the services.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_servicesStopped(self, services, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceObserver._op_servicesStopped.begin(self, ((services, ), _response, _ex, _sent, context))
"""
Receives the names of the services that were stopped.
Arguments:
services -- The names of the services.
"""
def end_servicesStopped(self, _r):
return _M_IceBox.ServiceObserver._op_servicesStopped.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceBox.ServiceObserverPrx.ice_checkedCast(proxy, '::IceBox::ServiceObserver', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceBox.ServiceObserverPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceBox::ServiceObserver'
_M_IceBox._t_ServiceObserverPrx = IcePy.defineProxy('::IceBox::ServiceObserver', ServiceObserverPrx)
_M_IceBox.ServiceObserverPrx = ServiceObserverPrx
del ServiceObserverPrx
_M_IceBox.ServiceObserver = Ice.createTempClass()
class ServiceObserver(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceBox::ServiceObserver')
def ice_id(self, current=None):
return '::IceBox::ServiceObserver'
@staticmethod
def ice_staticId():
return '::IceBox::ServiceObserver'
def servicesStarted(self, services, current=None):
"""
Receives the names of the services that were started.
Arguments:
services -- The names of the services.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'servicesStarted' not implemented")
def servicesStopped(self, services, current=None):
"""
Receives the names of the services that were stopped.
Arguments:
services -- The names of the services.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'servicesStopped' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceBox._t_ServiceObserverDisp)
__repr__ = __str__
_M_IceBox._t_ServiceObserverDisp = IcePy.defineClass('::IceBox::ServiceObserver', ServiceObserver, (), None, ())
ServiceObserver._ice_type = _M_IceBox._t_ServiceObserverDisp
ServiceObserver._op_servicesStarted = IcePy.Operation('servicesStarted', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_StringSeq, False, 0),), (), None, ())
ServiceObserver._op_servicesStopped = IcePy.Operation('servicesStopped', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_Ice._t_StringSeq, False, 0),), (), None, ())
_M_IceBox.ServiceObserver = ServiceObserver
del ServiceObserver
_M_IceBox._t_ServiceManager = IcePy.defineValue('::IceBox::ServiceManager', Ice.Value, -1, (), False, True, None, ())
if 'ServiceManagerPrx' not in _M_IceBox.__dict__:
_M_IceBox.ServiceManagerPrx = Ice.createTempClass()
class ServiceManagerPrx(Ice.ObjectPrx):
"""
Returns the checksums for the IceBox Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def getSliceChecksums(self, context=None):
return _M_IceBox.ServiceManager._op_getSliceChecksums.invoke(self, ((), context))
"""
Returns the checksums for the IceBox Slice definitions.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def getSliceChecksumsAsync(self, context=None):
return _M_IceBox.ServiceManager._op_getSliceChecksums.invokeAsync(self, ((), context))
"""
Returns the checksums for the IceBox Slice definitions.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_getSliceChecksums(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceManager._op_getSliceChecksums.begin(self, ((), _response, _ex, _sent, context))
"""
Returns the checksums for the IceBox Slice definitions.
Arguments:
Returns: A dictionary mapping Slice type ids to their checksums.
"""
def end_getSliceChecksums(self, _r):
return _M_IceBox.ServiceManager._op_getSliceChecksums.end(self, _r)
"""
Start an individual service.
Arguments:
service -- The service name.
context -- The request context for the invocation.
Throws:
AlreadyStartedException -- If the service is already running.
NoSuchServiceException -- If no service could be found with the given name.
"""
def startService(self, service, context=None):
return _M_IceBox.ServiceManager._op_startService.invoke(self, ((service, ), context))
"""
Start an individual service.
Arguments:
service -- The service name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def startServiceAsync(self, service, context=None):
return _M_IceBox.ServiceManager._op_startService.invokeAsync(self, ((service, ), context))
"""
Start an individual service.
Arguments:
service -- The service name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_startService(self, service, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceManager._op_startService.begin(self, ((service, ), _response, _ex, _sent, context))
"""
Start an individual service.
Arguments:
service -- The service name.
Throws:
AlreadyStartedException -- If the service is already running.
NoSuchServiceException -- If no service could be found with the given name.
"""
def end_startService(self, _r):
return _M_IceBox.ServiceManager._op_startService.end(self, _r)
"""
Stop an individual service.
Arguments:
service -- The service name.
context -- The request context for the invocation.
Throws:
AlreadyStoppedException -- If the service is already stopped.
NoSuchServiceException -- If no service could be found with the given name.
"""
def stopService(self, service, context=None):
return _M_IceBox.ServiceManager._op_stopService.invoke(self, ((service, ), context))
"""
Stop an individual service.
Arguments:
service -- The service name.
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def stopServiceAsync(self, service, context=None):
return _M_IceBox.ServiceManager._op_stopService.invokeAsync(self, ((service, ), context))
"""
Stop an individual service.
Arguments:
service -- The service name.
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_stopService(self, service, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceManager._op_stopService.begin(self, ((service, ), _response, _ex, _sent, context))
"""
Stop an individual service.
Arguments:
service -- The service name.
Throws:
AlreadyStoppedException -- If the service is already stopped.
NoSuchServiceException -- If no service could be found with the given name.
"""
def end_stopService(self, _r):
return _M_IceBox.ServiceManager._op_stopService.end(self, _r)
"""
Registers a new observer with the ServiceManager.
Arguments:
observer -- The new observer
context -- The request context for the invocation.
"""
def addObserver(self, observer, context=None):
return _M_IceBox.ServiceManager._op_addObserver.invoke(self, ((observer, ), context))
"""
Registers a new observer with the ServiceManager.
Arguments:
observer -- The new observer
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def addObserverAsync(self, observer, context=None):
return _M_IceBox.ServiceManager._op_addObserver.invokeAsync(self, ((observer, ), context))
"""
Registers a new observer with the ServiceManager.
Arguments:
observer -- The new observer
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_addObserver(self, observer, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceManager._op_addObserver.begin(self, ((observer, ), _response, _ex, _sent, context))
"""
Registers a new observer with the ServiceManager.
Arguments:
observer -- The new observer
"""
def end_addObserver(self, _r):
return _M_IceBox.ServiceManager._op_addObserver.end(self, _r)
"""
Shut down all services. This causes stop to be invoked on all configured services.
Arguments:
context -- The request context for the invocation.
"""
def shutdown(self, context=None):
return _M_IceBox.ServiceManager._op_shutdown.invoke(self, ((), context))
"""
Shut down all services. This causes stop to be invoked on all configured services.
Arguments:
context -- The request context for the invocation.
Returns: A future object for the invocation.
"""
def shutdownAsync(self, context=None):
return _M_IceBox.ServiceManager._op_shutdown.invokeAsync(self, ((), context))
"""
Shut down all services. This causes stop to be invoked on all configured services.
Arguments:
_response -- The asynchronous response callback.
_ex -- The asynchronous exception callback.
_sent -- The asynchronous sent callback.
context -- The request context for the invocation.
Returns: An asynchronous result object for the invocation.
"""
def begin_shutdown(self, _response=None, _ex=None, _sent=None, context=None):
return _M_IceBox.ServiceManager._op_shutdown.begin(self, ((), _response, _ex, _sent, context))
"""
Shut down all services. This causes stop to be invoked on all configured services.
Arguments:
"""
def end_shutdown(self, _r):
return _M_IceBox.ServiceManager._op_shutdown.end(self, _r)
@staticmethod
def checkedCast(proxy, facetOrContext=None, context=None):
return _M_IceBox.ServiceManagerPrx.ice_checkedCast(proxy, '::IceBox::ServiceManager', facetOrContext, context)
@staticmethod
def uncheckedCast(proxy, facet=None):
return _M_IceBox.ServiceManagerPrx.ice_uncheckedCast(proxy, facet)
@staticmethod
def ice_staticId():
return '::IceBox::ServiceManager'
_M_IceBox._t_ServiceManagerPrx = IcePy.defineProxy('::IceBox::ServiceManager', ServiceManagerPrx)
_M_IceBox.ServiceManagerPrx = ServiceManagerPrx
del ServiceManagerPrx
_M_IceBox.ServiceManager = Ice.createTempClass()
class ServiceManager(Ice.Object):
def ice_ids(self, current=None):
return ('::Ice::Object', '::IceBox::ServiceManager')
def ice_id(self, current=None):
return '::IceBox::ServiceManager'
@staticmethod
def ice_staticId():
return '::IceBox::ServiceManager'
def getSliceChecksums(self, current=None):
"""
Returns the checksums for the IceBox Slice definitions.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'getSliceChecksums' not implemented")
def startService(self, service, current=None):
"""
Start an individual service.
Arguments:
service -- The service name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AlreadyStartedException -- If the service is already running.
NoSuchServiceException -- If no service could be found with the given name.
"""
raise NotImplementedError("servant method 'startService' not implemented")
def stopService(self, service, current=None):
"""
Stop an individual service.
Arguments:
service -- The service name.
current -- The Current object for the invocation.
Returns: A future object for the invocation.
Throws:
AlreadyStoppedException -- If the service is already stopped.
NoSuchServiceException -- If no service could be found with the given name.
"""
raise NotImplementedError("servant method 'stopService' not implemented")
def addObserver(self, observer, current=None):
"""
Registers a new observer with the ServiceManager.
Arguments:
observer -- The new observer
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'addObserver' not implemented")
def shutdown(self, current=None):
"""
Shut down all services. This causes stop to be invoked on all configured services.
Arguments:
current -- The Current object for the invocation.
Returns: A future object for the invocation.
"""
raise NotImplementedError("servant method 'shutdown' not implemented")
def __str__(self):
return IcePy.stringify(self, _M_IceBox._t_ServiceManagerDisp)
__repr__ = __str__
_M_IceBox._t_ServiceManagerDisp = IcePy.defineClass('::IceBox::ServiceManager', ServiceManager, (), None, ())
ServiceManager._ice_type = _M_IceBox._t_ServiceManagerDisp
ServiceManager._op_getSliceChecksums = IcePy.Operation('getSliceChecksums', Ice.OperationMode.Idempotent, Ice.OperationMode.Nonmutating, False, None, (), (), (), ((), _M_Ice._t_SliceChecksumDict, False, 0), ())
ServiceManager._op_startService = IcePy.Operation('startService', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceBox._t_AlreadyStartedException, _M_IceBox._t_NoSuchServiceException))
ServiceManager._op_stopService = IcePy.Operation('stopService', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), IcePy._t_string, False, 0),), (), None, (_M_IceBox._t_AlreadyStoppedException, _M_IceBox._t_NoSuchServiceException))
ServiceManager._op_addObserver = IcePy.Operation('addObserver', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (((), _M_IceBox._t_ServiceObserverPrx, False, 0),), (), None, ())
ServiceManager._op_shutdown = IcePy.Operation('shutdown', Ice.OperationMode.Normal, Ice.OperationMode.Normal, False, None, (), (), (), None, ())
_M_IceBox.ServiceManager = ServiceManager
del ServiceManager
# End of module IceBox
Ice.sliceChecksums["::IceBox::AlreadyStartedException"] = "d5b097af3221b37482d5f175502abf62"
Ice.sliceChecksums["::IceBox::AlreadyStoppedException"] = "281d493a84d674b3a4335d6afc2c16"
Ice.sliceChecksums["::IceBox::NoSuchServiceException"] = "5957f1c582d9aebad557cbdb7820d4"
Ice.sliceChecksums["::IceBox::ServiceManager"] = "df3a42670c3ce4ef67d6125a5d04d4c"
Ice.sliceChecksums["::IceBox::ServiceObserver"] = "f657781cda7438532a6c33e95988479c" | zeroc-ice | /zeroc_ice-3.7.9.1-cp311-cp311-macosx_13_0_x86_64.whl/IceBox/IceBox_ice.py | IceBox_ice.py |
The Ice Certificate Utilities package includes the iceca command line utility and a small Python library to allow creating certificates for Ice clients or servers.
It relies on PyOpenSSL for the creation of certificates. The Java KeyStore files are created with the keytool utility. The Java BouncyCastle provider is required to create BouncyCastle KeyStore files.
Installation
============
We recommend using ``pip`` or ``easy_install`` to install this package.
Package Contents
================
The iceca command line utility
------------------------------
The iceca utility provides a small certificate authority to allow creating certificates for use with Ice client and servers. It supports commands for initialization of the CA database, certification creation and export.
Usage:
::
usage: iceca [--verbose --help --capass <pass>] init create list show export
The iceca command manages a small certificate authority to create and sign
certificates for Ice clients or servers.
Commands:
init Initialize the certificate authority database
create Create and sign a certificate/key pair
list List the created certificates
show Show a given certificate
export Export a given certificate
Usage of the ``init`` subcommand:
::
usage: init [--overwrite --no-capass]
Initializes the certificate authority database.
Options:
--overwrite Overwrite the existing CA database
--no-capass Don't protect the CA with a password
Usage of the ``create`` subcommand:
::
usage: create [--ip=<ip>] [--dns=<dns>] <alias> [<common-name>]
Creates and signs a certificate. A certificate is identified by its alias. If no
common name is specified, the alias is used as the common name.
Options:
--ip Optional IP subject alternative name field
--dns Optional DNS subject alternative name field
Usage of the ``list`` subcommand:
::
usage: list
List aliases for the certificates created with this CA.
Usage of the ``show`` subcommand:
::
usage: show <alias>
Print out the certificate associated to the given alias.
Usage of the ``export`` subcommand:
::
usage: export [--password <password>] [--alias <alias>] path
Export a certificate from the CA to the given file path. If --alias isn't
specified, the filename indicates which certificate to export. The file
extension also specifies the export format for the certificate. Supported
formats are:
PKCS12 (.p12, .pfx)
PEM (.pem)
DER (.der, .cer, .crt)
JKS (.jks, requires keytool to be in the PATH)
BKS (.bks, requires keytool and support for the BouncyCastle provider)
Options:
--password The password to use for protecting the exported certificate
--alias The alias of the certificate to export
The IceCertUtils module
-----------------------
Here's an example on how to create a server and client certificate with the IceCertUtils module:
::
import IceCertUtils
#
# Create the certicate factory
#
factory = IceCertUtils.CertificateFactory(cn = "My CA")
# Get the CA certificate and save it to PEM/DER and JKS files
factory.getCA().save("cacert.pem").save("cacert.der").save("cacert.jks")
#
# Create a client certificate
#
client = factory.create("client", cn = "Client")
# Save the client certificate to the PKCS12 format
client.save("client.p12")
# Save the client certificate to the JKS format and also include the CA
certificate in the keystore with the alias "cacert"
client.save("client.jks", caalias="cacert")
#
# Create the server certificate, include IP and DNS subject alternative names.
#
server = factory.create("server", cn = "Server", ip="127.0.0.1", dns="server.foo.com")
# Save the server certificate to the PKCS12 format
server.save("server.p12")
# Save the server certificate to the JKS format
server.save("server.jks", caalias="cacert")
# Save the client and server certificates to the BKS format. If the BKS
# provider is not installed this will throw.
try:
client.save("client.bks", caalias="cacert")
server.save("server.bks", caalias="cacert")
except Exception as ex:
print("warning: couldn't generate BKS certificates:\n" + str(ex))
factory.destroy()
| zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/README.rst | README.rst |
import os, random, tempfile, glob, datetime
from IceCertUtils.CertificateUtils import DistinguishedName, Certificate, CertificateFactory, b, d, read, write
from OpenSSL import crypto
def setSubject(dn, subj):
for k in [ "CN", "OU", "O", "L", "ST", "C", "emailAddress"]:
if hasattr(dn, k):
v = getattr(dn, k)
if v:
setattr(subj, k, v)
class PyOpenSSLCertificate(Certificate):
def __init__(self, *args):
Certificate.__init__(self, *args)
self.pem = os.path.join(self.parent.home, self.alias + ".pem")
self.key = os.path.join(self.parent.home, self.alias + "_key.pem")
self.pkey = None
self.x509 = None
def exists(self):
return os.path.exists(self.pem)
def init(self, pkey, x509):
self.pkey = pkey
self.x509 = x509
self.generateKEY()
self.generatePEM()
return self
def load(self):
cert = crypto.load_certificate(crypto.FILETYPE_PEM, read(self.pem))
key = crypto.load_privatekey(crypto.FILETYPE_PEM, read(self.key), b(self.parent.password))
subject = cert.get_subject()
self.dn = DistinguishedName(subject.commonName,
subject.organizationalUnitName,
subject.organizationName,
subject.localityName,
subject.stateOrProvinceName,
subject.countryName,
subject.emailAddress)
return self.init(key, cert)
def toText(self):
s = """Version: %s
Serial Number: %s
Signature Algorithm: %s
Issuer: %s
Validity:
Not before: %s
Not after: %s
Subject: %s
Subject Public Key Size: %s
X509v3 extensions:""" % (self.x509.get_version() + 1,
self.x509.get_serial_number(),
self.x509.get_signature_algorithm(),
str(self.x509.get_issuer()).replace("<X509Name object '", "").replace("'>", ""),
datetime.datetime.strptime(d(self.x509.get_notBefore()), "%Y%m%d%H%M%SZ"),
datetime.datetime.strptime(d(self.x509.get_notAfter()), "%Y%m%d%H%M%SZ"),
str(self.x509.get_subject()).replace("<X509Name object '", "").replace("'>", ""),
str(self.x509.get_pubkey().bits()))
for i in range(0, self.x509.get_extension_count()):
ext = self.x509.get_extension(i)
s += "\n " + d(ext.get_short_name()).strip() + ":\n " + str(ext).replace("\n", "\n ")
return s
def getSubjectHash(self):
return format(self.x509.subject_name_hash(), 'x')
def saveKey(self, path, password=None):
(_, ext) = os.path.splitext(path)
type = crypto.FILETYPE_PEM
if ext == ".der" or ext == ".crt" or ext == ".cer":
type = crypto.FILETYPE_ASN1
if password:
write(path, crypto.dump_privatekey(type, self.pkey, self.parent.cipher, b(password)))
else:
write(path, crypto.dump_privatekey(type, self.pkey))
return self
def savePKCS12(self, path, password=None, chain=True, root=False, addkey=None):
if addkey is None:
addkey = self != self.parent.cacert
p12 = crypto.PKCS12()
p12.set_certificate(self.x509)
p12.set_friendlyname(b(self.alias))
if addkey:
p12.set_privatekey(self.pkey)
if chain:
certs = []
parent = self.parent
while parent if root else parent.parent:
certs.append(parent.cacert.x509)
parent = parent.parent
p12.set_ca_certificates(certs)
write(path, p12.export(b(password or "password")))
return self
def savePEM(self, path, chain=True, root=False):
text = crypto.dump_certificate(crypto.FILETYPE_PEM, self.x509)
if chain:
parent = self.parent
while parent if root else parent.parent:
text += crypto.dump_certificate(crypto.FILETYPE_PEM, parent.cacert.x509)
parent = parent.parent
write(path, text)
return self
def saveDER(self, path):
write(path, crypto.dump_certificate(crypto.FILETYPE_ASN1, self.x509))
return self
def generateKEY(self):
if not os.path.exists(self.key):
write(self.key, crypto.dump_privatekey(crypto.FILETYPE_PEM, self.pkey, self.parent.cipher,
b(self.parent.password)))
def destroy(self):
Certificate.destroy(self)
if os.path.exists(self.key):
os.remove(self.key)
class PyOpenSSLCertificateFactory(CertificateFactory):
def __init__(self, *args, **kargs):
CertificateFactory.__init__(self, *args, **kargs)
if not self.parent:
self.keyalg = crypto.TYPE_RSA if self.keyalg == "rsa" else crypto.TYPE_DSA
self.cipher = "DES-EDE3-CBC" # Cipher used to encode the private key
if not self.cacert.exists():
# Generate the CA certificate
key = crypto.PKey()
key.generate_key(self.keyalg, self.keysize)
req = crypto.X509Req()
setSubject(self.cacert.dn, req.get_subject())
req.set_pubkey(key)
req.sign(key, self.sigalg)
x509 = crypto.X509()
x509.set_version(0x02)
x509.set_serial_number(random.getrandbits(64))
x509.gmtime_adj_notBefore(0)
x509.gmtime_adj_notAfter(60 * 60 * 24 * self.validity)
x509.set_subject(req.get_subject())
x509.set_pubkey(req.get_pubkey())
extensions = [
crypto.X509Extension(b('basicConstraints'), False, b('CA:true')),
crypto.X509Extension(b('subjectKeyIdentifier'), False, b('hash'), subject=x509),
]
subjectAltName = self.cacert.getAlternativeName()
if subjectAltName:
extensions.append(crypto.X509Extension(b('subjectAltName'), False, b(subjectAltName)))
if self.parent:
extensions.append(crypto.X509Extension(b('authorityKeyIdentifier'), False,
b('keyid:always,issuer:always'), issuer=self.parent.cacert.x509))
if self.parent.cacert.getAlternativeName():
extensions.append(crypto.X509Extension(b('issuerAltName'), False, b("issuer:copy"),
issuer=self.parent.cacert.x509))
x509.add_extensions(extensions)
if self.parent:
x509.set_issuer(self.parent.cacert.x509.get_subject())
x509.sign(self.parent.cacert.pkey, self.sigalg)
else:
x509.set_issuer(req.get_subject())
x509.sign(key, self.sigalg)
self.cacert.init(key, x509)
def _createFactory(self, *args, **kargs):
return PyOpenSSLCertificateFactory(*args, **kargs)
def _createChild(self, *args):
return PyOpenSSLCertificate(self, *args)
def _generateChild(self, cert, serial, validity):
key = crypto.PKey()
key.generate_key(self.keyalg, self.keysize)
req = crypto.X509Req()
setSubject(cert.dn, req.get_subject())
req.set_pubkey(key)
req.sign(key, self.sigalg)
x509 = crypto.X509()
x509.set_version(0x02)
x509.set_serial_number(serial or random.getrandbits(64))
if validity is None or validity > 0:
x509.gmtime_adj_notBefore(0)
x509.gmtime_adj_notAfter(60 * 60 * 24 * (validity or self.validity))
else:
x509.gmtime_adj_notBefore(60 * 60 * 24 * validity)
x509.gmtime_adj_notAfter(0)
x509.set_issuer(self.cacert.x509.get_subject())
x509.set_subject(req.get_subject())
x509.set_pubkey(req.get_pubkey())
extensions = [
crypto.X509Extension(b('subjectKeyIdentifier'), False, b('hash'), subject=x509),
crypto.X509Extension(b('authorityKeyIdentifier'), False, b('keyid:always,issuer:always'),
issuer=self.cacert.x509),
crypto.X509Extension(b('keyUsage'), False, b('nonRepudiation, digitalSignature, keyEncipherment'))
]
subAltName = cert.getAlternativeName()
if subAltName:
extensions.append(crypto.X509Extension(b('subjectAltName'), False, b(subAltName)))
if self.cacert.getAlternativeName():
extensions.append(crypto.X509Extension(b('issuerAltName'), False, b("issuer:copy"),issuer=self.cacert.x509))
extendedKeyUsage = cert.getExtendedKeyUsage()
if extendedKeyUsage:
extensions.append(crypto.X509Extension(b('extendedKeyUsage'),False,b(extendedKeyUsage)))
x509.add_extensions(extensions)
x509.sign(self.cacert.pkey, self.sigalg)
return cert.init(key, x509)
def list(self):
return [os.path.basename(a).replace("_key.pem","") for a in glob.glob(os.path.join(self.home, "*_key.pem"))] | zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/IceCertUtils/PyOpenSSLCertificateUtils.py | PyOpenSSLCertificateUtils.py |
import os, subprocess, glob, tempfile
from IceCertUtils.CertificateUtils import DistinguishedName, Certificate, CertificateFactory, b, d, read, opensslSupport, write
class KeyToolCertificate(Certificate):
def __init__(self, *args):
Certificate.__init__(self, *args)
self.jks = os.path.join(self.parent.home, self.alias + ".jks")
def exists(self):
return os.path.exists(self.jks)
def load(self):
subject = self.toText()
self.dn = DistinguishedName.parse(subject[subject.find(":") + 1:subject.find("\n")].strip())
return self
def toText(self):
return d(self.parent.keyTool("printcert", "-v", stdin = self.keyTool("exportcert")))
def saveKey(self, path, password=None):
if not opensslSupport:
raise RuntimeError("No openssl support, add openssl to your PATH to export private keys")
#
# Write password to safe temporary file
#
passpath = None
if password:
(f, passpath) = tempfile.mkstemp()
os.write(f, b(password))
os.close(f)
try:
pem = self.parent.run("openssl", "pkcs12", "-nocerts", "-nodes", "-in " + self.generatePKCS12(),
passin="file:" + self.parent.passpath,
passout=("file:" + passpath) if passpath else None)
(_, ext) = os.path.splitext(path)
outform = "DER" if ext == ".der" or ext == ".crt" or ext == ".cer" else "PEM"
self.parent.run("openssl", "pkcs8", "-nocrypt -topk8", outform=outform, out=path, stdin=pem)
finally:
if passpath:
os.remove(passpath)
return self
def saveJKS(self, *args, **kargs):
return Certificate.saveJKS(self, src=self.jks, *args, **kargs)
def saveBKS(self, *args, **kargs):
return Certificate.saveBKS(self, src=self.jks, *args, **kargs)
def savePKCS12(self, path, password = None, chain=True, root=True, addkey=None):
if not chain or not root:
raise RuntimeError("can only export PKCS12 chain with root certificate")
self.exportToKeyStore(path, password, addkey=addkey, src=self.jks)
return self
def savePEM(self, path, chain=True, root=False):
text = self.keyTool("exportcert", "-rfc")
if chain:
parent = self.parent
while parent if root else parent.parent:
text += parent.cacert.keyTool("exportcert", "-rfc")
parent = parent.parent
write(path, text)
return self
def saveDER(self, path):
self.keyTool("exportcert", file=path)
return self
def keyTool(self, *args, **kargs):
return self.parent.keyTool(cert=self, *args, **kargs)
def destroy(self):
Certificate.destroy(self)
if self.jks and os.path.exists(self.jks):
os.remove(self.jks)
class KeyToolCertificateFactory(CertificateFactory):
def __init__(self, *args, **kargs):
CertificateFactory.__init__(self, *args, **kargs)
# Transform key/signature algorithm to suitable values for keytool
if not self.parent:
self.keyalg = self.keyalg.upper()
self.sigalg = self.sigalg.upper() + "with" + self.keyalg;
# Create the CA self-signed certificate
if not self.cacert.exists():
cacert = self.cacert
subAltName = cacert.getAlternativeName()
issuerAltName = self.parent.cacert.getAlternativeName() if self.parent else None
ext = "-ext bc:c" + \
((" -ext san=" + subAltName) if subAltName else "") + \
((" -ext ian=" + issuerAltName) if issuerAltName else "")
if not self.parent:
cacert.keyTool("genkeypair", ext, validity=self.validity, sigalg=self.sigalg)
else:
self.cacert = self.parent.cacert
cacert.keyTool("genkeypair")
pem = cacert.keyTool("gencert", ext, validity = self.validity, stdin=cacert.keyTool("certreq"))
chain = ""
parent = self.parent
while parent:
chain += d(read(parent.cacert.pem))
parent = parent.parent
cacert.keyTool("importcert", stdin=chain + d(pem))
self.cacert = cacert
self.cacert.generatePEM()
def _createFactory(self, *args, **kargs):
