filename
stringlengths 7
140
| content
stringlengths 0
76.7M
|
---|---|
code/sorting/src/comb_sort/comb_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// comb_sort.swift
// Created by DaiPei on 2017/10/15.
//
import Foundation
func combSort(_ array: inout [Int]) {
let shrinkFactor = 0.8
var gap = array.count
var swapped = true
while gap >= 1 || swapped {
swapped = false
if gap >= 1 {
gap = Int(Double(gap) * shrinkFactor)
}
for i in stride(from: 0, to: array.count - gap, by: 1) {
if array[i] > array[i + gap] {
swap(&array, at: i, and: i + gap)
swapped = true
}
}
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/counting_sort/Counting_sort.php | <?php
// function for counting sort
function countingsort(&$Array, $n) {
$max = 0;
//find largest element in the Array
for ($i=0; $i<$n; $i++) {
if($max < $Array[$i]) {
$max = $Array[$i];
}
}
//Create a freq array to store number of occurrences of
//each unique elements in the given array
for ($i=0; $i<$max+1; $i++) {
$freq[$i] = 0;
}
for ($i=0; $i<$n; $i++) {
$freq[$Array[$i]]++;
}
//sort the given array using freq array
for ($i=0, $j=0; $i<=$max; $i++) {
while($freq[$i]>0) {
$Array[$j] = $i;
$j++;
$freq[$i]--;
}
}
}
// function to print array
function PrintArray($Array, $n) {
for ($i = 0; $i < $n; $i++)
echo $Array[$i]." ";
echo "\n";
}
// test the code
$MyArray = array(9, 1, 2, 5, 9, 9, 2, 1, 3, 3);
$n = sizeof($MyArray);
echo "Original Array\n";
PrintArray($MyArray, $n);
countingsort($MyArray, $n);
echo "\nSorted Array\n";
PrintArray($MyArray, $n);
?>
|
code/sorting/src/counting_sort/README.md | # Counting Sort
Counting sort is a a very time-efficient (and somewhat space-inefficient) algorithm based on keys between a specific range. It works by counting the number of objects having distinct key values (kind of hashing)and then doing some arithmetic to calculate the position of each object in the output sequence.
## Explanation
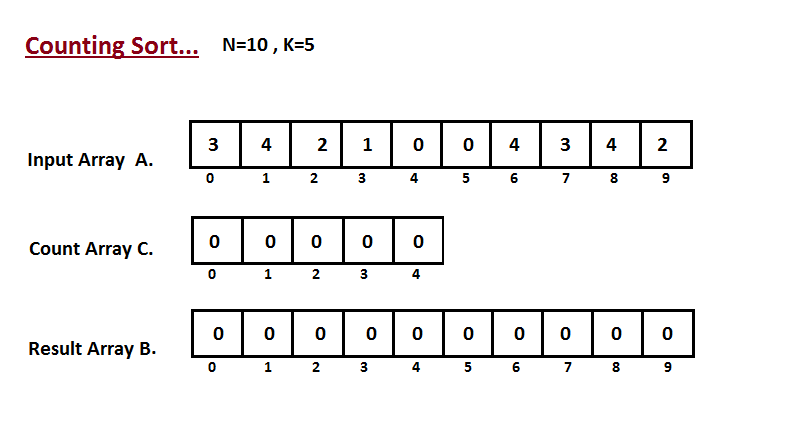
> Image credits: https://efficientalgorithms.blogspot.in/
## Algorithm
```
Counting_sort(A, k)
n = length[A]
create array B[n] & C[k+1]
for i=0 to k
C[i] = 0
for i = 1 to n
C[A[i]]++
for i = 1 to k
C[i] = C[i] + C[i - 1]
for i = n to 1
B[C[A[i]]] = A[i]
C[A[i]]--
```
## Complexity
**Time complexity**
**O(n+k)** where **n** is the number of elements in input array and **k** is the range of input.
**Space Comlexity**: `O(n+k)`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/counting_sort/counting_sort.c | #include <stdio.h>
#include <string.h>
#define RANGE 255
// Part of Cosmos by OpenGenus Foundation
// The main function that sort the given string arr[] in
// alphabatical order
void countSort(char arr[])
{
// The output character array that will have sorted arr
char output[strlen(arr)];
// Create a count array to store count of inidividul
// characters and initialize count array as 0
int count[RANGE + 1], i;
memset(count, 0, sizeof(count));
// Store count of each character
for(i = 0; arr[i]; ++i)
++count[arr[i]];
// Change count[i] so that count[i] now contains actual
// position of this character in output array
for (i = 1; i <= RANGE; ++i)
count[i] += count[i-1];
// Build the output character array
for (i = 0; arr[i]; ++i)
{
output[count[arr[i]]-1] = arr[i];
--count[arr[i]];
}
// Copy the output array to arr, so that arr now
// contains sorted characters
for (i = 0; arr[i]; ++i)
arr[i] = output[i];
}
// Driver program to test above function
int main()
{
char arr[] = "opengenus";//"applepp";
countSort(arr);
printf("Sorted character array is %sn", arr);
return 0;
}
|
code/sorting/src/counting_sort/counting_sort.cpp | #include <iostream>
#include <vector>
#include <climits>
/*
* Part of Cosmos by OpenGenus Foundation
*/
using namespace std;
void countingSort(vector<int> arr, vector<int>& sortedA)
{
int m = INT_MIN;
for (size_t i = 0; i < arr.size(); i++)
if (arr[i] > m)
m = arr[i];
int freq[m + 1]; //m is the maximum number in the array
for (int i = 0; i <= m; i++)
freq[i] = 0;
for (size_t i = 0; i < arr.size(); i++)
freq[arr[i]]++;
int j = 0;
for (int i = 0; i <= m; i++)
{
int tmp = freq[i];
while (tmp--)
{
sortedA[j] = i;
j++;
}
}
}
int main()
{
vector<int> arr{1, 4, 12, 34, 16, 11, 9, 1, 3, 33, 5};
vector<int> sortedA(arr.size() + 1); // stores sorted array
countingSort(arr, sortedA);
cout << "Sorted Array: ";
for (size_t i = 0; i < arr.size(); i++)
cout << sortedA[i] << " ";
return 0;
}
|
code/sorting/src/counting_sort/counting_sort.cs | using System;
using System.Collections.Generic;
namespace CS
{
/* Part of Cosmos by OpenGenus Foundation */
class CountingSort
{
static int[] Sort(int[] arr)
{
// Count the frequency of each number in the array
// The keys in this dictionary are the items themselves, the values are their frequencies
SortedDictionary<int, int> frequency = new SortedDictionary<int, int>();
foreach(var num in arr)
{
if(!frequency.ContainsKey(num))
{
frequency.Add(num, 0);
}
frequency[num]++;
}
// Create a new array for the output
var sorted = new int[arr.Length];
int index = 0;
foreach(var entry in frequency)
{
// Put each item (the key) from the dictionary into the new array as many times, as was the frequency (the value) of the item
for(int i = 0; i < entry.Value; i++)
{
sorted[index++] = entry.Key;
}
}
// Return the constructed array
return sorted;
}
static void Main(string[] args)
{
// Create an array of 10 random numbers
int[] numbers = new int[10];
var rand = new Random();
for(int i = 0; i < 10; i++)
{
numbers[i] = rand.Next(10);
}
Console.WriteLine("Initial ordering:");
foreach(var num in numbers)
{
Console.Write(num + " ");
}
// Store the sorted result in a separate variable and print it out
var sorted = Sort(numbers);
Console.WriteLine("\nSorted:");
foreach(var num in sorted)
{
Console.Write(num + " ");
}
}
}
}
|
code/sorting/src/counting_sort/counting_sort.go | package main
// Part of Cosmos by OpenGenus Foundation
import "fmt"
const MaxUint = ^uint(0)
const MaxInt = int(MaxUint >> 1)
const MinInt = -MaxInt - 1
var list = []int{-5, 12, 3, 4, 1, 2, 3, 5, 42, 34, 61, 2, 3, 5}
func countingSort(list []int) {
maxNumber := MinInt
minNumber := MaxInt
for _, v := range list {
if v > maxNumber {
maxNumber = v
}
if v < minNumber {
minNumber = v
}
}
count := make([]int, maxNumber-minNumber+1)
for _, x := range list {
count[x-minNumber]++
}
index := 0
for i, c := range count {
for ; c > 0; c-- {
list[index] = i + minNumber
index++
}
}
}
func main() {
fmt.Println("before sorting", list)
countingSort(list)
fmt.Println("after sorting", list)
}
|
code/sorting/src/counting_sort/counting_sort.java | // Java implementation of Counting Sort
// Part of Cosmos by OpenGenus Foundation
class CountingSort {
private void sort(char arr[]) {
int n = arr.length;
// The output character array that will have sorted arr
char output[] = new char[n];
// Create a count array to store count of inidividul
// characters and initialize count array as 0
int count[] = new int[256];
for (int i=0; i<256; ++i)
count[i] = 0;
// store count of each character
for (char anArr1 : arr) ++count[anArr1];
// Change count[i] so that count[i] now contains actual
// position of this character in output array
for (int i=1; i<=255; ++i)
count[i] += count[i-1];
// Build the output character array
for (char anArr : arr) {
output[count[anArr] - 1] = anArr;
--count[anArr];
}
// Copy the output array to arr, so that arr now
// contains sorted characters
System.arraycopy(output, 0, arr, 0, n);
}
// Driver method
public static void main(String args[]) {
CountingSort ob = new CountingSort();
char arr[] = {'g', 'e', 'e', 'k', 's', 'f', 'o',
'r', 'g', 'e', 'e', 'k', 's'
};
ob.sort(arr);
System.out.print("Sorted character array is ");
for (char anArr : arr) System.out.print(anArr);
}
}
|
code/sorting/src/counting_sort/counting_sort.js | // Part of Cosmos by OpenGenus Foundation
function countingSort(arr, min, max) {
var i,
z = 0,
count = [];
for (i = min; i <= max; i++) {
count[i] = 0;
}
for (i = 0; i < arr.length; i++) {
count[arr[i]]++;
}
for (i = min; i <= max; i++) {
while (count[i]-- > 0) {
arr[z++] = i;
}
}
return arr;
}
var arra = [3, 0, 2, 5, 4, 1];
console.log(arra.length);
console.log("Original Array Elements");
console.log(arra);
console.log("Sorted Array Elements");
console.log(countingSort(arra, 0, 5));
|
code/sorting/src/counting_sort/counting_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// counting_sort.m
// Created by DaiPei on 2017/10/15.
//
#import <Foundation/Foundation.h>
@interface CountingSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation CountingSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
// find the max and min value
NSNumber *max = @(INTMAX_MIN);
NSNumber *min = @(INTMAX_MAX);
for (NSNumber *val in array) {
if ([max compare:val] == NSOrderedAscending) {
max = val;
}
if ([min compare:val] == NSOrderedDescending) {
min = val;
}
}
// calculate every element position
int range = max.intValue - min.intValue + 1;
int *position = calloc(range, sizeof(int));
for (NSNumber *val in array) {
position[val.intValue - min.intValue]++;
}
for (int i = 1; i < range; i++) {
position[i] += position[i - 1];
}
// put every element to right position
int j = 0;
for (int i = 0; i < range; i++) {
while (j < position[i]) {
array[j] = @(i + min.intValue);
j++;
}
}
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray arrayWithCapacity:10];
for (int i = 0; i < 10; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
CountingSort *cs = [[CountingSort alloc] init];
[cs sort:array];
NSLog(@"after: %@", array);
}
return 0;
}
|
code/sorting/src/counting_sort/counting_sort.py | # Part of Cosmos by OpenGenus Foundation
# Python program for counting sort
# The main function that sort the given string arr[] in
# alphabetical order
def count_sort(arr):
# The output character array that will have sorted arr
output = [0 for i in range(256)]
# Create a count array to store count of inidividul
# characters and initialize count array as 0
count = [0 for i in range(256)]
# For storing the resulting answer since the
# string is immutable
ans = ["" for _ in arr]
# Store count of each character
for i in arr:
count[ord(i)] += 1
# Change count[i] so that count[i] now contains actual
# position of this character in output array
for i in range(256):
count[i] += count[i - 1]
# Build the output character array
for i in range(len(arr)):
output[count[ord(arr[i])] - 1] = arr[i]
count[ord(arr[i])] -= 1
# Copy the output array to arr, so that arr now
# contains sorted characters
for i in range(len(arr)):
ans[i] = output[i]
return ans
# Driver program to test above function
if __name__ == "__main__":
arr = "opengenus"
ans = count_sort(arr)
print("Sorted character array is %s" % ("".join(ans)))
|
code/sorting/src/counting_sort/counting_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// counting_sort.swift
// Created by DaiPei on 2017/10/15.
//
import Foundation
func countingSort(_ array: inout [Int]) {
// find max and min value in array
var max = Int.min
var min = Int.max
for item in array {
if item > max {
max = item
}
if item < min {
min = item
}
}
// calculate every element position
let range = max - min + 1
var position = [Int](repeatElement(0, count: range))
for item in array {
position[item - min] += 1
}
for i in 1..<position.count {
position[i] += position[i - 1]
}
// put every element to right position
var index = 0
for i in 0..<range {
while index < position[i] {
array[index] = i + min
index += 1
}
}
}
|
code/sorting/src/cycle_sort/README.md | # Cycle Sort
Cycle sort is an in-place, unstable sorting algorithm, a comparison sort that is theoretically optimal in terms of the total number of writes to the original array, unlike any other in-place sorting algorithm.
It is based on the idea that array to be sorted can be divided into cycles. Cycles can be visualized as a graph. We have n nodes and an edge directed from node i to node j if the element at i-th index must be present at j-th index in the sorted array.
