title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
[Java/Python 3] From O(n ^ (1 / 3)) to O(log(n)), easy codes w/ brief explanation & analysis. | minimum-garden-perimeter-to-collect-enough-apples | 1 | 1 | **Method 1:**\n\nDivide into 3 parts: axis, corner and other:\n\nUse `i`, `corner` and `other` to denote the number of apples on each half axis, each corner and other parts, respectively.\n\nObviously, for any given `i`, each half axis has `i` apples; each of the 4 corners has `2 * i` apples, and the rest part (quadrant) has two times of `(i + 1) + (i + 2) + (i + 3) + ... + (2 * i - 1)` apples, which sums to `((i + 1) + (2 * i - 1)) * ((2 * i - 1) - (i + 1) + 1) / 2 * 2 = 3 * i * (i - 1)`.\n\nSee the below for reference.\n\n----\ni, i + 1, i + 2, ..., 2 * i\n.............................\n.............................\n..........................i + 2\n..........................i + 1\n.............................i\n```java\n public long minimumPerimeter(long neededApples) {\n long perimeter = 0;\n for (long i = 1, apples = 0; apples < neededApples; ++i) {\n long corner = 2 * i, other = 3 * i * (i - 1);\n apples += 4 * (i + corner + other);\n perimeter = 8 * i;\n }\n return perimeter;\n }\n```\n```python\n def minimumPerimeter(self, neededApples: int) -> int:\n apples = i = 0\n while apples < neededApples:\n i += 1\n corner, other = 2 * i, 3 * i * (i - 1)\n apples += 4 * (corner + i + other)\n return 8 * i\n```\n\nSince `4 * (i + corner + other) = 4 * (i + 2 * i + 3 * i * (i - 1)) = 4 * (3 * i * i) = 12 * i * i`, the above codes can be simplified as follows: \n```java\n public long minimumPerimeter(long neededApples) {\n long i = 0;\n while (neededApples > 0) {\n neededApples -= 12 * ++i * i;\n }\n return 8 * i;\n }\n```\n```python\n def minimumPerimeter(self, neededApples: int) -> int:\n i = 0\n while neededApples > 0:\n i += 1\n neededApples -= 12 * i * i\n return 8 * i\n```\n\n\n**Analysis:**\n\nTime: `O(n ^ (1 / 3))`, space: `O(1)`, where `n = neededApples`.\n\n----\n\n**Method 2: Binary Search**\n\n\nThe codes in method 1 actually accumulate `12` times of `i ^ 2`. There is a formula: `sum(1 ^ 2 + 2 ^ 2 + ... + i ^ 2) = i * (i + 1) * (2 * i + 1) / 6`, the `12` times of which is \n\n```\n2 * i * (i + 1) * (2 * i + 1)\n``` \nSince the input is in range `1 - 10 ^ 15`, we can observe that `10 ^ 15 >= 2 * i * (i + 1) * (2 * i + 1) > i ^ 3`. Obviously\n```\ni ^ 3 < 10 ^ 15\n```\nwe have\n```\ni < 10 ^ 5\n```\nTherefore, the range of `i` is `1 ~ 10 ^ 5`, and we can use binary search to dicide the `i`.\n\n```java\n public long minimumPerimeter(long neededApples) {\n long lo = 1, hi = 100_000;\n while (lo < hi) {\n long mid = lo + hi >> 1;\n if (2 * mid * (mid + 1) * (2 * mid + 1) < neededApples) {\n lo = mid + 1;\n }else {\n hi = mid;\n }\n }\n return 8 * hi;\n }\n```\n```python\n\n```\n**Analysis:**\n\nTime complexity : `O(log(n ^ (1 / 3))) = O((1 / 3) * log(n)) = O(log(n))`, Therefore\n\nTime: `O(log(n)), space: `O(1)`, where `n = neededApples`.\n\n | 10 | Given an array `nums` of integers, return how many of them contain an **even number** of digits.
**Example 1:**
**Input:** nums = \[12,345,2,6,7896\]
**Output:** 2
**Explanation:**
12 contains 2 digits (even number of digits).
345 contains 3 digits (odd number of digits).
2 contains 1 digit (odd number of digits).
6 contains 1 digit (odd number of digits).
7896 contains 4 digits (even number of digits).
Therefore only 12 and 7896 contain an even number of digits.
**Example 2:**
**Input:** nums = \[555,901,482,1771\]
**Output:** 1
**Explanation:**
Only 1771 contains an even number of digits.
**Constraints:**
* `1 <= nums.length <= 500`
* `1 <= nums[i] <= 105` | Find a formula for the number of apples inside a square with a side length L. Iterate over the possible lengths of the square until enough apples are collected. |
Easy python solution | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n ratio = 0\n while neededApples > 0:\n ratio += 1\n neededApples -= ((3 * ratio * ratio) * 4)\n\n return ratio * 8\n``` | 0 | In a garden represented as an infinite 2D grid, there is an apple tree planted at **every** integer coordinate. The apple tree planted at an integer coordinate `(i, j)` has `|i| + |j|` apples growing on it.
You will buy an axis-aligned **square plot** of land that is centered at `(0, 0)`.
Given an integer `neededApples`, return _the **minimum perimeter** of a plot such that **at least**_ `neededApples` _apples are **inside or on** the perimeter of that plot_.
The value of `|x|` is defined as:
* `x` if `x >= 0`
* `-x` if `x < 0`
**Example 1:**
**Input:** neededApples = 1
**Output:** 8
**Explanation:** A square plot of side length 1 does not contain any apples.
However, a square plot of side length 2 has 12 apples inside (as depicted in the image above).
The perimeter is 2 \* 4 = 8.
**Example 2:**
**Input:** neededApples = 13
**Output:** 16
**Example 3:**
**Input:** neededApples = 1000000000
**Output:** 5040
**Constraints:**
* `1 <= neededApples <= 1015` | We just need to replace every even positioned character with the character s[i] positions ahead of the character preceding it Get the position of the preceeding character in alphabet then advance it s[i] positions and get the character at that position |
Easy python solution | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n ratio = 0\n while neededApples > 0:\n ratio += 1\n neededApples -= ((3 * ratio * ratio) * 4)\n\n return ratio * 8\n``` | 0 | Given an array `nums` of integers, return how many of them contain an **even number** of digits.
**Example 1:**
**Input:** nums = \[12,345,2,6,7896\]
**Output:** 2
**Explanation:**
12 contains 2 digits (even number of digits).
345 contains 3 digits (odd number of digits).
2 contains 1 digit (odd number of digits).
6 contains 1 digit (odd number of digits).
7896 contains 4 digits (even number of digits).
Therefore only 12 and 7896 contain an even number of digits.
**Example 2:**
**Input:** nums = \[555,901,482,1771\]
**Output:** 1
**Explanation:**
Only 1771 contains an even number of digits.
**Constraints:**
* `1 <= nums.length <= 500`
* `1 <= nums[i] <= 105` | Find a formula for the number of apples inside a square with a side length L. Iterate over the possible lengths of the square until enough apples are collected. |
Python3 | Binary Search solution | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | \n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n i = 0\n l = 1;r = 10**15\n while l<r:\n m = (l+r)//2\n if neededApples<=check(m):\n r = m\n else:\n l = m+1\n return l*8\n\n\n\n\ndef check(n):\n total = (1+n)*n//2*(2*n+1)*4\n return total\n\n\n``` | 0 | In a garden represented as an infinite 2D grid, there is an apple tree planted at **every** integer coordinate. The apple tree planted at an integer coordinate `(i, j)` has `|i| + |j|` apples growing on it.
You will buy an axis-aligned **square plot** of land that is centered at `(0, 0)`.
Given an integer `neededApples`, return _the **minimum perimeter** of a plot such that **at least**_ `neededApples` _apples are **inside or on** the perimeter of that plot_.
The value of `|x|` is defined as:
* `x` if `x >= 0`
* `-x` if `x < 0`
**Example 1:**
**Input:** neededApples = 1
**Output:** 8
**Explanation:** A square plot of side length 1 does not contain any apples.
However, a square plot of side length 2 has 12 apples inside (as depicted in the image above).
The perimeter is 2 \* 4 = 8.
**Example 2:**
**Input:** neededApples = 13
**Output:** 16
**Example 3:**
**Input:** neededApples = 1000000000
**Output:** 5040
**Constraints:**
* `1 <= neededApples <= 1015` | We just need to replace every even positioned character with the character s[i] positions ahead of the character preceding it Get the position of the preceeding character in alphabet then advance it s[i] positions and get the character at that position |
Python3 | Binary Search solution | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | \n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n i = 0\n l = 1;r = 10**15\n while l<r:\n m = (l+r)//2\n if neededApples<=check(m):\n r = m\n else:\n l = m+1\n return l*8\n\n\n\n\ndef check(n):\n total = (1+n)*n//2*(2*n+1)*4\n return total\n\n\n``` | 0 | Given an array `nums` of integers, return how many of them contain an **even number** of digits.
**Example 1:**
**Input:** nums = \[12,345,2,6,7896\]
**Output:** 2
**Explanation:**
12 contains 2 digits (even number of digits).
345 contains 3 digits (odd number of digits).
2 contains 1 digit (odd number of digits).
6 contains 1 digit (odd number of digits).
7896 contains 4 digits (even number of digits).
Therefore only 12 and 7896 contain an even number of digits.
**Example 2:**
**Input:** nums = \[555,901,482,1771\]
**Output:** 1
**Explanation:**
Only 1771 contains an even number of digits.
**Constraints:**
* `1 <= nums.length <= 500`
* `1 <= nums[i] <= 105` | Find a formula for the number of apples inside a square with a side length L. Iterate over the possible lengths of the square until enough apples are collected. |
Fully Explained **MADE EASY** | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | \n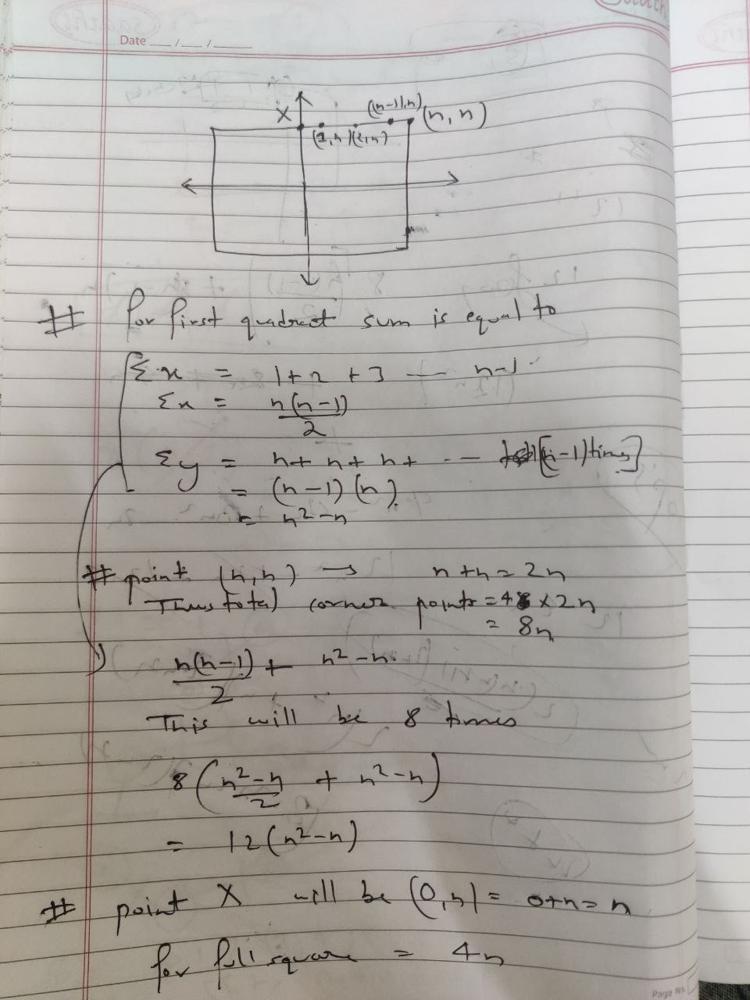\n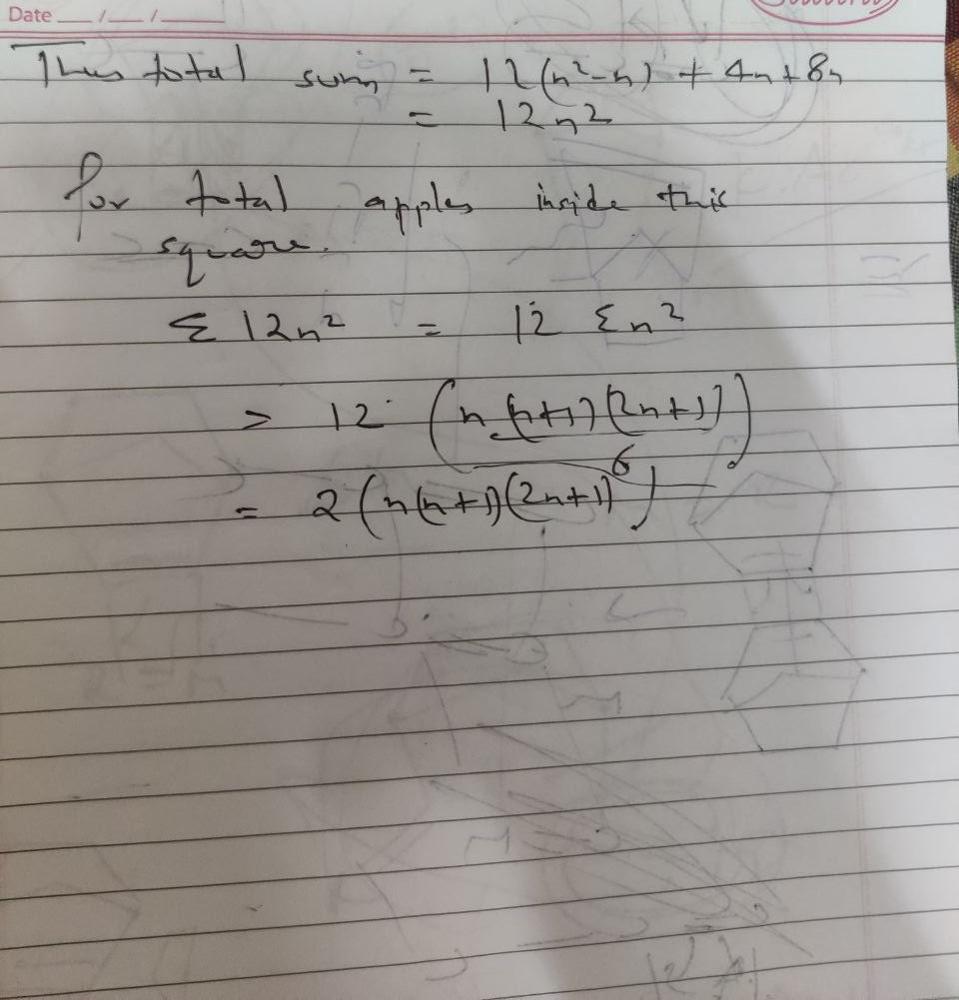\n\n\n# Complexity\n- Time complexity:\nO(neededapples)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n n = 0\n while True:\n f = 2*n*(n+1)*(2*n+1)\n if neededApples <= f:\n break\n n+=1\n return 8*n\n \n \n \n``` | 0 | In a garden represented as an infinite 2D grid, there is an apple tree planted at **every** integer coordinate. The apple tree planted at an integer coordinate `(i, j)` has `|i| + |j|` apples growing on it.
You will buy an axis-aligned **square plot** of land that is centered at `(0, 0)`.
Given an integer `neededApples`, return _the **minimum perimeter** of a plot such that **at least**_ `neededApples` _apples are **inside or on** the perimeter of that plot_.
The value of `|x|` is defined as:
* `x` if `x >= 0`
* `-x` if `x < 0`
**Example 1:**
**Input:** neededApples = 1
**Output:** 8
**Explanation:** A square plot of side length 1 does not contain any apples.
However, a square plot of side length 2 has 12 apples inside (as depicted in the image above).
The perimeter is 2 \* 4 = 8.
**Example 2:**
**Input:** neededApples = 13
**Output:** 16
**Example 3:**
**Input:** neededApples = 1000000000
**Output:** 5040
**Constraints:**
* `1 <= neededApples <= 1015` | We just need to replace every even positioned character with the character s[i] positions ahead of the character preceding it Get the position of the preceeding character in alphabet then advance it s[i] positions and get the character at that position |
Fully Explained **MADE EASY** | minimum-garden-perimeter-to-collect-enough-apples | 0 | 1 | \n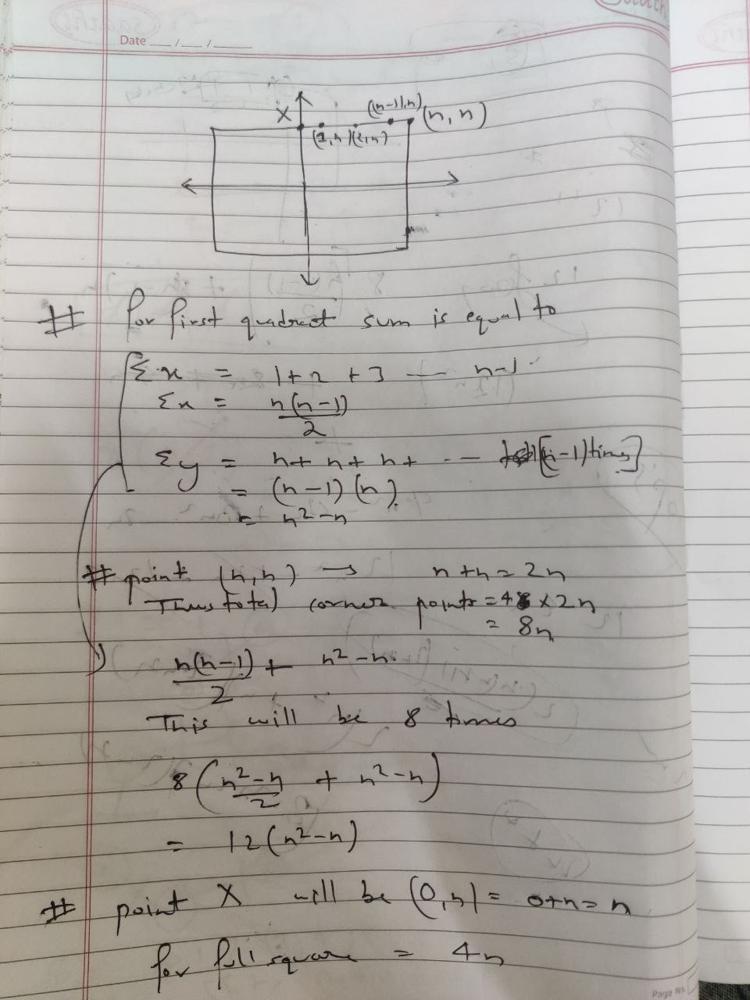\n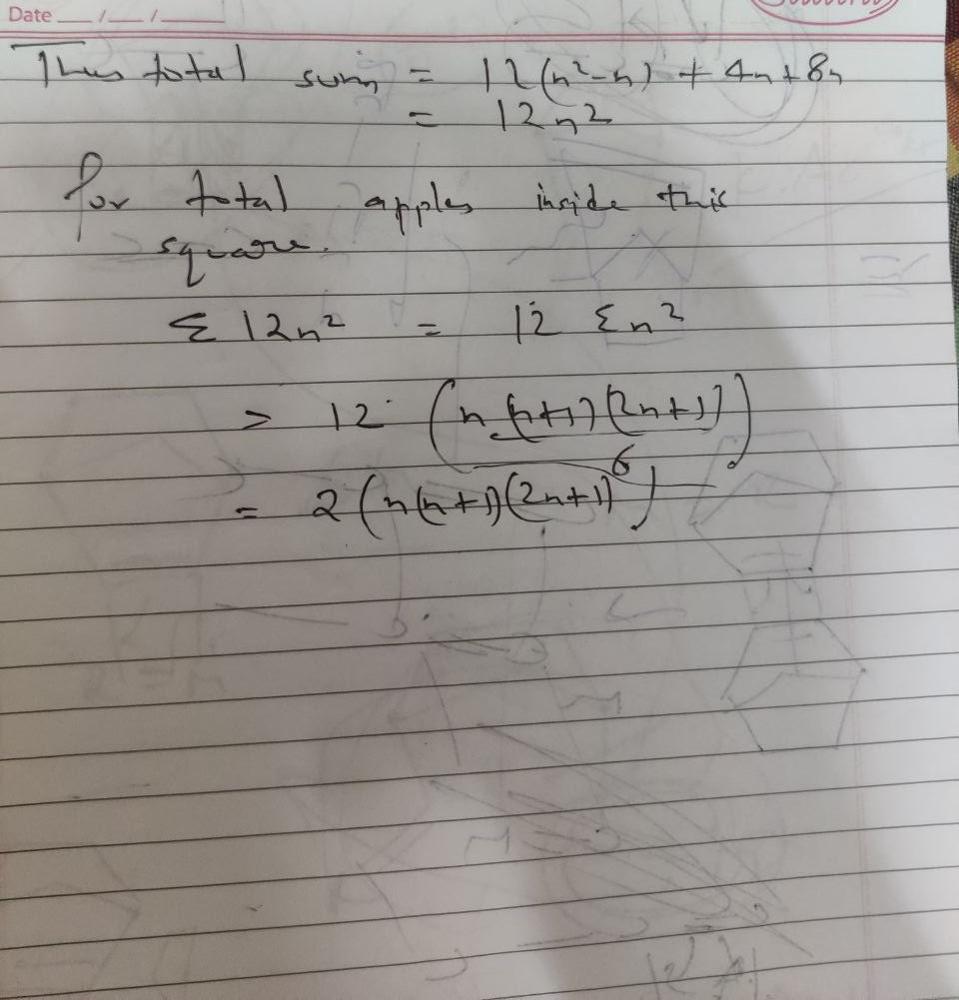\n\n\n# Complexity\n- Time complexity:\nO(neededapples)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def minimumPerimeter(self, neededApples: int) -> int:\n n = 0\n while True:\n f = 2*n*(n+1)*(2*n+1)\n if neededApples <= f:\n break\n n+=1\n return 8*n\n \n \n \n``` | 0 | Given an array `nums` of integers, return how many of them contain an **even number** of digits.
