question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
stone-game-vii | Python 3, today's one-liner | python-3-todays-one-liner-by-l1ne-x4dz | Explanation\n\nFairly typical Memo/DP problem, with a tricky bit on how you handle sums.\n\nYou can define a recursive function with arguments f(a, b) as the in | l1ne | NORMAL | 2021-06-11T20:21:57.488811+00:00 | 2021-06-11T20:21:57.488842+00:00 | 58 | false | # Explanation\n\nFairly typical Memo/DP problem, with a tricky bit on how you handle sums.\n\nYou can define a recursive function with arguments `f(a, b)` as the indexes in the array, inclusive:\n1. `f(a, b)` is `0` if `a == b`\n2. `f(a, b)` is max of:\n 1. `sum(a+1, b) - f(a+1, b)` - your score if you remove the left stone\n 2. `sum(a, b-1) - f(a, b-1)` - your score if you remove the right stone\n\nAlso remember that if we have `T = accumulate(A, initial=0)`, then `sum(a, b) == T[b+1] - T[a]`.\n* This makes computing a sum an `O(1)` time calculation instead of `O(n)` time, given we spend `O(n)` time and space up front.\n\nOk, we have all we need to write a memoized function!\n\n# Memoized (top-down)\n\nTime: `O(n^2)`\nSpace: `O(n^2)`\n\n**But it times out! :(**\n\n```python\nclass Solution:\n def stoneGameVII(self, A):\n T = list(accumulate(A, initial=0))\n \n @cache\n def f(a, b):\n return a < b and max(\n T[b+1] - T[a+1] - f(a+1, b),\n T[b] - T[a] - f(a, b-1)\n )\n \n return f(0, len(A) - 1)\n```\n\n# DP (bottom-up)\n\nMemoized times out, so we need a new approach.\n\nConvert it to DP... calculate the values bottom-up.\n\nTime: `O(n^2)`\nSpace: `O(n^2)`\n\n**It passes... ~6000 ms**\n\n```python\nclass Solution:\n def stoneGameVII(self, A):\n T = list(accumulate(A, initial=0))\n dp = defaultdict(int)\n for diff in range(1, len(A)):\n for a in range(len(A) - diff):\n b = a + diff\n dp[a, diff] = max(\n T[b+1] - T[a+1] - dp[a+1, diff-1],\n T[b] - T[a] - dp[a, diff-1]\n )\n return dp[0, len(A)-1]\n```\n\n# DP, optimized (bottom-up)\n\nIf we rethink things a little, we only need `O(n)` space instead of `O(n^2)` if we build it row by row.\n\nSwitch to a 1D array instead of a 2D array.\n\nTime: `O(n^2)`\nSpace: `O(n)`\n\n**Even better... 2700-3000 ms**\n\n```python\nclass Solution:\n def stoneGameVII(self, A):\n T = list(accumulate(A, initial=0))\n dp = [0] * len(A)\n for diff in range(1, len(A)):\n for a in range(len(A) - diff):\n b = a + diff\n dp[a] = max(\n T[b+1] - T[a+1] - dp[a+1],\n T[b] - T[a] - dp[a]\n )\n return dp[0]\n```\n\n# One line\n\nFinally, the reason you came here.\n\nIt\'s easy to build the DP array using a `reduce`, so lets do that.\n\nAlso wrap with an anonymous lamdba so we can pass in the `T` sums.\n\nTime & Space: same\n\n**Still 2700-3000 ms**\n\n```python\nclass Solution:\n def stoneGameVII(self, A):\n return (lambda *T: reduce(lambda dp,b: [max(T[a+b+1] - T[a+1] - dp[a+1], T[a+b] - T[a] - dp[a]) for a in range(len(A) - b)], range(1, len(A)), [0] * len(A))[0])(*accumulate(A, initial=0))\n```\n | 1 | 1 | [] | 0 |
stone-game-vii | Stone Game VII | Python | Self Explanatory Code | stone-game-vii-python-self-explanatory-c-rrxv | \nclass Solution:\n def dpFn(self, stones, total, left, right, dp):\n if dp[left][right] is None:\n if right == left:\n dp[l | akash3anup | NORMAL | 2021-06-11T18:40:53.488048+00:00 | 2022-03-11T14:39:58.365690+00:00 | 74 | false | ```\nclass Solution:\n def dpFn(self, stones, total, left, right, dp):\n if dp[left][right] is None:\n if right == left:\n dp[left][right] = 0\n if right - left == 1:\n dp[left][right] = max(stones[left], stones[right])\n if left < right:\n leftRemovedAliceScore = total - stones[left]\n bobScoreAfterAliceRemovedLeft = self.dpFn(stones, leftRemovedAliceScore, left + 1, right, dp)\n rightRemovedAliceScore = total - stones[right]\n bobScoreAfterAliceRemovedRight = self.dpFn(stones, rightRemovedAliceScore, left, right - 1, dp)\n dp[left][right] = max(leftRemovedAliceScore - bobScoreAfterAliceRemovedLeft,\n rightRemovedAliceScore - bobScoreAfterAliceRemovedRight)\n return dp[left][right]\n\n def stoneGameVII(self, stones: List[int]) -> int:\n n = len(stones)\n dp = [[None] * n for _ in range(n)]\n return self.dpFn(stones, sum(stones), 0, len(stones) - 1, dp)\n\n``` | 1 | 1 | [] | 0 |
stone-game-vii | Stone Game VII Time Limit Exceeded even after using memorization | stone-game-vii-time-limit-exceeded-even-hb0oa | This is the Solution i made using memorization. But still Iam getting Time Limit Error. Can anyone suggest Where am i Doing wrong?\n\n\n int helper(int left,int | rajkumar_darshanala | NORMAL | 2021-06-11T18:05:26.072161+00:00 | 2021-06-11T18:05:26.072207+00:00 | 82 | false | This is the Solution i made using memorization. But still Iam getting Time Limit Error. Can anyone suggest Where am i Doing wrong?\n\n```\n int helper(int left,int right,int sum,vector<int> stones,vector<vector<int>> &hm)\n {\n if(hm[left][right]!=-1)return hm[left][right];\n if(left==right)return stones[left];\n int left_res = helper(left+1,right,sum-stones[left],stones,hm); // selecting left most stone\n int right_res = helper(left,right-1,sum-stones[right],stones,hm); // selectin right most stone\n int sol = sum-max(left_res,right_res);\n hm[left][right]=sol;\n return (sol);\n }\n int stoneGameVII(vector<int>& stones) {\n int sum = 0;\n int size = stones.size();\n vector<vector<int>> hm(size,vector<int>(size,-1));\n for(int i:stones)\n sum+=i;\n return max(helper(1,size-1,sum-stones[0],stones,hm),helper(0,size-2,sum-stones[size-1],stones,hm));\n }\n``` | 1 | 0 | [] | 0 |
stone-game-vii | Stone Game VII || C++ || Bottom Up Approach || DP | stone-game-vii-c-bottom-up-approach-dp-b-49s7 | CODE :\n\nclass Solution {\npublic:\n int stoneGameVII(vector<int>& stones) {\n int n = stones.size();\n \n //precomputation \n i | naman_agr | NORMAL | 2021-06-11T12:01:38.476593+00:00 | 2021-06-11T12:01:38.476630+00:00 | 121 | false | **CODE :**\n```\nclass Solution {\npublic:\n int stoneGameVII(vector<int>& stones) {\n int n = stones.size();\n \n //precomputation \n int prefixSum[n];\n prefixSum[0] = stones[0];\n \n for(int i=1;i<n;i++)\n prefixSum[i] = stones[i] + prefixSum[i-1];\n \n // code\n int dp[n][n];\n for(int i=0;i<n-1;i++){\n dp[i][i+1] = max(stones[i], stones[i+1]);\n }\n for(int gap=2;gap<n;gap++)\n {\n for(int i=0;i+gap<n;i++){\n int j = i+gap;\n \n int sum = prefixSum[j];\n \n if(i>0)\n sum -= prefixSum[i-1];\n \n dp[i][j] = max(sum-stones[i]-dp[i+1][j], sum-stones[j]-dp[i][j-1]);\n }\n }\n return dp[0][n-1];\n }\n};\n```\nDO **UPVOTE** IF YOU LIKED IT !! | 1 | 0 | [] | 0 |
stone-game-vii | Detailed explanation, simple memoization using Java | detailed-explanation-simple-memoization-6o0d8 | Just think like a child. At every step there are 2 possibilities, \n\t1 .Picking the first stone.\n\t2. Picking the last stone.\n\nAt each step both of them wan | prakhar3agrwal | NORMAL | 2021-06-11T08:35:29.363239+00:00 | 2021-06-11T08:35:29.363312+00:00 | 67 | false | Just think like a child. At every step there are 2 possibilities, \n\t1 .Picking the first stone.\n\t2. Picking the last stone.\n\nAt each step both of them want to maximize the difference between their current score and the score that the other playes gets in the next step. This will be achieved by "maximizing the difference in the current score and the other player\'s score in the next step)\n\n**maximum(scoreOnRemovingFirstElement - score obtained on subsequent steps,**\n\t\t\t**scoreOnRemovingLastElement - score obtained on subsequent steps)**\n\n```\nclass Solution {\n \n int[][] dp = null;\n \n private int solve(int[] stones, int start, int end, int sum){\n if(start>=end){\n return 0;\n }\n if(dp[start][end] !=-1){\n return dp[start][end];\n }\n \n int scoreOnRemovingFirst = sum - stones[start];\n int scoreOnRemovingLast = sum - stones[end];\n \n dp[start][end] = Math.max(scoreOnRemovingFirst - solve(stones, start+1, end, scoreOnRemovingFirst),\n scoreOnRemovingLast - solve(stones, start, end-1, scoreOnRemovingLast));\n \n return dp[start][end];\n \n }\n \n public int stoneGameVII(int[] stones) {\n int n = stones.length;\n \n dp = new int[n][n];\n for(int i=0;i<n;i++){\n Arrays.fill(dp[i],-1);\n }\n \n int sum = 0;\n for(int num: stones){\n sum += num;\n }\n return solve(stones, 0, n-1, sum);\n \n }\n} | 1 | 0 | [] | 1 |
stone-game-vii | SImple C++ solution | O(n*n) | simple-c-solution-onn-by-abchandan11-fvpz | \nclass Solution {\npublic:\n \n int dfs(vector<int> &stones, int i, int j, int sum){\n \n //Because after removing you will not get anythin | abchandan11 | NORMAL | 2021-06-11T08:18:27.309000+00:00 | 2021-06-11T08:18:27.309044+00:00 | 93 | false | ```\nclass Solution {\npublic:\n \n int dfs(vector<int> &stones, int i, int j, int sum){\n \n //Because after removing you will not get anything because ntohing is left in the array.\n if(i==j) return 0;\n \n if(dp[i][j]) return dp[i][j];\n // dp[i][j] -> stores the difference\n int left_removed = sum - stones[i] - dfs(stones, i+1, j, sum - stones[i]);\n int right_removed = sum - stones[j] - dfs(stones, i, j-1, sum - stones[j]);\n return dp[i][j] = max(left_removed, right_removed);\n }\n \n int dp[1001][1001] = {};\n int stoneGameVII(vector<int>& stones) {\n int n = stones.size();\n int sum = 0;\n for(int i=0;i<n;i++){\n sum += stones[i];\n }\n \n return dfs(stones, 0,n-1,sum);\n }\n};\n``` | 1 | 1 | [] | 0 |
stone-game-vii | dp || recursion+memoisation | dp-recursionmemoisation-by-yaswanth0901-wdrb | ```\nclass Solution {\npublic:\n int dp[1001][1001];\n int helper(vector& v , int i , int j , int sum){\n if(i >= j or sum <= 0){\n retu | yaswanth0901 | NORMAL | 2021-06-10T09:51:48.466739+00:00 | 2021-06-10T09:51:48.466772+00:00 | 157 | false | ```\nclass Solution {\npublic:\n int dp[1001][1001];\n int helper(vector<int>& v , int i , int j , int sum){\n if(i >= j or sum <= 0){\n return 0;\n }\n if(dp[i][j] != -1){\n return dp[i][j];\n }\n int x = sum-v[i] - helper(v,i+1,j,sum-v[i]);\n int y = sum-v[j] - helper(v,i,j-1,sum-v[j]);\n return dp[i][j] = max(x,y);\n }\n int stoneGameVII(vector<int>& v) {\n int n = v.size();\n int s = 0;\n for(auto x : v){\n s+=x;\n }\n memset(dp , -1 , sizeof(dp));\n return helper(v,0,n-1,s);\n }\n}; | 1 | 0 | [] | 0 |
