licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1333 | function mapslices(f, x::DNDSparse, dims; name=nothing)
iterdims = setdiff([1:ndims(x);], map(d->keyindex(x, d), dims))
if iterdims != [1:length(iterdims);]
throw(ArgumentError("$dims must be the trailing dimensions of the table. You can use `permutedims` first to permute the dimensions."))
end
# Note: the key doesn't need to be put in a tuple, this is
# also bad for sortperm, but is required since DArrays aren't
# parameterized by the container type Columns
vals = isempty(dims) ? values(x) : (keys(x, (dims...,)), values(x))
tmp = ndsparse((keys(x, (iterdims...,)),), vals,
allowoverlap=false, closed=true)
cs = delayedmap(tmp.chunks) do c
ks = isempty(dims) ? columns(columns(keys(c))[1]) : IndexedTables.concat_cols(columns(keys(c))[1], columns(values(c))[1])
vs = isempty(dims) ? columns(values(c)) : columns(values(c))[2]
y = ndsparse(ks, vs)
mapslices(f, y, dims; name=name)
end
fromchunks(cs)
# cache_thunks(mapchunks(y -> mapslices(f, y, dims, name=name),
# t, keeplengths=false))
end
mapslices(f, x::DNDSparse, dims::Symbol; name=nothing) =
mapslices(f, x, (dims,); name=name)
function flatten(x::DIndexedTable, col)
fromchunks(delayedmap(t -> flatten(t, col), x.chunks))
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 2604 |
"""
t[idx...]
Returns a `DNDSparse` containing only the elements of `t` where the given indices (`idx`)
match. If `idx` has the same type as the index tuple of the `t`, then this is
considered a scalar indexing (indexing of a single value). In this case the value
itself is looked up and returned.
"""
function Base.getindex(t::DNDSparse{K}, idxs...) where K
if typeof(idxs) <: astuple(K)
_getindex_scalar(t, idxs)
else
_getindex(t, idxs)
end
end
function _getindex_scalar(t::DNDSparse{K,V}, idxs) where {K,V}
# scalar getindex
rng = map(x->[x], idxs)
t = collect(fromchunks(delayedmap(x->x[rng...], t.chunks)))
if isempty(t)
throw(KeyError(idxs))
else
first(t)
end
end
function _getindex(t::DNDSparse{K,V}, idxs) where {K,V}
if length(idxs) != ndims(t)
error("wrong number of indices")
end
for idx in idxs
isa(idx, AbstractVector) && (issorted(idx) || error("indices must be sorted for ranged/vector indexing"))
end
# Subset the chunks
# this is currently a linear search
mapchunks(t, keeplengths=false) do chunk
getindex(chunk, idxs...)
end |> cache_thunks
end
# update a given domain to include a new key
function update_domain(d::IndexSpace{<:NamedTuple}, key::Tuple)
knt = namedtuple(keys(first(d))...)(key)
IndexSpace(Interval(min(first(d.interval), knt),
max(last(d.interval), knt)),
Interval(map(min, first(d.interval), knt),
map(max, last(d.interval), knt)),
Nullable{Int}())
end
function update_domain(d::IndexSpace{<:Tuple}, key::Tuple)
IndexSpace(Interval(min(first(d.interval), key),
max(last(d.interval), key)),
Interval(map(min, first(d.interval), key),
map(max, last(d.interval), key)),
Nullable{Int}())
end
function insert_row!(x::DNDSparse{K,T}, idxs::Tuple, val) where {K,T}
perm = sortperm(x.domains, by=last)
cs = convert(Array{Any}, x.chunks[perm])
ds = x.domains[perm]
i = searchsortedfirst(astuple.(last.(x.domains)), idxs)
if i >= length(cs)
i = length(cs)
end
ds[i] = update_domain(ds[i], idxs)
tmp = cs[i]
cs[i] = compute(get_context(), delayed(x->(x[idxs...] = val; x))(cs[i]))
x.domains = ds
x.chunks = cs
end
function insert_row!(x::DNDSparse{K,T}, idxs::NamedTuple, val) where {K,T}
insert_row!(s, astuple(idxs), val)
end
function Base.setindex!(x::DNDSparse, val, idxs...)
insert_row!(x, idxs, val)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1886 | """
An interval type tailored specifically to store intervals of
indices of an NDSparse object. Some of the operations on this
like `in` or `<` may be controversial for a generic Interval type.
"""
struct Interval{T}
first::T
last::T
end
# desired properties:
Base.eltype(int::Interval{T}) where {T} = T
Base.first(int::Interval) = int.first
Base.last(int::Interval) = int.last
Base.isempty(int::Interval) = first(int) > last(int)
Base.isless(x::Interval, y::Interval) = x.last < y.first
Base.in(x, int::Interval) = first(int) <= x <= last(int)
Base.in(x::AbstractRange, int::Interval) = hasoverlap(Interval(first(x),last(x)), int)
Base.in(x::AbstractArray, int::Interval) = any(a in int for a in x)
Base.in(x::Colon, int::Interval) = true
Base.in(int::Interval, x::Union{AbstractArray, Colon}) = x in int
function hasoverlap(i1::Interval, i2::Interval)
(isempty(i1) || isempty(i2)) && return false
(first(i2) <= last(i1) && first(i1) <= last(i2)) ||
(first(i1) <= last(i2) && first(i2) <= last(i1))
end
boxintervals(i) = map(Interval, first(i), last(i))
function boxhasoverlap(a,b)
all(map(hasoverlap, boxintervals(a), boxintervals(b)))
end
function boxmerge(a, b)
c = map(merge, boxintervals(a), boxintervals(b))
Interval(map(first, c), map(last, c))
end
function Base.intersect(i1::Interval, i2::Interval)
Interval(max(first(i1), first(i2)), min(last(i1), last(i2)))
end
function Base.merge(i1::Interval, i2::Interval)
Interval(min(first(i1), first(i2)), max(last(i1), last(i2)))
end
function _map(f, i::Interval)
fst, lst = f(first(i)), f(last(i))
if lst < fst
throw(ArgumentError(
"map on $(typeof(i)) is only allowed on monotonically increasing functions"
)
)
end
Interval(fst, lst)
end
Base.show(io::IO, i::Interval) = (show(io, i.first); print(io, ".."); show(io, i.last))
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 10144 | const JULIADB_DIR = ".juliadb"
const JULIADB_FILECACHE = "csv_metadata"
const JULIADB_INDEXFILE = "juliadb_index"
function files_from_dir(dir)
dir = abspath(dir)
filter(isfile, [ joinpath(dir, f) for f in readdir(dir) if !startswith(f, ".") ])
end
function format_bytes(nb)
bytes, mb = Base.prettyprint_getunits(nb, length(Base._mem_units), Int64(1024))
if mb == 1
@sprintf("%d %s%s", bytes, Base._mem_units[mb], bytes==1 ? "" : "s")
else
@sprintf("%.3f %s", bytes, Base._mem_units[mb])
end
end
function offset_index!(x, o)
l = length(x)
copyto!(columns(x.index)[1], o:o+l-1)
x
end
function offset_index!(x::DNDSparse, o=1)
lengths = map(a->get(a.nrows), x.domains)
offs = [0; cumsum(lengths[1:end-1])] .+ 1
fromchunks(delayedmap(offset_index!, x.chunks, offs))
end
Base.@deprecate loadfiles(files, delim=','; opts...) loadndsparse(files; delim=delim, opts...)
"""
`loadtable(files::Union{AbstractVector,String}; <options>)`
Load a [table](@ref Table) from CSV files.
`files` is either a vector of file paths, or a directory name.
# Options:
- `output::AbstractString` -- directory name to write the table to. By default data is loaded directly to memory. Specifying this option will allow you to load data larger than the available memory.
- `indexcols::Vector` -- columns to use as primary key columns. (defaults to [])
- `datacols::Vector` -- non-indexed columns. (defaults to all columns but indexed columns). Specify this to only load a subset of columns. In place of the name of a column, you can specify a tuple of names -- this will treat any column with one of those names as the same column, but use the first name in the tuple. This is useful when the same column changes name between CSV files. (e.g. `vendor_id` and `VendorId`)
- `distributed::Bool` -- should the output dataset be loaded as a distributed table? If true, this will use all available worker processes to load the data. (defaults to true if workers are available, false if not)
- `chunks::Int` -- number of chunks to create when loading distributed. (defaults to number of workers)
- `delim::Char` -- the delimiter character. (defaults to `,`). Use `spacedelim=true` to split by spaces.
- `spacedelim::Bool`: parse space-delimited files. `delim` has no effect if true.
- `quotechar::Char` -- quote character. (defaults to `"`)
- `escapechar::Char` -- escape character. (defaults to `"`)
- `filenamecol::Union{Symbol, Pair}` -- create a column containing the file names from where each row came from. This argument gives a name to the column. By default, `basename(name)` of the name is kept, and ".csv" suffix will be stripped. To provide a custom function to apply on the names, use a `name => Function` pair. By default, no file name column will be created.
- `header_exists::Bool` -- does header exist in the files? (defaults to true)
- `colnames::Vector{String}` -- specify column names for the files, use this with (`header_exists=false`, otherwise first row is discarded). By default column names are assumed to be present in the file.
- `samecols` -- a vector of tuples of strings where each tuple contains alternative names for the same column. For example, if some files have the name "vendor_id" and others have the name "VendorID", pass `samecols=[("VendorID", "vendor_id")]`.
- `colparsers` -- either a vector or dictionary of data types or an [`AbstractToken` object](https://juliacomputing.com/TextParse.jl/stable/#Available-AbstractToken-types-1) from [TextParse](https://juliacomputing.com/TextParse.jl/stable) package. By default, these are inferred automatically. See `type_detect_rows` option below.
- `type_detect_rows`: number of rows to use to infer the initial `colparsers` defaults to 20.
- `nastrings::Vector{String}` -- strings that are to be considered missing values. (defaults to `TextParse.NA_STRINGS`)
- `skiplines_begin::Char` -- skip some lines in the beginning of each file. (doesn't skip by default)
- `usecache::Bool`: (vestigial)
"""
function loadtable(files::Union{AbstractVector,String}; opts...)
_loadtable(IndexedTable, files; opts...)
end
"""
`loadndsparse(files::Union{AbstractVector,String}; <options>)`
Load an [NDSparse](@ref) from CSV files.
`files` is either a vector of file paths, or a directory name.
# Options:
- `indexcols::Vector` -- columns to use as indexed columns. (by default a `1:n` implicit index is used.)
- `datacols::Vector` -- non-indexed columns. (defaults to all columns but indexed columns). Specify this to only load a subset of columns. In place of the name of a column, you can specify a tuple of names -- this will treat any column with one of those names as the same column, but use the first name in the tuple. This is useful when the same column changes name between CSV files. (e.g. `vendor_id` and `VendorId`)
All other options are identical to those in [`loadtable`](@ref)
"""
function loadndsparse(files::Union{AbstractVector,String}; opts...)
_loadtable(NDSparse, files; opts...)
end
# Can load both NDSparse and table
function _loadtable(T, files::Union{AbstractVector,String};
chunks=nothing,
output=nothing,
append=false,
indexcols=[],
distributed=chunks != nothing || length(procs()) > 1,
usecache=false,
opts...)
if isa(files, String)
if isdir(files)
files = files_from_dir(files)
elseif isfile(files)
files = [files]
else
throw(ArgumentError("Specified path is neither a file, " *
"nor a directory."))
end
else
for file in files
if !isfile(file)
throw(ArgumentError("No file named $file."))
end
end
end
if isempty(files)
throw(ArgumentError("Specify at least one file to load."))
end
if chunks === nothing && distributed
chunks = nworkers()
end
if !distributed
filegroups = [files]
else
if isa(chunks, Integer)
chunks = Dagger.split_range(1:length(files), chunks)
end
filegroups = filter(!isempty, map(x->files[x], chunks))
end
loadgroup = delayed() do group
_loadtable_serial(T, group; indexcols=indexcols, opts...)[1]
end
if output !== nothing && append
prevchunks = load(output).chunks
else
prevchunks = []
end
y = fromchunks(map(loadgroup, filegroups),
output=output, fnoffset=length(prevchunks))
x = fromchunks(vcat(prevchunks, y.chunks))
if output !== nothing
open(joinpath(output, JULIADB_INDEXFILE), "w") do io
serialize(io, x)
end
_makerelative!(x, output)
end
if x isa DNDSparse && isempty(indexcols)
# implicit index
x = offset_index!(x, 1)
end
if !distributed
return collect(x)
else
return x
end
end
Base.@deprecate ingest(files, output; kwargs...) loadndsparse(files; output=output, kwargs...)
Base.@deprecate ingest!(files, output; kwargs...) loadndsparse(files; output=output, append=true, kwargs...)
"""
`load(dir::AbstractString)`
Load a saved `DNDSparse` from `dir` directory. Data can be saved
using the `save` function.
"""
function load(f::AbstractString; procs=workers())
if isdir(f)
x = open(joinpath(f, JULIADB_INDEXFILE)) do io
deserialize(io)
end
_makerelative!(x, f)
_evenlydistribute!(x, procs)
x
elseif isfile(f)
MemPool.unwrap_payload(open(deserialize, f))
else
error("$f is not a file or directory")
end
end
"""
save(t::Union{DNDSparse, DIndexedTable}, destdir::AbstractString)
Saves a distributed dataset to disk in directory `destdir`. Saved data can be loaded with [`load`](@ref).
"""
function save(x::DDataset, output::AbstractString)
if !isempty(x.chunks)
y = fromchunks(x.chunks, output=output)
else
y = x
end
open(joinpath(output, JULIADB_INDEXFILE), "w") do io
serialize(io, y)
end
_makerelative!(y, output)
y
end
"""
save(t::Union{NDSparse, IndexedTable}, dest::AbstractString)
Save a dataset to disk as `dest`. Saved data can be loaded with [`load`](@ref).
"""
function save(data::Dataset, f::AbstractString)
sz = open(f, "w") do io
serialize(io, MemPool.MMWrap(data))
end
load(f)
end
function _makerelative!(t, dir::AbstractString)
foreach(t.chunks) do c
h = c.handle
if isa(h, FileRef)
c.handle = FileRef(joinpath(dir, h.file), h.size)
end
end
end
using MemPool
# Fix to load n/p number of files per process
function _evenlydistribute!(t, wrkrs)
for (r, w) in zip(t.chunks, Iterators.cycle(wrkrs))
if r.handle isa FileRef
r.handle.force_pid[] = w
end
end
end
deserialize(io::AbstractSerializer, DT::Type{DNDSparse{K,V}}) where {K,V} = _deser(io, DT)
deserialize(io::AbstractSerializer, DT::Type{DIndexedTable{T,K}}) where {T,K} = _deser(io, DT)
function _deser(io::AbstractSerializer, t)
nf = fieldcount(t)
x = ccall(:jl_new_struct_uninit, Any, (Any,), t)
t.mutable && Serialization.deserialize_cycle(io, x)
for i in 1:nf
tag = Int32(read(io.io, UInt8)::UInt8)
if tag != Serialization.UNDEFREF_TAG
ccall(:jl_set_nth_field, Cvoid, (Any, Csize_t, Any), x, i-1, Serialization.handle_deserialize(io, tag))
end
end
return x
end
function MemPool.mmwrite(io::AbstractSerializer, arr::StringArray)
Serialization.serialize_type(io, MemPool.MMSer{StringArray})
serialize(io, eltype(arr))
MemPool.mmwrite(io, arr.buffer)
MemPool.mmwrite(io, arr.offsets)
MemPool.mmwrite(io, arr.lengths)
return
end
function MemPool.mmread(::Type{StringArray}, io, mmap)
T = deserialize(io)
buffer = deserialize(io)
offsets = deserialize(io)
lengths = deserialize(io)
return StringArray{T, ndims(offsets)}(buffer, offsets, lengths)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 6761 | # Extract a column as a Dagger array
function Iterators.partition(t::DDataset, n::Integer)
PartitionIterator(t, n)
end
struct PartIteratorState{T}
chunkno::Int
chunk::T
used::Int
end
function iterate(p::PartitionIterator{<:DDataset})
state = if !isempty(p.c.chunks)
PartIteratorState(1, collect(p.c.chunks[1]), 0)
else
PartIteratorState(1, collect(p.c), 0)
end
return iterate(p, state)
end
function iterate(t::PartitionIterator{<:DDataset}, p::PartIteratorState)
if p.chunkno == length(t.c.chunks) && p.used >= length(p.chunk)
return nothing
end
if p.used + t.n <= length(p.chunk)
# easy
nextpart = subtable(p.chunk, p.used+1:(p.used+t.n))
return nextpart, PartIteratorState(p.chunkno, p.chunk, p.used + t.n)
else
part = subtable(p.chunk, p.used+1:length(p.chunk))
required = t.n - length(part)
r = required
chunkno = p.chunkno
used = length(p.chunk)
nextchunk = p.chunk
while r > 0
chunkno += 1
if chunkno > length(t.c.chunks)
# we're done, last chunk
return part, PartIteratorState(chunkno-1, nextchunk, used)
else
nextchunk = collect(t.c.chunks[chunkno])
if r > length(nextchunk)
part = _merge(part, nextchunk)
r -= length(nextchunk)
used = length(nextchunk)
else
part = _merge(part, subtable(nextchunk, 1:r))
used = r
r = 0
end
end
end
return part, PartIteratorState(chunkno, nextchunk, used)
end
end
Base.eltype(iter::PartitionIterator{<:DIndexedTable}) = IndexedTable
Base.eltype(iter::PartitionIterator{<:DNDSparse}) = NDSparse
function DColumns(arrays::Tup)
if length(arrays) == 0
error("""DColumns must be constructed with at least
one column.""")
end
i = findfirst(x->isa(x, ArrayOp), arrays)
wrap = isa(arrays, Tuple) ? tuple :
namedtuple(keys(arrays)...)∘tuple
if i == 0
error("""At least 1 array passed to
DColumns must be a DArray""")
end
darrays = asyncmap(arrays) do x
isa(x, ArrayOp) ? compute(get_context(), x) : x
end
dist = domainchunks(darrays[i])
darrays = map(darrays) do x
if isa(x, DArray)
domainchunks(x) == dist ?
x : error("Distribution incompatible")
else
Distribute(dist, x)
end
end
darrays = asyncmap(darrays) do x
compute(get_context(), x)
end
if length(darrays) == 1
cs = chunks(darrays[1])
chunkmatrix = reshape(cs, length(cs), 1)
else
chunkmatrix = reduce(hcat, map(chunks, darrays))
end
cs = mapslices(x -> delayed((c...) -> Columns(wrap(c...)))(x...), chunkmatrix, 2)[:]
T = isa(arrays, Tuple) ? Tuple{map(eltype, arrays)...} :
wrap{map(eltype, arrays)...}
DArray(T, domain(darrays[1]), domainchunks(darrays[1]), cs, dvcat)
end
function itable(keycols::DArray, valuecols::DArray)
cs = map(delayed(itable), chunks(keycols), chunks(valuecols))
cs1 = compute(get_context(),
delayed((xs...) -> [xs...]; meta=true)(cs...))
fromchunks(cs1)
end
function extractarray(t, f)
fromchunks(map(delayed(f), t.chunks))
end
# TODO: do this lazily after compute
# technically it's not necessary to communicate here
function Base.getindex(d::ColDict{<:DArray})
rows(table(d.columns...; names=d.names))
end
function columns(t::Union{DDataset, DArray})
cs = delayedmap(t.chunks) do c
x = columns(c)
if isa(x, AbstractArray)
tochunk(x)
elseif isa(x, Tup)
map(tochunk, IndexedTables.astuple(x))
else
# this should never happen
error("Columns $which could not be extracted")
end
end
tuples = collect(get_context(), treereduce(delayed(vcat), cs))
if length(cs) == 1
tuples = [tuples]
end
if isa(tuples[1], Tup)
arrays = map((xs...)->fromchunks([xs...]), tuples...)
if t isa DDataset
names = colnames(t)
else
names = fieldnames(eltype(t))
end
if all(x -> x isa Symbol, names)
IndexedTables.namedtuple(names...)(arrays)
else
arrays
end
else
fromchunks(tuples)
end
end
function columns(t::Union{DDataset, DArray}, which::Tuple)
columns(rows(t, which))
end
# TODO: make sure this is a DArray of Columns!!
Base.@pure IndexedTables.colnames(t::DArray{T}) where T<:Tup = fieldnames(T)
isarrayselect(x) = x isa AbstractArray || x isa Pair{<:Any, <:AbstractArray}
function dist_selector(t, f, which::Tup)
if any(isarrayselect, which)
refholder = []
t1 = compute(t)
w1 = map(which) do x
isarrayselect(x) ? distfor(t1, x, refholder) : x
end
# this repeats the non-chunks to all other chunks,
# then queries with the corresponding chunks
broadcast(t1.chunks, w1...) do x...
delayed((inp...)->f(inp[1], inp[2:end]))(x...)
end |> fromchunks
else
extractarray(t, x->f(x, which))
end
end
function dist_selector(t, f, which::AbstractArray)
which
end
function dist_selector(t, f, which)
extractarray(t, x->f(x,which))
end
function distfor(t, x::AbstractArray, refholder)
y = rows(t)
if length(y) != length(x)
error("Input column is not the same length as the table")
end
d = distribute(x, domainchunks(y))
push!(refholder, d)
d.chunks
end
function distfor(t, x::Pair{<:Any, <:AbstractArray}, refholder)
cs = distfor(t, x[2], refholder)
[delayed(c->x[1]=>c)(c) for c in cs]
end
function rows(t::Union{DDataset, DArray})
extractarray(t, rows)
end
function rows(t::Union{DDataset, DArray}, which)
dist_selector(t, rows, which)
end
function pkeys(t::DIndexedTable)
if isempty(t.pkey)
Columns((Base.OneTo(length(compute(t))),))
else
extractarray(t, pkeys)
end
end
pkeys(t::DNDSparse) = keys(t)
for f in [:keys, :values]
@eval function $f(t::DNDSparse)
extractarray(t, x -> $f(x))
end
@eval function $f(t::DNDSparse, which)
dist_selector(t, $f, which)
end
end
function column(t::DDataset, name)
extractarray(t, x -> column(x, name))
end
function column(t::DDataset, xs::AbstractArray)
# distribute(xs, rows(t).subdomains)
xs
end
function pairs(t::DNDSparse)
extractarray(t, x -> map(Pair, x.index, x.data))
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 11339 | ## Table
function rechunk_together(left, right, lkey, rkey,
lselect=excludecols(left, lkey), rselect=excludecols(right, rkey); chunks=nworkers())
# we will assume that right has to be aligned to left
l = reindex(left, lkey, lselect)
r = reindex(right, rkey, rselect)
if has_overlaps(l.domains, closed=true)
l = rechunk(l, chunks=chunks)
elseif !issorted(l.domains, by=first)
perm = sortperm(l.domains, by=first)
l = fromchunks(l.chunks[perm], domains=l.domains[perm])
end
splitters = map(last, l.domains)
r = rechunk(r, rkey, rselect,
splitters=splitters[1:end-1],
chunks_presorted=true,
#affinities=map(x->first(Dagger.affinity(x))[1].pid, l.chunks),
)
l, r
end
function Base.join(f, left::DDataset, right::DDataset;
how=:inner,
lkey=pkeynames(left), rkey=pkeynames(right),
lselect=left isa DNDSparse ? valuenames(left) : excludecols(left, lkey),
rselect=right isa DNDSparse ? valuenames(right) : excludecols(right, rkey),
broadcast=nothing,
chunks=nworkers(),
kwargs...)
cl = compute(left)
cr = compute(right)
if broadcast === :left
error("only broadcast = :right is supported at the moment")
elseif broadcast === :right
if !(how in [:inner, :left, :anti])
error("Can only do inner, left, or anti join with broadcast = :right")
end
if length(cr.chunks) == 1
right_ser = cr.chunks[1]
else
right_ser = collect(cr)
end
ps = map(cl.chunks) do c
a = Dagger.affinity(c)
first.(a[1:min(length(a), 1)])
end
tasks = [delayed(identity)(right_ser) for p in ps]
for (t, p) in zip(tasks, ps)
t.affinity = Nullable(Pair.(p, 1))
end
r = fromchunks(tasks)
l = cl
else
l, r = rechunk_together(left, right, lkey, rkey, lselect, rselect,
chunks=chunks)
end
i = 1
j = 1
cs = []
rempty = delayed(empty!∘copy)(r.chunks[end])
lempty = delayed(empty!∘copy)(l.chunks[end])
function joinchunks(x, y)
res = if broadcast !== nothing
join(f, x, y, lkey=lkey, rkey=rkey,
lselect=lselect, rselect=rselect,
how=how; kwargs...)
else
join(f, x, y, how=how; kwargs...)
end
end
while i <= length(l.chunks) && j <= length(r.chunks)
c1 = l.chunks[i]
c2 = r.chunks[j]
d1 = l.domains[i]
d2 = r.domains[j]
if hasoverlap(d1.interval, d2.interval)
i += 1
j += 1
elseif last(d1.interval) < last(d2.interval)
# this means there's no corresponding chunk on the right
c2 = rempty
i += 1
else
# this means there's no corresponding chunk on the left
c1 = lempty
j += 1
end
c = delayed(joinchunks)(c1,c2)
push!(cs, c)
end
while i <= length(l.chunks)
push!(cs, delayed(joinchunks)(l.chunks[i], rempty))
i+=1
end
while j <= length(r.chunks)
push!(cs, delayed(joinchunks)(lempty, r.chunks[j]))
j += 1
end
fromchunks(cs)
end
function Base.join(left::DDataset, right; how=:inner, kwargs...)
f = how === :anti ? ((x,y)->x) : IndexedTables.concat_tup
join(f, left, right; how=how, kwargs...)
end
function groupjoin(f, left::DDataset, right; how=:inner, kwargs...)
join(f, left, right; how=how, group=true, kwargs...)
end
function groupjoin(left::DDataset, right; how=:inner, kwargs...)
join(left, right; how=how, group=true, kwargs...)
end
function join(f, left::DDataset, right::IndexedTables.Dataset; how=:inner, kwargs...)
if how in [:inner, :left, :anti]
join(f, left, distribute(right, 1), broadcast=:right, how=how; kwargs...)
else
join(f, left, distribute(right, 1), how=how; kwargs...)
end
end
## NDSparse join
"""
naturaljoin(left::DNDSparse, right::DNDSparse, [op])
Returns a new `DNDSparse` containing only rows where the indices are present both in
`left` AND `right` tables. The data columns are concatenated.
"""
function naturaljoin(left::DNDSparse{I,D1}, right::DNDSparse{J,D2}) where {I,J,D1,D2}
naturaljoin(IndexedTables.concat_tup, left, right)
end
"""
naturaljoin(op, left::DNDSparse, right::DNDSparse, ascolumns=false)
Returns a new `DNDSparse` containing only rows where the indices are present both in
`left` AND `right` tables. The data columns are concatenated. The data of the matching
rows from `left` and `right` are combined using `op`. If `op` returns a tuple or
NamedTuple, and `ascolumns` is set to true, the output table will contain the tuple
elements as separate data columns instead as a single column of resultant tuples.
"""
function naturaljoin(op, left::DNDSparse, right::DNDSparse)
out_domains = Any[]
out_chunks = Any[]
# if the output data type is a tuple and `columns` arg is true,
# we want the output to be a Columns rather than an array of tuples
for i in 1:length(left.chunks)
lchunk = left.chunks[i]
subdomain = left.domains[i]
lbrect = subdomain.boundingrect
# for each chunk in `left`
# find all the overlapping chunks from `right`
overlapping = map(boundingrect.(right.domains)) do rbrect
boxhasoverlap(lbrect, rbrect)
end
overlapping_chunks = right.chunks[overlapping]
# each overlapping chunk from `right` should be joined
# with the chunk `lchunk`
joined_chunks = map(overlapping_chunks) do r
delayed(naturaljoin)(op, lchunk, r)
end
append!(out_chunks, joined_chunks)
overlapping_domains = map(r->intersect(subdomain, r),
right.domains[overlapping])
append!(out_domains, overlapping_domains)
end
return fromchunks(out_chunks, domains = out_domains)
end
Base.map(f, x::DNDSparse{I}, y::DNDSparse{I}) where {I} = naturaljoin(x, y, f)
# left join
"""
leftjoin(left::DNDSparse, right::DNDSparse, [op::Function])
Keeps only rows with indices in `left`. If rows of the same index are
present in `right`, then they are combined using `op`. `op` by default
picks the value from `right`.
"""
function leftjoin(op, left::DNDSparse{K,V}, right::DNDSparse,
joinwhen = boxhasoverlap,
chunkjoin = leftjoin) where {K,V}
out_chunks = Any[]
for i in 1:length(left.chunks)
lchunk = left.chunks[i]
subdomain = left.domains[i]
lbrect = subdomain.boundingrect
# for each chunk in `left`
# find all the overlapping chunks from `right`
overlapping = map(rbrect -> joinwhen(lbrect, rbrect),
boundingrect.(right.domains))
overlapping_chunks = right.chunks[overlapping]
if !isempty(overlapping_chunks)
push!(out_chunks, delayed(chunkjoin)(op, lchunk, treereduce(delayed(_merge), overlapping_chunks)))
else
emptyop = delayed() do op, t
empty = NDSparse(similar(keys(t), 0), similar(values(t), 0))
chunkjoin(op, t, empty)
end
push!(out_chunks, emptyop(op, lchunk))
end
end
cache_thunks(DNDSparse{K,V}(left.domains, out_chunks))
end
leftjoin(left::DNDSparse, right::DNDSparse) = leftjoin(IndexedTables.concat_tup, left, right)
function asofpred(lbrect, rbrect)
allbutlast(x::Interval) = Interval(first(x)[1:end-1],
last(x)[1:end-1])
all(boxhasoverlap(lbrect, rbrect)) ||
(all(boxhasoverlap(allbutlast(lbrect),
allbutlast(rbrect))) &&
!isless(last(lbrect), first(rbrect)))
end
function asofjoin(left::DNDSparse, right::DNDSparse)
leftjoin(IndexedTables.right, left, right, asofpred, (op, x,y)->asofjoin(x,y))
end
function merge(left::DNDSparse{I1,D1}, right::DNDSparse{I2,D2}; agg=IndexedTables.right) where {I1,I2,D1,D2}
I = promote_type(I1, I2) # output index type
D = promote_type(D1, D2) # output data type
t = DNDSparse{I,D}(vcat(left.domains, right.domains),
vcat(left.chunks, right.chunks))
overlap_merge(x, y) = merge(x, y, agg=agg)
if has_overlaps(t.domains)
t = rechunk(t,
merge=(x...)->_merge(overlap_merge, x...),
closed=agg!==nothing,
sortchunks=false)
end
return cache_thunks(t)
end
function merge(left::DIndexedTable, right::DIndexedTable; chunks=nworkers())
l, r = rechunk_together(left, right, pkeynames(left), pkeynames(right); chunks=chunks)
fromchunks(delayedmap(merge, l.chunks, r.chunks))
end
function subbox(i::Interval, idx)
Interval(i.first[idx], i.last[idx])
end
function bcast_narrow_space(d, idxs, fst, lst)
intv = Interval(
tuplesetindex(d.interval.first, fst, idxs),
tuplesetindex(d.interval.last, lst, idxs)
)
box = Interval(
tuplesetindex(d.boundingrect.first, fst, idxs),
tuplesetindex(d.boundingrect.last, lst, idxs)
)
IndexSpace(intv, box, Nullable{Int}())
end
function broadcast(f, A::DNDSparse, B::DNDSparse; dimmap=nothing)
if ndims(A) < ndims(B)
broadcast((x,y)->f(y,x), B, A; dimmap=dimmap)
end
if dimmap === nothing
dimmap = match_indices(A, B)
end
common_A = Iterators.filter(i->dimmap[i] > 0, 1:ndims(A)) |> collect
common_B = Iterators.filter(i -> i>0, dimmap) |> collect
#@assert length(common_B) == length(common_A)
# for every bounding box in A, take compare common_A
# bounding boxes vs. every common_B bounding box
out_chunks = []
innerbcast(a, b) = broadcast(f, a, b; dimmap=dimmap)
out_domains = []
for (dA, cA) in zip(A.domains, A.chunks)
for (dB, cB) in zip(B.domains, B.chunks)
boxA = subbox(dA.boundingrect, common_A)
boxB = subbox(dB.boundingrect, common_B)
fst = map(max, boxA.first, boxB.first)
lst = map(min, boxA.last, boxB.last)
dmn = bcast_narrow_space(dA, common_A, fst, lst)
if boxhasoverlap(boxA, boxB)
push!(out_chunks, delayed(innerbcast)(cA, cB))
push!(out_domains, dmn)
end
end
end
with_overlaps(out_domains, out_chunks) do chunks
treereduce(delayed(_merge), chunks)
end
end
broadcasted(f, A::DNDSparse, B::DNDSparse) = broadcast(f, A, B)
function match_indices(A::DNDSparse{K1},B::DNDSparse{K2}) where {K1,K2}
if K1 <: NamedTuple && K2 <: NamedTuple
Ap = dimlabels(A)
Bp = dimlabels(B)
else
Ap = K1.parameters
Bp = K2.parameters
end
IndexedTables.find_corresponding(Ap, Bp)
end
## Deprecation
Base.@deprecate naturaljoin(left::DNDSparse, right::DNDSparse, op::Function) naturaljoin(op, left::DNDSparse, right::DNDSparse)
Base.@deprecate leftjoin(left::DNDSparse, right::DNDSparse, op::Function) leftjoin(op, left, right)
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 5138 | module ML
using JuliaDB, Dagger, OnlineStats, PooledArrays, Statistics
import Dagger: ArrayOp, DArray, treereduce
import JuliaDB: Dataset, DDataset, nrows
import Base: merge
# Core schema types
schema(xs::AbstractArray) = nothing # catch-all
schema(xs::AbstractArray, ::Nothing) = nothing # catch-all
merge(::Nothing, ::Nothing) = nothing
width(::Nothing) = 0
featuremat!(A, ::Nothing, xs) = A
schema(xs::ArrayOp) = schema(compute(xs))
schema(xs::ArrayOp, T) = schema(compute(xs), T)
#-----------------------------------------------------------------------# Continuous
struct Continuous
stat::Variance
end
function schema(xs::AbstractArray, ::Type{Continuous})
Continuous(fit!(Variance(), xs))
end
function schema(xs::AbstractArray{<:Real})
schema(xs, Continuous)
end
width(::Continuous) = 1
function merge(c1::Continuous, c2::Continuous)
Continuous(merge(c1.stat, c2.stat))
end
Statistics.mean(c::Continuous)::Float64 = mean(c.stat)
Statistics.std(c::Continuous)::Float64 = std(c.stat)
function Base.show(io::IO, c::Continuous)
write(io, "Continous(μ=$(mean(c)), σ=$(std(c)))")
end
Base.@propagate_inbounds function featuremat!(A, c::Continuous, xs, dropmissing=Val(false))
m = mean(c)
s = std(c)
for i in 1:length(xs)
x = xs[i]
if dropmissing isa Val{true}
if ismissing(x)
continue
else
# A[i, 1] = (get(x) - m) / s
A[i, 1] = (x - m) / s
end
else
A[i, 1] = (x - m) / s
end
end
A
end
#-----------------------------------------------------------------------# Categorical
struct Categorical
stat::CountMap
end
function Categorical(xs::AbstractArray{T}) where {T}
Categorical(CountMap(Dict(zip(xs, 1:length(xs)))))
end
function schema(xs::AbstractArray{T}, ::Type{Categorical}) where {T}
Categorical(fit!(CountMap(T), xs))
end
function schema(xs::PooledArray)
schema(xs, Categorical)
end
Base.keys(c::Categorical) = keys(c.stat)
width(c::Categorical) = length(keys(c))
merge(c1::Categorical, c2::Categorical) = Categorical(merge(c1.stat, c2.stat))
function Base.show(io::IO, c::Categorical)
write(io, "Categorical($(collect(keys(c))))")
end
Base.@propagate_inbounds function featuremat!(A, c::Categorical, xs, dropmissing=Val(false))
ks = keys(c)
labeldict = Dict{eltype(ks), Int}(zip(ks, 1:length(ks)))
for i = 1:length(xs)
if dropmissing isa Val{true} && ismissing(xs[i])
continue
end
A[i, labeldict[xs[i]]] = one(eltype(A))
end
A
end
# distributed schema calculation
function schema(xs::DArray)
collect(treereduce(delayed(merge), delayedmap(x -> schema(x), xs.chunks)))
end
function schema(xs::DArray, ::Type{Continuous})
collect(treereduce(delayed(merge), delayedmap(x -> schema(x, Continuous), xs.chunks)))
end
function schema(xs::DArray, ::Type{Categorical})
collect(treereduce(delayed(merge), delayedmap(x -> schema(x, Categorical), xs.chunks)))
end
struct Maybe{T}
feature::T
end
function schema(xs::Vector{Union{Missing,S}}, T::Type) where {S}
Maybe(schema(collect(skipmissing(xs)), T))
end
schema(xs::Vector{Union{T,Missing}}) where {T} = Maybe(schema(collect(skipmissing(xs))))
width(c::Maybe) = width(c.feature) + 1
merge(m1::Maybe, m2::Maybe) = Maybe(merge(m1.feature, m2.feature))
nulls(xs) = map(ismissing, xs)
Base.@propagate_inbounds function featuremat!(A, c::Maybe, xs, dropmissing=Val(true))
copyto!(A, CartesianIndices((1:length(xs), 1:1)), reshape(nulls(xs), (length(xs), 1)), CartesianIndices((1:length(xs), 1:1)))
featuremat!(view(A, 1:length(xs), 2:size(A, 2)), c.feature, xs, Val(true))
A
end
# Schema inference
const Schema = Dict{Symbol,Any}
# vecTs: type of column vectors in each chunk
function schema(cols, names; hints=Dict())
d = Schema()
for (col, name) in zip(cols, names)
if haskey(hints, name)
d[name] = schema(col, hints[name])
else
d[name] = schema(col)
end
end
d
end
function schema(t::Union{Dataset, DDataset}; hints=Dict())
schema(collect(columns(t)), colnames(t), hints=hints)
end
width(sch::Schema) = sum(width(s) for s in values(sch))
function featuremat!(A, schemas::Schema, t::Dataset)
j = 0
for col in keys(schemas)
schema = schemas[col]
featuremat!(view(A, 1:length(t), j+1:j+width(schema)),
schema, column(t, col))
j += width(schema)
end
A
end
splitschema(xs::Schema, ks...) =
filter((k,v) -> k ∉ ks, xs),
filter((k,v) -> k ∈ ks, xs)
function featuremat(sch, xs)
featuremat!(zeros(Float32, length(xs), width(sch)), sch, xs)'
end
featuremat(t) = featuremat(schema(t), t)
function featuremat(s, t::DDataset)
t = compute(t)
w = width(s)
h = length(t)
lengths = get.(nrows.(t.domains))
domains = Dagger.DomainBlocks((1,1), ([w], cumsum(lengths)))
DArray(
Float32,
Dagger.ArrayDomain(w, sum(lengths)),
domains,
reshape(delayedmap(x->featuremat(s,x), t.chunks), (1, length(t.chunks)))
)
end
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 17716 | boundingrect(x::IndexSpace) = x.boundingrect
interval(x::IndexSpace) = x.interval
"""
DNDSparse{K,V} <: AbstractNDSparse
A distributed [NDSparse](@ref) datastructure. Can be constructed by:
- [`ndsparse`](@ref) from Julia objects
- [`loadndsparse`](@ref) from data on disk
- [`distribute`](@ref) from an [`NDSparse`](@ref) object
"""
mutable struct DNDSparse{K,V} <: AbstractNDSparse
domains::Vector{IndexSpace{K}}
chunks::Vector
end
const DDataset = Union{DIndexedTable, DNDSparse}
function ndsparse(::Val{:distributed}, ks::Tup,
vs::Union{Tup, AbstractArray};
agg=nothing,
allowoverlap=agg === nothing,
closed=true, chunks=nothing, kwargs...)
if chunks === nothing
# this means the vectors are distributed.
# pick the first distributed vector and distribute
# all others similarly
i = findfirst(x->isa(x, ArrayOp), ks)
if i != 0
darr = ks[i]
elseif vs isa Tup && any(x->isa(x, ArrayOp, vs))
darr = vs[findfirst(x->isa(x, ArrayOp), vs)]
elseif isa(vs, ArrayOp)
darr = vs
else
error("Don't know how to distribute. specify `chunks`")
end
chunks = domainchunks(compute(darr))
end
kdarrays = map(x->distribute(x, chunks), ks)
vdarrays = isa(vs, Tup) ? map(x->distribute(x, chunks), vs) : distribute(vs, chunks)
if isempty(kdarrays)
error("NDSparse must be constructed with at least one index column")
end
nchunks = length(kdarrays[1].chunks)
inames = isa(ks, NamedTuple) ? keys(ks) : nothing
ndims = length(ks)
dnames = isa(vs, NamedTuple) ? keys(vs) : nothing
iscols = isa(vs, Tup)
function makechunk(args...)
k = Columns(args[1:ndims], names=inames)
v = iscols ? Columns(args[ndims+1:end], names=dnames) : args[end]
ndsparse(k,v; agg=agg, kwargs...)
end
cs = Array{Any}(undef, nchunks)
for i = 1:nchunks
args = Any[map(x->x.chunks[i], kdarrays)...]
append!(args, isa(vs, Tup) ? [map(x->x.chunks[i], vdarrays)...] :
[vdarrays.chunks[i]])
cs[i] = delayed(makechunk)(args...)
end
fromchunks(cs, closed=closed,
merge=(x,y)->merge(x,y, agg=agg),
allowoverlap=allowoverlap)
end
function ndsparse(x::Dagger.DArray{<:Tup}, y; kwargs...)
ndsparse(columns(x), y; kwargs...)
end
function ndsparse(x::DNDSparse; kwargs...)
ndsparse(columns(x, pkeynames(x)), values(x); kwargs...)
end
Base.eltype(dt::DNDSparse{K,V}) where {K,V} = V
Base.keytype(dt::DNDSparse{K,V}) where {K,V} = IndexedTables.astuple(K)
IndexedTables.dimlabels(dt::DNDSparse{K}) where {K} = fieldnames(K)
Base.ndims(dt::DNDSparse{K}) where {K} = fieldcount(K)
keytype(dt::DNDSparse{K}) where {K} = astuple(K)
# TableLike API
Base.@pure function IndexedTables.colnames(t::DNDSparse{K,V}) where {K,V}
dnames = V<:Tup ? fieldnames(V) : (1,)
if dnames isa Tuple{Vararg{Integer}}
dnames = map(x->x+fieldcount(K), dnames)
end
(fieldnames(K)..., dnames...)
end
Base.@pure pkeynames(t::DNDSparse{K,V}) where {K, V} = (fieldnames(K)...,)
function IndexedTables.valuenames(t::DNDSparse{K,V}) where {K,V}
if V <: Tup
if V<:NamedTuple
(fieldnames(V)...,)
else
((ndims(t) .+ (1:fieldcount(V)))...,)
end
else
ndims(t) + 1
end
end
const compute_context = Ref{Union{Nothing, Context}}(nothing)
get_context() = compute_context[] == nothing ? Context() : compute_context[]
"""
compute(t::DNDSparse; allowoverlap, closed)
Computes any delayed-evaluations in the `DNDSparse`.
The computed data is left on the worker processes.
Subsequent operations on the results will reuse the chunks.
If `allowoverlap` is false then the computed data is re-sorted if required to have no
chunks with overlapping index ranges if necessary.
If `closed` is true then the computed data is re-sorted if required to have no
chunks with overlapping OR continuous boundaries.
See also [`collect`](@ref).
!!! warning
`compute(t)` requires at least as much memory as the size of the
result of the computing `t`. You usually don't need to do this for the whole dataset.
If the result is expected to be big, try `compute(save(t, "output_dir"))` instead.
See [`save`](@ref) for more.
"""
compute(t::DNDSparse; kwargs...) = compute(get_context(), t; kwargs...)
function compute(ctx, t::DNDSparse; allowoverlap=true, closed=false)
if any(Dagger.istask, t.chunks)
# we need to splat `thunks` so that Dagger knows the inputs
# are thunks and they need to be staged for scheduling
vec_thunk = delayed((refs...) -> [refs...]; meta=true)(t.chunks...)
cs = compute(ctx, vec_thunk) # returns a vector of Chunk objects
t1 = fromchunks(cs, allowoverlap=allowoverlap, closed=closed)
compute(t1)
else
map(Dagger.unrelease, t.chunks) # don't let this be freed
foreach(Dagger.persist!, t.chunks)
t
end
end
"""
collect(t::DNDSparse)
Gets distributed data in a DNDSparse `t` and merges it into
[NDSparse](#IndexedTables.NDSparse) object
!!! warning
`collect(t)` requires at least as much memory as the size of the
result of the computing `t`. If the result is expected to be big,
try `compute(save(t, "output_dir"))` instead. See [`save`](@ref) for more.
This data can be loaded later using [`load`](@ref).
"""
collect(t::DNDSparse) = collect(get_context(), t)
function Base.copy(x::DNDSparse{K,V}) where {K, V}
fromchunks(NDSparse,
delayedmap(copy, x.chunks),
domains=x.domains,
KV=(K,V))
end
function collect(ctx::Context, dt::DNDSparse{K,V}) where {K,V}
cs = dt.chunks
if length(cs) > 0
collect(ctx, treereduce(delayed(_merge), cs))
else
ndsparse(similar(IndexedTables.arrayof(K), 0),
similar(IndexedTables.arrayof(V), 0))
end
end
# Fast-path merge if the data don't overlap
function _merge(f, a::NDSparse, b::NDSparse)
if isempty(a)
b
elseif isempty(b)
a
elseif last(a.index) < first(b.index)
# can vcat
NDSparse(vcat(a.index, b.index), vcat(a.data, b.data),
presorted=true, copy=false)
elseif last(b.index) < first(a.index)
_merge(b, a)
else
f(a, b) # Keep equal index elements
end
end
_merge(f, x::NDSparse) = x
function _merge(f, x::NDSparse, y::NDSparse, ys::NDSparse...)
treereduce((a,b)->_merge(f, a, b), [x,y,ys...])
end
_merge(x::NDSparse, y::NDSparse...) = _merge((a,b) -> merge(a, b, agg=nothing), x, y...)
"""
map(f, t::DNDSparse)
Applies a function `f` on every element in the data of table `t`.
"""
Base.map(f, dt::DNDSparse) = mapchunks(c->map(f, c), dt)
struct EmptySpace{T} end
has_empty_values(domain::Pair{<:Any, <:EmptySpace}) = true
has_empty_values(domain::EmptySpace) = true
has_empty_values(domain) = false
# Teach dagger how to automatically figure out the
# metadata (in dagger parlance "domain") about an NDSparse chunk.
function Dagger.domain(nd::NDSparse)
T = eltype(keys(nd))
if isempty(nd)
return EmptySpace{T}()
end
wrap = T<:NamedTuple ? T∘tuple : tuple
interval = Interval(wrap(first(nd.index)...), wrap(last(nd.index)...))
# cs = astuple(nd.index.columns)
# extr = map(extrema, cs[2:end]) # we use first and last value of first column
# boundingrect = Interval(wrap(first(cs[1]), map(first, extr)...),
# wrap(last(cs[1]), map(last, extr)...))
return IndexSpace(interval, interval, Nullable{Int}(length(nd)))
end
function subindexspace(t::Union{NDSparse, IndexedTable}, r)
ks = pkeys(t)
T = eltype(typeof(ks))
wrap = T<:NamedTuple ? T∘tuple : tuple
if isempty(r)
return EmptySpace{T}()
end
interval = Interval(wrap(ks[first(r)]...), wrap(ks[last(r)]...))
cs = astuple(columns(ks))
extr = map(c -> extrema_range(c, r), cs[2:end])
boundingrect = Interval(wrap(cs[1][first(r)], map(first, extr)...),
wrap(cs[1][last(r)], map(last, extr)...))
return IndexSpace(interval, boundingrect, Nullable{Int}(length(r)))
end
Base.eltype(::IndexSpace{T}) where {T} = T
Base.eltype(::EmptySpace{T}) where {T} = T
Base.isempty(::EmptySpace) = true
Base.isempty(::IndexSpace) = false
nrows(td::IndexSpace) = td.nrows
nrows(td::EmptySpace) = Nullable(0)
Base.ndims(::IndexSpace{T}) where {T} = fieldcount(T)
Base.ndims(::EmptySpace{T}) where {T} = fieldcount(T)
Base.first(td::IndexSpace) = first(td.interval)
Base.last(td::IndexSpace) = last(td.interval)
mins(td::IndexSpace) = first(td.boundingrect)
maxes(td::IndexSpace) = last(td.boundingrect)
function Base.merge(d1::IndexSpace, d2::IndexSpace, collisions=true)
n = collisions || isnull(d1.nrows) || isnull(d2.nrows) ?
Nullable{Int}() :
Nullable(get(d1.nrows) + get(d2.nrows))
interval = merge(d1.interval, d2.interval)
boundingrect = boxmerge(d1.boundingrect, d2.boundingrect)
IndexSpace(interval, boundingrect, n)
end
Base.merge(d1::IndexSpace, d2::EmptySpace) = d1
Base.merge(d1::EmptySpace, d2::Union{IndexSpace, EmptySpace}) = d2
function Base.intersect(d1::IndexSpace, d2::IndexSpace)
interval = intersect(d1.interval, d2.interval)
boundingrect = intersect(d1.boundingrect, d2.boundingrect)
IndexSpace(interval, boundingrect, Nullable{Int}())
end
function Base.intersect(d1::EmptySpace, d2::Union{IndexSpace,EmptySpace})
d1
end
# given a chunks index constructed above, give an array of
# index spaces spanned by the chunks in the index
function index_spaces(t::NDSparse)
intervals = map(x-> Interval(map(first, x), map(last, x)), t.index)
boundingrects = map(x-> Interval(map(first, x), map(last, x)), columns(t.data).boundingrect)
map(IndexSpace, intervals, boundingrects, columns(t.data).length)
end
"""
The length of the `DNDSparse` if it can be computed. Will throw an error if not.
You can get the length of such tables after calling [`compute`](@ref) on them.
"""
function Base.length(t::DNDSparse)
l = trylength(t)
if isnull(l)
error("The length of the DNDSparse is not yet known since some of its parts are not yet computed. Call `compute` to compute them, and then call `length` on the result of `compute`.")
else
get(l)
end
end
function _has_overlaps(firsts, lasts, closed)
if !issorted(firsts)
p = sortperm(firsts)
firsts = firsts[p]
lasts = lasts[p]
end
for i = 1:length(firsts)
s_i = firsts[i]
j = searchsortedfirst(lasts, s_i)
# allow repeated indices between chunks
if j != i && j <= length(lasts) && isless(s_i, lasts[j])
return true
elseif closed && j != i && j <= length(lasts) && s_i == lasts[j]
return true
end
end
return false
end
function has_overlaps(domains; closed=true)
_has_overlaps(first.(domains), last.(domains), closed)
end
function has_overlaps(domains, dims::AbstractVector)
sub(x) = x[dims]
fs = sub.(first.(domains))
ls = sub.(last.(domains))
_has_overlaps(fs, ls, true)
end
with_overlaps(f, t::DDataset, closed=false) =
isempty(t.domains) ? t : with_overlaps(f, t.domains, t.chunks, closed)
function with_overlaps(f, domains::AbstractVector, chunks::AbstractVector, closed=false)
if !issorted(domains, by=first)
perm = sortperm(domains, by = first)
domains = domains[perm]
chunks = chunks[perm]
end
stack = [domains[1]]
groups = [[1]]
for i in 2:length(domains)
sub = domains[i]
if hasoverlap(stack[end].interval, sub.interval)
stack[end] = merge(stack[end], sub)
push!(groups[end], i)
else
push!(stack, sub)
push!(groups, [i])
end
end
cs = collect([f(chunks[group]) for group in groups])
fromchunks(cs)
end
function fromchunks(::Type{<:NDSparse}, chunks::AbstractArray;
domains::AbstractArray = map(domain, chunks),
KV = getkvtypes(chunks),
merge=_merge,
closed = false,
allowoverlap = true)
nzidxs = findall(!isempty, domains)
# work around #228, fixed in Julia 1.1
if isempty(nzidxs)
nzidxs = Int[]
end
domains = domains[nzidxs]
dt = DNDSparse{KV...}(domains, chunks[nzidxs])
if !allowoverlap && has_overlaps(domains, closed=closed)
return rechunk(dt, closed=closed, merge=merge)
else
return dt
end
end
function cache_thunks(t)
for c in t.chunks
if isa(c, Dagger.Thunk)
Dagger.cache_result!(c)
end
end
t
end
function getkvtypes(::Type{N}) where N<:NDSparse
eltype(N.parameters[3]), N.parameters[1]
end
_promote_type(T,S) = promote_type(T,S)
_promote_type(T::Type{<:IndexTuple}, S::Type{<:IndexTuple}) = map_params(_promote_type, T, S)
_promote_type(T::Type{<:IndexTuple}, ::Type{Union{}}) = T
_promote_type(::Type{Union{}}, S::Type{<:IndexTuple}) = S
function getkvtypes(xs::AbstractArray)
kvtypes = getkvtypes.(chunktype.(xs))
K, V = kvtypes[1]
for (Tk, Tv) in kvtypes[2:end]
K = _promote_type(Tk, K)
V = _promote_type(Tv, V)
end
(K, V)
end
### Distribute a NDSparse into a DNDSparse
"""
distribute(itable::NDSparse, rowgroups::AbstractArray)
Distributes an NDSparse object into a DNDSparse by splitting it up into chunks
of `rowgroups` elements. `rowgroups` is a vector specifying the number of
rows in the chunks.
Returns a `DNDSparse`.
"""
function distribute(nds::NDSparse{V}, rowgroups::AbstractArray;
allowoverlap = true, closed = false) where V
splits = cumsum([0; rowgroups])
if splits[end] != length(nds)
throw(ArgumentError("the row groups don't add up to total number of rows"))
end
ranges = map(UnitRange, splits[1:end-1].+1, splits[2:end])
# this works around locality optimizations in Dagger to make
# sure that the parts get distributed instead of being left on
# the master process - which would lead to all operations being serial.
chunks = map(r->delayed(identity)(subtable(nds, r)), ranges)
domains = map(r->subindexspace(nds, r), ranges)
realK = eltypes(typeof(columns(nds.index)))
cache_thunks(fromchunks(chunks, domains=domains, KV = (realK, V),
allowoverlap=allowoverlap, closed=closed))
end
distribute(t::DNDSparse, cs) = ndsparse(t, chunks=cs)
"""
distribute(itable::NDSparse, nchunks::Int=nworkers())
Distributes an NDSparse object into a DNDSparse of `nchunks` chunks
of approximately equal size.
Returns a `DNDSparse`.
"""
function distribute(nds::NDSparse, nchunks::Int=nworkers();
allowoverlap = true, closed = false)
N = length(nds)
q, r = divrem(N, nchunks)
nrows = vcat(collect(_repeated(q, nchunks)))
nrows[end] += r
distribute(nds, nrows; allowoverlap=allowoverlap, closed=closed)
end
"""
mapchunks(f, t::DNDSparse; keeplengths=true)
Applies a function to each chunk in `t`. Returns a new DNDSparse.
If `keeplength` is false, this means that the lengths of the output
chunks is unknown before [`compute`](@ref). This function is used
internally by many DNDSparse operations.
"""
function mapchunks(f, t::DNDSparse{K,V}; keeplengths=true) where {K,V}
chunks = map(delayed(f), t.chunks)
if keeplengths
DNDSparse{K, V}(t.domains, chunks)
else
DNDSparse{K, V}(map(null_length, t.domains), chunks)
end
end
function null_length(x::IndexSpace)
IndexSpace(x.interval, x.boundingrect, Nullable{Int}())
end
function subtable(t::DNDSparse{K,V}, idx) where {K, V}
if isnull(trylength(t))
t = compute(t)
end
if isempty(idx)
return DNDSparse{K, V}(similar(t.domains, 0), similar(t.chunks, 0))
end
ls = map(x->get(nrows(x)), t.domains)
cumls = cumsum(ls)
i = searchsortedlast(cumls, first(idx))
j = searchsortedfirst(cumls, last(idx))
# clip first and last chunks
strt = first(idx) - get(cumls, i-1, 0)
fin = cumls[j] - last(idx)
ds = t.domains[i:j]
cs = convert(Vector{Any}, t.chunks[i:j])
if i==j
cs[1] = delayed(x->subtable(x, strt:length(x)-fin))(cs[1])
i = ds[1]
ds[1] = IndexSpace(i.interval, i.boundingrect, Nullable(length(idx)))
else
cs[1] = delayed(x->subtable(x, strt:length(x)))(cs[1])
cs[end] = delayed(x->subtable(x, 1:length(x)-fin))(cs[end])
ds[1] = null_length(ds[1])
ds[end] = null_length(ds[2])
end
DNDSparse{K,V}(ds, cs)
end
function Base.show(io::IO, big::DNDSparse)
h, w = displaysize(io)
showrows = h - 5 # This will trigger an ellipsis when there's
# more to see than the screen fits
t = first(Iterators.partition(big, showrows))
if !(values(t) isa Columns)
cnames = colnames(keys(t))
eltypeheader = "$(eltype(t))"
else
cnames = colnames(t)
nf = fieldcount(eltype(t))
if eltype(t) <: NamedTuple
eltypeheader = "$(nf) field named tuples"
else
eltypeheader = "$(nf)-tuples"
end
end
len = trylength(big)
vals = isnull(len) ? "of" : "with $(get(len)) values"
header = "$(ndims(t))-d Distributed NDSparse $vals ($eltypeheader) in $(length(big.chunks)) chunks:"
showtable(io, t; header=header, cnames=cnames,
divider=ndims(t), ellipsis=:end)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1460 | #-----------------------------------------------------------------# partitionplot
@userplot struct PartitionPlot
args
end
@recipe function f(o::PartitionPlot; nparts=100, stat=Extrema(), by=nothing, dropmissing=false)
t = o.args[1]
sel = map(x -> lowerselection(t, x), o.args[2:end])
# Create the (Indexed)Partition object
o = if length(sel) == 1
Partition(stat, nparts)
elseif length(sel) == 2
T = typeof(collect(rows(t)[1])[sel[1]]) # Type of first selection
IndexedPartition(T, stat, nparts)
else
throw(ArgumentError("too many arguments for partitionplot"))
end
# Wrap the (Indexed)Partition in an FTSeries to possibly remove missing values
s = FTSeries(o; filter = dropmissing ? !IndexedTables._ismissing : x -> true)
if by === nothing
reduce(s, t; select=sel)
else
grp = groupreduce(s, t, by; select=sel)
grp = isa(t, DDataset) ? collect(grp) : grp
for row in rows(grp)
@series begin row[2] end
end
end
end
"""
partitionplot(table, y; stat=Extrema(), nparts=100, by=nothing, dropmissing=false)
partitionplot(table, x, y; stat=Extrema(), nparts=100, by=nothing, dropmissing=false)
Plot a summary of variable `y` against `x` (`1:length(y)` if not specified). Using `nparts`
approximately-equal sections along the x-axis, the data in `y` over each section is
summarized by `stat`.
"""
partitionplot
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 4365 | _merger(f) = f
_merger(f::OnlineStat) = merge
_merger(f::Tup) = map(_merger, f)
function reduce(f, t::DDataset; dims = nothing, cache = false, kw...)
if dims !== nothing
if !isa(t, DNDSparse)
error("`dims` only supported for NDSparse")
end
if !isempty(kw)
error("unsupported keyword arguments $kw")
end
return _reducedim(f, t, dims, cache)
end
if haskey(kw, :init)
return reduce_inited(f, t, kw.init, get(kw, :select, nothing))
end
select = get(kw, :select, valuenames(t))
xs = delayedmap(t.chunks) do x
f = isa(f, OnlineStat) ? copy(f) : f # required for > 1 chunks on the same proc
reduce(f, x; select=select)
end
if f isa Tup
g, _ = IndexedTables.init_funcs(f, false)
else
g = f
end
h = (a,b)->IndexedTables._apply(_merger(g), a,b)
collect(get_context(), treereduce(delayed(h), xs))
end
function reduce_inited(f, t::DDataset, v0, select)
xs = delayedmap(t.chunks) do x
f = isa(f, OnlineStat) ? copy(f) : f
reduce(f, x; select=select === nothing ? rows(x) : select)
end
g = isa(f, OnlineStat) ? merge : f
merge(collect(get_context(), treereduce(delayed(g), xs)), v0)
end
function groupreduce(f, t::DDataset, by=pkeynames(t); kwargs...)
@noinline function groupchunk(x)
groupreduce(f, x, by; kwargs...)
end
if f isa Tup || t isa DIndexedTable
g, _ = IndexedTables.init_funcs(f, false)
else
g = f
end
h = _merger(g)
if (f isa ApplyColwise) && f.functions isa Union{Function, Type}
mergef = (x,y) -> map(f.functions, x,y)
else
mergef = (x,y) -> IndexedTables._apply(h, x,y)
end
@noinline function mergechunk(x, y)
# use NDSparse's merge
if x isa IndexedTable
z = merge(_convert(NDSparse, x), _convert(NDSparse, y), agg=mergef)
_convert(IndexedTable, z)
else
merge(x,y, agg=mergef)
end
end
t1 = fromchunks(delayedmap(groupchunk, t.chunks))
with_overlaps(t1, true) do cs
treereduce(delayed(mergechunk), cs)
end
end
function groupby(f, t::DDataset, by=pkeynames(t);
select=(t isa DNDSparse ? valuenames(t) : Not(by)),
kwargs...)
by = lowerselection(t, by)
select = lowerselection(t, select)
if (by isa Tup) && isempty(by)
@noinline function _groupby(x)
groupby(f, x, by; select=select, kwargs...)
end
collect(get_context(), delayed(_groupby)(
treereduce(delayed(_merge), t.chunks)
)
)
elseif by != lowerselection(t, Keys()) || has_overlaps(t.domains, closed=true)
subsel = Tuple(setdiff(select, by))
# translate the new selection
t = rechunk(t, by, subsel)
newselect = map(select) do x
if x in by
findall(in(x), by)[1]
else
x1 = findall(in(x), subsel)
x1[1] + length(by)
end
end
return groupby(f, t; select=newselect, kwargs...)
else
@noinline function groupchunk(x)
groupby(f, x, by; select=select, kwargs...)
end
return fromchunks(delayedmap(groupchunk, t.chunks))
end
end
function _reducedim(f, x::DNDSparse, dims, cache)
keep = setdiff([1:ndims(x);], dims) # TODO: Allow symbols
if isempty(keep)
throw(ArgumentError("to remove all dimensions, use `reduce(f, A)`"))
end
groupreduce(f, x, (keep...,), select=valuenames(x), cache=cache)
end
_reducedim(f, x::DNDSparse, dims::Symbol, cache) = _reducedim(f, x, [dims], cache)
"""
reducedim_vec(f::Function, t::DNDSparse, dims)
Like `reducedim`, except uses a function mapping a vector of values to a scalar instead of a 2-argument scalar function.
See also [`reducedim`](@ref).
"""
function reducedim_vec(f, x::DNDSparse, dims)
keep = setdiff([1:ndims(x);], dims)
if isempty(keep)
throw(ArgumentError("to remove all dimensions, use `reduce(f, A)`"))
end
t = selectkeys(x, (keep...,); agg=nothing)
cache_thunks(groupby(f, t))
end
reducedim_vec(f, x::DNDSparse, dims::Symbol) = reducedim_vec(f, x, [dims])
Base.@deprecate aggregate_stats(s, t; by=pkeynames(t), with=valuenames(t)) groupreduce(s, t, by; select=with)
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1059 | function stack(t::DDataset, by = pkeynames(t); select = isa(t, DNDSparse) ? valuenames(t) : excludecols(t, by),
variable = :variable, value = :value)
function stackchunk(x)
stack(x, by; select=select, variable=variable, value=value)
end
return fromchunks(delayedmap(stackchunk, t.chunks))
end
function unstack(::Type{D}, ::Type{T}, key, val, cols::AbstractVector{S}) where {D <:DDataset, T, S}
D1 = D isa DNDSparse ? NDSparse : IndexedTable
function unstackchunk(x, y)
unstack(D1, T, x, y, cols)
end
fromchunks(delayedmap(unstackchunk, key.chunks, val.chunks))
end
function unstack(t::D, by = pkeynames(t); variable = :variable, value = :value) where {D<:DDataset}
tgrp = groupby((value => identity,), t, by, select = (variable, value))
S = eltype(colnames(t))
col = column(t, variable)
cols = S.(collect(Dagger.treereduce(delayed(union), delayedmap(unique, col.chunks))))
T = eltype(columns(t, value))
unstack(D, Base.nonmissingtype(T), pkeys(tgrp), columns(tgrp, value), cols)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 2861 | function Base.map(f, t::DDataset; select=nothing)
# TODO: fix when select has a user-supplied vector
delayedmap(t.chunks) do x
map(f, x; select=select)
end |> fromchunks
end
function dropmissing(t::DDataset, select=(colnames(t)...,))
delayedmap(t.chunks) do x
dropmissing(x, select)
end |> fromchunks
end
function convertmissing(t::DDataset, missingtype)
delayedmap(t.chunks) do x
convertmissing(x, missingtype)
end |> fromchunks
end
function Base.filter(f, t::DDataset; select=isa(f, Union{Tuple, Pair}) ? nothing : valuenames(t))
g = delayed(x -> filter(f, x; select=select))
cache_thunks(fromchunks(map(g, t.chunks)))
end
function selectkeys(x::DNDSparse, which; kwargs...)
ndsparse(rows(keys(x), which), values(x); kwargs...)
end
function selectvalues(x::DNDSparse, which; kwargs...)
ndsparse(keys(x), rows(values(x), which); kwargs...)
end
Base.@deprecate select(x::DNDSparse, conditions::Pair...) filter(conditions, x)
Base.@deprecate select(x::DNDSparse, which::DimName...; kwargs...) selectkeys(x, which; kwargs...)