## Explanation
Given an element a, we can find the index at which it will occur in the sorted list by simply counting the number of elements in the entire list that are smaller than a, in the following way:

> Image credits: wikipedia
## Algorithm
```
procedure cycle_sort
(var a: sort_array; n: array_size);
var i, j: array_size;
save, temp: array_element;
begin
for i := 1 to n do
begin
j := a[i].key;
if i ≠ j then
begin
save := a[i];
repeat
temp := a[j];
a[j] := save;
save := temp;
j := save.key;
until i = j { repeat };
a[i] := save;
end { if i ≠ j };
end {for i: = 1 to n }
end { procedure special_cycle_sort };
```
## Complexity
**Time complexity**
- Worst, average and best case time complexity: **O(n<sup>2</sup>)**
**Space complexity**
- Worst case: **O(n)** total
- Auxillary space: **O(1)**
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/cycle_sort/cycle_sort.c | #include <stdio.h>
void
swap(int *a, int *b)
{
int t = *b;
*b = *a;
*a = t;
}
void
cycleSort(int arr[], int n)
{
int cycle_start;
for (cycle_start = 0; cycle_start <= n - 2; ++cycle_start) {
int item = arr[cycle_start];
int pos = cycle_start;
int i;
for (i = cycle_start + 1; i < n; ++i)
if (arr[i] < item)
++pos;
if (pos == cycle_start)
continue;
while (item == arr[pos])
++pos;
if (pos != cycle_start)
swap(&item, &arr[pos]);
while (pos != cycle_start) {
pos = cycle_start;
for (i = cycle_start + 1; i < n; i++)
if (arr[i] < item)
++pos;
while (item == arr[pos])
++pos;
if (item != arr[pos])
swap(&item, &arr[pos]);
}
}
}
int
main()
{
int n;
printf("Enter size of the Array: ");
scanf("%d", &n);
int arr[n];
printf("Enter %d Integers:- \n", n);
int i;
for (i = 0; i < n; ++i)
scanf("%d", &arr[i]);
cycleSort(arr, n);
printf("Array after Sorting:- \n");
for (i = 0; i < n; ++i)
printf("%d ", arr[i]);
printf("\n");
return (0);
}
|
code/sorting/src/cycle_sort/cycle_sort.cpp | // C++ program to impleament cycle sort
#include <iostream>
#include <vector>
using namespace std;
// Part of Cosmos by OpenGenus Foundation
// Function sort the array using Cycle sort
void cycleSort (vector<int>& arr)
{
// traverse array elements and put it to on
// the right place
for (size_t cycle_start = 0; cycle_start <= arr.size() - 2; cycle_start++)
{
// initialize item as starting point
int item = arr[cycle_start];
// Find position where we put the item. We basically
// count all smaller elements on right side of item.
size_t pos = cycle_start;
for (size_t i = cycle_start + 1; i < arr.size(); i++)
if (arr[i] < item)
pos++;
// If item is already in correct position
if (pos == cycle_start)
continue;
// ignore all duplicate elements
while (item == arr[pos])
pos += 1;
// put the item to it's right position
if (pos != cycle_start)
swap(item, arr[pos]);
// Rotate rest of the cycle
while (pos != cycle_start)
{
pos = cycle_start;
// Find position where we put the element
for (size_t i = cycle_start + 1; i < arr.size(); i++)
if (arr[i] < item)
pos += 1;
// ignore all duplicate elements
while (item == arr[pos])
pos += 1;
// put the item to it's right position
if (item != arr[pos])
swap(item, arr[pos]);
}
}
}
// Main Function
int main()
{
vector<int> arr;
cout << "Enter the elements of the array: ";
int n;
while (cin >> n)
arr.push_back(n);
cycleSort(arr);
cout << "\nAfter sort : " << endl;
for (size_t i = 0; i < arr.size(); i++)
cout << arr[i] << " ";
return 0;
}
|
code/sorting/src/cycle_sort/cycle_sort.cs | using System;
using System.Linq;
/* Part of Cosmos by OpenGenus Foundation */
namespace OpenGenus
{
class Program
{
static void Main(string[] args)
{
var random = new Random();
var array = Enumerable.Range(1, 20).Select(x => random.Next(1,40)).ToArray();
Console.WriteLine("Unsorted");
Console.WriteLine(string.Join(",", array));
Console.WriteLine("\nSorted");
var writes = CycleSort(array);
Console.WriteLine(string.Join(",", array));
Console.WriteLine($"\nNumber of writes: {writes}");
Console.ReadLine();
}
// Adapted from Wikipedia CycleSort pseudocode
private static int CycleSort(int[] array)
{
// Sort an array in place and return the number of writes.
var writes = 0;
var cycleStart = 0;
var arrayLength = array.Length;
// Loop through the array to find cycles to rotate.
for (; cycleStart < arrayLength; cycleStart++) {
var item = array[cycleStart];
// Find where to put the item.
var position = cycleStart;
for (var i = cycleStart + 1; i < arrayLength; i++)
if (array[i] < item)
position++;
// If the item is already there, this is not a cycle.
if (position == cycleStart)
continue;
// Otherwise, put the item there or right after any duplicates.
while (item == array[position])
position++;
var tempSwapItem = array[position];
array[position] = item;
item = tempSwapItem;
writes++;
// Rotate the rest of the cycle.
while (position != cycleStart)
{
// Find where to put the item.
position = cycleStart;
for (var j = cycleStart + 1; j < arrayLength; j++)
if (array[j] < item)
position++;
// Put the item there or right after any duplicates.
while (item == array[position])
position++;
tempSwapItem = array[position];
array[position] = item;
item = tempSwapItem;
writes++;
}
}
return writes;
}
}
}
|
code/sorting/src/cycle_sort/cycle_sort.go | package main
import (
"fmt"
)
// Part of Cosmos by OpenGenus Foundation
func main() {
arr := []int{1, 6, 4, 7, 2, 8}
fmt.Printf("Before sort: %v\n", arr)
CycleSort(&arr)
fmt.Printf("After sort: %v\n", arr)
}
func CycleSort(arr *[]int) int {
writes := 0
// Loop through the array to find cycles to rotate.
for cycleStart, item := range *arr {
// Find where to put the item.
pos := cycleStart
for i := cycleStart + 1; i < len(*arr); i++ {
if (*arr)[i] < item {
pos++
}
}
// If the item is already there, this is not a cycle.
if pos != cycleStart {
// Put the item there or right after any duplicates.
for item == (*arr)[pos] {
pos++
}
(*arr)[pos], item = item, (*arr)[pos]
writes++
// Rotate the rest of the cycle
for pos != cycleStart {
// Find where to put the item
pos = cycleStart
for i := cycleStart + 1; i < len(*arr); i++ {
if (*arr)[i] < item {
pos++
}
}
// Put the item there or right after any duplicates.
for item == (*arr)[pos] {
pos++
}
(*arr)[pos], item = item, (*arr)[pos]
writes++
}
}
}
return writes
}
|
code/sorting/src/cycle_sort/cycle_sort.java | // Java program to impleament cycle sort
// Part of Cosmos by OpenGenus Foundation
import java.util.*;
import java.lang.*;
class CycleSort
{
// Function sort the array using Cycle sort
public static void cycleSort (int arr[], int n)
{
// count number of memory writes
int writes = 0;
// traverse array elements and put it on the right place
for (int cycle_start=0; cycle_start<n-1; cycle_start++)
{
// initialize item as starting point
int item = arr[cycle_start];
// Find position where we put the item. We basically
// count all smaller elements on right side of item.
int pos = cycle_start;
for (int i = cycle_start+1; i<n; i++)
if (arr[i] < item)
pos++;
// If item is already in correct position
if (pos == cycle_start)
continue;
// ignore all duplicate elements
while (item == arr[pos])
pos ++;
// put the item to it's right position
if (pos != cycle_start)
{
int temp = item;
item = arr[pos];
arr[pos] = temp;
writes++;
}
// Rotate rest of the cycle
while (pos != cycle_start)
{
pos = cycle_start;
// Find position where we put the element
for (int i = cycle_start+1; i<n; i++)
if (arr[i] < item)
pos += 1;
// ignore all duplicate elements
while (item == arr[pos])
pos += 1;
// put the item to it's right position
if (item != arr[pos])
{
int temp = item;
item = arr[pos];
arr[pos] = temp;
writes++;
}
}
}
}
// The Main function
public static void main(String[] args)
{
int arr[] = {1, 8, 3, 9, 10, 10, 2, 4 };
int n = arr.length;
cycleSort(arr, n) ;
System.out.println("After sort : ");
for (int i =0; i<n; i++)
System.out.print(arr[i] + " ");
}
}
|
code/sorting/src/cycle_sort/cycle_sort.js | /**
* Cycle Sort Implementation in JavaScript
*
* @author Ahmar Siddiqui <[email protected]>
* @github @ahhmarr
* @date 07/Oct/2017
* Part of Cosmos by OpenGenus Foundation
*/
const cycleSort = array => {
// last record will already be in place
for (let start = 0; start < array.length - 1; start++) {
let record = array[start];
// find where to put the record
let pos = start;
for (let i = start + 1; i < array.length; i++) {
if (array[i] < record) pos += 1;
}
// if the record is already there, this is not a cycle
if (pos == start) continue;
// otherwise, put the record there or right after any duplicates
while (record == array[pos]) {
pos++;
}
const swap = array[pos];
array[pos] = record;
record = swap;
// rotate the rest of the cycle
while (pos != start) {
// find where to put the record
pos = start;
for (let i = start + 1; i < array.length; i++) {
if (array[i] < record) pos += 1;
}
// put the record there or right after any duplicates
while (record == array[pos]) {
pos += 1;
}
const swap = array[pos];
array[pos] = record;
record = swap;
}
return array;
}
};
console.log(cycleSort([3, 2, 1, 4]));
|
code/sorting/src/cycle_sort/cycle_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// cycle_sort.m
// Created by DaiPei on 2017/10/15.
//
#import <Foundation/Foundation.h>
@interface CycleSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation CycleSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
for (int cycleStart = 0; cycleStart + 1 < array.count; cycleStart++) {
int pos = cycleStart;
NSNumber *value = array[cycleStart];
// count all smaller elements on the right side
for (int i = cycleStart + 1; i < array.count; i++) {
if ([value compare:array[i]] == NSOrderedDescending) {
pos++;
}
}
// continue when value in right position
if (pos == cycleStart) {
continue;
}
// ignore all duplicate elements
while ([value compare:array[pos]] == NSOrderedSame) {
pos++;
}
// put the value to the right position
[self swap:array at:pos and:cycleStart];
// cycle angain with the new value
while (pos != cycleStart) {
pos = cycleStart;
value = array[cycleStart];
// count all smaller elements on the right side of new value =
for (int i = cycleStart + 1; i < array.count; i++) {
if ([value compare:array[i]] == NSOrderedDescending) {
pos++;
}
}
// break when new value in right position
if (pos == cycleStart) {
break;
}
// ignore all duplicate elements
while ([value compare:array[pos]] == NSOrderedSame) {
pos++;
}
// put new value to the right position
[self swap:array at:pos and:cycleStart];
}
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray arrayWithCapacity:10];
for (int i = 0; i < 10; i++) {
int ran = arc4random() % 100 - 50;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
CycleSort *cs = [[CycleSort alloc] init];
[cs sort:array];
NSLog(@"after: %@", array);
}
return 0;
}
|
code/sorting/src/cycle_sort/cycle_sort.py | # Part of Cosmos by OpenGenus Foundation
def cycle_sort(arr):
writes = 0
# Loop through the array to find cycles
for cycle in range(0, len(arr) - 1):
item = arr[cycle]
# Find the location to place the item
pos = cycle
for i in range(cycle + 1, len(arr)):
if arr[i] < item:
pos += 1
# If item already present, not a cycle
if pos == cycle:
continue
# Else, put the item there or after any of its duplicates
while item == arr[pos]:
pos += 1
arr[pos], item = item, arr[pos]
writes += 1
# Rotate the rest of the cycle
while pos != cycle:
# Find location to put the item
pos = cycle
for i in range(cycle + 1, len(arr)):
if arr[i] < item:
pos += 1
# Put the item there or after any duplicates
while item == arr[pos]:
pos += 1
arr[pos], item = item, arr[pos]
writes += 1
return writes
if __name__ == "__main__":
arr = [1, 6, 4, 7, 2, 8]
print("Unsorted Array: ", arr)
cycle_sort(arr)
print("Sorted Array: ", arr)
|
code/sorting/src/cycle_sort/cycle_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// cycle_sort.swift
// Created by DaiPei on 2017/10/15.
//
import Foundation
func cycleSort(_ array: inout [Int]) {
for cycleStart in 0..<array.count {
var value = array[cycleStart]
var pos = cycleStart
// count all smaller elements on right side of item
for i in cycleStart + 1..<array.count {
if array[i] < value {
pos += 1
}
}
// if the value is already in right place
if pos == cycleStart {
continue
}
// ignore all duplicate elements
while array[pos] == value {
pos += 1
}
// put the value to the right place
swap(&array, at: pos, and: cycleStart)
// cycle again
while pos != cycleStart {
pos = cycleStart
value = array[cycleStart]
// count all smaller elements on right side of new value
for i in cycleStart + 1..<array.count {
if array[i] < value {
pos += 1
}
}
// break when there is not a element smaller than the value
if pos == cycleStart {
break
}
// ignore all duplicate elements
while array[pos] == value {
pos += 1
}
// put the value to the right place
swap(&array, at: pos, and: cycleStart)
}
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/flash_sort/README.md | # Flash sort
Flash sort is a distribution sorting algorithm. It has a usual performance of O(n) for uniformly distributed data sets. It has relatively less memory requirement.
The basic idea behind flashsort is that in a data set with a known distribution, it is easy to immediately estimate where an element should be placed after sorting when the range of the set is known.
|
code/sorting/src/flash_sort/flash_sort.c | // The flash sort algorithm
// The idea is simple
// If we know min max of arr is 0 and 100
// We know that 50 must be somewhere near the mid
#include <stdio.h>
#include <stdlib.h>
void myswap(int *a, int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
void flashSort(int *arr, int size)
{
// Phase 1
int min = arr[0];
int max = arr[0];
for (int i = 1; i < size; ++i)
{
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
}
if (min == max)
return;
int m = 0.43 * size; // Divide to m class
float c = (float)(m - 1) / (max - min);
int *L = (int *)calloc(m + 1, sizeof(int));
for (int i = 0; i < size; ++i)
{
int k = c * (arr[i] - min) + 1;
++L[k]; // L[k]: the number of class K
}
for (int k = 2; k <= m; ++k)
{
L[k] += L[k - 1]; // L[k]: upper bound of class K
}
// Phase 2
int move = 0; // number of move
int j = 0;
int k = m;
while (move < size - 1) // Only move size - 1 step
{
while (j >= L[k]) // arr[j] is in right class
{
++j;
k = c * (arr[j] - min) + 1;
}
int flash = arr[j]; // temp to hold the value
while (j < L[k]) // stop when arr[j] is changed
{
k = c * (flash - min) + 1;
myswap(&flash, &arr[--L[k]]);
++move;
}
}
// Phase 3
// Use Insertion sort for every class
// Don't sort the m class,
// Becuase the m class is all max
for (int k = 1; k < m; ++k)
{
for (int i = L[k] + 1; i < L[k + 1]; ++i)
{
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key)
{
arr[j + 1] = arr[j];
--j;
}
arr[j + 1] = key;
}
}
free(L);
}
int main()
{
int arr[] = {9, 1, 1, 8, 2, 2, 3, 6, 7, 12};
int size = sizeof(arr) / sizeof(arr[0]);
flashSort(arr, size);
for (int i = 0; i < size; ++i)
{
printf("%d ", arr[i]);
}
printf("\n");
}
|
code/sorting/src/flash_sort/flash_sort.js | "use strict";
function flash_sort(arr) {
let max = 0;
let min = arr[0];
const n = arr.length;
const m = ~~(0.45 * n);
const l = new Array(m);
for (var i = 1; i < n; ++i) {
if (arr[i] < min) {
min = arr[i];
}
if (arr[i] > arr[max]) {
max = i;
}
}
if (min === arr[max]) {
return arr;
}
const c1 = (m - 1) / (arr[max] - min);
for (var k = 0; k < m; k++) {
l[k] = 0;
}
for (var j = 0; j < n; ++j) {
k = ~~(c1 * (arr[j] - min));
++l[k];
}
for (let p = 1; p < m; ++p) {
l[p] = l[p] + l[p - 1];
}
let hold = arr[max];
arr[max] = arr[0];
arr[0] = hold;
//permutation
let move = 0;
let t;
let flash;
j = 0;
k = m - 1;
while (move < n - 1) {
while (j > l[k] - 1) {
++j;
k = ~~(c1 * (arr[j] - min));
}
if (k < 0) break;
flash = arr[j];
while (j !== l[k]) {
k = ~~(c1 * (flash - min));
hold = arr[(t = --l[k])];
arr[t] = flash;
flash = hold;
++move;
}
}
//insertion
for (j = 1; j < n; j++) {
hold = arr[j];
i = j - 1;
while (i >= 0 && arr[i] > hold) {
arr[i + 1] = arr[i--];
}
arr[i + 1] = hold;
}
return arr;
}
const arra = [3, 0, 2, 5, -1, 4, 1];
console.log(flash_sort(arra));
|
code/sorting/src/flash_sort/flash_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// flash_sort.m
// Created by DaiPei on 2017/10/17.