**Example 1:**
**Input:** nums = \[12,345,2,6,7896\]
**Output:** 2
**Explanation:**
12 contains 2 digits (even number of digits).
345 contains 3 digits (odd number of digits).
2 contains 1 digit (odd number of digits).
6 contains 1 digit (odd number of digits).
7896 contains 4 digits (even number of digits).
Therefore only 12 and 7896 contain an even number of digits.
**Example 2:**
**Input:** nums = \[555,901,482,1771\]
**Output:** 1
**Explanation:**
Only 1771 contains an even number of digits.
**Constraints:**
* `1 <= nums.length <= 500`
* `1 <= nums[i] <= 105` | Find a formula for the number of apples inside a square with a side length L. Iterate over the possible lengths of the square until enough apples are collected. |
Simple Python with comments. One pass O(n) with O(1) space | count-number-of-special-subsequences | 0 | 1 | ```\nclass Solution:\n def countSpecialSubsequences(self, nums: List[int]) -> int:\n total_zeros = 0 # number of subsequences of 0s so far\n total_ones = 0 # the number of subsequences of 0s followed by 1s so far\n total_twos = 0 # the number of special subsequences so far\n \n M = 1000000007\n \n for n in nums:\n if n == 0:\n # if we have found new 0 we can add it to any existing subsequence of 0s\n # or use only this 0\n total_zeros += (total_zeros + 1) % M\n elif n == 1:\n # if we have found new 1 we can add it to any existing subsequence of 0s or 0s and 1s\n # to get a valid subsequence of 0s and 1s\n total_ones += (total_zeros + total_ones) % M\n else:\n # if we have found new 2 we can add it to any existing subsequence of 0s and 1s 0r 0s,1s and 2s\n # to get a valid subsequence of 0s,1s and 2s\n total_twos += (total_ones + total_twos) % M\n \n return total_twos % M\n``` | 4 | A sequence is **special** if it consists of a **positive** number of `0`s, followed by a **positive** number of `1`s, then a **positive** number of `2`s.
* For example, `[0,1,2]` and `[0,0,1,1,1,2]` are special.
* In contrast, `[2,1,0]`, `[1]`, and `[0,1,2,0]` are not special.
Given an array `nums` (consisting of **only** integers `0`, `1`, and `2`), return _the **number of different subsequences** that are special_. Since the answer may be very large, **return it modulo** `109 + 7`.
A **subsequence** of an array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements. Two subsequences are **different** if the **set of indices** chosen are different.
**Example 1:**
**Input:** nums = \[0,1,2,2\]
**Output:** 3
**Explanation:** The special subsequences are bolded \[**0**,**1**,**2**,2\], \[**0**,**1**,2,**2**\], and \[**0**,**1**,**2**,**2**\].
**Example 2:**
**Input:** nums = \[2,2,0,0\]
**Output:** 0
**Explanation:** There are no special subsequences in \[2,2,0,0\].
**Example 3:**
**Input:** nums = \[0,1,2,0,1,2\]
**Output:** 7
**Explanation:** The special subsequences are bolded:
- \[**0**,**1**,**2**,0,1,2\]
- \[**0**,**1**,2,0,1,**2**\]
- \[**0**,**1**,**2**,0,1,**2**\]
- \[**0**,**1**,2,0,**1**,**2**\]
- \[**0**,1,2,**0**,**1**,**2**\]
- \[**0**,1,2,0,**1**,**2**\]
- \[0,1,2,**0**,**1**,**2**\]
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 2` | You need a data structure that maintains the states of the seats. This data structure should also allow you to get the first available seat and flip the state of a seat in a reasonable time. You can let the data structure contain the available seats. Then you want to be able to get the lowest element and erase an element, in a reasonable time. Ordered sets support these operations. |
Python (Simple Maths) | count-number-of-special-subsequences | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countSpecialSubsequences(self, nums):\n n = len(nums)\n\n zeros, zeros_ones, zeros_ones_twos = 0, 0, 0\n\n for i in range(n):\n if nums[i] == 0:\n zeros = 2*zeros + 1\n elif nums[i] == 1:\n zeros_ones = 2*zeros_ones + zeros\n else:\n zeros_ones_twos = 2*zeros_ones_twos + zeros_ones\n\n return zeros_ones_twos%(10**9+7)\n\n \n \n``` | 0 | A sequence is **special** if it consists of a **positive** number of `0`s, followed by a **positive** number of `1`s, then a **positive** number of `2`s.
* For example, `[0,1,2]` and `[0,0,1,1,1,2]` are special.
* In contrast, `[2,1,0]`, `[1]`, and `[0,1,2,0]` are not special.
Given an array `nums` (consisting of **only** integers `0`, `1`, and `2`), return _the **number of different subsequences** that are special_. Since the answer may be very large, **return it modulo** `109 + 7`.
A **subsequence** of an array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements. Two subsequences are **different** if the **set of indices** chosen are different.
**Example 1:**
**Input:** nums = \[0,1,2,2\]
**Output:** 3
**Explanation:** The special subsequences are bolded \[**0**,**1**,**2**,2\], \[**0**,**1**,2,**2**\], and \[**0**,**1**,**2**,**2**\].
**Example 2:**
**Input:** nums = \[2,2,0,0\]
**Output:** 0
**Explanation:** There are no special subsequences in \[2,2,0,0\].
**Example 3:**
**Input:** nums = \[0,1,2,0,1,2\]
**Output:** 7
**Explanation:** The special subsequences are bolded:
- \[**0**,**1**,**2**,0,1,2\]
- \[**0**,**1**,2,0,1,**2**\]
- \[**0**,**1**,**2**,0,1,**2**\]
- \[**0**,**1**,2,0,**1**,**2**\]
- \[**0**,1,2,**0**,**1**,**2**\]
- \[**0**,1,2,0,**1**,**2**\]
- \[0,1,2,**0**,**1**,**2**\]
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 2` | You need a data structure that maintains the states of the seats. This data structure should also allow you to get the first available seat and flip the state of a seat in a reasonable time. You can let the data structure contain the available seats. Then you want to be able to get the lowest element and erase an element, in a reasonable time. Ordered sets support these operations. |
Python easy to read and understand | dp | count-number-of-special-subsequences | 0 | 1 | ```\nclass Solution:\n def countSpecialSubsequences(self, nums: List[int]) -> int:\n t, mod = [0]*3, 10**9 + 7\n \n for num in nums:\n if num == 0:\n t[0] = t[0]*2 + 1\n elif num == 1:\n t[1] = t[1]*2 + t[0]\n else:\n t[2] = t[2]*2 + t[1]\n \n return t[2]%mod | 0 | A sequence is **special** if it consists of a **positive** number of `0`s, followed by a **positive** number of `1`s, then a **positive** number of `2`s.
* For example, `[0,1,2]` and `[0,0,1,1,1,2]` are special.
* In contrast, `[2,1,0]`, `[1]`, and `[0,1,2,0]` are not special.
Given an array `nums` (consisting of **only** integers `0`, `1`, and `2`), return _the **number of different subsequences** that are special_. Since the answer may be very large, **return it modulo** `109 + 7`.
A **subsequence** of an array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements. Two subsequences are **different** if the **set of indices** chosen are different.
**Example 1:**
**Input:** nums = \[0,1,2,2\]
**Output:** 3
**Explanation:** The special subsequences are bolded \[**0**,**1**,**2**,2\], \[**0**,**1**,2,**2**\], and \[**0**,**1**,**2**,**2**\].
**Example 2:**
**Input:** nums = \[2,2,0,0\]
**Output:** 0
**Explanation:** There are no special subsequences in \[2,2,0,0\].
**Example 3:**
**Input:** nums = \[0,1,2,0,1,2\]
**Output:** 7
**Explanation:** The special subsequences are bolded:
- \[**0**,**1**,**2**,0,1,2\]
- \[**0**,**1**,2,0,1,**2**\]
- \[**0**,**1**,**2**,0,1,**2**\]
- \[**0**,**1**,2,0,**1**,**2**\]
- \[**0**,1,2,**0**,**1**,**2**\]
- \[**0**,1,2,0,**1**,**2**\]
- \[0,1,2,**0**,**1**,**2**\]
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 2` | You need a data structure that maintains the states of the seats. This data structure should also allow you to get the first available seat and flip the state of a seat in a reasonable time. You can let the data structure contain the available seats. Then you want to be able to get the lowest element and erase an element, in a reasonable time. Ordered sets support these operations. |
python3 Solution | count-number-of-special-subsequences | 0 | 1 | \n```\nclass Solution:\n def countSpecialSubsequences(self, nums: List[int]) -> int:\n dp=[0]*3\n for num in nums:\n if num==0:\n dp[0]=2*dp[0]+1\n\n else:\n dp[num]=dp[num-1]+2*dp[num]\n\n mod=10**9+7\n return dp[2]%mod \n``` | 0 | A sequence is **special** if it consists of a **positive** number of `0`s, followed by a **positive** number of `1`s, then a **positive** number of `2`s.
* For example, `[0,1,2]` and `[0,0,1,1,1,2]` are special.
* In contrast, `[2,1,0]`, `[1]`, and `[0,1,2,0]` are not special.
Given an array `nums` (consisting of **only** integers `0`, `1`, and `2`), return _the **number of different subsequences** that are special_. Since the answer may be very large, **return it modulo** `109 + 7`.
A **subsequence** of an array is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements. Two subsequences are **different** if the **set of indices** chosen are different.
**Example 1:**
**Input:** nums = \[0,1,2,2\]
**Output:** 3
**Explanation:** The special subsequences are bolded \[**0**,**1**,**2**,2\], \[**0**,**1**,2,**2**\], and \[**0**,**1**,**2**,**2**\].
**Example 2:**
**Input:** nums = \[2,2,0,0\]
**Output:** 0
**Explanation:** There are no special subsequences in \[2,2,0,0\].
**Example 3:**
**Input:** nums = \[0,1,2,0,1,2\]
**Output:** 7
**Explanation:** The special subsequences are bolded:
- \[**0**,**1**,**2**,0,1,2\]
- \[**0**,**1**,2,0,1,**2**\]
- \[**0**,**1**,**2**,0,1,**2**\]
- \[**0**,**1**,2,0,**1**,**2**\]
- \[**0**,1,2,**0**,**1**,**2**\]
- \[**0**,1,2,0,**1**,**2**\]
- \[0,1,2,**0**,**1**,**2**\]
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 2` | You need a data structure that maintains the states of the seats. This data structure should also allow you to get the first available seat and flip the state of a seat in a reasonable time. You can let the data structure contain the available seats. Then you want to be able to get the lowest element and erase an element, in a reasonable time. Ordered sets support these operations. |
[Java/Python 3] Count, put into StringBuilder/list and join it. | delete-characters-to-make-fancy-string | 1 | 1 | Count consecutive same characters, if less than `3`, put into `StringBuilder/List`; Otherwise, ignore it.\n```java\n public String makeFancyString(String s) {\n StringBuilder sb = new StringBuilder();\n for (int i = 0, cnt = 0; i < s.length(); ++i) {\n if (i > 0 && s.charAt(i - 1) == s.charAt(i)) {\n ++cnt;\n }else {\n cnt = 1;\n }\n if (cnt < 3) {\n sb.append(s.charAt(i));\n }\n }\n return sb.toString();\n }\n```\n```python\n def makeFancyString(self, s: str) -> str:\n cnt, a = 0, []\n for i, c in enumerate(s):\n if i > 0 and c == s[i - 1]: \n cnt += 1 \n else:\n cnt = 1\n if cnt < 3:\n a.append(c)\n return \'\'.join(a)\n```\n\n**Analysis:**\nTime & space: `O(n)`, where `n = s.length()`. | 19 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
[Java/Python 3] Count, put into StringBuilder/list and join it. | delete-characters-to-make-fancy-string | 1 | 1 | Count consecutive same characters, if less than `3`, put into `StringBuilder/List`; Otherwise, ignore it.\n```java\n public String makeFancyString(String s) {\n StringBuilder sb = new StringBuilder();\n for (int i = 0, cnt = 0; i < s.length(); ++i) {\n if (i > 0 && s.charAt(i - 1) == s.charAt(i)) {\n ++cnt;\n }else {\n cnt = 1;\n }\n if (cnt < 3) {\n sb.append(s.charAt(i));\n }\n }\n return sb.toString();\n }\n```\n```python\n def makeFancyString(self, s: str) -> str:\n cnt, a = 0, []\n for i, c in enumerate(s):\n if i > 0 and c == s[i - 1]: \n cnt += 1 \n else:\n cnt = 1\n if cnt < 3:\n a.append(c)\n return \'\'.join(a)\n```\n\n**Analysis:**\nTime & space: `O(n)`, where `n = s.length()`. | 19 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Python | Easy Solution✅ | delete-characters-to-make-fancy-string | 0 | 1 | # Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n# Code\u2705\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n for letter in s:\n if len(stack) > 1 and letter == stack[-1] == stack[-2]:\n stack.pop()\n stack.append(letter)\n return \'\'.join(stack)\n\n``` | 10 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Python | Easy Solution✅ | delete-characters-to-make-fancy-string | 0 | 1 | # Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n# Code\u2705\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n for letter in s:\n if len(stack) > 1 and letter == stack[-1] == stack[-2]:\n stack.pop()\n stack.append(letter)\n return \'\'.join(stack)\n\n``` | 10 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Very simple solution. Runtime 99.41%!!! | delete-characters-to-make-fancy-string | 0 | 1 | \n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n\n ans, last, count = [], "", 0\n for ch in s:\n if ch == last:\n count += 1\n else:\n count = 0\n if count < 2:\n ans.append(ch)\n last = ch\n return "".join(ans)\n\n\n``` | 1 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Very simple solution. Runtime 99.41%!!! | delete-characters-to-make-fancy-string | 0 | 1 | \n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n\n ans, last, count = [], "", 0\n for ch in s:\n if ch == last:\n count += 1\n else:\n count = 0\n if count < 2:\n ans.append(ch)\n last = ch\n return "".join(ans)\n\n\n``` | 1 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
[Python3] stack | delete-characters-to-make-fancy-string | 0 | 1 | \n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n for ch in s: \n if len(stack) > 1 and stack[-2] == stack[-1] == ch: continue \n stack.append(ch)\n return "".join(stack)\n``` | 9 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
[Python3] stack | delete-characters-to-make-fancy-string | 0 | 1 | \n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n for ch in s: \n if len(stack) > 1 and stack[-2] == stack[-1] == ch: continue \n stack.append(ch)\n return "".join(stack)\n``` | 9 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
This my simple solution | delete-characters-to-make-fancy-string | 0 | 1 | ```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n if len(s) < 3:\n return s\n ans = \'\'\n ans += s[0]\n ans += s[1]\n for i in range(2,len(s)):\n if s[i] != ans[-1] or s[i] != ans[-2]:\n ans += s[i]\n return ans\n``` | 4 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
This my simple solution | delete-characters-to-make-fancy-string | 0 | 1 | ```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n if len(s) < 3:\n return s\n ans = \'\'\n ans += s[0]\n ans += s[1]\n for i in range(2,len(s)):\n if s[i] != ans[-1] or s[i] != ans[-2]:\n ans += s[i]\n return ans\n``` | 4 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Python count during traversal | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n cnt = 0\n res = []\n\n for i, c in enumerate(s):\n if i == 0:\n cnt += 1\n elif i > 0 and c == s[i - 1]:\n cnt += 1\n elif i > 0 and c != s[i - 1]:\n cnt = 1\n \n if cnt >= 3:\n continue\n res.append(c)\n \n return \'\'.join(res)\n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Python count during traversal | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n cnt = 0\n res = []\n\n for i, c in enumerate(s):\n if i == 0:\n cnt += 1\n elif i > 0 and c == s[i - 1]:\n cnt += 1\n elif i > 0 and c != s[i - 1]:\n cnt = 1\n \n if cnt >= 3:\n continue\n res.append(c)\n \n return \'\'.join(res)\n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Python stack (not that easy for me) | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n\n for c in s:\n if len(stack) >= 2 and stack[-1] == stack[-2] == c:\n stack.pop()\n \n stack.append(c)\n \n return \'\'.join(stack)\n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Python stack (not that easy for me) | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n stack = []\n\n for c in s:\n if len(stack) >= 2 and stack[-1] == stack[-2] == c:\n stack.pop()\n \n stack.append(c)\n \n return \'\'.join(stack)\n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
python | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n new_s = ""\n for i in range(len(s)-2):\n if s[i] == s[i+1] ==s[i+2]:\n pass\n else:\n new_s +=s[i]\n return new_s+s[-2:]\n \n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
python | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n new_s = ""\n for i in range(len(s)-2):\n if s[i] == s[i+1] ==s[i+2]:\n pass\n else:\n new_s +=s[i]\n return new_s+s[-2:]\n \n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Regular Expressions | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport re\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n n = 2\n regex = re.compile(rf"((.)\\2{{{n-1}}})\\2+") \n return regex.sub(r"\\1", s)\n\n\n\n\n \n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Regular Expressions | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport re\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n n = 2\n regex = re.compile(rf"((.)\\2{{{n-1}}})\\2+") \n return regex.sub(r"\\1", s)\n\n\n\n\n \n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Python & Rust Solutions | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```python []\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n result = ""\n for c in s:\n if result.endswith(c * 2):\n continue\n result += c\n return result\n```\n```rust []\nimpl Solution {\n pub fn make_fancy_string(s: String) -> String {\n let mut result = String::from("");\n for c in s.chars() {\n if result.ends_with(format!("{c}{c}").as_str()) {\n continue;\n }\n result.push(c);\n }\n result\n }\n}\n\n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Python & Rust Solutions | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```python []\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n result = ""\n for c in s:\n if result.endswith(c * 2):\n continue\n result += c\n return result\n```\n```rust []\nimpl Solution {\n pub fn make_fancy_string(s: String) -> String {\n let mut result = String::from("");\n for c in s.chars() {\n if result.ends_with(format!("{c}{c}").as_str()) {\n continue;\n }\n result.push(c);\n }\n result\n }\n}\n\n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Beats 91.63% of users with Python3 | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n # bruteforce solutions\n # 377ms\n # Beats 83.49% of users with Python3\n """\n from itertools import groupby\n ret = ""\n for k, v in groupby(s):\n n = len(list(v))\n if n > 2:\n ret += k * 2\n else:\n ret += k * n\n\n return ret\n """\n\n # refactoring version\n # 315ms\n # Beats 91.63% of users with Python3\n ret = [s[0]]\n cnt, prev = 1, s[0]\n for char in s[1:]:\n if prev == char:\n cnt += 1\n if cnt < 3:\n ret.append(char)\n else:\n ret.append(char)\n cnt = 1\n \n prev = char\n \n return "".join(ret)\n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
Beats 91.63% of users with Python3 | delete-characters-to-make-fancy-string | 0 | 1 | # Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n # bruteforce solutions\n # 377ms\n # Beats 83.49% of users with Python3\n """\n from itertools import groupby\n ret = ""\n for k, v in groupby(s):\n n = len(list(v))\n if n > 2:\n ret += k * 2\n else:\n ret += k * n\n\n return ret\n """\n\n # refactoring version\n # 315ms\n # Beats 91.63% of users with Python3\n ret = [s[0]]\n cnt, prev = 1, s[0]\n for char in s[1:]:\n if prev == char:\n cnt += 1\n if cnt < 3:\n ret.append(char)\n else:\n ret.append(char)\n cnt = 1\n \n prev = char\n \n return "".join(ret)\n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
python easy solution | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n temp=""\n if len(s)==1 or len(s)==2:\n return s\n elif len(s)>=3:\n temp=s[0:2]\n for i in range(2,len(s)):\n if s[i]!=s[i-1] or s[i-1]!=s[i-2]:\n temp=temp+s[i]\n return temp\n\n\n\n\n \n \n \n \n``` | 0 | A **fancy string** is a string where no **three** **consecutive** characters are equal.
