question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Using Hash Map || Easy || C++ | using-hash-map-easy-c-by-amit5446tiwari-w7c3 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | AMIT5446TIWARI | NORMAL | 2023-12-22T12:35:59.452218+00:00 | 2023-12-22T12:35:59.452241+00:00 | 30 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n int n1=nums1.size();\n int n2=nums2.size();\n unordered_map<long long,int> mp1;\n unordered_map<long long,int> mp2;\n for(int i=0;i<n1;i++){\n for(int j=i+1;j<n1;j++){\n mp1[(long long)nums1[i]*nums1[j]]++;\n }\n }\n for(int i=0;i<n2;i++){\n for(int j=i+1;j<n2;j++){\n mp2[(long long)nums2[i]*nums2[j]]++;\n }\n }\n int count=0;\n for(int i=0;i<n1;i++){\n int x=mp2[(long long)nums1[i]*nums1[i]];\n if(x>0) count+=x;\n }\n for(int i=0;i<n2;i++){\n int x=mp1[(long long)nums2[i]*nums2[i]];\n if(x>0) count+=x;\n }\n return count;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | C++11 STL std::unordered_map -- Map with Square as Key & Repeat Squares as Value | c11-stl-stdunordered_map-map-with-square-qn9d | Description\n\nEssentially, this solution breaks the problem into:\n1. Creating an unordered_map with squares as key and the value storing the number of times t | magnetrwn | NORMAL | 2023-12-07T21:58:33.422821+00:00 | 2023-12-07T21:58:33.422850+00:00 | 10 | false | # Description\n\nEssentially, this solution breaks the problem into:\n1. Creating an `unordered_map` with squares as key and the value storing the number of times the same square has been found (this is why we couldn\'t use an `unordered_set` here).\n2. Searching across the keys of the squares maps for matches with the product of two indexes inside of nums1 and nums2 in $$O( \\frac {n (n-1)} {2} )$$, since "**Note**" allows this instead of $$O(n^3)$$ if using three nested loops, or $$O(n^2 log \\space n)$$ using standard maps.\n\n**Note:** Using the `unordered_` flavor is important, as it allows $$O(1)$$ retrieval by employing hashing! A standard `map` could be slower due to $$O(log \\space n)$$ ordered retrieval time, which could add up for `.find()` occurring many times.\n\n# Code\n```\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n unordered_map<uint64_t, int> squares1, squares2;\n squares1.reserve(nums1.size());\n squares2.reserve(nums2.size());\n\n int found = 0;\n\n // (1)\n for (uint64_t bignum : nums1) {\n bignum *= bignum;\n squares1[bignum]++;\n }\n\n for (uint64_t bignum : nums2) {\n bignum *= bignum;\n squares2[bignum]++;\n }\n\n // (2)\n for (int i = 0; i < nums1.size() - 1; i++)\n for (int j = i + 1; j < nums1.size(); j++) {\n uint64_t bignum = static_cast<uint64_t>(nums1[i]) * static_cast<uint64_t>(nums1[j]);\n if (squares2.find(bignum) != squares2.end())\n found += squares2[bignum];\n }\n\n for (int i = 0; i < nums2.size() - 1; i++)\n for (int j = i + 1; j < nums2.size(); j++) {\n uint64_t bignum = static_cast<uint64_t>(nums2[i]) * static_cast<uint64_t>(nums2[j]);\n if (squares1.find(bignum) != squares1.end())\n found += squares1[bignum];\n }\n\n return found;\n }\n};\n``` | 0 | 0 | ['Math', 'Hash Function', 'C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | hash c solution | hash-c-solution-by-nameldi-ecq1 | \nint cal(int* nums1, int nums1Size, int* nums2, int nums2Size){\n int* hash2 = (int*)calloc(1e5+1, sizeof(int));\n int ans = 0;\n long long tmp;\n | nameldi | NORMAL | 2023-11-19T12:40:31.183051+00:00 | 2023-11-19T12:40:31.183067+00:00 | 0 | false | ```\nint cal(int* nums1, int nums1Size, int* nums2, int nums2Size){\n int* hash2 = (int*)calloc(1e5+1, sizeof(int));\n int ans = 0;\n long long tmp;\n for(int i = 0; i < nums2Size; i++){\n for(int j = 0; j < nums1Size; j++){\n tmp = nums1[j];\n tmp *= nums1[j];\n if(tmp % nums2[i] == 0){\n /* constraints: 1 <= nums1[i], nums2[i] <= 1e5 */\n ans += tmp / nums2[i] <= 1e5? hash2[tmp / nums2[i]] : 0;\n }\n }\n hash2[nums2[i]]++;\n }\n free(hash2);\n return ans;\n}\n\nint numTriplets(int* nums1, int nums1Size, int* nums2, int nums2Size) {\n return cal(nums1, nums1Size, nums2, nums2Size) + cal(nums2, nums2Size, nums1, nums1Size);\n}\n``` | 0 | 0 | ['C'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Map/Hash Table Solution in TS/JS | maphash-table-solution-in-tsjs-by-soccer-jch2 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nPrecalculated all the s | soccermaganti | NORMAL | 2023-11-05T19:43:56.311786+00:00 | 2023-11-05T19:43:56.311803+00:00 | 5 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nPrecalculated all the squared values and getting their frequencies. Then using two pointers iterated through both arrays checking for type 1 and type 2 and added the frequency count to the result value. If it returned undefined, it doesn\'t exist in the map. \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n^2)$$\n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n\n# Code\n```\nfunction numTriplets(nums1: number[], nums2: number[]): number {\n //type1\n const map1 = new Map();\n nums1.forEach(key => {\n let squared = Math.pow(key,2);\n if (!map1.has(squared)){\n map1.set(squared,1);\n } else {\n map1.set(squared, map1.get(squared) + 1);\n }\n });\n //type 2\n const map2 = new Map();\n nums2.forEach(key => {\n let squared = Math.pow(key,2);\n if (!map2.has(squared)){\n map2.set(squared,1);\n } else {\n map2.set(squared, map2.get(squared) + 1);\n }\n });\n \n let count = 0;\n for (let i = 0; i < nums1.length; i++){\n for (let j = 0; j < nums1.length; j++){\n if (i < j){\n let value = nums1[i] * nums1[j];\n if (map2.get(value) != undefined){\n count += map2.get(value);\n }\n }\n }\n }\n\n for (let i = 0; i < nums2.length; i++){\n for (let j = 0; j < nums2.length; j++){\n if (i < j){\n let value = nums2[i] * nums2[j];\n if (map1.get(value) != undefined){\n count += map1.get(value);\n }\n }\n }\n }\n return count;\n};\n``` | 0 | 0 | ['Array', 'Hash Table', 'Ordered Map', 'TypeScript'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | count the frequency of every product formed | easy to understand | count-the-frequency-of-every-product-for-9acr | Code\n\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) \n {\n int type1 = 0;\n unordered_map<long long in | akshat0610 | NORMAL | 2023-10-30T17:56:21.134271+00:00 | 2023-10-30T17:56:21.134291+00:00 | 25 | false | # Code\n```\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) \n {\n int type1 = 0;\n unordered_map<long long int,int>mp;\n for(int i = 0 ; i < nums1.size() ; i++) mp[1LL * nums1[i]*nums1[i]]++;\n for(int j = 0 ; j < nums2.size() ; j++)\n {\n for(int k = j+1 ; k < nums2.size() ; k++)\n {\n long long int product = (1LL*nums2[j]*nums2[k]);\n if(mp.find(product) != mp.end()) type1+=mp[product];\n }\n } \n \n mp.clear();\n int type2 = 0;\n for(int i = 0 ; i < nums2.size() ; i++) mp[1LL * nums2[i]*nums2[i]]++;\n for(int j = 0 ; j < nums1.size() ; j++)\n {\n for(int k = (j+1) ; k < nums1.size() ; k++)\n {\n long long int product = (1LL * nums1[j]*nums1[k]);\n if(mp.find(product) != mp.end()) type2+=mp[product];\n }\n }\n return (type1 + type2);\n }\n};\n``` | 0 | 0 | ['Array', 'Hash Table', 'Math', 'Two Pointers', 'C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | EASY CPP MAPPING | easy-cpp-mapping-by-divyanshojha99-53pa | Intuition\nThe problem requires finding the number of triplets that satisfy either Type 1 or Type 2 conditions. Type 1 triplets have the property that nums1[i]^ | divyanshojha99 | NORMAL | 2023-10-11T19:03:15.790517+00:00 | 2023-10-11T19:03:15.790534+00:00 | 11 | false | # Intuition\nThe problem requires finding the number of triplets that satisfy either Type 1 or Type 2 conditions. Type 1 triplets have the property that `nums1[i]^2` is equal to `nums2[j] * nums2[k]`, and Type 2 triplets have the property that `nums2[i]^2` is equal to `nums1[j] * nums1[k]`.\n\n# Approach\nTo solve this problem, we can iterate through both arrays and check for the conditions for Type 1 and Type 2 triplets.\n\n1. For each element in `nums1`, calculate `k` as the square of that element.\n2. Create an unordered map `mp` to store the counts of elements in `nums2`.\n3. Iterate through `nums2` and check if `k` is divisible by the current element `j`.\n - If it is divisible, calculate `d` as `k/j`.\n - Check if `d` is present in the map. If it is, increment the count `cnt` by the value associated with `d` in the map.\n - Update the count of the current element `j` in the map.\n4. Repeat the same process for `nums2` and `nums1` to handle Type 2 triplets.\n\nAfter both iterations, `cnt` will contain the total count of valid triplets.\n\n# Complexity\n- Time complexity: O(N*M), where N is the length of `nums1` and M is the length of `nums2`. We iterate through both arrays once.\n- Space complexity: O(M) for the unordered map to store counts of elements in `nums2`.\n\n# Code\n```cpp\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n int cnt = 0;\n for (long long i : nums1) {\n unordered_map<long long, long long> mp;\n long long k = i * i;\n for (auto j : nums2) {\n if (k % j == 0) {\n long long d = k / j;\n cnt += mp[d];\n }\n mp[j]++;\n }\n }\n for (long long i : nums2) {\n unordered_map<long long, long long> mp;\n long long k = i * i;\n for (auto j : nums1) {\n if (k % j == 0) {\n long long d = k / j;\n cnt += mp[d];\n }\n mp[j]++;\n }\n }\n return cnt;\n }\n};\n```\n\nThis code correctly counts the number of valid Type 1 and Type 2 triplets and returns the total count. | 0 | 0 | ['C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Java Easy Solution | java-easy-solution-by-madhurigali-wn6v | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | MadhuriGali | NORMAL | 2023-10-11T06:32:32.482829+00:00 | 2023-10-11T06:32:32.482849+00:00 | 25 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n2)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution {\n public int numTriplets(int[] nums1, int[] nums2) {\n if(nums1.length<=1 || nums2.length<=1 ) return 0;\n return cal(nums1,nums2)+cal(nums2,nums1);\n \n }public int cal(int[] nums1,int[] nums2){\n int count=0;\n HashMap<Integer,Integer> num1=new HashMap<>();\n \n for(int i:nums1){\n num1.put((i*i), num1.getOrDefault(i*i, 0)+1);\n }\n for(int i=0;i<nums2.length-1;i++){\n for(int j=i+1;j<nums2.length;j++){\n if(num1.get(nums2[i]*nums2[j])!=null) count+=(num1.get(nums2[i]*nums2[j]));\n }\n }\n return count; \n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Reducing arrays into a sum of the helper function results | reducing-arrays-into-a-sum-of-the-helper-17xz | Approach\nCreate a helper function counting possible products of a number in an array. Return the sum of both arrays being mapped to the power of 2 and reduced | melihov | NORMAL | 2023-09-15T09:00:03.808161+00:00 | 2023-09-15T09:13:25.226730+00:00 | 3 | false | # Approach\nCreate a helper function counting possible products of a number in an array. Return the sum of both arrays being mapped to the power of 2 and reduced with the helper function.\n\n# Code\n```\nclass Solution {\n\tfunc countProducts(of: Int, inArray: [Int]) -> Int {\n\t\tguard inArray.count > 1 else { return 0 }\n\n\t\tvar res = 0\n\n\t\tfor i in 0...(inArray.count - 2) {\n\t\t\tfor j in (i + 1)...(inArray.count - 1) {\n\t\t\t\tif inArray[i] * inArray[j] == of { res += 1 }\n\t\t\t}\n\t\t}\n\n\t\treturn res\n\t}\n\n\tfunc numTriplets(_ nums1: [Int], _ nums2: [Int]) -> Int {\n\t\tnums1.map { num in num * num }.reduce(0, { acc, num in\n\t\t\tacc + countProducts(of: num, inArray: nums2)\n\t\t}) + nums2.map { num in num * num }.reduce(0, { acc, num in\n\t\t\tacc + countProducts(of: num, inArray: nums1)\n\t\t})\n\t}\n}\n``` | 0 | 0 | ['Swift'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Two Pointer Approch Using HashMap | 100%Beats | two-pointer-approch-using-hashmap-100bea-2j1z | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Shree_Govind_Jee | NORMAL | 2023-09-13T17:46:07.256208+00:00 | 2023-09-13T17:46:07.256237+00:00 | 40 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(n2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n private int countways(int[] nums1, int []nums2){\n int count = 0;\n Map<Long, Integer> map = new HashMap<>();\n for (int n : nums2) {\n map.put((long) n * n, map.getOrDefault((long) n * n, 0) + 1);\n }\n\n for (int i = 0; i < nums1.length; i++) {\n for (int j = i + 1; j < nums1.length; j++) {\n long prod = (long) nums1[i] * (long) nums1[j];\n \n if (map.containsKey(prod)) count += map.get(prod);\n }\n }\n return count;\n }\n\n\n public int numTriplets(int[] nums1, int[] nums2) {\n Arrays.sort(nums1);\n Arrays.sort(nums2);\n \n return countways(nums1, nums2)+countways(nums2, nums1);\n }\n}\n``` | 0 | 0 | ['Array', 'Hash Table', 'Math', 'Two Pointers', 'Java'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Easy to understand JavaScript solution | easy-to-understand-javascript-solution-b-cbmn | Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n)\n\n# Code\n\nvar numTriplets = function(nums1, nums2) {\n const triplets = (numsA, numsB) | tzuyi0817 | NORMAL | 2023-08-27T06:22:19.836434+00:00 | 2023-08-27T06:23:23.795834+00:00 | 4 | false | # Complexity\n- Time complexity:\n$$O(n^2)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nvar numTriplets = function(nums1, nums2) {\n const triplets = (numsA, numsB) => {\n const squareMap = numsA.reduce((map, num) => {\n const square = num ** 2;\n const count = map.get(square) ?? 0;\n\n return map.set(square, count + 1);\n }, new Map());\n let result = 0;\n\n for (let a = 0; a < numsB.length; a++) {\n for (let b = a - 1; b >= 0; b--) {\n const product = numsB[a] * numsB[b];\n if (!squareMap.has(product)) continue;\n result += squareMap.get(product);\n }\n }\n return result;\n };\n\n return triplets(nums1, nums2) + triplets(nums2, nums1);\n};\n``` | 0 | 0 | ['JavaScript'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Only Using Map Simple Solution ✅✅ | only-using-map-simple-solution-by-nandun-hd8d | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | nandunk | NORMAL | 2023-08-25T14:40:06.652043+00:00 | 2023-08-25T14:40:06.652066+00:00 | 34 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(n2)$$ \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n unordered_map<long long,int>mp1,mp2;\n for(auto it:nums1){\n long long k=1LL*it*it;\n mp1[k]++;\n }\n for(auto it:nums2){\n long long k=1LL*it*it;\n mp2[k]++;\n }\n \n int cnt=0;\n for(int i=0;i<nums2.size()-1;i++){\n long long p=nums2[i];\n for(int j=i+1;j<nums2.size();j++){\n p=p*nums2[j];\n if(mp1.find(p)!=mp1.end()) cnt+=mp1[p];\n p=nums2[i];\n }\n\n }\n cout<<endl;\n for(int i=0;i<nums1.size()-1;i++){\n long long q=nums1[i];\n for(int j=i+1;j<nums1.size();j++){\n q=q*nums1[j];\n \n if(mp2.find(q)!=mp2.end()) cnt+=mp2[q];\n \n q=nums1[i];\n }\n\n }\n return cnt;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Python3 Two Pointer Solution | O(1) Space, O(n*m) Time with Indepth Explanation | python3-two-pointer-solution-o1-space-on-aqan | Intuition\n Describe your first thoughts on how to solve this problem. \nThere are two types of triples we\'re looking for: \n\n Type 1: Triplet (i, j, k) if | colewinfield | NORMAL | 2023-07-12T23:37:04.976835+00:00 | 2023-07-12T23:39:03.820890+00:00 | 55 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThere are two types of triples we\'re looking for: \n\n Type 1: Triplet (i, j, k) if nums1[i]^2 == nums2[j] * nums2[k] \n Type 2: Triplet (i, j, k) if nums2[i]^2 == nums1[j] * nums1[k]\n\nNotice the symmetry. It\'s saying we must look for triplets across both arrays. The best way to do this is by running the algorithm twice: once with nums1 as the target (the outer-loop) and again with nums2 as the target.\n\nTo do this without using any space (this can be done using a hashmap to count the instances where the conditions are true for both types), we can use two pointers like a 3Sum problem. In 3Sum, we investigate 1 number and then iterate our pointers over the remainder of a single array, squeezing the Left and Right pointers together. Then, iterate to the next number, shortening the remaining subarray. \n\nThis has a similar approach, except instead of a singular array, we use each number in Array1 `(a1)` and then search for a multiplicative pair in Array2 `(a2)` that would equal to the target of `a1[i]^2`. This works if `a2` is sorted. The time complexity for a sort is `O(nlogn)`, but this pales in comparison to the `O(mn)` runtime of the overall algorithm, so it\'s beneficial to do this. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe ```helper``` function will be used twice, once searching the `nums1` array against `nums2`, and then vice versa. \n\nIn the general case (with `a1 and a2`), sort `a2`, then go through each number in `a1`; our target number will be `a1[i]^2`. To optimally search for the pair in `a2`, we use 2 pointers, starting from the beginning and at the end: `l, r = 0, len(a2) - 1`. Then we iterate over them while `l < r`. \n\nThere are three conditions:\n* `a2[l] * a2[r] > target`: The pair is too large in comparison to our target. To mitigate this, we decrement `r`. This will move our pointer to a smaller number in the leftside (this is why we sorted), lowering our multiplicative result\n* `a2[l] * a2[r] < target`: Our pair isn\'t big enough, so we move our left pointer to the right where larger numbers are sorted, thus growing our multiplicative pair\n* `else: # we found our target`: This has 2 subconditions, when the left and right pointer are equal (i.e, `a2[l] == a2[r]`), and the other when they\'re not. \n * If they\'re equal, that means we shouldn\'t iterate over them to avoid recounting. So, we use the formula for counting pairs:`((n * (n - 1)) // 2)`. This is based on a combination formula, but for only 2 items. Get the size of the window, `n`, and use the formula. We `break` afterward because the numbers are equal, so there are no other pairs to consider between the two numbers.\n * Otherwise, they\'re not equal (but the pair is successful), so we count how many of each number there are and multiply the two together, `res += rightCount * leftCount`. \n\n\nRun this twice on both arrays. This would result in `O(2nm)` runtime, which reduces to `O(nm)`.\n\n# Complexity\n- Time complexity: `O(nm), where n == len(nums1) and m == len(nums2)`\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: `O(1)`\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int: \n def helper(a1, a2): # a1, a2: array1, array2; symmetry\n # sort the searchable array, a2, to make conditions possible\n a2.sort()\n res = 0\n for n in a1:\n target = n ** 2\n l, r = 0, len(a2) - 1\n while l < r: \n if a2[l] * a2[r] > target:\n r -= 1\n elif a2[l] * a2[r] < target:\n l += 1\n else:\n # if l, r are the same, use combination form and break\n if a2[l] == a2[r]:\n n = (r - l + 1)\n res += ((n * (n - 1))//2)\n break\n # count all the numbers at l and r\n # that are successful pairs\n left, right = a2[l], a2[r]\n leftCount, rightCount = 0, 0\n while l < len(a2) and a2[l] == left:\n leftCount += 1\n l += 1\n while r >= 0 and a2[r] == right:\n rightCount += 1\n r -= 1\n res += rightCount * leftCount\n \n return res\n \n # run it twice for the symmetry between type1/type2\n return helper(nums1, nums2) + helper(nums2, nums1)\n\n``` | 0 | 0 | ['Two Pointers', 'Python3'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Python3 solution || easy to understand || beginner's friendly | python3-solution-easy-to-understand-begi-tiv1 | Code\n\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n Map1 = Counter([n * n for n in nums1])\n Map2 = C | khushie45 | NORMAL | 2023-07-02T06:21:39.031739+00:00 | 2023-07-02T06:21:39.031765+00:00 | 27 | false | # Code\n```\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n Map1 = Counter([n * n for n in nums1])\n Map2 = Counter([n * n for n in nums2])\n\n res = 0\n for i in range(len(nums1) - 1):\n for j in range(i + 1, len(nums1)):\n res += Map2[nums1[i] * nums1[j]]\n\n for i in range(len(nums2) - 1):\n for j in range(i + 1, len(nums2)):\n res += Map1[nums2[i] * nums2[j]]\n\n return res\n``` | 0 | 0 | ['Hash Table', 'Python3'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Easy understand python solution | easy-understand-python-solution-by-peter-8o3c | Code\n\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n Count1 = Counter(nums1)\n Count2 = Counter(nums2) | petersing001 | NORMAL | 2023-06-21T09:52:40.480390+00:00 | 2023-06-21T09:52:40.480408+00:00 | 36 | false | # Code\n```\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n Count1 = Counter(nums1)\n Count2 = Counter(nums2)\n res = 0\n for i in Count1:\n db = Count2.copy()\n while db:\n item = db.popitem()\n Q, r = divmod(i*i, item[0])\n if r == 0 and Q in db:\n res += db[Q]*item[1]*Count1[i]\n db.pop(Q)\n elif r == 0 and Q == item[0]:\n res += comb(item[1],2)*Count1[i]\n \n for i in Count2:\n db = Count1.copy()\n while db:\n item = db.popitem()\n Q, r = divmod(i*i, item[0])\n if r == 0 and Q in db:\n res += db[Q]*item[1]*Count2[i]\n db.pop(Q)\n elif r == 0 and Q == item[0]:\n res += comb(item[1],2)*Count2[i]\n\n return res\n \n\n``` | 0 | 0 | ['Python3'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | unique and easy solution | unique-and-easy-solution-by-adityaclearz-vm2r | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | adityaclearzultra | NORMAL | 2023-06-21T07:06:10.386036+00:00 | 2023-06-21T07:06:10.386064+00:00 | 41 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\nlong mod = 1e9+7;\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n\n map<long long ,long long>mp1;\n for(long long i : nums1){\n long long n = (i)%mod*(i)%mod;\n n=n%mod;\n\n\n mp1[n]++;\n }\n map<long long ,long long>mp2;\n for(long long i : nums2){\n long long n = (i)%mod * (i)%mod;\n n=n%mod;\n\n mp2[n]++;\n }\n int res = 0;\n\n int i = 0;\n while(i<nums2.size()){\n\n int j = i+1;\n while(j<nums2.size()){\n\n long long n = (nums2[i])%mod*(nums2[j])%mod;\n n=n%mod;\n\n if(mp1.find(n)!=mp1.end()){\n\n res = res + mp1[n];\n }\n\n\n j++;\n }\n i++;\n }\n \n \n i = 0;\n while(i<nums1.size()){\n int j = i+1;\n while(j<nums1.size()){\n\n long long int n = (nums1[i])%mod*(nums1[j])%mod;\n n=n%mod;\n\n if(mp2.find(n)!=mp2.end()){\n res = res + mp2[n];\n }\n j++;\n }\n i++;\n }\n return res;\n \n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Python| pass with accumulate frequencys of nums^2 | python-pass-with-accumulate-frequencys-o-0z4n | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | MikeShieh | NORMAL | 2023-06-07T06:09:15.821123+00:00 | 2023-06-07T06:09:15.821167+00:00 | 15 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n# Code\n```\nclass Solution(object):\n def numTriplets(self, nums1, nums2):\n """\n :type nums1: List[int]\n :type nums2: List[int]\n :rtype: int\n """ \n square1 = map(lambda x : x* x, nums1)\n square2 = map(lambda x : x* x, nums2)\n #frequency of nums1^2 and nums2^2\n freq1, freq2 = defaultdict(int),defaultdict(int)\n m1, m2 = range(len(nums1)), range(len(nums2))\n for i in m1:\n freq1[square1[i]] += 1\n for i in m2:\n freq2[square2[i]] += 1\n \n Ans = 0\n #type1\n for item in freq1:\n for j in m2:\n if item % nums2[j] == 0: \n k = item / nums2[j]\n Ans += nums2[j+1:].count(k) * freq1[item]\n #type2\n for item in freq2:\n for j in m1:\n if item % nums1[j] == 0:\n k = item / nums1[j]\n Ans += nums1[j+1:].count(k) * freq2[item]\n\n return Ans\n``` | 0 | 0 | ['Python'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | C++ Solution || Easy Approach | c-solution-easy-approach-by-vedantk1003-fmoe | \nclass Solution {\npublic:\n #define ll long long int\n \n int count(vector<int> & A,vector<int>& B)\n {\n map<ll,int> m;\n int c=0;\ | vedantk1003 | NORMAL | 2023-05-25T17:09:43.842099+00:00 | 2023-05-25T17:09:43.842154+00:00 | 9 | false | ```\nclass Solution {\npublic:\n #define ll long long int\n \n int count(vector<int> & A,vector<int>& B)\n {\n map<ll,int> m;\n int c=0;\n for(auto a :A) m[(ll)a*a]++;\n\n for(int i=0;i<B.size()-1;i++)\n {\n for(int j{i+1};j<B.size();j++)\n {\n if(m.count((ll)B[i]*B[j]))\n c+=m[(ll)B[i]*B[j]];\n }\n }\n return c;\n }\n int numTriplets(vector<int>& nums1, vector<int>& nums2) {\n \n return count(nums1,nums2) + count(nums2,nums1);\n }\n};\n``` | 0 | 0 | ['C'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | Brute Force Slowish | brute-force-slowish-by-stolenfuture-zpe1 | Code\n\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n def count_triplets(nums1: List[int], nums2: List[int]) - | StolenFuture | NORMAL | 2023-05-19T00:42:22.922665+00:00 | 2023-05-19T00:42:22.922690+00:00 | 20 | false | # Code\n```\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n def count_triplets(nums1: List[int], nums2: List[int]) -> int:\n count = 0\n product_map = defaultdict(int)\n\n for i in range(len(nums1)):\n for j in range(i + 1, len(nums1)):\n product_map[nums1[i] * nums1[j]] += 1\n\n for i in range(len(nums2)):\n square = nums2[i] * nums2[i]\n count += product_map[square]\n\n return count\n\n return count_triplets(nums1, nums2) + count_triplets(nums2, nums1)\n\n``` | 0 | 0 | ['Python3'] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | PYTHON3 SIMPLEST SOLUTION. BEATS 96% USERS. SELF EXPLANATORY. | python3-simplest-solution-beats-96-users-y9fn | \nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n nums1SquareFrequency=defaultdict(int)\n nums2SquareFreq | pchakhilkumar | NORMAL | 2023-03-05T15:42:18.251667+00:00 | 2023-03-05T15:42:18.251704+00:00 | 8 | false | ```\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n nums1SquareFrequency=defaultdict(int)\n nums2SquareFrequency=defaultdict(int)\n for num in nums1:\n nums1SquareFrequency[num**2]+=1\n for num in nums2:\n nums2SquareFrequency[num**2]+=1\n ans=0\n for i in range(len(nums1)-1):\n for j in range(i+1,len(nums1)):\n ans+=nums2SquareFrequency[nums1[i]*nums1[j]]\n for i in range(len(nums2)-1):\n for j in range(i+1,len(nums2)):\n ans+=nums1SquareFrequency[nums2[i]*nums2[j]]\n return ans\n``` | 0 | 0 | [] | 0 |