#
# Intermediate CAs don't work well with keytool, they probably
# can but at this point we don't want to spend more time on
# this.
#
#return KeyToolCertificateFactory(*args, **kargs)
raise NotImplementedError("KeyTool implementation doesn't support intermediate CAs")
def _createChild(self, *args):
return KeyToolCertificate(self, *args)
def _generateChild(self, cert, serial, validity):
subAltName = cert.getAlternativeName()
issuerAltName = self.cacert.getAlternativeName()
extendedKeyUsage = cert.getExtendedKeyUsage()
# Generate a certificate/key pair
cert.keyTool("genkeypair")
# Create a certificate signing request
req = cert.keyTool("certreq")
ext = "-ext ku:c=dig,keyEnc" + \
((" -ext san=" + subAltName) if subAltName else "") + \
((" -ext ian=" + issuerAltName) if issuerAltName else "") + \
((" -ext eku=" + extendedKeyUsage) if extendedKeyUsage else "")
# Sign the certificate with the CA
if validity is None or validity > 0:
pem = cert.keyTool("gencert", ext, validity = (validity or self.validity), stdin=req)
else:
pem = cert.keyTool("gencert", ext, startdate = "{validity}d".format(validity=validity), validity=-validity,
stdin=req)
# Concatenate the CA and signed certificate and re-import it into the keystore
chain = []
parent = self
while parent:
chain.append(d(read(parent.cacert.pem)))
parent = parent.parent
cert.keyTool("importcert", stdin="".join(chain) + d(pem))
return cert
def list(self):
return [os.path.splitext(os.path.basename(a))[0] for a in glob.glob(os.path.join(self.home, "*.jks"))]
def keyTool(self, cmd, *args, **kargs):
command = "keytool -noprompt -{cmd}".format(cmd = cmd)
# Consume cert argument
cert = kargs.get("cert", None)
if cert: del kargs["cert"]
# Setup -keystore, -storepass and -alias arguments
if cmd in ["genkeypair", "exportcert", "certreq", "importcert"]:
command += " -alias {cert.alias} -keystore {cert.jks} -storepass:file {this.passpath}"
elif cmd in ["gencert"]:
command += " -alias {cacert.alias} -keystore {cacert.jks} -storepass:file {this.passpath}"
if cmd == "genkeypair":
command += " -keypass:file {this.passpath} -keyalg {this.keyalg} -keysize {this.keysize} -dname \"{cert.dn}\""
elif cmd == "certreq":
command += " -sigalg {this.sigalg}"
elif cmd == "gencert":
command += " -rfc"
command = command.format(cert = cert, cacert = self.cacert, this = self)
return self.run(command, *args, **kargs) | zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/IceCertUtils/KeyToolCertificateUtils.py | KeyToolCertificateUtils.py |
import os, random, tempfile, glob
from IceCertUtils.CertificateUtils import DistinguishedName, Certificate, CertificateFactory, b, d, read, write
def toDNSection(dn):
s = "[ dn ]\n"
for k, v in [ ("countryName", "C"),
("organizationalUnitName", "OU"),
("organizationName", "O"),
("localityName", "L"),
("stateOrProvinceName", "ST"),
("commonName", "CN"),
("emailAddress", "emailAddress")]:
if hasattr(dn, v):
value = getattr(dn, v)
if value:
s += "{k} = {v}\n".format(k = k, v = value)
return s
class OpenSSLCertificate(Certificate):
def __init__(self, *args):
Certificate.__init__(self, *args)
self.pem = os.path.join(self.parent.home, self.alias + ".pem")
self.key = os.path.join(self.parent.home, self.alias + "_key.pem")
def exists(self):
return os.path.exists(self.pem) and os.path.exists(self.key)
def load(self):
subject = d(self.openSSL("x509", "-noout", "-subject"))
if subject:
self.dn = DistinguishedName.parse(subject[subject.find("=") + 1:].replace("/", ","))
return self
def toText(self):
return d(self.openSSL("x509", "-noout", "-text"))
def getSubjectHash(self):
return d(self.openSSL("x509", "-noout", "-subject_hash"))
def saveKey(self, path, password=None):
(_, ext) = os.path.splitext(path)
outform = "PEM"
if ext == ".der" or ext == ".crt" or ext == ".cer":
outform = "DER"
#self.openSSL(self.parent.keyalg, outform=outform, out=path, password=password)
self.openSSL("pkcs8", "-nocrypt -topk8", outform=outform, out=path, password=password)
return self
def savePKCS12(self, path, password=None, chain=True, root=False, addkey=None):
if addkey is None:
addkey = self != self.parent.cacert
chainfile = None
if chain:
# Save the certificate chain to PKCS12
certs = ""
parent = self.parent
while parent if root else parent.parent:
certs += d(read(parent.cacert.pem))
parent = parent.parent
if len(certs) > 0:
(f, chainfile) = tempfile.mkstemp()
os.write(f, b(certs))
os.close(f)
key = "-inkey={0}".format(self.key) if addkey else "-nokeys"
try:
self.openSSL("pkcs12", out=path, inkey=self.key, certfile=chainfile, password=password or "password")
finally:
if chainfile:
os.remove(chainfile)
return self
def savePEM(self, path, chain=True, root=False):
text = self.openSSL("x509", outform="PEM")
if chain:
parent = self.parent
while parent if root else parent.parent:
text += parent.cacert.openSSL("x509", outform="PEM")
parent = parent.parent
write(path, text)
return self
def saveDER(self, path):
self.openSSL("x509", outform="DER", out=path)
return self
def destroy(self):
if os.path.exists(self.key):
os.remove(self.key)
Certificate.destroy(self)
def openSSL(self, *args, **kargs):
return self.parent.openSSL(cert=self, *args, **kargs)
class OpenSSLCertificateFactory(CertificateFactory):
def __init__(self, *args, **kargs):
CertificateFactory.__init__(self, *args, **kargs)
if self.keyalg == "dsa":
self.keyparams = os.path.join(self.home, "dsaparams.pem")
if not os.path.exists(self.keyparams):
self.run("openssl dsaparam -outform PEM -out {0} {1}".format(self.keyparams, self.keysize))
else:
self.keyparams = self.keysize
if not self.cacert.exists():
subAltName = self.cacert.getAlternativeName()
issuerAltName = self.parent.cacert.getAlternativeName() if self.parent else None
altName = (("\nsubjectAltName = " + subAltName) if subAltName else "") + \
(("\nissuerAltName = " + issuerAltName) if issuerAltName else "")
cacert = self.cacert
if not self.parent:
cacert.openSSL("req", "-x509", days = self.validity, config =
"""
[ req ]
x509_extensions = ext
distinguished_name = dn
prompt = no
[ ext ]
basicConstraints = CA:true
subjectKeyIdentifier = hash
authorityKeyIdentifier = keyid:always,issuer:always
{altName}
{dn}
""".format(dn=toDNSection(cacert.dn),altName=altName))
else:
self.cacert = self.parent.cacert
req = cacert.openSSL("req", config=
"""
[ req ]
distinguished_name = dn
prompt = no
{dn}
""".format(dn=toDNSection(cacert.dn)))
# Sign the certificate
cacert.openSSL("x509", "-req", set_serial=random.getrandbits(64), stdin=req, days = self.validity,
extfile=
"""
[ ext ]
basicConstraints = CA:true
subjectKeyIdentifier = hash
authorityKeyIdentifier = keyid:always,issuer:always
{altName}
""".format(altName=altName))
self.cacert = cacert
def _createFactory(self, *args, **kargs):
return OpenSSLCertificateFactory(*args, **kargs)
def _createChild(self, *args):
return OpenSSLCertificate(self, *args)
def _generateChild(self, cert, serial, validity):
subAltName = cert.getAlternativeName()
issuerAltName = self.cacert.getAlternativeName()
altName = (("\nsubjectAltName = " + subAltName) if subAltName else "") + \
(("\nissuerAltName = " + issuerAltName) if issuerAltName else "")
extendedKeyUsage = cert.getExtendedKeyUsage()
extendedKeyUsage = ("extendedKeyUsage = " + extendedKeyUsage) if extendedKeyUsage else ""
# Generate a certificate request
req = cert.openSSL("req", config=
"""
[ req ]
distinguished_name = dn
prompt = no
{dn}
""".format(dn=toDNSection(cert.dn)))
# Sign the certificate
cert.openSSL("x509", "-req", set_serial=serial or random.getrandbits(64), stdin=req,
days = validity or self.validity, extfile=
"""
[ ext ]
subjectKeyIdentifier = hash
authorityKeyIdentifier = keyid:always,issuer:always
keyUsage = nonRepudiation, digitalSignature, keyEncipherment
{extendedKeyUsage}
{altName}
""".format(altName=altName, extendedKeyUsage=extendedKeyUsage))
return cert
def list(self):
return [os.path.basename(a).replace("_key.pem","") for a in glob.glob(os.path.join(self.home, "*_key.pem"))]
def openSSL(self, cmd, *args, **kargs):
command = "openssl {cmd}".format(cmd = cmd)
# Consume cert argument
cert = kargs.get("cert", None)
if cert: del kargs["cert"]
# Consume config/extfile arguments
tmpfiles = []
for a in ["config", "extfile"]:
data = kargs.get(a, None)
if data:
del kargs[a]
(f, path) = tempfile.mkstemp()
os.write(f, b(data))
os.close(f)
command += " -{a} {path}".format(a=a, path=path)
tmpfiles.append(path)
# Consume password argument
password = kargs.get("password", None)
if password: del kargs["password"]
#
# Write password to safe temporary file
#
passpath = None
if password:
(f, passpath) = tempfile.mkstemp()
os.write(f, b(password))
os.close(f)
tmpfiles.append(passpath)
#
# Key creation and certificate request parameters
#
if cmd == "req":
command += " -keyform PEM -keyout {cert.key} -newkey {this.keyalg}:{this.keyparams}"
if "-x509" in args:
command += " -out {cert.pem} -passout file:\"{this.passpath}\"" # CA self-signed certificate
else:
command += " -passout file:\"{this.passpath}\""
#
# Signature parameters for "req -x509" (CA self-signed certificate) or
# "x509 -req" (signing certificate request)
#
if (cmd == "req" and "-x509" in args) or (cmd == "x509" and "-req" in args):
command += " -{this.sigalg}"
#
# Certificate request signature parameters
#
if cmd == "x509" and "-req" in args:
command += " -CA {cacert.pem} -CAkey {cacert.key} -passin file:\"{this.passpath}\" -extensions ext " \
"-out {cert.pem}"
#
# Export certificate parameters
#
if cmd == "x509" and not "-req" in args:
command += " -in {cert.pem}"
elif cmd == self.keyalg or cmd == "pkcs8":
command += " -in {cert.key} -passin file:\"{this.passpath}\""
if password:
command += " -passout file:\"{passpath}\""
elif cmd == "pkcs12":
command += " -in {cert.pem} -name {cert.alias} -export -passin file:\"{this.passpath}\""
command += " -passout file:\"{passpath}\""
command = command.format(cert = cert, cacert = self.cacert, this = self, passpath=passpath)
try:
return self.run(command, *args, **kargs)
finally:
for f in tmpfiles: # Remove temporary configuration files
os.remove(f) | zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/IceCertUtils/OpenSSLCertificateUtils.py | OpenSSLCertificateUtils.py |
import sys, os, shutil, subprocess, tempfile, random, re, atexit
try:
from subprocess import DEVNULL
except ImportError:
DEVNULL = open(os.devnull, 'wb')
def b(s):
return s if sys.version_info[0] == 2 else s.encode("utf-8") if isinstance(s, str) else s
def d(s):
return s if sys.version_info[0] == 2 else s.decode("utf-8") if isinstance(s, bytes) else s
def read(p):
with open(p, "r") as f: return f.read()
def write(p, data):
with open(p, "wb") as f: f.write(data)
#
# Make sure keytool is available
#
keytoolSupport = True
if subprocess.call("keytool", shell=True, stdout=DEVNULL, stderr=DEVNULL) != 0:
keytoolSupport = False
#
# Check if BouncyCastle support is available
#
bksSupport = False
if keytoolSupport:
bksProvider = "org.bouncycastle.jce.provider.BouncyCastleProvider"
p = subprocess.Popen("javap " + bksProvider, shell=True, stdout=DEVNULL, stderr=subprocess.PIPE)
stdout, stderr = p.communicate()
if p.wait() == 0 and d(stderr).find("Error:") == -1:
bksSupport = True
#
# Check if OpenSSL is available
#
opensslSupport = True
if subprocess.call("openssl version", shell=True, stdout=DEVNULL, stderr=DEVNULL) != 0:
opensslSupport = False
#
# Check if pyOpenSSL is available
#
pyopensslSupport = False
try:
import OpenSSL
v = re.match(r"([0-9]+)\.([0-9]+)", OpenSSL.__version__)
# Require pyOpenSSL >= 0.13
pyopensslSupport = (int(v.group(1)) * 100 + int(v.group(2))) >= 13
except:
pass
SPLIT_PATTERN = re.compile(r'''((?:[^,"']|"[^"]*"|'[^']*')+)''')
class DistinguishedName:
def __init__(self, CN, OU = None, O = None, L = None, ST = None, C = None, emailAddress = None, default = None):
self.CN = CN
self.OU = OU or (default.OU if default else "")
self.O = O or (default.O if default else "")
self.L = L or (default.L if default else "")
self.ST = ST or (default.ST if default else "")
self.C = C or (default.C if default else "")
self.emailAddress = emailAddress or (default.emailAddress if default else "")
def __str__(self):
return self.toString()
def toString(self, sep = ","):
s = ""
for k in ["CN", "OU", "O", "L", "ST", "C", "emailAddress"]:
if hasattr(self, k):
v = getattr(self, k)
if v:
v = v.replace(sep, "\\" + sep)
s += "{sep}{k}={v}".format(k = k, v = v, sep = "" if s == "" else sep)
return s
@staticmethod
def parse(str):
args = {}
for m in SPLIT_PATTERN.split(str)[1::2]:
p = m.find("=")
if p != -1:
v = m[p+1:].strip()
if v.startswith('"') and v.endswith('"'):
v = v[1:-1]
args[m[0:p].strip().upper()] = v
if "EMAILADDRESS" in args:
args["emailAddress"] = args["EMAILADDRESS"]
del args["EMAILADDRESS"]
return DistinguishedName(**args)
class Certificate:
def __init__(self, parent, alias, dn=None, altName=None, extendedKeyUsage=None):
self.parent = parent
self.dn = dn
self.altName = altName or {}
self.extendedKeyUsage = extendedKeyUsage
self.alias = alias
self.pem = None
def __str__(self):
return str(self.dn)
def exists(self):
return False
def generatePEM(self):
# Generate PEM for internal use
if not self.pem:
self.pem = os.path.join(self.parent.home, self.alias + ".pem")
if not os.path.exists(self.pem):
self.savePEM(self.pem)
def generatePKCS12(self, root=True):
# Generate PKCS12 for internal use
path = os.path.join(self.parent.home, self.alias + (".p12" if root else "-noroot.p12"))
if not os.path.exists(path):
self.savePKCS12(path, password=self.parent.password, chain=True, root=root, addkey=True)
return path
def save(self, path, *args, **kargs):
if os.path.exists(path):
os.remove(path)
(_, ext) = os.path.splitext(path)
if ext == ".p12" or ext == ".pfx":
return self.savePKCS12(path, *args, **kargs)
elif ext == ".jks":
return self.saveJKS(path, *args, **kargs)
elif ext == ".bks":
return self.saveBKS(path, *args, **kargs)
elif ext in [".der", ".cer", ".crt"]:
return self.saveDER(path)
elif ext == ".pem":
return self.savePEM(path)
else:
raise RuntimeError("unknown certificate extension `{0}'".format(ext))
def getSubjectHash(self):
raise NotImplementedError("getSubjectHash")
def saveKey(self, path, password=None):
raise NotImplementedError("saveKey")
def savePKCS12(self, path):
raise NotImplementedError("savePKCS12")
def savePEM(self, path):
raise NotImplementedError("savePEM")
def saveDER(self, path):
raise NotImplementedError("saveDER")
def saveJKS(self, *args, **kargs):
self.exportToKeyStore(*args, **kargs)
return self
def saveBKS(self, *args, **kargs):
if not bksSupport:
raise RuntimeError("No BouncyCastle support, you need to install the BouncyCastleProvider with your JDK")
self.exportToKeyStore(provider=bksProvider, *args, **kargs)
return self
def destroy(self):
for f in [os.path.join(self.parent.home, self.alias + ".pem"),
os.path.join(self.parent.home, self.alias + ".p12"),
os.path.join(self.parent.home, self.alias + "-noroot.p12")]:
if f and os.path.exists(f):
os.remove(f)
def exportToKeyStore(self, dest, password=None, alias=None, addkey=None, caalias=None, chain=True, root=False,
provider=None, src=None):
if addkey is None:
addkey = self != self.parent.cacert
if not keytoolSupport:
raise RuntimeError("No keytool support, add keytool from your JDK bin directory to your PATH")
def getType(path):
(_, ext) = os.path.splitext(path)
return { ".jks" : "JKS", ".bks": "BKS", ".p12": "PKCS12", ".pfx": "PKCS12" }[ext]
# Write password to safe temporary file
(f, passpath) = tempfile.mkstemp()
os.write(f, b(password or "password"))
os.close(f)
try:
#
# Add the CA certificate as a trusted certificate if requests
#
if caalias:
args = {
"dest": dest,
"desttype": getType(dest),
"pass": passpath,
"caalias": caalias,
"cert": self.parent.cacert,
"factory": self.parent
}
command = "keytool -noprompt -importcert -file {cert.pem} -alias {caalias}"
command += " -keystore {dest} -storepass:file {pass} -storetype {desttype}"
self.parent.run(command.format(**args), provider=provider)
if not src:
src = self.generatePKCS12(root)
if addkey:
args = {
"src": src,
"srctype" : getType(src),
"dest": dest,
"desttype": getType(dest),
"destalias": alias or self.alias,
"pass": passpath,
"cert": self,
"factory": self.parent,
}
command = "keytool -noprompt -importkeystore -srcalias {cert.alias} "
command += " -srckeystore {src} -srcstorepass:file {factory.passpath} -srcstoretype {srctype}"
command += " -destkeystore {dest} -deststorepass:file {pass} -destkeypass:file {pass} "
command += " -destalias {destalias} -deststoretype {desttype}"
else:
args = {
"dest": dest,
"destalias": alias or self.alias,
"desttype": getType(dest),
"pass": passpath,
"cert": self,
"factory": self.parent
}
command = "keytool -noprompt -importcert -file {cert.pem} -alias {destalias}"
command += " -keystore {dest} -storepass:file {pass} -storetype {desttype} "
self.parent.run(command.format(**args), provider=provider)
finally:
os.remove(passpath)
def getAlternativeName(self):
items = []
for k, v in self.altName.items():
items.append("{0}:{1}".format(k, v))
return ",".join(items) if len(items) > 0 else None
def getExtendedKeyUsage(self):
return self.extendedKeyUsage
defaultDN = DistinguishedName("ZeroC IceCertUtils CA", "Ice", "ZeroC, Inc.", "Jupiter", "Florida", "US",
emailAddress="[email protected]")
def getDNAndAltName(alias, defaultDN, dn=None, altName=None, **kargs):
def consume(kargs, keys):
args = {}
for k in keys:
if k in kargs:
args[k] = kargs[k]
del kargs[k]
elif k.upper() in kargs:
args[k] = kargs[k.upper()]
del kargs[k.upper()]
elif k.lower() in kargs:
args[k] = kargs[k.lower()]
del kargs[k.lower()]
if k in args and args[k] is None:
del args[k]
return (kargs, args)
if not altName:
# Extract alternative name arguments
(kargs, altName) = consume(kargs, ["IP", "DNS", "email", "URI"])
if not dn:
# Extract distinguished name arguments
(kargs, dn) = consume(kargs, ["CN", "OU", "O", "L", "ST", "C", "emailAddress"])
if len(dn) > 0:
dn = DistinguishedName(default=defaultDN, **dn)
else:
for k in ["ip", "dns", "email"]:
if k in altName:
dn = DistinguishedName(altName[k], default=defaultDN)
break
else:
dn = DistinguishedName(alias, default=defaultDN)
return (kargs, dn, altName)
class CertificateFactory:
def __init__(self, home=None, debug=None, validity=None, keysize=None, keyalg=None, sigalg=None, password=None,
parent=None, *args, **kargs):
(kargs, dn, altName) = getDNAndAltName("ca", defaultDN, **kargs)
if len(kargs) > 0:
raise TypeError("unexpected arguments")
self.parent = parent;
# Certificate generate parameters
self.validity = validity or (parent.validity if parent else 825)
self.keysize = keysize or (parent.keysize if parent else 2048)
self.keyalg = keyalg or (parent.keyalg if parent else "rsa")
self.sigalg = sigalg or (parent.sigalg if parent else "sha256")
# Temporary directory for storing intermediate files
self.rmHome = home is None
self.home = home or tempfile.mkdtemp();
self.certs = {}
self.factories = {}
# The password used to protect keys and key stores from the factory home directory
self.password = password or parent.password if parent else "password"
if parent:
self.passpath = parent.passpath
else:
(f, self.passpath) = tempfile.mkstemp()
os.write(f, b(self.password))
os.close(f)
@atexit.register
def rmpass():
if os.path.exists(self.passpath):
os.remove(self.passpath)
self.debug = debug or (parent.debug if parent else False)
if self.debug:
print("[debug] using %s implementation" % self.__class__.__name__)
# Load the CA certificate if it exists
self.cacert = self._createChild("ca", dn or defaultDN, altName)
self.certs["ca"] = self.cacert
if self.cacert.exists():
self.cacert.load()
def __str__(self):
return str(self.cacert)
def create(self, alias, serial=None, validity=None, extendedKeyUsage=None, *args, **kargs):
cert = self.get(alias)
if cert:
cert.destroy() # Remove previous certificate
(kargs, dn, altName) = getDNAndAltName(alias, self.cacert.dn, **kargs)
if len(args) > 0 or len(kargs) > 0:
raise TypeError("unexpected arguments")
cert = self._createChild(alias, dn, altName, extendedKeyUsage)
self._generateChild(cert, serial, validity)
self.certs[alias] = cert
return cert
def get(self, alias):
if alias in self.certs:
return self.certs[alias]
cert = self._createChild(alias)
if cert.exists():
self.certs[alias] = cert.load()
return cert
else:
return None
def getCA(self):
return self.cacert
def createIntermediateFactory(self, alias, *args, **kargs):
factory = self.getIntermediateFactory(alias)
if factory:
factory.destroy(force = True)
home = os.path.join(self.home, alias)
os.mkdir(home)
(kargs, dn, altName) = getDNAndAltName(alias, self.cacert.dn, **kargs)
if len(args) > 0 or len(kargs) > 0:
raise TypeError("unexpected arguments")
factory = self._createFactory(home = home, dn = dn, altName=altName, parent = self)
self.factories[alias] = factory
return factory
def getIntermediateFactory(self, alias):
if alias in self.factories:
return self.factories[alias]
home = os.path.join(self.home, alias)
if not os.path.isdir(home):
return None
factory = self._createFactory(home = home, parent = self)
self.factories[alias] = factory
return factory
def destroy(self, force=False):
if self.rmHome:
# Cleanup temporary directory
shutil.rmtree(self.home)
elif force:
if os.path.exists(self.passpath):
os.remove(self.passpath)
for (a,c) in self.certs.items():
c.destroy()
def run(self, cmd, *args, **kargs):
# Consume stdin argument
stdin = kargs.get("stdin", None)
if stdin : del kargs["stdin"]
for a in args:
cmd += " {a}".format(a = a)
for (key, value) in kargs.items():
if not value and value != "":
continue
value = str(value)
if value == "" or value.find(' ') >= 0:
cmd += " -{key} \"{value}\"".format(key=key, value=value)
else:
cmd += " -{key} {value}".format(key=key, value=value)
if self.debug:
print("[debug] %s" % cmd)
p = subprocess.Popen(cmd,
shell = True,
stdin = subprocess.PIPE if stdin else None,
stdout = subprocess.PIPE,
stderr = subprocess.PIPE,
bufsize = 0)
stdout, stderr = p.communicate(b(stdin))
if p.wait() != 0:
raise Exception("command failed: " + cmd + "\n" + d(stderr or stdout))
return stdout
def getDefaultImplementation():
if pyopensslSupport:
from IceCertUtils.PyOpenSSLCertificateUtils import PyOpenSSLCertificateFactory
return PyOpenSSLCertificateFactory
elif opensslSupport:
from IceCertUtils.OpenSSLCertificateUtils import OpenSSLCertificateFactory
return OpenSSLCertificateFactory
elif keytoolSupport:
from IceCertUtils.KeyToolCertificateUtils import KeyToolCertificateFactory
return KeyToolCertificateFactory
else:
raise ImportError("couldn't find a certificate utility to generate certificates. If you have a JDK installed, please add the JDK bin directory to your PATH, if you have openssl installed make sure it's in your PATH. You can also install the pyOpenSSL package from the Python package repository if you don't have OpenSSL or a JDK installed.") | zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/IceCertUtils/CertificateUtils.py | CertificateUtils.py |
import os, sys, getopt, tempfile, getpass, shutil, socket, uuid, IceCertUtils
def usage():
print("usage: " + sys.argv[0] + " [--verbose --help --capass <pass>] init create list show export")
print("")
print("The iceca command manages a small certificate authority to create and sign")
print("certificates for Ice clients or servers.")
