question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
ambiguous-coordinates | Java Backtracking Approach | java-backtracking-approach-by-kavin_kuma-cln7 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Kavin_Kumaran_Mad | NORMAL | 2024-05-05T12:02:51.001269+00:00 | 2024-05-05T12:02:51.001287+00:00 | 55 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport java.util.regex.Matcher;\nimport java.util.regex.Pattern;\nclass Solution {\n public List<String> ambiguousCoordinates(String s1) {\n String s = s1.substring(1,s1.length() - 1);\n\t boolean[] x = new boolean[2];\n\t boolean[] y = new boolean[2];\n\t x[0] = true;\n\t x[1] = true;\n\t y[0] = false;\n\t y[1] = true;\n List<String> list = new ArrayList<>();\n\t func("" + s.charAt(0),s,x,y,1,list,0);\n\t return list;\n\t}\n\t static void func(String p,String up,boolean[] x,boolean[] y,int i,List<String> list,int comma){\n\t if(i == up.length() - 1){\n\t if(x[0] == false && y[0] == true && y[1] == true){\n\t String dummy = p.substring(comma) + "." + up.charAt(i);\n\t //System.out.println(dummy);\n\t if(isValid(dummy)){\n\t list.add("(" + p + "." + up.charAt(i) + ")");\n\t }\n\t }\n\t if(x[0] == false && y[0] == true){\n\t String dummy = p.substring(comma) + up.charAt(i);\n\t if(isValid(dummy)){\n\t list.add("(" + p + up.charAt(i) + ")");\n\t }\n\t }\n\t if(x[0] == true){\n\t if(isValid(p)){\n\t list.add("(" + p + ", " + up.charAt(i) + ")");\n\t }\n\t }\n\t return;\n\t }\n if(x[0] == true && x[1] == true){\n x[1] = false;\n func(p + "." + up.charAt(i),up,x,y,i + 1,list,comma);\n x[1] = true;\n }\n if(x[0] == false && y[0] == true && y[1] == true){\n y[1] = false;\n func(p + "." + up.charAt(i),up,x,y,i + 1,list,comma);\n y[1] = true;\n }\n func(p + up.charAt(i),up,x,y,i + 1,list,comma);\n if(x[0] == true && y[0] == false){\n x[0] = false;\n y[0] = true;\n if(isValid(p)){\n func(p + ", " + up.charAt(i),up,x,y,i + 1,list,p.length() + 2);\n }\n x[0] = true;\n y[0] = false;\n }\n\t}\n\tstatic boolean isValid(String str){\n\t if(str.contains(".")){\n String[] parts=str.split("\\\\.");\n // if(parts[1].endsWith("0")) return false;\n // if(parts[0].equals("0")) return true;\n // if(parts[0].startsWith("0")) return false;\n // return true;\n if(!parts[0].equals("0") && parts[0].startsWith("0"))\n return false;\n else \n return !parts[1].endsWith("0");\n }\n else{\n if(str.equals("0"))\n return true;\n return !str.startsWith("0");\n }\n }\n}\n``` | 0 | 0 | ['Backtracking', 'Java'] | 0 |
ambiguous-coordinates | 816. Ambiguous Coordinates.cpp | 816-ambiguous-coordinatescpp-by-202021ga-2fex | Code\n\nclass Solution {\npublic:\n vector<string> ambiguousCoordinates(string S) {\n for (int i = 2; i < S.size() - 1; i++) {\n string str | 202021ganesh | NORMAL | 2024-04-14T09:26:07.980038+00:00 | 2024-04-14T09:26:07.980059+00:00 | 4 | false | **Code**\n```\nclass Solution {\npublic:\n vector<string> ambiguousCoordinates(string S) {\n for (int i = 2; i < S.size() - 1; i++) {\n string strs[2] = {S.substr(1,i-1), S.substr(i,S.size()-i-1)};\n xPoss.clear();\n for (int j = 0; j < 2; j++)\n if (xPoss.size() || !j) parse(strs[j], j);\n }\n return ans;\n }\nprivate:\n vector<string> ans, xPoss;\n void parse(string str, int xy) {\n if (str.size() == 1 || str.front() != \'0\')\n process(str, xy);\n if (str.size() > 1 && str.back() != \'0\')\n process(str.substr(0,1) + "." + str.substr(1), xy);\n if (str.size() > 2 && str.front() != \'0\' && str.back() != \'0\')\n for (int i = 2; i < str.size(); i++)\n process(str.substr(0,i) + "." + str.substr(i), xy);\n }\n void process(string str, int xy) {\n if (xy)\n for (auto x : xPoss)\n ans.push_back("(" + x + ", " + str + ")");\n else xPoss.push_back(str);\n }\n};\n``` | 0 | 0 | ['C'] | 0 |
ambiguous-coordinates | dry-hard impl | dry-hard-impl-by-wangcai20-bpvb | Intuition\n Describe your first thoughts on how to solve this problem. \nSplit string to pair combinations (1d list) - O(s length -1)\nFurther break down each p | wangcai20 | NORMAL | 2024-04-06T02:17:15.535989+00:00 | 2024-04-06T02:17:15.536028+00:00 | 10 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSplit string to pair combinations (1d list) - O(s length -1)\nFurther break down each pair to varition combinations (2d list) - O((left len -1)*(right len) -1)\n~ O(m^3) m is the length of s\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(m^3) m is the length of s\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<String> ambiguousCoordinates(String s) {\n // split string to pair combinations (1d list) - O(s length -1)\n // further break down each pair to varition combinations (2d list) - O((left len\n // -1)*(right len) -1)\n // ~ O(m^3) m is the length of s\n List<String[]> pairs = new LinkedList<>();\n for (int i = 2; i < s.length() - 1; i++) {\n String s1 = s.substring(1, i);\n String s2 = s.substring(i, s.length() - 1);\n if (s1.length() > 1 && Integer.valueOf(s1) == 0 || s2.length() > 1 && Integer.valueOf(s2) == 0)\n continue;\n pairs.add(new String[] { s1, s2 });\n }\n //pairs.forEach(x -> System.out.println(Arrays.toString(x)));\n List<String> res = new LinkedList<>();\n for (String[] pair : pairs) {\n List<String> left = mutate(pair[0]);\n List<String> right = mutate(pair[1]);\n for (String sLeft : left)\n for (String sRight : right)\n res.add("(" + sLeft + ", " + sRight + ")");\n }\n return res;\n }\n\n List<String> mutate(String s) {\n List<String> list = new LinkedList<>();\n if (s.length() == 1 || s.charAt(0) != \'0\')\n list.add(s);\n for (int i = 1; i < s.length(); i++) {\n String s1 = s.substring(0, i), s2 = s.substring(i, s.length());\n //System.out.println(s1 + "|" + s2);\n if (s1.length() > 1 && s1.charAt(0) == \'0\' || s2.charAt(s2.length() - 1) == \'0\')\n continue;\n list.add(s1 + "." + s2);\n }\n return list;\n }\n\n}\n``` | 0 | 0 | ['Java'] | 0 |
ambiguous-coordinates | Easy to understand C# solution - Beats 94.74% | easy-to-understand-c-solution-beats-9474-wkdd | Intuition\nThe problem can be approached by splitting the input string into two parts and then generating all possible combinations of valid numbers for each pa | zeeabutt | NORMAL | 2024-03-28T07:16:56.473031+00:00 | 2024-03-28T07:16:56.473065+00:00 | 5 | false | # Intuition\nThe problem can be approached by splitting the input string into two parts and then generating all possible combinations of valid numbers for each part. To generate valid numbers, we iterate through each possible split point and consider all valid integer and fractional parts. Finally, we construct valid coordinates by combining valid numbers from both parts.\n\n# Approach\n1. Remove the parentheses from the input string to get the numeric string.\n2. Iterate through all possible split points in the numeric string.\n3. Generate all possible valid numbers for the left and right parts using the `GenerateValidNumbers` function.\n4. Construct valid coordinates by combining valid numbers from both parts.\n5. Add the valid coordinates to the result list.\n6. Return the list of valid coordinates.\n\n# Complexity\n- Time complexity: O(n^3), where n is the length of the input string. \n - The overall time complexity is dominated by the nested loops used to iterate through all possible split points and generate valid numbers for each part.\n- Space complexity: O(n^2)\n - The space complexity is dominated by the result list containing all valid coordinates, which can have a maximum of O(n^2) elements (for all possible combinations of valid numbers).\n\n\n# Code\n```\nusing System;\nusing System.Collections.Generic;\n\npublic class Solution {\n public IList<string> AmbiguousCoordinates(string s) {\n IList<string> result = new List<string>();\n \n s = s.Substring(1, s.Length - 2); // Remove parentheses\n \n for (int i = 1; i < s.Length; i++) {\n string left = s.Substring(0, i);\n string right = s.Substring(i);\n \n foreach (string x in GenerateValidNumbers(left)) {\n foreach (string y in GenerateValidNumbers(right)) {\n result.Add($"({x}, {y})");\n }\n }\n }\n \n return result;\n }\n \n private IList<string> GenerateValidNumbers(string s) {\n IList<string> numbers = new List<string>();\n \n if (s.Length == 1 || !s.StartsWith("0")) { // Single digit or non-zero single digit\n numbers.Add(s);\n }\n \n for (int i = 1; i < s.Length; i++) {\n string integerPart = s.Substring(0, i);\n string fractionPart = s.Substring(i);\n \n if ((!integerPart.StartsWith("0") || integerPart == "0") && !fractionPart.EndsWith("0")) { // Avoid leading or trailing zeros\n numbers.Add($"{integerPart}.{fractionPart}");\n }\n }\n \n return numbers;\n }\n}\n\n``` | 0 | 0 | ['C#'] | 0 |