Given a string `s`, delete the **minimum** possible number of characters from `s` to make it **fancy**.
Return _the final string after the deletion_. It can be shown that the answer will always be **unique**.
**Example 1:**
**Input:** s = "leeetcode "
**Output:** "leetcode "
**Explanation:**
Remove an 'e' from the first group of 'e's to create "leetcode ".
No three consecutive characters are equal, so return "leetcode ".
**Example 2:**
**Input:** s = "aaabaaaa "
**Output:** "aabaa "
**Explanation:**
Remove an 'a' from the first group of 'a's to create "aabaaaa ".
Remove two 'a's from the second group of 'a's to create "aabaa ".
No three consecutive characters are equal, so return "aabaa ".
**Example 3:**
**Input:** s = "aab "
**Output:** "aab "
**Explanation:** No three consecutive characters are equal, so return "aab ".
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists only of lowercase English letters. | Is there a way to sort the queries so it's easier to search the closest room larger than the size? Use binary search to speed up the search time. |
python easy solution | delete-characters-to-make-fancy-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeFancyString(self, s: str) -> str:\n temp=""\n if len(s)==1 or len(s)==2:\n return s\n elif len(s)>=3:\n temp=s[0:2]\n for i in range(2,len(s)):\n if s[i]!=s[i-1] or s[i-1]!=s[i-2]:\n temp=temp+s[i]\n return temp\n\n\n\n\n \n \n \n \n``` | 0 | Given the `root` of a binary tree, return _the sum of values of its deepest leaves_.
**Example 1:**
**Input:** root = \[1,2,3,4,5,null,6,7,null,null,null,null,8\]
**Output:** 15
**Example 2:**
**Input:** root = \[6,7,8,2,7,1,3,9,null,1,4,null,null,null,5\]
**Output:** 19
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `1 <= Node.val <= 100` | What's the optimal way to delete characters if three or more consecutive characters are equal? If three or more consecutive characters are equal, keep two of them and delete the rest. |
Python 3 || 11 lines, w/ comments || T/M: 95% / 99% | check-if-move-is-legal | 0 | 1 | Pretty much the same eight-direction approach as other posted solutions, but with one additional twist. We construct a border of`["."]`cells around the`board`. Having this border reduces the number of states to be tested to determine whether a specific direction yields a legal move.\n```\nclass Solution:\n def checkMove(self, board: List[List[str]], rMove: int, cMove: int, color: str) -> bool:\n\n board = [[\'.\']*10] + [ # <-- border the board with [\'.\'] cells\n [\'.\']+row+[\'.\'] for row in board]+ [ \n [\'.\']*10]\n\n other = \'B\' if color == \'W\' else \'W\'\n\n dir = ((-1,-1), (-1,0), (-1, 1), # <-- The eight directions\n ( 0,-1), ( 0, 1),\n ( 1,-1), ( 1,0), ( 1, 1))\n\n for dr, dc in dir:\n\n r, c = rMove + 1 + dr, cMove + 1 + dc # <-- First, check whether cell adj to \n if board[r][c] != other: continue # move cell is the other color\n\n while board[r][c] == other: # <-- Second, iterate through any \n r+= dr # other-color cells\n c+= dc\n\n if board[r][c] == color: return True # <-- Third, if the cell after the string \n # of other-color cells is a color cell\n return False\n```\n[https://leetcode.com/problems/check-if-move-is-legal/submissions/1007185467/]()\n\n\n\nI could be wrong, but I think that time complexity is *O*(1) and space complexity is *O*(1).\n | 3 | You are given a **0-indexed** `8 x 8` grid `board`, where `board[r][c]` represents the cell `(r, c)` on a game board. On the board, free cells are represented by `'.'`, white cells are represented by `'W'`, and black cells are represented by `'B'`.
Each move in this game consists of choosing a free cell and changing it to the color you are playing as (either white or black). However, a move is only **legal** if, after changing it, the cell becomes the **endpoint of a good line** (horizontal, vertical, or diagonal).
A **good line** is a line of **three or more cells (including the endpoints)** where the endpoints of the line are **one color**, and the remaining cells in the middle are the **opposite color** (no cells in the line are free). You can find examples for good lines in the figure below:
Given two integers `rMove` and `cMove` and a character `color` representing the color you are playing as (white or black), return `true` _if changing cell_ `(rMove, cMove)` _to color_ `color` _is a **legal** move, or_ `false` _if it is not legal_.
**Example 1:**
**Input:** board = \[\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ "W ", "B ", "B ", ". ", "W ", "W ", "W ", "B "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\]\], rMove = 4, cMove = 3, color = "B "
**Output:** true
**Explanation:** '.', 'W', and 'B' are represented by the colors blue, white, and black respectively, and cell (rMove, cMove) is marked with an 'X'.
The two good lines with the chosen cell as an endpoint are annotated above with the red rectangles.
**Example 2:**
**Input:** board = \[\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", "B ", ". ", ". ", "W ", ". ", ". ", ". "\],\[ ". ", ". ", "W ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", "B ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", "B ", "W ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", "W ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", "B "\]\], rMove = 4, cMove = 4, color = "W "
**Output:** false
**Explanation:** While there are good lines with the chosen cell as a middle cell, there are no good lines with the chosen cell as an endpoint.
**Constraints:**
* `board.length == board[r].length == 8`
* `0 <= rMove, cMove < 8`
* `board[rMove][cMove] == '.'`
* `color` is either `'B'` or `'W'`. | null |
[Java/Python 3]Check 8 directions. | check-if-move-is-legal | 1 | 1 | **Q & A**\n\nQ1: Why `size` is initialized to `2`?\nA1: We actually check the cells starting from the `2nd` one, and the first one is the cell we are allowed to change color. \n\n**End of Q & A**\n\n----\n\nStarting from the given cell, check if any of the 8 directions has a good line.\n\nFor each direction:\nCheck if any of the neighbors is empty, if yes, break; otherwise, keep checking till encounter a cell of same color, return true if size no less than `3`, otherwise break.\n\n```java\n private static final int[][] d = {{0, 1}, {0, -1}, {1, 0}, {-1, 0}, {1, 1}, {-1, -1}, {1, -1}, {-1, 1}};\n public boolean checkMove(char[][] board, int r, int c, char color) {\n for (int k = 0; k < 8; ++k) {\n for (int i = r + d[k][0], j = c + d[k][1], size = 2; 0 <= i && i < 8 && 0 <= j && j < 8; i += d[k][0], j += d[k][1], ++size) {\n if (board[i][j] == \'.\' || size < 3 && board[i][j] == color) {\n break;\n } \n if (board[i][j] == color) {\n return true;\n }\n }\n }\n return false;\n }\n```\n```python\n def checkMove(self, board: List[List[str]], r: int, c: int, color: str) -> bool:\n for dr, dc in (0, 1), (0, -1), (1, 0), (-1, 0), (1, 1), (-1, -1), (1, -1), (-1, 1):\n i, j = r + dr, c + dc\n size = 2\n while 8 > i >= 0 <= j < 8:\n if board[i][j] == \'.\'or size < 3 and board[i][j] == color:\n break \n if board[i][j] == color:\n return True \n i += dr\n j += dc\n size += 1\n return False\n``` | 14 | You are given a **0-indexed** `8 x 8` grid `board`, where `board[r][c]` represents the cell `(r, c)` on a game board. On the board, free cells are represented by `'.'`, white cells are represented by `'W'`, and black cells are represented by `'B'`.
Each move in this game consists of choosing a free cell and changing it to the color you are playing as (either white or black). However, a move is only **legal** if, after changing it, the cell becomes the **endpoint of a good line** (horizontal, vertical, or diagonal).
A **good line** is a line of **three or more cells (including the endpoints)** where the endpoints of the line are **one color**, and the remaining cells in the middle are the **opposite color** (no cells in the line are free). You can find examples for good lines in the figure below:
Given two integers `rMove` and `cMove` and a character `color` representing the color you are playing as (white or black), return `true` _if changing cell_ `(rMove, cMove)` _to color_ `color` _is a **legal** move, or_ `false` _if it is not legal_.
**Example 1:**
**Input:** board = \[\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ "W ", "B ", "B ", ". ", "W ", "W ", "W ", "B "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\]\], rMove = 4, cMove = 3, color = "B "
**Output:** true
**Explanation:** '.', 'W', and 'B' are represented by the colors blue, white, and black respectively, and cell (rMove, cMove) is marked with an 'X'.
The two good lines with the chosen cell as an endpoint are annotated above with the red rectangles.
**Example 2:**
**Input:** board = \[\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", "B ", ". ", ". ", "W ", ". ", ". ", ". "\],\[ ". ", ". ", "W ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", "B ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", "B ", "W ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", "W ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", "B "\]\], rMove = 4, cMove = 4, color = "W "
**Output:** false
**Explanation:** While there are good lines with the chosen cell as a middle cell, there are no good lines with the chosen cell as an endpoint.
**Constraints:**
* `board.length == board[r].length == 8`
* `0 <= rMove, cMove < 8`
* `board[rMove][cMove] == '.'`
* `color` is either `'B'` or `'W'`. | null |
[Python3] check 8 directions | check-if-move-is-legal | 0 | 1 | \n```\nclass Solution:\n def checkMove(self, board: List[List[str]], rMove: int, cMove: int, color: str) -> bool:\n for di, dj in (0, 1), (1, 1), (1, 0), (1, -1), (0, -1), (-1, -1), (-1, 0), (-1, 1): \n i, j = rMove+di, cMove+dj\n step = 0\n while 0 <= i < 8 and 0 <= j < 8: \n if board[i][j] == color and step: return True \n if board[i][j] == "." or board[i][j] == color and not step: break \n i, j = i+di, j+dj\n step += 1\n return False\n``` | 8 | You are given a **0-indexed** `8 x 8` grid `board`, where `board[r][c]` represents the cell `(r, c)` on a game board. On the board, free cells are represented by `'.'`, white cells are represented by `'W'`, and black cells are represented by `'B'`.
Each move in this game consists of choosing a free cell and changing it to the color you are playing as (either white or black). However, a move is only **legal** if, after changing it, the cell becomes the **endpoint of a good line** (horizontal, vertical, or diagonal).
A **good line** is a line of **three or more cells (including the endpoints)** where the endpoints of the line are **one color**, and the remaining cells in the middle are the **opposite color** (no cells in the line are free). You can find examples for good lines in the figure below:
Given two integers `rMove` and `cMove` and a character `color` representing the color you are playing as (white or black), return `true` _if changing cell_ `(rMove, cMove)` _to color_ `color` _is a **legal** move, or_ `false` _if it is not legal_.
**Example 1:**
**Input:** board = \[\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ "W ", "B ", "B ", ". ", "W ", "W ", "W ", "B "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\]\], rMove = 4, cMove = 3, color = "B "
**Output:** true
**Explanation:** '.', 'W', and 'B' are represented by the colors blue, white, and black respectively, and cell (rMove, cMove) is marked with an 'X'.
The two good lines with the chosen cell as an endpoint are annotated above with the red rectangles.
**Example 2:**
**Input:** board = \[\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", "B ", ". ", ". ", "W ", ". ", ". ", ". "\],\[ ". ", ". ", "W ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", "B ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", "B ", "W ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", "W ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", "B "\]\], rMove = 4, cMove = 4, color = "W "
**Output:** false
**Explanation:** While there are good lines with the chosen cell as a middle cell, there are no good lines with the chosen cell as an endpoint.
**Constraints:**
* `board.length == board[r].length == 8`
* `0 <= rMove, cMove < 8`
* `board[rMove][cMove] == '.'`
* `color` is either `'B'` or `'W'`. | null |
Recursive Solution | check-if-move-is-legal | 0 | 1 | # Code\n```\nclass Solution:\n def checkMove(self, board: List[List[str]], rMove: int, cMove: int, color: str) -> bool:\n nrows = len(board)\n ncols = len(board[0])\n directions = [[1,0],[1,1],[0,1],[-1,1],[-1,0],[-1,-1],[0,-1],[1,-1]]\n \n def dfs(r,c,d,endpoint,length):\n if r<0 or r>=nrows or c<0 or c>=ncols:\n return False\n\n if board[r][c] == \'.\':\n return False\n\n if board[r][c] == endpoint:\n return length>2\n\n return dfs(r+d[0],c+d[1],d,endpoint,length+1)\n\n for d in directions:\n if dfs(rMove+d[0],cMove+d[1],d,color,2):\n return True\n \n return False\n\n``` | 0 | You are given a **0-indexed** `8 x 8` grid `board`, where `board[r][c]` represents the cell `(r, c)` on a game board. On the board, free cells are represented by `'.'`, white cells are represented by `'W'`, and black cells are represented by `'B'`.
Each move in this game consists of choosing a free cell and changing it to the color you are playing as (either white or black). However, a move is only **legal** if, after changing it, the cell becomes the **endpoint of a good line** (horizontal, vertical, or diagonal).
A **good line** is a line of **three or more cells (including the endpoints)** where the endpoints of the line are **one color**, and the remaining cells in the middle are the **opposite color** (no cells in the line are free). You can find examples for good lines in the figure below:
Given two integers `rMove` and `cMove` and a character `color` representing the color you are playing as (white or black), return `true` _if changing cell_ `(rMove, cMove)` _to color_ `color` _is a **legal** move, or_ `false` _if it is not legal_.
**Example 1:**
**Input:** board = \[\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\],\[ "W ", "B ", "B ", ". ", "W ", "W ", "W ", "B "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "B ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", ". ", ". ", ". ", ". "\]\], rMove = 4, cMove = 3, color = "B "
**Output:** true
**Explanation:** '.', 'W', and 'B' are represented by the colors blue, white, and black respectively, and cell (rMove, cMove) is marked with an 'X'.
The two good lines with the chosen cell as an endpoint are annotated above with the red rectangles.
**Example 2:**
**Input:** board = \[\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", "B ", ". ", ". ", "W ", ". ", ". ", ". "\],\[ ". ", ". ", "W ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", "W ", "B ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", "B ", "W ", ". ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", "W ", ". "\],\[ ". ", ". ", ". ", ". ", ". ", ". ", ". ", "B "\]\], rMove = 4, cMove = 4, color = "W "
**Output:** false
**Explanation:** While there are good lines with the chosen cell as a middle cell, there are no good lines with the chosen cell as an endpoint.
**Constraints:**
* `board.length == board[r].length == 8`
* `0 <= rMove, cMove < 8`
* `board[rMove][cMove] == '.'`
* `color` is either `'B'` or `'W'`. | null |
[Python3] dp | minimum-total-space-wasted-with-k-resizing-operations | 0 | 1 | \n```\nclass Solution:\n def minSpaceWastedKResizing(self, nums: List[int], k: int) -> int:\n \n @cache\n def fn(i, k): \n """Return min waste from i with k ops."""\n if i == len(nums): return 0\n if k < 0: return inf \n ans = inf\n rmx = rsm = 0\n for j in range(i, len(nums)): \n rmx = max(rmx, nums[j])\n rsm += nums[j]\n ans = min(ans, rmx*(j-i+1) - rsm + fn(j+1, k-1))\n return ans \n \n return fn(0, k)\n``` | 14 | You are currently designing a dynamic array. You are given a **0-indexed** integer array `nums`, where `nums[i]` is the number of elements that will be in the array at time `i`. In addition, you are given an integer `k`, the **maximum** number of times you can **resize** the array (to **any** size).
The size of the array at time `t`, `sizet`, must be at least `nums[t]` because there needs to be enough space in the array to hold all the elements. The **space wasted** at time `t` is defined as `sizet - nums[t]`, and the **total** space wasted is the **sum** of the space wasted across every time `t` where `0 <= t < nums.length`.
Return _the **minimum** **total space wasted** if you can resize the array at most_ `k` _times_.
**Note:** The array can have **any size** at the start and does **not** count towards the number of resizing operations.
**Example 1:**
**Input:** nums = \[10,20\], k = 0
**Output:** 10
**Explanation:** size = \[20,20\].
We can set the initial size to be 20.
The total wasted space is (20 - 10) + (20 - 20) = 10.
**Example 2:**
**Input:** nums = \[10,20,30\], k = 1
**Output:** 10
**Explanation:** size = \[20,20,30\].
We can set the initial size to be 20 and resize to 30 at time 2.
The total wasted space is (20 - 10) + (20 - 20) + (30 - 30) = 10.
**Example 3:**
**Input:** nums = \[10,20,15,30,20\], k = 2
**Output:** 15
**Explanation:** size = \[10,20,20,30,30\].
We can set the initial size to 10, resize to 20 at time 1, and resize to 30 at time 3.
The total wasted space is (10 - 10) + (20 - 20) + (20 - 15) + (30 - 30) + (30 - 20) = 15.