print("")
print("Commands:")
print("init Initialize the certificate authority database")
print("create Create and sign a certificate/key pair")
print("list List the created certificates")
print("show Show a given certificate")
print("export Export a given certificate")
print("")
sys.exit(1)
def b(s):
return s if sys.version_info[0] == 2 else s.encode("utf-8") if isinstance(s, str) else s
def question(message, expected = None):
sys.stdout.write(message)
sys.stdout.write(' ')
sys.stdout.flush()
choice = sys.stdin.readline().strip()
if expected:
return choice in expected
else:
return choice
def parseArgs(script, min, max, shortopts, longopts, usage):
try:
opts, args = getopt.getopt(sys.argv[script+1:], shortopts, longopts)
except getopt.GetoptError:
print("usage: " + sys.argv[script] + " " + usage)
sys.exit(1)
if len(args) < min or len(args) > max:
print("usage: " + sys.argv[script] + " " + usage)
sys.exit(1)
options = {}
for o, a in opts:
options[o[(2 if o.startswith("--") else 1):]] = a
return (options, args)
def getCertificateAuthority(home, cafile, capass, verbose):
if not os.path.exists(cafile):
print(sys.argv[0] + ": the CA is not initialized, run iceca init")
sys.exit(1)
if not capass:
if os.path.exists(os.path.join(home, "capass")):
with open(os.path.join(home, "capass")) as f: capass = f.read()
else:
capass = getpass.getpass("Enter the CA passphrase:")
return IceCertUtils.CertificateFactory(home=home, debug=verbose, password=capass)
def init(script, home, cafile, capass, verbose):
opts, _ = parseArgs(script, 0, 0, "", ["overwrite", "no-capass"], "[--overwrite --no-capass]\n"
"\n"
"Initializes the certificate authority database.\n"
"\nOptions:\n"
"--overwrite Overwrite the existing CA database\n"
"--no-capass Don't protect the CA with a password\n")
print("This script will initialize your organization's Certificate Authority.")
print('The CA database will be created in "%s"' % home)
if "overwrite" in opts:
# If the CA exists then destroy it.
if os.path.exists(cafile):
if not question("Warning: running this command will destroy your existing CA setup!\n"
"Do you want to continue? (y/n)", ['y', 'Y']):
sys.exit(1)
shutil.rmtree(home)
if os.path.exists(os.path.join(home, "capass")):
os.remove(os.path.join(home, "capass"))
#
# Check that the cafile doesn't exist
#
if os.path.exists(cafile):
print(sys.argv[0] + ": CA has already been initialized.")
print("Use the --overwrite option to re-initialize the database.")
sys.exit(1)
try:
os.makedirs(home)
except OSError:
pass
# Construct the DN for the CA certificate.
DNelements = {
'C': "Country name",
'ST':"State or province name",
'L': "Locality",
'O': "Organization name",
'OU':"Organizational unit name",
'CN':"Common name",
'emailAddress': "Email address"
}
dn = IceCertUtils.DistinguishedName("Ice CertUtils CA")
while True:
print("")
print("The subject name for your CA will be " + str(dn))
print("")
if question("Do you want to keep this as the CA subject name? (y/n) [y]", ['n', 'N']):
for k,v in DNelements.items():
v = question(v + ": ")
if k == 'C' and len(v) > 2:
print("The contry code can't be longer than 2 characters")
continue
setattr(dn, k, v)
else:
break
if "no-capass" in opts:
# If the user doesn't want a password, we save a random password under the CA home directory.
capass = str(uuid.uuid4())
with open(os.path.join(home, "capass"), "wb") as f: f.write(b(capass))
elif not capass:
capass = ""
while True:
capass = getpass.getpass("Enter the passphrase to protect the CA:")
if len(capass) < 6:
print("The CA passphrase must be at least 6 characters long")
else:
break
IceCertUtils.CertificateFactory(home=home, debug=verbose, dn=dn, password=capass)
print("The CA is initialized in " + home)
def create(script, factory):
opts, args = parseArgs(script, 1, 2, "", ["ip=", "dns="], "[--ip=<ip>] [--dns=<dns>] <alias> [<common-name>]\n"
"\n"
"Creates and signs a certificate. A certificate is identified by its alias. If no\n"
"common name is specified, the alias is used as the common name.\n"
"\nOptions:\n"
"--ip Optional IP subject alternative name field\n"
"--dns Optional DNS subject alternative name field\n"
"--eku Optional Extended Key Usage\n")
alias = args[0]
commonName = len(args) == 2 and args[1] or alias
cert = factory().create(alias, cn=commonName, ip=opts.get("ip", None), dns=opts.get("dns", None))
print("Created `%s' certificate `%s'" % (alias, str(cert)))
def export(script, factory):
opts, args = parseArgs(script, 1, 1, "", ["password=", "alias="], "[--password <password>] [--alias <alias>] path\n"
"\n"
"Export a certificate from the CA to the given file path. If --alias isn't\n"
"specified, the filename indicates which certificate to export. The file\n"
"extension also specifies the export format for the certificate. Supported\n"
"formats are:\n\n"
" PKCS12 (.p12, .pfx)\n"
" PEM (.pem)\n"
" DER (.der, .cer, .crt)\n"
" JKS (.jks, requires keytool to be in the PATH)\n"
" BKS (.bks, requires keytool and support for the BouncyCastle provider)\n"
"\nOptions:\n"
"--password The password to use for protecting the exported certificate\n"
"--alias The alias of the certificate to export\n")
path = args[0]
alias = opts.get("alias", os.path.splitext(os.path.basename(path))[0])
passphrase = opts.get("password", None)
if not passphrase and os.path.splitext(os.path.basename(path))[1] in [".p12", ".jks", ".bks"]:
passphrase = getpass.getpass("Enter the export passphrase:")
cert = factory().get(alias)
if cert:
cert.save(path, password=passphrase)
print("Exported certificate `{alias}' to `{path}'".format(alias=alias, path=path))
else:
print("Couldn't find certificate `%s'" % alias)
def list(script, factory):
opts, args = parseArgs(script, 0, 0, "", [], "\n"
"\n"
"List aliases for the certificates created with this CA.\n")
print("Certificates: %s" % factory().list())
def show(script, factory):
opts, args = parseArgs(script, 1, 1, "", [], "<alias>\n"
"\n"
"Print out the certificate associated to the given alias.\n")
alias = args[0]
cert = factory().get(alias)
if cert:
print("Certificate `%s':\n%s" % (alias, cert.toText()))
else:
print("Couldn't find certificate `%s'" % alias)
def main():
if len(sys.argv) == 1:
usage()
home = os.getenv("ICE_CA_HOME")
if home is None:
if sys.platform == "win32" or sys.platform[:6] == "cygwin":
home = os.getenv("LOCALAPPDATA")
else:
home = os.getenv("HOME")
if home is None:
print("Set ICE_CA_HOME to specify the location of the CA database")
sys.exit(1)
home = os.path.join(home, ".iceca")
home = os.path.normpath(home)
cafile = os.path.join(home, "ca.pem")
#
# Work out the position of the script.
#
script = 1
while script < len(sys.argv) and sys.argv[script].startswith("--"):
script = script + 1
if script >= len(sys.argv):
usage()
#
# Parse the global options.
#
try:
opts, args = getopt.getopt(sys.argv[1:script], "", [ "verbose", "help", "capass="])
except getopt.GetoptError:
usage()
verbose = False
capass = None
for o, a in opts:
if o == "--verbose":
verbose = True
if o == "--help":
usage()
elif o == "--capass":
capass = a
try:
if sys.argv[script] == "init":
init(script, home, cafile, capass, verbose)
sys.exit(0)
factory = lambda: getCertificateAuthority(home, cafile, capass, verbose)
if sys.argv[script] == "create":
create(script, factory)
elif sys.argv[script] == "export":
export(script, factory)
elif sys.argv[script] == "list":
list(script, factory)
elif sys.argv[script] == "show":
show(script, factory)
else:
usage()
except RuntimeError as err:
print("Error: {0}".format(err))
return 0
if __name__ == '__main__':
sys.exit(main()) | zeroc-icecertutils | /zeroc-icecertutils-1.0.4.tar.gz/zeroc-icecertutils-1.0.4/IceCertUtils/IceCaUtil.py | IceCaUtil.py |
The icehashpassword package provides the icehashpassword script which can be used to create passwords compatible with the Glacier2 crypt permissions verifier.
Installation
============
We recommend using ``pip`` or ``easy_install`` to install this
package.
Home Page
=========
Visit `ZeroC's home page <https://zeroc.com>`_ for the latest news
and information about Ice.
Package Contents
================
The package provides the icehashpassword utility.
Usage:
::
Usage: icehashpassword [options]
OPTIONS
-d MESSAGE_DIGEST_ALGORITHM, --digest=MESSAGE_DIGEST_ALGORITHM
The message digest algorithm to use with PBKDF2, valid values are (sha1, sha256, sha512).
-s SALT_SIZE, --salt=SALT_SIZE
Optional number of bytes to use when generating new salts.
-r ROUNDS, --rounds=ROUNDS
Optional number of rounds to use.
-h, --help
Show this message.
Support
=======
Join us on our `user forums <https://zeroc.com/forums/forum.php>`_ if you have questions
about this package.
| zeroc-icehashpassword | /zeroc-icehashpassword-1.0.2.tar.gz/zeroc-icehashpassword-1.0.2/README.rst | README.rst |
import sys, getopt, passlib.hash, passlib.hosts, getpass
usePBKDF2 = any(sys.platform == p for p in ["win32", "darwin", "cygwin"])
useCryptExt = any(sys.platform.startswith(p) for p in ["linux", "freebsd", "gnukfreebsd"])
def usage():
print("Usage: icehashpassword [options]")
print("")
print("OPTIONS")
if usePBKDF2:
print("")
print(" -d MESSAGE_DIGEST_ALGORITHM, --digest=MESSAGE_DIGEST_ALGORITHM")
print(" The message digest algorithm to use with PBKDF2, valid values are (sha1, sha256, sha512).")
print("")
print(" -s SALT_SIZE, --salt=SALT_SIZE")
print(" Optional number of bytes to use when generating new salts.")
print("")
elif useCryptExt:
print(" -d MESSAGE_DIGEST_ALGORITHM, --digest=MESSAGE_DIGEST_ALGORITHM")
print(" The message digest algorithm to use with crypt function, valid values are (sha256, sha512).")
print("")
if usePBKDF2 or useCryptExt:
print(" -r ROUNDS, --rounds=ROUNDS")
print(" Optional number of rounds to use.")
print("")
print(" -h, --help" )
print(" Show this message.")
print("")
def main():
digestAlgorithms = ()
shortArgs = "h"
longArgs = ["help"]
if usePBKDF2:
shortArgs += "d:s:r:"
longArgs += ["digest=", "salt=", "rounds="]
digestAlgorithms = ("sha1", "sha256", "sha512")
elif useCryptExt:
shortArgs += "d:r:"
longArgs += ["digest=", "rounds="]
digestAlgorithms = ("sha256", "sha512")
try:
opts, args = getopt.getopt(sys.argv[1:], shortArgs, longArgs)
except getopt.GetoptError as err:
print("")
print(str(err))
usage()
return 2
digest = None
salt = None
rounds = None
for o, a in opts:
if o in ("-h", "--help"):
usage()
return 0
elif o in ("-d", "--digest"):
if a in digestAlgorithms:
digest = a
else:
print("Unknown digest algorithm `" + a + "'")
return 2
elif o in ("-s", "--salt"):
try:
salt = int(a)
except ValueError as err:
print("Invalid salt size. Value must be an integer")
usage()
return 2
elif o in ("-r", "--rounds"):
try:
rounds = int(a)
except ValueError as err:
print("Invalid number of rounds. Value must be an integer")
usage()
return 2
passScheme = None
if usePBKDF2:
passScheme = passlib.hash.pbkdf2_sha256
if digest == "sha1":
passScheme = passlib.hash.pbkdf2_sha1
elif digest == "sha512":
passScheme = passlib.hash.pbkdf2_sha512
elif useCryptExt:
passScheme = passlib.hash.sha512_crypt
if digest == "sha256":
passScheme = passlib.hash.sha256_crypt
else:
#
# Fallback is the OS crypt function
#
passScheme = passlib.hosts.host_context
if rounds:
if not passScheme.min_rounds <= rounds <= passScheme.max_rounds:
print("Invalid number rounds for the digest algorithm. Value must be an integer between %s and %s" %
(passScheme.min_rounds, passScheme.max_rounds))
usage()
return 2
if salt:
if not passScheme.min_salt_size <= salt <= passScheme.max_salt_size:
print("Invalid salt size for the digest algorithm. Value must be an integer between %s and %s" %
(passScheme.min_salt_size, passScheme.max_salt_size))
usage()
return 2
# passlib 1.7 renamed encrypt to hash
encryptfn = passScheme.hash if hasattr(passScheme, "hash") else passScheme.encrypt
args = []
if sys.stdout.isatty():
args.append(getpass.getpass("Password: "))
else:
args.append(sys.stdin.readline().strip())
opts = {}
if salt:
opts["salt_size"] = salt
if rounds:
opts["rounds"] = rounds
print(encryptfn(*args, **opts))
return 0
if __name__ == '__main__':
sys.exit(main()) | zeroc-icehashpassword | /zeroc-icehashpassword-1.0.2.tar.gz/zeroc-icehashpassword-1.0.2/lib/icehashpassword.py | icehashpassword.py |
import ssl
import hmac
import json
import time
import hashlib
import requests
import threading
from websockets.sync.client import connect
class ZerocapWebsocketClient:
def __init__(self, api_key: str, api_secret: str, envion: str='prod'):
self.api_key = api_key
self.api_secret = api_secret
self.websocket = None
if envion == 'development':
self.base_url = "wss://dma-ws.defi.wiki/v2"
self.http_url = "https://dma-api.defi.wiki/v2/orders"
elif envion == 'uat':
self.base_url = "wss://dma-uat-ws.defi.wiki/v2"
self.http_url = "https://dma-uat-api.defi.wiki/v2/orders"
else:
self.base_url = "*"
self.http_url = "*"
self.signature = self.hashing()
# self.verify_identity()
def verify_identity(self):
headers = {'Content-Type': 'application/json'}
data = {"api_key": self.api_key, "signature": self.signature}
url = f"{self.http_url}/api_key_signature_valid"
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code != 200 or response.json().get('status_code') != 200:
raise Exception("Authentication failed")
def hashing(self):
return hmac.new(
self.api_secret.encode("utf-8"), self.api_key.encode("utf-8"), hashlib.sha256
).hexdigest()
def close(self):
try:
if self.websocket:
self.websocket.close()
except:
pass
return
def recv(self, websocket):
return json.loads(websocket.__next__())
def send(self, message: json):
try:
self.websocket.send(json.dumps(message))
except Exception as e:
raise Exception(e)
def test_send_heartbeat(self):
while True:
time.sleep(5)
if not self.websocket:
continue
try:
self.websocket.send(json.dumps({"type": "message", "message": "ping"}))
except Exception as e:
raise Exception(e)
return
def create_connection(self):
try:
threading.Thread(target=self.test_send_heartbeat).start()
with connect(self.base_url,
additional_headers={"api-key": self.api_key, "signature": self.signature}
) as self.websocket:
while True:
yield self.websocket.recv()
except Exception as e:
self.close()
raise Exception(e)
class ZerocapRestClient:
def __init__(self, api_key: str, api_secret: str, envion: str='prod'):
self.api_key = api_key
self.api_secret = api_secret
# signature = self.encryption_api_key()
if envion == 'development':
self.base_url = "https://dma-api.defi.wiki/v2/orders"
elif envion == 'uat':
self.base_url = "https://dma-uat-api.defi.wiki/v2/orders"
else:
self.base_url = ''
# url = f"{self.base_url}/api_key_signature_valid"
# headers = {
# 'Content-Type': 'application/json',
# }
# data = {
# "api_key": self.api_key,
# "signature": signature,
# }
# response = requests.post(url, data=json.dumps(data), headers=headers)
# check_pass = False
#
# if response.status_code == 200:
# result = response.json()
# if result["status_code"] ==200:
# check_pass = True
#
# if not check_pass:
# raise Exception("ZerocapRestClient init fail")
def hashing(self):
return hmac.new(
self.api_secret.encode("utf-8"), self.api_key.encode("utf-8"), hashlib.sha256
).hexdigest()
def encryption_api_key(self):
signature = self.hashing()
return signature
def create_order(
self,
symbol: str,
side: str,
type: str,
amount: str,
coinroutes_customer_id: str="",
price: str = "0",
client_order_id: str = "",
):
signature = self.encryption_api_key()
if signature == "fail":
raise Exception("Create Order Api Key error")
url = f"{self.base_url}/create_order"
headers = {
'Content-Type': 'application/json',
'api-key': self.api_key,
'signature': signature,
}
data = {
"symbol": symbol,
"side": side,
"type": type,
"amount": amount,
"price": price,
'coinroutes_customer_id': coinroutes_customer_id,
"client_order_id": client_order_id
}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
res = response.json()
return res
else:
raise Exception(response.text)
def fetch_order(
self, id: str
):
signature = self.encryption_api_key()
if signature == "fail":
raise Exception("Fetch Order Api Key error")
url = f"{self.base_url}/fetch_order"
headers = {
'api-key': self.api_key,
'signature': signature,
}
data = {
"id": id,
}
response = requests.get(url, params=data, headers=headers)
if response.status_code == 200:
res = response.json()
return res
else:
raise Exception(response.text)
def fetch_orders(
self,
start_datetime: int=0,
end_datetime: int=0,
symbol: str='',
page: int=1,
limit: int=500,
ids: str="",
status: str="",
sort_order: str="desc",
order_type: str="",
side: str="",
):
signature = self.encryption_api_key()
if signature == "fail":
return "Fetch Orders Api Key error"
url = f"{self.base_url}/fetch_orders"
headers = {
'Content-Type': 'application/json',
'api-key': self.api_key,
'signature': signature,
}
data = {
"symbol": symbol,
"start_datetime": start_datetime,
"end_datetime": end_datetime,
"page": page,
"ids": ids,
"status": status,
"sort_order": sort_order,
"order_type": order_type,
"side": side,
"limit": limit,
}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
res = response.json()
return res
else:
raise Exception(response.text) | zerocap-api-new-test | /zerocap_api_new_test-0.1.30.tar.gz/zerocap_api_new_test-0.1.30/zerocap_api_new_test/main.py | main.py |
install order
::
pip install zerocap-api-test -i https://www.pypi.org/simple/
rest_api_demo
::
from zerocap_api_test import ZerocapRestClient
api_key = ""
api_secret = ""
client = ZerocapRestClient(api_key, api_secret)
result = client.create_order(symbol, side, type, amount, price, client_order_id, note, third_identity_id)
result = client.fetch_order(id, note=None, third_identity_id=None)
result = client.fetch_orders(symbol=None, since=None, limit=None, note=None, third_identity_id=None)
websocket_demo
::
from zerocap_api_test import ZerocapRestClient
api_key = ""
api_secret = ""
websocket_client = ZerocapWebsocketTest(api_key, api_secret)
market_connect = websocket_client.get_market()
orders_connect = websocket_client.get_orders()
while True:
# Get subscription messages
message = websocket.get_message(market_connect)
print(f"Receiving message from server: \n{message}")
if not message:
print("Connection close")
break
| zerocap-api-test | /zerocap_api_test-0.1.4.tar.gz/zerocap_api_test-0.1.4/README.rst | README.rst |
import hmac
import json
import hashlib
import requests
from websocket import create_connection
class ZerocapWebsocketClient:
def __init__(self, api_key, api_secret):
self.api_key = api_key
self.api_secret = api_secret
self.market_websocket = None
self.order_websocket = None
self.base_url = "wss://dma-api.defi.wiki/ws"
self.http_url = "https://dma-api.defi.wiki/orders"
self.signature = self.hashing()
self.verify_identity()
def verify_identity(self):
headers = {'Content-Type': 'application/json'}
data = {"api_key": self.api_key, "signature": self.signature}
url = f"{self.http_url}/api_key_signature_valid"
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code != 200 or response.json().get('status_code') != 200:
raise Exception("Authentication failed")
def hashing(self):
return hmac.new(
self.api_secret.encode("utf-8"), self.api_key.encode("utf-8"), hashlib.sha256
).hexdigest()
def get_params(self, channel):
data_type = ""
if channel == "orders":
data_type = "order,trader"
elif channel == "market":
data_type = "price"
return {
"api_key": self.api_key,
"signature": self.signature,
"data_type": data_type,
}
def close(self, channel=None):
try:
if channel == "orders" and self.order_websocket:
self.order_websocket.close()
elif channel == "market" and self.market_websocket:
self.market_websocket.close()
else:
if self.order_websocket:
self.order_websocket.close()
if self.market_websocket:
self.market_websocket.close()
except:
pass
return
def get_message(self, ws_recv):
return ws_recv.__next__()
def get_orders(self):
try:
params = self.get_params(channel="orders")
wss_url = f'{self.base_url}/GetOrdersInfo?api_key={params["api_key"]}&signature={params["signature"]}&data_type={params["data_type"]}'
self.order_websocket = create_connection(wss_url)
while True:
message = self.order_websocket.recv()
yield message
except Exception as e:
self.close(channel="orders")
raise Exception(e)
def get_market(self):
try:
params = self.get_params(channel="market")
wss_url = f'{self.base_url}/GetMarket?api_key={params["api_key"]}&signature={params["signature"]}&data_type={params["data_type"]}'
self.market_websocket = create_connection(wss_url)
while True:
message = self.market_websocket.recv()
yield message
except Exception as e:
self.close(channel="market")
raise Exception(e)
class ZerocapRestClient:
def __init__(self, api_key, api_secret):
self.api_key = api_key
self.api_secret = api_secret
signature = self.encryption_api_key()
self.base_url = "https://dma-api.defi.wiki/orders"
url = f"{self.base_url}/api_key_signature_valid"
headers = {
'Content-Type': 'application/json',
}
data = {
"api_key": self.api_key,
"signature": signature,
}
response = requests.post(url, data=json.dumps(data), headers=headers)
check_pass = False
if response.status_code == 200:
result = response.json()
if result["status_code"] ==200:
check_pass = True
if not check_pass:
raise Exception("ZerocapRestClient init fail")
def hashing(self):
return hmac.new(
self.api_secret.encode("utf-8"), self.api_key.encode("utf-8"), hashlib.sha256
).hexdigest()
def encryption_api_key(self):
signature = self.hashing()
return signature
def create_order(self, symbol, side, type, amount, price, client_order_id, note, third_identity_id):
signature = self.encryption_api_key()
if signature == "fail":
return "Create Order Api Key error"
url = f"{self.base_url}/create_order"
headers = {
'Content-Type': 'application/json',
}
data = {
"symbol": symbol,
"side": side,
"type": type,
"amount": amount,
"price": price,
"client_order_id": client_order_id,
"account_vault": {
"third_identity_id": third_identity_id,
"api_key": self.api_key,
"signature": signature,
"note": note,
}
}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
res = response.json()
return res["data"]
else:
raise Exception(response.text)
def fetch_order(self, id, note='', third_identity_id=''):
signature = self.encryption_api_key()
if signature == "fail":
return "Fetch Order Api Key error"
url = f"{self.base_url}/fetch_order"
headers = {
'Content-Type': 'application/json',
}
data = {
"id": id,
"account_vault": {
"third_identity_id": third_identity_id,
"api_key": self.api_key,
"signature": signature,
"note": note,
}
}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
res = response.json()
return res["data"]
else:
raise Exception(response.text)
def fetch_orders(self, symbol: str='', since: int='', limit: int=0, note: str='', third_identity_id:str=''):
signature = self.encryption_api_key()
if signature == "fail":
return "Fetch Orders Api Key error"
url = f"{self.base_url}/fetch_orders"
headers = {
'Content-Type': 'application/json',
}
data = {
"symbol": symbol,
"since": since,
"limit": limit,
"account_vault": {
"third_identity_id": third_identity_id,
"api_key": self.api_key,
"signature": signature,
"note": note,
}
}
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code == 200:
res = response.json()
return res["data"]
else:
raise Exception(response.text) | zerocap-api-test | /zerocap_api_test-0.1.4.tar.gz/zerocap_api_test-0.1.4/zerocap_api_test/main.py | main.py |
.. _pip-install-zerocap-websocket-test--i-httpswwwpypiorgsimple:
pip install zerocap-websocket-test -i https://www.pypi.org/simple/
==================================================================
demo:
from zerocap_websocket_test import ZerocapWebsocketTest
API key and secret required
===========================
apiKey = ""
apiSecret = ""
websocket = ZerocapWebsocketTest(apiKey, apiSecret)
Subscribe to Market data
========================
market_connect = websocket.get_market()
Subscription order updates and transaction records
==================================================
orders_connect = websocket.get_orders()
while True:
::
# Get subscription messages
message = websocket.get_message(market_connect)
print(f"Receiving message from server: \n{message}")
if not message:
print("Connection close")
break
| zerocap-websocket-test | /zerocap_websocket_test-1.0.9.tar.gz/zerocap_websocket_test-1.0.9/README.rst | README.rst |
import hmac
import json
import hashlib
import requests
from websocket import create_connection, WebSocketException
class ZerocapWebsocketTest:
def __init__(self, apiKey, apiSecret, ):
# TODO add your own API key and secret
self.apiKey = apiKey
self.apiSecret = apiSecret
self.market_websocket = None
self.order_websocket = None
self.base_url = "wss://dma-api.defi.wiki/ws"
self.http_url = "https://dma-api.defi.wiki/orders"
self.signature = self.hashing()
self.verify_identity()
def verify_identity(self):
headers = {'Content-Type': 'application/json'}
data = {"api_key": self.apiKey, "signature": self.signature}
url = f"{self.http_url}/api_key_signature_valid"
response = requests.post(url, data=json.dumps(data), headers=headers)
if response.status_code != 200 or response.json().get('status_code') != 200:
raise Exception("Authentication failed")
def hashing(self):
return hmac.new(
self.apiSecret.encode("utf-8"), self.apiKey.encode("utf-8"), hashlib.sha256
).hexdigest()
def get_params(self, channel):
'''
Get request parameters
:param channel:
:return: params
'''
data_type = ""
if channel == "orders":
data_type = "order,trader"
elif channel == "market":
data_type = "price"
return {
"api_key": self.apiKey,
"signature": self.signature,
"data_type": data_type,
}
def close(self, channel=None):
if channel == "orders" and self.order_websocket:
self.order_websocket.close()
elif channel == "market" and self.market_websocket:
self.market_websocket.close()
else:
if self.order_websocket:
self.order_websocket.close()
if self.market_websocket:
self.market_websocket.close()
return
def get_message(self, ws_recv):
return ws_recv.__next__()
def get_orders(self):
try:
params = self.get_params(channel="orders")
wss_url = f'{self.base_url}/GetOrdersInfo?api_key={params["api_key"]}&signature={params["signature"]}&data_type={params["data_type"]}'
self.order_websocket = create_connection(wss_url)
while True:
message = self.order_websocket.recv()
yield message
except Exception as e:
self.close(channel="orders")
raise WebSocketException(500, f'{e}')
def get_market(self):
try:
params = self.get_params(channel="market")
wss_url = f'{self.base_url}/GetMarket?api_key={params["api_key"]}&signature={params["signature"]}&data_type={params["data_type"]}'
self.market_websocket = create_connection(wss_url)
while True:
message = self.market_websocket.recv()
yield message
except Exception as e:
self.close(channel="market")
raise WebSocketException(500, f'{e}') | zerocap-websocket-test | /zerocap_websocket_test-1.0.9.tar.gz/zerocap_websocket_test-1.0.9/zerocap_websocket_test/main.py | main.py |
Copyright 2003 Paul Scott-Murphy, 2014 William McBrine
This module provides a framework for the use of DNS Service Discovery
using IP multicast.