//
#import <Foundation/Foundation.h>
@interface FlashSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation FlashSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
// find the max and min item in array
NSInteger max = INTMAX_MIN;
NSInteger min = INTMAX_MAX;
for (NSNumber *item in array) {
if (item.integerValue > max) {
max = item.integerValue;
}
if (item.integerValue < min) {
min = item.integerValue;
}
}
if (min == max) {
return ;
}
// divide to m class
NSInteger m = (NSInteger)(0.43 * array.count);
double c = (double)(m - 1) / (double)(max - min);
// after this loop pClass[k] point to under bound of class k, it means every element is moved to the right class
int *pClass = (int *)calloc(m + 1, sizeof(int));
for (NSNumber *item in array) {
NSInteger k = (NSInteger)(c * (item.integerValue - min)) + 1;
pClass[k]++;
}
for (int k = 1; k <= m; k++) {
pClass[k] += pClass[k - 1];
}
// move every element to right class
NSUInteger move = 0;
NSUInteger j = 0;
NSInteger k = m;
while (move < array.count) {
while (j >= pClass[k]) {
j++;
k = (NSInteger)(c * (array[j].integerValue - min)) + 1;
}
NSInteger flash = array[j].integerValue;
while (j < pClass[k]) {
k = (NSInteger)(c * (flash - min)) + 1;
pClass[k]--;
NSInteger tmp = array[pClass[k]].integerValue;
array[pClass[k]] = @(flash);
flash = tmp;
move++;
}
}
// sort every class
for (int i = 1; i < m; i++) {
[self sortCore:array from:pClass[i] to:pClass[i + 1]];
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
- (void)sortCore:(NSMutableArray<NSNumber *> *)array from:(NSUInteger)a to:(NSUInteger)b {
if (a >= b) {
return ;
}
for (NSUInteger i = a; i + 1 < b; i++) {
NSUInteger p = i;
for (NSUInteger j = i + 1; j < b; j++) {
if ([array[p] compare:array[j]] == NSOrderedDescending) {
p = j;
}
}
[self swap:array at:p and:i];
}
}
@end
@interface SortTester : NSObject
+ (BOOL)isSorted:(NSArray<NSNumber *> *)array;
@end
@implementation SortTester
+ (BOOL)isSorted:(NSArray<NSNumber *> *)array {
for (int i = 1; i < array.count; i++) {
if ([array[i] compare:array[i - 1]] == NSOrderedAscending) {
return NO;
}
}
return YES;
}
@end
int main(int argc, const char * argv[]) {
for (int j = 0; j < 100; j++) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray array];
for (int i = 0; i < 20; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
FlashSort *fs = [[FlashSort alloc] init];
[fs sort:array];
NSLog(@"after: %@", array);
assert([SortTester isSorted:array]);
}
}
return 0;
}
|
code/sorting/src/flash_sort/flash_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// flash_sort.swift
// Created by DaiPei on 2017/10/17.
//
import Foundation
func flashSort(_ array: inout [Int]) {
// find the max and min item
var max = Int.min
var min = Int.max
for item in array {
if item > max {
max = item
}
if item < min {
min = item
}
}
if max == min {
return
}
// divide to m class
let m = Int(0.43 * Double(array.count))
let c = Double(m - 1) / Double(max - min)
// save every class pointer
var pClass = [Int](repeatElement(0, count: m + 1))
for item in array {
let k = Int(c * Double(item - min)) + 1
pClass[k] += 1
}
for k in 1...m {
pClass[k] += pClass[k - 1] // pClass[k]: point to upper bound of class k
}
// move every element to right class
var move = 0
var j = 0
var k = m
// after this loop pClass[k] point to under bound of class k, it means every element is moved to the right class
while move < array.count {
while j >= pClass[k] {
j += 1
k = Int(c * Double(array[j] - min)) + 1
}
var flash = array[j]
while j < pClass[k] {
k = Int(c * Double(flash - min)) + 1
pClass[k] -= 1
swap(&flash, &array[pClass[k]])
move += 1
}
}
// sort every class
for i in 1..<m {
sortCore(&array, from: pClass[i], to: pClass[i + 1])
}
}
private func swap(_ array: inout [Int], at a: Int, and b: Int) {
let tmp = array[a]
array[a] = array[b]
array[b] = tmp
}
private func sortCore(_ array: inout [Int], from a: Int, to b: Int) {
if a >= b {
return
}
for i in a..<b-1 {
var p = i
for j in i+1..<b {
if array[j] < array[p] {
p = j
}
}
swap(&array, at: p, and: i)
}
}
|
code/sorting/src/gnome_sort/README.md | # Gnome Sort
Gnome sort (or Stupid sort) is a sorting algorithm which is similar to insertion sort, except that moving an element to its proper place is accomplished by a series of swaps, as in bubble sort.
Gnome Sort is based on the technique used by the standard Dutch Garden Gnome, i.e., how he sorts a line of flower pots. Basically, he looks at the flower pot next to him and the previous one; if they are in the right order he steps one pot forward, otherwise he swaps them and steps one pot backwards. Boundary conditions: if there is no previous pot, he steps forwards; if there is no pot next to him, he is done.
## Explanation
Consider the given array:
`arr = [34 2 10 -9]`
1. Underlined elements are the pair under consideration.
2. **Red** colored are the pair which needs to be swapped.
3. Result of the swapping is colored as **blue**.

> Image credits: geeksforgeeks
## Algorithm
```
procedure gnomeSort(a[]):
pos := 0
while pos < length(a):
if (pos == 0 or a[pos] >= a[pos-1]):
pos := pos + 1
else:
swap a[pos] and a[pos-1]
pos := pos - 1
```
## Complexity
**Time complexity**
- Worst Case: **O(n<sup>2</sup>)**
- Average Case: **O(n<sup>2</sup>)**
- Best Case: **O(n)**
**Space complexity**: **O(1)** auxillary space
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/gnome_sort/gnome_sort.c | #include <stdio.h>
void
swap(int *a, int *b)
{
int t = *a;
*a = *b;
*b = t;
}
void
gnomeSort(int arr[], int n)
{
int index = 0;
while (index < n) {
if (index == 0)
++index;
if (arr[index] >= arr[index - 1])
++index;
else {
swap(&arr[index], &arr[index - 1]);
--index;
}
}
return;
}
int
main()
{
int n;
printf("Enter size of Array \n");
scanf("%d", &n);
int arr[n];
printf("Enter %d Integers \n", n);
int i;
for (i = 0; i < n; ++i)
scanf("%d", &arr[i]);
gnomeSort(arr, n);
printf("Array after sorting: \n");
for (i = 0; i < n; ++i)
printf("%d ", arr[i]);
printf("\n");
return (0);
}
|
code/sorting/src/gnome_sort/gnome_sort.cpp | // A C++ Program to implement Gnome Sort
#include <iostream>
using namespace std;
// A function to sort the algorithm using gnome sort
void gnomeSort(int arr[], int n)
{
int index = 0;
while (index < n)
{
if (index == 0)
index++;
if (arr[index] >= arr[index - 1])
index++;
else
{
swap(arr[index], arr[index - 1]);
index--;
}
}
return;
}
// A utility function ot print an array of size n
void printArray(int arr[], int n)
{
cout << "Sorted sequence after Gnome sort: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << "\n";
}
// Driver program to test above functions.
int main()
{
int arr[] = {34, 2, 10, -9};
int n = sizeof(arr) / sizeof(arr[0]);
gnomeSort(arr, n);
printArray(arr, n);
return 0;
}
|
code/sorting/src/gnome_sort/gnome_sort.go | package main
import (
"fmt"
)
// Gnome Sort in Golang
// Part of Cosmos by OpenGenus Foundation
// GnomeSort sorts the given slice
func GnomeSort(a []int) {
pos := 0
for pos < len(a) {
if pos == 0 || a[pos] >= a[pos-1] {
pos++
} else {
a[pos], a[pos-1] = swap(a[pos], a[pos-1])
pos--
}
}
}
func swap(x int, y int) (int, int) {
return y, x
}
func main() {
randomSlice := []int{5, 2, 8, 9, 10, 1, 3}
fmt.Println("Unsorted Slice: ", randomSlice)
GnomeSort(randomSlice)
// should receive [ 1, 2, 3, 5, 8, 9, 10 ]
fmt.Println("Sorted Slice: ", randomSlice)
}
|
code/sorting/src/gnome_sort/gnome_sort.java | // Java Program to implement Gnome Sort
import java.util.Arrays;
public class GnomeSort {
private static void gnomeSort(int arr[]) {
int index = 0;
while (index < arr.length) {
if ((index == 0) || (arr[index - 1] <= arr[index])) {
index++;
} else {
int temp = arr[index];
arr[index] = arr[index - 1];
arr[index - 1] = temp;
index--;
}
}
}
// Driver program to test above functions.
public static void main(String[] args) {
int arr[] = {34, 2, 10, -9};
System.out.println("Before: " + Arrays.toString(arr));
gnomeSort(arr);
System.out.println("After: " + Arrays.toString(arr));
}
} |
code/sorting/src/gnome_sort/gnome_sort.js | function gnomeSort(arr) {
let index = 0;
while (index <= arr.length) {
if (index > 0 && arr[index - 1] > arr[index]) {
let tmp = arr[index];
arr[index] = arr[index - 1];
arr[index - 1] = tmp;
--index;
} else {
--index;
}
}
}
// test case
const a = [3, 6, 2, 9, 8, 1, 4, 0, 3, 7, 7];
console.log(gnomeSort(a));
|
code/sorting/src/gnome_sort/gnome_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// gnome_sort.m
// Created by DaiPei on 2017/10/16.
//
#import <Foundation/Foundation.h>
@interface GnomeSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation GnomeSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
if (array.count <= 1) {
return ;
}
int index = 0;
while (index < array.count) {
if (index == 0) {
index++;
}
if ([array[index] compare:array[index - 1]] == NSOrderedAscending) {
[self swap:array at:index and:index - 1];
index--;
} else {
index++;
}
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray arrayWithCapacity:10];
for (int i = 0; i < 10; i++) {
int ran = arc4random() % 20;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
GnomeSort *gs = [[GnomeSort alloc] init];
[gs sort:array];
NSLog(@"after: %@", array);
}
return 0;
}
|
code/sorting/src/gnome_sort/gnome_sort.py | # Python program to implement Gnome Sort
# A function to sort the given list using Gnome sort
def gnome_sort(arr, n):
index = 0
while index < n:
if index == 0:
index = index + 1
if arr[index] >= arr[index - 1]:
index = index + 1
else:
arr[index], arr[index - 1] = arr[index - 1], arr[index]
index = index - 1
return arr
|
code/sorting/src/gnome_sort/gnome_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// gnome_sort.swift
// Created by DaiPei on 2017/10/16.
//
import Foundation
func gnomeSort(_ array: inout [Int]) {
if array.count <= 1 {
return
}
var index = 1
while index < array.count {
if index == 0 {
index += 1
}
if array[index] >= array[index - 1] {
index += 1
} else {
swap(&array, at: index, and: index - 1)
index -= 1
}
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/heap_sort/README.md | # Heap Sort
Heap sort is a comparison based, simple and efficient sorting algorithm. There are two main parts to the process:
* A heap is built from the unsorted data. The algorithm separates the unsorted data from the sorted data.
* The largest element from the unsorted data is moved into an array
Thus, Heap Sort uses a heap to sort the given elements.
## Explanation
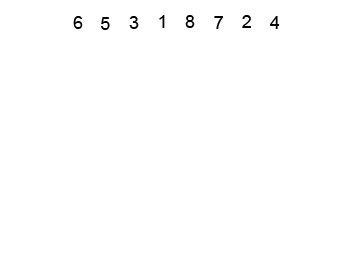
> Image credits: https://www.codingeek.com/
## Algorithm
```
Heapify (A, i)
l ← left [i]
r ← right [i]
if l ≤ heap-size [A] and A[l] > A[i]
then largest ← l
else largest ← i
if r ≤ heap-size [A] and A[i] > A[largest]
then largest ← r
if largest ≠ i
then exchange A[i] ↔ A[largest]
Heapify (A, largest)
```
## Complexity
**Time Complexity**:
Worst, average and best case time complexity: **O(n * log n)**
**Space complexity**: `O(1)`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/heap_sort/heap_sort.c | // C implementation of Heap Sort
// Part of Cosmos by OpenGenus Foundation
#include <stdio.h>
#include <stdlib.h>
// A heap has current size and array of elements
struct MaxHeap
{
int size;
int* array;
};
// A utility function to swap to integers
void swap(int* a, int* b) { int t = *a; *a = *b; *b = t; }
// The main function to heapify a Max Heap. The function
// assumes that everything under given root (element at
// index idx) is already heapified
void maxHeapify(struct MaxHeap* maxHeap, int idx)
{
int largest = idx; // Initialize largest as root
int left = (idx << 1) + 1; // left = 2*idx + 1
int right = (idx + 1) << 1; // right = 2*idx + 2
// See if left child of root exists and is greater than
// root
if (left < maxHeap->size &&
maxHeap->array[left] > maxHeap->array[largest])
largest = left;
// See if right child of root exists and is greater than
// the largest so far
if (right < maxHeap->size &&
maxHeap->array[right] > maxHeap->array[largest])
largest = right;
// Change root, if needed
if (largest != idx)
{
swap(&maxHeap->array[largest], &maxHeap->array[idx]);
maxHeapify(maxHeap, largest);
}
}
// A utility function to create a max heap of given capacity
struct MaxHeap* createAndBuildHeap(int *array, int size)
{
int i;
struct MaxHeap* maxHeap =
(struct MaxHeap*) malloc(sizeof(struct MaxHeap));
maxHeap->size = size; // initialize size of heap
maxHeap->array = array; // Assign address of first element of array
// Start from bottommost and rightmost internal mode and heapify all
// internal modes in bottom up way
for (i = (maxHeap->size - 2) / 2; i >= 0; --i)
maxHeapify(maxHeap, i);
return maxHeap;
}
// The main function to sort an array of given size
void heapSort(int* array, int size)
{
// Build a heap from the input data.
struct MaxHeap* maxHeap = createAndBuildHeap(array, size);
// Repeat following steps while heap size is greater than 1.
// The last element in max heap will be the minimum element
while (maxHeap->size > 1)
{
// The largest item in Heap is stored at the root. Replace
// it with the last item of the heap followed by reducing the
// size of heap by 1.
swap(&maxHeap->array[0], &maxHeap->array[maxHeap->size - 1]);
--maxHeap->size; // Reduce heap size
// Finally, heapify the root of tree.