**Constraints:**
* `1 <= nums.length <= 200`
* `1 <= nums[i] <= 106`
* `0 <= k <= nums.length - 1` | The grid is at a maximum 100 x 100, so it is clever to assume that the robot's initial cell is grid[101][101] Run a DFS from the robot's position to make sure that you can reach the target, otherwise you should return -1. Now that you are sure you can reach the target and that you know the grid, run Dijkstra to find the minimum cost. |
✔ Python3 Solution | DP | Clean & Concise | minimum-total-space-wasted-with-k-resizing-operations | 0 | 1 | # Complexity\n- Time complexity: $$O(K * N ^ 2)$$\n- Space complexity: $$O(N)$$\n\n# Code\n```Python\nclass Solution:\n def minSpaceWastedKResizing(self, A, K):\n N = len(A)\n dp = [0] + [float(\'inf\')] * N\n for k in range(K + 1):\n for i in range(N - 1, k - 1, -1):\n csum = cmax = 0\n for j in range(i, k - 1, -1):\n csum += A[j]\n cmax = max(cmax, A[j])\n dp[i + 1] = min(dp[i + 1], dp[j] + cmax * (i - j + 1) - csum)\n return dp[-1]\n``` | 2 | You are currently designing a dynamic array. You are given a **0-indexed** integer array `nums`, where `nums[i]` is the number of elements that will be in the array at time `i`. In addition, you are given an integer `k`, the **maximum** number of times you can **resize** the array (to **any** size).
The size of the array at time `t`, `sizet`, must be at least `nums[t]` because there needs to be enough space in the array to hold all the elements. The **space wasted** at time `t` is defined as `sizet - nums[t]`, and the **total** space wasted is the **sum** of the space wasted across every time `t` where `0 <= t < nums.length`.
Return _the **minimum** **total space wasted** if you can resize the array at most_ `k` _times_.
**Note:** The array can have **any size** at the start and does **not** count towards the number of resizing operations.
**Example 1:**
**Input:** nums = \[10,20\], k = 0
**Output:** 10
**Explanation:** size = \[20,20\].
We can set the initial size to be 20.
The total wasted space is (20 - 10) + (20 - 20) = 10.
**Example 2:**
**Input:** nums = \[10,20,30\], k = 1
**Output:** 10
**Explanation:** size = \[20,20,30\].
We can set the initial size to be 20 and resize to 30 at time 2.
The total wasted space is (20 - 10) + (20 - 20) + (30 - 30) = 10.
**Example 3:**
**Input:** nums = \[10,20,15,30,20\], k = 2
**Output:** 15
**Explanation:** size = \[10,20,20,30,30\].
We can set the initial size to 10, resize to 20 at time 1, and resize to 30 at time 3.
The total wasted space is (10 - 10) + (20 - 20) + (20 - 15) + (30 - 30) + (30 - 20) = 15.
**Constraints:**
* `1 <= nums.length <= 200`
* `1 <= nums[i] <= 106`
* `0 <= k <= nums.length - 1` | The grid is at a maximum 100 x 100, so it is clever to assume that the robot's initial cell is grid[101][101] Run a DFS from the robot's position to make sure that you can reach the target, otherwise you should return -1. Now that you are sure you can reach the target and that you know the grid, run Dijkstra to find the minimum cost. |
Python DP Top Down Solution | minimum-total-space-wasted-with-k-resizing-operations | 0 | 1 | ```\nclass Solution:\n def minSpaceWastedKResizing(self, nums: List[int], k: int) -> int:\n memo = {}\n\n def f(index,k):\n if (index,k) in memo: return memo[(index,k)]\n if index >= len(nums): return 0\n \n res = float("inf")\n if k > 0:\n cur_max = 0\n cur_total = 0\n q = 0\n for i in range(index,len(nums)):\n cur_max = max(cur_max,nums[i])\n cur_total += nums[i]\n q += 1\n res = min(res,f(i+1,k-1) + (cur_max*q)-cur_total)\n \n memo[(index,k)] = res\n \n return memo[(index,k)]\n\n return f(0,k+1)\n \n\n``` | 0 | You are currently designing a dynamic array. You are given a **0-indexed** integer array `nums`, where `nums[i]` is the number of elements that will be in the array at time `i`. In addition, you are given an integer `k`, the **maximum** number of times you can **resize** the array (to **any** size).
The size of the array at time `t`, `sizet`, must be at least `nums[t]` because there needs to be enough space in the array to hold all the elements. The **space wasted** at time `t` is defined as `sizet - nums[t]`, and the **total** space wasted is the **sum** of the space wasted across every time `t` where `0 <= t < nums.length`.
Return _the **minimum** **total space wasted** if you can resize the array at most_ `k` _times_.
**Note:** The array can have **any size** at the start and does **not** count towards the number of resizing operations.
**Example 1:**
**Input:** nums = \[10,20\], k = 0
**Output:** 10
**Explanation:** size = \[20,20\].
We can set the initial size to be 20.
The total wasted space is (20 - 10) + (20 - 20) = 10.
**Example 2:**
**Input:** nums = \[10,20,30\], k = 1
**Output:** 10
**Explanation:** size = \[20,20,30\].
We can set the initial size to be 20 and resize to 30 at time 2.
The total wasted space is (20 - 10) + (20 - 20) + (30 - 30) = 10.
**Example 3:**
**Input:** nums = \[10,20,15,30,20\], k = 2
**Output:** 15
**Explanation:** size = \[10,20,20,30,30\].
We can set the initial size to 10, resize to 20 at time 1, and resize to 30 at time 3.
The total wasted space is (10 - 10) + (20 - 20) + (20 - 15) + (30 - 30) + (30 - 20) = 15.
**Constraints:**
* `1 <= nums.length <= 200`
* `1 <= nums[i] <= 106`
* `0 <= k <= nums.length - 1` | The grid is at a maximum 100 x 100, so it is clever to assume that the robot's initial cell is grid[101][101] Run a DFS from the robot's position to make sure that you can reach the target, otherwise you should return -1. Now that you are sure you can reach the target and that you know the grid, run Dijkstra to find the minimum cost. |
Python DP Faster than 86% | minimum-total-space-wasted-with-k-resizing-operations | 0 | 1 | wasted[i][j] means the wasted space for nums[i] to nums[j].\ndp[k][i] means the minimum wasted space for nums[0] to nums[i] with k resize operations.\nThe subproblem can be represent as \n\ndp[k][i] = min(dp[k-1][l-1] + wasted[l][i]), where l is from k to i\n\n\n```\nclass Solution:\n def minSpaceWastedKResizing(self, nums: List[int], k: int) -> int:\n n = len(nums)\n \n if k == 0:\n mx = max(nums)\n return sum(list(map(lambda x: mx-x, nums)))\n if k >= n-1:\n return 0\n \n dp = [[math.inf for i in range(n)] for j in range(k+1)]\n wasted = [[0 for i in range(n)] for j in range(n)]\n \n for i in range(0, n):\n prev_max = nums[i]\n for j in range(i, n):\n if prev_max >= nums[j]:\n wasted[i][j] = wasted[i][j-1] + prev_max - nums[j]\n else:\n diff = nums[j] - prev_max\n wasted[i][j] = diff * (j - i) + wasted[i][j-1]\n prev_max = nums[j]\n \n for i in range(n):\n dp[0][i] = wasted[0][i]\n \n for j in range(1, k+1):\n for i in range(j, n):\n for l in range(j, i+1):\n dp[j][i] = min(dp[j][i], dp[j-1][l-1] + wasted[l][i])\n\n return dp[k][n-1]\n``` | 2 | You are currently designing a dynamic array. You are given a **0-indexed** integer array `nums`, where `nums[i]` is the number of elements that will be in the array at time `i`. In addition, you are given an integer `k`, the **maximum** number of times you can **resize** the array (to **any** size).
The size of the array at time `t`, `sizet`, must be at least `nums[t]` because there needs to be enough space in the array to hold all the elements. The **space wasted** at time `t` is defined as `sizet - nums[t]`, and the **total** space wasted is the **sum** of the space wasted across every time `t` where `0 <= t < nums.length`.
Return _the **minimum** **total space wasted** if you can resize the array at most_ `k` _times_.
**Note:** The array can have **any size** at the start and does **not** count towards the number of resizing operations.
**Example 1:**
**Input:** nums = \[10,20\], k = 0
**Output:** 10
**Explanation:** size = \[20,20\].
We can set the initial size to be 20.
The total wasted space is (20 - 10) + (20 - 20) = 10.
**Example 2:**
**Input:** nums = \[10,20,30\], k = 1
**Output:** 10
**Explanation:** size = \[20,20,30\].
We can set the initial size to be 20 and resize to 30 at time 2.
The total wasted space is (20 - 10) + (20 - 20) + (30 - 30) = 10.
**Example 3:**
**Input:** nums = \[10,20,15,30,20\], k = 2
**Output:** 15
**Explanation:** size = \[10,20,20,30,30\].
We can set the initial size to 10, resize to 20 at time 1, and resize to 30 at time 3.
The total wasted space is (10 - 10) + (20 - 20) + (20 - 15) + (30 - 30) + (30 - 20) = 15.
**Constraints:**
* `1 <= nums.length <= 200`
* `1 <= nums[i] <= 106`
* `0 <= k <= nums.length - 1` | The grid is at a maximum 100 x 100, so it is clever to assume that the robot's initial cell is grid[101][101] Run a DFS from the robot's position to make sure that you can reach the target, otherwise you should return -1. Now that you are sure you can reach the target and that you know the grid, run Dijkstra to find the minimum cost. |
[python3] DP with memoization, beat 100% python submissions | minimum-total-space-wasted-with-k-resizing-operations | 0 | 1 | ```\nclass Solution:\n def minSpaceWastedKResizing(self, A: List[int], K: int) -> int:\n def waste(i, j, h):\n sumI = sums[i-1] if i > 0 else 0\n return (j-i+1)*h - sums[j] + sumI\n \n def dp(i, k):\n if i <= k:\n return 0\n if k < 0:\n return MAX\n if (i, k) in memoize:\n return memoize[(i, k)]\n \n _max = A[i]\n r = MAX\n for j in range(i-1, -2, -1):\n r = min(r, dp(j, k-1) + waste(j+1, i, _max))\n _max = max(_max, A[j])\n\n memoize[(i, k)] = r\n return r\n \n sums = list(accumulate(A))\n n = len(A)\n MAX = 10**6*200\n memoize = {}\n\n return dp(n-1, K) | 1 | You are currently designing a dynamic array. You are given a **0-indexed** integer array `nums`, where `nums[i]` is the number of elements that will be in the array at time `i`. In addition, you are given an integer `k`, the **maximum** number of times you can **resize** the array (to **any** size).
The size of the array at time `t`, `sizet`, must be at least `nums[t]` because there needs to be enough space in the array to hold all the elements. The **space wasted** at time `t` is defined as `sizet - nums[t]`, and the **total** space wasted is the **sum** of the space wasted across every time `t` where `0 <= t < nums.length`.
Return _the **minimum** **total space wasted** if you can resize the array at most_ `k` _times_.
**Note:** The array can have **any size** at the start and does **not** count towards the number of resizing operations.
**Example 1:**
**Input:** nums = \[10,20\], k = 0
**Output:** 10
**Explanation:** size = \[20,20\].
We can set the initial size to be 20.
The total wasted space is (20 - 10) + (20 - 20) = 10.
**Example 2:**
**Input:** nums = \[10,20,30\], k = 1
**Output:** 10
**Explanation:** size = \[20,20,30\].
We can set the initial size to be 20 and resize to 30 at time 2.
The total wasted space is (20 - 10) + (20 - 20) + (30 - 30) = 10.
**Example 3:**
**Input:** nums = \[10,20,15,30,20\], k = 2
**Output:** 15
**Explanation:** size = \[10,20,20,30,30\].
We can set the initial size to 10, resize to 20 at time 1, and resize to 30 at time 3.
The total wasted space is (10 - 10) + (20 - 20) + (20 - 15) + (30 - 30) + (30 - 20) = 15.
**Constraints:**
* `1 <= nums.length <= 200`
* `1 <= nums[i] <= 106`
* `0 <= k <= nums.length - 1` | The grid is at a maximum 100 x 100, so it is clever to assume that the robot's initial cell is grid[101][101] Run a DFS from the robot's position to make sure that you can reach the target, otherwise you should return -1. Now that you are sure you can reach the target and that you know the grid, run Dijkstra to find the minimum cost. |
[Python3] Manacher | maximum-product-of-the-length-of-two-palindromic-substrings | 0 | 1 | \n```\nclass Solution:\n def maxProduct(self, s: str) -> int:\n n = len(s)\n \n # Manacher\'s algo\n hlen = [0]*n # half-length\n center = right = 0 \n for i in range(n): \n if i < right: hlen[i] = min(right - i, hlen[2*center - i])\n while 0 <= i-1-hlen[i] and i+1+hlen[i] < len(s) and s[i-1-hlen[i]] == s[i+1+hlen[i]]: \n hlen[i] += 1\n if right < i+hlen[i]: center, right = i, i+hlen[i]\n \n prefix = [0]*n\n suffix = [0]*n\n for i in range(n): \n prefix[i+hlen[i]] = max(prefix[i+hlen[i]], 2*hlen[i]+1)\n suffix[i-hlen[i]] = max(suffix[i-hlen[i]], 2*hlen[i]+1)\n \n for i in range(1, n): \n prefix[~i] = max(prefix[~i], prefix[~i+1]-2)\n suffix[i] = max(suffix[i], suffix[i-1]-2)\n \n for i in range(1, n): \n prefix[i] = max(prefix[i-1], prefix[i])\n suffix[~i] = max(suffix[~i], suffix[~i+1])\n \n return max(prefix[i-1]*suffix[i] for i in range(1, n))\n``` | 4 | You are given a **0-indexed** string `s` and are tasked with finding two **non-intersecting palindromic** substrings of **odd** length such that the product of their lengths is maximized.
More formally, you want to choose four integers `i`, `j`, `k`, `l` such that `0 <= i <= j < k <= l < s.length` and both the substrings `s[i...j]` and `s[k...l]` are palindromes and have odd lengths. `s[i...j]` denotes a substring from index `i` to index `j` **inclusive**.
Return _the **maximum** possible product of the lengths of the two non-intersecting palindromic substrings._
A **palindrome** is a string that is the same forward and backward. A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "ababbb "
**Output:** 9
**Explanation:** Substrings "aba " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Example 2:**
**Input:** s = "zaaaxbbby "
**Output:** 9
**Explanation:** Substrings "aaa " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Constraints:**
* `2 <= s.length <= 105`
* `s` consists of lowercase English letters. | Iterate over the string and mark each character as found (using a boolean array, bitmask, or any other similar way). Check if the number of found characters equals the alphabet length. |
Python (Simple Manacher's algorithm) | maximum-product-of-the-length-of-two-palindromic-substrings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxProduct(self, s):\n n = len(s)\n\n dp, before, after = [0]*n, [0]*n, [0]*n\n\n c, r = -1, -1\n\n for i in range(n):\n k = min(dp[2*c-i],r-i) if i<=r else 0\n p, q = i-k, i+k\n\n while p>=0 and q<n and s[p] == s[q]:\n before[q] = max(before[q],q-p+1)\n after[p] = max(after[p],q-p+1)\n p -= 1\n q += 1\n\n dp[i] = q-i-1\n\n if q-1 > r: c, r = i, q-1\n\n for i in range(1,n):\n before[i] = max(before[i-1],before[i])\n\n for i in range(n-2,-1,-1):\n after[i] = max(after[i+1],after[i])\n\n return max([before[i-1]*after[i] for i in range(1,n)])\n\n\n\n \n\n\n\n\n\n\n\n\n\n\n\n \n \n``` | 0 | You are given a **0-indexed** string `s` and are tasked with finding two **non-intersecting palindromic** substrings of **odd** length such that the product of their lengths is maximized.
More formally, you want to choose four integers `i`, `j`, `k`, `l` such that `0 <= i <= j < k <= l < s.length` and both the substrings `s[i...j]` and `s[k...l]` are palindromes and have odd lengths. `s[i...j]` denotes a substring from index `i` to index `j` **inclusive**.
Return _the **maximum** possible product of the lengths of the two non-intersecting palindromic substrings._
A **palindrome** is a string that is the same forward and backward. A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "ababbb "
**Output:** 9
**Explanation:** Substrings "aba " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Example 2:**
**Input:** s = "zaaaxbbby "
**Output:** 9
**Explanation:** Substrings "aaa " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Constraints:**
* `2 <= s.length <= 105`
* `s` consists of lowercase English letters. | Iterate over the string and mark each character as found (using a boolean array, bitmask, or any other similar way). Check if the number of found characters equals the alphabet length. |
Premium Limited Explained Solution | maximum-product-of-the-length-of-two-palindromic-substrings | 1 | 1 | \n```\nclass Solution\n{\npublic:\n long long maxProduct(string s)\n {\n\n int n = s.size();\n vector<int> d1 = vector<int>(s.size(), 0);\n for (int i = 0, l = 0, r = -1; i < n; i++)\n {\n int k = (i > r) ? 1 : min(d1[l + r - i], r - i + 1);\n while (0 <= i - k && i + k < n && s[i - k] == s[i + k])\n {\n k++;\n }\n d1[i] = k--;\n if (i + k > r)\n {\n l = i - k;\n r = i + k;\n }\n }\n\n vector<int> maxL = vector<int>(s.size(), 1);\n auto lQueue = set<pair<int, int>>();\n for (int i = 0; i < s.size(); i++)\n {\n if (i > 0)\n maxL[i] = max(maxL[i], maxL[i - 1]);\n\n lQueue.insert({i, d1[i]});\n\n while (lQueue.begin()->first + lQueue.begin()->second - 1 < i)\n {\n lQueue.erase(lQueue.begin());\n }\n\n maxL[i] = max(maxL[i], ((i - lQueue.begin()->first) * 2) + 1);\n }\n\n vector<int> maxR = vector<int>(s.size(), 1);\n auto rQueue = priority_queue<pair<int, int>>();\n\n for (int i = s.size() - 1; i >= 0; i--)\n {\n if (i < s.size() - 1)\n maxR[i] = max(maxR[i], maxR[i + 1]);\n\n rQueue.push({i, d1[i]});\n\n while (rQueue.top().first - (rQueue.top().second - 1) > i)\n {\n rQueue.pop();\n }\n\n maxR[i] = max(maxR[i], ((rQueue.top().first - i) * 2) + 1);\n }\n\n long long res = 0;\n for (int i = 0; i < s.size() - 1; i++)\n {\n res = max(res, (long long) maxL[i] * (long long)maxR[i + 1]);\n }\n return res;\n }\n};\n``` | 0 | You are given a **0-indexed** string `s` and are tasked with finding two **non-intersecting palindromic** substrings of **odd** length such that the product of their lengths is maximized.
More formally, you want to choose four integers `i`, `j`, `k`, `l` such that `0 <= i <= j < k <= l < s.length` and both the substrings `s[i...j]` and `s[k...l]` are palindromes and have odd lengths. `s[i...j]` denotes a substring from index `i` to index `j` **inclusive**.