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301
USA
"""
# Import the base module to patch
import zeroconf
# Import all components of the module to re-export
from zeroconf import *
# Import exceptions which aren't in __all__
from zeroconf import IncomingDecodeError, NonUniqueNameException
from zeroconf import NamePartTooLongException, AbstractMethodException
from zeroconf import BadTypeInNameException
# Import additional definitions required in the method below
from zeroconf import _HAS_A_TO_Z, _HAS_ONLY_A_TO_Z_NUM_HYPHEN, _HAS_ASCII_CONTROL_CHARS
from zeroconf import _HAS_ONLY_A_TO_Z_NUM_HYPHEN_UNDERSCORE
# This file exists because we need to over-ride the original implementation in
# python-zeroconf, where specifications break the RFC6763 rules on length
def service_type_name(type_: str, *, allow_underscores: bool = False) -> str:
"""
Validate a fully qualified service name, instance or subtype. [rfc6763]
Returns fully qualified service name.
Domain names used by mDNS-SD take the following forms:
<sn> . <_tcp|_udp> . local.
<Instance> . <sn> . <_tcp|_udp> . local.
<sub>._sub . <sn> . <_tcp|_udp> . local.
1) must end with 'local.'
This is true because we are implementing mDNS and since the 'm' means
multi-cast, the 'local.' domain is mandatory.
2) local is preceded with either '_udp.' or '_tcp.'
3) service name <sn> precedes <_tcp|_udp>
The rules for Service Names [RFC6335] state that they may be no more
than fifteen characters long (not counting the mandatory underscore),
consisting of only letters, digits, and hyphens, must begin and end
with a letter or digit, must not contain consecutive hyphens, and
must contain at least one letter.
The instance name <Instance> and sub type <sub> may be up to 63 bytes.
The portion of the Service Instance Name is a user-
friendly name consisting of arbitrary Net-Unicode text [RFC5198]. It
MUST NOT contain ASCII control characters (byte values 0x00-0x1F and
0x7F) [RFC20] but otherwise is allowed to contain any characters,
without restriction, including spaces, uppercase, lowercase,
punctuation -- including dots -- accented characters, non-Roman text,
and anything else that may be represented using Net-Unicode.
:param type_: Type, SubType or service name to validate
:return: fully qualified service name (eg: _http._tcp.local.)
"""
if not (type_.endswith('._tcp.local.') or type_.endswith('._udp.local.')):
raise BadTypeInNameException("Type '%s' must end with '._tcp.local.' or '._udp.local.'" % type_)
remaining = type_[: -len('._tcp.local.')].split('.')
name = remaining.pop()
if not name:
raise BadTypeInNameException("No Service name found")
if len(remaining) == 1 and len(remaining[0]) == 0:
raise BadTypeInNameException("Type '%s' must not start with '.'" % type_)
if name[0] != '_':
raise BadTypeInNameException("Service name (%s) must start with '_'" % name)
# remove leading underscore
name = name[1:]
# The following check was commented in order to disable name length validation
# if len(name) > 15:
# raise BadTypeInNameException("Service name (%s) must be <= 15 bytes" % name)
if '--' in name:
raise BadTypeInNameException("Service name (%s) must not contain '--'" % name)
if '-' in (name[0], name[-1]):
raise BadTypeInNameException("Service name (%s) may not start or end with '-'" % name)
if not _HAS_A_TO_Z.search(name):
raise BadTypeInNameException("Service name (%s) must contain at least one letter (eg: 'A-Z')" % name)
allowed_characters_re = (
_HAS_ONLY_A_TO_Z_NUM_HYPHEN_UNDERSCORE if allow_underscores else _HAS_ONLY_A_TO_Z_NUM_HYPHEN
)
if not allowed_characters_re.search(name):
raise BadTypeInNameException(
"Service name (%s) must contain only these characters: "
"A-Z, a-z, 0-9, hyphen ('-')%s" % (name, ", underscore ('_')" if allow_underscores else "")
)
if remaining and remaining[-1] == '_sub':
remaining.pop()
if len(remaining) == 0 or len(remaining[0]) == 0:
raise BadTypeInNameException("_sub requires a subtype name")
if len(remaining) > 1:
remaining = ['.'.join(remaining)]
if remaining:
length = len(remaining[0].encode('utf-8'))
if length > 63:
raise BadTypeInNameException("Too long: '%s'" % remaining[0])
if _HAS_ASCII_CONTROL_CHARS.search(remaining[0]):
raise BadTypeInNameException(
"Ascii control character 0x00-0x1F and 0x7F illegal in '%s'" % remaining[0]
)
return '_' + name + type_[-len('._tcp.local.') :]
try:
zeroconf.service_type_name = service_type_name
except AttributeError:
pass | zeroconf-monkey | /zeroconf_monkey-1.0.1-py3-none-any.whl/zeroconf_monkey.py | zeroconf_monkey.py |
import time
import json
import atexit
import socket
from collections import namedtuple, defaultdict
### zeroconf ###
from zeroconf import IPVersion, ServiceBrowser, Zeroconf, ServiceListener, ServiceInfo
SERVICES = defaultdict(list)
CAPABILITIES = defaultdict(list)
OWN_SERVICES = defaultdict(list)
Info = namedtuple("info", ("properties", "addresses", "service_info"))
def get_ip_address():
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.connect(("8.8.8.8", 80))
return s.getsockname()[0]
def better_listener_args(func):
def listener_method_proxy(self, zc, type_, name):
service_info = zc.get_service_info(type_, name)
if service_info:
try:
properties = {}
for k, v in service_info.properties.items():
k = k.decode()
if not v:
properties[k] = v
continue
try:
v = json.loads(v.decode().replace("'", '"'))
except:
v = v.decode()
properties[k] = v
except:
properties = {}
addresses = [
(socket.inet_ntoa(addr), service_info.port)
for addr in service_info.addresses
]
info = Info(properties, addresses, service_info)
else:
info = Info(None, None, None)
name = name.rstrip(type_)
func(self, zc, info, type_, name)
return listener_method_proxy
class CustomListener(ServiceListener):
@better_listener_args
def add_service(self, zc, info, type_, name):
if info.properties.get("application", None) == "TSM":
SERVICES[name].append(info)
capabilities = info.properties.get("capabilities", [])
for cap in capabilities:
CAPABILITIES[cap].append(info)
@better_listener_args
def remove_service(self, zc, info, type_, name):
if name in SERVICES:
del SERVICES[name]
@better_listener_args
def update_service(self, zc, info, type_, name):
if info.properties.get("application", None) == "TSM":
try:
SERVICES[name].remove(info)
except:
pass
SERVICES[name].append(info)
capabilities = info.properties.get("capabilities", [])
for cap in capabilities:
try:
CAPABILITIES[cap].remove(info)
except:
pass
CAPABILITIES[cap].append(info)
def get_service_by_name(name, block=True):
while True:
result = SERVICES.get(name, None)
if not block or result:
return (
list(sorted(result, key=lambda x: x.service_info.priority))
if result
else None
)
time.sleep(0.5)
def get_service_by_capability(capabilities, block=True):
# TODO: Implement properly
# Behavior:
# Search each service against the desired capabilities
# We may return more than one service if capabilities are only available split across them
# For each desired capability, we create a list of services who have that capability
# Within each list, we sort them by the closeness of the match to the desired capabilities
# For example, if we desire [gps, imud] and we have two services:
# S1: [gps]
# S2: [gps, imud]
# Then S2 would rank before S1
# Return Value:
# {cap: [services] for cap in capabilities}
# TODO: Should block until all capabilities can be filled
capabilities = set(capabilities)
filled_capabilities = set()
selected_services = {}
while True:
if filled_capabilities == capabilities:
return selected_services
for cap in capabilities - filled_capabilities:
result = CAPABILITIES.get(cap)
if result:
selected_services[cap] = sorted(
result, key=lambda x: x[1], reverse=True
)[0]
filled_capabilities.add(cap)
if not block:
return selected_services
time.sleep(0.5)
def advertise_service(
name,
application,
capabilities,
device_uuid,
port,
backend_uuid=None,
address=None,
type_="_http._tcp.local.",
block=True,
):
info = ServiceInfo(
f"{type_}",
f"{name}.{type_}",
addresses=[socket.inet_aton(address or get_ip_address())],
port=port,
properties={
"application": application,
"capabilities": capabilities,
"device_uuid": device_uuid,
"backend_uuid": backend_uuid,
},
)
OWN_SERVICES[name].append(info)
ZC.register_service(info)
while block:
time.sleep(1.0)
def unadvertise_service(name):
for service in OWN_SERVICES[name]:
ZC.unregister_service(service)
def cleanup():
ZC.close()
atexit.register(cleanup)
ZC = Zeroconf(ip_version=IPVersion.V4Only)
BROWSER = ServiceBrowser(ZC, ["_http._tcp.local."], listener=CustomListener()) | zeroconf-service | /zeroconf_service-2.2.0-py3-none-any.whl/zeroconf_service/utils.py | utils.py |
import json # noqa: F401
import socket
import asyncio
import threading
import traceback
from abc import abstractmethod, ABC
### Logging ###
from logzero import logger
### Data Handling ###
import websockets
from compress_pickle import dumps
### zeroconf ###
from zeroconf import Zeroconf, ServiceInfo, IPVersion
def get_ip_address():
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.connect(("8.8.8.8", 80))
return s.getsockname()[0]
class PullWorker(threading.Thread, ABC):
def __init__(self, **kwargs):
super(PullWorker, self).__init__(**kwargs)
self.data = {}
self.last_data = {}
self.stop_flag = False
self.name = type(self).__name__
def run(self):
logger.info(f"Starting {self.name}")
try:
self.execute()
except Exception as e:
logger.error(f"Encountered exception in {self.name}: {e}")
traceback.print_exc()
logger.info(f"Stopped {self.name}")
@abstractmethod
def execute(self):
pass
def get(self, ignore_cache=False):
if ignore_cache and self.data is not None:
return self.data
elif self.data is not None and self.last_data is not self.data:
self.last_data = self.data
return self.data
return None
class PushWorker(threading.Thread, ABC):
SERVICE_NAME = None
SERVICE_PORT = None
SERVICE_IP = None
SERVICE_APPLICATION = None
SERVICE_CAPABILITIES = []
SERVICE_DEVICE_UUID = None
SERVICE_BACKEND_UUID = None
def __init__(self, loop, **kwargs):
super(PushWorker, self).__init__(**kwargs)
self.connected = set()
self.loop = loop
self.data = {}
self.last_data = {}
self.stop_flag = False
self.name = type(self).__name__
self.service_info = None
if (
self.SERVICE_NAME
and self.SERVICE_PORT
and self.SERVICE_APPLICATION
and self.SERVICE_CAPABILITIES
):
self.zeroconf = Zeroconf(ip_version=IPVersion.V4Only)
self.service_info = ServiceInfo(
"_http._tcp.local.",
f"{self.SERVICE_NAME}._http._tcp.local.",
addresses=[socket.inet_aton(self.SERVICE_IP or get_ip_address())],
port=self.SERVICE_PORT,
properties={
"application": self.SERVICE_APPLICATION,
"capabilities": self.SERVICE_CAPABILITIES,
"device_uuid": self.SERVICE_DEVICE_UUID,
"backend_uuid": self.SERVICE_BACKEND_UUID,
},
)
def run(self):
logger.info(f"Starting {self.name}")
if self.service_info:
logger.info(f"Registering service {self.SERVICE_NAME}")
self.zeroconf.register_service(self.service_info)
try:
self.execute()
except Exception as e:
logger.error(f"Encountered exception in {self.name}: {e}")
traceback.print_exc()
finally:
if self.service_info:
logger.info(f"Unregistering service {self.SERVICE_NAME}")
self.zeroconf.unregister_service(self.service_info)
self.zeroconf.close()
logger.info(f"Stopped {self.name}")
@abstractmethod
def execute(self):
pass
async def handler(self, websocket, path):
self.connected.add(websocket)
try:
await websocket.recv()
except websockets.exceptions.ConnectionClosed:
pass
finally:
self.connected.remove(websocket)
def send_data(self, data, compress=True, use_json=True):
if compress:
dts = dumps(data, compression="lz4", protocol=4)
elif use_json:
dts = json.dumps(data).encode()
else:
dts = data
for websocket in self.connected.copy():
coro = websocket.send(dts)
_ = asyncio.run_coroutine_threadsafe(coro, self.loop) | zeroconf-service | /zeroconf_service-2.2.0-py3-none-any.whl/zeroconf_service/workers.py | workers.py |
python-zeroconf
===============
.. image:: https://github.com/jstasiak/python-zeroconf/workflows/CI/badge.svg
:target: https://github.com/jstasiak/python-zeroconf?query=workflow%3ACI+branch%3Amaster
.. image:: https://img.shields.io/pypi/v/zeroconf.svg
:target: https://pypi.python.org/pypi/zeroconf
.. image:: https://codecov.io/gh/jstasiak/python-zeroconf/branch/master/graph/badge.svg
:target: https://codecov.io/gh/jstasiak/python-zeroconf
`Documentation <https://python-zeroconf.readthedocs.io/en/latest/>`_.
This is fork of pyzeroconf, Multicast DNS Service Discovery for Python,
originally by Paul Scott-Murphy (https://github.com/paulsm/pyzeroconf),
modified by William McBrine (https://github.com/wmcbrine/pyzeroconf).
The original William McBrine's fork note::
This fork is used in all of my TiVo-related projects: HME for Python
(and therefore HME/VLC), Network Remote, Remote Proxy, and pyTivo.
Before this, I was tracking the changes for zeroconf.py in three
separate repos. I figured I should have an authoritative source.
Although I make changes based on my experience with TiVos, I expect that
they're generally applicable. This version also includes patches found
on the now-defunct (?) Launchpad repo of pyzeroconf, and elsewhere
around the net -- not always well-documented, sorry.
Compatible with:
* Bonjour
* Avahi
Compared to some other Zeroconf/Bonjour/Avahi Python packages, python-zeroconf:
* isn't tied to Bonjour or Avahi
* doesn't use D-Bus
* doesn't force you to use particular event loop or Twisted (asyncio is used under the hood but not required)
* is pip-installable
* has PyPI distribution
Python compatibility
--------------------
* CPython 3.7+
* PyPy3.7 7.3+
Versioning
----------
This project's versions follow the following pattern: MAJOR.MINOR.PATCH.
* MAJOR version has been 0 so far
* MINOR version is incremented on backward incompatible changes
* PATCH version is incremented on backward compatible changes
Status
------
This project is actively maintained.
Traffic Reduction
-----------------
Before version 0.32, most traffic reduction techniques described in https://datatracker.ietf.org/doc/html/rfc6762#section-7
where not implemented which could lead to excessive network traffic. It is highly recommended that version 0.32 or later
is used if this is a concern.
IPv6 support
------------
IPv6 support is relatively new and currently limited, specifically:
* `InterfaceChoice.All` is an alias for `InterfaceChoice.Default` on non-POSIX
systems.
* Dual-stack IPv6 sockets are used, which may not be supported everywhere (some
BSD variants do not have them).
* Listening on localhost (`::1`) does not work. Help with understanding why is
appreciated.
How to get python-zeroconf?
===========================
* PyPI page https://pypi.python.org/pypi/zeroconf
* GitHub project https://github.com/jstasiak/python-zeroconf
The easiest way to install python-zeroconf is using pip::
pip install zeroconf
How do I use it?
================
Here's an example of browsing for a service:
.. code-block:: python
from zeroconf import ServiceBrowser, ServiceListener, Zeroconf
class MyListener(ServiceListener):
def update_service(self, zc: Zeroconf, type_: str, name: str) -> None:
print(f"Service {name} updated")
def remove_service(self, zc: Zeroconf, type_: str, name: str) -> None:
print(f"Service {name} removed")
def add_service(self, zc: Zeroconf, type_: str, name: str) -> None:
info = zc.get_service_info(type_, name)
print(f"Service {name} added, service info: {info}")
zeroconf = Zeroconf()
listener = MyListener()
browser = ServiceBrowser(zeroconf, "_http._tcp.local.", listener)
try:
input("Press enter to exit...\n\n")
finally:
zeroconf.close()
.. note::
Discovery and service registration use *all* available network interfaces by default.
If you want to customize that you need to specify ``interfaces`` argument when
constructing ``Zeroconf`` object (see the code for details).
If you don't know the name of the service you need to browse for, try:
.. code-block:: python
from zeroconf import ZeroconfServiceTypes
print('\n'.join(ZeroconfServiceTypes.find()))
See examples directory for more.
Changelog
=========
0.39.3
======
* Fix port changes not being seen by ServiceInfo (#1100) @bdraco
0.39.2
======
* Performance improvements for parsing incoming packet data (#1095) (#1097) @bdraco
0.39.1
======
* Performance improvements for constructing outgoing packet data (#1090) @bdraco
0.39.0
======
Technically backwards incompatible:
* Switch to using async_timeout for timeouts (#1081) @bdraco
Significantly reduces the number of asyncio tasks that are created when
using `ServiceInfo` or `AsyncServiceInfo`
0.38.7
======
* Performance improvements for parsing incoming packet data (#1076) @bdraco
0.38.6
======
* Performance improvements for fetching ServiceInfo (#1068) @bdraco
0.38.5
======
* Fix ServiceBrowsers not getting ServiceStateChange.Removed callbacks on PTR record expire (#1064) @bdraco
ServiceBrowsers were only getting a `ServiceStateChange.Removed` callback
when the record was sent with a TTL of 0. ServiceBrowsers now correctly
get a `ServiceStateChange.Removed` callback when the record expires as well.
* Fix missing minimum version of python 3.7 (#1060) @stevencrader
0.38.4
======
* Fix IP Address updates when hostname is uppercase (#1057) @bdraco
ServiceBrowsers would not callback updates when the ip address changed
if the hostname contained uppercase characters
0.38.3
======
Version bump only, no changes from 0.38.2
0.38.2
======
* Make decode errors more helpful in finding the source of the bad data (#1052) @bdraco
0.38.1
======
* Improve performance of query scheduler (#1043) @bdraco
* Avoid linear type searches in ServiceBrowsers (#1044) @bdraco
0.38.0
======
* Handle Service types that end with another service type (#1041) @apworks1
Backwards incompatible:
* Dropped Python 3.6 support (#1009) @bdraco
0.37.0
======
Technically backwards incompatible:
* Adding a listener that does not inherit from RecordUpdateListener now logs an error (#1034) @bdraco
* The NotRunningException exception is now thrown when Zeroconf is not running (#1033) @bdraco
Before this change the consumer would get a timeout or an EventLoopBlocked
exception when calling `ServiceInfo.*request` when the instance had already been shutdown
or had failed to startup.
* The EventLoopBlocked exception is now thrown when a coroutine times out (#1032) @bdraco
Previously `concurrent.futures.TimeoutError` would have been raised
instead. This is never expected to happen during normal operation.
0.36.13
=======
* Unavailable interfaces are now skipped during socket bind (#1028) @bdraco
* Downgraded incoming corrupt packet logging to debug (#1029) @bdraco
Warning about network traffic we have no control over is confusing
to users as they think there is something wrong with zeroconf
0.36.12
=======
* Prevented service lookups from deadlocking if time abruptly moves backwards (#1006) @bdraco
The typical reason time moves backwards is via an ntp update
0.36.11
=======
No functional changes from 0.36.10. This release corrects an error in the README.rst file
that prevented the build from uploading to PyPI
0.36.10
=======
* scope_id is now stripped from IPv6 addresses if given (#1020) @StevenLooman
cpython 3.9 allows a suffix %scope_id in IPv6Address. This caused an error
with the existing code if it was not stripped
* Optimized decoding labels from incoming packets (#1019) @bdraco
0.36.9
======
* Ensure ServiceInfo orders newest addresses first (#1012) @bdraco
This change effectively restored the behavior before 1s cache flush
expire behavior described in rfc6762 section 10.2 was added for callers that rely on this.
0.36.8
======
* Fixed ServiceBrowser infinite loop when zeroconf is closed before it is canceled (#1008) @bdraco
0.36.7
======
* Improved performance of responding to queries (#994) (#996) (#997) @bdraco
* Improved log message when receiving an invalid or corrupt packet (#998) @bdraco
0.36.6
======
* Improved performance of sending outgoing packets (#990) @bdraco
0.36.5
======
* Reduced memory usage for incoming and outgoing packets (#987) @bdraco
0.36.4
======
* Improved performance of constructing outgoing packets (#978) (#979) @bdraco
* Deferred parsing of incoming packets when it can be avoided (#983) @bdraco
0.36.3
======
* Improved performance of parsing incoming packets (#975) @bdraco
0.36.2
======
* Include NSEC records for non-existent types when responding with addresses (#972) (#971) @bdraco
Implements RFC6762 sec 6.2 (http://datatracker.ietf.org/doc/html/rfc6762#section-6.2)
0.36.1
======
* Skip goodbye packets for addresses when there is another service registered with the same name (#968) @bdraco
If a ServiceInfo that used the same server name as another ServiceInfo
was unregistered, goodbye packets would be sent for the addresses and
would cause the other service to be seen as offline.
* Fixed equality and hash for dns records with the unique bit (#969) @bdraco
These records should have the same hash and equality since
the unique bit (cache flush bit) is not considered when adding or removing
the records from the cache.
0.36.0
======
Technically backwards incompatible:
* Fill incomplete IPv6 tuples to avoid WinError on windows (#965) @lokesh2019
Fixed #932
0.35.1
======
* Only reschedule types if the send next time changes (#958) @bdraco
When the PTR response was seen again, the timer was being canceled and
rescheduled even if the timer was for the same time. While this did
not cause any breakage, it is quite inefficient.
* Cache DNS record and question hashes (#960) @bdraco
The hash was being recalculated every time the object
was being used in a set or dict. Since the hashes are
effectively immutable, we only calculate them once now.