ambiguous-coordinates | Java Solution | java-solution-by-ndsjqwbbb-14na | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ndsjqwbbb | NORMAL | 2024-03-25T20:57:17.848219+00:00 | 2024-03-25T20:57:17.848242+00:00 | 14 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<String> ambiguousCoordinates(String s) {\n List<String> result = new ArrayList<>();\n s = s.substring(1, s.length() - 1);\n for(int i = 1; i < s.length(); i++){\n helper(result, s.substring(0, i), s.substring(i));\n }\n return result;\n }\n private void helper(List<String> result, String x, String y){\n List<String> coordinatex = breakstring(x);\n List<String> coordinatey = breakstring(y);\n for(String dx : coordinatex){\n if(valid(dx)){\n for(String dy : coordinatey){\n if(valid(dy)){\n result.add("(" + dx + ", " + dy + ")");\n }\n }\n }\n }\n }\n private List<String> breakstring(String x){\n List<String> list = new ArrayList<>();\n list.add(x);\n for(int i = 1; i < x.length(); i++){\n list.add(x.substring(0, i) + "." + x.substring(i));\n }\n return list;\n }\n private boolean valid(String s){\n if(s.contains(".")){\n String[] substring = s.split("\\\\.");\n if(!substring[0].equals("0") && substring[0].startsWith("0")){\n return false;\n }\n else{\n return !substring[1].endsWith("0");\n }\n }\n else{\n if(s.equals("0")){\n return true;\n }\n else{\n return !s.startsWith("0");\n }\n }\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
ambiguous-coordinates | Ambiguous Coordinates || Backtracking || C# || Beats 94.44% || | ambiguous-coordinates-backtracking-c-bea-nkwr | Rules to Consider.\n1. Has Two parts and each of these two parts contains at most 1 decimal\n2. Any non decimal part will not start with 0, if the part itsel is | diptesh308 | NORMAL | 2024-03-23T16:13:22.920143+00:00 | 2024-03-23T16:17:45.501587+00:00 | 2 | false | # Rules to Consider.\n1. Has Two parts and each of these two parts contains at most 1 decimal\n2. Any non decimal part will not start with 0, if the part itsel isn\'t 0. (Example : (**005**, 4) is not allowed because 005 starts with 0, but (0, 4) is allowed)\n3. Decimal strings **can not end with a 0**, so **0.10, 0.0, 0.0000** these all are invalid.\n4. String like (**5.**, 7) , (**50.**, 9.8) are not valid.\n\n# Approach\nBreak the string into two string. Now handle the first part(or first axis or cordinate), take decimal in it and without decimal as well. In code flag=0 is handling the first axis of the coordinate. and flag=1 is handling the y axis or last part of the coordinate or last string.\n\nCheck code comment for handling the edge cases.\n\n# Code\n```\npublic class Solution {\n IList<string> result= new List<string>();\n public void AmbiguousCoordinatesHelper(string first, string last,bool firstHasDec, bool lasHasDec, int flag)\n {\n if (first == "" || last=="")\n {\n return;\n }\n if (flag >= 2)\n {\n if ((first[0]!=\'.\' && first[first.Length-1]!=\'.\') && (last[0] != \'.\' && last[last.Length - 1] != \'.\'))\n {\n result.Add("("+first+","+" "+last+")");\n }\n return;\n }\n if (flag == 0)\n {\n for(int i=0;i<first.Length; i++)\n {\n string temp = first;\n if (i - 1 >= 0)\n {\n first = first.Insert(i, ".");\n firstHasDec = true;\n }\n \n if (first.Length > 1 && first[0] == \'0\' && first[1] != \'.\') /*To handle cases like 005, 004, 005.6 */\n {\n first = temp;\n continue;\n }\n if (first.Length > 1 && first[0] == \'0\' && first[1] == \'.\') /*Handle test case like 0.0 or 0.0000*/\n {\n decimal n = Convert.ToDecimal(first);\n if (n == 0)\n {\n first = temp;\n continue;\n }\n \n }\n if (firstHasDec && first[first.Length-1]!=\'.\' && first[first.Length - 1] == \'0\') /*Handle test case like 1.0, 1.5000, 4.020 (string is contained decimal to trigger this test case) */\n {\n first = temp;\n continue;\n }\n AmbiguousCoordinatesHelper(first, last, firstHasDec, lasHasDec, flag + 1);\n first = temp;\n firstHasDec = false;\n }\n }\n if (flag == 1)\n {\n for (int i = 0; i < last.Length; i++)\n {\n string temp = last;\n if (i - 1 >= 0)\n {\n last = last.Insert(i, ".");\n lasHasDec = true;\n }\n \n if (last.Length > 1 && last[0] == \'0\' && last[1] != \'.\')\n {\n last = temp;\n continue;\n }\n if (last.Length > 1 && last[0] == \'0\' && last[1] == \'.\')\n {\n decimal n=Convert.ToDecimal(last);\n if (n == 0)\n {\n last = temp;\n continue;\n }\n \n }\n if (lasHasDec && last[last.Length - 1] != \'.\' && last[last.Length - 1] == \'0\')\n {\n last = temp;\n continue;\n }\n AmbiguousCoordinatesHelper(first, last, firstHasDec, lasHasDec,flag + 1);\n last = temp;\n lasHasDec = false;\n }\n }\n }\n public IList<string> AmbiguousCoordinates(string s) {\n s = s.Substring(1,s.Length-2);\n for(int i = 0; i < s.Length; i++) \n {\n string left=s.Substring(0,i+1);\n string right=s.Substring(i+1);\n AmbiguousCoordinatesHelper(left, right,false,false, 0);\n }\n return result;\n }\n}\n``` | 0 | 0 | ['C#'] | 0 |
ambiguous-coordinates | C# simple coordinates parsing | 90ms 100% | c-simple-coordinates-parsing-90ms-100-by-jx5h | Code\n\npublic class Solution {\n public IList<string> AmbiguousCoordinates(string s) \n {\n var chars = s.ToCharArray();\n\n var r = new Li | Legon2k | NORMAL | 2024-03-16T05:37:57.917422+00:00 | 2024-03-16T05:49:04.678144+00:00 | 1 | false | # Code\n```\npublic class Solution {\n public IList<string> AmbiguousCoordinates(string s) \n {\n var chars = s.ToCharArray();\n\n var r = new List<string>();\n\n for(var i = 2; i < s.Length - 1; i++)\n {\n var l2 = GetCoordinates(s, chars, i, s.Length-1);\n\n if(l2.Any())\n {\n foreach(var d1 in GetCoordinates(s, chars, 1, i))\n foreach(var d2 in l2)\n r.Add($"({d1}, {d2})");\n }\n }\n\n return r;\n }\n\n List<string> GetCoordinates(string s, char[] chars, int l, int r)\n {\n List<string> list = new ();\n\n var len = r - l;\n\n if(len == 1) // there is only one integer variant with length = 1\n {\n list.Add(s[l..r]);\n\n return list;\n }\n\n if(s[l] != \'0\') // wide integers should not start with zero\n {\n list.Add(s[l..r]);\n }\n\n if(s[r-1] != \'0\') // decimal should not end with zero\n {\n if(s[l] == \'0\') // for leading zero is only one decimal variant\n {\n list.Add("0." + s[(l+1)..r]);\n }\n else\n {\n var ch = chars[l..(r+1)]; // get extra space at the end for \'.\'\n\n ch[^1] = \'.\'; // will swap \'.\' towards head and create variants\n\n for(var i=ch.Length-1; i>1; i--)\n {\n (ch[i], ch[i-1]) = (ch[i-1], ch[i]);\n\n list.Add(new string(ch));\n }\n }\n }\n\n return list;\n }\n}\n``` | 0 | 0 | ['C#'] | 0 |
