prompt
stringlengths 19
879k
| completion
stringlengths 3
53.8k
| api
stringlengths 8
59
|
---|---|---|
# Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing,
# software distributed under the License is distributed on an
# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
# KIND, either express or implied. See the License for the
# specific language governing permissions and limitations
# under the License.
"""
This module provides infrastructure to verify the correctness of
the command stream produced.
Currently it will invoke vela to generate a vela-optimized tflite
in which the command stream is contained as a custom operator.
This class include methods to parse the custom operator to extract
the command stream and perform an equivalency check for single operator
test cases.
"""
from typing import List
import os
import struct
import numpy
from enum import IntEnum
from ethosu.vela.register_command_stream_generator import CmdMode
from ethosu.vela.register_command_stream_generator import cmd0
from ethosu.vela.register_command_stream_generator import cmd1
import tvm
from tvm import relay
import tvm.relay.backend.contrib.ethosu.op as ethosu_ops
from tvm.topi.nn.utils import get_pad_tuple
from tests.python.relay.aot.aot_test_utils import (
AOTCompiledTestModel,
AOTDataLinkage,
AOTTestModel,
AOTTestRunner,
compile_models,
run_and_check,
)
class AttachType(IntEnum):
kGroupRoot = 1
kInline = 2
kInlinedAlready = 3
kScope = 4
kScanUpdate = 5
class VelaArtifacts:
def __init__(self):
self.cs = dict()
self.flash = dict()
self.sram = dict()
self.npu_ops = set()
def parse_relay_tflite_model(tflite_model, input_tensor, input_shape, input_dtype):
mod_, params_ = relay.frontend.from_tflite(
tflite_model,
shape_dict={input_tensor: input_shape},
dtype_dict={input_tensor: input_dtype},
)
return mod_, params_
def parse_tflite_model(model_file):
try:
import tflite
return tflite.Model.GetRootAsModel(model_file, 0)
except AttributeError:
import tflite.Model
return tflite.Model.Model.GetRootAsModel(model_file, 0)
def print_payload(payload):
cmds = deserialize_command_stream(payload)
for cmd_val in cmds:
cmd, val = parse_cmd(cmd_val)
s = str(cmd)
s = s.ljust(40)
s += str(val)
print(s)
def parse_cmd(binary_cmd):
code = binary_cmd[0] & 0x0000FFFF # lower 16 bits
param = binary_cmd[0] >> 16 # higher 16 bits
payload_mode = CmdMode(code & CmdMode.Mask)
if payload_mode == CmdMode.Payload32:
command = cmd1(code & CmdMode.CmdOpMask)
value = binary_cmd[1]
else:
command = cmd0(code & CmdMode.CmdOpMask)
value = param
return command, value
def check_cmms_equivalency(vela_cmd, vela_value, tvm_value, ignore_cmds=None):
if ignore_cmds is None:
ignore_cmds = []
if vela_value != tvm_value and vela_cmd not in ignore_cmds:
raise RuntimeError(
"ValueMismatch :: vela={}, tvm={} for command:{}".format(
vela_value, tvm_value, vela_cmd
)
)
def verify_cmms(cmms_tvm_blob, cmms_vela_blob):
vela_cmm = deserialize_command_stream(cmms_vela_blob)
tvm_cmm = deserialize_command_stream(cmms_tvm_blob)
cmms_zip = zip(vela_cmm, tvm_cmm)
first_ifm_found = False
last_ofm_found = False
ignore_commands = (
cmd1.NPU_SET_DMA0_SRC,
cmd1.NPU_SET_DMA0_DST,
cmd1.NPU_SET_WEIGHT_BASE,
cmd1.NPU_SET_OFM_BASE0,
cmd1.NPU_SET_IFM_BASE0,
cmd1.NPU_SET_SCALE_BASE,
)
ofm_region_params = []
ofm_bases = []
for vela_cmm, tvm_cmm in cmms_zip:
vela_cmd, vela_value = parse_cmd(vela_cmm)
tvm_cmd, tvm_value = parse_cmd(tvm_cmm)
assert vela_cmd == tvm_cmd
# The first IFM region could be different, but it needs to be 1 and 3.
if vela_cmd == cmd0.NPU_SET_IFM_REGION and not first_ifm_found:
if vela_value == 1 and tvm_value == 3:
first_ifm_found = True
continue
if vela_cmd == cmd1.NPU_SET_IFM_BASE0 and not first_ifm_found:
if tvm_value != 0:
raise RuntimeError("ValueError :: tvm primary ifm base should be zero")
continue
# OFM regions should be cached to be checked later
if vela_cmd == cmd0.NPU_SET_OFM_REGION:
ofm_region_params.append((vela_value, tvm_value))
continue
# OFM bases should be cached to be checked later
if vela_cmd == cmd1.NPU_SET_OFM_BASE0:
ofm_bases.append((vela_value, tvm_value))
continue
check_cmms_equivalency(vela_cmd, vela_value, tvm_value, ignore_commands)
# The last OFM region could be different but it should be 1 and 4.
last_vela_ofm_region, last_tvm_ofm_region = ofm_region_params.pop(-1)
if not (last_vela_ofm_region == 1 and last_tvm_ofm_region == 4):
raise RuntimeError(
"ValueMismatch :: vela={}, tvm={} for last ofm region it should be 1 and 4 respectively".format(
last_vela_ofm_region, last_tvm_ofm_region
)
)
# The rest of the OFM regions should be the same.
for vela_value, tvm_value in ofm_region_params:
check_cmms_equivalency(vela_cmd, vela_value, tvm_value, ignore_commands)
# The last OFM base should be zero for tvm
_, last_tvm_ofm_base = ofm_bases.pop(-1)
if not last_tvm_ofm_base == 0:
raise RuntimeError("ValueError :: tvm primary ofm base should be zero")
def deserialize_command_stream(blob):
assert isinstance(blob, bytes)
payload_bytes = struct.unpack("<{0}I".format(len(blob) // 4), blob)
cmms = []
# remove_header
payload_bytes = payload_bytes[8:]
idx = 0
while idx < len(payload_bytes):
cmd = []
code = payload_bytes[idx]
idx += 1
cmd.append(code)
payload_mode = CmdMode(code & CmdMode.Mask)
if payload_mode == CmdMode.Payload32:
value = payload_bytes[idx]
idx += 1
cmd.append(value)
cmms.append(cmd)
return cmms
def _create_test_runner(accel):
file_dir = os.path.dirname(os.path.abspath(__file__))
test_root = os.path.join(file_dir, "reference_system")
ethosu_macs = accel[accel.rfind("-") + 1 :]
return AOTTestRunner(
makefile="corstone300",
prologue="""
uart_init();
EthosuInit();
""",
includes=["uart.h", "ethosu_55.h", "ethosu_mod.h", "hard_fault.h"],
parameters={"ETHOSU_TEST_ROOT": test_root, "NPU_VARIANT": ethosu_macs},
pass_config={
"relay.ext.ethosu.options": {
"accelerator_config": accel,
}
},
)
def build_source(module, inputs, outputs, accel="ethos-u55-256", output_tolerance=0):
test_runner = _create_test_runner(accel)
return compile_models(
models=AOTTestModel(
module=module,
inputs=inputs,
outputs=outputs,
output_tolerance=output_tolerance,
extra_memory_in_bytes=16 * 1024 * 1024,
),
interface_api="c",
use_unpacked_api=True,
workspace_byte_alignment=16,
pass_config=test_runner.pass_config,
)
def verify_source(
models: List[AOTCompiledTestModel],
accel="ethos-u55-256",
):
"""
This method verifies the generated source from an NPU module by building it and running on an FVP.
"""
interface_api = "c"
test_runner = _create_test_runner(accel)
run_and_check(
models,
test_runner,
interface_api,
workspace_byte_alignment=16,
data_linkage=AOTDataLinkage(section="ethosu_scratch", alignment=16),
)
def flatten_numpy_data(data):
"""Flatten the numpy tensor to be single dimensional"""
total_elements = data.size
reshaped_data = | numpy.reshape(data, [total_elements]) | numpy.reshape |
import numpy as np
from astropy.table import Table
from astropy.io import ascii
from astropy import units as u
from sys import argv
import sys
import randhom as rh
#___________________________________
# Load settings
from configparser import ConfigParser
filename = rh.check_file(argv)
config = ConfigParser()
config.read(filename)
#___________________________________
# Prepare experiment
p = rh.load_parameters(config._sections)
from os import listdir
xi_files = listdir(p.input_dir)
rh.test_r_len(p.input_dir + xi_files[0], p.nr)
#___________________________________
# compute
xi0_rs, xi2_rs, xi4_rs = [], [], []
for xi_file in xi_files[:p.nfiles_max]:
cnames=['xi_0','xi_2','xi_4','xi_6']
xil_rs = ascii.read(p.input_dir + xi_file,names=cnames)
xi0_rs.append( xil_rs['xi_0'].data )
xi2_rs.append( xil_rs['xi_2'].data )
xi4_rs.append( xil_rs['xi_4'].data )
mean_xi0_rs = np.mean(xi0_rs, axis=0)
mean_xi2_rs = | np.mean(xi2_rs, axis=0) | numpy.mean |
import mmcv
import numpy as np
import os.path as osp
import cv2
import matplotlib.pyplot as plt
import random
import argparse
import cv2
import torch
from torch.utils.data import Dataset
import numpy as np
# from tensorboardX import SummaryWritter
from pysot.core.config import cfg
from pysot.models.model_builder import ModelBuilder
from pysot.tracker.tracker_builder import build_tracker
import datetime
import time
import pickle
from multiprocessing import Pool
from torch import multiprocessing
_TIMESTAMP_BIAS = 600
_TIMESTAMP_START = 840 # 60*14min
_TIMESTAMP_END = 1860 # 60*31min
_FPS = 30
torch.set_num_threads(0)
parser = argparse.ArgumentParser(description='tracking demo')
parser.add_argument('--config', type=str, help='config file')
parser.add_argument('--snapshot', type=str, help='model name')
parser.add_argument('--video_name', default='', type=str,
help='videos or image files')
args = parser.parse_args()
cfg.merge_from_file(args.config)
cfg.CUDA = True
# device = torch.device('cuda')
# random.seed(1)
# Firstly Load Proposal
random.seed(0)
np.random.seed(0)
torch.manual_seed(0)
torch.cuda.manual_seed(0)
torch.backends.cudnn.deterministic = True
torch.backends.cudnn.benchmark = False
class Tracking_Proposal(object):
def __init__(self,
img_prefix,
proposal_path,
video_stat_file,
new_length=32,
new_step=2,
with_pysot=True,
with_shuffle=False,
num_gpus=1,
num_workers=1):
self.img_prefix = img_prefix
self.new_step = new_step
self.new_length = new_length
self.proposal_dict, self.org_proposal_dict = self.load_proposal(proposal_path)
self.video_stats = dict([tuple(x.strip().split(' ')) for x in open(video_stat_file)])
self.with_model = False
self.with_pysot = with_pysot
self.with_shuffle = with_shuffle
self.result_dict = {}
self.num_gpus = num_gpus
self.num_workers = num_workers
def load_proposal(self, path):
proposal_dict = mmcv.load(path)
convert_dict = {}
for key, value in proposal_dict.items():
video_id, frame = key.split(',')
if convert_dict.get(video_id,None) is None:
convert_dict[video_id] = {}
elif convert_dict[video_id].get(frame, None) is None:
convert_dict[video_id][frame] = {}
convert_dict[video_id][frame] = value
return convert_dict, proposal_dict
def _load_image(self, directory, image_tmpl, modality, idx):
if modality in ['RGB', 'RGBDiff']:
return mmcv.imread(osp.join(directory, image_tmpl.format(idx)))
elif modality == 'Flow':
x_imgs = mmcv.imread(
osp.join(directory, image_tmpl.format('x', idx)),
flag='grayscale')
y_imgs = mmcv.imread(
osp.join(directory, image_tmpl.format('y', idx)),
flag='grayscale')
return [x_imgs, y_imgs]
else:
raise ValueError(
'Not implemented yet; modality should be '
'["RGB", "RGBDiff", "Flow"]')
def spilit_proposal_dict(self):
keys = list(self.proposal_dict.keys())
if self.with_shuffle:
random.shuffle(keys)
shuffle_dict = [(key, self.proposal_dict[key]) for key in keys]
video_per_gpu = int(len(shuffle_dict) // (self.num_gpus*self.num_workers))
self.sub_shuffle_dict_list = [shuffle_dict[i * video_per_gpu:(i + 1) * video_per_gpu] if i != self.num_gpus - 1
else shuffle_dict[i * video_per_gpu:]
for i in range(self.num_gpus*self.num_workers)]
self.shuffle_dict = shuffle_dict
def spilit_org_proposal_dict(self):
keys = list(self.org_proposal_dict.keys())
if self.with_shuffle:
random.shuffle(keys)
shuffle_dict = [(key, self.org_proposal_dict[key]) for key in keys if True or '-5KQ66BBWC' in key and 900<=int(key[-4:])<=950]
video_per_gpu = int(len(shuffle_dict) // (self.num_gpus*self.num_workers))
self.sub_shuffle_dict_list = [shuffle_dict[i * video_per_gpu:(i + 1) * video_per_gpu] if i != (self.num_gpus*self.num_workers) - 1
else shuffle_dict[i * video_per_gpu:]
for i in range(self.num_gpus*self.num_workers)]
# [print((i*video_per_gpu,(i+1)*video_per_gpu)) if i != (self.num_gpus*self.num_workers-1) else print(i*video_per_gpu,len(shuffle_dict)) for i in range(self.num_gpus*self.num_workers)]
self.shuffle_dict = shuffle_dict
def tracking(self, index):
# self.spilit_dict()
self.index = index
self.spilit_org_proposal_dict()
if self.with_pysot:
self.init_tracker()
# for video_id, frame_info in self.sub_shuffle_dict_list[index]:
# cnt = 0
len_proposal = sum([len(proposals) for frame_info,proposals in self.sub_shuffle_dict_list[index]])
print("Process:{},proposal lenght:{}".format(self.index, len_proposal))
cnt_time = 0
cnt_proposal = 0
begin = time.time()
cnt = 0
for id_frame, (frame_info, proposals) in enumerate(self.sub_shuffle_dict_list[index]):
video_id, timestamp = frame_info.split(',')
indice = _FPS * (int(timestamp) - _TIMESTAMP_START) + 1
image_tmpl = 'img_{:05}.jpg'
# forward tracking
self.result_dict[frame_info] = []
for ik, proposal in enumerate(proposals):
new_proposals = []
# begin = time.time()
# if ik != 9:
# continue
width, height = [int(ll) for ll in self.video_stats[video_id].split('x')]
ROI = np.array([int(x) for x in (proposal * np.array([
width, height, width, height, 1
]))[:4]])
track_window = tuple(np.concatenate([ROI[:2],ROI[-2:]-ROI[:2]],axis=0).tolist())
ann_frame = self._load_image(osp.join(self.img_prefix,
video_id),
image_tmpl, 'RGB', indice)
if False or frame_info == '-5KQ66BBWC4,0934' and False:
plt.imshow(ann_frame[:,:,::-1])
color = (random.random(), random.random(), random.random())
rect = plt.Rectangle((track_window[0],track_window[1]),
track_window[2],
track_window[3], fill=False,
edgecolor=color, linewidth=5)
plt.gca().add_patch(rect)
plt.show()
self.init_frame(ann_frame, track_window)
# Forcasting Tracking
p = indice - self.new_step
for i, ind in enumerate(
range(-2, -(self.new_length+1), -self.new_step)):
unann_frame = self._load_image(osp.join(self.img_prefix,
video_id),
image_tmpl, 'RGB', p)
if self.with_pysot:
track_window = self.pysot_tracking_roi(track_window,
key_frame=ann_frame,
tracked_frame=unann_frame, vis= frame_info == '-5KQ66BBWC4,0934' and False)
else:
track_window = self.cv2_tracking_roi(track_window,
org_frame=ann_frame,
tracked_frame=unann_frame)
new_proposals = [np.array(track_window) / np.array([width, height, width, height])] + new_proposals
# self.result_dict['{},{},{},{}'.format(video_id, '{:04d}'.format(int(timestamp)), ik, ind)] = np.array(track_window) / np.array([width, height, width, height])
ann_frame = unann_frame.copy()
p -= self.new_step
if frame_info == '-5KQ66BBWC4,0934' and False:
print(self.index, np.array(ROI) / np.array([width, height, width, height]), np.array(track_window) / np.array([width, height, width, height]))
track_window = tuple(np.concatenate([ROI[:2], ROI[-2:] - ROI[:2]], axis=0).tolist())
ann_frame = self._load_image(osp.join(self.img_prefix,
video_id),
image_tmpl, 'RGB', indice)
# self.result_dict['{},{},{},{}'.format(video_id, '{:04d}'.format(int(timestamp)), proposal,
new_proposals.append(np.array(ROI) / | np.array([width, height, width, height]) | numpy.array |
import numpy as np
from experiments import data_source
from experiments import data_transformation
from savigp.kernel import ExtRBF
from savigp.likelihood import UnivariateGaussian, SoftmaxLL
from savigp import Savigp
from sklearn.metrics import accuracy_score
from sklearn.decomposition import PCA
# define an automatic obtain kernel function.
def get_kernels(input_dim, num_latent_proc, variance = 1, lengthscale = None, ARD = False):
return [ExtRBF(
input_dim, variance = variance,
lengthscale=np.array((1.,)) if lengthscale is None else lengthscale,
ARD=ARD
)
for _ in range(num_latent_proc)]
# Load the boston dataset.
dataS = data_source.mnist_data()[0]
dim = dataS['train_outputs'].shape[1]
data_pca = {}
comp_dims = 20
print("compressed with PCA")
pca = PCA(comp_dims)
pca.fit(dataS["train_inputs"])
data_pca["train_inputs"] = pca.transform(dataS["train_inputs"])
data_pca["test_inputs"] = pca.transform(dataS["test_inputs"])
data_pca["train_outputs"] = dataS["train_outputs"]
data_pca["test_outputs"] = dataS["test_outputs"]
# data_pca["train_outputs"] = np.argmax(dataS["train_outputs"], axis=1).reshape(-1,1)
# data_pca["test_outputs"] = np.argmax(dataS["test_outputs"], axis=1).reshape(-1,1)
data = data_pca
print(data["train_inputs"][0])
print(data["train_outputs"][0])
# print(dim)
# data = data_source.boston_data()[0]
# print(data['test_outputs'])
# print(np.argmax(data['test_outputs'], axis = 1))
SF = 0.01
# Define a univariate Gaussian likelihood function with a variance of 1.
print("Define the softmax likelihood.")
# likelihood = UnivariateGaussian(np.array([1.0]))
likelihood = SoftmaxLL(dim)
# Define a radial basis kernel with a variance of 5, lengthscale of and ARD disabled.
print("Define a kernel")
kernel = get_kernels(data["train_inputs"].shape[1], dim, variance=5, lengthscale=np.array((2.,)))
# Set the number of inducing points to be half of the training data.
num_inducing = int(SF * data['train_inputs'].shape[0])
# Transform the data before training.
print("data transformation")
transform = data_transformation.IdentityTransformation(data['train_inputs'], data['train_outputs'])
train_inputs = transform.transform_X(data['train_inputs'])
train_outputs = transform.transform_Y(data['train_outputs'])
test_inputs = transform.transform_X(data['test_inputs'])
# Initialize the model.
print("initialize the model")
gp = Savigp(
likelihood=likelihood,
kernels=kernel,
num_inducing=num_inducing,
# random_inducing=True,
partition_size=3000,
debug_output=True
)
# Now fit the model to our training data.
print("fitting")
gp.fit(
train_inputs,
train_outputs,
num_threads=6,
optimization_config={'hyp': 15, "mog": 60, "inducing": 15},
max_iterations=300,
optimize_stochastic=False
)
# Make predictions. The third output is NLPD which is set to None unless test outputs are also
# provided.
print("make a prediction")
train_pred, _, _ = gp.predict(data['train_inputs'])
# predicted_mean, predicted_var, _ = gp.predict(data['test_inputs'])
predicted_mean, _, _ = gp.predict(data['test_inputs'])
# Untransform the results.
predicted_mean = transform.untransform_Y(predicted_mean)
train_pred = transform.untransform_Y(train_pred)
# predicted_var = transform.untransform_Y_var(predicted_var)
test_outputs = data['test_outputs']
# Print the accuracy
# train_acc = accuracy_score(np.argmax(train_pred, axis=1), data["train_outputs"])
train_acc = accuracy_score( | np.argmax(train_pred, axis=1) | numpy.argmax |
import numpy as np
import matplotlib.pyplot as plt
p_arr = np.linspace(0.9, 0.999, 6)
L = 10000
s = np.arange(1, L + 1)
nsp = lambda s, p: (1 - p) ** 2 * p ** s
for p in p_arr:
plt.loglog(-s * | np.log(p) | numpy.log |
'''Implementation of the Regularized Convolutional Neural Fitted Q-Iteration
(RC-NFQ) algorithm.
'''
import numpy as np
import time
from keras.models import Sequential, Graph
from keras.layers.core import Dense, Activation, Flatten, Dropout
from keras.layers.convolutional import Convolution2D
from keras.optimizers import RMSprop
from keras.callbacks import RemoteMonitor
from keras.callbacks import ModelCheckpoint
class NFQ:
"""Regularized Convolutional Neural Fitted Q-Iteration (RC-NFQ)
References:
- <NAME>. "Neural fitted Q iteration-first experiences
with a data efficient neural reinforcement learning method." Machine
Learning: ECML 2005. Springer Berlin Heidelberg, 2005. 317-328.
- <NAME> al. "Human-level control through deep
reinforcement learning." Nature 518.7540 (2015): 529-533.
- <NAME>. "Self-improving reactive agents based on reinforcement
learning, planning and teaching." Machine learning 8.3-4 (1992):
293-321.
- <NAME> (2016). "Regularized Convolutional Neural Fitted
Q-Iteration." Manuscript in preparation.
"""
def __init__(self,
state_dim,
nb_actions,
terminal_states,
convolutional=False,
mlp_layers=[20, 20],
discount_factor=0.99,
separate_target_network=False,
target_network_update_freq=None,
lr=0.01,
max_iters=20000):
"""Create an instance of the NFQ algorithm for a particular agent and
environment.
Parameters
----------
state_dim : The state dimensionality. An integer if
convolutional = False, a 2D tuple otherwise.
nb_actions : The number of possible actions
terminal_states : The integer indices of the terminal states
convolutional : Boolean. When True, uses convolutional neural networks
and dropout regularization. Otherwise, uses a simple
MLP.
mlp_layers : A list consisting of an integer number of neurons for each
hidden layer. Default = [20, 20]. For convolutional =
False.
discount_factor : The discount factor for Q-learning.
separate_target_network: boolean - If True, then it will use a separate
Q-network for computing the targets for the
Q-learning updates, and the target network
will be updated with the parameters of the
main Q-network every
target_network_update_freq iterations.
target_network_update_freq : The frequency at which to update the
target network.
lr : The learning rate for the RMSprop gradient descent algorithm.
max_iters : The maximum number of iterations that will be performed.
Used to allocate memory for NumPy arrays. Default = 20000.
max_q_predicted : The maximum number of Q-values that will be predicted.
Used to allocate memory for NumPy arrays. Default =
100000.
"""
self.convolutional = convolutional
self.separate_target_network = separate_target_network
self.k = 0 # Keep track of the number of iterations
self.discount_factor = discount_factor
self.nb_actions = nb_actions
self.state_dim = state_dim
self.lr = lr
self._loss_history = np.zeros((max_iters))
self._loss_history_test = np.zeros((max_iters))
self._q_predicted = np.empty((max_q_predicted), dtype=np.float32)
self._q_predicted[:] = np.NAN
self._q_predicted_counter = 0
self.terminal_states = terminal_states
if self.convolutional:
self.Q = self._init_convolutional_NFQ()
else:
self.Q = self._init_MLP(mlp_layers=mlp_layers)
if self.separate_target_network:
assert target_network_update_freq is not None
if self.convolutional:
self.Q_target = self._init_convolutional_NFQ()
else:
self.Q_target = self._init_MLP(mlp_layers=mlp_layers)
# Copy the initial weights from the Q network
self.Q_target.set_weights(self.Q.get_weights())
self.target_network_update_freq = target_network_update_freq
def __str__(self):
"""Print the current Q function and value function."""
string = ""
if self.convolutional:
string += 'Tabular values not available for NFQ with a ' + \
'Convolutional Neural Network function approximator.'
else:
for s in np.arange(self.state_dim):
for a in | np.arange(self.nb_actions) | numpy.arange |
import os
import time
from collections import OrderedDict
from contextlib import contextmanager
from functools import partial
from typing import *
import imageio
import mltk
import numpy as np
import tensorflow as tf
import tfsnippet as spt
from tensorflow.contrib.framework import add_arg_scope
from tensorflow.python.framework.errors_impl import ResourceExhaustedError
from ood_regularizer.experiment.datasets.celeba import load_celeba
from .global_config import global_config
__all__ = [
'TensorLike',
'get_activation_fn', 'get_kernel_regularizer', 'create_optimizer',
'get_scope_name', 'GraphNodes',
'batch_norm_2d', 'get_z_moments', 'unit_ball_regularization_loss',
'conv2d_with_output_shape', 'deconv2d_with_output_shape',
'NetworkLogger', 'NetworkLoggers', 'DummyNetworkLogger',
'get_network_logger', 'Timer',
'save_images_collection', 'find_largest_batch_size',
]
TensorLike = Union[tf.Tensor, spt.StochasticTensor]
def get_activation_fn():
activation_functions = {
'leaky_relu': tf.nn.leaky_relu,
'relu': tf.nn.relu,
}
return activation_functions[global_config.activation_fn]
def get_kernel_regularizer():
return spt.layers.l2_regularizer(global_config.kernel_l2_reg)
def create_optimizer(name: str,
learning_rate: Union[float, TensorLike]
) -> tf.train.Optimizer:
optimizer_cls = {
'Adam': tf.train.AdamOptimizer,
}[name]
return optimizer_cls(learning_rate)
def get_scope_name(default_name: str,
name: Optional[str] = None,
obj: Optional[Any] = None):
return spt.utils.get_default_scope_name(name or default_name, obj)
class GraphNodes(Dict[str, TensorLike]):
"""A dict that maps name to TensorFlow graph nodes."""
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
for k, v in self.items():
if not spt.utils.is_tensor_object(v):
raise TypeError(f'The value of `{k}` is not a tensor: {v!r}.')
def eval(self,
session: tf.Session = None,
feed_dict: Dict[tf.Tensor, Any] = None) -> Dict[str, Any]:
"""
Evaluate all the nodes with the specified `session`.
Args:
session: The TensorFlow session.
feed_dict: The feed dict.
Returns:
The node evaluation outputs.
"""
if session is None:
session = spt.utils.get_default_session_or_error()
keys = list(self)
tensors = [self[key] for key in keys]
outputs = session.run(tensors, feed_dict=feed_dict)
return dict(zip(keys, outputs))
def add_prefix(self, prefix: str) -> 'GraphNodes':
"""
Add a common prefix to all metrics in this collection.
Args:
prefix: The common prefix.
"""
return GraphNodes({f'{prefix}{k}': v for k, v in self.items()})
@add_arg_scope
def batch_norm_2d(input: TensorLike,
scope: str,
training: Union[bool, TensorLike] = False) -> tf.Tensor:
"""
Apply batch normalization on 2D convolutional layer.
Args:
input: The input tensor.
scope: TensorFlow variable scope.
training: Whether or not the model is under training stage?
Returns:
The normalized tensor.
"""
with tf.variable_scope(scope):
input, s1, s2 = spt.ops.flatten_to_ndims(input, ndims=4)
output = tf.layers.batch_normalization(
input,
axis=-1,
training=training,
name='norm'
)
output = spt.ops.unflatten_from_ndims(output, s1, s2)
return output
def get_z_moments(z: TensorLike,
value_ndims: int,
name: Optional[str] = None) -> Tuple[tf.Tensor, tf.Tensor]:
value_ndims = spt.utils.validate_enum_arg(
'value_ndims', value_ndims, [1, 3])
if value_ndims == 1:
z = spt.utils.InputSpec(shape=['...', '*']).validate('z', z)
else:
z = spt.utils.InputSpec(shape=['...', '?', '?', '*']).validate('z', z)
with tf.name_scope(name, default_name='get_z_moments', values=[z]):
rank = len(spt.utils.get_static_shape(z))
if value_ndims == 1:
axes = list(range(0, rank - 1))
else:
axes = list(range(0, rank - 3))
mean, variance = tf.nn.moments(z, axes=axes)
return mean, variance
def unit_ball_regularization_loss(mean: TensorLike,
var: TensorLike,
desired_var: Optional[float] = 1.,
name: Optional[str] = None) -> tf.Tensor:
mean = tf.convert_to_tensor(mean)
var = tf.convert_to_tensor(var)
with tf.name_scope(name,
default_name='unit_ball_regularization_loss',
values=[mean, var]):
loss = tf.reduce_mean(tf.square(mean))
if desired_var is not None:
loss += tf.reduce_mean(tf.square(var - desired_var))
return loss
def get_strides_for_conv(input_size, output_size, kernel_size):
input_size = int(input_size)
output_size = int(output_size)
if output_size > input_size:
raise ValueError('`output_size` must <= `input_size`: output_size {} '
'vs input_size {}'.format(output_size, input_size))
for j in range(1, input_size + 1):
out_size = (input_size + j - 1) // j
if out_size == output_size:
return j
raise ValueError('No strides can transform input_size {} into '
'output_size {} with a convolution operation'.
format(input_size, output_size))
def get_strides_for_deconv(input_size, output_size, kernel_size):
input_size = int(input_size)
output_size = int(output_size)
if output_size < input_size:
raise ValueError('`output_size` must >= `input_size`: output_size {} '
'vs input_size {}'.format(output_size, input_size))
for j in range(1, output_size + 1):
in_size = (output_size + j - 1) // j
if in_size == input_size:
return j
raise ValueError('No strides can transform input_size {} into '
'output_size {} with a deconvolution operation'.
format(input_size, output_size))
def conv2d_with_output_shape(conv_fn,
input: TensorLike,
output_shape: Iterable[int],
kernel_size: Union[int, Tuple[int, int]],
network_logger: Optional['NetworkLogger'] = None,
**kwargs) -> tf.Tensor:
"""
2D convolutional layer, with `strides` determined by the input shape and
output shape.
Args:
conv_fn: The convolution function.
input: The input tensor, at least 4-d, `(...,?,H,W,C)`.
output_shape: The output shape, `(H,W,C)`.
kernel_size: Kernel size over spatial dimensions.
network_logger: If specified, log the transformation.
\\**kwargs: Other named arguments passed to `conv_fn`.
Returns:
The output tensor.
"""
input = (spt.utils.InputSpec(shape=['...', '?', '*', '*', '*']).
validate('input', input))
input_shape = spt.utils.get_static_shape(input)[-3:]
output_shape = tuple(output_shape)
assert (len(output_shape) == 3 and None not in output_shape)
# compute the strides
strides = [
get_strides_for_conv(input_shape[i], output_shape[i], kernel_size)
for i in [0, 1]
]
# wrap network_logger if necessary
if network_logger is not None:
fn = partial(network_logger.log_apply, conv_fn, input)
else:
fn = partial(conv_fn, input)
# derive output by convolution
ret = fn(
out_channels=output_shape[-1],
kernel_size=kernel_size,
strides=strides,
channels_last=True,
**kwargs
)
# validate the output shape
spt.utils.InputSpec(shape=('...', '?') + output_shape).validate('ret', ret)
return ret
def deconv2d_with_output_shape(deconv_fn,
input: TensorLike,
output_shape: Iterable[int],
kernel_size: Union[int, Tuple[int, int]],
network_logger: Optional['NetworkLogger'] = None,
**kwargs) -> tf.Tensor:
"""
2D deconvolutional layer, with `strides` determined by the input shape and
output shape.
Args:
deconv_fn: The deconvolution function.
input: The input tensor, at least 4-d, `(...,?,H,W,C)`.
output_shape: The output shape, `(H,W,C)`.
kernel_size: Kernel size over spatial dimensions.
network_logger: If specified, log the transformation.
\\**kwargs: Other named arguments passed to `conv_fn`.
Returns:
The output tensor.
"""
input = (spt.utils.InputSpec(shape=['...', '?', '*', '*', '*']).
validate('input', input))
input_shape = spt.utils.get_static_shape(input)[-3:]
output_shape = tuple(output_shape)
assert (len(output_shape) == 3 and None not in output_shape)
# compute the strides
strides = [
get_strides_for_deconv(input_shape[i], output_shape[i], kernel_size)
for i in [0, 1]
]
# wrap network_logger if necessary
if network_logger is not None:
fn = partial(network_logger.log_apply, deconv_fn, input)
else:
fn = partial(deconv_fn, input)
# derive output by convolution
ret = fn(
out_channels=output_shape[-1],
output_shape=output_shape[:-1],
kernel_size=kernel_size,
strides=strides,
channels_last=True,
**kwargs
)
# validate the output shape
spt.utils.InputSpec(shape=('...', '?') + output_shape).validate('ret', ret)
return ret
class NetworkLogger(object):
"""
Class to log the high-level human readable network structures.
Although TensorFlow computation graph has enough information to figure out
what exactly the network architecture is, it is too verbose, and is not a
very easy tool to diagnose the network structure. Thus we have this class
to log some high-level human readable network structures.
"""
def __init__(self):
"""Construct a new :class:`NetworkLogger`."""
self._logs = []
@property
def logs(self) -> List[str]:
return self._logs
def log(self, input: TensorLike, transform: str, output: TensorLike):
"""
Log a transformation.
Args:
input: The input tensor.
transform: The transformation description.
output: The output tensor.
"""
self._logs.append((input, str(transform), output))
def log_apply(self,
fn: Callable[..., tf.Tensor],
input: TensorLike,
log_arg_names_: Iterable[str] = (
'units', # for dense
'strides', 'kernel_size', # for conv & deconv
'shortcut_kernel_size', # for resnet
'vertical_kernel_size',
'horizontal_kernel_size', # for pixelcnn
'name', 'scope', # for general layers
'ndims', 'shape', # for reshape_tail
'rate', # for dropout
),
*args, **kwargs) -> tf.Tensor:
"""
Do a transformation and log it.
Args:
fn: The transformation function, with accepts `input` as its
first argument.
input: The input tensor.
log_arg_names_: Names of the arguments in
`kwargs` to be logged.
*args: The arguments.
\\**kwargs: The named arguments.
"""
fn_name = fn.__name__ or repr(fn)
if log_arg_names_:
fn_args = []
log_arg_names_ = tuple(log_arg_names_)
for key in log_arg_names_:
if key in kwargs:
fn_args.append('{}={!r}'.format(key, kwargs[key]))
if fn_args:
fn_name = fn_name + '({})'.format(','.join(fn_args))
ret = fn(input, *args, **kwargs)
self.log(input, fn_name, ret)
return ret
class DummyNetworkLogger(NetworkLogger):
"""
A dummy network logger that logs nothing.
"""
def log(self, input: TensorLike, transform: str, output: TensorLike):
pass
def log_apply(self, fn, input, log_arg_names_=(), *args, **kwargs):
return fn(input, *args, **kwargs)
class NetworkLoggers(object):
"""Class to maintain a collection of :class:`NetworkLogger` instances."""
def __init__(self):
self._loggers = OrderedDict()
def get_logger(self, name: str) -> NetworkLogger:
"""
Get a :class:`NetworkLogger` with specified `name`.
Args:
name: Name of the network.
Returns:
The network logger instance.
"""
if name not in self._loggers:
self._loggers[name] = NetworkLogger()
return self._loggers[name]
def format_logs(self, title: str = 'Network Structure') -> str:
"""
Format the network structure log.
Args:
title: Title of the log.
Returns:
The formatted log.
"""
def format_shape(t):
if isinstance(t, spt.layers.PixelCNN2DOutput):
shape = spt.utils.get_static_shape(t.horizontal)
else:
shape = spt.utils.get_static_shape(t)
shape = ['?' if s is None else str(s) for s in shape]
return '(' + ','.join(shape) + ')'
table = spt.utils.ConsoleTable(3, col_align=['<', '^', '>'])
table.add_title(title)
table.add_hr('=')
for key in self._loggers:
logger = self._loggers[key]
table.add_skip()
table.add_title('{} Structure'.format(key))
table.add_row(['x', 'y=f(x)', 'y'])
table.add_hr('-')
if len(logger.logs) > 0:
for x, transform, y in logger.logs:
table.add_row([format_shape(x), transform, format_shape(y)])
else:
table.add_row(['', '(null)', ''])
return str(table)
def print_logs(self, title: str = 'Network Structure'):
"""
Print the network structure log.
Args:
title: Title of the log.
"""
print(self.format_logs(title))
@contextmanager
def as_default(self) -> Generator['NetworkLoggers', None, None]:
"""Push this object to the top of context stack."""
try:
_network_loggers_stack.push(self)
yield self
finally:
_network_loggers_stack.pop()
_network_loggers_stack = spt.utils.ContextStack()
def get_network_logger(name: str) -> NetworkLogger:
"""
Get a :class:`NetworkLogger` from the default loggers collection.
Args:
name: Name of the network.
Returns:
The network logger instance.
"""
if _network_loggers_stack.top() is None:
return DummyNetworkLogger()
else:
return _network_loggers_stack.top().get_logger(name)
class Timer(object):
"""A very simple timer."""
def __init__(self):
self._time_ticks = []
self.restart()
def timeit(self, tag):
self._time_ticks.append((tag, time.time()))
def print_logs(self):
if len(self._time_ticks) > 1:
print(
mltk.format_key_values(
[
(tag, spt.utils.humanize_duration(stop - start))
for (_, start), (tag, stop) in zip(
self._time_ticks[:-1], self._time_ticks[1:]
)
],
title='Time Consumption',
)
)
print('')
def restart(self):
self._time_ticks = [(None, time.time())]
def save_images_collection(images: np.ndarray,
filename: str,
grid_size: Tuple[int, int],
border_size: int = 0):
"""
Save a collection of images as a large image, arranged in grid.
Args:
images: The images collection. Each element should be a Numpy array,
in the shape of ``(H, W)``, ``(H, W, C)`` (if `channels_last` is
:obj:`True`) or ``(C, H, W)``.
filename: The target filename.
grid_size: The ``(rows, columns)`` of the grid.
border_size: Size of the border, for separating images.
(default 0, no border)
"""
# check the arguments
def validate_image(img):
if len(img.shape) == 2:
img = | np.reshape(img, img.shape + (1,)) | numpy.reshape |
"""
Most codes from https://github.com/carpedm20/DCGAN-tensorflow
"""
from __future__ import division
import math
import random
import pprint
import scipy.misc
import numpy as np
from time import gmtime, strftime
from six.moves import xrange
import matplotlib.pyplot as plt
import os, gzip
import tensorflow as tf
import tensorflow.contrib.slim as slim
from PIL import Image
from scipy.misc import imread
def load_fonts():
data_dir = os.path.join("/develop/data", "fonts")
splits_file = os.path.join(data_dir, "splits.txt")
labels_file = os.path.join(data_dir, "numlabels.txt")
images_dir = os.path.join(data_dir, "images28")
with open(splits_file) as f:
lines = f.readlines()
split_lines = [l.rstrip().split() for l in lines]
train_files = [s[0] for s in split_lines if s[1] == "0"]
# valid_files = [s[0] for s in split_lines if s[1] == "1"]
# test_files = [s[0] for s in split_lines if s[1] == "2"]
labels = {}
with open(splits_file) as f:
lines = f.readlines()
split_lines = [l.rstrip().split() for l in lines]
for s in split_lines:
labels[s[0]] = int(s[1])
trX = []
trY = []
for t in train_files:
trX.append(imread(os.path.join(images_dir, t)))
trY.append(labels[t])
num_train = len(train_files)
trX = np.asarray(trX).reshape(num_train, 28, 28, 1)
trY = np.asarray(trY).reshape(num_train).astype(np.int)
# teX = []
# teY = []
# for t in valid_files:
# teX.append(imread(t))
# teY.append(labels[t])
# num_valid = len(valid_files)
# teX = np.array(teX).reshape(num_valid, 28, 28, 1)
# teY = np.array(teY).reshape(num_valid)
X = trX
y = trY
# X = np.concatenate((trX, teX), axis=0)
# y = np.concatenate((trY, teY), axis=0).astype(np.int)
# print("----------> SAVING")
# for i in range(len(X)):
# img_name = f'data/mnist/images/{(i+1):05d}.png'
# im = Image.fromarray((X[i]).reshape(28,28).astype(np.uint8))
# im = im.convert('L')
# im.save(img_name)
# with open('data/mnist/labels.txt', 'w+') as the_file:
# for i in range(len(y)):
# the_file.write(f'{(i+1):05d}.png\t{y[i]}\n')
# with open('data/mnist/splits.txt', 'w+') as the_file:
# for i in range(len(y)):
# if (i < 50000):
# split = 0
# elif (i < 60000):
# split = 1
# else:
# split = 2
# the_file.write(f'{(i+1):05d}.png\t{split}\n')
seed = 547
np.random.seed(seed)
np.random.shuffle(X)
np.random.seed(seed)
np.random.shuffle(y)
y_vec = np.zeros((len(y), 62), dtype=np.float)
for i, label in enumerate(y):
y_vec[i, y[i]] = 1.0
return X / 255., y_vec
def load_mnist(dataset_name):
data_dir = os.path.join("./data", dataset_name)
def extract_data(filename, num_data, head_size, data_size):
with gzip.open(filename) as bytestream:
bytestream.read(head_size)
buf = bytestream.read(data_size * num_data)
data = np.frombuffer(buf, dtype=np.uint8).astype(np.float)
return data
data = extract_data(data_dir + '/train-images-idx3-ubyte.gz', 60000, 16, 28 * 28)
trX = data.reshape((60000, 28, 28, 1))
data = extract_data(data_dir + '/train-labels-idx1-ubyte.gz', 60000, 8, 1)
trY = data.reshape((60000))
data = extract_data(data_dir + '/t10k-images-idx3-ubyte.gz', 10000, 16, 28 * 28)
teX = data.reshape((10000, 28, 28, 1))
data = extract_data(data_dir + '/t10k-labels-idx1-ubyte.gz', 10000, 8, 1)
teY = data.reshape((10000))
trY = np.asarray(trY)
teY = np.asarray(teY)
X = | np.concatenate((trX, teX), axis=0) | numpy.concatenate |
import matplotlib
matplotlib.use('TkAgg')
import matplotlib.pyplot as plt
import numpy as np
import sys
sys.path.append('/home/groups/ZuckermanLab/copperma/cell/celltraj')
import celltraj
import h5py
import pickle
import os
import subprocess
import time
sys.path.append('/home/groups/ZuckermanLab/copperma/msmWE/BayesianBootstrap')
import bootstrap
import umap
import pyemma.coordinates as coor
import scipy
modelList=['PBS_17nov20','EGF_17nov20','HGF_17nov20','OSM_17nov20','BMP2_17nov20','IFNG_17nov20','TGFB_17nov20']
nmodels=len(modelList)
modelSet=[None]*nmodels
for i in range(nmodels):
modelName=modelList[i]
objFile=modelName+'_coords.obj'
objFileHandler=open(objFile,'rb')
modelSet[i]=pickle.load(objFileHandler)
objFileHandler.close()
wctm=celltraj.cellTraj()
fileSpecifier='/home/groups/ZuckermanLab/copperma/cell/live_cell/mcf10a/batch_17nov20/*_17nov20.h5'
print('initializing...')
wctm.initialize(fileSpecifier,modelName)
nfeat=modelSet[0].Xf.shape[1]
Xf=np.zeros((0,nfeat))
indtreatment=np.array([])
indcellSet=np.array([])
for i in range(nmodels):
Xf=np.append(Xf,modelSet[i].Xf,axis=0)
indtreatment=np.append(indtreatment,i*np.ones(modelSet[i].Xf.shape[0]))
indcellSet=np.append(indcellSet,modelSet[i].cells_indSet)
indtreatment=indtreatment.astype(int)
indcellSet=indcellSet.astype(int)
Xpca,pca=wctm.get_pca_fromdata(Xf,var_cutoff=.9)
wctm.Xpca=Xpca
wctm.pca=pca
for i in range(nmodels):
indsf=np.where(indtreatment==i)[0]
modelSet[i].Xpca=Xpca[indsf,:]
all_trajSet=[None]*nmodels
for i in range(nmodels):
modelSet[i].get_unique_trajectories()
all_trajSet[i]=modelSet[i].trajectories.copy()
self=wctm
for trajl in [8]: #,2,3,4,5,6,7,8,9,10,12,14,16,18,20,25,30,26]:
wctm.trajl=trajl
Xpcat=np.zeros((0,pca.ndim*trajl))
indtreatment_traj=np.array([])
indstack_traj=np.array([])
indframes_traj=np.array([])
cellinds0_traj=np.array([])
cellinds1_traj=np.array([])
for i in range(nmodels):
modelSet[i].trajectories=all_trajSet[i].copy()
modelSet[i].trajl=trajl
modelSet[i].traj=modelSet[i].get_traj_segments(trajl)
data=modelSet[i].Xpca[modelSet[i].traj,:]
data=data.reshape(modelSet[i].traj.shape[0],modelSet[i].Xpca.shape[1]*trajl)
Xpcat=np.append(Xpcat,data,axis=0)
indtreatment_traj=np.append(indtreatment_traj,i*np.ones(data.shape[0]))
indstacks=modelSet[i].cells_imgfileSet[modelSet[i].traj[:,0]]
indstack_traj=np.append(indstack_traj,indstacks)
indframes=modelSet[i].cells_frameSet[modelSet[i].traj[:,0]]
indframes_traj=np.append(indframes_traj,indframes)
cellinds0=modelSet[i].traj[:,0]
cellinds0_traj=np.append(cellinds0_traj,cellinds0)
cellinds1=modelSet[i].traj[:,-1]
cellinds1_traj=np.append(cellinds1_traj,cellinds1)
cellinds0_traj=cellinds0_traj.astype(int)
cellinds1_traj=cellinds1_traj.astype(int)
for neigen in [2]: #[1,2,3,4,5]:
reducer=umap.UMAP(n_neighbors=200,min_dist=0.1, n_components=neigen, metric='euclidean')
trans = reducer.fit(Xpcat)
x=trans.embedding_
indst=np.arange(x.shape[0]).astype(int)
wctm.Xtraj=x.copy()
wctm.indst=indst.copy()
indconds=np.array([[0,1],[2,3],[4,5]]).astype(int)
ncond=3
fl=12
fu=96
nbinstotal=15.*15.
indstw=np.where(np.logical_and(indframes_traj[indst]<fu,indframes_traj[indst]>fl))[0]
probSet=[None]*nmodels
avoverlapSet=np.zeros(ncond)
prob1,edges=np.histogramdd(x[indstw,:],bins=int(np.ceil(nbinstotal**(1./neigen))),density=True)
for icond in range(ncond):
imf1=indconds[icond,0]
imf2=indconds[icond,1]
inds_imf1=np.where(indstack_traj==imf1)[0]
inds_imf2=np.where(indstack_traj==imf2)[0]
inds_cond=np.append(inds_imf1,inds_imf2)
for imf in range(nmodels):
indstm=np.where(indtreatment_traj==imf)[0]
indstm_cond=np.intersect1d(indstm,inds_cond)
xt=x[indstm_cond,0:neigen]
indg=np.where(np.logical_not(np.logical_or(np.isnan(xt[:,0]),np.isinf(xt[:,0]))))[0]
xt=xt[indg]
prob,edges2=np.histogramdd(xt,bins=edges,density=True) #for d=1
prob=prob/np.sum(prob)
probSet[imf]=prob.copy()
poverlapMatrix=np.zeros((nmodels,nmodels))
for i in range(nmodels):
for j in range(nmodels):
probmin=np.minimum(probSet[i],probSet[j])
poverlapMatrix[i,j]=np.sum(probmin)
avoverlapSet[icond]=np.mean(poverlapMatrix[np.triu_indices(nmodels,1)])
sys.stdout.write('avoverlap: '+str(avoverlapSet[0])+' '+str(avoverlapSet[1])+' '+str(avoverlapSet[2])+'\n')
#np.savetxt('avoverlapSet_UMAP_trajl'+str(trajl)+'_ndim'+str(neigen)+'_19feb21.dat',avoverlapSet)
for i in range(nmodels):
modelSet[i].trajectories=all_trajSet[i].copy()
indconds=np.array([[0,1],[2,3],[4,5]]).astype(int)
ncond=3
probSet=[None]*nmodels
sigdxSet=np.zeros(ncond)
sigxSet=np.zeros(ncond)
dxSet=np.zeros(ncond)
x0=np.zeros((0,neigen))
x1= | np.zeros((0,neigen)) | numpy.zeros |
import os
from os.path import join
import numpy as np
from numpy.testing import (assert_equal, assert_allclose, assert_array_equal,
assert_raises)
import pytest
from numpy.random import (Generator, MT19937, ThreeFry, PCG32, PCG64,
Philox, Xoshiro256, Xoshiro512, RandomState)
from numpy.random.common import interface
try:
import cffi # noqa: F401
MISSING_CFFI = False
except ImportError:
MISSING_CFFI = True
try:
import ctypes # noqa: F401
MISSING_CTYPES = False
except ImportError:
MISSING_CTYPES = False
pwd = os.path.dirname(os.path.abspath(__file__))
def assert_state_equal(actual, target):
for key in actual:
if isinstance(actual[key], dict):
assert_state_equal(actual[key], target[key])
elif isinstance(actual[key], np.ndarray):
assert_array_equal(actual[key], target[key])
else:
assert actual[key] == target[key]
def uniform32_from_uint64(x):
x = np.uint64(x)
upper = np.array(x >> np.uint64(32), dtype=np.uint32)
lower = np.uint64(0xffffffff)
lower = np.array(x & lower, dtype=np.uint32)
joined = np.column_stack([lower, upper]).ravel()
out = (joined >> np.uint32(9)) * (1.0 / 2 ** 23)
return out.astype(np.float32)
def uniform32_from_uint53(x):
x = np.uint64(x) >> np.uint64(16)
x = np.uint32(x & np.uint64(0xffffffff))
out = (x >> np.uint32(9)) * (1.0 / 2 ** 23)
return out.astype(np.float32)
def uniform32_from_uint32(x):
return (x >> np.uint32(9)) * (1.0 / 2 ** 23)
def uniform32_from_uint(x, bits):
if bits == 64:
return uniform32_from_uint64(x)
elif bits == 53:
return uniform32_from_uint53(x)
elif bits == 32:
return uniform32_from_uint32(x)
else:
raise NotImplementedError
def uniform_from_uint(x, bits):
if bits in (64, 63, 53):
return uniform_from_uint64(x)
elif bits == 32:
return uniform_from_uint32(x)
def uniform_from_uint64(x):
return (x >> np.uint64(11)) * (1.0 / 9007199254740992.0)
def uniform_from_uint32(x):
out = np.empty(len(x) // 2)
for i in range(0, len(x), 2):
a = x[i] >> 5
b = x[i + 1] >> 6
out[i // 2] = (a * 67108864.0 + b) / 9007199254740992.0
return out
def uniform_from_dsfmt(x):
return x.view(np.double) - 1.0
def gauss_from_uint(x, n, bits):
if bits in (64, 63):
doubles = uniform_from_uint64(x)
elif bits == 32:
doubles = uniform_from_uint32(x)
else: # bits == 'dsfmt'
doubles = uniform_from_dsfmt(x)
gauss = []
loc = 0
x1 = x2 = 0.0
while len(gauss) < n:
r2 = 2
while r2 >= 1.0 or r2 == 0.0:
x1 = 2.0 * doubles[loc] - 1.0
x2 = 2.0 * doubles[loc + 1] - 1.0
r2 = x1 * x1 + x2 * x2
loc += 2
f = np.sqrt(-2.0 * np.log(r2) / r2)
gauss.append(f * x2)
gauss.append(f * x1)
return gauss[:n]
class Base(object):
dtype = np.uint64
data2 = data1 = {}
@classmethod
def setup_class(cls):
cls.bit_generator = Xoshiro256
cls.bits = 64
cls.dtype = np.uint64
cls.seed_error_type = TypeError
cls.invalid_seed_types = []
cls.invalid_seed_values = []
@classmethod
def _read_csv(cls, filename):
with open(filename) as csv:
seed = csv.readline()
seed = seed.split(',')
seed = [int(s.strip(), 0) for s in seed[1:]]
data = []
for line in csv:
data.append(int(line.split(',')[-1].strip(), 0))
return {'seed': seed, 'data': np.array(data, dtype=cls.dtype)}
def test_raw(self):
bit_generator = self.bit_generator(*self.data1['seed'])
uints = bit_generator.random_raw(1000)
assert_equal(uints, self.data1['data'])
bit_generator = self.bit_generator(*self.data1['seed'])
uints = bit_generator.random_raw()
assert_equal(uints, self.data1['data'][0])
bit_generator = self.bit_generator(*self.data2['seed'])
uints = bit_generator.random_raw(1000)
assert_equal(uints, self.data2['data'])
def test_random_raw(self):
bit_generator = self.bit_generator(*self.data1['seed'])
uints = bit_generator.random_raw(output=False)
assert uints is None
uints = bit_generator.random_raw(1000, output=False)
assert uints is None
def test_gauss_inv(self):
n = 25
rs = RandomState(self.bit_generator(*self.data1['seed']))
gauss = rs.standard_normal(n)
assert_allclose(gauss,
gauss_from_uint(self.data1['data'], n, self.bits))
rs = RandomState(self.bit_generator(*self.data2['seed']))
gauss = rs.standard_normal(25)
assert_allclose(gauss,
gauss_from_uint(self.data2['data'], n, self.bits))
def test_uniform_double(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
vals = uniform_from_uint(self.data1['data'], self.bits)
uniforms = rs.random(len(vals))
assert_allclose(uniforms, vals)
assert_equal(uniforms.dtype, np.float64)
rs = Generator(self.bit_generator(*self.data2['seed']))
vals = uniform_from_uint(self.data2['data'], self.bits)
uniforms = rs.random(len(vals))
assert_allclose(uniforms, vals)
assert_equal(uniforms.dtype, np.float64)
def test_uniform_float(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
vals = uniform32_from_uint(self.data1['data'], self.bits)
uniforms = rs.random(len(vals), dtype=np.float32)
assert_allclose(uniforms, vals)
assert_equal(uniforms.dtype, np.float32)
rs = Generator(self.bit_generator(*self.data2['seed']))
vals = uniform32_from_uint(self.data2['data'], self.bits)
uniforms = rs.random(len(vals), dtype=np.float32)
assert_allclose(uniforms, vals)
assert_equal(uniforms.dtype, np.float32)
def test_seed_float(self):
# GH #82
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(self.seed_error_type, rs.bit_generator.seed, np.pi)
assert_raises(self.seed_error_type, rs.bit_generator.seed, -np.pi)
def test_seed_float_array(self):
# GH #82
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([np.pi]))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([-np.pi]))
assert_raises(ValueError, rs.bit_generator.seed,
np.array([np.pi, -np.pi]))
assert_raises(TypeError, rs.bit_generator.seed, np.array([0, np.pi]))
assert_raises(TypeError, rs.bit_generator.seed, [np.pi])
assert_raises(TypeError, rs.bit_generator.seed, [0, np.pi])
def test_seed_out_of_range(self):
# GH #82
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(ValueError, rs.bit_generator.seed,
2 ** (2 * self.bits + 1))
assert_raises(ValueError, rs.bit_generator.seed, -1)
def test_seed_out_of_range_array(self):
# GH #82
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(ValueError, rs.bit_generator.seed,
[2 ** (2 * self.bits + 1)])
assert_raises(ValueError, rs.bit_generator.seed, [-1])
def test_repr(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
assert 'Generator' in repr(rs)
assert '{:#x}'.format(id(rs)).upper().replace('X', 'x') in repr(rs)
def test_str(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
assert 'Generator' in str(rs)
assert str(self.bit_generator.__name__) in str(rs)
assert '{:#x}'.format(id(rs)).upper().replace('X', 'x') not in str(rs)
def test_pickle(self):
import pickle
bit_generator = self.bit_generator(*self.data1['seed'])
state = bit_generator.state
bitgen_pkl = pickle.dumps(bit_generator)
reloaded = pickle.loads(bitgen_pkl)
reloaded_state = reloaded.state
assert_array_equal(Generator(bit_generator).standard_normal(1000),
Generator(reloaded).standard_normal(1000))
assert bit_generator is not reloaded
assert_state_equal(reloaded_state, state)
def test_invalid_state_type(self):
bit_generator = self.bit_generator(*self.data1['seed'])
with pytest.raises(TypeError):
bit_generator.state = {'1'}
def test_invalid_state_value(self):
bit_generator = self.bit_generator(*self.data1['seed'])
state = bit_generator.state
state['bit_generator'] = 'otherBitGenerator'
with pytest.raises(ValueError):
bit_generator.state = state
def test_invalid_seed_type(self):
bit_generator = self.bit_generator(*self.data1['seed'])
for st in self.invalid_seed_types:
with pytest.raises(TypeError):
bit_generator.seed(*st)
def test_invalid_seed_values(self):
bit_generator = self.bit_generator(*self.data1['seed'])
for st in self.invalid_seed_values:
with pytest.raises(ValueError):
bit_generator.seed(*st)
def test_benchmark(self):
bit_generator = self.bit_generator(*self.data1['seed'])
bit_generator._benchmark(1)
bit_generator._benchmark(1, 'double')
with pytest.raises(ValueError):
bit_generator._benchmark(1, 'int32')
@pytest.mark.skipif(MISSING_CFFI, reason='cffi not available')
def test_cffi(self):
bit_generator = self.bit_generator(*self.data1['seed'])
cffi_interface = bit_generator.cffi
assert isinstance(cffi_interface, interface)
other_cffi_interface = bit_generator.cffi
assert other_cffi_interface is cffi_interface
@pytest.mark.skipif(MISSING_CTYPES, reason='ctypes not available')
def test_ctypes(self):
bit_generator = self.bit_generator(*self.data1['seed'])
ctypes_interface = bit_generator.ctypes
assert isinstance(ctypes_interface, interface)
other_ctypes_interface = bit_generator.ctypes
assert other_ctypes_interface is ctypes_interface
def test_getstate(self):
bit_generator = self.bit_generator(*self.data1['seed'])
state = bit_generator.state
alt_state = bit_generator.__getstate__()
assert_state_equal(state, alt_state)
class TestXoshiro256(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = Xoshiro256
cls.bits = 64
cls.dtype = np.uint64
cls.data1 = cls._read_csv(
join(pwd, './data/xoshiro256-testset-1.csv'))
cls.data2 = cls._read_csv(
join(pwd, './data/xoshiro256-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = [('apple',), (2 + 3j,), (3.1,)]
cls.invalid_seed_values = [(-2,), (np.empty((2, 2), dtype=np.int64),)]
class TestXoshiro512(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = Xoshiro512
cls.bits = 64
cls.dtype = np.uint64
cls.data1 = cls._read_csv(
join(pwd, './data/xoshiro512-testset-1.csv'))
cls.data2 = cls._read_csv(
join(pwd, './data/xoshiro512-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = [('apple',), (2 + 3j,), (3.1,)]
cls.invalid_seed_values = [(-2,), (np.empty((2, 2), dtype=np.int64),)]
class TestThreeFry(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = ThreeFry
cls.bits = 64
cls.dtype = np.uint64
cls.data1 = cls._read_csv(
join(pwd, './data/threefry-testset-1.csv'))
cls.data2 = cls._read_csv(
join(pwd, './data/threefry-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = []
cls.invalid_seed_values = [(1, None, 1), (-1,), (2 ** 257 + 1,),
(None, None, 2 ** 257 + 1)]
def test_set_key(self):
bit_generator = self.bit_generator(*self.data1['seed'])
state = bit_generator.state
keyed = self.bit_generator(counter=state['state']['counter'],
key=state['state']['key'])
assert_state_equal(bit_generator.state, keyed.state)
class TestPhilox(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = Philox
cls.bits = 64
cls.dtype = np.uint64
cls.data1 = cls._read_csv(
join(pwd, './data/philox-testset-1.csv'))
cls.data2 = cls._read_csv(
join(pwd, './data/philox-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = []
cls.invalid_seed_values = [(1, None, 1), (-1,), (2 ** 257 + 1,),
(None, None, 2 ** 257 + 1)]
def test_set_key(self):
bit_generator = self.bit_generator(*self.data1['seed'])
state = bit_generator.state
keyed = self.bit_generator(counter=state['state']['counter'],
key=state['state']['key'])
assert_state_equal(bit_generator.state, keyed.state)
class TestPCG64(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = PCG64
cls.bits = 64
cls.dtype = np.uint64
cls.data1 = cls._read_csv(join(pwd, './data/pcg64-testset-1.csv'))
cls.data2 = cls._read_csv(join(pwd, './data/pcg64-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = [(np.array([1, 2]),), (3.2,),
(None, np.zeros(1))]
cls.invalid_seed_values = [(-1,), (2 ** 129 + 1,), (None, -1),
(None, 2 ** 129 + 1)]
def test_seed_float_array(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([np.pi]))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([-np.pi]))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([np.pi, -np.pi]))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
np.array([0, np.pi]))
assert_raises(self.seed_error_type, rs.bit_generator.seed, [np.pi])
assert_raises(self.seed_error_type, rs.bit_generator.seed, [0, np.pi])
def test_seed_out_of_range_array(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
assert_raises(self.seed_error_type, rs.bit_generator.seed,
[2 ** (2 * self.bits + 1)])
assert_raises(self.seed_error_type, rs.bit_generator.seed, [-1])
def test_advance_symmetry(self):
rs = Generator(self.bit_generator(*self.data1['seed']))
state = rs.bit_generator.state
step = -0x9e3779b97f4a7c150000000000000000
rs.bit_generator.advance(step)
val_neg = rs.integers(10)
rs.bit_generator.state = state
rs.bit_generator.advance(2**128 + step)
val_pos = rs.integers(10)
rs.bit_generator.state = state
rs.bit_generator.advance(10 * 2**128 + step)
val_big = rs.integers(10)
assert val_neg == val_pos
assert val_big == val_pos
class TestPCG32(TestPCG64):
@classmethod
def setup_class(cls):
cls.bit_generator = PCG32
cls.bits = 32
cls.dtype = np.uint32
cls.data1 = cls._read_csv(join(pwd, './data/pcg32-testset-1.csv'))
cls.data2 = cls._read_csv(join(pwd, './data/pcg32-testset-2.csv'))
cls.seed_error_type = TypeError
cls.invalid_seed_types = [(np.array([1, 2]),), (3.2,),
(None, np.zeros(1))]
cls.invalid_seed_values = [(-1,), (2 ** 129 + 1,), (None, -1),
(None, 2 ** 129 + 1)]
class TestMT19937(Base):
@classmethod
def setup_class(cls):
cls.bit_generator = MT19937
cls.bits = 32
cls.dtype = np.uint32
cls.data1 = cls._read_csv(join(pwd, './data/mt19937-testset-1.csv'))
cls.data2 = cls._read_csv(join(pwd, './data/mt19937-testset-2.csv'))
cls.seed_error_type = ValueError
cls.invalid_seed_types = []
cls.invalid_seed_values = [(-1,), np.array([2 ** 33])]
def test_seed_out_of_range(self):
# GH #82
rs = Generator(self.bit_generator(*self.data1['seed']))
| assert_raises(ValueError, rs.bit_generator.seed, 2 ** (self.bits + 1)) | numpy.testing.assert_raises |
import matplotlib.pyplot as plt
import seaborn as sns
import torch.nn as nn
import numpy as np
import torch
import os
from collections import Counter
from tasks import FreeRecall
# from models import CRPLSTM as Agent
from models import GRU as Agent
from models import compute_a2c_loss, compute_returns
from utils import to_sqpth, to_pth, to_np, to_sqnp, make_log_fig_dir, rm_dup, int2onehot
from stats import compute_stats, compute_recall_order, lag2index
from vis import plot_learning_curve
from sklearn.linear_model import RidgeClassifier
sns.set(style='white', palette='colorblind', context='talk')
subj_id = 0
# for subj_id in range(6):
np.random.seed(subj_id)
torch.manual_seed(subj_id)
print(subj_id)
# init task
n = 40
n_std_tr = 10
n_std_te = 10
v_tr = 3
v_te = 0
reward = 1
penalty = -.5
dim_hidden = 128
beta = 1
# noise_level = 0.025
noise_level = 0
task = FreeRecall(n_std=n_std_te, n=n, v=v_te, reward=reward, penalty=penalty)
dim_input = task.x_dim * 2 + 1
dim_output = task.x_dim + 1
# make log dirs
epoch_trained = 100000
exp_name = f'n-{n}-n_std-{n_std_tr}-v-{v_tr}/h-{dim_hidden}/sub-{subj_id}'
log_path, fig_path = make_log_fig_dir(exp_name, makedirs=False)
# reload the weights
fname = f'wts-{epoch_trained}.pth'
agent = Agent(dim_input, dim_hidden, dim_output, beta)
agent.load_state_dict(torch.load(os.path.join(log_path, fname)))
# testing
n_test = 2000
len_test_phase = task.n_std + v_tr + 1
# log_r = np.zeros((n_test, n_std_te))
log_r = [0 for i in range(n_test)]
log_a = np.full((n_test, len_test_phase), np.nan)
log_std_items = np.zeros((n_test, n_std_te))
log_h_std = np.full((n_test, task.n_std, dim_hidden), np.nan)
log_h_tst = np.full((n_test, len_test_phase, dim_hidden), np.nan)
i=0
for i in range(n_test):
# re-sample studied items
X = task.sample(to_pytorch=True)
log_std_items[i] = task.studied_item_ids
# study phase
r_t = torch.zeros(1)
hc_t = agent.get_zero_states()
for t, x_t_std in enumerate(X):
hc_t = agent.add_normal_noise(hc_t, scale=noise_level)
x_t = torch.cat([x_t_std, torch.zeros(task.x_dim), r_t])
[_, _, _, hc_t], _ = agent.forward(x_t, hc_t)
log_h_std[i, t] = to_sqnp(hc_t)
# recall phase
log_r_i = []
x_t_tst = torch.zeros(task.x_dim)
for t in range(len_test_phase):
x_t = torch.cat([torch.zeros(task.x_dim), x_t_tst, r_t.view(1)])
[a_t, pi_a_t, v_t, hc_t], _ = agent.forward(x_t, hc_t)
r_t = task.get_reward(a_t)
log_r_i.append(to_np(r_t))
# log
log_a[i, t] = a_t
log_h_tst[i, t] = to_sqnp(hc_t)
# if the model choose the stop, break
if int(to_np(a_t)) == task.n:
break
# make a onehot that represent the recalled item at time t
x_t_tst = int2onehot(a_t, task.n)
# collect the reward
log_r[i] = log_r_i
'''figures'''
# select the last n_test trials to analyze
targ = log_std_items
resp = log_a
'''compute error info'''
# compute number of lures for each trials
n_lures = np.zeros(n_test)
stop_times = np.full(n_test, np.nan)
for i in range(n_test):
stop_time = np.where(resp[i] == n)
# trim the trial at the stop point
if len(stop_time[0]) > 0:
stop_times[i] = int(stop_time[0])
resp_i = resp[i][:int(stop_time[0])]
else:
# stop_times[i] = len_test_phase
resp_i = resp[i]
# count the number of items that are not in the targets
for resp_it in resp_i:
if resp_it not in targ[i]:
n_lures[i] +=1
n_lures_mu, n_lures_se = compute_stats(n_lures)
stop_times_mu = np.nanmean(stop_times)
# compute number of repeats for each trials
repeats = np.zeros(n_test)
for i in range(n_test):
recalled_items_i = log_a[i][~np.isnan(log_a[i])]
# count the number of redundent items
repeats[i] = len(recalled_items_i) - len(np.unique(recalled_items_i))
repeats_mu, repeats_se = compute_stats(repeats)
print(f'Lures : mu = %.2f, se = %.2f' % (n_lures_mu, n_lures_se))
print(f'Repeats : mu = %.2f, se = %.2f' % (repeats_mu, repeats_se))
print(f'Stop time : mu = %.2f' % (stop_times_mu))
'''compute recall order '''
# compute the recall order given target and responses
order = compute_recall_order(targ, resp)
# compute the tally for actual and possible responses
tally = np.zeros((n_test, (n_std_te - 1)* 2))
tally_poss = np.zeros((n_test, (n_std_te - 1)* 2))
lags = []
for i in range(n_test):
order_i = order[i]
# order_i_rmnan = order_i[~np.isnan(order_i)]
order_i_rmnan = order_i[~np.isnan(order_i)]
order_i_rmnan = rm_dup(order_i_rmnan)
# order_i_rmnan = list(dict.fromkeys(order_i_rmnan))
for j in range(len(order_i_rmnan) - 1):
lag = int(order_i_rmnan[j+1] - order_i_rmnan[j])
if lag != 0:
lag_index = lag2index(lag, n_std_te)
# increment the count of the corresponding lag index
tally[i, lag_index] +=1
lags.append(lag)
for j in range(len(order_i_rmnan) - 1):
for o in range(n_std_te):
lag_poss = int(o - order_i_rmnan[j])
if lag_poss != 0:
lag_index = lag2index(lag_poss, n_std_te)
tally_poss[i, lag_index] +=1
assert np.all(tally_poss >= tally), 'possible count must >= actual count'
# compute conditional response probability
crp = np.divide(tally, tally_poss, out=np.zeros_like(tally), where=tally_poss!=0)
# crp = tally / tally.sum(axis=1)[:,None]
p_mu, p_se = compute_stats(crp, axis=0)
xticklabels = np.concatenate((np.arange(-(n_std_te - 1), 0), np.arange(1, n_std_te)))
f, ax = plt.subplots(1,1, figsize=(6,4))
ax.errorbar(x=np.arange(n_std_te - 1), y=p_mu[:n_std_te - 1], yerr = p_se[:n_std_te - 1],color='k')
ax.errorbar(x=np.arange(n_std_te - 1) + (n_std_te - 1), y=p_mu[-(n_std_te - 1):], yerr = p_se[-(n_std_te - 1):], color='k')
ax.set_ylim([0, None])
ax.set_xticks(np.arange((n_std_te - 1)*2))
ax.set_xticklabels(xticklabels)
ax.set_xlabel('Lag')
ax.set_ylabel('p')
ax.set_title('Conditional response probability')
sns.despine()
''' compute recall probabiliy arranged according to the studied order'''
# order = order[:,0]
# plot the serial position curve
unique_recalls = np.concatenate([np.unique(order[i]) for i in range(n_test)])
counter = Counter(unique_recalls)
recalls = np.array([counter[i] for i in range(n_std_te)])
spc_x = range(n_std_te)
spc_y = recalls / n_test
f, ax = plt.subplots(1,1, figsize=(6, 4))
ax.plot(spc_y)
ax.set_ylim([0, 1])
ax.set_xlabel('Position')
ax.set_ylabel('p')
ax.set_title('Recall probability')
sns.despine()
f, ax = plt.subplots(1,1, figsize=(6, 4))
ax.plot(spc_y)
ax.set_xlabel('Position')
ax.set_ylabel('p')
ax.set_title('Recall probability')
sns.despine()
''' compute p(1st recall) '''
counter = Counter(order[:,0])
recalls_1st = np.array([counter[i] for i in range(n_std_te)])
f, ax = plt.subplots(1,1, figsize=(6, 4))
ax.plot(recalls_1st / np.sum(recalls_1st))
# ax.set_ylim([None, 1])
ax.set_ylim([0, None])
ax.set_xlabel('Position')
ax.set_ylabel('p')
ax.set_title('The distribution of the 1st recall')
sns.despine()
f, ax = plt.subplots(1,1, figsize=(6, 4))
ax.plot(recalls_1st / np.sum(recalls_1st))
ax.set_xlabel('Position')
ax.set_ylabel('p')
ax.set_title('The distribution of the 1st recall')
sns.despine()
''' compute mean performance '''
n_items_recalled = np.empty(n_test, )
for i in range(n_test):
order_i = np.unique(order[i])
n_items_recalled[i] = len(order_i[~np.isnan(order_i)])
prop_item_recalled = n_items_recalled / n_std_te
pir_mu, pir_se = compute_stats(prop_item_recalled)
print(f'%% items recalled = %.2f, se = %.2f' % (pir_mu, pir_se))
mean_r_all_trials = [np.mean(log_r_) for log_r_ in log_r]
r_mu, r_se = compute_stats(mean_r_all_trials)
print(f'Average reward = %.2f, se = %.2f' % (r_mu, r_se))
'''MVPA decoding'''
n_tr = 1000
n_te = n_test - n_tr
y_te_std_hat = np.zeros((n, n_tr, n_std_te))
y_te_std = np.zeros((n, n_tr, n_std_te))
dec_acc = np.zeros(n)
# reshape X
log_h_std_rs = np.reshape(log_h_std, (-1, dim_hidden))
ridge_clfs = [None for _ in range(n)]
# train/test the clf on the study phase
for std_item_id in range(n):
# make y - whether item i has been presented in the past
pres_time_item_i = log_std_items == std_item_id
inwm_time_item_i = np.zeros_like(pres_time_item_i)
for i in range(n_test):
if np.any(pres_time_item_i[i, :]):
t = int(np.where(pres_time_item_i[i, :])[0])
inwm_time_item_i[i, t:] = 1
# reshape y for the classifier for the study phase
inwm_time_item_i_rs = | np.reshape(inwm_time_item_i, (-1,)) | numpy.reshape |
import abc
import sys
import time
from collections import OrderedDict
from functools import reduce
import numba
import numpy as np
from shapely.geometry import Polygon
from second.core import box_np_ops_JRDB
from second.core.geometry import (points_in_convex_polygon_3d_jit,
points_in_convex_polygon_jit)
import copy
class BatchSampler:
def __init__(self, sampled_list, name=None, epoch=None, shuffle=True, drop_reminder=False):
self._sampled_list = sampled_list
self._indices = np.arange(len(sampled_list))
if shuffle:
np.random.shuffle(self._indices)
self._idx = 0
self._example_num = len(sampled_list)
self._name = name
self._shuffle = shuffle
self._epoch = epoch
self._epoch_counter = 0
self._drop_reminder = drop_reminder
def _sample(self, num):
if self._idx + num >= self._example_num:
ret = self._indices[self._idx:].copy()
self._reset()
else:
ret = self._indices[self._idx:self._idx + num]
self._idx += num
return ret
def _reset(self):
if self._name is not None:
print("reset", self._name)
if self._shuffle:
np.random.shuffle(self._indices)
self._idx = 0
def sample(self, num):
indices = self._sample(num)
return [self._sampled_list[i] for i in indices]
# return np.random.choice(self._sampled_list, num)
class DataBasePreprocessing:
def __call__(self, db_infos):
return self._preprocess(db_infos)
@abc.abstractclassmethod
def _preprocess(self, db_infos):
pass
class DBFilterByDifficulty(DataBasePreprocessing):
def __init__(self, removed_difficulties):
self._removed_difficulties = removed_difficulties
print(removed_difficulties)
def _preprocess(self, db_infos):
new_db_infos = {}
for key, dinfos in db_infos.items():
new_db_infos[key] = [
info for info in dinfos
if info["difficulty"] not in self._removed_difficulties
]
return new_db_infos
class DBFilterByMinNumPoint(DataBasePreprocessing):
def __init__(self, min_gt_point_dict):
self._min_gt_point_dict = min_gt_point_dict
print(min_gt_point_dict)
def _preprocess(self, db_infos):
for name, min_num in self._min_gt_point_dict.items():
if min_num > 0:
filtered_infos = []
for info in db_infos[name]:
if info["num_points_in_gt"] >= min_num:
filtered_infos.append(info)
db_infos[name] = filtered_infos
return db_infos
class DataBasePreprocessor:
def __init__(self, preprocessors):
self._preprocessors = preprocessors
def __call__(self, db_infos):
for prepor in self._preprocessors:
db_infos = prepor(db_infos)
return db_infos
def random_crop_frustum(bboxes,
rect,
Trv2c,
P2,
max_crop_height=1.0,
max_crop_width=0.9):
num_gt = bboxes.shape[0]
crop_minxy = np.random.uniform(
[1 - max_crop_width, 1 - max_crop_height], [0.3, 0.3],
size=[num_gt, 2])
crop_maxxy = np.ones([num_gt, 2], dtype=bboxes.dtype)
crop_bboxes = np.concatenate([crop_minxy, crop_maxxy], axis=1)
left = np.random.choice([False, True], replace=False, p=[0.5, 0.5])
if left:
crop_bboxes[:, [0, 2]] -= crop_bboxes[:, 0:1]
# crop_relative_bboxes to real bboxes
crop_bboxes *= np.tile(bboxes[:, 2:] - bboxes[:, :2], [1, 2])
crop_bboxes += np.tile(bboxes[:, :2], [1, 2])
C, R, T = box_np_ops_JRDB.projection_matrix_to_CRT_kitti(P2)
frustums = box_np_ops_JRDB.get_frustum_v2(crop_bboxes, C)
frustums -= T
# frustums = np.linalg.inv(R) @ frustums.T
frustums = np.einsum('ij, akj->aki', np.linalg.inv(R), frustums)
frustums = box_np_ops_JRDB.camera_to_lidar(frustums, rect, Trv2c)
return frustums
def filter_gt_box_outside_range(gt_boxes, limit_range):
"""remove gtbox outside training range.
this function should be applied after other prep functions
Args:
gt_boxes ([type]): [description]
limit_range ([type]): [description]
"""
gt_boxes_bv = box_np_ops_JRDB.center_to_corner_box2d(
gt_boxes[:, [0, 1]], gt_boxes[:, [3, 3 + 1]], gt_boxes[:, 6])
bounding_box = box_np_ops_JRDB.minmax_to_corner_2d(
np.asarray(limit_range)[np.newaxis, ...])
ret = points_in_convex_polygon_jit(
gt_boxes_bv.reshape(-1, 2), bounding_box)
return np.any(ret.reshape(-1, 4), axis=1)
def filter_gt_box_outside_range_by_center(gt_boxes, limit_range):
"""remove gtbox outside training range.
this function should be applied after other prep functions
Args:
gt_boxes ([type]): [description]
limit_range ([type]): [description]
"""
gt_box_centers = gt_boxes[:, :2]
bounding_box = box_np_ops_JRDB.minmax_to_corner_2d(
np.asarray(limit_range)[np.newaxis, ...])
ret = points_in_convex_polygon_jit(gt_box_centers, bounding_box)
return ret.reshape(-1)
def filter_gt_low_points(gt_boxes,
points,
num_gt_points,
point_num_threshold=2):
points_mask = np.ones([points.shape[0]], np.bool)
gt_boxes_mask = | np.ones([gt_boxes.shape[0]], np.bool) | numpy.ones |
# -*- coding: utf-8 -*-
""" Lots of functions for drawing and plotting visiony things """
# TODO: New naming scheme
# viz_<funcname> should clear everything. The current axes and fig: clf, cla.
# # Will add annotations
# interact_<funcname> should clear everything and start user interactions.
# show_<funcname> should always clear the current axes, but not fig: cla #
# Might # add annotates? plot_<funcname> should not clear the axes or figure.
# More useful for graphs draw_<funcname> same as plot for now. More useful for
# images
import logging
import itertools as it
import utool as ut # NOQA
import matplotlib as mpl
import matplotlib.pyplot as plt
try:
from mpl_toolkits.axes_grid1 import make_axes_locatable
except ImportError as ex:
ut.printex(
ex,
'try pip install mpl_toolkits.axes_grid1 or something. idk yet',
iswarning=False,
)
raise
# import colorsys
import pylab
import warnings
import numpy as np
from os.path import relpath
try:
import cv2
except ImportError as ex:
print('ERROR PLOTTOOL CANNOT IMPORT CV2')
print(ex)
from wbia.plottool import mpl_keypoint as mpl_kp
from wbia.plottool import color_funcs as color_fns
from wbia.plottool import custom_constants
from wbia.plottool import custom_figure
from wbia.plottool import fig_presenter
DEBUG = False
(print, rrr, profile) = ut.inject2(__name__)
logger = logging.getLogger('wbia')
def is_texmode():
return mpl.rcParams['text.usetex']
# Bring over moved functions that still have dependants elsewhere
TAU = np.pi * 2
distinct_colors = color_fns.distinct_colors
lighten_rgb = color_fns.lighten_rgb
to_base255 = color_fns.to_base255
DARKEN = ut.get_argval(
'--darken', type_=float, default=(0.7 if ut.get_argflag('--darken') else None)
)
# logger.info('DARKEN = %r' % (DARKEN,))
all_figures_bring_to_front = fig_presenter.all_figures_bring_to_front
all_figures_tile = fig_presenter.all_figures_tile
close_all_figures = fig_presenter.close_all_figures
close_figure = fig_presenter.close_figure
iup = fig_presenter.iup
iupdate = fig_presenter.iupdate
present = fig_presenter.present
reset = fig_presenter.reset
update = fig_presenter.update
ORANGE = custom_constants.ORANGE
RED = custom_constants.RED
GREEN = custom_constants.GREEN
BLUE = custom_constants.BLUE
YELLOW = custom_constants.YELLOW
BLACK = custom_constants.BLACK
WHITE = custom_constants.WHITE
GRAY = custom_constants.GRAY
LIGHTGRAY = custom_constants.LIGHTGRAY
DEEP_PINK = custom_constants.DEEP_PINK
PINK = custom_constants.PINK
FALSE_RED = custom_constants.FALSE_RED
TRUE_GREEN = custom_constants.TRUE_GREEN
TRUE_BLUE = custom_constants.TRUE_BLUE
DARK_GREEN = custom_constants.DARK_GREEN
DARK_BLUE = custom_constants.DARK_BLUE
DARK_RED = custom_constants.DARK_RED
DARK_ORANGE = custom_constants.DARK_ORANGE
DARK_YELLOW = custom_constants.DARK_YELLOW
PURPLE = custom_constants.PURPLE
LIGHT_BLUE = custom_constants.LIGHT_BLUE
UNKNOWN_PURP = custom_constants.UNKNOWN_PURP
TRUE = TRUE_BLUE
FALSE = FALSE_RED
figure = custom_figure.figure
gca = custom_figure.gca
gcf = custom_figure.gcf
get_fig = custom_figure.get_fig
save_figure = custom_figure.save_figure
set_figtitle = custom_figure.set_figtitle
set_title = custom_figure.set_title
set_xlabel = custom_figure.set_xlabel
set_xticks = custom_figure.set_xticks
set_ylabel = custom_figure.set_ylabel
set_yticks = custom_figure.set_yticks
VERBOSE = ut.get_argflag(('--verbose-df2', '--verb-pt'))
# ================
# GLOBALS
# ================
TMP_mevent = None
plotWidget = None
def show_was_requested():
"""
returns True if --show is specified on the commandline or you are in
IPython (and presumably want some sort of interaction
"""
return not ut.get_argflag(('--noshow')) and (
ut.get_argflag(('--show', '--save')) or ut.inIPython()
)
# return ut.show_was_requested()
class OffsetImage2(mpl.offsetbox.OffsetBox):
"""
TODO: If this works reapply to mpl
"""
def __init__(
self,
arr,
zoom=1,
cmap=None,
norm=None,
interpolation=None,
origin=None,
filternorm=1,
filterrad=4.0,
resample=False,
dpi_cor=True,
**kwargs
):
mpl.offsetbox.OffsetBox.__init__(self)
self._dpi_cor = dpi_cor
self.image = mpl.offsetbox.BboxImage(
bbox=self.get_window_extent,
cmap=cmap,
norm=norm,
interpolation=interpolation,
origin=origin,
filternorm=filternorm,
filterrad=filterrad,
resample=resample,
**kwargs
)
self._children = [self.image]
self.set_zoom(zoom)
self.set_data(arr)
def set_data(self, arr):
self._data = np.asarray(arr)
self.image.set_data(self._data)
self.stale = True
def get_data(self):
return self._data
def set_zoom(self, zoom):
self._zoom = zoom
self.stale = True
def get_zoom(self):
return self._zoom
# def set_axes(self, axes):
# self.image.set_axes(axes)
# martist.Artist.set_axes(self, axes)
# def set_offset(self, xy):
# """
# set offset of the container.
# Accept : tuple of x,y coordinate in disokay units.
# """
# self._offset = xy
# self.offset_transform.clear()
# self.offset_transform.translate(xy[0], xy[1])
def get_offset(self):
"""
return offset of the container.
"""
return self._offset
def get_children(self):
return [self.image]
def get_window_extent(self, renderer):
"""
get the bounding box in display space.
"""
import matplotlib.transforms as mtransforms
w, h, xd, yd = self.get_extent(renderer)
ox, oy = self.get_offset()
return mtransforms.Bbox.from_bounds(ox - xd, oy - yd, w, h)
def get_extent(self, renderer):
# FIXME dpi_cor is never used
if self._dpi_cor: # True, do correction
# conversion (px / pt)
dpi_cor = renderer.points_to_pixels(1.0)
else:
dpi_cor = 1.0 # NOQA
zoom = self.get_zoom()
data = self.get_data()
# Data width and height in pixels
ny, nx = data.shape[:2]
# w /= dpi_cor
# h /= dpi_cor
# import utool
# if self.axes:
# Hack, find right axes
ax = self.figure.axes[0]
ax.get_window_extent()
# bbox = mpl.transforms.Bbox.union([ax.get_window_extent()])
# xmin, xmax = ax.get_xlim()
# ymin, ymax = ax.get_ylim()
# https://www.mail-archive.com/<EMAIL>/msg25931.html
fig = self.figure
# dpi = fig.dpi # (pt / in)
fw_in, fh_in = fig.get_size_inches()
# divider = make_axes_locatable(ax)
# fig_ppi = dpi * dpi_cor
# fw_px = fig_ppi * fw_in
# fh_px = fig_ppi * fh_in
# bbox.width
# transforms data to figure coordinates
# pt1 = ax.transData.transform_point([nx, ny])
pt1 = ax.transData.transform_point([1, 20])
pt2 = ax.transData.transform_point([0, 0])
w, h = pt1 - pt2
# zoom_factor = max(fw_px, )
# logger.info('fw_px = %r' % (fw_px,))
# logger.info('pos = %r' % (pos,))
# w = h = .2 * fw_px * pos[2]
# .1 * fig_dpi * fig_size[0] / data.shape[0]
# logger.info('zoom = %r' % (zoom,))
w, h = w * zoom, h * zoom
return w, h, 0, 0
# return 30, 30, 0, 0
def draw(self, renderer):
"""
Draw the children
"""
self.image.draw(renderer)
# bbox_artist(self, renderer, fill=False, props=dict(pad=0.))
self.stale = False
def overlay_icon(
icon,
coords=(0, 0),
coord_type='axes',
bbox_alignment=(0, 0),
max_asize=None,
max_dsize=None,
as_artist=True,
):
"""
Overlay a species icon
References:
http://matplotlib.org/examples/pylab_examples/demo_annotation_box.html
http://matplotlib.org/users/annotations_guide.html
/usr/local/lib/python2.7/dist-packages/matplotlib/offsetbox.py
Args:
icon (ndarray or str): image icon data or path
coords (tuple): (default = (0, 0))
coord_type (str): (default = 'axes')
bbox_alignment (tuple): (default = (0, 0))
max_dsize (None): (default = None)
CommandLine:
python -m wbia.plottool.draw_func2 --exec-overlay_icon --show --icon zebra.png
python -m wbia.plottool.draw_func2 --exec-overlay_icon --show --icon lena.png
python -m wbia.plottool.draw_func2 --exec-overlay_icon --show --icon lena.png --artist
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> pt.plot2(np.arange(100), np.arange(100))
>>> icon = ut.get_argval('--icon', type_=str, default='lena.png')
>>> coords = (0, 0)
>>> coord_type = 'axes'
>>> bbox_alignment = (0, 0)
>>> max_dsize = None # (128, None)
>>> max_asize = (60, 40)
>>> as_artist = not ut.get_argflag('--noartist')
>>> result = overlay_icon(icon, coords, coord_type, bbox_alignment,
>>> max_asize, max_dsize, as_artist)
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
# from mpl_toolkits.axes_grid.anchored_artists import AnchoredAuxTransformBox
import vtool as vt
ax = gca()
if isinstance(icon, str):
# hack because icon is probably a url
icon_url = icon
icon = vt.imread(ut.grab_file_url(icon_url))
if max_dsize is not None:
icon = vt.resize_to_maxdims(icon, max_dsize)
icon = vt.convert_colorspace(icon, 'RGB', 'BGR')
# imagebox = OffsetImage2(icon, zoom=.3)
if coord_type == 'axes':
xlim = ax.get_xlim()
ylim = ax.get_ylim()
xy = [
xlim[0] * (1 - coords[0]) + xlim[1] * (coords[0]),
ylim[0] * (1 - coords[1]) + ylim[1] * (coords[1]),
]
else:
raise NotImplementedError('')
# ab = AnchoredAuxTransformBox(ax.transData, loc=2)
# ab.drawing_area.add_artist(imagebox)
# *xycoords* and *textcoords* are strings that indicate the
# coordinates of *xy* and *xytext*, and may be one of the
# following values:
# 'figure points' #'figure pixels' #'figure fraction' #'axes points'
# 'axes pixels' #'axes fraction' #'data' #'offset points' #'polar'
if as_artist:
# Hack while I am trying to get constant size images working
if ut.get_argval('--save'):
# zoom = 1.0
zoom = 1.0
else:
zoom = 0.5
zoom = ut.get_argval('--overlay-zoom', default=zoom)
if False:
# TODO: figure out how to make axes fraction work
imagebox = mpl.offsetbox.OffsetImage(icon)
imagebox.set_width(1)
imagebox.set_height(1)
ab = mpl.offsetbox.AnnotationBbox(
imagebox,
xy,
xybox=(0.0, 0.0),
xycoords='data',
boxcoords=('axes fraction', 'data'),
# boxcoords="offset points",
box_alignment=bbox_alignment,
pad=0.0,
)
else:
imagebox = mpl.offsetbox.OffsetImage(icon, zoom=zoom)
ab = mpl.offsetbox.AnnotationBbox(
imagebox,
xy,
xybox=(0.0, 0.0),
xycoords='data',
# xycoords='axes fraction',
boxcoords='offset points',
box_alignment=bbox_alignment,
pad=0.0,
)
ax.add_artist(ab)
else:
img_size = vt.get_size(icon)
logger.info('img_size = %r' % (img_size,))
if max_asize is not None:
dsize, ratio = vt.resized_dims_and_ratio(img_size, max_asize)
width, height = dsize
else:
width, height = img_size
logger.info('width, height= %r, %r' % (width, height))
x1 = xy[0] + width * bbox_alignment[0]
y1 = xy[1] + height * bbox_alignment[1]
x2 = xy[0] + width * (1 - bbox_alignment[0])
y2 = xy[1] + height * (1 - bbox_alignment[1])
ax = plt.gca()
prev_aspect = ax.get_aspect()
# FIXME: adjust aspect ratio of extent to match the axes
logger.info('icon.shape = %r' % (icon.shape,))
logger.info('prev_aspect = %r' % (prev_aspect,))
extent = [x1, x2, y1, y2]
logger.info('extent = %r' % (extent,))
ax.imshow(icon, extent=extent)
logger.info('current_aspect = %r' % (ax.get_aspect(),))
ax.set_aspect(prev_aspect)
logger.info('current_aspect = %r' % (ax.get_aspect(),))
# x - width // 2, x + width // 2,
# y - height // 2, y + height // 2])
def update_figsize():
"""updates figsize based on command line"""
figsize = ut.get_argval('--figsize', type_=list, default=None)
if figsize is not None:
# Enforce inches and DPI
fig = gcf()
figsize = [eval(term) if isinstance(term, str) else term for term in figsize]
figw, figh = figsize[0], figsize[1]
logger.info('get_size_inches = %r' % (fig.get_size_inches(),))
logger.info('fig w,h (inches) = %r, %r' % (figw, figh))
fig.set_size_inches(figw, figh)
# logger.info('get_size_inches = %r' % (fig.get_size_inches(),))
def udpate_adjust_subplots():
"""
DEPRICATE
updates adjust_subplots based on command line
"""
adjust_list = ut.get_argval('--adjust', type_=list, default=None)
if adjust_list is not None:
# --adjust=[.02,.02,.05]
keys = ['left', 'bottom', 'wspace', 'right', 'top', 'hspace']
if len(adjust_list) == 1:
# [all]
vals = adjust_list * 3 + [1 - adjust_list[0]] * 2 + adjust_list
elif len(adjust_list) == 3:
# [left, bottom, wspace]
vals = adjust_list + [1 - adjust_list[0], 1 - adjust_list[1], adjust_list[2]]
elif len(adjust_list) == 4:
# [left, bottom, wspace, hspace]
vals = adjust_list[0:3] + [
1 - adjust_list[0],
1 - adjust_list[1],
adjust_list[3],
]
elif len(adjust_list) == 6:
vals = adjust_list
else:
raise NotImplementedError(
(
'vals must be len (1, 3, or 6) not %d, adjust_list=%r. '
'Expects keys=%r'
)
% (len(adjust_list), adjust_list, keys)
)
adjust_kw = dict(zip(keys, vals))
logger.info('**adjust_kw = %s' % (ut.repr2(adjust_kw),))
adjust_subplots(**adjust_kw)
def render_figure_to_image(fig, **savekw):
import io
import cv2
import wbia.plottool as pt
# Pop save kwargs from kwargs
# save_keys = ['dpi', 'figsize', 'saveax', 'verbose']
# Write matplotlib axes to an image
axes_extents = pt.extract_axes_extents(fig)
# assert len(axes_extents) == 1, 'more than one axes'
# if len(axes_extents) == 1:
# extent = axes_extents[0]
# else:
extent = mpl.transforms.Bbox.union(axes_extents)
with io.BytesIO() as stream:
# This call takes 23% - 15% of the time depending on settings
fig.savefig(stream, bbox_inches=extent, **savekw)
stream.seek(0)
data = np.fromstring(stream.getvalue(), dtype=np.uint8)
image = cv2.imdecode(data, 1)
return image
class RenderingContext(object):
def __init__(self, **savekw):
self.image = None
self.fig = None
self.was_interactive = None
self.savekw = savekw
def __enter__(self):
import wbia.plottool as pt
tmp_fnum = -1
import matplotlib as mpl
self.fig = pt.figure(fnum=tmp_fnum)
self.was_interactive = mpl.is_interactive()
if self.was_interactive:
mpl.interactive(False)
return self
def __exit__(self, type_, value, trace):
if trace is not None:
# logger.info('[util_time] Error in context manager!: ' + str(value))
return False # return a falsey value on error
# Ensure that this figure will not pop up
import wbia.plottool as pt
self.image = pt.render_figure_to_image(self.fig, **self.savekw)
pt.plt.close(self.fig)
if self.was_interactive:
mpl.interactive(self.was_interactive)
def extract_axes_extents(fig, combine=False, pad=0.0):
"""
CommandLine:
python -m wbia.plottool.draw_func2 extract_axes_extents
python -m wbia.plottool.draw_func2 extract_axes_extents --save foo.jpg
Notes:
contour does something weird to axes
with contour:
axes_extents = Bbox([[-0.839827203337, -0.00555555555556], [7.77743055556, 6.97227277762]])
without contour
axes_extents = Bbox([[0.0290607810781, -0.00555555555556], [7.77743055556, 5.88]])
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> import matplotlib.gridspec as gridspec
>>> import matplotlib.pyplot as plt
>>> pt.qtensure()
>>> fig = plt.figure()
>>> gs = gridspec.GridSpec(17, 17)
>>> specs = [
>>> gs[0:8, 0:8], gs[0:8, 8:16],
>>> gs[9:17, 0:8], gs[9:17, 8:16],
>>> ]
>>> rng = np.random.RandomState(0)
>>> X = (rng.rand(100, 2) * [[8, 8]]) + [[6, -14]]
>>> x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
>>> y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
>>> xx, yy = np.meshgrid(np.arange(x_min, x_max), np.arange(y_min, y_max))
>>> yynan = np.full(yy.shape, fill_value=np.nan)
>>> xxnan = np.full(yy.shape, fill_value=np.nan)
>>> cmap = plt.cm.RdYlBu
>>> norm = plt.Normalize(vmin=0, vmax=1)
>>> for count, spec in enumerate(specs):
>>> fig.add_subplot(spec)
>>> plt.plot(X.T[0], X.T[1], 'o', color='r', markeredgecolor='w')
>>> Z = rng.rand(*xx.shape)
>>> plt.contourf(xx, yy, Z, cmap=cmap, norm=norm, alpha=1.0)
>>> plt.title('full-nan decision point')
>>> plt.gca().set_aspect('equal')
>>> gs = gridspec.GridSpec(1, 16)
>>> subspec = gs[:, -1:]
>>> cax = plt.subplot(subspec)
>>> sm = plt.cm.ScalarMappable(cmap=cmap)
>>> sm.set_array(np.linspace(0, 1))
>>> plt.colorbar(sm, cax)
>>> cax.set_ylabel('ColorBar')
>>> fig.suptitle('SupTitle')
>>> subkw = dict(left=.001, right=.9, top=.9, bottom=.05, hspace=.2, wspace=.1)
>>> plt.subplots_adjust(**subkw)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
import wbia.plottool as pt
# Make sure we draw the axes first so we can
# extract positions from the text objects
fig.canvas.draw()
# Group axes that belong together
atomic_axes = []
seen_ = set([])
for ax in fig.axes:
div = pt.get_plotdat(ax, DF2_DIVIDER_KEY, None)
if div is not None:
df2_div_axes = pt.get_plotdat_dict(ax).get('df2_div_axes', [])
seen_.add(ax)
seen_.update(set(df2_div_axes))
atomic_axes.append([ax] + df2_div_axes)
# TODO: pad these a bit
else:
if ax not in seen_:
atomic_axes.append([ax])
seen_.add(ax)
hack_axes_group_row = ut.get_argflag('--grouprows')
if hack_axes_group_row:
groupid_list = []
for axs in atomic_axes:
for ax in axs:
groupid = ax.colNum
groupid_list.append(groupid)
groupxs = ut.group_indices(groupid_list)[1]
new_groups = ut.lmap(ut.flatten, ut.apply_grouping(atomic_axes, groupxs))
atomic_axes = new_groups
# [[(ax.rowNum, ax.colNum) for ax in axs] for axs in atomic_axes]
# save all rows of each column
dpi_scale_trans_inv = fig.dpi_scale_trans.inverted()
axes_bboxes_ = [axes_extent(axs, pad) for axs in atomic_axes]
axes_extents_ = [extent.transformed(dpi_scale_trans_inv) for extent in axes_bboxes_]
# axes_extents_ = axes_bboxes_
if combine:
if True:
# Grab include extents of figure text as well
# FIXME: This might break on OSX
# http://stackoverflow.com/questions/22667224/bbox-backend
renderer = fig.canvas.get_renderer()
for mpl_text in fig.texts:
bbox = mpl_text.get_window_extent(renderer=renderer)
extent_ = bbox.expanded(1.0 + pad, 1.0 + pad)
extent = extent_.transformed(dpi_scale_trans_inv)
# extent = extent_
axes_extents_.append(extent)
axes_extents = mpl.transforms.Bbox.union(axes_extents_)
else:
axes_extents = axes_extents_
# if True:
# axes_extents.x0 = 0
# # axes_extents.y1 = 0
return axes_extents
def axes_extent(axs, pad=0.0):
"""
Get the full extent of a group of axes, including axes labels, tick labels,
and titles.
"""
def axes_parts(ax):
yield ax
for label in ax.get_xticklabels():
if label.get_text():
yield label
for label in ax.get_yticklabels():
if label.get_text():
yield label
xlabel = ax.get_xaxis().get_label()
ylabel = ax.get_yaxis().get_label()
for label in (xlabel, ylabel, ax.title):
if label.get_text():
yield label
# def axes_parts2(ax):
# yield ('ax', ax)
# for c, label in enumerate(ax.get_xticklabels()):
# if label.get_text():
# yield ('xtick{}'.format(c), label)
# for label in ax.get_yticklabels():
# if label.get_text():
# yield ('ytick{}'.format(c), label)
# xlabel = ax.get_xaxis().get_label()
# ylabel = ax.get_yaxis().get_label()
# for key, label in (('xlabel', xlabel), ('ylabel', ylabel),
# ('title', ax.title)):
# if label.get_text():
# yield (key, label)
# yield from ax.lines
# yield from ax.patches
items = it.chain.from_iterable(axes_parts(ax) for ax in axs)
extents = [item.get_window_extent() for item in items]
# mpl.transforms.Affine2D().scale(1.1)
extent = mpl.transforms.Bbox.union(extents)
extent = extent.expanded(1.0 + pad, 1.0 + pad)
return extent
def save_parts(fig, fpath, grouped_axes=None, dpi=None):
"""
FIXME: this works in mpl 2.0.0, but not 2.0.2
Args:
fig (?):
fpath (str): file path string
dpi (None): (default = None)
Returns:
list: subpaths
CommandLine:
python -m wbia.plottool.draw_func2 save_parts
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> import matplotlib as mpl
>>> import matplotlib.pyplot as plt
>>> def testimg(fname):
>>> return plt.imread(mpl.cbook.get_sample_data(fname))
>>> fnames = ['grace_hopper.png', 'ada.png'] * 4
>>> fig = plt.figure(1)
>>> for c, fname in enumerate(fnames, start=1):
>>> ax = fig.add_subplot(3, 4, c)
>>> ax.imshow(testimg(fname))
>>> ax.set_title(fname[0:3] + str(c))
>>> ax.set_xticks([])
>>> ax.set_yticks([])
>>> ax = fig.add_subplot(3, 1, 3)
>>> ax.plot(np.sin(np.linspace(0, np.pi * 2)))
>>> ax.set_xlabel('xlabel')
>>> ax.set_ylabel('ylabel')
>>> ax.set_title('title')
>>> fpath = 'test_save_parts.png'
>>> adjust_subplots(fig=fig, wspace=.3, hspace=.3, top=.9)
>>> subpaths = save_parts(fig, fpath, dpi=300)
>>> fig.savefig(fpath)
>>> ut.startfile(subpaths[0])
>>> ut.startfile(fpath)
"""
if dpi:
# Need to set figure dpi before we draw
fig.dpi = dpi
# We need to draw the figure before calling get_window_extent
# (or we can figure out how to set the renderer object)
# if getattr(fig.canvas, 'renderer', None) is None:
fig.canvas.draw()
# Group axes that belong together
if grouped_axes is None:
grouped_axes = []
for ax in fig.axes:
grouped_axes.append([ax])
subpaths = []
_iter = enumerate(grouped_axes, start=0)
_iter = ut.ProgIter(list(_iter), label='save subfig')
for count, axs in _iter:
subpath = ut.augpath(fpath, chr(count + 65))
extent = axes_extent(axs).transformed(fig.dpi_scale_trans.inverted())
savekw = {}
savekw['transparent'] = ut.get_argflag('--alpha')
if dpi is not None:
savekw['dpi'] = dpi
savekw['edgecolor'] = 'none'
fig.savefig(subpath, bbox_inches=extent, **savekw)
subpaths.append(subpath)
return subpaths
def quit_if_noshow():
import utool as ut
saverequest = ut.get_argval('--save', default=None)
if not (saverequest or ut.get_argflag(('--show', '--save')) or ut.inIPython()):
raise ut.ExitTestException('This should be caught gracefully by ut.run_test')
def show_if_requested(N=1):
"""
Used at the end of tests. Handles command line arguments for saving figures
Referencse:
http://stackoverflow.com/questions/4325733/save-a-subplot-in-matplotlib
"""
if ut.NOT_QUIET:
logger.info('[pt] ' + str(ut.get_caller_name(range(3))) + ' show_if_requested()')
# Process figures adjustments from command line before a show or a save
# udpate_adjust_subplots()
adjust_subplots(use_argv=True)
update_figsize()
dpi = ut.get_argval('--dpi', type_=int, default=custom_constants.DPI)
SAVE_PARTS = ut.get_argflag('--saveparts')
fpath_ = ut.get_argval('--save', type_=str, default=None)
if fpath_ is None:
fpath_ = ut.get_argval('--saveparts', type_=str, default=None)
SAVE_PARTS = True
if fpath_ is not None:
from os.path import expanduser
fpath_ = expanduser(fpath_)
logger.info('Figure save was requested')
arg_dict = ut.get_arg_dict(
prefix_list=['--', '-'], type_hints={'t': list, 'a': list}
)
# import sys
from os.path import basename, splitext, join, dirname
import wbia.plottool as pt
import vtool as vt
# HACK
arg_dict = {
key: (val[0] if len(val) == 1 else '[' + ']['.join(val) + ']')
if isinstance(val, list)
else val
for key, val in arg_dict.items()
}
fpath_ = fpath_.format(**arg_dict)
fpath_ = ut.remove_chars(fpath_, ' \'"')
dpath, gotdpath = ut.get_argval(
'--dpath', type_=str, default='.', return_specified=True
)
fpath = join(dpath, fpath_)
if not gotdpath:
dpath = dirname(fpath_)
logger.info('dpath = %r' % (dpath,))
fig = pt.gcf()
fig.dpi = dpi
fpath_strict = ut.truepath(fpath)
CLIP_WHITE = ut.get_argflag('--clipwhite')
if SAVE_PARTS:
# TODO: call save_parts instead, but we still need to do the
# special grouping.
# Group axes that belong together
atomic_axes = []
seen_ = set([])
for ax in fig.axes:
div = pt.get_plotdat(ax, DF2_DIVIDER_KEY, None)
if div is not None:
df2_div_axes = pt.get_plotdat_dict(ax).get('df2_div_axes', [])
seen_.add(ax)
seen_.update(set(df2_div_axes))
atomic_axes.append([ax] + df2_div_axes)
# TODO: pad these a bit
else:
if ax not in seen_:
atomic_axes.append([ax])
seen_.add(ax)
hack_axes_group_row = ut.get_argflag('--grouprows')
if hack_axes_group_row:
groupid_list = []
for axs in atomic_axes:
for ax in axs:
groupid = ax.colNum
groupid_list.append(groupid)
groups = ut.group_items(atomic_axes, groupid_list)
new_groups = ut.emap(ut.flatten, groups.values())
atomic_axes = new_groups
# [[(ax.rowNum, ax.colNum) for ax in axs] for axs in atomic_axes]
# save all rows of each column
subpath_list = save_parts(
fig=fig, fpath=fpath_strict, grouped_axes=atomic_axes, dpi=dpi
)
absfpath_ = subpath_list[-1]
fpath_list = [relpath(_, dpath) for _ in subpath_list]
if CLIP_WHITE:
for subpath in subpath_list:
# remove white borders
pass
vt.clipwhite_ondisk(subpath, subpath)
else:
savekw = {}
# savekw['transparent'] = fpath.endswith('.png') and not noalpha
savekw['transparent'] = ut.get_argflag('--alpha')
savekw['dpi'] = dpi
savekw['edgecolor'] = 'none'
savekw['bbox_inches'] = extract_axes_extents(
fig, combine=True
) # replaces need for clipwhite
absfpath_ = ut.truepath(fpath)
fig.savefig(absfpath_, **savekw)
if CLIP_WHITE:
# remove white borders
fpath_in = fpath_out = absfpath_
vt.clipwhite_ondisk(fpath_in, fpath_out)
# img = vt.imread(absfpath_)
# thresh = 128
# fillval = [255, 255, 255]
# cropped_img = vt.crop_out_imgfill(img, fillval=fillval, thresh=thresh)
# logger.info('img.shape = %r' % (img.shape,))
# logger.info('cropped_img.shape = %r' % (cropped_img.shape,))
# vt.imwrite(absfpath_, cropped_img)
# if dpath is not None:
# fpath_ = ut.unixjoin(dpath, basename(absfpath_))
# else:
# fpath_ = fpath
fpath_list = [fpath_]
# Print out latex info
default_caption = '\n% ---\n' + basename(fpath).replace('_', ' ') + '\n% ---\n'
default_label = splitext(basename(fpath))[0] # [0].replace('_', '')
caption_list = ut.get_argval('--caption', type_=str, default=default_caption)
if isinstance(caption_list, str):
caption_str = caption_list
else:
caption_str = ' '.join(caption_list)
# caption_str = ut.get_argval('--caption', type_=str,
# default=basename(fpath).replace('_', ' '))
label_str = ut.get_argval('--label', type_=str, default=default_label)
width_str = ut.get_argval('--width', type_=str, default='\\textwidth')
width_str = ut.get_argval('--width', type_=str, default='\\textwidth')
logger.info('width_str = %r' % (width_str,))
height_str = ut.get_argval('--height', type_=str, default=None)
caplbl_str = label_str
if False and ut.is_developer() and len(fpath_list) <= 4:
if len(fpath_list) == 1:
latex_block = (
'\\ImageCommand{'
+ ''.join(fpath_list)
+ '}{'
+ width_str
+ '}{\n'
+ caption_str
+ '\n}{'
+ label_str
+ '}'
)
else:
width_str = '1'
latex_block = (
'\\MultiImageCommandII'
+ '{'
+ label_str
+ '}'
+ '{'
+ width_str
+ '}'
+ '{'
+ caplbl_str
+ '}'
+ '{\n'
+ caption_str
+ '\n}'
'{' + '}{'.join(fpath_list) + '}'
)
# HACK
else:
RESHAPE = ut.get_argval('--reshape', type_=tuple, default=None)
if RESHAPE:
def list_reshape(list_, new_shape):
for dim in reversed(new_shape):
list_ = list(map(list, zip(*[list_[i::dim] for i in range(dim)])))
return list_
newshape = (2,)
unflat_fpath_list = ut.list_reshape(fpath_list, newshape, trail=True)
fpath_list = ut.flatten(ut.list_transpose(unflat_fpath_list))
caption_str = '\\caplbl{' + caplbl_str + '}' + caption_str
figure_str = ut.util_latex.get_latex_figure_str(
fpath_list,
label_str=label_str,
caption_str=caption_str,
width_str=width_str,
height_str=height_str,
)
# import sys
# logger.info(sys.argv)
latex_block = figure_str
latex_block = ut.latex_newcommand(label_str, latex_block)
# latex_block = ut.codeblock(
# r'''
# \newcommand{\%s}{
# %s
# }
# '''
# ) % (label_str, latex_block,)
try:
import os
import psutil
import pipes
# import shlex
# TODO: separate into get_process_cmdline_str
# TODO: replace home with ~
proc = psutil.Process(pid=os.getpid())
home = os.path.expanduser('~')
cmdline_str = ' '.join(
[pipes.quote(_).replace(home, '~') for _ in proc.cmdline()]
)
latex_block = (
ut.codeblock(
r"""
\begin{comment}
%s
\end{comment}
"""
)
% (cmdline_str,)
+ '\n'
+ latex_block
)
except OSError:
pass
# latex_indent = ' ' * (4 * 2)
latex_indent = ' ' * (0)
latex_block_ = ut.indent(latex_block, latex_indent)
ut.print_code(latex_block_, 'latex')
if 'append' in arg_dict:
append_fpath = arg_dict['append']
ut.write_to(append_fpath, '\n\n' + latex_block_, mode='a')
if ut.get_argflag(('--diskshow', '--ds')):
# show what we wrote
ut.startfile(absfpath_)
# Hack write the corresponding logfile next to the output
log_fpath = ut.get_current_log_fpath()
if ut.get_argflag('--savelog'):
if log_fpath is not None:
ut.copy(log_fpath, splitext(absfpath_)[0] + '.txt')
else:
logger.info('Cannot copy log file because none exists')
if ut.inIPython():
import wbia.plottool as pt
pt.iup()
# elif ut.get_argflag('--cmd'):
# import wbia.plottool as pt
# pt.draw()
# ut.embed(N=N)
elif ut.get_argflag('--cmd'):
# cmd must handle show I think
pass
elif ut.get_argflag('--show'):
if ut.get_argflag('--tile'):
if ut.get_computer_name().lower() in ['hyrule']:
fig_presenter.all_figures_tile(percent_w=0.5, monitor_num=0)
else:
fig_presenter.all_figures_tile()
if ut.get_argflag('--present'):
fig_presenter.present()
for fig in fig_presenter.get_all_figures():
fig.set_dpi(80)
plt.show()
def distinct_markers(num, style='astrisk', total=None, offset=0):
r"""
Args:
num (?):
CommandLine:
python -m wbia.plottool.draw_func2 --exec-distinct_markers --show
python -m wbia.plottool.draw_func2 --exec-distinct_markers --mstyle=star --show
python -m wbia.plottool.draw_func2 --exec-distinct_markers --mstyle=polygon --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> style = ut.get_argval('--mstyle', type_=str, default='astrisk')
>>> marker_list = distinct_markers(10, style)
>>> x_data = np.arange(0, 3)
>>> for count, (marker) in enumerate(marker_list):
>>> pt.plot(x_data, [count] * len(x_data), marker=marker, markersize=10, linestyle='', label=str(marker))
>>> pt.legend()
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
num_sides = 3
style_num = {'astrisk': 2, 'star': 1, 'polygon': 0, 'circle': 3}[style]
if total is None:
total = num
total_degrees = 360 / num_sides
marker_list = [
(num_sides, style_num, total_degrees * (count + offset) / total)
for count in range(num)
]
return marker_list
def get_all_markers():
r"""
CommandLine:
python -m wbia.plottool.draw_func2 --exec-get_all_markers --show
References:
http://matplotlib.org/1.3.1/examples/pylab_examples/line_styles.html
http://matplotlib.org/api/markers_api.html#matplotlib.markers.MarkerStyle.markers
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> marker_dict = get_all_markers()
>>> x_data = np.arange(0, 3)
>>> for count, (marker, name) in enumerate(marker_dict.items()):
>>> pt.plot(x_data, [count] * len(x_data), marker=marker, linestyle='', label=name)
>>> pt.legend()
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
marker_dict = {
0: u'tickleft',
1: u'tickright',
2: u'tickup',
3: u'tickdown',
4: u'caretleft',
5: u'caretright',
6: u'caretup',
7: u'caretdown',
# None: u'nothing',
# u'None': u'nothing',
# u' ': u'nothing',
# u'': u'nothing',
u'*': u'star',
u'+': u'plus',
u',': u'pixel',
u'.': u'point',
u'1': u'tri_down',
u'2': u'tri_up',
u'3': u'tri_left',
u'4': u'tri_right',
u'8': u'octagon',
u'<': u'triangle_left',
u'>': u'triangle_right',
u'D': u'diamond',
u'H': u'hexagon2',
u'^': u'triangle_up',
u'_': u'hline',
u'd': u'thin_diamond',
u'h': u'hexagon1',
u'o': u'circle',
u'p': u'pentagon',
u's': u'square',
u'v': u'triangle_down',
u'x': u'x',
u'|': u'vline',
}
# marker_list = marker_dict.keys()
# marker_list = ['.', ',', 'o', 'v', '^', '<', '>', '1', '2', '3', '4', '8', 's', 'p', '*',
# 'h', 'H', '+', 'x', 'D', 'd', '|', '_', 'TICKLEFT', 'TICKRIGHT', 'TICKUP',
# 'TICKDOWN', 'CARETLEFT', 'CARETRIGHT', 'CARETUP', 'CARETDOWN']
return marker_dict
def get_pnum_func(nRows=1, nCols=1, base=0):
assert base in [0, 1], 'use base 0'
offst = 0 if base == 1 else 1
def pnum_(px):
return (nRows, nCols, px + offst)
return pnum_
def pnum_generator(nRows=1, nCols=1, base=0, nSubplots=None, start=0):
r"""
Args:
nRows (int): (default = 1)
nCols (int): (default = 1)
base (int): (default = 0)
nSubplots (None): (default = None)
Yields:
tuple : pnum
CommandLine:
python -m wbia.plottool.draw_func2 --exec-pnum_generator --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> nRows = 3
>>> nCols = 2
>>> base = 0
>>> pnum_ = pnum_generator(nRows, nCols, base)
>>> result = ut.repr2(list(pnum_), nl=1, nobr=True)
>>> print(result)
(3, 2, 1),
(3, 2, 2),
(3, 2, 3),
(3, 2, 4),
(3, 2, 5),
(3, 2, 6),
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> nRows = 3
>>> nCols = 2
>>> pnum_ = pnum_generator(nRows, nCols, start=3)
>>> result = ut.repr2(list(pnum_), nl=1, nobr=True)
>>> print(result)
(3, 2, 4),
(3, 2, 5),
(3, 2, 6),
"""
pnum_func = get_pnum_func(nRows, nCols, base)
total_plots = nRows * nCols
# TODO: have the last pnums fill in the whole figure
# when there are less subplots than rows * cols
# if nSubplots is not None:
# if nSubplots < total_plots:
# pass
for px in range(start, total_plots):
yield pnum_func(px)
def make_pnum_nextgen(nRows=None, nCols=None, base=0, nSubplots=None, start=0):
r"""
Args:
nRows (None): (default = None)
nCols (None): (default = None)
base (int): (default = 0)
nSubplots (None): (default = None)
start (int): (default = 0)
Returns:
iterator: pnum_next
CommandLine:
python -m wbia.plottool.draw_func2 --exec-make_pnum_nextgen --show
GridParams:
>>> param_grid = dict(
>>> nRows=[None, 3],
>>> nCols=[None, 3],
>>> nSubplots=[None, 9],
>>> )
>>> combos = ut.all_dict_combinations(param_grid)
GridExample:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> base, start = 0, 0
>>> pnum_next = make_pnum_nextgen(nRows, nCols, base, nSubplots, start)
>>> pnum_list = list( (pnum_next() for _ in it.count()) )
>>> print((nRows, nCols, nSubplots))
>>> result = ('pnum_list = %s' % (ut.repr2(pnum_list),))
>>> print(result)
"""
import functools
nRows, nCols = get_num_rc(nSubplots, nRows, nCols)
pnum_gen = pnum_generator(
nRows=nRows, nCols=nCols, base=base, nSubplots=nSubplots, start=start
)
pnum_next = functools.partial(next, pnum_gen)
return pnum_next
def get_num_rc(nSubplots=None, nRows=None, nCols=None):
r"""
Gets a constrained row column plot grid
Args:
nSubplots (None): (default = None)
nRows (None): (default = None)
nCols (None): (default = None)
Returns:
tuple: (nRows, nCols)
CommandLine:
python -m wbia.plottool.draw_func2 get_num_rc
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> cases = [
>>> dict(nRows=None, nCols=None, nSubplots=None),
>>> dict(nRows=2, nCols=None, nSubplots=5),
>>> dict(nRows=None, nCols=2, nSubplots=5),
>>> dict(nRows=None, nCols=None, nSubplots=5),
>>> ]
>>> for kw in cases:
>>> print('----')
>>> size = get_num_rc(**kw)
>>> if kw['nSubplots'] is not None:
>>> assert size[0] * size[1] >= kw['nSubplots']
>>> print('**kw = %s' % (ut.repr2(kw),))
>>> print('size = %r' % (size,))
"""
if nSubplots is None:
if nRows is None:
nRows = 1
if nCols is None:
nCols = 1
else:
if nRows is None and nCols is None:
from wbia.plottool import plot_helpers
nRows, nCols = plot_helpers.get_square_row_cols(nSubplots)
elif nRows is not None:
nCols = int(np.ceil(nSubplots / nRows))
elif nCols is not None:
nRows = int(np.ceil(nSubplots / nCols))
return nRows, nCols
def fnum_generator(base=1):
fnum = base - 1
while True:
fnum += 1
yield fnum
def make_fnum_nextgen(base=1):
import functools
fnum_gen = fnum_generator(base=base)
fnum_next = functools.partial(next, fnum_gen)
return fnum_next
BASE_FNUM = 9001
def next_fnum(new_base=None):
global BASE_FNUM
if new_base is not None:
BASE_FNUM = new_base
BASE_FNUM += 1
return BASE_FNUM
def ensure_fnum(fnum):
if fnum is None:
return next_fnum()
return fnum
def execstr_global():
execstr = ['global' + key for key in globals().keys()]
return execstr
def label_to_colors(labels_):
"""
returns a unique and distinct color corresponding to each label
"""
unique_labels = list(set(labels_))
unique_colors = distinct_colors(len(unique_labels))
label2_color = dict(zip(unique_labels, unique_colors))
color_list = [label2_color[label] for label in labels_]
return color_list
# def distinct_colors(N, brightness=.878, shuffle=True):
# """
# Args:
# N (int): number of distinct colors
# brightness (float): brightness of colors (maximum distinctiveness is .5) default is .878
# Returns:
# RGB_tuples
# Example:
# >>> from wbia.plottool.draw_func2 import * # NOQA
# """
# # http://blog.jianhuashao.com/2011/09/generate-n-distinct-colors.html
# sat = brightness
# val = brightness
# HSV_tuples = [(x * 1.0 / N, sat, val) for x in range(N)]
# RGB_tuples = list(map(lambda x: colorsys.hsv_to_rgb(*x), HSV_tuples))
# if shuffle:
# ut.deterministic_shuffle(RGB_tuples)
# return RGB_tuples
def add_alpha(colors):
return [list(color) + [1] for color in colors]
def get_axis_xy_width_height(ax=None, xaug=0, yaug=0, waug=0, haug=0):
"""gets geometry of a subplot"""
if ax is None:
ax = gca()
autoAxis = ax.axis()
xy = (autoAxis[0] + xaug, autoAxis[2] + yaug)
width = (autoAxis[1] - autoAxis[0]) + waug
height = (autoAxis[3] - autoAxis[2]) + haug
return xy, width, height
def get_axis_bbox(ax=None, **kwargs):
"""
# returns in figure coordinates?
"""
xy, width, height = get_axis_xy_width_height(ax=ax, **kwargs)
return (xy[0], xy[1], width, height)
def draw_border(ax, color=GREEN, lw=2, offset=None, adjust=True):
"""draws rectangle border around a subplot"""
if adjust:
xy, width, height = get_axis_xy_width_height(ax, -0.7, -0.2, 1, 0.4)
else:
xy, width, height = get_axis_xy_width_height(ax)
if offset is not None:
xoff, yoff = offset
xy = [xoff, yoff]
height = -height - yoff
width = width - xoff
rect = mpl.patches.Rectangle(xy, width, height, lw=lw)
rect = ax.add_patch(rect)
rect.set_clip_on(False)
rect.set_fill(False)
rect.set_edgecolor(color)
return rect
TAU = np.pi * 2
def rotate_plot(theta=TAU / 8, ax=None):
r"""
Args:
theta (?):
ax (None):
CommandLine:
python -m wbia.plottool.draw_func2 --test-rotate_plot
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> # build test data
>>> ax = gca()
>>> theta = TAU / 8
>>> plt.plot([1, 2, 3, 4, 5], [1, 2, 3, 2, 2])
>>> # execute function
>>> result = rotate_plot(theta, ax)
>>> # verify results
>>> print(result)
>>> show_if_requested()
"""
import vtool as vt
if ax is None:
ax = gca()
# import vtool as vt
xy, width, height = get_axis_xy_width_height(ax)
bbox = [xy[0], xy[1], width, height]
M = mpl.transforms.Affine2D(vt.rotation_around_bbox_mat3x3(theta, bbox))
propname = 'transAxes'
# propname = 'transData'
T = getattr(ax, propname)
T.transform_affine(M)
# T = ax.get_transform()
# Tnew = T + M
# ax.set_transform(Tnew)
# setattr(ax, propname, Tnew)
iup()
def cartoon_stacked_rects(xy, width, height, num=4, shift=None, **kwargs):
"""
pt.figure()
xy = (.5, .5)
width = .2
height = .2
ax = pt.gca()
ax.add_collection(col)
"""
if shift is None:
shift = np.array([-width, height]) * (0.1 / num)
xy = np.array(xy)
rectkw = dict(
ec=kwargs.pop('ec', None),
lw=kwargs.pop('lw', None),
linestyle=kwargs.pop('linestyle', None),
)
patch_list = [
mpl.patches.Rectangle(xy + shift * count, width, height, **rectkw)
for count in reversed(range(num))
]
col = mpl.collections.PatchCollection(patch_list, **kwargs)
return col
def make_bbox(
bbox,
theta=0,
bbox_color=None,
ax=None,
lw=2,
alpha=1.0,
align='center',
fill=None,
**kwargs
):
if ax is None:
ax = gca()
(rx, ry, rw, rh) = bbox
# Transformations are specified in backwards order.
trans_annotation = mpl.transforms.Affine2D()
if align == 'center':
trans_annotation.scale(rw, rh)
elif align == 'outer':
trans_annotation.scale(rw + (lw / 2), rh + (lw / 2))
elif align == 'inner':
trans_annotation.scale(rw - (lw / 2), rh - (lw / 2))
trans_annotation.rotate(theta)
trans_annotation.translate(rx + rw / 2, ry + rh / 2)
t_end = trans_annotation + ax.transData
bbox = mpl.patches.Rectangle((-0.5, -0.5), 1, 1, lw=lw, transform=t_end, **kwargs)
bbox.set_fill(fill if fill else None)
bbox.set_alpha(alpha)
# bbox.set_transform(trans)
bbox.set_edgecolor(bbox_color)
return bbox
# TODO SEPARTE THIS INTO DRAW BBOX AND DRAW_ANNOTATION
def draw_bbox(
bbox,
lbl=None,
bbox_color=(1, 0, 0),
lbl_bgcolor=(0, 0, 0),
lbl_txtcolor=(1, 1, 1),
draw_arrow=True,
theta=0,
ax=None,
lw=2,
):
if ax is None:
ax = gca()
(rx, ry, rw, rh) = bbox
# Transformations are specified in backwards order.
trans_annotation = mpl.transforms.Affine2D()
trans_annotation.scale(rw, rh)
trans_annotation.rotate(theta)
trans_annotation.translate(rx + rw / 2, ry + rh / 2)
t_end = trans_annotation + ax.transData
bbox = mpl.patches.Rectangle((-0.5, -0.5), 1, 1, lw=lw, transform=t_end)
bbox.set_fill(False)
# bbox.set_transform(trans)
bbox.set_edgecolor(bbox_color)
ax.add_patch(bbox)
# Draw overhead arrow indicating the top of the ANNOTATION
if draw_arrow:
arw_xydxdy = (-0.5, -0.5, 1.0, 0.0)
arw_kw = dict(head_width=0.1, transform=t_end, length_includes_head=True)
arrow = mpl.patches.FancyArrow(*arw_xydxdy, **arw_kw)
arrow.set_edgecolor(bbox_color)
arrow.set_facecolor(bbox_color)
ax.add_patch(arrow)
# Draw a label
if lbl is not None:
ax_absolute_text(
rx,
ry,
lbl,
ax=ax,
horizontalalignment='center',
verticalalignment='center',
color=lbl_txtcolor,
backgroundcolor=lbl_bgcolor,
)
def plot(*args, **kwargs):
yscale = kwargs.pop('yscale', 'linear')
xscale = kwargs.pop('xscale', 'linear')
logscale_kwargs = kwargs.pop('logscale_kwargs', {}) # , {'nonposx': 'clip'})
plot = plt.plot(*args, **kwargs)
ax = plt.gca()
yscale_kwargs = logscale_kwargs if yscale in ['log', 'symlog'] else {}
xscale_kwargs = logscale_kwargs if xscale in ['log', 'symlog'] else {}
ax.set_yscale(yscale, **yscale_kwargs)
ax.set_xscale(xscale, **xscale_kwargs)
return plot
def plot2(
x_data,
y_data,
marker='o',
title_pref='',
x_label='x',
y_label='y',
unitbox=False,
flipx=False,
flipy=False,
title=None,
dark=None,
equal_aspect=True,
pad=0,
label='',
fnum=None,
pnum=None,
*args,
**kwargs
):
"""
don't forget to call pt.legend
Kwargs:
linewidth (float):
"""
if x_data is None:
warnstr = '[df2] ! Warning: x_data is None'
logger.info(warnstr)
x_data = np.arange(len(y_data))
if fnum is not None or pnum is not None:
figure(fnum=fnum, pnum=pnum)
do_plot = True
# ensure length
if len(x_data) != len(y_data):
warnstr = '[df2] ! Warning: len(x_data) != len(y_data). Cannot plot2'
warnings.warn(warnstr)
draw_text(warnstr)
do_plot = False
if len(x_data) == 0:
warnstr = '[df2] ! Warning: len(x_data) == 0. Cannot plot2'
warnings.warn(warnstr)
draw_text(warnstr)
do_plot = False
# ensure in ndarray
if isinstance(x_data, list):
x_data = np.array(x_data)
if isinstance(y_data, list):
y_data = np.array(y_data)
ax = gca()
if do_plot:
ax.plot(x_data, y_data, marker, label=label, *args, **kwargs)
min_x = x_data.min()
min_y = y_data.min()
max_x = x_data.max()
max_y = y_data.max()
min_ = min(min_x, min_y)
max_ = max(max_x, max_y)
if equal_aspect:
# Equal aspect ratio
if unitbox is True:
# Just plot a little bit outside the box
set_axis_limit(-0.01, 1.01, -0.01, 1.01, ax)
# ax.grid(True)
else:
set_axis_limit(min_, max_, min_, max_, ax)
# aspect_opptions = ['auto', 'equal', num]
ax.set_aspect('equal')
else:
ax.set_aspect('auto')
if pad > 0:
ax.set_xlim(min_x - pad, max_x + pad)
ax.set_ylim(min_y - pad, max_y + pad)
# ax.grid(True, color='w' if dark else 'k')
if flipx:
ax.invert_xaxis()
if flipy:
ax.invert_yaxis()
use_darkbackground = dark
if use_darkbackground is None:
import wbia.plottool as pt
use_darkbackground = pt.is_default_dark_bg()
if use_darkbackground:
dark_background(ax)
else:
# No data, draw big red x
draw_boxedX()
presetup_axes(x_label, y_label, title_pref, title, ax=None)
def pad_axes(pad, xlim=None, ylim=None):
ax = gca()
if xlim is None:
xlim = ax.get_xlim()
if ylim is None:
ylim = ax.get_ylim()
min_x, max_x = xlim
min_y, max_y = ylim
ax.set_xlim(min_x - pad, max_x + pad)
ax.set_ylim(min_y - pad, max_y + pad)
def presetup_axes(
x_label='x',
y_label='y',
title_pref='',
title=None,
equal_aspect=False,
ax=None,
**kwargs
):
if ax is None:
ax = gca()
set_xlabel(x_label, **kwargs)
set_ylabel(y_label, **kwargs)
if title is None:
title = x_label + ' vs ' + y_label
set_title(title_pref + ' ' + title, ax=None, **kwargs)
if equal_aspect:
ax.set_aspect('equal')
def postsetup_axes(use_legend=True, bg=None):
import wbia.plottool as pt
if bg is None:
if pt.is_default_dark_bg():
bg = 'dark'
if bg == 'dark':
dark_background()
if use_legend:
legend()
def adjust_subplots(
left=None,
right=None,
bottom=None,
top=None,
wspace=None,
hspace=None,
use_argv=False,
fig=None,
):
"""
Kwargs:
left (float): left side of the subplots of the figure
right (float): right side of the subplots of the figure
bottom (float): bottom of the subplots of the figure
top (float): top of the subplots of the figure
wspace (float): width reserved for blank space between subplots
hspace (float): height reserved for blank space between subplots
"""
kwargs = dict(
left=left, right=right, bottom=bottom, top=top, wspace=wspace, hspace=hspace
)
kwargs = {k: v for k, v in kwargs.items() if v is not None}
if fig is None:
fig = gcf()
subplotpars = fig.subplotpars
adjust_dict = subplotpars.__dict__.copy()
if 'validate' in adjust_dict:
del adjust_dict['validate']
if '_validate' in adjust_dict:
del adjust_dict['_validate']
adjust_dict.update(kwargs)
if use_argv:
# hack to take args from commandline
adjust_dict = ut.parse_dict_from_argv(adjust_dict)
fig.subplots_adjust(**adjust_dict)
# =======================
# TEXT FUNCTIONS
# TODO: I have too many of these. Need to consolidate
# =======================
def upperleft_text(txt, alpha=0.6, color=None):
txtargs = dict(
horizontalalignment='left',
verticalalignment='top',
backgroundcolor=(0, 0, 0, alpha),
color=ORANGE if color is None else color,
)
relative_text((0.02, 0.02), txt, **txtargs)
def upperright_text(txt, offset=None, alpha=0.6):
txtargs = dict(
horizontalalignment='right',
verticalalignment='top',
backgroundcolor=(0, 0, 0, alpha),
color=ORANGE,
offset=offset,
)
relative_text((0.98, 0.02), txt, **txtargs)
def lowerright_text(txt):
txtargs = dict(
horizontalalignment='right',
verticalalignment='bottom',
backgroundcolor=(0, 0, 0, 0.6),
color=ORANGE,
)
relative_text((0.98, 0.92), txt, **txtargs)
def absolute_lbl(x_, y_, txt, roffset=(-0.02, -0.02), alpha=0.6, **kwargs):
"""alternative to relative text"""
txtargs = dict(
horizontalalignment='right',
verticalalignment='top',
backgroundcolor=(0, 0, 0, alpha),
color=ORANGE,
)
txtargs.update(kwargs)
ax_absolute_text(x_, y_, txt, roffset=roffset, **txtargs)
def absolute_text(pos, text, ax=None, **kwargs):
x, y = pos
ax_absolute_text(x, y, text, ax=ax, **kwargs)
def relative_text(pos, text, ax=None, offset=None, **kwargs):
"""
Places text on axes in a relative position
Args:
pos (tuple): relative xy position
text (str): text
ax (None): (default = None)
offset (None): (default = None)
**kwargs: horizontalalignment, verticalalignment, roffset, ha, va,
fontsize, fontproperties, fontproperties, clip_on
CommandLine:
python -m wbia.plottool.draw_func2 relative_text --show
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> x = .5
>>> y = .5
>>> txt = 'Hello World'
>>> pt.figure()
>>> ax = pt.gca()
>>> family = 'monospace'
>>> family = 'CMU Typewriter Text'
>>> fontproperties = mpl.font_manager.FontProperties(family=family,
>>> size=42)
>>> result = relative_text((x, y), txt, ax, halign='center',
>>> fontproperties=fontproperties)
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
if pos == 'lowerleft':
pos = (0.01, 0.99)
kwargs['halign'] = 'left'
kwargs['valign'] = 'bottom'
elif pos == 'upperleft':
pos = (0.01, 0.01)
kwargs['halign'] = 'left'
kwargs['valign'] = 'top'
x, y = pos
if ax is None:
ax = gca()
if 'halign' in kwargs:
kwargs['horizontalalignment'] = kwargs.pop('halign')
if 'valign' in kwargs:
kwargs['verticalalignment'] = kwargs.pop('valign')
xy, width, height = get_axis_xy_width_height(ax)
x_, y_ = ((xy[0]) + x * width, (xy[1] + height) - y * height)
if offset is not None:
xoff, yoff = offset
x_ += xoff
y_ += yoff
absolute_text((x_, y_), text, ax=ax, **kwargs)
def parse_fontkw(**kwargs):
r"""
Kwargs:
fontsize, fontfamilty, fontproperties
"""
from matplotlib.font_manager import FontProperties
if 'fontproperties' not in kwargs:
size = kwargs.get('fontsize', 14)
weight = kwargs.get('fontweight', 'normal')
fontname = kwargs.get('fontname', None)
if fontname is not None:
# TODO catch user warning
'/usr/share/fonts/truetype/'
'/usr/share/fonts/opentype/'
fontpath = mpl.font_manager.findfont(fontname, fallback_to_default=False)
font_prop = FontProperties(fname=fontpath, weight=weight, size=size)
else:
family = kwargs.get('fontfamilty', 'monospace')
font_prop = FontProperties(family=family, weight=weight, size=size)
else:
font_prop = kwargs['fontproperties']
return font_prop
def ax_absolute_text(x_, y_, txt, ax=None, roffset=None, **kwargs):
"""Base function for text
Kwargs:
horizontalalignment in ['right', 'center', 'left'],
verticalalignment in ['top']
color
"""
kwargs = kwargs.copy()
if ax is None:
ax = gca()
if 'ha' in kwargs:
kwargs['horizontalalignment'] = kwargs['ha']
if 'va' in kwargs:
kwargs['verticalalignment'] = kwargs['va']
if 'fontproperties' not in kwargs:
if 'fontsize' in kwargs:
fontsize = kwargs['fontsize']
font_prop = mpl.font_manager.FontProperties(
family='monospace',
# weight='light',
size=fontsize,
)
kwargs['fontproperties'] = font_prop
else:
kwargs['fontproperties'] = mpl.font_manager.FontProperties(family='monospace')
# custom_constants.FONTS.relative
if 'clip_on' not in kwargs:
kwargs['clip_on'] = True
if roffset is not None:
xroff, yroff = roffset
xy, width, height = get_axis_xy_width_height(ax)
x_ += xroff * width
y_ += yroff * height
return ax.text(x_, y_, txt, **kwargs)
def fig_relative_text(x, y, txt, **kwargs):
kwargs['horizontalalignment'] = 'center'
kwargs['verticalalignment'] = 'center'
fig = gcf()
# xy, width, height = get_axis_xy_width_height(ax)
# x_, y_ = ((xy[0]+width)+x*width, (xy[1]+height)-y*height)
fig.text(x, y, txt, **kwargs)
def draw_text(text_str, rgb_textFG=(0, 0, 0), rgb_textBG=(1, 1, 1)):
ax = gca()
xy, width, height = get_axis_xy_width_height(ax)
text_x = xy[0] + (width / 2)
text_y = xy[1] + (height / 2)
ax.text(
text_x,
text_y,
text_str,
horizontalalignment='center',
verticalalignment='center',
color=rgb_textFG,
backgroundcolor=rgb_textBG,
)
# def convert_keypress_event_mpl_to_qt4(mevent):
# global TMP_mevent
# TMP_mevent = mevent
# # Grab the key from the mpl.KeyPressEvent
# key = mevent.key
# logger.info('[df2] convert event mpl -> qt4')
# logger.info('[df2] key=%r' % key)
# # dicts modified from backend_qt4.py
# mpl2qtkey = {'control': Qt.Key_Control, 'shift': Qt.Key_Shift,
# 'alt': Qt.Key_Alt, 'super': Qt.Key_Meta,
# 'enter': Qt.Key_Return, 'left': Qt.Key_Left, 'up': Qt.Key_Up,
# 'right': Qt.Key_Right, 'down': Qt.Key_Down,
# 'escape': Qt.Key_Escape, 'f1': Qt.Key_F1, 'f2': Qt.Key_F2,
# 'f3': Qt.Key_F3, 'f4': Qt.Key_F4, 'f5': Qt.Key_F5,
# 'f6': Qt.Key_F6, 'f7': Qt.Key_F7, 'f8': Qt.Key_F8,
# 'f9': Qt.Key_F9, 'f10': Qt.Key_F10, 'f11': Qt.Key_F11,
# 'f12': Qt.Key_F12, 'home': Qt.Key_Home, 'end': Qt.Key_End,
# 'pageup': Qt.Key_PageUp, 'pagedown': Qt.Key_PageDown}
# # Reverse the control and super (aka cmd/apple) keys on OSX
# if sys.platform == 'darwin':
# mpl2qtkey.update({'super': Qt.Key_Control, 'control': Qt.Key_Meta, })
# # Try to reconstruct QtGui.KeyEvent
# type_ = QtCore.QEvent.Type(QtCore.QEvent.KeyPress) # The type should always be KeyPress
# text = ''
# # Try to extract the original modifiers
# modifiers = QtCore.Qt.NoModifier # initialize to no modifiers
# if key.find(u'ctrl+') >= 0:
# modifiers = modifiers | QtCore.Qt.ControlModifier
# key = key.replace(u'ctrl+', u'')
# logger.info('[df2] has ctrl modifier')
# text += 'Ctrl+'
# if key.find(u'alt+') >= 0:
# modifiers = modifiers | QtCore.Qt.AltModifier
# key = key.replace(u'alt+', u'')
# logger.info('[df2] has alt modifier')
# text += 'Alt+'
# if key.find(u'super+') >= 0:
# modifiers = modifiers | QtCore.Qt.MetaModifier
# key = key.replace(u'super+', u'')
# logger.info('[df2] has super modifier')
# text += 'Super+'
# if key.isupper():
# modifiers = modifiers | QtCore.Qt.ShiftModifier
# logger.info('[df2] has shift modifier')
# text += 'Shift+'
# # Try to extract the original key
# try:
# if key in mpl2qtkey:
# key_ = mpl2qtkey[key]
# else:
# key_ = ord(key.upper()) # Qt works with uppercase keys
# text += key.upper()
# except Exception as ex:
# logger.info('[df2] ERROR key=%r' % key)
# logger.info('[df2] ERROR %r' % ex)
# raise
# autorep = False # default false
# count = 1 # default 1
# text = str(text) # The text is somewhat arbitrary
# # Create the QEvent
# logger.info('----------------')
# logger.info('[df2] Create event')
# logger.info('[df2] type_ = %r' % type_)
# logger.info('[df2] text = %r' % text)
# logger.info('[df2] modifiers = %r' % modifiers)
# logger.info('[df2] autorep = %r' % autorep)
# logger.info('[df2] count = %r ' % count)
# logger.info('----------------')
# qevent = QtGui.QKeyEvent(type_, key_, modifiers, text, autorep, count)
# return qevent
# def test_build_qkeyevent():
# import draw_func2 as df2
# qtwin = df2.QT4_WINS[0]
# # This reconstructs an test mplevent
# canvas = df2.figure(1).canvas
# mevent = mpl.backend_bases.KeyEvent('key_press_event', canvas, u'ctrl+p', x=672, y=230.0)
# qevent = df2.convert_keypress_event_mpl_to_qt4(mevent)
# app = qtwin.backend.app
# app.sendEvent(qtwin.ui, mevent)
# #type_ = QtCore.QEvent.Type(QtCore.QEvent.KeyPress) # The type should always be KeyPress
# #text = str('A') # The text is somewhat arbitrary
# #modifiers = QtCore.Qt.NoModifier # initialize to no modifiers
# #modifiers = modifiers | QtCore.Qt.ControlModifier
# #modifiers = modifiers | QtCore.Qt.AltModifier
# #key_ = ord('A') # Qt works with uppercase keys
# #autorep = False # default false
# #count = 1 # default 1
# #qevent = QtGui.QKeyEvent(type_, key_, modifiers, text, autorep, count)
# return qevent
def show_histogram(data, bins=None, **kwargs):
"""
CommandLine:
python -m wbia.plottool.draw_func2 --test-show_histogram --show
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> # build test data
>>> data = np.array([1, 24, 0, 0, 3, 4, 5, 9, 3, 0, 0, 0, 0, 2, 2, 2, 0, 0, 1, 1, 0, 0, 0, 3,])
>>> bins = None
>>> # execute function
>>> result = show_histogram(data, bins)
>>> # verify results
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
logger.info('[df2] show_histogram()')
dmin = int(np.floor(data.min()))
dmax = int(np.ceil(data.max()))
if bins is None:
bins = dmax - dmin
fig = figure(**kwargs)
ax = gca()
ax.hist(data, bins=bins, range=(dmin, dmax))
# dark_background()
use_darkbackground = None
if use_darkbackground is None:
use_darkbackground = not ut.get_argflag('--save')
if use_darkbackground:
dark_background(ax)
return fig
# help(np.bincount)
# fig.show()
def show_signature(sig, **kwargs):
fig = figure(**kwargs)
plt.plot(sig)
fig.show()
def draw_stems(
x_data=None,
y_data=None,
setlims=True,
color=None,
markersize=None,
bottom=None,
marker=None,
linestyle='-',
):
"""
Draws stem plot
Args:
x_data (None):
y_data (None):
setlims (bool):
color (None):
markersize (None):
bottom (None):
References:
http://exnumerus.blogspot.com/2011/02/how-to-quickly-plot-multiple-line.html
CommandLine:
python -m wbia.plottool.draw_func2 --test-draw_stems --show
python -m wbia.plottool.draw_func2 --test-draw_stems
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> x_data = np.append(np.arange(1, 10), np.arange(1, 10))
>>> rng = np.random.RandomState(0)
>>> y_data = sorted(rng.rand(len(x_data)) * 10)
>>> # y_data = np.array([ut.get_nth_prime(n) for n in x_data])
>>> setlims = False
>>> color = [1.0, 0.0, 0.0, 1.0]
>>> markersize = 2
>>> marker = 'o'
>>> bottom = None
>>> result = draw_stems(x_data, y_data, setlims, color, markersize, bottom, marker)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
if y_data is not None and x_data is None:
x_data = np.arange(len(y_data))
pass
if len(x_data) != len(y_data):
logger.info('[df2] WARNING plot_stems(): len(x_data)!=len(y_data)')
if len(x_data) == 0:
logger.info('[df2] WARNING plot_stems(): len(x_data)=len(y_data)=0')
x_data_ = np.array(x_data)
y_data_ = np.array(y_data)
y_data_sortx = y_data_.argsort()[::-1]
x_data_sort = x_data_[y_data_sortx]
y_data_sort = y_data_[y_data_sortx]
if color is None:
color = [1.0, 0.0, 0.0, 1.0]
OLD = False
if not OLD:
if bottom is None:
bottom = 0
# Faster way of drawing stems
# with ut.Timer('new stem'):
stemlines = []
ax = gca()
x_segments = ut.flatten([[thisx, thisx, None] for thisx in x_data_sort])
if linestyle == '':
y_segments = ut.flatten([[thisy, thisy, None] for thisy in y_data_sort])
else:
y_segments = ut.flatten([[bottom, thisy, None] for thisy in y_data_sort])
ax.plot(x_segments, y_segments, linestyle, color=color, marker=marker)
else:
with ut.Timer('old stem'):
markerline, stemlines, baseline = pylab.stem(
x_data_sort, y_data_sort, linefmt='-', bottom=bottom
)
if markersize is not None:
markerline.set_markersize(markersize)
pylab.setp(markerline, 'markerfacecolor', 'w')
pylab.setp(stemlines, 'markerfacecolor', 'w')
if color is not None:
for line in stemlines:
line.set_color(color)
pylab.setp(baseline, 'linewidth', 0) # baseline should be invisible
if setlims:
ax = gca()
ax.set_xlim(min(x_data) - 1, max(x_data) + 1)
ax.set_ylim(min(y_data) - 1, max(max(y_data), max(x_data)) + 1)
def plot_sift_signature(sift, title='', fnum=None, pnum=None):
"""
Plots a SIFT descriptor as a histogram and distinguishes different bins
into different colors
Args:
sift (ndarray[dtype=np.uint8]):
title (str): (default = '')
fnum (int): figure number(default = None)
pnum (tuple): plot number(default = None)
Returns:
AxesSubplot: ax
CommandLine:
python -m wbia.plottool.draw_func2 --test-plot_sift_signature --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import vtool as vt
>>> sift = vt.demodata.testdata_dummy_sift(1, np.random.RandomState(0))[0]
>>> title = 'test sift histogram'
>>> fnum = None
>>> pnum = None
>>> ax = plot_sift_signature(sift, title, fnum, pnum)
>>> result = ('ax = %s' % (str(ax),))
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
fnum = ensure_fnum(fnum)
figure(fnum=fnum, pnum=pnum)
ax = gca()
plot_bars(sift, 16)
ax.set_xlim(0, 128)
ax.set_ylim(0, 256)
space_xticks(9, 16)
space_yticks(5, 64)
set_title(title, ax=ax)
# dark_background(ax)
use_darkbackground = None
if use_darkbackground is None:
use_darkbackground = not ut.get_argflag('--save')
if use_darkbackground:
dark_background(ax)
return ax
def plot_descriptor_signature(vec, title='', fnum=None, pnum=None):
"""
signature general for for any descriptor vector.
