filename
stringlengths 7
140
| content
stringlengths 0
76.7M
|
---|---|
code/sorting/src/merge_sort/merge_sort_extension.swift | /* Part of Cosmos by OpenGenus Foundation */
import Foundation
extension Array {
mutating func mergeSort(compareWith less: (Element, Element) -> Bool) {
mergeSort(compareWith: less, low: 0, high: self.count - 1)
}
private mutating func mergeSort(compareWith less: (Element, Element) -> Bool, low: Int, high: Int) {
if low < high {
let mid = (low + high) / 2
mergeSort(compareWith: less, low: low, high: mid)
mergeSort(compareWith: less, low: mid + 1, high: high)
merge(compareWith: less, low: low, mid: mid, high: high)
}
}
private mutating func merge(compareWith less: (Element, Element) -> Bool, low: Int, mid: Int, high: Int) {
var tmpArray = self
var i = low, j = mid + 1, k = low
while i <= mid && j <= high {
if less(tmpArray[i], tmpArray[j]) {
self[k] = tmpArray[i]
i += 1
} else {
self[k] = tmpArray[j]
j += 1
}
k += 1
}
while i <= mid {
self[k] = tmpArray[i]
i += 1
k += 1
}
while j <= mid {
self[k] = tmpArray[j]
j += 1
k += 1
}
}
}
|
code/sorting/src/merge_sort/merge_sort_linked_list.c | // Program to mergesort linked list
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} node;
node* insert(node *root, int data)
{
node *tail = malloc(sizeof(node));
tail->data = data;
tail->next = NULL;
if (!root)
{
root = tail;
return root;
}
node *temp = root;
while (temp->next)
{
temp = temp->next;
}
temp->next = tail;
return root;
}
void print(node *root)
{
node *temp = root;
while (temp)
{
printf("%d ", temp->data);
temp = temp->next;
}
}
node* merge(node *lroot, node *rroot)
{
node *root, *temp;
if (lroot->data <= rroot->data)
{
root = temp = lroot;
lroot = lroot->next;
}
else
{
root = temp = rroot;
rroot = rroot->next;
}
while (lroot && rroot)
{
if (lroot->data <= rroot->data)
{
temp->next = lroot;
temp = temp->next;
lroot = lroot->next;
}
else
{
temp->next = rroot;
temp = temp->next;
rroot = rroot->next;
}
}
while (lroot)
{
temp->next = lroot;
temp = temp->next;
lroot = lroot->next;
}
while (rroot)
{
temp->next = rroot;
temp = temp->next;
rroot = rroot->next;
}
return root;
}
node* merge_sort(node *root, int len)
{
node *temp = root, *prev;
int llen = 0;
if (len == 1)
{
return root;
}
while (llen < len / 2)
{
prev = temp;
temp = temp->next;
llen++;
}
prev->next = NULL;
node *lroot = merge_sort(root, llen);
node *rroot = merge_sort(temp, len - llen);
temp = merge(lroot, rroot);
return temp;
}
int main()
{
node *root = NULL, *temp;
int len = 0;
root = insert(root, 2);
root = insert(root, 1);
root = insert(root, 13);
root = insert(root, 42);
root = insert(root, -2);
root = insert(root, 67);
root = insert(root, 22);
root = insert(root, 3);
root = insert(root, 2);
root = insert(root, 12);
print(root);
temp = root;
while (temp)
{
temp = temp->next;
len++;
}
printf("\n");
root = merge_sort(root, len);
print(root);
return 0;
}
|
code/sorting/src/merge_sort/merge_sort_linked_list.cpp | /*
Here ListNode is class containing 'next' for next node, and value for
value at node.
*/
ListNode* mergeSort(ListNode* head)
{
if (head==NULL||head->next==NULL)
{
return head;
}
ListNode* mid = getMid(head);
ListNode* leftHalf = mergeSort(head);
ListNode* rightHalf = mergeSort(mid);
return merge(leftHalf, rightHalf);
}
ListNode* getMid(ListNode* head)
{
ListNode* prev = NULL;
ListNode *s_ptr = head;
ListNode* f_ptr = head;
while (f_ptr != NULL && f_ptr->next != NULL)
{
prev = s_ptr;
s_ptr = s_ptr->next;
f_ptr = f_ptr->next->next;
}
prev->next = NULL;
return s_ptr;
}
ListNode* merge(ListNode* l1, ListNode* l2)
{
ListNode* n_head = new ListNode(0);
ListNode* rear = n_head;
while (l1!=NULL && l2!=NULL)
{
if (l1->value < l2->value)
{
rear->next = l1;
l1 = l1->next;
}
else
{
rear->next = l2;
l2 = l2->next;
}
rear = rear->next;
}
if (l1 != NULL)
{
rear->next = l1;
}
if (l2!= NULL)
{
rear->next = l2;
}
return n_head->next;
}
|
code/sorting/src/pancake_sort/pancake_sort.cpp | #include <iostream>
#include <vector>
#include <algorithm>
template <typename T>
void pancakeSort(std::vector<T>& container)
{
for (int size = container.size(); size > 1; --size)
{
// Find position of max element
int maxPos = std::max_element(container.begin(), container.begin() + size) - container.begin();
// Reverse the vector from begin to maxPos (max element becomes first element)
std::reverse(container.begin(), container.begin() + maxPos + 1);
// Reverse the vector from begin to size (max element to correct position)
std::reverse(container.begin(), container.begin() + size);
}
}
template <typename T>
void printVector(std::vector<T> v)
{
for (auto i : v)
std::cout << i << " ";
std::cout << std::endl;
}
int main()
{
// Sample vector of ints
std::vector<int> v1 = {72, 23, 9, 31, 6, 8};
// Sort vector using pancake sort
pancakeSort(v1);
// Print sorted vector
printVector(v1);
// Sample vector of doubles
std::vector<double> v2 = {9.82, 7.36, 13.87, 2.28, 6.15, 45.56};
// Sort vector using pancake sort
pancakeSort(v2);
// Print sorted vector
printVector(v2);
// Sample vector of floats
std::vector<float> v3 = {74.25, 13.32, 25.71, 36.82, 10.11, 47.00};
// Sort vector using pancake sort
pancakeSort(v3);
// Print sorted vector
printVector(v3);
return 0;
}
|
code/sorting/src/pigeonhole_sort/README.md | # Pigeonhole Sort
The Pigeonhole sort is a sorting technique that is used when the range of keys is relatively small.
An array of pigeonholes (buckets, chunks of RAM) is reserved for each possible key value. The records from the unsorted list are scanned and copied into their respective pigeonholes based on their key values. Then, the contents of the pigeonholes are copied in sequence to the final destination.
## Explanation
Pigeonhole sort works in the following way:
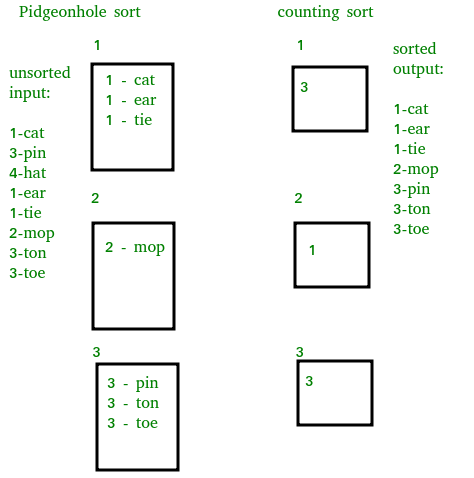
> Image credits: geeksforgeeks
It is similar to counting sort, but differs in that it "moves items twice: once to the bucket array and again to the final destination [whereas] counting sort builds an auxiliary array then uses the array to compute each item's final destination and move the item there".
## Algorithm
1. Find minimum and maximum values in array. Let the minimum and maximum values be ‘min’ and ‘max’ respectively. Also find range as ‘max-min-1’.
2. Set up an array of initially empty “pigeonholes” with the same size as that of the range.
3. Visit each element of the array and then put each element in its pigeonhole. An element `arr[i]` is put in hole at index `arr[i]` – min.
4. Start the loop all over the pigeonhole array in order and put the elements from non- empty holes back into the original array.
## Complexity
**Time complexity**: `O(N + n)`
where **N** is the range of key values and **n** is the input size
**Space complexity**: `O(N + n)`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/pigeonhole_sort/pigeonhole_sort.c | #include <stdio.h>
#include <stdlib.h>
// Part of Cosmos by OpenGenus Foundation
int main(){
printf("enter the size of array to be sorted:\n");
int n; scanf("%d", &n);
int pigeon[n];
printf("now enter the elements of the array:\n");
for(int i=0;i<n;++i){
scanf("%d", &pigeon[i]);
}
int max, min;
max = pigeon[0]; min = pigeon[0];
for(int i=0;i<n;++i){
if(min > pigeon[i]) min = pigeon[i];
if(max < pigeon[i]) max = pigeon[i];
}
//printf("%d\n %d\n",max, min );
int range = max +1;
int hole[range+1];
for(int i=0;i<range+1;++i){
hole[i] = 0;
}
for(int i=0;i<n;++i){
hole[pigeon[i]]++;
}
//for(int i=0;i<range+1;++i){
// printf("%d ",hole[i] );
//}
printf("\n");
for(int i=0;i<range+1;++i){
while(hole[i] > 0){
printf("%d ", i);
hole[i]--;
}
}
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.cpp | #include <vector>
#include <iostream>
using namespace std;
/* Sorts the array using pigeonhole algorithm */
// Part of Cosmos by OpenGenus Foundation
void pigeonholeSort(vector<int>& arr)
{
// Find minimum and maximum values in arr[]
int min = arr[0], max = arr[0];
for (size_t i = 1; i < arr.size(); i++)
{
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
}
int range = max - min + 1; // Find range
// Create an array of vectors. Size of array
// range. Each vector represents a hole that
// is going to contain matching elements.
vector<int> holes[range];
// Traverse through input array and put every
// element in its respective hole
for (size_t i = 0; i < arr.size(); i++)
holes[arr[i] - min].push_back(arr[i]);
// Traverse through all holes one by one. For
// every hole, take its elements and put in
// array.
int index = 0; // index in sorted array
for (int i = 0; i < range; i++)
{
vector<int>::iterator it;
for (it = holes[i].begin(); it != holes[i].end(); ++it)
arr[index++] = *it;
}
}
// Driver program to test the above function
int main()
{
vector<int> arr{25, 0, 2, 9, 1, 4, 8};
pigeonholeSort(arr);
cout << "Sorted order is : ";
for (size_t i = 0; i < arr.size(); i++)
cout << arr[i] << " ";
cout << "\n";
return 0;
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.cs | // Part of Cosmos by OpenGenus Foundation //
using System;
using System.Collections.Generic;
public class Program
{
//Main entrypoint and test setup
public static void Main()
{
int[] arr = new int[] {12, 11, 6, 2, 16, 7, 9, 18};
PigeonholeSort(ref arr);
Console.Write("Sorted order is: ");
for (int i = 0; i < arr.Length; i++)
Console.Write(arr[i] + " ");
}
// PigeonholeSort - Sorts the array using the pigeonhole algorithm.
// ref int[] arr - Pass a reference to an integer array to be sorted.
public static void PigeonholeSort(ref int[] arr)
{
// Initialise min and max to the same value
int min = arr[0];
int max = arr[0];
// Then get the min and max values of the array
for (int i = 1; i < arr.Length; i++)
{
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
}
// Range is our total valuespace
int range = max - min + 1;
// Create our array of lists, and initialise them
List<int>[] holes = new List<int>[range];
for(int i = 0; i < holes.Length; i++) { holes[i] = new List<int>(); }
// For every value in the array, put it in the corresponding pigeonhole
for (int i = 0; i < arr.Length; i++)
holes[arr[i]-min].Add(arr[i]);
// Finally, replace the values in the array with the values from each
// pigeonhole in order
int index = 0;
for (int i = 0; i < range; i++)
{
for (int j = 0; j < holes[i].Count; j++)
arr[index++] = holes[i][j];
}
}
} |
code/sorting/src/pigeonhole_sort/pigeonhole_sort.go | package main
import (
"fmt"
)
// Pigeonhole Sort in Golang
// Part of Cosmos by OpenGenus Foundation
// PigeonholeSort sorts the given slice
func PigeonholeSort(a []int) {
arrayLength := len(a)
// this is used to handle for the edge case where there is nothing to sort
if arrayLength == 0 {
return
}
min := a[0]
max := a[0]
// get the min and max values of the array
for _, value := range a {
if value < min {
min = value
}
if value > max {
max = value
}
}
size := max - min + 1
// create the pigeon holes with the initial values
holes := make(map[int][]int, size)
for i := 0; i < size; i++ {
holes[i] = []int{}
}
// for every value in the array, put it in the corresponding pigeonhole
for _, value := range a {
holes[value-min] = append(holes[value-min], value)
}
// finally, replace the values in the array with the values from each
// pigeonhole in order
j := 0
for i := 0; i < size; i++ {
for _, value := range holes[i] {
a[j] = value
j++
}
}
}
func main() {
randomSlice := []int{5, 2, 8, 9, 10, 1, 3}
fmt.Println("Unsorted Slice: ", randomSlice)
PigeonholeSort(randomSlice)
// should receive [ 1, 2, 3, 5, 8, 9, 10 ]
fmt.Println("Sorted Slice: ", randomSlice)
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.java | /* Part of Cosmos by OpenGenus Foundation */
/* Pigeonhole sorting is a sorting algorithm that is suitable for sorting lists
of elements where the number of elements and the number of possible key values
are approximately the same. In principle, it resembles Counting Sort.
While Counting Sort is a recursive algorithm, Pigeonhole Sort completes the sort
in a single iteration. It uses O(n) space and time.
*/
import java.util.ArrayList;
public class PigeonHoleSort {
public static void pigeonholeSort(int[] arr, int n) {
int min = arr[0];
int max = arr[0];
int range = 0;
// Obtain the range of the elements, to set bounds for the #pigeonholes
for(int i = 1;i<n;i++) {
if(arr[i]>max)
max = arr[i];
else if(arr[i]<min)
min = arr[i];
}
range = max-min+1;
// For each value in the range, create one pigeonhole
ArrayList<Integer>[] holes = new ArrayList[range];
for(int i = 0;i<range;i++) {
holes[i] = new ArrayList<Integer>();
}
// Traverse the list to be sorted. Each element is places in the hole matching its value.
for(int i = 0; i < n;i++) {
holes[arr[i]-min].add(arr[i]);
}
// Repopulate the original array picking up elements from the pigeonholes in order
int index = 0;
for(int i = 0;i<range;i++) {
for(int num : holes[i]) {
arr[index++] = num;
}
}
}
public static void main(String args[]) {
int arr[] = {3, 5, 8, 5};
pigeonholeSort(arr, arr.length);
for(int num : arr) {
System.out.println(num);
}
}
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.js | /**
* Perform a pigeonhole sort on an array of ints.