"""
convertdim(x::DNDSparse, d::DimName, xlate; agg::Function, name)
Apply function or dictionary `xlate` to each index in the specified dimension.
If the mapping is many-to-one, `agg` is used to aggregate the results.
`name` optionally specifies a name for the new dimension. `xlate` must be a
monotonically increasing function.
See also [`reduce`](@ref)
"""
function convertdim(t::DNDSparse{K,V}, d::DimName, xlat;
agg=nothing, vecagg=nothing, name=nothing) where {K,V}
if isa(d, Symbol)
dn = findfirst(dimlabels(t), d)
if dn == 0
throw(ArgumentError("table has no dimension \"$d\""))
end
d = dn
end
chunkf(c) = convertdim(c, d, xlat; agg=agg, vecagg=nothing, name=name)
chunks = map(delayed(chunkf), t.chunks)
xlatdim(intv, d) = Interval(tuplesetindex(first(intv), xlat(first(intv)[d]), d),
tuplesetindex(last(intv), xlat(last(intv)[d]), d))
# TODO: handle name kwarg
# apply xlat to bounding rectangles
domains = map(t.domains) do space
nrows = agg === nothing ? space.nrows : Nullable{Int}()
IndexSpace(xlatdim(space.interval, d), xlatdim(space.boundingrect, d), nrows)
end
t1 = DNDSparse{eltype(domains[1]),V}(domains, chunks)
if agg !== nothing && has_overlaps(domains)
overlap_merge(x, y) = merge(x, y, agg=agg)
chunk_merge(ts...) = _merge(overlap_merge, ts...)
cache_thunks(rechunk(t1, merge=chunk_merge, closed=true))
elseif vecagg != nothing
groupby(vecagg, t1) # already cached
else
cache_thunks(t1)
end
end
keyindex(t::DNDSparse, i::Int) = i
keyindex(t::DNDSparse{K}, i::Symbol) where {K} = findfirst(x->x===i, fieldnames(K))
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 2480 | #-----------------------------------------------------------------------# DataValueArray
function mmwrite(io::AbstractSerializer, xs::DataValueArray)
Serialization.serialize_type(io, MMSer{DataValueArray})
mmwrite(io, BitArray(xs.isna))
mmwrite(io, xs.values)
end
function mmread(::Type{DataValueArray}, io, mmap)
isnull = deserialize(io)
vals = deserialize(io)
DataValueArray(vals, isnull)
end
#-----------------------------------------------------------------------# PooledArray
function mmwrite(io::AbstractSerializer, xs::PooledArray)
Serialization.serialize_type(io, MMSer{PooledArray})
mmwrite(io, xs.refs)
mmwrite(io, xs.invpool)
end
function mmread(::Type{PooledArray}, io, mmap)
refs = deserialize(io)
invpool = deserialize(io)
PooledArray(PooledArrays.RefArray(refs), invpool)
end
#-----------------------------------------------------------------------# Columns
function mmwrite(io::AbstractSerializer, xs::Columns)
Serialization.serialize_type(io, MMSer{Columns})
if eltype(xs) <: NamedTuple
fnames = fieldnames(eltype(xs))
else
fnames = length(columns(xs))
end
serialize(io, fnames)
for c in columns(xs)
mmwrite(io, c)
end
end
function mmread(::Type{Columns}, io, mmap)
fnames = deserialize(io)
if isa(fnames, Int)
cols = [deserialize(io) for i=1:fnames]
Columns(Tuple(cols))
else
cols = [deserialize(io) for i=1:length(fnames)]
Columns(Tuple(cols); names=fnames)
end
end
#-----------------------------------------------------------------------# NDSparse
function mmwrite(io::AbstractSerializer, xs::NDSparse)
Serialization.serialize_type(io, MMSer{NDSparse})
flush!(xs)
mmwrite(io, xs.index)
mmwrite(io, xs.data)
end
function mmread(::Type{NDSparse}, io, mmap)
idx = deserialize(io)
data = deserialize(io)
NDSparse(idx, data, presorted=true, copy=false)
end
#-----------------------------------------------------------------------# IndexedTable
function mmwrite(io::AbstractSerializer, xs::IndexedTable)
Serialization.serialize_type(io, MMSer{IndexedTable})
#flush!(xs)
mmwrite(io, rows(xs))
mmwrite(io, xs.pkey)
mmwrite(io, xs.perms)
end
function mmread(::Type{IndexedTable}, io, mmap)
data = deserialize(io)
pkey = deserialize(io)
perms = deserialize(io)
table(data, pkey=pkey, perms=perms, presorted=true, copy=false)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 3870 | function reindex(t::DDataset, by=pkeynames(t), select=excludecols(t, by); kwargs...)
@noinline function _rechunk(c)
reindex(c, by, select; kwargs...)
end
fromchunks(delayedmap(_rechunk, t.chunks))
end
"""
`rechunk(t::Union{DNDSparse, DNDSparse}[, by[, select]]; <options>)`
Reindex and sort a distributed dataset by keys selected by `by`.
Optionally `select` specifies which non-indexed fields are kept. By default this is all fields not mentioned in `by` for Table and the value columns for NDSparse.
# Options:
- `chunks` -- how to distribute the data. This can be:
1. An integer -- number of chunks to create
2. An vector of `k` integers -- number of elements in each of the `k` chunks. `sum(k)` must be same as `length(t)`
3. The distribution of another array. i.e. `vec.subdomains` where `vec` is a distributed array.
- `merge::Function` -- a function which merges two sub-table or sub-ndsparse into one NDSparse. They may have overlaps in their indices.
- `splitters::AbstractVector` -- specify keys to split by. To create `n` chunks you would need to pass `n-1` splitters and also the `chunks=n` option.
- `chunks_sorted::Bool` -- are the chunks sorted locally? If true, this skips sorting or re-indexing them.
- `affinities::Vector{<:Integer}` -- which processes (Int pid) should each output chunk be created on. If unspecified all workers are used.
- `closed::Bool` -- if true, the same key will not be present in multiple chunks (although sorted). `true` by default.
- `nsamples::Integer` -- number of keys to randomly sample from each chunk to estimate splitters in the sorting process. (See [samplesort](https://en.wikipedia.org/wiki/Samplesort)). Defaults to 2000.
- `batchsize::Integer` -- how many chunks at a time from the input should be loaded into memory at any given time. This will essentially sort in batches of `batchsize` chunks.
"""
function rechunk(dt::DDataset,
by=pkeynames(dt),
select=dt isa DIndexedTable ? excludecols(dt, by) : valuenames(dt);
merge=_merge,
splitters=nothing,
chunks_presorted=false,
affinities=workers(),
chunks=nworkers(),
closed=true,
sortchunks=true,
nsamples=2000,
batchsize=max(2, nworkers()))
if sortchunks
perm = sortperm(dt.domains, by=first)
cs = dt.chunks[perm]
else
cs = dt.chunks
end
function sortandsample(data, nsamples, presorted)
r = sample(1:length(data), min(length(data), nsamples),
replace=false, ordered=true)
sorted = !presorted ? reindex(data, by, select) : data
chunk = !presorted ? tochunk(sorted) : nothing
(chunk, pkeys(sorted)[r])
end
dsort_chunks(cs, chunks, nsamples,
batchsize=batchsize,
sortandsample=sortandsample,
affinities=affinities,
splitters=splitters,
chunks_presorted=chunks_presorted,
merge=merge,
by=pkeys,
sub=subtable) |> fromchunks
end
### Permutedims
function Base.permutedims(t::DNDSparse{K,V}, p::AbstractVector) where {K,V}
if !(length(p) == ndims(t) && isperm(p))
throw(ArgumentError("argument to permutedims must be a valid permutation"))
end
permuteintv(intv,d) = Interval(first(intv)[d],
last(intv)[d])
idxs = map(t.domains) do dmn
IndexSpace(permuteintv(dmn.interval, p),
permuteintv(dmn.boundingrect, p),
dmn.nrows,
)
end
chunks = map(delayed(c -> permutedims(c, p)), t.chunks)
t1 = DNDSparse{eltype(idxs[1]), V}(idxs, chunks)
cache_thunks(rechunk(t1))
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 7885 | const IndexTuple = Union{Tuple, NamedTuple}
"""
IndexSpace(interval, boundingrect, nrows)
Metadata about an chunk.
- `interval`: An `Interval` object with the first and the last index tuples.
- `boundingrect`: An `Interval` object with the lowest and the highest indices as tuples.
- `nrows`: A `Nullable{Int}` of number of rows in the NDSparse, if knowable.
"""
struct IndexSpace{T<:IndexTuple}
interval::Interval{T}
boundingrect::Interval{T}
nrows::Nullable{Int}
end
"""
A distributed table
"""
mutable struct DIndexedTable{T,K} <: AbstractIndexedTable
# primary key columns
pkey::Vector{Int}
# extent of values in the pkeys
domains::Vector{IndexSpace}
chunks::Vector
end
noweakref(w::WeakRefString) = string(w)
noweakref(x) = x
function Dagger.domain(t::IndexedTable)
ks = pkeys(t)
T = eltype(ks)
if isempty(t)
return t.pkey => EmptySpace{T}()
end
wrap = T<:NamedTuple ? IndexedTables.namedtuple(fieldnames(T)...)∘tuple : tuple
interval = Interval(map(noweakref, first(ks)), map(noweakref, last(ks)))
cs = astuple(columns(ks))
extr = map(extrema, cs[2:end]) # we use first and last value of first column
boundingrect = Interval(wrap(noweakref(first(cs[1])), noweakref.(map(first, extr))...),
wrap(noweakref(last(cs[1])), noweakref.(map(last, extr))...))
return t.pkey => IndexSpace(interval, boundingrect, Nullable{Int}(length(t)))
end
# if one of the input vectors is a Dagger operation / array
# chose the distributed implementation.
IndexedTables._impl(::Val, ::Dagger.ArrayOp, x...) = Val(:distributed)
function table(::Val{:distributed}, tup::Tup; chunks=nothing, kwargs...)
if chunks === nothing
# this means the vectors are distributed.
# pick the first distributed vector and distribute
# all others similarly
idx = findfirst(x->isa(x, ArrayOp), tup)
if idx == 0
error("Don't know how to distribute. specify `chunks`")
end
darr = compute(tup[idx])
chunks = domainchunks(darr)
end
darrays = map(x->distribute(x, chunks), tup)
if isempty(darrays)
error("Table must be constructed with at least one column")
end
nchunks = length(darrays[1].chunks)
cs = Array{Any}(undef, nchunks)
names = isa(tup, NamedTuple) ? [keys(tup)...] : nothing
f = delayed((cs...) -> table(cs...; names=names, kwargs...))
for i = 1:nchunks
cs[i] = f(map(x->x.chunks[i], darrays)...)
end
fromchunks(cs)
end
# Copying constructor
function table(t::Union{IndexedTable, DIndexedTable};
columns=columns(t),
pkey=t.pkey,
presorted=false,
copy=true, kwargs...)
table(columns;
pkey=pkey,
presorted=presorted,
copy=copy, kwargs...)
end
Base.eltype(dt::DIndexedTable{T}) where {T} = T
function colnames(t::DIndexedTable{T}) where T
fieldnames(T)
end
function trylength(t)::Nullable{Int}
len = 0
for l in map(x->x.nrows, t.domains)
if !isnull(l)
len = len + get(l)
else
return nothing
end
end
return len
end
function Base.length(t::DIndexedTable)
l = trylength(t)
if isnull(l)
error("The length of the DNDSparse is not yet known since some of its parts are not yet computed. Call `compute` to compute them, and then call `length` on the result of `compute`.")
else
get(l)
end
end
"""
fromchunks(cs)
Construct a distributed object from chunks. Calls `fromchunks(T, cs)`
where T is the type of the data in the first chunk. Computes any thunks.
"""
function fromchunks(cs::AbstractArray, args...; output=nothing, fnoffset=0, kwargs...)
if output !== nothing
if !isdir(output)
mkdir(output)
end
cs = Any[(
fn = lpad(idx+fnoffset, 5, "0");
delayed(Dagger.savechunk, get_result=true)(
c, output, fn);
) for (idx, c) in enumerate(cs)]
vec_thunk = treereduce(delayed(vcat, meta=true), cs)
if length(cs) == 1
cs = [compute(get_context(), vec_thunk)]
else
cs = compute(get_context(), vec_thunk)
end
return fromchunks(cs)
elseif any(x->isa(x, Thunk), cs)
vec_thunk = delayed((refs...) -> [refs...]; meta=true)(cs...)
cs = compute(get_context(), vec_thunk)
end
T = chunktype(first(cs))
fromchunks(T, cs, args...; kwargs...)
end
function fromchunks(::Type{<:AbstractVector},
cs::AbstractArray, args...; kwargs...)
lengths = length.(domain.(cs))
dmnchunks = DomainBlocks((1,), (cumsum(lengths),))
T = promote_eltype_chunktypes(cs)
DArray(T, ArrayDomain(1:sum(lengths)),
dmnchunks, cs, dvcat)
end
function fromchunks(::Type{<:IndexedTable}, chunks::AbstractArray;
domains::AbstractArray = last.(domain.(chunks)),
pkey=first(domain(first(chunks))),
K = promote_eltypes(domains),
T = promote_eltype_chunktypes(chunks))
nzidxs = findall(!isempty, domains)
domains = domains[nzidxs]
DIndexedTable{T, K}(pkey, domains, chunks[nzidxs])
end
function promote_eltypes(ts::AbstractArray)
nonempty_domains = Iterators.filter(!has_empty_values, ts)
mapreduce(eltype, _promote_type, nonempty_domains, init = Union{})
end
function promote_eltype_chunktypes(ts::AbstractArray)
nonempty_chunks = Iterators.filter(!has_empty_values∘domain, ts)
mapreduce(eltype∘chunktype, _promote_type, nonempty_chunks, init = Union{})
end
"""
distribute(t::Table, chunks)
Distribute a table in `chunks` pieces. Equivalent to `table(t, chunks=chunks)`.
"""
distribute(t::IndexedTable, chunks) = table(t, chunks=chunks, copy=false)
compute(t::DIndexedTable; kwargs...) = compute(get_context(), t; kwargs...)
function compute(ctx, t::DIndexedTable; output=nothing)
if any(Dagger.istask, t.chunks)
fromchunks(IndexedTable, cs, output=output)
else
map(Dagger.unrelease, t.chunks) # don't let this be freed
foreach(Dagger.persist!, t.chunks)
t
end
end
distribute(t::DIndexedTable, cs) = table(t, chunks=cs)
collect(t::DIndexedTable) = collect(get_context(), t)
function collect(ctx::Context, dt::DIndexedTable{T}) where T
cs = dt.chunks
if length(cs) > 0
collect(ctx, treereduce(delayed(_merge), cs))
else
table(similar(IndexedTables.arrayof(T), 0), pkey=dt.pkey)
end
end
# merging two tables
function _merge(f, a::IndexedTable, b::IndexedTable)
if isempty(a.pkey) && isempty(b.pkey)
return table(vcat(rows(a), rows(b)))
end
@assert a.pkey == b.pkey
ia = pkeys(a)
ib = pkeys(b)
if isempty(a)
b
elseif isempty(b)
a
elseif last(ia) < first(ib)
# can vcat
table(map(vcat, columns(a), columns(b)), pkey=a.pkey, presorted=true, copy=false)
elseif last(ib) < first(ia)
_merge(f, b, a)
else
f(a,b)
end
end
_merge(f, x::IndexedTable) = x
function _merge(f, x::IndexedTable, y::IndexedTable, ys::IndexedTable...)
treereduce((a,b)->_merge(f, a, b), [x,y,ys...])
end
_merge(x::IndexedTable, y::IndexedTable...) = _merge((a,b) -> merge(a, b), x, y...)
function Base.show(io::IO, big::DIndexedTable)
h, w = displaysize(io)
showrows = h - 5 # This will trigger an ellipsis when there's
# more to see than the screen fits
t = first(Iterators.partition(big, showrows))
len = trylength(big)
vals = isnull(len) ? "" : " with $(get(len)) rows"
header = "Distributed Table$vals in $(length(big.chunks)) chunks:"
cstyle = Dict(i=>:bold for i in t.pkey)
showtable(io, t; header=header, ellipsis=:end, cstyle=cstyle)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 8585 | # treereduce method without v0 is in Dagger. Why does this live here?
function treereduce(f, xs, v0)
length(xs) == 0 && return v0
length(xs) == 1 && return xs[1]
l = length(xs)
m = div(l, 2)
f(treereduce(f, xs[1:m]), treereduce(f, xs[m+1:end]))
end
dvcat(x...; dims) = vcat(x...)
function subtable(nds::NDSparse, r)
NDSparse(keys(nds)[r], values(nds)[r], presorted=true, copy=false)
end
function subtable(t::IndexedTable, r)
t[r]
end
function extrema_range(x::AbstractArray{T}, r::UnitRange) where T
return first(x), last(x)
if !(1 <= first(r) && last(r) <= length(x))
throw(BoundsError(x, r))
end
isempty(r) && return extrema(x[r])
mn = x[first(r)]
mx = x[first(r)]
@inbounds @simd for i in r
mn = min(x[i], mn)
mx = max(x[i], mx)
end
mn, mx
end
# Data loading utilities
Base.@deprecate load_table(args...;kwargs...) loadndsparse(args...; distributed=false, kwargs...)
function prettify_filename(f)
f = basename(f)
if endswith(lowercase(f), ".csv")
f = f[1:end-4]
end
return f
end
function _loadtable_serial(T, file::Union{IO, AbstractString, AbstractArray};
delim=',',
indexcols=[],
datacols=nothing,
filenamecol=nothing,
agg=nothing,
presorted=false,
copy=false,
csvread=TextParse.csvread,
kwargs...)
#println("LOADING ", file)
count = Int[]
samecols = nothing
if indexcols !== nothing
if indexcols isa Union{Int, Symbol}
indexcols = (indexcols,)
end
samecols = collect(Iterators.filter(x->isa(x, Union{Tuple, AbstractArray}),
indexcols))
end
if datacols !== nothing
if datacols isa Union{Int, Symbol}
datacols = (datacols,)
end
append!(samecols, collect(Iterators.filter(x->isa(x, Union{Tuple, AbstractArray}),
datacols)))
end
if samecols !== nothing
samecols = map(x->map(string, x), samecols)
end
if isa(file, AbstractArray)
cols, header, count = csvread(file, delim;
samecols=samecols,
kwargs...)
else
cols, header = csvread(file, delim; kwargs...)
end
header = map(string, header)
if filenamecol !== nothing
# mimick a file name column
if filenamecol isa Pair
filenamecol, f = filenamecol
else
f = prettify_filename
end
if isa(file, AbstractArray)
namecol = reduce(vcat, fill.(f.(file), count))
else
namecol = fill(f(file), length(cols[1]))
end
cols = (namecol, cols...)
if !isempty(header)
pushfirst!(header, string(filenamecol))
end
end
if isempty(cols)
error("File contains no columns!")
end
n = length(first(cols))
implicitindex = false
## Construct Index
_indexcols = collect(map(x->lookupbyheader(header, x), indexcols))
if isempty(_indexcols)
implicitindex = true
index = Columns(([1:n;],))
else
indexcolnames = map(indexcols, _indexcols) do name, i
if i === nothing
error("Cannot index by unknown column $name")
else
isa(name, Int) ? canonical_name(header[name]) : canonical_name(name)
end
end
indexvecs = cols[_indexcols]
nullableidx = findall(x->eltype(x) <: Union{DataValue,Nullable} || Missing <: eltype(x), indexvecs)
if !isempty(nullableidx)
badcol_names = header[_indexcols[nullableidx]]
@warn("Indexed columns may contain Nullables or NAs. Column(s) with nullables: $(join(badcol_names, ", ", " and ")). This will result in wrong sorting.")
end
index = Columns(Tuple(indexvecs); names=indexcolnames)
end
## Construct Data
if datacols === nothing
_datacols = setdiff(1:length(cols), _indexcols)
datacols = header[_datacols]
else
_datacols = map(x->lookupbyheader(header, x), datacols)
end
if isempty(_datacols)
error("""You must specify at least one data column.
Either all columns in the file were indexed, or datacols was explicitly set to an empty array.""")
end
datacolnames = map(datacols, _datacols) do name, i
if i === nothing
if isa(name, Int)
error("Unknown column numbered $name specified in datacols")
else
return canonical_name(name) # use provided name for missing column
end
else
isa(name, Int) ? canonical_name(header[name]) : canonical_name(name)
end
end
datavecs = map(_datacols) do i
if i === nothing
# DataValueArray{Union{}}(n) # missing column
fill(missing, n)
else
cols[i]
end
end
data = Columns(Tuple(datavecs); names=datacolnames)
if T<:IndexedTable && implicitindex
table(data, copy = copy), true
else
convert(T, index, data, copy = copy), implicitindex
end
end
function lookupbyheader(header, key)
if isa(key, Symbol)
return lookupbyheader(header, string(key))
elseif isa(key, String)
return findfirst(x->x==key, header)
elseif isa(key, Int)
return 0 < key <= length(header) ? key : nothing
elseif isa(key, Tuple) || isa(key, Vector)
for k in key
x = lookupbyheader(header, k)
x != 0 && return x
end
return nothing
end
end
canonical_name(n::Symbol) = n
canonical_name(n::String) = Symbol(replace(n, r"\s" => "_"))
canonical_name(n::Union{Tuple, Vector}) = canonical_name(first(n))
function _repeated(x, n)
Iterators.repeated(x,n)
end
function approx_size(cs::Columns)
sum(map(approx_size, astuple(columns(cs))))
end
function approx_size(t::NDSparse)
approx_size(t.data) + approx_size(t.index)
end
function approx_size(pa::PooledArray)
approx_size(pa.refs) + approx_size(pa.pool) * 2
end
function approx_size(t::IndexedTable)
approx_size(rows(t))
end
function approx_size(x::StringArray)
approx_size(x.buffer) + approx_size(x.offsets) + approx_size(x.lengths)
end
# The following is not inferable, this is OK because the only place we use
# this doesn't need it.
function _map_params(f, T, S)
(f(_tuple_type_head(T), _tuple_type_head(S)), _map_params(f, _tuple_type_tail(T), _tuple_type_tail(S))...)
end
_map_params(f, T::Type{Tuple{}},S::Type{Tuple{}}) = ()
map_params(f, ::Type{T}, ::Type{S}) where {T,S} = f(T,S)
@inline _tuple_type_head(::Type{T}) where {T<:Tuple} = Base.tuple_type_head(T)
@inline _tuple_type_tail(::Type{T}) where {T<:Tuple} = Base.tuple_type_tail(T)
#function map_params{N}(f, T::Type{T} where T<:Tuple{Vararg{Any,N}}, S::Type{S} where S<: Tuple{Vararg{Any,N}})
Base.@pure function map_params(f, ::Type{T}, ::Type{S}) where {T<:Tuple,S<:Tuple}
if fieldcount(T) != fieldcount(S)
MethodError(map_params, (typeof(f), T,S))
end
Tuple{_map_params(f, T,S)...}
end
_tuple_type_head(T::Type{NT}) where {NT<: NamedTuple} = fieldtype(NT, 1)
Base.@pure function _tuple_type_tail(T::Type{NamedTuple{n,ts}}) where {n,ts}
_tuple_type_tail(ts)
end
Base.@pure @generated function map_params(f, ::Type{T}, ::Type{S}) where {T<:NamedTuple,S<:NamedTuple}
if fieldnames(T) != fieldnames(S)
MethodError(map_params, (T,S))
end
:(NamedTuple{$(fieldnames(T)), Tuple{_map_params(f, T, S)...}})
end
function randomsample(n, r::AbstractRange)
k = 0
taken = Set{eltype(r)}()
output = eltype(r)[]
while k < n && k < length(r)
x = rand(r)
if !(x in taken)
push!(taken, x)
push!(output, x)
k += 1
end
end
return output
end
function tuplesetindex(x::Tuple{Vararg{Any,N}}, v, i) where N
ntuple(Val(N)) do j
i == j ? v : x[j]
end
end
@inline tuplesetindex(x::NamedTuple, v, i::Symbol) = (; x..., i => v)
@inline function tuplesetindex(x::NamedTuple{N}, v, i::Int) where N
tuplesetindex(x, v, getfield(N, i))
end
function tuplesetindex(x::Union{NamedTuple, Tuple}, v::Tuple, i::Tuple)
reduce((t, j)->tuplesetindex(t, v[j], i[j]), 1:length(i), init=x)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 533 | using Distributed
include("testenv.jl")
addprocs_with_testenv(2)
using JuliaDB, Test, TextParse, IndexedTables, PooledArrays, Dagger, OnlineStats,
Statistics, MemPool, Random, Serialization, Dagger, Dates, WeakRefStrings, DataValues
include("test_iteration.jl")
include("test_util.jl")
include("test_table.jl")
include("test_query.jl")
include("test_join.jl")
include("test_misc.jl")
include("test_rechunk.jl")
include("test_readwrite.jl")
include("test_onlinestats.jl")
include("test_ml.jl")
include("test_weakrefstrings.jl")
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1436 | @testset "iteration" begin
x = distribute(NDSparse(Columns(a=[1,1], b=[1,2]), Columns(c=[3,4])), 2)
y = distribute(NDSparse(Columns(a=[1,1], b=[1,2]), [3,4]), 2)
@test column(x, :a) == [1,1]
@test column(x, :b) == [1,2]
@test column(x, :c) == [3,4]
@test column(x, 3) == [3,4]
@test column(y, 3) == [3,4]
@test columns(x) == (a=[1,1], b=[1,2], c=[3,4])
@test columns(x, (:a,:c)) == (a=[1,1], c=[3,4])
@test columns(y, (1, 3)) == ([1,1], [3,4])
@test rows(x) == [(a=1,b=1,c=3), (a=1,b=2,c=4)]
@test rows(x, :b) == [1, 2]
@test rows(x, (:b, :c)) == [(b=1,c=3), (b=2,c=4)]
#@test rows(x, (:c, as(-, :b, :x))) == [(c=3, x=-1),(c=4, x=-2)]
@test keys(x) == [(a=1,b=1), (a=1,b=2)]
@test keys(x, :a) == [1, 1]
#@test keys(x, (:a, :b, 2)) == [(1,1,1), (1,2,2)]
@test values(x) == [(c=3,), (c=4,)]
@test values(x,1) == [3,4]
#@test values(x,(1,1)) == [(3,3), (4,4)]
@test values(y) == [3, 4]
@test values(y,1) == [3,4]
#@test values(y,as(x->compute(sort(x, rev=true)), 1, :x)) == [4, 3]
#@test values(y,(as(-, 1, :x),)) == [(x=-3,), (x=-4,)]
@test collect(pairs(x)) == [(a=1,b=1)=>(c=3,), (a=1,b=2)=>(c=4,)]
@test collect(pairs(y)) == [(a=1,b=1)=>3, (a=1,b=2)=>4]
x = ndsparse(([1,2], [3,4]), (x=[0,1],), chunks=2)
@test ndsparse(keys(x), transform(values(x), :y => [1,2])) == ndsparse(([1,2], [3,4]), (x=[0,1], y=[1,2]))
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 2600 | @testset "join" begin
t1 = NDSparse(Columns(([1,1,2,2], [1,2,1,2])), [1,2,3,4])
t2 = NDSparse(Columns(([0,2,2,3], [1,1,2,2])), [1,2,3,4])
j1 = innerjoin(t1,t2)
j2 = innerjoin(+, t1,t2)
lj1 = leftjoin(t1,t2)
lj2 = leftjoin(+, t1,t2)
mj1 = merge(t1,t2)
mj2 = merge(t2,t1)
for n=1:5
d1 = distribute(t1, n)
for n2 = 1:5
d2 = distribute(t2, n2)
@test isequal(collect(innerjoin(d1, d2)), j1)
@test isequal(collect(innerjoin(+, d1, d2)), j2)
@test isequal(collect(leftjoin(d1, d2)), lj1)
@test isequal(collect(leftjoin(+, d1, d2)), lj2)
@test isequal(collect(merge(d1, d2)), mj1)
@test isequal(collect(merge(d2, d1)), mj2)
end
end
t1 = NDSparse([:msft,:ibm,:ge], [1,3,4], [100,200,150])
t2 = NDSparse([:ibm,:msft,:aapl,:ibm], [0,0,0,2], [100,99,101,98])
aj = asofjoin(t1,t2)
for n=1:5
d1 = distribute(t1, n)
for n2 = 1:5
d2 = distribute(t2, n2)
@test collect(asofjoin(d1, d2)) == aj
end
end
end
@testset "broadcast" begin
t1 = NDSparse(Columns(([1,2,3,4],[1,1,2,2])), [5,6,7,8])
t2 = NDSparse(Columns(([0,1,2,4],)),[0,10,100,10000])
for n=1:4
for m=1:4
d1 = distribute(t1, n)
d2 = distribute(t2, m)
@test collect(d1 .+ d2) == t1 .+ t2
@test collect(broadcast(+, d1, d2, dimmap=(0,1))) == broadcast(+, t1, t2, dimmap=(0,1))
end
end
end
@testset "join with missing" begin
y = rand(10)
z = rand(10)
# DIndexedTable
t = table((x=1:10, y=y), pkey=:x, chunks=2)
t2 = table((x=1:2:20, z=z), pkey=:x, chunks=2)
# DNDSparse
nd = ndsparse((x=1:10,), (y=y,), chunks=2)
nd2 = ndsparse((x=1:2:20,), (z=z,), chunks=2)
@testset "how = :left" begin
# Missing
z_left = Union{Float64,Missing}[missing for i in 1:10]
z_left[1:2:9] = z[1:5]
t_left = table((x = 1:10, y = y, z = z_left))
nd_left = ndsparse((x=1:10,), (y=y, z=z_left))
@test isequal(join(t, t2; how=:left), t_left)
@test isequal(join(nd, nd2; how=:left), nd_left)
# DataValue
z_left2 = [DataValue{Float64}() for i in 1:10]
z_left2[1:2:9] = z[1:5]
t_left2 = table((x=1:10, y = y, z = z_left2))
nd_left2 = ndsparse((x=1:10,), (y=y, z=z_left2))
@test isequal(join(t, t2, how=:left, missingtype=DataValue), t_left2)
@test isequal(join(nd, nd2, how=:left, missingtype=DataValue), nd_left2)
end
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 2780 | @testset "setindex!" begin
x = ndsparse(([1,2],[2,3]), [4,5], chunks=2)
x[2,3] = 6
@test x == ndsparse(([1,2], [2,3]), [4,6])
x[2,4] = 7
@test x == ndsparse(([1,2,2], [2,3,4]), [4,6,7])
x = ndsparse((x=[1,2],y=[2,3]), [4,5], chunks=2)
x[2,3] = 6
@test x == ndsparse((x=[1,2], y=[2,3]), [4,6])
x[2,4] = 7
@test x == ndsparse((x=[1,2,2], y=[2,3,4]), [4,6,7])
end
@testset "extractarray" begin
t = NDSparse(Columns(a=[1,1,1,2,2], b=[1,2,3,1,2]),
Columns(c=[1,2,3,4,5], d=[5,4,3,2,1]))
for i=[2, 3, 5]
d = compute(distribute(t, i))
dist = map(get, map(JuliaDB.nrows, d.domains))
@test map(length, Dagger.domainchunks(keys(d, 1))) == dist
@test collect(keys(d, 2)) == columns(t.index)[2]
@test collect( values(d, 2)) == columns(t.data)[2]
end
end
@testset "printing" begin
x = distribute(NDSparse([1], [1]), 1)
@test repr(x) == """
1-d Distributed NDSparse with 1 values (Int64) in 1 chunks:
1 │
──┼──
1 │ 1"""
end
import JuliaDB: chunks, index_spaces, has_overlaps
@testset "has_overlaps" begin
t = NDSparse(Columns(([1,1,2,2,2,3], [1,2,1,1,2,1])), [1,2,3,4,5,6])
d = distribute(t, [2,3,1])
i = d.domains
@test !has_overlaps(i, closed=false)
@test !has_overlaps(i)
d = distribute(t, [2,2,2])
i = d.domains
@test !has_overlaps(i, closed=false)
@test !has_overlaps(i)
d = distribute(t, [2,1,3])
i = d.domains
@test !has_overlaps(i, closed=false)
@test has_overlaps(i)
end
import JuliaDB: with_overlaps, delayed
@testset "with_overlaps" begin
t = NDSparse([1,1,2,2,2,3], [1,2,3,4,5,6])
d = distribute(t, [2,2,2])
group_count = 0
t1 = with_overlaps(d) do cs
if length(cs) > 1
group_count += 1
@test length(cs) == 2
@test collect(cs[1]) == NDSparse([2,2], [3,4])
@test collect(cs[2]) == NDSparse([2,3], [5,6])
return delayed((x,y) -> merge(x,y,agg=nothing))(cs...)
else
cs[1]
end
end
@test collect(groupreduce(+, t1)) == groupreduce(+, t)
@test group_count == 1
end
import JuliaDB: subtable
@testset "subtable" begin
t = NDSparse([1,2,3,4], [5,6,7,8])
d = distribute(t, 3)
for i=1:4
@test collect(subtable(d, 1:i)) == subtable(t, 1:i)
if i>=2
@test collect(subtable(d, 2:i)) == subtable(t, 2:i)
end
end
end
@testset "Iterators.partition" begin
t = compute(distribute(NDSparse([1:7;],[8:14;]), [3,2,2]))
parts = map(NDSparse, Iterators.partition([1:7;], 2) |> collect,
Iterators.partition([8:14;], 2) |> collect)
@test [p for p in Iterators.partition(t, 2)] == parts
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1920 | import JuliaDB.ML
@testset "feature extraction" begin
@testset "schema" begin
@test ML.schema(1:10).stat == fit!(Variance(), 1:10)
x = repeat([1,2], inner=5)
@test ML.schema(x, ML.Categorical).stat == fit!(CountMap(Int), x) == ML.schema(PooledArray(x)).stat
m = ML.schema(Vector{Union{Int,Missing}}(x))
@test m isa ML.Maybe
@test m.feature.stat == ML.schema(x).stat
t = table(PooledArray([1,2,1,2]), [1,2,3,4], names=[:x, :y])
sch = ML.schema(t)
@test sch[:x].stat == ML.schema(PooledArray([1,2,1,2])).stat
@test sch[:y].stat == ML.schema([1,2,3,4]).stat
end
@testset "featuremat" begin
@test ML.featuremat([1,3,5]) == [-1.0, 0, 1.0]'
Δ = 0.5 / std([1,2])
@test ML.featuremat([1,2,missing]) ≈ [0 0 1; -Δ Δ 0]
@test isempty(ML.featuremat(["x","y","x"]))
@test ML.featuremat(PooledArray(["x","y","x"])) == [1 0 1; 0 1 0]
t = table(PooledArray([1,1,2,2]), [1,2,3,4], [1,2,3,4], ["x", "y", "z", "a"], names=[:a,:b,:c,:d])
@test ML.featuremat(t) == collect(ML.featuremat(distribute(t, 2)))
x = randn(100)
@test ML.featuremat(x) ≈ ((x .- mean(x)) ./ std(x))'
end
@testset "Issue 149" begin
x = rand(10), rand(1:4, 10), rand(1:4, 10)
t = table(x..., names = [:x1, :x2, :x3])
td = table(x..., names = [:x1, :x2, :x3], chunks = 2)
sch = ML.schema(t, hints=Dict(
:x1 => ML.Continuous,
:x2 => ML.Categorical,
:x3 => ML.Categorical,
)
)
schd = ML.schema(td, hints=Dict(
:x1 => ML.Continuous,
:x2 => ML.Categorical,
:x3 => ML.Categorical,
)
)
@test schd[:x1].stat.σ2 ≈ sch[:x1].stat.σ2
@test schd[:x2].stat == sch[:x2].stat
@test schd[:x3].stat == sch[:x3].stat
end
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 735 | @testset "online stats" begin
global dt
t = NDSparse(Columns(([1,1,1,2,2,2,3,3,3], [1,2,3,1,2,3,1,2,3])), [0.5,1,1.5,1,2,3,2,3,4])
dt = distribute(t,[3,2,4])
@test value(reduce(Mean(), t))[1] ≈ 2
@test value(reduce(Mean(), dt))[1] ≈ 2
means = groupreduce(Series(Mean()), dt, 1)
@test collect(values(map(x->x.stats[1].μ, means))) == [1.0, 2.0, 3.0]
# @test length(means.chunks) == 2
# @test Series((keys(dt), values(dt)), LinReg(2)).stats[1].β |> string == string([0.615385, 0.448718])
# regs = aggregate_stats(Series(LinReg(2)), keys(dt, 1), keys(dt), values(dt))
# @test isapprox(values(map(x->coef(x.stats[1]), regs)) |> collect, [[0, 0.5], [0.0, 1.0], [0.3333333, 1.0]], rtol=10e-5) |> all
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 4049 | @testset "map & reduce" begin
t = NDSparse(Columns(([1,1,2,2], [1,2,1,2])), [1,2,3,4])
d = distribute(t, 2)
@test collect(map(-, d)) == map(-, t)
@test reduce(+, d) == 10
end
@testset "getindex" begin
t = NDSparse(Columns(x=[1,1,1,2,2], y=[1,2,3,1,2]), [1,2,3,4,5])
for n=1:5
d = distribute(t, n)
@test d[1,1] == t[1,1]
@test d[1,3] == t[1,3]
@test d[2,2] == t[2,2]
@test collect(d[1:1, 1:1]) == t[1:1, 1:1]
@test collect(d[1:2, 2:3]) == t[1:2, 2:3]
@test collect(d[:, 3]) == t[:, 3]
end
end
@testset "select" begin
t = NDSparse(Columns(a=[1,1,1,2,2], b=[1,2,3,1,2]), [1,2,3,4,5])
for i=[1, 3, 5]
d = distribute(t, i)
res = filter((1=>x->true, 2=>x->x%2 == 0), t)
@test collect(filter((1=>x->true, 2=>x->x%2 == 0), d)) == res
@test collect(filter((:a=>x->true, :b => x->x%2 == 0), d)) == res
# check empty, #228
res = filter((1=>x->false, 2=>x->x%2 == 0), t)
@test collect(filter((1=>x->false, 2=>x->x%2 == 0), d)) == res
@test collect(filter((:a=>x->false, :b => x->x%2 == 0), d)) == res
end
end
function Base.isapprox(x::NDSparse, y::NDSparse)
flush!(x); flush!(y)
all(map(isapprox, columns(x.data), columns(y.data)))
end
@testset "convertdim" begin
t = NDSparse(Columns(a=[1,1,1,2,2], b=[1,2,3,1,2]),
Columns(c=[1,2,3,4,5], d=[5,4,3,2,1]))
_plus(x,y) = map(+,x, y)
for i=[2, 3, 5]
d = distribute(t, i)
@test collect(convertdim(d, 2, x->x>=2)) == convertdim(t, 2, x->x>=2)
@test collect(convertdim(d, 2, x->x>=2, agg=_plus)) == convertdim(t, 2, x->x>=2, agg=_plus)
@test collect(convertdim(d, 2, x->x>=2, vecagg=length)) ==
convertdim(t, 2, x->x>=2, vecagg=length)
end
end
@testset "reduce" begin
t1 = NDSparse(Columns(([1,1,2,2], [1,2,1,2])), [1,2,3,4])
rd1 = reduce(+, t1, dims=1)
rd2 = reduce(+, t1, dims=2)
rdv1 = reducedim_vec(length, t1, 1)
rdv2 = reducedim_vec(length, t1, 2)
for n=1:5
d1 = distribute(t1, n, allowoverlap=true)
@test collect(reduce(+, d1, dims=1)) == rd1
@test collect(reduce(+, d1, dims=2)) == rd2
@test collect(reducedim_vec(length, d1, 1)) == rdv1
@test collect(reducedim_vec(length, d1, 2)) == rdv2
end
end
@testset "select" begin
t1 = NDSparse(Columns(([1,2,3,4], [2,1,1,2])), [1,2,3,4])
d1 = distribute(t1, 2)
@test collect(selectkeys(d1, 2, agg=+)) == selectkeys(t1, 2, agg=+)
end
@testset "permutedims" begin
t = NDSparse(Columns(([1,1,2,2], ["a","b","a","b"])), [1,2,3,4])
for n=1:5
d = distribute(t, n)
@test collect(permutedims(d, [2,1])) == permutedims(t, [2,1])
end
end
@testset "mapslices" begin
t = NDSparse(Columns(x=[1,1,2,2], y=[1,2,3,4]), [1,2,3,4])
for (dist,) in zip(Any[1, 2, [1,3], [3,1], [1,2,1]])
d = distribute(t, dist)
res = mapslices(collect, d, :y)
@test collect(res) == mapslices(collect, t, 2)
f = x->IndexedTables.NDSparse(IndexedTables.Columns(z=[1,2]), [3,4])
res2 = mapslices(f, d, 2)
@test collect(res2) == mapslices(f, t, 2)
end
t = ndsparse([1,2,3],[4,5,6], chunks=2)
@test mapslices(t, ()) do x
IndexedTables.ndsparse([1,2], first.([x,x]))
end == ndsparse(([1,1,2,2,3,3],[1,2,1,2,1,2],), [4,4,5,5,6,6])
end
@testset "flatten" begin
x = table([1,2], [[3,4], [5,6]], names=[:x, :y], chunks=2)
@test flatten(x, 2) == table([1,1,2,2], [3,4,5,6], names=[:x,:y])
x = table([1,2], [table([3,4],[5,6], names=[:a,:b]), table([7,8], [9,10], names=[:a,:b])], names=[:x, :y], chunks=2)
@test flatten(x, :y) == table([1,1,2,2], [3,4,7,8], [5,6,9,10], names=[:x,:a, :b])
t = table([1,1,2,2], [3,4,5,6], names=[:x,:y], chunks=2)
@test groupby((:normy => x->Iterators.repeated(mean(x), length(x)),),
t, :x, select=:y, flatten=true) == table([1,1,2,2], [3.5,3.5,5.5,5.5], names=[:x, :normy])
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 5737 | function roundtrip(x, eq=(==), io=IOBuffer())
mmwrite(Serializer(io), x)
@test eq(deserialize(seekstart(io)), x)
end
@testset "PooledArray/Vector{Union{T,Missing}}" begin
roundtrip(PooledArray([randstring(rand(1:10)) for i in 1:4]))
roundtrip([rand(Bool) ? rand() : missing for i in 1:50], isequal)
end
@testset "Columns" begin
roundtrip(Columns(([1,2], ["x","y"])))
roundtrip(Columns(x=[1,2], y=["x","y"]))
end
@testset "ndsparse" begin
ndsparse(Columns(([1,2], ["x","y"])),
Columns(x=[1,2], y=["x","y"])) |> roundtrip
end
@testset "table" begin
table([1,2], ["x","y"]) |> roundtrip
table(Columns(x=[1,2], y=["x","y"])) |> roundtrip
end
path = joinpath(dirname(@__FILE__), "..","test", "sample")
files = glob("*.csv", path)
const spdata_dist = loadndsparse(files, type_detect_rows=4,
indexcols=1:2, usecache=false, chunks=2)
const spdata_dist_path = loadndsparse(path, type_detect_rows=4,
indexcols=1:2, usecache=false, chunks=2)
loadndsparse(files[1:2], chunks=4)
_readstr(f) = open(f) do fh
readline(fh)
readstring(fh)
end
const spdata = loadndsparse(files;
distributed=false,
header_exists=true,
indexcols=1:2)
files = glob("*.csv", "sample")
shuffle_files = shuffle(files)
const spdata_unordered = loadndsparse(shuffle_files;
distributed=false,
indexcols=[])
ingest_output = tempname()
spdata_ingest = loadndsparse(files, output=ingest_output, indexcols=1:2, chunks=2)
ingest_output_unordered = tempname()
# note: this will result in a different table if files[3:end] is ingested first
spdata_ingest_unordered = loadndsparse(shuffle_files, output=ingest_output_unordered,
indexcols=[], chunks=2)
# spdata_ingest_unordered = loadndsparse(shuffle_files[4:end], output=ingest_output_unordered,
# append=true, indexcols=[])
# this should also test appending new files
import Dagger: Chunk
@testset "Load" begin
@test loadtable("missingcols/t1.csv") == table([0,0,0], [1,2,3], names=[:a,:x])
cache = joinpath(JuliaDB.JULIADB_DIR, JuliaDB.JULIADB_FILECACHE)
if isfile(cache)
rm(cache)
end
missingcoltbl = loadndsparse(joinpath(@__DIR__, "missingcols"), datacols=[:a, :x, :y], usecache=false, chunks=2)
@test eltype(missingcoltbl) == NamedTuple{(:a,:x,:y),Tuple{Int, Union{Missing,Int}, Union{Missing,Float64}}}
@test collect(loadtable(shuffle_files,chunks=2)) == table(spdata_unordered.data)
# file name as a column:
@test unique(keys(loadndsparse(path, indexcols=[:year, :date, :ticker],filenamecol=:year, usecache=false, chunks=2), :year)|> collect) == string.(2010:2015)
@test unique(keys(loadndsparse(path, indexcols=[:year, :date, :ticker],filenamecol=:year=>(x->x[3:4])∘basename, usecache=false, chunks=2), :year)|> collect) == string.(10:15)
@test collect(spdata_dist) == spdata
@test collect(spdata_dist_path) == spdata
@test collect(spdata_ingest) == spdata
@test collect(load(ingest_output)) == spdata
@test collect(load(ingest_output_unordered)) == spdata_unordered
@test issorted(collect(keys(load(ingest_output_unordered), 1)))
c = first(load(ingest_output).chunks)
#@test isa(c.handle, FileRef)
#@test collect(dt[["blah"], :,:]) == spdata
dt = loadndsparse(files, indexcols=[("date", "dummy"), ("dummy", "ticker")], usecache=false, chunks=2)
nds=collect(dt)
@test haskey(columns(nds.index), :date)
@test haskey(columns(nds.index), :dummy)
@test !haskey(columns(nds.index), :ticker)
@test length(columns(nds.index)) == 2
@test keys(columns(nds.data)) == (:open, :high, :low, :close, :volume)
@test length(columns(nds.data)) == 5
dt = loadndsparse(shuffle_files, usecache=false, chunks=2)
@test collect(dt) == spdata_unordered
@test issorted(collect(keys(dt, 1)))
# reuses csv read cache:
dt = loadndsparse(shuffle_files, indexcols=[], chunks=4, usecache=false)
@test collect(dt) == spdata_unordered
dt = loadndsparse(shuffle_files, indexcols=[], chunks=4) # cache test
@test collect(dt) == spdata_unordered
# test specifying column names
dt = loadndsparse(files[1:2], indexcols=[:a,:b], colnames=[:a,:b,:c,:d,:e,:f,:g], usecache=false, header_exists=false, chunks=2)
nds = collect(dt)
@test haskey(columns(nds.index), :a)
@test haskey(columns(nds.index), :b)
@test keys(columns(nds.data)) == (:c,:d,:e,:f,:g)
end
@testset "save" begin
t = NDSparse([1,2,3,4], [1,2,3,4])
n = tempname()
x = JuliaDB.save(distribute(t, 4), n)
t1 = load(n)
@test collect(t1) == collect(x)
@test !any(c->isempty(Dagger.affinity(c.handle)), t1.chunks)
rm(n, recursive=true)
t = table([1,2,3,4], [1,2,3,4], chunks=2)
n = tempname()
x = JuliaDB.save(t, n)
t1 = load(n)
@test collect(t1) == collect(t)
@test !any(c->isempty(Dagger.affinity(c.handle)), t1.chunks)
rm(n, recursive=true)
end
rm(ingest_output, recursive=true)
rm(ingest_output_unordered, recursive=true)
@testset "load and save with missings" begin
nm1, nm2 = tempname(), tempname()
# Create a csv with one missing value and a column with all missings
open(nm1, "w") do io
write(io, "A,B,C\n1,1,missing\n1,missing,missing\n")
end
t1 = loadtable(nm1, delim = ',')
save(t1, nm2)
t2 = load(nm2)
@test all([all(collect(c1 .=== c2)) for (c1, c2) in zip(columns(t1), columns(t2))])
# Clean up
rm(nm1, recursive=true)
rm(nm2, recursive=true)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 683 | @testset "rechunk" begin
t = NDSparse([1:10;], ones(10), ones(10))
d = distribute(t, 2)
d2 = compute(distribute(d, [2,3,4,1]))
@test get.(map(JuliaDB.nrows, d2.domains)) == [2,3,4,1]
end
@testset "rechunk by" begin
t = distribute(NDSparse(Columns(([1,1,1,2,2,2], [1,1,2,2,3,3])), [1:6;]), [2,2,2])
t1=rechunk(t, (1, 2=>x->x.>=2), closed=true, chunks=3)
@test collect(t1) == selectkeys(collect(t), (1,2=>x->x>=2))
@test collect(t1.chunks[1]) == NDSparse(Columns(([1,1],[false,false])), [1,2])
# @test collect(t1.chunks[2]) == NDSparse(Columns(([1],[2])), [3])
# @test collect(t1.chunks[3]) == NDSparse(Columns(([2,2,2],[2,3,3])), [4,5,6])
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 17261 | @everywhere using Statistics
import JuliaDB: pkeynames, pkeys, excludecols, select, transform
@testset "table" begin
t = table([1, 1, 1, 2, 2, 2], [1, 1, 2, 2, 1, 1], [1, 2, 3, 4, 5, 6], names=[:x, :y, :z], pkey=(:x, :y), chunks=2)
@test groupreduce(+, t, :x, select=:z) == table([1, 2], [6, 15], names=Symbol[:x, :+])
@test groupreduce(((x, y)->if x isa Int
(y = x + y,)
else
(y = x.y + y,)
end), t, :x, select=:z) == table([1, 2], [6, 15], names=Symbol[:x, :y])
@test groupreduce(:y => (+), t, :x, select=:z) == table([1, 2], [6, 15], names=Symbol[:x, :y])
x = ndsparse(["a", "b"], [3, 4], chunks=2)
@test (keytype(x), eltype(x)) == (Tuple{String}, Int64)
x = ndsparse((date = Date.(2014:2017),), [4:7;], chunks=2)
@test x[Date("2015-01-01")] == 5
@test (keytype(x), eltype(x)) == (Tuple{Date}, Int64)
x = ndsparse((["a", "b"], [3, 4]), [5, 6], chunks=2)
@test (keytype(x), eltype(x)) == (Tuple{String,Int64}, Int64)
@test x["a", 3] == 5
x = ndsparse((["a", "b"], [3, 4]), ([5, 6], [7.0, 8.0]), chunks=2)
x = ndsparse((x = ["a", "a", "b"], y = [3, 4, 4]), (p = [5, 6, 7], q = [8.0, 9.0, 10.0]), chunks=2)
@test (keytype(x), eltype(x)) == (Tuple{String,Int64}, NamedTuple{(:p,:q),Tuple{Int64,Float64}})
@test x["a", :] == ndsparse((y = [3, 4],), Columns((p = [5, 6], q = [8.0, 9.0])))
x = ndsparse([1, 2], [3, 4], chunks=2)
@test pkeynames(x) == (1,)
x = ndsparse((t = [0.01, 0.05],), (x = [1, 2], y = [3, 4]), chunks=2)
manh = map((row->row.x + row.y), x)
vx = map((row->row.x / row.t), x, select=(:t, :x))
polar = map((p->(r = hypot(p.x + p.y), θ = atan(p.y, p.x))), x)
#@test map(sin, polar, select=:θ) == ndsparse((t = [0.01, 0.05],), [0.948683, 0.894427])
a = table([1, 2, 3], [4, 5, 6], chunks=2)
b = table([1, 2, 3], [4, 5, 6], names=[:x, :y], chunks=2)
@test table(([1, 2, 3], [4, 5, 6])) == a
@test table((x = [1, 2, 3], y = [4, 5, 6])) == b
@test table(Columns(([1, 2, 3], [4, 5, 6]))) == a
@test table(Columns(x=[1, 2, 3], y=[4, 5, 6])) == b
@test b == table(b)
b = table([2, 3, 1], [4, 5, 6], names=[:x, :y], pkey=:x, chunks=2)
b = table([2, 1, 2, 1], [2, 3, 1, 3], [4, 5, 6, 7], names=[:x, :y, :z], pkey=(:x, :y), chunks=2)
t = table([1, 2], [3, 4], chunks=2)
@test pkeynames(t) == ()
t = table([1, 2], [3, 4], pkey=1, chunks=2)
@test pkeynames(t) == (1,)
t = table([2, 1], [1, 3], [4, 5], names=[:x, :y, :z], pkey=(1, 2), chunks=2)
@test pkeys(collect(t)) == Columns((x = [1, 2], y = [3, 1]))
@test pkeys(a) == Columns((Base.OneTo(3),))
a = table(["a", "b"], [3, 4], pkey=1, chunks=2)
@test pkeys(a) == Columns((String["a", "b"],))
t = table([2, 1], [1, 3], [4, 5], names=[:x, :y, :z], pkey=(1, 2), chunks=2)
@test excludecols(t, (:x,)) == (2, 3)
@test excludecols(t, (2,)) == (1, 3)
@test excludecols(t, pkeynames(t)) == (3,)
@test excludecols([1, 2, 3], (1,)) == ()
@test convert(IndexedTable, Columns(x=[1, 2], y=[3, 4]), Columns(z=[1, 2]), presorted=true) == table([1, 2], [3, 4], [1, 2], names=Symbol[:x, :y, :z])
@test colnames([1, 2, 3]) == (1,)
@test colnames(Columns(([1, 2, 3], [3, 4, 5]))) == (1, 2)
@test colnames(table([1, 2, 3], [3, 4, 5])) == (1, 2)
@test colnames(Columns(x=[1, 2, 3], y=[3, 4, 5])) == (:x, :y)
@test colnames(table([1, 2, 3], [3, 4, 5], names=[:x, :y])) == (:x, :y)
@test colnames(ndsparse(Columns(x=[1, 2, 3]), Columns(y=[3, 4, 5]))) == (:x, :y)
@test colnames(ndsparse(Columns(x=[1, 2, 3]), [3, 4, 5])) == (:x, 2)
@test colnames(ndsparse(Columns(x=[1, 2, 3]), [3, 4, 5])) == (:x, 2)
@test colnames(ndsparse(Columns(([1, 2, 3], [4, 5, 6])), Columns(x=[6, 7, 8]))) == (1, 2, :x)
@test colnames(ndsparse(Columns(x=[1, 2, 3]), Columns(([3, 4, 5], [6, 7, 8])))) == (:x, 2, 3)
t = table([1, 2], [3, 4], names=[:x, :y], chunks=2)
@test columns(t) == (x = [1, 2], y = [3, 4])
@test columns(t, :x) == [1, 2]
@test columns(t, (:x,)) == (x = [1, 2],)
@test columns(t, (:y, :x => (-))) == (y = [3, 4], x = [-1, -2])
t = table([1, 2], [3, 4], names=[:x, :y], chunks=2)
@test rows(t) == Columns((x = [1, 2], y = [3, 4]))
@test rows(t, :x) == [1, 2]
@test rows(t, (:x,)) == Columns((x = [1, 2],))
@test rows(t, (:y, :x => (-))) == Columns((y = [3, 4], x = [-1, -2]))
t = table([1, 2], [3, 4], names=[:x, :y], chunks=2)
@test transform(t, 2 => [5, 6]) == table([1, 2], [5, 6], names=Symbol[:x, :y])
@test transform(t, :x => :x => (x->1 / x)) == table([1.0, 0.5], [3, 4], names=Symbol[:x, :y])
t = table([0.01, 0.05], [1, 2], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
t2 = transform(t, :t => [0.1, 0.05])
@test t2 == table([0.05, 0.1], [2,1], [4,3], names=[:t,:x,:y])
t = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test transform(t, :z => [1 // 2, 3 // 4]) == table([0.01, 0.05], [2, 1], [3, 4], Rational{Int64}[1//2, 3//4], names=Symbol[:t, :x, :y, :z])
t = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test select(t, Not(:x)) == table([0.01, 0.05], [3, 4], names=Symbol[:t, :y])
t = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test insertcols(t, 2, :w => [0, 1]) == table([0.01, 0.05], [0, 1], [2, 1], [3, 4], names=Symbol[:t, :w, :x, :y])
t = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test insertcolsafter(t, :t, :w => [0, 1]) == table([0.01, 0.05], [0, 1], [2, 1], [3, 4], names=Symbol[:t, :w, :x, :y])
t = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test insertcolsbefore(t, :x, :w => [0, 1]) == table([0.01, 0.05], [0, 1], [2, 1], [3, 4], names=Symbol[:t, :w, :x, :y])
t = table([0.01, 0.05], [2, 1], names=[:t, :x], chunks=2)
@test rename(t, :t => :time) == table([0.01, 0.05], [2, 1], names=Symbol[:time, :x])
l = table([1, 1, 2, 2], [1, 2, 1, 2], [1, 2, 3, 4], names=[:a, :b, :c], pkey=(:a, :b), chunks=2)
r = table([0, 1, 1, 3], [1, 1, 2, 2], [1, 2, 3, 4], names=[:a, :b, :d], pkey=(:a, :b), chunks=2)
@test join(l, r) == table([1, 1], [1, 2], [1, 2], [2, 3], names=[:a, :b, :c, :d])
@test isequal(
join(l, r, how=:left),
table([1, 1, 2, 2], [1, 2, 1, 2], [1, 2, 3, 4], [2, 3, missing, missing], names=[:a, :b, :c, :d])
)
@test isequal(
join(l, r, how=:outer),
table([0, 1, 1, 2, 2, 3], [1, 1, 2, 1, 2, 2], [missing, 1, 2, 3, 4, missing], [1, 2, 3, missing, missing, 4], names=Symbol[:a, :b, :c, :d])
)
@test join(l, r, how=:anti) == table([2, 2], [1, 2], [3, 4], names=Symbol[:a, :b, :c])
l1 = table([1, 2, 2, 3], [1, 2, 3, 4], names=[:x, :y], chunks=2)
r1 = table([2, 2, 3, 3], [5, 6, 7, 8], names=[:x, :z], chunks=2)
@test join(l1, r1, lkey=:x, rkey=:x) == table([2, 2, 2, 2, 3, 3], [2, 2, 3, 3, 4, 4], [5, 6, 5, 6, 7, 8], names=Symbol[:x, :y, :z])
@test isequal(
join(l, r, lkey=:a, rkey=:a, lselect=:b, rselect=:d, how=:outer),
table((a=[0, 1, 1, 1, 1, 2, 2, 3], b=[missing, 1, 1, 2, 2, 1, 2, missing], d=[1, 2, 3, 2, 3, missing, missing, 4]))
)
l = table([1, 1, 1, 2], [1, 2, 2, 1], [1, 2, 3, 4], names=[:a, :b, :c], pkey=(:a, :b), chunks=2)
r = table([0, 1, 1, 2], [1, 2, 2, 1], [1, 2, 3, 4], names=[:a, :b, :d], pkey=(:a, :b), chunks=2)
@test groupjoin(l, r) == table([1, 2], [2, 1], [Columns((c = [2, 2, 3, 3], d = [2, 3, 2, 3])), Columns((c = [4], d = [4]))], names=Symbol[:a, :b, :groups])
@test groupjoin(l, r, how=:left) == table([1, 1, 2], [1, 2, 1], [Columns((c = [], d = [])), Columns((c = [2, 2, 3, 3], d = [2, 3, 2, 3])), Columns((c = [4], d = [4]))], names=Symbol[:a, :b, :groups])
@test groupjoin(l, r, how=:outer) == table([0, 1, 1, 2], [1, 1, 2, 1], [Columns((c = [], d = [])), Columns((c = [], d = [])), Columns((c = [2, 2, 3, 3], d = [2, 3, 2, 3])), Columns((c = [4], d = [4]))], names=Symbol[:a, :b, :groups])
@test groupjoin(l, r, lkey=:a, rkey=:a, lselect=:c, rselect=:d, how=:outer) == table([0, 1, 2], [Columns((c = [], d = [])), Columns((c = [1, 1, 2, 2, 3, 3], d = [2, 3, 2, 3, 2, 3])), Columns((c = [4], d = [4]))], names=Symbol[:a, :groups])
x = ndsparse((["ko", "ko", "xrx", "xrx"], Date.(["2017-11-11", "2017-11-12", "2017-11-11", "2017-11-12"])), [1, 2, 3, 4], chunks=2)
y = ndsparse((["ko", "ko", "xrx", "xrx"], Date.(["2017-11-12", "2017-11-13", "2017-11-10", "2017-11-13"])), [5, 6, 7, 8], chunks=2)
@test asofjoin(x, y) == ndsparse((String["ko", "ko", "xrx", "xrx"], Date.(["2017-11-11", "2017-11-12", "2017-11-11", "2017-11-12"])), [1, 5, 7, 7])
a = table([1, 3, 5], [1, 2, 3], names=[:x, :y], pkey=:x, chunks=2)
b = table([2, 3, 4], [1, 2, 3], names=[:x, :y], pkey=:x, chunks=2)
@test merge(a, b) == table([1, 2, 3, 3, 4, 5], [1, 1, 2, 2, 3, 3], names=Symbol[:x, :y])
a = ndsparse([1, 3, 5], [1, 2, 3], chunks=2)
b = ndsparse([2, 3, 4], [1, 2, 3], chunks=2)
@test merge(a, b) == ndsparse(([1, 2, 3, 4, 5],), [1, 1, 2, 3, 3])
@test merge(a, b, agg=+) == ndsparse(([1, 2, 3, 4, 5],), [1, 1, 4, 3, 3])
a = ndsparse(([1, 1, 2, 2], [1, 2, 1, 2]), [1, 2, 3, 4], chunks=2)
b = ndsparse([1, 2], [1 / 1, 1 / 2], chunks=2)
@test broadcast(*, a, b) == ndsparse(([1, 1, 2, 2], [1, 2, 1, 2]), [1.0, 2.0, 1.5, 2.0])
@test a .* b == ndsparse(([1, 1, 2, 2], [1, 2, 1, 2]), [1.0, 2.0, 1.5, 2.0])
@test broadcast(*, a, b, dimmap=(0, 1)) == ndsparse(([1, 1, 2, 2], [1, 2, 1, 2]), [1.0, 1.0, 3.0, 2.0])
t = table([0.1, 0.5, 0.75], [0, 1, 2], names=[:t, :x], chunks=2)
@test reduce(+, t, select=:t) == 1.35
@test reduce(((a, b)->(t = a.t + b.t, x = a.x + b.x)), t) == (t = 1.35, x = 3)
@test value(reduce(Mean(), t, select=:t)) == 0.45
y = reduce((min, max), t, select=:x)
@test y.max == 2
@test y.min == 0
y = reduce((sum = (+), prod = (*)), t, select=:x)
y = reduce((Mean(), Variance()), t, select=:t)
@test value(y.Mean) == 0.45
@test value(y.Variance) == 0.10749999999999998
@test reduce((xsum = (:x => (+)), negtsum = ((:t => (-)) => (+))), t) == (xsum = 3, negtsum = -1.35)
t = table([1, 1, 1, 2, 2, 2], [1, 1, 2, 2, 1, 1], [1, 2, 3, 4, 5, 6], names=[:x, :y, :z], chunks=2)
@test groupreduce(+, t, :x, select=:z) == table([1, 2], [6, 15], names=Symbol[:x, :+])
@test groupreduce(+, t, (:x, :y), select=:z) == table([1, 1, 2, 2], [1, 2, 1, 2], [3, 3, 11, 4], names=Symbol[:x, :y, :+])
@test groupreduce((+, min, max), t, (:x, :y), select=:z) == table([1, 1, 2, 2], [1, 2, 1, 2], [3, 3, 11, 4], [1, 3, 5, 4], [2, 3, 6, 4], names=Symbol[:x, :y, :+, :min, :max])
@test groupreduce((zsum = (+), zmin = min, zmax = max), t, (:x, :y), select=:z) == table([1, 1, 2, 2], [1, 2, 1, 2], [3, 3, 11, 4], [1, 3, 5, 4], [2, 3, 6, 4], names=Symbol[:x, :y, :zsum, :zmin, :zmax])
@test groupreduce((xsum = :z => +, negysum = (:y => -) => +), t, :x) == table([1, 2], [6, 15], [-4, -4], names=Symbol[:x, :xsum, :negysum])
t = table([1, 1, 1, 2, 2, 2], [1, 1, 2, 2, 1, 1], [1, 2, 3, 4, 5, 6], names=[:x, :y, :z], chunks=2)
@test groupby(mean, t, :x, select=:z) == table([1, 2], [2.0, 5.0], names=Symbol[:x, :mean])
@test groupby(identity, t, (:x, :y), select=:z) == table([1, 1, 2, 2], [1, 2, 1, 2], [[1, 2], [3], [5, 6], [4]], names=Symbol[:x, :y, :identity])
@test groupby(mean, t, (:x, :y), select=:z) == table([1, 1, 2, 2], [1, 2, 1, 2], [1.5, 3.0, 5.5, 4.0], names=Symbol[:x, :y, :mean])
#@test groupby((mean, std, var), t, :y, select=:z) == table([1, 2], [3.5, 3.5], [2.38048, 0.707107], [5.66667, 0.5], names=Symbol[:y, :mean, :std, :var])
@test groupby((q25 = (z->quantile(z, 0.25)), q50 = median, q75 = (z->quantile(z, 0.75))), t, :y, select=:z) == table([1, 2], [1.75, 3.25], [3.5, 3.5], [5.25, 3.75], names=Symbol[:y, :q25, :q50, :q75])
#@test groupby((xmean = (:z => mean), ystd = ((:y => (-)) => std)), t, :x) == table([1, 2], [2.0, 5.0], [0.57735, 0.57735], names=Symbol[:x, :xmean, :ystd])
@test groupby(identity, t, :x, select = (:y, :z), flatten = true) == rechunk(t, :x)
@test groupby((xmean = (:z => mean),), t, :x) == table([1, 2], [2.0, 5.0], names=Symbol[:x, :xmean])
t = table(1:4, [1, 4, 9, 16], [1, 8, 27, 64], names = [:x, :xsquare, :xcube], pkey = :x, chunks = 2)
long = stack(t; variable = :var, value = :val)
@test long == table([1, 1, 2, 2, 3, 3, 4, 4],
[:xsquare, :xcube, :xsquare, :xcube, :xsquare, :xcube, :xsquare, :xcube],
[1, 1, 4, 8, 9, 27, 16, 64];
names = [:x, :var, :val], pkey = :x)
@test unstack(long; variable = :var, value = :val) == t
x = ndsparse((x = [1, 1, 1, 2, 2, 2], y = [1, 2, 2, 1, 2, 2], z = [1, 1, 2, 1, 1, 2]), [1, 2, 3, 4, 5, 6], chunks=2)
@test reduce(+, x, dims=1) == ndsparse((y = [1, 2, 2], z = [1, 1, 2]), [5, 7, 9])
@test reduce(+, x, dims=(1, 3)) == ndsparse((y = [1, 2],), [5, 16])
tbl = table([0.01, 0.05], [2, 1], [3, 4], names=[:t, :x, :y], pkey=:t, chunks=2)
@test select(tbl, 2) == [2, 1]
@test select(tbl, :t) == [0.01, 0.05]
@test select(tbl, :t => (t->1 / t)) == [100.0, 20.0]
@test select(tbl, [3, 4]) == [3, 4]
@test select(tbl, (2, 1)) == table([2, 1], [0.01, 0.05], names=Symbol[:x, :t])
vx = select(tbl, (:x, :t) => (p->p.x / p.t))
@test select(tbl, (:x, :t => (-))) == table([1, 2], [-0.05, -0.01], names=Symbol[:x, :t])
@test select(tbl, (:x, :t, [3, 4])) == table([2, 1], [0.01, 0.05], [3, 4], names=[1, 2, 3])
@test select(tbl, (:x, :t, :z => [3, 4])) == table([2, 1], [0.01, 0.05], [3, 4], names=Symbol[:x, :t, :z])
@test select(tbl, (:x, :t, :minust => (:t => (-)))) == table([2, 1], [0.01, 0.05], [-0.01, -0.05], names=Symbol[:x, :t, :minust])
@test select(tbl, (:x, :t, :vx => ((:x, :t) => (p->p.x / p.t)))) == table([2, 1], [0.01, 0.05], [200.0, 20.0], names=Symbol[:x, :t, :vx])
t = table([2, 1], [1, 3], [4, 5], names=[:x, :y, :z], pkey=(1, 2), chunks=2)
@test reindex(t, (:y, :z)) == table([1, 3], [4, 5], [2, 1], names=Symbol[:y, :z, :x])
@test pkeynames(t) == (:x, :y)
#@test reindex(t, (:w => [4, 5], :z)) == table([4, 5], [5, 4], [1, 2], [3, 1], names=Symbol[:w, :z, :x, :y])
@test pkeynames(t) == (:x, :y)
t = table([0.01, 0.05], [1, 2], [3, 4], names=[:t, :x, :y], chunks=2)
manh = map((row->row.x + row.y), t)
polar = map((p->(r = hypot(p.x + p.y), θ = atan(p.y, p.x))), t)
vx = map((row->row.x / row.t), t, select=(:t, :x))
#@test collect(map(sin, polar, select=:θ)) ≈ [0.948683, 0.894427]
t = table([0.1, 0.5, missing, 0.7], [2, missing, 4, 5], [missing, 6, missing, 7], names=[:t, :x, :y], chunks=2)
@test dropmissing(t) == table([0.7], [5], [7], names=[:t, :x, :y])
@test isequal(
dropmissing(t, :y),
table([0.5, 0.7], [missing, 5], [6, 7], names=[:t, :x, :y], chunks=2)
)
@test isequal(
dropmissing(t, (:t, :x)),
table([.1,.7], [2,5], [missing,7], names=[:t,:x,:y], chunks=2)
)
t2 = table([.1, .5, NA, .7], [2, NA, 4, 5], [NA, 6, NA, 7], names=[:t, :x, :y], chunks=2)
@test dropmissing(t2) == dropmissing(t)
@test isequal(
dropmissing(t2, :y),
table([0.5, 0.7], [NA, 5], [6, 7], names=[:t, :x, :y], chunks=2)
)
@test isequal(
dropmissing(t2, (:t, :x)),
table([.1,.7], [2,5], [NA,7], names=[:t,:x,:y], chunks=2)
)
@test isequal(convertmissing(t, DataValue), t2)
@test isequal(convertmissing(t2, Missing), t)
@test typeof(column(dropmissing(t, :x), :x)) <: Dagger.DArray{Int64,1}
t = table(["a", "b", "c"], [0.01, 0.05, 0.07], [2, 1, 0], names=[:n, :t, :x], chunks=2)
@test filter((p->p.x / p.t < 100), t) == table(String["b", "c"], [0.05, 0.07], [1, 0], names=Symbol[:n, :t, :x])
x = ndsparse((n = ["a", "b", "c"], t = [0.01, 0.05, 0.07]), [2, 1, 0], chunks=2)
@test filter((y->y < 2), x) == ndsparse((n = String["b", "c"], t = [0.05, 0.07]), [1, 0])
@test filter(iseven, t, select=:x) == table(String["a", "c"], [0.01, 0.07], [2, 0], names=Symbol[:n, :t, :x])
@test filter((p->p.x / p.t < 100), t, select=(:x, :t)) == table(String["b", "c"], [0.05, 0.07], [1, 0], names=Symbol[:n, :t, :x])
@test filter((p->p[2] / p[1] < 100), x, select=(:t, 3)) == ndsparse((n = String["b", "c"], t = [0.05, 0.07]), [1, 0])
@test filter((:x => iseven, :t => (a->a > 0.01)), t) == table(String["c"], [0.07], [0], names=Symbol[:n, :t, :x])
@test filter((3 => iseven, :t => (a->a > 0.01)), x) == ndsparse((n = String["c"], t = [0.07]), [0])
a = table([1,3,5], [2,2,2], names = [:x, :y], chunks = 2)
@test summarize((mean, std), a) ==
(x_mean = 3.0, y_mean = 2.0, x_std = 2.0, y_std = 0.0)
@test summarize((mean, std), a, select = :x) == (mean = 3.0, std = 2.0)
@test summarize((m = mean, s = std), a) ==
(x_m = 3.0, y_m = 2.0, x_s = 2.0, y_s = 0.0)
b = table(["a","a","b","b"], [1,3,5,7], [2,2,2,2], names = [:x, :y, :z], pkey = :x, chunks = 2)
@test summarize(mean, b) == table(["a","b"], [2.0,6.0], [2.0,2.0], names = [:x, :y, :z], pkey = :x)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1143 | import JuliaDB: tuplesetindex
@testset "Utilities" begin
@testset "tuplesetindex" begin
@test tuplesetindex((1,2,3), 4, 2) == (1,4,3)
@test tuplesetindex((x=1,y=2,z=3), 4, 2) == (x=1,y=4,z=3)
@test tuplesetindex((x=1,y=2,z=3), 4, :y) == (x=1,y=4,z=3)
end
end
import JuliaDB: Interval, hasoverlap
@testset "Interval" begin
@testset "hasoverlap" begin
@test hasoverlap(Interval(0,2), Interval(1,2))
@test !(hasoverlap(Interval(2,1), Interval(1,2)))
@test hasoverlap(Interval(0,2), Interval(0,2))
@test hasoverlap(Interval(0,2), Interval(-1,2))
@test hasoverlap(Interval(0,2), Interval(2,3))
@test !hasoverlap(Interval(0,2), Interval(4,5))
end
@testset "in" begin
@test 1 in Interval(0, 2)
@test !(1 in Interval(2, 1))
@test !(3 in Interval(0, 2))
@test !(-1 in Interval(0, 2))
end
end
@testset "vcat PooledArray Vector{Union{T,Missing}}" begin
a = PooledArray(["x"])
b = Vector{Union{String,Missing}}(["y"])
c = vcat(a, b)
@test eltype(c) == Union{String,Missing}
@test c == ["x", "y"]
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 414 | @testset "WeakRefStrings" begin
# fix #198, #199
db = table(WeakRefStrings.StringVector(["A", "B", "A", "B"]), Dagger.distribute(ones(4), 2), names = [:x, :y])
z = groupreduce(+, db, :x, select = :y)
@test fieldtype(typeof(z).parameters[1], 1) == String
@test fieldtype(typeof(z).parameters[2], 1) == String
@test fieldtype(typeof(z.chunks[1].domain.second).parameters[1], 1) == String
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | code | 1398 | # This file is a part of Julia. License is MIT: https://julialang.org/license