Args:
vec (ndarray):
title (str): (default = '')
fnum (int): figure number(default = None)
pnum (tuple): plot number(default = None)
Returns:
AxesSubplot: ax
CommandLine:
python -m wbia.plottool.draw_func2 --test-plot_descriptor_signature --show
SeeAlso:
plot_sift_signature
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import vtool as vt
>>> vec = ((np.random.RandomState(0).rand(258) - .2) * 4)
>>> title = 'test sift histogram'
>>> fnum = None
>>> pnum = None
>>> ax = plot_descriptor_signature(vec, title, fnum, pnum)
>>> result = ('ax = %s' % (str(ax),))
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
fnum = ensure_fnum(fnum)
figure(fnum=fnum, pnum=pnum)
ax = gca()
plot_bars(vec, vec.size // 8)
ax.set_xlim(0, vec.size)
ax.set_ylim(vec.min(), vec.max())
# space_xticks(9, 16)
# space_yticks(5, 64)
set_title(title, ax=ax)
use_darkbackground = None
if use_darkbackground is None:
use_darkbackground = not ut.get_argflag('--save')
if use_darkbackground:
dark_background(ax)
return ax
def dark_background(ax=None, doubleit=False, force=False):
r"""
Args:
ax (None): (default = None)
doubleit (bool): (default = False)
CommandLine:
python -m wbia.plottool.draw_func2 --exec-dark_background --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> fig = pt.figure()
>>> pt.dark_background()
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
def is_using_style(style):
style_dict = mpl.style.library[style]
return len(ut.dict_isect(style_dict, mpl.rcParams)) == len(style_dict)
# is_using_style('classic')
# is_using_style('ggplot')
# HARD_DISABLE = force is not True
HARD_DISABLE = False
if not HARD_DISABLE and force:
# Should use mpl style dark background instead
bgcolor = BLACK * 0.9
if ax is None:
ax = gca()
from mpl_toolkits.mplot3d import Axes3D
if isinstance(ax, Axes3D):
ax.set_axis_bgcolor(bgcolor)
ax.tick_params(colors='white')
return
xy, width, height = get_axis_xy_width_height(ax)
if doubleit:
halfw = (doubleit) * (width / 2)
halfh = (doubleit) * (height / 2)
xy = (xy[0] - halfw, xy[1] - halfh)
width *= doubleit + 1
height *= doubleit + 1
rect = mpl.patches.Rectangle(xy, width, height, lw=0, zorder=0)
rect.set_clip_on(True)
rect.set_fill(True)
rect.set_color(bgcolor)
rect.set_zorder(-99999999999)
rect = ax.add_patch(rect)
def space_xticks(nTicks=9, spacing=16, ax=None):
if ax is None:
ax = gca()
ax.set_xticks(np.arange(nTicks) * spacing)
small_xticks(ax)
def space_yticks(nTicks=9, spacing=32, ax=None):
if ax is None:
ax = gca()
ax.set_yticks(np.arange(nTicks) * spacing)
small_yticks(ax)
def small_xticks(ax=None):
for tick in ax.xaxis.get_major_ticks():
tick.label.set_fontsize(8)
def small_yticks(ax=None):
for tick in ax.yaxis.get_major_ticks():
tick.label.set_fontsize(8)
def plot_bars(y_data, nColorSplits=1):
width = 1
nDims = len(y_data)
nGroup = nDims // nColorSplits
ori_colors = distinct_colors(nColorSplits)
x_data = np.arange(nDims)
ax = gca()
for ix in range(nColorSplits):
xs = np.arange(nGroup) + (nGroup * ix)
color = ori_colors[ix]
x_dat = x_data[xs]
y_dat = y_data[xs]
ax.bar(x_dat, y_dat, width, color=color, edgecolor=np.array(color) * 0.8)
def append_phantom_legend_label(label, color, type_='circle', alpha=1.0, ax=None):
"""
adds a legend label without displaying an actor
Args:
label (?):
color (?):
loc (str):
CommandLine:
python -m wbia.plottool.draw_func2 --test-append_phantom_legend_label --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> label = 'some label'
>>> color = 'b'
>>> loc = 'upper right'
>>> fig = pt.figure()
>>> ax = pt.gca()
>>> result = append_phantom_legend_label(label, color, loc, ax=ax)
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.quit_if_noshow()
>>> pt.show_phantom_legend_labels(ax=ax)
>>> pt.show_if_requested()
"""
# pass
# , loc=loc
if ax is None:
ax = gca()
_phantom_legend_list = getattr(ax, '_phantom_legend_list', None)
if _phantom_legend_list is None:
_phantom_legend_list = []
setattr(ax, '_phantom_legend_list', _phantom_legend_list)
if type_ == 'line':
phantom_actor = plt.Line2D((0, 0), (1, 1), color=color, label=label, alpha=alpha)
else:
phantom_actor = plt.Circle((0, 0), 1, fc=color, label=label, alpha=alpha)
# , prop=custom_constants.FONTS.legend)
# legend_tups = []
_phantom_legend_list.append(phantom_actor)
# ax.legend(handles=[phantom_actor], framealpha=.2)
# plt.legend(*zip(*legend_tups), framealpha=.2)
def show_phantom_legend_labels(ax=None, **kwargs):
if ax is None:
ax = gca()
_phantom_legend_list = getattr(ax, '_phantom_legend_list', None)
if _phantom_legend_list is None:
_phantom_legend_list = []
setattr(ax, '_phantom_legend_list', _phantom_legend_list)
# logger.info(_phantom_legend_list)
legend(handles=_phantom_legend_list, ax=ax, **kwargs)
# ax.legend(handles=_phantom_legend_list, framealpha=.2)
LEGEND_LOCATION = {
'upper right': 1,
'upper left': 2,
'lower left': 3,
'lower right': 4,
'right': 5,
'center left': 6,
'center right': 7,
'lower center': 8,
'upper center': 9,
'center': 10,
}
# def legend(loc='upper right', fontproperties=None):
def legend(
loc='best', fontproperties=None, size=None, fc='w', alpha=1, ax=None, handles=None
):
r"""
Args:
loc (str): (default = 'best')
fontproperties (None): (default = None)
size (None): (default = None)
CommandLine:
python -m wbia.plottool.draw_func2 --exec-legend --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> loc = 'best'
>>> import wbia.plottool as pt
>>> xdata = np.linspace(-6, 6)
>>> ydata = np.sin(xdata)
>>> pt.plot(xdata, ydata, label='sin')
>>> fontproperties = None
>>> size = None
>>> result = legend(loc, fontproperties, size)
>>> print(result)
>>> import wbia.plottool as pt
>>> pt.show_if_requested()
"""
assert loc in LEGEND_LOCATION or loc == 'best', 'invalid loc. try one of %r' % (
LEGEND_LOCATION,
)
if ax is None:
ax = gca()
if fontproperties is None:
prop = {}
if size is not None:
prop['size'] = size
# prop['weight'] = 'normal'
# prop['family'] = 'sans-serif'
else:
prop = fontproperties
legendkw = dict(loc=loc)
if prop:
legendkw['prop'] = prop
if handles is not None:
legendkw['handles'] = handles
legend = ax.legend(**legendkw)
if legend:
legend.get_frame().set_fc(fc)
legend.get_frame().set_alpha(alpha)
def plot_histpdf(data, label=None, draw_support=False, nbins=10):
freq, _ = plot_hist(data, nbins=nbins)
from wbia.plottool import plots
plots.plot_pdf(data, draw_support=draw_support, scale_to=freq.max(), label=label)
def plot_hist(data, bins=None, nbins=10, weights=None):
if isinstance(data, list):
data = np.array(data)
dmin = data.min()
dmax = data.max()
if bins is None:
bins = dmax - dmin
ax = gca()
freq, bins_, patches = ax.hist(data, bins=nbins, weights=weights, range=(dmin, dmax))
return freq, bins_
def variation_trunctate(data):
ax = gca()
data = np.array(data)
if len(data) == 0:
warnstr = '[df2] ! Warning: len(data) = 0. Cannot variation_truncate'
warnings.warn(warnstr)
return
trunc_max = data.mean() + data.std() * 2
trunc_min = np.floor(data.min())
ax.set_xlim(trunc_min, trunc_max)
# trunc_xticks = np.linspace(0, int(trunc_max),11)
# trunc_xticks = trunc_xticks[trunc_xticks >= trunc_min]
# trunc_xticks = np.append([int(trunc_min)], trunc_xticks)
# no_zero_yticks = ax.get_yticks()[ax.get_yticks() > 0]
# ax.set_xticks(trunc_xticks)
# ax.set_yticks(no_zero_yticks)
# _----------------- HELPERS ^^^ ---------
def scores_to_color(
score_list,
cmap_='hot',
logscale=False,
reverse_cmap=False,
custom=False,
val2_customcolor=None,
score_range=None,
cmap_range=(0.1, 0.9),
):
"""
Other good colormaps are 'spectral', 'gist_rainbow', 'gist_ncar', 'Set1',
'Set2', 'Accent'
# TODO: plasma
Args:
score_list (list):
cmap_ (str): defaults to hot
logscale (bool):
cmap_range (tuple): restricts to only a portion of the cmap to avoid extremes
Returns:
<class '_ast.ListComp'>
SeeAlso:
python -m wbia.plottool.color_funcs --test-show_all_colormaps --show --type "Perceptually Uniform Sequential"
CommandLine:
python -m wbia.plottool.draw_func2 scores_to_color --show
Example:
>>> # ENABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> ut.exec_funckw(pt.scores_to_color, globals())
>>> score_list = np.array([-1, -2, 1, 1, 2, 10])
>>> # score_list = np.array([0, .1, .11, .12, .13, .8])
>>> # score_list = np.linspace(0, 1, 100)
>>> cmap_ = 'plasma'
>>> colors = pt.scores_to_color(score_list, cmap_)
>>> import vtool as vt
>>> imgRGB = vt.atleast_nd(np.array(colors)[:, 0:3], 3, tofront=True)
>>> imgRGB = imgRGB.astype(np.float32)
>>> imgBGR = vt.convert_colorspace(imgRGB, 'BGR', 'RGB')
>>> pt.imshow(imgBGR)
>>> pt.show_if_requested()
Example:
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> score_list = np.array([-1, -2, 1, 1, 2, 10])
>>> cmap_ = 'hot'
>>> logscale = False
>>> reverse_cmap = True
>>> custom = True
>>> val2_customcolor = {
... -1: UNKNOWN_PURP,
... -2: LIGHT_BLUE,
... }
"""
assert len(score_list.shape) == 1, 'score must be 1d'
if len(score_list) == 0:
return []
def apply_logscale(scores):
scores = np.array(scores)
above_zero = scores >= 0
scores_ = scores.copy()
scores_[above_zero] = scores_[above_zero] + 1
scores_[~above_zero] = scores_[~above_zero] - 1
scores_ = np.log2(scores_)
return scores_
if logscale:
# Hack
score_list = apply_logscale(score_list)
# if loglogscale
# score_list = np.log2(np.log2(score_list + 2) + 1)
# if isinstance(cmap_, str):
cmap = plt.get_cmap(cmap_)
# else:
# cmap = cmap_
if reverse_cmap:
cmap = reverse_colormap(cmap)
# if custom:
# base_colormap = cmap
# data = score_list
# cmap = customize_colormap(score_list, base_colormap)
if score_range is None:
min_ = score_list.min()
max_ = score_list.max()
else:
min_ = score_range[0]
max_ = score_range[1]
if logscale:
min_, max_ = apply_logscale([min_, max_])
if cmap_range is None:
cmap_scale_min, cmap_scale_max = 0.0, 1.0
else:
cmap_scale_min, cmap_scale_max = cmap_range
extent_ = max_ - min_
if extent_ == 0:
colors = [cmap(0.5) for fx in range(len(score_list))]
else:
if False and logscale:
# hack
def score2_01(score):
return np.log2(
1
+ cmap_scale_min
+ cmap_scale_max * (float(score) - min_) / (extent_)
)
score_list = np.array(score_list)
# rank_multiplier = score_list.argsort() / len(score_list)
# normscore = np.array(list(map(score2_01, score_list))) * rank_multiplier
normscore = np.array(list(map(score2_01, score_list)))
colors = list(map(cmap, normscore))
else:
def score2_01(score):
return cmap_scale_min + cmap_scale_max * (float(score) - min_) / (extent_)
colors = [cmap(score2_01(score)) for score in score_list]
if val2_customcolor is not None:
colors = [
np.array(val2_customcolor.get(score, color))
for color, score in zip(colors, score_list)
]
return colors
def customize_colormap(data, base_colormap):
unique_scalars = np.array(sorted(np.unique(data)))
max_ = unique_scalars.max()
min_ = unique_scalars.min()
extent_ = max_ - min_
bounds = np.linspace(min_, max_ + 1, extent_ + 2)
# Get a few more colors than we actually need so we don't hit the bottom of
# the cmap
colors_ix = np.concatenate((np.linspace(0, 1.0, extent_ + 2), (0.0, 0.0, 0.0, 0.0)))
colors_rgba = base_colormap(colors_ix)
# TODO: parametarize
val2_special_rgba = {
-1: UNKNOWN_PURP,
-2: LIGHT_BLUE,
}
def get_new_color(ix, val):
if val in val2_special_rgba:
return val2_special_rgba[val]
else:
return colors_rgba[ix - len(val2_special_rgba) + 1]
special_colors = [get_new_color(ix, val) for ix, val in enumerate(bounds)]
cmap = mpl.colors.ListedColormap(special_colors)
norm = mpl.colors.BoundaryNorm(bounds, cmap.N)
sm = mpl.cm.ScalarMappable(cmap=cmap, norm=norm)
sm.set_array([])
# sm.set_clim(-0.5, extent_ + 0.5)
# colorbar = plt.colorbar(sm)
return cmap
def unique_rows(arr):
"""
References:
http://stackoverflow.com/questions/16970982/find-unique-rows-in-numpy-array
"""
rowblocks = np.ascontiguousarray(arr).view(
np.dtype((np.void, arr.dtype.itemsize * arr.shape[1]))
)
_, idx = np.unique(rowblocks, return_index=True)
unique_arr = arr[idx]
return unique_arr
def scores_to_cmap(scores, colors=None, cmap_='hot'):
if colors is None:
colors = scores_to_color(scores, cmap_=cmap_)
scores = np.array(scores)
colors = np.array(colors)
sortx = scores.argsort()
sorted_colors = colors[sortx]
# Make a listed colormap and mappable object
listed_cmap = mpl.colors.ListedColormap(sorted_colors)
return listed_cmap
DF2_DIVIDER_KEY = '_df2_divider'
def ensure_divider(ax):
"""Returns previously constructed divider or creates one"""
from wbia.plottool import plot_helpers as ph
divider = ph.get_plotdat(ax, DF2_DIVIDER_KEY, None)
if divider is None:
divider = make_axes_locatable(ax)
ph.set_plotdat(ax, DF2_DIVIDER_KEY, divider)
orig_append_axes = divider.append_axes
def df2_append_axes(
divider, position, size, pad=None, add_to_figure=True, **kwargs
):
"""override divider add axes to register the divided axes"""
div_axes = ph.get_plotdat(ax, 'df2_div_axes', [])
new_ax = orig_append_axes(
position, size, pad=pad, add_to_figure=add_to_figure, **kwargs
)
div_axes.append(new_ax)
ph.set_plotdat(ax, 'df2_div_axes', div_axes)
return new_ax
ut.inject_func_as_method(
divider, df2_append_axes, 'append_axes', allow_override=True
)
return divider
def get_binary_svm_cmap():
# useful for svms
return reverse_colormap(plt.get_cmap('bwr'))
def reverse_colormap(cmap):
"""
References:
http://nbviewer.ipython.org/github/kwinkunks/notebooks/blob/master/Matteo_colourmaps.ipynb
"""
if isinstance(cmap, mpl.colors.ListedColormap):
return mpl.colors.ListedColormap(cmap.colors[::-1])
else:
reverse = []
k = []
for key, channel in cmap._segmentdata.items():
data = []
for t in channel:
data.append((1 - t[0], t[1], t[2]))
k.append(key)
reverse.append(sorted(data))
cmap_reversed = mpl.colors.LinearSegmentedColormap(
cmap.name + '_reversed', dict(zip(k, reverse))
)
return cmap_reversed
def interpolated_colormap(color_frac_list, resolution=64, space='lch-ab'):
"""
http://stackoverflow.com/questions/12073306/customize-colorbar-in-matplotlib
CommandLine:
python -m wbia.plottool.draw_func2 interpolated_colormap --show
Example:
>>> # DISABLE_DOCTEST
>>> from wbia.plottool.draw_func2 import * # NOQA
>>> import wbia.plottool as pt
>>> color_frac_list = [
>>> (pt.TRUE_BLUE, 0),
>>> #(pt.WHITE, .5),
>>> (pt.YELLOW, .5),
>>> (pt.FALSE_RED, 1.0),
>>> ]
>>> color_frac_list = [
>>> (pt.RED, 0),
>>> (pt.PINK, .1),
>>> (pt.ORANGE, .2),
>>> (pt.GREEN, .5),
>>> (pt.TRUE_BLUE, .7),
>>> (pt.PURPLE, 1.0),
>>> ]
>>> color_frac_list = [
>>> (pt.RED, 0/6),
>>> (pt.YELLOW, 1/6),
>>> (pt.GREEN, 2/6),
>>> (pt.CYAN, 3/6),
>>> (pt.BLUE, 4/6), # FIXME doesn't go in correct direction
>>> (pt.MAGENTA, 5/6),
>>> (pt.RED, 6/6),
>>> ]
>>> color_frac_list = [
>>> ((1, 0, 0, 0), 0/6),
>>> ((1, 0, .001/255, 0), 6/6), # hack
>>> ]
>>> space = 'hsv'
>>> color_frac_list = [
>>> (pt.BLUE, 0.0),
>>> (pt.GRAY, 0.5),
>>> (pt.YELLOW, 1.0),
>>> ]
>>> color_frac_list = [
>>> (pt.GREEN, 0.0),
>>> (pt.GRAY, 0.5),
>>> (pt.RED, 1.0),
>>> ]
>>> space = 'lab'
>>> #resolution = 16 + 1
>>> resolution = 256 + 1
>>> cmap = interpolated_colormap(color_frac_list, resolution, space)
>>> import wbia.plottool as pt
>>> pt.quit_if_noshow()
>>> a = np.linspace(0, 1, resolution).reshape(1, -1)
>>> pylab.imshow(a, aspect='auto', cmap=cmap, interpolation='nearest') # , origin="lower")
>>> plt.grid(False)
>>> pt.show_if_requested()
"""
import colorsys
if len(color_frac_list[0]) != 2:
color_frac_list = list(
zip(color_frac_list, np.linspace(0, 1, len(color_frac_list)))
)
colors = ut.take_column(color_frac_list, 0)
fracs = ut.take_column(color_frac_list, 1)
# resolution = 17
basis = np.linspace(0, 1, resolution)
fracs = np.array(fracs)
indices = | np.searchsorted(fracs, basis) | numpy.searchsorted |
# coding: utf-8
# # Packages
# In[2]:
import pickle
import numpy as np
import cv2
import glob
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
get_ipython().run_line_magic('matplotlib', 'inline')
#%matplotlib qt
# # Reading and Plotting Images
# In[3]:
image = mpimg.imread('test_images/straight_lines2.jpg')
image123 = mpimg.imread('test_images/test5.jpg')
print('This image is:', type(image), 'with dimensions:', image.shape)
plt.imshow(image)
print('\nThis image is distorted')
# # Do camera calibration given object points and image points
# In[4]:
# prepare object points, like (0,0,0), (1,0,0), (2,0,0) ....,(6,5,0)
objp = np.zeros((6*9,3), np.float32)
objp[:,:2] = np.mgrid[0:9,0:6].T.reshape(-1,2)
# Arrays to store object points and image points from all the images.
objpoints = [] # 3d points in real world space
imgpoints = [] # 2d points in image plane.
# Make a list of calibration images
images = glob.glob('camera_cal/calibration*.jpg')
# Step through the list and search for chessboard corners
for fname in images:
img = cv2.imread(fname)
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
# Find the chessboard corners
ret, corners = cv2.findChessboardCorners(gray, (9,6),None)
# If found, add object points, image points
if ret == True:
objpoints.append(objp)
imgpoints.append(corners)
# Draw and display the corners
img = cv2.drawChessboardCorners(img, (9,6), corners, ret)
cv2.imshow('img',img)
cv2.waitKey(500)
cv2.destroyAllWindows()
# # Undistorting an image
# In[5]:
undist_array = []
def cal_undistort(img, objpoints, imgpoints):
# Use cv2.calibrateCamera() and cv2.undistort()
ret, mtx, dist, rvecs, tvecs = cv2.calibrateCamera(objpoints, imgpoints, img.shape[1::-1], None, None)
undist = cv2.undistort(img, mtx, dist, None, mtx)
return undist
test_images = glob.glob('test_images/test*.jpg')
#test_images_straight = glob.glob('test_images/straight_lines*.jpg')
#undistorted = cal_undistort(image, objpoints, imgpoints)
for fname in test_images:
image1 = mpimg.imread(fname)
undistorted = cal_undistort(image1, objpoints, imgpoints)
undist_array.append(undistorted)
plt.figure()
plt.imshow(undistorted)
print('\nThese images are undistorted')
# # Undistorted images to Binary
# In[6]:
binary_image_array = []
for image in undist_array:
#image = undistorted
#gray = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
hls = cv2.cvtColor(image, cv2.COLOR_RGB2HLS)
s_channel = hls[:,:,2]
gray = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
sobelx = cv2.Sobel(gray, cv2.CV_64F, 1, 0) # Take the derivative in x
abs_sobelx = np.absolute(sobelx) # Absolute x derivative to accentuate lines away from horizontal
scaled_sobel = np.uint8(255*abs_sobelx/np.max(abs_sobelx))
thresh_min = 20
thresh_max = 100
sxbinary = np.zeros_like(scaled_sobel)
sxbinary[(scaled_sobel >= thresh_min) & (scaled_sobel <= thresh_max)] = 1
s_thresh_min = 170
s_thresh_max = 255
s_binary = np.zeros_like(s_channel)
s_binary[(s_channel >= s_thresh_min) & (s_channel <= s_thresh_max)] = 1
color_binary = np.dstack(( np.zeros_like(sxbinary), sxbinary, s_binary)) * 255
combined_binary = np.zeros_like(sxbinary)
combined_binary[(s_binary == 1) | (sxbinary == 1)] = 1
binary_image_array.append(combined_binary)
plt.figure()
plt.imshow(combined_binary, cmap='gray')
# # Define important Parameters
# In[7]:
nx = 9 # the number of inside corners in x
ny = 6 # the number of inside corners in y
img_size = (image.shape[1], image.shape[0])
src = np.float32([[590,450],[687,450],[1100,720],[200,720]])
dst = np.float32([[300,0],[900,0],[900,720],[300,720]])
M = cv2.getPerspectiveTransform(src, dst)
M_inverse = cv2.getPerspectiveTransform(dst, src)
# # Perspective Transform on binary images
# In[8]:
def warper(img, src, dst):
# Compute and apply perpective transform
img_size = (img.shape[1], img.shape[0])
M = cv2.getPerspectiveTransform(src, dst)
warped = cv2.warpPerspective(img, M, img_size, flags=cv2.INTER_NEAREST) # keep same size as input image
return warped
# In[9]:
binary_warped_images = []
for img in binary_image_array:
warped_image = warper(img, src, dst)
#warped_image_gray = cv2.cvtColor(warped_image,cv2.COLOR_BGR2GRAY)
plt.figure()
plt.imshow(warped_image, cmap='gray')
binary_warped_images.append(warped_image)
# # Finding Lane Pixels and Sliding window
# In[10]:
def find_lane_pixels(binary_warped):
# Take a histogram of the bottom half of the image
histogram = np.sum(binary_warped[binary_warped.shape[0]//2:,:], axis=0)
# Create an output image to draw on and visualize the result
out_img = np.dstack((binary_warped, binary_warped, binary_warped))
# Find the peak of the left and right halves of the histogram
# These will be the starting point for the left and right lines
midpoint = np.int(histogram.shape[0]//2)
leftx_base = np.argmax(histogram[:midpoint])
rightx_base = np.argmax(histogram[midpoint:]) + midpoint
# HYPERPARAMETERS
# Choose the number of sliding windows
nwindows = 9
# Set the width of the windows +/- margin
margin = 100
# Set minimum number of pixels found to recenter window
minpix = 50
# Set height of windows - based on nwindows above and image shape
window_height = np.int(binary_warped.shape[0]//nwindows)
# Identify the x and y positions of all nonzero pixels in the image
nonzero = binary_warped.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
# Current positions to be updated later for each window in nwindows
leftx_current = leftx_base
rightx_current = rightx_base
# Create empty lists to receive left and right lane pixel indices
left_lane_inds = []
right_lane_inds = []
# Step through the windows one by one
for window in range(nwindows):
# Identify window boundaries in x and y (and right and left)
win_y_low = binary_warped.shape[0] - (window+1)*window_height
win_y_high = binary_warped.shape[0] - window*window_height
### TO-DO: Find the four below boundaries of the window ###
win_xleft_low = leftx_current - margin
win_xleft_high = leftx_current + margin
win_xright_low = rightx_current - margin
win_xright_high = rightx_current + margin
# Draw the windows on the visualization image
cv2.rectangle(out_img,(win_xleft_low,win_y_low),
(win_xleft_high,win_y_high),(0,255,0), 2)
cv2.rectangle(out_img,(win_xright_low,win_y_low),
(win_xright_high,win_y_high),(0,255,0), 2)
### TO-DO: Identify the nonzero pixels in x and y within the window ###
good_left_inds = ((nonzeroy >= win_y_low) & (nonzeroy < win_y_high) &
(nonzerox >= win_xleft_low) & (nonzerox < win_xleft_high)).nonzero()[0]
good_right_inds = ((nonzeroy >= win_y_low) & (nonzeroy < win_y_high) &
(nonzerox >= win_xright_low) & (nonzerox < win_xright_high)).nonzero()[0]
# Append these indices to the lists
left_lane_inds.append(good_left_inds)
right_lane_inds.append(good_right_inds)
### TO-DO: If you found > minpix pixels, recenter next window ###
### (`right` or `leftx_current`) on their mean position ###
if len(good_left_inds) > minpix:
leftx_current = np.int(np.mean(nonzerox[good_left_inds]))
if len(good_right_inds) > minpix:
rightx_current = np.int(np.mean(nonzerox[good_right_inds]))
# Concatenate the arrays of indices (previously was a list of lists of pixels)
try:
left_lane_inds = np.concatenate(left_lane_inds)
right_lane_inds = np.concatenate(right_lane_inds)
except ValueError:
# Avoids an error if the above is not implemented fully
pass
# Extract left and right line pixel positions
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
return leftx, lefty, rightx, righty, out_img
def fit_polynomial(binary_warped):
# Find our lane pixels first
leftx, lefty, rightx, righty, out_img = find_lane_pixels(binary_warped)
# Fit a second order polynomial to each using `np.polyfit`
left_fit = np.polyfit(lefty, leftx, 2)
right_fit = np.polyfit(righty, rightx, 2)
# Generate x and y values for plotting
ploty = np.linspace(0, binary_warped.shape[0]-1, binary_warped.shape[0] )
try:
left_fitx = left_fit[0]*ploty**2 + left_fit[1]*ploty + left_fit[2]
right_fitx = right_fit[0]*ploty**2 + right_fit[1]*ploty + right_fit[2]
except TypeError:
# Avoids an error if `left` and `right_fit` are still none or incorrect
print('The function failed to fit a line!')
left_fitx = 1*ploty**2 + 1*ploty
right_fitx = 1*ploty**2 + 1*ploty
## Visualization ##
# Colors in the left and right lane regions
out_img[lefty, leftx] = [255, 0, 0]
out_img[righty, rightx] = [0, 0, 255]
# Plots the left and right polynomials on the lane lines
plt.plot(left_fitx, ploty, color='yellow')
plt.plot(right_fitx, ploty, color='yellow')
return out_img
#out_img = fit_polynomial(binary_warped_images[0])
#plt.imshow(out_img)
for img in binary_warped_images:
result = fit_polynomial(img)
plt.imshow(result)
plt.figure()
# # Radius of curvature
# In[11]:
def measure_radius_of_curvature(x_values, binary_warped):
num_rows = binary_warped.shape[0]
ym_per_pix = 30/720 # meters per pixel in y dimension
xm_per_pix = 3.7/700 # meters per pixel in x dimension
# If no pixels were found return None
y_points = np.linspace(0, num_rows-1, num_rows)
y_eval = np.max(y_points)
# Fit new polynomials to x,y in world space
fit_cr = np.polyfit(y_points*ym_per_pix, x_values*xm_per_pix, 2)
curverad = ((1 + (2*fit_cr[0]*y_eval*ym_per_pix + fit_cr[1])**2)**1.5) / np.absolute(2*fit_cr[0])
return curverad
# # Finding the Lines
# In[12]:
final_lane_images = []
left_fit = np.array([ 2.13935315e-04, -3.77507980e-01, 4.76902175e+02])
right_fit = np.array([4.17622148e-04, -4.93848953e-01, 1.11806170e+03])
def fit_poly(img_shape, leftx, lefty, rightx, righty):
### TO-DO: Fit a second order polynomial to each with np.polyfit() ###
left_fit = np.polyfit(lefty, leftx, 2)
right_fit = np.polyfit(righty, rightx, 2)
# Generate x and y values for plotting
ploty = np.linspace(0, img_shape[0]-1, img_shape[0])
### TO-DO: Calc both polynomials using ploty, left_fit and right_fit ###
left_fitx = left_fit[0]*ploty**2 + left_fit[1]*ploty + left_fit[2]
right_fitx = right_fit[0]*ploty**2 + right_fit[1]*ploty + right_fit[2]
return left_fitx, right_fitx, ploty
def search_around_poly(binary_warped):
# HYPERPARAMETER
# Choose the width of the margin around the previous polynomial to search
# The quiz grader expects 100 here, but feel free to tune on your own!
margin = 100
# Grab activated pixels
nonzero = binary_warped.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
### TO-DO: Set the area of search based on activated x-values ###
### within the +/- margin of our polynomial function ###
### Hint: consider the window areas for the similarly named variables ###
### in the previous quiz, but change the windows to our new search area ###
left_lane_inds = ((nonzerox > (left_fit[0]*(nonzeroy**2) + left_fit[1]*nonzeroy +
left_fit[2] - margin)) & (nonzerox < (left_fit[0]*(nonzeroy**2) +
left_fit[1]*nonzeroy + left_fit[2] + margin)))
right_lane_inds = ((nonzerox > (right_fit[0]*(nonzeroy**2) + right_fit[1]*nonzeroy +
right_fit[2] - margin)) & (nonzerox < (right_fit[0]*(nonzeroy**2) +
right_fit[1]*nonzeroy + right_fit[2] + margin)))
# Again, extract left and right line pixel positions
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
# Fit new polynomials
left_fitx, right_fitx, ploty = fit_poly(binary_warped.shape, leftx, lefty, rightx, righty)
left_curve_radius = measure_radius_of_curvature(left_fitx, binary_warped)
right_curve_radius = measure_radius_of_curvature(right_fitx, binary_warped)
average_curve_radius = (left_curve_radius + right_curve_radius)/2
curvature_string = "Radius of curvature: %.2f m" % average_curve_radius
print(curvature_string)
road_center = (right_fitx[719] + left_fitx[719])/2
xm_per_pix = 3.7/700 # meters per pixel in x dimension
center_offset_pixels = abs(img_size[0]/2 - road_center)
center_offset_meters = xm_per_pix*center_offset_pixels
offset = "Center offset: %.2f m" % center_offset_meters
print(offset)
## Visualization ##
# Create an image to draw on and an image to show the selection window
out_img = np.dstack((binary_warped, binary_warped, binary_warped))*255
window_img = np.zeros_like(out_img)
# Color in left and right line pixels
out_img[nonzeroy[left_lane_inds], nonzerox[left_lane_inds]] = [255, 0, 0]
out_img[nonzeroy[right_lane_inds], nonzerox[right_lane_inds]] = [0, 0, 255]
# Generate a polygon to illustrate the search window area
# And recast the x and y points into usable format for cv2.fillPoly()
left_line_window1 = np.array([np.transpose(np.vstack([left_fitx-margin, ploty]))])
left_line_window2 = np.array([np.flipud(np.transpose(np.vstack([left_fitx+margin,
ploty])))])
left_line_pts = np.hstack((left_line_window1, left_line_window2))
right_line_window1 = np.array([np.transpose(np.vstack([right_fitx-margin, ploty]))])
right_line_window2 = np.array([np.flipud(np.transpose(np.vstack([right_fitx+margin,
ploty])))])
right_line_pts = np.hstack((right_line_window1, right_line_window2))
# Draw the lane onto the warped blank image
cv2.fillPoly(window_img, np.int_([left_line_pts]), (0,255, 0))
cv2.fillPoly(window_img, np.int_([right_line_pts]), (0,255, 0))
result = cv2.addWeighted(out_img, 1, window_img, 0.3, 0)
# Plot the polynomial lines onto the image
plt.plot(left_fitx, ploty, color='yellow')
plt.plot(right_fitx, ploty, color='yellow')
## End visualization steps ##
return result
# Run image through the pipeline
# Note that in your project, you'll also want to feed in the previous fits
for image in binary_warped_images:
result = search_around_poly(image)
final_lane_images.append(result)
plt.imshow(result)
plt.figure()
# # Calculating the radius and offset
# In[13]:
def radius_and_offset(image):
margin = 100
nonzero = image.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
left_lane_inds = ((nonzerox > (left_fit[0]*(nonzeroy**2) + left_fit[1]*nonzeroy +
left_fit[2] - margin)) & (nonzerox < (left_fit[0]*(nonzeroy**2) +
left_fit[1]*nonzeroy + left_fit[2] + margin)))
right_lane_inds = ((nonzerox > (right_fit[0]*(nonzeroy**2) + right_fit[1]*nonzeroy +
right_fit[2] - margin)) & (nonzerox < (right_fit[0]*(nonzeroy**2) +
right_fit[1]*nonzeroy + right_fit[2] + margin)))
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
left_fitx, right_fitx, ploty = fit_poly(image.shape, leftx, lefty, rightx, righty)
left_curve_radius = measure_radius_of_curvature(left_fitx, image)
right_curve_radius = measure_radius_of_curvature(right_fitx, image)
average_curve_radius = (left_curve_radius + right_curve_radius)/2
curvature_string = "Radius of curvature: %.2f m" % average_curve_radius
#print(curvature_string)
road_center = (right_fitx[719] + left_fitx[719])/2
xm_per_pix = 3.7/700 # meters per pixel in x dimension
center_offset_pixels = abs(img_size[0]/2 - road_center)
center_offset_meters = xm_per_pix*center_offset_pixels
offset = "Center offset: %.2f m" % center_offset_meters
#print(offset)
return curvature_string, offset
# # Lane Area over final image
# In[14]:
for image in binary_warped_images:
margin = 100
nonzero = image.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
left_lane_inds = ((nonzerox > (left_fit[0]*(nonzeroy**2) + left_fit[1]*nonzeroy +
left_fit[2] - margin)) & (nonzerox < (left_fit[0]*(nonzeroy**2) +
left_fit[1]*nonzeroy + left_fit[2] + margin)))
right_lane_inds = ((nonzerox > (right_fit[0]*(nonzeroy**2) + right_fit[1]*nonzeroy +
right_fit[2] - margin)) & (nonzerox < (right_fit[0]*(nonzeroy**2) +
right_fit[1]*nonzeroy + right_fit[2] + margin)))
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
# Fit new polynomials
left_fitx, right_fitx, ploty = fit_poly(image.shape, leftx, lefty, rightx, righty)
warp_zero = np.zeros_like(image).astype(np.uint8)
color_warp = np.dstack((warp_zero, warp_zero, warp_zero))
# Recast the x and y points into usable format for cv2.fillPoly()
pts_left = np.array([np.transpose(np.vstack([left_fitx, ploty]))])
pts_right = np.array([np.flipud(np.transpose(np.vstack([right_fitx, ploty])))])
pts = np.hstack((pts_left, pts_right))
# Draw the lane onto the warped blank image
cv2.fillPoly(color_warp, np.int_([pts]), (0,255, 0))
# Warp the blank back to original image space using inverse perspective matrix (Minv)
newwarp = cv2.warpPerspective(color_warp, M_inverse, img_size)
# Combine the result with the original image
result = cv2.addWeighted(undistorted, 1, newwarp, 0.3, 0)
plt.imshow(result)
# In[15]:
from moviepy.editor import VideoFileClip
from IPython.display import HTML
# # Pipeline
# In[16]:
def process_image(image):
# TODO: put your pipeline here
initial_image = np.copy(image)
undistorted = cal_undistort(initial_image, objpoints, imgpoints)
#gray = cv2.cvtColor(undistorted, cv2.COLOR_RGB2GRAY)
hls = cv2.cvtColor(undistorted, cv2.COLOR_RGB2HLS)
s_channel = hls[:,:,2]
gray = cv2.cvtColor(undistorted, cv2.COLOR_RGB2GRAY)
sobelx = cv2.Sobel(gray, cv2.CV_64F, 1, 0) # Take the derivative in x
abs_sobelx = np.absolute(sobelx) # Absolute x derivative to accentuate lines away from horizontal
scaled_sobel = np.uint8(255*abs_sobelx/np.max(abs_sobelx))
thresh_min = 20
thresh_max = 100
sxbinary = np.zeros_like(scaled_sobel)
sxbinary[(scaled_sobel >= thresh_min) & (scaled_sobel <= thresh_max)] = 1
s_thresh_min = 170
s_thresh_max = 255
s_binary = np.zeros_like(s_channel)
s_binary[(s_channel >= s_thresh_min) & (s_channel <= s_thresh_max)] = 1
color_binary = np.dstack(( np.zeros_like(sxbinary), sxbinary, s_binary)) * 255
combined_binary = np.zeros_like(sxbinary)
combined_binary[(s_binary == 1) | (sxbinary == 1)] = 1
warped_image = warper(combined_binary, src, dst)
#result = fit_polynomial(warped_image)
#result = search_around_poly(warped_image)
curvature_string, offset = radius_and_offset(warped_image)
#print(curvature_string)
#print(offset)
margin = 100
nonzero = warped_image.nonzero()
nonzeroy = np.array(nonzero[0])
nonzerox = np.array(nonzero[1])
left_lane_inds = ((nonzerox > (left_fit[0]*(nonzeroy**2) + left_fit[1]*nonzeroy +
left_fit[2] - margin)) & (nonzerox < (left_fit[0]*(nonzeroy**2) +
left_fit[1]*nonzeroy + left_fit[2] + margin)))
right_lane_inds = ((nonzerox > (right_fit[0]*(nonzeroy**2) + right_fit[1]*nonzeroy +
right_fit[2] - margin)) & (nonzerox < (right_fit[0]*(nonzeroy**2) +
right_fit[1]*nonzeroy + right_fit[2] + margin)))
leftx = nonzerox[left_lane_inds]
lefty = nonzeroy[left_lane_inds]
rightx = nonzerox[right_lane_inds]
righty = nonzeroy[right_lane_inds]
left_fitx, right_fitx, ploty = fit_poly(warped_image.shape, leftx, lefty, rightx, righty)
warp_zero = np.zeros_like(warped_image).astype(np.uint8)
color_warp = np.dstack((warp_zero, warp_zero, warp_zero))
pts_left = np.array([np.transpose(np.vstack([left_fitx, ploty]))])
pts_right = np.array([np.flipud(np.transpose( | np.vstack([right_fitx, ploty]) | numpy.vstack |
"""CBMA methods from the multilevel kernel density analysis (MKDA) family."""
import logging
import multiprocessing as mp
import numpy as np
from scipy import special
from statsmodels.sandbox.stats.multicomp import multipletests
from tqdm.auto import tqdm
from ... import references
from ...due import due
from ...stats import null_to_p, one_way, two_way
from ...transforms import p_to_z
from ...utils import use_memmap, vox2mm
from ..kernel import KDAKernel, MKDAKernel
from .base import CBMAEstimator, PairwiseCBMAEstimator
LGR = logging.getLogger(__name__)
@due.dcite(references.MKDA, description="Introduces MKDA.")
class MKDADensity(CBMAEstimator):
r"""Multilevel kernel density analysis- Density analysis.
Parameters
----------
kernel_transformer : :obj:`nimare.meta.kernel.KernelTransformer`, optional
Kernel with which to convolve coordinates from dataset. Default is
:class:`nimare.meta.kernel.MKDAKernel`.
null_method : {"approximate", "montecarlo"}, optional
Method by which to determine uncorrected p-values.
"approximate" is faster, but slightly less accurate.
"montecarlo" can be much slower, and is only slightly more accurate.
n_iters : int, optional
Number of iterations to use to define the null distribution.
This is only used if ``null_method=="montecarlo"``.
Default is 10000.
n_cores : :obj:`int`, optional
Number of cores to use for parallelization.
This is only used if ``null_method=="montecarlo"``.
If <=0, defaults to using all available cores.
Default is 1.
**kwargs
Keyword arguments. Arguments for the kernel_transformer can be assigned
here, with the prefix '\kernel__' in the variable name.
Notes
-----
The MKDA density algorithm is also implemented in MATLAB at
https://github.com/canlab/Canlab_MKDA_MetaAnalysis.
Available correction methods: :func:`MKDADensity.correct_fwe_montecarlo`
References
----------
* Wager, <NAME>., <NAME>, and <NAME>. "Meta-analysis
of functional neuroimaging data: current and future directions." Social
cognitive and affective neuroscience 2.2 (2007): 150-158.
https://doi.org/10.1093/scan/nsm015
"""
def __init__(
self,
kernel_transformer=MKDAKernel,
null_method="approximate",
n_iters=10000,
n_cores=1,
**kwargs,
):
if not (isinstance(kernel_transformer, MKDAKernel) or kernel_transformer == MKDAKernel):
LGR.warning(
f"The KernelTransformer being used ({kernel_transformer}) is not optimized "
f"for the {type(self).__name__} algorithm. "
"Expect suboptimal performance and beware bugs."
)
# Add kernel transformer attribute and process keyword arguments
super().__init__(kernel_transformer=kernel_transformer, **kwargs)
self.null_method = null_method
self.n_iters = n_iters
self.n_cores = n_cores
self.dataset = None
self.results = None
def _compute_weights(self, ma_values):
"""Determine experiment-wise weights per the conventional MKDA approach."""
# TODO: Incorporate sample-size and inference metadata extraction and
# merging into df.
# This will need to be distinct from the kernel_transformer-based kind
# done in CBMAEstimator._preprocess_input
ids_df = self.inputs_["coordinates"].groupby("id").first()
n_exp = len(ids_df)
# Default to unit weighting for missing inference or sample size
if "inference" not in ids_df.columns:
ids_df["inference"] = "rfx"
if "sample_size" not in ids_df.columns:
ids_df["sample_size"] = 1.0
n = ids_df["sample_size"].astype(float).values
inf = ids_df["inference"].map({"ffx": 0.75, "rfx": 1.0}).values
weight_vec = n_exp * ((np.sqrt(n) * inf) / np.sum(np.sqrt(n) * inf))
weight_vec = weight_vec[:, None]
assert weight_vec.shape[0] == ma_values.shape[0]
return weight_vec
def _compute_summarystat(self, ma_values):
# Note: .dot should be faster, but causes multiprocessing to stall
# on some (Mac) architectures. If this is ever resolved, we can
# replace with the commented line.
# return ma_values.T.dot(self.weight_vec_).ravel()
weighted_ma_vals = ma_values * self.weight_vec_
return weighted_ma_vals.sum(0)
def _determine_histogram_bins(self, ma_maps):
"""Determine histogram bins for null distribution methods.
Parameters
----------
ma_maps
Notes
-----
This method adds one entry to the null_distributions_ dict attribute: "histogram_bins".
"""
if isinstance(ma_maps, list):
ma_values = self.masker.transform(ma_maps)
elif isinstance(ma_maps, np.ndarray):
ma_values = ma_maps.copy()
else:
raise ValueError(f"Unsupported data type '{type(ma_maps)}'")
prop_active = ma_values.mean(1)
self.null_distributions_["histogram_bins"] = np.arange(len(prop_active) + 1, step=1)
def _compute_null_approximate(self, ma_maps):
"""Compute uncorrected null distribution using approximate solution.
Parameters
----------
ma_maps : list of imgs or numpy.ndarray
MA maps.
Notes
-----
This method adds two entries to the null_distributions_ dict attribute:
"histogram_bins" and "histogram_weights".
"""
if isinstance(ma_maps, list):
ma_values = self.masker.transform(ma_maps)
elif isinstance(ma_maps, np.ndarray):
ma_values = ma_maps.copy()
else:
raise ValueError(f"Unsupported data type '{type(ma_maps)}'")
# MKDA maps are binary, so we only have k + 1 bins in the final
# histogram, where k is the number of studies. We can analytically
# compute the null distribution by convolution.
prop_active = ma_values.mean(1)
ss_hist = 1.0
for exp_prop in prop_active:
ss_hist = np.convolve(ss_hist, [1 - exp_prop, exp_prop])
self.null_distributions_["histogram_bins"] = np.arange(len(prop_active) + 1, step=1)
self.null_distributions_["histweights_corr-none_method-approximate"] = ss_hist
@due.dcite(references.MKDA, description="Introduces MKDA.")
class MKDAChi2(PairwiseCBMAEstimator):
r"""Multilevel kernel density analysis- Chi-square analysis.
.. versionchanged:: 0.0.8
* [REF] Use saved MA maps, when available.
Parameters
----------
kernel_transformer : :obj:`nimare.meta.kernel.KernelTransformer`, optional
Kernel with which to convolve coordinates from dataset. Default is
:class:`nimare.meta.kernel.MKDAKernel`.
prior : float, optional
Uniform prior probability of each feature being active in a map in
the absence of evidence from the map. Default: 0.5
**kwargs
Keyword arguments. Arguments for the kernel_transformer can be assigned
here, with the prefix '\kernel__' in the variable name.
Notes
-----
The MKDA Chi-square algorithm was originally implemented as part of the Neurosynth Python
library (https://github.com/neurosynth/neurosynth).
Available correction methods: :func:`MKDAChi2.correct_fwe_montecarlo`,
:obj:`MKDAChi2.correct_fdr_bh`
References
----------
* Wager, <NAME>., <NAME>, and <NAME>. "Meta-analysis
of functional neuroimaging data: current and future directions." Social
cognitive and affective neuroscience 2.2 (2007): 150-158.
https://doi.org/10.1093/scan/nsm015
"""
def __init__(self, kernel_transformer=MKDAKernel, prior=0.5, **kwargs):
if not (isinstance(kernel_transformer, MKDAKernel) or kernel_transformer == MKDAKernel):
LGR.warning(
f"The KernelTransformer being used ({kernel_transformer}) is not optimized "
f"for the {type(self).__name__} algorithm. "
"Expect suboptimal performance and beware bugs."
)
# Add kernel transformer attribute and process keyword arguments
super().__init__(kernel_transformer=kernel_transformer, **kwargs)
self.prior = prior
@use_memmap(LGR, n_files=2)
def _fit(self, dataset1, dataset2):
self.dataset1 = dataset1
self.dataset2 = dataset2
self.masker = self.masker or dataset1.masker
self.null_distributions_ = {}
ma_maps1 = self._collect_ma_maps(
maps_key="ma_maps1",
coords_key="coordinates1",
fname_idx=0,
)
ma_maps2 = self._collect_ma_maps(
maps_key="ma_maps2",
coords_key="coordinates2",
fname_idx=1,
)
# Calculate different count variables
n_selected = ma_maps1.shape[0]
n_unselected = ma_maps2.shape[0]
n_mappables = n_selected + n_unselected
n_selected_active_voxels = np.sum(ma_maps1, axis=0)
n_unselected_active_voxels = np.sum(ma_maps2, axis=0)
# Remove large arrays
del ma_maps1, ma_maps2
# Nomenclature for variables below: p = probability,
# F = feature present, g = given, U = unselected, A = activation.
# So, e.g., pAgF = p(A|F) = probability of activation
# in a voxel if we know that the feature is present in a study.
pF = n_selected / n_mappables
pA = np.array(
(n_selected_active_voxels + n_unselected_active_voxels) / n_mappables
).squeeze()
# Conditional probabilities
pAgF = n_selected_active_voxels / n_selected
pAgU = n_unselected_active_voxels / n_unselected
pFgA = pAgF * pF / pA
# Recompute conditionals with uniform prior
pAgF_prior = self.prior * pAgF + (1 - self.prior) * pAgU
pFgA_prior = pAgF * self.prior / pAgF_prior
# One-way chi-square test for consistency of activation
pAgF_chi2_vals = one_way(np.squeeze(n_selected_active_voxels), n_selected)
pAgF_p_vals = special.chdtrc(1, pAgF_chi2_vals)
pAgF_sign = np.sign(n_selected_active_voxels - np.mean(n_selected_active_voxels))
pAgF_z = p_to_z(pAgF_p_vals, tail="two") * pAgF_sign
# Two-way chi-square for specificity of activation
cells = np.squeeze(
np.array(
[
[n_selected_active_voxels, n_unselected_active_voxels],
[
n_selected - n_selected_active_voxels,
n_unselected - n_unselected_active_voxels,
],
]
).T
)
pFgA_chi2_vals = two_way(cells)
pFgA_p_vals = special.chdtrc(1, pFgA_chi2_vals)
pFgA_p_vals[pFgA_p_vals < 1e-240] = 1e-240
pFgA_sign = np.sign(pAgF - pAgU).ravel()
pFgA_z = p_to_z(pFgA_p_vals, tail="two") * pFgA_sign
images = {
"prob_desc-A": pA,
"prob_desc-AgF": pAgF,
"prob_desc-FgA": pFgA,
("prob_desc-AgF_given_pF=%0.2f" % self.prior): pAgF_prior,
("prob_desc-FgA_given_pF=%0.2f" % self.prior): pFgA_prior,
"z_desc-consistency": pAgF_z,
"z_desc-specificity": pFgA_z,
"chi2_desc-consistency": pAgF_chi2_vals,
"chi2_desc-specificity": pFgA_chi2_vals,
"p_desc-consistency": pAgF_p_vals,
"p_desc-specificity": pFgA_p_vals,
}
return images
def _run_fwe_permutation(self, params):
iter_df1, iter_df2, iter_xyz1, iter_xyz2 = params
iter_xyz1 = np.squeeze(iter_xyz1)
iter_xyz2 = np.squeeze(iter_xyz2)
iter_df1[["x", "y", "z"]] = iter_xyz1
iter_df2[["x", "y", "z"]] = iter_xyz2
temp_ma_maps1 = self.kernel_transformer.transform(
iter_df1, self.masker, return_type="array"
)
n_selected = temp_ma_maps1.shape[0]
n_selected_active_voxels = np.sum(temp_ma_maps1, axis=0)
del temp_ma_maps1
temp_ma_maps2 = self.kernel_transformer.transform(
iter_df2, self.masker, return_type="array"
)
n_unselected = temp_ma_maps2.shape[0]
n_unselected_active_voxels = | np.sum(temp_ma_maps2, axis=0) | numpy.sum |
import numpy
import pyaudio
import threading
class SwhRecorder:
"""Simple, cross-platform class to record from the microphone."""
MAX_FREQUENCY = 5000 # sounds above this are just annoying
MIN_FREQUENCY = 16 # can't hear anything less than this
def __init__(self, buckets=300, min_freq=16, max_freq=5000):
"""minimal garb is executed when class is loaded."""
self.buckets = buckets
self.MIN_FREQUENCY = min_freq
self.MAX_FREQUENCY = max_freq
self.p = pyaudio.PyAudio()
self.input_device = self.p.get_default_input_device_info()
self.secToRecord = 0.08
self.RATE = int(self.input_device['defaultSampleRate'])
self.BUFFERSIZE = int(self.secToRecord * self.RATE) # should be a power of 2 and at least double buckets
self.threadsDieNow = False
self.newData = False
self.buckets_within_frequency = (self.MAX_FREQUENCY * self.BUFFERSIZE) / self.RATE
self.buckets_per_final_bucket = max(int(self.buckets_within_frequency / buckets), 1)
self.buckets_below_frequency = int((self.MIN_FREQUENCY * self.BUFFERSIZE) / self.RATE)
self.buffersToRecord = int(self.RATE * self.secToRecord / self.BUFFERSIZE)
if self.buffersToRecord == 0:
self.buffersToRecord = 1
def setup(self):
"""initialize sound card."""
self.inStream = self.p.open(
format=pyaudio.paInt16,
channels=1,
rate=self.RATE,
input=True,
frames_per_buffer=self.BUFFERSIZE,
input_device_index=self.input_device['index'])
self.audio = numpy.empty((self.buffersToRecord * self.BUFFERSIZE), dtype=numpy.int16)
def close(self):
"""cleanly back out and release sound card."""
self.continuousEnd()
self.inStream.stop_stream()
self.inStream.close()
self.p.terminate()
def getAudio(self):
"""get a single buffer size worth of audio."""
audioString = self.inStream.read(self.BUFFERSIZE)
return numpy.fromstring(audioString, dtype=numpy.int16)
def record(self, forever=True):
"""record secToRecord seconds of audio."""
while True:
if self.threadsDieNow:
break
for i in range(self.buffersToRecord):
try:
audio = self.getAudio()
self.audio[i * self.BUFFERSIZE:(i + 1) * self.BUFFERSIZE] = audio
except: #OSError input overflowed
print('OSError: input overflowed')
self.newData = True
if forever is False:
break
def continuousStart(self):
"""CALL THIS to start running forever."""
self.t = threading.Thread(target=self.record)
self.t.start()
def continuousEnd(self):
"""shut down continuous recording."""
self.threadsDieNow = True
if hasattr(self, 't') and self.t:
self.t.join()
def fft(self):
if not self.newData:
return None
data = self.audio.flatten()
self.newData = False
left, right = numpy.split(numpy.abs(numpy.fft.fft(data)), 2)
ys = numpy.add(left, right[::-1]) # don't lose power, add negative to positive
ys = ys[self.buckets_below_frequency:]
# Shorten to requested number of buckets within MAX_FREQUENCY
final = numpy.copy(ys[::self.buckets_per_final_bucket])
final_size = len(final)
for i in range(1, self.buckets_per_final_bucket):
data_to_combine = numpy.copy(ys[i::self.buckets_per_final_bucket])
data_to_combine.resize(final_size)
final = | numpy.add(final, data_to_combine) | numpy.add |
#!/usr/bin/env python
# -*- coding: utf-8 -*-
"""
Chromatic Adaptation Transforms
===============================
Defines various chromatic adaptation transforms (CAT) and objects to
calculate the chromatic adaptation matrix between two given *CIE XYZ*
colourspace matrices:
- :attr:`XYZ_SCALING_CAT`: *XYZ Scaling* CAT [1]_
- :attr:`BRADFORD_CAT`: *Bradford* CAT [1]_
- :attr:`VON_KRIES_CAT`: *Von Kries* CAT [1]_
- :attr:`FAIRCHILD_CAT`: *Fairchild* CAT [2]_
- :attr:`CAT02_CAT`: *CAT02* CAT [3]_
See Also
--------
`Chromatic Adaptation Transforms IPython Notebook
<http://nbviewer.ipython.org/github/colour-science/colour-ipython/blob/master/notebooks/adaptation/cat.ipynb>`_ # noqa
References
----------
.. [1] http://brucelindbloom.com/Eqn_ChromAdapt.html
.. [2] http://rit-mcsl.org/fairchild//files/FairchildYSh.zip
.. [3] http://en.wikipedia.org/wiki/CIECAM02#CAT02
"""
from __future__ import division, unicode_literals
import numpy as np
from colour.utilities import CaseInsensitiveMapping
__author__ = 'Colour Developers'
__copyright__ = 'Copyright (C) 2013 - 2014 - Colour Developers'
__license__ = 'New BSD License - http://opensource.org/licenses/BSD-3-Clause'
__maintainer__ = 'Colour Developers'
__email__ = '<EMAIL>'
__status__ = 'Production'
__all__ = ['XYZ_SCALING_CAT',
'BRADFORD_CAT',
'VON_KRIES_CAT',
'FAIRCHILD_CAT',
'CAT02_CAT',
'CAT02_INVERSE_CAT',
'CHROMATIC_ADAPTATION_METHODS',
'chromatic_adaptation_matrix']
XYZ_SCALING_CAT = np.array(np.identity(3)).reshape((3, 3))
"""
*XYZ Scaling* chromatic adaptation transform. [1]_
XYZ_SCALING_CAT : array_like, (3, 3)
"""
BRADFORD_CAT = np.array(
[[0.8951000, 0.2664000, -0.1614000],
[-0.7502000, 1.7135000, 0.0367000],
[0.0389000, -0.0685000, 1.0296000]])
"""
*Bradford* chromatic adaptation transform. [1]_
BRADFORD_CAT : array_like, (3, 3)
"""
VON_KRIES_CAT = np.array(
[[0.4002400, 0.7076000, -0.0808100],
[-0.2263000, 1.1653200, 0.0457000],
[0.0000000, 0.0000000, 0.9182200]])
"""
*<NAME>* chromatic adaptation transform. [1]_
VON_KRIES_CAT : array_like, (3, 3)
"""
FAIRCHILD_CAT = np.array(
[[.8562, .3372, -.1934],
[-.8360, 1.8327, .0033],
[.0357, -.0469, 1.0112]])
"""
*Fairchild* chromatic adaptation transform. [2]_
FAIRCHILD_CAT : array_like, (3, 3)
"""
CAT02_CAT = np.array(
[[0.7328, 0.4296, -0.1624],
[-0.7036, 1.6975, 0.0061],
[0.0030, 0.0136, 0.9834]])
"""
*CAT02* chromatic adaptation transform. [3]_
CAT02_CAT : array_like, (3, 3)
"""
CAT02_INVERSE_CAT = np.linalg.inv(CAT02_CAT)
"""
Inverse *CAT02* chromatic adaptation transform. [3]_
CAT02_INVERSE_CAT : array_like, (3, 3)
"""
CHROMATIC_ADAPTATION_METHODS = CaseInsensitiveMapping(
{'XYZ Scaling': XYZ_SCALING_CAT,
'Bradford': BRADFORD_CAT,
'<NAME>': VON_KRIES_CAT,
'Fairchild': FAIRCHILD_CAT,
'CAT02': CAT02_CAT})
"""
Supported chromatic adaptation transform methods.
CHROMATIC_ADAPTATION_METHODS : dict
('XYZ Scaling', 'Bradford', '<NAME>', 'Fairchild, 'CAT02')
"""
def chromatic_adaptation_matrix(XYZ1, XYZ2, method='CAT02'):
"""
Returns the *chromatic adaptation* matrix from given source and target
*CIE XYZ* colourspace *array_like* variables.
Parameters
----------
XYZ1 : array_like, (3,)
*CIE XYZ* source *array_like* variable.
XYZ2 : array_like, (3,)
*CIE XYZ* target *array_like* variable.
method : unicode, optional
('XYZ Scaling', 'Bradford', '<NAME>', 'Fairchild, 'CAT02'),
Chromatic adaptation method.
Returns
-------
ndarray, (3, 3)
Chromatic adaptation matrix.
Raises
------
KeyError
If chromatic adaptation method is not defined.
References
----------
.. [4] http://brucelindbloom.com/Eqn_ChromAdapt.html
(Last accessed 24 February 2014)
Examples
--------
>>> XYZ1 = np.array([1.09923822, 1.000, 0.35445412])
>>> XYZ2 = np.array([0.96907232, 1.000, 1.121792157])
>>> chromatic_adaptation_matrix(XYZ1, XYZ2) # doctest: +ELLIPSIS
array([[ 0.8714561..., -0.1320467..., 0.4039483...],
[-0.0963880..., 1.0490978..., 0.160403... ],
[ 0.0080207..., 0.0282636..., 3.0602319...]])
Using *Bradford* method:
>>> XYZ1 = np.array([1.09923822, 1.000, 0.35445412])
>>> XYZ2 = np.array([0.96907232, 1.000, 1.121792157])
>>> method = 'Bradford'
>>> chromatic_adaptation_matrix(XYZ1, XYZ2, method) # doctest: +ELLIPSIS
array([[ 0.8518131..., -0.1134786..., 0.4124804...],
[-0.1277659..., 1.0928930..., 0.1341559...],
[ 0.0845323..., -0.1434969..., 3.3075309...]])
"""
method_matrix = CHROMATIC_ADAPTATION_METHODS.get(method)
if method_matrix is None:
raise KeyError(
'"{0}" chromatic adaptation method is not defined! Supported '
'methods: "{1}".'.format(method,
CHROMATIC_ADAPTATION_METHODS.keys()))
XYZ1, XYZ2 = np.ravel(XYZ1), | np.ravel(XYZ2) | numpy.ravel |
import numpy as np
import pandas as pd
import pytest
from sklearn.feature_selection import SelectKBest, chi2 as sk_chi2
from inz.utils import chi2, select_k_best, split, train_test_split
def test_split_list_int():
ints = list(range(7))
want = [[0, 1, 2], [3, 4, 5], [6]]
get = list(split(ints, 3))
assert len(get) == len(want)
assert get == want
def test_split_int():
ints = range(7)
want = [[0, 1, 2], [3, 4, 5], [6]]
get = list(split(ints, 3))
assert len(get) == len(want)
assert get == want
def test_split_list_int_greater_width():
ints = list(range(3))
want = [[0, 1, 2]]
get = list(split(ints, 4))
assert len(get) == len(want)
assert get == want
def test_split_list_str():
strings = list(map(str, range(6)))
want = [['0', '1'], ['2', '3'], ['4', '5']]
get = list(split(strings, 2))
assert len(get) == len(want)
assert get == want
def test_str():
string = ''.join(map(str, range(6)))
want = [['0', '1'], ['2', '3'], ['4', '5']]
get = list(split(string, 2))
assert len(get) == len(want)
assert get == want
def test_split_ndarray_int():
array = np.arange(10, dtype=int).reshape(-1, 2)
want = [np.array([[0, 1], [2, 3]]),
np.array([[4, 5], [6, 7]]),
np.array([[8, 9]])]
get = list(split(array, 2))
assert len(get) == len(want)
for i, j in zip(get, want):
assert type(i) == type(j)
assert np.array_equal(i, j)
def test_split_generator_str():
strings = map(str, range(6))
want = [['0', '1'], ['2', '3'], ['4', '5']]
get = list(split(strings, 2))
assert len(get) == len(want)
assert get == want
def test_split_list_int_not_allow():
ints = list(range(7))
want = [[0, 1, 2], [3, 4, 5]]
get = list(split(ints, 3, False))
assert len(get) == len(want)
assert get == want
def test_split_list_int_greater_width_not_allow():
ints = list(range(3))
want = []
get = list(split(ints, 4, False))
assert len(get) == len(want)
assert get == want
def test_split_list_str_not_allow():
strings = list(map(str, range(6)))
want = [['0', '1'], ['2', '3'], ['4', '5']]
get = list(split(strings, 2, False))
assert len(get) == len(want)
assert get == want
def test_split_ndarray_int_not_allow():
array = np.arange(10, dtype=int).reshape(-1, 2)
want = [np.array([[0, 1], [2, 3]]),
np.array([[4, 5], [6, 7]])]
get = list(split(array, 2, False))
assert len(get) == len(want)
for i, j in zip(get, want):
assert type(i) == type(j)
assert np.array_equal(i, j)
def test_split_generator_str_not_allow():
strings = map(str, range(6))
want = [['0', '1'], ['2', '3'], ['4', '5']]
get = list(split(strings, 2, False))
assert len(get) == len(want)
assert get == want
@pytest.fixture
def data():
X = pd.read_csv('../../data/data.csv')
y = X.pop('Choroba')
return X.values, y.values
def test_chi2(data):
X, y = data
sk_val, _ = sk_chi2(X, y)
my_val = chi2(X, y)
np.testing.assert_equal(sk_val, my_val)
def test_select_k_best(data):
X, y = data
for i in range(1, 31):
sk_sup1 = SelectKBest(sk_chi2, i).fit(X, y).get_support()
sk_sup2 = SelectKBest(sk_chi2, i).fit(X, y).get_support(True)
my_sup1 = select_k_best(X, y, k=i)
my_sup2 = select_k_best(X, y, k=i, indices=True)
np.testing.assert_equal(sk_sup1, my_sup1, str(i))
np.testing.assert_equal(sk_sup2, sorted(my_sup2), str(i))
def test_train_test_split():
x = np.arange(10)
get = train_test_split(x, shuffle=False)
want = [np.arange(7), np.arange(7, 10)]
for i in zip(get, want):
np.testing.assert_equal(*i)
def test_train_test_split5():
x = np.arange(10)
get = train_test_split(x, test_size=.5, shuffle=False)
want = [np.arange(5), | np.arange(5, 10) | numpy.arange |
#!/usr/bin/env python
# MIT License
#
# Copyright (c) 2019 <NAME>
#
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
#
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
#
# This file is a part of the CIRN Interaction Language.
from __future__ import print_function, division
import logging
from os import path
import math
import numpy as np
from .reservation_reader import ReservationReader
from .observer_reader import ObserverReader
from .spectrum_grid import SpectrumGrid
from .usage_reader import UsageReader
from .geodata_reader import GeodataReader
class SpecEval(object):
"""
Creates statistics on spectrum usage.
"""
def __init__(self):
self.log = logging.getLogger('spec_eval')
# for the observer data
self.observer_dir = None
self.observer_config = None
self.observer_grid = None
self.observer_threshold = None
# for the CIL reports
self.gateway_pcap = None
self.gateway_stats = None
self.measured_voxels = []
self.forecast_voxels = []
# for the spec-val tool
self.gdf_file_name = None
self.gdf_file_report = None
self.gdf_file_shapes = None
# bounds of the spectrum region
self.time_start = None
self.time_stop = None
self.time_bins = None
self.freq_start = None
self.freq_stop = None
self.freq_bins = None
# numpy matrixes with duty cycles for each bin
self.observer_duty = None # from observer passband data
self.observer_quantized = None # from observer passband data, quantized to be true or false
self.measured_duty = None # from CIL past measurements
self.measured_quantized = None # from CIL past measurements, quantized to be true or false
self.forecast_duty = None # from CIL future forecasts
self.gdf_file_duty = None # from the spec-val tool
self.forecast_quantized = None # from CIL future forecasts, quantized to be true or false
# numpy matrices with quantized errors for each bin
self.measured_v_observer = None
self.forecast_v_observer = None
def read_observer_data(self, observer_dir):
self.log.info('Reading observer data from %s',
path.basename(observer_dir))
reader = ObserverReader(observer_dir)
reader.read_state_change()
reader.read_configs()
reader.read_pass_band_rf()
self.observer_dir = observer_dir
self.observer_config = reader.config
self.observer_grid = reader.grid
def read_usage_data(self, gateway_pcap, gateway_srn=None, incumbent_srn=None):
self.log.info('Reading usage data from %s',
path.basename(gateway_pcap))
reader = UsageReader(gateway_pcap, gateway_srn, incumbent_srn)
reader.read_messages()
self.gateway_pcap = gateway_pcap
self.gateway_stats = reader.stats
self.measured_voxels = reader.measured_past_voxels
self.forecast_voxels = reader.forecast_future_voxels
def read_gdf_data(self, gdf_file_name, rf_start_time):
self.log.info('Reading geodata frames from %s', gdf_file_name)
reader = GeodataReader(gdf_file_name, rf_start_time)
self.gdf_file_name = gdf_file_name
self.gdf_file_report = {
'filename': reader.stats['filename'],
'bbox': reader.stats['bbox'],
'geometry_types': reader.stats['geometry_types'],
}
self.gdf_file_shapes = reader.select_shapes()
def set_bounds(self, time_start, time_stop, time_bins, time_step,
freq_start, freq_stop, freq_bins, freq_step):
# use the bounds from the observer if not specified
time_start = time_start or self.observer_grid.time_start
time_stop = time_stop or self.observer_grid.time_stop
freq_start = freq_start or self.observer_grid.freq_start
freq_stop = freq_stop or self.observer_grid.freq_stop
# make sure that we have proper ranges
time_start = min(max(time_start, self.observer_grid.time_start),
self.observer_grid.time_stop)
time_stop = min(max(time_stop, self.observer_grid.time_start),
self.observer_grid.time_stop)
freq_start = min(max(freq_start, self.observer_grid.freq_start),
self.observer_grid.freq_stop)
freq_stop = min(max(freq_stop, self.observer_grid.freq_start),
self.observer_grid.freq_stop)
# override bins if step is specified
if time_step and (time_stop - time_start) > time_step > 0:
time_stop = time_start + \
math.floor((time_stop - time_start) / time_step) * time_step
time_bins = int(round((time_stop - time_start) / time_step))
if freq_step and (freq_stop - freq_start) > freq_step > 0:
freq_stop = freq_start + \
math.floor((freq_stop - freq_start) / freq_step) * freq_step
freq_bins = int(round((freq_stop - freq_start) / freq_step))
# save everything
self.time_start = time_start
self.time_stop = time_stop
self.time_bins = time_bins
self.freq_start = freq_start
self.freq_stop = freq_stop
self.freq_bins = freq_bins
self.log.info('Selected times: start %s stop %s',
time_start, time_stop)
self.log.info('Selected bins: time %s freq %s', time_bins, freq_bins)
def calculate_observer_duty_cylces(self, threshold):
self.log.info('Calculating observer duty cycles')
grid = self.observer_grid.threshold(threshold)
grid = grid.resample(
self.time_start, self.time_stop, self.time_bins,
self.freq_start, self.freq_stop, self.freq_bins)
self.observer_threshold = threshold
self.observer_duty = grid.data
self.observer_quantized = self.observer_grid.resample( self.time_start, self.time_stop, self.time_bins, self.freq_start, self.freq_stop, self.freq_bins, take_max=True ).data
self.observer_quantized[self.observer_quantized >= threshold] = 1
self.observer_quantized[self.observer_quantized < 1] = 0
def calculate_spectrum_usage_duty_cycles(self):
self.log.info('Calculating measured spectrum duty cycles')
grid = SpectrumGrid(
self.time_start, self.time_stop, self.time_bins,
self.freq_start, self.freq_stop, self.freq_bins)
for voxel in self.measured_voxels:
grid.add_voxel(voxel)
self.measured_duty = grid.data
self.measured_quantized = grid.data
self.measured_quantized[self.measured_quantized>0] = 1
self.log.info('Calculating forecast spectrum duty cycles')
grid = SpectrumGrid(
self.time_start, self.time_stop, self.time_bins,
self.freq_start, self.freq_stop, self.freq_bins)
for voxel in self.forecast_voxels:
grid.add_voxel(voxel)
self.forecast_duty = grid.data
self.forecast_quantized = grid.data ;
self.forecast_quantized[self.forecast_quantized>0] = 1
def calculate_gdf_file_duty_cycles(self):
self.log.info('Calculating geodata spectrum duty cycles')
grid = SpectrumGrid(
self.time_start, self.time_stop, self.time_bins,
self.freq_start, self.freq_stop, self.freq_bins)
for shape in self.gdf_file_shapes:
grid.add_shape(shape)
self.gdf_file_duty = grid.data
def get_diff_stat(self, diff):
return {
'above': float(np.average(np.maximum(diff, 0))),
'below': float(np.average(np.maximum(-diff, 0))),
'total': float(np.average(np.abs(diff)))
}
def get_diff_quant_stat(self, a, b):
above = a-b ;
below = -above ;
total = np.abs(a-b) ;
above[above<0] = 0 ;
below[below<0] = 0 ;
return {
'above': float(np.sum(above)/np.sum(b)),
'below': float(np.sum(below)/np.sum(b)),
'total': float(np.sum(total)/np.sum(b)),
}
def create_report(self):
self.log.info('Creating reports')
self.measured_v_observer = -1*self.measured_quantized - (self.observer_quantized*-5)
self.forecast_v_observer = -1*self.forecast_quantized - (self.observer_quantized*-5)
# fraction of spectrum where there is anything
observer_occupied = np.array(self.observer_duty > 0.0, dtype=float)
measured_occupied = np.array(self.measured_duty > 0.0, dtype=float)
forecast_occupied = np.array(self.forecast_duty > 0.0, dtype=float)
report = {
'bounds': {
'time_start': self.time_start,
'time_stop': self.time_stop,
'time_bins': self.time_bins,
'time_step': (self.time_stop - self.time_start) / self.time_bins,
'freq_start': self.freq_start,
'freq_stop': self.freq_stop,
'freq_bins': self.freq_bins,
'freq_step': (self.freq_stop - self.freq_start) / self.freq_bins,
},
'observer': {
'directory': path.basename(self.observer_dir),
'center_frequency': self.observer_config.get('center_frequency'),
'rf_bandwidth': self.observer_config.get('rf_bandwidth'),
'real_frame_len': self.observer_config.get('real_frame_len'),
'threshold': self.observer_threshold,
},
'gateway': {
'filename': path.basename(self.gateway_pcap),
'forecast_future_voxels': self.gateway_stats.get('forecast_future_voxels'),
'forecast_past_voxels': self.gateway_stats.get('forecast_past_voxels'),
'measured_future_voxels': self.gateway_stats.get('measured_future_voxels'),
'measured_past_voxels': self.gateway_stats.get('measured_past_voxels'),
'measured_past_duplicates': self.gateway_stats.get('measured_past_duplicates'),
},
'gdf-file': self.gdf_file_report,
'duty_cycles': {
'observer': float( | np.average(self.observer_duty) | numpy.average |
#!/usr/bin/env python
"""Acquisition script for Keysight E4990A."""
import argparse
import collections
import configparser
import datetime
import functools
import numbers
import pathlib
import shutil
import subprocess
import sys
import time
import traceback
import matplotlib.pyplot as pyplot
import numpy
import pyvisa
import scipy.io as scio
FILE_EXT = '.mat'
CONFIG_FILENAME_DEFAULT = 'e4990a.ini'
program_version = None
time_now = None
__version__ = '2.6'
class E4990AError(Exception):
"""Exception class for all errors raised in this module.
The `main` function has an exception handler for this class.
"""
def to_number(f, s):
"""Convert string to a number with format specified by `f`."""
if s is None:
return s
if isinstance(s, numbers.Number):
return f(float(s))
if ',' in s: # comma-separated values
return [f(float(i.strip())) for i in s.strip().split(',')]
return f(float(s.strip()))
def to_int(s):
"""Convert string to an integer."""
return to_number(int, s)
def to_float(s, precision=None):
"""Convert string to a float."""
if precision is not None:
f = functools.partial(round, ndigits=precision)
else:
f = lambda x: x
return to_number(f, s)
def resource_path(file_name):
"""Resolve filename within the PyInstaller executable."""
try:
base_path = pathlib.Path(sys._MEIPASS)
except AttributeError:
base_path = pathlib.Path('.').resolve()
return base_path.joinpath(file_name)
def acquire(filename, config_filename, fixture_compensation):
"""Read the configuration file, initiate communication with the
instrument and execute the sweep or fixture compensation.
"""
cfg = read_config(config_filename)
rm = pyvisa.ResourceManager()
print(rm.visalib)
if cfg.ip_address:
resource_name = f'TCPIP::{cfg.ip_address}::INSTR'
else:
resources = rm.list_resources('USB?*INSTR')
if not resources:
raise E4990AError("No USB instruments found")
if len(resources) > 1:
msg = "Multiple USB instruments found:\n"
for r in resources:
msg += ('\t' + r)
raise E4990AError(msg)
resource_name = resources[0]
print(f"Opening resource: {resource_name}")
try:
inst = rm.open_resource(resource_name)
except pyvisa.errors.VisaIOError as e:
raise E4990AError(f"{e}") from e
# Timeout must be longer than sweep interval.
inst.timeout = 15000
try:
if fixture_compensation:
run_fixture_compensation(inst, cfg)
else:
try:
run_sweep(inst, filename, cfg)
finally:
inst.write(':SOUR:BIAS:STAT OFF')
if cfg.plotting_enabled:
input("Press [ENTER] to exit\n")
finally:
inst.close()
rm.close()
def read_config(config_filename):
"""Parse the configuration file and return a named tuple of
configuration data.
"""
parser = configparser.ConfigParser()
ConfigBase = collections.namedtuple('ConfigBase', [
'ip_address',
'start_frequency',
'stop_frequency',
'number_of_points',
'segments',
'measurement_speed',
'number_of_sweep_averages',
'number_of_point_averages',
'oscillator_voltage',
'bias_voltage',
'number_of_intervals',
'interval_period',
'plotting_enabled'
])
class Configuration(ConfigBase):
"""Named tuple of configuration parameters."""
def print(self):
"""Print the configuration parameters."""
print("Acquisition parameters:")
if self.ip_address is not None:
print(f"\tIP address: {self.ip_address}")
if self.start_frequency is not None:
print(f"\tStart frequency: {self.start_frequency / 1e3:.3e} kHz")
if self.stop_frequency is not None:
print(f"\tStop frequency: {self.stop_frequency / 1e3:.3e} kHz")
if self.number_of_points is not None:
print(f"\tNumber of points: {self.number_of_points}")
if self.segments is not None:
print(f"\tSegments: {self.segments}")
print(f"\tMeasurement speed: {self.measurement_speed}")
print(f"\tNumber of sweep averages: {self.number_of_sweep_averages}")
print(f"\tNumber of point averages: {self.number_of_point_averages}")
print(f"\tOscillator voltage: {self.oscillator_voltage} Volts")
print(f"\tBias voltage: {self.bias_voltage} Volts")
print(f"\tNumber of intervals: {self.number_of_intervals}")
print(f"\tInterval period: {self.interval_period} seconds")
print(f"\tPlotting enabled: {self.plotting_enabled}")
parser.read(config_filename)
sweep_section = parser['sweep']
cfg = Configuration(
parser.get('resource', 'ip_address', fallback=None),
to_int(sweep_section.getfloat('start_frequency')),
to_int(sweep_section.getfloat('stop_frequency')),
sweep_section.getint('number_of_points'),
sweep_section.get('segments'),
sweep_section.getint('measurement_speed', fallback=1),
sweep_section.getint('number_of_sweep_averages', fallback=1),
sweep_section.getint('number_of_point_averages', fallback=1),
to_float(sweep_section.getfloat('oscillator_voltage'), 3),
to_float(sweep_section.getfloat('bias_voltage'), 3),
sweep_section.getint('number_of_intervals'),
sweep_section.getfloat('interval_period'),
parser.getboolean('plotting', 'enabled', fallback=True)
)
linear_sweep_params = \
(cfg.start_frequency, cfg.stop_frequency, cfg.number_of_points)
if cfg.segments and any(linear_sweep_params):
raise E4990AError(
"Configuration contains segmented and linear sweep parameters.\n"
"Define only segments or "
"start_frequency/stop_frequency/number_of_points.")
return cfg
def run_sweep(inst, filename, cfg):
"""Execute the sweep acquisition and save data to a MAT file."""
print(f"Acquisition program version: {program_version}")
idn = inst.query('*IDN?').strip()
print(idn)
opt = inst.query('*OPT?').strip()
print('Options installed:', opt)
cfg.print()
inst.write('*CLS')
def print_status(st):
return "ON" if st else "OFF"
fixture = inst.query(':SENS:FIXT:SEL?').strip()
print(f"Fixture: {fixture}")
print("Fixture compensation status:")
open_cmp_status = to_int(inst.query(':SENS1:CORR2:OPEN?'))
print(f"\tOpen fixture compensation: {print_status(open_cmp_status)}")
short_cmp_status = to_int(inst.query(':SENS1:CORR2:SHOR?'))
print(f"\tShort fixture compensation: {print_status(short_cmp_status)}")
query = functools.partial(inst.query_ascii_values, separator=',',
container=numpy.array)
number_of_points = configure_sweep_parameters(inst, cfg)
x = query(':SENS1:FREQ:DATA?')
# Check that compensation is valid for the sweep frequency range.
# Check the frequencies for the open compensation and assume that
# frequencies for the short compensation are the same.
fix_cmp_frequencies = query(':SENS1:CORR2:ZME:OPEN:FREQ?')
fix_cmp_number_of_points = to_int(inst.query(':SENS1:CORR2:ZME:OPEN:POIN?'))
if number_of_points != fix_cmp_number_of_points or \
not | numpy.array_equal(x, fix_cmp_frequencies) | numpy.array_equal |
"""
A module providing some utility functions regarding bezier path manipulation.
"""
import numpy as np
from math import sqrt
from matplotlib.path import Path
from operator import xor
import warnings
# some functions
def get_intersection(cx1, cy1, cos_t1, sin_t1,
cx2, cy2, cos_t2, sin_t2):
""" return a intersecting point between a line through (cx1, cy1)
and having angle t1 and a line through (cx2, cy2) and angle t2.
"""
# line1 => sin_t1 * (x - cx1) - cos_t1 * (y - cy1) = 0.
# line1 => sin_t1 * x + cos_t1 * y = sin_t1*cx1 - cos_t1*cy1
line1_rhs = sin_t1 * cx1 - cos_t1 * cy1
line2_rhs = sin_t2 * cx2 - cos_t2 * cy2
# rhs matrix
a, b = sin_t1, -cos_t1
c, d = sin_t2, -cos_t2
ad_bc = a*d-b*c
if ad_bc == 0.:
raise ValueError("Given lines do not intersect")
#rhs_inverse
a_, b_ = d, -b
c_, d_ = -c, a
a_, b_, c_, d_ = [k / ad_bc for k in [a_, b_, c_, d_]]
x = a_* line1_rhs + b_ * line2_rhs
y = c_* line1_rhs + d_ * line2_rhs
return x, y
def get_normal_points(cx, cy, cos_t, sin_t, length):
"""
For a line passing through (*cx*, *cy*) and having a angle *t*,
return locations of the two points located along its perpendicular line at the distance of *length*.
"""
if length == 0.:
return cx, cy, cx, cy
cos_t1, sin_t1 = sin_t, -cos_t
cos_t2, sin_t2 = -sin_t, cos_t
x1, y1 = length*cos_t1 + cx, length*sin_t1 + cy
x2, y2 = length*cos_t2 + cx, length*sin_t2 + cy
return x1, y1, x2, y2
## BEZIER routines
# subdividing bezier curve
# http://www.cs.mtu.edu/~shene/COURSES/cs3621/NOTES/spline/Bezier/bezier-sub.html
def _de_casteljau1(beta, t):
next_beta = beta[:-1] * (1-t) + beta[1:] * t
return next_beta
def split_de_casteljau(beta, t):
"""split a bezier segment defined by its controlpoints *beta*
into two separate segment divided at *t* and return their control points.
"""
beta = np.asarray(beta)
beta_list = [beta]
while True:
beta = _de_casteljau1(beta, t)
beta_list.append(beta)
if len(beta) == 1:
break
left_beta = [beta[0] for beta in beta_list]
right_beta = [beta[-1] for beta in reversed(beta_list)]
return left_beta, right_beta
def find_bezier_t_intersecting_with_closedpath(bezier_point_at_t, inside_closedpath,
t0=0., t1=1., tolerence=0.01):
""" Find a parameter t0 and t1 of the given bezier path which
bounds the intersecting points with a provided closed
path(*inside_closedpath*). Search starts from *t0* and *t1* and it
uses a simple bisecting algorithm therefore one of the end point
must be inside the path while the orther doesn't. The search stop
when |t0-t1| gets smaller than the given tolerence.
value for
- bezier_point_at_t : a function which returns x, y coordinates at *t*
- inside_closedpath : return True if the point is insed the path
"""
# inside_closedpath : function
start = bezier_point_at_t(t0)
end = bezier_point_at_t(t1)
start_inside = inside_closedpath(start)
end_inside = inside_closedpath(end)
if not xor(start_inside, end_inside):
raise ValueError("the segment does not seemed to intersect with the path")
while 1:
# return if the distance is smaller than the tolerence
if (start[0]-end[0])**2 + (start[1]-end[1])**2 < tolerence**2:
return t0, t1
# calculate the middle point
middle_t = 0.5*(t0+t1)
middle = bezier_point_at_t(middle_t)
middle_inside = inside_closedpath(middle)
if xor(start_inside, middle_inside):
t1 = middle_t
end = middle
end_inside = middle_inside
else:
t0 = middle_t
start = middle
start_inside = middle_inside
class BezierSegment:
"""
A simple class of a 2-dimensional bezier segment
"""
# Highrt order bezier lines can be supported by simplying adding
# correcponding values.
_binom_coeff = {1:np.array([1., 1.]),
2:np.array([1., 2., 1.]),
3:np.array([1., 3., 3., 1.])}
def __init__(self, control_points):
"""
*control_points* : location of contol points. It needs have a
shpae of n * 2, where n is the order of the bezier line. 1<=
n <= 3 is supported.
"""
_o = len(control_points)
self._orders = np.arange(_o)
_coeff = BezierSegment._binom_coeff[_o - 1]
_control_points = np.asarray(control_points)
xx = _control_points[:,0]
yy = _control_points[:,1]
self._px = xx * _coeff
self._py = yy * _coeff
def point_at_t(self, t):
"evaluate a point at t"
one_minus_t_powers = np.power(1.-t, self._orders)[::-1]
t_powers = np.power(t, self._orders)
tt = one_minus_t_powers * t_powers
_x = sum(tt * self._px)
_y = sum(tt * self._py)
return _x, _y
def split_bezier_intersecting_with_closedpath(bezier,
inside_closedpath,
tolerence=0.01):
"""
bezier : control points of the bezier segment
inside_closedpath : a function which returns true if the point is inside the path
"""
bz = BezierSegment(bezier)
bezier_point_at_t = bz.point_at_t
t0, t1 = find_bezier_t_intersecting_with_closedpath(bezier_point_at_t,
inside_closedpath,
tolerence=tolerence)
_left, _right = split_de_casteljau(bezier, (t0+t1)/2.)
return _left, _right
def find_r_to_boundary_of_closedpath(inside_closedpath, xy,
cos_t, sin_t,
rmin=0., rmax=1., tolerence=0.01):
"""
Find a radius r (centered at *xy*) between *rmin* and *rmax* at
which it intersect with the path.
inside_closedpath : function
cx, cy : center
cos_t, sin_t : cosine and sine for the angle
rmin, rmax :
"""
cx, cy = xy
def _f(r):
return cos_t*r + cx, sin_t*r + cy
find_bezier_t_intersecting_with_closedpath(_f, inside_closedpath,
t0=rmin, t1=rmax, tolerence=tolerence)
## matplotlib specific
def split_path_inout(path, inside, tolerence=0.01, reorder_inout=False):
""" divide a path into two segment at the point where inside(x, y)
becomes False.
"""
path_iter = path.iter_segments()
ctl_points, command = path_iter.next()
begin_inside = inside(ctl_points[-2:]) # true if begin point is inside
bezier_path = None
ctl_points_old = ctl_points
concat = np.concatenate
iold=0
i = 1
for ctl_points, command in path_iter:
iold=i
i += len(ctl_points)/2
if inside(ctl_points[-2:]) != begin_inside:
bezier_path = concat([ctl_points_old[-2:], ctl_points])
break
ctl_points_old = ctl_points
if bezier_path is None:
raise ValueError("The path does not seem to intersect with the patch")
bp = zip(bezier_path[::2], bezier_path[1::2])
left, right = split_bezier_intersecting_with_closedpath(bp,
inside,
tolerence)
if len(left) == 2:
codes_left = [Path.LINETO]
codes_right = [Path.MOVETO, Path.LINETO]
elif len(left) == 3:
codes_left = [Path.CURVE3, Path.CURVE3]
codes_right = [Path.MOVETO, Path.CURVE3, Path.CURVE3]
elif len(left) == 4:
codes_left = [Path.CURVE4, Path.CURVE4, Path.CURVE4]
codes_right = [Path.MOVETO, Path.CURVE4, Path.CURVE4, Path.CURVE4]
else:
raise ValueError()
verts_left = left[1:]
verts_right = right[:]
#i += 1
if path.codes is None:
path_in = Path(concat([path.vertices[:i], verts_left]))
path_out = Path(concat([verts_right, path.vertices[i:]]))
else:
path_in = Path(concat([path.vertices[:iold], verts_left]),
concat([path.codes[:iold], codes_left]))
path_out = Path(concat([verts_right, path.vertices[i:]]),
concat([codes_right, path.codes[i:]]))
if reorder_inout and begin_inside == False:
path_in, path_out = path_out, path_in
return path_in, path_out
def inside_circle(cx, cy, r):
r2 = r**2
def _f(xy):
x, y = xy
return (x-cx)**2 + (y-cy)**2 < r2
return _f
# quadratic bezier lines
def get_cos_sin(x0, y0, x1, y1):
dx, dy = x1-x0, y1-y0
d = (dx*dx + dy*dy)**.5
return dx/d, dy/d
def check_if_parallel(dx1, dy1, dx2, dy2, tolerence=1.e-5):
""" returns
* 1 if two lines are parralel in same direction
* -1 if two lines are parralel in opposite direction
* 0 otherwise
"""
theta1 = np.arctan2(dx1, dy1)
theta2 = np.arctan2(dx2, dy2)
dtheta = np.abs(theta1 - theta2)
if dtheta < tolerence:
return 1
elif | np.abs(dtheta - np.pi) | numpy.abs |
from enum import Enum
from types import SimpleNamespace
from typing import Any, Dict, List, Literal, Optional, Set, Tuple
import json
import matplotlib.pyplot as plt #type: ignore
import numpy as np
# ===============================================
# Colorama Filler
# ===============================================
try:
from colorama import Fore # type: ignore
except:
colors = ["RED", "YELLOW", "GREEN", "BLUE", "ORANGE", "MAGENTA", "CYAN"]
kwargs = {}
for col in colors:
kwargs[col] = ""
kwargs[f"LIGHT{col}_EX"]
Fore = SimpleNamespace(RESET="", **kwargs)
# ===============================================
# Data Types
# ===============================================
class DataType(Enum):
DISTRIBUTION = "distribution"
SEQUENTIAL = "sequential"
MAP = "map"
DISTRIBUTION_2D = "distribution_2d"
# ===============================================
# Metrics
# ===============================================
class Metrics:
def __init__(self) -> None:
self.metrics: Dict[str, Dict[str, Any]] = {}
self._auto_bins: List[str] = []
def new_data(self, name: str, type: DataType, auto_bins: bool = False, **kwargs):
entry = {
"type": type.value,
"data": [],
**kwargs
}
self.metrics[name] = entry
if auto_bins:
self._auto_bins.append(name)
def add(self, name: str, data: Any):
self.metrics[name]["data"].append(data)
def save(self, path: str = "metrics.json"):
for name in self._auto_bins:
self.auto_bins(name)
with open(path, "w") as fd:
json.dump(self.metrics, fd)
def auto_bins(self, name: str):
mini = min(self.metrics[name]["data"])
maxi = max(self.metrics[name]["data"])
self.metrics[name]["bins"] = list(range(mini, maxi + 2))
# ===============================================
# Globasl for Plotting
# ===============================================
StoredDataKey = Literal["data", "orientation", "type", "bins", "labels", "measure"]
StoredData = Dict[StoredDataKey, Any]
__OPTIONS_STR__ = "@"
__GRAPH_STR__ = "+"
__KWARGS_STR__ = "$"
__ALLOWED_KWARGS__ = ["logx", "logy", "loglog", "exp"]
__OPTIONS__ = ["sharex", "sharey", "sharexy", "grid"]
# ===============================================
# Utils for plotting
# ===============================================
def __optional_split__(text: str, split: str) -> List[str]:
if split in text:
return text.split(split)
return [text]
def __find_metrics_for__(metrics: Dict[str, Dict[StoredDataKey, Any]], prefix: str) -> List[str]:
candidates = list(metrics.keys())
for i, l in enumerate(prefix):
if l == "*":
return candidates
candidates = [cand for cand in candidates if len(
cand) > i and cand[i] == l]
if len(candidates) == 1:
return candidates
for cand in candidates:
if len(cand) == len(prefix):
return [cand]
return candidates
def __to_nice_name__(name: str) -> str:
return name.replace("_", " ").replace(".", " ").title()
def layout_for_subplots(nplots: int, screen_ratio: float = 16 / 9) -> Tuple[int, int]:
"""
Find a good number of rows and columns for organizing the specified number of subplots.
Parameters
-----------
- **nplots**: the number of subplots
- **screen_ratio**: the ratio width/height of the screen
Return
-----------
[nrows, ncols] for the sublopt layout.
It is guaranteed that ```nplots <= nrows * ncols```.
"""
nrows = int(np.floor(np.sqrt(nplots / screen_ratio)))
ncols = int(np.floor(nrows * screen_ratio))
# Increase size so that everything can fit
while nrows * ncols < nplots:
if nrows < ncols:
nrows += 1
elif ncols < nrows:
ncols += 1
else:
if screen_ratio >= 1:
ncols += 1
else:
nrows += 1
# If we can reduce the space, reduce it
while (nrows - 1) * ncols >= nplots and (ncols - 1) * nrows >= nplots:
if nrows > ncols:
nrows -= 1
elif nrows < ncols:
ncols -= 1
else:
if screen_ratio < 1:
ncols -= 1
else:
nrows -= 1
while (nrows - 1) * ncols >= nplots:
nrows -= 1
while (ncols - 1) * nrows >= nplots:
ncols -= 1
return nrows, ncols
# ===============================================
# Main function called
# ===============================================
def interactive_plot(metrics: Dict[str, Dict[StoredDataKey, Any]]):
while True:
print("Available data:", ", ".join([Fore.LIGHTYELLOW_EX + s + Fore.RESET for s in metrics.keys()]))
try:
query = input("Which metric do you want to plot?\n>" + Fore.LIGHTGREEN_EX)
print(Fore.RESET, end=" ")
except EOFError:
break
global_options = __optional_split__(query.lower().strip(), __OPTIONS_STR__)
choice = global_options.pop(0)
elements = __optional_split__(choice, __GRAPH_STR__)
things_with_options = [__get_graph_options__(x) for x in elements]
metrics_with_str_options = [(__find_metrics_for__(metrics, x), y) for x,y in things_with_options]
metrics_with_options = [(x, __parse_kwargs__(y))
for x, y in metrics_with_str_options if len(x) > 0]
if len(metrics_with_options) == 0:
print(Fore.RED + "No metrics found matching query:\'" +
Fore.LIGHTRED_EX + query + Fore.RED + "\'" + Fore.RESET)
continue
correctly_expanded_metrics_with_otpions: List[Tuple[List[str], Dict]] = []
for x, y in metrics_with_options:
if y["exp"]:
for el in x:
correctly_expanded_metrics_with_otpions.append(([el], y))
else:
correctly_expanded_metrics_with_otpions.append((x, y))
plt.figure()
__plot_all__(metrics, correctly_expanded_metrics_with_otpions, global_options)
plt.show()
# ===============================================
# Plot a list of metrics on the same graph
# ===============================================
def __plot_sequential__(metrics: Dict[str, Dict[StoredDataKey, Any]], metrics_name: List[str], logx: bool = False, logy: bool = False):
plt.xlabel("Time")
if logx:
if logy:
plt.loglog()
else:
plt.semilogx()
elif logy:
plt.semilogy()
for name in metrics_name:
nice_name = __to_nice_name__(name)
plt.plot(metrics[name]["data"], label=nice_name)
if len(metrics_name) > 1:
plt.legend()
else:
plt.ylabel(nice_name)
def __plot_distribution__(metrics: Dict[str, Dict[StoredDataKey, Any]], metrics_name: List[str], logx: bool = False, logy: bool = False):
# This may also contain maps
# We should convert distributions to maps
new_metrics: Dict[str, Dict[StoredDataKey, Any]] = {}
for name in metrics_name:
info = metrics[name]
# If a MAP no need to convert
if info["type"] == DataType.MAP:
new_metrics[name] = metrics[name]
continue
# Compute histogram
bins = info.get("bins", None) or "auto"
hist, edges = | np.histogram(metrics[name]["data"], bins=bins, density=True) | numpy.histogram |
'''plotting functions for Validation
input -- one or more Single_TractorCat() objects
-- ref is reference Single_TractorCat()
test is test ...
'''
import matplotlib
matplotlib.use('Agg') #display backend
import matplotlib.pyplot as plt
import os
import sys
import numpy as np
from scipy import stats as sp_stats
# Globals
class PlotKwargs(object):
def __init__(self):
self.ax= dict(fontweight='bold',fontsize='medium')
self.text=dict(fontweight='bold',fontsize='medium',va='top',ha='left')
self.leg=dict(frameon=True,fontsize='x-small')
self.save= dict(bbox_inches='tight',dpi=150)
kwargs= PlotKwargs()
##########
# Helpful functions for making plots
def bin_up(data_bin_by,data_for_percentile, bin_minmax=(18.,26.),nbins=20):
'''bins "data_for_percentile" into "nbins" using "data_bin_by" to decide how indices are assigned to bins
returns bin center,N,q25,50,75 for each bin
'''
bin_edges= np.linspace(bin_minmax[0],bin_minmax[1],num= nbins+1)
N= np.zeros(nbins)+np.nan
q25,q50,q75= N.copy(),N.copy(),N.copy()
for i,low,hi in zip(range(nbins-1), bin_edges[:-1],bin_edges[1:]):
keep= np.all((low <= data_bin_by,data_bin_by < hi),axis=0)
if np.where(keep)[0].size > 0:
N[i]= np.where(keep)[0].size
q25[i]= np.percentile(data_for_percentile[keep],q=25)
q50[i]= np.percentile(data_for_percentile[keep],q=50)
q75[i]= np.percentile(data_for_percentile[keep],q=75)
else:
# already initialized to nan
pass
return (bin_edges[1:]+bin_edges[:-1])/2.,N,q25,q50,q75
# Main plotting functions
def nobs(obj, name=''):
'''make histograms of nobs so can compare depths of g,r,z between the two catalogues
obj -- Single_TractorCat()'''
hi= np.max(obj.t['decam_nobs'][:,[1,2,4]])
fig,ax= plt.subplots(3,1)
for i, band,iband in zip(range(3),['g','r','z'],[1,2,4]):
ax[i].hist(obj.t['decam_nobs'][:,iband],\
bins=hi+1,normed=True,cumulative=True,align='mid')
xlab=ax[i].set_xlabel('nobs %s' % band, **kwargs.ax)
ylab=ax[i].set_ylabel('CDF', **kwargs.ax)
plt.savefig(os.path.join(obj.outdir,'nobs_%s.png' % name), \
bbox_extra_artists=[xlab,ylab], **kwargs.save)
plt.close()
def radec(obj,name=''):
'''ra,dec distribution of objects
obj -- Single_TractorCat()'''
plt.scatter(obj.t['ra'], obj.t['dec'], \
edgecolor='b',c='none',lw=1.)
xlab=plt.xlabel('RA', **kwargs.ax)
ylab=plt.ylabel('DEC', **kwargs.ax)
plt.savefig(os.path.join(obj.outdir,'radec_%s.png' % name), \
bbox_extra_artists=[xlab,ylab], **kwargs.save)
plt.close()
def hist_types(obj, name=''):
'''number of psf,exp,dev,comp, etc
obj -- Single_TractorCat()'''
types= ['PSF','SIMP','EXP','DEV','COMP']
# the x locations for the groups
ind = np.arange(len(types))
# the width of the bars
width = 0.35
ht= np.zeros(len(types),dtype=int)
for cnt,typ in enumerate(types):
# Mask to type desired
ht[cnt]= obj.number_not_masked(['current',typ.lower()])
# Plot
fig, ax = plt.subplots()
rects = ax.bar(ind, ht, width, color='b')
ylab= ax.set_ylabel("counts")
ax.set_xticks(ind + width)
ax.set_xticklabels(types)
plt.savefig(os.path.join(obj.outdir,'hist_types_%s.png' % name), \
bbox_extra_artists=[ylab], **kwargs.save)
plt.close()
def sn_vs_mag(obj, mag_minmax=(18.,26.),name=''):
'''plots Signal to Noise vs. mag for each band
obj -- Single_TractorCat()'''
min,max= mag_minmax
# Bin up SN values
bin_SN={}
for band,iband in zip(['g','r','z'],[1,2,4]):
bin_SN[band]={}
bin_SN[band]['binc'],N,bin_SN[band]['q25'],bin_SN[band]['q50'],bin_SN[band]['q75']=\
bin_up(obj.t['decam_mag'][:,iband], \
obj.t['decam_flux'][:,iband]*np.sqrt(obj.t['decam_flux_ivar'][:,iband]),\
bin_minmax=mag_minmax)
#setup plot
fig,ax=plt.subplots(1,3,figsize=(9,3),sharey=True)
plt.subplots_adjust(wspace=0.25)
#plot SN
for cnt,band,color in zip(range(3),['g','r','z'],['g','r','m']):
#horiz line at SN = 5
ax[cnt].plot([mag_minmax[0],mag_minmax[1]],[5,5],'k--',lw=2)
#data
ax[cnt].plot(bin_SN[band]['binc'], bin_SN[band]['q50'],c=color,ls='-',lw=2)
ax[cnt].fill_between(bin_SN[band]['binc'],bin_SN[band]['q25'],bin_SN[band]['q75'],color=color,alpha=0.25)
#labels
for cnt,band in zip(range(3),['g','r','z']):
ax[cnt].set_yscale('log')
xlab=ax[cnt].set_xlabel('%s' % band, **kwargs.ax)
ax[cnt].set_ylim(1,100)
ax[cnt].set_xlim(mag_minmax)
ylab=ax[0].set_ylabel('S/N', **kwargs.ax)
ax[2].text(26,5,'S/N = 5 ',**kwargs.text)
plt.savefig(os.path.join(obj.outdir,'sn_%s.png' % name), \
bbox_extra_artists=[xlab,ylab], **kwargs.save)
plt.close()
def create_confusion_matrix(ref_obj,test_obj):
'''compares MATCHED reference (truth) to test (prediction)
ref_obj,test_obj -- reference,test Single_TractorCat()
return 5x5 confusion matrix and colum/row names'''
cm=np.zeros((5,5))-1
types=['PSF','SIMP','EXP','DEV','COMP']
for i_ref,ref_type in enumerate(types):
cut_ref= np.where(ref_obj.t['type'] == ref_type)[0]
#n_ref= ref_obj.number_not_masked(['current',ref_type.lower()])
for i_test,test_type in enumerate(types):
n_test= np.where(test_obj.t['type'][ cut_ref ] == test_type)[0].size
if cut_ref.size > 0: cm[i_ref,i_test]= float(n_test)/cut_ref.size
else: cm[i_ref,i_test]= np.nan
return cm,types
def confusion_matrix(ref_obj,test_obj, name='',\
ref_name='ref',test_name='test'):
'''plot confusion matrix
ref_obj,test_obj -- reference,test Single_TractorCat()'''
cm,ticknames= create_confusion_matrix(ref_obj,test_obj)
plt.imshow(cm, interpolation='nearest', cmap=plt.cm.Blues,vmin=0,vmax=1)
cbar=plt.colorbar()
plt.xticks(range(len(ticknames)), ticknames)
plt.yticks(range(len(ticknames)), ticknames)
ylab=plt.ylabel('True (%s)' % ref_name, **kwargs.ax)
xlab=plt.xlabel('Predicted (%s)' % test_name, **kwargs.ax)
for row in range(len(ticknames)):
for col in range(len(ticknames)):
if np.isnan(cm[row,col]):
plt.text(col,row,'n/a',va='center',ha='center')
elif cm[row,col] > 0.5:
plt.text(col,row,'%.2f' % cm[row,col],va='center',ha='center',color='yellow')
else:
plt.text(col,row,'%.2f' % cm[row,col],va='center',ha='center',color='black')
plt.savefig(os.path.join(ref_obj.outdir,'confusion_matrix_%s.png' % name), \
bbox_extra_artists=[xlab,ylab], **kwargs.save)
plt.close()
def matched_dist(obj,dist, name=''):
'''dist -- array of distances in degress between matched objects'''
pixscale=dict(decam=0.25,bass=0.45)
# Plot
fig,ax=plt.subplots()
ax.hist(dist*3600,bins=50,color='b',align='mid')
ax2 = ax.twiny()
ax2.hist(dist*3600./pixscale['bass'],bins=50,color='g',align='mid',\
visible=False)
xlab= ax.set_xlabel("arcsec")
xlab= ax2.set_xlabel("pixels [BASS]")
ylab= ax.set_ylabel("Matched")
plt.savefig(os.path.join(obj.outdir,"separation_hist_%s.png" % name), \
bbox_extra_artists=[xlab,ylab], **kwargs.save)
plt.close()
def chi_v_gaussian(ref_obj,test_obj, low=-8.,hi=8., name=''):
# Compute Chi
chi={}
for band,iband in zip(['g','r','z'],[1,2,4]):
chi[band]= (ref_obj.t['decam_flux'][:,iband]-test_obj.t['decam_flux'][:,iband])/\
np.sqrt( | np.power(ref_obj.t['decam_flux_ivar'][:,iband],-1) | numpy.power |
# -*- coding: utf-8 -*-
"""
Created on Thu Aug 22 15:58:31 2019
@author: DaniJ
"""
import numpy as np
import scipy as sp
from bvp import solve_bvp
from scipy import linalg
#from four_layer_model_2try_withFixSpeciesOption_Scaling import four_layer_model_2try_withFixSpeciesOption_Scaling as flm
from matplotlib import pyplot as plt
'''
In this first try we will assume that the vector of unknowns is composed in the following order:
'''
def PB_and_fourlayermodel (T, X_guess, A, Z, log_k, idx_Aq, pos_psi_S1_vec, pos_psi_S2_vec, temp, sS1, aS1, sS2, aS2, e, CapacitancesS1, CapacitancesS2, d0,df, idx_fix_species = None, zel=1, tolerance_NR = 1e-6, max_iterations = 100,scalingRC = True, tol_PB=1e-6):
counter_iterations = 0
abs_err = tolerance_NR + 1
if idx_fix_species != None:
X_guess [idx_fix_species] = T [idx_fix_species]
tempv = (np.linspace(d0,df,100));
y_0 = np.zeros((2,tempv.shape[0]))
bvp_class = [y_0]
while abs_err>tolerance_NR and counter_iterations < max_iterations:
# Calculate Y
[Y, T, bvp_class] = func_NR_FLM (X_guess, A, log_k, temp, idx_Aq, sS1, aS1, sS2, aS2, e, CapacitancesS1, CapacitancesS2, T, Z, zel, pos_psi_S1_vec, pos_psi_S2_vec, d0,df, bvp_class, idx_fix_species,tol_PB)
# Calculate Z
J = Jacobian_NR_FLM (X_guess, A, log_k, temp, idx_Aq, sS1, aS1, sS2, aS2, e, CapacitancesS1, CapacitancesS2, T, Z, zel, pos_psi_S1_vec, pos_psi_S2_vec, d0,df, bvp_class, idx_fix_species,tol_PB)
# Scaling technique is the RC technique from "Thermodynamic Equilibrium Solutions Through a Modified Newton Raphson Method"-<NAME>, <NAME>, <NAME>, and <NAME> (2016)
if scalingRC == True:
D1 = diagonal_row(J)
D2 = diagonal_col(J)
J_new = np.matmul(D1,np.matmul(J, D2))
Y_new = np.matmul(D1, Y)
delta_X_new = linalg.solve(J_new,-Y_new)
delta_X = np.matmul(D2, delta_X_new)
else:
# Calculating the diff, Delta_X
delta_X = linalg.solve(J,-Y)
#print(delta_X))
# Relaxation factor borrow from <NAME> to avoid negative values
max_1 = 1
max_2 =np.amax(-2*np.multiply(delta_X, 1/X_guess))
Max_f = np.amax([max_1, max_2])
Del_mul = 1/Max_f
X_guess=X_guess + Del_mul*delta_X
#print(X_guess)
Xmod=X_guess.copy()
for i in range(len(X_guess)):
if X_guess[i]<=0:
Xmod[i]=1
log_C = log_k + np.matmul(A,np.log10(Xmod))
# transf
C = 10**(log_C)
u = np.matmul(A.transpose(),C)
#print(C)
# Vector_error
d = u-T
print(d)
if idx_fix_species != None:
d[idx_fix_species] =0
abs_err = max(abs(d))
counter_iterations += 1
if counter_iterations >= max_iterations:
raise ValueError('Max number of iterations surpassed.')
#return X_guess, C
# Speciation - mass action law
Xmod=X_guess.copy()
for i in range(len(X_guess)):
if X_guess[i]<=0:
Xmod[i]=1
log_C = log_k + np.matmul(A,np.log10(Xmod))
# transf
C = 10**(log_C)
return X_guess, C
def func_NR_FLM (X, A, log_k, temp, idx_Aq, sS1, aS1, sS2, aS2, e, CapacitancesS1, CapacitancesS2, T, Z, zel, pos_psi_S1_vec, pos_psi_S2_vec, d0,df, bvp_solver, idx_fix_species=None, tol_PB=1e-6):
"""
This function is supossed to be linked to the four_layer_two_surface_speciation function.
It just gave the evaluated vector of Y, and T for the Newton-raphson procedure.
The formulation of Westall (1980) is followed.
FLM = four layer model
"""
# Speciation - mass action law
Xmod=X.copy()
for i in range(len(X)):
if X[i]<=0:
Xmod[i]=1
log_C = log_k + np.matmul(A, | np.log10(Xmod) | numpy.log10 |
from lib.utils.config import cfg
from lib.utils.base_utils import PoseTransformer, read_pose, read_pickle, save_pickle
import os
import numpy as np
from transforms3d.quaternions import mat2quat
import glob
from PIL import Image
from scipy import stats
import OpenEXR
import Imath
from multiprocessing.dummy import Pool
import struct
import scipy.io as sio
class DataStatistics(object):
# world_to_camera_pose = np.array([[-1.19209304e-07, 1.00000000e+00, -2.98023188e-08, 1.19209304e-07],
# [-8.94069672e-08, 2.22044605e-16, -1.00000000e+00, 8.94069672e-08],
# [-1.00000000e+00, -8.94069672e-08, 1.19209304e-07, 1.00000000e+00]])
world_to_camera_pose = np.array([[-1.00000024e+00, -8.74227979e-08, -5.02429621e-15, 8.74227979e-08],
[5.02429621e-15, 1.34358856e-07, -1.00000012e+00, -1.34358856e-07],
[8.74227979e-08, -1.00000012e+00, 1.34358856e-07, 1.00000012e+00]])
def __init__(self, class_type):
self.class_type = class_type
self.mask_path = os.path.join(cfg.LINEMOD,'{}/mask/*.png'.format(class_type))
self.dir_path = os.path.join(cfg.LINEMOD_ORIG,'{}/data'.format(class_type))
dataset_pose_dir_path = os.path.join(cfg.DATA_DIR, 'dataset_poses')
os.system('mkdir -p {}'.format(dataset_pose_dir_path))
self.dataset_poses_path = os.path.join(dataset_pose_dir_path, '{}_poses.npy'.format(class_type))
blender_pose_dir_path = os.path.join(cfg.DATA_DIR, 'blender_poses')
os.system('mkdir -p {}'.format(blender_pose_dir_path))
self.blender_poses_path = os.path.join(blender_pose_dir_path, '{}_poses.npy'.format(class_type))
os.system('mkdir -p {}'.format(blender_pose_dir_path))
self.pose_transformer = PoseTransformer(class_type)
def get_proper_crop_size(self):
mask_paths = glob.glob(self.mask_path)
widths = []
heights = []
for mask_path in mask_paths:
mask = Image.open(mask_path).convert('1')
mask = np.array(mask).astype(np.int32)
row_col = np.argwhere(mask == 1)
min_row, max_row = np.min(row_col[:, 0]), np.max(row_col[:, 0])
min_col, max_col = np.min(row_col[:, 1]), np.max(row_col[:, 1])
width = max_col - min_col
height = max_row - min_row
widths.append(width)
heights.append(height)
widths = np.array(widths)
heights = np.array(heights)
print('min width: {}, max width: {}'.format(np.min(widths), np.max(widths)))
print('min height: {}, max height: {}'.format(np.min(heights), np.max(heights)))
def get_quat_translation(self, object_to_camera_pose):
object_to_camera_pose = np.append(object_to_camera_pose, [[0, 0, 0, 1]], axis=0)
world_to_camera_pose = np.append(self.world_to_camera_pose, [[0, 0, 0, 1]], axis=0)
object_to_world_pose = np.dot(np.linalg.inv(world_to_camera_pose), object_to_camera_pose)
quat = mat2quat(object_to_world_pose[:3, :3])
translation = object_to_world_pose[:3, 3]
return quat, translation
def get_dataset_poses(self):
if os.path.exists(self.dataset_poses_path):
poses = np.load(self.dataset_poses_path)
return poses[:, :3], poses[:, 3:]
eulers = []
translations = []
train_set = np.loadtxt(os.path.join(cfg.LINEMOD, '{}/training_range.txt'.format(self.class_type)),np.int32)
for idx in train_set:
rot_path = os.path.join(self.dir_path, 'rot{}.rot'.format(idx))
tra_path = os.path.join(self.dir_path, 'tra{}.tra'.format(idx))
pose = read_pose(rot_path, tra_path)
euler = self.pose_transformer.orig_pose_to_blender_euler(pose)
eulers.append(euler)
translations.append(pose[:, 3])
eulers = np.array(eulers)
translations = | np.array(translations) | numpy.array |
# The # %% lines denote cells, allowing this file to be run using
# the interactive mode of the Python VS Code extension. But you
# can also run it simply as a normal python script.
# %%
import matplotlib.pyplot as plt
import numpy as np
import os
import seaborn as sns
from scipy.signal import correlate2d
from e2cnn.diffops.utils import discretize_homogeneous_polynomial as dp
from e2cnn import nn
from e2cnn import gspaces
# %%
sns.set_theme(context="paper")
os.makedirs("fig", exist_ok=True)
# %%
x = np.linspace(0, np.pi, 128)
xx, yy = np.meshgrid(x[32:], x[:64])
zz_x = np.sin(xx + yy) + 0.5 * (xx - 2)
zz_y = np.cos(xx - yy) + 0.5 * (yy - 2)
zz_mag = np.sqrt(zz_x**2 + zz_y**2)
plt.figure(figsize=(6, 4))
plt.gcf().set_facecolor((0.95, 0.95, 0.95))
plt.quiver(zz_x[::8, ::8], zz_y[::8, ::8], zz_mag[::8, ::8])
plt.axis("equal")
plt.axis("off")
plt.gcf().tight_layout()
plt.savefig("fig/vector_input.pdf", bbox_inches="tight", facecolor=plt.gcf().get_facecolor())
# %%
# the order is like that ... for reasons
# y_grad, x_grad = np.gradient(zz)
# gradient_mag = np.sqrt(x_grad**2 + y_grad**2)
# Laplacian of the divergence:
x_filter = dp([-2, -1, 0, 1, 2], np.array([0, 1, 0, 1])).reshape(5, 5)
# curl:
x_filter += dp([-2, -1, 0, 1, 2], np.array([1, 0])).reshape(5, 5)
y_filter = dp([-2, -1, 0, 1, 2], np.array([1, 0, 1, 0])).reshape(5, 5)
y_filter += dp([-2, -1, 0, 1, 2], | np.array([0, -1]) | numpy.array |
#Loading dependencies
import numpy as np
from collections import Counter
from .BaseMRRPlan import *
class MRRTwoActions(BaseMRRPlan):
def __init__(self, **params):
super().__init__(**params)
self.start = params.pop(start)
action_duration = params.pop('action_duration')
self.mrr_duration = {BINAR: action_duration}
self.randomize_mrr()
def randomize_mrr(self):
'''Randomly initialize the mrr'''
self.mrr = | np.random.randint(2, size=(self.settings.n_elements, self.settings.n_steps)) | numpy.random.randint |
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import copy
from glob import glob
import matplotlib.pyplot as plt
import multiprocessing
import numpy as np
import os
import pandas as pd
import shutil
from .tileserver_helpers import create_hf
from .tileserver_helpers import collect_coord_info
from .tileserver_helpers import check_par_jobs
from .tileserver_scraper import scraper
from .tileserver_stitcher import stitcher
class TileServer:
def __init__(
self,
metadata_path,
geometry="polygone",
download_url="https://mapseries-tilesets.s3.amazonaws.com/1inch_2nd_ed/{z}/{x}/{y}.png",
):
"""Initialize TileServer class and read in metadata
self.metadata is a dictionary that contains info about the maps to be downloaded.
Each key in this dict should have
["properties"]["IMAGEURL"]
["geometry"]["coordinates"]
["properties"]["IMAGE"]
Args:
metadata_path: path to a metadata file downloaded from a tileserver.
This file contains information about one series of maps stored on the tileserver.
Some example metadata files can be found in `MapReader/mapreader/persistent_data`
geometry (str, optional): Geometry that defines the boundaries. Defaults to "polygone".
download_url (str, optional): Base URL to download the maps. Defaults to "https://mapseries-tilesets.s3.amazonaws.com/1inch_2nd_ed/{z}/{x}/{y}.png".
"""
# initialize variables
self.detected_rect_boundary = False
self.found_queries = None
self.geometry = geometry
self.download_url = download_url
if isinstance(metadata_path, str) and os.path.isfile(metadata_path):
# Read a metada file
json_fio = open(metadata_path, "r")
json_f = json_fio.readlines()[0]
# change metadata to a dictionary:
metadata_all = eval(json_f)
metadata = metadata_all["features"]
elif isinstance(metadata_path, dict):
metadata = metadata_all["features"]
elif isinstance(metadata_path, list):
metadata = metadata_path[:]
else:
raise ValueError(f"Could not find or load: {metadata_path}")
# metadata contains the "features" of metadata_all
self.metadata = metadata
print(self.__str__())
def create_info(self):
"""
Extract information from metadata and create metadata_info_list and metadata_coord_arr.
This is a helper function for other methods in this class
"""
metadata_info_list = []
metadata_coord_arr = []
for one_item in self.metadata:
min_lon, max_lon, min_lat, max_lat = self.detect_rectangle_boundary(
one_item["geometry"]["coordinates"][0][0]
)
# XXX hardcoded: the file path is hardcoded to map_<IMAGE>.png
metadata_info_list.append(
[
one_item["properties"]["IMAGEURL"],
"map_" + one_item["properties"]["IMAGE"] + ".png",
]
)
# Collect boundaries for fast queries
metadata_coord_arr.append([min_lon, max_lon, min_lat, max_lat])
self.metadata_info_list = metadata_info_list
self.metadata_coord_arr = np.array(metadata_coord_arr).astype(np.float)
self.detected_rect_boundary = True
def modify_metadata(self, remove_image_ids=[], only_keep_image_ids=[]):
"""Modify metadata using metadata[...]["properties"]["IMAGE"]
Parameters
----------
remove_image_ids : list, optional
Image IDs to be removed from metadata variable
"""
print(f"[INFO] #images (before modification): {len(self.metadata)}")
one_edit = False
if len(remove_image_ids) != 0:
for i in range(len(self.metadata) - 1, -1, -1):
if self.metadata[i]["properties"]["IMAGE"] in remove_image_ids:
print(f'Removing {self.metadata[i]["properties"]["IMAGE"]}')
del self.metadata[i]
one_edit = True
if len(only_keep_image_ids) != 0:
for i in range(len(self.metadata) - 1, -1, -1):
if self.metadata[i]["properties"]["IMAGE"] not in only_keep_image_ids:
print(f'Removing {self.metadata[i]["properties"]["IMAGE"]}')
del self.metadata[i]
one_edit = True
if one_edit:
print("[INFO] run .create_info")
self.create_info()
print(f"[INFO] #images (after modification): {len(self.metadata)}")
def query_point(self, latlon_list, append=False):
"""Query maps from a list of lats/lons using metadata file
Args:
latlon_list (list): a list that contains lats/lons: [lat, lon] or [[lat1, lon1], [lat2, lon2], ...]
append (bool, optional): If True, append the new query to the list of queries. Defaults to False.