* @param {Array} - array of ints
* @returns {Array} - sorted array of ints
*/
function pigeonholeSort(array) {
// Figure out the range. This part isn't really the algorithm, it's
// figuring out the parameters for it.
const max = Math.max(...array);
const min = Math.min(...array);
const numHoles = max - min + 1;
// Create pigeonholes
let holes = Array(numHoles).fill(0);
// Populate pigeonholes with items
for (let item of array) {
holes[item - min] += 1;
}
let sortedArray = [];
for (let i = 0; i < holes.length; i++) {
while (holes[i] > 0) {
sortedArray.push(i + min);
holes[i] -= 1;
}
}
return sortedArray;
}
/* For example:
let array = [5, 2, 8, 9, 10, 1, 3];
console.log(pigeonholeSort(array));
> [ 1, 2, 3, 5, 8, 9, 10 ]
*/
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// pigeonhole_sort.m
// Created by DaiPei on 2017/10/19.
//
#import <Foundation/Foundation.h>
@interface PigeonholeSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation PigeonholeSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
// find the max and min value in array
NSNumber *max = @(INTMAX_MIN);
NSNumber *min = @(INTMAX_MAX);
for (NSNumber *item in array) {
if ([max compare:item] == NSOrderedAscending) {
max = item;
}
if ([min compare:item] == NSOrderedDescending) {
min = item;
}
}
// put element to right hole
int range = max.intValue - min.intValue + 1;
NSMutableArray<NSMutableArray *> *holes = [NSMutableArray array];
for (int i = 0; i < range; i++) {
[holes addObject:[NSMutableArray array]];
}
for (NSNumber *item in array) {
[holes[item.intValue - min.intValue] addObject:item];
}
// use holes to make sorted array
int index = 0;
for (NSMutableArray *hole in holes) {
for (NSNumber *item in hole) {
array[index++] = item;
}
}
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray arrayWithCapacity:10];
for (int i = 0; i < 10; i++) {
int ran = arc4random() % 20 - 10;
[array addObject:@(ran)];
}
NSLog(@"before: %@", array);
PigeonholeSort *ps = [[PigeonholeSort alloc] init];
[ps sort:array];
NSLog(@"after: %@", array);
}
return 0;
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.php | <?php
/* Part of Cosmos by OpenGenus Foundation */
/* Example Usage:
print_r(pigeonholeSort([9, 2, 17, 5, 16]));
*/
function pigeonholeSort($array) {
$min = min($array);
$max = max($array);
$numHoles = $max - $min + 1;
$holes = array_fill(0, $numHoles, 0);
foreach ($array as $item) {
$holes[$item - $min] += 1;
}
$sortedArray = [];
for ($i = 0; $i < count($holes); $i++) {
while ($holes[$i] > 0) {
array_push($sortedArray, $i + $min);
$holes[$i] -= 1;
}
}
return $sortedArray;
}
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.py | # Part of Cosmos by OpenGenus Foundation
def pigeonhole_sort(a):
# size of range of values in the list
m_min = min(a)
m_max = max(a)
size = m_max - m_min + 1
# our list of pigeonholes
holes = [0] * size
# Populating the pigeonholes.
for x in a:
assert type(x) is int, "integers only"
holes[x - m_min] += 1
# Putting the elements back into the array in order
i = 0
for count in xrange(size):
while holes[count] > 0:
holes[count] -= 1
a[i] = count + m_min
i += 1
|
code/sorting/src/pigeonhole_sort/pigeonhole_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// pigeonhole_sort.swift
// Created by DaiPei on 2017/10/18.
//
import Foundation
func pigeonholeSort(_ array: inout [Int]) {
// find max and min value in array
var max = Int.min
var min = Int.max
for item in array {
if item > max {
max = item
}
if item < min {
min = item
}
}
// put element to right hole
let range = max - min + 1
var holes = [[Int]](repeatElement([Int](), count: range))
for item in array {
holes[item - min].append(item)
}
// use holes to make sorted array
var index = 0
for hole in holes {
for item in hole {
array[index] = item
index += 1
}
}
}
|
code/sorting/src/pigeonhole_sort/pigeonholesort.scala | /* Part of Cosmos by OpenGenus Foundation */
import scala.collection.mutable.ArrayBuffer
object PigeonHoleSort {
def sort(list: List[Int]): List[Int] = {
val min = list.min
val max = list.max
val buffer: ArrayBuffer[Boolean] = ArrayBuffer((min to max).map(_ => false): _ *)
list.foreach(x => buffer(x - min) = true)
Stream.from(min).zip(buffer).collect {
case (value, hole) if hole => value
}.toList
}
def main(args: Array[String]): Unit = {
print(PigeonHoleSort.sort(List(-1,2,1,10,15,13)))
}
}
|
code/sorting/src/postmans_sort/postmans_sort.c | // C Program to Implement Postman Sort Algorithm
#include <stdio.h>
int main()
{
int arr[100], arr1[100];
int i, j, maxdigits = 0;
printf("Enter size of array :");
int count;
scanf("%d", & count);
printf("Enter elements into array :");
for (i = 0; i < count; ++i)
{
printf("Element - %d : ", i);
scanf("%d", & arr[i]);
arr1[i] = arr[i];
}
//Finding the longest digits in the array and no of elements in that digit
for (i = 0; i < count; ++i)
{
int numdigits = 0;
int t = arr[i]; /*first element in t */
while (t > 0)
{
numdigits++; //Counting the no of elements of a digit
t /= 10;
}
if (maxdigits < numdigits)
maxdigits = numdigits; //Storing the length of longest digit
}
int n = 1;
while (--maxdigits)
n *= 10;
for (i = 0; i < count; ++i)
{
int max = arr[i] / n; //Dividing by a particular base
int t = i;
for (j = i + 1; j < count; ++j)
{
if (max > (arr[j] / n))
{
max = arr[j] / n;
t = j;
}
}
int temp = arr1[t];
arr1[t] = arr1[i];
arr1[i] = temp;
temp = arr[t];
arr[t] = arr[i];
arr[i] = temp;
}
while (n >= 1)
{
for (i = 0; i < count;)
{
int t1 = arr[i] / n;
for (j = i + 1; t1 == (arr[j] / n); ++j);
int j1 = j; //Storing the end position to perform arrangement in the array
int i1 = i; //Storing the Start position to perform arrangement in the array
//Arranging the array
for (i1 = i; i1 < j1 - 1; ++i1)
{
for (j = i1 + 1; j < j1; ++j)
{
if (arr1[i1] > arr1[j])
{
int temp = arr1[i1];
arr1[i1] = arr1[j];
arr1[j] = temp;
temp = (arr[i1] % 10);
arr[i1] = (arr[j] % 10);
arr[j] = temp;
}
}
}
i = j;
}
n /= 10;
}
// Printing the arranged array
printf("\nSorted Array (Postman sort) :");
for (i = 0; i < count; ++i)
printf("%d ", arr1[i]);
printf("\n");
return 0;
}
/* Enter the Size of the array
6
Enter the elements of the array
Element - 0 : 43
Element - 1 : 35
Element - 2 : 75
Element - 3 : 1
Element - 4 : 68
Element - 5 : 453
Sorted Array
1 35 43 68 75 453 */
|
code/sorting/src/postmans_sort/postmans_sort.cpp | #include <stdio.h>
void arrange(int,int);
int array[100], array1[100];
int i, j, temp, max, count, maxdigits = 0, c = 0;
int main()
{
int t1, t2, k, t, n = 1;
printf("Enter size of array :");
scanf("%d", &count);
printf("Enter elements into array :");
// inputing array elements
for (i = 0; i < count; i++)
{
scanf("%d", &array[i]);
array1[i] = array[i];
}
//determining significant digits in all the elements
for (i = 0; i < count; i++)
{
t = array[i];
while(t > 0)
{
c++;
t = t / 10;
}
//maximium significant digits
if (maxdigits < c)
maxdigits = c;
c = 0;
}
//setting the base
while(--maxdigits)
n = n * 10;
// elements arranged on the basis of MSD
for (i = 0; i < count; i++)
{
max = array[i] / n;
t = i;
for (j = i + 1; j < count;j++)
{
if (max > (array[j] / n))
{
max = array[j] / n;
t = j;
}
}
temp = array1[t];
array1[t] = array1[i];
array1[i] = temp;
temp = array[t];
array[t] = array[i];
array[i] = temp;
}
//elements arranged according to the next MSD
while (n >= 1)
{
for (i = 0; i < count;)
{
t1 = array[i] / n;
for (j = i + 1; t1 == (array[j] / n); j++);
arrange(i, j);
i = j;
}
n = n / 10;
}
printf("\nSorted Array (Postman sort) :");
for (i = 0; i < count; i++)
printf("%d ", array1[i]);
printf("\n");
return 0;
}
// function to arrange the intergers having same bases
void arrange(int k,int n)
{
for (i = k; i < n - 1; i++)
{
for (j = i + 1; j < n; j++)
{
if (array1[i] > array1[j])
{
temp = array1[i];
array1[i] = array1[j];
array1[j] = temp;
temp = (array[i] % 10);
array[i] = (array[j] % 10);
array[j] = temp;
}
}
}
}
|
code/sorting/src/quick_sort/README.md | # Quicksort
Quicksort is a fast sorting algorithm that takes a **divide-and-conquer approach** for sorting lists. It picks one element as a pivot element and partitions the array around it such that: left side of pivot contains all the elements that are less than the pivot element and right side contains all elements greater than the pivot.
## Explanation
Following example explains how the pivot value is found in an array.

> Image credits: tutorialspoint
The pivot value divides the list into two parts and recursively, we find the pivot for each sub-lists until all lists contains only one element.
## Algorithm
```
procedure partition(A, start, end) is
pivot := A[end]
i := start - 1
for j := start to end - 1 do
if A[j] < pivot then
i := i + 1
swap A[i] with A[j]
swap A[i + 1] with A[end]
return i + 1
procedure quicksort(A, start, end) is
if start < end then
p := partition(A, start, end)
quicksort(A, start, p - 1 )
quicksort(A, p + 1, end)
```
The entire array is sorted by `quicksort(A, 0, length(A)-1)`.
## Complexity
**Time complexity**
- Worst case: **O(n<sup>2</sup>)**
- Average case: **O(n * log n)**
- Best case:
- **O(n * log n)** for simple partition
- **O(n)** for three-way partition and equal keys
**Space complexity**
- Worst case: **O(n)** auxiliary (naive)
- Average case: **O(log n)** auxiliary
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/quick_sort/dutch_national_flag.cpp | /* Part of Cosmos by OpenGenus Foundation */
#include <vector>
#include <stdlib.h>
#include <iostream>
using namespace std;
// Dutch National Flag Sort for array items 0,1,2
void flagSort(vector<int> &v)
{
int lo = 0;
int hi = v.size() - 1;
int mid = 0;
while (mid <= hi)
switch (v[mid])
{
case 0:
swap(v[lo++], v[mid++]);
break;
case 1:
mid++;
break;
case 2:
swap(v[mid], v[hi--]);
break;
}
}
/* UTILITY FUNCTIONS */
void swap(int &a, int &b)
{
int temp = a;
a = b;
b = temp;
}
void printarr(vector<int> &v)
{
for (size_t i = 0; i < v.size(); ++i)
cout << v[i] << " ";
cout << endl;
}
void flagFill(vector<int> &v)
{
for (size_t i = 0; i < v.size(); ++i)
v[i] = rand() % 3;
}
// Driver program
int main()
{
int size = 10;
cout << "Input test array size: ";
cin >> size;
vector<int> v(size);
flagFill(v);
cout << "Unsorted: ";
printarr(v);
flagSort(v);
cout << "Sorted: ";
printarr(v);
return 0;
}
|
code/sorting/src/quick_sort/quick_sort.c | /*Part of Cosmos by OpenGenus Foundation*/
#include <stdio.h>
void swap(int *p, int *q)
{
int temp = *p;
*p = *q;
*q = temp;
}
//Last element is used a spivot
//Places elements smaller than pivot to its left side
//Places elements larger than pivot to its right side
int partition(int a[], int low, int high)
{
int pivot = a[high];
int i = (low - 1);
for (int j = low; j <= high- 1; j++)
{
if (a[j] <= pivot)
{
i++;
swap(&a[i], &a[j]);
}
}
swap(&a[i + 1], &a[high]);
return (i + 1);
}
void quickSort(int a[], int low, int high)
{
if (low < high)
{
int k = partition(a, low, high);
//k is pivot index
quickSort(a, low, k - 1);
quickSort(a, k + 1, high);
}
}
void print(int a[], int size)
{
int i;
for (i = 0; i < size; i++)
printf("%d ", a[i]);
printf("\n");
}
int main()
{
int n, i;
printf("What is the size of the array?\n");
scanf("%d",&n);
int a[n];
printf("Enter elements of the array one by one\n");
for(i = 0; i < n; i++){
scanf("\n%d",&a[i]);
}
quickSort(a, 0, n - 1);
printf("Sorted array: ");
print(a, n);
return 0;
}
|
code/sorting/src/quick_sort/quick_sort.cpp | /*
* Part of Cosmos by OpenGenus Foundation
*
* quick sort synopsis
*
* namespace quick_sort_impl {
* struct quick_sort_tag {};
* struct iterative_quick_sort_tag :quick_sort_tag {};
* struct recursive_quick_sort_tag :quick_sort_tag {};
*
* template<typename _Random_Acccess_Iter, typename _Compare>
* _Random_Acccess_Iter
* partition(_Random_Acccess_Iter first, _Random_Acccess_Iter last, _Compare comp);
*
* template<typename _Random_Acccess_Iter, typename _Compare>
* void
* quickSortImpl(_Random_Acccess_Iter first,
* _Random_Acccess_Iter last,
* _Compare comp,
* std::random_access_iterator_tag,
* recursive_quick_sort_tag);
*
* template<typename _Random_Acccess_Iter, typename _Compare>
* void
* quickSortImpl(_Random_Acccess_Iter first,
* _Random_Acccess_Iter last,
* _Compare comp,
* std::random_access_iterator_tag,
* iterative_quick_sort_tag);
* } // quick_sort_impl
*
* template<typename _Random_Acccess_Iter, typename _Compare>
* void
* quickSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end, _Compare comp);
*
* template<typename _Random_Acccess_Iter>
* void
* quickSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end);
*/
#include <stack>
#include <functional>
namespace quick_sort_impl {
struct quick_sort_tag {};
struct iterative_quick_sort_tag : quick_sort_tag {};
struct recursive_quick_sort_tag : quick_sort_tag {};
template<typename _Random_Acccess_Iter, typename _Compare>
_Random_Acccess_Iter
partition(_Random_Acccess_Iter first, _Random_Acccess_Iter last, _Compare comp)
{
_Random_Acccess_Iter i = first, j = last + 1;
while (true)
{
while (i + 1 <= last && comp(*++i, *first))
;
while (j - 1 >= first && comp(*first, *--j))
;
if (i >= j)
break;
std::swap(*i, *j);
}
std::swap(*first, *j);
return j;
}
template<typename _Random_Acccess_Iter, typename _Compare>
void
