title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Easy solution with clear approach explanation 98.22% beats | maximum-alternating-subsequence-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIn the problem statement it was clearly mentioned that we need to take maximum altering sum. so we have to take one max element and then a min element and that too in order.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nso iterate through the loop in list and take the two numbers if nums[i]>nums[i+1].\nlast item we need to take everytime. if you think why the answer is it was a odd indices number any way so we need to add it to result to get maximum list. \n\n# Complexity\n- Time complexity:\n- O(n)\n- we iterate through the list so it takes n time.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(1)\n- we used a single variable ans so 1 space required.\n- please upvote me if you like the answer,it will gives me motivation.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxAlternatingSum(self, nums: List[int]) -> int:\n ans = 0\n for i in range(len(nums) - 1):\n if nums[i] > nums[i + 1]:\n ans += nums[i] - nums[i + 1]\n ans += nums[-1]\n return ans\n``` | 3 | The **alternating sum** of a **0-indexed** array is defined as the **sum** of the elements at **even** indices **minus** the **sum** of the elements at **odd** indices.
* For example, the alternating sum of `[4,2,5,3]` is `(4 + 5) - (2 + 3) = 4`.
Given an array `nums`, return _the **maximum alternating sum** of any subsequence of_ `nums` _(after **reindexing** the elements of the subsequence)_.
A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the remaining elements' relative order. For example, `[2,7,4]` is a subsequence of `[4,2,3,7,2,1,4]` (the underlined elements), while `[2,4,2]` is not.
**Example 1:**
**Input:** nums = \[4,2,5,3\]
**Output:** 7
**Explanation:** It is optimal to choose the subsequence \[4,2,5\] with alternating sum (4 + 5) - 2 = 7.
**Example 2:**
**Input:** nums = \[5,6,7,8\]
**Output:** 8
**Explanation:** It is optimal to choose the subsequence \[8\] with alternating sum 8.
**Example 3:**
**Input:** nums = \[6,2,1,2,4,5\]
**Output:** 10
**Explanation:** It is optimal to choose the subsequence \[6,1,5\] with alternating sum (6 + 5) - 1 = 10.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 105` | Try thinking about the problem as if the array is empty. Then you only need to form goal using elements whose absolute value is <= limit. You can greedily set all of the elements except one to limit or -limit, so the number of elements you need is ceil(abs(goal)/ limit). You can "normalize" goal by offsetting it by the sum of the array. For example, if the goal is 5 and the sum is -3, then it's exactly the same as if the goal is 8 and the array is empty. The answer is ceil(abs(goal-sum)/limit) = (abs(goal-sum)+limit-1) / limit. |
📌 4 lines || 96% faster || Easy-approach 🐍 | maximum-alternating-subsequence-sum | 0 | 1 | ## IDEA:\n\uD83D\uDC49 Maximize the max_diff.\n\uD83D\uDC49 Minimize the min_diff.\nGiven an alternating sequence (a0, a1... ak), the change in value after appending an element x depends only on whether we have an even or odd number of elements so far:\n\nIf we have even # of elements, we add x; otherwise, we subtract x. So, tracking the best subsequences of odd and even sizes gives an extremely simple update formula.\n\n\'\'\'\n\n\tclass Solution:\n def maxAlternatingSum(self, nums: List[int]) -> int:\n \n ma=0\n mi=0\n for num in nums:\n ma=max(ma,num-mi)\n mi=min(mi,num-ma)\n \n return ma | 9 | The **alternating sum** of a **0-indexed** array is defined as the **sum** of the elements at **even** indices **minus** the **sum** of the elements at **odd** indices.
* For example, the alternating sum of `[4,2,5,3]` is `(4 + 5) - (2 + 3) = 4`.
Given an array `nums`, return _the **maximum alternating sum** of any subsequence of_ `nums` _(after **reindexing** the elements of the subsequence)_.
A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the remaining elements' relative order. For example, `[2,7,4]` is a subsequence of `[4,2,3,7,2,1,4]` (the underlined elements), while `[2,4,2]` is not.
**Example 1:**
**Input:** nums = \[4,2,5,3\]
**Output:** 7
**Explanation:** It is optimal to choose the subsequence \[4,2,5\] with alternating sum (4 + 5) - 2 = 7.
**Example 2:**
**Input:** nums = \[5,6,7,8\]
**Output:** 8
**Explanation:** It is optimal to choose the subsequence \[8\] with alternating sum 8.
**Example 3:**
**Input:** nums = \[6,2,1,2,4,5\]
**Output:** 10
**Explanation:** It is optimal to choose the subsequence \[6,1,5\] with alternating sum (6 + 5) - 1 = 10.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 105` | Try thinking about the problem as if the array is empty. Then you only need to form goal using elements whose absolute value is <= limit. You can greedily set all of the elements except one to limit or -limit, so the number of elements you need is ceil(abs(goal)/ limit). You can "normalize" goal by offsetting it by the sum of the array. For example, if the goal is 5 and the sum is -3, then it's exactly the same as if the goal is 8 and the array is empty. The answer is ceil(abs(goal-sum)/limit) = (abs(goal-sum)+limit-1) / limit. |
Python Easy memoization | maximum-alternating-subsequence-sum | 0 | 1 | ```\nclass Solution(object):\n def maxAlternatingSum(self, nums):\n dp = {}\n def max_sum(arr,i,add = False):\n if i>=len(arr):\n return 0\n if (i,add) in dp:\n return dp[(i,add)]\n nothing = max_sum(arr,i+1, add)\n if not add:\n added = max_sum(arr,i+1,True) + arr[i]\n dp[(i,add)] = max(added,nothing)\n elif add:\n subs = max_sum(arr,i+1, False) -arr[i]\n dp[(i,add)] = max(nothing, subs)\n return dp[(i,add)]\n return max_sum(nums,0)\n``` | 1 | The **alternating sum** of a **0-indexed** array is defined as the **sum** of the elements at **even** indices **minus** the **sum** of the elements at **odd** indices.
* For example, the alternating sum of `[4,2,5,3]` is `(4 + 5) - (2 + 3) = 4`.
Given an array `nums`, return _the **maximum alternating sum** of any subsequence of_ `nums` _(after **reindexing** the elements of the subsequence)_.
A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the remaining elements' relative order. For example, `[2,7,4]` is a subsequence of `[4,2,3,7,2,1,4]` (the underlined elements), while `[2,4,2]` is not.
**Example 1:**
**Input:** nums = \[4,2,5,3\]
**Output:** 7
**Explanation:** It is optimal to choose the subsequence \[4,2,5\] with alternating sum (4 + 5) - 2 = 7.
**Example 2:**
**Input:** nums = \[5,6,7,8\]
**Output:** 8
**Explanation:** It is optimal to choose the subsequence \[8\] with alternating sum 8.
**Example 3:**
**Input:** nums = \[6,2,1,2,4,5\]
**Output:** 10
**Explanation:** It is optimal to choose the subsequence \[6,1,5\] with alternating sum (6 + 5) - 1 = 10.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 105` | Try thinking about the problem as if the array is empty. Then you only need to form goal using elements whose absolute value is <= limit. You can greedily set all of the elements except one to limit or -limit, so the number of elements you need is ceil(abs(goal)/ limit). You can "normalize" goal by offsetting it by the sum of the array. For example, if the goal is 5 and the sum is -3, then it's exactly the same as if the goal is 8 and the array is empty. The answer is ceil(abs(goal-sum)/limit) = (abs(goal-sum)+limit-1) / limit. |
Python 3 | DP, O(N) | Explanation | maximum-alternating-subsequence-sum | 0 | 1 | ### Explanation\n- Only 2 different states: even sum or odd sum\n- Like the `hint` section, you can keep 2 variables and track the mamimum along the way\n- See below for more explanation\n### Implementation\n```\nclass Solution:\n def maxAlternatingSum(self, nums: List[int]) -> int:\n # even: max alternating sum of an even-length subsequence\n # odd: max alternating sum of an odd-length subsequence\n even = odd = 0 \n for num in nums:\n even, odd = max(even, odd-num), max(odd, even+num)\n return max(even, odd)\n``` | 4 | The **alternating sum** of a **0-indexed** array is defined as the **sum** of the elements at **even** indices **minus** the **sum** of the elements at **odd** indices.
* For example, the alternating sum of `[4,2,5,3]` is `(4 + 5) - (2 + 3) = 4`.
Given an array `nums`, return _the **maximum alternating sum** of any subsequence of_ `nums` _(after **reindexing** the elements of the subsequence)_.
A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the remaining elements' relative order. For example, `[2,7,4]` is a subsequence of `[4,2,3,7,2,1,4]` (the underlined elements), while `[2,4,2]` is not.
**Example 1:**
**Input:** nums = \[4,2,5,3\]
**Output:** 7
**Explanation:** It is optimal to choose the subsequence \[4,2,5\] with alternating sum (4 + 5) - 2 = 7.
**Example 2:**
**Input:** nums = \[5,6,7,8\]
**Output:** 8
**Explanation:** It is optimal to choose the subsequence \[8\] with alternating sum 8.
**Example 3:**
**Input:** nums = \[6,2,1,2,4,5\]
**Output:** 10
**Explanation:** It is optimal to choose the subsequence \[6,1,5\] with alternating sum (6 + 5) - 1 = 10.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 105` | Try thinking about the problem as if the array is empty. Then you only need to form goal using elements whose absolute value is <= limit. You can greedily set all of the elements except one to limit or -limit, so the number of elements you need is ceil(abs(goal)/ limit). You can "normalize" goal by offsetting it by the sum of the array. For example, if the goal is 5 and the sum is -3, then it's exactly the same as if the goal is 8 and the array is empty. The answer is ceil(abs(goal-sum)/limit) = (abs(goal-sum)+limit-1) / limit. |
[Python3] priority queues | design-movie-rental-system | 0 | 1 | \n```\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.avail = {}\n self.price = {}\n self.movies = defaultdict(list)\n for s, m, p in entries: \n heappush(self.movies[m], (p, s))\n self.avail[s, m] = True # unrented \n self.price[s, m] = p\n self.rented = []\n \n\n def search(self, movie: int) -> List[int]:\n if movie not in self.movies: return []\n ans, temp = [], []\n while len(ans) < 5 and self.movies[movie]: \n p, s = heappop(self.movies[movie])\n temp.append((p, s))\n if self.avail[s, movie]: ans.append((p, s))\n for p, s in temp: heappush(self.movies[movie], (p, s))\n return [x for _, x in ans]\n \n\n def rent(self, shop: int, movie: int) -> None:\n self.avail[shop, movie] = False\n p = self.price[shop, movie]\n heappush(self.rented, (p, shop, movie))\n \n\n def drop(self, shop: int, movie: int) -> None:\n self.avail[shop, movie] = True \n \n\n def report(self) -> List[List[int]]:\n ans = []\n while len(ans) < 5 and self.rented: \n p, s, m = heappop(self.rented)\n if not self.avail[s, m] and (not ans or ans[-1] != (p, s, m)): \n ans.append((p, s, m))\n for p, s, m in ans: heappush(self.rented, (p, s, m))\n return [[s, m] for _, s, m in ans]\n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n```\n\nAdding `SortedList` implementation \n```\nfrom sortedcontainers import SortedList \n\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.avail = {}\n self.price = {}\n for shop, movie, price in entries: \n self.price[shop, movie] = price \n self.avail.setdefault(movie, SortedList()).add((price, shop))\n self.rented = SortedList()\n\n \n def search(self, movie: int) -> List[int]:\n return [x for _, x in self.avail.get(movie, [])[:5]]\n \n\n def rent(self, shop: int, movie: int) -> None:\n price = self.price[shop, movie]\n self.avail[movie].remove((price, shop))\n self.rented.add((price, shop, movie))\n\n def drop(self, shop: int, movie: int) -> None:\n price = self.price[shop, movie]\n self.avail[movie].add((price, shop))\n self.rented.remove((price, shop, movie))\n\n def report(self) -> List[List[int]]:\n return [[x, y] for _, x, y in self.rented[:5]]\n``` | 9 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
[Python] beat 100% Time 100% Space with a Top5HeapSet | design-movie-rental-system | 0 | 1 | # Code\n```\nclass Top5HeapSet:\n def __init__(self):\n self._top5 = []\n self._heap = []\n self._to_remove = set()\n\n def top5(self):\n return self._top5\n\n def add(self, val):\n if val in self._to_remove:\n self._to_remove.remove(val)\n else:\n bisect.insort(self._top5, val)\n if len(self._top5) > 5:\n heapq.heappush(self._heap, self._top5.pop())\n\n def _can_pop(self):\n while self._heap and self._heap[0] in self._to_remove:\n self._to_remove.remove(heapq.heappop(self._heap))\n\n return len(self._heap) > 0\n\n def remove(self, val):\n if val in self._top5:\n self._top5.remove(val)\n if self._can_pop():\n self._top5.append(heapq.heappop(self._heap))\n else:\n self._to_remove.add(val)\n \n \n\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.movies_by_price = collections.defaultdict(Top5HeapSet)\n self.movies_by_shop = collections.defaultdict(dict)\n for shop, movie, price in entries:\n self.movies_by_price[movie].add((price, shop))\n self.movies_by_shop[movie][shop] = price\n self.rented_movies_by_price = Top5HeapSet()\n\n def search(self, movie: int) -> List[int]:\n return [shop for price, shop in self.movies_by_price[movie].top5()]\n\n def rent(self, shop: int, movie: int) -> None:\n price = self.movies_by_shop[movie][shop]\n self.movies_by_price[movie].remove((price, shop))\n self.rented_movies_by_price.add((price, shop, movie))\n\n def drop(self, shop: int, movie: int) -> None:\n price = self.movies_by_shop[movie][shop]\n self.movies_by_price[movie].add((price, shop))\n self.rented_movies_by_price.remove((price, shop, movie))\n\n def report(self) -> List[List[int]]:\n return [[shop, movie] for price, shop, movie in self.rented_movies_by_price.top5()]\n \n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
Python solution using dictionaries and set | design-movie-rental-system | 0 | 1 | The way it handles availability could be improved. It is still faster than other Python solutions. Possible reason is the version change in Python. \n\n# Code\n```\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n\n movies = defaultdict(list)\n \n for shop, movie, price in entries:\n movies[movie].append((price, shop))\n \n for lst in movies.values():\n lst.sort()\n \n self.shop_index = {\n movie: {\n shop:i for i, (_, shop) in enumerate(lst)\n } \n for movie, lst in movies.items()\n }\n \n self.available = {movie: [True]*len(lst) for movie, lst in movies.items()}\n \n self.movies = dict(movies)\n self.rented = set()\n\n def search(self, movie: int) -> List[int]:\n entries = self.movies.get(movie, [])\n available = self.available.get(movie, [])\n result = []\n i = 0\n while i < len(entries) and len(result) < 5:\n\n if available[i]:\n price, shop = entries[i]\n result.append(shop)\n \n i += 1\n \n return result\n \n\n def rent(self, shop: int, movie: int) -> None:\n available = self.available[movie]\n idx = self.shop_index[movie][shop]\n available[idx] = False\n price, shop = self.movies[movie][idx]\n report_entry = (price, shop, movie)\n self.rented.add(report_entry)\n\n\n def drop(self, shop: int, movie: int) -> None:\n available = self.available[movie]\n idx = self.shop_index[movie][shop]\n available[idx] = True\n price, _ = self.movies[movie][idx]\n report_entry = (price, shop, movie)\n self.rented.discard(report_entry)\n \n def report(self) -> List[List[int]]: \n five_cheapest = heapq.nsmallest(5, self.rented)\n return [(shop, movie) for _, shop, movie in five_cheapest]\n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
SortedDict python | design-movie-rental-system | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom sortedcontainers import SortedDict\nclass MovieRentingSystem:\n\n def __init__(self, n: int, e: List[List[int]]):\n self.reps=SortedDict()\n self.prices=dict()\n self.shops=dict()\n self.reports=SortedDict()\n for i in e:\n if not i[1] in self.prices:self.prices[i[1]]=SortedDict()\n self.prices[i[1]][(i[2],i[0])]=(i[0],i[1]) \n if not i[1] in self.shops:self.shops[i[1]]=dict()\n self.shops[i[1]][i[0]]=i[2]\n \n def search(self, movie: int) -> List[int]:\n res=[]\n if not movie in self.prices or len(self.prices[movie])==0:return []\n for count,i in enumerate(self.prices[movie].keys()):\n res.append(self.prices[movie][i][0])\n if count==4:break\n return res\n def rent(self, shop: int, movie: int) -> None:\n \n self.reports[(self.shops[movie][shop],shop,movie)]=""\n self.reps[(shop,movie)]=self.shops[movie][shop]\n self.prices[movie].pop((self.shops[movie][shop],shop))\n self.shops[movie].pop(shop)\n \n\n def drop(self, shop: int, movie: int) -> None:\n \n pop_elem=(self.reps[(shop,movie)],shop,movie)\n return_reps=self.reps[(shop,movie)]\n self.prices[movie][(return_reps,shop)]=(shop,movie)\n self.shops[movie][shop]=return_reps\n self.reps.pop((shop,movie))\n self.reports.pop(pop_elem)\n \n def report(self) -> List[List[int]]:\n res=[]\n for count,i in enumerate(self.reports.keys()):\n res.append([int(i[1]),int(i[2])])\n if count==4:break\n return res\n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
python | design-movie-rental-system | 0 | 1 | # Code\n```\nfrom sortedcontainers import SortedList\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.movies = defaultdict(SortedList)\n self.shop = {}\n \n for s, m, p in entries:\n self.shop[(s, m)] = p\n self.movies[m].add((p, s))\n \n self.rented = SortedList()\n\n def search(self, movie: int) -> List[int]:\n if movie in self.movies:\n \n return [v for _, v in self.movies[movie][:5]] \n\n def rent(self, shop: int, movie: int) -> None:\n p = self.shop[(shop, movie)]\n self.movies[movie].remove((p, shop))\n self.rented.add((p, shop, movie))\n \n def drop(self, shop: int, movie: int) -> None:\n p = self.shop[(shop, movie)]\n self.rented.remove((p, shop, movie))\n self.movies[movie].add((p, shop))\n\n def report(self) -> List[List[int]]:\n return [[u, v] for _, u, v in self.rented[:5]]\n\n\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
Python Easy Solution Using SortedList | Faster than 99% | | design-movie-rental-system | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom sortedcontainers import SortedList\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.arr = defaultdict(SortedList)\n self.map = {}\n for i,j,k in entries:\n self.map[(i,j)] = k\n self.arr[j].add([k,i])\n self.rented = SortedList()\n \n\n def search(self, movie: int) -> List[int]:\n return [i for k,i in self.arr[movie][:5]] \n\n def rent(self, shop: int, movie: int) -> None:\n price = self.map[(shop, movie)]\n self.arr[movie].discard([price, shop])\n self.rented.add((price, shop, movie))\n\n def drop(self, shop: int, movie: int) -> None:\n price = self.map[(shop, movie)]\n self.arr[movie].add([price, shop])\n self.rented.discard((price, shop, movie))\n\n\n def report(self) -> List[List[int]]:\n return [[j,k] for i,j,k in self.rented[:5]]\n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
Python (BEATS 100%) Solution with heaps and memoization | design-movie-rental-system | 0 | 1 | Previous `search()` and `report()` calls are stored and returned if no updates have been made yet.\n\n# Code\n```\nfrom heapq import heappush\nclass MovieRentingSystem:\n\n def __init__(self, n: int, entries: List[List[int]]):\n self.movies = {}\n self.prices = {}\n self.rented = set()\n self.prev_search = {}\n self.prev_report = None\n for entry in entries:\n if entry[1] not in self.movies:\n self.movies[entry[1]] = set()\n self.movies[entry[1]].add((entry[2], entry[0],))\n \n if entry[0] in self.prices:\n self.prices[entry[0]][entry[1]] = entry[2]\n else:\n self.prices[entry[0]] = {entry[1]: entry[2]}\n\n def search(self, movie: int) -> List[int]:\n if movie in self.prev_search and self.prev_search[movie]:\n return self.prev_search[movie]\n if movie in self.movies:\n res = list(self.movies[movie])\n heapify(res)\n ans = [heappop(res)[1] for i in range(min(len(res), 5))]\n else:\n ans = []\n self.prev_search[movie] = ans\n return ans\n \n\n def rent(self, shop: int, movie: int) -> None:\n self.prev_search[movie] = None\n self.prev_report = None\n price = self.prices[shop][movie]\n self.movies[movie].remove((price, shop,))\n self.rented.add((price, shop, movie,))\n\n def drop(self, shop: int, movie: int) -> None:\n self.prev_search[movie] = None\n self.prev_report = None\n price = self.prices[shop][movie]\n self.movies[movie].add((price, shop,))\n self.rented.remove((price, shop, movie,))\n\n def report(self) -> List[List[int]]:\n if self.prev_report:\n return self.prev_report\n res = list(self.rented)\n heapify(res)\n ans = [list(heappop(res)[1:]) for i in range(min(len(res), 5))]\n self.prev_report = ans\n return ans\n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
Python sortedlist solution | design-movie-rental-system | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n- Used sorted sets to handle rented and unrented movies\n- Also need map of {shop: {movie: price}} to keep track of movie prices\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nnlogn init, rest are log n and constant\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n# Code\n```\nfrom sortedcontainers import SortedList\n\nclass MovieRentingSystem:\n\n from collections import defaultdict\n\n # O(nlogn)\n def __init__(self, n: int, entries: List[List[int]]):\n # shop: dict{movies: price}\n self.entries = defaultdict(lambda: defaultdict(int))\n\n # movie : [(price, shop)]\n self.unrented_movies = {}\n\n for shop, movie, price in entries:\n\n if movie not in self.unrented_movies:\n self.unrented_movies[movie] = SortedList()\n self.unrented_movies[movie].add((price, shop))\n\n self.entries[shop][movie] = price\n\n \n self.rented_movies = SortedList()\n \n # O(1)\n def search(self, movie: int) -> List[int]:\n if movie not in self.unrented_movies:\n return []\n movie_prices = self.unrented_movies[movie][:5]\n return [shop for (price, shop) in movie_prices]\n\n # O(log n)\n def rent(self, shop: int, movie: int) -> None:\n price = self.entries[shop][movie]\n self.unrented_movies[movie].discard((price, shop))\n self.rented_movies.add((price, shop, movie))\n \n # O(log n)\n def drop(self, shop: int, movie: int) -> None:\n price = self.entries[shop][movie]\n self.unrented_movies[movie].add((price, shop))\n self.rented_movies.discard((price, shop, movie))\n \n # O(1)\n def report(self) -> List[List[int]]:\n rented_movies = self.rented_movies[:5]\n return [[shop, movie] for (price, shop, movie) in rented_movies]\n \n\n\n# Your MovieRentingSystem object will be instantiated and called as such:\n# obj = MovieRentingSystem(n, entries)\n# param_1 = obj.search(movie)\n# obj.rent(shop,movie)\n# obj.drop(shop,movie)\n# param_4 = obj.report()\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
Python (Simple Maths) | design-movie-rental-system | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom sortedcontainers import SortedList\n\nclass MovieRentingSystem:\n\n def __init__(self, n, entries):\n self.dict1 = defaultdict(SortedList)\n self.dict2 = {}\n self.dict3 = SortedList()\n\n for s,m,p in entries:\n self.dict1[m].add((p,s))\n self.dict2[(s,m)] = p\n\n def search(self, movie):\n return [i[1] for i in self.dict1[movie][:5]]\n\n def rent(self, shop, movie):\n price = self.dict2[(shop,movie)]\n self.dict1[movie].remove((price,shop))\n self.dict3.add((price,shop,movie))\n\n def drop(self, shop, movie):\n price = self.dict2[(shop,movie)]\n self.dict1[movie].add((price,shop))\n self.dict3.remove((price,shop,movie))\n\n def report(self):\n return [[i[1],i[2]] for i in self.dict3[:5]]\n \n\n``` | 0 | You have a movie renting company consisting of `n` shops. You want to implement a renting system that supports searching for, booking, and returning movies. The system should also support generating a report of the currently rented movies.
Each movie is given as a 2D integer array `entries` where `entries[i] = [shopi, moviei, pricei]` indicates that there is a copy of movie `moviei` at shop `shopi` with a rental price of `pricei`. Each shop carries **at most one** copy of a movie `moviei`.
The system should support the following functions:
* **Search**: Finds the **cheapest 5 shops** that have an **unrented copy** of a given movie. The shops should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopi` should appear first. If there are less than 5 matching shops, then all of them should be returned. If no shop has an unrented copy, then an empty list should be returned.
* **Rent**: Rents an **unrented copy** of a given movie from a given shop.
* **Drop**: Drops off a **previously rented copy** of a given movie at a given shop.
* **Report**: Returns the **cheapest 5 rented movies** (possibly of the same movie ID) as a 2D list `res` where `res[j] = [shopj, moviej]` describes that the `jth` cheapest rented movie `moviej` was rented from the shop `shopj`. The movies in `res` should be sorted by **price** in ascending order, and in case of a tie, the one with the **smaller** `shopj` should appear first, and if there is still tie, the one with the **smaller** `moviej` should appear first. If there are fewer than 5 rented movies, then all of them should be returned. If no movies are currently being rented, then an empty list should be returned.
Implement the `MovieRentingSystem` class:
* `MovieRentingSystem(int n, int[][] entries)` Initializes the `MovieRentingSystem` object with `n` shops and the movies in `entries`.
* `List search(int movie)` Returns a list of shops that have an **unrented copy** of the given `movie` as described above.
* `void rent(int shop, int movie)` Rents the given `movie` from the given `shop`.
* `void drop(int shop, int movie)` Drops off a previously rented `movie` at the given `shop`.
* `List> report()` Returns a list of cheapest **rented** movies as described above.
**Note:** The test cases will be generated such that `rent` will only be called if the shop has an **unrented** copy of the movie, and `drop` will only be called if the shop had **previously rented** out the movie.