stone-game-vii | C++ | Priority Queue + DP | c-priority-queue-dp-by-420__iq-xid1 | \nclass Solution {\npublic:\n int maxResult(vector<int>& nums, int k) {\n priority_queue<pair<int,int>> dq;\n int n = nums.size();\n vec | 420__iq | NORMAL | 2021-06-09T13:53:34.244236+00:00 | 2021-06-09T13:53:34.244332+00:00 | 104 | false | ```\nclass Solution {\npublic:\n int maxResult(vector<int>& nums, int k) {\n priority_queue<pair<int,int>> dq;\n int n = nums.size();\n vector<int> dp(n+5,0);\n for(int i=0 ; i<n ; i++){\n if(dq.empty()){\n dq.push(make_pair(nums[i],i));\n dp[i] = nums[i];\n }\n else{\n while(dq.top().second<(i-k)){\n dq.pop();\n }\n dp[i] = dq.top().first+nums[i];\n dq.push(make_pair(dp[i],i));\n }\n cout << dp[i] << endl;\n }\n return dp[n-1];\n }\n};\n``` | 1 | 2 | [] | 0 |
stone-game-vii | Easy Code Explantion in Java. From- Recursion, Memoization, 2D(n*n) DP to 1D(n) DP | easy-code-explantion-in-java-from-recurs-nx8i | Anybody who have chance can pick from the starting or the ending. So, the maximum differentce Alice can achieve is by picking the 1st stone or last stone. We ne | MongoDB24 | NORMAL | 2021-06-06T08:41:32.338887+00:00 | 2021-06-06T08:46:00.413623+00:00 | 98 | false | Anybody who have chance can pick from the starting or the ending. So, the maximum differentce Alice can achieve is by picking the 1st stone or last stone. We need to consider both of the cases and we\'ll choose the maximum difference that Alice can get from the two choices.\nWe\'ll be adding current sum of the array (sum) - the element which is discarded(start or end) from consideration as the current score of the player.\nAnd then using the remaining array as the new array to further problems\n\n--RECURSION--\n```\nclass Solution {\n public int stoneGameVII(int[] stones) {\n int n = stones.length;\n int sum = 0;\n for (int i=0; i<n; i++)\n sum += stones[i];\n return helper(stones, 0, n-1, sum);\n }\n public int helper(int[] stones, int start, int end, int sum) {\n if(start>=end)\n return 0;\n int a = sum - stones[end];\n int b = sum - stones[start];\n return Math.max(a-helper(stones, start, end-1, a),\n b-helper(stones, start+1, end, b));\n }\n}\n```\n--MEMOIZATION--\n```\nclass Solution {\n public int stoneGameVII(int[] stones) {\n int n = stones.length;\n int[][] dp = new int[n][n];\n for(int[] row: dp)\n Arrays.fill(row, -1);\n int sum = 0;\n for (int i=0; i<n; i++)\n sum += stones[i];\n return helper(stones, 0, n-1, dp, sum);\n }\n public int helper(int[] stones, int start, int end, int[][] dp, int sum) {\n if(start>=end)\n return 0;\n if(dp[start][end]!=-1)\n return dp[start][end];\n int a = sum - stones[end];\n int b = sum - stones[start];\n return dp[start][end] = Math.max(a-helper(stones, start, end-1, dp, a),\n b-helper(stones, start+1, end, dp, b));\n }\n}\n```\n\n--DYNAMIC PROGRAMMING USING 2D--\n```\nclass Solution {\n public int stoneGameVII(int[] stones) {\n int n = stones.length;\n int[][] dp = new int[n][n];\n for(int i = n-1; i>=0; i--) {\n int sum = stones[i];\n for(int j = i; j<n; j++) {\n if(i==j)\n dp[i][j] = 0;\n else{\n sum += stones[j];\n int a = sum - stones[j];\n int b = sum - stones[i];\n dp[i][j] = Math.max(a-dp[i][j-1], b-dp[i+1][j]);\n }\n }\n }\n return dp[0][n-1];\n }\n}\n```\n\n--DYNAMIC PROGRAMMING USING 1D DP--\n```\nclass Solution {\n public int stoneGameVII(int[] stones) {\n int n = stones.length;\n int[] dp = new int[n];\n for(int i = n-1; i>=0; i--) {\n int prev = 0;\n int sum = stones[i];\n for (int j=i; j<n; j++) {\n if(i==j)\n dp[j] = 0;\n else {\n sum += stones[j];\n int a = sum - stones[j];\n int b = sum - stones[i];\n dp[j] = Math.max(a-prev, b-dp[j]);\n }\n prev = dp[j];\n } \n }\n return dp[n-1];\n }\n}\n```\n | 1 | 0 | [] | 0 |
stone-game-vii | C++ Tabulation Clean Code | c-tabulation-clean-code-by-rishabh-malho-rbd6 | \n int stoneGameVII(vector<int>& stones) {\n int n = stones.size();\n vector<int> prefixSum(n+1,0);\n for (int i = 1; i <= n; i++)\n pr | Rishabh-malhotraa | NORMAL | 2021-05-19T11:40:06.715067+00:00 | 2021-05-19T11:40:06.715101+00:00 | 197 | false | ```\n int stoneGameVII(vector<int>& stones) {\n int n = stones.size();\n vector<int> prefixSum(n+1,0);\n for (int i = 1; i <= n; i++)\n prefixSum[i] = prefixSum[i-1] + stones[i-1];\n\n vector<vector<int>> dp(n, vector<int>(n,0));\n\n for (int gap = 1 ; gap < n ; gap++ ){\n for (int i = 0, j = gap; j < n ; j++, i++){\n int start = prefixSum[j + 1] - prefixSum[i+1];\n int end = prefixSum[j] - prefixSum[i];\n \n dp[i][j] = max( start - dp[i+1][j] , end - dp[i][j-1]); \n }\n }\n return dp[0][n-1]; \n }\n``` | 1 | 0 | ['Dynamic Programming', 'C'] | 0 |
stone-game-vii | Java Top Down dp | java-top-down-dp-by-jalwal-4p9r | \nclass Solution {\n \n int dp[][] = new int[1003][1003];\n \n public int stoneGameVII(int[] S) {\n int sum = 0;\n for(int v : S) sum+ | jalwal | NORMAL | 2021-04-30T05:08:45.018604+00:00 | 2021-04-30T05:08:45.018647+00:00 | 91 | false | ```\nclass Solution {\n \n int dp[][] = new int[1003][1003];\n \n public int stoneGameVII(int[] S) {\n int sum = 0;\n for(int v : S) sum+= v;\n return solve(S , 0 , S.length - 1 , sum);\n }\n \n public int solve(int[] S , int strt , int end , int sum) {\n if(strt >= end) return 0;\n \n if(dp[strt][end] != 0) return dp[strt][end];\n \n int l = sum - S[strt] - solve(S , strt + 1 , end , sum - S[strt]);\n int r = sum - S[end] - solve(S , strt , end - 1 , sum - S[end]);\n \n return dp[strt][end] = Math.max(l , r);\n }\n}\n``` | 1 | 0 | [] | 0 |
stone-game-vii | c++ recursion + memoization | c-recursion-memoization-by-the_expandabl-1g4u | class Solution {\npublic: \n int dp[1001][1001];\n int solve(vector<int>&a,int i,int j,int sum)\n {\n //base case\n if(i>j)return 0;\n | the_expandable | NORMAL | 2021-04-04T04:24:39.463206+00:00 | 2021-04-04T04:24:39.463234+00:00 | 153 | false | ```class Solution {\npublic: \n int dp[1001][1001];\n int solve(vector<int>&a,int i,int j,int sum)\n {\n //base case\n if(i>j)return 0;\n if(dp[i][j]!=-1)return dp[i][j];\n return dp[i][j]= max(sum-a[i]-solve(a,i+1,j,sum-a[i]) , sum - a[j] - solve(a,i,j-1,sum-a[j]));\n return dp[i][j];\n }\n int stoneGameVII(vector<int>& a) {\n int n=a.size();\n // int lsum[n] , rsum[n];\n int sum = 0 ;\n for(int i=0;i<n;i++)\n sum += a[i];\n memset(dp,-1,sizeof(dp));\n return solve(a,0,n-1,sum);\n }\n};``` | 1 | 1 | [] | 0 |
stone-game-vii | Recurring DP Solution with memoization | recurring-dp-solution-with-memoization-b-i22d | \nclass Solution {\npublic:\n \n int dp[1001][1001];\n \n int recur(vector<int>& stones, int l, int r, int sum)\n {\n \n if(l==r) r | alone18 | NORMAL | 2021-03-15T19:27:36.011287+00:00 | 2021-03-15T19:27:36.011331+00:00 | 126 | false | ```\nclass Solution {\npublic:\n \n int dp[1001][1001];\n \n int recur(vector<int>& stones, int l, int r, int sum)\n {\n \n if(l==r) return 0;\n \n if(dp[l][r]!=-1) return dp[l][r];\n \n dp[l][r] = max(abs(sum-stones[l] - recur(stones,l+1,r,sum-stones[l])),\n abs(sum-stones[r] - recur(stones,l,r-1,sum-stones[r])));\n \n return dp[l][r];\n \n }\n \n int stoneGameVII(vector<int>& stones) {\n \n memset(dp,-1,sizeof(dp));\n \n int sum=0;\n \n for(auto xx:stones) sum+=xx;\n \n return recur(stones,0,stones.size()-1,sum);\n \n }\n};\n``` | 1 | 0 | [] | 1 |
stone-game-vii | Digest of DP solution | digest-of-dp-solution-by-puru_cat-ir57 | Conclusion\nStrategy of Alice and Bob is the same, so they can share the same DP.\n\n## Definition\ndp[i][j] := max diff of score of A if stones[i:j] can be cho | puru_cat | NORMAL | 2021-01-02T02:21:17.967300+00:00 | 2021-01-02T02:22:54.678389+00:00 | 168 | false | ## Conclusion\nStrategy of Alice and Bob is the same, so they can share the same DP.\n\n## Definition\ndp[i][j] := max diff of score of A if stones[i:j] can be chose by A firstly.\n\n## Explanation\n\n```\ndp[i][j] = max(Alice_score_[i,j] - Bob_score_[i,j]) // *1 (use later).\n // This is Alice\'s turn,because "Alice want to maximize the difference".\n // dp[i][j] is always non-negative by optimal choose, because all elements of stones is positive.\n= sum[i+1:j] + max(Alice_score_[i+1,j] - Bob_score_[i+1,j]) // Suppose i-th is chosen by Alice.\n= sum[i+1:j] - max(Bob_score_[i+1,j] - Alice_score_[i+1,j])\n= sum[i+1:j] - dp[i+1][j] // By *1. \n// dp[i+1][j] represents Bob\'s turn.\n// Because if Bob maximize dp[i+1][j], then "Bob can minimize the score\'s difference".\n```\n | 1 | 0 | ['Dynamic Programming', 'Memoization'] | 0 |
stone-game-vii | [Java] <O(n^2), O(n)> DP with clean code beats 100% | java-on2-on-dp-with-clean-code-beats-100-jz72 | Even though Bob always loses the game, his goal to minimize the final score difference can be seen as trying to maximize the score difference in his turns i.e. | shk10 | NORMAL | 2020-12-30T22:35:32.524231+00:00 | 2020-12-30T22:35:32.524265+00:00 | 195 | false | Even though Bob always loses the game, his goal to minimize the final score difference can be seen as trying to maximize the score difference in his turns i.e. score(B) - score(A). Alice\'s goal is also the same, maximize score difference in his turns i.e. score(A) - score(B).\n\nLet ```dp[i][j] = maximum value of score(current player) - score(other player) using stones[i..j]```.\n\n- If current player removes ```stones[i]```:\n\t- ```score(current player)``` increases by ```sum of stones[i+1..j]``` thereby also increasing the score difference by same amount.\n\t- game continues on ```stones[i+1..j]``` where other player becomes the current player so ```dp[i+1][j]``` represents the score difference from other player\'s point of view so to use it in our recurrence relation, we need to negate it.\n- Similar reasoning applies to removing ```stones[j]```\n\nSo, ```dp[i][j] = max(sum of stones[i+1..j] - dp[i+1][j], sum of stones[i..j-1] - dp[i][j-1])```\n\nSums of stones in a range can be computed faster using prefix sums or on the fly if you\'re clever ;)\n\nSo, ```dp[i][j] = max(sum[i][j] - stones[i] - dp[i+1][j], sum[i][j] - stones[j] - dp[i][j-1])```\n\n```\n// 35 ms. 96.66%\npublic int stoneGameVII(int[] stones) {\n int n = stones.length;\n int[][] dp = new int[n][n];\n for(int i = n - 1; i >= 0; i--) {\n for(int j = i + 1, sum = stones[i]; j < n; j++) {\n sum += stones[j];\n dp[i][j] = Math.max(sum - stones[i] - dp[i + 1][j], sum - stones[j] - dp[i][j - 1]);\n }\n }\n return dp[0][n - 1];\n}\n```\n\nTime complexity: O(n^2)\nSpace complexity: O(n^2)\n\nSince answer for R range dp values (R = j - i + 1) depends only on R-1 range dp values, we can reduce space complexity by only keeping last range values.\n\n```\n// 16 ms. 100%\npublic int stoneGameVII(int[] stones) {\n int n = stones.length;\n int[] dp = new int[n];\n for(int i = n - 1; i >= 0; i--) {\n for(int j = i + 1, sum = stones[i]; j < n; j++) {\n sum += stones[j];\n dp[j] = Math.max(sum - stones[i] - dp[j], sum - stones[j] - dp[j - 1]);\n }\n }\n return dp[n - 1];\n}\n```\n\nTime complexity: O(n^2)\nSpace complexity: O(n) | 1 | 0 | ['Java'] | 0 |