quickSortImpl(_Random_Acccess_Iter first,
_Random_Acccess_Iter last,
_Compare comp,
std::random_access_iterator_tag,
recursive_quick_sort_tag)
{
if (first < last)
{
// first is pivot
auto mid = quick_sort_impl::partition(first, last, comp);
quickSortImpl(first,
mid - 1,
comp,
std::random_access_iterator_tag(),
recursive_quick_sort_tag());
quickSortImpl(mid + 1,
last,
comp,
std::random_access_iterator_tag(),
recursive_quick_sort_tag());
}
}
template<typename _Random_Acccess_Iter, typename _Compare>
void
quickSortImpl(_Random_Acccess_Iter first,
_Random_Acccess_Iter last,
_Compare comp,
std::random_access_iterator_tag,
iterative_quick_sort_tag)
{
if (first < last)
{
std::stack<std::pair<_Random_Acccess_Iter, _Random_Acccess_Iter>> st;
st.push(std::make_pair(first, last));
while (!st.empty())
{
_Random_Acccess_Iter left, right, i, j;
left = st.top().first;
right = st.top().second;
st.pop();
// ignore if only one elem
if (left >= right)
continue;
auto mid = quick_sort_impl::partition(left, right, comp);
st.push(std::make_pair(left, mid - 1));
st.push(std::make_pair(mid + 1, right));
}
}
}
} // quick_sort_impl
template<typename _Random_Acccess_Iter, typename _Compare>
void
quickSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end, _Compare comp)
{
using namespace quick_sort_impl;
if (begin == end)
return;
--end;
auto category = typename std::iterator_traits<_Random_Acccess_Iter>::iterator_category();
return quickSortImpl(begin, end, comp, category, iterative_quick_sort_tag());
}
template<typename _Random_Acccess_Iter>
void
quickSort(_Random_Acccess_Iter begin, _Random_Acccess_Iter end)
{
using value_type = typename std::iterator_traits<_Random_Acccess_Iter>::value_type;
return quickSort(begin, end, std::less<value_type>());
}
|
code/sorting/src/quick_sort/quick_sort.cs | using System;
using System.Collections.Generic;
/*
* Part of Cosmos by OpenGenus Foundation
*/
namespace ConsoleApplicationQSort
{
class Program
{
static void Main(string[] args)
{
var A = new int[] { 9, 8, 7, 6, 5, 4, 3, 2, 1 };
var sorter = new QSort<int>(A);
sorter.Sort();
foreach (var i in sorter.A)
Console.WriteLine(i);
Console.Read();
}
}
class QSort<T> where T:IComparable
{
public IList<T> A;
public QSort(IList<T> A)
{
this.A = A;
}
public int Partition(int L, int U)
{
int s = U;
int p = L;
while (s != p)
{
if (A[p].CompareTo(A[s]) <= 0)
{
p++;
}
else
{
Swap(p, s);
Swap(p, s - 1);
s--;
}
}
return p;
}
private void Swap(int p, int s)
{
T tmp = A[p];
A[p] = A[s];
A[s] = tmp;
}
public void Sort(int L, int U)
{
if (L >= U) return;
int p = Partition(L, U);
Sort(L, p-1);
Sort(p + 1, U);
}
public void Sort()
{
Sort(0, A.Count - 1);
}
}
}
|
code/sorting/src/quick_sort/quick_sort.elm | module QuickSort exposing (sort)
sort : List a -> (a -> a -> Order) -> List a
sort list order =
case list of
[] ->
[]
x :: xs ->
let
less =
List.filter (order x >> (==) GT) xs
greater =
List.filter (order x >> (/=) GT) xs
in
sort less order ++ [ x ] ++ sort greater order
|
code/sorting/src/quick_sort/quick_sort.go | // Quick Sort in Golang
// Part of Cosmos by OpenGenus Foundation
package main
import (
"fmt"
"math/rand"
)
// QuickSort sorts the given slice
func QuickSort(a []int) []int {
if len(a) < 2 {
return a
}
left, right := 0, len(a) - 1
pivot := rand.Int() % len(a)
a[pivot], a[right] = a[right], a[pivot]
for i, _ := range a {
if a[i] < a[right] {
a[left], a[i] = a[i], a[left]
left++
}
}
a[left], a[right] = a[right], a[left]
QuickSort(a[:left])
QuickSort(a[left + 1:])
return a
}
func main() {
randomSlice := []int{ 1, 6, 2, 4, 9, 0, 5, 3, 7, 8 }
fmt.Println("Unsorted Slice: ", randomSlice)
QuickSort(randomSlice)
fmt.Println("Sorted Slice: ", randomSlice)
}
|
code/sorting/src/quick_sort/quick_sort.hs | -- Part of Cosmos by OpenGenus Foundation
quicksort :: (Ord a) => [a] -> [a]
quicksort [] = []
quicksort (x:xs) = let less = filter (<x) xs in
let greater = filter (>=x) xs in
(quicksort less) ++ [x] ++ (quicksort greater) |
code/sorting/src/quick_sort/quick_sort.java | // Part of Cosmos by OpenGenus Foundation
class QuickSort {
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // last element is the pivot
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) { // if j'th element is less than or equal to the pivot
i++; // then swap the i'th element with the j'th element
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// swap arr[i+1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1; // return position of the pivot
}
void sort(int arr[], int low, int high) {
if (low < high) {
// after the following function call elemnt at positon pi
// is at it's correct poition in the sorted array
int piv = partition(arr, low, high);
sort(arr, low, piv - 1); // recursively sort
sort(arr, piv + 1, high); // rest of the array
}
}
static void printArray(int arr[]) {
int n = arr.length;
for (int i = 0; i < n; ++i) {
System.out.print(arr[i] + " ");
}
System.out.println();
}
public static void main(String args[]) {
int arr[] = {5, 7, 11, 56, 12, 1, 9};
int n = arr.length;
QuickSort qso = new QuickSort();
qso.sort(arr, 0, n - 1);
System.out.println("sorted array");
printArray(arr);
}
}
|
code/sorting/src/quick_sort/quick_sort.js | /*Part of Cosmos by OpenGenus Foundation*/
//here a is the array name
/*low is the initial index for sorting
i.e. 0 for sorting whole array*/
/*high is the ending index for sorting
i.e. array's length for sorting whole array*/
/*expected call for the function is as follows:
quickSort(array, 0, array.length -1);*/
function quickSort(a, low, high) {
var i = low;
var j = high;
var temp;
var k = (low + high) / 2; //k is pivot index
var pivot = parseInt(
a[k.toFixed()]
); /*k may be a fraction, toFixed takes it to the nearest integer*/
while (i <= j) {
while (parseInt(a[i]) < pivot) i++;
while (parseInt(a[j]) > pivot) j--;
if (i <= j) {
temp = a[i];
a[i] = a[j];
a[j] = temp;
i++;
j--;
}
}
if (low < j) quickSort(a, low, j);
if (i < high) quickSort(a, i, high);
return a;
}
|
code/sorting/src/quick_sort/quick_sort.lua | function quicksort(t, start, endi)
start, endi = start or 1, endi or #t
--partition w.r.t. first element
if(endi - start < 1) then return t end
local pivot = start
for i = start + 1, endi do
if t[i] <= t[pivot] then
if i == pivot + 1 then
t[pivot], t[pivot + 1] = t[pivot + 1], t[pivot]
else
t[pivot], t[pivot + 1], t[i] = t[i], t[pivot], t[pivot + 1]
end
pivot = pivot + 1
end
end
t = quicksort(t, start, pivot - 1)
return quicksort(t, pivot + 1, endi)
end
|
code/sorting/src/quick_sort/quick_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// quick_sort.m
// Created by DaiPei on 2017/10/10.
//
#import <Foundation/Foundation.h>
@interface QuickSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation QuickSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
[self sort:array low:0 high:array.count - 1];
}
- (void)sort:(NSMutableArray<NSNumber *> *)array low:(NSUInteger)low high:(NSUInteger)high {
if (low < high) {
NSUInteger div = [self partition:array low:low high:high];
if (div != 0) {
[self sort:array low:low high:div - 1];
}
[self sort:array low:div + 1 high:high];
}
}
- (NSUInteger)partition:(NSMutableArray<NSNumber *> *)array low:(NSUInteger)low high:(NSUInteger)high {
NSUInteger mid = (low + high) / 2;
[self swap:array at:mid and:high];
NSUInteger p1 = low, p2 = low;
while (p2 < high) {
if ([array[p2] compare:array[high]] == NSOrderedAscending) {
[self swap:array at:p1 and:p2];
p1++;
}
p2++;
}
[self swap:array at:p1 and:high];
return p1;
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSMutableArray *array = [NSMutableArray arrayWithCapacity:100];
for (int i = 0; i < 100; i++) {
uint32_t ran = arc4random() % 1000;
[array addObject:@(ran)];
}
NSLog(@"Enter elements of the array one by one:");
QuickSort *qs = [[QuickSort alloc] init];
[qs sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/quick_sort/quick_sort.ml | (* Part of Cosmos by OpenGenus Foundation *)
let rec quick_sort = function
| [] -> []
| hd :: tl ->
let lower, higher = List.partition (fun x -> x < hd) tl in
quick_sort lower @ (hd :: quick_sort higher);;
|
code/sorting/src/quick_sort/quick_sort.py | """
Part of Cosmos by OpenGenus Foundation
"""
def quick_sort(arr):
quick_sort_helper(arr, 0, len(arr) - 1)
def quick_sort_helper(arr, first, last):
if first < last:
splitpoint = partition(arr, first, last)
quick_sort_helper(arr, first, splitpoint - 1)
quick_sort_helper(arr, splitpoint + 1, last)
def partition(arr, first, last):
pivot = arr[first]
left = first + 1
right = last
done = False
while not done:
while left <= right and arr[left] <= pivot:
left = left + 1
while arr[right] >= pivot and right >= left:
right = right - 1
if right < left:
done = True
else:
temp = arr[left]
arr[left] = arr[right]
arr[right] = temp
temp = arr[first]
arr[first] = arr[right]
arr[right] = temp
return right
|
code/sorting/src/quick_sort/quick_sort.rb | # Part of Cosmos by OpenGenus Foundation
def quick_sort(array, beg_index, end_index)
if beg_index < end_index
pivot_index = partition(array, beg_index, end_index)
quick_sort(array, beg_index, pivot_index - 1)
quick_sort(array, pivot_index + 1, end_index)
end
array
end
# returns an index of where the pivot ends up
def partition(array, beg_index, end_index)
# current_index starts the subarray with larger numbers than the pivot
current_index = beg_index
i = beg_index
while i < end_index
if array[i] <= array[end_index]
swap(array, i, current_index)
current_index += 1
end
i += 1
end
# after this swap all of the elements before the pivot will be smaller and
# after the pivot larger
swap(array, end_index, current_index)
current_index
end
def swap(array, first_element, second_element)
temp = array[first_element]
array[first_element] = array[second_element]
array[second_element] = temp
end
puts quick_sort([2, 3, 1, 5], 0, 3).inspect # will return [1, 2, 3, 5]
|
code/sorting/src/quick_sort/quick_sort.rs | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
/* Part of Cosmos by OpenGenus Foundation */
// This algorithm taken from https://github.com/servo/rust-quicksort
//! In-place sorting.
#![cfg_attr(not(test), no_std)]
#[cfg(test)]
extern crate std as core;
use core::cmp::Ordering;
fn quicksort_helper<T, F>(arr: &mut [T], left: isize, right: isize, compare: &F)
where F: Fn(&T, &T) -> Ordering {
if right <= left {
return
}
let mut i: isize = left - 1;
let mut j: isize = right;
let mut p: isize = i;
let mut q: isize = j;
unsafe {
let v: *mut T = &mut arr[right as usize];
loop {
i += 1;
while compare(&arr[i as usize], &*v) == Ordering::Less {
i += 1
}
j -= 1;
while compare(&*v, &arr[j as usize]) == Ordering::Less {
if j == left {
break
}
j -= 1;
}
if i >= j {
break
}
arr.swap(i as usize, j as usize);
if compare(&arr[i as usize], &*v) == Ordering::Equal {
p += 1;
arr.swap(p as usize, i as usize)
}
if compare(&*v, &arr[j as usize]) == Ordering::Equal {
q -= 1;
arr.swap(j as usize, q as usize)
}
}
}
arr.swap(i as usize, right as usize);
j = i - 1;
i += 1;
let mut k: isize = left;
while k < p {
arr.swap(k as usize, j as usize);
k += 1;
j -= 1;
assert!(k < arr.len() as isize);
}
k = right - 1;
while k > q {
arr.swap(i as usize, k as usize);
k -= 1;
i += 1;
assert!(k != 0);
}
quicksort_helper(arr, left, j, compare);
quicksort_helper(arr, i, right, compare);
}
/// An in-place quicksort.
///
/// The algorithm is from Sedgewick and Bentley, "Quicksort is Optimal":
/// http://www.cs.princeton.edu/~rs/talks/QuicksortIsOptimal.pdf
pub fn quicksort_by<T, F>(arr: &mut [T], compare: F) where F: Fn(&T, &T) -> Ordering {
if arr.len() <= 1 {
return
}
let len = arr.len();
quicksort_helper(arr, 0, (len - 1) as isize, &compare);
}
/// An in-place quicksort for ordered items.
#[inline]
pub fn quicksort<T>(arr: &mut [T]) where T: Ord {
quicksort_by(arr, |a, b| a.cmp(b))
}
#[cfg(test)]
extern crate rand;
#[cfg(test)]
pub mod test {
use rand::{self, Rng};
use super::quicksort;
#[test]
pub fn random() {
let mut rng = rand::thread_rng();
for _ in 0u32 .. 50000u32 {
let len: usize = rng.gen();
let mut v: Vec<isize> = rng.gen_iter::<isize>().take((len % 32) + 1).collect();
quicksort(&mut v);
for i in 0 .. v.len() - 1 {
assert!(v[i] <= v[i + 1])
}
}
}
}
|
code/sorting/src/quick_sort/quick_sort.scala | /* Part of Cosmos by OpenGenus Foundation */
object QuickSort extends App {
def quickSort[A <% Ordered[A]](input: List[A]): List[A] = {
input match {
case Nil => Nil
case head :: tail =>
val (l, g) = tail partition (_ < head)
quickSort(l) ::: head :: quickSort(g)
}
}
val input = (0 to 1000) map (_ => scala.util.Random.nextInt(1000))
val output = quickSort(input.toList)
Console.println(output)
} |
code/sorting/src/quick_sort/quick_sort.sh | #!/bin/bash
# Lomuto partition scheme.
# Part of Cosmos by OpenGenus Foundation
declare -a array
ARRAYSZ=10
create_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
array[${i}]=${RANDOM}
i=$((i+1))
done
}
print_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
echo ${array[${i}]}
i=$((i+1))
done
}
verify_sort() {
i=1
while [ $i -lt $ARRAYSZ ]; do
if [ ${array[$i]} -lt ${array[$((i-1))]} ]; then
echo "Array did not sort, see elements $((i-1)) and $i."
exit 1
fi
i=$((i+1))
done
echo "Array sorted correctly."