**Example 1:**
**Input**
\[ "MovieRentingSystem ", "search ", "rent ", "rent ", "report ", "drop ", "search "\]
\[\[3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]\], \[1\], \[0, 1\], \[1, 2\], \[\], \[1, 2\], \[2\]\]
**Output**
\[null, \[1, 0, 2\], null, null, \[\[0, 1\], \[1, 2\]\], null, \[0, 1\]\]
**Explanation**
MovieRentingSystem movieRentingSystem = new MovieRentingSystem(3, \[\[0, 1, 5\], \[0, 2, 6\], \[0, 3, 7\], \[1, 1, 4\], \[1, 2, 7\], \[2, 1, 5\]\]);
movieRentingSystem.search(1); // return \[1, 0, 2\], Movies of ID 1 are unrented at shops 1, 0, and 2. Shop 1 is cheapest; shop 0 and 2 are the same price, so order by shop number.
movieRentingSystem.rent(0, 1); // Rent movie 1 from shop 0. Unrented movies at shop 0 are now \[2,3\].
movieRentingSystem.rent(1, 2); // Rent movie 2 from shop 1. Unrented movies at shop 1 are now \[1\].
movieRentingSystem.report(); // return \[\[0, 1\], \[1, 2\]\]. Movie 1 from shop 0 is cheapest, followed by movie 2 from shop 1.
movieRentingSystem.drop(1, 2); // Drop off movie 2 at shop 1. Unrented movies at shop 1 are now \[1,2\].
movieRentingSystem.search(2); // return \[0, 1\]. Movies of ID 2 are unrented at shops 0 and 1. Shop 0 is cheapest, followed by shop 1.
**Constraints:**
* `1 <= n <= 3 * 105`
* `1 <= entries.length <= 105`
* `0 <= shopi < n`
* `1 <= moviei, pricei <= 104`
* Each shop carries **at most one** copy of a movie `moviei`.
* At most `105` calls **in total** will be made to `search`, `rent`, `drop` and `report`. | Run a Dijkstra from node numbered n to compute distance from the last node. Consider all edges [u, v] one by one and direct them such that distance of u to n > distance of v to n. If both u and v are at the same distance from n, discard this edge. Now this problem reduces to computing the number of paths from 1 to n in a DAG, a standard DP problem. |
【Video】Give me 5 minutes - How we think about a solution | maximum-product-difference-between-two-pairs | 1 | 1 | # Intuition\r\nUse the two biggest numbers and the two smallest numbers\r\n\r\n---\r\n\r\n# Solution Video\r\n\r\nhttps://youtu.be/DMzedO_tGr8\r\n\r\n\u25A0 Timeline of the video\r\n\r\n`0:05` Explain solution formula\r\n`0:47` Talk about the first smallest and the second smallest\r\n`3:32` Talk about the first biggest and the second biggest\r\n`5:11` coding\r\n`8:10` Time Complexity and Space Complexity\r\n`8:26` Step by step algorithm of my solution code\r\n\r\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\r\n\r\n**\u25A0 Subscribe URL**\r\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\r\n\r\nSubscribers: 3,518\r\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\r\nThank you for your support!\r\n\r\n---\r\n\r\n# Approach\r\n\r\n## How we think about a solution\r\n\r\nConstraints says `1 <= nums[i] <= 104`, so simply maximum product difference should be\r\n\r\n```\r\nfirst biggest * second biggest - first smallest * second smallest\r\n```\r\n\r\nIf we use `sort` and then take the first two numbers and the last two numbers, we can solve this question. But that is $$O(nlogn)$$. It\'s a boring answer. Let\'s think about $$O(n)$$ solution.\r\n\r\nLet\'s think about 4 cases.\r\n\r\n---\r\n\r\n- First smallest\r\n- Second smallest\r\n- First biggest\r\n- Second biggest\r\n\r\n---\r\nBasically we iterate through all numbers one by one. The numbers are compared in the order specified above.\r\n\r\n#### First smallest\r\n\r\nIf current number is smaller than the current smallest number,\r\n\r\n---\r\n\r\n\u2B50\uFE0F Points\r\n\r\nthe current number will be the first smallest number.\r\nthe current first smallest number will be the second smallest number.\r\n\r\n---\r\n\r\n#### Second smallest\r\n\r\nNext, we check the second smallest. If current number is smaller than the current second smallest number,\r\n\r\n---\r\n\r\n\u2B50\uFE0F Points\r\n\r\nWe can just update the second smallest number with current number, because we already compared current number with the first smallest number, so we are sure that the current number is \r\n\r\n```\r\nthe first smallest < current < the second smallest\r\n```\r\n\r\n---\r\n\r\nSo, current number will be the second smallest number.\r\n\r\nRegarding biggest numbers, we apply the same idea. Let me explain them quickly.\r\n\r\n#### First biggest\r\n\r\nIf current number is bigger than the current biggest number,\r\n\r\n---\r\n\r\n\u2B50\uFE0F Points\r\n\r\nthe current number will be the first biggest number.\r\nthe current first biggest number will be the second biggest number.\r\n\r\n---\r\n\r\n#### Second biggest\r\n\r\nIf current number is bigger than the current second bigger number,\r\n\r\n---\r\n\r\n\u2B50\uFE0F Points\r\n\r\nWe are sure\r\n\r\n```\r\nthe first biggest > current > the second biggest\r\n```\r\n\r\nBecause we already compared current number with the first biggest number.\r\n\r\n---\r\n\r\nSo, current number will be the second biggest number.\r\n\r\nEasy!\uD83D\uDE04\r\nLet\'s see a real algorithm!\r\n\r\n---\r\n\r\n# Complexity\r\n- Time complexity: $$O(n)$$\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity: $$O(1)$$\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n```python []\r\nclass Solution:\r\n def maxProductDifference(self, nums: List[int]) -> int:\r\n first_big = second_big = 0\r\n first_small = second_small = float("inf")\r\n\r\n for n in nums:\r\n if n < first_small:\r\n second_small, first_small = first_small, n \r\n elif n < second_small: \r\n second_small = n \r\n\r\n if n > first_big:\r\n second_big, first_big = first_big, n\r\n elif n > second_big:\r\n second_big = n \r\n\r\n return first_big * second_big - first_small * second_small\r\n```\r\n```javascript []\r\n/**\r\n * @param {number[]} nums\r\n * @return {number}\r\n */\r\nvar maxProductDifference = function(nums) {\r\n let firstBig = secondBig = 0;\r\n let firstSmall = secondSmall = Infinity;\r\n\r\n for (const n of nums) {\r\n if (n < firstSmall) {\r\n [secondSmall, firstSmall] = [firstSmall, n];\r\n } else if (n < secondSmall) {\r\n secondSmall = n;\r\n }\r\n\r\n if (n > firstBig) {\r\n [secondBig, firstBig] = [firstBig, n];\r\n } else if (n > secondBig) {\r\n secondBig = n;\r\n }\r\n }\r\n\r\n return firstBig * secondBig - firstSmall * secondSmall; \r\n};\r\n```\r\n```java []\r\nclass Solution {\r\n public int maxProductDifference(int[] nums) {\r\n int firstBig = 0, secondBig = 0;\r\n int firstSmall = Integer.MAX_VALUE, secondSmall = Integer.MAX_VALUE;\r\n\r\n for (int n : nums) {\r\n if (n < firstSmall) {\r\n secondSmall = firstSmall;\r\n firstSmall = n;\r\n } else if (n < secondSmall) {\r\n secondSmall = n;\r\n }\r\n\r\n if (n > firstBig) {\r\n secondBig = firstBig;\r\n firstBig = n;\r\n } else if (n > secondBig) {\r\n secondBig = n;\r\n }\r\n }\r\n\r\n return firstBig * secondBig - firstSmall * secondSmall; \r\n }\r\n}\r\n```\r\n```C++ []\r\nclass Solution {\r\npublic:\r\n int maxProductDifference(vector<int>& nums) {\r\n int firstBig = 0, secondBig = 0;\r\n int firstSmall = INT_MAX, secondSmall = INT_MAX;\r\n\r\n for (int n : nums) {\r\n if (n < firstSmall) {\r\n secondSmall = firstSmall;\r\n firstSmall = n;\r\n } else if (n < secondSmall) {\r\n secondSmall = n;\r\n }\r\n\r\n if (n > firstBig) {\r\n secondBig = firstBig;\r\n firstBig = n;\r\n } else if (n > secondBig) {\r\n secondBig = n;\r\n }\r\n }\r\n\r\n return firstBig * secondBig - firstSmall * secondSmall; \r\n }\r\n};\r\n```\r\n\r\n## Step by step algorithm\r\n\r\n1. **Initialize Variables:**\r\n ```python\r\n first_big = second_big = 0\r\n first_small = second_small = float("inf")\r\n ```\r\n - `first_big`, `second_big`: Initialize variables to keep track of the two largest numbers.\r\n - `first_small`, `second_small`: Initialize variables to keep track of the two smallest numbers. `float("inf")` is used to represent positive infinity.\r\n\r\n2. **Iterate Through the Numbers:**\r\n ```python\r\n for n in nums:\r\n ```\r\n - Loop through each number in the input list `nums`.\r\n\r\n3. **Update Variables for Smallest Numbers:**\r\n ```python\r\n if n < first_small:\r\n second_small, first_small = first_small, n\r\n elif n < second_small:\r\n second_small = n\r\n ```\r\n - If the current number `n` is smaller than the current smallest number (`first_small`), update both smallest numbers accordingly.\r\n - If `n` is smaller than the second smallest number (`second_small`) but not the smallest, update only the second smallest.\r\n\r\n4. **Update Variables for Largest Numbers:**\r\n ```python\r\n if n > first_big:\r\n second_big, first_big = first_big, n\r\n elif n > second_big:\r\n second_big = n\r\n ```\r\n - If the current number `n` is larger than the current largest number (`first_big`), update both largest numbers accordingly.\r\n - If `n` is larger than the second largest number (`second_big`) but not the largest, update only the second largest.\r\n\r\n5. **Calculate and Return Result:**\r\n ```python\r\n return first_big * second_big - first_small * second_small\r\n ```\r\n - Calculate the product difference between the two largest and two smallest numbers.\r\n - Return the result.\r\n\r\nThe algorithm efficiently finds the two largest and two smallest numbers in the list and computes their product difference.\r\n\r\n---\r\n\r\nThank you for reading my post.\r\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\r\n\r\n\u25A0 Subscribe URL\r\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\r\n\r\n\u25A0 Twitter\r\nhttps://twitter.com/CodingNinjaAZ\r\n\r\n### My next daily coding challenge post and video.\r\n\r\npost\r\nhttps://leetcode.com/problems/image-smoother/solutions/4423391/video-give-me-10-minutes-how-we-think-about-a-solution/\r\n\r\nvideo\r\nhttps://youtu.be/eCHajvYReSg\r\n\r\n\u25A0 Timeline of the video\r\n\r\n`0:03` Explain how we solve Image Smoother\r\n`1:13` Check Top side\r\n`2:18` Check Bottom side\r\n`4:11` Check left and right side\r\n`3:32` Talk about the first biggest and the second biggest\r\n`6:26` coding\r\n`9:24` Time Complexity and Space Complexity\r\n`9:45` Step by step algorithm of my solution code\r\n\r\n### My previous daily coding challenge post and video.\r\n\r\npost\r\nhttps://leetcode.com/problems/design-a-food-rating-system/solutions/4414691/video-give-me-5-minutes-how-we-think-about-a-solution/\r\n\r\nvideo\r\nhttps://youtu.be/F6RAuglUOSg\r\n\r\n\u25A0 Timeline of the video\r\n\r\n`0:04` Explain constructor\r\n`1:21` Why -rating instead of rating?\r\n`4:09` Talk about changeRating\r\n`5:19` Time Complexity and Space Complexity\r\n`6:36` Step by step algorithm of my solution code\r\n`6:39` Python Tips - SortedList\r\n | 36 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
1-line vs 2 priority_queues vs loop||11 ms Beats 99.06% | maximum-product-difference-between-two-pairs | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nUse sort 1-line by Python\nC++ sort.\nOther approaches are\n- Heap solution\n- Loop with conditional clause\n# Approach\n<!-- Describe your approach to solving the problem. -->\n[https://youtu.be/I2VRYiBjK1k?si=UkDt91Fhu3wAh1K2](https://youtu.be/I2VRYiBjK1k?si=UkDt91Fhu3wAh1K2)\n\nSince the question is easy, try other approach by using priority_queue. Keep the size below 3 for 2 priority_queues with different orderings. \n\nAt final 1 priority_queue contains 2 biggest numbers, & other priority_queue contains 2 smallest numbers.\n\nThis method is not slow runs in 19ms & beats 83.95%.\n\nA loop with if-else clause is also implemented which runs in 11 ms &beats 99.06%. This is a a standard solution which is explained as follows\n\nVariable Initialization:\n`int max0=1, max1=1, min1=INT_MAX, min0=INT_MAX;`: Initializes four variables (max0, max1, min1, min0) to specific values. max0 and max1 are initialized to 1, and min1 and min0 are initialized to the maximum representable integer value (INT_MAX).\nLoop Over Input Vector:\n\n`for(int x: nums) { ... }`: Iterates over each element x in the nums vector.\nInside the loop, there are conditional statements to update the maximum and minimum values:\nIf x is greater than max0, update max1 and max0 accordingly.\nIf x is less than min0, update min1 and min0 accordingly.\n\n\n`return max0*max1-min0*min1;`: Computes the result, which is the product of the two largest elements (max0 and max1) minus the product of the two smallest elements (min0 and min1), and returns it.\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n\\log n)$$\n2 priority_queues: $O(n)$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n2 priority_queues: $O(n)$\n# Python 1-line Code\n```\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n return (X:=sorted(nums))[-1]*X[-2]-X[1]*X[0]\n```\n# C++ using sort\n```\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n sort(nums.begin(), nums.end());\n int n=nums.size();\n return nums[n-1]*nums[n-2]-nums[1]*nums[0];\n \n }\n};\n```\n# C++ using 2 priority_queues||19ms Beats 83.95%\n```\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n priority_queue<int> pq0;\n priority_queue<int, vector<int>, greater<int>> pq1;\n for(int x: nums){\n pq0.push(x);\n if (pq0.size()>2){\n int y=pq0.top();\n pq0.pop();\n pq1.push(y);\n }\n if (pq1.size()>2)\n pq1.pop();\n }\n int w=pq0.top();\n pq0.pop();\n w*=pq0.top();\n int z=pq1.top();\n pq1.pop();\n z*=pq1.top();\n return z-w;\n }\n};\n```\n# C++ loop with if-else runs in 11 ms Beats 99.06%\n\n```\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n int max0=1, max1=1, min1=INT_MAX, min0=INT_MAX;\n for(int x: nums){\n if (x>max0){\n max1=max0;\n max0=x; \n }\n else max1=max(max1, x);\n if (x<min0) {\n min1=min0;\n min0=x;\n }\n else min1=min(min1, x);\n }\n return max0*max1-min0*min1;\n }\n};\n```\n | 8 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
🔥 Beats 100% | 2 Approaches | Easiest code with explaination 🚀😎 | maximum-product-difference-between-two-pairs | 1 | 1 | # Intuition\r\nThe goal is to maximize the product difference between two pairs. To achieve this, we want to select the two largest numbers for the first pair and the two smallest numbers for the second pair. By sorting the array and choosing the extremes, we can maximize the product difference.\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n1. First calculate the maximum number store it and then erase it.\r\n2. Similarly, we need one more number follow the same procedure.\r\n3. For minimum numbers also steps are similar.\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity: O(N)\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity: O(1)\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\n// 1. Using Sorting\r\nclass Solution {\r\n\r\npublic:\r\n\r\n int maxProductDifference(vector<int>& nums) {\r\n\r\n ios_base::sync_with_stdio(false);\r\n\r\n cout.tie(0);\r\n\r\n cin.tie(0);\r\n\r\n sort(nums.begin(),nums.end());\r\n\r\n int n = nums.size();\r\n\r\n return ((nums[n-1] * nums[n-2]) - (nums[0] * nums[1]));\r\n\r\n }\r\n\r\n};\r\n\r\n// 2. Using STL\r\nclass Solution {\r\npublic:\r\n int maxProductDifference(vector<int>& nums) {\r\n ios_base::sync_with_stdio(false);\r\n cout.tie(0);\r\n cin.tie(0);\r\n \r\n // code\r\n auto it = max_element(nums.begin(),nums.end());\r\n int index = it - nums.begin();\r\n int max1 = nums[index];\r\n nums.erase(nums.begin()+index);\r\n\r\n it = max_element(nums.begin(),nums.end());\r\n index = it - nums.begin();\r\n int max2 = nums[index];\r\n nums.erase(nums.begin()+index);\r\n cout << max1 << " " << max2 << endl;\r\n\r\n it = min_element(nums.begin(),nums.end());\r\n index = it - nums.begin();\r\n int min1 = nums[index];\r\n nums.erase(nums.begin()+index);\r\n\r\n it = min_element(nums.begin(),nums.end());\r\n index = it - nums.begin();\r\n int min2 = nums[index];\r\n nums.erase(nums.begin()+index);\r\n \r\n return (max1 * max2 - min1 * min2);\r\n }\r\n};\r\n``` | 4 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
Leetcode daily problems 🔥 | Easy Explanation | C++✔ | JAVA✔ | PYTHON✔ | C ✔| | maximum-product-difference-between-two-pairs | 1 | 1 | # ***Please upvote , if you find it a little helpful*\uD83D\uDE22**\n\n---\n\n\n# Approach 1 : Using sorting\n# Intuition\nThe goal is to find the maximum product difference between two pairs of distinct indices.\n\n# Approach\nThe algorithm utilizes sorting to find the maximum product difference between two pairs of distinct indices.\n\n1. **Sorting:**\n - Sort the `nums` array in ascending order.\n\n2. **Calculation:**\n - Calculate the product difference using the formula:\n - `(nums[n-1] * nums[n-2]) - (nums[0] * nums[1])`\n - where `n` is the size of the array.\n\n\n# Complexity\n- **Time complexity**: O(n log n), where n is the size of the array due to the sorting operation.\n\n- **Space complexity:** O(1)\n\n<iframe src="https://leetcode.com/playground/5AF2rnnq/shared" frameBorder="0" width="400" height="300"></iframe>\n\n### Python code\n```PYTHON\n#python code\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n nums.sort()\n n = len(nums)\n return (nums[n - 1] * nums[n - 2]) - (nums[0] * nums[1])\n\n```\n---\n\n\n---\n\n\n\n# APPROACH 2 : Optimised approach\n# Intuition\nThe goal is to find the maximum product difference between two pairs of distinct indices.\n\n# Approach\nWe can maintain the smallest and largest values encountered during the iteration through the array. By keeping track of the two smallest and two largest elements, we can compute the product difference efficiently.\n\nThe algorithm initializes four variables (`smallest1`, `smallest2`, `largest1`, and `largest2`) to store the two smallest and two largest elements.\n\n1. **Initialization:**\n - `smallest1` = `smallest2` = INT_MAX\n - `largest1` = `largest2` = INT_MIN\n\n2. **Iteration:**\n - Iterate through the array, updating variables based on the current element.\n - If `num` <= `smallest1`, update `smallest2` and `smallest1`.\n - If `num` <= `smallest2`, update only `smallest2`.\n - If `num` >= `largest1`, update `largest2` and `largest1`.\n - If `num` >= `largest2`, update only `largest2`.\n\n3. **Calculation:**\n - After the iteration, calculate the product difference:\n - `(largest1 * largest2) - (smallest1 * smallest2)`.\n\n\n# Complexity\n- **Time complexity**: The algorithm iterates through the array once, making it a linear time complexity, i.e., O(n), where n is the size of the array.\n\n- **Space complexity:** The algorithm uses a constant amount of space for the variables, resulting in O(1) space complexity.\n\n\n<iframe src="https://leetcode.com/playground/8Pc5KWrd/shared" frameBorder="0" width="400" height="300"></iframe>\n\n### Python code\n```PYTHON \n#python code\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n smallest1, smallest2 = float(\'inf\'), float(\'inf\')\n largest1, largest2 = float(\'-inf\'), float(\'-inf\')\n\n for num in nums:\n if num <= smallest1:\n smallest2, smallest1 = smallest1, num\n elif num <= smallest2:\n smallest2 = num\n\n if num >= largest1:\n largest2, largest1 = largest1, num\n elif num >= largest2:\n largest2 = num\n\n return (largest1 * largest2) - (smallest1 * smallest2)\n\n\n```\n | 4 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
Python3 Solution | maximum-product-difference-between-two-pairs | 0 | 1 | \n```\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n nums.sort()\n return (nums[-1]*nums[-2])-(nums[0]*nums[1])\n``` | 3 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | maximum-product-difference-between-two-pairs | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n*(Also explained in the code)*\n\n#### ***Approach 1(with Sort)***\n1. sorts the input array `nums` in ascending order.\n1. Computes the difference between the product of the last two elements and the product of the first two elements in the sorted array.\n 1. Returns the calculated difference between the maximum product (of the last two elements) and the minimum product (of the first two elements) in the array.\n\n# Complexity\n- Time complexity:\n $$O(nlogn)$$\n \n\n- Space complexity:\n $$O(logn)$$ or $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n sort(nums.begin(),nums.end());\n int n =nums.size();\n\n return nums[n-2]*nums[n-1]-nums[0]*nums[1];\n }\n};\n\n\n```\n\n```Java []\nclass Solution {\n public int maxProductDifference(int[] nums) {\n Arrays.sort(nums);\n return nums[nums.length - 1] * nums[nums.length - 2] - nums[0] * nums[1];\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n nums.sort()\n return nums[-1] * nums[-2] - nums[0] * nums[1]\n\n\n```\n```javascript []\nfunction maxProductDifference(nums) {\n nums.sort((a, b) => a - b);\n const n = nums.length;\n\n return nums[n - 2] * nums[n - 1] - nums[0] * nums[1];\n}\n\n\n\n```\n---\n#### ***Approach 2(Keeping track of 2 largest and 2 smallest)***\n1. `maxProductDifference` finds the maximum difference between the product of the largest and second-largest numbers and the product of the smallest and second-smallest numbers in the given array `nums`.\n1. It iterates through the array, keeping track of the largest, second-largest, smallest, and second-smallest numbers encountered.\n1. Finally, it computes the product difference of these values and returns the result.\n\n# Complexity\n- Time complexity:\n $$O(n)$$\n \n\n- Space complexity:\n $$O(1)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n // Initialize variables to hold the largest, second-largest, smallest, and second-smallest numbers\n int biggest = 0;\n int secondBiggest = 0;\n int smallest = INT_MAX;\n int secondSmallest = INT_MAX;\n \n // Iterate through the input array \'nums\'\n for (int num : nums) {\n // Check if \'num\' is greater than the current \'biggest\'\n if (num > biggest) {\n // Update \'secondBiggest\' and \'biggest\' accordingly\n secondBiggest = biggest;\n biggest = num;\n } else {\n // If \'num\' is not the largest, update \'secondBiggest\' if necessary\n secondBiggest = max(secondBiggest, num);\n }\n \n // Check if \'num\' is smaller than the current \'smallest\'\n if (num < smallest) {\n // Update \'secondSmallest\' and \'smallest\' accordingly\n secondSmallest = smallest;\n smallest = num;\n } else {\n // If \'num\' is not the smallest, update \'secondSmallest\' if necessary\n secondSmallest = min(secondSmallest, num);\n }\n }\n \n // Calculate the product difference of the largest and smallest values and return the result\n return biggest * secondBiggest - smallest * secondSmallest;\n }\n};\n\n\n\n```\n```Java []\nclass Solution {\n public int maxProductDifference(int[] nums) {\n int biggest = 0;\n int secondBiggest = 0;\n int smallest = Integer.MAX_VALUE;\n int secondSmallest = Integer.MAX_VALUE;\n \n for (int num : nums) {\n if (num > biggest) {\n secondBiggest = biggest;\n biggest = num;\n } else {\n secondBiggest = Math.max(secondBiggest, num);\n }\n \n if (num < smallest) {\n secondSmallest = smallest;\n smallest = num;\n } else {\n secondSmallest = Math.min(secondSmallest, num);\n }\n }\n \n return biggest * secondBiggest - smallest * secondSmallest;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n biggest = 0\n second_biggest = 0\n smallest = inf\n second_smallest = inf\n \n for num in nums:\n if num > biggest:\n second_biggest = biggest\n biggest = num\n else:\n second_biggest = max(second_biggest, num)\n \n if num < smallest:\n second_smallest = smallest\n smallest = num\n else:\n second_smallest = min(second_smallest, num)\n \n return biggest * second_biggest - smallest * second_smallest\n\n\n```\n```javascript []\n\n\n\n```\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 10 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