stone-game-vii | java Solution | java-solution-by-solo007-b1nw | \nclass Solution {\n int dp[][];\n public int stoneGameVII(int[] stones) {\n dp=new int[stones.length][stones.length];\n for (int arr[]:dp)\ | solo007 | NORMAL | 2020-12-25T12:17:48.408515+00:00 | 2020-12-25T12:17:48.408554+00:00 | 76 | false | ```\nclass Solution {\n int dp[][];\n public int stoneGameVII(int[] stones) {\n dp=new int[stones.length][stones.length];\n for (int arr[]:dp)\n {\n Arrays.fill(arr,-1);\n }\n int prefix[]=new int[stones.length];\n prefix[0]=stones[0];\n int n=stones.length;\n for (int i=1;i<n;i++)\n {\n prefix[i]=prefix[i-1]+stones[i];\n }\n return helper(stones,0,stones.length-1,prefix);\n\n }\n int helper(int arr[],int i,int j,int prefix[])\n {\n if (i>=j)\n {\n return 0;\n }\n else\n {\n if (dp[i][j]!=-1)\n {\n return dp[i][j];\n }\n int res1=prefix[j]-prefix[i]-helper(arr,i+1,j,prefix);\n int res2=prefix[j-1]-(((i-1>=0))?prefix[i-1]:0)-helper(arr,i,j-1,prefix);\n return dp[i][j]=Math.max(res1,res2);\n }\n }\n}\n``` | 1 | 0 | [] | 0 |
stone-game-vii | Most detailed explanation in O(n*n) | most-detailed-explanation-in-onn-by-user-kgen | A very similar problem arrived at "atcoder educational dp contest" named deque.\n\nBoth the problems as just a small changeand things will work fine. Please ref | user1637u | NORMAL | 2020-12-25T08:05:43.656459+00:00 | 2020-12-25T08:06:41.038597+00:00 | 287 | false | A very similar problem arrived at **"atcoder educational dp contest"** named deque.\n\nBoth the problems as just a small changeand things will work fine. Please refer this problem **ATcoder L Deque**.\n\nIt\'s full detailed solution in on [CP-central App](https://play.google.com/store/apps/details?id=com.central.cp&hl=en_US&gl=US://) (along with other atcoder dp contest editorials).\n\n```\nint F(int L, int R,int player) {\n //..base case when there is only one element\n if (L == R) {\n return 0; //..nothing to add to the score of current player\n }\n\n //..if value already exists return directly\n if (dp[L][R][player] != -1) return dp[L][R][player];\n\n\n int val = 0;\n if(player == 0){ //..if it\'s player 0\'s turn\n int sum = pre[R] -((L == 0) ? 0 :pre[L-1]);\n int left = F(L+1,R,1) + (sum - a[L]); <----------\n int right = F(L,R-1,1) + (sum - a[R]); <----------\n val = Math.max(left, right);\n }\n else{\n int sum = pre[R] - ((L == 0) ? 0 :pre[L-1]);\n int left = F(L+1,R,0) - (sum - a[L]); <----------\n int right = F(L,R-1,0) - (sum - a[R]); <----------\n val = Math.min(left, right);\n }\n\n dp[L][R][player] = val;\n return val;\n }\n```\n\n# The only difference is we add the stone\'s value to player\'s score in Atcoder problem where as in this problem we add the sum of rest of the stones excluding the one he selected\n | 1 | 0 | [] | 1 |
reverse-degree-of-a-string | Beats 98% | SImple to understand | beats-98-simple-to-understand-by-pariksh-aigi | Code | parikshit_ | NORMAL | 2025-03-29T17:18:32.802727+00:00 | 2025-03-29T17:18:32.802727+00:00 | 124 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
lookup = {}
j = 71 #diff between ascii of "a" to get 26
for c in range(97, 123, 1):
lookup[chr(c)] = 97 - j
j += 1
sm = 0
for i in range(len(s)):
sm += lookup[s[i]] * (i + 1)
return sm
``` | 4 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Iterate and sum | iterate-and-sum-by-kreakemp-6rsd | Code
Here is an article of my last interview experience - A Journey to FAANG Company, I recomand you to go through this to know which all resources I have used | kreakEmp | NORMAL | 2025-03-29T16:33:47.723442+00:00 | 2025-03-29T16:33:47.723442+00:00 | 741 | false |
# Code
```cpp []
int reverseDegree(string s) {
int ans = 0;
for(int i = 0; i < s.size(); ++i) ans += (26 - (s[i] - 'a'))*(i+1);
return ans;
}
```
```Java []
int reverseDegree(String s) {
int ans = 0;
for(int i = 0; i < s.length(); ++i) ans += (26 - (s.charAt(i) - 'a'))*(i+1);
return ans;
}
```
---
<span style="color:green">
<b>Here is an article of my last interview experience - A Journey to FAANG Company, I recomand you to go through this to know which all resources I have used & how I cracked interview at Amazon: </b> </span>
https://leetcode.com/discuss/interview-experience/3171859/Journey-to-a-FAANG-Company-Amazon-or-SDE2-(L5)-or-Bangalore-or-Oct-2022-Accepted
---
| 4 | 0 | ['C++', 'Java'] | 0 |
reverse-degree-of-a-string | 100% Beats || One line of Code | 100-beats-one-line-of-code-by-kdhakal-chxn | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | kdhakal | NORMAL | 2025-04-09T13:29:00.106596+00:00 | 2025-04-09T13:29:00.106596+00:00 | 95 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for(int i = 0; i < s.length(); i++) {
sum += (i + 1) * (97 + 26 - (int) s.charAt(i));
}
return sum;
}
}
``` | 3 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Python Elegant & Short | Enumeration | python-elegant-short-enumeration-by-kyry-vsjd | Complexity
Time complexity: O(n)
Space complexity: O(1)
Code | Kyrylo-Ktl | NORMAL | 2025-03-31T06:35:35.613702+00:00 | 2025-03-31T06:35:35.613702+00:00 | 264 | false | # Complexity
- Time complexity: $$O(n)$$
- Space complexity: $$O(1)$$
# Code
```python3 []
class Solution:
def reverseDegree(self, string: str) -> int:
degree = 0
for idx, char in enumerate(string, start=1):
degree += idx * (26 - ord(char) + ord('a'))
return degree
``` | 3 | 0 | ['String', 'Enumeration', 'Python', 'Python3'] | 0 |
reverse-degree-of-a-string | One Linear | one-linear-by-charnavoki-uswl | null | charnavoki | NORMAL | 2025-03-31T06:26:38.660784+00:00 | 2025-03-31T06:26:38.660784+00:00 | 80 | false | ```javascript []
const reverseDegree = s =>
[...s].reduce((s, c, i) => s + (123 - c.charCodeAt()) * (i + 1), 0);
``` | 3 | 0 | ['JavaScript'] | 0 |
reverse-degree-of-a-string | 💢Faster✅💯 Lesser C++✅Python3🐍✅Java✅C✅Python🐍✅C#✅💥🔥💫Explained☠💥🔥 Beats 💯 | faster-lesser-cpython3javacpythoncexplai-b7jo | IntuitionApproach
JavaScript Code --> https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590864475
C++ Code --> https://leetcode.com/problems | Edwards310 | NORMAL | 2025-03-30T08:47:53.144004+00:00 | 2025-03-30T08:47:53.144004+00:00 | 286 | false | 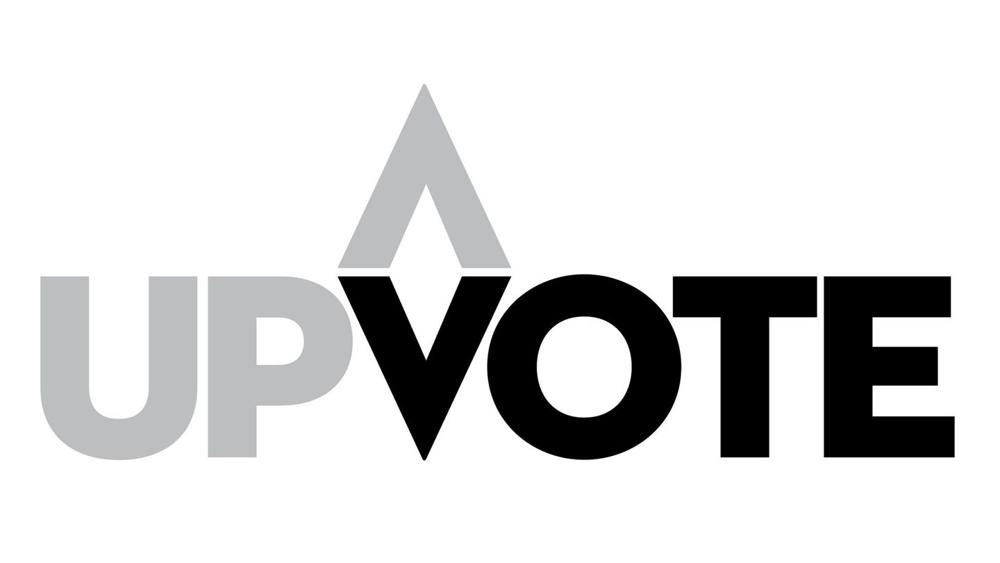
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your first thoughts on how to solve this problem. -->
- ***JavaScript Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590864475
- ***C++ Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590851456
- ***Python3 Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590854477
- ***Java Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590847690
- ***C Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590858122
- ***Python Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590851972
- ***C# Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590860308
- ***Go Code -->*** https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590866438
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(N)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
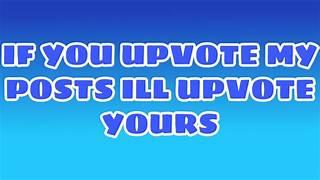 | 3 | 0 | ['Math', 'String', 'C', 'String Matching', 'Python', 'C++', 'Java', 'Go', 'Python3', 'JavaScript'] | 0 |
reverse-degree-of-a-string | Simple, easy || O(n) | simple-easy-on-by-im_obid-cxmv | Code | im_obid | NORMAL | 2025-04-08T16:46:09.312324+00:00 | 2025-04-08T16:46:09.312324+00:00 | 55 | false |
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
int i = 1;
for (char ch : s.toCharArray()) {
sum += i * (26 - (ch - 'a'));
i++;
}
return sum;
}
}
``` | 2 | 0 | ['Java'] | 1 |
reverse-degree-of-a-string | Reverse Degree of a String | Reverse Degree of a String Solution | CPP | reverse-degree-of-a-string-reverse-degre-kw5q | IntuitionThe problem asks us to calculate a custom score for a string where:
Each character’s score is based on its position in the reversed English alphabet.
T | Checkmatecoder | NORMAL | 2025-04-05T09:45:14.007451+00:00 | 2025-04-05T09:45:14.007451+00:00 | 78 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem asks us to calculate a custom score for a string where:
Each character’s score is based on its position in the reversed English alphabet.
That means:
'a' = 26, 'b' = 25, ..., 'z' = 1
Multiply this reversed position with the character's 1-based index in the string and sum all such values for the final result.
So we’re combining two concepts:
Character encoding (a to z) & Positional weight
# Approach
<!-- Describe your approach to solving the problem. -->
1. Initialize a variable total to 0 for storing the final score.
2. Traverse the string character by character using a loop.
3. For each character:
Get its 1-based position in the string: i + 1
Calculate its reversed alphabet index using:
26 - (s[i] - 'a')
4. Multiply the reversed index with the position.
5. Add the result to the total score.
6. After the loop ends, return the final value.
# Complexity
- Time complexity: O(n) where n is the length of the string
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1) constant space.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int total = 0;
for (int i = 0; i < s.length(); ++i) {
int position = i + 1;
int reversePos = 26 - (s[i] - 'a');
total += reversePos * position;
}
return total;
}
};
``` | 2 | 0 | ['String', 'Simulation', 'C++'] | 0 |
reverse-degree-of-a-string | 🔥 Very Easy Detailed Solution || 🎯 JAVA || 🎯 C++ | very-easy-detailed-solution-java-c-by-ch-ve0l | Approach
The given function computes a "reverse degree" for a string, where each character's contribution is calculated based on its reverse position in the al | chaturvedialok44 | NORMAL | 2025-03-30T15:40:41.151931+00:00 | 2025-03-30T15:40:41.151931+00:00 | 167 | false | # Approach
<!-- Describe your approach to solving the problem. -->
1. The given function computes a "reverse degree" for a string, where each character's contribution is calculated based on its reverse position in the alphabet.
2. reversedValue = 26 - (s.charAt(i) - 'a'): This gives the reverse alphabetical position of a character ('a' becomes 26, 'b' becomes 25, ..., 'z' becomes 1).