}
partition() { #lo is $1, hi is $2
pivot=${array[$2]}
i=$(($1-1))
j=$1
while [ $j -lt $2 ]; do
if [ ${array[$j]} -lt $pivot ]; then
i=$((i+1))
tmp=${array[$i]}
array[$i]=${array[$j]}
array[$j]=$tmp
fi
j=$((j+1))
done
if [ ${array[$2]} -lt ${array[$((i+1))]} ]; then
tmp=${array[$((i+1))]}
array[$((i+1))]=${array[$2]}
array[$2]=$tmp
fi
partition_ret=$((i+1))
}
quicksort() { #lo is $1, hi is $2
if [ $1 -lt $2 ]; then
partition $1 $2
local pret=$partition_ret
quicksort $1 $((pret - 1))
quicksort $((pret + 1)) $2
fi
}
create_array
print_array
quicksort 0 $((ARRAYSZ-1))
echo
print_array
verify_sort
|
code/sorting/src/quick_sort/quick_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// quick_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func quickSort(_ array: inout [Int]) {
quickSort(&array, low: 0, high: array.count - 1)
}
private func quickSort(_ array: inout [Int], low: Int, high: Int) {
if low < high {
let div = partition(&array, low: low, high: high)
quickSort(&array, low: low, high: div - 1)
quickSort(&array, low: div + 1, high: high)
}
}
private func partition(_ array: inout [Int], low: Int, high: Int) -> Int {
var div = low
var p = low
let mid = (low + high) / 2
swap(&array, at: mid, and: high)
while p < high {
if array[p] < array[high] {
swap(&array, at: p, and: div)
div += 1
}
p += 1
}
swap(&array, at: high, and: div)
return div
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/quick_sort/quick_sort.ts | // Part of Cosmos by OpenGenus Foundation
function quicksort(A: number[]): number[] {
let stack = [{ lo: 0, hi: A.length - 1 }];
while (stack.length > 0) {
let { lo, hi } = stack.pop();
if (lo >= hi) {
continue;
}
let pivot = A[lo];
let i = lo;
let j = hi;
let p: number;
while (true) {
while (A[i] <= pivot && i <= j) { i++; }
while (A[j] >= pivot && j >= i) { j--; }
if (j <= i) {
let tmp = A[lo];
A[lo] = A[j];
A[j] = A[lo];
p = j;
break;
}
let tmp = A[i];
A[i] = A[j];
A[j] = A[i];
i++;
j--;
}
stack.push({ lo: lo, hi: p - 1});
stack.push({ lo: p + 1, hi: hi });
}
return A;
}
|
code/sorting/src/quick_sort/quick_sort_extension.swift | /* Part of Cosmos by OpenGenus Foundation */
import Foundation
private func swap<T>(_ array: inout [T], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
extension Array {
mutating func quickSort(compareWith less: (Element, Element) -> Bool) {
quickSort(compareWith: less, low: 0, high: self.count - 1)
}
private mutating func quickSort(compareWith less: (Element, Element) -> Bool, low: Int, high: Int) {
if low < high {
let div = partition(compareWith: less, low: low, high: high)
quickSort(compareWith: less, low: low, high: div - 1)
quickSort(compareWith: less, low: div + 1, high: high)
}
}
private mutating func partition(compareWith less: (Element, Element) -> Bool, low: Int, high: Int) -> Int {
var div = low
var p = low
let mid = (low + high) / 2
swap(&self, at: mid, and: high)
while p < high {
if less(self[p], self[high]) {
swap(&self, at: p, and: div)
div += 1
}
p += 1
}
swap(&self, at: high, and: div)
return div
}
}
|
code/sorting/src/quick_sort/quick_sort_in_place.scala | import annotation.tailrec
import collection.mutable.{IndexedSeq, IndexedSeqLike}
object QuicksortInPlace {
def sort[T](data: IndexedSeqLike[T, _])(implicit ord: Ordering[T]): Unit = {
sort(data, 0, data.length)
}
// start inclusive, end exclusive
def sort[T](data: IndexedSeqLike[T, _], start: Int, end: Int)(implicit ord: Ordering[T]): Unit = {
val runLength = end - start
if (runLength < 2) {
return
}
else if (runLength == 2) {
if (ord.gt(data(start), data(start + 1))) {
val t = data(start)
data(start) = data(start + 1)
data(start + 1) = t
}
return
}
else {
val pivot = {
val midIndex = start + (end - start) / 2
val (a, b, c) = (data(start), data(midIndex), data(end - 1))
if (ord.lt(a, b)) {
if (ord.lt(b, c)) b
else ord.max(a, c)
} else {
if (ord.lt(c, b)) b
else ord.min(a, c)
}
}
// Dutch flag version
var low = start
var mid = start
var high = end - 1
while (mid <= high) {
if (ord.lt(data(mid), pivot)) {
val t = data(mid)
data(mid) = data(low)
data(low) = t
low += 1
mid += 1
}
else if (ord.equiv(data(mid), pivot)) {
mid += 1
}
else {
// mid is higher than pivot
val t = data(mid)
data(mid) = data(high)
data(high) = t
high -= 1
}
}
sort(data, start, low)
sort(data, mid, end)
}
}
}
object Main {
def main(args: Array[String]): Unit = {
val a = IndexedSeq((0 until 100000) map (_ => scala.util.Random.nextInt(100000)):_*)
val s = System.currentTimeMillis()
QuicksortInPlace.sort(a)
val e = System.currentTimeMillis()
println(a.toList == a.toList.sorted)
println((e - s) + " ms")
}
}
|
code/sorting/src/quick_sort/quick_sort_median_of_medians.c | #include<stdio.h>
#define SIZE 10000
int a[SIZE], b[SIZE];
int count = 0;
void swap(int *a,int *b)
{
*a = *a + *b;
*b = *a - *b;
*a = *a - *b;
}
int median(int x, int y, int z)
{
if((x > y && x < z) || (x > z && x < y)) return 1;
else if((y > x && y < z) || (y < x && y > z)) return 2;
else return 3;
}
void qsort(int l, int r)
{
if(l >= r)
return;
count += (r - l);
int pvt, i, j, temp;
temp = median(a[l], a[(r + l) / 2], a[r]);
switch(temp)
{
case 1: break;
case 2: swap(&a[l], &a[(r+l)/2]); break;
case 3: swap(&a[l], &a[r]);
}
pvt = a[l];
for(i = l + 1, j = l + 1; j <= r; j++)
if(a[j] < pvt)
{
temp = a[j]; a[j] = a[i]; a[i] = temp; i++;
}
temp = a[l]; a[l] = a[i - 1]; a[i - 1] = temp;
qsort(l, i - 2);
qsort(i, r);
}
int main()
{
int i;
for(i = 0; i < SIZE; i++)
scanf("%d", a + i);
qsort(0, SIZE - 1);
printf("\n%d\n", count);
return 0;
}
|
code/sorting/src/quick_sort/quick_sort_three_way.cpp | /* Part of Cosmos by OpenGenus Foundation */
#include <vector>
#include <stdlib.h>
#include <iostream>
using namespace std;
/* UTILITY FUNCTIONS */
void swap(int &a, int &b)
{
int temp = a;
a = b;
b = temp;
}
void printarr(vector<int> &v)
{
for (size_t i = 0; i < v.size(); ++i)
cout << v[i] << " ";
cout << endl;
}
void fill(vector<int> &v, int max)
{
for (size_t i = 0; i < v.size(); ++i)
v[i] = rand() % max + 1;
}
// three-way-partioning
void partition(vector<int> &v, int low, int high, int &i, int &j)
{
if (high - low <= 1)
{
if (v[high] < v[low])
swap(v[high], v[low]);
i = low;
j = high;
return;
}
int mid = low;
int pivot = v[high];
while (mid <= high)
{
if (v[mid] < pivot)
swap(v[low++], v[mid++]);
else if (v[mid] == pivot)
mid++;
else if (v[mid] > pivot)
swap(v[mid], v[high--]);
}
i = low - 1;
j = mid;
}
void quicksort(vector<int> &v, int low, int high)
{
if (low >= high)
return;
int i, j;
partition(v, low, high, i, j);
// Recursively sort two halves
quicksort(v, low, i);
quicksort(v, j, high);
}
// Driver program
int main()
{
int size = 10;
int maxRand = 10;
cout << "Input test array size: ";
cin >> size;
vector<int> v(size);
cout << "Maximum random number: ";
cin >> maxRand;
fill(v, maxRand);
cout << "Unsorted: ";
printarr(v);
quicksort(v, 0, size - 1);
cout << "Sorted: ";
printarr(v);
return 0;
}
|
code/sorting/src/radix_sort/README.md | # Radix Sort
Radix sort is a **non-comparative integer sorting algorithm**.
It works by doing digit by digit sort starting from least significant digit to most significant digit. Radix sort uses counting sort as a subroutine to sort the digits in each place value. This means that for a three-digit number in base 10, counting sort will be called to sort the 1's place, then it will be called to sort the 10's place, and finally, it will be called to sort the 100's place, resulting in a completely sorted list.
## Explanation
Consider the following example:
Original, unsorted list:
```170, 45, 75, 90, 802, 2, 24, 66```
Sorting by least significant digit (1s place) gives:
```170, 90, 802, 2, 24, 45, 75, 66```
Notice that we keep 802 before 2, because 802 occurred before 2 in the original list, and similarly for pairs 170 & 90 and 45 & 75.
Sorting by next digit (10s place) gives:
```802, 2, 24, 45, 66, 170, 75, 90```
Notice that 802 again comes before 2 as 802 comes before 2 in the previous list.
Sorting by most significant digit (100s place) gives:
```2, 24, 45, 66, 75, 90, 170, 802```
It is important to realize that each of the above steps requires just a single pass over the data, since each item can be placed in its correct bucket without having to be compared with other items.
Some radix sort implementations allocate space for buckets by first counting the number of keys that belong in each bucket before moving keys into those buckets. The number of times that each digit occurs is stored in an array. Consider the previous list of keys viewed in a different way:
```170, 045, 075, 090, 002, 024, 802, 066```
The first counting pass starts on the least significant digit of each key, producing an array of bucket sizes:
```
2 (bucket size for digits of 0: 170, 090)
2 (bucket size for digits of 2: 002, 802)
1 (bucket size for digits of 4: 024)
2 (bucket size for digits of 5: 045, 075)
1 (bucket size for digits of 6: 066)
```
A second counting pass on the next more significant digit of each key will produce an array of bucket sizes:
```
2 (bucket size for digits of 0: 002, 802)
1 (bucket size for digits of 2: 024)
1 (bucket size for digits of 4: 045)
1 (bucket size for digits of 6: 066)
2 (bucket size for digits of 7: 170, 075)
1 (bucket size for digits of 9: 090)
```
A third and final counting pass on the most significant digit of each key will produce an array of bucket sizes:
```
6 (bucket size for digits of 0: 002, 024, 045, 066, 075, 090)
1 (bucket size for digits of 1: 170)
1 (bucket size for digits of 8: 802)
```
At least one LSD radix sort implementation now counts the number of times that each digit occurs in each column for all columns in a single counting pass. Other LSD radix sort implementations allocate space for buckets dynamically as the space is needed.
## Algorithm
```
Radix-Sort (list, n)
shift = 1
for loop = 1 to keysize do
for entry = 1 to n do
bucketnumber = (list[entry].key / shift) mod 10
append (bucket[bucketnumber], list[entry])
list = combinebuckets()
shift = shift * 10
```
## Complexity
**Time complexity**
- Worst, average and best case time complexity: `O(nk)`
Where, **n** is the input numbers with maximum **k** digits.
If the numbers are of finite size, the algorithm runs in `O(n)` asymptotic time.
**Space complexity**: `O(n + k)`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/radix_sort/radix_sort.c | #include <stdio.h>
#include <stdlib.h>
// Part of Cosmos by OpenGenus Foundation
// Initial code by GelaniNijraj
// Debug by adeen-s
int find_max(int* number, int length){
int i, max = number[0];
for(i = 0; i < length; i++)
max = number[i] > max ? number[i] : max;
return max;
}
int* sort(int* number, int length){
int i, j;
int k = 0, n = 1, max, lsd;
int** temp = (int **)malloc(sizeof(int) * length);
int* order;
max = find_max(number, length);
for(i = 0; i < length; i++)
temp[i] = (int *)calloc(length, sizeof(int));
order = (int *)calloc(length, sizeof(int));
while(n <= max){
for(i = 0; i < length; i++){
lsd = (number[i] / n) % 10;
temp[lsd][order[lsd]] = number[i];
order[lsd] += 1;
}
for(i = 0; i < length; i++){
if(order[i] != 0){
for(j = 0; j < order[i]; j++){
number[k] = temp[i][j];
k += 1;
}
}
order[i] = 0;
}
n *= 10;
k = 0;
}
return number;
}
void display(int* number, int length){
int i;
for(i = 0; i < length; i++)
printf("%d ", number[i]);
printf("\n");
}
int main(int argc, char** argv){
int arr[] = {34,3212,51,52,612,456,12,31,412,123,1,3};
int length = 12;
printf("Before : ");
display(arr, length);
sort(arr, length);
printf("After : ");
display(arr, length);
return 0;
}
|
code/sorting/src/radix_sort/radix_sort.cpp | /* Part of Cosmos by OpenGenus Foundation */
#include <iostream>
#include <vector>
#include <cmath>
void radix_sort(std::vector<int> &data)
{
using namespace std;
vector<int> tmp[10]; //store 0~9;
int max_data = *(max(std::begin(data), std::end(data)));
int n = 1;
while (n <= max_data)
{
for (auto v : data)
{
int lsd = (v / n) % 10;
tmp[lsd].emplace_back(v);
}
int k = 0;
for (auto &v: tmp) //vector
{
if (v.size() <= 0)
continue;
for (auto num: v)
data[k++] = num;
v.clear();
}
n *= 10;
}
}
int main()
{
using namespace std;
vector<int> data = {34, 12, 51, 52, 612, 456, 12, 31, 412, 123, 1, 3};
cout << "before sorting" << endl;
for (auto v: data)
cout << v << " ";
cout << endl;
radix_sort(data);
cout << "after sorting" << endl;
for (auto v: data)
cout << v << " ";
cout << endl;
}
|
code/sorting/src/radix_sort/radix_sort.go | /* Part of Cosmos by OpenGenus Foundation */
package main
import "fmt"
const MaxUint = ^uint(0)
const MaxInt = int(MaxUint >> 1)
const MinInt = -MaxInt - 1
func radix_sort(data []int) {
maxNumber := MinInt
for _, v := range data {
if v > maxNumber {
maxNumber = v
}
}
n := 1
bucket := make([][]int, 10)
for n <= maxNumber {
for _, v := range data {
bucket[(v/n)%10] = append(bucket[(v/n)%10], v)
}
n *= 10
k := 0
for i, v := range bucket {
for _, d := range v {
data[k] = d
k++
}
bucket[i] = bucket[i][:0]
}
}
}
func main() {
data := []int{43, 123, 51, 5, 1, 4, 7, 234, 6123, 5123, 11, 23, 5123}
fmt.Println("Before sorting", data)
radix_sort(data)
fmt.Println("Adfter sorting", data)
}
|
code/sorting/src/radix_sort/radix_sort.hs | module Radix where
import Data.List
import Data.Bits
-- Part of Cosmos by OpenGenus Foundation
-- intoBuckets separates the input list into two lists, one where a bit
-- is set, and one where the bit is not set.
intoBuckets' odds evens bit [] = (reverse odds, reverse evens)
intoBuckets' odds evens bit (x:xs)
| x `testBit` bit = intoBuckets' (x:odds) evens bit xs
intoBuckets' odds evens bit (x:xs) = intoBuckets' odds (x:evens) bit xs
intoBuckets :: (Num a, Integral a, Bits a) => Int -> [a] -> ([a], [a])
intoBuckets = intoBuckets' [] []
-- radix' sorts by each binary digit from 0 to max
radix' 0 _ list = list
radix' n max list = radix' (n - 1) max $ lows ++ highs
where
(highs, lows) = intoBuckets (max - n) list
-- radix simply calculates the number of passes required for a full
-- run of radix sort, and then applies radix' that many times.