maxHeapify(maxHeap, 0);
}
}
// A utility function to print a given array of given size
void printArray(int* arr, int size)
{
int i;
for (i = 0; i < size; ++i)
printf("%d ", arr[i]);
}
/* Driver program to test above functions */
int main()
{
int arr[] = {12, 11, 13, 5, 6, 7};
int size = sizeof(arr)/sizeof(arr[0]);
heapSort(arr, size);
printf("\nSorted array is \n");
printArray(arr, size);
return 0;
}
|
code/sorting/src/heap_sort/heap_sort.cpp | /* Part of Cosmos by OpenGenus Foundation */
#include <vector>
#include <iostream>
using namespace std;
void heapify (vector<int> &v, int n, int i)
{
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && v[l] > v[largest])
largest = l;
if (r < n && v[r] > v[largest])
largest = r;
if (largest != i)
{
swap(v[i], v[largest]);
heapify(v, n, largest);
}
}
// The heap sort function that should be called
void heap_sort(vector<int> &v)
{
int n = v.size();
// Build heap (rearrange array)
for (int i = n / 2 - 1; i >= 0; --i)
heapify(v, n, i);
// Extract element from heap 1 by 1
for (int i = n - 1; i >= 0; --i)
{
swap(v[0], v[i]);
heapify(v, i, 0);
}
}
// Testing the heap sort implementation
int main()
{
vector<int> v = {2, 3, 6, 7, 4, 1};
heap_sort(v);
for (size_t i = 0; i < v.size(); ++i)
cout << v[i] << " ";
return 0;
}
|
code/sorting/src/heap_sort/heap_sort.cs | using System;
using System.Collections.Generic;
namespace CS
{
/* Part of Cosmos by OpenGenus Foundation */
class MergeSort
{
private static int ParentIndex(int index)
{
return (index - 1) / 2;
}
private static int LeftChildIndex(int index)
{
return 2 * index + 1;
}
private static int RightChildIndex(int index)
{
return 2 * index + 2;
}
private static void SiftDown(int[] arr, int start, int end)
{
int root = start;
int child;
while ((child = LeftChildIndex(root)) <= end)
{
int swap = root;
if (arr[swap] < arr[child])
{
swap = child;
}
if (child + 1 <= end && arr[swap] < arr[child + 1])
{
swap = child + 1;
}
if (swap == root)
{
return;
}
else
{
int temp = arr[root];
arr[root] = arr[swap];
arr[swap] = temp;
root = swap;
}
}
}
static int[] Sort(int[] arr)
{
// Heapify
for (int i = ParentIndex(arr.Length - 1); i >= 0; i--)
{
SiftDown(arr, i, arr.Length - 1);
}
// Sort
int end = arr.Length - 1;
while (end >= 0)
{
int temp = arr[end];
arr[end] = arr[0];
arr[0] = temp;
end--;
SiftDown(arr, 0, end);
}
return arr;
}
static void Main(string[] args)
{
// Create an array of 10 random numbers
int[] numbers = new int[10];
var rand = new Random();
for (int i = 0; i < 10; i++)
{
numbers[i] = rand.Next(10);
}
Console.WriteLine("Initial ordering:");
foreach (var num in numbers)
{
Console.Write(num + " ");
}
// Store the sorted result in a separate variable and print it out
var sorted = Sort(numbers);
Console.WriteLine("\nSorted:");
foreach (var num in sorted)
{
Console.Write(num + " ");
}
}
}
}
|
code/sorting/src/heap_sort/heap_sort.go | /* Part of Cosmos by OpenGenus Foundation */
package main
import "fmt"
type MaxHeap struct {
data []int
}
func swap(x, y int) (int, int) {
return y, x
}
func (h *MaxHeap) Insert(value int) {
h.data = append(h.data, value)
index := len(h.data) - 1
parent := (index - 1) / 2
for index > 0 && h.data[index] < h.data[parent] {
h.data[index], h.data[parent] = swap(h.data[index], h.data[parent])
index = parent
parent = (index - 1) / 2
}
}
func (h *MaxHeap) Top() int {
return h.data[0]
}
func (h *MaxHeap) ExtractMax() int {
index := len(h.data) - 1
max := h.Top()
h.data[0], h.data[index] = swap(h.data[0], h.data[index])
h.data = h.data[:index]
h.heapify(0)
return max
}
func (h *MaxHeap) heapify(index int) {
left := (index * 2) + 1
right := left + 1
target := index
if left < len(h.data) && h.data[left] < h.data[index] {
target = left
}
if right < len(h.data) && h.data[right] < h.data[target] {
target = right
}
if target != index {
h.data[index], h.data[target] = swap(h.data[index], h.data[target])
h.heapify(target)
}
}
func (h *MaxHeap) Empty() bool {
return len(h.data) == 0
}
func HeapSort(data []int) {
var h MaxHeap
for _, v := range data {
h.Insert(v)
}
i := 0
for !h.Empty() {
data[i] = h.Top()
h.ExtractMax()
i++
}
}
func main() {
data := []int{45, 6, 4, 3, 2, 72, 34, 12, 456, 29, 312}
fmt.Println("Beforew heap sort")
fmt.Println(data)
HeapSort(data)
fmt.Println("After heap sort ")
fmt.Println(data)
}
|
code/sorting/src/heap_sort/heap_sort.java | import java.util.*;
/*
* Part of Cosmos by OpenGenus Foundation
*/
public class HeapSort {
public static void main(String[] args) {
// array used for testing
int[] a = {5, 11, 1234, 2, 6};
System.out.println(Arrays.toString(a));
sort(a);
System.out.println(Arrays.toString(a));
}
public static void sort(int[] a) {
int n = a.length;
// create the heap
for (int i = n / 2 - 1; i >= 0; i--)
heapify(a, n, i);
// extract element from the heap
for (int i=n-1; i>=0; i--)
{
int temp = a[0];
a[0] = a[i];
a[i] = temp;
// rebuild the heap
heapify(a, i, 0);
}
}
public static void heapify(int arr[], int n, int i)
{
int largest = i;
int l = 2*i + 1;
int r = 2*i + 2;
// If left child is larger than root
if (l < n && arr[l] > arr[largest])
largest = l;
// If right child is larger than largest so far
if (r < n && arr[r] > arr[largest])
largest = r;
// If largest is not root
if (largest != i)
{
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
// Recursively heapify the affected sub-tree
heapify(arr, n, largest);
}
}
}
|
code/sorting/src/heap_sort/heap_sort.js | /*
* Part of Cosmos by OpenGenus Foundation
*/
var arrayLength;
function heap_root(inputArray, i) {
var left = 2 * i + 1;
var right = 2 * i + 2;
var max = i;
if (left < arrayLength && inputArray[left] > inputArray[max]) {
max = left;
}
if (right < arrayLength && inputArray[right] > inputArray[max]) {
max = right;
}
if (max != i) {
swap(inputArray, i, max);
heap_root(inputArray, max);
}
}
function swap(inputArray, index_A, index_B) {
var temp = inputArray[index_A];
inputArray[index_A] = inputArray[index_B];
inputArray[index_B] = temp;
}
function heapSort(inputArray) {
arrayLength = inputArray.length;
for (var i = Math.floor(arrayLength / 2); i >= 0; i -= 1) {
heap_root(inputArray, i);
}
for (i = inputArray.length - 1; i > 0; i--) {
swap(inputArray, 0, i);
arrayLength--;
heap_root(inputArray, 0);
}
}
|
code/sorting/src/heap_sort/heap_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// heap_sort.m
// Created by DaiPei on 2017/10/9.
//
#import <Foundation/Foundation.h>
@interface HeapSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation HeapSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
int n = (int)array.count;
// create the heap
for (int i = n / 2; i >= 0; i--) {
[self sink:array at:i to:n - 1];
}
// sort core
for (int i = n - 1; i > 0; i--) {
[self swap:array at:i and:0];
[self sink:array at:0 to:i - 1];
}
}
- (void)sink:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA to:(NSUInteger)indexB {
NSUInteger pMax;
while ((indexA + 1) * 2 - 1 <= indexB) {
NSUInteger l = (indexA + 1) * 2 - 1;
NSUInteger r = (indexA + 1) * 2;
if (r <= indexB && [array[l] compare:array[r]] == NSOrderedAscending) {
pMax = r;
} else {
pMax = l;
}
if ([array[indexA] compare:array[pMax]] == NSOrderedAscending) {
[self swap:array at:indexA and:pMax];
indexA = pMax;
} else {
break;
}
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray array];
for (int i = 0; i < 20; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
HeapSort *hs = [[HeapSort alloc] init];
[hs sort:array];
NSLog(@"after: %@", array);
}
return 0;
}
|
code/sorting/src/heap_sort/heap_sort.py | # Part of Cosmos by OpenGenus Foundation
import math
def heap_root(arr, i, length):
left = 2 * i + 1
right = 2 * i + 2
max_index = i
if left < length and arr[left] > arr[max_index]:
max_index = left
if right < length and arr[right] > arr[max_index]:
max_index = right
if max_index != i:
swap(arr, max_index, i)
heap_root(arr, max_index, length)
def swap(arr, a, b):
arr[a], arr[b] = arr[b], arr[a]
# sorting is in the ascending order
def heap_sort(arr):
# form a max heap
for i in range(math.floor(len(arr) / 2), -1, -1):
heap_root(arr, i, len(arr))
length = len(arr)
# remove the root and reform the heap
for i in range(len(arr) - 1, 0, -1):
swap(arr, 0, i)
length -= 1
heap_root(arr, 0, length)
|
code/sorting/src/heap_sort/heap_sort.rb | # Part of Cosmos by OpenGenus Foundation
class Array
def heap_sort
heapify
the_end = length - 1
while the_end > 0
self[the_end], self[0] = self[0], self[the_end]
the_end -= 1
sift_down(0, the_end)
end
self
end
def heapify
# the_start is the last parent node
the_start = (length - 2) / 2
while the_start >= 0
sift_down(the_start, length - 1)
the_start -= 1
end
self
end
def sift_down(the_start, the_end)
root = the_start
while root * 2 + 1 <= the_end
child = root * 2 + 1
swap = root
swap = child if self[swap] < self[child]
swap = child + 1 if child + 1 <= the_end && self[swap] < self[child + 1]
if swap != root
self[root], self[swap] = self[swap], self[root]
root = swap
else
return
end
end
end
end
random_names = File.readlines('randomnames.txt')
puts random_names.heap_sort
|
code/sorting/src/heap_sort/heap_sort.rs | // Part of Cosmos by OpenGenus Foundation
fn heapify(arr: &mut [i32], n: usize, i: usize){
let mut largest = i; //largest as root
let l = 2*i + 1; //left
let r = 2*i + 2; //right
//if child larger than root
if l < n && arr[l as usize] > arr[largest as usize]{
largest = l;
}
//if child larger than largest
if r < n && arr[r as usize] > arr[largest as usize]{
largest = r;
}
//if largest is not root
if largest != i{
let temp = arr[i as usize];
arr[i as usize] = arr[largest as usize];
arr[largest as usize] = temp;
//recursively heapify
heapify(arr, n, largest);
}
}
fn heap_sort(arr: &mut [i32], n: usize){
//build heap
for i in (0..n / 2 - 1).rev(){
heapify(arr, n, i);
}
for i in (0..n - 1).rev(){
//move current root to end
let temp = arr[i as usize];
arr[i as usize] = arr[0];
arr[0] = temp;
heapify(arr, i, 0);
}
}
fn print_array(arr: &mut [i32], n: usize){
for i in (0..n).rev(){
print!("{} ", arr[i as usize]);
}
println!("");
}
//test heap sort
fn main() {
let mut arr: [i32; 8] = [1, 2, 3, 4, 5, 6, 7, 8];
let n: usize = arr.len();
heap_sort(&mut arr, n);
println!("Sorted Array:");
print_array(&mut arr, n);
}
|
code/sorting/src/heap_sort/heap_sort.sc | object heapsort {
def left(i: Int) = (2*i) + 1
def right(i: Int) = left(i) + 1
def parent(i: Int) = (i - 1) / 2
def swap(a: Array[Int], i: Int, j: Int): Unit = {
val t = a(i)
a(i) = a(j)
a(j) = t
}
def maxHeap (a: Array[Int], i: Int, size: Int): Unit = {
val l = left(i)
val r = right(i)
var m = -1
m = if (l < size && a(l) > a(i)) {l} else {i}
m = if (r < size && a(r) > a(m)) {r} else {m}
if (m != i) {
swap(a, i, m)
maxHeap(a, m, size)
}
}
def buildMaxHeap (a: Array[Int], size: Int): Unit = {
println(s"$a -> $size")
val hs = size / 2
for (i <- 0 to hs) {
println(s"i -> $i")
maxHeap(a, hs - i, size)
}
}
def heapSort (a: Array[Int]) {
buildMaxHeap(a, a.length)
for (i <- (0 until a.length).reverse) {
swap(a, 0, i)
maxHeap(a, 0, i)
}
}
val data = Array (1,0,2,9,3,8,4,7,5,6)
heapSort (data)
data
}
|
code/sorting/src/heap_sort/heap_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// heap_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func heapSort(_ array: inout [Int]) {
// create heap
for i in stride(from: array.count / 2, to: -1, by: -1) {
sink(array: &array, at: i, to: array.count - 1)
}
// sort core
for i in stride(from: array.count - 1, to: 0, by: -1) {
swap(&array, at: i, and: 0)
sink(array: &array, at: 0, to: i - 1)
}
}
private func sink(array: inout [Int], at indexA: Int, to indexB: Int) {
var i = indexA
while (i + 1) * 2 - 1 <= indexB {
let leftChild = (i + 1) * 2 - 1
let rightChild = (i + 1) * 2
let pMax: Int
if rightChild <= indexB && array[leftChild] < array[rightChild] {
pMax = rightChild
} else {
pMax = leftChild
}
if (array[pMax] > array[i]) {
swap(&array, at: pMax, and: i)
i = pMax
} else {
break
}
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/heap_sort/heapsort.go | package main
import "fmt"
import "math/rand"
import "time"
type MaxHeap struct {
slice []int
heapSize int
}
func BuildMaxHeap(slice []int) MaxHeap {
h := MaxHeap{slice: slice, heapSize: len(slice)}
for i := len(slice) / 2; i >= 0; i-- {
h.MaxHeapify(i)
}
return h
}
func (h MaxHeap) MaxHeapify(i int) {
l, r := 2*i+1, 2*i+2
max := i
if l < h.size() && h.slice[l] > h.slice[max] {
max = l
}
if r < h.size() && h.slice[r] > h.slice[max] {
max = r
}
//log.Printf("MaxHeapify(%v): l,r=%v,%v; max=%v\t%v\n", i, l, r, max, h.slice)
if max != i {
h.slice[i], h.slice[max] = h.slice[max], h.slice[i]
h.MaxHeapify(max)
}
}
func (h MaxHeap) size() int { return h.heapSize } // ???
func heapSort(slice []int) []int {
h := BuildMaxHeap(slice)
//log.Println(slice)
for i := len(h.slice) - 1; i >= 1; i-- {
h.slice[0], h.slice[i] = h.slice[i], h.slice[0]
h.heapSize--
h.MaxHeapify(0)
if i == len(h.slice)-1 || i == len(h.slice)-3 || i == len(h.slice)-5 {
element := (i - len(h.slice)) * -1
fmt.Println("Heap after removing ", element, " elements")
fmt.Println(h.slice)
}
}
return h.slice
}
func main() {
s1 := rand.NewSource(time.Now().UnixNano())
r1 := rand.New(s1)
s := make([]int, 20)
for index, _ := range s {
s[index] = r1.Intn(400)
}
fmt.Println("Randomly generated array:")
fmt.Println(s)
h := BuildMaxHeap(s)
fmt.Println("\nInital Heap: ")
fmt.Println(h.slice, "\n")
s = heapSort(s)
fmt.Println("\nFinal List:")
fmt.Println(s)
}
|
code/sorting/src/insertion_sort/README.md | # Insertion Sort
Insertion sort iterates through an array by taking one input element at each iteration, and grows a sorted output. With each iteration, insertion sorts removes the element at the current index, finds its correct position in the sorted output list, and inserts it there. This is repeated until no elements remain.
It is highly recommended to use Insertion Sort for sorting small or nearly sorted lists.
## Explanation

> Image credits: Wikimedia Commons
## Algorithm
1. Get unsorted array/list
2. Set 'marker' for sorted section after first element in array/list
3. Select first unsorted number
4. Swap number with left elements until it is in correct position in sorted sub-array
5. Move sorted section marker over to the right one position
6. Repeat steps 3 through 5 until no more elements exist in unsorted sub-array.
## Complexity
**Time Complexity:**
- Worst case: **O(n<sup>2</sup>)**
- Average: **O(n<sup>2</sup>)**
- Best case: **O(n)**
**Space Complexity:**
- Average: **O(n)**
<p align="center">
Refer to <a href="https://iq.opengenus.org/insertion-sort-analysis/">Insertion Sort Analysis</a> for more explanation.