# This includes a few helper variables and functions that provide information about the
# test environment (command line flags, current module, etc).
# This file can be included multiple times in the same module if necessary,
# which can happen with unisolated test runs.
if !isdefined(@__MODULE__, :testenv_defined)
const testenv_defined = true
if haskey(ENV, "JULIA_TEST_EXEFLAGS")
const test_exeflags = `$(Base.shell_split(ENV["JULIA_TEST_EXEFLAGS"]))`
else
inline_flag = Base.JLOptions().can_inline == 1 ? `` : `--inline=no`
cov_flag = ``
if Base.JLOptions().code_coverage == 1
cov_flag = `--code-coverage=user`
elseif Base.JLOptions().code_coverage == 2
cov_flag = `--code-coverage=all`
end
const test_exeflags = `$cov_flag $inline_flag --check-bounds=yes --startup-file=no --depwarn=error`
end
if haskey(ENV, "JULIA_TEST_EXENAME")
const test_exename = `$(Base.shell_split(ENV["JULIA_TEST_EXENAME"]))`
elseif haskey(ENV, "JRUN_NAME")
const test_exename = "/opt/julia-0.6/bin/julia"
else
const test_exename = `$(joinpath(Sys.BINDIR, Base.julia_exename()))`
end
addprocs_with_testenv(X; kwargs...) = addprocs(X; exename=test_exename, exeflags=test_exeflags, kwargs...)
end
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 1375 | ## v0.7.0
- **(breaking)** `groupby` and `groupreduce` now select all but the grouped columns (as opposed to all columns) (#120)
- **(feature)** `usekey=true` keyword argument to `groupby` will cause the grouping function to be called with two arguments: the grouping key, and the selected subset of records. (#120)
- **(breaking)** leftjoin and outerjoin, operations don't speculatively create `DataValueArray` anymore. It will be created if there are some keys which do not have a corresponding match in the other table. (#121)
- **(feature)** `Not`, `Join`, `Between` and `Function` selectors have been added.
## v0.8.0
- **(breaking)** Uses new redisigned version of OnlineStats
- **(breaking)** Does not wrap OnlineStats in Series wrapper. (IndexedTables.jl#149) this means `m = reduce(Mean(), t, select=:x)` will return a `Mean` object rather than a `Series(Mean())` object. Also `value(m) == 0.45` for example, rather than `value(m) == (0.45,)`
- **(feature)** - `view` works with logical indexes now (IndexedTables#134)
## v0.9.0
- **(breaking)** Missing values represented as `Union{T,Missing}` rather than `DataValue`.
## v0.10.0
- **(breaking)** Support for both `Missing` and `DataValue` representations of missing data.
- Defaults to `Missing`. functions that can create missing values have been given a `missingtype = Missing` keyword argument. | JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 1509 | <p align="center"><a href="http://juliadb.org" target="_blank"><img width="128" src="https://user-images.githubusercontent.com/25916/36773410-843e61b0-1c7f-11e8-818b-3edb08da8f41.png" alt="JuliaDB logo"></a></p>
<p align="center">
<a href="https://juliadata.github.io/JuliaDB.jl/latest/" target="_blank"><img src="https://img.shields.io/badge/docs-latest-blue.svg" alt="Latest documentation"></a>
<a href="https://travis-ci.org/JuliaData/JuliaDB.jl" target="_blank"><img src="https://camo.githubusercontent.com/bd3887b7ff22081a1ab567075dc99f23a082cb94/68747470733a2f2f7472617669732d63692e6f72672f4a756c6961436f6d707574696e672f4a756c696144422e6a6c2e7376673f6272616e63683d6d6173746572" alt="Travis CI"></a>
<a href="https://codecov.io/gh/JuliaData/JuliaDB.jl" target="_blank"><img src="https://codecov.io/gh/JuliaData/JuliaDB.jl/branch/master/graph/badge.svg" alt="Code Coverage"></a>
</p>
### JuliaDB is a package for working with large persistent data sets
We recognized the need for an all-Julia, end-to-end tool that can
- Load multi-dimensional datasets quickly and incrementally.
- Index the data and perform filter, aggregate, sort and join operations.
- Save results and load them efficiently later.
- Use Julia's built-in parallelism to fully utilize any machine or cluster.
We built JuliaDB to fill this void.
### Get Started
Introduce yourself to JuliaDB's features at [juliadb.org](https://juliadb.org) or jump into the documentation [here](https://juliadata.github.io/JuliaDB.jl/latest/)!
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 73 | # API
```@index
```
```@autodocs
Modules = [JuliaDB, IndexedTables]
``` | JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 4658 | ```@setup basics
using JuliaDB
```
# Basics
JuliaDB offers two main data structures as well as distributed counterparts. This allows
you to easily scale up an analysis, as operations that work on non-distributed tables
either work out of the box or are easy to transition for distributed tables.
Here is a high level overview of tables in JuliaDB:
- Tables store data in **columns**.
- Tables are typed.
- Changing a table in some way therefore requires returning a **new** table (underlying data is not copied).
- JuliaDB has few mutating operations because a new table is necessary in most cases.
### Data for examples:
```@example basics
x = 1:10
y = vcat(fill('a', 4), fill('b', 6))
z = randn(10);
```
## [`IndexedTable`](@ref)
An [`IndexedTable`](@ref) is wrapper around a (named) tuple of Vectors, but it behaves like
a Vector of (named) tuples. You can choose to sort the table by any number of primary
keys (in this case columns `:x` and `:y`).
An `IndexedTable` is created with data in Julia via the [`table`](@ref) function or with
data on disk via the [`loadtable`](@ref) function.
```@repl basics
t = table((x=x, y=y, z=z); pkey = [:x, :y])
t[1]
t[end]
```
## [`NDSparse`](@ref)
An [`NDSparse`](@ref) has a similar underlying structure to [`IndexedTable`](@ref), but it
behaves like a sparse array with arbitrary indices. The keys of an `NDSparse` are sorted,
much like the primary keys of an `IndexedTable`.
An `NDSparse` is created with data in Julia via the [`ndsparse`](@ref) function or with
data on disk via the [`loadndsparse`](@ref) function.
```@repl basics
nd = ndsparse((x=x, y=y), (z=z,))
nd[1, 'a']
nd[10, 'j'].z
nd[1, :]
```
## Selectors
JuliaDB has a variety of ways to select columns. These selection methods get used across
many JuliaDB's functions: [`select`](@ref), [`reduce`](@ref), [`groupreduce`](@ref),
[`groupby`](@ref), [`join`](@ref), [`transform`](@ref), [`reindex`](@ref), and more.
To demonstrate selection, we'll use the [`select`](@ref) function. A selection can be any
of the following types:
1. `Integer` -- returns the column at this position.
2. `Symbol` -- returns the column with this name.
3. `Pair{Selection => Function}` -- selects and maps a function over the selection, returns the result.
4. `AbstractArray` -- returns the array itself. This must be the same length as the table.
5. `Tuple` of `Selection` -- returns a table containing a column for every selector in the tuple.
6. `Regex` -- returns the columns with names that match the regular expression.
7. `Type` -- returns columns with elements of the given type.
8. `Not(Selection)` -- returns columns that are not included in the selection.
9. `Between(first, last)` -- returns columns between `first` and `last`.
10. `Keys()` -- return the primary key columns.
```@example basics
t = table(1:10, randn(10), rand(Bool, 10); names = [:x, :y, :z])
```
#### select the :x vector
```@repl basics
select(t, 1)
select(t, :x)
```
#### map a function to the :y vector
```@repl basics
select(t, 2 => abs)
select(t, :y => x -> x > 0 ? x : -x)
```
#### select the table of :x and :z
```@repl basics
select(t, (:x, :z))
select(t, r"(x|z)")
```
#### map a function to the table of :x and :y
```@repl basics
select(t, (:x, :y) => row -> row[1] + row[2])
select(t, (1, :y) => row -> row.x + row.y)
```
#### select columns that are subtypes of Integer
```@repl basics
select(t, Integer)
```
#### select columns that are not subtypes of Integer
```@repl basics
select(t, Not(Integer))
```
## Loading and Saving
```@setup loadsave
using Pkg
Pkg.add("RDatasets")
```
### Loading Data From CSV
Loading a CSV file (or multiple files) into one of JuliaDB's tabular data structures is accomplished via the [`loadtable`](@ref) and [`loadndsparse`](@ref) functions.
```@example loadsave
using JuliaDB, DelimitedFiles
x = rand(10, 2)
writedlm("temp.csv", x, ',')
t = loadtable("temp.csv")
```
!!! note
`loadtable` and `loadndsparse` use `Missing` to represent missing values. To load a CSV that instead uses `DataValue`, see [CSVFiles.jl](https://github.com/queryverse/CSVFiles.jl). For more information on missing value representations, see [Missing Values](@ref).
### Converting From Other Data Structures
```@example loadsave
using JuliaDB, RDatasets
df = dataset("datasets", "iris") # load data as DataFrame
table(df) # Convert DataFrame to IndexedTable
```
### Save Table into Binary Format
A table can be saved to disk (for fast, efficient reloading) via the [`save`](@ref) function.
### Load Table from Binary Format
Tables that have been `save`-ed can be loaded efficiently via [`load`](@ref).
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 1626 | ```@raw html
<center>
<img src="https://user-images.githubusercontent.com/25916/36773410-843e61b0-1c7f-11e8-818b-3edb08da8f41.png" width=200>
</center>
```
# Overview
**JuliaDB is a package for working with persistent data sets.**
We recognized the need for an all-Julia, end-to-end tool that can
1. Load multi-dimensional datasets quickly and incrementally.
2. Index the data and perform filter, aggregate, sort and join operations.
3. Save results and load them efficiently later.
4. Readily use Julia's built-in [parallelism](https://docs.julialang.org/en/v1/manual/parallel-computing/) to fully utilize any machine or cluster.
We built JuliaDB to fill this void.
JuliaDB provides distributed table and array datastructures with convenient functions to load data from CSV. JuliaDB is Julia all the way down. This means queries can be composed with Julia code that may use a vast ecosystem of packages.
## Quickstart
```julia
using Pkg
Pkg.add("JuliaDB")
using JuliaDB
# Create a table where the first column is the "primary key"
t = table(rand(Bool, 10), rand(10), pkey=1)
```
## Parallelism
The parallel/distributed features of JuliaDB are available by either:
1. Starting Julia with `N` workers: `julia -p N`
2. Calling `addprocs(N)` before `using JuliaDB`
!!! note
Multiple processes may not be beneficial for datasets with less than a few million rows.
## Additional Resources
- [#juliadb Channel in the JuliaLang Slack](https://julialang.slack.com/messages/C86LDBEBD/)
- [JuliaLang Discourse](https://discourse.julialang.org)
- [Issue Tracker](https://github.com/JuliaComputing/JuliaDB.jl/issues)
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 373 | ```@setup join
using JuliaDB
```
# Joins
## Table Joins
Table joins are accomplished through the [`join`](@ref) function.
## Appending Tables with the Same Columns
The [`merge`](@ref) function will combine tables while maintaining the sorting of the
primary key(s).
```@repl join
t1 = table(1:5, rand(5); pkey=1)
t2 = table(6:10, rand(5); pkey=1)
merge(t1, t2)
``` | JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 881 | ```@setup dv
using JuliaDB, Pkg
Pkg.add("DataValues")
```
# Missing Values
Julia has several different ways of representing missing data. If a column of data may contain missing values, JuliaDB supports both missing value representations of `Union{T, Missing}` and `DataValue{T}`.
While `Union{T, Missing}` is the default representation, functions that generate missing values ([`join`](@ref)) have a `missingtype = Missing` keyword argument that can be set to `DataValue`.
- The [`convertmissing`](@ref) function is used to switch the representation of missing values.
```@repl dv
using DataValues
convertmissing(table([1, NA]), Missing)
convertmissing(table([1, missing]), DataValue)
```
- The [`dropmissing`](@ref) function will remove rows that contain `Missing` or missing `DataValue`s.
```@repl dv
dropmissing(table([1, NA]))
dropmissing(table([1, missing]))
```
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 5604 | # Feature Extraction
Machine learning models are composed of mathematical operations on matrices of numbers. However, data in the real world is often in tabular form containing more than just numbers. Hence, the first step in applying machine learning is to turn such tabular non-numeric data into a matrix of numbers. Such matrices are called "feature matrices". JuliaDB contains an `ML` module which has helper functions to extract feature matrices.
In this document, we will turn the [titanic dataset from Kaggle](https://www.kaggle.com/c/titanic) into numeric form and apply a machine learning model on it.
```@example titanic
using JuliaDB
download("https://raw.githubusercontent.com/agconti/"*
"kaggle-titanic/master/data/train.csv", "train.csv")
train_table = loadtable("train.csv", escapechar='"')
select(train_table, Not((:Name, :Ticket, :Cabin))) # hide
```
## ML.schema
Schema is a programmatic description of the data in each column. It is a dictionary which maps each column (by name) to its schema type (mainly `Continuous`, and `Categorical`).
- `ML.Continuous`: data is drawn from the real number line (e.g. Age)
- `ML.Categorical`: data is drawn from a fixed set of values (e.g. Sex)
`ML.schema(train_table)` will go through the data and infer the types and distribution of data. Let's try it without any arguments on the titanic dataset:
```@example titanic
using JuliaDB: ML
ML.schema(train_table)
```
Here is how the schema was inferred:
- Numeric fields were inferred to be `Continuous`, their mean and standard deviations were computed. This will later be used in normalizing the column in the feature matrix using the formula `((value - mean) / standard_deviation)`. This will bring all columns to the same "scale" making the training more effective.
- Some string columns are inferred to be `Categorical` (e.g. Sex, Embarked) - this means that the column is a [PooledArray](https://github.com/JuliaComputing/PooledArrays.jl), and is drawn from a small "pool" of values. For example Sex is either "male" or "female"; Embarked is one of "Q", "S", "C" or ""
- Some string columns (e.g. Name) get the schema `nothing` -- such columns usually contain unique identifying data, so are not useful in machine learning.
- The age column was inferred as `Maybe{Continuous}` -- this means that there are missing values in the column. The mean and standard deviation computed are for the non-missing values.
You may note that `Survived` column contains only 1s and 0s to denote whether a passenger survived the disaster or not. However, our schema inferred the column to be `Continuous`. To not be overly presumptive `ML.schema` will assume all numeric columns are continuous by default. We can give the hint that the Survived column is categorical by passing the `hints` arguemnt as a dictionary of column name to schema type. Further, we will also treat `Pclass` (passenger class) as categorical and suppress `Parch` and `SibSp` fields.
```@example titanic
sch = ML.schema(train_table, hints=Dict(
:Pclass => ML.Categorical,
:Survived => ML.Categorical,
:Parch => nothing,
:SibSp => nothing,
:Fare => nothing,
)
)
```
## Split schema into input and output
In a machine learning model, a subset of fields act as the input to the model, and one or more fields act as the output (predicted variables). For example, in the titanic dataset, you may want to predict whether a person will survive or not. So "Survived" field will be the output column. Using the `ML.splitschema` function, you can split the schema into input and output schema.
```@example titanic
input_sch, output_sch = ML.splitschema(sch, :Survived)
```
## Extracting feature matrix
Once the schema has been created, you can extract the feature matrix according to the given schema using `ML.featuremat`:
```@example titanic
train_input = ML.featuremat(input_sch, train_table)
```
```@example titanic
train_output = ML.featuremat(output_sch, train_table)
```
## Learning
Let us create a simple neural network to learn whether a passenger will survive or not using the [Flux](https://fluxml.github.io/) framework.
`ML.width(schema)` will give the number of features in the `schema` we will use this in specifying the model size:
```@example titanic
using Flux
model = Chain(
Dense(ML.width(input_sch), 32, relu),
Dense(32, ML.width(output_sch)),
softmax)
loss(x, y) = Flux.mse(model(x), y)
opt = Flux.ADAM(Flux.params(model))
evalcb = Flux.throttle(() -> @show(loss(first(data)...)), 2);
```
Train the data in 10 iterations
```@example titanic
data = [(train_input, train_output)]
for i = 1:10
Flux.train!(loss, data, opt, cb = evalcb)
end
```
`data` given to the model is a vector of batches of input-output matrices. In this case we are training with just 1 batch.
## Prediction
Now let's load some testing data to use the model we learned to predict survival.
```@example titanic
download("https://raw.githubusercontent.com/agconti/"*
"kaggle-titanic/master/data/test.csv", "test.csv")
test_table = loadtable("test.csv", escapechar='"')
test_input = ML.featuremat(input_sch, test_table) ;
```
Run the model on one observation:
```@example titanic
model(test_input[:, 1])
```
The output has two numbers which add up to 1: the probability of not surviving vs that of surviving. It seems, according to our model, that this person is unlikely to survive on the titanic.
You can also run the model on all observations by simply passing the whole feature matrix to `model`.
```@example titanic
model(test_input)
```
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 1806 | ```@setup onlinestats
using OnlineStats
```
# OnlineStats Integration
[OnlineStats](https://github.com/joshday/OnlineStats.jl) is a package for calculating statistics and models with online (one observation at a time) parallelizable algorithms. This integrates tightly with JuliaDB's distributed data structures to calculate statistics on large datasets. The full documentation for OnlineStats [is available here](https://joshday.github.io/OnlineStats.jl/latest/).
## Basics
OnlineStats' objects can be updated with more data and also merged together. The image below demonstrates what goes on under the hood in JuliaDB to compute a statistic `s` in parallel.
```@raw html
<img src="https://user-images.githubusercontent.com/8075494/32748459-519986e8-c88a-11e7-89b3-80dedf7f261b.png" width=400>
```
OnlineStats integration is available via the [`reduce`](@ref) and [`groupreduce`](@ref) functions. An OnlineStat acts differently from a normal reducer:
- Normal reducer `f`: `val = f(val, row)`
- OnlineStat reducer `o`: `fit!(o, row)`
```@repl onlinestats
using JuliaDB, OnlineStats
t = table(1:100, rand(Bool, 100), randn(100));
reduce(Mean(), t; select = 3)
grp = groupreduce(Mean(), t, 2; select=3)
select(grp, (1, 2 => value))
```
!!! note
The `OnlineStats.value` function extracts the value of the statistic. E.g. `value(Mean())`.
### Calculating Statistics on Multiple Columns.
The `OnlineStats.Group` type is used for calculating statistics on multiple data streams. A `Group` that computes the same `OnlineStat` can be created through integer multiplication:
```@example onlinestats
reduce(3Mean(), t)
```
Alternatively, a `Group` can be created by providing a collection of `OnlineStat`s.
```@example onlinestats
reduce(Group(Extrema(Int), CountMap(Bool), Mean()), t)
``` | JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 465 | ```@setup ops
using JuliaDB, OnlineStats
```
# Table Operations
```@contents
Pages = ["operations.md"]
Depth = 2
```
## Column Operations
- [`transform`](@ref)
- [`insertcols`](@ref)
- [`insertcolsafter`](@ref)
- [`insertcolsbefore`](@ref)
- [`rename`](@ref)
## [`filter`](@ref)
## [`flatten`](@ref)
## [`groupby`](@ref)
## [`reduce`](@ref) and [`groupreduce`](@ref)
## [`transform`](@ref)
## [`stack`](@ref) and [`unstack`](@ref)
## [`summarize`](@ref)
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 2843 | # Out-of-core processing
JuliaDB can load data that is too big to fit in memory (RAM) as well as run a subset of operations on big tables. In particular, [OnlineStats Integration](@ref) works with [`reduce`](@ref) and [`groupreduce`](@ref) for running statistical analyses that traditionally would not be possible!
## Processing Scheme
- Data is loaded into a distributed dataset containing "chunks" that safely fit in memory.
- Data is processed `Distributed.nworkers()` chunks at a time (each worker processes a chunk and then moves onto the next chunk).
- Note: This means `Distributed.nworkers() * avg_size_of_chunk` will be in RAM simultaneously.
- Output data is accumulated in-memory.
The limitations of this processing scheme is that only certain operations work out-of-core:
- [`loadtable`](@ref)
- [`loadndsparse`](@ref)
- [`load`](@ref)
- [`reduce`](@ref)
- [`groupreduce`](@ref)
- [`join`](@ref) (see [Join to Big Table](@ref))
## Loading Data
The [`loadtable`](@ref) and [`loadndsparse`](@ref) functions accept the keyword arguments `output` and `chunks` that specify the directory to save the data into and the number of chunks to be generated from the input files, respectively.
Here's an example:
```
loadtable(glob("*.csv"), output="bin", chunks=100; kwargs...)
```
Suppose there are 800 `.csv` files in the current directory. They will be read into 100 chunks (8 files per chunk). Each worker process will load 8 files into memory, save the chunk into a single binary file in the `bin` directory, and move onto the next 8 files.
!!! note
`Distributed.nworkers() * (number_of_csvs / chunks)` needs to fit in memory simultaneously.
Once data has been loaded in this way, you can reload the dataset (extremely fast) via
```
tbl = load("bin")
```
## [`reduce`](@ref) and [`groupreduce`](@ref) Operations
`reduce` is the simplest out-of-core operation since it works pair-wise. You can also perform group-by operations with a reducer via `groupreduce`.
```@example outofcore
using JuliaDB, OnlineStats
x = rand(Bool, 100)
y = x + randn(100)
t = table((x=x, y=y))
groupreduce(+, t, :x; select = :y)
```
You can replace the reducer with any `OnlineStat` object (see [OnlineStats Integration](@ref) for more details):
```@example outofcore
groupreduce(Sum(), t, :x; select = :y)
```
## Join to Big Table
[`join`](@ref) operations have limited out-of-core support. Specifically,
```
join(bigtable, smalltable; broadcast=:right, how=:inner|:left|:anti)
```
Here `bigtable` can be larger than memory, while `Distributed.nworkers()` copies of `smalltable` must fit in memory. Note that only `:inner`, `:left`, and `:anti` joins are supported (no `:outer` joins). In this operation, `smalltable` is first broadcast to all processors and `bigtable` is joined `Distributed.nworkers()` chunks at a time.
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 3006 | # Plotting
```@setup plot
using Pkg, Random
Pkg.add("StatsPlots")
Pkg.add("GR")
using StatsPlots
ENV["GKSwstype"] = "100"
gr()
Random.seed!(1234) # set random seed to get consistent plots
```
## StatsPlots
JuliaDB has all access to all the power and flexibility of [Plots](https://github.com/JuliaPlots/Plots.jl)
via [StatsPlots](https://github.com/JuliaPlots/StatsPlots.jl) and the `@df` macro.
```@example plot
using JuliaDB, StatsPlots
t = table((x = randn(100), y = randn(100)))
@df t scatter(:x, :y)
savefig("statplot.png"); nothing # hide
```

## Plotting Big Data
For large datasets, it isn't feasible to render every data point. The OnlineStats package provides a number of [data structures for big data visualization](http://joshday.github.io/OnlineStats.jl/latest/visualizations.html) that can be created via the [`reduce`](@ref) and [`groupreduce`](@ref) functions.
- Example data:
```@example plot
using JuliaDB, Plots, OnlineStats
x = randn(10^6)
y = x + randn(10^6)
z = x .> 1
z2 = (x .+ y) .> 0
t = table((x=x, y=y, z=z, z2=z2))
```
### Mosaic Plots
A [mosaic plot](https://en.wikipedia.org/wiki/Mosaic_plot) visualizes the bivariate distribution of two categorical variables.
```@example plot
o = reduce(Mosaic(Bool, Bool), t; select = (3, 4))
plot(o)
png("mosaic.png"); nothing # hide
```

### Histograms
```@example plot
grp = groupreduce(Hist(-5:.5:5), t, :z, select = :x)
plot(plot.(select(grp, 2))...; link=:all)
png("hist.png"); nothing # hide
```

```@example plot
grp = groupreduce(KHist(20), t, :z, select = :x)
plot(plot.(select(grp, 2))...; link = :all)
png("hist2.png"); nothing # hide
```

### Partition and IndexedPartition
- `Partition(stat, n)` summarizes a univariate data stream.
- The `stat` is fitted over `n` approximately equal-sized pieces.
- `IndexedPartition(T, stat, n)` summarizes a bivariate data stream.
- The `stat` is fitted over `n` pieces covering the domain of another variable of type `T`.
```@example plot
o = reduce(Partition(KHist(10), 50), t; select=:y)
plot(o)
png("partition.png"); nothing # hide
```

```@example plot
o = reduce(IndexedPartition(Float64, KHist(10), 50), t; select=(:x, :y))
plot(o)
png("partition2.png"); nothing # hide
```

### GroupBy
```@example plot
o = reduce(GroupBy{Bool}(KHist(20)), t; select = (:z, :x))
plot(o)
png("groupby.png"); nothing # hide
```

### Convenience function for Partition and IndexedPartition
You can also use the [`partitionplot`](@ref) function, a slightly less verbose way of plotting `Partition` and `IndexedPartition` objects.
```@example plot
# x by itself
partitionplot(t, :x, stat = Extrema())
savefig("partitionplot1.png"); nothing # hide
```

```@example plot
# y by x, grouped by z
partitionplot(t, :x, :y, stat = Extrema(), by = :z)
savefig("partitionplot2.png"); nothing # hide
```
 | JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"MIT"
] | 0.13.1 | 97f24d428f00f0e8c662d2aa52a389f3fcc08897 | docs | 21226 | # Tutorial
## Introduction
This is a port of a well known [tutorial](https://rpubs.com/justmarkham/dplyr-tutorial) for the [JuliaDB](http://juliadb.org/latest/) package. This tutorial is available as a Jupyter notebook [here](https://github.com/piever/JuliaDBTutorial/blob/master/hflights.ipynb).
## Getting the data
The flights dataset for the tutorial is [here](https://raw.githubusercontent.com/piever/JuliaDBTutorial/master/hflights.csv). Alternatively, run the following in Julia:
```julia
download("https://raw.githubusercontent.com/piever/JuliaDBTutorial/master/hflights.csv")
```
## Loading the data
Loading a csv file is straightforward with JuliaDB:
```julia
using JuliaDB
flights = loadtable("hflights.csv")
```
Of course, replace the path with the location of the dataset you have just downloaded.
## Filtering the data
In order to select only rows matching certain criteria, use the `filter` function:
```julia
filter(i -> (i.Month == 1) && (i.DayofMonth == 1), flights)
```
To test if one of two conditions is verified:
```julia
filter(i -> (i.UniqueCarrier == "AA") || (i.UniqueCarrier == "UA"), flights)
# in this case, you can simply test whether the `UniqueCarrier` is in a given list:
filter(i -> i.UniqueCarrier in ["AA", "UA"], flights)
```
## Select: pick columns by name
You can use the `select` function to select a subset of columns:
```julia
select(flights, (:DepTime, :ArrTime, :FlightNum))
```
Table with 227496 rows, 3 columns:
DepTime ArrTime FlightNum
───────────────────────────
1400 1500 428
1401 1501 428
1352 1502 428
1403 1513 428
1405 1507 428
1359 1503 428
1359 1509 428
1355 1454 428
1443 1554 428
1443 1553 428
1429 1539 428
1419 1515 428
⋮
1939 2119 124
556 745 280
1026 1208 782
1611 1746 1050
758 1051 201
1307 1600 471
1818 2111 1191
2047 2334 1674
912 1031 127
656 812 621
1600 1713 1597
Let's select all columns between `:Year` and `:Month` as well as all columns containing "Taxi" or "Delay" in their name. `Between` selects columns between two specified extremes, passing a function filters column names by that function and `All` takes the union of all selectors (or all columns, if no selector is specified).
```julia
select(flights, All(Between(:Year, :DayofMonth), i -> occursin("Taxi", string(i)), i -> occursin("Delay", string(i))))
```
Table with 227496 rows, 7 columns:
Year Month DayofMonth TaxiIn TaxiOut ArrDelay DepDelay
────────────────────────────────────────────────────────────
2011 1 1 7 13 -10 0
2011 1 2 6 9 -9 1
2011 1 3 5 17 -8 -8
2011 1 4 9 22 3 3
2011 1 5 9 9 -3 5
2011 1 6 6 13 -7 -1
2011 1 7 12 15 -1 -1
2011 1 8 7 12 -16 -5
2011 1 9 8 22 44 43
2011 1 10 6 19 43 43
2011 1 11 8 20 29 29
2011 1 12 4 11 5 19
⋮
2011 12 6 4 15 14 39
2011 12 6 13 9 -10 -4
2011 12 6 4 12 -12 1
2011 12 6 3 9 -9 16
2011 12 6 3 10 -4 -2
2011 12 6 5 10 0 7
2011 12 6 5 11 -9 8
2011 12 6 4 9 4 7
2011 12 6 4 14 -4 -3
2011 12 6 3 9 -13 -4
2011 12 6 3 11 -12 0
The same could be achieved more concisely using regular expressions:
```julia
select(flights, All(Between(:Year, :DayofMonth), r"Taxi|Delay"))
```
## Applying several operations
If one wants to apply several operations one after the other, there are two main approaches:
- nesting
- piping
Let's assume we want to select `UniqueCarrier` and `DepDelay` columns and filter for delays over 60 minutes. Since the `DepDelay` column has missing data, we also need to filter out `missing` values via `!ismissing`. The nesting approach would be:
```julia
filter(i -> !ismissing(i.DepDelay > 60), select(flights, (:UniqueCarrier, :DepDelay)))
```
Table with 224591 rows, 2 columns:
UniqueCarrier DepDelay
───────────────────────
"AA" 0
"AA" 1
"AA" -8
"AA" 3
"AA" 5
"AA" -1
"AA" -1
"AA" -5
"AA" 43
"AA" 43
⋮
"WN" 1
"WN" 16
"WN" -2
"WN" 7
"WN" 8
"WN" 7
"WN" -3
"WN" -4
"WN" 0
For piping, we'll use the excellent [Lazy](https://github.com/MikeInnes/Lazy.jl) package.
```julia
import Lazy
Lazy.@as x flights begin
select(x, (:UniqueCarrier, :DepDelay))
filter(i -> !ismissing(i.DepDelay > 60), x)
end
```
Table with 224591 rows, 2 columns:
UniqueCarrier DepDelay
───────────────────────
"AA" 0
"AA" 1
"AA" -8
"AA" 3
"AA" 5
"AA" -1
"AA" -1
"AA" -5
"AA" 43
"AA" 43
⋮
"WN" 1
"WN" 16
"WN" -2
"WN" 7
"WN" 8
"WN" 7
"WN" -3
"WN" -4
"WN" 0
where the variable `x` denotes our data at each stage. At the beginning it is `flights`, then it only has the two relevant columns and, at the last step, it is filtered.
## Reorder rows
Select `UniqueCarrier` and `DepDelay` columns and sort by `DepDelay`:
```julia
sort(flights, :DepDelay, select = (:UniqueCarrier, :DepDelay))
```
Table with 227496 rows, 2 columns:
UniqueCarrier DepDelay
───────────────────────
"OO" -33
"MQ" -23
"XE" -19
"XE" -19
"CO" -18
"EV" -18
"XE" -17
"CO" -17
"XE" -17
"MQ" -17
"XE" -17
"DL" -17
⋮
"US" missing
"US" missing
"US" missing
"WN" missing
"WN" missing
"WN" missing
"WN" missing
"WN" missing
"WN" missing
"WN" missing
"WN" missing
or, in reverse order:
```julia
sort(flights, :DepDelay, select = (:UniqueCarrier, :DepDelay), rev = true)
```
## Apply a function row by row
To apply a function row by row, use `map`: the first argument is the anonymous function, the second is the dataset.
```julia
speed = map(i -> i.Distance / i.AirTime * 60, flights)
```
227496-element Array{Union{Missing, Float64},1}:
336.0
298.6666666666667
280.0
344.61538461538464
305.45454545454544
298.6666666666667
312.55813953488376
336.0
327.8048780487805
298.6666666666667
320.0
⋮
473.7931034482758
479.30232558139534
496.6265060240964
468.59999999999997
478.1632653061224
483.0927835051546
498.5106382978723
445.57377049180326
424.6875
460.6779661016949
## Add new variables
Use the `transform` function to add a column to an existing dataset:
```julia
transform(flights, :Speed => speed)
```
If you need to add the new column to the existing dataset:
```julia
flights = transform(flights, :Speed => speed)
```
## Reduce variables to values
To get the average delay, we first filter away datapoints where `ArrDelay` is missing, then group by `:Dest`, select `:ArrDelay` and compute the mean:
```julia
using Statistics
groupby(mean ∘ skipmissing, flights, :Dest, select = :ArrDelay)
```
Table with 116 rows, 2 columns:
Dest avg_delay
────────────────
"ABQ" 7.22626
"AEX" 5.83944
"AGS" 4.0
"AMA" 6.8401
"ANC" 26.0806
"ASE" 6.79464
"ATL" 8.23325
"AUS" 7.44872
"AVL" 9.97399
"BFL" -13.1988
"BHM" 8.69583
"BKG" -16.2336
⋮
"SJU" 11.5464
"SLC" 1.10485
"SMF" 4.66271
"SNA" 0.35801
"STL" 7.45488
"TPA" 4.88038
"TUL" 6.35171
"TUS" 7.80168
"TYS" 11.3659
"VPS" 12.4572
"XNA" 6.89628
## Performance tip
If you'll group often by the same variable, you can sort your data by that variable at once to optimize future computations.
```julia
sortedflights = reindex(flights, :Dest)
```
Table with 227496 rows, 22 columns:
Columns:
# colname type
────────────────────────────────────────────────────
1 Dest String
2 Year Int64
3 Month Int64
4 DayofMonth Int64
5 DayOfWeek Int64
6 DepTime DataValues.DataValue{Int64}
7 ArrTime DataValues.DataValue{Int64}
8 UniqueCarrier String
9 FlightNum Int64
10 TailNum String
11 ActualElapsedTime DataValues.DataValue{Int64}
12 AirTime DataValues.DataValue{Int64}
13 ArrDelay DataValues.DataValue{Int64}
14 DepDelay DataValues.DataValue{Int64}
15 Origin String
16 Distance Int64
17 TaxiIn DataValues.DataValue{Int64}
18 TaxiOut DataValues.DataValue{Int64}
19 Cancelled Int64
20 CancellationCode String
21 Diverted Int64
22 Speed DataValues.DataValue{Float64}
```julia
using BenchmarkTools
println("Presorted timing:")
@benchmark groupby(mean ∘ skipmissing, sortedflights, select = :ArrDelay)
```
Presorted timing:
BenchmarkTools.Trial:
memory estimate: 31.23 MiB
allocs estimate: 1588558
--------------
minimum time: 39.565 ms (8.03% GC)
median time: 44.401 ms (9.83% GC)
mean time: 44.990 ms (10.36% GC)
maximum time: 57.016 ms (15.96% GC)
--------------
samples: 112
evals/sample: 1
```julia
println("Non presorted timing:")
@benchmark groupby(mean ∘ skipmissing, flights, select = :ArrDelay)
```
Non presorted timing:
BenchmarkTools.Trial:
memory estimate: 1.81 KiB
allocs estimate: 30
--------------
minimum time: 195.095 μs (0.00% GC)
median time: 212.309 μs (0.00% GC)
mean time: 230.878 μs (0.20% GC)
maximum time: 4.859 ms (95.04% GC)
--------------
samples: 10000
evals/sample: 1
Using `summarize`, we can summarize several columns at the same time:
```julia
summarize(mean ∘ skipmissing, flights, :Dest, select = (:Cancelled, :Diverted))
# For each carrier, calculate the minimum and maximum arrival and departure delays:
cols = Tuple(findall(i -> occursin("Delay", string(i)), colnames(flights)))
summarize((min = minimum∘skipmissing, max = maximum∘skipmissing), flights, :UniqueCarrier, select = cols)
```
Table with 15 rows, 5 columns:
UniqueCarrier ArrDelay_min DepDelay_min ArrDelay_max DepDelay_max
─────────────────────────────────────────────────────────────────────
"AA" -39 -15 978 970
"AS" -43 -15 183 172
"B6" -44 -14 335 310
"CO" -55 -18 957 981
"DL" -32 -17 701 730
"EV" -40 -18 469 479
"F9" -24 -15 277 275
"FL" -30 -14 500 507
"MQ" -38 -23 918 931
"OO" -57 -33 380 360
"UA" -47 -11 861 869
"US" -42 -17 433 425
"WN" -44 -10 499 548
"XE" -70 -19 634 628
"YV" -32 -11 72 54
For each day of the year, count the total number of flights and sort in descending order:
```julia
Lazy.@as x flights begin
groupby(length, x, :DayofMonth)
sort(x, :length, rev = true)
end
```
Table with 31 rows, 2 columns:
DayofMonth length
──────────────────
28 7777
27 7717
21 7698
14 7694
7 7621
18 7613
6 7606
20 7599
11 7578
13 7546
10 7541
17 7537
⋮
25 7406
16 7389
8 7366
12 7301
4 7297
19 7295
24 7234
5 7223
30 6728
29 6697
31 4339
For each destination, count the total number of flights and the number of distinct planes that flew there
```julia
groupby((flight_count = length, plane_count = length∘union), flights, :Dest, select = :TailNum)
```
Table with 116 rows, 3 columns:
Dest flight_count plane_count
────────────────────────────────
"ABQ" 2812 716
"AEX" 724 215
"AGS" 1 1
"AMA" 1297 158
"ANC" 125 38
"ASE" 125 60
"ATL" 7886 983
"AUS" 5022 1015
"AVL" 350 142
"BFL" 504 70
"BHM" 2736 616
"BKG" 110 63
⋮
"SJU" 391 115
"SLC" 2033 368
"SMF" 1014 184
"SNA" 1661 67
"STL" 2509 788
"TPA" 3085 697
"TUL" 2924 771
"TUS" 1565 226
"TYS" 1210 227
"VPS" 880 224
"XNA" 1172 177
## Window functions
In the previous section, we always applied functions that reduced a table or vector to a single value.
Window functions instead take a vector and return a vector of the same length, and can also be used to
manipulate data. For example we can rank, within each `UniqueCarrier`, how much
delay a given flight had and figure out the day and month with the two greatest delays:
```julia
using StatsBase
fc = dropmissing(flights, :DepDelay)
gfc = groupby(fc, :UniqueCarrier, select = (:Month, :DayofMonth, :DepDelay), flatten = true) do dd
rks = ordinalrank(column(dd, :DepDelay), rev = true)
sort(dd[rks .<= 2], by = i -> i.DepDelay, rev = true)
end
```
Table with 30 rows, 4 columns:
UniqueCarrier Month DayofMonth DepDelay
──────────────────────────────────────────
"AA" 12 12 970
"AA" 11 19 677
"AS" 2 28 172
"AS" 7 6 138
"B6" 10 29 310
"B6" 8 19 283
"CO" 8 1 981
"CO" 1 20 780
"DL" 10 25 730
"DL" 4 5 497
"EV" 6 25 479
"EV" 1 5 465
⋮
"OO" 4 4 343
"UA" 6 21 869
"UA" 9 18 588
"US" 4 19 425
"US" 8 26 277
"WN" 4 8 548
"WN" 9 29 503
"XE" 12 29 628
"XE" 12 29 511
"YV" 4 22 54
"YV" 4 30 46
Though in this case, it would have been simpler to use Julia partial sorting:
```julia
groupby(fc, :UniqueCarrier, select = (:Month, :DayofMonth, :DepDelay), flatten = true) do dd
partialsort(dd, 1:2, by = i -> i.DepDelay, rev = true)
end
```
Table with 30 rows, 4 columns:
UniqueCarrier Month DayofMonth DepDelay
──────────────────────────────────────────
"AA" 12 12 970
"AA" 11 19 677
"AS" 2 28 172
"AS" 7 6 138
"B6" 10 29 310
"B6" 8 19 283
"CO" 8 1 981
"CO" 1 20 780
"DL" 10 25 730
"DL" 4 5 497
"EV" 6 25 479
"EV" 1 5 465
⋮
"OO" 4 4 343
"UA" 6 21 869
"UA" 9 18 588
"US" 4 19 425
"US" 8 26 277
"WN" 4 8 548
"WN" 9 29 503
"XE" 12 29 628
"XE" 12 29 511
"YV" 4 22 54
"YV" 4 30 46
For each month, calculate the number of flights and the change from the previous month
```julia
using ShiftedArrays
y = groupby(length, flights, :Month)
lengths = columns(y, :length)
transform(y, :change => lengths .- lag(lengths))
```
Table with 12 rows, 3 columns:
Month length change
─────────────────────
1 18910 missing
2 17128 -1782
3 19470 2342
4 18593 -877
5 19172 579
6 19600 428
7 20548 948
8 20176 -372
9 18065 -2111
10 18696 631
11 18021 -675
12 19117 1096
## Visualizing your data
The [StatsPlots](https://github.com/JuliaPlots/StatsPlots.jl) and [GroupedErrors](https://github.com/piever/GroupedErrors.jl) package as well as native plotting recipes from JuliaDB using [OnlineStats](https://github.com/joshday/OnlineStats.jl) make a rich set of visualizations possible with an intuitive syntax.
Use the `@df` macro to be able to refer to columns simply by their name. You can work with these symobls as if they are regular vectors. Here for example, we split data according to whether the distance is smaller or bigger than `1000`.
```julia
using StatsPlots
gr(fmt = :png) # choose the fast GR backend and set format to png: svg would probably crash with so many points
@df flights scatter(:DepDelay, :ArrDelay, group = :Distance .> 1000, layout = 2, legend = :topleft)
```
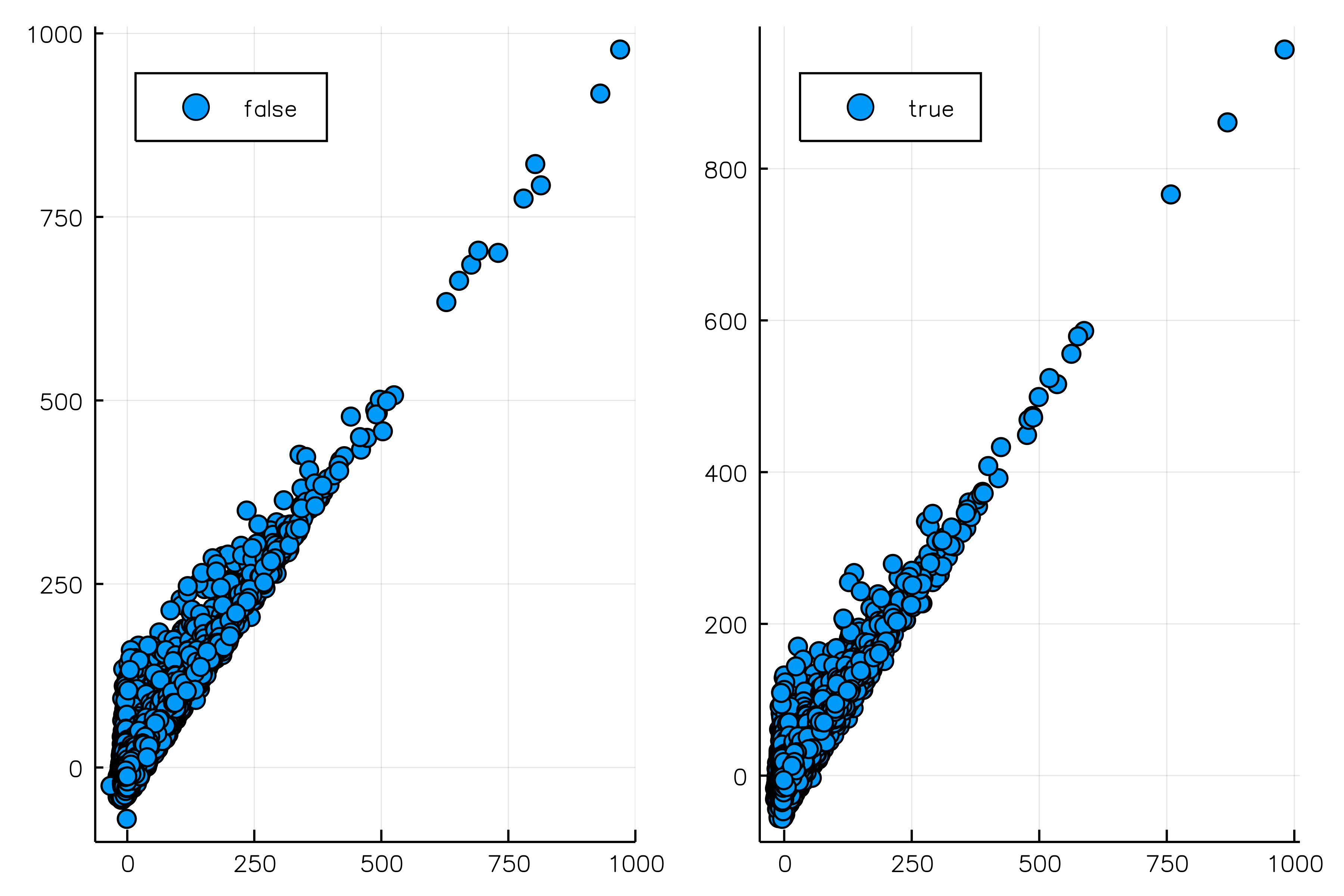
## Online statistics
For large datasets, summary statistics can be computed using efficient online algorithms implemnted in OnlineStats. Here we will use an online algorithm to compute the `mean` traveled distance split across month of the year.
```julia
using OnlineStats
grpred = groupreduce(Mean(), flights, :Month; select = :Distance)
```
Table with 12 rows, 2 columns:
Month Mean
────────────────────────────────────
1 Mean: n=18910 | value=760.804
2 Mean: n=17128 | value=763.909
3 Mean: n=19470 | value=782.788
4 Mean: n=18593 | value=783.845
5 Mean: n=19172 | value=789.66
6 Mean: n=19600 | value=797.869
7 Mean: n=20548 | value=798.52
8 Mean: n=20176 | value=793.727
9 Mean: n=18065 | value=790.444
10 Mean: n=18696 | value=788.256
11 Mean: n=18021 | value=790.691
12 Mean: n=19117 | value=809.024
Extract the values of the OnlineStat objects with the `value` function.
```julia
select(grpred, (:Month, :Mean => value))
```
Table with 12 rows, 2 columns:
Month Mean
──────────────
1 760.804
2 763.909
3 782.788
4 783.845
5 789.66
6 797.869
7 798.52
8 793.727
9 790.444
10 788.256
11 790.691
12 809.024
## Interfacing with online datasets
JuliaDB can also smoothly interface online datasets using packages from the [JuliaDatabases](https://github.com/JuliaDatabases) organization. Here's how it would work with a MySQL dataset:
```julia
using MySQL, JuliaDB
```
```julia
conn = MySQL.connect(host::String, user::String, passwd::String; db::String = "") # edit as needed for your dataset
MySQL.query(conn, "SELECT Name, Salary FROM Employee;") |> table # execute the query and collect as a table
MySQL.disconnect(conn)
```
| JuliaDB | https://github.com/JuliaData/JuliaDB.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 201 | using Documenter, SemanticAST
makedocs(sitename="SemanticAST",
pages = [
"index.md",
"Public interface" => "interface.md",
])
deploydocs(
repo = "github.com/BenChung/SemanticAST.jl.git"
)
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 385 | module SemanticAST
using MLStyle
using MLStyle.AbstractPatterns
using MLStyle.AbstractPatterns.BasicPatterns
import JuliaSyntax: @K_str, @KSet_str
using JuliaSyntax
const SN = JuliaSyntax.SyntaxNode
const SH = JuliaSyntax.SyntaxHead
include("util.jl")
include("ast_defns.jl")
include("mlstyle_helpers.jl")
include("analyzer.jl")
export expand_forms, expand_toplevel, ExpandCtx
end
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 52661 | "The error reporting mode to use"
abstract type ErrorReporting end
"Create an ASTException when an issue is encountered and continue with the fallback."
struct ExceptionErrorReporting <: ErrorReporting end
"Do nothing when an issue is encountered and continue with the fallback."
struct SilentErrorReporting <: ErrorReporting end
"""
The abstract type for macro expansion contexts.
There are two macro extension points in the analyzer: `resolve_toplevel_macro(ast::SyntaxNode, ::MacroContext, ::Val{Symbol}, args::Vector{SyntaxNode}, ctx::ExpandCtx)::ToplevelStmts` and `resolve_macro(ast, ::MacroContext, ::Val{Symbol}, args, ctx)::Expression`.
Both share the same signature:
* `ast`: The SyntaxNode root of the macro invocation.
* `MacroContext`: The context with which to resolve macros in. Implement a new `MacroContext` by implementing `resolve_toplevel_macro` and `resolve_macro` that accept it; the provided one is `DefaultMacroContext`.
* `Val{Symbol}`: The macro's name (in `Val` form).
* `args`: The SyntaxNodes that represent the arguments to the macro.
* `ctx`: The expansion context inside which the analysis is being done.
They differ in that `resolve_toplevel_macro` returns a `TopLevelStmts` (a statement that can only exist at the top level), while `resolve_macro` returns an `Expression`. An example of how to write a macro analyzer
can be seen in the default implementation for `@inline` and `@noinline`:
```
resolve_macro(ast, ::DefaultMacroContext, ::Union{Val{Symbol("@inline")}, Val{Symbol("@noinline")}, Val{Symbol("@inbounds")}}, args, ctx) = expand_forms(args[1], ctx)
```
From the perspective of the default analyzer `@inline` and `@noinline` are no-ops, so analysis continues by simply calling back into `expand_forms` on the first
argument.
"""
abstract type MacroContext end
"""
The default macro analysis context.
Currently implements top-level support for:
* @doc
* @inline, @noinline
* @assume_effects, @constprop
Expression-level support is provided for:
* @doc
* @inline, @noinline
* @eval
* @generated
* @assume_effects
"""
struct DefaultMacroContext <: MacroContext end
"""
ExpandCtx(is_toplevel::Bool=false, is_loop::Bool= false; macro_context=DefaultMacroContext(), error_context=ExceptionErrorReporting())
The context in which to perform expansion from SyntaxNodes to ASTNodes.
* `is_toplevel`: is the analyzed expression at the top level or not?
* `is_loop`: is the expression in a loop or not (used for determining if `break` or `continue` are valid)?
* `macro_context`: The context with which to expand macros. See the discussion in readme.
* `error_context`: The error reporting context. By default, two are provided ([ExceptionErrorReporting](@ref) and [SilentErrorReporting](@ref)), with exceptions being the default.
"""
struct ExpandCtx{ER <: ErrorReporting, MC <: MacroContext}
is_toplevel::Bool
is_loop::Bool
macro_context::MC
error_context::ER
ExpandCtx(is_toplevel::Bool=false, is_loop::Bool=false;
macro_context::MC=DefaultMacroContext(), error_context::ER=ExceptionErrorReporting()) where {ER, MC} =
new{ER, MC}(is_toplevel, is_loop, macro_context, error_context)
ExpandCtx(base::ExpandCtx{ER, MC};
is_toplevel::Union{Bool, Nothing}=nothing,
is_loop::Union{Bool, Nothing}=nothing) where {ER, MC}= new{ER, MC}(
isnothing(is_toplevel) ? false : is_toplevel,
isnothing(is_loop) ? base.is_loop : is_loop,
base.macro_context, base.error_context)
end
handle_error(context::ExpandCtx, node::JuliaSyntax.SyntaxNode, reporting::ExceptionErrorReporting, message::String, continuation::Union{Function, Nothing}) = throw(ASTException(node, message))
handle_error(context::ExpandCtx, node::JuliaSyntax.SyntaxNode, reporting::SilentErrorReporting, message::String, continuation::Union{Function, Nothing}) = if !isnothing(continuation) continuation() else nothing end
isdecl(ast) = @match ast begin
SN(SH(K"::", _), _) => true
_ => false
end
isassignment(ast) = @match ast begin
SN(SH(K"=", _), _) => true
_ => false
end
iskwarg(ast) = @match ast begin
SN(SH(K"=", _), _) => true
_ => false
end
identifier_name(ast) = @match ast begin
SN(SH(K"Identifier", _), _) => ast
_ => nothing
end
eventually_call = @λ begin
SN(SH(K"call", _), _) => true
SN(SH(K"where", _), [name, _...]) => eventually_call(name)
SN(SH(K"::", _), [name, _]) => eventually_call(name)
_ => false
end
isdotop_named(ast) = let idname = identifier_name(ast); !isnothing(idname) && JuliaSyntax.is_dotted(JuliaSyntax.head(idname)) end
check_dotop(ctx, ast) =
if isdotop_named(ast)
handle_error(ctx, ast, ctx.error_context, "Invalid function name", nothing)
else
@match ast begin
SN(SH(K".", _), [rec, name]) => check_dotop(ctx, name)
SN(SH(K"quote" || K"break", _), [body]) => check_dotop(ctx, body)
_ => nothing
end;
ast
end
kindof(ex) = JuliaSyntax.kind(JuliaSyntax.head(ex))
childof = JuliaSyntax.child
just_argslist(args) = kindof(args[1]) ∈ KSet"tuple block ..." || (kindof(args[1]) == K"where" && just_argslist(args[1]))
function flatten_where_expr(ex, ctx)
vars = Any[]
if kindof(ex) != K"where"
throw(ASTException(ex, "Invalid expression (internal error: flattening non-where)")) # using direct throw due to fatal error
end
while kindof(ex) == K"where"
@match ex begin
SN(SH(K"where", _), [body, SN(SH(K"braces", _), [current_vars...])]) => begin
push!.((vars, ), current_vars)
ex = body
end
SN(SH(K"where", _), [body, var]) => begin
push!(vars, var)
ex = body
end
_ => return handle_error(ctx, ex, ctx.error_context, "Invalid use of where", () -> (ex, vars))
end
end
return (ex, vars)
end
function analyze_typevar(ex, ctx)
check_sym(s) = (kindof(s) == K"Identifier" ? Expr(s) : handle_error(ctx, s, ctx.error_context, "Invalid type variable (non-symbol)", () -> TyVar(gensym(), nothing, nothing, ex)))
@match ex begin
ex && GuardBy(isatom) => TyVar(check_sym(ex), nothing, nothing, ex)
SN(SH(K"Identifier", _), _) => TyVar(check_sym(ex), nothing, nothing, ex)
SN(SH(K"comparison", _), [a, SN(SH(K"<:", _), _), b, SN(SH(K"<:", _), _), c]) => TyVar(check_sym(b), expand_forms(c, ctx), expand_forms(a, ctx), ex)
SN(SH(K"<:", _), [a, b]) => TyVar(check_sym(a), expand_forms(b, ctx), nothing, ex)
SN(SH(K">:", _), [a, b]) => TyVar(check_sym(a), nothing, expand_forms(b, ctx), ex)
_ => handle_error(ctx, ex, ctx.error_context, "invalid variable expression in \"where\"", () -> TyVar(gensym(), nothing, nothing, ex))
end
end
analyze_tuple_assignment(expr, ctx) = @match expr begin
SN(SH(K"Identifier", _), _) && name => IdentifierAssignment(Expr(name), expr)
SN(SH(K"::", _), [name, type]) => TypedAssignment(IdentifierAssignment(Expr(name)), expand_forms(type, ctx), expr)
_ => handle_error(ctx, expr, ctx.error_context, "invalid assignment location", () -> IdentifierAssignment(gensym(), expr))
end
unquote = @λ begin
SN(SH(K"quote", _), [body]) => Expr(body)
end
analyze_lvalue(expr, ctx; is_for=false) = @match expr begin
SN(SH(K"Identifier", _), _) && id =>
if Expr(id) ∈ [:ccall, :global]
handle_error(ctx, expr, ctx.error_context, "invalid assignment location", () -> IdentifierAssignment(gensym(), expr))
else
IdentifierAssignment(Expr(id), expr)
end
SN(SH(K"where", _), ()) => IdentifierAssignment(:where, expr) # why is this an empty tuple? I don't know!