radix :: (Num a, Ord a, Integral a, Bits a) => [a] -> [a]
radix list = radix' (m) m list
where
m = floor ((log (fromIntegral (foldl max 0 list)) / log 2) + 1)
-- Here's a testcase:
test :: [Integer]
test = [539, 581, 943, 94, 189, 928, 481, 343, 487, 34, 230, 775, 524, 97, 347, 357, 109, 935, 542, 298, 943, 921, 696, 306, 727, 995, 148, 413, 986, 154, 934, 495, 664, 33, 484, 808, 770, 976, 705, 52, 521, 909, 491, 530, 466, 741, 121, 321, 174, 851, 639, 418, 589, 721, 982, 131, 91, 741, 268, 10, 432, 171, 14, 919, 807, 57, 201, 973, 638, 279, 526, 656, 867, 332, 45, 567, 505, 349, 898, 239, 151, 317, 397, 91, 400, 456, 260, 352, 649, 438, 627, 789, 748, 440, 416, 823, 61, 71, 693, 559]
testSorted = [10, 14, 33, 34, 45, 52, 57, 61, 71, 91, 91, 94, 97, 109, 121, 131, 148, 151, 154, 171, 174, 189, 201, 230, 239, 260, 268, 279, 298, 306, 317, 321, 332, 343, 347, 349, 352, 357, 397, 400, 413, 416, 418, 432, 438, 440, 456, 466, 481, 484, 487, 491, 495, 505, 521, 524, 526, 530, 539, 542, 559, 567, 581, 589, 627, 638, 639, 649, 656, 664, 693, 696, 705, 721, 727, 741, 741, 748, 770, 775, 789, 807, 808, 823, 851, 867, 898, 909, 919, 921, 928, 934, 935, 943, 943, 973, 976, 982, 986, 995]
-- A very simple test driver
main = print (radix test == testSorted)
|
code/sorting/src/radix_sort/radix_sort.java | /* Part of Cosmos by OpenGenus Foundation */
import java.util.ArrayList;
import java.util.List;
public class RadixSort
{
public static void main(String[] args)
{
int[] nums = {100, 5, 100, 19, 320000, 0, 67, 542, 10, 222};
radixSort(nums);
printArray(nums);
}
/**
* This method sorts a passed array of positive integers using radix sort
* @param input: the array to be sorted
*/
public static void radixSort(int[] input)
{
List<Integer>[] buckets = new ArrayList[10];
for(int i = 0; i < buckets.length; i++)//initialize buckets
buckets[i] = new ArrayList<Integer>();
int divisor = 1;
String s = Integer.toString(findMax(input));
int count = s.length();//count is the number of digits of the largest number
for(int i = 0; i < count; divisor*=10, i++)
{
for(Integer num : input)
{
assert(num >= 0);
int temp = num / divisor;
buckets[temp % 10].add(num);
}
//Load buckets back into the input array
int j = 0;
for (int k = 0; k < 10; k++)
{
for (Integer x : buckets[k])
{
input[j++] = x;
}
buckets[k].clear();
}
}
}
/**
* This method returns the maximum integer in an integer array.
* @param input: the array
* @return the maximum integer in input
*/
public static int findMax(int[] input)
{
assert(input.length != 0);
int max = input[0];
for(int i = 1; i < input.length; i++)
if(input[i] > max) max = input[i];
return max;
}
/**
* This method prints a passed array
* @param array: the array to be printed
*/
public static void printArray(int[] array)
{
System.out.print("[ ");
for(Integer i : array)
System.out.print(i+" ");
System.out.print("]");
}
}
|
code/sorting/src/radix_sort/radix_sort.js | // Part of Cosmos by OpenGenus Foundation
// Implementation of radix sort in JavaScript
var testArray = [331, 454, 230, 34, 343, 45, 59, 453, 345, 231, 9];
function radixBucketSort(arr) {
var idx1, idx2, idx3, len1, len2, radix, radixKey;
var radices = {},
buckets = {},
num,
curr;
var currLen, radixStr, currBucket;
len1 = arr.length;
len2 = 10; // radix sort uses ten buckets
// find the relevant radices to process for efficiency
for (idx1 = 0; idx1 < len1; idx1++) {
radices[arr[idx1].toString().length] = 0;
}
// loop for each radix. For each radix we put all the items
// in buckets, and then pull them out of the buckets.
for (radix in radices) {
// put each array item in a bucket based on its radix value
len1 = arr.length;
for (idx1 = 0; idx1 < len1; idx1++) {
curr = arr[idx1];
// item length is used to find its current radix value
currLen = curr.toString().length;
// only put the item in a radix bucket if the item
// key is as long as the radix
if (currLen >= radix) {
// radix starts from beginning of key, so need to
// adjust to get redix values from start of stringified key
radixKey = curr.toString()[currLen - radix];
// create the bucket if it does not already exist
if (!buckets.hasOwnProperty(radixKey)) {
buckets[radixKey] = [];
}
// put the array value in the bucket
buckets[radixKey].push(curr);
} else {
if (!buckets.hasOwnProperty("0")) {
buckets["0"] = [];
}
buckets["0"].push(curr);
}
}
// for current radix, items are in buckets, now put them
// back in the array based on their buckets
// this index moves us through the array as we insert items
idx1 = 0;
// go through all the buckets
for (idx2 = 0; idx2 < len2; idx2++) {
// only process buckets with items
if (buckets[idx2] != null) {
currBucket = buckets[idx2];
// insert all bucket items into array
len1 = currBucket.length;
for (idx3 = 0; idx3 < len1; idx3++) {
arr[idx1++] = currBucket[idx3];
}
}
}
buckets = {};
}
}
radixBucketSort(testArray);
console.dir(testArray);
|
code/sorting/src/radix_sort/radix_sort.py | # Part of Cosmos by OpenGenus Foundation
def radix_sort(number, maxNumber):
length = len(number)
k = 0
n = 1
temp = []
for i in range(length):
temp.append([0] * length)
order = [0] * length
while n <= maxNumber:
for i in range(length):
lsd = (number[i] // n) % 10
temp[lsd][order[lsd]] = number[i]
order[lsd] += 1
for i in range(length):
if order[i] != 0:
for j in range(order[i]):
number[k] = temp[i][j]
k += 1
order[i] = 0
n *= 10
k = 0
number = [34, 3212, 51, 52, 612, 456, 12, 31, 412, 123, 1, 3]
print("before radix_sorting", number)
radix_sort(number, max(number))
print("after radix_sorting", number)
|
code/sorting/src/radix_sort/radix_sort.rs | /* Part of Cosmos by OpenGenus Foundation */
fn main() {
let mut arr: Vec<u32> = vec![34, 3212, 51, 52, 612, 456, 12, 31, 412, 123, 1, 3];
println!("Unsorted: {:?}", arr);
radix_sort(&mut arr);
println!("Sorted: {:?}", arr);
}
fn radix_sort(input: &mut [u32]) {
if input.len() == 0 {
return;
}
// initialize buckets
let mut buckets: Vec<Vec<u32>> = Vec::with_capacity(10);
for _ in 0..10 {
buckets.push(Vec::new());
}
// count how many digits the longest number has
// unwrap is safe here because we return in case of an empty input
let count: usize = input.iter()
.max()
.unwrap()
.to_string()
.len();
let mut divisor: u32 = 1;
for _ in 0..count {
for num in input.iter() {
let temp = (num / divisor) as usize;
buckets[temp % 10].push(*num);
}
let mut j: usize = 0;
for k in 0..10 {
for x in buckets[k].iter() {
input[j] = *x;
j += 1;
}
buckets[k].clear();
}
divisor *= 10;
}
}
|
code/sorting/src/radix_sort/radix_sort.sh | #!/bin/bash
read -p "Enter the array size you want: " size
i=0
declare -a arrayname
while [ $i -lt $size ]
do
read -p "Enter the $(($i+1)) element of array: " arrayname[i]
i=$[$i+1]
done
echo "Input array is: ${arrayname[@]}"
max=0
for el in ${arrayname[@]}
do
if [ $el -gt $max ]
then
max=$el
fi
done
echo "Max element is found!!!: ${max}"
declare -a oarr #output array
declare -a carr #count array
#note that -ge is for greater than or equal to and gt is only for greater than
exp=1
z=1
while [ $(($max/$exp)) -gt 0 ]
do
#echo "Iteration number ${z} with exp value ${exp}"
#echo "Initializing all the elements of count array to 0"
i=0
#assuming d=10 number of digits max
while [ $i -lt 10 ]
do
carr[i]=0
i=$[$i+1]
done
#echo "printing the count array after initialization"
#echo "${carr[@]}"
i=0
while [ $i -lt $size ]
do
k=$(((${arrayname[i]}/$exp)%10))
carr[k]=$[${carr[k]}+1]
i=$[$i+1]
done
i=1
while [ $i -lt 10 ]
do
k=$[$i-1]
carr[i]=$((${carr[i]}+${carr[k]}))
i=$[$i+1]
done
i=$[$size-1]
while [ $i -ge 0 ]
do
k=$(((${arrayname[i]}/$exp)%10))
oarr[$((${carr[k]}-1))]=${arrayname[i]}
carr[k]=$((${carr[k]}-1))
i=$[$i-1]
done
#echo "Printing the sorted array for current bit iteration"
#echo ${oarr[@]}
for (( i=0; i<$size; i++ )); do
arrayname[i]=${oarr[i]}
done
z=$[$z+1]
exp=$[$exp*10]
done
echo "Printing final sorted array"
echo ${arrayname[@]}
|
code/sorting/src/selection_sort/README.md | # Selection Sort
Selection sort is a simple sorting algorithm. It is an in-place comparison-based algorithm in which the list is divided into two parts, the sorted part at the left end and the unsorted part at the right end. Initially, the sorted part is empty and the unsorted part is the entire list.
The smallest element is selected from the unsorted array and swapped with the leftmost element, and that element becomes a part of the sorted array. This process continues moving unsorted array boundary by one element to the right.
This algorithm is not suitable for large data sets as its average and worst case complexity is **Ο(n * n)**, where **n** is the number of items.
## Explanation
Consider the following example:
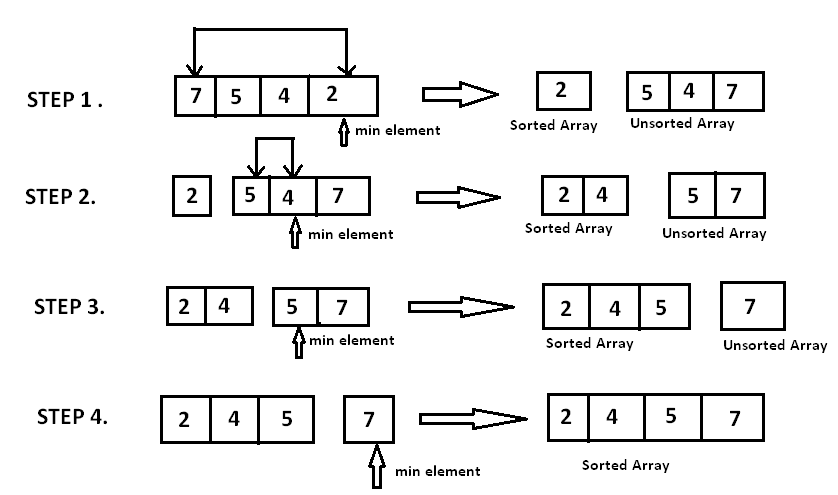
> Image credits: Hackerearth
Input: `[7 5 4 2]`
Output: `[2 4 5 7]`
## Pseudocode
```
SelectionSort(A):
for j ← 1 to n-1
smallest ← j
for i ← j + 1 to n
if A[i] < A[smallest]
smallest ← i
Swap A[j] ↔ A[smallest]
```
## Complexity
**Time complexity**
- Worst case: **O(n<sup>2</sup>)**
- Average case: **θ(n<sup>2</sup>)**
- Best case: **Ω(n<sup>2</sup>)**
**Space complexity**: **O(1)** auxillary
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/selection_sort/SELCTION_SORT.ASM | ; Author: SYEED MOHD AMEEN
; Email: [email protected]
;---------------------------------------------------------------;
; SELECTION SORT SUBROUTINE ;
;---------------------------------------------------------------;
;----------------------------------------------------------------;
; FUNCTION PARAMETERS ;
;----------------------------------------------------------------;
; 1. push number of element in array ;
; 2. push base address of Array ;
;----------------------------------------------------------------;
SELSORT:
POP AX ;POP RET ADDRESS OF SUBROUTINE
POP SI ;BASE ADDRESS OF ARRAY
POP CX ;COUNTER REGISTER
PUSH AX ;PUSH RET ADDRESS OF SUBROUTINE
COUNTER_SELSORT: EQU 0X4500
DPTR_SELSORT: EQU 0X4510
MOV ES:[COUNTER_SELSORT],CX
MOV ES:[COUNTER_SELSORT+2],CX
MOV ES:[DPTR_SELSORT],SI
XOR BX,BX ;CLEAR INDEX REGISTER
REPEAT2_SELSORT:
MOV AH,[SI+BX] ;MOVE INITIAL ELEMENT AND COMPARE IN ENTIRE ARRAY
REPEAT1_SELSORT:
CMP AH,[SI+BX+1]
JC NOSWAP_SELSORT
XCHG AH,[SI+BX+1]
NOSWAP_SELSORT:
INC SI ;INCREMENT INDEX REGISTER
LOOP REPEAT1_SELSORT ;REPEAT UNTIL COUNTER != 0
MOV SI,ES:[DPTR_SELSORT] ;MOVE BASE ADDRESS OF ARRAY
DEC ES:[COUNTER_SELSORT+2]
MOV CX,ES:[COUNTER_SELSORT+2] ;MOVE COUNTER INTO CX REG.