number-of-ways-where-square-of-number-is-equal-to-product-of-two-numbers | [LC-1577-M | Python3] A Plain Solution | lc-1577-m-python3-a-plain-solution-by-dr-equi | Use collections.Counter and iterations to count.\n\npython3 []\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n | drsv | NORMAL | 2023-02-18T02:01:14.716064+00:00 | 2023-02-18T02:11:23.839462+00:00 | 26 | false | Use `collections.Counter` and iterations to count.\n\n```python3 []\nclass Solution:\n def numTriplets(self, nums1: List[int], nums2: List[int]) -> int:\n sq_cnter1 = Counter([num**2 for num in nums1])\n sq_cnter2 = Counter([num**2 for num in nums2])\n\n def count(nums, sq_cnter):\n n = len(nums)\n cnt = 0\n for i in range(n-1):\n for j in range(i+1, n):\n cnt += sq_cnter[nums[i]*nums[j]]\n return cnt\n \n return count(nums1, sq_cnter2) + count(nums2, sq_cnter1)\n``` | 0 | 0 | ['Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | [Python3, Java, C++] Easy Sliding Window O(n) | python3-java-c-easy-sliding-window-on-by-eyf1 | Intuition: \nWhenever you are faced with a circular array question, you can just append the array to itself to get rid of the circular array part of the proble | tojuna | NORMAL | 2022-01-09T04:01:51.703446+00:00 | 2022-01-09T09:12:54.378181+00:00 | 17,196 | false | *Intuition*: \nWhenever you are faced with a circular array question, you can just append the array to itself to get rid of the circular array part of the problem\n\n***Explanation***:\n* Count number of ones in nums, let the number of ones be stored in the variable `ones`\n* Append nums to nums because we have to look at it as a circular array\n* Find the maximum number of ones in a window of size `ones` in the new array\n* Number of swaps = `ones` - maximum number of ones in a window of size `ones`\n<iframe src="https://leetcode.com/playground/kCYY9jmQ/shared" frameBorder="0" width="600" height="300"></iframe>\n\nWe can also solve the same in O(1) space. Loop through the array twice:\n```\ndef minSwaps(self, nums: List[int]) -> int:\n\tones, n = nums.count(1), len(nums)\n\tx, onesInWindow = 0, 0\n\tfor i in range(n * 2):\n\t\tif i >= ones and nums[i % n - ones]: x -= 1\n\t\tif nums[i % n] == 1: x += 1\n\t\tonesInWindow = max(x, onesInWindow)\n\treturn ones - onesInWindow\n``` | 262 | 6 | ['C', 'Java', 'Python3'] | 25 |
minimum-swaps-to-group-all-1s-together-ii | Same Concept of Sliding Window || O(N) in 3 Langs. || Margin(94%) | same-concept-of-sliding-window-on-in-3-l-ms67 | \n# Intuition Behind the Code\n### Fools yell from bot accounts, we just need to move on.. This is my soln with submission history attached below..After Several | Aim_High_212 | NORMAL | 2024-08-02T00:32:52.087485+00:00 | 2024-08-02T03:55:56.604735+00:00 | 35,104 | false | \n# Intuition Behind the Code\n### Fools yell from bot accounts, we just need to move on.. This is my soln with submission history attached below..After Several attempts was able to provide soln with acceptable margin\n\n1. Counting 1s (`k`):\n - First, the code calculates the total number of 1s in the array, denoted by `k`. This is because the task is to find the smallest window (or segment) of size `k` that contains the maximum number of 1s.\n\n2. Initial Window Calculation:\n - The code then computes the number of 1s in the first window of size `k`. This is achieved by summing up the first `k` elements of the array. This sum is stored in the variable `cnt` and also in `mx` (as the initial maximum count of 1s in any window).\n\n3. Sliding Window Technique:\n - The core part of the solution involves a sliding window approach to move through the array and calculate the number of 1s in each window of size `k`.\n - For each step from `i = k` to `i = n + k - 1` (where `n` is the length of the array):\n - The code updates the `cnt` by adding the next element in the window (`nums[i % n]`) and removing the element that is sliding out of the window (`nums[(i - k + n) % n]`).\n - The maximum number of 1s found in any window of size `k` is updated in `mx`.\n\n4. Result Calculation:\n - Finally, the result is derived as `k - mx`, which represents the minimum number of swaps needed. This is because `k` is the total number of 1s, and `mx` is the maximum number of 1s found in any window of size `k`. Therefore, the difference `k - mx` gives the minimum number of 0s that need to be swapped with 1s to achieve the desired grouping of 1s.\n\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n\n```python []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n k = nums.count(1)\n mx = cnt = sum(nums[:k])\n n = len(nums)\n for i in range(k, n + k):\n cnt += nums[i % n]\n cnt -= nums[(i - k + n) % n]\n mx = max(mx, cnt)\n return k - mx\n```\n```java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int k = Arrays.stream(nums).sum();\n int n = nums.length;\n int cnt = 0;\n for (int i = 0; i < k; ++i) {\n cnt += nums[i];\n }\n int mx = cnt;\n for (int i = k; i < n + k; ++i) {\n cnt += nums[i % n] - nums[(i - k + n) % n];\n mx = Math.max(mx, cnt);\n }\n return k - mx;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int k = accumulate(nums.begin(), nums.end(), 0);\n int n = nums.size();\n int cnt = accumulate(nums.begin(), nums.begin() + k, 0);\n int mx = cnt;\n for (int i = k; i < n + k; ++i) {\n cnt += nums[i % n] - nums[(i - k + n) % n];\n mx = max(mx, cnt);\n }\n return k - mx;\n }\n};\n```\n\n# Code built after many attempts:\n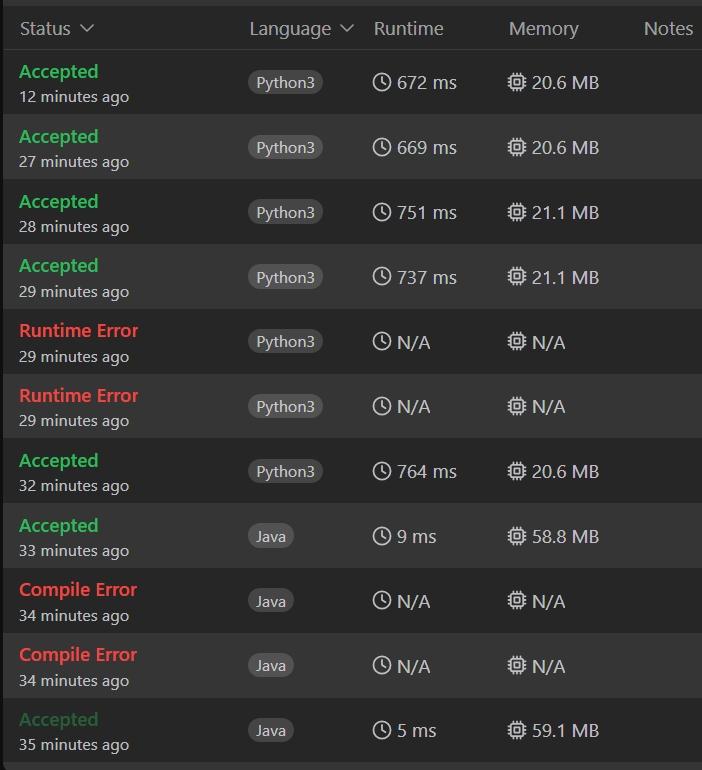\n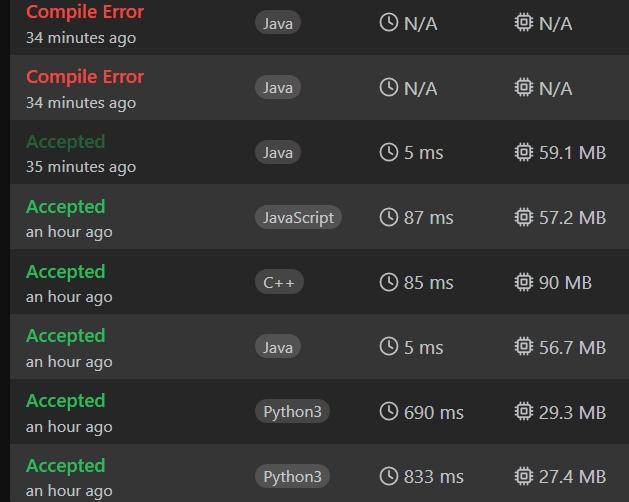\n\n | 123 | 3 | ['Array', 'Sliding Window', 'Python', 'C++', 'Java', 'Python3'] | 24 |
minimum-swaps-to-group-all-1s-together-ii | Sliding Window | sliding-window-by-votrubac-b335 | We move the fixed-size sliding window, wrapping it around the array. The lenght of the sliding window is the number of ones.\n\nWe track and return the minimum | votrubac | NORMAL | 2022-01-09T04:02:09.487035+00:00 | 2022-01-09T06:51:08.297220+00:00 | 11,269 | false | We move the fixed-size sliding window, wrapping it around the array. The lenght of the sliding window is the number of `ones`.\n\nWe track and return the minimum number of "holes" in our sliding window. Each of those "holes" needs to be swapped.\n\n**Java**\n```java\npublic int minSwaps(int[] nums) {\n int ones = Arrays.stream(nums).sum(), n = nums.length, res = nums.length;\n for (int i = 0, j = 0, cnt = 0; i < n; ++i) {\n while (j - i < ones)\n cnt += nums[j++ % n];\n res = Math.min(res, ones - cnt);\n cnt -= nums[i];\n }\n return res;\n}\n```\n**C++**\n```cpp\nint minSwaps(vector<int>& nums) {\n int ones = count(begin(nums), end(nums), 1), n = nums.size(), res = n;\n for (int i = 0, j = 0, cnt = 0; i < n; ++i) {\n while (j - i < ones)\n cnt += nums[j++ % n];\n res = min(res, ones - cnt);\n cnt -= nums[i];\n }\n return res;\n}\n``` | 98 | 6 | ['C', 'Java'] | 16 |
minimum-swaps-to-group-all-1s-together-ii | O(n) | Sliding Window | 2-ms Beats 100% Java | C++ | Python | Rust | Go | JavaScript | on-sliding-window-2-ms-beats-100-java-c-r0xy5 | Appraoch 1\n# Intuition\n\nThis problem of finding the minimum number of swaps to group all 1\'s in a circular binary array presents a unique challenge due to i | kartikdevsharma_ | NORMAL | 2024-08-02T04:22:06.594261+00:00 | 2024-08-20T06:50:09.856272+00:00 | 14,093 | false | # Appraoch 1\n# Intuition\n\nThis problem of finding the minimum number of swaps to group all 1\'s in a circular binary array presents a unique challenge due to its circular nature. The key insight is to recognize that we\'re essentially looking for a contiguous subarray of a specific length (equal to the total number of 1\'s) that contains the maximum number of 1\'s.\n\nGiven the circular nature of the array, we can visualize it as a ring. The optimal solution will be a segment of this ring that contains the most 1\'s. The number of swaps needed will be the difference between the total number of 1\'s and the number of 1\'s already in this optimal segment.\n\nThis problem can be efficiently solved using a sliding window technique, adapted for a circular array. The circular property allows us to consider all possible contiguous segments without explicitly handling different starting positions.\n\n# Approach\n\nThe solution employs a two-pointer technique combined with a sliding window, adapted for a circular array.\n1. **Count Total Ones**: \n - We start by iterating through the array to count the total number of 1\'s.\n - This count serves two purposes:\n a) It helps us handle edge cases efficiently.\n b) It determines the size of our sliding window.\n - The count is crucial because it represents the size of the group we\'re trying to form.\n\n2. **Handle Edge Cases**:\n - If there are no 1\'s in the array, or if all elements are 1\'s, no swaps are needed.\n - We can immediately return 0 in these cases, saving unnecessary computations.\n\n3. **Initialize Window**:\n - We create an initial window of size `totalOnes`.\n - We count the number of 1\'s in this initial window.\n - This window represents our first potential segment where all 1\'s could be grouped.\n\n4. **Slide the Window**:\n - We use two pointers to slide the window around the circular array.\n - The sliding process involves:\n a) Removing the element at the start of the window.\n b) Adding the next element at the end of the window.\n - We use the modulo operator to wrap around the array, simulating its circular nature.\n - This clever use of the modulo operator allows us to handle the circular property without creating a duplicate array or using extra space.\n\n5. **Track Maximum**:\n - As we slide the window, we keep track of the maximum number of 1\'s seen in any window configuration.\n - This maximum represents the largest group of 1\'s that already exist contiguously in the array.\n - Updating this maximum is done in constant time at each step.\n\n6. **Calculate Minimum Swaps**:\n - After sliding the window through all positions, we know the maximum number of 1\'s that exist contiguously in the array.\n - The minimum number of swaps is the difference between the total number of 1\'s and this maximum.\n - This difference represents the number of 1\'s that need to be moved to create a contiguous group of all 1\'s.\n\n\n# Complexity\n\n- **Time complexity: O(n)**\n - We perform two main passes through the array:\n 1. First pass to count the total number of 1\'s: O(n)\n 2. Second pass to slide the window: O(n)\n - All operations inside the loops are constant time.\n - Therefore, the overall time complexity is O(n), where n is the length of the input array.\n - This linear time complexity is optimal for this problem, as we need to examine each element at least once.\n\n- **Space complexity: O(1)**\n - We only use a constant amount of extra space:\n - `totalOnes`: integer\n - `currentOnes`: integer\n - `maxOnes`: integer\n - Loop variables\n - No additional data structures are used.\n - The space complexity remains constant regardless of the input size.\n - This constant space usage is a significant advantage of this approach, making it memory-efficient even for very large inputs.\n\n### Code\n```Java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length;\n int totalOnes = 0;\n\n // Count total number of 1\'s\n for (int num : nums) {\n totalOnes += num;\n }\n\n // Edge cases\n if (totalOnes == 0 || totalOnes == n) return 0;\n\n int currentOnes = 0;\n\n // Count 1\'s in the first window of size totalOnes\n for (int i = 0; i < totalOnes; i++) {\n currentOnes += nums[i];\n }\n\n int maxOnes = currentOnes;\n\n // Use two pointers to slide the window\n for (int i = 0; i < n; i++) {\n currentOnes -= nums[i];\n currentOnes += nums[(i + totalOnes) % n];\n maxOnes = Math.max(maxOnes, currentOnes);\n }\n\n return totalOnes - maxOnes;\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int totalOnes = 0;\n \n // Count total number of 1\'s\n for (int num : nums) {\n totalOnes += num;\n }\n \n // Edge cases\n if (totalOnes == 0 || totalOnes == n) return 0;\n \n int currentOnes = 0;\n \n // Count 1\'s in the first window of size totalOnes\n for (int i = 0; i < totalOnes; i++) {\n currentOnes += nums[i];\n }\n \n int maxOnes = currentOnes;\n \n // Use two pointers to slide the window\n for (int i = 0; i < n; i++) {\n currentOnes -= nums[i];\n currentOnes += nums[(i + totalOnes) % n];\n maxOnes = max(maxOnes, currentOnes);\n }\n \n return totalOnes - maxOnes;\n }\n};\n\n```\n```Python []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n = len(nums)\n total_ones = sum(nums)\n \n # Edge cases\n if total_ones == 0 or total_ones == n:\n return 0\n \n current_ones = sum(nums[:total_ones])\n max_ones = current_ones\n \n # Use two pointers to slide the window\n for i in range(n):\n current_ones -= nums[i]\n current_ones += nums[(i + total_ones) % n]\n max_ones = max(max_ones, current_ones)\n \n return total_ones - max_ones\n\n```\n```Go []\nfunc minSwaps(nums []int) int {\n n := len(nums)\n totalOnes := 0\n \n // Count total number of 1\'s\n for _, num := range nums {\n totalOnes += num\n }\n \n // Edge cases\n if totalOnes == 0 || totalOnes == n {\n return 0\n }\n \n currentOnes := 0\n \n // Count 1\'s in the first window of size totalOnes\n for i := 0; i < totalOnes; i++ {\n currentOnes += nums[i]\n }\n \n maxOnes := currentOnes\n \n // Use two pointers to slide the window\n for i := 0; i < n; i++ {\n currentOnes -= nums[i]\n currentOnes += nums[(i + totalOnes) % n]\n if currentOnes > maxOnes {\n maxOnes = currentOnes\n }\n }\n \n return totalOnes - maxOnes\n}\n\n```\n```Rust []\nimpl Solution {\n pub fn min_swaps(nums: Vec<i32>) -> i32 {\n let n = nums.len();\n let total_ones = nums.iter().sum::<i32>();\n \n // Edge cases\n if total_ones == 0 || total_ones as usize == n {\n return 0;\n }\n \n let mut current_ones = nums.iter().take(total_ones as usize).sum::<i32>();\n let mut max_ones = current_ones;\n \n // Use two pointers to slide the window\n for i in 0..n {\n current_ones -= nums[i];\n current_ones += nums[(i + total_ones as usize) % n];\n max_ones = max_ones.max(current_ones);\n }\n \n total_ones - max_ones\n }\n}\n```\n```JavaScript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minSwaps = function(nums) {\n const n = nums.length;\n const totalOnes = nums.reduce((sum, num) => sum + num, 0);\n \n // Edge cases\n if (totalOnes === 0 || totalOnes === n) return 0;\n \n let currentOnes = nums.slice(0, totalOnes).reduce((sum, num) => sum + num, 0);\n let maxOnes = currentOnes;\n \n // Use two pointers to slide the window\n for (let i = 0; i < n; i++) {\n currentOnes -= nums[i];\n currentOnes += nums[(i + totalOnes) % n];\n maxOnes = Math.max(maxOnes, currentOnes);\n }\n \n return totalOnes - maxOnes;\n};\n\n```\n\n\n\n---\n\n\n\n# Approach 2\n\n# Intuition\n\nThe circular nature of the array suggests that we need to consider all possible contiguous subarrays, including those that wrap around the end of the array.\n\nThe key insight is that if we can efficiently calculate the number of 1\'s in any contiguous subarray of a given length, we can determine the minimum number of swaps needed. This leads us to consider using a cumulative sum approach, which allows for constant-time queries of subarray sums.\n\n# Approach\n\n1. **Handling Circularity**: To address the circular nature of the array, we create a doubled array by concatenating the original array with itself. This allows us to consider all possible contiguous subarrays, including those that wrap around the end of the original array.\n\n2. **Cumulative Sum Array**: We construct a cumulative sum array, where each element represents the sum of all elements up to that point in the doubled array. This allows us to calculate the sum of any subarray in constant time.\n\n3. **Window Size**: The optimal grouping of 1\'s will have a size equal to the total number of 1\'s in the array. We calculate this total as our window size.\n\n4. **Checking All Windows**: We iterate through all possible windows of the calculated size, using the cumulative sum array to efficiently determine the number of 1\'s in each window.\n\n5. **Calculating Swaps**: The number of swaps needed for each window is the difference between the total number of 1\'s and the number of 1\'s already in the window. The minimum of these differences across all windows is our answer.\n\nStep-by-step breakdown of the algorithm:\n\n1. Count the total number of 1\'s in the input array.\n2. Create a doubled array by concatenating the input array with itself.\n3. Construct a cumulative sum array from the doubled array.\n4. Iterate through all possible windows of size equal to the total number of 1\'s.\n5. For each window, calculate the number of 1\'s it contains using the cumulative sum array.\n6. Keep track of the maximum number of 1\'s found in any window.\n7. Return the difference between the total number of 1\'s and the maximum found in any window.\n\n# Complexity\n\n- **Time complexity: O(n)**\n - Counting the total number of 1\'s: O(n)\n - Creating the doubled array: O(n)\n - Constructing the cumulative sum array: O(n)\n - Iterating through all windows: O(n)\n - All other operations are constant time.\n \n Where n is the length of the input array. Despite the nested loops, we only perform a constant number of operations for each element of the original array.\n\n- **Space complexity: O(n)**\n - The doubled array: O(n)\n - The cumulative sum array: O(n)\n - All other variables use constant space.\n \n Where n is the length of the input array. We use linear extra space primarily for the doubled array and the cumulative sum array.\n\n\n### Code \n\n\n```Java []\n\nclass Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length;\n int totalOnes = 0;\n\n // Count total number of 1\'s and create a doubled array\n int[] doubledNums = new int[2 * n];\n for (int i = 0; i < n; i++) {\n totalOnes += nums[i];\n doubledNums[i] = doubledNums[i + n] = nums[i];\n }\n\n // Edge cases\n if (totalOnes == 0 || totalOnes == n) return 0;\n\n // Create cumulative sum array\n int[] cumulativeSum = new int[2 * n + 1];\n for (int i = 0; i < 2 * n; i++) {\n cumulativeSum[i + 1] = cumulativeSum[i] + doubledNums[i];\n }\n\n int maxOnesInWindow = 0;\n\n // Check all possible windows of size totalOnes\n for (int i = 0; i <= n; i++) {\n int onesInWindow = cumulativeSum[i + totalOnes] - cumulativeSum[i];\n maxOnesInWindow = Math.max(maxOnesInWindow, onesInWindow);\n }\n\n return totalOnes - maxOnesInWindow;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int totalOnes = 0;\n \n // Count total number of 1\'s and create a doubled array\n vector<int> doubledNums(2 * n);\n for (int i = 0; i < n; i++) {\n totalOnes += nums[i];\n doubledNums[i] = doubledNums[i + n] = nums[i];\n }\n \n // Edge cases\n if (totalOnes == 0 || totalOnes == n) return 0;\n \n // Create cumulative sum array\n vector<int> cumulativeSum(2 * n + 1, 0);\n for (int i = 0; i < 2 * n; i++) {\n cumulativeSum[i + 1] = cumulativeSum[i] + doubledNums[i];\n }\n \n int maxOnesInWindow = 0;\n \n // Check all possible windows of size totalOnes\n for (int i = 0; i <= n; i++) {\n int onesInWindow = cumulativeSum[i + totalOnes] - cumulativeSum[i];\n maxOnesInWindow = max(maxOnesInWindow, onesInWindow);\n }\n \n return totalOnes - maxOnesInWindow;\n }\n};\n\n```\n```Python []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n = len(nums)\n total_ones = sum(nums)\n \n # Edge cases\n if total_ones == 0 or total_ones == n:\n return 0\n \n # Create doubled array and cumulative sum\n doubled_nums = nums + nums\n cumulative_sum = [0] + list(accumulate(doubled_nums))\n \n max_ones_in_window = 0\n \n # Check all possible windows of size total_ones\n for i in range(n + 1):\n ones_in_window = cumulative_sum[i + total_ones] - cumulative_sum[i]\n max_ones_in_window = max(max_ones_in_window, ones_in_window)\n \n return total_ones - max_ones_in_window\n\n\n```\n```Go []\nfunc minSwaps(nums []int) int {\n n := len(nums)\n totalOnes := 0\n \n // Count total number of 1\'s and create a doubled array\n doubledNums := make([]int, 2*n)\n for i := 0; i < n; i++ {\n totalOnes += nums[i]\n doubledNums[i] = nums[i]\n doubledNums[i+n] = nums[i]\n }\n \n // Edge cases\n if totalOnes == 0 || totalOnes == n {\n return 0\n }\n \n // Create cumulative sum array\n cumulativeSum := make([]int, 2*n+1)\n for i := 0; i < 2*n; i++ {\n cumulativeSum[i+1] = cumulativeSum[i] + doubledNums[i]\n }\n \n maxOnesInWindow := 0\n \n // Check all possible windows of size totalOnes\n for i := 0; i <= n; i++ {\n onesInWindow := cumulativeSum[i+totalOnes] - cumulativeSum[i]\n if onesInWindow > maxOnesInWindow {\n maxOnesInWindow = onesInWindow\n }\n }\n \n return totalOnes - maxOnesInWindow\n}\n\n```\n```Rust []\nimpl Solution {\n pub fn min_swaps(nums: Vec<i32>) -> i32 {\n let n = nums.len();\n let total_ones = nums.iter().sum::<i32>();\n \n // Edge cases\n if total_ones == 0 || total_ones == n as i32 {\n return 0;\n }\n \n // Create doubled array and cumulative sum\n let doubled_nums: Vec<i32> = nums.iter().chain(nums.iter()).cloned().collect();\n let mut cumulative_sum = vec![0; 2 * n + 1];\n for i in 0..2*n {\n cumulative_sum[i + 1] = cumulative_sum[i] + doubled_nums[i];\n }\n \n let mut max_ones_in_window = 0;\n \n // Check all possible windows of size total_ones\n for i in 0..=n {\n let ones_in_window = cumulative_sum[i + total_ones as usize] - cumulative_sum[i];\n max_ones_in_window = max_ones_in_window.max(ones_in_window);\n }\n \n total_ones - max_ones_in_window\n }\n}\n\n```\n```JavaScript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minSwaps = function(nums) {\n const n = nums.length;\n const totalOnes = nums.reduce((sum, num) => sum + num, 0);\n \n // Edge cases\n if (totalOnes === 0 || totalOnes === n) return 0;\n \n // Create doubled array and cumulative sum\n const doubledNums = [...nums, ...nums];\n const cumulativeSum = [0];\n for (let i = 0; i < 2 * n; i++) {\n cumulativeSum[i + 1] = cumulativeSum[i] + doubledNums[i];\n }\n \n let maxOnesInWindow = 0;\n \n // Check all possible windows of size totalOnes\n for (let i = 0; i <= n; i++) {\n const onesInWindow = cumulativeSum[i + totalOnes] - cumulativeSum[i];\n maxOnesInWindow = Math.max(maxOnesInWindow, onesInWindow);\n }\n \n return totalOnes - maxOnesInWindow;\n};\n\n``` | 72 | 0 | ['Array', 'Sliding Window', 'C++', 'Java', 'Go', 'Python3', 'Rust', 'JavaScript'] | 11 |
minimum-swaps-to-group-all-1s-together-ii | Easy understanding C++ code with approach | easy-understanding-c-code-with-approach-4ie5x | We are given a binary circular array (0s and 1s) and we are required to group all the 1s together with minimum no. of swaps.\n\nFirst we count the total no. of | venkatasaitanish | NORMAL | 2022-01-10T08:20:37.346382+00:00 | 2022-01-10T09:58:32.716288+00:00 | 4,606 | false | We are given a binary circular array (0s and 1s) and we are required to group all the 1s together with minimum no. of swaps.\n\nFirst we count the total no. of 1s in the given array. If the no. of 1s in the array is less than or equal to 1, this means all the 1s are grouped together, so no swaps are required and we return 0.\n\nWe are required to minimise the no. of swaps, so we find the ***sub array having maximum no. of ones in all sub arrays with length equal to total no. of ones.*** This makes the no. of 0s in the sub array low and swaps are reduced. \nSo now the answer is no. of 0s present in the subarray we found as we have to swap those 0s with the remaining 1s. i.e, the answer is **no. of zeroes in the sub array found = (total length of sub array - ones in that sub array).**\nFor finding the sub array having maximum ones of length k equal to total no. of ones , it looks like a ***fixed length sliding window problem*** which could be solved in O(n) time.\n\nBut now the question also mentions a specific point that it is a **circular array**. So the last element in the given array is followed with the first element. So we have n sub arrays for any length (n represents no. of elements in the given array). So while sliding the window, now we iterate upto (n + sub array length) to cover all sub arrays in the circular pattern.\n\n**Time Complexity:** O(n), **Space Complexity:** O(1)\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int ones = 0; // total no. of ones\n for(int i=0;i<n;i++){\n if(nums[i]==1) ones++;\n }\n if(ones<=1) return 0;\n \n int k = ones; // sliding window length\n int maxOnes = 0; // maxOnes in sub array of length k \n int cnt = 0;\n for(int i=0;i<k;i++){\n if(nums[i]==1) cnt++;\n }\n maxOnes = cnt;\n for(int i=k;i<n+k;i++){\n if(nums[i-k]==1) cnt--; // if element removing from window is 1, then decrease count.\n if(nums[i%n]==1) cnt++; // if element adding to window is 1, then increase count.\n maxOnes = max(maxOnes,cnt); // maintaing maxOnes for all sub arrays of length k.\n }\n return(k - maxOnes); // (total length of subarray - ones in the sub array found) \n }\n};\n```\n\n***Upvote if it helps.*** | 57 | 1 | ['C', 'Sliding Window', 'C++'] | 7 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Sliding window || O(n) || Full exaplanation | c-sliding-window-on-full-exaplanation-by-mste | 1)Count the total number of 1s. Let m be that number\n2)Find contiguous region of length m that has the most 1s in it\n3)The number of 0s in this region is the | abhi_ak1012 | NORMAL | 2022-01-09T05:28:05.004852+00:00 | 2022-01-09T05:51:51.708530+00:00 | 2,973 | false | 1)Count the total number of 1s. Let **m** be that number\n2)Find **contiguous region** of length **m** that has the most 1s in it\n3)The number of **0s** in this region is the minimum required swaps.*Each swap will move one 1 into the region and **one 0** to the remainder.*\n4)Finally we will use modulo operation for handling the case of circular array.\n\nImplementation:\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int count_1=0;\n int n=nums.size();\n \n if(n==1)return 0;\n \n for(int i=0;i<n;i++){\n if(nums[i]==1)\n count_1++;\n }\n \n int windowsize=count_1; // window size for counting maximum no. of 1s\n count_1=0;\n \n for(int i=0;i<windowsize;i++){\n if(nums[i]==1)count_1++;\n }\n \n int mx=count_1; //for storing maximum count of 1s in a window\n for(int i=windowsize;i<n+windowsize;i++){\n if(nums[i%n]==1)\n count_1++;\n if(nums[(i-windowsize)%n]==1)\n count_1--;\n mx=max(count_1,mx);\n }\n \n return windowsize-mx;\n \n }\n};\n```\n\n | 26 | 1 | ['Array', 'C', 'Sliding Window'] | 5 |
minimum-swaps-to-group-all-1s-together-ii | Sliding window with comments, Python | sliding-window-with-comments-python-by-k-xrd8 | First, by appending nums to nums, you can ignore the effect of split case.\nThen, you look at the window whose width is width. Here, width = the number of 1\'s | kryuki | NORMAL | 2022-01-09T06:05:02.445830+00:00 | 2022-01-17T07:38:56.491118+00:00 | 2,032 | false | First, by appending nums to nums, you can ignore the effect of split case.\nThen, you look at the window whose width is `width`. Here, `width` = the number of 1\'s in the original nums. This is because you have to gather all 1\'s in this window at the end of some swap operations. Therefore, the number of swap operation is the number of 0\'s in this window. \nThe final answer should be the minimum value of this.\n\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n width = sum(num == 1 for num in nums) #width of the window\n nums += nums\n res = width\n curr_zeros = sum(num == 0 for num in nums[:width]) #the first window is nums[:width]\n \n for i in range(width, len(nums)):\n curr_zeros -= (nums[i - width] == 0) #remove the leftmost 0 if exists\n curr_zeros += (nums[i] == 0) #add the rightmost 0 if exists\n res = min(res, curr_zeros) #update if needed\n \n return res\n``` | 23 | 1 | ['Sliding Window', 'Python', 'Python3'] | 4 |