3. The character's contribution to the final sum is weighted by its 1-based position in the string: sum += reversedValue * position.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
$O(n)$
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
$O(1)$
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
int reversedValue = 26 - (s.charAt(i) - 'a');
int position = i + 1;
sum += reversedValue * position;
}
return sum;
}
}
```
```C++ []
class Solution {
public:
int reverseDegree(string s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
int reversedValue = 26 - (s[i] - 'a');
int position = i + 1;
sum += reversedValue * position;
}
return sum;
}
};
``` | 2 | 0 | ['Math', 'String', 'String Matching', 'Simulation', 'C++', 'Java'] | 2 |
reverse-degree-of-a-string | Easy to understand | Beginner friendly | easy-to-understand-beginner-friendly-rev-nvdq | IntuitionGet the ascii value of z and for each character in a string ==> substract z_ascii with current char which will give alphabets value in reverse order. M | alishershaesta | NORMAL | 2025-03-29T17:20:37.100205+00:00 | 2025-03-29T17:26:33.214258+00:00 | 166 | false | # Intuition
Get the ascii value of z and for each character in a string ==> substract `z_ascii` with current `char` which will give alphabets value in reverse order. Multiply this value with current index and store in result.
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
i = 1
result = 0
z_ascii = ord('z')
for c in s:
result += (z_ascii - ord(c) + 1) * i
i += 1
return result
``` | 2 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | EASIEST SOLUTION | BEGINNER FRIENDLY | 0ms Runtime | Beats 100 % 🥷🏻🗡️☄️✨ | easiest-solution-beginner-friendly-0ms-r-wqb2 | IntuitionApproachComplexity
Time complexity: O(n);
Space complexity:O(1)
Code | tyagideepti9 | NORMAL | 2025-03-29T16:54:07.214794+00:00 | 2025-03-29T16:54:07.214794+00:00 | 140 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(n);
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```csharp []
public class Solution {
public int ReverseDegree(string s) {
int ans = 0;
int i=1;
foreach(char ch in s){
ans += Math.Abs( (ch-'a')-26) * i;
i++;
}
return ans;
}
}
``` | 2 | 0 | ['Java', 'C#', 'MS SQL Server'] | 0 |
reverse-degree-of-a-string | easiest solution with explanation | easiest-solution-with-explanation-by-par-ip10 | IntuitionThis function calculates a custom value for a string s by iterating through each character and computing a weighted sum. The weight for each character | paragbansal120 | NORMAL | 2025-04-08T18:02:47.361709+00:00 | 2025-04-08T18:02:47.361709+00:00 | 26 | false | # Intuition
This function calculates a custom value for a string s by iterating through each character and computing a weighted sum. The weight for each character is determined by its reversed alphabetical position ('a' being 26, 'b' being 25, and so on) and a sequential multiplier k. The result aggregates these weighted values.
The main intuition is to compute a score based on reversing the alphabetical order of characters and applying an increasing positional weight.
# Approach
Initialization: Start with a multiplier k initialized to 1.
Initialize ans to 0, which will store the final computed result.
Iterate Through the String: Convert the string into a character array using toCharArray().
For each character ch: Calculate its reversed alphabetical value using the expression (26 - (ch - 'a')).
Multiply this value by the current multiplier k and add it to ans.
Increment the multiplier k for the next character.
Return the Result: Once the iteration is complete, return the calculated value stored in ans.
# Complexity
- Time Complexity: $$O(n)$$, where n is the length of the string. This is because each character is processed exactly once in a single loop.
- Space Complexity: $$O(1)$$, as the computation only uses a few integer variables and no additional data structures.
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int k=1;
int ans=0;
for(char ch : s.toCharArray())
{
ans+=(26-(ch-'a'))*k++;
}
return ans;
}
}
``` | 1 | 0 | ['String', 'Simulation', 'Java'] | 0 |
reverse-degree-of-a-string | Simple code - Try this! | simple-code-try-this-by-sairangineeni-3816 | IntuitionWe are asked to compute a weighted sum where each character's weight is determined by its position in the reversed alphabet and its index in the origin | Sairangineeni | NORMAL | 2025-04-08T01:08:08.017360+00:00 | 2025-04-08T01:08:08.017360+00:00 | 30 | false | # Intuition
We are asked to compute a weighted sum where each character's weight is determined by its position in the reversed alphabet and its index in the original string.
Since the weights depend on both the character and its position, we can either compute them directly or reverse the string and adjust positions accordingly.
---
# Approach
Reverse the string using StringBuilder to match the order described in the problem.
For each character in the reversed string:
Compute its reversed alphabetical value: 'z' - ch + 1.
Compute its original position in the input string: s.length() - i.
Multiply both and add to the running total.
Return the total sum.
# Code
```java []
class Solution {
public static int reverseDegree(String s) {
StringBuilder sb = new StringBuilder(s);
sb.reverse();
int sum = 0;
for (int i = 0; i < sb.length(); i++) {
int val = sb.charAt(i);
int reverse = 'z'-val+1;
int position = s.length() - i;
sum += reverse * position;
}
return sum;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | 3 Line Solution || 100% Beats 🚀 | 3-line-solution-100-beats-by-aryaman123-zkf7 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | aryaman123 | NORMAL | 2025-04-06T15:45:00.148990+00:00 | 2025-04-06T15:45:00.148990+00:00 | 66 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for(int i = 0; i<s.length() ; i++){
sum+= (26-((int)(s.charAt(i)-'a')))*(i+1);
}
return sum;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | 0ms 100% O(N) | 0ms-100-on-by-michelusa-jbks | Parse the string, applying the reverse degree formula.Time complexity: O(N)
Space complexity: O(1)Code | michelusa | NORMAL | 2025-04-04T10:37:14.505615+00:00 | 2025-04-04T10:37:14.505615+00:00 | 36 | false |
Parse the string, applying the reverse degree formula.
Time complexity: O(N)
Space complexity: O(1)
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int reverse_degree = 0;
const int n = s.size();
for (int i = 0; i < n; ++i) {
reverse_degree += (i + 1) * ('z' - s[i] + 1);
}
return reverse_degree;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | 🚀 Fast C++ Solution (Beats 100%!) ⚡ | fast-c-solution-beats-100-by-sohamkhanna-yduj | IntuitionThe problem requires us to compute a specific numerical value based on the given string. Observing the formula used in the implementation, we can infer | sohamkhanna | NORMAL | 2025-04-02T15:12:21.796675+00:00 | 2025-04-02T15:12:21.796675+00:00 | 41 | false | # Intuition
The problem requires us to compute a specific numerical value based on the given string. Observing the formula used in the implementation, we can infer that it processes each character of the string by using its ASCII value and a weighted position. The goal is to generate a unique numeric representation for a given string.
# Approach
1. Initialize a variable `count` to store the computed result.
2. Iterate over each character in the string `s`, considering its index `i`.
3. For each character `s[i]`, calculate `(i + 1) * (123 - s[i])`, where:
- `i + 1` gives a 1-based index weight.
- `123 - s[i]` computes a transformation of the ASCII value.
4. Sum these computed values into `count`.
5. Return `count` as the final result.
# Complexity
- **Time Complexity:** $$O(n)$$, where $$n$$ is the length of the string. The algorithm iterates through the string once, performing constant-time operations per character.
- **Space Complexity:** $$O(1)$$, as only a single integer variable (`count`) is used for computation, independent of the input size.
# Code
```cpp
class Solution {
public:
int reverseDegree(string s) {
int count = 0;
for(int i = 0; i < s.size(); i++) {
count += (i + 1) * (123 - s[i]);
}
return count;
}
};
| 1 | 0 | ['String', 'Simulation', 'C++'] | 0 |
reverse-degree-of-a-string | Beginner-Friendly Python Solution | Reverse Alphabet Weighted Sum | beginner-friendly-python-solution-revers-q66q | IntuitionThe problem involves computing a "reverse degree" for a given string. The idea is to map each character to its "reverse position" in the alphabet (i.e. | NRSingh | NORMAL | 2025-04-02T04:19:53.827587+00:00 | 2025-04-02T04:19:53.827587+00:00 | 39 | false | # Intuition
The problem involves computing a "reverse degree" for a given string. The idea is to map each character to its "reverse position" in the alphabet (i.e., 'a' → 26, 'b' → 25, ..., 'z' → 1) and then multiply it by its position in the string.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
1. Convert the string into a list of characters.
2. Iterate through each character in the string:
* Compute its reverse position in the alphabet using:
reverse=26−(ASCII value of character−ASCII value of ’a’)
* Multiply this value by its (1-based) index in the string.
* Accumulate the result in total.
3. Return the final computed total.
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
O(n), since the loop iterates through the length of s once.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
O(1), as no extra data structures (except for a few variables) are used.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
total = 0
for i in range(len(s)):
reverse = 26-(ord(s[i])-ord("a"))
total+=reverse*(i+1)
return total
``` | 1 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | ☑️ Reversing Degree of a String.☑️ | reversing-degree-of-a-string-by-abdusalo-i78b | Code | Abdusalom_16 | NORMAL | 2025-03-30T17:47:59.350206+00:00 | 2025-03-30T17:47:59.350206+00:00 | 13 | false | # Code
```dart []
class Solution {
int reverseDegree(String s) {
int sum = 0;
List<String> lowercaseLetters = [
'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o',
'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z'
];
for(int i = 0; i < s.length; i++){
sum += (lowercaseLetters.indexOf(s[i])-26).abs() * (i+1);
}
return sum;
}
}
``` | 1 | 0 | ['Dart'] | 0 |
reverse-degree-of-a-string | <<BEAT 100% SOLUTIONS>> | beat-100-solutions-by-dakshesh_vyas123-oczl | PLEASE UPVOTE MECode | Dakshesh_vyas123 | NORMAL | 2025-03-30T17:34:59.708512+00:00 | 2025-03-30T17:34:59.708512+00:00 | 23 | false | # PLEASE UPVOTE ME
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int ans=0;
for(int i=0;i<s.size();i++){
int a=(int)s[i];
ans+=((i+1)*(26-(a-97)));}
return ans;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | Simple and Concise Pythonic Solution | simple-and-concise-pythonic-solution-by-5f67i | IntuitionSimple iteration through the input string, manipulate the index (of iteration) and value of the chars to compute the reverse degree.ApproachMake use of | vyomthakkar | NORMAL | 2025-03-30T13:34:10.592122+00:00 | 2025-03-30T13:34:10.592122+00:00 | 22 | false | # Intuition
Simple iteration through the input string, manipulate the index (of iteration) and value of the chars to compute the reverse degree.
# Approach
Make use of the ord function provided by python to convert current char to numeric form and then find offset/distance from the numeric form of "z" and multiply that with index of iteration to obtain reverse degree.
# Complexity
- Time complexity:
O(n)
- Space complexity:
O(1)
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
reverse_degree = 0
for i,c in enumerate(s):
reverse_idx = (ord("z") - ord(c))+1
reverse_degree += ((i+1)*reverse_idx)
return reverse_degree
``` | 1 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Nice and Easy | O(1) space Complexity | good solution | beats 100% | nice-and-easy-o1-space-complexity-good-s-ao2w | Intuition-71 for reaching 25 then -((ch-91) * 2) for reversing the indexing
just use pen and papperComplexity
Time complexity:
O(n)
Space complexity:
O(1)
C | hirensolanki9697 | NORMAL | 2025-03-30T05:05:45.861035+00:00 | 2025-03-30T05:05:45.861035+00:00 | 28 | false | # Intuition
-71 for reaching 25 then -((ch-91) * 2) for reversing the indexing
just use pen and papper
# Complexity
- Time complexity:
$$O(n)$$
- Space complexity:
$$O(1)$$
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int n = s.length();
int degree = 0;
for(int i=0;i<n;i++){
char ch = s.charAt(i);
int val = ch - 71-( (ch-97) * 2 );
degree = degree + (i+1)*val;
}
return degree;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Fairly Easy Hashmap Solution (Python 3) | fairly-easy-hashmap-solution-python-3-by-vhbl | IntuitionI saw that we were assigning values to char's, and then if you read the constraints we only need to worry about lowercase. So we don't need to do some | tommyfeeley | NORMAL | 2025-03-30T04:22:40.658583+00:00 | 2025-03-30T04:22:40.658583+00:00 | 8 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
I saw that we were assigning values to char's, and then if you read the constraints we only need to worry about lowercase. So we don't need to do some weird .lower() stuff in our logic somewhere. To me it seemed most obvious to just make a hashmap then, and assign the values(keys) the problem tells you. So 26 for A, 25 for B, ... 1 for Z.
Then we've just gotta somehow add the value from the keys in our hash map, and after re reading I only noticed how we have to multiply by the index. And the 1-index thing just means we're starting with index=1, where it would normally be index=0. So we'll have to do a little something to accomodate that but nothing crazy.
# Approach
<!-- Describe your approach to solving the problem. -->
So we just make our hashmap which is pretty annoying to setup but also makes it really obvious what you're doing at least.
Then we create an int to add our values multiplied by our index to for our answer. So we just want this set to 0 so we can += to it easily.