0.35.0
======
* Reduced chance of accidental synchronization of ServiceInfo requests (#955) @bdraco
* Sort aggregated responses to increase chance of name compression (#954) @bdraco
Technically backwards incompatible:
* Send unicast replies on the same socket the query was received (#952) @bdraco
When replying to a QU question, we do not know if the sending host is reachable
from all of the sending sockets. We now avoid this problem by replying via
the receiving socket. This was the existing behavior when `InterfaceChoice.Default`
is set.
This change extends the unicast relay behavior to used with `InterfaceChoice.Default`
to apply when `InterfaceChoice.All` or interfaces are explicitly passed when
instantiating a `Zeroconf` instance.
Fixes #951
0.34.3
======
* Fix sending immediate multicast responses (#949) @bdraco
0.34.2
======
* Coalesce aggregated multicast answers (#945) @bdraco
When the random delay is shorter than the last scheduled response,
answers are now added to the same outgoing time group.
This reduces traffic when we already know we will be sending a group of answers
inside the random delay window described in
datatracker.ietf.org/doc/html/rfc6762#section-6.3
* Ensure ServiceInfo requests can be answered inside the default timeout with network protection (#946) @bdraco
Adjust the time windows to ensure responses that have triggered the
protection against against excessive packet flooding due to
software bugs or malicious attack described in RFC6762 section 6
can respond in under 1350ms to ensure ServiceInfo can ask two
questions within the default timeout of 3000ms
0.34.1
======
* Ensure multicast aggregation sends responses within 620ms (#942) @bdraco
Responses that trigger the protection against against excessive
packet flooding due to software bugs or malicious attack described
in RFC6762 section 6 could cause the multicast aggregation response
to be delayed longer than 620ms (The maximum random delay of 120ms
and 500ms additional for aggregation).
Only responses that trigger the protection are delayed longer than 620ms
0.34.0
======
* Implemented Multicast Response Aggregation (#940) @bdraco
Responses are now aggregated when possible per rules in RFC6762
section 6.4
Responses that trigger the protection against against excessive
packet flooding due to software bugs or malicious attack described
in RFC6762 section 6 are delayed instead of discarding as it was
causing responders that implement Passive Observation Of Failures
(POOF) to evict the records.
Probe responses are now always sent immediately as there were cases
where they would fail to be answered in time to defend a name.
0.33.4
======
* Ensure zeroconf can be loaded when the system disables IPv6 (#933) @che0
0.33.3
======
* Added support for forward dns compression pointers (#934) @bdraco
* Provide sockname when logging a protocol error (#935) @bdraco
0.33.2
======
* Handle duplicate goodbye answers in the same packet (#928) @bdraco
Solves an exception being thrown when we tried to remove the known answer
from the cache when the second goodbye answer in the same packet was processed
Fixed #926
* Skip ipv6 interfaces that return ENODEV (#930) @bdraco
0.33.1
======
* Version number change only with less restrictive directory permissions
Fixed #923
0.33.0
======
This release eliminates all threading locks as all non-threadsafe operations
now happen in the event loop.
* Let connection_lost close the underlying socket (#918) @bdraco
The socket was closed during shutdown before asyncio's connection_lost
handler had a chance to close it which resulted in a traceback on
windows.
Fixed #917
Technically backwards incompatible:
* Removed duplicate unregister_all_services code (#910) @bdraco
Calling Zeroconf.close from same asyncio event loop zeroconf is running in
will now skip unregister_all_services and log a warning as this a blocking
operation and is not async safe and never has been.
Use AsyncZeroconf instead, or for legacy code call async_unregister_all_services before Zeroconf.close
0.32.1
======
* Increased timeout in ServiceInfo.request to handle loaded systems (#895) @bdraco
It can take a few seconds for a loaded system to run the `async_request`
coroutine when the event loop is busy, or the system is CPU bound (example being
Home Assistant startup). We now add an additional `_LOADED_SYSTEM_TIMEOUT` (10s)
to the `run_coroutine_threadsafe` calls to ensure the coroutine has the total
amount of time to run up to its internal timeout (default of 3000ms).
Ten seconds is a bit large of a timeout; however, it is only used in cases
where we wrap other timeouts. We now expect the only instance the
`run_coroutine_threadsafe` result timeout will happen in a production
circumstance is when someone is running a `ServiceInfo.request()` in a thread and
another thread calls `Zeroconf.close()` at just the right moment that the future
is never completed unless the system is so loaded that it is nearly unresponsive.
The timeout for `run_coroutine_threadsafe` is the maximum time a thread can
cleanly shut down when zeroconf is closed out in another thread, which should
always be longer than the underlying thread operation.
0.32.0
======
This release offers 100% line and branch coverage.
* Made ServiceInfo first question QU (#852) @bdraco
We want an immediate response when requesting with ServiceInfo
by asking a QU question; most responders will not delay the response
and respond right away to our question. This also improves compatibility
with split networks as we may not have been able to see the response
otherwise. If the responder has not multicast the record recently,
it may still choose to do so in addition to responding via unicast
Reduces traffic when there are multiple zeroconf instances running
on the network running ServiceBrowsers
If we don't get an answer on the first try, we ask a QM question
in the event, we can't receive a unicast response for some reason
This change puts ServiceInfo inline with ServiceBrowser which
also asks the first question as QU since ServiceInfo is commonly
called from ServiceBrowser callbacks
* Limited duplicate packet suppression to 1s intervals (#841) @bdraco
Only suppress duplicate packets that happen within the same
second. Legitimate queriers will retry the question if they
are suppressed. The limit was reduced to one second to be
in line with rfc6762
* Made multipacket known answer suppression per interface (#836) @bdraco
The suppression was happening per instance of Zeroconf instead
of per interface. Since the same network can be seen on multiple
interfaces (usually and wifi and ethernet), this would confuse the
multi-packet known answer supression since it was not expecting
to get the same data more than once
* New ServiceBrowsers now request QU in the first outgoing when unspecified (#812) @bdraco
https://datatracker.ietf.org/doc/html/rfc6762#section-5.4
When we start a ServiceBrowser and zeroconf has just started up, the known
answer list will be small. By asking a QU question first, it is likely
that we have a large known answer list by the time we ask the QM question
a second later (current default which is likely too low but would be
a breaking change to increase). This reduces the amount of traffic on
the network, and has the secondary advantage that most responders will
answer a QU question without the typical delay answering QM questions.
* IPv6 link-local addresses are now qualified with scope_id (#343) @ibygrave
When a service is advertised on an IPv6 address where
the scope is link local, i.e. fe80::/64 (see RFC 4007)
the resolved IPv6 address must be extended with the
scope_id that identifies through the "%" symbol the
local interface to be used when routing to that address.
A new API `parsed_scoped_addresses()` is provided to
return qualified addresses to avoid breaking compatibility
on the existing parsed_addresses().
* Network adapters that are disconnected are now skipped (#327) @ZLJasonG
* Fixed listeners missing initial packets if Engine starts too quickly (#387) @bdraco
When manually creating a zeroconf.Engine object, it is no longer started automatically.
It must manually be started by calling .start() on the created object.
The Engine thread is now started after all the listeners have been added to avoid a
race condition where packets could be missed at startup.
* Fixed answering matching PTR queries with the ANY query (#618) @bdraco
* Fixed lookup of uppercase names in the registry (#597) @bdraco
If the ServiceInfo was registered with an uppercase name and the query was
for a lowercase name, it would not be found and vice-versa.
* Fixed unicast responses from any source port (#598) @bdraco
Unicast responses were only being sent if the source port
was 53, this prevented responses when testing with dig:
dig -p 5353 @224.0.0.251 media-12.local
The above query will now see a response
* Fixed queries for AAAA records not being answered (#616) @bdraco
* Removed second level caching from ServiceBrowsers (#737) @bdraco
The ServiceBrowser had its own cache of the last time it
saw a service that was reimplementing the DNSCache and
presenting a source of truth problem that lead to unexpected
queries when the two disagreed.
* Fixed server cache not being case-insensitive (#731) @bdraco
If the server name had uppercase chars and any of the
matching records were lowercase, and the server would not be
found
* Fixed cache handling of records with different TTLs (#729) @bdraco
There should only be one unique record in the cache at
a time as having multiple unique records will different
TTLs in the cache can result in unexpected behavior since
some functions returned all matching records and some
fetched from the right side of the list to return the
newest record. Instead we now store the records in a dict
to ensure that the newest record always replaces the same
unique record, and we never have a source of truth problem
determining the TTL of a record from the cache.
* Fixed ServiceInfo with multiple A records (#725) @bdraco
If there were multiple A records for the host, ServiceInfo
would always return the last one that was in the incoming
packet, which was usually not the one that was wanted.
* Fixed stale unique records expiring too quickly (#706) @bdraco
Records now expire 1s in the future instead of instant removal.
tools.ietf.org/html/rfc6762#section-10.2
Queriers receiving a Multicast DNS response with a TTL of zero SHOULD
NOT immediately delete the record from the cache, but instead record
a TTL of 1 and then delete the record one second later. In the case
of multiple Multicast DNS responders on the network described in
Section 6.6 above, if one of the responders shuts down and
incorrectly sends goodbye packets for its records, it gives the other
cooperating responders one second to send out their own response to
"rescue" the records before they expire and are deleted.
* Fixed exception when unregistering a service multiple times (#679) @bdraco
* Added an AsyncZeroconfServiceTypes to mirror ZeroconfServiceTypes to zeroconf.asyncio (#658) @bdraco
* Fixed interface_index_to_ip6_address not skiping ipv4 adapters (#651) @bdraco
* Added async_unregister_all_services to AsyncZeroconf (#649) @bdraco
* Fixed services not being removed from the registry when calling unregister_all_services (#644) @bdraco
There was a race condition where a query could be answered for a service
in the registry, while goodbye packets which could result in a fresh record
being broadcast after the goodbye if a query came in at just the right
time. To avoid this, we now remove the services from the registry right
after we generate the goodbye packet
* Fixed zeroconf exception on load when the system disables IPv6 (#624) @bdraco
* Fixed the QU bit missing from for probe queries (#609) @bdraco
The bit should be set per
datatracker.ietf.org/doc/html/rfc6762#section-8.1
* Fixed the TC bit missing for query packets where the known answers span multiple packets (#494) @bdraco
* Fixed packets not being properly separated when exceeding maximum size (#498) @bdraco
Ensure that questions that exceed the max packet size are
moved to the next packet. This fixes DNSQuestions being
sent in multiple packets in violation of:
datatracker.ietf.org/doc/html/rfc6762#section-7.2
Ensure only one resource record is sent when a record
exceeds _MAX_MSG_TYPICAL
datatracker.ietf.org/doc/html/rfc6762#section-17
* Fixed PTR questions asked in uppercase not being answered (#465) @bdraco
* Added Support for context managers in Zeroconf and AsyncZeroconf (#284) @shenek
* Implemented an AsyncServiceBrowser to compliment the sync ServiceBrowser (#429) @bdraco
* Added async_get_service_info to AsyncZeroconf and async_request to AsyncServiceInfo (#408) @bdraco
* Implemented allowing passing in a sync Zeroconf instance to AsyncZeroconf (#406) @bdraco
* Fixed IPv6 setup under MacOS when binding to "" (#392) @bdraco
* Fixed ZeroconfServiceTypes.find not always cancels the ServiceBrowser (#389) @bdraco
There was a short window where the ServiceBrowser thread
could be left running after Zeroconf is closed because
the .join() was never waited for when a new Zeroconf
object was created
* Fixed duplicate packets triggering duplicate updates (#376) @bdraco
If TXT or SRV records update was already processed and then
received again, it was possible for a second update to be
called back in the ServiceBrowser
* Fixed ServiceStateChange.Updated event happening for IPs that already existed (#375) @bdraco
* Fixed RFC6762 Section 10.2 paragraph 2 compliance (#374) @bdraco
* Reduced length of ServiceBrowser thread name with many types (#373) @bdraco
* Fixed empty answers being added in ServiceInfo.request (#367) @bdraco
* Fixed ServiceInfo not populating all AAAA records (#366) @bdraco
Use get_all_by_details to ensure all records are loaded
into addresses.
Only load A/AAAA records from the cache once in load_from_cache
if there is a SRV record present
Move duplicate code that checked if the ServiceInfo was complete
into its own function
* Fixed a case where the cache list can change during iteration (#363) @bdraco
* Return task objects created by AsyncZeroconf (#360) @nocarryr
Traffic Reduction:
* Added support for handling QU questions (#621) @bdraco
Implements RFC 6762 sec 5.4:
Questions Requesting Unicast Responses
datatracker.ietf.org/doc/html/rfc6762#section-5.4
* Implemented protect the network against excessive packet flooding (#619) @bdraco
* Additionals are now suppressed when they are already in the answers section (#617) @bdraco
* Additionals are no longer included when the answer is suppressed by known-answer suppression (#614) @bdraco
* Implemented multi-packet known answer supression (#687) @bdraco
Implements datatracker.ietf.org/doc/html/rfc6762#section-7.2
* Implemented efficient bucketing of queries with known answers (#698) @bdraco
* Implemented duplicate question suppression (#770) @bdraco
http://datatracker.ietf.org/doc/html/rfc6762#section-7.3
Technically backwards incompatible:
* Update internal version check to match docs (3.6+) (#491) @bdraco
Python version earlier then 3.6 were likely broken with zeroconf
already, however, the version is now explicitly checked.
* Update python compatibility as PyPy3 7.2 is required (#523) @bdraco
Backwards incompatible:
* Drop oversize packets before processing them (#826) @bdraco
Oversized packets can quickly overwhelm the system and deny
service to legitimate queriers. In practice, this is usually due to broken mDNS
implementations rather than malicious actors.
* Guard against excessive ServiceBrowser queries from PTR records significantly lowerthan recommended (#824) @bdraco
We now enforce a minimum TTL for PTR records to avoid
ServiceBrowsers generating excessive queries refresh queries.
Apple uses a 15s minimum TTL, however, we do not have the same
level of rate limit and safeguards, so we use 1/4 of the recommended value.
* RecordUpdateListener now uses async_update_records instead of update_record (#419, #726) @bdraco
This allows the listener to receive all the records that have
been updated in a single transaction such as a packet or
cache expiry.
update_record has been deprecated in favor of async_update_records
A compatibility shim exists to ensure classes that use
RecordUpdateListener as a base class continue to have
update_record called, however, they should be updated
as soon as possible.
A new method async_update_records_complete is now called on each
listener when all listeners have completed processing updates
and the cache has been updated. This allows ServiceBrowsers
to delay calling handlers until they are sure the cache
has been updated as its a common pattern to call for
ServiceInfo when a ServiceBrowser handler fires.
The async\_ prefix was chosen to make it clear that these
functions run in the eventloop and should never do blocking
I/O. Before 0.32+ these functions ran in a select() loop and
should not have been doing any blocking I/O, but it was not
clear to implementors that I/O would block the loop.
* Pass both the new and old records to async_update_records (#792) @bdraco
Pass the old_record (cached) as the value and the new_record (wire)
to async_update_records instead of forcing each consumer to
check the cache since we will always have the old_record
when generating the async_update_records call. This avoids
the overhead of multiple cache lookups for each listener.
0.31.0
======
* Separated cache loading from I/O in ServiceInfo and fixed cache lookup (#356),
thanks to J. Nick Koston.
The ServiceInfo class gained a load_from_cache() method to only fetch information
from Zeroconf cache (if it exists) with no IO performed. Additionally this should
reduce IO in cases where cache lookups were previously incorrectly failing.
0.30.0
======
* Some nice refactoring work including removal of the Reaper thread,
thanks to J. Nick Koston.
* Fixed a Windows-specific The requested address is not valid in its context regression,
thanks to Timothee ‘TTimo’ Besset and J. Nick Koston.
* Provided an asyncio-compatible service registration layer (in the zeroconf.asyncio module),
thanks to J. Nick Koston.
0.29.0
======
* A single socket is used for listening on responding when `InterfaceChoice.Default` is chosen.
Thanks to J. Nick Koston.
Backwards incompatible:
* Dropped Python 3.5 support
0.28.8
======
* Fixed the packet generation when multiple packets are necessary, previously invalid
packets were generated sometimes. Patch thanks to J. Nick Koston.
0.28.7
======
* Fixed the IPv6 address rendering in the browser example, thanks to Alexey Vazhnov.
* Fixed a crash happening when a service is added or removed during handle_response
and improved exception handling, thanks to J. Nick Koston.
0.28.6
======
* Loosened service name validation when receiving from the network this lets us handle
some real world devices previously causing errors, thanks to J. Nick Koston.
0.28.5
======
* Enabled ignoring duplicated messages which decreases CPU usage, thanks to J. Nick Koston.
* Fixed spurious AttributeError: module 'unittest' has no attribute 'mock' in tests.
0.28.4
======
* Improved cache reaper performance significantly, thanks to J. Nick Koston.
* Added ServiceListener to __all__ as it's part of the public API, thanks to Justin Nesselrotte.
0.28.3
======
* Reduced a time an internal lock is held which should eliminate deadlocks in high-traffic networks,
thanks to J. Nick Koston.
0.28.2
======
* Stopped asking questions we already have answers for in cache, thanks to Paul Daumlechner.
* Removed initial delay before querying for service info, thanks to Erik Montnemery.
0.28.1
======
* Fixed a resource leak connected to using ServiceBrowser with multiple types, thanks to
J. Nick Koston.
0.28.0
======
* Improved Windows support when using socket errno checks, thanks to Sandy Patterson.
* Added support for passing text addresses to ServiceInfo.
* Improved logging (includes fixing an incorrect logging call)
* Improved Windows compatibility by using Adapter.index from ifaddr, thanks to PhilippSelenium.
* Improved Windows compatibility by stopping using socket.if_nameindex.
* Fixed an OS X edge case which should also eliminate a memory leak, thanks to Emil Styrke.
Technically backwards incompatible:
* ``ifaddr`` 0.1.7 or newer is required now.
0.27.1
------
* Improved the logging situation (includes fixing a false-positive "packets() made no progress
adding records", thanks to Greg Badros)
0.27.0
------
* Large multi-resource responses are now split into separate packets which fixes a bad
mdns-repeater/ChromeCast Audio interaction ending with ChromeCast Audio crash (and possibly
some others) and improves RFC 6762 compliance, thanks to Greg Badros
* Added a warning presented when the listener passed to ServiceBrowser lacks update_service()
callback
* Added support for finding all services available in the browser example, thanks to Perry Kunder
Backwards incompatible:
* Removed previously deprecated ServiceInfo address constructor parameter and property
0.26.3
------
* Improved readability of logged incoming data, thanks to Erik Montnemery
* Threads are given unique names now to aid debugging, thanks to Erik Montnemery
* Fixed a regression where get_service_info() called within a listener add_service method
would deadlock, timeout and incorrectly return None, fix thanks to Erik Montnemery, but
Matt Saxon and Hmmbob were also involved in debugging it.
0.26.2
------
* Added support for multiple types to ServiceBrowser, thanks to J. Nick Koston
* Fixed a race condition where a listener gets a message before the lock is created, thanks to
J. Nick Koston
0.26.1
------
* Fixed a performance regression introduced in 0.26.0, thanks to J. Nick Koston (this is close in
spirit to an optimization made in 0.24.5 by the same author)
0.26.0
------
* Fixed a regression where service update listener wasn't called on IP address change (it's called
on SRV/A/AAAA record changes now), thanks to Matt Saxon
Technically backwards incompatible:
* Service update hook is no longer called on service addition (service added hook is still called),
this is related to the fix above
0.25.1
------
* Eliminated 5s hangup when calling Zeroconf.close(), thanks to Erik Montnemery
0.25.0
------
* Reverted uniqueness assertions when browsing, they caused a regression
Backwards incompatible:
* Rationalized handling of TXT records. Non-bytes values are converted to str and encoded to bytes
using UTF-8 now, None values mean value-less attributes. When receiving TXT records no decoding
is performed now, keys are always bytes and values are either bytes or None in value-less
attributes.
0.24.5
------
* Fixed issues with shared records being used where they shouldn't be (TXT, SRV, A records are
unique now), thanks to Matt Saxon
* Stopped unnecessarily excluding host-only interfaces from InterfaceChoice.all as they don't
forbid multicast, thanks to Andreas Oberritter
* Fixed repr() of IPv6 DNSAddress, thanks to Aldo Hoeben
* Removed duplicate update messages sent to listeners, thanks to Matt Saxon
* Added support for cooperating responders, thanks to Matt Saxon
* Optimized handle_response cache check, thanks to J. Nick Koston
* Fixed memory leak in DNSCache, thanks to J. Nick Koston
0.24.4
------
* Fixed resetting TTL in DNSRecord.reset_ttl(), thanks to Matt Saxon
* Improved various DNS class' string representations, thanks to Jay Hogg
0.24.3
------
* Fixed import-time "TypeError: 'ellipsis' object is not iterable." on CPython 3.5.2
0.24.2
------
* Added support for AWDL interface on macOS (needed and used by the opendrop project but should be
useful in general), thanks to Milan Stute
* Added missing type hints
0.24.1
------
* Applied some significant performance optimizations, thanks to Jaime van Kessel for the patch and
to Ghostkeeper for performance measurements
* Fixed flushing outdated cache entries when incoming record is unique, thanks to Michael Hu
* Fixed handling updates of TXT records (they'd not get recorded previously), thanks to Michael Hu
0.24.0
------
* Added IPv6 support, thanks to Dmitry Tantsur
* Added additional recommended records to PTR responses, thanks to Scott Mertz
* Added handling of ENOTCONN being raised during shutdown when using Eventlet, thanks to Tamás Nepusz
* Included the py.typed marker in the package so that type checkers know to use type hints from the
source code, thanks to Dmitry Tantsur
0.23.0
------
* Added support for MyListener call getting updates to service TXT records, thanks to Matt Saxon
* Added support for multiple addresses when publishing a service, getting/setting single address
has become deprecated. Change thanks to Dmitry Tantsur
Backwards incompatible:
* Dropped Python 3.4 support
0.22.0
------
* A lot of maintenance work (tooling, typing coverage and improvements, spelling) done, thanks to Ville Skyttä
* Provided saner defaults in ServiceInfo's constructor, thanks to Jorge Miranda
* Fixed service removal packets not being sent on shutdown, thanks to Andrew Bonney
* Added a way to define TTL-s through ServiceInfo contructor parameters, thanks to Andrew Bonney
Technically backwards incompatible:
* Adjusted query intervals to match RFC 6762, thanks to Andrew Bonney
* Made default TTL-s match RFC 6762, thanks to Andrew Bonney
0.21.3
------
* This time really allowed incoming service names to contain underscores (patch released
as part of 0.21.0 was defective)
0.21.2
------
* Fixed import-time typing-related TypeError when older typing version is used
0.21.1
------
* Fixed installation on Python 3.4 (we use typing now but there was no explicit dependency on it)
0.21.0
------
* Added an error message when importing the package using unsupported Python version
* Fixed TTL handling for published service
* Implemented unicast support
* Fixed WSL (Windows Subsystem for Linux) compatibility
* Fixed occasional UnboundLocalError issue
* Fixed UTF-8 multibyte name compression
* Switched from netifaces to ifaddr (pure Python)
* Allowed incoming service names to contain underscores
0.20.0
------
* Dropped support for Python 2 (this includes PyPy) and 3.3
* Fixed some class' equality operators
* ServiceBrowser entries are being refreshed when 'stale' now
* Cache returns new records first now instead of last
0.19.1
------
* Allowed installation with netifaces >= 0.10.6 (a bug that was concerning us
got fixed)
0.19.0
------
* Technically backwards incompatible - restricted netifaces dependency version to
work around a bug, see https://github.com/jstasiak/python-zeroconf/issues/84 for
details
0.18.0
------
* Dropped Python 2.6 support
* Improved error handling inside code executed when Zeroconf object is being closed
0.17.7
------
* Better Handling of DNS Incoming Packets parsing exceptions
* Many exceptions will now log a warning the first time they are seen
* Catch and log sendto() errors
* Fix/Implement duplicate name change
* Fix overly strict name validation introduced in 0.17.6
* Greatly improve handling of oversized packets including:
- Implement name compression per RFC1035
- Limit size of generated packets to 9000 bytes as per RFC6762
- Better handle over sized incoming packets
* Increased test coverage to 95%
0.17.6
------
* Many improvements to address race conditions and exceptions during ZC()
startup and shutdown, thanks to: morpav, veawor, justingiorgi, herczy,
stephenrauch
* Added more test coverage: strahlex, stephenrauch
* Stephen Rauch contributed:
- Speed up browser startup
- Add ZeroconfServiceTypes() query class to discover all advertised service types
- Add full validation for service names, types and subtypes
- Fix for subtype browsing
- Fix DNSHInfo support
0.17.5
------
* Fixed OpenBSD compatibility, thanks to Alessio Sergi
* Fixed race condition on ServiceBrowser startup, thanks to gbiddison
* Fixed installation on some Python 3 systems, thanks to Per Sandström
* Fixed "size change during iteration" bug on Python 3, thanks to gbiddison
0.17.4
------
* Fixed support for Linux kernel versions < 3.9 (thanks to Giovanni Harting
and Luckydonald, GitHub pull request #26)
0.17.3
------
* Fixed DNSText repr on Python 3 (it'd crash when the text was longer than
10 bytes), thanks to Paulus Schoutsen for the patch, GitHub pull request #24
0.17.2
------
* Fixed installation on Python 3.4.3+ (was failing because of enum34 dependency
which fails to install on 3.4.3+, changed to depend on enum-compat instead;
thanks to Michael Brennan for the original patch, GitHub pull request #22)
0.17.1
------
* Fixed EADDRNOTAVAIL when attempting to use dummy network interfaces on Windows,
thanks to daid
0.17.0
------
* Added some Python dependencies so it's not zero-dependencies anymore
* Improved exception handling (it'll be quieter now)
* Messages are listened to and sent using all available network interfaces
by default (configurable); thanks to Marcus Müller
* Started using logging more freely
* Fixed a bug with binary strings as property values being converted to False
(https://github.com/jstasiak/python-zeroconf/pull/10); thanks to Dr. Seuss
* Added new ``ServiceBrowser`` event handler interface (see the examples)
* PyPy3 now officially supported
* Fixed ServiceInfo repr on Python 3, thanks to Yordan Miladinov
0.16.0
------
* Set up Python logging and started using it
* Cleaned up code style (includes migrating from camel case to snake case)
0.15.1
------
* Fixed handling closed socket (GitHub #4)
0.15
----
* Forked by Jakub Stasiak
* Made Python 3 compatible
* Added setup script, made installable by pip and uploaded to PyPI
* Set up Travis build
* Reformatted the code and moved files around
* Stopped catching BaseException in several places, that could hide errors
* Marked threads as daemonic, they won't keep application alive now
0.14
----
* Fix for SOL_IP undefined on some systems - thanks Mike Erdely.