</p>
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/sorting/src/insertion_sort/insertion_sort.c | /* Part of Cosmos by OpenGenus Foundation */
#include <stdio.h>
typedef int bool;
void insertionSort(int*, int, bool);
void printArray(int*, int);
int
main()
{
int n;
/* Taking number of array elements as input from user */
printf("Enter size of array:\n");
scanf("%d", &n);
int arr[n];
/* Taking array elements as input from user */
printf("Enter array elements:\n");
for(int i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
bool order;
/* Input order of sorting. 1 for Ascending, 0 for Descending */
printf("Enter order of sorting (1: Ascending; 0: Descending)\n");
scanf("%d", &order);
/* If user inputs order besides 1 or 0 */
if (order != 0 && order != 1) {
printf("Undefined sorting order.\n");
return 1;
}
/* Sort array */
insertionSort(arr, n, order);
/* Print sorted array */
printArray(arr, n);
return (0);
}
/* Insertion sort function */
void
insertionSort(int arr[], int n, bool order)
{
int key;
int j;
/* Order 1 corresponds to Ascending order */
if (order == 1) {
for(int i = 1; i < n; i++) {
key = arr[i];
j = i - 1;
/*
* Shift elements of arr[0..i-1], that are
* greater than key, to one position ahead
* of their current position
*/
while(j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
/* Order 0 corresponds to Descending order */
else if (order == 0) {
for(int i = 1; i < n; i++) {
key = arr[i];
j = i - 1;
/*
* Shift elements of arr[0..i-1], that are
* lesser than key, to one position ahead
* of their current position
*/
while(j >= 0 && arr[j] < key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
/* If order not 0 or 1, undefined */
else {
printf("Undefind sorting order.\n");
}
}
/* To print array */
void
printArray(int arr[], int n)
{
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
|
code/sorting/src/insertion_sort/insertion_sort.cpp | /*
* Part of Cosmos by OpenGenus Foundation
*
* insertion sort synopsis
*
* template<typename _Bidirectional_Iter, typename _Compare>
* void
* insertionSort(_Bidirectional_Iter begin, _Bidirectional_Iter end, _Compare compare);
*
* template<typename _Bidirectional_Iter>
* void
* insertionSort(_Bidirectional_Iter begin, _Bidirectional_Iter end);
*/
#include <functional>
template<typename _Bidirectional_Iter, typename _Compare>
void
insertionSort(_Bidirectional_Iter begin, _Bidirectional_Iter end, _Compare compare)
{
if (begin != end)
{
auto backOfBegin = begin;
--backOfBegin;
auto curr = begin;
for (++curr; curr != end; ++curr)
{
auto pivot = *curr;
auto backward = curr;
auto nextOfBackward = curr;
while (--backward != backOfBegin && compare(pivot, *backward))
{
*nextOfBackward = *backward;
--nextOfBackward;
}
*nextOfBackward = pivot;
}
}
}
template<typename _Bidirectional_Iter>
void
insertionSort(_Bidirectional_Iter begin, _Bidirectional_Iter end)
{
using value_type = typename std::iterator_traits<_Bidirectional_Iter>::value_type;
insertionSort(begin, end, std::less<value_type>());
}
|
code/sorting/src/insertion_sort/insertion_sort.cs | /*
* C# Program to Perform Insertion Sort
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
int[] arr = new int[5] { 83, 12, 3, 34, 60 };
int i;
Console.WriteLine("The Array is :");
for (i = 0; i < 5; i++)
{
Console.WriteLine(arr[i]);
}
InsertionSort(arr, 5);
Console.WriteLine("The Sorted Array is :");
for (i = 0; i < 5; i++)
Console.WriteLine(arr[i]);
Console.ReadLine();
}
static void InsertionSort(int[] data, int n)
{
int i, j;
for (i = 1; i < n; i++)
{
int item = data[i];
int ins = 0;
/* Move elements of data[0..i-1],
that are greater than item,
to one position ahead of
their current position */
for (j = i - 1; j >= 0 && ins != 1; )
{
if (item < data[j])
{
data[j + 1] = data[j];
j--;
data[j + 1] = item;
}
else ins = 1;
}
}
}
}
}
|
code/sorting/src/insertion_sort/insertion_sort.dart | main() {
print(insertionSort([8, 9, 4, 2, 6, 10, 12]));
}
List<int> insertionSort(List<int> list) {
for (int j = 1; j < list.length; j++) {
int key = list[j];
int i = j - 1;
while (i >= 0 && list[i] > key) {
list[i + 1] = list[i];
i = i - 1;
list[i + 1] = key;
}
}
return list;
}
|
code/sorting/src/insertion_sort/insertion_sort.go | package main
import (
"fmt"
)
// Part of Cosmos by OpenGenus Foundation
func main() {
array := []int{9, 6, 7, 3, 2, 4, 5, 1}
insertion(array)
}
func insertion(array []int) {
lenght := len(array)
for i := 1; i < lenght; i++ {
j := i
for j > 0 && array[j-1] > array[j] {
array[j-1], array[j] = array[j], array[j-1]
j = j - 1
}
}
fmt.Println(array)
}
|
code/sorting/src/insertion_sort/insertion_sort.hs | {-
Part of Cosmos by OpenGenus Foundation
-}
insertion_sort :: Ord a => [a] -> [a]
insertion_sort [] = []
insertion_sort [x] = [x]
insertion_sort (x:arrx) = insert $ insertion_sort arrx
where insert [] = [x]
insert (y:arry)
| x < y = x : y : arry
| otherwise = y : insert arry
main = do
let arr = [3,5,12,56,92,123,156,190,201,222]
putStrLn("Sorted Array: "
++ show(insertion_sort(arr)))
|
code/sorting/src/insertion_sort/insertion_sort.java | // Part of Cosmos by OpenGenus Foundation
class InsertionSort {
void sort(int arr[]) { // sort() performs the insertion sort on the array which is passed as argument from main()
for (int i = 0; i < arr.length; ++i) {
for (int j = i; j > 0 && arr[j - 1] > arr[j]; j--) { // swapping of elements if j-1th element is greater than jth element
int temp = arr[j];
arr[j] = arr[j - 1];
arr[j - 1] = temp;
}
}
}
// Driver method
public static void main(String args[]) {
int arr[] = {8, 2, 111, 3, 15, 61, 17}; // array to be sorted
System.out.println("Before: " + Arrays.toString(arr)); // printing array before sorting
InsertionSort ob = new InsertionSort(); // object of class InsertionSort is created
ob.sort(arr); // arr is passed as argument to the function sort() is called
System.out.println("After:" + Arrays.toString(arr)); // printing array after insertion sort is applied
}
}
|
code/sorting/src/insertion_sort/insertion_sort.js | // Part of Cosmos by OpenGenus Foundation
function insertionSort(array) {
var length = array.length;
for (var i = 1; i < length; i++) {
var temp = array[i];
for (var j = i - 1; j >= 0 && array[j] > temp; j--) {
array[j + 1] = array[j];
}
array[j + 1] = temp;
}
return array;
}
// insertionSort([1,2,3,4,58,99,1])
|
code/sorting/src/insertion_sort/insertion_sort.kt | import java.util.*
fun <T : Comparable<T>> insertionsort(items : MutableList<T>) : List<T>
{
if (items.isEmpty())
{
return items
}
for (count in 1..items.count() - 1)
{
val item = items[count]
var i = count
while (i>0 && item < items[i - 1])
{
items[i] = items[i - 1]
i -= 1
}
items[i] = item
}
return items
}
fun main(args: Array<String>)
{
val names = mutableListOf("one", "Two", "Zack", "Daniel", "Adam")
println(names)
println(insertionsort(names))
}
|
code/sorting/src/insertion_sort/insertion_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// insertion_sort.m
// Created by DaiPei on 2017/10/9.
//
#import <Foundation/Foundation.h>
@interface InsertionSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *) array;
@end
@implementation InsertionSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
for (int i = 1; i < array.count; i++) {
NSNumber *k = array[i];
int j;
for (j = i; j > 0 && [k compare:array[j - 1]] == NSOrderedAscending; j--) {
array[j] = array[j - 1];
}
array[j] = k;
}
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
int n = 0;
NSLog(@"What is the size of the array?");
scanf("%d", &n);
NSMutableArray *array = [NSMutableArray arrayWithCapacity:n];
NSLog(@"Enter elements of the array one by one:");
for (int i = 0; i < n; i++) {
int tmp;
scanf("%d", &tmp);
[array addObject:@(tmp)];
}
InsertionSort *is = [[InsertionSort alloc] init];
[is sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/insertion_sort/insertion_sort.ml | (* Part of Cosmos by OpenGenus Foundation *)
let rec sort = function
| [] -> []
| hd :: tl -> insert hd (sort tl)
and insert x = function
| [] -> [x]
| hd :: tl -> if x < hd then x :: hd :: tl else hd :: insert x tl
;;
sort [];;
sort [1];;
sort [1; 2];;
sort [2; 1];;
sort [4; 3; 2; 1];;
sort [2; 5; 4; 3; 1];;
|
code/sorting/src/insertion_sort/insertion_sort.php | <?php
// Part of Cosmos by OpenGenus Foundation
$array = array(0,1,6,7,6,3,4,2);
function insert_sort($array)
{
for($i = 0; $i < count($array); $i++)
{
$val = $array[$i];
$j = $i-1;
while($j >= 0 && $array[$j] > $val)
{
$array[$j+1] = $array[$j];
$j--;
}
$array[$j+1] = $val;
}
return $array;
}
print 'unsorted array';
echo "\n";
print_r($array);
print 'sorted array';
echo "\n";
print_r(insert_sort($array));
?>
|
code/sorting/src/insertion_sort/insertion_sort.py | """
Part of Cosmos by OpenGenus Foundation
Program for InsertionSort sorting
"""
def insertion_sort(L):
length = len(L)
for i in range(length):
key = L[i]
j = i - 1
while j >= 0 and key < L[j]:
L[j + 1] = L[j]
j -= 1
L[j + 1] = key
return L
if __name__ == "__main__":
# creating a random array of length 100
L = np.random.random_integers(100, size=(100,)).tolist()
print("Given array : \n", L)
print("Sorted array : \n", insertion_sort(L))
|
code/sorting/src/insertion_sort/insertion_sort.rb | # Part of Cosmos by OpenGenus Foundation
def insertion_sort(arr)
n = arr.length
i = 1
while i < n
key = arr[i]
j = i - 1
while (j >= 0) && (key < arr[j])
arr[j + 1] = arr[j]
j -= 1
end
arr[j + 1] = key
i += 1
end
arr
end
arr = [3, 1, 5, 8, 11, 10, 23, 24, -1]
puts "Unsorted Array: #{arr}"
puts "Sorted Array after Insertion Sort: #{insertion_sort(arr)}"
|
code/sorting/src/insertion_sort/insertion_sort.re | let rec sort =
fun
| [] => []
| [hd, ...tl] => insert(hd, sort(tl))
and insert = x =>
fun
| [] => [x]
| [hd, ...tl] =>
if (x < hd) {
[x, hd, ...tl];
} else {
[hd, ...insert(x, tl)];
};
|
code/sorting/src/insertion_sort/insertion_sort.rs | // Part of Cosmos by OpenGenus Foundation
fn insertion_sort(array_A: &mut [int, ..6]) {
// Implementation of the traditional insertion sort algorithm in Rust
// The idea is to both learn the language and re visit classic (and not so much)
// algorithms.
// This algorithm source has been extracted from "Introduction to Algorithms" - Cormen
let mut i: int;
//utility variable. Ugly hack to avoid casting back and forward from int to uint
let mut i_u: uint;
let mut key: int;
// It is not necessary to explicitly declare j
for j in range(1u, array_A.len()) { //arrays only work with uint (unsigned)
key = array_A[j];
i_u = j - 1;
i = i_u as int;
while i >= 0 && array_A[i_u] > key {
array_A[i_u + 1] = array_A[i_u];
i = i - 1;
i_u = i as uint;
};
array_A[i_u + 1] = key;
};
}
fn main() {
//This should be replaced eventually by the unit test framework.
let mut array_A = [31i, 41, 59, 26, 41, 58];
// let mut array_A = [5i,2,4,6,1,3];
// Need to pass a reference, so the sorting function can modify the
// array being sorted (this is very similar to C lang).
insertion_sort(&mut array_A);
//Just for printing purposes
for j in range(0u, array_A.len()) {
println!("{}", array_A[j]);
};
}
|
code/sorting/src/insertion_sort/insertion_sort.sh | #!/bin/bash
# Part of Cosmos by OpenGenus Foundation
declare -a array
ARRAYSZ=10
create_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
array[${i}]=${RANDOM}
i=$((i+1))
done
}
print_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
echo ${array[${i}]}
i=$((i+1))
done
}
verify_sort() {
i=1
while [ $i -lt $ARRAYSZ ]; do
if [ ${array[$i]} -lt ${array[$((i-1))]} ]; then
echo "Array did not sort, see elements $((i-1)) and $i."
exit 1
fi
i=$((i+1))
done
echo "Array sorted correctly."
}
insertion_sort() {
i=1
while [ $i -lt ${ARRAYSZ} ]; do
j=$i
while [ $j -gt 0 ] && [ ${array[$((j-1))]} -gt ${array[$j]} ]; do
tmp=${array[$((j-1))]}
array[$((j-1))]=${array[$j]}
array[$j]=$tmp;
j=$((j-1))
done
i=$((i+1))
done
}
create_array
print_array
insertion_sort
echo
print_array
verify_sort
|
code/sorting/src/insertion_sort/insertion_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// insertion_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func insertionSort(_ array: inout [Int]) {
for i in 1..<array.count {
var j = Int(i)
let key = array[j]
while j >= 1 && key < array[j - 1] {
array[j] = array[j - 1]
j -= 1
}
array[j] = key
}
}
|
code/sorting/src/insertion_sort/insertion_sort_extension.swift | /* Part of Cosmos by OpenGenus Foundation */
import Foundation
extension Array {
mutating func insertionSort(compareWith less: (Element, Element) -> Bool) {
if self.count <= 1 {
return
}
for i in 1..<self.count {
var j = Int(i)
let key = self[j]
while j >= 1 && less(key, self[j - 1]) {
self[j] = self[j - 1]
j -= 1
}
self[j] = key
}
}
}
|
code/sorting/src/intro_sort/README.md | # Intro Sort
Introsort or introspective sort is the best sorting algorithm around. It is a **hybrid sorting algorithm** and thus uses three sorting algorithm to minimise the running time, i.e., **Quicksort, Heapsort and Insertion Sort**.
## Explanation
Introsort begins with quicksort and if the recursion depth goes more than a particular limit it switches to Heapsort to avoid Quicksort’s worse case **O(n<sup>2</sup>)** time complexity. It also uses insertion sort when the number of elements to sort is quite less.
Thus, it first creates a partition, and 3 cases arrive from here:
1. If the partition size is such that there is a possibility to exceed the maximum depth limit then, the Introsort switches to Heapsort. We define the maximum depth limit as 2 * logn.
2. If the partition size is too small then Quicksort decays to Insertion Sort. We define this cutoff as 16 (due to research). So if the partition size is less than 16 then we will do insertion sort.
3. If the partition size is under the limit and not too small (i.e., between 16 and `(2 * log(N))`, then it performs a simple quicksort.