minimum-swaps-to-group-all-1s-together-ii | Sliding window||45ms Beats 99.61% | sliding-window45ms-beats-9961-by-anwende-ird1 | Intuition\n Describe your first thoughts on how to solve this problem. \nUse sliding window. Since the array is circular, the right pointer r should go to til n | anwendeng | NORMAL | 2024-08-02T01:57:01.885902+00:00 | 2024-08-02T12:13:17.327589+00:00 | 5,936 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse sliding window. Since the array is circular, the right pointer r should go to til `n+n0` or `n+n1` to find the circular subinterval of length `n0` (`n1`) all 1s (0s) with minimal swaps.\n\nLater try optimization. 2nd C++ tries to reduce the number of if-branches & use `n0=count(nums, 0)`.\n# Approach\n[Please turn English subtitles if necessary]\n[https://youtu.be/KZHcVDVmJLY?si=5RVFUxbQ4HW1TfBl](https://youtu.be/KZHcVDVmJLY?si=5RVFUxbQ4HW1TfBl)\n<!-- Describe your approach to solving the problem. -->\n1. Use count to count how many 1 are in `nums` & store in `n1`. Set `mswap=INT_MAX`, `cnt1=0`.\n2. Use loop from l=0, r=0 while `r<n+n1` with step r++ do the following\n```\ncnt1+= nums[r%n];\nif (r-l+1 > n1) \n cnt1-=nums[l++ % n];\n \nif (r-l+1 == n1) // change all into 1 for nums[l+1..r]\n mswap = min(mswap, n1-cnt1);\n```\n3. `mswap` is the answer\n4. 2nd approach tries to reduce the number if-branches.\n5. The initial 2 pointers are set l=0, r=n0. cnt1 is initialized with `count(nums[0..n0-1], 1)` so that if-branches are removed.\n# Real RUN for testcase\nConsider the testcase=`[1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1,0,1]`\nAdd some output in the 2nd C++, one has\n\n```\nnums:\n[1010101010101010101010101010101010101010101]\nUse cnt1 times swaps to obtain the following form:\n[0000000000000000000001111111111111111111111]\tcnt1=11 mswap=11\n[1000000000000000000000111111111111111111111]\tcnt1=10 mswap=10\n[1100000000000000000000011111111111111111111]\tcnt1=11 mswap=10\n[1110000000000000000000001111111111111111111]\tcnt1=10 mswap=10\n[1111000000000000000000000111111111111111111]\tcnt1=11 mswap=10\n[1111100000000000000000000011111111111111111]\tcnt1=10 mswap=10\n[1111110000000000000000000001111111111111111]\tcnt1=11 mswap=10\n[1111111000000000000000000000111111111111111]\tcnt1=10 mswap=10\n[1111111100000000000000000000011111111111111]\tcnt1=11 mswap=10\n[1111111110000000000000000000001111111111111]\tcnt1=10 mswap=10\n[1111111111000000000000000000000111111111111]\tcnt1=11 mswap=10\n[1111111111100000000000000000000011111111111]\tcnt1=10 mswap=10\n[1111111111110000000000000000000001111111111]\tcnt1=11 mswap=10\n[1111111111111000000000000000000000111111111]\tcnt1=10 mswap=10\n[1111111111111100000000000000000000011111111]\tcnt1=11 mswap=10\n[1111111111111110000000000000000000001111111]\tcnt1=10 mswap=10\n[1111111111111111000000000000000000000111111]\tcnt1=11 mswap=10\n[1111111111111111100000000000000000000011111]\tcnt1=10 mswap=10\n[1111111111111111110000000000000000000001111]\tcnt1=11 mswap=10\n[1111111111111111111000000000000000000000111]\tcnt1=10 mswap=10\n[1111111111111111111100000000000000000000011]\tcnt1=11 mswap=10\n[1111111111111111111110000000000000000000001]\tcnt1=10 mswap=10\n[1111111111111111111111000000000000000000000]\tcnt1=11 mswap=10\n[0111111111111111111111100000000000000000000]\tcnt1=11 mswap=10\n[0011111111111111111111110000000000000000000]\tcnt1=11 mswap=10\n[0001111111111111111111111000000000000000000]\tcnt1=11 mswap=10\n[0000111111111111111111111100000000000000000]\tcnt1=11 mswap=10\n[0000011111111111111111111110000000000000000]\tcnt1=11 mswap=10\n[0000001111111111111111111111000000000000000]\tcnt1=11 mswap=10\n[0000000111111111111111111111100000000000000]\tcnt1=11 mswap=10\n[0000000011111111111111111111110000000000000]\tcnt1=11 mswap=10\n[0000000001111111111111111111111000000000000]\tcnt1=11 mswap=10\n[0000000000111111111111111111111100000000000]\tcnt1=11 mswap=10\n[0000000000011111111111111111111110000000000]\tcnt1=11 mswap=10\n[0000000000001111111111111111111111000000000]\tcnt1=11 mswap=10\n[0000000000000111111111111111111111100000000]\tcnt1=11 mswap=10\n[0000000000000011111111111111111111110000000]\tcnt1=11 mswap=10\n[0000000000000001111111111111111111111000000]\tcnt1=11 mswap=10\n[0000000000000000111111111111111111111100000]\tcnt1=11 mswap=10\n[0000000000000000011111111111111111111110000]\tcnt1=11 mswap=10\n[0000000000000000001111111111111111111111000]\tcnt1=11 mswap=10\n[0000000000000000000111111111111111111111100]\tcnt1=11 mswap=10\n[0000000000000000000011111111111111111111110]\tcnt1=11 mswap=10\n[0000000000000000000001111111111111111111111]\tcnt1=11 mswap=10\n```\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code||C++45ms Beats 99.61%\n```\nclass Solution {\npublic:\n static int minSwaps(vector<int>& nums) {\n const int n=nums.size();\n const int n1=count(nums.begin(), nums.end(), 1);\n int mswap=INT_MAX, cnt1=0;\n\n for (int l=0, r=0; r < n+n1; r++) {\n cnt1+= nums[r%n];\n if (r-l+1 > n1) \n cnt1-=nums[l++ % n];\n \n if (r-l+1 == n1) \n mswap = min(mswap, n1-cnt1);\n }\n return mswap;\n }\n};\n\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n\n```\n# 2nd C++ reducing if-branches||44ms Beats 99.61%\n```\nclass Solution {\npublic:\n static int minSwaps(vector<int>& nums) {\n const int n=nums.size();\n const int n1=count(nums.begin(), nums.end(), 1), n0=n-n1;\n int mswap;\n int cnt1=mswap=count(nums.begin(), nums.begin()+n0, 1);\n for (int l=0, r=n0; r < n+n0; r++, l++) {\n cnt1+= nums[r%n]-nums[l];\n mswap=min(mswap, cnt1);//change all to 0 for nums[l+1..r]\n }\n return mswap;\n }\n};\n\n\n\nauto init = []() {\n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n```\n# Python solution in the video\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n=len(nums)\n n1=sum(nums)\n n0=n-n1\n mswap=cnt1=sum(nums[0:n0])\n for l in range(n):\n r=l+n0\n cnt1+=nums[r%n]-nums[l]\n mswap=min(mswap, cnt1)\n return mswap\n \n``` | 18 | 1 | ['Array', 'Sliding Window', 'C++', 'Python3'] | 10 |
minimum-swaps-to-group-all-1s-together-ii | Java - Sliding window - O(n) - Easy understanding | java-sliding-window-on-easy-understandin-6w4z | To solve this problem lets understand the basic sliding window technique :\n\t\n\t1. We should go for sliding window when we want to find the minimum or maximum | Surendaar | NORMAL | 2022-01-09T05:30:44.900299+00:00 | 2022-01-09T05:32:16.709408+00:00 | 2,900 | false | To solve this problem lets understand the basic sliding window technique :\n\t\n\t1. We should go for sliding window when we want to find the minimum or maximum range in a given array\n\t2. Sliding window has 2 methods as fixed and dynamic range\n\nLets understand the problem more clearly:\n\t1. first we need to group 1\'s inorder to do that we need to first find the number of 1\'s available\n\t2. If there are no one\'s or all one\'s we can directly return zero as we don\'t have to process anything\n\t3. After knowning the 1\'s we just have to find the number of zero\'s from 0 to n for each window of size equal to one. (since we know the number of one this problem is fixed sliding window)\n\t4. now initial number of zeroes is found when i==0 then we can just see the element leaving the sliding window i-1 and element adding into the sliding window i+totalOne-1\n\t5. If both the elements are 0 or 1 then the count of 0\'s remain same, if element added into sliding window is 0 and leaving sliding window is 1 then count of 0 is increasing and vice versa\n\t6. And one last thing to note is -> since it is given is circular array we have to modulo by len so that for later part where i+totalOne-1>len it automatically go within the range of len.\n\nHope its easy to understand, please upvote if its helpful.. Happy learning :)\n```\n\tpublic int minSwaps(int[] nums) {\n\t\tint len = nums.length;\n\t\tint totalOne = 0;\n\n\t\tfor (int i = 0; i < len; i++) {\n\t\t\tif (nums[i] == 1)\n\t\t\t\ttotalOne++;\n\t\t}\n\t\tif (len == totalOne || totalOne == 0)\n\t\t\treturn 0;\n\t\tint min = Integer.MAX_VALUE;\n\t\tint i = 0;\n\t\tint count = 0;\n\t\tfor (int k = i; k < totalOne; k++) {\n\t\t\tif (nums[k] == 0)\n\t\t\t\tcount++;\n\t\t}\n\t\tmin = Math.min(min, count);\n\t\ti++;\n\t\tfor (; i < len; i++) {\n\t\t\tint j = (i + totalOne - 1) % len;\n\t\t\tif (nums[j] == 0 && nums[i - 1] == 1)\n\t\t\t\tcount++;\n\t\t\telse if (nums[j] == 1 && nums[i - 1] == 0)\n\t\t\t\tcount--;\n\t\t\tmin = Math.min(min, count);\n\t\t}\n\t\treturn min;\n\t}\n\t\t | 18 | 1 | ['Sliding Window', 'Java'] | 8 |
minimum-swaps-to-group-all-1s-together-ii | Easy Solution Beats 100% | easy-solution-beats-100-by-sachinonly-rcnf | Sliding Window\nPython []\nclass Solution(object):\n def minSwaps(self, nums):\n total = sum(nums) # Total number of 1\'s in the array\n n = l | Sachinonly__ | NORMAL | 2024-08-02T02:00:59.880435+00:00 | 2024-08-02T09:58:14.926300+00:00 | 4,140 | false | # Sliding Window\n```Python []\nclass Solution(object):\n def minSwaps(self, nums):\n total = sum(nums) # Total number of 1\'s in the array\n n = len(nums)\n \n # If there are no 1\'s, no swaps are needed\n if total == 0:\n return 0\n \n # Extend the array to handle the circular nature\n nums *= 2\n \n # Initialize the sliding window\n window_sum = sum(nums[:total])\n max_ones = window_sum\n swaps = total - max_ones\n \n for i in range(1, n):\n # Update the number of 1\'s in the current window by sliding the window\n window_sum += nums[i + total - 1] - nums[i - 1]\n max_ones = max(max_ones, window_sum)\n swaps = min(swaps, total - max_ones)\n \n return swaps\n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(std::vector<int>& nums) {\n int total = std::accumulate(nums.begin(), nums.end(), 0); // Total number of 1\'s in the array\n int n = nums.size();\n \n // If there are no 1\'s, no swaps are needed\n if (total == 0) {\n return 0;\n }\n \n // Extend the array to handle the circular nature\n nums.insert(nums.end(), nums.begin(), nums.end());\n \n // Initialize the sliding window\n int window_sum = std::accumulate(nums.begin(), nums.begin() + total, 0);\n int max_ones = window_sum;\n int swaps = total - max_ones;\n \n for (int i = 1; i < n; ++i) {\n // Update the number of 1\'s in the current window by sliding the window\n window_sum += nums[i + total - 1] - nums[i - 1];\n max_ones = std::max(max_ones, window_sum);\n swaps = std::min(swaps, total - max_ones);\n }\n \n return swaps;\n }\n};\n```\n```Java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int total = 0;\n for (int num : nums) {\n total += num; // Total number of 1\'s in the array\n }\n int n = nums.length;\n \n // If there are no 1\'s, no swaps are needed\n if (total == 0) {\n return 0;\n }\n \n // Extend the array to handle the circular nature\n int[] extendedNums = new int[n * 2];\n System.arraycopy(nums, 0, extendedNums, 0, n);\n System.arraycopy(nums, 0, extendedNums, n, n);\n \n // Initialize the sliding window\n int windowSum = 0;\n for (int i = 0; i < total; i++) {\n windowSum += extendedNums[i];\n }\n int maxOnes = windowSum;\n int swaps = total - maxOnes;\n \n for (int i = 1; i < n; i++) {\n // Update the number of 1\'s in the current window by sliding the window\n windowSum += extendedNums[i + total - 1] - extendedNums[i - 1];\n maxOnes = Math.max(maxOnes, windowSum);\n swaps = Math.min(swaps, total - maxOnes);\n }\n \n return swaps;\n }\n}\n```\n\n# Prefix Sum\n```python []\nclass Solution(object):\n def minSwaps(self, nums):\n n = len(nums)\n total = sum(nums)\n nums *= 2\n prefix = [0]\n for x in nums:\n prefix.append(prefix[-1] + x) \n\n biggest_ones = 0\n for i in range(n):\n biggest_ones = max(biggest_ones, prefix[i + total] - prefix[i])\n return total - biggest_ones \n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(std::vector<int>& nums) {\n int n = nums.size();\n int total = std::accumulate(nums.begin(), nums.end(), 0);\n nums.insert(nums.end(), nums.begin(), nums.end());\n \n std::vector<int> prefix(1, 0);\n for (int x : nums) {\n prefix.push_back(prefix.back() + x);\n }\n \n int biggest_ones = 0;\n for (int i = 0; i < n; ++i) {\n biggest_ones = std::max(biggest_ones, prefix[i + total] - prefix[i]);\n }\n \n return total - biggest_ones;\n }\n};\n```\n```Java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length;\n int total = 0;\n for (int num : nums) {\n total += num;\n }\n\n int[] extendedNums = new int[2 * n];\n System.arraycopy(nums, 0, extendedNums, 0, n);\n System.arraycopy(nums, 0, extendedNums, n, n);\n \n int[] prefix = new int[2 * n + 1];\n for (int i = 0; i < 2 * n; i++) {\n prefix[i + 1] = prefix[i] + extendedNums[i];\n }\n\n int biggestOnes = 0;\n for (int i = 0; i < n; i++) {\n biggestOnes = Math.max(biggestOnes, prefix[i + total] - prefix[i]);\n }\n \n return total - biggestOnes;\n }\n}\n\n```\n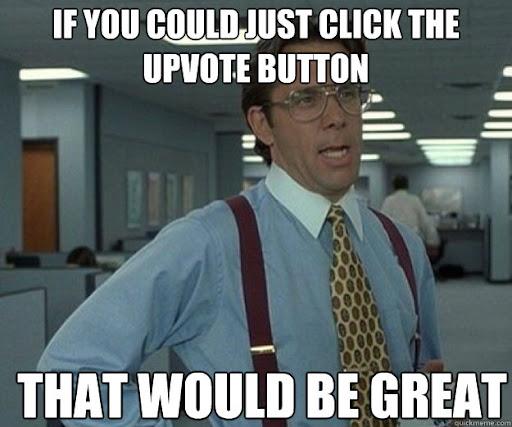\n\n | 16 | 1 | ['Array', 'Sliding Window', 'Prefix Sum', 'Python', 'C++', 'Java'] | 6 |
minimum-swaps-to-group-all-1s-together-ii | ✅💯🔥Simple Code🚀📌|| 🔥✔️Easy to understand🎯 || 🎓🧠Beats 100%🔥|| Beginner friendly💀💯 | simple-code-easy-to-understand-beats-100-7ugx | Beats 100%\n\n\n# Code for Java\n\nclass Solution {\n public int minSwaps(int[] nums) {\n int s = nums.length;\n int ones = 0;\n for (in | atishayj4in | NORMAL | 2024-08-02T07:35:41.772274+00:00 | 2024-08-02T07:45:08.882925+00:00 | 1,555 | false | # Beats 100%\n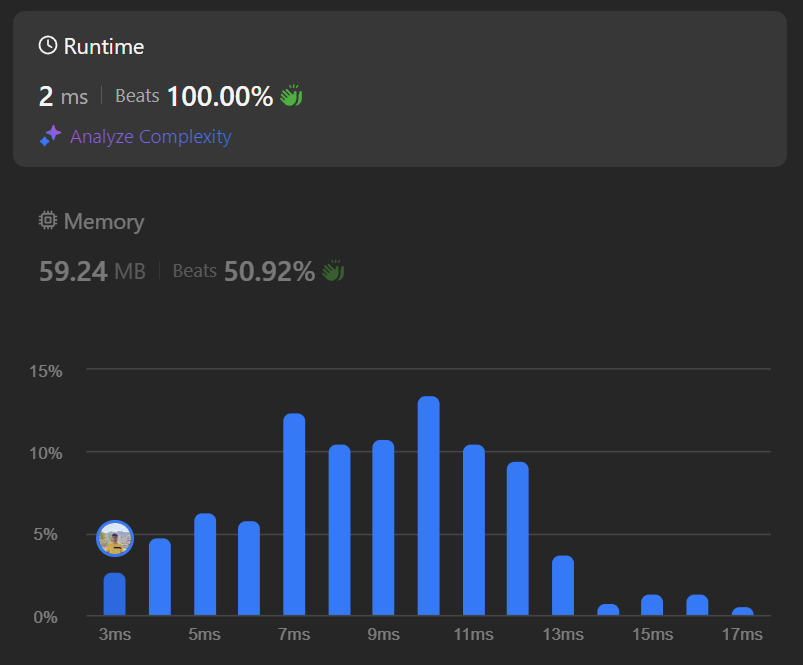\n\n# Code for Java\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int s = nums.length;\n int ones = 0;\n for (int n: nums) {\n ones+=n;\n }\n if (ones==0 || ones==s){\n return 0;\n }\n int curr = 0;\n for(int i=0; i<ones; i++) {\n curr+=nums[i];\n }\n int maxi = curr;\n for (int i=0; i<s; i++) {\n curr-=nums[i];\n curr+=nums[(i+ones)%s];\n maxi=Math.max(maxi, curr);\n }\n return ones-maxi;\n }\n}\n```\n# Code for C++\n```\n#include <iostream>\n#include <vector>\nusing namespace std;\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int s = nums.size();\n int ones = 0;\n for (int n : nums) {\n ones += n;\n }\n if (ones == 0 || ones == s) {\n return 0;\n }\n int curr = 0;\n for (int i = 0; i < ones; i++) {\n curr += nums[i];\n }\n int maxi = curr;\n for (int i = 0; i < s; i++) {\n curr -= nums[i];\n curr += nums[(i + ones) % s];\n maxi = max(maxi, curr);\n }\n return ones - maxi;\n }\n};\n```\n# Code for Python\n```\ndef min_swaps(nums):\n n = len(nums)\n ones = sum(nums)\n if ones==0 or ones==n:\n return 0\n curr = sum(nums[:ones])\n maxi = curr\n for i in range(n):\n curr-=nums[i]\n curr+=nums[(i+ones)%n]\n maxi=max(maxi, curr)\n return ones-maxi\n\n```\n# Code for JavaScript\n```\nfunction minSwaps(nums) {\n const s = nums.length;\n let ones = 0;\n for (let n of nums) {\n ones += n;\n }\n if (ones === 0 || ones === s) {\n return 0;\n }\n let curr = 0;\n for (let i = 0; i < ones; i++) {\n curr += nums[i];\n }\n let maxi = curr;\n for (let i = 0; i < s; i++) {\n curr -= nums[i];\n curr += nums[(i + ones) % s];\n maxi = Math.max(maxi, curr);\n }\n return ones - maxi;\n}\n```\n# Code for C\n```\n#include <stdio.h>\nint minSwaps(int nums[], int s) {\n int ones = 0;\n for (int i = 0; i < s; i++) {\n ones += nums[i];\n }\n if (ones == 0 || ones == s) {\n return 0;\n }\n int curr = 0;\n for (int i = 0; i < ones; i++) {\n curr += nums[i];\n }\n int maxi = curr;\n for (int i = 0; i < s; i++) {\n curr -= nums[i];\n curr += nums[(i + ones) % s];\n maxi = (maxi > curr) ? maxi : curr;\n }\n return ones - maxi;\n}\n```\n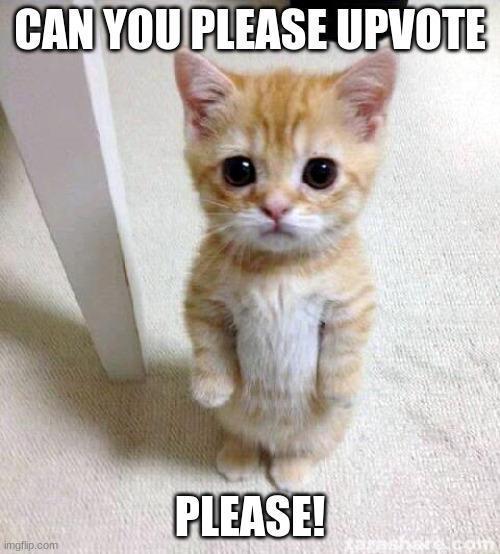 | 15 | 0 | ['Array', 'C', 'Sliding Window', 'Python', 'C++', 'Java', 'JavaScript'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | C++ || PYTHON || Sliding Window (approach) || Easy to understand || O(1) space | c-python-sliding-window-approach-easy-to-k5tx | Intuition - It\'s a circular array problem, but to handle circular array contraint just append the array to itself or use modulo for this purpose and it will be | typical_soul | NORMAL | 2022-01-09T05:30:54.413025+00:00 | 2022-01-25T05:29:27.719028+00:00 | 1,217 | false | **Intuition - It\'s a circular array problem, but to handle circular array contraint just append the array to itself or use modulo for this purpose and it will become a linear array type problem.**\n\n**-** find the total number of 1\'s in whole array. like **totalOnes** in the below code.\n**-** count of number of 1\'s in window of constant size **totalOnes** and keep moving this window by one index to get maximum count of 1\'s in all windows.\n**-** in the window with maximum of number of 1\'s, we will swap all of its 0\'s with other 1\'s which are not in this window, so our anser will be **(totalOnes - maxOnesInWindow)**.\n**-** When i >= n we will use modulo to make it work like a circular array.\n# **C++**\n```\nclass Solution{\npublic:\n int minSwaps(vector<int> &nums){\n int totalOnes = 0, n = nums.size();\n //here totalOnes is the number of 1\'s present in array\n for (auto it : nums)\n if (it == 1) totalOnes++;\n\t\t\n //now we will count the maximum number of 1\'s in any window of size totalOnes\n int maxOnesInWindow = 0, onesInCurrWindow = 0, i = 0;\n for (i = 0; i < totalOnes; i++)\n if (nums[i] == 1) maxOnesInWindow++;\n \n //onesInCurrWindow is the count of 1\'s in the current window\n\t\tonesInCurrWindow = maxOnesInWindow;\n\t\t\n //Now we will move the array with a constant window size of totalOnes\n for (; i < n + totalOnes; i++){\n //In this step we are moving window forward by one step\n //if nums[i%n] is 1 then add 1 to onesInCurrWindow\n //if nums[i - totalOnes] is 1 then subtrct 1 from onesInCurrWindow\n onesInCurrWindow += (nums[i % n] - nums[i - totalOnes]);\n maxOnesInWindow = max(onesInCurrWindow, maxOnesInWindow);\n }\n return totalOnes - maxOnesInWindow;\n }\n};\n```\n# **PYTHON**\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n\t #here totalOnes is the number of 1\'s present in array\n totalOnes = sum(i == 1 for i in nums)\n\t\t\n\t\t#onesInCurrWindow is the count of 1\'s in the current window\n\t\tonesInCurrWindow = sum(i == 1 for i in nums[:totalOnes])\n \n\t\t#now we will count the maximum number of 1\'s in any window of size totalOnes\n\t\tmaxOnesInWindow = onesInCurrWindow\n\t\t\n #Now we will move the array with a constant window size of totalOnes\n for i in range(totalOnes, len(nums) + totalOnes):\n\t\t #In this step we are moving window forward by one step\n #if nums[i%n] is 1 then add 1 to onesInCurrWindow\n #if nums[i - totalOnes] is 1 then subtrct 1 from onesInCurrWindow\n onesInCurrWindow += (nums[i % len(nums)] - nums[i - totalOnes]);\n maxOnesInWindow = max(maxOnesInWindow, onesInCurrWindow)\n \n return totalOnes - maxOnesInWindow\n```\n\n**Time complexity :- O(n)\nSpace complexity :- O(1)**\n\n**Question - why we are traversing only upto i < (n + totalOnes) ?**\n**Answer** - because our window size is totalOnes so, the last window we need to check will be (last one element + (totalOnes-1) elements from starting)....\nand we are using i as rightmost index of every window, so the largest value i will attain inside for loop is (n+totalOne-1).\n**Question - What is logic of this line ```onesInCurrWindow += (nums[i % n] - nums[i - totalOnes])``` or why are we adding when ```nums[i%n]==1``` and subtracting when ```nums[i-totalOnes]==1``` ?**\n**Answer** - actually here in each iteration we are moving our window of constant size totalOnes by one index, and counting number of 1\'s in that new window....\ntake this example num = [1, 0, 0, 1, 0, 1, 0 , 1]\nhere totalOnes = 4,\n\nwhen i = 3, our window is [nums[0], nums[1], nums[2], nums[3]] or [1,0,0,1] and number of 1\'s in this window is 2. so, onesInCurrWindow = 2.\n\nnow move forward by one index ,\ni = 4, and now our current window will be [nums[1], nums[2], nums[3], nums[4]]....\nnow what\'s the difference in this new window and previous window,,, you will see that the first element of previous window(which is nums[i-totalOnes] or nums[0]) is removed and we added a new element(which is nums[i]) and this is new window.\nso, how to update the value of onesInCurrWindow, as said above we have removed nums[i-totalValues] so, check whether that nums[i-totalOnes] == 1, if yes then subtract 1 from onesInCurrWindow because that element is no longer part of our current window hence we need to remove it\'s 1 count from onesInCurrWindow,,, and we have added a new element nums[i] in current window so, check whether nums[i] is 1 or not, if it is 1 then add add +1 in onesInCurrWindow.\n\n**If you find this useful please UPVOTE, or have any suggection then please share it in comments** | 14 | 0 | ['Sliding Window'] | 6 |
minimum-swaps-to-group-all-1s-together-ii | [Java/Python 3] Sliding Window O(n) code w/ brief explanation and analysis. | javapython-3-sliding-window-on-code-w-br-wqoh | Denote as winWidth the total number of 1\'s in the input array, then the goal of swaps is to get a window of size winWidth full of 1\'s. Therefore, we can maint | rock | NORMAL | 2022-01-09T04:32:18.550171+00:00 | 2022-01-12T16:00:38.518308+00:00 | 1,219 | false | Denote as `winWidth` the total number of `1`\'s in the input array, then the goal of swaps is to get a window of size `winWidth` full of `1`\'s. Therefore, we can maintain a sliding window of size `winWidth` to find the maximum `1`\'s inside, and accordingly the minimum number of `0`\'s inside the sliding window is the solution.\n\n```java\n public int minSwaps(int[] nums) {\n int winWidth = 0;\n for (int n : nums) {\n winWidth += n;\n }\n int mx = 0;\n for (int lo = -1, hi = 0, onesInWin = 0, n = nums.length; hi < 2 * n; ++hi) {\n onesInWin += nums[hi % n];\n if (hi - lo > winWidth) {\n onesInWin -= nums[++lo % n];\n }\n mx = Math.max(mx, onesInWin);\n }\n return winWidth - mx;\n }\n```\n```python\n def minSwaps(self, nums: List[int]) -> int:\n win_width = sum(nums)\n lo, mx, ones_in_win = -1, 0, 0\n n = len(nums)\n for hi in range(2 * n):\n ones_in_win += nums[hi % n]\n if hi - lo > win_width:\n lo += 1\n ones_in_win -= nums[lo % n]\n mx = max(mx, ones_in_win) \n return win_width - mx\n```\n\n**Analysis:**\n\nTime: `O(n)`, space: `O(1)`, where `n = nums.length`. | 14 | 6 | ['Java', 'Python3'] | 3 |
minimum-swaps-to-group-all-1s-together-ii | O(N) || EASY- EXPLAINED ||JAVA C++ PYTHON | on-easy-explained-java-c-python-by-abhis-91dk | HEY , IF THE HELPS YOU THEN PLS UPVOTE IT . THANKS @aryan43 for C++ code. Hope to see your knight badge soon.\n\n### Approach\n\n1. Count the total number of 1s | Abhishekkant135 | NORMAL | 2024-08-02T00:28:50.444047+00:00 | 2024-08-02T00:28:50.444075+00:00 | 3,871 | false | # HEY , IF THE HELPS YOU THEN PLS UPVOTE IT . THANKS @aryan43 for C++ code. Hope to see your knight badge soon.\n\n### Approach\n\n1. **Count the total number of 1s** in the array. This count will be the size of the window we need to consider.\n2. **Form an extended array**: To handle the circular nature, concatenate the array to itself. This allows us to simulate the circular behavior by considering subarrays that wrap around the end to the beginning.\n3. **Sliding Window Technique**: Use a sliding window of size equal to the count of 1s to find the subarray with the minimum number of 0s. This gives the minimum swaps needed.\n\n### Detailed Steps\n\n1. **Initial Setup**:\n - Count the total number of 1s in the array.\n - If there are no 1s, no swaps are needed.\n\n2. **Extended Array**:\n - Concatenate the array with itself to handle the circular nature.\n\n3. **Sliding Window**:\n - Initialize a window of size equal to the number of 1s.\n - Count the number of 0s in the initial window.\n - Slide the window across the extended array and update the count of 0s.\n - Track the minimum count of 0s in any window of the required size.\n\n4. **Result**:\n - The minimum count of 0s found in any window is the minimum number of swaps needed.\n\n### Python Solution\n\n```python\nclass Solution(object):\n def minSwaps(self, nums):\n """\n :type nums: List[int]\n :rtype: int\n """\n n = len(nums)\n count_ones = nums.count(1)\n if count_ones == 0:\n return 0\n\n # Extend the array to handle the circular nature\n extended_nums = nums + nums\n\n # Initialize the number of zeroes in the first window\n current_zeroes = extended_nums[:count_ones].count(0)\n min_zeroes = current_zeroes\n\n # Slide the window\n for i in range(count_ones, len(extended_nums)):\n if extended_nums[i] == 0:\n current_zeroes += 1\n if extended_nums[i - count_ones] == 0:\n current_zeroes -= 1\n\n min_zeroes = min(min_zeroes, current_zeroes)\n\n return min_zeroes\n\n# Test the solution\nsol = Solution()\nnums = [1, 0, 1, 0, 1, 0, 0, 1]\nprint(sol.minSwaps(nums)) # Expected output should consider the circular nature\n```\n\n### C++ Solution\n\n```cpp\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int count_ones = count(nums.begin(), nums.end(), 1);\n if (count_ones == 0) return 0;\n\n // Extend the array to handle the circular nature\n vector<int> extended_nums(nums.begin(), nums.end());\n extended_nums.insert(extended_nums.end(), nums.begin(), nums.end());\n\n // Initialize the number of zeroes in the first window\n int current_zeroes = count(extended_nums.begin(), extended_nums.begin() + count_ones, 0);\n int min_zeroes = current_zeroes;\n\n // Slide the window\n for (int i = count_ones; i < extended_nums.size(); ++i) {\n if (extended_nums[i] == 0) current_zeroes++;\n if (extended_nums[i - count_ones] == 0) current_zeroes--;\n\n min_zeroes = min(min_zeroes, current_zeroes);\n }\n\n return min_zeroes;\n }\n};\n\n// Test the solution\nint main() {\n Solution sol;\n vector<int> nums = {1, 0, 1, 0, 1, 0, 0, 1};\n cout << sol.minSwaps(nums) << endl; // Expected output should consider the circular nature\n return 0;\n}\n```\n\n### Java Solution\n\n```java\nclass Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length;\n int countOnes = 0;\n for (int num : nums) {\n if (num == 1) countOnes++;\n }\n if (countOnes == 0) return 0;\n\n // Extend the array to handle the circular nature\n int[] extendedNums = new int[2 * n];\n System.arraycopy(nums, 0, extendedNums, 0, n);\n System.arraycopy(nums, 0, extendedNums, n, n);\n\n // Initialize the number of zeroes in the first window\n int currentZeroes = 0;\n for (int i = 0; i < countOnes; i++) {\n if (extendedNums[i] == 0) currentZeroes++;\n }\n int minZeroes = currentZeroes;\n\n // Slide the window\n for (int i = countOnes; i < extendedNums.length; i++) {\n if (extendedNums[i] == 0) currentZeroes++;\n if (extendedNums[i - countOnes] == 0) currentZeroes--;\n\n minZeroes = Math.min(minZeroes, currentZeroes);\n }\n\n return minZeroes;\n }\n\n // Test the solution\n public static void main(String[] args) {\n Solution sol = new Solution();\n int[] nums = {1, 0, 1, 0, 1, 0, 0, 1};\n System.out.println(sol.minSwaps(nums)); // Expected output should consider the circular nature\n }\n}\n```\n\n### Explanation\n\n1. **Count the number of 1s**: The initial step counts how many `1`s are in the array.\n2. **Extended Array**: We duplicate the array to handle its circular nature.\n3. **Sliding Window Initialization**: The window is initialized to the size of the number of `1`s, and the count of `0`s in the window is calculated.\n4. **Sliding the Window**: As the window slides across the extended array, the count of `0`s is updated dynamically by adding the new element and removing the old one.\n5. **Tracking Minimum Zeroes**: The minimum count of `0`s found in any window is the result, as it represents the fewest swaps needed to group all `1`s together.\n\n**Time Complexity**: O(n), where n is the number of elements in the original array. This is because we are iterating over the array multiple times in linear time.\n**Space Complexity**: O(n), since we are using an extended array that is twice the size of the original array. | 12 | 1 | ['Array', 'Sliding Window', 'Python', 'C++', 'Java'] | 7 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Sliding Window | c-sliding-window-by-abhay5349singh-tizg | Connect with me on LinkedIn: https://www.linkedin.com/in/abhay5349singh/\n\nApproach: let count of 1s be \'k\', so after rearranging, we will be having a window | abhay5349singh | NORMAL | 2022-01-09T04:07:23.980364+00:00 | 2023-07-14T04:07:11.097329+00:00 | 1,628 | false | **Connect with me on LinkedIn**: https://www.linkedin.com/in/abhay5349singh/\n\n**Approach**: let count of 1s be \'k\', so after rearranging, we will be having a window of size \'k\'\n \n```\nclass Solution {\npublic:\n\n int minSwaps(vector<int> &a) {\n int n=a.size();\n \n int k=0;\n for(int i=0;i<n;i++) k += (a[i]==1);\n \n a.insert(a.end(), a.begin(), a.end()); // doubling the size of array as it is circular\n \n int ans=n;\n \n int i=0, j=0, one=0;\n while(j<2*n){\n one += (a[j]==1);\n \n if(j-i+1 == k){\n ans = min(ans,k-one); // k-one will number of swaps required to make all elements of window sized \'k\' as 1\n one -= (a[i]==1);\n \n i++;\n }\n j++;\n }\n \n return (ans==n ? 0:ans);\n }\n};\n```\n\n**Do Upvote If It Helps** | 11 | 1 | ['C++'] | 5 |