ambiguous-coordinates | Overly complicated custom iterator solution | overly-complicated-custom-iterator-solut-krdv | Code\n\nimpl Solution {\n pub fn ambiguous_coordinates(s: String) -> Vec<String> {\n let mut ans = vec![];\n let s = &s[1..s.len() - 1];\n | BitUnWise | NORMAL | 2024-03-09T04:25:23.507565+00:00 | 2024-03-09T04:25:23.507582+00:00 | 3 | false | # Code\n```\nimpl Solution {\n pub fn ambiguous_coordinates(s: String) -> Vec<String> {\n let mut ans = vec![];\n let s = &s[1..s.len() - 1];\n for i in 1..s.len() {\n let (left, right) = s.split_at(i);\n let left: SeperatorIterator = left.into();\n let right: SeperatorIterator = right.into();\n for (l, r) in left.flat_map(|l| right.clone().map(move |r| (l.clone(), r))) {\n ans.push(format!("({l}, {r})"));\n }\n }\n ans\n }\n}\n\n#[derive(Clone, Debug)]\nenum SeperatorIterator<\'a> {\n ZeroStart(Option<String>),\n ZeroEnd(Option<String>),\n Regular(SeperatorCounter<\'a>),\n Invalid,\n}\n\nimpl<\'a> From<&\'a str> for SeperatorIterator<\'a> {\n fn from(s: &\'a str) -> Self {\n if s.len() == 1 {\n return Self::Regular(SeperatorCounter::new(s));\n }\n let b = s.as_bytes();\n if b[0] == b\'0\' {\n if *b.last().unwrap() != b\'0\' {\n let mut res = String::with_capacity(s.len() + 1);\n res.push(s.chars().next().unwrap());\n res.push(\'.\');\n res.extend(s.chars().skip(1));\n return Self::ZeroStart(Some(res));\n } else {\n return Self::Invalid;\n }\n }\n if *b.last().unwrap() == b\'0\' {\n return Self::ZeroEnd(Some(s.to_owned()));\n }\n Self::Regular(SeperatorCounter::new(s))\n }\n}\n\nimpl<\'a> Iterator for SeperatorIterator<\'a> {\n type Item = String;\n\n fn next(&mut self) -> Option<String> {\n match self {\n Self::ZeroStart(s) => s.take(),\n Self::ZeroEnd(s) => s.take(),\n Self::Regular(x) => x.next(),\n Self::Invalid => None\n }\n }\n}\n\n#[derive(Clone, Debug)]\nstruct SeperatorCounter<\'a> {\n s: &\'a str,\n index: usize,\n}\n\nimpl<\'a> SeperatorCounter<\'a> {\n fn new(s: &\'a str) -> Self {\n Self {\n s,\n index: 0,\n }\n }\n}\n\nimpl<\'a> Iterator for SeperatorCounter<\'a> {\n type Item = String;\n\n fn next(&mut self) -> Option<String> {\n if self.index == 0 {\n self.index += 1;\n return Some(self.s.to_owned());\n }\n if self.index >= self.s.len() {\n return None;\n }\n let (left, right) = self.s.split_at(self.index);\n let mut res = String::with_capacity(self.s.len() + 1);\n res.push_str(left);\n res.push(\'.\');\n res.push_str(right);\n self.index += 1;\n Some(res)\n }\n}\n\n``` | 0 | 0 | ['Rust'] | 0 |
ambiguous-coordinates | Short Go Solution | short-go-solution-by-quoderat-iabx | \nimport "fmt"\n\nfunc ambiguousCoordinates(s string) []string {\n\tresults := []string{}\n\ts = s[1 : len(s)-1]\n\tfor c := 1; c < len(s); c++ {\n\t\tp2s := am | quoderat | NORMAL | 2024-02-17T01:58:36.017245+00:00 | 2024-02-17T01:58:36.017263+00:00 | 4 | false | ```\nimport "fmt"\n\nfunc ambiguousCoordinates(s string) []string {\n\tresults := []string{}\n\ts = s[1 : len(s)-1]\n\tfor c := 1; c < len(s); c++ {\n\t\tp2s := ambiguousNumber(s[c:])\n\t\tfor _, p1 := range ambiguousNumber(s[:c]) {\n\t\t\tfor _, p2 := range p2s {\n\t\t\t\tresults = append(results, fmt.Sprintf("(%s, %s)", p1, p2))\n\t\t\t}\n\t\t}\n\t}\n\treturn results\n}\n\nfunc ambiguousNumber(s string) []string {\n results := []string{}\n\tif len(s) == 1 || s[0] != \'0\' {\n\t\tresults = append(results, s)\n\t}\n\tfor c := 1; c < len(s); c++ {\n\t\tp1, p2 := s[:c], s[c:]\n\t\tif p2[len(p2)-1] == \'0\' || (p1[0] == \'0\' && len(p1) > 1) {\n\t\t\tcontinue\n\t\t}\n\t\tresults = append(results, fmt.Sprintf("%s.%s", p1, p2))\n\t}\n\treturn results\n}\n\n``` | 0 | 0 | ['Go'] | 0 |
ambiguous-coordinates | Not a good question. Intuitive solution. | not-a-good-question-intuitive-solution-b-61ok | Intuition\nThis is the worst kind of questions on Leetcode. It has nothing to do with any algorithm or data structure worth practicing, but adds layers and laye | trunkunala | NORMAL | 2024-02-02T00:45:23.421559+00:00 | 2024-02-02T00:45:23.421580+00:00 | 9 | false | # Intuition\nThis is the worst kind of questions on Leetcode. It has nothing to do with any algorithm or data structure worth practicing, but adds layers and layers of difficulties that are tricky to resolve. \n\nSo basically the idea is to split the string into two parts, convert each part into an acceptable form and combine. For example, for "123456" we can split the string into ```1,23456```, ```12,3456```, ```123,456```,```1234,56```, ```12345,6```. Then we start to add decimal points on left and right part. \n\n# Approach\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<String> ambiguousCoordinates(String s) {\n return helper(s.substring(1, s.length()-1));\n }\n\n List<String> helper(String s){\n\n List<String> res = new ArrayList();\n\n for(int i=1 ; i<s.length(); i++){\n\n String leftPart = s.substring(0, i);\n String rightPart = s.substring(i);\n\n if(consecZero(leftPart)||consecZero(rightPart)) continue;\n \n List<String> left = addDot(leftPart);\n List<String> right = addDot(rightPart);\n\n for(String l: left){\n for(String r: right){\n \n // System.out.println(l+",,,"+r);\n String newString = "("+l+", "+r+")";\n\n res.add(newString);\n\n }\n }\n\n }\n\n return res;\n\n }\n\n boolean consecZero(String s){\n if(s.length()==1) return false;\n\n for(int i=0; i<s.length(); i++){\n\n if(s.charAt(i)!=\'0\') return false;\n\n }\n return true;\n }\n\n List<String> addDot(String s){\n // System.out.println(s+"...");\n if(s.length()==1) {\n List<String> res= new ArrayList();\n res.add(s);\n return res; \n }\n\n List<String> res = new ArrayList();\n \n\n if(s.startsWith("0")) {\n String ele = s.substring(0, 1)+"."+s.substring(1);\n if(isValid(ele))\n res.add(ele);\n return res;\n } else {\n res.add(s);\n // System.out.println("ff");\n for(int i=1; i<s.length(); i++){\n String ele = s.substring(0, i)+"."+s.substring(i);\n // System.out.println("..ele"+ele);\n if(isValid(ele))\n res.add(ele);\n }\n\n }\n return res;\n }\n\n boolean isValid(String s){\n return s.charAt(s.length()-1)!=\'0\';\n }\n}\n``` | 0 | 0 | ['Java'] | 0 |
ambiguous-coordinates | TS Solution | ts-solution-by-gennadysx-zxqp | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | GennadySX | NORMAL | 2024-01-25T08:37:07.659227+00:00 | 2024-01-25T08:37:07.659246+00:00 | 4 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfunction ambiguousCoordinates(s: string): string[] {\n const res: string[] = [];\n const s2: string = s.substr(1, s.length - 2);\n const n: number = s2.length;\n \n for (let i = 1; i < n; i++) {\n const first: string[] = getNumbers(s2.substr(0, i));\n const second: string[] = getNumbers(s2.substr(i));\n \n for (let j = 0; j < first.length; j++) {\n for (let k = 0; k < second.length; k++) {\n res.push("(" + first[j] + ", " + second[k] + ")");\n }\n }\n }\n return res;\n}\n\nfunction getNumbers(num: string): string[] {\n const res: string[] = [];\n const n: number = num.length;\n \n if (n == 1) \n return [num];\n \n if (num[0] != \'0\') \n res.push(num);\n \n if (num[0] == \'0\') {\n if (num[num.length - 1] == \'0\') return [];\n return ["0." + num.substr(1)];\n }\n \n for (let i = 1; i < n; i++) {\n if (num.substr(i).substr(-1) == \'0\') continue;\n res.push(num.substr(0, i) + "." + num.substr(i));\n }\n \n return res;\n}\n``` | 0 | 0 | ['TypeScript'] | 0 |
ambiguous-coordinates | Python Medium | python-medium-by-lucasschnee-vv0o | \nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n t = ""\n for char in s:\n if char not in "()":\n | lucasschnee | NORMAL | 2024-01-19T17:20:17.589150+00:00 | 2024-01-19T17:20:17.589176+00:00 | 15 | false | ```\nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n t = ""\n for char in s:\n if char not in "()":\n t += char\n \n \n s = t\n \n N = len(s)\n \n self.options = set()\n \n def calc(index, left, right):\n\n if index == N:\n if left == "" or right == "":\n return\n \n \n b1 = any(char not in ".0" for char in left) \n b2 = any(char not in ".0" for char in right) \n \n if not b1 and len(left) > 1:\n return \n \n if not b2 and len(right) > 1:\n return\n \n if "." in left and left[0] == "0" and left[1] != ".":\n return\n \n if "." in right and right[0] == "0" and right[1] != ".":\n return\n \n if len(left) > 1 and left[0] == "0" and left[1] != ".":\n return\n \n if len(right) > 1 and right[0] == "0" and right[1] != ".":\n return\n \n if "." in left:\n index = left.index(".")\n index += 1\n check = True\n while index < len(left):\n if left[index] != "0":\n check = False\n break\n index += 1\n \n if check:\n return\n \n if left[-1] == "0":\n return\n \n if "." in right:\n index = right.index(".")\n \n index += 1\n check = True\n while index < len(right):\n if right[index] != "0":\n check = False\n break\n index += 1\n \n if check:\n return\n \n if right[-1] == "0":\n return\n \n \n \n \n self.options.add((left, right))\n \n return\n \n if not right:\n calc(index + 1, left + s[index], right)\n \n if left != "" and "." not in left:\n calc(index + 1, left + "." + s[index], right)\n \n calc(index + 1, left, right + s[index])\n \n if right != "" and "." not in right:\n calc(index + 1, left, right + "." + s[index])\n \n \n \n \n \n \n calc(0, "", "")\n \n arr = []\n \n for a, b in self.options:\n arr.append("(" + a + ", " + b + ")")\n \n return arr\n``` | 0 | 0 | ['Python3'] | 0 |