## Algorithm
```
procedure sort(A : array):
let maxdepth = ⌊log(length(A))⌋ × 2
introsort(A, maxdepth)
procedure introsort(A, maxdepth):
n ← length(A)
if n ≤ 1:
return // base case
else if maxdepth = 0:
heapsort(A)
else:
p ← partition(A)
introsort(A[0:p], maxdepth - 1)
introsort(A[p+1:n], maxdepth - 1)
```
## Complexity
**Time complexity**
Worst, average and best case time complexity: **O(n * logn)**
**Space complexity**
**O(log N)** auxiliary space
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/intro_sort/intro_sort.cpp | #include <cmath>
#include <algorithm>
#include <iostream>
using namespace std;
void introsort_r(int a[], int first, int last, int depth);
void _introsort(int a[], int n);
int _partition(int a[], int first, int last);
void _insertion(int a[], int n);
void _swap(int *a, int *b);
void _doheap(int a[], int begin, int end);
void _heapsort(int a[], int begin, int end);
bool _isSorted(int a[], int end);
void introsort_r(int a[], int first, int last, int depth)
{
while (last - first > 0)
{
if (depth == 0)
_heapsort(&a[first], first, last - first + 1 );
else
{
int pivot;
if (_isSorted(a, last))
break;
pivot = _partition(a, first, last);
introsort_r(a, pivot + 1, last, depth - 1);
last = pivot - 1;
}
}
}
void _introsort(int a[], int n)
{
introsort_r ( a, 0, n - 1, (int(2 * log(double(n)))));
_insertion(a, n);
}
int _partition ( int a[], int first, int last)
{
int pivot, mid = (first + last) / 2;
pivot = a[first] > a[mid] ? first : mid;
if (a[pivot] > a[last])
pivot = last;
_swap( &a[pivot], &a[first] );
pivot = first;
while (first < last)
{
if (a[first] <= a[last])
_swap( &a[pivot++], &a[first] );
++first;
}
_swap (&a[pivot], &a[last]);
return pivot;
}
void _insertion ( int a[], int n )
{
int i;
for (i = 1; i < n; i++)
{
int j, save = a[i];
for (j = i; j >= 1 && a[j - 1] > save; j--)
a[j] = a[j - 1];
a[j] = save;
}
}
void _swap(int *a, int *b)
{
int save = *a;
*a = *b;
*b = save;
}
void _doheap(int a[], int begin, int end )
{
int save = a[begin];
while (begin <= end / 2)
{
int k = 2 * begin;
while (k < end && a[k] < a[k + 1])
++k;
if (save >= a[k])
break;
a[begin] = a[k];
begin = k;
}
a[begin] = save;
}
void _heapsort(int a[], int begin, int end )
{
int i;
for (int i = (end - 1) / 2; i >= begin; i--)
_doheap( a, i, end - 1);
for (i = end - 1; i > begin; i--)
{
_swap( &a[i], &a[begin]);
_doheap(a, begin, i - 1);
}
}
bool _isSorted(int a[], int end)
{
for (int i = 0; i < end; i++)
{
if (a[i] > a[i + 1])
return false;
else
return true;
}
return true;
}
int main()
{
int arrayNum[50], t;
cout << "Enter Number of Elements:";
cin >> t;
for (int y = 0; y < t; y++)
cin >> arrayNum[y];
_introsort(arrayNum, t);
for (int x = 0; x < t; x++)
std::cout << x << ":" << arrayNum[x] << std::endl;
cin.get();
return 0;
}
|
code/sorting/src/intro_sort/intro_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// intro_sort.m
// Created by DaiPei on 2017/10/22.
//
#import <Foundation/Foundation.h>
@interface IntroSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation IntroSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
[self sortCore:array from:0 to:(int)array.count - 1 depth:(int)(log((double)array.count))];
}
- (void)sortCore:(NSMutableArray<NSNumber *> *)array from:(int)a to:(int)b depth:(int)depth {
if (a < b) {
if (depth == 0) {
[self heapSort:array from:a to:b];
} else {
if ([self isSorted:array from:a to:b]) {
return ;
}
int div = [self partition:array low:a high:b];
[self sortCore:array from:a to:div - 1 depth:depth - 1];
[self sortCore:array from:div + 1 to:b depth:depth - 1];
}
}
}
// quick sort core
- (int)partition:(NSMutableArray<NSNumber *> *)array low:(int)low high:(int)high {
int mid = (low + high) / 2;
[self swap:array at:mid and:high];
int p1 = low, p2 = low;
while (p2 < high) {
if ([array[p2] compare:array[high]] == NSOrderedAscending) {
[self swap:array at:p1 and:p2];
p1++;
}
p2++;
}
[self swap:array at:p1 and:high];
return p1;
}
// heap sort part
- (void)heapSort:(NSMutableArray<NSNumber *> *)array from:(int)a to:(int)b {
// create the heap
for (int i = (a + b) / 2; i >= a; i--) {
[self sink:array at:i from:a to:b];
}
// sort core
for (int i = b; i > a; i--) {
[self swap:array at:i and:a];
[self sink:array at:a from:a to:i - 1];
}
}
- (void)sink:(NSMutableArray<NSNumber *> *)array at:(int)x from:(int)a to:(int)b {
int b1 = b - a + 1;
int i = x;
int i1 = i - a + 1;
while (i1 * 2 <= b1) {
int l1 = 2 * i1;
int r1 = 2 * i1 + 1;
int l = l1 + a - 1;
int r = r1 + a - 1;
int p;
if (r <= b && [array[r] compare:array[l]] == NSOrderedDescending) {
p = r;
} else {
p = l;
}
if ([array[i] compare:array[p]] == NSOrderedAscending) {
[self swap:array at:i and:p];
i = p;
i1 = i - a + 1;
} else {
break;
}
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
- (BOOL)isSorted:(NSMutableArray<NSNumber *> *)array from:(int)a to:(int)b {
for (int i = a + 1; i <= b; i++) {
if ([array[i] compare:array[i - 1]] == NSOrderedAscending) {
return NO;
}
}
return YES;
}
@end
int main(int argc, const char * argv[]) {
for (int j = 0; j < 100; j++) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray array];
for (int i = 0; i < 20; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
IntroSort *is = [[IntroSort alloc] init];
[is sort:array];
NSLog(@"after: %@", array);
if (![is isSorted:array from:0 to:(int)array.count - 1]) {
NSLog(@"ATTENTION!!!!!!!!!!!!!!");
@throw [NSException exceptionWithName:@"array is not sorted" reason:nil userInfo:nil];
}
}
}
return 0;
}
|
code/sorting/src/intro_sort/intro_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// intro_sort.swift
// Created by DaiPei on 2017/10/21.
//
import Foundation
func introSort(_ array: inout [Int]) {
introSortCore(&array, from: 0, to: array.count - 1, depth: Int(2 * log(Double(array.count))));
}
private func introSortCore(_ array: inout [Int], from a: Int, to b: Int, depth: Int) {
if a < b {
if depth == 0 {
heapSort(&array, from: a, to: b)
} else {
if isSorted(&array, from: a, to: b) {
return
}
let div = partition(&array, low: a, high: b)
introSortCore(&array, from: a, to: div - 1, depth: depth - 1)
introSortCore(&array, from: div + 1, to: b, depth: depth - 1)
}
}
}
// quick sort core
private func partition(_ array: inout [Int], low: Int, high: Int) -> Int {
var div = low
var p = low
let mid = (low + high) / 2
swap(&array, at: mid, and: high)
while p < high {
if array[p] < array[high] {
swap(&array, at: p, and: div)
div += 1
}
p += 1
}
swap(&array, at: high, and: div)
return div
}
// heap sort part
private func heapSort(_ array: inout [Int], from a: Int, to b: Int) {
// create heap
for i in stride(from: (a + b) / 2, to: a - 1, by: -1) {
sink(&array, at: i, from: a, to: b)
}
// sort core
for i in stride(from: b, to: a, by: -1) {
swap(&array, at: i, and: a)
sink(&array, at: a, from: a, to: i - 1)
}
}
private func sink(_ array: inout [Int], at x: Int, from a: Int, to b: Int) {
let b1 = b - a + 1
var i = x
var i1 = i - a + 1
while 2 * i1 <= b1 {
let l1 = 2 * i1
let r1 = 2 * i1 + 1
let l = l1 + a - 1
let r = r1 + a - 1
let p: Int
if r <= b && array[l] < array[r] {
p = r
} else {
p = l
}
if array[p] > array[i] {
swap(&array, at: p, and: i)
i = p
i1 = i - a + 1
} else {
break
}
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
private func isSorted(_ array: inout [Int], from a: Int, to b: Int) -> Bool {
for i in a+1...b {
if array[i] < array[i - 1] {
return false
}
}
return true
}
|
code/sorting/src/median_sort/median_sort.cpp | #include <iostream>
using namespace std;
// Part of Cosmos by OpenGenus Foundation
void swap(int *a, int *b)
{
int temp;
temp = *a;
*a = *b;
*b = temp;
}
int Partition(int a[], int low, int high)
{
int pivot, index, i;
index = low;
pivot = high;
for (i = low; i < high; i++)
if (a[i] < a[pivot])
{
swap(&a[i], &a[index]);
index++;
}
swap(&a[pivot], &a[index]);
return index;
}
int QuickSort(int a[], int low, int high)
{
int pindex;
if (low < high)
{
pindex = Partition(a, low, high);
QuickSort(a, low, pindex - 1);
QuickSort(a, pindex + 1, high);
}
return 0;
}
int main()
{
int n, m, bi, ai, i, k;
double median;
cout << "Enter the number of element in the first data set: ";
cin >> n;
int a[n];
for (i = 0; i < n; i++)
{
cout << "Enter " << i + 1 << "th element: ";
cin >> a[i];
}
cout << "\nEnter the number of element in the second data set: ";
cin >> m;
int b[m];
for (i = 0; i < m; i++)
{
cout << "Enter " << i + 1 << "th element: ";
cin >> b[i];
}
QuickSort(a, 0, n - 1);
QuickSort(b, 0, m - 1);
cout << "\tThe Median from these data set is: ";
ai = 0;
bi = 0;
if ((m + n) % 2 == 1)
{
k = (n + m) / 2 + 1;
while (k > 0)
{
if (a[ai] <= b[bi] && ai < n)
{
k--;
if (k == 0)
cout << a[ai];
ai++;
}
else if (a[ai] > b[bi] && bi < m)
{
k--;
if (k == 0)
cout << b[bi];
bi++;
}
}
}
else
{
k = (n + m) / 2 + 1;
while (k > 0)
{
if (a[ai] <= b[bi] && ai < n)
{
k--;
if (k <= 1)
median += a[ai];
ai++;
}
else if (a[ai] > b[bi] && bi < m)
{
k--;
if (k <= 1)
median += b[bi];
bi++;
}
}
cout << median / 2;
}
}
|
code/sorting/src/median_sort/median_sort.cs | /* Part of Cosmos by OpenGenus Foundation */
using System;
namespace CS
{
public class MedianSort
{
static void swap(ref int a, ref int b)
{
int temp = a;
a = b;
b = temp;
}
static int SelectPivotIndex(int[] arr, int right, int left) {
int mid = (left + right) / 2;
if (arr[left] > arr[mid]) {
if (arr[left] > arr[right]) {
if (arr[right] > arr[mid])
return right;
else
return mid;
} else {
return left;
}
}
else
{
if (arr[mid] > arr[right]) {
if (arr[left] > arr[right])
return left;
else
return right;
} else {
return mid;
}
}
}
static int Partition(ref int[] arr, int left, int right, int pivot) {
swap(ref arr[pivot], ref arr[right]);
int store = left;
for (int i = left; i < right; i++) {
if(arr[i] <= arr[right]) {
swap(ref arr[i], ref arr[store]);
store++;
}
}
swap(ref arr[store], ref arr[right]);
return store;
}
static int SelectKth(ref int[] arr, int k, int left, int right) {
int idx = SelectPivotIndex(arr, left, right);
int pivot = Partition(ref arr, left, right, idx);
int kth = left + k - 1;
if (kth == pivot)
return pivot;
if (kth < pivot)
return SelectKth(ref arr, k, left, pivot - 1);
else
return SelectKth(ref arr, kth - pivot, pivot + 1, right);
}
static void Sort(ref int[] arr, int left, int right) {
if (left >= right)
return;
int medianIdx = SelectKth(ref arr, 1 + (right - left) / 2, left, right);
int mid = (right + left) / 2;
swap(ref arr[mid], ref arr[medianIdx]);
int k = mid + 1;
for (int i = left; i < mid; i++)
{
if (arr[i] > arr[mid])
{
while (arr[k] > arr[mid])
k++;
swap(ref arr[i], ref arr[k]);
}
}
Sort(ref arr, left, mid - 1);
Sort(ref arr, mid + 1, right);
}
static void Main(string[] args)
{
const int numbers = 25;
int[] arr = new int[numbers];
var rand = new Random();
for (int i = 0; i < numbers; i++)
{
arr[i] = rand.Next(99);
}
Console.WriteLine("Before Sorting");
foreach (var num in arr)
{
Console.Write(num + " ");
}
Sort(ref arr, 0, arr.Length-1);
Console.WriteLine("\nSorted:");
foreach (var num in arr)
{
Console.Write(num + " ");
}
}
}
}
|
code/sorting/src/median_sort/median_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// median_sort.m
// Created by DaiPei on 2017/10/27.
//
#import <Foundation/Foundation.h>
@interface MedianSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation MedianSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
[self recursiveSort:array left:0 right:array.count - 1];
}
- (void)recursiveSort:(NSMutableArray<NSNumber *> *)array left:(NSUInteger)left right:(NSUInteger)right {
if (left < right) {
NSUInteger pivot = [self pivot:array left:left right:right];
pivot = [self partition:array left:left right:right pivot:pivot];
if (pivot != 0) {
[self recursiveSort:array left:left right:pivot - 1];
}
[self recursiveSort:array left:pivot + 1 right:right];
}
}
- (NSUInteger)pivot:(NSMutableArray<NSNumber *> *)array left:(NSUInteger)left right:(NSUInteger)right {
NSUInteger count = right - left + 1;
if (count < 6) {
return [self median5:array left:left right:right];
}
for (NSUInteger subLeft = left; subLeft < right; subLeft += 5) {
NSUInteger subRight = subLeft + 4;
if (subRight > right) {
subRight = right;
}
NSUInteger median = [self median5:array left:subLeft right:subRight];
[self swap:array at:median and:left + (subLeft - left) / 5];
}
return [self select:array left:left right:left + (count - 1) / 5 ofK:left + count / 10];
}
- (NSUInteger)median5:(NSMutableArray<NSNumber *> *)array left:(NSUInteger)left right:(NSUInteger)right {
NSUInteger mid = (left + right) / 2;
for (NSUInteger i = left; i < right; i++) {
NSUInteger p = i;
for (NSUInteger j = i + 1; j <= right; j++) {
if ([array[p] compare:array[j]] == NSOrderedDescending) {
p = j;
}
}
[self swap:array at:i and:p];
if (i >= mid) {
break;
}
}
return mid;
}
- (NSUInteger)select:(NSMutableArray<NSNumber *> *)array left:(NSUInteger)left right:(NSUInteger)right ofK:(NSUInteger)k {
if (left == right) {
return left;
}
while (YES) {
NSUInteger piv = [self pivot:array left:left right:right];
piv = [self partition:array left:left right:right pivot:piv];
if (piv == k) {
break;
} else if (piv < k) {
left = piv + 1;
} else {
right = piv - 1;
}
}
return k;
}
- (NSUInteger)partition:(NSMutableArray<NSNumber *> *)array left:(NSUInteger)left right:(NSUInteger)right pivot:(NSUInteger)pivot {
NSUInteger p1 = left;
NSUInteger p2 = left;
[self swap:array at:pivot and:right];
while (p1 < right) {
if ([array[right] compare:array[p1]] == NSOrderedDescending) {
[self swap:array at:p1 and:p2];
p2++;
}
p1++;
}
[self swap:array at:right and:p2];
return p2;
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
@interface SortTester : NSObject
+ (BOOL)isSorted:(NSArray<NSNumber *> *)array;
@end
@implementation SortTester
+ (BOOL)isSorted:(NSArray<NSNumber *> *)array {
for (int i = 1; i < array.count; i++) {
if ([array[i] compare:array[i - 1]] == NSOrderedAscending) {
return NO;
}
}
return YES;
}
@end
int main(int argc, const char * argv[]) {
for (int j = 0; j < 100; j++) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray array];
for (int i = 0; i < 20; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
MedianSort *ms = [[MedianSort alloc] init];
[ms sort:array];
NSLog(@"after: %@", array);
assert([SortTester isSorted:array]);
}
}
return 0;
}
|
code/sorting/src/median_sort/median_sort.py | # Part of Cosmos by OpenGenus Foundation
def median_sort(list):
data = sorted(list)
n = len(data)
if n == 0:
return None
if n % 2 == 1:
return data[n // 2]
else:
i = n // 2
return (data[i - 1] + data[i]) / 2
# list = [0,2,3,4,5,6,7,8,9]
# print calculateMedian(list)
# uncomment the above to see how does python median sort works
|
code/sorting/src/median_sort/median_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// median_sort.swift
// Created by DaiPei on 2017/10/25.