minimum-swaps-to-group-all-1s-together-ii | 🔥 Java O(N) Explanation with Diagrams ✍🏻 | java-on-explanation-with-diagrams-by-yas-kj36 | \uD83D\uDCCC Sliding Window \n\n\n\n\n\n\n\n\nTime Complexity : O(N) || Space Complexity : O(N)\n\n\n public int minSwaps(int[] nums) {\n \n in | Yash_kr | NORMAL | 2022-03-09T10:33:57.301327+00:00 | 2022-03-12T16:21:39.199004+00:00 | 635 | false | # \uD83D\uDCCC Sliding Window \n<img width="50%" src="https://assets.leetcode.com/users/images/74c6717f-82e1-49ef-ac59-0def9f630ae6_1646821665.903593.png">\n<img width="50%" src="https://assets.leetcode.com/users/images/55496339-0d86-4821-b376-f7c1eb100d99_1646821665.8353672.png">\n<img width="50%" src="https://assets.leetcode.com/users/images/f3e7c571-5148-4f2f-849d-0031f7d37041_1646821665.8760197.png">\n<img width="50%" src="https://assets.leetcode.com/users/images/d98142ea-c40c-4865-9be9-9c7908e0d702_1646821664.4522345.png">\n\n[](http://)\n[](http://)\n\n**Time Complexity : O(N) || Space Complexity : O(N)**\n\n```\n public int minSwaps(int[] nums) {\n \n int l = nums.length;\n\t\t//prefix array : storing ones count upto ith index\n int[] ones = new int[l];\n ones[0] = nums[0]==1 ? 1 : 0;\n for(int i=1;i<l;i++){\n\t\t if(nums[i] == 1) ones[i] = ones[i-1] + 1;\n\t\t else ones[i] = ones[i-1];\n }\n \n if(ones[l-1]==l || ones[l-1]==0) return 0; // either all ones or no ones\n \n int ws = ones[l-1]; //window size = total no of ones in the array\n int minSwaps = Integer.MAX_VALUE;\n int si = 0, ei;\n\t\t\n while(si<nums.length)\n {\n ei = (si+ws-1)%l;\n\t\t\tint totalones;\n\t\t\t\n if(ei>=si) totalones = ones[ei]-( si==0 ? 0 : ones[si-1]); //normal window\n else totalones = ones[ei] + (ones[l-1]-ones[si-1]); //circular window\n\t\t\t\n\t\t\tint swapsreq = ws-totalones; // swaps req. = no. of zeroes in the window\n if(swapsreq < minSwaps) minSwaps = swapsreq;\n \n si++;\n }\n \n return minSwaps;\n }\n```\n\n***\uD83D\uDCCC UPVOTE If you liked the explanation \uD83D\uDE04\uD83D\uDE0A*** | 10 | 1 | ['Sliding Window'] | 5 |
minimum-swaps-to-group-all-1s-together-ii | [C++] Sliding window | c-sliding-window-by-sanjeev1709912-aexu | This problem is extension of previous problem Minimum Swaps to Group All 1\'s Together\nSo, I treat it in same way i treat previous problem using sliding windo | sanjeev1709912 | NORMAL | 2022-01-09T04:03:21.426102+00:00 | 2022-01-09T04:38:53.347707+00:00 | 379 | false | This problem is extension of previous problem **[Minimum Swaps to Group All 1\'s Together](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together/)**\nSo, I treat it in same way i treat previous problem using sliding window.\n\nQ1. What must we find?\nAns. we must find portion in which the number of one is maximum.\n\nQ2. How to decide portion size? -> protion_size==window_size\nAns. Count number of one present in given array one.\n\t\tExample \n\t\t1 0 1 0 1\n\t\tHere size of window is 3.\n\nQ3. How to tackle circular constrain?\nAns. This is my favourite part most of time when I find this kind of statement, I prefer to append same array twice\uD83D\uDE05.\n\t Sorry for that it came with time you will find multiple question follow same approach.\n\n<iframe src="https://leetcode.com/playground/RuH79KjM/shared" frameBorder="0" width="800" height="600"></iframe> | 9 | 6 | [] | 2 |
minimum-swaps-to-group-all-1s-together-ii | EASY AND SIMPLE APPROACH | easy-and-simple-approach-by-abb333-dqr9 | Complexity\n- Time complexity: O(N)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(1)\n Add your space complexity here, e.g. O(n) \n\n# Co | abb333 | NORMAL | 2024-08-02T05:26:53.565047+00:00 | 2024-08-02T05:26:53.565079+00:00 | 1,299 | false | # Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& v) {\n int ones = 0;\n for (auto& x : v) {\n if (x == 1) {\n ones++;\n }\n }\n \n if (ones == 0 || ones == 1) {\n return 0;\n }\n \n int n = v.size();\n int windowSum = accumulate(v.begin(), v.begin() + ones, 0);\n int maxOnesInWindow = windowSum;\n \n for (int i = 0; i < n; ++i) {\n int end = (i + ones) % n; \n int start = i;\n \n windowSum -= v[start];\n windowSum += v[end];\n \n maxOnesInWindow = max(maxOnesInWindow, windowSum);\n }\n \n return ones - maxOnesInWindow;\n }\n};\n\n``` | 8 | 0 | ['Array', 'Sliding Window', 'C++'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Python 3 || 7 lines, extend , zip || T/S: 99% / 27% | python-3-7-lines-extend-zip-ts-99-27-by-i4bkj | \nclass Solution:\n def minSwaps(self, nums: list[int]) -> int:\n\n n, k = len(nums), sum(nums)\n mx = tally = sum(nums[:k])\n nums.exte | Spaulding_ | NORMAL | 2024-08-02T01:40:39.672844+00:00 | 2024-08-02T01:41:20.713420+00:00 | 56 | false | ```\nclass Solution:\n def minSwaps(self, nums: list[int]) -> int:\n\n n, k = len(nums), sum(nums)\n mx = tally = sum(nums[:k])\n nums.extend(nums[:k])\n \n for num1, num2 in zip(nums[k:], nums):\n tally+= num1 - num2\n if tally > mx: mx = tally\n \n return k - mx\n```\n[https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together-ii/submissions/1341324911/](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together-ii/submissions/1341324911/)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(1), in which *N* ~ `len(nums). | 8 | 0 | ['Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Classic Sliding Window Question | classic-sliding-window-question-by-heman-pgh4 | Basic Idea: Find the total no. of ones in the given array. Now make a sliding window of that size. Window with maximum no. of ones will require the minimum numb | hemantsingh_here | NORMAL | 2024-08-02T15:52:27.592382+00:00 | 2024-08-02T15:52:27.592412+00:00 | 135 | false | **Basic Idea:** Find the total no. of ones in the given array. Now make a sliding window of that size. Window with maximum no. of ones will require the minimum number of swaps.\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int count_1 = count(nums.begin(), nums.end(), 1);\n\n if (count_1 == 0 || count_1 == n) {\n return 0;\n }\n\n\n int current_ones = 0;\n for (int i = 0; i < count_1; i++) {\n current_ones += nums[i];\n }\n int max_ones = current_ones;\n\n for (int i = 1; i < n; i++) {\n current_ones = current_ones - nums[i - 1] + nums[(i + count_1 - 1) % n];\n max_ones = max(max_ones, current_ones);\n }\n\n int min_swaps_needed = count_1 - max_ones;\n\n return min_swaps_needed;\n }\n};\n``` | 7 | 0 | ['C'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Sliding Window | O(n) | C++ | Easy approach | Simple | sliding-window-on-c-easy-approach-simple-4u5t | Intuition\n Describe your first thoughts on how to solve this problem. \nI started thinking that, eventually, all the ones in the array will be grouped together | gavnish_kumar | NORMAL | 2024-08-02T05:32:01.975843+00:00 | 2024-08-02T05:32:01.975904+00:00 | 947 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI started thinking that, eventually, all the ones in the array will be grouped together to form a subarray whose size is equal to the total number of ```1\'s``` in the array. From this, I considered how to handle a subarray of that specific size.\n\nThis led me to the sliding window approach. By traversing the array with a fixed window size equal to the total number of ```1\'s```, I can calculate the maximum number of ```1\'s``` in each window. The answer will then be the number of ```0\'s``` in the subarray with the maximum number of ```1\'s```.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int totalOnes= accumulate(nums.begin(),nums.end(),0);\n int n= nums.size(),l=0,r=0,currOnes=0;\n while(r<totalOnes){\n if(nums[r]==1) currOnes++;\n r++;\n }\n r=r%n;\n int maxOnes=currOnes;\n while(l<n){\n if(nums[l]==1) currOnes--;\n if(nums[r]==1) currOnes++;\n maxOnes= max(maxOnes,currOnes);\n r= (r+1)%n;\n l++;\n }\n return totalOnes-maxOnes;\n }\n};\n``` | 7 | 0 | ['C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Easy Sliding window | with vid explanation | easy-sliding-window-with-vid-explanation-3t70 | Vid Explanation\nhttps://youtu.be/xsH9DvzkRPY\n# Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem requires finding the minim | Atharav_s | NORMAL | 2024-08-02T05:40:18.416124+00:00 | 2024-08-02T05:40:18.416158+00:00 | 565 | false | # Vid Explanation\nhttps://youtu.be/xsH9DvzkRPY\n# Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires finding the minimum number of swaps to group all 1s together in a circular array. Intuitively, we can focus on a window of size equal to the total number of 1s. We need to find the minimum number of zeros within this window as that\'s directly related to the number of swaps required.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe\'ll use a sliding window approach:\n\n1. Calculate the total number of 1s (window size).\n2. Count the number of zeros within the initial window.\n3. Slide the window and update the count of zeros.\n4. Keep track of the minimum number of zeros encountered.\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\n int window = 0;\n\n for(auto num: nums){\n\n if (num == 1) window++;\n \n }\n\n int n = nums.size();\n int zero = 0;\n\n for(int i=0;i<window;i++){\n\n if(nums[i]==0) zero++;\n \n }\n\n int swap = zero;\n\n for(int i=window;i<n + window;i++){\n\n if(nums[i % n] == 0) zero++;\n\n if(nums[i-window] == 0) zero--;\n\n swap = min(swap,zero);\n \n }\n\n return swap;\n \n }\n};\n``` | 6 | 1 | ['C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Sliding Window || easy to understand | c-sliding-window-easy-to-understand-by-v-iiic | Just check the number of zeroes in each window of size equal to the number of 1\'s present in the array.\n\n\nclass Solution {\npublic:\n int minSwaps(vector | VineetKumar2023 | NORMAL | 2022-01-09T04:02:08.686352+00:00 | 2022-01-09T04:02:08.686398+00:00 | 521 | false | Just check the number of zeroes in each window of size equal to the number of 1\'s present in the array.\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int count=0;\n for(int i=0;i<nums.size();i++)\n if(nums[i]==1)\n count++;\n int result=0;\n \n for(int i=0;i<count;i++)\n {\n if(nums[i]==0)\n result++;\n }\n \n int ans=result;\n for(int i=1;i<nums.size();i++)\n {\n int j=(i+count-1)%nums.size();\n if(nums[i-1]==0)\n result--;\n if(nums[j]==0)\n result++;\n ans=min(ans,result);\n }\n return ans;\n }\n};\n``` | 6 | 0 | ['C', 'Sliding Window'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Easy C++ Solution || Sliding Window | easy-c-solution-sliding-window-by-jitena-jisx | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | jitenagarwal20 | NORMAL | 2024-08-02T05:28:14.793776+00:00 | 2024-08-02T05:28:14.793807+00:00 | 75 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int ans=INT_MAX,cnt0=0,cnt1=0,n=nums.size();\n for(auto it:nums){\n if(it==1)\n cnt1++;\n }\n for(int i=0;i<cnt1;i++){\n if(nums[i]==0)\n cnt0++;\n }\n ans=min(cnt0,ans);\n for(int i=cnt1;i<2*n;i++){\n if(nums[i%n]==0)\n cnt0++;\n if(nums[(i-cnt1)%n]==0)\n cnt0--;\n ans=min(ans,cnt0);\n }\n return ans;\n }\n};\n``` | 5 | 0 | ['C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Best Approach in Easy words (Javascript, C++, Java and Python) | best-approach-in-easy-words-javascript-c-hg0l | Approach\nSince we have to group all 1s in the nums, we will first calculate the total number of 1s. Which will be the windowSize.\n\nAfter this we will count t | Nabeel7801 | NORMAL | 2024-08-02T00:39:41.475974+00:00 | 2024-08-02T13:21:23.171211+00:00 | 1,516 | false | # Approach\nSince we have to group all 1s in the nums, we will first calculate the total number of 1s. Which will be the `windowSize`.\n\nAfter this we will count the 1s in that sliding window. And then iterate through the entire length of the nums array. By doing this we can get the maximum number of 1s in the window `max1s`\n\nOnce we have the max1s, we can simply substract the max number of 1s from total number of 1s to get the minimum swaps (converted from 0 to 1).\n\n# Complexity\n- Time complexity:\nO(n)\n\n\n- Space complexity:\nO(n)\n\n# Code\n```javascript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minSwaps = function(nums) {\n const n = nums.length;\n \n const windowSize = nums.reduce((sum, num) => sum + num, 0);\n \n let slideWindow = nums.slice(0, windowSize);\n\n let slide1s = slideWindow.reduce((sum, num) => sum + num, 0);\n let max1s = slide1s;\n for (let i = 0; i < nums.length; i++) {\n if (nums[(windowSize+i) % n] === 1) {\n slide1s++; // Adding New one\n }\n\n if (nums[i] === 1) {\n slide1s--; // Removing old one\n }\n\n max1s = Math.max(max1s, slide1s);\n }\n\n return windowSize - max1s; // Minimum swaps;\n};\n```\n```C++ []\n#include <vector>\n#include <numeric>\n#include <algorithm>\n\nint minSwaps(std::vector<int>& nums) {\n int n = nums.size();\n \n int windowSize = std::accumulate(nums.begin(), nums.end(), 0);\n \n std::vector<int> slideWindow(nums.begin(), nums.begin() + windowSize);\n\n int slide1s = std::accumulate(slideWindow.begin(), slideWindow.end(), 0);\n int max1s = slide1s;\n for (int i = 0; i < n; ++i) {\n if (nums[(windowSize + i) % n] == 1) {\n slide1s++; // Adding new one\n }\n\n if (nums[i] == 1) {\n slide1s--; // Removing old one\n }\n\n max1s = std::max(max1s, slide1s);\n }\n\n return windowSize - max1s; // Minimum swaps\n}\n```\n```Java []\nimport java.util.*;\n\npublic class Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length;\n \n int windowSize = Arrays.stream(nums).sum();\n \n int slide1s = 0;\n for (int i = 0; i < windowSize; i++) {\n slide1s += nums[i];\n }\n int max1s = slide1s;\n for (int i = 0; i < n; i++) {\n if (nums[(windowSize + i) % n] == 1) {\n slide1s++; // Adding new one\n }\n\n if (nums[i] == 1) {\n slide1s--; // Removing old one\n }\n\n max1s = Math.max(max1s, slide1s);\n }\n\n return windowSize - max1s; // Minimum swaps\n }\n}\n```\n```python []\ndef minSwaps(nums):\n n = len(nums)\n \n windowSize = sum(nums)\n \n slideWindow = nums[:windowSize]\n \n slide1s = sum(slideWindow)\n max1s = slide1s\n for i in range(n):\n if nums[(windowSize + i) % n] == 1:\n slide1s += 1 # Adding new one\n\n if nums[i] == 1:\n slide1s -= 1 # Removing old one\n\n max1s = max(max1s, slide1s)\n\n return windowSize - max1s # Minimum swaps\n\n```\n\n\n---\n\n\n\n**If my solution was helpful do give it a UpVote so that others can get this easy solution as well.**\n\n\n | 5 | 0 | ['Array', 'Sliding Window', 'Python', 'C++', 'Java', 'JavaScript'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Sliding Window + Comments | sliding-window-comments-by-xxvvpp-fksb | We just want to get all the 1\'s together irrespective of the places where we put them, we just want them together.\n\n Maintain a window of size of number of o | xxvvpp | NORMAL | 2022-01-09T04:42:23.664787+00:00 | 2022-01-09T07:04:31.007469+00:00 | 574 | false | We just want to get all the 1\'s together irrespective of the places where we put them, we just want them together.\n\n* Maintain a window of size of number of ones in whole array.\n* Now check in every consecutive window of same size,keep on counting number of zeroes present.\n* Number of Zeroes present in the current window will be the minimum replacement swap.\n\n# Twist: \nThe window needs to run in a circular manner.\n`r=(r+1)%n` -> For getting circular window.\n\n# C++: \n \n int minSwaps(vector<int>& nums){ \n int n=size(nums),mn=n;\n \n int ones= count(begin(nums),end(nums),1);\n \n //if no one then no swap possible\n if(ones==0) return 0;\n \n //For maintaining the number of one\'s zeroes\n int curr_zero=0;\n \n int left=0,right=0;\n \n while(right<=ones-2){ \n if(nums[right]==0) curr_zero++;\n right++;\n }\n \n if(nums[right]==0) curr_zero++;\n \n \n //Keep on shrinking window size\n while(left<n){\n \n //Number of Minimum Swaps in current window is equal to no. of places occulped by zeroes.\n mn= min(mn,curr_zero); \n \n //Shrink window from left\n if(nums[left++]==0) curr_zero--;\n \n right=(right+1)%n; //circular array\n \n if(nums[right]==0) curr_zero++; //get count of last element in new window\n }\n \n return mn;\n \n } | 5 | 0 | ['C'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | ✅ One Line Solution | one-line-solution-by-mikposp-731w | (Disclaimer: this is not an example to follow in a real project - it is written for fun and training mostly)\n\nTime complexity: O(n). Space complexity: O(1).\n | MikPosp | NORMAL | 2024-08-02T09:38:27.100381+00:00 | 2024-08-02T09:38:27.100416+00:00 | 497 | false | (Disclaimer: this is not an example to follow in a real project - it is written for fun and training mostly)\n\nTime complexity: $$O(n)$$. Space complexity: $$O(1)$$.\n```\nclass Solution:\n def minSwaps(self, a: List[int]) -> int:\n return (n:=len(a),o:=sum(a),w:=sum(a[:o])) and o-max(w:=w-a[i]+a[(i+o)%n] for i in range(n))\n``` | 4 | 0 | ['Array', 'Sliding Window', 'Python', 'Python3'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | C++ | Efficient Minimum Swaps to Group All 1's Together II | 49ms Beats [99.02%] | c-efficient-minimum-swaps-to-group-all-1-q2i7 | Intuition\nTo minimize the number of swaps needed to group all 1\'s in a circular binary array, we need to find the optimal position where all 1\'s can be group | user4612MW | NORMAL | 2024-08-02T03:05:23.362198+00:00 | 2024-08-02T03:05:23.362255+00:00 | 18 | false | # Intuition\nTo minimize the number of swaps needed to group all $$1$$\'s in a circular binary array, we need to find the optimal position where all $$1$$\'s can be grouped together with the fewest swaps. The challenge is to consider the circular nature of the array, which means that the end of the array is adjacent to the beginning.\n\n# Approach\nFirst, we count the total number of $$1$$\'s in the array. Then, we use a sliding window approach to find the maximum number of $$1$$\'s that can be grouped together in any window of length equal to the total count of $$1$$\'s. This is done by extending the array to simulate the circular property. For each possible window, we track the number of $$1$$\'s in the current window and update the maximum number of $$1$$\'s found. Finally, the minimum swaps required are calculated as the difference between the total number of $$1$$\'s and the maximum number of $$1$$\'s that can be grouped together.\n\n# Complexity\n- **Time complexity** $$(O(n))$$, where $$(n)$$ is the length of the array. We traverse the array and its extended version once.\n- **Space complexity** $$(O(1))$$. We use a constant amount of extra space for variables.\n\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int ones = count(nums.begin(), nums.end(), 1), maxOnes{0}, winOnes{0}, n = nums.size();\n if (ones == 0) return 0;\n for (int i{0}; i < 2 * n; ++i) {\n winOnes += nums[i % n];\n if (i >= ones) winOnes -= nums[(i - ones) % n];\n maxOnes = max(maxOnes, winOnes);\n }\n return ones - maxOnes;\n }\n};\n\n```\n\n## **If you found this solution helpful, please upvote! \uD83D\uDE0A** | 4 | 0 | ['C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Best for beginners✅ | best-for-beginners-by-ratneshh-rm8t | Intuition\n\n Describe your first thoughts on how to solve this problem. \nUnderstanding the Problem: Recognize that we need to rearrange the array such that al | ratneshh | NORMAL | 2024-08-02T02:09:04.093550+00:00 | 2024-08-02T02:09:04.093575+00:00 | 1,262 | false | # Intuition\n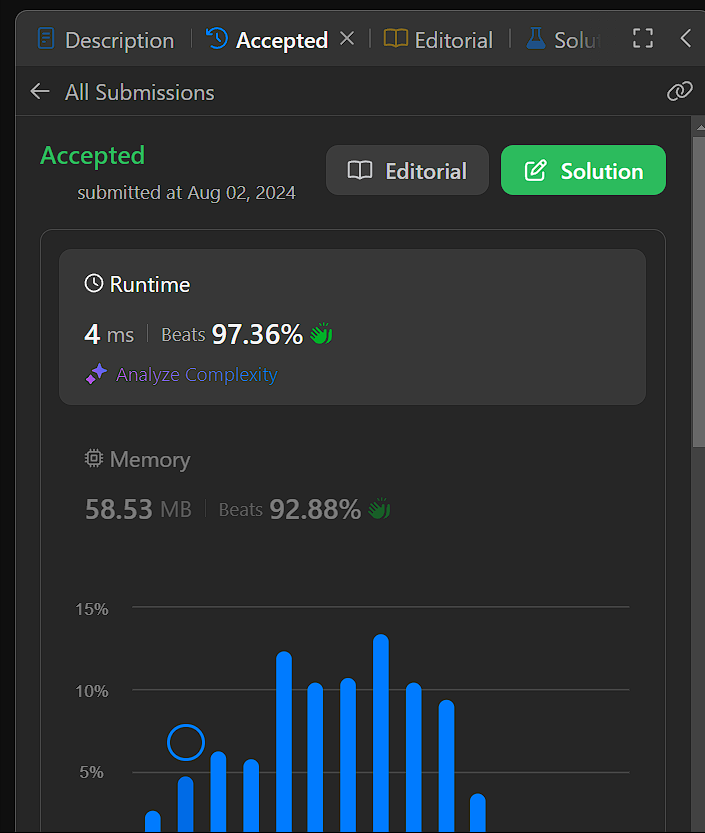\n<!-- Describe your first thoughts on how to solve this problem. -->\nUnderstanding the Problem: Recognize that we need to rearrange the array such that all 1s are grouped together, and we want to do this with the minimum number of swaps. The array is circular, meaning the first and last elements are considered adjacent.\n\nCounting 1s: Start by counting the total number of 1s (oneCount) in the array. If oneCount is 0 or 1, no swaps are needed (return 0).\n\nSliding Window Approach: Utilize a sliding window of size oneCount to evaluate each possible segment of the array where all 1s could potentially be grouped together.\n\nCalculating Swaps: For each position of the sliding window:\n\nCalculate the number of 1s in the current window.\nDetermine the number of 0s that would need to be swapped to move all 1s into a contiguous block in this window.\nCircular Consideration: Since the array is circular, after reaching the end of the array, continue counting from the beginning. This requires careful handling to ensure all possible positions are evaluated.\n\nMinimize Swaps: Track the minimum number of swaps required across all valid window positions.\n\nSteps to Implement:\nStep 1: Count the total number of 1s in the array (oneCount).\nStep 2: Handle edge cases where oneCount is 0 or 1.\nStep 3: Initialize a sliding window to calculate the initial number of swaps required for the first segment of size oneCount.\nStep 4: Slide the window across the array, updating the count of 1s and calculating the corresponding swaps for each window position.\nStep 5: Use modulo arithmetic to handle the circular nature of the array.\nStep 6: Return the minimum number of swaps found across all window positions.\nBy following these steps, we can efficiently determine the minimum number of swaps required to group all 1s together in the circular array nums. This approach ensures that we consider all possible ways to rearrange the array while minimizing computational complexity.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n->Count 1s: First, count the total number of 1s (oneCount) in the array. If oneCount is less than 2, return 0 because no swaps are needed.\n\n->Initial Window Sum: Use a sliding window of size oneCount to calculate the sum of 1s in the initial window of the array.\n\n->Calculate Initial Swaps: Compute the number of 0s in the initial window that need to be swapped to group all 1s together initially.\n\nSliding Window Technique:\n\nMove the window across the array to simulate different starting positions where all 1s can be grouped together.\nFor each new position of the window:\nSubtract the contribution of the element that is sliding out of the window.\nAdd the contribution of the element that is sliding into the window.\nCalculate the number of swaps required to group all 1s together for the current window position.\nUpdate the minimum number of swaps required (minSwaps).\nCircular Consideration:\n\nSince the array is circular, after reaching the end of the array, continue counting from the beginning to maintain the circular nature.\nReturn Result: After iterating through all possible positions, minSwaps will contain the minimum number of swaps required to group all 1s together optimally in the circular array nums.\n\n# Complexity\n- Time complexity:O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int oneCount = 0;\n int n = nums.length;\n for (int num : nums) {\n if (num == 1)oneCount++;\n }\n if (oneCount <= 1)\n return 0;\n int minSwaps = Integer.MAX_VALUE;\n int windowSum = 0;\n for (int i = 0; i < oneCount; i++) {\n windowSum += nums[i];\n }\n minSwaps = oneCount - windowSum;\n for (int i = 1; i <= n; i++) {\n windowSum -= nums[i - 1];\n windowSum += nums[(i + oneCount - 1) % n];\n int swapsNeeded = oneCount - windowSum;\n minSwaps = Math.min(minSwaps, swapsNeeded);\n }\n \n return minSwaps;\n }\n}\n\n``` | 4 | 0 | ['Array', 'Sliding Window', 'Java'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Fixed sliding Window✔️✔️✅ | fixed-sliding-window-by-dixon_n-tw3j | Code\n\nclass Solution {\n public int minSwaps(int[] nums) {\n int ones = 0;\n for (int num : nums) {\n ones += num;\n }\n\n | Dixon_N | NORMAL | 2024-06-06T13:28:35.450205+00:00 | 2024-06-06T13:28:35.450236+00:00 | 211 | false | # Code\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int ones = 0;\n for (int num : nums) {\n ones += num;\n }\n\n int n = nums.length;\n int res = nums.length;\n int left = 0, right = 0, cnt = 0;\n\n // Create a sliding window of size \'ones\'\n while (right < ones) {\n cnt += nums[right % n];\n right++;\n }\n\n // Calculate initial "holes" in the window\n res = ones - cnt;\n\n // Move the sliding window across the array\n while (right < n + ones) {\n cnt += nums[right % n];\n cnt -= nums[left % n];\n right++;\n left++;\n res = Math.min(res, ones - cnt);\n }\n\n return res;\n }\n}\n\n```\n\n## Sliding window All 4 patterns covered\n\n[3. Longest Substring Without Repeating Characters](https://leetcode.com/problems/longest-substring-without-repeating-characters/solutions/5174962/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[1004. Max Consecutive Ones III](https://leetcode.com/problems/max-consecutive-ones-iii/solutions/5174824/dig-deep-into-sliding-window-pattern-two-pointers/)\n[1493. Longest Subarray of 1\'s After Deleting One Element](https://leetcode.com/problems/longest-subarray-of-1s-after-deleting-one-element/solutions/5182014/sliding-window-and-two-pointers/)\n[2348. Number of Zero-Filled Subarrays](https://leetcode.com/problems/number-of-zero-filled-subarrays/solutions/5185486/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[2110. Number of Smooth Descent Periods of a Stock](https://leetcode.com/problems/number-of-smooth-descent-periods-of-a-stock/solutions/5185520/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[904. Fruit Into Baskets](https://leetcode.com/problems/fruit-into-baskets/solutions/5175513/deep-understanding-of-two-pointer-and-intuitive-problems/) **sliding window + Hashmap**\n[1358. Number of Substrings Containing All Three Characters](https://leetcode.com/problems/number-of-substrings-containing-all-three-characters/solutions/5180267/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[424. Longest Repeating Character Replacement](https://leetcode.com/problems/longest-repeating-character-replacement/solutions/5175013/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[930. Binary Subarrays With Sum](https://leetcode.com/problems/binary-subarrays-with-sum/solutions/5180407/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[1248. Count Number of Nice Subarrays](https://leetcode.com/problems/count-number-of-nice-subarrays/solutions/5175648/dig-deep-into-sliding-window-pattern-two-pointers/) **count SubArray payyern**\n[2537. Count the Number of Good Subarrays](https://leetcode.com/problems/count-the-number-of-good-subarrays/solutions/5185714/deep-understanding-of-two-pointer-and-intuitive-problems/)\n[992. Subarrays with K Different Integers](https://leetcode.com/problems/subarrays-with-k-different-integers/solutions/5175637/dig-deep-into-sliding-window-pattern-two-pointers/) **Very important Solution Must Read**\n[560. Subarray Sum Equals K](https://leetcode.com/problems/subarray-sum-equals-k/solutions/4416842/simple-as-that-important-pattern-must-read/)\n[713. Subarray Product Less Than K](https://leetcode.com/problems/subarray-product-less-than-k/solutions/5182026/sliding-window-two-pointers/)\n[2302. Count Subarrays With Score Less Than K](https://leetcode.com/problems/count-subarrays-with-score-less-than-k/solutions/5185326/sliding-window-two-pointers-pattern/)\n[209. Minimum Size Subarray Sum](https://leetcode.com/problems/minimum-size-subarray-sum/solutions/5184815/sliding-window-two-pointers-pattern-4-minimum-shortest-valid-length/) **-- Read**\n[1234. Replace the Substring for Balanced String](https://leetcode.com/problems/replace-the-substring-for-balanced-string/solutions/5184770/sliding-window-and-two-pointers-pattern-4-shortest-minimum-valid-length/)\n[76. Minimum Window Substring](https://leetcode.com/problems/minimum-window-substring/solutions/5217464/sliding-window-two-pointers/)\n[567. Permutation in String](https://leetcode.com/problems/permutation-in-string/solutions/5181748/dig-into-deep-understanding/)\n[438. Find All Anagrams in a String](https://leetcode.com/problems/find-all-anagrams-in-a-string/solutions/5269110/must-read-5-types-of-patterns-sliding-window-and-two-pointers/)\n[239. Sliding Window Maximum](https://leetcode.com/problems/sliding-window-maximum/solutions/5175931/nge-pattern/)\n[1838. Frequency of the Most Frequent Element](https://leetcode.com/problems/frequency-of-the-most-frequent-element/solutions/5208112/understanding-sliding-window-and-two-pointers-intuition/)\n[1888. Minimum Number of Flips to Make the Binary String Alternating](https://leetcode.com/problems/minimum-number-of-flips-to-make-the-binary-string-alternating/solutions/5208326/minflips/)\n[1208. Get Equal Substrings Within Budget](https://leetcode.com/problems/get-equal-substrings-within-budget/solutions/5182062/sliding-window-two-pointers-pattern/)\n[1695. Maximum Erasure Value](https://leetcode.com/problems/maximum-erasure-value/solutions/5182119/sliding-window-two-pointers-pattern/)\n[2024. Maximize the Confusion of an Exam](https://leetcode.com/problems/maximize-the-confusion-of-an-exam/solutions/5218273/sliding-window-and-two-pointer-pattern/)\n\n\n | 4 | 0 | ['Java'] | 4 |