SN(SH(K".", _), [a,b]) => FieldAssignment(expand_forms(a, ctx), unquote(b), expr)
SN(SH(K"tuple", _), [_, args..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, expr, ctx.error_context, "invalid assignment location", () -> IdentifierAssignment(gensym(), expr))
SN(SH(K"tuple", _), [SN(SH(K"parameters", _), params)]) =>
NamedTupleAssignment(map(x -> analyze_tuple_assignment(x, ctx), params), expr)
SN(SH(K"tuple", _), [args...]) => begin
has_varargs = false
assignment_args = LValue[]
for arg in args
@match arg begin
SN(SH(K"...", _), body) => begin
if has_varargs
push!(assignment_args, handle_error(ctx, arg, ctx.error_context, "multiple \"...\" on lhs of assignment", () -> IdentifierAssignment(gensym(), expr)))
end
has_varargs = true
push!(assignment_args, VarargAssignment(length(body) > 0 ? analyze_lvalue(body[1], ctx) : nothing, arg))
end
arg => push!(assignment_args, analyze_lvalue(arg, ctx))
end
end
TupleAssignment(assignment_args, expr)
end
SN(SH(K"typed_hcat", _), _) => handle_error(ctx, expr, ctx.error_context, "invalid spacing in left side of indexed assignment", () -> IdentifierAssignment(gensym(), expr))
SN(SH(K"typed_vcat" || K"typed_ncat", _), _) => handle_error(ctx, expr, ctx.error_context, "unexpected \";\" in left side of indexed assignment", () -> IdentifierAssignment(gensym(), expr))
SN(SH(K"ref", _), [a, idxs...]) => RefAssignment(expand_forms(a, ctx), split_arguments(idxs, ctx)..., expr)
SN(SH(K"::", _), [typ]) => handle_error(ctx, expr, ctx.error_context, "invalid assignment location", () -> IdentifierAssignment(gensym(), expr))
SN(SH(K"::", _), [call && GuardBy(eventually_call), T]) && funspec => FunctionAssignment(expand_function_name(funspec, ctx)..., expr)
SN(SH(K"::", _), [x, T]) => TypedAssignment(analyze_lvalue(x, ctx), expand_forms(T, ctx), expr)
SN(SH(K"vcat" || K"ncat", _), _) => handle_error(ctx, expr, ctx.error_context, "use \"(a, b) = ...\" to assign multiple values", () -> IdentifierAssignment(gensym(), expr))
SN(SH(K"call" || K"where", _), _) && name => FunctionAssignment(expand_function_name(name, ctx)..., expr)
SN(SH(K"curly", _), [name, tyargs...]) => length(tyargs) == 0 ? handle_error(ctx, expr, ctx.error_context, "empty type parameter list", () -> analyze_lvalue(name, ctx)) : UnionAllAssignment(analyze_lvalue(name, ctx), analyze_typevar.(tyargs, (ctx, )), expr)
SN(SH(K"outer", _), [SN(SH(K"Identifier", _), _) && id]) =>
if is_for
OuterIdentifierAssignment(Expr(id), expr)
else
handle_error(ctx, expr, ctx.error_context, "no outer local variable declaration exists", () -> IdentifierAssignment(Expr(id), expr))
end
_ => handle_error(ctx, expr, ctx.error_context, "invalid assignment location", () -> IdentifierAssignment(gensym(), expr))
end
expr_contains_p(pred, expr, filter = x -> true) =
filter(expr) && (pred(expr) || @match expr begin
SN(SH(K"quote", _), _) => false
SN(_, args) => any(expr_contains_p.(pred, args, filter))
e => throw("unhandled $e")
end)
isparam = @λ begin SN(SH(K"parameters", _), _) => true; _ => false end
contains_destructuring = @λ begin
SN(SH(K"::" || K"=", _), _) => true
_ => false
end
is_return = @λ begin
SN(SH(K"return", _), _) => true
_ => false
end
contains_return(e) = expr_contains_p(is_return, e)
check_recursive_assignment(expr) =
if expr_contains_p(contains_destructuring, expr)
false
else
true
end
analyze_argument(expr, is_kw, ctx) = @match (expr, is_kw) begin
(SN(SH(K"tuple", _), _), false) => (FnArg(analyze_lvalue(expr, ctx), nothing, nothing, expr), false)
(SN(SH(K"::", _), [binding, typ]), false) => check_recursive_assignment(binding) ?
(FnArg(analyze_lvalue(binding, ctx), nothing, expand_forms(typ, ctx), expr), false) :
(handle_error(ctx, expr, ctx.error_context, "invalid recursive destructuring syntax", () -> FnArg(IdentifierAssignment(gensym(), expr), nothing, expand_forms(typ, ctx), expr)), false)
(SN(SH(K"::", _), [typ]), false) => (FnArg(nothing, nothing, expand_forms(typ, ctx), expr), false)
(SN(SH(K"=", _), [head, default]), is_kw) => (let (base, _) = analyze_argument(head, false, ctx); FnArg(base.binding, expand_forms(default, ctx), base.type, expr) end, true)
(SN(SH(K"...", _), [bound]), false) => (FnArg(VarargAssignment(analyze_lvalue(bound, ctx), expr), nothing, nothing, expr), false)
(SN(SH(K"...", _), []), false) => (FnArg(VarargAssignment(nothing, expr), nothing, nothing, expr), false)
(_, false) => (FnArg(analyze_lvalue(expr, ctx), nothing, nothing, expr), false)
(_, true) => (handle_error(ctx, expr, ctx.error_context, "optional positional arguments must occur at end", () -> first(analyze_argument(expr, false, ctx))), true)
(_, _) => (handle_error(ctx, expr, ctx.error_context, "invalid argument syntax", () -> FnArg(IdentifierAssignment(gensym(), expr), nothing, nothing, expr)), is_kw)
end
analyze_kwargs(expr, ctx) = @match expr begin
SN(SH(K"parameters", _), _) => handle_error(ctx, expr, ctx.error_context, "more than one semicolon in argument list", () -> KwArg(gensym(), nothing, nothing, expr))
SN(SH(K"=", _), [SN(SH(K"::", _), [SN(SH(K"Identifier", _), ) && name, type]), body]) => begin
KwArg(Expr(name), expand_forms(type, ctx), expand_forms(body, ctx), expr)
end
SN(SH(K"=", _), [SN(SH(K"Identifier", _), _) && name, body]) => KwArg(Expr(name), nothing, expand_forms(body, ctx), expr)
SN(SH(K"...", _), [SN(SH(K"Identifier", _), _) && name]) => KwArg(Expr(name), nothing, nothing, expr, true)
SN(SH(K"=", _), [SN(SH(K"::", _), [name, type]), body]) => handle_error(ctx, expr, ctx.error_context, "is not a valid function argument name", () -> KwArg(gensym(), expand_forms(type, ctx), expand_forms(body, ctx), expr))
SN(SH(K"=", _), [name, body]) => handle_error(ctx, expr, ctx.error_context, "is not a valid function argument name", () -> KwArg(Expr(name), nothing, expand_forms(body, ctx), expr))
SN(SH(K"...", _), [name]) => handle_error(ctx, expr, ctx.error_context, "is not a valid function argument name", () -> KwArg(gensym(), nothing, nothing, expr))
SN(SH(K"Identifier", _), _) && ident => KwArg(Expr(ident), nothing, nothing, expr)
SN(SH(K"::", _), [SN(SH(K"Identifier", _), _) && ident, type]) => KwArg(Expr(ident), expand_forms(type, ctx), nothing, expr)
other => handle_error(ctx, expr, ctx.error_context, "invalid keyword argument syntax", () -> KwArg(gensym(), nothing, nothing, expr))
end
hasduplicates(array) = length(unique(array)) != length(array)
unpack_fn_name(e, ctx) = @match e begin
SN(SH(K".", _), [rec, SN(SH(K"quote", _), [field && SN(SH(K"Identifier", _), _)])]) => push!(unpack_fn_name(rec, ctx), Expr(field))
SN(SH(K"Identifier", _), _) && name => [Expr(name)]
expr => handle_error(ctx, expr, ctx.error_context, "invalid function name", () -> [gensym()])
end
parse_func_name(ctx, receiver, args, e) = TypeFuncName(expand_forms(receiver, ctx), [let param = extract_implicit_whereparam(arg, ctx); isnothing(param) ? expand_forms(arg, ctx) : param end for arg in args], e)
resolve_function_name(e, ctx) = @match e begin
SN(SH(K"Identifier", _), _) && name => ResolvedName([Expr(name)], e) # symbols are valid
SN(SH(K".", _), _) && e => ResolvedName(unpack_fn_name(e, ctx), e)
SN(SH(K"::", _), [name, type]) => DeclName(analyze_lvalue(name, ctx), expand_forms(type, ctx), e)
SN(SH(K"::", _), [type]) => DeclName(nothing, expand_forms(type, ctx), e)
SN(SH(K"curly", _), [receiver, args..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, e, ctx.error_context, "unexpected semicolon", () -> parse_func_name(ctx, receiver, args, e))
SN(SH(K"curly", _), [receiver, args...]) =>
if any(iskwarg, args)
handle_error(ctx, e, ctx.error_context, "unexpected semicolon", () -> parse_func_name(ctx, receiver, filter(a -> !iskwarg(a), args), e))
else
parse_func_name(ctx, receiver, args, e)
end
SN(GuardBy(JuliaSyntax.is_operator) && GuardBy((!) ∘ JuliaSyntax.is_dotted), ()) && name => ResolvedName([Expr(name)], e) # so are non-dotted operators
nothing => AnonFuncName(e)
_ => handle_error(ctx, e, ctx.error_context, "invalid function name", () -> ResolvedName([gensym()], e))
end
function analyze_call(call, name, args, raw_typevars, rett, ctx; is_macro=false)
sparams = analyze_typevar.(raw_typevars, (ctx, ))
# todo: nospecialize
is_kw = false
args_stmts = [
begin
(result, is_kw) = analyze_argument(expr, is_kw, ctx)
result
end for expr in filter((!) ∘ isparam, args)]
params = filter(isparam, args)
kwargs_stmts = []
if length(params) == 1
kwargs_stmts = @match params[1] begin
SN(SH(K"parameters", _), [params...]) =>
if !is_macro
map(x -> analyze_kwargs(x, ctx), params)
else
map(x -> handle_error(ctx, x, ctx.error_context, "macros cannot accept keyword arguments", () -> analyze_kwargs(x, ctx)), params)
end
end
elseif length(params) > 1
handle_error(ctx, call, ctx.error_context, "more than one semicolon in argument list", nothing)
end
if !isnothing(name)
if is_macro
@match name begin
SN(SH(K"Identifier", _), _) => nothing
_ => handle_error(ctx, call, ctx.error_context, "invalid macro definition", nothing)
end
end
name = check_dotop(ctx, name)
end
varargs_indices = findall(arg -> arg.binding isa VarargAssignment, args_stmts)
if !isempty(varargs_indices) && last(varargs_indices) != length(args_stmts)
handle_error(ctx, call, ctx.error_context, "invalid \"...\" on non-final argument", nothing)
end
get_fnarg_name = @λ begin
FnArg(IdentifierAssignment(name, _) || TypedAssignment(IdentifierAssignment(name, _), _, _,), _, _, _) => [name]
expr => begin [] end
end
argnames = vcat(collect(Iterators.flatten(map(get_fnarg_name, args_stmts))), map(x -> x.name, kwargs_stmts))
staticnames = map(x -> x.name, sparams)
if !isempty(argnames) && hasduplicates(argnames)
handle_error(ctx, call, ctx.error_context, "function argument name not unique", nothing)
elseif !isempty(staticnames) && hasduplicates(staticnames)
handle_error(ctx, call, ctx.error_context, "function static parameter names not unique", nothing)
elseif !isempty(argnames) && !isempty(staticnames) && (argnames ⊆ staticnames || staticnames ⊆ argnames)
handle_error(ctx, call, ctx.error_context, "function argument and static parameter names must be distinct", nothing)
end
return resolve_function_name(name, ctx), args_stmts, kwargs_stmts, sparams, (isnothing(rett) ? nothing : expand_forms(rett, ctx))
end
function destructure_function_head(name, ctx)
(name, raw_typevars) = @match name begin
SN(SH(K"::", _), [nexpr, SN(SH(K"where", _), _) && clause]) => begin
return (nexpr, flatten_where_expr(clause, ctx)...)
end
SN(SH(K"where", _), _) => flatten_where_expr(name, ctx)
_ => (name, [])
end # this control flow is very cursed sorry
rett, name = @match name begin
SN(SH(K"::", _), [true_name, typ]) => (typ, true_name)
_ => (nothing, name) # todo return a valid thingie
end
return name, rett, raw_typevars
end
function expand_function_name(name, ctx; is_macro = false)
orig_expr = name
name, rett, raw_typevars = destructure_function_head(name, ctx)
return @match name begin
SN(SH(K"call", _), [name, args...]) => analyze_call(orig_expr, name, args, raw_typevars, rett, ctx; is_macro = is_macro)
SN(SH(K"tuple", _), [args...]) => analyze_call(orig_expr, nothing, args, raw_typevars, rett, ctx; is_macro=is_macro)
SN(SH(K"Identifier", _), _) => (ResolvedName([Expr(name)], name), [], KwArg[], TyVar[], nothing)
_ => (handle_error(ctx, orig_expr, ctx.error_context, "invalid assignment location name $name", () -> ResolvedName([gensym()], orig_expr)), [], KwArg[], TyVar[], nothing)
end
end
function expand_anon_function(spec, ctx)
orig_expr = spec
spec, rett, raw_typevars = destructure_function_head(spec, ctx)
return @match spec begin
SN(SH(K"tuple", _), [args...]) => analyze_call(orig_expr, nothing, args, raw_typevars, rett, ctx)
SN(SH(K"Identifier", _), _) && arg => analyze_call(orig_expr, nothing, [arg], raw_typevars, rett, ctx)
_ => (handle_error(ctx, orig_expr, ctx.error_context, "invalid assignment location name $name", () -> ResolvedName([gensym()], orig_expr)), [], KwArg[], TyVar[], nothing)
end
end
function analyze_let_eq(expr)
return @match expr begin
FunctionAssignment(ResolvedName([_], _), _, _, _, _, _) && fun => fun
FunctionAssignment(name, a, b, c, d, e) && fun => handle_error(ctx, name.location.basenode, ctx.error_context, "invalid let syntax", () -> FunctionAssignment(ResolvedName(gensym, name.location.basenode), a, b, c, d, e))
TupleAssignment(_, _) && tup => tup
IdentifierAssignment(_, _) && id => id
TypedAssignment(_, _, _) && ty => ty
_ => handle_error(ctx, expr.location.basenode, ctx.error_context, "invalid let syntax", () -> IdentifierAssignment(gensym(), expr.location.basenode))
end
end
analyze_let_binding(expr, ctx) = @match expr begin
SN(SH(K"Identifier", _), _) && ident => Expr(ident)
SN(SH(K"=", _), [a, b]) => (analyze_let_eq(analyze_lvalue(a, ctx))) => expand_forms(b, ctx)
_ => begin handle_error(ctx, expr, ctx.error_context, "invalid let syntax", nothing); nothing end
end
function expand_macro_name(name, ctx)
name, args_stmts, kwargs_stmts, sparams, rett = expand_function_name(name, ctx; is_macro = true)
return name, args_stmts, sparams, rett
end
eventually_decl(expr) = @match expr begin
SN(SH(K"Identifier", _), _) => true
SN(SH(K"::" || K"const" || K"=", _), [name, _...]) => eventually_decl(name)
_ => false
end
analyze_type_sig = @λ begin
SN(SH(K"Identifier", _), _) && name => (name, [], nothing)
SN(SH(K"curly", _), [SN(SH(K"Identifier", _), _) && name, params...]) => (name, params, nothing)
SN(SH(K"<:", _), [SN(SH(K"Identifier", _), _) && name, super]) => (name, [], super)
SN(SH(K"<:", _), [SN(SH(K"curly", _), [SN(SH(K"Identifier", _), _) && name, params...]), super]) => (name, params, super)
_ && invalid => nothing
end
node_to_bool = @λ begin
SN(SH(K"true",_), _) => true
SN(SH(K"false",_), _) => false
expr => throw(ASTException(expr, "boolean used in non-boolean context")) # left due to being a fatal internal error
end
unpack_attrs(expr, attrs, mutable, ctx) = @match (expr, mutable) begin
(SN(SH(K"=", _), [lhs && GuardBy(eventually_decl), value]), _) => handle_error(ctx, expr, ctx.error_context, "operator = inside type definition is reserved", () -> StructField(gensym(), nothing, attrs, expr))
(SN(SH(K"::", _), [SN(SH(K"Identifier", _), _) && name, typ]), _) => StructField(Expr(name), expand_forms(typ, ctx), attrs, expr)
(SN(SH(K"Identifier", _), _) && name, _) => StructField(Expr(name), nothing, attrs, expr)
(SN(SH(K"const", _), [ex]), true) => unpack_attrs(ex, attrs | FIELD_CONST, mutable, ctx)
(SN(SH(K"const", _), [ex]), false) => handle_error(ctx, expr, ctx.error_context, "invalid field attribute const for immutable struct", nothing)
_ => throw("invalid field attribute")
end
quoted = @λ begin
SN(SH(K"quote", _), _) => true
_ => false
end
effect_free = @λ begin
SN(SH(K"true" || K"false", _), _) => true
SN(SH(K".", _), [SH(SN(K"Identifier", _), _), _]) => true # sym-dot?
GuardBy(quoted) => true
_ => false
end
is_string = @λ begin
SN(SH(K"string" || K"String", _), _) => true
_ => false
end
unzip(a) = map(x->getfield.(a, x), fieldnames(eltype(a)))
decompose_decl(e) = @match e begin
SN(SH(K"::", _), [name, typ]) => (name, typ)
ex => (ex, nothing)
end
flatten_blocks(e) = @match e begin
SN(SH(K"block", _), [contents...]) => vcat((flatten_blocks.(contents))...)
expr => [expr]
end
function expand_struct_def(expr, mut, sig, fields, ctx)
analyzed = analyze_type_sig(sig)
if isnothing(analyzed)
handle_error(ctx, expr, ctx.error_context, "invalid type signature", nothing)
return StructDefStmt(gensym(), [], nothing, [], [], expr)
else
name, params, super = analyzed
end
bounds = analyze_typevar.(params, (ctx, ))
params = getfield.(bounds, :name)
fields = flatten_blocks(fields)
fieldspecs = map(fld -> unpack_attrs(fld, FIELD_NONE, mut, ctx), filter(eventually_decl, fields))
defs = filter((!) ∘ eventually_decl, fields)
defs = filter(d -> !(effect_free(d) || is_string(d)), defs)
cstrs = expand_forms.(defs, (ctx, )) # need to eventually do some transformations here?
return StructDefStmt(Expr(name), params, isnothing(super) ? nothing : expand_forms(super, ctx), fieldspecs, cstrs, expr)
end
function expand_abstract_def(expr, sig, ctx)
analyzed = analyze_type_sig(sig)
if isnothing(analyzed)
handle_error(ctx, expr, ctx.error_context, "invalid type signature", nothing)
return AbstractDefStmt(gensym(), [], nothing, expr)
else
name, params, super = analyzed
end
bounds = analyze_typevar.(params, (ctx, ))
params = getfield.(bounds, :name)
return AbstractDefStmt(Expr(name), params, isnothing(super) ? nothing : expand_forms(super, ctx), expr)
end
function expand_primitive_def(expr, sig, size, ctx)
analyzed = analyze_type_sig(sig)
if isnothing(analyzed)
handle_error(ctx, expr, ctx.error_context, "invalid type signature", nothing)
return PrimitiveDefStmt(gensym(), [], nothing, Literal(0, expr), expr)
else
name, params, super = analyzed
end
bounds = analyze_typevar.(params, (ctx, ))
params = getfield.(bounds, :name)
return PrimitiveDefStmt(Expr(name), params, isnothing(super) ? nothing : expand_forms(super, ctx), expand_forms(size, ctx), expr)
end
extract_implicit_whereparam(expr, ctx) = @match expr begin
SN(SH(K"<:", _), [bound]) => TyVar(nothing, expand_forms(bound, ctx), nothing, expr)
SN(SH(K">:", _), [bound]) => TyVar(nothing, nothing, expand_forms(bound, ctx), expr)
_ => nothing
end
isatom(ast) = @match ast begin
SH(K"Float" || K"Integer" || K"String" || K"Char" || K"true" || K"false", _) => true
_ => false
end
ifnotfalse(ast, then) = @match ast begin
SN(SH(K"false",_ ), _) => nothing
_ => then(ast)
end
analyze_kw_param(expr, ctx) = @match expr begin
SN(SH(K"parameters", _), [params...]) => handle_error(ctx, expr, ctx.error_context, "more than one semicolon in argument list", () -> KeywordArg(gensym(), nothing, expr))
SN(SH(K"=", _), [name && SN(SH(K"Identifier", _), _), value]) => KeywordArg(Expr(name), expand_forms(value, ctx), expr)
SN(SH(K"Identifier", _), _) && name => KeywordArg(Expr(name), expand_forms(name, ctx), expr)
SN(SH(K".", _), [_, SN(SH(K"quote", _), [field && SN(SH(K"Identifier", _), _)])]) => KeywordArg(Expr(field), expand_forms(expr, ctx), expr)
SN(SH(K"...", _), [splat]) => SplatArg(expand_forms(splat, ctx), expr)
_ => throw("inexhaustive $expr")
end
analyze_kw_call(params, ctx) = map(x -> analyze_kw_param(x, ctx), params)
function split_arguments(args, ctx; down=expand_forms)
pos_args = []; kw_args = []
has_parameters = false
for arg in args
@match arg begin
SN(SH(K"parameters", _), [params...]) =>
if !has_parameters
append!(kw_args, analyze_kw_call(params, ctx))
has_parameters = true
else
handle_error(ctx, arg, ctx.error_context, "more than one semicolon in argument list", nothing)
end
SN(SH(K"=", _), [SN(SH(K"Identifier", _), _) && name, value]) => push!(kw_args, KeywordArg(Expr(name), down(value, ctx), arg))
SN(SH(K"...", _), [arg]) => push!(pos_args, SplatArg(down(arg, ctx), arg))
expr => push!(pos_args, PositionalArg(down(expr, ctx), arg))
end
end
return pos_args, kw_args
end
function split_op_arguments(args, ctx; down=expand_forms)
pos_args = []; kw_args = []
for arg in args
push!(pos_args, PositionalArg(down(arg, ctx), arg))
end
return pos_args, kw_args
end
expand_broadcast(e) = dot_to_fuse(e)
convert_decltype = @λ begin
K"const" => DECL_CONST
K"local" => DECL_LOCAL
K"global" => DECL_GLOBAL
end
expand_declaration(decltype, expr, ctx) = @match expr begin
SN(SH(K"Identifier", _), _) =>
if decltype != DECL_CONST
VarDecl(IdentifierAssignment(Expr(expr), expr), nothing, decltype, expr)
else
handle_error(ctx, expr, ctx.error_context, "expected assignment after \"const\"", () -> VarDecl(IdentifierAssignment(Expr(expr), expr), nothing, DECL_NONE, expr))
end
SN(SH(K"::", _), _) => VarDecl(analyze_lvalue(expr, ctx), nothing, decltype, expr)
SN(SH(GuardBy(JuliaSyntax.is_prec_assignment), _), [lhs, rhs]) => VarDecl(analyze_lvalue(lhs, ctx), expand_forms(rhs, ctx), decltype, expr)
end
expand_named_tuple_arg(expr, ctx) = @match expr begin
SN(SH(K"=", _), [SN(SH(K"Identifier", _), _) && name, rhs]) => NamedValue(Expr(name), expand_forms(rhs, ctx), expr)
SN(SH(K"=", _), [lhs, rhs]) => handle_error(ctx, expr, ctx.error_context, "invalid name", () -> NamedValue(gensym(), expand_forms(rhs, ctx), expr))
SN(SH(K"Identifier", _), _) && name => NamedValue(Expr(name), expand_forms(name, ctx), expr)
SN(SH(K".", _), [rec, SN(SH(K"quote", _), [field && SN(SH(K"Identifier", _), _)])]) && ast => NamedValue(Expr(field), expand_forms(ast, ctx), expr)
SN(SH(K"call", GuardBy(JuliaSyntax.is_infix_op_call)), [lhs, SN(SH(K"=>", _), _), rhs]) => ComputedNamedValue(expand_forms(lhs, ctx), expand_forms(rhs, ctx), expr)
SN(SH(K"...", _), [splat]) => SplattedNamedValue(expand_forms(splat, ctx), expr)
expr => begin handle_error(ctx, expr, ctx.error_context, "invalid named tuple argument", nothing); nothing end
end
function expand_named_tuple(args, ctx)
tupleargs = []
for arg in args
expanded = expand_named_tuple_arg(arg, ctx)
if !isnothing(expanded)
push!(tupleargs, expanded)
end
end
return tupleargs
end
string_interpoland(expr, ctx) = @match expr begin
SN(SH(K"String", _), _) && str => Expr(str)
other => expand_forms(other, ctx)
end
expand_if_clause(expr, clauses, ctx) = @match clauses begin
[cond, then, SN(SH(K"elseif", _), elsif)] => pushfirst!(expand_if_clause(expr, elsif, ctx), IfClause(expand_forms(cond, ctx), expand_forms(then, ctx), expr))
[cond, then, els] => [IfClause(expand_forms(cond, ctx), expand_forms(then, ctx), expr), IfClause(nothing, expand_forms(els, ctx), expr)]
[cond, then] => [IfClause(expand_forms(cond, ctx), expand_forms(then, ctx), expr)]
end
expand_if(expr, params, ctx) = IfStmt(expand_if_clause(expr, params, ctx), expr)
analyze_iterspec(expr, ctx) = @match expr begin
SN(SH(K"=", _), [var, iter]) => analyze_lvalue(var, ctx; is_for=true) => expand_forms(iter, ctx)
end
is_update(kw) = kw ∈ KSet"+= -= *= /= //= \= ^= ÷= |= &= ⊻= <<= >>= >>>= %= $= ⩴ ≕"
expand_hcat_arg(expr, ctx) = @match expr begin
GuardBy(isassignment) => begin handle_error(ctx, expr, ctx.error_context, "misplaced assignment statement", nothing); nothing end
GuardBy(isparam) => begin handle_error(ctx, expr, ctx.error_context, "unexpected semicolon in array expression", nothing); nothing end
SN(SH(K"...", _), [body]) => Splat(expand_forms(body, ctx), expr)
e => expand_forms(e, ctx)
end
expand_row(el, toplevel, ctx) = @match el begin
GuardBy(isassignment) => begin handle_error(ctx, el, ctx.error_context, "misplaced assignment statement", nothing); nothing end
GuardBy(isparam) => begin handle_error(ctx, el, ctx.error_context, "unexpected semicolon in array expression", nothing); nothing end
SN(SH(K"row", _), elems) => toplevel ?
Row(filter((!) ∘ isnothing, expand_row.(elems, false, (ctx, ))), el) :
begin handle_error(ctx, el, ctx.error_context, "nested rows not supported", nothing); nothing end
SN(SH(K"...", _), [body]) => Splat(expand_forms(body, ctx), el)
e => expand_forms(e, ctx)
end
expand_nrow(expr, ctx) = @match expr begin
GuardBy(isassignment) => begin handle_error(ctx, expr, ctx.error_context, "misplaced assignment statement", nothing); nothing end
GuardBy(isparam) => begin handle_error(ctx, expr, ctx.error_context, "unexpected semicolon in array expression", nothing); nothing end
SN(SH(K"nrow", flgs), elems) => NRow(JuliaSyntax.numeric_flags(flgs), filter((!) ∘ isnothing, expand_nrow.(elems, (ctx, ))), expr)
SN(SH(K"...", _), body) => begin handle_error(ctx, expr, ctx.error_context, "Splatting ... in an hvncat with multiple dimensions is not supported", nothing); nothing end
e => expand_forms(e, ctx)
end
expand_iterator(expr, ctx)::Iterspec = @match expr begin
SN(SH(K"cartesian_iterator", _), iters) => Cartesian(expand_iterator.(iters, (ctx, )), expr)
SN(SH(K"=", _), [lhs, rhs]) => IterEq(analyze_lvalue(lhs, ctx), expand_forms(rhs, ctx), expr)
SN(SH(K"filter", _), [iters..., cond]) => Filter(expand_iterator.(iters, (ctx,)), expand_forms(cond, ctx), expr)
end
expand_generator(expr, ctx)::Expression = @match expr begin
SN(SH(K"generator", _), [expr, iters...]) => Generator(expand_forms(expr, ctx), Iterspec[expand_iterator(iter, ctx) for iter in iters], expr)
end
expand_import_source(ctx, expr) = @match expr begin
SN(SH(K"importpath", _), path) => expand_import_path(ctx,path)
end
expand_import_clause(ctx, expr; allow_relative=true) = @match expr begin
SN(SH(K"importpath", _), path) => Dep(expand_import_path(ctx, path; allow_relative=allow_relative), expr)
SN(SH(K"as", _), [SN(SH(K"importpath", _), path), SN(SH(K"Identifier", _), _) && alias]) => AliasDep(expand_import_path(ctx, path; allow_relative=allow_relative), Expr(alias), expr)
end
function expand_import_path(ctx, src_path; allow_relative=true)
path = nothing
for path_el in src_path
path = @match (path_el, path) begin
(SN(SH(K"Identifier" || K"MacroName", _), _) && id, nothing) => ImportId(Expr(id), path_el)
(SN(SH(K"Identifier" || K"MacroName", _), _) && id, path) => ImportField(path, Expr(id), path_el)
(SN(SH(K".", _), _), nothing) => allow_relative ? ImportRelative(1, path_el) : handle_error(ctx, path_el, ctx.error_context, "invalid path: \".\" in identifier path", () -> ImportRelative(1, path_el))
(SN(SH(K".", _), _), ImportRelative(lvl, _)) => allow_relative ? ImportRelative(lvl+1, path_el) : handle_error(ctx, path_el, ctx.error_context, "invalid path: \".\" in identifier path", () -> ImportRelative(lvl+1, path_el))
(SN(SH(K".", _), _), _) => handle_error(ctx, path_el, ctx.error_context, "invalid path: \".\" in identifier path", () -> ImportRelative(1, path_el))
(_, _) => handle_error(ctx, path_el, ctx.error_context, "invalid path", () -> ImportRelative(1, path_el))
end
end
return path
end
expand_tuple_arg(expr, ctx) = @match expr begin
SN(SH(K"...", _), [ex]) => Splat(expand_forms(ex, ctx), expr)
_ => expand_forms(expr, ctx)
end
expand_docstring(expand, args, ast, ctx) = @match args begin
[docs, SN(SH(K"::", _), [SN(SH(K"call" || K"where", _), _) && fn, typ])] => let (name, args, kwargs, sparams, rett) = expand_function_name(fn,ctx); CallDocstring(expand_forms(docs, ctx), name, args, kwargs, sparams, expand_forms(typ, ctx), ast) end
[docs, SN(SH(K"call" || K"where", _), _) && fn] => CallDocstring(expand_forms(docs, ctx), expand_function_name(fn, ctx)..., ast)
[docs, inner_ast] => Docstring(expand_forms(docs, ctx), expand(inner_ast, ctx), ast)
end
resolve_toplevel_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@doc")}, args, ctx) = MacroExpansionStmt(expand_docstring(expand_toplevel, args, ast, ctx), ast)
resolve_toplevel_macro(ast, ::DefaultMacroContext, ::Union{Val{Symbol("@inline")}, Val{Symbol("@noinline")}}, args, ctx) = expand_toplevel(args[1], ctx)
resolve_toplevel_macro(ast, ::DefaultMacroContext, ::Union{Val{Symbol("@assume_effects")}, Val{Symbol("@constprop")}}, args, ctx) = expand_toplevel(args[2], ctx)
resolve_toplevel_macro(ast, ::DefaultMacroContext, ::Val{mc}, args, ctx) where mc = handle_error(ctx, ast, ctx.error_context, "Unrecognized macro $mc $(typeof(mc))", () -> MacroExpansionStmt(Literal(:unknown, ast), ast))
resolve_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@doc")}, args, ctx) = MacroExpansion(expand_docstring(expand_forms, args, ast, ctx), ast)
resolve_macro(ast, ::DefaultMacroContext, ::Union{Val{Symbol("@inline")}, Val{Symbol("@noinline")}, Val{Symbol("@inbounds")}}, args, ctx) = expand_forms(args[1], ctx)
resolve_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@eval")}, args, ctx) = Literal(:(), ast)
resolve_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@horner")}, args, ctx) = FunCall(Variable(:evalpoly, ast), PositionalArg.([expand_forms(args[1], ctx), TupleExpr(expand_forms.(args[2:end], (ctx, )), ast)], (ast, )), Vector{Union{KeywordArg, SplatArg}}[], ast)
resolve_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@generated")}, args, ctx) = Literal(true, ast)
resolve_macro(ast, ::DefaultMacroContext, ::Val{Symbol("@assume_effects")}, args, ctx) = expand_forms(args[2], ctx)
resolve_macro(ast, ::DefaultMacroContext, ::Val{mc}, args, ctx) where mc = handle_error(ctx, ast, ctx.error_context, "Unrecognized macro $mc", () -> MacroExpansion(Literal(:unknown, ast), ast))
next_ctx(head, ctx) = ExpandCtx(ctx)
"""
expand_toplevel(ast::JuliaSyntax.SyntaxNode)::ToplevelStmts
expand_toplevel(ast::JuliaSyntax.SyntaxNode, ctx::ExpandCtx)::ToplevelStmts
Takes a SyntaxNode representing an entire file and lowers it to a [ToplevelStmts](@ref).
Toplevel vs. expression can be thought of generally as "where would it make sense for a
`module` or `using` statement to exist?". As an example, the contents of a file are usually
at the top level as is the arugment to an `eval` call. In constrast, a method body will not be
at the top level as neither module nor using statements are valid inside of it.
For most use cases this is the reccomended entry point. Use `expand_forms` if you need to expand
a specific expression.
"""
expand_toplevel(ast::JuliaSyntax.SyntaxNode) = expand_toplevel(ast, ExpandCtx(true, false))
expand_toplevel(ast::JuliaSyntax.SyntaxNode, ctx::ExpandCtx) = @match ast begin
SN(SH(K"using", _), [SN(SH(K":", _), [mod, terms...])]) => SourceUsingStmt(expand_import_source(ctx, mod), expand_import_clause.((ctx,), terms; allow_relative=false), ast)
SN(SH(K"using", _), [terms...]) => UsingStmt(expand_import_source.((ctx,), terms), ast)
SN(SH(K"import", _), [SN(SH(K":", _), [mod, terms...])]) => SourceImportStmt(expand_import_source(ctx, mod), expand_import_clause.((ctx,), terms; allow_relative=false), ast)
SN(SH(K"import", _), [terms...]) => ImportStmt(expand_import_clause.((ctx,), terms), ast)
SN(SH(K"export", _), syms) => ExportStmt(Expr.(syms), ast)
SN(SH(K"module", flags), [name, SN(SH(K"block", _), stmts)]) => ModuleStmt(!JuliaSyntax.has_flags(flags, JuliaSyntax.BARE_MODULE_FLAG), Expr(name), expand_toplevel.(stmts, (ctx, )), ast)
SN(SH(K"abstract", _), [sig]) => expand_abstract_def(ast, sig, ctx)
SN(SH(K"struct", flags), [sig, fields]) => expand_struct_def(ast, JuliaSyntax.has_flags(flags, JuliaSyntax.MUTABLE_FLAG), sig, fields, ctx)
SN(SH(K"primitive", _), [sig, size]) => expand_primitive_def(ast, sig, size, ctx)
SN(SH(K"toplevel", _), exprs) => ToplevelStmt(expand_toplevel.(exprs, (ctx, )), ast)
SN(SH(K"doc", _), [args...]) => resolve_toplevel_macro(ast, ctx.macro_context, Val{Symbol("@doc")}(), args, ctx)
SN(SH(K"macrocall", _), [name, args...]) => resolve_toplevel_macro(ast, ctx.macro_context, Val{Symbol(name)}(), args, ctx)
expr => ExprStmt(expand_forms(expr, JuliaSyntax.head(expr), JuliaSyntax.children(expr), ExpandCtx(ctx; is_toplevel = true)), ast)
end
expand_curly_internal(receiver, ctx, args, ast) =
CallCurly(expand_forms(receiver, ctx), [let param = extract_implicit_whereparam(arg, ctx); isnothing(param) ? expand_forms(arg, ctx) : param end for arg in args], ast)
expand_curly(receiver, ctx, args, ast) =
any(iskwarg, args) ?
handle_error(ctx, ast, ctx.error_context, "misplaced assignment statement", () -> expand_curly_internal(receiver, ctx, filter((!) ∘ isnothing, args), ast)) :
expand_curly_internal(receiver, ctx, args, ast)
expand_vect(ctx, ast, elems) = any(isassignment, elems) ?
handle_error(ctx, ast, ctx.error_context, "misplaced assignment statement", () -> Vect(filter((!) ∘ isnothing, expand_hcat_arg.(filter((!) ∘ isnothing, elems), (ctx, ))), ast)) :
Vect(filter((!) ∘ isnothing, expand_hcat_arg.(elems, (ctx, ))), ast)
function expand_try_catch(ctx, block, clauses, ast)
expanded_block = expand_forms(block, ctx)
catch_var = nothing
catch_body = nothing
else_body = nothing
finally_body = nothing
for clause in clauses
@match clause begin
SN(SH(K"catch", _), [SN(SH(K"false", _), _), body]) => begin catch_body = expand_forms(body, ctx) end
SN(SH(K"catch", _), [var, body]) => begin catch_var = Expr(var); catch_body = expand_forms(body, ctx) end
SN(SH(K"else", _), [body]) => begin else_body = expand_forms(body, ctx) end
SN(SH(K"finally", _), [body]) => begin finally_body = expand_forms(body, ctx) end
end
end
TryCatch(expanded_block, catch_var, catch_body, else_body, finally_body, ast)
end
"""
expand_forms(ast::JuliaSyntax.SyntaxNode, ctx::ExpandCtx)
Expands an expression (such as a method body) from a SyntaxNode to an Expression with respect to a [ExpandCtx](@ref). See [expand_toplevel](@ref) for
more discussion.
"""
expand_forms(ast::JuliaSyntax.SyntaxNode, ctx::ExpandCtx) = expand_forms(ast, JuliaSyntax.head(ast), JuliaSyntax.children(ast), next_ctx(JuliaSyntax.head(ast), ctx))
expand_forms(ast, head, children, ctx) = @match (head, children) begin
(SH(K"Float" || K"Float32" || K"Integer" || K"String" || K"Char" || K"char" || K"true" || K"false" || K"HexInt" || K"OctInt" || K"BinInt", _), _) => Literal(Expr(ast), ast)
(SH(K"quote",_), _) => Quote(ast, ast) # need to port bits of macroexpansion into this
(SH(K"function", _), [name, body]) => FunctionDef(expand_function_name(name, ctx)..., expand_forms(body, ctx), ast)
(SH(K"function", _), [name]) => FunctionDef(expand_function_name(name, ctx)..., nothing, ast)
(SH(K"->", _), [name, body]) => FunctionDef(expand_anon_function(name, ctx)..., expand_forms(body, ctx), ast)
(SH(K"let", _), [SN(SH(K"block", _), bindings), body]) => LetBinding(filter((!) ∘ isnothing, analyze_let_binding.(bindings, (ctx, ))), expand_forms(body, ctx), ast)
(SH(K"let", _), [binding, body]) => LetBinding(filter((!) ∘ isnothing,[analyze_let_binding(binding, ctx)]), expand_forms(body, ctx), ast)
(SH(K"macro", _), [SN(SH(K"call", _), _) && name, body]) => @match expand_macro_name(name, ctx) begin
(ResolvedName(nm, _) && name, args_stmts, sparams, rett) => MacroDef(name, args_stmts, sparams, rett, expand_forms(body, ctx), ast)
(nm, args_stmts, sparams, rett) => handle_error(ctx, nm.location.basenode, ctx.error_context, "Invalid macro name", () -> MacroDef(ResolvedName([gensym()], ast), args_stmts, sparams, rett, expand_forms(body, ctx), ast))
end
(SH(K"macro", _), [SN(SH(K"Identifier", _), _) && name]) => MacroDef(expand_macro_name(name, ctx)..., nothing, ast)
(SH(K"macro", _), _) => handle_error(ctx, ast, ctx.error_context, "invalid macro definition", () -> MacroDef(ResolvedName([gensym()], ast), nothing, ast))
(SH(K"try", _), [block, clauses...]) => expand_try_catch(ctx, block, clauses, ast)
(SH(K"block", _), stmts) => Block(expand_forms.(stmts, (ctx, )), ast)
(SH(K".", _), [op]) => Broadcast(expand_forms(op, ctx), ast)
(SH(K".", _), [f, SN(SH(K"quote", _), [x && SN(SH(K"Identifier", _), _)])]) => GetProperty(expand_forms(f, ctx), Expr(x), ast)
(SH(K".", _), [f, SN(SH(K"quote", _), [x]) && lit]) => GetProperty(expand_forms(f, ctx), Literal(Expr(x), lit), ast)
(SH(K".", _), [f, SN(SH(K"tuple", _), args)]) => FunCall(Broadcast(expand_forms(f, ctx), ast), split_arguments(args, ctx)..., ast)
(SH(K"comparison", _), [initial, args...]) => Comparison(expand_forms(initial, ctx), map((op, value) -> expand_forms(op, ctx) => expand_forms(value, ctx), args[1:2:end], args[2:2:end]), ast)
(SH(K"&&", _ && GuardBy(JuliaSyntax.is_dotted)), [l, r]) => FunCall(Broadcast(Variable(:(&&), ast), ast), split_op_arguments([l, r], ctx)..., ast)
(SH(K"||", _ && GuardBy(JuliaSyntax.is_dotted)), [l, r]) => FunCall(Broadcast(Variable(:(||), ast), ast), split_op_arguments([l, r], ctx)..., ast)
(SH(K"=", _ && GuardBy(JuliaSyntax.is_dotted)), [lhs, rhs]) => BroadcastAssignment(expand_forms(lhs, ctx), expand_forms(rhs, ctx), ast)
(SH(K"<:", _), [a,b]) => FunCall(Variable(:(<:), ast), split_arguments([a, b], ctx)..., ast)
(SH(K">:", _), [a,b]) => FunCall(Variable(:(>:), ast), split_arguments([a, b], ctx)..., ast)
(SH(K"-->", _), _) => FunCall(Variable(:(-->)), split_arguments([a, b], ctx)..., ast)
(SH(K"where", _), [typ, SN(SH(K"braces", _), vars)]) => WhereType(expand_forms(typ, ctx), analyze_typevar.(vars, (ctx, )), ast)
(SH(K"where", _), [typ, var]) => WhereType(expand_forms(typ, ctx), [analyze_typevar(var, ctx)], ast)
(SH((K"const" || K"local" || K"global") && decltype, _), decls) => Declaration(expand_declaration.(convert_decltype(decltype), decls, (ctx, )), ast)
(SH(K"=", _), [lhs, rhs]) => Assignment(analyze_lvalue(lhs, ctx), expand_forms(rhs, ctx), ast)
(SH(K"ref", _), [arr, idxes..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, ast, ctx.error_context, "unexpected semicolon in array expression", () -> GetIndex(expand_forms(arr, ctx), map(el->PositionalArg(expand_forms(el, ctx), el), idxes), ast))
(SH(K"ref", _), [arr, idxes...]) => GetIndex(expand_forms(arr, ctx), map(el->PositionalArg(expand_forms(el, ctx), el), idxes), ast)
(SH(K"curly", _), [receiver, args..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, ast, ctx.error_context, "unexpected semicolon", () -> expand_curly(receiver, ctx, args, ast))
(SH(K"curly", _), [receiver, args...]) => expand_curly(receiver, ctx, args, ast)
(SH(K"call", GuardBy(JuliaSyntax.is_infix_op_call)), [arg1, f, arg2...]) && call => FunCall(expand_forms(f, ctx), split_op_arguments([arg1; arg2], ctx)..., ast) # todo Global method definition needs to be placed at the toplevel
(SH(K"call", GuardBy(JuliaSyntax.is_postfix_op_call)), [arg, f]) => FunCall(expand_forms(f, ctx), split_op_arguments([arg], ctx)..., ast)
(SH(K"call", GuardBy(JuliaSyntax.is_prefix_op_call)), [f, arg]) => FunCall(expand_forms(f, ctx), [PositionalArg(expand_forms(arg, ctx), ast)], [], ast)
(SH(K"call", _), [f, args...]) => FunCall(expand_forms(f, ctx), split_arguments(args, ctx)..., ast)
(SH(K"juxtapose", _), [a, b]) => FunCall(Variable(:(*), ast), [PositionalArg(expand_forms(a, ctx), ast), PositionalArg(expand_forms(b, ctx), ast)], [], ast)
(SH(K"dotcall", GuardBy(JuliaSyntax.is_infix_op_call)), [arg1, f, arg2...]) => FunCall(Broadcast(expand_forms(f, ctx), ast), split_op_arguments([arg1; arg2], ctx)..., ast)
(SH(K"dotcall", GuardBy(JuliaSyntax.is_postfix_op_call)), [arg, f]) => FunCall(Broadcast(expand_forms(f, ctx), ast), split_op_arguments([arg], ctx)..., ast)
(SH(K"dotcall", GuardBy(JuliaSyntax.is_prefix_op_call)), [f, arg]) => FunCall(Broadcast(expand_forms(f, ctx), ast), [PositionalArg(expand_forms(arg, ctx), ast)], [], ast)
(SH(K"dotcall", _), [f, args...]) => FunCall(Broadcast(expand_forms(f, ctx), ast), split_arguments(args, ctx)..., ast)
(SH(K"do", _), [call, args, body]) =>
let
(_, args, _, _, _) = expand_anon_function(args, ctx);
DoStatement(expand_forms(call, ctx), args, expand_forms(body, ctx), ast)
end
(SH(K"tuple", _), [arg1, args..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, ast, ctx.error_context, "unexpected semicolon in tuple", () -> TupleExpr(expand_tuple_arg.([arg1; args], (ctx, )), ast))
(SH(K"tuple", _), [SN(SH(K"parameters", _), params)]) => NamedTupleExpr(expand_named_tuple(params, ctx), ast)
(SH(K"tuple", _), args) => any(isassignment, args) ? NamedTupleExpr(expand_named_tuple(args, ctx), ast) : TupleExpr(expand_tuple_arg.(args, (ctx, )), ast)
(SH(K"braces", _), _) => handle_error(ctx, ast, ctx.error_context, "{ } vector syntax is discontinued", () -> Literal(nothing, ast))
(SH(K"bracescat", _), _) => handle_error(ctx, ast, ctx.error_context, "{ } matrix syntax is discontinued", () -> Literal(nothing, ast))
(SH(K"string", _), args) => StringInterpolate(string_interpoland.(args, (ctx, )), ast)
(SH(K"::", _), [exp]) => handle_error(ctx, ast, ctx.error_context, "invalid \"::\" syntax", () -> expand_forms(exp, ctx))
(SH(K"::", _), [val, typ]) => TypeAssert(expand_forms(val, ctx), expand_forms(typ, ctx), ast)
(SH(K"if", _), params) => expand_if(ast, params, ctx)
(SH(K"while", _), [cond, body]) => let ictx = ExpandCtx(ctx; is_loop=true); WhileStmt(expand_forms(cond, ictx), expand_forms(body, ictx), ast) end
(SH(K"break", _), _) => if ctx.is_loop BreakStmt(ast) else handle_error(ctx, ast, ctx.error_context, "break or continue outside loop", () -> Literal(nothing, ast)) end
(SH(K"continue", _), _) => if ctx.is_loop ContinueStmt(ast) else handle_error(ctx, ast, ctx.error_context, "break or continue outside loop", () -> Literal(nothing, ast)) end
(SH(K"return", _), []) => ReturnStmt(nothing, ast)
(SH(K"return", _), [val]) => ReturnStmt(expand_forms(val, ctx), ast)
(SH(K"for", _), [SN(SH(K"=", _), _) && iterspec, body]) => let ictx = ExpandCtx(ctx; is_loop=true); ForStmt(Pair{LValue, Expression}[analyze_iterspec(iterspec, ictx)], expand_forms(body, ictx), ast) end
(SH(K"for", _), [SN(SH(K"cartesian_iterator", _), iterspecs), body]) => let ictx = ExpandCtx(ctx; is_loop=true); ForStmt(Pair{LValue, Expression}[analyze_iterspec(iterspec, ictx) for iterspec in iterspecs], expand_forms(body, ictx), ast) end
(SH(K"&&", _), [l, r]) => FunCall(Variable(:(&&), ast), split_op_arguments([l, r], ctx)..., ast)
(SH(K"||", _), [l, r]) => FunCall(Variable(:(||), ast), split_op_arguments([l, r], ctx)..., ast)
(SH(upd && GuardBy(is_update), _ && GuardBy(JuliaSyntax.is_dotted)), [lhs, rhs]) => BroadcastUpdate(Symbol(upd), expand_forms(lhs,ctx), expand_forms(rhs, ctx), ast)
(SH(upd && GuardBy(is_update), _), [lhs, rhs]) => Update(Symbol(upd), analyze_lvalue(lhs, ctx), expand_forms(rhs, ctx), ast)
(SH(K"$", _), _) => handle_error(ctx, ast, ctx.error_context, "\"\$\" expression outside quote", () -> Literal(nothing, ast))
(SH(K"...", _), _) => handle_error(ctx, ast, ctx.error_context, "\"...\" expression outside call", () -> Literal(nothing, ast))
(SH(K"vect", _), [elems..., SN(SH(K"parameters", _), _)]) => handle_error(ctx, ast, ctx.error_context, "unexpected semicolon in array expression", () -> expand_vect(ctx, ast, elems))
(SH(K"vect", _), elems) => expand_vect(ctx, ast, elems)
(SH(K"hcat", _), elems && GuardBy(x -> any(isassignment, x))) => handle_error(ctx, ast, ctx.error_context, "misplaced assignment statement", () -> HCat(nothing, filter((!) ∘ isnothing, expand_hcat_arg.(filter((!) ∘ isassignment, elems), (ctx, ))), ast))
(SH(K"hcat", _), elems) => HCat(nothing, filter((!) ∘ isnothing, expand_hcat_arg.(elems, (ctx, ))), ast)
(SH(K"typed_hcat", _), elems && GuardBy(x -> any(isassignment, x))) => handle_error(ctx, ast, ctx.error_context, "misplaced assignment statement", () -> HCat(expand_forms(type, ctx), filter((!) ∘ isnothing, expand_hcat_arg.(filter((!) ∘ isassignment, elems), (ctx, ))), ast))
(SH(K"typed_hcat", _), [type, elems...]) => HCat(expand_forms(type, ctx), filter((!) ∘ isnothing, expand_hcat_arg.(elems, (ctx, ))), ast)
(SH(K"vcat", _), params) => VCat(nothing, filter((!) ∘ isnothing, expand_row.(params, true, (ctx, ))), ast)
(SH(K"typed_vcat", _), [type, params...]) => VCat(expand_forms(type, ctx), filter((!) ∘ isnothing, expand_row.(params, true, (ctx, ))), ast)
(SH(K"ncat", flags), elems) => NCat(nothing, JuliaSyntax.numeric_flags(flags), filter((!) ∘ isnothing, expand_nrow.(elems, (ctx, ))), ast)
(SH(K"typed_ncat", flags), [type, elems...]) => NCat(expand_forms(type, ctx), JuliaSyntax.numeric_flags(flags), filter((!) ∘ isnothing, expand_nrow.(elems, (ctx, ))), ast)
(SH(K"generator", _), elems && GuardBy(x -> any(contains_return, x))) => handle_error(ctx, ast, ctx.error_context, "\"return\" not allowed inside comprehension or generator", () -> expand_generator(ast, ctx))
(SH((K"generator" || K"cartesian_iterator") && head, _), [expr, iters...]) => expand_generator(ast, ctx)
(SH(K"comprehension", _), [generator]) => Comprehension(nothing, expand_generator(generator, ctx), ast)
(SH(K"typed_comprehension", _), [type, generator]) => Comprehension(expand_forms(type, ctx), expand_generator(generator, ctx), ast)
(SH(K"Identifier", _), _) => Variable(Expr(ast), ast)
(SH(K"doc", _), [args...]) => resolve_macro(ast, ctx.macro_context, Val{Symbol(Symbol("@doc"))}(), args, ctx)
(SH(K"macrocall", _), [mcro, args...]) => resolve_macro(ast, ctx.macro_context, Val{Symbol(mcro)}(), args, ctx)
(SH(K"?", _), [cond, then, els]) => Ternary(expand_forms(cond, ctx), expand_forms(then, ctx), expand_forms(els, ctx), ast)
(SH(K"using" || K"import" || K"export" || K"module" || K"abstract" || K"struct" || K"primitive", _), _) => handle_error(ctx, ast, ctx.error_context, "must be at top level", () -> Literal(nothing, ast))
(SH(op && GuardBy(JuliaSyntax.is_operator), _ && GuardBy(JuliaSyntax.is_dotted)), _) => Broadcast(Variable(Symbol(string(op)), ast), ast)
(SH(K"&", _), [var]) => handle_error(ctx, ast, ctx.error_context, "Invalid &", () -> expand_forms(var, ctx))
(SH(GuardBy(JuliaSyntax.is_operator), _), _) => try Variable(Expr(ast), ast) catch e println(ast); handle_error(ctx, ast, ctx.error_context, "invalid expression", () -> Literal(nothing, ast)) end
(head, args) => throw("Unimplemented $head")
end
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 11235 | "An ASTNode is the parent type of all SemanticAST AST elements. It's used when the object could be literally anything. ASTNodes will have a `.location` field of type SourcePosition, however."
abstract type ASTNode end
"`Expression`s are non-toplevel ASTNodes (that is, `1+1` is an `Expression` while `using Test` is not)."
abstract type Expression <: ASTNode end
"Represents the original location and range an AST element came from, currently represented as the originating
`JuliaSyntax.SyntaxNode` it was analyzed from."
struct SourcePosition
basenode::JuliaSyntax.SyntaxNode
end
Base.show(io::IO, pos::SourcePosition) = begin print(io, "SourcePosition") end
#"A type variable with name name, upper bound ub, and lower bound lb"
@ast_node struct TyVar <: ASTNode
name::Union{Symbol, Nothing}
ub::Any
lb::Any
end
export TyVar
"LValues are expressions that can appear on the left-hand-side of an assignment expression. For instance, in the expression `x[foo] = 3` the part `x[foo]` is an lvalue. The language of lvalues is limited beyond the general expression language and they are represented independently by SemanticAST."
abstract type LValue <: ASTNode end
#"A function argument declaration, consisting of a binding (an LValue or nothing, in case of a wildcard), a default value if provided, and a type ascription if provided."
@ast_node struct FnArg <: ASTNode
binding::Union{LValue, Nothing}
default_value::Union{ASTNode, Nothing}
type::Union{Expression, Nothing}
end
export FnArg, LValue
"A keyword argument declaration. A keyword argument must have a name and may have a type, a default value, may be a vararg"
struct KwArg <: ASTNode
name::Symbol
type::Union{ASTNode, Nothing}
default_value::Union{ASTNode, Nothing}
is_vararg::Bool
location::Union{SourcePosition, Nothing}
KwArg(name, type, default_value, basenode, is_vararg = false) = new(name, type, default_value, is_vararg, isnothing(basenode) ? nothing : SourcePosition(basenode))
end
@as_record KwArg
Base.:(==)(a::KwArg, b::KwArg) = a.name == b.name && a.type == b.type && a.default_value == b.default_value && a.is_vararg == b.is_vararg
export KwArg
@enum FieldAttributes FIELD_NONE=0 FIELD_CONST=1
Base.show(io::IO, d::FieldAttributes) = print(io, d)
Base.:(|)(a::FieldAttributes, b::FieldAttributes) = FieldAttributes(Int(a) | Int(b))
@ast_node struct StructField <: ASTNode
name::Symbol
type::Union{Expression, Nothing}
attributes::FieldAttributes
end
export StructField, FieldAttributes
@ast_data CallArg <: ASTNode begin
PositionalArg(body::Expression)
KeywordArg(name::Symbol, value::Expression)
SplatArg(body::Union{Expression, Nothing})
end
export CallArg, PositionalArg, KeywordArg, SplatArg
const PositionalArgs = Union{PositionalArg, SplatArg}
export PositionalArgs
@enum DeclAttributes DECL_NONE=0 DECL_CONST=1 DECL_LOCAL=2 DECL_GLOBAL=4
Base.show(io::IO, d::DeclAttributes) = print(io, d)
@ast_node struct VarDecl <: ASTNode
binding::LValue
value::Union{Expression, Nothing}
flags::DeclAttributes
end
export VarDecl
@ast_data NamedTupleBody <: ASTNode begin
NamedValue(name::Symbol, value::Expression)
ComputedNamedValue(name::Expression, value::Expression)
SplattedNamedValue(splat::Expression)
end
export NamedTupleBody, NamedValue, ComputedNamedValue, SplattedNamedValue
@ast_node struct IfClause <: ASTNode
cond::Union{Expression, Nothing}
body::Expression
end
export IfClause
@ast_data Rows <: ASTNode begin
Splat(body::Expression)
Row(elems::Vector{Union{Expression, Splat}})
NRow(dim::Int, elems::Vector{Union{NRow, Expression}})
end
export Rows, Splat, Row, NRow
@ast_data Iterspec <: ASTNode begin
IterEq(lhs::LValue, rhs::Expression)
Filter(inner::Vector{Iterspec}, cond::Expression)
Cartesian(dims::Vector{Iterspec})
end
export Iterspec, IterEq, Filter
@ast_data ImportPath <: ASTNode begin
ImportField(source::ImportPath, name::Symbol)
ImportId(name::Symbol)
ImportRelative(levels::Int)
end
export ImportPath, ImportField, ImportId, ImportRelative
@ast_data DepClause <: ASTNode begin
Dep(path::ImportPath)
AliasDep(path::ImportPath, alias::Symbol)
end
export DepClause, Dep, AliasDep
@ast_data FunctionName <: ASTNode begin
ResolvedName(path::Vector{Symbol})
DeclName(binding::Union{LValue, Nothing}, typ::Expression)
TypeFuncName(receiver::Expression, args::Vector{Union{Expression, TyVar}})
AnonFuncName()
end
export FunctionName, ResolvedName, DeclName, TypeFuncName, AnonFuncName
@ast_data ToplevelStmts <: ASTNode begin
StructDefStmt(name::Symbol, params::Vector{Symbol}, super::Union{Expression, Nothing}, fields::Vector{StructField}, cstrs::Vector{Expression})
AbstractDefStmt(name::Symbol, params::Vector{Symbol}, super::Union{Expression, Nothing})
PrimitiveDefStmt(name::Symbol, params::Vector{Symbol}, super::Union{Expression, Nothing}, size::Expression)
UsingStmt(mods::Vector{ImportPath})
ImportStmt(mods::Vector{DepClause})
SourceUsingStmt(sourcemod::ImportPath, stmts::Vector{DepClause})
SourceImportStmt(sourcemod::ImportPath, stmts::Vector{DepClause})
ExportStmt(syms::Vector{Symbol})
ModuleStmt(std_imports::Bool, name::Symbol, body::Vector{ToplevelStmts})
ExprStmt(expr::Expression)
ToplevelStmt(exprs::Vector{ToplevelStmts})
MacroExpansionStmt(value::Any)
end
export ToplevelStmts, StructDefStmt, AbstractDefStmt, PrimitiveDefStmt, UsingStmt, ImportStmt, SourceUsingStmt, SourceImportStmt, ExportStmt, ModuleStmt, ExprStmt, ToplevelStmt, MacroExpansionStmt
@ast_data Expression <: ASTNode begin
Literal(expr::Any)
Variable(name::Symbol)
FunctionDef(name::FunctionName, arguments::Vector{FnArg}, kw_args::Vector{KwArg}, sparams::Vector{TyVar}, rett::Union{Expression, Nothing}, body::Union{Expression, Nothing})
MacroDef(name::ResolvedName, arguments::Vector{FnArg}, sparams::Vector{TyVar}, rett::Union{Expression, Nothing}, body::Union{Expression, Nothing})
Block(exprs::Vector{Expression})
LetBinding(bindings::Vector{Union{Pair{<:LValue, <:Expression}, Symbol}}, body::Expression)
TryCatch(body::Expression, catch_var::Union{Symbol, Nothing}, catch_body::Union{Expression, Nothing}, else_body::Union{Expression, Nothing}, finally_body::Union{Expression, Nothing})
CallCurly(receiver::Expression, args::Vector{Union{Expression, TyVar}})
Comparison(first::Expression, clauses::Vector{Pair{Expression, Expression}})
Broadcast(op::Expression)
FunCall(receiver::Expression, pos_args::Vector{PositionalArgs}, kw_args::Vector{Union{KeywordArg, SplatArg}})
GetIndex(arr::Expression, arguments::Vector{PositionalArgs})
GetProperty(rec::Expression, prop::Union{Symbol, Literal})
Assignment(lhs::LValue, rhs::Expression)
BroadcastAssignment(lhs::Expression, rhs::Expression)
Update(op::Symbol, lhs::LValue, rhs::Expression)
BroadcastUpdate(op::Symbol, lhs::Expression, rhs::Expression)
WhereType(type::Expression, vars::Vector{TyVar})
Declaration(vars::Vector{VarDecl})
DoStatement(func::Expression, arguments::Vector{FnArg}, body::Expression)
TupleExpr(exprs::Vector{Union{Expression, Splat}})
NamedTupleExpr(exprs::Vector{NamedTupleBody})
StringInterpolate(components::Vector{Union{String, Expression}})
TypeAssert(val::Expression, typ::Expression)
IfStmt(clauses::Vector{IfClause})
WhileStmt(cond::Expression, body::Expression)
BreakStmt()
ContinueStmt()
ReturnStmt(retval::Union{Expression, Nothing})
ForStmt(iters::Vector{Pair{LValue, Expression}}, body::Expression)
Vect(elems::Vector{Union{Expression, Splat}})
HCat(type::Union{Expression, Nothing}, elems::Vector{Union{Expression, Splat}})
VCat(type::Union{Expression, Nothing}, rows::Vector{Union{Row, Expression, Splat}})
NCat(type::Union{Expression, Nothing}, dim::Int, rows::Vector{Union{NRow, Expression}})
Generator(expr::Expression, iterators::Vector{Iterspec})
Comprehension(type::Union{Expression, Nothing}, gen::Generator)
Quote(ast::JuliaSyntax.SyntaxNode)
MacroExpansion(value::Any)
Ternary(cond::Expression, then::Expression, els::Expression)
end
export Expression, Literal, Variable, FunctionDef, MacroDef, Block, LetBinding, TryCatch, CallCurly, Comparison, Broadcast, FunCall, GetIndex, GetProperty, Assignment, Update, WhereType, Declaration, DoStatement, TupleExpr, NamedTupleExpr, StringInterpolate, TypeAssert, IfStmt, WhileStmt, BreakStmt, ContinueStmt, ReturnStmt, ForStmt, Vect, HCat, VCat, NCat, Generator, Comprehension, Quote, MacroExpansion, Ternary
@ast_data LValueImpl <: LValue begin
IdentifierAssignment(id::Symbol)
OuterIdentifierAssignment(id::Symbol)
FieldAssignment(obj::Expression, name::Symbol)
TupleAssignment(params::Vector{LValue})
RefAssignment(arr::Expression, arguments::Vector{PositionalArgs}, kwargs::Vector{KeywordArg})
VarargAssignment(tgt::Union{Nothing, LValue})
TypedAssignment(lhs::LValue, type::Expression)
NamedTupleAssignment(params::Vector{Union{IdentifierAssignment, TypedAssignment}})
FunctionAssignment(name::FunctionName, args_stmts::Vector{FnArg}, kwargs_stmts::Vector{KwArg}, sparams::Vector{TyVar}, rett::Union{Expression, Nothing})
UnionAllAssignment(name::LValue, tyargs::Vector{TyVar})
end
export LValueImpl, IdentifierAssignment, OuterIdentifierAssignment, FieldAssignment, TupleAssignment, RefAssignment, VarargAssignment, TypedAssignment, NamedTupleAssignment, FunctionAssignment, BroadcastAssignment, UnionAllAssignment
@ast_data Docstrings <: ASTNode begin
Docstring(doc::Expression, defn::Union{Expression, ToplevelStmts})
CallDocstring(doc::Expression, name::FunctionName, arguments::Vector{FnArg}, kw_args::Vector{KwArg}, sparams::Vector{TyVar}, rett::Union{Expression, Nothing})
end
export Docstring, CallDocstring
@generated function Base.show(io::IO, a::T) where T<:Union{Docstrings, Expression, VarDecl, NamedTupleBody, IfClause, Rows, Iterspec, ImportPath, DepClause, FunctionName, ToplevelStmts, LValueImpl, CallArg, FnArg, TyVar, KwArg, StructField}
outbody = []
fldnames = filter(x -> x != :location, fieldnames(T))
for fld in fldnames[1:end-1]
push!(outbody, :(show(io, a.$fld)))
push!(outbody, :(print(io, ", ")))
end
if length(fldnames) > 0
push!(outbody, :(show(io, a.$(fldnames[end]))))
end
return quote
print(io, string(nameof(T)))
print(io, "(")
$(outbody...)
print(io, ")")
end
end
const ASTNodes = Union{Docstrings, Expression, VarDecl, NamedTupleBody, IfClause, Rows, Iterspec, ImportPath, DepClause, FunctionName, ToplevelStmts, LValueImpl, CallArg, FnArg, TyVar, LValue, KwArg, StructField}
function Base.show(io::IO, v::Vector{T}) where T<:Union{ASTNodes, Tuple{Vararg{ASTNodes}}, Pair{X, Y} where {X<:ASTNodes,Y<:ASTNodes}, Union{String, Y} where Y <: ASTNodes}
print(io, "[")
for el in v[1:end-1]
show(io, el)
print(io, ", ")
end
if length(v) > 0
show(io, v[end])
end
print(io, "]")
end
function Base.show(io::IO, (l, r)::Pair{N1, N2}) where {N1 <: ASTNodes, N2 <: ASTNodes}
show(io, l)
print(io, " => ")
show(io, r)
end
@generated function Base.:(==)(a::T, b::T) where T<:Union{Docstrings, Expression, VarDecl, NamedTupleBody, IfClause, Rows, Iterspec, ImportPath, DepClause, FunctionName, ToplevelStmts, LValueImpl, CallArg, FnArg}
if length(fieldnames(T)) == 0
return true
end
return :(Base.:(&)($(map(x->:(a.$x == b.$x), filter(x->x != :location, fieldnames(T)))...)))