"""
# Detect the boundaries of maps
if not self.detected_rect_boundary:
self.create_info()
if not type(latlon_list[0]) == list:
latlon_list = [latlon_list]
if append and (self.found_queries != None):
found_queries = copy.deepcopy(self.found_queries)
else:
found_queries = []
for one_q in latlon_list:
lat_q, lon_q = one_q
indx_q = np.where(
(self.metadata_coord_arr[:, 2] <= lat_q)
& (lat_q <= self.metadata_coord_arr[:, 3])
& (self.metadata_coord_arr[:, 0] <= lon_q)
& (lon_q <= self.metadata_coord_arr[:, 1])
)[0]
if len(indx_q) == 0:
print(f"Could not find any candidates for {one_q}")
continue
indx_q = indx_q[0]
already_in_candidates = False
# Check if the query is already in the list
if len(found_queries) > 0:
for one_in_candidates in found_queries:
if self.metadata_info_list[indx_q][0] == one_in_candidates[0]:
already_in_candidates = True
print(f"Already in the query list: {one_in_candidates[0]}")
break
if not already_in_candidates:
found_queries.append(copy.deepcopy(self.metadata_info_list[indx_q]))
found_queries[-1].extend(
[copy.deepcopy(self.metadata_coord_arr[indx_q]), indx_q]
)
self.found_queries = found_queries
def print_found_queries(self):
"""Print found queries"""
print(12 * "-")
print(f"Found items:")
print(12 * "-")
if not self.found_queries:
print("[]")
else:
for one_item in self.found_queries:
print(f"URL: \t{one_item[0]}")
print(f"filepath:\t{one_item[1]}")
print(f"coords: \t{one_item[2]}")
print(f"index: \t{one_item[3]}")
print(20 * "=")
def detect_rectangle_boundary(self, coords):
"""Detect rectangular boundary given a set of coordinates"""
if self.geometry == "polygone":
coord_arr = np.array(coords)
# if len(coord_arr) != 5:
# raise ValueError(f"[ERROR] expected length of coordinate list is 5. coords: {coords}")
min_lon = np.min(coord_arr[:, 0])
max_lon = np.max(coord_arr[:, 0])
min_lat = np.min(coord_arr[:, 1])
max_lat = np.max(coord_arr[:, 1])
### # XXX this method results in smaller rectangles (compared to the original polygone) particularly
### # if the map is strongly tilted
### min_lon = np.sort(coord_arr[:-1, 0])[1]
### max_lon = np.sort(coord_arr[:-1, 0])[2]
### min_lat = np.sort(coord_arr[:-1, 1])[1]
### max_lat = np.sort(coord_arr[:-1, 1])[2]
return min_lon, max_lon, min_lat, max_lat
def create_metadata_query(self):
"""
Create a metadata type variable out of all queries.
This will be later used in download_tileserver method
"""
self.metadata_query = []
for one_item in self.found_queries:
self.metadata_query.append(copy.deepcopy(self.metadata[one_item[3]]))
def minmax_latlon(self):
"""Method to return min/max of lats/lons"""
if not self.detected_rect_boundary:
self.create_info()
print(
f"Min/Max Lon: {np.min(self.metadata_coord_arr[:, 0])}, { | np.max(self.metadata_coord_arr[:, 1]) | numpy.max |
#!/usr/bin/env python
# -*- coding: latin-1 -*-
import numpy as np
from .TriaMesh import TriaMesh
def import_fssurf(infile):
"""
Load triangle mesh from FreeSurfer surface geometry file
:return: TriaMesh
"""
verbose = 1
if verbose > 0:
print("--> FS Surf format ... ")
try:
# here we use our copy to also support surfaces from dev (and maybe v7*?)
# these have an empty line and mess up Nibabel
# once this is fixed in nibabel we can switch back
from .read_geometry import read_geometry
surf = read_geometry(infile, read_metadata=True)
except IOError:
print("[file not found or not readable]\n")
return
return TriaMesh(surf[0], surf[1], fsinfo=surf[2])
def import_off(infile):
"""
Load triangle mesh from OFF txt file
:return: TriaMesh
"""
verbose = 1
if verbose > 0:
print("--> OFF format ... ")
try:
f = open(infile, 'r')
except IOError:
print("[file not found or not readable]\n")
return
line = f.readline()
while line[0] == '#':
line = f.readline()
if not line.startswith("OFF"):
print("[OFF keyword not found] --> FAILED\n")
f.close()
return
# expect tria and vertex sizes after OFF line:
line = f.readline()
larr = line.split()
pnum = int(larr[0])
tnum = int(larr[1])
# print(" tnum: {} pnum: {}".format(tnum,pnum))
# read points as chunch
v = np.fromfile(f, 'float32', 3 * pnum, ' ')
v.shape = (pnum, 3)
# read trias as chunch
t = np.fromfile(f, 'int', 4 * tnum, ' ')
t.shape = (tnum, 4)
# print(" t0: {} ".format(t[0, :]))
# make sure first column is equal to 3 (trias)
# max0 = np.amax(t[:, 0])
# print(" max: {}".format(max0))
if np.amax(t[:, 0]) != 3:
print("[no triangle data] --> FAILED\n")
f.close()
return
t = t[:, 1:]
f.close()
print(" --> DONE ( V: " + str(v.shape[0]) + " , T: " + str(t.shape[0]) + " )\n")
return TriaMesh(v, t)
def import_vtk(infile):
"""
Load triangle mesh from VTK txt file
:return: TriaMesh
"""
verbose = 1
if verbose > 0:
print("--> VTK format ... ")
try:
f = open(infile, 'r')
except IOError:
print("[file not found or not readable]\n")
return
# skip comments
line = f.readline()
while line[0] == '#':
line = f.readline()
# search for ASCII keyword in first 5 lines:
count = 0
while count < 5 and not line.startswith("ASCII"):
line = f.readline()
# print line
count = count + 1
if not line.startswith("ASCII"):
print("[ASCII keyword not found] --> FAILED\n")
return
# expect Dataset Polydata line after ASCII:
line = f.readline()
if not line.startswith("DATASET POLYDATA") and not line.startswith("DATASET UNSTRUCTURED_GRID"):
print("[read: " + line + " expected DATASET POLYDATA or DATASET UNSTRUCTURED_GRID] --> FAILED\n")
return
# read number of points
line = f.readline()
larr = line.split()
if larr[0] != "POINTS" or (larr[2] != "float" and larr[2] != "double"):
print("[read: " + line + " expected POINTS # float or POINTS # double ] --> FAILED\n")
return
pnum = int(larr[1])
# read points as chunk
v = np.fromfile(f, 'float32', 3 * pnum, ' ')
v.shape = (pnum, 3)
# expect polygon or tria_strip line
line = f.readline()
larr = line.split()
if larr[0] == "POLYGONS" or larr[0] == "CELLS":
tnum = int(larr[1])
ttnum = int(larr[2])
npt = float(ttnum) / tnum
if npt != 4.0:
print("[having: " + str(npt) + " data per tria, expected trias 3+1] --> FAILED\n")
return
t = np.fromfile(f, 'int', ttnum, ' ')
t.shape = (tnum, 4)
if t[tnum - 1][0] != 3:
print("[can only read triangles] --> FAILED\n")
return
t = np.delete(t, 0, 1)
elif larr[0] == "TRIANGLE_STRIPS":
tnum = int(larr[1])
# ttnum = int(larr[2])
tt = []
for i in range(tnum):
larr = f.readline().split()
if len(larr) == 0:
print("[error reading triangle strip (i)] --> FAILED\n")
return
n = int(larr[0])
if len(larr) != n + 1:
print("[error reading triangle strip (ii)] --> FAILED\n")
return
# create triangles from strip
# note that larr tria info starts at index 1
for ii in range(2, n):
if ii % 2 == 0:
tria = [larr[ii - 1], larr[ii], larr[ii + 1]]
else:
tria = [larr[ii], larr[ii - 1], larr[ii + 1]]
tt.append(tria)
t = | np.array(tt) | numpy.array |
import numpy as np
from PIL import Image
from nets.srgan import build_generator
from utils.utils import preprocess_input, cvtColor
class SRGAN(object):
#-----------------------------------------#
# 注意修改model_path
#-----------------------------------------#
_defaults = {
#-----------------------------------------------#
# model_path指向logs文件夹下的权值文件
#-----------------------------------------------#
"model_path" : 'model_data/Generator_SRGAN.h5',
#-----------------------------------------------#
# 上采样的倍数,和训练时一样
#-----------------------------------------------#
"scale_factor" : 4,
}
#---------------------------------------------------#
# 初始化SRGAN
#---------------------------------------------------#
def __init__(self, **kwargs):
self.__dict__.update(self._defaults)
for name, value in kwargs.items():
setattr(self, name, value)
self.generate()
#---------------------------------------------------#
# 创建生成模型
#---------------------------------------------------#
def generate(self):
self.net = build_generator([None, None, 3], self.scale_factor)
self.net.load_weights(self.model_path)
print('{} model loaded.'.format(self.model_path))
def generate_1x1_image(self, image):
#---------------------------------------------------------#
# 在这里将图像转换成RGB图像,防止灰度图在预测时报错。
# 代码仅仅支持RGB图像的预测,所有其它类型的图像都会转化成RGB
#---------------------------------------------------------#
image = cvtColor(image)
#---------------------------------------------------------#
# 添加上batch_size维度,并进行归一化
#---------------------------------------------------------#
image_data = np.expand_dims(preprocess_input( | np.array(image, dtype='float32') | numpy.array |
"""Test application services
"""
import numpy as np
import pytest
import xarray as xr
from xesmf.data import wave_smooth
from xesmf.util import grid_global
from xclim.sdba.adjustment import QuantileDeltaMapping
from dodola.services import (
bias_correct,
build_weights,
rechunk,
regrid,
remove_leapdays,
clean_cmip6,
downscale,
correct_wet_day_frequency,
train_qdm,
apply_qdm,
train_aiqpd,
apply_aiqpd,
)
import dodola.repository as repository
def _datafactory(x, start_time="1950-01-01", variable_name="fakevariable"):
"""Populate xr.Dataset with synthetic data for testing"""
start_time = str(start_time)
if x.ndim != 1:
raise ValueError("'x' needs dim of one")
time = xr.cftime_range(
start=start_time, freq="D", periods=len(x), calendar="standard"
)
out = xr.Dataset(
{variable_name: (["time", "lon", "lat"], x[:, np.newaxis, np.newaxis])},
coords={
"index": time,
"time": time,
"lon": (["lon"], [1.0]),
"lat": (["lat"], [1.0]),
},
)
return out
def _gcmfactory(x, gcm_variable="fakevariable", start_time="1950-01-01"):
"""Populate xr.Dataset with synthetic GCM data for testing
that includes extra dimensions and leap days to be removed.
"""
start_time = str(start_time)
if x.ndim != 1:
raise ValueError("'x' needs dim of one")
time = xr.cftime_range(
start=start_time, freq="D", periods=len(x), calendar="standard"
)
out = xr.Dataset(
{
gcm_variable: (
["time", "lon", "lat", "member_id"],
x[:, np.newaxis, np.newaxis, np.newaxis],
)
},
coords={
"index": time,
"time": time,
"bnds": [0, 1],
"lon": (["lon"], [1.0]),
"lat": (["lat"], [1.0]),
"member_id": (["member_id"], [1.0]),
"height": (["height"], [1.0]),
"time_bnds": (["time", "bnds"], np.ones((len(x), 2))),
},
)
return out
@pytest.fixture
def domain_file(request):
"""Creates a fake domain Dataset for testing"""
lon_name = "lon"
lat_name = "lat"
domain = grid_global(request.param, request.param)
domain[lat_name] = np.unique(domain[lat_name].values)
domain[lon_name] = np.unique(domain[lon_name].values)
return domain
def test_apply_qdm(tmpdir):
"""Test to apply a trained QDM to input data and read the output.
This is an integration test between train_qdm, apply_qdm.
"""
# Setup input data.
target_variable = "fakevariable"
variable_kind = "additive"
n_histdays = 10 * 365 # 10 years of daily historical.
n_simdays = 50 * 365 # 50 years of daily simulation.
model_bias = 2
ts_ref = np.ones(n_histdays, dtype=np.float64)
ts_sim = np.ones(n_simdays, dtype=np.float64)
hist = _datafactory(ts_ref + model_bias, variable_name=target_variable)
ref = _datafactory(ts_ref, variable_name=target_variable)
sim = _datafactory(ts_sim + model_bias, variable_name=target_variable)
# Load up a fake repo with our input data in the place of big data and cloud
# storage.
qdm_key = "memory://test_apply_qdm/qdm.zarr"
hist_key = "memory://test_apply_qdm/hist.zarr"
ref_key = "memory://test_apply_qdm/ref.zarr"
sim_key = "memory://test_apply_qdm/sim.zarr"
# Writes NC to local disk, so dif format here:
sim_adjusted_key = tmpdir.join("sim_adjusted.nc")
repository.write(sim_key, sim)
repository.write(hist_key, hist)
repository.write(ref_key, ref)
target_year = 1995
train_qdm(
historical=hist_key,
reference=ref_key,
out=qdm_key,
variable=target_variable,
kind=variable_kind,
)
apply_qdm(
simulation=sim_key,
qdm=qdm_key,
year=target_year,
variable=target_variable,
out=sim_adjusted_key,
)
adjusted_ds = xr.open_dataset(str(sim_adjusted_key))
assert target_variable in adjusted_ds.variables
@pytest.mark.parametrize("kind", ["multiplicative", "additive"])
def test_train_qdm(kind):
"""Test that train_qdm outputs store giving sdba.adjustment.QuantileDeltaMapping
Checks that output is consistent if we do "additive" or "multiplicative"
QDM kinds.
"""
# Setup input data.
n_years = 10
n = n_years * 365
model_bias = 2
ts = np.sin(np.linspace(-10 * 3.14, 10 * 3.14, n)) * 0.5
hist = _datafactory(ts + model_bias)
ref = _datafactory(ts)
output_key = "memory://test_train_qdm/test_output.zarr"
hist_key = "memory://test_train_qdm/hist.zarr"
ref_key = "memory://test_train_qdm/ref.zarr"
# Load up a fake repo with our input data in the place of big data and cloud
# storage.
repository.write(hist_key, hist)
repository.write(ref_key, ref)
train_qdm(
historical=hist_key,
reference=ref_key,
out=output_key,
variable="fakevariable",
kind=kind,
)
assert QuantileDeltaMapping.from_dataset(repository.read(output_key))
@pytest.mark.parametrize(
"method, expected_head, expected_tail",
[
pytest.param(
"QDM",
np.array(
[6.6613381e-16, 8.6090365e-3, 1.7215521e-2, 2.58169e-2, 3.4410626e-2]
),
np.array(
[
-3.4410626e-2,
-2.58169e-2,
-1.7215521e-2,
-8.6090365e-3,
-1.110223e-15,
]
),
id="QDM head/tail",
),
],
)
def test_bias_correct_basic_call(method, expected_head, expected_tail):
"""Simple integration test of bias_correct service"""
# Setup input data.
n_years = 10
n = n_years * 365 # need daily data...
# Our "biased model".
model_bias = 2
ts = np.sin(np.linspace(-10 * np.pi, 10 * np.pi, n)) * 0.5
x_train = _datafactory((ts + model_bias))
# True "observations".
y_train = _datafactory(ts)
# Yes, we're testing and training on the same data...
x_test = x_train.copy(deep=True)
output_key = "memory://test_bias_correct_basic_call/test_output.zarr"
training_model_key = "memory://test_bias_correct_basic_call/x_train.zarr"
training_obs_key = "memory://test_bias_correct_basic_call/y_train.zarr"
forecast_model_key = "memory://test_bias_correct_basic_call/x_test.zarr"
# Load up a fake repo with our input data in the place of big data and cloud
# storage.
repository.write(training_model_key, x_train)
repository.write(training_obs_key, y_train)
repository.write(forecast_model_key, x_test)
bias_correct(
forecast_model_key,
x_train=training_model_key,
train_variable="fakevariable",
y_train=training_obs_key,
out=output_key,
out_variable="fakevariable",
method=method,
)
# We can't just test for removal of bias here since quantile mapping
# and adding in trend are both components of bias correction,
# so testing head and tail values instead
np.testing.assert_almost_equal(
repository.read(output_key)["fakevariable"].squeeze(drop=True).values[:5],
expected_head,
)
np.testing.assert_almost_equal(
repository.read(output_key)["fakevariable"].squeeze(drop=True).values[-5:],
expected_tail,
)
@pytest.mark.parametrize(
"domain_file, regrid_method",
[pytest.param(1.0, "bilinear"), pytest.param(1.0, "conservative")],
indirect=["domain_file"],
)
def test_build_weights(domain_file, regrid_method, tmpdir):
"""Test that services.build_weights produces a weights file"""
# Output to tmp dir so we cleanup & don't clobber existing files...
weightsfile = tmpdir.join("a_file_path_weights.nc")
# Make fake input data.
ds_in = grid_global(30, 20)
ds_in["fakevariable"] = wave_smooth(ds_in["lon"], ds_in["lat"])
domain_file_url = "memory://test_regrid_methods/a/domainfile/path.zarr"
repository.write(domain_file_url, domain_file)
url = "memory://test_build_weights/a_file_path.zarr"
repository.write(url, ds_in)
build_weights(url, regrid_method, domain_file_url, outpath=weightsfile)
# Test that weights file is actually created where we asked.
assert weightsfile.exists()
def test_rechunk():
"""Test that rechunk service rechunks"""
chunks_goal = {"time": 4, "lon": 1, "lat": 1}
test_ds = xr.Dataset(
{"fakevariable": (["time", "lon", "lat"], np.ones((4, 4, 4)))},
coords={
"time": [1, 2, 3, 4],
"lon": (["lon"], [1.0, 2.0, 3.0, 4.0]),
"lat": (["lat"], [1.5, 2.5, 3.5, 4.5]),
},
)
in_url = "memory://test_rechunk/input_ds.zarr"
out_url = "memory://test_rechunk/output_ds.zarr"
repository.write(in_url, test_ds)
rechunk(
in_url,
target_chunks=chunks_goal,
out=out_url,
)
actual_chunks = repository.read(out_url)["fakevariable"].data.chunksize
assert actual_chunks == tuple(chunks_goal.values())
@pytest.mark.parametrize(
"domain_file, regrid_method, expected_shape",
[
pytest.param(
1.0,
"bilinear",
(180, 360),
id="Bilinear regrid",
),
pytest.param(
1.0,
"conservative",
(180, 360),
id="Conservative regrid",
),
],
indirect=["domain_file"],
)
def test_regrid_methods(domain_file, regrid_method, expected_shape):
"""Smoke test that services.regrid outputs with different regrid methods
The expected shape is the same, but change in methods should not error.
"""
# Make fake input data.
ds_in = grid_global(30, 20)
ds_in["fakevariable"] = wave_smooth(ds_in["lon"], ds_in["lat"])
in_url = "memory://test_regrid_methods/an/input/path.zarr"
domain_file_url = "memory://test_regrid_methods/a/domainfile/path.zarr"
out_url = "memory://test_regrid_methods/an/output/path.zarr"
repository.write(in_url, ds_in)
repository.write(domain_file_url, domain_file)
regrid(in_url, out=out_url, method=regrid_method, domain_file=domain_file_url)
actual_shape = repository.read(out_url)["fakevariable"].shape
assert actual_shape == expected_shape
@pytest.mark.parametrize(
"domain_file, expected_dtype",
[
pytest.param(
2.0,
"float64",
id="Cast output to float64",
),
pytest.param(
2.0,
"float32",
id="Cast output to float32",
),
],
indirect=["domain_file"],
)
def test_regrid_dtype(domain_file, expected_dtype):
"""Tests that services.regrid casts output to different dtypes"""
# Make fake input data.
ds_in = grid_global(5, 10)
ds_in["fakevariable"] = wave_smooth(ds_in["lon"], ds_in["lat"])
in_url = "memory://test_regrid_dtype/an/input/path.zarr"
domain_file_url = "memory://test_regrid_dtype/a/domainfile/path.zarr"
out_url = "memory://test_regrid_dtype/an/output/path.zarr"
repository.write(in_url, ds_in)
repository.write(domain_file_url, domain_file)
regrid(
in_url,
out=out_url,
method="bilinear",
domain_file=domain_file_url,
astype=expected_dtype,
)
actual_dtype = repository.read(out_url)["fakevariable"].dtype
assert actual_dtype == expected_dtype
@pytest.mark.parametrize(
"domain_file, expected_shape",
[
pytest.param(
1.0,
(180, 360),
id="Regrid to domain file grid",
),
pytest.param(
2.0,
(90, 180),
id="Regrid to global 2.0° x 2.0° grid",
),
],
indirect=["domain_file"],
)
def test_regrid_resolution(domain_file, expected_shape):
"""Smoke test that services.regrid outputs with different grid resolutions
The expected shape is the same, but change in methods should not error.
"""
# Make fake input data.
ds_in = grid_global(30, 20)
ds_in["fakevariable"] = wave_smooth(ds_in["lon"], ds_in["lat"])
in_url = "memory://test_regrid_resolution/an/input/path.zarr"
domain_file_url = "memory://test_regrid_resolution/a/domainfile/path.zarr"
out_url = "memory://test_regrid_resolution/an/output/path.zarr"
repository.write(in_url, ds_in)
repository.write(domain_file_url, domain_file)
regrid(in_url, out=out_url, method="bilinear", domain_file=domain_file_url)
actual_shape = repository.read(out_url)["fakevariable"].shape
assert actual_shape == expected_shape
@pytest.mark.parametrize(
"domain_file", [pytest.param(1.0, id="Regrid to domain file grid")], indirect=True
)
def test_regrid_weights_integration(domain_file, tmpdir):
"""Test basic integration between service.regrid and service.build_weights"""
expected_shape = (180, 360)
# Output to tmp dir so we cleanup & don't clobber existing files...
weightsfile = tmpdir.join("a_file_path_weights.nc")
# Make fake input data.
ds_in = grid_global(30, 20)
ds_in["fakevariable"] = wave_smooth(ds_in["lon"], ds_in["lat"])
in_url = "memory://test_regrid_weights_integration/an/input/path.zarr"
domain_file_url = "memory://test_regrid_weights_integration/a/domainfile/path.zarr"
out_url = "memory://test_regrid_weights_integration/an/output/path.zarr"
repository.write(in_url, ds_in)
repository.write(domain_file_url, domain_file)
# First, use service to pre-build regridding weights files, then read-in to regrid.
build_weights(
in_url, method="bilinear", domain_file=domain_file_url, outpath=weightsfile
)
regrid(
in_url,
out=out_url,
method="bilinear",
weights_path=weightsfile,
domain_file=domain_file_url,
)
actual_shape = repository.read(out_url)["fakevariable"].shape
assert actual_shape == expected_shape
def test_clean_cmip6():
"""Tests that cmip6 cleanup removes extra dimensions on dataset"""
# Setup input data
n = 1500 # need over four years of daily data
ts = np.sin(np.linspace(-10 * np.pi, 10 * np.pi, n)) * 0.5
ds_gcm = _gcmfactory(ts, start_time="1950-01-01")
in_url = "memory://test_clean_cmip6/an/input/path.zarr"
out_url = "memory://test_clean_cmip6/an/output/path.zarr"
repository.write(in_url, ds_gcm)
clean_cmip6(in_url, out_url, leapday_removal=True)
ds_cleaned = repository.read(out_url)
assert "height" not in ds_cleaned.coords
assert "member_id" not in ds_cleaned.coords
assert "time_bnds" not in ds_cleaned.coords
@pytest.mark.parametrize(
"gcm_variable", [pytest.param("tasmax"), pytest.param("tasmin"), pytest.param("pr")]
)
def test_cmip6_precip_unitconversion(gcm_variable):
"""Tests that precip units are converted in CMIP6 cleanup if variable is precip"""
# Setup input data
n = 1500 # need over four years of daily data
ts = np.sin( | np.linspace(-10 * np.pi, 10 * np.pi, n) | numpy.linspace |
import numpy as np
import matplotlib.pylab as plt
import obspy.signal.cross_correlation as cc
import obspy
from obspy.core.stream import Stream
from obspy.core.trace import Trace
import os
import pylab
class Misfit:
def __init__(self, directory):
self.save_dir = directory
def get_RMS(self, data_obs, data_syn):
N = data_syn.__len__() # Normalization factor
RMS = np.sqrt(np.sum(data_obs - data_syn) ** 2 / N)
return RMS
def norm(self, data_obs, data_syn):
norm = np.linalg.norm(data_obs - data_syn)
return norm
def L2_stream(self, p_obs, p_syn, s_obs, s_syn, or_time, var):
dt = s_obs[0].meta.delta
misfit = np.array([])
time_shift = np.array([], dtype=int)
# S - correlations:
for i in range(len(s_obs)):
cc_obspy = cc.correlate(s_obs[i].data, s_syn[i].data, int(0.25* len(s_obs[i].data)))
shift, CC_s = cc.xcorr_max(cc_obspy)
s_syn_shift = self.shift(s_syn[i].data, -shift)
time_shift = np.append(time_shift, shift)
# d_obs_mean = np.mean(s_obs[i].data)
# var_array = var * d_obs_mean
var_array = np.var(s_obs[i].data)
# var_array = var**2
misfit = np.append(misfit, np.matmul((s_obs[i].data - s_syn_shift).T, (s_obs[i].data - s_syn_shift)) / (
2 * (var_array)))
# time = -time_shift * dt # Relatively, the s_wave arrives now time later or earlier than it originally did
# plt.plot(s_syn_shift,label='s_shifted',linewidth=0.3)
# plt.plot(s_syn[i], label='s_syn',linewidth=0.3)
# plt.plot(s_obs[i], label='s_obs',linewidth=0.3)
# plt.legend()
# plt.savefig()
# plt.close()
# P- correlation
for i in range(len(p_obs)):
cc_obspy = cc.correlate(s_obs[i].data, s_syn[i].data, int(0.25 * len(p_obs[i].data)))
shift, CC_p = cc.xcorr_max(cc_obspy)
p_syn_shift = self.shift(p_syn[i].data, -shift)
time_shift = np.append(time_shift, shift)
# d_obs_mean = np.mean(p_obs[i].data)
# var_array = var * d_obs_mean
var_array = np.var(p_obs[i].data)
misfit = np.append(misfit, np.matmul((p_obs[i].data - p_syn_shift).T, (p_obs[i].data - p_syn_shift)) / (
2 * (var_array)))
# time = -time_shift + len(s_obs)] * dt # Relatively, the s_wave arrives now time later or earlier than it originally did
# plt.plot(p_syn_shift, label='p_shifted')
# plt.plot(p_syn[i], label='p_syn')
# plt.plot(p_obs[i], label='p_obs')
# plt.legend()
# # plt.show()
# plt.close()
sum_misfit = np.sum(misfit)
return sum_misfit, time_shift
def CC_stream(self, p_obs, p_syn, s_obs,s_syn,p_start_obs,p_start_syn):
dt = s_obs[0].meta.delta
misfit = np.array([])
misfit_obs = np.array([])
time_shift = np.array([], dtype=int)
amplitude = np.array([])
amp_obs = p_obs.copy()
amp_obs.trim(p_start_obs, p_start_obs + 25)
amp_syn = p_syn.copy()
amp_syn.trim(p_start_syn, p_start_syn + 25)
# S - correlations:
for i in range(len(s_obs)):
cc_obspy = cc.correlate(s_obs[i].data, s_syn[i].data, int(0.25*len(s_obs[i].data)))
shift, CC_s = cc.xcorr_max(cc_obspy, abs_max=False)
s_syn_shift_obspy = self.shift(s_syn[i].data, -shift)
D_s = 1 - CC_s # Decorrelation
time_shift = np.append(time_shift, shift)
misfit = np.append(misfit, ((CC_s - 0.95) ** 2) / (2 * (0.1) ** 2))# + np.abs(shift))
# misfit = np.append(misfit,((CC - 0.95) ** 2) / (2 * (0.1) ** 2))
# plt.plot(self.zero_to_nan(s_syn_shift_obspy),label='s_syn_shifted_obspy',linewidth=0.3)
# plt.plot(self.zero_to_nan(s_syn[i]),label = 's_syn',linewidth=0.3)
# plt.plot(self.zero_to_nan(s_obs[i]),label = 's_obs',linestyle = ":",linewidth=0.3)
# plt.legend()
# plt.tight_layout()
# plt.savefig(self.save_dir + '/S_%s.pdf' % s_obs.traces[i].meta.channel)
plt.close()
# P- correlation
for i in range(len(p_obs)):
cc_obspy = cc.correlate(p_obs[i].data, p_syn[i].data, int( 0.25*len(p_obs[i].data)))
shift, CC_p = cc.xcorr_max(cc_obspy ,abs_max=False)
# time = -shift * p_syn[i].meta.delta
p_syn_shift_obspy = self.shift(p_syn[i].data, -shift)
D_p = 1 - CC_p # Decorrelation
time_shift = np.append(time_shift, shift)
misfit = np.append(misfit, ((CC_p - 0.95) ** 2) / (2 * (0.1) ** 2) )#+ np.abs(shift))
A = (np.dot(amp_obs.traces[i],amp_syn.traces[i])/np.dot(amp_obs.traces[i],amp_obs.traces[i]))
amplitude = np.append(amplitude,abs(A))
# plt.plot(p_obs.traces[i].data[0:200],label='P_obs',linewidth=0.3)
# plt.plot(p_syn.traces[i].data[0:200],label = 'p_syn',linewidth=0.3)
# plt.legend()
# plt.tight_layout()
# plt.show()
# plt.savefig(self.save_dir + '/P_%s.pdf' % s_obs.traces[i].meta.channel)
# plt.close()
sum_misfit = np.sum(misfit)
return misfit, time_shift, amplitude
def shift(self, np_array, time_shift):
new_array = np.zeros_like(np_array)
if time_shift < 0:
new_array[-time_shift:] = np_array[:time_shift]
elif time_shift == 0:
new_array[:] = np_array[:]
else:
new_array[:-time_shift] = np_array[time_shift:]
return new_array
def SW_CC(self, SW_env_obs, SW_env_syn):
# I suppose that your observed traces and synthetic traces are filtered with same bandwidths in same order!
R_dict = {}
misfit = np.array([])
for i in range((len(SW_env_obs))):
dt = SW_env_obs[i].meta.delta
cc_obspy = cc.correlate(SW_env_obs[i].data, SW_env_syn[i].data, int(0.25*len(SW_env_obs[i].data)))
shift, CC = cc.xcorr_max(cc_obspy)
SW_syn_shift_obspy = self.shift(SW_env_syn[i].data, -shift)
D = 1 - CC # Decorrelation
misfit = np.append(misfit, ((CC - 0.95) ** 2) / (2 * (0.1) ** 2) + np.abs(shift))
R_dict.update({'%s' % SW_env_obs.traces[i].stats.channel: {'misfit': misfit[i], 'time_shift': shift}})
sum_misfit = np.sum(misfit)
return sum_misfit
def SW_L2(self, SW_env_obs, SW_env_syn, var, amplitude):
misfit = | np.array([]) | numpy.array |
import math
from collections import OrderedDict
import numpy as np
import os, pickle as pkl
import json
import qelos_core as q
from torch import nn
import torch
EPS = 1e-6
class WordVecBase(object):
masktoken = "<MASK>"
raretoken = "<RARE>"
def __init__(self, worddic, **kw):
"""
Takes a worddic and provides word id lookup and vector retrieval interface
"""
super(WordVecBase, self).__init__(**kw)
self.D = OrderedDict() if worddic is None else worddic
# region NON-BLOCK API :::::::::::::::::::::::::::::::::::::
def getindex(self, word):
return self.D[word] if word in self.D else self.D[self.raretoken] if self.raretoken in self.D else -1
def __mul__(self, other):
""" given word (string), returns index (integer) based on dictionary """
return self.getindex(other)
def __contains__(self, word):
return word in self.D
def getvector(self, word):
raise NotImplemented()
def __getitem__(self, word):
""" given word (string or index), returns vector """
return self.getvector(word)
@property
def shape(self):
raise NotImplemented()
def cosine(self, A, B):
return | np.dot(A, B) | numpy.dot |
import multiprocessing
import numpy as np
import pyrosetta
import re
from itertools import permutations
from rif.eigen_types import X3
from rif.geom.ray_hash import RayToRay4dHash
from rif.hash import XformHash_bt24_BCC6_X3f
# NOTE(onalant): change below to desired ray pair provider
from privileged_residues.chemical import catpi
from privileged_residues.geometry import coords_to_transform
from privileged_residues.util import models_from_pdb, numpy_to_rif
dt = [("hash", "u8"), ("residue", "S3"), ("transform", "u8")]
attr = [("cart_resl", 0.1),
("ori_resl", 2.0),
("cart_bound", 16.0)]
attr = dict(attr)
cart_resl = attr["cart_resl"]
ori_resl = attr["ori_resl"]
cart_bound = attr["cart_bound"]
select = pyrosetta.rosetta.core.select.residue_selector.TrueResidueSelector()
lattice = XformHash_bt24_BCC6_X3f(cart_resl, ori_resl, cart_bound)
# WARN(onalant): constants recommended by <NAME>; do not change unless /absolutely/ sure
LEVER = 10
BOUND = 1000
raygrid = RayToRay4dHash(ori_resl, LEVER, bound=BOUND)
# NOTE(onalant): change as necessary
target_pair = (2, 5)
assert(2 == len(target_pair))
def write_records_from_structure(pipe, posefile):
print("[WORKER-%s]" % (re.match(".*(\d+)", multiprocessing.current_process().name).group(1)), posefile)
for pose in models_from_pdb(posefile):
for (a, b) in permutations(target_pair):
raypair = catpi.rays_from_residue(pose.residue(a))
hashed_rays = raygrid.get_keys(*(numpy_to_rif(r) for r in raypair)).item()
fxA = catpi.rsd_to_fxnl_grp[pose.residue(a).name3()]
fxB = catpi.rsd_to_fxnl_grp[pose.residue(b).name3()]
xyzA = np.array([pose.residue(a).xyz(atom) for atom in fxA.atoms])
xyzB = np.array([pose.residue(b).xyz(atom) for atom in fxB.atoms])
xfA = coords_to_transform(xyzA)
xfB = coords_to_transform(xyzB)
xfrel = xfB @ np.linalg.inv(xfA)
transform = lattice.get_key(xfrel.astype("f4").flatten().view(X3)).item()
record = | np.array([(hashed_rays, fxB.grp, transform)], dtype=dt) | numpy.array |
from pprint import pprint
import numpy as np
import cv2
import os
import matplotlib.pyplot as plt
from keras.models import Model
from keras.layers import BatchNormalization, LeakyReLU, Conv2D
from keras.layers import Conv2DTranspose, Input, Concatenate
from keras.applications import MobileNetV2
import keras.backend as K
from mask_to_contours import find_contours, draw_contours
def show_performance(model):
""" This function is to show some examples from validation data """
val_image_ids_ = [i for i in val_image_ids]
np.random.shuffle(val_image_ids_)
df_val = area_filter(val_image_ids_, val_coco)
image_id = df_val['image_id'].iloc[0]
annotation_ids = df_val[df_val['image_id'] == image_id]['annotation_id'].tolist()
image_json = val_coco.loadImgs([image_id])[0]
raw_image = cv2.imread(os.path.join("{}/{}/{}".format(data_dir, val_type, image_json['file_name'])))
height, width, _ = raw_image.shape
# decode the mask, using annotation id created at the group by above
binary_mask = process_mask(val_coco, annotation_ids, width, height)
# preprocess input and mask (resize to 128, scale to [0, 1))
input_image, input_mask = preprocess(raw_image, binary_mask)
input_mask = | np.expand_dims(input_mask, axis=-1) | numpy.expand_dims |
"""
Test Surrogates Overview
========================
"""
# Author: <NAME> <<EMAIL>>
# License: new BSD
from PIL import Image
import numpy as np
import scripts.surrogates_overview as exo
import scripts.image_classifier as imgclf
import sklearn.datasets
import sklearn.linear_model
SAMPLES = 10
BATCH = 50
SAMPLE_IRIS = False
IRIS_SAMPLES = 50000
def test_bilmey_image():
"""Tests surrogate image bLIMEy."""
# Load the image
doggo_img = Image.open('surrogates_overview/img/doggo.jpg')
doggo_array = np.array(doggo_img)
# Load the classifier
clf = imgclf.ImageClassifier()
explain_classes = [('tennis ball', 852),
('golden retriever', 207),
('Labrador retriever', 208)]
# Configure widgets to select occlusion colour, segmentation granularity
# and explained class
colour_selection = {
i: i for i in ['mean', 'black', 'white', 'randomise-patch', 'green']
}
granularity_selection = {'low': 13, 'medium': 30, 'high': 50}
# Generate explanations
blimey_image_collection = {}
for gran_name, gran_number in granularity_selection.items():
blimey_image_collection[gran_name] = {}
for col_name in colour_selection:
blimey_image_collection[gran_name][col_name] = \
exo.build_image_blimey(
doggo_array,
clf.predict_proba,
explain_classes,
explanation_size=5,
segments_number=gran_number,
occlusion_colour=col_name,
samples_number=SAMPLES,
batch_size=BATCH,
random_seed=42)
exp = []
for gran_ in blimey_image_collection:
for col_ in blimey_image_collection[gran_]:
exp.append(blimey_image_collection[gran_][col_]['surrogates'])
assert len(exp) == len(EXP_IMG)
for e, E in zip(exp, EXP_IMG):
assert sorted(list(e.keys())) == sorted(list(E.keys()))
for key in e.keys():
assert e[key]['name'] == E[key]['name']
assert len(e[key]['explanation']) == len(E[key]['explanation'])
for e_, E_ in zip(e[key]['explanation'], E[key]['explanation']):
assert e_[0] == E_[0]
assert np.allclose(e_[1], E_[1], atol=.001, equal_nan=True)
def test_bilmey_tabular():
"""Tests surrogate tabular bLIMEy."""
# Load the iris data set
iris = sklearn.datasets.load_iris()
iris_X = iris.data # [:, :2] # take the first two features only
iris_y = iris.target
iris_labels = iris.target_names
iris_feature_names = iris.feature_names
label2class = {lab: i for i, lab in enumerate(iris_labels)}
# Fit the classifier
logreg = sklearn.linear_model.LogisticRegression(C=1e5)
logreg.fit(iris_X, iris_y)
# explained class
_dtype = iris_X.dtype
explained_instances = {
'setosa': np.array([5, 3.5, 1.5, 0.25]).astype(_dtype),
'versicolor': np.array([5.5, 2.75, 4.5, 1.25]).astype(_dtype),
'virginica': np.array([7, 3, 5.5, 2.25]).astype(_dtype)
}
petal_length_idx = iris_feature_names.index('petal length (cm)')
petal_length_bins = [1, 2, 3, 4, 5, 6, 7]
petal_width_idx = iris_feature_names.index('petal width (cm)')
petal_width_bins = [0, .5, 1, 1.5, 2, 2.5]
discs_ = []
for i, ix in enumerate(petal_length_bins): # X-axis
for iix in petal_length_bins[i + 1:]:
for j, jy in enumerate(petal_width_bins): # Y-axis
for jjy in petal_width_bins[j + 1:]:
discs_.append({
petal_length_idx: [ix, iix],
petal_width_idx: [jy, jjy]
})
for inst_i in explained_instances:
for cls_i in iris_labels:
for disc_i, disc in enumerate(discs_):
inst = explained_instances[inst_i]
cls = label2class[cls_i]
exp = exo.build_tabular_blimey(
inst, cls, iris_X, iris_y, logreg.predict_proba, disc,
IRIS_SAMPLES, SAMPLE_IRIS, 42)
key = '{}&{}&{}'.format(inst_i, cls, disc_i)
exp_ = EXP_TAB[key]
assert exp['explanation'].shape[0] == exp_.shape[0]
assert np.allclose(
exp['explanation'], exp_, atol=.001, equal_nan=True)
EXP_IMG = [
{207: {'explanation': [(13, -0.24406872165780585),
(11, -0.20456180387430317),
(9, -0.1866779131424261),
(4, 0.15001224157793785),
(3, 0.11589480417160983)],
'name': 'golden retriever'},
208: {'explanation': [(13, -0.08395966359346249),
(0, -0.0644986107387837),
(9, 0.05845584633658977),
(1, 0.04369763085720947),
(11, -0.035958188394941866)],
'name': '<NAME>'},
852: {'explanation': [(13, 0.3463529698715463),
(11, 0.2678050131923326),
(4, -0.10639863421417416),
(6, 0.08345792378117327),
(9, 0.07366945242386444)],
'name': '<NAME>'}},
{207: {'explanation': [(13, -0.0624167912596456),
(7, 0.06083359545295548),
(3, 0.0495953943686462),
(11, -0.04819787147412231),
(2, -0.03858823761391199)],
'name': '<NAME>'},
208: {'explanation': [(13, -0.08408428146916162),
(7, 0.07704235920590158),
(3, 0.06646468388122273),
(11, -0.0638326572126609),
(2, -0.052621478002380796)],
'name': '<NAME>'},
852: {'explanation': [(11, 0.35248212611685886),
(13, 0.2516925608037859),
(2, 0.13682853028454384),
(9, 0.12930134856644754),
(6, 0.1257747954095489)],
'name': '<NAME>'}},
{207: {'explanation': [(3, 0.21351937934930917),
(10, 0.16933456312772083),
(11, -0.13447244552856766),
(8, 0.11058919217055371),
(2, -0.06269239798368743)],
'name': '<NAME>'},
208: {'explanation': [(8, 0.05995551486884414),
(9, -0.05375302972380482),
(11, -0.051997353324246445),
(6, 0.04213181405953071),
(2, -0.039169895361928275)],
'name': '<NAME>'},
852: {'explanation': [(7, 0.31382219776986503),
(11, 0.24126214884275987),
(13, 0.21075924370226598),
(2, 0.11937652039885377),
(8, -0.11911265319329697)],
'name': '<NAME>'}},
{207: {'explanation': [(3, 0.39254403293049134),
(9, 0.19357165018747347),
(6, 0.16592079671652987),
(0, 0.14042059731407297),
(1, 0.09793027079765507)],
'name': '<NAME>'},
208: {'explanation': [(9, -0.19351859273276703),
(1, -0.15262967987262344),
(3, 0.12205127112235375),
(2, 0.11352141032313934),
(6, -0.11164209893429898)],
'name': '<NAME>'},
852: {'explanation': [(7, 0.17213007100844877),
(0, -0.1583030948868859),
(3, -0.13748574615069775),
(5, 0.13273283867075436),
(11, 0.12309551170070354)],
'name': '<NAME>'}},
{207: {'explanation': [(3, 0.4073533182995105),
(10, 0.20711667988142463),
(8, 0.15360813290032324),
(6, 0.1405424759832785),
(1, 0.1332920685413575)],
'name': '<NAME>'},
208: {'explanation': [(9, -0.14747910525112617),
(1, -0.13977061235228924),
(2, 0.10526833898161611),
(6, -0.10416022118399552),
(3, 0.09555992655161764)],
'name': '<NAME>'},
852: {'explanation': [(11, 0.2232260929107954),
(7, 0.21638443149433054),
(5, 0.21100464215582274),
(13, 0.145614853795006),
(1, -0.11416523431311262)],
'name': '<NAME>'}},
{207: {'explanation': [(1, 0.14700178977744183),
(0, 0.10346667279328238),
(2, 0.10346667279328238),
(7, 0.10346667279328238),
(8, 0.10162900633690726)],
'name': '<NAME>'},
208: {'explanation': [(10, -0.10845134816658476),
(8, -0.1026920429226184),
(6, -0.10238154733842847),
(18, 0.10094164937411244),
(16, 0.08646888450232793)],
'name': '<NAME>'},
852: {'explanation': [(18, -0.20542297091894474),
(13, 0.2012751176130666),
(8, -0.19194747162742365),
(20, 0.14686930696710473),
(15, 0.11796990086271067)],
'name': '<NAME>'}},
{207: {'explanation': [(13, 0.12446259821701779),
(17, 0.11859084421095789),
(15, 0.09690553833007137),
(12, -0.08869743701731962),
(4, 0.08124900427893789)],
'name': '<NAME>'},
208: {'explanation': [(10, -0.09478194981909983),
(20, -0.09173392507039077),
(9, 0.08768898801254493),
(17, -0.07553994244536394),
(4, 0.07422905503397653)],
'name': '<NAME>'},
852: {'explanation': [(21, 0.1327882942965061),
(1, 0.1238236573086363),
(18, -0.10911712271717902),
(19, 0.09707191051320978),
(6, 0.08593672504338913)],
'name': '<NAME>'}},
{207: {'explanation': [(6, 0.14931728779865114),
(14, 0.14092073957103526),
(1, 0.11071480021464616),
(4, 0.10655287976934531),
(8, 0.08705404649152573)],
'name': '<NAME>'},
208: {'explanation': [(8, -0.12242580400886727),
(9, 0.12142729544158742),
(14, -0.1148252787068248),
(16, -0.09562322208795092),
(4, 0.09350160975513132)],
'name': '<NAME>'},
852: {'explanation': [(6, 0.04227675072263027),
(9, -0.03107924340879173),
(14, 0.028007115650713045),
(13, 0.02771190348545554),
(19, 0.02640441416071482)],
'name': '<NAME>'}},
{207: {'explanation': [(19, 0.14313680656283245),
(18, 0.12866508562342843),
(8, 0.11809779264185447),
(0, 0.11286255403442104),
(2, 0.11286255403442104)],
'name': '<NAME>'},
208: {'explanation': [(9, 0.2397917428082761),
(14, -0.19435572812170654),
(6, -0.1760894833446507),
(18, -0.12243333818399058),
(15, 0.10986343675377105)],
'name': '<NAME>'},
852: {'explanation': [(14, 0.15378038774613365),
(9, -0.14245940635481966),
(6, 0.10213601012183973),
(20, 0.1009180838986786),
(3, 0.09780065767815548)],
'name': '<NAME>'}},
{207: {'explanation': [(15, 0.06525850448807077),
(9, 0.06286791243851698),
(19, 0.055189970374185854),
(8, 0.05499197604401475),
(13, 0.04748220842936177)],
'name': '<NAME>'},
208: {'explanation': [(6, -0.31549091899770765),
(5, 0.1862302670824446),
(8, -0.17381478451341995),
(10, -0.17353516098662508),
(14, -0.13591542421754205)],
'name': '<NAME>'},
852: {'explanation': [(14, 0.2163853942943355),
(6, 0.17565046338282214),
(1, 0.12446193028474549),
(9, -0.11365789839746396),
(10, 0.09239073691962967)],
'name': '<NAME>'}},
{207: {'explanation': [(19, 0.1141207265647932),
(36, -0.08861425922625768),
(30, 0.07219209872026074),
(9, -0.07150939547859836),
(38, -0.06988288637544438)],
'name': '<NAME>'},
208: {'explanation': [(29, 0.10531073909547647),
(13, 0.08279642208039652),
(34, -0.0817952443980797),
(33, -0.08086848205765082),
(12, 0.08086848205765082)],
'name': '<NAME>'},
852: {'explanation': [(13, -0.1330452414595897),
(4, 0.09942366413042845),
(12, -0.09881995683190645),
(33, 0.09881995683190645),
(19, -0.09596925317560831)],
'name': '<NAME>'}},
{207: {'explanation': [(37, 0.08193926967758253),
(35, 0.06804043021426347),
(15, 0.06396269230810163),
(11, 0.062255657227065296),
(8, 0.05529200233091672)],
'name': '<NAME>'},
208: {'explanation': [(19, 0.05711957286614678),
(27, -0.050230108135410824),
(16, -0.04743034616549999),
(5, -0.046717346734255705),
(9, -0.04419100026638039)],
'name': '<NAME>'},
852: {'explanation': [(3, -0.08390967998497496),
(30, -0.07037680222442452),
(22, 0.07029819368543713),
(8, -0.06861396187180349),
(37, -0.06662511956402824)],
'name': '<NAME>'}},
{207: {'explanation': [(19, 0.048418845359024805),
(9, -0.0423869575883795),
(30, 0.04012650790044438),
(36, -0.03787242980067195),
(10, 0.036557999380695635)],
'name': '<NAME>'},
208: {'explanation': [(10, 0.12120686823129677),
(17, 0.10196564232230493),
(7, 0.09495133975425854),
(25, -0.0759657891182803),
(2, -0.07035244568286837)],
'name': '<NAME>'},
852: {'explanation': [(3, -0.0770578003457272),
(28, 0.0769372258280398),
(6, -0.06044725989272927),
(22, 0.05550155775286349),
(31, -0.05399028046597057)],
'name': '<NAME>'}},
{207: {'explanation': [(14, 0.05371383110181226),
(0, -0.04442539316084218),
(18, 0.042589475382826494),
(19, 0.04227647855354252),
(17, 0.041685661662754295)],
'name': '<NAME>'},
208: {'explanation': [(29, 0.14419601354489464),
(17, 0.11785174500536676),
(36, 0.1000501679652906),
(10, 0.09679790134851017),
(35, 0.08710376081189208)],
'name': '<NAME>'},
852: {'explanation': [(8, -0.02486237985832769),
(3, -0.022559886154747102),
(11, -0.021878686669239856),
(36, 0.021847953817988534),
(19, -0.018317598300716522)],
'name': '<NAME>'}},
{207: {'explanation': [(37, 0.08098729255605368),
(35, 0.06639102704982619),
(15, 0.06033721190370432),
(34, 0.05826267856117829),
(28, 0.05549505160798173)],
'name': '<NAME>'},
208: {'explanation': [(17, 0.13839012042250542),
(10, 0.11312187488346881),
(7, 0.10729071207480922),
(25, -0.09529127965797404),
(11, -0.09279834572979286)],
'name': '<NAME>'},
852: {'explanation': [(3, -0.028385651836694076),
(22, 0.023364702783498722),
(8, -0.023097812578270233),
(30, -0.022931236620034406),
(37, -0.022040170736525342)],
'name': '<NAME>'}}
]
EXP_TAB = {
'setosa&0&0': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&1': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&2': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&3': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&4': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&5': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&6': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&7': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&8': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&9': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&10': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&11': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&12': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&13': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&14': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&15': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&16': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&17': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&18': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&19': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&20': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&21': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&22': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&23': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&24': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&25': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&26': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&27': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&28': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&29': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&30': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&31': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&32': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&33': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&34': np.array([0.7974072911132786, 0.006894018772033576]),
'setosa&0&35': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&36': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&37': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&38': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&39': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&40': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&41': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&42': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&43': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&44': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&45': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&46': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&47': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&48': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&49': np.array([0.4656481363306145, 0.007982539480288167]),
'setosa&0&50': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&51': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&52': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&53': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&54': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&55': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&56': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&57': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&58': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&59': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&60': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&61': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&62': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&63': np.array([0.13694026920485936, 0.36331091829858003]),
'setosa&0&64': np.array([0.3094460464703627, 0.11400643817329122]),
'setosa&0&65': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&66': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&67': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&68': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&69': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&70': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&71': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&72': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&73': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&74': np.array([0.13694026920485936, 0.36331091829858003]),
'setosa&0&75': np.array([0.0, 0.95124502153736]),
'setosa&0&76': np.array([0.0, 0.9708703761803881]),
'setosa&0&77': np.array([0.0, 0.5659706098422994]),
'setosa&0&78': np.array([0.0, 0.3962828716108186]),
'setosa&0&79': np.array([0.0, 0.2538069363248767]),
'setosa&0&80': np.array([0.0, 0.95124502153736]),
'setosa&0&81': np.array([0.0, 0.95124502153736]),
'setosa&0&82': np.array([0.0, 0.95124502153736]),
'setosa&0&83': np.array([0.0, 0.95124502153736]),
'setosa&0&84': np.array([0.0, 0.9708703761803881]),
'setosa&0&85': np.array([0.0, 0.9708703761803881]),
'setosa&0&86': np.array([0.0, 0.9708703761803881]),
'setosa&0&87': np.array([0.0, 0.5659706098422994]),
'setosa&0&88': np.array([0.0, 0.5659706098422994]),
'setosa&0&89': np.array([0.0, 0.3962828716108186]),
'setosa&0&90': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&91': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&92': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&93': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&94': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&95': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&96': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&97': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&98': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&99': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&100': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&101': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&102': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&103': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&104': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&105': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&106': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&107': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&108': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&109': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&110': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&111': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&112': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&113': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&114': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&115': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&116': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&117': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&118': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&119': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&120': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&121': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&122': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&123': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&124': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&125': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&126': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&127': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&128': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&129': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&130': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&131': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&132': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&133': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&134': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&135': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&136': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&137': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&138': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&139': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&140': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&141': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&142': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&143': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&144': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&145': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&146': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&147': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&148': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&149': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&150': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&151': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&152': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&153': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&154': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&155': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&156': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&157': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&158': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&159': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&160': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&161': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&162': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&163': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&164': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&165': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&166': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&167': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&168': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&169': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&170': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&171': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&172': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&173': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&174': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&175': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&176': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&177': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&178': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&179': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&180': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&181': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&182': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&183': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&184': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&185': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&186': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&187': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&188': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&189': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&190': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&191': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&192': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&193': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&194': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&195': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&196': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&197': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&198': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&199': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&200': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&201': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&202': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&203': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&204': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&205': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&206': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&207': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&208': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&209': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&210': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&211': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&212': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&213': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&214': np.array([0.9706534384443797, 0.007448195602953232]),
'setosa&0&215': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&216': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&217': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&218': np.array([0.7431524521056113, 0.24432235603856345]),
'setosa&0&219': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&220': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&221': np.array([0.4926091071260067, 0.49260910712601286]),
'setosa&0&222': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&223': np.array([0.9550700362273441, 0.025428672111930138]),
'setosa&0&224': np.array([0.9672121512728677, 0.012993005706020341]),
'setosa&0&225': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&226': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&227': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&228': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&229': np.array([0.7974072911132786, 0.006894018772033576]),
'setosa&0&230': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&231': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&232': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&233': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&234': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&235': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&236': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&237': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&238': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&239': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&240': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&241': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&242': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&243': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&244': np.array([0.7974072911132786, 0.006894018772033576]),
'setosa&0&245': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&246': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&247': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&248': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&249': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&250': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&251': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&252': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&253': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&254': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&255': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&256': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&257': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&258': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&259': np.array([0.7974072911132786, 0.006894018772033576]),
'setosa&0&260': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&261': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&262': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&263': np.array([0.19685199412911678, 0.7845879230594391]),
'setosa&0&264': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&265': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&266': np.array([0.07476043598366156, 0.9062715528547001]),
'setosa&0&267': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&268': np.array([0.7770298852793471, 0.0294434304771479]),
'setosa&0&269': np.array([0.7936433456054741, 0.01258375207649658]),
'setosa&0&270': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&271': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&272': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&273': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&274': np.array([0.4656481363306145, 0.007982539480288167]),
'setosa&0&275': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&276': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&277': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&278': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&279': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&280': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&281': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&282': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&283': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&284': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&285': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&286': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&287': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&288': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&289': np.array([0.4656481363306145, 0.007982539480288167]),
'setosa&0&290': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&291': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&292': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&293': np.array([0.050316962184345455, 0.9292276112117481]),
'setosa&0&294': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&295': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&296': np.array([0.0171486447659196, 0.9632117581295891]),
'setosa&0&297': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&298': np.array([0.06151571389390039, 0.524561199322281]),
'setosa&0&299': np.array([0.4329463382004908, 0.057167210150691136]),
'setosa&0&300': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&301': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&302': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&303': np.array([0.13694026920485936, 0.36331091829858003]),
'setosa&0&304': np.array([0.3094460464703627, 0.11400643817329122]),
'setosa&0&305': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&306': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&307': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&308': np.array([0.029402442458921055, 0.9481684282717416]),
'setosa&0&309': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&310': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&311': np.array([0.00988785935411159, 0.9698143912008228]),
'setosa&0&312': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&313': np.array([0.009595083643662688, 0.5643652067423869]),
'setosa&0&314': np.array([0.13694026920485936, 0.36331091829858003]),
'setosa&1&0': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&1': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&2': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&3': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&4': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&5': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&6': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&7': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&8': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&9': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&10': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&11': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&12': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&13': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&14': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&15': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&16': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&17': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&18': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&19': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&20': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&21': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&22': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&23': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&24': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&25': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&26': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&27': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&28': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&29': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&30': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&31': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&32': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&33': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&34': np.array([-0.26192650167775977, 0.33491141590339474]),
'setosa&1&35': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&36': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&37': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&38': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&39': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&40': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&41': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&42': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&43': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&44': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&45': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&46': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&47': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&48': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&49': np.array([0.4079256832347186, 0.038455640985860955]),
'setosa&1&50': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&51': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&52': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&53': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&54': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&55': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&56': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&57': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&58': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&59': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&60': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&61': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&62': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&63': np.array([0.13717260713320106, 0.3627779907901665]),
'setosa&1&64': np.array([0.3093950298647913, 0.1140298206733954]),
'setosa&1&65': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&66': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&67': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&68': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&69': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&70': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&71': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&72': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&73': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&74': np.array([0.13717260713320106, 0.3627779907901665]),
'setosa&1&75': np.array([0.0, -0.4756207622944677]),
'setosa&1&76': np.array([0.0, -0.4854334805210761]),
'setosa&1&77': np.array([0.0, 0.16885577975809635]),
'setosa&1&78': np.array([0.0, 0.395805885538554]),
'setosa&1&79': np.array([0.0, 0.2538072707138344]),
'setosa&1&80': np.array([0.0, -0.4756207622944677]),
'setosa&1&81': np.array([0.0, -0.4756207622944677]),
'setosa&1&82': np.array([0.0, -0.4756207622944677]),
'setosa&1&83': np.array([0.0, -0.4756207622944677]),
'setosa&1&84': np.array([0.0, -0.4854334805210761]),
'setosa&1&85': np.array([0.0, -0.4854334805210761]),
'setosa&1&86': np.array([0.0, -0.4854334805210761]),
'setosa&1&87': np.array([0.0, 0.16885577975809635]),
'setosa&1&88': np.array([0.0, 0.16885577975809635]),
'setosa&1&89': np.array([0.0, 0.395805885538554]),
'setosa&1&90': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&91': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&92': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&93': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&94': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&95': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&96': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&97': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&98': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&99': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&100': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&101': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&102': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&103': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&104': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&105': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&106': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&107': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&108': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&109': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&110': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&111': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&112': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&113': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&114': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&115': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&116': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&117': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&118': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&119': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&120': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&121': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&122': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&123': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&124': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&125': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&126': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&127': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&128': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&129': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&130': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&131': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&132': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&133': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&134': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&135': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&136': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&137': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&138': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&139': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&140': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&141': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&142': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&143': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&144': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&145': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&146': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&147': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&148': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&149': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&150': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&151': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&152': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&153': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&154': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&155': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&156': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&157': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&158': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&159': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&160': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&161': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&162': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&163': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&164': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&165': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&166': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&167': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&168': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&169': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&170': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&171': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&172': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&173': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&174': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&175': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&176': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&177': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&178': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&179': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&180': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&181': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&182': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&183': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&184': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&185': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&186': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&187': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&188': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&189': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&190': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&191': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&192': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&193': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&194': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&195': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&196': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&197': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&198': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&199': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&200': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&201': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&202': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&203': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&204': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&205': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&206': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&207': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&208': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&209': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&210': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&211': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&212': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&213': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&214': np.array([-0.4964962439921071, 0.3798215458387346]),
'setosa&1&215': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&216': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&217': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&218': np.array([-0.37157553889555184, -0.1221600832023858]),
'setosa&1&219': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&220': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&221': np.array([-0.2463036871609408, -0.24630368716093934]),
'setosa&1&222': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&223': np.array([-0.9105775730167809, 0.6842162738602727]),
'setosa&1&224': np.array([-0.6718337295341267, 0.6620422637360075]),
'setosa&1&225': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&226': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&227': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&228': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&229': np.array([-0.26192650167775977, 0.33491141590339474]),
'setosa&1&230': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&231': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&232': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&233': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&234': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&235': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&236': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&237': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&238': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&239': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&240': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&241': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&242': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&243': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&244': np.array([-0.26192650167775977, 0.33491141590339474]),
'setosa&1&245': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&246': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&247': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&248': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&249': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&250': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&251': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&252': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&253': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&254': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&255': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&256': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&257': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&258': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&259': np.array([-0.26192650167775977, 0.33491141590339474]),
'setosa&1&260': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&261': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&262': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&263': np.array([0.32199975656257585, -0.748229355246375]),
'setosa&1&264': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&265': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&266': np.array([0.43843349141088417, -0.8642740701867918]),
'setosa&1&267': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&268': np.array([-0.7141739659554724, 0.6619819140152877]),
'setosa&1&269': np.array([-0.4446001433508151, 0.6107546840046902]),
'setosa&1&270': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&271': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&272': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&273': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&274': np.array([0.4079256832347186, 0.038455640985860955]),
'setosa&1&275': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&276': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&277': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&278': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&279': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&280': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&281': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&282': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&283': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&284': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&285': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&286': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&287': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&288': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&289': np.array([0.4079256832347186, 0.038455640985860955]),
'setosa&1&290': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&291': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&292': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&293': np.array([0.7749499208750121, -0.814718944080443]),
'setosa&1&294': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&295': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&296': np.array([0.80403091954169, -0.844515250413482]),
'setosa&1&297': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&298': np.array([0.5826506963750848, -0.22335655671229107]),
'setosa&1&299': np.array([0.33108168891715983, 0.13647816746351163]),
'setosa&1&300': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&301': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&302': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&303': np.array([0.13717260713320106, 0.3627779907901665]),
'setosa&1&304': np.array([0.3093950298647913, 0.1140298206733954]),
'setosa&1&305': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&306': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&307': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&308': np.array([0.4933316375690333, -0.5272416708629277]),
'setosa&1&309': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&310': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&311': np.array([0.5041830043657418, -0.5392782673950876]),
'setosa&1&312': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&313': np.array([0.25657760110071476, 0.12592645350389123]),
'setosa&1&314': np.array([0.13717260713320106, 0.3627779907901665]),
'setosa&2&0': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&1': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&2': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&3': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&4': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&5': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&6': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&7': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&8': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&9': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&10': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&11': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&12': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&13': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&14': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&15': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&16': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&17': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&18': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&19': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&20': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&21': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&22': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&23': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&24': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&25': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&26': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&27': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&28': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&29': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&30': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&31': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&32': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&33': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&34': np.array([-0.5354807894355184, -0.3418054346754283]),
'setosa&2&35': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&36': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&37': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&38': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&39': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&40': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&41': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&42': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&43': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&44': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&45': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&46': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&47': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&48': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&49': np.array([-0.8735738195653328, -0.046438180466149094]),
'setosa&2&50': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&51': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&52': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&53': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&54': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&55': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&56': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&57': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&58': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&59': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&60': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&61': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&62': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&63': np.array([-0.2741128763380603, -0.7260889090887469]),
'setosa&2&64': np.array([-0.6188410763351541, -0.22803625884668638]),
'setosa&2&65': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&66': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&67': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&68': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&69': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&70': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&71': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&72': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&73': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&74': np.array([-0.2741128763380603, -0.7260889090887469]),
'setosa&2&75': np.array([0.0, -0.47562425924289314]),
'setosa&2&76': np.array([0.0, -0.48543689565931186]),
'setosa&2&77': np.array([0.0, -0.7348263896003956]),
'setosa&2&78': np.array([0.0, -0.7920887571493729]),
'setosa&2&79': np.array([0.0, -0.507614207038711]),
'setosa&2&80': np.array([0.0, -0.47562425924289314]),
'setosa&2&81': np.array([0.0, -0.47562425924289314]),
'setosa&2&82': np.array([0.0, -0.47562425924289314]),
'setosa&2&83': np.array([0.0, -0.47562425924289314]),
'setosa&2&84': np.array([0.0, -0.48543689565931186]),
'setosa&2&85': np.array([0.0, -0.48543689565931186]),
'setosa&2&86': np.array([0.0, -0.48543689565931186]),
'setosa&2&87': np.array([0.0, -0.7348263896003956]),
'setosa&2&88': np.array([0.0, -0.7348263896003956]),
'setosa&2&89': np.array([0.0, -0.7920887571493729]),
'setosa&2&90': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&91': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&92': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&93': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&94': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&95': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&96': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&97': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&98': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&99': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&100': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&101': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&102': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&103': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&104': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&105': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&106': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&107': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&108': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&109': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&110': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&111': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&112': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&113': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&114': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&115': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&116': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&117': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&118': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&119': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&120': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&121': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&122': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&123': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&124': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&125': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&126': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&127': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&128': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&129': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&130': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&131': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&132': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&133': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&134': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&135': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&136': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&137': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&138': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&139': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&140': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&141': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&142': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&143': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&144': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&145': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&146': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&147': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&148': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&149': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&150': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&151': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&152': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&153': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&154': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&155': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&156': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&157': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&158': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&159': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&160': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&161': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&162': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&163': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&164': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&165': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&166': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&167': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&168': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&169': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&170': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&171': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&172': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&173': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&174': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&175': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&176': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&177': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&178': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&179': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&180': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&181': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&182': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&183': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&184': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&185': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&186': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&187': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&188': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&189': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&190': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&191': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&192': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&193': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&194': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&195': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&196': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&197': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&198': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&199': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&200': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&201': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&202': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&203': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&204': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&205': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&206': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&207': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&208': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&209': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&210': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&211': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&212': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&213': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&214': np.array([-0.47415719445227245, -0.38726974144168774]),
'setosa&2&215': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&216': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&217': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&218': np.array([-0.3715769132100501, -0.12216227283618744]),
'setosa&2&219': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&220': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&221': np.array([-0.24630541996506924, -0.24630541996506994]),
'setosa&2&222': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&223': np.array([-0.044492463210563125, -0.7096449459722027]),
'setosa&2&224': np.array([-0.29537842173874096, -0.6750352694420283]),
'setosa&2&225': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&226': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&227': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&228': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&229': np.array([-0.5354807894355184, -0.3418054346754283]),
'setosa&2&230': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&231': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&232': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&233': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&234': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&235': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&236': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&237': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&238': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&239': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&240': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&241': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&242': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&243': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&244': np.array([-0.5354807894355184, -0.3418054346754283]),
'setosa&2&245': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&246': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&247': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&248': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&249': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&250': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&251': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&252': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&253': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&254': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&255': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&256': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&257': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&258': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&259': np.array([-0.5354807894355184, -0.3418054346754283]),
'setosa&2&260': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&261': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&262': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&263': np.array([-0.5188517506916893, -0.036358567813067795]),
'setosa&2&264': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&265': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&266': np.array([-0.513193927394545, -0.041997482667908786]),
'setosa&2&267': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&268': np.array([-0.06285591932387405, -0.6914253444924359]),
'setosa&2&269': np.array([-0.34904320225465857, -0.6233384360811872]),
'setosa&2&270': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&271': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&272': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&273': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&274': np.array([-0.8735738195653328, -0.046438180466149094]),
'setosa&2&275': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&276': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&277': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&278': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&279': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&280': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&281': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&282': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&283': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&284': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&285': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&286': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&287': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&288': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&289': np.array([-0.8735738195653328, -0.046438180466149094]),
'setosa&2&290': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&291': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&292': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&293': np.array([-0.8252668830593567, -0.11450866713130638]),
'setosa&2&294': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&295': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&296': np.array([-0.8211795643076093, -0.1186965077161071]),
'setosa&2&297': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&298': np.array([-0.6441664102689847, -0.3012046426099901]),
'setosa&2&299': np.array([-0.7640280271176497, -0.19364537761420375]),
'setosa&2&300': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&301': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&302': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&303': np.array([-0.2741128763380603, -0.7260889090887469]),
'setosa&2&304': np.array([-0.6188410763351541, -0.22803625884668638]),
'setosa&2&305': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&306': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&307': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&308': np.array([-0.5227340800279542, -0.42092675740881474]),
'setosa&2&309': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&310': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&311': np.array([-0.5140708637198534, -0.43053612380573514]),
'setosa&2&312': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&313': np.array([-0.2661726847443776, -0.6902916602462779]),
'setosa&2&314': np.array([-0.2741128763380603, -0.7260889090887469]),
'versicolor&0&0': np.array([-0.7431524521056113, -0.24432235603856345]),
'versicolor&0&1': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&2': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&3': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&4': np.array([-0.9706534384443797, 0.007448195602953232]),
'versicolor&0&5': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&6': np.array([-0.967167257194905, -0.011919414234523772]),
'versicolor&0&7': np.array([-0.953200964337313, -0.027163424176667752]),
'versicolor&0&8': np.array([-0.8486399726113752, -0.13537345771621853]),
'versicolor&0&9': np.array([-0.9658161779555727, -0.01446062269877741]),
'versicolor&0&10': np.array([-0.9493506964095418, -0.0312186903717912]),
'versicolor&0&11': np.array([-0.7870031444780577, -0.1952404625292782]),
'versicolor&0&12': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&13': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&14': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&15': np.array([-0.7431524521056113, -0.24432235603856345]),
'versicolor&0&16': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&17': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&18': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&19': np.array([-0.9706534384443797, 0.007448195602953232]),
'versicolor&0&20': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&21': np.array([-0.967167257194905, -0.011919414234523772]),
'versicolor&0&22': np.array([-0.953200964337313, -0.027163424176667752]),
'versicolor&0&23': np.array([-0.8486399726113752, -0.13537345771621853]),
'versicolor&0&24': np.array([-0.9658161779555727, -0.01446062269877741]),
'versicolor&0&25': np.array([-0.9493506964095418, -0.0312186903717912]),
'versicolor&0&26': np.array([-0.7870031444780577, -0.1952404625292782]),
'versicolor&0&27': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&28': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&29': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&30': np.array([-0.19685199412911655, -0.7845879230594393]),
'versicolor&0&31': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&32': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&33': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&34': np.array([-0.7974072911132788, 0.006894018772033604]),
'versicolor&0&35': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&36': np.array([-0.7779663027946229, -0.2981599980028888]),
'versicolor&0&37': np.array([-0.6669876551417979, -0.2911996622134135]),
'versicolor&0&38': np.array([-0.3355030348883163, -0.6305271339971502]),
'versicolor&0&39': np.array([-0.7658431164447598, -0.3248317507526541]),
'versicolor&0&40': np.array([-0.6459073168288453, -0.31573292128613833]),
'versicolor&0&41': np.array([-0.2519677855687844, -0.7134447168661863]),
'versicolor&0&42': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&43': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&44': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&45': np.array([0.05031696218434577, -0.929227611211748]),
'versicolor&0&46': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&47': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&48': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&49': np.array([0.4656481363306145, 0.007982539480288167]),
'versicolor&0&50': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&51': np.array([0.6614632074748169, -0.6030419328583525]),
'versicolor&0&52': np.array([0.5519595359123358, -0.6434192906054143]),
'versicolor&0&53': np.array([0.14241819268815753, -0.8424615476000691]),
'versicolor&0&54': np.array([0.667423576348749, -0.6594086777766442]),
'versicolor&0&55': np.array([0.5429872243487625, -0.6697888833280774]),
'versicolor&0&56': np.array([0.1140907502997574, -0.8737800276630269]),
'versicolor&0&57': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&58': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&59': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&60': np.array([0.029402442458921384, -0.9481684282717414]),
'versicolor&0&61': np.array([0.009887859354111524, -0.9698143912008228]),
'versicolor&0&62': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&63': np.array([0.13694026920485936, 0.36331091829858003]),
'versicolor&0&64': np.array([0.3094460464703627, 0.11400643817329122]),
'versicolor&0&65': np.array([0.009887859354111524, -0.9698143912008228]),
'versicolor&0&66': np.array([0.42809266524335826, -0.40375108595117376]),
'versicolor&0&67': np.array([0.45547700380103057, -0.6083463409799501]),
'versicolor&0&68': np.array([0.19002455311770447, -0.8848597943731074]),
'versicolor&0&69': np.array([0.436966114193701, -0.4638042290788281]),
'versicolor&0&70': np.array([0.45424510803217066, -0.6425314361631614]),
'versicolor&0&71': np.array([0.1746467870122951, -0.9073062742839755]),
'versicolor&0&72': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&73': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&74': np.array([0.13694026920485936, 0.36331091829858003]),
'versicolor&0&75': np.array([0.0, -0.95124502153736]),
'versicolor&0&76': np.array([0.0, -0.9708703761803881]),
'versicolor&0&77': np.array([0.0, 0.5659706098422994]),
'versicolor&0&78': np.array([0.0, 0.3962828716108186]),
'versicolor&0&79': np.array([0.0, 0.2538069363248767]),
'versicolor&0&80': np.array([0.0, -0.9708703761803881]),
'versicolor&0&81': np.array([0.0, -0.3631376646911367]),
'versicolor&0&82': np.array([0.0, -0.5804857652839247]),
'versicolor&0&83': np.array([0.0, -0.8943993997517804]),
'versicolor&0&84': np.array([0.0, -0.4231275527222919]),
'versicolor&0&85': np.array([0.0, -0.6164235822373675]),
'versicolor&0&86': np.array([0.0, -0.9166476163222441]),
'versicolor&0&87': np.array([0.0, 0.5659706098422994]),
'versicolor&0&88': np.array([0.0, 0.5659706098422994]),
'versicolor&0&89': np.array([0.0, 0.3962828716108186]),
'versicolor&0&90': np.array([-0.7431524521056113, -0.24432235603856345]),
'versicolor&0&91': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&92': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&93': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&94': np.array([-0.9706534384443797, 0.007448195602953232]),
'versicolor&0&95': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&96': np.array([-0.967167257194905, -0.011919414234523772]),
'versicolor&0&97': np.array([-0.953200964337313, -0.027163424176667752]),
'versicolor&0&98': np.array([-0.8486399726113752, -0.13537345771621853]),
'versicolor&0&99': np.array([-0.9658161779555727, -0.01446062269877741]),
'versicolor&0&100': np.array([-0.9493506964095418, -0.0312186903717912]),
'versicolor&0&101': np.array([-0.7870031444780577, -0.1952404625292782]),
'versicolor&0&102': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&103': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&104': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&105': np.array([-0.19685199412911655, -0.7845879230594393]),
'versicolor&0&106': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&107': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&108': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&109': np.array([-0.7974072911132788, 0.006894018772033604]),
'versicolor&0&110': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&111': np.array([-0.7779663027946229, -0.2981599980028888]),
'versicolor&0&112': np.array([-0.6669876551417979, -0.2911996622134135]),
'versicolor&0&113': np.array([-0.3355030348883163, -0.6305271339971502]),
'versicolor&0&114': np.array([-0.7658431164447598, -0.3248317507526541]),
'versicolor&0&115': np.array([-0.6459073168288453, -0.31573292128613833]),
'versicolor&0&116': np.array([-0.2519677855687844, -0.7134447168661863]),
'versicolor&0&117': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&118': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&119': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&120': np.array([-0.05855179950109871, -0.9211684729232403]),
'versicolor&0&121': np.array([-0.020067537725011863, -0.960349531159508]),
'versicolor&0&122': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&123': np.array([-0.6813845327458135, 0.6599725404733693]),
'versicolor&0&124': np.array([-0.5182062652425321, 0.3958533237517639]),
'versicolor&0&125': np.array([-0.020067537725011863, -0.960349531159508]),
'versicolor&0&126': np.array([-0.5107107533700952, 0.0075507123577884866]),
'versicolor&0&127': np.array([-0.1464063320531759, -0.4788055402156298]),
'versicolor&0&128': np.array([-0.061109248092233844, -0.8620287767000373]),
'versicolor&0&129': np.array([-0.4706137753079746, -0.057389625790424635]),
'versicolor&0&130': np.array([-0.06804620923037683, -0.5677904519730453]),
'versicolor&0&131': np.array([-0.020216773196675246, -0.9057119888626176]),
'versicolor&0&132': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&133': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&134': np.array([-0.6813845327458135, 0.6599725404733693]),
'versicolor&0&135': np.array([-0.19684482070614498, -0.7845939961595055]),
'versicolor&0&136': np.array([-0.07475231751447156, -0.9062785678426409]),
'versicolor&0&137': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&138': np.array([-0.7694171988675237, 0.276633135028249]),
'versicolor&0&139': np.array([-0.8063011502229427, 0.4134300066735808]),
'versicolor&0&140': np.array([-0.07475231751447156, -0.9062785678426409]),
'versicolor&0&141': np.array([-0.7985789197998611, 0.0026209054759345337]),
'versicolor&0&142': np.array([-0.7182275903095532, -0.11963032135457498]),
'versicolor&0&143': np.array([-0.2798927835773098, -0.6581136857450849]),
'versicolor&0&144': np.array([-0.7920119433269182, -0.0142751249964083]),
'versicolor&0&145': np.array([-0.6943081428778407, -0.14852813120265815]),
'versicolor&0&146': np.array([-0.16106555563262584, -0.777621649099753]),
'versicolor&0&147': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&148': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&149': np.array([-0.7694171988675237, 0.276633135028249]),
'versicolor&0&150': np.array([-0.7431524521056113, -0.24432235603856345]),
'versicolor&0&151': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&152': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&153': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&154': np.array([-0.9706534384443797, 0.007448195602953232]),
'versicolor&0&155': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&156': np.array([-0.967167257194905, -0.011919414234523772]),
'versicolor&0&157': np.array([-0.953200964337313, -0.027163424176667752]),
'versicolor&0&158': np.array([-0.8486399726113752, -0.13537345771621853]),
'versicolor&0&159': np.array([-0.9658161779555727, -0.01446062269877741]),
'versicolor&0&160': np.array([-0.9493506964095418, -0.0312186903717912]),
'versicolor&0&161': np.array([-0.7870031444780577, -0.1952404625292782]),
'versicolor&0&162': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&163': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&164': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&165': np.array([-0.19685199412911655, -0.7845879230594393]),
'versicolor&0&166': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&167': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&168': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&169': np.array([-0.7974072911132788, 0.006894018772033604]),
'versicolor&0&170': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&171': np.array([-0.7779663027946229, -0.2981599980028888]),
'versicolor&0&172': np.array([-0.6669876551417979, -0.2911996622134135]),
'versicolor&0&173': np.array([-0.3355030348883163, -0.6305271339971502]),
'versicolor&0&174': np.array([-0.7658431164447598, -0.3248317507526541]),
'versicolor&0&175': np.array([-0.6459073168288453, -0.31573292128613833]),
'versicolor&0&176': np.array([-0.2519677855687844, -0.7134447168661863]),
'versicolor&0&177': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&178': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&179': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&180': np.array([-0.05855179950109871, -0.9211684729232403]),
'versicolor&0&181': np.array([-0.020067537725011863, -0.960349531159508]),
'versicolor&0&182': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&183': np.array([-0.6813845327458135, 0.6599725404733693]),
'versicolor&0&184': np.array([-0.5182062652425321, 0.3958533237517639]),
'versicolor&0&185': np.array([-0.020067537725011863, -0.960349531159508]),
'versicolor&0&186': np.array([-0.5107107533700952, 0.0075507123577884866]),
'versicolor&0&187': np.array([-0.1464063320531759, -0.4788055402156298]),
'versicolor&0&188': np.array([-0.061109248092233844, -0.8620287767000373]),
'versicolor&0&189': np.array([-0.4706137753079746, -0.057389625790424635]),
'versicolor&0&190': np.array([-0.06804620923037683, -0.5677904519730453]),
'versicolor&0&191': np.array([-0.020216773196675246, -0.9057119888626176]),
'versicolor&0&192': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&193': np.array([-0.5775164514598086, 0.6278692602817483]),
'versicolor&0&194': np.array([-0.6813845327458135, 0.6599725404733693]),
'versicolor&0&195': np.array([-0.19684482070614498, -0.7845939961595055]),
'versicolor&0&196': np.array([-0.07475231751447156, -0.9062785678426409]),
'versicolor&0&197': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&198': np.array([-0.7694171988675237, 0.276633135028249]),
'versicolor&0&199': np.array([-0.8063011502229427, 0.4134300066735808]),
'versicolor&0&200': np.array([-0.07475231751447156, -0.9062785678426409]),
'versicolor&0&201': np.array([-0.7985789197998611, 0.0026209054759345337]),
'versicolor&0&202': np.array([-0.7182275903095532, -0.11963032135457498]),
'versicolor&0&203': np.array([-0.2798927835773098, -0.6581136857450849]),
'versicolor&0&204': np.array([-0.7920119433269182, -0.0142751249964083]),
'versicolor&0&205': np.array([-0.6943081428778407, -0.14852813120265815]),
'versicolor&0&206': np.array([-0.16106555563262584, -0.777621649099753]),
'versicolor&0&207': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&208': np.array([-0.6782037543706109, 0.2956007367698983]),
'versicolor&0&209': np.array([-0.7694171988675237, 0.276633135028249]),
'versicolor&0&210': np.array([-0.7431524521056113, -0.24432235603856345]),
'versicolor&0&211': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&212': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&213': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&214': np.array([-0.9706534384443797, 0.007448195602953232]),
'versicolor&0&215': np.array([-0.4926091071260067, -0.49260910712601286]),
'versicolor&0&216': np.array([-0.967167257194905, -0.011919414234523772]),
'versicolor&0&217': np.array([-0.953200964337313, -0.027163424176667752]),
'versicolor&0&218': np.array([-0.8486399726113752, -0.13537345771621853]),
'versicolor&0&219': np.array([-0.9658161779555727, -0.01446062269877741]),
'versicolor&0&220': np.array([-0.9493506964095418, -0.0312186903717912]),
'versicolor&0&221': np.array([-0.7870031444780577, -0.1952404625292782]),
'versicolor&0&222': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&223': np.array([-0.9550700362273441, 0.025428672111930138]),
'versicolor&0&224': np.array([-0.9672121512728677, 0.012993005706020341]),
'versicolor&0&225': np.array([-0.04777085826693217, -0.931704979630315]),
'versicolor&0&226': np.array([-0.016252316132452975, -0.9640854286687816]),
'versicolor&0&227': np.array([-0.44101924439572626, 0.5583264842761904]),
'versicolor&0&228': np.array([-0.5844994389588399, 0.5715208832363579]),
'versicolor&0&229': np.array([-0.46216647196120714, 0.35468591243823655]),
'versicolor&0&230': np.array([-0.016252316132452975, -0.9640854286687816]),
'versicolor&0&231': np.array([-0.3707180757031537, -0.1977196581472426]),
'versicolor&0&232': np.array([-0.1043459833293615, -0.5233314327065356]),
'versicolor&0&233': np.array([-0.049289647556763364, -0.8736084405111605]),
'versicolor&0&234': np.array([-0.34078174031874375, -0.25874482325965437]),
'versicolor&0&235': np.array([-0.050841051273783675, -0.5877587283589205]),
'versicolor&0&236': np.array([-0.0161720977425142, -0.9096817855236822]),
'versicolor&0&237': np.array([-0.44101924439572626, 0.5583264842761904]),
'versicolor&0&238': np.array([-0.44101924439572626, 0.5583264842761904]),
'versicolor&0&239': np.array([-0.5844994389588399, 0.5715208832363579]),
'versicolor&0&240': np.array([-0.11329659732608087, -0.8671819100849522]),
'versicolor&0&241': np.array([-0.040390637135858574, -0.9402832917474078]),
'versicolor&0&242': np.array([-0.5276460255602035, 0.28992233541586077]),
'versicolor&0&243': np.array([-0.6392402874163683, 0.24114611970435948]),
'versicolor&0&244': np.array([-0.6814868825686854, 0.35066801608083215]),
'versicolor&0&245': np.array([-0.040390637135858574, -0.9402832917474078]),
'versicolor&0&246': np.array([-0.6425009695928476, -0.24851992476830956]),
'versicolor&0&247': np.array([-0.5151243662384031, -0.3255567772442641]),
'versicolor&0&248': np.array([-0.16157511199607094, -0.7754323813403634]),
'versicolor&0&249': np.array([-0.6300442788906601, -0.28361140069713875]),
'versicolor&0&250': np.array([-0.4875864856121089, -0.3614122096616301]),
'versicolor&0&251': np.array([-0.08968204532514226, -0.8491191210330045]),
'versicolor&0&252': np.array([-0.5276460255602035, 0.28992233541586077]),
'versicolor&0&253': np.array([-0.5276460255602035, 0.28992233541586077]),
'versicolor&0&254': np.array([-0.6392402874163683, 0.24114611970435948]),
'versicolor&0&255': np.array([-0.19685199412911655, -0.7845879230594393]),
'versicolor&0&256': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&257': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&258': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&259': np.array([-0.7974072911132788, 0.006894018772033604]),
'versicolor&0&260': np.array([-0.07476043598366228, -0.9062715528546994]),
'versicolor&0&261': np.array([-0.7779663027946229, -0.2981599980028888]),
'versicolor&0&262': np.array([-0.6669876551417979, -0.2911996622134135]),
'versicolor&0&263': np.array([-0.3355030348883163, -0.6305271339971502]),
'versicolor&0&264': np.array([-0.7658431164447598, -0.3248317507526541]),
'versicolor&0&265': np.array([-0.6459073168288453, -0.31573292128613833]),
'versicolor&0&266': np.array([-0.2519677855687844, -0.7134447168661863]),
'versicolor&0&267': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&268': np.array([-0.7770298852793476, 0.029443430477147536]),
'versicolor&0&269': np.array([-0.7936433456054744, 0.012583752076496493]),
'versicolor&0&270': np.array([0.05031696218434577, -0.929227611211748]),
'versicolor&0&271': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&272': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&273': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&274': np.array([0.4656481363306145, 0.007982539480288167]),
'versicolor&0&275': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&276': np.array([0.6614632074748169, -0.6030419328583525]),
'versicolor&0&277': np.array([0.5519595359123358, -0.6434192906054143]),
'versicolor&0&278': np.array([0.14241819268815753, -0.8424615476000691]),
'versicolor&0&279': np.array([0.667423576348749, -0.6594086777766442]),
'versicolor&0&280': np.array([0.5429872243487625, -0.6697888833280774]),
'versicolor&0&281': np.array([0.1140907502997574, -0.8737800276630269]),
'versicolor&0&282': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&283': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&284': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&285': np.array([0.05031696218434577, -0.929227611211748]),
'versicolor&0&286': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&287': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&288': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&289': np.array([0.4656481363306145, 0.007982539480288167]),
'versicolor&0&290': np.array([0.017148644765919676, -0.9632117581295891]),
'versicolor&0&291': np.array([0.6614632074748169, -0.6030419328583525]),
'versicolor&0&292': np.array([0.5519595359123358, -0.6434192906054143]),
'versicolor&0&293': np.array([0.14241819268815753, -0.8424615476000691]),
'versicolor&0&294': np.array([0.667423576348749, -0.6594086777766442]),
'versicolor&0&295': np.array([0.5429872243487625, -0.6697888833280774]),
'versicolor&0&296': np.array([0.1140907502997574, -0.8737800276630269]),
'versicolor&0&297': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&298': np.array([0.06151571389390039, 0.524561199322281]),
'versicolor&0&299': np.array([0.4329463382004908, 0.057167210150691136]),
'versicolor&0&300': np.array([0.029402442458921384, -0.9481684282717414]),
'versicolor&0&301': np.array([0.009887859354111524, -0.9698143912008228]),
'versicolor&0&302': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&303': np.array([0.13694026920485936, 0.36331091829858003]),
'versicolor&0&304': np.array([0.3094460464703627, 0.11400643817329122]),
'versicolor&0&305': np.array([0.009887859354111524, -0.9698143912008228]),
'versicolor&0&306': np.array([0.42809266524335826, -0.40375108595117376]),
'versicolor&0&307': np.array([0.45547700380103057, -0.6083463409799501]),
'versicolor&0&308': np.array([0.19002455311770447, -0.8848597943731074]),
'versicolor&0&309': np.array([0.436966114193701, -0.4638042290788281]),
'versicolor&0&310': np.array([0.45424510803217066, -0.6425314361631614]),
'versicolor&0&311': np.array([0.1746467870122951, -0.9073062742839755]),
'versicolor&0&312': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&313': np.array([0.009595083643662688, 0.5643652067423869]),
'versicolor&0&314': np.array([0.13694026920485936, 0.36331091829858003]),
'versicolor&1&0': np.array([0.37157553889555184, 0.1221600832023858]),
'versicolor&1&1': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&2': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&3': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&4': np.array([0.4964962439921071, 0.3798215458387346]),
'versicolor&1&5': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&6': np.array([0.2805345936193346, 0.6595182922149835]),
'versicolor&1&7': np.array([0.08302493125394889, 0.6186280682763334]),
'versicolor&1&8': np.array([0.22125635302655813, 0.2925832702358638]),
'versicolor&1&9': np.array([0.2365788606456636, 0.7120007179768731]),
'versicolor&1&10': np.array([0.022347126801293967, 0.6718013300441928]),
'versicolor&1&11': np.array([0.10063786451829529, 0.4085974066833644]),
'versicolor&1&12': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&13': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&14': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&15': np.array([0.37157553889555184, 0.1221600832023858]),
'versicolor&1&16': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&17': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&18': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&19': np.array([0.4964962439921071, 0.3798215458387346]),
'versicolor&1&20': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&21': np.array([0.2805345936193346, 0.6595182922149835]),
'versicolor&1&22': np.array([0.08302493125394889, 0.6186280682763334]),
'versicolor&1&23': np.array([0.22125635302655813, 0.2925832702358638]),
'versicolor&1&24': np.array([0.2365788606456636, 0.7120007179768731]),
'versicolor&1&25': np.array([0.022347126801293967, 0.6718013300441928]),
'versicolor&1&26': np.array([0.10063786451829529, 0.4085974066833644]),
'versicolor&1&27': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&28': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&29': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&30': np.array([-0.32199975656257646, 0.7482293552463756]),
'versicolor&1&31': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&32': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&33': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&34': np.array([0.2619265016777598, 0.33491141590339474]),
'versicolor&1&35': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&36': np.array([0.20183015430619713, 0.7445346002055082]),
'versicolor&1&37': np.array([-0.05987874887638573, 0.6927937290176818]),
'versicolor&1&38': np.array([-0.2562642052727569, 0.6920266972283227]),
'versicolor&1&39': np.array([0.1736438124560164, 0.7898174616442941]),
'versicolor&1&40': np.array([-0.10114089899940126, 0.7326610366533243]),
'versicolor&1&41': np.array([-0.34479806250338163, 0.7789143553916729]),
'versicolor&1&42': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&43': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&44': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&45': np.array([0.7749499208750119, 0.8147189440804429]),
'versicolor&1&46': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&47': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&48': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&49': np.array([0.4079256832347186, 0.038455640985860955]),
'versicolor&1&50': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&51': np.array([0.18555813792691386, 0.6940923833143309]),
'versicolor&1&52': np.array([0.32639262064172164, 0.6296083447134281]),
'versicolor&1&53': np.array([0.6964303997553315, 0.7444536452136676]),
'versicolor&1&54': np.array([0.18216358701833335, 0.747615101407194]),
'versicolor&1&55': np.array([0.33549445287370383, 0.6526039763053625]),
'versicolor&1&56': np.array([0.7213651642695392, 0.7718874443854203]),
'versicolor&1&57': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&58': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&59': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&60': np.array([0.4933316375690332, 0.5272416708629276]),
'versicolor&1&61': np.array([0.5041830043657418, 0.5392782673950876]),
'versicolor&1&62': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&63': np.array([0.13717260713320106, 0.3627779907901665]),
'versicolor&1&64': np.array([0.3093950298647913, 0.1140298206733954]),
'versicolor&1&65': np.array([0.5041830043657418, 0.5392782673950876]),
'versicolor&1&66': np.array([0.1413116283690917, 0.7479856297394165]),
'versicolor&1&67': np.array([0.189773257421942, 0.6552150653012478]),
'versicolor&1&68': np.array([0.40694846236352233, 0.5109051764198169]),
'versicolor&1&69': np.array([0.1390424906594644, 0.7991613016301518]),
'versicolor&1&70': np.array([0.1945777487290197, 0.6743932844312892]),
'versicolor&1&71': np.array([0.415695226122737, 0.5230815102377903]),
'versicolor&1&72': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&73': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&74': np.array([0.13717260713320106, 0.3627779907901665]),
'versicolor&1&75': np.array([0.0, 0.4756207622944677]),
'versicolor&1&76': np.array([0.0, 0.4854334805210761]),
'versicolor&1&77': np.array([0.0, 0.16885577975809635]),
'versicolor&1&78': np.array([0.0, 0.395805885538554]),
'versicolor&1&79': np.array([0.0, 0.2538072707138344]),
'versicolor&1&80': np.array([0.0, 0.4854334805210761]),
'versicolor&1&81': np.array([0.0, 0.7613919530844643]),
'versicolor&1&82': np.array([0.0, 0.6668230985485095]),
'versicolor&1&83': np.array([0.0, 0.4904755652105692]),
'versicolor&1&84': np.array([0.0, 0.8121046082359693]),
'versicolor&1&85': np.array([0.0, 0.6855766903749089]),
'versicolor&1&86': np.array([0.0, 0.5008471974438506]),
'versicolor&1&87': np.array([0.0, 0.16885577975809635]),
'versicolor&1&88': np.array([0.0, 0.16885577975809635]),
'versicolor&1&89': np.array([0.0, 0.395805885538554]),
'versicolor&1&90': np.array([0.37157553889555184, 0.1221600832023858]),
'versicolor&1&91': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&92': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&93': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&94': np.array([0.4964962439921071, 0.3798215458387346]),
'versicolor&1&95': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&96': np.array([0.2805345936193346, 0.6595182922149835]),
'versicolor&1&97': np.array([0.08302493125394889, 0.6186280682763334]),
'versicolor&1&98': np.array([0.22125635302655813, 0.2925832702358638]),
'versicolor&1&99': np.array([0.2365788606456636, 0.7120007179768731]),
'versicolor&1&100': np.array([0.022347126801293967, 0.6718013300441928]),
'versicolor&1&101': np.array([0.10063786451829529, 0.4085974066833644]),
'versicolor&1&102': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&103': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&104': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&105': np.array([-0.32199975656257646, 0.7482293552463756]),
'versicolor&1&106': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&107': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&108': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&109': np.array([0.2619265016777598, 0.33491141590339474]),
'versicolor&1&110': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&111': np.array([0.20183015430619713, 0.7445346002055082]),
'versicolor&1&112': np.array([-0.05987874887638573, 0.6927937290176818]),
'versicolor&1&113': np.array([-0.2562642052727569, 0.6920266972283227]),
'versicolor&1&114': np.array([0.1736438124560164, 0.7898174616442941]),
'versicolor&1&115': np.array([-0.10114089899940126, 0.7326610366533243]),
'versicolor&1&116': np.array([-0.34479806250338163, 0.7789143553916729]),
'versicolor&1&117': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&118': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&119': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&120': np.array([0.8224435822504677, 0.05315271528828394]),
'versicolor&1&121': np.array([0.820222886307464, 0.055413714884152906]),
'versicolor&1&122': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&123': np.array([0.8282924295054531, 0.0752641855714259]),
'versicolor&1&124': np.array([0.8476206690613984, 0.02146454924522743]),
'versicolor&1&125': np.array([0.820222886307464, 0.055413714884152906]),
'versicolor&1&126': np.array([0.69362517791403, 0.2579390890424607]),
'versicolor&1&127': np.array([0.7261791877801502, 0.16248655642013624]),
'versicolor&1&128': np.array([0.8190416077589757, 0.05661509439536992]),
'versicolor&1&129': np.array([0.6654762076749751, 0.2949291633432878]),
'versicolor&1&130': np.array([0.7118161070185614, 0.17683644094125878]),
'versicolor&1&131': np.array([0.8165214253946836, 0.059175619390630096]),
'versicolor&1&132': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&133': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&134': np.array([0.8282924295054531, 0.0752641855714259]),
'versicolor&1&135': np.array([0.5188109114552927, 0.03638964581864269]),
'versicolor&1&136': np.array([0.5131478569192371, 0.04203387599862816]),
'versicolor&1&137': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&138': np.array([0.5965042032375719, 0.48856644624972617]),
'versicolor&1&139': np.array([0.5436097000280874, 0.1461891067488832]),
'versicolor&1&140': np.array([0.5131478569192371, 0.04203387599862816]),
'versicolor&1&141': np.array([0.32513442685780247, 0.6124765483184536]),
'versicolor&1&142': np.array([0.1812883360919208, 0.5504982486874137]),
'versicolor&1&143': np.array([0.4788153032824012, 0.08625929936974323]),
'versicolor&1&144': np.array([0.28490718210609345, 0.6650298146522879]),
'versicolor&1&145': np.array([0.1313204067730033, 0.597079642504441]),
'versicolor&1&146': np.array([0.46583127837967303, 0.09875847161509169]),
'versicolor&1&147': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&148': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&149': np.array([0.5965042032375719, 0.48856644624972617]),
'versicolor&1&150': np.array([0.37157553889555184, 0.1221600832023858]),
'versicolor&1&151': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&152': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&153': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&154': np.array([0.4964962439921071, 0.3798215458387346]),
'versicolor&1&155': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&156': np.array([0.2805345936193346, 0.6595182922149835]),
'versicolor&1&157': np.array([0.08302493125394889, 0.6186280682763334]),
'versicolor&1&158': np.array([0.22125635302655813, 0.2925832702358638]),
'versicolor&1&159': np.array([0.2365788606456636, 0.7120007179768731]),
'versicolor&1&160': np.array([0.022347126801293967, 0.6718013300441928]),
'versicolor&1&161': np.array([0.10063786451829529, 0.4085974066833644]),
'versicolor&1&162': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&163': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&164': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&165': np.array([-0.32199975656257646, 0.7482293552463756]),
'versicolor&1&166': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&167': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&168': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&169': np.array([0.2619265016777598, 0.33491141590339474]),
'versicolor&1&170': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&171': np.array([0.20183015430619713, 0.7445346002055082]),
'versicolor&1&172': np.array([-0.05987874887638573, 0.6927937290176818]),
'versicolor&1&173': np.array([-0.2562642052727569, 0.6920266972283227]),
'versicolor&1&174': np.array([0.1736438124560164, 0.7898174616442941]),
'versicolor&1&175': np.array([-0.10114089899940126, 0.7326610366533243]),
'versicolor&1&176': np.array([-0.34479806250338163, 0.7789143553916729]),
'versicolor&1&177': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&178': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&179': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&180': np.array([0.8224435822504677, 0.05315271528828394]),
'versicolor&1&181': np.array([0.820222886307464, 0.055413714884152906]),
'versicolor&1&182': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&183': np.array([0.8282924295054531, 0.0752641855714259]),
'versicolor&1&184': np.array([0.8476206690613984, 0.02146454924522743]),
'versicolor&1&185': np.array([0.820222886307464, 0.055413714884152906]),
'versicolor&1&186': np.array([0.69362517791403, 0.2579390890424607]),
'versicolor&1&187': np.array([0.7261791877801502, 0.16248655642013624]),
'versicolor&1&188': np.array([0.8190416077589757, 0.05661509439536992]),
'versicolor&1&189': np.array([0.6654762076749751, 0.2949291633432878]),
'versicolor&1&190': np.array([0.7118161070185614, 0.17683644094125878]),
'versicolor&1&191': np.array([0.8165214253946836, 0.059175619390630096]),
'versicolor&1&192': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&193': np.array([0.8393089066702096, 0.0788980157959197]),
'versicolor&1&194': np.array([0.8282924295054531, 0.0752641855714259]),
'versicolor&1&195': np.array([0.5188109114552927, 0.03638964581864269]),
'versicolor&1&196': np.array([0.5131478569192371, 0.04203387599862816]),
'versicolor&1&197': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&198': np.array([0.5965042032375719, 0.48856644624972617]),
'versicolor&1&199': np.array([0.5436097000280874, 0.1461891067488832]),
'versicolor&1&200': np.array([0.5131478569192371, 0.04203387599862816]),
'versicolor&1&201': np.array([0.32513442685780247, 0.6124765483184536]),
'versicolor&1&202': np.array([0.1812883360919208, 0.5504982486874137]),
'versicolor&1&203': np.array([0.4788153032824012, 0.08625929936974323]),
'versicolor&1&204': np.array([0.28490718210609345, 0.6650298146522879]),
'versicolor&1&205': np.array([0.1313204067730033, 0.597079642504441]),
'versicolor&1&206': np.array([0.46583127837967303, 0.09875847161509169]),
'versicolor&1&207': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&208': np.array([0.73294627367007, 0.4610490766898855]),
'versicolor&1&209': np.array([0.5965042032375719, 0.48856644624972617]),
'versicolor&1&210': np.array([0.37157553889555184, 0.1221600832023858]),
'versicolor&1&211': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&212': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&213': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&214': np.array([0.4964962439921071, 0.3798215458387346]),
'versicolor&1&215': np.array([0.2463036871609408, 0.24630368716093934]),
'versicolor&1&216': np.array([0.2805345936193346, 0.6595182922149835]),
'versicolor&1&217': np.array([0.08302493125394889, 0.6186280682763334]),
'versicolor&1&218': np.array([0.22125635302655813, 0.2925832702358638]),
'versicolor&1&219': np.array([0.2365788606456636, 0.7120007179768731]),
'versicolor&1&220': np.array([0.022347126801293967, 0.6718013300441928]),
'versicolor&1&221': np.array([0.10063786451829529, 0.4085974066833644]),
'versicolor&1&222': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&223': np.array([0.9105775730167809, 0.6842162738602727]),
'versicolor&1&224': np.array([0.6718337295341267, 0.6620422637360075]),
'versicolor&1&225': np.array([0.6253337666017573, 0.21983620140147825]),
'versicolor&1&226': np.array([0.6178968870349187, 0.22747652768125623]),
'versicolor&1&227': np.array([0.7245803616608639, 0.18141483095066183]),
'versicolor&1&228': np.array([0.6762617119303499, 0.19305674697949574]),
'versicolor&1&229': np.array([0.7182033715159247, 0.0970420677941148]),
'versicolor&1&230': np.array([0.6178968870349187, 0.22747652768125623]),
'versicolor&1&231': np.array([0.4976586558055923, 0.5393318265947251]),
'versicolor&1&232': np.array([0.4361093214026388, 0.4279491486345008]),
'versicolor&1&233': np.array([0.613985959011319, 0.23148898930908424]),
'versicolor&1&234': np.array([0.46747697713468217, 0.586607956360002]),
'versicolor&1&235': np.array([0.41044950174869577, 0.45415985894965977]),
'versicolor&1&236': np.array([0.6057447478066579, 0.23993389556303918]),
'versicolor&1&237': np.array([0.7245803616608639, 0.18141483095066183]),
'versicolor&1&238': np.array([0.7245803616608639, 0.18141483095066183]),
'versicolor&1&239': np.array([0.6762617119303499, 0.19305674697949574]),
'versicolor&1&240': np.array([0.056623968925773045, 0.43360725859686644]),
'versicolor&1&241': np.array([0.020169511418752378, 0.47015948158260334]),
'versicolor&1&242': np.array([0.5806365328450954, 0.47262706807712623]),
'versicolor&1&243': np.array([0.4146290154471569, 0.4964318942067898]),
'versicolor&1&244': np.array([0.3351719071445682, 0.20616862401308342]),
'versicolor&1&245': np.array([0.020169511418752378, 0.47015948158260334]),
'versicolor&1&246': np.array([0.24022705822940116, 0.7185371033867092]),
'versicolor&1&247': np.array([0.010447231513465048, 0.6616528865917504]),
'versicolor&1&248': np.array([0.024556360933646205, 0.4723948285969902]),
'versicolor&1&249': np.array([0.21321406009810842, 0.7648907754638917]),
'versicolor&1&250': np.array([-0.027450681014480036, 0.6999336015080245]),
'versicolor&1&251': np.array([-0.0164329511444131, 0.5132208276383963]),
'versicolor&1&252': np.array([0.5806365328450954, 0.47262706807712623]),
'versicolor&1&253': np.array([0.5806365328450954, 0.47262706807712623]),
'versicolor&1&254': np.array([0.4146290154471569, 0.4964318942067898]),
'versicolor&1&255': np.array([-0.32199975656257646, 0.7482293552463756]),
'versicolor&1&256': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&257': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&258': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&259': np.array([0.2619265016777598, 0.33491141590339474]),
'versicolor&1&260': np.array([-0.43843349141088417, 0.8642740701867917]),
'versicolor&1&261': np.array([0.20183015430619713, 0.7445346002055082]),
'versicolor&1&262': np.array([-0.05987874887638573, 0.6927937290176818]),
'versicolor&1&263': np.array([-0.2562642052727569, 0.6920266972283227]),
'versicolor&1&264': np.array([0.1736438124560164, 0.7898174616442941]),
'versicolor&1&265': np.array([-0.10114089899940126, 0.7326610366533243]),
'versicolor&1&266': np.array([-0.34479806250338163, 0.7789143553916729]),
'versicolor&1&267': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&268': np.array([0.7141739659554727, 0.6619819140152878]),
'versicolor&1&269': np.array([0.44460014335081516, 0.6107546840046902]),
'versicolor&1&270': np.array([0.7749499208750119, 0.8147189440804429]),
'versicolor&1&271': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&272': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&273': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&274': np.array([0.4079256832347186, 0.038455640985860955]),
'versicolor&1&275': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&276': np.array([0.18555813792691386, 0.6940923833143309]),
'versicolor&1&277': np.array([0.32639262064172164, 0.6296083447134281]),
'versicolor&1&278': np.array([0.6964303997553315, 0.7444536452136676]),
'versicolor&1&279': np.array([0.18216358701833335, 0.747615101407194]),
'versicolor&1&280': np.array([0.33549445287370383, 0.6526039763053625]),
'versicolor&1&281': np.array([0.7213651642695392, 0.7718874443854203]),
'versicolor&1&282': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&283': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&284': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&285': np.array([0.7749499208750119, 0.8147189440804429]),
'versicolor&1&286': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&287': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&288': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&289': np.array([0.4079256832347186, 0.038455640985860955]),
'versicolor&1&290': np.array([0.8040309195416899, 0.8445152504134819]),
'versicolor&1&291': np.array([0.18555813792691386, 0.6940923833143309]),
'versicolor&1&292': np.array([0.32639262064172164, 0.6296083447134281]),
'versicolor&1&293': np.array([0.6964303997553315, 0.7444536452136676]),
'versicolor&1&294': np.array([0.18216358701833335, 0.747615101407194]),
'versicolor&1&295': np.array([0.33549445287370383, 0.6526039763053625]),
'versicolor&1&296': np.array([0.7213651642695392, 0.7718874443854203]),
'versicolor&1&297': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&298': np.array([0.5826506963750848, -0.22335655671229107]),
'versicolor&1&299': np.array([0.33108168891715983, 0.13647816746351163]),
'versicolor&1&300': np.array([0.4933316375690332, 0.5272416708629276]),
'versicolor&1&301': np.array([0.5041830043657418, 0.5392782673950876]),
'versicolor&1&302': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&303': np.array([0.13717260713320106, 0.3627779907901665]),
'versicolor&1&304': np.array([0.3093950298647913, 0.1140298206733954]),
'versicolor&1&305': np.array([0.5041830043657418, 0.5392782673950876]),
'versicolor&1&306': np.array([0.1413116283690917, 0.7479856297394165]),
'versicolor&1&307': np.array([0.189773257421942, 0.6552150653012478]),
'versicolor&1&308': np.array([0.40694846236352233, 0.5109051764198169]),
'versicolor&1&309': np.array([0.1390424906594644, 0.7991613016301518]),
'versicolor&1&310': np.array([0.1945777487290197, 0.6743932844312892]),
'versicolor&1&311': np.array([0.415695226122737, 0.5230815102377903]),
'versicolor&1&312': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&313': np.array([0.25657760110071476, 0.12592645350389123]),
'versicolor&1&314': np.array([0.13717260713320106, 0.3627779907901665]),
'versicolor&2&0': np.array([0.37157691321004915, 0.12216227283618836]),
'versicolor&2&1': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&2': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&3': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&4': np.array([0.4741571944522723, -0.3872697414416878]),
'versicolor&2&5': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&6': np.array([0.68663266357557, -0.6475988779804592]),
'versicolor&2&7': np.array([0.8701760330833639, -0.5914646440996656]),
'versicolor&2&8': np.array([0.6273836195848199, -0.15720981251964872]),
'versicolor&2&9': np.array([0.7292373173099087, -0.6975400952780954]),
'versicolor&2&10': np.array([0.9270035696082471, -0.640582639672401]),
'versicolor&2&11': np.array([0.6863652799597699, -0.21335694415409426]),
'versicolor&2&12': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&13': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&14': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&15': np.array([0.37157691321004915, 0.12216227283618836]),
'versicolor&2&16': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&17': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&18': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&19': np.array([0.4741571944522723, -0.3872697414416878]),
'versicolor&2&20': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&21': np.array([0.68663266357557, -0.6475988779804592]),
'versicolor&2&22': np.array([0.8701760330833639, -0.5914646440996656]),
'versicolor&2&23': np.array([0.6273836195848199, -0.15720981251964872]),
'versicolor&2&24': np.array([0.7292373173099087, -0.6975400952780954]),
'versicolor&2&25': np.array([0.9270035696082471, -0.640582639672401]),
'versicolor&2&26': np.array([0.6863652799597699, -0.21335694415409426]),
'versicolor&2&27': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&28': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&29': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&30': np.array([0.5188517506916897, 0.036358567813067386]),
'versicolor&2&31': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&32': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&33': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&34': np.array([0.5354807894355184, -0.3418054346754283]),
'versicolor&2&35': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&36': np.array([0.5761361484884252, -0.44637460220261904]),
'versicolor&2&37': np.array([0.7268664040181829, -0.40159406680426807]),
'versicolor&2&38': np.array([0.5917672401610737, -0.061499563231173816]),
'versicolor&2&39': np.array([0.5921993039887428, -0.46498571089163954]),
'versicolor&2&40': np.array([0.7470482158282458, -0.4169281153671854]),
'versicolor&2&41': np.array([0.5967658480721675, -0.06546963852548916]),
'versicolor&2&42': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&43': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&44': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&45': np.array([-0.8252668830593566, 0.11450866713130668]),
'versicolor&2&46': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&47': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&48': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&49': np.array([-0.8735738195653328, -0.046438180466149094]),
'versicolor&2&50': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&51': np.array([-0.8470213454017305, -0.0910504504559782]),
'versicolor&2&52': np.array([-0.8783521565540571, 0.01381094589198601]),
'versicolor&2&53': np.array([-0.8388485924434891, 0.09800790238640067]),
'versicolor&2&54': np.array([-0.8495871633670822, -0.08820642363054954]),
'versicolor&2&55': np.array([-0.8784816772224661, 0.017184907022714958]),
'versicolor&2&56': np.array([-0.835455914569297, 0.10189258327760495]),
'versicolor&2&57': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&58': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&59': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&60': np.array([-0.5227340800279543, 0.4209267574088147]),
'versicolor&2&61': np.array([-0.5140708637198534, 0.4305361238057349]),
'versicolor&2&62': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&63': np.array([-0.2741128763380603, -0.7260889090887469]),
'versicolor&2&64': np.array([-0.6188410763351541, -0.22803625884668638]),
'versicolor&2&65': np.array([-0.5140708637198534, 0.4305361238057349]),
'versicolor&2&66': np.array([-0.56940429361245, -0.3442345437882425]),
'versicolor&2&67': np.array([-0.6452502612229726, -0.04686872432129788]),
'versicolor&2&68': np.array([-0.596973015481227, 0.37395461795328944]),
'versicolor&2&69': np.array([-0.5760086048531655, -0.3353570725513232]),
'versicolor&2&70': np.array([-0.6488228567611906, -0.03186184826812757]),
'versicolor&2&71': np.array([-0.5903420131350324, 0.384224764046184]),
'versicolor&2&72': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&73': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&74': np.array([-0.2741128763380603, -0.7260889090887469]),
'versicolor&2&75': np.array([0.0, 0.47562425924289314]),
'versicolor&2&76': np.array([0.0, 0.4854368956593117]),
'versicolor&2&77': np.array([0.0, -0.7348263896003956]),
'versicolor&2&78': np.array([0.0, -0.7920887571493729]),
'versicolor&2&79': np.array([0.0, -0.507614207038711]),
'versicolor&2&80': np.array([0.0, 0.4854368956593117]),
'versicolor&2&81': np.array([0.0, -0.3982542883933272]),
'versicolor&2&82': np.array([0.0, -0.08633733326458487]),
'versicolor&2&83': np.array([0.0, 0.4039238345412103]),
'versicolor&2&84': np.array([0.0, -0.38897705551367706]),
'versicolor&2&85': np.array([0.0, -0.06915310813754129]),
'versicolor&2&86': np.array([0.0, 0.41580041887839214]),
'versicolor&2&87': np.array([0.0, -0.7348263896003956]),
'versicolor&2&88': np.array([0.0, -0.7348263896003956]),
'versicolor&2&89': np.array([0.0, -0.7920887571493729]),
'versicolor&2&90': np.array([0.37157691321004915, 0.12216227283618836]),
'versicolor&2&91': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&92': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&93': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&94': np.array([0.4741571944522723, -0.3872697414416878]),
'versicolor&2&95': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&96': np.array([0.68663266357557, -0.6475988779804592]),
'versicolor&2&97': np.array([0.8701760330833639, -0.5914646440996656]),
'versicolor&2&98': np.array([0.6273836195848199, -0.15720981251964872]),
'versicolor&2&99': np.array([0.7292373173099087, -0.6975400952780954]),
'versicolor&2&100': np.array([0.9270035696082471, -0.640582639672401]),
'versicolor&2&101': np.array([0.6863652799597699, -0.21335694415409426]),
'versicolor&2&102': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&103': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&104': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&105': np.array([0.5188517506916897, 0.036358567813067386]),
'versicolor&2&106': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&107': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&108': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&109': np.array([0.5354807894355184, -0.3418054346754283]),
'versicolor&2&110': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&111': np.array([0.5761361484884252, -0.44637460220261904]),
'versicolor&2&112': np.array([0.7268664040181829, -0.40159406680426807]),
'versicolor&2&113': np.array([0.5917672401610737, -0.061499563231173816]),
'versicolor&2&114': np.array([0.5921993039887428, -0.46498571089163954]),
'versicolor&2&115': np.array([0.7470482158282458, -0.4169281153671854]),
'versicolor&2&116': np.array([0.5967658480721675, -0.06546963852548916]),
'versicolor&2&117': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&118': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&119': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&120': np.array([-0.7638917827493686, 0.868015757634957]),
'versicolor&2&121': np.array([-0.8001553485824509, 0.9049358162753539]),
'versicolor&2&122': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&123': np.array([-0.14690789675963867, -0.7352367260447958]),
'versicolor&2&124': np.array([-0.32941440381886555, -0.4173178729969913]),
'versicolor&2&125': np.array([-0.8001553485824509, 0.9049358162753539]),
'versicolor&2&126': np.array([-0.18291442454393395, -0.2654898014002494]),
'versicolor&2&127': np.array([-0.5797728557269727, 0.3163189837954924]),
'versicolor&2&128': np.array([-0.7579323596667402, 0.8054136823046655]),
'versicolor&2&129': np.array([-0.1948624323669993, -0.23753953755286383]),
'versicolor&2&130': np.array([-0.6437698977881832, 0.3909540110317858]),
'versicolor&2&131': np.array([-0.7963046521980063, 0.846536369471985]),
'versicolor&2&132': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&133': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&134': np.array([-0.14690789675963867, -0.7352367260447958]),
'versicolor&2&135': np.array([-0.3219660907491514, 0.7482043503408669]),
'versicolor&2&136': np.array([-0.43839553940476644, 0.8642446918440131]),
'versicolor&2&137': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&138': np.array([0.17291299562995102, -0.7651995812779756]),
'versicolor&2&139': np.array([0.2626914501948546, -0.5596191134224637]),
'versicolor&2&140': np.array([-0.43839553940476644, 0.8642446918440131]),
'versicolor&2&141': np.array([0.4734444929420575, -0.6150974537943872]),
'versicolor&2&142': np.array([0.5369392542176313, -0.430867927332838]),
'versicolor&2&143': np.array([-0.19892251970509112, 0.5718543863753405]),
'versicolor&2&144': np.array([0.5071047612208237, -0.6507546896558788]),
'versicolor&2&145': np.array([0.5629877361048359, -0.4485515113017818]),
'versicolor&2&146': np.array([-0.3047657227470458, 0.6788631774846587]),
'versicolor&2&147': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&148': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&149': np.array([0.17291299562995102, -0.7651995812779756]),
'versicolor&2&150': np.array([0.37157691321004915, 0.12216227283618836]),
'versicolor&2&151': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&152': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&153': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&154': np.array([0.4741571944522723, -0.3872697414416878]),
'versicolor&2&155': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&156': np.array([0.68663266357557, -0.6475988779804592]),
'versicolor&2&157': np.array([0.8701760330833639, -0.5914646440996656]),
'versicolor&2&158': np.array([0.6273836195848199, -0.15720981251964872]),
'versicolor&2&159': np.array([0.7292373173099087, -0.6975400952780954]),
'versicolor&2&160': np.array([0.9270035696082471, -0.640582639672401]),
'versicolor&2&161': np.array([0.6863652799597699, -0.21335694415409426]),
'versicolor&2&162': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&163': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&164': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&165': np.array([0.5188517506916897, 0.036358567813067386]),
'versicolor&2&166': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&167': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&168': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&169': np.array([0.5354807894355184, -0.3418054346754283]),
'versicolor&2&170': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&171': np.array([0.5761361484884252, -0.44637460220261904]),
'versicolor&2&172': np.array([0.7268664040181829, -0.40159406680426807]),
'versicolor&2&173': np.array([0.5917672401610737, -0.061499563231173816]),
'versicolor&2&174': np.array([0.5921993039887428, -0.46498571089163954]),
'versicolor&2&175': np.array([0.7470482158282458, -0.4169281153671854]),
'versicolor&2&176': np.array([0.5967658480721675, -0.06546963852548916]),
'versicolor&2&177': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&178': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&179': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&180': np.array([-0.7638917827493686, 0.868015757634957]),
'versicolor&2&181': np.array([-0.8001553485824509, 0.9049358162753539]),
'versicolor&2&182': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&183': np.array([-0.14690789675963867, -0.7352367260447958]),
'versicolor&2&184': np.array([-0.32941440381886555, -0.4173178729969913]),
'versicolor&2&185': np.array([-0.8001553485824509, 0.9049358162753539]),
'versicolor&2&186': np.array([-0.18291442454393395, -0.2654898014002494]),
'versicolor&2&187': np.array([-0.5797728557269727, 0.3163189837954924]),
'versicolor&2&188': np.array([-0.7579323596667402, 0.8054136823046655]),
'versicolor&2&189': np.array([-0.1948624323669993, -0.23753953755286383]),
'versicolor&2&190': np.array([-0.6437698977881832, 0.3909540110317858]),
'versicolor&2&191': np.array([-0.7963046521980063, 0.846536369471985]),
'versicolor&2&192': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&193': np.array([-0.26179245521040034, -0.7067672760776678]),
'versicolor&2&194': np.array([-0.14690789675963867, -0.7352367260447958]),
'versicolor&2&195': np.array([-0.3219660907491514, 0.7482043503408669]),
'versicolor&2&196': np.array([-0.43839553940476644, 0.8642446918440131]),
'versicolor&2&197': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&198': np.array([0.17291299562995102, -0.7651995812779756]),
'versicolor&2&199': np.array([0.2626914501948546, -0.5596191134224637]),
'versicolor&2&200': np.array([-0.43839553940476644, 0.8642446918440131]),
'versicolor&2&201': np.array([0.4734444929420575, -0.6150974537943872]),
'versicolor&2&202': np.array([0.5369392542176313, -0.430867927332838]),
'versicolor&2&203': np.array([-0.19892251970509112, 0.5718543863753405]),
'versicolor&2&204': np.array([0.5071047612208237, -0.6507546896558788]),
'versicolor&2&205': np.array([0.5629877361048359, -0.4485515113017818]),
'versicolor&2&206': np.array([-0.3047657227470458, 0.6788631774846587]),
'versicolor&2&207': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&208': np.array([-0.05474251929945989, -0.7566498134597841]),
'versicolor&2&209': np.array([0.17291299562995102, -0.7651995812779756]),
'versicolor&2&210': np.array([0.37157691321004915, 0.12216227283618836]),
'versicolor&2&211': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&212': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&213': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&214': np.array([0.4741571944522723, -0.3872697414416878]),
'versicolor&2&215': np.array([0.24630541996506908, 0.24630541996506994]),
'versicolor&2&216': np.array([0.68663266357557, -0.6475988779804592]),
'versicolor&2&217': np.array([0.8701760330833639, -0.5914646440996656]),
'versicolor&2&218': np.array([0.6273836195848199, -0.15720981251964872]),
'versicolor&2&219': np.array([0.7292373173099087, -0.6975400952780954]),
'versicolor&2&220': np.array([0.9270035696082471, -0.640582639672401]),
'versicolor&2&221': np.array([0.6863652799597699, -0.21335694415409426]),
'versicolor&2&222': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&223': np.array([0.04449246321056282, -0.709644945972203]),
'versicolor&2&224': np.array([0.2953784217387408, -0.6750352694420283]),
'versicolor&2&225': np.array([-0.5775629083348267, 0.7118687782288384]),
'versicolor&2&226': np.array([-0.6016445709024666, 0.7366089009875252]),
'versicolor&2&227': np.array([-0.28356111726513855, -0.739741315226852]),
'versicolor&2&228': np.array([-0.0917622729715107, -0.7645776302158537]),
'versicolor&2&229': np.array([-0.25603689955471853, -0.451727980232351]),
'versicolor&2&230': np.array([-0.6016445709024666, 0.7366089009875252]),
'versicolor&2&231': np.array([-0.1269405801024398, -0.34161216844748166]),
'versicolor&2&232': np.array([-0.33176333807327857, 0.09538228407203546]),
'versicolor&2&233': np.array([-0.564696311454556, 0.6421194512020755]),
'versicolor&2&234': np.array([-0.12669523681593967, -0.32786313310034665]),
'versicolor&2&235': np.array([-0.35960845047491363, 0.1335988694092619]),
'versicolor&2&236': np.array([-0.589572650064144, 0.6697478899606418]),
'versicolor&2&237': np.array([-0.28356111726513855, -0.739741315226852]),
'versicolor&2&238': np.array([-0.28356111726513855, -0.739741315226852]),
'versicolor&2&239': np.array([-0.0917622729715107, -0.7645776302158537]),
'versicolor&2&240': np.array([0.05667262840030629, 0.4335746514880877]),
'versicolor&2&241': np.array([0.0202211257171063, 0.470123810164804]),
'versicolor&2&242': np.array([-0.052990507284891984, -0.7625494034929868]),
'versicolor&2&243': np.array([0.22461127196921116, -0.7375780139111495]),
'versicolor&2&244': np.array([0.3463149754241171, -0.5568366400939154]),
'versicolor&2&245': np.array([0.0202211257171063, 0.470123810164804]),
'versicolor&2&246': np.array([0.4022739113634462, -0.4700171786183992]),
'versicolor&2&247': np.array([0.5046771347249378, -0.33609610934748635]),
'versicolor&2&248': np.array([0.1370187510624256, 0.30303755274337163]),
'versicolor&2&249': np.array([0.41683021879255133, -0.4812793747667524]),
'versicolor&2&250': np.array([0.5150371666265885, -0.33852139184639396]),
'versicolor&2&251': np.array([0.10611499646955676, 0.33589829339460586]),
'versicolor&2&252': np.array([-0.052990507284891984, -0.7625494034929868]),
'versicolor&2&253': np.array([-0.052990507284891984, -0.7625494034929868]),
'versicolor&2&254': np.array([0.22461127196921116, -0.7375780139111495]),
'versicolor&2&255': np.array([0.5188517506916897, 0.036358567813067386]),
'versicolor&2&256': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&257': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&258': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&259': np.array([0.5354807894355184, -0.3418054346754283]),
'versicolor&2&260': np.array([0.5131939273945454, 0.04199748266790813]),
'versicolor&2&261': np.array([0.5761361484884252, -0.44637460220261904]),
'versicolor&2&262': np.array([0.7268664040181829, -0.40159406680426807]),
'versicolor&2&263': np.array([0.5917672401610737, -0.061499563231173816]),
'versicolor&2&264': np.array([0.5921993039887428, -0.46498571089163954]),
'versicolor&2&265': np.array([0.7470482158282458, -0.4169281153671854]),
'versicolor&2&266': np.array([0.5967658480721675, -0.06546963852548916]),
'versicolor&2&267': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&268': np.array([0.06285591932387405, -0.6914253444924359]),
'versicolor&2&269': np.array([0.34904320225465857, -0.6233384360811872]),
'versicolor&2&270': np.array([-0.8252668830593566, 0.11450866713130668]),
'versicolor&2&271': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&272': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&273': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&274': np.array([-0.8735738195653328, -0.046438180466149094]),
'versicolor&2&275': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&276': np.array([-0.8470213454017305, -0.0910504504559782]),
'versicolor&2&277': np.array([-0.8783521565540571, 0.01381094589198601]),
'versicolor&2&278': np.array([-0.8388485924434891, 0.09800790238640067]),
'versicolor&2&279': np.array([-0.8495871633670822, -0.08820642363054954]),
'versicolor&2&280': np.array([-0.8784816772224661, 0.017184907022714958]),
'versicolor&2&281': np.array([-0.835455914569297, 0.10189258327760495]),
'versicolor&2&282': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&283': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&284': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&285': np.array([-0.8252668830593566, 0.11450866713130668]),
'versicolor&2&286': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&287': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&288': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&289': np.array([-0.8735738195653328, -0.046438180466149094]),
'versicolor&2&290': np.array([-0.8211795643076095, 0.11869650771610692]),
'versicolor&2&291': np.array([-0.8470213454017305, -0.0910504504559782]),
'versicolor&2&292': np.array([-0.8783521565540571, 0.01381094589198601]),
'versicolor&2&293': np.array([-0.8388485924434891, 0.09800790238640067]),
'versicolor&2&294': np.array([-0.8495871633670822, -0.08820642363054954]),
'versicolor&2&295': np.array([-0.8784816772224661, 0.017184907022714958]),
'versicolor&2&296': np.array([-0.835455914569297, 0.10189258327760495]),
'versicolor&2&297': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&298': np.array([-0.6441664102689847, -0.3012046426099901]),
'versicolor&2&299': np.array([-0.7640280271176497, -0.19364537761420375]),
'versicolor&2&300': np.array([-0.5227340800279543, 0.4209267574088147]),
'versicolor&2&301': np.array([-0.5140708637198534, 0.4305361238057349]),
'versicolor&2&302': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&303': np.array([-0.2741128763380603, -0.7260889090887469]),
'versicolor&2&304': np.array([-0.6188410763351541, -0.22803625884668638]),
'versicolor&2&305': np.array([-0.5140708637198534, 0.4305361238057349]),
'versicolor&2&306': np.array([-0.56940429361245, -0.3442345437882425]),
'versicolor&2&307': np.array([-0.6452502612229726, -0.04686872432129788]),
'versicolor&2&308': np.array([-0.596973015481227, 0.37395461795328944]),
'versicolor&2&309': np.array([-0.5760086048531655, -0.3353570725513232]),
'versicolor&2&310': np.array([-0.6488228567611906, -0.03186184826812757]),
'versicolor&2&311': np.array([-0.5903420131350324, 0.384224764046184]),
'versicolor&2&312': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&313': np.array([-0.2661726847443776, -0.6902916602462779]),
'versicolor&2&314': np.array([-0.2741128763380603, -0.7260889090887469]),
'virginica&0&0': np.array([-0.7431524521056113, -0.24432235603856345]),
'virginica&0&1': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&2': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&3': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&4': np.array([-0.9706534384443797, 0.007448195602953232]),
'virginica&0&5': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&6': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&7': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&8': np.array([-0.8486399726113752, -0.13537345771621853]),
'virginica&0&9': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&10': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&11': np.array([-0.7870031444780577, -0.1952404625292782]),
'virginica&0&12': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&13': np.array([-0.9569238464170641, -0.02354905845282574]),
'virginica&0&14': np.array([-0.9677320606992984, -0.012432557482778654]),
'virginica&0&15': np.array([-0.7431524521056113, -0.24432235603856345]),
'virginica&0&16': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&17': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&18': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&19': np.array([-0.9706534384443797, 0.007448195602953232]),
'virginica&0&20': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&21': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&22': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&23': np.array([-0.8486399726113752, -0.13537345771621853]),
'virginica&0&24': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&25': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&26': np.array([-0.7870031444780577, -0.1952404625292782]),
'virginica&0&27': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&28': np.array([-0.9569238464170641, -0.02354905845282574]),
'virginica&0&29': np.array([-0.9677320606992984, -0.012432557482778654]),
'virginica&0&30': np.array([-0.19685199412911655, -0.7845879230594393]),
'virginica&0&31': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&32': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&33': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&34': np.array([-0.7974072911132788, 0.006894018772033604]),
'virginica&0&35': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&36': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&37': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&38': np.array([-0.3355030348883163, -0.6305271339971502]),
'virginica&0&39': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&40': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&41': np.array([-0.2519677855687844, -0.7134447168661863]),
'virginica&0&42': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&43': np.array([-0.7799744386472778, -0.026476616324402506]),
'virginica&0&44': np.array([-0.7942342242967624, -0.0119572163963601]),
'virginica&0&45': np.array([-0.05031696218434577, -0.929227611211748]),
'virginica&0&46': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&47': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&48': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&49': np.array([-0.4656481363306145, 0.007982539480288167]),
'virginica&0&50': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&51': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&52': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&53': np.array([-0.14241819268815753, -0.8424615476000691]),
'virginica&0&54': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&55': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&56': np.array([-0.1140907502997574, -0.8737800276630269]),
'virginica&0&57': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&58': np.array([-0.14198277461566922, -0.4577720226157396]),
'virginica&0&59': np.array([-0.4385442121294165, -0.05333645823279597]),
'virginica&0&60': np.array([0.029402442458921384, -0.9481684282717414]),
'virginica&0&61': np.array([0.009887859354111524, -0.9698143912008228]),
'virginica&0&62': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&63': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&64': np.array([0.3094460464703627, 0.11400643817329122]),
'virginica&0&65': np.array([0.009887859354111524, -0.9698143912008228]),
'virginica&0&66': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&67': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&68': np.array([0.19002455311770447, -0.8848597943731074]),
'virginica&0&69': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&70': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&71': np.array([0.1746467870122951, -0.9073062742839755]),
'virginica&0&72': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&73': np.array([0.11200181312407695, -0.5330612470996793]),
'virginica&0&74': np.array([0.19998284600732558, -0.3489062419702088]),
'virginica&0&75': np.array([0.0, -0.95124502153736]),
'virginica&0&76': np.array([0.0, -0.9708703761803881]),
'virginica&0&77': np.array([0.0, -0.5659706098422994]),
'virginica&0&78': np.array([0.0, -0.3962828716108186]),
'virginica&0&79': np.array([0.0, 0.2538069363248767]),
'virginica&0&80': np.array([0.0, -0.9708703761803881]),
'virginica&0&81': np.array([0.0, -0.5659706098422994]),
'virginica&0&82': np.array([0.0, -0.3962828716108186]),
'virginica&0&83': np.array([0.0, -0.8943993997517804]),
'virginica&0&84': np.array([0.0, -0.5659706098422994]),
'virginica&0&85': np.array([0.0, -0.3962828716108186]),
'virginica&0&86': np.array([0.0, -0.9166476163222441]),
'virginica&0&87': np.array([0.0, -0.3962828716108186]),
'virginica&0&88': np.array([0.0, -0.5466925844560601]),
'virginica&0&89': np.array([0.0, -0.38529908946531777]),
'virginica&0&90': np.array([-0.7431524521056113, -0.24432235603856345]),
'virginica&0&91': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&92': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&93': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&94': np.array([-0.9706534384443797, 0.007448195602953232]),
'virginica&0&95': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&96': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&97': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&98': np.array([-0.8486399726113752, -0.13537345771621853]),
'virginica&0&99': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&100': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&101': np.array([-0.7870031444780577, -0.1952404625292782]),
'virginica&0&102': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&103': np.array([-0.9569238464170641, -0.02354905845282574]),
'virginica&0&104': np.array([-0.9677320606992984, -0.012432557482778654]),
'virginica&0&105': np.array([-0.19685199412911655, -0.7845879230594393]),
'virginica&0&106': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&107': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&108': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&109': np.array([-0.7974072911132788, 0.006894018772033604]),
'virginica&0&110': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&111': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&112': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&113': np.array([-0.3355030348883163, -0.6305271339971502]),
'virginica&0&114': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&115': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&116': np.array([-0.2519677855687844, -0.7134447168661863]),
'virginica&0&117': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&118': np.array([-0.7799744386472778, -0.026476616324402506]),
'virginica&0&119': np.array([-0.7942342242967624, -0.0119572163963601]),
'virginica&0&120': np.array([-0.05031696218434577, -0.929227611211748]),
'virginica&0&121': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&122': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&123': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&124': np.array([-0.4656481363306145, 0.007982539480288167]),
'virginica&0&125': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&126': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&127': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&128': np.array([-0.14241819268815753, -0.8424615476000691]),
'virginica&0&129': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&130': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&131': np.array([-0.1140907502997574, -0.8737800276630269]),
'virginica&0&132': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&133': np.array([-0.14198277461566922, -0.4577720226157396]),
'virginica&0&134': np.array([-0.4385442121294165, -0.05333645823279597]),
'virginica&0&135': np.array([-0.19684482070614498, -0.7845939961595055]),
'virginica&0&136': np.array([-0.07475231751447156, -0.9062785678426409]),
'virginica&0&137': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&138': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&139': np.array([-0.8063011502229427, 0.4134300066735808]),
'virginica&0&140': np.array([-0.07475231751447156, -0.9062785678426409]),
'virginica&0&141': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&142': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&143': np.array([-0.2798927835773098, -0.6581136857450849]),
'virginica&0&144': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&145': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&146': np.array([-0.16106555563262584, -0.777621649099753]),
'virginica&0&147': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&148': np.array([-0.6898990333725056, -0.2534947697713122]),
'virginica&0&149': np.array([-0.769491694075929, -0.22884642137519118]),
'virginica&0&150': np.array([-0.7431524521056113, -0.24432235603856345]),
'virginica&0&151': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&152': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&153': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&154': np.array([-0.9706534384443797, 0.007448195602953232]),
'virginica&0&155': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&156': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&157': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&158': np.array([-0.8486399726113752, -0.13537345771621853]),
'virginica&0&159': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&160': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&161': np.array([-0.7870031444780577, -0.1952404625292782]),
'virginica&0&162': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&163': np.array([-0.9569238464170641, -0.02354905845282574]),
'virginica&0&164': np.array([-0.9677320606992984, -0.012432557482778654]),
'virginica&0&165': np.array([-0.19685199412911655, -0.7845879230594393]),
'virginica&0&166': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&167': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&168': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&169': np.array([-0.7974072911132788, 0.006894018772033604]),
'virginica&0&170': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&171': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&172': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&173': np.array([-0.3355030348883163, -0.6305271339971502]),
'virginica&0&174': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&175': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&176': np.array([-0.2519677855687844, -0.7134447168661863]),
'virginica&0&177': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&178': np.array([-0.7799744386472778, -0.026476616324402506]),
'virginica&0&179': np.array([-0.7942342242967624, -0.0119572163963601]),
'virginica&0&180': np.array([-0.05031696218434577, -0.929227611211748]),
'virginica&0&181': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&182': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&183': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&184': np.array([-0.4656481363306145, 0.007982539480288167]),
'virginica&0&185': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&186': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&187': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&188': np.array([-0.14241819268815753, -0.8424615476000691]),
'virginica&0&189': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&190': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&191': np.array([-0.1140907502997574, -0.8737800276630269]),
'virginica&0&192': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&193': np.array([-0.14198277461566922, -0.4577720226157396]),
'virginica&0&194': np.array([-0.4385442121294165, -0.05333645823279597]),
'virginica&0&195': np.array([-0.19684482070614498, -0.7845939961595055]),
'virginica&0&196': np.array([-0.07475231751447156, -0.9062785678426409]),
'virginica&0&197': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&198': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&199': np.array([-0.8063011502229427, 0.4134300066735808]),
'virginica&0&200': np.array([-0.07475231751447156, -0.9062785678426409]),
'virginica&0&201': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&202': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&203': np.array([-0.2798927835773098, -0.6581136857450849]),
'virginica&0&204': np.array([-0.6782037543706109, -0.29560073676989834]),
'virginica&0&205': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&206': np.array([-0.16106555563262584, -0.777621649099753]),
'virginica&0&207': np.array([-0.7694171988675237, -0.276633135028249]),
'virginica&0&208': np.array([-0.6898990333725056, -0.2534947697713122]),
'virginica&0&209': np.array([-0.769491694075929, -0.22884642137519118]),
'virginica&0&210': np.array([-0.7431524521056113, -0.24432235603856345]),
'virginica&0&211': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&212': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&213': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&214': np.array([-0.9706534384443797, 0.007448195602953232]),
'virginica&0&215': np.array([-0.4926091071260067, -0.49260910712601286]),
'virginica&0&216': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&217': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&218': np.array([-0.8486399726113752, -0.13537345771621853]),
'virginica&0&219': np.array([-0.9550700362273441, -0.025428672111930138]),
'virginica&0&220': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&221': np.array([-0.7870031444780577, -0.1952404625292782]),
'virginica&0&222': np.array([-0.9672121512728677, -0.012993005706020504]),
'virginica&0&223': np.array([-0.9569238464170641, -0.02354905845282574]),
'virginica&0&224': np.array([-0.9677320606992984, -0.012432557482778654]),
'virginica&0&225': np.array([-0.05031696218434577, -0.929227611211748]),
'virginica&0&226': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&227': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&228': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&229': np.array([-0.4656481363306145, 0.007982539480288167]),
'virginica&0&230': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&231': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&232': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&233': np.array([-0.14241819268815753, -0.8424615476000691]),
'virginica&0&234': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&235': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&236': np.array([-0.1140907502997574, -0.8737800276630269]),
'virginica&0&237': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&238': np.array([-0.14198277461566922, -0.4577720226157396]),
'virginica&0&239': np.array([-0.4385442121294165, -0.05333645823279597]),
'virginica&0&240': np.array([-0.11329659732608087, -0.8671819100849522]),
'virginica&0&241': np.array([-0.040390637135858574, -0.9402832917474078]),
'virginica&0&242': np.array([-0.5276460255602035, -0.28992233541586077]),
'virginica&0&243': np.array([-0.6392402874163683, -0.24114611970435948]),
'virginica&0&244': np.array([-0.6814868825686854, 0.35066801608083215]),
'virginica&0&245': np.array([-0.040390637135858574, -0.9402832917474078]),
'virginica&0&246': np.array([-0.5276460255602035, -0.28992233541586077]),
'virginica&0&247': np.array([-0.6392402874163683, -0.24114611970435948]),
'virginica&0&248': np.array([-0.16157511199607094, -0.7754323813403634]),
'virginica&0&249': np.array([-0.5276460255602035, -0.28992233541586077]),
'virginica&0&250': np.array([-0.6392402874163683, -0.24114611970435948]),
'virginica&0&251': np.array([-0.08968204532514226, -0.8491191210330045]),
'virginica&0&252': np.array([-0.6392402874163683, -0.24114611970435948]),
'virginica&0&253': np.array([-0.544626974647221, -0.24972982107967573]),
'virginica&0&254': np.array([-0.6426355680762406, -0.20016519137103667]),
'virginica&0&255': np.array([-0.19685199412911655, -0.7845879230594393]),
'virginica&0&256': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&257': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&258': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&259': np.array([-0.7974072911132788, 0.006894018772033604]),
'virginica&0&260': np.array([-0.07476043598366228, -0.9062715528546994]),
'virginica&0&261': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&262': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&263': np.array([-0.3355030348883163, -0.6305271339971502]),
'virginica&0&264': np.array([-0.7770298852793477, -0.029443430477147373]),
'virginica&0&265': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&266': np.array([-0.2519677855687844, -0.7134447168661863]),
'virginica&0&267': np.array([-0.7936433456054744, -0.012583752076496493]),
'virginica&0&268': np.array([-0.7799744386472778, -0.026476616324402506]),
'virginica&0&269': np.array([-0.7942342242967624, -0.0119572163963601]),
'virginica&0&270': np.array([-0.04201361383207032, -0.9372571358382161]),
'virginica&0&271': np.array([-0.014237661899709955, -0.9660323357290304]),
'virginica&0&272': np.array([-0.04813346258022244, -0.5416229439456887]),
'virginica&0&273': np.array([-0.3109532939139045, -0.22759134703604383]),
'virginica&0&274': np.array([-0.4167677904879879, 0.22207334821665425]),
'virginica&0&275': np.array([-0.014237661899709955, -0.9660323357290304]),
'virginica&0&276': np.array([-0.04813346258022244, -0.5416229439456887]),
'virginica&0&277': np.array([-0.3109532939139045, -0.22759134703604383]),
'virginica&0&278': np.array([-0.07857689135903215, -0.8696882596532965]),
'virginica&0&279': np.array([-0.04813346258022244, -0.5416229439456887]),
'virginica&0&280': np.array([-0.3109532939139045, -0.22759134703604383]),
'virginica&0&281': np.array([-0.05160969201296555, -0.9000166344885441]),
'virginica&0&282': np.array([-0.3109532939139045, -0.22759134703604383]),
'virginica&0&283': np.array([-0.0766197045034485, -0.5080325256323984]),
'virginica&0&284': np.array([-0.32767091750230254, -0.19689316772421933]),
'virginica&0&285': np.array([-0.05031696218434577, -0.929227611211748]),
'virginica&0&286': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&287': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&288': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&289': np.array([-0.4656481363306145, 0.007982539480288167]),
'virginica&0&290': np.array([-0.017148644765919676, -0.9632117581295891]),
'virginica&0&291': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&292': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&293': np.array([-0.14241819268815753, -0.8424615476000691]),
'virginica&0&294': np.array([-0.061515713893900315, -0.524561199322281]),
'virginica&0&295': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&296': np.array([-0.1140907502997574, -0.8737800276630269]),
'virginica&0&297': np.array([-0.4329463382004908, -0.057167210150691136]),
'virginica&0&298': np.array([-0.14198277461566922, -0.4577720226157396]),
'virginica&0&299': np.array([-0.4385442121294165, -0.05333645823279597]),
'virginica&0&300': np.array([0.029402442458921384, -0.9481684282717414]),
'virginica&0&301': np.array([0.009887859354111524, -0.9698143912008228]),
'virginica&0&302': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&303': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&304': np.array([0.3094460464703627, 0.11400643817329122]),
'virginica&0&305': np.array([0.009887859354111524, -0.9698143912008228]),
'virginica&0&306': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&307': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&308': np.array([0.19002455311770447, -0.8848597943731074]),
'virginica&0&309': np.array([0.009595083643662688, -0.5643652067423869]),
'virginica&0&310': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&311': np.array([0.1746467870122951, -0.9073062742839755]),
'virginica&0&312': np.array([0.13694026920485936, -0.36331091829858003]),
'virginica&0&313': np.array([0.11200181312407695, -0.5330612470996793]),
'virginica&0&314': np.array([0.19998284600732558, -0.3489062419702088]),
'virginica&1&0': np.array([0.37157553889555184, 0.1221600832023858]),
'virginica&1&1': np.array([0.2463036871609408, 0.24630368716093934]),
'virginica&1&2': np.array([0.9105775730167809, -0.6842162738602727]),
'virginica&1&3': np.array([0.6718337295341265, -0.6620422637360074]),
'virginica&1&4': np.array([0.4964962439921071, 0.3798215458387346]),
'virginica&1&5': np.array([0.2463036871609408, 0.24630368716093934]),
'virginica&1&6': np.array([0.9105775730167809, -0.6842162738602727]),
'virginica&1&7': np.array([0.6718337295341265, -0.6620422637360074]),
'virginica&1&8': np.array([0.22125635302655813, 0.2925832702358638]),
'virginica&1&9': np.array([0.9105775730167809, -0.6842162738602727]),
'virginica&1&10': np.array([0.6718337295341265, -0.6620422637360074]),
'virginica&1&11': np.array([0.10063786451829529, 0.4085974066833644]),
'virginica&1&12': np.array([0.6718337295341265, -0.6620422637360074]),
'virginica&1&13': np.array([0.8441748651745272, -0.6057436494968107]),
'virginica&1&14': np.array([0.6453274192140858, -0.6334259878992301]),
'virginica&1&15': | np.array([0.37157553889555184, 0.1221600832023858]) | numpy.array |
import asyncio
import json
import os
import time
import warnings
import wave
from queue import Queue
from typing import Callable
import numpy as np
import pvporcupine
import sounddevice as sd
import vosk
from fluxhelper import osInterface
from speech_recognition import AudioFile, Recognizer, UnknownValueError
from tensorflow.keras.models import load_model
from vosk import SetLogLevel
RATE = 16000
DURATION = 0.5
CHANNELS = 1
CHUNK = 512
MAX_FREQ = 18
# Disable logging
warnings.filterwarnings("ignore")
os.environ["TF_CPP_MIN_LOG_LEVEL"] = "3"
SetLogLevel(-1)
class VAD:
"""
Main Voice activity detection class.