* Cleaned up examples.
* Lowercased module name.
0.13
----
* Various minor changes; see git for details.
* No longer compatible with Python 2.2. Only tested with 2.5-2.7.
* Fork by William McBrine.
0.12
----
* allow selection of binding interface
* typo fix - Thanks A. M. Kuchlingi
* removed all use of word 'Rendezvous' - this is an API change
0.11
----
* correction to comments for addListener method
* support for new record types seen from OS X
- IPv6 address
- hostinfo
* ignore unknown DNS record types
* fixes to name decoding
* works alongside other processes using port 5353 (e.g. on Mac OS X)
* tested against Mac OS X 10.3.2's mDNSResponder
* corrections to removal of list entries for service browser
0.10
----
* Jonathon Paisley contributed these corrections:
- always multicast replies, even when query is unicast
- correct a pointer encoding problem
- can now write records in any order
- traceback shown on failure
- better TXT record parsing
- server is now separate from name
- can cancel a service browser
* modified some unit tests to accommodate these changes
0.09
----
* remove all records on service unregistration
* fix DOS security problem with readName
0.08
----
* changed licensing to LGPL
0.07
----
* faster shutdown on engine
* pointer encoding of outgoing names
* ServiceBrowser now works
* new unit tests
0.06
----
* small improvements with unit tests
* added defined exception types
* new style objects
* fixed hostname/interface problem
* fixed socket timeout problem
* fixed add_service_listener() typo bug
* using select() for socket reads
* tested on Debian unstable with Python 2.2.2
0.05
----
* ensure case insensitivty on domain names
* support for unicast DNS queries
0.04
----
* added some unit tests
* added __ne__ adjuncts where required
* ensure names end in '.local.'
* timeout on receiving socket for clean shutdown
License
=======
LGPL, see COPYING file for details.
| zeroconf | /zeroconf-0.39.3.tar.gz/zeroconf-0.39.3/README.rst | README.rst |
python-zeroconf (Fork of last Python 2.x supported version)
===============
For original:
* PyPI page https://pypi.python.org/pypi/zeroconf
* GitHub project https://github.com/jstasiak/python-zeroconf
Changelog
=========
0.19.2
------
* Bugfix: allow underscores in instance name prefix (RFC 6763 - 4.1.1)
0.19.1
------
* Allowed installation with netifaces >= 0.10.6 (a bug that was concerning us
got fixed)
0.19.0
------
* Technically backwards incompatible - restricted netifaces dependency version to
work around a bug, see https://github.com/jstasiak/python-zeroconf/issues/84 for
details
0.18.0
------
* Dropped Python 2.6 support
* Improved error handling inside code executed when Zeroconf object is being closed
0.17.7
------
* Better Handling of DNS Incoming Packets parsing exceptions
* Many exceptions will now log a warning the first time they are seen
* Catch and log sendto() errors
* Fix/Implement duplicate name change
* Fix overly strict name validation introduced in 0.17.6
* Greatly improve handling of oversized packets including:
- Implement name compression per RFC1035
- Limit size of generated packets to 9000 bytes as per RFC6762
- Better handle over sized incoming packets
* Increased test coverage to 95%
0.17.6
------
* Many improvements to address race conditions and exceptions during ZC()
startup and shutdown, thanks to: morpav, veawor, justingiorgi, herczy,
stephenrauch
* Added more test coverage: strahlex, stephenrauch
* Stephen Rauch contributed:
- Speed up browser startup
- Add ZeroconfServiceTypes() query class to discover all advertised service types
- Add full validation for service names, types and subtypes
- Fix for subtype browsing
- Fix DNSHInfo support
0.17.5
------
* Fixed OpenBSD compatibility, thanks to Alessio Sergi
* Fixed race condition on ServiceBrowser startup, thanks to gbiddison
* Fixed installation on some Python 3 systems, thanks to Per Sandström
* Fixed "size change during iteration" bug on Python 3, thanks to gbiddison
0.17.4
------
* Fixed support for Linux kernel versions < 3.9 (thanks to Giovanni Harting
and Luckydonald, GitHub pull request #26)
0.17.3
------
* Fixed DNSText repr on Python 3 (it'd crash when the text was longer than
10 bytes), thanks to Paulus Schoutsen for the patch, GitHub pull request #24
0.17.2
------
* Fixed installation on Python 3.4.3+ (was failing because of enum34 dependency
which fails to install on 3.4.3+, changed to depend on enum-compat instead;
thanks to Michael Brennan for the original patch, GitHub pull request #22)
0.17.1
------
* Fixed EADDRNOTAVAIL when attempting to use dummy network interfaces on Windows,
thanks to daid
0.17.0
------
* Added some Python dependencies so it's not zero-dependencies anymore
* Improved exception handling (it'll be quieter now)
* Messages are listened to and sent using all available network interfaces
by default (configurable); thanks to Marcus Müller
* Started using logging more freely
* Fixed a bug with binary strings as property values being converted to False
(https://github.com/jstasiak/python-zeroconf/pull/10); thanks to Dr. Seuss
* Added new ``ServiceBrowser`` event handler interface (see the examples)
* PyPy3 now officially supported
* Fixed ServiceInfo repr on Python 3, thanks to Yordan Miladinov
0.16.0
------
* Set up Python logging and started using it
* Cleaned up code style (includes migrating from camel case to snake case)
0.15.1
------
* Fixed handling closed socket (GitHub #4)
0.15
----
* Forked by Jakub Stasiak
* Made Python 3 compatible
* Added setup script, made installable by pip and uploaded to PyPI
* Set up Travis build
* Reformatted the code and moved files around
* Stopped catching BaseException in several places, that could hide errors
* Marked threads as daemonic, they won't keep application alive now
0.14
----
* Fix for SOL_IP undefined on some systems - thanks Mike Erdely.
* Cleaned up examples.
* Lowercased module name.
0.13
----
* Various minor changes; see git for details.
* No longer compatible with Python 2.2. Only tested with 2.5-2.7.
* Fork by William McBrine.
0.12
----
* allow selection of binding interface
* typo fix - Thanks A. M. Kuchlingi
* removed all use of word 'Rendezvous' - this is an API change
0.11
----
* correction to comments for addListener method
* support for new record types seen from OS X
- IPv6 address
- hostinfo
* ignore unknown DNS record types
* fixes to name decoding
* works alongside other processes using port 5353 (e.g. on Mac OS X)
* tested against Mac OS X 10.3.2's mDNSResponder
* corrections to removal of list entries for service browser
0.10
----
* Jonathon Paisley contributed these corrections:
- always multicast replies, even when query is unicast
- correct a pointer encoding problem
- can now write records in any order
- traceback shown on failure
- better TXT record parsing
- server is now separate from name
- can cancel a service browser
* modified some unit tests to accommodate these changes
0.09
----
* remove all records on service unregistration
* fix DOS security problem with readName
0.08
----
* changed licensing to LGPL
0.07
----
* faster shutdown on engine
* pointer encoding of outgoing names
* ServiceBrowser now works
* new unit tests
0.06
----
* small improvements with unit tests
* added defined exception types
* new style objects
* fixed hostname/interface problem
* fixed socket timeout problem
* fixed add_service_listener() typo bug
* using select() for socket reads
* tested on Debian unstable with Python 2.2.2
0.05
----
* ensure case insensitivty on domain names
* support for unicast DNS queries
0.04
----
* added some unit tests
* added __ne__ adjuncts where required
* ensure names end in '.local.'
* timeout on receiving socket for clean shutdown
License
=======
LGPL, see COPYING file for details.
| zeroconf2 | /zeroconf2-0.19.2.tar.gz/zeroconf2-0.19.2/README.rst | README.rst |
from __future__ import (
absolute_import, division, print_function, unicode_literals)
""" Multicast DNS Service Discovery for Python, v0.14-wmcbrine
Copyright 2003 Paul Scott-Murphy, 2014 William McBrine
This module provides a framework for the use of DNS Service Discovery
using IP multicast.
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301
USA
"""
import enum
import errno
import logging
import re
import select
import socket
import struct
import sys
import threading
import time
from functools import reduce
import netifaces
from six import binary_type, indexbytes, int2byte, iteritems, text_type
from six.moves import xrange
__author__ = 'Paul Scott-Murphy, William McBrine'
__maintainer__ = 'Jakub Stasiak <[email protected]>'
__version__ = '0.19.2'
__license__ = 'LGPL'
__all__ = [
"__version__",
"Zeroconf", "ServiceInfo", "ServiceBrowser",
"Error", "InterfaceChoice", "ServiceStateChange",
]
log = logging.getLogger(__name__)
log.addHandler(logging.NullHandler())
if log.level == logging.NOTSET:
log.setLevel(logging.WARN)
# Some timing constants
_UNREGISTER_TIME = 125
_CHECK_TIME = 175
_REGISTER_TIME = 225
_LISTENER_TIME = 200
_BROWSER_TIME = 500
# Some DNS constants
_MDNS_ADDR = '224.0.0.251'
_MDNS_PORT = 5353
_DNS_PORT = 53
_DNS_TTL = 60 * 60 # one hour default TTL
_MAX_MSG_TYPICAL = 1460 # unused
_MAX_MSG_ABSOLUTE = 8966
_FLAGS_QR_MASK = 0x8000 # query response mask
_FLAGS_QR_QUERY = 0x0000 # query
_FLAGS_QR_RESPONSE = 0x8000 # response
_FLAGS_AA = 0x0400 # Authoritative answer
_FLAGS_TC = 0x0200 # Truncated
_FLAGS_RD = 0x0100 # Recursion desired
_FLAGS_RA = 0x8000 # Recursion available
_FLAGS_Z = 0x0040 # Zero
_FLAGS_AD = 0x0020 # Authentic data
_FLAGS_CD = 0x0010 # Checking disabled
_CLASS_IN = 1
_CLASS_CS = 2
_CLASS_CH = 3
_CLASS_HS = 4
_CLASS_NONE = 254
_CLASS_ANY = 255
_CLASS_MASK = 0x7FFF
_CLASS_UNIQUE = 0x8000
_TYPE_A = 1
_TYPE_NS = 2
_TYPE_MD = 3
_TYPE_MF = 4
_TYPE_CNAME = 5
_TYPE_SOA = 6
_TYPE_MB = 7
_TYPE_MG = 8
_TYPE_MR = 9
_TYPE_NULL = 10
_TYPE_WKS = 11
_TYPE_PTR = 12
_TYPE_HINFO = 13
_TYPE_MINFO = 14
_TYPE_MX = 15
_TYPE_TXT = 16
_TYPE_AAAA = 28
_TYPE_SRV = 33
_TYPE_ANY = 255
# Mapping constants to names
_CLASSES = {_CLASS_IN: "in",
_CLASS_CS: "cs",
_CLASS_CH: "ch",
_CLASS_HS: "hs",
_CLASS_NONE: "none",
_CLASS_ANY: "any"}
_TYPES = {_TYPE_A: "a",
_TYPE_NS: "ns",
_TYPE_MD: "md",
_TYPE_MF: "mf",
_TYPE_CNAME: "cname",
_TYPE_SOA: "soa",
_TYPE_MB: "mb",
_TYPE_MG: "mg",
_TYPE_MR: "mr",
_TYPE_NULL: "null",
_TYPE_WKS: "wks",
_TYPE_PTR: "ptr",
_TYPE_HINFO: "hinfo",
_TYPE_MINFO: "minfo",
_TYPE_MX: "mx",
_TYPE_TXT: "txt",
_TYPE_AAAA: "quada",
_TYPE_SRV: "srv",
_TYPE_ANY: "any"}
_HAS_A_TO_Z = re.compile(r'[A-Za-z]')
_HAS_ONLY_A_TO_Z_NUM_HYPHEN = re.compile(r'^[A-Za-z0-9_\-]+$')
_HAS_ASCII_CONTROL_CHARS = re.compile(r'[\x00-\x1f\x7f]')
@enum.unique
class InterfaceChoice(enum.Enum):
Default = 1
All = 2
@enum.unique
class ServiceStateChange(enum.Enum):
Added = 1
Removed = 2
HOST_ONLY_NETWORK_MASK = '255.255.255.255'
# utility functions
def current_time_millis():
"""Current system time in milliseconds"""
return time.time() * 1000
def service_type_name(type_):
"""
Validate a fully qualified service name, instance or subtype. [rfc6763]
Returns fully qualified service name.
Domain names used by mDNS-SD take the following forms:
<sn> . <_tcp|_udp> . local.
<Instance> . <sn> . <_tcp|_udp> . local.
<sub>._sub . <sn> . <_tcp|_udp> . local.
1) must end with 'local.'
This is true because we are implementing mDNS and since the 'm' means
multi-cast, the 'local.' domain is mandatory.
2) local is preceded with either '_udp.' or '_tcp.'
3) service name <sn> precedes <_tcp|_udp>
The rules for Service Names [RFC6335] state that they may be no more
than fifteen characters long (not counting the mandatory underscore),
consisting of only letters, digits, and hyphens, must begin and end
with a letter or digit, must not contain consecutive hyphens, and
must contain at least one letter.
The instance name <Instance> and sub type <sub> may be up to 63 bytes.
The portion of the Service Instance Name is a user-
friendly name consisting of arbitrary Net-Unicode text [RFC5198]. It
MUST NOT contain ASCII control characters (byte values 0x00-0x1F and
0x7F) [RFC20] but otherwise is allowed to contain any characters,
without restriction, including spaces, uppercase, lowercase,
punctuation -- including dots -- accented characters, non-Roman text,
and anything else that may be represented using Net-Unicode.
:param type_: Type, SubType or service name to validate
:return: fully qualified service name (eg: _http._tcp.local.)
"""
if not (type_.endswith('._tcp.local.') or type_.endswith('._udp.local.')):
raise BadTypeInNameException(
"Type '%s' must end with '._tcp.local.' or '._udp.local.'" %
type_)
remaining = type_[:-len('._tcp.local.')].split('.')
name = remaining.pop()
if not name:
raise BadTypeInNameException("No Service name found")
if len(remaining) == 1 and len(remaining[0]) == 0:
raise BadTypeInNameException(
"Type '%s' must not start with '.'" % type_)
if name[0] != '_':
raise BadTypeInNameException(
"Service name (%s) must start with '_'" % name)
# remove leading underscore
name = name[1:]
if len(name) > 15:
raise BadTypeInNameException(
"Service name (%s) must be <= 15 bytes" % name)
if '--' in name:
raise BadTypeInNameException(
"Service name (%s) must not contain '--'" % name)
if '-' in (name[0], name[-1]):
raise BadTypeInNameException(
"Service name (%s) may not start or end with '-'" % name)
if not _HAS_A_TO_Z.search(name):
raise BadTypeInNameException(
"Service name (%s) must contain at least one letter (eg: 'A-Z')" %
name)
if not _HAS_ONLY_A_TO_Z_NUM_HYPHEN.search(name):
raise BadTypeInNameException(
"Service name (%s) must contain only these characters: "
"A-Z, a-z, 0-9, hyphen ('-')" % name)
if remaining and remaining[-1] == '_sub':
remaining.pop()
if len(remaining) == 0 or len(remaining[0]) == 0:
raise BadTypeInNameException(
"_sub requires a subtype name")
if len(remaining) > 1:
remaining = ['.'.join(remaining)]
if remaining:
length = len(remaining[0].encode('utf-8'))
if length > 63:
raise BadTypeInNameException("Too long: '%s'" % remaining[0])
if _HAS_ASCII_CONTROL_CHARS.search(remaining[0]):
raise BadTypeInNameException(
"Ascii control character 0x00-0x1F and 0x7F illegal in '%s'" %
remaining[0])
return '_' + name + type_[-len('._tcp.local.'):]
# Exceptions
class Error(Exception):
pass
class IncomingDecodeError(Error):
pass
class NonUniqueNameException(Error):
pass
class NamePartTooLongException(Error):
pass
class AbstractMethodException(Error):
pass
class BadTypeInNameException(Error):
pass
# implementation classes
class QuietLogger(object):
_seen_logs = {}
@classmethod
def log_exception_warning(cls, logger_data=None):
exc_info = sys.exc_info()
exc_str = str(exc_info[1])
if exc_str not in cls._seen_logs:
# log at warning level the first time this is seen
cls._seen_logs[exc_str] = exc_info
logger = log.warning
else:
logger = log.debug
if logger_data is not None:
logger(*logger_data)
logger('Exception occurred:', exc_info=exc_info)
@classmethod
def log_warning_once(cls, *args):
msg_str = args[0]
if msg_str not in cls._seen_logs:
cls._seen_logs[msg_str] = 0
logger = log.warning
else:
logger = log.debug
cls._seen_logs[msg_str] += 1
logger(*args)
class DNSEntry(object):
"""A DNS entry"""
def __init__(self, name, type_, class_):
self.key = name.lower()
self.name = name
self.type = type_
self.class_ = class_ & _CLASS_MASK
self.unique = (class_ & _CLASS_UNIQUE) != 0
def __eq__(self, other):
"""Equality test on name, type, and class"""
return (isinstance(other, DNSEntry) and
self.name == other.name and
self.type == other.type and
self.class_ == other.class_)
def __ne__(self, other):
"""Non-equality test"""
return not self.__eq__(other)
@staticmethod
def get_class_(class_):
"""Class accessor"""
return _CLASSES.get(class_, "?(%s)" % class_)
@staticmethod
def get_type(t):
"""Type accessor"""
return _TYPES.get(t, "?(%s)" % t)
def to_string(self, hdr, other):
"""String representation with additional information"""
result = "%s[%s,%s" % (hdr, self.get_type(self.type),
self.get_class_(self.class_))
if self.unique:
result += "-unique,"
else:
result += ","
result += self.name
if other is not None:
result += ",%s]" % other
else:
result += "]"
return result
class DNSQuestion(DNSEntry):
"""A DNS question entry"""
def __init__(self, name, type_, class_):
DNSEntry.__init__(self, name, type_, class_)
def answered_by(self, rec):
"""Returns true if the question is answered by the record"""
return (self.class_ == rec.class_ and
(self.type == rec.type or self.type == _TYPE_ANY) and
self.name == rec.name)
def __repr__(self):
"""String representation"""
return DNSEntry.to_string(self, "question", None)
class DNSRecord(DNSEntry):
"""A DNS record - like a DNS entry, but has a TTL"""
def __init__(self, name, type_, class_, ttl):
DNSEntry.__init__(self, name, type_, class_)
self.ttl = ttl
self.created = current_time_millis()
def __eq__(self, other):
"""Abstract method"""
raise AbstractMethodException
def suppressed_by(self, msg):
"""Returns true if any answer in a message can suffice for the
information held in this record."""
for record in msg.answers:
if self.suppressed_by_answer(record):
return True
return False
def suppressed_by_answer(self, other):
"""Returns true if another record has same name, type and class,
and if its TTL is at least half of this record's."""
return self == other and other.ttl > (self.ttl / 2)
def get_expiration_time(self, percent):
"""Returns the time at which this record will have expired
by a certain percentage."""
return self.created + (percent * self.ttl * 10)
def get_remaining_ttl(self, now):
"""Returns the remaining TTL in seconds."""
return max(0, (self.get_expiration_time(100) - now) / 1000.0)
def is_expired(self, now):
"""Returns true if this record has expired."""
return self.get_expiration_time(100) <= now
def is_stale(self, now):
"""Returns true if this record is at least half way expired."""
return self.get_expiration_time(50) <= now
def reset_ttl(self, other):
"""Sets this record's TTL and created time to that of
another record."""
self.created = other.created
self.ttl = other.ttl
def write(self, out):
"""Abstract method"""
raise AbstractMethodException
def to_string(self, other):
"""String representation with additional information"""
arg = "%s/%s,%s" % (
self.ttl, self.get_remaining_ttl(current_time_millis()), other)
return DNSEntry.to_string(self, "record", arg)
class DNSAddress(DNSRecord):
"""A DNS address record"""
def __init__(self, name, type_, class_, ttl, address):
DNSRecord.__init__(self, name, type_, class_, ttl)
self.address = address
def write(self, out):
"""Used in constructing an outgoing packet"""
out.write_string(self.address)
def __eq__(self, other):
"""Tests equality on address"""
return isinstance(other, DNSAddress) and self.address == other.address
def __repr__(self):
"""String representation"""
try:
return str(socket.inet_ntoa(self.address))
except Exception: # TODO stop catching all Exceptions
return str(self.address)
class DNSHinfo(DNSRecord):
"""A DNS host information record"""
def __init__(self, name, type_, class_, ttl, cpu, os):
DNSRecord.__init__(self, name, type_, class_, ttl)
try:
self.cpu = cpu.decode('utf-8')
except AttributeError:
self.cpu = cpu
try:
self.os = os.decode('utf-8')
except AttributeError:
self.os = os
def write(self, out):
"""Used in constructing an outgoing packet"""
out.write_character_string(self.cpu.encode('utf-8'))
out.write_character_string(self.os.encode('utf-8'))
def __eq__(self, other):
"""Tests equality on cpu and os"""
return (isinstance(other, DNSHinfo) and
self.cpu == other.cpu and self.os == other.os)
def __repr__(self):
"""String representation"""
return self.cpu + " " + self.os
class DNSPointer(DNSRecord):
"""A DNS pointer record"""
def __init__(self, name, type_, class_, ttl, alias):
DNSRecord.__init__(self, name, type_, class_, ttl)
self.alias = alias
def write(self, out):
"""Used in constructing an outgoing packet"""
out.write_name(self.alias)
def __eq__(self, other):
"""Tests equality on alias"""
return isinstance(other, DNSPointer) and self.alias == other.alias
def __repr__(self):
"""String representation"""
return self.to_string(self.alias)
class DNSText(DNSRecord):
"""A DNS text record"""
def __init__(self, name, type_, class_, ttl, text):
assert isinstance(text, (bytes, type(None)))
DNSRecord.__init__(self, name, type_, class_, ttl)
self.text = text
def write(self, out):
"""Used in constructing an outgoing packet"""
out.write_string(self.text)
def __eq__(self, other):
"""Tests equality on text"""
return isinstance(other, DNSText) and self.text == other.text
def __repr__(self):
"""String representation"""
if len(self.text) > 10:
return self.to_string(self.text[:7]) + "..."
else:
return self.to_string(self.text)
class DNSService(DNSRecord):
"""A DNS service record"""
def __init__(self, name, type_, class_, ttl,
priority, weight, port, server):
DNSRecord.__init__(self, name, type_, class_, ttl)
self.priority = priority
self.weight = weight
self.port = port
self.server = server
def write(self, out):
"""Used in constructing an outgoing packet"""
out.write_short(self.priority)
out.write_short(self.weight)
out.write_short(self.port)
out.write_name(self.server)
def __eq__(self, other):
"""Tests equality on priority, weight, port and server"""
return (isinstance(other, DNSService) and
self.priority == other.priority and
self.weight == other.weight and
self.port == other.port and
self.server == other.server)
def __repr__(self):
"""String representation"""
return self.to_string("%s:%s" % (self.server, self.port))
class DNSIncoming(QuietLogger):
"""Object representation of an incoming DNS packet"""
def __init__(self, data):
"""Constructor from string holding bytes of packet"""
self.offset = 0
self.data = data
self.questions = []
self.answers = []
self.id = 0
self.flags = 0
self.num_questions = 0
self.num_answers = 0
self.num_authorities = 0
self.num_additionals = 0
self.valid = False
try:
self.read_header()
self.read_questions()
self.read_others()
self.valid = True
except (IndexError, struct.error, IncomingDecodeError):
self.log_exception_warning((
'Choked at offset %d while unpacking %r', self.offset, data))
def unpack(self, format_):
length = struct.calcsize(format_)
info = struct.unpack(
format_, self.data[self.offset:self.offset + length])
self.offset += length
return info
def read_header(self):
"""Reads header portion of packet"""
(self.id, self.flags, self.num_questions, self.num_answers,
self.num_authorities, self.num_additionals) = self.unpack(b'!6H')
def read_questions(self):
"""Reads questions section of packet"""
for i in xrange(self.num_questions):
name = self.read_name()
type_, class_ = self.unpack(b'!HH')
question = DNSQuestion(name, type_, class_)
self.questions.append(question)
# def read_int(self):
# """Reads an integer from the packet"""
# return self.unpack(b'!I')[0]
def read_character_string(self):
"""Reads a character string from the packet"""
length = indexbytes(self.data, self.offset)
self.offset += 1
return self.read_string(length)
def read_string(self, length):
"""Reads a string of a given length from the packet"""
info = self.data[self.offset:self.offset + length]
self.offset += length
return info
def read_unsigned_short(self):
"""Reads an unsigned short from the packet"""
return self.unpack(b'!H')[0]
def read_others(self):
"""Reads the answers, authorities and additionals section of the
packet"""
n = self.num_answers + self.num_authorities + self.num_additionals
for i in xrange(n):
domain = self.read_name()
type_, class_, ttl, length = self.unpack(b'!HHiH')
rec = None
if type_ == _TYPE_A:
rec = DNSAddress(
domain, type_, class_, ttl, self.read_string(4))
elif type_ == _TYPE_CNAME or type_ == _TYPE_PTR:
rec = DNSPointer(
domain, type_, class_, ttl, self.read_name())
elif type_ == _TYPE_TXT:
rec = DNSText(
domain, type_, class_, ttl, self.read_string(length))
elif type_ == _TYPE_SRV:
rec = DNSService(
domain, type_, class_, ttl,
self.read_unsigned_short(), self.read_unsigned_short(),
self.read_unsigned_short(), self.read_name())
elif type_ == _TYPE_HINFO:
rec = DNSHinfo(
domain, type_, class_, ttl,
self.read_character_string(), self.read_character_string())
elif type_ == _TYPE_AAAA:
rec = DNSAddress(
domain, type_, class_, ttl, self.read_string(16))
else:
# Try to ignore types we don't know about
# Skip the payload for the resource record so the next
# records can be parsed correctly
self.offset += length
if rec is not None:
self.answers.append(rec)
def is_query(self):
"""Returns true if this is a query"""
return (self.flags & _FLAGS_QR_MASK) == _FLAGS_QR_QUERY
def is_response(self):
"""Returns true if this is a response"""
return (self.flags & _FLAGS_QR_MASK) == _FLAGS_QR_RESPONSE
def read_utf(self, offset, length):
"""Reads a UTF-8 string of a given length from the packet"""
return text_type(self.data[offset:offset + length], 'utf-8', 'replace')
def read_name(self):
"""Reads a domain name from the packet"""
result = ''
off = self.offset
next_ = -1
first = off
while True:
length = indexbytes(self.data, off)
off += 1
if length == 0:
break
t = length & 0xC0
if t == 0x00:
result = ''.join((result, self.read_utf(off, length) + '.'))