Return _the **maximum** possible product of the lengths of the two non-intersecting palindromic substrings._
A **palindrome** is a string that is the same forward and backward. A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "ababbb "
**Output:** 9
**Explanation:** Substrings "aba " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Example 2:**
**Input:** s = "zaaaxbbby "
**Output:** 9
**Explanation:** Substrings "aaa " and "bbb " are palindromes with odd length. product = 3 \* 3 = 9.
**Constraints:**
* `2 <= s.length <= 105`
* `s` consists of lowercase English letters. | Iterate over the string and mark each character as found (using a boolean array, bitmask, or any other similar way). Check if the number of found characters equals the alphabet length. |
[Python3] move along s | check-if-string-is-a-prefix-of-array | 0 | 1 | \n```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n i = 0\n for word in words: \n if s[i:i+len(word)] != word: return False \n i += len(word)\n if i == len(s): return True \n return False \n```\n\nThe solutions for weekly 255 can be found in this [commit](https://github.com/gaosanyong/leetcode/commit/312849ed66e5ad76fa278cbb34dd57772fb3ceeb). | 24 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
python3 code | check-if-string-is-a-prefix-of-array | 0 | 1 | # Code\n```\nclass Solution:\n def isPrefixString(self, s: str, words):\n subresult = [\'\'.join(words[:i+1]) for i in range(len(words))]\n for substr in subresult:\n if substr == s: return True\n return False\n```\n\n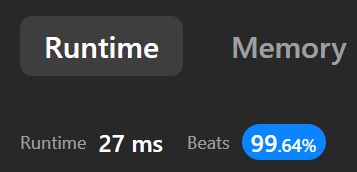 | 4 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python - Most Simple Solution - One Liner! | check-if-string-is-a-prefix-of-array | 0 | 1 | **Solution**:\n```\nclass Solution:\n def isPrefixString(self, s, words):\n return s in accumulate(words)\n``` | 5 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python Easy | check-if-string-is-a-prefix-of-array | 0 | 1 | ```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n \n a = \'\'\n \n for i in words:\n \n a += i\n \n if a == s:\n return True\n if not s.startswith(a):\n break\n \n return False \n``` | 11 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python solution for beginners by beginner. | check-if-string-is-a-prefix-of-array | 0 | 1 | \nRuntime: 36 ms, faster than 91.08% of Python3 online submissions for Check If String Is a Prefix of Array.\nMemory Usage: 13.8 MB, less than 97.53% of Python3 online submissions for Check If String Is a Prefix of Array.\n\n```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n ans = \'\'\n for i in words:\n ans += i\n if ans == s :\n return True\n return False\n``` | 3 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python easy to understand | check-if-string-is-a-prefix-of-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n\n combinedWords = ""\n\n for word in words:\n combinedWords += word\n if s == combinedWords:\n return True\n\n return False\n``` | 0 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
[ Python3] Simple Brute Force - Beginner | check-if-string-is-a-prefix-of-array | 0 | 1 | **Brute Force Solution**\n```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n #Initialize empty string\n\t\tpr = \'\'\n\t\t#Store the length of string\n l = len(s)\n for i in words:\n\t\t\t#keep concatenating the elements in words to pr and check if it is equal to s\n pr += i\n if pr == s:\n return True\n\t\t\t# If the len of pr is more than that of s and the previous condition is not satisfied, then its not a prefix string\n elif len(pr) > l:\n return False\n``` | 0 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python | check-if-string-is-a-prefix-of-array | 0 | 1 | # Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(n)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)\n# Code\n```\nclass Solution:\n def isPrefixString(self, s: str, words: List[str]) -> bool:\n word = \'\'\n\n for i in range(len(words)):\n word += words[i]\n\n if word == s:\n return True\n\n return False\n``` | 0 | Given a string `s` and an array of strings `words`, determine whether `s` is a **prefix string** of `words`.
A string `s` is a **prefix string** of `words` if `s` can be made by concatenating the first `k` strings in `words` for some **positive** `k` no larger than `words.length`.
Return `true` _if_ `s` _is a **prefix string** of_ `words`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "iloveleetcode ", words = \[ "i ", "love ", "leetcode ", "apples "\]
**Output:** true
**Explanation:**
s can be made by concatenating "i ", "love ", and "leetcode " together.
**Example 2:**
**Input:** s = "iloveleetcode ", words = \[ "apples ", "i ", "love ", "leetcode "\]
**Output:** false
**Explanation:**
It is impossible to make s using a prefix of arr.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 20`
* `1 <= s.length <= 1000`
* `words[i]` and `s` consist of only lowercase English letters. | It is always optimal to buy the least expensive ice cream bar first. Sort the prices so that the cheapest ice cream bar comes first. |
Python - Priority Queue - Beats 90% | remove-stones-to-minimize-the-total | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nUse a max heap to retrieve the max element every time. We will then run k operations until we have our minimum sum. We must push a negative value because heapq in python is default to minheap.\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n max_heap = []\n\n for pile in piles:\n heapq.heappush(max_heap, -pile)\n\n while k != 0:\n stone = heapq.heappop(max_heap)\n stone = math.ceil(stone * -1 / 2)\n heapq.heappush(max_heap, -stone)\n k -= 1\n\n return -sum(max_heap)\n``` | 4 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
[Python] 90% -- unusual -- use dict and iterate from max stones | remove-stones-to-minimize-the-total | 0 | 1 | # Summary \nThe solution is fast due to the use of a dictionary that uses hashing and due to the fact that we do not move the piles in any way (we just change the number of piles in the dict). But we use a lot of memory for this.\n\n\n\u2764\uFE0F **Please upvote if you liked this unusual approach**\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n*Same comments in the code*\n\n1) Make a dictionary where the element\'s key - `piles[i]` and value - number of piles\n2) Find **max** stones in piles\n3) Iterate from **max** to **0**\n 1) Find pile with this number of stones\n 2) Remove stones (while there are the piles and k is not 0) and move new piles (changing the number of piles in the dict)\n 3) Break if `k==0`\n4) Count the amount\n\n\n# Complexity\n- Time complexity: $$O(max(stonesInPiles))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n+k)$$ \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n # 1) Make a dictionary where \n # the element\'s key - `piles[i]` and value - number of piles\n dictPiles = {}\n for p in piles:\n if p in dictPiles:\n dictPiles[p] += 1\n else:\n dictPiles[p] = 1\n \n # 2) Find max stones in piles\n maxp = max(piles) \n\n # 3) Iterate from max to 0\n for stones in range(maxp,0,-1):\n # 3.a) Find pile with this number of stones\n if stones in dictPiles:\n # 3.b) Remove stones (while there are the piles \n # and k is not 0) and move new piles (changing \n # the number of piles in the dict)\n while dictPiles[stones] and k:\n dictPiles[stones] -= 1\n # move the pile after deletion\n t = stones - (stones // 2)\n if t in dictPiles:\n dictPiles[t] += 1\n else:\n dictPiles[t] = 1\n k -= 1\n\n # 3.c) Break if k==0\n if not k:\n break\n \n # 4) Count the amount\n sumS = 0\n for k,v in dictPiles.items():\n sumS += k*v\n\n return sumS\n``` | 3 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Max Heap Solution | remove-stones-to-minimize-the-total | 0 | 1 | ```\nimport heapq\n\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n maxHeap = []\n \n for i in range(len(piles)):\n heapq.heappush(maxHeap, -piles[i])\n\n i = k\n while i > 0:\n temp = heapq.heappop(maxHeap)\n j = temp//2\n heapq.heappush(maxHeap,j)\n i -= 1\n \n return -sum(maxHeap)\n``` | 3 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Python | O(n + max) w/o Heap | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\n\nSince the piles are relatively small, there are more efficient ways to maintain a priority queue.\n\n# Approach\n\nUse a frequency table to handle duplicate pile sizes: `cnt[size]` is the number of piles with given `size`. \n\nAfter building the table, loop from largest to smallest pile size and batch process all piles of this size. Namely, if there are `cnt[pile]` piles of the current size and some `operations` remaining, then we perform a batch of `min(cnt[pile], operations)` operations. \n\nNotice how batch processing removes $$k$$ from the complexity entirely.\n\n# Complexity\n\n- Time complexity: $$O(n + \\max)$$. $$O(n)$$ to build the frequency table, $$O(\\max)$$ to process it.\n\n- Space complexity: $$O(\\max)$$ to store the frequency table.\n\n# Code\n\n```python\nclass Solution:\n def minStoneSum(self, piles: list[int], operations: int) -> int:\n max_pile = max(piles)\n\n cnt = [0] * (max_pile + 1)\n for pile in piles:\n cnt[pile] += 1\n\n for pile in range(max_pile, 0, -1):\n take = min(cnt[pile], operations)\n cnt[pile] -= take\n cnt[pile - pile // 2] += take\n\n operations -= take\n if not operations:\n break\n\n return sum(pile * cnt[pile] for pile in range(max_pile + 1))\n\n``` | 2 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
[Python] Hash table, beats 100% | remove-stones-to-minimize-the-total | 0 | 1 | # Description\nHere\'s something a bit different from the typical heap solution.\n\nObserve that the *entries* in the input actually have an upper bound that is *below* the maximum input length, which makes hash table an efficient choice of data structure. The keys are integers, so we can use an array as the hash table, which also lets us find the largest pile in constant time - as long as we remember to remove the empty piles from the top.\n\n# Complexity\n- Time complexity: O(n + k)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(max(piles))\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n\n # Construct frequency table\n count = [0 for x in range(max(piles)+1)]\n for x in piles: count[x] += 1\n\n # Perform pile-halving\n for _ in range(k):\n count[-1] -= 1\n count[len(count) // 2] += 1\n\n # Remove empty piles from top, to ensure top pile has any stones\n while count[-1] == 0: count.pop()\n \n # Count remainder\n return sum(i * count[i] for i in range(len(count)))\n\n``` | 2 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Python3 Heap | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIn each iteration, we need to \n1. pop the maximum elements in piles\n2. do the operation \n3. put the new element back to piles\n\nWe could simply implement it by sort piles, get the maximum, do the operation and put it back. However, it exceeds time limit. \n\nIntuitively, we think of using heap. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nPython has ready to use heap API, [heapq](https://docs.python.org/3/library/heapq.html). However, it is a min heap which pops the minimum item while we need a max heap which pops the maximum item. \n\nA easy way to change a min heap to a max heap is to convert all elements to negative. \n\n\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n negativePiles = [-p for p in piles]\n total = sum(piles)\n heapq.heapify(negativePiles)\n for _ in range(k):\n maxi = heapq.heappop(negativePiles)\n decrement = math.floor(-maxi / 2)\n total -= decrement\n heapq.heappush(negativePiles, maxi + decrement)\n \n return total\n\n\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Easy Python Solution using maxHeap | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\nEach operation involves selecting a pile of stones with the maximum number of stones, removing half of the stones, and putting the remaining stones back into the pile. The code then returns the maximum number of stones that can be left in the piles after all operations are performed.\n\n# Approach\nThe code first creates a list of negative integers representing the piles, and uses the heapq module to turn it into a max heap. It then repeatedly pops the maximum element from the heap, halves it, and puts it back into the heap until the desired number of operations have been performed. Finally, it calculates the sum of the remaining elements in the heap, converts it back to a positive value, and returns it as the result.\n\n# Complexity\n- Time complexity:\nO(k log n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n\n maxPiles = [-i for i in piles]\n heapq.heapify(maxPiles)\n for i in range(k):\n temp = -heapq.heappop(maxPiles)\n val = temp // 2\n heapq.heappush(maxPiles, -(temp - val))\n return -sum(maxPiles)\n \n \n\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Max heap|| with intuition and approach || with picture explanation || Ceil not Floor | remove-stones-to-minimize-the-total | 0 | 1 | # Approach\n- We have been given a pile of stones and from these we have to remove \nceil(piles[i]/2) stones\n- we can perform above operattion of removing stone on the same pile more than once\n- k -> it is the no of times you have to perform above operation\n- And we need to return minimum no of stones left after performing k operations\n---\n1. Make a max heap of the given list of piles\n2. as you know we can only make min heap in python ,so multiple the with elements with "-" , heapify your heap your max will be ready ...!!!\n3. for atmost k times\n4. we pop the top element perform operation i.e., ceil(piles[i]/2) \n5. and check whether it is greater than 0 or not if so then we\'ll again push that element back into the heap after performing step 2\n6. from above steps we are done with one operation , so decrease the k by 1\n7. now return the sum of elements of max_heap and multiply it with "-"\n8. you\'ll get your minimum no of stones\n\n# Dry Run\n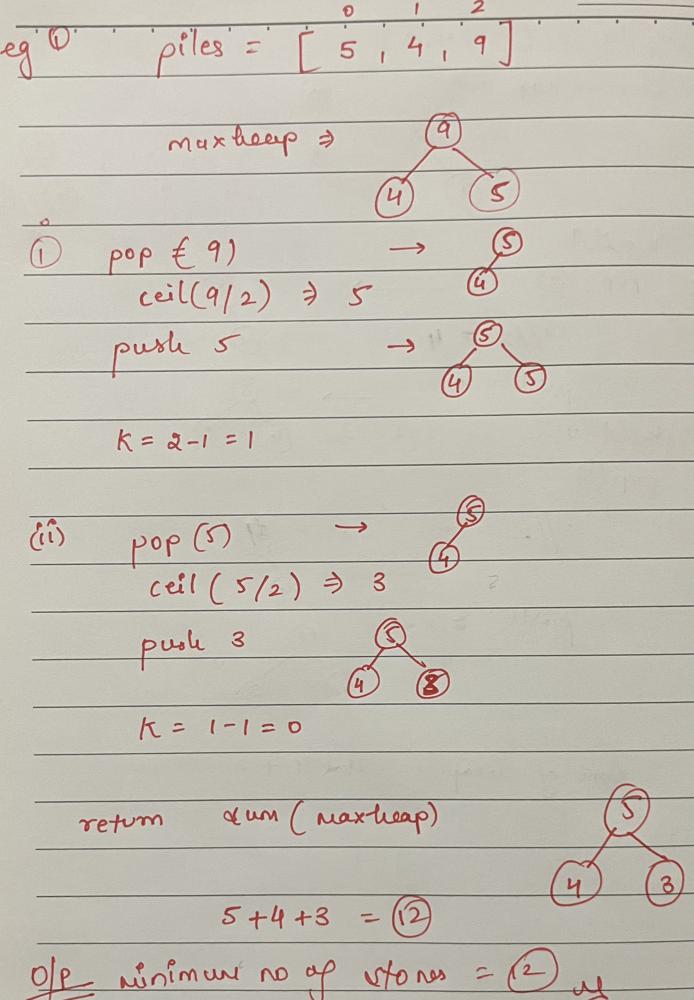\n\n\n\n\n# Code\n```\n#Test cases are given according to ceil not floor\n#so while solving the question you should consider ceil value of operation instead of floor value\n\nimport math\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n #first of all make a max heap of piles\n max_heap=[-x for x in piles]\n #heapify it\n heapq.heapify(max_heap)\n for i in range(k):\n #pop the element from max heap\n first_pile= -heapq.heappop(max_heap)\n #then perform the operation as stated in the ques\n first_pile= math.ceil(first_pile/2)\n #then if it is greater than 0 push it back to the max heap\n if first_pile > 0:\n heapq.heappush(max_heap,-first_pile)\n #decrease the no of operation i.e., k \n k-=1\n #finally return the sum of elements of max heap and don\'t forget to multiply the ans with "-"..lol..!!\n return (-(sum(max_heap)))\n \n\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Python3 | 1400 ms (100% faster code) | O(max(n*ln(n), k)) | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst of all, sort given array in non-decreasing order. Time complexity $$O(n * ln(n))$$. \nThen we do the following steps:\n- we find the maximum value between the last element in the array and the first element in the queue\n- pop this element\n- add element // to queue\n\nTime complexity of this is $$O(k)$$\n\nAnd total time complexity is $$O(max(n*ln(n), k))$$\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(max(n*ln(n), k))$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n piles.sort()\n q = deque()\n\n for i in range(k):\n p1 = 0\n p2 = 0\n if piles:\n p1 = piles[-1]\n if q:\n p2 = q[0]\n if p1 > p2:\n p = piles.pop()\n else:\n p = q.popleft()\n q.append(math.ceil(p / 2))\n\n return sum(piles) + sum(q)\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
python3, sortedcontainers.SortedList() | remove-stones-to-minimize-the-total | 0 | 1 | https://leetcode.com/submissions/detail/866817274/\nRuntime: 3083 ms \nMemory Usage: 29 MB \n```\nfrom sortedcontainers import SortedList\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n l = SortedList()\n l.update(piles)\n remained = sum(piles)\n for _ in range(k):\n p = l.pop(-1)\n r = p//2\n if r==0: break\n remained -= r\n l.add(p-r)\n return remained\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Python | Max Heap | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nNeed to greedily figureout the maximum element of the pile.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse a max heap to store the piles. Pop the heap, modify the pile and then push it back in the heap.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n + klog(n))$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ can be $$O(1)$$ if heap is created via the input list.\n\n# Code\n```\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n max_heap = []\n for pile in piles:\n heapq.heappush(max_heap, -pile)\n\n for _ in range(k):\n pile = heapq.heappop(max_heap)\n pile = math.ceil(-pile/2)\n heapq.heappush(max_heap, -pile)\n \n return -sum(max_heap)\n\n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Easy python solution using min heap :) 🔥 | remove-stones-to-minimize-the-total | 0 | 1 | # Intuition\nwe need to reduce the maximum element in array so we need to keep track of max elememt :) so heap comes in our way.\n\n# Approach\nI used heapq to heapify the given array by multiplying each value by -1 to get a max heap :)\n\n# Complexity\n- Time complexity: O(n + log(n))\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport heapq\nclass Solution:\n def minStoneSum(self, piles: List[int], k: int) -> int:\n \n total = sum(piles)\n\n piles = [-i for i in piles]\n\n heapq.heapify(piles)\n\n while k > 0:\n temp = heapq.heappop(piles)*-1\n\n total -= temp//2\n temp -= temp//2\n heapq.heappush(piles, temp*-1)\n\n k -= 1\n return total\n \n\n\n \n``` | 1 | You are given a **0-indexed** integer array `piles`, where `piles[i]` represents the number of stones in the `ith` pile, and an integer `k`. You should apply the following operation **exactly** `k` times:
* Choose any `piles[i]` and **remove** `floor(piles[i] / 2)` stones from it.
**Notice** that you can apply the operation on the **same** pile more than once.
Return _the **minimum** possible total number of stones remaining after applying the_ `k` _operations_.
`floor(x)` is the **greatest** integer that is **smaller** than or **equal** to `x` (i.e., rounds `x` down).
**Example 1:**
**Input:** piles = \[5,4,9\], k = 2
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[5,4,5\].
- Apply the operation on pile 0. The resulting piles are \[3,4,5\].
The total number of stones in \[3,4,5\] is 12.
**Example 2:**
**Input:** piles = \[4,3,6,7\], k = 3
**Output:** 12
**Explanation:** Steps of a possible scenario are:
- Apply the operation on pile 2. The resulting piles are \[4,3,3,7\].
- Apply the operation on pile 3. The resulting piles are \[4,3,3,4\].
- Apply the operation on pile 0. The resulting piles are \[2,3,3,4\].
The total number of stones in \[2,3,3,4\] is 12.