This uses deep learning to predict whether a piece of audio is considered a speech or not a speech.
Parameters
----------
`modelPath` : str
path to the model.h5 file.
`sensitivity : float
how sensitive the detection is.
Methods
-------
`isSpeech(stream: bytes)` :
returns True if the classified stream is a voice and False if not.
"""
def __init__(self, modelPath: str, sensitivity: float = 0.90):
self.model = load_model(modelPath)
self.buffer = []
self.sensitivity = sensitivity
async def _formatPredictions(self, predictions) -> list:
"""
Format the predictions into a more readable and easy to traverse format.
"""
predictions = [[i, float(r)] for i, r in enumerate(predictions)]
predictions.sort(key=lambda x: x[1], reverse=True)
return predictions
async def isSpeech(self, stream: bytes) -> bool:
"""
Makes a prediction from the given stream bytes.
Parameters
----------
`stream` : bytes
raw bytes stream (usually retrieved from pyaudio's .read function or sounddevice)
Returns True if the classified stream is a voice and False if not.
"""
# Convert the raw streams into a numpy array and get the decibels
arr = np.frombuffer(stream, dtype=np.int16)
db = 20 * np.log10(np.abs(np.fft.rfft(arr[:2048])))
# Collect decibel values from relevent frequencies (MAX_FREQ)
features = list( | np.round(db[3:MAX_FREQ], 2) | numpy.round |
from math import *
import sys, os, argparse, time
from re import split as regexp_split
import numpy as np
import numpy.ma as ma
import general_scripts as gs
import general_maths as gm
import transforms3d.quaternions as qops
import transforms3d_supplement as qs
import fitting_Ct_functions as fitCt
import spectral_densities as sd
from scipy.optimize import fmin_powell
# There may be minor mistakes in the selection text. Try to identify what is wrong.
def confirm_seltxt(ref, Hseltxt, Xseltxt):
bError=False
indH = mol.topology.select(Hseltxt)
indX = mol.topology.select(Xseltxt)
numH = len(indH) ; numX = len(numX)
if numH == 0:
bError=True
t1 = mol.topology.select('name H')
t2 = mol.topology.select('name HN')
t3 = mol.topology.select('name HA')
print( " .... ERROR: The 'name H' selects %i atoms, 'name HN' selects %i atoms, and 'name HA' selects %i atoms." % (t1, t2, t3) )
if numX == 0:
bError=True
t1 = mol.topology.select('name N')
t2 = mol.topology.select('name NT')
print( " .... ERROR: The 'name N' selects %i atoms, and 'name NT' selects %i atoms." % (t1, t2) )
resH = [ mol.topology.atom(x).residue.resSeq for x in indH ]
resX = [ mol.topology.atom(x).residue.resSeq for x in indX ]
if resX != resH:
bError=True
print( " .... ERROR: The residue lists are not the name between the two selections:" )
print( " .... Count for X (%i)" % numX, resX )
print( " .... Count for H (%i)" % numH, resH )
if bError:
sys.exit(1)
return indH, indX, resX
def extract_vectors_from_structure( pdbFile, Hseltxt='name H', Xsel='name N and not resname PRO', trjFile=None ):
print( "= = = Using vectors as found directly in the coordinate files, via MDTraj module." )
print( "= = = NOTE: no fitting is conducted." )
import mdtraj as md
if not trjFile is None:
mol = md.load(trjFile, top=pdbFile)
print( "= = = PDB file %s and trajectory file %s loaded." % (pdbFile, trjFile) )
else:
omol = md.load(pdbFile)
print( "= = = PDB file %s loaded." % pdbFile )
indH, indX, resXH = confirm_seltxt(ref, Hsel, Xsel)
# Extract submatrix of vector trajectory
vecXH = np.take(mol.xyz, indH, axis=1) - np.take(mol.xyz, indX, axis=1)
vecXH = qs.vecnorm_NDarray(vecXH, axis=2)
# = = = Check shape and switch to match.
if vecXH.shape[0] == 1:
return resXH, vecXH[0]
else:
return resXH, np.swapaxes(vecXH,0,1)
def sanity_check_two_list(listA, listB, string, bVerbose=False):
if not gm.list_identical( listA, listB ):
print( "= = ERROR: Sanity checked failed for %s!" % string )
if bVerbose:
print( listA )
print( listB )
else:
print( " ...first residues:", listA[0], listB[0] )
print( " ...set intersection (unordered):", set(listA).intersection(set(listB)) )
sys.exit(1)
return
def _obtain_Jomega(RObj, nSites, S2, consts, taus, vecXH, weights=None):
"""
The inputs vectors have dimensions (nSites, nSamples, 3) or just (nSites, 3)
the datablock being returned has dimensions:
- (nFrequencies, nSites) of there is no uncertainty calculations. 5 being the five frequencies J(0), J(wN) J(wH+wN), J(wH), J(wH-wN)
- (nFrequencies, nSites, 2) if there is uncertainty calculations.
"""
nFrequencies= len(RObj.omega)
if RObj.rotdifModel.name == 'rigid_sphere':
datablock=np.zeros((5,nSites), dtype=np.float32)
for i in range(nSites):
J = sd.J_combine_isotropic_exp_decayN(RObj.omega, 1.0/(6.0*RObj.rotdifModel.D), S2[i], consts[i], taus[i])
datablock[:,i]=J
return datablock
elif RObj.rotdifModel.name == 'rigid_symmtop':
# Automatically use the vector-form of function.
if len(vecXH.shape) > 2:
# An ensemble of vectors at each site. Measure values for all of them then average with/without weights.
datablock=np.zeros( (nFrequencies, nSites, 2), dtype=np.float32)
npts=vecXH.shape[1]
for i in range(nSites):
# = = = Calculate at each residue and sum over Jmat( nSamples, 2)
Jmat = sd.J_combine_symmtop_exp_decayN(RObj.omega, vecXH[i], RObj.rotdifModel.D[0], RObj.rotdifModel.D[1], S2[i], consts[i], taus[i])
if weights is None:
datablock[:,i,0] = np.mean(Jmat, axis=0)
datablock[:,i,1] = np.std(Jmat, axis=0)
else:
datablock[:,i,0] = np.average(Jmat, axis=0, weights=weights[i])
datablock[:,i,1] = np.sqrt( np.average( (Jmat - datablock[:,i,0])**2.0, axis=0, weights=weights[i]) )
return datablock
else:
#Single XH vector at each site. Ignore weights, as they should not exist.
datablock=np.zeros((5,nSites), dtype=np.float32)
for i in range(nSites):
Jmat = sd.J_combine_symmtop_exp_decayN(RObj.omega, vecXH[i], RObj.rotdifModel.D[0], RObj.rotdifModel.D[1], S2[i], consts[i], taus[i])
datablock[:,i]=Jmat
return datablock
# = = Should only happen with fully anisotropic models.
print( "= = ERROR: Unknown rotdifModel in the relaxation object used in calculations!", file=sys.stderr )
return []
def _obtain_R1R2NOErho(RObj, nSites, S2, consts, taus, vecXH, weights=None, CSAvaluesArray=None):
"""
The inputs vectors have dimensions (nSites, nSamples, 3) or just (nSites, 3)
the datablock being returned has dimensions:
- ( 4, nSites) of there is no uncertainty calculations. 4 corresponding each to R1, R2, NOE, and rho.
- ( 4, nSites, 2) if there is uncertainty calculations.
"""
if CSAvaluesArray is None:
CSAvaluesArray = np.repeat(CSAvaluesArray, nSites)
if RObj.rotdifModel.name == 'direct_transform':
datablock=np.zeros((4,nSites), dtype=np.float32)
for i in range(nSites):
J = sd.J_direct_transform(RObj.omega, consts[i], taus[i])
R1, R2, NOE = RObj.get_relax_from_J( J, CSAvalue=CSAvaluesArray[i] )
rho = RObj.get_rho_from_J( J )
datablock[:,i]=[R1,R2,NOE,rho]
return datablock
elif RObj.rotdifModel.name == 'rigid_sphere':
datablock=np.zeros((4,nSites), dtype=np.float32)
for i in range(nSites):
J = sd.J_combine_isotropic_exp_decayN(RObj.omega, 1.0/(6.0*RObj.rotdifModel.D), S2[i], consts[i], taus[i])
R1, R2, NOE = RObj.get_relax_from_J( J, CSAvalue=CSAvaluesArray[i] )
rho = RObj.get_rho_from_J( J )
datablock[:,i]=[R1,R2,NOE,rho]
return datablock
elif RObj.rotdifModel.name == 'rigid_symmtop':
# Automatically use the vector-form of function.
if len(vecXH.shape) > 2:
# An ensemble of vectors for each site.
datablock=np.zeros((4,nSites,2), dtype=np.float32)
npts=vecXH.shape[1]
#tmpR1 = np.zeros(npts) ; tmpR2 = np.zeros(npts) ; tmpNOE = np.zeros(npts)
#tmprho = np.zeros(npts)
for i in range(nSites):
Jmat = sd.J_combine_symmtop_exp_decayN(RObj.omega, vecXH[i], RObj.rotdifModel.D[0], RObj.rotdifModel.D[1], S2[i], consts[i], taus[i])
# = = = Calculate relaxation values from the entire sample of vectors before any averagins is to be done
tmpR1, tmpR2, tmpNOE = RObj.get_relax_from_J_simd( Jmat, CSAvalue=CSAvaluesArray[i] )
tmprho = RObj.get_rho_from_J_simd( Jmat )
#for j in range(npts):
# tmpR1[j], tmpR2[j], tmpNOE[j] = RObj.get_relax_from_J( Jmat[j] )
# tmprho[j] = RObj.get_rho_from_J( Jmat[j] )
if weights is None:
R1 = np.mean(tmpR1) ; R2 = np.mean(tmpR2) ; NOE = np.mean(tmpNOE)
R1sig = np.std(tmpR1); R2sig = np.std(tmpR2) ; NOEsig = np.std(tmpNOE)
rho = np.mean(tmprho); rhosig = np.std(tmprho)
datablock[:,i]=[[R1,R1sig],[R2,R2sig],[NOE,NOEsig],[rho,rhosig]]
else:
datablock[0,i]=gm.weighted_average_stdev(tmpR1, weights[i])
datablock[1,i]=gm.weighted_average_stdev(tmpR2, weights[i])
datablock[2,i]=gm.weighted_average_stdev(tmpNOE, weights[i])
datablock[3,i]=gm.weighted_average_stdev(tmprho, weights[i])
return datablock
else:
#Single XH vector for each site.
datablock=np.zeros((4,nSites), dtype=np.float32)
for i in range(nSites):
Jmat = sd.J_combine_symmtop_exp_decayN(RObj.omega, vecXH[i], RObj.rotdifModel.D[0], RObj.rotdifModel.D[1], S2[i], consts[i], taus[i])
if len(Jmat.shape) == 1:
# A single vector was given.
R1, R2, NOE = RObj.get_relax_from_J( Jmat, CSAvalue=CSAvaluesArray[i] )
rho = RObj.get_rho_from_J( Jmat )
datablock[:,i]=[R1,R2,NOE,rho]
return datablock
# = = Should only happen with fully anisotropic models.
print( "= = ERROR: Unknown rotdifModel in the relaxation object used in calculations!", file=sys.stderr )
return []
def optfunc_R1R2NOE_inner(datablock, expblock):
if len(datablock.shape)==3 and len(expblock.shape)==3 :
chisq = np.square(datablock[...,0] - expblock[...,0])
sigsq = np.square(datablock[...,1]) + | np.square(expblock[...,1]) | numpy.square |
from solver import Solver
from task_scheduler import TaskScheduler
from DAG_scheduler import DAGScheduler
from data_loader import DataLoader
import numpy as np
import logging
import time
def DepthBasedBaseline(file_path="../ToyData.xlsx"):
"""
Depth Based Baseline, implemented by <NAME>
"""
f = open('depthbased_baseline.txt', 'w')
f.close()
start_time = time.time()
task_scheduler = TaskScheduler(file_path=file_path)
solver = Solver(file_path=file_path)
cur_depth = 0
max_depth = task_scheduler.max_depth
data_center = None
placement = None
finish_time = None
task_set = None
task_set_new = None
final_placement = []
final_time = 0
while(cur_depth<=max_depth):
if cur_depth ==0:
task_set = task_scheduler.get_taskset(cur_depth)
data_center = task_scheduler.get_datacenter()
placement, finish_time = solver.get_placement(task_set, data_center)
time_cost = np.max(finish_time)
with open('depthbased_baseline.txt', 'a') as f:
f.write("depth %d cost time %f\n"%(cur_depth, time_cost))
f.close()
print("time cost:", time_cost)
final_time += time_cost
cur_depth += 1
final_placement.append(placement)
continue
task_set_new = task_scheduler.get_taskset(cur_depth)
data_center = solver.update_datacenter(placement, task_set_new, task_set, data_center)
placement, finish_time = solver.get_placement(task_set_new, data_center)
final_placement.append(placement)
time_cost = np.max(finish_time)
print("time cost:", time_cost)
with open('depthbased_baseline.txt', 'a') as f:
f.write("depth %d cost time %f\n"%(cur_depth, time_cost))
f.close()
final_time += time_cost
task_set = task_set_new
cur_depth += 1
final_placement_show_depthbased(final_placement, task_scheduler)
print("-----Depth Based Baseline Finish. Final Time:%f----\n"%final_time)
end_time = time.time()
print("-----Execution Time of Depth Based Baseline:-----",end_time-start_time)
with open('depthbased_baseline.txt', 'a') as f:
f.write("-----Depth Based Baseline Finish. Final Time:%f----\n"%final_time)
f.write("-----Execution Time of Depth Based Baseline: %f----\n"%(end_time-start_time))
f.close()
def JobStepBasedBaseline(file_path="../ToyData.xlsx", threshold=5):
"""
Job Step Based Baseline, implemented by <NAME>
"""
f = open('jobbased_baseline.txt', 'w')
f.close()
start_time = time.time()
print('Job Step Based Baseline, threshold = %d '%threshold)
task_scheduler = TaskScheduler(file_path=file_path, threshold=threshold)
solver = Solver(file_path=file_path)
cur_step = 0
max_step = task_scheduler.max_step
data_center = None
placement = None
finish_time = None
task_set = None
task_set_new = None
final_time = 0
final_placement = []
while(cur_step<=max_step):
if cur_step ==0:
task_set = task_scheduler.get_taskset_jobbased(cur_step)
data_center = task_scheduler.get_datacenter()
placement, finish_time = solver.get_placement(task_set, data_center)
time_cost = np.max(finish_time)
with open('jobbased_baseline.txt', 'a') as f:
f.write("step %d cost time %f\n"%(cur_step, time_cost))
f.close()
final_time += time_cost
cur_step += 1
final_placement.append(placement)
continue
task_set_new = task_scheduler.get_taskset_jobbased(cur_step)
new_data_center = solver.update_datacenter(placement, task_set_new, task_set, data_center)
placement, finish_time = solver.get_placement(task_set_new, new_data_center)
time_cost = np.max(finish_time)
with open('jobbased_baseline.txt', 'a') as f:
f.write("step %d cost time %f\n"%(cur_step, time_cost))
# print("time cost:", time_cost)
final_time += time_cost
task_set = task_set_new
final_placement.append(placement)
cur_step += 1
print("-----Job Step Based Baseline Finish. Final Time:%f----\n"%final_time)
## show result
final_placement_show_jobbased(final_placement, task_scheduler)
end_time = time.time()
print("-----Execution Time of Job Based Baseline: %f-------\n"%(end_time-start_time))
with open('jobbased_baseline.txt', 'a') as f:
f.write("-----Job Step Based Baseline Finish. Final Time:%f----\n"%final_time)
f.write("-----Execution Time of Job Based Baseline: %f-------\n"%(end_time-start_time))
f.close()
return final_placement
def final_placement_show_jobbased(final_placement, task_scheduler):
"""
Input: list of several placements in several step
Output: show the placement
"""
f = open('jobbased_baseline.txt', 'a')
for i in range(len(final_placement)):
f.write("Step%d:\n"%i)
print("Step%d:"%i)
placement = final_placement[i]
task_set = task_scheduler.get_taskset_jobbased(i)
for id in range(len(task_set)):
task = task_set[id]
job_id = int(ord(task.task_name[1]) - ord('A'))
task_id = int(task.task_name[2]) - 1
try:
dc_id = np.where(placement[job_id][task_id]==1)[0] + 1
except:
dc_id = np.where(placement[job_id][task_id]==1)[0][0] + 1
f.write(str(task) + 'place in DC' + str(dc_id)+'\n')
print(task, 'place in DC', dc_id)
f.close()
def final_placement_show_depthbased(final_placement, task_scheduler):
"""
Input: list of several placements in several step
Output: show the placement
"""
f = open('depthbased_baseline.txt', 'a')
for i in range(task_scheduler.max_depth):
f.write("Depth%d:\n"%i)
print("Depth%d:"%i)
placement = final_placement[i]
task_set = task_scheduler.get_taskset(i)
for id in range(len(task_set)):
task = task_set[id]
job_id = int(ord(task.task_name[1]) - ord('A'))
task_id = int(task.task_name[2]) - 1
try:
dc_id = np.where(placement[job_id][task_id]==1)[0] + 1
except:
dc_id = | np.where(placement[job_id][task_id]==1) | numpy.where |
# Databricks notebook source
# MAGIC %md
# MAGIC ## Problem formulation
# MAGIC A common problem in computer vision is estimating the fundamental matrix based on a image pair. The fundamental matrix relates corresponding points in stereo geometry, and is useful as a pre-processing step for example when one wants to perform reconstruction of a captured scene. In this small project we use a scalable distributed algorithm to compute fundamental matrices between a large set of images.
# MAGIC
# MAGIC #### Short theory section
# MAGIC Assume that we want to link points in some image taken by camera <img src="https://latex.codecogs.com/svg.latex?&space;P_1" /> to points in an image taken by another camera <img src="https://latex.codecogs.com/svg.latex?&space;P_2" />. Let <img src="https://latex.codecogs.com/svg.latex?&space;x_i" /> and <img src="https://latex.codecogs.com/svg.latex?&space;x_i'" /> denote the projections of global point <img src="https://latex.codecogs.com/svg.latex?&space;X_i" /> onto the cameras <img src="https://latex.codecogs.com/svg.latex?&space;P_1" /> and <img src="https://latex.codecogs.com/svg.latex?&space;P_2" />, respectivly. Then the points are related as follows
# MAGIC
# MAGIC <img src="https://latex.codecogs.com/svg.latex?&space;\begin{cases}\lambda_i x_i = P_1X_i \\ \lambda_i' x_i' = P_2X_i
# MAGIC \end{cases} \Leftrightarrow \quad \begin{cases}\lambda_i x_i = P_1HH^{-1}X_i \\ \lambda_i' x_i' = P_2HH^{-1}X_i
# MAGIC \end{cases} \Leftrightarrow \quad \begin{cases}\lambda_i x_i = \tilde{P_1}\tilde{X_i} \\ \lambda_i' x_i' = \tilde{P_2}\tilde{X_i}
# MAGIC \end{cases}" />
# MAGIC
# MAGIC where <img src="https://latex.codecogs.com/svg.latex?&space;\lambda, \lambda'" /> are scale factors. Since we always can apply a projective transformation <img src="https://latex.codecogs.com/svg.latex?&space;H" /> to set one of the cameras to <img src="https://latex.codecogs.com/svg.latex?&space;P_1 = [I \quad 0]" /> and the other to some <img src="https://latex.codecogs.com/svg.latex?&space;P_2 = [A \quad t]" /> we can parametrize the global point <img src="https://latex.codecogs.com/svg.latex?&space;X_i" /> by
# MAGIC <img src="https://latex.codecogs.com/svg.latex?&space;X_i(\lambda) = [\lambda x_i \quad 1]^T" />. Thus the projected point onto camera <img src="https://latex.codecogs.com/svg.latex?&space;P_2" /> is represented by the line <img src="https://latex.codecogs.com/svg.latex?&space;P_2X_i(\lambda) = \lambda Ax_i + t " />. This line is called the epipolar line to the point <img src="https://latex.codecogs.com/svg.latex?&space;x_i" /> in epipolar geomtry, and descirbes how the point <img src="https://latex.codecogs.com/svg.latex?&space;x_i" /> in image 1 is related to points on in image 2. Since
# MAGIC all scene points that can project to <img src="https://latex.codecogs.com/svg.latex?&space;x_i" /> are on the viewing ray, all points in the second image that can
# MAGIC correspond <img src="https://latex.codecogs.com/svg.latex?&space;x_i" /> have to be on the epipolar line. This condition is called the epipolar constraint.
# MAGIC
# MAGIC 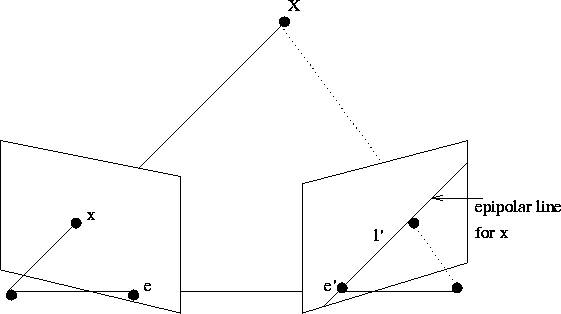
# MAGIC
# MAGIC Taking two points on this line (one of them being <img src="https://latex.codecogs.com/svg.latex?&space;e'" /> using <img src="https://latex.codecogs.com/svg.latex?&space;\lambda = 0" />), (add what is e_2)
# MAGIC we can derive an expression of this line <img src="https://latex.codecogs.com/svg.latex?&space;\ell" />, as any point x on the line <img src="https://latex.codecogs.com/svg.latex?&space;\ell" /> must fulfill <img src="https://latex.codecogs.com/svg.latex?&space;\ell^Tx = 0" />. Thus the line is thus given by
# MAGIC
# MAGIC
# MAGIC <img src="https://latex.codecogs.com/svg.latex?&space;\ell = t
# MAGIC \times (Ax +t ) = t \times (Ax) = e' \times Ax_i.\\" />
# MAGIC
# MAGIC Let <img src="https://latex.codecogs.com/svg.latex?&space;F = e' \times A " />, this is called the fundamental matrix. The fundamental matrix thus is a mathematical formulation which links points in image 1 to lines in image 2 (and vice versa). If <img src="https://latex.codecogs.com/svg.latex?&space;x'" /> corresponds to <img src="https://latex.codecogs.com/svg.latex?&space;x" /> then the epipolar constraint can be written
# MAGIC
# MAGIC <img src="https://latex.codecogs.com/svg.latex?&space;x'^T\ell = x'^T F x = 0 " />
# MAGIC
# MAGIC F is a 3x 3 matrix with 9 entiers and has 7 degrees of freedom. It can be estimated using 7 points using the 7-point algorithm.
# MAGIC
# MAGIC
# MAGIC Before we have assumed the the correspndeces between points in the imagaes are known, however these are found by first extracting features in the images using some form of feature extractor (e.g. SIFT) and subsequently finding matches using some mathcing criterion/algorithm (e.g. using Lowes criterion or in our case FLANN based matcher)
# MAGIC #### SIFT
# MAGIC Scale-invariant feature transform (SIFT) is a feature detection algorithm which detect and describe local features in images, see examples of detected SIFT features in the two images (a) and (b). SIFT finds local features present in the image and compute desriptors and locations of these features. Next we need to link the features present in image 1 to the features in image 2, which can be done using e.g. a FLANN (Fast Library for Approximate Nearest Neighbors) based matcher. In short the features in the images are compared and the matches are found using a nearest neighbor search. After a matching algorithm is used we have correspandence between the detected points in image 1 and image 2, see example in image (c) below. Note that there is still a high probaility that some of these matches are incorrect.
# MAGIC
# MAGIC 
# MAGIC
# MAGIC ### RANSAC
# MAGIC Some matches found by the FLANN may be incorrect, and a common robust method used for reducing the influence of these outliers in the estimation of F is RANSAC (RANdom SAmpling Consensus). In short, it relies on the fact that the inliers will tend to a consesus regarding the correct estimation, whereas the outlier estimation will show greater variation. By sampling random sets of points with size corresponding to the degrees of freedom of the model, calculating their corresponding estimations, and grouping all estimations with a difference below a set threshold, the largest consesus group is found. This set is then lastly used for the final estimate of F.
# COMMAND ----------
# MAGIC %md
# MAGIC ###For a more joyful presentation of the theory, listed to The Fundamental Matrix Song! (link)
# MAGIC [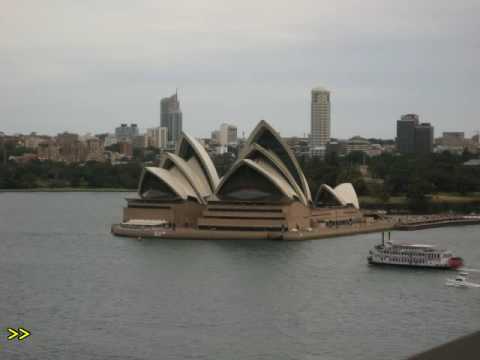](https://www.youtube.com/watch?v=DgGV3l82NTk)
# COMMAND ----------
# MAGIC %md
# MAGIC OpenCV is an well-known open-source library for computer vision, machine learning, and image processing tasks. In this project we will use it for feature extraction (SIFT), feature matching (FLANN) and the estimation of the fundamental matrix (using the 7-point algorithm). Let us install opencv
# COMMAND ----------
# MAGIC %pip install opencv-python
# COMMAND ----------
# MAGIC %md
# MAGIC Also we need to download a dataset that we can work with, this dataset is collected by <NAME> from LTH.
# MAGIC This is achieved by the bash shell script below.
# MAGIC The dataset is placed in the /tmp folder using the -P "prefix"
# COMMAND ----------
# MAGIC %sh
# MAGIC rm -r /tmp/0019
# MAGIC rm -r /tmp/eglise_int1.zip
# MAGIC
# MAGIC wget -P /tmp vision.maths.lth.se/calledataset/eglise_int/eglise_int1.zip
# MAGIC unzip /tmp/eglise_int1.zip -d /tmp/0019/
# MAGIC rm -r /tmp/eglise_int1.zip
# COMMAND ----------
# MAGIC %sh
# MAGIC rm -r /tmp/eglise_int2.zip
# MAGIC
# MAGIC wget -P /tmp vision.maths.lth.se/calledataset/eglise_int/eglise_int2.zip
# MAGIC unzip /tmp/eglise_int2.zip -d /tmp/0019/
# MAGIC rm -r /tmp/eglise_int2.zip
# COMMAND ----------
# MAGIC %sh
# MAGIC rm -r /tmp/eglise_int3.zip
# MAGIC
# MAGIC wget -P /tmp vision.maths.lth.se/calledataset/eglise_int/eglise_int3.zip
# MAGIC unzip /tmp/eglise_int3.zip -d /tmp/0019/
# MAGIC rm -r /tmp/eglise_int3.zip
# COMMAND ----------
# MAGIC %sh
# MAGIC cd /tmp/0019/
# MAGIC for f in *; do mv "$f" "eglise_$f"; done
# MAGIC cd /databricks/driver
# COMMAND ----------
# MAGIC %sh
# MAGIC rm -r /tmp/gbg.zip
# MAGIC
# MAGIC wget -P /tmp vision.maths.lth.se/calledataset/gbg/gbg.zip
# MAGIC unzip /tmp/gbg.zip -d /tmp/0019/
# MAGIC rm -r /tmp/gbg.zip
# COMMAND ----------
# MAGIC %scala
# MAGIC
# MAGIC import sys.process._
# MAGIC
# MAGIC //"wget -P /tmp vision.maths.lth.se/calledataset/door/door.zip" !!
# MAGIC //"unzip /tmp/door.zip -d /tmp/door/"!!
# MAGIC
# MAGIC //move downloaded dataset to dbfs
# MAGIC
# MAGIC val localpath="file:/tmp/0019/"
# MAGIC
# MAGIC dbutils.fs.rm("dbfs:/datasets/0019/mixedimages", true) // the boolean is for recursive rm
# MAGIC
# MAGIC dbutils.fs.mkdirs("dbfs:/datasets/0019/mixedimages")
# MAGIC
# MAGIC dbutils.fs.cp(localpath, "dbfs:/datasets/0019/mixedimages", true)
# COMMAND ----------
# MAGIC %sh
# MAGIC rm -r /tmp/0019
# COMMAND ----------
# MAGIC %scala
# MAGIC
# MAGIC display(dbutils.fs.ls("dbfs:/datasets/0019/mixedimages"))
# COMMAND ----------
#Loading one image from the dataset for testing
import numpy as np
import cv2
import matplotlib.pyplot as plt
def plot_img(figtitle,img):
#create figure with std size
fig = plt.figure(figtitle, figsize=(10, 5))
plt.imshow(img)
display(plt.show())
img1 = cv2.imread("/dbfs/datasets/0019/mixedimages/eglise_DSC_0133.JPG")
#img2 = cv2.imread("/dbfs/datasets/0019/mixedimages/DSC_0133.JPG")
plot_img("eglise", img1)
#plot_img("gbg", img2)
# COMMAND ----------
# MAGIC %md
# MAGIC
# MAGIC Read Image Dataset
# COMMAND ----------
import glob
import numpy as np
import cv2
import os
dataset_path = "/dbfs/datasets/0019/mixedimages/"
#get all filenames in folder
files = glob.glob(os.path.join(dataset_path,"*.JPG"))
dataset = []
#load all images
for i, file in enumerate(files): # Alex: changed
# Load an color image
#img = cv2.imread(file)
#add image and image name as a tupel to the list
dataset.append((file))
if i >= 150: # Alex: changed
break
# COMMAND ----------
# MAGIC %md
# MAGIC
# MAGIC Define maps
# COMMAND ----------
import glob
import numpy as np
import cv2
import matplotlib.pyplot as plt
max_features = 1000
def plot_img(figtitle,s):
img = cv2.imread(s)
#create figure with std size
fig = plt.figure(figtitle, figsize=(10, 5))
plt.imshow(img)
display(plt.show())
def extract_features(s):
"""
"""
img = cv2.imread(s) # Johan : here we load the images on the executor from dbfs into memory
#convert to gray scale
gray= cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
sift = cv2.SIFT_create(max_features)
#extract sift features and descriptors
kp, des = sift.detectAndCompute(gray, None)
#convert keypoint class to list of feature locations (for serialization)
points=[]
for i in range(len(kp)):
points.append(kp[i].pt)
#return a tuple of image name, image, feature points, descriptors, called a feature tuple
return (s, points, des) # Johan : here we don't send the images
def estimate_fundamental_matrix(s):
"""
"""
# s[0] is a feature tuple for the first image, s[1] is the same for the second image
a = s[0]
b = s[1]
# unpacks the tuples
name1, kp1, desc1 = a
name2, kp2, desc2 = b
# Create FLANN matcher object
FLANN_INDEX_KDTREE = 0
indexParams = dict(algorithm=FLANN_INDEX_KDTREE,
trees=5)
searchParams = dict(checks=50)
flann = cv2.FlannBasedMatcher(indexParams,
searchParams)
# matches the descriptors, for each query descriptor it finds the two best matches among the train descriptors
matches = flann.knnMatch(desc1, desc2, k=2)
goodMatches = []
pts1 = []
pts2 = []
# compares the best with the second best match and only adds those where the best match is significantly better than the next best.
for i,(m,n) in enumerate(matches):
if m.distance < 0.8*n.distance:
goodMatches.append([m.queryIdx, m.trainIdx])
pts2.append(kp2[m.trainIdx])
pts1.append(kp1[m.queryIdx])
pts1 = np.array(pts1, dtype=np.float32)
pts2 = np.array(pts2, dtype=np.float32)
# finds the fundamental matrix using ransac:
# selects minimal sub-set of the matches,
# estimates the fundamental matrix,
# checks how many of the matches satisfy the epipolar geometry (the inlier set)
# iterates this for a number of iterations,
# returns the fundamental matrix and mask with the largest number of inliers.
F, mask = cv2.findFundamentalMat(pts1, pts2, cv2.FM_RANSAC)
inlier_matches = []
# removes all matches that are not inliers
if mask is not None:
for i, el in enumerate(mask):
if el == 1:
inlier_matches.append(goodMatches[i])
# returns a tuple containing the feature tuple of image one and image two, the fundamental matrix and the inlier matches
return (a, b, F, inlier_matches)
def display_data(data):
for el in data:
print(el[2])
print("#######################################################")
# COMMAND ----------
# MAGIC %md
# MAGIC Perform Calculations
# COMMAND ----------
# creates an rdd from the loaded images (im_name, image)
rdd = sc.parallelize(dataset,20)
print("num partitions: ",rdd.getNumPartitions())
# applys the feature extraction to the images
rdd_features = rdd.map(extract_features) # Alex: we could leave the name but remove the image in a and b
print("num partitions: ",rdd_features.getNumPartitions())
# forms pairs of images by applying the cartisian product and filtering away the identity pair
rdd_pairs = rdd_features.cartesian(rdd_features).filter(lambda s: s[0][0] != s[1][0])
print("num partitions: ",rdd_pairs.getNumPartitions())
# applys the fundamental matrix estimation function on the pairs formed in the previous step and filters away all pairs with a low inlier set.
rdd_fundamental_matrix = rdd_pairs.map(estimate_fundamental_matrix).filter(lambda s: len(s[3]) > 50)
print("num partitions: ",rdd_fundamental_matrix.getNumPartitions())
# collects the result from the nodes
data = rdd_fundamental_matrix.collect()
# displays the fundamental matrices
display_data(data)
# COMMAND ----------
# MAGIC %md
# MAGIC Now we have computed the fundamental matrices, let us have a look at them by present the epipolar lines.
# COMMAND ----------
import random
def drawlines(img1,img2,lines,pts1,pts2):
#from opencv tutorial
''' img1 - image on which we draw the epilines for the points in img2
lines - corresponding epilines '''
r,c,_ = img1.shape
for r,pt1,pt2 in zip(lines,pts1,pts2):
color = tuple(np.random.randint(0,255,3).tolist())
x0,y0 = map(int, [0, -r[2]/r[1] ])
x1,y1 = map(int, [c, -(r[2]+r[0]*c)/r[1] ])
img1 = cv2.line(img1, (x0,y0), (x1,y1), color,3)
img1 = cv2.circle(img1,tuple(pt1),10,color,-1)
img2 = cv2.circle(img2,tuple(pt2),10,color,-1)
return img1,img2
# draws a random subset of the data
sampling = random.choices(data, k=4)
#plotts the inlier features in the first image and the corresponding epipolar lines in the second image
i = 0
fig, axs = plt.subplots(1, 8, figsize=(25, 5))
for el in sampling:
a, b, F, matches = el;
if F is None:
continue
name1, kp1, desc1 = a
name2, kp2, desc2 = b
im1 = cv2.imread(name1)
im2 = cv2.imread(name2)
pts1 = []
pts2 = []
for m in matches:
pts1.append(kp1[m[0]]);
pts2.append(kp2[m[1]]);
pts1 = np.array(pts1, dtype=np.float32)
pts2 = | np.array(pts2, dtype=np.float32) | numpy.array |
"""
This script identifies the time between the fist two action potentials
from a ramp protocol in order to quantify burstiness.
"""
import pyabf
import glob
import numpy as np
import matplotlib.pyplot as plt
def getListOfProtocol(protocol="0111", folderPath="X:/Data/SD/OXT-Subiculum/abfs"):
matchingPaths = []
for path in glob.glob(folderPath + "/*.abf"):
abf = pyabf.ABF(path, loadData=False)
if (abf.adcUnits[0] != "mV"):
print("bad units: " + path)
continue
if abf.protocol.startswith("0111"):
matchingPaths.append(path)
return matchingPaths
with open("paths-all.txt") as f:
abfPaths = f.readlines()
abfPaths = [x.strip() for x in abfPaths]
abfPaths = [x for x in abfPaths if ".abf" in x]
abfPaths = [x for x in abfPaths if not "#" in x]
def getFullTrace(abf: pyabf.ABF):
sweeps = [None] * abf.sweepCount
for sweepIndex in range(abf.sweepCount):
abf.setSweep(sweepIndex)
sweeps[sweepIndex] = abf.sweepY
return | np.concatenate(sweeps) | numpy.concatenate |
import numpy as np
from scipy.optimize import minimize
from scipy import optimize
# array operations
class OrthAE(object):
def __init__(self, views, latent_spaces, x = None, knob = 0):
# x: input, column-wise
# y: output, column-wise
# h: hidden layer
# views and latent_spaces: specify number of neurons in each view and latent space respectively
# params: initial weights of the orthogonal autoencoders
self.x = x
self.knob = knob
self.views = views
self.latent_spaces = latent_spaces
# sets of weights
self.w1 = np.zeros((np.sum(latent_spaces), np.sum(views) + 1))
self.w2 = np.zeros((np.sum(views), np.sum(latent_spaces) + 1))
# add orthogonal constraints
start_idx_hidden = np.cumsum(self.latent_spaces)
start_idx_input = np.cumsum(np.append([0],self.views))
# public latent space has fully connection
self.w1[0:start_idx_hidden[0],:] = 1
# private latent spaces only connect to respective views
for i in range(latent_spaces.size-1):
self.w1[start_idx_hidden[i]: start_idx_hidden[i+1], start_idx_input[i]: start_idx_input[i+1]] = 1
self.w2[:, :-1] = np.transpose(self.w1[:, :-1])
self.w2[:, -1] = 1
# index of effective weigths
self.index1 = np.where( self.w1 != 0 )
self.index2 = np.where( self.w2 != 0 )
# # randomly initialize weights
# self.w1[self.index1] = randInitWeights(self.w1.shape)[self.index1]
# self.w2[self.index2] = randInitWeights(self.w2.shape)[self.index2]
# print self.w1
# print self.w2
def feedforward(self):
instance_count = self.x.shape[1]
bias = np.ones((1, instance_count))
# a's are with bias
self.a1 = np.concatenate(( self.x, bias ))
# before activation
self.h = np.dot(self.w1, self.a1)
self.a2 = np.concatenate(( activate(self.h), bias ))
self.y = np.dot(self.w2, self.a2)
self.a3 = activate(self.y)
return self.a3
def backpropogateT(self, weights, y):
self.weightSplit(weights)
self.feedforward()
return self.backpropogate(y)
def backpropogate(self, y):
# knob is to prevent over-fitting
instance_count = self.x.shape[1]
delta = (self.a3 - y)/instance_count * activateGradient(self.y)
self.w2_gradient = np.dot(delta, np.transpose(self.a2))
# regularization
self.w2_gradient[:, :-1] += np.dot(self.knob, self.w2[:, :-1])/instance_count
delta = np.dot(np.transpose(self.w2[:, :-1]), delta) * activateGradient(self.h)
self.w1_gradient = np.dot(delta, np.transpose(self.a1))
# regularization
self.w1_gradient[:, :-1] += np.dot(self.knob, self.w1[:, :-1])/instance_count
return np.concatenate(( self.w1_gradient[self.index1].flatten(), self.w2_gradient[self.index2].flatten() ), axis=1)
def costT(self, weights, y):
self.weightSplit(weights)
self.feedforward()
return self.cost(y)
def cost(self, y):
instance_count = self.x.shape[1]
result = np.sum(np.square(self.w1)) + np.sum(np.square(self.w2))
result = np.sum(np.square(self.a3 - y)) + | np.dot(self.knob, result) | numpy.dot |
# (c) <NAME>, 2010-2022. MIT License. Based on own private work over the years.
import time
import warnings
from functools import partial
from typing import Any, Optional
import numpy as np
import pandas as pd
from scipy.stats import chi2, f
from sklearn.base import BaseEstimator, TransformerMixin
from sklearn.cross_decomposition import PLSRegression as PLS_sklearn
from sklearn.decomposition import PCA as PCA_sklearn
from sklearn.utils.validation import check_is_fitted
from .plots import loadings_plot, score_plot, spe_plot, t2_plot
epsqrt = np.sqrt(np.finfo(float).eps)
class SpecificationWarning(UserWarning):
"""Parent warning class."""
pass
class MCUVScaler(BaseEstimator, TransformerMixin):
"""
Create our own mean centering and scaling to unit variance (MCUV) class
The default scaler in sklearn does not handle small datasets accurately, with ddof.
"""
def __init__(self):
pass
def fit(self, X, y=None):
self.center_ = pd.DataFrame(X).mean()
# this is the key difference with "preprocessing.StandardScaler"
self.scale_ = pd.DataFrame(X).std(ddof=1)
self.scale_[self.scale_ == 0] = 1.0 # columns with no variance are left as-is.
return self
def transform(self, X):
check_is_fitted(self, "center_")
check_is_fitted(self, "scale_")
X = pd.DataFrame(X).copy()
return (X - self.center_) / self.scale_
def inverse_transform(self, X):
check_is_fitted(self, "center_")
check_is_fitted(self, "scale_")
X = pd.DataFrame(X).copy()
return X * self.scale_ + self.center_
class PCA(PCA_sklearn):
def __init__(
self,
n_components=None,
*,
copy=True,
whiten=False,
svd_solver="auto",
tol=0.0,
iterated_power="auto",
random_state=None,
# Own extra inputs, for the case when there is missing data
missing_data_settings: Optional[dict] = None,
):
super().__init__(
n_components=n_components,
copy=copy,
whiten=whiten,
svd_solver=svd_solver,
tol=tol,
iterated_power=iterated_power,
random_state=random_state,
)
self.n_components: int = n_components
self.missing_data_settings = missing_data_settings
self.has_missing_data = False
def fit(self, X, y=None) -> PCA_sklearn:
"""
Fits a principal component analysis (PCA) model to the data.
Parameters
----------
X : array-like, shape (n_samples, n_features)
Training data, where `n_samples` is the number of samples (rows)
and `n_features` is the number of features (columns).
y : Not used; by default None
Returns
-------
PCA
Model object.
"""
if not isinstance(X, pd.DataFrame):
X = pd.DataFrame(X)
self.N, self.K = X.shape
# Check if number of components is supported against maximum requested
min_dim = int(min(self.N, self.K))
self.A: int = min_dim if self.n_components is None else int(self.n_components)
if self.A > min_dim:
warn = (
"The requested number of components is more than can be "
"computed from data. The maximum number of components is "
f"the minimum of either the number of rows ({self.N}) or "
f"the number of columns ({self.K})."
)
warnings.warn(warn, SpecificationWarning)
self.A = self.n_components = min_dim
if np.any(X.isna()):
# If there are missing data, then the missing data settings apply. Defaults are:
#
# md_method = "pmp"
# md_tol = np.sqrt(np.finfo(float).eps)
# md_max_iter = 1000
default_mds = dict(md_method="tsr", md_tol=epsqrt, md_max_iter=1000)
if isinstance(self.missing_data_settings, dict):
default_mds.update(self.missing_data_settings)
self.missing_data_settings = default_mds
self = PCA_missing_values(
n_components=self.n_components,
missing_data_settings=self.missing_data_settings,
)
self.N, self.K = X.shape
self.A = self.n_components
self.fit(X)
else:
self = super().fit(X)
self.x_loadings = self.components_.T
self.extra_info = {}
self.extra_info["timing"] = np.zeros((1, self.A)) * np.nan
self.extra_info["iterations"] = np.zeros((1, self.A)) * np.nan
# We have now fitted the model. Apply some convenience shortcuts for the user.
self.A = self.n_components
self.N = self.n_samples_
self.K = self.n_features_
self.loadings = pd.DataFrame(self.x_loadings.copy())
self.loadings.index = X.columns
component_names = [a + 1 for a in range(self.A)]
self.loadings.columns = component_names
self.scaling_factor_for_scores = pd.Series(
np.sqrt(self.explained_variance_),
index=component_names,
name="Standard deviation per score",
)
self.Hotellings_T2 = pd.DataFrame(
np.zeros(shape=(self.N, self.A)),
columns=component_names,
index=X.index,
# name="Hotelling's T^2 statistic, per component",
)
if self.has_missing_data:
self.x_scores = pd.DataFrame(
self.x_scores_, columns=component_names, index=X.index
)
self.squared_prediction_error = pd.DataFrame(
self.squared_prediction_error_,
columns=component_names,
index=X.index.copy(),
)
self.R2 = pd.Series(
self.R2_,
index=component_names,
name="Model's R^2, per component",
)
self.R2cum = pd.Series(
self.R2cum_,
index=component_names,
name="Cumulative model's R^2, per component",
)
self.R2X_cum = pd.DataFrame(
self.R2X_cum_,
columns=component_names,
index=X.columns,
# name ="Per variable R^2, per component"
)
else:
self.x_scores = pd.DataFrame(
super().fit_transform(X), columns=component_names, index=X.index
)
self.squared_prediction_error = pd.DataFrame(
np.zeros((self.N, self.A)),
columns=component_names,
index=X.index.copy(),
)
self.R2 = pd.Series(
np.zeros(shape=(self.A,)),
index=component_names,
name="Model's R^2, per component",
)
self.R2cum = pd.Series(
np.zeros(shape=(self.A,)),
index=component_names,
name="Cumulative model's R^2, per component",
)
self.R2X_cum = pd.DataFrame(
np.zeros(shape=(self.K, self.A)),
columns=component_names,
index=X.columns,
# name ="Per variable R^2, per component"
)
if not self.has_missing_data:
Xd = X.copy()
prior_SSX_col = ssq(Xd.values, axis=0)
base_variance = np.sum(prior_SSX_col)
for a in range(self.A):
Xd -= self.x_scores.iloc[:, [a]] @ self.loadings.iloc[:, [a]].T
# These are the Residual Sums of Squares (RSS); i.e X-X_hat
row_SSX = ssq(Xd.values, axis=1)
col_SSX = ssq(Xd.values, axis=0)
# Don't use a check correction factor. Define SPE simply as the sum of squares of
# the errors, then take the square root, so it is interpreted like a standard error.
# If the user wants to normalize it, then this is a clean base value to start from.
self.squared_prediction_error.iloc[:, a] = np.sqrt(row_SSX)
# TODO: some entries in prior_SSX_col can be zero and leads to nan's in R2X_cum
self.R2X_cum.iloc[:, a] = 1 - col_SSX / prior_SSX_col
# R2 and cumulative R2 value for the whole block
self.R2cum.iloc[a] = 1 - sum(row_SSX) / base_variance
if a > 0:
self.R2.iloc[a] = self.R2cum.iloc[a] - self.R2cum.iloc[a - 1]
else:
self.R2.iloc[a] = self.R2cum.iloc[a]
# end: has no missing data
for a in range(self.A):
self.Hotellings_T2.iloc[:, a] = (
self.Hotellings_T2.iloc[:, max(0, a - 1)]
+ (self.x_scores.iloc[:, a] / self.scaling_factor_for_scores.iloc[a])
** 2
)
# Replace `self.loadings` with self.x_loadings
self.x_loadings = self.loadings
self.ellipse_coordinates = partial(
ellipse_coordinates,
n_components=self.n_components,
scaling_factor_for_scores=self.scaling_factor_for_scores,
n_rows=self.N,
)
self.T2_limit = partial(T2_limit, n_components=self.n_components, n_rows=self.N)
self.SPE_plot = partial(spe_plot, model=self)
self.T2_plot = partial(t2_plot, model=self)
self.loadings_plot = partial(loadings_plot, model=self, loadings_type="p")
self.score_plot = partial(score_plot, model=self)
return self
def fit_transform(self, X, y=None):
self.fit(X)
assert False, "Still do the transform part"
def SPE_limit(self, conf_level=0.95) -> float:
check_is_fitted(self, "squared_prediction_error")
return spe_calculation(
spe_values=self.squared_prediction_error.iloc[:, self.A - 1],
conf_level=conf_level,
)
def predict(self, X):
"""
Using the PCA model on new data coming in matrix X.
"""
class State(object):
"""Class object to hold the prediction results together."""
pass
state = State()
state.N, state.K = X.shape
assert (
self.K == state.K
), "Prediction data must have same number of columns as training data."
state.x_scores = X @ self.x_loadings
# TODO: handle the missing data version here still
for a in range(self.A):
pass
# p = self.x_loadings.iloc[:, [a]]
# temp = X @ self.x_loadings.iloc[:, [a]]
# X_mcuv -= temp @ p.T
# state.x_scores[:, [a]] = temp
# Scores are calculated, now do the rest
state.Hotellings_T2 = np.sum(
np.power((state.x_scores / self.scaling_factor_for_scores.values), 2), 1
)
# Calculate SPE-residuals (sum over rows of the errors)
X_mcuv = X.copy()
X_mcuv -= state.x_scores @ self.x_loadings.T
state.squared_prediction_error = np.sqrt(np.power(X_mcuv, 2).sum(axis=1))
return state
class PCA_missing_values(BaseEstimator, TransformerMixin):
"""
Create our PCA class if there is even a single missing data value in the X input array.
The default method to impute missing values is the TSR algorithm (`md_method="tsr"`).
Missing data method options are:
* 'pmp' Projection to Model Plane
* 'scp' Single Component Projection
* 'nipals' Same as 'scp': non-linear iterative partial least squares.
* 'tsr': TODO
* Other options? See papers by <NAME> and also:
https://analyticalsciencejournals.onlinelibrary.wiley.com/doi/abs/10.1002/cem.750
"""
valid_md_methods = ["pmp", "scp", "nipals", "tsr"]
def __init__(
self,
n_components=None,
copy: bool = True,
missing_data_settings=dict,
):
self.n_components = n_components
self.missing_data_settings = missing_data_settings
self.has_missing_data = True
self.missing_data_settings["md_max_iter"] = int(
self.missing_data_settings["md_max_iter"]
)
assert (
self.missing_data_settings["md_tol"] < 10
), "Tolerance should not be too large"
assert (
self.missing_data_settings["md_tol"] > epsqrt**1.95
), "Tolerance must exceed machine precision"
assert self.missing_data_settings["md_method"] in self.valid_md_methods, (
f"Missing data method is not recognized. Must be one of {self.valid_md_methods}.",
)
def fit(self, X, y=None):
# Force input to NumPy array:
self.data = np.asarray(X)
# Other setups:
self.n_components = self.A
self.n_samples_ = self.N
self.n_features_ = self.K
self.x_loadings = np.zeros((self.K, self.A))
self.x_scores_ = np.zeros((self.N, self.A))
self.R2_ = np.zeros(shape=(self.A,))
self.R2cum_ = np.zeros(shape=(self.A,))
self.R2X_cum_ = np.zeros(shape=(self.K, self.A))
self.squared_prediction_error_ = np.zeros((self.N, self.A))
# Perform MD algorithm here
if self.missing_data_settings["md_method"].lower() == "pmp":
self._fit_pmp(X)
elif self.missing_data_settings["md_method"].lower() in ["scp", "nipals"]:
self._fit_nipals_pca(settings=self.missing_data_settings)
elif self.missing_data_settings["md_method"].lower() in ["tsr"]:
self._fit_tsr(settings=self.missing_data_settings)
# Additional calculations, which can be done after the missing data method is complete.
self.explained_variance_ = np.diag(self.x_scores_.T @ self.x_scores_) / (
self.N - 1
)
return self
def transform(self, X):
check_is_fitted(self, "blah")
X = X.copy()
return X
def inverse_transform(self, X):
check_is_fitted(self, "blah")
X = X.copy()
return X
def _fit_nipals_pca(self, settings):
"""
Internal method to fit the PCA model using the NIPALS algorithm.
"""
# NIPALS algorithm
K, A = self.K, self.A
# Create direct links to the data
Xd = np.asarray(self.data)
base_variance = ssq(Xd)
# Initialize storage:
self.extra_info = {}
self.extra_info["timing"] = np.zeros(A) * np.nan
self.extra_info["iterations"] = np.zeros(A) * np.nan
for a in np.arange(A):
# Timers and housekeeping
start_time = time.time()
itern = 0
start_SS_col = ssq(Xd, axis=0)
if sum(start_SS_col) < epsqrt:
emsg = (
"There is no variance left in the data array: cannot "
f"compute any more components beyond component {a}."
)
raise RuntimeError(emsg)
# Initialize t_a with random numbers, or carefully select a column
# from X. <-- Don't do this anymore.
# Rather: Pick a column from X as the initial guess instead.
t_a_guess = Xd[:, [0]] # .reshape(self.N, 1)
t_a_guess[np.isnan(t_a_guess)] = 0
t_a = t_a_guess + 1.0
p_a = np.zeros((K, 1))
while not (
terminate_check(t_a_guess, t_a, iterations=itern, settings=settings)
):
# 0: Richardson's acceleration, or any numerical acceleration
# method for PCA where there is slow convergence?
# 0: starting point for convergence checking on next loop
t_a_guess = t_a.copy()
# 1: Regress the score, t_a, onto every column in X, compute the
# regression coefficient and store in p_a
# p_a = X.T * t_a / (t_a.T * t_a)
# p_a = (X.T)(t_a) / ((t_a.T)(t_a))
# p_a = np.dot(X.T, t_a) / ssq(t_a)
p_a = quick_regress(Xd, t_a)
# 2: Normalize p_a to unit length
p_a /= np.sqrt(ssq(p_a))
# 3: Now regress each row in X on the p_a vector, and store the
# regression coefficient in t_a
# t_a = X * p_a / (p_a.T * p_a)
# t_a = (X)(p_a) / ((p_a.T)(p_a))
# t_a = np.dot(X, p_a) / ssq(p_a)
t_a = quick_regress(Xd, p_a)
itern += 1
self.extra_info["timing"][a] = time.time() - start_time
self.extra_info["iterations"][a] = itern
# Loop terminated! Now deflate the X-matrix
Xd -= np.dot(t_a, p_a.T)
# These are the Residual Sums of Squares (RSS); i.e X-X_hat
row_SSX = ssq(Xd, axis=1)
col_SSX = ssq(Xd, axis=0)
self.squared_prediction_error_.iloc[:, a] = np.sqrt(row_SSX)
# TODO: some entries in start_SS_col can be zero and leads to nan's in R2X_cum
self.R2X_cum_.iloc[:, a] = 1 - col_SSX / start_SS_col
# R2 and cumulative R2 value for the whole block
self.R2cum_.iloc[a] = 1 - sum(row_SSX) / base_variance
if a > 0:
self.R2_.iloc[a] = self.R2cum_.iloc[a] - self.R2cum_.iloc[a - 1]
else:
self.R2_.iloc[a] = self.R2cum_.iloc[a]
# VIP value (only calculated for X-blocks); only last column is useful
# self.VIP_a = np.zeros((self.K, self.A))
# self.VIP = np.zeros(self.K)
# Store results
# -------------
# Flip the signs of the column vectors in P so that the largest
# magnitude element is positive
# (<NAME>, Geladi, PCA, CILS, 1987, p 42)
# http://dx.doi.org/10.1016/0169-7439(87)80084-9
max_el_idx = np.argmax(np.abs(p_a))
if np.sign(p_a[max_el_idx]) < 1:
p_a *= -1.0
t_a *= -1.0
# Store the loadings and scores
self.x_loadings.iloc[:, a] = p_a.flatten()
self.x_scores_.iloc[:, a] = t_a.flatten()
# end looping on A components
def _fit_tsr(self, settings):
delta = 1e100
Xd = np.asarray(self.data)
N, K, A = self.N, self.K, self.A
mmap = np.isnan(Xd)
# Could impute missing values per row, but leave it at zero, assuming the data are MCUV.
Xd[mmap] = 0.0
itern = 0
while (itern < settings["md_max_iter"]) and (delta > settings["md_tol"]):
itern += 1
missing_X = Xd[mmap]
mean_X = np.mean(Xd, axis=0)
S = np.cov(Xd, rowvar=False, ddof=1)
Xc = Xd - mean_X
if N > K:
_, _, V = np.linalg.svd(Xc, full_matrices=False)
else:
V, _, _ = np.linalg.svd(Xc.T, full_matrices=False)
V = V.T[:, 0:A] # transpose first
for n in range(N):
# If there are missing values (mis) in the n-th row. Compared to obs-erved values.
row_mis = mmap[n, :]
row_obs = ~row_mis
if np.any(row_mis):
# Form the so-called key-matrix, L
L = V[row_obs, 0 : min(A, sum(row_obs))]
S11 = S[row_obs, :][:, row_obs]
S21 = S[row_mis, :][:, row_obs]
z2 = (S21 @ L) @ np.linalg.pinv(L.T @ S11 @ L) @ L.T
Xc[n, row_mis] = z2 @ Xc[n, row_obs]
Xd = Xc + mean_X
delta = np.mean((Xd[mmap] - missing_X) ** 2)
# All done: return the results in `self`
S = np.cov(Xd, rowvar=False, ddof=1)
_, _, V = np.linalg.svd(S, full_matrices=False)
self.x_loadings = (V[0:A, :]).T # transpose result to the right shape: K x A
self.x_scores_ = (Xd - np.mean(Xd, axis=0)) @ self.x_loadings
self.data = Xd
class PLS(PLS_sklearn):
def __init__(
self,
n_components: int = 2,
*,
scale: bool = True,
max_iter: int = 1000,
tol: float = epsqrt,
copy: bool = True,
# Own extra inputs, for the case when there is missing data
missing_data_settings: Optional[dict] = None,
):
super().__init__(
n_components=n_components,
scale=scale,
max_iter=max_iter,
tol=tol,
copy=copy,
)
self.n_components: int = n_components
self.missing_data_settings = missing_data_settings
self.has_missing_data = False
def fit(self, X, Y) -> PLS_sklearn:
"""
Fits a projection to latent structures (PLS) or Partial Least Square (PLS) model to the
data.
Parameters
----------
X : array-like, shape (n_samples, n_features)
Training data, where `n_samples` is the number of samples (rows)
and `n_features` is the number of features (columns).
Y : array-like, shape (n_samples, n_targets)
Training data, where `n_samples` is the number of samples (rows)
and `n_targets` is the number of target outputs (columns).
Returns
-------
PLS
Model object.
References
----------
Abdi, "Partial least squares regression and projection on latent structure
regression (PLS Regression)", 2010, DOI: 10.1002/wics.51
"""
self.N, self.K = X.shape
self.Ny, self.M = Y.shape
assert self.Ny == self.N, (
f"The X and Y arrays must have the same number of rows: X has {self.N} and "
f"Y has {self.Ny}."
)
# Check if number of components is supported against maximum requested
min_dim = min(self.N, self.K)
self.A = min_dim if self.n_components is None else int(self.n_components)
if self.A > min_dim:
warn = (
"The requested number of components is more than can be "
"computed from data. The maximum number of components is "
f"the minimum of either the number of rows ({self.N}) or "
f"the number of columns ({self.K})."
)
warnings.warn(warn, SpecificationWarning)
self.A = self.n_components = min_dim
if np.any(Y.isna()) or np.any(X.isna()):
# If there are missing data, then the missing data settings apply. Defaults are:
#
# md_method = "tsr"
# md_tol = np.sqrt(np.finfo(float).eps)
# md_max_iter = self.max_iter
default_mds = dict(
md_method="tsr", md_tol=epsqrt, md_max_iter=self.max_iter
)
if isinstance(self.missing_data_settings, dict):
default_mds.update(self.missing_data_settings)
self.missing_data_settings = default_mds
self = PLS_missing_values(
n_components=self.n_components,
missing_data_settings=self.missing_data_settings,
)
self.N, self.K = X.shape
self.Ny, self.M = Y.shape
self.A = self.n_components
# Call the sub-function to do the PLS fit when missing data are present
self.fit(X, Y)
else:
self = super().fit(X, Y)
# x_scores #T: N x A
# y_scores # U: N x A
# x_weights # W: K x A
# y_weights # identical values to y_loadings. So this is redundant then.
# x_loadings # P: K x A
# y_loadings # C: M x A
self.extra_info = {}
self.extra_info["timing"] = np.zeros(self.A) * np.nan
self.extra_info["iterations"] = np.array(self.n_iter_)
# We have now fitted the model. Apply some convenience shortcuts for the user.
self.A = self.n_components
# Further convenience calculations
# "direct_weights" is matrix R = W(P'W)^{-1} [R is KxA matrix]; useful since T = XR
direct_weights = self.x_weights_ @ np.linalg.inv(
self.x_loadings_.T @ self.x_weights_
)
# beta = RC' [KxA by AxM = KxM]: the KxM matrix gives the direct link from the k-th (input)
# X variable, to the m-th (output) Y variable.
beta_coefficients = direct_weights @ self.y_loadings_.T
# Initialize storage:
component_names = [a + 1 for a in range(self.A)]
self.x_scores = pd.DataFrame(
self.x_scores_, index=X.index, columns=component_names
)
self.y_scores = pd.DataFrame(
self.y_scores_, index=Y.index, columns=component_names
)
self.x_weights = pd.DataFrame(
self.x_weights_, index=X.columns, columns=component_names
)
self.y_weights = pd.DataFrame(
self.y_weights_, index=Y.columns, columns=component_names
)
self.x_loadings = pd.DataFrame(
self.x_loadings_, index=X.columns, columns=component_names
)
self.y_loadings = pd.DataFrame(
self.y_loadings_, index=Y.columns, columns=component_names
)
self.predictions = pd.DataFrame(
self.x_scores @ self.y_loadings.T, index=Y.index, columns=Y.columns
)
self.direct_weights = pd.DataFrame(
direct_weights, index=X.columns, columns=component_names
)
self.beta_coefficients = pd.DataFrame(
beta_coefficients, index=X.columns, columns=Y.columns
)
self.explained_variance = np.diag(self.x_scores.T @ self.x_scores) / (
self.N - 1
)
self.scaling_factor_for_scores = pd.Series(
np.sqrt(self.explained_variance),
index=component_names,
name="Standard deviation per score",
)
self.Hotellings_T2 = pd.DataFrame(
np.zeros(shape=(self.N, self.A)),
columns=component_names,
index=X.index.copy(),
)
self.Hotellings_T2.index.name = "Hotelling's T^2 statistic, per component (col)"
self.squared_prediction_error = pd.DataFrame(
np.zeros((self.N, self.A)),
columns=component_names,
index=X.index.copy(),
)
self.R2 = pd.Series(
np.zeros(shape=(self.A)),
index=component_names,
name="Output R^2, per component (col)",
)
self.R2cum = pd.Series(
np.zeros(shape=(self.A)),
index=component_names,
name="Output cumulative R^2, per component (col)",
)
self.R2X_cum = pd.DataFrame(
np.zeros(shape=(self.K, self.A)),
index=X.columns.copy(),
columns=component_names,
)
self.R2X_cum.index.name = "R^2 per variable (row), per component (col)"
self.R2Y_cum = pd.DataFrame(
np.zeros(shape=(self.M, self.A)),
index=Y.columns.copy(),
columns=component_names,
)
self.R2Y_cum.index.name = "R^2 per variable (row), per component (col)"
self.RMSE = pd.DataFrame(
np.zeros(shape=(self.M, self.A)),
index=Y.columns.copy(),
columns=component_names,
)
self.RMSE.index.name = (
"Root mean square error per variable (row), per component (col)"
)
Xd = X.copy()
Yd = Y.copy()
prior_SSX_col = ssq(Xd.values, axis=0)
prior_SSY_col = ssq(Yd.values, axis=0)
base_variance_Y = np.sum(prior_SSY_col)
for a in range(self.A):
self.Hotellings_T2.iloc[:, a] = (
self.Hotellings_T2.iloc[:, max(0, a - 1)]
+ (self.x_scores.iloc[:, a] / self.scaling_factor_for_scores.iloc[a])
** 2
)
Xd -= self.x_scores.iloc[:, [a]] @ self.x_loadings.iloc[:, [a]].T
y_hat = (
self.x_scores.iloc[:, 0 : (a + 1)]
@ self.y_loadings.iloc[:, 0 : (a + 1)].T
)
# These are the Residual Sums of Squares (RSS); i.e X-X_hat
row_SSX = ssq(Xd.values, axis=1)
col_SSX = ssq(Xd.values, axis=0)
row_SSY = ssq(y_hat.values, axis=1)
col_SSY = ssq(y_hat.values, axis=0)
# R2 and cumulative R2 value for the whole block
self.R2cum.iloc[a] = sum(row_SSY) / base_variance_Y
if a > 0:
self.R2.iloc[a] = self.R2cum.iloc[a] - self.R2cum.iloc[a - 1]
else:
self.R2.iloc[a] = self.R2cum.iloc[a]
# Don't use a check correction factor. Define SPE simply as the sum of squares of
# the errors, then take the square root, so it is interpreted like a standard error.
# If the user wants to normalize it, then this is a clean base value to start from.
self.squared_prediction_error.iloc[:, a] = np.sqrt(row_SSX)
# TODO: some entries in prior_SSX_col can be zero and leads to nan's in R2X_cum
self.R2X_cum.iloc[:, a] = 1 - col_SSX / prior_SSX_col
self.R2Y_cum.iloc[:, a] = col_SSY / prior_SSY_col
self.RMSE.iloc[:, a] = (Yd.values - y_hat).pow(2).mean().pow(0.5)
self.ellipse_coordinates = partial(
ellipse_coordinates,
n_components=self.n_components,
scaling_factor_for_scores=self.scaling_factor_for_scores,
n_rows=self.N,
)
self.T2_limit = partial(T2_limit, n_components=self.n_components, n_rows=self.N)
self.SPE_plot = partial(spe_plot, model=self)
self.T2_plot = partial(t2_plot, model=self)
self.loadings_plot = partial(loadings_plot, model=self)
self.score_plot = partial(score_plot, model=self)
return self
def predict(self, X):
"""
Using the PLS model on new data coming in matrix X.
"""
class State(object):
"""Class object to hold the prediction results together."""
pass
state = State()
state.N, state.K = X.shape
assert (
self.K == state.K
), "Prediction data must have same number of columns as training data."
state.x_scores = X @ self.direct_weights
# TODO: handle the missing data version here still
for a in range(self.A):
pass
# p = self.x_loadings.iloc[:, [a]]
# w = self.x_weights.iloc[:, [a]]
# temp = X @ self.direct_weights.iloc[:, [a]]
# X_mcuv -= temp @ p.T
# state.x_scores[:, [a]] = temp
# Scores are calculated, now do the rest
state.Hotellings_T2 = np.sum(
np.power((state.x_scores / self.scaling_factor_for_scores.values), 2), 1
)
# Calculate SPE-residuals (sum over rows of the errors)
X_mcuv = X.copy()
X_mcuv -= state.x_scores @ self.x_loadings.T
state.squared_prediction_error = np.sqrt(np.power(X_mcuv, 2).sum(axis=1))
# Predicted values from the model (user still has to un-preprocess these predictions!)
state.y_hat = state.x_scores @ self.y_loadings.T
return state
def SPE_limit(self, conf_level=0.95) -> float:
check_is_fitted(self, "squared_prediction_error")
return spe_calculation(
spe_values=self.squared_prediction_error.iloc[:, self.A - 1],
conf_level=conf_level,
)
class PLS_missing_values(BaseEstimator, TransformerMixin):
"""
Create our PLS class if there is even a single missing data value in the X input array.
The default method to impute missing values is the TSR algorithm (`md_method="tsr"`).
Missing data method options are:
* 'pmp' Projection to Model Plane
* 'scp' Single Component Projection
* 'nipals' Same as 'scp': non-linear iterative partial least squares.
* 'tsr': Trimmed score regression
* Other options? See papers by <NAME> and also:
https://analyticalsciencejournals.onlinelibrary.wiley.com/doi/abs/10.1002/cem.750
"""
valid_md_methods = ["pmp", "scp", "nipals", "tsr"]
def __init__(
self,
n_components=None,
copy: bool = True,
missing_data_settings=dict,
):
self.n_components = n_components
self.missing_data_settings = missing_data_settings
self.has_missing_data = True
self.missing_data_settings["md_max_iter"] = int(
self.missing_data_settings["md_max_iter"]
)
assert (
self.missing_data_settings["md_tol"] < 10
), "Tolerance should not be too large"
assert (
self.missing_data_settings["md_tol"] > epsqrt**1.95
), "Tolerance must exceed machine precision"
assert self.missing_data_settings["md_method"] in self.valid_md_methods, (
f"Missing data method is not recognized. Must be one of {self.valid_md_methods}.",
)
def fit(self, X, Y):
"""
Fits a PLS latent variable model between `X` and `Y` data arrays, accounting for missing
values (nan's) in either or both arrays.
1. Höskuldsson, PLS regression methods, Journal of Chemometrics, 2(3), 211-228, 1998,
http://dx.doi.org/10.1002/cem.1180020306
"""
# Force input to NumPy array:
self.Xd = np.asarray(X)
self.Yd = np.asarray(Y)
if np.any(np.sum(self.Yd, axis=1) == 0):
raise Warning(
(
"Cannot handle the case yet where the entire observation in Y-matrix is "
"missing. Please remove those rows and refit model."
)
)
# Other setups:
self.n_components = self.A
self.n_samples_ = self.N
self.n_features_ = self.K
# self.M ?
self.x_scores_ = np.zeros((self.N, self.A)) # T: N x A
self.y_scores_ = np.zeros((self.N, self.A)) # U: N x A
self.x_weights_ = np.zeros((self.K, self.A)) # W: K x A
self.y_weights_ = None
self.x_loadings_ = np.zeros((self.K, self.A)) # P: K x A
self.y_loadings_ = np.zeros((self.M, self.A)) # C: M x A
self.R2 = np.zeros(shape=(self.A,))
self.R2cum = np.zeros(shape=(self.A,))
self.R2X_cum = np.zeros(shape=(self.K, self.A))
self.R2Y_cum = np.zeros(shape=(self.M, self.A))
self.squared_prediction_error = np.zeros((self.N, self.A))
# Perform MD algorithm here
if self.missing_data_settings["md_method"].lower() == "pmp":
self._fit_pmp_pls(X)
elif self.missing_data_settings["md_method"].lower() in ["scp", "nipals"]:
self._fit_nipals_pls(settings=self.missing_data_settings)
elif self.missing_data_settings["md_method"].lower() in ["tsr"]:
self._fit_tsr_pls(settings=self.missing_data_settings)
# Additional calculations, which can be done after the missing data method is complete.
# self.explained_variance_ = np.diag(self.x_scores.T @ self.x_scores) / (self.N - 1)
return self
def _fit_nipals_pls(self, settings):
"""
Internal method to fit the PLS model using the NIPALS algorithm.
"""
# NIPALS algorithm
A = self.A
# Initialize storage:
self.extra_info = {}
self.extra_info["timing"] = np.zeros(A) * np.nan
self.extra_info["iterations"] = np.zeros(A) * np.nan
for a in np.arange(A):
# Timers and housekeeping
start_time = time.time()
itern = 0
start_SSX_col = ssq(self.Xd, axis=0)
start_SSY_col = ssq(self.Yd, axis=0)
if sum(start_SSX_col) < epsqrt:
emsg = (
"There is no variance left in the data array for X: cannot "
f"compute any more components beyond component {a}."
)
raise RuntimeError(emsg)
if sum(start_SSY_col) < epsqrt:
emsg = (
"There is no variance left in the data array for Y: cannot "
f"compute any more components beyond component {a}."
)
raise RuntimeError(emsg)
# Initialize t_a with random numbers, or carefully select a column from X or Y?
# Find a column with the largest variance as t1_start; replace missing with zeros
# All columns have the same variance if the data have been scaled to unit variance!
u_a_guess = self.Yd[:, [0]]
u_a_guess[np.isnan(u_a_guess)] = 0
u_a = u_a_guess + 1.0
while not (
terminate_check(u_a_guess, u_a, iterations=itern, settings=settings)
):
# 0: starting point for convergence checking on next loop
u_a_guess = u_a.copy()
# 1: Regress the score, u_a, onto every column in X, compute the
# regression coefficient and store in w_a
# w_a = X.T * u_a / (u_a.T * u_a)
w_a = quick_regress(self.Xd, u_a)
# 2: Normalize w_a to unit length
w_a /= np.sqrt(ssq(w_a))
# 3: Now regress each row in X on the w_a vector, and store the
# regression coefficient in t_a
# t_a = X * w_a / (w_a.T * w_a)
t_a = quick_regress(self.Xd, w_a)
# 4: Now regress score, t_a, onto every column in Y, compute the
# regression coefficient and store in c_a
# c_a = Y * t_a / (t_a.T * t_a)
c_a = quick_regress(self.Yd, t_a)
# 5: Now regress each row in Y on the c_a vector, and store the
# regression coefficient in u_a
# u_a = Y * c_a / (c_a.T * c_a)
#
# TODO(KGD): % Still handle case when entire row in Y is missing
u_a = quick_regress(self.Yd, c_a)
itern += 1
self.extra_info["timing"][a] = time.time() - start_time
self.extra_info["iterations"][a] = itern
if itern > settings["md_max_iter"]:
Warning(
"PLS missing data [SCP method]: maximum number of iterations reached!"
)
# Loop terminated!
# 6: Now deflate the X-matrix. To do that we need to calculate loadings for the
# X-space. Regress columns of t_a onto each column in X and calculate loadings, p_a.
# Use this p_a to deflate afterwards.
p_a = quick_regress(self.Xd, t_a) # Note the similarity with step 4!
self.Xd -= np.dot(t_a, p_a.T) # and that similarity helps understand
self.Yd -= np.dot(t_a, c_a.T) # the deflation process.
## VIP value (only calculated for X-blocks); only last column is useful
# self.stats.VIP_a = np.zeros((self.K, self.A))
# self.stats.VIP = np.zeros(self.K)
# Store results
# -------------
# Flip the signs of the column vectors in P so that the largest
# magnitude element is positive (Wold, Esbensen, Geladi, PCA,
# CILS, 1987, p 42)
max_el_idx = np.argmax(np.abs(p_a))
if | np.sign(p_a[max_el_idx]) | numpy.sign |
# Copyright (c) 2011, <NAME> [see LICENSE.txt]
# This software is funded in part by NIH Grant P20 RR016454.
# Python 2 to 3 workarounds
import sys
if sys.version_info[0] == 2:
_strobj = str
_xrange = xrange
elif sys.version_info[0] == 3:
_strobj = str
_xrange = range
import unittest
import warnings
import os
import numpy as np
from pyvttbl import DataFrame
from pyvttbl.plotting import interaction_plot
from pyvttbl.misc.support import *
class Test_interaction_plot(unittest.TestCase):
def test0(self):
"""no error bars specified"""
R = {'aggregate': None,
'clevels': [1],
'fname': 'output\\interaction_plot(WORDS~AGE_X_CONDITION).png',
'maintitle': 'WORDS by AGE * CONDITION',
'numcols': 1,
'numrows': 1,
'rlevels': [1],
'subplot_titles': [''],
'xmaxs': [1.5],
'xmins': [-0.5],
'y': [[[11.0, 14.8],
[7.0, 6.5],
[13.4, 17.6],
[12.0, 19.3],
[6.9, 7.6]]],
'yerr': [[]],
'ymin': 0.0,
'ymax': 27.183257964740832}
# a simple plot
df=DataFrame()
df.TESTMODE=True
df.read_tbl('data/words~ageXcondition.csv')
D=df.interaction_plot('WORDS','AGE',
seplines='CONDITION',
output_dir='output')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,
np.array(R['y']).flat):
self.assertAlmostEqual(d,r)
for d,r in zip(np.array(D['yerr']).flat,
np.array(R['yerr']).flat):
self.assertAlmostEqual(d,r)
def test01(self):
"""confidence interval error bars specified"""
R = {'aggregate': 'ci',
'clevels': [1],
'fname': 'output\\interaction_plot(WORDS~AGE_X_CONDITION,yerr=95% ci).png',
'maintitle': 'WORDS by AGE * CONDITION',
'numcols': 1,
'numrows': 1,
'rlevels': [1],
'subplot_titles': [''],
'xmaxs': [1.5],
'xmins': [-0.5],
'y': [[[11.0, 14.8],
[7.0, 6.5],
[13.4, 17.6],
[12.0, 19.3],
[6.9, 7.6]]],
'yerr': [[]],
'ymin': 0.0,
'ymax': 27.183257964740832}
# a simple plot
df=DataFrame()
df.TESTMODE=True
df.read_tbl('data/words~ageXcondition.csv')
D=df.interaction_plot('WORDS','AGE',
seplines='CONDITION',
output_dir='output',
yerr='ci')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,
np.array(R['y']).flat):
self.assertAlmostEqual(d,r)
for d,r in zip(np.array(D['yerr']).flat,
np.array(R['yerr']).flat):
self.assertAlmostEqual(d,r)
def test02(self):
"""using loftus and masson error bars"""
# a simple plot
df=DataFrame()
df.read_tbl('data/words~ageXcondition.csv')
aov = df.anova('WORDS', wfactors=['AGE','CONDITION'])
aov.plot('WORDS','AGE', seplines='CONDITION',
errorbars='ci', output_dir='output')
def test1(self):
R = {'aggregate': None,
'clevels': ['M1', 'M2', 'M3'],
'fname': 'output\\interaction_plot(ERROR~TIMEOFDAY_X_COURSE_X_MODEL,yerr=1.0).png',
'maintitle': 'ERROR by TIMEOFDAY * COURSE * MODEL',
'numcols': 3,
'numrows': 1,
'rlevels': [1],
'subplot_titles': ['M1', 'M2', 'M3'],
'xmaxs': [1.5, 1.5, 1.5],
'xmins': [-0.5, -0.5, -0.5],
'y': [[[ 9. , 4.33333333],
[ 8.66666667, 3.66666667],
[ 4.66666667, 1.66666667]],
[[ 7.5 , 2.66666667],
[ 6. , 2.66666667],
[ 5. , 1.66666667]],
[[ 5. , 2.66666667],
[ 3.5 , 2.33333333],
[ 2.33333333, 1.33333333]]],
'yerr': [[1.0, 1.0],
[1.0, 1.0],
[1.0, 1.0]],
'ymax': 11.119188627248182,
'ymin': 0.0}
# specify yerr
df=DataFrame()
df.TESTMODE = True
df.read_tbl('data/error~subjectXtimeofdayXcourseXmodel_MISSING.csv')
D=df.interaction_plot('ERROR','TIMEOFDAY',
seplines='COURSE',
sepxplots='MODEL',
yerr=1.,
output_dir='output')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,
np.array(R['y']).flat):
self.assertAlmostEqual(d,r)
for d,r in zip(np.array(D['yerr']).flat,
np.array(R['yerr']).flat):
self.assertAlmostEqual(d,r)
def test2(self):
R = {'aggregate': 'ci',
'clevels': [1],
'fname': 'output\\interaction_plot(SUPPRESSION~CYCLE_X_AGE_X_PHASE,yerr=95% ci).png',
'maintitle': 'SUPPRESSION by CYCLE * AGE * PHASE',
'numcols': 1,
'numrows': 2,
'rlevels': ['I', 'II'],
'subplot_titles': ['I', 'II'],
'xmaxs': [4.1749999999999998, 4.1749999999999998],
'xmins': [0.32499999999999996, 0.32499999999999996],
'y': [[[ 17.33333333, 22.41666667, 22.29166667, 20.75 ],
[ 7.34166667, 9.65 , 9.70833333, 9.10833333]],
[[ 26.625 , 38.70833333, 39.08333333, 40.83333333],
[ 10.24166667, 12.575 , 13.19166667, 12.79166667]]],
'yerr': [[ 1.81325589, 1.44901936, 1.60883063, 1.57118871],
[ 2.49411239, 1.34873573, 1.95209851, 1.35412572]],
'ymax': 64.8719707118471,
'ymin': 0.0}
# generate yerr
df=DataFrame()
df.TESTMODE = True
df.read_tbl('data\suppression~subjectXgroupXageXcycleXphase.csv')
D = df.interaction_plot('SUPPRESSION','CYCLE',
seplines='AGE',
sepyplots='PHASE',
yerr='ci',
output_dir='output')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,np.array(R['y']).flat):
self.assertAlmostEqual(d,r)
for d,r in zip(np.array(D['yerr']).flat,np.array(R['yerr']).flat):
self.assertAlmostEqual(d,r)
def test3(self):
R = {'aggregate': 'ci',
'clevels': ['I', 'II'],
'fname': 'output\\whereGROUPnotLAB.png',
'maintitle': 'SUPPRESSION by CYCLE * AGE * PHASE * GROUP',
'numcols': 2,
'numrows': 2,
'rlevels': ['AA', 'AB'],
'subplot_titles': ['GROUP = AA, PHASE = AA',
'GROUP = AA, PHASE = AA',
'GROUP = AB, PHASE = AB',
'GROUP = AB, PHASE = AB'],
'xmaxs': [4.1500000000000004,
4.1500000000000004,
4.1500000000000004,
4.1500000000000004],
'xmins': [0.84999999999999998,
0.84999999999999998,
0.84999999999999998,
0.84999999999999998],
'y': [[[ 17.75 , 22.375, 23.125, 20.25 ],
[ 8.675, 10.225, 10.5 , 9.925]],
[[ 20.875, 28.125, 20.75 , 24.25 ],
[ 8.3 , 10.25 , 9.525, 11.1 ]],
[[ 12.625, 23.5 , 20. , 15.625],
[ 5.525, 8.825, 9.125, 7.75 ]],
[[ 22.75 , 41.125, 46.125, 51.75 ],
[ 8.675, 13.1 , 14.475, 12.85 ]]],
'ymax': 64.8719707118471,
'ymin': 0.0}
# separate y plots and separate x plots
df=DataFrame()
df.TESTMODE = True
df.read_tbl('data\suppression~subjectXgroupXageXcycleXphase.csv')
D = df.interaction_plot('SUPPRESSION','CYCLE',
seplines='AGE',
sepxplots='PHASE',
sepyplots='GROUP',yerr='ci',
where=[('GROUP','not in',['LAB'])],
fname='whereGROUPnotLAB.png',
output_dir='output')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,np.array(R['y']).flat):
self.assertAlmostEqual(d,r)
def test31(self):
# separate y plots and separate x plots
df=DataFrame()
df.TESTMODE = True
df.read_tbl('data\suppression~subjectXgroupXageXcycleXphase.csv')
D = df.interaction_plot('SUPPRESSION','CYCLE',
seplines='AGE',
sepxplots='GROUP',
sepyplots='PHASE',
yerr='sem',
output_dir='output')
# the code for when seplines=None is in a different branch
# these test that code
def test4(self):
R = {'aggregate': None,
'clevels': ['adjective',
'counting',
'imagery',
'intention',
'rhyming'],
'fname': 'output\\interaction_plot(WORDS~AGE_X_CONDITION).png',
'maintitle': 'WORDS by AGE * CONDITION',
'numcols': 5,
'numrows': 1,
'rlevels': [1],
'subplot_titles': ['adjective',
'counting',
'imagery',
'intention',
'rhyming'],
'xmaxs': [1.5, 1.5, 1.5, 1.5, 1.5],
'xmins': [-0.5, -0.5, -0.5, -0.5, -0.5],
'y': [[ 11. , 14.8],
[ 7. , 6.5],
[ 13.4, 17.6],
[ 12. , 19.3],
[ 6.9, 7.6]],
'yerr': [[], [], [], [], []],
'ymax': 27.183257964740832,
'ymin': 0.0}
# a simple plot
df=DataFrame()
df.TESTMODE = True
df.read_tbl('data/words~ageXcondition.csv')
D = df.interaction_plot('WORDS','AGE',
sepxplots='CONDITION',
output_dir='output')
self.assertEqual(D['aggregate'], R['aggregate'])
self.assertEqual(D['clevels'], R['clevels'])
self.assertEqual(D['rlevels'], R['rlevels'])
self.assertEqual(D['numcols'], R['numcols'])
self.assertEqual(D['numrows'], R['numrows'])
self.assertEqual(D['fname'], R['fname'])
self.assertEqual(D['maintitle'], R['maintitle'])
self.assertEqual(D['subplot_titles'], R['subplot_titles'])
self.assertAlmostEqual(D['ymin'], R['ymin'])
self.assertAlmostEqual(D['ymax'], R['ymax'])
for d,r in zip(np.array(D['y']).flat,
| np.array(R['y']) | numpy.array |
import time
import numpy as np
from scipy import signal
import matplotlib.pyplot as plt
def fft_window(tnum, nfft, window, overlap):
# IN : full length of time series, nfft, window name, overlap ratio
# OUT : bins, 1 x nfft window function
# use overlapping
bins = int(np.fix((int(tnum/nfft) - overlap)/(1.0 - overlap)))
# window function
if window == 'rectwin': # overlap = 0.5
win = np.ones(nfft)
elif window == 'hann': # overlap = 0.5
win = np.hanning(nfft)
elif window == 'hamm': # overlap = 0.5
win = np.hamming(nfft)
elif window == 'kaiser': # overlap = 0.62
win = np.kaiser(nfft, beta=30)
elif window == 'HFT248D': # overlap = 0.84
z = 2*np.pi/nfft*np.arange(0,nfft)
win = 1 - 1.985844164102*np.cos(z) + 1.791176438506*np.cos(2*z) - 1.282075284005*np.cos(3*z) + \
0.667777530266*np.cos(4*z) - 0.240160796576*np.cos(5*z) + 0.056656381764*np.cos(6*z) - \
0.008134974479*np.cos(7*z) + 0.000624544650*np.cos(8*z) - 0.000019808998*np.cos(9*z) + \
0.000000132974*np.cos(10*z)
return bins, win
def fftbins(x, dt, nfft, window, overlap, detrend=0, full=0):
# IN : 1 x tnum data
# OUT : bins x faxis fftdata
tnum = len(x)
bins, win = fft_window(tnum, nfft, window, overlap)
win_factor = np.mean(win**2) # window factors
# make an x-axis #
ax = np.fft.fftfreq(nfft, d=dt) # full 0~fN -fN~-f1
if np.mod(nfft, 2) == 0: # even nfft
ax = np.hstack([ax[0:int(nfft/2)], -(ax[int(nfft/2)]), ax[int(nfft/2):nfft]])
if full == 1: # full shift to -fN ~ 0 ~ fN
ax = np.fft.fftshift(ax)
else: # half 0~fN
ax = ax[0:int(nfft/2+1)]
# make fftdata
if full == 1: # full shift to -fN ~ 0 ~ fN
if np.mod(nfft, 2) == 0: # even nfft
fftdata = np.zeros((bins, nfft+1), dtype=np.complex_)
else: # odd nfft
fftdata = np.zeros((bins, nfft), dtype=np.complex_)
else: # half 0 ~ fN
fftdata = np.zeros((bins, int(nfft/2+1)), dtype=np.complex_)
for b in range(bins):
idx1 = int(b*np.fix(nfft*(1 - overlap)))
idx2 = idx1 + nfft
sx = x[idx1:idx2]
if detrend == 0:
sx = signal.detrend(sx, type='constant') # subtract mean
elif detrend == 1:
sx = signal.detrend(sx, type='linear')
sx = sx * win # apply window function
# get fft
SX = np.fft.fft(sx, n=nfft)/nfft # divide by the length
if np.mod(nfft, 2) == 0: # even nfft
SX = np.hstack([SX[0:int(nfft/2)], np.conj(SX[int(nfft/2)]), SX[int(nfft/2):nfft]])
if full == 1: # shift to -fN ~ 0 ~ fN
SX = np.fft.fftshift(SX)
else: # half 0 ~ fN
SX = SX[0:int(nfft/2+1)]
fftdata[b,:] = SX
return ax, fftdata, win_factor
def cwt(x, dt, df, detrend=0, full=1):
# detrend signal
if detrend == 0:
x = signal.detrend(x, type='constant') # subtract mean
elif detrend == 1:
x = signal.detrend(x, type='linear')
# make a t-axis
tnum = len(x)
nfft = nextpow2(tnum) # power of 2
t = np.arange(nfft)*dt
# make a f-axis with constant df
s0 = 2.0*dt # the smallest scale
ax = np.arange(0.0, 1.0/(1.03*s0), df) # 1.03 for the Morlet wavelet function
# scales
old_settings = np.seterr(divide='ignore')
sj = 1.0/(1.03*ax)
np.seterr(**old_settings)
dj = np.log2(sj/s0) / np.arange(len(sj)) # dj; necessary for reconstruction
sj[0] = tnum*dt/2.0
dj[0] = 0 # remove infinity point due to fmin = 0
# Morlet wavelet function (unnormalized)
omega0 = 6.0 # nondimensional wavelet frequency
wf0 = lambda eta: np.pi**(-1.0/4) * np.exp(1.0j*omega0*eta) * np.exp(-1.0/2*eta**2)
ts = np.sqrt(2)*sj # e-folding time for Morlet wavelet with omega0 = 6; significance level
# FFT of signal
X = np.fft.fft(x, n=nfft)/nfft
# calculate CWT
snum = len(sj)
cwtdata = np.zeros((nfft, snum), dtype=np.complex_)
for j, s in enumerate(sj):
# nondimensional time axis at time scale s
eta = t/s
# FFT of the normalized wavelet function
W = np.fft.fft( np.conj( wf0(eta - np.mean(eta))*np.sqrt(dt/s) ) )
# Wavelet transform at scae s for all n time
cwtdata[:,j] = np.conj(np.fft.fftshift(np.fft.ifft(X * W) * nfft)) # phase direction correct
# full size
if full == 1:
cwtdata = np.hstack([np.fliplr(np.conj(cwtdata)), cwtdata[:,1:]]) # real x only
ax = np.hstack([-ax[::-1], ax[1:]])
return ax, cwtdata[0:tnum,:], dj, ts
def cross_power(XX, YY, win_factor):
Pxy = np.mean(XX * np.conjugate(YY), 0)
Pxy = np.abs(Pxy).real / win_factor
return Pxy
def coherence(XX, YY):
# normalization outside loop
# Pxy = np.mean(XX * np.conjugate(YY), 0)
# Pxx = np.mean(XX * np.conjugate(XX), 0).real
# Pyy = np.mean(YY * np.conjugate(YY), 0).real
# Gxy = np.abs(Pxy).real / np.sqrt(Pxx * Pyy)
# normalization inside loop
bins = XX.shape[0]
val = np.zeros(XX.shape, dtype=np.complex_)
for i in range(bins):
X = XX[i,:]
Y = YY[i,:]
Pxx = X * np.matrix.conjugate(X)
Pyy = Y * np.matrix.conjugate(Y)
val[i,:] = X*np.matrix.conjugate(Y) / np.sqrt(Pxx*Pyy)
# average over bins
Gxy = np.mean(val, 0)
Gxy = np.abs(Gxy).real
return Gxy
def cross_phase(XX, YY):
Pxy = np.mean(XX * np.conjugate(YY), 0)
Axy = np.arctan2(Pxy.imag, Pxy.real).real
return Axy
def correlation(XX, YY, win_factor):
bins = XX.shape[0]
nfreq = XX.shape[1]
val = np.zeros(XX.shape, dtype=np.complex_)
for b in range(bins):
X = XX[b,:]
Y = YY[b,:]
val[b,:] = np.fft.ifftshift(X*np.matrix.conjugate(Y) / win_factor)
val[b,:] = np.fft.ifft(val[b,:], n=nfreq)*nfreq
val[b,:] = np.fft.fftshift(val[b,:])
val[b,:] = np.flip(val[b,:], axis=0)
# average over bins; return real value
Cxy = np.mean(val, 0)
return Cxy.real
def corr_coef(XX, YY):
bins = XX.shape[0]
nfreq = XX.shape[1]
val = np.zeros(XX.shape, dtype=np.complex_)
for b in range(bins):
X = XX[b,:]
Y = YY[b,:]
x = np.fft.ifft(np.fft.ifftshift(X), n=nfreq)*nfreq
Rxx = np.mean(x**2)
y = np.fft.ifft(np.fft.ifftshift(Y), n=nfreq)*nfreq
Ryy = np.mean(y**2)
val[b,:] = np.fft.ifftshift(X*np.matrix.conjugate(Y))
val[b,:] = np.fft.ifft(val[b,:], n=nfreq)*nfreq
val[b,:] = np.fft.fftshift(val[b,:])
val[b,:] = np.flip(val[b,:], axis=0)/np.sqrt(Rxx*Ryy)
# average over bins; return real value
cxy = np.mean(val, 0)
return cxy.real
def xspec(XX, YY, win_factor):
val = np.abs(XX * np.conjugate(YY)).real
return val
def bicoherence(XX, YY):
# ax1 = self.Dlist[dtwo].ax # full -fN ~ fN
# ax2 = np.fft.ifftshift(self.Dlist[dtwo].ax) # full 0 ~ fN, -fN ~ -f1
# ax2 = ax2[0:int(nfft/2+1)] # half 0 ~ fN
bins = XX.shape[0]
full = XX.shape[1]
half = int(full/2+1) # half length
# calculate bicoherence
B = np.zeros((full, half), dtype=np.complex_)
P12 = np.zeros((full, half))
P3 = np.zeros((full, half))
val = np.zeros((full, half))
for b in range(bins):
X = XX[b,:] # full -fN ~ fN
Y = YY[b,:] # full -fN ~ fN
Xhalf = np.fft.ifftshift(X) # full 0 ~ fN, -fN ~ -f1
Xhalf = Xhalf[0:half] # half 0 ~ fN
X1 = np.transpose(np.tile(X, (half, 1)))
X2 = np.tile(Xhalf, (full, 1))
X3 = np.zeros((full, half), dtype=np.complex_)
for j in range(half):
if j == 0:
X3[0:, j] = Y[j:]
else:
X3[0:(-j), j] = Y[j:]
B = B + X1 * X2 * np.matrix.conjugate(X3) # complex bin average
P12 = P12 + (np.abs(X1 * X2).real)**2 # real average
P3 = P3 + (np.abs(X3).real)**2 # real average
# val = np.log10(np.abs(B)**2) # bispectrum
old_settings = np.seterr(invalid='ignore')
val = (np.abs(B)**2) / P12 / P3 # bicoherence
np.seterr(**old_settings)
# summation over pairs
sum_val = np.zeros(full)
for i in range(half):
if i == 0:
sum_val = sum_val + val[:,i]
else:
sum_val[i:] = sum_val[i:] + val[:-i,i]
N = np.array([i+1 for i in range(half)] + [half for i in range(full-half)])
sum_val = sum_val / N # element wise division
return val, sum_val
def ritz_nonlinear(XX, YY):
bins = XX.shape[0]
full = XX.shape[1]
kidx = get_kidx(full)
Aijk = np.zeros((full, full), dtype=np.complex_) # Xo1 Xo2 cXo
cAijk = np.zeros((full, full), dtype=np.complex_) # cXo1 cXo2 Xo
Bijk = np.zeros((full, full), dtype=np.complex_) # Yo cXo1 cXo2
Aij = np.zeros((full, full)) # |Xo1 Xo2|^2
Ak = np.zeros(full) # Xo cXo
Bk = np.zeros(full, dtype=np.complex_) # Yo cXo
for b in range(bins):
X = XX[b,:] # full -fN ~ fN
Y = YY[b,:] # full -fN ~ fN
# make Xi and Xj
Xi = np.transpose(np.tile(X, (full, 1))) # columns of (-fN ~ fN)
Xj = np.tile(X, (full, 1)) # rows of (-fN ~ fN)
# make Xk and Yk
Xk = np.zeros((full, full), dtype=np.complex_)
Yk = np.zeros((full, full), dtype=np.complex_)
for k in range(full):
idx = kidx[k]
for n, ij in enumerate(idx):
Xk[ij] = X[k]
Yk[ij] = Y[k]
# do ensemble average
Aijk = Aijk + Xi * Xj * np.matrix.conjugate(Xk) / bins
cAijk = cAijk + np.matrix.conjugate(Xi) * np.matrix.conjugate(Xj) * Xk / bins
Bijk = Bijk + np.matrix.conjugate(Xi) * np.matrix.conjugate(Xj) * Yk / bins
Aij = Aij + (np.abs(Xi * Xj).real)**2 / bins
Ak = Ak + (np.abs(X).real)**2 / bins
Bk = Bk + Y * np.matrix.conjugate(X) / bins
# Linear transfer function ~ growth rate
Lk = np.zeros(full, dtype=np.complex_)
bsum = np.zeros(full, dtype=np.complex_)
asum = np.zeros(full)
for k in range(full):
idx = kidx[k]
for n, ij in enumerate(idx):
bsum[k] = bsum[k] + Aijk[ij] * Bijk[ij] / Aij[ij]
asum[k] = asum[k] + (np.abs(Aijk[ij]).real)**2 / Aij[ij]
Lk = (Bk - bsum) / (Ak - asum)
# Quadratic transfer function ~ nonlinear energy transfer rate
Lkk = np.zeros((full, full), dtype=np.complex_)
for k in range(full):
idx = kidx[k]
for n, ij in enumerate(idx):
Lkk[ij] = Lk[k]
Qijk = (Bijk - Lkk * cAijk) / Aij
return Lk, Qijk, Bk, Aijk
def wit_nonlinear(XX, YY):
bins = XX.shape[0]
full = XX.shape[1]
kidx = get_kidx(full)
Lk = np.zeros(full, dtype=np.complex_) # Linear
Qijk = np.zeros((full, full), dtype=np.complex_) # Quadratic
print('For stable calculations, bins ({0}) >> full/2 ({1})'.format(bins, full/2))
for k in range(full):
idx = kidx[k]
# construct equations for each k
U = np.zeros((bins, len(idx)+1), dtype=np.complex_) # N (number of ensembles) x P (number of pairs + 1)
V = np.zeros(bins, dtype=np.complex_) # N x 1
for b in range(bins):
U[b,0] = XX[b,k]
for n, ij in enumerate(idx):
U[b,n+1] = XX[b, ij[0]]*XX[b, ij[1]]
V[b] = YY[b,k]
# solution for each k
H = np.matmul(np.linalg.pinv(U), V)
Lk[k] = H[0]
for n, ij in enumerate(idx):
Qijk[ij] = H[n+1]
# calculate others for the rates
Aijk = np.zeros((full, full), dtype=np.complex_) # Xo1 Xo2 cXo
Bk = np.zeros(full, dtype=np.complex_) # Yo cXo
# print('DO NOT CALCULATE RATES')
for b in range(bins):
X = XX[b,:] # full -fN ~ fN
Y = YY[b,:] # full -fN ~ fN
# make Xi and Xj
Xi = np.transpose(np.tile(X, (full, 1))) # columns of (-fN ~ fN)
Xj = np.tile(X, (full, 1)) # rows of (-fN ~ fN)
# make Xk and Yk
Xk = np.zeros((full, full), dtype=np.complex_)
for k in range(full):
idx = kidx[k]
for n, ij in enumerate(idx):
Xk[ij] = X[k]
# do ensemble average
Aijk = Aijk + Xi * Xj * np.matrix.conjugate(Xk) / bins
Bk = Bk + Y * np.matrix.conjugate(X) / bins
return Lk, Qijk, Bk, Aijk
def local_coherency(XX, YY, Lk, Qijk, Aijk):
bins = XX.shape[0]
full = XX.shape[1]
# calculate Glin
Pxx = np.mean(XX * np.conjugate(XX), 0).real
Pyy = np.mean(YY * np.conjugate(YY), 0).real
Glin = np.abs(Lk)**2 * Pxx / Pyy
# calculate Aijij
kidx = get_kidx(full) # kidx
Aijij = | np.zeros((full, full)) | numpy.zeros |
#!/usr/bin/env python
'''
Miscellaneous utilities that are extremely helpful but cannot be clubbed
into other modules.
'''
import torch
# Scientific computing
import numpy as np
import scipy.linalg as lin
from scipy import io
# Plotting
import cv2
import matplotlib.pyplot as plt
def nextpow2(x):
'''
Return smallest number larger than x and a power of 2.
'''
logx = np.ceil(np.log2(x))
return pow(2, logx)
def normalize(x, fullnormalize=False):
'''
Normalize input to lie between 0, 1.
Inputs:
x: Input signal
fullnormalize: If True, normalize such that minimum is 0 and
maximum is 1. Else, normalize such that maximum is 1 alone.
Outputs:
xnormalized: Normalized x.
'''
if x.sum() == 0:
return x
xmax = x.max()
if fullnormalize:
xmin = x.min()
else:
xmin = 0
xnormalized = (x - xmin)/(xmax - xmin)
return xnormalized
def asnr(x, xhat, compute_psnr=False):
'''
Compute affine SNR, which accounts for any scaling and shift between two
signals
Inputs:
x: Ground truth signal(ndarray)
xhat: Approximation of x
Outputs:
asnr_val: 20log10(||x||/||x - (a.xhat + b)||)
where a, b are scalars that miminize MSE between x and xhat
'''
mxy = (x*xhat).mean()
mxx = (xhat*xhat).mean()
mx = xhat.mean()
my = x.mean()
a = (mxy - mx*my)/(mxx - mx*mx)
b = my - a*mx
if compute_psnr:
return psnr(x, a*xhat + b)
else:
return rsnr(x, a*xhat + b)
def rsnr(x, xhat):
'''
Compute reconstruction SNR for a given signal and its reconstruction.
Inputs:
x: Ground truth signal (ndarray)
xhat: Approximation of x
Outputs:
rsnr_val: RSNR = 20log10(||x||/||x-xhat||)
'''
xn = lin.norm(x.reshape(-1))
en = lin.norm((x-xhat).reshape(-1)) + 1e-12
rsnr_val = 20* | np.log10(xn/en) | numpy.log10 |
import sys
import cftime
import numpy as np
import pandas as pd
import pytest
import xarray as xr
# Import from directory structure if coverage test, or from installed
# packages otherwise
if "--cov" in str(sys.argv):
from src.geocat.comp import anomaly, climatology, month_to_season, calendar_average, climatology_average
else:
from geocat.comp import anomaly, climatology, month_to_season, calendar_average, climatology_average
dset_a = xr.tutorial.open_dataset("rasm")
dset_b = xr.tutorial.open_dataset("air_temperature")
dset_c = dset_a.copy().rename({"time": "Times"})
dset_encoded = xr.tutorial.open_dataset("rasm", decode_cf=False)
def get_fake_dataset(start_month, nmonths, nlats, nlons):
"""Returns a very simple xarray dataset for testing.
Data values are equal to "month of year" for monthly time steps.
"""
# Create coordinates
months = pd.date_range(start=pd.to_datetime(start_month),
periods=nmonths,
freq="MS")
lats = np.linspace(start=-90, stop=90, num=nlats, dtype="float32")
lons = np.linspace(start=-180, stop=180, num=nlons, dtype="float32")
# Create data variable. Construct a 3D array with time as the first
# dimension.
month_values = np.expand_dims(np.arange(start=1, stop=nmonths + 1),
axis=(1, 2))
var_values = np.tile(month_values, (1, nlats, nlons))
ds = xr.Dataset(
data_vars={
"my_var": (("time", "lat", "lon"), var_values.astype("float32")),
},
coords={
"time": months,
"lat": lats,
"lon": lons
},
)
return ds
def _get_dummy_data(start_date,
end_date,
freq,
nlats,
nlons,
calendar='standard'):
"""Returns a simple xarray dataset to test with.
Data can be hourly, daily, or monthly.
"""
# Coordinates
time = xr.cftime_range(start=start_date,
end=end_date,
freq=freq,
calendar=calendar)
lats = np.linspace(start=-90, stop=90, num=nlats, dtype='float32')
lons = np.linspace(start=-180, stop=180, num=nlons, dtype='float32')
# Create data variable
values = np.expand_dims(np.arange(len(time)), axis=(1, 2))
data = np.tile(values, (1, nlats, nlons))
ds = xr.Dataset(data_vars={'data': (('time', 'lat', 'lon'), data)},
coords={
'time': time,
'lat': lats,
'lon': lons
})
return ds
def test_climatology_invalid_freq():
with pytest.raises(ValueError):
climatology(dset_a, "hourly")
def test_climatology_encoded_time():
with pytest.raises(ValueError):
climatology(dset_encoded, "monthly")
@pytest.mark.parametrize("dataset", [dset_a, dset_b, dset_c["Tair"]])
@pytest.mark.parametrize("freq", ["day", "month", "year", "season"])
def test_climatology_setup(dataset, freq):
computed_dset = climatology(dataset, freq)
assert type(dataset) == type(computed_dset)
@pytest.mark.parametrize("dataset", [dset_a, dset_b, dset_c["Tair"]])
@pytest.mark.parametrize("freq", ["day", "month", "year", "season"])
def test_anomaly_setup(dataset, freq):
computed_dset = anomaly(dataset, freq)
assert type(dataset) == type(computed_dset)
ds1 = get_fake_dataset(start_month="2000-01", nmonths=12, nlats=1, nlons=1)
# Create another dataset for the year 2001.
ds2 = get_fake_dataset(start_month="2001-01", nmonths=12, nlats=1, nlons=1)
# Create a dataset that combines the two previous datasets, for two
# years of data.
ds3 = xr.concat([ds1, ds2], dim="time")
# Create a dataset with the wrong number of months.
partial_year_dataset = get_fake_dataset(start_month="2000-01",
nmonths=13,
nlats=1,
nlons=1)
# Create a dataset with a custom time coordinate.
custom_time_dataset = get_fake_dataset(start_month="2000-01",
nmonths=12,
nlats=1,
nlons=1)
custom_time_dataset = custom_time_dataset.rename({"time": "my_time"})
# Create a more complex dataset just to verify that get_fake_dataset()
# is generally working.
complex_dataset = get_fake_dataset(start_month="2001-01",
nmonths=12,
nlats=10,
nlons=10)
@pytest.mark.parametrize("dataset, season, expected", [(ds1, "JFM", 2.0),
(ds1, "JJA", 7.0)])
def test_month_to_season_returns_middle_month_value(dataset, season, expected):
season_ds = month_to_season(dataset, season)
np.testing.assert_equal(season_ds["my_var"].data, expected)
def test_month_to_season_bad_season_exception():
with pytest.raises(KeyError):
month_to_season(ds1, "TEST")
def test_month_to_season_partial_years_exception():
with pytest.raises(ValueError):
month_to_season(partial_year_dataset, "JFM")
@pytest.mark.parametrize("dataset, season, expected", [(ds1, "NDJ", 11.5)])
def test_month_to_season_final_season_returns_2month_average(
dataset, season, expected):
season_ds = month_to_season(dataset, season)
np.testing.assert_equal(season_ds["my_var"].data, expected)
@pytest.mark.parametrize(
"season",
[
"DJF",
"JFM",
"FMA",
"MAM",
"AMJ",
"MJJ",
"JJA",
"JAS",
"ASO",
"SON",
"OND",
"NDJ",
],
)
def test_month_to_season_returns_one_point_per_year(season):
nyears_of_data = ds3.sizes["time"] / 12
season_ds = month_to_season(ds3, season)
assert season_ds["my_var"].size == nyears_of_data
@pytest.mark.parametrize(
"dataset, time_coordinate, var_name, expected",
[
(custom_time_dataset, "my_time", "my_var", 2.0),
(dset_c.isel(x=110, y=200), None, "Tair", [-10.56, -8.129, -7.125]),
],
)
def test_month_to_season_custom_time_coordinate(dataset, time_coordinate,
var_name, expected):
season_ds = month_to_season(dataset, "JFM", time_coord_name=time_coordinate)
np.testing.assert_almost_equal(season_ds[var_name].data,
expected,
decimal=1)
# Test Datasets For calendar_average() and climatology_average()
minute = _get_dummy_data('2020-01-01', '2021-12-31 23:30:00', '30min', 1, 1)
hourly = _get_dummy_data('2020-01-01', '2021-12-31 23:00:00', 'H', 1, 1)
daily = _get_dummy_data('2020-01-01', '2021-12-31', 'D', 1, 1)
monthly = _get_dummy_data('2020-01-01', '2021-12-01', 'MS', 1, 1)
# Computational Tests for calendar_average()
hour_avg = np.arange(0.5, 35088.5, 2).reshape((365 + 366) * 24, 1, 1)
hour_avg_time = xr.cftime_range('2020-01-01 00:30:00',
'2021-12-31 23:30:00',
freq='H')
min_2_hour_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), hour_avg)},
coords={
'time': hour_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(minute, min_2_hour_avg)])
def test_30min_to_hourly_calendar_average(dset, expected):
result = calendar_average(dset, freq='hour')
xr.testing.assert_equal(result, expected)
day_avg = np.arange(11.5, 17555.5, 24).reshape(366 + 365, 1, 1)
day_avg_time = xr.cftime_range('2020-01-01 12:00:00',
'2021-12-31 12:00:00',
freq='D')
hour_2_day_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), day_avg)},
coords={
'time': day_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(hourly, hour_2_day_avg)])
def test_hourly_to_daily_calendar_average(dset, expected):
result = calendar_average(dset, freq='day')
xr.testing.assert_equal(result, expected)
month_avg = np.array([
15, 45, 75, 105.5, 136, 166.5, 197, 228, 258.5, 289, 319.5, 350, 381, 410.5,
440, 470.5, 501, 531.5, 562, 593, 623.5, 654, 684.5, 715
]).reshape(24, 1, 1)
month_avg_time = xr.cftime_range('2020-01-01', '2022-01-01', freq='MS')
month_avg_time = xr.DataArray(np.vstack((month_avg_time[:-1], month_avg_time[1:])).T,
dims=['time', 'nbd']) \
.mean(dim='nbd')
day_2_month_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), month_avg)},
coords={
'time': month_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(daily, day_2_month_avg)])
def test_daily_to_monthly_calendar_average(dset, expected):
result = calendar_average(dset, freq='month')
xr.testing.assert_equal(result, expected)
season_avg = np.array([29.5, 105.5, 197.5, 289, 379.5, 470.5, 562.5, 654,
715]).reshape(9, 1, 1)
season_avg_time = xr.cftime_range('2019-12-01', '2022-03-01', freq='QS-DEC')
season_avg_time = xr.DataArray(np.vstack((season_avg_time[:-1], season_avg_time[1:])).T,
dims=['time', 'nbd']) \
.mean(dim='nbd')
day_2_season_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), season_avg)},
coords={
'time': season_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
season_avg = np.array(
[0.483333333, 3, 6.010869565, 9, 11.96666667, 15, 18.01086957, 21,
23]).reshape(9, 1, 1)
month_2_season_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), season_avg)},
coords={
'time': season_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(daily, day_2_season_avg),
(monthly, month_2_season_avg)])
def test_daily_monthly_to_seasonal_calendar_average(dset, expected):
result = calendar_average(dset, freq='season')
xr.testing.assert_allclose(result, expected)
year_avg_time = [
cftime.datetime(2020, 7, 2),
cftime.datetime(2021, 7, 2, hour=12)
]
day_2_year_avg = [[[182.5]], [[548]]]
day_2_year_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), day_2_year_avg)},
coords={
'time': year_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
month_2_year_avg = [[[5.513661202]], [[17.5260274]]]
month_2_year_avg = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), month_2_year_avg)},
coords={
'time': year_avg_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(daily, day_2_year_avg),
(monthly, month_2_year_avg)])
def test_daily_monthly_to_yearly_calendar_average(dset, expected):
result = calendar_average(dset, freq='year')
xr.testing.assert_allclose(result, expected)
# Computational Tests for climatology_average()
hour_clim = np.concatenate([np.arange(8784.5, 11616.5, 2),
np.arange(2832.5, 2880.5, 2),
np.arange(11640.5, 26328.5, 2)])\
.reshape(8784, 1, 1)
hour_clim_time = xr.cftime_range('2020-01-01 00:30:00',
'2020-12-31 23:30:00',
freq='H')
min_2_hourly_clim = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), hour_clim)},
coords={
'time': hour_clim_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(minute, min_2_hourly_clim)])
def test_30min_to_hourly_climatology_average(dset, expected):
result = climatology_average(dset, freq='hour')
xr.testing.assert_allclose(result, expected)
day_clim = np.concatenate([np.arange(4403.5, 5819.5, 24),
[1427.5],
np.arange(5831.5, 13175.5, 24)]) \
.reshape(366, 1, 1)
day_clim_time = xr.cftime_range('2020-01-01 12:00:00',
'2020-12-31 12:00:00',
freq='24H')
hour_2_day_clim = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), day_clim)},
coords={
'time': day_clim_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(hourly, hour_2_day_clim)])
def test_hourly_to_daily_climatology_average(dset, expected):
result = climatology_average(dset, freq='day')
xr.testing.assert_equal(result, expected)
month_clim = np.array([
198, 224.5438596, 257.5, 288, 318.5, 349, 379.5, 410.5, 441, 471.5, 502,
532.5
]).reshape(12, 1, 1)
month_clim_time = xr.cftime_range('2020-01-01', '2021-01-01', freq='MS')
month_clim_time = xr.DataArray(np.vstack(
(month_clim_time[:-1], month_clim_time[1:])).T,
dims=['time', 'nbd']).mean(dim='nbd')
day_2_month_clim = xr.Dataset(
data_vars={'data': (('time', 'lat', 'lon'), month_clim)},
coords={
'time': month_clim_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(daily, day_2_month_clim)])
def test_daily_to_monthly_climatology_average(dset, expected):
result = climatology_average(dset, freq='month')
xr.testing.assert_allclose(result, expected)
season_clim = np.array([320.9392265, 380, 288, 471.5]).reshape(4, 1, 1)
season_clim_time = ['DJF', 'JJA', 'MAM', 'SON']
day_2_season_clim = xr.Dataset(
data_vars={'data': (('season', 'lat', 'lon'), season_clim)},
coords={
'season': season_clim_time,
'lat': [-90.0],
'lon': [-180.0]
})
season_clim = np.array([10.04972376, 12.01086957, 9, 15]).reshape(4, 1, 1)
month_2_season_clim = xr.Dataset(
data_vars={'data': (('season', 'lat', 'lon'), season_clim)},
coords={
'season': season_clim_time,
'lat': [-90.0],
'lon': [-180.0]
})
@pytest.mark.parametrize('dset, expected', [(daily, day_2_season_clim),
(monthly, month_2_season_clim)])
def test_daily_monthly_to_seasonal_climatology_average(dset, expected):
result = climatology_average(dset, freq='season')
xr.testing.assert_allclose(result, expected)
# Argument Tests for climatology_average() and calendar_average()
@pytest.mark.parametrize('freq', ['TEST', None])
def test_invalid_freq_climatology_average(freq):
with pytest.raises(KeyError):
climatology_average(monthly, freq=freq)
@pytest.mark.parametrize('freq', ['TEST', None])
def test_invalid_freq_calendar_average(freq):
with pytest.raises(KeyError):
calendar_average(monthly, freq=freq)
time_dim = 'my_time'
custom_time = daily.rename({'time': time_dim})
custom_time_expected = day_2_month_clim.rename({'time': time_dim})
@pytest.mark.parametrize('dset, expected, time_dim',
[(custom_time, custom_time_expected, time_dim)])
def test_custom_time_coord_climatology_average(dset, expected, time_dim):
result = climatology_average(dset, freq='month', time_dim=time_dim)
xr.testing.assert_allclose(result, expected)
custom_time_expected = day_2_month_avg.rename({'time': time_dim})
@pytest.mark.parametrize('dset, expected, time_dim',
[(custom_time, custom_time_expected, time_dim)])
def test_custom_time_coord_calendar_average(dset, expected, time_dim):
result = calendar_average(dset, freq='month', time_dim=time_dim)
xr.testing.assert_allclose(result, expected)
array = daily['data']
array_expected = day_2_month_clim['data']
@pytest.mark.parametrize('da, expected', [(array, array_expected)])
def test_xr_DataArray_support_climatology_average(da, expected):
result = climatology_average(da, freq='month')
xr.testing.assert_allclose(result, expected)
array_expected = day_2_month_avg['data']
@pytest.mark.parametrize('da, expected', [(array, array_expected)])
def test_xr_DataArray_support_calendar_average(da, expected):
result = calendar_average(da, freq='month')
xr.testing.assert_equal(result, expected)
dset_encoded = xr.tutorial.open_dataset("air_temperature", decode_cf=False)
def test_non_datetime_like_objects_climatology_average():
with pytest.raises(ValueError):
climatology_average(dset_encoded, 'month')
def test_non_datetime_like_objects_calendar_average():
with pytest.raises(ValueError):
calendar_average(dset_encoded, 'month')
time = pd.to_datetime(['2020-01-01', '2020-01-02', '2020-01-04'])
non_uniform = xr.Dataset(data_vars={'data': (('time'), np.arange(3))},
coords={'time': time})
def test_non_uniformly_spaced_data_climatology_average():
with pytest.raises(ValueError):
climatology_average(non_uniform, freq='day')
def test_non_uniformly_spaced_data_calendar_average():
with pytest.raises(ValueError):
calendar_average(non_uniform, freq='day')
julian_daily = _get_dummy_data('2020-01-01',
'2021-12-31',
'D',
1,
1,
calendar='julian')
noleap_daily = _get_dummy_data('2020-01-01',
'2021-12-31',
'D',
1,
1,
calendar='noleap')
all_leap_daily = _get_dummy_data('2020-01-01',
'2021-12-31',
'D',
1,
1,
calendar='all_leap')
day_360_daily = _get_dummy_data('2020-01-01',
'2021-12-30',
'D',
1,
1,
calendar='360_day')
# Daily -> Monthly Climatologies for Julian Calendar
julian_month_clim = np.array([198, 224.54385965, 257.5, 288, 318.5, 349,
379.5, 410.5, 441, 471.5, 502, 532.5])\
.reshape(12, 1, 1)
julian_month_clim_time = xr.cftime_range('2020-01-01',
'2021-01-01',
freq='MS',
calendar='julian')
julian_month_clim_time = xr.DataArray( | np.vstack((julian_month_clim_time[:-1], julian_month_clim_time[1:])) | numpy.vstack |
#K-means Clustering
#%reset -f
#Importing Libraries
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.cluster import DBSCAN
from pyclustertend import hopkins
from sklearn.cluster import MeanShift, estimate_bandwidth
from sklearn.metrics import silhouette_score
#Importing Dataset
df = pd.read_csv('appdata10.csv')
df_user = pd.DataFrame(np.arange(0,len(df)), columns=['user'])
df = pd.concat([df_user, df], axis=1)
df.info()
df.head()
df.tail()
df.columns.values
#Converting columns to Datatime
df['Timestamp'] = pd.to_datetime(df['Timestamp'])
time_new = df['Timestamp'].iloc[0]
df['Hour'] = df['Timestamp'].apply(lambda time_new: time_new.hour)
df['Month'] = df['Timestamp'].apply(lambda time_new: time_new.month)
df['Day'] = df['Timestamp'].apply(lambda time_new: time_new.dayofweek)
df["hour"] = df.hour.str.slice(1, 3).astype(int)
#Data analysis
statistical = df.describe()
#Verifying null values
sns.heatmap(df.isnull(), yticklabels=False, cbar=False, cmap='viridis')
df.isna().any()
df.isna().sum()
#Define X
features = ['tipo_de_negociacao','percentual_venda', 'quantas_correcoes',
'quantos_pontos_avancou', 'quantos_pontos_retornados', 'amplitude']
X = df[features]
#Taking care of missing data
'''
from sklearn.preprocessing import Imputer
imputer = Imputer(missing_values='NaN', strategy='mean', axis=0)
imputer = imputer.fit(X[:, 1:3])
X[:, 1:3] = imputer.transform(X[:, 1:3] )
'''
#Encoding categorical data
'''
from sklearn.preprocessing import LabelEncoder, OneHotEncoder
labelenconder_x = LabelEncoder()
X.iloc[:, 1] = labelenconder_x.fit_transform(X.iloc[:, 1])
onehotencoder_x = OneHotEncoder(categorical_features=[1])
X2 = pd.DataFrame(onehotencoder_x.fit_transform(X).toarray())
y = pd.DataFrame(labelenconder_x.fit_transform(y))
#Dummies Trap
X2 = X2.iloc[:, 1:]
X2 = X2.iloc[:,[0,1,2]]
X2 = X2.rename(columns={1:'pay_schedule_1', 2:'pay_schedule_2', 3:'pay_schedule_3'})
X = pd.concat([X,X2], axis=1)
X = X.drop(['pay_schedule'], axis=1)
'''
#Visualizing data
sns.pairplot(data=df, hue='target_names', vars= ['mean radius', 'mean texture', 'mean area', 'mean perimeter', 'mean smoothness'])
sns.countplot(x='target_names', data=df, label='Count')
sns.scatterplot(x='mean area', y='mean smoothness',hue='target_names', data=df)
plt.figure(figsize=(20,10))
sns.heatmap(data=df.corr(), annot=True, cmap='viridis')
# Hopkins Test
'''
the null hypothesis (no meaningfull cluster) happens when the hopkins test is
around 0.5 and the hopkins test tends to 0 when meaningful cluster exists in
the space. Usually, we can believe in the existence of clusters when the
hopkins score is bellow 0.25.
Here the value of the hopkins test is quite high but one could think there is
cluster in our subspace. BUT the hopkins test is highly influenced by outliers,
let's try once again with normalised data.
'''
hopkins(X, X.shape[0])
# Construção do modelo DBSCAN
dbscan = DBSCAN(eps = 0.2, min_samples = 5, metric = 'euclidean')
y_pred = dbscan.fit_predict(X)
# Construção do modelo mean shift
# bandwidth = Comprimento da Interação entre os exemplos, também conhecido como a largura de banda do algoritmo.
bandwidth = estimate_bandwidth(X, quantile = .1, n_samples = 500)
mean_shift = MeanShift(bandwidth = bandwidth, bin_seeding = True)
mean_shift.fit(X)
#Using the Elbow Method to find the optimal number of clusters
from sklearn.cluster import KMeans
wcss = []
for i in range(1,11):
kmeans = KMeans(n_clusters=i, init='k-means++', n_init=10, max_iter=300, random_state=0) #n_init e max_iter são padrões.
kmeans.fit(X)
wcss.append(kmeans.inertia_)
plt.plot(range(1,11), wcss)
plt.title('The Elbow Method')
plt.xlabel('Number of Clusters')
plt.ylabel('WCSS')
plt.show() #Quando parar de cair exageradamente no gráfico, este será o número de cluster. Neste caso serão 5 cluesters
#Applying the K-means to Dataset
kmeans = KMeans(n_clusters=5, init='k-means++', n_init=10, max_iter=300, random_state=0)
kmeans.fit(X)
print(90*'_')
print("\nCount of features in each cluster")
print(90*'_')
pd.value_counts(kmeans.labels_, sort=False)
# Silhouette Score
labels = modelo_v1.labels_
silhouette_score(pca, labels, metric = 'euclidean')
# Function that creates a DataFrame with a column for Cluster Number
def pd_centers(featuresUsed, centers):
colNames = list(featuresUsed)
colNames.append('prediction')
# Zip with a column called 'prediction' (index)
Z = [np.append(A, index) for index, A in enumerate(centers)]
# Convert to pandas data frame for plotting
P = pd.DataFrame(Z, columns=colNames)
P['prediction'] = P['prediction'].astype(int)
return P
# Function that creates Parallel Plots
from itertools import cycle, islice
from pandas.plotting import parallel_coordinates
def parallel_plot(data):
my_colors = list(islice(cycle(['b', 'r', 'g', 'y', 'k']), None, len(data)))
plt.figure(figsize=(15,8)).gca().axes.set_ylim([-3,+3])
parallel_coordinates(data, 'prediction', color = my_colors, marker='o')
P = pd_centers(featuresUsed=features, centers=kmeans.cluster_centers_)
P
parallel_plot(P)
y_kmeans = kmeans.fit_predict(X)
#Visualising the clusters
plt.scatter(np.array(X)[y_kmeans == 0, 0], np.array(X)[y_kmeans == 0, 1], s = 100, c = 'red', label = 'Careful')
plt.scatter(np.array(X)[y_kmeans == 1, 0], np.array(X)[y_kmeans == 1, 1], s = 100, c = 'blue', label = 'Standard')
plt.scatter( | np.array(X) | numpy.array |
# -*- coding: utf-8 -*-
# Visualizzazione dell'andamento della funzione di errore quadratico nella regressione
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import seaborn as sns
# +
plt.style.use('fivethirtyeight')
plt.rcParams['font.family'] = 'sans-serif'
plt.rcParams['font.serif'] = 'Ubuntu'
plt.rcParams['font.monospace'] = 'Ubuntu Mono'
plt.rcParams['font.size'] = 10
plt.rcParams['axes.labelsize'] = 10
plt.rcParams['axes.labelweight'] = 'bold'
plt.rcParams['axes.titlesize'] = 10
plt.rcParams['xtick.labelsize'] = 8
plt.rcParams['ytick.labelsize'] = 8
plt.rcParams['legend.fontsize'] = 10
plt.rcParams['figure.titlesize'] = 12
plt.rcParams['image.cmap'] = 'jet'
plt.rcParams['image.interpolation'] = 'none'
plt.rcParams['figure.figsize'] = (16, 8)
plt.rcParams['lines.linewidth'] = 2
plt.rcParams['lines.markersize'] = 8
colors = ['xkcd:pale orange', 'xkcd:sea blue', 'xkcd:pale red', 'xkcd:sage green', 'xkcd:terra cotta', 'xkcd:dull purple', 'xkcd:teal', 'xkcd:goldenrod', 'xkcd:cadet blue',
'xkcd:scarlet']
# -
# definisce un vettore di colori
colors = sns.color_palette("husl", 4)
# dichiara alcune proprietà grafiche della figura
sns.set(style="darkgrid", context='paper', palette=colors, rc={"figure.figsize": (16, 8),'image.cmap': 'jet', 'lines.linewidth':.7})
# legge i dati in dataframe pandas
data = pd.read_csv("../dataset/cars.csv", delimiter=',', header=0, names=['X','y'])
# calcola dimensione dei dati
n = len(data)
# visualizza dati mediante scatter
fig = plt.figure()
fig.patch.set_facecolor('white')
ax = fig.gca()
ax.scatter(data['X'], data['y'], s=40,c='r', marker='o', alpha=.5)
plt.xlabel(u'Velocità in mph', fontsize=14)
plt.ylabel('Distanza di arresto in ft', fontsize=14)
plt.show()
# Estrae dal dataframe l'array X delle features e aggiunge ad esso una colonna di 1
X=np.array(data['X']).reshape(-1,1)
X = np.column_stack((np.ones(n), X))
# Estrae dal dataframe l'array t dei valori target
t=np.array(data['y']).reshape(-1,1)
# mostra distribuzione dell'errore quadratico medio al variare dei coefficienti
# insieme dei valori considerati per i coefficienti
w0_list = np.linspace(-100, 100, 100)
w1_list = np.linspace(-100, 100, 100)
# crea una griglia di coppie di valori
w0, w1 = np.meshgrid(w0_list, w1_list)
# definisce la funzione da calcolare in ogni punto della griglia
def error(v1, v2):
theta = | np.array((v1, v2)) | numpy.array |
# This code is part of Qiskit.
#
# (C) Copyright IBM 2017, 2020.
#
# This code is licensed under the Apache License, Version 2.0. You may
# obtain a copy of this license in the LICENSE.txt file in the root directory
# of this source tree or at http://www.apache.org/licenses/LICENSE-2.0.
#
# Any modifications or derivative works of this code must retain this
# copyright notice, and modified files need to carry a notice indicating
# that they have been altered from the originals.
"""Tests for PauliList class."""
import unittest
from test import combine
import itertools
import numpy as np
from ddt import ddt
from scipy.sparse import csr_matrix
from qiskit import QiskitError
from qiskit.circuit.library import (
CXGate,
CYGate,
CZGate,
HGate,
IGate,
SdgGate,
SGate,
SwapGate,
XGate,
YGate,
ZGate,
)
from qiskit.quantum_info.operators import (
Clifford,
Operator,
Pauli,
PauliList,
PauliTable,
StabilizerTable,
)
from qiskit.quantum_info.random import random_clifford, random_pauli_list
from qiskit.test import QiskitTestCase
from .test_pauli import pauli_group_labels
def pauli_mat(label):
"""Return Pauli matrix from a Pauli label"""
mat = np.eye(1, dtype=complex)
if label[0:2] == "-i":
mat *= -1j
label = label[2:]
elif label[0] == "-":
mat *= -1
label = label[1:]
elif label[0] == "i":
mat *= 1j
label = label[1:]
for i in label:
if i == "I":
mat = np.kron(mat, np.eye(2, dtype=complex))
elif i == "X":
mat = np.kron(mat, np.array([[0, 1], [1, 0]], dtype=complex))
elif i == "Y":
mat = np.kron(mat, np.array([[0, -1j], [1j, 0]], dtype=complex))
elif i == "Z":
mat = np.kron(mat, np.array([[1, 0], [0, -1]], dtype=complex))
else:
raise QiskitError(f"Invalid Pauli string {i}")
return mat
class TestPauliListInit(QiskitTestCase):
"""Tests for PauliList initialization."""
def test_array_init(self):
"""Test array initialization."""