Then the weirdest part probably is we need to define our index, outside of the loop, which feels kind of wrong in python lol. And since we're 1-indexed as it told us, we just set the index to 1 — i=1
Now we loop through our string for each character in it. For each character, we add to total: the value associated with our key (char from string) multiplied by the index where we found that key.
Add one to the index count each time we go through a character from the string to keep index properly updated. Since we're using it to get the value we're adding to the total, everything will be busted otherwise.
Finally we can return the total, which is returned after multiplying the key's value by the key's index, for every character/key in the string.
# Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
hashy = {'a': 26, 'b':25, 'c': 24,'d': 23, 'e': 22, 'f':21, 'g':20 , 'h':19 ,'i':18 ,'j':17 ,'k':16 ,'l':15 ,'m':14 ,'n':13 ,'o':12 ,'p':11 ,'q':10 ,'r':9 ,'s':8 ,'t':7 ,'u': 6,'v': 5,'w': 4, 'x': 3, 'y': 2, 'z': 1}
total = 0
i = 1
for x in s:
total += hashy[x] * i
i+= 1
return total
``` | 1 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | 🔄🔢Reverse Weighted Alphabet Sum Calculation | Simulating |Optimal | Greedy Approach 🚀 | reverse-weighted-alphabet-sum-calculatio-yuvm | IntuitionThe problem involves computing a specific "reverse degree" for a given string, where each character's contribution is based on its position and its dis | franesh | NORMAL | 2025-03-30T02:01:02.752082+00:00 | 2025-03-30T02:01:02.752082+00:00 | 21 | false | ### **Intuition**
The problem involves computing a specific "reverse degree" for a given string, where each character's contribution is based on its position and its distance from the letter 'a'.
### **Approach**
1. We iterate through each character of the string.
2. For each character `s[i]`, calculate its **reverse distance** from 'a':
\[
26 - (s[i] - 'a')
\]
- This formula ensures that 'a' contributes 26, 'b' contributes 25, ..., 'z' contributes 1.
3. Multiply this value by its **1-based position** in the string (`i + 1`).
4. Accumulate the values in a `count` variable.
5. Return `count` as the final result.
---
### **Complexity Analysis**
- **Time Complexity:** \(O(n)\)
- We traverse the string once, performing constant-time operations at each step.
- **Space Complexity:** \(O(1)\)
- Only a few integer variables are used; no extra space is required.
---
### **Example Walkthrough**
#### **Example 1**
**Input:** `"abc"`
**Steps:**
- 'a': \((26 - (0)) \times 1 = 26\)
- 'b': \((26 - (1)) \times 2 = 50\)
- 'c': \((26 - (2)) \times 3 = 72\)
**Total:** \(26 + 50 + 72 = 148\)
**Output:** `148`
---
### **Code Explanation**
```cpp
class Solution {
public:
int reverseDegree(string s) {
int count = 0;
int n = s.size();
for (int i = 0; i < n; i++) {
count += (26 - (s[i] - 'a')) * (i + 1);
}
return count;
}
};
```
**Why This Works:**
- `s[i] - 'a'` gives the zero-based index of the character in the alphabet.
- `26 - (s[i] - 'a')` gives the reverse distance.
- Multiplying by `(i + 1)` incorporates the positional weight.
# Upvote For the Effort🚀 | 1 | 0 | ['Math', 'String', 'Greedy', 'String Matching', 'Simulation', 'Counting', 'C++'] | 0 |
reverse-degree-of-a-string | Time O(n) space O(1) 100% beat | time-on-space-o1-100-beat-by-vishal1002-gjz5 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | vishal1002 | NORMAL | 2025-03-29T22:21:22.118599+00:00 | 2025-03-29T22:21:22.118599+00:00 | 12 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int ans=0;
for(int i=0;i<s.size();i++){
ans+=(i+1)*('a'+26-s[i]);
}
return ans;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | BEAT 100% | beat-100-by-runningfalcon-zpfu | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | runningfalcon | NORMAL | 2025-03-29T21:27:57.109308+00:00 | 2025-03-29T21:27:57.109308+00:00 | 27 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int ans = 0;
for (int i = 0; i < s.length(); i++) {
char x = s.charAt(i);
int val = 'z' - x + 1;
//System.out.println(val);
ans += val * (i + 1);
}
return ans;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | c#, Linq | c-linq-by-bytchenko-g6uw | IntuitionWe can compute the required value explicitly.ApproachLINQ queryComplexity
Time complexity:
O(n)
Space complexity:
O(1)Code | bytchenko | NORMAL | 2025-03-29T20:50:06.797087+00:00 | 2025-03-29T20:50:06.797087+00:00 | 5 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
We can compute the required value explicitly.
# Approach
<!-- Describe your approach to solving the problem. -->
LINQ query
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
$$O(n)$$
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
$$O(1)$$
# Code
```csharp []
public class Solution {
public int ReverseDegree(string s) => s
.Select((c, i) => (i + 1) * ('z' - c + 1))
.Sum();
}
``` | 1 | 0 | ['C#'] | 0 |
reverse-degree-of-a-string | EASY C++ 100% O(N) | easy-c-100-on-by-hnmali-b8bs | Code | hnmali | NORMAL | 2025-03-29T18:39:15.361387+00:00 | 2025-03-29T18:39:15.361387+00:00 | 55 | false | # Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int sum = 0;
for(int i = 0; i < s.size(); i++)
sum += (26 - s[i] + 'a') * (i+1);
return sum;
}
};
``` | 1 | 0 | ['Math', 'String', 'C++'] | 0 |
reverse-degree-of-a-string | Simple C++ Solution | Strings | O(n) | simple-c-solution-strings-on-by-ipriyans-achc | IntuitionComplexity
Time complexity:
O(n)
Space complexity:
O(1)
Code | ipriyanshi | NORMAL | 2025-03-29T17:18:42.037199+00:00 | 2025-03-29T17:18:55.748718+00:00 | 49 | false | # Intuition
https://youtu.be/eY5WeKfq3js
# Complexity
- Time complexity:
$$O(n)$$
- Space complexity:
$$O(1)$$
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int n = s.length();
int result = 0;
for(int i = 0; i < n; i++){
result += ('z' - s[i] + 1) * (i+1);
}
return result;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | AAAAAAAAAAAAAAAAAAAAAA | aaaaaaaaaaaaaaaaaaaaaa-by-danisdeveloper-xaju | Code | DanisDeveloper | NORMAL | 2025-03-29T17:16:26.912399+00:00 | 2025-03-29T17:16:26.912399+00:00 | 17 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
res = 0
for i, c in enumerate(s):
res += (26 - ord(c) + ord('a')) * (i + 1)
return res
``` | 1 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Reverse abc | beats 100% | easy to understand | python3 | unique solution 🥷🏼🤺🍻 | reverse-abc-beats-100-easy-to-understand-mzmb | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | aman-sagar | NORMAL | 2025-03-29T16:59:00.327762+00:00 | 2025-03-29T16:59:00.327762+00:00 | 60 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
d = {x: i+1 for i,x in enumerate("zyxwvutsrqponmlkjihgfedcba")}
sum_ = 0
for i in range(len(s)):
sum_ += d[s[i]]*(i+1)
return sum_
``` | 1 | 0 | ['Python3'] | 2 |
reverse-degree-of-a-string | cpp 🔥 | cpp-by-varuntyagig-fjne | Code | varuntyagig | NORMAL | 2025-03-29T16:53:02.287254+00:00 | 2025-03-29T16:53:02.287254+00:00 | 15 | false | # Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
string str = "zyxwvutsrqponmlkjihgfedcba";
int reverseDegree = 0;
for (int i = 0; i < s.length(); ++i) {
int x = str.find(s[i]) + 1, y = (i + 1);
reverseDegree += (x * y);
}
return reverseDegree;
}
};
``` | 1 | 0 | ['String', 'C++'] | 0 |
reverse-degree-of-a-string | Java Solution for problem 1 | java-solution-for-problem-1-by-mukundan_-rkoh | IntuitionApproachComplexity
Time complexity:O(N)
Space complexity:O(1)
Code | Mukundan_13 | NORMAL | 2025-03-29T16:47:24.493145+00:00 | 2025-03-29T16:47:24.493145+00:00 | 35 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum=0,prod=1;
for(int i=0;i<s.length();i++)
{
prod=26-(s.charAt(i)-'a');
sum+=prod*(i+1);
}
return sum;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Python3 || iteration || T/S: 99% / 90% | python3-iteration-ts-99-90-by-spaulding-t2tb | https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590244511/I could be wrong, but I think that time complexity is O(N) and space complexity | Spaulding_ | NORMAL | 2025-03-29T16:31:46.937148+00:00 | 2025-03-29T16:38:34.508562+00:00 | 24 | false | ```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
ans, idx = 0, 1
for ch in s:
ans+= (123 - ord(ch)) * idx
idx+= 1
return ans
```
```cpp []
class Solution {
public:
int reverseDegree(const std::string& s) {
int ans = 0, idx = 1;
for (char ch : s) {
ans += (123 - static_cast<int>(ch)) * idx;
idx++;}
return ans;}
};
```
```java []
class Solution {
public int reverseDegree(String s) {
int ans = 0, idx = 1;
for (char ch : s.toCharArray()) {
ans += (123 - (int) ch) * idx;
idx++;}
return ans;}
}
```
[https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590244511/](https://leetcode.com/problems/reverse-degree-of-a-string/submissions/1590244511/)
I could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(1), in which *N* ~ `len(s)`. | 1 | 0 | ['C++', 'Java', 'Python3'] | 0 |
reverse-degree-of-a-string | Almost a one liner || C++ || beats 100%🚀💯 | almost-a-one-liner-c-beats-100-by-codewi-l86l | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | CodeWithMithun | NORMAL | 2025-03-29T16:18:49.342670+00:00 | 2025-03-29T16:20:51.532353+00:00 | 43 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(n)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int n=s.length(),sum=0;
for(int i=0;i<n;i++){
// index number * reverse ascii of the character
sum += (i+1)*(26 - (s[i]-'a'));
}
return sum;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | BEST OPTIMAL SOLUTION✅✅ | best-optimal-solution-by-gaurav_sk-l540 | Complexity
Time complexity: O(N)
Space complexity: O(1)
Code | Gaurav_SK | NORMAL | 2025-03-29T16:09:46.702306+00:00 | 2025-03-29T16:09:46.702306+00:00 | 27 | false |
# Complexity
- Time complexity: O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int getRevAlpha(char ch){
ch = tolower(ch);
return (ch >= 'a' && ch <= 'z')? (26 - (ch - 'a')) : 0;
}
int reverseDegree(string s) {
int sum = 0;
for (int i=0; i<s.length(); i++){
int revPos = getRevAlpha(s[i]);
int indexString = i + 1;
sum += revPos * indexString;
}
return sum;
}
};
``` | 1 | 0 | ['String', 'String Matching', 'Enumeration', 'C++'] | 0 |
reverse-degree-of-a-string | ||✅ Easiest Solution ✅ || | easiest-solution-by-coding_with_star-xn6c | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Coding_With_Star | NORMAL | 2025-03-29T16:08:22.587695+00:00 | 2025-03-29T16:08:22.587695+00:00 | 20 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int ans = 0;
for (int i = 0; i < s.length(); i++) {
int revIndex = 26 - (s[i] - 'a'); // Get reversed alphabet position
ans += revIndex * (i + 1); // Multiply with 1-based index
}
return ans;
}
};
``` | 1 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | BRITE FORCE EASY || JAVA | brite-force-easy-java-by-akshay_kadamm-ljea | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Akshay_Kadamm | NORMAL | 2025-03-29T16:06:12.609879+00:00 | 2025-03-29T16:06:12.609879+00:00 | 34 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int ans=0;
for(int i=0;i<s.length();i++){
int c= s.charAt(i)-'a';
ans+= (26- c)*(i+1);
}
return ans;
}
}
``` | 1 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | 1 ms Beats 100%!! simple easy java code | 1-ms-beats-100-simple-easy-java-code-by-7kqpi | IntuitionApproachComplexity
Time complexity: O(n)
Space complexity: O(1)
Code | Md_Ziyad_Hussain | NORMAL | 2025-03-29T16:03:57.511133+00:00 | 2025-03-29T16:03:57.511133+00:00 | 28 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: $$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int ans = 0;
for(int i = 0; i < s.length(); i++){
char c = s.charAt(i);
ans += (26 - (c - 'a')) * (i + 1);
}
return ans;
}
}
``` | 1 | 0 | ['String', 'Java'] | 0 |
reverse-degree-of-a-string | Simple and Easily Understandable with 0(1) Space Complexity | simple-and-easily-understandable-sol-by-ey7w5 | IntuitionWith ASCII ValuesApproachComplexity
Time complexity:O(n)
Space complexity:O(1)
Code | varun_cns | NORMAL | 2025-03-29T16:02:13.528831+00:00 | 2025-03-29T16:03:58.526810+00:00 | 10 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
With ASCII Values
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int n = s.length();
int sum = 0;
for (int i = 0; i < n; i++) {
char ch = s.charAt(i);
int ascii = ch;
sum+= (123-ch)*(i+1);
}
return sum;
}
}
``` | 1 | 0 | ['Math', 'String', 'Java'] | 0 |
reverse-degree-of-a-string | Easy python solution | easy-python-solution-by-vigneshkanna108-09yf | Code | vigneshkanna108 | NORMAL | 2025-03-29T16:02:11.372452+00:00 | 2025-03-29T16:02:11.372452+00:00 | 36 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
res = 0
for i in range(len(s)):
char = s[i]
pos = 26 - (ord(char) - ord('a'))
res += ((i+1)*pos)
return res
``` | 1 | 0 | ['Simulation', 'Python3'] | 0 |
reverse-degree-of-a-string | Reverse Degree String | reverse-degree-string-by-nimi_sharma-gctx | IntuitionThe goal is to calculate a weighted score for a string where each character is given a "reverse alphabetical value" (i.e., 'a' = 26, 'b' = 25, ..., 'z' | nimi_sharma | NORMAL | 2025-04-12T09:20:09.705736+00:00 | 2025-04-12T09:20:09.705736+00:00 | 1 | false | # Intuition
The goal is to calculate a weighted score for a string where each character is given a "reverse alphabetical value" (i.e., 'a' = 26, 'b' = 25, ..., 'z' = 1), and that value is multiplied by its 1-based position in the string. This creates a kind of "reverse weighted degree" for the string.