✅ One Line Solution | maximum-product-difference-between-two-pairs | 0 | 1 | # Code #1 - Fast\nTime complexity: $$O(n)$$. Space complexity: $$O(1)$$.\n```\nclass Solution:\n def maxProductDifference(self, A: List[int]) -> int:\n return prod(nlargest(2,A)) - prod(nsmallest(2,A))\n```\n\n# Code #2 - Slow\nTime complexity: $$O(n*log(n))$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def maxProductDifference(self, A: List[int]) -> int:\n return prod((A:=sorted(A))[-2:]) - prod(A[:2])\n``` | 4 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
Simple and Easy Code to understand ||Beginner Friendly||C++||java||python | maximum-product-difference-between-two-pairs | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n log n), where n is the number of elements in the input array. This is because sorting the array takes O(n log n) time.\n\n- Space complexity:\no(1)\n```c++ []\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n sort(nums.begin(),nums.end());\n int n1=(nums[nums.size()-1]) * (nums[nums.size()-2]);\n int n2=nums[0]*nums[1];\n return n1-n2;\n }\n};\n```\n```python []\nclass Solution:\n def maxProductDifference(self, nums):\n nums.sort()\n n1 = nums[-1] * nums[-2]\n n2 = nums[0] * nums[1]\n return n1 - n2\n\n```\n```java []\nclass Solution {\n public int maxProductDifference(int[] nums) {\n Arrays.sort(nums);\n int n1 = nums[nums.length - 1] * nums[nums.length - 2];\n int n2 = nums[0] * nums[1];\n return n1 - n2;\n }\n}\n```\n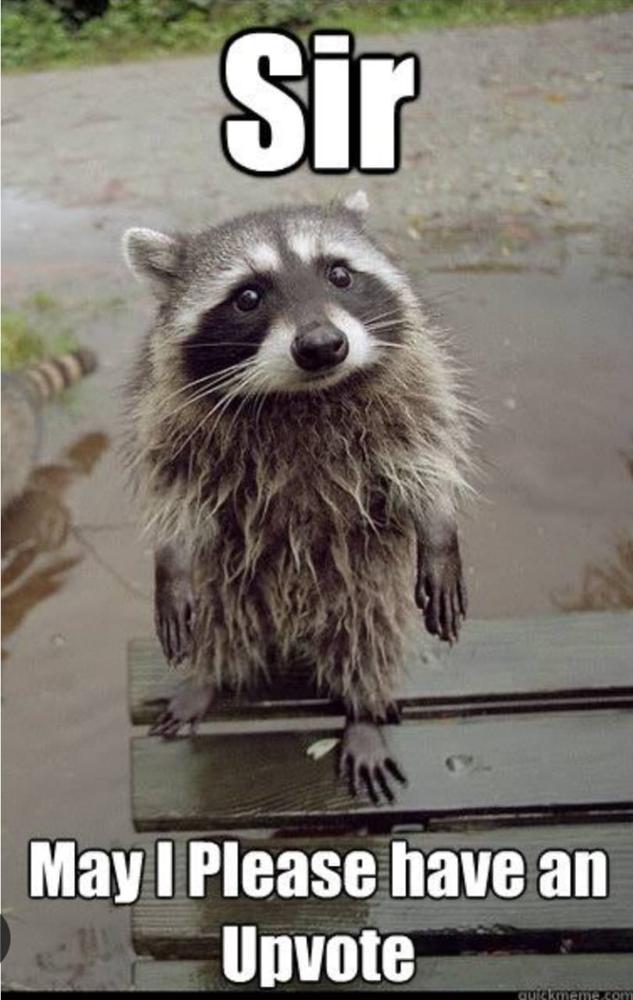\n | 8 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
Beats 99% || [Java/C++/Python3] || Beginner Friendly Solution | maximum-product-difference-between-two-pairs | 1 | 1 | # Intuition\n1. Product difference will be maximum when we will, subtract of two smallest number from the product of two greatest number.\n2. We have to find the greatest two number and smallest two number.\n\n# Approach\n1. **Initialization:**\n - Set `min1` to the first element in the array (nums[0]).\n - Set `min2` to the second element in the array (nums[1]).\n - Set `max1` to the larger of the first two elements.\n - Set `max2` to the smaller of the first two elements.\n2. Iterative Update:\n - Iterate through the array starting from the third element (`i=2`).\n - Update `min1` and `min2` if the current element is smaller than both `min1` and `min2`.\n - Update `max1` and `max2` if the current element is larger than both `max1` and `max2`.\n3. **Calculation of Result:**\n - After the iteration, calculate the result by subtracting the product of the two smallest elements (`min1` `*` `min2`) from the product of the two largest elements (`max1` `*` `max2`).\n \n*The code essentially identifies the two smallest and two largest elements in the array and computes the maximum product difference using these values. It\'s an efficient way to find the desired result in a single pass through the array.*\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` Java []\nclass Solution {\n public int maxProductDifference(int[] nums) {\n if(nums.length <= 2) {\n return 0;\n }\n\n int min1 = nums[0]; //Smallest\n int min2 = nums[1]; //Second Smallest\n int max1 = nums[1]; //Largest\n int max2 = nums[0]; //Second Largest\n if(nums[0] > nums[1]){\n min1 = nums[1];\n min2 = nums[0];\n max1 = nums[0];\n max2 = nums[1];\n }\n\n for(int i=2; i<nums.length; i++) {\n if(nums[i] < min2) {\n if(nums[i] < min1) {\n min2 = min1;\n min1 = nums[i];\n }\n else {\n min2 = nums[i];\n }\n }\n\n if(nums[i] > max2) {\n if(nums[i] > max1) {\n max2 = max1;\n max1 = nums[i];\n }\n else {\n max2 = nums[i];\n }\n }\n }\n return max1*max2 - min1*min2;\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int maxProductDifference(vector<int>& nums) {\n if (nums.size() <= 2) {\n return 0;\n }\n\n int min1 = nums[0]; // Smallest\n int min2 = nums[1]; // Second Smallest\n int max1 = nums[1]; // Largest\n int max2 = nums[0]; // Second Largest\n if (nums[0] > nums[1]) {\n min1 = nums[1];\n min2 = nums[0];\n max1 = nums[0];\n max2 = nums[1];\n }\n\n for (int i = 2; i < nums.size(); i++) {\n if (nums[i] < min2) {\n if (nums[i] < min1) {\n min2 = min1;\n min1 = nums[i];\n } else {\n min2 = nums[i];\n }\n }\n\n if (nums[i] > max2) {\n if (nums[i] > max1) {\n max2 = max1;\n max1 = nums[i];\n } else {\n max2 = nums[i];\n }\n }\n }\n return max1 * max2 - min1 * min2;\n }\n};\n```\n``` Python3 []\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n if len(nums) <= 2:\n return 0\n\n min1 = min(nums[0], nums[1]) # Smallest\n min2 = max(nums[0], nums[1]) # Second Smallest\n max1 = max(nums[0], nums[1]) # Largest\n max2 = min(nums[0], nums[1]) # Second Largest\n\n for i in range(2, len(nums)):\n if nums[i] < min2:\n if nums[i] < min1:\n min2 = min1\n min1 = nums[i]\n else:\n min2 = nums[i]\n\n if nums[i] > max2:\n if nums[i] > max1:\n max2 = max1\n max1 = nums[i]\n else:\n max2 = nums[i]\n\n return max1 * max2 - min1 * min2\n``` | 1 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
simple and easy to understand solution using java and python | maximum-product-difference-between-two-pairs | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem involves finding the maximum product difference between pairs of elements in an array. To maximize the product difference, we should select the largest and second-largest elements and the smallest and second-smallest elements. Sorting the array allows us to easily access these elements.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Sort the array in ascending order.\n- Calculate the product difference using the two largest elements and the two smallest elements.\n- Return the result.\n\n# Complexity\n- Time complexity: O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n\n``` python3 []\nclass Solution:\n def maxProductDifference(self, nums: List[int]) -> int:\n nums.sort()\n return (nums[-1] * nums[-2]) - (nums[0] * nums[1])\n \n```\n``` java []\nimport java.util.Arrays;\nclass Solution {\n public int maxProductDifference(int[] nums) {\n Arrays.sort(nums);\n return ((nums[nums.length-2]*nums[nums.length-1])-(nums[0]*nums[1]));\n }\n}\n``` | 1 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
O(n) solution | 1 pass ✅🏁 | maximum-product-difference-between-two-pairs | 0 | 1 | # Intuition\r\nWe can get the $$Biggest$$ $$Difference$$ when we find the difference b/w the products of the highest two integers and the lowest two integers.\r\n\r\n**Why?**\r\nProduct of the lowest two integers gives the lowest possible product\r\nAnd the product between the two highest integers gives the highest possible product in the given array.\r\nSo, when we subtract the largest number from the smallest -> we get the biggest difference possible.\r\n\r\n# Approach\r\nHere, we will go for an O(n) approach and ditch the sorting of the array.\r\n\uD83D\uDCA1Remember the problem where we had to find the highest and the second highest numbers in an array?\r\n\u2705Exactly! This is what we\'ll be using to solve this problem.\r\nLet\'s begin\r\n\r\n1. Initialise 4 variables to store the highest,second highest, smallest and the second smallest numbers.\r\n2. Iterate from 0 to n, where n is the length of the given array\r\n a. `If we come across an element greater than the current value of highest -> this highest becomes the second highest element so far and the new element becomes the highest`\r\n b. `If the element is smaller than the current highest but greater than the second_highest, the new element simply becomes the second_highest`\r\n c. ` If we encounter an element smaller than the current smallest, the current smallest becomes the second smallest and the new element becomes the smallest`\r\n d. `If the current element is greater than the current smallest but smaller than the second_smallest, the new element simply becomes the second_smallest`\r\n\r\n\r\n# Complexity\r\n- Time complexity: O(n)\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity: O(1)\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def maxProductDifference(self, nums: List[int]) -> int:\r\n \r\n smallest,second_smallest = float(\'inf\'),float(\'inf\')\r\n highest,second_highest = float(\'-inf\'),float(\'-inf\')\r\n \r\n for i in nums:\r\n if i > highest:\r\n second_highest = highest\r\n highest = i\r\n else:\r\n if i > second_highest:\r\n second_highest = i\r\n\r\n if i < smallest:\r\n second_smallest = smallest\r\n smallest = i\r\n else:\r\n if i < second_smallest:\r\n second_smallest = i\r\n\r\n return (highest*second_highest)-(smallest*second_smallest)\r\n \r\n\r\n``` | 1 | The **product difference** between two pairs `(a, b)` and `(c, d)` is defined as `(a * b) - (c * d)`.
* For example, the product difference between `(5, 6)` and `(2, 7)` is `(5 * 6) - (2 * 7) = 16`.
Given an integer array `nums`, choose four **distinct** indices `w`, `x`, `y`, and `z` such that the **product difference** between pairs `(nums[w], nums[x])` and `(nums[y], nums[z])` is **maximized**.
Return _the **maximum** such product difference_.
**Example 1:**
**Input:** nums = \[5,6,2,7,4\]
**Output:** 34
**Explanation:** We can choose indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4).
The product difference is (6 \* 7) - (2 \* 4) = 34.
**Example 2:**
**Input:** nums = \[4,2,5,9,7,4,8\]
**Output:** 64
**Explanation:** We can choose indices 3 and 6 for the first pair (9, 8) and indices 1 and 5 for the second pair (2, 4).
The product difference is (9 \* 8) - (2 \* 4) = 64.
**Constraints:**
* `4 <= nums.length <= 104`
* `1 <= nums[i] <= 104` | Let's note that for the XOR of all segments with size K to be equal to zeros, nums[i] has to be equal to nums[i+k] Basically, we need to make the first K elements have XOR = 0 and then modify them. |
Python 3 || 12 lines, each layer as 1D array || T/M: 98% / 96% | cyclically-rotating-a-grid | 0 | 1 | ```\r\nclass Solution:\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n \r\n m, n = len(grid), len(grid[0])\r\n MN = min(m, n)//2\r\n \r\n for i in range(MN):\r\n\r\n XO, X1, YO, Y1 = i, m-i-1, i, n-i-1\r\n rot = k%(2*(X1+Y1-XO-YO)) \r\n\r\n layerXY = list(chain( [(XO, y) for y in range(YO, Y1)],\r\n [(x, Y1) for x in range(XO, X1)],\r\n [(X1, y) for y in range(YO+1,Y1+1)][::-1],\r\n [(x, YO) for x in range(XO+1,X1+1)][::-1]))\r\n\r\n layer = [grid[a][b] for (a,b) in layerXY]\r\n layer = zip(layer[rot:]+layer[:rot], layerXY)\r\n\r\n for num, (a,b) in layer: grid[a][b] = num\r\n\r\n return grid\r\n```\r\n[https://leetcode.com/problems/cyclically-rotating-a-grid/submissions/915814775/](http://)\r\n\r\nI could be wrong, but I think that time complexity is *O*(*MN*) and space complexity is *O*(*M*+*N*). | 3 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
Python 3 || 12 lines, each layer as 1D array || T/M: 98% / 96% | cyclically-rotating-a-grid | 0 | 1 | ```\r\nclass Solution:\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n \r\n m, n = len(grid), len(grid[0])\r\n MN = min(m, n)//2\r\n \r\n for i in range(MN):\r\n\r\n XO, X1, YO, Y1 = i, m-i-1, i, n-i-1\r\n rot = k%(2*(X1+Y1-XO-YO)) \r\n\r\n layerXY = list(chain( [(XO, y) for y in range(YO, Y1)],\r\n [(x, Y1) for x in range(XO, X1)],\r\n [(X1, y) for y in range(YO+1,Y1+1)][::-1],\r\n [(x, YO) for x in range(XO+1,X1+1)][::-1]))\r\n\r\n layer = [grid[x][y] for x, y in layerXY]\r\n layer = zip(layer[rot:]+layer[:rot], layerXY)\r\n\r\n for num, (x, y) in layer: grid[x][y] = num\r\n\r\n return grid\r\n```\r\n[https://leetcode.com/problems/cyclically-rotating-a-grid/submissions/915814775/](http://)\r\n\r\nI could be wrong, but I think that time complexity is *O*(*MN*) and space complexity is *O*(*M*+*N*). | 3 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
[Python3] brute-force | cyclically-rotating-a-grid | 0 | 1 | \n```\nclass Solution:\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\n m, n = len(grid), len(grid[0]) # dimensions \n \n for r in range(min(m, n)//2): \n i = j = r\n vals = []\n for jj in range(j, n-j-1): vals.append(grid[i][jj])\n for ii in range(i, m-i-1): vals.append(grid[ii][n-j-1])\n for jj in range(n-j-1, j, -1): vals.append(grid[m-i-1][jj])\n for ii in range(m-i-1, i, -1): vals.append(grid[ii][j])\n \n kk = k % len(vals)\n vals = vals[kk:] + vals[:kk]\n \n x = 0 \n for jj in range(j, n-j-1): grid[i][jj] = vals[x]; x += 1\n for ii in range(i, m-i-1): grid[ii][n-j-1] = vals[x]; x += 1\n for jj in range(n-j-1, j, -1): grid[m-i-1][jj] = vals[x]; x += 1\n for ii in range(m-i-1, i, -1): grid[ii][j] = vals[x]; x += 1\n return grid\n``` | 26 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
📌 Simple-Approach || Well-explained || 95% faster 🐍 | cyclically-rotating-a-grid | 0 | 1 | ## IDEA: \n\uD83D\uDC49 keep the boundary matrix in a list\n\uD83D\uDC49 Then rotate by len % k\n\uD83D\uDC49 again store in the matrix\n\'\'\'\n\n\tclass Solution:\n def rotateGrid(self, mat: List[List[int]], k: int) -> List[List[int]]:\n \n top = 0\n bottom = len(mat)-1\n left = 0\n right = len(mat[0])-1\n res = []\n\t\t\n # storing in res all the boundry matrix elements.\n while left<right and top<bottom:\n local=[]\n for i in range(left,right+1):\n local.append(mat[top][i])\n top+=1\n\t\t\t\n for i in range(top,bottom+1):\n local.append(mat[i][right])\n right-=1\n\t\t\t\n for i in range(right,left-1,-1):\n local.append(mat[bottom][i])\n bottom-=1\n\t\t\t\n for i in range(bottom,top-1,-1):\n local.append(mat[i][left])\n left+=1\n \n res.append(local)\n\t\t\t\n\t\t# rotating the elements by k.\n for ele in res:\n l=len(ele)\n r=k%l\n ele[::]=ele[r:]+ele[:r]\n \n\t\t# Again storing in the matrix. \n top = 0\n bottom = len(mat)-1\n left = 0\n right = len(mat[0])-1\n while left<right and top<bottom:\n local=res.pop(0)\n for i in range(left,right+1):\n mat[top][i] = local.pop(0)\n top+=1\n\t\t\t\n for i in range(top,bottom+1):\n mat[i][right] = local.pop(0)\n right-=1\n\t\t\t\n for i in range(right,left-1,-1):\n mat[bottom][i] = local.pop(0)\n bottom-=1\n\t\t\t\n for i in range(bottom,top-1,-1):\n mat[i][left] = local.pop(0)\n left+=1\n \n return mat\n\t\t\nIf you got helped ...... Please **upvote !!** \uD83E\uDD1E\nif any doubt feel free to ask \uD83E\uDD17 | 7 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
Rotate in-place. Space O(1). Time O(n). | cyclically-rotating-a-grid | 0 | 1 | ```\r\nclass Solution:\r\n def translate(self, i, a, bx, by):\r\n dx, dy = bx-a-1, by-a-1\r\n if i <= dy:\r\n return (a, i+a)\r\n i -= dy\r\n if i <= dx:\r\n return (i+a, by-1)\r\n i -= dx\r\n if i <= dy:\r\n return (bx-1, by-i-1)\r\n i -= dy\r\n return (bx-i-1, a)\r\n\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n c, n, m = 0, len(grid), len(grid[0])\r\n while c < n-c and c < m-c:\r\n l = (n+m-c*4-2)*2\r\n i, start = 0, 0\r\n for _ in range(l):\r\n if i == start:\r\n start += 1\r\n i = start\r\n x, y = self.translate(i, c, n-c, m-c)\r\n curr_num = grid[x][y]\r\n temp = curr_num\r\n i = (l+i-k)%l\r\n x, y = self.translate(i, c, n-c, m-c)\r\n curr_num = grid[x][y]\r\n grid[x][y] = temp\r\n c += 1\r\n return grid\r\n``` | 0 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
[Python3] - Layerwise Modulo Rotation - O(m+n) Space - O(m*n) Time | cyclically-rotating-a-grid | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\nFirst thing is to understand that its way better to compute the updated position of an element in O(1) other than simulating every of the k shifts.\r\n\r\nWe then need a function that computes the rx and cx for every position in one layer. I called that function next_position. With that, one can compute row index and column index for a given layer, while the position is a counter clockwise linear index.\r\n\r\nE.G. for a 4x6 matrix outer layer\r\n0 15 14 13 12 11\r\n1 10\r\n2 9\r\n3 4 5 6 7 8\r\n\r\nAt first, I wanted to do the rotation also in place but it was too complicated for me to directly do, so I introduced an auxiliary array for each layer, which I first fill with the values and then place those values back into the original matrix.\r\n\r\nThis makes it easier for me to keep track of the changes.\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\nLayer wise rotation and linear indexing.\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\nO(m*n) as we need to touch every element in the matrix once.\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\nO(m+n) in the worst case for the auxiliary array of the outermost layer.\r\n# Code\r\n```\r\nclass Solution:\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n\r\n # we can do the rotation by rotating every layer (write a function\r\n # for that) also we can save time by computing the modulo and \r\n # rotation direction\r\n\r\n # save the dimensions of the grid\r\n m = len(grid)\r\n n = len(grid[0])\r\n\r\n # make a function to compute the next position in terms of rows\r\n # and columns\r\n def next_position(position, layer):\r\n\r\n # compute how many numbers we need to rotate in this layer\r\n # (two times the number of elements in a row\r\n # plus the elements in the column while subtracting elements\r\n # that are already counted by the row)\r\n counter = 2*(m-2*layer) + 2*(n-2*layer-2)\r\n\r\n # compute the modulo of the position so we do not encounter\r\n # shady positions\r\n position = position % counter\r\n\r\n if position < (m-2*layer-1):\r\n # print(\'left\')\r\n return layer+position, layer\r\n elif position < (m-2*layer-1)+(n-2*layer-1):\r\n # print(\'down\')\r\n return m-layer-1, layer + position - (m-2*layer) + 1\r\n elif position < 2*(m-2*layer-1)+(n-2*layer-1):\r\n # print(\'right\')\r\n return (m-layer-1) - (position - (m-2*layer-1) - (n-2*layer-1)), n-layer-1\r\n else:\r\n # print(\'up\')\r\n return layer, (n-layer-1) - (position - 2*(m-2*layer-1) - (n-2*layer-1))\r\n\r\n # first write a function that rotates a layer by x positions\r\n def rotate_layer(layer):\r\n\r\n # compute how many numbers we need to rotate in this layer\r\n # (two times the number of elements in a row\r\n # plus the elements in the column while subtracting elements\r\n # that are already counted by the row)\r\n counter = 2*(m-2*layer) + 2*(n-2*layer-2)\r\n \r\n # make an array to fill the numbers (we could do it in place\r\n # but that is to complicated for me currently)\r\n new_elements = [0]*counter\r\n\r\n # go through the matrix and rotate by position\r\n for idx in range(0, counter):\r\n\r\n # get the indices where we need to look for the current number\r\n rx, cx = next_position(idx, layer)\r\n # print(\'Layer\', layer, \'Position\', idx, \'Coordinates\', rx, cx)\r\n\r\n # compute the new index\r\n nx = (idx+k) % counter\r\n\r\n # fill in the array\r\n new_elements[nx] = grid[rx][cx]\r\n \r\n # go through the original array and fill in the values\r\n for idx in range(0, counter):\r\n\r\n # get the indices where we need to look for the current number\r\n rx, cx = next_position(idx, layer)\r\n\r\n # fill in the array\r\n grid[rx][cx] = new_elements[idx]\r\n \r\n # go through each layer and make the rotation\r\n for lay in range(min(m, n)//2):\r\n rotate_layer(lay)\r\n return grid\r\n``` | 0 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
[Python3] Solving like Spiral Matrix | cyclically-rotating-a-grid | 0 | 1 | # Code\r\n```\r\nclass Solution:\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n m = len(grid)\r\n n = len(grid[0])\r\n rDir = [0, 1, 0, -1]\r\n cDir = [1, 0, -1, 0]\r\n\r\n dir = 0\r\n l = m*n\r\n\r\n r = 0\r\n c = 0\r\n\r\n lst = []\r\n cur = []\r\n\r\n for i in range(l):\r\n cur.append(grid[r][c])\r\n grid[r][c] = -1000\r\n\r\n newR = r + rDir[dir]\r\n newC = c + cDir[dir]\r\n\r\n if newR < 0 or newR >= m or newC < 0 or newC >= n or grid[newR][newC] == -1000:\r\n dir += 1\r\n if dir == 4:\r\n dir = 0\r\n\r\n tmp = []\r\n for e in cur:\r\n tmp.append(e)\r\n lst.append(tmp)\r\n cur = []\r\n\r\n r += rDir[dir]\r\n c += cDir[dir]\r\n \r\n lst.append(cur)\r\n\r\n dir = 0\r\n r = 0\r\n c = 0\r\n\r\n level = 0\r\n idx = k % len(lst[level])\r\n\r\n seen = [[False for i in range(n)] for j in range(m)]\r\n for i in range(l):\r\n if idx == len(lst[level]):\r\n idx = 0\r\n grid[r][c] = lst[level][idx]\r\n idx += 1\r\n seen[r][c] = True\r\n\r\n newR = r + rDir[dir]\r\n newC = c + cDir[dir]\r\n\r\n if newR < 0 or newR >= m or newC < 0 or newC >= n or seen[newR][newC]:\r\n dir += 1\r\n \r\n if dir == 4: \r\n dir = 0\r\n level += 1\r\n\r\n if len(lst[level]) == 0:\r\n break\r\n idx = k % len(lst[level])\r\n\r\n r += rDir[dir]\r\n c += cDir[dir]\r\n\r\n return grid\r\n``` | 0 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
[Spaghetti Code] what did i write..... | cyclically-rotating-a-grid | 0 | 1 | # Intuition\r\nI know this hurt your eyes.. buy i really want to post it out......\r\n**DO NOT LEARN FROM THIS !@#!**\r\n\r\n\r\n# Readability\r\nNone, zero, written by idiot\r\n\r\n# Approach\r\ngroup by layer --> reshape layer in clockwise manner --> add offset and transform back to traditional array\r\n\r\n# Complexity\r\n- Time complexity:\r\nO(M*N)\r\n\r\n- Space complexity:\r\nO(M*N)\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def rotateGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:\r\n\r\n #element ordered in row manner, grouped by layer\r\n rows=len(grid)\r\n cols=len(grid[0])\r\n layers=min(rows//2,cols//2)\r\n sliced_grid=[[] for _ in range(layers)]\r\n for i in range(rows):\r\n layers_in_this_row=min(i+1,rows-i)\r\n for j in range(cols):\r\n sliced_grid[min(min(j,cols-j-1),layers_in_this_row-1)].append(grid[i][j])\r\n print(sliced_grid)\r\n\r\n #reshape layer, element ordered in clockwise manner\r\n reshaped=[[0 for __ in range(len(slice)) ] for slice in sliced_grid]\r\n for layer,layer_item in enumerate(sliced_grid):\r\n back_trace_id=-1\r\n back=True\r\n top_row_element_count=cols-layer*2\r\n for idx,item in enumerate(layer_item):\r\n if idx<top_row_element_count:\r\n reshaped[layer][idx]=item\r\n elif idx<len(layer_item)-top_row_element_count and back:\r\n reshaped[layer][back_trace_id]=item\r\n back=not back\r\n back_trace_id-=1\r\n elif idx<len(layer_item)-top_row_element_count and not back: \r\n reshaped[layer][idx+back_trace_id+1]=item\r\n back=not back\r\n else:\r\n reshaped[layer][back_trace_id]=item\r\n back_trace_id-=1\r\n print(reshaped)\r\n\r\n #reconstruct to traditional row format, rotate by offset\r\n offset=k\r\n reconstruct=[[0 for _ in range(cols)] for _ in range(rows)]\r\n offset_count=[[0,len(reshaped[layer])-1,True] for layer in range(layers)]\r\n for i in range(rows):\r\n layers_in_this_row=min(i+1,rows-i)\r\n for j in range(cols):\r\n layer=min(min(j,cols-j-1),layers_in_this_row-1)\r\n top_row_element_count=cols-layer*2\r\n if offset_count[layer][0]<top_row_element_count:\r\n reconstruct[i][j]=reshaped[layer][(offset+offset_count[layer][0])%len(reshaped[layer])]\r\n offset_count[layer][0]+=1\r\n elif offset_count[layer][0]<len(reshaped[layer])-top_row_element_count and offset_count[layer][2]:\r\n reconstruct[i][j]=reshaped[layer][(offset+offset_count[layer][1])%len(reshaped[layer])]\r\n offset_count[layer][0]+=1\r\n offset_count[layer][1]-=1\r\n offset_count[layer][2] = not offset_count[layer][2]\r\n elif offset_count[layer][0]<len(reshaped[layer])-top_row_element_count and not offset_count[layer][2]: \r\n reconstruct[i][j]=reshaped[layer][(offset+offset_count[layer][0]-(len(reshaped[layer])-offset_count[layer][1]-1))%len(reshaped[layer])]\r\n offset_count[layer][0]+=1\r\n offset_count[layer][2] = not offset_count[layer][2]\r\n else: \r\n reconstruct[i][j]=reshaped[layer][(offset+offset_count[layer][1])%len(reshaped[layer])]\r\n offset_count[layer][1]-=1\r\n\r\n print(reconstruct)\r\n return reconstruct\r\n\r\n\r\n\r\n``` | 0 | You are given an `m x n` integer matrix `grid`, where `m` and `n` are both **even** integers, and an integer `k`.