end
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 1535 |
function MLStyle.pattern_uncall(::Type{JuliaSyntax.SyntaxNode}, self, type_params, type_args, args)
t = JuliaSyntax.SyntaxNode
tcons(_...) = t
comp = PComp("$t", tcons;)
function extract(sub::Any, i::Int, ::Any, ::Any)
if i == 1
return :(JuliaSyntax.head($sub))
elseif i == 2
return :(JuliaSyntax.children($sub))
end
end
return decons(comp, extract, map(self, args))
end
function MLStyle.pattern_uncall(::Type{JuliaSyntax.SyntaxHead}, self, type_params, type_args, args)
t = JuliaSyntax.SyntaxHead
tcons(_...) = t
comp = PComp("$t", tcons;)
function extract(sub::Any, i::Int, ::Any, ::Any)
if i == 1
return :($sub.kind)
elseif i == 2
return :($sub.flags)
end
end
return decons(comp, extract, map(self, args))
end
struct SyntaxFlags
flags::JuliaSyntax.RawFlags
end
flag_assignment = Dict{Symbol, UInt16}(
:TRIVIA => JuliaSyntax.TRIVIA_FLAG,
:INFIX => JuliaSyntax.INFIX_FLAG,
:DOTOP => JuliaSyntax.DOTOP_FLAG,
:TRIPLE_STRING => JuliaSyntax.TRIPLE_STRING_FLAG,
:RAW_STRING => JuliaSyntax.RAW_STRING_FLAG,
:SUFFIXED => JuliaSyntax.SUFFIXED_FLAG
)
@active InfixHead(x) begin
return JuliaSyntax.is_infix(x)
end
@active DottedHead(x) begin
return JuliaSyntax.is_dotted(x)
end
function MLStyle.pattern_uncall(::Type{SyntaxFlags}, self, type_params, type_args, args)
if any((!).(haskey.((flag_assignment,), args)))
throw("invalid flag in $args")
end
flag_match = reduce(|, getindex.((flag_assignment,), args); init=0)
end
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 5251 | struct ASTException <: Exception
source_node::JuliaSyntax.SyntaxNode
reason::String
end
first_byte(e::ASTException) = e.source_node.position
last_byte(e::ASTException) = e.source_node.position + JuliaSyntax.span(e.source_node) - 1
global ast_id::Int = 0
function make_id()
old = ast_id
ast_id += 1
return ast_id
end
# stolen from JuliaSyntax in most part
function Base.show(io::IO, exception::ASTException)
source = exception.source_node.source
color,prefix = (:light_red, "Error")
line, col = JuliaSyntax.source_location(source, first_byte(exception))
linecol = "$line:$col"
filename = source.filename
if !isnothing(filename)
locstr = "$filename:$linecol"
if get(io, :color, false)
# Also add hyperlinks in color terminals
url = "file://$(abspath(filename))#$linecol"
locstr = "\e]8;;$url\e\\$locstr\e]8;;\e\\"
end
else
locstr = "line $linecol"
end
print(io, prefix, ": ")
printstyled(io, exception.reason, color=color)
printstyled(io, "\n", "@ $locstr", color=:light_black)
print(io, "\n")
p = first_byte(exception)
q = last_byte(exception)
text = JuliaSyntax.sourcetext(source)
if q < p || (p == q && source[p] == '\n')
# An empty or invisible range! We expand it symmetrically to make it
# visible.
p = max(firstindex(text), prevind(text, p))
q = min(lastindex(text), nextind(text, q))
end
# p and q mark the start and end of the diagnostic range. For context,
# buffer these out to the surrouding lines.
a,b = JuliaSyntax.source_line_range(source, p, context_lines_before=2, context_lines_after=1)
c,d = JuliaSyntax.source_line_range(source, q, context_lines_before=1, context_lines_after=2)
hicol = (100,40,40)
# TODO: show line numbers on left
print(io, source[a:prevind(text, p)])
# There's two situations, either
if b >= c
# The diagnostic range is compact and we show the whole thing
# a...............
# .....p...q......
# ...............b
JuliaSyntax._printstyled(io, source[p:q]; bgcolor=hicol)
else
# Or large and we trucate the code to show only the region around the
# start and end of the error.
# a...............
# .....p..........
# ...............b
# (snip)
# c...............
# .....q..........
# ...............d
JuliaSyntax._printstyled(io, source[p:b]; bgcolor=hicol)
println(io, "…")
JuliaSyntax._printstyled(io, source[c:q]; bgcolor=hicol)
end
print(io, source[nextind(text,q):d])
println(io)
end
macro ast_data(name, body)
@assert body isa Expr && body.head == :block
outbody = []
supertype = nothing
@match name begin
:($sname <: $_) => begin supertype = sname end
_ => nothing
end
for elem in body.args
@match elem begin
:($casename($(params...))) => begin
push!(outbody, :(@ast_node struct $casename <: $supertype; $(params...) end))
visit_body = []
for param in params
@match param begin
:($fldname::$fldtype) && fldspec => begin
push!(visit_body, :(visit(enter, exit, arg.$fldname)))
end
end
end
end
lnn :: LineNumberNode => push!(outbody, lnn)
end
end
return quote
abstract type $name end
$(outbody...)
end
end
macro ast_node(defn)
@match defn begin
:(struct $prop <: $super; $(fields...); end) => begin
output_body = []
hash_body = []
eq_body = []
init_fields = []
for field in fields
@match field begin
:($fldname::$fldtype) && fldspec => begin
if fldname != :location
push!(init_fields, fldname => fldtype)
else
throw("Location specified explicitly; ast node locations are implicit!")
end
push!(output_body, fldspec)
push!(hash_body, :(h = hash(node.$fldname, h)))
push!(eq_body, :(self.$fldname == other.$fldname))
end
_::LineNumberNode => nothing
end
end
eq_check = foldl((exp, el) -> :($exp && $el), eq_body; init = :(self.location == other.location))
out = quote
struct $prop <: $super;
$(output_body...);
location::Union{$SemanticAST.SourcePosition, Nothing};
$prop($((:($argname) for (argname, argtype) in init_fields)...), basenode::Union{$JuliaSyntax.SyntaxNode, Nothing}) =
new($((argname for (argname, argtype) in init_fields)...), isnothing(basenode) ? nothing : $SemanticAST.SourcePosition(basenode))
end;
function $(esc(:visit))(enter, exit, arg::$(esc(prop))) enter(arg); $((:(visit(enter, exit, arg.$argname)) for (argname, argtype) in init_fields)...); exit(arg) end
function Base.hash(node::$(esc(prop)), h::UInt)
$(hash_body...)
h = hash(node.location, h)
return h
end
Base.:(==)(self::$(esc(prop)), other::$(esc(prop))) = $eq_check
$MLStyle.@as_record $(esc(prop))
end
return out
end
end
end
visit(enter, exit, elems::Vector{T}) where T = for elem in elems visit(enter, exit,elem) end
visit(enter, exit, elem::Pair{T, U}) where {T, U} = begin visit(enter, exit, elem[1]); visit(enter, exit, elem[2]) end
visit(enter, exit, alt) = begin enter(alt); exit(alt) end | SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | code | 49720 | using SemanticAST
import SemanticAST: just_argslist, flatten_where_expr, analyze_typevar, ASTException
using Test
import JuliaSyntax
import JuliaSyntax: @K_str, @KSet_str
const SN = JuliaSyntax.SyntaxNode
const SH = JuliaSyntax.SyntaxHead
childof = JuliaSyntax.child
kindof(ex) = JuliaSyntax.kind(JuliaSyntax.head(ex))
@testset "visit" begin
y = 0
enter = (x) -> y = 1
exit = (x) -> begin @test y == 1; y = 2 end
SemanticAST.visit(enter, exit, SemanticAST.StringInterpolate([], nothing))
@test y == 2
end
af1 = JuliaSyntax.parseall(SN, "function () end")
af2 = JuliaSyntax.parseall(SN, "function (x) end")
af3 = JuliaSyntax.parseall(SN, "function (x,y) end")
af4 = JuliaSyntax.parseall(SN, "function (x,y) where T end")
nf1 = JuliaSyntax.parseall(SN, "function f() end")
nf2 = JuliaSyntax.parseall(SN, "function f(x) end")
nf3 = JuliaSyntax.parseall(SN, "function f(x, y) end")
nf4 = JuliaSyntax.parseall(SN, "function f(x, y) where T end")
@testset "just_argslist" begin
@test just_argslist(childof(af1, 1))
@test just_argslist(childof(af2, 1))
@test just_argslist(childof(af3, 1))
@test just_argslist(childof(af4, 1))
@test !just_argslist(childof(nf1, 1))
@test !just_argslist(childof(nf2, 1))
@test !just_argslist(childof(nf3, 1))
@test !just_argslist(childof(nf4, 1))
end
wex1 = JuliaSyntax.parseall(SN, "x where T")
wex2 = JuliaSyntax.parseall(SN, "x where T where S")
wex3 = JuliaSyntax.parseall(SN, "x where T <: I")
wex4 = JuliaSyntax.parseall(SN, "x where T <: I where I")
wex5 = JuliaSyntax.parseall(SN, "x where {T, V}")
@testset "flatten_where_expr" begin
ctx = ExpandCtx(true, false)
@test sprint(show, flatten_where_expr(childof(wex1, 1), ctx)) == "(x, Any[T])"
@test sprint(show, flatten_where_expr(childof(wex2, 1), ctx)) == "(x, Any[S, T])"
@test sprint(show, flatten_where_expr(childof(wex3, 1), ctx)) == "(x, Any[(<: T I)])"
@test sprint(show, flatten_where_expr(childof(wex4, 1), ctx)) == "(x, Any[I, (<: T I)])"
@test sprint(show, flatten_where_expr(childof(wex5, 1), ctx)) == "(x, Any[T, V])"
end
atv1 = JuliaSyntax.parseall(SN, "A <: B")
atv2 = JuliaSyntax.parseall(SN, "A >: B")
atv3 = JuliaSyntax.parseall(SN, "A <: B <: C")
atv4 = JuliaSyntax.parseall(SN, "A >: B >: C")
atv5 = JuliaSyntax.parseall(SN, "A + 2 <: B")
@testset "analyze_typevar" begin
@test string(analyze_typevar(childof(atv1, 1), ExpandCtx())) == "TyVar(:A, Variable(:B), nothing)"
@test string(analyze_typevar(childof(atv2, 1), ExpandCtx())) == "TyVar(:A, nothing, Variable(:B))"
@test string(analyze_typevar(childof(atv3, 1), ExpandCtx())) == "TyVar(:B, Variable(:C), Variable(:A))"
@test_throws ASTException analyze_typevar(childof(atv4, 1), ExpandCtx())
@test_throws ASTException analyze_typevar(childof(atv5, 1), ExpandCtx())
end
struct ErrorResult end
expr_tests() = [
:function => [
"function foo() end" => "FunctionDef(ResolvedName([:foo]), [], [], [], nothing, Block([]))",
"function foo(a) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [], [], nothing, Block([]))",
"function foo(::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(nothing, nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function foo(a::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function foo(a::Int, b::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function foo(a::Int, b::Int, c=2) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:c), Literal(2), nothing)], [], [], nothing, Block([]))",
"function foo(a::Int, b::Int, c=2, d::Int) end" => ErrorResult(),
"function foo(; c) end" => "FunctionDef(ResolvedName([:foo]), [], [KwArg(:c, nothing, nothing, false)], [], nothing, Block([]))",
"function foo(; c=2) end" => "FunctionDef(ResolvedName([:foo]), [], [KwArg(:c, nothing, Literal(2), false)], [], nothing, Block([]))",
"function foo(; c::Int=2) end" => "FunctionDef(ResolvedName([:foo]), [], [KwArg(:c, Variable(:Int), Literal(2), false)], [], nothing, Block([]))",
"function foo(a; c::Int=2) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [KwArg(:c, Variable(:Int), Literal(2), false)], [], nothing, Block([]))",
"function foo(a; c::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [KwArg(:c, Variable(:Int), nothing, false)], [], nothing, Block([]))",
"function foo(a; c::Int, d) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [KwArg(:c, Variable(:Int), nothing, false), KwArg(:d, nothing, nothing, false)], [], nothing, Block([]))",
"function foo((a,b), c) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(TupleAssignment([IdentifierAssignment(:a), IdentifierAssignment(:b)]), nothing, nothing), FnArg(IdentifierAssignment(:c), nothing, nothing)], [], [], nothing, Block([]))",
"function foo((a,b)...) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(VarargAssignment(TupleAssignment([IdentifierAssignment(:a), IdentifierAssignment(:b)])), nothing, nothing)], [], [], nothing, Block([]))",
"function foo(a, b..., c) end" => ErrorResult(),
"function foo(; (a,b)::Tuple{Int, Int} = (1, 2)) end" => ErrorResult(),
"function foo(; (a,b)) end" => ErrorResult(),
"function foo((;a,b)) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(NamedTupleAssignment([IdentifierAssignment(:a), IdentifierAssignment(:b)]), nothing, nothing)], [], [], nothing, Block([]))",
"function foo((;a=2,b)) end" => ErrorResult(),
"function foo((;a,b)::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(NamedTupleAssignment([IdentifierAssignment(:a), IdentifierAssignment(:b)]), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function foo() :: Int end" => "FunctionDef(ResolvedName([:foo]), [], [], [], Variable(:Int), Block([]))",
"function foo() :: Int where T end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, nothing, nothing)], Variable(:Int), Block([]))",
"function foo() :: Int where {T, V} end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, nothing, nothing), TyVar(:V, nothing, nothing)], Variable(:Int), Block([]))",
"function foo() :: Int where {T <: V, V} end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, Variable(:V), nothing), TyVar(:V, nothing, nothing)], Variable(:Int), Block([]))",
"function foo() :: Int where {T} where V end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:V, nothing, nothing), TyVar(:T, nothing, nothing)], Variable(:Int), Block([]))",
"function foo() :: Int where {T} where {U, V} end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:U, nothing, nothing), TyVar(:V, nothing, nothing), TyVar(:T, nothing, nothing)], Variable(:Int), Block([]))",
"function foo()::T where T end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, nothing, nothing)], Variable(:T), Block([]))",
"function foo() where {T} where {U, V} end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:U, nothing, nothing), TyVar(:V, nothing, nothing), TyVar(:T, nothing, nothing)], nothing, Block([]))",
"function foo() where Int <: T <: Float32 end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, Variable(:Float32), Variable(:Int))], nothing, Block([]))",
"function foo()::T where Int <: T <: Float32 end" => "FunctionDef(ResolvedName([:foo]), [], [], [TyVar(:T, Variable(:Float32), Variable(:Int))], Variable(:T), Block([]))",
"evalpoly(x::T, p::T)::T where T<:Number = 2" => "Assignment(FunctionAssignment(ResolvedName([:evalpoly]), [FnArg(IdentifierAssignment(:x), nothing, Variable(:T)), FnArg(IdentifierAssignment(:p), nothing, Variable(:T))], [], [TyVar(:T, Variable(:Number), nothing)], Variable(:T)), Literal(2))",
"function foo(a) where {T} where {U, V} end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [], [TyVar(:U, nothing, nothing), TyVar(:V, nothing, nothing), TyVar(:T, nothing, nothing)], nothing, Block([]))",
"function foo(a; b::T) where {T} where {U, V} end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [KwArg(:b, Variable(:T), nothing, false)], [TyVar(:U, nothing, nothing), TyVar(:V, nothing, nothing), TyVar(:T, nothing, nothing)], nothing, Block([]))",
"function foo(a, a) end" => ErrorResult(),
"function foo(a::Int, a::Int) end" => ErrorResult(),
"function foo((a,b), b::Int) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(TupleAssignment([IdentifierAssignment(:a), IdentifierAssignment(:b)]), nothing, nothing), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function foo(; a=1, a=2) end" => ErrorResult(),
"function foo(a; a=1) end" => ErrorResult(),
"function foo() where {T, T} end" => ErrorResult(),
"function foo() where T where T end" => ErrorResult(),
"function foo(T) where T end" => ErrorResult(),
"function () end" => "FunctionDef(AnonFuncName(), [], [], [], nothing, Block([]))",
"function (a) end" => "FunctionDef(AnonFuncName(), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [], [], nothing, Block([]))",
"function (a::Int) end" => "FunctionDef(AnonFuncName(), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function (a::Int, b::Int) end" => "FunctionDef(AnonFuncName(), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int))], [], [], nothing, Block([]))",
"function (a::Int, b::Int, c=2) end" => "FunctionDef(AnonFuncName(), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:c), Literal(2), nothing)], [], [], nothing, Block([]))",
"function (a::Int, b::Int, c=2, d::Int) end" => ErrorResult(),
"function foo end" => "FunctionDef(ResolvedName([:foo]), [], [], [], nothing, nothing)",
"function (::Type{T})(x) end" => "FunctionDef(DeclName(nothing, CallCurly(Variable(:Type), [Variable(:T)])), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], nothing, Block([]))",
"function A.f() end" => "FunctionDef(ResolvedName([:A, :f]), [], [], [], nothing, Block([]))",
"function f{T}() end" => "FunctionDef(TypeFuncName(Variable(:f), [Variable(:T)]), [], [], [], nothing, Block([]))",
"function f()::T end" => "FunctionDef(ResolvedName([:f]), [], [], [], Variable(:T), Block([]))",
"function f()::g(T) end" => "FunctionDef(ResolvedName([:f]), [], [], [], FunCall(Variable(:g), [PositionalArg(Variable(:T))], []), Block([]))",
"(2+2)(x,y) = x" => ErrorResult(),
"function foo() where 2+T end" => ErrorResult(),
"function foo((x::Int, y)::String) end" => ErrorResult(),
"function foo(;x ; y) end" => ErrorResult(),
"function foo(;(x,y)...) end" => ErrorResult(),
"function foo{; a}() end" => ErrorResult(),
"function foo{a=y}() end" => ErrorResult(),
"function foo(a::b = 9) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), Literal(9), Variable(:b))], [], [], nothing, Block([]))",
"function foo(a::b = 9, b::c = 17) end" => "FunctionDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), Literal(9), Variable(:b)), FnArg(IdentifierAssignment(:b), Literal(17), Variable(:c))], [], [], nothing, Block([]))",
"function -(a, b) end" => "FunctionDef(ResolvedName([:-]), [FnArg(IdentifierAssignment(:a), nothing, nothing), FnArg(IdentifierAssignment(:b), nothing, nothing)], [], [], nothing, Block([]))",
"function .-(a, b) end" => ErrorResult(),
"function \$x(a, b) end" => ErrorResult(),
"function f(;x...) end" => "FunctionDef(ResolvedName([:f]), [], [KwArg(:x, nothing, nothing, true)], [], nothing, Block([]))",
"function f(a, b, c...) end" => "FunctionDef(ResolvedName([:f]), [FnArg(IdentifierAssignment(:a), nothing, nothing), FnArg(IdentifierAssignment(:b), nothing, nothing), FnArg(VarargAssignment(IdentifierAssignment(:c)), nothing, nothing)], [], [], nothing, Block([]))"
],
:macro => [
"macro foo end" => "MacroDef(ResolvedName([:foo]), [], [], nothing, nothing)",
"macro foo.bar() end" => ErrorResult(),
"macro foo{2}() end" => ErrorResult(),
"macro foo() end" => "MacroDef(ResolvedName([:foo]), [], [], nothing, Block([]))",
"macro foo(a) end" => "MacroDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, nothing)], [], nothing, Block([]))",
"macro foo(a::Int) end" => "MacroDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int))], [], nothing, Block([]))",
"macro foo(a::Int, b::Int) end" => "MacroDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int))], [], nothing, Block([]))",
"macro foo(a::Int, b::Int, c=2) end" => "MacroDef(ResolvedName([:foo]), [FnArg(IdentifierAssignment(:a), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:b), nothing, Variable(:Int)), FnArg(IdentifierAssignment(:c), Literal(2), nothing)], [], nothing, Block([]))",
"macro foo(a::Int, b::Int, c=2, d::Int) end" => ErrorResult(),
"macro foo(; x) end" => ErrorResult()
],
:weirdwhere => [
"where = 3" => "Assignment(IdentifierAssignment(:where), Literal(3))",
"func(where) = 12" => "Assignment(FunctionAssignment(ResolvedName([:func]), [FnArg(IdentifierAssignment(:where), nothing, nothing)], [], [], nothing), Literal(12))",
"func(where::Int) = 12" => "Assignment(FunctionAssignment(ResolvedName([:func]), [FnArg(IdentifierAssignment(:where), nothing, Variable(:Int))], [], [], nothing), Literal(12))"
],
:try => [
"try \n x \n catch e \n y \n finally \n z end" => "TryCatch(Block([Variable(:x)]), :e, Block([Variable(:y)]), nothing, Block([Variable(:z)]))",
"try \n x \n catch e \n y \n else z finally \n w end" => "TryCatch(Block([Variable(:x)]), :e, Block([Variable(:y)]), Block([Variable(:z)]), Block([Variable(:w)]))",
"try x catch end" => "TryCatch(Block([Variable(:x)]), nothing, Block([]), nothing, nothing)",
"try x catch ; y end" => "TryCatch(Block([Variable(:x)]), nothing, Block([Variable(:y)]), nothing, nothing)",
"try x catch \n y end" => "TryCatch(Block([Variable(:x)]), nothing, Block([Variable(:y)]), nothing, nothing)",
"try x catch e y end" => "TryCatch(Block([Variable(:x)]), :e, Block([Variable(:y)]), nothing, nothing)",
"try x finally y end" => "TryCatch(Block([Variable(:x)]), nothing, nothing, nothing, Block([Variable(:y)]))",
"try catch ; else end" => "TryCatch(Block([]), nothing, Block([]), Block([]), nothing)"
],
:curly => [
"a{;a}" => ErrorResult(),
"a{a=2}" => ErrorResult(),
"a{}" => "CallCurly(Variable(:a), [])",
"a{b}" => "CallCurly(Variable(:a), [Variable(:b)])",
"a{<:T}" => "CallCurly(Variable(:a), [TyVar(nothing, Variable(:T), nothing)])",
"a{>:T}" => "CallCurly(Variable(:a), [TyVar(nothing, nothing, Variable(:T))])"
],
:(.) => [
"a.f" => "GetProperty(Variable(:a), :f)",
"a.\"f\"" => "GetProperty(Variable(:a), Literal(\"f\"))",
"a.(f)" => "FunCall(Broadcast(Variable(:a)), [PositionalArg(Variable(:f))], [])",
"a.(f, x=9, z)" => "FunCall(Broadcast(Variable(:a)), [PositionalArg(Variable(:f)), PositionalArg(Variable(:z))], [KeywordArg(:x, Literal(9))])",
"a.(f.(x))" => "FunCall(Broadcast(Variable(:a)), [PositionalArg(FunCall(Broadcast(Variable(:f)), [PositionalArg(Variable(:x))], []))], [])",
"a.(2 .+ 2)" => "FunCall(Broadcast(Variable(:a)), [PositionalArg(FunCall(Broadcast(Variable(:+)), [PositionalArg(Literal(2)), PositionalArg(Literal(2))], []))], [])",
"a .&& b" => "FunCall(Broadcast(Variable(:&&)), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])",
"a .|| b" => "FunCall(Broadcast(Variable(:||)), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])",
"a .= b" => "BroadcastAssignment(Variable(:a), Variable(:b))",
"a[5] .= b" => "BroadcastAssignment(GetIndex(Variable(:a), [PositionalArg(Literal(5))]), Variable(:b))",
"a[5, 6] .= b" => "BroadcastAssignment(GetIndex(Variable(:a), [PositionalArg(Literal(5)), PositionalArg(Literal(6))]), Variable(:b))",
"x+y .= y" => "BroadcastAssignment(FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], []), Variable(:y))",
"x+y .+= y" => "BroadcastUpdate(:+=, FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], []), Variable(:y))",
".!f" => "FunCall(Broadcast(Variable(:!)), [PositionalArg(Variable(:f))], [])"
],
:call => [
"a(x)" => "FunCall(Variable(:a), [PositionalArg(Variable(:x))], [])",
"a(x,y)" => "FunCall(Variable(:a), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], [])",
"a(x,y; y=2, z=3)" => "FunCall(Variable(:a), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], [KeywordArg(:y, Literal(2)), KeywordArg(:z, Literal(3))])",
"a(x, y...; z=9)" => "FunCall(Variable(:a), [PositionalArg(Variable(:x)), SplatArg(Variable(:y))], [KeywordArg(:z, Literal(9))])",
"a - b - c" => "FunCall(Variable(:-), [PositionalArg(FunCall(Variable(:-), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])), PositionalArg(Variable(:c))], [])",
"a + b + c" => "FunCall(Variable(:+), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b)), PositionalArg(Variable(:c))], [])",
"a + b .+ c" => "FunCall(Broadcast(Variable(:+)), [PositionalArg(FunCall(Variable(:+), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])), PositionalArg(Variable(:c))], [])",
"[x +y]" => "HCat(nothing, [Variable(:x), FunCall(Variable(:+), [PositionalArg(Variable(:y))], [])])",
"[x+y +z]" => "HCat(nothing, [FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], []), FunCall(Variable(:+), [PositionalArg(Variable(:z))], [])])",
"[x +₁y]" => "Vect([FunCall(Variable(:+₁), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], [])])",
"[x+y+z]" => "Vect([FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y)), PositionalArg(Variable(:z))], [])])",
"[x+y + z]" => "Vect([FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y)), PositionalArg(Variable(:z))], [])])",
"a +₁ b +₁ c" => "FunCall(Variable(:+₁), [PositionalArg(FunCall(Variable(:+₁), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])), PositionalArg(Variable(:c))], [])",
"a .+ b .+ c" => "FunCall(Broadcast(Variable(:+)), [PositionalArg(FunCall(Broadcast(Variable(:+)), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])), PositionalArg(Variable(:c))], [])",
"f(a).g(b)" => "FunCall(GetProperty(FunCall(Variable(:f), [PositionalArg(Variable(:a))], []), :g), [PositionalArg(Variable(:b))], [])",
"foo(;x ; y)" => ErrorResult(),
"f(;x...)" => "FunCall(Variable(:f), [], [SplatArg(Variable(:x))])",
"+(x=1)" => "FunCall(Variable(:+), [PositionalArg(Assignment(IdentifierAssignment(:x), Literal(1)))], [])"
],
:unionall => [
"A where B" => "WhereType(Variable(:A), [TyVar(:B, nothing, nothing)])",
"A where {}" => "WhereType(Variable(:A), [])",
"A where B where C" => "WhereType(WhereType(Variable(:A), [TyVar(:B, nothing, nothing)]), [TyVar(:C, nothing, nothing)])",
"A where B<:C" => "WhereType(Variable(:A), [TyVar(:B, Variable(:C), nothing)])",
"A where Z<:W<:X" => "WhereType(Variable(:A), [TyVar(:W, Variable(:X), Variable(:Z))])",
"A where {Z<:W<:X, K}" => "WhereType(Variable(:A), [TyVar(:W, Variable(:X), Variable(:Z)), TyVar(:K, nothing, nothing)])"
],
:const => [
"const a = 1" => "Declaration([VarDecl(IdentifierAssignment(:a), Literal(1), DECL_CONST)])",
"const a::Int = 1" => "Declaration([VarDecl(TypedAssignment(IdentifierAssignment(:a), Variable(:Int)), Literal(1), DECL_CONST)])"
],
:local => [
"local a" => "Declaration([VarDecl(IdentifierAssignment(:a), nothing, DECL_LOCAL)])",
"local a::Int" => "Declaration([VarDecl(TypedAssignment(IdentifierAssignment(:a), Variable(:Int)), nothing, DECL_LOCAL)])",
"local a::Int, (b=4), c::String" => "Declaration([VarDecl(TypedAssignment(IdentifierAssignment(:a), Variable(:Int)), nothing, DECL_LOCAL), VarDecl(IdentifierAssignment(:b), Literal(4), DECL_LOCAL), VarDecl(TypedAssignment(IdentifierAssignment(:c), Variable(:String)), nothing, DECL_LOCAL)])"
],
:global => [
"global a" => "Declaration([VarDecl(IdentifierAssignment(:a), nothing, DECL_GLOBAL)])",
"global a::Int" => "Declaration([VarDecl(TypedAssignment(IdentifierAssignment(:a), Variable(:Int)), nothing, DECL_GLOBAL)])",
"global a::Int, (b=4), c::String" => "Declaration([VarDecl(TypedAssignment(IdentifierAssignment(:a), Variable(:Int)), nothing, DECL_GLOBAL), VarDecl(IdentifierAssignment(:b), Literal(4), DECL_GLOBAL), VarDecl(TypedAssignment(IdentifierAssignment(:c), Variable(:String)), nothing, DECL_GLOBAL)])"
],
:(=) => [
"a = 2" => "Assignment(IdentifierAssignment(:a), Literal(2))",
"a = b = 2" => "Assignment(IdentifierAssignment(:a), Assignment(IdentifierAssignment(:b), Literal(2)))",
"a.b = 2" => "Assignment(FieldAssignment(Variable(:a), :b), Literal(2))",
"a.b.c = 5" => "Assignment(FieldAssignment(GetProperty(Variable(:a), :b), :c), Literal(5))",
"a.b[3] = 9" => "Assignment(RefAssignment(GetProperty(Variable(:a), :b), [PositionalArg(Literal(3))], []), Literal(9))",
"a.b[3, 4] = 9" => "Assignment(RefAssignment(GetProperty(Variable(:a), :b), [PositionalArg(Literal(3)), PositionalArg(Literal(4))], []), Literal(9))",
"a.b[3; x = 0] = 9" => ErrorResult(),
"a.b[3 9] = 9" => ErrorResult(),
"(a.b, c) = 2" => "Assignment(TupleAssignment([FieldAssignment(Variable(:a), :b), IdentifierAssignment(:c)]), Literal(2))",
"y :: Int = 3" => "Assignment(TypedAssignment(IdentifierAssignment(:y), Variable(:Int)), Literal(3))",
"{ faulty } = 9" => ErrorResult(),
"(x..., y..., z) = (1,2)" => ErrorResult(),
"[a; b] = 2" => ErrorResult(),
"[a b] = 2" => ErrorResult(),
"[a ;; b] = 2" => ErrorResult(),
"f() = 3" => "Assignment(FunctionAssignment(ResolvedName([:f]), [], [], [], nothing), Literal(3))",
"f(x) = x" => "Assignment(FunctionAssignment(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], nothing), Variable(:x))",
"f(x)::Int = x" => "Assignment(FunctionAssignment(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], Variable(:Int)), Variable(:x))",
"f(x::T) where T = x" => "Assignment(FunctionAssignment(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, Variable(:T))], [], [TyVar(:T, nothing, nothing)], nothing), Variable(:x))",
"f(x::T) where T <: V = x" => "Assignment(FunctionAssignment(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, Variable(:T))], [], [TyVar(:T, Variable(:V), nothing)], nothing), Variable(:x))",
"f()::T where T = x" => "Assignment(FunctionAssignment(ResolvedName([:f]), [], [], [TyVar(:T, nothing, nothing)], Variable(:T)), Variable(:x))",
"x{y} = 2" => "Assignment(UnionAllAssignment(IdentifierAssignment(:x), [TyVar(:y, nothing, nothing)]), Literal(2))",
"x{} = 2" => ErrorResult()
],
:comparison => [
"a < b < c" => "Comparison(Variable(:a), [Variable(:<) => Variable(:b), Variable(:<) => Variable(:c)])",
"a <= b < c" => "Comparison(Variable(:a), [Variable(:<=) => Variable(:b), Variable(:<) => Variable(:c)])",
"a <: b <: c" => "Comparison(Variable(:a), [Variable(:<:) => Variable(:b), Variable(:<:) => Variable(:c)])",
"a .< b == c" => "Comparison(Variable(:a), [Broadcast(Variable(:<)) => Variable(:b), Variable(:(==)) => Variable(:c)])"
],
:ref => [
"x[2]" => "GetIndex(Variable(:x), [PositionalArg(Literal(2))])",
"x[2,3]" => "GetIndex(Variable(:x), [PositionalArg(Literal(2)), PositionalArg(Literal(3))])",
"x[2,x=3; z=9]" => ErrorResult(),
"x[2,z...; y=0]" => ErrorResult(),
"x[y=3]" => "GetIndex(Variable(:x), [PositionalArg(Assignment(IdentifierAssignment(:y), Literal(3)))])"
],
:do => [
"f() do ; end" => "DoStatement(FunCall(Variable(:f), [], []), [], Block([]))",
"f() do ; body end" => "DoStatement(FunCall(Variable(:f), [], []), [], Block([Variable(:body)]))",
"f() do x, y; body end" => "DoStatement(FunCall(Variable(:f), [], []), [FnArg(IdentifierAssignment(:x), nothing, nothing), FnArg(IdentifierAssignment(:y), nothing, nothing)], Block([Variable(:body)]))",
"f(x) do y body end" => "DoStatement(FunCall(Variable(:f), [PositionalArg(Variable(:x))], []), [FnArg(IdentifierAssignment(:y), nothing, nothing)], Block([Variable(:body)]))"
],
:tuple => [
"(1,2,3)" => "TupleExpr([Literal(1), Literal(2), Literal(3)])",
"(a=1, b=2)" => "NamedTupleExpr([NamedValue(:a, Literal(1)), NamedValue(:b, Literal(2))])",
"(a=1, b=2; c=3)" => ErrorResult(),
"(a=1, )" => "NamedTupleExpr([NamedValue(:a, Literal(1))])",
"(;a=2, b=2)" => "NamedTupleExpr([NamedValue(:a, Literal(2)), NamedValue(:b, Literal(2))])",
"(2=3, a=9)" => ErrorResult(),
"((x+y) => 3, b=3)" => "NamedTupleExpr([ComputedNamedValue(FunCall(Variable(:+), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], []), Literal(3)), NamedValue(:b, Literal(3))])",
"(;x, y)" => "NamedTupleExpr([NamedValue(:x, Variable(:x)), NamedValue(:y, Variable(:y))])",
"(;x.x, x.y)" => "NamedTupleExpr([NamedValue(:x, GetProperty(Variable(:x), :x)), NamedValue(:y, GetProperty(Variable(:x), :y))])",
"(x..., )" => "TupleExpr([Splat(Variable(:x))])",
"(x=y, z...)" => "NamedTupleExpr([NamedValue(:x, Variable(:y)), SplattedNamedValue(Variable(:z))])"
],
:string => [
"\"hello \$world today\"" => "StringInterpolate([\"hello \", Variable(:world), \" today\"])",
"\"hello \$(\"inner \$string here \$bar\") today\"" => "StringInterpolate([\"hello \", StringInterpolate([\"inner \", Variable(:string), \" here \", Variable(:bar)]), \" today\"])"
],
:(::) => [
"::Int" => ErrorResult(),
"a::Int" => "TypeAssert(Variable(:a), Variable(:Int))",
"(a,b)::Tuple{Int, Int}" => "TypeAssert(TupleExpr([Variable(:a), Variable(:b)]), CallCurly(Variable(:Tuple), [Variable(:Int), Variable(:Int)]))"
],
:if => [
"if a; b end" => "IfStmt([IfClause(Variable(:a), Block([Variable(:b)]))])",
"if a; end" => "IfStmt([IfClause(Variable(:a), Block([]))])",
"if a; b else c end" => "IfStmt([IfClause(Variable(:a), Block([Variable(:b)])), IfClause(nothing, Block([Variable(:c)]))])",
"if a; b elseif c; d end" => "IfStmt([IfClause(Variable(:a), Block([Variable(:b)])), IfClause(Variable(:c), Block([Variable(:d)]))])",
"if a; b elseif c; d else f end" => "IfStmt([IfClause(Variable(:a), Block([Variable(:b)])), IfClause(Variable(:c), Block([Variable(:d)])), IfClause(nothing, Block([Variable(:f)]))])"
],
:while => [
"while true end" => "WhileStmt(Literal(true), Block([]))",
"while true 2+3 end" => "WhileStmt(Literal(true), Block([FunCall(Variable(:+), [PositionalArg(Literal(2)), PositionalArg(Literal(3))], [])]))",
],
:break => [
"break" => ErrorResult(),
"while true; break end" => "WhileStmt(Literal(true), Block([BreakStmt()]))",
"for i=1:10; break end" => "ForStmt([IdentifierAssignment(:i) => FunCall(Variable(:(:)), [PositionalArg(Literal(1)), PositionalArg(Literal(10))], [])], Block([BreakStmt()]))",
"for i=1:10; if true break end end" => "ForStmt([IdentifierAssignment(:i) => FunCall(Variable(:(:)), [PositionalArg(Literal(1)), PositionalArg(Literal(10))], [])], Block([IfStmt([IfClause(Literal(true), Block([BreakStmt()]))])]))",
"while break; 2 end" => "WhileStmt(BreakStmt(), Block([Literal(2)]))",
"for i in [break;] 2 end" => "ForStmt([IdentifierAssignment(:i) => VCat(nothing, [BreakStmt()])], Block([Literal(2)]))"
],
:iterators => [
"1:10" => "FunCall(Variable(:(:)), [PositionalArg(Literal(1)), PositionalArg(Literal(10))], [])",
"1:10:5" => "FunCall(Variable(:(:)), [PositionalArg(Literal(1)), PositionalArg(Literal(10)), PositionalArg(Literal(5))], [])",
],
:continue => [
"continue" => ErrorResult()
"while true; continue end" => "WhileStmt(Literal(true), Block([ContinueStmt()]))"
],
:for => [
"for x in y; end" => "ForStmt([IdentifierAssignment(:x) => Variable(:y)], Block([]))",
"for x in y; w end" => "ForStmt([IdentifierAssignment(:x) => Variable(:y)], Block([Variable(:w)]))",
"for x in y, z in l; w end" => "ForStmt([IdentifierAssignment(:x) => Variable(:y), IdentifierAssignment(:z) => Variable(:l)], Block([Variable(:w)]))",
"for outer x in y; w end" => "ForStmt([OuterIdentifierAssignment(:x) => Variable(:y)], Block([Variable(:w)]))",
"for outer x in y, z in l; w end" => "ForStmt([OuterIdentifierAssignment(:x) => Variable(:y), IdentifierAssignment(:z) => Variable(:l)], Block([Variable(:w)]))"
],
:update => [
"x += y" => "Update(:+=, IdentifierAssignment(:x), Variable(:y))",
"x %= y" => "Update(:%=, IdentifierAssignment(:x), Variable(:y))",
"x \$= y" => "Update(:\$=, IdentifierAssignment(:x), Variable(:y))",
"x ≕ y" => "Update(:≕, IdentifierAssignment(:x), Variable(:y))",
"x ⩴ y" => "Update(:⩴, IdentifierAssignment(:x), Variable(:y))",
"(x, y) += z" => "Update(:+=, TupleAssignment([IdentifierAssignment(:x), IdentifierAssignment(:y)]), Variable(:z))",
"x .+= y" => "BroadcastUpdate(:+=, Variable(:x), Variable(:y))",
"(x, y) .+= z" => "BroadcastUpdate(:+=, TupleExpr([Variable(:x), Variable(:y)]), Variable(:z))",
"x.y += z" => "Update(:+=, FieldAssignment(Variable(:x), :y), Variable(:z))"
],
:vect => [
"[1,2,3]" => "Vect([Literal(1), Literal(2), Literal(3)])",
"[1,2,x...]" => "Vect([Literal(1), Literal(2), Splat(Variable(:x))])",
"[1,2,x...,5]" => "Vect([Literal(1), Literal(2), Splat(Variable(:x)), Literal(5)])",
"[a=1, 2]" => ErrorResult(),
"[1, 2; 3]" => ErrorResult(),
"[1, 2; x=y]" => ErrorResult()
],
:vcat => [
"[1 2;4 5]" => "VCat(nothing, [Row([Literal(1), Literal(2)]), Row([Literal(4), Literal(5)])])",
"[1 2;4 x=5]" => ErrorResult(),
"[1 2;4 x...]" => "VCat(nothing, [Row([Literal(1), Literal(2)]), Row([Literal(4), Splat(Variable(:x))])])"
],
:hcat => [
"[1 2 3]" => "HCat(nothing, [Literal(1), Literal(2), Literal(3)])",
"[1 2 x...]" => "HCat(nothing, [Literal(1), Literal(2), Splat(Variable(:x))])",
"[1 2 x... 5]" => "HCat(nothing, [Literal(1), Literal(2), Splat(Variable(:x)), Literal(5)])",
"[a=1 2]" => ErrorResult(),
"[1 2; x=y]" => ErrorResult()
],
:ncat => [
"[1 ;; 2 ;; 3]" => "NCat(nothing, 2, [Literal(1), Literal(2), Literal(3)])",
"[1 ;; 2 ;; 3 ; x = 5]" => ErrorResult(),
"[1 ;; 2 ;; 3 ; x...]" => ErrorResult(),
"[1 ; 2 ;; 3 ; 4 ;; 5 ; 6]" => "NCat(nothing, 2, [NRow(1, [Literal(1), Literal(2)]), NRow(1, [Literal(3), Literal(4)]), NRow(1, [Literal(5), Literal(6)])])",
"[1 ; 2 ;; 3 ; 4 ;;; 5 ; 6 ;; 7 ; 8 ;;; 9 ; 10 ;; 11 ; 12]" => "NCat(nothing, 3, [NRow(2, [NRow(1, [Literal(1), Literal(2)]), NRow(1, [Literal(3), Literal(4)])]), NRow(2, [NRow(1, [Literal(5), Literal(6)]), NRow(1, [Literal(7), Literal(8)])]), NRow(2, [NRow(1, [Literal(9), Literal(10)]), NRow(1, [Literal(11), Literal(12)])])])"
],
:typed_vcat => [
"x[1 2;4 5]" => "VCat(Variable(:x), [Row([Literal(1), Literal(2)]), Row([Literal(4), Literal(5)])])"
],
:typed_hcat => [
"x[1 2 3]" => "HCat(Variable(:x), [Literal(1), Literal(2), Literal(3)])"
],
:typed_ncat => [
"x[1 ;; 2 ;; 3]" => "NCat(Variable(:x), 2, [Literal(1), Literal(2), Literal(3)])"
],
:comprehension => [
"[x for x in y]" => "Comprehension(nothing, Generator(Variable(:x), [IterEq(IdentifierAssignment(:x), Variable(:y))]))",
"[x for x in y if z]" => "Comprehension(nothing, Generator(Variable(:x), [Filter([IterEq(IdentifierAssignment(:x), Variable(:y))], Variable(:z))]))",
"Int[x for x in z, w in l]" => "Comprehension(Variable(:Int), Generator(Variable(:x), [Cartesian([IterEq(IdentifierAssignment(:x), Variable(:z)), IterEq(IdentifierAssignment(:w), Variable(:l))])]))"
],
:generator => [
"(return x for x in y)" => ErrorResult(),
"(x for a in as)" => "Generator(Variable(:x), [IterEq(IdentifierAssignment(:a), Variable(:as))])",
"(x for a = as for b = bs if cond1 for c = cs if cond2)" => "Generator(Variable(:x), [IterEq(IdentifierAssignment(:a), Variable(:as)), Filter([IterEq(IdentifierAssignment(:b), Variable(:bs))], Variable(:cond1)), Filter([IterEq(IdentifierAssignment(:c), Variable(:cs))], Variable(:cond2))])",
"(x for a = as if begin cond2 end)" => "Generator(Variable(:x), [Filter([IterEq(IdentifierAssignment(:a), Variable(:as))], Block([Variable(:cond2)]))])",
"(a for x in xs if cond)" => "Generator(Variable(:a), [Filter([IterEq(IdentifierAssignment(:x), Variable(:xs))], Variable(:cond))])",
"(xy for x in xs for y in ys)" => "Generator(Variable(:xy), [IterEq(IdentifierAssignment(:x), Variable(:xs)), IterEq(IdentifierAssignment(:y), Variable(:ys))])",
"(xy for x in xs, y in ys)" => "Generator(Variable(:xy), [Cartesian([IterEq(IdentifierAssignment(:x), Variable(:xs)), IterEq(IdentifierAssignment(:y), Variable(:ys))])])",
"(xy for x in xs for y in ys for z in zs)" => "Generator(Variable(:xy), [IterEq(IdentifierAssignment(:x), Variable(:xs)), IterEq(IdentifierAssignment(:y), Variable(:ys)), IterEq(IdentifierAssignment(:z), Variable(:zs))])",
"(x for x in xs, y in ys if w)" => "Generator(Variable(:x), [Filter([Cartesian([IterEq(IdentifierAssignment(:x), Variable(:xs)), IterEq(IdentifierAssignment(:y), Variable(:ys))])], Variable(:w))])",
],
:struct => [
"struct Foo end" => ErrorResult()
],
:abstract => [
"abstract type Foo end" => ErrorResult()
],
:primitive => [
"primitive type A 32 end" => ErrorResult()
],
:import => [
"import Foo" => ErrorResult()
],
:using => [
"using Foo" => ErrorResult()
],
:export => [
"export foo" => ErrorResult()
],
:module => [
"module Foo end" => ErrorResult()
],
:let => [
"let x = y; z end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[IdentifierAssignment(:x) => Variable(:y)], Block([Variable(:z)]))",
"let ; z end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[], Block([Variable(:z)]))",
"let 2+2; 34 end" => ErrorResult(),
"let x() = 3; x() end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[FunctionAssignment(ResolvedName([:x]), [], [], [], nothing) => Literal(3)], Block([FunCall(Variable(:x), [], [])]))",
"let x; 2 end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[:x], Block([Literal(2)]))",
"let (x, y) = 2; 2 end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[TupleAssignment([IdentifierAssignment(:x), IdentifierAssignment(:y)]) => Literal(2)], Block([Literal(2)]))",
"let x::Real = 2; x end" => "LetBinding(Union{Symbol, Pair{<:LValue, <:Expression}}[TypedAssignment(IdentifierAssignment(:x), Variable(:Real)) => Literal(2)], Block([Variable(:x)]))",
],
:docstring => [
"""
\"\"\"
Foo Bar Baz
\"\"\"
function x() end
""" => "MacroExpansion(Docstring(StringInterpolate([\"Foo Bar Baz\\n\"]), FunctionDef(ResolvedName([:x]), [], [], [], nothing, Block([]))))"
],
:boolops => [
"a && b" => "FunCall(Variable(:&&), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])",
"x && (y = w)" => "FunCall(Variable(:&&), [PositionalArg(Variable(:x)), PositionalArg(Assignment(IdentifierAssignment(:y), Variable(:w)))], [])",
"a || b" => "FunCall(Variable(:||), [PositionalArg(Variable(:a)), PositionalArg(Variable(:b))], [])"
],
:? => [
"x ? y : z" => "Ternary(Variable(:x), Variable(:y), Variable(:z))"
],
:return => [
"return" => "ReturnStmt(nothing)",
"return 2" => "ReturnStmt(Literal(2))"
],
:literals => [
"true" => "Literal(true)",
"false" => "Literal(false)",
"'c'" => "Literal('c')",
"3" => "Literal(3)",
],
:typerel => [
"x <: y" => "FunCall(Variable(:<:), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], [])",
"x >: y" => "FunCall(Variable(:>:), [PositionalArg(Variable(:x)), PositionalArg(Variable(:y))], [])"
],
:transpose => [
"x'" => "FunCall(Variable(Symbol(\"'\")), [PositionalArg(Variable(:x))], [])"
],
:juxtapose => [
"2x" => "FunCall(Variable(:*), [PositionalArg(Literal(2)), PositionalArg(Variable(:x))], [])",
"2x + 2y" => "FunCall(Variable(:+), [PositionalArg(FunCall(Variable(:*), [PositionalArg(Literal(2)), PositionalArg(Variable(:x))], [])), PositionalArg(FunCall(Variable(:*), [PositionalArg(Literal(2)), PositionalArg(Variable(:y))], []))], [])"
],
:literal => [
"2" => "Literal(2)",
"2.0" => "Literal(2.0)",
"0b1010" => "Literal(0x0a)",
"0x10" => "Literal(0x10)",
"0o10" => "Literal(0x08)",
"2.f0" => "Literal(2.0f0)",
"'h'" => "Literal('h')",
#"`h`" => "FunCall(Variable(:*), [PositionalArg(Literal(2)), PositionalArg(Variable(:x))], [])" TODO
]
]
toplevel_tests() = [
:struct => [
"struct Foo end" => "StructDefStmt(:Foo, Symbol[], nothing, [], [])",
"struct Foo <: Bar end" => "StructDefStmt(:Foo, Symbol[], Variable(:Bar), [], [])",
"struct Foo{X} <: Bar end" => "StructDefStmt(:Foo, [:X], Variable(:Bar), [], [])",
"struct Foo{X} <: Bar{X} end" => "StructDefStmt(:Foo, [:X], CallCurly(Variable(:Bar), [Variable(:X)]), [], [])",
"struct Foo{X<:Int} <: Bar{X} end" => "StructDefStmt(:Foo, [:X], CallCurly(Variable(:Bar), [Variable(:X)]), [], [])",
"struct Foo{X, Y} <: Baz{Y} end" => "StructDefStmt(:Foo, [:X, :Y], CallCurly(Variable(:Baz), [Variable(:Y)]), [], [])",
"struct Foo x end" => "StructDefStmt(:Foo, Symbol[], nothing, [StructField(:x, nothing, FIELD_NONE)], [])",
"struct Foo x; y end" => "StructDefStmt(:Foo, Symbol[], nothing, [StructField(:x, nothing, FIELD_NONE), StructField(:y, nothing, FIELD_NONE)], [])",
"struct Foo x::Int end" => "StructDefStmt(:Foo, Symbol[], nothing, [StructField(:x, Variable(:Int), FIELD_NONE)], [])",
"struct Foo x::Int; y::String end" => "StructDefStmt(:Foo, Symbol[], nothing, [StructField(:x, Variable(:Int), FIELD_NONE), StructField(:y, Variable(:String), FIELD_NONE)], [])",
"struct Foo const x::Int end" => ErrorResult(),
"struct Foo x=3 end" => ErrorResult(),
"mutable struct Foo x::Int; const y::String end" => "StructDefStmt(:Foo, Symbol[], nothing, [StructField(:x, Variable(:Int), FIELD_NONE), StructField(:y, Variable(:String), FIELD_CONST)], [])"
],
:abstract => [
"abstract type Foo end" => "AbstractDefStmt(:Foo, Symbol[], nothing)",
"abstract type Foo <: Bar end" => "AbstractDefStmt(:Foo, Symbol[], Variable(:Bar))",
"abstract type Foo{X} <: Bar end" => "AbstractDefStmt(:Foo, [:X], Variable(:Bar))",
"abstract type Foo{X} <: Bar{X} end" => "AbstractDefStmt(:Foo, [:X], CallCurly(Variable(:Bar), [Variable(:X)]))",
"abstract type Foo{X<:Int} <: Bar{X} end" => "AbstractDefStmt(:Foo, [:X], CallCurly(Variable(:Bar), [Variable(:X)]))",
"abstract type Foo{X, Y} <: Baz{Y} end" => "AbstractDefStmt(:Foo, [:X, :Y], CallCurly(Variable(:Baz), [Variable(:Y)]))",
"abstract type A end" => "AbstractDefStmt(:A, Symbol[], nothing)",
"abstract type A ; end" => "AbstractDefStmt(:A, Symbol[], nothing)",
"abstract type A <: B end" => "AbstractDefStmt(:A, Symbol[], Variable(:B))",
"abstract type A <: B{T,S} end" => "AbstractDefStmt(:A, Symbol[], CallCurly(Variable(:B), [Variable(:T), Variable(:S)]))",
"abstract type A < B end" => ErrorResult()
],
:primitive => [
"primitive type A 32 end" => "PrimitiveDefStmt(:A, Symbol[], nothing, Literal(32))",
"primitive type A 32 ; end" => "PrimitiveDefStmt(:A, Symbol[], nothing, Literal(32))",
"primitive type A <: B \n 8 \n end" => "PrimitiveDefStmt(:A, Symbol[], Variable(:B), Literal(8))"
],
:import => [
"import Foo" => "ImportStmt([Dep(ImportId(:Foo))])",
"import Foo: bar" => "SourceImportStmt(ImportId(:Foo), [Dep(ImportId(:bar))])",
"import Foo.bar: baz" => "SourceImportStmt(ImportField(ImportId(:Foo), :bar), [Dep(ImportId(:baz))])",
"import Foo.bar" => "ImportStmt([Dep(ImportField(ImportId(:Foo), :bar))])",
"import Foo: baz, bing" => "SourceImportStmt(ImportId(:Foo), [Dep(ImportId(:baz)), Dep(ImportId(:bing))])",
"import ..Foo" => "ImportStmt([Dep(ImportField(ImportRelative(2), :Foo))])",
"import ..Foo, ..Baz" => "ImportStmt([Dep(ImportField(ImportRelative(2), :Foo)), Dep(ImportField(ImportRelative(2), :Baz))])",
"import ..Foo: Baz" => "SourceImportStmt(ImportField(ImportRelative(2), :Foo), [Dep(ImportId(:Baz))])",
"import Foo: baz as Bar, b.bar" => "SourceImportStmt(ImportId(:Foo), [AliasDep(ImportId(:baz), :Bar), Dep(ImportField(ImportId(:b), :bar))])",
"import Foo as Bar" => "ImportStmt([AliasDep(ImportId(:Foo), :Bar)])",
"import Foo as Bar, Bar as Baz" => "ImportStmt([AliasDep(ImportId(:Foo), :Bar), AliasDep(ImportId(:Bar), :Baz)])",
"import Foo.Bar + 2" => ErrorResult(),
"import Foo.@baz" => "ImportStmt([Dep(ImportField(ImportId(:Foo), Symbol(\"@baz\")))])",
"import Foo: @baz" => "SourceImportStmt(ImportId(:Foo), [Dep(ImportId(Symbol(\"@baz\")))])",
"import Foo.Bar: @baz" => "SourceImportStmt(ImportField(ImportId(:Foo), :Bar), [Dep(ImportId(Symbol(\"@baz\")))])",
"import Foo: ..Bar" => ErrorResult(),
"import Foo: ..Bar as Baz" => ErrorResult()
],
:using => [
"using Foo" => "UsingStmt([ImportId(:Foo)])",
"using Foo, Bar" => "UsingStmt([ImportId(:Foo), ImportId(:Bar)])",
"using Foo, ..Bar" => "UsingStmt([ImportId(:Foo), ImportField(ImportRelative(2), :Bar)])",
"using Foo: bar" => "SourceUsingStmt(ImportId(:Foo), [Dep(ImportId(:bar))])",
"using Foo.bar: baz" => "SourceUsingStmt(ImportField(ImportId(:Foo), :bar), [Dep(ImportId(:baz))])",
"using Foo.bar" => "UsingStmt([ImportField(ImportId(:Foo), :bar)])",
"using Foo: baz, bing" => "SourceUsingStmt(ImportId(:Foo), [Dep(ImportId(:baz)), Dep(ImportId(:bing))])",
"using ..Foo" => "UsingStmt([ImportField(ImportRelative(2), :Foo)])",
"using Foo: baz as Bar, b.bar" => "SourceUsingStmt(ImportId(:Foo), [AliasDep(ImportId(:baz), :Bar), Dep(ImportField(ImportId(:b), :bar))])",
"using Foo: ..Bar" => ErrorResult(),
"using Foo: ..Bar as Baz" => ErrorResult(),
"using ..Foo, ..Bar" => "UsingStmt([ImportField(ImportRelative(2), :Foo), ImportField(ImportRelative(2), :Bar)])"
],
:export => [
"export foo" => "ExportStmt([:foo])",
"export foo, bar, baz" => "ExportStmt([:foo, :bar, :baz])"
],
:module => [
"module Foo end" => "ModuleStmt(true, :Foo, [])",
"module Foo module Bar end end" => "ModuleStmt(true, :Foo, [ModuleStmt(true, :Bar, [])])",
"function f() module Foo module Bar end end end" => ErrorResult(),
"baremodule Foo end" => "ModuleStmt(false, :Foo, [])"
],
:inline => [
"@inline function foobarbaz() end" => "ExprStmt(FunctionDef(ResolvedName([:foobarbaz]), [], [], [], nothing, Block([])))"
"function foo() @inline function bar() end end" => "ExprStmt(FunctionDef(ResolvedName([:foo]), [], [], [], nothing, Block([FunctionDef(ResolvedName([:bar]), [], [], [], nothing, Block([]))])))"
],
:noinline => [
"@noinline function foobarbaz() end" => "ExprStmt(FunctionDef(ResolvedName([:foobarbaz]), [], [], [], nothing, Block([])))"
"function foo() @noinline function bar() end end" => "ExprStmt(FunctionDef(ResolvedName([:foo]), [], [], [], nothing, Block([FunctionDef(ResolvedName([:bar]), [], [], [], nothing, Block([]))])))"
],
:other_macros => [
"@assume_effects :consistent function foobarbaz() end" => "ExprStmt(FunctionDef(ResolvedName([:foobarbaz]), [], [], [], nothing, Block([])))"
"if @generated; 2 else 3 end" => "ExprStmt(IfStmt([IfClause(Literal(true), Block([Literal(2)])), IfClause(nothing, Block([Literal(3)]))]))"
],
:docstring => [
"""
\"\"\"
Foo Bar Baz
\"\"\"
function x() end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"Foo Bar Baz\\n\"]), ExprStmt(FunctionDef(ResolvedName([:x]), [], [], [], nothing, Block([])))))",
"""
\"\"\"
...
\"\"\"
const a = 1
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(Declaration([VarDecl(IdentifierAssignment(:a), Literal(1), DECL_CONST)]))))",
"""
\"\"\"
...
\"\"\"
global a = 1
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(Declaration([VarDecl(IdentifierAssignment(:a), Literal(1), DECL_GLOBAL)]))))",
"""
\"\"\"
...
\"\"\"
baremodule M end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ModuleStmt(false, :M, [])))",
"""
\"\"\"
...
\"\"\"
module M end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ModuleStmt(true, :M, [])))",
"""
\"\"\"
...
\"\"\"
abstract type T1 end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), AbstractDefStmt(:T1, Symbol[], nothing)))",
"""
\"\"\"
...
\"\"\"
mutable struct T2 end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), StructDefStmt(:T2, Symbol[], nothing, [], [])))",
"""
\"\"\"
...
\"\"\"
struct T3 end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), StructDefStmt(:T3, Symbol[], nothing, [], [])))",
"""
\"\"\"
...
\"\"\"
macro m(x) end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(MacroDef(ResolvedName([:m]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], nothing, Block([])))))",
"""
\"\"\"
...
\"\"\"
f(x) = x
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(Assignment(FunctionAssignment(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], nothing), Variable(:x)))))",
"""
\"\"\"
...
\"\"\"
function f(x)
x
end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(FunctionDef(ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], nothing, Block([Variable(:x)])))))",
"""
\"\"\"
...
\"\"\"
f(x)
""" => "MacroExpansionStmt(CallDocstring(StringInterpolate([\"...\\n\"]), ResolvedName([:f]), [FnArg(IdentifierAssignment(:x), nothing, nothing)], [], [], nothing))",
"""
\"\"\"
...
\"\"\"
function f end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"...\\n\"]), ExprStmt(FunctionDef(ResolvedName([:f]), [], [], [], nothing, nothing))))",
"""
\"\"\"
... \$testme end
\"\"\"
function f end
""" => "MacroExpansionStmt(Docstring(StringInterpolate([\"... \", Variable(:testme), \" end\\n\"]), ExprStmt(FunctionDef(ResolvedName([:f]), [], [], [], nothing, nothing))))",
"""
\"\"\"
... \$testme end
\"\"\"
foo(bar::baz)::bing
""" => "MacroExpansionStmt(CallDocstring(StringInterpolate([\"... \", Variable(:testme), \" end\\n\"]), ResolvedName([:foo]), [FnArg(IdentifierAssignment(:bar), nothing, Variable(:baz))], [], [], Variable(:bing)))",
"""
\"\"\"
... \$testme end
\"\"\"
foo(bar::baz)::bing where Bar
""" => "MacroExpansionStmt(CallDocstring(StringInterpolate([\"... \", Variable(:testme), \" end\\n\"]), ResolvedName([:foo]), [FnArg(IdentifierAssignment(:bar), nothing, Variable(:baz))], [], [], WhereType(Variable(:bing), [TyVar(:Bar, nothing, nothing)])))",
"""
\"\"\"
... testme end
\"\"\"
foo(bar; baz::Int = 2)
""" => "MacroExpansionStmt(CallDocstring(StringInterpolate([\"... testme end\\n\"]), ResolvedName([:foo]), [FnArg(IdentifierAssignment(:bar), nothing, nothing)], [KwArg(:baz, Variable(:Int), Literal(2), false)], [], nothing))",
"""
\"\"\"
... testme end
\"\"\"
foo(bar; baz::Int = 2)::Int
""" => "MacroExpansionStmt(CallDocstring(StringInterpolate([\"... testme end\\n\"]), ResolvedName([:foo]), [FnArg(IdentifierAssignment(:bar), nothing, nothing)], [KwArg(:baz, Variable(:Int), Literal(2), false)], [], Variable(:Int)))"
]
]
@testset "Statement tests" begin
@testset "$head" for (head, test_specs) in toplevel_tests()
@testset "$input" for (input, output) in test_specs
if output isa ErrorResult
@test_throws ASTException expand_toplevel(childof(JuliaSyntax.parseall(SN, input), 1), ExpandCtx(true, false))
else
@test string(expand_toplevel(childof(JuliaSyntax.parseall(SN, input), 1), ExpandCtx(true, false))) == output
end
end
end
end
@testset "Expression tests" begin
@testset "$head" for (head, test_specs) in expr_tests()
@testset "$input" for (input, output) in test_specs
if output isa ErrorResult
@test_throws ASTException expand_forms(childof(JuliaSyntax.parseall(SN, input), 1), ExpandCtx(true, false))
@test string(expand_forms(childof(JuliaSyntax.parseall(SN, input), 1), ExpandCtx(true, false; error_context=SemanticAST.SilentErrorReporting()))) != nothing
else
ast = expand_forms(childof(JuliaSyntax.parseall(SN, input), 1), ExpandCtx(true, false))
SemanticAST.visit((x) -> nothing, y->nothing, ast)
@test string(ast) == output
end
end
end
end
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | docs | 6719 | # SemanticAST
[](https://benchung.github.io/SemanticAST.jl/dev)
SemanticAST.jl aims to provide a unified view of Julia's AST by reproducing much of the logic that the [lowering](https://docs.julialang.org/en/v1/devdocs/eval/#dev-parsing) phase does.
Many of the more structural errors that Julia produces (for example how a toplevel `::Int` declaration begets `syntax: invalid "::" syntax`) are not actually produced by the parser
but instead by a later lowering phase. In effect, lowering (in conjunction with macro expansion) defines all of the Julia AST forms that can be successfully compiled to executable runtime code.
If it can be lowered, it will at least *attempt* to execute.
`Expr` ASTs are notoriously annoying to deal with to the point that packages like
[ExprTools](https://github.com/invenia/ExprTools.jl) are widely used to analyze what's actually there. `Expr`s will come in all sorts of wild and wonderful forms and having a library
to deal with all of the possible cases is a great help. However, ExprTools only goes so far: it solely provides a consistent representation of function definitions. SemanticAST aims to
go further.
SemanticAST aims to extend this sort of "rationalization" to all of Julia's syntax; it provides a single set of AST definitions that explicitly declare every parameter and are descriptive
about what their fields mean. Moreover, SemanticAST's types try to be complete --- that is, you can use the type of a field in a SemanticAST struct to determine precisely what any possible
member might be. Additionally, by sitting on top of JuliaSyntax, SemanticAST provides detailed source location information both in the output AST and in the lowering-equivalent error messages
that SemanticAST produces The objective, then, is to eliminate the surprise inherent to working with Julia's AST forms, allowing easier development of static analysis tooling for Julia.
The implementation strategy for SemanticAST is to bring together lowering, parts of toplevel evaluation, and parts of the Julia runtime itself to identify as many errors as early as possible.
Ideally, SemanticAST will accept any program that is "runnable" by Julia, even if it might encounter a dynamic error. Errors produced by SemanticAST aim to parallel errors produced by Julia
itself as it goes to compile a given `Expr` to IR, as well as parts of evaluation of that IR.
# Use
SemanticAST's entry points are through the `expand_forms` and `expand_toplevel` functions; given a
`JuliaSyntax.SyntaxNode` and a `ExpandCtx` they will attempt to unpack a `SyntaxNode` into a `SemanticAST.Expression` and `SemanticAST.ToplevelStmts`, respectively.
Note that SemanticAST AST forms all contain a `location` field that's implicitly inserted by the `@ast_node` and `@ast_data` macros that is of type `SemanticAST.SourcePosition`.
As an example, suppose we wanted to analyze the program `hello_world.jl` that looked like
```
function hello_world()
println("hello world!")
end
```
the complete analysis program would look like:
```
using JuliaSyntax, SemanticAST
filename = "hello_world.jl"
source_tree = parsestmt(SyntaxNode, read(filename, String), filename=filename)
semantic_tree = expand_toplevel(source_tree)
```
that's it!
## Limitation: Macros
At present SemanticAST tries to cover much of "basic" Julia - but there's a major fly in the ointment: macros.
Macros are challenging for the SemanticAST approach because they can accept non-standard Julia `Expr`s and then both
produce unusual `Expr` forms as well as use a wide range of forms not otherwise produced by parsing. As SemanticAST sits
on top of JuliaSyntax and only understands JuliaSyntax `SyntaxNode`s it cannot practically run macros directly as while a
`SyntaxNode` can be converted to an `Expr` vice versa is not possible without loss of information. Thus, at present,
macro invocations are simply replaced with a placeholder. There's limited hardcoded support for a small list of built-in macros
and an extension mechanism, but no more.