# Approach
Iterate through the string character by character. For each character:
- Convert it into its reverse alphabetical value using: `26 - (ch - 'a')`.
- Multiply this value by the character's 1-based index in the string.
- Accumulate the result in a running sum.
# Complexity
- Time complexity:
O(n)
- Space complexity:
O(1)
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int n = s.length();
int productSum = 0;
int i = 1;
for(char ch:s)
{
productSum += (i*(26-(ch-'a')));
i++;
}
return productSum;
}
};
``` | 0 | 0 | ['String', 'C', 'Simulation', 'C++', 'Java'] | 0 |
reverse-degree-of-a-string | Very Easy Understandable sol || 3 line code | very-easy-understandable-sol-3-line-code-y90z | Complexity
Time complexity: O(n)
Space complexity: O(1)
Code | sumo25 | NORMAL | 2025-04-12T05:25:32.205005+00:00 | 2025-04-12T05:25:32.205005+00:00 | 2 | false |
# Complexity
- Time complexity: $$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum=0,i=0;
for(char ch:s.toCharArray()){
sum+=((26-(ch-'a'))*++i);
}
return sum;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | My first solution and also one same with optimized approach using ASCII characters. | my-first-solution-and-also-one-same-with-0hbq | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | RChowdary | NORMAL | 2025-04-11T21:29:43.850625+00:00 | 2025-04-11T21:29:43.850625+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for(int i = 0; i < s.length(); i++){
if(s.charAt(i) == 'a'){
sum += 26 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'b'){
sum += 25 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'c'){
sum += 24 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'd'){
sum += 23 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'e'){
sum += 22 *(i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'f'){
sum += 21 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'g'){
sum += 20 * (i+1);
System.out.println(sum);
}
if(s.charAt(i) == 'h')
sum += 19 * (i+1);
if(s.charAt(i) == 'i')
sum += 18 * (i+1);
if(s.charAt(i) == 'j')
sum += 17 * (i+1);
if(s.charAt(i) == 'k')
sum += 16 * (i+1);
if(s.charAt(i) == 'l')
sum += 15 * (i+1);
if(s.charAt(i) == 'm')
sum += 14 * (i+1);
if(s.charAt(i) == 'n')
sum += 13 * (i+1);
if(s.charAt(i) == 'o')
sum += 12 * (i+1);
if(s.charAt(i) == 'p')
sum += 11 * (i+1);
if(s.charAt(i) == 'q')
sum += 10 * (i+1);
if(s.charAt(i) == 'r')
sum += 9 * (i+1);
if(s.charAt(i) == 's')
sum += 8 * (i+1);
if(s.charAt(i) == 't')
sum += 7 * (i+1);
if(s.charAt(i) == 'u')
sum += 6 * (i+1);
if(s.charAt(i) == 'v')
sum += 5 * (i+1);
if(s.charAt(i) == 'w')
sum += 4 * (i+1);
if(s.charAt(i) == 'x')
sum += 3 * (i+1);
if(s.charAt(i) == 'y')
sum += 2 * (i+1);
if(s.charAt(i) == 'z')
sum += 1 * (i+1);
}
return sum;
}
}
##################################
//Optimed One
public int reverseDegree( String s){
int sum = 0;
for( int i = 0; i< s.length(); i++){
sum += (i+1) * (97 + 26 - (int)s.charAt(i));
}
return sum;
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Simple string methods with Ascii using java | simple-string-methods-with-ascii-using-j-ugjx | Code | vijayakumar-1728 | NORMAL | 2025-04-11T16:09:09.284794+00:00 | 2025-04-11T16:09:09.284794+00:00 | 1 | false |
# Code
```java []
class Solution {
public int reverseDegree(String s) {
String s1="zyxwvutsrqponmlkjihgfedcba";
int sum=0,a=0,b=0;
for(int i=0;i<s.length();i++){
a=(int)(s.charAt(i)); // get ASCII
b=s1.indexOf(s.charAt(i)); // get reverse order no
int mul=(i+1)*(b+1); // index +1 multiply reverse no +1
sum+=mul;
}
return sum;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | READ AT YOUR OWN RISK !!! || Simplified Approach || Beats 100 %🔥😊 | read-at-your-own-risk-simplified-approac-rloo | IntuitionThe main thing to figure out here , how can i make my a = 26 and so on ....ApproachWell first thing we can know is that i have to add 26 to my ans
mea | 47_BOT | NORMAL | 2025-04-11T13:37:20.526046+00:00 | 2025-04-11T13:37:20.526046+00:00 | 1 | false | # Intuition
The main thing to figure out here , how can i make my a = 26 and so on ....
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
Well first thing we can know is that i have to add 26 to my ans
meaning , you might be asking why ? well **let me Ask you how can you make a = 26**
u can't do stuff like "Ans = z - a - c - d - 5 - 26 + 100"
or start doing like
int a = 26 ;
int b = 25 ;
int c = 24 ;
et cetra lol
```
Result += 26 ;
```
*now i also have to multiply that value with my index* so it's quite simple as stated in the question
```
Result += (st + 1) * 26 ;
```
Why ? Add 1 in our Result because index starting index is 0
whereas i have to start multiplying with 1 so i hope you understand till now
**How to Add a and other values now? from the string?**
Let's see I know i have to add 26 for 'a' so ho can i make that 0 when i get it in the string
```
Result += (st + 1) * (26 - (s[i] - 'a')) ;
lets check it in the simple way not the hard one :)
for :
i = 0 ; // 'a'
Result += (0 + 1) * (26 - ('a' - 'a')) ; // = 26
what if i encounter b in the start or at any index then what ?
lets see that
Result += (0 + 1) * (26 - ('b' - 'a')) ; // = 25
similarly for c = 24 and so till z = 1
```
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(N)
- Time complexity is O(N) as we havee to have to check for every value in the given string and add that value to our ans
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
- Since the their is only one variable we are using and that is our Result
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int end = s.length() - 1 ;
int Result = 0 ;
for(int i = 0 ; i <= end ; i += 1)
{
Result += (i + 1) * (26 - (s[i] - 'a')) ;
// Damn Bro even a small bracket mistake makes the whole question go wrong lol
}
return Result ;
}
};
``` | 0 | 0 | ['Math', 'C++'] | 0 |
reverse-degree-of-a-string | very short and optimized answer | very-short-and-optimized-answer-by-sjais-zgvl | null | SJaiShu | NORMAL | 2025-04-11T06:15:59.045884+00:00 | 2025-04-11T06:15:59.045884+00:00 | 3 | false | ```cpp []
class Solution {
public:
int reverseDegree(string s){
int n = s.size(), ans = 0;
for(int i = 0; i < n; i++){
int val = 'z' - s[i] + 1;
ans += val * (i + 1);
}
return ans;
}
};
``` | 0 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | Simple solution | simple-solution-by-g112000-roeq | Code | g112000 | NORMAL | 2025-04-10T11:40:18.723406+00:00 | 2025-04-10T11:40:18.723406+00:00 | 3 | false |
# Code
```golang []
func reverseDegree(s string) int {
var result int
for i, char := range s {
result += int(rune('z')+1-char) * (i+1)
}
return result
}
``` | 0 | 0 | ['Go'] | 0 |
reverse-degree-of-a-string | Python | Easiest solution | python-easiest-solution-by-mitali615-m0fc | Code | mitali615 | NORMAL | 2025-04-10T07:50:29.361205+00:00 | 2025-04-10T07:50:29.361205+00:00 | 1 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
sum = 0
for i in range(len(s)):
sum += (i + 1) * (97 + 26 - ord(s[i]))
return sum
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | i using map and manual mapping | i-using-map-and-manual-mapping-by-sebase-8idc | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Sebasers | NORMAL | 2025-04-10T01:13:56.773111+00:00 | 2025-04-10T01:13:56.773111+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```golang []
func reverseDegree(s string) (r int) {
x := "abcdefghijklmnopqrstuvwxyz"
t := ""
for i := len(x) - 1; i >= 0; i-- {
t += string(x[i])
}
m := map[string]int{}
for i, v := range t {
m[string(v)] = i + 1
}
for i := 0; i < len(s); i++{
r += m[string(s[i])] * (i + 1)
}
return
}
``` | 0 | 0 | ['Go'] | 0 |
reverse-degree-of-a-string | Reverse Degree of a String - HashMap approach | reverse-degree-of-a-string-hashmap-appro-nyxf | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | ChaithraDee | NORMAL | 2025-04-09T15:11:08.993287+00:00 | 2025-04-09T15:11:08.993287+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
n = 26
mpp = {}
for i in range(97, 123):
mpp[chr(i)] = n
n-= 1
sm = 0
for index, letter in enumerate(s):
sm += (index + 1) * (mpp[letter])
return sm
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Using string manipulation | using-string-manipulation-by-kunal_1310-2mke | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | kunal_1310 | NORMAL | 2025-04-09T08:21:25.462314+00:00 | 2025-04-09T08:21:25.462314+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int result = 0;
for (int i = 0; i < s.size(); i++) {
int temp = '{' - s[i];
int pro = temp * (i+1);
result += pro;
}
return result;
}
};
``` | 0 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | School level Code | school-level-code-by-aashish_bedi-jdex | Code | Aashish_Bedi | NORMAL | 2025-04-09T07:35:26.916476+00:00 | 2025-04-09T07:35:26.916476+00:00 | 2 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
my_dict = {
'a' : 26,
'b' : 25,
'c' : 24,
'd' : 23,
'e' : 22,
'f' : 21,
'g' : 20,
'h' : 19,
'i' : 18,
'j' : 17,
'k' : 16,
'l' : 15,
'm' : 14,
'n' : 13,
'o' : 12,
'p' : 11,
'q' : 10,
'r' : 9,
's' : 8,
't' : 7,
'u' : 6,
'v' : 5,
'w' : 4,
'x' : 3,
'y' : 2,
'z' : 1
}
res = 0
for i in range(len(s)):
res += (my_dict[s[i]]*(i+1))
return res
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Beats 100%. | beats-100-by-srirammente-bt6l | IntuitionThe problem asks us to calculate the "reverse degree" of a string, where the reverse degree is determined by the position of each character in the reve | srirammente | NORMAL | 2025-04-09T06:50:32.486269+00:00 | 2025-04-09T06:50:32.486269+00:00 | 2 | false | # Intuition
The problem asks us to calculate the "reverse degree" of a string, where the reverse degree is determined by the position of each character in the reversed alphabet (i.e., 'a' = 26, 'b' = 25, ..., 'z' = 1). We need to multiply each character's reverse alphabet value with its position in the string (1-indexed) and then sum up these products to get the final result.
# Approach
1. Reverse Alphabet Mapping: First, we need to map each character to its reverse alphabetical value.
This can be done by calculating:
- `reverse_val(c) = 26 - (c - 'a')` For example, for 'a', `reverse_val('a') = 26 - (0) = 26`, for 'b', `reverse_val('b') = 26 - (1) = 25`, and so on.
1. Iterating Over String: We then iterate over the string, taking each character and its corresponding position in the string. For each character:
- Calculate its reverse value as described.
- Multiply this reverse value by its 1-indexed position in the string.
- Add the result to a running sum.
1. Return the Sum: After iterating over all characters in the string, we return the accumulated sum as the reverse degree.
# Complexity
- Time complexity:
The algorithm iterates over each character in the string exactly once, so the time complexity is 𝑂(𝑛), where 𝑛 is the length of the string.
- Space complexity:
Space Complexity: The algorithm uses a constant amount of extra space, so the space complexity is O(1).