**Constraints:**
* `1 <= piles.length <= 105`
* `1 <= piles[i] <= 104`
* `1 <= k <= 105` | To simulate the problem we first need to note that if at any point in time there are no enqueued tasks we need to wait to the smallest enqueue time of a non-processed element We need a data structure like a min-heap to support choosing the task with the smallest processing time from all the enqueued tasks |
Beginner-friendly || Simple stack-simulation in Python3 | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | # Intuition\nLets clarify the description:\n- our task is to validate sequence of **brackets**, that consists **exactly** of pairs `[` and `]` (50% / 50%)\n- the final answer will represent the **count** of swaps that\'re needed to perform in order to make a sequence **valid**\n \n```py\n# Example 1\nseq = \'[[]]\'\n# no swaps are required\nswaps = 0\n\n# Example 2\nseq = \']][[\'\n# one of the possible variants\n# swap 0 and 3\nsteps = 1\n```\n### One of the possible solutions is to swap the current bracket with one, that\'s close to the `end`.\n\n---\n\n\nThis is where the [Stack DS](https://en.wikipedia.org/wiki/Stack_(abstract_data_type)) is come from!\n\n# Approach\n1. initialize an `end` variable to store the last index of a sequence `s`\n2. simulate the `stack` as a **number** to reduce **space complexity**\n3. initialize an `ans` variable to store a number of swaps\n4. iterate before `end`\n5. at each step check the current bracket\n6. if it\'s an **open bracket**, increment a `stack`\n7. else check the `stack` and **decrement** amount of **open brackets** inside\n8. otherwise try to find **the nearest closing bracket** and reduce `end`, and increment an `ans`\n9. return the number of swaps `ans` \n\n# Complexity\n- Time complexity: **O(n)** in worst case scenario, because at each `while` we **reduce** the `end` index\n\n- Space complexity: **O(1)**, we don\'t use extra space.\n\n# Code\n```\nclass Solution:\n def minSwaps(self, s: str) -> int:\n end = len(s) - 1\n stack = 0\n ans = 0\n\n for start in range(end):\n if s[start] == \'[\':\n stack += 1\n elif stack:\n stack -= 1\n else:\n ans += 1\n\n while s[end] != \'[\':\n end -= 1\n\n stack += 1\n end -= 1\n\n return ans\n``` | 1 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
O(n) time with O(1) space - Counting problem | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | # Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution:\n def minSwaps(self, s: str) -> int:\n\n\n # stack = []\n count = 0\n res = 0\n for i in s:\n if i == \'[\':\n count += 1\n else:\n if count>0:\n count -= 1\n continue\n else:\n res += 1\n return ceil(res/2)\n\n``` | 1 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
[Python 3] Stack - Simple | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(N)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(N)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minSwaps(self, s: str) -> int:\n cnt = 0\n stack = []\n for p in s:\n if p == "[": stack.append("[")\n else:\n if not stack: \n stack.append("]")\n cnt += 1\n else: \n stack.pop()\n return cnt\n``` | 8 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
[Java/Python 3] Space O(1) time O(n) codes w/ brief explanation. | minimum-number-of-swaps-to-make-the-string-balanced | 1 | 1 | Traverse the input `s`, remove all matched `[]`\'s. Since the number of `[`\'s and `]`\'s are same, the remaining must be `]]]...[[[`. Otherwise, **if left half had any `[`, there would be at least a matched `[]` among them.**\n\n1. For every `2` pairs of square brackets, a swap will make them matched;\n2. If only `1` pair not matched, we need a swap.\nTherefore, we need at least `(pairs + 1) / 2` swaps.\n\n----\n**Method 1: Stack**\nOnly store open square brackets.\n```java\n public int minSwaps(String s) {\n Deque<Character> stk = new ArrayDeque<>();\n for (char c : s.toCharArray()) {\n if (!stk.isEmpty() && c == \']\') {\n stk.pop();\n }else if (c == \'[\') {\n stk.push(c);\n }\n }\n return (stk.size() + 1) / 2;\n }\n```\n```python\n def minSwaps(self, s: str) -> int:\n stk = []\n for c in s:\n if stk and c == \']\':\n stk.pop()\n elif c == \'[\':\n stk.append(c)\n return (len(stk) + 1) // 2\n```\n**Analysis:**\nTime & space: `O(n)`, where `n = s.length()`.\n\n----\n\nThere are only `2` types of characters in `s`, so we can get rid of stack in above codes and use two counters, `openBracket` and `closeBracket`, to memorize the unmatched open and close square brackets: - Credit to **@saksham66** for suggestion of variables naming.\n```java\n public int minSwaps(String s) {\n int openBracket = 0;\n for (int i = 0, closeBracket = 0; i < s.length(); ++i) {\n char c = s.charAt(i);\n if (openBracket > 0 && c == \']\') {\n --openBracket;\n }else if (c == \'[\') {\n ++openBracket;\n }else {\n ++closeBracket;\n }\n }\n return (openBracket + 1) / 2;\n }\n```\n```python\n def minSwaps(self, s: str) -> int:\n open_bracket = close_bracket = 0\n for c in s:\n if open_bracket > 0 and c == \']\':\n open_bracket -= 1\n elif c == \'[\':\n open_bracket += 1\n else:\n close_bracket += 1 \n return (open_bracket + 1) // 2\n```\nSince the occurrences of `[`\'s and `]`\'s are same, we actually only need to count `openBracket`, and `closeBracket` is redundant. Credit to **@fishballLin**\n\n```java\n public int minSwaps(String s) {\n int openBracket = 0;\n for (int i = 0; i < s.length(); ++i) {\n char c = s.charAt(i);\n if (openBracket > 0 && c == \']\') {\n --openBracket;\n }else if (c == \'[\') {\n ++openBracket;\n }\n }\n return (openBracket + 1) / 2;\n }\n```\n\n```python\n def minSwaps(self, s: str) -> int:\n open_bracket = 0\n for c in s:\n if open_bracket > 0 and c == \']\':\n open_bracket -= 1\n elif c == \'[\':\n open_bracket += 1\n return (open_bracket + 1) // 2\n```\n\n**Analysis:**\nTime: `O(n)`, space: `O(1)`, where `n = s.length()`.\n | 47 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
Two Pointers | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | We go left to right (`i`), and track open and closed brackets in `bal`. When `bal` goes negative, we have an orphan `]`. \n\nTo balance it out, we need to find an orphaned `[` and swap those two. So, we go right-to-left (`j`), and we can search for the orphaned `[`. \n\nWhy is this strategy optimal? Because we have to fix all orphaned `]` and `[` brackets. The optimal strategy is to fix two brackets with one swap.\n\n#### Alternative Solution\nSee the original solution for additional details. To find the number of swaps, we can just count all orphaned `]` (or `[`) in `cnt`. The answer is `(cnt + 1) / 2` to fix the string, since one swap matches 4 brackest (or two in the last swap - if `cnt` is odd.\n\nThis solution was inspired by our conversation with [digvijay91](https://leetcode.com/digvijay91/) (see comments for additional details).\n\n**C++**\n```cpp\nint minSwaps(string s) {\n int bal = 0, cnt = 0;\n for (auto ch : s)\n if (bal == 0 && ch == \']\')\n ++cnt;\n else\n bal += ch == \'[\' ? 1 : -1;\n return (cnt + 1) / 2;\n}\n```\n\n#### Original Solution\n**C++**\n```cpp\nint minSwaps(string s) {\n int res = 0, bal = 0;\n for (int i = 0, j = s.size() - 1; i < j; ++i) {\n bal += s[i] == \'[\' ? 1 : -1;\n if (bal < 0) {\n for (int bal1 = 0; bal1 >= 0; --j)\n bal1 += s[j] == \']\' ? 1 : -1;\n swap(s[i], s[j + 1]);\n ++res;\n bal = 1;\n }\n }\n return res;\n}\n```\n**Python 3**\n```python\nclass Solution:\n def minSwaps(self, s: str) -> int:\n res, bal = 0, 0\n for ch in s:\n bal += 1 if ch == \'[\' else -1\n if bal == -1:\n res += 1\n bal = 1\n return res\n``` | 23 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
✔ Python3 Solution | Clean & Concise | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Code\n```\nclass Solution:\n def minSwaps(self, s):\n cur, ans = 0, 0\n for i in s:\n if i == \']\' and cur == 0: ans += 1\n if i == \'[\' or cur == 0: cur += 1\n else: cur -= 1\n return ans\n``` | 2 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
Easy and Intuitive Python Solution With Images and Walkthrough | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | ## Hi guys, I hope my post can help you in understanding this problem. ##\n\n### First, we need to understand what is the "**balanced**" scenario. There are 3 types of balanced scenario as shown below: ###\n<img src = "https://assets.leetcode.com/users/images/cd21075c-57a8-46b0-bd0e-911d7e13e3b1_1649990481.5305083.png" width = "700" height = "250"> \n\n### This is the "**worst**" scenario where everything is not in order: ###\n<img src = "https://assets.leetcode.com/users/images/6d0b6637-419a-4c6d-a0ad-8f9fbd8496bc_1649990610.3125339.png" width = "600" height = "200">\n\n### This is the half-good half bad scenario. Some brackets are balanced, some are not. The highlighted brackets are balanced. ###\n<img src = "https://assets.leetcode.com/users/images/38fbb1f8-f14e-49bd-bf6c-b38ddbf3d80b_1649990736.5708444.png" width = "700" height = "300">\n\n### Note that the following is a **NON**-efficient way in swapping. We are swapping more than needed. We are swapping 4 times in this case. ### \n<img src = "https://assets.leetcode.com/users/images/fa8c4ba5-8cb0-46d3-8031-109aa7f690e5_1649991599.0594234.png" width = "700" height = "300"> )\n\n### The most efficient way to swap the brackets to become balanced is by using the following trick. We only swap 2 times in this case. Swap brackets 3 and 6 followed with 1 and 8. Note that this is for even numbers of pairs of unbalanced brackets. In this case, we have 4 pairs of unbalanced brackets which is an even numbers of pairs of unbalanced brackets. ###\n<img src = "https://assets.leetcode.com/users/images/b119c713-0bac-428c-9586-95d5b6351294_1649991848.5251782.png" width = "700" height = "250" >\n\n### Question: What if we have an odd number of pairs of unbalanced brackets? How many times do we need to switch? Is it the same as even number of unbalanced brackets? In the image below, we have 3 pairs of unbalanced brackets which is an odd pair. ###\n<img src = "https://assets.leetcode.com/users/images/9809a53a-5b93-4496-879c-f568a90b353d_1649992024.3351176.png" width = "700" height = "100">\n\n<img src = "https://assets.leetcode.com/users/images/8c99ca9b-57ed-4ddc-ad08-2cfad70d15fa_1649992325.9850376.png" width = "700" height = "250">\n\n### We can have other methods to switch the brackets to make it balanced. To find the minimum number of swaps to make the string balanced, we have to use the most effective swapping technique which is shown in the image below. In this case we need to switch 2 times. You can try out other possible methods to check it yourself.\n<img src = "https://assets.leetcode.com/users/images/2d6c9d51-e8b9-40c7-b597-0e638b2f441a_1649992386.3647358.png" width = "700" height = "250">\n\n### Notice!!! We can ignore balanced brackets in the middle of the given string if there is any! For example, take a look at the image below. ###\n\n<img src="https://assets.leetcode.com/users/images/209111b1-2f2a-4c8d-8380-665c6f38ed24_1649993867.3050117.png" width = "700" height = "300">\n\n\n### After cancelling the highlighted part, we are left with the simple case of either odd pairs of unbalanced brackets or an even case of unbalanced brackets. ###\n\n### In conclusion, for both even number or odd number of unbalanced brackets, the minimum swaps needed is (n + 1) // 2 since that is the most efficient way to perform the minimum number of swaps. The (n + 1) is used to account for the odd case. For example, in the previous example, we have 3 odd pairs of brackets so minimum number of swaps needed = (3 + 1) // 2 = 2. \n\n\n### \n### Question: How do we know whether a string is balanced or not? We can use a counter to keep track of the number of opening " [ " and number \n### of " ] " bracket. Whenever we encounter " [ " we will add one to our counter and we will only decrement the counter if and only if the counter is positive and the current bracket is a closing one (" ] " ). \n\n### ...\n### ...\n### ...\n\n### Why? Let\'s take a look at the image below!\n<img src = "https://assets.leetcode.com/users/images/9e94ae78-20b2-4afd-951f-b0a13b0a8b82_1649994288.6538506.png" width = "700" height = "150">\n\n### For this normal case, the result will be zero after we reached the end of the string. The steps would be +1 + 1 -1 + 1 - 1 - 1 = 0\n\n### Special case !!! ###\n<img src = "https://assets.leetcode.com/users/images/937ba7ed-93b6-4c85-9197-397fe939a26a_1649994520.1879165.png" width = "700" height = "150">\n\n### The image above will result in a count of 0 ( -1 + 1 -1 + 1). Does it mean it is balanced? No, it is not. To tackle this special case, we will increase the counter value by one if and only if it is an opening bracket (" [ " ) and decrease the counter value by one if and only if it is a closing bracket (" ] " ) and the counter is positive. Reason? This is because we are finding the number of unbalanced pairs of brackets. Whenever we encounter an opening bracket, there must be a closing bracket waiting after the opening bracket to make it balanced. So for the case like the one in the image here: \n\n<img src = "https://assets.leetcode.com/users/images/937ba7ed-93b6-4c85-9197-397fe939a26a_1649994520.1879165.png" width = "700" height = "150">\n\n### We will skip through the first bracket and do nothing to our counter even if it is a closing one and move to the next bracket. The reason we are allowed to skip this bracket is although we know that it is an unbalanced bracket is because we always know that there is an extra opening bracket waiting for this closing bracket somewhere in the future that makes this pair an unbalanced one. We can increment the counter when we find this leftover opening bracket later in the string afterwards. ###\n\n# Detailed walkthrough of the changes in counter value: \n### Step 1: \n### We will not decrement our counter since counter is initially zero. Move to the next bracket.\n### Step 2:\n### It is an opening bracket so add one to our counter. This is because this signifies a possibility of an (one) unbalanced bracket. Now, counter = 1. Move to the next bracket.\n### Step 3:\n### It is a closing bracket and since counter is greater than zero, we can decrease our counter value. We found a matching pair so it is logical and reasonable to decrement our value by one to indicate that the possibility of an (one) unbalanced bracket has decrease by one. Move to the next bracket.\n### Step 4:\n### It is an opening bracket so increase counter value by one. Now here, counter = 1 and the loops stop. ### That means We found one pair of unbalanced bracket and well where does this come from? This is a result of the mismatched( unbalanced bracket we encounter at start remember???) Hence, that is the reason why we do not need to decrease the counter at step one. The fact is we will always find a pair of opening bracket and opening bracket. If the counter is not positive and we encounter a closing bracket, we will skip it and not decrement the counter and move on and we know that we will find its counter (opening bracket in the future) and we can add one to the counter later on in the future.\n\n\n```\nclass Solution:\n def minSwaps(self, s: str) -> int:\n count = 0\n for i in s:\n if i == "[":\n count += 1 # increment only if we encounter an open bracket. \n else:\n if count > 0: #decrement only if count is positive. Else do nothing and move on. This is because for the case " ] [ [ ] " we do not need to in\n count -= 1\n return (count + 1) // 2\n \n```\n\n\n\n\n\n\n\n\n | 18 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
pyton easy soution understadble | minimum-number-of-swaps-to-make-the-string-balanced | 0 | 1 | * 1. class Solution:\n* def minSwaps(self, s: str) -> int:\n* c=0\n* for i in s:\n* if i=="[":\n* c=c+1\n* elif c>0:\n* c=c-1\n* return (c+1)//2 | 1 | You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
A string is called **balanced** if and only if:
* It is the empty string, or
* It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
* It can be written as `[C]`, where `C` is a **balanced** string.
You may swap the brackets at **any** two indices **any** number of times.
Return _the **minimum** number of swaps to make_ `s` _**balanced**_.
**Example 1:**
**Input:** s = "\]\[\]\[ "
**Output:** 1
**Explanation:** You can make the string balanced by swapping index 0 with index 3.
The resulting string is "\[\[\]\] ".
**Example 2:**
**Input:** s = "\]\]\]\[\[\[ "
**Output:** 2
**Explanation:** You can do the following to make the string balanced:
- Swap index 0 with index 4. s = "\[\]\]\[\]\[ ".
- Swap index 1 with index 5. s = "\[\[\]\[\]\] ".
The resulting string is "\[\[\]\[\]\] ".
**Example 3:**
**Input:** s = "\[\] "
**Output:** 0
**Explanation:** The string is already balanced.
**Constraints:**
* `n == s.length`
* `2 <= n <= 106`
* `n` is even.
* `s[i]` is either `'['` or `']'`.