//
import Foundation
func medianSort(_ array: inout [Int]) {
recursiveMediaSort(&array, left: 0, right: array.count - 1)
}
private func recursiveMediaSort(_ array: inout [Int], left: Int, right: Int) {
if left < right {
var piv = pivot(&array, left: left, right: right)
piv = partition(&array, left: left, right: right, pivot: piv)
recursiveMediaSort(&array, left: left, right: piv - 1)
recursiveMediaSort(&array, left: piv + 1, right: right)
}
}
// find a good pivot for quick sort
private func pivot(_ array: inout [Int], left: Int, right: Int) -> Int {
let range = right - left + 1
if range < 6 {
return median5(&array, left: left, right: right)
}
for subLeft in stride(from: left, to: right + 1, by: 5) {
var subRight = subLeft + 4
if subRight > right {
subRight = right
}
let medianIndex = median5(&array, left: subLeft, right: subRight)
swap(&array, at: medianIndex, and: Int(floor(Double(subLeft - left) / 5)) + left)
}
return select(&array, left: left, right: left + (range - 1) / 5, of: left + range / 10)
}
// find median in 5 number
private func median5(_ array: inout [Int], left: Int, right: Int) -> Int {
let mid = (left + right) / 2
if left < right {
for i in left..<right {
var p = i
for j in i + 1...right {
if array[j] < array[p] {
p = j
}
}
swap(&array, at: p, and: i)
if i >= mid {
break
}
}
}
return mid
}
// select the rank k number in array
private func select(_ array: inout [Int], left: Int, right: Int, of k: Int) -> Int {
if (left == right) {
return left
}
var piv: Int
var r = right
var l = left
repeat {
piv = pivot(&array, left: l, right: r)
piv = partition(&array, left: l, right: r, pivot: piv)
if piv == k {
break
} else if piv > k {
r = piv - 1
} else {
l = piv + 1
}
} while piv != k
return k
}
// normal partition method in quick sort
private func partition(_ array: inout [Int], left: Int, right: Int, pivot: Int) -> Int {
var p1 = left
var p2 = left
swap(&array, at: pivot, and: right)
while p2 < right {
if array[p2] < array[right] {
swap(&array, at: p2, and: p1)
p1 += 1
}
p2 += 1
}
swap(&array, at: right, and: p1)
return p1
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/median_sort/median_sort_fast.cpp | #include <vector>
using namespace std;
// Part of Cosmos by OpenGenus Foundation
int selectPivotIndex(const vector<int>& A, const int left, const int right)
{
const int mid = (left + right) / 2; // median of three
if (A[left] > A[mid]) // this is really insertion sort
{
if (A[left] > A[right])
{
if (A[right] > A[mid])
return right;
else
return mid;
}
else
return left;
}
else
{
if (A[mid] > A[right])
{
if (A[left] > A[right])
return left;
else
return right;
}
else
return mid;
}
}
int partition(vector<int>& A, const int left, const int right,
const int pivot)
{
swap(A[pivot], A[right]);
int store = left;
for (int i = left; i < right; i++)
if (A[i] <= A[right])
{
swap(A[i], A[store]); store++;
}
swap(A[store], A[right]);
return store;
}
int selectKth(vector<int>& A, const int k, const int left, const int right)
{
const int idx = selectPivotIndex(A, left, right); // pivot around element
const int pivot = partition(A, left, right, idx);
const int kth = left + k - 1;
if (kth == pivot)
return pivot;
if (kth < pivot)
return selectKth(A, k, left, pivot - 1);
else
return selectKth(A, kth - pivot, pivot + 1, right);
}
void medianSort(vector<int>& A, const int left, const int right)
{
if (left >= right)
return;
const int medianIdx = selectKth(A, 1 + (right - left) / 2, left, right);
const int mid = (right + left) / 2;
swap(A[mid], A[medianIdx]); // median valued element is now the middle
int k = mid + 1;
for (int i = left; i < mid; i++)
if (A[i] > A[mid])
{
while (A[k] > A[mid])
k++; // find element on right to move left of mid
swap(A[i], A[k]);
}
medianSort(A, left, mid - 1);
medianSort(A, mid + 1, right);
}
void sort(vector<int>& A)
{
medianSort(A, 0, A.size() - 1);
}
|
code/sorting/src/merge_sort/README.md | # Merge Sort
The merge sort is a comparison-based sorting algorithm.
This algorithm works by dividing the unsorted list into n sublists; each one containing one element (which
is considered sorted, since it only has one element).
Then it merges the sublists to produce new sorted sublists, this is repeated until there's only one sublist remaining, which is the resulting sorted list.
## Explanation
The following illustrates complete merge sort process for the given array:
`{38, 27, 43, 3, 9, 82, 10}`
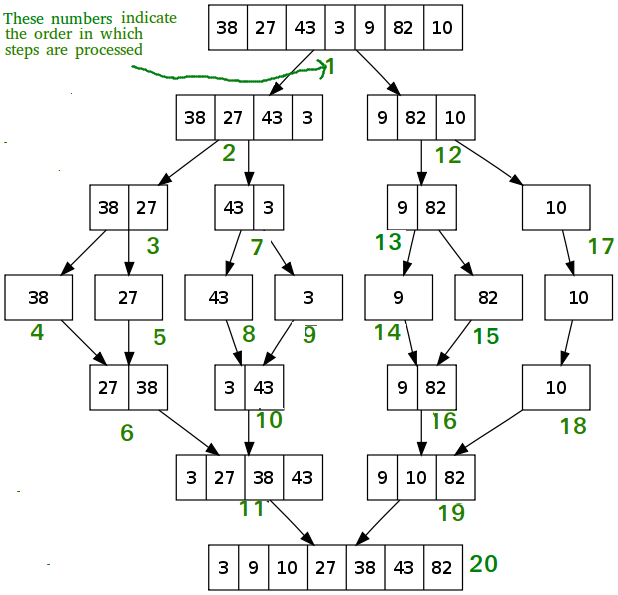
> Image credits: geeksforgeeks
## Algorithm
```
procedure mergesort( var a as array )
if ( n == 1 ) return a
var l1 as array = a[0] ... a[n/2]
var l2 as array = a[n/2+1] ... a[n]
l1 = mergesort( l1 )
l2 = mergesort( l2 )
return merge( l1, l2 )
end procedure
procedure merge( var a as array, var b as array )
var c as array
while ( a and b have elements )
if ( a[0] > b[0] )
add b[0] to the end of c
remove b[0] from b
else
add a[0] to the end of c
remove a[0] from a
end if
end while
while ( a has elements )
add a[0] to the end of c
remove a[0] from a
end while
while ( b has elements )
add b[0] to the end of c
remove b[0] from b
end while
return c
end procedure
```
## Complexity
**Time complexity**
Worst, average and best case time complexity: **O(n * logn)**
**Space Complexity**
Auxillary space: **O(n)**
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/merge_sort/merge_sort.c | /*
* Part of Cosmos by OpenGenus Foundation
*/
#include <stdio.h>
typedef int bool;
/* Merges two subhalves of a[].
First sub-half is a[low..mid]
Second sub-half is a[mid+1..high]
*/
void
merging (int a[], int b[], int low, int mid, int high, bool order)
{
int l1, l2, i;
/* Order 1 for sorting in Ascending order */
if (order == 1) {
/* Merge the two sub-halves into array b in sorted manner */
for (l1 = low, l2 = mid + 1, i = low; l1 <= mid && l2 <= high; i++) {
if (a[l1] <= a[l2]) {
b[i] = a[l1++];
}
else {
b[i] = a[l2++];
}
}
/* copy the remaining elements of the first sub-array (low-mid) , if any */
while (l1 <= mid) {
b[i++] = a[l1++];
}
/* copy the remaining elements of the second sub-array (mid+1-high), if any */
while (l2 <= high) {
b[i++] = a[l2++];
}
/* copy the entire modified array b back into the array a */
for(i = low; i <= high; i++) {
a[i] = b[i];
}
}
/* Order 0 for sorting in Descending order */
else if (order == 0) {
/* Merge the two sub-halves into array b in sorted manner */
for (l1 = low, l2 = mid + 1, i = low; l1 <= mid && l2 <= high; i++) {
if (a[l1] >= a[l2]) {
b[i] = a[l1++];
}
else {
b[i] = a[l2++];
}
}
/* copy the remaining elements of the first sub-array (low-mid) , if any */
while (l1 <= mid) {
b[i++] = a[l1++];
}
/* copy the remaining elements of the second sub-array (mid+1-high), if any */
while (l2 <= high) {
b[i++] = a[l2++];
}
/* copy the entire modified array b back into the array a */
for(i = low; i <= high; i++) {
a[i] = b[i];
}
}
/* If order not 0 or 1, sorting order undefined */
else {
printf("Undefined sorting order.\n");
}
}
/* low is for left index and high is right index of the
sub-array of a to be sorted */
void
sort(int a[], int b[], int low, int high, bool order)
{
int mid;
if (low < high) {
mid = (low + high) / 2;
//sort first and second halves
sort(a, b, low, mid, order);
sort(a, b, mid+1, high, order);
merging(a, b, low, mid, high, order);
}
else {
return;
}
}
int
main()
{
int n;
/* Take number of elements as input */
printf("Enter number of elements:\n");
scanf("%d", &n);
int a[n];
/* Take array elements as input */
printf("Enter array elements:\n");
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
/* Declare new array to store sorted array */
int b[n];
bool order;
/* Take sorting order as input */
printf("Enter order of sorting (1: Ascending; 0:Descending)\n");
scanf("%d", &order);
/* If user does not enter 0 or 1 for order */
if (order != 1 && order != 0) {
printf("Undefined sorting order.\n");
return 1;
}
sort(a, b, 0, n, order);
/* Print final sorted array */
for(int i = 0; i < n; i++) {
printf("%d ", a[i]);
}
printf("\n");
return (0);
}
|
code/sorting/src/merge_sort/merge_sort.cpp | /*
* Part of Cosmos by OpenGenus Foundation
*
* merge sort synopsis
*
* namespace merge_sort_impl {
* template<typename _Random_Acccess_Iter>
* _Random_Acccess_Iter
* advance(_Random_Acccess_Iter it, std::ptrdiff_t n);
* } // merge_sort_impl
*
* template<typename _Random_Acccess_Iter, typename _Compare>
* void mergeSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end, _Compare comp);
*
* template<typename _Random_Acccess_Iter>
* void mergeSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end);
*/
#include <list>
#include <iterator>
#include <cstddef>
namespace merge_sort_impl {
template<typename _Random_Acccess_Iter>
_Random_Acccess_Iter
advance(_Random_Acccess_Iter it, std::ptrdiff_t n)
{
std::advance(it, n);
return it;
}
template<typename _Random_Acccess_Iter, typename _Compare>
void merge(_Random_Acccess_Iter begin,
_Random_Acccess_Iter end,
_Compare comp)
{
using value_type = typename std::iterator_traits<_Random_Acccess_Iter>::value_type;
auto end1 = begin;
std::advance(end1, std::distance(begin, end) >> 1);
auto begin1 = begin,
begin2 = end1,
end2 = end;
std::list<value_type> tmp{};
while (begin1 < end1 && begin2 < end2)
tmp.push_back(comp(*begin1, *begin2) ? *begin1++ : *begin2++);
while (begin1 < end1)
*--begin2 = *--end1;
while (!tmp.empty())
{
*--begin2 = tmp.back();
tmp.pop_back();
}
}
} // merge_sort_impl
template<typename _Random_Acccess_Iter, typename _Compare>
void
mergeSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end, _Compare comp)
{
size_t dist = std::distance(begin, end);
if (dist > 1)
{
auto mid = begin;
std::advance(mid, dist >> 1);
mergeSort(begin, mid, comp);
mergeSort(mid, end, comp);
merge_sort_impl::merge(begin, end, comp);
}
}
template<typename _Random_Acccess_Iter>
void
mergeSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end)
{
using value_type = typename std::iterator_traits<_Random_Acccess_Iter>::value_type;
mergeSort(begin, end, std::less<value_type>());
}
|
code/sorting/src/merge_sort/merge_sort.cs | using System;
using System.Collections.Generic;
namespace CS
{
/* Part of Cosmos by OpenGenus Foundation */
class MergeSort
{
private static int[] SortInternal(int[] arr, int start, int count)
{
// Base case - 0 or 1 elements
if (count == 1)
{
return new int[] { arr[start] };
}
if (count == 0)
{
return new int[0];
}
// Split
int[] part1 = SortInternal(arr, start, count / 2);
int[] part2 = SortInternal(arr, start + count / 2, count - count / 2);
// Assemble stage output
int[] result = new int[count];
for (int i1 = 0, i2 = 0, j = 0; j < count; j++)
{
if (i1 < part1.Length && i2 < part2.Length)
{
if (part1[i1] < part2[i2])
{
result[j] = part1[i1];
i1++;
}
else
{
result[j] = part2[i2];
i2++;
}
}
else if (i1 < part1.Length)
{
result[j] = part1[i1];
i1++;
}
else
{
result[j] = part2[i2];
i2++;
}
}
// Return result
return result;
}
static int[] Sort(int[] arr)
{
return SortInternal(arr, 0, arr.Length);
}
static void Main(string[] args)
{
// Create an array of 10 random numbers
int[] numbers = new int[10];
var rand = new Random();
for (int i = 0; i < 10; i++)
{
numbers[i] = rand.Next(10);
}
Console.WriteLine("Initial ordering:");
foreach (var num in numbers)
{
Console.Write(num + " ");
}
// Store the sorted result in a separate variable and print it out
var sorted = Sort(numbers);
Console.WriteLine("\nSorted:");
foreach (var num in sorted)
{
Console.Write(num + " ");
}
}
}
}
|
code/sorting/src/merge_sort/merge_sort.fs | //Part of Cosmos by OpenGenus Foundation
open System
open System.Collections.Generic
let rec merge (left:IComparable list) (right:IComparable list): IComparable list =
match (left,right) with
| (_,[]) -> left
| ([],_) -> right
| (l::ls,r::rs) when l < r -> l::merge ls right
| (l::ls,r::rs) -> r::merge left rs
let rec merge_sort=
function
| [x]-> [x]
| x -> let (left,right) = List.splitAt (x.Length/2) x
merge (merge_sort left) (merge_sort right)
[<EntryPoint>]
let main(argv) =
printfn "%A" <| merge_sort [10;22;41;2;33;12;52]
0
|
code/sorting/src/merge_sort/merge_sort.go | package main
import (
"fmt"
)
func main() {
A := []int{3, 5, 1, 6, 1, 7, 2, 4, 5}
fmt.Println(sort(A))
}
// top-down approach
func sort(A []int) []int {
if len(A) <= 1 {
return A
}
left, right := split(A)
left = sort(left)
right = sort(right)
return merge(left, right)
}
// split array into two
func split(A []int) ([]int, []int) {
return A[0:len(A) / 2], A[len(A) / 2:]
}
// assumes that A and B are sorted
func merge(A, B []int) []int {
arr := make([]int, len(A) + len(B))
// index j for A, k for B
j, k := 0, 0
for i := 0; i < len(arr); i++ {
// fix for index out of range without using sentinel
if j >= len(A) {
arr[i] = B[k]
k++
continue
} else if k >= len(B) {
arr[i] = A[j]
j++
continue
}
// default loop condition
if A[j] > B[k] {
arr[i] = B[k]
k++
} else {
arr[i] = A[j]
j++
}
}
return arr
}
|
code/sorting/src/merge_sort/merge_sort.hs | -- Part of Cosmos by OpenGenus Foundation
split_list::[a]->([a],[a])
split_list x = splitAt (div (length x) $ 2) x
merge_sort::Ord a=>[a]->[a]
merge_sort x
| length x == 0 = []
| length x == 1 = x
| length x > 1 = merge (merge_sort left) (merge_sort right)
where (left,right)=split_list x
merge::Ord a=>[a]->[a]->[a]
merge [] r = r
merge l [] = l
merge l@(l':ls) r@(r':rs)
| l' < r' = l' : merge ls r
| otherwise = r' : merge rs l
main = do
print $ merge_sort [9,6,4,3,2,1,2,5]
|
code/sorting/src/merge_sort/merge_sort.java | /* Java program for Merge Sort */
// Part of Cosmos by OpenGenus Foundation
class MergeSort {
// Merges two subarrays of arr[].