# Matrix array initialization
with self.subTest(msg="bool array"):
z = np.array([[False], [True]])
x = np.array([[False], [True]])
pauli_list = PauliList.from_symplectic(z, x)
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg="bool array no copy"):
z = np.array([[False], [True]])
x = np.array([[True], [True]])
pauli_list = PauliList.from_symplectic(z, x)
z[0, 0] = not z[0, 0]
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
def test_string_init(self):
"""Test string initialization."""
# String initialization
with self.subTest(msg='str init "I"'):
pauli_list = PauliList("I")
z = np.array([[False]])
x = np.array([[False]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "X"'):
pauli_list = PauliList("X")
z = np.array([[False]])
x = np.array([[True]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "Y"'):
pauli_list = PauliList("Y")
z = np.array([[True]])
x = np.array([[True]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "Z"'):
pauli_list = PauliList("Z")
z = np.array([[True]])
x = np.array([[False]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "iZ"'):
pauli_list = PauliList("iZ")
z = np.array([[True]])
x = np.array([[False]])
phase = np.array([3])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
np.testing.assert_equal(pauli_list.phase, phase)
with self.subTest(msg='str init "-Z"'):
pauli_list = PauliList("-Z")
z = np.array([[True]])
x = np.array([[False]])
phase = np.array([2])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
np.testing.assert_equal(pauli_list.phase, phase)
with self.subTest(msg='str init "-iZ"'):
pauli_list = PauliList("-iZ")
z = np.array([[True]])
x = np.array([[False]])
phase = np.array([1])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
np.testing.assert_equal(pauli_list.phase, phase)
with self.subTest(msg='str init "IX"'):
pauli_list = PauliList("IX")
z = np.array([[False, False]])
x = np.array([[True, False]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "XI"'):
pauli_list = PauliList("XI")
z = np.array([[False, False]])
x = np.array([[False, True]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "YZ"'):
pauli_list = PauliList("YZ")
z = np.array([[True, True]])
x = np.array([[False, True]])
np.testing.assert_equal(pauli_list.z, z)
np.testing.assert_equal(pauli_list.x, x)
with self.subTest(msg='str init "iZY"'):
pauli_list = PauliList("iZY")
z = np.array([[True, True]])
x = np.array([[True, False]])
phase = np.array([3])
| np.testing.assert_equal(pauli_list.z, z) | numpy.testing.assert_equal |
'''
episodestats.py
implements statistic that are used in producing employment statistics for the
lifecycle model
'''
import h5py
import numpy as np
import numpy_financial as npf
import matplotlib.pyplot as plt
import matplotlib as mpl
import seaborn as sns
from scipy.stats import norm
#import locale
from tabulate import tabulate
import pandas as pd
import scipy.optimize
from tqdm import tqdm_notebook as tqdm
from . empstats import Empstats
from scipy.stats import gaussian_kde
#locale.setlocale(locale.LC_ALL, 'fi_FI')
def modify_offsettext(ax,text):
'''
For y axis
'''
x_pos = 0.0
y_pos = 1.0
horizontalalignment='left'
verticalalignment='bottom'
offset = ax.yaxis.get_offset_text()
#value=offset.get_text()
# value=float(value)
# if value>=1e12:
# text='biljoonaa'
# elif value>1e9:
# text=str(value/1e9)+' miljardia'
# elif value==1e9:
# text=' miljardia'
# elif value>1e6:
# text=str(value/1e6)+' miljoonaa'
# elif value==1e6:
# text='miljoonaa'
# elif value>1e3:
# text=str(value/1e3)+' tuhatta'
# elif value==1e3:
# text='tuhatta'
offset.set_visible(False)
ax.text(x_pos, y_pos, text, transform=ax.transAxes,
horizontalalignment=horizontalalignment,
verticalalignment=verticalalignment)
class Labels():
def get_labels(self,language='English'):
labels={}
if language=='English':
labels['osuus tilassa x']='Proportion in state {} [%]'
labels['age']='Age [y]'
labels['ratio']='Proportion [%]'
labels['unemp duration']='Length of unemployment [y]'
labels['scaled freq']='Scaled frequency'
labels['probability']='probability'
labels['telp']='Employee pension premium'
labels['sairausvakuutus']='Health insurance'
labels['työttömyysvakuutusmaksu']='Unemployment insurance'
labels['puolison verot']='Partners taxes'
labels['taxes']='Taxes'
labels['asumistuki']='Housing benefit'
labels['toimeentulotuki']='Supplementary benefit'
labels['tyottomyysturva']='Unemployment benefit'
labels['paivahoito']='Daycare'
labels['elake']='Pension'
labels['tyollisyysaste']='Employment rate'
labels['tyottomien osuus']='Proportion of unemployed'
labels['havainto']='Observation'
labels['tyottomyysaste']='Unemployment rate [%]'
labels['tyottomien osuus']='Proportion of unemployed [%]'
labels['tyollisyysaste %']='Employment rate [%]'
labels['ero osuuksissa']='Difference in proportions [%]'
labels['osuus']='proportion'
labels['havainto, naiset']='data, women'
labels['havainto, miehet']='data, men'
labels['palkkasumma']='Palkkasumma [euroa]'
labels['Verokiila %']='Verokiila [%]'
labels['Työnteko [hlö/htv]']='Työnteko [hlö/htv]'
labels['Työnteko [htv]']='Työnteko [htv]'
labels['Työnteko [hlö]']='Työnteko [hlö]'
labels['Työnteko [miljoonaa hlö/htv]']='Työnteko [miljoonaa hlö/htv]'
labels['Työnteko [miljoonaa htv]']='Työnteko [miljoonaa htv]'
labels['Työnteko [miljoonaa hlö]']='Työnteko [miljoonaa hlö]'
labels['Osatyönteko [%-yks]']='Osa-aikatyössä [%-yks]'
labels['Muut tulot [euroa]']='Muut tulot [euroa]'
labels['Henkilöitä']='Henkilöitä'
labels['Verot [euroa]']='Verot [euroa]'
labels['Verot [[miljardia euroa]']='Verot [[miljardia euroa]'
labels['Verokertymä [euroa]']='Verokertymä [euroa]'
labels['Verokertymä [miljardia euroa]']='Verokertymä [miljardia euroa]'
labels['Muut tarvittavat tulot [euroa]']='Muut tarvittavat tulot [euroa]'
labels['Muut tarvittavat tulot [miljardia euroa]']='Muut tarvittavat tulot [miljardia euroa]'
labels['malli']='Life cycle model'
else:
labels['osuus tilassa x']='Osuus tilassa {} [%]'
labels['age']='Ikä [v]'
labels['ratio']='Osuus tilassa [%]'
labels['unemp duration']='työttömyysjakson pituus [v]'
labels['scaled freq']='skaalattu taajuus'
labels['probability']='todennäköisyys'
labels['telp']='TEL-P'
labels['sairausvakuutus']='Sairausvakuutus'
labels['työttömyysvakuutusmaksu']='Työttömyysvakuutusmaksu'
labels['puolison verot']='puolison verot'
labels['taxes']='Verot'
labels['asumistuki']='Asumistuki'
labels['toimeentulotuki']='Toimeentulotuki'
labels['tyottomyysturva']='Työttömyysturva'
labels['paivahoito']='Päivähoito'
labels['elake']='Eläke'
labels['tyollisyysaste']='työllisyysaste'
labels['tyottomien osuus']='työttömien osuus'
labels['havainto']='havainto'
labels['tyottomyysaste']='Työttömyysaste [%]'
labels['tyottomien osuus']='Työttömien osuus väestöstö [%]'
labels['tyollisyysaste %']='Työllisyysaste [%]'
labels['ero osuuksissa']='Ero osuuksissa [%]'
labels['osuus']='Osuus'
labels['havainto, naiset']='havainto, naiset'
labels['havainto, miehet']='havainto, miehet'
labels['palkkasumma']='Palkkasumma [euroa]'
labels['Verokiila %']='Verokiila [%]'
labels['Työnteko [hlö/htv]']='Työnteko [hlö/htv]'
labels['Työnteko [htv]']='Työnteko [htv]'
labels['Työnteko [hlö]']='Työnteko [hlö]'
labels['Työnteko [miljoonaa hlö/htv]']='Työnteko [miljoonaa hlö/htv]'
labels['Työnteko [miljoonaa htv]']='Työnteko [miljoonaa htv]'
labels['Työnteko [miljoonaa hlö]']='Työnteko [miljoonaa hlö]'
labels['Osatyönteko [%-yks]']='Osa-aikatyössä [%-yks]'
labels['Muut tulot [euroa]']='Muut tulot [euroa]'
labels['Henkilöitä']='Henkilöitä'
labels['Verot [euroa]']='Verot [euroa]'
labels['Verot [[miljardia euroa]']='Verot [[miljardia euroa]'
labels['Verokertymä [euroa]']='Verokertymä [euroa]'
labels['Verokertymä [miljardia euroa]']='Verokertymä [miljardia euroa]'
labels['Muut tarvittavat tulot [euroa]']='Muut tarvittavat tulot [euroa]'
labels['Muut tarvittavat tulot [miljardia euroa]']='Muut tarvittavat tulot [miljardia euroa]'
labels['malli']='elinkaarimalli'
return labels
class EpisodeStats():
def __init__(self,timestep,n_time,n_emps,n_pop,env,minimal,min_age,max_age,min_retirementage,year=2018,version=3,params=None,gamma=0.92,lang='English'):
self.version=version
self.gamma=gamma
self.params=params
self.lab=Labels()
self.reset(timestep,n_time,n_emps,n_pop,env,minimal,min_age,max_age,min_retirementage,year,params=params,lang=lang)
print('version',version)
def reset(self,timestep,n_time,n_emps,n_pop,env,minimal,min_age,max_age,min_retirementage,year,version=None,params=None,lang=None,dynprog=False):
self.min_age=min_age
self.max_age=max_age
self.min_retirementage=min_retirementage
self.minimal=minimal
if params is not None:
self.params=params
if lang is None:
self.language='English'
else:
self.language=lang
if version is not None:
self.version=version
self.setup_labels()
self.n_employment=n_emps
self.n_time=n_time
self.timestep=timestep # 0.25 = 3kk askel
self.inv_timestep=int(np.round(1/self.timestep)) # pitää olla kokonaisluku
self.n_pop=n_pop
self.year=year
self.env=env
self.reaalinen_palkkojenkasvu=0.016
self.palkkakerroin=(0.8*1+0.2*1.0/(1+self.reaalinen_palkkojenkasvu))**self.timestep
self.elakeindeksi=(0.2*1+0.8*1.0/(1+self.reaalinen_palkkojenkasvu))**self.timestep
self.dynprog=dynprog
if self.minimal:
self.version=0
if self.version in set([0,101]):
self.n_groups=1
else:
self.n_groups=6
self.empstats=Empstats(year=self.year,max_age=self.max_age,n_groups=self.n_groups,timestep=self.timestep,n_time=self.n_time,
min_age=self.min_age)
self.init_variables()
def init_variables(self):
n_emps=self.n_employment
self.empstate=np.zeros((self.n_time,n_emps))
self.gempstate=np.zeros((self.n_time,n_emps,self.n_groups))
self.deceiced=np.zeros((self.n_time,1))
self.alive=np.zeros((self.n_time,1))
self.galive=np.zeros((self.n_time,self.n_groups))
self.rewstate=np.zeros((self.n_time,n_emps))
self.poprewstate=np.zeros((self.n_time,self.n_pop))
self.salaries_emp=np.zeros((self.n_time,n_emps))
#self.salaries=np.zeros((self.n_time,self.n_pop))
self.actions=np.zeros((self.n_time,self.n_pop))
self.popempstate=np.zeros((self.n_time,self.n_pop))
self.popunemprightleft=np.zeros((self.n_time,self.n_pop))
self.popunemprightused=np.zeros((self.n_time,self.n_pop))
self.tyoll_distrib_bu=np.zeros((self.n_time,self.n_pop))
self.unemp_distrib_bu=np.zeros((self.n_time,self.n_pop))
self.siirtyneet=np.zeros((self.n_time,n_emps))
self.siirtyneet_det=np.zeros((self.n_time,n_emps,n_emps))
self.pysyneet=np.zeros((self.n_time,n_emps))
self.aveV=np.zeros((self.n_time,self.n_pop))
self.time_in_state=np.zeros((self.n_time,n_emps))
self.stat_tyoura=np.zeros((self.n_time,n_emps))
self.stat_toe=np.zeros((self.n_time,n_emps))
self.stat_pension=np.zeros((self.n_time,n_emps))
self.stat_paidpension=np.zeros((self.n_time,n_emps))
self.out_of_work=np.zeros((self.n_time,n_emps))
self.stat_unemp_len=np.zeros((self.n_time,self.n_pop))
self.stat_wage_reduction=np.zeros((self.n_time,n_emps))
self.stat_wage_reduction_g=np.zeros((self.n_time,n_emps,self.n_groups))
self.infostats_group=np.zeros((self.n_pop,1))
self.infostats_taxes=np.zeros((self.n_time,1))
self.infostats_wagetaxes=np.zeros((self.n_time,1))
self.infostats_taxes_distrib=np.zeros((self.n_time,n_emps))
self.infostats_etuustulo=np.zeros((self.n_time,1))
self.infostats_etuustulo_group=np.zeros((self.n_time,self.n_groups))
self.infostats_perustulo=np.zeros((self.n_time,1))
self.infostats_palkkatulo=np.zeros((self.n_time,1))
self.infostats_palkkatulo_eielakkeella=np.zeros((self.n_time,1))
self.infostats_palkkatulo_group=np.zeros((self.n_time,self.n_groups))
self.infostats_palkkatulo_eielakkeella_group=np.zeros((self.n_time,1))
self.infostats_ansiopvraha=np.zeros((self.n_time,1))
self.infostats_ansiopvraha_group=np.zeros((self.n_time,self.n_groups))
self.infostats_asumistuki=np.zeros((self.n_time,1))
self.infostats_asumistuki_group=np.zeros((self.n_time,self.n_groups))
self.infostats_valtionvero=np.zeros((self.n_time,1))
self.infostats_valtionvero_group=np.zeros((self.n_time,self.n_groups))
self.infostats_kunnallisvero=np.zeros((self.n_time,1))
self.infostats_kunnallisvero_group=np.zeros((self.n_time,self.n_groups))
self.infostats_valtionvero_distrib=np.zeros((self.n_time,n_emps))
self.infostats_kunnallisvero_distrib=np.zeros((self.n_time,n_emps))
self.infostats_ptel=np.zeros((self.n_time,1))
self.infostats_tyotvakmaksu=np.zeros((self.n_time,1))
self.infostats_tyoelake=np.zeros((self.n_time,1))
self.infostats_kokoelake=np.zeros((self.n_time,1))
self.infostats_opintotuki=np.zeros((self.n_time,1))
self.infostats_isyyspaivaraha=np.zeros((self.n_time,1))
self.infostats_aitiyspaivaraha=np.zeros((self.n_time,1))
self.infostats_kotihoidontuki=np.zeros((self.n_time,1))
self.infostats_sairauspaivaraha=np.zeros((self.n_time,1))
self.infostats_toimeentulotuki=np.zeros((self.n_time,1))
self.infostats_tulot_netto=np.zeros((self.n_time,1))
self.infostats_pinkslip=np.zeros((self.n_time,n_emps))
self.infostats_pop_pinkslip=np.zeros((self.n_time,self.n_pop))
self.infostats_chilren18_emp=np.zeros((self.n_time,n_emps))
self.infostats_chilren7_emp=np.zeros((self.n_time,n_emps))
self.infostats_chilren18= | np.zeros((self.n_time,1)) | numpy.zeros |
"""
Example distributions.
"""
from __future__ import absolute_import, division, print_function
import collections
from typing import Callable, Optional
from scipy.stats import multivariate_normal, ortho_group
import tensorflow_probability as tfp
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
from config import TF_FLOATS
tfd = tfp.distributions
TF_FLOAT = TF_FLOATS[tf.keras.backend.floatx()]
# NP_FLOAT = [tf.keras.backend.floatx()]
# pylint:disable=invalid-name
# pylint:disable=unused-argument
def w1(z):
"""Transformation."""
return tf.math.sin(2. * np.pi * z[0] / 4.)
def w2(z):
"""Transformation."""
return 3. * tf.exp(-0.5 * (((z[0] - 1.) / 0.6)) ** 2)
def w3(z):
"""Transformation."""
return 3. * (1 + tf.exp(-(z[0] - 1.) / 0.3)) ** (-1)
def plot_samples2D(
samples: np.ndarray,
title: str = None,
fig: Optional[plt.Figure] = None,
ax: Optional[plt.Axes] = None,
**kwargs,
):
"""Plot collection of 2D samples.
NOTE: **kwargs are passed to `ax.plot(...)`.
"""
if fig is None and ax is None:
fig, ax = plt.subplots()
_ = ax.plot(samples[:, 0], samples[:, 1], **kwargs)
if title is not None:
_ = ax.set_title(title, fontsize='x-large')
return fig, ax
def meshgrid(x, y=None):
"""Create a mesgrid of dtype 'float32'."""
if y is None:
y = x
[gx, gy] = np.meshgrid(x, y, indexing='ij')
gx, gy = np.float32(gx), np.float32(gy)
grid = np.concatenate([gx.ravel()[None, :], gy.ravel()[None, :]], axis=0)
return grid.T.reshape(x.size, y.size, 2)
# pylint:disable=too-many-arguments
def contour_potential(
potential_fn: Callable,
ax: Optional[plt.Axes] = None,
title: Optional[str] = None,
xlim: Optional[float] = 5.,
ylim: Optional[float] = 5.,
cmap: Optional[str] = 'inferno',
dtype: Optional[str] = 'float32'
):
"""Plot contours of `potential_fn`."""
if isinstance(xlim, (tuple, list)):
x0, x1 = xlim
else:
x0 = -xlim
x1 = xlim
if isinstance(ylim, (tuple, list)):
y0, y1 = ylim
else:
y0 = -ylim
y1 = ylim
grid = np.mgrid[x0:x1:100j, y0:y1:100j]
# grid_2d = meshgrid(np.arange(x0, x1, 0.05), np.arange(y0, y1, 0.05))
grid_2d = grid.reshape(2, -1).T
cmap = plt.get_cmap(cmap)
if ax is None:
_, ax = plt.subplots()
try:
pdf1e = np.exp(-potential_fn(grid_2d))
except Exception as e:
pdf1e = np.exp(-potential_fn(tf.cast(grid_2d, dtype)))
z = pdf1e.reshape(100, 100)
_ = ax.contourf(grid[0], grid[1], z, cmap=cmap, levels=8)
if title is not None:
ax.set_title(title, fontsize='x-large')
plt.tight_layout()
return ax
def two_moons_potential(z):
"""two-moons like potential."""
z = tf.transpose(z)
term1 = 0.5 * ((tf.linalg.norm(z, axis=0) - 2.) / 0.4) ** 2
logterm1 = tf.exp(-0.5 * ((z[0] - 2.) / 0.6) ** 2)
logterm2 = tf.exp(-0.5 * ((z[0] + 2.) / 0.6) ** 2)
output = term1 - tf.math.log(logterm1 + logterm2)
return output
def sin_potential(z):
"""Sin-like potential."""
z = tf.transpose(z)
x = z[0]
y = z[1]
# x, y = z
return 0.5 * ((y - w1(z)) / 0.4) ** 2 + 0.1 * tf.math.abs(x)
def sin_potential1(z):
"""Modified sin potential."""
z = tf.transpose(z)
logterm1 = tf.math.exp(-0.5 * ((z[1] - w1(z)) / 0.35) ** 2)
logterm2 = tf.math.exp(-0.5 * ((z[1] - w1(z) + w2(z)) / 0.35) ** 2)
term3 = 0.1 * tf.math.abs(z[0])
output = -1. * tf.math.log(logterm1 + logterm2) + term3
return output
def sin_potential2(z):
"""Modified sin potential."""
z = tf.transpose(z)
logterm1 = tf.math.exp(-0.5 * ((z[1] - w1(z)) / 0.4) ** 2)
logterm2 = tf.math.exp(-0.5 * ((z[1] - w1(z) + w3(z)) / 0.35) ** 2)
term3 = 0.1 * tf.math.abs(z[0])
output = -1. * tf.math.log(logterm1 + logterm2) + term3
return output
def quadratic_gaussian(x, mu, S):
"""Simple quadratic Gaussian (normal) distribution."""
x = tf.cast(x, dtype=TF_FLOAT)
return tf.linalg.diag_part(0.5 * ((x - mu) @ S) @ tf.transpose((x - mu)))
def random_tilted_gaussian(dim, log_min=-2., log_max=2.):
"""Implements a randomly tilted Gaussian (Normal) distribution."""
mu = np.zeros((dim,))
R = ortho_group.rvs(dim)
sigma = np.diag(
np.exp(np.log(10.) * np.random.uniform(log_min, log_max, size=(dim,)))
)
S = R.T.dot(sigma).dot(R)
return Gaussian(mu, S)
def gen_ring(r=1., var=1., nb_mixtures=2):
"""Generate a ring of Gaussian distributions."""
base_points = []
for t in range(nb_mixtures):
c = np.cos(2 * np.pi * t / nb_mixtures)
s = np.sin(2 * np.pi * t / nb_mixtures)
base_points.append(np.array([r * c, r * s]))
# v = np.array(base_points)
sigmas = [var * np.eye(2) for t in range(nb_mixtures)]
pis = [1. / nb_mixtures] * nb_mixtures
pis[0] += 1. - sum(pis)
return GMM(base_points, sigmas, pis)
def distribution_arr(x_dim, num_distributions):
"""Create array describing likelihood of drawing from distributions."""
if num_distributions > x_dim:
pis = [1. / num_distributions] * num_distributions
pis[0] += 1 - sum(pis)
if x_dim == num_distributions:
big_pi = round(1.0 / num_distributions, x_dim)
pis = num_distributions * [big_pi]
else:
big_pi = (1.0 / num_distributions) - x_dim * 1e-16
pis = num_distributions * [big_pi]
small_pi = (1. - sum(pis)) / (x_dim - num_distributions)
pis.extend((x_dim - num_distributions) * [small_pi])
# return np.array(pis)#, dtype=NP_FLOAT)
return np.array(pis)
def ring_of_gaussians(num_modes, sigma, r=1.):
"""Create ring of Gaussians for GaussianMixtureModel.
Args:
num_modes (int): Number of modes for distribution.
sigma (float): Standard deviation of each mode.
x_dim (int): Spatial dimensionality in which the distribution exists.
radius (float): Radius from the origin along which the modes are
located.
Returns:
distribution (GMM object): Gaussian mixture distribution.
mus (np.ndarray): Array of the means of the distribution.
covs (np.array): Covariance matrices.
distances (np.ndarray): Array of the differences between different
modes.
"""
distribution = gen_ring(r=r, var=sigma, nb_mixtures=num_modes)
mus = np.array(distribution.mus)
covs = np.array(distribution.sigmas)
diffs = mus[1:] - mus[:-1, :]
distances = [np.sqrt(np.dot(d, d.T)) for d in diffs]
return distribution, mus, covs, distances
def lattice_of_gaussians(num_modes, sigma, x_dim=2, size=None):
"""Create lattice of Gaussians for GaussianMixtureModel.
Args:
num_modes (int): Number of modes for distribution.
sigma (float): Standard deviation of each mode.
x_dim (int): Spatial dimensionality in which the distribution exists.
size (int): Spatial extent of lattice.
Returns:
distribution (GMM object): Gaussian mixture distribution.
covs (np.array): Covariance matrices.
mus (np.ndarray): Array of the means of the distribution.
pis (np.ndarray): Array of relative probabilities for each mode. Must
sum to 1.
"""
if size is None:
size = int(np.sqrt(num_modes))
mus = np.array([(i, j) for i in range(size) for j in range(size)])
covs = np.array([sigma * np.eye(x_dim) for _ in range(num_modes)])
pis = [1. / num_modes] * num_modes
pis[0] += 1. - sum(pis)
distribution = GMM(mus, covs, pis)
return distribution, mus, covs, pis
class Gaussian:
"""Implements a standard Gaussian distribution."""
def __init__(self, mu, sigma):
self.mu = mu
self.sigma = sigma
self.i_sigma = np.linalg.inv(np.copy(sigma))
def get_energy_function(self):
"""Return the potential energy function of the Gaussian."""
def fn(x, *args, **kwargs):
S = tf.constant(tf.cast(self.i_sigma, TF_FLOAT))
mu = tf.constant(tf.cast(self.mu, TF_FLOAT))
return quadratic_gaussian(x, mu, S)
return fn
def get_samples(self, n):
"""Get `n` samples from the distribution."""
C = np.linalg.cholesky(self.sigma)
x = np.random.randn(n, self.sigma.shape[0])
return x.dot(C.T)
def log_density(self, x):
"""Return the log_density of the distribution."""
return multivariate_normal(mean=self.mu, cov=self.sigma).logpdf(x)
class TiltedGaussian(Gaussian):
"""Implements a tilted Gaussian."""
def __init__(self, dim, log_min, log_max):
self.R = ortho_group.rvs(dim)
rand_unif = np.random.uniform(log_min, log_max, size=(dim,))
self.diag = np.diag(np.exp(np.log(10.) * rand_unif))
S = self.R.T.dot(self.diag).dot(self.R)
self.dim = dim
Gaussian.__init__(self, np.zeros((dim,)), S)
def get_samples(self, n):
"""Get `n` samples from the distribution."""
x = np.random.randn(200, self.dim)
x = x.dot(np.sqrt(self.diag))
x = x.dot(self.R)
return x
class RoughWell:
"""Implements a rough well distribution."""
def __init__(self, dim, eps, easy=False):
self.dim = dim
self.eps = eps
self.easy = easy
def get_energy_function(self):
"""Return the potential energy function of the distribution."""
def fn(x, *args, **kwargs):
n = tf.reduce_sum(tf.square(x), 1)
eps2 = self.eps * self.eps
if not self.easy:
out = (0.5 * n
+ self.eps * tf.reduce_sum(tf.math.cos(x/eps2), 1))
else:
out = (0.5 * n
+ self.eps * tf.reduce_sum(tf.cos(x / self.eps), 1))
return out
return fn
def get_samples(self, n):
"""Get `n` samples from the distribution."""
# we can approximate by a gaussian for eps small enough
return np.random.randn(n, self.dim)
class GaussianFunnel:
"""Gaussian funnel distribution."""
def __init__(self, dim=2, clip=6., sigma=2.):
self.dim = dim
self.sigma = sigma
self.clip = 4 * self.sigma
def get_energy_function(self):
"""Returns the (potential) energy function of the distribution."""
def fn(x):
v = x[:, 0]
log_p_v = tf.square(v / self.sigma)
s = tf.exp(v)
sum_sq = tf.reduce_sum(tf.square(x[:, 1:]), axis=1)
n = tf.cast(tf.shape(x)[1] - 1, TF_FLOAT)
E = 0.5 * (log_p_v + sum_sq / s + n * tf.math.log(2.0 * np.pi * s))
s_min = tf.exp(-self.clip)
s_max = tf.exp(self.clip)
E_safe1 = 0.5 * (
log_p_v + sum_sq / s_max + n * tf.math.log(2. * np.pi * s_max)
)
E_safe2 = 0.5 * (
log_p_v + sum_sq / s_min + n * tf.math.log(2.0 * np.pi * s_min)
)
# E_safe = tf.minimum(E_safe1, E_safe2)
E_ = tf.where(tf.greater(v, self.clip), E_safe1, E)
E_ = tf.where(tf.greater(-self.clip, v), E_safe2, E_)
return E_
return fn
def get_samples(self, n):
"""Get `n` samples from the distribution."""
samples = np.zeros((n, self.dim))
for t in range(n):
v = self.sigma * np.random.randn()
s = np.exp(v / 2)
samples[t, 0] = v
samples[t, 1:] = s * np.random.randn(self.dim-1)
return samples
def log_density(self, x):
"""Return the log density of the distribution."""
v = x[:, 0]
log_p_v = np.square(v / self.sigma)
s = np.exp(v)
sum_sq = np.square(x[:, 1:]).sum(axis=1)
n = tf.shape(x)[1] - 1
return 0.5 * (
log_p_v + sum_sq / s + (n / 2) * tf.math.log(2 * np.pi * s)
)
class GMM:
"""Implements a Gaussian Mixutre Model distribution."""
def __init__(self, mus, sigmas, pis):
assert len(mus) == len(sigmas)
assert sum(pis) == 1.0
self.mus = mus
self.sigmas = sigmas
self.pis = pis
self.nb_mixtures = len(pis)
self.k = mus[0].shape[0]
self.i_sigmas = []
self.constants = []
for i, sigma in enumerate(sigmas):
self.i_sigmas.append(tf.cast(np.linalg.inv(sigma), TF_FLOAT))
det = np.sqrt((2 * np.pi) ** self.k * np.linalg.det(sigma))
det = tf.cast(det, TF_FLOAT)
self.constants.append(tf.cast(pis[i] / det, dtype=TF_FLOAT))
def get_energy_function(self):
"""Get the energy function of the distribution."""
def fn(x):
V = tf.concat([
tf.expand_dims(-quadratic_gaussian(x, self.mus[i],
self.i_sigmas[i])
+ tf.math.log(self.constants[i]), axis=1)
for i in range(self.nb_mixtures)
], axis=1)
return -1.0 * tf.math.reduce_logsumexp(V, axis=1)
return fn
def get_samples(self, n):
"""Get `n` samples from the distribution."""
categorical = | np.random.choice(self.nb_mixtures, size=(n,), p=self.pis) | numpy.random.choice |
# This module has been generated automatically from space group information
# obtained from the Computational Crystallography Toolbox
#
"""
Space groups
This module contains a list of all the 230 space groups that can occur in
a crystal. The variable space_groups contains a dictionary that maps
space group numbers and space group names to the corresponding space
group objects.
.. moduleauthor:: <NAME> <<EMAIL>>
"""
#-----------------------------------------------------------------------------
# Copyright (C) 2013 The Mosaic Development Team
#
# Distributed under the terms of the BSD License. The full license is in
# the file LICENSE.txt, distributed as part of this software.
#-----------------------------------------------------------------------------
import numpy as N
class SpaceGroup(object):
"""
Space group
All possible space group objects are created in this module. Other
modules should access these objects through the dictionary
space_groups rather than create their own space group objects.
"""
def __init__(self, number, symbol, transformations):
"""
:param number: the number assigned to the space group by
international convention
:type number: int
:param symbol: the Hermann-Mauguin space-group symbol as used
in PDB and mmCIF files
:type symbol: str
:param transformations: a list of space group transformations,
each consisting of a tuple of three
integer arrays (rot, tn, td), where
rot is the rotation matrix and tn/td
are the numerator and denominator of the
translation vector. The transformations
are defined in fractional coordinates.
:type transformations: list
"""
self.number = number
self.symbol = symbol
self.transformations = transformations
self.transposed_rotations = N.array([N.transpose(t[0])
for t in transformations])
self.phase_factors = N.exp(N.array([(-2j*N.pi*t[1])/t[2]
for t in transformations]))
def __repr__(self):
return "SpaceGroup(%d, %s)" % (self.number, repr(self.symbol))
def __len__(self):
"""
:return: the number of space group transformations
:rtype: int
"""
return len(self.transformations)
def symmetryEquivalentMillerIndices(self, hkl):
"""
:param hkl: a set of Miller indices
:type hkl: Scientific.N.array_type
:return: a tuple (miller_indices, phase_factor) of two arrays
of length equal to the number of space group
transformations. miller_indices contains the Miller
indices of each reflection equivalent by symmetry to the
reflection hkl (including hkl itself as the first element).
phase_factor contains the phase factors that must be applied
to the structure factor of reflection hkl to obtain the
structure factor of the symmetry equivalent reflection.
:rtype: tuple
"""
hkls = N.dot(self.transposed_rotations, hkl)
p = N.multiply.reduce(self.phase_factors**hkl, -1)
return hkls, p
space_groups = {}
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(1, 'P 1', transformations)
space_groups[1] = sg
space_groups['P 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(2, 'P -1', transformations)
space_groups[2] = sg
space_groups['P -1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(3, 'P 1 2 1', transformations)
space_groups[3] = sg
space_groups['P 1 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(4, 'P 1 21 1', transformations)
space_groups[4] = sg
space_groups['P 1 21 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(5, 'C 1 2 1', transformations)
space_groups[5] = sg
space_groups['C 1 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(6, 'P 1 m 1', transformations)
space_groups[6] = sg
space_groups['P 1 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(7, 'P 1 c 1', transformations)
space_groups[7] = sg
space_groups['P 1 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(8, 'C 1 m 1', transformations)
space_groups[8] = sg
space_groups['C 1 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(9, 'C 1 c 1', transformations)
space_groups[9] = sg
space_groups['C 1 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(10, 'P 1 2/m 1', transformations)
space_groups[10] = sg
space_groups['P 1 2/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(11, 'P 1 21/m 1', transformations)
space_groups[11] = sg
space_groups['P 1 21/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(12, 'C 1 2/m 1', transformations)
space_groups[12] = sg
space_groups['C 1 2/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(13, 'P 1 2/c 1', transformations)
space_groups[13] = sg
space_groups['P 1 2/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(14, 'P 1 21/c 1', transformations)
space_groups[14] = sg
space_groups['P 1 21/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(15, 'C 1 2/c 1', transformations)
space_groups[15] = sg
space_groups['C 1 2/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(16, 'P 2 2 2', transformations)
space_groups[16] = sg
space_groups['P 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(17, 'P 2 2 21', transformations)
space_groups[17] = sg
space_groups['P 2 2 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(18, 'P 21 21 2', transformations)
space_groups[18] = sg
space_groups['P 21 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(19, 'P 21 21 21', transformations)
space_groups[19] = sg
space_groups['P 21 21 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(20, 'C 2 2 21', transformations)
space_groups[20] = sg
space_groups['C 2 2 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(21, 'C 2 2 2', transformations)
space_groups[21] = sg
space_groups['C 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(22, 'F 2 2 2', transformations)
space_groups[22] = sg
space_groups['F 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(23, 'I 2 2 2', transformations)
space_groups[23] = sg
space_groups['I 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(24, 'I 21 21 21', transformations)
space_groups[24] = sg
space_groups['I 21 21 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(25, 'P m m 2', transformations)
space_groups[25] = sg
space_groups['P m m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(26, 'P m c 21', transformations)
space_groups[26] = sg
space_groups['P m c 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(27, 'P c c 2', transformations)
space_groups[27] = sg
space_groups['P c c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(28, 'P m a 2', transformations)
space_groups[28] = sg
space_groups['P m a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(29, 'P c a 21', transformations)
space_groups[29] = sg
space_groups['P c a 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(30, 'P n c 2', transformations)
space_groups[30] = sg
space_groups['P n c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(31, 'P m n 21', transformations)
space_groups[31] = sg
space_groups['P m n 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(32, 'P b a 2', transformations)
space_groups[32] = sg
space_groups['P b a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(33, 'P n a 21', transformations)
space_groups[33] = sg
space_groups['P n a 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(34, 'P n n 2', transformations)
space_groups[34] = sg
space_groups['P n n 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(35, 'C m m 2', transformations)
space_groups[35] = sg
space_groups['C m m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(36, 'C m c 21', transformations)
space_groups[36] = sg
space_groups['C m c 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(37, 'C c c 2', transformations)
space_groups[37] = sg
space_groups['C c c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(38, 'A m m 2', transformations)
space_groups[38] = sg
space_groups['A m m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(39, 'A b m 2', transformations)
space_groups[39] = sg
space_groups['A b m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(40, 'A m a 2', transformations)
space_groups[40] = sg
space_groups['A m a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(41, 'A b a 2', transformations)
space_groups[41] = sg
space_groups['A b a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(42, 'F m m 2', transformations)
space_groups[42] = sg
space_groups['F m m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,1,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,1,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(43, 'F d d 2', transformations)
space_groups[43] = sg
space_groups['F d d 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(44, 'I m m 2', transformations)
space_groups[44] = sg
space_groups['I m m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(45, 'I b a 2', transformations)
space_groups[45] = sg
space_groups['I b a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(46, 'I m a 2', transformations)
space_groups[46] = sg
space_groups['I m a 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(47, 'P m m m', transformations)
space_groups[47] = sg
space_groups['P m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(48, 'P n n n :2', transformations)
space_groups[48] = sg
space_groups['P n n n :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(49, 'P c c m', transformations)
space_groups[49] = sg
space_groups['P c c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(50, 'P b a n :2', transformations)
space_groups[50] = sg
space_groups['P b a n :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(51, 'P m m a', transformations)
space_groups[51] = sg
space_groups['P m m a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(52, 'P n n a', transformations)
space_groups[52] = sg
space_groups['P n n a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(53, 'P m n a', transformations)
space_groups[53] = sg
space_groups['P m n a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(54, 'P c c a', transformations)
space_groups[54] = sg
space_groups['P c c a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(55, 'P b a m', transformations)
space_groups[55] = sg
space_groups['P b a m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(56, 'P c c n', transformations)
space_groups[56] = sg
space_groups['P c c n'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(57, 'P b c m', transformations)
space_groups[57] = sg
space_groups['P b c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(58, 'P n n m', transformations)
space_groups[58] = sg
space_groups['P n n m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(59, 'P m m n :2', transformations)
space_groups[59] = sg
space_groups['P m m n :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(60, 'P b c n', transformations)
space_groups[60] = sg
space_groups['P b c n'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(61, 'P b c a', transformations)
space_groups[61] = sg
space_groups['P b c a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(62, 'P n m a', transformations)
space_groups[62] = sg
space_groups['P n m a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(63, 'C m c m', transformations)
space_groups[63] = sg
space_groups['C m c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(64, 'C m c a', transformations)
space_groups[64] = sg
space_groups['C m c a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(65, 'C m m m', transformations)
space_groups[65] = sg
space_groups['C m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(66, 'C c c m', transformations)
space_groups[66] = sg
space_groups['C c c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(67, 'C m m a', transformations)
space_groups[67] = sg
space_groups['C m m a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(68, 'C c c a :2', transformations)
space_groups[68] = sg
space_groups['C c c a :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(69, 'F m m m', transformations)
space_groups[69] = sg
space_groups['F m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,3,3])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,0,3])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(70, 'F d d d :2', transformations)
space_groups[70] = sg
space_groups['F d d d :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(71, 'I m m m', transformations)
space_groups[71] = sg
space_groups['I m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(72, 'I b a m', transformations)
space_groups[72] = sg
space_groups['I b a m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(73, 'I b c a', transformations)
space_groups[73] = sg
space_groups['I b c a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(74, 'I m m a', transformations)
space_groups[74] = sg
space_groups['I m m a'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(75, 'P 4', transformations)
space_groups[75] = sg
space_groups['P 4'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(76, 'P 41', transformations)
space_groups[76] = sg
space_groups['P 41'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(77, 'P 42', transformations)
space_groups[77] = sg
space_groups['P 42'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(78, 'P 43', transformations)
space_groups[78] = sg
space_groups['P 43'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(79, 'I 4', transformations)
space_groups[79] = sg
space_groups['I 4'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(80, 'I 41', transformations)
space_groups[80] = sg
space_groups['I 41'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(81, 'P -4', transformations)
space_groups[81] = sg
space_groups['P -4'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(82, 'I -4', transformations)
space_groups[82] = sg
space_groups['I -4'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(83, 'P 4/m', transformations)
space_groups[83] = sg
space_groups['P 4/m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(84, 'P 42/m', transformations)
space_groups[84] = sg
space_groups['P 42/m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(85, 'P 4/n :2', transformations)
space_groups[85] = sg
space_groups['P 4/n :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(86, 'P 42/n :2', transformations)
space_groups[86] = sg
space_groups['P 42/n :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(87, 'I 4/m', transformations)
space_groups[87] = sg
space_groups['I 4/m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-3,-3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,5,5])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(88, 'I 41/a :2', transformations)
space_groups[88] = sg
space_groups['I 41/a :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(89, 'P 4 2 2', transformations)
space_groups[89] = sg
space_groups['P 4 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(90, 'P 4 21 2', transformations)
space_groups[90] = sg
space_groups['P 4 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(91, 'P 41 2 2', transformations)
space_groups[91] = sg
space_groups['P 41 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(92, 'P 41 21 2', transformations)
space_groups[92] = sg
space_groups['P 41 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(93, 'P 42 2 2', transformations)
space_groups[93] = sg
space_groups['P 42 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(94, 'P 42 21 2', transformations)
space_groups[94] = sg
space_groups['P 42 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,3])
trans_den = N.array([1,1,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(95, 'P 43 2 2', transformations)
space_groups[95] = sg
space_groups['P 43 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(96, 'P 43 21 2', transformations)
space_groups[96] = sg
space_groups['P 43 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(97, 'I 4 2 2', transformations)
space_groups[97] = sg
space_groups['I 4 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(98, 'I 41 2 2', transformations)
space_groups[98] = sg
space_groups['I 41 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(99, 'P 4 m m', transformations)
space_groups[99] = sg
space_groups['P 4 m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(100, 'P 4 b m', transformations)
space_groups[100] = sg
space_groups['P 4 b m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(101, 'P 42 c m', transformations)
space_groups[101] = sg
space_groups['P 42 c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(102, 'P 42 n m', transformations)
space_groups[102] = sg
space_groups['P 42 n m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(103, 'P 4 c c', transformations)
space_groups[103] = sg
space_groups['P 4 c c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(104, 'P 4 n c', transformations)
space_groups[104] = sg
space_groups['P 4 n c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(105, 'P 42 m c', transformations)
space_groups[105] = sg
space_groups['P 42 m c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(106, 'P 42 b c', transformations)
space_groups[106] = sg
space_groups['P 42 b c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(107, 'I 4 m m', transformations)
space_groups[107] = sg
space_groups['I 4 m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(108, 'I 4 c m', transformations)
space_groups[108] = sg
space_groups['I 4 c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(109, 'I 41 m d', transformations)
space_groups[109] = sg
space_groups['I 41 m d'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(110, 'I 41 c d', transformations)
space_groups[110] = sg
space_groups['I 41 c d'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(111, 'P -4 2 m', transformations)
space_groups[111] = sg
space_groups['P -4 2 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(112, 'P -4 2 c', transformations)
space_groups[112] = sg
space_groups['P -4 2 c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(113, 'P -4 21 m', transformations)
space_groups[113] = sg
space_groups['P -4 21 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(114, 'P -4 21 c', transformations)
space_groups[114] = sg
space_groups['P -4 21 c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(115, 'P -4 m 2', transformations)
space_groups[115] = sg
space_groups['P -4 m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(116, 'P -4 c 2', transformations)
space_groups[116] = sg
space_groups['P -4 c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(117, 'P -4 b 2', transformations)
space_groups[117] = sg
space_groups['P -4 b 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(118, 'P -4 n 2', transformations)
space_groups[118] = sg
space_groups['P -4 n 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(119, 'I -4 m 2', transformations)
space_groups[119] = sg
space_groups['I -4 m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(120, 'I -4 c 2', transformations)
space_groups[120] = sg
space_groups['I -4 c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(121, 'I -4 2 m', transformations)
space_groups[121] = sg
space_groups['I -4 2 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,3])
trans_den = N.array([2,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,5])
trans_den = N.array([1,2,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(122, 'I -4 2 d', transformations)
space_groups[122] = sg
space_groups['I -4 2 d'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(123, 'P 4/m m m', transformations)
space_groups[123] = sg
space_groups['P 4/m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(124, 'P 4/m c c', transformations)
space_groups[124] = sg
space_groups['P 4/m c c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(125, 'P 4/n b m :2', transformations)
space_groups[125] = sg
space_groups['P 4/n b m :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(126, 'P 4/n n c :2', transformations)
space_groups[126] = sg
space_groups['P 4/n n c :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(127, 'P 4/m b m', transformations)
space_groups[127] = sg
space_groups['P 4/m b m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(128, 'P 4/m n c', transformations)
space_groups[128] = sg
space_groups['P 4/m n c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(129, 'P 4/n m m :2', transformations)
space_groups[129] = sg
space_groups['P 4/n m m :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(130, 'P 4/n c c :2', transformations)
space_groups[130] = sg
space_groups['P 4/n c c :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(131, 'P 42/m m c', transformations)
space_groups[131] = sg
space_groups['P 42/m m c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(132, 'P 42/m c m', transformations)
space_groups[132] = sg
space_groups['P 42/m c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(133, 'P 42/n b c :2', transformations)
space_groups[133] = sg
space_groups['P 42/n b c :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(134, 'P 42/n n m :2', transformations)
space_groups[134] = sg
space_groups['P 42/n n m :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(135, 'P 42/m b c', transformations)
space_groups[135] = sg
space_groups['P 42/m b c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(136, 'P 42/m n m', transformations)
space_groups[136] = sg
space_groups['P 42/m n m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(137, 'P 42/n m c :2', transformations)
space_groups[137] = sg
space_groups['P 42/n m c :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(138, 'P 42/n c m :2', transformations)
space_groups[138] = sg
space_groups['P 42/n c m :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(139, 'I 4/m m m', transformations)
space_groups[139] = sg
space_groups['I 4/m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(140, 'I 4/m c m', transformations)
space_groups[140] = sg
space_groups['I 4/m c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-3,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-3,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,5,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,5])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,5,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,3,5])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(141, 'I 41/a m d :2', transformations)
space_groups[141] = sg
space_groups['I 41/a m d :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-3,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-3,-3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,5,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,5])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,5,5])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,-1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(142, 'I 41/a c d :2', transformations)
space_groups[142] = sg
space_groups['I 41/a c d :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(143, 'P 3', transformations)
space_groups[143] = sg
space_groups['P 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(144, 'P 31', transformations)
space_groups[144] = sg
space_groups['P 31'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(145, 'P 32', transformations)
space_groups[145] = sg
space_groups['P 32'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(146, 'R 3 :H', transformations)
space_groups[146] = sg
space_groups['R 3 :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(147, 'P -3', transformations)
space_groups[147] = sg
space_groups['P -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(148, 'R -3 :H', transformations)
space_groups[148] = sg
space_groups['R -3 :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(149, 'P 3 1 2', transformations)
space_groups[149] = sg
space_groups['P 3 1 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(150, 'P 3 2 1', transformations)
space_groups[150] = sg
space_groups['P 3 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(151, 'P 31 1 2', transformations)
space_groups[151] = sg
space_groups['P 31 1 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(152, 'P 31 2 1', transformations)
space_groups[152] = sg
space_groups['P 31 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(153, 'P 32 1 2', transformations)
space_groups[153] = sg
space_groups['P 32 1 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(154, 'P 32 2 1', transformations)
space_groups[154] = sg
space_groups['P 32 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(155, 'R 3 2 :H', transformations)
space_groups[155] = sg
space_groups['R 3 2 :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(156, 'P 3 m 1', transformations)
space_groups[156] = sg
space_groups['P 3 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(157, 'P 3 1 m', transformations)
space_groups[157] = sg
space_groups['P 3 1 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(158, 'P 3 c 1', transformations)
space_groups[158] = sg
space_groups['P 3 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(159, 'P 3 1 c', transformations)
space_groups[159] = sg
space_groups['P 3 1 c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(160, 'R 3 m :H', transformations)
space_groups[160] = sg
space_groups['R 3 m :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(161, 'R 3 c :H', transformations)
space_groups[161] = sg
space_groups['R 3 c :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(162, 'P -3 1 m', transformations)
space_groups[162] = sg
space_groups['P -3 1 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(163, 'P -3 1 c', transformations)
space_groups[163] = sg
space_groups['P -3 1 c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(164, 'P -3 m 1', transformations)
space_groups[164] = sg
space_groups['P -3 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(165, 'P -3 c 1', transformations)
space_groups[165] = sg
space_groups['P -3 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(166, 'R -3 m :H', transformations)
space_groups[166] = sg
space_groups['R -3 m :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,7])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,2,2])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,2,1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,5])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([2,1,1])
trans_den = N.array([3,3,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,-1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,-1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([2,1,-1])
trans_den = N.array([3,3,6])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(167, 'R -3 c :H', transformations)
space_groups[167] = sg
space_groups['R -3 c :H'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(168, 'P 6', transformations)
space_groups[168] = sg
space_groups['P 6'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(169, 'P 61', transformations)
space_groups[169] = sg
space_groups['P 61'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(170, 'P 65', transformations)
space_groups[170] = sg
space_groups['P 65'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(171, 'P 62', transformations)
space_groups[171] = sg
space_groups['P 62'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(172, 'P 64', transformations)
space_groups[172] = sg
space_groups['P 64'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(173, 'P 63', transformations)
space_groups[173] = sg
space_groups['P 63'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(174, 'P -6', transformations)
space_groups[174] = sg
space_groups['P -6'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(175, 'P 6/m', transformations)
space_groups[175] = sg
space_groups['P 6/m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(176, 'P 63/m', transformations)
space_groups[176] = sg
space_groups['P 63/m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(177, 'P 6 2 2', transformations)
space_groups[177] = sg
space_groups['P 6 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(178, 'P 61 2 2', transformations)
space_groups[178] = sg
space_groups['P 61 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,5])
trans_den = N.array([1,1,6])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(179, 'P 65 2 2', transformations)
space_groups[179] = sg
space_groups['P 65 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(180, 'P 62 2 2', transformations)
space_groups[180] = sg
space_groups['P 62 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,2])
trans_den = N.array([1,1,3])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(181, 'P 64 2 2', transformations)
space_groups[181] = sg
space_groups['P 64 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(182, 'P 63 2 2', transformations)
space_groups[182] = sg
space_groups['P 63 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(183, 'P 6 m m', transformations)
space_groups[183] = sg
space_groups['P 6 m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(184, 'P 6 c c', transformations)
space_groups[184] = sg
space_groups['P 6 c c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(185, 'P 63 c m', transformations)
space_groups[185] = sg
space_groups['P 63 c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(186, 'P 63 m c', transformations)
space_groups[186] = sg
space_groups['P 63 m c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(187, 'P -6 m 2', transformations)
space_groups[187] = sg
space_groups['P -6 m 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(188, 'P -6 c 2', transformations)
space_groups[188] = sg
space_groups['P -6 c 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(189, 'P -6 2 m', transformations)
space_groups[189] = sg
space_groups['P -6 2 m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(190, 'P -6 2 c', transformations)
space_groups[190] = sg
space_groups['P -6 2 c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(191, 'P 6/m m m', transformations)
space_groups[191] = sg
space_groups['P 6/m m m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(192, 'P 6/m c c', transformations)
space_groups[192] = sg
space_groups['P 6/m c c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(193, 'P 63/m c m', transformations)
space_groups[193] = sg
space_groups['P 63/m c m'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,1,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,1,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,-1,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,-1,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(194, 'P 63/m m c', transformations)
space_groups[194] = sg
space_groups['P 63/m m c'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(195, 'P 2 3', transformations)
space_groups[195] = sg
space_groups['P 2 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(196, 'F 2 3', transformations)
space_groups[196] = sg
space_groups['F 2 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(197, 'I 2 3', transformations)
space_groups[197] = sg
space_groups['I 2 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(198, 'P 21 3', transformations)
space_groups[198] = sg
space_groups['P 21 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(199, 'I 21 3', transformations)
space_groups[199] = sg
space_groups['I 21 3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(200, 'P m -3', transformations)
space_groups[200] = sg
space_groups['P m -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(201, 'P n -3 :2', transformations)
space_groups[201] = sg
space_groups['P n -3 :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(202, 'F m -3', transformations)
space_groups[202] = sg
space_groups['F m -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,3,3])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,3,3])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,3,3])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([3,0,3])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([3,0,3])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,3])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,0,3])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([4,1,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,-1,1])
trans_den = N.array([4,4,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([3,3,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([3,3,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,3,1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([3,1,1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([3,3,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([4,2,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([4,4,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(203, 'F d -3 :2', transformations)
space_groups[203] = sg
space_groups['F d -3 :2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(204, 'I m -3', transformations)
space_groups[204] = sg
space_groups['I m -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,-1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,-1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(205, 'P a -3', transformations)
space_groups[205] = sg
space_groups['P a -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([-1,0,0])
trans_den = N.array([2,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(206, 'I a -3', transformations)
space_groups[206] = sg
space_groups['I a -3'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(207, 'P 4 3 2', transformations)
space_groups[207] = sg
space_groups['P 4 3 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(208, 'P 42 3 2', transformations)
space_groups[208] = sg
space_groups['P 42 3 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(209, 'F 4 3 2', transformations)
space_groups[209] = sg
space_groups['F 4 3 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,-1,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,-1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,0,0,1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,-1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,1,0,0,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,0,0,-1,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,-1,0,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,-1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,-1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,0,-1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,-1,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,0,1,0,-1,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,0,1,0,-1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,-1,0,1,0,1,0,0])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,-1,0,1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,1,0,-1,0,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,3,3])
trans_den = N.array([4,4,4])
transformations.append((rot, trans_num, trans_den))
rot = N.array([0,0,1,1,0,0,0,1,0])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = | N.array([0,1,0,0,0,1,1,0,0]) | numpy.array |
from ..regions import GenomicRegions
import numpy as np
import pypipegraph as ppg
import pandas as pd
from pathlib import Path
from mbf_externals.util import lazy_method
_exon_regions_overlapping_cache = {}
_genes_per_genome_singletons = {}
class Genes(GenomicRegions):
def __new__(cls, genome, alternative_load_func=None, *args, **kwargs):
"""Make sure that Genes for a full genome (ie. before filtering) are singletonic. Ie.
you can always safely call Genes(my_genome), without worrying about duplicate objects"""
if alternative_load_func is None:
if ppg.util.global_pipegraph:
if not hasattr(
ppg.util.global_pipegraph, "_genes_per_genome_singletons"
):
ppg.util.global_pipegraph._genes_per_genome_singletons = {}
singleton_dict = ppg.util.global_pipegraph._genes_per_genome_singletons
else:
singleton_dict = _genes_per_genome_singletons
if not genome in singleton_dict:
singleton_dict[genome] = GenomicRegions.__new__(cls)
return singleton_dict[genome]
else:
return GenomicRegions.__new__(cls)
def __init__(
self,
genome,
alternative_load_func=None,
name=None,
dependencies=None,
result_dir=None,
sheet_name=None,
vid=None,
):
if hasattr(self, "_already_inited"):
if (
alternative_load_func is not None
and alternative_load_func != self.genes_load_func
): # pragma: no cover -
# this can only happen iff somebody starts to singletonize the Genes
# with loading_functions - otherwise the duplicate object
# checker will kick in first
raise ValueError(
"Trying to define Genes(%s) twice with different loading funcs"
% self.name
)
pass
else:
if name is None:
if alternative_load_func:
raise ValueError(
"If you pass in an alternative_load_func you also need to specify a name"
)
name = "Genes_%s" % genome.name
self.top_level = True
else:
self.top_level = False
if alternative_load_func is None:
load_func = lambda: genome.df_genes.reset_index() # noqa: E731
else:
load_func = alternative_load_func
self.genes_load_func = load_func
if result_dir:
pass
elif sheet_name:
result_dir = result_dir or Path("results") / "Genes" / sheet_name / name
else:
result_dir = result_dir or Path("results") / "Genes" / name
result_dir = Path(result_dir).absolute()
self.column_properties = {
"chr": {
"user_visible": False,
"priority": -997,
"description": "On which chromosome (or contig) the gene is loacted",
},
"start": {
"user_visible": False,
"priority": -997,
"description": "Left most position of this gene",
},
"stop": {
"user_visible": False,
"priority": -997,
"description": "Right most position of this gene",
},
"tss": {
"user_visible": False,
"priority": -997,
"description": "Position of Transcription start site on the chromosome",
},
"tes": {
"user_visible": False,
"priority": -997,
"description": "Position of the annotated end of transcription",
},
"gene_stable_id": {
"user_visible": True,
"priority": -1000,
"index": True,
"description": "Unique identification name for this gene",
},
"name": {
"user_visible": True,
"priority": -999,
"index": True,
"description": "Offical name for this gene",
"nocase": True,
},
"strand": {
"user_visible": True,
"priority": -998,
"description": "Which is the coding strand (1 forward, -1 reverse)",
},
"description": {
"user_visible": True,
"priority": -998.5,
"description": "A short description of the gene",
},
}
GenomicRegions.__init__(
self,
name,
self._load,
dependencies if dependencies is not None else [],
genome,
on_overlap="ignore",
result_dir=result_dir,
sheet_name=sheet_name,
summit_annotator=False,
)
if self.load_strategy.build_deps:
deps = [genome.download_genome()]
# function invariant for _load is done by GR.__init__
deps.append(genome.job_genes())
deps.append(genome.job_transcripts())
self.load_strategy.load().depends_on(deps)
self._already_inited = True
self.vid = vid
def __str__(self):
return "Genes(%s)" % self.name
def __repr__(self):
return "Genes(%s)" % self.name
def register(self):
pass
def _load(self):
"""Load func
"""
if hasattr(self, "df"): # pragma: no cover
return
df = self.genes_load_func()
if not isinstance(df, pd.DataFrame):
raise ValueError(
"GenomicRegion.loading_function must return a DataFrame, was: %s"
% type(df)
)
for col in self.get_default_columns():
if not col in df.columns:
func_filename = self.genes_load_func.__code__.co_filename
func_line_no = self.genes_load_func.__code__.co_firstlineno
raise ValueError(
"%s not in dataframe returned by GenomicRegion.loading_function %s %s - it did return %s"
% (col, func_filename, func_line_no, df.columns)
)
allowed_chromosomes = set(self.genome.get_chromosome_lengths().keys())
if len(df):
for chr in df["chr"]:
if not chr in allowed_chromosomes:
raise ValueError(
"Invalid chromosome found when loading %s: '%s', expected one of: %s\nLoading func was %s"
% (
self.name,
chr,
sorted(allowed_chromosomes),
self.genes_load_func,
)
)
if not np.issubdtype(df["tss"].dtype, np.integer):
raise ValueError(
"tss needs to be an integer, was: %s" % df["tss"].dtype
)
if not np.issubdtype(df["tes"].dtype, np.integer):
raise ValueError(
"tes needs to be an integer, was: %s" % df["tes"].dtype
)
# df = self.handle_overlap(df) Genes don't care about overlap
if not "start" in df.columns:
df = df.assign(start=np.min([df["tss"], df["tes"]], 0))
if not "stop" in df.columns:
df = df.assign(stop=np.max([df["tss"], df["tes"]], 0))
else:
df = df.assign(
start=np.array([], dtype=np.int32), stop= | np.array([], dtype=np.int32) | numpy.array |
import ast
import matplotlib.pyplot as plt
import numpy as np
from scipy.stats import wilcoxon
from matplotlib.ticker import FormatStrFormatter
import matplotlib
from tabulate import tabulate
text_dir = 'data/qa_example/'
counterfactual_dir = 'counterfactuals/qa_example/model_dist_1layer/'
probe_type = 'model_dist'
test_layers = [i for i in range(1, 25)]
layer_offset = test_layers[0]
# For a single sentence, plot the distribution over start probabilities for the original and updated embeddings
# as well as just the deltas.
def plot_sentence_probs(sentence_idx):
fig, (ax1, ax2) = plt.subplots(nrows=2, figsize=(10, 10))
fig.suptitle("Absolute and Relative Start Token Probabilities")
x_axis = [i + 1 for i in range(len(original_start_probs[sentence_idx]))]
# Plot the absolute probability values
ax1.set_title("Start probabilities for layer " + str(layer) + " and sentence " + str(sentence_idx))
ax1.set_xlabel('Token idx')
ax1.errorbar(x_axis, original_start_probs[sentence_idx], linestyle='--', color='green', marker='s', label='Original')
ax1.errorbar(x_axis, nn1_parse_updated_start_probs[sentence_idx], color='red', marker='s', label='Conj. Parse')
ax1.errorbar(x_axis, nn2_parse_updated_start_probs[sentence_idx], color='blue', marker='s', label='NN2 Parse')
ax1.legend(loc="upper left")
ax2.set_title("Changes in start probabilities for layer " + str(layer) + " and sentence " + str(sentence_idx))
ax2.set_xlabel('Token idx')
nn1_delta = [nn1_parse_updated_start_probs[sentence_idx][i] - original_start_probs[sentence_idx][i] for i in range(len(original_start_probs[sentence_idx]))]
nn2_delta = [nn2_parse_updated_start_probs[sentence_idx][i] - original_start_probs[sentence_idx][i] for i in range(len(original_start_probs[sentence_idx]))]
ax2.errorbar(x_axis, nn1_delta, color='red', marker='s', label='Conj. Parse')
ax2.errorbar(x_axis, nn2_delta, color='blue', marker='s', label='NN2 Parse')
ax2.legend(loc='upper left')
plt.show()
# Read in the other question info as well
corpus_types = []
answer_lengths = []
start_likelihoods = []
contexts = []
questions = []
answers = []
with open(text_dir + 'setup.txt', 'r') as setup_file:
for line_idx, line in enumerate(setup_file):
split_line = line.split('\t')
corpus_types.append(split_line[0])
answer_lengths.append(int(split_line[1]))
start_likelihoods.append(float(split_line[2]))
contexts.append(split_line[3])
questions.append(split_line[4])
answers.append(split_line[5])
# Read in the token id data. We care about probability changes at specific locations, which were stored way back
# when the corpus was generated in token_idxs.txt.
# We care about 4 locations (determiner and noun) x (location 1 and location 2)
det1_token_idxs = []
nn1_token_idxs = []
det2_token_idxs = []
nn2_token_idxs = []
all_token_idxs = [det1_token_idxs, nn1_token_idxs, det2_token_idxs, nn2_token_idxs]
with open(text_dir + 'token_idxs.txt', 'r') as token_file:
for line_idx, line in enumerate(token_file):
if line_idx % 2 == 0:
continue # Have twice as many token lines as needed because the sentence were duplicated.
split_line = line.split('\t')
det1_token_idxs.append(int(split_line[0]))
nn1_token_idxs.append(int(split_line[1]))
det2_token_idxs.append(int(split_line[2]))
nn2_token_idxs.append(int(split_line[3]))
all_layers_original_starts = []
all_layers_nn1_parse_starts = []
all_layers_nn2_parse_starts = []
for layer in test_layers:
# Read in how the probabilities got updated.
original_start_probs = []
nn1_parse_updated_start_probs = []
nn2_parse_updated_start_probs = []
with open(counterfactual_dir + probe_type + str(layer) + '/updated_probs.txt', 'r') as results_file:
for line_idx, line in enumerate(results_file):
split_line = line.split('\t')
if line_idx == 0: # The first line has some cruft based on how files are generated.
continue
if line_idx % 2 == 1:
original_start_probs.append([ast.literal_eval(data)[0] for data in split_line])
nn1_parse_updated_start_probs.append([ast.literal_eval(data)[2] for data in split_line])
else:
nn2_parse_updated_start_probs.append([ast.literal_eval(data)[2] for data in split_line])
# Now we have the data, so if you want to plot probabilities for a single sentence, you can.
# Plot stuff for just a single sentence.
# for i in range(1):
# plot_sentence_probs(i)
# Dump the layer-specific data into an aggregator.
all_layers_original_starts.append(original_start_probs)
all_layers_nn1_parse_starts.append(nn1_parse_updated_start_probs)
all_layers_nn2_parse_starts.append(nn2_parse_updated_start_probs)
def get_token_idx_start_update(token_idxs):
nn1_updates = []
nn1_updates_std = []
nn2_updates = []
nn2_updates_std = []
nn1_all = []
nn2_all = []
for layer in test_layers:
layer_specific_nn1_updates = []
layer_specific_nn2_updates = []
for sentence_idx, token_idx in enumerate(token_idxs):
if token_idx == -1:
print("Invalid token, skipping")
layer_specific_nn1_updates.append(0)
layer_specific_nn2_updates.append(0)
continue
original_prob = all_layers_original_starts[layer - layer_offset][sentence_idx][token_idx]
nn1_parse_prob = all_layers_nn1_parse_starts[layer - layer_offset][sentence_idx][token_idx]
nn2_parse_prob = all_layers_nn2_parse_starts[layer - layer_offset][sentence_idx][token_idx]
layer_specific_nn1_updates.append(nn1_parse_prob - original_prob)
layer_specific_nn2_updates.append(nn2_parse_prob - original_prob)
nn1_updates.append(np.mean(layer_specific_nn1_updates))
nn1_updates_std.append(np.std(layer_specific_nn1_updates))
nn2_updates.append(np.mean(layer_specific_nn2_updates))
nn2_updates_std.append(np.std(layer_specific_nn2_updates))
nn1_all.append(layer_specific_nn1_updates)
nn2_all.append(layer_specific_nn2_updates)
return nn1_updates, nn1_updates_std, nn2_updates, nn2_updates_std, nn1_all, nn2_all
def plot_start_updates():
x_axis = [i for i in test_layers]
fig, axes = plt.subplots(nrows=4, figsize=(10, 20))
for i in range(4):
tokens = all_token_idxs[i]
_, _, _, _, nn1_all, nn2_all =\
get_token_idx_start_update(tokens)
# Now do the plotting
ax = axes[i]
ax.set_title("Start prob deltas for token " + str(i))
ax.set_xlabel('Layer idx')
ax.errorbar(x_axis, np.mean(nn1_all, axis=1), color='red', marker='s', label='NP1 Parse')
ax.errorbar(x_axis, np.mean(nn2_all, axis=1), color='blue', marker='s', label='NP2 Parse')
ax.axhline()
ax.legend(loc='upper left')
plt.savefig(counterfactual_dir + probe_type + '_token_updates.png')
plt.show()
# Plot aggregate data.
plot_start_updates()
_, _, _, _, p1_tok0, p2_tok0 = get_token_idx_start_update(all_token_idxs[0])
_, _, _, _, p1_tok1, p2_tok1 = get_token_idx_start_update(all_token_idxs[1])
_, _, _, _, p1_tok2, p2_tok2 = get_token_idx_start_update(all_token_idxs[2])
_, _, _, _, p1_tok3, p2_tok3 = get_token_idx_start_update(all_token_idxs[3])
def calculate_stats(p1_tokens, p2_tokens, string_label):
p1 = np.asarray(p1_tokens[0])
for p1_idx, p1_tokens_entry in enumerate(p1_tokens):
if p1_idx == 0:
continue
p1 = p1 + np.asarray(p1_tokens_entry)
p2 = np.asarray(p2_tokens[0])
for p2_idx, p2_tokens_entry in enumerate(p2_tokens):
if p2_idx == 0:
continue
p2 = p2 + np.asarray(p2_tokens_entry)
for layer in range(p1.shape[0]):
stat, p = wilcoxon(p1[layer], p2[layer], alternative='greater')
_, less_p = wilcoxon(p1[layer], p2[layer], alternative='less')
if p < 0.01:
print("Sig. greater:\t", string_label, "for layer", layer + layer_offset)
continue
if less_p < 0.01:
print("Sig. less:\t", string_label, "for layer", layer + layer_offset)
continue
print("Not significant for layer", layer + layer_offset)
print()
calculate_stats((p1_tok0, p1_tok1), (p2_tok0, p2_tok1), "NP1")
calculate_stats((p1_tok2, p1_tok3), (p2_tok2, p2_tok3), "NP2")
parse1_np1_delta = np.asarray(p1_tok0) + np.asarray(p1_tok1)
parse1_np2_delta = (np.asarray(p1_tok2) + np.asarray(p1_tok3))
parse2_np1_delta = np.asarray(p2_tok0) + np.asarray(p2_tok1)
parse2_np2_delta = (np.asarray(p2_tok2) + np.asarray(p2_tok3))
calculate_stats((parse1_np1_delta, -1 * parse1_np2_delta),
(parse2_np1_delta, -1 * parse2_np2_delta),
"Overall shift")
calculate_stats((p1_tok0, p1_tok1), (np.zeros_like(p2_tok2), np.zeros_like(p2_tok3)), "Parse1 NP1 vs. 0")
calculate_stats((p1_tok2, p1_tok3), (np.zeros_like(p2_tok2), np.zeros_like(p2_tok3)), "Parse1 NP2 vs. 0")
calculate_stats((p2_tok2, p2_tok3), (np.zeros_like(p2_tok2), np.zeros_like(p2_tok3)), "Parse2 NP2 vs. 0")
calculate_stats((p2_tok0, p2_tok1), (np.zeros_like(p2_tok2), np.zeros_like(p2_tok3)), "Parse2 NP1 vs. 0")
# Plot the net shift in probability mass between tokens from the different noun phrases, plotting one line per
# parse.
def plot_aggregate_delta():
p1_net = parse1_np1_delta - parse1_np2_delta
p2_net = parse2_np1_delta - parse2_np2_delta
x_axis = [i for i in test_layers]
fig, ax = plt.subplots(figsize=(10, 5))
ax.errorbar(x_axis, np.mean(p1_net, axis=1), color='red', marker='s', label='Parse 1')
ax.errorbar(x_axis, | np.mean(p2_net, axis=1) | numpy.mean |
import numpy as np
from scipy.sparse import csr_matrix
from feature_mining.em_base import ExpectationMaximization
class ExpectationMaximizationVector(ExpectationMaximization):
"""
Vectorized implementation of EM algorithm.
"""
def __init__(self, dump_path="../tests/data/em_01/"):
print(type(self).__name__, '- init...')
ExpectationMaximization.__init__(self, dump_path=dump_path)
# Parameters for matrix result interpretation
self.features_map = {}
self.words_map = {}
self.words_list = {}
# TODO: one more optimization place
self.topic_model_sentence_matrix = None
self.iv = None # identity array of v size
# TODO: Remove these after testing validated
# Parameters for temporary import transformation
self.reviews_matrix = np.array([])
self.pi_matrix = np.array([])
self.topic_model_matrix = ()
self.reviews_binary = np.array([])
self.previous_pi_matrix = None
self.expose_sentence_sum_for_testing = None
self.denom = 0.0
self.nom = 0.0
self.m_sum = None
def import_data(self):
"""
Needed data structures could be further transformed here.
Input:
please see import_data_temporary (for now)
Output:
- self.reviews_matrix: matrix representation of Reviews
- self.topic_model_matrix: matrix representation of TopicModel
- self.pi_matrix: matrix representation of PI
- self.m: number of sections (sentences)
- self.v: number of words in vocabulary
- self.f: number of features/aspects
- self.iv: identity vector of b length
- self.features_map: used for testing - maps features to id-s
- self.words_map: used for testing - maps words to id-s
- self.words_list: list of words (inverse of words_map)
- self.background_probability = background_probability_vector
:return:
"""
print(type(self).__name__, '- import data...')
self.import_data_temporary() # TODO: deactivate this when our data is available
def import_data_temporary(self):
"""
Transforms data read from a snapshot of Santu's data, after 1 execution of E-M steps.
Input:
- Reviews.npy: np dump of the Reviews structure
- TopicModel.npy: np dump of TopicModel
- BackgroundProbability: np dump of BackgroundProbability
- HP: np dump of hidden parameters
- PI: np dump of pi (important for testing purposes, since this is randomly generated)
:return:
"""
# TODO: this should be deleted once the implementation is safe
print(type(self).__name__, '- import data ********temporary********...')
self.reviews = np.load(self.dump_path + "Reviews.npy")
self.topic_model = np.load(self.dump_path + 'TopicModel.npy').item()
self.background_probability = np.load(self.dump_path + 'BackgroundProbability.npy').item()
self.hidden_parameters = np.load(self.dump_path + "HP.npy")
self.hidden_parameters_background = np.load(self.dump_path + "HPB.npy")
self.pi = np.load(self.dump_path + "PI.npy")
"""
Prepare data for testing vectorised solution.
Want to convert the photo of Santu's data for vectorised needs.
:return:
"""
m = 0 # number of sentences (lines) in all reviews - 8799
nw = 0 # number of words in vocabulary - 7266
na = 0 # number of aspects - 9
for reviewNum in range(0, len(self.reviews)):
for lineNum in range(0, len(self.reviews[reviewNum])):
m += 1
for feature in self.topic_model:
na += 1
words_dict = {}
for feature in self.topic_model.keys():
for word in self.topic_model[feature]:
words_dict[word] = True
nw = len(words_dict.keys()) # 7266
word_list = sorted(words_dict.keys())
words_map = {}
for word_id in range(0, len(word_list)):
words_map[word_list[word_id]] = word_id
# initialize reviews with zeros
reviews_matrix = | np.zeros(m * nw) | numpy.zeros |
import numpy as np
from scipy import interpolate
from cemc.wanglandau.wltools import convert_array, adapt_array
import sqlite3 as sq
import time
class Histogram( object ):
"""
Class for tracking the WL histtogram and related quantities
"""
def __init__( self, Nbins, Emin, Emax, logger ):
self.Nbins = Nbins
self.Emin = Emin
self.Emax = Emax
self.histogram = np.zeros(self.Nbins, dtype=np.int32)
self.logdos = np.zeros( self.Nbins )
self.growth_variance = np.zeros( self.Nbins )
self.logger = logger
self.largest_energy_ever = -np.inf
self.smallest_energy_ever = np.inf
self.tot_number = 0
self.number_of_converged = 0
self.known_state = np.zeros(self.Nbins,dtype=np.uint8)
# Assume that both the maximum state is found by some algorithm that
# ensures that there exists states
self.known_state[0] = 1
self.known_state[-1] = 1
def get_energy( self, indx ):
"""
Returns the energy corresponding to one bin
"""
return self.Emin + (self.Emax-self.Emin )*indx/self.Nbins
def get_bin( self, energy ):
"""
Returns the bin corresponding to one energy
"""
return int( (energy-self.Emin)*self.Nbins/(self.Emax-self.Emin) )
def update( self, selected_bin, mod_factor ):
"""
Updates all quantities
"""
self.known_state[selected_bin] = 1
self.histogram[selected_bin] += 1
self.logdos[selected_bin] += mod_factor
self.growth_variance += self.Nbins**(-2)
self.growth_variance[selected_bin] += (1.0 - 2.0/self.Nbins)
def update_range( self ):
"""
Updates the range of the histogram to better fit the required energies
"""
upper = self.Nbins
for i in range(len(self.histogram)-1,0,-1):
if ( self.histogram[i] > 0 ):
upper = i
break
lower = 0
for i in range(len(self.histogram)):
if ( self.histogram[i] > 0 ):
lower = i
break
Emin = self.get_energy(lower)
Emax = self.get_energy(upper)
if ( Emax != self.largest_energy_ever and self.largest_energy_ever!=-np.inf):
Emax = self.largest_energy_ever
if ( Emin != self.smallest_energy_ever and self.smallest_energy_ever!=np.inf):
Emin = self.smallest_energy_ever
if ( Emin >= Emax ):
Emax = Emin+10.0
if ( Emin != self.Emin or Emax != self.Emax ):
self.redistribute_hist(Emin,Emax)
def redistribute_hist( self, Emin, Emax ):
"""
Redistributes the histogram to better fit the new energies
"""
if ( Emin > Emax ):
Emin = Emax-10.0
eps = 1E-8
Emax += eps
old_E = np.linspace( self.Emin, self.Emax, self.Nbins )
new_E = np.linspace( Emin, Emax, self.Nbins )
interp_hist = interpolate.interp1d( old_E, self.histogram, bounds_error=False, fill_value=0 )
new_hist = interp_hist(new_E)
interp_logdos = interpolate.interp1d( old_E, self.logdos, bounds_error=False, fill_value=0 )
new_logdos = interp_logdos(new_E)
interp_var = interpolate.interp1d( old_E, self.growth_variance, bounds_error=False, fill_value="extrapolate" )
self.growth_variance = interp_var( new_E )
# Scale
if ( np.sum(new_hist) > 0 ):
new_hist *= np.sum(self.histogram)/np.sum(new_hist)
if ( np.sum(new_logdos) > 0 ):
new_logdos *= np.sum(self.logdos)/np.sum(new_logdos)
self.histogram = np.floor(new_hist).astype(np.int32)
self.logdos = new_logdos
for i in range(len(self.histogram)):
if ( self.histogram[i] == 0 ):
# Set the DOS to 1 if the histogram indicates that it has never been visited
# This just an artifact of the interpolation and setting it low will make
# sure that these parts of the space gets explored
self.logdos[i] = 0.0
self.Emin = Emin
self.Emax = Emax
def get_growth_fluctuation( self ):
"""
Returns the fluctuation of the growth term
"""
N = np.sum(self.histogram)
if ( N <= 1 ):
return None
std = | np.sqrt( self.growth_variance/N ) | numpy.sqrt |
# "Lorenz-95" (or 96) model.
#
# A summary for the purpose of DA is provided in
# section 3.5 of thesis found at
# ora.ox.ac.uk/objects/uuid:9f9961f0-6906-4147-a8a9-ca9f2d0e4a12
#
# A more detailed summary is given in Chapter 11 of
# Majda, Harlim: Filtering Complex Turbulent Systems"
#
# Note: implementation is ndim-agnostic.
#
# Note: the model integration is unstable (--> infinity)
# in the presence of large peaks in amplitude,
# Example: x = [0,-30,0,30]; step(x,dt=0.05,recursion=4).
# This may be occasioned by the Kalman analysis update,
# especially if the system is only partially observed.
# Is this effectively a CFL condition? Could be addressed by:
# - post-processing,
# - modifying the step() function, e.g.:
# - crop amplitude
# - or lowering dt
# - using an implicit time stepping scheme instead of rk4
import numpy as np
from scipy.linalg import circulant
try:
from tools.math import rk4, integrate_TLM, is1d
except:
from DAPPER.tools.math import rk4, integrate_TLM, is1d
Force = 8.0
prevent_blow_up = False
def dxdt(x):
a = x.ndim-1
s = lambda x,n: np.roll(x,-n,axis=a)
return (s(x,1)-s(x,-2))*s(x,-1) - x + Force
def step(x0, t, dt):
#if prevent_blow_up:
#clip = abs(x0)>30
#x0[clip] *= 0.1
return rk4(lambda t,x: dxdt(x), x0, np.nan, dt)
def TLM(x):
"""Tangent linear model"""
assert is1d(x)
m = len(x)
TLM = | np.zeros((m,m)) | numpy.zeros |
# Copyright 2021 NREL
# Licensed under the Apache License, Version 2.0 (the "License"); you may not
# use this file except in compliance with the License. You may obtain a copy of
# the License at http://www.apache.org/licenses/LICENSE-2.0
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations under
# the License.
from datetime import timedelta as td
from itertools import product
import numpy as np
import pandas as pd
from floris.utilities import wrap_360
from . import utilities as fsut
def df_movingaverage(
df_in,
cols_angular,
window_width=td(seconds=60),
min_periods=1,
center=True,
calc_median_min_max_std=False,
return_index_mapping=False,
):
# Copy and ensure dataframe is indexed by time
df = df_in.copy()
if "time" in df.columns:
df = df.set_index("time")
# Find non-angular columns
if isinstance(cols_angular, bool):
if cols_angular:
cols_angular = [c for c in df.columns]
else:
cols_angular = []
cols_regular = [c for c in df.columns if c not in cols_angular]
# Now calculate cos and sin components for angular columns
sin_cols = ["{:s}_sin".format(c) for c in cols_angular]
cos_cols = ["{:s}_cos".format(c) for c in cols_angular]
df[sin_cols] = np.sin(df[cols_angular] * np.pi / 180.0)
df[cos_cols] = np.cos(df[cols_angular] * np.pi / 180.0)
# Drop angular columns
df = df.drop(columns=cols_angular)
# Now calculate rolling (moving) average
df_roll = df.rolling(
window_width,
center=center,
axis=0,
min_periods=min_periods
)
# First calculate mean values of non-angular columns
df_ma = df_roll[cols_regular].mean().copy()
# Now add mean values of angular columns
df_ma[cols_angular] = wrap_360(
np.arctan2(
df_roll[sin_cols].mean().values,
df_roll[cos_cols].mean().values
) * 180.0 / np.pi
)
# Figure out which indices/data points belong to each window
if (return_index_mapping or calc_median_min_max_std):
df_tmp = df_ma[[]].copy().reset_index(drop=False)
df_tmp["tmp"] = 1
df_tmp = df_tmp.rolling(window_width, center=center, axis=0, on="time")["tmp"]
# Grab index of first and last time entry for each window
windows_min = list(df_tmp.apply(lambda x: x.index[0]).astype(int))
windows_max = list(df_tmp.apply(lambda x: x.index[-1]).astype(int))
# Now create a large array that contains the array of indices, with
# the values in each row corresponding to the indices upon which that
# row's moving/rolling average is based. Note that we purposely create
# a larger matrix than necessary, since some rows/windows rely on more
# data (indices) than others. This is the case e.g., at the start of
# the dataset, at the end, and when there are gaps in the data. We fill
# the remaining matrix entries with "-1".
dn = int(np.ceil(window_width/fsut.estimate_dt(df_in["time"]))) + 5
data_indices = -1 * np.ones((df_ma.shape[0], dn), dtype=int)
for ii in range(len(windows_min)):
lb = windows_min[ii]
ub = windows_max[ii]
ind = np.arange(lb, ub + 1, dtype=int)
data_indices[ii, ind - lb] = ind
# Calculate median, min, max, std if necessary
if calc_median_min_max_std:
# Append all current columns with "_mean"
df_ma.columns = ["{:s}_mean".format(c) for c in df_ma.columns]
# Add statistics for regular columns
funs = ["median", "min", "max", "std"]
cols_reg_stats = ["_".join(i) for i in product(cols_regular, funs)]
df_ma[cols_reg_stats] = df_roll[cols_regular].agg(funs).copy()
# Add statistics for angular columns
# Firstly, create matrix with indices for the mean values
data_indices_mean = np.tile(np.arange(0, df_ma.shape[0]), (dn, 1)).T
# Grab raw and mean data and format as numpy arrays
D = df_in[cols_angular].values
M = df_ma[["{:s}_mean".format(c) for c in cols_angular]].values
# Add NaN row as last row. This corresponds to the -1 indices
# that we use as placeholders. This way, those indices do not
# count towards the final statistics (median, min, max, std).
D = np.vstack([D, np.nan * np.ones(D.shape[1])])
M = np.vstack([M, np.nan * np.ones(M.shape[1])])
# Now create a 3D matrix containing all values. The three dimensions
# come from:
# > [0] one dimension containing the rolling windows,
# > [1] one with the raw data underlying each rolling window,
# > [2] one for each angular column within the dataset
values = D[data_indices, :]
values_mean = M[data_indices_mean, :]
# Center values around values_mean
values[values > (values_mean + 180.0)] += -360.0
values[values < (values_mean - 180.0)] += 360.0
# Calculate statistical properties and wrap to [0, 360)
values_median = wrap_360(np.nanmedian(values, axis=1))
values_min = wrap_360(np.nanmin(values, axis=1))
values_max = wrap_360(np.nanmax(values, axis=1))
values_std = wrap_360(np.nanstd(values, axis=1))
# Save to dataframe
df_ma[["{:s}_median".format(c) for c in cols_angular]] = values_median
df_ma[["{:s}_min".format(c) for c in cols_angular]] = values_min
df_ma[["{:s}_max".format(c) for c in cols_angular]] = values_max
df_ma[["{:s}_std".format(c) for c in cols_angular]] = values_std
if return_index_mapping:
return df_ma, data_indices
return df_ma
def df_downsample(
df_in,
cols_angular,
window_width=td(seconds=60),
min_periods=1,
center=False,
calc_median_min_max_std=False,
return_index_mapping=False,
):
# Copy and ensure dataframe is indexed by time
df = df_in.copy()
if "time" in df.columns:
df = df.set_index("time")
# Find non-angular columns
cols_regular = [c for c in df.columns if c not in cols_angular]
# Now calculate cos and sin components for angular columns
sin_cols = ["{:s}_sin".format(c) for c in cols_angular]
cos_cols = ["{:s}_cos".format(c) for c in cols_angular]
df[sin_cols] = np.sin(df[cols_angular] * np.pi / 180.0)
df[cos_cols] = np.cos(df[cols_angular] * np.pi / 180.0)
# Drop angular columns
df = df.drop(columns=cols_angular)
# Add _N for each variable to keep track of n.o. data points
cols_all = df.columns
cols_N = ["{:s}_N".format(c) for c in cols_all]
df[cols_N] = 1 - df[cols_all].isna().astype(int)
# Now calculate downsampled dataframe, automatically
# mark by label on the right (i.e., "past 10 minutes").
df_resample = df.resample(window_width, label="right", axis=0)
# First calculate mean values of non-angular columns
df_out = df_resample[cols_regular].mean().copy()
# Now add mean values of angular columns
df_out[cols_angular] = wrap_360(
np.arctan2(
df_resample[sin_cols].mean().values,
df_resample[cos_cols].mean().values
) * 180.0 / np.pi
)
# Check if we have enough samples for every measurement
if min_periods > 1:
N_counts = df_resample[cols_N].sum()
df_out[N_counts < min_periods] = None # Remove data relying on too few samples
# Figure out which indices/data points belong to each window
if (return_index_mapping or calc_median_min_max_std):
df_tmp = df[[]].copy().reset_index()
df_tmp["tmp"] = 1
df_tmp = df_tmp.resample(window_width, on="time", label="right", axis=0)["tmp"]
# Grab index of first and last time entry for each window
def get_first_index(x):
if len(x) <= 0:
return -1
else:
return x.index[0]
def get_last_index(x):
if len(x) <= 0:
return -1
else:
return x.index[-1]
windows_min = list(df_tmp.apply(get_first_index).astype(int))
windows_max = list(df_tmp.apply(get_last_index).astype(int))
# Now create a large array that contains the array of indices, with
# the values in each row corresponding to the indices upon which that
# row's moving/rolling average is based. Note that we purposely create
# a larger matrix than necessary, since some rows/windows rely on more
# data (indices) than others. This is the case e.g., at the start of
# the dataset, at the end, and when there are gaps in the data. We fill
# the remaining matrix entries with "-1".
dn = int(np.ceil(window_width/fsut.estimate_dt(df_in["time"]))) + 5
data_indices = -1 * np.ones((df_out.shape[0], dn), dtype=int)
for ii in range(len(windows_min)):
lb = windows_min[ii]
ub = windows_max[ii]
if not ((lb == -1) | (ub == -1)):
ind = np.arange(lb, ub + 1, dtype=int)
data_indices[ii, ind - lb] = ind
# Calculate median, min, max, std if necessary
if calc_median_min_max_std:
# Append all current columns with "_mean"
df_out.columns = ["{:s}_mean".format(c) for c in df_out.columns]
# Add statistics for regular columns
funs = ["median", "min", "max", "std"]
cols_reg_stats = ["_".join(i) for i in product(cols_regular, funs)]
df_out[cols_reg_stats] = df_resample[cols_regular].agg(funs).copy()
# Add statistics for angular columns
# Firstly, create matrix with indices for the mean values
data_indices_mean = np.tile(np.arange(0, df_out.shape[0]), (dn, 1)).T
# Grab raw and mean data and format as numpy arrays
D = df_in[cols_angular].values
M = df_out[["{:s}_mean".format(c) for c in cols_angular]].values
# Add NaN row as last row. This corresponds to the -1 indices
# that we use as placeholders. This way, those indices do not
# count towards the final statistics (median, min, max, std).
D = np.vstack([D, np.nan * np.ones(D.shape[1])])
M = np.vstack([M, np.nan * np.ones(M.shape[1])])
# Now create a 3D matrix containing all values. The three dimensions
# come from:
# > [0] one dimension containing the rolling windows,
# > [1] one with the raw data underlying each rolling window,
# > [2] one for each angular column within the dataset
values = D[data_indices, :]
values_mean = M[data_indices_mean, :]
# Center values around values_mean
values[values > (values_mean + 180.0)] += -360.0
values[values < (values_mean - 180.0)] += 360.0
# Calculate statistical properties and wrap to [0, 360)
values_median = wrap_360(np.nanmedian(values, axis=1))
values_min = wrap_360(np.nanmin(values, axis=1))
values_max = wrap_360(np.nanmax(values, axis=1))
values_std = wrap_360(np.nanstd(values, axis=1))
# Save to dataframe
df_out[["{:s}_median".format(c) for c in cols_angular]] = values_median
df_out[["{:s}_min".format(c) for c in cols_angular]] = values_min
df_out[["{:s}_max".format(c) for c in cols_angular]] = values_max
df_out[["{:s}_std".format(c) for c in cols_angular]] = values_std
if center:
# Shift time column towards center of the bin
df_out.index = df_out.index - window_width / 2.0
if return_index_mapping:
return df_out, data_indices
return df_out
def df_resample_by_interpolation(
df,
time_array,
circular_cols,
interp_method='linear',
max_gap=None,
verbose=True
):
# Copy with properties but no actual data
df_res = df.head(0).copy()
# Remove timezones, if any
df = df.copy()
time_array = [pd.to_datetime(t).tz_localize(None) for t in time_array]
time_array = np.array(time_array, dtype='datetime64')
df["time"] = df["time"].dt.tz_localize(None)
# Fill with np.nan values and the correct time array (without tz)
df_res['time'] = time_array
t0 = time_array[0]
df_t = np.array(df['time'] - t0, dtype=np.timedelta64)
xp = df_t/np.timedelta64(1, 's') # Convert to regular seconds
xp = np.array(xp, dtype=float)
# Normalize time variables
time_array = np.array([t - t0 for t in time_array], dtype=np.timedelta64)
x = time_array/ | np.timedelta64(1, 's') | numpy.timedelta64 |
import numpy as np
import pandas as pd
import numpy.random as npr
from operator import xor
import seaborn as sns
import matplotlib.pyplot as plt
sns.set(font_scale = 1.3)
sns.set_style('white')
def pns(z, y):
y_giv_z = np.sum(z * y) / np.sum(z)
y_giv_nz = np.sum((1-z) * y) / np.sum(1-z)
lb = np.maximum(0, y_giv_z - y_giv_nz)
ub = np.minimum(y_giv_z, 1-y_giv_nz)
return lb, ub
def cond_pns(z0, y, cond):
# cond is the column index of z1 this is being flipped; all other columns are being held fixed
z_full = z0.copy()
nz_full = z0.copy()
nz_full[:,cond] = 1 - nz_full[:,cond]
z = np.prod(z_full, axis=1)
nz = np.prod(nz_full, axis=1)
y_giv_z = np.sum(z * y) / np.sum(z)
y_giv_nz = np.sum((nz) * y) / np.sum(nz)
lb = np.maximum(0, y_giv_z - y_giv_nz)
ub = np.minimum(y_giv_z, 1-y_giv_nz)
return lb, ub
def pn(z, y):
y_giv_z = np.sum(z * y) / np.sum(z)
pns_lb, pns_ub = pns(z,y)
lb = pns_lb / y_giv_z
ub = pns_ub / y_giv_z
return lb, ub
def ps(z, y):
ny_giv_nz = 1 - np.sum((1-z) * y) / np.sum(1-z)
pns_lb, pns_ub = pns(z,y)
lb = pns_lb / ny_giv_nz
ub = pns_ub / ny_giv_nz
return lb, ub
def eval_pns_pn_ps(x, y, lower=True):
pns_l_y, pns_u_y = pns(x,y)
pn_l_y, pn_u_y = pn(x,y)
ps_l_y, ps_u_y = ps(x,y)
if lower:
return pns_l_y, pn_l_y, ps_l_y
else:
return pns_u_y, pn_u_y, ps_u_y
def eval_cond_pns(x, y, lower=True):
pns_l_ys, pns_u_ys = [], []
for i in range(x.shape[1]):
pns_l_y, pns_u_y = cond_pns(x,y,i)
pns_l_ys.append(pns_l_y)
pns_u_ys.append(pns_u_y)
if lower:
return pns_l_ys
else:
return pns_u_ys
def gen_z(p, noise_p, n_samples):
z1 = npr.binomial(1, p=p, size=n_samples)
z2 = z1^(npr.binomial(1, p=noise_p, size=n_samples))
# print("correlation z1, z2\n", np.corrcoef(z1, z2))
# as noise_p increase, z1 and z2 are less highly correlated.
return z1, z2
def gen_y(z1, z2, noise_y, n_samples):
y1 = z1^(npr.binomial(1, p=noise_y, size=n_samples))
y2 = (z1 * z2)^(npr.binomial(1, p=noise_y, size=n_samples))
# z1 is necessary and sufficient for y1.
# z1 is necessary but insufficient for y2.
# z2 is neither necessary nor sufficient for y1.
# z2 is necessary but insufficient for y2.
# z1 * z2 is necessary and sufficient for y2.
return y1, y2
# params = {'p': 0.5, # p(z1=1)
# 'noise_p': 0.2, # p(z2|z1)
# 'noise_y': 0.1, # p(y|z1,z2)
# 'n_samples': 1000,
# 'n_trials': 100}
def calc_all(p, noise_p, noise_y, n_samples, n_trials=100):
res_all = []
params = {'p': p, # p(z1=1)
'noise_p': noise_p, # p(z2|z1)
'noise_y': noise_y, # p(y|z1,z2)
'n_samples': n_samples,
'n_trials': 100}
for _ in range(params['n_trials']):
res = pd.DataFrame(params, index=[0])
z1, z2 = gen_z(params['p'], params['noise_p'], params['n_samples'])
y1, y2 = gen_y(z1, z2, params['noise_y'], params['n_samples'])
params['z1_z2_corr'] = | np.corrcoef(z1, z2) | numpy.corrcoef |
import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
from torch.utils.data import DataLoader, SubsetRandomSampler
from torch.utils.tensorboard import SummaryWriter
from tqdm import tqdm
from pathlib import Path
import itertools
import sys
from datasets import lits17_no_slice, brats20_no_slice, kits21_no_slice
from losses import MultiTverskyLoss
from UNet3D import Encoder, Decoder
from scipy.stats import entropy
from matplotlib import colors
from mpl_toolkits.axes_grid1 import make_axes_locatable
from evaluations import eval_uncertainty, eval_dice
def forward(encoder, decoder_mean, decoder_var, x):
out, context_1, context_2, context_3, context_4 = encoder(x)
mean = decoder_mean(out, context_1, context_2, context_3, context_4)
var = decoder_var(out, context_1, context_2, context_3, context_4)
return mean, var
def predict(encoder, decoder_mean, decoder_var, x, T=10):
mean, var = forward(encoder, decoder_mean, decoder_var, x)
running_x = torch.zeros(var.shape).to(x.device)
noise = torch.randn(var.shape).to(x.device)
for i in range(T):
x = mean + var * noise
running_x += F.softmax(x, dim=1)
return running_x / T
# returns aleatoric uncertainty map, yhat
def aleatoric_uncertainty_density_model(encoder, decoder_mean, decoder_var, x, T=10):
encoder = encoder.eval()
decoder_mean = decoder_mean.eval()
decoder_var = decoder_var.eval()
with torch.no_grad():
yhat = predict(encoder, decoder_mean, decoder_var, x, T=T)
return entropy(yhat.cpu().numpy()[0], axis=0), yhat[0, 1].cpu().numpy()
# returns epistemic uncertainty map, yhat
def epistemic_uncertainty_mc_dropout(encoder, decoder_mean, decoder_var, x, K=10):
encoder = encoder.eval()
decoder_mean = decoder_mean.eval()
decoder_var = decoder_var.eval()
enable_dropout(encoder)
enable_dropout(decoder_mean)
yhat = []
for k in range(K):
with torch.no_grad():
yhat_mean, yhat_var = forward(encoder, decoder_mean, decoder_var, x)
yhat_mean = F.softmax(yhat_mean, dim=1).cpu().numpy()
yhat.append(yhat_mean)
yhat = np.stack(yhat).mean(axis=0)
epis = entropy(yhat, axis=1)
return epis[0], yhat[0, 1]
def enable_dropout(model):
for m in model.modules():
if m.__class__.__name__.startswith('Dropout'):
m.train()
ROOT = Path('/scratch/zc2357/cv/final/nyu-cv2271-final/baseline/runs/')
tasks = [
{
'name': 'lits17_baseline',
'dataset': lits17_no_slice,
'in_channels': 1,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec12_04-00-51_gr017.nyu.cluster_lits17_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'lits17_epoch_55_step_5824_encoder.pth',
'decoder_mean_path': 'lits17_epoch_55_epoch_55_loss_0.04442_decoder_mean.pth',
'decoder_var_path': 'lits17_epoch_55_epoch_55_loss_0.04442_decoder_var.pth',
},
{
'name': 'lits17_cotraining',
'dataset': lits17_no_slice,
'in_channels': 1,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec11_19-20-25_gr011.nyu.cluster_lits17_brats20_kits21_cotraining_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'lits17_epoch_59_step_6241_encoder.pth',
'decoder_mean_path': 'lits17_epoch_59_epoch_59_loss_0.04083_decoder_mean.pth',
'decoder_var_path': 'lits17_epoch_59_epoch_59_loss_0.04083_decoder_var.pth',
},
{
'name': 'brats20_baseline',
'dataset': brats20_no_slice,
'in_channels': 4,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec11_14-53-08_gr011.nyu.cluster_brats20_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'brats20_epoch_21_step_6490_encoder.pth',
'decoder_mean_path': 'brats20_epoch_21_epoch_21_loss_0.09565_decoder_mean.pth',
'decoder_var_path': 'brats20_epoch_21_epoch_21_loss_0.09565_decoder_var.pth',
},
{
'name': 'brats20_cotraining',
'dataset': brats20_no_slice,
'in_channels': 4,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec11_19-20-25_gr011.nyu.cluster_lits17_brats20_kits21_cotraining_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'brats20_epoch_14_step_4596_encoder.pth',
'decoder_mean_path': 'brats20_epoch_14_epoch_14_loss_0.09117_decoder_mean.pth',
'decoder_var_path': 'brats20_epoch_14_epoch_14_loss_0.09117_decoder_var.pth',
},
{
'name': 'kits21_baseline',
'dataset': kits21_no_slice,
'in_channels': 1,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec11_05-24-43_gr038.nyu.cluster_kits21_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'kits21_epoch_25_step_6240_encoder.pth',
'decoder_mean_path': 'kits21_epoch_25_epoch_25_loss_0.05592_decoder_mean.pth',
'decoder_var_path': 'kits21_epoch_25_epoch_25_loss_0.05592_decoder_var.pth',
},
{
'name': 'kits21_cotraining',
'dataset': kits21_no_slice,
'in_channels': 1,
'n_classes': 2,
'enabled': True,
'root': ROOT / 'Dec11_19-20-25_gr011.nyu.cluster_lits17_brats20_kits21_cotraining_baseline_lr1.0e-04_weightDecay1.0e-02',
'encoder_path': 'kits21_epoch_25_step_6241_encoder.pth',
'decoder_mean_path': 'kits21_epoch_25_epoch_25_loss_0.05474_decoder_mean.pth',
'decoder_var_path': 'kits21_epoch_25_epoch_25_loss_0.05474_decoder_var.pth',
},
]
# DO NOT CHANGE; CHANGING THESE BREAKS REPLICATION
SEED = 42
TRAIN_VAL_SPLIT = 0.8 # 80% training
# /DO NOT CHANGE
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
for i in range(len(tasks)):
task = tasks[i]
if task['enabled']:
n = len(task['dataset'])
idxrange = | np.arange(n) | numpy.arange |
"""
Draw Figures - Chapter 6
This script generates all of the figures that appear in Chapter 6 of the textbook.
Ported from MATLAB Code
<NAME>
25 March 2021
"""
import utils
import matplotlib.pyplot as plt
import numpy as np
import seaborn as sns
def make_all_figures(close_figs=False):
"""
Call all the figure generators for this chapter
:close_figs: Boolean flag. If true, will close all figures after generating them; for batch scripting.
Default=False
:return: List of figure handles
"""
# Find the output directory
prefix = utils.init_output_dir('chapter6')
# Activate seaborn for prettier plots
sns.set()
# Generate all figures
fig1 = make_figure_1(prefix)
fig2 = make_figure_2(prefix)
fig3 = make_figure_3(prefix)
fig4 = make_figure_4(prefix)
figs = [fig1, fig2, fig3, fig4]
if close_figs:
for fig in figs:
plt.close(fig)
return None
else:
plt.show()
return figs
def make_figure_1(prefix=None):
"""
Figure 1, Bayesian Example
Ported from MATLAB Code
<NAME>
25 March 2021
:param prefix: output directory to place generated figure
:return: figure handle
"""
d_lam_narrow_beam = 4
num_array_elements = 10
psi_0 = 135*np.pi/180
# psi_0 = 95*np.pi/180
# Narrow Beam (Marginal Distribution)
def element_pattern(psi):
return np.absolute(np.cos(psi-np.pi/2))**1.2
def array_function_narrow(psi):
numerator = np.sin(np.pi*d_lam_narrow_beam*num_array_elements*(np.cos(psi)-np.cos(psi_0)))
denominator = np.sin(np.pi*d_lam_narrow_beam*(np.cos(psi)-np.cos(psi_0)))
# Throw in a little error handling; division by zero throws a runtime warning
limit_mask = denominator == 0
valid_mask = np.logical_not(limit_mask)
valid_result = np.absolute(numerator[valid_mask] / denominator[valid_mask])/num_array_elements
return np.piecewise(x=psi, condlist=[limit_mask],
funclist=[1, valid_result])
# Wide Beam (Prior Distribution)
d_lam_wide_beam = .5
def array_function_wide(psi):
numerator = np.sin(np.pi*d_lam_wide_beam*num_array_elements*(np.cos(psi)-np.cos(psi_0)))
denominator = np.sin(np.pi*d_lam_wide_beam*(np.cos(psi)-np.cos(psi_0)))
# Throw in a little error handling; division by zero throws a runtime warning
limit_mask = denominator == 0
valid_mask = np.logical_not(limit_mask)
valid_result = np.absolute(numerator[valid_mask] / denominator[valid_mask])/num_array_elements
return np.piecewise(x=psi, condlist=[limit_mask],
funclist=[1, valid_result])
fig1 = plt.figure()
psi_vec = np.arange(start=0, step=np.pi/1000, stop=np.pi)
plt.plot(psi_vec, element_pattern(psi_vec)*array_function_narrow(psi_vec), label='Narrow Beam (marginal)')
plt.plot(psi_vec, array_function_wide(psi_vec), label='Wide Beam (prior)')
plt.xlabel(r'$\psi$')
plt.legend(loc='upper left')
# Save figure
if prefix is not None:
plt.savefig(prefix + 'fig1.svg')
plt.savefig(prefix + 'fig1.png')
return fig1
def make_figure_2(prefix=None):
"""
Figure 2, Convex Optimization Example
Ported from MATLAB Code
<NAME>
25 March 2021
:param prefix: output directory to place generated figure
:return: figure handle
"""
# True position
x0 = np.array([1, .5])
# Grid
xx = np.expand_dims(np.arange(start=-5, step=.01, stop=5), axis=1)
yy = np.expand_dims(np.arange(start=-3, step=.01, stop=3), axis=0)
# Broadcast xx and yy
out_shp = np.broadcast(xx, yy)
xx_full = np.broadcast_to(xx, out_shp.shape)
yy_full = np.broadcast_to(yy, out_shp.shape)
# Crude cost function; the different weights force an elliptic shape
f = 1.*(xx-x0[0])**2 + 5.*(yy-x0[1])**2
# Iterative estimates
x_est = np.array([[-3, 2],
[2, -1.5],
[1, 2.2],
[1, .6]])
# Plot results
fig2 = plt.figure()
plt.contour(xx_full, yy_full, f)
plt.scatter(x0[0], x0[1], marker='^', label='True Minimum')
plt.plot(x_est[:, 0], x_est[:, 1], linestyle='--', marker='+', label='Estimate')
plt.text(-3, 2.1, 'Initial Estimate', fontsize=10)
plt.legend(loc='lower left')
if prefix is not None:
plt.savefig(prefix + 'fig2.svg')
plt.savefig(prefix + 'fig2.png')
return fig2
def make_figure_3(prefix=None):
"""
Figure 3, Tracker Example
Ported from MATLAB Code
<NAME>
25 March 2021
:param prefix: output directory to place generated figure
:return: figure handle
"""
# Measurements
y = np.array([1, 1.1, 1.3, 1.4, 1.35, 1.3, .7, .75])
# Estimates
x = np.array([1, 1.05, 1.2, 1.35, 1.45, 1.35, 1.2, .8])
# Confidence Intervals
s2 = np.array([.8, .5, .4, .3, .3, .2, .2, .6])
num_updates = y.size
# Plot result
fig3 = plt.figure()
for i in np.arange(start=1, stop=s2.size):
plt.fill(i + np.array([.2, .2, -.2, -.2, .2]),
x[i] + s2[i-1]*np.array([-1, 1, 1, -1, -1]),
color=(.8, .8, .8), label=None)
x_vec = np.arange(num_updates)
plt.scatter(x_vec, y, marker='x', label='Measurement', zorder=10)
plt.plot(x_vec, x, linestyle='-.', marker='o', label='Estimate')
plt.legend(loc='upper left')
plt.xlabel('Time')
plt.ylabel(r'Parameter ($\theta$)')
if prefix is not None:
plt.savefig(prefix + 'fig3.svg')
plt.savefig(prefix + 'fig3.png')
return fig3
def make_figure_4(prefix=None):
"""
Figure 4, Angle Error Variance
Ported from MATLAB Code
<NAME>
25 March 2021
:param prefix: output directory to place generated figure
:return: figure handle
"""
# Sensor Coordinates
x0 = np.array([0, 0])
# xs = np.array([2, 1.4])
# Bearing and confidence interval
aoa = 40
aoa_rad = | np.deg2rad(aoa) | numpy.deg2rad |
import numpy as np
import oddt
from oddt.spatial import distance
from oddt.docking.internal import vina_ligand, get_children, get_close_neighbors, num_rotors_pdbqt
from oddt.scoring.functions import nnscore, rfscore, ri_score
class CustomEngine(object):
def __init__(self, rec, lig=None, scoring_func=None, box=None, box_size=1.):
self.box_size = box_size
self.scoring_func = scoring_func
if rec:
if isinstance(rec, str):
receptor_format = rec.split('.')[-1]
try:
self.receptor = next(oddt.toolkit.readfile(receptor_format, rec))
except ValueError:
raise Exception('Unsupported receptor file format.')
elif isinstance(rec, oddt.toolkit.Molecule):
self.receptor = rec
else:
raise Exception('Unsupported protein format.')
self.receptor.protein = True
self.receptor.removeh()
self.set_protein(self.receptor)
if lig:
if isinstance(lig, oddt.toolkit.Molecule):
self.ligand = lig
else:
raise Exception('Unsupported ligand format.')
self.ligand.removeh()
self.set_ligand(self.ligand)
self.prepare_scoring_function()
self.set_box(box)
self.mask_inter = {}
self.mask_intra = {}
def set_box(self, box):
if box is not None:
self.box = np.array(box)
# delete unused atoms
r = self.rec_dict['coords']
# X, Y, Z within box and cutoff
mask = (self.box[0][0] - 8 <= r[:, 0]) & (r[:, 0] <= self.box[1][0] + 8)
mask *= (self.box[0][1] - 8 <= r[:, 1]) & (r[:, 1] <= self.box[1][1] + 8)
mask *= (self.box[0][2] - 8 <= r[:, 2]) & (r[:, 2] <= self.box[1][2] + 8)
self.rec_dict = self.rec_dict # [mask]
else:
self.box = box
def set_protein(self, rec):
"""Prepare protein to docking."""
if rec is None:
self.rec_dict = None
self.mask_inter = {}
else:
self.rec_dict = rec.atom_dict[rec.atom_dict['atomicnum'] != 1].copy()
self.mask_inter = {}
def set_ligand(self, lig):
"""Prepare ligand to docking."""
lig_hvy_mask = (lig.atom_dict['atomicnum'] != 1)
self.lig_dict = lig.atom_dict[lig_hvy_mask].copy()
self.num_rotors = num_rotors_pdbqt(lig)
self.mask_inter = {}
self.mask_intra = {}
# Find distant members (min 3 consecutive bonds)
mask = np.vstack([~get_close_neighbors(lig, i, num_bonds=3)
for i in range(len(lig.atoms))])
mask = mask[lig_hvy_mask[np.newaxis, :] * lig_hvy_mask[:, np.newaxis]]
self.lig_distant_members = mask.reshape(lig_hvy_mask.sum(), lig_hvy_mask.sum())
# prepare rotors dictionary
self.rotors = []
for b in lig.bonds:
if b.isrotor:
a2 = int(b.atoms[0].idx0)
a3 = int(b.atoms[1].idx0)
for n in b.atoms[0].neighbors:
if a3 != int(n.idx0) and n.atomicnum != 1:
a1 = int(n.idx0)
break
for n in b.atoms[1].neighbors:
if a2 != int(n.idx0) and n.atomicnum != 1:
a4 = int(n.idx0)
break
rot_mask = get_children(lig, a3, a2)[lig_hvy_mask]
# translate atom indicies to lig_dict indicies (heavy only)
a1 = np.argwhere(self.lig_dict['id'] == a1).flatten()[0]
a2 = np.argwhere(self.lig_dict['id'] == a2).flatten()[0]
a3 = np.argwhere(self.lig_dict['id'] == a3).flatten()[0]
a4 = np.argwhere(self.lig_dict['id'] == a4).flatten()[0]
# rotate smaller part of the molecule
if rot_mask.sum() > len(rot_mask):
rot_mask = -rot_mask
a4, a3, a2, a1 = a1, a2, a3, a4
self.rotors.append({'atoms': (a1, a2, a3, a4), 'mask': rot_mask})
# Setup cached ligand coords
self.lig = vina_ligand(self.lig_dict['coords'].copy(), len(self.rotors), self, self.box_size)
def prepare_scoring_function(self):
"""Initiate scoring functions based on AI/ML methods."""
sf = None
if self.scoring_func == 'nnscore':
sf = nnscore.load()
sf.set_protein(self.receptor)
elif self.scoring_func == 'rfscore':
sf = rfscore.load()
sf.set_protein(self.receptor)
self.trained_scorer = sf
def score(self, coords=None):
"""Score given coordinates."""
if coords is None:
coords = self.lig_dict['coords']
self.ligand.coords = coords
if self.trained_scorer: # nnscore/rfscore
return self.trained_scorer.predict([self.ligand])[0]
elif self.scoring_func == 'ri_score':
return ri_score(self.ligand, self.receptor)
elif self.scoring_func == 'interaction_energy':
return np.sum(self.score_inter(coords) + self.score_intra(coords))
else:
raise Exception('Unsupported scoring function.')
def score_inter(self, coords):
"""Calculate inter-molecular energy between protein and ligand."""
# Inter-molecular
r = distance(self.rec_dict['coords'], coords)
d = (r - self.rec_dict['radius'][:, np.newaxis] - self.lig_dict['radius'][np.newaxis, :])
mask = r < 8
inter = []
# Gauss 1
inter.append( | np.exp(-(d[mask] / 0.5)**2) | numpy.exp |
# -*- coding: utf-8 -*-
"""
Container for the primary EMUS routines.
"""
import numpy as np
from scipy.special import logsumexp
try:
import usample.linalg as lm
import usample.autocorrelation as autocorrelation
from .usutils import unpackNbrs
except ImportError:
import linalg as lm
import autocorrelation as autocorrelation
from usutils import unpackNbrs
def calculate_obs(psis,z,f1data,f2data=None):
"""Estimates the value of an observable or ratio of observables.
Parameters
----------
psis : 3D data structure
Data structure containing psi values. See documentation for a detailed explanation.
z : 1D array
Array containing the normalization constants
f1data : 2D data structure
Trajectory of observable in the numerator. First dimension corresponds to the umbrella index and the second to the point in the trajectory.
f2data : 2D data structure, optional
Trajectory of observable in the denominator.
Returns
-------
avg : float
The estimate of <f_1>/<f_2>.
"""
f1avg = 0
f2avg = 0
for i,psi_i in enumerate(psis):
psi_xi = np.array(psi_i)
psi_i_sum = np.sum(psi_xi,axis=1)
f1_i = | np.array(f1data[i]) | numpy.array |
import sys
import gzip
import ubjson
import pickle
import numpy as np
import pprint
pp = pprint.PrettyPrinter()
from time import sleep
from copy import deepcopy
from functools import partial
from parser.reader import Game
from sklearn import svm
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.decomposition import PCA
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LSTM
##########################################################
# Utils
##########################################################
def get_next_game(f):
pgn = f.readline()
while True:
if "EOF" in pgn: return None
new_line = f.readline()
pgn += new_line
if new_line[:2] == "1.": break
return pgn
def serialize_data(src, data):
with gzip.open(src, "wb") as f:
ubjson.dump(data, f)
def deserialize_data(src):
with gzip.open(src, "rb") as f:
return ubjson.load(f)
##########################################################
# Extractors
##########################################################
def extract_pieces(game):
game.go_to_move(len(game.moves))
a, b = game.num_pieces()
out = np.zeros(256)
out[(a-1)*16 + (b-1)] = 1
return out
def extract_num_pieces_over_n_moves(game, n=50):
n_moves_vector = []
for i in range(1, n+1):
try:
game.go_to_move(i)
next_num_pieces = game.num_pieces()[i%2]
except Exception:
next_num_pieces = 0
n_moves_vector.append(next_num_pieces)
return np.array(n_moves_vector)
def generate_coefficient_matrix_for_extract_pieces(clf):
'''
Generate a 16 x 16 coefficient matrix from a 256-long vector of
coefficients (from a trained classifier)
Probably should only be used for the above extractor `extract_pieces`
'''
coeff_matrix = np.zeros((16, 16))
for a in range(16):
for b in range(16):
coeff_matrix[a][b] = round(clf.coef_[0][a*16 + b], 2)
return coeff_matrix
def extract_coords_n_moves(game, n=15):
return game.vectorize_moves(n)
def extract_sparse_vector(game):
full_game_vector = np.array([])
next_state = game.board_state(0)
for i in range(1, 36):
try: next_state = game.board_state(i)
except Exception: pass
full_game_vector = np.concatenate((full_game_vector, next_state))
return full_game_vector
def extract_sparse_vector_n_moves_even(game, n=14):
n_moves_vector = np.array([])
next_state = game.board_state(0)
for i in range(1, n+1):
try: next_state = game.board_state(i)
except Exception: pass
if i % 2 == 0:
n_moves_vector = | np.concatenate((n_moves_vector, next_state)) | numpy.concatenate |
import numpy as np
import glob
import os
import matplotlib.pyplot as plt
import corner
def load_summary(filename):
dtype=[('minr', 'f8'),
('maxr', 'f8'),
('ca_ratio', 'f8'),
('ba_ratio', 'f8'),
('a', 'f8'),
('center', 'f8'),
('width', 'f8'),
('mu', 'f8')]
summary = np.loadtxt(filename, dtype=dtype)
return summary
def load_experiment(input_path="../data/mstar_selected_summary/vmax_sorted/", n_sat=11, full_data=False):
files = glob.glob(input_path+"M31_group_*_nsat_{}.dat".format(n_sat))
group_id = []
for f in files:
i = int(f.split("_")[-3])
if i not in group_id:
group_id.append(i)
#print(group_id, len(group_id))
n_groups = len(group_id)
fields = ['width','mu', 'a', 'ba_ratio', 'ca_ratio']
M31_all = {}
MW_all = {}
if full_data:
for field in fields:
M31_all[field] = np.empty((0))
MW_all[field] = np.empty((0))
M31_all[field+'_random'] = np.empty((0))
MW_all[field+'_random'] = np.empty((0))
else:
for field in fields:
M31_all[field] = | np.ones(n_groups) | numpy.ones |
# code to calculate fundamental stellar parameters and distances using
# a "direct method", i.e. adopting a fixed reddening map and bolometric
# corrections
import astropy.units as units
from astropy.coordinates import SkyCoord
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from scipy.interpolate import RegularGridInterpolator
import pdb
def distance_likelihood(plx, plxe, ds):
"""Distance Likelihood
Likelihood of distance given measured parallax
Args:
plx (float): parallax
plxe (float): parallax uncertainty
ds (array): distance in parsecs
Returns:
array: likelihood (not log-likelihood)
"""
lh = ((1.0/(np.sqrt(2.0*np.pi)*plxe))
* np.exp( (-1.0/(2.0*plxe**2))*(plx - 1.0/ds)**2))
return lh
def distance_prior(ds, L):
"""Distance prior
Exponetial decreasing vol density prior
Returns:
array: prior probability (not log-prior)
"""
prior = ds**2/(2.0*L**3.0)*np.exp(-ds/L)
return prior
def stparas(input, dnumodel=-99, bcmodel=-99, dustmodel=-99, dnucor=-99,
useav=-99, plot=0, band='k', ext=-99):
# IAU XXIX Resolution, Mamajek et al. (2015)
r_sun = 6.957e10
gconst = 6.67408e-8
gm = 1.3271244e26
m_sun = gm/gconst
rho_sun = m_sun/(4./3.*np.pi*r_sun**3)
g_sun = gconst*m_sun/r_sun**2.
# solar constants
numaxsun = 3090.
dnusun = 135.1
teffsun = 5777.
Msun = 4.74 # NB this is fixed to MESA BCs!