There are two macro extension points in the analyzer: `resolve_toplevel_macro(ast::SyntaxNode, ::MacroContext, ::Val{Symbol}, args::Vector{SyntaxNode}, ctx::ExpandCtx)::ToplevelStmts` and `resolve_macro(ast, ::MacroContext, ::Val{Symbol}, args, ctx)::Expression`.
Both share the same signature:
* `ast`: The SyntaxNode root of the macro invocation.
* `MacroContext`: The context with which to resolve macros in. Implement a new `MacroContext` by implementing `resolve_toplevel_macro` and `resolve_macro` that accept it; the provided one is `DefaultMacroContext`.
* `Val{Symbol}`: The macro's name (in `Val` form).
* `args`: The SyntaxNodes that represent the arguments to the macro.
* `ctx`: The expansion context inside which the analysis is being done.
They differ in that `resolve_toplevel_macro` returns a `TopLevelStmts` (a statement that can only exist at the top level), while `resolve_macro` returns an `Expression`. An example of how to write a macro analyzer
can be seen in the default implementation for `@inline` and `@noinline`:
```
resolve_macro(ast, ::DefaultMacroContext, ::Union{Val{Symbol("@inline")}, Val{Symbol("@noinline")}, Val{Symbol("@inbounds")}}, args, ctx) = expand_forms(args[1], ctx)
```
From the perspective of the default analyzer `@inline` and `@noinline` are no-ops, so analysis continues by simply calling back into `expand_forms` on the first
argument.
## Limitation: Quoting
While SemanticAST will not *choke* on quotes, it won't interpret them intelligently. Quotes are seen as a big blob of `Expr`,
*including unquotes*.
# State
SemanticAST is part of the implementation of [my thesis on typing Julia](https://benchung.github.io/papers/thesis.pdf) and its current implementation
was designed specifically to fit the needs of a type system. As a result, it is missing a number of features that might be interesting. Two that I think
would be interesting are:
* The ability to analyze normal `Exprs` and not only JuliaSyntax nodes. This could be accomplished by extending the custom patterns to support `Expr`s, and would allow SemanticAST to be used after macro expansion (or as part of normal Julia macro implementations) when combined with
* Support for semantic forms that don't result from parsing. My goal was to support parser output but macros are allowed to use a larger `Expr` language. As a result, analyzing post-expansion `Expr`s is not possible.
Be warned, this project is currently academic software. It might break, have obvious bugs, and otherwise have weird and unexpected issues. Moreover,
the `ASTNode` language is not stable: it may change unexpectedly in future releases.
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | docs | 5005 | # SemanticAST
SemanticAST.jl aims to provide a unified view of Julia's AST by reproducing much of the logic that the [lowering](https://docs.julialang.org/en/v1/devdocs/eval/#dev-parsing) phase does.
Many of the more structural errors that Julia produces (for example how a toplevel `::Int` declaration begets `syntax: invalid "::" syntax`) are not actually produced by the parser
but instead by a later lowering phase. In effect, lowering (in conjunction with macro expansion) defines all of the Julia AST forms that can be successfully compiled to executable runtime code.
If it can be lowered, it will at least *attempt* to execute.
`Expr` ASTs are notoriously annoying to deal with to the point that packages like
[ExprTools](https://github.com/invenia/ExprTools.jl) are widely used to analyze what's actually there. `Expr`s will come in all sorts of wild and wonderful forms and having a library
to deal with all of the possible cases is a great help. However, ExprTools only goes so far: it solely provides a consistent representation of function definitions. SemanticAST aims to
go further.
SemanticAST aims to extend this sort of "rationalization" to all of Julia's syntax; it provides a single set of AST definitions that explicitly declare every parameter and are descriptive
about what their fields mean. Moreover, SemanticAST's types try to be complete --- that is, you can use the type of a field in a SemanticAST struct to determine precisely what any possible
member might be. Additionally, by sitting on top of JuliaSyntax, SemanticAST provides detailed source location information both in the output AST and in the lowering-equivalent error messages
that SemanticAST produces The objective, then, is to eliminate the surprise inherent to working with Julia's AST forms, allowing easier development of static analysis tooling for Julia.
The implementation strategy for SemanticAST is to bring together lowering, parts of toplevel evaluation, and parts of the Julia runtime itself to identify as many errors as early as possible.
Ideally, SemanticAST will accept any program that is "runnable" by Julia, even if it might encounter a dynamic error. Errors produced by SemanticAST aim to parallel errors produced by Julia
itself as it goes to compile a given `Expr` to IR, as well as parts of evaluation of that IR.
```@contents
```
# Use
SemanticAST's entry points are through the `expand_forms` and `expand_toplevel` functions; given a
`JuliaSyntax.SyntaxNode` and a `ExpandCtx` they will attempt to unpack a `SyntaxNode` into a `SemanticAST.Expression` and `SemanticAST.ToplevelStmts`, respectively.
Note that SemanticAST AST forms all contain a `location` field that's implicitly inserted by the `@ast_node` and `@ast_data` macros that is of type `SemanticAST.SourcePosition`.
As an example, suppose we wanted to analyze the program `hello_world.jl` that looked like
```
function hello_world()
println("hello world!")
end
```
the complete analysis program would look like:
```
using JuliaSyntax, SemanticAST
filename = "hello_world.jl"
source_tree = parsestmt(SyntaxNode, read(filename, String), filename=filename)
semantic_tree = expand_toplevel(source_tree)
```
that's it!
## Limitation: Macros
At present SemanticAST tries to cover much of "basic" Julia - but there's a major fly in the ointment: macros.
Macros are challenging for the SemanticAST approach because they can accept non-standard Julia `Expr`s and then both
produce unusual `Expr` forms as well as use a wide range of forms not otherwise produced by parsing. As SemanticAST sits
on top of JuliaSyntax and only understands JuliaSyntax `SyntaxNode`s it cannot practically run macros directly as while a
`SyntaxNode` can be converted to an `Expr` vice versa is not possible without loss of information. Thus, at present,
macro invocations are simply replaced with a placeholder. There's limited hardcoded support for a small list of built-in macros
and an extension mechanism, but no more.
## Limitation: Quoting
While SemanticAST will not *choke* on quotes, it won't interpret them intelligently. Quotes are seen as a big blob of `Expr`,
*including unquotes*.
# State
SemanticAST is part of the implementation of [my thesis on typing Julia](https://benchung.github.io/papers/thesis.pdf) and its current implementation
was designed specifically to fit the needs of a type system. As a result, it is missing a number of features that might be interesting. Two that I think
would be interesting are:
* The ability to analyze normal `Exprs` and not only JuliaSyntax nodes. This could be accomplished by extending the custom patterns to support `Expr`s, and would allow SemanticAST to be used after macro expansion (or as part of normal Julia macro implementations) when combined with
* Support for semantic forms that don't result from parsing. My goal was to support parser output but macros are allowed to use a larger `Expr` language. As a result, analyzing post-expansion `Expr`s is not possible.
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"Apache-2.0"
] | 0.1.1 | c408f08bc3bf6f86c5297a357e743276c7e69db7 | docs | 407 | SemanticAST parses a `SyntaxNode` as provided by JuliaSyntax into its internal `ASTNode` structures for use by downstream analyses. This process is performed by one of two methods: `expand_forms` and `expand_toplevel`.
```@meta
CurrentModule = SemanticAST
```
```@docs
expand_forms
expand_toplevel
ExpandCtx
ErrorReporting
ExceptionErrorReporting
SilentErrorReporting
MacroContext
DefaultMacroContext
```
| SemanticAST | https://github.com/BenChung/SemanticAST.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1174 | using Documenter
using Literate
using AutomotiveSimulator
# generate tutorials and how-to guides using Literate
src = joinpath(@__DIR__, "src")
lit = joinpath(@__DIR__, "lit")
notebooks = joinpath(src, "notebooks")
for (root, _, files) in walkdir(lit), file in files
splitext(file)[2] == ".jl" || continue
ipath = joinpath(root, file)
opath = splitdir(replace(ipath, lit=>src))[1]
Literate.markdown(ipath, opath, documenter = true)
Literate.notebook(ipath, notebooks, execute = false)
end
makedocs(
modules = [AutomotiveSimulator],
format = Documenter.HTML(),
sitename="AutomotiveSimulator.jl",
pages=[
"Home" => "index.md",
"Tutorials" => [
"tutorials/straight_roadway.md",
"tutorials/stadium.md",
"tutorials/intersection.md",
"tutorials/crosswalk.md",
"tutorials/sidewalk.md",
"tutorials/feature_extraction.md"
],
"Manual" => [
"Roadways.md",
"actions.md",
"states.md",
"agent_definitions.md",
"behaviors.md",
"simulation.md",
"collision_checkers.md",
"feature_extraction.md"
],
"Internals" =>[
"Vec.md"
]
]
)
deploydocs(
repo = "github.com/sisl/AutomotiveSimulator.jl.git",
)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 5229 | # # Crosswalk
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/crosswalk.ipynb)
# In this notebook we demonstrate how to define a crosswalk with pedestrians using `AutomotiveSimulator`.
# To do this, we define a crosswalk area as well as a pedestrian agent type.
# ## Generate a crosswalk environment
# We define a new concrete type that will contain the roadway (where cars drive)
# and the crosswalk definition which is just a regular lane.
using AutomotiveSimulator
using AutomotiveVisualization
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
using Random
struct CrosswalkEnv
roadway::Roadway{Float64}
crosswalk::Lane{Float64}
end
# The crosswalk lane consists of a straight road segment perpendicular to the road.
# We will define the roadway just as a straight road.
# geometry parameters
roadway_length = 50.
crosswalk_length = 20.
crosswalk_width = 6.0
crosswalk_pos = roadway_length/2
# Generate a straight 2-lane roadway and a crosswalk lane
roadway = gen_straight_roadway(2, roadway_length)
crosswalk_start = VecE2(crosswalk_pos, -crosswalk_length/2)
crosswalk_end = VecE2(crosswalk_pos, crosswalk_length/2)
crosswalk_lane = gen_straight_curve(crosswalk_start, crosswalk_end, 2)
crosswalk = Lane(LaneTag(2,1), crosswalk_lane, width = crosswalk_width)
cw_segment = RoadSegment(2, [crosswalk])
push!(roadway.segments, cw_segment) # append it to the roadway
# initialize crosswalk environment
env = CrosswalkEnv(roadway, crosswalk)
#md nothing # hide
# **Render the crosswalk**
# We will define a new method to render this new environment.
# The roadway part is just rendered regularly, we add specific instuction
# for the crosswalk part that will display the white stripes.
using Cairo
function AutomotiveVisualization.add_renderable!(rendermodel::RenderModel, env::CrosswalkEnv)
## render the road without the crosswalk
roadway = gen_straight_roadway(2, roadway_length)
add_renderable!(rendermodel, roadway)
## render crosswalk
curve = env.crosswalk.curve
n = length(curve)
pts = Array{Float64}(undef, 2, n)
for (i,pt) in enumerate(curve)
pts[1,i] = pt.pos.x
pts[2,i] = pt.pos.y
end
add_instruction!(
rendermodel, render_dashed_line,
(pts, colorant"white", env.crosswalk.width, 1.0, 1.0, 0.0, Cairo.CAIRO_LINE_CAP_BUTT)
)
return rendermodel
end
snapshot = render([env])
#md write("crosswalk.svg", snapshot) # hide
#md # 
# ### Navigate the crosswalk example
# Cars will be navigating in the roadway just as before.
# For the pedestrian we can define a new vehicle definition where we specify
# the size of the bounding box represented by the pedestrian.
# field of the VehicleDef type
fieldnames(VehicleDef)
## Agent.Class is from AutomotiveSimulator
const PEDESTRIAN_DEF = VehicleDef(AgentClass.PEDESTRIAN, 1.0, 1.0)
#md nothing # hide
# Car definition
car_initial_state = VehicleState(VecSE2(5.0, 0., 0.), roadway.segments[1].lanes[1],roadway, 8.0)
car = Entity(car_initial_state, VehicleDef(), :car)
# Pedestrian definition using our new Vehicle definition
ped_initial_state = VehicleState(VecSE2(+24.5,-7.0,π/2), env.crosswalk, roadway, 0.5)
ped = Entity(ped_initial_state, PEDESTRIAN_DEF, :pedestrian)
scene = Scene([car, ped])
# visualize the initial state
snapshot = render([env, scene])
#md write("crosswalk_initial.svg", snapshot) # hide
#md # 
# ### Simulate the scenario
# As before, associate a driver model to each vehicle in the scene.
# We will use the model defined in the intersection example for both agents.
mutable struct LinearDriver <: DriverModel{LaneFollowingAccel}
a::LaneFollowingAccel
p::Float64 # confidence on the pedestrian intention
k::Float64 # gain
end
function AutomotiveSimulator.observe!(model::LinearDriver, scene::Scene, roadway::Roadway, egoid)
model.a = LaneFollowingAccel(model.k*model.p)
## change the confidence based on some policy
## you can get the position of the pedestrian from the scene
model.p = 100.0
end
Base.rand(rng::AbstractRNG, model::LinearDriver) = model.a
timestep = 0.1
nticks = 50
## define a model for each entities present in the scene
models = Dict{Symbol, DriverModel}()
## Constant speed model
models[:car] = LinearDriver(LaneFollowingAccel(0.0), 20.0, -0.02)
models[:pedestrian] = IntelligentDriverModel(v_des=1.0)
## execute the simulation
scenes = simulate(scene, roadway, models, nticks, timestep)
#md nothing # hide
# ## Generate a video with Reel.jl
using Reel
function animate_record(scenes::Vector{Scene{E}},dt::Float64, env::CrosswalkEnv) where {E<:Entity}
duration = length(scenes)*dt::Float64
fps = Int(1/dt)
function render_rec(t, dt)
frame_index = Int(floor(t/dt)) + 1
return render([env, scenes[frame_index]])
end
return duration, fps, render_rec
end
duration, fps, render_hist = animate_record(scenes, timestep, env)
film = roll(render_hist, fps=fps, duration=duration)
#md write("crosswalk_animated.gif", film) # hide
#md nothing # hide
#md # 
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 3146 | # # Feature Extraction
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/feature_extraction.ipynb)
# In this example we demonstrate how to extract feature from trajectories using AutomotiveSimulator
# **Load a Dataset**
# First let's create a synthetic dataset.
using AutomotiveSimulator
using AutomotiveVisualization
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
using Random
roadway = roadway = gen_straight_roadway(3, 1000.0)
veh_state = VehicleState(Frenet(roadway[LaneTag(1,2)], 0.0), roadway, 10.)
veh1 = Entity(veh_state, VehicleDef(), "bob")
veh_state = VehicleState(Frenet(roadway[LaneTag(1,2)], 20.0), roadway, 2.)
veh2 = Entity(veh_state, VehicleDef(), "alice")
dt = 0.5
n_steps = 10
models = Dict{String, DriverModel}()
models["bob"] = Tim2DDriver(mlane=MOBIL())
set_desired_speed!(models["bob"], 10.0)
models["alice"] = Tim2DDriver(mlane=MOBIL())
set_desired_speed!(models["alice"], 2.0)
scene = Scene([veh1, veh2])
scenes = simulate(scene, roadway, models, n_steps, dt)
camera = SceneFollowCamera()
update_camera!(camera, scene)
snapshot = render([roadway, scene], camera=camera)
#md write("feature_initial.svg", snapshot) # hide
#md # 
#md nothing # hide
# One can also load the data from the Stadium tutorial
#md load data from stadium tutorial
#md scenes = open("2Dstadium_listrec.txt", "r") do io
#md read(io, Vector{EntityScene{VehicleState, VehicleDef, String}})
#md end
# **Extract features from a recorded trajectory**
# Recorded trajectories are expected to be vectors of `Scene`s where each element correspond to one time step.
# To extract features, one can use the `extract_features` function which takes as input
# a list of feature we want to extract and the list of vehicle ids for which we want those features.
# For this example, let's first query two features, the longitudinal and lateral position of Bob, and whether or not Bob is colliding:
dfs = extract_features((posfs, posft, iscolliding), roadway, scenes, ["bob"])
dfs["bob"]
# To query features for all traffic participants we can just add their ID to the list:
dfs = extract_features((posfs, posft, iscolliding), roadway, scenes, ["bob", "alice"])
dfs["alice"]
# The output is a dictionary mapping ID to dataframes. To learn more about DataFrames visit [DataFrames.jl](https://github.com/JuliaData/DataFrames.jl).
# For the list of all possible features available see the documentation.
# Features are generally just function. AutomotiveSimulator provides some convenience
# to automatically generate feature function like `distance_to_$x`
# The `distance_to` function takes as input a vehicle ID and returns a function to extract
# the distance between the queried vehicle and the vehicle given to `distance_to`
distance_to("alice")
# we can use this newly generated funciton in the feature extraction pipeline
dfs = extract_features((distance_to_alice, posfs), roadway, scenes, ["bob"])
dfs["bob"].distance_to_alice[1] # distance between Bob and Alice in the first scene.
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 7817 | # # Intersection
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/intersection.ipynb)
# In this example we demonstrate how to define a T-shape intersection with `AutomotiveSimulator`.
# You will also learn how to define your own custom action type and driver model type.
# ## Generate a T-Shape intersection
# In order to generate the road network, we first initialize a Roadway object.
using AutomotiveSimulator
using AutomotiveVisualization
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
using Random
roadway = Roadway()
#md nothing # hide
# Define coordinates of the entry and exit points to the intersection
r = 5.0 # turn radius
w = DEFAULT_LANE_WIDTH
A = VecSE2(0.0,w,-π)
B = VecSE2(0.0,0.0,0.0)
C = VecSE2(r,-r,-π/2)
D = VecSE2(r+w,-r,π/2)
E = VecSE2(2r+w,0,0)
F = VecSE2(2r+w,w,-π)
#md nothing # hide
# The next step consists in appending all the lanes to the road network.
# We can define a helper function to add a new lane to the roadway.
function append_to_curve!(target::Curve, newstuff::Curve)
s_end = target[end].s
for c in newstuff
push!(target, CurvePt(c.pos, c.s+s_end, c.k, c.kd))
end
return target
end
#md nothing # hide
# Example of a lane that consists of 3 road segments, a straight curve
# (from the left to the center), a turning part (right turn) and a final
# straight curve.
# Append right turn coming from the left
curve = gen_straight_curve(convert(VecE2, B+VecE2(-100,0)), convert(VecE2, B), 2)
append_to_curve!(curve, gen_bezier_curve(B, C, 0.6r, 0.6r, 51)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, C), convert(VecE2, C+VecE2(0,-50.0)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
# visualize the current lane constellation
snapshot = render([roadway])
#md write("partial_intersection.svg", snapshot) # hide
#md # 
# Let's repeat the process and complete the T-shape intersection
# Append straight left
curve = gen_straight_curve(convert(VecE2, B+VecE2(-100,0)), convert(VecE2, B), 2)
append_to_curve!(curve, gen_straight_curve(convert(VecE2, B), convert(VecE2, E), 2)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, E), convert(VecE2, E+VecE2(50,0)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
# Append straight right
curve = gen_straight_curve(convert(VecE2, F+VecE2(50,0)), convert(VecE2, F), 2)
append_to_curve!(curve, gen_straight_curve(convert(VecE2, F), convert(VecE2, A), 2)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, A), convert(VecE2, A+VecE2(-100,0)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
# Append left turn coming from the right
curve = gen_straight_curve(convert(VecE2, F+VecE2(50,0)), convert(VecE2, F), 2)
append_to_curve!(curve, gen_bezier_curve(F, C, 0.9r, 0.9r, 51)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, C), convert(VecE2, C+VecE2(0,-50)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
# Append right turn coming from below
curve = gen_straight_curve(convert(VecE2, D+VecE2(0,-50)), convert(VecE2, D), 2)
append_to_curve!(curve, gen_bezier_curve(D, E, 0.6r, 0.6r, 51)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, E), convert(VecE2, E+VecE2(50,0)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
# Append left turn coming from below
curve = gen_straight_curve(convert(VecE2, D+VecE2(0,-50)), convert(VecE2, D), 2)
append_to_curve!(curve, gen_bezier_curve(D, A, 0.9r, 0.9r, 51)[2:end])
append_to_curve!(curve, gen_straight_curve(convert(VecE2, A), convert(VecE2, A+VecE2(-100,0)), 2))
lane = Lane(LaneTag(length(roadway.segments)+1,1), curve)
push!(roadway.segments, RoadSegment(lane.tag.segment, [lane]))
snapshot = render([roadway])
#md write("intersection.svg", snapshot) # hide
#md # 
# We can identify each lane thanks to the following user-defined functions.
# We define a `LaneOverlay` object that indicate the lane to highlight.
# One could implement any custom type to display other information on the lane.
# We then add a new method to the `add_renderable!` function that execute the specific
# action (coloring in blue). Look at `AutomotiveVisualization.jl` for more detail on the function
# `add_renderable!`.
# The following animation iterates over the individual lanes of the intersection
# layout and highlights them:
struct LaneOverlay
roadway::Roadway
lane::Lane
color::Colorant
end
function AutomotiveVisualization.add_renderable!(rendermodel::RenderModel, overlay::LaneOverlay)
add_renderable!(rendermodel, overlay.lane, overlay.roadway, color_asphalt=overlay.color)
end
using Reel
animation = roll(fps=1.0, duration=length(roadway.segments)) do t, dt
i = Int(floor(t/dt)) + 1
renderables = [
roadway,
LaneOverlay(roadway, roadway[LaneTag(i,1)], RGBA(0.0,0.0,1.0,0.5))
]
render(renderables)
end;
#md write("highlighted_lanes.gif", animation) # hide
#md nothing # hide
#md # 
# ## Navigation in the new road network
# Let's populate the intersection
vs0 = VehicleState(B + polar(50.0,-π), roadway, 8.0) # initial state of the vehicle
scene = Scene([Entity(vs0, VehicleDef(), 1)])
snapshot = render([roadway, scene])
#md write("intersection_populated.svg", snapshot) # hide
#md # 
# We will use lateral and longitudinal acceleration to control a car in the intersection. The first step is to define a corresponding action type that will contain the acceleration inputs.
struct LaneSpecificAccelLatLon
a_lat::Float64
a_lon::Float64
end
# Next, add a method to the propagate function to update the state using our new action type.
function AutomotiveSimulator.propagate(veh::Entity, action::LaneSpecificAccelLatLon, roadway::Roadway, Δt::Float64)
lane_tag_orig = veh.state.posF.roadind.tag
state = propagate(veh, LatLonAccel(action.a_lat, action.a_lon), roadway, Δt)
roadproj = proj(state.posG, roadway[lane_tag_orig], roadway, move_along_curves=false)
retval = VehicleState(Frenet(roadproj, roadway), roadway, state.v)
return retval
end
# **Driver Model:**
# We define a driver model,
# which can be seen as a distribution over actions. # TODO
# Here we will define the simplest model, which is to repeat the same action.
struct InterDriver <: DriverModel{LaneSpecificAccelLatLon}
a::LaneSpecificAccelLatLon
end
AutomotiveSimulator.observe!(model::InterDriver, scene::Scene, roadway::Roadway, egoid::Int64) = model
Base.rand(::AbstractRNG, model::InterDriver) = model.a
# **Simulate:**
# First associate a model to each driver in the scene using a dictionary.
# Here we only have one driver identified by its ID: 1.
# Then everything is ready to run the `simulate!` function.
using Reel
timestep = 0.1
nticks = 100
vs0 = VehicleState(B + polar(50.0,-π), roadway, 8.0)
scene = Scene([Entity(vs0, VehicleDef(), 1)])
models = Dict(1 => InterDriver(LaneSpecificAccelLatLon(0.0,0.0)))
scenes = simulate(scene, roadway, models, nticks, timestep)
animation = roll(fps=1.0/timestep, duration=nticks*timestep) do t, dt
i = Int(floor(t/dt)) + 1
renderables = [roadway, scenes[i]]
render(renderables)
end
#md write("animated_intersection.gif", animation) # hide
#md # 
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 6241 | # # Sidewalk
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/sidewalk.ipynb)
# In this notebook, we will be creating a sidewalk environment in which
# pedestrians can walk along the sidewalk and cross the street as cars pass.
using Parameters
using AutomotiveSimulator
using AutomotiveVisualization
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
using Cairo
# Define sidewalk IDs
const TOP = 1
const BOTTOM = 2
#md nothing # hide
# ### Creating the Environment
# Here, we create a new type of environment called SidewalkEnv. It consists of a roadway, crosswalk, and sidewalk. A sidewalk is a Vector of Lanes that run alongside the road.
@with_kw mutable struct SidewalkEnv
roadway::Roadway
crosswalk::Lane
sidewalk::Vector{Lane}
end;
#md nothing # hide
# #### Defining the Sidewalk
# We define the sidewalk's parameters.
## Geometry parameters
roadway_length = 100.
crosswalk_length = 15.
crosswalk_width = 6.0
crosswalk_pos = roadway_length/2
sidewalk_width = 3.0
sidewalk_pos = crosswalk_length/2 - sidewalk_width / 2
#md nothing # hide
# Now we create the sidewalk environment.
# Our environment will consist of:
# * 1-way road with 2 lanes
# * Unsignalized zebra crosswalk perpendicular to the road
# * Sidewalks above and below the road
# Generate straight roadway of length roadway_length with 2 lanes.
# Returns a Roadway type (Array of segments).
# There is already a method to generate a simple straight roadway, which we use here.
roadway = gen_straight_roadway(2, roadway_length)
# Generate the crosswalk.
# Our crosswalk does not have a predefined method for generation, so we define it with a LaneTag and a curve.
n_samples = 2 # for curve generation
crosswalk = Lane(LaneTag(2,1), gen_straight_curve(VecE2(crosswalk_pos, -crosswalk_length/2),
VecE2(crosswalk_pos, crosswalk_length/2),
n_samples), width = crosswalk_width)
cw_segment = RoadSegment(2, [crosswalk])
push!(roadway.segments, cw_segment) # Append the crosswalk to the roadway
## Generate the sidewalk
top_sidewalk = Lane(LaneTag(3, TOP), gen_straight_curve(VecE2(0., sidewalk_pos),
VecE2(roadway_length, sidewalk_pos),
n_samples), width = sidewalk_width)
bottom_sidewalk = Lane(LaneTag(3, BOTTOM), gen_straight_curve(VecE2(0., -(sidewalk_pos - sidewalk_width)),
VecE2(roadway_length, -(sidewalk_pos - sidewalk_width)),
n_samples), width = sidewalk_width)
# Note: we subtract the sidewalk_width from the sidewalk position so that the edge is flush with the road.
sw_segment = RoadSegment(3, [top_sidewalk, bottom_sidewalk])
push!(roadway.segments, sw_segment)
# Initialize crosswalk environment
env = SidewalkEnv(roadway, crosswalk, [top_sidewalk, bottom_sidewalk]);
#md nothing # hide
# Since there is no defined `add_renderable!` method for the crosswalk and the sidewalk, we must define it ourselves.
function AutomotiveVisualization.add_renderable!(rendermodel::RenderModel, env::SidewalkEnv)
## Render sidewalk
for sw in env.sidewalk
curve = sw.curve
n = length(curve)
pts = Array{Float64}(undef, 2, n)
for (i,pt) in enumerate(curve)
pts[1,i] = pt.pos.x
pts[2,i] = pt.pos.y
end
add_instruction!(rendermodel, render_line, (pts, colorant"grey", sw.width, Cairo.CAIRO_LINE_CAP_BUTT))
end
## Render roadway
roadway = gen_straight_roadway(2, roadway_length)
add_renderable!(rendermodel, roadway)
## Render crosswalk
curve = env.crosswalk.curve
n = length(curve)
pts = Array{Float64}(undef, 2, n)
for (i,pt) in enumerate(curve)
pts[1,i] = pt.pos.x
pts[2,i] = pt.pos.y
end
## We can add render instructions from AutomotiveVisualization.
## Here we want the crosswalk to appear as a white-striped zebra crossing rather than a road.
add_instruction!(rendermodel, render_dashed_line, (pts, colorant"white", env.crosswalk.width, 1.0, 1.0, 0.0, Cairo.CAIRO_LINE_CAP_BUTT))
return rendermodel
end
snapshot = render([env])
#md write("sidewalk.svg", snapshot) # hide
#md # 
# Now we can define our pedestrian.
# We define its class and the dimensions of its bounding box.
const PEDESTRIAN_DEF = VehicleDef(AgentClass.PEDESTRIAN, 1.0, 1.0)
#md nothing # hide
# We assign models to each agent in the scene.
timestep = 0.1
# Crossing pedestrian definition
ped_init_state = VehicleState(VecSE2(49.0,-3.0,0.), env.sidewalk[BOTTOM], roadway, 1.3)
ped = Entity(ped_init_state, PEDESTRIAN_DEF, :pedestrian)
# Car definition
car_initial_state = VehicleState(VecSE2(0.0, 0., 0.), roadway.segments[1].lanes[1],roadway, 8.0)
car = Entity(car_initial_state, VehicleDef(), :car)
scene = Scene([ped, car])
# Define a model for each entity present in the scene
models = Dict{Symbol, DriverModel}(
:pedestrian => SidewalkPedestrianModel(
timestep=timestep, crosswalk=env.crosswalk,
sw_origin = env.sidewalk[BOTTOM], sw_dest = env.sidewalk[TOP]
),
:car => LatLonSeparableDriver( # produces LatLonAccels
ProportionalLaneTracker(), # lateral model
IntelligentDriverModel(), # longitudinal model
)
)
#md nothing # hide
### Simulate
# Finally, we simulate and visualize the scene.
using Reel
nticks = 300
scenes = simulate(scene, roadway, models, nticks, timestep)
animation = roll(fps=1.0/timestep, duration=nticks*timestep) do t, dt
i = Int(floor(t/dt)) + 1
render([env, scenes[i]])
end;
#md write("sidewalk_animation.gif", animation); # hide
#md # 
# We can use a slider to scroll through each scene in the simulation. This usually takes less time than rendering a video.
#md using Interact
#md using Reel
#md using Blink
#md
#md w = Window()
#md viz = @manipulate for i in 1 : length(scenes)
#md render([env, scenes[i]])
#md end
#md body!(w, viz)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 4079 | # # Driving in a Stadium
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/stadium.ipynb)
# This example demonstrates a 2D driving simulation where cars drive around a three-lane stadium.
# The entities are defined by the types:
#
# - `S` - `VehicleState`, containing the vehicle position (both globally and relative to the lane) and speed
# - `D` - `VehicleDef`, containing length, width, and class
# - `I` - `Symbol`, a unique label for each vehicle
#
# The environment is represented by a `Roadway` object which
# allows to define roads consisting of multiple lanes based on the RNDF format.
# We load relevant modules and generate a 3-lane stadium roadway:
using AutomotiveSimulator
using AutomotiveVisualization
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
using Distributions
roadway = gen_stadium_roadway(3)
snapshot = render([roadway])
#md write("stadium.svg", snapshot) # hide
#md # 
# As a next step, let's populate a scene with vehicles
w = DEFAULT_LANE_WIDTH
scene = Scene([
Entity(VehicleState(VecSE2(10.0, -w, 0.0), roadway, 29.0), VehicleDef(), :alice),
Entity(VehicleState(VecSE2(40.0, 0.0, 0.0), roadway, 22.0), VehicleDef(), :bob),
Entity(VehicleState(VecSE2(30.0, -2w, 0.0), roadway, 27.0), VehicleDef(), :charlie),
])
car_colors = get_pastel_car_colors(scene)
renderables = [
roadway,
(FancyCar(car=veh, color=car_colors[veh.id]) for veh in scene)...
]
snapshot = render(renderables)
#md write("stadium_with_cars.svg", snapshot) # hide
#md # 
# We can assign driver models to each agent and simulate the scenario.
timestep = 0.1
nticks = 300
models = Dict{Symbol, DriverModel}(
:alice => LatLonSeparableDriver( # produces LatLonAccels
ProportionalLaneTracker(), # lateral model
IntelligentDriverModel(), # longitudinal model
),
:bob => Tim2DDriver(
mlane = MOBIL(),
),
:charlie => StaticDriver{AccelTurnrate, MvNormal}(
MvNormal([0.0,0.0], [1.0,0.1])
)
)
set_desired_speed!(models[:alice], 12.0)
set_desired_speed!(models[:bob], 10.0)
set_desired_speed!(models[:charlie], 8.0)
scenes = simulate(scene, roadway, models, nticks, timestep)
# md nothing # hide
# An animation of the simulation can be rendered using the `Reel` package
using Reel
using Printf
camera = TargetFollowCamera(:alice; zoom=10.)
animation = roll(fps=1.0/timestep, duration=nticks*timestep) do t, dt
i = Int(floor(t/dt)) + 1
update_camera!(camera, scenes[i])
renderables = [
roadway,
(FancyCar(car=veh, color=car_colors[veh.id]) for veh in scenes[i])...,
IDOverlay(x_off=-2, y_off=1, scene=scenes[i]),
TextOverlay(text=[@sprintf("time: %.1fs", t)], pos=VecE2(40,40), font_size=24)
]
render(renderables, camera=camera)
end
#md write("animated_stadium.gif", animation) # hide
#md # 
# Alternatively, one can also use the `Interact` framework to inspect the simulation record interactively.
#md using Interact
#md using Reel
#md using Blink
#md
#md w = Window()
#md viz = @manipulate for step in 1 : length(scenes)
#md render([roadway, scenes[step]])
#md end
#md body!(w, viz)
# The simulation results can be saved to a text file. Only entities using `VehicleState` and `VehicleDef` are supported out of the box.
# If you wish to do IO operation with different states and definition types you have to implement `Base.write` and `Base.read` for those new types.
open("2Dstadium_listrec.txt", "w") do io
write(io, scenes)
end
# The trajectory data file can be loaded in a similar way. You need to specify the output type.
loaded_scenes = open("2Dstadium_listrec.txt", "r") do io
read(io, Vector{EntityScene{VehicleState, VehicleDef, String}})
end
render([roadway, loaded_scenes[1]])
#md write("stadium_with_cars_loaded.svg", snapshot) # hide
#md # 
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 3724 | # # Driving on a Straight Roadway
#md # [](@__NBVIEWER_ROOT_URL__/notebooks/straight_roadway.ipynb)
# This example demonstrates a simple, one-dimensional driving simulation in which
# cars drive along a straight roadway.
# The vehicles are represented using the `Entity` type with the followin attributes:
#
# - `state` - `VehicleState`, containing the vehicle position and speed
# - `def` - `VehicleDef`, containing length, width, and class
# - `id` - `Int`, a unique label for each vehicle`
#
# A driving situation with different vehicles at a given time is referred to as a scene. It is represented by the `Scene` object.
# A `Scene` can be thought of as a vector of vehicles. However, in addition to simple vectors it allows to query vehicles by ID using the `get_by_id` function.
# We use a straight roadway with 1 lane as the environment.
using AutomotiveSimulator
using AutomotiveVisualization # for rendering
AutomotiveVisualization.colortheme["background"] = colorant"white"; # hide
roadway = gen_straight_roadway(1, 2000.0) # 200m long straight roadway with 1 lane
scene = Scene([
Entity(VehicleState(VecSE2(10.0,0.0,0.0), roadway, 8.0), VehicleDef(), 1),
Entity(VehicleState(VecSE2(50.0,0.0,0.0), roadway, 12.5), VehicleDef(), 2),
Entity(VehicleState(VecSE2(150.0,0.0,0.0), roadway, 6.0), VehicleDef(), 3),
])
veh_1 = get_by_id(scene, 1) # note that the order of the vehicles in the scene does not necessarily match the id
camera = StaticCamera(position=VecE2(100.0,0.0), zoom=4.75, canvas_height=100)
snapshot = render([roadway, scene], camera=camera)
#md write("straight_roadway.svg", snapshot) # hide
#md # 
# In this call to the `render` function, we use the default rendering behavior for
# entities. More advanced examples will show how the rendering of entities can be customized.
# We can add an overlay that displays the car id:
idoverlay = IDOverlay(scene=scene, color=colorant"black", font_size=20, y_off=1.)
snapshot = render([roadway, scene, idoverlay], camera=camera)
#md write("straight_roadway_with_id.svg", snapshot) # hide
#md # 
# To run a simulation we need driving models that produce actions.
# For this we will use `LaneFollowingDriver`s that produce `LaneFollowingAccel`s.
# For this demo, we will give each car a different model.
models = Dict{Int, LaneFollowingDriver}(
1 => StaticLaneFollowingDriver(0.0), # always produce zero acceleration
2 => IntelligentDriverModel(v_des=12.0), # default IDM with a desired speed of 12 m/s
3 => PrincetonDriver(v_des = 10.0), # default Princeton driver with a desired speed of 10m/s
)
nticks = 100
timestep = 0.1
scenes = simulate(scene, roadway, models, nticks, timestep)
#md nothing # hide
# We can visualize the simulation as a sequence of images, for example using the
# `Reel` package
using Reel
animation = roll(fps=1.0/timestep, duration=nticks*timestep) do t, dt
i = Int(floor(t/dt)) + 1
idoverlay.scene = scenes[i]
renderables = [roadway, scenes[i], idoverlay]
render(renderables, camera=camera)
end
#md write("straight_roadway_animated.gif", animation) # hide
#md nothing # hide
#md # 
# In order to inspect the simulation interactively, we can use the `Interact` package
#md using Interact
#md using Blink
#md using ElectronDisplay
#md
#md w = Window()
#md viz = @manipulate for step in 1 : length(scenes)
#md renderables = [roadway, scenes[step], IDOverlay(scene=scenes[step], roadway=roadway)]
#md render(renderables, camera=camera)
#md end
#md body!(w, viz)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 5973 | module AutomotiveSimulator
using Printf
using LinearAlgebra
using Parameters
using StaticArrays
using Distributions
using Reexport
using Random
using DataFrames
using Tricks: static_hasmethod
include(joinpath(@__DIR__, "Vec", "Vec.jl"))
@reexport using .Vec
# Roadways
export CurvePt,
Curve,
CurveIndex,
CurveProjection,
CURVEINDEX_START,
get_lerp_time,
index_closest_to_point,
get_curve_index,
curveindex_end
include("roadways/curves.jl")
export
LaneTag,
LaneBoundary,
Lane,
LaneConnection,
SpeedLimit,
RoadSegment,
Roadway,
RoadProjection,
RoadIndex,
NULL_BOUNDARY,
NULL_LANETAG,
NULL_ROADINDEX,
DEFAULT_SPEED_LIMIT,
DEFAULT_LANE_WIDTH,
is_in_exits,
is_in_entrances,
is_at_curve_end,
is_between_segments_lo,
is_between_segments_hi,
is_between_segments,
has_segment,
has_lanetag,
has_next,
has_prev,
next_lane,
prev_lane,
next_lane_point,
prev_lane_point,
connect!,
move_along,
n_lanes_left,
leftlane,
n_lanes_right,
rightlane,
lanes,
lanetags,
read
include("roadways/roadways.jl")
export
Frenet,
NULL_FRENET
include("roadways/frenet.jl")
export
gen_straight_curve,
gen_straight_segment,
gen_straight_roadway,
gen_stadium_roadway,
gen_bezier_curve
include("roadways/roadway_generation.jl")
## agent definitions
export
AbstractAgentDefinition,
AgentClass,
VehicleDef,
BicycleModel,
length,
width,
class
include("agent-definitions/agent_definitions.jl")
export
Entity,
Scene,
EntityScene,
capacity,
id2index,
get_by_id,
get_first_available_id,
posf,
posg,
vel,
velf,
velg,
VehicleState,
get_center,
get_footpoint,
get_front,
get_rear,
get_lane
include("states/entities.jl")
include("states/scenes.jl")
include("states/interface.jl")
include("states/vehicle_state.jl")
## Collision Checkers
export
ConvexPolygon,
CPAMemory,
CollisionCheckResult,
to_oriented_bounding_box!,
get_oriented_bounding_box,
is_colliding,
is_potentially_colliding,
get_collision_time,
get_first_collision,
is_collision_free,
get_distance,
get_edge,
collision_checker,
polygon
include("collision-checkers/minkowski.jl")
include("collision-checkers/parallel_axis.jl")
## Feature Extraction
export AbstractFeature,
EntityFeature,
SceneFeature,
TemporalFeature,
extract_features,
extract_feature,
featuretype,
# provided feature functions
posgx,
posgy,
posgθ,
posfs,
posft,
posfϕ,
vel,
velfs,
velft,
velgx,
velgy,
time_to_crossing_right,
time_to_crossing_left,
estimated_time_to_lane_crossing,
iswaiting,
iscolliding,
distance_to,
acc,
accfs,
accft,
jerk,
jerkft,
turn_rate_g,
turn_rate_f,
isbraking,
isaccelerating
include("feature-extraction/features.jl")
export lane_width,
markerdist_left,
markerdist_right,
road_edge_dist_left,
road_edge_dist_right,
lane_offset_left,
lane_offset_right,
has_lane_left,
has_lane_right,
lane_curvature
include("feature-extraction/lane_features.jl")
export
VehicleTargetPoint,
VehicleTargetPointFront,
VehicleTargetPointCenter,
VehicleTargetPointRear,
targetpoint_delta,
find_neighbor,
NeighborLongitudinalResult,
FrenetRelativePosition,
get_frenet_relative_position,
dist_to_front_neighbor,
front_neighbor_speed,
time_to_collision
include("feature-extraction/neighbors_features.jl")
export
LidarSensor,
nbeams,
observe!,
RoadlineLidarSensor,
nlanes,
LanePortion,
RoadwayLidarCulling,
ensure_leaf_in_rlc!,
get_lane_portions
include("feature-extraction/lidar_sensor.jl")
## Actions
export
propagate,
EntityAction,
LaneFollowingAccel,
AccelTurnrate,
AccelDesang,
LatLonAccel,
AccelSteeringAngle,
PedestrianLatLonAccel
include("actions/interface.jl")
include("actions/lane_following_accel.jl")
include("actions/accel_turnrate.jl")
include("actions/accel_desang.jl")
include("actions/lat_lon_accel.jl")
include("actions/accel_steering.jl")
include("actions/pedestrian_lat_lon_accel.jl")
export
DriverModel,
StaticDriver,
action_type,
set_desired_speed!,
observe!,
reset_hidden_state!,
reset_hidden_states!
include("behaviors/interface.jl")
export
LaneFollowingDriver,
StaticLaneFollowingDriver,
PrincetonDriver,
IntelligentDriverModel,
ProportionalSpeedTracker,
track_longitudinal!,
LateralDriverModel,
ProportionalLaneTracker,
LatLonSeparableDriver,
Tim2DDriver,
SidewalkPedestrianModel,
LaneChangeChoice,
LaneChangeModel,
get_lane_offset,
DIR_RIGHT,
DIR_MIDDLE,
DIR_LEFT,
MOBIL,
TimLaneChanger
include("behaviors/lane_following_drivers.jl")
include("behaviors/princeton_driver.jl")
include("behaviors/speed_trackers.jl")
include("behaviors/intelligent_driver_model.jl")
include("behaviors/lateral_driver_models.jl")
include("behaviors/lane_change_models.jl")
include("behaviors/MOBIL.jl")
include("behaviors/tim_lane_changer.jl")
include("behaviors/lat_lon_separable_driver.jl")
include("behaviors/tim_2d_driver.jl")
include("behaviors/sidewalk_pedestrian_model.jl")
export
simulate,
simulate!,
run_callback,
CollisionCallback,
observe_from_history!,
simulate_from_history!,
simulate_from_history,
maximum_entities
include("simulation/simulation.jl")
include("simulation/callbacks.jl")
include("simulation/simulation_from_history.jl")
end # AutomotiveSimulator
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2333 | module Vec
using StaticArrays
using LinearAlgebra
using Printf
import LinearAlgebra: ⋅, ×
export
AbstractVec,
VecE, # an abstract euclidean-group vector
VecSE, # an abstract special euclidean-group element
VecE2, # two-element Float64 vector, {x, y}
VecE3, # three-element Float64 vector, {x, y, z}
VecSE2, # point in special euclidean group of order 2, {x, y, θ}
polar, # construct a polar vector with (r,θ)
proj, # vector projection
# proj(a::vec, b::vec, ::Type{Float64}) will do scalar projection of a onto b
# proj(a::vec, b::vec, ::Type{Vec}) will do vector projection of a onto b
lerp, # linear interpolation between two vec's
invlerp,
lerp_angle,
normsquared, # see docstrings below
scale_euclidean,
clamp_euclidean,
normalize_euclidian,
dist, # scalar distance between two vec's
dist2, # squared scalar distance between two vec's
rot, # rotate the vector, always using the Right Hand Rule
rot_normalized, # like rot, but assumes axis is normalized
deltaangle, # signed delta angle
angledist, # distance between two angles
inertial2body,
body2inertial,
orientation,
are_collinear,
get_intersection,
Circ,
AABB,
OBB
abstract type AbstractVec{N, R} <: FieldVector{N, R} end
abstract type VecE{N, R} <: AbstractVec{N, R} end
abstract type VecSE{N, R} <: AbstractVec{N, R} end
lerp(a::Real, b::Real, t::Real) = a + (b-a)*t
invlerp(a::Real, b::Real, c::Real) = (c - a)/(b-a)
"The L2 norm squared."
normsquared(a::AbstractVec) = sum(x^2 for x in a)
"Scale the euclidean part of the vector by a factor b while leaving the orientation part unchanged."
scale_euclidean(a::VecE, b::Real) = b.*a
"Clamp each element of the euclidean part of the vector while leaving the orientation part unchanged."
clamp_euclidean(a::VecE, lo::Real, hi::Real) = clamp.(a, lo, hi)
"Normalize the euclidean part of the vector while leaving the orientation part unchanged."
normalize_euclidian(a::VecE, p::Real=2) = normalize(a, p)
include("common.jl")
include("vecE2.jl")
include("vecE3.jl")
include("vecSE2.jl")
include("geom/geom.jl")
include("coordinate_transforms.jl")
include("quat.jl")
Base.vec(v::Union{AbstractVec, Quat, RPY}) = convert(Vector{Float64}, v)
end # module
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 600 | const DUMMY_PRECISION = 1e-12
Base.isfinite(a::AbstractVec) = all(isfinite, a)
Base.isinf(a::AbstractVec) = any(isinf, a)
Base.isnan(a::AbstractVec) = any(isnan, a)
function StaticArrays.similar_type(::Type{V}, ::Type{F}, size::Size{N}) where {V<:AbstractVec, F<:AbstractFloat, N}
if size == Size(V) && eltype(V) == F
return V
else # convert back to SArray
return SArray{Tuple{N...},F,length(size),prod(size)}
end
end
Base.abs(a::AbstractVec) = error("abs(v::AbstractVec) has been removed. Use norm(v) to get the norm; abs.(v) to get the element-wise absolute value.")
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 12614 | export
AbstractCoordinate,
LatLonAlt,
ECEF,
UTM,
ENU,
NED,
WGS_84,
INTERNATIONAL,
check_is_in_radians,
ensure_lon_between_pies,
deg_min_sec_to_degrees,
degrees_to_deg_min_sec,
get_earth_radius,
GeodeticDatum,
eccentricity
###################
"""
Defines a reference ellipsoid used to map a position on the Earth
relative to said ellipsoid
"""
struct GeodeticDatum
a::Float64 # equitorial radius
b::Float64 # polar radius
end
const WGS_84 = GeodeticDatum(6378137.0, 6356752.314245)
const INTERNATIONAL = GeodeticDatum(6378388.0, 6356911.94613)
eccentricity_sq(datum::GeodeticDatum) = 1.0 - datum.b^2 / datum.a^2
eccentricity(datum::GeodeticDatum) = sqrt(eccentricity_sq(datum))
function radius_of_curvature_in_the_meridian(ϕ::Float64, datum::GeodeticDatum)
# ϕ = meridian angle (latitude)
a = datum.a
e = eccentricity(datum)
e² = e*e
sin²ϕ = sin(ϕ)^2
ρ = a*(1-e²) / ((1-e²*sin²ϕ)^1.5)
ρ
end
function radius_of_curvature_in_the_prime_vertical(ϕ::Float64, datum::GeodeticDatum=WGS_84)
# ϕ = meridian angle (latitude)
a = datum.a
e = eccentricity(datum)
e² = e*e
sin²ϕ = sin(ϕ)^2
ν = a / sqrt(1 - e²*sin²ϕ)
ν
end
############################################################################
#
#
#
#
############################################################################
abstract type AbstractCoordinate end
"""
The latitude, longitude, altitude coordinate system
"""
struct LatLonAlt <: AbstractCoordinate
lat::Float64 # [rad] ϕ, angle between the equatorial plane and the straight line that passes through the point and through the center of the Earth
lon::Float64 # [rad] λ, angle (typically east) from a reference meridian to another meridian that passes through that point
alt::Float64 # [m] above reference ellipsoid
end
Base.print(io::IO, lla::LatLonAlt) = @printf(io, "%6.2f %7.2f %10.2f", rad2deg(lla.lat), rad2deg(lla.lon), lla.alt)
function check_is_in_radians(lla::LatLonAlt)
if abs(lla.lat) > π/2 || abs(lla.lon) > π
warn("lat and lon may not be in radians!")
end
nothing
end
function ensure_lon_between_pies(lla::LatLonAlt)
# lan ∈ [-π/2, π/2]
# lon ∈ [-π, π]
lon = lla.lon
while lon ≤ -π
lon += 2π
end
while lon ≥ π
lon -= 2π
end
LatLonAlt(lla.lat, lon, lla.alt)
end
deg_min_sec_to_degrees(deg::Real, min::Real, sec::Real) = convert(Float64, deg + min/60.0 + sec/3600.0)
function degrees_to_deg_min_sec(degrees::Real)
degrees = convert(Float64, degrees)
deg, remainder = divrem(degrees, 1.0)
min, remainder = divrem(remainder*60.0, 1.0)
sec = remainder * 60.0
(convert(Int, deg), convert(Int, min), sec)
end
function get_earth_radius(lat::Float64, datum::GeodeticDatum=WGS_84)
a, b = datum.a, datum.b
c, s = cos(lat), sin(lat)
return sqrt(((a^2*c)^2 + (b^2*s)^2)/((a*c)^2 + (b*s)^2))
end
Base.convert(::Type{VecE3}, lla::LatLonAlt) = VecE3(lla.lat, lla.lon, lla.alt)
Base.convert(::Type{LatLonAlt}, p::VecE3) = LatLonAlt(p.x, p.y, p.z)
"""
The Earth-centered, Earth-fixed coordinate system
"""
struct ECEF <: AbstractCoordinate
x::Float64 # [m]
y::Float64 # [m]
z::Float64 # [m]
end
Base.convert(::Type{VecE3}, p::ECEF) = VecE3(p.x, p.y, p.z)
Base.convert(::Type{ECEF}, p::VecE3) = ECEF(p.x, p.y, p.z)
const UTM_LATITUDE_LIMIT_NORTH = deg2rad(84)
const UTM_LATITUDE_LIMIT_SOUTH = deg2rad(-80)
"""
Universal Transverse Mercator coordinate system
"""
struct UTM <: AbstractCoordinate
e::Float64 # [m]
n::Float64 # [m]
u::Float64 # [m] above reference ellipsoid
zone::Int # UTM zone (∈ 1:60)
end
Base.convert(::Type{VecE3}, p::UTM) = VecE3(p.e, p.n, p.u)
function Base.:+(a::UTM, b::UTM)
@assert(a.zone == b.zone)
UTM(a.e+b.e, a.n+b.n, a.u+b.u, a.zone)
end
function Base.:-(a::UTM, b::UTM)
@assert(a.zone == b.zone)
UTM(a.e-b.e, a.n-b.n, a.u-b.u, a.zone)
end
"""
East North Up coordinate system
relative to some point on the surface of the earth
"""
struct ENU <: AbstractCoordinate
e::Float64 # [m]
n::Float64 # [m]
u::Float64 # [m] above reference location
end
Base.convert(::Type{VecE3}, p::ENU) = VecE3(p.e, p.n, p.u)
Base.convert(::Type{ENU}, p::VecE3) = ENU(p.x, p.y, p.z)
Base.:+(a::ENU, b::ENU) = ENU(a.e+b.e, a.n+b.n, a.u+b.u)
Base.:-(a::ENU, b::ENU) = ENU(a.e-b.e, a.n-b.n, a.u-b.u)
"""
North East Down coordinate system
"""
struct NED <: AbstractCoordinate
n::Float64 # [m]
e::Float64 # [m]
d::Float64 # [m] below reference location
end
Base.convert(::Type{VecE3}, p::NED) = VecE3(p.n, p.e, p.d)
Base.:+(a::NED, b::NED) = NED(a.n+b.n, a.e+b.e, a.d+b.d)
Base.:-(a::NED, b::NED) = NED(a.n-b.n, a.e-b.e, a.d-b.d)
###################
function Base.convert(::Type{ECEF}, lla::LatLonAlt, datum::GeodeticDatum=WGS_84)
ϕ = lla.lat
λ = lla.lon
h = lla.alt
e² = eccentricity_sq(datum)
sϕ = sin(ϕ)
cϕ = cos(ϕ)
sλ = sin(λ)
cλ = cos(λ)
Rₙ = radius_of_curvature_in_the_prime_vertical(ϕ, datum)
x = (Rₙ + h)*cϕ*cλ
y = (Rₙ + h)*cϕ*sλ
z = (Rₙ*(1.0-e²)+ h)*sϕ
ECEF(x,y,z)
end
function Base.convert(::Type{LatLonAlt}, ecef::ECEF, datum::GeodeticDatum=WGS_84)
# https://microem.ru/files/2012/08/GPS.G1-X-00006.pdf
#=
Convert Earth-Centered-Earth-Fixed (ECEF) to lat, Lon, Altitude
Input is x, y, z in meters
Returns lat and lon in radians, and altitude in meters
correction for altitude near poles left out.
=#
a = datum.a
b = datum.b
e² = eccentricity_sq(datum)
x = ecef.x
y = ecef.y
z = ecef.z
λ = atan(y, x)
p = sqrt(x*x + y*y)
θ = atan(z*a, (p*b))
h = 0.0
ϕ = atan(z,p*(1.0-e²))
# the equation diverges at the poles
if isapprox(abs(θ), π/2, atol=0.1)
Rₙ = radius_of_curvature_in_the_prime_vertical(ϕ, datum)
ϕₛ = sin(ϕ)
L = z + e²*Rₙ*ϕₛ
h = L / ϕₛ - Rₙ
else
Δh = Inf
while Δh > 1e-4
N = a / sqrt(1-e²*sin(ϕ)^2)
h₂ = p/cos(ϕ) - N
ϕ = atan(z, p*(1-e²*N/(N + h₂)))
Δh = abs(h - h₂)
h = h₂
end
end
ensure_lon_between_pies(LatLonAlt(ϕ, λ, h))
end
function Base.convert(::Type{UTM}, lla::LatLonAlt, datum::GeodeticDatum=WGS_84, zone::Integer=-1)
# see DMATM 8358.2 by the Army
# code verified using their examples
check_is_in_radians(lla)
lla = ensure_lon_between_pies(lla)
lat = lla.lat
lon = lla.lon
if lat > UTM_LATITUDE_LIMIT_NORTH || lat < UTM_LATITUDE_LIMIT_SOUTH
error("latitude $(rad2deg(lat)) is out of limits!")
end
FN = 0.0 # false northing, zero in the northern hemisphere
FE = 500000.0 # false easting
ko = 0.9996 # central scale factor
zone_centers = collect(0:59).*(6.0*π/180) .- (177.0*π/180) # longitudes of the zone centers
if zone == -1
zone = argmin(map(x->abs(lon - x), zone_centers)) # index of min zone center
end
long0 = zone_centers[zone]
s = sin(lat)
c = cos(lat)
t = tan(lat)
a = datum.a
b = datum.b
n = (a-b)/(a+b)
e₁ = sqrt((a^2-b^2)/a^2) # first eccentricity
ep = sqrt((a^2-b^2)/b^2) # second eccentricity
nu = a/(1-e₁^2*s^2)^0.5 # radius of curvature in the prime vertical
dh = lon - long0 # longitudinal distance from central meridian
A = a*(1 - n + 5.0/4*(n^2-n^3) + 81.0/64*(n^4-n^5))
B = 3.0/2*a*(n-n^2 + 7.0/8*(n^3-n^4) + 55.0/64*n^5)
C = 15.0/16*a*(n^2-n^3 + 3.0/4*(n^4-n^5))
D = 35.0/48*a*(n^3-n^4 + 11.0/16.0*n^5)
E = 315.0/512*a*(n^4-n^5)
S = A*lat - B*sin(2*lat) + C*sin(4*lat) - D*sin(6*lat) + E*sin(8*lat)
T1 = S*ko
T2 = nu*s*c*ko/2
T3 = nu*s*c^3*ko/24 * (5 - t^2 + 9*ep^2*c^2 + 4*ep^4*c^4)
T4 = nu*s*c^5*ko/720 * (61 - 58*t^2 + t^4 + 270*ep^2*c^2 - 330*(t*ep*c)^2
+ 445*ep^4*c^4 + 324*ep^6*c^6 - 680*t^2*ep^4*c^4
+ 88*ep^8*c^8 - 600*t^2*ep^6*c^6 - 192*t^2*ep^8*c^8)
T5 = nu*s*c^7*ko/40320 * (1385 - 3111*t^2 + 543*t^4 - t^6)
T6 = nu*c*ko
T7 = nu*c^3*ko/6 * (1 - t^2 + ep^2*c^2)
T8 = nu*c^5*ko/120 * (5 - 18*t^2 + t^4 + 14*ep^2*c^2 - 58*t^2*ep^2*c^2 + 13*ep^4*c^4
+ 4*ep^6*c^6 - 64*t^2*ep^4*c^4 - 24*t^2*ep^6*c^6)
T9 = nu*c^7*ko/5040 * (61 - 479*t^2 + 179*t^4 - t^6)
E = FE + (dh*T6 + dh^3*T7 + dh^5*T8 + dh^7*T9) # easting [m]
N = FN + (T1 + dh^2*T2 + dh^4*T3 + dh^6*T4 + dh^8*T5) # northing [m]
U = lla.alt
UTM(E, N, U, zone)
end
function Base.convert(::Type{LatLonAlt}, utm::UTM, datum::GeodeticDatum=WGS_84)
# see DMATM 8358.2 by the Army
# code verified using their examples
zone = utm.zone
@assert(1 ≤ zone ≤ 60)
λₒ = deg2rad(6.0*zone - 183) # longitude of the origin of the projection
kₒ = 0.9996 # central scale factor
a = datum.a
b = datum.b
e = eccentricity(datum)
e² = e*e
e⁴ = e²*e²
e⁶ = e⁴*e²
eₚ = sqrt((a^2-b^2)/b^2) # second eccentricity
eₚ² = eₚ*eₚ
eₚ⁴ = eₚ²*eₚ²
eₚ⁶ = eₚ⁴*eₚ²
eₚ⁸ = eₚ⁴*eₚ⁴
N = utm.n
arc = N / kₒ
μ = arc / (a * (1 - e²/4 - 3*e⁴/64 - 5*e⁶/256))
ei = (1 - sqrt(1 - e²)) / (1 + sqrt(1 - e²))
ca = 3*ei/2 - 27*ei^3 / 32.0
cb = 21 * ei^2 / 16 - 55*ei^4 / 32
cc = 151 * ei^3 / 96
cd = 1097 * ei^4 / 512
ϕₚ = μ + ca * sin(2μ) + cb*sin(4μ) + cc*sin(6μ) + cd*sin(8μ)
# const FN = 0.0 # false northing, zero in the northern hemisphere
E = utm.e
FE = 500000.0 # false easting
ΔE = E - FE
ΔE² = ΔE*ΔE
ΔE³ = ΔE²*ΔE
ΔE⁴ = ΔE²*ΔE²
ΔE⁵ = ΔE³*ΔE²
ΔE⁶ = ΔE³*ΔE³
ΔE⁷ = ΔE⁴*ΔE³
ΔE⁸ = ΔE⁴*ΔE⁴
tanϕₚ = tan(ϕₚ)
tan²ϕₚ = tanϕₚ*tanϕₚ
tan⁴ϕₚ = tan²ϕₚ*tan²ϕₚ
tan⁶ϕₚ = tan⁴ϕₚ*tan²ϕₚ
cosϕₚ = cos(ϕₚ)
cos²ϕₚ = cosϕₚ*cosϕₚ
cos⁴ϕₚ = cos²ϕₚ*cos²ϕₚ
cos⁶ϕₚ = cos⁴ϕₚ*cos²ϕₚ
cos⁸ϕₚ = cos⁴ϕₚ*cos⁴ϕₚ
kₒ² = kₒ*kₒ
kₒ³ = kₒ²*kₒ
kₒ⁴ = kₒ²*kₒ²
kₒ⁵ = kₒ³*kₒ²
kₒ⁶ = kₒ³*kₒ³
kₒ⁷ = kₒ⁴*kₒ³
kₒ⁸ = kₒ⁴*kₒ⁴
ν = radius_of_curvature_in_the_prime_vertical(ϕₚ, datum)
ν² = ν*ν
ν³ = ν²*ν
ν⁴ = ν²*ν²
ν⁵ = ν³*ν²
ν⁶ = ν³*ν³
ν⁷ = ν⁴*ν³
ν⁸ = ν⁴*ν⁴
ρ = radius_of_curvature_in_the_meridian(ϕₚ, datum)
T10 = tanϕₚ/(2ρ*ν*kₒ²)
T11 = tanϕₚ/(24ρ*ν³*kₒ⁴) * (5.0 + 3*tan²ϕₚ + eₚ²*cos²ϕₚ - 4*eₚ⁴*cosϕₚ - 9*tan²ϕₚ*eₚ²*cos²ϕₚ)
T12 = tanϕₚ/(720ρ*ν⁵*kₒ⁶) * (61.0 + 90*tan²ϕₚ + 46.0*eₚ²*cos²ϕₚ + 45*tan⁴ϕₚ - 252*tan²ϕₚ*eₚ²*cos²ϕₚ -
3*eₚ⁴*cos⁴ϕₚ + 100*eₚ⁶*cos⁶ϕₚ - 66*tan²ϕₚ*eₚ⁴*cos⁴ϕₚ -
90*tan⁴ϕₚ*eₚ²*cos²ϕₚ + 88*eₚ⁸*cos⁸ϕₚ + 225*tan⁴ϕₚ*eₚ⁴*cos⁴ϕₚ +
84*tan²ϕₚ*eₚ⁶*cos⁶ϕₚ - 192*tan²ϕₚ*eₚ⁸*cos⁸ϕₚ)
T13 = tanϕₚ/(40320ρ*ν⁷*kₒ⁸) * (1385.0 + 3633*tan²ϕₚ + 4095*tan⁴ϕₚ + 1575*tan⁶ϕₚ)
T14 = 1.0/(ν*cosϕₚ*kₒ)
T15 = (1.0 + 2*tan²ϕₚ + eₚ²*cos²ϕₚ) / (6*(ν³*cosϕₚ*kₒ³))
T16 = (5.0 + 6*eₚ²*cos²ϕₚ + 28*tan²ϕₚ - 3*eₚ⁴*cos⁴ϕₚ + 8*tan²ϕₚ*eₚ²*cos²ϕₚ +
24*tan⁴ϕₚ - 4*eₚ⁶*cos⁶ϕₚ + 4*tan²ϕₚ*eₚ⁴*cos⁴ϕₚ + 24*tan²ϕₚ*eₚ⁶*cos⁶ϕₚ) /
(120*(ν⁵*cosϕₚ*kₒ⁵))
T17 = (61.0 + 662*tan²ϕₚ + 1320*tan⁴ϕₚ + 720*tan⁶ϕₚ)/(5040*(ν⁷*cosϕₚ*kₒ⁷))
ϕ = ϕₚ - ΔE²*T10 + ΔE⁴*T11 - ΔE⁶*T12 + ΔE⁸*T13
λ = λₒ + ΔE*T14 - ΔE³*T15 + ΔE⁵*T16 - ΔE⁷*T17
alt = utm.u
LatLonAlt(ϕ, λ, alt)
end
function Base.convert(::Type{ECEF}, enu::ENU, reference::LatLonAlt)
# http://www.navipedia.net/index.php/Transformations_between_ECEF_and_ENU_coordinates
ϕ = reference.lat
λ = reference.lon
sϕ, cϕ = sin(ϕ), cos(ϕ)
sλ, cλ = sin(λ), cos(λ)
R = [-sλ -cλ*sϕ cλ*cϕ;
cλ -sλ*sϕ sλ*cϕ;
0.0 cϕ sϕ]
p = [enu.e, enu.n, enu.u]
ecef = R*p
ref_ecef = convert(ECEF, reference)
ECEF(ecef[1] + ref_ecef.x, ecef[2] + ref_ecef.y, ecef[3] + ref_ecef.z)
end
function Base.convert(::Type{ENU}, ecef::ECEF, refLLA::LatLonAlt, refECEF::ECEF=convert(ECEF, refLLA))
# http://www.navipedia.net/index.php/Transformations_between_ECEF_and_ENU_coordinates
ϕ = refLLA.lat
λ = refLLA.lon
a = refLLA.alt
sϕ, cϕ = sin(ϕ), cos(ϕ)
sλ, cλ = sin(λ), cos(λ)
R = [-sλ cλ 0.0;
-cλ*sϕ -sλ*sϕ cϕ;
cλ*cϕ sλ*cϕ sϕ]
p = [ecef.x - refECEF.x, ecef.y - refECEF.y, ecef.z - refECEF.z]
enu = R*p
ENU(enu[1], enu[2], enu[3])
end
Base.convert(::Type{NED}, enu::ENU) = NED(enu.n, enu.e, -enu.u)
Base.convert(::Type{ENU}, ned::NED) = ENU(ned.e, ned.n, -ned.d)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 5664 | export
Quat,
quat_for_a2b,
get_axis,
get_rotation_angle,
RPY
struct Quat <: FieldVector{4, Float64}
x::Float64
y::Float64
z::Float64
w::Float64
end
Quat(v::VecE3, w::Float64) = Quat(v.x, v.y, v.z, w)
Quat(w::Float64, v::VecE3) = Quat(v.x, v.y, v.z, w)
Base.show(io::IO, q::Quat) = @printf(io, "Quat({%6.3f, %6.3f, %6.3f}, %6.3f)", q.x, q.y, q.z, q.w)
"""
Conjugate of a quaternion
"""
Base.conj(q::Quat) = Quat(-q.x,-q.y,-q.z, q.w)
"""
A VecE3 of the imaginary part (x,y,z)
"""
Base.imag(q::Quat) = VecE3(q.x, q.y, q.z)
"""
Return the multiplicative inverse of q.