minimum-swaps-to-group-all-1s-together-ii | [JAVA] O(N) Sliding Window Solution || Read the commented code if you have time. | java-on-sliding-window-solution-read-the-4236 | \n public int minSwaps(int[] arr) {\n int count = 0;\n int n=arr.length;\n for (int i = 0; i < n; ++i)\n count+=arr[i];\n\n | bharghavsaip | NORMAL | 2022-05-04T17:54:34.973398+00:00 | 2022-05-04T18:15:01.517326+00:00 | 597 | false | \n public int minSwaps(int[] arr) {\n int count = 0;\n int n=arr.length;\n for (int i = 0; i < n; ++i)\n count+=arr[i];\n\n // Find unwanted elements in current\n // window of size \'count\'\n int bad = 0;\n for (int i = 0; i < count; ++i)\n if (arr[i] == 0)\n ++bad;\n\n // Initialize answer with \'bad\' value of\n // current window\n int ans = bad;\n for (int i = 0, j = count; j < 2*n; ++i, ++j) {\n\n // Decrement count of previous window\n if (arr[i%n] == 0)\n --bad;\n\n // Increment count of current window\n if (arr[j%n] == 0)\n ++bad;\n\n // Update ans if count of \'bad\'\n // is less in current window\n ans = Math.min(ans, bad);\n }\n return ans;\n }\n | 4 | 0 | ['Array', 'Sliding Window', 'Java'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | C++ solution(sliding window) | c-solutionsliding-window-by-sethiyashris-lzjt | Here we use the concept of sliding window..we count the number of 1\'s in vector and make a window of that size...and we once traverse in the window of that siz | sethiyashristi20 | NORMAL | 2022-03-27T14:41:25.782675+00:00 | 2022-03-27T14:42:29.752148+00:00 | 176 | false | **Here we use the concept of sliding window..we count the number of 1\'s in vector and make a window of that size...and we once traverse in the window of that size and count no of 1\'s in that window(count)...and assign maxcount as count and noww we simply traverse the complete vector in that window size(k) and decrease the size of count if the last element was 1 and increase the count by one if the new element is 1\nAnd then return (window size(k)-maxcount) **\n\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\t\n int count=0,maxcount=0,k=0;\n for(int i=0;i<nums.size();i++)\n {\n if(nums[i]==1) k++;\n }\n if(k<=1) return 0;\n for(int i=0;i<k;i++)\n {\n if(nums[i]==1)\n count++;\n }\n maxcount=count;\n for(int i=k;i<nums.size()+k;i++)\n {\n if(nums[i-k]==1)count--;\n if(nums[i%nums.size()]==1)count++;\n maxcount=max(maxcount,count);\n }\n return (k-maxcount);\n }\n};\n(please do upvote if you like it) | 4 | 0 | ['Sliding Window'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | C++ Simple and Clean Sliding-Window Solution, Detailed Explanation | c-simple-and-clean-sliding-window-soluti-11u5 | Idea:\nWe want to find the "window" of size ones with the most ones.\nFirst, we count the ones in the original array.\nNow, because the array is circular, the e | yehudisk | NORMAL | 2022-01-13T14:18:05.036676+00:00 | 2022-01-13T14:18:05.036728+00:00 | 344 | false | **Idea:**\nWe want to find the "window" of size `ones` with the most ones.\nFirst, we count the ones in the original array.\nNow, because the array is circular, the easiest way is to concatenate two arrays and then we can search the window regularly.\nWe count the ones in the initial window in the left - from index 0 to `ones`.\nThen, in each iteration, we move the window one step to the right.\nIf the number we removed in the left is a one - we decrement `ones_in_window`.\nIf the number we added in the right is a one - we increment `ones_in_window`.\nWe keep track of the `mx`.\nOur result will be `ones - mx` because we need the number of **zeros** to swap in the window.\n\n**Time Complexity:** O(n)\n**Space Complexity:** O(n) (Because we are doubling the array)\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int ones = count(nums.begin(), nums.end(), 1), n = nums.size();\n nums.insert(nums.end(), nums.begin(), nums.end());\n \n int ones_in_window = 0, mx = 0;\n for (int i = 0; i < ones; i++)\n ones_in_window += nums[i];\n\n mx = ones_in_window;\n \n for (int i = ones; i < n + ones; i++) {\n if (nums[i - ones] == 1) ones_in_window--;\n if (nums[i] == 1) ones_in_window++;\n mx = max(mx, ones_in_window);\n }\n\n return ones - mx;\n }\n};\n```\n**Like it? please upvote!** | 4 | 0 | ['C'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Intuition and Thought Process with code explained | intuition-and-thought-process-with-code-dq17t | The questions asks for the minimum number of swaps to group all the 1\'s together at any location.\n\n\nThought process:\n\n I initially started thinking in ter | khsquare | NORMAL | 2022-01-09T09:09:58.133268+00:00 | 2022-01-09T09:09:58.133312+00:00 | 426 | false | The questions asks for the minimum number of swaps to group all the 1\'s together at **any location**.\n\n\nThought process:\n\n* I initially started thinking in terms of sorting the array in non-increasing order (**this meant brining all the 1\'s to the front of the array**). I then thought of using xor (**with bitset in c++**) to see the number of positions at which the original array and the sorted array differ. The number of positions at which they differ(i.e. the count of set bits in result of xor) will be equal to the number of swaps needed. This thought process gave me the realisation that sorting the array to bring all 1\'s to front of the array is just one possibility of how all the 1\'s can be grouped together. This can be better understood with an example. \n* Consider nums = [0,0, 1, 0, 1, 1,] => nums_modified= [1, 1, 1, 0, 0, 0]. It is clear from the earlier observation that nums_modified array is just one possible form of rearrangement to group all the 1\'s together. The other forms of rearrangement that group all 1\'s together include [0, 1, 1, 1, 0, 0] or [0,0,1,1,1,0] or [1, 0, 0, 0, 1, 1] ....so on. It is important to note that all of these rearrangements just differ in the position at which the group of 1\'s begins.\n* Now the ultimate aim would be to check which of these possible rearrangements of the original array will result in minimum number of swaps.\n* To break down the problem into simpler sub-problems, I wanted to first find out the number of swaps needed to convert the original array to any of the possible rearrangements. In a logical sense, it is easy to observe that the number of 0\'s that overlap with 1\'s is the number of swaps needed for the conversion.\n* The only part left is finding the number of swaps needed to convert the original array to each of the possible rearrangement and return the minimum number of swaps. This observation helped me in identifying the crux of the problem which is **Sliding window.**\n* The size of the window would be equal to the number of 1\'s and the number of swaps will be equal to the number of 0\'s in each of the window\'s of size k.\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int k = count(nums.begin(), nums.end(), 1);\n \n if(k <= 1 || k == n) return 0; //some minor optimizations\n \n //the window [i......j] is of size k\n\t\tint ans = INT_MAX, i = 0, j = 0, count = 0;\n \n while(i < n){\n\t\t\n\t\t//increement swaps count if 0 is encountered in the window\n if(nums[j] == 0)\n ++count;\n \n //when the window size becomes k, increement i(i.e. sliding the window)\n\t\t\tif((j - i + 1 + n)%n == k){\n ans = min(ans, count);\n if(nums[i] == 0)\n count--;\n ++i;\n }\n \n j = (j + 1)%n; \n }\n return ans; \n }\n};\n```\n\n | 4 | 0 | ['C', 'Sliding Window', 'C++'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Prefix Sum | c-prefix-sum-by-kapooryash713-c4ff | Approach:\n1- We will calculate the number of 1\'s in the array.\n2- We will create Prefix sum array.\n3- Since, we know we can swap any distinct elements of an | kapooryash713 | NORMAL | 2022-01-09T07:55:49.492852+00:00 | 2022-01-09T07:57:23.107986+00:00 | 219 | false | Approach:\n1- We will calculate the number of 1\'s in the array.\n2- We will create Prefix sum array.\n3- Since, we know we can swap any distinct elements of any indices. Example : [1,0,1,0,0,1] . Here if we swap the 1 index with the last index. array becomes [1,1,1,0,0,0]. So we know that that we need to check the required swaps if we substract the total ones with the number of one\'s in that window. Example- if the array is [1,0,0,1,1,1,1,0,0,1],\nwe will check the number of one\'s in the window of total ones. Say total ones are- 6. Size of array 10\nSo we will check in the window of six. First window is 1,0,0,1,1,1. So, number of ones in this window is 4 and total ones are 6. so required swaps in this window is 6-4=2.\nAs in the question it is mentioned that array is **circular** so if the index in the array is such that index+count>=size of array. We can actually mod the index. Lets say we are at the last index of array. window is [1] and also the mod is (9+6-1)%10 = 4. So we will consider index 9,0,1,2,3,4.\nSo we will simply count the minimum among the ans.\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int maxi = INT_MAX;\n int c=(nums[0]==1)?1:0;\n for(int i=1;i<nums.size();i++){\n if(nums[i]==1) c++;\n nums[i]+=nums[i-1]; \n }\n for(int i=0;i<nums.size();i++){\n int lastSum = (i-1>=0)?nums[i-1]:0;\n int nextSum = (i+c-1<nums.size())?nums[i+c-1]:(nums[nums.size()-1]+nums[(i+c-1)%nums.size()]);\n maxi = min(maxi,c-(nextSum-lastSum));\n }\n return maxi;\n }\n};\n``` | 4 | 1 | [] | 1 |
minimum-swaps-to-group-all-1s-together-ii | ✅ [C++] Easy Code with inline Explaination. O(n) | c-easy-code-with-inline-explaination-on-9hgbq | \nint minSwaps(vector<int>& nums) {\n int one=0,n=nums.size(),ans=INT_MAX;\n for(int i=0;i<n;i++) if(nums[i]) one++;\n int start=0,end=0,cn | Yogaboi | NORMAL | 2022-01-09T04:02:36.851319+00:00 | 2022-01-09T04:33:10.759012+00:00 | 380 | false | ```\nint minSwaps(vector<int>& nums) {\n int one=0,n=nums.size(),ans=INT_MAX;\n for(int i=0;i<n;i++) if(nums[i]) one++;\n int start=0,end=0,cnt=0; //cnt -> keep count of one\'s inside window //Window look like this [start ,,,....,,, end] \n while(end<n+one){ // check until window slide complete array + circular \n if(nums[end%n])cnt++; //update count of one\'s // mod (%) to keep variable < size of array\n if(one==(end-start+1)%n){ //if window size is equal to total one\'s\n ans=min(ans,one-cnt); // keep track of minimun\n if(nums[start%n])cnt--; //update count of one\'s \n start++; //move window 1 step forword\n }\n end++; //move window 1 step forword\n }\n return ans==INT_MAX?0:ans;\n }\n```\n\t\n\t**Upvote if you appreciate** | 4 | 1 | ['C', 'Sliding Window', 'C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Minimum Swaps to Group All 1's together - sliding window technique | minimum-swaps-to-group-all-1s-together-s-96c4 | Intuition\n Describe your first thoughts on how to solve this problem. \nTo solve the problem of finding the minimum number of swaps required to group all 1s in | sudharshm2005 | NORMAL | 2024-08-02T16:23:11.157912+00:00 | 2024-08-02T16:23:11.157943+00:00 | 22 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo solve the problem of finding the minimum number of swaps required to group all 1s in a binary array, we need to find the smallest window size containing the maximum number of 1s and then calculate how many 0s are in that window. This number will be the minimum number of swaps needed.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- **Count the Number of 1s:** Calculate the total number of 1s in the array, denoted as k.\n- **Special Cases:** If there are no 1s or only one 1, no swaps are needed.\n- **Extend the Array:** To handle the circular nature of the problem, create a doubled array which is the concatenation of the array with itself.\n- **Sliding Window Technique:** Use a sliding window of size k to find the maximum number of 1s in any such window within the doubled array.\n- **Calculate Swaps:** The minimum swaps required will be k (total number of 1s) minus the maximum number of 1s found in any window of size k.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$, where n is the length of the array. This is because the sliding window operation traverses the array linearly.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ due to the space needed for the doubled array.\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n k = sum(nums) # Total number of 1\'s in the array\n\n if k == 0 or k == 1:\n return 0 # No swaps needed if there are no 1\'s or just one 1\n\n doubled_arr = nums + nums # Extend the array to handle circular nature\n\n current_ones = sum(doubled_arr[:k]) # Number of 1\'s in first window\n max_ones_in_window = current_ones # Initialize max ones in any windows\n\n for i in range(1, len(nums)): # slide the windows \n current_ones = current_ones - doubled_arr[i - 1] + doubled_arr[i + k - 1]\n max_ones_in_window = max(max_ones_in_window, current_ones)\n\n return k - max_ones_in_window # Calculate minimum swaps required\n\n```\n\n## I\u2019m glad you found this useful! If it made a difference, an upvote would be greatly appreciated. Wishing you lots of success on your coding journey! | 3 | 0 | ['Array', 'Sliding Window', 'Python3'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Full Explanation(image) easy 8 line with Bonus code.....👻 | full-explanationimage-easy-8-line-with-b-47oc | Bonus Code at the end of explanation\n\n\nProblem Understanding\nThe goal is to find the minimum number of swaps required to group all 1s together in a circular | Kunj_2803 | NORMAL | 2024-08-02T13:06:38.242444+00:00 | 2024-08-02T13:50:39.211762+00:00 | 307 | false | **Bonus Code at the end of explanation**\n\n\n**Problem Understanding**\nThe goal is to find the minimum number of swaps required to group all 1s together in a circular array.\n\n**Solution :**\n**1.Get targeted ones window size**\n by `window=nums.count(1)` or `window=sum(nums)`\n\n**2.ans and count_1 to store answer and initial results** \n ans and count_1 are initialized to the sum of the first window elements of nums.\nn is the length of the array nums.\n\n**3. Sliding Window Approach**\nThe goal is to find the maximum number of 1s in any window of size window in the circular array. This is done using a sliding window technique with modulo.\n\n**4.In loop**\n this loop updates ans as maximum ones in window of size window.\n**5.return Statement**\n As minimum swaps are difference between total ones we want to get together and the maximum ones initaily availabale in size of window in nums array.\n**Summary**\nThe algorithm uses a sliding window approach to find the maximum number of 1s in any subarray of size window within a circular array. The minimum number of swaps is then the difference between the total number of 1s and this maximum value. This ensures that all 1s can be grouped together with the least number of swaps.\n\n**Example**\nConsider the array `nums = [1, 0, 1, 0, 1, 1, 0, 0]`\n\n1. Calculate window :\nwindow = sum(nums) = 1 + 0 + 1 + 0 + 1 + 1 + 0 + 0 = 4\n\n2. Initialize the first window:\nFor the first window of size k (first 4 elements):\n\n`Window 1: [1, 0, 1, 0]`\nCount of 1s: 2\n\n**Slide the window and update the count of 1s:**\n\nMove the window one position to the right, considering the circular nature of the array.\n\n`Window 2: [0, 1, 0, 1]`\nCount of 1s: 2\n\n`Window 3: [1, 0, 1, 1]`\nCount of 1s: 3\n\n`Window 4: [0, 1, 1, 0]`\nCount of 1s: 2\n\n**Fifth Window (Wrap around the array):**\n\n\n`Window 5: [1, 1, 0, 0]`\nCount of 1s: 2\n\n**Sixth Window (Wrap around the array):**\n\n`Window 6: [1, 0, 0, 1]`\nCount of 1s: 2\n\n**Maximum count of 1s in any window:** \nThe maximum count of 1s in any window is 3.\n\nCalculate the minimum swaps needed:\nMinimum swaps = Total 1s - Maximum count of 1s in any window = 4 - 3 = 1\n\nVisual Representation\nLet\'s visualize these windows and their counts of 1s:\n\n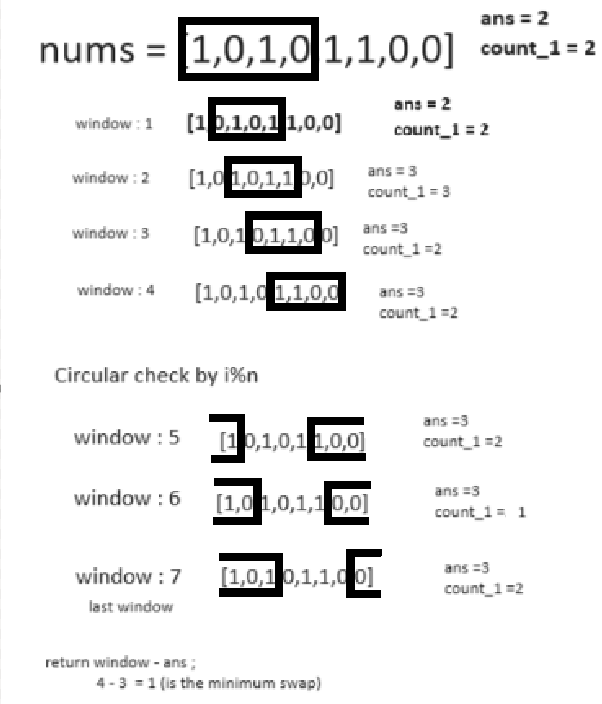\n\nFrom this, we see that the maximum count of 1s in any window of size 4 is 3. Thus, the minimum number of swaps needed to group all 1s together is 1.\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python3 []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n # we want to group all ones together as the total 1 is the target window we want to make \n window = sum(nums)\n # initialize the total ones in first window\n ans = count_1 = sum(nums[:window])\n n=len(nums)\n # loop to first to last window (using modulo to encounter circular array...)\n for i in range(window,n+window):\n # next element if 1 +1 else +0\n count_1 += nums[i%n]\n # windows left most element if 1 +1 else 0\n count_1 -= nums[(i-window+n)%n]\n # updating ans as maximum one_s in that window\n ans = max(ans,count_1)\n # returning window(targeted ones together) - maximum ones we get = so it will return minimum 0 (as 0 are swaps) \n return window - ans\n```\n```C++ []\nclass Solution {\npublic:\n int minSwaps(std::vector<int>& nums) {\n int k = std::accumulate(nums.begin(), nums.end(), 0);\n int n = nums.size();\n int ans = 0, count_1 = 0;\n \n for (int i = 0; i < k; i++) {\n count_1 += nums[i];\n }\n \n ans = count_1;\n \n for (int i = k; i < n + k; i++) {\n count_1 += nums[i % n];\n count_1 -= nums[(i - k + n) % n];\n ans = std::max(ans, count_1);\n }\n\n return k - ans;\n }\n};\n```\n```java []\nclass Solution {\n public int minSwaps(List<Integer> nums) {\n int k = 0;\n for (int num : nums) {\n k += num;\n }\n\n int n = nums.size();\n int ans = 0, count_1 = 0;\n \n for (int i = 0; i < k; i++) {\n count_1 += nums.get(i);\n }\n \n ans = count_1;\n \n for (int i = k; i < n + k; i++) {\n count_1 += nums.get(i % n);\n count_1 -= nums.get((i - k + n) % n);\n ans = Math.max(ans, count_1);\n }\n\n return k - ans;\n }\n}\n```\n**Bonus Code:**\n if you can\'t understand concept of modulo (%) operation append window sized nums to the last of nums array to check circularly \n(time and space complexity is slithly increased)\n```Python3 []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n k = sum(nums)\n ans = count_1 = sum(nums[:k])\n nums = nums + nums[:k]\n n=len(nums)\n for i in range(k,n):\n count_1 += nums[i]\n count_1 -= nums[i-k]\n ans = max(ans,count_1)\n return k - ans\n```\n\n\n***Upvote if you like it**\n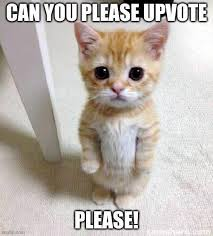\n\n | 3 | 0 | ['Sliding Window', 'C++', 'Java', 'Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Basic yet highly efficient solution. | basic-yet-highly-efficient-solution-by-n-750x | Complexity\n- Time complexity: (O(n)+O(windowSize)+O(n))~O(n)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:O(1)\n Add your space complexity | NehaSinghal032415 | NORMAL | 2024-08-02T11:36:49.882124+00:00 | 2024-08-02T11:36:49.882146+00:00 | 68 | false | # Complexity\n- Time complexity: (O(n)+O(windowSize)+O(n))~O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int windowSize=0;\n int minimumSwap=0;\n int currentZero=0;\n int n=nums.length;\n for(int num: nums){\n windowSize+=num;\n }\n for(int i=0;i<windowSize;i++){\n if(nums[i]==0)currentZero++;\n }\n int start=0;\n int end=windowSize-1;\n minimumSwap=currentZero;\n while(start<n){\n if(nums[start]==0){\n currentZero--;\n }\n start++;\n end++;\n if(nums[end%n]==0){\n currentZero++;\n }\n minimumSwap=Math.min(minimumSwap,currentZero);\n }\n return minimumSwap;\n }\n} \n | 3 | 0 | ['Array', 'Sliding Window', 'Java'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | simple and easy C++ solution 😍❤️🔥 | simple-and-easy-c-solution-by-shishirrsi-afjw | \n# if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n###### Let\'s Connect on LinkedIn: www.linkedin.com/in/shishirrsiam\n###### Let\'s Connect on Facebook | shishirRsiam | NORMAL | 2024-08-02T10:00:22.016691+00:00 | 2024-08-02T10:00:22.016720+00:00 | 524 | false | \n# if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n###### Let\'s Connect on LinkedIn: www.linkedin.com/in/shishirrsiam\n###### Let\'s Connect on Facebook: www.fb.com/shishirrsiam\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& oky) \n {\n vector<int> nums = oky;\n int oneCount = 0, n = nums.size();\n for(int i = 0; i < n; i++)\n {\n oneCount += nums[i];\n nums.push_back(oky[i]);\n }\n\n int ans = INT_MAX, i = 0, j = 0, windowOneCount = 0 ;\n while(j < oneCount)\n windowOneCount += nums[j++];\n ans = min(ans, oneCount - windowOneCount);\n\n while(j < n + n)\n {\n if(nums[j++]) windowOneCount++;\n if(nums[i++]) windowOneCount--;\n ans = min(ans, oneCount - windowOneCount);\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['Array', 'Sliding Window', 'C++'] | 3 |
minimum-swaps-to-group-all-1s-together-ii | Python | Sliding Window | python-sliding-window-by-khosiyat-ycqe | see the Successfully Accepted Submission\n\n# Code\n\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n = len(nums)\n k = sum(n | Khosiyat | NORMAL | 2024-08-02T08:21:59.305345+00:00 | 2024-08-02T08:21:59.305372+00:00 | 543 | false | [see the Successfully Accepted Submission](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together-ii/submissions/1341619703/?envType=daily-question&envId=2024-08-02)\n\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n = len(nums)\n k = sum(nums) \n if k == 0 or k == n:\n return 0 \n\n extended_nums = nums + nums[:k-1]\n current_zeros = sum(1 for i in range(k) if extended_nums[i] == 0)\n min_zeros = current_zeros\n \n for i in range(1, n):\n if extended_nums[i-1] == 0:\n current_zeros -= 1\n if extended_nums[i+k-1] == 0:\n current_zeros += 1\n min_zeros = min(min_zeros, current_zeros)\n \n return min_zeros\n \n```\n\n\n## Steps:\n\n### 1. Count the Total Number of 1s:\n- First, we need to count the total number of 1s in the array. Let\'s call this count `k`.\n\n### 2. Initial Window Setup:\n- We\'ll use a sliding window of size `k` to traverse the array. This window will help us count the number of 0s in any window of size `k`.\n\n### 3. Calculate Initial Number of 0s in the First Window:\n- Set up the initial window and count how many 0s are in this window.\n\n### 4. Slide the Window:\n- Move the window one position at a time. For each move, adjust the count of 0s based on the elements that are entering and leaving the window.\n- Track the minimum number of 0s found in any window of size `k`.\n\n### 5. Handle Circular Nature:\n- Since the array is circular, extend the array by appending the first `k-1` elements at the end of the array. This allows the sliding window to naturally wrap around the end of the array.\n\n### 6. Result:\n- The result will be the minimum number of 0s found in any window of size `k`, as this represents the fewest swaps needed to group all 1s together.\n\n## Explanation:\n\n### Counting 1s (`k`):\n- We count the total number of 1s to determine the window size.\n\n### Sliding Window:\n- By extending the array, we handle the circular nature seamlessly.\n\n### Tracking Minimum 0s:\n- As we slide the window, we keep track of the minimum number of 0s within any window of size `k`, which corresponds to the minimum swaps needed.\n\n\n\n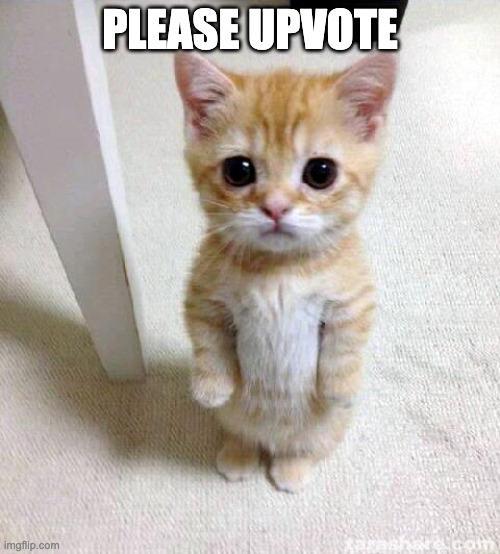 | 3 | 0 | ['Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Use Silding Window And Find the Window Needing Min. Swaps | Java | C++ | [Vidoeo Solution] | use-silding-window-and-find-the-window-n-xube | Intuition, approach, and complexity discussed in video solution in detail\nhttps://youtu.be/LRjOvAslzCg\n# Code\nJava\n\nclass Solution {\n public int minSwa | Lazy_Potato_ | NORMAL | 2024-08-02T05:49:44.219180+00:00 | 2024-08-02T05:49:44.219207+00:00 | 393 | false | # Intuition, approach, and complexity discussed in video solution in detail\nhttps://youtu.be/LRjOvAslzCg\n# Code\nJava\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n return Math.min(findMinSwaps(nums, 0), findMinSwaps(nums, 1));\n }\n private int findMinSwaps(int nums[], int val){\n int valCnt = 0, len = nums.length;\n for(var num : nums)if(num == val)valCnt++;\n int left = 0, right = 0, windowSize = valCnt;\n int oppValsInCurrWindow = 0, opsVal = (val == 1) ? 0 : 1;\n while(right < windowSize){\n if(nums[right++] == opsVal)oppValsInCurrWindow++;\n }\n int minOppValsInWindowsTillNow = oppValsInCurrWindow;\n while(right < (len)){\n if(nums[left++] == opsVal)oppValsInCurrWindow--;\n if(nums[right++] == opsVal) oppValsInCurrWindow++;\n minOppValsInWindowsTillNow = Math.min(minOppValsInWindowsTillNow, oppValsInCurrWindow);\n }\n return minOppValsInWindowsTillNow;\n }\n}\n```\nC++\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n return min(findMinSwaps(nums, 0), findMinSwaps(nums, 1));\n }\n\nprivate:\n int findMinSwaps(vector<int>& nums, int val) {\n int valCnt = 0, len = nums.size();\n for(auto num : nums) if(num == val) valCnt++;\n \n int left = 0, right = 0, windowSize = valCnt;\n int oppValsInCurrWindow = 0, opsVal = (val == 1) ? 0 : 1;\n \n while(right < windowSize) {\n if(nums[right++] == opsVal) oppValsInCurrWindow++;\n }\n \n int minOppValsInWindowsTillNow = oppValsInCurrWindow;\n \n while(right < len) {\n if(nums[left++] == opsVal) oppValsInCurrWindow--;\n if(nums[right++] == opsVal) oppValsInCurrWindow++;\n minOppValsInWindowsTillNow = min(minOppValsInWindowsTillNow, oppValsInCurrWindow);\n }\n \n return minOppValsInWindowsTillNow;\n }\n};\n\n``` | 3 | 0 | ['Array', 'Sliding Window', 'C++', 'Java'] | 15 |