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
int flag = 1;
for (char c : s.toCharArray()) {
int a = (26 + 'a' - c) * flag;
sum += a;
flag++;
}
return sum;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | My stupid solution | my-stupid-solution-by-kernelk14-xw1n | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | kernelk14 | NORMAL | 2025-04-09T04:18:59.551253+00:00 | 2025-04-09T04:18:59.551253+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python []
class Solution(object):
def reverseDegree(self, s):
"""
:type s: str
:rtype: int
"""
self.alphas = {
'a': 26,
'b': 25,
'c': 24,
'd': 23,
'e': 22,
'f': 21,
'g': 20,
'h': 19,
'i': 18,
'j': 17,
'k': 16,
'l': 15,
'm': 14,
'n': 13,
'o': 12,
'p': 11,
'q': 10,
'r': 9,
's': 8,
't': 7,
'u': 6,
'v': 5,
'w': 4,
'x': 3,
'y': 2,
'z': 1,
}
ctr = 0
for index, letters in enumerate(list(s.lower())):
ctr += (self.alphas[letters] * (index + 1))
print(ctr)
return ctr
``` | 0 | 0 | ['Python'] | 0 |
reverse-degree-of-a-string | just use math (easy java solution) | just-use-math-easy-java-solution-by-dpas-sfdz | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | dpasala | NORMAL | 2025-04-09T04:07:34.582863+00:00 | 2025-04-09T04:07:34.582863+00:00 | 0 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++)
sum += ((26 - ((int)(s.charAt(i) - 'a'))) * (i + 1));
return sum;
}
}
``` | 0 | 0 | ['Math', 'String', 'Java'] | 0 |
reverse-degree-of-a-string | just use math (easy java solution) | just-use-math-easy-java-solution-by-dpas-jzgo | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | dpasala | NORMAL | 2025-04-09T04:07:32.673412+00:00 | 2025-04-09T04:07:32.673412+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++)
sum += ((26 - ((int)(s.charAt(i) - 'a'))) * (i + 1));
return sum;
}
}
``` | 0 | 0 | ['Math', 'String', 'Java'] | 0 |
reverse-degree-of-a-string | 98% 1 LINE Solution | 98-1-line-solution-by-soramicha-v4cl | Complexity
Time complexity: O(n)
Space complexity: O(n)
Code | soramicha | NORMAL | 2025-04-09T00:09:12.202747+00:00 | 2025-04-09T00:09:12.202747+00:00 | 2 | false | # Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(n)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
return sum((27 - (ord(c) - 96)) * (index + 1) for index, c in enumerate(s))
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Simple solution -> Beats 100.00% | simple-solution-beats-10000-by-developer-62us | IntuitionApproachComplexity
Time complexity:
O(n)
Space complexity:
O(1)
Code | DevelopersUsername | NORMAL | 2025-04-08T18:42:17.335279+00:00 | 2025-04-08T18:42:17.335279+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
O(n)
- Space complexity:
O(1)
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int ans = 0;
char[] chars = s.toCharArray();
for (int i = 0; i < chars.length; i++)
ans += (i + 1) * (26 - (chars[i] - 'a'));
return ans;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | C# Solution [Runtime 1 ms Beats 100.00% & Memory 41.86 MB Beats 90.52%] | c-solution-runtime-1-ms-beats-10000-memo-x32j | IntuitionWe just have to use the hint, it said "Simulate the operations as described", which is useful.Normally, the problem is clear without a hint.
I just loo | RachidBelouche | NORMAL | 2025-04-08T13:17:09.589488+00:00 | 2025-04-08T13:17:09.589488+00:00 | 6 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
We just have to use the hint, it said "Simulate the operations as described", which is useful.
Normally, the problem is clear without a hint.
I just look in the hint hoping for 0 ms runtime tip, but there's nothing, I don't know what can I do to reach 0 ms.
But this solution I reach, it's time complexity O(n) with space complexity of O(1).
We have to iterate over all characters, and then we have to use the number 123 (why because z have to be one, while z have ascii code of 122, so 123 - 122 = 1 and a have ascci code of 97, so 123 - 97 = 26), so we don't need to use 'z' - s[i] + 1.
I optimize it by using iteration from 0 until s.Length, then we pre-increment i in the calculation of i (better than use i + 1 and increment i after that for the next iteration).
# Approach
<!-- Describe your approach to solving the problem. -->
1. Initialize the result variable.
```
int res = 0;
```
2. Loop over all characters and calculate the result.
```
for(int i = 0; i < s.Length;){
res += (123 - s[i]) * ++i;
}
```
3. Return the result.
```
return res;
```
# Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
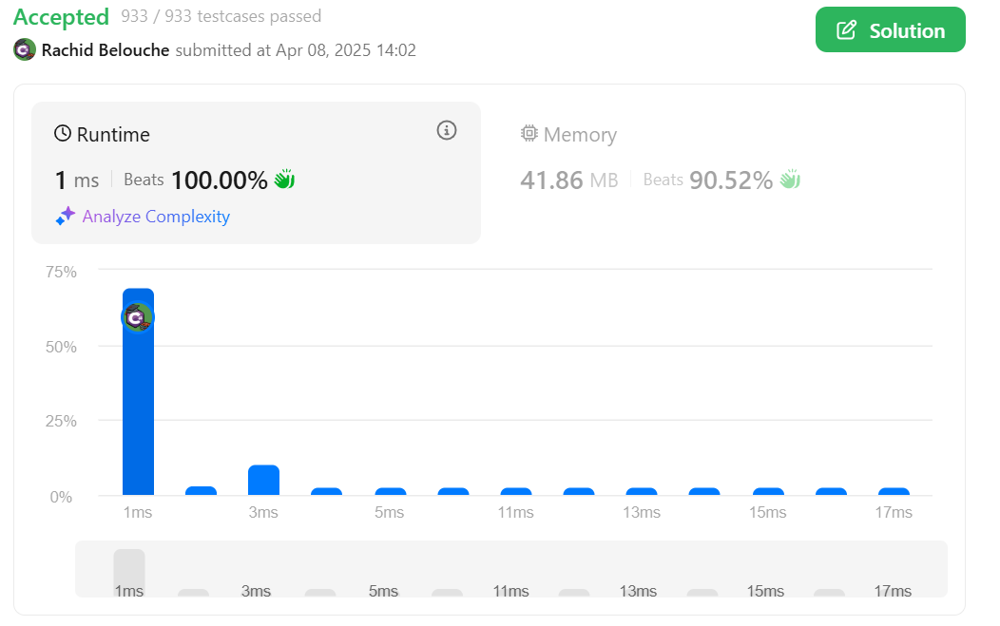
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
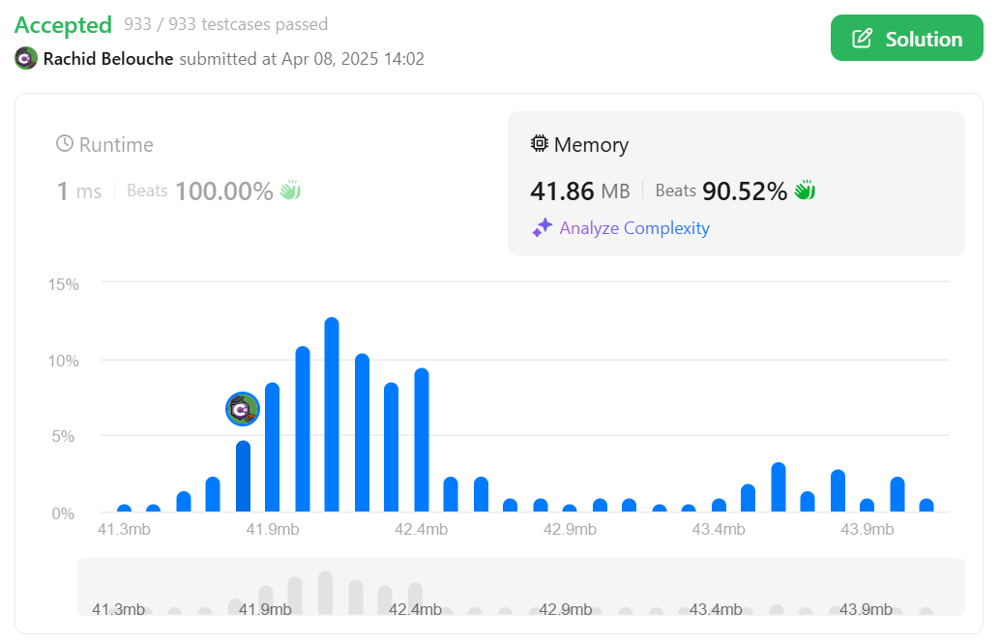
# Code
```csharp []
public class Solution {
public int ReverseDegree(string s) {
int res = 0;
for(int i = 0; i < s.Length;){
res += (123 - s[i]) * ++i;
}
return res;
}
}
``` | 0 | 0 | ['Math', 'String', 'Simulation', 'C#'] | 0 |
reverse-degree-of-a-string | Javascript Solution O(n) | javascript-solution-on-by-kamalverma1207-csep | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | kamalverma1207 | NORMAL | 2025-04-08T07:10:32.941009+00:00 | 2025-04-08T07:10:32.941009+00:00 | 6 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```javascript []
/**
* @param {string} s
* @return {number}
*/
var reverseDegree = function (s) {
let sum = 0;
for (let i = 0; i < s.length; i++) {
sum = sum + (123 - s[i].charCodeAt()) * (i + 1);
}
return sum;
};
``` | 0 | 0 | ['JavaScript'] | 0 |
reverse-degree-of-a-string | Swift💯 1 liner | swift-1-liner-by-upvotethispls-9bvg | One-Liner, terse (accepted answer) | UpvoteThisPls | NORMAL | 2025-04-08T06:15:13.163688+00:00 | 2025-04-08T06:15:13.163688+00:00 | 2 | false | **One-Liner, terse (accepted answer)**
```
class Solution {
func reverseDegree(_ s: String) -> Int {
zip(s.utf8.lazy.map{123-Int($0)},1...).map(*).reduce(0,+)
}
}
``` | 0 | 0 | ['Swift'] | 0 |
reverse-degree-of-a-string | Java Solution | java-solution-by-a_shekhar-uzzx | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | a_shekhar | NORMAL | 2025-04-07T16:10:39.079427+00:00 | 2025-04-07T16:10:39.079427+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int degree = 0;
for(int i = 0; i < s.length(); i++) {
degree += (i+1) * (123 - (int) s.charAt(i));
}
return degree;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | 3498. Reverse Degree of a String | 3498-reverse-degree-of-a-string-by-pgmre-y38r | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | pgmreddy | NORMAL | 2025-04-07T09:38:05.519903+00:00 | 2025-04-07T09:38:05.519903+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```javascript []
var reverseDegree = function (s) {
const reversePosition = (ch) => 26 - (ch.charCodeAt(0) - 'a'.charCodeAt(0))
let pos = 0
let sum = 0
for (let ch of s) {
pos++
let rpos = reversePosition(ch)
sum += rpos * pos
}
return sum
};
``` | 0 | 0 | ['JavaScript'] | 0 |
reverse-degree-of-a-string | Simple solution in Typescript | simple-solution-in-typescript-by-i6ugo4p-r8u2 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | i6UGo4pbBE | NORMAL | 2025-04-07T09:05:09.211721+00:00 | 2025-04-07T09:05:09.211721+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```typescript []
function reverseDegree(s: string): number {
let alphabet = new Map()
let value = 26
let total = 0
for (let i = 97; i <= 122; i++) {
alphabet.set(String.fromCharCode(i), value--);
}
for (let j = 0; j < s.length; j++) {
total += (j + 1) * alphabet.get(s[j]);
}
return total
};
``` | 0 | 0 | ['TypeScript'] | 0 |
reverse-degree-of-a-string | Something Interesting | something-interesting-by-shakhob-1l92 | Code | Shakhob | NORMAL | 2025-04-07T07:35:50.001646+00:00 | 2025-04-07T07:35:50.001646+00:00 | 2 | false | # Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
summa = 0
for i in range(len(s)):
summa += (26 - (ord(s[i])- 97)) * (i + 1)
return summa
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Optimized simple solution - beats 100%🔥 | optimized-simple-solution-beats-100-by-c-6z41 | Complexity
Time complexity: O(N)
Space complexity: O(1)
Code | cyrusjetson | NORMAL | 2025-04-06T15:59:11.923634+00:00 | 2025-04-06T15:59:11.923634+00:00 | 3 | false | # Complexity
- Time complexity: O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int res = 0;
int i = 1;
for (char c : s.toCharArray()) {
int t1 = 26 - (c - 'a');
res += t1 * i++;
}
return res;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Fast and short solution | fast-and-short-solution-by-angielf-sv5m | Approach
ord(c) gives the ASCII value of the character c.
ord('z') gives the ASCII value of 'z'.
The difference ord('z') - ord(c) gives the distance from 'z', | angielf | NORMAL | 2025-04-06T08:39:41.840911+00:00 | 2025-04-06T08:39:41.840911+00:00 | 6 | false | # Approach
1. ord(c) gives the ASCII value of the character c.
- ord('z') gives the ASCII value of 'z'.