The matrix is composed of several layers, which is shown in the below image, where each color is its own layer:
A cyclic rotation of the matrix is done by cyclically rotating **each layer** in the matrix. To cyclically rotate a layer once, each element in the layer will take the place of the adjacent element in the **counter-clockwise** direction. An example rotation is shown below:
Return _the matrix after applying_ `k` _cyclic rotations to it_.
**Example 1:**
**Input:** grid = \[\[40,10\],\[30,20\]\], k = 1
**Output:** \[\[10,20\],\[40,30\]\]
**Explanation:** The figures above represent the grid at every state.
**Example 2:**
**Input:** grid = \[\[1,2,3,4\],\[5,6,7,8\],\[9,10,11,12\],\[13,14,15,16\]\], k = 2
**Output:** \[\[3,4,8,12\],\[2,11,10,16\],\[1,7,6,15\],\[5,9,13,14\]\]
**Explanation:** The figures above represent the grid at every state.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `2 <= m, n <= 50`
* Both `m` and `n` are **even** integers.
* `1 <= grid[i][j] <= 5000`
* `1 <= k <= 109` | null |
Python Solution | Detailed Explenation | number-of-wonderful-substrings | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n- We want to iterate through all substrings and check if each one has at most 1 oddly occurring letter\r\n- Tracking odd/even counts directly for all letters would be complicated\r\n- Instead, we can encode the state as a bitmask to track odd/even\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n- Use a mask to encode 10 letter odd/even counts in binary\r\n- Flip the bit when we see the letter to change odd/even count\r\n- Check if mask is seen before to get all valid substrings with that state\r\n- Dynamic programming to store state counts and reuse\r\n# Complexity\r\n## Time complexity : O(N)\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n- Iterate string of length N\r\n- Checking mask counts is O(1) with array\r\n## Space complexity: O(1)\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n- cnt array stores 1024 state combinations\r\n- Not dependent on input size\r\n- Constant extra space\r\n# Code\r\n```\r\nclass Solution:\r\n def wonderfulSubstrings(self, word: str) -> int:\r\n cnt = [0] * 1024\r\n cnt[0] = 1\r\n \r\n ans = 0\r\n mask = 0\r\n \r\n chars = list(word)\r\n for c in chars:\r\n idx = ord(c) - ord(\'a\')\r\n mask ^= 1 << idx\r\n ans += cnt[mask]\r\n \r\n for i in range(10):\r\n lookFor = mask ^ (1 << i)\r\n ans += cnt[lookFor]\r\n \r\n cnt[mask] += 1\r\n \r\n return ans\r\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
[Python 3] Bitmask - O(n), O(1) | number-of-wonderful-substrings | 0 | 1 | \n# Code\n```\nclass Solution:\n def wonderfulSubstrings(self, word: str) -> int:\n seen = defaultdict(int)\n seen[0] += 1\n ans = 0\n mask = 0\n for c in word:\n mask ^= (1 << (ord(c) - ord(\'a\')))\n ans += seen[mask]\n for i in range(ord(\'j\') - ord(\'a\') + 1):\n ans += seen[mask ^ (1 << i)]\n seen[mask] += 1\n return ans\n``` | 1 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
[Python3] freq table w. mask | number-of-wonderful-substrings | 0 | 1 | \n```\nclass Solution:\n def wonderfulSubstrings(self, word: str) -> int:\n ans = mask = 0\n freq = defaultdict(int, {0: 1})\n for ch in word: \n mask ^= 1 << ord(ch)-97\n ans += freq[mask]\n for i in range(10): ans += freq[mask ^ 1 << i]\n freq[mask] += 1\n return ans \n``` | 11 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Python O(n) solution with comments, Prefix sum, bit mask | number-of-wonderful-substrings | 0 | 1 | explaination for this `res+=dp[curr ^(1<<i)]`.\nIntuition :\nThis was similar to solving the problem of finding the subarray of sum k. This can be solved using prefix sum concept . For example given an array a= [ 2, 3 ,4 ,5 ,10] and k=9, we can calculate running sum and store Its prefix sum array as dp=[2,5,9,14,24]. Here for a running sum "rs =14 " which occured at index j=3, we can check if "rs - k=14-9=5" is available in dp array. let\'s say "rs - k=5" was available at index i=1 (i<j) then subarray a[i+1 : j ]=a[2:3] will sum upto k=9.\n\nNow coming to this problem, let\'s say we want a subarray which gives 1000000000 and we have a "curr" value( similar to running sum mentioned above ) so we should check if dp[ curr - 1000000000] . But since these are binary numbers dp[ curr - 1000000000] is equivalent to dp[ curr ^ 1000000000] --> dp[ curr^(1<<pos)] here pos=10. \nThe reason for checking ``curr^1000000000`` was `curr ^ (curr^1000000000) = 1000000000 `.\nSimilarily we want to check all other possibilities like 0100000000, 0010000000,... etc. All these cases corresponds to having one character with odd count and others with even count\n\n\n```\nclass Solution:\n def wonderfulSubstrings(self, word: str) -> int:\n \n # we are representing each char with a bit, 0 for count being even and 1 for odd\n # 10 char from a to j\n # array to store 2^10 numbers\n dp=[0]*1024\n \n # jihgfedcba -> 0000000000\n curr=0 # 000..(0-> 10 times) \n \n # since we are starting with curr as 0 make dp[0]=1\n dp[0]=1\n \n # result\n res=0\n \n for c in word:\n # 1<<i sets i th bit to 1 and else to 0\n # xor will toggle the bit\n curr^= (1<<(ord(c)-ord(\'a\')))\n \n # if curr occurred earlier at j and now at i then [j+1: i] has all zeroes\n # this was to count all zeroes case\n res+=dp[curr]\n \n # now to check if these 100000..,010000..,001.. cases can be acheived using brute force\n # we want to see if curr ^ delta = 10000.. or 010000.. etc\n # curr^delta =1000... then\n # curr ^ 1000.. = delta\n \n for i in range(10):\n res+=dp[curr ^(1<<i)] \n \n dp[curr]+=1 \n \n \n \n return res\n``` | 6 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Easiest Python Solution using bit manipulation | number-of-wonderful-substrings | 0 | 1 | # Code\r\n```\r\nclass Solution:\r\n def wonderfulSubstrings(self, word: str) -> int:\r\n ans=mask=0 #consider mask be 0\r\n freq=defaultdict(int,{0:1}) #for counting freq if that mask may already appear or not\r\n\r\n for i in word:\r\n mask^=1<<ord(i)-97 # mask it \r\n #doing as follows:\r\n # let a = 001\r\n # let b = 010\r\n # let c = 100\r\n # let d = 1000 and so on \r\n \r\n ans+=freq[mask] # in this step checking and adding mask already appear or not \r\n # for even occurence\r\n # example let s="ababa"\r\n # mask value of each char 13201\r\n # see in this test case XOR till second last char will be 0 as all pairs it will also consider WOnderful \r\n #but see at last char mask is 1 therefore their is anthore Wonderfiul substring i.e baba \r\n\r\n for j in range(10):\r\n\r\n ans+=freq[mask^1<<j] #for odd no Wonderful substring of atleast 1 we will check mask for each char as \'a\' to \'j\' \r\n #we will XOR mask with each value \r\n #if mask become zero that means it is appearing only once as XOR of 0 ^0=0 and 1^1=0\r\n \r\n freq[mask]+=1 #adding to dict \r\n return ans\r\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Python Solution | number-of-wonderful-substrings | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def wonderfulSubstrings(self, word: str) -> int:\r\n mask = {}\r\n base = 1\r\n for i in range(ord(\'a\'), ord(\'j\')+1):\r\n a = chr(i)\r\n mask[a] = base\r\n base = base << 1\r\n\r\n prefix = 0\r\n prefix_dict = defaultdict(int)\r\n prefix_dict[prefix] = 1\r\n ret = 0\r\n for w in word:\r\n prefix = prefix ^ mask[w]\r\n ret += prefix_dict[prefix]\r\n for a in mask:\r\n ret += prefix_dict[prefix ^ mask[a]]\r\n prefix_dict[prefix] += 1\r\n return ret\r\n\r\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Counting Wonderful Substrings: An Efficient Algorithm Using Bit Manipulation | number-of-wonderful-substrings | 0 | 1 | # Intuition\r\nThe problem is about counting the number of "wonderful" substrings in a given word. A "wonderful" substring is one in which at most one character occurs an odd number of times. The first thought is to check every possible substring, but that would be inefficient. A better way might be to use bitwise manipulation to keep track of odd and even occurrences of characters in the substrings.\r\n\r\n## Approach\r\n1. **Initialize Variables**: Use a `mask` variable to keep track of the odd/even occurrence of each character in the substring. Use a `freq_counter` dictionary to keep track of the frequency of each mask value, which helps in counting the number of "wonderful" substrings efficiently.\r\n2. **Iterate Through the String**: Iterate through the string, updating the `mask` at each step.\r\n3. **Counting Substrings**: Utilize the updated `mask` and `freq_counter` to count the number of "wonderful" substrings that end at the current character.\r\n4. **Special Case**: Special consideration is given to substrings that have exactly one character with an odd frequency, by toggling each bit one at a time and checking if that \'flipped\' mask has appeared before.\r\n\r\n## Complexity\r\n\r\n- **Time Complexity**: The time complexity is $O(n)$ where $ n $ is the length of the word. We go through each character once, and for each character, we perform constant-time operations.\r\n \r\n- **Space Complexity**: The space complexity is $O(1)$ as we only use a constant amount of extra space. The maximum number of unique masks is $2^{10} = 1024$, which is a constant number because the input word consists of the first ten lowercase English letters (\'a\' through \'j\').\r\n\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def wonderfulSubstrings(self, word: str) -> int:\r\n count = 0\r\n mask = 0\r\n freq_counter = {0: 1} # To account for empty substring\r\n \r\n for char in word:\r\n # Update the mask for the current character\r\n mask ^= 1 << (ord(char) - ord(\'a\'))\r\n \r\n # Count "wonderful" substrings ending at this character\r\n count += freq_counter.get(mask, 0)\r\n \r\n # Check for "wonderful" substrings that have exactly one character with odd frequency\r\n for i in range(10): # Since there are only 10 different letters (\'a\' to \'j\')\r\n count += freq_counter.get(mask ^ (1 << i), 0)\r\n \r\n # Update frequency counter\r\n freq_counter[mask] = freq_counter.get(mask, 0) + 1\r\n \r\n return count\r\n\r\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Python Solution using bit-masking and find pair which have xor equal to target in O(n*10) and O(1) | number-of-wonderful-substrings | 0 | 1 | # Code\n```\nclass Solution:\n def wonderfulSubstrings(self, word: str) -> int:\n s=ans=0\n d={0:1}\n for val in word:\n s^=(1<<(ord(val)-97))\n for item in [0,1,2,4,8,16,32,64,128,256,512]:\n if(item^s in d):\n ans+=d[item^s]\n if(s in d):\n d[s]+=1\n else:\n d[s]=1\n return ans\n \n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Bitwise Masking for Efficiently Counting Wonderful Substring (91% beats) | number-of-wonderful-substrings | 0 | 1 | # Intuition\r\nGiven a word, our objective is to find out how many of its substrings are "wonderful". A substring is considered "wonderful" if at most one of its characters appears an odd number of times. The direct approach would be to generate all substrings and then check the frequency of each character in every substring, but this method is inefficient for longer words. Instead, using bitwise operations, we can track the odd and even occurrences of each character in an efficient manner. A binary mask works well for this, where each bit in the mask signifies the odd/even state for each character in the word.\r\n\r\n# Approach\r\n\r\n1. **Mask Representation**: Each of the 10 lowercase English letters can be represented using a single bit. If the bit is `1`, it indicates that the character has been seen an odd number of times so far; if `0`, it\'s been seen an even number of times.\r\n2. **Iterate Over the Word**: As we iterate over each character in the word, we update our mask to reflect the new state (odd/even) of the characters seen so far.\r\n3. **Counting Wonderful Substrings**: We maintain a count of how often each possible mask has appeared. As we iterate over the word, for each mask, we look at the previous counts of the same mask and the counts of masks that differ from the current one by just one bit.\r\n4. **Return the Result**: Sum up all the counts to get the total number of "wonderful" substrings.\r\n\r\n# Complexity\r\n- Time complexity: `O(n)`, where `n` is the length of the word. We iterate over the word once, and for each character, we perform constant-time operations.\r\n\r\n- Space complexity: : `O(1)`, since the size of the count array is constant (1025 elements, regardless of the word\'s length).\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def wonderfulSubstrings(self, word: str) -> int:\r\n mask, count = 0, [1] + [0] * 1024\r\n res = 0\r\n for ch in word:\r\n mask ^= 1 << (ord(ch) - ord(\'a\'))\r\n res += count[mask]\r\n for i in range(10):\r\n res += count[mask ^ (1 << i)]\r\n count[mask] += 1\r\n return res\r\n\r\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Python | Bitmask | O(10n) | number-of-wonderful-substrings | 0 | 1 | # Code\n```\nfrom collections import defaultdict\nclass Solution:\n def wonderfulSubstrings(self, word: str) -> int:\n d = defaultdict(int)\n mask = 0\n d[mask] = 1\n res = 0\n for c in word:\n mask ^= 1 << (ord(c)-ord(\'a\'))\n res += d[mask]\n for i in range(10):\n res += d[mask ^ (1 << i)]\n d[mask] += 1\n return res\n\n\n``` | 0 | A **wonderful** string is a string where **at most one** letter appears an **odd** number of times.
* For example, `"ccjjc "` and `"abab "` are wonderful, but `"ab "` is not.
Given a string `word` that consists of the first ten lowercase English letters (`'a'` through `'j'`), return _the **number of wonderful non-empty substrings** in_ `word`_. If the same substring appears multiple times in_ `word`_, then count **each occurrence** separately._
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** word = "aba "
**Output:** 4
**Explanation:** The four wonderful substrings are underlined below:
- "**a**ba " -> "a "
- "a**b**a " -> "b "
- "ab**a** " -> "a "
- "**aba** " -> "aba "
**Example 2:**
**Input:** word = "aabb "
**Output:** 9
**Explanation:** The nine wonderful substrings are underlined below:
- "**a**abb " -> "a "
- "**aa**bb " -> "aa "
- "**aab**b " -> "aab "
- "**aabb** " -> "aabb "
- "a**a**bb " -> "a "
- "a**abb** " -> "abb "
- "aa**b**b " -> "b "
- "aa**bb** " -> "bb "
- "aab**b** " -> "b "
**Example 3:**
**Input:** word = "he "
**Output:** 2
**Explanation:** The two wonderful substrings are underlined below:
- "**h**e " -> "h "
- "h**e** " -> "e "
**Constraints:**
* `1 <= word.length <= 105`
* `word` consists of lowercase English letters from `'a'` to `'j'`. | The answer is false if the number of nonequal positions in the strings is not equal to 0 or 2. Check that these positions have the same set of characters. |
Recursive Python Solution | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\nSince we need to build certain rooms before others, it is clear we need to use topological sorting to find a valid combination.\r\n\r\nBut how do we get all combinations that are valid? Let\'s think in simpler cases:\r\n1. If the tree consists of one path, there is only one valid combination (See the first example)\r\n2. If the tree has two branches. The left branch has nodes (a1,a2,a3,a4) in topo sorted order. The right branch has nodes (b1,b2,b3,b4) in topo sorted order. Then, the valid combinations would be any mix of the sequence (a1,a2,a3,a4) interspersed with the sequence (b1,b2,b3,b4), such as (a1,a2,b1,b2,b3,a3,a4,b4) for example. The number of possible combinations like this is described by the **stars and bars** combinatorics problem. Specifically for this problem we can do (len(sequence1)+len(sequence2)) Choose (min(len(sequence1),len(sequence2))) to get the total number of combinations.\r\n3. If the tree has >2 branches, then we can split our problem into chunks: first determine the valid combinations of the first pair of sequences. Then, treating the first pair of sequences as one combined sequence, find the number of combinations between this combined sequence and the next branch, multiplying the result to the current answer. Repeat this until all branches have been explored.\r\n\r\n# Approach\r\nIt will be much easier to do this problem recursively using DFS. At each call, we return the number of nodes in the current tree as this is the number of items in the sequence of a subtree.\r\n\r\n# Complexity\r\n- Time complexity:\r\nO(n) time for tree traversal (though this inaccurately assumes the time to calculate combinations is O(1))\r\n\r\n- Space complexity:\r\nO(n)\r\n\r\n# Code\r\n```\r\nfrom math import comb\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n modulo = 10**9+7\r\n ingoing = defaultdict(set)\r\n outgoing = defaultdict(set)\r\n for i in range(1,len(prevRoom)):\r\n ingoing[i].add(prevRoom[i])\r\n outgoing[prevRoom[i]].add(i)\r\n ans = [1]\r\n def r(i):\r\n if len(outgoing[i]) == 0:\r\n return 1 # just self\r\n nodes_in_tree = 0\r\n for v in outgoing[i]:\r\n cn = r(v)\r\n if nodes_in_tree != 0:\r\n ans[0] *= comb(nodes_in_tree+cn,cn)\r\n ans[0] %= modulo\r\n nodes_in_tree += cn\r\n \r\n return nodes_in_tree + 1\r\n \r\n r(0)\r\n \r\n return ans[0] % modulo\r\n``` | 1 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
Recursive Python Solution | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\nSince we need to build certain rooms before others, it is clear we need to use topological sorting to find a valid combination.\r\n\r\nBut how do we get all combinations that are valid? Let\'s think in simpler cases:\r\n1. If the tree consists of one path, there is only one valid combination (See the first example)\r\n2. If the tree has two branches. The left branch has nodes (a1,a2,a3,a4) in topo sorted order. The right branch has nodes (b1,b2,b3,b4) in topo sorted order. Then, the valid combinations would be any mix of the sequence (a1,a2,a3,a4) interspersed with the sequence (b1,b2,b3,b4), such as (a1,a2,b1,b2,b3,a3,a4,b4) for example. The number of possible combinations like this is described by the **stars and bars** combinatorics problem. Specifically for this problem we can do (len(sequence1)+len(sequence2)) Choose (min(len(sequence1),len(sequence2))) to get the total number of combinations.\r\n3. If the tree has >2 branches, then we can split our problem into chunks: first determine the valid combinations of the first pair of sequences. Then, treating the first pair of sequences as one combined sequence, find the number of combinations between this combined sequence and the next branch, multiplying the result to the current answer. Repeat this until all branches have been explored.\r\n\r\n# Approach\r\nIt will be much easier to do this problem recursively using DFS. At each call, we return the number of nodes in the current tree as this is the number of items in the sequence of a subtree.\r\n\r\n# Complexity\r\n- Time complexity:\r\nO(n) time for tree traversal (though this inaccurately assumes the time to calculate combinations is O(1))\r\n\r\n- Space complexity:\r\nO(n)\r\n\r\n# Code\r\n```\r\nfrom math import comb\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n modulo = 10**9+7\r\n ingoing = defaultdict(set)\r\n outgoing = defaultdict(set)\r\n for i in range(1,len(prevRoom)):\r\n ingoing[i].add(prevRoom[i])\r\n outgoing[prevRoom[i]].add(i)\r\n ans = [1]\r\n def r(i):\r\n if len(outgoing[i]) == 0:\r\n return 1 # just self\r\n nodes_in_tree = 0\r\n for v in outgoing[i]:\r\n cn = r(v)\r\n if nodes_in_tree != 0:\r\n ans[0] *= comb(nodes_in_tree+cn,cn)\r\n ans[0] %= modulo\r\n nodes_in_tree += cn\r\n \r\n return nodes_in_tree + 1\r\n \r\n r(0)\r\n \r\n return ans[0] % modulo\r\n``` | 1 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
Explanation of Another Users Solution | Comments, Explanation, Example | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\nAll credit for intution goes to user solution at https://leetcode.com/problems/count-ways-to-build-rooms-in-an-ant-colony/solutions/1299545/python3-post-order-dfs/\r\n\r\nI include comments, explanation and example walkthrough for completeness\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\nThe solution works by first building the tree of index connections indicated by previous room. \r\n\r\nOnce this is done, based on a node_ids neighbors the tree can be traversed to determine the number of combinations and builds. The key insight is that a leaf has 1 node, and 1 way to build it (they are the end of the DFS) \r\n\r\nFrom this, the tree can now map the rooms that have no previous id as these are necessarily the leafs \r\n\r\nFrom here, we can kick off the dfs from root 0 and determine the values via a consideration of number of rooms and their permutation impact on the number of ways to build the ant colony \r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n # make a tree map of the previous rooms by enumeration of prevRoom \r\n tree = collections.defaultdict(list)\r\n for index, room in enumerate(prevRoom) : tree[room].append(index)\r\n\r\n # dfs based on node id, where if node id is not recorded, there are 1 nodes and 1 way to build a leaf node\r\n def dfs(node_id) : \r\n if not tree[node_id] : return 1, 1\r\n else : \r\n # otherwise, we start with 0 num nodes and 1 way to build \r\n num_nodes, ways_to_build = 0, 1 \r\n # and recursively consider all neighbor nodes of this node in the tree \r\n for neighbors_id in tree[node_id] : \r\n # where for each neighbor, we conduct this same dfs \r\n neighbors_num_nodes, neighbors_ways = dfs(neighbors_id)\r\n # updating our number of nodes by incrementation \r\n num_nodes += neighbors_num_nodes\r\n # then calculating ways to build as the multiplication of self with n choose k where \r\n # n is number of nodes inclusive of current neighbors and k is neighbors num nodes \r\n # this represents how many ways we can build neighbors num nodes based on the current num nodes \r\n # and then multiply by neighbors ways for each of the ways the neighbors can build \r\n # this then in total represents the ways to build n choose k * multiple of neighbors methods \r\n # this increases as num_nodes increases, and is bound by modulo as well internally. Could be bound externally \r\n ways_to_build = (ways_to_build * math.comb(num_nodes, neighbors_num_nodes) * neighbors_ways) % 1_000_000_007\r\n # at end, return num nodes + 1 and ways to build \r\n return num_nodes + 1, ways_to_build\r\n\r\n # recursive walk through of Example 2 \r\n \'\'\'\r\n previousRooms generates Tree as \r\n Tree -> \r\n -1 : [0]\r\n 0 : [1, 2]\r\n 1 : [3]\r\n 2 : [4] \r\n \r\n Call dfs using 0 as node_id \r\n 0 is in tree, so we continue \r\n num nodes = 0, ways to build = 1 \r\n for neighbors_id in tree[0] -> for neighbors in [1, 2] \r\n neighbors_id = 1 \r\n neighbors_num_nodes, neighbors_ways = dfs(1)\r\n dfs(1) -> 1 in tree, so continue \r\n num_nodes_1, ways_to_build_1 = 0, 1 \r\n for neighbors_id in [3] \r\n neighbors_num_nodes, neighbors_ways = 1, 1 (3 is a leaf) \r\n num_nodes_1 = 1 \r\n ways_to_build_1 = (1 * 1 C 1 * 1) % mod = 1 \r\n return 1+1, 1 \r\n neighbors_num_nodes, neighbors_ways = 2, 1 \r\n num_nodes = 2\r\n ways_to_build = (1 * 2 C 2 * 1) % mod = 1 \r\n neighbors_id = 2 \r\n dfs(2) -> will return 2, 1 \r\n num_nodes = 4 \r\n ways to build = (1 * 4 C 2 * 1) % mod = 6 \r\n 4 C 2 is six as from [a, b, c, d] you have [a, [b, c, d]], [b, [c, d]], [c, d], where the second [] means choose one from\r\n return then 5, 6 (5 nodes, 6 ways to build)\r\n \'\'\'\r\n # return the number of ways to build the rooms \r\n return dfs(0)[1]\r\n\r\n``` | 1 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