ambiguous-coordinates | C# Fast & Clean Solution Time: 107 ms (100.00%), Space: 61.4 MB (33.33%) | c-fast-clean-solution-time-107-ms-10000-1s182 | \npublic class Solution \n{\n private bool IsLegal(string num)\n {\n //\'1\' \'.\'\n if(num.Length == 1) return num != ".";\n \n | sighyu | NORMAL | 2023-12-21T23:12:28.545102+00:00 | 2023-12-21T23:12:28.545124+00:00 | 4 | false | ```\npublic class Solution \n{\n private bool IsLegal(string num)\n {\n //\'1\' \'.\'\n if(num.Length == 1) return num != ".";\n \n //\'00\' \'001\' \'00.01\'\n if(num[0] == \'0\' && num[1] == \'0\') return false;\n \n int decimalPointIdx = num.IndexOf(\'.\');\n \n //01.2\n if(num[0] == \'0\' && decimalPointIdx != 1) return false;\n \n //\'.1\'\n if(decimalPointIdx == 0) return false;\n \n //\'123\' \'01\'\n if(decimalPointIdx == -1) return num[0] != \'0\';\n \n //\'0.0\' \'0.00\' \'1.0\'\n return num.Last() != \'0\';\n }\n \n private List<string> GetAllDecimals(string num)\n {\n var list = new List<string>();\n \n if(IsLegal(num)) list.Add(num);\n \n for(int i = 1; i < num.Length; i++)\n {\n var currNum = num.Insert(i, ".");\n if(IsLegal(currNum)) list.Add(currNum);\n }\n\t\t\n return list;\n }\n \n public IList<string> AmbiguousCoordinates(string s) \n {\n var input = s.Substring(1, s.Length-2);\n var output = new List<string>();\n var firstHalf = new StringBuilder();\n \n for(int i = 0; i < input.Length-1; i++)\n {\n firstHalf.Append(input[i]);\n var secondHalf = input.Substring(i+1);\n \n var firstList = GetAllDecimals(firstHalf.ToString());\n var secondList = GetAllDecimals(secondHalf);\n \n foreach(var firstNum in firstList)\n secondList.ForEach(secondNum => output.Add("(" + firstNum + ", " + secondNum + ")"));\n }\n \n return output;\n }\n}\n``` | 0 | 0 | [] | 0 |
ambiguous-coordinates | Why the downvotes? Clean code - Python/Java | why-the-downvotes-clean-code-pythonjava-gu3fq | Intuition\n\nThis question definitely doesn\'t deserve so many downvotes. The edge cases may seem bad initially, but in code it\'s only a couple if elif conditi | katsuki-bakugo | NORMAL | 2023-12-15T02:41:34.608930+00:00 | 2023-12-17T00:55:43.579535+00:00 | 30 | false | > # Intuition\n\nThis question definitely doesn\'t deserve so many downvotes. The edge cases may seem bad initially, but in code it\'s only a couple `if elif` conditionals to make this pass.\n\nMy initial thoughts with this question were [Leetcode 22](https://leetcode.com/problems/generate-parentheses/description/). But we don\'t actually need recursion at all to solve this problem, and the key to it is realising we can `exhaust the comma separations` by just using loops. See comments in-line:\n\n---\n> # Solutions\n\n```python []\nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n #trim parantheses and create res array\n string,res = s[1:-1],[]\n\n #exhaust all possible left and right partitions of s\n for i in range(1,len(string)):\n #exhaust all possible spaces we can place the decimal for each partition\n leftPartition = self._makeDecimals(string[:i])\n rightPartition = self._makeDecimals(string[i:])\n\n if leftPartition and rightPartition:\n #go through every combination we found\n for lPartition in leftPartition:\n for rPartition in rightPartition:\n #and add the result\n res.append(f"({lPartition}, {rPartition})")\n return res\n\n def _makeDecimals(self,currentPartition):\n #holds the original number\n partitions = [currentPartition]\n\n #if it\'s a length of 1 it\'s value is irrelevant, whether it\'s just\n #a 0, or another integer, we always want it\n if len(currentPartition) == 1:\n return partitions\n \n #if it begins with a 0\n elif currentPartition[0] == "0":\n #and ends with a 0\n if currentPartition[-1] == "0":\n #we can\'t have 0.4430, 0.10 etc, so return []\n return []\n #otherwise we know it\'ll be 0. something, so return the rest of curr partition\n return [f"0.{currentPartition[1:]}"]\n #say we have a case where (10,0) and (1.0,0)\n elif currentPartition[-1] == "0":\n #we\'d want to keep the 10, and discard the 1.0 since 1.0 is invalid\n #so we can perform a final check to see if we end in 0, after passing the previous conditionals\n #if we do, we know it\'ll be something akin to 2.3330 3.0 4.560 etc.\n return partitions\n \n #if we\'ve made it this far we\'re valid\n for i in range(1,len(currentPartition)):\n #so compute all decimal combinations\n partitions.append(currentPartition[:i]+"."+currentPartition[i:])\n return partitions\n```\n```Java []\nclass Solution{\n public Solution(){};\n\n public List<String> ambiguousCoordinates(String s){\n String string = s.substring(1, s.length()-1);\n List<String> res = new ArrayList<>();\n\n for (int i = 1; i < string.length();i++){\n List<String> leftPartition = makeDecimals(string.substring(0, i));\n List<String> rightPartition = makeDecimals(string.substring(i, string.length()));\n \n if (!leftPartition.isEmpty() && !rightPartition.isEmpty()){\n for (String lPartition: leftPartition){\n for (String rPartition : rightPartition){\n res.add("(" + lPartition + ", " + rPartition + ")");\n }\n }\n }\n }\n return res;\n }\n\n private List<String> makeDecimals(String currentPartition){\n List<String> partitions = new ArrayList<>();\n partitions.add(currentPartition);\n \n if (currentPartition.length()==1){\n return partitions;\n }else if (currentPartition.charAt(0) == \'0\'){\n if (currentPartition.charAt(currentPartition.length()-1)==\'0\'){\n return new ArrayList<>();\n }\n List<String> curr = new ArrayList<>();\n curr.add("0."+currentPartition.substring(1, currentPartition.length()));\n return curr;\n }else if (currentPartition.charAt(currentPartition.length()-1)==\'0\'){\n return partitions;\n }\n for (int i = 1; i < currentPartition.length();i++){\n partitions.add(currentPartition.substring(0,i)+"."+currentPartition.substring(i, currentPartition.length()));\n }\n return partitions;\n }\n}\n``` | 0 | 0 | ['String', 'Python', 'Java', 'Python3'] | 0 |
ambiguous-coordinates | Ambiguous Coordinates || JAVASCRIPT || Solution by Bharadwaj | ambiguous-coordinates-javascript-solutio-nq2a | Approach\nBrute Force\n\n# Complexity\n- Time complexity:\nO(n^4)\n\n- Space complexity:\nO(n^2)\n\n# Code\n\nvar ambiguousCoordinates = function(s) {\n let a | Manu-Bharadwaj-BN | NORMAL | 2023-11-27T11:48:56.358182+00:00 | 2023-11-27T11:48:56.358203+00:00 | 2 | false | # Approach\nBrute Force\n\n# Complexity\n- Time complexity:\nO(n^4)\n\n- Space complexity:\nO(n^2)\n\n# Code\n```\nvar ambiguousCoordinates = function(s) {\n let ans = [];\n for(let i = 2; i < s.length - 1; i++){\n let left = s.slice(1, i);\n let right = s.slice(i, s.length - 1);\n\n let ls = [left], rs = [right];\n for(let i = 1; i < left.length; i++){\n let temp1 = left.slice(0, i);\n let temp2 = left.slice(i);\n ls.push(`${temp1}.${temp2}`);\n }\n for(let i = 1; i < right.length; i++){\n let temp1 = right.slice(0, i);\n let temp2 = right.slice(i, right.length);\n rs.push(`${temp1}.${temp2}`);\n }\n for(let l of ls){\n if(!/^([1-9]\\d*|0\\.\\d*[1-9]|[1-9]\\d*\\.\\d*[1-9]|0)$/i.test(l)) continue;\n for(let r of rs){\n if(!/^([1-9]\\d*|0\\.\\d*[1-9]|[1-9]\\d*\\.\\d*[1-9]|0)$/i.test(r)) continue;\n ans.push(`(${l}, ${r})`);\n }\n }\n }\n return ans;\n};\n``` | 0 | 0 | ['JavaScript'] | 0 |