# assumed uncertainty in bolometric corrections
err_bc=0.02
# assumed uncertainty in extinction
err_ext=0.02
# object containing output values
out = resdata()
## extinction coefficients
extfactors=ext
if (len(band) == 4):
bd=band[0:1]
else:
bd=band[0:2]
######################################
# case 1: input is parallax + colors #
######################################
#with h5py.File(bcmodel,'r') as h5:
teffgrid = bcmodel['teffgrid'][:]
logggrid = bcmodel['logggrid'][:]
fehgrid = bcmodel['fehgrid'][:]
avgrid = bcmodel['avgrid'][:]
bc_band = bcmodel['bc_'+bd][:]
if ((input.plx > 0.)):
# load up bolometric correction grid
# only K-band for now
points = (teffgrid,logggrid,fehgrid,avgrid)
values = bc_band
interp = RegularGridInterpolator(points,values)
### Monte Carlo starts here
# number of samples
nsample = int(1e5)
# length scale for exp decreasing vol density prior in pc
L = 1350.0
# maximum distance to sample (in pc)
maxdis = 1e5
# get a rough maximum and minimum distance
tempdis = 1.0/input.plx
tempdise = input.plxe/input.plx**2
maxds = tempdis + 5.0*tempdise
minds = tempdis - 5.0*tempdise
ds = np.arange(1.0, maxdis, 1.0)
lh = distance_likelihood(input.plx, input.plxe, ds)
prior = distance_prior(ds, L)
dis = lh*prior
dis2 = dis/np.sum(dis)
norm = dis2/np.max(dis2)
# Deal with negative and positive parallaxes differently:
if tempdis > 0:
# Determine maxds based on posterior:
um = np.where((ds > tempdis) & (norm < 0.001))[0]
# Determine minds just like maxds:
umin = np.where((ds < tempdis) & (norm < 0.001))[0]
else:
# Determine maxds based on posterior, taking argmax
# instead of tempdis which is wrong:
um = np.where((ds > np.argmax(norm)) & (norm < 0.001))[0]
# Determine minds just like maxds:
umin = np.where((ds < np.argmax(norm)) & (norm < 0.001))[0]
if (len(um) > 0):
maxds = np.min(ds[um])
else:
maxds = 1e5
if (len(umin) > 0):
minds = np.max(ds[umin])
else:
minds = 1.0
print('using max distance:', maxds)
print('using min distance:', minds)
ds = np.linspace(minds,maxds,nsample)
lh = distance_likelihood(input.plx, input.plxe, ds)
prior = distance_prior(ds, L)
dis = lh*prior
dis2=dis/np.sum(dis)
# sample distances following the discrete distance posterior
np.random.seed(seed=10)
dsamp = np.random.choice(ds, p=dis2, size=nsample)
# interpolate dustmodel dataframe to determine values of reddening.
if (isinstance(dustmodel,pd.DataFrame) == False):
ebvs = np.zeros(len(dsamp))
avs = ebvs
else:
xp = np.concatenate(
([0.0], np.array(dustmodel.columns[2:].str[3:], dtype='float'))
)
fp = np.concatenate(([0.0], | np.array(dustmodel.iloc[0][2:]) | numpy.array |
import tensorflow as tf
from sklearn.preprocessing import MinMaxScaler
from sklearn.model_selection import train_test_split
import math
import numpy as np
class DataGenerator:
def __init__(self, config, features, labels):
self.config = config
self.features_train, self.features_test, self.labels_train, self.labels_test = train_test_split(features, labels, test_size=self.config.test_size, shuffle=False)
self.features_scaler = MinMaxScaler()
self.features_scaler.fit(self.features_train)
self.labels_scaler = MinMaxScaler()
self.labels_scaler.fit(self.labels_train)
self.X_train, self.Y_train = self.sliding_window(self.features_scaler.transform(self.features_train), self.labels_scaler.transform(self.labels_train), self.config.sequence_length)
self.X_test, self.Y_test = self.sliding_window(self.features_scaler.transform(self.features_test), self.labels_scaler.transform(self.labels_test), self.config.sequence_length)
self.num_iter_per_epoch = math.ceil(len(self.X_train) / self.config.batch_size)
def sliding_window(self, features, labels, sequence_length, step=1):
X = []
Y = []
for i in range(0, len(features) - sequence_length, step):
X.append(features[i:i + sequence_length])
Y.append(labels[i + sequence_length])
X = np.array(X)
Y = | np.array(Y) | numpy.array |
import os
if not os.path.exists("temp"):
os.mkdir("temp")
def add_pi_obj_func_test():
import os
import pyemu
pst = os.path.join("utils","dewater_pest.pst")
pst = pyemu.optimization.add_pi_obj_func(pst,out_pst_name=os.path.join("temp","dewater_pest.piobj.pst"))
print(pst.prior_information.loc["pi_obj_func","equation"])
#pst._update_control_section()
assert pst.control_data.nprior == 1
def fac2real_test():
import os
import numpy as np
import pyemu
# pp_file = os.path.join("utils","points1.dat")
# factors_file = os.path.join("utils","factors1.dat")
# pyemu.utils.gw_utils.fac2real(pp_file,factors_file,
# out_file=os.path.join("utils","test.ref"))
pp_file = os.path.join("utils", "points2.dat")
factors_file = os.path.join("utils", "factors2.dat")
pyemu.geostats.fac2real(pp_file, factors_file,
out_file=os.path.join("temp", "test.ref"))
arr1 = np.loadtxt(os.path.join("utils","fac2real_points2.ref"))
arr2 = np.loadtxt(os.path.join("temp","test.ref"))
#print(np.nansum(np.abs(arr1-arr2)))
#print(np.nanmax(np.abs(arr1-arr2)))
nmax = np.nanmax(np.abs(arr1-arr2))
assert nmax < 0.01
# import matplotlib.pyplot as plt
# diff = (arr1-arr2)/arr1 * 100.0
# diff[np.isnan(arr1)] = np.nan
# p = plt.imshow(diff,interpolation='n')
# plt.colorbar(p)
# plt.show()
def vario_test():
import numpy as np
import pyemu
contribution = 0.1
a = 2.0
for const in [pyemu.utils.geostats.ExpVario,pyemu.utils.geostats.GauVario,
pyemu.utils.geostats.SphVario]:
v = const(contribution,a)
h = v._h_function(np.array([0.0]))
assert h == contribution
h = v._h_function(np.array([a*1000]))
assert h == 0.0
v2 = const(contribution,a,anisotropy=2.0,bearing=90.0)
print(v2._h_function(np.array([a])))
def aniso_test():
import pyemu
contribution = 0.1
a = 2.0
for const in [pyemu.utils.geostats.ExpVario,pyemu.utils.geostats.GauVario,
pyemu.utils.geostats.SphVario]:
v = const(contribution,a)
v2 = const(contribution,a,anisotropy=2.0,bearing=90.0)
v3 = const(contribution,a,anisotropy=2.0,bearing=0.0)
pt0 = (0,0)
pt1 = (1,0)
assert v.covariance(pt0,pt1) == v2.covariance(pt0,pt1)
pt0 = (0,0)
pt1 = (0,1)
assert v.covariance(pt0,pt1) == v3.covariance(pt0,pt1)
def geostruct_test():
import pyemu
v1 = pyemu.utils.geostats.ExpVario(0.1,2.0)
v2 = pyemu.utils.geostats.GauVario(0.1,2.0)
v3 = pyemu.utils.geostats.SphVario(0.1,2.0)
g = pyemu.utils.geostats.GeoStruct(0.2,[v1,v2,v3])
pt0 = (0,0)
pt1 = (0,0)
print(g.covariance(pt0,pt1))
assert g.covariance(pt0,pt1) == 0.5
pt0 = (0,0)
pt1 = (1.0e+10,0)
assert g.covariance(pt0,pt1) == 0.2
def struct_file_test():
import os
import pyemu
structs = pyemu.utils.geostats.read_struct_file(
os.path.join("utils","struct.dat"))
#print(structs[0])
pt0 = (0,0)
pt1 = (0,0)
for s in structs:
assert s.covariance(pt0,pt1) == s.nugget + \
s.variograms[0].contribution
with open(os.path.join("utils","struct_out.dat"),'w') as f:
for s in structs:
s.to_struct_file(f)
structs1 = pyemu.utils.geostats.read_struct_file(
os.path.join("utils","struct_out.dat"))
for s in structs1:
assert s.covariance(pt0,pt1) == s.nugget + \
s.variograms[0].contribution
def covariance_matrix_test():
import os
import pandas as pd
import pyemu
pts = pd.read_csv(os.path.join("utils","points1.dat"),delim_whitespace=True,
header=None,names=["name","x","y"],usecols=[0,1,2])
struct = pyemu.utils.geostats.read_struct_file(
os.path.join("utils","struct.dat"))[0]
struct.variograms[0].covariance_matrix(pts.x,pts.y,names=pts.name)
print(struct.covariance_matrix(pts.x,pts.y,names=pts.name).x)
def setup_ppcov_simple():
import os
import platform
exe_file = os.path.join("utils","ppcov.exe")
print(platform.platform())
if not os.path.exists(exe_file) or not platform.platform().lower().startswith("win"):
print("can't run ppcov setup")
return
pts_file = os.path.join("utils","points1_test.dat")
str_file = os.path.join("utils","struct_test.dat")
args1 = [pts_file,'0.0',str_file,"struct1",os.path.join("utils","ppcov.struct1.out"),'','']
args2 = [pts_file,'0.0',str_file,"struct2",os.path.join("utils","ppcov.struct2.out"),'','']
args3 = [pts_file,'0.0',str_file,"struct3",os.path.join("utils","ppcov.struct3.out"),'','']
for args in [args1,args2,args3]:
in_file = os.path.join("utils","ppcov.in")
with open(in_file,'w') as f:
f.write('\n'.join(args))
os.system(exe_file + '<' + in_file)
def ppcov_simple_test():
import os
import numpy as np
import pandas as pd
import pyemu
pts_file = os.path.join("utils","points1_test.dat")
str_file = os.path.join("utils","struct_test.dat")
mat1_file = os.path.join("utils","ppcov.struct1.out")
mat2_file = os.path.join("utils","ppcov.struct2.out")
mat3_file = os.path.join("utils","ppcov.struct3.out")
ppc_mat1 = pyemu.Cov.from_ascii(mat1_file)
ppc_mat2 = pyemu.Cov.from_ascii(mat2_file)
ppc_mat3 = pyemu.Cov.from_ascii(mat3_file)
pts = pd.read_csv(pts_file,header=None,names=["name","x","y"],usecols=[0,1,2],
delim_whitespace=True)
struct1,struct2,struct3 = pyemu.utils.geostats.read_struct_file(str_file)
print(struct1)
print(struct2)
print(struct3)
for mat,struct in zip([ppc_mat1,ppc_mat2,ppc_mat3],[struct1,struct2,struct3]):
str_mat = struct.covariance_matrix(x=pts.x,y=pts.y,names=pts.name)
print(str_mat.row_names)
delt = mat.x - str_mat.x
assert np.abs(delt).max() < 1.0e-7
def setup_ppcov_complex():
import os
import platform
exe_file = os.path.join("utils","ppcov.exe")
print(platform.platform())
if not os.path.exists(exe_file) or not platform.platform().lower().startswith("win"):
print("can't run ppcov setup")
return
pts_file = os.path.join("utils","points1_test.dat")
str_file = os.path.join("utils","struct_complex.dat")
args1 = [pts_file,'0.0',str_file,"struct1",os.path.join("utils","ppcov.complex.struct1.out"),'','']
args2 = [pts_file,'0.0',str_file,"struct2",os.path.join("utils","ppcov.complex.struct2.out"),'','']
for args in [args1,args2]:
in_file = os.path.join("utils","ppcov.in")
with open(in_file,'w') as f:
f.write('\n'.join(args))
os.system(exe_file + '<' + in_file)
def ppcov_complex_test():
import os
import numpy as np
import pandas as pd
import pyemu
pts_file = os.path.join("utils","points1_test.dat")
str_file = os.path.join("utils","struct_complex.dat")
mat1_file = os.path.join("utils","ppcov.complex.struct1.out")
mat2_file = os.path.join("utils","ppcov.complex.struct2.out")
ppc_mat1 = pyemu.Cov.from_ascii(mat1_file)
ppc_mat2 = pyemu.Cov.from_ascii(mat2_file)
pts = pd.read_csv(pts_file,header=None,names=["name","x","y"],usecols=[0,1,2],
delim_whitespace=True)
struct1,struct2 = pyemu.utils.geostats.read_struct_file(str_file)
print(struct1)
print(struct2)
for mat,struct in zip([ppc_mat1,ppc_mat2],[struct1,struct2]):
str_mat = struct.covariance_matrix(x=pts.x,y=pts.y,names=pts.name)
delt = mat.x - str_mat.x
print(mat.x[:,0])
print(str_mat.x[:,0])
print(np.abs(delt).max())
assert np.abs(delt).max() < 1.0e-7
#break
def pp_to_tpl_test():
import os
import pyemu
pp_file = os.path.join("utils","points1.dat")
pp_df = pyemu.pp_utils.pilot_points_to_tpl(pp_file,name_prefix="test_")
print(pp_df.columns)
def tpl_to_dataframe_test():
import os
import pyemu
pp_file = os.path.join("utils","points1.dat")
pp_df = pyemu.pp_utils.pilot_points_to_tpl(pp_file,name_prefix="test_")
df_tpl = pyemu.pp_utils.pp_tpl_to_dataframe(pp_file+".tpl")
assert df_tpl.shape[0] == pp_df.shape[0]
# def to_mps_test():
# import os
# import pyemu
# jco_file = os.path.join("utils","dewater_pest.jcb")
# jco = pyemu.Jco.from_binary(jco_file)
# #print(jco.x)
# pst = pyemu.Pst(jco_file.replace(".jcb",".pst"))
# #print(pst.nnz_obs_names)
# oc_dict = {oc:"l" for oc in pst.nnz_obs_names}
# obj_func = {name:1.0 for name in pst.par_names}
#
# #pyemu.optimization.to_mps(jco=jco_file)
# #pyemu.optimization.to_mps(jco=jco_file,obs_constraint_sense=oc_dict)
# #pyemu.optimization.to_mps(jco=jco_file,obj_func="h00_00")
# decision_var_names = pst.parameter_data.loc[pst.parameter_data.pargp=="q","parnme"].tolist()
# pyemu.optimization.to_mps(jco=jco_file,obj_func=obj_func,decision_var_names=decision_var_names,
# risk=0.975)
def setup_pp_test():
import os
import pyemu
try:
import flopy
except:
return
model_ws = os.path.join("..","examples","Freyberg","extra_crispy")
ml = flopy.modflow.Modflow.load("freyberg.nam",model_ws=model_ws,check=False)
pp_dir = os.path.join("utils")
#ml.export(os.path.join("temp","test_unrot_grid.shp"))
sr = pyemu.helpers.SpatialReference().from_namfile(
os.path.join(ml.model_ws, ml.namefile),
delc=ml.dis.delc, delr=ml.dis.delr)
sr.rotation = 0.
par_info_unrot = pyemu.pp_utils.setup_pilotpoints_grid(sr=sr, prefix_dict={0: "hk1",1:"hk2"},
every_n_cell=2, pp_dir=pp_dir, tpl_dir=pp_dir,
shapename=os.path.join("temp", "test_unrot.shp"),
)
#print(par_info_unrot.parnme.value_counts())
gs = pyemu.geostats.GeoStruct(variograms=pyemu.geostats.ExpVario(a=1000,contribution=1.0))
ok = pyemu.geostats.OrdinaryKrige(gs,par_info_unrot)
ok.calc_factors_grid(sr)
sr2 = pyemu.helpers.SpatialReference.from_gridspec(
os.path.join(ml.model_ws, "test.spc"), lenuni=2)
par_info_drot = pyemu.pp_utils.setup_pilotpoints_grid(sr=sr2, prefix_dict={0: ["hk1_", "sy1_", "rch_"]},
every_n_cell=2, pp_dir=pp_dir, tpl_dir=pp_dir,
shapename=os.path.join("temp", "test_unrot.shp"),
)
ok = pyemu.geostats.OrdinaryKrige(gs, par_info_unrot)
ok.calc_factors_grid(sr2)
par_info_mrot = pyemu.pp_utils.setup_pilotpoints_grid(ml,prefix_dict={0:["hk1_","sy1_","rch_"]},
every_n_cell=2,pp_dir=pp_dir,tpl_dir=pp_dir,
shapename=os.path.join("temp","test_unrot.shp"))
ok = pyemu.geostats.OrdinaryKrige(gs, par_info_unrot)
ok.calc_factors_grid(ml.sr)
sr.rotation = 15
#ml.export(os.path.join("temp","test_rot_grid.shp"))
#pyemu.gw_utils.setup_pilotpoints_grid(ml)
par_info_rot = pyemu.pp_utils.setup_pilotpoints_grid(sr=sr,every_n_cell=2, pp_dir=pp_dir, tpl_dir=pp_dir,
shapename=os.path.join("temp", "test_rot.shp"))
ok = pyemu.geostats.OrdinaryKrige(gs, par_info_unrot)
ok.calc_factors_grid(sr)
print(par_info_unrot.x)
print(par_info_drot.x)
print(par_info_mrot.x)
print(par_info_rot.x)
def read_hob_test():
import os
import pyemu
hob_file = os.path.join("utils","HOB.txt")
df = pyemu.gw_utils.modflow_hob_to_instruction_file(hob_file)
print(df.obsnme)
def read_pval_test():
import os
import pyemu
pval_file = os.path.join("utils", "meras_trEnhance.pval")
pyemu.gw_utils.modflow_pval_to_template_file(pval_file)
def pp_to_shapefile_test():
import os
import pyemu
try:
import shapefile
except:
print("no pyshp")
return
pp_file = os.path.join("utils","points1.dat")
shp_file = os.path.join("temp","points1.dat.shp")
pyemu.pp_utils.write_pp_shapfile(pp_file)
def write_tpl_test():
import os
import pyemu
tpl_file = os.path.join("utils","test_write.tpl")
in_file = os.path.join("temp","tpl_test.dat")
par_vals = {"q{0}".format(i+1):12345678.90123456 for i in range(7)}
pyemu.pst_utils.write_to_template(par_vals,tpl_file,in_file)
def read_pestpp_runstorage_file_test():
import os
import pyemu
rnj_file = os.path.join("utils","freyberg.rnj")
#rnj_file = os.path.join("..", "..", "verification", "10par_xsec", "master_opt1","pest.rnj")
p1,o1 = pyemu.helpers.read_pestpp_runstorage(rnj_file)
p2,o2 = pyemu.helpers.read_pestpp_runstorage(rnj_file,9)
diff = p1 - p2
diff.sort_values("parval1",inplace=True)
def smp_to_ins_test():
import os
import pyemu
smp = os.path.join("utils","TWDB_wells.smp")
ins = os.path.join('temp',"test.ins")
try:
pyemu.pst_utils.smp_to_ins(smp,ins)
except:
pass
else:
raise Exception("should have failed")
pyemu.smp_utils.smp_to_ins(smp,ins,True)
def master_and_workers():
import shutil
import pyemu
worker_dir = os.path.join("..","verification","10par_xsec","template_mac")
master_dir = os.path.join("temp","master")
if not os.path.exists(master_dir):
os.mkdir(master_dir)
assert os.path.exists(worker_dir)
pyemu.helpers.start_workers(worker_dir,"pestpp","pest.pst",1,
worker_root="temp",master_dir=master_dir)
#now try it from within the master dir
base_cwd = os.getcwd()
os.chdir(master_dir)
pyemu.helpers.start_workers(os.path.join("..","..",worker_dir),
"pestpp","pest.pst",3,
master_dir='.')
os.chdir(base_cwd)
def first_order_pearson_regul_test():
import os
from pyemu import Schur
from pyemu.utils.helpers import first_order_pearson_tikhonov,zero_order_tikhonov
w_dir = "la"
sc = Schur(jco=os.path.join(w_dir,"pest.jcb"))
pt = sc.posterior_parameter
zero_order_tikhonov(sc.pst)
first_order_pearson_tikhonov(sc.pst,pt,reset=False)
print(sc.pst.prior_information)
sc.pst.rectify_pi()
assert sc.pst.control_data.pestmode == "regularization"
sc.pst.write(os.path.join('temp','test.pst'))
def zero_order_regul_test():
import os
import pyemu
pst = pyemu.Pst(os.path.join("pst","inctest.pst"))
pyemu.helpers.zero_order_tikhonov(pst)
print(pst.prior_information)
assert pst.control_data.pestmode == "regularization"
pst.write(os.path.join('temp','test.pst'))
pyemu.helpers.zero_order_tikhonov(pst,reset=False)
assert pst.prior_information.shape[0] == pst.npar_adj * 2
def kl_test():
import os
import numpy as np
import pandas as pd
import pyemu
import matplotlib.pyplot as plt
try:
import flopy
except:
print("flopy not imported...")
return
model_ws = os.path.join("..","verification","Freyberg","extra_crispy")
ml = flopy.modflow.Modflow.load("freyberg.nam",model_ws=model_ws,check=False)
str_file = os.path.join("..","verification","Freyberg","structure.dat")
arr_tru = np.loadtxt(os.path.join("..","verification",
"Freyberg","extra_crispy",
"hk.truth.ref")) + 20
basis_file = os.path.join("utils","basis.jco")
tpl_file = os.path.join("utils","test.tpl")
factors_file = os.path.join("temp","factors.dat")
num_eig = 100
prefixes = ["hk1"]
df = pyemu.utils.helpers.kl_setup(num_eig=num_eig, sr=ml.sr,
struct=str_file,
factors_file=factors_file,
basis_file=basis_file,
prefixes=prefixes,islog=False)
basis = pyemu.Matrix.from_binary(basis_file)
basis = basis[:,:num_eig]
arr_tru = np.atleast_2d(arr_tru.flatten()).transpose()
proj = np.dot(basis.T.x,arr_tru)[:num_eig]
#proj.autoalign = False
back = np.dot(basis.x, proj)
back = back.reshape(ml.nrow,ml.ncol)
df.parval1 = proj
arr = pyemu.geostats.fac2real(df,factors_file,out_file=None)
fig = plt.figure(figsize=(10, 10))
ax1, ax2 = plt.subplot(121),plt.subplot(122)
mn,mx = arr_tru.min(),arr_tru.max()
print(arr.max(), arr.min())
print(back.max(),back.min())
diff = np.abs(back - arr)
print(diff.max())
assert diff.max() < 1.0e-5
def ok_test():
import os
import pandas as pd
import pyemu
str_file = os.path.join("utils","struct_test.dat")
pts_data = pd.DataFrame({"x":[1.0,2.0,3.0],"y":[0.,0.,0.],"name":["p1","p2","p3"]})
gs = pyemu.utils.geostats.read_struct_file(str_file)[0]
ok = pyemu.utils.geostats.OrdinaryKrige(gs,pts_data)
interp_points = pts_data.copy()
kf = ok.calc_factors(interp_points.x,interp_points.y)
#for ptname in pts_data.name:
for i in kf.index:
assert len(kf.loc[i,"inames"])== 1
assert kf.loc[i,"ifacts"][0] == 1.0
assert sum(kf.loc[i,"ifacts"]) == 1.0
print(kf)
def ok_grid_test():
try:
import flopy
except:
return
import numpy as np
import pandas as pd
import pyemu
nrow,ncol = 10,5
delr = np.ones((ncol)) * 1.0/float(ncol)
delc = np.ones((nrow)) * 1.0/float(nrow)
num_pts = 0
ptx = np.random.random(num_pts)
pty = np.random.random(num_pts)
ptname = ["p{0}".format(i) for i in range(num_pts)]
pts_data = pd.DataFrame({"x":ptx,"y":pty,"name":ptname})
pts_data.index = pts_data.name
pts_data = pts_data.loc[:,["x","y","name"]]
sr = flopy.utils.SpatialReference(delr=delr,delc=delc)
pts_data.loc["i0j0", :] = [sr.xcentergrid[0,0],sr.ycentergrid[0,0],"i0j0"]
pts_data.loc["imxjmx", :] = [sr.xcentergrid[-1, -1], sr.ycentergrid[-1, -1], "imxjmx"]
str_file = os.path.join("utils","struct_test.dat")
gs = pyemu.utils.geostats.read_struct_file(str_file)[0]
ok = pyemu.utils.geostats.OrdinaryKrige(gs,pts_data)
kf = ok.calc_factors_grid(sr,verbose=False,var_filename=os.path.join("temp","test_var.ref"),minpts_interp=1)
ok.to_grid_factors_file(os.path.join("temp","test.fac"))
def ok_grid_zone_test():
try:
import flopy
except:
return
import numpy as np
import pandas as pd
import pyemu
nrow,ncol = 10,5
delr = np.ones((ncol)) * 1.0/float(ncol)
delc = np.ones((nrow)) * 1.0/float(nrow)
num_pts = 0
ptx = np.random.random(num_pts)
pty = np.random.random(num_pts)
ptname = ["p{0}".format(i) for i in range(num_pts)]
pts_data = pd.DataFrame({"x":ptx,"y":pty,"name":ptname})
pts_data.index = pts_data.name
pts_data = pts_data.loc[:,["x","y","name"]]
sr = flopy.utils.SpatialReference(delr=delr,delc=delc)
pts_data.loc["i0j0", :] = [sr.xcentergrid[0,0],sr.ycentergrid[0,0],"i0j0"]
pts_data.loc["imxjmx", :] = [sr.xcentergrid[-1, -1], sr.ycentergrid[-1, -1], "imxjmx"]
pts_data.loc[:,"zone"] = 1
pts_data.zone.iloc[1] = 2
print(pts_data.zone.unique())
str_file = os.path.join("utils","struct_test.dat")
gs = pyemu.utils.geostats.read_struct_file(str_file)[0]
ok = pyemu.utils.geostats.OrdinaryKrige(gs,pts_data)
zone_array = np.ones((nrow,ncol))
zone_array[0,0] = 2
kf = ok.calc_factors_grid(sr,verbose=False,
var_filename=os.path.join("temp","test_var.ref"),
minpts_interp=1,zone_array=zone_array)
ok.to_grid_factors_file(os.path.join("temp","test.fac"))
def ppk2fac_verf_test():
import os
import numpy as np
import pyemu
try:
import flopy
except:
return
ws = os.path.join("..","verification","Freyberg")
gspc_file = os.path.join(ws,"grid.spc")
pp_file = os.path.join(ws,"pp_00_pp.dat")
str_file = os.path.join(ws,"structure.complex.dat")
ppk2fac_facfile = os.path.join(ws,"ppk2fac_fac.dat")
pyemu_facfile = os.path.join("temp","pyemu_facfile.dat")
sr = flopy.utils.SpatialReference.from_gridspec(gspc_file)
ok = pyemu.utils.OrdinaryKrige(str_file,pp_file)
ok.calc_factors_grid(sr,maxpts_interp=10)
ok.to_grid_factors_file(pyemu_facfile)
zone_arr = np.loadtxt(os.path.join(ws,"extra_crispy","ref","ibound.ref"))
pyemu_arr = pyemu.utils.fac2real(pp_file,pyemu_facfile,out_file=None)
ppk2fac_arr = pyemu.utils.fac2real(pp_file,ppk2fac_facfile,out_file=None)
pyemu_arr[zone_arr == 0] = np.NaN
pyemu_arr[zone_arr == -1] = np.NaN
ppk2fac_arr[zone_arr == 0] = np.NaN
ppk2fac_arr[zone_arr == -1] = np.NaN
diff = np.abs(pyemu_arr - ppk2fac_arr)
print(diff)
assert np.nansum(diff) < 1.0e-6,np.nansum(diff)
# def opt_obs_worth():
# import os
# import pyemu
# wdir = os.path.join("utils")
# os.chdir(wdir)
# pst = pyemu.Pst(os.path.join("supply2_pest.fosm.pst"))
# zero_weight_names = [n for n,w in zip(pst.observation_data.obsnme,pst.observation_data.weight) if w == 0.0]
# #print(zero_weight_names)
# #for attr in ["base_jacobian","hotstart_resfile"]:
# # pst.pestpp_options[attr] = os.path.join(wdir,pst.pestpp_options[attr])
# #pst.template_files = [os.path.join(wdir,f) for f in pst.template_files]
# #pst.instruction_files = [os.path.join(wdir,f) for f in pst.instruction_files]
# #print(pst.template_files)
# df = pyemu.optimization.get_added_obs_importance(pst,obslist_dict={"zeros":zero_weight_names})
# os.chdir("..")
# print(df)
def mflist_budget_test():
import pyemu
import os
import pandas as pd
try:
import flopy
except:
print("no flopy...")
return
model_ws = os.path.join("..","examples","Freyberg_transient")
ml = flopy.modflow.Modflow.load("freyberg.nam",model_ws=model_ws,check=False,load_only=[])
list_filename = os.path.join(model_ws,"freyberg.list")
assert os.path.exists(list_filename)
df = pyemu.gw_utils.setup_mflist_budget_obs(list_filename,start_datetime=ml.start_datetime)
print(df)
times = df.loc[df.index.str.startswith('vol_wells')].index.str.split(
'_', expand=True).get_level_values(2)[::100]
times = pd.to_datetime(times, yearfirst=True)
df = pyemu.gw_utils.setup_mflist_budget_obs(
list_filename, start_datetime=ml.start_datetime, specify_times=times)
flx, vol = pyemu.gw_utils.apply_mflist_budget_obs(
list_filename, 'flux.dat', 'vol.dat', start_datetime=ml.start_datetime,
times='budget_times.config'
)
assert (flx.index == vol.index).all()
assert (flx.index == times).all()
def mtlist_budget_test():
import pyemu
import pandas as pd
import os
try:
import flopy
except:
print("no flopy...")
return
list_filename = os.path.join("utils","mt3d.list")
assert os.path.exists(list_filename)
frun_line,ins_files, df = pyemu.gw_utils.setup_mtlist_budget_obs(
list_filename,start_datetime='1-1-1970')
assert len(ins_files) == 2
frun_line,ins_files, df = pyemu.gw_utils.setup_mtlist_budget_obs(
list_filename,start_datetime='1-1-1970', gw_prefix='')
assert len(ins_files) == 2
frun_line, ins_files, df = pyemu.gw_utils.setup_mtlist_budget_obs(
list_filename, start_datetime=None)
assert len(ins_files) == 2
list_filename = os.path.join("utils", "mt3d_imm_sor.lst")
assert os.path.exists(list_filename)
frun_line, ins_files, df = pyemu.gw_utils.setup_mtlist_budget_obs(
list_filename, start_datetime='1-1-1970')
def geostat_prior_builder_test():
import os
import numpy as np
import pyemu
pst_file = os.path.join("pst","pest.pst")
pst = pyemu.Pst(pst_file)
# print(pst.parameter_data)
tpl_file = os.path.join("utils", "pp_locs.tpl")
str_file = os.path.join("utils", "structure.dat")
cov = pyemu.helpers.geostatistical_prior_builder(pst_file,{str_file:tpl_file})
d1 = np.diag(cov.x)
df = pyemu.pp_utils.pp_tpl_to_dataframe(tpl_file)
df.loc[:,"zone"] = np.arange(df.shape[0])
gs = pyemu.geostats.read_struct_file(str_file)
cov = pyemu.helpers.geostatistical_prior_builder(pst_file,{gs:df},
sigma_range=4)
nnz = np.count_nonzero(cov.x)
assert nnz == pst.npar_adj
d2 = np.diag(cov.x)
assert np.array_equiv(d1, d2)
pst.parameter_data.loc[pst.par_names[1:10], "partrans"] = "tied"
pst.parameter_data.loc[pst.par_names[1:10], "partied"] = pst.par_names[0]
cov = pyemu.helpers.geostatistical_prior_builder(pst, {gs: df},
sigma_range=4)
nnz = np.count_nonzero(cov.x)
assert nnz == pst.npar_adj
ttpl_file = os.path.join("temp", "temp.dat.tpl")
with open(ttpl_file, 'w') as f:
f.write("ptf ~\n ~ temp1 ~\n")
pst.add_parameters(ttpl_file, ttpl_file.replace(".tpl", ""))
pst.parameter_data.loc["temp1", "parubnd"] = 1.1
pst.parameter_data.loc["temp1", "parlbnd"] = 0.9
cov = pyemu.helpers.geostatistical_prior_builder(pst, {str_file: tpl_file})
assert cov.shape[0] == pst.npar_adj
def geostat_draws_test():
import os
import numpy as np
import pyemu
pst_file = os.path.join("pst","pest.pst")
pst = pyemu.Pst(pst_file)
print(pst.parameter_data)
tpl_file = os.path.join("utils", "pp_locs.tpl")
str_file = os.path.join("utils", "structure.dat")
pe = pyemu.helpers.geostatistical_draws(pst_file,{str_file:tpl_file})
assert (pe.shape == pe.dropna().shape)
pst.parameter_data.loc[pst.par_names[1:10], "partrans"] = "tied"
pst.parameter_data.loc[pst.par_names[1:10], "partied"] = pst.par_names[0]
pe = pyemu.helpers.geostatistical_draws(pst, {str_file: tpl_file})
assert (pe.shape == pe.dropna().shape)
df = pyemu.pp_utils.pp_tpl_to_dataframe(tpl_file)
df.loc[:,"zone"] = np.arange(df.shape[0])
gs = pyemu.geostats.read_struct_file(str_file)
pe = pyemu.helpers.geostatistical_draws(pst_file,{gs:df},
sigma_range=4)
ttpl_file = os.path.join("temp", "temp.dat.tpl")
with open(ttpl_file, 'w') as f:
f.write("ptf ~\n ~ temp1 ~\n")
pst.add_parameters(ttpl_file, ttpl_file.replace(".tpl", ""))
pst.parameter_data.loc["temp1", "parubnd"] = 1.1
pst.parameter_data.loc["temp1", "parlbnd"] = 0.9
pst.parameter_data.loc[pst.par_names[1:10],"partrans"] = "tied"
pst.parameter_data.loc[pst.par_names[1:10], "partied"] = pst.par_names[0]
pe = pyemu.helpers.geostatistical_draws(pst, {str_file: tpl_file})
assert (pe.shape == pe.dropna().shape)
# def linearuniversal_krige_test():
# try:
# import flopy
# except:
# return
#
# import numpy as np
# import pandas as pd
# import pyemu
# nrow,ncol = 10,5
# delr = np.ones((ncol)) * 1.0/float(ncol)
# delc = np.ones((nrow)) * 1.0/float(nrow)
#
# num_pts = 0
# ptx = np.random.random(num_pts)
# pty = np.random.random(num_pts)
# ptname = ["p{0}".format(i) for i in range(num_pts)]
# pts_data = pd.DataFrame({"x":ptx,"y":pty,"name":ptname})
# pts_data.index = pts_data.name
# pts_data = pts_data.loc[:,["x","y","name"]]
#
#
# sr = flopy.utils.SpatialReference(delr=delr,delc=delc)
# pts_data.loc["i0j0", :] = [sr.xcentergrid[0,0],sr.ycentergrid[0,0],"i0j0"]
# pts_data.loc["imxjmx", :] = [sr.xcentergrid[-1, -1], sr.ycentergrid[-1, -1], "imxjmx"]
# pts_data.loc["i0j0","value"] = 1.0
# pts_data.loc["imxjmx","value"] = 0.0
#
# str_file = os.path.join("utils","struct_test.dat")
# gs = pyemu.utils.geostats.read_struct_file(str_file)[0]
# luk = pyemu.utils.geostats.LinearUniversalKrige(gs,pts_data)
# df = luk.estimate_grid(sr,verbose=True,
# var_filename=os.path.join("utils","test_var.ref"),
# minpts_interp=1)
def gslib_2_dataframe_test():
import os
import pyemu
gslib_file = os.path.join("utils","ch91pt.shp.gslib")
df = pyemu.geostats.gslib_2_dataframe(gslib_file)
print(df)
def sgems_to_geostruct_test():
import os
import pyemu
xml_file = os.path.join("utils", "ch00")
gs = pyemu.geostats.read_sgems_variogram_xml(xml_file)
def load_sgems_expvar_test():
import os
import numpy as np
#import matplotlib.pyplot as plt
import pyemu
dfs = pyemu.geostats.load_sgems_exp_var(os.path.join("utils","ch00_expvar"))
xmn,xmx = 1.0e+10,-1.0e+10
for d,df in dfs.items():
xmn = min(xmn,df.x.min())
xmx = max(xmx,df.x.max())
xml_file = os.path.join("utils", "ch00")
gs = pyemu.geostats.read_sgems_variogram_xml(xml_file)
v = gs.variograms[0]
#ax = gs.plot(ls="--")
#plt.show()
#x = np.linspace(xmn,xmx,100)
#y = v.inv_h(x)
#
#plt.plot(x,y)
#plt.show()
def read_hydmod_test():
import os
import numpy as np
import pandas as pd
import pyemu
try:
import flopy
except:
return
df, outfile = pyemu.gw_utils.modflow_read_hydmod_file(os.path.join('utils','freyberg.hyd.bin'),
os.path.join('temp','freyberg.hyd.bin.dat'))
df = pd.read_csv(os.path.join('temp', 'freyberg.hyd.bin.dat'), delim_whitespace=True)
dftrue = pd.read_csv(os.path.join('utils', 'freyberg.hyd.bin.dat.true'), delim_whitespace=True)
assert np.allclose(df.obsval.values, dftrue.obsval.values)
def make_hydmod_insfile_test():
import os
import shutil
import pyemu
try:
import flopy
except:
return
shutil.copy2(os.path.join('utils','freyberg.hyd.bin'),os.path.join('temp','freyberg.hyd.bin'))
pyemu.gw_utils.modflow_hydmod_to_instruction_file(os.path.join('temp','freyberg.hyd.bin'))
#assert open(os.path.join('utils','freyberg.hyd.bin.dat.ins'),'r').read() == open('freyberg.hyd.dat.ins', 'r').read()
assert os.path.exists(os.path.join('temp','freyberg.hyd.bin.dat.ins'))
def plot_summary_test():
import os
import pandas as pd
import pyemu
try:
import matplotlib.pyplot as plt
except:
return
par_df = pd.read_csv(os.path.join("utils","freyberg_pp.par.usum.csv"),
index_col=0)
idx = list(par_df.index.map(lambda x: x.startswith("HK")))
par_df = par_df.loc[idx,:]
ax = pyemu.plot_utils.plot_summary_distributions(par_df,label_post=True)
plt.savefig(os.path.join("temp","hk_par.png"))
plt.close()
df = os.path.join("utils","freyberg_pp.pred.usum.csv")
figs,axes = pyemu.plot_utils.plot_summary_distributions(df,subplots=True)
#plt.show()
for i,fig in enumerate(figs):
plt.figure(fig.number)
plt.savefig(os.path.join("temp","test_pred_{0}.png".format(i)))
plt.close(fig)
df = os.path.join("utils","freyberg_pp.par.usum.csv")
figs, axes = pyemu.plot_utils.plot_summary_distributions(df,subplots=True)
for i,fig in enumerate(figs):
plt.figure(fig.number)
plt.savefig(os.path.join("temp","test_par_{0}.png".format(i)))
plt.close(fig)
def hds_timeseries_test():
import os
import shutil
import numpy as np
import pandas as pd
try:
import flopy
except:
return
import pyemu
model_ws =os.path.join("..","examples","Freyberg_transient")
org_hds_file = os.path.join(model_ws, "freyberg.hds")
hds_file = os.path.join("temp", "freyberg.hds")
org_cbc_file = org_hds_file.replace(".hds",".cbc")
cbc_file = hds_file.replace(".hds", ".cbc")
shutil.copy2(org_hds_file, hds_file)
shutil.copy2(org_cbc_file, cbc_file)
m = flopy.modflow.Modflow.load("freyberg.nam", model_ws=model_ws, check=False)
kij_dict = {"test1": [0, 0, 0], "test2": (1, 1, 1), "test": (0, 10, 14)}
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, include_path=True)
# m.change_model_ws("temp",reset_external=True)
# m.write_input()
# pyemu.os_utils.run("mfnwt freyberg.nam",cwd="temp")
cmd, df1 = pyemu.gw_utils.setup_hds_timeseries(cbc_file, kij_dict, include_path=True, prefix="stor",
text="storage", fill=0.0)
cmd,df2 = pyemu.gw_utils.setup_hds_timeseries(cbc_file, kij_dict, model=m, include_path=True, prefix="stor",
text="storage",fill=0.0)
print(df1)
d = np.abs(df1.obsval.values - df2.obsval.values)
print(d.max())
assert d.max() == 0.0,d
try:
pyemu.gw_utils.setup_hds_timeseries(cbc_file, kij_dict, model=m, include_path=True, prefix="consthead",
text="constant head")
except:
pass
else:
raise Exception("should have failed")
try:
pyemu.gw_utils.setup_hds_timeseries(cbc_file, kij_dict, model=m, include_path=True, prefix="consthead",
text="JUNK")
except:
pass
else:
raise Exception("should have failed")
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, include_path=True,prefix="hds")
m = flopy.modflow.Modflow.load("freyberg.nam",model_ws=model_ws,load_only=[],check=False)
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict,model=m,include_path=True)
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, model=m, include_path=True,prefix="hds")
org_hds_file = os.path.join("utils", "MT3D001.UCN")
hds_file = os.path.join("temp", "MT3D001.UCN")
shutil.copy2(org_hds_file, hds_file)
kij_dict = {"test1": [0, 0, 0], "test2": (1, 1, 1)}
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, include_path=True)
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, include_path=True, prefix="hds")
m = flopy.modflow.Modflow.load("freyberg.nam", model_ws=model_ws, load_only=[], check=False)
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, model=m, include_path=True)
pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, model=m, include_path=True, prefix="hds")
# df1 = pd.read_csv(out_file, delim_whitespace=True)
# pyemu.gw_utils.apply_hds_obs(hds_file)
# df2 = pd.read_csv(out_file, delim_whitespace=True)
# diff = df1.obsval - df2.obsval
def grid_obs_test():
import os
import shutil
import numpy as np
import pandas as pd
try:
import flopy
except:
return
import pyemu
m_ws = os.path.join("..", "examples", "freyberg_sfr_update")
org_hds_file = os.path.join("..","examples","Freyberg_Truth","freyberg.hds")
org_multlay_hds_file = os.path.join(m_ws, "freyberg.hds") # 3 layer version
org_ucn_file = os.path.join(m_ws, "MT3D001.UCN") # mt example
hds_file = os.path.join("temp","freyberg.hds")
multlay_hds_file = os.path.join("temp", "freyberg_3lay.hds")
ucn_file = os.path.join("temp", "MT3D001.UCN")
out_file = hds_file+".dat"
multlay_out_file = multlay_hds_file+".dat"
ucn_out_file = ucn_file+".dat"
shutil.copy2(org_hds_file,hds_file)
shutil.copy2(org_multlay_hds_file, multlay_hds_file)
shutil.copy2(org_ucn_file, ucn_file)
pyemu.gw_utils.setup_hds_obs(hds_file)
df1 = pd.read_csv(out_file,delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(hds_file)
df2 = pd.read_csv(out_file,delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert abs(diff.max()) < 1.0e-6, abs(diff.max())
pyemu.gw_utils.setup_hds_obs(multlay_hds_file)
df1 = pd.read_csv(multlay_out_file,delim_whitespace=True)
assert len(df1) == 3*len(df2), "{} != 3*{}".format(len(df1), len(df2))
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file,delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval,df2.obsval), abs(diff.max())
pyemu.gw_utils.setup_hds_obs(hds_file,skip=-999)
df1 = pd.read_csv(out_file,delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(hds_file)
df2 = pd.read_csv(out_file,delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert diff.max() < 1.0e-6
pyemu.gw_utils.setup_hds_obs(ucn_file, skip=1.e30, prefix='ucn')
df1 = pd.read_csv(ucn_out_file, delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(ucn_file)
df2 = pd.read_csv(ucn_out_file, delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
# skip = lambda x : x < -888.0
skip = lambda x: x if x > -888.0 else np.NaN
pyemu.gw_utils.setup_hds_obs(hds_file,skip=skip)
df1 = pd.read_csv(out_file,delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(hds_file)
df2 = pd.read_csv(out_file,delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert diff.max() < 1.0e-6
kperk_pairs = (0,0)
pyemu.gw_utils.setup_hds_obs(hds_file,kperk_pairs=kperk_pairs,
skip=skip)
df1 = pd.read_csv(out_file,delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(hds_file)
df2 = pd.read_csv(out_file,delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert diff.max() < 1.0e-6
kperk_pairs = [(0, 0), (0, 1), (0, 2)]
pyemu.gw_utils.setup_hds_obs(multlay_hds_file, kperk_pairs=kperk_pairs,
skip=skip)
df1 = pd.read_csv(multlay_out_file, delim_whitespace=True)
assert len(df1) == 3*len(df2), "{} != 3*{}".format(len(df1), len(df2))
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file, delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
kperk_pairs = [(0, 0), (0, 1), (0, 2), (2, 0), (2, 1), (2, 2)]
pyemu.gw_utils.setup_hds_obs(multlay_hds_file, kperk_pairs=kperk_pairs,
skip=skip)
df1 = pd.read_csv(multlay_out_file, delim_whitespace=True)
assert len(df1) == 2 * len(df2), "{} != 2*{}".format(len(df1), len(df2))
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file, delim_whitespace=True)
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
m = flopy.modflow.Modflow.load("freyberg.nam", model_ws=m_ws, load_only=["BAS6"],forgive=False,verbose=True)
kperk_pairs = [(0, 0), (0, 1), (0, 2)]
skipmask = m.bas6.ibound.array
pyemu.gw_utils.setup_hds_obs(multlay_hds_file, kperk_pairs=kperk_pairs,
skip=skipmask)
df1 = pd.read_csv(multlay_out_file, delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file, delim_whitespace=True)
assert len(df1) == len(df2) == np.abs(skipmask).sum(), \
"array skip failing, expecting {0} obs but returned {1}".format(np.abs(skipmask).sum(), len(df1))
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
kperk_pairs = [(0, 0), (0, 1), (0, 2), (2, 0), (2, 1), (2, 2)]
skipmask = m.bas6.ibound.array[0]
pyemu.gw_utils.setup_hds_obs(multlay_hds_file, kperk_pairs=kperk_pairs,
skip=skipmask)
df1 = pd.read_csv(multlay_out_file, delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file, delim_whitespace=True)
assert len(df1) == len(df2) == 2 * m.nlay * np.abs(skipmask).sum(), "array skip failing"
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
kperk_pairs = [(0, 0), (0, 1), (0, 2), (2, 0), (2, 1), (2, 2)]
skipmask = m.bas6.ibound.array
pyemu.gw_utils.setup_hds_obs(multlay_hds_file, kperk_pairs=kperk_pairs,
skip=skipmask)
df1 = pd.read_csv(multlay_out_file, delim_whitespace=True)
pyemu.gw_utils.apply_hds_obs(multlay_hds_file)
df2 = pd.read_csv(multlay_out_file, delim_whitespace=True)
assert len(df1) == len(df2) == 2 * np.abs(skipmask).sum(), "array skip failing"
diff = df1.obsval - df2.obsval
assert np.allclose(df1.obsval, df2.obsval), abs(diff.max())
def postprocess_inactive_conc_test():
import os
import shutil
import numpy as np
import pandas as pd
try:
import flopy
except:
return
import pyemu
bd = os.getcwd()
model_ws = os.path.join("..", "examples", "Freyberg_transient")
org_hds_file = os.path.join("utils", "MT3D001.UCN")
hds_file = os.path.join("temp", "MT3D001.UCN")
shutil.copy2(org_hds_file, hds_file)
kij_dict = {"test1": [0, 0, 0], "test2": (1, 1, 1), "inact": [0, 81, 35]}
m = flopy.modflow.Modflow.load("freyberg.nam", model_ws=model_ws, load_only=[], check=False)
frun_line, df = pyemu.gw_utils.setup_hds_timeseries(hds_file, kij_dict, model=m, include_path=True, prefix="hds",
postprocess_inact=1E30)
os.chdir("temp")
df0 = pd.read_csv("{0}_timeseries.processed".format(os.path.split(hds_file)[-1]), delim_whitespace=True).T
df1 = pd.read_csv("{0}_timeseries.post_processed".format(os.path.split(hds_file)[-1]), delim_whitespace=True).T
eval(frun_line)
df2 = pd.read_csv("{0}_timeseries.processed".format(os.path.split(hds_file)[-1]), delim_whitespace=True).T
df3 = pd.read_csv("{0}_timeseries.post_processed".format(os.path.split(hds_file)[-1]), delim_whitespace=True).T
assert np.allclose(df0, df2)
assert np.allclose(df2.test1, df3.test1)
assert np.allclose(df2.test2, df3.test2)
assert np.allclose(df3, df1)
os.chdir(bd)
def gw_sft_ins_test():
import os
import pyemu
sft_outfile = os.path.join("utils","test_sft.out")
#pyemu.gw_utils.setup_sft_obs(sft_outfile)
#pyemu.gw_utils.setup_sft_obs(sft_outfile,start_datetime="1-1-1970")
df = pyemu.gw_utils.setup_sft_obs(sft_outfile, start_datetime="1-1-1970",times=[10950.00])
#print(df)
def sfr_helper_test():
import os
import shutil
import pandas as pd
import pyemu
import flopy
#setup the process
m = flopy.modflow.Modflow.load("supply2.nam",model_ws="utils",check=False,verbose=True,forgive=False,load_only=["dis","sfr"])
sd = m.sfr.segment_data[0].copy()
sd["flow"] = 1.0
sd["pptsw"] = 1.0
m.sfr.segment_data = {k:sd.copy() for k in range(m.nper)}
df_sfr = pyemu.gw_utils.setup_sfr_seg_parameters(
m, include_temporal_pars=['hcond1', 'flow'])
print(df_sfr)
os.chdir("utils")
# change the name of the sfr file that will be created
pars = {}
with open("sfr_seg_pars.config") as f:
for line in f:
line = line.strip().split()
pars[line[0]] = line[1]
pars["sfr_filename"] = "test.sfr"
with open("sfr_seg_pars.config", 'w') as f:
for k, v in pars.items():
f.write("{0} {1}\n".format(k, v))
# change some hcond1 values
df = pd.read_csv("sfr_seg_temporal_pars.dat", delim_whitespace=False, index_col=0)
df.loc[:, "flow"] = 10.0
df.to_csv("sfr_seg_temporal_pars.dat", sep=',')
sd1 = pyemu.gw_utils.apply_sfr_seg_parameters().segment_data
m1 = flopy.modflow.Modflow.load("supply2.nam", load_only=["sfr"], check=False)
os.chdir("..")
for kper,sd in m1.sfr.segment_data.items():
#print(sd["flow"],sd1[kper]["flow"])
for i1,i2 in zip(sd["flow"],sd1[kper]["flow"]):
assert i1 * 10 == i2,"{0},{1}".format(i1,i2)
df_sfr = pyemu.gw_utils.setup_sfr_seg_parameters("supply2.nam", model_ws="utils", include_temporal_pars=True)
os.chdir("utils")
# change the name of the sfr file that will be created
pars = {}
with open("sfr_seg_pars.config") as f:
for line in f:
line = line.strip().split()
pars[line[0]] = line[1]
pars["sfr_filename"] = "test.sfr"
with open("sfr_seg_pars.config", 'w') as f:
for k, v in pars.items():
f.write("{0} {1}\n".format(k, v))
# change some hcond1 values
df = pd.read_csv("sfr_seg_pars.dat", delim_whitespace=False,index_col=0)
df.loc[:, "hcond1"] = 1.0
df.to_csv("sfr_seg_pars.dat", sep=',')
# make sure the hcond1 mult worked...
sd1 = pyemu.gw_utils.apply_sfr_seg_parameters().segment_data[0]
m1 = flopy.modflow.Modflow.load("supply2.nam", load_only=["sfr"], check=False)
sd2 = m1.sfr.segment_data[0]
sd1 = pd.DataFrame.from_records(sd1)
sd2 = pd.DataFrame.from_records(sd2)
# print(sd1.hcond1)
# print(sd2.hcond2)
assert sd1.hcond1.sum() == sd2.hcond1.sum()
# change some hcond1 values
df = pd.read_csv("sfr_seg_pars.dat",delim_whitespace=False,index_col=0)
df.loc[:,"hcond1"] = 0.5
df.to_csv("sfr_seg_pars.dat",sep=',')
#change the name of the sfr file that will be created
pars = {}
with open("sfr_seg_pars.config") as f:
for line in f:
line = line.strip().split()
pars[line[0]] = line[1]
pars["sfr_filename"] = "test.sfr"
with open("sfr_seg_pars.config",'w') as f:
for k,v in pars.items():
f.write("{0} {1}\n".format(k,v))
#make sure the hcond1 mult worked...
sd1 = pyemu.gw_utils.apply_sfr_seg_parameters().segment_data[0]
m1 = flopy.modflow.Modflow.load("supply2.nam",load_only=["sfr"],check=False)
sd2 = m1.sfr.segment_data[0]
sd1 = pd.DataFrame.from_records(sd1)
sd2 = pd.DataFrame.from_records(sd2)
#print(sd1.hcond1)
#print(sd2.hcond2)
os.chdir("..")
assert (sd1.hcond1 * 2.0).sum() == sd2.hcond1.sum()
def sfr_obs_test():
import os
import pyemu
import flopy
sfr_file = os.path.join("utils","freyberg.sfr.out")
pyemu.gw_utils.setup_sfr_obs(sfr_file)
pyemu.gw_utils.setup_sfr_obs(sfr_file,seg_group_dict={"obs1":[1,4],"obs2":[16,17,18,19,22,23]})
m = flopy.modflow.Modflow.load("freyberg.nam",model_ws="utils",load_only=[],check=False)
pyemu.gw_utils.setup_sfr_obs(sfr_file,model=m)
pyemu.gw_utils.apply_sfr_obs()
pyemu.gw_utils.setup_sfr_obs(sfr_file, seg_group_dict={"obs1": [1, 4], "obs2": [16, 17, 18, 19, 22, 23]},model=m)
def sfr_reach_obs_test():
import os
import pyemu
import flopy
import pandas as pd
import numpy as np
sfr_file = os.path.join("utils","freyberg.sfr.out")
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, seg_reach=[[1, 2], [4, 1], [2, 2]])
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, seg_reach=np.array([[1, 2], [4, 1], [2, 2]]))
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file)
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*40 # (nper*nobs)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file,seg_reach={"obs1": [1, 2], "obs2": [4, 1]})
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
seg_reach_df = pd.DataFrame.from_dict({"obs1": [1, 2], "obs2": [4, 1]}, columns=['segment', 'reach'], orient='index')
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, seg_reach=seg_reach_df)
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
m = flopy.modflow.Modflow.load("freyberg.nam", model_ws="utils", load_only=[], check=False)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, model=m)
pyemu.gw_utils.apply_sfr_reach_obs()
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*40 # (nper*nobs)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, seg_reach={"obs1": [1, 2], "obs2": [4, 1], "blah": [2, 1]}, model=m)
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
pyemu.gw_utils.setup_sfr_reach_obs(sfr_file, model=m, seg_reach=seg_reach_df)
proc = pd.read_csv("{0}.reach_processed".format(sfr_file), sep=' ')
assert proc.shape[0] == 3*2 # (nper*nobs)
def gage_obs_test():
import os
import pyemu
import numpy as np
bd = os.getcwd()
os.chdir("utils")
gage_file = "RmSouth_pred_7d.gage1.go"
gage = pyemu.gw_utils.setup_gage_obs(gage_file, start_datetime='2007-04-11')
if gage is not None:
print(gage[1], gage[2])
times = np.concatenate(([0], np.arange(7., 7. * 404, 7.)))
gage = pyemu.gw_utils.setup_gage_obs(gage_file, start_datetime='2007-04-11', times=times)
if gage is not None:
print(gage[1], gage[2])
pyemu.gw_utils.apply_gage_obs()
os.chdir(bd)
def pst_from_parnames_obsnames_test():
import pyemu
import os
parnames = ['param1','par2','p3']
obsnames = ['obervation1','ob2','o6']
pst = pyemu.helpers.pst_from_parnames_obsnames(parnames, obsnames)
pst.write('simpletemp.pst')
newpst = pyemu.Pst('simpletemp.pst')
assert newpst.nobs == len(obsnames)
assert newpst.npar == len(parnames)
def write_jactest_test():
import os
import pyemu
pst = pyemu.Pst(os.path.join("pst", "5.pst"))
print(pst.parameter_data)
#return
df = pyemu.helpers.build_jac_test_csv(pst,num_steps=5)
print(df)
df = pyemu.helpers.build_jac_test_csv(pst, num_steps=5,par_names=["par1"])
print(df)
df = pyemu.helpers.build_jac_test_csv(pst, num_steps=5,forward=False)
print(df)
df.to_csv(os.path.join("temp","sweep_in.csv"))
print(pst.parameter_data)
pst.write(os.path.join("temp","test.pst"))
#pyemu.helpers.run("sweep test.pst",cwd="temp")
def plot_id_bar_test():
import pyemu
import matplotlib.pyplot as plt
w_dir = "la"
ev = pyemu.ErrVar(jco=os.path.join(w_dir, "pest.jcb"))
id_df = ev.get_identifiability_dataframe(singular_value=15)
pyemu.plot_utils.plot_id_bar(id_df)
#plt.show()
def jco_from_pestpp_runstorage_test():
import os
import pyemu
jco_file = os.path.join("utils","pest.jcb")
jco = pyemu.Jco.from_binary(jco_file)
rnj_file = jco_file.replace(".jcb",".rnj")
pst_file = jco_file.replace(".jcb",".pst")
jco2 = pyemu.helpers.jco_from_pestpp_runstorage(rnj_file,pst_file)
diff = (jco - jco2).to_dataframe()
print(diff)
def hfb_test():
import os
try:
import flopy
except:
return
import pyemu
org_model_ws = os.path.join("..", "examples", "freyberg_sfr_update")
nam_file = "freyberg.nam"
m = flopy.modflow.Modflow.load(nam_file, model_ws=org_model_ws, check=False)
try:
pyemu.gw_utils.write_hfb_template(m)
except:
pass
else:
raise Exception()
hfb_data = []
jcol1, jcol2 = 14,15
for i in range(m.nrow):
hfb_data.append([0,i,jcol1,i,jcol2,0.001])
flopy.modflow.ModflowHfb(m,0,0,len(hfb_data),hfb_data=hfb_data)
m.change_model_ws("temp")
m.write_input()
m.exe_name = "mfnwt"
try:
m.run_model()
except:
pass
tpl_file,df = pyemu.gw_utils.write_hfb_template(m)
assert os.path.exists(tpl_file)
assert df.shape[0] == m.hfb6.hfb_data.shape[0]
def hfb_zn_mult_test():
import os
try:
import flopy
except:
return
import pyemu
import pandas as pd
org_model_ws = os.path.join("..", "examples", "freyberg_sfr_update")
nam_file = "freyberg.nam"
m = flopy.modflow.Modflow.load(
nam_file, model_ws=org_model_ws, check=False)
try:
pyemu.gw_utils.write_hfb_template(m)
except:
pass
else:
raise Exception()
hfb_data = []
jcol1, jcol2 = 14, 15
for i in range(m.nrow)[:11]:
hfb_data.append([0, i, jcol1, i, jcol2, 0.001])
for i in range(m.nrow)[11:21]:
hfb_data.append([0, i, jcol1, i, jcol2, 0.002])
for i in range(m.nrow)[21:]:
hfb_data.append([0, i, jcol1, i, jcol2, 0.003])
flopy.modflow.ModflowHfb(m, 0, 0, len(hfb_data), hfb_data=hfb_data)
orig_len = len(m.hfb6.hfb_data)
m.change_model_ws("temp")
m.write_input()
m.exe_name = "mfnwt"
try:
m.run_model()
except:
pass
orig_vals, tpl_file = pyemu.gw_utils.write_hfb_zone_multipliers_template(m)
assert os.path.exists(tpl_file)
hfb_pars = pd.read_csv(os.path.join(m.model_ws, 'hfb6_pars.csv'))
hfb_tpl_contents = open(tpl_file, 'r').readlines()
mult_str = ''.join(hfb_tpl_contents[1:]).replace(
'~ hbz_0000 ~', '0.1').replace(
'~ hbz_0001 ~', '1.0').replace(
'~ hbz_0002 ~', '10.0')
with open(hfb_pars.mlt_file.values[0], 'w') as mfp:
mfp.write(mult_str)
pyemu.gw_utils.apply_hfb_pars(os.path.join(m.model_ws, 'hfb6_pars.csv'))
with open(hfb_pars.mlt_file.values[0], 'r') as mfp:
for i, line in enumerate(mfp):
pass
mhfb = flopy.modflow.ModflowHfb.load(hfb_pars.model_file.values[0], m)
assert i-1 == orig_len == len(mhfb.hfb_data)
def read_runstor_test():
import os
import numpy as np
import pandas as pd
import pyemu
d = os.path.join("utils","runstor")
pst = pyemu.Pst(os.path.join(d,"pest.pst"))
par_df,obs_df = pyemu.helpers.read_pestpp_runstorage(os.path.join(d,"pest.rns"),"all")
par_df2 = pd.read_csv(os.path.join(d,"sweep_in.csv"),index_col=0)
obs_df2 = pd.read_csv(os.path.join(d,"sweep_out.csv"),index_col=0)
obs_df2.columns = obs_df2.columns.str.lower()
obs_df2 = obs_df2.loc[:,obs_df.columns]
par_df2 = par_df2.loc[:,par_df.columns]
pdif = np.abs(par_df.values - par_df2.values).max()
odif = np.abs(obs_df.values - obs_df2.values).max()
print(pdif,odif)
assert pdif < 1.0e-6,pdif
assert odif < 1.0e-6,odif
try:
pyemu.helpers.read_pestpp_runstorage(os.path.join(d, "pest.rns"), "junk")
except:
pass
else:
raise Exception()
def smp_test():
import os
from pyemu.utils import smp_to_dataframe, dataframe_to_smp, \
smp_to_ins
from pyemu.pst.pst_utils import parse_ins_file
smp_filename = os.path.join("misc", "gainloss.smp")
df = smp_to_dataframe(smp_filename)
print(df.dtypes)
dataframe_to_smp(df, smp_filename + ".test")
smp_to_ins(smp_filename)
obs_names = parse_ins_file(smp_filename + ".ins")
print(len(obs_names))
smp_filename = os.path.join("misc", "sim_hds_v6.smp")
df = smp_to_dataframe(smp_filename)
print(df.dtypes)
dataframe_to_smp(df, smp_filename + ".test")
smp_to_ins(smp_filename)
obs_names = parse_ins_file(smp_filename + ".ins")
print(len(obs_names))
def smp_dateparser_test():
import os
import pyemu
from pyemu.utils import smp_to_dataframe, dataframe_to_smp, \
smp_to_ins
smp_filename = os.path.join("misc", "gainloss.smp")
df = smp_to_dataframe(smp_filename, datetime_format="%d/%m/%Y %H:%M:%S")
print(df.dtypes)
dataframe_to_smp(df, smp_filename + ".test")
smp_to_ins(smp_filename)
obs_names = pyemu.pst_utils.parse_ins_file(smp_filename + ".ins")
print(len(obs_names))
smp_filename = os.path.join("misc", "sim_hds_v6.smp")
df = smp_to_dataframe(smp_filename)
print(df.dtypes)
dataframe_to_smp(df, smp_filename + ".test")
smp_to_ins(smp_filename)
obs_names = pyemu.pst_utils.parse_ins_file(smp_filename + ".ins")
print(len(obs_names))
def fieldgen_dev():
import shutil
import numpy as np
import pandas as pd
try:
import flopy
except:
return
import pyemu
org_model_ws = os.path.join("..", "examples", "freyberg_sfr_update")
nam_file = "freyberg.nam"
m = flopy.modflow.Modflow.load(nam_file, model_ws=org_model_ws, check=False)
flopy.modflow.ModflowRiv(m, stress_period_data={0: [[0, 0, 0, 30.0, 1.0, 25.0],
[0, 0, 1, 31.0, 1.0, 25.0],
[0, 0, 1, 31.0, 1.0, 25.0]]})
org_model_ws = "temp"
m.change_model_ws(org_model_ws)
m.write_input()
new_model_ws = "temp_fieldgen"
ph = pyemu.helpers.PstFromFlopyModel(nam_file, new_model_ws=new_model_ws,
org_model_ws=org_model_ws,
grid_props=[["upw.hk", 0], ["rch.rech", 0]],
remove_existing=True,build_prior=False)
v = pyemu.geostats.ExpVario(1.0,1000,anisotropy=10,bearing=45)
gs = pyemu.geostats.GeoStruct(nugget=0.0,variograms=v,name="aniso")
struct_dict = {gs:["hk","ss"]}
df = pyemu.helpers.run_fieldgen(m,10,struct_dict,cwd=new_model_ws)
import matplotlib.pyplot as plt
i = df.index.map(lambda x: int(x.split('_')[0]))
j = df.index.map(lambda x: int(x.split('_')[1]))
arr = np.zeros((m.nrow,m.ncol))
arr[i,j] = df.iloc[:,0]
plt.imshow(arr)
plt.show()
def ok_grid_invest():
try:
import flopy
except:
return
import numpy as np
import pandas as pd
import pyemu
nrow,ncol = 50,50
delr = np.ones((ncol)) * 1.0/float(ncol)
delc = np.ones((nrow)) * 1.0/float(nrow)
num_pts = 100
ptx = np.random.random(num_pts)
pty = np.random.random(num_pts)
ptname = ["p{0}".format(i) for i in range(num_pts)]
pts_data = pd.DataFrame({"x":ptx,"y":pty,"name":ptname})
pts_data.index = pts_data.name
pts_data = pts_data.loc[:,["x","y","name"]]
sr = flopy.utils.SpatialReference(delr=delr,delc=delc)
pts_data.loc["i0j0", :] = [sr.xcentergrid[0,0],sr.ycentergrid[0,0],"i0j0"]
pts_data.loc["imxjmx", :] = [sr.xcentergrid[-1, -1], sr.ycentergrid[-1, -1], "imxjmx"]
str_file = os.path.join("utils","struct_test.dat")
gs = pyemu.utils.geostats.read_struct_file(str_file)[0]
ok = pyemu.utils.geostats.OrdinaryKrige(gs,pts_data)
kf = ok.calc_factors_grid(sr,verbose=False,var_filename=os.path.join("temp","test_var.ref"),minpts_interp=1)
kf2 = ok.calc_factors_grid(sr, verbose=False, var_filename=os.path.join("temp", "test_var.ref"), minpts_interp=1,num_threads=10)
ok.to_grid_factors_file(os.path.join("temp","test.fac"))
diff = (kf.err_var - kf2.err_var).apply(np.abs).sum()
assert diff < 1.0e-10
def specsim_test():
try:
import flopy
except:
return
import numpy as np
import pandas as pd
import pyemu
num_reals = 100
nrow,ncol = 40,20
a = 2500
contrib = 1.0
nugget = 0
delr = np.ones((ncol)) * 250
delc = np.ones((nrow)) * 250
variograms = [pyemu.geostats.ExpVario(contribution=contrib,a=a,anisotropy=1,bearing=10)]
gs = pyemu.geostats.GeoStruct(variograms=variograms,transform="none",nugget=nugget)
broke_delr = delr.copy()
broke_delr[0] = 0.0
broke_delc = delc.copy()
broke_delc[0] = 0.0
try:
ss = pyemu.geostats.SpecSim2d(geostruct=gs,delx=broke_delr,dely=delc)
except Exception as e:
pass
else:
raise Exception("should have failed")
variograms = [pyemu.geostats.ExpVario(contribution=contrib, a=a, anisotropy=1, bearing=00)]
gs = pyemu.geostats.GeoStruct(variograms=variograms, transform="none", nugget=nugget)
try:
ss = pyemu.geostats.SpecSim2d(geostruct=gs,delx=broke_delr,dely=delc)
except Exception as e:
pass
else:
raise Exception("should have failed")
try:
ss = pyemu.geostats.SpecSim2d(geostruct=gs,delx=delr,dely=broke_delc)
except Exception as e:
pass
else:
raise Exception("should have failed")
bd = os.getcwd()
try:
variograms = [pyemu.geostats.ExpVario(contribution=contrib, a=a, anisotropy=10, bearing=0)]
gs = pyemu.geostats.GeoStruct(variograms=variograms, transform="log", nugget=nugget)
np.random.seed(1)
ss = pyemu.geostats.SpecSim2d(geostruct=gs, delx=delr, dely=delc)
mean_value = 15.0
reals = ss.draw_arrays(num_reals=num_reals, mean_value=mean_value)
assert reals.shape == (num_reals, nrow, ncol),reals.shape
reals = np.log10(reals)
mean_value = np.log10(mean_value)
var = np.var(reals, axis=0).mean()
mean = reals.mean()
theo_var = ss.geostruct.sill
print(var, theo_var)
print(mean, mean_value)
assert np.abs(var - theo_var) < 0.1
assert np.abs(mean - mean_value) < 0.1
np.random.seed(1)
variograms = [pyemu.geostats.ExpVario(contribution=contrib, a=a, anisotropy=10, bearing=0)]
gs = pyemu.geostats.GeoStruct(variograms=variograms, transform="none", nugget=nugget)
ss = pyemu.geostats.SpecSim2d(geostruct=gs, delx=delr, dely=delc)
mean_value = 25.0
reals = ss.draw_arrays(num_reals=num_reals,mean_value=mean_value)
assert reals.shape == (num_reals,nrow,ncol)
var = | np.var(reals,axis=0) | numpy.var |
import os
import itertools
import numpy as np
from skimage import data_dir
from skimage.io import imread
from skimage.transform import radon, iradon, iradon_sart, rescale
from skimage._shared import testing
from skimage._shared.testing import test_parallel
from skimage._shared._warnings import expected_warnings
PHANTOM = imread(os.path.join(data_dir, "phantom.png"),
as_gray=True)[::2, ::2]
PHANTOM = rescale(PHANTOM, 0.5, order=1,
mode='constant', anti_aliasing=False, multichannel=False)
def _debug_plot(original, result, sinogram=None):
from matplotlib import pyplot as plt
imkwargs = dict(cmap='gray', interpolation='nearest')
if sinogram is None:
plt.figure(figsize=(15, 6))
sp = 130
else:
plt.figure(figsize=(11, 11))
sp = 221
plt.subplot(sp + 0)
plt.imshow(sinogram, aspect='auto', **imkwargs)
plt.subplot(sp + 1)
plt.imshow(original, **imkwargs)
plt.subplot(sp + 2)
plt.imshow(result, vmin=original.min(), vmax=original.max(), **imkwargs)
plt.subplot(sp + 3)
plt.imshow(result - original, **imkwargs)
plt.colorbar()
plt.show()
def _rescale_intensity(x):
x = x.astype(float)
x -= x.min()
x /= x.max()
return x
def check_radon_center(shape, circle):
# Create a test image with only a single non-zero pixel at the origin
image = np.zeros(shape, dtype=np.float)
image[(shape[0] // 2, shape[1] // 2)] = 1.
# Calculate the sinogram
theta = np.linspace(0., 180., max(shape), endpoint=False)
sinogram = radon(image, theta=theta, circle=circle)
# The sinogram should be a straight, horizontal line
sinogram_max = np.argmax(sinogram, axis=0)
print(sinogram_max)
assert np.std(sinogram_max) < 1e-6
shapes_for_test_radon_center = [(16, 16), (17, 17)]
circles_for_test_radon_center = [False, True]
@testing.parametrize("shape, circle",
itertools.product(shapes_for_test_radon_center,
circles_for_test_radon_center))
def test_radon_center(shape, circle):
check_radon_center(shape, circle)
rectangular_shapes = [(32, 16), (33, 17)]
@testing.parametrize("shape", rectangular_shapes)
def test_radon_center_rectangular(shape):
check_radon_center(shape, False)
def check_iradon_center(size, theta, circle):
debug = False
# Create a test sinogram corresponding to a single projection
# with a single non-zero pixel at the rotation center
if circle:
sinogram = np.zeros((size, 1), dtype=np.float)
sinogram[size // 2, 0] = 1.
else:
diagonal = int(np.ceil(np.sqrt(2) * size))
sinogram = np.zeros((diagonal, 1), dtype=np.float)
sinogram[sinogram.shape[0] // 2, 0] = 1.
maxpoint = np.unravel_index(np.argmax(sinogram), sinogram.shape)
print('shape of generated sinogram', sinogram.shape)
print('maximum in generated sinogram', maxpoint)
# Compare reconstructions for theta=angle and theta=angle + 180;
# these should be exactly equal
reconstruction = iradon(sinogram, theta=[theta], circle=circle)
reconstruction_opposite = iradon(sinogram, theta=[theta + 180],
circle=circle)
print('rms deviance:',
np.sqrt(np.mean((reconstruction_opposite - reconstruction)**2)))
if debug:
import matplotlib.pyplot as plt
imkwargs = dict(cmap='gray', interpolation='nearest')
plt.figure()
plt.subplot(221)
plt.imshow(sinogram, **imkwargs)
plt.subplot(222)
plt.imshow(reconstruction_opposite - reconstruction, **imkwargs)
plt.subplot(223)
plt.imshow(reconstruction, **imkwargs)
plt.subplot(224)
plt.imshow(reconstruction_opposite, **imkwargs)
plt.show()
assert np.allclose(reconstruction, reconstruction_opposite)
sizes_for_test_iradon_center = [16, 17]
thetas_for_test_iradon_center = [0, 90]
circles_for_test_iradon_center = [False, True]
@testing.parametrize("size, theta, circle",
itertools.product(sizes_for_test_iradon_center,
thetas_for_test_iradon_center,
circles_for_test_radon_center))
def test_iradon_center(size, theta, circle):
check_iradon_center(size, theta, circle)
def check_radon_iradon(interpolation_type, filter_type):
debug = False
image = PHANTOM
reconstructed = iradon(radon(image, circle=False), filter=filter_type,
interpolation=interpolation_type, circle=False)
delta = np.mean(np.abs(image - reconstructed))
print('\n\tmean error:', delta)
if debug:
_debug_plot(image, reconstructed)
if filter_type in ('ramp', 'shepp-logan'):
if interpolation_type == 'nearest':
allowed_delta = 0.03
else:
allowed_delta = 0.025
else:
allowed_delta = 0.05
assert delta < allowed_delta
filter_types = ["ramp", "shepp-logan", "cosine", "hamming", "hann"]
interpolation_types = ['linear', 'nearest']
radon_iradon_inputs = list(itertools.product(interpolation_types,
filter_types))
# cubic interpolation is slow; only run one test for it
radon_iradon_inputs.append(('cubic', 'shepp-logan'))
@testing.parametrize("interpolation_type, filter_type",
radon_iradon_inputs)
def test_radon_iradon(interpolation_type, filter_type):
check_radon_iradon(interpolation_type, filter_type)
def test_iradon_angles():
"""
Test with different number of projections
"""
size = 100
# Synthetic data
image = np.tri(size) + | np.tri(size) | numpy.tri |
import unittest
import numpy
import pytest
try:
import scipy.sparse
import scipy.stats
scipy_available = True
except ImportError:
scipy_available = False
import cupy
from cupy import testing
from cupy.testing import condition
from cupyx.scipy import sparse
@testing.parameterize(*testing.product({
'dtype': [numpy.float32, numpy.float64],
}))
@unittest.skipUnless(scipy_available, 'requires scipy')
class TestLsqr(unittest.TestCase):
def setUp(self):
rvs = scipy.stats.randint(0, 15).rvs
self.A = scipy.sparse.random(50, 50, density=0.2, data_rvs=rvs)
self.b = numpy.random.randint(15, size=50)
def test_size(self):
for xp, sp in ((numpy, scipy.sparse), (cupy, sparse)):
A = sp.csr_matrix(self.A, dtype=self.dtype)
b = xp.array(numpy.append(self.b, [1]), dtype=self.dtype)
with pytest.raises(ValueError):
sp.linalg.lsqr(A, b)
def test_shape(self):
for xp, sp in ((numpy, scipy.sparse), (cupy, sparse)):
A = sp.csr_matrix(self.A, dtype=self.dtype)
b = xp.array( | numpy.tile(self.b, (2, 1)) | numpy.tile |
"""OA
"""
import numpy as np
from NNBlocks import tanh,sigm,relu,iden,isNone,expo,lrelu
def boxcars(periods):
periods = np.array(periods,dtype=int)
phases = np.zeros(periods.shape,dtype=int)
def filt(v,ph=phases,pd=periods,stored=[None]):
if isNone(stored[0]):
stored[0] = np.zeros(periods.shape+v.shape)
stored[0][ph==0] = 0
stored[0] += v
ph += 1
f = ph>=pd
ph[f] = 0
return stored[0],f
return filt
REGULARIZATION_FACTOR = 0.01
MAX_GRADIENT_STEP = 0.1
class NN:
one = np.array([1])
def __init__(self,shape=[1,8],af=tanh,history=4):
self.hist = history
self.hist_ind = 0
try:
len(af)
self.af = af
except:
self.af = [af]*(len(shape)-1)
self.shape = shape
self.weights = [np.zeros((shape[i+1],shape[i]+1),dtype=float) for i in range(len(shape)-1)]
self.gd_deltas = [np.copy(w) for w in self.weights]
self.vals = [np.zeros((history,s),dtype=float) for s in shape]
self.fvals = [np.zeros((history,s),dtype=float) for s in shape]
def reset_mem(self):
for i in range(len(self.vals)):
self.vals[i].fill(0)
self.fvals[i].fill(0)
def scramble(self,keep=0,mag=1):
for w in self.weights:
w *= keep
w += np.random.normal(w)*mag
def len(self):
return self.shape[-1]
def hi(self,o=0):
return (self.hist_ind+o)%self.hist
def __call__(self,inp=None):
if not isNone(inp):
h = self.hist_ind = self.hi(1)
self.fvals[0][h][:]= inp
for i in range(len(self.weights)):
self.vals[i+1][h][:]=self.weights[i]@ | np.concatenate((self.fvals[i][h],NN.one)) | numpy.concatenate |
#!/usr/bin/env python
"""Module with class for single video"""
__author__ = '<NAME>'
__date__ = 'August 2018'
from collections import Counter
import numpy as np
import math as m
import os
from os.path import join
from ute.utils.arg_pars import opt
from ute.utils.logging_setup import logger
from ute.utils.util_functions import dir_check
from ute.viterbi_utils.viterbi_w_lenth import Viterbi
from ute.viterbi_utils.grammar import Grammar, SingleTranscriptGrammar
from ute.viterbi_utils.length_model import PoissonModel
class Video(object):
"""Single video with respective for the algorithm parameters"""
def __init__(self, path, K, *, gt=[], name='', start=0, with_bg=False, frame_sampling=1):
"""
Args:
path (str): path to video representation
K (int): number of subactivities in current video collection
reset (bool): necessity of holding features in each instance
gt (arr): ground truth labels
gt_with_0 (arr): ground truth labels with SIL (0) label
name (str): short name without any extension
start (int): start index in mask for the whole video collection
"""
self.iter = 0
self.path = path
self._K = K
self.name = name
self._frame_sampling = frame_sampling
self._likelihood_grid = None
self._valid_likelihood = None
self._theta_0 = 0.1
self._subact_i_mask = np.eye(self._K)
self.n_frames = 0
self._features = None
self.global_start = start
self.global_range = None
self.gt = gt
self._gt_unique = np.unique(self.gt)
self.features()
self._check_gt()
# counting of subactivities np.array
self.a = np.zeros(self._K)
# ordering, init with canonical ordering
self._pi = list(range(self._K))
self.inv_count_v = np.zeros(self._K - 1)
# subactivity per frame
self._z = []
self._z_idx = []
self._init_z_framewise()
# temporal labels
self.temp = None
self._init_temporal_labels()
# background
self._with_bg = with_bg
self.fg_mask = np.ones(self.n_frames, dtype=bool)
if self._with_bg:
self._init_fg_mask()
self._subact_count_update()
self.segmentation = {'gt': (self.gt, None)}
def features(self):
"""Load features given path if haven't do it before"""
if self._features is None:
if opt.ext == 'npy':
self._features = np.load(self.path)
else:
self._features = np.loadtxt(self.path)
######################################
# fixed.order._coffee_mlp_!pose_full_vae0_time10.0_epochs60_embed20_n1_!ordering_gmm1_one_!gt_lr0.0001_lr_zeros_b0_v1_l0_c1_.pth.tar
# if self._features.shape[-1] == 65 and opt.feature_dim == 64:
# self._features = self._features[1:, 1:]
# np.savetxt(self.path, self._features)
# if opt.data_type == 0 and opt.dataset == 'fs':
# self._features = self._features.T
if opt.f_norm: # normalize features
mask = np.ones(self._features.shape[0], dtype=bool)
for rdx, row in enumerate(self._features):
if np.sum(row) == 0:
mask[rdx] = False
z = self._features[mask] - np.mean(self._features[mask], axis=0)
z = z / | np.std(self._features[mask], axis=0) | numpy.std |
"""
Generate input data based on Section 7 in Kelly 2007
"""
import numpy as np
from scipy.stats.distributions import rv_continuous
__all__ = ['simulation_kelly']
class simulation_dist(rv_continuous):
def _pdf(self, x):
# eq 110
return 0.796248 * np.exp(x) / (1 + np.exp(2.75 * x))
def simulation_kelly(size=50, low=-10, high=10, alpha=1, beta=0.5,
epsilon=(0, 0.75), scalex=1, scaley=1, multidim=1):
"""
Data simulator from Kelly 2007
Parameters
==========
size
low
high
alpha
beta
epsilon
scalex
scaley
multidim
"""
eps = np.random.normal(epsilon[0], scale=epsilon[1], size=size)
dist = simulation_dist(a=low, b=high)
# I'm sorry about ksi, but it's less ambigous than having
# xi for greek and xi for vector x_i
ksi = dist.rvs(size=(multidim, size))
# eq 1
beta = np.atleast_1d(beta)
eta = alpha + np.dot(beta, ksi) + eps
tau = | np.var(ksi) | numpy.var |
from py4j.clientserver import ClientServer, JavaParameters, PythonParameters;
import sys;
import os;
import numpy;
import io;
import re;
import time;
class DOCoMETRe(object):
#global experiments;
global jvmMode;
def __init__(self, gateway):
self.gateway = gateway;
self.experiments = dict();
if(jvmMode): gateway.jvm.System.out.println("In __init__ gateway");
def shutDownServer(self, object):
if(jvmMode): self.gateway.jvm.System.out.println("In shutdown server");
pass;
def toString(self):
if(jvmMode): self.gateway.jvm.System.out.println("In toString");
return "This is DOCoMETRe Python Entry Point";
def loadData(self, dataType, loadName, dataFilesList, sessionsProperties):
if(dataType == "DOCOMETRE"):
self.loadDataDocometre(loadName, dataFilesList, sessionsProperties);
elif(dataType == "OPTITRACK_TYPE_1"):
self.loadDataOptitrackType1(loadName, dataFilesList);
else:
pass;
def loadDataDocometre(self, loadName, dataFilesList, sessionsProperties):
if(jvmMode): self.gateway.jvm.System.out.println("In loadDataDocometre");
if ".sau" in dataFilesList:
if(jvmMode): self.gateway.jvm.System.out.println("For now, sau files are not handled with Python");
elif ".samples" in dataFilesList:
self.loadDataDocometreSAMPLES(loadName, dataFilesList, sessionsProperties);
elif ".adw" in dataFilesList:
if(jvmMode): self.gateway.jvm.System.out.println("For now, ADW files are not handled with Python");
else:
if(jvmMode): self.gateway.jvm.System.out.println("Data files format not hanled with Python");
def loadDataOptitrackType1(self, loadName, dataFilesAbsolutePath):
if(jvmMode): self.gateway.jvm.System.out.println("In loadDataOptitrackType1");
createdCategories = dict();
createdChannelsNames = list();
channelNames = list();
nbSamples = 0;
firstTime = True;
files = dataFilesAbsolutePath.split(";");
nbFiles = len(files);
nbTrials = nbFiles;
for n in range(0, nbFiles):
fileName = os.path.basename(files[n]);
fileName = os.path.splitext(fileName)[0];
fileNameSplitted = fileName.split("_");
criteria = fileNameSplitted[1];
if criteria in createdCategories:
trialsList = createdCategories[criteria];
append = False;
if isinstance(trialsList, numpy.ndarray):
if n+1 not in trialsList:
append = True;
if append:
trialsList = | numpy.append(trialsList, n+1) | numpy.append |
import numpy as np
from dqn_agent import DQNAgent
from mix_ttn_agent_online_offline import TTNAgent_online_offline_mix
import os
import time
# import matplotlib.pyplot as plt
from collections import OrderedDict
import itertools
import torch as T
import argparse
# import datetime as date
from datetime import datetime
from replay_memory import ReplayBuffer
from training3 import train_offline_online, train_online, train_offline
import training3
np_load_old = np.load
# modify the default parameters of np.load
np.load = lambda *a, **k: np_load_old(*a, allow_pickle=True)
import gym
def main(alg_type, hyper_num, data_length_num, mem_size, num_rep, offline, fqi_rep_num, num_step_ratio_mem, en,
dirfeature, feature, num_epoch ,batch_size, replace_target_cnt,target_separate, method_sarsa, data_dir, learning_feat):
data_lengths = [mem_size, 6000, 10000, 20000, 30000]
data_length = data_lengths[data_length_num]
fqi_reps = [1, 10, 50, 100, 300]
fqi_rep = fqi_reps[fqi_rep_num]
if feature == 'tc':
tilecoding = 1
else:
tilecoding = 0
alg = alg_type
gamma = 0.99
rand_seed = num_rep * 32 # 332
num_steps = num_step_ratio_mem # 200000
## normolize states
def process_state(state, normalize=True):
# states = np.array([state['position'], state['vel']])
if normalize:
if en == "Acrobot":
states = np.array([state[0], state[1], state[2], state[3], state[4], state[5]])
states[0] = (states[0] + 1) / (2)
states[1] = (states[1] + 1) / (2)
states[2] = (states[2] + 1) / (2)
states[3] = (states[3] + 1) / (2)
states[4] = (states[4] + (4 * np.pi)) / (2 * 4 * np.pi)
states[5] = (states[5] + (9 * np.pi)) / (2 * 4 * np.pi)
elif en == "LunarLander":
states = np.array([state[0], state[1], state[2], state[3], state[4], state[5], state[6], state[7]])
mean = [0, 0.9, 0, -0.6, 0, 0, 0, 0]
deviation = [0.35, 0.6, 0.7, 0.6, 0.5, 0.5, 1.0, 1.0]
states[0] = (states[0] - mean[0]) / (deviation[0])
states[1] = (states[1] - mean[1]) / (deviation[1])
states[2] = (states[2] - mean[2]) / (deviation[2])
states[3] = (states[3] - mean[3]) / (deviation[3])
states[4] = (states[4] - mean[4]) / (deviation[4])
states[5] = (states[5] - mean[5]) / (deviation[5])
elif en == "cartpole":
states = np.array([state[0], state[1], state[2], state[3]])
states[0] = states[0]
states[1] = states[1]
states[2] = states[2]
states[3] = states[3]
elif en == "Mountaincar":
states = np.array([state[0], state[1]])
states[0] = (states[0] + 1.2) / (0.6 + 1.2)
states[1] = (states[1] + 0.07) / (0.07 + 0.07)
elif en == "catcher":
states = np.array([state['player_x'], state['player_vel'], state['fruit_x'], state['fruit_y']])
states[0] = (states[0] - 25.5) / 26
states[1] = states[1] / 10
states[2] = (states[2] - 30) / 22
states[3] = (states[3] - 18.5) / 47
return states
ple_rewards = {
"positive": 1.0,
"negative": -1.0,
"tick": 0.0,
"loss": -0.0,
"win": 5.0
}
## select environment
if en == "Mountaincar":
env = gym.make('MountainCar-v0')
input_dim = env.observation_space.shape[0]
num_act = 3
elif en == "Acrobot":
env = gym.make('Acrobot-v1')
input_dim = env.observation_space.shape[0]
num_act = 3
elif en == "LunarLander":
env = gym.make('LunarLander-v2')
input_dim = env.observation_space.shape[0]
num_act = 4
elif en == "cartpole":
env = gym.make('CartPole-v0')
input_dim = env.observation_space.shape[0]
num_act = 2
env.seed(rand_seed)
T.manual_seed(rand_seed)
np.random.seed(rand_seed)
# dqn:
hyper_sets_DQN = OrderedDict([("nn_lr", np.power(10, [-3.25, -3.5, -3.75, -4.0, -4.25])),
("eps_decay_steps", [10000, 20000, 40000]),
])
## DQN
q_nnet_params = {"update_fqi_steps": 50000,
"num_actions": num_act,
"eps_init": 1.0,
"eps_final": 0.01,
"batch_size": 32, # TODO try big batches
"replay_memory_size": mem_size,
"replay_init_size": 5000,
"update_target_net_steps": 1000
}
## TTN
nnet_params = {"loss_features": 'semi_MSTDE', # "next_reward_state", next_state, semi_MSTDE
"beta1": 0.0,
"beta2": 0.99,
"eps_init": 1.0,
"eps_final": 0.01,
"num_actions": num_act,
"replay_memory_size": mem_size, # 200000 if using ER
"replay_init_size": 5000,
"batch_size": 32,
"fqi_reg_type": "prev", # "l2" or "prev"
}
## TTN
hyper_sets_lstdq = OrderedDict([("nn_lr", np.power(10, [-4.0])), # [-2.0, -2.5, -3.0, -3.5, -4.0]
("reg_A",
[10, 20, 30, 50, 70, 100, 2, 3, 5, 8, 1, 0, 0.01, 0.002, 0.0003, 0.001, 0.05]),
# [0.0, -1.0, -2.0, -3.0] can also do reg towards previous weights
("eps_decay_steps", [1]),
("update_freq", [1000]),
("data_length", [data_length]),
("fqi_rep", [fqi_rep]),
])
files_name = "Data//{}_{}".format(en, mem_size)
if rnd:
files_name = "Data//{}_rnd_{}".format(en, mem_size)
if alg == 'fqi':
log_file = files_name+str(alg)
if alg == 'dqn':
log_file = files_name+str(alg)
if alg in ("fqi"):
hyper_sets = hyper_sets_lstdq
TTN = True
elif alg == "dqn":
hyper_sets = hyper_sets_DQN
TTN = False
#
hyperparams_all = list(itertools.product(*list(hyper_sets.values())))
hyperparams = hyperparams_all
with open(log_file + ".txt", 'w') as f:
print("Start! Seed: {}".format(rand_seed), file=f)
times = []
start_time = time.perf_counter()
# num_steps = 200000
prev_action_flag = 1
num_repeats = num_rep # 10
# TTN = True
# for hyper in hyperparams:
# run the algorithm
hyper = hyperparams[hyper_num]
hyperparam_returns = []
hyperparam_values = []
hyperparam_episodes = []
hyperparam_losses = []
data = dict([('state', []), ('action', []), ('reward', []), ('nstate', []), ('naction', []), ('done', [])])
if alg in ('lstdq', 'fqi'):
params = OrderedDict([("nn_lr", hyper[0]),
("reg_A", hyper[1]),
("eps_decay_steps", hyper[2]),
("update_freq", hyper[3]),
("data_length", hyper[4]),
("fqi_rep", hyper[5]),
])
elif alg in ("dqn"):
params = OrderedDict([("nn_lr", hyper[0]),
("eps_decay_steps", hyper[1])])
if TTN:
nn = TTNAgent_online_offline_mix(gamma, nnet_params=nnet_params, other_params=params,
input_dims=input_dim, num_units_rep=128, dir=dir, offline=offline,
num_tiling=16, num_tile=4, method_sarsa=method_sarsa,
tilecoding=tilecoding, replace_target_cnt=replace_target_cnt, target_separate=target_separate)
else:
nn = DQNAgent(gamma, q_nnet_params, params, input_dims=input_dim)
def generate_data():
for rep in range(1):
with open(log_file + ".txt", 'a') as f:
print("new run for the current setting".format(rep), file=f)
# saver = tf.train.Saver(keep_checkpoint_every_n_hours=2)
# populate the replay buffer with some number of transitions
if alg == 'dqn' or (TTN and nnet_params['replay_memory_size'] > 0):
print("initialize buffer")
frame_history = []
prev_state = None
prev_action = None
done = 0
state_unnormal = env.reset()
state = process_state(state_unnormal)
# populate the replay buffer
while nn.memory.mem_cntr < mem_size: #nnet_params["replay_init_size"]:
if done:
state_unnormal = env.reset()
state = process_state(state_unnormal)
# get action
action = np.random.randint(0, num_act)
# add to buffer
if prev_state is not None:
if prev_action_flag == 1:
nn.memory.store_transition_withnewaction(prev_state, np.squeeze(prev_action), reward, state,
np.squeeze(action), int(done))
else:
nn.memory.store_transition(prev_state, np.squeeze(prev_action), reward, state, int(done))
# do one step in the environment
new_state_unnormal, reward, done, info = env.step(np.squeeze(action)) # action is a 1x1 matrix
new_state = process_state(new_state_unnormal)
# update saved states
prev_state = state
prev_action = action
state = new_state
#############################################
prev_state = None
prev_action = None
run_returns = []
run_episodes = []
run_episode_length = []
run_values = []
run_losses = []
start_run_time = time.perf_counter()
episode_length = 0
done = 0
val = 0.0
ret = 0.0
episodes = 0
ctr = 0
ch = 0
state_unnormal = env.reset()
state = process_state(state_unnormal)
i = 0
for ij in range(num_steps):
episode_length += 1
i += 1
if done:
run_episodes.append(episodes)
run_returns.append(ret)
run_episode_length.append(episode_length)
with open(log_file + ".txt", 'a') as f:
print('time:', datetime.today().strftime("%H_%M_%S"), 'return', '{}'.format(int(val)).ljust(4),
i,
len(run_returns),
"\t{:.2f}".format(np.mean(run_returns[-100:])), file=f)
if TTN:
print(episodes, ij, round(val, 2), round(ret, 2), round(np.mean(run_returns[-100:]), 3), np.mean(run_returns),
episode_length) # nn.lin_values.data
else:
print(episodes, ij, round(val, 2), round(ret, 2), round(np.mean(run_returns[-100:]), 3), np.mean(run_returns),
episode_length)
# avg_returns.append(np.mean(all_returns[-100:]))
print("episode length is:", episode_length, "return is:", ret)
val = 0.0
ret = 0.0
i = 0
episodes += 1
episode_length = 0
state_unnormal = env.reset()
state = process_state(state_unnormal)
## Get action
if TTN:
action = nn.choose_action(state)
q_values = nn.lin_values
else: # DQN
action, q_values = nn.choose_action(state)
# run_values.append(T.mean(q_values.data, axis=1)[0].detach())
# update parameters
if prev_state is not None:
if prev_action_flag == 1:
if reward > 0:
for ii in range(1):
nn.memory.store_transition_withnewaction(prev_state, | np.squeeze(prev_action) | numpy.squeeze |
"""This module defines the replay buffers. A replay buffer is a data structure that stores
transitions coming from the environment, and allows sampling. This module provides a base class
BaseReplayBuffer that defines the minimal interface that any replay buffer implementation should
provide.
Contents:
Base classes:
- BaseReplayBuffer
Implementations:
-FIFOReplayBuffer
"""
import collections
import random
import abc
import numbers
import numpy as np
# --------------------------------------- REPLAY BUFFERS ------------------------------------------#
class BaseReplayBuffer(abc.ABC, collections.UserList):
"""The base class for replay buffers.
Any derived replay buffer must present an Iterable interface, therefore allowing iteration,
sampling, etc.
"""
def add_iterable(self, iterable):
for i in iterable:
self.remember(i)
def __add__(self, e):
if hasattr(e, '__iter__'):
return self.add_iterable(e)
else:
return self.remember(e)
def append(self, e):
self.remember(e)
def extend(self, e):
return self.add_iterable(e)
@abc.abstractmethod
def remember(self, transition, *args, **kwargs):
"""Remember the given transition.
Args:
transition (tuple): A transition in the form (s, a, r, s', *info). After s' any
additional information can be passed.
"""
pass
@abc.abstractmethod
def sample(self, size, *args, **kwargs):
"""Sampling operation on the replay buffer.
Args:
size (int): Number of transitions to sample.
Returns:
A tuple (transitions, info) where transitions is a list of sampled transitions, and
info is a dictionary with additional information.
"""
pass
class FIFOReplayBuffer(BaseReplayBuffer):
"""Defines a simple fixed-size, FIFO evicted replay buffer, that stores transitions and allows
sampling.
Transitions are tuples in the form (s, a, r, s', ...), where after s' any additional
information can be stored.
"""
def __init__(self, maxlen):
"""Instantiate the replay buffer.
Args:
maxlen (int): Maximum number of transitions to be stored.
"""
super().__init__()
self.buffer = collections.deque(maxlen=maxlen)
def remember(self, transition, *args, **kwargs):
"""Store the given transition
Args:
transition (list): List in the form [s, a, r, s', ...]. Note that s' should be None
if the episode ended. After s' any additional information can be passed.
Raises:
AssertionError if given shapes do not match with those declared at initialization.
"""
self.buffer.append(transition)
def sample(self, size, *args, **kwargs):
"""Sample uniformly from the replay buffer.
Args:
size (int): Number of transitions to sample.
Returns:
A tuple (transitions, info). transitions is a list with the sampled transitions.
info is an empty dictionary.
"""
if size > len(self.buffer):
raise ValueError(
'Trying to sample ' + str(size) + ' items when buffer has only ' +
str(len(self.buffer)) + ' items.'
)
indices = np.arange(len(self.buffer))
sampled_indices = np.random.choice(a=indices, size=size, replace=False)
return [self.buffer[i] for i in sampled_indices], {} # Empty dict for compatibility
class PrioritizedReplayBuffer(FIFOReplayBuffer):
"""Implementation of a prioritized replay buffer.
This replay buffer stores transitions (s, a, r, s', w) where w is the weight. The sampling is
done by sampling a chunk of the given chunk_size, and performing a weighted sampling on it.
This allows sampling to be done in constant time. The probability of a transition i is given
by w_i^alpha / sum w_k^alpha. If exceeding the maximum length, samples are evicted with a
FIFO policy.
"""
def __init__(self, maxlen, alpha=0.8, chunk_size=2000):
"""Instantiate the replay buffer.
Args:
maxlen (int): Maximum number of transitions that the replay buffer will keep.
alpha (float): Level of prioritization, between 0 and 1.
chunk_size (int): Dimension of the random chunk from which transitions will be sampled.
"""
super().__init__(maxlen=maxlen)
self.alpha = alpha
self.chunk_size = chunk_size
self.avg_td_error = 0
def remember(self, transition, *args, **kwargs):
"""Add the transition to the buffer.
Args:
transition (list): Transition in the form [s, a, r, s', w, ...] where w is the
un-normalized weight of the transition.
"""
assert len(transition) >= 5, 'The given transition must be [s, a, r, s\', w, ...].'
super().remember(transition)
def sample(self, size, *args, **kwargs):
"""Sample the given number of transitions with probability proportional to the weights.
Args:
size (int): Number of transitions to sample.
Returns:
A tuple (transitions, info) where transitions is a list of the sampled transitions and
info is a dictionary {'weights': weights} with weights being the normalized weights
of the sampled transitions.
"""
if size > len(self.buffer):
raise ValueError(
'Trying to sample ' + str(size) + ' items when buffer has only ' +
str(len(self.buffer))
)
chunk = np.random.choice(a=np.arange(len(self.buffer)), size=self.chunk_size, replace=False)
td_errors = np.array([self.buffer[i][4] + 1e-6 for i in chunk])
self.avg_td_error = td_errors.mean() # Update statistics
# Compute probabilities and sample
probabilities = | np.power(td_errors, self.alpha) | numpy.power |
# Copyright (c) 2020 Mitsubishi Electric Research Laboratories (MERL). All rights reserved. The software, documentation and/or data in this file is provided on an "as is" basis, and MERL has no obligations to provide maintenance, support, updates, enhancements or modifications. MERL specifically disclaims any warranties, including, but not limited to, the implied warranties of merchantability and fitness for any particular purpose. In no event shall MERL be liable to any party for direct, indirect, special, incidental, or consequential damages, including lost profits, arising out of the use of this software and its documentation, even if MERL has been advised of the possibility of such damages. As more fully described in the license agreement that was required in order to download this software, documentation and/or data, permission to use, copy and modify this software without fee is granted, but only for educational, research and non-commercial purposes.
import numpy as np
import os
from nuscenes.utils.data_classes import Box
from pyquaternion import Quaternion
from numba import njit
import time
def point_in_hull_slow(point, hull, tolerance=1e-12):
"""
Check if a point lies in a convex hull. This implementation is slow.
:param point: nd.array (1 x d); d: point dimension
:param hull: The scipy ConvexHull object
:param tolerance: Used to compare to a small positive constant because of issues of numerical precision
(otherwise, you may find that a vertex of the convex hull is not in the convex hull)
"""
return all((np.dot(eq[:-1], point) + eq[-1] <= tolerance) for eq in hull.equations)
def point_in_hull_fast(points: np.array, bounding_box: Box):
"""
Check if a point lies in a bounding box. We first rotate the bounding box to align with axis. Meanwhile, we
also rotate the whole point cloud. Finally, we just check the membership with the aid of aligned axis.
This implementation is fast.
:param points: nd.array (N x d); N: the number of points, d: point dimension
:param bounding_box: the Box object
return: The membership of points within the bounding box
"""
# Make sure it is a unit quaternion
bounding_box.orientation = bounding_box.orientation.normalised
# Rotate the point clouds
pc = bounding_box.orientation.inverse.rotation_matrix @ points.T
pc = pc.T
orientation_backup = Quaternion(bounding_box.orientation) # Deep clone it
bounding_box.rotate(bounding_box.orientation.inverse)
corners = bounding_box.corners()
# Test if the points are in the bounding box
idx = np.where((corners[0, 7] <= pc[:, 0]) & (pc[:, 0] <= corners[0, 0]) &
(corners[1, 1] <= pc[:, 1]) & (pc[:, 1] <= corners[1, 0]) &
(corners[2, 2] <= pc[:, 2]) & (pc[:, 2] <= corners[2, 0]))[0]
# recover
bounding_box.rotate(orientation_backup)
return idx
def calc_displace_vector(points: np.array, curr_box: Box, next_box: Box):
"""
Calculate the displacement vectors for the input points.
This is achieved by comparing the current and next bounding boxes. Specifically, we first rotate
the input points according to the delta rotation angle, and then translate them. Finally we compute the
displacement between the transformed points and the input points.
:param points: The input points, (N x d). Note that these points should be inside the current bounding box.
:param curr_box: Current bounding box.
:param next_box: The future next bounding box in the temporal sequence.
:return: Displacement vectors for the points.
"""
assert points.shape[1] == 3, "The input points should have dimension 3."
# Make sure the quaternions are normalized
curr_box.orientation = curr_box.orientation.normalised
next_box.orientation = next_box.orientation.normalised
delta_rotation = curr_box.orientation.inverse * next_box.orientation
rotated_pc = (delta_rotation.rotation_matrix @ points.T).T
rotated_curr_center = np.dot(delta_rotation.rotation_matrix, curr_box.center)
delta_center = next_box.center - rotated_curr_center
rotated_tranlated_pc = rotated_pc + delta_center
pc_displace_vectors = rotated_tranlated_pc - points
return pc_displace_vectors
def get_static_and_moving_cells(batch_disp_field_gt, upper_thresh=0.1, frame_skip=3):
"""
Get the indices/masks of static and moving cells. Ths speed of static cells is bounded by upper_thresh.
In particular, for a given cell, if its displacement over the past 1 second (about 20 sample data) is in the
range [0, upper_thresh], we consider it as static cell, otherwise as moving cell.
:param batch_disp_field_gt: Batch of ground-truth displacement fields. numpy array, shape (seq len, h, w)
:param upper_thresh: The speed upper bound
:param frame_skip: The number of skipped frame in the sweep sequence. This is used for computing the upper bound
for defining static objects
"""
upper_bound = (frame_skip + 1) / 20 * upper_thresh
disp_norm = np.linalg.norm(batch_disp_field_gt, ord=2, axis=-1)
static_cell_mask = disp_norm <= upper_bound
static_cell_mask = np.all(static_cell_mask, axis=0) # along the sequence axis
moving_cell_mask = np.logical_not(static_cell_mask)
return static_cell_mask, moving_cell_mask
def voxelize(pts, voxel_size, extents=None, num_T=35, seed: float = None):
"""
Voxelize the input point cloud. Code modified from https://github.com/Yc174/voxelnet
Voxels are 3D grids that represent occupancy info.
The input for the voxelization is expected to be a PointCloud
with N points in 4 dimension (x,y,z,i). Voxel size is the quantization size for the voxel grid.
voxel_size: I.e. if voxel size is 1 m, the voxel space will be
divided up within 1m x 1m x 1m space. This space will be -1 if free/occluded and 1 otherwise.
min_voxel_coord: coordinates of the minimum on each axis for the voxel grid
max_voxel_coord: coordinates of the maximum on each axis for the voxel grid
num_divisions: number of grids in each axis
leaf_layout: the voxel grid of size (numDivisions) that contain -1 for free, 0 for occupied
:param pts: Point cloud as N x [x, y, z, i]
:param voxel_size: Quantization size for the grid, vd, vh, vw
:param extents: Optional, specifies the full extents of the point cloud.
Used for creating same sized voxel grids. Shape (3, 2)
:param num_T: Number of points in each voxel after sampling/padding
:param seed: The random seed for fixing the data generation.
"""
# Check if points are 3D, otherwise early exit
if pts.shape[1] < 3 or pts.shape[1] > 4:
raise ValueError("Points have the wrong shape: {}".format(pts.shape))
if extents is not None:
if extents.shape != (3, 2):
raise ValueError("Extents are the wrong shape {}".format(extents.shape))
filter_idx = np.where((extents[0, 0] < pts[:, 0]) & (pts[:, 0] < extents[0, 1]) &
(extents[1, 0] < pts[:, 1]) & (pts[:, 1] < extents[1, 1]) &
(extents[2, 0] < pts[:, 2]) & (pts[:, 2] < extents[2, 1]))[0]
pts = pts[filter_idx]
# Discretize voxel coordinates to given quantization size
discrete_pts = np.floor(pts[:, :3] / voxel_size).astype(np.int32)
# Use Lex Sort, sort by x, then y, then z
x_col = discrete_pts[:, 0]
y_col = discrete_pts[:, 1]
z_col = discrete_pts[:, 2]
sorted_order = np.lexsort((z_col, y_col, x_col))
# Save original points in sorted order
points = pts[sorted_order]
discrete_pts = discrete_pts[sorted_order]
# Format the array to c-contiguous array for unique function
contiguous_array = np.ascontiguousarray(discrete_pts).view(
np.dtype((np.void, discrete_pts.dtype.itemsize * discrete_pts.shape[1])))
# The new coordinates are the discretized array with its unique indexes
_, unique_indices = np.unique(contiguous_array, return_index=True)
# Sort unique indices to preserve order
unique_indices.sort()
voxel_coords = discrete_pts[unique_indices]
# Number of points per voxel, last voxel calculated separately
num_points_in_voxel = np.diff(unique_indices)
num_points_in_voxel = np.append(num_points_in_voxel, discrete_pts.shape[0] - unique_indices[-1])
# Compute the minimum and maximum voxel coordinates
if extents is not None:
min_voxel_coord = np.floor(extents.T[0] / voxel_size)
max_voxel_coord = np.ceil(extents.T[1] / voxel_size) - 1
else:
min_voxel_coord = np.amin(voxel_coords, axis=0)
max_voxel_coord = np.amax(voxel_coords, axis=0)
# Get the voxel grid dimensions
num_divisions = ((max_voxel_coord - min_voxel_coord) + 1).astype(np.int32)
# Bring the min voxel to the origin
voxel_indices = (voxel_coords - min_voxel_coord).astype(int)
# Padding the points within each voxel
padded_voxel_points = np.zeros([unique_indices.shape[0], num_T, pts.shape[1] + 3], dtype=np.float32)
padded_voxel_points = padding_voxel(padded_voxel_points, unique_indices, num_points_in_voxel, points, num_T, seed)
return padded_voxel_points, voxel_indices, num_divisions
@njit
def padding_voxel(padded_voxel_points, unique_indices, num_points_in_voxel, points, num_T, seed):
if seed is not None:
np.random.seed(seed)
for i, v in enumerate(zip(unique_indices, num_points_in_voxel)):
if v[1] < num_T:
padded_voxel_points[i, :v[1], :4] = points[v[0]:v[0] + v[1], :]
middle_points_x = np.mean(points[v[0]:v[0] + v[1], 0])
middle_points_y = np.mean(points[v[0]:v[0] + v[1], 1])
middle_points_z = np.mean(points[v[0]:v[0] + v[1], 2])
padded_voxel_points[i, :v[1], 4] = padded_voxel_points[i, :v[1], 0] - middle_points_x
padded_voxel_points[i, :v[1], 5] = padded_voxel_points[i, :v[1], 1] - middle_points_y
padded_voxel_points[i, :v[1], 6] = padded_voxel_points[i, :v[1], 2] - middle_points_z
else:
inds = np.random.choice(v[1], num_T)
padded_voxel_points[i, :, :4] = points[v[0] + inds, :]
middle_points_x = np.mean(points[v[0] + inds, 0])
middle_points_y = np.mean(points[v[0] + inds, 1])
middle_points_z = np.mean(points[v[0] + inds, 2])
padded_voxel_points[i, :, 4] = padded_voxel_points[i, :, 0] - middle_points_x
padded_voxel_points[i, :, 5] = padded_voxel_points[i, :, 1] - middle_points_y
padded_voxel_points[i, :, 6] = padded_voxel_points[i, :, 2] - middle_points_z
return padded_voxel_points
def gen_2d_grid_gt(data_dict: dict, grid_size: np.array, extents: np.array = None,
frame_skip: int = 0, reordered: bool = False, proportion_thresh: float = 0.5,
category_num: int = 5, one_hot_thresh: float = 0.8, h_flip: bool = False,
min_point_num_per_voxel: int = -1,
return_past_2d_disp_gt: bool = False,
return_instance_map: bool = False):
"""
Generate the 2d grid ground-truth for the input point cloud.
The ground-truth is: the displacement vectors of the occupied pixels in BEV image.
The displacement is computed w.r.t the current time and the future time
:param data_dict: The dictionary containing the data information
:param grid_size: The size of each pixel
:param extents: The extents of the point cloud on the 2D xy plane. Shape (3, 2)
:param frame_skip: The number of sample frames that need to be skipped
:param reordered: Whether need to reorder the results, so that the first element corresponds to the oldest past
record. This option is only effective when return_past_2d_disp_gt = True.
:param proportion_thresh: Within a given pixel, only when the proportion of foreground points exceeds this threshold
will we compute the displacement vector for this pixel.
:param category_num: The number of categories for points.
:param one_hot_thresh: When the proportion of the majority points within a cell exceeds this threshold, we
compute the (hard) one-hot category vector for this cell, otherwise compute the soft category vector.
:param h_flip: Flip the point clouds horizontally
:param min_point_num_per_voxel: Minimum point number inside each voxel (cell). If smaller than this threshold, we
do not compute the displacement vector for this voxel (cell), and set the displacement to zero.
:param return_past_2d_disp_gt: Whether to compute the ground-truth displacement filed for the past sweeps.
:param return_instance_map: Whether to return the instance id map.
:return: The ground-truth displacement field. Shape (num_sweeps, image height, image width, 2).
"""
num_sweeps = data_dict['num_sweeps']
times = data_dict['times']
num_past_sweeps = len(np.where(times >= 0)[0])
num_future_sweeps = len(np.where(times < 0)[0])
assert num_past_sweeps + num_future_sweeps == num_sweeps, "The number of sweeps is incorrect!"
pc_list = []
for i in range(num_sweeps):
pc = data_dict['pc_' + str(i)]
if h_flip:
pc[0, :] = -pc[0, :] # flip x coordinate
pc_list.append(pc.T)
# Retrieve the instance boxes
num_instances = data_dict['num_instances']
instance_box_list = list()
instance_cat_list = list() # for instance categories
for i in range(num_instances):
instance = data_dict['instance_boxes_' + str(i)]
category = data_dict['category_' + str(i)]
instance_box_list.append(instance)
instance_cat_list.append(category)
# ----------------------------------------------------
# Filter and sort the reference point cloud
refer_pc = pc_list[0]
refer_pc = refer_pc[:, 0:3]
if extents is not None:
if extents.shape != (3, 2):
raise ValueError("Extents are the wrong shape {}".format(extents.shape))
filter_idx = np.where((extents[0, 0] < refer_pc[:, 0]) & (refer_pc[:, 0] < extents[0, 1]) &
(extents[1, 0] < refer_pc[:, 1]) & (refer_pc[:, 1] < extents[1, 1]) &
(extents[2, 0] < refer_pc[:, 2]) & (refer_pc[:, 2] < extents[2, 1]))[0]
refer_pc = refer_pc[filter_idx]
# -- Discretize pixel coordinates to given quantization size
discrete_pts = np.floor(refer_pc[:, 0:2] / grid_size).astype(np.int32)
# -- Use Lex Sort, sort by x, then y
x_col = discrete_pts[:, 0]
y_col = discrete_pts[:, 1]
sorted_order = np.lexsort((y_col, x_col))
refer_pc = refer_pc[sorted_order]
discrete_pts = discrete_pts[sorted_order]
contiguous_array = np.ascontiguousarray(discrete_pts).view(
np.dtype((np.void, discrete_pts.dtype.itemsize * discrete_pts.shape[1])))
# -- The new coordinates are the discretized array with its unique indexes
_, unique_indices = np.unique(contiguous_array, return_index=True)
# -- Sort unique indices to preserve order
unique_indices.sort()
pixel_coords = discrete_pts[unique_indices]
# -- Number of points per voxel, last voxel calculated separately
num_points_in_pixel = np.diff(unique_indices)
num_points_in_pixel = np.append(num_points_in_pixel, discrete_pts.shape[0] - unique_indices[-1])
# -- Compute the minimum and maximum voxel coordinates
if extents is not None:
min_pixel_coord = np.floor(extents.T[0, 0:2] / grid_size)
max_pixel_coord = np.ceil(extents.T[1, 0:2] / grid_size) - 1
else:
min_pixel_coord = np.amin(pixel_coords, axis=0)
max_pixel_coord = np.amax(pixel_coords, axis=0)
# -- Get the voxel grid dimensions
num_divisions = ((max_pixel_coord - min_pixel_coord) + 1).astype(np.int32)
# -- Bring the min voxel to the origin
pixel_indices = (pixel_coords - min_pixel_coord).astype(int)
# ----------------------------------------------------
# ----------------------------------------------------
# Get the point cloud subsets, which are inside different instance bounding boxes
refer_box_list = list()
refer_pc_idx_per_bbox = list()
points_category = np.zeros(refer_pc.shape[0], dtype=np.int) # store the point categories
pixel_instance_id = np.zeros(pixel_indices.shape[0], dtype=np.uint8)
points_instance_id = np.zeros(refer_pc.shape[0], dtype=np.int)
for i in range(num_instances):
instance_cat = instance_cat_list[i]
instance_box = instance_box_list[i]
instance_box_data = instance_box[0]
assert not np.isnan(instance_box_data).any(), "In the keyframe, there should not be NaN box annotation!"
if h_flip:
tmp_quad = instance_box_data[6:].copy()
tmp_quad[2] *= -1 # y
tmp_quad[3] *= -1 # z
tmp_quad = Quaternion(tmp_quad)
tmp_center = instance_box_data[0:3].copy()
tmp_center[0] = -tmp_center[0]
tmp_box = Box(center=tmp_center, size=instance_box_data[3:6], orientation=Quaternion(tmp_quad))
else:
tmp_box = Box(center=instance_box_data[:3], size=instance_box_data[3:6],
orientation=Quaternion(instance_box_data[6:]))
idx = point_in_hull_fast(refer_pc[:, 0:3], tmp_box)
refer_pc_idx_per_bbox.append(idx)
refer_box_list.append(tmp_box)
points_category[idx] = instance_cat
points_instance_id[idx] = i + 1 # object id starts from 1, background has id 0
assert np.max(points_instance_id) <= 255, "The instance id exceeds uint8 max."
if len(refer_pc_idx_per_bbox) > 0:
refer_pc_idx_inside_box = np.concatenate(refer_pc_idx_per_bbox).tolist()
else:
refer_pc_idx_inside_box = []
refer_pc_idx_outside_box = set(range(refer_pc.shape[0])) - set(refer_pc_idx_inside_box)
refer_pc_idx_outside_box = list(refer_pc_idx_outside_box)
# Compute pixel (cell) categories
pixel_cat = np.zeros([unique_indices.shape[0], category_num], dtype=np.float32)
most_freq_info = []
for h, v in enumerate(zip(unique_indices, num_points_in_pixel)):
pixel_elements_categories = points_category[v[0]:v[0] + v[1]]
elements_freq = np.bincount(pixel_elements_categories, minlength=category_num)
assert np.sum(elements_freq) == v[1], "The frequency count is incorrect."
elements_freq = elements_freq / float(v[1])
most_freq_cat, most_freq = np.argmax(elements_freq), np.max(elements_freq)
most_freq_info.append([most_freq_cat, most_freq])
most_freq_elements_idx = np.where(pixel_elements_categories == most_freq_cat)[0]
pixel_elements_instance_ids = points_instance_id[v[0]:v[0] + v[1]]
most_freq_instance_id = pixel_elements_instance_ids[most_freq_elements_idx[0]]
if most_freq >= one_hot_thresh:
one_hot_cat = np.zeros(category_num, dtype=np.float32)
one_hot_cat[most_freq_cat] = 1.0
pixel_cat[h] = one_hot_cat
pixel_instance_id[h] = most_freq_instance_id
else:
pixel_cat[h] = elements_freq # use soft category probability vector.
pixel_cat_map = np.zeros((num_divisions[0], num_divisions[1], category_num), dtype=np.float32)
pixel_cat_map[pixel_indices[:, 0], pixel_indices[:, 1]] = pixel_cat[:]
pixel_instance_map = np.zeros((num_divisions[0], num_divisions[1]), dtype=np.uint8)
pixel_instance_map[pixel_indices[:, 0], pixel_indices[:, 1]] = pixel_instance_id[:]
# Set the non-zero pixels to 1.0, which will be helpful for loss computation
# Note that the non-zero pixels correspond to both the foreground and background objects
non_empty_map = np.zeros((num_divisions[0], num_divisions[1]), dtype=np.float32)
non_empty_map[pixel_indices[:, 0], pixel_indices[:, 1]] = 1.0
# Ignore the voxel/pillar which contains number of points less than min_point_num_per_voxel; only for fg points
cell_pts_num = np.zeros((num_divisions[0], num_divisions[1]), dtype=np.float32)
cell_pts_num[pixel_indices[:, 0], pixel_indices[:, 1]] = num_points_in_pixel[:]
tmp_pixel_cat_map = np.argmax(pixel_cat_map, axis=2)
ignore_mask = np.logical_and(cell_pts_num <= min_point_num_per_voxel, tmp_pixel_cat_map != 0)
ignore_mask = np.logical_not(ignore_mask)
ignore_mask = np.expand_dims(ignore_mask, axis=2)
# Compute the displacement vectors w.r.t. the other sweeps
all_disp_field_gt_list = list()
all_valid_pixel_maps_list = list() # valid pixel map will be used for masking the computation of loss
# -- Skip some frames if necessary
past_part = list(range(0, num_past_sweeps, frame_skip + 1))
future_part = list(range(num_past_sweeps + frame_skip, num_sweeps, frame_skip + 1))
if return_past_2d_disp_gt:
zero_disp_field = np.zeros((num_divisions[0], num_divisions[1], 2), dtype=np.float32)
all_disp_field_gt_list.append(zero_disp_field) # append once, which corresponds to the current frame
all_valid_pixel_maps_list.append(non_empty_map)
frame_considered = np.asarray(past_part + future_part)
frame_considered = frame_considered[1:]
else:
frame_considered = np.asarray(future_part)
for i in frame_considered:
curr_disp_vectors = np.zeros_like(refer_pc, dtype=np.float32)
curr_disp_vectors.fill(np.nan)
curr_disp_vectors[refer_pc_idx_outside_box,] = 0.0
# First, for each instance, compute the corresponding points displacement.
for j in range(num_instances):
instance_box = instance_box_list[j]
instance_box_data = instance_box[i] # This is for the i-th sweep
if np.isnan(instance_box_data).any(): # It is possible that in this sweep there is no annotation
continue
if h_flip:
tmp_quad = instance_box_data[6:].copy()
tmp_quad[2] *= -1 # y
tmp_quad[3] *= -1 # z
tmp_quad = Quaternion(tmp_quad)
tmp_center = instance_box_data[0:3].copy()
tmp_center[0] = -tmp_center[0]
tmp_box = Box(center=tmp_center, size=instance_box_data[3:6], orientation=Quaternion(tmp_quad))
else:
tmp_box = Box(center=instance_box_data[:3], size=instance_box_data[3:6],
orientation=Quaternion(instance_box_data[6:]))
pc_in_bbox_idx = refer_pc_idx_per_bbox[j]
disp_vectors = calc_displace_vector(refer_pc[pc_in_bbox_idx], refer_box_list[j], tmp_box)
curr_disp_vectors[pc_in_bbox_idx] = disp_vectors[:]
# Second, compute the mean displacement vector and category for each non-empty pixel
disp_field = np.zeros([unique_indices.shape[0], 2], dtype=np.float32) # we only consider the 2D field
# We only compute loss for valid pixels where there are corresponding box annotations between two frames
valid_pixels = np.zeros(unique_indices.shape[0], dtype=np.bool)
for h, v in enumerate(zip(unique_indices, num_points_in_pixel)):
# Only when the number of majority points exceeds predefined proportion, we compute
# the displacement vector for this pixel. Otherwise, We consider it is background (possibly ground plane)
# and has zero displacement.
pixel_elements_categories = points_category[v[0]:v[0] + v[1]]
most_freq_cat, most_freq = most_freq_info[h]
if most_freq >= proportion_thresh:
most_freq_cat_idx = np.where(pixel_elements_categories == most_freq_cat)[0]
most_freq_cat_disp_vectors = curr_disp_vectors[v[0]:v[0] + v[1], :3]
most_freq_cat_disp_vectors = most_freq_cat_disp_vectors[most_freq_cat_idx]
if np.isnan(most_freq_cat_disp_vectors).any(): # contains invalid disp vectors
valid_pixels[h] = 0.0
else:
mean_disp_vector = np.mean(most_freq_cat_disp_vectors, axis=0)
disp_field[h] = mean_disp_vector[0:2] # ignore the z direction
valid_pixels[h] = 1.0
# Finally, assemble to a 2D image
disp_field_sparse = np.zeros((num_divisions[0], num_divisions[1], 2), dtype=np.float32)
disp_field_sparse[pixel_indices[:, 0], pixel_indices[:, 1]] = disp_field[:]
disp_field_sparse = disp_field_sparse * ignore_mask
valid_pixel_map = np.zeros((num_divisions[0], num_divisions[1]), dtype=np.float32)
valid_pixel_map[pixel_indices[:, 0], pixel_indices[:, 1]] = valid_pixels[:]
all_disp_field_gt_list.append(disp_field_sparse)
all_valid_pixel_maps_list.append(valid_pixel_map)
all_disp_field_gt_list = np.stack(all_disp_field_gt_list, axis=0)
all_valid_pixel_maps_list = np.stack(all_valid_pixel_maps_list, axis=0)
if reordered and return_past_2d_disp_gt:
num_past = len(past_part)
all_disp_field_gt_list[0:num_past] = all_disp_field_gt_list[(num_past - 1)::-1]
all_valid_pixel_maps_list[0:num_past] = all_valid_pixel_maps_list[(num_past - 1)::-1]
if return_instance_map:
return all_disp_field_gt_list, all_valid_pixel_maps_list, non_empty_map, pixel_cat_map, pixel_indices, pixel_instance_map,instance_box_list,instance_cat_list
else:
return all_disp_field_gt_list, all_valid_pixel_maps_list, non_empty_map, pixel_cat_map, pixel_indices,instance_box_list,instance_cat_list
def voxelize_occupy(pts, voxel_size, extents=None, return_indices=False):
"""
Voxelize the input point cloud. We only record if a given voxel is occupied or not, which is just binary indicator.
The input for the voxelization is expected to be a PointCloud
with N points in 4 dimension (x,y,z,i). Voxel size is the quantization size for the voxel grid.
voxel_size: I.e. if voxel size is 1 m, the voxel space will be
divided up within 1m x 1m x 1m space. This space will be 0 if free/occluded and 1 otherwise.
min_voxel_coord: coordinates of the minimum on each axis for the voxel grid
max_voxel_coord: coordinates of the maximum on each axis for the voxel grid
num_divisions: number of grids in each axis
leaf_layout: the voxel grid of size (numDivisions) that contain -1 for free, 0 for occupied
:param pts: Point cloud as N x [x, y, z, i]
:param voxel_size: Quantization size for the grid, vd, vh, vw
:param extents: Optional, specifies the full extents of the point cloud.
Used for creating same sized voxel grids. Shape (3, 2)
:param return_indices: Whether to return the non-empty voxel indices.
"""
# Function Constants
VOXEL_EMPTY = 0
VOXEL_FILLED = 1
# Check if points are 3D, otherwise early exit
if pts.shape[1] < 3 or pts.shape[1] > 4:
raise ValueError("Points have the wrong shape: {}".format(pts.shape))
if extents is not None:
if extents.shape != (3, 2):
raise ValueError("Extents are the wrong shape {}".format(extents.shape))
filter_idx = np.where((extents[0, 0] < pts[:, 0]) & (pts[:, 0] < extents[0, 1]) &
(extents[1, 0] < pts[:, 1]) & (pts[:, 1] < extents[1, 1]) &
(extents[2, 0] < pts[:, 2]) & (pts[:, 2] < extents[2, 1]))[0]
pts = pts[filter_idx]
# Discretize voxel coordinates to given quantization size
discrete_pts = np.floor(pts[:, :3] / voxel_size).astype(np.int32)
# Use Lex Sort, sort by x, then y, then z
x_col = discrete_pts[:, 0]
y_col = discrete_pts[:, 1]
z_col = discrete_pts[:, 2]
sorted_order = np.lexsort((z_col, y_col, x_col))
# Save original points in sorted order
discrete_pts = discrete_pts[sorted_order]
# Format the array to c-contiguous array for unique function
contiguous_array = np.ascontiguousarray(discrete_pts).view(
np.dtype((np.void, discrete_pts.dtype.itemsize * discrete_pts.shape[1])))
# The new coordinates are the discretized array with its unique indexes
_, unique_indices = np.unique(contiguous_array, return_index=True)
# Sort unique indices to preserve order
unique_indices.sort()
voxel_coords = discrete_pts[unique_indices]
# Compute the minimum and maximum voxel coordinates
if extents is not None:
min_voxel_coord = np.floor(extents.T[0] / voxel_size)
max_voxel_coord = np.ceil(extents.T[1] / voxel_size) - 1
else:
min_voxel_coord = np.amin(voxel_coords, axis=0)
max_voxel_coord = np.amax(voxel_coords, axis=0)
# Get the voxel grid dimensions
num_divisions = ((max_voxel_coord - min_voxel_coord) + 1).astype(np.int32)
# Bring the min voxel to the origin
voxel_indices = (voxel_coords - min_voxel_coord).astype(int)
# Create Voxel Object with -1 as empty/occluded
leaf_layout = VOXEL_EMPTY * np.ones(num_divisions.astype(int), dtype=np.float32)
# Fill out the leaf layout
leaf_layout[voxel_indices[:, 0],
voxel_indices[:, 1],
voxel_indices[:, 2]] = VOXEL_FILLED
if return_indices:
return leaf_layout, voxel_indices
else:
return leaf_layout
def voxelize_pillar_indices(pts, voxel_size, extents=None):
"""
Voxelize the input point cloud into pillars. We only return the indices
The input for the voxelization is expected to be a PointCloud
with N points in 4 dimension (x,y,z,i). Voxel size is the quantization size for the voxel grid.
voxel_size: I.e. if voxel size is 1 m, the voxel space will be
divided up within 1m x 1m x 1m space. This space will be 0 if free/occluded and 1 otherwise.
min_voxel_coord: coordinates of the minimum on each axis for the voxel grid
max_voxel_coord: coordinates of the maximum on each axis for the voxel grid
num_divisions: number of grids in each axis
leaf_layout: the voxel grid of size (numDivisions) that contain -1 for free, 0 for occupied
:param pts: Point cloud as N x [x, y, z, i]
:param voxel_size: Quantization size for the grid, vh, vw
:param extents: Optional, specifies the full extents of the point cloud.
Used for creating same sized voxel grids. Shape (3, 2)
"""
# Check if points are 3D, otherwise early exit
if pts.shape[1] < 3 or pts.shape[1] > 4:
raise ValueError("Points have the wrong shape: {}".format(pts.shape))
if extents is not None:
if extents.shape != (3, 2):
raise ValueError("Extents are the wrong shape {}".format(extents.shape))
filter_idx = np.where((extents[0, 0] < pts[:, 0]) & (pts[:, 0] < extents[0, 1]) &
(extents[1, 0] < pts[:, 1]) & (pts[:, 1] < extents[1, 1]) &
(extents[2, 0] < pts[:, 2]) & (pts[:, 2] < extents[2, 1]))[0]
pts = pts[filter_idx]
# Discretize voxel coordinates to given quantization size
discrete_pts = np.floor(pts[:, :2] / voxel_size).astype(np.int32)
# Use Lex Sort, sort by x, then y
x_col = discrete_pts[:, 0]
y_col = discrete_pts[:, 1]
sorted_order = np.lexsort((y_col, x_col))
# Save original points in sorted order
points = pts[sorted_order]
discrete_pts = discrete_pts[sorted_order]
# Format the array to c-contiguous array for unique function
contiguous_array = np.ascontiguousarray(discrete_pts).view(
np.dtype((np.void, discrete_pts.dtype.itemsize * discrete_pts.shape[1])))
# The new coordinates are the discretized array with its unique indexes
_, unique_indices = np.unique(contiguous_array, return_index=True)
# Sort unique indices to preserve order
unique_indices.sort()
voxel_coords = discrete_pts[unique_indices]
# Number of points per voxel, last voxel calculated separately
num_points_in_pillar = np.diff(unique_indices)
num_points_in_pillar = np.append(num_points_in_pillar, discrete_pts.shape[0] - unique_indices[-1])
# Compute the minimum and maximum voxel coordinates
if extents is not None:
min_voxel_coord = np.floor(extents.T[0, 0:2] / voxel_size)
else:
min_voxel_coord = np.amin(voxel_coords, axis=0)
# Bring the min voxel to the origin
voxel_indices = (voxel_coords - min_voxel_coord).astype(int)
return points, voxel_indices, num_points_in_pillar
def voxelize_point_pillar(pts, grid_size, extents=None, num_points=100, num_pillars=2500, seed=None,
is_padded_pillar=False):
"""
Discretize the input point cloud into pillars.
The input for the voxelization is expected to be a PointCloud
with N points in 4 dimension (x,y,z,i). Grid size is the quantization size for the 2d grid.
grid_size: I.e. if grid size is 1 m, the grid space will be
divided up within 1m x 1m space. This space will be -1 if free/occluded and 1 otherwise.
min_grid_coord: coordinates of the minimum on each axis for the 2d grid
max_grid_coord: coordinates of the maximum on each axis for the 2d grid
num_divisions: number of grids in each axis
leaf_layout: the 2d grid of size (numDivisions) that contain -1 for free, 0 for occupied
:param pts: Point cloud as N x [x, y, z, i]
:param grid_size: Quantization size for the grid, (vh, vw)
:param extents: Optional, specifies the full extents of the point cloud.
Used for creating same sized voxel grids. Shape (3, 2)
:param num_points: Number of points in each pillar after sampling/padding
:param num_pillars: Number of pillars after sampling/padding
:param seed: Random seed for fixing data generation.
:param is_padded_pillar: Whether need to pad/sample the pillar
"""
if seed is not None:
np.random.seed(seed)
# Check if points are 3D, otherwise early exit
if pts.shape[1] < 3 or pts.shape[1] > 4:
raise ValueError("Points have the wrong shape: {}".format(pts.shape))
if extents is not None:
if extents.shape != (3, 2):
raise ValueError("Extents are the wrong shape {}".format(extents.shape))
filter_idx = np.where((extents[0, 0] < pts[:, 0]) & (pts[:, 0] < extents[0, 1]) &
(extents[1, 0] < pts[:, 1]) & (pts[:, 1] < extents[1, 1]) &
(extents[2, 0] < pts[:, 2]) & (pts[:, 2] < extents[2, 1]))[0]
pts = pts[filter_idx]
# Discretize point coordinates to given 2d quantization size
discrete_pts = np.floor(pts[:, :2] / grid_size).astype(np.int32)
# Use Lex Sort, sort by x, then y. We do not care about z
x_col = discrete_pts[:, 0]
y_col = discrete_pts[:, 1]
sorted_order = np.lexsort((y_col, x_col))
# Save original points in sorted order
points = pts[sorted_order]
discrete_pts = discrete_pts[sorted_order]
# Format the array to c-contiguous array for unique function
contiguous_array = np.ascontiguousarray(discrete_pts).view(
np.dtype((np.void, discrete_pts.dtype.itemsize * discrete_pts.shape[1])))
# The new coordinates are the discretized array with its unique indexes
_, unique_indices = np.unique(contiguous_array, return_index=True)
# Sort unique indices to preserve order
unique_indices.sort()
grid_coords = discrete_pts[unique_indices]
# Number of points per voxel, last voxel calculated separately
num_points_in_pillar = np.diff(unique_indices)
num_points_in_pillar = np.append(num_points_in_pillar, discrete_pts.shape[0] - unique_indices[-1])
# Compute the minimum and maximum voxel coordinates
if extents is not None:
min_grid_coord = np.floor(extents.T[0, 0:2] / grid_size)
max_grid_coord = np.ceil(extents.T[1, 0:2] / grid_size) - 1
else:
min_grid_coord = np.amin(grid_coords, axis=0)
max_grid_coord = np.amax(grid_coords, axis=0)
# Get the voxel grid dimensions
num_divisions = ((max_grid_coord - min_grid_coord) + 1).astype(np.int32)
# Bring the min voxel to the origin
pixel_indices = (grid_coords - min_grid_coord).astype(int)
# Padding the points within each voxel
x_offset = grid_size[0] / 2.0 + extents[0, 0]
y_offset = grid_size[1] / 2.0 + extents[1, 0]
padded_grid_points = np.zeros([unique_indices.shape[0], num_points, pts.shape[1] + 3 + 2], dtype=np.float32)
padded_pillar = np.zeros([num_pillars, num_points, pts.shape[1] + 3 + 2], dtype=np.float32)
padded_pixel_indices = np.zeros([num_pillars, pixel_indices.shape[1]], dtype=np.int64)
padded_grid_points = padding_point_pillar(padded_grid_points, unique_indices, num_points, num_points_in_pillar,
points, pixel_indices, grid_size, x_offset, y_offset, seed)
if is_padded_pillar:
# Padding or sampling the pillars TODO: early sampling to avoid unnecessary computation
if unique_indices.shape[0] < num_pillars:
padded_pillar[:unique_indices.shape[0], :, :] = padded_grid_points[:]
padded_pixel_indices[:unique_indices.shape[0], :] = pixel_indices[:]
else:
pillar_inds = np.random.choice(unique_indices.shape[0], num_pillars)
padded_pillar[:, :, :] = padded_grid_points[pillar_inds, :, :]
padded_pixel_indices[:, :] = pixel_indices[pillar_inds, :]
else:
padded_pillar = padded_grid_points
padded_pixel_indices = pixel_indices
return padded_pillar, padded_pixel_indices, num_divisions
@njit
def padding_point_pillar(padded_grid_points, unique_indices, num_points, num_points_in_pillar,
points, pixel_indices, grid_size, x_offset, y_offset, seed):
if seed is not None:
np.random.seed(seed)
for i, v in enumerate(zip(unique_indices, num_points_in_pillar)):
if v[1] < num_points:
padded_grid_points[i, :v[1], :4] = points[v[0]:v[0] + v[1], :]
middle_points_x = np.mean(points[v[0]:v[0] + v[1], 0])
middle_points_y = np.mean(points[v[0]:v[0] + v[1], 1])
middle_points_z = np.mean(points[v[0]:v[0] + v[1], 2])
padded_grid_points[i, :v[1], 4] = padded_grid_points[i, :v[1], 0] - middle_points_x
padded_grid_points[i, :v[1], 5] = padded_grid_points[i, :v[1], 1] - middle_points_y
padded_grid_points[i, :v[1], 6] = padded_grid_points[i, :v[1], 2] - middle_points_z
center_offsets = np.zeros((v[1], 2), dtype=np.float32)
center_offsets[:, 0] = padded_grid_points[i, :v[1], 0] - (pixel_indices[i, 0] * grid_size[0] + x_offset)
center_offsets[:, 1] = padded_grid_points[i, :v[1], 1] - (pixel_indices[i, 1] * grid_size[1] + y_offset)
padded_grid_points[i, :v[1], 7:] = center_offsets[:]
else:
inds = np.random.choice(v[1], num_points)
padded_grid_points[i, :, :4] = points[v[0] + inds, :]
middle_points_x = np.mean(points[v[0] + inds, 0])
middle_points_y = np.mean(points[v[0] + inds, 1])
middle_points_z = np.mean(points[v[0] + inds, 2])
padded_grid_points[i, :, 4] = padded_grid_points[i, :, 0] - middle_points_x
padded_grid_points[i, :, 5] = padded_grid_points[i, :, 1] - middle_points_y
padded_grid_points[i, :, 6] = padded_grid_points[i, :, 2] - middle_points_z
center_offsets = np.zeros((num_points, 2), dtype=np.float32)
center_offsets[:, 0] = padded_grid_points[i, :, 0] - (pixel_indices[i, 0] * grid_size[0] + x_offset)
center_offsets[:, 1] = padded_grid_points[i, :, 1] - (pixel_indices[i, 1] * grid_size[1] + y_offset)
padded_grid_points[i, :, 7:] = center_offsets[:]
return padded_grid_points
def compute_ratio_cat_and_motion(dataset_root=None, frame_skip=3, voxel_size=(0.4, 0.4, 0.4), split='train',
area_extents=np.array([[-30., 30.], [-30., 30.], [-2., 2.]]), num_obj_cat=5,
num_motion_cat=3):
"""
Compute the ratios between foreground and background (and static and moving) cells. The ratios will be used for
non-uniform weighting to mitigate the class imbalance during training.
:param dataset_root: The path to the dataset
:param frame_skip: The number of frame skipped in a sample sequence
:param voxel_size: Voxel size, which determines the "image" resolution
:param split: The data split
:param area_extents: The area of interest for point cloud
:param num_obj_cat: The number of object categories.
:param num_motion_cat: The number of motion categories. Currently it is 2 (ie, static and moving).