Explanation of Another Users Solution | Comments, Explanation, Example | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\nAll credit for intution goes to user solution at https://leetcode.com/problems/count-ways-to-build-rooms-in-an-ant-colony/solutions/1299545/python3-post-order-dfs/\r\n\r\nI include comments, explanation and example walkthrough for completeness\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\nThe solution works by first building the tree of index connections indicated by previous room. \r\n\r\nOnce this is done, based on a node_ids neighbors the tree can be traversed to determine the number of combinations and builds. The key insight is that a leaf has 1 node, and 1 way to build it (they are the end of the DFS) \r\n\r\nFrom this, the tree can now map the rooms that have no previous id as these are necessarily the leafs \r\n\r\nFrom here, we can kick off the dfs from root 0 and determine the values via a consideration of number of rooms and their permutation impact on the number of ways to build the ant colony \r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n # make a tree map of the previous rooms by enumeration of prevRoom \r\n tree = collections.defaultdict(list)\r\n for index, room in enumerate(prevRoom) : tree[room].append(index)\r\n\r\n # dfs based on node id, where if node id is not recorded, there are 1 nodes and 1 way to build a leaf node\r\n def dfs(node_id) : \r\n if not tree[node_id] : return 1, 1\r\n else : \r\n # otherwise, we start with 0 num nodes and 1 way to build \r\n num_nodes, ways_to_build = 0, 1 \r\n # and recursively consider all neighbor nodes of this node in the tree \r\n for neighbors_id in tree[node_id] : \r\n # where for each neighbor, we conduct this same dfs \r\n neighbors_num_nodes, neighbors_ways = dfs(neighbors_id)\r\n # updating our number of nodes by incrementation \r\n num_nodes += neighbors_num_nodes\r\n # then calculating ways to build as the multiplication of self with n choose k where \r\n # n is number of nodes inclusive of current neighbors and k is neighbors num nodes \r\n # this represents how many ways we can build neighbors num nodes based on the current num nodes \r\n # and then multiply by neighbors ways for each of the ways the neighbors can build \r\n # this then in total represents the ways to build n choose k * multiple of neighbors methods \r\n # this increases as num_nodes increases, and is bound by modulo as well internally. Could be bound externally \r\n ways_to_build = (ways_to_build * math.comb(num_nodes, neighbors_num_nodes) * neighbors_ways) % 1_000_000_007\r\n # at end, return num nodes + 1 and ways to build \r\n return num_nodes + 1, ways_to_build\r\n\r\n # recursive walk through of Example 2 \r\n \'\'\'\r\n previousRooms generates Tree as \r\n Tree -> \r\n -1 : [0]\r\n 0 : [1, 2]\r\n 1 : [3]\r\n 2 : [4] \r\n \r\n Call dfs using 0 as node_id \r\n 0 is in tree, so we continue \r\n num nodes = 0, ways to build = 1 \r\n for neighbors_id in tree[0] -> for neighbors in [1, 2] \r\n neighbors_id = 1 \r\n neighbors_num_nodes, neighbors_ways = dfs(1)\r\n dfs(1) -> 1 in tree, so continue \r\n num_nodes_1, ways_to_build_1 = 0, 1 \r\n for neighbors_id in [3] \r\n neighbors_num_nodes, neighbors_ways = 1, 1 (3 is a leaf) \r\n num_nodes_1 = 1 \r\n ways_to_build_1 = (1 * 1 C 1 * 1) % mod = 1 \r\n return 1+1, 1 \r\n neighbors_num_nodes, neighbors_ways = 2, 1 \r\n num_nodes = 2\r\n ways_to_build = (1 * 2 C 2 * 1) % mod = 1 \r\n neighbors_id = 2 \r\n dfs(2) -> will return 2, 1 \r\n num_nodes = 4 \r\n ways to build = (1 * 4 C 2 * 1) % mod = 6 \r\n 4 C 2 is six as from [a, b, c, d] you have [a, [b, c, d]], [b, [c, d]], [c, d], where the second [] means choose one from\r\n return then 5, 6 (5 nodes, 6 ways to build)\r\n \'\'\'\r\n # return the number of ways to build the rooms \r\n return dfs(0)[1]\r\n\r\n``` | 1 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
[Python3] post-order dfs | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | \n```\nclass Solution:\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\n tree = defaultdict(list)\n for i, x in enumerate(prevRoom): tree[x].append(i)\n \n def fn(n): \n """Return number of nodes and ways to build sub-tree."""\n if not tree[n]: return 1, 1 # leaf \n c, m = 0, 1\n for nn in tree[n]: \n cc, mm = fn(nn)\n c += cc\n m = (m * comb(c, cc) * mm) % 1_000_000_007\n return c+1, m\n \n return fn(0)[1] \n```\n\n```\nMOD = 1_000_000_007\n\nclass Solution:\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\n tree = defaultdict(list)\n for i, x in enumerate(prevRoom): tree[x].append(i)\n \n N = 100001\n fact, ifact, inv = [1] * N, [1] * N, [1] * N\n for i in range(1, N): \n if i >= 2: inv[i] = (MOD - MOD//i) * inv[MOD % i] % MOD; \n fact[i] = fact[i-1] * i % MOD \n ifact[i] = ifact[i-1] * inv[i] % MOD \n \n def fn(n): \n """Return number of nodes and ways to build sub-tree."""\n if not tree[n]: return 1, 1 # leaf \n c, m = 0, 1\n for nn in tree[n]: \n cc, mm = fn(nn)\n c += cc\n m = m * mm * ifact[cc] % MOD\n return c+1, m * fact[c] % MOD\n \n return fn(0)[1]\n``` | 10 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
[Python3] post-order dfs | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | \n```\nclass Solution:\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\n tree = defaultdict(list)\n for i, x in enumerate(prevRoom): tree[x].append(i)\n \n def fn(n): \n """Return number of nodes and ways to build sub-tree."""\n if not tree[n]: return 1, 1 # leaf \n c, m = 0, 1\n for nn in tree[n]: \n cc, mm = fn(nn)\n c += cc\n m = (m * comb(c, cc) * mm) % 1_000_000_007\n return c+1, m\n \n return fn(0)[1] \n```\n\n```\nMOD = 1_000_000_007\n\nclass Solution:\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\n tree = defaultdict(list)\n for i, x in enumerate(prevRoom): tree[x].append(i)\n \n N = 100001\n fact, ifact, inv = [1] * N, [1] * N, [1] * N\n for i in range(1, N): \n if i >= 2: inv[i] = (MOD - MOD//i) * inv[MOD % i] % MOD; \n fact[i] = fact[i-1] * i % MOD \n ifact[i] = ifact[i-1] * inv[i] % MOD \n \n def fn(n): \n """Return number of nodes and ways to build sub-tree."""\n if not tree[n]: return 1, 1 # leaf \n c, m = 0, 1\n for nn in tree[n]: \n cc, mm = fn(nn)\n c += cc\n m = m * mm * ifact[cc] % MOD\n return c+1, m * fact[c] % MOD\n \n return fn(0)[1]\n``` | 10 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
Python 3 solution | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n kMod = 1_000_000_007\r\n graph = collections.defaultdict(list)\r\n\r\n for i, prev in enumerate(prevRoom):\r\n graph[prev].append(i)\r\n\r\n def dfs(node: int) -> Tuple[int, int]:\r\n if not graph[node]:\r\n return 1, 1\r\n\r\n ans = 1\r\n l = 0\r\n\r\n for child in graph[node]:\r\n temp, r = dfs(child)\r\n ans = (ans * temp * math.comb(l + r, r)) % kMod\r\n l += r\r\n\r\n return ans, l + 1\r\n\r\n return dfs(0)[0]\r\n\r\n``` | 0 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
Python 3 solution | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom: List[int]) -> int:\r\n kMod = 1_000_000_007\r\n graph = collections.defaultdict(list)\r\n\r\n for i, prev in enumerate(prevRoom):\r\n graph[prev].append(i)\r\n\r\n def dfs(node: int) -> Tuple[int, int]:\r\n if not graph[node]:\r\n return 1, 1\r\n\r\n ans = 1\r\n l = 0\r\n\r\n for child in graph[node]:\r\n temp, r = dfs(child)\r\n ans = (ans * temp * math.comb(l + r, r)) % kMod\r\n l += r\r\n\r\n return ans, l + 1\r\n\r\n return dfs(0)[0]\r\n\r\n``` | 0 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
Python (Simple Maths) | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom):\r\n dict1 = collections.defaultdict(list)\r\n\r\n for i in range(len(prevRoom)):\r\n dict1[prevRoom[i]].append(i)\r\n\r\n def dfs(n):\r\n if not dict1[n]:\r\n return 1, 1\r\n\r\n count, ways = 0, 1\r\n\r\n for i in dict1[n]:\r\n c, w = dfs(i)\r\n count += c\r\n ways = (ways*math.comb(count,c)*w)%(10**9+7)\r\n return count + 1, ways\r\n\r\n return dfs(0)[1]\r\n\r\n\r\n\r\n\r\n \r\n\r\n\r\n``` | 0 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
Python (Simple Maths) | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nclass Solution:\r\n def waysToBuildRooms(self, prevRoom):\r\n dict1 = collections.defaultdict(list)\r\n\r\n for i in range(len(prevRoom)):\r\n dict1[prevRoom[i]].append(i)\r\n\r\n def dfs(n):\r\n if not dict1[n]:\r\n return 1, 1\r\n\r\n count, ways = 0, 1\r\n\r\n for i in dict1[n]:\r\n c, w = dfs(i)\r\n count += c\r\n ways = (ways*math.comb(count,c)*w)%(10**9+7)\r\n return count + 1, ways\r\n\r\n return dfs(0)[1]\r\n\r\n\r\n\r\n\r\n \r\n\r\n\r\n``` | 0 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
[Python] Beats [94.74%, 40.35%] with easy simple structure | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | ```\nimport math\nclass Solution:\n def waysToBuildRooms(self, p: List[int]) -> int:\n def aux(l):\n sum_l = sum(l)\n l.sort(reverse=True)\n i = l[0]\n a = 1\n for j in range(1, len(l)):\n for k in range(l[j]):\n a *= (i+k+1)\n a = a//math.factorial(l[j])\n i += l[j]\n a = a%(10**9+7)\n return a\n \n n = len(p)\n if n == 2:\n return 1\n d_chil = {} # dict of children \n for i in range(1, n):\n if p[i] in d_chil.keys():\n d_chil[p[i]] += [i]\n else:\n d_chil[p[i]] = [i]\n \n d_n_chil = {}# dict of how many children a node has + 1(itself)\n def find_n_chil(idx):\n if idx not in d_chil.keys():\n d_n_chil[idx] = 1\n return 1\n a = 0\n for i in range(len(d_chil[idx])):\n a += find_n_chil(d_chil[idx][i])\n d_n_chil[idx] = a+1\n return a+1\n \n find_n_chil(0)\n \n def count_ways(idx):\n if idx not in d_chil.keys():\n return 1\n l = d_chil[idx]\n if len(l) == 1:\n return count_ways(l[0])\n else:\n a = 1\n s = []\n for i in l:\n a *= count_ways(i)\n a = a%(10**9+7)\n s += [d_n_chil[i]]\n a *= aux(s)\n return a%(10**9+7)\n return count_ways(0)\n``` | 1 | You are an ant tasked with adding `n` new rooms numbered `0` to `n-1` to your colony. You are given the expansion plan as a **0-indexed** integer array of length `n`, `prevRoom`, where `prevRoom[i]` indicates that you must build room `prevRoom[i]` before building room `i`, and these two rooms must be connected **directly**. Room `0` is already built, so `prevRoom[0] = -1`. The expansion plan is given such that once all the rooms are built, every room will be reachable from room `0`.
You can only build **one room** at a time, and you can travel freely between rooms you have **already built** only if they are **connected**. You can choose to build **any room** as long as its **previous room** is already built.
Return _the **number of different orders** you can build all the rooms in_. Since the answer may be large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** prevRoom = \[-1,0,1\]
**Output:** 1
**Explanation:** There is only one way to build the additional rooms: 0 -> 1 -> 2
**Example 2:**
**Input:** prevRoom = \[-1,0,0,1,2\]
**Output:** 6
**Explanation:**
The 6 ways are:
0 -> 1 -> 3 -> 2 -> 4
0 -> 2 -> 4 -> 1 -> 3
0 -> 1 -> 2 -> 3 -> 4
0 -> 1 -> 2 -> 4 -> 3
0 -> 2 -> 1 -> 3 -> 4
0 -> 2 -> 1 -> 4 -> 3
**Constraints:**
* `n == prevRoom.length`
* `2 <= n <= 105`
* `prevRoom[0] == -1`
* `0 <= prevRoom[i] < n` for all `1 <= i < n`
* Every room is reachable from room `0` once all the rooms are built. | The center is the only node that has more than one edge. The center is also connected to all other nodes. Any two edges must have a common node, which is the center. |
[Python] Beats [94.74%, 40.35%] with easy simple structure | count-ways-to-build-rooms-in-an-ant-colony | 0 | 1 | ```\nimport math\nclass Solution:\n def waysToBuildRooms(self, p: List[int]) -> int:\n def aux(l):\n sum_l = sum(l)\n l.sort(reverse=True)\n i = l[0]\n a = 1\n for j in range(1, len(l)):\n for k in range(l[j]):\n a *= (i+k+1)\n a = a//math.factorial(l[j])\n i += l[j]\n a = a%(10**9+7)\n return a\n \n n = len(p)\n if n == 2:\n return 1\n d_chil = {} # dict of children \n for i in range(1, n):\n if p[i] in d_chil.keys():\n d_chil[p[i]] += [i]\n else:\n d_chil[p[i]] = [i]\n \n d_n_chil = {}# dict of how many children a node has + 1(itself)\n def find_n_chil(idx):\n if idx not in d_chil.keys():\n d_n_chil[idx] = 1\n return 1\n a = 0\n for i in range(len(d_chil[idx])):\n a += find_n_chil(d_chil[idx][i])\n d_n_chil[idx] = a+1\n return a+1\n \n find_n_chil(0)\n \n def count_ways(idx):\n if idx not in d_chil.keys():\n return 1\n l = d_chil[idx]\n if len(l) == 1:\n return count_ways(l[0])\n else:\n a = 1\n s = []\n for i in l:\n a *= count_ways(i)\n a = a%(10**9+7)\n s += [d_n_chil[i]]\n a *= aux(s)\n return a%(10**9+7)\n return count_ways(0)\n``` | 1 | We are given a list `nums` of integers representing a list compressed with run-length encoding.
Consider each adjacent pair of elements `[freq, val] = [nums[2*i], nums[2*i+1]]` (with `i >= 0`). For each such pair, there are `freq` elements with value `val` concatenated in a sublist. Concatenate all the sublists from left to right to generate the decompressed list.
Return the decompressed list.
**Example 1:**
**Input:** nums = \[1,2,3,4\]
**Output:** \[2,4,4,4\]
**Explanation:** The first pair \[1,2\] means we have freq = 1 and val = 2 so we generate the array \[2\].
The second pair \[3,4\] means we have freq = 3 and val = 4 so we generate \[4,4,4\].
At the end the concatenation \[2\] + \[4,4,4\] is \[2,4,4,4\].
**Example 2:**
**Input:** nums = \[1,1,2,3\]
**Output:** \[1,3,3\]
**Constraints:**
* `2 <= nums.length <= 100`
* `nums.length % 2 == 0`
* `1 <= nums[i] <= 100` | Use dynamic programming. Let dp[i] be the number of ways to solve the problem for the subtree of node i. Imagine you are trying to fill an array with the order of traversal, dp[i] equals the multiplications of the number of ways to distribute the subtrees of the children of i on the array using combinatorics, multiplied bu their dp values. |
Python3 and C++ Solutions | build-array-from-permutation | 0 | 1 | # Solution in C++\n```\nclass Solution {\npublic:\n vector<int> buildArray(vector<int>& nums) {\n vector<int> answer;\n for (int i = 0; i < nums.size(); i++) {\n answer.push_back(nums[nums[i]]);\n }\n return answer;\n }\n};\n```\n# Solution in Python3\n```\nclass Solution:\n def buildArray(self, nums: List[int]) -> List[int]:\n answer = []\n for i in range(len(nums)):\n answer.append(nums[nums[i]])\n \n return answer\n``` | 2 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Simple python solution | build-array-from-permutation | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def buildArray(self, nums: List[int]) -> List[int]:\n ans=[]\n for i in nums:\n ans.append(nums[i])\n return ans\n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Simple Brute Force Method | build-array-from-permutation | 0 | 1 | # Code\n```\nclass Solution:\n def buildArray(self, nums: List[int]) -> List[int]:\n ans = []\n for i in range(len(nums)):\n ans.append(nums[nums[i]])\n return ans\n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
beginner friendly in python with memory beats of 100% | build-array-from-permutation | 0 | 1 | \nclass Solution:\n def buildArray(self, nums: List[int]) -> List[int]:\n lst=[]\n for i in range(len(nums)):\n lst.append(nums[nums[i]])\n return lst\n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Python one line simple solution | build-array-from-permutation | 0 | 1 | **Python :**\n\n```\ndef buildArray(self, nums: List[int]) -> List[int]:\n\treturn [nums[i] for i in nums]\n```\n\n**Like it ? please upvote !** | 48 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
【Video】Give me 10 minutes - O(n) solution - How we think about a solution | eliminate-maximum-number-of-monsters | 1 | 1 | # Intuition\nWe can calculate number of turns to arrive at the city\n\n# Approach\n\n---\n\n# Solution Video\n\nhttps://youtu.be/2tUBAJEn-S8\n\n\u25A0 Timeline of the video\n\n`0:04` Heap solution I came up with at first\n`2:38` Two key points with O(n) solution for Eliminate Maximum Number of Monsters\n`2:59` Explain the first key point\n`5:08` Explain the second key point\n`6:19` How we can eliminate monsters that are out of bounds\n`8:48` Let\'s see another case\n`9:52` How we can calculate eliminated numbers\n`10:56` Coding\n`13:03` Time Complexity and Space Complexity\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,966\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n---\n\n## How we think about a solution\n\n\nFirst of all, I came up with heap solution like this.\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n heap = []\n for i in range(len(dist)):\n heapq.heappush(heap, dist[i] / speed[i])\n \n res = 0\n\n while heap:\n if heapq.heappop(heap) <= res:\n break\n res += 1\n \n return res \n```\n\nThe heap has `number of turns` to arrive at city and we can eliminate monsters one by one that arrive at the city earlier, so `res` should be `number of monsters we can eliminate`, but it is considered as `chances` to try to eliminate them so far.\n\nSo if minimum turn in heap is less than or equal to `res`, monsters will arrive at the city before they are eliminated. The number of turns to arrive must be greater than or equal to `res`(chance) if you want to eliminate them.\n\nFor example\n```\nInput: dist = [1,2,2,3], speed = [1,1,1,1]\n```\n\nHeap has\n```\n[1.0, 2.0, 2.0, 3.0]\n```\nIn that case, we can eliminate `1.0` and one of `2.0` monsters. So now `res` should be `2`. \n\nLook at index `3`. there is another monster arriving at the city with `2` turns. But we elimiante `2.0` at index `2` when `res = 2`, so `2.0` at index `3` will reach the city before we elimiante it.\n\n```\noutput: 2\n```\n\nTime complexity is $$O(n log n)$$. I thought it was not bad. However result is slow. Actually I\'m not sure why. Is there special case?\n\nThat\'s how I try to think about $$O(n)$$ solution.\n\n\n---\n\n## O(n) solution\n\nWe have two points from the solution above.\n\n---\n\n\u2B50\uFE0F Points\n\n- We can calculate number of turns to arrive at the city.\n\n- We can eliminate monsters if number of turns to arrive is greater than or equal to chance to eliminate them.\n\n---\n\nLet me explain one by one.\n\n- **We can calculate number of turns to arrive at the city**\n\n```\nInput: dist = [1,3,4], speed = [1,1,1]\n```\n\nIn this case, There are 3 monsters, so create `monsters` list which is length of `3` with all `0` values.\n\n```\nmonsters = [0,0,0]\n\nIndex number is arrival(number of turns to arrive at the city)\nValue is number of monsters.\n```\n\n\nThen calculate each arrival\n```\nmath.ceil(dist[i] / speed[i])\n```\n\n- Why do we need `math.ceil`?\n\nBecause there are cases where we get float from `dist[i] / speed[i]`. Let\'s say we get `1.5`(dist = `3`, speed = `2`). In that case, the monster need `2` turns to arrive at the city. That\'s why we need to round it up. \n\nIf dist = `2` and speed = `2`, the monster need only `1` turn to arrive at the city.\n\nIn the end, we will have `monsters` list like this\n\n```\nmonsters = [0,1,0]\n\nmath.ceil(1 / 1) = 1\nmath.ceil(3 / 1) = 3 (out of bounds)\nmath.ceil(4 / 1) = 4 (out of bounds)\n```\n\nThat means there is one monster will arrive at the city with `1` turn. Wait! Somebody is wondering where the other monsters are. They are out of bounds(`3/1` and `4/1`).\n\nNo problem, we can count them. Let me explain it later.\n\n---\n\n\n- **We can eliminate monsters if number of turns to arrive is greater than or equal to chance to eliminate them**\n\nIt\'s time to calculate the number of monsters we can eliminate. Let\'s iteration through `monsters` list\n\n```\nmonsters = [0,1,0]\neliminated = 0 (0 monsters at index 0)\ni = 0 (coming from len(dist) or len(speed))\n```\n\n```\nmonsters = [0,1,0]\neliminated = 1 (`1 monster at index 1, we can eliminate the monster)\ni = 1\n```\n```\nmonsters = [0,1,0]\neliminated = 1 (0 monsters at index 2)\ni = 2\n```\nSo return value is `1`? That is wrong. We still have two monsters at index `3` and `4`. They are out of bounds.\n\n```\nmonsters = [0,1,0] (solution code)\nmonsters = [0,1,0,1,1] (including monsters which are out of bounds) \n```\nBut we don\'t have to care about monsters which are out of bounds, because since `monsters` list is created by `len(dist)` or `len(speed)`, we know that length of mosters list is `number of monsters` approaching to the city.\n\n---\n\n\u2B50\uFE0F Points\n\nWe reach the end of `mosters` list, that means we can eliminate all monsters including monsters which are out of bounds. Because we have a change to elimiate a monster every turns.\n\nIn other words, `index number of monsters list` is `chances` to eliminate them.\n\nDo you remember the second key point? It says "We can eliminate monsters if number of turns to arrive is greater than or equal to chance to eliminate them."\n\nWe can definitely eliminate monsters that are out of bounds when we reach end of `monsters` list.\n\nTo prove that,\n\nWhen we reach the end of monsters list, we have `3` chances(length of `monsters` list) and we use `1` change for a monster at index `1`.