ambiguous-coordinates | ✅simple iterative solution || python | simple-iterative-solution-python-by-dark-3l72 | \n# Code\n\nclass Solution:\n\n def check(self,s):\n i=0\n j=len(s)-1\n if(s[i]==\'(\'):i+=1\n elif(s[j]==\')\'):j-=1\n s= | darkenigma | NORMAL | 2023-09-30T09:23:34.881389+00:00 | 2023-09-30T09:23:34.881413+00:00 | 23 | false | \n# Code\n```\nclass Solution:\n\n def check(self,s):\n i=0\n j=len(s)-1\n if(s[i]==\'(\'):i+=1\n elif(s[j]==\')\'):j-=1\n s=s[i:j+1]\n k=s.find(".")\n if(k!=-1):\n if(1<k and s[0]==\'0\'):return 0 \n if(s[len(s)-1]==\'0\'):return 0\n return 1\n elif( str(int(s))==s):return 1 \n return 0\n\n\n def ambiguousCoordinates(self, s: str) -> List[str]:\n l=[]\n for i in range(1,len(s)-2):\n fp=s[:i+1]\n sp=s[i+1:]\n fl=[]\n sl=[]\n if((self.check(fp))):fl.append(fp)\n if((self.check(sp))):sl.append(sp)\n for j in range(1,len(fp)-1):\n temp=fp[:j+1]+"."+fp[j+1:]\n if((self.check(temp))):fl.append(temp)\n for j in range(0,len(sp)-2):\n temp=sp[:j+1]+"."+sp[j+1:]\n if((self.check(temp))):sl.append(temp)\n for j in fl:\n for k in sl:\n l.append(j+", "+k)\n\n n=len(l)\n return l\n``` | 0 | 0 | ['String', 'Python3'] | 0 |
ambiguous-coordinates | C# annoying validation 😒 | c-annoying-validation-by-valtss-hql3 | Intuition\n Describe your first thoughts on how to solve this problem. \nBrute force with validation of generated numbers.\n\n# Approach\n Describe your approac | valtss | NORMAL | 2023-09-25T20:33:25.341871+00:00 | 2023-09-25T20:33:54.514186+00:00 | 13 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nBrute force with validation of generated numbers.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Split input into 2 parts (left & right) process each in seperation.\n- Combine results in nested loop.\n- Call function to validate if generated number looks reasonable.\n\n# Complexity\n- Time complexity:\nSeems like a $$O(n^3)$$ in the worst case.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nSeems like a $$O(n^2)$$ in the worst case.\n\n# Code\n```\npublic class Solution {\n\n bool reasonable(string s)\n {\n// use a hack to simplofy\n if (s.IndexOf(\'.\') < 0)\n {\n return $"{int.Parse(s)}" == s;\n }\n \n// only cases with floating point left here - can\'t end with a 0\n if (s.Last() == \'0\') \n return false; \n\n// floating point that starts with a 0, means must have a . as 2nd\n if (s[0] == \'0\')\n {\n if (s.Length < 3)\n return false;\n\n if (s[1] != \'.\')\n {\n return false;\n }\n }\n \n return true;\n }\n// generate possible cases out of supplied digits\n IList<string> generate(string s)\n {\n var a = new List<string>();\n a.Add(s);\n for (int i = 1; i < s.Length; i++)\n {\n a.Add($"{s.Substring(0,i)}.{s.Substring(i, s.Length-i)}");\n }\n return a;\n }\n\n public IList<string> AmbiguousCoordinates(string s) {\n\n var st = s.Substring(1, s.Length-2);\n var answer = new List<string>();\n // slide possible position of , \n for (int i = 1; i < st.Length; i++)\n {\n var ls = st.Substring(0, i);\n var rs = st.Substring(i, st.Length-i);\n\n foreach(var lc in generate(ls))\n {\n if (!reasonable(lc))\n continue;\n\n foreach(var rc in generate(rs))\n {\n if (reasonable(rc))\n answer.Add($"({lc}, {rc})");\n }\n }\n } \n return answer;\n }\n}\n``` | 0 | 0 | ['C#'] | 0 |
ambiguous-coordinates | Go solution with comments | go-solution-with-comments-by-dchooyc-81nr | \nfunc ambiguousCoordinates(s string) []string {\n res := []string{}\n // remove parenthesis\n s = s[1:len(s) - 1]\n\n for i := 1; i < len(s); i++ { | dchooyc | NORMAL | 2023-09-15T19:02:45.169967+00:00 | 2023-09-15T19:02:45.169993+00:00 | 13 | false | ```\nfunc ambiguousCoordinates(s string) []string {\n res := []string{}\n // remove parenthesis\n s = s[1:len(s) - 1]\n\n for i := 1; i < len(s); i++ {\n // generate possible x coordinates based on split\n lefts := gen(s, 0, i)\n // generate possible y coordinates based on split\n rights := gen(s, i, len(s))\n\n // for each possible pair of coordinates\n for _, left := range lefts {\n for _, right := range rights {\n val := "(" + left + ", " + right + ")"\n res = append(res, val)\n }\n }\n }\n\n return res\n}\n\nfunc gen(s string, start, end int) []string {\n res := []string{}\n\n // partitioning for whole number and decimal part\n for p := 1; p <= end - start; p++ {\n // whole number part\n w := s[start:start + p]\n // decimal number part\n d := s[start + p:end]\n\n // either whole number part is a zero or it cannot start with zero\n // and either decimal part doesn\'t exist or it cannot end with a zero\n if (w == "0" || w[0] != \'0\') && (len(d) == 0 || d[len(d) - 1] != \'0\') {\n if len(d) == 0 {\n // decimal part does not exist\n res = append(res, w)\n } else {\n // decimal exists\n res = append(res, w + "." + d)\n }\n }\n }\n\n return res\n}\n``` | 0 | 0 | ['Go'] | 0 |
ambiguous-coordinates | [Python] Solution + explanation | python-solution-explanation-by-ngrishano-0u1x | We can split this problem into two subproblems:\n1. Get all the possible values for coordinates substring (102 -> [1.02, 10.2, 102], 00 -> [])\n2. Get all the p | ngrishanov | NORMAL | 2023-09-10T20:12:44.970072+00:00 | 2023-09-10T20:12:44.970092+00:00 | 27 | false | We can split this problem into two subproblems:\n1. Get all the possible values for coordinates substring (`102` -> `[1.02, 10.2, 102]`, `00` -> `[]`)\n2. Get all the possible combinations for two coordinates substrings\n\nFor the first subproblem, refer to `variants` function. It should be self-explanatory: we iterate through coordinate substring and split it into whole part and fractional part. Then we validate each part according to problem description. If both parts are valid, we add it to our answer.\n\nFor the second subproblem we iterate through input string, split it into two parts, call `variants` function and use `itertools.product` to generate all the possible combinations.\n\n# Code\n```\nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n s = s[1:len(s) - 1]\n\n @functools.cache\n def variants(val):\n vals = []\n\n for i in range(1, len(val) + 1):\n whole = val[:i]\n fractional = val[i:]\n\n if whole[0] == "0" and len(whole) > 1:\n continue\n\n if fractional:\n if fractional[-1] == "0":\n continue\n\n vals.append(f"{whole}.{fractional}")\n else:\n vals.append(whole) \n\n return vals\n\n result = []\n\n for i in range(1, len(s)):\n result.extend(\n f"({a}, {b})" \n for a, b in itertools.product(variants(s[:i]), variants(s[i:]))\n )\n\n return result\n \n``` | 0 | 0 | ['Python3'] | 0 |
ambiguous-coordinates | Easiest Solution | easiest-solution-by-kunal7216-2eai | \n\n# Code\njava []\n// the constraints are really small so we can use brute force , rest of the code is self explanatory\n\nclass Solution {\n public List<S | Kunal7216 | NORMAL | 2023-09-08T13:18:05.426823+00:00 | 2023-09-08T13:18:05.426840+00:00 | 31 | false | \n\n# Code\n```java []\n// the constraints are really small so we can use brute force , rest of the code is self explanatory\n\nclass Solution {\n public List<String> ambiguousCoordinates(String s) {\n String str = s.substring(1,s.length()-1); // remove brackets from the expression to make it easier to process \n List<String> list = new ArrayList();\n for(int i =0;i<str.length()-1;i++){\n String s1 = str.substring(0,i+1); // first coordinate \n String s2 = str.substring(i+1); // second coordinate \n if(isValid(s1)&&isValid(s2)){ // if both are valid numbers , then we will add this to our result \n String ans = "("+s1+", "+s2+")";\n list.add(ans);\n }\n // now we form two lists l1 and l2 , l1 consists of all possible decimal numbers that can be formed from s1 , l2 consists of all possible decimal numbers that can be formed from s2 \n List<String> l1 = new ArrayList();\n List<String> l2 = new ArrayList();\n fillList(s1,l1);\n fillList(s2,l2);\n for(String ss1 : l1){\n for(String ss2 : l2){\n String ans = "("+ss1+", "+ss2+")"; // adding all (decimal , decimal) pairs to answer\n list.add(ans);\n }\n }\n if(isValid(s1)){ // add all (integer , decimal) pairs to answer if s1 is a valid integer\n for(String ss2 : l2){\n String ans = "("+s1+", "+ss2+")";\n list.add(ans);\n }\n }\n if(isValid(s2)){ // add all (decimal , integer) pairs to answer if s2 is a valid integer \n for(String ss1 : l1){\n String ans = "("+ss1+", "+s2+")";\n list.add(ans);\n }\n }\n \n }\n return list;\n }\n public boolean isValid(String s){ // this is used to check if an integer is valid and it is also used to check if part of number before decimal is valid \n if(s.length()==1) return true; // always valid \n return s.charAt(0)==\'0\'?false:true; // form : 0000....... invalid \n }\n public boolean isSecondValid(String s){ // this is used to check if part of number after decimal is valid \n boolean found = false; // found == true if >=\'1\' found \n for(int i = 0;i<s.length();i++){\n char ch = s.charAt(i);\n if(ch>=\'1\') found = true;\n else{\n if(found){ // if >=\'1\' found check if after this do we have only zeroes , because 0.5000000 is invalid \n boolean allZeroes = true;\n for(int j = i+1;j<s.length();j++){\n if(s.charAt(j)>=\'1\') allZeroes = false;\n }\n if(allZeroes) return false;\n }\n }\n }\n return found; // found becomes true when >=\'1\' is found so if we have something like 0.0000000 its invalid and we return false in that case \n }\n \n public void fillList(String s , List<String> list){ // fills list with all decimal numbers possible from given string s\n for(int i = 0;i<s.length()-1;i++){\n String s1 = s.substring(0,i+1);\n String s2 = s.substring(i+1);\n if(isValid(s1)&&isSecondValid(s2)){\n String ans = s1+"."