Note that in most cases, i.e., if you simply want the opposite rotation,
and/or the quaternion is normalized, then it is enough to use the conjugate.
Based on Eigen's inverse.
Returns the zero Quat if q is zero.
"""
function Base.inv(q::Quat)
n2 = q.x^2 + q.y^2 + q.z^2 + q.w^2 # squared norm
if n2 > 0
return Quat(conj(q) / n2)
else
# return an invalid result to flag the error
return Quat(0.0,0.0,0.0,0.0)
end
end
"""
Rotate a vector by a quaternion.
If you are going to rotate a bunch of vectors, covert q to a rotation matrix first.
Based on the Eigen implementation for _transformVector
"""
function rot(q::Quat, v::VecE3)
uv = imag(q)×v
uv += uv
return v + q.w*uv + imag(q)×uv;
end
"""
Quaternion multiplication
"""
function Base.:*(a::Quat, b::Quat)
r1, v1 = a.w, imag(a)
r2, v2 = b.w, imag(b)
return Quat(r1*v2 + r2*v1 + v1×v2, r1*r2 - v1⋅v2)
end
Base.:*(v::VecE3, b::Quat) = Quat(0.0, v) * b
Base.:*(a::Quat, v::VecE3) = a * Quat(0.0, v)
"""
The angle (in radians) between two rotations
"""
function angledist(a::Quat, b::Quat)
d = a * conj(b)
return 2*atan(LinearAlgebra.norm(imag(d)), abs(d.w))
end
"""
The quaternion that transforms a into b through a rotation
Based on Eigen's implementation for setFromTwoVectors
"""
function quat_for_a2b(a::VecE3, b::VecE3)
v0 = LinearAlgebra.normalize(a)
v1 = LinearAlgebra.normalize(b)
c = v1⋅v0
# if dot == -1, vectors are nearly opposites
# => accurately compute the rotation axis by computing the
# intersection of the two planes. This is done by solving:
# x^T v0 = 0
# x^T v1 = 0
# under the constraint:
# ||x|| = 1
# which yields a singular value problem
if c < -1.0 + DUMMY_PRECISION
c = max(c, -1.0)
M = hcat(convert(Vector{Float64}, v0),
convert(Vector{Float64}, v1))'
s = LinearAlgebra.svdfact(M, thin=false)
axis = convert(VecE3, s[:V][:,2])
w2 = (1.0 + c)*0.5
return Quat(axis * sqrt(1.0 - w2), sqrt(w2))
else
axis = v0×v1
s = sqrt((1.0+c)*2.0)
invs = 1.0/s
return Quat(axis * invs, s * 0.5)
end
end
"""
Returns the spherical linear interpolation between the two quaternions
a and b for t ∈ [0,1].
Based on the Eigen implementation for slerp.
"""
function lerp(a::Quat, b::Quat, t::Float64)
d = a⋅b
absD = abs(d)
if(absD ≥ 1.0 - eps())
scale0, scale1 = 1.0 - t, t
else
# θ is the angle between the 2 quaternions
θ = acos(absD)
sinθ = sin(θ)
scale0, scale1 = sin( ( 1.0 - t ) * θ) / sinθ,
sin( ( t * θ) ) / sinθ
end
if d < 0
scale1 = -scale1
end
return Quat(scale0*a + scale1*b)
end
"""
The rotation axis for a quaternion.
"""
function get_axis(q::Quat)
θ = 2*atan(sqrt(q.x*q.x + q.y*q.y + q.z*q.z), q.w)
h = sin(θ/2)
x = q.x / h
y = q.y / h
z = q.z / h
VecE3(x, y, z)
end
"""
The rotation angle for a quaternion.
"""
get_rotation_angle(q::Quat) = 2.0*acos(q.w)
"""
Draw a random unit quaternion following a uniform distribution law on SO(3).
Based on the Eigen implementation for UnitRandom.
"""
function Base.rand(::Type{Quat})
u1 = rand()
u2 = 2π*rand()
u3 = 2π*rand()
a = sqrt(1 - u1)
b = sqrt(u1)
return Quat(a*sin(u2), a*cos(u2), b*sin(u3), b*cos(u3))
end
"""
Roll Pitch Yaw
"""
struct RPY <: FieldVector{3, Float64}
r::Float64
p::Float64
y::Float64
end
function Base.convert(::Type{RPY}, q::Quat)
q2 = LinearAlgebra.normalize(q)
x = q2[1]
y = q2[2]
z = q2[3]
w = q2[4]
# roll (x-axis rotation)
sinr = 2(w * x + y * z)
cosr = 1.0 - 2(x * x + y * y)
roll = atan(sinr, cosr)
# pitch (y-axis rotation)
sinp = 2(w * y - z * x)
if (abs(sinp) >= 1)
pitch = π/2 * sign(sinp) # use 90 degrees if out of range
else
pitch = asin(sinp)
end
# yaw (z-axis rotation)
siny = 2(w * z + x * y)
cosy = 1.0 - 2(y * y + z * z)
yaw = atan(siny, cosy)
RPY(roll, pitch, yaw)
end
function Base.convert(::Type{Matrix{Float64}}, quat::Quat)
# convert it to a rotation matrix
w = quat.w
x = quat.x
y = quat.y
z = quat.z
n = w * w + x * x + y * y + z * z
s = (n == 0.0) ? 0.0 : 2.0 / n
wx = s * w * x; wy = s * w * y; wz = s * w * z
xx = s * x * x; xy = s * x * y; xz = s * x * z
yy = s * y * y; yz = s * y * z; zz = s * z * z
[ 1 - (yy + zz) xy - wz xz + wy;
xy + wz 1 - (xx + zz) yz - wx;
xz - wy yz + wx 1 - (xx + yy) ]
end
function Base.convert(::Type{Quat}, rpy::RPY)
t0 = cos(rpy.y/2)
t1 = sin(rpy.y/2)
t2 = cos(rpy.r/2)
t3 = sin(rpy.r/2)
t4 = cos(rpy.p/2)
t5 = sin(rpy.p/2)
qw = t0 * t2 * t4 + t1 * t3 * t5
qx = t0 * t3 * t4 - t1 * t2 * t5
qy = t0 * t2 * t5 + t1 * t3 * t4
qz = t1 * t2 * t4 - t0 * t3 * t5
return Quat(qx, qy, qz, qw)
end | AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1158 | #=
VecE2: a 2d euclidean vector
=#
struct VecE2{R<:Real} <: VecE{2,R}
x::R
y::R
end
VecE2() = VecE2(0.0,0.0)
VecE2(x::Integer, y::Integer) = VecE2(float(x), float(y))
VecE2(t::Tuple) = VecE2(promote(t...)...)
polar(r::Real, θ::Real) = VecE2(r*cos(θ), r*sin(θ))
Base.show(io::IO, a::VecE2) = @printf(io, "VecE2(%.3f, %.3f)", a.x, a.y)
Base.atan(a::VecE2) = atan(a.y, a.x)
dist(a::VecE2, b::VecE2) = hypot(a.x-b.x, a.y-b.y)
function dist2(a::VecE2, b::VecE2)
Δx = a.x-b.x
Δy = a.y-b.y
Δx*Δx + Δy*Δy
end
proj(a::VecE2, b::VecE2, ::Type{Float64}) = (a.x*b.x + a.y*b.y) / hypot(b.x, b.y) # dot(a,b) / |b|
function proj(a::VecE2, b::VecE2, ::Type{VecE2})
# dot(a,b) / dot(b,b) ⋅ b
s = (a.x*b.x + a.y*b.y) / (b.x*b.x + b.y*b.y)
VecE2(s*b.x, s*b.y)
end
lerp(a::VecE2, b::VecE2, t::Real) = VecE2(a.x + (b.x-a.x)*t, a.y + (b.y-a.y)*t)
Base.rot180(a::VecE2) = VecE2(-a.x, -a.y)
Base.rotl90(a::VecE2) = VecE2(-a.y, a.x)
Base.rotr90(a::VecE2) = VecE2( a.y, -a.x)
function rot(a::VecE2, θ::Float64)
#=
Rotates counter-clockwise about origin
=#
c = cos(θ)
s = sin(θ)
VecE2(a.x*c - a.y*s, a.x*s+a.y*c)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2235 | #=
VecE3: a 3d euclidean vector
=#
struct VecE3{R<:Real} <: VecE{3,R}
x::R
y::R
z::R
end
VecE3() = VecE3(0.0,0.0,0.0)
VecE3(x::Integer, y::Integer, z::Integer) = VecE3(float(x), float(y), float(z))
VecE3(t::Tuple) = VecE3(promote(t...)...)
Base.show(io::IO, a::VecE3) = @printf(io, "VecE3(%.3f, %.3f, %.3f)", a.x, a.y, a.z)
function dist(a::VecE3, b::VecE3)
Δx = a.x-b.x
Δy = a.y-b.y
Δz = a.z-b.z
sqrt(Δx*Δx + Δy*Δy + Δz*Δz)
end
function dist2(a::VecE3, b::VecE3)
Δx = a.x-b.x
Δy = a.y-b.y
Δz = a.z-b.z
Δx*Δx + Δy*Δy + Δz*Δz
end
proj(a::VecE3, b::VecE3, ::Type{Float64}) = (a.x*b.x + a.y*b.y + a.z*b.z) / sqrt(b.x*b.x + b.y*b.y + b.z*b.z) # dot(a,b) / |b|
function proj(a::VecE3, b::VecE3, ::Type{VecE3})
# dot(a,b) / dot(b,b) ⋅ b
s = (a.x*b.x + a.y*b.y + a.z*b.z) / (b.x*b.x + b.y*b.y + b.z*b.z)
VecE3(s*b.x, s*b.y, s*b.z)
end
lerp(a::VecE3, b::VecE3, t::Real) = VecE3(a.x + (b.x-a.x)*t, a.y + (b.y-a.y)*t, a.z + (b.z-a.z)*t)
function rot(a::VecE3, axis::VecE3, θ::Real)
#=
rotate the point a: (x,y,z) about the axis: (u,v,w) by the angle θ following the
right hand rule
=#
x,y,z = a.x, a.y, a.z
u,v,w = axis.x, axis.y, axis.z
u² = u*u
v² = v*v
w² = w*w
m = u² + v² + w²
c = cos(θ)
s = sin(θ)
mc = 1.0 - c
rtms = sqrt(m)*s
magc = m*c
ux_vy_wz_mc = (u*x + v*y + w*z)*mc
new_x = (u*ux_vy_wz_mc + x*magc + rtms*(-w*y + v*z)) / m
new_y = (v*ux_vy_wz_mc + y*magc + rtms*( w*x - u*z)) / m
new_z = (w*ux_vy_wz_mc + z*magc + rtms*(-v*x + u*y)) / m
VecE3(new_x, new_y, new_z)
end
function rot_normalized(a::VecE3, axis::VecE3, θ::Real)
#=
rotate the point a: (x,y,z) about the axis: (u,v,w) by the angle θ following the
right hand rule
Here we assume axis is normalized
=#
x,y,z = a.x, a.y, a.z
u,v,w = axis.x, axis.y, axis.z
u² = u*u
v² = v*v
w² = w*w
m = u² + v² + w²
c = cos(θ)
s = sin(θ)
mc = 1.0 - c
ux_vy_wz_mc = (u*x + v*y + w*z)*mc
new_x = u*ux_vy_wz_mc + x*c + (-w*y + v*z)*s
new_y = v*ux_vy_wz_mc + y*c + ( w*x - u*z)*s
new_z = w*ux_vy_wz_mc + z*c + (-v*x + u*y)*s
VecE3(new_x, new_y, new_z)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2671 | #=
VecSE2: a 2d euclidean vector with an orientation
=#
struct VecSE2{R<:Real} <: VecSE{3,R}
x::R
y::R
θ::R
end
VecSE2() = VecSE2(0.0,0.0,0.0)
VecSE2(x::Real, y::Real) = VecSE2(x,y,0.0)
VecSE2(a::VecE2, θ::Real=0.0) = VecSE2(a.x, a.y, θ)
VecSE2(t::Tuple) = VecSE2(promote(t...)...)
Base.convert(::Type{VecE2}, a::VecSE2) = VecE2(a.x, a.y)
VecE2(a::VecSE2) = VecE2(a.x, a.y)
polar(r::Real, ϕ::Real, θ::Real) = VecSE2(r*cos(ϕ), r*sin(ϕ), θ)
Base.show(io::IO, a::VecSE2) = @printf(io, "VecSE2({%.3f, %.3f}, %.3f)", a.x, a.y, a.θ)
Base.:+(a::VecSE2, b::VecE2) = VecSE2(a.x+b.x, a.y+b.y, a.θ)
Base.:+(a::VecE2, b::VecSE2) = VecSE2(a.x+b.x, a.y+b.y, b.θ)
Base.:-(a::VecSE2, b::VecE2) = VecSE2(a.x-b.x, a.y-b.y, a.θ)
Base.:-(a::VecE2, b::VecSE2) = VecSE2(a.x-b.x, a.y-b.y, -b.θ)
op_overload_error = """
Operator overloading for Real and VecSE2 has been removed.
To add or subtract, use VecSE2(x,y,θ) + b*VecE2(1.0, 1.0).
To multiply or divide, use scale_euclidean(VecSE2(x,y,θ), b)
"""
Base.:-(b::Real, a::VecSE2) = error(op_overload_error)
Base.:-(a::VecSE2, b::Real) = error(op_overload_error)
Base.:+(b::Real, a::VecSE2) = error(op_overload_error)
Base.:+(a::VecSE2, b::Real) = error(op_overload_error)
Base.:*(b::Real, a::VecSE2) = error(op_overload_error)
Base.:*(a::VecSE2, b::Real) = error(op_overload_error)
Base.:/(a::VecSE2, b::Real) = error(op_overload_error)
Base.:^(a::VecSE2, b::Integer) = error(op_overload_error)
Base.:^(a::VecSE2, b::AbstractFloat) = error(op_overload_error)
Base.:%(a::VecSE2, b::Real) = error(op_overload_error)
scale_euclidean(a::VecSE2, b::Real) = VecSE2(b*a.x, b*a.y, a.θ)
clamp_euclidean(a::VecSE2, lo::Real, hi::Real) = VecSE2(clamp(a.x, lo, hi), clamp(a.y, lo, hi), a.θ)
norm(a::VecSE2, p::Real=2) = error("norm is not defined for VecSE2 - use norm(VecE2(v)) to get the norm of the Euclidean part")
normsquared(a::VecSE2) = norm(a)^2 # this will correctly throw an error
normalize(a::VecSE2, p::Real=2) = error("normalize is not defined for VecSE2. Use normalize_euclidean(v) to normalize the euclidean part of the vector")
function normalize_euclidian(a::VecSE2, p::Real=2)
n = norm(VecE2(a))
return VecSE2(a.x/n, a.y/n, a.θ)
end
Base.atan(a::VecSE2) = atan(a.y, a.x)
function lerp(a::VecSE2, b::VecSE2, t::Real)
x = a.x + (b.x-a.x)*t
y = a.y + (b.y-a.y)*t
θ = lerp_angle(a.θ, b.θ, t)
VecSE2(x, y, θ)
end
Base.rot180(a::VecSE2) = VecSE2(a.x, a.y, a.θ+π)
Base.rotl90(a::VecSE2) = VecSE2(a.x, a.y, a.θ+0.5π)
Base.rotr90(a::VecSE2) = VecSE2(a.x, a.y, a.θ-0.5π)
rot(a::VecSE2, Δθ::Float64) = VecSE2(a.x, a.y, a.θ+Δθ)
Base.mod2pi(a::VecSE2) = VecSE2(a.x, a.y, mod2pi(a.θ))
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 829 | export
LineSegment1D
"""
A line segment in one dimension with a ≤ b
"""
struct LineSegment1D
a::Float64
b::Float64
LineSegment1D(a::Real, b::Real) = new(convert(Float64, min(a,b)), convert(Float64, max(a,b)))
end
Base.:+(seg::LineSegment1D, v::Real) = LineSegment1D(seg.a + v, seg.b + v)
Base.:-(seg::LineSegment1D, v::Real) = LineSegment1D(seg.a - v, seg.b - v)
"""
The distance between the line segment and the point P
"""
function get_distance(seg::LineSegment1D, P::Real)::Float64
if P < seg.a
return seg.a - P
elseif P > seg.b
return P - seg.b
else
return 0.0
end
end
Base.in(v::Float64, P::LineSegment1D) = P.a ≤ v ≤ P.b
Base.in(Q::LineSegment1D, P::LineSegment1D) = Q.a ≥ P.a && Q.b ≤ P.b
intersects(P::LineSegment1D, Q::LineSegment1D) = P.b ≥ Q.a && Q.b ≥ P.a
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2802 | #=
Geometry in 2d euclidean space
=#
"""
deltaangle(a::Real, b::Real)
Return the minimum δ such that
a + δ = mod(b, 2π)
"""
deltaangle(a::Real, b::Real) = atan(sin(b-a), cos(b-a))
"""
Distance between two angles
"""
angledist( a::Real, b::Real ) = abs(deltaangle(a,b))
"""
Linear interpolation between angles
"""
lerp_angle(a::Real, b::Real, t::AbstractFloat) = a + deltaangle(a, b)*t
"""
True if the values are collinear within a tolerance
"""
function are_collinear(a::AbstractVec, b::AbstractVec, c::AbstractVec, tol::Float64=1e-8)
# http://mathworld.wolfram.com/Collinear.html
# if val = 0 then they are collinear
val = a.x*(b.y-c.y) + b.x*(c.y-a.y)+c.x*(a.y-b.y)
abs(val) < tol
end
"""
To find orientation of ordered triplet (p, q, r).
The function returns following values
0 --> p, q and r are colinear
1 --> Clockwise
2 --> Counterclockwise
"""
function orientation(P::VecE2, Q::VecE2, R::VecE2)
val = (Q.y - P.y)*(R.x - Q.x) - (Q.x - P.x)*(R.y - Q.y)
if val ≈ 0
return 0 # colinear
end
return (val > 0) ? 1 : 2
end
function inertial2body(point::VecE2, reference::VecSE2)
#=
Convert a point in an inertial cartesian coordinate frame
to be relative to a body's coordinate frame
The body's position is given relative to the same inertial coordinate frame
=#
s, c = sin(reference.θ), cos(reference.θ)
Δx = point.x - reference.x
Δy = point.y - reference.y
VecE2(c*Δx + s*Δy, c*Δy - s*Δx)
end
function inertial2body(point::VecSE2, reference::VecSE2)
#=
Convert a point in an inertial cartesian coordinate frame
to be relative to a body's coordinate frame
The body's position is given relative to the same inertial coordinate frame
=#
s, c = sin(reference.θ), cos(reference.θ)
Δx = point.x - reference.x
Δy = point.y - reference.y
VecSE2(c*Δx + s*Δy, c*Δy - s*Δx, point.θ - reference.θ)
end
function body2inertial(point::VecE2, reference::VecSE2)
#=
Convert a point in a body-relative cartesian coordinate frame
to be relative to a the inertial coordinate frame the body is described by
=#
c, s = cos(reference.θ), sin(reference.θ)
VecE2(c*point.x -s*point.y + reference.x, s*point.x +c*point.y + reference.y)
end
function body2inertial(point::VecSE2, reference::VecSE2)
#=
Convert a point in a body-relative cartesian coordinate frame
to be relative to a the inertial coordinate frame the body is described by
=#
c, s = cos(reference.θ), sin(reference.θ)
VecSE2(c*point.x -s*point.y + reference.x, s*point.x +c*point.y + reference.y, reference.θ + point.θ)
end
include("1d.jl")
include("lines.jl")
include("line_segments.jl")
include("rays.jl")
include("projectiles.jl")
include("solids.jl")
include("hyperplanes.jl") | AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2389 | export
Plane3,
get_signed_distance
"""
Plane3
A hyperplane is an affine subspace of dimension n-1 in a space of dimension n.
For example, a hyperplane in a plane is a line; a hyperplane in 3-space is a plane.
This class represents an hyperplane in 3D space. It is the zero set of
n⋅x + d = 0 where n is a unit normal vector of the plane (linear part)
and d is the distance (offset) to the origin.
"""
struct Plane3
normal::VecE3 # should always be normalized
offset::Float64
Plane3() = new(VecE3(1.0,0.0,0.0), 0.0)
"""
Constructs a plane from its normal n and distance to the origin d
such that the algebraic equation of the plane is n⋅x + d = 0.
"""
Plane3(normal::VecE3, offset::Real) = new(LinearAlgebra.normalize(normal), convert(Float64, offset))
"""
Construct a plane from its normal and a point on the plane.
"""
function Plane3(normal::VecE3, P::VecE3)
n = LinearAlgebra.normalize(normal)
offset = -n⋅P
return new(n, offset)
end
end
"""
Constructs a plane passing through the three points.
"""
function Plane3(p0::VecE3, p1::VecE3, p2::VecE3)
v0 = p2 - p0
v1 = p1 - p0
normal = v0×v1
nnorm = LinearAlgebra.norm(normal)
if nnorm <= LinearAlgebra.norm(v0)*LinearAlgebra.norm(v1)*eps()
M = hcat(convert(Vector{Float64}, v0),
convert(Vector{Float64}, v1))'
s = LinearAlgebra.svdfact(M, thin=false)
normal = convert(VecE3, s[:V][:,2])
else
normal = LinearAlgebra.normalize(normal)
end
offset = -p0⋅normal
return Plane3(normal, offset)
end
"""
Returns the signed distance between the plane and a point
"""
get_signed_distance(plane::Plane3, A::VecE3) = plane.normal⋅A + plane.offset
"""
Returns the absolute distance between the plane and a point
"""
get_distance(plane::Plane3, A::VecE3) = abs(get_signed_distance(plane, A))
"""
Returns the projection of a point onto the plane
"""
proj(A::VecE3, plane::Plane3) = A - get_signed_distance(plane, A) * plane.normal
"""
What side of the plane you are on
Is -1 if on the negative side, 1 if on the positive side, and 0 if on the plane
"""
function get_side(plane::Plane3, A::VecE3, ε::Float64=DUMMY_PRECISION)
signed_dist = get_signed_distance(plane, A)
if abs(signed_dist) < ε
return 0
else
return sign(signed_dist)
end
end | AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2893 | export
LineSegment,
parallel
struct LineSegment
A::VecE2
B::VecE2
end
Base.:+(seg::LineSegment, V::VecE2) = LineSegment(seg.A + V, seg.B + V)
Base.:-(seg::LineSegment, V::VecE2) = LineSegment(seg.A - V, seg.B - V)
Base.convert(::Type{Line}, seg::LineSegment) = Line(seg.A, seg.B)
Base.convert(::Type{LineSegment}, line::Line) = LineSegment(line.A, line.B)
get_polar_angle(seg::LineSegment) = mod2pi(atan(seg.B.y - seg.A.y, seg.B.x - seg.A.x))
"""
The distance between the line segment and the point P
"""
function get_distance(seg::LineSegment, P::VecE2)
ab = seg.B - seg.A
pb = P - seg.A
denom = normsquared(ab)
if denom == 0.0
return 0.0
end
r = (ab⋅pb)/denom
if r ≤ 0.0
LinearAlgebra.norm(P - seg.A)
elseif r ≥ 1.0
LinearAlgebra.norm(P - seg.B)
else
LinearAlgebra.norm(P - (seg.A + r*ab))
end
end
"""
What side of the line you are on, based on A → B
"""
get_side(seg::LineSegment, p::VecE2) = sign((seg.B.x-seg.A.x) * (p.y-seg.A.y) - (seg.B.y-seg.A.y) * (p.x-seg.A.x))
"""
The angular distance between the two line segments
"""
function angledist(segA::LineSegment, segB::LineSegment)
u = segA.B - segA.A
v = segB.B - segB.A
sqdenom = (u⋅u)*(v⋅v)
if isapprox(sqdenom, 0.0, atol=1e-10)
return NaN
end
return acos((u⋅v) / sqrt(sqdenom))
end
"""
True if the two segments are parallel
"""
function parallel(segA::LineSegment, segB::LineSegment, ε::Float64=1e-10)
θ = angledist(segA, segB)
return isapprox(θ, 0.0, atol=ε)
end
"""
Given P colinear with seg, the function checks if
point P lies on the line segment.
"""
function on_segment(P::VecE2, seg::LineSegment)
return P.x ≤ max(seg.A.x, seg.B.x) && P.x ≥ min(seg.A.x, seg.B.x) &&
P.y ≤ max(seg.A.y, seg.B.y) && P.y ≥ min(seg.A.y, seg.B.y)
end
on_segment(A::VecE2, P::VecE2, B::VecE2) = on_segment(P, LineSegment(A,B))
"""
returns true if line segments segP and segQ intersect
"""
function intersects(segP::LineSegment, segQ::LineSegment)
p1 = segP.A
q1 = segP.B
p2 = segQ.A
q2 = segQ.B
# Find the four orientations needed for general and
# special cases
o1 = orientation(p1, q1, p2)
o2 = orientation(p1, q1, q2)
o3 = orientation(p2, q2, p1)
o4 = orientation(p2, q2, q1)
# Special Cases
if (o1 != o2 && o3 != o4) || # General case
(o1 == 0 && on_segment(p1, p2, q1)) || # p1, q1 and p2 are colinear and p2 lies on segment p1q1
(o2 == 0 && on_segment(p1, q2, q1)) || # p1, q1 and p2 are colinear and q2 lies on segment p1q1
(o3 == 0 && on_segment(p2, p1, q2)) || # p2, q2 and p1 are colinear and p1 lies on segment p2q2
(o4 == 0 && on_segment(p2, q1, q2)) # p2, q2 and q1 are colinear and q1 lies on segment p2q2
return true
end
return false # Doesn't fall in any of the above cases
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1165 | export
Line,
get_polar_angle,
get_distance,
get_side
struct Line
C::VecE2
θ::Float64
end
Line(A::VecE2, B::VecE2) = Line((A+B)/2, atan(B - A))
Base.:-(line::Line, V::VecE2) = Line(line.C - V, line.θ)
Base.:+(line::Line, V::VecE2) = Line(line.C + V, line.θ)
get_polar_angle(line::Line) = line.θ
rot(line::Line, Δθ::Float64) = Line(line.C, line.θ+Δθ)
Base.rot180(line::Line) = rot(line, 1π)
Base.rotl90(line::Line) = rot(line, π/2)
Base.rotr90(line::Line) = rot(line, -π/2)
"""
The distance between the line and the point P
"""
function get_distance(line::Line, P::VecE2)
ab = polar(1.0, line.θ)
pb = P - line.C
denom = normsquared(ab)
if denom ≈ 0.0
return 0.0
end
r = (ab⋅pb)/denom
return LinearAlgebra.norm(P - (line.C + r*ab))
end
"""
What side of the line you are on
Is 1 if on the left, -1 if on the right, and 0 if on the line
"""
function get_side(line::Line, p::VecE2, ε::Float64=1e-10)
ab = polar(1.0, line.θ)
signed_dist = ab.x*(p.y-line.C.y) - ab.y*(p.x-line.C.x)
if abs(signed_dist) < ε
return 0
else
return convert(Int, sign(signed_dist))
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 4636 | export
Projectile,
propagate,
closest_time_of_approach,
closest_approach_distance,
closest_time_of_approach_and_distance,
get_intersection_time
struct Projectile
pos::VecSE2 # position (x,y,θ)
v::Float64 # speed
end
propagate(P::Projectile, Δt::Float64) = Projectile(P.pos + polar(P.v*Δt, P.pos.θ), P.v)
"""
The time at which two projectiles are closest to one another
A negative value indicates that this occurred in the past.
see: http://geomalgorithms.com/a07-_distance.html
"""
function closest_time_of_approach(A::Projectile, B::Projectile)
W = convert(VecE2, A.pos - B.pos)
Δ = polar(A.v, A.pos.θ) - polar(B.v, B.pos.θ)
aΔ = normsquared(Δ)
if aΔ ≈ 0.0
return 0.0
else
return -(W⋅Δ) / aΔ
end
end
function closest_approach_distance(A::Projectile, B::Projectile, t_CPA::Float64=closest_time_of_approach(A, B))
Aₜ = convert(VecE2, propagate(A, t_CPA).pos)
Bₜ = convert(VecE2, propagate(B, t_CPA).pos)
return LinearAlgebra.norm(Aₜ - Bₜ)
end
function closest_time_of_approach_and_distance(A::Projectile, B::Projectile)
t_CPA = closest_time_of_approach(A, B)
d_CPA = closest_approach_distance(A, B, t_CPA)
return (t_CPA, d_CPA)
end
function get_intersection_time(A::Projectile, seg::LineSegment)
o = VecE2(A.pos)
v₁ = o - seg.A
v₂ = seg.B - seg.A
v₃ = polar(1.0, A.pos.θ + π/2)
denom = (v₂⋅v₃)
if !isapprox(denom, 0.0, atol=1e-10)
d₁ = (v₂×v₁) / denom # time for projectile (0 ≤ t₁)
t₂ = (v₁⋅v₃) / denom # time for segment (0 ≤ t₂ ≤ 1)
if 0.0 ≤ d₁ && 0.0 ≤ t₂ ≤ 1.0
return d₁/A.v
end
else
# denom is zero if the segment and the projectile are parallel
# only collide if they are perfectly aligned
if are_collinear(A.pos, seg.A, seg.B)
dist_a = normsquared(seg.A - o)
dist_b = normsquared(seg.B - o)
return sqrt(min(dist_a, dist_b)) / A.v
end
end
return Inf
end
function closest_time_of_approach_and_distance(P::Projectile, seg::LineSegment, if_no_col_skip_eval::Bool=false)
o = VecE2(P.pos)
v₁ = o - seg.A
v₂ = seg.B - seg.A
v₃ = polar(1.0, P.pos.θ + π/2)
denom = (v₂⋅v₃)
if !isapprox(denom, 0.0, atol=1e-10)
d₁ = (v₂×v₁) / denom # time for projectile (0 ≤ d₁)
t₂ = (v₁⋅v₃) / denom # time for segment (0 ≤ t₂ ≤ 1)
if 0.0 ≤ d₁ && 0.0 ≤ t₂ ≤ 1.0
t = d₁/P.v
return (t, 0.0)
else
# no collision, get time of closest approach to each endpoint
if !if_no_col_skip_eval
r = polar(1.0, P.pos.θ)
projA = proj(seg.A - o, r, VecE2)
projB = proj(seg.B - o, r, VecE2)
tA = max(LinearAlgebra.norm(projA)/P.v * sign(r⋅projA), 0.0)
tB = max(LinearAlgebra.norm(projB)/P.v * sign(r⋅projB), 0.0)
pA = VecE2(propagate(P, tA).pos)
pB = VecE2(propagate(P, tB).pos)
distA = normsquared(seg.A - pA)
distB = normsquared(seg.B - pB)
if distA < distB
return (tA, sqrt(distA))
else
return (tB, sqrt(distB))
end
else
return (Inf, Inf)
end
end
else
# denom is zero if the segment and the projectile are parallel
# only collide if they are perfectly aligned
if are_collinear(P.pos, seg.A, seg.B)
# either closest now, will be to A, or will be to B
dist_a = normsquared(seg.A - o)
dist_b = normsquared(seg.B - o)
t = sqrt(min(dist_a, dist_b)) / P.v
return (t, 0.0)
else
# not colinear
if !if_no_col_skip_eval
# either closest now, will be to A, or will be to B
r = polar(1.0, P.pos.θ)
projA = proj(seg.A - o, r, VecE2)
projB = proj(seg.B - o, r, VecE2)
tA = max(LinearAlgebra.norm(projA)/P.v * sign(r⋅projA), 0.0)
tB = max(LinearAlgebra.norm(projB)/P.v * sign(r⋅projB), 0.0)
pA = VecE2(propagate(P, tA).pos)
pB = VecE2(propagate(P, tB).pos)
distA = normsquared(seg.A - pA)
distB = normsquared(seg.B - pB)
if distA < distB
return (tA, distA)
else
return (tB, distB)
end
else
return (Inf,Inf)
end
end
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 3421 | export
Ray,
intersects # true if A and B intersect
const Ray = VecSE2 # has an origin and an orientation
function intersects(A::Ray, B::Ray)
as = convert(VecE2, A)
bs = convert(VecE2, B)
ad = polar(1.0, A.θ)
bd = polar(1.0, B.θ)
dx = bs.x - as.x
dy = bs.y - as.y
det = bd.x * ad.y - bd.y * ad.x
if det == 0.0
return false
end
u = (dy * bd.x - dx * bd.y) / det
v = (dy * ad.x - dx * ad.y) / det
return u > 0.0 && v > 0.0
end
function intersects(ray::Ray, line::Line;
ε::Float64 = 1e-10,
)
v₁ = VecE2(ray) - line.C
v₂ = polar(1.0, line.θ)
v₃ = polar(1.0, ray.θ + π/2)
denom = v₂⋅v₃
if !(denom ≈ 0.0)
t₁ = (v₂×v₁) / denom # time for ray (0 ≤ t₁)
return -ε ≤ t₁
else
# denom is zero if the line and the ray are parallel
# only collide if they are perfectly aligned
return isapprox(angledist(ray, line), 0.0, atol=ε)
end
end
function intersects(ray::Ray, seg::LineSegment)
R = VecE2(ray)
v₁ = R - seg.A
v₂ = seg.B - seg.A
v₃ = polar(1.0, ray.θ + π/2)
denom = v₂⋅v₃
if !isapprox(denom, 0.0, atol=1e-10)
t₁ = (v₂×v₁) / denom # time for ray (0 ≤ t₁)
t₂ = (v₁⋅v₃) / denom # time for segment (0 ≤ t₂ ≤ 1)
return 0 ≤ t₁ && 0 ≤ t₂ ≤ 1
else
# denom is zero if the segment and the ray are parallel
# only collide if they are perfectly aligned
# must ensure that at least one point is in the positive ray direction
r = polar(1.0, ray.θ)
return are_collinear(R, seg.A, seg.B) &&
(r⋅(seg.A - R) ≥ 0 || r⋅(seg.B - R) ≥ 0)
end
end
"""
returns VecE2 of where intersection occurs, and VecE2(NaN,NaN) otherwise
"""
function Base.intersect(A::VecSE2, B::VecSE2)
as = convert(VecE2, A)
bs = convert(VecE2, B)
ad = polar(1.0, A.θ)
bd = polar(1.0, B.θ)
dx = bs.x - as.x
dy = bs.y - as.y
det = bd.x * ad.y - bd.y * ad.x
if !(det ≈ 0.0) # no intersection
u = (dy * bd.x - dx * bd.y) / det
v = (dy * ad.x - dx * ad.y) / det
if u > 0.0 && v > 0.0
return as + u*ad
end
end
# TODO - if det == 0 could be the case that they are colinear, and the first point of intersection should be taken
return VecE2(NaN,NaN) # no intersection
end
function Base.intersect(ray::Ray, seg::LineSegment)
R = VecE2(ray)
v₁ = VecE2(R) - seg.A
v₂ = seg.B - seg.A
v₃ = polar(1.0, ray.θ + π/2)
denom = v₂⋅v₃
if !isapprox(denom, 0.0, atol=1e-10)
t₁ = (v₂×v₁) / denom # time for ray (0 ≤ t₁)
t₂ = (v₁⋅v₃) / denom # time for segment (0 ≤ t₂ ≤ 1)
if 0 ≤ t₁ && 0 ≤ t₂ ≤ 1
return R + polar(t₁, ray.θ)
end
else
# denom is zero if the segment and the ray are parallel
# only collide if they are perfectly aligned
# must ensure that at least one point is in the positive ray direction
r = polar(1.0, ray.θ)
if are_collinear(R, seg.A, seg.B) &&
(r⋅(seg.A - R) ≥ 0 || r⋅(seg.B - R) ≥ 0)
return R
end
end
return VecE2(NaN,NaN) # no intersection
end
"""
What side of the ray you are on
Is -1 if on the left, 1 if on the right, and 0 if on the ray
"""
function get_side(ray::Ray, p::VecE2)
ab = polar(1.0, ray.θ)
return sign(ab.x*(p.y-ray.y) - ab.y*(p.x-ray.x))
end | AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1892 | export
Circ,
AABB,
AABB_center_length_width,
OBB
struct Circ{V<:AbstractVec}
c::V # center
r::Float64 # radius
end
Circ(x::Real, y::Real, r::Real) = Circ{VecE2}(VecE2(x, y), r)
Circ(x::Real, y::Real, z::Real, r::Real) = Circ{VecE3}(VecE3(x, y, z), r)
Base.:+(circ::Circ{VecE2}, v::VecE2) = Circ{VecE2}(circ.c + v, circ.r)
Base.:-(circ::Circ{VecE2}, v::VecE2) = Circ{VecE2}(circ.c - v, circ.r)
Base.in(p::V, circ::Circ{V}) where {V} = normsquared(circ.c - p) ≤ circ.r*circ.r
inertial2body(circ::Circ{VecE2}, reference::VecSE2) = Circ{VecE2}(inertial2body(circ.c, reference), circ.r)
# Axis-Aligned Bounding Box
struct AABB
center::VecE2
len::Float64 # length along x axis
wid::Float64 # width along y axis
end
function AABB(bot_left::VecE2, top_right::VecE2)
center = (bot_left + top_right)/2
Δ = top_right - bot_left
return AABB(center, abs(Δ.x), abs(Δ.y))
end
Base.:+(box::AABB, v::VecE2) = AABB(box.center + v, box.len, box.wid)
Base.:-(box::AABB, v::VecE2) = AABB(box.center - v, box.len, box.wid)
function Base.in(P::VecE2, box::AABB)
-box.len/2 ≤ P.x - box.center.x ≤ box.len/2 &&
-box.wid/2 ≤ P.y - box.center.y ≤ box.wid/2
end
# Oriented Bounding Box
struct OBB
aabb::AABB
θ::Float64
end
function OBB(center::VecE2, len::Float64, wid::Float64, θ::Float64)
del = VecE2(len/2, wid/2)
return OBB(AABB(center, len, wid), θ)
end
OBB(center::VecSE2, len::Float64, wid::Float64) = OBB(convert(VecE2, center), len, wid, center.θ)
Base.:+(box::OBB, v::VecE2) = OBB(box.aabb+v, box.θ)
Base.:-(box::OBB, v::VecE2) = OBB(box.aabb-v, box.θ)
function Base.in(P::VecE2, box::OBB)
C = box.aabb.center
in(rot(P-C, -box.θ)+C, box.aabb)
end
function inertial2body(box::OBB, reference::VecSE2)
c = VecSE2(box.aabb.center, box.θ)
c′ = inertial2body(c, reference)
OBB(c′, box.aabb.len, box.aabb.wid)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1700 | """
AccelDesang
An action type with a longitudinal acceleration and a desired heading angle
# Fields
- `a::Float64` longitudinal acceleration [m/s^2]
- `ϕdes::Float64` desired heading angle
"""
struct AccelDesang
a::Float64 # accel [m/s²]
ϕdes::Float64 # Desired heading angle
end
Base.show(io::IO, a::AccelDesang) = @printf(io, "AccelDesang(%6.3f,%6.3f)", a.a, a.ϕdes)
Base.length(::Type{AccelDesang}) = 2
"""
Base.convert(::Type{AccelDesang}, v::Vector{Float64})
Convert a vector containing the acceleration and desired heading angle
into AccelDesang type
"""
Base.convert(::Type{AccelDesang}, v::Vector{Float64}) = AccelDesang(v[1], v[2])
"""
Base.copyto!(v::Vector{Float64}, a::AccelDesang)
Extract the AccelDesang components into a vector and return the vector
"""
function Base.copyto!(v::Vector{Float64}, a::AccelDesang)
v[1] = a.a
v[2] = a.ϕdes
v
end
"""
propagate(veh::Entity{VehicleState,D,I}, action::AccelDesang, roadway::Roadway, Δt::Float64; n_integration_steps::Int=4) where {D,I}
Propagate vehicle forward in time using a desired acceleration
and heading angle
"""
function propagate(veh::Entity{VehicleState,D,I}, action::AccelDesang, roadway::Roadway, Δt::Float64; n_integration_steps::Int=4) where {D,I}
a = action.a # accel
ϕdes = action.ϕdes # desired heading angle
x, y, θ = posg(veh.state)
v = vel(veh.state)
δt = Δt/n_integration_steps
for i in 1 : n_integration_steps
posF = Frenet(VecSE2(x, y, θ), roadway)
ω = ϕdes - posF.ϕ
x += v*cos(θ)*δt
y += v*sin(θ)*δt
θ += ω*δt
v += a*δt
end
posG = VecSE2(x, y, θ)
VehicleState(posG, roadway, v)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1892 | """
AccelSteeringAngle
Allows driving the car in a circle based on the steering angle
If steering angle less than threshold 0.01 radian, just drives straight
# Fields
- `a::Float64` longitudinal acceleration [m/s^2]
- `δ::Float64` Steering angle [rad]
"""
struct AccelSteeringAngle
a::Float64 # accel [m/s²]
δ::Float64 # steering angle [rad]
end
Base.show(io::IO, a::AccelSteeringAngle) = @printf(io, "AccelSteeringAngle(%6.3f,%6.3f)", a.a, a.δ)
Base.length(::Type{AccelSteeringAngle}) = 2
"""
Take a vector containing acceleration and desired steering angle and
convert to AccelSteeringAngle
"""
Base.convert(::Type{AccelSteeringAngle}, v::Vector{Float64}) = AccelSteeringAngle(v[1], v[2])
"""
Extract acceleration and steering angle components from AccelSteeringAngle
and return them into a vector
"""
function Base.copyto!(v::Vector{Float64}, a::AccelSteeringAngle)
v[1] = a.a
v[2] = a.δ
v
end
"""
propagate vehicle forward in time given a desired acceleration and
steering angle. If steering angle higher than 0.1 radian, the vehicle
drives in a circle
"""
function propagate(veh::Entity{VehicleState, BicycleModel, Int}, action::AccelSteeringAngle, roadway::Roadway, Δt::Float64)
L = veh.def.a + veh.def.b
l = -veh.def.b
a = action.a # accel [m/s²]
δ = action.δ # steering wheel angle [rad]
x, y, θ = posg(veh.state)
v = vel(veh.state)
s = v*Δt + a*Δt*Δt/2 # distance covered
v′ = v + a*Δt
if abs(δ) < 0.01 # just drive straight
posG = posg(veh.state) + polar(s, θ)
else # drive in circle
R = L/tan(δ) # turn radius
β = s/R
xc = x - R*sin(θ) + l*cos(θ)
yc = y + R*cos(θ) + l*sin(θ)
θ′ = mod(θ+β, 2π)
x′ = xc + R*sin(θ+β) - l*cos(θ′)
y′ = yc - R*cos(θ+β) - l*sin(θ′)
posG = VecSE2(x′, y′, θ′)
end
VehicleState(posG, roadway, v′)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1314 | """
AccelTurnrate
An action type with a longitudinal acceleration and a turn rate
# Fields
- `a::Float64` longitudinal acceleration [m/s^2]
- `ω::Float64` desired turn rate [rad/sec]
"""
struct AccelTurnrate
a::Float64
ω::Float64
end
Base.show(io::IO, a::AccelTurnrate) = @printf(io, "AccelTurnrate(%6.3f,%6.3f)", a.a, a.ω)
Base.length(::Type{AccelTurnrate}) = 2
"""
Convert an input vector containing desired acceleration and turn rate
into the AccelTurnrate type
"""
Base.convert(::Type{AccelTurnrate}, v::Vector{Float64}) = AccelTurnrate(v[1], v[2])
"""
Return a vector containing the desired acceleration and turn rate
"""
function Base.copyto!(v::Vector{Float64}, a::AccelTurnrate)
v[1] = a.a
v[2] = a.ω
v
end
"""
Propagate vehicle forward in time using a desired acceleration and
turn rate
"""
function propagate(veh::Entity{VehicleState,D,I}, action::AccelTurnrate, roadway::Roadway, Δt::Float64; n_integration_steps::Int=4) where {D,I}
a = action.a # accel
ω = action.ω # turnrate
x, y, θ = posg(veh.state)
v = vel(veh.state)
δt = Δt / n_integration_steps
for i in 1 : n_integration_steps
x += v*cos(θ)*δt
y += v*sin(θ)*δt
θ += ω*δt
v += a*δt
end
posG = VecSE2(x, y, θ)
VehicleState(posG, roadway, v)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1379 | """
propagate(veh::Entity{S,D,I}, action::A, roadway::R, Δt::Float64) where {S,D,I,A,R}
Take an entity of type {S,D,I} and move it over Δt seconds to produce a new
entity based on the action on the given roadway.
"""
propagate(veh::Entity{S,D,I}, action::A, roadway::R, Δt::Float64) where {S,D,I,A,R} = error("propagate not implemented for Entity{$S, $D, $I}, actions $A, and roadway $R")
propagate(veh::Entity{S,D,I}, state::S, roadway::R, Δt::Float64) where {S,D,I,R} = state
"""
Mapping from actions to entity ids. The main use case is for keeping track of the action history
in the same way as the scene history in the `simulate!` function.
Initialize as
EntityAction(a, id)
where `a` is an action which can be used to propagate entities. `id` is the entity identifier.
"""
struct EntityAction{A,I}
action::A
id::I
end
function Base.findfirst(id, scene::Scene{A}) where {A<:EntityAction}
for am_index in 1 : scene.n
am = scene.entities[am_index]
if am.id == id
return am_index
end
end
return nothing
end
function id2index(scene::Scene{A}, id) where {A<:EntityAction}
entity_index = findfirst(id, scene)
if (entity_index === nothing)
throw(BoundsError(scene, [id]))
end
return entity_index
end
get_by_id(scene::Scene{A}, id) where {A<:EntityAction} = scene[id2index(scene, id)]
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 757 | """
LaneFollowingAccel
Longitudinal acceleration.
The resulting vehicle velocity is capped below at 0 (i.e. standstill). Negative velocities are not allowed.
# Fields
- `a::Float64` longitudinal acceleration [m/s^2]
"""
struct LaneFollowingAccel
a::Float64
end
function propagate(veh::Entity{VehicleState,D,I}, action::LaneFollowingAccel, roadway::Roadway, ΔT::Float64) where {D,I}
a_lon = action.a
ds = vel(veh.state)
ΔT² = ΔT*ΔT
Δs = ds*ΔT + 0.5*a_lon*ΔT²
v₂ = max(ds + a_lon*ΔT, 0.) # no negative velocities
roadind = move_along(posf(veh.state).roadind, roadway, Δs)
posG = roadway[roadind].pos
posF = Frenet(roadind, roadway, t=posf(veh.state).t, ϕ=posf(veh.state).ϕ)
VehicleState(posG, posF, v₂)
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1502 | """
LatLonAccel
Acceleration in the frenet frame
# Fields
- `a_lat::Float64` Lateral acceleration [m/s^2]
- `a_lon::Float64` Longitudinal acceleration [m/s^2]
"""
struct LatLonAccel
a_lat::Float64
a_lon::Float64
end
LatLonAccel(alat::Float64, alon::LaneFollowingAccel) = LatLonAccel(alat, alon.a)
Base.show(io::IO, a::LatLonAccel) = @printf(io, "LatLonAccel(%6.3f, %6.3f)", a.a_lat, a.a_lon)
Base.length(::Type{LatLonAccel}) = 2
Base.convert(::Type{LatLonAccel}, v::Vector{Float64}) = LatLonAccel(v[1], v[2])
function Base.copyto!(v::Vector{Float64}, a::LatLonAccel)
v[1] = a.a_lat
v[2] = a.a_lon
v
end
function propagate(veh::Entity{VehicleState, D, I}, action::LatLonAccel, roadway::Roadway, ΔT::Float64) where {D, I}
a_lat = action.a_lat
a_lon = action.a_lon
v = vel(veh.state)
ϕ = posf(veh.state).ϕ
ds = v*cos(ϕ)
t = posf(veh.state).t
dt = v*sin(ϕ)
ΔT² = ΔT*ΔT
Δs = ds*ΔT + 0.5*a_lon*ΔT²
Δt = dt*ΔT + 0.5*a_lat*ΔT²
ds₂ = ds + a_lon*ΔT
dt₂ = dt + a_lat*ΔT
speed₂ = sqrt(dt₂*dt₂ + ds₂*ds₂)
v₂ = sqrt(dt₂*dt₂ + ds₂*ds₂) # v is the magnitude of the velocity vector
ϕ₂ = atan(dt₂, ds₂)
roadind = move_along(posf(veh.state).roadind, roadway, Δs)
footpoint = roadway[roadind]
posG = VecE2{Float64}(footpoint.pos.x,footpoint.pos.y) + polar(t + Δt, footpoint.pos.θ + π/2)
posG = VecSE2{Float64}(posG.x, posG.y, footpoint.pos.θ + ϕ₂)
veh = VehicleState(posG, roadway, v₂)
return veh
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 988 | """
PedestrianLatLonAccel
Pedestrian walking action. Acceleration in the Frenet frame, along with
desired lane after crossing the street.
# Fields
- `a_lat::Float64` lateral acceleration [m/s^2]
- `a_lon::Float64` longitudinal accelaration [m/s^2]
- `lane_des::Lane` desired lane to move to
"""
struct PedestrianLatLonAccel
a_lat::Float64
a_lon::Float64
lane_des::Lane
end
function AutomotiveSimulator.propagate(ped::Entity{VehicleState, D, I},
action::PedestrianLatLonAccel,
roadway::Roadway,
ΔT::Float64=0.1
) where {D,I}
state = propagate(ped, LatLonAccel(action.a_lat, action.a_lon), roadway, ΔT)
roadproj = proj(posg(state), roadway[action.lane_des.tag], roadway, move_along_curves=false)
retval = VehicleState(Frenet(roadproj, roadway), roadway, vel(state))
return retval
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 3032 | """
AbstractAgentDefinition
An Agent definition represents static parameters characterizing an agent,
such as its physical dimensions.
"""
abstract type AbstractAgentDefinition end
"""
length(def::AbstractAgentDefinition)
return the length of the vehicle
"""
Base.length(def::AbstractAgentDefinition) = error("length not implemented for agent definition of type $(typeof(def))")
"""
width(def::AbstractAgentDefinition)
return the width of the vehicle
"""
width(def::AbstractAgentDefinition) = error("width not implemented for agent definition of type $(typeof(def))")
"""
class(def::AbstractAgentDefinition)
return the class of the vehicle
"""
class(def::AbstractAgentDefinition) = error("class not implemented for agent definition of type $(typeof(def))")
"""
A module to represent the different class of agents:
- Motorcycle
- Car
- Truck
- Pedestrian
"""
module AgentClass
const MOTORCYCLE = 1
const CAR = 2
const TRUCK = 3
const PEDESTRIAN = 4
end
"""
VehicleDef(;class::Float64, length::Float64, width::Float64)
Vehicle definition which contains a class and a bounding box.
"""
struct VehicleDef <: AbstractAgentDefinition
class::Int64 # ∈ AgentClass
length::Float64
width::Float64
end
function VehicleDef(;class::Int64 = AgentClass.CAR,
length::Float64 = 4.0,
width::Float64 = 1.8)
return VehicleDef(class, length, width)
end
Base.length(d::VehicleDef) = d.length
width(d::VehicleDef) = d.width
class(d::VehicleDef) = d.class
const NULL_VEHICLEDEF = VehicleDef(AgentClass.CAR, NaN, NaN)
function Base.show(io::IO, d::VehicleDef)
class = d.class == AgentClass.CAR ? "CAR" :
d.class == AgentClass.MOTORCYCLE ? "MOTORCYCLE" :
d.class == AgentClass.TRUCK ? "TRUCK" :
d.class == AgentClass.PEDESTRIAN ? "PEDESTRIAN" :
"UNKNOWN"
@printf(io, "VehicleDef(%s, %.3f, %.3f)", class, d.length, d.width)
end
Base.write(io::IO, def::VehicleDef) = @printf(io, "%d %.16e %.16e", def.class, def.length, def.width)
function Base.read(io::IO, ::Type{VehicleDef})
tokens = split(strip(readline(io)), ' ')
class = parse(Int, tokens[1])
length = parse(Float64, tokens[2])
width = parse(Float64, tokens[3])
return VehicleDef(class, length, width)
end
"""
BicycleModel
BicycleModel(def::VehicleDef; a::Float64 = 1.5, b::Float64 = 1.5)
Vehicle definition representing the bicycle model
# Fields
- `def::VehicleDef`
- `a::Float64` distance between cg and front axle [m]
- `b::Float64` distance between cg and rear axle [m]
"""
struct BicycleModel <: AbstractAgentDefinition
def::VehicleDef
a::Float64 # distance between cg and front axle [m]
b::Float64 # distance between cg and rear axle [m]
end
function BicycleModel(def::VehicleDef;
a::Float64 = 1.5,
b::Float64 = 1.5,
)
return BicycleModel(def, a, b)
end
Base.length(d::BicycleModel) = d.def.length
width(d::BicycleModel) = d.def.width
class(d::BicycleModel) = d.def.class
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 7551 | """
MOBIL
See Treiber & Kesting, 'Modeling Lane-Changing Decisions with MOBIL'
# Constructor
`MOBIL(;kwargs...)`
The keyword arguments are the fields highlighted below.
# Fields
- `dir::Int = DIR_MIDDLE`
- `mlon::LaneFollowingDriver=IntelligentDriverModel()`
- `safe_decel::Float64=2.0`
- `politeness::Float64=0.35`
- `advantage_threshold::Float64=0.1`
"""
@with_kw mutable struct MOBIL <: LaneChangeModel{LaneChangeChoice}
dir::Int64 = DIR_MIDDLE
mlon::LaneFollowingDriver = IntelligentDriverModel()
safe_decel::Float64 = 2.0 # safe deceleration (positive value)
politeness::Float64 = 0.35 # politeness factor (suggested p ∈ [0.2,0.5])
advantage_threshold::Float64 = 0.1 # Δaₜₕ
end
"""
Set the desired speed of the longitudinal model within MOBIL
"""
function set_desired_speed!(model::MOBIL, v_des::Float64)
set_desired_speed!(model.mlon, v_des)
model
end
function observe!(model::MOBIL, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
vehicle_index = findfirst(egoid, scene)
veh_ego = scene[vehicle_index]
v = vel(veh_ego.state)
egostate_M = veh_ego.state
ego_lane = get_lane(roadway, veh_ego)
fore_M = find_neighbor(scene, roadway, veh_ego, targetpoint_ego=VehicleTargetPointFront(), targetpoint_neighbor=VehicleTargetPointRear())
rear_M = find_neighbor(scene, roadway, veh_ego, rear=true, targetpoint_ego=VehicleTargetPointRear(), targetpoint_neighbor=VehicleTargetPointFront())
# accel if we do not make a lane change
accel_M_orig = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, egoid)).a
model.dir = DIR_MIDDLE
advantage_threshold = model.advantage_threshold
if n_lanes_left(roadway, ego_lane) > 0
rear_L = find_neighbor(scene, roadway, veh_ego,
lane=leftlane(roadway, veh_ego),
rear=true,
targetpoint_ego=VehicleTargetPointRear(),
targetpoint_neighbor=VehicleTargetPointFront())
# candidate position after lane change is over
footpoint = get_footpoint(veh_ego)
lane = get_lane(roadway, veh_ego)
lane_L = roadway[LaneTag(lane.tag.segment, lane.tag.lane + 1)]
roadproj = proj(footpoint, lane_L, roadway)
frenet_L = Frenet(RoadIndex(roadproj), roadway)
egostate_L = VehicleState(frenet_L, roadway, vel(veh_ego.state))
Δaccel_n = 0.0
passes_safety_criterion = true
if rear_L.ind != nothing
id = scene[rear_L.ind].id
accel_n_orig = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# update ego state in scene
scene[vehicle_index] = Entity(veh_ego, egostate_L)
accel_n_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
body = inertial2body(get_rear(scene[vehicle_index]), get_front(scene[rear_L.ind])) # project ego to be relative to target
s_gap = body.x
# restore ego state
scene[vehicle_index] = veh_ego
passes_safety_criterion = accel_n_test ≥ -model.safe_decel && s_gap ≥ 0
Δaccel_n = accel_n_test - accel_n_orig
end
if passes_safety_criterion
Δaccel_o = 0.0
if rear_M.ind != nothing
id = scene[rear_M.ind].id
accel_o_orig = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# update ego state in scene
scene[vehicle_index] = Entity(veh_ego, egostate_L)
accel_o_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# restore ego state
scene[vehicle_index] = veh_ego
Δaccel_o = accel_o_test - accel_o_orig
end
# update ego state in scene
scene[vehicle_index] = Entity(veh_ego, egostate_L)
accel_M_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, egoid)).a
# restore ego state
scene[vehicle_index] = veh_ego
Δaccel_M = accel_M_test - accel_M_orig
Δaₜₕ = Δaccel_M + model.politeness*(Δaccel_n + Δaccel_o)
if Δaₜₕ > advantage_threshold
model.dir = DIR_LEFT
advantage_threshold = Δaₜₕ
end
end
end
if n_lanes_right(roadway, ego_lane) > 0
rear_R = find_neighbor(scene, roadway, veh_ego, lane=rightlane(roadway, veh_ego), targetpoint_ego=VehicleTargetPointRear(), targetpoint_neighbor=VehicleTargetPointFront())
# candidate position after lane change is over
footpoint = get_footpoint(veh_ego)
lane = roadway[veh_ego.state.posF.roadind.tag]
lane_R = roadway[LaneTag(lane.tag.segment, lane.tag.lane - 1)]
roadproj = proj(footpoint, lane_R, roadway)
frenet_R = Frenet(RoadIndex(roadproj), roadway)
egostate_R = VehicleState(frenet_R, roadway, vel(veh_ego.state))
Δaccel_n = 0.0
passes_safety_criterion = true
if rear_R.ind != nothing
id = scene[rear_R.ind].id
accel_n_orig = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# update ego vehicle in scene
scene[vehicle_index] = Entity(veh_ego, egostate_R)
accel_n_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
body = inertial2body(get_rear(scene[vehicle_index]), get_front(scene[rear_R.ind])) # project ego to be relative to target
s_gap = body.x
# restore ego vehicle state
scene[vehicle_index] = veh_ego
passes_safety_criterion = accel_n_test ≥ -model.safe_decel && s_gap ≥ 0
Δaccel_n = accel_n_test - accel_n_orig
end
if passes_safety_criterion
Δaccel_o = 0.0
if rear_M.ind != nothing
id = scene[rear_M.ind].id
accel_o_orig = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# update ego vehicle in scene
scene[vehicle_index] = Entity(veh_ego, egostate_R)
accel_o_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, id)).a
# restore ego vehicle state
scene[vehicle_index] = veh_ego
Δaccel_o = accel_o_test - accel_o_orig
end
# update ego vehicle in scene
scene[vehicle_index] = Entity(veh_ego, egostate_R)
accel_M_test = rand(observe!(reset_hidden_state!(model.mlon), scene, roadway, egoid)).a
# restor ego vehicle state
scene[vehicle_index] = veh_ego
Δaccel_M = accel_M_test - accel_M_orig
Δaₜₕ = Δaccel_M + model.politeness*(Δaccel_n + Δaccel_o)
if Δaₜₕ > advantage_threshold
model.dir = DIR_RIGHT
advantage_threshold = Δaₜₕ
elseif Δaₜₕ == advantage_threshold
# in case of tie, if we are accelerating we go left, else, right
if Δaccel_M > model.politeness*(Δaccel_n + Δaccel_o)
model.dir = DIR_LEFT
else
model.dir = DIR_RIGHT
end
end
end
end
model
end
Base.rand(rng::AbstractRNG, model::MOBIL) = LaneChangeChoice(model.dir)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 3654 | """
IntelligentDriverModel <: LaneFollowingDriver
The Intelligent Driver Model. A rule based driving model that is governed by parameter
settings. The output is an longitudinal acceleration.
Here, we have extended IDM to the errorable IDM. If a standard deviation parameter is
specified, then the output is a longitudinal acceleration sampled from a normal distribution
around the non-errorable IDM output.
# Fields
- `a::Float64 = NaN` the predicted acceleration i.e. the output of the model
- `σ::Float64 = NaN` allows errorable IDM, optional stdev on top of the model, set to zero or NaN for deterministic behavior
- `k_spd::Float64 = 1.0` proportional constant for speed tracking when in freeflow [s⁻¹]
- `δ::Float64 = 4.0` acceleration exponent
- `T::Float64 = 1.5` desired time headway [s]
- `v_des::Float64 = 29.0` desired speed [m/s]
- `s_min::Float64 = 5.0` minimum acceptable gap [m]
- `a_max::Float64 = 3.0` maximum acceleration ability [m/s²]
- `d_cmf::Float64 = 2.0` comfortable deceleration [m/s²] (positive)
- `d_max::Float64 = 9.0` maximum deceleration [m/s²] (positive)
"""
@with_kw mutable struct IntelligentDriverModel <: LaneFollowingDriver
a::Float64 = NaN # predicted acceleration
σ::Float64 = NaN # optional stdev on top of the model, set to zero or NaN for deterministic behavior
k_spd::Float64 = 1.0 # proportional constant for speed tracking when in freeflow [s⁻¹]
δ::Float64 = 4.0 # acceleration exponent [-]
T::Float64 = 1.5 # desired time headway [s]
v_des::Float64 = 29.0 # desired speed [m/s]
s_min::Float64 = 5.0 # minimum acceptable gap [m]
a_max::Float64 = 3.0 # maximum acceleration ability [m/s²]
d_cmf::Float64 = 2.0 # comfortable deceleration [m/s²] (positive)
d_max::Float64 = 9.0 # maximum deceleration [m/s²] (positive)
end
function set_desired_speed!(model::IntelligentDriverModel, v_des::Float64)
model.v_des = v_des
model
end
function track_longitudinal!(model::IntelligentDriverModel, v_ego::Float64, v_oth::Float64, headway::Float64)
if !isnan(v_oth)
@assert !isnan(headway)
if headway < 0.0
@debug("IntelligentDriverModel Warning: IDM received a negative headway $headway"*
", a collision may have occured.")
model.a = -model.d_max
else
Δv = v_oth - v_ego
s_des = model.s_min + v_ego*model.T - v_ego*Δv / (2*sqrt(model.a_max*model.d_cmf))
v_ratio = model.v_des > 0.0 ? (v_ego/model.v_des) : 1.0
model.a = model.a_max * (1.0 - v_ratio^model.δ - (s_des/headway)^2)
end
else
# no lead vehicle, just drive to match desired speed
Δv = model.v_des - v_ego
model.a = Δv*model.k_spd # predicted accel to match target speed
end
@assert !isnan(model.a)
model.a = clamp(model.a, -model.d_max, model.a_max)
return model
end
reset_hidden_state!(model::IntelligentDriverModel) = model
function Base.rand(rng::AbstractRNG, model::IntelligentDriverModel)
if isnan(model.σ) || model.σ ≤ 0.0
return LaneFollowingAccel(model.a)
else
LaneFollowingAccel(rand(rng, Normal(model.a, model.σ)))
end
end
function Distributions.pdf(model::IntelligentDriverModel, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
return a == model.a ? Inf : 0.
else
return pdf(Normal(model.a, model.σ), a.a)
end
end
function Distributions.logpdf(model::IntelligentDriverModel, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
return a == model.a ? Inf : 0.
else
return logpdf(Normal(model.a, model.σ), a.a)
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2564 | """
DriverModel{DriveAction}
A DriverModel represents a specific driving behavior.
It specifies the action taken by the agent at a given scene.
The ation will be of type `DriveAction`.
It can be interpreted as a distribution, the likelihood of taking a certain action
in a given scene.
The DriverModel type is an abstract type! Custom driver models should inherit from it.
"""
abstract type DriverModel{DriveAction} end
"""
action_type(::DriverModel{A}) where {A}
returns the type of the actions that are sampled from the model
"""
action_type(::DriverModel{A}) where {A} = A
"""
set_desired_speed!(model::DriverModel, v_des::Float64)
Sets a desired speed.
This method is relevant for models like IDM where the vehicle tracks a nominal speed.
"""
function set_desired_speed! end
"""
reset_hidden_state!(model::DriverModel)
Resets the hidden states of the model.
"""
function reset_hidden_state! end
"""
reset_hidden_states!(models::Dict{I,M}) where {M<:DriverModel}
reset hidden states of all driver models in `models`
"""
function reset_hidden_states!(models::Dict{I,M}) where {I, M<:DriverModel}
for model in values(models)
reset_hidden_state!(model)
end
return models
end
"""
observe!(model::DriverModel, scene, roadway, egoid)
Observes the scene and updates the model states accordingly.
"""
function observe! end
"""
rand(model::DriverModel)
rand(rng::AbstractRNG, model::DriverModel)
Samples an action from the model.