off += length
elif t == 0xC0:
if next_ < 0:
next_ = off + 1
off = ((length & 0x3F) << 8) | indexbytes(self.data, off)
if off >= first:
raise IncomingDecodeError(
"Bad domain name (circular) at %s" % (off,))
first = off
else:
raise IncomingDecodeError("Bad domain name at %s" % (off,))
if next_ >= 0:
self.offset = next_
else:
self.offset = off
return result
class DNSOutgoing(object):
"""Object representation of an outgoing packet"""
def __init__(self, flags, multicast=True):
self.finished = False
self.id = 0
self.multicast = multicast
self.flags = flags
self.names = {}
self.data = []
self.size = 12
self.state = self.State.init
self.questions = []
self.answers = []
self.authorities = []
self.additionals = []
def __repr__(self):
return '<DNSOutgoing:{%s}>' % ', '.join([
'multicast=%s' % self.multicast,
'flags=%s' % self.flags,
'questions=%s' % self.questions,
'answers=%s' % self.answers,
'authorities=%s' % self.authorities,
'additionals=%s' % self.additionals,
])
class State(enum.Enum):
init = 0
finished = 1
def add_question(self, record):
"""Adds a question"""
self.questions.append(record)
def add_answer(self, inp, record):
"""Adds an answer"""
if not record.suppressed_by(inp):
self.add_answer_at_time(record, 0)
def add_answer_at_time(self, record, now):
"""Adds an answer if it does not expire by a certain time"""
if record is not None:
if now == 0 or not record.is_expired(now):
self.answers.append((record, now))
def add_authorative_answer(self, record):
"""Adds an authoritative answer"""
self.authorities.append(record)
def add_additional_answer(self, record):
""" Adds an additional answer
From: RFC 6763, DNS-Based Service Discovery, February 2013
12. DNS Additional Record Generation
DNS has an efficiency feature whereby a DNS server may place
additional records in the additional section of the DNS message.
These additional records are records that the client did not
explicitly request, but the server has reasonable grounds to expect
that the client might request them shortly, so including them can
save the client from having to issue additional queries.
This section recommends which additional records SHOULD be generated
to improve network efficiency, for both Unicast and Multicast DNS-SD
responses.
12.1. PTR Records
When including a DNS-SD Service Instance Enumeration or Selective
Instance Enumeration (subtype) PTR record in a response packet, the
server/responder SHOULD include the following additional records:
o The SRV record(s) named in the PTR rdata.
o The TXT record(s) named in the PTR rdata.
o All address records (type "A" and "AAAA") named in the SRV rdata.
12.2. SRV Records
When including an SRV record in a response packet, the
server/responder SHOULD include the following additional records:
o All address records (type "A" and "AAAA") named in the SRV rdata.
"""
self.additionals.append(record)
def pack(self, format_, value):
self.data.append(struct.pack(format_, value))
self.size += struct.calcsize(format_)
def write_byte(self, value):
"""Writes a single byte to the packet"""
self.pack(b'!c', int2byte(value))
def insert_short(self, index, value):
"""Inserts an unsigned short in a certain position in the packet"""
self.data.insert(index, struct.pack(b'!H', value))
self.size += 2
def write_short(self, value):
"""Writes an unsigned short to the packet"""
self.pack(b'!H', value)
def write_int(self, value):
"""Writes an unsigned integer to the packet"""
self.pack(b'!I', int(value))
def write_string(self, value):
"""Writes a string to the packet"""
assert isinstance(value, bytes)
self.data.append(value)
self.size += len(value)
def write_utf(self, s):
"""Writes a UTF-8 string of a given length to the packet"""
utfstr = s.encode('utf-8')
length = len(utfstr)
if length > 64:
raise NamePartTooLongException
self.write_byte(length)
self.write_string(utfstr)
def write_character_string(self, value):
assert isinstance(value, bytes)
length = len(value)
if length > 256:
raise NamePartTooLongException
self.write_byte(length)
self.write_string(value)
def write_name(self, name):
"""
Write names to packet
18.14. Name Compression
When generating Multicast DNS messages, implementations SHOULD use
name compression wherever possible to compress the names of resource
records, by replacing some or all of the resource record name with a
compact two-byte reference to an appearance of that data somewhere
earlier in the message [RFC1035].
"""
# split name into each label
parts = name.split('.')
if not parts[-1]:
parts.pop()
# construct each suffix
name_suffices = ['.'.join(parts[i:]) for i in range(len(parts))]
# look for an existing name or suffix
for count, sub_name in enumerate(name_suffices):
if sub_name in self.names:
break
else:
count += 1
# note the new names we are saving into the packet
for suffix in name_suffices[:count]:
self.names[suffix] = self.size + len(name) - len(suffix) - 1
# write the new names out.
for part in parts[:count]:
self.write_utf(part)
# if we wrote part of the name, create a pointer to the rest
if count != len(name_suffices):
# Found substring in packet, create pointer
index = self.names[name_suffices[count]]
self.write_byte((index >> 8) | 0xC0)
self.write_byte(index & 0xFF)
else:
# this is the end of a name
self.write_byte(0)
def write_question(self, question):
"""Writes a question to the packet"""
self.write_name(question.name)
self.write_short(question.type)
self.write_short(question.class_)
def write_record(self, record, now):
"""Writes a record (answer, authoritative answer, additional) to
the packet"""
if self.state == self.State.finished:
return 1
start_data_length, start_size = len(self.data), self.size
self.write_name(record.name)
self.write_short(record.type)
if record.unique and self.multicast:
self.write_short(record.class_ | _CLASS_UNIQUE)
else:
self.write_short(record.class_)
if now == 0:
self.write_int(record.ttl)
else:
self.write_int(record.get_remaining_ttl(now))
index = len(self.data)
# Adjust size for the short we will write before this record
self.size += 2
record.write(self)
self.size -= 2
length = sum((len(d) for d in self.data[index:]))
# Here is the short we adjusted for
self.insert_short(index, length)
# if we go over, then rollback and quit
if self.size > _MAX_MSG_ABSOLUTE:
while len(self.data) > start_data_length:
self.data.pop()
self.size = start_size
self.state = self.State.finished
return 1
return 0
def packet(self):
"""Returns a string containing the packet's bytes
No further parts should be added to the packet once this
is done."""
overrun_answers, overrun_authorities, overrun_additionals = 0, 0, 0
if self.state != self.State.finished:
for question in self.questions:
self.write_question(question)
for answer, time_ in self.answers:
overrun_answers += self.write_record(answer, time_)
for authority in self.authorities:
overrun_authorities += self.write_record(authority, 0)
for additional in self.additionals:
overrun_additionals += self.write_record(additional, 0)
self.state = self.State.finished
self.insert_short(0, len(self.additionals) - overrun_additionals)
self.insert_short(0, len(self.authorities) - overrun_authorities)
self.insert_short(0, len(self.answers) - overrun_answers)
self.insert_short(0, len(self.questions))
self.insert_short(0, self.flags)
if self.multicast:
self.insert_short(0, 0)
else:
self.insert_short(0, self.id)
return b''.join(self.data)
class DNSCache(object):
"""A cache of DNS entries"""
def __init__(self):
self.cache = {}
def add(self, entry):
"""Adds an entry"""
self.cache.setdefault(entry.key, []).append(entry)
def remove(self, entry):
"""Removes an entry"""
try:
list_ = self.cache[entry.key]
list_.remove(entry)
except (KeyError, ValueError):
pass
def get(self, entry):
"""Gets an entry by key. Will return None if there is no
matching entry."""
try:
list_ = self.cache[entry.key]
for cached_entry in list_:
if entry.__eq__(cached_entry):
return cached_entry
except (KeyError, ValueError):
return None
def get_by_details(self, name, type_, class_):
"""Gets an entry by details. Will return None if there is
no matching entry."""
entry = DNSEntry(name, type_, class_)
return self.get(entry)
def entries_with_name(self, name):
"""Returns a list of entries whose key matches the name."""
try:
return self.cache[name.lower()]
except KeyError:
return []
def current_entry_with_name_and_alias(self, name, alias):
now = current_time_millis()
for record in self.entries_with_name(name):
if (record.type == _TYPE_PTR and
not record.is_expired(now) and
record.alias == alias):
return record
def entries(self):
"""Returns a list of all entries"""
if not self.cache:
return []
else:
# avoid size change during iteration by copying the cache
values = list(self.cache.values())
return reduce(lambda a, b: a + b, values)
class Engine(threading.Thread):
"""An engine wraps read access to sockets, allowing objects that
need to receive data from sockets to be called back when the
sockets are ready.
A reader needs a handle_read() method, which is called when the socket
it is interested in is ready for reading.
Writers are not implemented here, because we only send short
packets.
"""
def __init__(self, zc):
threading.Thread.__init__(self, name='zeroconf-Engine')
self.daemon = True
self.zc = zc
self.readers = {} # maps socket to reader
self.timeout = 5
self.condition = threading.Condition()
self.start()
def run(self):
while not self.zc.done:
with self.condition:
rs = self.readers.keys()
if len(rs) == 0:
# No sockets to manage, but we wait for the timeout
# or addition of a socket
self.condition.wait(self.timeout)
if len(rs) != 0:
try:
rr, wr, er = select.select(rs, [], [], self.timeout)
if not self.zc.done:
for socket_ in rr:
reader = self.readers.get(socket_)
if reader:
reader.handle_read(socket_)
except (select.error, socket.error) as e:
# If the socket was closed by another thread, during
# shutdown, ignore it and exit
if e.args[0] != socket.EBADF or not self.zc.done:
raise
def add_reader(self, reader, socket_):
with self.condition:
self.readers[socket_] = reader
self.condition.notify()
def del_reader(self, socket_):
with self.condition:
del self.readers[socket_]
self.condition.notify()
class Listener(QuietLogger):
"""A Listener is used by this module to listen on the multicast
group to which DNS messages are sent, allowing the implementation
to cache information as it arrives.
It requires registration with an Engine object in order to have
the read() method called when a socket is available for reading."""
def __init__(self, zc):
self.zc = zc
self.data = None
def handle_read(self, socket_):
try:
data, (addr, port) = socket_.recvfrom(_MAX_MSG_ABSOLUTE)
except Exception:
self.log_exception_warning()
return
log.debug('Received from %r:%r: %r ', addr, port, data)
self.data = data
msg = DNSIncoming(data)
if not msg.valid:
pass
elif msg.is_query():
# Always multicast responses
if port == _MDNS_PORT:
self.zc.handle_query(msg, _MDNS_ADDR, _MDNS_PORT)
# If it's not a multicast query, reply via unicast
# and multicast
elif port == _DNS_PORT:
self.zc.handle_query(msg, addr, port)
self.zc.handle_query(msg, _MDNS_ADDR, _MDNS_PORT)
else:
self.zc.handle_response(msg)
class Reaper(threading.Thread):
"""A Reaper is used by this module to remove cache entries that
have expired."""
def __init__(self, zc):
threading.Thread.__init__(self, name='zeroconf-Reaper')
self.daemon = True
self.zc = zc
self.start()
def run(self):
while True:
self.zc.wait(10 * 1000)
if self.zc.done:
return
now = current_time_millis()
for record in self.zc.cache.entries():
if record.is_expired(now):
self.zc.update_record(now, record)
self.zc.cache.remove(record)
class Signal(object):
def __init__(self):
self._handlers = []
def fire(self, **kwargs):
for h in list(self._handlers):
h(**kwargs)
@property
def registration_interface(self):
return SignalRegistrationInterface(self._handlers)
class SignalRegistrationInterface(object):
def __init__(self, handlers):
self._handlers = handlers
def register_handler(self, handler):
self._handlers.append(handler)
return self
def unregister_handler(self, handler):
self._handlers.remove(handler)
return self
class ServiceBrowser(threading.Thread):
"""Used to browse for a service of a specific type.
The listener object will have its add_service() and
remove_service() methods called when this browser
discovers changes in the services availability."""
def __init__(self, zc, type_, handlers=None, listener=None):
"""Creates a browser for a specific type"""
assert handlers or listener, 'You need to specify at least one handler'
if not type_.endswith(service_type_name(type_)):
raise BadTypeInNameException
threading.Thread.__init__(
self, name='zeroconf-ServiceBrowser_' + type_)
self.daemon = True
self.zc = zc
self.type = type_
self.services = {}
self.next_time = current_time_millis()
self.delay = _BROWSER_TIME
self._handlers_to_call = []
self._service_state_changed = Signal()
self.done = False
if hasattr(handlers, 'add_service'):
listener = handlers
handlers = None
handlers = handlers or []
if listener:
def on_change(zeroconf, service_type, name, state_change):
args = (zeroconf, service_type, name)
if state_change is ServiceStateChange.Added:
listener.add_service(*args)
elif state_change is ServiceStateChange.Removed:
listener.remove_service(*args)
else:
raise NotImplementedError(state_change)
handlers.append(on_change)
for h in handlers:
self.service_state_changed.register_handler(h)
self.start()
@property
def service_state_changed(self):
return self._service_state_changed.registration_interface
def update_record(self, zc, now, record):
"""Callback invoked by Zeroconf when new information arrives.
Updates information required by browser in the Zeroconf cache."""
def enqueue_callback(state_change, name):
self._handlers_to_call.append(
lambda zeroconf: self._service_state_changed.fire(
zeroconf=zeroconf,
service_type=self.type,
name=name,
state_change=state_change,
))
if record.type == _TYPE_PTR and record.name == self.type:
expired = record.is_expired(now)
service_key = record.alias.lower()
try:
old_record = self.services[service_key]
except KeyError:
if not expired:
self.services[service_key] = record
enqueue_callback(ServiceStateChange.Added, record.alias)
else:
if not expired:
old_record.reset_ttl(record)
else:
del self.services[service_key]
enqueue_callback(ServiceStateChange.Removed, record.alias)
return
expires = record.get_expiration_time(75)
if expires < self.next_time:
self.next_time = expires
def cancel(self):
self.done = True
self.zc.remove_listener(self)
self.join()
def run(self):
self.zc.add_listener(self, DNSQuestion(self.type, _TYPE_PTR, _CLASS_IN))
while True:
now = current_time_millis()
if len(self._handlers_to_call) == 0 and self.next_time > now:
self.zc.wait(self.next_time - now)
if self.zc.done or self.done:
return
now = current_time_millis()
if self.next_time <= now:
out = DNSOutgoing(_FLAGS_QR_QUERY)
out.add_question(DNSQuestion(self.type, _TYPE_PTR, _CLASS_IN))
for record in self.services.values():
if not record.is_expired(now):
out.add_answer_at_time(record, now)
self.zc.send(out)
self.next_time = now + self.delay
self.delay = min(20 * 1000, self.delay * 2)
if len(self._handlers_to_call) > 0 and not self.zc.done:
handler = self._handlers_to_call.pop(0)
handler(self.zc)
class ServiceInfo(object):
"""Service information"""
def __init__(self, type_, name, address=None, port=None, weight=0,
priority=0, properties=None, server=None):
"""Create a service description.
type_: fully qualified service type name
name: fully qualified service name
address: IP address as unsigned short, network byte order
port: port that the service runs on
weight: weight of the service
priority: priority of the service
properties: dictionary of properties (or a string holding the
bytes for the text field)
server: fully qualified name for service host (defaults to name)"""
if not type_.endswith(service_type_name(name)):
raise BadTypeInNameException
self.type = type_
self.name = name
self.address = address
self.port = port
self.weight = weight
self.priority = priority
if server:
self.server = server
else:
self.server = name
self._properties = {}
self._set_properties(properties)
@property
def properties(self):
return self._properties
def _set_properties(self, properties):
"""Sets properties and text of this info from a dictionary"""
if isinstance(properties, dict):
self._properties = properties
list_ = []
result = b''
for key, value in iteritems(properties):
if isinstance(key, text_type):
key = key.encode('utf-8')
if value is None:
suffix = b''
elif isinstance(value, text_type):
suffix = value.encode('utf-8')
elif isinstance(value, binary_type):
suffix = value
elif isinstance(value, int):
if value:
suffix = b'true'
else:
suffix = b'false'
else:
suffix = b''
list_.append(b'='.join((key, suffix)))
for item in list_:
result = b''.join((result, int2byte(len(item)), item))
self.text = result
else:
self.text = properties
def _set_text(self, text):
"""Sets properties and text given a text field"""
self.text = text
result = {}
end = len(text)
index = 0
strs = []
while index < end:
length = indexbytes(text, index)
index += 1
strs.append(text[index:index + length])
index += length
for s in strs:
parts = s.split(b'=', 1)
try:
key, value = parts
except ValueError:
# No equals sign at all
key = s
value = False
else:
if value == b'true':
value = True
elif value == b'false' or not value:
value = False
# Only update non-existent properties
if key and result.get(key) is None:
result[key] = value
self._properties = result
def get_name(self):
"""Name accessor"""
if self.type is not None and self.name.endswith("." + self.type):
return self.name[:len(self.name) - len(self.type) - 1]
return self.name
def update_record(self, zc, now, record):
"""Updates service information from a DNS record"""
if record is not None and not record.is_expired(now):
if record.type == _TYPE_A:
# if record.name == self.name:
if record.name == self.server:
self.address = record.address
elif record.type == _TYPE_SRV:
if record.name == self.name:
self.server = record.server
self.port = record.port
self.weight = record.weight
self.priority = record.priority
# self.address = None
self.update_record(
zc, now, zc.cache.get_by_details(
self.server, _TYPE_A, _CLASS_IN))
elif record.type == _TYPE_TXT:
if record.name == self.name:
self._set_text(record.text)
def request(self, zc, timeout):
"""Returns true if the service could be discovered on the
network, and updates this object with details discovered.
"""
now = current_time_millis()
delay = _LISTENER_TIME
next_ = now + delay
last = now + timeout
record_types_for_check_cache = [
(_TYPE_SRV, _CLASS_IN),
(_TYPE_TXT, _CLASS_IN),
]
if self.server is not None:
record_types_for_check_cache.append((_TYPE_A, _CLASS_IN))
for record_type in record_types_for_check_cache:
cached = zc.cache.get_by_details(self.name, *record_type)
if cached:
self.update_record(zc, now, cached)
if None not in (self.server, self.address, self.text):
return True
try:
zc.add_listener(self, DNSQuestion(self.name, _TYPE_ANY, _CLASS_IN))
while None in (self.server, self.address, self.text):
if last <= now:
return False
if next_ <= now:
out = DNSOutgoing(_FLAGS_QR_QUERY)
out.add_question(
DNSQuestion(self.name, _TYPE_SRV, _CLASS_IN))
out.add_answer_at_time(
zc.cache.get_by_details(
self.name, _TYPE_SRV, _CLASS_IN), now)
out.add_question(
DNSQuestion(self.name, _TYPE_TXT, _CLASS_IN))
out.add_answer_at_time(
zc.cache.get_by_details(
self.name, _TYPE_TXT, _CLASS_IN), now)
if self.server is not None:
out.add_question(
DNSQuestion(self.server, _TYPE_A, _CLASS_IN))
out.add_answer_at_time(
zc.cache.get_by_details(
self.server, _TYPE_A, _CLASS_IN), now)
zc.send(out)
next_ = now + delay
delay *= 2
zc.wait(min(next_, last) - now)
now = current_time_millis()
finally:
zc.remove_listener(self)
return True
def __eq__(self, other):
"""Tests equality of service name"""
return isinstance(other, ServiceInfo) and other.name == self.name
def __ne__(self, other):
"""Non-equality test"""
return not self.__eq__(other)
def __repr__(self):
"""String representation"""
return '%s(%s)' % (
type(self).__name__,
', '.join(
'%s=%r' % (name, getattr(self, name))
for name in (
'type', 'name', 'address', 'port', 'weight', 'priority',
'server', 'properties',
)
)
)
class ZeroconfServiceTypes(object):
"""
Return all of the advertised services on any local networks
"""
def __init__(self):
self.found_services = set()
def add_service(self, zc, type_, name):
self.found_services.add(name)
def remove_service(self, zc, type_, name):
pass
@classmethod
def find(cls, zc=None, timeout=5, interfaces=InterfaceChoice.All):
"""
Return all of the advertised services on any local networks.
:param zc: Zeroconf() instance. Pass in if already have an
instance running or if non-default interfaces are needed
:param timeout: seconds to wait for any responses
:return: tuple of service type strings
"""
local_zc = zc or Zeroconf(interfaces=interfaces)
listener = cls()
browser = ServiceBrowser(
local_zc, '_services._dns-sd._udp.local.', listener=listener)
# wait for responses
time.sleep(timeout)
# close down anything we opened
if zc is None:
local_zc.close()
else:
browser.cancel()
return tuple(sorted(listener.found_services))
def get_all_addresses(address_family):
return list(set(
addr['addr']
for iface in netifaces.interfaces()
for addr in netifaces.ifaddresses(iface).get(address_family, [])
if addr.get('netmask') != HOST_ONLY_NETWORK_MASK
))
def normalize_interface_choice(choice, address_family):
if choice is InterfaceChoice.Default:
choice = ['0.0.0.0']
elif choice is InterfaceChoice.All:
choice = get_all_addresses(address_family)
return choice
def new_socket():
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
# SO_REUSEADDR should be equivalent to SO_REUSEPORT for
# multicast UDP sockets (p 731, "TCP/IP Illustrated,
# Volume 2"), but some BSD-derived systems require
# SO_REUSEPORT to be specified explicity. Also, not all
# versions of Python have SO_REUSEPORT available.
# Catch OSError and socket.error for kernel versions <3.9 because lacking
# SO_REUSEPORT support.
try:
reuseport = socket.SO_REUSEPORT
except AttributeError:
pass
else:
try:
s.setsockopt(socket.SOL_SOCKET, reuseport, 1)
except (OSError, socket.error) as err:
# OSError on python 3, socket.error on python 2
if not err.errno == errno.ENOPROTOOPT:
raise
# OpenBSD needs the ttl and loop values for the IP_MULTICAST_TTL and
# IP_MULTICAST_LOOP socket options as an unsigned char.
ttl = struct.pack(b'B', 255)
s.setsockopt(socket.IPPROTO_IP, socket.IP_MULTICAST_TTL, ttl)
loop = struct.pack(b'B', 1)
s.setsockopt(socket.IPPROTO_IP, socket.IP_MULTICAST_LOOP, loop)
s.bind(('', _MDNS_PORT))
return s
def get_errno(e):
assert isinstance(e, socket.error)
return e.args[0]
class Zeroconf(QuietLogger):
"""Implementation of Zeroconf Multicast DNS Service Discovery
Supports registration, unregistration, queries and browsing.
"""
def __init__(
self,
interfaces=InterfaceChoice.All,
):
"""Creates an instance of the Zeroconf class, establishing
multicast communications, listening and reaping threads.