minimum-swaps-to-group-all-1s-together-ii | Swift💯fast🛝🪟 | swiftfast-by-upvotethispls-5bpf | Sliding Window (accepted answer)\n\nclass Solution {\n func minSwaps(_ nums: [Int]) -> Int {\n let windowSize = nums.reduce(0,+)\n var onesInWi | UpvoteThisPls | NORMAL | 2024-08-02T00:46:20.793526+00:00 | 2024-08-02T01:41:19.936519+00:00 | 104 | false | **Sliding Window (accepted answer)**\n```\nclass Solution {\n func minSwaps(_ nums: [Int]) -> Int {\n let windowSize = nums.reduce(0,+)\n var onesInWindow = nums.prefix(windowSize).reduce(0,+)\n let maxOnesInWindow = nums.indices.reduce(0) { maxOnes, i in\n onesInWindow -= nums[i] // slide trailing edge\n onesInWindow += nums[(i+windowSize) % nums.count] // slide leading edge\n return max(maxOnes, onesInWindow)\n }\n return windowSize - maxOnesInWindow\n }\n}\n```\n1) Create a window (window size = 1\'s count). \n2) Slide window across all elements of array. Determine which window position has max 1\'s present\n3) Subtract max 1\'s from window size and that\'s the minimum swap count to return.\n | 3 | 0 | ['Swift', 'Sliding Window'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Python sliding window solution | python-sliding-window-solution-by-vincen-dr4u | \ndef minSwaps(self, nums: List[int]) -> int:\n\tk, n = sum(nums), len(nums)\n\ts, mx = sum(nums[:k]), 0\n\tfor i in range(k, 2*n):\n\t\ts += (nums[i%n]-nums[i% | vincent_great | NORMAL | 2022-08-16T20:35:47.161154+00:00 | 2022-08-16T20:35:47.161179+00:00 | 295 | false | ```\ndef minSwaps(self, nums: List[int]) -> int:\n\tk, n = sum(nums), len(nums)\n\ts, mx = sum(nums[:k]), 0\n\tfor i in range(k, 2*n):\n\t\ts += (nums[i%n]-nums[i%n-k])\n\t\tmx = max(s, mx)\n\treturn k-mx\n``` | 3 | 0 | [] | 2 |
minimum-swaps-to-group-all-1s-together-ii | 2 Solutions | O(1) Space | O(N) Time | Similar to Minimum Swaps to Group All 1's Together | 2-solutions-o1-space-on-time-similar-to-mdn67 | Count the minimum swaps required to group all ones in non circular Array. Question: https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together/\n2. Si | rahul_107 | NORMAL | 2022-01-31T18:41:33.120289+00:00 | 2022-01-31T18:50:48.160511+00:00 | 341 | false | 1. Count the minimum swaps required to group all ones in non circular Array. Question: https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together/\n2. Similarly, Count the minimum swaps required to group all zeros in non circular Array.\n3. Return minimum of both. (By seeing the example attached with code we can verify that the result will always either contains all 0s or 1s together in the array. \n4. To calculate 1st point. We will first find total ones in array. Then fix the size of window as total. Find the maximum no. of ones that can exist in any window of size \'total\' in array. Result = Total - max Size. Because we only need to swap \'Result\' number of values from that particular window.\n5. To calculate 2nd pointjust replace 1s with 0s.\n\n**Approach1: Using Sliding Window O(1) Space, O(N) Time**\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int op1 = minSwapsHelper(nums, 0);\n int op2 = minSwapsHelper(nums, 1);\n return Math.min(op1, op2);\n }\n\t// Minimum swaps required to grup all \'val\' in non circular array.\n public int minSwapsHelper(int[] data, int val) {\n int len = data.length;\n int total = 0;\n for (int i = len - 1; i >= 0; i--) {\n if (data[i] == val) total++;\n }\n if (total == 0 || total == len) return 0;\n int i = 0, j = 0;\n int maxWindowCount = 0, curWindowCount = 0;\n while (j < total) {\n if (data[j++] == val) curWindowCount++;\n }\n maxWindowCount = Math.max(maxWindowCount, curWindowCount);\n while (j < len) {\n if (data[i++] == val) curWindowCount--;\n if (data[j++] == val) curWindowCount++;\n maxWindowCount = Math.max(maxWindowCount, curWindowCount);\n }\n return total - maxWindowCount;\n }\n}\n/*\n[0,1,1,1,0,0,1,1,0]\n\n111110000\n011111000\n001111100\n000111110\n000011111\n100001111\n110000111\n111000011\n111100001\n*/\n```\n\n**Approach2: Using Suffix Sum, O(N) Space, O(N) Time**\n```\nclass Solution {\n public int minSwaps(int[] nums) {\n int op1 = minSwapsHelper(nums, 0);\n int op2 = minSwapsHelper(nums, 1);\n return Math.min(op1, op2);\n }\n public int minSwapsHelper(int[] data, int val) {\n int len = data.length;\n int[] right = new int[len + 1];\n for (int i = len - 1; i >= 0; i--) {\n right[i] = right[i + 1];\n if (data[i] == (val^1)) right[i]++;\n }\n int total = right[0];\n int curCount = 0;\n int res = total - right[len - total];\n for (int i = 0; i < total; i++) {\n if (data[i] == (val^1)) curCount++;\n int rem = (total - i - 1);\n int ans = ((i + 1) - curCount) + (rem - right[len - rem]);\n res = Math.min(res, ans);\n }\n return res;\n }\n}\n/*\nFor Non Circular array,\n[1,0,1,0,1,0,0,1,1,0,1]\n 0 1 1 2 2 3 4 4 4 5 5\n 5 5 4 4 3 3 2 1 1 1 0\n \n11111100000 = 3\n01111110000 = 1 + 3\n00111111000 = 1 + 2\n00011111100 = 2 + 1\n00001111110 = 2 + 1\n00000111111 = 3\nmin = 3\n*/\n``` | 3 | 0 | ['Sliding Window', 'Prefix Sum'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | a few solutions | a-few-solutions-by-claytonjwong-7685 | Let K be the quantity of 1s in the input array A. Then we can append A onto itself (to consider the wrap-around case) and use a sliding window i..j of size K t | claytonjwong | NORMAL | 2022-01-15T21:31:33.786840+00:00 | 2022-01-15T21:36:20.997289+00:00 | 113 | false | Let `K` be the quantity of 1s in the input array `A`. Then we can append `A` onto itself (to consider the wrap-around case) and use a sliding window `i..j` of size `K` to count the 1s within the window. The "cost" of swapping 1s into the 0s within the window is then the size of the window minus the count of 1s within the window, ie. `K - x`, which is the quantity of 0s within the window. Thus it is a trivial optimization to count 0s within the window rather than using inversion to calculate it.\n\n---\n\n*Kotlin*\n```\nclass Solution {\n fun minSwaps(A_: IntArray): Int {\n var (N, K) = Pair(A_.size, A_.sum()!!)\n var A = intArrayOf(*A_.copyOf(), *A_.copyOf()) // \u2B50\uFE0F to consider wrap-around\n var x = A.slice(0 until K).sum()!!\n var best = K - x\n var (i, j) = Pair(0, K)\n while (j < 2 * N) {\n if (A[i++] == 1) --x\n if (A[j++] == 1) ++x\n best = Math.min(best, K - x)\n }\n return best\n }\n}\n```\n\n*Javascript*\n```\nlet minSwaps = A => {\n let N = A.length,\n K = _.sum(A);\n A.push(...A); // \u2B50\uFE0F to consider wrap-around\n let x = _.sum(A.slice(0, K)),\n best = K - x;\n let i = 0,\n j = K;\n while (j < 2 * N) {\n if (A[i++]) --x;\n if (A[j++]) ++x;\n best = Math.min(best, K - x);\n }\n return best;\n};\n```\n\n*Python3*\n```\nclass Solution:\n def minSwaps(self, A: List[int]) -> int:\n N, K = len(A), sum(A)\n A.extend(A[:]) # \u2B50\uFE0F to consider wrap-around\n x = sum(A[:K])\n best = K - x\n i = 0\n j = K\n while j < 2 * N:\n if A[i]: x -= 1\n if A[j]: x += 1\n best = min(best, K - x)\n i += 1; j += 1\n return best\n```\n\n*Rust*\n```\nimpl Solution {\n pub fn min_swaps(A_: Vec<i32>) -> i32 {\n let N = A_.len();\n let K: i32 = A_.iter().sum();\n let mut A = Vec::new();\n for i in 1..=2 {\n A.extend_from_slice(&A_); // \u2B50\uFE0F to consider wrap-around\n }\n let mut x: i32 = *&A[..K as usize].iter().sum();\n let mut best = K - x;\n let mut i: usize = 0;\n let mut j: usize = K as usize;\n while j < 2 * N {\n if A[i] == 1 { x -= 1; }\n if A[j] == 1 { x += 1; }\n best = std::cmp::min(best, K - x);\n i += 1; j += 1;\n }\n return best;\n }\n}\n```\n\n*C++*\n```\nclass Solution {\npublic:\n using VI = vector<int>;\n int minSwaps(VI& A) {\n int N = A.size(),\n K = accumulate(A.begin(), A.end(), 0);\n A.insert(A.end(), A.begin(), A.end()); // \u2B50\uFE0F to consider wrap-around\n auto x = accumulate(A.begin(), A.begin() + K, 0),\n best = K - x;\n auto i = 0,\n j = K;\n while (j < 2 * N) {\n if (A[i++]) --x;\n if (A[j++]) ++x;\n best = min(best, K - x);\n }\n return best;\n } \n};\n``` | 3 | 0 | [] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Counting Ones || Sliding Window Approach || C++ Clean Code | counting-ones-sliding-window-approach-c-3o71c | Code: \n\n\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int cntOnes = 0;\n int n = nums.size();\n \n for(auto | i_quasar | NORMAL | 2022-01-09T06:14:12.151873+00:00 | 2022-01-09T06:14:12.151915+00:00 | 145 | false | # Code: \n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int cntOnes = 0;\n int n = nums.size();\n \n for(auto& num : nums) if(num) cntOnes++;\n \n if(cntOnes == 0 || cntOnes == n) return 0;\n \n int maxOnes = 0, ones = 0;\n int window = cntOnes;\n \n for(int i=0; i<window; i++) {\n if(nums[i])\n ones++;\n }\n \n maxOnes = max(maxOnes, ones);\n \n for(int i=1; i<n; i++) {\n if(nums[i - 1]) ones--;\n if(nums[(i + window - 1) % n]) ones++;\n maxOnes = max(maxOnes, ones);\n }\n \n return cntOnes - maxOnes;\n }\n};\n```\n\n**Complexity :** \n\n* Time : `O(N)` , N is length of `nums` array\n* Space : `O(1)\n\n***If you find this helpful, do give it a like :)*** | 3 | 0 | ['Sliding Window', 'Counting'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | C++ | SLIDING WINDOW | 0-1 COUNT | c-sliding-window-0-1-count-by-chikzz-5mct | PLEASE UPVOTE IF U LIKE MY SOLUTION\n\n\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n=nums.size();\n int co=0,minn=n+1 | chikzz | NORMAL | 2022-01-09T05:26:55.412895+00:00 | 2022-01-09T05:26:55.412940+00:00 | 175 | false | **PLEASE UPVOTE IF U LIKE MY SOLUTION**\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n=nums.size();\n int co=0,minn=n+1;\n for(auto &x:nums)\n {if(x==1)co++;}\n int cz=n-co;\n int coo=0,czz=0;\n for(int i=0;i<co;i++)\n {\n if(nums[i]==1)coo++;\n }\n for(int i=0;i<=n-co;i++)\n {\n if(i!=0)\n {\n if(nums[i-1]==1)coo--;\n if(nums[i+co-1]==1)coo++;\n }\n minn=min(minn,co-coo);\n }\n for(int i=0;i<cz;i++)\n {\n if(nums[i]==0)czz++;\n }\n for(int i=0;i<=n-cz;i++)\n {\n if(i!=0)\n {\n if(nums[i-1]==0)czz--;\n if(nums[i+cz-1]==0)czz++;\n }\n minn=min(minn,cz-czz);\n }\n return minn;\n }\n};\n``` | 3 | 1 | [] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Python Solution Sliding Window with Explaination | python-solution-sliding-window-with-expl-y9jf | After swaps , the length of the sequence of 1 is equal to the total number of 1. Use sliding window with the size of the total number of 1 to find the interval | yuyingji | NORMAL | 2022-01-09T04:16:13.875605+00:00 | 2022-01-09T05:34:39.456483+00:00 | 181 | false | After swaps , the length of the sequence of 1 is equal to the total number of 1. Use sliding window with the size of the total number of 1 to find the interval which has the most number of 1. The number of 0 in this interval will be the minimum swap needed.\n\nk is the number of 1.\ns represent the number of 1 in [i-k + 1: i + 1]\n\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n k = sum(nums)\n if len(nums) ==1:\n return 0\n s = collections.defaultdict(int)\n s[0] = sum(nums[-k + 1:])+ nums[0]\n for i in range(1, len(nums)):\n s[i] = s[i-1] - nums[i -1 -k + 1] + nums[i]\n print(s)\n return k - max(s.values()) | 3 | 1 | ['Sliding Window'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | BEATS 98% || 4STEPS XPLANATION || JAVA|| EASY BEGINNER | beats-98-4steps-xplanation-java-easy-beg-odt5 | BOISS N PRETTIES UPVOTE!! KRDE NA BRO ;() TUJHE MOMOS KI KASAM.\n\n1. Prefix Sum Calculation:\n - Well No explanation for this.\n\n2. Initial Conditions:\n | Abhishekkant135 | NORMAL | 2024-08-26T19:21:33.106345+00:00 | 2024-08-26T19:21:33.106374+00:00 | 12 | false | # BOISS N PRETTIES UPVOTE!! KRDE NA BRO ;() TUJHE MOMOS KI KASAM.\n\n1. **Prefix Sum Calculation:**\n - Well No explanation for this.\n\n2. **Initial Conditions:**\n - `i` and `j` are initialized to 0 and `totalOnes`.\n - `totalOnes` represents the total number of 1s in the array.\n\n3. **Sliding Window and Swaps:**\n - The `while` loop will run till `i` reaches the end of the array.\n - Inside the loop (PAY ATTENTION):\n - If `j` is within the valid range (less than or equal to `totalOnes`), the minimum swaps are calculated using the prefix sum and the current window size (`j - i`).\n - If `j` is outside the valid range, the minimum swaps are calculated considering the wrap-around of the array.\n - `i` and `j` are incremented to move the sliding window.\n - If `j` reaches the end of the array, it is reset to 1 to handle the circular nature.\n - Look if you are not able to get this create a dry run and you will notice it happening say for nums=1,0,1,0,0,1.\n\n4. **Return Minimum Swaps:**\n - The final `ans` variable holds the minimum number of swaps required. The code returns this value YEAH BBY!.\n\n\n\n# Code\n```java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int [] prefixSum=new int [nums.length+1];\n for(int i=1;i<=nums.length;i++){\n prefixSum[i]=prefixSum[i-1]+nums[i-1];\n }\n int i=0;\n int totalOnes=prefixSum[nums.length];\n int j=totalOnes;\n int ans=Integer.MAX_VALUE;\n while(i<prefixSum.length){\n if(j>=totalOnes){\n ans=Math.min(ans,totalOnes-(prefixSum[j]-prefixSum[i]));\n }\n else{\n ans=Math.min(ans,totalOnes-(prefixSum[prefixSum.length-1]+prefixSum[j]-prefixSum[i]));\n }\n i++;\n j++;\n if(j==prefixSum.length){\n j=1;\n }\n }\n return ans;\n }\n}\n``` | 2 | 0 | ['Sliding Window', 'Java'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Easiest Explanation You Will Find | easiest-explanation-you-will-find-by-hri-ywzy | Approach\nIf you have premium account, solve this problem first: 1151. Minimum Swaps to Group All 1\'s Together. This is a similar problem where the array is no | hridoy100 | NORMAL | 2024-08-03T19:53:49.858060+00:00 | 2024-08-03T19:53:49.858090+00:00 | 14 | false | # Approach\nIf you have premium account, solve this problem first: [1151. Minimum Swaps to Group All 1\'s Together](https://leetcode.com/problems/minimum-swaps-to-group-all-1s-together/). This is a similar problem where the array is not circular.\n\nTo solve that problem you will use a basic sliding window technique:\n\n``` java []\npublic int minSwaps(int[] data) {\n int window=Arrays.stream(data).sum();\n int maxOnesInWindow=0;\n int onesInCurWindow=0;\n for(int right=0; right<data.length; right++) {\n onesInCurWindow+=data[right];\n if(right > window-1) {\n onesInCurWindow-=data[right-window];\n }\n maxOnesInWindow = Math.max(maxOnesInWindow, onesInCurWindow);\n }\n return window - maxOnesInWindow;\n}\n```\nFor circular array, we will use this same function. The circular property of the array opens up more possible groupings to consider compared to a linear array. So, we will also need to consider **zeroGroups**. When zeroes are grouped, ones are also grouped. And when we swap a zero, we will swap it with a one. So, here\'s the solution:\n\n# Code\n``` Java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int ones = Arrays.stream(nums).sum();\n // swaps required to group zeroes\n // if zeroes are grouped, so are the ones...\n int zeroGroups = slidingWindow(nums, 0, nums.length-ones);\n // swaps required to group ones\n int oneGroups = slidingWindow(nums, 1, ones);\n // minimum swaps required\n return Math.min(zeroGroups, oneGroups);\n }\n\n int slidingWindow(int[] nums, int val, int window) {\n int valsInWindow = 0;\n int maxValsInWindow = 0;\n for(int i=0; i<nums.length; i++) {\n // our val can be 0 or 1\n // if current number matches val, then increment valsInWindow\n valsInWindow += (nums[i] == val? 1: 0);\n if(i>window-1) {\n // let windowIndexBfrStart = i-window,\n // if the value in nums[windowIndexBfrStart] == val,\n // decrement it from valsInWindow\n valsInWindow -= (nums[i-window]==val? 1 : 0);\n }\n maxValsInWindow = Math.max(maxValsInWindow, valsInWindow);\n }\n return window - maxValsInWindow;\n }\n}\n```\n\n\n# Complexity\n- Time complexity: $$O(n)$$\nEach time traversing the loop only once. So, total $2n = n$ loop traversals.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\nNo extra array, map or any other datastructure.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n | 2 | 0 | ['Array', 'Sliding Window', 'Java'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Easy Solution || Beats 96.66% || Sliding Window || O(n) Time complexity || O(1) Space Complexity | easy-solution-beats-9666-sliding-window-0uo0q | Intuition\n\n####### SLIDING WINDOW ###########\nPLEASE upVOTE \uD83D\uDE4F\uD83D\uDE4F\n\n\n# Approach\n\nApproach of this question is so simple, just w | vector_08 | NORMAL | 2024-08-02T17:53:36.695358+00:00 | 2024-08-02T17:53:36.695388+00:00 | 48 | false | # Intuition\n```\n####### SLIDING WINDOW ###########\nPLEASE upVOTE \uD83D\uDE4F\uD83D\uDE4F\n```\n\n# Approach\n```\nApproach of this question is so simple, just we have to think more about circular array and subarrays.\nStep 1 : Declare a variable totalOnes and store total number of ones in it.\nStep 2 : Declare a variable currOnes then store the number of ones in it from 0th index till totalSum th index.(note that the window will be constant size of as same as totalSum value)\nStep 3 : Declare variable maxOnes and give it the value of currOnes for 1st window of size totalSum.\nStep 4 : Now at last to second step run loop from totalSum\'th index uptill (array size + totalOnes) and add the new value of further index of window and subtract the prevoius start index of previous window. And store the max number of ones into the maxOnes variable at each index.\nStep 5 : At the end of the day we have to return totalOnes-maxOnes for our ans.****\n```\n\n# Complexity\n- Time complexity:\n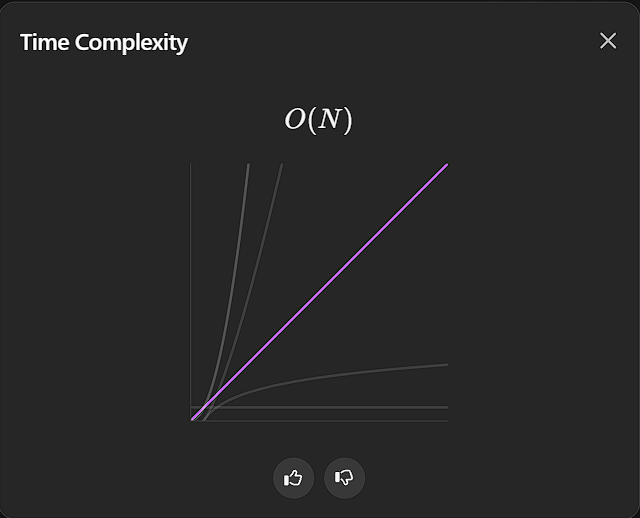\n\n\n- Space complexity:\n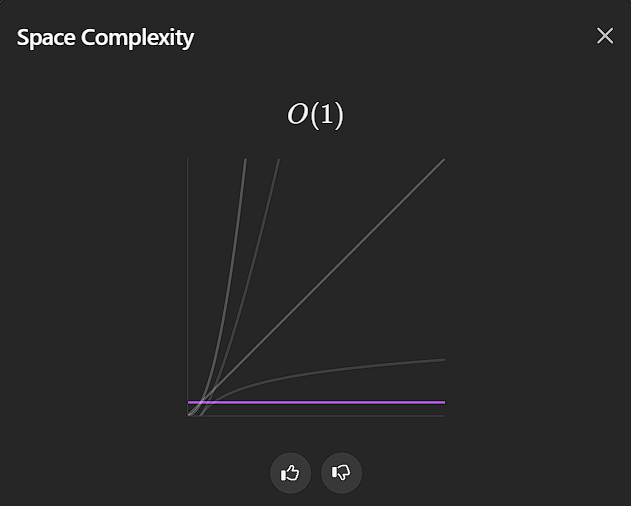\n\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int totalOnes = 0;\n int currOnes = 0;\n for(int i=0;i<nums.size();i++){\n if(nums[i]==1){\n totalOnes++;\n }\n }\n for(int i=0;i<totalOnes;i++){\n if(nums[i]==1){\n currOnes++;\n }\n }\n int maxOnes = currOnes;\n for(int i=totalOnes;i<totalOnes+nums.size();i++){\n currOnes = currOnes + nums[i%nums.size()] - nums[(i-totalOnes+nums.size())%nums.size()];\n maxOnes = max(maxOnes, currOnes);\n }\n return totalOnes-maxOnes;\n }\n};\n``` | 2 | 0 | ['Array', 'Sliding Window', 'C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Answer lelo!! Sliding window approach for the code | answer-lelo-sliding-window-approach-for-he5nc | \n# Approach\n Describe your approach to solving the problem. \nInitialization:\n\n- k is the total number of 1s in the array. This is because we want to group | AmanShukla07 | NORMAL | 2024-08-02T14:18:05.364488+00:00 | 2024-08-02T16:29:46.267628+00:00 | 61 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\n**Initialization:**\n\n- k is the total number of 1s in the array. This is because we want to group all 1s together.\n- mx (maximum number of 1s in any window of length k) and cnt (current count of 1s in the first window) are initialized to the number of 1s in the first window of size k.\n\n**Sliding Window:**\n\n- We use a sliding window technique to count the number of 1s in every possible window of size k as we slide the window from the beginning of the list to the end.\n\n- for i in range(k, n + k): This loop is used to slide the window from the beginning of the list to the end, wrapping around the end of the list to simulate a circular array.\n\n- cnt += nums[i % n]: Adds the value of the new element entering the window from the right side.\n\n- cnt -= nums[(i - k + n) % n]: Subtracts the value of the element leaving the window from the left side.\n\n- mx = max(mx, cnt): Updates the maximum count of 1s found in any window of size k.\n\n**Result Calculation:**\n\n- Finally, return k - mx calculates the minimum number of swaps required. This is because k is the total number of 1s, and mx is the maximum number of 1s we can group together without any swap. Therefore, k - mx gives the number of 1s that are not in the best position, which equals the number of swaps needed.\n\n# Example\n nums = [0,1,0,1,1,0,0]\n\n1. Count of 1s (k):\n\n- k = 3 (there are three 1s in the list).\n\n2. Initial Window Calculation:\n\n- The initial window of size k (first 3 elements): [0,1,0].\n- Number of 1s in this window: cnt = 1.\n- mx is initialized to 1.\n\n**Sliding Window:**\n\n- Slide the window and calculate the number of 1s for each window of size k:\n 1. Window [1,0,1] (indices 1 to 3): cnt = 2, update mx to 2.\n 2. Window [0,1,1] (indices 2 to 4): cnt = 2, no change to mx.\n 3. Window [1,1,0] (indices 3 to 5): cnt = 2, no change to mx.\n 4. Window [1,0,0] (indices 4 to 6): cnt = 1, no change to mx.\n\n**Calculate Minimum Swaps:**\n\n- k - mx = 3 - 2 = 1.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n k = nums.count(1)\n mx = cnt = sum(nums[:k])\n n = len(nums)\n for i in range(k, n + k):\n cnt += nums[i % n]\n cnt -= nums[(i - k + n) % n]\n mx = max(mx, cnt)\n return k - mx\n``` | 2 | 1 | ['Array', 'Sliding Window', 'Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | easy C++ O(n) approach | easy-c-on-approach-by-aayush8910sh-avur | \n\n# Approach\n1. Count the number of 1s in the array:\n\n2. Traverse the array to count the total number of 1s, denoted as k.\nIf k is 0 or equal to the lengt | aayush8910sh | NORMAL | 2024-08-02T12:25:25.588810+00:00 | 2024-08-02T12:25:25.588844+00:00 | 125 | false | \n\n# Approach\n1. Count the number of 1s in the array:\n\n2. Traverse the array to count the total number of 1s, denoted as k.\nIf k is 0 or equal to the length of the array (n), return 0 because no swaps are needed.\n3. Initialize the first window:\n\n4. Use a sliding window of size k to count the number of 0s in the first window (the first k elements of the array).\nThis count is stored in current_0s.\n5. Sliding window: Iterate through the array from the second element to the end, adjusting the window by one element at each step.\n6. For each new position of the window: Subtract the count of 0s for the element that is sliding out of the window (i.e., the element at index i-1). Add the count of 0s for the new element that is entering the window (i.e., the element at index (i + k - 1) % n to handle circularity).\n\n7. Update minimum count: Track and update the minimum number of 0s found in any window of size k.\n\nThis minimum number represents the minimum swaps needed since each 0 in the window corresponds to a necessary swap to group all 1s together.\n\n\nExample\nLet\'s walk through an example:\n\nGiven nums = [1, 0, 1, 0, 1, 0, 0, 1].\n\nCount the number of 1s:\n\nk = 4 (since there are four 1s in the array).\nInitialize the first window:\n\nThe first window of size k is [1, 0, 1, 0].\nNumber of 0s in this window is 2.\nSliding window:\n\nSlide the window one position to the right:\nNew window: [0, 1, 0, 1].\nAdjust current_0s by removing the element that slid out (1 at index 0) and adding the element that slid in (0 at index 4).\nNumber of 0s in this window is 2.\nContinue sliding:\nNext window: [1, 0, 1, 0], number of 0s is 2.\nNext window: [0, 1, 0, 0], number of 0s is 3.\nNext window: [1, 0, 0, 1], number of 0s is 2.\nNext window: [0, 0, 1, 1], number of 0s is 2.\nNext window: [0, 1, 1, 1], number of 0s is 1.\nUpdate minimum count:\n\nThe minimum number of 0s found in any window of size k is 1.\nHence, the minimum number of swaps needed to group all 1s together is \n\n# Complexity\n- Time complexity:\nO(n)\n\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int k = 0;\n\n // Count the number of 1s in the array\n for(int num : nums) {\n if(num == 1) k++;\n }\n\n // If there are no 1s or the array is all 1s, no swaps needed\n if(k == 0 || k == n) return 0;\n\n // Initialize the number of 0s in the first window of size k\n int current_0s = 0;\n for(int i = 0; i < k; i++) {\n if(nums[i] == 0) current_0s++;\n }\n\n int min_0s = current_0s;\n\n // Use sliding window to find the minimum number of 0s in any window of size k\n for(int i = 1; i < n; i++) {\n // Remove the element that is sliding out of the window\n if(nums[i - 1] == 0) current_0s--;\n // Add the element that is sliding into the window\n if(nums[(i + k - 1) % n] == 0) current_0s++;\n\n // Update the minimum number of 0s found\n if(current_0s < min_0s) min_0s = current_0s;\n }\n\n return min_0s;\n }\n};\n\n``` | 2 | 0 | ['C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | 💢☠💫Easiest👾Faster✅💯 Lesser🧠 🎯 C++✅Python3🐍✅Java✅C✅Python🐍✅C#✅💥🔥💫Explained☠💥🔥 Beats 💯 | easiestfaster-lesser-cpython3javacpython-tqc8 | Intuition\n\n\n\n\n\n\n\njavascript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minSwaps = function (nums) {\n let n = nums.length, one | Edwards310 | NORMAL | 2024-08-02T11:33:31.336516+00:00 | 2024-08-02T11:33:31.336539+00:00 | 232 | false | # Intuition\n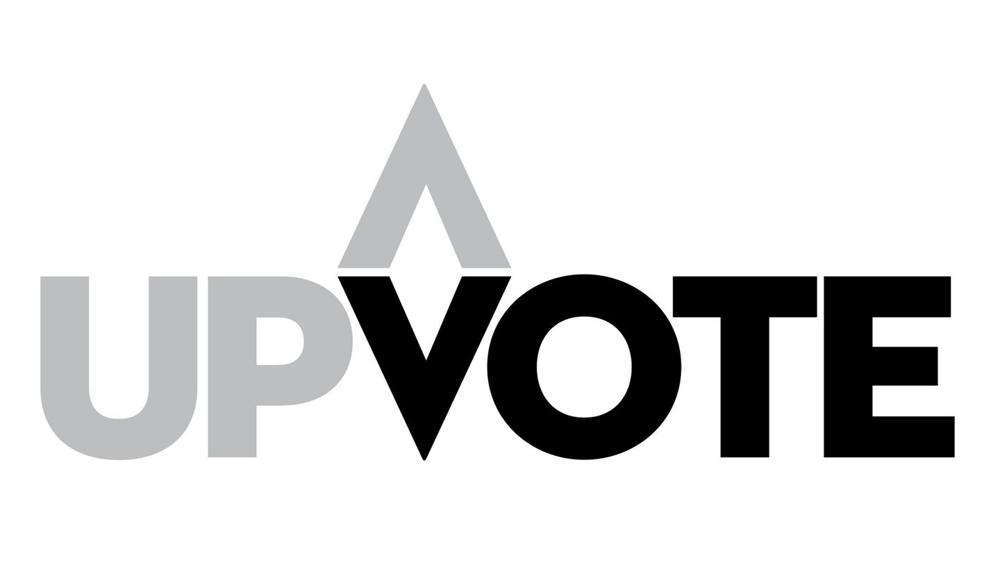\n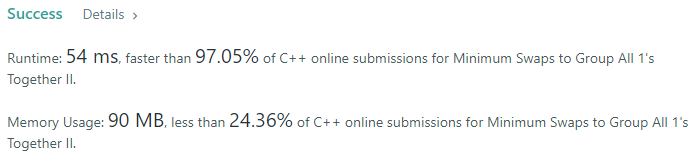\n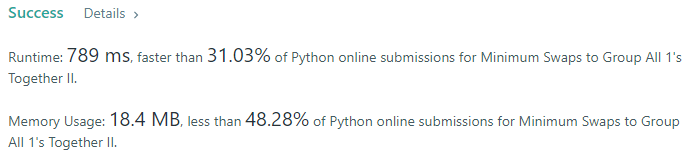\n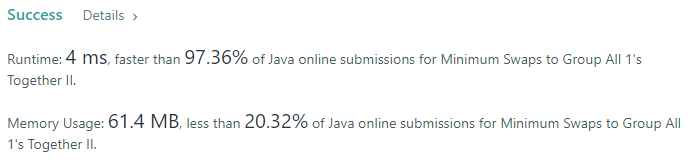\n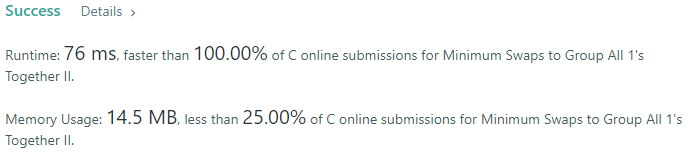\n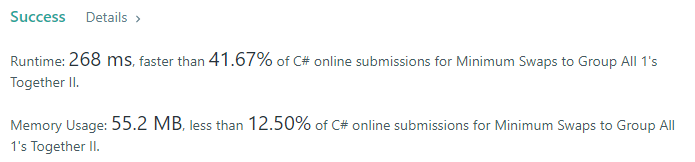\n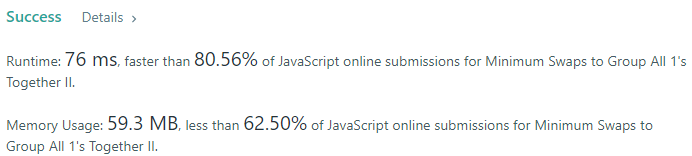\n```javascript []\n/**\n * @param {number[]} nums\n * @return {number}\n */\nvar minSwaps = function (nums) {\n let n = nums.length, ones = 0;\n let circular = Array(2 * n).fill(0);\n for (let u = 0; u < n; ++u) {\n circular[u] = circular[u + n] = nums[u];\n ones += nums[u];\n }\n var maxOnes = 0;\n var currOnes = 0, i = 0, j = 0;\n for (j = 0; j < ones; ++j)\n currOnes += circular[j];\n maxOnes = Math.max(maxOnes, currOnes);\n while (j < circular.length) {\n currOnes = currOnes - circular[i] + circular[j];\n if (maxOnes < currOnes)\n maxOnes = currOnes;\n ++j;\n ++i;\n }\n\n return ones - maxOnes;\n};\n```\n```C++ []\n// Start Of Edwards310\'s Template...