"""
if dataset_root is None:
dataset_root = '/homes/pwu/_drives/cv0/data/homes/pwu/preprocessed_pc'
scene_dirs = [d for d in os.listdir(dataset_root) if os.path.isdir(os.path.join(dataset_root, d))]
if split == 'train':
scene_dirs = scene_dirs[:len(scene_dirs) // 2]
else:
scene_dirs = scene_dirs[len(scene_dirs) // 2:]
sample_seq_files = []
for s_dir in scene_dirs:
sample_dir = os.path.join(dataset_root, s_dir)
sample_files = [os.path.join(sample_dir, f) for f in os.listdir(sample_dir)
if os.path.isfile(os.path.join(sample_dir, f))]
sample_seq_files += sample_files
num_sample_seqs = len(sample_seq_files)
obj_cat_cnt = np.zeros(num_obj_cat, dtype=np.int64)
motion_cat_cnt = np.zeros(num_motion_cat, dtype=np.int64)
for idx in range(num_sample_seqs):
sample_file = sample_seq_files[idx]
all_disp_field_gt, all_valid_pixel_maps, non_empty_map, pixel_cat_map_gt \
= gen_2d_grid_gt(sample_file, grid_size=voxel_size[0:2], reordered=True,
extents=area_extents, frame_skip=frame_skip)
# -- Compute speed level ground truth
motion_status_gt = compute_speed_level(all_disp_field_gt, frame_skip=frame_skip)
# -- Count the object category number
max_prob = np.amax(pixel_cat_map_gt, axis=-1)
filter_mask = max_prob == 1.0
pixel_cat_map = np.argmax(pixel_cat_map_gt, axis=-1) + 1 # category starts from 1 (background), etc
pixel_cat_map = (pixel_cat_map * non_empty_map * filter_mask).astype(np.int)
for i in range(num_obj_cat):
curr_cat_mask = pixel_cat_map == (i + 1)
curr_cat_num = np.sum(curr_cat_mask)
obj_cat_cnt[i] += curr_cat_num
# -- Count the motion category number
motion_cat_map = np.argmax(motion_status_gt, axis=-1) + 1 # category starts from 1 (static), etc
motion_cat_map = (motion_cat_map * non_empty_map * filter_mask).astype(np.int)
for i in range(num_motion_cat):
curr_motion_mask = motion_cat_map == (i + 1)
curr_motion_num = np.sum(curr_motion_mask)
motion_cat_cnt[i] += curr_motion_num
print("Finish {}".format(idx))
print("The category numbers: \n{}".format(obj_cat_cnt))
print("The motion numbers: \n{}\n".format(motion_cat_cnt))
# Convert to ratio
obj_cat_ratio = obj_cat_cnt / np.sum(obj_cat_cnt)
motion_cat_ratio = motion_cat_cnt / np.sum(motion_cat_cnt)
print("The category ratios: \n{}".format(obj_cat_ratio))
print("The motion ratios: \n{}\n".format(motion_cat_ratio))
# Convert to inverse ratio
obj_cat_ratio_inverse = np.exp(-obj_cat_ratio)
motion_cat_ratio_inverse = np.exp(-motion_cat_ratio)
print("The category reverse ratios: \n{}".format(obj_cat_ratio_inverse))
print("The motion reverse ratios: \n{}\n".format(motion_cat_ratio_inverse))
def compute_speed_level(all_disp_field_gt, total_future_sweeps=20, frame_skip=3):
speed_intervals = np.array([[0, 5.0], [5.0, 20.0], [20.0, np.inf]]) # unit: m/s
selected_future_sweeps = np.arange(0, total_future_sweeps + 1, frame_skip + 1)
selected_future_sweeps = selected_future_sweeps[1:]
last_future_sweep_id = selected_future_sweeps[-1]
distance_intervals = speed_intervals * (last_future_sweep_id / 20.0)
speed_level = np.zeros((all_disp_field_gt.shape[1], all_disp_field_gt.shape[2],
speed_intervals.shape[0]), dtype=np.float32)
last_frame_disp_norm = np.linalg.norm(all_disp_field_gt, ord=2, axis=-1)
last_frame_disp_norm = last_frame_disp_norm[-1, :, :]
for s, d in enumerate(distance_intervals):
mask = np.logical_and(d[0] <= last_frame_disp_norm, last_frame_disp_norm < d[1])
one_hot_vector = np.zeros(speed_intervals.shape[0], dtype=np.float32)
one_hot_vector[s] = 1.0
speed_level[mask] = one_hot_vector[:]
return speed_level
def compute_speed_level_with_static(all_disp_field_gt, total_future_sweeps=20, frame_skip=3):
speed_intervals = np.array([[0.0, 0.0], [0, 5.0], [5.0, 20.0], [20.0, np.inf]]) # unit: m/s
# First, compute the static and moving cell masks
all_disp_field_gt_norm = np.linalg.norm(all_disp_field_gt, ord=2, axis=-1)
upper_thresh = 0.2
upper_bound = (frame_skip + 1) / 20 * upper_thresh
selected_future_sweeps = np.arange(0, total_future_sweeps + 1, frame_skip + 1)
selected_future_sweeps = selected_future_sweeps[1:]
future_sweeps_disp_field_gt_norm = all_disp_field_gt_norm[-len(selected_future_sweeps):, ...]
static_cell_mask = future_sweeps_disp_field_gt_norm <= upper_bound
static_cell_mask = np.all(static_cell_mask, axis=0) # along the sequence axis
moving_cell_mask = | np.logical_not(static_cell_mask) | numpy.logical_not |
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon Jul 13 09:58:50 2020
@author: duttar
Description: Solving the problem A = Bx
A is the timeseries stack of InSAR pixel wise
B is matrix including time and ADDT
x is a vector containing seasonal and overall subsidence
"""
import os
import numpy as np
import matplotlib.pyplot as plt
import h5py
from datetime import datetime as dt
import multiprocessing
from joblib import Parallel, delayed
from functools import partial
import scipy.io as sio
def datenum(d):
'''
Serial date number
used for SBI_Year
'''
return 366 + d.toordinal() + (d - dt.fromordinal(d.toordinal())).total_seconds()/(24*60*60)
def SBI_Year(imd):
'''
A simple script that takes in numbers on the format '19990930'
and changes to a number format 1999.7590
imd - numpy (n,1) Vector with dates in strings
out - numpy (n,1) Vector with dates in int
created by <NAME>
'''
dstr = imd
nd = imd.shape[0]
out = np.zeros((nd,1))
for k in range(nd):
# get first day of year, minus 1/2 a day:
d1 = dt.strptime(dstr[k][0][0:4]+'0101', '%Y%m%d')
dn1 = datenum(d1) - 0.5
# get last day of year, plus 0.5
d2 = dt.strptime(dstr[k][0][0:4]+'1231', '%Y%m%d')
dne = datenum(d2) + 0.5
# get number of days in that year:
ndays = dne - dn1
# get day of year:
d3 = dt.strptime(dstr[k][0], '%Y%m%d')
doy = datenum(d3) - dn1
# get fractional year:
fracyr = doy/ndays
out[k] = int(dstr[k][0][0:4])+ fracyr
return out
# work directory
proj_dir = os.path.expanduser('/data/not_backed_up/rdtta/Permafrost/Alaska/North_slope/DT102/Stack/timeseries')
# file in geo coordinates
geom_file = os.path.join(proj_dir, 'geo/geo_geometryRadar.h5')
ts_file = os.path.join(proj_dir, 'geo/geo_timeseries_ramp_demErr.h5')
maskfile = os.path.join(proj_dir, 'geo/geo_maskTempCoh.h5')
with h5py.File(ts_file, "r") as f:
# read the timeseries file
a_group_key = list(f.keys())[2]
ts_data = list(f[a_group_key])
a_group_key = list(f.keys())[1]
dates = list(f[a_group_key])
with h5py.File(geom_file, "r") as f:
# read the geometry file
a_group_key = list(f.keys())[0]
azim_angle = list(f[a_group_key])
a_group_key = list(f.keys())[1]
height = list(f[a_group_key])
a_group_key = list(f.keys())[2]
inc_angle = list(f[a_group_key])
a_group_key = list(f.keys())[3]
latitude = list(f[a_group_key])
a_group_key = list(f.keys())[4]
longitude = list(f[a_group_key])
with h5py.File(maskfile, "r") as f:
a_group_key = list(f.keys())[0]
maskbool = list(f[a_group_key])
maskbool = np.array(maskbool)
# convert dates from type 'bytes' to string
numdates = np.size(dates)
datesn = np.empty([numdates, 1], dtype="<U10")
dates_int = np.zeros((numdates,1))
for i in range(numdates):
datesn[i] = dates[i].decode("utf-8")
dates_int[i] = int(dates[i].decode("utf-8"))
dates_frac = SBI_Year(datesn)
# select the dates to put in matrix A
inddates = np.zeros((numdates,1))
for i in range(numdates):
dates_i = dates_frac[i]
frac_part = dates_i - np.floor(dates_i)
if frac_part < .41506849 :
inddates[i] = 0
elif frac_part > .81506849 :
inddates[i] = 0
else:
inddates[i] = 1
include_dates = np.where(inddates == 1)[0]
print('included dates for estimation are: \n', datesn[include_dates])
dates_frac_included = dates_frac[include_dates]
# load the addt files
dates_floor = np.floor(dates_frac_included)
for i in range(include_dates.shape[0]-1):
if i == 0:
years_incl = dates_floor[i]
if dates_floor[i+1] != dates_floor[i]:
years_incl = np.concatenate((years_incl, dates_floor[i+1]), axis=0)
a_dictionary = {}
mat_c1 = np.empty(())
for years in years_incl:
varmat_load = 'data_temp_addt/ds633/addt' + str(np.int(years)) + '.mat'
mat_c1 = sio.loadmat(varmat_load)
lonlat = mat_c1['lonlat']
var_addt = 'addt' + str(np.int(years))
a_dictionary["addt_%s" %np.int(years)] = mat_c1[var_addt]
a_dictionary["detailsaddt_%s" %np.int(years)] = mat_c1['details_addt']
# get the timeseries data attributes
ifglen = np.shape(longitude)[0]
ifgwid = np.shape(longitude)[1]
ts_data = np.array(ts_data)
longitude = np.array(longitude)
latitude = np.array(latitude)
numpixels = ifglen*ifgwid
lonvals_addt = lonlat[:,0] - 360
latvals_addt = lonlat[:,1]
# normalize the addt values with the overall maximum value
maxaddt = np.zeros((years_incl.shape[0], 1))
i = 0
for years in years_incl:
varaddt = "addt_" + str(np.int(years))
maxaddt[i] = np.max(a_dictionary[varaddt])
i = i+1
maxaddtall = np.max(maxaddt) # maximum addt value
addt_pixelwise = np.zeros((include_dates.shape[0], ifglen, ifgwid))
for i in range(numpixels):
if np.mod(i, 50000) == 0:
print('loops completed: ', i)
ind_len = np.mod(i+1, ifglen) - 1
if np.mod(i+1, ifglen) == 0:
ind_len = ifglen -1
ind_wid = np.int(np.floor((i+1)/ifglen)) - 1
if maskbool[ind_len, ind_wid] == False:
continue
# get the latitude and longitude at the index
lon_valind = longitude[ind_len, ind_wid]
lat_valind = latitude[ind_len, ind_wid]
# find the closest lonlat of the addt values
abs_dist_lon = np.abs(lonvals_addt - lon_valind)
abs_dist_lat = np.abs(latvals_addt - lat_valind)
ind_close1 = np.where(abs_dist_lon == np.min(abs_dist_lon))
ind_close2 = np.where(abs_dist_lat == np.min(abs_dist_lat))
indcommon = np.intersect1d(ind_close1, ind_close2)
if indcommon.shape[0] > 1:
indcommon = indcommon[0]
ind_tsdate = 0
# go through the time series dates and find the corresponding addt values
for day in dates_frac_included:
if np.mod(np.floor(day),4) > 0:
leapdays = 365
else:
leapdays = 366
dayindex = (day - np.floor(day))* leapdays + .5
varaddt1 = "detailsaddt_" + str(np.int(np.floor(day)[0]))
firstday_addt = a_dictionary[varaddt1][indcommon, 1]
varaddt2 = "addt_" + str(np.int( | np.floor(day) | numpy.floor |
import unittest
import numpy as np
import fastpli.objects
import fastpli.tools
class MainTest(unittest.TestCase):
def setUp(self):
self.fiber = fastpli.objects.Fiber([[0, 0, 0, 1], [1, 1, 1, 2]])
self.fiber_bundle = fastpli.objects.FiberBundle(self.fiber.copy())
self.fiber_bundles = fastpli.objects.FiberBundles(self.fiber.copy())
def test_init(self):
fastpli.objects.FiberBundle()
fastpli.objects.FiberBundles()
a = np.array([0, 0, 0, 0])
_ = fastpli.objects.Fiber([[0, 0, 0, 1], [0, 0, 1, 2]])
f = fastpli.objects.Fiber(a)
self.assertTrue(isinstance(f, fastpli.objects.Fiber))
f = fastpli.objects.Fiber(f)
self.assertTrue(isinstance(f, fastpli.objects.Fiber))
fb = fastpli.objects.FiberBundle([a])
self.assertTrue(isinstance(fb, fastpli.objects.FiberBundle))
fb = fastpli.objects.FiberBundle(f)
self.assertTrue(isinstance(fb, fastpli.objects.FiberBundle))
fb = fastpli.objects.FiberBundle(fb)
self.assertTrue(isinstance(fb, fastpli.objects.FiberBundle))
fbs = fastpli.objects.FiberBundles([[a]])
self.assertTrue(isinstance(fbs, fastpli.objects.FiberBundles))
fbs = fastpli.objects.FiberBundles(f)
self.assertTrue(isinstance(fbs, fastpli.objects.FiberBundles))
fbs = fastpli.objects.FiberBundles([f, f])
self.assertTrue(isinstance(fbs, fastpli.objects.FiberBundles))
fbs = fastpli.objects.FiberBundles(fbs)
self.assertTrue(isinstance(fbs, fastpli.objects.FiberBundles))
fb = fastpli.objects.FiberBundle([[[0, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]],
[[1, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]]])
for f in fb:
self.assertTrue(isinstance(f, fastpli.objects.Fiber))
self.assertTrue(isinstance(f._data, np.ndarray))
fbs = fastpli.objects.FiberBundles([[[[0, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]],
[[1, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]]],
[[[0, 1, 2, 3], [1, 2, 3, 4],
[2, 4, 5, 5]],
[[1, 1, 2, 3], [1, 2, 3, 4],
[2, 4, 5, 5]],
[[1, 1, 2, 3], [1, 2, 3, 4],
[2, 4, 5, 5]]]])
for fb in fbs:
self.assertTrue(isinstance(fb, fastpli.objects.FiberBundle))
for f in fb:
self.assertTrue(isinstance(f, fastpli.objects.Fiber))
self.assertTrue(isinstance(f._data, np.ndarray))
def test_type(self):
self.assertTrue(isinstance(self.fiber[:], np.ndarray))
self.assertTrue(self.fiber[:].dtype == float)
self.assertTrue(
fastpli.objects.Fiber([[1, 1, 1, 1]], np.float32).dtype ==
np.float32)
def test_layers(self):
fastpli.objects.FiberBundle(self.fiber_bundle,
[(0.333, -0.004, 10, 'p'),
(0.666, 0, 5, 'b'), (1.0, 0.004, 1, 'r')])
fastpli.objects.FiberBundles(self.fiber_bundles,
[[(0.333, -0.004, 10, 'p'),
(0.666, 0, 5, 'b'),
(1.0, 0.004, 1, 'r')]])
fb = fastpli.objects.FiberBundle([[[0, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]],
[[1, 0, 0, 1], [1, 1, 1, 1],
[2, 2, 2, 1]]])
fb = fastpli.objects.FiberBundle(fb, [(0.333, -0.004, 10, 'p'),
(0.666, 0, 5, 'b'),
(1.0, 0.004, 1, 'r')])
fbs = [[[[0, 0, 0, 1], [1, 1, 1, 1], [2, 2, 2, 1]],
[[1, 0, 0, 1], [1, 1, 1, 1], [2, 2, 2, 1]]],
[[[0, 1, 2, 3], [1, 2, 3, 4], [2, 4, 5, 5]],
[[1, 1, 2, 3], [1, 2, 3, 4], [2, 4, 5, 5]],
[[1, 1, 2, 3], [1, 2, 3, 4], [2, 4, 5, 5]]]]
fbs = fastpli.objects.FiberBundles(fbs,
[[(0.333, -0.004, 10, 'p'),
(0.666, 0, 5, 'b'),
(1.0, 0.004, 1, 'r')]] * len(fbs))
def test_resize(self):
fiber = self.fiber.scale(10)
self.assertTrue(np.array_equal(fiber[:], self.fiber[:] * 10))
fb = self.fiber_bundle.scale(10)
for f in fb:
self.assertTrue(np.array_equal(f[:], self.fiber[:] * 10))
fbs = self.fiber_bundles.scale(10)
for fb in fbs:
for f in fb:
self.assertTrue(np.array_equal(f[:], self.fiber[:] * 10))
fiber = self.fiber.scale(10, mode='points')
self.assertTrue(np.array_equal(fiber[:, :-2], self.fiber[:, :-2] * 10))
self.assertTrue(np.array_equal(fiber[:, -1], self.fiber[:, -1]))
fiber = self.fiber.scale(10, mode='radii')
self.assertTrue(np.array_equal(fiber[:, :-2], self.fiber[:, :-2]))
self.assertTrue(np.array_equal(fiber[:, -1], self.fiber[:, -1] * 10))
def test_rotation(self):
fiber = self.fiber.rotate(fastpli.tools.rotation.x(0))
self.assertTrue(np.array_equal(self.fiber[:], fiber[:]))
fiber = self.fiber.rotate(fastpli.tools.rotation.x(np.deg2rad(90)))
self.assertTrue(
np.allclose(fiber[:], np.array([[0, 0, 0, 1], [1, -1, 1, 2]])))
fiber = self.fiber.rotate(fastpli.tools.rotation.x(np.deg2rad(90)),
[1, 1, 1])
self.assertTrue(
np.allclose(fiber[:], np.array([[0, 2, 0, 1], [1, 1, 1, 2]])))
fiber_bundle = self.fiber_bundle.rotate(
fastpli.tools.rotation.x(np.deg2rad(90)), [1, 1, 1])
self.assertTrue(len(fiber_bundle) == len(self.fiber_bundle))
for f in fiber_bundle:
self.assertTrue(
np.allclose(f[:], np.array([[0, 2, 0, 1], [1, 1, 1, 2]])))
for fb in self.fiber_bundles:
for f in fb:
fiber = f.rotate(fastpli.tools.rotation.x(np.deg2rad(90)),
[1, 1, 1])
self.assertTrue(
np.allclose(fiber[:], np.array([[0, 2, 0, 1], [1, 1, 1,
2]])))
def test_translate(self):
fiber = self.fiber.translate([1, 1, 1])
self.assertTrue(
np.array_equal(fiber[:, :3],
self.fiber[:, :3] + np.array([1, 1, 1])))
self.assertTrue(np.array_equal(fiber[:, -1], self.fiber[:, -1]))
fiber_bundle = self.fiber_bundle.translate([1, 1, 1])
self.assertTrue(len(fiber_bundle) == len(self.fiber_bundle))
for f in fiber_bundle:
self.assertTrue(
np.array_equal(fiber[:, :3],
self.fiber[:, :3] + np.array([1, 1, 1])))
self.assertTrue(np.array_equal(f[:, -1], self.fiber[:, -1]))
for fb in self.fiber_bundles:
for f in fb:
fiber = f.translate([1, 1, 1])
self.assertTrue(
np.array_equal(fiber[:, :3],
self.fiber[:, :3] + np.array([1, 1, 1])))
self.assertTrue(np.array_equal(f[:, -1], self.fiber[:, -1]))
def test_apply(self):
# Fiber
fiber = fastpli.objects.Fiber([[0, 0, 0, 1], [1, 1, 1, 2]], dtype=float)
fiber_ = fiber.apply(lambda x: x + 1)
self.assertTrue(isinstance(fiber_, fastpli.objects.Fiber))
self.assertTrue(np.array_equal(fiber[:] + 1, fiber_[:]))
fiber_ = fiber.apply_to_points(lambda x: x + 1)
self.assertTrue(isinstance(fiber_, fastpli.objects.Fiber))
self.assertTrue(np.array_equal(fiber[:, :-1] + 1, fiber_[:, :-1]))
self.assertTrue( | np.array_equal(fiber[:, -1], fiber_[:, -1]) | numpy.array_equal |
import numpy as np
from numpy import pi
import logging
import h5py
from numpy import pi
import logging, os
from .Diagnostics import *
from .Saving import *
class Model(object):
""" Python class that represents the barotropic quasigeostrophic
pseudospectral model in a doubly periodic domain. Physical parameters
observe SI units.
Parameters
-----------
nx: integer (optional)
Number of grid points in the x-direction.
The number of modes is nx/2+1.
ny: integer (optional)
Number of grid points in the y-direction.
If None, then ny=nx.
L: float (optional)
Domain size.
dt: float (optional)
Time step for time integration.
twrite: integer (optional)
Print model status to screen every twrite time steps.
tmax: float (optional)
Total time of simulation.
U: float (optional)
Uniform zonal flow
use_filter: bool (optional)
If True, then uses exponential spectral filter.
nu4: float (optional)
Fouth-order hyperdiffusivity of potential vorticity.
nu: float (optional)
Diffusivity of potential vorticity.
mu: float (optional)
Linear drag of potential vorticity.
passive_scalar: bool (optional)
If True, then calculates passive scalar solution.
nu4c: float (optional)
Fouth-order hyperdiffusivity of passive scalar.
nuc: float (optional)
Diffusivity of passive scalar.
muc: float (optional)
Linear drag of passive scalar.
dealias: bool (optional)
If True, then dealias solution using 2/3 rule.
save_to_disk: bool (optional)
If True, then save parameters and snapshots to disk.
overwrite: bool (optional)
If True, then overwrite extant files.
tsave_snapshots: integer (optional)
Save snapshots every tsave_snapshots time steps.
tdiags: integer (optional)
Calculate diagnostics every tdiags time steps.
path: string (optional)
Location for saving output files.
"""
def __init__(
self,
nx=128,
ny=None,
L=5e5,
dt=10000.,
twrite=1000,
tswrite=10,
tmax=250000.,
use_filter = True,
U = .0,
nu4=5.e9,
nu = 0,
mu = 0,
beta = 0,
passive_scalar = False,
nu4c = 5.e9,
nuc = 0,
muc = 0,
dealias = False,
save_to_disk=False,
overwrite=True,
tsave_snapshots=10,
tdiags = 10,
path = 'output/',
use_mkl=False,
nthreads=1):
self.nx = nx
self.ny = nx
self.L = L
self.W = L
self.dt = dt
self.twrite = twrite
self.tswrite = tswrite
self.tmax = tmax
self.tdiags = tdiags
self.passive_scalar = passive_scalar
self.dealias = dealias
self.U = U
self.beta = beta
self.nu4 = nu4
self.nu = nu
self.mu = mu
self.nu4c = nu4c
self.nuc = nuc
self.muc = muc
self.save_to_disk = save_to_disk
self.overwrite = overwrite
self.tsnaps = tsave_snapshots
self.path = path
self.use_filter = use_filter
self.use_mkl = use_mkl
self.nthreads = nthreads
self._initialize_logger()
self._initialize_grid()
self._allocate_variables()
self._initialize_filter()
self._initialize_etdrk4()
self._initialize_time()
initialize_save_snapshots(self, self.path)
save_setup(self, )
self.cflmax = .5
self._initialize_fft()
self._initialize_diagnostics()
def _allocate_variables(self):
""" Allocate variables so that variable addresses are close in memory.
"""
self.dtype_real = np.dtype('float64')
self.dtype_cplx = np.dtype('complex128')
self.shape_real = (self.ny, self.nx)
self.shape_cplx = (self.ny, self.nx//2+1)
# vorticity
self.q = np.zeros(self.shape_real, self.dtype_real)
self.qh = np.zeros(self.shape_cplx, self.dtype_cplx)
self.qh0 = np.zeros(self.shape_cplx, self.dtype_cplx)
self.qh1 = np.zeros(self.shape_cplx, self.dtype_cplx)
# stream function
self.p = np.zeros(self.shape_real, self.dtype_real)
self.ph = np.zeros(self.shape_cplx, self.dtype_cplx)
def run_with_snapshots(self, tsnapstart=0., tsnapint=432000.):
""" Run the model for prescribed time and yields to user code.
Parameters
----------
tsnapstart : float
The timestep at which to begin yielding.
tstapint : int (number of time steps)
The interval at which to yield.
"""
tsnapints = np.ceil(tsnapint/self.dt)
while(self.t < self.tmax):
self._step_forward()
if self.t>=tsnapstart and (self.tc%tsnapints)==0:
yield self.t
return
def run(self):
""" Run the model until the end (`tmax`).
The algorithm is:
1) Save snapshots (i.e., save the initial condition).
2) Take a tmax/dt steps forward.
3) Save diagnostics.
"""
# save initial conditions
if self.save_to_disk:
if self.passive_scalar:
save_snapshots(self,fields=['t','q','c'])
else:
save_snapshots(self,fields=['t','q'])
# run the model
while(self.t < self.tmax):
self._step_forward()
# save diagnostics
if self.save_to_disk:
save_diagnostics(self)
def _step_forward(self):
""" Step solutions forwards. The algorithm is:
1) Take one time step with ETDRK4 scheme.
2) Incremente diagnostics.
3) Print status.
4) Save snapshots.
"""
self._step_etdrk4()
increment_diagnostics(self,)
self._print_status()
save_snapshots(self,fields=['t','q','c'])
def _initialize_time(self):
""" Initialize model clock and other time variables.
"""
self.t=0 # time
self.tc=0 # time-step number
### initialization routines, only called once at the beginning ###
def _initialize_grid(self):
""" Create spatial and spectral grids and normalization constants.
"""
self.x,self.y = np.meshgrid(
np.arange(0.5,self.nx,1.)/self.nx*self.L,
np.arange(0.5,self.ny,1.)/self.ny*self.W )
self.dk = 2.*pi/self.L
self.dl = 2.*pi/self.L
# wavenumber grids
self.nl = self.ny
self.nk = self.nx//2+1
self.ll = self.dl*np.append( np.arange(0.,self.nx/2),
np.arange(-self.nx/2,0.) )
self.kk = self.dk*np.arange(0.,self.nk)
self.k, self.l = np.meshgrid(self.kk, self.ll)
self.ik = 1j*self.k
self.il = 1j*self.l
# physical grid spacing
self.dx = self.L / self.nx
self.dy = self.W / self.ny
# constant for spectral normalizations
self.M = self.nx*self.ny
# isotropic wavenumber^2 grid
# the inversion is not defined at kappa = 0
self.wv2 = self.k**2 + self.l**2
self.wv = np.sqrt( self.wv2 )
self.wv4 = self.wv2**2
iwv2 = self.wv2 != 0.
self.wv2i = np.zeros_like(self.wv2)
self.wv2i[iwv2] = self.wv2[iwv2]**-1
def _initialize_background(self):
raise NotImplementedError(
'needs to be implemented by Model subclass')
def _initialize_inversion_matrix(self):
raise NotImplementedError(
'needs to be implemented by Model subclass')
def _initialize_forcing(self):
raise NotImplementedError(
'needs to be implemented by Model subclass')
def _initialize_filter(self):
"""Set up spectral filter or dealiasing."""
if self.use_filter:
cphi=0.65*pi
wvx=np.sqrt((self.k*self.dx)**2.+(self.l*self.dy)**2.)
self.filtr = np.exp(-23.6*(wvx-cphi)**4.)
self.filtr[wvx<=cphi] = 1.
self.logger.info(' Using filter')
elif self.dealias:
self.filtr = np.ones_like(self.wv2)
self.filtr[self.nx/3:2*self.nx/3,:] = 0.
self.filtr[:,self.ny/3:2*self.ny/3] = 0.
self.logger.info(' Dealiasing with 2/3 rule')
else:
self.filtr = np.ones_like(self.wv2)
self.logger.info(' No dealiasing; no filter')
def _do_external_forcing(self):
pass
def _initialize_logger(self):
""" Initialize logger.
"""
self.logger = logging.getLogger(__name__)
fhandler = logging.StreamHandler()
formatter = logging.Formatter('%(levelname)s: %(message)s')
fhandler.setFormatter(formatter)
if not self.logger.handlers:
self.logger.addHandler(fhandler)
self.logger.setLevel(10)
# this prevents the logger from propagating into the ipython notebook log
self.logger.propagate = False
self.logger.info(' Logger initialized')
def _step_etdrk4(self):
""" Take one step forward using an exponential time-dfferencing method
with a Runge-Kutta 4 scheme.
Rereferences
------------
See Cox and Matthews, J. Comp. Physics., 176(2):430-455, 2002.
Kassam and Trefethen, IAM J. Sci. Comput., 26(4):1214-233, 2005.
"""
self.qh0 = self.qh.copy()
Fn0 = -self.jacobian_psi_q()
self.qh = (self.expch_h*self.qh0 + Fn0*self.Qh)*self.filtr
self.qh1 = self.qh.copy()
if self.passive_scalar:
self.ch0 = self.ch.copy()
Fn0c = -self.jacobian_psi_c()
self.ch = (self.expch_hc*self.ch0 + Fn0c*self.Qhc)*self.filtr
self.ch1 = self.ch.copy()
self._calc_derived_fields()
c1 = self._calc_ep_c()
self._invert()
k1 = self._calc_ep_psi()
Fna = -self.jacobian_psi_q()
self.qh = (self.expch_h*self.qh0 + Fna*self.Qh)*self.filtr
if self.passive_scalar:
Fnac = -self.jacobian_psi_c()
self.ch = (self.expch_hc*self.ch0 + Fnac*self.Qhc)*self.filtr
self._calc_derived_fields()
c2 = self._calc_ep_c()
self._invert()
k2 = self._calc_ep_psi()
Fnb = -self.jacobian_psi_q()
self.qh = (self.expch_h*self.qh1 + ( 2.*Fnb - Fn0 )*self.Qh)*self.filtr
if self.passive_scalar:
Fnbc = -self.jacobian_psi_c()
self.ch = (self.expch_hc*self.ch1 + ( 2.*Fnbc - Fn0c )*self.Qhc)*self.filtr
self._calc_derived_fields()
c3 = self._calc_ep_c()
self._invert()
k3 = self._calc_ep_psi()
Fnc = -self.jacobian_psi_q()
self.qh = (self.expch*self.qh0 + Fn0*self.f0 + 2.*(Fna+Fnb)*self.fab\
+ Fnc*self.fc)*self.filtr
if self.passive_scalar:
Fncc = -self.jacobian_psi_c()
self.ch = (self.expchc*self.ch0 + Fn0c*self.f0c+ 2.*(Fnac+Fnbc)*self.fabc\
+ Fncc*self.fcc)*self.filtr
self._calc_derived_fields()
c4 = self._calc_ep_c()
self.cvar += self.dt*(c1 + 2*(c2+c3) + c4)/6.
# invert
self._invert()
# calcuate q
self.q = self.ifft(self.qh).real
if self.passive_scalar:
self.c = self.ifft(self.ch).real
k4 = self._calc_ep_psi()
self.Ke += self.dt*(k1 + 2*(k2+k3) + k4)/6.
def _initialize_etdrk4(self):
""" Compute coefficients of the exponential time-dfferencing method
with a Runge-Kutta 4 scheme.
Rereferences
------------
See Cox and Matthews, J. Comp. Physics., 176(2):430-455, 2002.
Kassam and Trefethen, IAM J. Sci. Comput., 26(4):1214-233, 2005.
"""
#
# coefficients for q-equation
#
# the exponent for the linear part
c = np.zeros((self.nl,self.nk),self.dtype_cplx)
c += -self.nu4*self.wv4 - self.nu*self.wv2 - self.mu - 1j*self.k*self.U
c += self.beta*self.ik*self.wv2i
ch = c*self.dt
self.expch = np.exp(ch)
self.expch_h = np.exp(ch/2.)
self.expch2 = np.exp(2.*ch)
M = 32. # number of points for line integral in the complex plane
rho = 1. # radius for complex integration
r = rho*np.exp(2j*np.pi*((np.arange(1.,M+1))/M)) # roots for integral
LR = ch[...,np.newaxis] + r[np.newaxis,np.newaxis,...]
LR2 = LR*LR
LR3 = LR2*LR
self.Qh = self.dt*(((np.exp(LR/2.)-1.)/LR).mean(axis=-1))
self.f0 = self.dt*( ( ( -4. - LR + ( np.exp(LR)*( 4. - 3.*LR + LR2 ) ) )/ LR3 ).mean(axis=-1) )
self.fab = self.dt*( ( ( 2. + LR + np.exp(LR)*( -2. + LR ) )/ LR3 ).mean(axis=-1) )
self.fc = self.dt*( ( ( -4. -3.*LR - LR2 + np.exp(LR)*(4.-LR) )/ LR3 ).mean(axis=-1) )
if self.passive_scalar:
#
# coefficients for c-equation
#
# the exponent for the linear part
c = np.zeros((self.nl,self.nk),self.dtype_cplx)
c += -self.nu4c*self.wv4 - self.nuc*self.wv2 - self.muc
ch = c*self.dt
self.expchc = np.exp(ch)
self.expch_hc = np.exp(ch/2.)
self.expch2c = np.exp(2.*ch)
r = rho*np.exp(2j*np.pi*((np.arange(1.,M+1))/M)) # roots for integral
LR = ch[...,np.newaxis] + r[np.newaxis,np.newaxis,...]
LR2 = LR*LR
LR3 = LR2*LR
self.Qhc = self.dt*(((np.exp(LR/2.)-1.)/LR).mean(axis=-1))
self.f0c = self.dt*( ( ( -4. - LR + ( np.exp(LR)*( 4. - 3.*LR + LR2 ) ) )/ LR3 ).mean(axis=-1) )
self.fabc = self.dt*( ( ( 2. + LR + np.exp(LR)*( -2. + LR ) )/ LR3 ).mean(axis=-1) )
self.fcc = self.dt*( ( ( -4. -3.*LR - LR2 + np.exp(LR)*(4.-LR) )/ LR3 ).mean(axis=-1) )
def jacobian_psi_q(self):
""" Compute the advective term–––the Jacobian between psi and q.
Returns
-------
complex array of floats
The Fourier transform of Jacobian(psi,q)
"""
self.u, self.v = self.ifft(-self.il*self.ph).real, self.ifft(self.ik*self.ph).real
q = self.ifft(self.qh).real
return self.ik*self.fft(self.u*q) + self.il*self.fft(self.v*q)
def jacobian_psi_c(self):
""" Compute the advective term of the passive scalar equation–––the
Jacobian between psi and c.
Returns
-------
complex array of floats
The Fourier transform of Jacobian(psi,c)
"""
self.c = self.ifft(self.ch).real
return self.ik*self.fft(self.u*self.c) + self.il*self.fft(self.v*self.c)
def _invert(self):
""" Calculate the streamfunction given the potential vorticity.
"""
# invert for psi
self.ph = -self.wv2i*(self.qh)
# physical space
self.p = self.ifft(self.ph)
def set_q(self,q):
""" Initialize the potential vorticity.
Parameters
----------
q: an array of floats of dimension (nx,ny):
The potential vorticity in physical space.
"""
self.q = q
self.qh = self.fft(self.q)
self._invert()
self.Ke = self._calc_ke_qg()
def set_c(self,c):
""" Initialize the potential vorticity.
Parameters
----------
c: an array of floats of dimension (nx,ny):
The passive scalar in physical space.
"""
self.c = c
self.ch = self.fft(self.c)
self.cvar = self.spec_var(self.ch)
def _initialize_fft(self):
""" Define the two-dimensional FFT methods.
"""
# need to fix bug in mkl_fft.irfft2
if self.use_mkl:
#import mkl
#mkl.set_num_threads(self.nthreads)
#import mkl_fft
#self.fft = (lambda x : mkl_fft.rfft2(x))
#self.ifft = (lambda x : mkl_fft.irfft2(x))
pass
else:
pass
self.fft = (lambda x : np.fft.rfft2(x))
self.ifft = (lambda x : np.fft.irfft2(x))
def _print_status(self):
""" Print out the the model status.
Step: integer
Number of time steps completed
Time: float
The elapsed time.
P: float
The percentage of simulation completed.
Ke: float
The geostrophic kinetic energy.
CFL: float
The CFL number.
"""
self.tc += 1
self.t += self.dt
if (self.tc % self.twrite)==0:
self.ke = self._calc_ke_qg()
self.cfl = self._calc_cfl()
self.logger.info('Step: %i, Time: %4.3e, P: %4.3e , Ke: %4.3e, CFL: %4.3f'
, self.tc,self.t, self.t/self.tmax, self.ke, self.cfl )
assert self.cfl<self.cflmax, self.logger.error('CFL condition violated')
def _calc_ke_qg(self):
""" Compute geostrophic kinetic energy, Ke. """
return 0.5*self.spec_var(self.wv*self.ph)
def _calc_ens(self):
""" Compute geostrophic potential enstrophy. """
return 0.5*self.spec_var(self.qh)
def _calc_ep_psi(self):
""" Compute dissipation of Ke """
lap2psi = self.ifft(self.wv4*self.ph)
lapq = self.ifft(-self.wv2*self.qh)
return self.nu4*(self.q*lap2psi).mean() - self.nu*(self.p*lapq).mean()\
+ self.mu*(self.p*self.q).mean()
def _calc_ep_c(self):
""" Compute dissipation of C2 """
return -2*self.nu4c*(self.lapc**2).mean() - 2*self.nu*self.gradC2\
- 2*self.muc*self.C2
def _calc_chi_c(self):
""" Compute dissipation of gradC2 """
lap2c = self.ifft(self.wv4*self.ch)
return 2*self.nu4c*(lap2c*self.lapc).mean() - 2*self.nu*(self.lapc**2).mean()\
- 2*self.muc*self.gradC2
def _calc_chi_q(self):
"""" Calculates dissipation of geostrophic potential
enstrophy, S. """
return -self.nu4*self.spec_var(self.wv2*self.qh)
def spec_var(self, ph):
""" Compute variance of a variable `p` from its Fourier transform `ph` """
var_dens = 2. * | np.abs(ph) | numpy.abs |
from __future__ import print_function
from __future__ import absolute_import
from __future__ import division
import sys
from numpy import array
from numpy import eye
from numpy import zeros
from numpy import float64
from numpy.linalg import cond
from scipy.linalg import solve
from scipy.linalg import lstsq
from compas.numerical import normrow
from compas.numerical import normalizerow
from compas.geometry import midpoint_point_point_xy
__all__ = [
'update_q_from_qind',
'update_form_from_force'
]
EPS = 1 / sys.float_info.epsilon
def update_q_from_qind(E, q, dep, ind):
"""Update the full set of force densities using the values of the independent edges.
Parameters
----------
E : sparse csr matrix
The equilibrium matrix.
q : array
The force densities of the edges.
dep : list
The indices of the dependent edges.
ind : list
The indices of the independent edges.
Returns
-------
None
The force densities are modified in-place.
Examples
--------
>>>
"""
m = E.shape[0] - len(dep)
qi = q[ind]
Ei = E[:, ind]
Ed = E[:, dep]
if m > 0:
Edt = Ed.transpose()
A = Edt.dot(Ed).toarray()
b = Edt.dot(Ei).dot(qi)
else:
A = Ed.toarray()
b = Ei.dot(qi)
if | cond(A) | numpy.linalg.cond |
# This module has been generated automatically from space group information
# obtained from the Computational Crystallography Toolbox
#
"""
Space groups
This module contains a list of all the 230 space groups that can occur in
a crystal. The variable space_groups contains a dictionary that maps
space group numbers and space group names to the corresponding space
group objects.
.. moduleauthor:: <NAME> <<EMAIL>>
"""
#-----------------------------------------------------------------------------
# Copyright (C) 2013 The Mosaic Development Team
#
# Distributed under the terms of the BSD License. The full license is in
# the file LICENSE.txt, distributed as part of this software.
#-----------------------------------------------------------------------------
import numpy as N
class SpaceGroup(object):
"""
Space group
All possible space group objects are created in this module. Other
modules should access these objects through the dictionary
space_groups rather than create their own space group objects.
"""
def __init__(self, number, symbol, transformations):
"""
:param number: the number assigned to the space group by
international convention
:type number: int
:param symbol: the Hermann-Mauguin space-group symbol as used
in PDB and mmCIF files
:type symbol: str
:param transformations: a list of space group transformations,
each consisting of a tuple of three
integer arrays (rot, tn, td), where
rot is the rotation matrix and tn/td
are the numerator and denominator of the
translation vector. The transformations
are defined in fractional coordinates.
:type transformations: list
"""
self.number = number
self.symbol = symbol
self.transformations = transformations
self.transposed_rotations = N.array([N.transpose(t[0])
for t in transformations])
self.phase_factors = N.exp(N.array([(-2j*N.pi*t[1])/t[2]
for t in transformations]))
def __repr__(self):
return "SpaceGroup(%d, %s)" % (self.number, repr(self.symbol))
def __len__(self):
"""
:return: the number of space group transformations
:rtype: int
"""
return len(self.transformations)
def symmetryEquivalentMillerIndices(self, hkl):
"""
:param hkl: a set of Miller indices
:type hkl: Scientific.N.array_type
:return: a tuple (miller_indices, phase_factor) of two arrays
of length equal to the number of space group
transformations. miller_indices contains the Miller
indices of each reflection equivalent by symmetry to the
reflection hkl (including hkl itself as the first element).
phase_factor contains the phase factors that must be applied
to the structure factor of reflection hkl to obtain the
structure factor of the symmetry equivalent reflection.
:rtype: tuple
"""
hkls = N.dot(self.transposed_rotations, hkl)
p = N.multiply.reduce(self.phase_factors**hkl, -1)
return hkls, p
space_groups = {}
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(1, 'P 1', transformations)
space_groups[1] = sg
space_groups['P 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(2, 'P -1', transformations)
space_groups[2] = sg
space_groups['P -1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(3, 'P 1 2 1', transformations)
space_groups[3] = sg
space_groups['P 1 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(4, 'P 1 21 1', transformations)
space_groups[4] = sg
space_groups['P 1 21 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(5, 'C 1 2 1', transformations)
space_groups[5] = sg
space_groups['C 1 2 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(6, 'P 1 m 1', transformations)
space_groups[6] = sg
space_groups['P 1 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(7, 'P 1 c 1', transformations)
space_groups[7] = sg
space_groups['P 1 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(8, 'C 1 m 1', transformations)
space_groups[8] = sg
space_groups['C 1 m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(9, 'C 1 c 1', transformations)
space_groups[9] = sg
space_groups['C 1 c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(10, 'P 1 2/m 1', transformations)
space_groups[10] = sg
space_groups['P 1 2/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,0])
trans_den = N.array([1,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(11, 'P 1 21/m 1', transformations)
space_groups[11] = sg
space_groups['P 1 21/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(12, 'C 1 2/m 1', transformations)
space_groups[12] = sg
space_groups['C 1 2/m 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(13, 'P 1 2/c 1', transformations)
space_groups[13] = sg
space_groups['P 1 2/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,-1,-1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(14, 'P 1 21/c 1', transformations)
space_groups[14] = sg
space_groups['P 1 21/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,-1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,-1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(15, 'C 1 2/c 1', transformations)
space_groups[15] = sg
space_groups['C 1 2/c 1'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(16, 'P 2 2 2', transformations)
space_groups[16] = sg
space_groups['P 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(17, 'P 2 2 21', transformations)
space_groups[17] = sg
space_groups['P 2 2 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(18, 'P 21 21 2', transformations)
space_groups[18] = sg
space_groups['P 21 21 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(19, 'P 21 21 21', transformations)
space_groups[19] = sg
space_groups['P 21 21 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,1])
trans_den = N.array([1,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,1])
trans_den = N.array([2,2,2])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(20, 'C 2 2 21', transformations)
space_groups[20] = sg
space_groups['C 2 2 21'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
sg = SpaceGroup(21, 'C 2 2 2', transformations)
space_groups[21] = sg
space_groups['C 2 2 2'] = sg
transformations = []
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,0,0])
trans_den = N.array([1,1,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([0,1,1])
trans_den = N.array([1,2,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([-1,0,0,0,-1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,0,1])
trans_den = N.array([2,1,2])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,1,0,0,0,1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = N.array([2,2,1])
transformations.append((rot, trans_num, trans_den))
rot = N.array([1,0,0,0,-1,0,0,0,-1])
rot.shape = (3, 3)
trans_num = N.array([1,1,0])
trans_den = | N.array([2,2,1]) | numpy.array |
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# =============================================================================
# Created By : <NAME>
# Date Last Modified: Aug 1 2021
# =============================================================================
import numpy as np
import matplotlib.pyplot as plt
import warnings
from astropy.utils.exceptions import AstropyWarning
warnings.simplefilter('ignore', category=AstropyWarning)
warnings.filterwarnings('ignore')
import random
import seaborn as sns
sns.set_theme()
# Function that describes the period distribution of a particular type of
# variable star in the LMC
def get_period_distribution(type, ogle_dir):
# Read in OGLE catalog of variables stars
dt = np.dtype([('id', 'U40'), ('I', float), ('V', float), ('P', float),
('eP', float), ('t0', float), ('Iamp', float), ('R21', float),
('phi21', float), ('R31', float), ('phi31', float)])
if type == 'CEP':
fo_vars = np.loadtxt(ogle_dir+'ccep/cep1O.dat', dtype=dt)
fu_vars = np.loadtxt(ogle_dir+'ccep/cepF.dat', dtype=dt)
bins = np.linspace(0.2, 40.2, 401)
elif type == 'AC':
fo_vars = np.loadtxt(ogle_dir+'acep/acep1O.dat', dtype=dt)
fu_vars = np.loadtxt(ogle_dir+'acep/acepF.dat', dtype=dt)
bins = np.linspace(0.3, 3.0, 28)
elif type == 'T2C':
t2c = np.loadtxt(ogle_dir+'t2cep/t2cep.dat', dtype=dt)
dt2 = np.dtype([('id', 'U40'), ('type', 'U10')])
t2c2 = np.loadtxt(ogle_dir+'t2cep/ident.dat', dtype=dt2, usecols=(0,1))
fo_vars = t2c[t2c2['type'] == 'BLHer']
fu_vars = t2c[t2c2['type'] == 'WVir']
bins = np.linspace(1.0, 40.0, 79)
elif type == 'RRL':
fu_vars = np.loadtxt(ogle_dir+'rrl/RRab.dat', dtype=dt)
fo_vars = np.loadtxt(ogle_dir+'rrl/RRc.dat', dtype=dt)
bins = np.linspace(0.2, 1.0, 81)
bins_centered = (bins[:-1] + bins[1:]) / 2
bin_size = bins[1] - bins[0]
fig, ax = plt.subplots(1,1)
sns.histplot(fu_vars['P'], bins=bins, color='xkcd:rose')
sns.histplot(fo_vars['P'], bins=bins, color='xkcd:steel blue')
n_fu, _ = np.histogram(fu_vars['P'], bins=bins,
density=True)
n_fo, _ = np.histogram(fo_vars['P'], bins=bins,
density=True)
ax.set_xlabel('Period')
ax.set_ylabel('Normalized N')
plt.title('{} simulated period distribution'.format(type))
plt.show()
# probability of each period bin
p_prob_fu = n_fu*bin_size
p_prob_fo = n_fo*bin_size
return bins_centered, p_prob_fo, p_prob_fu
# Function that describes the amplitude distribution of a particular type of
# variable star in the LMC
def get_amp_distribution(type, ogle_dir):
# Read in OGLE catalog of variables stars
dt = np.dtype([('id', 'U40'), ('I', float), ('V', float), ('P', float),
('eP', float), ('t0', float), ('Iamp', float), ('R21', float),
('phi21', float), ('R31', float), ('phi31', float)])
if type == 'CEP':
fo_vars = np.loadtxt(ogle_dir+'ccep/cep1O.dat', dtype=dt)
fu_vars = np.loadtxt(ogle_dir+'ccep/cepF.dat', dtype=dt)
elif type == 'AC':
fo_vars = np.loadtxt(ogle_dir+'acep/acep1O.dat', dtype=dt)
fu_vars = np.loadtxt(ogle_dir+'acep/acepF.dat', dtype=dt)
elif type == 'T2C':
t2c = np.loadtxt(ogle_dir+'t2cep/t2cep.dat', dtype=dt)
dt2 = np.dtype([('id', 'U40'), ('type', 'U10')])
t2c2 = np.loadtxt(ogle_dir+'t2cep/ident.dat', dtype=dt2, usecols=(0,1))
fo_vars = t2c[t2c2['type'] == 'BLHer']
fu_vars = t2c[t2c2['type'] == 'WVir']
elif type == 'RRL':
fu_vars = np.loadtxt(ogle_dir+'rrl/RRab.dat', dtype=dt)
fo_vars = np.loadtxt(ogle_dir+'rrl/RRc.dat', dtype=dt)
if (type == 'RRL') | (type == 'CEP'):
bins = np.linspace(0.0, 1.0, 101)
else:
bins = np.linspace(0.0, 1.0, 11)
bins_centered = (bins[:-1] + bins[1:]) / 2
bin_size = bins[1] - bins[0]
fig, ax = plt.subplots(1,1)
sns.histplot(fu_vars['Iamp'], bins=bins, color='xkcd:rose')
sns.histplot(fo_vars['Iamp'], bins=bins, color='xkcd:steel blue')
n_fu, _ = np.histogram(fu_vars['Iamp'], bins=bins,
density=True)
n_fo, _ = np.histogram(fo_vars['Iamp'], bins=bins,
density=True)
ax.set_xlabel('Amplitude')
ax.set_ylabel('Normalized N')
plt.title('{} simulated amp distribution'.format(type))
plt.show()
# probability of each amplitude bin
amp_prob_fu = n_fu*bin_size
amp_prob_fo = n_fo*bin_size
return bins_centered, amp_prob_fo, amp_prob_fu
# Generates the parameters of simulated stars
def simulate_params(var_types, num_stars, ogle_dir, id_offset=0, seed=0):
dt = np.dtype([('id', int), ('type', 'U3'), ('mode', 'U2'),
('period', float), ('mag', float), ('amp', float)])
sim_data = np.zeros(num_stars*2*len(var_types), dtype=dt)
for i in range(len(var_types)):
type = var_types[i]
### First, pick period
bins, prob_fo, prob_fu = get_period_distribution(type, ogle_dir)
# pick approximate periods from distribution
if seed != 0:
np.random.seed(seed)
periods_fu = np.random.choice(bins, num_stars, p=prob_fu)
periods_fo = np.random.choice(bins, num_stars, p=prob_fo)
bin_size = bins[1] - bins[0]
periods_fu += np.random.normal(0, bin_size, num_stars)
periods_fo += np.random.normal(0, bin_size, num_stars)
# put in hard limits for periods of certain types
# NOTE: for T2C, fo/fu is actually BL Her/W Virginis
if type == 'T2C':
for j in range(num_stars):
# this causes a bit of weirdness, but ok for now
periods_fo[j] = np.max([periods_fo[j], 1.0])
periods_fo[j] = np.min([periods_fo[j], 4.0])
periods_fu[j] = np.max([periods_fu[j], 4.0])
### Now do amplitudes
if type == 'RRL':
# Use period-amplitude relation to assign amplitudes
# period-amplitude relaiton is defined by OGLE IV LMC RRL
PA_coeff_fu = np.array([-1.58, -2.99, -0.08])
PA_coeff_fo = np.array([-4.11, -3.94, -0.66])
PA_fu_sig = 0.14
PA_fo_sig = 0.06
fu_amp = PA_coeff_fu[0]*np.log10(periods_fu)**2 + \
PA_coeff_fu[1]*np.log10(periods_fu) + PA_coeff_fu[2]
fo_amp = PA_coeff_fo[0]*np.log10(periods_fo)**2 + \
PA_coeff_fo[1]*np.log10(periods_fo) + PA_coeff_fo[2]
fu_amp += np.random.normal(0, PA_fu_sig, num_stars)
fo_amp += np.random.normal(0, PA_fo_sig, num_stars)
# no other variables follow a period-amplitude relation, so
# randomly assign an amplitude
else:
# Pick amplitudes from probability distribution
bins, prob_fo, prob_fu = get_amp_distribution(type, ogle_dir)
fu_amp = np.random.choice(bins, num_stars, p=prob_fu)
fo_amp = np.random.choice(bins, num_stars, p=prob_fo)
# perturb the amplitudes
bin_size = bins[1] - bins[0]
fu_amp += np.random.normal(0, bin_size, num_stars)
fo_amp += np.random.normal(0, bin_size, num_stars)
### Now assign mean magnitudes
if type == 'RRL':
# Use I band PL relation to assign magnitudes from periods
fu_pl_sig = 0.15
fo_pl_sig = 0.16
fo_mag = -2.014*np.log10(periods_fo) + 17.743
fu_mag = -1.889*np.log10(periods_fu) + 18.164
# add some noise
fo_mag += np.random.normal(0, fo_pl_sig, num_stars)
fu_mag += np.random.normal(0, fu_pl_sig, num_stars)
if type == 'CEP':
fu_pl_sig = 0.15
fo_pl_sig = 0.16
fo_mag = -3.311*(np.log10(periods_fo)-1.0) + 12.897
fu_mag = -2.912*(np.log10(periods_fu)-1.0) + 13.741
fo_mag += np.random.normal(0, fo_pl_sig, num_stars)
fu_mag += np.random.normal(0, fu_pl_sig, num_stars)
if type == 'AC':
fu_pl_sig = 0.23
fo_pl_sig = 0.16
fo_mag = -3.302*np.log10(periods_fo) + 16.656
fu_mag = -2.962*np.log10(periods_fu) + 17.368
fo_mag += np.random.normal(0, fo_pl_sig, num_stars)
fu_mag += np.random.normal(0, fu_pl_sig, num_stars)
if type == 'T2C':
fo_pl_sig = 0.40
fu_pl_sig = 0.40
fo_mag = -2.033*np.log10(periods_fo) + 18.015
fu_mag = -2.033*np.log10(periods_fu) + 18.015
fo_mag += np.random.normal(0, fo_pl_sig, num_stars)
fu_mag += np.random.normal(0, fu_pl_sig, num_stars)
start_fo = i*num_stars*2
start_fu = i*num_stars + (i+1)*num_stars
stop_fu = (i+1)*num_stars*2
sim_data['id'] = np.arange(num_stars*2*len(var_types))+1+id_offset
sim_data['type'][start_fo:stop_fu] = type
sim_data['mode'][start_fo:start_fu] = 'FO'
sim_data['mode'][start_fu:stop_fu] = 'FU'
sim_data['period'][start_fo:start_fu] = periods_fo
sim_data['period'][start_fu:stop_fu] = periods_fu
sim_data['mag'][start_fo:start_fu] = fo_mag
sim_data['mag'][start_fu:stop_fu] = fu_mag
sim_data['amp'][start_fo:start_fu] = fo_amp
sim_data['amp'][start_fu:stop_fu] = fu_amp
return sim_data
# Generates the lcv files of simulated stars, which can be run through your
# fitting algorithm.
# Will create true_params.txt, as well as .fitlc files for each of your simulated
# stars.
def make_simulated_lcv(sim_data, mjds, filters, exptimes, mu, scatter_array, error_key,
error_array, output_dir, period_search_range=[0.1, 3.0], append=False, rrl_cutoff=99,
template_file = 'var_templates.txt', seed=0):
if seed != 0:
np.random.seed(seed)
num_stars = len(sim_data['id'])
if append == False:
params = open(output_dir+'true_params.txt', 'w')
params.write('#id type mode temp period t0 mag1 amp1 sig1 mag2 amp2 sig2\n')
else:
params = open(output_dir+'true_params.txt', 'a')
# transform parameters to desired filters
# first define shift in zero point according to the approximate distance modulus
zp_shift = mu - 18.477 # estimated target distance modulus - LMC distance modulus
# Now transform magnitudes into desired filters using period color relations
# and amplitudes using amplitude ratios
filters_unique = np.unique(filters)
num_filters = len(filters_unique)
mags = np.zeros((num_stars, num_filters))
amps = np.zeros((num_stars, num_filters))
for i in range(num_filters):
# need to add in other colors!
filter = filters_unique[i]
if filter == 'B':
rrl = sim_data['type'] == 'RRL'
if len(sim_data['id'][rrl]) > 0:
logP = np.log10(sim_data['period'][rrl])
fo = sim_data['mode'][rrl] == 'FO'
logP[fo] += 0.127
color = 1.072 + 1.325*logP # CRRP (B-I)
color += np.random.normal(0, 0.10, len(logP))
mags[rrl,i] = sim_data['mag'][rrl] + zp_shift + color
amps[rrl,i] = sim_data['amp'][rrl]*2.06 # amp ratio from CRRP RRL
cep = ~rrl
if len(sim_data['id'][cep]) > 0:
P = sim_data['period'][cep]
fo = sim_data['mode'][cep] == 'FO'
logP = np.log10(sim_data['period'][cep])
color = 0.636*logP + 0.685 # Sandage 2009
color += np.random.normal(0, 0.10, len(logP))
mags[cep,i] = sim_data['mag'][cep] + zp_shift + color
amps[cep,i] = sim_data['amp'][cep]*2.06 # amp ratio from CRRP RRL
if filter == 'V':
rrl = sim_data['type'] == 'RRL'
if len(sim_data['id'][rrl]) > 0:
logP = np.log10(sim_data['period'][rrl])
fo = sim_data['mode'][rrl] == 'FO'
logP[fo] += 0.127
color = 0.606 + 0.676*logP # CRRP (V-I)
color += np.random.normal(0, 0.05, len(logP))
mags[rrl,i] = sim_data['mag'][rrl] + zp_shift + color
amps[rrl,i] = sim_data['amp'][rrl]*1.57 # amp ratio from CRRP RRL
cep = ~rrl
if len(sim_data['id'][cep]) > 0:
P = sim_data['period'][cep]
fo = sim_data['mode'][cep] == 'FO'
logP = np.log10(sim_data['period'][cep])
color = 0.276*logP + 0.450 # Sandage 2009 - apply to all types of cepheids
color += np.random.normal(0, 0.08, len(logP))
mags[cep,i] = sim_data['mag'][cep] + zp_shift + color
amps[cep,i] = sim_data['amp'][cep]*1.57 # amp ratio from CRRP RRL
# if filter == 'R':
if filter == 'I':
mags[:,i] = sim_data['mag'] + zp_shift
amps[:,i] = sim_data['amp']
# if filter == 'J':
# if filter == 'H':
# if filter == 'K':
# if filter == '[3.6]':
# if filter == '[4.5]':
# force amplitude to be positive
amps = np.abs(amps)
fo_temps = np.array([6])
fu_temps = np.array([0, 1, 2,3, 4, 5, 7, 8])
template_number = np.zeros(num_stars)
template_number[sim_data['mode'] == 'FO'] = np.random.choice(fo_temps, int(num_stars/2))
template_number[sim_data['mode'] == 'FU'] = np.random.choice(fu_temps, int(num_stars/2))
mjd_range = np.array([np.min(mjds), np.max(mjds)])
t0 = np.random.uniform(low=mjd_range[0], high=mjd_range[1], size=num_stars)
# Get template light curves
dt = np.dtype([('phase', float), ('ab1', float), ('ab2', float),
('ab3', float), ('ab4', float), ('ab5', float), ('ab6', float),
('c', float), ('ab7', float), ('ab8', float)])
templates = np.loadtxt(template_file, dtype=dt)
templates_new = np.c_[templates['ab1'], templates['ab2'], templates['ab3'],
templates['ab4'], templates['ab5'], templates['ab6'], templates['c'],
templates['ab7'], templates['ab8']]
templates_mean = np.mean(templates_new, axis=0)
for i in range(num_stars):
ph = templates['phase']# + np.random.uniform()
#ncycles = 50 ### make smarter
# calculate how many pulsation cycles we need for this star
mjd_window = mjd_range[1] - mjd_range[0]
ncycles = int(np.ceil(mjd_window/sim_data['period'][i]))*2
mags_all = np.zeros(len(mjds))
errs_all = np.zeros(len(mjds))
xx = int(template_number[i])
lcv_scatter = np.zeros(num_filters)
for j in range(num_filters):
# build template with correct period, amp, mean mag
mag_temp = (templates_new[:,xx]-templates_mean[xx])*amps[i,j] + mags[i,j]
t_one_cycle = ph*sim_data['period'][i] + t0[i]
t_repeated = []
for k in np.arange(0,ncycles):
t_repeated = np.append(t_repeated, t_one_cycle+(k-ncycles/2)*sim_data['period'][i])
mag_repeated = np.tile(mag_temp, ncycles)
# sample template at proper mjds
mjds_in_filter = mjds[filters == filters_unique[j]]
num_obs_in_filter = len(mjds_in_filter)
mag_sim = np.interp(mjds_in_filter, t_repeated, mag_repeated)
# add in scatter to light curve
# scatter is a function of magnitude, as described in inputs
# to this functiion
if scatter_array.ndim == 2:
lcv_scatter[j] = np.random.normal(scatter_array[j,0], scatter_array[j,1])
lcv_scatter[j] = np.abs(lcv_scatter[j])
elif scatter_array.ndim == 3:
temp_scatter = np.interp(mags[i,j], scatter_array[j,:,0], scatter_array[j,:,1])
temp_sig = np.interp(mags[i,j], scatter_array[j,:,0], scatter_array[j,:,2])
lcv_scatter[j] = np.random.normal(temp_scatter, temp_sig, 1)
lcv_scatter[j] = np.abs(lcv_scatter[j])
mag_sim += np.random.normal(0, lcv_scatter[j], num_obs_in_filter)
mags_all[filters == filters_unique[j]] = mag_sim
# assign photometric uncertainty to each point
# photometric uncertainty is also a function of magnitude
if error_array.ndim == 3:
err_sim = np.zeros(len(mag_sim))
for k in range(num_obs_in_filter):
this_exptime = exptimes[filters == filters_unique[j]][k]
# find right row in error_array for this filter and exptime
row = (error_key['filter'] == filters_unique[j]) & \
(error_key['exptime'] == this_exptime)
temp_err = np.interp(mag_sim[k], error_array[row,:,0][0], error_array[row,:,1][0])
temp_sig = np.interp(mag_sim[k], error_array[row,:,0][0], error_array[row,:,2][0])
# put in safeguard to avoid negative numbers
err_sim[k] = np.random.normal(temp_err, temp_sig, 1)
err_sim[k] = np.abs(err_sim[k])
# put in error floor so we don't get unrealisticaly low numbers
if err_sim[k] < 0.005:
err_sim[k] = 0.005
elif error_array.ndim == 2:
err_sim = np.random.normal(error_array[j,0], error_array[j,1],
len(mag_sim))
err_sim = np.abs(err_sim)
errs_all[filters == filters_unique[j]] = err_sim
# save simulated light curve into file
filters_int = np.zeros(len(filters))
for j in range(num_filters):
filters_int[filters == filters_unique[j]] = j
data_save = np.c_[filters_int, mjds, mags_all, errs_all]
f = open(output_dir+'sim_{}.fitlc'.format(sim_data['id'][i]), 'w')
if rrl_cutoff < 99:
if mags[i,-1] > rrl_cutoff:
f.write('amoeba\nperst=0.1\npered=1.0\n')
else:
f.write('amoeba\nperst={}\npered={}\n'.format(period_search_range[0],
period_search_range[1]))
else:
f.write('amoeba\nperst={}\npered={}\n'.format(period_search_range[0],
period_search_range[1]))
np.savetxt(f, data_save, fmt='%2i %12.6f %7.4f %6.4f')
f.close()
format_1 = '{:4} {:4} {:4} {:4} {:8.5f} {:10.4f}'
string_1 = format_1.format(int(sim_data['id'][i]), sim_data['type'][i],
sim_data['mode'][i], int(template_number[i]), sim_data['period'][i],
t0[i])
string_2 = ''
for j in range(num_filters):
format_2 = ' {:6.3f} {:4.2f} {:4.2f}'
string_2 += format_2.format(mags[i,j], amps[i,j], lcv_scatter[j])
line = string_1 + string_2 + '\n'
params.write(line)
params.close()
def make_simulated_lcv_single_snr(sim_data, mjds, filters, exptimes, mu,
scatter_mean_snr, scatter_std_snr, error_mean_snr, error_std_snr, output_dir,
period_search_range=[0.1, 1.0], append=False, rrl_cutoff=99,
template_file='var_templates.txt'):
num_stars = len(sim_data['id'])
if append == False:
params = open(output_dir+'true_params.txt', 'w')
params.write('#id type mode temp period t0 mag1 amp1 sig1 mag2 amp2 sig2\n')
else:
params = open(output_dir+'true_params.txt', 'a')
# transform parameters to desired filters
# first transform from I band LMC magnitude to I band target magnitude
zp_shift = mu - 18.477 # estimated target distance modulus - LMC distance modulus
# Now transform magnitudes into desired filters using period color relations
# and amplitudes using amplitude ratios
filters_unique = np.unique(filters)
num_filters = len(filters_unique)
mags = np.zeros((num_stars, num_filters))
amps = np.zeros((num_stars, num_filters))
for i in range(num_filters):
# need to add in other colors!
filter = filters_unique[i]
if filter == 'B':
rrl = sim_data['type'] == 'RRL'
if len(sim_data['id'][rrl]) > 0:
logP = np.log10(sim_data['period'][rrl])
fo = sim_data['mode'][rrl] == 'FO'
logP[fo] += 0.127
color = 1.072 + 1.325*logP # CRRP (B-I)
color += np.random.normal(0, 0.10, len(logP))
mags[rrl,i] = sim_data['mag'][rrl] + zp_shift + color
amps[rrl,i] = sim_data['amp'][rrl]*2.06
cep = ~rrl
if len(sim_data['id'][cep]) > 0:
P = sim_data['period'][cep]
fo = sim_data['mode'][cep] == 'FO'
logP = np.log10(sim_data['period'][cep])
color = 0.636*logP + 0.685 # Sandage 2009
color += np.random.normal(0, 0.10, len(logP))
mags[cep,i] = sim_data['mag'][cep] + zp_shift + color
amps[cep,i] = sim_data['amp'][cep]*2.06
if filter == 'V':
rrl = sim_data['type'] == 'RRL'
if len(sim_data['id'][rrl]) > 0:
logP = np.log10(sim_data['period'][rrl])
fo = sim_data['mode'][rrl] == 'FO'
logP[fo] += 0.127
color = 0.606 + 0.676*logP # CRRP (V-I) ### something is off here
color += np.random.normal(0, 0.05, len(logP))
mags[rrl,i] = sim_data['mag'][rrl] + zp_shift + color
amps[rrl,i] = sim_data['amp'][rrl]*1.57
cep = ~rrl
if len(sim_data['id'][cep]) > 0:
P = sim_data['period'][cep]
fo = sim_data['mode'][cep] == 'FO'
logP = np.log10(sim_data['period'][cep])
color = 0.276*logP + 0.450 # Sandage 2009 - apply to all types of cepheids
color += np.random.normal(0, 0.08, len(logP))
mags[cep,i] = sim_data['mag'][cep] + zp_shift + color
amps[cep,i] = sim_data['amp'][cep]*1.57
# if filter == 'R':
if filter == 'I':
mags[:,i] = sim_data['mag'] + zp_shift
amps[:,i] = sim_data['amp']
# if filter == 'J':
# if filter == 'H':
# if filter == 'K':
# if filter == '[3.6]':
# if filter == '[4.5]':
# force amplitude to be positive
amps = np.abs(amps)
fo_temps = np.array([6])
fu_temps = np.array([0, 1, 2,3, 4, 5, 7, 8])
template_number = np.zeros(num_stars)
template_number[sim_data['mode'] == 'FO'] = np.random.choice(fo_temps, int(num_stars/2))
template_number[sim_data['mode'] == 'FU'] = np.random.choice(fu_temps, int(num_stars/2))
mjd_range = np.array([np.min(mjds), np.max(mjds)])
t0 = np.random.uniform(low=mjd_range[0], high=mjd_range[1], size=num_stars)
# Get template light curves
dt = np.dtype([('phase', float), ('ab1', float), ('ab2', float),
('ab3', float), ('ab4', float), ('ab5', float), ('ab6', float),
('c', float), ('ab7', float), ('ab8', float)])
templates = np.loadtxt(template_file, dtype=dt)
templates_new = np.c_[templates['ab1'], templates['ab2'], templates['ab3'],
templates['ab4'], templates['ab5'], templates['ab6'], templates['c'],
templates['ab7'], templates['ab8']]
templates_mean = np.mean(templates_new, axis=0)
for i in range(num_stars):
ph = templates['phase']# + np.random.uniform()
#ncycles = 50 ### make smarter
# calculate how many pulsation cycles we need for this star
mjd_window = mjd_range[1] - mjd_range[0]
ncycles = int(np.ceil(mjd_window/sim_data['period'][i]))*2
mags_all = np.zeros(len(mjds))
errs_all = np.zeros(len(mjds))
xx = int(template_number[i])
lcv_scatter = np.zeros(num_filters)
for j in range(num_filters):
# build template with correct period, amp, mean mag
mag_temp = (templates_new[:,xx]-templates_mean[xx])*amps[i,j] + mags[i,j]
t_one_cycle = ph*sim_data['period'][i] + t0[i]
t_repeated = []
for k in np.arange(0,ncycles):
t_repeated = | np.append(t_repeated, t_one_cycle+(k-ncycles/2)*sim_data['period'][i]) | numpy.append |
import argparse
import cv2
import json
import numpy as np
import os
import pickle
import torch
from argparse import Namespace
from scipy.special import softmax
from sklearn.externals import joblib
from pyquaternion import Quaternion
from tqdm import tqdm
from network import CameraBranch
class Camera_Branch_Inference():
def __init__(self, cfg, device):
self.cfg = cfg
# img preprocess
self.img_input_shape = tuple([int(_) for _ in cfg.img_resize.split('x')])
self.img_mean = np.load(cfg.img_mean)
# device
self.device = device
# Model
self.model = CameraBranch(cfg)
self.model = torch.nn.DataParallel(self.model)
self.model = self.model.to(device)
self.model.load_state_dict(torch.load(cfg.model_weight))
self.model = self.model.eval()
self.model = self.model.to(device)
# bin -> vectors
self.kmeans_trans = joblib.load(cfg.kmeans_trans_path)
self.kmeans_rots = joblib.load(cfg.kmeans_rots_path)
def inference(self, img1_path, img2_path):
img1 = cv2.imread(img1_path)
img2 = cv2.imread(img2_path)
img1 = cv2.resize(img1, self.img_input_shape) - self.img_mean
img2 = cv2.resize(img2, self.img_input_shape) - self.img_mean
img1 = np.transpose(img1, (2, 0, 1))
img2 = | np.transpose(img2, (2, 0, 1)) | numpy.transpose |
from typing import Union
import pandas as pd
import numpy as np
from pandas import Series, DataFrame
from pandas.core.arrays import ExtensionArray
from sklearn import preprocessing
import time
def convert_date_2_timestamp(date_str):
time_array = time.strptime(date_str, "%Y%m%d")
return int(time.mktime(time_array));
def normalization(data):
_range = np.max(data) - | np.min(data) | numpy.min |
''' All DUT alignment functions in space and time are listed here plus additional alignment check functions'''
from __future__ import division
import logging
import sys
import os
from collections import Iterable
import math
import tables as tb
import numpy as np
import scipy
from matplotlib.backends.backend_pdf import PdfPages
from tqdm import tqdm
from beam_telescope_analysis.telescope.telescope import Telescope
from beam_telescope_analysis.tools import analysis_utils
from beam_telescope_analysis.tools import plot_utils
from beam_telescope_analysis.tools import geometry_utils
from beam_telescope_analysis.tools import data_selection
from beam_telescope_analysis.track_analysis import find_tracks, fit_tracks, line_fit_3d, _fit_tracks_kalman_loop
from beam_telescope_analysis.result_analysis import calculate_residuals, histogram_track_angle, get_angles
from beam_telescope_analysis.tools.storage_utils import save_arguments
default_alignment_parameters = ["translation_x", "translation_y", "translation_z", "rotation_alpha", "rotation_beta", "rotation_gamma"]
default_cluster_shapes = [1, 3, 5, 13, 14, 7, 11, 15]
kfa_alignment_descr = np.dtype([('translation_x', np.float64),
('translation_y', np.float64),
('translation_z', np.float64),
('rotation_alpha', np.float64),
('rotation_beta', np.float64),
('rotation_gamma', np.float64),
('translation_x_err', np.float64),
('translation_y_err', np.float64),
('translation_z_err', np.float64),
('rotation_alpha_err', np.float64),
('rotation_beta_err', np.float64),
('rotation_gamma_err', np.float64),
('translation_x_delta', np.float64),
('translation_y_delta', np.float64),
('translation_z_delta', np.float64),
('rotation_alpha_delta', np.float64),
('rotation_beta_delta', np.float64),
('rotation_gamma_delta', np.float64),
('annealing_factor', np.float64)])
@save_arguments
def apply_alignment(telescope_configuration, input_file, output_file=None, local_to_global=True, align_to_beam=False, chunk_size=1000000):
'''Convert local to global coordinates and vice versa.
Note:
-----
This function cannot be easily made faster with multiprocessing since the computation function (apply_alignment_to_chunk) does not
contribute significantly to the runtime (< 20 %), but the copy overhead for not shared memory needed for multipgrocessing is higher.
Also the hard drive IO can be limiting (30 Mb/s read, 20 Mb/s write to the same disk)
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_file : string
Filename of the input file (merged or tracks file).
output_file : string
Filename of the output file with the converted coordinates (merged or tracks file).
local_to_global : bool
If True, convert from local to global coordinates.
align_to_beam : bool
If True, use telescope alignment to align to the beam (beam along z axis).
chunk_size : uint
Chunk size of the data when reading from file.
Returns
-------
output_file : string
Filename of the output file with new coordinates.
'''
telescope = Telescope(telescope_configuration)
n_duts = len(telescope)
logging.info('=== Apply alignment to %d DUTs ===', n_duts)
if output_file is None:
output_file = os.path.splitext(input_file)[0] + ('_global_coordinates.h5' if local_to_global else '_local_coordinates.h5')
def convert_data(dut, dut_index, node, conv, data):
if isinstance(dut, Telescope):
data['x_dut_%d' % dut_index], data['y_dut_%d' % dut_index], data['z_dut_%d' % dut_index] = conv(
x=data['x_dut_%d' % dut_index],
y=data['y_dut_%d' % dut_index],
z=data['z_dut_%d' % dut_index],
translation_x=dut.translation_x,
translation_y=dut.translation_y,
translation_z=dut.translation_z,
rotation_alpha=dut.rotation_alpha,
rotation_beta=dut.rotation_beta,
rotation_gamma=dut.rotation_gamma)
else:
data['x_dut_%d' % dut_index], data['y_dut_%d' % dut_index], data['z_dut_%d' % dut_index] = conv(
x=data['x_dut_%d' % dut_index],
y=data['y_dut_%d' % dut_index],
z=data['z_dut_%d' % dut_index])
if "Tracks" in node.name:
format_strings = ['offset_{dimension}_dut_{dut_index}']
if "DUT%d" % dut_index in node.name:
format_strings.extend(['offset_{dimension}'])
for format_string in format_strings:
if format_string.format(dimension='x', dut_index=dut_index) in node.dtype.names:
data[format_string.format(dimension='x', dut_index=dut_index)], data[format_string.format(dimension='y', dut_index=dut_index)], data[format_string.format(dimension='z', dut_index=dut_index)] = conv(
x=data[format_string.format(dimension='x', dut_index=dut_index)],
y=data[format_string.format(dimension='y', dut_index=dut_index)],
z=data[format_string.format(dimension='z', dut_index=dut_index)],
translation_x=dut.translation_x,
translation_y=dut.translation_y,
translation_z=dut.translation_z,
rotation_alpha=dut.rotation_alpha,
rotation_beta=dut.rotation_beta,
rotation_gamma=dut.rotation_gamma)
format_strings = ['slope_{dimension}_dut_{dut_index}']
if "DUT%d" % dut_index in node.name:
format_strings.extend(['slope_{dimension}'])
for format_string in format_strings:
if format_string.format(dimension='x', dut_index=dut_index) in node.dtype.names:
data[format_string.format(dimension='x', dut_index=dut_index)], data[format_string.format(dimension='y', dut_index=dut_index)], data[format_string.format(dimension='z', dut_index=dut_index)] = conv(
x=data[format_string.format(dimension='x', dut_index=dut_index)],
y=data[format_string.format(dimension='y', dut_index=dut_index)],
z=data[format_string.format(dimension='z', dut_index=dut_index)],
# no translation for the slopes
translation_x=0.0,
translation_y=0.0,
translation_z=0.0,
rotation_alpha=dut.rotation_alpha,
rotation_beta=dut.rotation_beta,
rotation_gamma=dut.rotation_gamma)
format_strings = ['{dimension}_err_dut_{dut_index}']
for format_string in format_strings:
if format_string.format(dimension='x', dut_index=dut_index) in node.dtype.names:
data[format_string.format(dimension='x', dut_index=dut_index)], data[format_string.format(dimension='y', dut_index=dut_index)], data[format_string.format(dimension='z', dut_index=dut_index)] = np.abs(conv(
x=data[format_string.format(dimension='x', dut_index=dut_index)],
y=data[format_string.format(dimension='y', dut_index=dut_index)],
z=data[format_string.format(dimension='z', dut_index=dut_index)],
# no translation for the errors
translation_x=0.0,
translation_y=0.0,
translation_z=0.0,
rotation_alpha=dut.rotation_alpha,
rotation_beta=dut.rotation_beta,
rotation_gamma=dut.rotation_gamma))
# Looper over the hits of all DUTs of all hit tables in chunks and apply the alignment
with tb.open_file(input_file, mode='r') as in_file_h5:
with tb.open_file(output_file, mode='w') as out_file_h5:
for node in in_file_h5.root: # Loop over potential hit tables in data file
logging.info('== Apply alignment to node %s ==', node.name)
hits_aligned_table = out_file_h5.create_table(
where=out_file_h5.root,
name=node.name,
description=node.dtype,
title=node.title,
filters=tb.Filters(
complib='blosc',
complevel=5,
fletcher32=False))
pbar = tqdm(total=node.shape[0], ncols=80)
for data_chunk, index in analysis_utils.data_aligned_at_events(node, chunk_size=chunk_size): # Loop over the hits
for dut_index, dut in enumerate(telescope): # Loop over the DUTs
if local_to_global:
conv = dut.local_to_global_position
else:
conv = dut.global_to_local_position
if align_to_beam and not local_to_global:
convert_data(dut=telescope, dut_index=dut_index, node=node, conv=conv, data=data_chunk)
convert_data(dut=dut, dut_index=dut_index, node=node, conv=conv, data=data_chunk)
if align_to_beam and local_to_global:
convert_data(dut=telescope, dut_index=dut_index, node=node, conv=conv, data=data_chunk)
hits_aligned_table.append(data_chunk)
pbar.update(data_chunk.shape[0])
pbar.close()
return output_file
def prealign(telescope_configuration, input_correlation_file, output_telescope_configuration=None, select_duts=None, select_reference_dut=0, reduce_background=True, use_location=False, plot=True):
'''Deduce a pre-alignment from the correlations, by fitting the correlations with a straight line (gives offset, slope, but no tild angles).
The user can define cuts on the fit error and straight line offset in an interactive way.
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_correlation_file : string
Filename of the input correlation file.
output_telescope_configuration : string
Filename of the output telescope configuration file.
select_duts : iterable
List of duts for which the prealignment is done. If None, prealignment is done for all duts.
select_reference_dut : uint
DUT index of the reference plane. Default is DUT 0.
reduce_background : bool
If True, use correlation histograms with reduced background (by applying SVD method to the correlation matrix).
plot : bool
If True, create additional output plots.
'''
telescope = Telescope(telescope_configuration)
n_duts = len(telescope)
logging.info('=== Pre-alignment of %d DUTs ===' % n_duts)
if output_telescope_configuration is None:
output_telescope_configuration = os.path.splitext(telescope_configuration)[0] + '_prealigned.yaml'
elif output_telescope_configuration == telescope_configuration:
raise ValueError('Output telescope configuration file must be different from input telescope configuration file.')
# remove reference DUT from list of all DUTs
if select_duts is None:
select_duts = list(set(range(n_duts)) - set([select_reference_dut]))
else:
select_duts = list(set(select_duts) - set([select_reference_dut]))
if plot is True:
output_pdf = PdfPages(os.path.splitext(input_correlation_file)[0] + '_prealigned.pdf', keep_empty=False)
else:
output_pdf = None
with tb.open_file(input_correlation_file, mode="r") as in_file_h5:
# loop over DUTs for pre-alignment
for actual_dut_index in select_duts:
actual_dut = telescope[actual_dut_index]
logging.info("== Pre-aligning %s ==" % actual_dut.name)
x_global_pixel, y_global_pixel, z_global_pixel = [], [], []
for column in range(1, actual_dut.n_columns + 1):
global_positions = actual_dut.index_to_global_position(
column=[column] * actual_dut.n_rows,
row=range(1, actual_dut.n_rows + 1))
x_global_pixel = np.hstack([x_global_pixel, global_positions[0]])
y_global_pixel = np.hstack([y_global_pixel, global_positions[1]])
z_global_pixel = np.hstack([z_global_pixel, global_positions[2]])
# calculate rotation matrix for later rotation corrections
rotation_alpha = actual_dut.rotation_alpha
rotation_beta = actual_dut.rotation_beta
rotation_gamma = actual_dut.rotation_gamma
R = geometry_utils.rotation_matrix(
alpha=rotation_alpha,
beta=rotation_beta,
gamma=rotation_gamma)
select = None
# loop over x- and y-axis
for x_direction in [True, False]:
if reduce_background:
node = in_file_h5.get_node(in_file_h5.root, 'Correlation_%s_%d_%d_reduced_background' % ('x' if x_direction else 'y', select_reference_dut, actual_dut_index))
else:
node = in_file_h5.get_node(in_file_h5.root, 'Correlation_%s_%d_%d' % ('x' if x_direction else 'y', select_reference_dut, actual_dut_index))
dut_name = actual_dut.name
ref_name = telescope[select_reference_dut].name
pixel_size = actual_dut.column_size if x_direction else actual_dut.row_size
logging.info('Pre-aligning data from %s', node.name)
bin_size = node.attrs.resolution
ref_hist_extent = node.attrs.ref_hist_extent
ref_hist_size = (ref_hist_extent[1] - ref_hist_extent[0])
dut_hist_extent = node.attrs.dut_hist_extent
dut_hist_size = (dut_hist_extent[1] - dut_hist_extent[0])
# retrieve data
data = node[:]
# Calculate the positions on the x axis
dut_pos = np.linspace(start=dut_hist_extent[0] + bin_size / 2.0, stop=dut_hist_extent[1] - bin_size / 2.0, num=data.shape[0], endpoint=True)
# calculate maximum per column
max_select = np.argmax(data, axis=1)
hough_data = np.zeros_like(data)
hough_data[np.arange(data.shape[0]), max_select] = 1
# transpose for correct angle
hough_data = hough_data.T
accumulator, theta, rho, theta_edges, rho_edges = analysis_utils.hough_transform(hough_data, theta_res=0.1, rho_res=1.0, return_edges=True)
def largest_indices(ary, n):
''' Returns the n largest indices from a numpy array.
https://stackoverflow.com/questions/6910641/how-to-get-indices-of-n-maximum-values-in-a-numpy-array
'''
flat = ary.flatten()
indices = np.argpartition(flat, -n)[-n:]
indices = indices[np.argsort(-flat[indices])]
return np.unravel_index(indices, ary.shape)
# finding correlation
# check for non-zero values to improve speed
count_nonzero = np.count_nonzero(accumulator)
indices = np.vstack(largest_indices(accumulator, count_nonzero)).T
for index in indices:
rho_idx, th_idx = index[0], index[1]
rho_val, theta_val = rho[rho_idx], theta[th_idx]
slope_idx, offset_idx = -np.cos(theta_val) / np.sin(theta_val), rho_val / np.sin(theta_val)
slope = slope_idx
offset = offset_idx * bin_size + ref_hist_extent[0] + 0.5 * bin_size
# check for proper slope
if np.isclose(slope, 1.0, rtol=0.0, atol=0.1) or np.isclose(slope, -1.0, rtol=0.0, atol=0.1):
break
else:
raise RuntimeError('Cannot find %s correlation between %s and %s' % ("X" if x_direction else "Y", telescope[select_reference_dut].name, actual_dut.name))
# offset in the center of the pixel matrix
offset_center = offset + slope * (0.5 * dut_hist_size - 0.5 * bin_size)
# calculate offset for local frame
offset_plot = offset - slope * dut_pos[0]
# find loactions where the max. correlation is close to expected value
x_list = find_inliers(
x=dut_pos[max_select != 0],
y=(max_select[max_select != 0] * bin_size - ref_hist_size / 2.0 + bin_size / 2.0),
m=slope,
c=offset_plot,
threshold=pixel_size * np.sqrt(12) * 2)
# 1-dimensional clustering of calculated locations
kernel = scipy.stats.gaussian_kde(x_list)
densities = kernel(dut_pos)
max_density = np.max(densities)
# calculate indices where value is close to max. density
indices = np.where(densities > max_density * 0.5)
# get locations from indices
x_list = dut_pos[indices]
# calculate range where correlation exists
dut_pos_limit = [np.min(x_list), np.max(x_list)]
plot_utils.plot_hough(
dut_pos=dut_pos,
data=hough_data,
accumulator=accumulator,
offset=offset_plot,
slope=slope,
dut_pos_limit=dut_pos_limit,
theta_edges=theta_edges,
rho_edges=rho_edges,
ref_hist_extent=ref_hist_extent,
dut_hist_extent=dut_hist_extent,
ref_name=ref_name,
dut_name=dut_name,
x_direction=x_direction,
reduce_background=reduce_background,
output_pdf=output_pdf)
if select is None:
select = np.ones_like(x_global_pixel, dtype=np.bool)
if x_direction:
select &= (x_global_pixel >= dut_pos_limit[0]) & (x_global_pixel <= dut_pos_limit[1])
if slope < 0.0:
R = np.linalg.multi_dot([geometry_utils.rotation_matrix_y(beta=np.pi), R])
translation_x = offset_center
else:
select &= (y_global_pixel >= dut_pos_limit[0]) & (y_global_pixel <= dut_pos_limit[1])
if slope < 0.0:
R = np.linalg.multi_dot([geometry_utils.rotation_matrix_x(alpha=np.pi), R])
translation_y = offset_center
# Setting new parameters
# Only use new limits if they are narrower
# Convert from global to local coordinates
local_coordinates = actual_dut.global_to_local_position(
x=x_global_pixel[select],
y=y_global_pixel[select],
z=z_global_pixel[select])
if actual_dut.x_limit is None:
actual_dut.x_limit = (min(local_coordinates[0]), max(local_coordinates[0]))
else:
actual_dut.x_limit = (max((min(local_coordinates[0]), actual_dut.x_limit[0])), min((max(local_coordinates[0]), actual_dut.x_limit[1])))
if actual_dut.y_limit is None:
actual_dut.y_limit = (min(local_coordinates[1]), max(local_coordinates[1]))
else:
actual_dut.y_limit = (max((min(local_coordinates[1]), actual_dut.y_limit[0])), min((max(local_coordinates[1]), actual_dut.y_limit[1])))
# Setting geometry
actual_dut.translation_x = translation_x
actual_dut.translation_y = translation_y
rotation_alpha, rotation_beta, rotation_gamma = geometry_utils.euler_angles(R=R)
actual_dut.rotation_alpha = rotation_alpha
actual_dut.rotation_beta = rotation_beta
actual_dut.rotation_gamma = rotation_gamma
telescope.save_configuration(configuration_file=output_telescope_configuration)
if output_pdf is not None:
output_pdf.close()
return output_telescope_configuration
def find_inliers(x, y, m, c, threshold=1.0):
''' Find inliers.
Parameters
----------
x : list
X coordinates.
y : list
Y coordinates.
threshold : float
Maximum distance of the data points for inlier selection.
Returns
-------
x_list : array
X coordianates of inliers.
'''
# calculate distance to reference hit
dist = np.abs(m * x + c - y)
sel = dist < threshold
return x[sel]
def find_line_model(points):
""" find a line model for the given points
:param points selected points for model fitting
:return line model
"""
# [WARNING] vertical and horizontal lines should be treated differently
# here we just add some noise to avoid division by zero
# find a line model for these points
m = (points[1, 1] - points[0, 1]) / (points[1, 0] - points[0, 0] + sys.float_info.epsilon) # slope (gradient) of the line
c = points[1, 1] - m * points[1, 0] # y-intercept of the line
return m, c
def find_intercept_point(m, c, x0, y0):
""" find an intercept point of the line model with
a normal from point (x0,y0) to it
:param m slope of the line model
:param c y-intercept of the line model
:param x0 point's x coordinate
:param y0 point's y coordinate
:return intercept point
"""
# intersection point with the model
x = (x0 + m * y0 - m * c) / (1 + m**2)
y = (m * x0 + (m**2) * y0 - (m**2) * c) / (1 + m**2) + c
return x, y
def find_ransac(x, y, iterations=100, threshold=1.0, ratio=0.5):
''' RANSAC implementation
Note
----
Implementation from <NAME>,
https://salzis.wordpress.com/2014/06/10/robust-linear-model-estimation-using-ransac-python-implementation/
Parameters
----------
x : list
X coordinates.
y : list
Y coordinates.
iterations : int
Maximum number of iterations.
threshold : float
Maximum distance of the data points for inlier selection.
ratio : float
Break condition for inliers.
Returns
-------
model_ratio : float
Ration of inliers to outliers.
model_m : float
Slope.
model_c : float
Offset.
model_x_list : array
X coordianates of inliers.
model_y_list : array
Y coordianates of inliers.
'''
data = np.column_stack((x, y))
n_samples = x.shape[0]
model_ratio = 0.0
model_m = 0.0
model_c = 0.0
# perform RANSAC iterations
for it in range(iterations):
all_indices = np.arange(n_samples)
np.random.shuffle(all_indices)
indices_1 = all_indices[:2] # pick up two random points
indices_2 = all_indices[2:]
maybe_points = data[indices_1, :]
test_points = data[indices_2, :]
# find a line model for these points
m, c = find_line_model(maybe_points)
x_list = []
y_list = []
num = 0
# find orthogonal lines to the model for all testing points
for ind in range(test_points.shape[0]):
x0 = test_points[ind, 0]
y0 = test_points[ind, 1]
# find an intercept point of the model with a normal from point (x0,y0)
x1, y1 = find_intercept_point(m, c, x0, y0)
# distance from point to the model
dist = math.sqrt((x1 - x0)**2 + (y1 - y0)**2)
# check whether it's an inlier or not
if dist < threshold:
x_list.append(x0)
y_list.append(y0)
num += 1
# in case a new model is better - cache it
if num / float(n_samples) > model_ratio:
model_ratio = num / float(n_samples)
model_m = m
model_c = c
model_x_list = np.array(x_list)
model_y_list = np.array(y_list)
# we are done in case we have enough inliers
if num > n_samples * ratio:
break
return model_ratio, model_m, model_c, model_x_list, model_y_list
def align(telescope_configuration, input_merged_file, output_telescope_configuration=None, select_duts=None, alignment_parameters=None, select_telescope_duts=None, select_extrapolation_duts=None, select_fit_duts=None, select_hit_duts=None, max_iterations=3, max_events=None, fit_method='fit', beam_energy=None, particle_mass=None, scattering_planes=None, track_chi2=10.0, cluster_shapes=None, quality_distances=(250.0, 250.0), isolation_distances=(500.0, 500.0), use_limits=True, plot=True, chunk_size=1000000):
''' This function does an alignment of the DUTs and sets translation and rotation values for all DUTs.
The reference DUT defines the global coordinate system position at 0, 0, 0 and should be well in the beam and not heavily rotated.
To solve the chicken-and-egg problem that a good dut alignment needs hits belonging to one track, but good track finding needs a good dut alignment this
function work only on already prealigned hits belonging to one track. Thus this function can be called only after track finding.
These steps are done
1. Take the found tracks and revert the pre-alignment
2. Take the track hits belonging to one track and fit tracks for all DUTs
3. Calculate the residuals for each DUT
4. Deduce rotations from the residuals and apply them to the hits
5. Deduce the translation of each plane
6. Store and apply the new alignment
repeat step 3 - 6 until the total residual does not decrease (RMS_total = sqrt(RMS_x_1^2 + RMS_y_1^2 + RMS_x_2^2 + RMS_y_2^2 + ...))
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_merged_file : string
Filename of the input merged file.
output_telescope_configuration : string
Filename of the output telescope configuration file.
select_duts : iterable or iterable of iterable
The combination of duts that are algined at once. One should always align the high resolution planes first.
E.g. for a telesope (first and last 3 planes) with 2 devices in the center (3, 4):
select_duts=[[0, 1, 2, 5, 6, 7], # align the telescope planes first
[4], # align first DUT
[3]] # align second DUT
alignment_parameters : list of lists of strings
The list of alignment parameters for each align_dut. Valid parameters:
- translation_x: horizontal axis
- translation_y: vertical axis
- translation_z: beam axis
- rotation_alpha: rotation around x-axis
- rotation_beta: rotation around y-axis
- rotation_gamma: rotation around z-axis (beam axis)
If None, all paramters will be selected.
select_telescope_duts : iterable
The given DUTs will be used to align the telescope along the z-axis.
Usually the coordinates of these DUTs are well specified.
At least 2 DUTs need to be specified. The z-position of the selected DUTs will not be changed by default.
select_extrapolation_duts : list
The given DUTs will be used for track extrapolation for improving track finding efficiency.
In some rare cases, removing DUTs with a coarse resolution might improve track finding efficiency.
If None, select all DUTs.
If list is empty or has a single entry, disable extrapolation (at least 2 DUTs are required for extrapolation to work).
select_fit_duts : iterable or iterable of iterable
Defines for each select_duts combination wich devices to use in the track fit.
E.g. To use only the telescope planes (first and last 3 planes) but not the 2 center devices
select_fit_duts=[0, 1, 2, 5, 6, 7]
select_hit_duts : iterable or iterable of iterable
Defines for each select_duts combination wich devices must have a hit to use the track for fitting. The hit
does not have to be used in the fit itself! This is useful for time reference planes.
E.g. To use telescope planes (first and last 3 planes) + time reference plane (3)
select_hit_duts = [0, 1, 2, 4, 5, 6, 7]
max_iterations : uint
Maximum number of iterations of calc residuals, apply rotation refit loop until constant result is expected.
Usually the procedure converges rather fast (< 5 iterations).
Non-telescope DUTs usually require 2 itearations.
max_events: uint
Radomly select max_events for alignment. If None, use all events, which might slow down the alignment.
fit_method : string
Available methods are 'kalman', which uses a Kalman Filter for track calculation, and 'fit', which uses a simple
straight line fit for track calculation.
beam_energy : float
Energy of the beam in MeV, e.g., 2500.0 MeV for ELSA beam. Only used for the Kalman Filter.
particle_mass : float
Mass of the particle in MeV, e.g., 0.511 MeV for electrons. Only used for the Kalman Filter.
scattering_planes : list or dict
Specifies additional scattering planes in case of DUTs which are not used or additional material in the way of the tracks.
The list must contain dictionaries containing the following keys:
material_budget: material budget of the scattering plane
translation_x/translation_y/translation_z: x/y/z position of the plane (in um)
rotation_alpha/rotation_beta/rotation_gamma: alpha/beta/gamma angle of scattering plane (in radians)
The material budget is defined as the thickness devided by the radiation length.
If scattering_planes is None, no scattering plane will be added.
track_chi2 : float or list
Setting the limit on the track chi^2. If None or 0.0, no cut will be applied.
A smaller value reduces the number of tracks for the alignment.
A large value increases the number of tracks but at the cost of alignment efficiency bacause of potentially bad tracks.
A good start value is 5.0 to 10.0 for high energy beams and 15.0 to 50.0 for low energy beams.
cluster_shapes : iterable or iterable of iterables
List of cluster shapes (unsigned integer) for each DUT. Only the selected cluster shapes will be used for the alignment.
Cluster shapes have impact on precision of the alignment. Larger clusters and certain cluster shapes can have a significant uncertainty for the hit position.
If None, use default cluster shapes [1, 3, 5, 13, 14, 7, 11, 15], i.e. 1x1, 2x1, 1x2, 3-pixel cluster, 4-pixel cluster. If empty list, all cluster sizes will be used.
The cluster shape can be calculated with the help of beam_telescope_analysis.tools.analysis_utils.calculate_cluster_array/calculate_cluster_shape.
quality_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the quality flag. The selected track and corresponding hit
must have a smaller distance to have the quality flag to be set to 1.
The purpose of quality_distances is to find good tracks for the alignment.
A good start value is 1-2x the pixel pitch for large pixels and high-energy beams and 5-10x the pixel pitch for small pixels and low-energy beams.
A too small value will remove good tracks, a too large value will allow bad tracks to contribute to the alignment.
If None, set distance to infinite.
isolation_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the isolated track/hit flag. Any other occurence of tracks or hits from the same event
within this distance will prevent the flag from beeing set.
The purpose of isolation_distances is to find good tracks for the alignment. Hits and tracks which are too close to each other should be removed.
The value given by isolation_distances should be larger than the quality_distances value to be effective,
A too small value will remove almost no tracks, a too large value will remove good tracks.
If None, set distance to 0.
isolation_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the quality flag. Any other occurence of tracks or hits from the same event
within this distance will reject the quality flag.
The purpose of isolation_distances is to remove tracks from alignment that could be potentially fake tracks (noisy detector / high beam density).
If None, use infinite distance.
use_limits : bool
If True, use column and row limits from pre-alignment for selecting the data.
plot : bool
If True, create additional output plots.
chunk_size : uint
Chunk size of the data when reading from file.
'''
telescope = Telescope(telescope_configuration)
n_duts = len(telescope)
logging.info('=== Alignment of %d DUTs ===' % len(set(np.unique(np.hstack(np.array(select_duts))).tolist())))
# Create list with combinations of DUTs to align
if select_duts is None: # If None: align all DUTs
select_duts = list(range(n_duts))
# Check for value errors
if not isinstance(select_duts, Iterable):
raise ValueError("Parameter select_duts is not an iterable.")
elif not select_duts: # empty iterable
raise ValueError("Parameter select_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_duts)):
select_duts = [select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_duts)):
raise ValueError("Not all items in parameter select_duts are iterable.")
# Finally check length of all iterables in iterable
for dut in select_duts:
if not dut: # check the length of the items
raise ValueError("Item in parameter select_duts has length 0.")
# Check if some DUTs will not be aligned
non_select_duts = set(range(n_duts)) - set(np.unique(np.hstack(np.array(select_duts))).tolist())
if non_select_duts:
logging.info('These DUTs will not be aligned: %s' % ", ".join(telescope[dut_index].name for dut_index in non_select_duts))
# Create list
if alignment_parameters is None:
alignment_parameters = [[None] * len(duts) for duts in select_duts]
# Check for value errors
if not isinstance(alignment_parameters, Iterable):
raise ValueError("Parameter alignment_parameters is not an iterable.")
elif not alignment_parameters: # empty iterable
raise ValueError("Parameter alignment_parameters has no items.")
# Finally check length of all arrays
if len(alignment_parameters) != len(select_duts): # empty iterable
raise ValueError("Parameter alignment_parameters has the wrong length.")
for index, alignment_parameter in enumerate(alignment_parameters):
if alignment_parameter is None:
alignment_parameters[index] = [None] * len(select_duts[index])
if len(alignment_parameters[index]) != len(select_duts[index]): # check the length of the items
raise ValueError("Item in parameter alignment_parameter has the wrong length.")
# Create track, hit selection
if select_hit_duts is None: # If None: use all DUTs
select_hit_duts = []
# copy each item
for duts in select_duts:
select_hit_duts.append(duts[:]) # require a hit for each fit DUT
# Check iterable and length
if not isinstance(select_hit_duts, Iterable):
raise ValueError("Parameter select_hit_duts is not an iterable.")
elif not select_hit_duts: # empty iterable
raise ValueError("Parameter select_hit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_hit_duts)):
select_hit_duts = [select_hit_duts[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_hit_duts)):
raise ValueError("Not all items in parameter select_hit_duts are iterable.")
# Finally check length of all arrays
if len(select_hit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_hit_duts has the wrong length.")
for hit_dut in select_hit_duts:
if len(hit_dut) < 2: # check the length of the items
raise ValueError("Item in parameter select_hit_duts has length < 2.")
# Create track, hit selection
if select_fit_duts is None: # If None: use all DUTs
select_fit_duts = []
# copy each item from select_hit_duts
for hit_duts in select_hit_duts:
select_fit_duts.append(hit_duts[:]) # require a hit for each fit DUT
# Check iterable and length
if not isinstance(select_fit_duts, Iterable):
raise ValueError("Parameter select_fit_duts is not an iterable.")
elif not select_fit_duts: # empty iterable
raise ValueError("Parameter select_fit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_fit_duts)):
select_fit_duts = [select_fit_duts[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_fit_duts)):
raise ValueError("Not all items in parameter select_fit_duts are iterable.")
# Finally check length of all arrays
if len(select_fit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_fit_duts has the wrong length.")
for index, fit_dut in enumerate(select_fit_duts):
if len(fit_dut) < 2: # check the length of the items
raise ValueError("Item in parameter select_fit_duts has length < 2.")
if set(fit_dut) - set(select_hit_duts[index]): # fit DUTs are required to have a hit
raise ValueError("DUT in select_fit_duts is not in select_hit_duts.")
# Create chi2 array
if not isinstance(track_chi2, Iterable):
track_chi2 = [track_chi2] * len(select_duts)
# Finally check length
if len(track_chi2) != len(select_duts):
raise ValueError("Parameter track_chi2 has the wrong length.")
# expand dimensions
# Check iterable and length for each item
for index, chi2 in enumerate(track_chi2):
# Check if non-iterable
if not isinstance(chi2, Iterable):
track_chi2[index] = [chi2] * len(select_duts[index])
# again check for consistency
for index, chi2 in enumerate(track_chi2):
# Check iterable and length
if not isinstance(chi2, Iterable):
raise ValueError("Item in parameter track_chi2 is not an iterable.")
if len(chi2) != len(select_duts[index]): # empty iterable
raise ValueError("Item in parameter track_chi2 has the wrong length.")
# Create cluster shape selection
if cluster_shapes is None: # If None: set default value for all DUTs
cluster_shapes = [cluster_shapes] * len(select_duts)
# Check iterable and length
if not isinstance(cluster_shapes, Iterable):
raise ValueError("Parameter cluster_shapes is not an iterable.")
# elif not cluster_shapes: # empty iterable
# raise ValueError("Parameter cluster_shapes has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable) and val is not None, cluster_shapes)):
cluster_shapes = [cluster_shapes[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, cluster_shapes)):
raise ValueError("Not all items in parameter cluster_shapes are iterable or None.")
# Finally check length of all arrays
if len(cluster_shapes) != len(select_duts): # empty iterable
raise ValueError("Parameter cluster_shapes has the wrong length.")
# expand dimensions
# Check iterable and length for each item
for index, shapes in enumerate(cluster_shapes):
# Check if only non-iterable in iterable
if shapes is None:
cluster_shapes[index] = [shapes] * len(select_duts[index])
elif all(map(lambda val: not isinstance(val, Iterable) and val is not None, shapes)):
cluster_shapes[index] = [shapes[:] for _ in select_duts[index]]
# again check for consistency
for index, shapes in enumerate(cluster_shapes):
# Check iterable and length
if not isinstance(shapes, Iterable):
raise ValueError("Item in parameter cluster_shapes is not an iterable.")
elif not shapes: # empty iterable
raise ValueError("Item in parameter cluster_shapes has no items.")
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, shapes)):
raise ValueError("Not all items of item in cluster_shapes are iterable or None.")
if len(shapes) != len(select_duts[index]): # empty iterable
raise ValueError("Item in parameter cluster_shapes has the wrong length.")
# Create quality distance
if isinstance(quality_distances, tuple) or quality_distances is None:
quality_distances = [quality_distances] * n_duts
# Check iterable and length
if not isinstance(quality_distances, Iterable):
raise ValueError("Parameter quality_distances is not an iterable.")
elif not quality_distances: # empty iterable
raise ValueError("Parameter quality_distances has no items.")
# Finally check length of all arrays
if len(quality_distances) != n_duts: # empty iterable
raise ValueError("Parameter quality_distances has the wrong length.")
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, quality_distances)):
raise ValueError("Not all items in parameter quality_distances are iterable or None.")
# Finally check length of all arrays
for distance in quality_distances:
if distance is not None and len(distance) != 2: # check the length of the items
raise ValueError("Item in parameter quality_distances has length != 2.")
# Create reject quality distance
if isinstance(isolation_distances, tuple) or isolation_distances is None:
isolation_distances = [isolation_distances] * n_duts
# Check iterable and length
if not isinstance(isolation_distances, Iterable):
raise ValueError("Parameter isolation_distances is no iterable.")
elif not isolation_distances: # empty iterable
raise ValueError("Parameter isolation_distances has no items.")
# Finally check length of all arrays
if len(isolation_distances) != n_duts: # empty iterable
raise ValueError("Parameter isolation_distances has the wrong length.")
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, isolation_distances)):
raise ValueError("Not all items in Parameter isolation_distances are iterable or None.")
# Finally check length of all arrays
for distance in isolation_distances:
if distance is not None and len(distance) != 2: # check the length of the items
raise ValueError("Item in parameter isolation_distances has length != 2.")
if not isinstance(max_iterations, Iterable):
max_iterations = [max_iterations] * len(select_duts)
# Finally check length of all arrays
if len(max_iterations) != len(select_duts): # empty iterable
raise ValueError("Parameter max_iterations has the wrong length.")
if not isinstance(max_events, Iterable):
max_events = [max_events] * len(select_duts)
# Finally check length
if len(max_events) != len(select_duts):
raise ValueError("Parameter max_events has the wrong length.")
if output_telescope_configuration is None:
if 'prealigned' in telescope_configuration:
output_telescope_configuration = telescope_configuration.replace('prealigned', 'aligned')
else:
output_telescope_configuration = os.path.splitext(telescope_configuration)[0] + '_aligned.yaml'
elif output_telescope_configuration == telescope_configuration:
raise ValueError('Output telescope configuration file must be different from input telescope configuration file.')
if os.path.isfile(output_telescope_configuration):
logging.info('Output telescope configuration file already exists. Keeping telescope configuration file.')
aligned_telescope = Telescope(configuration_file=output_telescope_configuration)
# For the case where not all DUTs are aligned,
# only revert the alignment for the DUTs that will be aligned.
for align_duts in select_duts:
for dut in align_duts:
aligned_telescope[dut] = telescope[dut]
aligned_telescope.save_configuration()
else:
telescope.save_configuration(configuration_file=output_telescope_configuration)
prealigned_track_candidates_file = os.path.splitext(input_merged_file)[0] + '_track_candidates_prealigned_tmp.h5'
# clean up remaining files
if os.path.isfile(prealigned_track_candidates_file):
os.remove(prealigned_track_candidates_file)
for index, align_duts in enumerate(select_duts):
# Find pre-aligned tracks for the 1st step of the alignment.
# This file can be used for different sets of alignment DUTs,
# so keep the file and remove later.
if not os.path.isfile(prealigned_track_candidates_file):
logging.info('= Alignment step 1: Finding pre-aligned tracks =')
find_tracks(
telescope_configuration=telescope_configuration,
input_merged_file=input_merged_file,
output_track_candidates_file=prealigned_track_candidates_file,
select_extrapolation_duts=select_extrapolation_duts,
align_to_beam=True,
max_events=None)
logging.info('== Aligning %d DUTs: %s ==', len(align_duts), ", ".join(telescope[dut_index].name for dut_index in align_duts))
_duts_alignment(
output_telescope_configuration=output_telescope_configuration, # aligned configuration
merged_file=input_merged_file,
prealigned_track_candidates_file=prealigned_track_candidates_file,
align_duts=align_duts,
alignment_parameters=alignment_parameters[index],
select_telescope_duts=select_telescope_duts,
select_extrapolation_duts=select_extrapolation_duts,
select_fit_duts=select_fit_duts[index],
select_hit_duts=select_hit_duts[index],
max_iterations=max_iterations[index],
max_events=max_events[index],
fit_method=fit_method,
beam_energy=beam_energy,
particle_mass=particle_mass,
scattering_planes=scattering_planes,
track_chi2=track_chi2[index],
cluster_shapes=cluster_shapes[index],
quality_distances=quality_distances,
isolation_distances=isolation_distances,
use_limits=use_limits,
plot=plot,
chunk_size=chunk_size)
if os.path.isfile(prealigned_track_candidates_file):
os.remove(prealigned_track_candidates_file)
return output_telescope_configuration
def align_kalman(telescope_configuration, input_merged_file, output_telescope_configuration=None, output_alignment_file=None, select_duts=None, alignment_parameters=None, select_telescope_duts=None, select_extrapolation_duts=None, select_fit_duts=None, select_hit_duts=None, max_events=None, beam_energy=None, particle_mass=None, scattering_planes=None, track_chi2=10.0, cluster_shapes=None, annealing_factor=10000, annealing_tracks=5000, max_tracks=10000, alignment_parameters_errors=None, use_limits=True, plot=True, chunk_size=1000):
''' This function does an alignment of the DUTs and sets translation and rotation values for all DUTs.
The reference DUT defines the global coordinate system position at 0, 0, 0 and should be well in the beam and not heavily rotated.
To solve the chicken-and-egg problem that a good dut alignment needs hits belonging to one track, but good track finding needs a good dut alignment this
function work only on already prealigned hits belonging to one track. Thus this function can be called only after track finding.
These steps are done
1. Take the found tracks and revert the pre-alignment
2. Take the track hits belonging to one track and fit tracks for all DUTs
3. Calculate the residuals for each DUT
4. Deduce rotations from the residuals and apply them to the hits
5. Deduce the translation of each plane
6. Store and apply the new alignment
repeat step 3 - 6 until the total residual does not decrease (RMS_total = sqrt(RMS_x_1^2 + RMS_y_1^2 + RMS_x_2^2 + RMS_y_2^2 + ...))
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_merged_file : string
Filename of the input merged file.
output_telescope_configuration : string
Filename of the output telescope configuration file.
select_duts : iterable or iterable of iterable
The combination of duts that are algined at once. One should always align the high resolution planes first.
E.g. for a telesope (first and last 3 planes) with 2 devices in the center (3, 4):
select_duts=[[0, 1, 2, 5, 6, 7], # align the telescope planes first
[4], # align first DUT
[3]] # align second DUT
alignment_parameters : list of lists of strings
The list of alignment parameters for each align_dut. Valid parameters:
- translation_x: horizontal axis
- translation_y: vertical axis
- translation_z: beam axis
- rotation_alpha: rotation around x-axis
- rotation_beta: rotation around y-axis
- rotation_gamma: rotation around z-axis (beam axis)
If None, all paramters will be selected.
select_telescope_duts : iterable
The given DUTs will be used to align the telescope along the z-axis.
Usually the coordinates of these DUTs are well specified.
At least 2 DUTs need to be specified. The z-position of the selected DUTs will not be changed by default.
select_extrapolation_duts : list
The given DUTs will be used for track extrapolation for improving track finding efficiency.
In some rare cases, removing DUTs with a coarse resolution might improve track finding efficiency.
If None, select all DUTs.
If list is empty or has a single entry, disable extrapolation (at least 2 DUTs are required for extrapolation to work).
select_fit_duts : iterable or iterable of iterable
Defines for each select_duts combination wich devices to use in the track fit.
E.g. To use only the telescope planes (first and last 3 planes) but not the 2 center devices
select_fit_duts=[0, 1, 2, 5, 6, 7]
select_hit_duts : iterable or iterable of iterable
Defines for each select_duts combination wich devices must have a hit to use the track for fitting. The hit
does not have to be used in the fit itself! This is useful for time reference planes.
E.g. To use telescope planes (first and last 3 planes) + time reference plane (3)
select_hit_duts = [0, 1, 2, 4, 5, 6, 7]
max_iterations : uint
Maximum number of iterations of calc residuals, apply rotation refit loop until constant result is expected.
Usually the procedure converges rather fast (< 5 iterations).
Non-telescope DUTs usually require 2 itearations.
max_events: uint
Radomly select max_events for alignment. If None, use all events, which might slow down the alignment.
fit_method : string
Available methods are 'kalman', which uses a Kalman Filter for track calculation, and 'fit', which uses a simple
straight line fit for track calculation.
beam_energy : float
Energy of the beam in MeV, e.g., 2500.0 MeV for ELSA beam. Only used for the Kalman Filter.
particle_mass : float
Mass of the particle in MeV, e.g., 0.511 MeV for electrons. Only used for the Kalman Filter.
scattering_planes : list or dict
Specifies additional scattering planes in case of DUTs which are not used or additional material in the way of the tracks.
The list must contain dictionaries containing the following keys:
material_budget: material budget of the scattering plane
translation_x/translation_y/translation_z: x/y/z position of the plane (in um)
rotation_alpha/rotation_beta/rotation_gamma: alpha/beta/gamma angle of scattering plane (in radians)
The material budget is defined as the thickness devided by the radiation length.
If scattering_planes is None, no scattering plane will be added.
track_chi2 : float or list
Setting the limit on the track chi^2. If None or 0.0, no cut will be applied.
A smaller value reduces the number of tracks for the alignment.
A large value increases the number of tracks but at the cost of alignment efficiency bacause of potentially bad tracks.
A good start value is 5.0 to 10.0 for high energy beams and 15.0 to 50.0 for low energy beams.
cluster_shapes : iterable or iterable of iterables
List of cluster shapes (unsigned integer) for each DUT. Only the selected cluster shapes will be used for the alignment.
Cluster shapes have impact on precision of the alignment. Larger clusters and certain cluster shapes can have a significant uncertainty for the hit position.
If None, use default cluster shapes [1, 3, 5, 13, 14, 7, 11, 15], i.e. 1x1, 2x1, 1x2, 3-pixel cluster, 4-pixel cluster. If empty list, all cluster sizes will be used.
The cluster shape can be calculated with the help of beam_telescope_analysis.tools.analysis_utils.calculate_cluster_array/calculate_cluster_shape.
quality_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the quality flag. The selected track and corresponding hit
must have a smaller distance to have the quality flag to be set to 1.
The purpose of quality_distances is to find good tracks for the alignment.
A good start value is 1-2x the pixel pitch for large pixels and high-energy beams and 5-10x the pixel pitch for small pixels and low-energy beams.
A too small value will remove good tracks, a too large value will allow bad tracks to contribute to the alignment.
If None, set distance to infinite.
isolation_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the isolated track/hit flag. Any other occurence of tracks or hits from the same event
within this distance will prevent the flag from beeing set.
The purpose of isolation_distances is to find good tracks for the alignment. Hits and tracks which are too close to each other should be removed.
The value given by isolation_distances should be larger than the quality_distances value to be effective,
A too small value will remove almost no tracks, a too large value will remove good tracks.
If None, set distance to 0.
isolation_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the quality flag. Any other occurence of tracks or hits from the same event
within this distance will reject the quality flag.
The purpose of isolation_distances is to remove tracks from alignment that could be potentially fake tracks (noisy detector / high beam density).
If None, use infinite distance.
use_limits : bool
If True, use column and row limits from pre-alignment for selecting the data.
plot : bool
If True, create additional output plots.
chunk_size : uint
Chunk size of the data when reading from file.
'''
telescope = Telescope(telescope_configuration)
n_duts = len(telescope)
logging.info('=== Alignment of %d DUTs ===' % len(set(np.unique(np.hstack(np.array(select_duts))).tolist())))
# Create list with combinations of DUTs to align
if select_duts is None: # If None: align all DUTs
select_duts = list(range(n_duts))
# Check for value errors
if not isinstance(select_duts, Iterable):
raise ValueError("Parameter select_duts is not an iterable.")
elif not select_duts: # empty iterable
raise ValueError("Parameter select_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_duts)):
select_duts = [select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_duts)):
raise ValueError("Not all items in parameter select_duts are iterable.")
# Finally check length of all iterables in iterable
for dut in select_duts:
if not dut: # check the length of the items
raise ValueError("Item in parameter select_duts has length 0.")
# Check if some DUTs will not be aligned
non_select_duts = set(range(n_duts)) - set(np.unique(np.hstack(np.array(select_duts))).tolist())
if non_select_duts:
logging.info('These DUTs will not be aligned: %s' % ", ".join(telescope[dut_index].name for dut_index in non_select_duts))
# Create list
if alignment_parameters is None:
alignment_parameters = [[None] * len(duts) for duts in select_duts]
# Check for value errors
if not isinstance(alignment_parameters, Iterable):
raise ValueError("Parameter alignment_parameters is not an iterable.")
elif not alignment_parameters: # empty iterable
raise ValueError("Parameter alignment_parameters has no items.")
# Finally check length of all arrays
if len(alignment_parameters) != len(select_duts): # empty iterable
raise ValueError("Parameter alignment_parameters has the wrong length.")
# for index, alignment_parameter in enumerate(alignment_parameters):
# if alignment_parameter is None:
# alignment_parameters[index] = [None] * len(select_duts[index])
# if len(alignment_parameters[index]) != len(select_duts[index]): # check the length of the items
# raise ValueError("Item in parameter alignment_parameter has the wrong length.")
# Create track, hit selection
if select_hit_duts is None: # If None: use all DUTs
select_hit_duts = []
# copy each item
for duts in select_duts:
select_hit_duts.append(duts[:]) # require a hit for each fit DUT
# Check iterable and length
if not isinstance(select_hit_duts, Iterable):
raise ValueError("Parameter select_hit_duts is not an iterable.")
elif not select_hit_duts: # empty iterable
raise ValueError("Parameter select_hit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_hit_duts)):
select_hit_duts = [select_hit_duts[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_hit_duts)):
raise ValueError("Not all items in parameter select_hit_duts are iterable.")
# Finally check length of all arrays
if len(select_hit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_hit_duts has the wrong length.")
for hit_dut in select_hit_duts:
if len(hit_dut) < 2: # check the length of the items
raise ValueError("Item in parameter select_hit_duts has length < 2.")
# Create track, hit selection
if select_fit_duts is None: # If None: use all DUTs
select_fit_duts = []
# copy each item from select_hit_duts
for hit_duts in select_hit_duts:
select_fit_duts.append(hit_duts[:]) # require a hit for each fit DUT
# Check iterable and length
if not isinstance(select_fit_duts, Iterable):
raise ValueError("Parameter select_fit_duts is not an iterable.")
elif not select_fit_duts: # empty iterable
raise ValueError("Parameter select_fit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable), select_fit_duts)):
select_fit_duts = [select_fit_duts[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_fit_duts)):
raise ValueError("Not all items in parameter select_fit_duts are iterable.")
# Finally check length of all arrays
if len(select_fit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_fit_duts has the wrong length.")
for index, fit_dut in enumerate(select_fit_duts):
if len(fit_dut) < 2: # check the length of the items
raise ValueError("Item in parameter select_fit_duts has length < 2.")
if set(fit_dut) - set(select_hit_duts[index]): # fit DUTs are required to have a hit
raise ValueError("DUT in select_fit_duts is not in select_hit_duts.")
# Create cluster shape selection
if cluster_shapes is None: # If None: set default value for all DUTs
cluster_shapes = [cluster_shapes] * len(select_duts)
# Check iterable and length
if not isinstance(cluster_shapes, Iterable):
raise ValueError("Parameter cluster_shapes is not an iterable.")
# elif not cluster_shapes: # empty iterable
# raise ValueError("Parameter cluster_shapes has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable) and val is not None, cluster_shapes)):
cluster_shapes = [cluster_shapes[:] for _ in select_duts]
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, cluster_shapes)):
raise ValueError("Not all items in parameter cluster_shapes are iterable or None.")
# Finally check length of all arrays
if len(cluster_shapes) != len(select_duts): # empty iterable
raise ValueError("Parameter cluster_shapes has the wrong length.")
# expand dimensions
# Check iterable and length for each item
for index, shapes in enumerate(cluster_shapes):
# Check if only non-iterable in iterable
if shapes is None:
cluster_shapes[index] = [shapes] * len(select_duts[index])
elif all(map(lambda val: not isinstance(val, Iterable) and val is not None, shapes)):
cluster_shapes[index] = [shapes[:] for _ in select_duts[index]]
# again check for consistency
for index, shapes in enumerate(cluster_shapes):
# Check iterable and length
if not isinstance(shapes, Iterable):
raise ValueError("Item in parameter cluster_shapes is not an iterable.")
elif not shapes: # empty iterable
raise ValueError("Item in parameter cluster_shapes has no items.")
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable) or val is None, shapes)):
raise ValueError("Not all items of item in cluster_shapes are iterable or None.")
if len(shapes) != len(select_duts[index]): # empty iterable
raise ValueError("Item in parameter cluster_shapes has the wrong length.")
if not isinstance(max_events, Iterable):
max_events = [max_events] * len(select_duts)
# Finally check length
if len(max_events) != len(select_duts):
raise ValueError("Parameter max_events has the wrong length.")
if not isinstance(max_tracks, Iterable):
max_tracks = [max_tracks] * len(select_duts)
# Finally check length
if len(max_tracks) != len(select_duts):
raise ValueError("Parameter max_tracks has the wrong length.")
if output_telescope_configuration is None:
if 'prealigned' in telescope_configuration:
output_telescope_configuration = telescope_configuration.replace('prealigned', 'aligned_kalman')
else:
output_telescope_configuration = os.path.splitext(telescope_configuration)[0] + '_aligned_kalman.yaml'
elif output_telescope_configuration == telescope_configuration:
raise ValueError('Output telescope configuration file must be different from input telescope configuration file.')
if os.path.isfile(output_telescope_configuration):
logging.info('Output telescope configuration file already exists. Keeping telescope configuration file.')
aligned_telescope = Telescope(configuration_file=output_telescope_configuration)
# For the case where not all DUTs are aligned,
# only revert the alignment for the DUTs that will be aligned.
for align_duts in select_duts:
for dut in align_duts:
aligned_telescope[dut] = telescope[dut]
aligned_telescope.save_configuration()
else:
telescope.save_configuration(configuration_file=output_telescope_configuration)
if output_alignment_file is None:
output_alignment_file = os.path.splitext(input_merged_file)[0] + '_KFA_alignment.h5'
else:
output_alignment_file = output_alignment_file
for index, align_duts in enumerate(select_duts):
# Find pre-aligned tracks for the 1st step of the alignment.
# This file can be used for different sets of alignment DUTs,
# so keep the file and remove later.
prealigned_track_candidates_file = os.path.splitext(input_merged_file)[0] + '_track_candidates_prealigned_%i_tmp.h5' % index
find_tracks(
telescope_configuration=telescope_configuration,
input_merged_file=input_merged_file,
output_track_candidates_file=prealigned_track_candidates_file,
select_extrapolation_duts=select_extrapolation_duts,
align_to_beam=True,
max_events=max_events[index])
logging.info('== Aligning %d DUTs: %s ==', len(align_duts), ", ".join(telescope[dut_index].name for dut_index in align_duts))
_duts_alignment_kalman(
telescope_configuration=output_telescope_configuration, # aligned configuration
output_alignment_file=output_alignment_file,
input_track_candidates_file=prealigned_track_candidates_file,
select_duts=align_duts,
alignment_parameters=alignment_parameters[index],
select_telescope_duts=select_telescope_duts,
select_fit_duts=select_fit_duts[index],
select_hit_duts=select_hit_duts[index],
beam_energy=beam_energy,
particle_mass=particle_mass,
scattering_planes=scattering_planes,
track_chi2=track_chi2[index],
annealing_factor=annealing_factor,
annealing_tracks=annealing_tracks,
max_tracks=max_tracks[index],
alignment_parameters_errors=alignment_parameters_errors,
use_limits=use_limits,
plot=plot,
chunk_size=chunk_size,
iteration_index=index)
return output_telescope_configuration
def _duts_alignment(output_telescope_configuration, merged_file, align_duts, prealigned_track_candidates_file, alignment_parameters, select_telescope_duts, select_extrapolation_duts, select_fit_duts, select_hit_duts, max_iterations, max_events, fit_method, beam_energy, particle_mass, scattering_planes, track_chi2, cluster_shapes, quality_distances, isolation_distances, use_limits, plot=True, chunk_size=100000): # Called for each list of DUTs to align
alignment_duts = "_".join(str(dut) for dut in align_duts)
aligned_telescope = Telescope(configuration_file=output_telescope_configuration)
output_track_candidates_file = None
iteration_steps = range(max_iterations)
for iteration_step in iteration_steps:
# aligning telescope DUTs to the beam axis (z-axis)
if set(align_duts) & set(select_telescope_duts):
align_telescope(
telescope_configuration=output_telescope_configuration,
select_telescope_duts=list(set(align_duts) & set(select_telescope_duts)))
actual_align_duts = align_duts
actual_fit_duts = select_fit_duts
# reqire hits in each DUT that will be aligned
actual_hit_duts = [list(set(select_hit_duts) | set([dut_index])) for dut_index in actual_align_duts]
actual_quality_duts = actual_hit_duts
fit_quality_distances = np.zeros_like(quality_distances)
for index, item in enumerate(quality_distances):
if index in align_duts:
fit_quality_distances[index, 0] = np.linspace(item[0] * 1.08447**max_iterations, item[0], max_iterations)[iteration_step]
fit_quality_distances[index, 1] = np.linspace(item[1] * 1.08447**max_iterations, item[1], max_iterations)[iteration_step]
else:
fit_quality_distances[index, 0] = item[0]
fit_quality_distances[index, 1] = item[1]
fit_quality_distances = fit_quality_distances.tolist()
if iteration_step > 0:
logging.info('= Alignment step 1 - iteration %d: Finding tracks for %d DUTs =', iteration_step, len(align_duts))
# remove temporary file
if output_track_candidates_file is not None:
os.remove(output_track_candidates_file)
output_track_candidates_file = os.path.splitext(merged_file)[0] + '_track_candidates_aligned_duts_%s_tmp_%d.h5' % (alignment_duts, iteration_step)
find_tracks(
telescope_configuration=output_telescope_configuration,
input_merged_file=merged_file,
output_track_candidates_file=output_track_candidates_file,
select_extrapolation_duts=select_extrapolation_duts,
align_to_beam=True,
max_events=max_events)
# The quality flag of the actual align DUT depends on the alignment calculated
# in the previous iteration, therefore this step has to be done every time
logging.info('= Alignment step 2 - iteration %d: Fitting tracks for %d DUTs =', iteration_step, len(align_duts))
output_tracks_file = os.path.splitext(merged_file)[0] + '_tracks_aligned_duts_%s_tmp_%d.h5' % (alignment_duts, iteration_step)
fit_tracks(
telescope_configuration=output_telescope_configuration,
input_track_candidates_file=prealigned_track_candidates_file if iteration_step == 0 else output_track_candidates_file,
output_tracks_file=output_tracks_file,
max_events=None if iteration_step > 0 else max_events,
select_duts=actual_align_duts,
select_fit_duts=actual_fit_duts,
select_hit_duts=actual_hit_duts,
exclude_dut_hit=False, # for biased residuals
select_align_duts=actual_align_duts, # correct residual offset for align DUTs
method=fit_method,
beam_energy=beam_energy,
particle_mass=particle_mass,
scattering_planes=scattering_planes,
quality_distances=quality_distances,
isolation_distances=isolation_distances,
use_limits=use_limits,
plot=plot,
chunk_size=chunk_size)
logging.info('= Alignment step 3a - iteration %d: Selecting tracks for %d DUTs =', iteration_step, len(align_duts))
output_selected_tracks_file = os.path.splitext(merged_file)[0] + '_tracks_aligned_selected_tracks_duts_%s_tmp_%d.h5' % (alignment_duts, iteration_step)
# generate query for select_tracks
# generate default selection of cluster shapes: 1x1, 2x1, 1x2, 3-pixel cluster, 4-pixel cluster
for index, shapes in enumerate(cluster_shapes):
if shapes is None:
cluster_shapes[index] = default_cluster_shapes
query_string = [((('(track_chi_red < %f)' % track_chi2[index]) if track_chi2[index] else '') + (' & ' if (track_chi2[index] and cluster_shapes[index]) else '') + (('(' + ' | '.join([('(cluster_shape_dut_{0} == %d)' % cluster_shape) for cluster_shape in cluster_shapes[index]]).format(dut_index) + ')') if cluster_shapes[index] else '')) for index, dut_index in enumerate(actual_align_duts)]
data_selection.select_tracks(
telescope_configuration=output_telescope_configuration,
input_tracks_file=output_tracks_file,
output_tracks_file=output_selected_tracks_file,
select_duts=actual_align_duts,
select_hit_duts=actual_hit_duts,
select_quality_duts=actual_quality_duts,
select_isolated_track_duts=actual_quality_duts,
select_isolated_hit_duts=actual_quality_duts,
query=query_string,
max_events=None,
chunk_size=chunk_size)
# if fit DUTs were aligned, update telescope alignment
if set(align_duts) & set(select_fit_duts):
logging.info('= Alignment step 3b - iteration %d: Aligning telescope =', iteration_step)
output_track_angles_file = os.path.splitext(merged_file)[0] + '_tracks_angles_aligned_selected_tracks_duts_%s_tmp_%d.h5' % (alignment_duts, iteration_step)
histogram_track_angle(
telescope_configuration=output_telescope_configuration,
input_tracks_file=output_selected_tracks_file,
output_track_angle_file=output_track_angles_file,
select_duts=actual_align_duts,
n_bins=100,
plot=plot)
# Read and store beam angle to improve track finding
if (set(align_duts) & set(select_fit_duts)):
with tb.open_file(output_track_angles_file, mode="r") as in_file_h5:
if not np.isnan(in_file_h5.root.Global_alpha_track_angle_hist.attrs.mean) and not np.isnan(in_file_h5.root.Global_beta_track_angle_hist.attrs.mean):
aligned_telescope = Telescope(configuration_file=output_telescope_configuration)
aligned_telescope.rotation_alpha = in_file_h5.root.Global_alpha_track_angle_hist.attrs.mean
aligned_telescope.rotation_beta = in_file_h5.root.Global_beta_track_angle_hist.attrs.mean
aligned_telescope.save_configuration()
else:
logging.warning("Cannot read track angle histograms, track finding might be spoiled")
os.remove(output_track_angles_file)
if plot:
logging.info('= Alignment step 3c - iteration %d: Calculating residuals =', iteration_step)
output_residuals_file = os.path.splitext(merged_file)[0] + '_residuals_aligned_selected_tracks_%s_tmp_%d.h5' % (alignment_duts, iteration_step)
calculate_residuals(
telescope_configuration=output_telescope_configuration,
input_tracks_file=output_selected_tracks_file,
output_residuals_file=output_residuals_file,
select_duts=actual_align_duts,
use_limits=use_limits,
plot=True,
chunk_size=chunk_size)
os.remove(output_residuals_file)
logging.info('= Alignment step 4 - iteration %d: Calculating transformation matrix for %d DUTs =', iteration_step, len(align_duts))
calculate_transformation(
telescope_configuration=output_telescope_configuration,
input_tracks_file=output_selected_tracks_file,
select_duts=actual_align_duts,
select_alignment_parameters=[(["translation_x", "translation_y", "rotation_alpha", "rotation_beta", "rotation_gamma"] if (dut_index in select_telescope_duts and (alignment_parameters is None or alignment_parameters[i] is None)) else (default_alignment_parameters if (alignment_parameters is None or alignment_parameters[i] is None) else alignment_parameters[i])) for i, dut_index in enumerate(actual_align_duts)],
use_limits=use_limits,
max_iterations=100,
chunk_size=chunk_size)
# Delete temporary files
os.remove(output_tracks_file)
os.remove(output_selected_tracks_file)
# Delete temporary files
if output_track_candidates_file is not None:
os.remove(output_track_candidates_file)
def _duts_alignment_kalman(telescope_configuration, output_alignment_file, input_track_candidates_file, alignment_parameters, select_telescope_duts, select_duts=None, select_hit_duts=None, select_fit_duts=None, min_track_hits=None, beam_energy=2500, particle_mass=0.511, scattering_planes=None, track_chi2=25.0, use_limits=True, iteration_index=0, exclude_dut_hit=False, annealing_factor=10000, annealing_tracks=5000, max_tracks=10000, alignment_parameters_errors=None, plot=True, chunk_size=1000):
'''Calculate tracks and set tracks quality flag for selected DUTs.
Two methods are available to generate tracks: a linear fit (method="fit") and a Kalman Filter (method="kalman").
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_track_candidates_file : string
Filename of the input track candidate file.
output_tracks_file : string
Filename of the output tracks file.
max_events : uint
Maximum number of randomly chosen events. If None, all events are taken.
select_duts : list
Specify the fit DUTs for which tracks will be fitted and a track array will be generated.
If None, for all DUTs are selected.
select_hit_duts : list or list of lists
Specifying DUTs that are required to have a hit for each selected DUT.
If None, no DUT is required to have a hit.
select_fit_duts : list or list of lists
Specifying DUTs that are used for the track fit for each selected DUT.
If None, all DUTs are used for the track fit.
Note: This parameter needs to be set correctly. Usually not all available DUTs should be used for track fitting.
The list usually only contains DUTs, which are part of the telescope.
min_track_hits : uint or list
Minimum number of track hits for each selected DUT.
If None or list item is None, the minimum number of track hits is the length of select_fit_duts.
exclude_dut_hit : bool or list
Decide whether or not to use hits in the actual fit DUT for track fitting (for unconstrained residuals).
If False (default), use all DUTs as specified in select_fit_duts and use them for track fitting if hits are available (potentially constrained residuals).
If True, do not use hits form the actual fit DUT for track fitting, even if specified in select_fit_duts (unconstrained residuals).
method : string
Available methods are 'kalman', which uses a Kalman Filter for track calculation, and 'fit', which uses a simple
straight line fit for track calculation.
beam_energy : float
Energy of the beam in MeV, e.g., 2500.0 MeV for ELSA beam. Only used for the Kalman Filter.
particle_mass : float
Mass of the particle in MeV, e.g., 0.511 MeV for electrons. Only used for the Kalman Filter.
scattering_planes : list of Dut objects
Specifies additional scattering planes in case of DUTs which are not used or additional material in the way of the tracks.
Scattering planes must contain the following attributes:
name: name of the scattering plane
material_budget: material budget of the scattering plane
translation_x/translation_y/translation_z: x/y/z position of the plane (in um)
rotation_alpha/rotation_beta/rotation_gamma: alpha/beta/gamma angle of scattering plane (in radians)
The material budget is defined as the thickness devided by the radiation length.
If scattering_planes is None, no scattering plane will be added. Only available when using the Kalman Filter.
See the example on how to create scattering planes in the example script folder.
quality_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the quality flag. The selected track and corresponding hit
must have a smaller distance to have the quality flag to be set to 1.
If None, set distance to infinite.
isolation_distances : 2-tuple or list of 2-tuples
X and y distance (in um) for each DUT to calculate the isolated track/hit flag. Any other occurence of tracks or hits from the same event
within this distance will prevent the flag from beeing set.
If None, set distance to 0.
use_limits : bool
If True, use column and row limits from pre-alignment for selecting the data.
keep_data : bool
Keep all track candidates in data and add track info only to fitted tracks. Necessary for purity calculations.
full_track_info : bool
If True, the track vector and position of all DUTs is appended to track table in order to get the full track information.
If False, only the track vector and position of the actual fit DUT is appended to track table.
chunk_size : uint
Chunk size of the data when reading from file.
Returns
-------
output_tracks_file : string
Filename of the output tracks file.
'''
def _store_alignment_data(alignment_values, n_tracks_processed, chi2s, chi2s_probs, deviation_cuts):
''' Helper function to write alignment data to output file.
'''
# Do not forget to save configuration to .yaml file.
telescope.save_configuration()
# Store alignment results in file
for dut_index, _ in enumerate(telescope):
try: # Check if table exists already, then append data
alignment_table = out_file_h5.get_node('/Alignment_DUT%i' % dut_index)
except tb.NoSuchNodeError: # Table does not exist, thus create new
alignment_table = out_file_h5.create_table(
where=out_file_h5.root,
name='Alignment_DUT%i' % dut_index,
description=alignment_values[dut_index].dtype,
title='Alignment_DUT%i' % dut_index,
filters=tb.Filters(
complib='blosc',
complevel=5,
fletcher32=False))
alignment_table.append(alignment_values[dut_index])
alignment_table.attrs.deviation_cuts = deviation_cuts
alignment_table.attrs.n_tracks_processed = n_tracks_processed[dut_index]
alignment_table.flush()
# Store chi2 values
try: # Check if table exists already, then append data
out_chi2s = out_file_h5.get_node('/TrackChi2')
out_chi2s_probs = out_file_h5.get_node('/TrackpValue')
except tb.NoSuchNodeError: # Table does not exist, thus create new
out_chi2s = out_file_h5.create_earray(
where=out_file_h5.root,
name='TrackChi2',
title='Track Chi2',
atom=tb.Atom.from_dtype(chi2s.dtype),
shape=(0,),
filters=tb.Filters(
complib='blosc',
complevel=5,
fletcher32=False))
out_chi2s_probs = out_file_h5.create_earray(
where=out_file_h5.root,
name='TrackpValue',
title='Track pValue',
atom=tb.Atom.from_dtype(chi2s.dtype),
shape=(0,),
filters=tb.Filters(
complib='blosc',
complevel=5,
fletcher32=False))
out_chi2s.append(chi2s)
out_chi2s.flush()
out_chi2s_probs.append(chi2s_probs)
out_chi2s_probs.flush()
out_chi2s.attrs.max_track_chi2 = track_chi2
def _alignment_loop(actual_align_state, actual_align_cov, initial_rotation_matrix, initial_position_vector):
''' Helper function which loops over track chunks and performs the alignnment.
'''
# Init progressbar
n_tracks = in_file_h5.root.TrackCandidates.shape[0]
pbar = tqdm(total=n_tracks, ncols=80)
# Number of processed tracks for every DUT
n_tracks_processed = np.zeros(shape=(len(telescope)), dtype=np.int)
# Number of tracks fulfilling hit requirement
total_n_tracks_valid_hits = 0
# Maximum allowed relative change for each alignment parameter. Can be adjusted.
deviation_cuts = [0.05, 0.05, 0.05, 0.05, 0.05, 0.05]
alpha = np.zeros(shape=len(telescope), dtype=np.float64) # annealing factor
# Loop in chunks over tracks. After each chunk, alignment values are stored.
for track_candidates_chunk, index_chunk in analysis_utils.data_aligned_at_events(in_file_h5.root.TrackCandidates, chunk_size=10000):
# Select only tracks for which hit requirement is fulfilled
track_candidates_chunk_valid_hits = track_candidates_chunk[track_candidates_chunk['hit_flag'] & dut_hit_mask == dut_hit_mask]
total_n_tracks_valid_hits_chunk = track_candidates_chunk_valid_hits.shape[0]
total_n_tracks_valid_hits += total_n_tracks_valid_hits_chunk
# Per chunk variables
chi2s = np.zeros(shape=(total_n_tracks_valid_hits_chunk), dtype=np.float64) # track chi2s
chi2s_probs = np.zeros(shape=(total_n_tracks_valid_hits_chunk), dtype=np.float64) # track pvalues
alignment_values = np.full(shape=(len(telescope), total_n_tracks_valid_hits_chunk), dtype=kfa_alignment_descr, fill_value=np.nan) # alignment values
# Loop over tracks in chunk
for track_index, track in enumerate(track_candidates_chunk_valid_hits):
track_hits = np.full((1, n_duts, 6), fill_value=np.nan, dtype=np.float64)
# Compute aligned position and apply the alignment
for dut_index, dut in enumerate(telescope):
# Get local track hits
track_hits[:, dut_index, 0] = track['x_dut_%s' % dut_index]
track_hits[:, dut_index, 1] = track['y_dut_%s' % dut_index]
track_hits[:, dut_index, 2] = track['z_dut_%s' % dut_index]
track_hits[:, dut_index, 3] = track['x_err_dut_%s' % dut_index]
track_hits[:, dut_index, 4] = track['y_err_dut_%s' % dut_index]
track_hits[:, dut_index, 5] = track['z_err_dut_%s' % dut_index]
# Calculate new alignment (takes initial alignment and actual *change* of parameters)
new_rotation_matrix, new_position_vector = _update_alignment(initial_rotation_matrix[dut_index], initial_position_vector[dut_index], actual_align_state[dut_index])
# Get euler angles from rotation matrix
alpha_average, beta_average, gamma_average = geometry_utils.euler_angles(R=new_rotation_matrix)
# Set new alignment to DUT
dut._translation_x = float(new_position_vector[0])
dut._translation_y = float(new_position_vector[1])
dut._translation_z = float(new_position_vector[2])
dut._rotation_alpha = float(alpha_average)
dut._rotation_beta = float(beta_average)
dut._rotation_gamma = float(gamma_average)
alignment_values[dut_index, track_index]['translation_x'] = dut.translation_x
alignment_values[dut_index, track_index]['translation_y'] = dut.translation_y
alignment_values[dut_index, track_index]['translation_z'] = dut.translation_z
alignment_values[dut_index, track_index]['rotation_alpha'] = dut.rotation_alpha
alignment_values[dut_index, track_index]['rotation_beta'] = dut.rotation_beta
alignment_values[dut_index, track_index]['rotation_gamma'] = dut.rotation_gamma
C = actual_align_cov[dut_index]
alignment_values[dut_index, track_index]['translation_x_err'] = np.sqrt(C[0, 0])
alignment_values[dut_index, track_index]['translation_y_err'] = np.sqrt(C[1, 1])
alignment_values[dut_index, track_index]['translation_z_err'] = np.sqrt(C[2, 2])
alignment_values[dut_index, track_index]['rotation_alpha_err'] = np.sqrt(C[3, 3])
alignment_values[dut_index, track_index]['rotation_beta_err'] = np.sqrt(C[4, 4])
alignment_values[dut_index, track_index]['rotation_gamma_err'] = np.sqrt(C[5, 5])
# Calculate deterministic annealing (scaling factor for covariance matrix) in order to take into account misalignment
alpha[dut_index] = _calculate_annealing(k=n_tracks_processed[dut_index], annealing_factor=annealing_factor, annealing_tracks=annealing_tracks)
# Store annealing factor
alignment_values[dut_index, track_index]['annealing_factor'] = alpha[dut_index]
# Run Kalman Filter
try:
offsets, slopes, chi2s_reg, chi2s_red, chi2s_prob, x_err, y_err, cov, cov_obs, obs_mat = _fit_tracks_kalman_loop(track_hits, telescope, fit_duts, beam_energy, particle_mass, scattering_planes, alpha)
except Exception as e:
print(e, 'TRACK FITTING')
continue
# Store chi2 and pvalue
chi2s[track_index] = chi2s_red
chi2s_probs[track_index] = chi2s_prob
# Data quality check I: Check chi2 of track
if chi2s_red > track_chi2:
continue
# Actual track states
p0 = np.column_stack((offsets[0, :, 0], offsets[0, :, 1],
slopes[0, :, 0], slopes[0, :, 1]))
# Covariance matrix (x, y, dx, dy) of track estimates
C0 = cov[0, :, :, :]
# Covariance matrix (x, y, dx, dy) of observations
V = cov_obs[0, :, :, :]
# Measurement matrix
H = obs_mat[0, :, :, :]
# Actual alignment parameters and its covariance
a0 = actual_align_state.copy()
E0 = actual_align_cov.copy()
# Updated alignment parameters and its covariance
E1 = np.zeros_like(E0)
a1 = np.zeros_like(a0)
# Update all alignables
actual_align_state, actual_align_cov, alignment_values, n_tracks_processed = _update_alignment_parameters(
telescope, H, V, C0, p0, a0, E0, track_hits, a1, E1,
alignment_values, deviation_cuts,
actual_align_state, actual_align_cov, n_tracks_processed, track_index)
# Reached number of max. specified tracks. Stop alignment
if n_tracks_processed.min() > max_tracks:
pbar.update(track_index)
pbar.write('Processed {0} tracks (per DUT) out of {1} tracks'.format(n_tracks_processed, total_n_tracks_valid_hits))
pbar.close()
logging.info('Maximum number of tracks reached! Stopping alignment...')