* The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`. | Think about (a&b) ^ (a&c). Can you simplify this expression? It is equal to a&(b^c). Then, (arr1[i]&arr2[0])^(arr1[i]&arr2[1]).. = arr1[i]&(arr2[0]^arr2[1]^arr[2]...). Let arr2XorSum = (arr2[0]^arr2[1]^arr2[2]...), arr1XorSum = (arr1[0]^arr1[1]^arr1[2]...) so the final answer is (arr2XorSum&arr1[0]) ^ (arr2XorSum&arr1[1]) ^ (arr2XorSum&arr1[2]) ^ ... = arr2XorSum & arr1XorSum. |
Python | Binary Search | Easy to Understand | Beats 99% | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | Simple Upper Bound and Binary Search\n\n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n stack,ans=[],[]\n \n for i in obstacles:\n br=bisect_right(stack,i)\n if br==len(stack):\n stack.append(i)\n else:\n stack[br]=i\n ans.append(br+1)\n \n return ans | 9 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
Binary search easy solution | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | # Code\n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n\n def bisearch(lis, height):\n l, r = 0, len(lis)\n while l < r:\n m = (l + r) // 2\n if height < lis[m]:\n r = m\n if height >= lis[m]:\n l = m + 1\n return l\n\n ans = [1] * len(obstacles)\n lis = []\n\n for i, height in enumerate(obstacles):\n idx = bisearch(lis, height)\n if idx == len(lis):\n lis.append(height)\n else:\n lis[idx] = height\n ans[i] = idx + 1\n return ans\n\n``` | 1 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
python 3 - Binary search | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | # Intuition\n(this question becomes a piece of cake if you use python :P)\n\nTo do this question, you must know the technique in solving 300. Longest Increasing Subsequence. This question is an advance version of Q300.\n\nThis question ask you to find the longest increasing subseqence for each i.\n\nYou can\'t use SortedList because order matters.\n\n\n# Approach\nBinary search\n\n# Complexity\n- Time complexity:\nO(nlogn) -> do bs on each i\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n\n import bisect\n cur = list()\n ans = list()\n \n for ob in obstacles:\n if not cur:\n cur.append(ob)\n ans.append(1)\n else:\n inserti = bisect.bisect(cur, ob)\n ans.append(inserti + 1)\n if inserti == len(cur): # at the end of cur\n cur.append(ob)\n else: # can replace\n cur[inserti] = ob\n return ans\n``` | 1 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
Patience Sort - Same as Longest Increasing Subsequence | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | \n# Code\n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n arr, res = [], [0] * len(obstacles)\n for i, x in enumerate(obstacles):\n k = bisect_right(arr, x)\n if k == len(arr): arr.append(x)\n else: arr[k] = x\n res[i] = k + 1\n return res\n``` | 1 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
python3 Solution | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | \n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n mono=[]\n ans=[]\n for obstacl in obstacles:\n i=bisect.bisect(mono,obstacl)\n ans.append(i+1)\n if i==len(mono):\n mono.append(0)\n\n mono[i]=obstacl\n\n return ans \n``` | 1 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
Easy Understand | Simple Method | Binary Search + Stack | Java | Python | C++✅ | find-the-longest-valid-obstacle-course-at-each-position | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nEvery time a number is traversed, if:\n- If the current number is greater than or equal to the number on the top of the stack, it will be directly pushed onto the stack\n- If the current number is less than the number on the top of the stack, use binary search to find the first number in the stack that is greater than the current number and replace it\n\nThen how to consider must choose the i-th obstacle:\nOnly need to check at the end of each loop, what is the number to be replaced (or added), and then the final result will come out\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Example\nComsider the example `[1,2,3,1,2,3]`\n- Initially `stack` is `[0, 0, 0, 0, 0, 0]` and `res` is `[0, 0, 0, 0, 0, 0]`\n- When `i = 0`, `obstacles[i] = 1`\n - `top == -1` and we go to `if` branch\n - `stack[0] = 1`, `res[0] = 1`\n- When `i = 1`, `obstacles[i] = 2`\n - `top=0` and stack is `[1, 0, 0, 0, 0, 0]`\n - `obstacles[i] >= stack[top]` and we go to `if` branch\n - `stack[1] = 2`, `res[1] = 2`\n- When `i = 2`, `obstacles[i] = 3`\n - ...\n- When `i = 3`, `obstacles[i] = 1` notice!\n - Now stack is `[1, 2, 3, 0, 0, 0]`\n - We go to `else` branch\n - We need use binary search to find the first number that larger than `obstacles[i]` from 0 to top=2 in the stack\n - `r = 1`, `stack[r] = obstacles[i]`, `res[3] = r+1`\n- When `i = 4`, `obstacles[i] = 2`\n - `top=2`, and stack is `[1, 1, 3, 0, 0, 0]`\n - `obstacles[i] < stack[top]` and we go to `else` branch\n - Find first number larger than `obstacles[i]` from 0 to top=2\n - `r = 2`, `stack[r] = obstacles[i]`, `res[4] = r+1`\n- Now `stack` is `[1, 1, 2, 0, 0, 0]` and `res` is `[1, 2, 3, 2, 3, 0]`\n- When `i = 5`, `obstacles[i] = 3`\n - `obstacles[i] >= stack[top]` and we go to `if` branch\n - `top++=3`, `stack[top] = 3`, `res[5] = top+1`\n- Finally, \n - Stack is `[1, 1, 2, 3, 0, 0]`\n - res is `[1, 2, 3, 2, 3, 4]`\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(nlogn)$$\n\n# Code\n``` java []\nclass Solution {\n public int[] longestObstacleCourseAtEachPosition(int[] obstacles) {\n int[] stack = new int[obstacles.length];\n int top = -1;\n int[] res = new int[obstacles.length];\n for (int i = 0; i < obstacles.length; i++) {\n if (top == -1 || obstacles[i] >= stack[top]) {\n stack[++top] = obstacles[i];\n res[i] = top + 1;\n } else {\n int l = 0, r = top, m;\n while (l < r) {\n m = l + (r - l) / 2;\n if (stack[m] <= obstacles[i]) {\n l = m + 1;\n } else {\n r = m;\n }\n }\n stack[r] = obstacles[i];\n res[i] = r + 1;\n }\n }\n return res;\n }\n}\n```\n``` python []\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n lst,ans=[],[]\n for i in obstacles:\n b=bisect_right(lst,i)\n if b==len(lst):\n lst.append(i)\n else:\n lst[b]=i\n ans.append(b+1)\n return ans\n```\n``` C++ []\nclass Solution \n{\npublic:\n vector<int> longestObstacleCourseAtEachPosition(vector<int>& obstacles) \n {\n vector<int> a;\n vector<int> res;\n for (auto x : obstacles)\n {\n int idx = upper_bound(a.begin(), a.end(), x) - a.begin();\n if (idx == a.size())\n a.push_back(x);\n else\n a[idx] = x;\n res.push_back(idx + 1);\n }\n\n return res;\n }\n};\n```\n\n**Please upvate if helpful!!**\n\n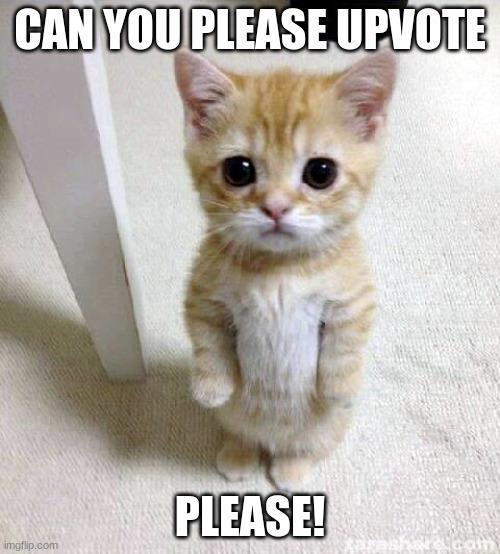 | 27 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
python3 solution beats 98.48% 🚀🚀🔥 || quibler7 | find-the-longest-valid-obstacle-course-at-each-position | 0 | 1 | # Code\n```\nclass Solution:\n def longestObstacleCourseAtEachPosition(self, obstacles: List[int]) -> List[int]:\n n = len(obstacles)\n res = n*[1]\n v = []\n for i in range(n):\n tr = bisect_right(v, obstacles[i])\n if tr == len(v): v.append(obstacles[i])\n else: v[tr] = obstacles[i]\n res[i] = tr + 1\n return res\n```\n\n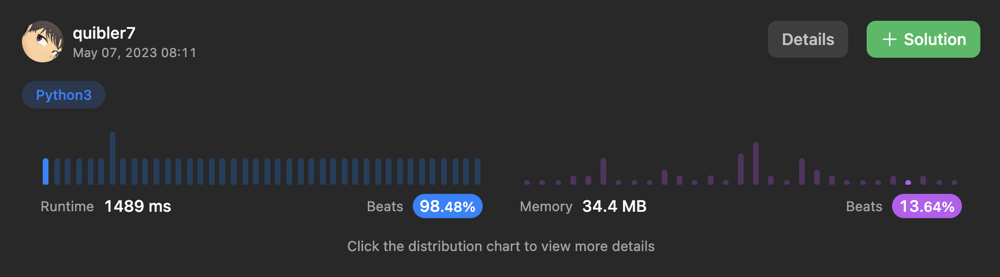\n | 1 | You want to build some obstacle courses. You are given a **0-indexed** integer array `obstacles` of length `n`, where `obstacles[i]` describes the height of the `ith` obstacle.
For every index `i` between `0` and `n - 1` (**inclusive**), find the length of the **longest obstacle course** in `obstacles` such that:
* You choose any number of obstacles between `0` and `i` **inclusive**.
* You must include the `ith` obstacle in the course.
* You must put the chosen obstacles in the **same order** as they appear in `obstacles`.
* Every obstacle (except the first) is **taller** than or the **same height** as the obstacle immediately before it.
Return _an array_ `ans` _of length_ `n`, _where_ `ans[i]` _is the length of the **longest obstacle course** for index_ `i` _as described above_.
**Example 1:**
**Input:** obstacles = \[1,2,3,2\]
**Output:** \[1,2,3,3\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[1\], \[1\] has length 1.
- i = 1: \[1,2\], \[1,2\] has length 2.
- i = 2: \[1,2,3\], \[1,2,3\] has length 3.
- i = 3: \[1,2,3,2\], \[1,2,2\] has length 3.
**Example 2:**
**Input:** obstacles = \[2,2,1\]
**Output:** \[1,2,1\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[2\], \[2\] has length 1.
- i = 1: \[2,2\], \[2,2\] has length 2.
- i = 2: \[2,2,1\], \[1\] has length 1.
**Example 3:**
**Input:** obstacles = \[3,1,5,6,4,2\]
**Output:** \[1,1,2,3,2,2\]
**Explanation:** The longest valid obstacle course at each position is:
- i = 0: \[3\], \[3\] has length 1.
- i = 1: \[3,1\], \[1\] has length 1.
- i = 2: \[3,1,5\], \[3,5\] has length 2. \[1,5\] is also valid.
- i = 3: \[3,1,5,6\], \[3,5,6\] has length 3. \[1,5,6\] is also valid.
- i = 4: \[3,1,5,6,4\], \[3,4\] has length 2. \[1,4\] is also valid.
- i = 5: \[3,1,5,6,4,2\], \[1,2\] has length 2.
**Constraints:**
* `n == obstacles.length`
* `1 <= n <= 105`
* `1 <= obstacles[i] <= 107` | null |
[Python3] 1-line | number-of-strings-that-appear-as-substrings-in-word | 0 | 1 | \n```\nclass Solution:\n def numOfStrings(self, patterns: List[str], word: str) -> int:\n return sum(x in word for x in patterns)\n```\n\nYou can find the solutions to weekly 254 in this [repo](https://github.com/gaosanyong/leetcode/commit/dcc452fea7d159bd4fae8318c14d546f17783894). | 23 | Given an array of strings `patterns` and a string `word`, return _the **number** of strings in_ `patterns` _that exist as a **substring** in_ `word`.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** patterns = \[ "a ", "abc ", "bc ", "d "\], word = "abc "
**Output:** 3
**Explanation:**
- "a " appears as a substring in "abc ".
- "abc " appears as a substring in "abc ".
- "bc " appears as a substring in "abc ".
- "d " does not appear as a substring in "abc ".
3 of the strings in patterns appear as a substring in word.
**Example 2:**
**Input:** patterns = \[ "a ", "b ", "c "\], word = "aaaaabbbbb "
**Output:** 2
**Explanation:**
- "a " appears as a substring in "aaaaabbbbb ".
- "b " appears as a substring in "aaaaabbbbb ".
- "c " does not appear as a substring in "aaaaabbbbb ".
2 of the strings in patterns appear as a substring in word.
**Example 3:**
**Input:** patterns = \[ "a ", "a ", "a "\], word = "ab "
**Output:** 3
**Explanation:** Each of the patterns appears as a substring in word "ab ".
**Constraints:**
* `1 <= patterns.length <= 100`
* `1 <= patterns[i].length <= 100`
* `1 <= word.length <= 100`
* `patterns[i]` and `word` consist of lowercase English letters. | Start from each 'a' and find the longest beautiful substring starting at that index. Based on the current character decide if you should include the next character in the beautiful substring. |
C++ / Python (1 line) simple solution | number-of-strings-that-appear-as-substrings-in-word | 0 | 1 | **C++ :**\n\n```\nint numOfStrings(vector<string>& patterns, string word) {\n int counter = 0;\n \n for(auto p: patterns)\n if(word.find(p) != std::string::npos)\n ++counter;\n \n return counter;\n }\n```\n\n**Python :**\n\n```\ndef numOfStrings(self, patterns: List[str], word: str) -> int:\n\treturn len([p for p in patterns if p in word])\n```\n\n**Like it ? please upvote !** | 16 | Given an array of strings `patterns` and a string `word`, return _the **number** of strings in_ `patterns` _that exist as a **substring** in_ `word`.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** patterns = \[ "a ", "abc ", "bc ", "d "\], word = "abc "
**Output:** 3
**Explanation:**
- "a " appears as a substring in "abc ".
- "abc " appears as a substring in "abc ".
- "bc " appears as a substring in "abc ".
- "d " does not appear as a substring in "abc ".
3 of the strings in patterns appear as a substring in word.
**Example 2:**
**Input:** patterns = \[ "a ", "b ", "c "\], word = "aaaaabbbbb "
**Output:** 2
**Explanation:**
- "a " appears as a substring in "aaaaabbbbb ".
- "b " appears as a substring in "aaaaabbbbb ".
- "c " does not appear as a substring in "aaaaabbbbb ".
2 of the strings in patterns appear as a substring in word.
**Example 3:**
**Input:** patterns = \[ "a ", "a ", "a "\], word = "ab "
**Output:** 3
**Explanation:** Each of the patterns appears as a substring in word "ab ".
**Constraints:**
* `1 <= patterns.length <= 100`
* `1 <= patterns[i].length <= 100`
* `1 <= word.length <= 100`
* `patterns[i]` and `word` consist of lowercase English letters. | Start from each 'a' and find the longest beautiful substring starting at that index. Based on the current character decide if you should include the next character in the beautiful substring. |
smallest solution in python by smarthood | number-of-strings-that-appear-as-substrings-in-word | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numOfStrings(self, patterns: List[str], word: str) -> int:\n count=0\n for i in patterns:\n if i in word:\n count+=1\n return count\n``` | 2 | Given an array of strings `patterns` and a string `word`, return _the **number** of strings in_ `patterns` _that exist as a **substring** in_ `word`.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** patterns = \[ "a ", "abc ", "bc ", "d "\], word = "abc "
**Output:** 3
**Explanation:**
- "a " appears as a substring in "abc ".
- "abc " appears as a substring in "abc ".
- "bc " appears as a substring in "abc ".
- "d " does not appear as a substring in "abc ".
3 of the strings in patterns appear as a substring in word.
**Example 2:**
**Input:** patterns = \[ "a ", "b ", "c "\], word = "aaaaabbbbb "
**Output:** 2
**Explanation:**
- "a " appears as a substring in "aaaaabbbbb ".
- "b " appears as a substring in "aaaaabbbbb ".
- "c " does not appear as a substring in "aaaaabbbbb ".
2 of the strings in patterns appear as a substring in word.
**Example 3:**
**Input:** patterns = \[ "a ", "a ", "a "\], word = "ab "
**Output:** 3
**Explanation:** Each of the patterns appears as a substring in word "ab ".
**Constraints:**
* `1 <= patterns.length <= 100`
* `1 <= patterns[i].length <= 100`
* `1 <= word.length <= 100`
* `patterns[i]` and `word` consist of lowercase English letters. | Start from each 'a' and find the longest beautiful substring starting at that index. Based on the current character decide if you should include the next character in the beautiful substring. |
Python one-liner | number-of-strings-that-appear-as-substrings-in-word | 0 | 1 | ```\nclass Solution:\n def numOfStrings(self, patterns: List[str], word: str) -> int:\n return len([pattern for pattern in patterns if pattern in word])\n``` | 1 | Given an array of strings `patterns` and a string `word`, return _the **number** of strings in_ `patterns` _that exist as a **substring** in_ `word`.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** patterns = \[ "a ", "abc ", "bc ", "d "\], word = "abc "
**Output:** 3
**Explanation:**
- "a " appears as a substring in "abc ".
- "abc " appears as a substring in "abc ".
- "bc " appears as a substring in "abc ".
- "d " does not appear as a substring in "abc ".
3 of the strings in patterns appear as a substring in word.
**Example 2:**
**Input:** patterns = \[ "a ", "b ", "c "\], word = "aaaaabbbbb "
**Output:** 2
**Explanation:**
- "a " appears as a substring in "aaaaabbbbb ".
- "b " appears as a substring in "aaaaabbbbb ".
- "c " does not appear as a substring in "aaaaabbbbb ".
2 of the strings in patterns appear as a substring in word.
**Example 3:**
**Input:** patterns = \[ "a ", "a ", "a "\], word = "ab "
**Output:** 3
**Explanation:** Each of the patterns appears as a substring in word "ab ".
**Constraints:**
* `1 <= patterns.length <= 100`
* `1 <= patterns[i].length <= 100`
* `1 <= word.length <= 100`
* `patterns[i]` and `word` consist of lowercase English letters. | Start from each 'a' and find the longest beautiful substring starting at that index. Based on the current character decide if you should include the next character in the beautiful substring. |
Swap & One Loop || Python 3 | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | # Intuition\nI found it a very mathematical based question rather than using an algorithm for this. Though there are various sorting algorithm but you can solve it using normal swaps.\nkeep your window of size 3 and check is the window is sorted in any manner (reverse included) it is so then swap any two elements and move forward. You will get you ans ;)\n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n left = 0\n while left < len(nums) - 2:\n if nums[left: left + 3] == sorted(nums[left: left + 3]) or nums[left: left + 3] == sorted(nums[left: left + 3], reverse= True):\n nums[left + 1], nums[left + 2] = nums[left + 2], nums[left + 1]\n left += 1\n return nums\n\n``` | 1 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
python3 unknown approach ??? o(n) | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n nums.sort()\n res = []\n i= 0 \n j=len(nums)-1\n while i<j:\n res.append(nums[i])\n res.append(nums[j])\n i+=1\n j-=1\n if len(res) != len(nums):\n res.append(nums[i])\n return res\n``` | 1 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Clean and simple O(N) and O(1) python solution | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | \n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n #Time - O(N) and space - O(1)\n # This is in place replacement\n # Step 1: Even place i has to be less than i-1\n # Step 2: odd place i has to be greater than i-1\n # Step 1 and 2 can be interchanged\n for i in range(len(nums)):\n if i%2:\n if nums[i]>nums[i-1]:\n nums[i], nums[i-1] = nums[i-1], nums[i]\n else:\n if nums[i]<nums[i-1]:\n nums[i], nums[i-1] = nums[i-1], nums[i]\n return nums\n``` | 1 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
PYTHON EASY SOLUTION | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | The only way the average of the neighbors can give us the number is if one of them is greater and the other is smaller than the middle number.\n\nTo make the number different from the average of its neighbors, we just have to first sort the array and then take one number from the start then from the end. If we do the same process by picking a number from the front then from the end we can achieve what we want. \n\n\n```class Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n nums.sort()\n start, end = 0, len(nums)-1\n answer = []\n \n while start <= end:\n answer.append(nums[start])\n answer.append(nums[end])\n start+=1\n end-=1\n if len(nums) %2 != 0:\n answer.pop()\n return answer\n\t\t``` | 1 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Beats 99% - Easy to Understand - Wiggle Sort! | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | # Intuition\n- Exact solution to wiggle sort\n- If two neighbours are both higher -> middle element can\'t be average\n- If two neighbours are both lower -> middle element can\'t be average\n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n def swap(i,j):\n t = nums[i]\n nums[i] = nums[j]\n nums[j] = t\n\n increasing = True\n n = len(nums)\n for i in range(1, n):\n if(increasing and nums[i] > nums[i-1] or\n not increasing and nums[i] < nums[i-1]\n ):\n increasing = not increasing\n continue\n else:\n swap(i, i-1)\n increasing = not increasing\n \n return nums\n``` | 0 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Unproven theory but beats 90%+ in cpu and memory | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | # Intuition\nReduce the chances that your resultin array will fail by rearranging the numers as follows:\n1. sort `nums`\n2. take the array of every other element from the sorted `nums` and...\n3. reverse that sub-array\n4. put it back in place\n5. The center element is now still your most likely \'problem\' element since it has the same neighbors as it did in the sorted list. So take the center element and move it to the end of the array\n\nThis passes all tests but I have no proof that shows that this cannot be defeated.\n\n# Complexity\nO(n log(n) ) due to sorting the array. But it seems you make up for it against other algos that \'guess + check + repeat\' by shuffling etc. since those O(n) algorithms typically have to repeat so many times they lose to this algorithm.\n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n nums.sort()\n nums[::2]=(nums[::2])[::-1]\n to_delete = (len(nums)-1)//2\n nums.append(nums[to_delete])\n del nums[to_delete]\n return nums\n\n``` | 0 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Sorted - Interleaving - O(nlogn) | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | # Complexity\n```\n- Time complexity: O(nlogn)\n- Space complexity: O(n)\n```\n# Code\n```python\nclass Solution:\n def rearrangeArray(self, A: List[int]) -> List[int]:\n B = sorted(A); A[1::2], A[::2] = B[:len(B) // 2], B[len(B) // 2:]\n return A\n``` | 0 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Python easy sol.. | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | \n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n nums.sort()\n print(nums)\n res=[]\n f,r=0,len(nums)-1\n while f<r:\n res.append(nums[f])\n res.append(nums[r])\n f+=1\n r-=1\n if f==r:\n res.append(nums[f])\n return res\n``` | 0 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
Python Two Pointers with Explanation | array-with-elements-not-equal-to-average-of-neighbors | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def rearrangeArray(self, nums: List[int]) -> List[int]:\n # Time --> O(n)\n # Space --> O(1) as output as is not counted\n\n # Logic:\n # First, sort the list, then use two-pointer concept, keep two pointers, left and right.\n # left = 0 and right = len(nums)-1\n # Place the elements alternatively, so that the output array will have elements of left and right alternatively\n # With this, the average of two consecutive left elements can\'t be equals to the right element.\n\n\n nums.sort() # sort the list\n left,right = 0, len(nums)-1 # using two pointers\n result = [] # output list\n while left <= right:\n # if left pointer == right pointer, then only append once\n if left == right:\n result.append(nums[left])\n left += 1\n right -= 1\n else:\n # else append left and right in this order and shift the pointers\n result.append(nums[left])\n left += 1\n result.append(nums[right])\n right -= 1\n return result # returning the result\n``` | 0 | You are given a **0-indexed** array `nums` of **distinct** integers. You want to rearrange the elements in the array such that every element in the rearranged array is **not** equal to the **average** of its neighbors.