\n#pragma GCC optimize("O2")\n#include <bits/stdc++.h>\n\nusing namespace std;\n#define FOR(a, b, c) for (int a = b; a < c; a++)\n#define FOR1(a, b, c) for (int a = b; a <= c; ++a)\n#define Rep(i, n) FOR(i, 0, n)\n#define Rep1(i, n) FOR1(i, 1, n)\n#define RepA(ele, nums) for (auto& ele : nums)\n#define WHL(i, n) while (i < n)\n\n// end of Edwards310\'s Template\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size(), ones = 0;\n vi circular(n * 2, 0);\n Rep(i, n) {\n circular[i] = circular[i + n] = nums[i];\n ones += nums[i];\n }\n int maxOnes = 0, currOnes = 0, i = 0, j = 0;\n for (j = 0; j < ones; ++j)\n currOnes += circular[j];\n\n maxOnes = fmax(maxOnes, currOnes);\n WHL(j, circular.size()) {\n currOnes = currOnes - circular[i] + circular[j];\n maxOnes = fmax(maxOnes, currOnes);\n ++j, ++i;\n }\n return ones - maxOnes;\n }\n};\n```\n```Python3 []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n, ones = len(nums), 0\n\n res = [0] * (2 * n)\n for i in range(n):\n res[i] = res[i + n] = nums[i]\n ones += nums[i]\n\n maxOnes = currOnes = i = j = 0\n\n while j < ones:\n currOnes += res[j]\n j += 1\n\n maxOnes = max(maxOnes, currOnes)\n while j < len(res):\n currOnes = currOnes - res[i] + res[j]\n maxOnes = max(maxOnes, currOnes)\n j += 1\n i += 1\n return ones - maxOnes\n\n```\n```Java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int n = nums.length, ones = 0;\n int[] circular = new int[n * 2];\n for (int i = 0; i < n; ++i) {\n circular[i] = circular[i + n] = nums[i];\n ones += nums[i];\n }\n\n // create a window of size ones\n int maxOnes = 0, curOnes = 0, i = 0, j = 0;\n for (j = 0; j < ones; ++j) {\n curOnes += circular[j];\n }\n\n if (maxOnes < curOnes) {\n maxOnes = curOnes;\n }\n\n while (j < circular.length) {\n curOnes = curOnes - circular[i] + circular[j];\n if (maxOnes < curOnes)\n maxOnes = curOnes;\n ++j;\n ++i;\n }\n\n return ones - maxOnes;\n }\n}\n```\n```C []\nint minSwaps(int* nums, int numsSize) {\n int n = numsSize, ones = 0;\n int circular[2 * n] = {};\n for (int i = 0; i < n; ++i) {\n circular[i] = circular[i + n] = nums[i];\n ones += nums[i];\n }\n\n // create a window of size ones\n int maxOnes = 0, curOnes = 0, i = 0, j = 0;\n for (j = 0; j < ones; ++j) {\n curOnes += circular[j];\n }\n\n if (maxOnes < curOnes)\n maxOnes = curOnes;\n\n while (j < (2 * n)) {\n curOnes = curOnes - circular[i] + circular[j];\n if (maxOnes < curOnes)\n maxOnes = curOnes;\n ++j;\n ++i;\n }\n\n return ones - maxOnes;\n}\n```\n```Python []\nclass Solution(object):\n def minSwaps(self, nums):\n """\n :type nums: List[int]\n :rtype: int\n """\n n , ones = len(nums) , 0\n \n res = [0] * (2 * n)\n for i in range(n):\n res[i] = res[i + n] = nums[i]\n ones += nums[i]\n \n maxOnes = currOnes = i = j = 0\n \n while j < ones:\n currOnes += res[j]\n j += 1\n \n maxOnes = max(maxOnes, currOnes)\n while j < len(res):\n currOnes = currOnes - res[i] + res[j]\n maxOnes = max(maxOnes, currOnes)\n j += 1\n i += 1\n return ones - maxOnes\n```\n```C# []\npublic class Solution {\n public int MinSwaps(int[] nums) {\n int n = nums.Length, ones = 0;\n int[] circular = new int[n * 2];\n for (int u = 0; u < n; ++u) {\n circular[u] = circular[u + n] = nums[u];\n ones += nums[u];\n }\n int maxOnes = 0, curOnes = 0, i = 0, j = 0;\n for (j = 0; j < ones; ++j)\n curOnes += circular[j];\n maxOnes = Math.Max(maxOnes, curOnes);\n\n while (j < circular.Length) {\n curOnes = curOnes - circular[i] + circular[j];\n maxOnes = Math.Max(maxOnes, curOnes);\n ++j;\n ++i;\n }\n\n return ones - maxOnes;\n }\n}\n```\n\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(2 * n)\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n n , ones = len(nums) , 0\n \n res = [0] * (2 * n)\n for i in range(n):\n res[i] = res[i + n] = nums[i]\n ones += nums[i]\n \n maxOnes = currOnes = i = j = 0\n \n while j < ones:\n currOnes += res[j]\n j += 1\n \n maxOnes = max(maxOnes, currOnes)\n while j < len(res):\n currOnes = currOnes - res[i] + res[j]\n maxOnes = max(maxOnes, currOnes)\n j += 1\n i += 1\n return ones - maxOnes\n```\n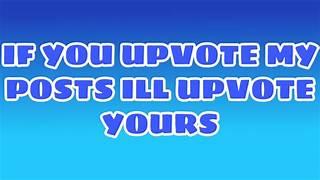\n | 2 | 0 | ['Array', 'C', 'Sliding Window', 'Python', 'C++', 'Java', 'TypeScript', 'Python3', 'JavaScript', 'C#'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Java Clean Solution | Daily Challenges | java-clean-solution-daily-challenges-by-xg63k | Complexity\n- Time complexity:O(n*X)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:O(1)\n Add your space complexity here, e.g. O(n) \n\n# Co | Shree_Govind_Jee | NORMAL | 2024-08-02T07:44:32.012874+00:00 | 2024-08-02T07:44:32.012900+00:00 | 204 | false | # Complexity\n- Time complexity:$$O(n*X)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n// class Solution {\n// public int minSwaps(int[] nums) {\n// int one = 0;\n// for (int num : nums) {\n// one += num;\n// }\n\n// int cnt_one = 0, window = 0;\n// for (int i = 0; i < nums.length + one; i++) {\n// if (i >= one && nums[i - one] == 1) {\n// cnt_one--;\n// }\n// if (nums[i % nums.length] == 1) {\n// cnt_one++;\n// }\n\n// window = Math.max(cnt_one, window);\n// }\n// return one - window;\n// }\n// }\n\n\n\n// SLIDING WINDOW....\nclass Solution {\n public int minSwaps(int[] nums) {\n int one = 0;\n for (int num : nums) {\n one += num;\n }\n\n int res = nums.length;\n for (int i = 0, j = 0, cnt = 0; i < nums.length; i++) {\n while (j - i < one) {\n cnt += nums[j % nums.length];\n j++;\n }\n\n res = Math.min(res, one - cnt);\n cnt -= nums[i];\n }\n return res;\n }\n}\n``` | 2 | 0 | ['Array', 'Math', 'Greedy', 'Sliding Window', 'Java'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Kotlin. Beats 100% (355 ms). Doubled array (System.arraycopy) + sliding window | kotlin-beats-100-355-ms-doubled-array-sy-4mxq | \n\n# Code\n\nclass Solution {\n fun minSwaps(nums: IntArray): Int {\n var ones = 0\n for (n in nums) {\n ones += n\n }\n\n | mobdev778 | NORMAL | 2024-08-02T07:36:44.898248+00:00 | 2024-08-02T15:24:49.020291+00:00 | 37 | false | \n\n# Code\n```\nclass Solution {\n fun minSwaps(nums: IntArray): Int {\n var ones = 0\n for (n in nums) {\n ones += n\n }\n\n var windowOnes = 0\n for (i in 0 until ones) {\n windowOnes += nums[i]\n }\n if (windowOnes == ones) return 0\n\n var maxWindowOnes = windowOnes\n\n val bigArray = IntArray(nums.size shl 1)\n System.arraycopy(nums, 0, bigArray, 0, nums.size)\n System.arraycopy(nums, 0, bigArray, nums.size, nums.size)\n\n var left = 0\n var right = ones\n while (left < nums.size) {\n windowOnes -= bigArray[left++]\n windowOnes += bigArray[right++]\n maxWindowOnes = Math.max(maxWindowOnes, windowOnes)\n }\n return ones - maxWindowOnes\n }\n}\n```\n\n# Approach\n\nTo avoid unnecessary fiddling around with a looping array, I simply decided to make an array of size "x2" and search for a solution on this doubled array using a sliding window algorithm.\nThis will save time on the extra check for the transition of the right border of the window to the left border.\n\nTo copy the array I use the System.arraycopy() operation - this is the fastest that the virtual machine can offer for copying the contents of arrays.\n\nAfter the array is created, we no longer need to bother with possible indexes going beyond the "nums.size", so we can focus entirely on finding the result.\n\nWe use the sliding window algorithm. To find a solution, first count all the "1" numbers inside the array and write it to the variable "ones". Next, I define a window of size "ones" and start moving it inside the array "nums".\n\nThe best window will be one that collects the maximum possible number of "1" numbers from "nums".\n\nThe minimum number of swap operations will be the difference between the total number of 1s and the maximum number of 1s that got into the window.\n\nExample 1:\n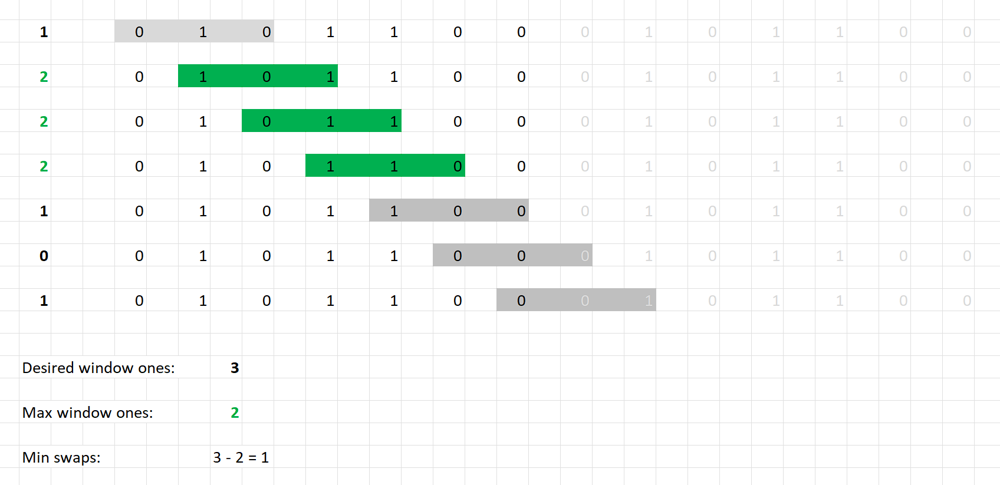\n\nExample 2:\n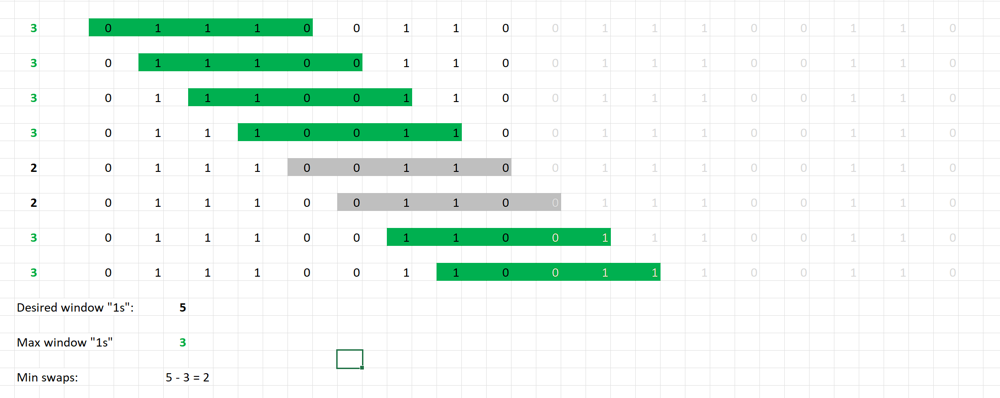\n\nOne more attempt (one pointer sliding window):\n\n\n\n```\nclass Solution {\n fun minSwaps(nums: IntArray): Int {\n var ones = 0\n for (n in nums) {\n ones += n\n }\n\n var windowOnes = 0\n for (i in 0 until ones) {\n windowOnes += nums[i]\n }\n if (windowOnes == ones) return 0\n\n var maxWindowOnes = windowOnes\n\n val bigArray = IntArray(nums.size shl 1)\n System.arraycopy(nums, 0, bigArray, 0, nums.size)\n System.arraycopy(nums, 0, bigArray, nums.size, nums.size)\n\n for (i in 0 until nums.size) {\n windowOnes -= bigArray[i]\n if (bigArray[i + ones] == 1) {\n maxWindowOnes = Math.max(maxWindowOnes, ++windowOnes)\n }\n }\n return ones - maxWindowOnes\n }\n}\n```\n | 2 | 0 | ['Sliding Window', 'Kotlin'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Simple C++ solution | Sliding Window | simple-c-solution-sliding-window-by-saur-dvzf | Intuition\nSince we need to find the minimum swaps, we need to count the zeros in a fixed window of size equal to the total number of ones.\n\n# Approach\n1. Co | saurabhdamle11 | NORMAL | 2024-08-02T05:34:57.401928+00:00 | 2024-08-02T05:34:57.401961+00:00 | 379 | false | # Intuition\nSince we need to find the minimum swaps, we need to count the zeros in a fixed window of size equal to the total number of ones.\n\n# Approach\n1. Count the number of ones which will be the size of the sliding window.\n2. Append the array to itself at the end as it is a circular array.\n3. Count the number of zeros in the window.\n4. Move the sliding window till the end and find the minimum count of the zeros.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int m = nums.size();\n int ones = 0;\n for(int i=0;i<m;++i){if(nums[i]==1){++ones;}}\n if(ones<=1){return 0;}\n for(int i=0;i<m;++i){nums.push_back(nums[i]);}\n m=nums.size();\n int left = 0;int right = ones-1;int swaps = 0;\n for(int i=left;i<=right;++i){if(nums[i]==0){++swaps;}}\n int ans = swaps;\n while(right<m-1){\n if(nums[++right]==0){++swaps;}\n if(nums[left++]==0){--swaps;}\n ans=min(ans,swaps);\n }\n return ans;\n }\n};\n```\n\nPlease upvote if you found it useful!! | 2 | 0 | ['Array', 'Sliding Window', 'C++'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | 93% Beats in C++ || Easy understandable Sliding window code 💯✅ | 93-beats-in-c-easy-understandable-slidin-ok63 | \n\n\n# Approach\n Describe your approach to solving the problem. \nWe can observe that after grouping the number of ones in the group is number of ones in the | srimukheshsuru | NORMAL | 2024-08-02T05:17:18.851453+00:00 | 2024-08-02T05:17:18.851481+00:00 | 63 | false | 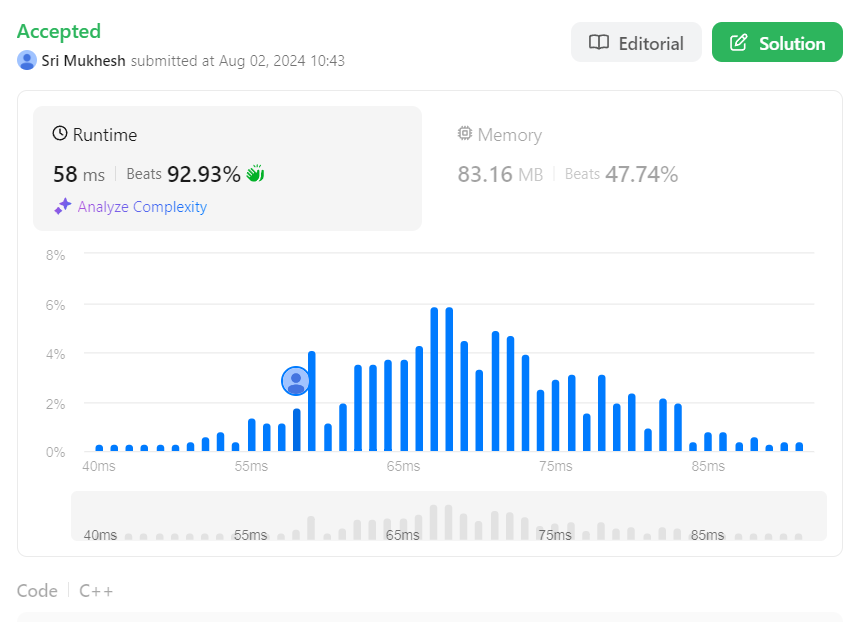\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can observe that after grouping the number of ones in the group is number of ones in the complete vector. now apply a sliding window and check for all subarrays of the size number of ones that how many ones are still required for making that subarray a group of all ones,So min of all that subarrays will give us the answer \n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n ios_base::sync_with_stdio(false);\n cin.tie(NULL);\n int n = nums.size();\n int ones= 0;\n for(int i=0;i<n;i++){\n if(nums[i]==1) ones++;\n }\n int numones=0;\n for(int i=0;i<ones;i++){\n if(nums[i] == 1) {\n numones++;\n }\n }\n int ans = ones-numones;\n for(int i=1;i<n;i++){\n if(nums[i-1] == 1){\n numones--;\n }\n if(nums[(ones+i-1)%n] ==1){\n numones++;\n }\n ans = min(ans , ones-numones);\n }\n return ans;\n\n }\n};\n``` | 2 | 0 | ['Array', 'Sliding Window', 'C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Easy to understand C++ solution || Sliding window || 🔥Beats 91%, O(n) | easy-to-understand-c-solution-sliding-wi-eaqa | Intuition\nProblem can be approached using a sliding window technique to find the segment with the maximum number of 1\'s, as this will result in the minimum sw | omkarsalunkhe3597 | NORMAL | 2024-08-02T05:14:25.887441+00:00 | 2024-08-02T05:14:25.887466+00:00 | 158 | false | # Intuition\nProblem can be approached using a sliding window technique to find the segment with the maximum number of 1\'s, as this will result in the minimum swaps required to group all the 1\'s together.\n\n\n# Approach\n1. **Calculate Total Ones**:\n - Count the total number of 1\'s in the array, which determines the window size for the sliding window approach.\n\n2. **Initialize Window**:\n - Calculate the number of 1\'s in the initial window of size equal to `total_ones`.\n\n3. **Sliding Window Technique**:\n - Use two pointers `i` and `j` to represent the start and end of the current window.\n - As the window slides over the array, adjust the count of `current_ones` by decrementing when leaving a 1 and incrementing when entering a 1.\n - Compute the minimum swaps needed by comparing `total_ones - current_ones` for each window position.\n\n4. **Circular Array Handling**:\n - To account for the circular nature of the array, use the `modulo` operation to wrap the window around the array when the end is reached.\n\n# Complexity\n- Time complexity:\n$$O(N)$$, where $$N$$ is the length of the input array. The array is traversed twice: once to count the 1\'s and once for the sliding window.\n- Space complexity:\n$$O(1)$$, as no additional space proportional to the input size is used.\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int n = nums.size();\n int total_ones = 0;\n\n // Calculate the total number of ones in the array\n for (int i = 0; i < n; i++) {\n if (nums[i] == 1) total_ones++;\n }\n\n // Count ones in the initial window\n int current_ones = 0;\n for (int i = 0; i < total_ones; i++) {\n if (nums[i] == 1) current_ones++;\n }\n\n // Initialize variables for sliding window\n int i = 0, j = total_ones - 1, minSwaps = INT_MAX;\n\n // Slide the window over the circular array\n while (i < n) {\n // Update the minimum number of swaps needed\n minSwaps = min(minSwaps, total_ones - current_ones);\n\n // Slide the window to the right\n if (nums[i++] == 1) current_ones--;\n j++;\n\n // Handle wrap-around using modulo operation\n int index = j;\n if (j >= n) index = j - n;\n if (nums[index] == 1) current_ones++;\n }\n\n return minSwaps;\n }\n};\n```\n## \uD83D\uDE80 Upvote Alert! \uD83D\uDE80\nHey, awesome coder! If you found this explanation and solution helpful, please hit that upvote button! It\'ll make my day brighter than a perfectly optimized algorithm and keep me motivated to churn out more helpful content. \uD83C\uDF1F\n\nWhy upvote, you ask?\n\nYou\'ll make me smile \uD83D\uDE0A\nIt gives me the energy to write solutions for more problems \uD83D\uDCAA\nYou\'ll be spreading good coding karma in the community \u2728\nSo go ahead, smash that upvote button! You know you want to! \uD83D\uDD3C\n | 2 | 0 | ['Array', 'Sliding Window', 'C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | ✅✅👌👌GREAT SOLUTION ,UNDERSTAND BETTER, EASY APPROACH💯💯 | great-solution-understand-better-easy-ap-3b0a | PRETTY EASY APPROACH FOR BETTER UNDERSTANDING THE SLIDING WINDOW CONCEPT (BEGINNER FRIENDLY)\n\n\npublic int minSwaps(int[] a) {\n\tint windowSize = 0;\n\tfor(i | _dc | NORMAL | 2024-08-02T04:49:52.898535+00:00 | 2024-08-02T12:55:42.740359+00:00 | 86 | false | ***_PRETTY EASY APPROACH FOR BETTER UNDERSTANDING THE SLIDING WINDOW CONCEPT (BEGINNER FRIENDLY)_***\n\n```\npublic int minSwaps(int[] a) {\n\tint windowSize = 0;\n\tfor(int x : a){\n\t\twindowSize = x == 1 ? windowSize+1 : windowSize; \n\t} \n\tif (windowSize ==a.length)return 0;\n\n\tint back= 0 ;int front=windowSize - 1 ;\n\tint initialCount =0;\n\tfor(int i =0 ; i<= front; i++){\n\t if(a[i]==0) initialCount++;\n\t}\n\tback++;\n\tfront++; \n\tint ans = initialCount;\n\twhile(back<a.length){\n\t\t if(front==a.length)front=0;\n\t\tinitialCount = giveMeCounts(a,back,front,initialCount);\n\t\t ans = Math.min(ans , initialCount);\n\t\tback++; front++;\n\t}\n\treturn ans;\n} \npublic int giveMeCounts(int []a , int b, int f,int initialCount){\n\n\tinitialCount = a[b-1]==0 ? initialCount - 1 :initialCount;\n\tinitialCount = a[f]== 0 ? initialCount+1:initialCount;\n // System.out.println(b + " "+ f + "-" + initialCount);\n\t return initialCount;\n}\n}\n```\n\n***PLEASE UPVOTE IF U LIKE***\n\n | 2 | 1 | ['Java'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Sliding Window || Fixed Size || C++ | sliding-window-fixed-size-c-by-oreocraz_-wpra | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | oreocraz_273 | NORMAL | 2024-08-02T04:43:45.602849+00:00 | 2024-08-02T04:43:45.602883+00:00 | 183 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n \n int one=0;\n for(auto& x: nums)\n if(x==1)\n one++;\n if(one<=1)\n return 0;\n vector<int> a=nums;\n for(auto& x:nums)\n {\n a.push_back(x);\n }\n int n=a.size();\n int ans=n;\n int i=0,j=0;\n int count=0;\n for(;j<n;j++)\n {\n if(a[j]==0)\n count++;\n while(i<j && (j-i+1)>one)\n {\n if(a[i]==0)\n count--;\n i++; \n }\n if((j-i+1)==one)\n ans=min(ans,count);\n }\n\n return ans;\n }\n};\n``` | 2 | 0 | ['Sliding Window', 'C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | ✅💪🔥 Simplest Python code || Sliding Window method || Beats 93% 🔥 || Easy understanding | simplest-python-code-sliding-window-meth-iu8m | Intuition\nAdd the same array and use sliding window approach.\n\n# Approach\n- Count the total number of 1\'s: We count how many 1\'s are in the original array | 1MB | NORMAL | 2024-08-02T04:17:28.822723+00:00 | 2024-08-02T04:17:28.822757+00:00 | 10 | false | # Intuition\nAdd the same array and use sliding window approach.\n\n# Approach\n- **Count the total number of 1\'s**: We count how many 1\'s are in the original array. This count determines the size of the window we need to consider for grouping all 1\'s together. **Edge case :** If the count is equal to the length of the array or if the number of ones are zero then return 0 swaps.\n\n- **Extend the array**: To handle the circular nature of the array, we extend the array by appending it to itself. This way, we can easily simulate the circular behavior using a simple linear traversal.\n\n```\n total_ones = nums.count(1)\n if total_ones == 0 or total_ones == len(nums):\n return 0\n extended_nums = nums + nums\n```\n\n- **Initialize the sliding window:** We start by calculating the number of 1\'s in the first window (of size equal to the total number of 1\'s).\n\n- **Slide the window:** We slide the window across the extended array and keep track of the maximum number of 1\'s found in any window of the given size.\n\n```\n current_ones = sum(extended_nums[:total_ones])\n max_ones = current_ones\n for i in range(1, len(nums)):\n current_ones = current_ones - extended_nums[i - 1] + extended_nums[i + total_ones - 1]\n max_ones = max(max_ones, current_ones)\n```\n\n- **Calculate the minimum swaps:** The minimum number of swaps required to group all 1\'s together is the total number of 1\'s minus the maximum number of 1\'s found in any window.\n\n```\n min_swaps = total_ones - max_ones\n return min_swaps\n```\n\n# Complexity\n- Time complexity:\n`O(N)`\n\n- Space complexity:\n`O(N)`\n\n\n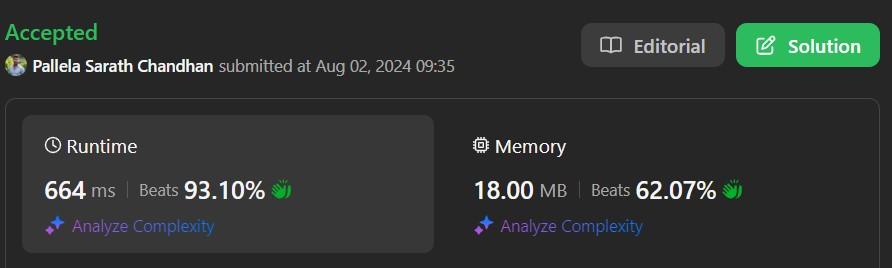\n\n\n\n# Code\n```\nclass Solution(object):\n def minSwaps(self, nums):\n total_ones = nums.count(1)\n if total_ones == 0 or total_ones == len(nums):\n return 0\n extended_nums = nums + nums\n current_ones = sum(extended_nums[:total_ones])\n max_ones = current_ones\n for i in range(1, len(nums)):\n current_ones = current_ones - extended_nums[i - 1] + extended_nums[i + total_ones - 1]\n max_ones = max(max_ones, current_ones)\n min_swaps = total_ones - max_ones\n return min_swaps\n\n \n``` | 2 | 0 | ['Array', 'Sliding Window', 'Python'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | 2 min read Python solution || Beginner friendly | 2-min-read-python-solution-beginner-frie-00t7 | Intuition\n Describe your first thoughts on how to solve this problem. \nWe will solve this problem using a sliding window approach.\n\n# Approach\n Describe yo | satwika-55 | NORMAL | 2024-08-02T04:03:59.105178+00:00 | 2024-08-02T04:04:42.207907+00:00 | 592 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe will solve this problem using a sliding window approach.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSince the question describes a circular array, we create a new array doubling the original array.\n```\n arr = nums + nums\n```\nNow we will create a window of size of total number of ones.\n```\nl = 0\nr = total_ones - 1\n```\nAt each step,move the window one step forwards, and subtract the size of window from the length of window.\n```\nwhile r < len(arr)-1:\n sm -= arr[l]\n sm += arr[r+1]\n l += 1\n r += 1\n```\nAnd finally,at each step take the minimum and return it. \n\nTake a look at the code and you will understand.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n0(2n) == 0(N)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n0(2n) == 0(N)\n\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n total_ones = sum(nums)\n arr = nums + nums\n ans = float(\'inf\')\n l = 0\n r = total_ones - 1\n sm = sum(arr[l:r+1])\n ans = min(ans,r-l+1-sm)\n while r < len(arr)-1:\n sm -= arr[l]\n sm += arr[r+1]\n l += 1\n r += 1\n ans = min(ans,r-l+1-sm)\n return ans\n\n \n``` | 2 | 0 | ['Python3'] | 3 |
minimum-swaps-to-group-all-1s-together-ii | "Optimal Code | TC O(n) | SC O(1) | Beats 93% | Easy to understand" | optimal-code-tc-on-sc-o1-beats-93-easy-t-fdau | Intuition\n Describe your first thoughts on how to solve this problem. \nThe goal is to find the minimum number of swaps required to group all the 1s together i | coder_for_sure | NORMAL | 2024-08-02T03:09:48.253492+00:00 | 2024-08-02T03:19:02.863527+00:00 | 32 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe goal is to find the minimum number of swaps required to group all the 1s together in a given binary array. The approach revolves around the idea of using a sliding window to find the position where the group of 1s can be moved to minimize the number of swaps.\n\n**Intuition Behind the Code**\n\n**Counting the 1s (once):** This tells us the target size of the window that should contain all the 1s after the optimal arrangement.\n**Sliding Window:** The sliding window technique is used to efficiently calculate the number of 1s in each possible window of size once by maintaining a running count (ow) and updating it as the window slides. This avoids recalculating the sum from scratch for each new position of the window.\n**Maximizing 1s in the Window (max1):** By keeping track of the maximum number of 1s in any window, we determine the best case scenario with the least number of swaps needed.\n**Final Calculation (once - max1):** This gives the number of 0s that need to be swapped out to achieve the optimal window with all 1s grouped together.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Count the Total Number of 1s (once):\n\n The total number of 1s in the array (once) determines the size of the window that needs to be created, where ideally all 1s should be grouped together. This is because if there are k 1s in the array, the resulting group of 1s after swaps should also have a window of size k.\n\n2. Calculate the Initial Number of 1s in the First Window (ow):\n\n Start by calculating the number of 1s in the first window of size once starting from the beginning of the array. This helps establish a baseline for the number of 1s we can expect to find in any window of this size.\n\n3. Sliding Window to Find Maximum Number of 1s in Any Window of Size once:\n\n Slide the window across the array to check each possible group of once elements. For each shift, update the count of 1s in the current window by:\n Adding 1 if the element entering the window (from the right) is 1.\n Subtracting 1 if the element exiting the window (from the left) is 1.\n Keep track of the maximum number of 1s (max1) found in any window of this size.\n\n4. Calculate the Minimum Number of Swaps:\n\n The minimum number of swaps required to group all 1s together is given by the difference between the total number of 1s (once) and the maximum number of 1s found in any window (max1). This is because the difference represents the number of 0s in the optimal window, which need to be swapped out with 1s from outside the window.\n\nFor maintaining the circular array case, we can use "%" of len(arr).\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)\n\n\n# Code\n```\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n once=nums.count(1)\n ow=sum(nums[:once])\n n=len(nums)\n if ow==n:\n return 0\n max1=0\n for i in range(len(nums)):\n if nums[i%n]==0 and nums[(i+once)%n] ==1:\n ow+=1\n elif nums[i%n]==1 and nums[(i+once)%n] ==0:\n ow-=1\n max1=max(max1,ow)\n return once-max1\n \n``` | 2 | 0 | ['Sliding Window', 'Python3'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Easy Sliding Window Solution | Golang | easy-sliding-window-solution-golang-by-j-xzse | Intuition\n Describe your first thoughts on how to solve this problem. \nWe can use a sliding window to check the number of ones missing from each interval. The | jonalfarlinga | NORMAL | 2024-08-02T02:32:27.399160+00:00 | 2024-08-02T02:32:27.399199+00:00 | 91 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can use a sliding window to check the number of ones missing from each interval. The fact that the list is circular, means that we need to ensure we check the window starting before index `0`.\n\nThe size of the window, $$k$$, is the number of ones in the array. In any given window of length $$k$$, the number of zeroes in the window is the number of swaps needed. \n\nThen we only need to check each window, remembering the minimum number of swaps to return. \n \n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Count and store the number of `ones` in `nums` - this will define the length of the window\n2. For the final `ones` in `nums`, count the `swaps` needed, and set `min_swaps` equal to `swaps`\n3. Starting at index `0`\n - If the current index is a zero, increment `swaps`\n - Set the previous index at `ones` places behind `i`, adding `len(nums)` if it is below zero\n - If the previous index is a zero, decrement `swaps`\n - If `swaps` is less than `min_swaps`, set the new minimum\n4. Return `min_swaps`\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. -->\nCounting ones is n-time, and setting the initial window is k-time, where k is the total number of ones. Checking each window is n-time. So the total time complexity is $$O(2n + k)$$, which simplifies to $$O(n)$$.\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThere is no need for additional data structures, so space complexity is constant.