:type interfaces: :class:`InterfaceChoice` or sequence of ip addresses
"""
# hook for threads
self._GLOBAL_DONE = False
self._listen_socket = new_socket()
interfaces = normalize_interface_choice(interfaces, socket.AF_INET)
self._respond_sockets = []
for i in interfaces:
log.debug('Adding %r to multicast group', i)
try:
self._listen_socket.setsockopt(
socket.IPPROTO_IP, socket.IP_ADD_MEMBERSHIP,
socket.inet_aton(_MDNS_ADDR) + socket.inet_aton(i))
except socket.error as e:
if get_errno(e) == errno.EADDRINUSE:
log.info(
'Address in use when adding %s to multicast group, '
'it is expected to happen on some systems', i,
)
elif get_errno(e) == errno.EADDRNOTAVAIL:
log.info(
'Address not available when adding %s to multicast '
'group, it is expected to happen on some systems', i,
)
continue
else:
raise
respond_socket = new_socket()
respond_socket.setsockopt(
socket.IPPROTO_IP, socket.IP_MULTICAST_IF, socket.inet_aton(i))
self._respond_sockets.append(respond_socket)
self.listeners = []
self.browsers = {}
self.services = {}
self.servicetypes = {}
self.cache = DNSCache()
self.condition = threading.Condition()
self.engine = Engine(self)
self.listener = Listener(self)
self.engine.add_reader(self.listener, self._listen_socket)
self.reaper = Reaper(self)
self.debug = None
@property
def done(self):
return self._GLOBAL_DONE
def wait(self, timeout):
"""Calling thread waits for a given number of milliseconds or
until notified."""
with self.condition:
self.condition.wait(timeout / 1000.0)
def notify_all(self):
"""Notifies all waiting threads"""
with self.condition:
self.condition.notify_all()
def get_service_info(self, type_, name, timeout=3000):
"""Returns network's service information for a particular
name and type, or None if no service matches by the timeout,
which defaults to 3 seconds."""
info = ServiceInfo(type_, name)
if info.request(self, timeout):
return info
def add_service_listener(self, type_, listener):
"""Adds a listener for a particular service type. This object
will then have its update_record method called when information
arrives for that type."""
self.remove_service_listener(listener)
self.browsers[listener] = ServiceBrowser(self, type_, listener)
def remove_service_listener(self, listener):
"""Removes a listener from the set that is currently listening."""
if listener in self.browsers:
self.browsers[listener].cancel()
del self.browsers[listener]
def remove_all_service_listeners(self):
"""Removes a listener from the set that is currently listening."""
for listener in [k for k in self.browsers]:
self.remove_service_listener(listener)
def register_service(self, info, ttl=_DNS_TTL, allow_name_change=False):
"""Registers service information to the network with a default TTL
of 60 seconds. Zeroconf will then respond to requests for
information for that service. The name of the service may be
changed if needed to make it unique on the network."""
self.check_service(info, allow_name_change)
self.services[info.name.lower()] = info
if info.type in self.servicetypes:
self.servicetypes[info.type] += 1
else:
self.servicetypes[info.type] = 1
now = current_time_millis()
next_time = now
i = 0
while i < 3:
if now < next_time:
self.wait(next_time - now)
now = current_time_millis()
continue
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
out.add_answer_at_time(
DNSPointer(info.type, _TYPE_PTR, _CLASS_IN, ttl, info.name), 0)
out.add_answer_at_time(
DNSService(info.name, _TYPE_SRV, _CLASS_IN,
ttl, info.priority, info.weight, info.port,
info.server), 0)
out.add_answer_at_time(
DNSText(info.name, _TYPE_TXT, _CLASS_IN, ttl, info.text), 0)
if info.address:
out.add_answer_at_time(
DNSAddress(info.server, _TYPE_A, _CLASS_IN,
ttl, info.address), 0)
self.send(out)
i += 1
next_time += _REGISTER_TIME
def unregister_service(self, info):
"""Unregister a service."""
try:
del self.services[info.name.lower()]
if self.servicetypes[info.type] > 1:
self.servicetypes[info.type] -= 1
else:
del self.servicetypes[info.type]
except Exception as e: # TODO stop catching all Exceptions
log.exception('Unknown error, possibly benign: %r', e)
now = current_time_millis()
next_time = now
i = 0
while i < 3:
if now < next_time:
self.wait(next_time - now)
now = current_time_millis()
continue
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
out.add_answer_at_time(
DNSPointer(info.type, _TYPE_PTR, _CLASS_IN, 0, info.name), 0)
out.add_answer_at_time(
DNSService(info.name, _TYPE_SRV, _CLASS_IN, 0,
info.priority, info.weight, info.port, info.name), 0)
out.add_answer_at_time(
DNSText(info.name, _TYPE_TXT, _CLASS_IN, 0, info.text), 0)
if info.address:
out.add_answer_at_time(
DNSAddress(info.server, _TYPE_A, _CLASS_IN, 0,
info.address), 0)
self.send(out)
i += 1
next_time += _UNREGISTER_TIME
def unregister_all_services(self):
"""Unregister all registered services."""
if len(self.services) > 0:
now = current_time_millis()
next_time = now
i = 0
while i < 3:
if now < next_time:
self.wait(next_time - now)
now = current_time_millis()
continue
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
for info in self.services.values():
out.add_answer_at_time(DNSPointer(
info.type, _TYPE_PTR, _CLASS_IN, 0, info.name), 0)
out.add_answer_at_time(DNSService(
info.name, _TYPE_SRV, _CLASS_IN, 0,
info.priority, info.weight, info.port, info.server), 0)
out.add_answer_at_time(DNSText(
info.name, _TYPE_TXT, _CLASS_IN, 0, info.text), 0)
if info.address:
out.add_answer_at_time(DNSAddress(
info.server, _TYPE_A, _CLASS_IN, 0,
info.address), 0)
self.send(out)
i += 1
next_time += _UNREGISTER_TIME
def check_service(self, info, allow_name_change):
"""Checks the network for a unique service name, modifying the
ServiceInfo passed in if it is not unique."""
# This is kind of funky because of the subtype based tests
# need to make subtypes a first class citizen
service_name = service_type_name(info.name)
if not info.type.endswith(service_name):
raise BadTypeInNameException
instance_name = info.name[:-len(service_name) - 1]
next_instance_number = 2
now = current_time_millis()
next_time = now
i = 0
while i < 3:
# check for a name conflict
while self.cache.current_entry_with_name_and_alias(
info.type, info.name):
if not allow_name_change:
raise NonUniqueNameException
# change the name and look for a conflict
info.name = '%s-%s.%s' % (
instance_name, next_instance_number, info.type)
next_instance_number += 1
service_type_name(info.name)
next_time = now
i = 0
if now < next_time:
self.wait(next_time - now)
now = current_time_millis()
continue
out = DNSOutgoing(_FLAGS_QR_QUERY | _FLAGS_AA)
self.debug = out
out.add_question(DNSQuestion(info.type, _TYPE_PTR, _CLASS_IN))
out.add_authorative_answer(DNSPointer(
info.type, _TYPE_PTR, _CLASS_IN, _DNS_TTL, info.name))
self.send(out)
i += 1
next_time += _CHECK_TIME
def add_listener(self, listener, question):
"""Adds a listener for a given question. The listener will have
its update_record method called when information is available to
answer the question."""
now = current_time_millis()
self.listeners.append(listener)
if question is not None:
for record in self.cache.entries_with_name(question.name):
if question.answered_by(record) and not record.is_expired(now):
listener.update_record(self, now, record)
self.notify_all()
def remove_listener(self, listener):
"""Removes a listener."""
try:
self.listeners.remove(listener)
self.notify_all()
except Exception as e: # TODO stop catching all Exceptions
log.exception('Unknown error, possibly benign: %r', e)
def update_record(self, now, rec):
"""Used to notify listeners of new information that has updated
a record."""
for listener in self.listeners:
listener.update_record(self, now, rec)
self.notify_all()
def handle_response(self, msg):
"""Deal with incoming response packets. All answers
are held in the cache, and listeners are notified."""
now = current_time_millis()
for record in msg.answers:
expired = record.is_expired(now)
if record in self.cache.entries():
if expired:
self.cache.remove(record)
else:
entry = self.cache.get(record)
if entry is not None:
entry.reset_ttl(record)
else:
self.cache.add(record)
for record in msg.answers:
self.update_record(now, record)
def handle_query(self, msg, addr, port):
"""Deal with incoming query packets. Provides a response if
possible."""
out = None
# Support unicast client responses
#
if port != _MDNS_PORT:
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA, multicast=False)
for question in msg.questions:
out.add_question(question)
for question in msg.questions:
if question.type == _TYPE_PTR:
if question.name == "_services._dns-sd._udp.local.":
for stype in self.servicetypes.keys():
if out is None:
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
out.add_answer(msg, DNSPointer(
"_services._dns-sd._udp.local.", _TYPE_PTR,
_CLASS_IN, _DNS_TTL, stype))
for service in self.services.values():
if question.name == service.type:
if out is None:
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
out.add_answer(msg, DNSPointer(
service.type, _TYPE_PTR,
_CLASS_IN, _DNS_TTL, service.name))
else:
try:
if out is None:
out = DNSOutgoing(_FLAGS_QR_RESPONSE | _FLAGS_AA)
# Answer A record queries for any service addresses we know
if question.type in (_TYPE_A, _TYPE_ANY):
for service in self.services.values():
if service.server == question.name.lower():
out.add_answer(msg, DNSAddress(
question.name, _TYPE_A,
_CLASS_IN | _CLASS_UNIQUE,
_DNS_TTL, service.address))
service = self.services.get(question.name.lower(), None)
if not service:
continue
if question.type in (_TYPE_SRV, _TYPE_ANY):
out.add_answer(msg, DNSService(
question.name, _TYPE_SRV, _CLASS_IN | _CLASS_UNIQUE,
_DNS_TTL, service.priority, service.weight,
service.port, service.server))
if question.type in (_TYPE_TXT, _TYPE_ANY):
out.add_answer(msg, DNSText(
question.name, _TYPE_TXT, _CLASS_IN | _CLASS_UNIQUE,
_DNS_TTL, service.text))
if question.type == _TYPE_SRV:
out.add_additional_answer(DNSAddress(
service.server, _TYPE_A, _CLASS_IN | _CLASS_UNIQUE,
_DNS_TTL, service.address))
except Exception: # TODO stop catching all Exceptions
self.log_exception_warning()
if out is not None and out.answers:
out.id = msg.id
self.send(out, addr, port)
def send(self, out, addr=_MDNS_ADDR, port=_MDNS_PORT):
"""Sends an outgoing packet."""
packet = out.packet()
if len(packet) > _MAX_MSG_ABSOLUTE:
self.log_warning_once("Dropping %r over-sized packet (%d bytes) %r",
out, len(packet), packet)
return
log.debug('Sending %r (%d bytes) as %r...', out, len(packet), packet)
for s in self._respond_sockets:
if self._GLOBAL_DONE:
return
try:
bytes_sent = s.sendto(packet, 0, (addr, port))
except Exception: # TODO stop catching all Exceptions
# on send errors, log the exception and keep going
self.log_exception_warning()
else:
if bytes_sent != len(packet):
self.log_warning_once(
'!!! sent %d out of %d bytes to %r' % (
bytes_sent, len(packet)), s)
def close(self):
"""Ends the background threads, and prevent this instance from
servicing further queries."""
if not self._GLOBAL_DONE:
self._GLOBAL_DONE = True
# remove service listeners
self.remove_all_service_listeners()
self.unregister_all_services()
# shutdown recv socket and thread
self.engine.del_reader(self._listen_socket)
self._listen_socket.close()
self.engine.join()
# shutdown the rest
self.notify_all()
self.reaper.join()
for s in self._respond_sockets:
s.close() | zeroconf2 | /zeroconf2-0.19.2.tar.gz/zeroconf2-0.19.2/zeroconf.py | zeroconf.py |
# zeroconnect
[](https://pypi.org/project/zeroconnect)
[](https://pypi.org/project/zeroconnect)
Use zeroconf to automatically connect devices via TCP on a LAN.
I can hardly believe this doesn't exist already, but after searching for an hour, in despair I resign myself to write my own, and patch the glaring hole in existence.
-----
**Table of Contents**
- [Installation](#installation)
- [Usage](#usage)
- [License](#license)
- [Tips](#tips)
- [Bonus!](#bonus)
## Installation
```console
pip install zeroconnect
```
## Usage
One or more servers, and one or more clients, run connected to the same LAN. (Wifi or ethernet.)
### Most basic
Service:
```python
from zeroconnect import ZeroConnect
def rxMessageConnection(messageSock, nodeId, serviceId):
print(f"got message connection from {nodeId}")
data = messageSock.recvMsg()
print(data)
messageSock.sendMsg(b"Hello from server")
ZeroConnect().advertise(rxMessageConnection, "YOUR_SERVICE_ID_HERE")
```
Client:
```python
from zeroconnect import ZeroConnect
messageSock = ZeroConnect().connectToFirst("YOUR_SERVICE_ID_HERE")
messageSock.sendMsg(b"Hello from client")
data = messageSock.recvMsg()
print(data)
```
### Less basic
Service:
```python
from zeroconnect import ZeroConnect
SERVICE_ID = "YOUR_SERVICE_ID_HERE"
zc = ZeroConnect("NODE_ID")
def rxMessageConnection(messageSock, nodeId, serviceId):
print(f"got message connection from {nodeId}")
# If you also want to spontaneously send messages, pass the socket to e.g. another thread.
while True:
data = messageSock.recvMsg()
print(data)
if data == b'enable jimjabber':
print(f"ENABLE JIMJABBER")
elif data == b'save msg:':
toSave = messageSock.recvMsg()
print(f"SAVE MESSAGE {toSave}")
elif data == b'marco':
messageSock.sendMsg(b'polo')
print(f"PING PONGED")
elif data == None:
print(f"Connection closed from {nodeId}")
messageSock.close()
return
else:
print(f"Unhandled message: {data}")
# Use messageSock.sock for e.g. sock.getsockname()
# I recommend messageSock.close() after you're done with it - but it'll get closed on zc.close(), at least
zc.advertise(rxMessageConnection, SERVICE_ID) # Implicit mode=SocketMode.Messages
try:
input("Press enter to exit...\n\n")
finally:
zc.close()
```
Client:
```python
from zeroconnect import ZeroConnect, SocketMode
SERVICE_ID = "YOUR_SERVICE_ID_HERE"
zc = ZeroConnect("NODE_ID") # Technically the nodeId is optional; it'll assign you a random UUID
ads = zc.scan(SERVICE_ID, time=5)
# OR: ads = zc.scan(SERVICE_ID, NODE_ID)
# An `Ad` contains a `serviceId` and `nodeId` etc.; see `Ad` for details
messageSock = zc.connect(ads[0], mode=SocketMode.Messages) # Send and receive messages; the default mode
# OR: messageSock = zc.connectToFirst(SERVICE_ID)
# OR: messageSock = zc.connectToFirst(nodeId=NODE_ID)
# OR: messageSock = zc.connectToFirst(SERVICE_ID, NODE_ID, timeout=10)
messageSock.sendMsg(b"enable jimjabber")
messageSock.sendMsg(b"save msg:")
messageSock.sendMsg(b"i love you")
messageSock.sendMsg(b"marco")
print(f"rx: {messageSock.recvMsg()}")
# ...
zc.close()
```
You can also get raw sockets rather than MessageSockets, if you prefer:
Server:
```python
from zeroconnect import ZeroConnect, SocketMode
SERVICE_ID = "YOUR_SERVICE_ID_HERE"
zc = ZeroConnect("NODE_ID")
def rxRawConnection(sock, nodeId, serviceId):
print(f"got raw connection from {nodeId}")
data = sock.recv(1024)
print(data)
sock.sendall(b"Hello from server\n")
# sock is a plain socket; use accordingly
zc.advertise(rxRawConnection, SERVICE_ID, mode=SocketMode.Raw)
try:
input("Press enter to exit...\n\n")
finally:
zc.close()
```
Client:
```python
from zeroconnect import ZeroConnect, SocketMode
SERVICE_ID = "YOUR_SERVICE_ID_HERE"
zc = ZeroConnect("NODE_ID") # Technically the nodeId is optional; it'll assign you a random UUID
ads = zc.scan(SERVICE_ID, time=5)
# OR: ads = zc.scan(SERVICE_ID, NODE_ID)
# An `Ad` contains a `serviceId` and `nodeId` etc.; see `Ad` for details
sock = zc.connect(ads[0], mode=SocketMode.Raw) # Get the raw streams
# OR: sock = zc.connectToFirst(SERVICE_ID, mode=SocketMode.Raw)
# OR: sock = zc.connectToFirst(nodeId=NODE_ID, mode=SocketMode.Raw)
# OR: sock = zc.connectToFirst(SERVICE_ID, NODE_ID, mode=SocketMode.Raw, timeout=10)
sock.sendall(b"Hello from client\n")
data = sock.recv(1024)
print(f"rx: {data}")
# ...
zc.close()
```
There's a few other functions you might find useful. Look at the source code.
Here; I'll paste the declaration of all the public `ZeroConnect` methods here.
```python
def __init__(self, localId=None):
def advertise(self, callback, serviceId, port=0, host="0.0.0.0", mode=SocketMode.Messages):
def scan(self, serviceId=None, nodeId=None, time=30):
def scanGen(self, serviceId=None, nodeId=None, time=30):
def connectToFirst(self, serviceId=None, nodeId=None, localServiceId="", mode=SocketMode.Messages, timeout=30):
def connect(self, ad, localServiceId="", mode=SocketMode.Messages):
def broadcast(self, message, serviceId=None, nodeId=None):
def getConnections(self):
def close(self):
```
## Tips
Be careful not to have two nodes recv from each other at the same time, or they'll deadlock.
However, you CAN have them send at the same time (at least according to my tests).
`ZeroConnect` is intended to be manipulated via its methods, but it probably won't immediately explode if you
read the data in the fields.
Note that some computers/networks block zeroconf, or external connection attempts, etc.
Calling `broadcast` will automatically clean up dead connections.
If you close your socket immediately after sending a message, the data may not finish sending. Not my fault; blame socket.
`broadcast` uses MessageSockets, so if you're using a raw socket, be aware the message will be prefixed with a header, currently
an 8 byte unsigned long representing the length of the subsequent message. See `MessageSocket`.
See logging.py to see logging settings, or do like so:
```python
from zeroconnect.logging import *
setLogLevel(-1) # 4+ for everything current, -1 for nothing except uncaught exceptions
# It also contains some presets; ERROR/WARN/INFO/VERBOSE/DEBUG atm.
# Also, you can move all the logging to stderr with `setLogType(2)`.
```
## Bonus!
Also includes zcat, like ncat/nc/netcat. Use as follows:
RX:
```bash
python -m zeroconnect.zcat -l SERVICE_ID [NODE_ID] > FILE
```
TX:
```bash
cat FILE | python -m zeroconnect.zcat SERVICE_ID [NODE_ID]
```
## License
`zeroconnect` is distributed under the terms of the [MIT](https://spdx.org/licenses/MIT.html) license.
## TODO
ssl
lower timeouts?
connect to all, forever?
connection callback
maybe some automated tests?
.advertiseSingle to get one connection? for quick stuff?
| zeroconnect | /zeroconnect-1.0.1.tar.gz/zeroconnect-1.0.1/README.md | README.md |
import binascii
import click
import logging
import sys
import os.path
import six
from IPython import embed
from functools import update_wrapper
import ZODB.FileStorage
from zerodb import DB
from zerodb.permissions import base
from zerodbext.server.cert import generate_cert
logging.basicConfig()
_username = None
_passphrase = None
_sock = None
_server_certificate = None
_server_key = None
_user_certificate = None
ZEO_TEMPLATE = """<zeo>
address {sock}
<ssl>
certificate {certificate}
key {key}
authenticate DYNAMIC
</ssl>
</zeo>
<filestorage>
path {dbfile}
pack-gc false
</filestorage>"""
@click.group()
def cli():
pass
# XXX make this more convenient, e.g.
# Generate server certificate [Y/n] etc
def _auth_options(f, confirm_passphrase=True):
"""Decorator to enable username, passphrase and sock options to command"""
@click.option(
"--server-certificate", prompt="Server certificate",
type=click.STRING, default="",
help="Server certificate file path (.pem)")
@click.option(
"--server-key", prompt="Server key",
type=click.STRING, default="",
help="Server certificate key file path (.pem)")
@click.option(
"--username", prompt="Username", default="root", type=click.STRING,
help="Admin username")
@click.option(
"--passphrase", prompt="Passphrase", hide_input=True,
confirmation_prompt=confirm_passphrase, type=click.STRING,
default="", help="Admin passphrase")
@click.option(
"--sock", prompt="Sock", default="localhost:8001",
type=click.STRING, help="Storage server socket (TCP or UNIX)")
@click.option(
"--user-certificate", prompt="User certificate",
type=click.STRING, default="",
help="User certificate file path (.pem)")
@click.pass_context
def auth_func(ctx, server_certificate, server_key,
username, passphrase, sock, user_certificate,
*args, **kw):
global _username
global _passphrase
global _sock
global _server_certificate
global _server_key
global _user_certificate
_username = username
_passphrase = passphrase or None
_server_certificate = server_certificate or None
_server_key = server_key or None
_user_certificate = user_certificate or None
if sock.startswith("/"):
_sock = sock
else:
sock = sock.split(":")
_sock = (str(sock[0]), int(sock[1]))
ctx.invoke(f, *args, **kw)
return update_wrapper(auth_func, f)
def signup_options(f):
return _auth_options(f, confirm_passphrase=True)
def auth_options(f):
return _auth_options(f, confirm_passphrase=False)
@cli.command()
@auth_options
def console():
"""
Console for managing users (add, remove, change password)
"""
# XXX redo all of these!!
def useradd(username, pubkey):
storage.add_user(username, binascii.unhexlify(pubkey))
def userdel(username):
storage.del_user(username)
def chkey(username, pubkey):
storage.change_key(username, binascii.unhexlify(pubkey))
banner = "\n".join([
"Usage:",
"========",
"useradd(username, pubkey) - add user",
"userdel(username) - remove user",
"chkey(username, pubkey) - change pubkey",
"get_pubkey(username, password) - get public key from passphrase",
"exit() or ^D - exit"])
db = DB(_sock, username=_username, password=_passphrase)
storage = db._storage
sys.path.append(".")
embed(banner1=banner)
@cli.command()
@click.option("--path", default=None, type=click.STRING,
help="Path to db and configs")
@click.option("--absolute-path/--no-absolute-path", default=False,
help="Use absolute paths in configs")
@signup_options
def init_db(path, absolute_path):
"""
Initialize database if doesn't exist.
Creates conf/ directory with config files and db/ with database files
"""
global _server_key
global _server_certificate
global _user_certificate
if path:
if not os.path.exists(path):
raise IOError("Path provided doesn't exist")
else:
path = os.getcwd()
if absolute_path:
dbfile_path = os.path.join(path, "db", "db.fs")
else:
dbfile_path = os.path.join("db", "db.fs")
conf_dir = os.path.join(path, "conf")
db_dir = os.path.join(path, "db")
server_conf = os.path.join(conf_dir, "server.conf")
if os.path.exists(server_conf):
raise IOError("Config files already exist, remove them or edit")
if not os.path.exists(conf_dir):
os.mkdir(conf_dir)
if not os.path.exists(db_dir):
os.mkdir(db_dir)
if not _server_key:
key_pem, cert_pem = generate_cert()
_server_key = os.path.join(conf_dir, "server_key.pem")
_server_certificate = os.path.join(conf_dir, "server.pem")
with open(_server_key, "w") as f:
f.write(key_pem)
with open(_server_certificate, "w") as f:
f.write(cert_pem)
server_content = ZEO_TEMPLATE.format(
sock=_sock
if isinstance(_sock, six.string_types) else "{0}:{1}".format(*_sock),
dbfile=dbfile_path,
certificate=_server_certificate,
key=_server_key,
)
with open(server_conf, "w") as f:
f.write(server_content)
if not _user_certificate and not _passphrase:
_user_certificate = os.path.join(conf_dir, "user.pem")
_user_key = os.path.join(conf_dir, "user_key.pem")
key_pem, cert_pem = generate_cert()
with open(_user_key, "w") as f:
f.write(key_pem)
with open(_user_certificate, "w") as f:
f.write(cert_pem)
if _user_certificate:
with open(_user_certificate) as f:
pem_data = f.read()
else:
pem_data = None
base.init_db(ZODB.FileStorage.FileStorage(dbfile_path),
uname=_username, password=_passphrase,
pem_data=pem_data)
click.echo("Config files created, you can start zerodb-server")
@cli.command()
def clear():
"""
Remove all database files (including auth db)
"""
for f in os.listdir("db"):
if f.startswith("db.fs"):
os.remove(os.path.join("db", f))
for f in os.listdir("conf"):
if f.startswith("authdb.db"):
os.remove(os.path.join("conf", f))
click.echo("Database removed")
if __name__ == "__main__":
cli() | zerodb-server | /zerodb-server-0.2.0a1.tar.gz/zerodb-server-0.2.0a1/zerodbext/server/manage.py | manage.py |
Installing :mod:`repoze.catalog`
================================
How To Install
--------------
You will need `Python <http://python.org>`_ version 2.4 or better to
run :mod:`repoze.catalog`. Development of :mod:`repoze.catalog` is
done primarily under Python 2.6, so that version is recommended.
:mod:`repoze.catalog` also runs under Python 2.4 and 2.5 with limited
functionality. It does *not* run under Python 3.X.
.. warning:: To succesfully install :mod:`repoze.catalog`, you will need an
environment capable of compiling Python C code. See the
documentation about installing, e.g. ``gcc`` and ``python-devel``
for your system. You will also need :term:`setuptools` installed
within your Python system in order to run the ``easy_install``
command.
It is advisable to install :mod:`repoze.catalog` into a
:term:`virtualenv` in order to obtain isolation from any "system"
packages you've got installed in your Python version (and likewise, to
prevent :mod:`repoze.catalog` from globally installing versions of
packages that are not compatible with your system Python).
After you've got the requisite dependencies installed, you may install
:mod:`repoze.catalog` into your Python environment using the following
command::
$ easy_install repoze.catalog
What Gets Installed
-------------------
When you ``easy_install`` :mod:`repoze.catalog`, various Zope
libraries and ZODB are installed.
| zerodbext.catalog | /zerodbext.catalog-0.8.4.tar.gz/zerodbext.catalog-0.8.4/docs/install.rst | install.rst |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.