\n\nWe still have `2` chances at index `2`, the number of monsters that are out of bounds is `2` and they are coming from idnex `3` and `4`. So, we can elimaite all monsters.\n\nIn reality, \n```\nwhen index is 0(the first turn), elimiate a monster at index 1\nwhen index is 1(the second turn), elimiate a monster at index 3\nwhen index is 2(the thrid turn), elimiate a monster at index 4\n```\n---\n\nIn that case, we can simply return `len(dist)` or `len(speed)`.\n```\nreturn 3\n```\n\nLet\'s see one more case quickly.\n\n```\nInput: dist = [1,2,2,3], speed = [1,1,1,1]\n```\n`monsters` list should be\n```\nmonsters = [0,1,2,1]\n\nmath.ceil(dist[i] / speed[i])\nmath.ceil(1 / 1)\nmath.ceil(2 / 1)\nmath.ceil(2 / 1)\nmath.ceil(3 / 1)\n```\n\n```\nmonsters = [0,1,2,1]\neliminated = 0 (0 monsters at index 0)\ni = 0 (coming from len(dist) or len(speed))\n```\n```\nmonsters = [0,1,2,1]\neliminated = 1 (1 monster at index 1)\ni = 1\n```\n```\nmonsters = [0,1,2,1]\neliminated = 2 (2 monster at index 2, we can eliminete one monster)\ni = 2\n```\nWe can\'t eliminate two monsters at the same time, so\n```\nreturn 2(= eliminated)\n```\n\n- How we can calculate `eliminated` in solution code?\n\n`i` is number of chance to eliminate monsters. So, at each index, if total number of monsters is greater than index number, some monsters reach the city, that\'s why we return current index `i` because we can eliminte number of `i` monsters.\n\nTotal number of monsters so far should be `eliminated monsters` + `monsters from current index`\n```\nif eliminated + monsters[i] > i:\n return i\n```\n\nAs you can see in the first example, if we reach end of monsters list, that means we can elimiate all monsters including out of bounds. So we can eliminate `n` monster. `n` is length of `dist` or `speed`.\n\nI hope you understand main idea now. I spent 2 hours to write this. lol\nLet\'s see a real algorithm!\n\n---\n\n\n\n### Algorithm Overview:\n\nThe code is designed to determine how many monsters can be eliminated before they reach the target position based on their distances and speeds.\n\n### Detailed Explanation:\n\n1. Initialize necessary variables:\n - `n` is assigned the length of the `dist` list, representing the number of monsters.\n\n - `monsters` is initialized as a list of zeros with a length of `n`. This list will keep track of the number of monsters arriving at each time step.\n\n2. Iterate through the monsters:\n - A loop runs from 0 to `n-1`, representing each monster.\n\n - For each monster, calculate the `arrival` time, which is determined by dividing the monster\'s distance by its speed and rounding up using `math.ceil()`. This gives the time it will take for the monster to arrive at the target position.\n\n - If the `arrival` time is less than `n`, increment the corresponding element in the `monsters` list to count the number of monsters arriving at that time.\n\n3. Eliminate monsters:\n - Initialize the variable `eliminated` to 0. It will keep track of the number of eliminated monsters.\n\n - Iterate through the elements of the `monsters` list.\n\n - For each element, check if the current count of eliminated monsters (`eliminated`) plus the number of monsters arriving at this time step is greater than or equal to the time step `i`.\n\n - If this condition is met, return `i` as the result, indicating that the maximum number of monsters that can be eliminated before reaching their target position is at time `i`.\n\n - Update `eliminated` by adding the number of monsters arriving at this time step.\n \n4. If no return statement is executed within the loop, it means that all monsters have arrived before being eliminated. In this case, return `n`, indicating that all monsters have reached their target positions without being eliminated.\n\nThe algorithm calculates the maximum number of monsters that can be eliminated based on their arrival times and provides the earliest time when the elimination limit is reached.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n1. The code first iterates through the \'dist\' and \'speed\' lists to calculate the arrival time for each monster and increments the corresponding bucket in the \'monsters\' list. This step has a time complexity of $$O(n)$$, where \'n\' is the length of the input lists.\n\n2. The code then iterates through the \'monsters\' list to check if the monsters can be eliminated at each time step. This step also has a time complexity of $$O(n)$$.\n\nTherefore, the overall time complexity of the code is $$O(n)$$.\n\n- Space complexity: $$O(n)$$\n\n1. The code uses an additional list \'monsters\' of the same size as the input lists, which has a space complexity of $$O(n)$$ to store the counts of monsters arriving at each time step.\n\n3. 2. There are a few integer variables used, but their space complexity is constant $$O(1)$$.\n\nTherefore, the overall space complexity of the code is $$O(n)$$ due to the \'monsters\' list.\n\n```python []\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n n = len(dist)\n monsters = [0] * n\n\n for i in range(n):\n arrival = math.ceil(dist[i] / speed[i])\n\n if arrival < n:\n monsters[arrival] += 1\n\n eliminated = 0\n for i in range(n):\n if eliminated + monsters[i] > i:\n return i\n\n eliminated += monsters[i]\n\n return n \n```\n```javascript []\nvar eliminateMaximum = function(dist, speed) {\n const n = dist.length;\n const monsters = new Array(n).fill(0);\n\n for (let i = 0; i < n; i++) {\n const arrival = Math.ceil(dist[i] / speed[i]);\n\n if (arrival < n) {\n monsters[arrival]++;\n }\n }\n\n let eliminated = 0;\n for (let i = 0; i < n; i++) {\n if (eliminated + monsters[i] > i) {\n return i;\n }\n\n eliminated += monsters[i];\n }\n\n return n; \n};\n```\n```java []\nclass Solution {\n public int eliminateMaximum(int[] dist, int[] speed) {\n int n = dist.length;\n int[] monsters = new int[n];\n\n for (int i = 0; i < n; i++) {\n int arrival = (int) Math.ceil((double) dist[i] / speed[i]);\n\n if (arrival < n) {\n monsters[arrival]++;\n }\n }\n\n int eliminated = 0;\n for (int i = 0; i < n; i++) {\n if (eliminated + monsters[i] > i) {\n return i;\n }\n\n eliminated += monsters[i];\n }\n\n return n; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int eliminateMaximum(vector<int>& dist, vector<int>& speed) {\n int n = dist.size();\n vector<int> monsters(n, 0);\n\n for (int i = 0; i < n; i++) {\n int arrival = ceil(static_cast<double>(dist[i]) / speed[i]);\n\n if (arrival < n) {\n monsters[arrival]++;\n }\n }\n\n int eliminated = 0;\n for (int i = 0; i < n; i++) {\n if (eliminated + monsters[i] > i) {\n return i;\n }\n\n eliminated += monsters[i];\n }\n\n return n; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/determine-if-a-cell-is-reachable-at-a-given-time/solutions/4262613/video-give-me-5-minutes-o1-time-and-space-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/7nxGK97CmIw\n\n\u25A0 Timeline of the video\n\n`0:04` How we can calculate x and y distances\n`0:55` We need to consider 2 cases and explain what if start and finish cell are the same position\n`2:20` Explain case 2, what if start and finish cell are different positions\n`4:53` Coding\n`6:18` Time Complexity and Space Complexity\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/seat-reservation-manager/solutions/4256920/video-give-me-5-minutes-bests-9879-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/rgdtMOI5AKA\n\n\u25A0 Timeline of the video\n\n`0:04` Difficulty of Seat Reservation Manager\n`0:52` Two key points to solve Seat Reservation Manager\n`3:25` Coding\n`5:18` Time Complexity and Space Complexity\n | 49 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Python3 Solution | eliminate-maximum-number-of-monsters | 0 | 1 | \n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n n=len(dist)\n time=[]\n for i in range(n):\n time.append((dist[i]/speed[i]))\n time.sort()\n for i in range(n):\n if i>=time[i]:\n i-=1\n break\n return i+1 \n``` | 3 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | eliminate-maximum-number-of-monsters | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1(Sort by arrival time)***\n1. **Input Parameters:** The function `eliminateMaximum` takes two input vectors, `dist` and `speed`, representing the distance and speed of each character.\n\n1. **Arrival Time Calculation:**\n\n - It calculates the arrival time for each character and stores it in a vector called `arrival`. Arrival time is calculated by dividing the distance (`dist[i]`) by the speed (`speed[i]`). These arrival times represent the time it takes for each character to reach their destination.\n1. **Sorting Arrival Times:**\n\n - The `arrival` vector is sorted in non-decreasing order. This step is essential because it allows characters to be considered in the order of their arrival times.\n1. **Loop to Find Elimination:**\n\n - An integer variable `ans` is initialized to zero. This variable will store the number of characters that can reach their destination before any elimination occurs.\n\n - The code enters a loop that iterates through the `arrival` times.\n\n - For each character\'s arrival time, it checks if that character arrives at or before their index (`i`) in the sorted order. This check compares the arrival time with the character\'s position in the sorted order.\n - The loop breaks as soon as it finds a character whose arrival time is greater than their index in the sorted order. This indicates that the character arrives too late and cannot eliminate any characters before they reach their destinations.\n\n1. **Return:**\n\n- The function returns the value stored in the `ans` variable, which represents the maximum number of characters that can reach their destinations without getting eliminated.\n\n# Complexity\n- *Time complexity:*\n $$O(nlogn)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int eliminateMaximum(vector<int>& dist, vector<int>& speed) {\n vector<float> arrival;\n for (int i = 0; i < dist.size(); i++) {\n arrival.push_back((float) dist[i] / speed[i]);\n }\n \n sort(arrival.begin(), arrival.end());\n int ans = 0;\n \n for (int i = 0; i < arrival.size(); i++) {\n if (arrival[i] <= i) {\n break;\n }\n \n ans++;\n }\n \n return ans;\n }\n};\n\n```\n\n```C []\n\n\nint eliminateMaximum(int* dist, int* speed, int size) {\n float* arrival = (float*)malloc(size * sizeof(float));\n\n for (int i = 0; i < size; i++) {\n arrival[i] = (float)dist[i] / speed[i];\n }\n\n qsort(arrival, size, sizeof(float), compareFloat);\n\n int ans = 0;\n\n for (int i = 0; i < size; i++) {\n if (arrival[i] <= i) {\n break;\n }\n\n ans++;\n }\n\n free(arrival);\n return ans;\n}\n\nint compareFloat(const void* a, const void* b) {\n return (*(float*)a > *(float*)b) - (*(float*)a < *(float*)b);\n}\n\n\n\n\n\n\n```\n```Java []\nclass Solution {\n public int eliminateMaximum(int[] dist, int[] speed) {\n double[] arrival = new double[dist.length];\n for (int i = 0; i < dist.length; i++) {\n arrival[i] = (double) dist[i] / speed[i];\n }\n \n Arrays.sort(arrival);\n int ans = 0;\n \n for (int i = 0; i < arrival.length; i++) {\n if (arrival[i] <= i) {\n break;\n }\n \n ans++;\n }\n \n return ans;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n arrival = []\n for i in range(len(dist)):\n arrival.append(dist[i] / speed[i])\n \n arrival.sort()\n ans = 0\n\n for i in range(len(arrival)):\n if arrival[i] <= i:\n break\n \n ans += 1\n\n return ans\n\n\n```\n```javascript []\nfunction eliminateMaximum(dist, speed) {\n const arrival = [];\n for (let i = 0; i < dist.length; i++) {\n arrival.push(dist[i] / speed[i]);\n }\n\n arrival.sort((a, b) => a - b);\n\n let ans = 0;\n\n for (let i = 0; i < arrival.length; i++) {\n if (arrival[i] <= i) {\n break;\n }\n\n ans++;\n }\n\n return ans;\n}\n\n\n\n\n\n```\n\n\n---\n\n#### ***Approach 2(Heap)***\n1. The goal of this code is to determine how many characters can reach their destination before the time limit is reached.\n\n1. It takes two vectors as input: `dist` (representing the distances each character needs to travel) and `speed` (representing the speeds of each character).\n\n1. The code uses a `priority_queue` (min heap) of floating-point values to store the arrival times of each character. The `greater<float>` comparator ensures that smaller arrival times are at the top of the heap.\n\n1. It then iterates through the `dist` and `speed` vectors, calculates the arrival time for each character by dividing distance by speed, and pushes these arrival times onto the priority queue.\n\n1. The variable `ans` is initialized to 0, which will be used to count the number of characters that reach their destination before the time limit is exceeded.\n\n1. The code enters a loop, checking if the heap is not empty and if the arrival time of the character at the top of the heap is less than or equal to `ans`. If the condition is met, it means that the character can reach its destination before or exactly at the current time `ans`.\n\n1. In this case, `ans` is incremented to account for the character\'s arrival, and the character is removed from the heap using `heap.pop()`.\n\n1. The loop continues until a character is encountered whose arrival time exceeds the current `ans` or the heap becomes empty.\n\n1. Finally, the code returns the value of `ans`, which represents the maximum number of characters that can reach their destination before the time limit is reached.\n\n# Complexity\n- *Time complexity:*\n $$O(nlogn)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int eliminateMaximum(vector<int>& dist, vector<int>& speed) {\n priority_queue<float, vector<float>, greater<float>> heap;\n \n for (int i = 0; i < dist.size(); i++) {\n heap.push((float) dist[i] / speed[i]);\n }\n\n int ans = 0;\n while (!heap.empty()) {\n if (heap.top() <= ans) {\n break;\n }\n \n ans++;\n heap.pop();\n }\n \n return ans;\n }\n};\n\n```\n\n```C []\n\n\n// Function to create a min heap and push elements into it\nvoid push(float* heap, int* heapSize, float value) {\n heap[*heapSize] = value;\n int current = (*heapSize)++;\n while (current > 0) {\n int parent = (current - 1) / 2;\n if (heap[current] >= heap[parent]) {\n break;\n }\n float temp = heap[current];\n heap[current] = heap[parent];\n heap[parent] = temp;\n current = parent;\n }\n}\n\n// Function to pop the smallest element from the heap\nfloat pop(float* heap, int* heapSize) {\n float result = heap[0];\n heap[0] = heap[--(*heapSize)];\n int current = 0;\n while (true) {\n int child = 2 * current + 1;\n if (child >= (*heapSize)) {\n break;\n }\n if (child + 1 < (*heapSize) && heap[child] > heap[child + 1]) {\n child++;\n }\n if (heap[current] <= heap[child]) {\n break;\n }\n float temp = heap[current];\n heap[current] = heap[child];\n heap[child] = temp;\n current = child;\n }\n return result;\n}\n\nint eliminateMaximum(int* dist, int* speed, int distSize, int speedSize) {\n // Create a min heap to store arrival times\n float arrival[distSize];\n int arrivalSize = 0;\n\n // Push elements into the heap\n for (int i = 0; i < distSize; i++) {\n float arrivalTime = (float)dist[i] / speed[i];\n push(arrival, &arrivalSize, arrivalTime);\n }\n\n int ans = 0;\n\n while (arrivalSize > 0) {\n if (arrival[0] <= ans) {\n break;\n }\n ans++;\n pop(arrival, &arrivalSize);\n }\n\n return ans;\n}\n\n\n\n\n\n\n```\n```Java []\n\nclass Solution {\n public int eliminateMaximum(int[] dist, int[] speed) {\n PriorityQueue<Double> heap = new PriorityQueue();\n for (int i = 0; i < dist.length; i++) {\n heap.add ((double) dist[i] / speed[i]);\n }\n \n int ans = 0;\n while (!heap.isEmpty()) {\n if (heap.remove() <= ans) {\n break;\n }\n \n ans++;\n }\n \n return ans;\n }\n}\n\n```\n```python3 []\nimport heapq\n\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n heap = []\n for i in range(len(dist)):\n heap.append(dist[i] / speed[i])\n \n heapq.heapify(heap)\n ans = 0\n while heap:\n if heapq.heappop(heap) <= ans:\n break\n \n ans += 1\n \n return ans\n\n\n```\n```javascript []\n\nclass Solution {\n eliminateMaximum(dist, speed) {\n const arrival = [];\n \n for (let i = 0; i < dist.length; i++) {\n arrival.push(dist[i] / speed[i]);\n }\n \n arrival.sort((a, b) => a - b);\n let ans = 0;\n \n while (arrival.length > 0) {\n if (arrival[0] <= ans) {\n break;\n }\n \n ans++;\n arrival.shift();\n }\n \n return ans;\n }\n}\n\n\n\n```\n\n\n---\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
O(n) solve | count the monster | dp | prefix Sum | eliminate-maximum-number-of-monsters | 0 | 1 | \n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n \n #deducing the time to reach in the city for every monster\n for i in range(len(dist)): dist[i]/=speed[i] \n\n\n # number of monster have to kill before ith minute\n haveToKill = [0 for i in dist]\n\n for x in dist:\n\n if ceil(x)<len(dist):\n\n haveToKill[ceil(x)]+=1\n #have to kill this monster before the time of ceil(x)\n #if ceil(x)>=len(dist) or n that means it already been killed cz, you already fired n shots\n\n\n \n for i in range(len(speed)):\n\n #calculating the total monster that needed to be killed before i minute\n if i>0: haveToKill[i]+=haveToKill[i-1]\n\n # here ith time means you fired i+1 shot. if the number of monster exceed the number of shot, we have to return i\n if haveToKill[i]>i: return i\n \n return len(dist)\n\n\n``` | 2 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
🔥BEATS 100% | ✅[PY / Java / C++ / C / C# / JS / Swift / Ruby] | eliminate-maximum-number-of-monsters | 1 | 1 | \n```python []\nclass Solution:\n def eliminateMaximum(self, dist, speed):\n n = len(dist)\n time = [float(d) / s for d, s in zip(dist, speed)]\n time.sort()\n \n curr = 0\n for i in range(n):\n if time[i] <= i:\n break\n curr += 1\n \n return curr\n\n```\n```java []\nimport java.util.Arrays;\n\nclass Solution {\n public int eliminateMaximum(int[] dist, int[] speed) {\n int n = dist.length;\n double[] time = new double[n];\n \n for (int i = 0; i < n; i++) {\n time[i] = (double) dist[i] / speed[i];\n }\n \n Arrays.sort(time);\n \n int curr = 0;\n for (int i = 0; i < n; i++) {\n if (time[i] <= i) {\n break;\n }\n curr++;\n }\n \n return curr;\n }\n}\n\n```\n```C++ []\nclass Solution {\npublic:\n int eliminateMaximum(vector<int>& dist, vector<int>& speed) {\n int n = dist.size();\n vector<double> time(n);\n\n for (int i = 0; i < n; i++) {\n time[i] = static_cast<double>(dist[i]) / speed[i];\n }\n\n sort(time.begin(), time.end());\n \n int curr = 0;\n for (int i = 0; i < n; i++) {\n if (time[i] <= i) {\n break;\n }\n curr++;\n }\n\n return curr;\n }\n};\n```\n```Javascript []\nfunction eliminateMaximum(dist, speed) {\n const n = dist.length;\n const time = dist.map((d, i) => d / speed[i]);\n time.sort();\n\n let curr = 0;\n for (let i = 0; i < n; i++) {\n if (time[i] <= i) {\n break;\n }\n curr++;\n }\n\n return curr;\n}\n\n```\n```C []\nint eliminateMaximum(int dist[], int speed[], int n) {\n double time[n];\n for (int i = 0; i < n; i++) {\n time[i] = (double)dist[i] / speed[i];\n }\n\n qsort(time, n, sizeof(double), compare);\n\n int curr = 0;\n for (int i = 0; i < n; i++) {\n if (time[i] <= i) {\n break;\n }\n curr++;\n }\n\n return curr;\n}\n\n```\n```C# []\npublic class Solution {\n public int EliminateMaximum(int[] dist, int[] speed) {\n int n = dist.Length;\n double[] time = new double[n];\n \n for (int i = 0; i < n; i++) {\n time[i] = (double)dist[i] / speed[i];\n }\n \n Array.Sort(time);\n \n int curr = 0;\n for (int i = 0; i < n; i++) {\n if (time[i] <= i) {\n break;\n }\n curr++;\n }\n \n return curr;\n }\n}\n\n```\n```Swift []\nclass Solution {\n func eliminateMaximum(_ dist: [Int], _ speed: [Int]) -> Int {\n let n = dist.count\n var time = [Double](repeating: 0, count: n)\n \n for i in 0..<n {\n time[i] = Double(dist[i]) / Double(speed[i])\n }\n \n time.sort()\n \n var curr = 0\n for i in 0..<n {\n if time[i] <= Double(i) {\n break\n }\n curr += 1\n }\n \n return curr\n }\n}\n\n```\n```Ruby []\ndef eliminate_maximum(dist, speed)\n n = dist.length\n time = dist.zip(speed).map { |d, s| d.to_f / s }\n time.sort!\n\n curr = 0\n n.times do |i|\n if time[i] <= i\n break\n end\n curr += 1\n end\n\n return curr\nend\n\n```\n\n | 2 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