"""
Base.rand(model::DriverModel) = rand(Random.GLOBAL_RNG, model)
Base.rand(rng::AbstractRNG, model::DriverModel) = error("AutomotiveSimulatorError: Base.rand(::AbstractRNG, ::$(typeof(model))) not implemented")
####
"""
StaticDriver{A,P<:ContinuousMultivariateDistribution} <: DriverModel{A}
A driver model where actions are always sampled by the same distribution specified
by the field `distribution`.
# Fields
- `distribution::P`
"""
struct StaticDriver{A,P<:ContinuousMultivariateDistribution} <: DriverModel{A}
distribution::P
end
function Base.rand(rng::AbstractRNG, model::StaticDriver{A,P}) where {A,P}
a = rand(rng, model.distribution)
return convert(A, a)
end
Distributions.pdf(model::StaticDriver{A}, a::A) where {A} = pdf(model.distribution, convert(Vector{Float64}, a))
Distributions.logpdf(model::StaticDriver{A}, a::A) where {A} = logpdf(model.distribution, convert(Vector{Float64}, a))
set_desired_speed!(model::StaticDriver, v::Float64) = model
observe!(model::StaticDriver, scene::S, roadway::Roadway, egoid::I) where {S, I} = model
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 763 | """
LaneChangeChoice
A choice of whether to change lanes, and what direction to do it in
"""
const DIR_RIGHT = -1
const DIR_MIDDLE = 0
const DIR_LEFT = 1
struct LaneChangeChoice
dir::Int # -1, 0, 1
end
Base.show(io::IO, a::LaneChangeChoice) = @printf(io, "LaneChangeChoice(%d)", a.dir)
function get_lane_offset(a::LaneChangeChoice, scene::Scene, roadway::Roadway, vehicle_index::Int)
if a.dir == DIR_MIDDLE
return posf(scene[vehicle_index].state).t
elseif a.dir == DIR_LEFT
return lane_offset_left(roadway, scene[vehicle_index])
else
@assert(a.dir == DIR_RIGHT)
return lane_offset_right(roadway, scene[vehicle_index])
end
end
####################
abstract type LaneChangeModel{A} <: DriverModel{A} end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1304 | abstract type LaneFollowingDriver <: DriverModel{LaneFollowingAccel} end
track_longitudinal!(model::LaneFollowingDriver, v_ego::Float64, v_oth::Float64, headway::Float64) = model # do nothing by default
function observe!(model::LaneFollowingDriver, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
ego = get_by_id(scene, egoid)
fore = find_neighbor(scene, roadway, ego, targetpoint_ego=VehicleTargetPointFront(), targetpoint_neighbor=VehicleTargetPointRear())
v_ego = vel(ego)
v_oth = NaN
headway = NaN
if fore.ind != nothing
v_oth = vel(scene[fore.ind].state)
headway = fore.Δs
end
track_longitudinal!(model, v_ego, v_oth, headway)
return model
end
mutable struct StaticLaneFollowingDriver <: LaneFollowingDriver
a::LaneFollowingAccel
end
StaticLaneFollowingDriver() = StaticLaneFollowingDriver(LaneFollowingAccel(0.0))
StaticLaneFollowingDriver(a::Float64) = StaticLaneFollowingDriver(LaneFollowingAccel(a))
Base.rand(rng::AbstractRNG, model::StaticLaneFollowingDriver) = model.a
Distributions.pdf(model::StaticLaneFollowingDriver, a::LaneFollowingAccel) = isapprox(a.a, model.a.a) ? Inf : 0.0
Distributions.logpdf(model::StaticLaneFollowingDriver, a::LaneFollowingAccel) = isapprox(a.a, model.a.a) ? Inf : -Inf
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1039 | struct LatLonSeparableDriver{A} <: DriverModel{A}
mlat::LateralDriverModel
mlon::LaneFollowingDriver
end
LatLonSeparableDriver(mlat::LateralDriverModel, mlon::LaneFollowingDriver) = LatLonSeparableDriver{LatLonAccel}(mlat, mlon)
function set_desired_speed!(model::LatLonSeparableDriver, v_des::Float64)
set_desired_speed!(model.mlon, v_des)
model
end
function observe!(model::LatLonSeparableDriver, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
observe!(model.mlat, scene, roadway, egoid)
observe!(model.mlon, scene, roadway, egoid)
model
end
function Base.rand(rng::AbstractRNG, model::LatLonSeparableDriver{A}) where A
action_lat = rand(rng, model.mlat)
action_lon = rand(rng, model.mlon)
A(action_lat, action_lon)
end
Distributions.pdf(model::LatLonSeparableDriver{A}, a::A) where A = pdf(model.mlat, a.a_lat) * pdf(model.mlon, a.a_lon)
Distributions.logpdf(model::LatLonSeparableDriver{A}, a::A) where A = logpdf(model.mlat, a.a_lat) + logpdf(model.mlon, a.a_lon)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2102 | abstract type LateralDriverModel{A} <: DriverModel{A} end
# TODO: Why does this struct live in the `lateral_driver_models.jl` file
"""
ProportionalLaneTracker
A controller that executes the lane change decision made by the `lane change models`
# Constructors
ProportionalLaneTracker(;σ::Float64 = NaN,kp::Float64 = 3.0,kd::Float64 = 2.0)
# Fields
- `a::Float64 = NaN` predicted acceleration
- `σ::Float64 = NaN` optional stdev on top of the model, set to zero or NaN for deterministic behavior
- `kp::Float64 = 3.0` proportional constant for lane tracking
- `kd::Float64 = 2.0` derivative constant for lane tracking
"""
@with_kw mutable struct ProportionalLaneTracker <: LateralDriverModel{Float64}
a::Float64 = NaN # predicted acceleration
σ::Float64 = NaN # optional stdev on top of the model, set to zero or NaN for deterministic behavior
kp::Float64 = 3.0 # proportional constant for lane tracking
kd::Float64 = 2.0 # derivative constant for lane tracking
end
function track_lateral!(model::ProportionalLaneTracker, laneoffset::Float64, lateral_speed::Float64)
model.a = -laneoffset*model.kp - lateral_speed*model.kd
model
end
function observe!(model::ProportionalLaneTracker, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
ego_index = findfirst(egoid, scene)
veh_ego = scene[ego_index]
t = posf(veh_ego.state).t # lane offset
dt = velf(veh_ego.state).t # rate of change of lane offset
model.a = -t*model.kp - dt*model.kd
model
end
function Base.rand(rng::AbstractRNG, model::ProportionalLaneTracker)
if isnan(model.σ) || model.σ ≤ 0.0
model.a
else
rand(rng, Normal(model.a, model.σ))
end
end
function Distributions.pdf(model::ProportionalLaneTracker, a_lat::Float64)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
pdf(Normal(model.a, model.σ), a_lat)
end
end
function Distributions.logpdf(model::ProportionalLaneTracker, a_lat::Float64)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
logpdf(Normal(model.a, model.σ), a_lat)
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1768 | """
PrincetonDriver <: LaneFollowingDriver
A lane following driver model that controls longitudinal speed by following a front car.
# Fields
- `a::Float64`
- `σ::Float64 = NaN` optional stdev on top of the model, set to zero or NaN for deterministic behavior
- `k::Float64 = 1.0` proportional constant for speed tracking [s⁻¹]
- `v_des::Float64 = 29.0` desired speed [m/s]
"""
@with_kw mutable struct PrincetonDriver <: LaneFollowingDriver
a::Float64 = NaN # predicted acceleration
σ::Float64 = NaN# optional stdev on top of the model, set to zero or NaN for deterministic behavior
k::Float64 = 1.0 # proportional constant for speed tracking [s⁻¹]
v_des::Float64 = 29.0 # desired speed [m/s]
end
function set_desired_speed!(model::PrincetonDriver, v_des::Float64)
model.v_des = v_des
model
end
function track_longitudinal!(model::PrincetonDriver, v_ego::Float64, v_oth::Float64, headway::Float64)
v_des = model.v_des
k = model.k
if !isnan(v_oth)
v_des = min(v_oth*(1-exp(-k*headway/v_oth - 1)), v_des)
end
Δv = v_des - v_ego
model.a = Δv*k # predicted accel to match target speed
model
end
function Base.rand(rng::AbstractRNG, model::PrincetonDriver)
if isnan(model.σ) || model.σ ≤ 0.0
LaneFollowingAccel(model.a)
else
LaneFollowingAccel(rand(rng, Normal(model.a, model.σ)))
end
end
function Distributions.pdf(model::PrincetonDriver, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
pdf(Normal(model.a, model.σ), a.a)
end
end
function Distributions.logpdf(model::PrincetonDriver, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
logpdf(Normal(model.a, model.σ), a.a)
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 7483 | """
CrossingPhase
Crossing phases for SidewalkPedestrianModel.
For a crossing pedestrian, phases go: -2, -1, 0, 1
For a non-crossing pedestrian that pauses at the crosswalk, phases go: -2, -1, 1
For a non-crossing pedestrian that does not pause at the crosswalk, phases go: -2, 1
Model based on Feliciani et al (2017) - A simulation model for non-signalized pedestrian
crosswalks based on evidence from on field observation.
"""
struct CrossingPhase
phase::Int # -2, -1, 0, 1
end
const APPROACHING = -2 # Keep walking at constant speed
const APPRAISING = -1 # Decelerate until reach curbside
const CROSSING = 0 # Accelerate until desired speed
const LEAVING = 1 # Keep walking at constant speed
"""
SidewalkPedestrianModel
Walks along the sidewalk until approaching the crosswalk. Waits for the cars to pass, then crosses.
# Fields
- `timestep::Float64`
- `phase::Int = APPROACHING`
- `ttc_threshold::Float64 = clamp(rand(Normal(4.0, 2.5)), 1.0, Inf)`
- `crosswalk::Lane = Lane()`
- `sw_origin::Lane = Lane()`
- `sw_dest::Lane = Lane()`
- `a::PedestrianLatLonAccel = PedestrianLatLonAccel(0.0, 0.0, sw_origin)` makes you turn, left/right
- `σ::Float64 = NaN` optional stdev on top of the model, set to zero or NaN for deterministic
- `v_des_approach::Float64 = clamp(rand(Normal(1.28, 0.18)), 0.0, Inf)` Based on Feliciani et al. results
- `v_des_appraise::Float64 = clamp(rand(Normal(0.94, 0.21)), 0.0, Inf)`
- `v_des_cross::Float64 = clamp(rand(Normal(1.35, 0.18)), 0.0, Inf)`
- `ped_accel::Float64 = 0.30`
- `ped_decel::Float64 = -0.50`
"""
@with_kw mutable struct SidewalkPedestrianModel <: DriverModel{PedestrianLatLonAccel}
timestep::Float64
phase::Int = APPROACHING
ttc_threshold::Float64 = clamp(rand(Normal(4.0, 2.5)), 1.0, Inf)
crosswalk::Lane = Lane()
sw_origin::Lane = Lane()
sw_dest::Lane = Lane()
# conflict_lanes::Vector{Lane} = Lane[]
# Values from Feliciani
a::PedestrianLatLonAccel = PedestrianLatLonAccel(0.0, 0.0, sw_origin) # makes you turn, left/right
σ::Float64 = NaN # optional stdev on top of the model, set to zero or NaN for deterministic behavior
v_des_approach::Float64 = clamp(rand(Normal(1.28, 0.18)), 0.0, Inf) # Based on Feliciani et al. results
v_des_appraise::Float64 = clamp(rand(Normal(0.94, 0.21)), 0.0, Inf)
v_des_cross::Float64 = clamp(rand(Normal(1.35, 0.18)), 0.0, Inf)
ped_accel::Float64 = 0.30
ped_decel::Float64 = -0.50
# For graphing
pos_x = Float64[]
pos_y = Float64[]
velocities = Float64[]
phases = Int[]
end
Base.rand(rng::AbstractRNG, model::SidewalkPedestrianModel) = model.a
function AutomotiveSimulator.observe!(model::SidewalkPedestrianModel, scene::Scene{Entity{VehicleState, D, I}}, roadway::Roadway, egoid::I) where {D, I}
ped = scene[findfirst(egoid, scene)]
push!(model.pos_x, posg(ped.state).x)
push!(model.pos_y, posg(ped.state).y)
push!(model.velocities, vel(ped.state))
push!(model.phases, model.phase)
if model.phase == APPROACHING
update_approaching(ped, model, roadway, model.crosswalk, model.sw_origin)
elseif model.phase == APPRAISING
update_appraising(ped, scene, model, roadway, model.crosswalk, model.sw_origin)
elseif model.phase == CROSSING
update_crossing(ped, scene, model, roadway, model.crosswalk)
elseif model.phase == LEAVING
update_leaving(ped, scene, model, roadway, model.sw_dest)
else
# Model phase is out of bounds
end
end
function update_approaching(ped::Entity{S, D, I},
model::SidewalkPedestrianModel,
roadway::Roadway,
crosswalk::Lane,
sw_origin::Lane
) where {S, D, I}
# Keep walking at constant speed until close to crosswalk
# If waiting to cross, go to appraising. Else, go to leaving phase
Δs = get_distance_to_lane(ped, crosswalk, roadway)
if Δs < 3.0
model.phase = APPRAISING
end
end
function update_appraising(ped::Entity{S, D, I},
scene::Scene,
model::SidewalkPedestrianModel,
roadway::Roadway,
crosswalk::Lane,
sw_origin::Lane,
) where {S, D<:AbstractAgentDefinition, I}
Δs = get_distance_to_lane(ped, crosswalk, roadway)
if Δs < 0.1
set_inst_vel(ped, model, sw_origin, 0.0)
for veh in scene
if class(veh.def) == AgentClass.PEDESTRIAN
continue
end
# Check vehicle distance to the crosswalk
posG = posg(veh.state)
cw_posF = Frenet(posG, model.crosswalk, roadway)
lane = get_lane(roadway, veh)
Δs = get_distance_to_lane(veh, model.crosswalk, roadway)
ttc = Δs/vel(veh.state)
if 0.0 < ttc < model.ttc_threshold
return # Exit if any car is not within threshold
end
end
model.phase = CROSSING
else
# Decelerate to appraise speed
set_accel(ped, model, model.v_des_appraise, sw_origin)
end
end
function update_crossing(ped::Entity{S, D, I},
scene::Scene,
model::SidewalkPedestrianModel,
roadway::Roadway,
crosswalk::Lane
) where {S, D, I}
# Accelerate until desired speed
set_accel(ped, model, model.v_des_cross, crosswalk)
# If ped is at end of crosswalk, transition to leaving phase.
Δs = get_distance_to_lane(ped, model.sw_dest, roadway)
if Δs < 0.2
set_inst_vel(ped, model, model.crosswalk, 0.0)
model.phase = LEAVING
end
end
function update_leaving(ped::Entity{S, D, I},
scene::Scene,
model::SidewalkPedestrianModel,
roadway::Roadway,
sw_dest::Lane
) where {S, D, I}
# Keep walking at constant speed
set_accel(ped, model, model.v_des_approach, model.sw_dest)
end
function set_inst_vel(ped::Entity{VehicleState, D, I}, model::SidewalkPedestrianModel, lane_des::Lane, v_des::Float64) where {D, I}
model.a = PedestrianLatLonAccel(0.0, calc_a(vel(ped.state), v_des, model.timestep), lane_des)
end
function calc_a(v_init::Float64, v::Float64, Δt::Float64)
return (v - v_init)/Δt
end
function set_accel(ped::Entity{VehicleState, D, I}, model::SidewalkPedestrianModel, v_des::Float64, lane_des::Lane) where {D, I}
if vel(ped.state) < v_des
model.a = PedestrianLatLonAccel(0.0, model.ped_accel, lane_des)
elseif vel(ped.state) > v_des
model.a = PedestrianLatLonAccel(0.0, model.ped_decel, lane_des)
else
model.a = PedestrianLatLonAccel(0.0, 0.0, lane_des)
end
end
# Edited from AutomotivePOMDPs.jl - projects collision point onto the lane.
# Should work in any orientation.
function get_distance_to_lane(veh::Entity{VehicleState, D, I}, lane_des::Lane, roadway::Roadway) where {D, I}
vehlane = get_lane(roadway, veh)
lane_segment = LineSegment(lane_des.curve[1].pos, lane_des.curve[end].pos)
collision_point = intersect(Ray(posg(veh.state)), lane_segment)
lane_des_proj = Frenet(VecSE2(collision_point), vehlane, roadway)
Δs = lane_des_proj.s - posf(veh.state).s
return Δs
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 1755 | """
ProportionalSpeedTracker <: LaneFollowingDriver
Longitudinal proportional speed control.
# Fields
- `a::Float64 = NaN` predicted acceleration
- `σ::Float64 = NaN` optional stdev on top of the model, set to zero or NaN for deterministic behavior
- `k::Float64 = 1.0` proportional constant for speed tracking [s⁻¹]
- `v_des::Float64 = 29.0` desired speed [m/s]
"""
@with_kw mutable struct ProportionalSpeedTracker <: LaneFollowingDriver
a::Float64 = NaN # predicted acceleration
σ::Float64 = NaN# optional stdev on top of the model, set to zero or NaN for deterministic behavior
k::Float64 = 1.0# proportional constant for speed tracking [s⁻¹]
v_des::Float64 = 29.0 # desired speed [m/s]
end
function set_desired_speed!(model::ProportionalSpeedTracker, v_des::Float64)
model.v_des = v_des
model
end
function track_longitudinal!(model::ProportionalSpeedTracker, v_ego::Float64, v_oth::Float64, headway::Float64)
Δv = model.v_des - v_ego
model.a = Δv*model.k # predicted accel to match target speed
return model
end
reset_hidden_state!(model::ProportionalSpeedTracker) = model
function Base.rand(rng::AbstractRNG, model::ProportionalSpeedTracker)
if isnan(model.σ) || model.σ ≤ 0.0
LaneFollowingAccel(model.a)
else
LaneFollowingAccel(rand(rng, Normal(model.a, model.σ)))
end
end
function Distributions.pdf(model::ProportionalSpeedTracker, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
pdf(Normal(model.a, model.σ), a.a)
end
end
function Distributions.logpdf(model::ProportionalSpeedTracker, a::LaneFollowingAccel)
if isnan(model.σ) || model.σ ≤ 0.0
Inf
else
logpdf(Normal(model.a, model.σ), a.a)
end
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 2700 | """
Tim2DDriver
Driver that combines longitudinal driver and lateral driver into one model.
# Constructors
`Tim2DDriver(;kwargs...)`
The keywords argument are the fields described below.
# Fields
- `mlon::LaneFollowingDriver = IntelligentDriverModel()` Longitudinal driving model
- `mlat::LateralDriverModel = ProportionalLaneTracker()` Lateral driving model
- `mlane::LaneChangeModel =TimLaneChanger` Lane change model
"""
@with_kw mutable struct Tim2DDriver <: DriverModel{LatLonAccel}
mlon::LaneFollowingDriver = IntelligentDriverModel()
mlat::LateralDriverModel = ProportionalLaneTracker()
mlane::LaneChangeModel = TimLaneChanger()
end
function set_desired_speed!(model::Tim2DDriver, v_des::Float64)
set_desired_speed!(model.mlon, v_des)
set_desired_speed!(model.mlane, v_des)
model
end
function track_longitudinal!(driver::LaneFollowingDriver, scene::Scene{Entity{VehicleState, D, I}}, roadway::Roadway, vehicle_index::Int64, fore::NeighborLongitudinalResult) where {D, I}
v_ego = vel(scene[vehicle_index].state)
if fore.ind != nothing
headway, v_oth = fore.Δs, vel(scene[fore.ind].state)
else
headway, v_oth = NaN, NaN
end
return track_longitudinal!(driver, v_ego, v_oth, headway)
end
function observe!(driver::Tim2DDriver, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
observe!(driver.mlane, scene, roadway, egoid)
vehicle_index = findfirst(egoid, scene)
ego = scene[vehicle_index]
lane_change_action = rand(driver.mlane)
laneoffset = get_lane_offset(lane_change_action, scene, roadway, vehicle_index)
lateral_speed = velf(scene[vehicle_index]).t
if lane_change_action.dir == DIR_MIDDLE
target_lane = get_lane(roadway, ego)
elseif lane_change_action.dir == DIR_LEFT
target_lane = leftlane(roadway, ego)
else
@assert(lane_change_action.dir == DIR_RIGHT)
target_lane = rightlane(roadway, ego)
end
fore = find_neighbor(scene, roadway, ego,
lane=target_lane,
targetpoint_ego=VehicleTargetPointFront(),
targetpoint_neighbor=VehicleTargetPointRear())
track_lateral!(driver.mlat, laneoffset, lateral_speed)
track_longitudinal!(driver.mlon, scene, roadway, vehicle_index, fore)
driver
end
Base.rand(rng::AbstractRNG, driver::Tim2DDriver) = LatLonAccel(rand(rng, driver.mlat), rand(rng, driver.mlon).a)
Distributions.pdf(driver::Tim2DDriver, a::LatLonAccel) = pdf(driver.mlat, a.a_lat) * pdf(driver.mlon, a.a_lon)
Distributions.logpdf(driver::Tim2DDriver, a::LatLonAccel) = logpdf(driver.mlat, a.a_lat) * logpdf(driver.mlon, a.a_lon)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 4736 | """
TimLaneChanger
A simple lane change behavior that changes lanes whenever the lead car is going slower than our desired speed.
Lane changes are made when there is an available lane, fore/rear gaps exceed our thresholds, we are faster
than a rear vehicle in the target lane, and any lead vehicle in the target lane is faster than we can currently go.
Has not been published anywhere, so first use in a paper would have to describe this.
See MOBIL if you want a lane changer you can cite.
# Constructors
TimLaneChanger(v_des::Float64=29.0, threshold_fore::Float64 = 50.0,threshold_lane_change_gap_fore::Float64 = 10.0, threshold_lane_change_gap_rear::Float64 = 10.0,dir::Int=DIR_MIDDLE)
# Fields
- `dir::Int = DIR_MIDDLE` the desired lane to go to eg: left,middle (i.e. stay in same lane) or right
- `v_des::Float64 = 29.0` desired velocity
- `threshold_fore::Float64 = 50.0` Distance from lead vehicle
- `threshold_lane_change_gap_fore::Float64 = 10.0` Space in front
- `threshold_lane_change_gap_rear::Float64 = 10.0` Space rear
"""
@with_kw mutable struct TimLaneChanger <: LaneChangeModel{LaneChangeChoice}
dir::Int = DIR_MIDDLE
v_des::Float64 = 29.0
threshold_fore::Float64 = 50.0
threshold_lane_change_gap_fore::Float64 = 10.0
threshold_lane_change_gap_rear::Float64 = 10.0
end
function set_desired_speed!(model::TimLaneChanger, v_des::Float64)
model.v_des = v_des
model
end
function observe!(model::TimLaneChanger, scene::Scene{Entity{S, D, I}}, roadway::Roadway, egoid::I) where {S, D, I}
vehicle_index = findfirst(egoid, scene)
veh_ego = scene[vehicle_index]
v = vel(veh_ego.state)
left_lane_exists = has_lane_left(roadway, veh_ego)
right_lane_exists = has_lane_right(roadway, veh_ego)
fore_M = find_neighbor(scene, roadway, veh_ego,
targetpoint_ego = VehicleTargetPointFront(),
targetpoint_neighbor = VehicleTargetPointRear(),
max_distance = model.threshold_fore)
fore_L = find_neighbor(scene, roadway, veh_ego,
targetpoint_ego = VehicleTargetPointFront(),
targetpoint_neighbor = VehicleTargetPointRear(),
lane = leftlane(roadway, veh_ego)
)
fore_R = find_neighbor(scene, roadway, veh_ego,
targetpoint_ego = VehicleTargetPointFront(),
targetpoint_neighbor = VehicleTargetPointRear(),
lane = rightlane(roadway, veh_ego))
rear_L = find_neighbor(scene, roadway, veh_ego,
targetpoint_ego = VehicleTargetPointFront(),
targetpoint_neighbor = VehicleTargetPointRear(),
lane = leftlane(roadway, veh_ego), rear=true)
rear_R = find_neighbor(scene, roadway, veh_ego,
targetpoint_ego = VehicleTargetPointFront(),
targetpoint_neighbor = VehicleTargetPointRear(),
lane = rightlane(roadway, veh_ego), rear=true)
model.dir = DIR_MIDDLE
if fore_M.Δs < model.threshold_fore # there is a lead vehicle
veh_M = scene[fore_M.ind]
speed_M = vel(veh_M.state)
if speed_M ≤ min(model.v_des, v) # they are driving slower than we want
speed_ahead = speed_M
# consider changing to a different lane
if right_lane_exists &&
fore_R.Δs > model.threshold_lane_change_gap_rear && # there is space rear
rear_R.Δs > model.threshold_lane_change_gap_fore && # there is space fore
(rear_R.ind == nothing || vel(scene[rear_R.ind].state) ≤ v) && # we are faster than any follower
(fore_R.ind == nothing || vel(scene[fore_R.ind].state) > speed_ahead) # lead is faster than current speed
speed_ahead = fore_R.ind != nothing ? vel(scene[fore_R.ind].state) : Inf
model.dir = DIR_RIGHT
end
if left_lane_exists &&
fore_L.Δs > model.threshold_lane_change_gap_rear && # there is space rear
rear_L.Δs > model.threshold_lane_change_gap_fore && # there is space fore
(rear_L.ind == nothing || vel(scene[rear_L.ind].state) ≤ v) && # we are faster than any follower
(fore_L.ind == nothing || vel(scene[fore_L.ind].state) > speed_ahead) # lead is faster than current speed
speed_ahead = fore_L.ind != nothing ? vel(scene[fore_L.ind].state) : Inf
model.dir = DIR_LEFT
end
end
end
model
end
Base.rand(rng::AbstractRNG, model::TimLaneChanger) = LaneChangeChoice(model.dir)
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 328 | #=
TODO find an interface to keep both collision checkers around: Minkowski and Parallel Axis
abstract type CollisionChecker end
struct MinkowskiChecker <: CollisionChecker end
struct ParallelAxisChecker <: CollisionChecker end
is_colliding(vehA, vehB, collision_checker::AbstractCollisionChecker = ParallelAxisChecker())
=# | AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 17524 | # Collision Detection routines based on Minkowski techniques.
######################################
function cyclic_shift_left!(arr::AbstractVector, d::Int, n::Int)
#=
Perform a cyclic rotation of the elements in the array, in place
d = amount to shift
n = length of array (if we only want to work with first n elements)
=#
for i in 1 : gcd(d, n)
# move i-th values of blocks
temp = arr[i]
j = i
while true
k = j + d
if k > n
k = k - n
end
if k == i
break
end
arr[j] = arr[k]
j = k
end
arr[j] = temp
end
arr
end
######################################
function get_signed_area(pts::Vector{VecE2{Float64}}, npts::Int = length(pts))
# https://en.wikipedia.org/wiki/Shoelace_formula
# sign of -1 means clockwise, sign of 1 means counterclockwise
retval = pts[npts].x*pts[1].y - pts[1].x*pts[npts].y
for i in 1 : npts-1
retval += pts[i].x * pts[i+1].y
retval -= pts[i+1].x * pts[i].y
end
retval / 2
end
"""
get_edge(pts::Vector{VecE2{Float64}}, i::Int, npts::Int=length(pts))
returns the ith edge in pts
"""
function get_edge(pts::Vector{VecE2{Float64}}, i::Int, npts::Int=length(pts))
a = pts[i]
b = i+1 ≤ npts ? pts[i+1] : pts[1]
LineSegment(a,b)
end
######################################
"""
ConvexPolygon
ConvexPolygon(npts::Int)
ConvexPolygon(pts::Vector{VecE2{Float64}})
Mutable structure to represent a convex polygon. It is used by the Minkowski sum collision checker
# Fields
- `pts::Vector{VecE2{Float64}}`
- `npts::Int`
"""
mutable struct ConvexPolygon
pts::Vector{VecE2{Float64}} # ordered counterclockwise along polygon boundary s.t. first edge has minimum polar angle in [0,2π]
npts::Int # number of pts that are currently used (since we preallocate a longer array)
end
ConvexPolygon(npts::Int) = ConvexPolygon(Array{VecE2{Float64}}(undef, npts), 0)
ConvexPolygon(pts::Vector{VecE2{Float64}}) = ConvexPolygon(pts, length(pts))
function Base.iterate(poly::ConvexPolygon, i::Int=1)
if i > length(poly)
return nothing
end
return (poly.pts[i], i+1)
end
Base.length(poly::ConvexPolygon) = poly.npts
Base.isempty(poly::ConvexPolygon) = poly.npts == 0
get_edge(P::ConvexPolygon, i::Int) = get_edge(P.pts, i, P.npts)
get_signed_area(poly::ConvexPolygon) = get_signed_area(poly.pts, poly.npts)
function Base.empty!(poly::ConvexPolygon)
poly.npts = 0
poly
end
function Base.copyto!(dest::ConvexPolygon, src::ConvexPolygon)
dest.npts = src.npts
copyto!(dest.pts, 1, src.pts, 1, src.npts)
dest
end
function Base.push!(poly::ConvexPolygon, v::VecE2)
poly.pts[poly.npts+1] = v
poly.npts += 1
poly
end
function Base.in(v::VecE2, poly::ConvexPolygon)
# does NOT include pts on the physical boundary
previous_side = 0
for i in 1 : length(poly)
seg = get_edge(poly, i)
affine_segment = seg.B - seg.A
affine_point = v - seg.A
current_side = round(Int, sign(cross(affine_segment, affine_point))) # sign indicates side
if current_side == 0
# outside or over an edge
return false
elseif previous_side == 0
# first segment
previous_side = current_side
elseif previous_side != current_side
# all most be on the same side
return false
end
end
true
end
Base.in(v::VecSE2{Float64}, poly::ConvexPolygon) = in(convert(VecE2, v), poly)
function Base.show(io::IO, poly::ConvexPolygon)
@printf(io, "ConvexPolygon: len %d (max %d pts)\n", length(poly), length(poly.pts))
for i in 1 : length(poly)
print(io, "\t"); show(io, poly.pts[i])
print(io, "\n")
end
end
AutomotiveSimulator.get_center(poly::ConvexPolygon) = sum(poly.pts) / poly.npts
function Vec.get_distance(poly::ConvexPolygon, v::VecE2; solid::Bool=true)
if solid && in(v, poly)
0.0
else
min_dist = Inf
for i in 1 : length(poly)
seg = get_edge(poly, i)
min_dist = min(min_dist, get_distance(seg, v))
end
min_dist
end
end
function ensure_pts_sorted_by_min_polar_angle!(poly::ConvexPolygon, npts::Int=poly.npts)
@assert(npts ≥ 3)
@assert(sign(get_signed_area(poly)) == 1) # must be counter-clockwise
# ensure that edges are sorted by minimum polar angle in [0,2π]
angle_start = Inf
index_start = -1
for i in 1 : npts
seg = get_edge(poly.pts, i, npts)
θ = atan(seg.B.y - seg.A.y, seg.B.x - seg.A.x)
if θ < 0.0
θ += 2π
end
if θ < angle_start
angle_start = θ
index_start = i
end
end
if index_start != 1
cyclic_shift_left!(poly.pts, index_start-1, npts)
end
poly
end
function shift!(poly::ConvexPolygon, v::VecE2)
for i in 1 : length(poly)
poly.pts[i] += v
end
ensure_pts_sorted_by_min_polar_angle!(poly)
poly
end
function rotate!(poly::ConvexPolygon, θ::Float64)
for i in 1 : length(poly)
poly.pts[i] = Vec.rot(poly.pts[i], θ)
end
ensure_pts_sorted_by_min_polar_angle!(poly)
poly
end
function mirror!(poly::ConvexPolygon)
for i in 1 : length(poly)
poly.pts[i] = -poly.pts[i]
end
ensure_pts_sorted_by_min_polar_angle!(poly)
poly
end
function minkowksi_sum!(retval::ConvexPolygon, P::ConvexPolygon, Q::ConvexPolygon)
#=
For two convex polygons P and Q in the plane with m and n vertices, their Minkowski sum is a
convex polygon with at most m + n vertices and may be computed in time O (m + n) by a very simple procedure,
which may be informally described as follows.
Assume that the edges of a polygon are given and the direction, say, counterclockwise, along the polygon boundary.
Then it is easily seen that these edges of the convex polygon are ordered by polar angle.
Let us merge the ordered sequences of the directed edges from P and Q into a single ordered sequence S.
Imagine that these edges are solid arrows which can be moved freely while keeping them parallel to their original direction.
Assemble these arrows in the order of the sequence S by attaching the tail of the next arrow to the head of the previous arrow.
It turns out that the resulting polygonal chain will in fact be a convex polygon which is the Minkowski sum of P and Q.
=#
empty!(retval)
index_P = 1
index_Q = 1
θp = get_polar_angle(get_edge(P, index_P))
θq = get_polar_angle(get_edge(Q, index_Q))
while index_P ≤ length(P) || index_Q ≤ length(Q)
# select next edge with minimum polar angle
if θp == θq
seg_p = get_edge(P, index_P)
seg_q = get_edge(Q, index_Q)
O = isempty(retval) ? P.pts[1] + Q.pts[1] : retval.pts[retval.npts]
push!(retval, O + seg_p.B - seg_p.A + seg_q.B - seg_q.A)
index_P += 1
θp = index_P ≤ length(P) ? get_polar_angle(get_edge(P, index_P)) : Inf
index_Q += 1
θq = index_Q ≤ length(Q) ? get_polar_angle(get_edge(Q, index_Q)) : Inf
elseif θp ≤ θq
seg = get_edge(P, index_P)
O = isempty(retval) ? P.pts[1] + Q.pts[1] : retval.pts[retval.npts]
push!(retval, O + seg.B - seg.A)
index_P += 1
θp = index_P ≤ length(P) ? get_polar_angle(get_edge(P, index_P)) : Inf
else
seg = get_edge(Q, index_Q)
O = isempty(retval) ? P.pts[1] + Q.pts[1] : retval.pts[retval.npts]
push!(retval, O + seg.B - seg.A)
index_Q += 1
θq = index_Q ≤ length(Q) ? get_polar_angle(get_edge(Q, index_Q)) : Inf
end
end
ensure_pts_sorted_by_min_polar_angle!(retval)
retval
end
function minkowski_difference!(retval::ConvexPolygon, P::ConvexPolygon, Q::ConvexPolygon)
#=
The minkowski difference is what you get by taking the minkowski sum of a shape and the mirror of another shape.
So, your second shape gets flipped about the origin (all of its points are negated).
The idea is that you do a binary operation on two shapes to get a new shape,
and if the origin (the zero vector) is inside that shape, then they are colliding.
=#
minkowksi_sum!(retval, P, mirror!(Q))
end
function is_colliding(P::ConvexPolygon, Q::ConvexPolygon, temp::ConvexPolygon=ConvexPolygon(length(P) + length(Q)))
minkowski_difference!(temp, P, Q)
in(VecE2(0,0), temp)
end
function Vec.get_distance(P::ConvexPolygon, Q::ConvexPolygon, temp::ConvexPolygon=ConvexPolygon(length(P) + length(Q)))
minkowski_difference!(temp, P, Q)
get_distance(temp, VecE2(0,0))
end
"""
to_oriented_bounding_box!(retval::ConvexPolygon, center::VecSE2{Float64}, len::Float64, wid::Float64)
to_oriented_bounding_box!(retval::ConvexPolygon, veh::Entity{S,D,I}, center::VecSE2{Float64} = get_center(veh))
Fills in the vertices of `retval` according to the rectangle specification: center, length, width
"""
function to_oriented_bounding_box!(retval::ConvexPolygon, center::VecSE2{Float64}, len::Float64, wid::Float64)
@assert(len > 0)
@assert(wid > 0)
@assert(!isnan(center.θ))
@assert(!isnan(center.x))
@assert(!isnan(center.y))
x = polar(len/2, center.θ)
y = polar(wid/2, center.θ+π/2)
C = convert(VecE2,center)
retval.pts[1] = x - y + C
retval.pts[2] = x + y + C
retval.pts[3] = -x + y + C
retval.pts[4] = -x - y + C
retval.npts = 4
AutomotiveSimulator.ensure_pts_sorted_by_min_polar_angle!(retval)
retval
end
function to_oriented_bounding_box!(retval::ConvexPolygon, veh::Entity{S,D,I}, center::VecSE2{Float64} = get_center(veh)) where {S,D<:AbstractAgentDefinition,I}
# get an oriented bounding box at the vehicle's position
to_oriented_bounding_box!(retval, center, length(veh.def), width(veh.def))
retval
end
"""
get_oriented_bounding_box(center::VecSE2{Float64}, len::Float64, wid::Float64) = to_oriented_bounding_box!(ConvexPolygon(4), center, len, wid)
get_oriented_bounding_box(veh::Entity{S,D,I}, center::VecSE2{Float64} = get_center(veh)) where {S,D<:AbstractAgentDefinition,I}
Returns a ConvexPolygon representing a bounding rectangle of the size specified by center, length, width
"""
get_oriented_bounding_box(center::VecSE2{Float64}, len::Float64, wid::Float64) = to_oriented_bounding_box!(ConvexPolygon(4), center, len, wid)
function get_oriented_bounding_box(veh::Entity{S,D,I}, center::VecSE2{Float64} = get_center(veh)) where {S,D<:AbstractAgentDefinition,I}
return to_oriented_bounding_box!(ConvexPolygon(4), veh, center)
end
######################################
"""
is_colliding(ray::VecSE2{Float64}, poly::ConvexPolygon)
returns true if the ray collides with the polygon
"""
function is_colliding(ray::VecSE2{Float64}, poly::ConvexPolygon)
# collides if at least one of the segments collides with the ray
for i in 1 : length(poly)
seg = get_edge(poly, i)
if intersects(ray, seg)
return true
end
end
false
end
"""
get_collision_time(ray::VecSE2{Float64}, poly::ConvexPolygon, ray_speed::Float64)
returns the collision time between a ray and a polygon given `ray_speed`
"""
function get_collision_time(ray::VecSE2{Float64}, poly::ConvexPolygon, ray_speed::Float64)
min_col_time = Inf
for i in 1 : length(poly)
seg = get_edge(poly, i)
col_time = get_intersection_time(Projectile(ray, ray_speed), seg)
if !isnan(col_time) && col_time < min_col_time
min_col_time = col_time
end
end
min_col_time
end
######################################
"""
CPAMemory
A structure to cache the bounding boxes around vehicle. It is part of the internals of the
Minkowski collision checker.
# Fields
- `vehA::ConvexPolygon` bounding box for vehicle A
- `vehB::ConvexPolygon` bounding box for vehicle B
- `mink::ConvexPolygon` minkowski bounding box
"""
struct CPAMemory
vehA::ConvexPolygon # bounding box for vehicle A
vehB::ConvexPolygon # bounding box for vehicle B
mink::ConvexPolygon # minkowski bounding box
CPAMemory() = new(ConvexPolygon(4), ConvexPolygon(4), ConvexPolygon(8))
end
function _bounding_radius(veh::Entity{S,D,I}) where {S,D<:AbstractAgentDefinition,I}
return sqrt(length(veh.def)*length(veh.def)/4 + width(veh.def)*width(veh.def)/4)
end
"""
is_potentially_colliding(A::Entity{S,D,I}, B::Entity{S,D,I}) where {S,D<:AbstractAgentDefinition,I}
A fast collision check to remove things clearly not colliding
"""
function is_potentially_colliding(A::Entity{S,D,I}, B::Entity{S,D,I}) where {S, D<:AbstractAgentDefinition,I}
Δ² = normsquared(VecE2(posg(A.state) - posg(B.state)))
r_a = _bounding_radius(A)
r_b = _bounding_radius(B)
Δ² ≤ r_a*r_a + 2*r_a*r_b + r_b*r_b
end
"""
is_colliding(A::Entity{S,D,I}, B::Entity{S,D,I}, mem::CPAMemory=CPAMemory()) where {D<:AbstractAgentDefinition,I}
returns true if the vehicles A and B are colliding. It uses Minkowski sums.
is_colliding(mem::CPAMemory)
returns true if vehA and vehB in mem collides.
"""
function is_colliding(A::Entity{S,D,I}, B::Entity{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
if is_potentially_colliding(A, B)
to_oriented_bounding_box!(mem.vehA, A)
to_oriented_bounding_box!(mem.vehB, B)
return is_colliding(mem)
end
false
end
is_colliding(mem::CPAMemory) = is_colliding(mem.vehA, mem.vehB, mem.mink)
"""
Vec.get_distance(A::Entity{S,D,I}, B::Entity{S,D,I}, mem::CPAMemory=CPAMemory()) where {D<:AbstractAgentDefinition,I}
returns the euclidean distance between A and B.
"""
function Vec.get_distance(A::Entity{S,D,I}, B::Entity{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
to_oriented_bounding_box!(mem.vehA, A)
to_oriented_bounding_box!(mem.vehB, B)
get_distance(mem)
end
Vec.get_distance(mem::CPAMemory) = get_distance(mem.vehA, mem.vehB, mem.mink)
"""
CollisionCheckResult
A type to store the result of a collision checker
# Fields
- `is_colliding::Bool`
- `A::Int64` # index of 1st vehicle
- `B::Int64` # index of 2nd vehicle
"""
struct CollisionCheckResult
is_colliding::Bool
A::Int64 # index of 1st vehicle
B::Int64 # index of 2nd vehicle
# TODO: penetration vector?
end
"""
get_first_collision(scene::EntityScene{S,D,I}, target_index::Int, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
Loops through the scene and finds the first collision between a vehicle and scene[target_index]
"""
function get_first_collision(scene::EntityScene{S,D,I}, target_index::Int, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
A = target_index
vehA = scene[A]
to_oriented_bounding_box!(mem.vehA, vehA)
for (B,vehB) in enumerate(scene)
if B != A
to_oriented_bounding_box!(mem.vehB, vehB)
if is_potentially_colliding(vehA, vehB) && is_colliding(mem)
return CollisionCheckResult(true, A, B)
end
end
end
CollisionCheckResult(false, A, 0)
end
"""
get_first_collision(scene::EntityScene{S,D,I}, vehicle_indeces::AbstractVector{Int}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
Loops through the scene and finds the first collision between any two vehicles in `vehicle_indeces`.
"""
function get_first_collision(scene::EntityScene{S,D,I}, vehicle_indeces::AbstractVector{Int}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
N = length(vehicle_indeces)
for (a,A) in enumerate(vehicle_indeces)
vehA = scene[A]
to_oriented_bounding_box!(mem.vehA, vehA)
for b in a +1 : length(vehicle_indeces)
B = vehicle_indeces[b]
vehB = scene[B]
if is_potentially_colliding(vehA, vehB)
to_oriented_bounding_box!(mem.vehB, vehB)
if is_colliding(mem)
return CollisionCheckResult(true, A, B)
end
end
end
end
CollisionCheckResult(false, 0, 0)
end
"""
get_first_collision(scene::EntityScene{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
Loops through the scene and finds the first collision between any two vehicles
"""
get_first_collision(scene::EntityScene{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I} = get_first_collision(scene, 1:length(scene), mem)
"""
is_collision_free(scene::EntityScene{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
is_collision_free(scene::EntityScene{S,D,I}, vehicle_indeces::AbstractVector{Int}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I}
Check that there is no collisions between any two vehicles in `scene`
If `vehicle_indeces` is used, it only checks for vehicles within `scene[vehicle_indeces]`
"""
is_collision_free(scene::EntityScene{S,D,I}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I} = !(get_first_collision(scene, mem).is_colliding)
is_collision_free(scene::EntityScene{S,D,I}, vehicle_indeces::AbstractVector{Int}, mem::CPAMemory=CPAMemory()) where {S,D<:AbstractAgentDefinition,I} = get_first_collision(scene, vehicle_indeces, mem).is_colliding
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 5583 | """
collision_checker(veh_a::Entity, veh_b::Entity)
collision_checker(veh_a, veh_b, veh_a_def::AbstractAgentDefinition, veh_b_def::AbstractAgentDefinition)
return True if `veh_a` and `veh_b` collides.
Relies on the parallel axis theorem.
"""
function collision_checker(veh_a::Entity, veh_b::Entity)
return collision_checker(veh_a.state, veh_b.state, veh_a.def, veh_b.def)
end
function collision_checker(veh_a, veh_b, veh_a_def::AbstractAgentDefinition, veh_b_def::AbstractAgentDefinition)
center_a = posg(veh_a)
center_b = posg(veh_b)
l_a = length(veh_a_def)
w_a = width(veh_a_def)
l_b = length(veh_b_def)
w_b = width(veh_b_def)
# first fast check:
@fastmath begin
Δ = sqrt((posg(veh_a).x - posg(veh_b).x)^2 + (posg(veh_a).y - posg(veh_b).y)^2)
r_a = sqrt(l_a*l_a/4 + w_a*w_a/4)
r_b = sqrt(l_b*l_b/4 + w_b*w_b/4)
end
if Δ ≤ r_a + r_b
# fast check is true, run parallel axis theorem
Pa = polygon(center_a, veh_a_def)
Pb = polygon(center_b, veh_b_def)
return overlap(Pa, Pb)
end
return false
end
"""
collision_checker(scene::Scene{Entity{S,D,I}}, egoid::I) where {S, D<:AbstractAgentDefinition, I}
return true if any entity in the scene collides with the entity of id `egoid`.
"""
function collision_checker(scene::Scene{Entity{S,D,I}}, egoid::I) where {S, D<:AbstractAgentDefinition, I}
ego = get_by_id(scene, egoid)
for veh in scene
if veh.id != egoid
if collision_checker(veh, ego)
return true
end
end
end
return false
end
"""
polygon(pos::VecSE2{Float64}, veh_def::AbstractAgentDefinition)
polygon(x::Float64,y::Float64,theta::Float64, length::Float64, width::Float64)
returns a 4x2 static matrix corresponding to a rectangle around a car
centered at `pos` and of dimensions specified by `veh_def`
"""
function polygon(pos::VecSE2{Float64}, veh_def::AbstractAgentDefinition)
x, y ,θ = pos
return polygon(x, y, θ, length(veh_def), width(veh_def))
end
function polygon(x::Float64,y::Float64,theta::Float64, length::Float64, width::Float64)
l_ = length/2
w_ = width/2
# compute each vertex:
@fastmath begin
#top left
Ax = x + l_*cos(theta) - w_*sin(theta)
Ay = y + l_*sin(theta) + w_*cos(theta)
#top right
Bx = x + l_*cos(theta) + w_*sin(theta)
By = y + l_*sin(theta) - w_*cos(theta)
# bottom right
Cx = x - l_*cos(theta) + w_*sin(theta)
Cy = y - l_*sin(theta) - w_*cos(theta)
# bttom left
Dx = x - l_*cos(theta) - w_*sin(theta)
Dy = y - l_*sin(theta) + w_*cos(theta)
end
P = @SMatrix [Ax Ay;
Bx By;
Cx Cy;
Dx Dy]
return P
end
### POLYGON INTERSECTION USING PARALLEL AXIS THEOREM
"""
overlap(poly_a::SMatrix{4, 2, Float64}, poly_b::SMatrix{4, 2, Float64})
Check if two convex polygons overlap, using the parallel axis theorem
a polygon is a nx2 matrix where n in the number of verteces
http://gamemath.com/2011/09/detecting-whether-two-convex-polygons-overlap/
/!\\ edges needs to be ordered
"""
function overlap(poly_a::SMatrix{M, 2, Float64},
poly_b::SMatrix{N, 2, Float64}) where {M,N}
if find_separating_axis(poly_a, poly_b)
return false
end
if find_separating_axis(poly_b, poly_a)
return false
end
return true
end
"""
find_separating_axis(poly_a::SMatrix{4, 2, Float64}, poly_b::SMatrix{4, 2, Float64})
build a list of candidate separating axes from the edges of a
/!\\ edges needs to be ordered
"""
function find_separating_axis(poly_a::SMatrix{M, 2, Float64},
poly_b::SMatrix{N, 2, Float64}) where {M, N}
n_a = size(poly_a)[1]
n_b = size(poly_b)[1]
axis = zeros(2)
@views previous_vertex = poly_a[end,:]
for i=1:n_a # loop through all the edges n edges
@views current_vertex = poly_a[i,:]
# get edge vector
edge = current_vertex - previous_vertex
# rotate 90 degrees to get the separating axis
axis[1] = edge[2]
axis[2] = -edge[1]
# project polygons onto the axis
a_min,a_max = polygon_projection(poly_a, axis)
b_min,b_max = polygon_projection(poly_b, axis)
# check separation
if a_max < b_min
return true#,current_vertex,previous_vertex,edge,a_max,b_min
end
if b_max < a_min
return true#,current_vertex,previous_vertex,edge,a_max,b_min
end
@views previous_vertex = poly_a[i,:]
end
# no separation was found
return false
end
"""
polygon_projection(poly::SMatrix{4, 2, Float64}, axis::Vector{Float64})
return the projection interval for the polygon poly over the axis axis
"""
function polygon_projection(poly::SMatrix{N, 2, Float64},
axis::Vector{Float64}) where {N}
n_a = size(poly)[1]
@inbounds @fastmath @views d1 = dot(poly[1,:],axis)
@inbounds @fastmath @views d2 = dot(poly[2,:],axis)
# initialize min and max
if d1<d2
out_min = d1
out_max = d2
else
out_min = d2
out_max = d1
end
for i=1:n_a
@inbounds @fastmath @views d = dot(poly[i,:],axis)
if d < out_min
out_min = d
elseif d > out_max
out_max = d
end
end
return out_min,out_max
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 12337 | ## define features traits
# most general feature(roadway, scenes, actions, vehid)
abstract type AbstractFeature end
# feature(roadway, veh)
struct EntityFeature <: AbstractFeature end
# feature(roadway, scene, veh)
struct SceneFeature <: AbstractFeature end
# feature(roadway, scenes, veh)
struct TemporalFeature <: AbstractFeature end
# feature(actions, veh)
# struct TemporalActionFeature <: AbstractFeature end
# feature(action, veh)
# struct ActionFeature <: AbstractFeature end
"""
featuretype(f::Function)
function used for dispatching feature types depending on the method of the feature function
"""
function featuretype(f::Function)
if static_hasmethod(f, Tuple{Roadway, Entity})
return EntityFeature()
elseif static_hasmethod(f, Tuple{Roadway, Scene, Entity})
return SceneFeature()
elseif static_hasmethod(f, Tuple{Roadway, Vector{<:Scene}, Entity})
return TemporalFeature()
else
error(""""
unsupported feature type
try adding a new feature trait or implement one of the existing feature type supported
""")
end
return
end
## Feature extraction functions
# Top level extract several features
"""
extract_features(features, roadway::Roadway, scenes::Vector{<:Scene}, ids::Vector{I}) where I
Extract information from a list of scenes. It returns a dictionary of DataFrame objects.
# Inputs
- `features`: a tuple of feature functions. The feature types supported are `EntityFeature`, `SceneFeature` and `TemporalFeature`. Check the documentation for the list of available feature functions, and how to implement you own feature function.
- `roadway::Roadway` : the roadway associated to the scenes.
- `scenes::Vector{<:Scene}` : the simulation data from which we wish to extract information from. Each scene in the vector corresponds to one time step.
- `ids::Vector{I}`: a list of entity IDs for which we want to extract the information.
# Output
A dictionary mapping IDs to `DataFrame` objects. For a given ID, the DataFrame's columns correspond to the name of the input feature functions.
The row correspond to the feature value for each scene (time history).
# Example:
```julia
roadway = gen_straight_roadway(4, 100.0)
scene_0 = Scene([
Entity(VehicleState(VecSE2( 0.0,0.0,0.0), roadway, 10.0), VehicleDef(AgentClass.CAR, 5.0, 2.0), 1),
Entity(VehicleState(VecSE2(10.0,0.0,0.0), roadway, 10.0), VehicleDef(AgentClass.CAR, 5.0, 2.0), 2),
])
scene_1 = Scene([
Entity(VehicleState(VecSE2( 10.0,0.0,0.0), roadway, 10.0), VehicleDef(AgentClass.CAR, 5.0, 2.0), 1),
Entity(VehicleState(VecSE2(20.0,0.0,0.0), roadway, 10.0), VehicleDef(AgentClass.CAR, 5.0, 2.0), 2),
])
dfs = extract_features((posgx, iscolliding), roadway, [scene_0, scene_1], [1,2])
dfs[1].posgx # history of global x position for vehicle of ID 1
```
"""
function extract_features(features, roadway::Roadway, scenes::Vector{<:Scene}, ids::Vector{I}) where I
dfs_list = broadcast(f -> extract_feature(featuretype(f), f, roadway, scenes, ids), features)
# join all the dataframes
dfs = Dict{I, DataFrame}()
for id in ids
dfs[id] = DataFrame([Symbol(features[i]) => dfs_list[i][id] for i=1:length(features)])
end
return dfs
end
# feature is a general (temporal) feature, broadcast on id only
function extract_feature(ft::AbstractFeature, feature, roadway::Roadway, scenes::Vector{<:Scene}, ids)
values = feature.(Ref(roadway), Ref(scenes), ids) # vector of vectors
return Dict(zip(ids, values))
end
# feature is a scene feature, broadcast on ids and scenes
function extract_feature(ft::SceneFeature, feature, roadway::Roadway, scenes::Vector{<:Scene}, ids)
values = broadcast(ids) do id
veh_hist = get_by_id.(scenes, Ref(id))
feature.(Ref(roadway), scenes, veh_hist)
end
return Dict(zip(ids, values))
end
# feature is an entity feature, broadcast on ids and scenes
function extract_feature(ft::EntityFeature, feature, roadway::Roadway, scenes::Vector{<:Scene}, ids)
values = broadcast(ids) do id
veh_hist= get_by_id.(scenes, Ref(id))
feature.(Ref(roadway), veh_hist)
end
return Dict(zip(ids, values))
end
## feature functions
# vehicle only features
"""
posgx(roadway::Roadway, veh::Entity)
returns x position in the global frame
"""
posgx(roadway::Roadway, veh::Entity) = posg(veh).x
"""
posgy(roadway::Roadway, veh::Entity)
returns y position in the global frame
"""
posgy(roadway::Roadway, veh::Entity) = posg(veh).y
"""
posgθ(roadway::Roadway, veh::Entity)
returns heading in the global frame
"""
posgθ(roadway::Roadway, veh::Entity) = posg(veh).θ
"""
posfs(roadway::Roadway, veh::Entity)
returns longitudinal position in Frenet frame
"""
posfs(roadway::Roadway, veh::Entity) = posf(veh).s
"""
posft(roadway::Roadway, veh::Entity)
returns lateral position in Frenet frame
"""
posft(roadway::Roadway, veh::Entity) = posf(veh).t
"""
posfϕ(roadway::Roadway, veh::Entity)
returns heading relative to centerline of the lane
"""
posfϕ(roadway::Roadway, veh::Entity) = posf(veh).ϕ
"""
vel(roadway::Roadway, veh::Entity)
returns the velocity of `veh`
"""
vel(roadway::Roadway, veh::Entity) = vel(veh)
"""
velfs(roadway::Roadway, veh::Entity)
returns the longitudinal velocity in the Frenet frame
"""
velfs(roadway::Roadway, veh::Entity) = velf(veh).s
"""
velft(roadway::Roadway, veh::Entity)
returns the lateral velocity in the Frenet frame
"""
velft(roadway::Roadway, veh::Entity) = velf(veh).t
"""
velgx(roadway::Roadway, veh::Entity)
returns the velocity in the x direction of the global frame
"""
velgx(roadway::Roadway, veh::Entity) = velg(veh).x
"""
velgy(roadway::Roadway, veh::Entity)
returns the velocity in the y direction of the global frame
"""
velgy(roadway::Roadway, veh::Entity) = velg(veh).y
"""
time_to_crossing_right(roadway::Roadway, veh::Entity)
time before crossing the right lane marker assuming constant lateral speed.
"""
function time_to_crossing_right(roadway::Roadway, veh::Entity)
d_mr = markerdist_right(roadway, veh)
velf_t = velf(veh).t
if d_mr > 0.0 && velf_t < 0.0
return -d_mr / velf_t
else
return Inf
end
end
"""
time_to_crossing_left(roadway::Roadway, veh::Entity)
time before crossing the left lane marker assuming constant lateral speed.
"""
function time_to_crossing_left(roadway::Roadway, veh::Entity)
d_mr = markerdist_left(roadway, veh)
velf_t = velf(veh).t
if d_mr > 0.0 && velf_t > 0.0
return -d_mr / velf_t
else
return Inf
end
end
"""
estimated_time_to_lane_crossing(roadway::Roadway, veh::Entity)
minimum of the time to crossing left and time to crossing right.
"""
function estimated_time_to_lane_crossing(roadway::Roadway, veh::Entity)
ttcr_left = time_to_crossing_left(roadway, veh)
ttcr_right = time_to_crossing_right(roadway, veh)
return min(ttcr_left, ttcr_right)
end
"""
iswaiting(roadway::Roadway, veh::Entity)
returns true if the vehicle is waiting (if the velocity is 0.0)
"""
iswaiting(roadway::Roadway, veh::Entity) = vel(veh) ≈ 0.0
## scene features
"""
iscolliding(roadway::Roadway, scene::Scene, veh::Entity)
returns true if the vehicle is colliding with another vehicle in the scene.
"""
function iscolliding(roadway::Roadway, scene::Scene, veh::Entity)
return collision_checker(scene, veh.id)
end
"""
distance_to(egoid)
generate a feature function distance_to_\$egoid.
`distance_to_\$egoid(roadway, scene, veh)` returns the distance between veh and the vehicle of id egoid in the scene.
"""
function distance_to(egoid)
@eval begin
function ($(Symbol("distance_to_$(egoid)")))(roadway::Roadway, scene::Scene, veh::Entity)
ego = get_by_id(scene, $egoid)
return norm(VecE2(posg(ego) - posg(veh)))
end
export $(Symbol("distance_to_$(egoid)"))
$(Symbol("distance_to_$(egoid)"))
end
end
# temporal features
"""
acc(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the history of acceleration of the entity of id vehid using finite differences on the velocity.
The first element is `missing`.
"""
function acc(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
result = zeros(Union{Missing,Float64},length(scenes))
angles = broadcast(x->vel(get_by_id(x, vehid)), scenes)
result[1] = missing
result[2:end] .= diff(angles)
return result
end
"""
accfs(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the history of longitudinal acceleration in the Frenet frame of the entity of id vehid using finite differences on the velocity.
The first element is `missing`.
"""
function accfs(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
result = zeros(Union{Missing,Float64},length(scenes))
angles = broadcast(x->velf(get_by_id(x, vehid)).s, scenes)
result[1] = missing
result[2:end] .= diff(angles)
return result
end
"""
accft(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the history of lateral acceleration in the Frenet frame of the entity of id vehid using finite differences on the velocity.
The first element is `missing`.
"""
function accft(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
result = zeros(Union{Missing,Float64},length(scenes))
angles = broadcast(x->velf(get_by_id(x, vehid)).t, scenes)
result[1] = missing
result[2:end] .= diff(angles)
return result
end
"""
jerk(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the jerk history of the entity of id vehid using finite differences on acceleration (which uses finite differences on velocity).
The first two elements are missing.
"""
function jerk(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
return vcat(missing, diff(acc(roadway, scenes, vehid)))
end
"""
jerkfs(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the longitudinal jerk history in the Frenet frame of the entity of id vehid using finite differences on acceleration (which uses finite differences on velocity).
The first two elements are missing.
"""
function jerkfs(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
return vcat(missing, diff(accfs(roadway, scenes, vehid)))
end
"""
jerkft(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the lateral jerk history in the Frenet frame of the entity of id vehid using finite differences on acceleration (which uses finite differences on velocity).
The first two elements are missing.
"""
function jerkft(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
return vcat(missing, diff(accft(roadway, scenes, vehid)))
end
"""
turn_rate_g(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the turn rate history in the Frenet frame of the entity of id vehid using finite differences on global heading.
The first element is missing.
"""
function turn_rate_g(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
result = zeros(Union{Missing,Float64},length(scenes))
angles = broadcast(x->posg(get_by_id(x, vehid)).θ, scenes)
result[1] = missing
result[2:end] .= diff(angles)
return result
end
"""
turn_rate_g(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
returns the turn rate history in the Frenet frame of the entity of id vehid using finite differences on heading in the Frenet frame.
The first element is missing.
"""
function turn_rate_f(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
result = zeros(Union{Missing,Float64},length(scenes))
angles = broadcast(x->posf(get_by_id(x, vehid)).ϕ, scenes)
result[1] = missing
result[2:end] .= diff(angles)
return result
end
"""
isbraking(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
history of braking events: true when the acceleration is negative.
The first element is missing.
"""
function isbraking(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
return acc(roadway, scenes, vehid) .< 0.0
end
"""
isaccelerating(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
history of acceleration events: true when the acceleration is positive
The first element is missing.
"""
function isaccelerating(roadway::Roadway, scenes::Vector{<:Scene}, vehid)
return acc(roadway, scenes, vehid) .> 0.0
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
|
[
"MIT"
] | 0.1.2 | 8abb2a4050d43b49f41adcfb23ed82f136c76aea | code | 4501 |
"""
leftlane(roadway::Roadway, veh::Entity)
returns the lane to the left of the lane veh is currently in, returns nothing if it does not exists
"""
function leftlane(roadway::Roadway, veh::Entity)
return leftlane(roadway, get_lane(roadway, veh))
end
"""
rightlane(roadway::Roadway, veh::Entity)
returns the lane to the right of the lane veh is currently in, returns nothing if it does not exists
"""
function rightlane(roadway::Roadway, veh::Entity)
return rightlane(roadway, get_lane(roadway, veh))
end
"""
n_lanes_left(roadway::Roadway, veh::Entity)
return the numbers of lanes to the left of `veh`
"""
n_lanes_left(roadway::Roadway, veh::Entity) = n_lanes_left(roadway, get_lane(roadway, veh))
"""
n_lanes_right(roadway::Roadway, veh::Entity)
return the numbers of lanes to the right of `veh`
"""
n_lanes_right(roadway::Roadway, veh::Entity) = n_lanes_right(roadway, get_lane(roadway, veh))
"""
lane_width(roadway::Roadway, veh::Entity)
Returns the width of the lane where `veh` is.
"""
function lane_width(roadway::Roadway, veh::Entity)
lane = get_lane(roadway, veh.state)
if n_lanes_left(roadway, veh) > 0
footpoint = get_footpoint(veh)
lane_left = roadway[LaneTag(lane.tag.segment, lane.tag.lane + 1)]
return -proj(footpoint, lane_left, roadway).curveproj.t
else
return lane.width
end
end
"""
markerdist_left(roadway::Roadway, veh::Entity)
distance of `veh` to the left marker of the lane
"""
function markerdist_left(roadway::Roadway, veh::Entity)
t = posf(veh.state).t
lw = lane_width(roadway, veh)
return lw/2 - t
end
"""
markerdist_left(roadway::Roadway, veh::Entity)
distance of `veh` to the right marker of the lane
"""
function markerdist_right(roadway::Roadway, veh::Entity)
t = posf(veh.state).t
lw = lane_width(roadway, veh)
return lw/2 + t
end
"""
road_edge_dist_left(roadway::Roadway, veh::Entity)
feature function, extract the lateral distance to the left edge of the road
"""
function road_edge_dist_left(roadway::Roadway, veh::Entity)
offset = posf(veh).t
footpoint = get_footpoint(veh)
lane = get_lane(roadway, veh)
roadproj = proj(footpoint, lane, roadway)
curvept = roadway[RoadIndex(roadproj)]
lane = roadway[roadproj.tag]
return lane.width/2 + norm(VecE2(curvept.pos - footpoint)) - offset
end
"""
road_edge_dist_right(roadway::Roadway, veh::Entity)
feature function, extract the lateral distance to the right edge of the road
"""
function road_edge_dist_right(roadway::Roadway, veh::Entity)
offset = posf(veh).t
footpoint = get_footpoint(veh)
lane = get_lane(roadway, veh)
roadproj = proj(footpoint, lane, roadway)
curvept = roadway[RoadIndex(roadproj)]
lane = roadway[roadproj.tag]
return lane.width/2 + norm(VecE2(curvept.pos - footpoint)) + offset
end
"""
lane_offset_left(roadway::Roadway, veh::Entity)
returns the distance to the left lane border, if there are no left lane returns `missing`
"""
function lane_offset_left(roadway::Roadway, veh::Entity)
t = posf(veh).t
lane = get_lane(roadway, veh)
if n_lanes_left(roadway, lane) > 0
lane_offset = t - lane.width/2 - leftlane(roadway, veh).width/2
return lane_offset
else
missing
end
end
"""
lane_offset_right(roadway::Roadway, veh::Entity)
returns the distance to the right lane border, if there are no right lane returns `missing`
"""
function lane_offset_right(roadway::Roadway, veh::Entity)
t = posf(veh).t
lane = get_lane(roadway, veh)
if n_lanes_right(roadway, lane) > 0
lane_offset = t + lane.width/2 + rightlane(roadway, veh).width/2
return lane_offset
else
missing
end
end
"""
has_lane_right(roadway::Roadway, veh::Entity)
Return true if `veh` has a lane on its right.
"""
function has_lane_right(roadway::Roadway, veh::Entity)
return n_lanes_right(roadway, veh) > 0
end
"""
has_lane_left(roadway::Roadway, veh::Entity)
Return true if `veh` has a lane on its left.
"""
function has_lane_left(roadway::Roadway, veh::Entity)
return n_lanes_left(roadway, veh) > 0
end
"""
lane_curvature(roadway::Roadway, veh::Entity)
Return the curvature of the lane at `veh`'s position.
Return missing if the curvature is `NaN`
"""
function lane_curvature(roadway::Roadway, veh::Entity)
curvept = roadway[posf(veh.state).roadind]
val = curvept.k
if isnan(val)
return missing
end
return val
end
| AutomotiveSimulator | https://github.com/sisl/AutomotiveSimulator.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.