\n\n# Code\n```\nfunc minSwaps(nums []int) int {\n ones := 0\n for _, num := range nums {\n if num == 1 {\n ones++\n }\n }\n \n swaps := 0\n for i := len(nums)-ones; i < len(nums); i++ {\n if nums[i] == 0 {\n swaps += 1\n }\n }\n \n min_swaps := swaps\n for i := 0; i < len(nums); i++ {\n if nums[i] == 0 {\n swaps += 1\n }\n last := i-ones\n if last < 0 {\n last += len(nums)\n }\n if nums[last] == 0 {\n swaps -= 1\n }\n if swaps < min_swaps {\n min_swaps = swaps\n }\n }\n return min_swaps\n}\n``` | 2 | 0 | ['Array', 'Sliding Window', 'Go'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Easy Video Solution || Faster than 97% | c-easy-video-solution-faster-than-97-by-i4l8i | The video soltuion for the below code is\nhttps://youtu.be/ow8SNSXYepc\n\n# Code\n\nclass Solution {\nprivate:\n int minCount(vector<int>& nums, int check){\ | __SAI__NIVAS__ | NORMAL | 2024-08-02T02:26:17.708464+00:00 | 2024-08-02T02:26:17.708494+00:00 | 584 | false | The video soltuion for the below code is\nhttps://youtu.be/ow8SNSXYepc\n\n# Code\n```\nclass Solution {\nprivate:\n int minCount(vector<int>& nums, int check){\n int sz = nums.size();\n int windowSize = 0;\n for(auto &num : nums) windowSize += (num == check);\n int maxWindowCheckerCnt = INT_MIN; // Will have max size of checker numbers that are present in any window\n int currWindowCheckerCnt = 0;\n for(int i = 0 ; i < windowSize ; i++){\n currWindowCheckerCnt += (nums[i] == check);\n }\n maxWindowCheckerCnt = max(maxWindowCheckerCnt, currWindowCheckerCnt);\n for(int i = windowSize ; i < sz ; i++){\n currWindowCheckerCnt -= (nums[i - windowSize] == check);\n currWindowCheckerCnt += (nums[i] == check);\n maxWindowCheckerCnt = max(maxWindowCheckerCnt, currWindowCheckerCnt);\n }\n return windowSize - maxWindowCheckerCnt;\n }\npublic:\n int minSwaps(vector<int>& nums) {\n ios_base::sync_with_stdio(false);\n cin.tie(NULL);\n cout.tie(NULL);\n // 0 1 0 1 1 0 0\n // 0 0 1 1 1 0 0 -- 1st Way\n // 0 0 0 1 1 1 0 -- 2nd Way\n\n // 1 1 0 0 1 -- Linear array -- 1\n \n\n // Linear array\n // 1 0 0 0 0 1 0 1 1 0 1\n // All 5 ones should be grouped together\n // 1st window --> 1, 1 0 0 0 0\n // 2nd window --> 1, 0 0 0 0 1\n // 3rd window --> 1, 0 0 0 1 0\n // 4th window --> 2, 0 0 1 0 1 --> 3 ops\n // 5th window --> 3, 0 1 0 1 1\n // 6th window --> 3, 1 0 1 1 0 --> 2 ops\n // 7th window --> 3, 0 1 1 0 1 --> 2 ops\n\n // Intuition --> Linear --> Count number of ones present in the linear vector for all the windows with size total number of ones we will find the window which is having maximum number of ones and subract it with original window size.\n\n\n // Circular array\n // Instead of ones I will group all 0\'s\n // 0 1 1 1 1 0 1 0 0 1 0\n // 2 ops\n // <1>\'s<0\'s><1\'s>\n return min(minCount(nums, 1), minCount(nums, 0));\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | C# Solution for Minimum Swaps to Group All 1's Together II Problem | c-solution-for-minimum-swaps-to-group-al-otzq | Intuition\n Describe your first thoughts on how to solve this problem. \n1.\tCalculate Total Ones: Count the total number of 1\u2019s (totalOnes) in the array.\ | Aman_Raj_Sinha | NORMAL | 2024-08-02T00:57:04.956955+00:00 | 2024-08-02T00:57:04.956982+00:00 | 220 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n1.\tCalculate Total Ones: Count the total number of 1\u2019s (totalOnes) in the array.\n2.\tPrefix Sums: Use a prefix sum array to quickly calculate the number of 1\u2019s in any subarray. This helps in efficiently computing the number of 1\u2019s in any sliding window.\n3.\tFind Minimum Swaps: Using the prefix sum array, find the minimum number of swaps required to group all 1\u2019s together by checking all possible windows of size totalOnes.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1.\tCalculate Total Ones:\n\t\u2022\tLoop through the array to count the number of 1\u2019s (totalOnes).\n2.\tPrefix Sum Array:\n\t\u2022\tCreate a prefix sum array where prefixSum[i] contains the sum of elements from the start of the array to the i-1 index.\n\t\u2022\tThis allows quick calculation of the number of 1\u2019s in any subarray by taking the difference between two prefix sums.\n3.\tFind Minimum Swaps:\n\t\u2022\tLoop through the array and check all possible windows of size totalOnes to find the one with the maximum number of 1\u2019s.\n\t\u2022\tUse the prefix sum array to calculate the number of 1\u2019s in each window efficiently.\n\t\u2022\tThe minimum swaps required will be totalOnes - maxOnesInWindow.\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\u2022\tCalculating Total Ones: This takes O(n).\n\u2022\tPrefix Sum Array Calculation: This takes O(n).\n\u2022\tSliding Window Check: This takes O(n).\nThus, the total time complexity is O(n).\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\u2022\tPrefix Sum Array: This requires O(n) space.\n\u2022\tAdditional Variables: A few extra variables are used, which is O(1).\nTherefore, the space complexity is O(n).\n\n# Code\n```\npublic class Solution {\n public int MinSwaps(int[] nums) {\n int n = nums.Length;\n \n // Step 1: Calculate total number of 1\'s\n int totalOnes = 0;\n foreach (int num in nums) {\n if (num == 1) {\n totalOnes++;\n }\n }\n \n // Edge case: if there are no 1\'s or only one 1, no swaps needed\n if (totalOnes <= 1) {\n return 0;\n }\n \n // Step 2: Compute the prefix sum array\n int[] prefixSum = new int[n + 1];\n for (int i = 0; i < n; i++) {\n prefixSum[i + 1] = prefixSum[i] + nums[i];\n }\n \n // Step 3: Find the minimum number of swaps required\n int minSwaps = int.MaxValue;\n \n // Check all possible windows of size totalOnes\n for (int i = 0; i < n; i++) {\n int end = i + totalOnes - 1;\n int currentOnes;\n \n if (end < n) {\n currentOnes = prefixSum[end + 1] - prefixSum[i];\n } else {\n currentOnes = (prefixSum[n] - prefixSum[i]) + (prefixSum[end - n + 1]);\n }\n \n minSwaps = Math.Min(minSwaps, totalOnes - currentOnes);\n }\n \n return minSwaps;\n }\n}\n``` | 2 | 0 | ['C#'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Group all 1s and 0s together, Sliding Window | group-all-1s-and-0s-together-sliding-win-gbbn | Channel Link\n\n# Approach\nGroup all 1s together\nGroup all 0s together ( it will result in 1s placed at corners )\n\n# Complexity\n- Time complexity: O(n)\n A | cs_iitian | NORMAL | 2024-08-02T00:42:33.839589+00:00 | 2024-08-02T01:00:01.755349+00:00 | 914 | false | ## [Channel Link](https://www.youtube.com/channel/UCuxmikkhqbmBOUVxf-61hxw)\n\n# Approach\nGroup all 1s together\nGroup all 0s together ( it will result in 1s placed at corners )\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int countOfOnes = count(nums.begin(), nums.end(), 1);\n int n = nums.size();\n return min(solve(nums, n, 0, n - countOfOnes), solve(nums, n, 1, countOfOnes));\n }\n\nprivate:\n int solve(vector<int>& nums, int n, int g, int windowSize) {\n if (windowSize == 0) return 0;\n int swaps = 0;\n for (int i = 0; i < windowSize; i++) {\n if (nums[i] != g) swaps++;\n }\n int minSwaps = swaps;\n for (int i = windowSize; i < n; i++) {\n if (nums[i - windowSize] != g) swaps--;\n if (nums[i] != g) swaps++;\n minSwaps = min(minSwaps, swaps);\n }\n return minSwaps;\n }\n};\n```\n```java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int countOfOnes = 0;\n int n = nums.length;\n for(int x: nums) if(x == 1) countOfOnes++;\n return Math.min(\n solve(nums, n, 0, n - countOfOnes), // group all 0s together\n solve(nums, n, 1, countOfOnes) // group all 1s together\n );\n }\n\n public int solve(int[] nums, int n, int g, int windowSize) {\n if (windowSize == 0) return 0;\n int swaps = 0;\n for (int i = 0; i < windowSize; i++) {\n if (nums[i] != g) swaps++;\n }\n int minSwaps = swaps;\n for (int i = windowSize; i < n; i++) {\n if (nums[i - windowSize] != g) swaps--;\n if (nums[i] != g) swaps++;\n minSwaps = Math.min(minSwaps, swaps);\n }\n return minSwaps;\n }\n}\n```\n```Python3 []\nclass Solution:\n def minSwaps(self, nums: List[int]) -> int:\n countOfOnes = nums.count(1)\n n = len(nums)\n\n def solve(nums: List[int], n: int, g: int, windowSize: int) -> int:\n if windowSize == 0:\n return 0\n \n swaps = 0\n for i in range(windowSize):\n if nums[i] != g:\n swaps += 1\n \n minSwaps = swaps\n for i in range(windowSize, n):\n if nums[i - windowSize] != g:\n swaps -= 1\n if nums[i] != g:\n swaps += 1\n minSwaps = min(minSwaps, swaps)\n \n return minSwaps\n\n return min(solve(nums, n, 0, n - countOfOnes), solve(nums, n, 1, countOfOnes))\n``` | 2 | 0 | ['Java'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | JS | js-by-manu-bharadwaj-bn-2tbt | Code\n\nvar minSwaps = function (nums) {\n let n = nums.length;\n let x = nums.reduce((x, e) => x + e, 0);\n\n let max = -Infinity, y = 0;\n for (le | Manu-Bharadwaj-BN | NORMAL | 2024-03-28T06:01:26.873321+00:00 | 2024-03-28T06:01:26.873353+00:00 | 90 | false | # Code\n```\nvar minSwaps = function (nums) {\n let n = nums.length;\n let x = nums.reduce((x, e) => x + e, 0);\n\n let max = -Infinity, y = 0;\n for (let z = 0, a = -x; z < n + x; z++, a++) {\n y += nums[z % n];\n if (a >= 0) {\n y -= nums[a % n];\n }\n max = Math.max(max, y);\n }\n return x - max;\n};\n``` | 2 | 0 | ['JavaScript'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Easy implementation || beats 97.42% | easy-implementation-beats-9742-by-jeet_s-p7w3 | Intuition\n\n Describe your first thoughts on how to solve this problem. \nUse fixed size sliding window technique in which size of the window is total number o | jeet_sankala | NORMAL | 2024-02-03T11:58:06.680872+00:00 | 2024-02-03T12:02:42.382042+00:00 | 424 | false | # Intuition\n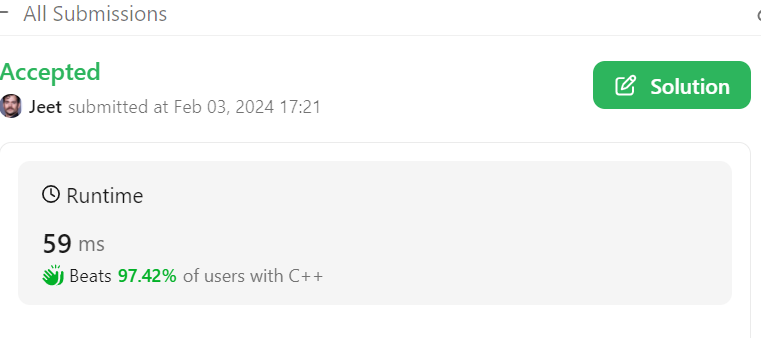\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse fixed size sliding window technique in which size of the window is total number of 1\'s(k) in the array , to avoid overhead of circular array we can append the first k elements to given array or whole array would also work here.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nJust go through the window and compute the minimum number of zeros in the window .\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n+k)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(k)\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int i=0,j=0,k=0;\n for(auto it:nums) if(it) k++;\n if(k==0) return 0;\n int ans=1e6,zero=0;\n for(int x=0;x<k;x++) nums.push_back(nums[x]);\n int n=nums.size(); \n while(j<n){\n if(!nums[j]) zero++;\n if(j-i+1<k) j++;\n else if(j-i+1==k){\n ans=min(ans,zero);\n if(!nums[i]) zero--;\n i++;\n j++;\n }\n } \n return ans;\n }\n};\n``` | 2 | 0 | ['Sliding Window', 'C++'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | Java/Python/Typescript Sliding window technique Time complexity: O(n)/Space complexity: O(1) | javapythontypescript-sliding-window-tech-gyk8 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\nThe approach to solving this problem involves using a sliding window tech | akashgond3112 | NORMAL | 2023-10-02T20:59:18.642390+00:00 | 2023-10-02T20:59:18.642412+00:00 | 54 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThe approach to solving this problem involves using a sliding window technique to find the minimum number of swaps required to group all the ones together in a circular binary array.\n\n1. First, count the total number of ones in the given array, which will help us determine the size of the window required to group all the ones together.\n\n2. Initialize variables:\n - `totalOnes`: The total number of ones in the array.\n - `n`: The length of the input array.\n - `startIndex` and `endIndex`: Pointers to define the current window.\n - `currentWindowOnes`: Count of ones in the current window.\n - `swaps`: Initialize it with the maximum possible swaps (`n`).\n\n3. Start a while loop with `endIndex` initially at 0. The loop will run until `endIndex` reaches `2 * n`, considering the circular property of the array.\n\n4. Inside the loop:\n - If `endIndex` is less than `totalOnes`, we are in the first window of size `totalOnes`. Check if the element at `nums[endIndex]` is 1. If it is, increment `currentWindowOnes`.\n - Increment `endIndex` to expand the current window.\n - Calculate the number of swaps required to move ones outside of the current window (`swaps = totalOnes - currentWindowOnes`).\n\n5. Once `endIndex` is greater than or equal to `totalOnes`, we start moving the window. In this part:\n - Check if the element at `nums[startIndex % n]` is 1. If it is, decrement `currentWindowOnes` as we are moving the window away from a 1.\n - Check if the element at `nums[endIndex % n]` is 1. If it is, increment `currentWindowOnes` as we are moving the window towards a 1.\n - Increment both `startIndex` and `endIndex` to move the window.\n - Update `swaps` with the minimum swaps required to group the ones within the current window using `Math.min(swaps, totalOnes - currentWindowOnes)`.\n\n6. The loop continues until `endIndex` reaches `2 * n`, evaluating swaps for each window along the way.\n\n7. Finally, return the minimum value of `swaps`, which represents the minimum number of swaps required to group all the ones together in the circular array.\n\n\n# Complexity\n- Time complexity:\nThe time complexity of the provided solution is O(n), where n is the length of the input array `nums`. Here\'s a breakdown of the time complexity:\n\n1. **Counting Ones:** In the initial loop where we count the total number of ones in the array, we iterate through the entire `nums` array once. This takes O(n) time.\n\n2. **Sliding Window:** The main part of the algorithm uses a sliding window approach to find the minimum swaps. This part iterates through the entire circular array once. The while loop runs until `endIndex` reaches `2 * n`. Since each iteration of the while loop processes one element, it runs in O(n) time as well.\n\nTherefore, the overall time complexity of the algorithm is dominated by the O(n) loop, making it O(n) in total.\n\n- Space complexity:\nThe space complexity of the provided solution is O(1), meaning it uses a constant amount of additional space regardless of the size of the input array. Here\'s why:\n\n1. **Constant Variables:** The algorithm uses a fixed number of variables to store information (e.g., `totalOnes`, `n`, `startIndex`, `endIndex`, `currentWindowOnes`, `swaps`). These variables do not depend on the size of the input array, so they contribute only a constant amount of space.\n\n2. **No Additional Data Structures:** The algorithm does not use any additional data structures like arrays, lists, or sets to store data. It only uses simple variables for calculations.\n\nSince the space used by the algorithm remains constant (it doesn\'t grow with the input size), the space complexity is O(1).\n\n# Code\n```java []\nclass Solution {\n public int minSwaps(int[] nums) {\n int totalOnes = 0;\n for (int one : nums) {\n if (one == 1) totalOnes++;\n }\n\n int n = nums.length,\n startIndex = 0,\n endIndex = 0,\n currentWindowOnes = 0,\n swaps = n;\n\n while (endIndex < 2 * n) {\n\n if (endIndex < totalOnes) {\n if (nums[endIndex] == 1) currentWindowOnes++;\n\n endIndex++;\n swaps = totalOnes - currentWindowOnes;\n\n } else {\n if (nums[startIndex % n] == 1) currentWindowOnes--;\n\n if (nums[endIndex % n] == 1) currentWindowOnes++;\n\n startIndex++;\n endIndex++;\n\n swaps = Math.min(swaps, totalOnes - currentWindowOnes);\n }\n\n }\n\n return swaps;\n }\n}\n```\n```python []\nclass Solution(object):\n def minSwaps(self, nums):\n """\n :type nums: List[int]\n :rtype: int\n """\n total_ones = 0\n for one in nums:\n if one == 1:\n total_ones += 1\n\n n = len(nums)\n start_index = 0\n end_index = 0\n current_window_ones = 0\n swaps = n\n\n while end_index < 2 * n:\n\n if end_index < total_ones:\n if nums[end_index] == 1:\n current_window_ones += 1\n\n end_index += 1\n swaps = total_ones - current_window_ones\n else:\n if nums[start_index % n] == 1:\n current_window_ones -= 1\n\n if nums[end_index % n] == 1:\n current_window_ones += 1\n\n start_index += 1\n end_index += 1\n\n swaps = min(swaps, total_ones - current_window_ones)\n\n return swaps\n```\n```typescript []\nfunction minSwaps(nums: number[]): number {\n let totalOnes: number = 0;\n for (const one of nums) {\n if (one == 1) totalOnes++;\n }\n\n let n = nums.length,\n startIndex = 0,\n endIndex = 0,\n currentWindowOnes = 0,\n swaps = n;\n\n while (endIndex < 2 * n) {\n\n if (endIndex < totalOnes) {\n if (nums[endIndex] == 1) currentWindowOnes++;\n\n endIndex++;\n swaps = totalOnes - currentWindowOnes;\n\n } else {\n if (nums[startIndex % n] == 1) currentWindowOnes--;\n\n if (nums[endIndex % n] == 1) currentWindowOnes++;\n\n startIndex++;\n endIndex++;\n\n swaps = Math.min(swaps, totalOnes - currentWindowOnes);\n }\n\n }\n\n return swaps;\n}\n```\n\n | 2 | 0 | ['Python', 'Java', 'TypeScript'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | C++ Solution | Sliding Window | Easy to Understand | c-solution-sliding-window-easy-to-unders-pw6z | \nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\n int n = nums.size();\n int target = 0;\n\n for(int i=0;i<n;i++) {\n | Adi_217 | NORMAL | 2023-08-03T14:06:58.315265+00:00 | 2023-08-03T14:06:58.315285+00:00 | 164 | false | ```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\n int n = nums.size();\n int target = 0;\n\n for(int i=0;i<n;i++) {\n if(nums[i]==1) {\n target++;\n }\n }\n\n if(target == 0) {\n return 0;\n }\n\n for(int i=0;i<n;i++) {\n nums.push_back(nums[i]);\n }\n\n n*=2;\n\n int ans = INT_MAX;\n\n int i=0,j=0;\n int cnt = 0;\n while(j<n) {\n if(j-i+1<target) {\n if(nums[j]==1) {\n cnt++;\n }\n j++;\n } else if(j-i+1==target) {\n if(nums[j]==1) {\n cnt++;\n }\n\n ans = min(ans, target-cnt);\n\n if(nums[i]==1) {\n cnt--;\n }\n i++;\n j++;\n }\n }\n return ans;\n\n }\n};\n``` | 2 | 0 | ['Two Pointers', 'Sliding Window', 'C++'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Sliding Window Easy , C++ Beats 90% ✅✅ | sliding-window-easy-c-beats-90-by-deepak-98lf | Approach\n Describe your approach to solving the problem. \nwhat do you think about Window Size.......???\n\nWindow Size = No. of 1 in the given array\nNow try | Deepak_5910 | NORMAL | 2023-06-26T07:53:10.387234+00:00 | 2023-06-26T07:53:47.367901+00:00 | 421 | false | # Approach\n<!-- Describe your approach to solving the problem. -->\nwhat do you think about Window Size.......???\n\n**Window Size = No. of 1 in the given array**\nNow try to find out the window size subarray that contains maximum 1.\n\n**Note:-to Handle the circular array problem append the array itself.**\n\n# Complexity\n- Time complexity:O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& arr) {\n int n = arr.size(),cnt0= 0,cnt1 = 0,ans = INT_MAX;\n for(int i = 0;i<n;i++)\n {\n if(arr[i]) cnt1++;\n arr.push_back(arr[i]); \n }\n \n for(int i = 0;i<cnt1;i++)\n if(!arr[i]) cnt0++;\n\n ans = min(ans,cnt0);\n for(int i = cnt1;i<arr.size();i++)\n {\n if(!arr[i]) cnt0++;\n if(!arr[i-cnt1]) cnt0--;\n\n ans = min(ans,cnt0);\n }\n return ans; \n }\n};\n```\n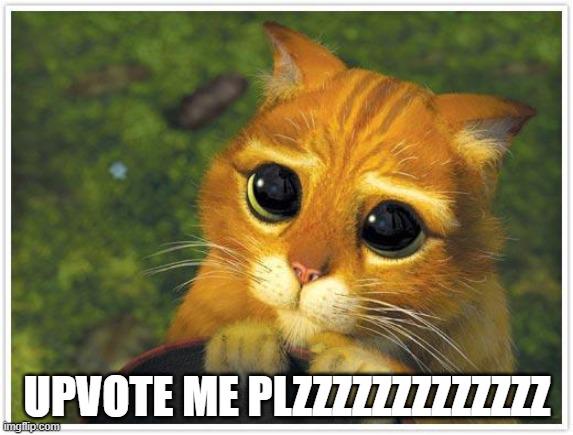\n | 2 | 0 | ['Sliding Window', 'C++'] | 2 |
minimum-swaps-to-group-all-1s-together-ii | ❇ Crisp n Clear👌 🏆O(N)❤️ Javascript❤️ Runtime👀90%🕕 Meaningful Vars✍️ 🔴❇ ✅ 👉 💪🙏 | crisp-n-clear-on-javascript-runtime90-me-1dyk | Intuition\n Describe your first thoughts on how to solve this problem. \nCount how many total ones are there(totalOnes)\n\n# Approach\n Describe your approach t | anurag-sindhu | NORMAL | 2023-01-08T15:22:15.319988+00:00 | 2023-01-08T15:22:15.320029+00:00 | 622 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nCount how many total ones are there(totalOnes)\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nselect first initial block and how many ones are there\nfind a block of totalOnes length which has maxOnesInBlock\nreturn totalOnes - maxOnesInBlock;\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nvar minSwaps = function (nums) {\n let totalOnes = nums.reduce(\n (accumulator, value) => (value && ++accumulator) || accumulator,\n 0\n );\n const block = nums.slice(0, totalOnes);\n let totalOnesInBlock = block.reduce(\n (accumulator, value) => (value && ++accumulator) || accumulator,\n 0\n );\n let maxOnesInBlock = totalOnesInBlock;\n for (let index = 0; index < nums.length; index++) {\n if (nums[(index + totalOnes) % nums.length] === 1) {\n totalOnesInBlock += 1;\n }\n if (nums[index % nums.length] === 1) {\n totalOnesInBlock -= 1;\n }\n if (maxOnesInBlock < totalOnesInBlock) {\n maxOnesInBlock = totalOnesInBlock;\n }\n }\n return totalOnes - maxOnesInBlock;\n};\n``` | 2 | 0 | ['JavaScript'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | Short & Concise Sliding window | C++ | short-concise-sliding-window-c-by-tushar-s94k | \nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int l = 0, s = 0, i = 0, ans = INT_MAX;\n for(int i : nums) l += i;\n | TusharBhart | NORMAL | 2022-10-14T16:25:20.587945+00:00 | 2022-10-14T16:25:20.587991+00:00 | 527 | false | ```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n int l = 0, s = 0, i = 0, ans = INT_MAX;\n for(int i : nums) l += i;\n nums.insert(nums.end(), nums.begin(), nums.end());\n \n for(int j=0; j<nums.size(); j++) {\n s += nums[j];\n if(j - i + 1 == l) {\n ans = min(ans, l - s);\n s -= nums[i++];\n }\n }\n return ans == INT_MAX ? 0 : ans;\n }\n};\n``` | 2 | 0 | ['Sliding Window', 'C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | 📌📌Sliding Window || Easy To Understand || C++ Code | sliding-window-easy-to-understand-c-code-e9s8 | Using Sliding Window\n\n Time Complexity :- O(N)\n\n Space Complexity :- O(N)\n\n\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\n i | __KR_SHANU_IITG | NORMAL | 2022-10-10T10:30:03.521165+00:00 | 2022-10-10T10:30:03.521202+00:00 | 754 | false | * ***Using Sliding Window***\n\n* ***Time Complexity :- O(N)***\n\n* ***Space Complexity :- O(N)***\n\n```\nclass Solution {\npublic:\n int minSwaps(vector<int>& nums) {\n\n int n = nums.size();\n\n // count the no. of ones in nums\n\n int count_1 = 0;\n\n for(int i = 0; i < n; i++)\n {\n if(nums[i])\n {\n count_1++;\n }\n }\n\n // if count of 1 is zero then return 0\n\n if(count_1 == 0)\n {\n return 0;\n }\n\n // make a double_arr by concatenating nums to itself, for handling the case of circular array\n\n vector<int> double_arr = nums;\n\n for(int i = 0; i < n; i++)\n {\n double_arr.push_back(nums[i]);\n }\n\n // now find the maximum no. of ones in count_1 sized subarray\n\n int maxi = 0;\n\n int count = 0;\n\n for(int i = 0; i < double_arr.size(); i++)\n {\n if(i < count_1)\n {\n if(double_arr[i])\n {\n count++;\n }\n }\n else\n {\n maxi = max(maxi, count);\n\n if(double_arr[i - count_1])\n {\n count--;\n }\n\n if(double_arr[i])\n {\n count++;\n }\n }\n }\n\n maxi = max(maxi, count);\n\n // count_1 - maxi will be required no. of swaps\n\n return count_1 - maxi;\n }\n};\n``` | 2 | 0 | ['C', 'Sliding Window', 'C++'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | Interview Ready Solution | Easy To Understand | Sliding Window | interview-ready-solution-easy-to-underst-yecg | I understand this might not be the best leetcode runtime or memory usuage solution but it is the most understandble for interview purpose.\n\n```\nclass Solutio | Sim1337 | NORMAL | 2022-07-23T15:38:27.333007+00:00 | 2022-07-23T15:38:27.333032+00:00 | 256 | false | I understand this might not be the best leetcode runtime or memory usuage solution but it is the most understandble for interview purpose.\n\n```\nclass Solution {\n //Time: O(n), where n represent the length of nums\n //Space: O(1), no need to store any data in any DS\n public int minSwaps(int[] nums) {\n //count the total numbers of ones\n int totalOnes = 0;\n for(int one : nums){\n if(one == 1) totalOnes++;\n }\n \n //initlize the length of given array, start/end of sliding window, current window ones, and swaps which holds length of given array\n int n = nums.length, \n start = 0,\n end =0, \n currentWindowOnes = 0, \n swaps = n;\n \n //count the ones of first window\n while(end < totalOnes){\n if(nums[end] == 1) currentWindowOnes++;\n \n end++;\n }\n //calculate the first window swaps required\n swaps = totalOnes - currentWindowOnes;\n \n //2 * n because of circular array\n while(end < 2 * n){\n //if the current start of circular array is 1 then decrease the current window ones\n if(nums[start % n] == 1) currentWindowOnes--;\n \n //if the current end of circular array is 1 then increase the current window ones\n if(nums[end % n] == 1) currentWindowOnes++;\n \n //increase both pointers\n start++;\n end++;\n \n //find the minmum swaps of current window\n swaps = Math.min(swaps, totalOnes - currentWindowOnes);\n }\n \n return swaps;\n }\n} | 2 | 0 | ['Sliding Window', 'Java'] | 1 |
minimum-swaps-to-group-all-1s-together-ii | JavaScript Solution - Sliding Window | javascript-solution-sliding-window-by-de-1ce1 | \nvar minSwaps = function(nums) {\n const MAX = Number.MAX_SAFE_INTEGER;\n \n let ones = nums.reduce((acc, bit) => acc + bit, 0);\n \n const doub | Deadication | NORMAL | 2022-05-30T02:49:22.212997+00:00 | 2022-05-30T02:51:12.827251+00:00 | 258 | false | ```\nvar minSwaps = function(nums) {\n const MAX = Number.MAX_SAFE_INTEGER;\n \n let ones = nums.reduce((acc, bit) => acc + bit, 0);\n \n const doubledNums = nums.concat(nums.slice(0, nums.length - 1));\n \n let minSwap = MAX;\n let left = 0;\n let onesWithinWindow = 0;\n \n for (let i = 0; i < doubledNums.length; ++i) {\n const rightBit = doubledNums[i];\n \n if (rightBit === 1) onesWithinWindow += 1;\n \n if (i >= ones) {\n const leftBit = doubledNums[left];\n \n if (leftBit === 1) onesWithinWindow -= 1;\n ++left;\n }\n \n if (i + 1 >= ones) minSwap = Math.min(minSwap, ones - onesWithinWindow);\n }\n \n return minSwap;\n};\n``` | 2 | 0 | ['Sliding Window', 'JavaScript'] | 0 |
minimum-swaps-to-group-all-1s-together-ii | C++ || Sliding Window approach || Removing circularity | c-sliding-window-approach-removing-circu-ti5d | ,,,\n\n int minSwaps(vector& nums) {\n \n int window=count(nums.begin(), nums.end(), 1); //determining window size by counting number of occu | mrigank_2003 | NORMAL | 2022-02-27T05:21:07.063692+00:00 | 2022-02-27T05:34:27.691457+00:00 | 107 | false | ,,,\n\n int minSwaps(vector<int>& nums) {\n \n int window=count(nums.begin(), nums.end(), 1); //determining window size by counting number of occurance of 1.\n nums.insert(nums.end(), nums.begin(), nums.end()); //in order to convert circular array to linear one appending the same array at the end of itself.\n int count=0, comp;\n for(int i=0; i<window; i++){ //now counting occurance of 1 in first window.\n if(nums[i]==1){\n count++;\n }\n }\n comp=count;\n \n for(int i=1; i<nums.size()-window+1; i++){ //checking for the the element just before the first element of the current window.\n if(nums[i-1]==1){ \n count--;\n }\n if(nums[i+window-1]==1){ //checking for last element of the window if 1 occurs then increment by 1.\n count++;\n }\n if(count>comp){ //if minimum swaps are required then in a particular window we require maximum occurance of 1 so as to have minimum number to zero, if occurance of 0 will be less then less number of swaps are required.\n comp=count;\n }\n }\n return (window-comp); \n }\n};\n\'\'\' | 2 | 0 | ['C', 'Sliding Window'] | 0 |
Subsets and Splits