+s2;\n list.add(ans);\n }\n }\n }\n}\n```\n```c++ []\nclass Solution {\npublic:\n \n bool valid(string t) { // is this coord valid? \n int i=1,n=t.size();\n if(n>=2 and t[0]==\'0\' and t[1]!=\'.\') // 01, 03... --> not valid\n return false;\n \n while(i<n and t[i]!=\'.\') // get position of the point\n i++;\n if(i!=n and t[n-1]==\'0\') // 234.0, 931.424320 --> not valid\n return false;\n i++;\n while(i<n and t[i]==\'0\') // if after the point are all zeros, not valid\n i++;\n if(i==n) return false;\n \n return true; // if here, coord is valid\n }\n \n void get_coord(string s1, string s2, vector<string>& res) {\n int n1=s1.size(), n2=s2.size();\n for(int i=0;i<n1;i++){ \n // get every possible partition, as before, but with point at the middle\n // es. s1 = 1234 -> (.1234), (1.234), (12.234), (123.4)\n string s11 = s1.substr(0,i);\n string s12 = s1.substr(i);\n string t1 = s11 + "." + s12;\n if(t1[0] == \'.\') // remove initial point if present\n t1=t1.substr(1);\n if(valid(t1)){ // if it is valid, look at the second string\n for(int i=0;i<n2;i++){\n string s21 = s2.substr(0,i);\n string s22 = s2.substr(i); \n string t2 = s21 + "." + s22;\n if(t2[0] == \'.\') // remove initial point if present\n t2=t2.substr(1);\n if(valid(t2)){ // if the second is valid too, add to res\n res.push_back("("+t1+", "+t2+")");\n }\n }\n }\n }\n } \n \n vector<string> ambiguousCoordinates(string s) {\n s = s.substr(1,s.size()-2); // string without ( )\n vector<string> res;\n int n=s.size();\n for(int i=0;i<n;i++) {\n // get every double partition of string\n // es. s = 1234 -> (, 1234), (1, 234), (12, 234), (123, 4)\n get_coord(s.substr(0,i), s.substr(i), res); \n }\n return res;\n }\n};\n``` | 0 | 0 | ['C++', 'Java'] | 0 |
ambiguous-coordinates | Very simple Intuition solution || C++ | very-simple-intuition-solution-c-by-rkku-ic39 | Complexity\n- Time complexity: O(N^3)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(N)\n Add your space complexity here, e.g. O(n) \n\n# | rkkumar421 | NORMAL | 2023-06-16T08:26:53.214302+00:00 | 2023-06-16T08:29:14.059038+00:00 | 70 | false | # Complexity\n- Time complexity: O(N^3)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n \n void solve(vector<string> &ans, string &a, string &b){\n // Here we have to validate and then add that to answer\n vector<string> tem;\n string t = "(";\n // putting dot on\n for(int i=0;i<a.length();i++){\n t = "(";\n if(i == 0) t += a;\n else{\n t += a.substr(0,i);\n t += \'.\';\n t += a.substr(i,a.length()-i);\n }\n // Now we got t\n // check for 00. or 000. 01.\n if(t[1] == \'0\'){\n // if first char is zero we must see if we got any other char after that or not if we got that we break\n if(t.length() > 2 && t[2] != \'.\') continue;\n }\n // check for .000 or .00\n if(i != 0 && t[t.length()-1] == \'0\') continue;\n tem.push_back(t);\n }\n t = "";\n for(int i=0;i<b.length();i++){\n if(i == 0) t = b;\n else{\n t = b.substr(0,i);\n t += \'.\';\n t += b.substr(i,b.length()-i);\n }\n // Now we got t\n // check for 00. or 000. 01.\n if(t[0] == \'0\'){\n // if first char is zero we must see if we got any other char after that or not if we got that we break\n if(t.length() > 1 && t[1] != \'.\') continue;\n }\n // check for .000 or .00\n if(i != 0 && t[t.length()-1] == \'0\') continue;\n for(auto s: tem){\n string n = s;\n n += ", ";\n n += t;\n n += \')\';\n ans.push_back(n);\n }\n }\n }\n \n vector<string> ambiguousCoordinates(string s) {\n vector<string> ans;\n string fi = "", se = "";\n // we are making first coordinate and second then try to add point in all and validate \n for(int j = 1;j<s.length()-2;j++){\n fi += s[j];\n se = s.substr(j+1,s.length()-1-(j+1));\n // Now we have to pass this\n solve(ans,fi,se);\n }\n return ans;\n }\n};\n``` | 0 | 0 | ['String', 'C++'] | 0 |
ambiguous-coordinates | Easy division | easy-division-by-xjpig-hsrf | Intuition\n Describe your first thoughts on how to solve this problem. \nsuppose s=pre+suf, the corresponding coordinates include the join of all possible divis | XJPIG | NORMAL | 2023-06-03T08:16:39.234528+00:00 | 2023-06-03T08:16:39.234571+00:00 | 52 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nsuppose s=pre+suf, the corresponding coordinates include the join of all possible divisions of pre/suf. Note that, for any pre/suf, it is not valid for division if the length of it is larger than 1 and both the front and end of it are \'0\'s.\n\n\n# Complexity\n- Time complexity: $$O(n^3)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n^3)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n unordered_map<string,vector<string>> mp;\n vector<string> helper(string&s){\n if(mp.count(s)) return mp[s];\n if(s.size()>1 and s[0]==\'0\' and s.back()==\'0\') return {};\n if(s.back()==\'0\'){\n mp[s]={s};\n return mp[s];\n }\n if(s[0]==\'0\'){\n string tmp=s.substr(0,1)+\'.\'+s.substr(1);\n mp[s]={tmp};\n return mp[s];\n }\n for(int i=1;i<s.size();i++){\n string tmp=s.substr(0,i)+\'.\'+s.substr(i);\n mp[s].emplace_back(tmp);\n }\n mp[s].emplace_back(s);\n return mp[s];\n }\npublic:\n vector<string> ambiguousCoordinates(string s) {\n vector<string> result;\n for(int i=1;i+2<s.size();i++){\n string pre=s.substr(1,i),suf=s.substr(i+1,s.size()-i-2);\n auto ss1=helper(pre);\n auto ss2=helper(suf);\n if(ss1.empty() or ss2.empty()) continue;\n for(auto&x:ss1) for(auto&y:ss2){\n string tmp="("+x+", "+y+")";\n result.emplace_back(tmp);\n }\n }\n return result;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
ambiguous-coordinates | Solution in C++ | solution-in-c-by-ashish_madhup-u90n | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ashish_madhup | NORMAL | 2023-05-29T10:01:55.859590+00:00 | 2023-05-29T10:01:55.859640+00:00 | 55 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<string> cases(string &&s)\n {\n if (s.size() == 1) \n return {s};\n if (s.front() == \'0\') \n { \n if (s.back() == \'0\') \n return\n {\n\n };\n return {"0." + s.substr(1)}; \n }\n if (s.back() == \'0\') \n return \n {\n s\n };\n vector<string> res\n {\n s\n }; \n for (int i = 1; i < s.size(); i++)\n res.emplace_back(s.substr(0, i) + "." + s.substr(i));\n return res;\n}\nvector<string> ambiguousCoordinates(string S)\n {\n vector<string> res;\n for (int i = 2; i < S.size() - 1; i++) \n for (auto &x : cases(S.substr(1, i - 1)))\n for (auto &y : cases(S.substr(i, S.size() - i - 1)))\n res.emplace_back("(" + x + ", " + y + ")");\n return res;\n }\n};\n``` | 0 | 0 | ['C++'] | 0 |
ambiguous-coordinates | Python3 - Ye Olde One Liner | python3-ye-olde-one-liner-by-godshiva-e7cr | Intuition\nCuz why not?\n\n# Code\n\nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n return [f"({x}, {y})" for d in [lambda n | godshiva | NORMAL | 2023-05-29T04:33:06.910105+00:00 | 2023-05-29T04:33:06.910141+00:00 | 39 | false | # Intuition\nCuz why not?\n\n# Code\n```\nclass Solution:\n def ambiguousCoordinates(self, s: str) -> List[str]:\n return [f"({x}, {y})" for d in [lambda n: [x for x in [f"{n[:i]}.{n[i:]}".strip(\'.\') for i in range(1, len(n)+1)] if (\'.\' not in x or x[-1] != \'0\') and x[:2] != \'00\' and (x[0] != \'0\' or x[:2]==\'0.\' or len(x) == 1)]] for i in range(2, len(s)-1) for x in d(s[1:i]) for y in d(s[i:-1])]\n\n\n\n``` | 0 | 0 | ['Python3'] | 0 |