// First subarray is arr[l..m]
// Second subarray is arr[m+1..r]
private void merge(int arr[], int l, int m, int r) {
// Find sizes of two subarrays to be merged
int n1 = m - l + 1;
int n2 = r - m;
/* Create temp arrays */
int L[] = new int [n1];
int R[] = new int [n2];
/*Copy data to temp arrays*/
System.arraycopy(arr, l + 0, L, 0, n1);
for (int j=0; j<n2; ++j)
R[j] = arr[m + 1+ j];
/* Merge the temp arrays */
// Initial indexes of first and second subarrays
int i = 0, j = 0;
// Initial index of merged subarry array
int k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
}
else {
arr[k] = R[j];
j++;
}
k++;
}
/* Copy remaining elements of L[] if any */
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
/* Copy remaining elements of R[] if any */
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
// Main function that sorts arr[l..r] using
// merge()
private void sort(int arr[], int l, int r) {
if (l < r) {
// Find the middle point
int m = (l+r)/2;
// Sort first and second halves
sort(arr, l, m);
sort(arr , m+1, r);
// Merge the sorted halves
merge(arr, l, m, r);
}
}
/* A utility function to print array of size n */
private static void printArray(int arr[]) {
int n = arr.length;
for (int anArr : arr) System.out.print(anArr + " ");
System.out.println();
}
// Driver method
public static void main(String args[]) {
int arr[] = {12, 11, 13, 5, 6, 7};
System.out.println("Given Array");
printArray(arr);
MergeSort ob = new MergeSort();
ob.sort(arr, 0, arr.length-1);
System.out.println("\nSorted array");
printArray(arr);
}
}
|
code/sorting/src/merge_sort/merge_sort.js | var a = [34, 203, 3, 746, 200, 984, 198, 764, 9];
// Part of Cosmos by OpenGenus Foundation
function mergeSort(arr) {
if (arr.length < 2) return arr;
var middle = parseInt(arr.length / 2);
var left = arr.slice(0, middle);
var right = arr.slice(middle, arr.length);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
var result = [];
while (left.length && right.length) {
if (left[0] <= right[0]) {
result.push(left.shift());
} else {
result.push(right.shift());
}
}
while (left.length) result.push(left.shift());
while (right.length) result.push(right.shift());
return result;
}
console.log(mergeSort(a));
|
code/sorting/src/merge_sort/merge_sort.kt | import java.util.*
fun <T : Comparable<T>>mergesort(items : MutableList<T>) : MutableList<T> {
if (items.isEmpty()) {
return items
}
fun merge(left : MutableList<T>, right : MutableList<T>) : MutableList<T> {
var merged : MutableList<T> = arrayListOf<T>()
while(!left.isEmpty() && !right.isEmpty()) {
val temp : T
if (left.first() < right.first()) {
temp = left.removeAt(0)
}
else {
temp = right.removeAt(0)
}
merged.add(temp)
}
if (!left.isEmpty()) merged.addAll(left)
if (!right.isEmpty()) merged.addAll(right)
return merged
}
val pivot = items.count()/2
var left = mergesort(items.subList(0, pivot))
var right = mergesort(items.subList(pivot, items.count() - 1))
return merge(left, right)
}
fun main(args : Array<String>) {
val names = mutableListOf("John", "Tim", "Zack", "Daniel", "Adam")
println(names)
println(mergesort(names))
}
|
code/sorting/src/merge_sort/merge_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// merge_sort.m
// Created by DaiPei on 2017/10/10.
//
#import <Foundation/Foundation.h>
@interface MergeSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation MergeSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
[self sort:array withLow:0 high:array.count - 1];
}
- (void)sort:(NSMutableArray<NSNumber *> *)array withLow:(NSUInteger)low high:(NSUInteger)high {
if (low < high) {
NSUInteger mid = (low + high) / 2;
[self sort:array withLow:low high:mid];
[self sort:array withLow:mid + 1 high:high];
[self merge:array withLow:low mid:mid high:high];
}
}
- (void)merge:(NSMutableArray<NSNumber *> *)array withLow:(NSUInteger)low mid:(NSUInteger)mid high:(NSUInteger)high {
NSArray *tmpArray = [array copy];
NSUInteger i = low, j = mid + 1, k = low;
while (i <= mid && j <= high) {
if ([tmpArray[i] compare:tmpArray[j]] == NSOrderedAscending) {
array[k] = tmpArray[i];
i++;
} else {
array[k] = tmpArray[j];
j++;
}
k++;
}
while (i <= mid) {
array[k] = tmpArray[i];
i++;
k++;
}
while (j <= high) {
array[k] = tmpArray[j];
j++;
k++;
}
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
int n = 0;
NSLog(@"What is the size of the array?");
scanf("%d", &n);
NSMutableArray *array = [NSMutableArray arrayWithCapacity:n];
NSLog(@"Enter elements of the array one by one:");
for (int i = 0; i < n; i++) {
int tmp;
scanf("%d", &tmp);
[array addObject:@(tmp)];
}
MergeSort *ms = [[MergeSort alloc] init];
[ms sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/merge_sort/merge_sort.php | <?php
function merge_sort($my_array){
if(count($my_array) == 1 ) return $my_array;
$mid = count($my_array) / 2;
$left = array_slice($my_array, 0, $mid);
$right = array_slice($my_array, $mid);
$left = merge_sort($left);
$right = merge_sort($right);
return merge($left, $right);
}
function merge($left, $right){
$res = array();
while (count($left) > 0 && count($right) > 0){
if($left[0] > $right[0]){
$res[] = $right[0];
$right = array_slice($right , 1);
}else{
$res[] = $left[0];
$left = array_slice($left, 1);
}
}
while (count($left) > 0){
$res[] = $left[0];
$left = array_slice($left, 1);
}
while (count($right) > 0){
$res[] = $right[0];
$right = array_slice($right, 1);
}
return $res;
}
$test_array = array(100, 54, 7, 2, 5, 4, 1);
echo "Original Array : ";
echo implode(', ',$test_array );
echo "\nSorted Array :";
echo implode(', ',merge_sort($test_array))."\n";
?> |
code/sorting/src/merge_sort/merge_sort.pl | %National University from Colombia
%Author:Andres Vargas, Jaime
%Gitub: https://github.com/jaavargasar
%webpage: https://jaavargasar.github.io/
%Language: Prolog
% Merge Sort
llist([],0).
llist([_|T],N):- llist(T,NT),N is NT +1.
divHalf(List,A,B) :- llsplit(List,List,A,B).
llsplit(S,[],[],S).
llsplit(S,[_],[],S).
llsplit([H|T],[_,_|T2],[H|A],S) :-llsplit(T,T2,A,S).
merge([], H, H).
merge(H, [], H).
merge([H|T], [Y|K], [H|S]) :- H =< Y, merge(T, [Y|K], S).
merge([H|T], [Y|K], [Y|S]) :- Y =< H, merge([H|T], K, S).
mergesort( [],[] ).
mergesort([H], [H]).
mergesort([H|T], S) :-
llist(T,LEN),
LEN > 0,
divHalf([H|T], Y, Z),
mergesort(Y, L1),
mergesort(Z, L2),
merge(L1, L2, S).
|
code/sorting/src/merge_sort/merge_sort.py | # Part of Cosmos by OpenGenus Foundation
# Split array in half, sort the 2 halves and merge them.
# When merging we pick the smallest element in the array.
# The "edge" case is when one of the arrays has no elements in it
# To avoid checking and breaking the look, finding which array has elements and then
# Starting another loop, we simply alias the arrays. This abstracts the issue without
# Creating a mess of code. When we alias, we decide with Xor which array to pick,
# Since we cover 0 ^ 0 case in the beginning of the loop,
# while bool(a) or bool(b) makes 0^0 is impossible to happen, Xor has 3 cases, 1 ^ 0, 0 ^ 1, 1^1 to evaluate.
# Since the 2 first cases are the edge we go inside the loop and alias the array that has elements in it.
# If it's 1^1 we simply pick the array with the smallest element.
from random import randint
def merge_sort(array):
if len(array) == 1:
return array
left = merge_sort(array[: (len(array) // 2)])
right = merge_sort(array[(len(array) // 2) :])
out = []
while bool(left) or bool(right):
if bool(left) ^ bool(right):
alias = left if left else right
else:
alias = left if left[0] < right[0] else right
out.append(alias[0])
alias.remove(alias[0])
return out
randomized: list = [randint(0, 1000) for x in range(10000)]
clone = [x for x in randomized]
assert sorted(clone) == merge_sort(randomized)
|
code/sorting/src/merge_sort/merge_sort.rb | # Part of Cosmos by OpenGenus Foundation
def merge(a, l, m, r)
n1 = m - l + 1
left = Array.new(n1) # Created temp array for storing left subarray
n2 = r - m
right = Array.new(n2) # Created temp array for storing right subarray
for i in 0...n1 # Copy values from left subarray to temp array
left[i] = a[l + i]
end
for i in 0...n2 # Copy values from Right subarray to temp array
right[i] = a[m + 1 + i]
end
i = 0
j = 0
# Comparing and merging two sub arrays
for k in l..r
if i >= n1
a[k] = right[j]
j += 1
elsif j >= n2
a[k] = left[i]
i += 1
elsif left[i] > right[j]
a[k] = right[j]
j += 1
else
a[k] = left[i]
i += 1
end
end
end
# Merge Sort
def merge_sort(a, l, r)
if l < r
m = l + (r - l) / 2
merge_sort(a, l, m)
merge_sort(a, m + 1, r)
merge(a, l, m, r)
end
end
|
code/sorting/src/merge_sort/merge_sort.rs | // Part of Cosmos by OpenGenus Foundation
/// A program that defines an unsorted vector,
/// and sorts it using a merge sort implementation.
fn main() {
let mut arr = vec![4, 5, 9, 1, 3, 0, 7, 2, 8];
merge_sort(&mut arr);
println!("Sorted array: {:?}", arr)
}
/// Sort the given array using merge sort.
/// The given array is `modified`.
fn merge_sort(arr: &mut Vec<i32>) {
let size = arr.len();
let mut buffer = vec![0; size];
merge_split(arr, 0, size, &mut buffer);
}
/// Initiate a merge sort by splitting the given `arr`.
/// The given vector is split recursively between `start` (inclusive) and
/// `end` (exclusive), and merged again in the proper order to sort the vector.
///
/// The `buffer` vector is used to temporarily move slices to, and has the same
/// size.
fn merge_split(arr: &mut Vec<i32>, start: usize, end: usize, buffer: &mut Vec<i32>) {
if end - start > 1 {
// Determine the split index
let split = (start + end) / 2;
// Split and merge sort
merge_split(arr, start, split, buffer);
merge_split(arr, split, end, buffer);
merge(arr, buffer, start, split, end);
merge_copy(buffer, arr, start, end);
}
}
/// Merges two slices called left and right from `src` into `dest` in the
/// correct order to sort them.
///
/// The slices are defined by their indices:
/// - Left: from `start` (inclusive) to `split` (exclusive).
/// - Right: from `split` (inclusive) to `end` (exclusive).
///
/// Only the `dest` vector is modified.
fn merge(src: &Vec<i32>, dest: &mut Vec<i32>, start: usize, split: usize, end: usize) {
// Define cursor indices for the left and right part that will be merged
let mut left = start;
let mut right = split;
// Merge the parts into the destination in the correct order
for i in start..end {
if left < split && (right >= end || src[left] <= src[right]) {
dest[i] = src[left];
left += 1;
} else {
dest[i] = src[right];
right += 1;
}
}
}
/// Copy elements from the `src` vector into `dest`.
/// Start at element `start` (included) and copy until element `end` (excluded).
///
/// Only the `dest` vector is modified.
fn merge_copy(src: &Vec<i32>, dest: &mut Vec<i32>, start: usize, end: usize) {
(start..end).for_each(|i| dest[i] = src[i]);
}
|
code/sorting/src/merge_sort/merge_sort.scala | object Main
{
def merge(left: List[Int], right: List[Int]): List[Int] =
{
if (left.isEmpty)
{
return right
}
else if (right.isEmpty)
{
return left
}
if (left.head < right.head)
{
return left.head :: merge(left.tail, right)
}
else
{
return right.head :: merge(left, right.tail)
}
}
def merge_sort(xs: List[Int]): List[Int] = {
if (xs.size == 1) {
return xs
}
merge(merge_sort(xs.slice(0, xs.size/2)), merge_sort(xs.slice(xs.size/2, xs.size)))
}
def main(args: Array[String]) =
{
println(merge(List(1,3,5), List(2,4,6)))
println(merge_sort(List(4,2,3,1,6,2,7,1,116,3,11,135)))
}
}
|
code/sorting/src/merge_sort/merge_sort.sh | #!/bin/bash
# code for merge sort in shell script.
declare -a array
ARRAYSZ=10
create_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
array[${i}]=${RANDOM}
i=$((i+1))
done
}
merge() {
local first=2
local second=$(( $1 + 2 ))
for i in ${@:2}
do
if [[ $first -eq $(( $1 + 2 )) ]]
then
echo ${@:$second:1} ; ((second += 1))
else
if [[ $second -eq $(( ${#@} + 1 )) ]]
then
echo ${@:$first:1} ; ((first += 1))
else
if [[ ${@:$first:1} -lt ${@:$second:1} ]]
then
echo ${@:$first:1} ; ((first += 1))
else
echo ${@:$second:1} ; ((second += 1))
fi
fi
fi
done
}
mergesort() {
if [[ $1 -ge 2 ]]
then
local med=$(($1 / 2))
local first=$(mergesort $med ${@:2:$med})
local second=$(mergesort $(( $1 - $med )) ${@:$(( $med + 2 )):$(( $1 - $med ))})
echo $(merge $med ${first[@]} ${second[@]})
else
echo $2
fi
}
create_array()
echo ${array[@]} ; echo $(mergesort 10 ${array[@]})
|
code/sorting/src/merge_sort/merge_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// merge_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func mergeSort(_ array: inout [Int]) {
mergeSort(&array, low:0, high: array.count - 1)
}
private func mergeSort(_ array: inout [Int], low: Int, high: Int) {
if low < high {
let mid = (low + high) / 2
mergeSort(&array, low:low, high:mid)
mergeSort(&array, low:mid + 1, high:high)
merge(&array, low:low, mid:mid, high:high)
}
}
private func merge(_ array: inout [Int], low: Int, mid: Int, high: Int) {
var tmpArray = [Int](array)
var i = low, j = mid + 1, k = low
while i <= mid && j <= high {
if tmpArray[i] < tmpArray[j] {
array[k] = tmpArray[i]
i += 1
} else {
array[k] = tmpArray[j]
j += 1
}
k += 1
}
while i <= mid {
array[k] = tmpArray[i]
i += 1
k += 1
}
while j <= mid {
array[k] = tmpArray[j]
j += 1
k += 1
}
}
|
code/sorting/src/merge_sort/merge_sort.ts | // Part of Cosmos by OpenGenus Foundation
export default function MergeSort(items: number[]): number[] {
return divide(items);
}
function divide(items: number[]): number[] {
var halfLength = Math.ceil(items.length / 2);
var low = items.slice(0, halfLength);
var high = items.slice(halfLength);
if (halfLength > 1) {
low = divide(low);
high = divide(high);
}
return combine(low, high);
}
function combine(low: number[], high: number[]): number[] {
var indexLow = 0;
var indexHigh = 0;
var lengthLow = low.length;
var lengthHigh = high.length;
var combined = [];
while (indexLow < lengthLow || indexHigh < lengthHigh) {
var lowItem = low[indexLow];
var highItem = high[indexHigh];
if (lowItem !== undefined) {
if (highItem === undefined) {
combined.push(lowItem);
indexLow++;
} else {
if (lowItem <= highItem) {
combined.push(lowItem);
indexLow++;
} else {
combined.push(highItem);
indexHigh++;
}
}
} else {
if (highItem !== undefined) {
combined.push(highItem);
indexHigh++;
}
}
}
return combined;
}
|
Subsets and Splits