C++ O(n) Prefix sum||52ms beats 100%||w pyplot fig | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nJust consider the arrival times of monsters.\nIn fact just consider how many monsters in the time iterval (t-1, t] will arrive.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n[Chinese spoken! Please turn English subtitles if necessary]\n[https://youtu.be/9rIFcLPTEAo?si=REhv_D9HRXOe4olA](https://youtu.be/9rIFcLPTEAo?si=REhv_D9HRXOe4olA)\nUse the array monsters_time[t] to store the monsters within the time interval (t-1, t]. When `t>n`, set `t=n`; the slow monsters can be easily handled.\n\nUse prefix sum\n`monsters+=monsters_time[shot-1];\n`\nwhen `shot<=monsters` return `shot-1`\n\nUse matplotlib.pyplot to show the testcase\n```\ndist = [3,5,7,4,5]\nspeed = [2,3,6,3,2]\n```\n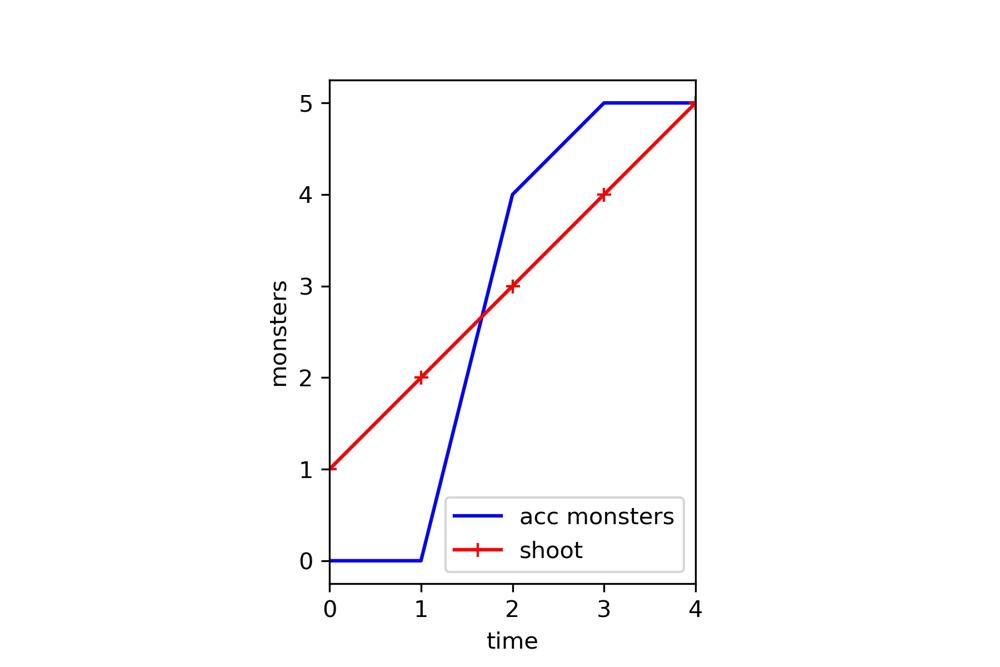\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n# Code\n```\nclass Solution {\npublic:\n int eliminateMaximum(vector<int>& dist, vector<int>& speed) {\n int n=dist.size();\n vector<int> monsters_time(n+1, 0);\n #pragma unroll\n for(int i=0; i<n; i++){\n int t=ceil((double)dist[i]/speed[i]);\n if (t>n) t=n;\n monsters_time[t]++;\n }\n int shot=1, monsters=0;\n #pragma unroll\n for (; shot<=n; shot++){\n monsters+=monsters_time[shot-1];\n // cout<<"shot="<<shot<<" monsters="<<monsters<<"\\n";\n if (shot<=monsters) break;\n }\n return --shot;\n }\n};\nauto init = []()\n{ \n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n```\n# Python Solution\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n n=len(dist)\n monsters_t=[0]*(n+1)\n\n for i in range(n):\n # the following is ceil function\n t=(dist[i]+speed[i]-1)//speed[i] \n if t>n: \n t=n\n monsters_t[t]+=1\n \n shot=1\n monsters=0\n while shot<=n:\n monsters+=monsters_t[shot-1]\n if shot<=monsters: \n break\n shot+=1\n shot-=1\n return shot\n \n \n```\n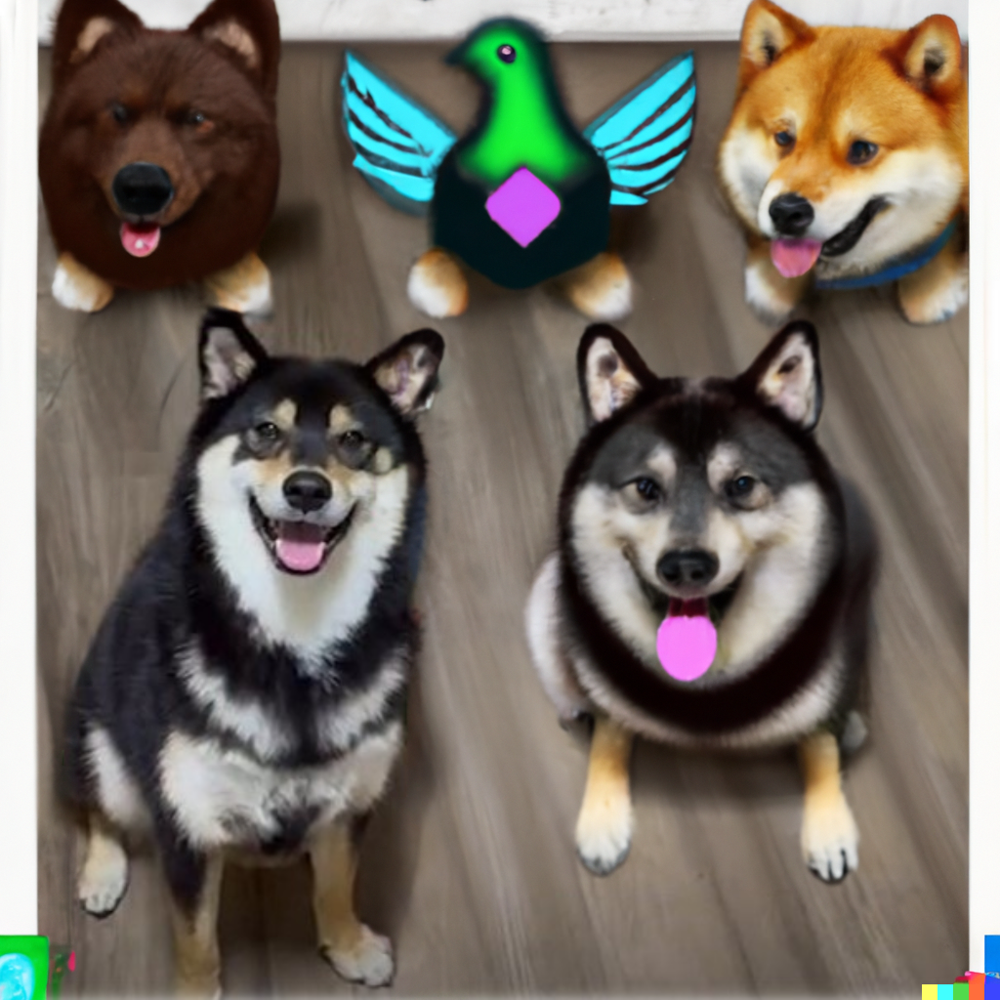\n | 24 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Python 3 | One line | Beats 99% | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n return next((i for i,t in enumerate(sorted(d/s for d,s in zip(dist, speed))) if i>=t), len(dist))\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Python one-liner | eliminate-maximum-number-of-monsters | 0 | 1 | It\'s just a simple Python one-liner, \'cause I haven\'t seen one.\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n return next((i for i, t in enumerate(sorted(d / s for d, s in zip(dist, speed))) if i >= t), len(dist))\n\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Space : O(1), Time : O(nlogn) Daily Challenge 7-Nov-2023, python3 | eliminate-maximum-number-of-monsters | 0 | 1 | \n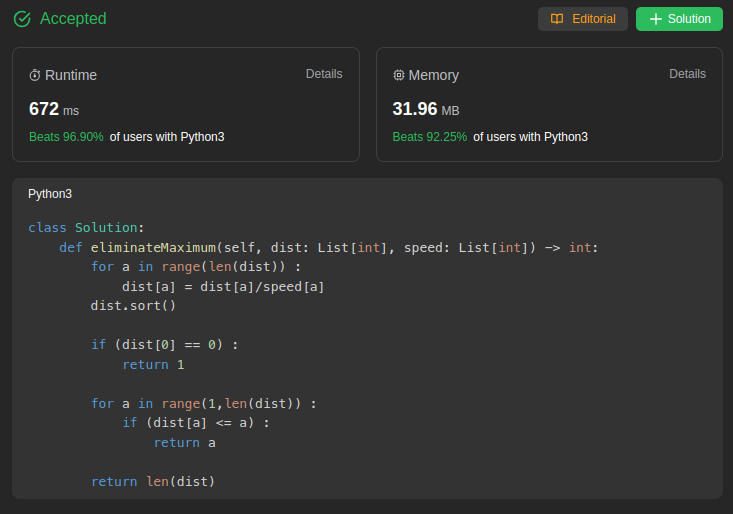\n\n# Approach\nCalculate the no.of steps well in advance and sort them. Check the no.of monsters that can be killed before they could approach the city\n\n# Complexity\n- Time complexity: $$O(nlogn)$$\nSorting makes the complexity $$O(nlogn)$$\n\n- Space complexity: $$O(1)$$\nSince the code is not using any extra auxillary space, complexity is $$O(1)$$\n\n# Code\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n for a in range(len(dist)) :\n dist[a] = dist[a]/speed[a]\n dist.sort()\n \n if (dist[0] == 0) :\n return 1\n\n for a in range(1,len(dist)) :\n if (dist[a] <= a) :\n return a\n\n return len(dist)\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Beats 99% Python3 | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nBy calculating arrival times of each monster, and knowing we can always kill first monster at t=0, we can have a go at counting the number of kills before one reaches the city. The first element in the sorted arrival times can never arrive before _t = 0_, so that\'s the one guaranteed kill. The index of the elements in the `arrivals` array corresponds to the time elapsed before it can be shot down, so as long as `arrivals[i]` doesn\'t outpace `i`, we can continue increasing the counter.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nComputing arrival times is just dividing distance by speed.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$, as we just have to iterate over the arrival times.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nWe need $$O(n)$$ space to store the arrivals.\n\n# Code\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n arrivals = [d/s for d,s in zip(dist,speed)]\n arrivals.sort()\n for i, t in enumerate(arrivals):\n if i >= t:\n return i\n return i + 1\n\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Easy python solution using greedy and sorting | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\nfind the `arrival time` of each monster and find out how many you can eliminate.\n\n# Approach\nsort the `arrival time` and check if arrival > `ith` min, increment `counter` else break.\n\nfinally return `counter`.\n\n# Complexity\n- Time complexity:\nO(nlogn)\n\n- Space complexity:\nO(n)\n\n# Code\n```py\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n arrival_time, counter = [], 1\n for i in range(len(dist)) : \n if dist[i]%speed[i] == 0 :\n arrival_time.append(dist[i]//speed[i])\n else : \n arrival_time.append(dist[i]//speed[i] + 1)\n\n arrival_time.sort()\n \n for i in range(1, len(arrival_time)) : \n if arrival_time[i] <= i : \n break\n counter += 1\n \n return counter \n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Python3 O(n) FT 99%: Ceil | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\n\nMost solutions sort by arrival time in $O(n \\log(n))$ time, and $O(N)$ extra space because a list of arrival times must exist to be sorted.\n\nThe idea is that for each cannon shot, we see if the next monster would arrive before, at, or on that time:\n* before the time: we lose\n* at the same time: we lose (see problem description)\n* after the time: we survive to maybe fire again\n\nSo sorting and getting a cumulative count of monsters up to each time is best.\n\nBut do we really need to sort?\n* we can safely ignore monsters that will arrive after time N-1 because we\'ll have time to shoot them before the end\n* we don\'t really need to sort the monsters, we just need to know that we have enough cannon shots to kill the number of monsters that arrive by each time\n\nSo instead of a normal sort, we count up the monsters at each time.\n\nThen we get the cumulative number of monsters at each time to make sure we have enough cannon shots to handle them before they arrive.\n\n# Approach\n\nSee the code for details.\n\nBriefly\n* we make a list of counts for monsters that arrive at time 0, times <= 1, times <= 2, etc.\n* we take the ceiling of the arrival times because anything that arrives in `(0, 1]` can be shot with the first cannon shot. Anything in `(1, 2]` can be shot with the second cannon shot. Etc.\n* then we get the cumulative counts. If the cumulative count is too high then we lose. We know how many monsters we shot: the time `t` at which we couldn\'t take the next shot; because we survived to make `t` shots at times `0..t-1`\n\n# Complexity\n- Time complexity: $O(N)$, counting sort and a left-to-right scan\n\n- Space complexity: $O(N)$ for counts\n\n# Code\n```\nfrom heapq import *\n\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n # we probably always want to kill monsters in order of earliest to latest arrival time\n # time starts at 0\n # each time we pop the monster with the next earliest arrival time\n\n # we can have 0 monsters arrive at or before time 0\n # at most 1 monster arrive at or before time 1\n # and so on\n N = len(dist)\n counts = [0]*N\n for d, s in zip(dist, speed):\n t = ceil(d/s)\n if t < N:\n counts[t] += 1\n # else t >= N, we have enough time to fire the cannon enough times\n\n total = 0\n for t in range(N):\n total += counts[t]\n if total > t:\n return t # fired the cannon at all t prior times\n\n return N # killed all monsters\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
✅ Python3 | 666ms Beats 99% | 4 Lines | Easy to Understand ✅ | eliminate-maximum-number-of-monsters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nThe best strategy is to kill the monsters in the order that they will arrive. With that in mind it becomes clear that we should sort the monsters by their arrival time, and then check if we have enough time to kill each monster, stopping when one reaches the city or we killed them all.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\nCreate a list of the times at which each monster will arrive, sorted in ascending order. Then iterate over that list, checking if we have enough time to kill all the monsters up until that point. If we don\'t then return how many we\'ve killed so far.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n$$O(n)$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n monsterArrivals = sorted([dist[i] / speed[i] for i in range(len(dist))])\n for total, monster in enumerate(monsterArrivals):\n if total >= monster: return total\n return total+1\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Beats 100% in python. O(n logn) - Time | eliminate-maximum-number-of-monsters | 0 | 1 | \n\n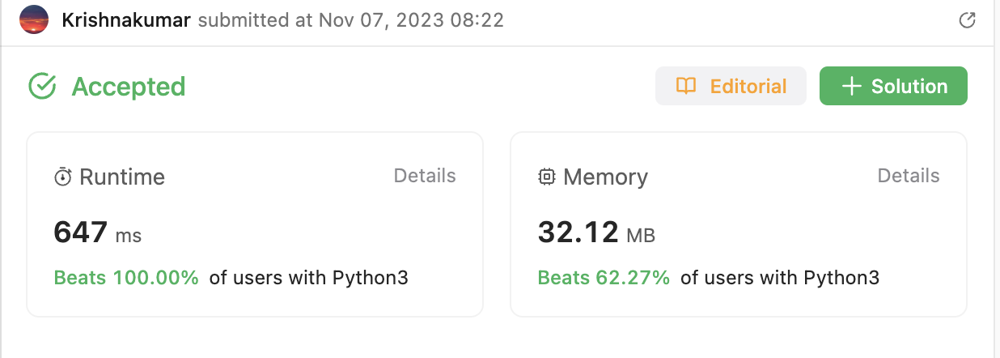\n\n\n# Intuition and Approach\n- Calculate the time and sort it.\n- The time here is the time the monster will take to reach the city. \n- We sort it because we have to kill the monster which will reach city early in time.\n\nFirst we try to kill monster at index 0, time[0], \nit can be killed if its value(`time[0]`) is > `0` minutes\n\nThen we try to kill monster at index 1, time[1], \nit can be killed if its value(`time[1]`) is > `1` minutes\n\nsimilarly, we try to kill monster at index i, time[i],\nit can be killed if its value(`time[i]`) is > `i` minutes\n\nat any point, if `time[i] <= i`, we are not able to kill the monster and the monster attacked the city. so far we have killed `i` monsters. so we return `i`\n\n\n# Complexity\n- Time complexity: $$O(nlogn)$$ for the sort operation\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$ . \nwe can make it $$O(1)$$ if we are allowed to modify any one of the input (dist or speed)\n\n# Solution 1 - python - sort\n```\nclass Solution:\n def eliminateMaximum(self, dist: List[int], speed: List[int]) -> int:\n time = list(map(truediv, dist, speed))\n time.sort()\n\n for i in range(len(time)):\n if time[i] <= i:\n return i\n return i+1\n``` | 1 | You are playing a video game where you are defending your city from a group of `n` monsters. You are given a **0-indexed** integer array `dist` of size `n`, where `dist[i]` is the **initial distance** in kilometers of the `ith` monster from the city.
The monsters walk toward the city at a **constant** speed. The speed of each monster is given to you in an integer array `speed` of size `n`, where `speed[i]` is the speed of the `ith` monster in kilometers per minute.
You have a weapon that, once fully charged, can eliminate a **single** monster. However, the weapon takes **one minute** to charge.The weapon is fully charged at the very start.
You lose when any monster reaches your city. If a monster reaches the city at the exact moment the weapon is fully charged, it counts as a **loss**, and the game ends before you can use your weapon.
Return _the **maximum** number of monsters that you can eliminate before you lose, or_ `n` _if you can eliminate all the monsters before they reach the city._
**Example 1:**
**Input:** dist = \[1,3,4\], speed = \[1,1,1\]
**Output:** 3
**Explanation:**
In the beginning, the distances of the monsters are \[1,3,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,2,3\]. You eliminate the second monster.
After a minute, the distances of the monsters are \[X,X,2\]. You eliminate the thrid monster.
All 3 monsters can be eliminated.
**Example 2:**
**Input:** dist = \[1,1,2,3\], speed = \[1,1,1,1\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[1,1,2,3\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,1,2\], so you lose.
You can only eliminate 1 monster.
**Example 3:**
**Input:** dist = \[3,2,4\], speed = \[5,3,2\]
**Output:** 1
**Explanation:**
In the beginning, the distances of the monsters are \[3,2,4\]. You eliminate the first monster.
After a minute, the distances of the monsters are \[X,0,2\], so you lose.
You can only eliminate 1 monster.
**Constraints:**
* `n == dist.length == speed.length`
* `1 <= n <= 105`
* `1 <= dist[i], speed[i] <= 105` | null |
Easy to understand code ,one liner. | count-good-numbers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n return (pow(5,(n + 1)//2,1000000007)*pow(4,n//2,1000000007))%1000000007\n\n``` | 1 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
Using exponentiation squaring | count-good-numbers | 0 | 1 | # Intuition\nI realized that the problem is basically finding the product of 5\\*4\\*5\\*4\\*... where the no. of numbers we are multiplying is n (given).\n\nSo I tried looking for ways to multiply a number repeatedly mod k (=10**9 + 7). \n\n# Approach\nFirst I tried using for loops, but that gave time limit exceeded.\n\nSo I used the idea that powers of numbers eventually loop when finding them modulo a number. E.g. if I want to find powers of 5 modulo 7, they are 5, 4, 6, 2, 3, 1, 5, 4, 6, 2, 3, 1 ... . Basically I stored the products in a python set (which is an example of a hash table) and when the product repeated, use that to find the rest of the product pretty quickly. But that gave memory limit exceeded, since I had to store all the products (there could be upto 10**9 + 7 in this problem) in a python set.\n\nSo the final approach which I have used here is "Exponentiation by Squaring" which I found on StackOverFlow (credit to users jason and Tom Smith). Link is here: https://stackoverflow.com/questions/2177781/how-to-calculate-modulus-of-large-numbers#:~:text=As%20an%20example%2C%20let\'s%20compute,is%20already%20reduced%20mod%20221%20.&text=Therefore%2C%205%5E55%20%3D%20112,b%20multiplications%20instead%20of%20b%20.\n\nIt\'s a very quick and powerful way to find (n**p)%k i.e. modulus of large powers.\n\n# Complexity\n- Time complexity: $$O(log_2(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(log_2(n))$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# a function to find n**p mod k as efficiently as possible (using exponentiation by squaring)\ndef power(n, p, k):\n n = n % k\n product = 1\n while p > 0:\n if p%2 == 1:\n product = (product*n)%k\n n = (n*n)%k\n p //= 2\n return product\n\nclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n no_of_prime_digits = n//2\n modulus = (10**9)+7\n product = power(20, no_of_prime_digits, modulus)\n if n%2 == 1:\n product = (product*5) % modulus\n return product\n``` | 1 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
Easy Iterative Binary exponentiation | Python | C++ | Golang | count-good-numbers | 0 | 1 | # Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(log n)$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n\n# Code\n```python []\nimport math\n\nclass Solution:\n MOD = int(1e9+7)\n\n @staticmethod\n def binexp(a, b, MOD):\n a %= MOD\n res = 1\n\n while b > 0:\n if b & 1:\n res = res * a % MOD\n a = a * a % MOD\n b >>= 1\n\n return res\n\n def countGoodNumbers(self, n: int) -> int:\n odds = math.floor(n/2)\n evens = math.ceil(n/2)\n return int(self.binexp(5, evens, self.MOD) * self.binexp(4, odds, self.MOD) % self.MOD)\n```\n\n``` C++ []\nclass Solution {\npublic:\n static constexpr int MOD = 1e9 + 7;\n\n static long long binexp(long long a, long long b, int MOD) {\n a %= MOD;\n int res = 1;\n\n while (b > 0) {\n if (b & 1) {\n res = (res * 1LL * a) % MOD;\n }\n a = (a * 1LL * a) % MOD;\n b >>= 1;\n }\n\n return res;\n }\n\n int countGoodNumbers(long long n) {\n long long odds = floor(n / 2.0);\n long long evens = ceil(n / 2.0);\n return (binexp(5, evens, MOD) * 1LL * binexp(4, odds, MOD)) % MOD;\n }\n};\n```\n\n``` Golang []\nconst MOD = int(1e9 + 7)\n\nfunc binexp(a, b, MOD int) int {\n\ta %= MOD\n\tres := 1\n\n\tfor b > 0 {\n\t\tif b&1 == 1 {\n\t\t\tres = (res * a) % MOD\n\t\t}\n\t\ta = (a * a) % MOD\n\t\tb >>= 1\n\t}\n\n\treturn res\n}\n\nfunc countGoodNumbers(n int64) int {\n\todds := int(n / 2)\n\tevens := int((n + 1) / 2)\n\treturn (binexp(5, evens, MOD) * binexp(4, odds, MOD)) % MOD\n}\n``` | 5 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
Python, recursion beats 81%, running time 44ms | count-good-numbers | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Spilt numbers of odd indexes and even indexes in separate variables.\n- Using binary exponentiation to calculate the `5**even_indexes and 4**odd_indexes`\n- Using Modulo at every multiplication to not let it overflow\n\n# Complexity\n1. Time complexity: `O(log\u2061n)`\n- At each recursive call we reduce `n` by half, so we will make only `log n`number of calls for the `binaryExp` function, and the multiplication of two numbers is considered as a constant time operation.\n- Thus, it will take overall `O(log\u2061 n)` time.\n\n1. Space complexity: `O(log\u2061n)`\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n- The recursive stack can use at most `O(log\u2061n)` space at any time.\n\n\n# Code\n```\nclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n mod = 1000000007\n odd = n//2\n even = n//2 + n%2\n return (self.binaryExp(5, even)%mod *self.binaryExp(4, odd)%mod)%mod\n \n def binaryExp(self, x, n):\n mod = 1000000007\n if n==0:\n return 1\n if n < 0:\n return 1/self.binaryExp(x, -n)\n \n if n%2==0:\n return self.binaryExp((x*x)%mod, n//2)\n else:\n return x * self.binaryExp((x*x)%mod, (n-1)//2)\n \n\n \n \n\n``` | 4 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
[Java/Python 3] Iterative O(logn) code, similar to LC50 Pow(x, n) w/ brief explanation and analysis. | count-good-numbers | 1 | 1 | 1. For each even index, there are 5 options: `0`, `2`, `4`, `6`, `8`;\n2. For each odd index, there are 4 options: `2`, `3`, `5`, `7`;\n3. If `n` is even, the solution is `(4 * 5) ^ (n / 2)`; if odd, `(4 * 5) ^ (n / 2) * 5`.\n\n**Method 1: Bruteforce**\n\nThe input range is as large as `10 ^ 15`, hence the following bruteforec codes will get `TLE` (Time Limit Exceeded) without any surprise.\n\n```java\n private static final int MOD = 1_000_000_007;\n public int countGoodNumbers(long n) {\n long good = n % 2 == 0 ? 1 : 5;\n for (long i = 0, x = 4 * 5; i < n / 2; ++i) {\n good = good * x % MOD; \n }\n return (int)good;\n }\n```\n```python\n def countGoodNumbers(self, n: int) -> int:\n good, x = 5 ** (n % 2), 4 * 5\n for _ in range(n // 2):\n good = good * x % (10 ** 9 + 7)\n return good\n```\n\n**Analysis:**\n\nTime: `O(n)`, space: `O(1)`.\n\n----\n\n**Method 2: fast power**\n\nOur main object is to compute `x ^ (n // 2)`. To deal with the large input of `10 ^ 15`, we can use fast power: \n\nDenote `n // 2` as `i`. \n1. If `i` is even, increase `x` to `x ^ 2` and divide `i` by `2`;\n2. If `i` is odd, multiply `good` by `x` and deduct `i` by `1`; now `i` is even, go to procedure `1`.\n\nRepeat the above till `i` decrease to `0`, and `good` is our solution. Please refer to the following recursive codes:\n\n```java\n private static final int MOD = 1_000_000_007;\n public int countGoodNumbers(long n) {\n return (int)(countGood(n / 2, 4 * 5) * (n % 2 == 0 ? 1 : 5) % MOD);\n }\n private long countGood(long power, long x) {\n if (power == 0) {\n return 1;\n }else if (power % 2 == 0) {\n return countGood(power / 2, x * x % MOD);\n } \n return x * countGood(power - 1, x) % MOD;\n }\n```\n```python\n def countGoodNumbers(self, n: int) -> int:\n \n def countGood(power: int, x: int) -> int:\n if power == 0:\n return 1 \n elif power % 2 == 0:\n return countGood(power // 2, x * x % MOD)\n return x * countGood(power - 1, x) % MOD\n\n MOD = 10 ** 9 + 7\n return 5 ** (n % 2) * countGood(n // 2, 4 * 5) % MOD\n```\n**Analysis:**\n\nTime & space: `O(logn)`.\n\nWe can further change the above recursive versions into iterative ones:\n```java\n private static final int MOD = 1_000_000_007;\n public int countGoodNumbers(long n) {\n long good = n % 2 == 0 ? 1 : 5;\n for (long i = n / 2, x = 4 * 5; i > 0; i /= 2, x = x * x % MOD) {\n if (i % 2 != 0) {\n good = good * x % MOD;\n }\n }\n return (int)good;\n }\n```\n```python\n def countGoodNumbers(self, n: int) -> int:\n MOD = 10 ** 9 + 7\n good, x, i = 5 ** (n % 2), 4 * 5, n // 2\n while i > 0:\n if i % 2 == 1:\n good = good * x % MOD\n x = x * x % MOD\n i //= 2\n return good\n```\n\n**Analysis:**\n\nTime: `O(logn)`, space: `O(1)`. | 21 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
Python Solution One Linear || Easy to understand | count-good-numbers | 0 | 1 | ```\nfrom math import ceil,floor\nclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n return (pow(5,ceil(n/2),1000_000_007) * pow(4,floor(n/2),1000_000_007))% 1000_000_007\n``` | 2 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
📌 Well-explained || 4 Lines || 97% faster || easily-understandable 🐍 | count-good-numbers | 0 | 1 | ## Idea :\n\uD83D\uDC49 *find number of odd and even places of from given n.*\n\uD83D\uDC49 *each even places can take (0,2,4,6,8) 5 different numbers.*\n\uD83D\uDC49 *each odd places can take (2,3,5,7) 4 different prime numbers.*\n\uD83D\uDC49 *return the total combination of both even and odd places.*\n\n### **pow(a,b,c) = (a^b)%c**\n\ne.g. : n = 4\n\n_ _ _ _ _ _\n\tne = 2 # n//2\n\tno = 2 # n//2\n\tte = (5**2)%MOD #te=25\n\tto = (4**2)%MOD # to=16\n\t\n\treturn (25*16)%MOD # 400\n\t\n\'\'\'\n\n\tclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n MOD = 10**9+7\n\t\t\n\t\t# No. of even places\n if n%2==0:\n ne=n//2\n else:\n ne=(n+1)//2\n # No. of odd places\n no=n//2\n \n te = pow(5,ne,MOD) #Total number of even places combinations.\n tp = pow(4,no,MOD) #Total number of odd/prime combinations.\n return (tp*te)%MOD\n\t\t\nIf you have any doubt feel free to ask. \uD83E\uDD17\nIf you got any help then DO **upvote!!** \uD83E\uDD1E\nThanks | 9 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
[Python3] Powermod hack, 3 lines | count-good-numbers | 0 | 1 | ---\n- ### the combinatorics part goes like this:\n- there are ceil(n/2) even positions and floor(n/2) odd positions\n- for each even positions the variations are (02,4,6,8). there are five variations for each even position.\n- for each odd position the variations are (2,3,5,7). there are four variations for each odd position.\n- thus, the total number of variations, aka the answer is 5^ceil(n/2) * 4^floor(n/2), up to the mod operation.\n- this leads to the commented out naive solution, which is not fast enough.\n---\n- ### speedup\n- there is a powermod operation that speeds the whole thing up.\n- powermod calculates the second power mod the number, fourth power mod the number, eighth power mod the number, etc.\n- then it converts the power into binary and simply multiply the powermod results where the power has a one bit.\n- for example, to compute the fifth power of x mod y, first calculate x mod y, x^2 mod y, x^4 mod y.\n- then realzing five in binary is \'101\' and multiply x^4 mod y with x mod y\n- this has logarithmic time and space complexity with regards to the power\n---\n- ### the hack\n- powermod is somewhat tricky to implement from scratch, especially during a contet\n- so .. realizing the modern language of python has a implicit powermod implemented in ```pow```, why don\'t we use that?\n\n```\nclass Solution:\n def countGoodNumbers(self, n: int) -> int:\n \'\'\'\n ans=1\n MOD=int(10**9+7)\n for i in range(n):\n if i%2==0:\n ans*=5\n else:\n ans*=4\n ans%=MOD\n return ans\n \'\'\'\n MOD=int(10**9+7)\n\n fives,fours=n//2+n%2,n//2\n # 5^fives*4^fours % MOD\n # = 5^fives % MOD * 4^fours % MOD\n return (pow(5,fives,MOD) * pow(4,fours,MOD)) % MOD\n``` | 5 | A digit string is **good** if the digits **(0-indexed)** at **even** indices are **even** and the digits at **odd** indices are **prime** (`2`, `3`, `5`, or `7`).
* For example, `"2582 "` is good because the digits (`2` and `8`) at even positions are even and the digits (`5` and `2`) at odd positions are prime. However, `"3245 "` is **not** good because `3` is at an even index but is not even.
Given an integer `n`, return _the **total** number of good digit strings of length_ `n`. Since the answer may be large, **return it modulo** `109 + 7`.
A **digit string** is a string consisting of digits `0` through `9` that may contain leading zeros.