More formally, the rearranged array should have the property such that for every `i` in the range `1 <= i < nums.length - 1`, `(nums[i-1] + nums[i+1]) / 2` is **not** equal to `nums[i]`.
Return _**any** rearrangement of_ `nums` _that meets the requirements_.
**Example 1:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** \[1,2,4,5,3\]
**Explanation:**
When i=1, nums\[i\] = 2, and the average of its neighbors is (1+4) / 2 = 2.5.
When i=2, nums\[i\] = 4, and the average of its neighbors is (2+5) / 2 = 3.5.
When i=3, nums\[i\] = 5, and the average of its neighbors is (4+3) / 2 = 3.5.
**Example 2:**
**Input:** nums = \[6,2,0,9,7\]
**Output:** \[9,7,6,2,0\]
**Explanation:**
When i=1, nums\[i\] = 7, and the average of its neighbors is (9+6) / 2 = 7.5.
When i=2, nums\[i\] = 6, and the average of its neighbors is (7+2) / 2 = 4.5.
When i=3, nums\[i\] = 2, and the average of its neighbors is (6+0) / 2 = 3.
**Constraints:**
* `3 <= nums.length <= 105`
* `0 <= nums[i] <= 105` | Is it possible to find the max height if given the height range of a particular building? You can find the height range of a restricted building by doing 2 passes from the left and right. |
[Python] One-liner solution with mathematical proof and explanation | minimum-non-zero-product-of-the-array-elements | 0 | 1 | To calculate the minimum non-zero product we always have to change the value such as it will provide the minimum multiplication value -\n\ni.e \nn = 3\n1 - 001\n2 - 010\n3 - 011\n4 - 100\n5 - 101\n6 - 110\n7 - 111\n\nHere we convert all the value **i** to **1** and **pow(2, n)-i-1** to **pow(2, n)-2** *(where i<pow(2, n-1))*\n\nBasically we convert the first half of the occurances to 1 which in return provide us minimum multiplication value.\n\nThe above explanation will lead us to the following deduction as -\n1 - 001\n2 - 001 (swapped with 5)\n3 - 001 (swapped with 4)\n4 - 110\n5 - 110\n6 - 110\n7 - 111\n\n\n...................................................................................................................................................................................................................................\n**Mathematical proof :**\n\nSuppose n = 3, it will have 2^3-1 = 7 non-zero occurances.\nNow we have \n2+5 = 7\n\nlet x = 2, y = 5, z = 7\n\nso, x+y = z -----------------------**{1}**\nx * y>x+y (if x>1 and y>1)\nx * y>x+y\nx * y > z --------------**(using {1})**\n**x * y > (z-1) * (1)**\nHence we can use this to formulate the minimum multiplication value.\n\nWe can do transition between the elements so that **upper half** become **1** and **lower half** become **2^n-2**. Also **last element can\'t be transformed as we are neglecting the zero valued element so we multiply it as it is**.\n\n\n\n\n--------------------------------------------------------------------------------------------------------------------------------\n\n\nAnother way to understand it as we push one element to attain minimum possible value (which is 1 here) and their counter part will increase accordingly.\n\nNow for 3, pairing it as (1, 6) (2, 5) (3, 4) (7).\nwe decrease the minimum value (suppose k) to 1 and increase the other value (suppose m) to m+k-1\n\nSo now the pairs are (1, 6) (1, 6) (1, 6) (7) which will give us minimum multiplication value.\n\n\nNow take n = 4, \nwe have pairs as (1, 14) (2, 13) (3, 12) (4, 11) (5, 10) (6, 9) (7, 8) (15) \nwhich we can reform as (1, 14) (1, 14) (1, 14) (1, 14) (1, 14) (1, 14) (1, 14) (15)\n\n\nHope you understand the approach. Queries are welcomed.\n\n**The code goes as -**\n\n\n```\ndef minNonZeroProduct(self, p: int) -> int:\n return (pow(2**p-2, (2**p-2)//2, 1000000007)*(2**p-1))%1000000007 | 6 | You are given a positive integer `p`. Consider an array `nums` (**1-indexed**) that consists of the integers in the **inclusive** range `[1, 2p - 1]` in their binary representations. You are allowed to do the following operation **any** number of times:
* Choose two elements `x` and `y` from `nums`.
* Choose a bit in `x` and swap it with its corresponding bit in `y`. Corresponding bit refers to the bit that is in the **same position** in the other integer.
For example, if `x = 1101` and `y = 0011`, after swapping the `2nd` bit from the right, we have `x = 1111` and `y = 0001`.
Find the **minimum non-zero** product of `nums` after performing the above operation **any** number of times. Return _this product_ _**modulo**_ `109 + 7`.
**Note:** The answer should be the minimum product **before** the modulo operation is done.
**Example 1:**
**Input:** p = 1
**Output:** 1
**Explanation:** nums = \[1\].
There is only one element, so the product equals that element.
**Example 2:**
**Input:** p = 2
**Output:** 6
**Explanation:** nums = \[01, 10, 11\].
Any swap would either make the product 0 or stay the same.
Thus, the array product of 1 \* 2 \* 3 = 6 is already minimized.
**Example 3:**
**Input:** p = 3
**Output:** 1512
**Explanation:** nums = \[001, 010, 011, 100, 101, 110, 111\]
- In the first operation we can swap the leftmost bit of the second and fifth elements.
- The resulting array is \[001, 110, 011, 100, 001, 110, 111\].
- In the second operation we can swap the middle bit of the third and fourth elements.
- The resulting array is \[001, 110, 001, 110, 001, 110, 111\].
The array product is 1 \* 6 \* 1 \* 6 \* 1 \* 6 \* 7 = 1512, which is the minimum possible product.
**Constraints:**
* `1 <= p <= 60` | We can see that the problem can be represented as a directed graph with an edge from each boy to the girl he invited. We need to choose a set of edges such that no to source points in the graph (i.e., boys) have an edge with the same endpoint (i.e., the same girl). The problem is maximum bipartite matching in the graph. |
[Python3] 2-line | minimum-non-zero-product-of-the-array-elements | 0 | 1 | \n```\nclass Solution:\n def minNonZeroProduct(self, p: int) -> int:\n x = (1 << p) - 1\n return pow(x-1, (x-1)//2, 1_000_000_007) * x % 1_000_000_007\n```\n\nYou can find the solutions to weekly 256 in this [repo](https://github.com/gaosanyong/leetcode/commit/dcc452fea7d159bd4fae8318c14d546f17783894). | 6 | You are given a positive integer `p`. Consider an array `nums` (**1-indexed**) that consists of the integers in the **inclusive** range `[1, 2p - 1]` in their binary representations. You are allowed to do the following operation **any** number of times:
* Choose two elements `x` and `y` from `nums`.
* Choose a bit in `x` and swap it with its corresponding bit in `y`. Corresponding bit refers to the bit that is in the **same position** in the other integer.
For example, if `x = 1101` and `y = 0011`, after swapping the `2nd` bit from the right, we have `x = 1111` and `y = 0001`.
Find the **minimum non-zero** product of `nums` after performing the above operation **any** number of times. Return _this product_ _**modulo**_ `109 + 7`.
**Note:** The answer should be the minimum product **before** the modulo operation is done.
**Example 1:**
**Input:** p = 1
**Output:** 1
**Explanation:** nums = \[1\].
There is only one element, so the product equals that element.
**Example 2:**
**Input:** p = 2
**Output:** 6
**Explanation:** nums = \[01, 10, 11\].
Any swap would either make the product 0 or stay the same.
Thus, the array product of 1 \* 2 \* 3 = 6 is already minimized.
**Example 3:**
**Input:** p = 3
**Output:** 1512
**Explanation:** nums = \[001, 010, 011, 100, 101, 110, 111\]
- In the first operation we can swap the leftmost bit of the second and fifth elements.
- The resulting array is \[001, 110, 011, 100, 001, 110, 111\].
- In the second operation we can swap the middle bit of the third and fourth elements.
- The resulting array is \[001, 110, 001, 110, 001, 110, 111\].
The array product is 1 \* 6 \* 1 \* 6 \* 1 \* 6 \* 7 = 1512, which is the minimum possible product.
**Constraints:**
* `1 <= p <= 60` | We can see that the problem can be represented as a directed graph with an edge from each boy to the girl he invited. We need to choose a set of edges such that no to source points in the graph (i.e., boys) have an edge with the same endpoint (i.e., the same girl). The problem is maximum bipartite matching in the graph. |
Python solution: get as many 1 as possible | minimum-non-zero-product-of-the-array-elements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs swaps cannot change total number of 1 and 0s in a bit, and the question asks for minimum NONZERO product, so it\'s all about getting as many 1 in the final state as possible. ($$1 = 0001, 14 = 1110, 2 = 0010, 13 = 1101$$, $$1 \\times 14 < 2 \\times 13$$) \n# Approach\n<!-- Describe your approach to solving the problem. -->\nAs shown in the Examples, we see there are $$2 ^ {p - 1}$$ 1s and $$2 ^ {p - 1} - 1$$ 0s at each bit. We know we cannot move the 1s out of $$2 ^ {p} - 1$$ (the largest number) (there are no where else to go), but we can turn the $$2 ^ {p - 1} - 1$$ numbers with 0th bit = 1 to become $$1$$, and in the process, we get $$2 ^ {p - 1} - 1$$ $$2 ^ {p} - 2$$s. \n\n# Code\n```\nclass Solution:\n def minNonZeroProduct(self, p: int) -> int:\n MOD = 1000000007\n largest = pow(2, p) - 1\n secondLargest = largest - 1\n numberOfTimes = pow(2, p - 1) - 1\n return (largest * pow(secondLargest, numberOfTimes, MOD)) % MOD\n``` | 0 | You are given a positive integer `p`. Consider an array `nums` (**1-indexed**) that consists of the integers in the **inclusive** range `[1, 2p - 1]` in their binary representations. You are allowed to do the following operation **any** number of times:
* Choose two elements `x` and `y` from `nums`.
* Choose a bit in `x` and swap it with its corresponding bit in `y`. Corresponding bit refers to the bit that is in the **same position** in the other integer.
For example, if `x = 1101` and `y = 0011`, after swapping the `2nd` bit from the right, we have `x = 1111` and `y = 0001`.
Find the **minimum non-zero** product of `nums` after performing the above operation **any** number of times. Return _this product_ _**modulo**_ `109 + 7`.
**Note:** The answer should be the minimum product **before** the modulo operation is done.
**Example 1:**
**Input:** p = 1
**Output:** 1
**Explanation:** nums = \[1\].
There is only one element, so the product equals that element.
**Example 2:**
**Input:** p = 2
**Output:** 6
**Explanation:** nums = \[01, 10, 11\].
Any swap would either make the product 0 or stay the same.
Thus, the array product of 1 \* 2 \* 3 = 6 is already minimized.
**Example 3:**
**Input:** p = 3
**Output:** 1512
**Explanation:** nums = \[001, 010, 011, 100, 101, 110, 111\]
- In the first operation we can swap the leftmost bit of the second and fifth elements.
- The resulting array is \[001, 110, 011, 100, 001, 110, 111\].
- In the second operation we can swap the middle bit of the third and fourth elements.
- The resulting array is \[001, 110, 001, 110, 001, 110, 111\].
The array product is 1 \* 6 \* 1 \* 6 \* 1 \* 6 \* 7 = 1512, which is the minimum possible product.
**Constraints:**
* `1 <= p <= 60` | We can see that the problem can be represented as a directed graph with an edge from each boy to the girl he invited. We need to choose a set of edges such that no to source points in the graph (i.e., boys) have an edge with the same endpoint (i.e., the same girl). The problem is maximum bipartite matching in the graph. |
[Python] | minimum-non-zero-product-of-the-array-elements | 0 | 1 | # Intuition\nYou have to match ones with largest possible numbers:\nSo we will have \n`000...01`\n`111...10`\ncoming in pairs.\nAnd there will be one remaining\n`111...11`\nYou have to multiply them all. For this is best to implement pow(x,y) function which calculates powers quickly.\n\n# Speed up\nSince there are only 60 possible inputs, you can precompute all the results and return the one it is asked for.\n\n# Code\n```\ndef minNonZeroProduct(self, p: int) -> int:\n mod = 1_000_000_007\n @cache\n def helper(p, n): # 1111111..110 ^ n\n if n == 0: return 1\n res = p if n & 1 else 1\n x = helper(p, n//2)\n res *= x\n res *= x\n return res % mod\n # onesAndZero = (2**p -2)\n # return ((2**p-1) * helper(2**(p-1)-1)) % mod\n\n # we can precompute all the results:\n # print(list(((2**z-1) * helper((2**z-2), 2**(z-1)-1)) % mod for z in range(1, 61)))\n precompResults = [0, 1, 6, 1512, 581202553, 202795991, 57405498, 316555604, 9253531, 857438053, 586669277, 647824153, 93512543, 391630296, 187678728, 431467833, 539112180, 368376380, 150112795, 484576688, 212293935, 828477683, 106294648, 618323081, 186692306, 513022074, 109245444, 821184946, 2043018, 26450314, 945196305, 138191773, 505517599, 861896614, 640964173, 112322054, 217659727, 680742062, 673217940, 945471045, 554966674, 190830260, 403329489, 305023508, 229675479, 865308368, 689473871, 161536946, 99452142, 720364340, 172386396, 198445540, 265347860, 504260931, 247773741, 65332879, 891336224, 221172799, 643213635, 926891661, 813987236]\n return precompResults[p]\n``` | 0 | You are given a positive integer `p`. Consider an array `nums` (**1-indexed**) that consists of the integers in the **inclusive** range `[1, 2p - 1]` in their binary representations. You are allowed to do the following operation **any** number of times:
* Choose two elements `x` and `y` from `nums`.
* Choose a bit in `x` and swap it with its corresponding bit in `y`. Corresponding bit refers to the bit that is in the **same position** in the other integer.
For example, if `x = 1101` and `y = 0011`, after swapping the `2nd` bit from the right, we have `x = 1111` and `y = 0001`.
Find the **minimum non-zero** product of `nums` after performing the above operation **any** number of times. Return _this product_ _**modulo**_ `109 + 7`.
**Note:** The answer should be the minimum product **before** the modulo operation is done.
**Example 1:**
**Input:** p = 1
**Output:** 1
**Explanation:** nums = \[1\].
There is only one element, so the product equals that element.
**Example 2:**
**Input:** p = 2
**Output:** 6
**Explanation:** nums = \[01, 10, 11\].
Any swap would either make the product 0 or stay the same.
Thus, the array product of 1 \* 2 \* 3 = 6 is already minimized.
**Example 3:**
**Input:** p = 3
**Output:** 1512
**Explanation:** nums = \[001, 010, 011, 100, 101, 110, 111\]
- In the first operation we can swap the leftmost bit of the second and fifth elements.
- The resulting array is \[001, 110, 011, 100, 001, 110, 111\].
- In the second operation we can swap the middle bit of the third and fourth elements.
- The resulting array is \[001, 110, 001, 110, 001, 110, 111\].
The array product is 1 \* 6 \* 1 \* 6 \* 1 \* 6 \* 7 = 1512, which is the minimum possible product.
**Constraints:**
* `1 <= p <= 60` | We can see that the problem can be represented as a directed graph with an edge from each boy to the girl he invited. We need to choose a set of edges such that no to source points in the graph (i.e., boys) have an edge with the same endpoint (i.e., the same girl). The problem is maximum bipartite matching in the graph. |
Maximise & Minimise Patterns | Very Descriptive | Greedy & Math | minimum-non-zero-product-of-the-array-elements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMathematics has sometimes been called a science of patterns (Resnik, 1981). In this particular problem you do not dare to do the `brute-force`(change all numbers to binary form and try to change the numbers in the binary representation bit by bit) it is simply inconvenient. Instead we will find some patterns and hence some math. Enjoy the show.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet\'s see one example: `p = 3`\nOur potential numbers will be in the inclusive range `[1, 2^(p)-1] = [1, 7]`. The binary representation of the numbers in the range:\n```\ninitial first second\n1 = 001 001 001\n2 = 010 110 110\n3 = 011 001 001\n\n4 = 100 100 110\n5 = 101 001 001\n6 = 110 110 110\n\n7 = 111 111 111\n```\n> In the first operation we can swap the leftmost bit of the second and fifth elements.\n - The resulting array is [001, 110, 011, 100, 001, 110, 111].\n\n> In the second operation we can swap the middle bit of the third and fourth elements.\n - The resulting array is [001, 110, 001, 110, 001, 110, 111].\n\n> The array product is 1 * 6 * 1 * 6 * 1 * 6 * 7 = 1512, which is the minimum possible product.\n\nWe have changed three numbers to `1` and another three numbers to `6` and leave the maximum `7` unchanged. So at the end the pattern becomes: there will be `mid = (2^(p)-1)//2` number of both `1`\'s and `2^(p)-2`\'s and one `maxm = 2^(p)-1`. Which means the product will become:\n> product = `1 * 1 * 1 ...[mid times]` `*` `maxm-1 * maxm-1 * maxm-1 ...[mid times]` `*` `maxm` \nproduct= `1 ^ mid` `*` `(maxm-1) ^ mid` `*` `maxm`\n\n# Complexity\n- Time complexity: $$O(log n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n> We will use modulo operator twice, one when computing the power and another when returning the result.\n```\nclass Solution:\n def minNonZeroProduct(self, p: int) -> int:\n maxm = (2**p)-1\n mid = maxm // 2\n mod = (10**9)+7\n return (pow(maxm-1, mid, mod) * maxm) % mod\n```\nI am sure you are UPVOTING, cause you like my description :) THANK YOU VERY MUCH. | 0 | You are given a positive integer `p`. Consider an array `nums` (**1-indexed**) that consists of the integers in the **inclusive** range `[1, 2p - 1]` in their binary representations. You are allowed to do the following operation **any** number of times:
* Choose two elements `x` and `y` from `nums`.
* Choose a bit in `x` and swap it with its corresponding bit in `y`. Corresponding bit refers to the bit that is in the **same position** in the other integer.
For example, if `x = 1101` and `y = 0011`, after swapping the `2nd` bit from the right, we have `x = 1111` and `y = 0001`.
Find the **minimum non-zero** product of `nums` after performing the above operation **any** number of times. Return _this product_ _**modulo**_ `109 + 7`.
**Note:** The answer should be the minimum product **before** the modulo operation is done.
**Example 1:**
**Input:** p = 1
**Output:** 1
**Explanation:** nums = \[1\].
There is only one element, so the product equals that element.
**Example 2:**
**Input:** p = 2
**Output:** 6
**Explanation:** nums = \[01, 10, 11\].
Any swap would either make the product 0 or stay the same.
Thus, the array product of 1 \* 2 \* 3 = 6 is already minimized.
**Example 3:**
**Input:** p = 3
**Output:** 1512
**Explanation:** nums = \[001, 010, 011, 100, 101, 110, 111\]
- In the first operation we can swap the leftmost bit of the second and fifth elements.
- The resulting array is \[001, 110, 011, 100, 001, 110, 111\].
- In the second operation we can swap the middle bit of the third and fourth elements.
- The resulting array is \[001, 110, 001, 110, 001, 110, 111\].
The array product is 1 \* 6 \* 1 \* 6 \* 1 \* 6 \* 7 = 1512, which is the minimum possible product.
**Constraints:**
* `1 <= p <= 60` | We can see that the problem can be represented as a directed graph with an edge from each boy to the girl he invited. We need to choose a set of edges such that no to source points in the graph (i.e., boys) have an edge with the same endpoint (i.e., the same girl). The problem is maximum bipartite matching in the graph. |
Subsets and Splits