ambiguous-coordinates | [Accepted] Swift | accepted-swift-by-vasilisiniak-3nn0 | \nclass Solution {\n func ambiguousCoordinates(_ s: String) -> [String] {\n\n func lr(_ s: String) -> [(String, String)] {\n (1..<s.count). | vasilisiniak | NORMAL | 2023-04-08T08:17:54.050494+00:00 | 2023-04-08T08:17:54.050533+00:00 | 50 | false | ```\nclass Solution {\n func ambiguousCoordinates(_ s: String) -> [String] {\n\n func lr(_ s: String) -> [(String, String)] {\n (1..<s.count).map { (String(s.prefix($0)), String(s.dropFirst($0))) }\n }\n\n func opts(_ s: String) -> [String] {\n guard !s.hasPrefix("00") || s.contains(where: { $0 != "0" }) else { return [] }\n guard !s.hasPrefix("0") || !s.hasSuffix("0") || s == "0" else { return [] }\n guard s.count > 1, !s.hasSuffix("0") else { return [s] }\n guard !s.hasPrefix("0") else { return ["\\(0).\\(s.dropFirst())"] }\n return [s] + lr(s).map { "\\($0).\\($1)" }\n }\n \n return lr(String(s.dropFirst().dropLast()))\n .map { (opts($0), opts($1)) }\n .filter { !$0.isEmpty && !$1.isEmpty }\n .flatMap { ls, rs in ls.flatMap { l in rs.map { r in "(\\(l), \\(r))" } } }\n }\n}\n``` | 0 | 0 | ['Swift'] | 0 |
ambiguous-coordinates | I ❤ Ruby | i-ruby-by-0x81-cmdx | ruby\ndef ambiguous_coordinates s\n f = -> s do\n r, z = [s], s.size\n return r if z == 1\n return (s[/^0/] ? [] : r) if s[/0$/]\n | 0x81 | NORMAL | 2023-03-29T13:30:05.921684+00:00 | 2023-03-29T13:30:05.921718+00:00 | 40 | false | ```ruby\ndef ambiguous_coordinates s\n f = -> s do\n r, z = [s], s.size\n return r if z == 1\n return (s[/^0/] ? [] : r) if s[/0$/]\n return [\'0.\' + s[1..]] if s[/^0/]\n (1...z).reduce(r) { _1 << s.clone.insert(_2, ?.) }\n end\n (1...(s = s[1..-2]).size).flat_map do | i |\n f.(s[...i]).product(f.(s[i..])).map do\n "(#{_1.first}, #{_1.last})"\n end\n end\nend\n``` | 0 | 0 | ['Ruby'] | 0 |
ambiguous-coordinates | C++ | c-by-tinachien-0xew | \nclass Solution {\nprivate:\n vector<string>splits(string s){\n if(s.size() == 0)\n return {} ;\n if(s.size() > 1 && s[0] == \'0\' | TinaChien | NORMAL | 2023-03-28T11:33:40.916237+00:00 | 2023-03-28T11:33:40.916280+00:00 | 54 | false | ```\nclass Solution {\nprivate:\n vector<string>splits(string s){\n if(s.size() == 0)\n return {} ;\n if(s.size() > 1 && s[0] == \'0\' && s.back() == \'0\')\n return {} ;\n if(s.back() == \'0\')\n return {s} ;\n if(s.front() == \'0\')\n return {"0." + s.substr(1)} ;\n \n vector<string>ret ;\n ret.push_back(s) ;\n for(int i = 1; i < s.size(); i++){\n ret.push_back(s.substr(0, i) + "." + s.substr(i)) ;\n }\n return ret ;\n }\npublic:\n vector<string> ambiguousCoordinates(string s) {\n vector<string>ret ;\n s = s.substr(1, s.size()-2) ;\n for(int i = 1; i < s.size(); i++){\n for(auto& x : splits(s.substr(0, i))){\n for(auto& y : splits(s.substr(i))){\n ret.push_back("(" + x + ", " + y +")" ) ;\n }\n }\n }\n return ret ;\n }\n};\n``` | 0 | 0 | [] | 0 |
find-the-number-of-good-pairs-i | Java EASY Solution for Beginners [ 99.96% ] [ 1ms ] | java-easy-solution-for-beginners-9996-1m-e36v | Approach\n1. Initialize Counter:\n - Create a variable count to store the number of valid pairs.\n\n2. Outer Loop:\n - Iterate through each element i in num | RajarshiMitra | NORMAL | 2024-05-29T16:47:09.898898+00:00 | 2024-06-16T06:43:26.825504+00:00 | 1,072 | false | # Approach\n1. **Initialize Counter**:\n - Create a variable `count` to store the number of valid pairs.\n\n2. **Outer Loop**:\n - Iterate through each element `i` in `nums1`.\n\n3. **Inner Loop**:\n - For each element `i` in `nums1`, iterate through each element `j` in `nums2`.\n\n4. **Check Condition**:\n - Check if `nums1[i] % (nums2[j] * k) == 0`.\n\n5. **Update Counter**:\n - If the condition is true, increment `count`.\n\n6. **Return Result**:\n - Return the value of `count`.\n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count=0;\n for(int i=0; i<nums1.length; i++){\n for(int j=0; j<nums2.length; j++){\n if(nums1[i]%(nums2[j]*k) == 0) count++;\n }\n }\n return count;\n }\n}\n```\n\n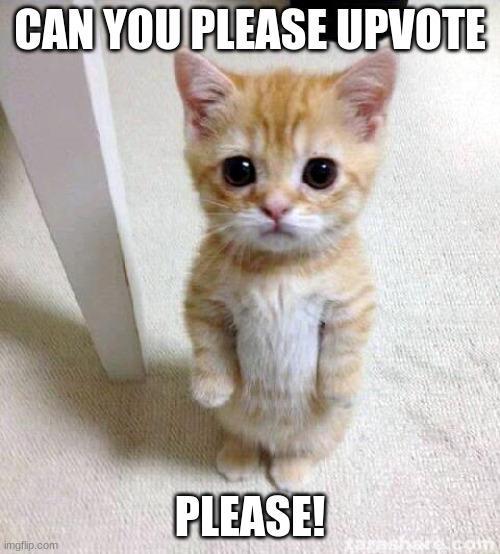\n | 13 | 0 | ['Java'] | 2 |
find-the-number-of-good-pairs-i | Python 3 || 4 lines, filter nums1 and count || T/S: 98% / 61% | python-3-4-lines-filter-nums1-and-count-ky3a1 | \nclass Solution:\n def numberOfPairs(self, nums1: List[int], \n nums2: List[int], k: int, ans = 0) -> int:\n\n nums1 = lis | Spaulding_ | NORMAL | 2024-05-26T04:10:36.402442+00:00 | 2024-05-31T05:47:14.472523+00:00 | 855 | false | ```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], \n nums2: List[int], k: int, ans = 0) -> int:\n\n nums1 = list(map(lambda x: x//k, filter(lambda x: x%k == 0, nums1)))\n \n for n1, n2 in product(nums1, nums2):\n \n if n1%n2 == 0: ans+= 1\n\n return ans\n```\n[https://leetcode.com/problems/find-the-number-of-good-pairs-i/submissions/1268155427/](https://leetcode.com/problems/find-the-number-of-good-pairs-i/submissions/1268155427/)\n\nI could be wrong, but I think that time complexity is *O*(*MN*) and space complexity is *O*(1), in which *M* ~ `len(nums1)` and *N* ~ `len(nums2)`. | 10 | 0 | ['Python3'] | 1 |
find-the-number-of-good-pairs-i | Simple java solution | simple-java-solution-by-siddhant_1602-heag | Complexity\n- Time complexity: O(n^2)\n\n- Space complexity: O(1)\n\n# Code\n\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) { | Siddhant_1602 | NORMAL | 2024-05-26T04:08:27.519795+00:00 | 2024-05-26T04:08:27.519815+00:00 | 894 | false | # Complexity\n- Time complexity: $$O(n^2)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count=0;\n for(int i=0;i<nums1.length;i++)\n {\n for(int j=0;j<nums2.length;j++)\n {\n if(nums1[i]%(nums2[j]*k)==0)\n {\n count++;\n }\n }\n }\n return count;\n }\n}\n``` | 9 | 2 | ['Java'] | 2 |
find-the-number-of-good-pairs-i | Simple And Easy Solution | simple-and-easy-solution-by-kg-profile-idda | Code\n\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n c = 0\n for i in range(len(nums1)):\n | KG-Profile | NORMAL | 2024-05-26T04:04:08.241513+00:00 | 2024-05-26T04:04:08.241546+00:00 | 1,710 | false | # Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n c = 0\n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if nums1[i] % (nums2[j] * k) == 0:\n c += 1\n return c\n``` | 9 | 0 | ['Python3'] | 1 |
find-the-number-of-good-pairs-i | Assembly with explanation and comparison with C | assembly-with-explanation-and-comparison-5wdf | \n# Rationale\n\nI\'ve solved this LeetCode problem using inline assembly in C. My goal isn\'t to showcase complexity, but rather to challenge myself and deepen | pcardenasb | NORMAL | 2024-06-13T23:46:58.410365+00:00 | 2024-06-13T23:46:58.410383+00:00 | 150 | false | \n# Rationale\n\nI\'ve solved this LeetCode problem using inline assembly in C. My goal isn\'t to showcase complexity, but rather to challenge myself and deepen my understanding of assembly language. \n\nAs a beginner in assembly, I welcome any improvements or comments on my code.\n\nAdditionally, I aim to share insights on practicing assembly on LeetCode.\n\n# Intuition\n\nIterate through all pair and check if `num1` is divisible by `(num2 * k)`\n\n# Approach\n\nMake two loops to iterate through all pairs. Use `mul` for multiplication and `div` for remainder. In this problem I have to use callee-saved register, so I have to push them to the stack. Use View [code](#assembly-code) comments for explanation.\n\n# Complexity\n- Time complexity: $$O(\\text{nums1Size}\\times\\text{nums2Size})$$. Fortunately, $$\\text{nums1Size}, \\text{nums2Size} \\le 50$$.\n- Space complexity: $$O(1)$$, I have to push 3 64bits register to stack.\n\n# Assembly Code\nThis mixed block shows the C code with inline assembly and the assembly code. View [Notes about assembly](#notes-about-assembly) for notes about the syntax used. Moreover, It shows the assembly code without comment for [comparision with C](#comparison-with-c). If you want to test this code you can [run locally](#run-locally).