- The difference ord('z') - ord(c) gives the distance from 'z', and adding 1 converts it to a 1-indexed system where 'a' corresponds to 26 and 'z' corresponds to 1.
2. The expression (i + 1) adjusts the index to be 1-indexed, as required by the problem statement.
# Complexity
- Time complexity:
$$O(n)$$, where n is the length of the string s. This is because the solution processes each character exactly once to compute the total.
- Space complexity:
$$O(1)$$ because the solution uses a constant amount of extra space.
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
return sum((ord('z') - ord(c) + 1) * (i + 1) for i, c in enumerate(s))
```
``` javascript []
/**
* @param {string} s
* @return {number}
*/
var reverseDegree = function(s) {
let total = 0;
for (let i = 0; i < s.length; i++) {
total += ('z'.charCodeAt(0) - s.charCodeAt(i) + 1) * (i + 1);
}
return total;
};
```
``` php []
class Solution {
/**
* @param String $s
* @return Integer
*/
function reverseDegree($s) {
$total = 0;
for ($i = 0; $i < strlen($s); $i++) {
$total += (ord('z') - ord($s[$i]) + 1) * ($i + 1);
}
return $total;
}
}
``` | 0 | 0 | ['Math', 'String', 'PHP', 'Python3', 'JavaScript'] | 0 |
reverse-degree-of-a-string | Easy simple | easy-simple-by-sachinab-ee2d | Code | sachinab | NORMAL | 2025-04-06T08:23:30.705786+00:00 | 2025-04-06T08:23:30.705786+00:00 | 3 | false |
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int res = 0;
for(int i=0; i<s.length(); i++){
res += ((i+1)*(26-(s.charAt(i)-'a')));
}
return res;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | Java easy solutions | java-easy-solutions-by-sanket_donga-s6ie | IntuitionThe problem seems to involve computing a weighted sum based on the reverse alphabetical position of characters in a string.ApproachFor each character i | sanket_donga | NORMAL | 2025-04-06T06:31:01.483767+00:00 | 2025-04-06T06:31:01.483767+00:00 | 1 | false | # Intuition
The problem seems to involve computing a weighted sum based on the reverse alphabetical position of characters in a string.
# Approach
For each character in the string:
- Calculate its reverse alphabetical index (i.e., 'z' - character + 1).
- Multiply it by its 1-based position in the string.
- Accumulate the result into a total sum.
# Complexity
- Time complexity: O(n), where n is the length of the string.
- Space complexity: O(1), constant extra space used.
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
int revIdx = 'z' - s.charAt(i);
revIdx++;
sum += (i + 1) * revIdx;
}
return sum;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | count each symbol | count-each-symbol-by-zemamba-lfst | null | zemamba | NORMAL | 2025-04-05T22:22:33.415712+00:00 | 2025-04-05T22:22:33.415712+00:00 | 1 | false | ```javascript []
/**
* @param {string} s
* @return {number}
*/
var reverseDegree = function(s) {
ans = 0
for (let i = 0; i < s.length; i++) {
ans += (i + 1) * (26 - (s.charCodeAt(i) - 97))
}
return ans
};
``` | 0 | 0 | ['JavaScript'] | 0 |
reverse-degree-of-a-string | Python Easy Solution | python-easy-solution-by-sumedh0706-rp3x | Code | Sumedh0706 | NORMAL | 2025-04-05T20:19:23.264937+00:00 | 2025-04-05T20:19:23.264937+00:00 | 1 | false | # Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
res=0
for i in range(len(s)):
res=res+((i+1)*(ord('z')-ord(s[i])+1))
return res
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | Python solution | python-solution-by-sivakumar-manoharan-hg9y | Code | sivakumar-manoharan | NORMAL | 2025-04-05T14:19:02.027668+00:00 | 2025-04-05T14:19:02.027668+00:00 | 1 | false |
# Code
```python3 []
class Solution:
def reverseOrder(self, c):
return ord('z') - ord(c) +1
def reverseDegree(self, s: str) -> int:
sum = 0
for i,c in enumerate(s):
index = self.reverseOrder(c)
index_in_s = i+1
sum += (index_in_s * index)
return sum
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | C++ 100% Time Complexity solution | c-100-time-complexity-solution-by-qc5pmh-tidx | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | qC5pmHTtDM | NORMAL | 2025-04-05T12:15:51.621892+00:00 | 2025-04-05T12:15:51.621892+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int output = 0;
for(int i = 0;i<s.length();i++){
output += (26-(s[i]-'a'))*(i+1);
}
return output;
}
};
``` | 0 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | Super Simple Python Solution for beginners!!! | super-simple-python-solution-for-beginne-5v5s | Code | rajsekhar5161 | NORMAL | 2025-04-05T04:34:09.477866+00:00 | 2025-04-05T04:34:09.477866+00:00 | 6 | false | # Code
```python []
class Solution(object):
def reverseDegree(self, s):
sums=0
dic={'a': 26, 'b': 25, 'c': 24, 'd': 23, 'e': 22, 'f': 21, 'g': 20,
'h': 19, 'i': 18, 'j': 17, 'k': 16, 'l': 15, 'm': 14, 'n': 13,
'o': 12, 'p': 11, 'q': 10, 'r': 9, 's': 8, 't': 7, 'u': 6, 'v': 5,
'w': 4, 'x': 3, 'y': 2, 'z': 1 }
for i in range(len(s)):
sums+= (dic[s[i]] * (i+1))
return sums
``` | 0 | 0 | ['String', 'Simulation', 'Python'] | 0 |
reverse-degree-of-a-string | 100% Efficient Solution | 100-efficient-solution-by-shashikumar_n_-0bm2 | Code | SHASHIKUMAR_N_T | NORMAL | 2025-04-04T17:27:44.432102+00:00 | 2025-04-04T17:27:44.432102+00:00 | 2 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
return sum((26 - (ord(c) - ord('a'))) * (i + 1) for i, c in enumerate(s))
```
```Java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
int reversePos = 26 - (s.charAt(i) - 'a'); // Reverse alphabet position
sum += reversePos * (i + 1); // Multiply by 1-indexed position
}
return sum;
}
}
```
```C []
int reverseDegree(char* s) {
int sum = 0;
for (int i = 0; s[i] != '\0'; i++) {
int reversePos = 26 - (s[i] - 'a');
sum += reversePos * (i + 1);
}
return sum;
}
```
```C++ []
class Solution {
public:
int reverseDegree(string s) {
int sum = 0;
for (int i = 0; s[i] != '\0'; i++) {
int reversePos = 26 - (s[i] - 'a');
sum += reversePos * (i + 1);
}
return sum;
}
};
``` | 0 | 0 | ['String', 'C', 'Simulation', 'C++', 'Java', 'Python3'] | 0 |
reverse-degree-of-a-string | 100% Efficient Solution | 100-efficient-solution-by-shashikumar_n_-ily9 | Code | SHASHIKUMAR_N_T | NORMAL | 2025-04-04T17:27:39.473046+00:00 | 2025-04-04T17:27:39.473046+00:00 | 3 | false |
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
return sum((26 - (ord(c) - ord('a'))) * (i + 1) for i, c in enumerate(s))
```
```Java []
class Solution {
public int reverseDegree(String s) {
int sum = 0;
for (int i = 0; i < s.length(); i++) {
int reversePos = 26 - (s.charAt(i) - 'a'); // Reverse alphabet position
sum += reversePos * (i + 1); // Multiply by 1-indexed position
}
return sum;
}
}
```
```C []
int reverseDegree(char* s) {
int sum = 0;
for (int i = 0; s[i] != '\0'; i++) {
int reversePos = 26 - (s[i] - 'a');
sum += reversePos * (i + 1);
}
return sum;
}
```
```C++ []
class Solution {
public:
int reverseDegree(string s) {
int sum = 0;
for (int i = 0; s[i] != '\0'; i++) {
int reversePos = 26 - (s[i] - 'a');
sum += reversePos * (i + 1);
}
return sum;
}
};
``` | 0 | 0 | ['String', 'C', 'Simulation', 'C++', 'Java', 'Python3'] | 0 |
reverse-degree-of-a-string | Reverse degree of a string | reverse-degree-of-a-string-by-harshitara-4t4t | IntuitionThe idea is to assign more weight to letters that are closer to the end of the alphabet (like 'z', 'y', etc.) and also to letters that appear later in | harshitarathod00 | NORMAL | 2025-04-04T16:39:38.989126+00:00 | 2025-04-04T16:39:38.989126+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The idea is to assign more weight to letters that are closer to the end of the alphabet (like 'z', 'y', etc.) and also to letters that appear later in the string.
- Normally, 'a' is 0, 'b' is 1, ..., 'z' is 25.
- We flip this by using 26 - (s[i] - 'a'), so 'a' becomes 26, 'b' becomes 25, ..., 'z' becomes 1.
Then, to emphasize the position in the string, we multiply this reversed value by (i + 1), giving more importance to characters appearing later in the string.
So, characters that are late in the alphabet and appear late in the string will contribute the most to the final value.
This creates a "reverse weighted sum" based on both character value and position.
# Approach
<!-- Describe your approach to solving the problem. -->
1. Initialize ans = 0.
2. Find the length n of the input string.
3. For each character at index i:
- Calculate its distance from 'a': s[i] - 'a'
- Subtract this from 26: 26 - diff
- Multiply it with its 1-based index: (i + 1)
- Add the result to ans.
4. Return the final result.
# Complexity
- Time complexity:O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```c []
int reverseDegree(char* s) {
int ans=0;
int n=strlen(s);
for(int i=0;i<n;i++){
int diff=s[i]-'a';
ans+=(26-diff)*(i+1);
}
return ans;
}
``` | 0 | 0 | ['String', 'C', 'Simulation'] | 0 |
reverse-degree-of-a-string | java code | java-code-by-shaima31-354d | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | shaima31 | NORMAL | 2025-04-04T15:41:49.506913+00:00 | 2025-04-04T15:41:49.506913+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int reverseDegree(String s) {
int ans=0;
for (int i=0;i<s.length();i++){
ans+=(26-(s.charAt(i)-'a'))*(i+1);
}
return ans;
}
}
``` | 0 | 0 | ['Java'] | 0 |
reverse-degree-of-a-string | golang | golang-by-marcdidom-enkf | Code | marcdidom | NORMAL | 2025-04-04T14:32:56.913095+00:00 | 2025-04-04T14:32:56.913095+00:00 | 6 | false |
# Code
```golang []
func reverseDegree(s string) int {
total := 0
for i, ch := range s {
// Calculate the reversed position of the character: 'a' = 26, 'b' = 25, ..., 'z' = 1.
reversedPos := int('z'-ch) + 1
// (i + 1) because positions are 1-indexed.
total += reversedPos * (i + 1)
}
return total
}
``` | 0 | 0 | ['Go'] | 0 |
reverse-degree-of-a-string | Beats 100% 0ms Easy to understand | beats-100-0ms-easy-to-understand-by-kara-gpzb | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Karan_mishra22 | NORMAL | 2025-04-04T14:20:04.079044+00:00 | 2025-04-04T14:20:04.079044+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s)
{
auto get_index = [](char c)
{
char ch = 'a';
for (int i = 26; i >= 1; i--)
{
if (ch == c)
{
return i;
}
// cout << i << " - " << ch << endl;
ch++;
}
return -1;
};
int ans = 0;
for (int i = 0; i < s.size(); i++)
{
// cout<<s[i]<<" "<<get_index(s[i])<<endl;;
ans = ans + (get_index(s[i]) * (i + 1));
}
return ans;
}
};
``` | 0 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | 0(n) | 0n-by-antoniojsp-l76o | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | antoniojsp | NORMAL | 2025-04-04T12:38:04.903770+00:00 | 2025-04-04T12:38:04.903770+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def reverseDegree(self, s: str) -> int:
result = 0
for i,j in enumerate(s, start=1):
position_char = ord(j)-96
reversal = 27 - position_char
result += (reversal*i)
return result
``` | 0 | 0 | ['Python3'] | 0 |
reverse-degree-of-a-string | O(n) | on-by-antoniojsp-yrjc | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | antoniojsp | NORMAL | 2025-04-04T12:36:58.184360+00:00 | 2025-04-04T12:36:58.184360+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int reverseDegree(string s) {
int result = 0;
for(auto i = 0;i<s.size();i++){
int ord = s[i] - 96;
result = result + ((27-ord)*(i+1));
}
return result;
}
};
``` | 0 | 0 | ['C++'] | 0 |
reverse-degree-of-a-string | Simple & Short || Beats 100% | simple-short-beats-100-by-meky20500-zskz | Code | meky20500 | NORMAL | 2025-04-03T21:42:35.060900+00:00 | 2025-04-03T21:42:35.060900+00:00 | 6 | false | # Code
```csharp []
public class Solution
{
public int ReverseDegree(string s)
{
int ans = 0;
for(int i = 0; i < s.Length; i++)
{
ans+= (i+1) * (26 - (s[i]-'a'));
}
return ans;
}
}
``` | 0 | 0 | ['String', 'Simulation', 'C#'] | 0 |
Subsets and Splits