**Example 1:**
**Input:** n = 1
**Output:** 5
**Explanation:** The good numbers of length 1 are "0 ", "2 ", "4 ", "6 ", "8 ".
**Example 2:**
**Input:** n = 4
**Output:** 400
**Example 3:**
**Input:** n = 50
**Output:** 564908303
**Constraints:**
* `1 <= n <= 1015` | null |
Python (Simple Binary Search) | longest-common-subpath | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def longestCommonSubpath(self, n, paths):\n def get_hash(path,k):\n h, base, ans = 0, 1<<17, set()\n\n for i in range(len(path)):\n h = h*base + path[i]\n\n if i >= k:\n h -= path[i-k]*pow(base,k,self.mod)\n\n h = h%self.mod\n\n if i >= k-1:\n ans.add(h)\n\n return ans\n\n self.mod = (1<<128)-159\n\n low, high = 0, min(len(p) for p in paths)\n\n while low <= high:\n mid = (low+high)//2\n tt = set.intersection(*[get_hash(p,mid) for p in paths])\n\n if len(tt):\n low = mid + 1\n else:\n high = mid - 1\n\n return high\n\n\n\n\n\n\n\n \n\n\n\n\n\n\n\n\n\n\n \n\n \n``` | 0 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
[Python3] rolling hash | longest-common-subpath | 0 | 1 | \n```\nclass RabinKarp: \n\n def __init__(self, s): \n """Calculate rolling hash of s"""\n self.m = 1_111_111_111_111_111_111\n self.pow = [1]\n self.roll = [0] # rolling hash \n\n p = 1_000_000_007\n for x in s: \n self.pow.append(self.pow[-1] * p % self.m)\n self.roll.append((self.roll[-1] * p + x) % self.m)\n\n def query(self, i, j): \n """Return rolling hash of s[i:j]"""\n return (self.roll[j] - self.roll[i] * self.pow[j-i]) % self.m\n\n\nclass Solution:\n def longestCommonSubpath(self, n: int, paths: List[List[int]]) -> int:\n rks = [RabinKarp(x) for x in paths]\n \n def fn(x):\n """Return True if a common subpath of length x is found."""\n seen = set()\n for rk, path in zip(rks, paths): \n vals = {rk.query(i, i+x) for i in range(len(path)-x+1)}\n if not seen: seen = vals\n else: seen &= vals\n if not seen: return False \n return True \n \n lo, hi = 0, len(paths[0])\n while lo < hi: \n mid = lo + hi + 1 >> 1\n if fn(mid): lo = mid \n else: hi = mid - 1\n return lo\n``` | 0 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
Easy python solution | count-square-sum-triples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport math\nclass Solution:\n def countTriples(self, n: int) -> int:\n count=0\n x=[x for x in range(1,n+1)]\n for i in range(1,n):\n for j in range(1,n):\n a=x[i]\n b=x[j]\n c=(a*a)+(b*b)\n k=math.sqrt(c)\n if k in x:\n count=count+1\n return count\n``` | 3 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Beats 95.86% || Count square sum triples | count-square-sum-triples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countTriples(self, n: int) -> int:\n count=0\n for i in range(1,n):\n for j in range(i+1,n):\n sqr=math.sqrt((i**2)+(j**2))\n if sqr<=n and int(sqr)==sqr:\n count+=2\n return count\n``` | 3 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Python simple solution | count-square-sum-triples | 0 | 1 | **Python :**\n\n```\ndef countTriples(self, n: int) -> int:\n\tres = 0\n\n\tfor i in range(1, n):\n\t\tfor j in range(i + 1, n):\n\t\t\ts = math.sqrt(i * i + j * j)\n\t\t\tif int(s) == s and s <= n:\n\t\t\t\tres += 2\n\n\treturn res\n```\n\n**Like it ? please upvote !** | 31 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
[Python 3] Brute force with optimisation || beats 93% || 145ms | count-square-sum-triples | 0 | 1 | ```python3 []\nclass Solution:\n def countTriples(self, n: int) -> int:\n res, limit = 0, n*n\n for i in range(1, n):\n for j in range(i+1, n):\n k = i*i + j*j\n if k > limit: break\n if sqrt(k) % 1 == 0:\n res += 2\n \n return res\n```\n\n | 4 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Easy Solution || PYTHON | count-square-sum-triples | 0 | 1 | ```\n```class Solution:\n def countTriples(self, n: int) -> int:\n count = 0\n sqrt = 0\n for i in range(1,n-1):\n for j in range(i+1, n):\n sqrt = ((i*i) + (j*j)) ** 0.5\n if sqrt % 1 == 0 and sqrt <= n:\n count += 2\n return (count)\n\t\t\n\n*Please Upvote if you like* | 2 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Easy Solution Beats 90% | count-square-sum-triples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countTriples(self, n: int) -> int:\n ans = 0\n for i in range(1,n+1):\n for j in range(i+1,n+1):\n k = math.sqrt(i*i + j*j)\n if int(k)==k and k<=n:\n ans+=2\n return ans\n``` | 1 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
count-square-sum-triples | count-square-sum-triples | 0 | 1 | # Code\n```\nclass Solution:\n def countTriples(self, n: int) -> int:\n l = []\n for i in range(1,n+1):\n l.append(pow(i,2))\n count = 0\n for i in range(len(l)-1,2,-1):\n for j in range(i):\n a = l[i] - l[j]\n if a in l[:i]:\n count+=1\n return count\n\n \n``` | 0 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
python simple solution | count-square-sum-triples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countTriples(self, n: int) -> int:\n c= 0\n for i in range(1,n+1):\n for j in range(i+1,n+1):\n for k in range(j+1,n+1):\n if i*i+j*j==k*k:\n c+=2\n return c\n \n``` | 0 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Python solution || BFS | nearest-exit-from-entrance-in-maze | 0 | 1 | \n# Code\n```\nfrom queue import Queue\n\nclass Solution:\n def nearestExit(self, maze: List[List[str]], entrance: List[int]) -> int:\n \n q = Queue()\n q.put((entrance[0] , entrance[1] , 0))\n t = 0\n ans =[]\n maze[entrance[0]][entrance[1]] = "+"\n \n while(not q.empty()) :\n s = q.qsize()\n for i in range(s) :\n node = q.get() \n # boundary conditions\n if node[0]==0 or node[0]==len(maze)-1 :\n if node[2]!=0 :\n return node[2]\n ans += [node[2]]\n elif node[1] == 0 or node[1]==len(maze[0])-1 :\n if node[2]!=0 :\n return node[2]\n ans += [node[2]]\n \n dx = [0 , -1 , 0 ,1 ]\n dy = [-1 , 0 , 1 , 0]\n\n for ind in range(4) :\n x = node[0] + dx[ind]\n y = node[1] + dy[ind] \n if x>=0 and x<len(maze) and y>=0 and y<len(maze[0]) and maze[x][y]!="+" :\n maze[x][y] = "+"\n q.put((x,y,node[2]+1))\n\n if len(ans) == 0:\n return -1 \n \n return -1 if max(ans)==0 else max(ans) \n``` | 1 | You are given an `m x n` matrix `maze` (**0-indexed**) with empty cells (represented as `'.'`) and walls (represented as `'+'`). You are also given the `entrance` of the maze, where `entrance = [entrancerow, entrancecol]` denotes the row and column of the cell you are initially standing at.
In one step, you can move one cell **up**, **down**, **left**, or **right**. You cannot step into a cell with a wall, and you cannot step outside the maze. Your goal is to find the **nearest exit** from the `entrance`. An **exit** is defined as an **empty cell** that is at the **border** of the `maze`. The `entrance` **does not count** as an exit.
Return _the **number of steps** in the shortest path from the_ `entrance` _to the nearest exit, or_ `-1` _if no such path exists_.
**Example 1:**
**Input:** maze = \[\[ "+ ", "+ ", ". ", "+ "\],\[ ". ", ". ", ". ", "+ "\],\[ "+ ", "+ ", "+ ", ". "\]\], entrance = \[1,2\]
**Output:** 1
**Explanation:** There are 3 exits in this maze at \[1,0\], \[0,2\], and \[2,3\].
Initially, you are at the entrance cell \[1,2\].
- You can reach \[1,0\] by moving 2 steps left.
- You can reach \[0,2\] by moving 1 step up.
It is impossible to reach \[2,3\] from the entrance.
Thus, the nearest exit is \[0,2\], which is 1 step away.
**Example 2:**
**Input:** maze = \[\[ "+ ", "+ ", "+ "\],\[ ". ", ". ", ". "\],\[ "+ ", "+ ", "+ "\]\], entrance = \[1,0\]
**Output:** 2
**Explanation:** There is 1 exit in this maze at \[1,2\].
\[1,0\] does not count as an exit since it is the entrance cell.
Initially, you are at the entrance cell \[1,0\].
- You can reach \[1,2\] by moving 2 steps right.
Thus, the nearest exit is \[1,2\], which is 2 steps away.
**Example 3:**
**Input:** maze = \[\[ ". ", "+ "\]\], entrance = \[0,0\]
**Output:** -1
**Explanation:** There are no exits in this maze.
**Constraints:**
* `maze.length == m`
* `maze[i].length == n`
* `1 <= m, n <= 100`
* `maze[i][j]` is either `'.'` or `'+'`.
* `entrance.length == 2`
* `0 <= entrancerow < m`
* `0 <= entrancecol < n`
* `entrance` will always be an empty cell. | null |
Python3 Breadth First Search Solution | nearest-exit-from-entrance-in-maze | 0 | 1 | \n# Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution:\n def nearestExit(self, maze: List[List[str]], entrance: List[int]) -> int:\n m = len(maze)\n n = len(maze[0])\n\n queue = collections.deque()\n queue.append((entrance[0], entrance[1], 0))\n visited = set()\n visited.add((entrance[0], entrance[1]))\n\n while queue:\n for _ in range(len(queue)):\n r, c, level = queue.popleft()\n\n if [r, c] != entrance:\n if r == 0 or r == m-1 or c == 0 or c == n-1:\n return level\n\n for nr, nc in [(r, c+1), (r, c-1), (r+1, c), (r-1, c)]:\n if 0 <= nr < m and 0 <= nc < n and (nr, nc) not in visited and maze[nr][nc] == \'.\':\n queue.append((nr, nc, level + 1))\n visited.add((nr, nc))\n\n return -1\n \n``` | 0 | You are given an `m x n` matrix `maze` (**0-indexed**) with empty cells (represented as `'.'`) and walls (represented as `'+'`). You are also given the `entrance` of the maze, where `entrance = [entrancerow, entrancecol]` denotes the row and column of the cell you are initially standing at.
In one step, you can move one cell **up**, **down**, **left**, or **right**. You cannot step into a cell with a wall, and you cannot step outside the maze. Your goal is to find the **nearest exit** from the `entrance`. An **exit** is defined as an **empty cell** that is at the **border** of the `maze`. The `entrance` **does not count** as an exit.
Return _the **number of steps** in the shortest path from the_ `entrance` _to the nearest exit, or_ `-1` _if no such path exists_.
**Example 1:**
**Input:** maze = \[\[ "+ ", "+ ", ". ", "+ "\],\[ ". ", ". ", ". ", "+ "\],\[ "+ ", "+ ", "+ ", ". "\]\], entrance = \[1,2\]
**Output:** 1
**Explanation:** There are 3 exits in this maze at \[1,0\], \[0,2\], and \[2,3\].
Initially, you are at the entrance cell \[1,2\].
- You can reach \[1,0\] by moving 2 steps left.
- You can reach \[0,2\] by moving 1 step up.
It is impossible to reach \[2,3\] from the entrance.
Thus, the nearest exit is \[0,2\], which is 1 step away.
**Example 2:**
**Input:** maze = \[\[ "+ ", "+ ", "+ "\],\[ ". ", ". ", ". "\],\[ "+ ", "+ ", "+ "\]\], entrance = \[1,0\]
**Output:** 2
**Explanation:** There is 1 exit in this maze at \[1,2\].
\[1,0\] does not count as an exit since it is the entrance cell.
Initially, you are at the entrance cell \[1,0\].
- You can reach \[1,2\] by moving 2 steps right.
Thus, the nearest exit is \[1,2\], which is 2 steps away.
**Example 3:**
**Input:** maze = \[\[ ". ", "+ "\]\], entrance = \[0,0\]
**Output:** -1
**Explanation:** There are no exits in this maze.
**Constraints:**
* `maze.length == m`
* `maze[i].length == n`
* `1 <= m, n <= 100`
* `maze[i][j]` is either `'.'` or `'+'`.
* `entrance.length == 2`
* `0 <= entrancerow < m`
* `0 <= entrancecol < n`
* `entrance` will always be an empty cell. | null |
Python 3 | Simple Math | Explanation | sum-game | 0 | 1 | ### Explanation\n- Intuition: Starting with the three examples, you will soon realize that this is essentially a Math problem.\n- Our goal is to see if left sum can equal to right sum, that is, `left_sum - right_sum == 0`. \n- To make it easier to understand, we can move digits to one side and `?` mark to the other side, for example, `?3295???`\n\t- Can be represented as `?329=5???` -> `3+2+9-5=???-?` -> `9=??`\n\t- Now, the original question becomes: Given `9=??` and Alice plays first, can we get this left & right not equal to each other?\n\t- The answer is NO. It doesn\'t matter what number `x` Alice gives, Bob only need to provide `9-x` to make sure the left equals to right.\n- Let\'s try out some other examples, based on the previous observation.\n\t- `8=??`, can Alice win? \n\t\t- Yes, if Alice plays 9 first\n\t- `9=???`, can Alice win?\n\t\t- Yes, since Alice can play 1 time more than Bob\n\t- `9=????`, can Alice win?\n\t\t- Yes, if the sum of Alice\'s 2 plays is greater than 9\n\t- `18=????`, can Alice win?\n\t\t- No, ame as `9=??`, doesn\'t matter what `x` Alice plays, Bob just need to play `9-x`\n\t- `18=??`, can Alice win?\n\t\t- Yes, unless Alice & Bob both play 9 (not optimal play, against the game rule)\n- I think now you should get the idea of the game. Let\'s say, for left side & right side, we move the smaller sum to the other side of the equal sign (we call the result `digit_sum`); for question mark, we move it to the opposite direction (we call the result `?_count`. After doing something Math, the only situation that Bob can win is that:\n\t- `?_count % 2 == 0 and digit_sum == ?_count // 2 * 9`. This basically saying that:\n\t\t- `?_count` has to be an even number\n\t\t- `digit_sum` is a multiple of 9\n\t\t- Half number of plays (or `?`) * 9 equals to `digit_sum`\n- In the following implementation:\n\t- `q_cnt_1`: **Q**uestion mark **c**ou**nt** for the **1**st half of `num`\n\t- `q_cnt_2`: **Q**uestion mark **c**ou**nt** for the **2**nd half of `num`\n\t- `s1`: **S**um of digits for the **1**st half of `num`\n\t- `s2`: **S**um of digits for the **2**nd half of `num`\n\t- `s_diff`: **S**um difference (we take the greater sum - the smaller sum)\n\t- `q_diff`: **Q**uestion mark difference (opposite direction to the digit sum move)\n\n\n### Implementation\n```\nclass Solution:\n def sumGame(self, num: str) -> bool:\n n = len(num)\n q_cnt_1 = s1 = 0\n for i in range(n//2): # get digit sum and question mark count for the first half of `num`\n if num[i] == \'?\':\n q_cnt_1 += 1\n else: \n s1 += int(num[i])\n q_cnt_2 = s2 = 0\t\t\t\t\n for i in range(n//2, n): # get digit sum and question mark count for the second half of `num`\n if num[i] == \'?\':\n q_cnt_2 += 1\n else: \n s2 += int(num[i])\n s_diff = s1 - s2 # calculate sum difference and question mark difference\n q_diff = q_cnt_2 - q_cnt_1\n return not (q_diff % 2 == 0 and q_diff // 2 * 9 == s_diff) # When Bob can\'t win, Alice wins\n``` | 25 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Python 3 || 3 lines, w/ example || T/M: 100% / 8% | sum-game | 0 | 1 | ```\nclass Solution:\n def sumGame(self, num: str) -> bool: # Example: num = "?2936 5??6?"\n\n n = len(num)//2 # n = 10//2 = 5\n num = [9 if ch==\'?\' else 2*int(ch) for ch in num] # num = [_,4,18,6,12, 10,_,_,12,_] <-- double the digits\n # = [9,4,18,6,12, 10,9,9,12,9] <-- add the 9s\n\n return sum(num[:n]) != sum(num[n:]) # return 49 != 49 <-- False\n\n```\n[https://leetcode.com/problems/sum-game/submissions/917654596/](http://)\n\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 4 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Python3 solution: top down DP: trim down the parameter set to get accepted | sum-game | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI thought of DP at once, and it will TLE if I keep both numbers of "?" on the two sides. It will be also be the case for the left and right sums.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe base case for DP is if we reach only 1 "?" left behind, Bob can only win if:\n1. It\'s his turn\n2. The sum Difference is on the opposite side of the "?". e.g. $$0 <= leftSum - rightSum <= 9$$ and the extra "?" is on the right.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ for diff\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n# Code\n```\n#\n# @lc app=leetcode id=1927 lang=python3\n#\n# [1927] Sum Game\n#\n\n# @lc code=start\nfrom functools import lru_cache\n\n\nclass Solution:\n \'\'\'\n The order of "?" does not matter. Only while side it is on and how many of them each side have\n And think about it... if it is "?21":"21?", if Alice put "121" into the left, Bob can always put "211" on the right to balance it out. Then it\'s the difference between number of l and r "?" that matters! \n let dp(countDiff, sumDiff, turn) = Alice will win given l - r = countDiff, and the leftSum and rightSum diffrence is given by lrDiff, and it\'s Alice\'s (0) or Bob\'s (1) turn\n \'\'\'\n @lru_cache(None)\n def dp(self, diff, lrDiff, turn):\n if diff == 1:\n if turn == 0: return True\n else: return not (-9 <= lrDiff <= 0)\n elif diff == -1:\n if turn == 0: return True\n else: return not (0 <= lrDiff <= 9) \n else:\n if turn == 0:\n result = False\n else:\n result = True\n if diff > 0:\n for j in range(10):\n if turn == 0:\n result = result or self.dp(diff - 1, lrDiff + int(j), 1 - turn)\n else:\n result = result and self.dp(diff - 1, lrDiff + int(j), 1 - turn)\n else:\n for j in range(10):\n if turn == 0:\n result = result or self.dp(diff + 1, lrDiff - int(j), 1- turn)\n else:\n result = result and self.dp(diff + 1, lrDiff - int(j), 1- turn)\n return result\n\n def sumGame(self, num: str) -> bool:\n n, l, r, leftSum, rightSum = len(num), 0, 0, 0, 0\n for i, c in enumerate(num):\n if i < n // 2:\n if c == "?":\n l += 1\n else:\n leftSum += int(c)\n else:\n if c == "?":\n r += 1\n else:\n rightSum += int(c)\n\n return self.dp(l - r, leftSum - rightSum, 0) \n \n# @lc code=end\n\n\n``` | 0 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Simple? Python3 Explanation | sum-game | 0 | 1 | # Intuition\nIf players play optimally, Alice will try to push the sides further apart to create an unwinnable scenario for Bob using 0\'s and 9\'s. Bob will counteract these moves also using 0\'s and 9s to keep the sides within 9 before his final move. Since one player will exclusively use 0\'s and the other 9\'s, the average value of a question mark is 4.5 (9/2).\n\nAlso, if there are an odd number of question marks Alice wins. This solution covers that case, no need to add additional complexity by checking.\n\n# Approach\n- Sum up left and right sides, using the value of 4.5 when a ? mark is encountered. \n- If leftsum == rightsum, Alice always wins. Otherwise Bob.\n\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(1)\n\n# Code\n```\nclass Solution:\n def sumGame(self, num: str) -> bool:\n \n n = len(num)\n h = n // 2\n\n leftsum = 0\n # iterate over first half\n for char in num[:h]:\n if char == "?":\n leftsum += 4.5\n else:\n leftsum += int(char)\n \n rightsum = 0\n # iterate over second half\n for char in num[h:]:\n if char == "?":\n rightsum += 4.5\n else:\n rightsum += int(char)\n \n return True if leftsum != rightsum else False\n``` | 0 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Basic Python Solution | sum-game | 0 | 1 | \n# Approach\nThis uses a lot of memory as I utilize a simple approach; define all the conditions and then do all of the math.\nI use the first couple of for loops to set my variables and then I solve using the information gathered\n\nBOB CAN ONLY WIN IF HE GOES LAST AND THE DIFFERENCE BETWEEN THE TWO SIDES IS 9.\nGUESSES ON OPPOSITE SIDES CANCEL OUT GUESSES ON THE SAME SIDE ARE THE ONES THAT MATTER\n\nFeel free to suggest improvements!\n\n# Code\n```\nclass Solution:\n def sumGame(self, num: str) -> bool:\n lis = list(num)\n first, sec, fq, sq = 0,0,0,0\n for i in range(int(len(lis)/2)):\n if lis[i] == \'?\':\n fq +=1\n else:\n first +=int(lis[i])\n for i in range(int(len(lis)/2),len(lis)):\n if lis[i] == \'?\':\n sq +=1\n else:\n sec +=int(lis[i])\n if ((fq-sq)%2==0):\n if(9/2*(fq-sq)==sec-first ):\n return False\n return True\n``` | 0 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Intuition on Necessary and Sufficient condition: Python3 | sum-game | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nFirstly, it should be quite obvious that Bob cannot win if the number of ? is odd, so we will assume that count("?") is even now.\n\nWe will abbreviate LHS and RHS for left and right hand side. This solution will explore the possible thought process for getting the answer.\n\n**Example 1**\n\nFirst we will consider a simple case: `??27` Why does Bob win here? Whatever number Alice places on the LHS, Bob can place 9 - that number to make the total 9 on the LHS. If the RHS had a sum that wasn\'t 9, Alice could make the LHS too small/too big for Bob to win. Clearly the number 9 is special here.\n\n**Example 2**\n\nNow consider the 3rd test case `?3295???`. The LHS has a sum of 14 and a ?, the RHS has a sum of 5 and 3 ?.\nIt turns out that Bob can always win. The key here is that `sum(LHS) - sum(RHS) == 9`, and `numberof?(RHS) - numberof?(LHS) == 2`. This will be important later.\n\nBob will simply let Alice place whatever number anywhere, and Bob can place that same number on the other side. For example, Alice may place a 9 in the first ?. Bob responds by placing a 9 on the other side. Alice could place a 0 on the RHS, Bob places a 0 on the LHS.\n\nThis will reduce the case down to something much like the first example, where the difference in the sums of the LHS and RHS is exactly 9 and we have 2 ? on the RHS. No matter what Alice places now, Bob simply responds with 9 - that number, balancing the sums.\n\n**Example 3**\n\nLets now consider a last example `????4545`. This has a sum of 18 on the RHS and 4 ? on the LHS. Bob can always win here. First, Alice places whatever, and Bob places 9 - whatever Alice placed. This uses up two ? and brings the total up to 9 on the LHS.\nThen Bob does the same thing again, resulting in a total of 18.\n\nIt should be quite clear that Bob has the power to increase the sum of one side by exactly 9 each time regardless of what Alice does, by just placing a number equal to 9 - whatever Alice placed last.\n\nNotice how `sum(LHS) - sum(RHS) == -18`, and `numberof?(RHS) - numberof?(LHS) == -4`.\n\nYou can run a few more examples through your head, but you might spot a pattern, and guess that Bob has a winning strategy whenever:\n```\nsum(LHS) - sum(RHS) == 9/2 * (numberof?(RHS) - numberof?(LHS))\n```\n\nThis expression encodes what conditions we have been talking about: the difference in the sums must be a multiple of 9, because Bob is able to increase the total by 9 for every two ?.\n\nNotice how all 3 examples above satisfy this condition. I will not prove mathematically that this is true but you can hopefully get the idea why this condition works.\n\nWe have roughly shown that\n`sum(LHS) - sum(RHS) == 9/2 * (numberof?(RHS) - numberof?(LHS))`\nIs a sufficient condition for Bob to win. Now, let\'s get some intuition on why this condition is necessary as well.\n\n**Example 4**\n\nConsider `??28`. Alice can easily win by adding a 0, making it impossible for Bob. Similarly, `??26` is also a win for Alice by just adding a 9.\n\n**Example 5**\n\nConsider now `?3294???`. I changed one number from the 3rd testcase. This is a win for Alice. Alice can simply place a 9 on the LHS and then the RHS needs to sum to 19 for Bob to win. But this is not possible, as Alice can just place 0\'s repeatedly and Bob cannot reach 19.\n\nSimilarly, `?3296???` is an Alice win. Alice places a 9 on the RHS repeatedly and 0\'s repeatedly on the LHS and Bob cannot do anything about it. Alice\'s strategy is to add 9\'s to the side which has the potential to become bigger (potential coming from unplaced numbers), and to add 0\'s to the other side.\n\nThe question marks ? seem to have a role in that a pair of them one one side is as good as a value 9 on the same side. It\'s like each ? has a intrinsic value of 9/2.\n\nAgain, I won\'t prove that the condition given above is necessary for Bob\'s victory, but some ideas should convince you that it is true.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\nThe condition\n\n`sum(LHS) - sum(RHS) == 9/2 * (numberof?(RHS) - numberof?(LHS))`\n\ncan be rearranged to be:\n\n`sum(LHS) + 9/2 * numberof?(LHS) == sum(RHS) + 9/2 * numberof?(RHS)`\n\nSimply loop through the first half of `nums` and sum up all the characters in it, with `?` counting as 4.5. Then loop through the second half, and instead subtract the sum. If the sum is 0, return `False`.\n\nYou can also double everything to avoid using floats like I did.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ where n is the length of `num`. Around 80%.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nDon\'t care.\n# Code\nNot optimised.\n```\nclass Solution:\n def sumGame(self, num: str) -> bool:\n t = 0\n for i in num[:len(num)//2]:\n if i == "?":\n t += 9\n else:\n t += 2*int(i)\n for i in num[len(num)//2:]:\n if i == "?":\n t -= 9\n else:\n t -= 2*int(i)\n return t != 0\n``` | 0 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Python 3 Solution | sum-game | 0 | 1 | # Code\n```\nclass Solution:\n def sumGame(self, num: str) -> bool:\n\n numL = list(num[:len(num)//2])\n numR = list(num[len(num)//2:])\n\n sumL = 0\n sumR = 0\n\n qmL = 0\n qmR = 0\n\n for x,y in zip(numL,numR):\n if x=="?":\n qmL += 1\n else:\n sumL += int(x)\n\n if y=="?":\n qmR += 1\n else:\n sumR += int(y)\n\n if (qmR + qmL)%2 == 1:\n return True\n\n if (sumR - sumL) == 9*(qmL - qmR)//2:\n return False\n\n return True\n\n\n \n``` | 0 | Alice and Bob take turns playing a game, with **Alice** **starting first**.
You are given a string `num` of **even length** consisting of digits and `'?'` characters. On each turn, a player will do the following if there is still at least one `'?'` in `num`:
1. Choose an index `i` where `num[i] == '?'`.
2. Replace `num[i]` with any digit between `'0'` and `'9'`.
The game ends when there are no more `'?'` characters in `num`.
For Bob to win, the sum of the digits in the first half of `num` must be **equal** to the sum of the digits in the second half. For Alice to win, the sums must **not be equal**.
* For example, if the game ended with `num = "243801 "`, then Bob wins because `2+4+3 = 8+0+1`. If the game ended with `num = "243803 "`, then Alice wins because `2+4+3 != 8+0+3`.
Assuming Alice and Bob play **optimally**, return `true` _if Alice will win and_ `false` _if Bob will win_.
**Example 1:**
**Input:** num = "5023 "
**Output:** false
**Explanation:** There are no moves to be made.
The sum of the first half is equal to the sum of the second half: 5 + 0 = 2 + 3.
**Example 2:**
**Input:** num = "25?? "
**Output:** true
**Explanation:** Alice can replace one of the '?'s with '9' and it will be impossible for Bob to make the sums equal.
**Example 3:**
**Input:** num = "?3295??? "
**Output:** false
**Explanation:** It can be proven that Bob will always win. One possible outcome is:
- Alice replaces the first '?' with '9'. num = "93295??? ".
- Bob replaces one of the '?' in the right half with '9'. num = "932959?? ".
- Alice replaces one of the '?' in the right half with '2'. num = "9329592? ".
- Bob replaces the last '?' in the right half with '7'. num = "93295927 ".
Bob wins because 9 + 3 + 2 + 9 = 5 + 9 + 2 + 7.
**Constraints:**
* `2 <= num.length <= 105`
* `num.length` is **even**.
* `num` consists of only digits and `'?'`. | It is fast enough to check all possible subarrays The end of each ascending subarray will be the start of the next |
Subsets and Splits