# Store alignment data
_store_alignment_data(alignment_values[:, :track_index + 1], n_tracks_processed, chi2s[:track_index + 1], chi2s_probs[:track_index + 1], deviation_cuts)
return
pbar.update(track_candidates_chunk.shape[0])
pbar.write('Processed {0} tracks (per DUT) out of {1} tracks'.format(n_tracks_processed, total_n_tracks_valid_hits))
# Store alignment data
_store_alignment_data(alignment_values, n_tracks_processed, chi2s, chi2s_probs, deviation_cuts)
pbar.close()
telescope = Telescope(telescope_configuration)
n_duts = len(telescope)
logging.info('= Alignment step 2 - Fitting tracks for %d DUTs =', len(select_duts))
if iteration_index == 0: # clean up before starting alignment. In case different sets of DUTs are aligned after each other only clean up once.
if os.path.exists(output_alignment_file):
os.remove(output_alignment_file)
logging.info('=== Fitting tracks of %d DUTs ===' % n_duts)
if not beam_energy:
raise ValueError('Beam energy not given (in MeV).')
if not particle_mass:
raise ValueError('Particle mass not given (in MeV).')
if select_duts is None:
select_duts = list(range(n_duts)) # standard setting: fit tracks for all DUTs
elif not isinstance(select_duts, Iterable):
select_duts = [select_duts]
# Check for duplicates
if len(select_duts) != len(set(select_duts)):
raise ValueError("Found douplicate in select_duts.")
# Check if any iterable in iterable
if any(map(lambda val: isinstance(val, Iterable), select_duts)):
raise ValueError("Item in parameter select_duts is iterable.")
# Create track, hit selection
if select_fit_duts is None: # If None: use all DUTs
select_fit_duts = list(range(n_duts))
# # copy each item
# for hit_duts in select_hit_duts:
# select_fit_duts.append(hit_duts[:]) # require a hit for each fit DUT
# Check iterable and length
if not isinstance(select_fit_duts, Iterable):
raise ValueError("Parameter select_fit_duts is not an iterable.")
elif not select_fit_duts: # empty iterable
raise ValueError("Parameter select_fit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable) and val is not None, select_fit_duts)):
select_fit_duts = [select_fit_duts[:] for _ in select_duts]
# if None use all DUTs
for index, item in enumerate(select_fit_duts):
if item is None:
select_fit_duts[index] = list(range(n_duts))
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_fit_duts)):
raise ValueError("Not all items in parameter select_fit_duts are iterable.")
# Finally check length of all arrays
if len(select_fit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_fit_duts has the wrong length.")
for index, fit_dut in enumerate(select_fit_duts):
if len(fit_dut) < 2: # check the length of the items
raise ValueError("Item in parameter select_fit_duts has length < 2.")
# Create track, hit selection
if select_hit_duts is None: # If None, require no hit
# select_hit_duts = list(range(n_duts))
select_hit_duts = []
# Check iterable and length
if not isinstance(select_hit_duts, Iterable):
raise ValueError("Parameter select_hit_duts is not an iterable.")
# elif not select_hit_duts: # empty iterable
# raise ValueError("Parameter select_hit_duts has no items.")
# Check if only non-iterable in iterable
if all(map(lambda val: not isinstance(val, Iterable) and val is not None, select_hit_duts)):
select_hit_duts = [select_hit_duts[:] for _ in select_duts]
# If None, require no hit
for index, item in enumerate(select_hit_duts):
if item is None:
select_hit_duts[index] = []
# Check if only iterable in iterable
if not all(map(lambda val: isinstance(val, Iterable), select_hit_duts)):
raise ValueError("Not all items in parameter select_hit_duts are iterable.")
# Finally check length of all arrays
if len(select_hit_duts) != len(select_duts): # empty iterable
raise ValueError("Parameter select_hit_duts has the wrong length.")
# Check iterable and length
if not isinstance(exclude_dut_hit, Iterable):
exclude_dut_hit = [exclude_dut_hit] * len(select_duts)
elif not exclude_dut_hit: # empty iterable
raise ValueError("Parameter exclude_dut_hit has no items.")
# Finally check length of all array
if len(exclude_dut_hit) != len(select_duts): # empty iterable
raise ValueError("Parameter exclude_dut_hit has the wrong length.")
# Check if only bools in iterable
if not all(map(lambda val: isinstance(val, (bool,)), exclude_dut_hit)):
raise ValueError("Not all items in parameter exclude_dut_hit are boolean.")
# Check iterable and length
if not isinstance(min_track_hits, Iterable):
min_track_hits = [min_track_hits] * len(select_duts)
# Finally check length of all arrays
if len(min_track_hits) != len(select_duts): # empty iterable
raise ValueError("Parameter min_track_hits has the wrong length.")
fitted_duts = []
with tb.open_file(input_track_candidates_file, mode='r') as in_file_h5:
with tb.open_file(output_alignment_file, mode='a') as out_file_h5:
for fit_dut_index, actual_fit_dut in enumerate(select_duts): # Loop over the DUTs where tracks shall be fitted for
# Test whether other DUTs have identical tracks
# if yes, save some CPU time and fit only once.
# This following list contains all DUT indices that will be fitted
# during this step of the loop.
if actual_fit_dut in fitted_duts:
continue
# calculate all DUTs with identical tracks to save processing time
actual_fit_duts = []
for curr_fit_dut_index, curr_fit_dut in enumerate(select_duts):
if (curr_fit_dut == actual_fit_dut or
(((exclude_dut_hit[curr_fit_dut_index] is False and exclude_dut_hit[fit_dut_index] is False and set(select_fit_duts[curr_fit_dut_index]) == set(select_fit_duts[fit_dut_index])) or
(exclude_dut_hit[curr_fit_dut_index] is False and exclude_dut_hit[fit_dut_index] is True and set(select_fit_duts[curr_fit_dut_index]) == (set(select_fit_duts[fit_dut_index]) - set([actual_fit_dut]))) or
(exclude_dut_hit[curr_fit_dut_index] is True and exclude_dut_hit[fit_dut_index] is False and (set(select_fit_duts[curr_fit_dut_index]) - set([curr_fit_dut])) == set(select_fit_duts[fit_dut_index])) or
(exclude_dut_hit[curr_fit_dut_index] is True and exclude_dut_hit[fit_dut_index] is True and (set(select_fit_duts[curr_fit_dut_index]) - set([curr_fit_dut])) == (set(select_fit_duts[fit_dut_index]) - set([actual_fit_dut])))) and
set(select_hit_duts[curr_fit_dut_index]) == set(select_hit_duts[fit_dut_index]) and
min_track_hits[curr_fit_dut_index] == min_track_hits[fit_dut_index])):
actual_fit_duts.append(curr_fit_dut)
# continue with fitting
logging.info('== Fit tracks for %s ==', ', '.join([telescope[curr_dut].name for curr_dut in actual_fit_duts]))
# select hit DUTs based on input parameters
# hit DUTs are always enforced
hit_duts = select_hit_duts[fit_dut_index]
dut_hit_mask = 0 # DUTs required to have hits
for dut_index in hit_duts:
dut_hit_mask |= ((1 << dut_index))
logging.info('Require hits in %d DUTs for track selection: %s', len(hit_duts), ', '.join([telescope[curr_dut].name for curr_dut in hit_duts]))
# select fit DUTs based on input parameters
# exclude actual DUTs from fit DUTs if exclude_dut_hit parameter is set (for, e.g., unbiased residuals)
fit_duts = list(set(select_fit_duts[fit_dut_index]) - set([actual_fit_dut])) if exclude_dut_hit[fit_dut_index] else select_fit_duts[fit_dut_index]
if min_track_hits[fit_dut_index] is None:
actual_min_track_hits = len(fit_duts)
else:
actual_min_track_hits = min_track_hits[fit_dut_index]
if actual_min_track_hits < 2:
raise ValueError('The number of required hits is smaller than 2. Cannot fit tracks for %s.', telescope[actual_fit_dut].name)
dut_fit_mask = 0 # DUTs to be used for the fit
for dut_index in fit_duts:
dut_fit_mask |= ((1 << dut_index))
if actual_min_track_hits > len(fit_duts):
raise RuntimeError("min_track_hits for DUT%d is larger than the number of fit DUTs" % (actual_fit_dut,))
logging.info('Require at least %d hits in %d DUTs for track selection: %s', actual_min_track_hits, len(fit_duts), ', '.join([telescope[curr_dut].name for curr_dut in fit_duts]))
if scattering_planes is not None:
logging.info('Adding the following scattering planes: %s', ', '.join([scp.name for scp in scattering_planes]))
# Actual *change* of alignment parameters and covariance
actual_align_state = np.zeros(shape=(len(telescope), 6), dtype=np.float64) # No change at beginning
actual_align_cov = np.zeros(shape=(len(telescope), 6, 6), dtype=np.float64)
# Calculate initial alignment
initial_rotation_matrix, initial_position_vector, actual_align_cov = _calculate_initial_alignment(telescope, select_duts, select_telescope_duts, alignment_parameters, actual_align_cov, alignment_parameters_errors)
# Loop over tracks in chunks and perform alignment.
_alignment_loop(actual_align_state, actual_align_cov, initial_rotation_matrix, initial_position_vector)
fitted_duts.extend(actual_fit_duts)
output_pdf_file = output_alignment_file[:-3] + '.pdf'
# Plot alignment result
plot_utils.plot_kf_alignment(output_alignment_file, telescope, output_pdf_file)
# Delete tmp track candidates file
os.remove(input_track_candidates_file)
def align_telescope(telescope_configuration, select_telescope_duts, reference_dut=None):
telescope = Telescope(telescope_configuration)
logging.info('= Beam-alignment of the telescope =')
logging.info('Use %d DUTs for beam-alignment: %s', len(select_telescope_duts), ', '.join([telescope[index].name for index in select_telescope_duts]))
telescope_duts_positions = np.full((len(select_telescope_duts), 3), fill_value=np.nan, dtype=np.float64)
for index, dut_index in enumerate(select_telescope_duts):
telescope_duts_positions[index, 0] = telescope[dut_index].translation_x
telescope_duts_positions[index, 1] = telescope[dut_index].translation_y
telescope_duts_positions[index, 2] = telescope[dut_index].translation_z
# the x and y translation for the reference DUT will be set to 0
if reference_dut is not None:
first_telescope_dut_index = reference_dut
else:
# calculate reference DUT, use DUT with the smallest z position
first_telescope_dut_index = select_telescope_duts[np.argmin(telescope_duts_positions[:, 2])]
offset, slope = line_fit_3d(positions=telescope_duts_positions)
first_telescope_dut = telescope[first_telescope_dut_index]
logging.info('Reference DUT for beam-alignment: %s', first_telescope_dut.name)
first_dut_translation_x = first_telescope_dut.translation_x
first_dut_translation_y = first_telescope_dut.translation_y
first_telescope_dut_intersection = geometry_utils.get_line_intersections_with_dut(
line_origins=offset[np.newaxis, :],
line_directions=slope[np.newaxis, :],
translation_x=first_telescope_dut.translation_x,
translation_y=first_telescope_dut.translation_y,
translation_z=first_telescope_dut.translation_z,
rotation_alpha=first_telescope_dut.rotation_alpha,
rotation_beta=first_telescope_dut.rotation_beta,
rotation_gamma=first_telescope_dut.rotation_gamma)
for actual_dut in telescope:
dut_intersection = geometry_utils.get_line_intersections_with_dut(
line_origins=offset[np.newaxis, :],
line_directions=slope[np.newaxis, :],
translation_x=actual_dut.translation_x,
translation_y=actual_dut.translation_y,
translation_z=actual_dut.translation_z,
rotation_alpha=actual_dut.rotation_alpha,
rotation_beta=actual_dut.rotation_beta,
rotation_gamma=actual_dut.rotation_gamma)
actual_dut.translation_x -= (dut_intersection[0, 0] - first_telescope_dut_intersection[0, 0] + first_dut_translation_x)
actual_dut.translation_y -= (dut_intersection[0, 1] - first_telescope_dut_intersection[0, 1] + first_dut_translation_y)
# set telescope alpha/beta rotation for a better beam alignment and track finding improvement
# this is compensating the previously made changes to the DUT coordinates
total_angles, alpha_angles, beta_angles = get_angles(
slopes=slope[np.newaxis, :],
xz_plane_normal=np.array([0.0, 1.0, 0.0]),
yz_plane_normal=np.array([1.0, 0.0, 0.0]),
dut_plane_normal=np.array([0.0, 0.0, 1.0]))
telescope.rotation_alpha -= alpha_angles[0]
telescope.rotation_beta -= beta_angles[0]
telescope.save_configuration()
def calculate_transformation(telescope_configuration, input_tracks_file, select_duts, select_alignment_parameters=None, use_limits=True, max_iterations=None, chunk_size=1000000):
'''Takes the tracks and calculates and stores the transformation parameters.
Parameters
----------
telescope_configuration : string
Filename of the telescope configuration file.
input_tracks_file : string
Filename of the input tracks file.
select_duts : list
Selecting DUTs that will be processed.
select_alignment_parameters : list
Selecting the transformation parameters that will be stored to the telescope configuration file for each selected DUT.
If None, all 6 transformation parameters will be calculated.
use_limits : bool
If True, use column and row limits from pre-alignment for selecting the data.
chunk_size : int
Chunk size of the data when reading from file.
'''
telescope = Telescope(telescope_configuration)
logging.info('== Calculating transformation for %d DUTs ==' % len(select_duts))
if select_alignment_parameters is None:
select_alignment_parameters = [default_alignment_parameters] * len(select_duts)
if len(select_duts) != len(select_alignment_parameters):
raise ValueError("Parameter select_alignment_parameters has the wrong length.")
for index, actual_alignment_parameters in enumerate(select_alignment_parameters):
if actual_alignment_parameters is None:
select_alignment_parameters[index] = default_alignment_parameters
else:
non_valid_paramters = set(actual_alignment_parameters) - set(default_alignment_parameters)
if non_valid_paramters:
raise ValueError("Found invalid values in parameter select_alignment_parameters: %s." % ", ".join(non_valid_paramters))
with tb.open_file(input_tracks_file, mode='r') as in_file_h5:
for index, actual_dut_index in enumerate(select_duts):
actual_dut = telescope[actual_dut_index]
node = in_file_h5.get_node(in_file_h5.root, 'Tracks_DUT%d' % actual_dut_index)
logging.info('= Calculate transformation for %s =', actual_dut.name)
logging.info("Modify alignment parameters: %s", ', '.join([alignment_paramter for alignment_paramter in select_alignment_parameters[index]]))
if use_limits:
limit_x_local = actual_dut.x_limit # (lower limit, upper limit)
limit_y_local = actual_dut.y_limit # (lower limit, upper limit)
else:
limit_x_local = None
limit_y_local = None
rotation_average = None
translation_average = None
# euler_angles_average = None
# calculate equal chunk size
start_val = max(int(node.nrows / chunk_size), 2)
while True:
chunk_indices = np.linspace(0, node.nrows, start_val).astype(np.int)
if np.all(np.diff(chunk_indices) <= chunk_size):
break
start_val += 1
chunk_index = 0
n_tracks = 0
total_n_tracks = 0
while chunk_indices[chunk_index] < node.nrows:
tracks_chunk = node.read(start=chunk_indices[chunk_index], stop=chunk_indices[chunk_index + 1])
# select good hits and tracks
selection = np.logical_and(~np.isnan(tracks_chunk['x_dut_%d' % actual_dut_index]), ~np.isnan(tracks_chunk['track_chi2']))
tracks_chunk = tracks_chunk[selection] # Take only tracks where actual dut has a hit, otherwise residual wrong
# Coordinates in global coordinate system (x, y, z)
hit_x_local, hit_y_local, hit_z_local = tracks_chunk['x_dut_%d' % actual_dut_index], tracks_chunk['y_dut_%d' % actual_dut_index], tracks_chunk['z_dut_%d' % actual_dut_index]
offsets = np.column_stack(actual_dut.local_to_global_position(
x=tracks_chunk['offset_x'],
y=tracks_chunk['offset_y'],
z=tracks_chunk['offset_z']))
slopes = np.column_stack(actual_dut.local_to_global_position(
x=tracks_chunk['slope_x'],
y=tracks_chunk['slope_y'],
z=tracks_chunk['slope_z'],
translation_x=0.0,
translation_y=0.0,
translation_z=0.0,
rotation_alpha=actual_dut.rotation_alpha,
rotation_beta=actual_dut.rotation_beta,
rotation_gamma=actual_dut.rotation_gamma))
if not np.allclose(hit_z_local, 0.0):
raise RuntimeError("Transformation into local coordinate system gives z != 0")
limit_xy_local_sel = np.ones_like(hit_x_local, dtype=np.bool)
if limit_x_local is not None and np.isfinite(limit_x_local[0]):
limit_xy_local_sel &= hit_x_local >= limit_x_local[0]
if limit_x_local is not None and np.isfinite(limit_x_local[1]):
limit_xy_local_sel &= hit_x_local <= limit_x_local[1]
if limit_y_local is not None and np.isfinite(limit_y_local[0]):
limit_xy_local_sel &= hit_y_local >= limit_y_local[0]
if limit_y_local is not None and np.isfinite(limit_y_local[1]):
limit_xy_local_sel &= hit_y_local <= limit_y_local[1]
hit_x_local = hit_x_local[limit_xy_local_sel]
hit_y_local = hit_y_local[limit_xy_local_sel]
hit_z_local = hit_z_local[limit_xy_local_sel]
hit_local = np.column_stack([hit_x_local, hit_y_local, hit_z_local])
slopes = slopes[limit_xy_local_sel]
offsets = offsets[limit_xy_local_sel]
n_tracks = | np.count_nonzero(limit_xy_local_sel) | numpy.count_nonzero |
import tensorflow_advanced_segmentation_models as tasm
import os
import cv2
import numpy as np
from time import time
import tensorflow as tf
import albumentations as A
import matplotlib.pyplot as plt
import tensorflow.keras.backend as K
from labelme.utils import lblsave as label_save
from common.utils import visualize_segmentation
from PIL import Image
DATA_DIR = "/home/minouei/Downloads/datasets/contract/version2"
x_train_dir = os.path.join(DATA_DIR, 'images/train')
y_train_dir = os.path.join(DATA_DIR, 'annotations/train')
x_valid_dir = os.path.join(DATA_DIR, 'images/val')
y_valid_dir = os.path.join(DATA_DIR, 'annotations/val')
x_test_dir = os.path.join(DATA_DIR, 'images/val')
y_test_dir = os.path.join(DATA_DIR, 'annotations/val')
"""### Helper Functions"""
# helper function for data visualization
def visualize(**images):
"""PLot images in one row."""
n = len(images)
plt.figure(figsize=(16, 5))
ns=''
for i, (name, image) in enumerate(images.items()):
plt.subplot(1, n, i + 1)
plt.xticks([])
plt.yticks([])
plt.title(' '.join(name.split('_')).title())
plt.imshow(image)
ns=f'{name}_{i}'
# plt.show()
plt.savefig(ns+'.png', bbox_inches='tight')
# helper function for data visualization
def denormalize(x):
"""Scale image to range 0..1 for correct plot"""
x_max = np.percentile(x, 98)
x_min = np.percentile(x, 2)
x = (x - x_min) / (x_max - x_min)
x = x.clip(0, 1)
return x
def round_clip_0_1(x, **kwargs):
return x.round().clip(0, 1)
"""## Data Augmentation Functions"""
# define heavy augmentations
def get_training_augmentation(height, width):
train_transform = [
A.PadIfNeeded(min_height=height, min_width=width, always_apply=True, border_mode=0),
A.Resize(height, width, always_apply=True)
]
return A.Compose(train_transform)
def get_validation_augmentation(height, width):
"""Add paddings to make image shape divisible by 32"""
test_transform = [
A.PadIfNeeded(height, width),
A.Resize(height, width, always_apply=True)
]
return A.Compose(test_transform)
def data_get_preprocessing(preprocessing_fn):
"""Construct preprocessing transform
Args:
preprocessing_fn (callbale): data normalization function
(can be specific for each pretrained neural network)
Return:
transform: albumentations.Compose
"""
_transform = [
A.Lambda(image=preprocessing_fn),
]
return A.Compose(_transform)
"""## Define some global variables"""
TOTAL_CLASSES = ['background', 'headerlogo', 'twocoltabel', 'recieveraddress', 'text', 'senderaddress', 'ortdatum',
'companyinfo', 'fulltabletyp1', 'fulltabletyp2', 'copylogo', 'footerlogo', 'footertext', 'signatureimage', 'fulltabletyp3', 'unlabelled']
MODEL_CLASSES = TOTAL_CLASSES
ALL_CLASSES = False
if MODEL_CLASSES == TOTAL_CLASSES:
MODEL_CLASSES = MODEL_CLASSES[:-1]
ALL_CLASSES = True
BATCH_SIZE = 1
N_CLASSES = 16
HEIGHT = 704
WIDTH = 704
BACKBONE_NAME = "efficientnetb3"
WEIGHTS = "imagenet"
WWO_AUG = False # train data with and without augmentation
"""### Functions to calculate appropriate class weights"""
################################################################################
# Class Weights
################################################################################
def get_dataset_counts(d):
pixel_count = np.array([i for i in d.values()])
sum_pixel_count = 0
for i in pixel_count:
sum_pixel_count += i
return pixel_count, sum_pixel_count
def get_dataset_statistics(pixel_count, sum_pixel_count):
pixel_frequency = np.round(pixel_count / sum_pixel_count, 4)
mean_pixel_frequency = np.round( | np.mean(pixel_frequency) | numpy.mean |
# -*- coding: utf-8 -*-
from context import matext as mx
import numpy as np
import time
import operator as op
def time_function(times, f, *args):
tic = time.time()
for i in range(times):
f(*args)
toc = time.time()
return toc - tic
def print_result (operation, matext_time, numpy_time, difference):
print('[{}]\t Matex runtime: {:.3f} sec,\
Numpy runtime: {:.3f} sec,\
Maximum elementwise difference: {}'\
.format(operation, matext_time, numpy_time, difference))
if __name__ == '__main__':
# Let's compare with numpy how fast the implementations are
times = 1
N = 400
M = 600
np_array1 = | np.random.rand(N,N) | numpy.random.rand |
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import tensorflow as tf
from lib.ops import *
import collections
import os
import math
import scipy.misc as sic
import numpy as np
# Define the dataloader
def data_loader(FLAGS):
with tf.device('/cpu:0'):
# Define the returned data batches
Data = collections.namedtuple('Data', 'paths_LR, paths_HR, inputs, targets, image_count, steps_per_epoch')
#Check the input directory
if (FLAGS.input_dir_LR == 'None') or (FLAGS.input_dir_HR == 'None'):
raise ValueError('Input directory is not provided')
if (not os.path.exists(FLAGS.input_dir_LR)) or (not os.path.exists(FLAGS.input_dir_HR)):
raise ValueError('Input directory not found')
image_list_LR = os.listdir(FLAGS.input_dir_LR)
image_list_LR = [_ for _ in image_list_LR if _.endswith('.png')]
if len(image_list_LR)==0:
raise Exception('No png files in the input directory')
image_list_LR_temp = sorted(image_list_LR)
image_list_LR = [os.path.join(FLAGS.input_dir_LR, _) for _ in image_list_LR_temp]
image_list_HR = [os.path.join(FLAGS.input_dir_HR, _) for _ in image_list_LR_temp]
image_list_LR_tensor = tf.convert_to_tensor(image_list_LR, dtype=tf.string)
image_list_HR_tensor = tf.convert_to_tensor(image_list_HR, dtype=tf.string)
with tf.variable_scope('load_image'):
# define the image list queue
# image_list_LR_queue = tf.train.string_input_producer(image_list_LR, shuffle=False, capacity=FLAGS.name_queue_capacity)
# image_list_HR_queue = tf.train.string_input_producer(image_list_HR, shuffle=False, capacity=FLAGS.name_queue_capacity)
#print('[Queue] image list queue use shuffle: %s'%(FLAGS.mode == 'Train'))
output = tf.train.slice_input_producer([image_list_LR_tensor, image_list_HR_tensor],
shuffle=False, capacity=FLAGS.name_queue_capacity)
# Reading and decode the images
reader = tf.WholeFileReader(name='image_reader')
image_LR = tf.read_file(output[0])
image_HR = tf.read_file(output[1])
input_image_LR = tf.image.decode_png(image_LR, channels=3)
input_image_HR = tf.image.decode_png(image_HR, channels=3)
input_image_LR = tf.image.convert_image_dtype(input_image_LR, dtype=tf.float32)
input_image_HR = tf.image.convert_image_dtype(input_image_HR, dtype=tf.float32)
assertion = tf.assert_equal(tf.shape(input_image_LR)[2], 3, message="image does not have 3 channels")
with tf.control_dependencies([assertion]):
input_image_LR = tf.identity(input_image_LR)
input_image_HR = tf.identity(input_image_HR)
# Normalize the low resolution image to [0, 1], high resolution to [-1, 1]
a_image = preprocessLR(input_image_LR)
b_image = preprocess(input_image_HR)
inputs, targets = [a_image, b_image]
# The data augmentation part
with tf.name_scope('data_preprocessing'):
with tf.name_scope('random_crop'):
# Check whether perform crop
if (FLAGS.random_crop is True) and FLAGS.mode == 'train':
print('[Config] Use random crop')
# Set the shape of the input image. the target will have 4X size
input_size = tf.shape(inputs)
target_size = tf.shape(targets)
offset_w = tf.cast(tf.floor(tf.random_uniform([], 0, tf.cast(input_size[1], tf.float32) - FLAGS.crop_size)),
dtype=tf.int32)
offset_h = tf.cast(tf.floor(tf.random_uniform([], 0, tf.cast(input_size[0], tf.float32) - FLAGS.crop_size)),
dtype=tf.int32)
if FLAGS.task == 'SRGAN' or FLAGS.task == 'SRResnet':
inputs = tf.image.crop_to_bounding_box(inputs, offset_h, offset_w, FLAGS.crop_size,
FLAGS.crop_size)
targets = tf.image.crop_to_bounding_box(targets, offset_h*4, offset_w*4, FLAGS.crop_size*4,
FLAGS.crop_size*4)
elif FLAGS.task == 'denoise':
inputs = tf.image.crop_to_bounding_box(inputs, offset_h, offset_w, FLAGS.crop_size,
FLAGS.crop_size)
targets = tf.image.crop_to_bounding_box(targets, offset_h, offset_w,
FLAGS.crop_size, FLAGS.crop_size)
# Do not perform crop
else:
inputs = tf.identity(inputs)
targets = tf.identity(targets)
with tf.variable_scope('random_flip'):
# Check for random flip:
if (FLAGS.flip is True) and (FLAGS.mode == 'train'):
print('[Config] Use random flip')
# Produce the decision of random flip
decision = tf.random_uniform([], 0, 1, dtype=tf.float32)
input_images = random_flip(inputs, decision)
target_images = random_flip(targets, decision)
else:
input_images = tf.identity(inputs)
target_images = tf.identity(targets)
if FLAGS.task == 'SRGAN' or FLAGS.task == 'SRResnet':
input_images.set_shape([FLAGS.crop_size, FLAGS.crop_size, 3])
target_images.set_shape([FLAGS.crop_size*4, FLAGS.crop_size*4, 3])
elif FLAGS.task == 'denoise':
input_images.set_shape([FLAGS.crop_size, FLAGS.crop_size, 3])
target_images.set_shape([FLAGS.crop_size, FLAGS.crop_size, 3])
if FLAGS.mode == 'train':
paths_LR_batch, paths_HR_batch, inputs_batch, targets_batch = tf.train.shuffle_batch([output[0], output[1], input_images, target_images],
batch_size=FLAGS.batch_size, capacity=FLAGS.image_queue_capacity+4*FLAGS.batch_size,
min_after_dequeue=FLAGS.image_queue_capacity, num_threads=FLAGS.queue_thread)
else:
paths_LR_batch, paths_HR_batch, inputs_batch, targets_batch = tf.train.batch([output[0], output[1], input_images, target_images],
batch_size=FLAGS.batch_size, num_threads=FLAGS.queue_thread, allow_smaller_final_batch=True)
steps_per_epoch = int(math.ceil(len(image_list_LR) / FLAGS.batch_size))
if FLAGS.task == 'SRGAN' or FLAGS.task == 'SRResnet':
inputs_batch.set_shape([FLAGS.batch_size, FLAGS.crop_size, FLAGS.crop_size, 3])
targets_batch.set_shape([FLAGS.batch_size, FLAGS.crop_size*4, FLAGS.crop_size*4, 3])
elif FLAGS.task == 'denoise':
inputs_batch.set_shape([FLAGS.batch_size, FLAGS.crop_size, FLAGS.crop_size, 3])
targets_batch.set_shape([FLAGS.batch_size, FLAGS.crop_size, FLAGS.crop_size, 3])
return Data(
paths_LR=paths_LR_batch,
paths_HR=paths_HR_batch,
inputs=inputs_batch,
targets=targets_batch,
image_count=len(image_list_LR),
steps_per_epoch=steps_per_epoch
)
# The test data loader. Allow input image with different size
def test_data_loader(FLAGS):
# Get the image name list
if (FLAGS.input_dir_LR == 'None') or (FLAGS.input_dir_HR == 'None'):
raise ValueError('Input directory is not provided')
if (not os.path.exists(FLAGS.input_dir_LR)) or (not os.path.exists(FLAGS.input_dir_HR)):
raise ValueError('Input directory not found')
image_list_LR_temp = os.listdir(FLAGS.input_dir_LR)
image_list_LR = [os.path.join(FLAGS.input_dir_LR, _) for _ in image_list_LR_temp if _.split('.')[-1] == 'png']
image_list_HR = [os.path.join(FLAGS.input_dir_HR, _) for _ in image_list_LR_temp if _.split('.')[-1] == 'png']
# Read in and preprocess the images
def preprocess_test(name, mode):
im = sic.imread(name, mode="RGB").astype(np.float32)
# check grayscale image
if im.shape[-1] != 3:
h, w = im.shape
temp = np.empty((h, w, 3), dtype=np.uint8)
temp[:, :, :] = im[:, :, np.newaxis]
im = temp.copy()
if mode == 'LR':
im = im / np.max(im)
elif mode == 'HR':
im = im / np.max(im)
im = im * 2 - 1
return im
image_LR = [preprocess_test(_, 'LR') for _ in image_list_LR]
image_HR = [preprocess_test(_, 'HR') for _ in image_list_HR]
# Push path and image into a list
Data = collections.namedtuple('Data', 'paths_LR, paths_HR, inputs, targets')
return Data(
paths_LR = image_list_LR,
paths_HR = image_list_HR,
inputs = image_LR,
targets = image_HR
)
# The inference data loader. Allow input image with different size
def inference_data_loader(FLAGS):
# Get the image name list
if (FLAGS.input_dir_LR == 'None'):
raise ValueError('Input directory is not provided')
if not os.path.exists(FLAGS.input_dir_LR):
raise ValueError('Input directory not found')
image_list_LR_temp = os.listdir(FLAGS.input_dir_LR)
image_list_LR = [os.path.join(FLAGS.input_dir_LR, _) for _ in image_list_LR_temp if _.split('.')[-1] == 'png']
# Read in and preprocess the images
def preprocess_test(name):
im = sic.imread(name, mode="RGB").astype(np.float32)
# check grayscale image
if im.shape[-1] != 3:
h, w = im.shape
temp = | np.empty((h, w, 3), dtype=np.uint8) | numpy.empty |
import glob
import logging
import os
import numpy as np
import pickle
import sys
import torch
from torch_geometric.data import Data
from torch_geometric.nn.pool import radius_graph
from tqdm import tqdm
from typing import Callable, List, Optional
from aegnn.utils.multiprocessing import TaskManager
from .utils.normalization import normalize_time
from .base.event_dm import EventDataModule
class Gen1(EventDataModule):
def __init__(self, batch_size: int = 64, shuffle: bool = True, num_workers: int = 8, pin_memory: bool = False,
transform: Optional[Callable[[Data], Data]] = None):
super(Gen1, self).__init__(img_shape=(304, 240), batch_size=batch_size, shuffle=shuffle,
num_workers=num_workers, pin_memory=pin_memory, transform=transform)
pre_processing_params = {"r": 3.0, "d_max": 128, "n_samples": 25000, "sampling": True}
self.save_hyperparameters({"preprocessing": pre_processing_params})
def _prepare_dataset(self, mode: str):
raw_files = self.raw_files(mode)
logging.debug(f"Found {len(raw_files)} raw files in dataset (mode = {mode})")
total_bbox_count = self._total_bbox_count(raw_files)
logging.debug(f"Total count of (filtered) bounding boxes = {total_bbox_count}")
task_manager = TaskManager(self.num_workers, queue_size=self.num_workers)
for rf in tqdm(raw_files):
task_manager.queue(self._processing, rf=rf, root=self.root, read_annotations=self._read_annotations,
read_label=self._read_label, buffer_to_data=self._buffer_to_data)
task_manager.join()
def _processing(self, rf: str, root: str, read_annotations, read_label, buffer_to_data):
data_loader = PSEELoader(rf)
params = self.hparams.preprocessing
bounding_boxes = read_annotations(rf)
labels = np.array(read_label(bounding_boxes))
for i, bbox in enumerate(bounding_boxes):
processed_dir = os.path.join(root, "processed")
processed_file = rf.replace(root, processed_dir).replace(".dat", f"{i}.pkl")
if os.path.exists(processed_file):
continue
# Determine temporal window around the current bounding box [t_start, t_end]. Add all of the
# bounding boxes within this window to the sample.
sample_dict = dict()
t_bbox = bbox[0]
t_start = t_bbox - 100000 # 100 ms
t_end = t_bbox + 300000 # 300 ms
bbox_mask = np.logical_and(t_start < bounding_boxes['ts'], bounding_boxes['ts'] < t_end)
sample_dict['bbox'] = torch.tensor(bounding_boxes[bbox_mask].tolist())
sample_dict['label'] = labels[bbox_mask]
sample_dict['raw_file'] = rf
# Load raw data around bounding box.
idx_start = data_loader.seek_time(t_start)
data = data_loader.load_delta_t(t_end - t_start)
sample_dict['raw'] = (idx_start, data.size) # offset and number of events
if data.size < 4000:
continue
# Normalize data to same number of events per sample. Therefore, either use subsampling to get
# a larger temporal window or do not to get a more connected graph.
num_samples = params["n_samples"]
if data.size <= num_samples:
sample_idx = np.arange(data.size)
elif params["sampling"]: # sub-sampling -> random N events
sample_idx = np.random.choice(np.arange(data.size), size=num_samples, replace=False)
else: # no sub-sampling -> first N events
sample_idx = np.arange(num_samples)
sample = buffer_to_data(data[sample_idx])
sample_dict['sample_idx'] = sample_idx
# Graph generation (edges w.r.t. sub-sampled graph).
device = torch.device(torch.cuda.current_device())
sample.pos[:, 2] = normalize_time(sample.pos[:, 2])
edge_index = radius_graph(sample.pos.to(device), r=params["r"], max_num_neighbors=params["d_max"])
sample_dict['edge_index'] = edge_index.cpu()
# Store resulting dictionary in file, however, the data only contains the data necessary
# to re-create the graph, not the raw data itself.
os.makedirs(os.path.dirname(processed_file), exist_ok=True)
with open(processed_file, 'wb') as f:
pickle.dump(sample_dict, f)
#########################################################################################################
# Processing ############################################################################################
#########################################################################################################
@staticmethod
def _read_annotations(raw_file: str, skip_ts=int(5e5), min_box_diagonal=60, min_box_side=20) -> np.ndarray:
boxes = np.load(raw_file.replace("_td.dat", "_bbox.npy"))
# Bounding box filtering to avoid dealing with too small or initial bounding boxes.
# See https://github.com/prophesee-ai/prophesee-automotive-dataset-toolbox/blob/master/src/io/box_filtering.py
# Format: (ts, x, y, w, h, class_id, confidence = 1, track_id)
ts = boxes['ts']
width = boxes['w']
height = boxes['h']
diagonal_square = width**2+height**2
mask = (ts > skip_ts)*(diagonal_square >= min_box_diagonal**2)*(width >= min_box_side)*(height >= min_box_side)
return boxes[mask]
@staticmethod
def _read_label(bounding_boxes: np.ndarray) -> List[str]:
class_id = bounding_boxes['class_id'].tolist()
label_dict = {0: "car", 1: "pedestrian"}
return [label_dict.get(cid, None) for cid in class_id]
@staticmethod
def _buffer_to_data(buffer: np.ndarray, **kwargs) -> Data:
x = torch.from_numpy(buffer['x'].astype(np.float32))
y = torch.from_numpy(buffer['y'].astype(np.float32))
t = torch.from_numpy(buffer['t'].astype(np.float32))
p = torch.from_numpy(buffer['p'].astype(np.float32)).view(-1, 1)
pos = torch.stack([x, y, t], dim=1)
return Data(x=p, pos=pos, **kwargs)
def _load_processed_file(self, f_path: str) -> Data:
with open(f_path, 'rb') as f:
data_dict = pickle.load(f)
data_loader = PSEELoader(data_dict['raw_file'])
raw_start, raw_num_events = data_dict['raw']
data_loader.seek_event(raw_start)
data = data_loader.load_n_events(raw_num_events)
data = data[data_dict['sample_idx']]
data = self._buffer_to_data(data, label=data_dict['label'], file_id=f_path)
data.bbox = data_dict['bbox'][:, 1:6].long() # (x, y, w, h, class_id)
data.y = data.bbox[:, -1]
data.pos[:, 2] = normalize_time(data.pos[:, 2]) # time normalization
data.edge_index = data_dict['edge_index']
return data
#########################################################################################################
# Files #################################################################################################
#########################################################################################################
def raw_files(self, mode: str) -> List[str]:
return glob.glob(os.path.join(self.root, mode, "*_td.dat"))
def processed_files(self, mode: str) -> List[str]:
processed_dir = os.path.join(self.root, "processed")
return glob.glob(os.path.join(processed_dir, mode, "*.pkl"))
def _total_bbox_count(self, raw_files: List[str]) -> int:
num_bbox = 0
for rf in raw_files:
bounding_boxes = self._read_annotations(rf)
num_bbox += bounding_boxes.size
return num_bbox
@property
def classes(self) -> List[str]:
return ["car", "pedestrian"]
#########################################################################################################
# Gen1 specific loader function from https://github.com/prophesee-ai/prophesee-automotive-dataset-toolbox
#########################################################################################################
EV_TYPE = [('t', 'u4'), ('_', 'i4')] # Event2D
EV_STRING = 'Event2D'
class PSEELoader(object):
"""
PSEELoader loads a dat or npy file and stream events
"""
def __init__(self, datfile):
"""
ctor
:param datfile: binary dat or npy file
"""
self._extension = datfile.split('.')[-1]
assert self._extension in ["dat", "npy"], 'input file path = {}'.format(datfile)
self._file = open(datfile, "rb")
self._start, self.ev_type, self._ev_size, self._size = _parse_header(self._file)
assert self._ev_size != 0
self._dtype = EV_TYPE
self._decode_dtype = []
for dtype in self._dtype:
if dtype[0] == '_':
self._decode_dtype += [('x', 'u2'), ('y', 'u2'), ('p', 'u1')]
else:
self._decode_dtype.append(dtype)
# size
self._file.seek(0, os.SEEK_END)
self._end = self._file.tell()
self._ev_count = (self._end - self._start) // self._ev_size
self.done = False
self._file.seek(self._start)
# If the current time is t, it means that next event that will be loaded has a
# timestamp superior or equal to t (event with timestamp exactly t is not loaded yet)
self.current_time = 0
self.duration_s = self.total_time() * 1e-6
def reset(self):
"""reset at beginning of file"""
self._file.seek(self._start)
self.done = False
self.current_time = 0
def event_count(self):
"""
getter on event_count
:return:
"""
return self._ev_count
def get_size(self):
""""(height, width) of the imager might be (None, None)"""
return self._size
def __repr__(self):
"""
prints properties
:return:
"""
wrd = ''
wrd += 'PSEELoader:' + '\n'
wrd += '-----------' + '\n'
if self._extension == 'dat':
wrd += 'Event Type: ' + str(EV_STRING) + '\n'
elif self._extension == 'npy':
wrd += 'Event Type: numpy array element\n'
wrd += 'Event Size: ' + str(self._ev_size) + ' bytes\n'
wrd += 'Event Count: ' + str(self._ev_count) + '\n'
wrd += 'Duration: ' + str(self.duration_s) + ' s \n'
wrd += '-----------' + '\n'
return wrd
def load_n_events(self, ev_count):
"""
load batch of n events
:param ev_count: number of events that will be loaded
:return: events
Note that current time will be incremented to reach the timestamp of the first event not loaded yet
"""
event_buffer = np.empty((ev_count + 1,), dtype=self._decode_dtype)
pos = self._file.tell()
count = (self._end - pos) // self._ev_size
if ev_count >= count:
self.done = True
ev_count = count
_stream_td_data(self._file, event_buffer, self._dtype, ev_count)
self.current_time = event_buffer['t'][ev_count - 1] + 1
else:
_stream_td_data(self._file, event_buffer, self._dtype, ev_count + 1)
self.current_time = event_buffer['t'][ev_count]
self._file.seek(pos + ev_count * self._ev_size)
return event_buffer[:ev_count]
def load_delta_t(self, delta_t):
"""
loads a slice of time.
:param delta_t: (us) slice thickness
:return: events
Note that current time will be incremented by delta_t.
If an event is timestamped at exactly current_time it will not be loaded.
"""
if delta_t < 1:
raise ValueError("load_delta_t(): delta_t must be at least 1 micro-second: {}".format(delta_t))
if self.done or (self._file.tell() >= self._end):
self.done = True
return np.empty((0,), dtype=self._decode_dtype)
final_time = self.current_time + delta_t
tmp_time = self.current_time
start = self._file.tell()
pos = start
nevs = 0
batch = 100000
event_buffer = []
# data is read by buffers until enough events are read or until the end of the file
while tmp_time < final_time and pos < self._end:
count = (min(self._end, pos + batch * self._ev_size) - pos) // self._ev_size
buffer = np.empty((count,), dtype=self._decode_dtype)
_stream_td_data(self._file, buffer, self._dtype, count)
tmp_time = buffer['t'][-1]
event_buffer.append(buffer)
nevs += count
pos = self._file.tell()
if tmp_time >= final_time:
self.current_time = final_time
else:
self.current_time = tmp_time + 1
assert len(event_buffer) > 0
idx = np.searchsorted(event_buffer[-1]['t'], final_time)
event_buffer[-1] = event_buffer[-1][:idx]
event_buffer = np.concatenate(event_buffer)
idx = len(event_buffer)
self._file.seek(start + idx * self._ev_size)
self.done = self._file.tell() >= self._end
return event_buffer
def seek_event(self, ev_count):
"""
seek in the file by ev_count events
:param ev_count: seek in the file after ev_count events
Note that current time will be set to the timestamp of the next event.
"""
if ev_count <= 0:
self._file.seek(self._start)
self.current_time = 0
elif ev_count >= self._ev_count:
# we put the cursor one event before and read the last event
# which puts the file cursor at the right place
# current_time is set to the last event timestamp + 1
self._file.seek(self._start + (self._ev_count - 1) * self._ev_size)
self.current_time = np.fromfile(self._file, dtype=self._dtype, count=1)['t'][0] + 1
else:
# we put the cursor at the *ev_count*nth event
self._file.seek(self._start + ev_count * self._ev_size)
# we read the timestamp of the following event (this change the position in the file)
self.current_time = np.fromfile(self._file, dtype=self._dtype, count=1)['t'][0]
# this is why we go back at the right position here
self._file.seek(self._start + ev_count * self._ev_size)
self.done = self._file.tell() >= self._end
def seek_time(self, final_time, term_criterion: int = 100000):
"""
go to the time final_time inside the file. This is implemented using a binary search algorithm
:param final_time: expected time
:param term_criterion: (nb event) binary search termination criterion
it will load those events in a buffer and do a numpy searchsorted so the result is always exact
"""
if final_time > self.total_time():
self._file.seek(self._end)
self.done = True
self.current_time = self.total_time() + 1
return
if final_time <= 0:
self.reset()
return
low = 0
high = self._ev_count
# binary search
while high - low > term_criterion:
middle = (low + high) // 2
self.seek_event(middle)
mid = np.fromfile(self._file, dtype=self._dtype, count=1)['t'][0]
if mid > final_time:
high = middle
elif mid < final_time:
low = middle + 1
else:
self.current_time = final_time
self.done = self._file.tell() >= self._end
return
# we now know that it is between low and high
self.seek_event(low)
final_buffer = np.fromfile(self._file, dtype=self._dtype, count=high - low)['t']
final_index = np.searchsorted(final_buffer, final_time)
self.seek_event(low + final_index)
self.current_time = final_time
self.done = self._file.tell() >= self._end
return low + final_index
def total_time(self):
"""
get total duration of video in mus, providing there is no overflow
:return:
"""
if not self._ev_count:
return 0
# save the state of the class
pos = self._file.tell()
current_time = self.current_time
done = self.done
# read the last event's timestamp
self.seek_event(self._ev_count - 1)
time = np.fromfile(self._file, dtype=self._dtype, count=1)['t'][0]
# restore the state
self._file.seek(pos)
self.current_time = current_time
self.done = done
return time
def __del__(self):
self._file.close()
def _parse_header(f):
"""
Parses the header of a dat file
Args:
- f file handle to a dat file
return :
- int position of the file cursor after the header
- int type of event
- int size of event in bytes
- size (height, width) tuple of int or None
"""
f.seek(0, os.SEEK_SET)
bod = None
end_of_header = False
header = []
num_comment_line = 0
size = [None, None]
# parse header
while not end_of_header:
bod = f.tell()
line = f.readline()
if sys.version_info > (3, 0):
first_item = line.decode("latin-1")[:2]
else:
first_item = line[:2]
if first_item != '% ':
end_of_header = True
else:
words = line.split()
if len(words) > 1:
if words[1] == 'Date':
header += ['Date', words[2] + ' ' + words[3]]
if words[1] == 'Height' or words[1] == b'Height': # compliant with python 3 (and python2)
size[0] = int(words[2])
header += ['Height', words[2]]
if words[1] == 'Width' or words[1] == b'Width': # compliant with python 3 (and python2)
size[1] = int(words[2])
header += ['Width', words[2]]
else:
header += words[1:3]
num_comment_line += 1
# parse data
f.seek(bod, os.SEEK_SET)
if num_comment_line > 0: # Ensure compatibility with previous files.
# Read event type
ev_type = np.frombuffer(f.read(1), dtype=np.uint8)[0]
# Read event size
ev_size = np.frombuffer(f.read(1), dtype=np.uint8)[0]
else:
ev_type = 0
ev_size = sum([int(n[-1]) for _, n in EV_TYPE])
bod = f.tell()
return bod, ev_type, ev_size, size
def _stream_td_data(file_handle, buffer, dtype, ev_count=-1):
"""
Streams data from opened file_handle
args :
- file_handle: file object
- buffer: pre-allocated buffer to fill with events
- dtype: expected fields
- ev_count: number of events
"""
dat = np.fromfile(file_handle, dtype=dtype, count=ev_count)
count = len(dat['t'])
for name, _ in dtype:
if name == '_':
buffer['x'][:count] = np.bitwise_and(dat["_"], 16383)
buffer['y'][:count] = np.right_shift(np.bitwise_and(dat["_"], 268419072), 14)
buffer['p'][:count] = np.right_shift( | np.bitwise_and(dat["_"], 268435456) | numpy.bitwise_and |
import random
import numpy as np
from sklearn.model_selection import StratifiedKFold
def run_neural_network(prepare_input_data, build_neural_network, evaluate_model):
"""
Performs cross validation for the clean classifier, using 5 splits.
:param prepare_input_data: callback to prepare input data
:param build_neural_network: callback to build the neural network
:param evaluate_model: callback to prepare and evaluate the model
:return:
"""
input_data = prepare_input_data()
random.shuffle(input_data)
images = [elem['data'] for elem in input_data]
labels = [elem['image_type'] for elem in input_data]
images = np.array(images)
labels = np.array(labels)
kfold = StratifiedKFold(n_splits=5, shuffle=True, random_state=None)
cvscores = []
cvlosses = []
i = 0
for train, test in kfold.split(images, labels):
i += 1
print("cross validation: ", i)
model = build_neural_network()
val_loss, val_acc = evaluate_model(model, images[test], labels[test], images[train], labels[train])
print('Loss value ' + str(val_loss))
print('Accuracy ' + str(val_acc))
cvscores.append(val_acc * 100)
cvlosses.append(val_loss)
print("%.2f%% (+/- %.2f%%)" % ( | np.mean(cvscores) | numpy.mean |
"""
Command line scripts for managing HDeepRM experiments.
"""
import argparse as ap
import csv
import json
import os
import os.path as path
import random as rnd
import subprocess as sp
import evalys.jobset as ej
import evalys.utils as eu
import evalys.visu.core as evc
import evalys.visu.gantt as evg
import evalys.visu.legacy as evleg
import evalys.visu.lifecycle as evl
import evalys.visu.series as evs
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
import numpy as np
from hdeeprm.util import generate_workload, generate_platform
def launch() -> None:
"""Utility for launching HDeepRM experiments.
It takes care of creating the Platform XML file, the Workload JSON file and the Resource Hierarcy.
It also runs both Batsim and PyBatsim.
Command line arguments:
| ``options_file`` - Options file in JSON.
| ``customworkload`` - (Optional) Path to the custom workload in case one is used.
| ``agent`` (Optional) File with the learning agent definition.
| ``inmodel`` - (Optional) Path for previous model loading.
| ``outmodel`` - (Optional) Path for saving new model.
| ``nbruns`` - (Optional) Number of train runs for the learning agent.
"""
parser = ap.ArgumentParser(description='Launches HDeepRM experiments')
parser.add_argument('options_file', type=str, help='Options file defining the experiment')
parser.add_argument('-cw', '--customworkload', type=str, help='Path for the custom workload')
parser.add_argument('-a', '--agent', type=str, help='File with the learning agent definition')
parser.add_argument('-im', '--inmodel', type=str, help='Path for previous model loading')
parser.add_argument('-om', '--outmodel', type=str, help='Path for saving new model')
parser.add_argument('-nr', '--nbruns', type=int, default=1,
help='Number of simulations to be run')
args = parser.parse_args()
# Load the options
print('Loading options')
with open(args.options_file, 'r') as in_f:
options = json.load(in_f)
options['pybatsim']['seed'] = options['seed']
if args.agent:
options['pybatsim']['agent']['file'] = path.abspath(args.agent)
if args.inmodel:
options['pybatsim']['agent']['input_model'] = path.abspath(args.inmodel)
if args.outmodel:
options['pybatsim']['agent']['output_model'] = path.abspath(args.outmodel)
# Set the seed for random libraries
print('Setting the random seed')
rnd.seed(options['seed'])
np.random.seed(options['seed'])
# Check if Platform, Resource Hierarchy, Workload and Job Limits are already present
files = ('platform.xml', 'res_hierarchy.pkl', 'workload.json', 'job_limits.pkl')
skipped = []
present = {}
for fil in files:
if path.isfile(f'./{fil}'):
present[fil] = True
else:
present[fil] = False
# Generate the Platform and Resource Hierarchy
new_reference_speed = False
if not present['platform.xml'] or not present['res_hierarchy.pkl']:
if not present['platform.xml']:
print('Generating platform XML')
else:
skipped.append('platform')
if not present['res_hierarchy.pkl']:
print('Generating resource hierarchy')
new_reference_speed = True
else:
skipped.append('res_hierarchy')
generate_platform(options['platform_file_path'],
gen_platform_xml=not present['platform.xml'],
gen_res_hierarchy=not present['res_hierarchy.pkl'])
print('Saved "platform.xml" and "res_hierarchy.pkl" in current directory')
else:
skipped.extend(('platform', 'res_hierarchy'))
# If a custom workload is provided, just calculate the operations with respect to the reference
# speed
if args.customworkload:
print(f'Utilizing {args.customworkload} as workload, adjusting operations')
generate_workload(options['workload_file_path'], options['nb_resources'],
options['nb_jobs'], custom_workload_path=args.customworkload)
print('Saved "workload.json" and "job_limits.pkl" in current directory')
# Generate the Workload
elif not present['workload.json'] or not present['job_limits.pkl'] or new_reference_speed:
print('Generating workload JSON and job limits')
generate_workload(options['workload_file_path'], options['nb_resources'],
options['nb_jobs'])
print('Saved "workload.json" and "job_limits.pkl" in current directory')
else:
skipped.extend(('workload', 'job_limits'))
for skip in skipped:
print(f'Skipping {skip} generation - Using file in current directory')
# Launch both PyBatsim and Batsim instances for running the simulation
for _ in range(args.nbruns):
with open('pybatsim.log', 'w+') as out_f:
pybs = sp.Popen(('pybatsim', '-o', json.dumps(options['pybatsim']),
path.join(path.dirname(path.realpath(__file__)),
'entrypoints/HDeepRMWorkloadManager.py')),
stdout=out_f, stderr=out_f)
sp.run(('batsim', '-E', '-w', 'workload.json', '-p', 'platform.xml'), check=True)
pybs.communicate()
def visual() -> None:
"""Utility for analysing stats from HDeepRM outcomes.
It utilizes `Evalys <https://gitlab.inria.fr/batsim/evalys>`_ for plotting useful information from
output files. Supports *queue_size*, *utilization*, *lifecycle*, *gantt*, *gantt_no_label*,
*core_bubbles*, *mem_bubbles*, *mem_bw_overutilization*, *losses*, *rewards*, *action_preferences*.
Command line arguments:
| ``visualization`` - Type of visualization to be printed.
| ``save`` - (Optional) Save the plot in the provided file path.
"""
parser = ap.ArgumentParser(description='Plots information about the simulation outcome')
parser.add_argument('visualization', type=str,
choices=('queue_size', 'utilization', 'lifecycle', 'gantt',
'gantt_no_label', 'core_bubbles', 'mem_bubbles',
'mem_bw_overutilization', 'losses', 'rewards', 'action_preferences'),
help='Statistic to visualise')
parser.add_argument('-s', '--save', type=str, help='Save the plot in the specified file path')
args = parser.parse_args()
# Obtain the title for the plots given the agent configuration
with open('./options.json', 'r') as in_f:
options = json.load(in_f)
agent_options = options['pybatsim']['agent']
env_options = options['pybatsim']['env']
if agent_options['type'] == 'CLASSIC':
job_sel, core_sels = tuple(
env_options["actions"]["selection"][0].items()
)[0]
title = f'{agent_options["type"]} {job_sel}-{core_sels[0]}'
elif agent_options['type'] == 'LEARNING':
title = (f'{agent_options["type"]} {agent_options["run"]} {agent_options["hidden"]} '
f'{agent_options["lr"]} {agent_options["gamma"]}')
else:
raise ValueError('Invalid agent type in "options.json"')
# Job visualizations
if args.visualization in ('queue_size', 'utilization', 'lifecycle', 'gantt', 'gantt_no_label'):
jobset = ej.JobSet.from_csv('./out_jobs.csv', resource_bounds=(0, options['nb_resources']))
if args.visualization == 'queue_size':
_fixed_plot_series(jobset, name='queue', title=f'Queue size over time for {title}',
legend_label='Queue size')
plt.xlabel('Simulation time')
plt.ylabel('Pending jobs')
elif args.visualization == 'utilization':
_fixed_plot_series(jobset, name='utilization', title=f'Utilization over time for {title}',
legend_label='Load')
plt.xlabel('Simulation time')
plt.ylabel('Active cores')
elif args.visualization == 'lifecycle':
evl.plot_lifecycle(jobset, title=f'Job lifecycle for {title}')
elif args.visualization == 'gantt':
evg.plot_gantt(jobset, title=f'Gantt chart for {title}')
plt.xlabel('Simulation time')
elif args.visualization == 'gantt_no_label':
evg.plot_gantt(jobset, title=f'Gantt chart for {title}', labeler=lambda _: '')
plt.xlabel('Simulation time')
# Over-utilization visualizations
elif args.visualization in ('core_bubbles', 'mem_bubbles', 'mem_bw_overutilization'):
with open('overutilizations.json', 'r') as in_f:
overutilizations = json.load(in_f)
with open('out_schedule.csv', 'r') as in_f:
_, values = [row for row in csv.reader(in_f, delimiter=',')]
makespan = float(values[2])
_, ax = plt.subplots()
ax.set_xlim(0, makespan)
ax.set_xlabel('Simulation time')
ax.grid(True)
if args.visualization == 'core_bubbles':
ax.set_title(f'Core bubbles for {title}')
ax.set_ylim(0, len(overutilizations['core']))
ax.plot(overutilizations['core'], range(len(overutilizations['core'])))
elif args.visualization == 'mem_bubbles':
ax.set_title(f'Memory bubbles for {title}')
ax.set_ylim(0, len(overutilizations['mem']))
ax.plot(overutilizations['mem'], range(len(overutilizations['mem'])))
elif args.visualization == 'mem_bw_overutilization':
ax.set_title(f'Mem BW overutilization spans for {title}')
ax.set_ylim(0, overutilizations['mem_bw']['nb_procs'])
ax.set_ylabel('Processors')
for proc_id in overutilizations['mem_bw']['procs']:
ax.broken_barh(overutilizations['mem_bw']['procs'][proc_id]['values'],
(int(proc_id), int(proc_id)))
# Learning visualizations
elif args.visualization in ('losses', 'rewards', 'action_preferences'):
_, ax = plt.subplots()
ax.set_xlabel('Episodes')
ax.grid(True)
if args.visualization == 'losses':
with open('losses.log', 'r') as in_f:
losses = in_f.readlines()
ax.set_title(f'Loss evolution for {title}')
ax.set_ylabel('Loss value')
ax.plot(tuple(range(len(losses))), tuple(map(float, losses)), 'r')
elif args.visualization == 'rewards':
with open('rewards.log', 'r') as in_f:
rewards = in_f.readlines()
ax.set_title(f'Reward evolution for {title}')
ax.set_ylabel('Reward')
ax.plot(tuple(range(len(rewards))), tuple(map(float, rewards)))
elif args.visualization == 'action_preferences':
ax.set_title(f'Action preferences for {title}')
ax.set_ylabel('Probability')
action_names = []
for sel in env_options['actions']['selection']:
for job_sel, core_sels in sel.items():
for core_sel in core_sels:
action_names.append(f'{job_sel}-{core_sel}')
with open('probs.json', 'r') as in_f:
all_probs = json.load(in_f)['probs']
for action_name, probs in zip(action_names, all_probs):
ax.plot(tuple(range(len(probs))), tuple(map(float, probs)), label=action_name)
ax.legend()
ax.set_ylim(bottom=0)
if args.save:
plt.savefig(args.save, format='svg', dpi=1200)
plt.show()
def metrics() -> None:
"""Utility for comparing metrics between simulation runs.
Plots a grid with different metrics resulting from *out_schedule.csv*.
Command line arguments:
| ``res1`` - *out_schedule.csv* file from run 1.
| ``res2`` - *out_schedule.csv* file from run 2.
"""
parser = ap.ArgumentParser(description='Compares metrics between simulation runs')
parser.add_argument('res1', type=str, help='out_schedule.csv file from run 1')
parser.add_argument('res2', type=str, help='out_schedule.csv file from run 2')
parser.add_argument('-n1', '--name1', type=str, default='A1',
help='Name of the first policy or agent')
parser.add_argument('-n2', '--name2', type=str, default='A2',
help='Name of the second policy or agent')
parser.add_argument('-s', '--save', type=str, help='Save the plot in the specified file path')
args = parser.parse_args()
with open(args.res1, 'r') as res1_f,\
open(args.res2, 'r') as res2_f:
res1_reader = csv.reader(res1_f, delimiter=',')
res2_reader = csv.reader(res2_f, delimiter=',')
names = ('Energy (joules)', 'Makespan', 'Max. slowdown', 'Max. turnaround time',
'Max. waiting time', 'Mean slowdown', 'Mean turnaround time', 'Mean waiting time')
_, res1_metrics = [row for row in res1_reader]
_, res2_metrics = [row for row in res2_reader]
_, axes = plt.subplots(nrows=2, ncols=4, constrained_layout=True, figsize=(12, 4))
for i, row in enumerate(axes):
for j, col in enumerate(row):
metric1 = float(res1_metrics[i * 4 + j + 1])
metric2 = float(res2_metrics[i * 4 + j + 1])
x = (1, 2)
bars = col.bar(x, (metric1, metric2), color=('green', 'blue'),
edgecolor='black', width=0.6)
col.set_xlim(0, 3)
col.set_ylim(0, max(1.0, 1.15 * max(metric1, metric2)))
col.set_xticks(x)
col.set_xticklabels((args.name1, args.name2))
col.yaxis.set_major_formatter(mtick.ScalarFormatter())
col.ticklabel_format(axis='y', style='sci', scilimits=(0, 3))
for rect in bars:
height = rect.get_height()
if height >= 1e7:
text = f'{height:.2e}'
else:
text = f'{int(height)}'
col.text(rect.get_x() + rect.get_width() / 2.0, height,
text, ha='center', va='bottom')
col.set_title(names[i * 4 + j])
if args.save:
plt.savefig(args.save, format='svg', dpi=1200)
plt.show()
def jobstat() -> None:
"""Utility for obtaining statistics about jobs in the workload trace.
It can calculate several statistics by parsing the *workload.json* file.
Command line arguments:
| ``workload`` - *workload.json* with the trace.
| ``datafield`` - Job data field from which to obtain information. One of *req_time*, *size*,
*mem* or *mem_bw*.
| ``statistic`` - Statistic to be calculated. One of *min*, *max*, *mean*, *median*, *p95* or
*p99*.
"""
parser = ap.ArgumentParser(description='Compares metrics between simulation runs')
parser.add_argument('workload', type=str, help='JSON workload file with the trace')
parser.add_argument('datafield', type=str, choices=('req_time', 'size', 'mem', 'mem_bw'),
help='Job data field from which to obtain information')
parser.add_argument('statistic', type=str,
choices=('min', 'max', 'mean', 'median', 'p95', 'p99'),
help='Statistic to be calculated')
args = parser.parse_args()
with open(args.workload, 'r') as in_f:
workload = json.load(in_f)
if args.datafield == 'size':
data = np.array(
[job['res'] for job in workload['jobs']]
)
else:
data = np.array(
[workload['profiles'][job['profile']][args.datafield] for job in workload['jobs']]
)
if args.statistic == 'min':
print( | np.min(data) | numpy.min |
# coding=utf-8
"""
Module containing definition of a gym_acnsim observation and factory
functions for different builtin observations.
See the SimObservation docstring for more information on the
SimObservation class.
Each factory function takes no arguments and returns an instance of type
SimObservation. Each factory function defines a space_function and and
an obs_function with the following signatures:
space_function: Callable[[GymInterface], spaces.Space]
obs_function: Callable[[GymInterface], np.ndarray]
The space_function gives a gym space for a given observation type.
The obs_function gives a gym observation for a given observation type.
The observation returned by obs_function is a point in the space
returned by space_function.
"""
from typing import Callable
import numpy as np
from gym import spaces
from acnportal.acnsim import EV
from ..interfaces import GymTrainedInterface
class SimObservation:
"""
Class representing an OpenAI Gym observation of an ACN-Sim
simulation.
An instance of SimObservation contains a space_function, which
generates a gym space from an input Interface using attributes and
functions of the input Interface, and an obs_function, which
generates a gym observation from an input Interface using attributes
and functions of the input Interface. Each instance also requires a
name (given as a string).
This class enables Simulation environments with customizable
observations, as a SimObservation object with user-defined or built
in space and obs functions can be input to a BaseSimEnv-like object
to enable a new observation without creating a new environment.
Each type of observation is the same type of object, but the details
of the space and obs functions are different. This was done because
space and obs functions are static, as observations of a specific
type do not have any attributes. However, each observation type
requires both a space and observation generating function, so a
wrapping data structure is required.
Attributes:
_space_function (Callable[[GymInterface], spaces.Space]):
Function that accepts a GymInterface and generates a gym
space in which all observations for this instance exist.
_obs_function (Callable[[GymInterface], np.ndarray]): Function
that accepts a GymInterface and generates a gym observation
based on the input interface.
name (str): Name of this observation. This attribute allows an
environment to distinguish between different types of
observation.
"""
_space_function: Callable[[GymTrainedInterface], spaces.Space]
_obs_function: Callable[[GymTrainedInterface], np.ndarray]
name: str
def __init__(
self,
space_function: Callable[[GymTrainedInterface], spaces.Space],
obs_function: Callable[[GymTrainedInterface], np.ndarray],
name: str,
) -> None:
"""
Args:
space_function (Callable[[GymInterface], spaces.Space]):
Function that accepts a GymInterface and generates a
gym space in which all observations for this instance
exist.
obs_function (Callable[[GymInterface], np.ndarray]):
Function that accepts a GymInterface and generates a
gym observation based on the input interface.
name (str): Name of this observation. This attribute allows
an environment to distinguish between different types of
observation.
Returns:
None.
"""
self._space_function = space_function
self._obs_function = obs_function
self.name = name
def get_space(self, interface: GymTrainedInterface) -> spaces.Space:
"""
Returns the gym space in which all observations for this
observation type exist. The characteristics of the interface
(for example, number of EVSEs if station demands are observed)
may change the dimensions of the returned space, so this method
requires a GymInterface as input.
Args:
interface (GymTrainedInterface): Interface to an ACN-Sim Simulation
that contains details of and functions to generate
details about the current Simulation.
Returns:
spaces.Space: A gym space in which all observations for this
observation type exist.
"""
return self._space_function(interface)
def get_obs(self, interface: GymTrainedInterface) -> np.ndarray:
"""
Returns a gym observation for the state of the simulation given
by interface. The exact observation depends on both the input
interface and the observation generating function obs_func with
which this object was initialized.
Args:
interface (GymTrainedInterface): Interface to an ACN-Sim Simulation
that contains details of and functions to generate
details about the current Simulation.
Returns:
np.ndarray: A gym observation generated by _obs_function
with this interface.
"""
return self._obs_function(interface)
# Per active EV observation factory functions. Note that all EV data
# is shifted up by 1, as 0's indicate no EV is plugged in.
def _ev_observation(
attribute_function: Callable[[GymTrainedInterface, EV], float], name: str
) -> SimObservation:
# noinspection PyMissingOrEmptyDocstring
def space_function(interface: GymTrainedInterface) -> spaces.Space:
return spaces.Box(
low=0, high=np.inf, shape=(len(interface.station_ids),), dtype="float"
)
# noinspection PyMissingOrEmptyDocstring
def obs_function(interface: GymTrainedInterface) -> np.ndarray:
attribute_values: dict = {station_id: 0 for station_id in interface.station_ids}
for ev in interface.active_evs:
attribute_values[ev.station_id] = attribute_function(interface, ev) + 1
return np.array(list(attribute_values.values()))
return SimObservation(space_function, obs_function, name=name)
def arrival_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe active EV arrivals.
Zeros in the output observation array indicate no EV is plugged in;
as such, all observations are shifted up by 1.
"""
return _ev_observation(lambda _, ev: ev.arrival, "arrivals")
def departure_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe active EV departures.
Zeros in the output observation array indicate no EV is plugged in;
as such, all observations are shifted up by 1.
"""
return _ev_observation(lambda _, ev: ev.departure, "departures")
def remaining_demand_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe active EV remaining energy demands in amp periods.
Zeros in the output observation array indicate no EV is plugged in;
as such, all observations are shifted up by 1.
"""
return _ev_observation(
lambda interface, ev: interface.remaining_amp_periods(ev), "demands"
)
# Network-wide observation factory functions.
def _constraints_observation(attribute: str, name: str) -> SimObservation:
# noinspection PyMissingOrEmptyDocstring
def space_function(interface: GymTrainedInterface) -> spaces.Space:
return spaces.Box(
low=-np.inf,
high=np.inf,
shape=getattr(interface.get_constraints(), attribute).shape,
dtype="float",
)
# noinspection PyMissingOrEmptyDocstring
def obs_function(interface: GymTrainedInterface) -> np.ndarray:
return getattr(interface.get_constraints(), attribute)
return SimObservation(space_function, obs_function, name=name)
def constraint_matrix_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe the network constraint matrix.
"""
return _constraints_observation("constraint_matrix", "constraint matrix")
def magnitudes_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe the network limiting current magnitudes in amps.
"""
return _constraints_observation("magnitudes", "magnitudes")
def phases_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe the network phases.
"""
# noinspection PyMissingOrEmptyDocstring
def space_function(interface: GymTrainedInterface) -> spaces.Space:
return spaces.Box(
low=-np.inf,
high=np.inf,
shape=interface.infrastructure_info().phases.shape,
dtype="float",
)
# noinspection PyMissingOrEmptyDocstring
def obs_function(interface: GymTrainedInterface) -> np.ndarray:
return interface.infrastructure_info().phases
return SimObservation(space_function, obs_function, name="phases")
def timestep_observation() -> SimObservation:
""" Generates a SimObservation instance that wraps functions to
observe the current timestep of the simulation, in periods.
To comply with the timesteps returned by arrival and departure
observations, the observed timestep is one greater than than that
returned by the simulation. Simulations thus start at timestep 1
from an RL agent's perspective.
"""
# noinspection PyUnusedLocal
# noinspection PyMissingOrEmptyDocstring
def space_function(interface: GymTrainedInterface) -> spaces.Space:
return spaces.Box(low=0, high=np.inf, shape=(1,), dtype="float")
# noinspection PyMissingOrEmptyDocstring
def obs_function(interface: GymTrainedInterface) -> np.ndarray:
return | np.array(interface.current_time + 1) | numpy.array |
import numpy as np
import icesatUtils as iu
def index_1d_pd(x, bins):
import pandas as pd
df = pd.DataFrame({'x': x})
edges = [b[0] for b in bins] + [bins[-1][1]]
df['x_range'] = pd.cut(df['x'], edges)
d = df.groupby('x_range').indices
x_index = []
for i, key in enumerate(d):
index = d[key]
if len(index) > 0:
x_index.append([i, index])
return x_index
def bin_linear(x, bins, loading_bar=False):
return index_1d(x, bins, loading_bar)
def index_1d(x, bins, loading_bar=False):
"""
Function to support variable binning in 1d.
Meant to be used in combination with bin_1d.
Bins are created with bin_1d, but useful bin
indices are made by this function.
Input:
x - array to be indexed
bins - array of start/end times/locations,
such as [[-1,1],[1,3],[3,5],...]
Output:
a bin index for every x value, so,
for instance, given bins
[[-1,1],[1,3],[3,5]], and x is
np.linspace(-1,5), then all x values
within [-1,1] will be given a value of
0, all x-values within [1,3] are given
a value of 1, and all x-values within
[3,5] are given a value of 2, such as
[0,0,0,...,1,1,1,...,2,2,2]
Example:
# print values of x within bins
# defined by A
import region_detect as rd
import numpy as np
A = [0,2,4,6,8]
x = np.linspace(0,10)
bins = rd.bin_1d(A,2)
index = rd.index_1d(x,bins)
for i in sorted(np.unique(index)):
if i != -1:
b = index == i
print(x[b])
Caveats:
For cases when x is exactly equal to
bin edges, the index will favor the
left side of the bin. i.e.,
import region_detect as rd
import numpy as np
A = [0,2,4,6,8]
x = np.arange(10)
bins = rd.bin_1d(A,2)
index = rd.index_1d(x,bins)
print(index)
print(x)
-1 is assigned to values of x that
do not fall in a bin
Function does not handle nans
"""
# import pandas as pd
# df = pd.DataFrame({'x': x})
# edges = [b[0] for b in bins] + [bins[-1][1]]
# df['x_range'] = pd.cut(df['x'], edges)
# d = df.groupby('x_range').indices
# x_index = []
# for i, key in enumerate(d):
# index = d[key]
# if len(index) > 0:
# x_index.append([i, index])
if np.isnan(x).any():
raise ValueError('nans cannot exist in x')
index_s = np.argsort(x)
index_s_rev = np.argsort(index_s)
x_s = x[index_s]
if ((x_s[index_s_rev] - x) != 0.0).any():
# check that x_s is increasing, and sorting
# is reversible
raise ValueError('indexing error')
n = len(x_s)
iterator = range(n)
if loading_bar:
from tqdm import tqdm
iterator = tqdm(range(n))
i_index_s = np.full(n,-1).astype(int)
in_reg = 0 # in region
i = 0 # bin index
for j in iterator:
if bins[i][0] <= x_s[j] <= bins[i][1]:
# i_index_s[index_s_rev[j]] = i
i_index_s[j] = i
in_reg = 1
else:
if in_reg:
in_reg = 0
if i < len(bins)-1:
i += 1
if bins[i][0] <= x_s[j] <= bins[i][1]:
# i_index_s[index_s_rev[j]] = i
i_index_s[j] = i
else:
break
else:
# skipped over region
while x_s[j] > bins[i][1]:
if i < len(bins)-1:
i += 1
else:
break
i_index = i_index_s[index_s_rev]
return i_index
def bin_reg(A, dx, safety_factor=1.5, check_overlap=False):
return bin_1d(A, dx, safety_factor, check_overlap)
def bin_1d(A, dx, safety_factor=1.5, check_overlap=False):
"""
Function to create variable 1d binning.
This works similar to find_region, except that
this bins exact lengths, based on the separation
in values of A. For example,
A = [0,2,4,6,8,...]
dx = 2 ,
bin_reg will output
[[-1,1],[1,3],[3,5],[5,7],[7,9],...]
The advantage is that, if values were unevenly
spaced (as in ATL08), then one could set
A = df_08['alongtrack']
dx = 100 # meters
bin_reg() will make 1d regions of nearly
100m length; dx is more for what happens
when values are truly spaced much further
than 100m
Input:
A - numpy array, already increasing
dx - some nominal change in A values
safety_factor - if a change in A far exceeds
the given dx, then the binning code
will simply bin that as if it were
a 1km ATL08 bin; we know that not
to be the case, but we know the
upper limit of 08 bins to be around
105m(?). So, one can set this
to specify what the error might be.
check_overlap - bins should never overlap (up to roundoff),
so this performs an additional check to
ensure this is the case, and notifies the
user otherwise
Output:
binned regions,
return bin_dim
"""
def check_bin_overlap(bins, tag, loading_bar=False, tol=1e-6):
print('checking bin overlap for %s..' % tag)
iterator = range(len(bins))
if loading_bar and tqdm_found:
from tqdm import tqdm
iterator = tqdm(range(len(bins)))
# for i, bin1 in enumerate(bins):
for i in iterator:
bin1 = bins[i]
for j, bin2 in enumerate(bins):
if i == j:
continue
# bin1, bin2 = list(np.round(bin1,8)), list(np.round(bin2,8))
reg = rd.inner_region(bin1, bin2, equal=False)
if reg != []:
if reg[1]-reg[0] < tol:
continue
print('warning: %s overlap' % tag)
print(i, bin1)
print(j, bin2)
print(reg)
iu.pause()
if (np.diff(A) < 0.0).any():
print('warning: x decreasing')
if dx <= 0:
print('warning: dx < 0')
A = np.append(min(A) - dx, A)
A = np.append(A, max(A) + dx)
dx_lim = safety_factor*dx
bin_dim = []
for j in range(1,len(A)-1):
da1 = A[j] - A[j-1]
da2 = A[j+1] - A[j]
if da1 > dx_lim:
da1 = dx_lim
if da2 > dx_lim:
da2 = dx_lim
bin_dim.append([A[j] - da1/2.0, A[j] + da2/2.0])
if check_overlap:
check_bin_overlap(bin_dim, 'debug', loading_bar=True, tol=1e-6)
return bin_dim
def calc_overlap(x1, x2, dx, dx_overlap=0.0, dx2=None):
"""
Find the inner overlaps between arrays x1 and x2
Input:
x1 - 1d array
x2 - 1d array
dx - change in x assigned to each point
dx_overlap - addition on either end of each region
dx2 - optional dx for x2, if sampling rates are different
i.e. overlaps in 03 and 08
Output
return regions_overlap
regions of start/end ttg
Example:
import region_detect as rd
# ... load in 03 data
dt0 = 1.0 / 10000 # change in time for ATL03
regions_overlap = rd.calc_overlap(t1, t2, 10*dt0, dt0)
"""
dt1 = dx
regions2, _ = find_region(x2, dx, dx_overlap)
regions2 = combine_region(regions2)
if type(dx2) == type(None):
dx2 = dx
regions1, _ = find_region(x1, dx2, dx_overlap)
regions1 = combine_region(regions1)
regions_overlap = []
for reg2 in regions2:
for reg1 in regions1:
reg = inner_region(reg2, reg1)
if reg != []:
regions_overlap.append(reg)
return regions_overlap
def filt_reg_x(x, regions, equal=False):
"""
Given a list of regions, this
filters the array x for only values
in those regions.
Input:
x - numpy array of values
regions - list of 1d start/end values
i.e., [[t0,t1], [t2,t3], ...]
equal - boolean to include end-points in 1d
regions or not
Output:
the filtered x values and corresponding
boolean indexing, which may concievably
be applied to some corresponding y values
return x_filt, jr
Example:
import numpy as np
import region_detect as rd
x = np.linspace(0,10)
y = np.linspace(10,20)
regions = [[0,3], [6,7]]
x_filt, jr = rd.filt_reg_x(x, regions)
y_filt = y[jr]
# x_filt contains values only within (0,3)
# and (6,7)
"""
if type(x) != np.ndarray:
x = | np.array(x) | numpy.array |
# -*- coding: utf-8 -*-
"""
Master Thesis <NAME>
Data File
"""
###############################################################################
## IMPORT PACKAGES & SCRIPTS ##
###############################################################################
### PACKAGES ###
import numpy as np
import pandas as pd
from scipy.stats import norm
from scipy.linalg import block_diag
import pickle as pkl
import os
### SCRIPTS ###
import param as pm
import forecast as fcst
###############################################################################
## FUNCTIONS DEFINITIONS ##
###############################################################################
### EXPORT / IMPORT SOLUTIONS TO PKL FILE ###
def sol_export(filename, data):
rltDir = 'rlt/case%s_t%s_loadVar%s_pvVar%s_%s_cc%s_drcc%s_flx%s_%s_bat%s/'\
%(pm.N_BUS,pm.T,pm.FLGVAR_LOAD,pm.FLGVAR_PV,pm.FCSTCASE[0],\
pm.FLGCC, pm.FLGDRCC,pm.FLGSHIFT,pm.UNBALANCE,pm.FLGBAT)
if os.path.exists(rltDir):
output = open(rltDir + filename + ".pkl", 'wb') # create output file
pkl.dump(data, output) # write data to output file
output.close() # close output file
else:
os.mkdir(rltDir) # create new directory
output = open(rltDir + filename + ".pkl", 'wb') # create output file
pkl.dump(data, output) # write data to output file
output.close() # close output file
def sol_import(filename):
rltDir = 'rlt/case%s_t%s_loadVar%s_pvVar%s_%s_cc%s_drcc%s_flx%s_%s_bat%s/'\
%(pm.N_BUS,pm.T,pm.FLGVAR_LOAD,pm.FLGVAR_PV,pm.FCSTCASE[0],\
pm.FLGCC, pm.FLGDRCC,pm.FLGSHIFT,pm.UNBALANCE,pm.FLGBAT)
file = open(rltDir + filename + ".pkl", 'rb') # open results file
tmp = pkl.load(file) # create arry from file
file.close() # close file
return tmp
### SYMMETRIC INPUT DATA FOR N PHASES ###
def phase_multiplication(data):
dim = len(data) # get dimension of input data
phase = np.ones((pm.N_PH)) # array to multiply input with number of phases
tmp = []
for i in range(dim):
tmp = np.append(tmp,data[i]*phase, axis=0)
return tmp
###############################################################################
## READ DATA FROM CSV FILES ##
###############################################################################
def read_data(src):
srcDir = 'src/case%s/'%pm.N_BUS
return pd.read_csv(srcDir + src +"Data.csv", delimiter=',')
busData = read_data('bus')
branchData = read_data('branch')
costData = read_data('cost')
impData = read_data('imp')
loadData = read_data('load')
batData = read_data('bat')
genData = read_data('gen')
invData = read_data('inv')
oltcData = read_data('oltc')
###############################################################################
## AUXILIARX PARAMETERS ##
###############################################################################
n = len(busData) # number of nodes
l = len(branchData) # number of branches
loadCase = len(pm.LOADCASE)
pvCase = len(pm.PVCASE)
vBase = busData.values[:,1] # base value voltage [kV]
zBase = (vBase*1e3)**2/(pm.S_BASE*1e6) # base value impedance [Ohm]
iBase = pm.S_BASE*1e6/(vBase[1:]*1e3) # base value current [A]
###############################################################################
## GRID DATA ##
###############################################################################
### VOLTAGES ###
class bus:
# reference voltage at slack node magnitude
vSlack = busData.values[0,4]
# voltage phasors
a = np.exp(1j*120*np.pi/180) # symmetrical components operator
if pm.N_PH == 3:
phasor_slack = np.array([1,a**2,a]) # slack voltage phasor
phasor_rot = np.array([1,a,a**2]) # rotation phasor
else:
phasor_slack = np.array([1]) # slack voltage phasor
phasor_rot = np.array([1]) # rotation phasor
# slack voltage real & imag part
vSlackRe = np.tile(vSlack*np.real(phasor_slack[0:pm.N_PH]),n)
vSlackIm = np.tile(vSlack*np.imag(phasor_slack[0:pm.N_PH]),n)
# rotation of voltage phasor real & imag part
rotRe = np.tile(np.real(phasor_rot[0:pm.N_PH]),n)
rotIm = np.tile(np.imag(phasor_rot[0:pm.N_PH]),n)
# VUF
vufMax = busData.values[:,6] # maximum vuf [-]
# bounds
vBus_ub = phase_multiplication(busData.values[:,3]) # upper bound
vBus_lb = phase_multiplication(busData.values[:,2]) # lower bound
### BRANCHES ###
class branch:
# stacked impedance matrix
def z_stack(config):
zBr = np.zeros((l,pm.N_PH,pm.N_PH), dtype=complex) # pre-allocate
length = branchData.values[:,5] # length of branch [km]
data = (impData.values[:,1:].astype(float)) # impedance [Ohm/km]
for k in range(l):
idx = int(np.where(impData.values[:,0] == config[k])[0])
tmp = data[idx:idx+pm.N_PH,:]/zBase[k+1] # array with R & X for branch k [p.u.]
zBr[k,:,:] = np.array([[tmp[i,j] + 1j*tmp[i,j+1] for j in range(0,2*pm.N_PH,2)]\
for i in range(pm.N_PH)])*length[k] # impedance
return zBr
fbus = branchData.values[:,2].astype(int) # from bus
tbus = branchData.values[:,3].astype(int) # to bus
zBrStacked = z_stack(branchData.values[:,1]) # stacked impedance matrix
zBr = block_diag(*zBrStacked) # (block) diagonal matrix with impedances
rBr = np.real(zBr) # diagonal matrix with resistances
xBr = np.imag(zBr) # diagonal matrix with reactances
# bounds
iBr_ub = phase_multiplication(branchData.values[:,4]/iBase) # thermal limit [p.u.]
### SETS OF NODES ###
class sets:
bat = list(np.where(batData.values[:,1]>0,1,0)) # battery node
flx = list(np.where(loadData.values[:,3]!=0,1,0)) # flexible loads
flxPhase = list(phase_multiplication(flx).astype(int)) # extended for n phases
ren = list(np.where(loadData.values[:,1]>0,1,0)) # renewable generators
# list with location of sets
def idx_list(data, rng):
tmp = [i for i in range(rng) if data[i] == 1]
return tmp
idxRen = idx_list(phase_multiplication(ren), n*pm.N_PH) # set of PV Buses
idxBat = idx_list(phase_multiplication(bat), n*pm.N_PH) # set of bat Buses
idxFlx = idx_list(phase_multiplication(flx), n*pm.N_PH) # set of flexible loads Buses
### LOADS ###
class load:
# normalize load profiles & assign to node
def load_profile(i):
profile = pd.read_csv('src/load_profiles/Load_profile_%s.csv'%i)
load_max = np.max(profile.values[:,1]) # maximum load
# normalized load profile
profile_norm = (profile.values[:,1]/load_max).astype(float)
# discretized load profile into T steps
nMeasure = int(24/pm.TIMESTEP)
profile_disc = np.array([np.mean(profile_norm[j*int(pm.TIMESTEP*60):\
(j+1)*int(pm.TIMESTEP*60)])\
for j in range(nMeasure)])
### TAKE VALUES FROM 12:00 +- T/2 ###
t_middle = 1/pm.TIMESTEP*12
t_start = int(t_middle - pm.T/2)
t_end = int(t_middle + pm.T/2)
# export
profile_load = profile_disc[t_start:t_end]
return profile_load
# index of load profile
profile = loadData.values[:,5].astype(int)
# peak load and power factor per node
sPeak = loadData.values[:,1]/(pm.S_BASE*1e3)
pf = loadData.values[:,2]
# active & reactive power demand [p.u]
pDem = np.zeros((n*pm.N_PH,pm.T,loadCase))
qDem = np.zeros((n*pm.N_PH,pm.T,loadCase))
for c in range(loadCase):
for i in range(n):
for j in range(pm.N_PH):
if pm.FLGLOAD == 1:
# active power demand
pDem[i*pm.N_PH+j,:,c] = pm.LOADCASE[c]*pm.LOADSHARE[j]*\
sPeak[i]*pf[i]*\
load_profile(profile[i])
# reactive power demand
qDem[i*pm.N_PH+j,:,c] = pm.LOADCASE[c]*pm.LOADSHARE[j]*\
sPeak[i]*np.sin(np.arccos(pf[i]))*\
load_profile(profile[i])
else:
pDem[i*pm.N_PH+j,:,c] = pm.LOADCASE[c]*pm.LOADSHARE[j]*\
sPeak[i]*pf[i]
qDem[i*pm.N_PH+j,:,c] = pm.LOADCASE[c]*pm.LOADSHARE[j]*\
sPeak[i]*np.sin(np.arccos(pf[i]))
# bounds
# max/min load shifting
sShift_ub = pm.FLGSHIFT*phase_multiplication(sets.flx*loadData.values[:,4])
sShift_lb = pm.FLGSHIFT*phase_multiplication(sets.flx*loadData.values[:,3])
# load shedding
pShed_ub = pm.FLGSHED*pDem
qShed_ub = pm.FLGSHED*qDem
### BESS ###
class bess:
icBat = pm.FLGBAT*batData.values[:,1]/pm.S_BASE # installed capacity [p.u.]
etaBat = batData.values[:,2] # efficiency
socMin = batData.values[:,3] # soc min
socMax = batData.values[:,4] # soc max
socInit = batData.values[:,5] # initial soc
e2p = batData.values[:,6] # energy-to-power ratio [MWh/MW]
# bounds
pCh_ub = pm.FLGBAT*(sets.bat*icBat/e2p) # battery charging
pDis_ub = pm.FLGBAT*(sets.bat*icBat/e2p) # battery discharging
eBat_ub = icBat*socMax # soc max
eBat_lb = icBat*socMin # soc min
### GENERATORS ###
class gen:
### IC PV EITHER FROM INPUT DATA OR FACTOR OF PEAK LOAD ###
if pm.FLGPV == 0:
# from input data - installed capacity [p.u.]
icPV = []
for i in range(loadCase):
for j in range(pvCase):
icPV.append(pm.PVCASE[j]*genData.values[:,1]/pm.S_BASE)
icPV = np.array(icPV).transpose()
else:
# dependent on load
icPV = [] # installed capacity [p.u.]
for i in range(loadCase):
for j in range(pvCase):
icPV.append(pm.PVCASE[j]*pm.LOADCASE[i]*load.sPeak)
# create array from list
icPV = np.array(icPV).transpose()
pfMax = phase_multiplication(genData.values[:,2]) # maximum power factor cos(phi)
pfMin = -phase_multiplication(genData.values[:,2]) # minimum power factor cos(phi)
prMax = np.sqrt((1-pfMax**2)/pfMax**2) # maximum power ratio gamma
prMin = -np.sqrt((1-np.square(pfMin))/np.square(pfMin)) # minimum power ratio gamma
### INVERTERS ###
class inverter:
def phase_selection(data,phase):
dim = len(data) # get dimension of input data
nPhase = np.ones((pm.N_PH)) # array to multiply input with number of phases
tmp = []
for i in range(dim):
if phase[i] == 3:
tmp = np.append(tmp,data[i]*nPhase/pm.N_PH, axis=0)
else:
tmp = np.append(tmp,np.zeros((pm.N_PH)), axis=0)
tmp[i*pm.N_PH + phase[i]] = data[i]
return tmp
phase_pv = invData.values[:,3].astype(int) # to which phases PV is connected to
phase_bat = invData.values[:,4].astype(int) # to which phases bat is connected to
# maximum renewable inverter capacity [p.u]
capPV = []
for c in range(pvCase*loadCase):
capPV.append(phase_selection(invData.values[:,1]*gen.icPV[:,c],phase_pv))
capPV = np.array(capPV).transpose()
# maximum bat inverter capacity [p.u.]
capBat = phase_selection(invData.values[:,2]*bess.icBat/bess.e2p,phase_bat)
### COSTS ###
class cost:
def cost_pu(data):
# calculate costs in [euro/p.u.] and per timestep
return data*pm.TIMESTEP*pm.S_BASE
curt = cost_pu(phase_multiplication(costData.values[:,1])) # active power curtailment
ren = cost_pu(phase_multiplication(costData.values[:,2])) # renewable energy source
bat = cost_pu(costData.values[:,3]) # battery
shed = cost_pu(phase_multiplication(costData.values[:,4])) # load shedding
shift = cost_pu(phase_multiplication(costData.values[:,5])) # load shifting
qSupport = cost_pu(phase_multiplication(costData.values[:,6])) # reactive power injection
loss = cost_pu(phase_multiplication(costData.values[:-1,7])) # active power losses
slackRev = cost_pu(costData.values[0,8]) # revenue for selling to upper level grid
slackCost = cost_pu(costData.values[0,9]) # active power from upper level grid
slackQ = cost_pu(costData.values[0,10]) # reactive power from upper level grid
### OLTC TRAFO ###
class oltc:
oltc_min = oltcData.values[:,1] # minimum value [p.u.]
oltc_max = oltcData.values[:,2] # maximum value [p.u.]
oltc_steps = oltcData.values[:,3] # number of steps [-]
oltcSum = int(oltcData.values[:,4]) # max number of shifts per time horizon [-]
symmetry = int(oltcData.values[:,5]) # symmetric = 1, asymmetric = 0
# voltage difference per shift [p.u.]
dV = float((oltc_max - oltc_min)/oltc_steps)
dVRe = dV*bus.vSlackRe # real part [p.u.]
dVIm = dV*bus.vSlackIm # imag part [p.u.]
# bound
tauMax = int(pm.FLGOLTC*(oltc_steps/2))
tauMin = int(pm.FLGOLTC*(-oltc_steps/2))
###############################################################################
## PV FORECAST ##
###############################################################################
class pv:
def pv_phase(data,phase):
dim = len(data) # get dimension of input data
nPhase = np.array(pm.PVSHARE) # array to multiply input with number of phases
tmp = []
for i in range(dim):
if phase[i] == 3:
tmp = np.append(tmp,data[i]*nPhase, axis=0)
else:
tmp = np.append(tmp,np.zeros((pm.N_PH)), axis=0)
tmp[i*pm.N_PH + phase[i]] = data[i]
return tmp
### CHECK IF FORECAST FILE EXISTS ###
fcstFile = 'src/fcst/forecastPV_v%s_%s_t%s.pkl'%(pm.V_FCST,pm.FCSTCASE[0],pm.T)
if os.path.exists(fcstFile):
### READ FCST FILE ###
file = open(fcstFile, 'rb') # open results file
pvFcst = pkl.load(file) # create arry from file
file.close() # close file
else:
### RUN FORECAST ###
print('Run forecasting script ...')
pvFcst = fcst.pv_fcst()
print('... done!')
nSamples = np.size(pvFcst[3],1) # number of samples
dataFcst = pvFcst[3] # all forecast data
# installed capacity per phase
icPhase = np.zeros((l*pm.N_PH,loadCase*pvCase))
for c in range(loadCase*pvCase):
icPhase[:,c] = pv_phase(gen.icPV[1:,c],inverter.phase_pv[1:])
# forecasted PV infeed per phase
pPV = np.zeros((n*pm.N_PH,pm.T,pvCase*loadCase))
for c in range(pvCase*loadCase):
pPV[:,:,c] = np.append(np.zeros((pm.N_PH,pm.T)),\
np.dot(icPhase[:,c].reshape(l*pm.N_PH,1),\
pvFcst[0].reshape(1,pm.T)),axis=0)
### COVARIANCE MATRIX ###
# covariance matrix for all timesteps
cov = np.zeros((l*pm.N_PH,l*pm.N_PH,pm.T,pvCase*loadCase))
for c in range(pvCase*loadCase):
for t in range(pm.T):
# full covariance matrix
cov[:,:,t,c] = np.cov(np.dot(icPhase[:,c].reshape(l*pm.N_PH,1),\
dataFcst[t,:].reshape(1,nSamples)))
# delete empty columnds and rows
rowDel = []
for i in range(l*pm.N_PH):
if np.all(cov[i,:,int(pm.T/2),:] == 0):
rowDel.append(i)
covRed = np.delete(cov,rowDel,1)
covRed = np.delete(covRed,rowDel,0)
# independent measurements
nMeas = np.size(covRed,axis=0)
covInd = np.zeros((nMeas,nMeas,pm.T,pvCase*loadCase))
for i in range(nMeas):
for j in range(nMeas):
for t in range(pm.T):
for c in range(pvCase*loadCase):
if i != j:
covInd[i,j,t,c] = 0
else:
covInd[i,j,t,c] = covRed[i,j,t,c]
# sqrt of covariance matrix
covSqrt = | np.sqrt(covInd) | numpy.sqrt |
from keras.layers import ZeroPadding2D, BatchNormalization, Input, MaxPooling2D, AveragePooling2D, Conv2D, LeakyReLU, Flatten, Conv2DTranspose, Activation, add, Lambda, GaussianNoise, merge, concatenate, Dropout
from keras_contrib.layers.normalization import InstanceNormalization
from keras.layers.core import Dense, Flatten, Reshape
from keras.models import Model, load_model
from keras.optimizers import Adam, adam
from keras.activations import tanh
from keras.regularizers import l2
import keras.backend as K
from keras.initializers import RandomNormal
import cv2
from tensorflow.contrib.kfac.python.ops import optimizer
from collections import OrderedDict
from time import localtime, strftime
from scipy.misc import imsave, toimage
import numpy as np
import json
import sys
import time
import datetime
sys.path.append('..')
import load_data
import os
import csv
class UNIT():
def __init__(self, lr = 1e-4, date_time_string_addition=''):
self.channels = 3 # 1 for grayscale 3 RGB
weight_decay = 0.0001/2
# Load data
nr_A_train_imgs = 1
nr_B_train_imgs = 1
nr_A_test_imgs = 1
nr_B_test_imgs = None
image_folder = 'dataset-name/'
# Fetch data during training instead of pre caching all images - might be necessary for large datasets
self.use_data_generator = False
if self.use_data_generator:
print('--- Using dataloader during training ---')
else:
print('--- Caching data ---')
sys.stdout.flush()
if self.use_data_generator:
self.data_generator = load_data.load_data(
self.channels, generator=True, subfolder=image_folder)
nr_A_train_imgs=2
nr_B_train_imgs=2
data = load_data.load_data(self.channels,
nr_A_train_imgs=nr_A_train_imgs,
nr_B_train_imgs=nr_B_train_imgs,
nr_A_test_imgs=nr_A_test_imgs,
nr_B_test_imgs=nr_B_test_imgs,
subfolder=image_folder)
self.A_train = data["trainA_images"]
self.B_train = data["trainB_images"]
self.A_test = data["testA_images"]
self.B_test = data["testB_images"]
self.testA_image_names = data["testA_image_names"]
self.testB_image_names = data["testB_image_names"]
self.img_width = self.A_train.shape[2]
self.img_height = self.A_train.shape[1]
self.latent_dim = (int(self.img_height / 4), int(self.img_width / 4), 256)
self.img_shape = (self.img_height, self.img_width, self.channels)
self.date_time = strftime("%Y%m%d-%H%M%S", localtime()) + date_time_string_addition
self.learning_rate = lr
self.beta_1 = 0.5
self.beta_2 = 0.999
self.lambda_0 = 10
self.lambda_1 = 0.1
self.lambda_2 = 100
self.lambda_3 = self.lambda_1 # cycle
self.lambda_4 = self.lambda_2 # cycle
# Optimizer
opt = Adam(self.learning_rate, self.beta_1, self.beta_2)
optStandAdam = Adam()
# Simple Model
self.superSimple = self.modelSimple()
self.superSimple.compile(optimizer=optStandAdam,
loss="mae")
# Discriminator
self.discriminatorA = self.modelMultiDiscriminator("discriminatorA")
self.discriminatorB = self.modelMultiDiscriminator("discriminatorB")
for layer in self.discriminatorA.layers:
if hasattr(layer, 'kernel_regularizer'):
layer.kernel_regularizer= l2(weight_decay)
layer.bias_regularizer = l2(weight_decay)
if hasattr(layer, 'kernel_initializer'):
layer.kernel_initializer = RandomNormal(mean=0.0, stddev=0.02)
layer.bias_initializer = RandomNormal(mean=0.0, stddev=0.02)
for layer in self.discriminatorB.layers:
if hasattr(layer, 'kernel_regularizer'):
layer.kernel_regularizer= l2(weight_decay)
layer.bias_regularizer = l2(weight_decay)
if hasattr(layer, 'kernel_initializer'):
layer.kernel_initializer = RandomNormal(mean=0.0, stddev=0.02)
layer.bias_initializer = RandomNormal(mean=0.0, stddev=0.02)
self.discriminatorA.compile(optimizer=opt,
loss=['binary_crossentropy',
'binary_crossentropy',
'binary_crossentropy'],
loss_weights=[self.lambda_0,
self.lambda_0,
self.lambda_0])
self.discriminatorB.compile(optimizer=opt,
loss=['binary_crossentropy',
'binary_crossentropy',
'binary_crossentropy'],
loss_weights=[self.lambda_0,
self.lambda_0,
self.lambda_0])
# Encoder
self.encoderA = self.modelEncoder("encoderA")
self.encoderB = self.modelEncoder("encoderB")
self.encoderShared = self.modelSharedEncoder("encoderShared")
self.decoderShared = self.modelSharedDecoder("decoderShared")
# Generator
self.generatorA = self.modelGenerator("generatorA")
self.generatorB = self.modelGenerator("generatorB")
# Input Encoder Decoder
imgA = Input(shape=(self.img_shape))
imgB = Input(shape=(self.img_shape))
encodedImageA = self.encoderA(imgA)
encodedImageB = self.encoderB(imgB)
sharedA = self.encoderShared(encodedImageA)
sharedB = self.encoderShared(encodedImageB)
outSharedA = self.decoderShared(sharedA)
outSharedB = self.decoderShared(sharedB)
# Input Generator
outAa = self.generatorA(outSharedA)
outBa = self.generatorA(outSharedB)
outAb = self.generatorB(outSharedA)
outBb = self.generatorB(outSharedB)
guess_outBa = self.discriminatorA(outBa)
guess_outAb = self.discriminatorB(outAb)
# Cycle
cycle_encodedImageA = self.encoderA(outBa)
cycle_encodedImageB = self.encoderB(outAb)
cycle_sharedA = self.encoderShared(cycle_encodedImageA)
cycle_sharedB = self.encoderShared(cycle_encodedImageB)
cycle_outSharedA = self.decoderShared(cycle_sharedA)
cycle_outSharedB = self.decoderShared(cycle_sharedB)
cycle_Ab_Ba = self.generatorA(cycle_outSharedB)
cycle_Ba_Ab = self.generatorB(cycle_outSharedA)
# Train only generators
self.discriminatorA.trainable = False
self.discriminatorB.trainable = False
self.encoderGeneratorModel = Model(inputs=[imgA, imgB],
outputs=[sharedA, sharedB,
cycle_sharedA, cycle_sharedB,
outAa, outBb,
cycle_Ab_Ba, cycle_Ba_Ab,
guess_outBa[0], guess_outAb[0],
guess_outBa[1], guess_outAb[1],
guess_outBa[2], guess_outAb[2]])
for layer in self.encoderGeneratorModel.layers:
if hasattr(layer, 'kernel_regularizer'):
layer.kernel_regularizer= l2(weight_decay)
layer.bias_regularizer = l2(weight_decay)
if hasattr(layer, 'kernel_initializer'):
layer.kernel_initializer = RandomNormal(mean=0.0, stddev=0.02)
layer.bias_initializer = RandomNormal(mean=0.0, stddev=0.02)
self.encoderGeneratorModel.compile(optimizer=opt,
loss=[self.vae_loss_CoGAN, self.vae_loss_CoGAN,
self.vae_loss_CoGAN, self.vae_loss_CoGAN,
'mae', 'mae',
'mae', 'mae',
'binary_crossentropy', 'binary_crossentropy',
'binary_crossentropy', 'binary_crossentropy',
'binary_crossentropy', 'binary_crossentropy'],
loss_weights=[self.lambda_1, self.lambda_1,
self.lambda_3, self.lambda_3,
self.lambda_2, self.lambda_2,
self.lambda_4, self.lambda_4,
self.lambda_0, self.lambda_0,
self.lambda_0, self.lambda_0,
self.lambda_0, self.lambda_0])
#===============================================================================
# Decide what to Run
self.trainFullModel()
#self.load_model_and_generate_synthetic_images("name_of_saved_model", epoch) # eg. "20180504-140511_test1", 180
#self.loadAllWeightsToModelsIncludeDisc("name_of_saved_model", epoch)
#===============================================================================
# Architecture functions
def resblk(self, x0, k):
# first layer
x = Conv2D(filters=k, kernel_size=3, strides=1, padding="same")(x0)
x = BatchNormalization(axis=3, momentum=0.9, epsilon=1e-05, center=True)(x, training=True)
x = Activation('relu')(x)
# second layer
x = Conv2D(filters=k, kernel_size=3, strides=1, padding="same")(x)
x = BatchNormalization(axis=3, momentum=0.9, epsilon=1e-05, center=True)(x, training=True)
x = Dropout(0.5)(x, training=True)
# merge
x = add([x, x0])
return x
#===============================================================================
# Loss function from PyTorch implementation from original article
def vae_loss_CoGAN(self, y_true, y_pred):
y_pred_2 = K.square(y_pred)
encoding_loss = K.mean(y_pred_2)
return encoding_loss
#===============================================================================
# Models
def modelMultiDiscriminator(self, name):
x1 = Input(shape=self.img_shape)
x2 = AveragePooling2D(pool_size=(2, 2), strides=None, padding='valid', data_format=None)(x1)
x4 = AveragePooling2D(pool_size=(2, 2), strides=None, padding='valid', data_format=None)(x2)
x1_out = self.modelDiscriminator(x1)
x2_out = self.modelDiscriminator(x2)
x4_out = self.modelDiscriminator(x4)
return Model(inputs=x1, outputs=[x1_out, x2_out, x4_out], name=name)
def modelDiscriminator(self, x):
# Layer 1
x = Conv2D(64, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 2
x = Conv2D(128, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 3
x = Conv2D(256, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 4
x = Conv2D(512, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 5
x = Conv2D(1, kernel_size=1, strides=1)(x)
prediction = Activation('sigmoid')(x)
return prediction
def modelEncoder(self, name):
inputImg = Input(shape=self.img_shape)
# Layer 1
x = ZeroPadding2D(padding=(3, 3))(inputImg)
x = Conv2D(64, kernel_size=7, strides=1, padding='valid')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 2
x = ZeroPadding2D(padding=(1, 1))(x)
x = Conv2D(128, kernel_size=3, strides=2, padding='valid')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 3
x = ZeroPadding2D(padding=(1, 1))(x)
x = Conv2D(256, kernel_size=3, strides=2, padding='valid')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 4: 2 res block
x = self.resblk(x, 256)
# Layer 5: 3 res block
x = self.resblk(x, 256)
# Layer 6: 3 res block
z = self.resblk(x, 256)
return Model(inputs=inputImg, outputs=z, name=name)
def modelSharedEncoder(self, name):
input = Input(shape=self.latent_dim)
x = self.resblk(input, 256)
z = GaussianNoise(stddev=1)(x, training=True)
return Model(inputs=input, outputs=z, name=name)
def modelSharedDecoder(self, name):
input = Input(shape=self.latent_dim)
x = self.resblk(input, 256)
return Model(inputs=input, outputs=x, name=name)
def modelGenerator(self, name):
inputImg = Input(shape=self.latent_dim)
# Layer 1: 1 res block
x = self.resblk(inputImg, 256)
# Layer 2: 2 res block
x = self.resblk(x, 256)
# Layer 3: 3 res block
x = self.resblk(x, 256)
# Layer 4:
x = Conv2DTranspose(128, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 5:
x = Conv2DTranspose(64, kernel_size=3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.01)(x)
# Layer 6
x = Conv2DTranspose(self.channels, kernel_size=1, strides=1, padding='valid')(x)
z = Activation("tanh")(x)
return Model(inputs=inputImg, outputs=z, name=name)
def modelSimple(self):
inputImg = Input(shape=self.img_shape)
x = Conv2D(256, kernel_size=1, strides=1, padding='same')(inputImg)
x = Activation('relu')(x)
prediction = Conv2D(1, kernel_size=5, strides=1, padding='same')(x)
return Model(input=inputImg, output=prediction)
#===============================================================================
# Training
def trainFullModel(self, epochs=100, batch_size=1, save_interval=1):
def run_training_iteration(loop_index, epoch_iterations, imgA, imgB):
# Flip was not done in article
# if np.random.rand(1) > 0.5:
# imgA = cv2.flip(imgA[0], 1)
# imgA = imgA[np.newaxis,:,:,:]
# if np.random.rand(1) > 0.5:
# imgB = cv2.flip(imgB[0], 1)
# imgB = imgB[np.newaxis,:,:,:]
# Generate fake images
encodedImageA = self.encoderA.predict(imgA)
encodedImageB = self.encoderB.predict(imgB)
sharedA = self.encoderShared.predict(encodedImageA)
sharedB = self.encoderShared.predict(encodedImageB)
outSharedA = self.decoderShared.predict(sharedA)
outSharedB = self.decoderShared.predict(sharedB)
outAa = self.generatorA.predict(outSharedA)
outBa = self.generatorA.predict(outSharedB)
outAb = self.generatorB.predict(outSharedA)
outBb = self.generatorB.predict(outSharedB)
# Train discriminator
dA_loss_real = self.discriminatorA.train_on_batch(imgA, real_labels)
dA_loss_fake = self.discriminatorA.train_on_batch(outBa, synthetic_labels)
dA_loss = np.add(dA_loss_real, dA_loss_fake)
dB_loss_real = self.discriminatorB.train_on_batch(imgB, real_labels)
dB_loss_fake = self.discriminatorB.train_on_batch(outAb, synthetic_labels)
dB_loss = np.add(dB_loss_real, dB_loss_fake)
# Train generator
g_loss = self.encoderGeneratorModel.train_on_batch([imgA, imgB],
[dummy, dummy,
dummy, dummy,
imgA, imgB,
imgA, imgB,
real_labels1, real_labels1,
real_labels2, real_labels2,
real_labels3, real_labels3])
# Store training data
epoch_list.append(epoch)
loop_index_list.append(loop_index)
# Discriminator loss
loss_dA_real_list.append(dA_loss_real[0])
loss_dA_fake_list.append(dA_loss_fake[0])
loss_dB_real_list.append(dB_loss_real[0])
loss_dB_fake_list.append(dB_loss_fake[0])
dA_sum_loss_list.append(dA_loss[0])
dB_sum_loss_list.append(dB_loss[0])
# Generator loss
loss_gen_list_1.append(g_loss[0])
loss_gen_list_2.append(g_loss[1])
loss_gen_list_3.append(g_loss[2])
loss_gen_list_4.append(g_loss[3])
loss_gen_list_5.append(g_loss[4])
loss_gen_list_6.append(g_loss[5])
loss_gen_list_7.append(g_loss[6])
loss_gen_list_8.append(g_loss[7])
loss_gen_list_9.append(g_loss[8])
loss_gen_list_10.append(g_loss[9])
loss_gen_list_11.append(g_loss[10])
print('----------------Epoch-------640x480---------', epoch, '/', epochs - 1)
print('----------------Loop index-----------', loop_index, '/', epoch_iterations - 1)
print('Discriminator TOTAL loss: ', dA_loss[0] + dB_loss[0])
print('Discriminator A loss total: ', dA_loss[0])
print('Discriminator B loss total: ', dB_loss[0])
print('Genarator loss total: ', g_loss[0])
print('----------------Discriminator loss----')
print('dA_loss_real: ', dA_loss_real[0])
print('dA_loss_fake: ', dA_loss_fake[0])
print('dB_loss_real: ', dB_loss_real[0])
print('dB_loss_fake: ', dB_loss_fake[0])
print('----------------Generator loss--------')
print('Shared A: ', g_loss[1])
print('Shared B: ', g_loss[2])
print('Cycle shared A: ', g_loss[3])
print('Cycle shared B: ', g_loss[4])
print('OutAa MAE: ', g_loss[5])
print('OutBb MAE: ', g_loss[6])
print('Cycle_Ab_Ba MAE: ', g_loss[7])
print('Cycle_Ba_Ab MAE: ', g_loss[8])
print('guess_outBa: ', g_loss[9])
print('guess_outAb: ', g_loss[10])
print('guess_outBa: ', g_loss[11])
print('guess_outAb: ', g_loss[12])
print('guess_outBa: ', g_loss[13])
print('guess_outAb: ', g_loss[14])
sys.stdout.flush()
if loop_index % 5 == 0:
# Save temporary images continously
self.save_tmp_images(imgA, imgB)
self.print_ETA(start_time, epoch, epoch_iterations, loop_index)
A_train = self.A_train
B_train = self.B_train
self.history = OrderedDict()
self.epochs = epochs
self.batch_size = batch_size
loss_dA_real_list = []
loss_dA_fake_list = []
loss_dB_real_list = []
loss_dB_fake_list = []
dA_sum_loss_list = []
dB_sum_loss_list = []
loss_gen_list_1 = []
loss_gen_list_2 = []
loss_gen_list_3 = []
loss_gen_list_4 = []
loss_gen_list_5 = []
loss_gen_list_6 = []
loss_gen_list_7 = []
loss_gen_list_8 = []
loss_gen_list_9 = []
loss_gen_list_10 = []
loss_gen_list_11 = []
epoch_list = []
loop_index_list = []
#dummy = []
#dummy = shape=self.latent_dim
#dummy = np.zeros(shape=self.latent_dim)
#dummy = np.expand_dims(dummy, 0)
dummy = np.zeros(shape = ((self.batch_size,) + self.latent_dim))
self.writeMetaDataToJSON()
self.saveImages('init', 1)
sys.stdout.flush()
# Start stopwatch for ETAs
start_time = time.time()
label_shape1 = (batch_size,) + self.discriminatorA.output_shape[0][1:]
label_shape2 = (batch_size,) + self.discriminatorA.output_shape[1][1:]
label_shape3 = (batch_size,) + self.discriminatorA.output_shape[2][1:]
real_labels1 = np.ones(label_shape1)
real_labels2 = np.ones(label_shape2)
real_labels3 = np.ones(label_shape3)
synthetic_labels1 = | np.zeros(label_shape1) | numpy.zeros |
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
from magpylib.source.magnet import Box,Cylinder
from magpylib import Collection, displaySystem, Sensor
from scipy.optimize import fsolve, least_squares
import matplotlib.animation as manimation
import random
import MDAnalysis
import MDAnalysis.visualization.streamlines_3D
import mayavi
from mayavi import mlab
import math, os, time
from multiprocessing import Pool, freeze_support
from pathlib import Path
all = True
iterations = 180
num_processes = 30
printouts = False
axis = 0
movie_path = '/home/letrend/Videos/magnetic_arrangements/more_sensors'
# def gen_magnets():
# magnets = [Box(mag=(0,0,2000),dim=(5,5,5),pos=(0,0,10))]
# return magnets
# define sensor
sensor_pos = [[-22.7,7.7,0],[-14.7,-19.4,0],[14.7,-19.4,0],[22.7,7.7,0]]#[[22.7,7.7,0],[14.7,-19.4,0],[-14.7,-19.4,0],[-22.7,7.7,0]]
# sensor_rot = [[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]],[0,[0,0,1]]]
sensors = []
for pos in sensor_pos:
# sensors.append(Sensor(pos=pos,angle=sensor_rot[i][0], axis=sensor_rot[i][1]))
s = Sensor(pos=pos,angle=90,axis=(0,0,1))
sensors.append(s)
# for i in np.linspace(0,360,10):
# # sensors.append(Sensor(pos=pos,angle=sensor_rot[i][0], axis=sensor_rot[i][1]))
# s = Sensor(pos=(math.sin(i)*15,math.cos(i)*15,15),angle=90,axis=(0,0,1))
# sensors.append(s)
# def gen_magnets():
# return [Box(mag=(500,0,0),dim=(10,10,10),pos=(0,12,0)), Box(mag=(0,500,0),dim=(10,10,10),pos=(10.392304845,-6,0),angle=60, axis=(0,0,1)), Box(mag=(0,0,500),dim=(10,10,10),pos=(-10.392304845,-6,0),angle=-60, axis=(0,0,1))]
cs = 5
dimx,dimy,dimz = 3,3,3
mag_pos = [4, 0, 1, 0, 0, 4, 1, 3, 5, 6, 0, 4, 6, 1, 6, 0, 6, 5, 5, 4, 5, 1, 5, 2, 3, 5, 1,]
field_strength = 1000
# # hallbach 0, works well
# def gen_magnets(mag_pos):
# magnets = []
# for i in range(0,dimx):
# for j in range(0,dimy):
# for k in range(0,dimz):
# if mag_pos[i*dimx+j*dimy+k*dimz]==1:
# magnets.append(Box(mag=(field_strength,0,0),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# if mag_pos[i*dimx+j*dimy+k*dimz]==2:
# magnets.append(Box(mag=(-field_strength,0,0),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# if mag_pos[i*dimx+j*dimy+k*dimz]==3:
# magnets.append(Box(mag=(0,field_strength,0),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# if mag_pos[i*dimx+j*dimy+k*dimz]==4:
# magnets.append(Box(mag=(0,-field_strength,0),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# if mag_pos[i*dimx+j*dimy+k*dimz]==5:
# magnets.append(Box(mag=(0,0,field_strength),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# if mag_pos[i*dimx+j*dimy+k*dimz]==6:
# magnets.append(Box(mag=(0,0,-field_strength),dim=(cs,cs,cs),pos=((i-dimx//2)*(cs+1),(j-dimy//2)*(cs+1),(k-dimz//2)*(cs+1))))
# return magnets
cs = 10
field_strenght = 1000
# # hallbach 0, works well
# def gen_magnets(mag_pos):
# magnets = []
# magnets.append(Box(mag=(field_strenght,0,0),dim=(cs,cs,cs),pos=(0,0,15)))
# # magnets.append(Box(mag=(0,-field_strenght,0),dim=(cs,cs,cs),pos=(-(cs+1),0,0)))
# # magnets.append(Box(mag=(0,field_strenght,0),dim=(cs,cs,cs),pos=(cs+1,0,0)))
# # magnets.append(Box(mag=(0,-field_strenght,0),dim=(cs,cs,cs),pos=(0,-(cs+1),0)))
# # magnets.append(Box(mag=(0,field_strenght,0),dim=(cs,cs,cs),pos=(0,cs+1,0)))
# # magnets.append(Box(mag=(0,-field_strenght,0),dim=(cs,cs,cs),pos=(-(cs+1),(cs+1),0)))
# # magnets.append(Box(mag=(0,field_strenght,0),dim=(cs,cs,cs),pos=(-(cs+1),-(cs+1),0)))
# # magnets.append(Box(mag=(0,0,field_strenght),dim=(cs,cs,cs),pos=((cs+1),-(cs+1),0)))
# # magnets.append(Box(mag=(0,field_strenght,0),dim=(cs,cs,cs),pos=((cs+1),(cs+1),0)))
#
# return magnets
# hallbach 0, works well
def gen_magnets(mag_pos):
magnets = []
magnets.append(Box(mag=(0,0,-500),dim=(5,5,5),pos=(0,0,0)))
magnets.append(Box(mag=(-500,0,0),dim=(5,5,5),pos=(-5,5,0)))
magnets.append(Box(mag=(-500,0,0),dim=(5,5,5),pos=(-5,0,0)))
magnets.append(Box(mag=(-500,0,0),dim=(5,5,5),pos=(-5,-5,0)))
magnets.append(Box(mag=(500,0,0),dim=(5,5,5),pos=(5,0,0)))
magnets.append(Box(mag=(500,0,0),dim=(5,5,5),pos=(5,-5,0)))
magnets.append(Box(mag=(0,-500,0),dim=(5,5,5),pos=(0,-5,0)))
magnets.append(Box(mag=(500,0,0),dim=(5,5,5),pos=(5,5,0)))
magnets.append(Box(mag=(0,500,0),dim=(5,5,5),pos=(0,5,0)))
return magnets
# def gen_magnets():
# magnets = []
# j = 0
# for i in range(0,360,15):
# if j%2==0:
# magnets.append(Box(mag=(0,500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# else:
# magnets.append(Box(mag=(500,0,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# if i!=60 and i!=240:
# if j%2==0:
# magnets.append(Box(mag=(0,0,-500),dim=(5,5,5),angle=-i,axis=(1,0,0),pos=(0,math.sin((i+30)/180.0*math.pi)*12,math.cos((i+30)/180.0*math.pi)*12)))
# else:
# magnets.append(Box(mag=(0,500,0),dim=(5,5,5),angle=-i,axis=(1,0,0),pos=(0,math.sin((i+30)/180.0*math.pi)*12,math.cos((i+30)/180.0*math.pi)*12)))
# j=j+1
# return magnets
# def gen_magnets():
# magnets = []
# j = 0
# k = 0
# for i in range(0,360,60):
# if j<3:
# magnets.append(Box(mag=(0,500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# else:
# magnets.append(Box(mag=(0,-500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# j = j+1
# if i!=60 and i!=240:
# if k<2:
# magnets.append(Box(mag=(0,0,-500),dim=(5,5,5),angle=-i-30,axis=(1,0,0),pos=(0,math.sin((i+30)/180.0*math.pi)*12,math.cos((i+30)/180.0*math.pi)*12)))
# else:
# magnets.append(Box(mag=(0,0,500),dim=(5,5,5),angle=-i-30,axis=(1,0,0),pos=(0,math.sin((i+30)/180.0*math.pi)*12,math.cos((i+30)/180.0*math.pi)*12)))
# k = k+1
# return magnets
# # alternating 2rings
# def gen_magnets():
# magnets = []
# j = 0
# k = 0
# for i in range(0,360,120):
# if j%2==0:
# magnets.append(Box(mag=(0,500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# else:
# magnets.append(Box(mag=(0,-500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# if j%2==0:
# magnets.append(Box(mag=(0,0,-500),dim=(5,5,5),angle=-i-90,axis=(1,0,0),pos=(0,math.sin((i+90)/180.0*math.pi)*12,math.cos((i+90)/180.0*math.pi)*12)))
# else:
# magnets.append(Box(mag=(0,0,500),dim=(5,5,5),angle=-i-90,axis=(1,0,0),pos=(0,math.sin((i+90)/180.0*math.pi)*12,math.cos((i+90)/180.0*math.pi)*12)))
# j = j+1
# return magnets
# #two rings not alterating
# def gen_magnets():
# magnets = []
# j= 0
# for i in range(0,360,120):
# if j==0:
# magnets.append(Box(mag=(0,500,0),dim=(5,5,5),angle=-i,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# elif j==1:
# magnets.append(Box(mag=(0,0,-500),dim=(5,5,5),angle=-i+j*10,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# else:
# magnets.append(Box(mag=(500,0,0),dim=(5,5,5),angle=-i+j*10,axis=(0,0,1),pos=(math.sin(i/180.0*math.pi)*12,math.cos(i/180.0*math.pi)*12,0)))
# if j!=1:
# magnets.append(Box(mag=(0,0,500),dim=(5,5,5),angle=j*10,axis=(1,0,0),pos=(0,math.sin((i)/180.0*math.pi)*12,math.cos((i)/180.0*math.pi)*12)))
# j = j+1
#
# return magnets
matplotlib.use('Agg')
mlab.options.offscreen = True
def func1(iter):
c = Collection(gen_magnets(mag_pos))
if axis==0:
rot = [iter-90,0,0]
if axis==1:
rot = [0,iter-90,0]
if axis==2:
rot = [0,0,iter-90]
c.rotate(rot[0],(1,0,0), anchor=(0,0,0))
c.rotate(rot[1],(0,1,0), anchor=(0,0,0))
c.rotate(rot[2],(0,0,1), anchor=(0,0,0))
x_lower,x_upper = -30, 30
y_lower,y_upper = -30, 30
z_lower,z_upper = -30, 30
grid_spacing_value = 2#0.25
xd = int((x_upper-x_lower)/grid_spacing_value)
yd = int((y_upper-y_lower)/grid_spacing_value)
zd = int((z_upper-z_lower)/grid_spacing_value)
x, y, z = np.mgrid[x_lower:x_upper:xd*1j,
y_lower:y_upper:yd*1j,
z_lower:z_upper:zd*1j]
xs = np.linspace(x_lower,x_upper,xd)
ys = np.linspace(y_lower,y_upper,yd)
zs = np.linspace(z_lower,z_upper,zd)
U = np.zeros((xd,yd,zd))
V = np.zeros((xd,yd,zd))
W = np.zeros((xd,yd,zd))
i = 0
if printouts:
print("simulating magnetic data")
for zi in zs:
POS = np.array([(x,y,zi) for x in xs for y in ys])
Bs = c.getB(POS).reshape(len(xs),len(ys),3)
U[:,:,i]=Bs[:,:,0]
V[:,:,i]=Bs[:,:,1]
W[:,:,i]=Bs[:,:,2]
i=i+1
if printouts:
print("(%d/%d)"%(i,zd))
i = 0
if printouts:
print("generating flow whole cube")
fig = mlab.figure(bgcolor=(1,1,1), size=(1500, 1500), fgcolor=(0, 0, 0))
fig.scene.disable_render = True
for xi in xs:
st = mlab.flow(x, y, z, U,V,W, line_width=0.1,
seedtype='plane', integration_direction='both',figure=fig,opacity=0.05)
st.streamline_type = 'tube'
st.seed.visible = False
st.tube_filter.radius = 0.1
st.seed.widget.origin = np.array([ xi, y_lower, z_upper])
st.seed.widget.point1 = np.array([ xi, y_upper, z_upper])
st.seed.widget.point2 = np.array([ xi, y_lower, z_lower])
st.seed.widget.resolution = 10#int(xs.shape[0])
st.seed.widget.enabled = True
st.seed.widget.handle_property.opacity = 0
st.seed.widget.plane_property.opacity = 0
if printouts:
print("(%d/%d)"%(i,xd))
i= i+1
fig.scene.disable_render = False
mayavi.mlab.view(azimuth=-50, elevation=70)
mlab.axes(extent = [x_lower, x_upper, y_lower, y_upper, z_lower, z_upper],figure=fig)
if axis==0:
mlab.savefig(movie_path+'/x-axis/movie001/'+'anim%05d.png'%(iter))
if axis==1:
mlab.savefig(movie_path+'/y-axis/movie001/'+'anim%05d.png'%(iter))
if axis==2:
mlab.savefig(movie_path+'/z-axis/movie001/'+'anim%05d.png'%(iter))
mlab.clf(fig)
fig = mlab.figure(bgcolor=(1,1,1), size=(1500, 1500), fgcolor=(0, 0, 0))
fig.scene.disable_render = True
if printouts:
print("generating flow sensor plane")
for zi in np.linspace(-1,1,10):
st = mlab.flow(x, y, z, U,V,W, line_width=0.1,
seedtype='plane', integration_direction='both',figure=fig,opacity=0.05)
st.streamline_type = 'tube'
st.seed.visible = False
st.tube_filter.radius = 0.1
st.seed.widget.origin = np.array([ x_lower, y_upper, zi])
st.seed.widget.point1 = np.array([ x_upper, y_upper, zi])
st.seed.widget.point2 = np.array([ x_lower, y_lower, zi])
st.seed.widget.resolution = 10#int(xs.shape[0])
st.seed.widget.enabled = True
st.seed.widget.handle_property.opacity = 0
st.seed.widget.plane_property.opacity = 0
if printouts:
print("(%d/%d)"%(i,xd))
i= i+1
fig.scene.disable_render = False
mayavi.mlab.view(azimuth=-50, elevation=70)
mlab.axes(extent = [x_lower, x_upper, y_lower, y_upper, z_lower, z_upper],figure=fig)
if axis==0:
mlab.savefig(movie_path+'/x-axis/movie002/'+'anim%05d.png'%(iter))
if axis==1:
mlab.savefig(movie_path+'/y-axis/movie002/'+'anim%05d.png'%(iter))
if axis==2:
mlab.savefig(movie_path+'/z-axis/movie002/'+'anim%05d.png'%(iter))
mlab.clf(fig)
angle_error = np.zeros(360)
b_field_error = np.zeros(360)
def func2(iter):
c = Collection(gen_magnets(mag_pos))
if axis==0:
rot = [iter-90,0,0]
if axis==1:
rot = [0,iter-90,0]
if axis==2:
rot = [0,0,iter-90]
c.rotate(rot[0],(1,0,0), anchor=(0,0,0))
c.rotate(rot[1],(0,1,0), anchor=(0,0,0))
c.rotate(rot[2],(0,0,1), anchor=(0,0,0))
b_target = []
for sens in sensors:
b_target.append(sens.getB(c))
# calculate B-field on a grid
xs = np.linspace(-30,30,33)
ys = | np.linspace(-30,30,44) | numpy.linspace |
import numpy as np
import pandas as pd
_EPSILON = 1e-6
# 交易日历
tradeDays = pd.read_hdf("emulator_v0/tradeDays.h5").iloc[22:]
tradeDays.reset_index(drop=True, inplace=True)
# 股票池
dataset_universe = pd.read_hdf("emulator_v0/universe_SH50.h5")
# 报价单
dataset_open = pd.read_hdf("emulator_v0/dataset_open.h5")
dataset_open.fillna(0, inplace=True)
dataset_close = pd.read_hdf("emulator_v0/dataset_close.h5")
dataset_close.fillna(0, inplace=True)
dataset_universe = pd.DataFrame(
data=-1, index=dataset_open.index,
columns=dataset_open.columns)
class Trading(object):
def __init__(self, buy_fee, sell_fee):
self.tradeDays = tradeDays
self.universe = dataset_universe
self.open = np.array(dataset_open)
self.close = np.array(dataset_close)
self.buy_fee = buy_fee
self.sell_fee = sell_fee
self.reset()
def reset(self):
self.portfolio = np.zeros_like(self.universe.iloc[0], dtype="float")
self.cash = 1e8
self.valuation = 0 # 持仓估值
self.total_value = self.cash + self.valuation
def step(self, order, universe, counter):
# 转换交易指令
day = self.tradeDays[counter]
order = order - 1
order = self.get_order(order, universe, day)
# 买卖操作信号
mask = np.sign(np.maximum(self.open[counter] - 1, 0))
buy_op = np.maximum(order, 0) * mask
sell_op = np.minimum(order, 0) * mask
# 卖买交易
self.sell(sell_op, counter)
self.buy(buy_op, counter)
# 当日估值
new_value = self.assess(counter)
reward = np.log(new_value / self.total_value)
self.total_value = new_value
# MAX EPISODE
if counter >= 468:
done = True
elif self.total_value < 1e8 * 0.2:
done = True
else:
done = False
return reward, done, self.total_value, self.portfolio
def get_order(self, order, universe, day):
self.universe.loc[day, universe] = order
return | np.array(dataset_universe.loc[day]) | numpy.array |
import numpy as np
from matplotlib import pyplot as plt
from mpl_toolkits.mplot3d import Axes3D # noqa: F401 unused import
import xml.etree.ElementTree as ET
from os.path import isfile, join
from os import getcwd
from scipy.spatial import distance
##############################
# MACROS
#################################
# # Geometry data
# A = -65
# B = 25
# YMAX = 20
# THICKNESS = -10 # negative to match equation
# # Mesh data
# VERTICAL_RES = 60
# N_LAYERS = 4 # from endo to epi, not including endo
# CIRCUNFERENTIAL_RES = 30
# Geo
A = -65
B = 25
H = 0
K = 0
YMAX = 20
_TYPE = 1
N_INTERNAL_LAYERS = 8 # Horizontal res --> will add two layers (internal and external)
N_NODES_PER_LAYER = 32 # Vertical res --> will add one or 2 nodes to fix top/bottom constrains
N_REVS = 36 # Circf. res --> need to be multiple of 3 and 2
##############################
# 2D Functions
#################################
class vector2d:
def __init__(self, p1, p2, has_normal=True):
self.p1 = p1
self.p2 = p2
self.vec = self.vector2d()
self.unit = self.unit_vector()
self.to_plot = [[p1[0], p2[0]], [p1[1],p2[1]]]
self.normal = vector2d([-self.vec[1], self.vec[0]], [self.vec[1], -self.vec[0]], has_normal=False) if has_normal else None
def __call__(self):
return self.vec
def __str__(self):
return "Vector2d: p1: {p1:} p2: {p2:}".format(p1=self.p1, p2=self.p2)
def vector2d(self):
return np.array([self.p2[a] - self.p1[a] for a in range(len(self.p1))])
def unit_vector(self):
return self.vec / np.linalg.norm(self.vec)
def rotate(self,theta):
rotation_matrix = np.array([[np.cos(theta), -np.sin(theta)], [np.sin(theta), np.cos(theta)]])
p2 = np.matmul(rotation_matrix, self.vec)
p2 += np.array(self.p1)
return vector2d(self.p1, p2)
class dataPoint:
def __init__(self, coord, l=-1, s=-1, rh=-1, n=-1):
self.coord = coord
self.layer = l
self.section = s
self.refH = rh
self.nodeNumber = n
def copy(self):
return dataPoint(self.coord, self.layer, self.section, self.refH, self.nodeNumber)
def vector2dFromP1(center, length, dir):
p1 = np.array(center)
p2 = np.array([length * dir[0], length * dir[1]]) + p1
return vector2d(p1,p2)
def angle_between(v1, v2):
""" Returns the angle in radians between vectors 'v1' and 'v2' """
return np.arccos(np.clip(np.dot(v1.unit, v2.unit), -1.0, 1.0))
def regress(xs,ys,deg):
coeffs = np.polyfit(xs,ys,deg)
# if _print == True:
# a = ['(x^'+str(len(coeffs)-(i+1))+") * "+str(y) if i+1 !=len(coeffs) else str(y) for i, y in enumerate(coeffs)]
# print("Coeffs: " + str(coeffs) + " | " + " + ".join(a)[:-1:])
# return lambda x: np.sum([(x**len(coeffs)-(i+1))*y if i+1 !=len(coeffs) else y for i, y in enumerate(coeffs)])
return np.poly1d(coeffs)
# Ellipse Functions
def ellipse(a, b, h=0, k=0, _type=0, ref=-1):
def eq(val):
if _type == 0: # solved for y (return y, given x)
return (a/b) * -ref * np.sqrt(b**2 - (val-h)**2) + k
# return np.sqrt((1 - (val - h)**2 ) /b**2) + k
elif _type == 1: # solved for x (return x, given y)
return (b/a) * ref * np.sqrt(a**2 - (val-k)**2) + h
return eq
def ellipse_focci(a,b,h=0,k=0):
c = np.sqrt(a**2 - b**2)
return np.array([h, k + c]), np.array([h, k - c])
def sctattered_ellipse(a,b,h,k, yrange, xrange, x_res, y_res):
# Define eq of elipse
y_ellpisis = ellipse(a,b,h,k,1)
x_ellpisis = ellipse(a,b,h,k,0)
# Get min and max values
ymin = np.min(yrange)
ymax = np.max(yrange)
xmin = np.min(xrange)
xmax = np.max(xrange)
# Calculate undistributed points
ys_ybased = np.linspace(ymin, ymax, x_res)
xs_ybased = np.array([y_ellpisis(y) for y in ys_ybased ])
xs_xbased = np.linspace(xmin, xmax, y_res)
ys_xbased = np.array([x_ellpisis(x) for x in xs_xbased ])
# Set points in a single array
xs = np.append(xs_ybased, xs_xbased)
ys = np.append(ys_ybased, ys_xbased)
# Sort points
s1 = np.zeros((len(xs), 2))
for i, x in enumerate(xs):
s1[i][0] = x
s1[i][1] = ys[i]
s1 = s1[np.argsort(s1[:, 1])]
s2 = np.zeros((2,len(s1)))
for i in range(len(s1)):
s2[0][i] = s1[i][0]
s2[1][i] = s1[i][1]
return s1, s2
def regressed_ellipse(a,b,h,k, yrange, xrange, yswitch=0.80, breakpoints=[0], res=100, deg=2, axis=1):
# Define min and max values
ymin = | np.min(yrange) | numpy.min |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.