INC BX ;INCREMENT INDEX REGISTER
CMP BX,ES:[COUNTER_SELSORT]
JNE SKIP_SELSORT ;IF BX == ES:[COUNTER_SELSORT] RET SUBROUTINE
RET ;RETURN SUBROUTINE
SKIP_SELSORT:
JMP REPEAT2_SELSORT
|
code/sorting/src/selection_sort/selection_sort.c | /* Part of Cosmos by OpenGenus Foundation */
#include <stdio.h>
typedef int bool;
/* Function to swap 2 array elements */
void
swap(int *p, int *q)
{
int temp = *p;
*p = *q;
*q = temp;
}
/* Function to sort an array using Selection sort */
void
selectionSort(int arr[], int n, bool order)
{
int idx;
/* Order 1 for AScending sort */
if (order == 1) {
for (int i = 0; i < n - 1; i++) {
/* Find smallest element in the unsorted subarray */
idx = i;
for (int j = i + 1; j < n; j++) {
if (arr[idx] > arr[j]) {
idx = j;
}
}
/* Move minimum element from unsorted subarray to sorted subarray */
if (idx != i) {
swap(&arr[i], &arr[idx]);
}
}
}
/* Order 0 for Descending sort */
else if (order == 0) {
for (int i = 0; i < n - 1; i++) {
/* Find largest element in the unsorted subarray */
idx = i;
for (int j = i + 1; j < n; j++) {
if (arr[idx] < arr[j]) {
idx = j;
}
}
/* Move maximum element from unsorted subarray to sorted subarray */
if (idx != i) {
swap(&arr[i], &arr[idx]);
}
}
}
/* If order not 0 or 1, sorting order undefined */
else {
printf("Undefined sorting order");
}
}
/* Function to print array */
void
printArray(int arr[], int n)
{
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int
main()
{
int n;
/* Input number of elements */
printf("Enter the number of Elements: \n");
scanf("%d",&n);
int arr[n];
/* Input array elements */
printf("Enter the array elements: \n");
for (int i = 0; i < n; i++) {
scanf("%d",&arr[i]);
}
bool order;
/* Input sorting order */
printf("Enter sorting order: (1: Ascending; 0: Descending): \n");
scanf("%d", &order);
/* If user inputs anything except 0 or 1 for order */
if (order != 1 && order != 0) {
printf("Undefined sorting order.\n");
return 1;
}
/* Sort array */
selectionSort(arr, n, order);
/*Print sorted array */
printf("Sorted array:\n");
printArray(arr, n);
return (0);
}
|
code/sorting/src/selection_sort/selection_sort.cpp | /*
* Part of Cosmos by OpenGenus Foundation
*
* selection sort synopsis
*
* template<typename _Input_Iter, typename _Compare>
* void
* selectionSort(_Input_Iter begin, _Input_Iter end, _Compare compare);
*
* template<typename _Input_Iter>
* void
* selectionSort(_Input_Iter begin, _Input_Iter end);
*/
#include <functional>
template<typename _Input_Iter, typename _Compare>
void
selectionSort(_Input_Iter begin, _Input_Iter end, _Compare compare)
{
if (begin != end)
for (auto curr = begin; curr != end; ++curr)
{
auto minimum = curr;
auto forward = curr;
while (++forward != end)
if (compare(*forward, *minimum))
minimum = forward;
std::iter_swap(minimum, curr);
}
}
template<typename _Input_Iter>
void
selectionSort(_Input_Iter begin, _Input_Iter end)
{
using value_type = typename std::iterator_traits<_Input_Iter>::value_type;
selectionSort(begin, end, std::less<value_type>());
}
|
code/sorting/src/selection_sort/selection_sort.cs | // Part of Cosmos by OpenGenus Foundation
public class SelectionSort
{
public static void Main(string[] args)
{
int[] items = new int[] {52, 62, 143, 73, 22, 26, 27, 14, 62, 84, 15 };
Sort(items);
System.Console.WriteLine("Sorted: ");
for (int i = 0; i < items.Length; i++)
System.Console.Write(items[i] + " ");
}
private static void Sort(int[] items)
{
for (int i = 0; i < items.Length - 1; i++)
{
int minIndex = i;
for (int j = i + 1; j < items.Length; j++)
if (items[j] < items[minIndex])
minIndex = j;
int temp = items[minIndex];
items[minIndex] = items[i];
items[i] = temp;
}
}
}
|
code/sorting/src/selection_sort/selection_sort.go | package main
// Selection Sort in Golang
// Part of Cosmos by OpenGenus Foundation
import "fmt"
func selectionSort(array [8]int) [8]int {
for i := 0; i < len(array); i++ {
min := i
for j := i + 1; j < len(array); j++ {
if array[j] < array[min] {
min = j
}
}
array[i], array[min] = array[min], array[i]
}
return array
}
func main() {
array := [8]int{5, 6, 1, 2, 7, 9, 8, 4}
sorted := selectionSort(array)
fmt.Printf("%v\n", sorted)
}
|
code/sorting/src/selection_sort/selection_sort.hs | -- Part of Cosmos by OpenGenus Foundation
selectionsort :: (Ord a) => [a] -> [a]
selectionsort [] = []
selectionsort l = let Just (m, i) = minIndex l in
m:selectionsort (take i l ++ drop (i+1) l)
minIndex :: (Ord a) => [a] -> Maybe (a, Int)
minIndex [] = Nothing
minIndex (x:xs) = case minIndex xs of
Nothing -> Just (x, 0)
Just (m, i) -> Just (if x < m then (x, 0) else (m, i+1))
|
code/sorting/src/selection_sort/selection_sort.java | /**
* Utility class for sorting an array using Selection Sort algorithm. Selection
* Sort is a basic algorithm for sorting with O(n^2) time complexity. Basic idea
* of this algorithm is to find a local minimum, which is the minimum value from
* (i+1) to length of the array [i+1, arr.length), and swap it with the current
* working index (i).
*
* Part of Cosmos by OpenGenus Foundation
*/
class SelectionSort {
/**
* Example usage.
*/
public static void main(String[] args) {
int[] arr = { 1, 5, 2, 5, 2, 9, 7 };
SelectionSort.sort(arr);
System.out.print(java.util.Arrays.toString(arr));
}
/**
* Sort an array using Selection Sort algorithm.
*
* @param arr
* is an array to be sorted
*/
public static void sort(int[] arr) {
for (int i = 0; i < arr.length; i++) {
int min = i;
for (int j = i + 1; j < arr.length; j++) {
/* find local min */
if (arr[j] < arr[min]) {
min = j;
}
}
swap(arr, i, min);
}
}
/**
* Utility method for swapping elements in an array.
*
* @param arr
* is an array to be swapped
* @param i
* is index of first element
* @param j
* is index of second element
*/
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
|
code/sorting/src/selection_sort/selection_sort.js | // Part of Cosmos by OpenGenus Foundation
function selectionSort(inputArray) {
var len = inputArray.length;
for (var i = 0; i < len; i++) {
var minAt = i;
for (var j = i + 1; j < len; j++) {
if (inputArray[j] < inputArray[minAt]) minAt = j;
}
if (minAt != i) {
var temp = inputArray[i];
inputArray[i] = inputArray[minAt];
inputArray[minAt] = temp;
}
}
return inputArray;
}
|
code/sorting/src/selection_sort/selection_sort.kt | // Part of Cosmos by OpenGenus Foundation
import java.util.*
fun <T : Comparable<T>> sort(array: Array<T>) {
for (i in array.indices) {
var min = i
for (j in i + 1 until array.size) {
/* find local min */
if (array[j] < array[min]) {
min = j
}
}
val temp = array[i]
array[i] = array[min]
array[min] = temp
}
}
fun main(args: Array<String>) {
val arr = arrayOf(1, 5, 2, 5, 2, 9, 7)
sort(arr)
print(Arrays.toString(arr))
} |
code/sorting/src/selection_sort/selection_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// selection_sort.m
// Created by DaiPei on 2017/10/9.
//
#import <Foundation/Foundation.h>
@interface SelectionSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation SelectionSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
for (int i = 0; i + 1 < array.count; i++) {
int p = i;
for (int j = i + 1; j < array.count; j++) {
if ([array[j] compare:array[p]] == NSOrderedAscending) {
p = j;
}
}
[self swap:array at:i and:p];
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
int n = 0;
NSLog(@"What is the size of the array?");
scanf("%d", &n);
NSMutableArray *array = [NSMutableArray arrayWithCapacity:n];
NSLog(@"Enter elements of the array one by one:");
for (int i = 0; i < n; i++) {
int tmp;
scanf("%d", &tmp);
[array addObject:@(tmp)];
}
SelectionSort *ss = [[SelectionSort alloc] init];
[ss sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/selection_sort/selection_sort.php | <?php
// Part of Cosmos by OpenGenus Foundation
function selection_sort($arr) {
$n = count($arr);
foreach ($arr as $k => $el) {
$min = $k;
for ($j = $k + 1; $j < $n; $j++) {
if ($arr[$j] < $arr[$min]) {
$min = $j;
}
}
$hold = $el;
$el = $arr[$min];
$arr[$min] = $hold;
}
return $arr;
}
$test = [1, 3, 43, 2, 14, 53, 12, 87, 33];
print_r($test);
$sorted = selection_sort($test);
print_r($sorted);
|
code/sorting/src/selection_sort/selection_sort.py | # Part of Cosmos by OpenGenus Foundation
def selection_sort(array):
for i in range(len(array) - 1):
minimumValue = i
for j in range(i + 1, len(array)):
if array[j] < array[minimumValue]:
minimumValue = j
temp = array[minimumValue]
array[minimumValue] = array[i]
array[i] = temp
return array
|
code/sorting/src/selection_sort/selection_sort.rb | # Part of Cosmos by OpenGenus Foundation
def SelectionSort(arr)
n = arr.length - 1
i = 0
while i <= n - 1
smallest = i
j = i + 1
while j <= n
smallest = j if arr[j] < arr[smallest]
j += 1
end
arr[i], arr[smallest] = arr[smallest], arr[i] if i != smallest
i += 1
end
end
arr = [95, 39.5, 35, 75, 85, 69].shuffle
SelectionSort(arr)
puts "After Sorting from Selection Sort.Sorted array is: #{arr.inspect}"
|
code/sorting/src/selection_sort/selection_sort.rs | // Part of Cosmos by OpenGenus Foundation
fn selection_sort(mut arr :Vec<i32>) -> Vec<i32> {
let len = arr.len();
for i in 0..len-1 {
let mut min = i;
for j in i+1..len {
if arr[j] < arr[min] {
min = j;
}
}
if min != i{
arr.swap(i, min);
}
}
arr
}
fn main() {
let arr = vec![1, 3, 43, 2, 14, 53, 12, 87, 33];
let arr = selection_sort(arr);
println!("Sorted array: {:?}", arr);
} |
code/sorting/src/selection_sort/selection_sort.sh | #!/bin/bash
# Part of Cosmos by OpenGenus Foundation
declare -a array
ARRAYSZ=10
create_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
array[${i}]=${RANDOM}
i=$((i+1))
done
}
print_array() {
i=0
while [ $i -lt $ARRAYSZ ]; do
echo ${array[${i}]}
i=$((i+1))
done
}
verify_sort() {
i=1
while [ $i -lt $ARRAYSZ ]; do
if [ ${array[$i]} -lt ${array[$((i-1))]} ]; then
echo "Array did not sort, see elements $((i-1)) and $i."
exit 1
fi
i=$((i+1))
done
echo "Array sorted correctly."
}
selection_sort() {
i=0
while [ $i -lt $((ARRAYSZ-1)) ]; do
minIdx=$i
for j in $( seq $((i+1)) $((ARRAYSZ-1)) ); do
if [ ${array[$minIdx]} -gt ${array[$j]} ]; then
minIdx=$j
fi
done
if [ $minIdx -ne $i ]; then
tmp=${array[$minIdx]}
array[$minIdx]=${array[$i]}
array[$i]=$tmp
fi
i=$((i+1))
done
}
create_array
print_array
selection_sort
echo
print_array
verify_sort
|
code/sorting/src/selection_sort/selection_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// selection_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func selectionSort(_ array: inout [Int]) {
for i in stride(from: 0, to: array.count - 1, by: 1) {
var min = i
for j in i+1..<array.count {
if array[j] < array[min] {
min = j
}
}
swap(&array, at: min, and: i)
}
}
private func swap(_ array: inout [Int], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
|
code/sorting/src/selection_sort/selection_sort.vb | Module selection_sort
' Part of Cosmos by OpenGenus Foundation
Sub Main()
' Example array
Dim arr() As Integer = {1, 5, 2, 5, 2, 9, 7}
selection_sort.Sort(arr)
' Print the array
For i = 0 To arr.Length - 1
Console.Write(arr(i))
If i < arr.Length - 1 Then
Console.Write(", ")
End If
Next
Console.ReadKey()
End Sub
Sub Sort(arr() As Integer)
For i = 0 To arr.Length - 1
Dim min As Integer = i
For j = i + 1 To arr.Length - 1
' Find local min
If arr(j) < arr(min) Then
min = j
End If
Next
selection_sort.Swap(arr, i, min)
Next
End Sub
' A basic swap method
Sub Swap(arr() As Integer, j As Integer, i As Integer)
Dim temp As Integer = arr(i)
arr(i) = arr(j)
arr(j) = temp
End Sub
End Module
|
code/sorting/src/selection_sort/selection_sort_extension.swift | /* Part of Cosmos by OpenGenus Foundation */
import Foundation
private func swap<T>(_ array: inout [T], at indexA: Int, and indexB: Int) {
let tmp = array[indexA]
array[indexA] = array[indexB]
array[indexB] = tmp
}
extension Array {
mutating func selectionSort(compareWith less: (Element, Element) -> Bool) {
for i in 0..<self.count {
var min = i
for j in i + 1..<self.count {
if less(self[j], self[min]) {
min = j
}
}
swap(&self, at: min, and: i)
}
}
}
|
code/sorting/src/shaker_sort/README.md | # Shaker Sort
Shakersort or cocktail sort is a _**bidirectional**_ variation of bubble sort.
In shaker sort, n elements are sorted in `n/2` phases. Each phase of shaker sort consists of a left to right bubbling pass followed by a right to left bubbling pass. In a bubbling pass pairs of adjacent elements are compared and swapped if they are out of order.
This algorithm differs from bubble sort in that it works in both directions.
In the first, the lightest element ascends to the end of the list and in the second heaviest element descends to the beginning of the list (or vice versa).
## Explanation
Example : Let us consider an example array: **(5 1 4 2 8 0 2)**
First Forward Pass:
```
(5 1 4 2 8 0 2) ? (1 5 4 2 8 0 2), Swap since 5 > 1
(1 5 4 2 8 0 2) ? (1 4 5 2 8 0 2), Swap since 5 > 4
(1 4 5 2 8 0 2) ? (1 4 2 5 8 0 2), Swap since 5 > 2
(1 4 2 5 8 0 2) ? (1 4 2 5 8 0 2)
(1 4 2 5 8 0 2) ? (1 4 2 5 0 8 2), Swap since 8 > 0
(1 4 2 5 0 8 2) ? (1 4 2 5 0 2 8), Swap since 8 > 2
```
After first forward pass, greatest element of the array will be present at the last index of array.
First Backward Pass:
```
(1 4 2 5 0 2 8) ? (1 4 2 5 0 2 8)
(1 4 2 5 0 2 8) ? (1 4 2 0 5 2 8), Swap since 5 > 0
(1 4 2 0 5 2 8) ? (1 4 0 2 5 2 8), Swap since 2 > 0
(1 4 0 2 5 2 8) ? (1 0 4 2 5 2 8), Swap since 4 > 0
(1 0 4 2 5 2 8) ? (0 1 4 2 5 2 8), Swap since 1 > 0
```
After first backward pass, smallest element of the array will be present at the first index of the array.
Second Forward Pass:
```(0 1 4 2 5 2 8) ? (0 1 4 2 5 2 8)
(0 1 4 2 5 2 8) ? (0 1 2 4 5 2 8), Swap since 4 > 2
(0 1 2 4 5 2 8) ? (0 1 2 4 5 2 8)
(0 1 2 4 5 2 8) ? (0 1 2 4 2 5 8), Swap since 5 > 2
```
Second Backward Pass:
```(0 1 2 4 2 5 8) ? (0 1 2 2 4 5 8), Swap since 4 > 2```
Now, the array is already sorted, but our algorithm doesn’t know if it is completed. The algorithm needs to complete this whole pass without any swap to know it is sorted.
```
(0 1 2 2 4 5 8) ? (0 1 2 2 4 5 8)
(0 1 2 2 4 5 8) ? (0 1 2 2 4 5 8)
```
## Algorithm
```
procedure cocktailShakerSort( A : list of sortable items ) defined as:
do
swapped := false
for each i in 0 to length( A ) - 2 do:
if A[ i ] > A[ i + 1 ] then // test whether the two elements are in the wrong order
swap( A[ i ], A[ i + 1 ] ) // let the two elements change places
swapped := true
end if
end for
if not swapped then
// we can exit the outer loop here if no swaps occurred.
break do-while loop
end if
swapped := false
for each i in length( A ) - 2 to 0 do:
if A[ i ] > A[ i + 1 ] then
swap( A[ i ], A[ i + 1 ] )
swapped := true
end if
end for
while swapped // if no elements have been swapped, then the list is sorted
end procedure
```
## Complexity
**Time complexity**
- Worst Case: **O(n<sup>2</sup>)**
- Average Case: **O(n<sup>2</sup>)**
- Best Case: **O(n)**
**Space complexity**: **O(n)** auxillary
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/sorting/src/shaker_sort/shaker_sort.c | /* Part of Cosmos by OpenGenus Foundation */
/*Added by arpank10 */
#include <stdio.h>
#include <stdbool.h>
/*
Cocktail Shaker Sort implementation in C
*/
void shaker_sort(int a[],int n)
{
int i=0,j;
bool swapped=false;
for(i=0;i<n/2;i++)
{
/*
* Indices of the inner loops result from an
* invariant of the algorithm. In 'i'th execution
* of the outer loop, it is guaranteed that the
* leftmost and rightmost i elements will be sorted.
* Hence, inner loops only check the others for
* inversions. That is why, n/2 iterations of the
* outer loop suffices to sort the array. After that
* many iterations, left and right halves are sorted.
*/
/* Traverse from left to right */
for(j=i;j<n-1-i;j++)
{
if(a[j+1]<a[j])
{
/* Inversion found - swap elements */
int temp=a[j];
a[j]=a[j+1];
a[j+1]=temp;
swapped=true;
}
}
/* Traverse from right to left - hence, bidirectional */
for(j=n-i-2;j>i;j--)
{
if(a[j-1]>a[j])
{
/* Inversion found - swap elements */
int temp=a[j];
a[j]=a[j-1];
a[j-1]=temp;
swapped=true;
}
}
/*If no elements are swapped in the iteration array is sorted */
if(!swapped)
break;
}
}
int main()
{
int n;
printf("Enter size of array:\n");
scanf("%d",&n);
printf("Enter the array elements:\n");
int a[n];
int i;
for(i=0;i<n;i++)
scanf("%d",&a[i]);
shaker_sort(a,n);
printf("Sorted Array:\n");
for(i=0;i<n;i++)
printf("%d ",a[i]);
printf("\n");
return 0;
}
|
code/sorting/src/shaker_sort/shaker_sort.cpp | /* Part of Cosmos by OpenGenus Foundation */
/*
* This is coctail shaker sort (bidirectional buble sort)
*/
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void shaker_sort(vector<int> &v)
{
bool swap = false;
for (unsigned i = 0; i < v.size() / 2; ++i)
{
for (unsigned j = i; j < v.size() - i - 1; ++j)
if (v[j] > v[j + 1])
{
int tmp = v[j];
v[j] = v[j + 1];
v[j + 1] = tmp;
swap = true;
}
for (unsigned j = v.size() - 2 - i; j > i; j--)
if (v[j] < v[j - 1])
{
int tmp = v[j];
v[j] = v[j - 1];
v[j - 1] = tmp;
swap = true;
}
if (!swap)
break; // The array is sorted when
// there are no elements have been swapped.
}
}
int main()
{
int size = 0;
cout << "Enter size of array: ";
cin >> size;
vector<int> source_array;
for (int i = 0; i < size; ++i)
source_array.push_back(rand() % 100 + 1);
cout << "Source array is: ";
for (int j : source_array)
cout << j << " ";
cout << endl;
shaker_sort(source_array);
cout << "Sorted array is: ";
for (int j : source_array)
cout << j << " ";
cout << endl;
return 0;
}
|
code/sorting/src/shaker_sort/shaker_sort.cs | using System;
using System.Linq;
/* Part of Cosmos by OpenGenus Foundation */
namespace OpenGenus
{
class Program
{
static void Main(string[] args)
{
var random = new Random();
var unsorted = Enumerable.Range(1, 20).Select(x => random.Next(1,40)).ToArray();
Console.WriteLine("Unsorted");
Console.WriteLine(string.Join(",", unsorted));
Console.WriteLine("\nSorted");
var sorted = ShakerSort(unsorted);
Console.WriteLine(string.Join(",", sorted));
Console.ReadLine();
}
private static int[] ShakerSort(int[] array)
{
var swap = false;
int tempSwap;
var arrayLen = array.Length;
for (var i = 0; i < arrayLen / 2; ++i)
{
// pass to right pushing the largest value along
for (var j = i; j < arrayLen - i - 1; ++j)
{
if (array[j] > array[j + 1])
{
tempSwap = array[j];
array[j] = array[j + 1];
array[j + 1] = tempSwap;
swap = true;
}
}
// pass back to left pushing the smallest down
for (var j = arrayLen - i - 2; j > i; j--)
{
if (array[j] < array[j - 1])
{
tempSwap = array[j];
array[j] = array[j - 1];
array[j - 1] = tempSwap;
swap = true;
}
}
// if nothing was swapped, then the array is sorted
if (!swap)
break;
}
return array;
}
}
}
|
code/sorting/src/shaker_sort/shaker_sort.go | // Part of Cosmos by OpenGenus Foundation
package main
import "fmt"
func shakerSort(sortMe []int) {
beginningIdx := 0
endIdx := len(sortMe) - 1
for beginningIdx <= endIdx {
newBeginningIdx := endIdx
newEndIdx := beginningIdx
swap := false
for i := beginningIdx; i < endIdx; i++ {
if sortMe[i] > sortMe[i+1] {
sortMe[i], sortMe[i+1] = sortMe[i+1], sortMe[i]
swap = true
newEndIdx = i
}
}
if !swap {
break
}
endIdx = newEndIdx
swap = false
for i := endIdx; i >= beginningIdx; i-- {
if sortMe[i] > sortMe[i+1] {
sortMe[i], sortMe[i+1] = sortMe[i+1], sortMe[i]
swap = true
newBeginningIdx = i
}
}
if !swap {
break
}
beginningIdx = newBeginningIdx
}
}
func main() {
sortMe := [10]int{6, 4, 9, 5, 3, 10, 8, 1, 7, 2}
fmt.Println(sortMe)
shakerSort(sortMe[0:10])
fmt.Println(sortMe)
}
|
code/sorting/src/shaker_sort/shaker_sort.java | /* Part of Cosmos by OpenGenus Foundation */
public class ShakerSort {
static void sort(int[] arr) {
boolean swapped;
int llim = 0;
int rlim = arr.length - 1;
int curr = llim;
int tmp;
while (llim <= rlim) {
swapped = false;
while (curr + 1 <= rlim) {
if (arr[curr] > arr[curr + 1]) {
tmp = arr[curr];
arr[curr] = arr[curr + 1];
arr[curr + 1] = tmp;
swapped = true;
}
curr += 1;
}
if (!swapped){
return;
}
rlim -= 1;
curr = rlim;
swapped = false;
while (curr - 1 >= llim) {
if (arr[curr] < arr[curr - 1]) {
tmp = arr[curr];
arr[curr] = arr[curr - 1];
arr[curr - 1] = tmp;
swapped = true;
}
curr -= 1;
}
if (!swapped){
return;
}
llim += 1;
curr = llim;
}
}
public static void main(String[] args) {
int[][] testCases = {
{},
{1},
{1, 2},
{2, 1},
{1, 2, 3},
{3, 1, 2},
{4, 3, 2, 1},
{4, 5, 2, 1, 3},
{2, 2, 3, 3, 1, 3},
};
for (int[] arr : testCases) {
sort(arr);
for (int a : arr)
System.out.print(a + " ");
System.out.println();
}
}
}
|
code/sorting/src/shaker_sort/shaker_sort.js | // Part of Cosmos by OpenGenus Foundation
// Sort a list using shaker sort (bidirectional bubble sort)
function shaker_sort(list) {
let swapped = false;
let length = list.length;
do {
// Testing from head to tail of list
for (let i = 0; i < length - 1; i++) {
if (list[i] > list[i + 1]) {
let aux = list[i];
list[i] = list[i + 1];
list[i + 1] = aux;
swapped = true;
}
}
if (swapped === false) break;
swapped = false;
// Testing from tail to head of list
for (let i = length - 2; i >= 0; i--) {
if (list[i] > list[i + 1]) {
let aux = list[i];
list[i] = list[i + 1];
list[i + 1] = aux;
swapped = true;
}
}
} while (swapped);
return list;
}
|
code/sorting/src/shaker_sort/shaker_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// shaker_sort.m
// Created by DaiPei on 2017/10/9.
//
#import <Foundation/Foundation.h>
@interface ShakerSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation ShakerSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
BOOL swapped = NO;
for (int i = 0; i < array.count / 2; i++) {
for (int j = i; j + 1 < array.count - i; j++) {
if ([array[j] compare:array[j + 1]] == NSOrderedDescending) {
[self swap:array at:j and:j + 1];
swapped = YES;
}
}
for (int j = (int)array.count - i - 2; j > i; j--) {
if ([array[j] compare:array[j - 1]] == NSOrderedAscending) {
[self swap:array at:j and:j - 1];
swapped = YES;
}
}
if (!swapped) {
break;
}
}
}
- (void)swap:(NSMutableArray<NSNumber *> *)array at:(NSUInteger)indexA and:(NSUInteger)indexB {
NSNumber *tmp = array[indexA];
array[indexA] = array[indexB];
array[indexB] = tmp;
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
int n = 0;
NSLog(@"What is the size of the array?");
scanf("%d", &n);
NSMutableArray *array = [NSMutableArray arrayWithCapacity:n];
NSLog(@"Enter elements of the array one by one:");
for (int i = 0; i < n; i++) {
int tmp;
scanf("%d", &tmp);
[array addObject:@(tmp)];
}
ShakerSort *ss = [[ShakerSort alloc] init];
[ss sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/shaker_sort/shaker_sort.php | <?php
/* Part of Cosmos by OpenGenus Foundation */
namespace OpenGenus;
trait ShakerSortTrait {
/**
* @param array $list
* @return array
*/
public function sort(array $list) : array
{
do {
$swapped = false;
$length = count($list);
for ($i = 0; $i < $length - 2; $i++) {
if ($list[$i] > $list[$i + 1]) {
$list = $this->swap($list, $i, $i + 1);
$swapped = true;
}
}
if (false === $swapped) {
break;
}
$swapped = false;
for ($i = $length - 2; $i > 0; $i--) {
if ($list[$i] > $list[$i + 1]) {
$list = $this->swap($list, $i, $i + 1);
$swapped = true;
}
}
} while(true === $swapped);
return $list;
}
/**
* @param array $input
* @param int $fromIndex
* @param int $toIndex
* @return array
*/
private function swap(array $input, int $fromIndex, int $toIndex) : array
{
$swapFrom = $input[$fromIndex];
$input[$fromIndex] = $input[$toIndex];
$input[$toIndex] = $swapFrom;
return $input;
}
} |
code/sorting/src/shaker_sort/shaker_sort.py | # Part of Cosmos by OpenGenus Foundation
import random
def shaker_sort(sort_list):
beginning_idx = 0
end_idx = len(sort_list) - 1
while beginning_idx <= end_idx:
swap = False
for i in range(beginning_idx, end_idx):
if sort_list[i] > sort_list[i + 1]:
sort_list[i], sort_list[i + 1] = sort_list[i + 1], sort_list[i]
swap = True
end_idx = i
if not swap:
break
swap = False
for i in reversed(range(beginning_idx, end_idx)):
if sort_list[i] > sort_list[i + 1]:
sort_list[i], sort_list[i + 1] = sort_list[i + 1], sort_list[i]
swap = True
beginning_idx = i
if not swap:
break
return sort_list
if __name__ == "__main__":
count = 20
source_list = []
random.seed()
for j in range(0, count):
source_list.append(random.randint(0, 100))
print("Input:", source_list)
shaker_sort(source_list)
print("Output:", source_list)
|
code/sorting/src/shaker_sort/shaker_sort.rs | /* Part of Cosmos by OpenGenus Foundation */
/* Added by Ondama */
/** Read a line of input **/
fn read () -> String {
let mut input = String::new();
std::io::stdin().read_line(&mut input).expect("failed to read from stdin");
input.trim().to_owned()
}
/** Display a message and parse input **/
fn prompt<T: std::str::FromStr + std::default::Default> (prompt: &str) -> T {
println!("{}", prompt);
let num = read();
match num.parse() {
Ok(i) => i,
Err(..) => {
eprintln!("'{}' is not valid, using default instead.", num);
T::default()
}
}
}
/** Sort array **/
fn shaker_sort<T: std::cmp::PartialOrd + std::fmt::Debug> (arr: &mut Vec<T>) {
let size = arr.len();
for i in 0..size/2 {
let mut swapped: bool = false;
let k = size - i - 1;
for j in i..k {
let p = j;
let q = p + 1;
if arr[p] < arr[q] {
arr.swap(p, q);
swapped = true;
}
}
for j in i..k {
let p = k - j;
let q = p - 1;
if arr[p] > arr[q] {
arr.swap(p, q);
swapped = true;
}
}
if !swapped {
break;
}
}
}
/** Entry point **/
fn main () {
// The size is a natural number
let size = prompt::<usize>(&"Enter size of array:");
// Fill array
let mut arr = Vec::new();
loop {
let item = prompt::<i32>(&"Next array element:");
arr.push(item);
if arr.len() == size {
break;
}
}
// Sort array
shaker_sort::<i32>(&mut arr);
// Print sorted array
println!("{:?}", arr);
}
|
code/sorting/src/shaker_sort/shaker_sort.swift | // Part of Cosmos by OpenGenus Foundation
import Foundation;
func shaker_sort(v: inout [UInt32]) {
let size = v.count;
var swap = false;
for i in 0 ... size / 2 - 1 {
for j in stride(from: i, to: size - i - 1, by: 1) {
if v[j] > v[j + 1] {
let tmp = v[j];
v[j] = v[j + 1];
v[j + 1] = tmp;
swap = true;
}
}
for j in stride(from: size - 2 - i, to: i, by: -1) {
if v[j] < v[j - 1] {
let tmp = v[j];
v[j] = v[j - 1];
v[j - 1] = tmp;
swap = true;
}
}
if (!swap) {
break;
}
}
}
func test() {
print("Size of array: ", terminator:"");
let size = Int(readLine()!)!;
var arr = [UInt32]();
for _ in 1 ... size {
arr.append(arc4random_uniform(100));
}
print("Original: ");
print(arr);
shaker_sort(v: &arr);
print("Sorted: ");
print(arr);
}
test();
|
code/sorting/src/shell_sort/README.md | # Shellsort
The Shellsort algorithm is a search algorithm. It's a generalization of the insertion sort that allows the exchange of items that are far apart.
The method starts by sorting pair of elements far apart from each other in the array, then progressively reducing the gap between the elements to be compared.
## Explanation
Consider the following example:

This list has nine items. If we use an increment of three, there are three sublists, each of which can be sorted by an insertion sort. After completing these sorts, we get the list as shown below.

Although this list is not completely sorted, something very interesting has happened. By sorting the sublists, we have moved the items closer to where they actually belong.
Figure below shows a final insertion sort using an increment of one; in other words, a standard insertion sort. Note that by performing the earlier sublist sorts, we have now reduced the total number of shifting operations necessary to put the list in its final order. For this case, we need only four more shifts to complete the process.

> Image credits: http://interactivepython.org
## Algorithm
```
procedure shellSort()
A : array of items
/* calculate interval*/
while interval < A.length /3 do:
interval = interval * 3 + 1
end while
while interval > 0 do:
for outer = interval; outer < A.length; outer ++ do:
/* select value to be inserted */
valueToInsert = A[outer]
inner = outer
/*shift element towards right*/
while inner > interval -1 && A[inner - interval] >= valueToInsert do:
A[inner] = A[inner - interval]
inner = inner - interval
end while
/* insert the number at hole position */
A[inner] = valueToInsert
end for
/* calculate interval*/
interval = (interval -1)/3
end while
end procedure
```
## Complexity
**Time complexity**
- Worst Case: **O(n<sup>2</sup>)**
- Average Case: depends on gap sequence
- Best Case: **O(n logn)**
**Space complexity**: **O(n)** total, **O(1)** auxillary
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
--- |
code/sorting/src/shell_sort/shell_sort.c | #include <stdio.h>
// Part of Cosmos by OpenGenus Foundation
void shell_sort(int *a, int n)
{
int i, j, gap, temp;
/* Assess the array in gaps starting at half the array size - Each gap is a sublist of 2 values */
for(gap = n/2 ; gap > 0 ; gap /= 2)
{
/* Index at the right value of each gap */
for(i = gap ; i < n ; i++)
{
/* Take a copy of the value under inspection */
temp = a[i];
for(j = i ; j >= gap ; j-= gap)
{
/* Compare the values in the sub lists and swap where necessary */
if(temp < a[j-gap])
a[j] = a[j-gap];
else
break;
}
a[j] = temp;
}
}
}
int main(void)
{
int i;
int a[10] = { 5 , 211 , 66 , 7 , 12 , 2, 76 , 134 , 99 , 9 };
printf("In: ");
for(i = 0 ; i < 10 ; i++)
{
printf("%d ", a[i]);
}
shell_sort(a, 10);
printf("\nOut: ");
for(i = 0 ; i < 10 ; i++)
{
printf("%d ", a[i]);
}
return 0;
}
|
code/sorting/src/shell_sort/shell_sort.cpp | #include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// Part of Cosmos by OpenGenus Foundation
void shellSort(vector<int> &ar)
{
size_t j;
for (size_t gap = ar.size() / 2; gap > 0; gap /= 2)
for (size_t i = gap; i < ar.size(); i++)
{
int temp = ar[i];
for (j = i; j >= gap && temp < ar[j - gap]; j -= gap)
ar[j] = ar[j - gap];
ar[j] = temp;
}
}
int main()
{
vector<int> inputArray;
cout << "Enter the elements of the array: ";
for (int i; cin >> i;)
inputArray.push_back(i);
shellSort(inputArray);
for (size_t i = 0; i < inputArray.size(); i++)
cout << inputArray[i] << " ";
cout << endl;
return 0;
}
|
code/sorting/src/shell_sort/shell_sort.go | package main
import (
"fmt"
)
func main() {
var n int
fmt.Println("Please enter the lenght of the array:")
fmt.Scan(&n)
X := make([]int, n)
fmt.Println("Now, enter the elements X0 X1 ... Xn-1")
for i := 0; i < n; i++ {
fmt.Scanln(&X[i])
}
fmt.Printf("Unsorted array: %v\n", X)
fmt.Printf("Sorted array: %v\n", BubbleSort(X))
}
func shellSort(array []int) {
h := 1
for h < len(array) {
h = 3 * h + 1
}
for h >= 1 {
for i := h; i < len(array); i++ {
for j := i; j >= h && array[j] < array[j - h]; j = j - h {
algoutils.Swap(array, j, j - h)
}
}
h = h/3
}
} |
code/sorting/src/shell_sort/shell_sort.java | // Java implementation of ShellSort
// Part of Cosmos by OpenGenus Foundation
class ShellSort
{
/* An utility function to print array of size n*/
static void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
/* function to sort arr using shellSort */
int sort(int arr[])
{
int n = arr.length;
// Start with a big gap, then reduce the gap
for (int gap = n/2; gap > 0; gap /= 2)
{
// Do a gapped insertion sort for this gap size.
// The first gap elements a[0..gap-1] are already
// in gapped order keep adding one more element
// until the entire array is gap sorted
for (int i = gap; i < n; i += 1)
{
// add a[i] to the elements that have been gap
// sorted save a[i] in temp and make a hole at
// position i
int temp = arr[i];
// shift earlier gap-sorted elements up until
// the correct location for a[i] is found
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap)
arr[j] = arr[j - gap];
// put temp (the original a[i]) in its correct
// location
arr[j] = temp;
}
}
return 0;
}
// Driver method
public static void main(String args[])
{
int arr[] = {12, 34, 54, 2, 3};
System.out.println("Array before sorting");
printArray(arr);
ShellSort ob = new ShellSort();
ob.sort(arr);
System.out.println("Array after sorting");
printArray(arr);
}
}
|
code/sorting/src/shell_sort/shell_sort.js | // Part of Cosmos by OpenGenus Foundation
function shellSort(a) {
for (var h = a.length; h > 0; h = parseInt(h / 2)) {
for (var i = h; i < a.length; i++) {
var k = a[i];
for (var j = i; j >= h && k < a[j - h]; j -= h) a[j] = a[j - h];
a[j] = k;
}
}
return a;
}
|
code/sorting/src/shell_sort/shell_sort.kt | import java.util.*
fun MutableList<Int>.swap(index1: Int, index2: Int) {
val tmp = this[index1]
this[index1] = this[index2]
this[index2] = tmp
}
fun MutableList<Int>.shellSort(): MutableList<Int> {
var sublistCount = count() / 2
while (sublistCount > 0) {
var index = 0
outer@while (index >= 0 && index < count()) {
if (index + sublistCount >= count()) break
if (this[index] > this[index + sublistCount]) {
swap(index, index + sublistCount)
}
if (sublistCount == 1 && index > 0) {
while (this[index - 1] > this[index] && index - 1 > 0) {
swap(index - 1, index)
index -= 1
}
++index
}
else {
++index
continue@outer
}
}
sublistCount /= 2
}
return this
}
fun main(args: Array<String>) {
var mutableListOfInt = mutableListOf(10, 4, 5, 2, 1, 3, 6, 9, 8, 7)
print(mutableListOfInt.shellSort().toString())
}
|
code/sorting/src/shell_sort/shell_sort.m | /* Part of Cosmos by OpenGenus Foundation */
//
// shell_sort.m
// Created by DaiPei on 2017/10/9.
//
#import <Foundation/Foundation.h>
@interface ShellSort : NSObject
- (void)sort:(NSMutableArray<NSNumber *> *)array;
@end
@implementation ShellSort
- (void)sort:(NSMutableArray<NSNumber *> *)array {
for (int h = (int)array.count / 2; h > 0; h /= 2) {
for (int i = h; i < array.count; i++) {
NSNumber *k = array[i];
int j;
for (j = i; j >= h && [k compare:array[j - h]] == NSOrderedAscending; j -= h) {
array[j] = array[j - h];
}
array[j] = k;
}
}
}
@end
int main(int argc, const char * argv[]) {
@autoreleasepool {
int n = 0;
NSLog(@"What is the size of the array?");
scanf("%d", &n);
NSMutableArray *array = [NSMutableArray arrayWithCapacity:n];
NSLog(@"Enter elements of the array one by one:");
for (int i = 0; i < n; i++) {
int tmp;
scanf("%d", &tmp);
[array addObject:@(tmp)];
}
ShellSort *ss = [[ShellSort alloc] init];
[ss sort:array];
NSLog(@"%@", array);
}
return 0;
}
|
code/sorting/src/shell_sort/shell_sort.py | # Part of Cosmos by OpenGenus Foundation
def shell_sort(alist):
sub_list_count = len(alist) // 2
while sub_list_count > 0:
for start in range(sub_list_count):
gap_insertion_sort(alist, start, sub_list_count)
sub_list_count = sub_list_count // 2
def gap_insertion_sort(alist, start, gap):
for i in range(start + gap, len(alist), gap):
currentvalue = alist[i]
position = i
while position >= gap and alist[position - gap] > currentvalue:
alist[position] = alist[position - gap]
position = position - gap
alist[position] = currentvalue
alist = [91, 91, 82, 45, 48, 35, 83, 70, 97]
print("Before: ", alist)
shell_sort(alist)
print("After: ", alist)
|
code/sorting/src/shell_sort/shell_sort.swift | /* Part of Cosmos by OpenGenus Foundation */
//
// shell_sort.swift
// Created by DaiPei on 2017/10/11.
//
import Foundation
func shellSort(_ array: inout [Int]) {
var h = array.count / 2
while h > 0 {
for i in h..<array.count {
let key = array[i]
var j = i
while j >= h && key < array[j - h] {
array[j] = array[j - h]
j -= h
}
array[j] = key
}
h /= 2
}
}
|
code/sorting/src/shell_sort/shellsort.go | package main
import "fmt"
import "math/rand"
func main() {
arr := RandomArray(10)
fmt.Println("Initial array is:", arr)
fmt.Println("")
for d := int(len(arr)/2); d > 0; d /= 2 {
for i := d; i < len(arr); i++ {
for j := i; j >= d && arr[j-d] > arr[j]; j -= d {
arr[j], arr[j-d] = arr[j-d], arr[j]
}
}
}
fmt.Println("Sorted array is: ", arr)
}
func RandomArray(n int) []int {
arr := make([]int, n)
for i := 0; i <= n - 1; i++ {
arr[i] = rand.Intn(n)
}
return arr
}
|
code/sorting/src/sleep_sort/README.md | # Sleep Sort
The **sleep sort** algorithm is closely related to the operating system. Each input is placed in a new thread which will sleep for a certain amount of time that is proportional to the value of its element. For example, a thread with a value of 10 will sleep longer than a thread with a value of 2. The thread with the least amount of sleep time gets printed first. When the next thread is done sleeping, that element is then printed. The output is thus sorted.
## Limitations
* Won’t work with negative values since the OS can’t sleep for a negative amount of time
* Huge amounts of elements will cause the algorithm to be slow
* Elements with large values will cause a slow algorithm.
|
code/sorting/src/sleep_sort/sleep_sort.c | /* Part of Cosmos by OpenGenus Foundation */
/* sleep sort works both in windows and linux*/
/* WINDOWS SPECIFIC CODE*/
#if defined(WIN32) || defined(_WIN32) || \
defined(__WIN32) && !defined(__CYGWIN__)
#include <process.h>
#include <windows.h>
void
routine(void *a)
{
int n = *(int *)a;
Sleep(n);
printf("%d ", n);
}
void
sleep_sort(int arr[], int n)
{
HANDLE threads[n];
int i;
for (i = 0; i < n; ++i) {
threads[i] = (HANDLE)_beginthread(&routine, 0, &arr[i]);
}
WaitForMultipleObjects(n, threads, TRUE, INFINITE);
}
/* LINUX SPECIFIC CODE*/
#elif defined(__linux__)
#include <assert.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
static void
fdpipe(FILE** read_fp, FILE** write_fp)
{
int fds[2];
int err = pipe(fds);
assert(err == 0);
*read_fp = fdopen(fds[0], "r");
*write_fp = fdopen(fds[1], "w");
}
void
sleep_sort(int* values, size_t cnt)
{
FILE *read_fp, *write_fp;
fdpipe(&read_fp, &write_fp);
size_t i;
for (i = 0; i < cnt; ++i) {
switch (fork()) {
case -1:
assert(0);
break;
case 0:
/* child process */
sleep(values[i]);
fprintf(write_fp, "%d\n", values[i]);
exit(0);
break;
default:
break;
}
}
fclose(write_fp);
int tmp;
for (i = 0; i < cnt; ++i) {
tmp = fscanf(read_fp, "%d\n", values + i);
assert(tmp == 1);
}
}
#endif
int
main()
{
int i;
int arr[] = {2, 1, 4, 3};
int n = sizeof(arr) / sizeof(arr[0]);
sleep_sort(arr, n);
/* print out the sorted elements*/
for (i = 0; i < n; ++i) {
printf("%d\n", arr[i]);
}
return (0);
}
|
code/sorting/src/sleep_sort/sleep_sort.cpp | /* Part of Cosmos by OpenGenus Foundation */
#include <iostream>
#include <chrono>
#include <vector>
#include <thread>
using namespace std;
int main(int argc, char** argv)
{
vector<thread> threads;
for (auto i = 1; i < argc; ++i)
{
threads.emplace_back(
[i, &argv]()
{
int arg = stoi(argv[i]);
this_thread::sleep_for(chrono::seconds(arg));
cout << argv[i] << endl;
}
);
}
for (auto &thread: threads)
thread.join();
return 0;
}
|
code/sorting/src/sleep_sort/sleep_sort.cs | /* Part of Cosmos by OpenGenus Foundation */
using System;
using System.Threading;
using System.Linq;
namespace dotnetcore
{
class Program
{
static void Main(string[] args)
{
SleepSort(new int[] {5, 4, 1, 2, 3});
}
static void SleepSort(int[] data)
{
data.AsParallel().WithDegreeOfParallelism(data.Length).Select(SleepAndReturn).ForAll(Console.WriteLine);
}
static int SleepAndReturn(int interval)
{
Thread.Sleep(interval*interval);
return interval;
}
}
}
|
code/sorting/src/sleep_sort/sleep_sort.go | /* Part of Cosmos by OpenGenus Foundation */
package main
import "fmt"
import "runtime"
import "time"
func sleepNumber(number int, results chan<- int) {
time.Sleep(time.Duration(number) * time.Second)
results <- number
}
func sleepSort(data []int) {
results := make(chan int, len(data))
for _, v := range data {
go sleepNumber(v, results)
}
for i, _ := range data {
data[i] = <-results
}
close(results)
}
func main() {
data := []int{8, 2, 4, 5, 6, 7, 1, 4, 10}
runtime.GOMAXPROCS(len(data))
fmt.Println("Before sorting", data)
sleepSort(data)
fmt.Println("After sorting", data)
}
|
code/sorting/src/sleep_sort/sleep_sort.java | /* Part of Cosmos by OpenGenus Foundation */
import java.util.concurrent.CountDownLatch;
public class SleepSort {
public static void sleepSortAndPrint(int[] nums) {
final CountDownLatch doneSignal = new CountDownLatch(nums.length);
for (final int num : nums) {
new Thread(new Runnable() {
public void run() {
doneSignal.countDown();
try {
doneSignal.await();
//using straight milliseconds produces unpredictable
//results with small numbers
//using 1000 here gives a nifty demonstration
Thread.sleep(num * 1000);
System.out.println(num);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
}
}
public static void main(String[] args) {
int[] nums = new int[args.length];
for (int i = 0; i < args.length; i++)
nums[i] = Integer.parseInt(args[i]);
sleepSortAndPrint(nums);
}
}
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.