\n\n```c [C (inline asm)]\nint numberOfPairs(int* nums1, int nums1Size, int* nums2, int nums2Size, int k) {\n\tint ret;\n\t__asm__(\n".intel_syntax noprefix\\n"\n"\t## rdi: nums1\\n"\n"\t## esi -> sil: 1 <=nums1Size <= 50\\n"\n"\t## rdx: nums2 # will be replaced by r14\\n"\n"\t## ecx -> cl: 1 <= nums2Size <= 50\\n"\n"\t## r8 -> r8b: 1 <= k <= 50\\n"\n"\txor r9, r9\t\t\t\t\t# loop counter for nums1\\n"\n"\txor r10, r10\t\t\t\t# loop counter for nums2\\n"\n"\tpush r12\t\t\t\t\t# temporal value that will be restored\\n"\n"\tpush r13\t\t\t\t\t# Counter of good pairs (would be RAX\\n"\n"\t\t\t\t\t\t\t\t# but will be overwriten by div)\\n"\n"\txor r13, r13\\n"\n"\t\\n"\n"\tpush r14\t\t\t\t\t# store nums2 because rdx is volatile\\n"\n"\t\t\t\t\t\t\t\t# when using div\\n"\n"\tmov r14, rdx\\n"\n"\\n"\n"\\n"\n"loop1:\\n"\n"\tmov r11b, BYTE PTR[rdi+r9*4] # Get the current num1 in R11b\\n"\n"\txor r10b, r10b\t\t\t\t # Reset the loop counter for num2\\n"\n"loop2:\\n"\n"\tmov al, BYTE PTR[r14+r10*4]\t# Get the current num2 in AL\\n"\n"\tmul r8b\t\t\t\t\t\t# multiply by k. Then AX = k*num2\\n"\n"\tmov r12w, ax\t\t\t\t# R12w = k*num2\\n"\n"\\n"\n"\tmovzx ax, r11b\t\t\t\t# Set AX = num1\\n"\n"\txor dx, dx\t\t\t\t\t# Reset DX to 0 for division\\n"\n"\tdiv r12w\t\t\t\t\t# Divide DX:AX by R12w (num1/(k*num2))\\n"\n"\\n"\n"\ttest dx, dx\t\t\t\t\t# Check if remainder (DX) is 0\\n"\n"\tjnz not_divisor\\n"\n"\tinc r13w\t\t\t\t\t# if remainder is 0, increase R13\\n"\n"not_divisor:\\n"\n"\tinc r10b\t\t\t\t\t# loop2 update\\n"\n"\tcmp r10b, cl\t\t\t\t# loop2 condition\\n"\n"\tjl loop2\t\t\t\t\t# jump if loop2 condition\\n"\n"\t\\n"\n"\tinc r9b\t\t\t\t\t\t# loop1 update\\n"\n"\tcmp r9b, sil\t\t\t\t# loop2 condition\\n"\n"\tjl loop1\t\t\t\t\t# jump if jump1 condition\\n"\n"\\n"\n"\tmovzx eax, r13w\t\t\t\t# Assign R13w to EAX to return\\n"\n"epilog:\\n"\n"\tpop r14\\n"\n"\tpop r13\\n"\n"\tpop r12\\n"\n".att_syntax\\n"\n\t\t: "=a"(ret)\n\t);\n\treturn ret;\n}\n```\n```assembly [ASM (with comments)]\n\t.intel_syntax noprefix\n\t.globl numberOfPairs\n\nnumberOfPairs:\n\t## rdi: nums1\n\t## esi -> sil: 1 <=nums1Size <= 50\n\t## rdx: nums2 ; will be replaced by r14\n\t## ecx -> cl: 1 <= nums2Size <= 50\n\t## r8 -> r8b: 1 <= k <= 50\n\txor r9, r9\t\t\t\t\t# loop counter for nums1\n\txor r10, r10\t\t\t\t# loop counter for nums2\n\tpush r12\t\t\t\t\t# temporal value that will be restored\n\tpush r13\t\t\t\t\t# Counter of good pairs (would be RAX\n\t\t\t\t\t\t\t\t# but will be overwriten by div)\n\txor r13, r13\n\t\n\tpush r14\t\t\t\t\t# store nums2 because rdx is volatile\n\t\t\t\t\t\t\t\t# when using div\n\tmov r14, rdx\n\n\nloop1:\n\tmov r11b, BYTE PTR[rdi+r9*4] # Get the current num1 in R11b\n\txor r10b, r10b\t\t\t\t # Reset the loop counter for num2\nloop2:\n\tmov al, BYTE PTR[r14+r10*4]\t# Get the current num2 in AL\n\tmul r8b\t\t\t\t\t\t# multiply by k. Then AX = k*num2\n\tmov r12w, ax\t\t\t\t# R12w = k*num2\n\n\tmovzx ax, r11b\t\t\t\t# Set AX = num1\n\txor dx, dx\t\t\t\t\t# Reset DX to 0 for division\n\tdiv r12w\t\t\t\t\t# Divide DX:AX by R12w (num1/(k*num2))\n\n\ttest dx, dx\t\t\t\t\t# Check if remainder (DX) is 0\n\tjnz not_divisor\n\tinc r13w\t\t\t\t\t# if remainder is 0, increase R13\nnot_divisor:\n\tinc r10b\t\t\t\t\t# loop2 update\n\tcmp r10b, cl\t\t\t\t# loop2 condition\n\tjl loop2\t\t\t\t\t# jump if loop2 condition\n\t\n\tinc r9b\t\t\t\t\t\t# loop1 update\n\tcmp r9b, sil\t\t\t\t# loop2 condition\n\tjl loop1\t\t\t\t\t# jump if jump1 condition\n\n\tmovzx eax, r13w\t\t\t\t# Assign R13w to EAX to return\nepilog:\n\tpop r14\n\tpop r13\n\tpop r12\n\tret\n```\n```assembly [ASM (without comments)]\n\t.intel_syntax noprefix\n\t.globl numberOfPairs\n\nnumberOfPairs:\n\n\n\n\n\n\txor r9, r9\n\txor r10, r10\n\tpush r12\n\tpush r13\n\n\txor r13, r13\n\t\n\tpush r14\n\n\tmov r14, rdx\n\n\nloop1:\n\tmov r11b, BYTE PTR[rdi+r9*4]\n\txor r10b, r10b\nloop2:\n\tmov al, BYTE PTR[r14+r10*4]\n\tmul r8b\n\tmov r12w, ax\n\n\tmovzx ax, r11b\n\txor dx, dx\n\tdiv r12w\n\n\ttest dx, dx\n\tjnz not_divisor\n\tinc r13w\nnot_divisor:\n\tinc r10b\n\tcmp r10b, cl\n\tjl loop2\n\t\n\tinc r9b\n\tcmp r9b, sil\n\tjl loop1\n\n\tmovzx eax, r13w\nepilog:\n\tpop r14\n\tpop r13\n\tpop r12\n\tret\n```\n```assembly [ASM (without comments)]\n\t.intel_syntax noprefix\n\t.globl numberOfPairs\nnumberOfPairs:\n\txor r9, r9\n\txor r10, r10\n\tpush r12\n\tpush r13\n\txor r13, r13\n\tpush r14\n\tmov r14, rdx\nloop1:\n\tmov r11b, BYTE PTR[rdi+r9*4]\n\txor r10b, r10b\nloop2:\n\tmov al, BYTE PTR[r14+r10*4]\n\tmul r8b\n\tmov r12w, ax\n\tmovzx ax, r11b\n\txor dx, dx\n\tdiv r12w\n\ttest dx, dx\n\tjnz not_divisor\n\tinc r13w\nnot_divisor:\n\tinc r10b\n\tcmp r10b, cl\n\tjl loop2\n\tinc r9b\n\tcmp r9b, sil\n\tjl loop1\n\tmovzx eax, r13w\nepilog:\n\tpop r14\n\tpop r13\n\tpop r12\n\tret\n```\n\n# Notes about assembly\n\n- This code is written Intel syntax using the `.intel_syntax noprefix` directive.\n- This code uses `#` for comments because GAS (GNU Assembler) uses this syntax and Leetcode uses GCC for compiling.\n- The reason I return from the "function" by jumping to the `.epilog` is that it\'s easier to remove everything after the `.epilog` to obtain the C code with inline assembly. I am aware that in assembly I can have multiple ret instructions in my "function", but in inline assembly I need to replace those `ret` instructions with a jump to the end of the inline assembly block.\n\n# Comparison with C\n\nThis my solution using C and the assembly code generated by `gcc -S`. I removed unnecesary lines with the following shell command. I also generated the code with optimization level `-O0`, `-O1`, `-O2`, `-Os`, `-Oz` for comparison.\n\n```bash\n$ gcc -O2 -fno-asynchronous-unwind-tables -masm=intel -S code.c -o- | \n sed \'/^\\(.LCOLD\\|.LHOT\\|\\s*\\(\\.file\\|\\.type\\|\\.text\\|\\.p2align\\|\\.size\\|\\.ident\\|\\.section\\)\\)/d\'\n```\n\nNote that the C code may use `restrict`s, `const`s and type castings in order to get optimized code. Moreover, after the generated assembly code, I have also included my solution using assembly without comments for comparison.\n\n```C [-C code]\nint numberOfPairs(const int* restrict nums1, const char nums1Size, const int* restrict nums2, const char nums2Size, const char k) {\n\tunsigned short res = 0;\n\tfor (char i = 0; i < nums1Size; i++) {\n\t\tchar x = nums1[i];\n\t\tfor (char j = 0; j < nums2Size; j++) {\n\t\t\tif (x % (((char) nums2[j]) * k) == 0) {\n\t\t\t\tres++;\n\t\t\t}\n\t\t}\n\t}\n\treturn res;\n}\n```\n```assembly [-gcc -O0]\n\t.intel_syntax noprefix\n\t.globl\tnumberOfPairs\nnumberOfPairs:\n\tpush\trbp\n\tmov\trbp, rsp\n\tmov\tQWORD PTR -24[rbp], rdi\n\tmov\tQWORD PTR -40[rbp], rdx\n\tmov\teax, ecx\n\tmov\tecx, r8d\n\tmov\tedx, esi\n\tmov\tBYTE PTR -28[rbp], dl\n\tmov\tBYTE PTR -32[rbp], al\n\tmov\teax, ecx\n\tmov\tBYTE PTR -44[rbp], al\n\tmov\tWORD PTR -2[rbp], 0\n\tmov\tBYTE PTR -5[rbp], 0\n\tjmp\t.L2\n.L6:\n\tmovsx\trax, BYTE PTR -5[rbp]\n\tlea\trdx, 0[0+rax*4]\n\tmov\trax, QWORD PTR -24[rbp]\n\tadd\trax, rdx\n\tmov\teax, DWORD PTR [rax]\n\tmov\tBYTE PTR -3[rbp], al\n\tmov\tBYTE PTR -4[rbp], 0\n\tjmp\t.L3\n.L5:\n\tmovsx\teax, BYTE PTR -3[rbp]\n\tmovsx\trdx, BYTE PTR -4[rbp]\n\tlea\trcx, 0[0+rdx*4]\n\tmov\trdx, QWORD PTR -40[rbp]\n\tadd\trdx, rcx\n\tmov\tedx, DWORD PTR [rdx]\n\tmovsx\tecx, dl\n\tmovsx\tedx, BYTE PTR -44[rbp]\n\tmov\tedi, edx\n\timul\tedi, ecx\n\tcdq\n\tidiv\tedi\n\tmov\tecx, edx\n\tmov\teax, ecx\n\ttest\teax, eax\n\tjne\t.L4\n\tmovzx\teax, WORD PTR -2[rbp]\n\tadd\teax, 1\n\tmov\tWORD PTR -2[rbp], ax\n.L4:\n\tmovzx\teax, BYTE PTR -4[rbp]\n\tadd\teax, 1\n\tmov\tBYTE PTR -4[rbp], al\n.L3:\n\tmovzx\teax, BYTE PTR -4[rbp]\n\tcmp\tal, BYTE PTR -32[rbp]\n\tjl\t.L5\n\tmovzx\teax, BYTE PTR -5[rbp]\n\tadd\teax, 1\n\tmov\tBYTE PTR -5[rbp], al\n.L2:\n\tmovzx\teax, BYTE PTR -5[rbp]\n\tcmp\tal, BYTE PTR -28[rbp]\n\tjl\t.L6\n\tmovzx\teax, WORD PTR -2[rbp]\n\tpop\trbp\n\tret\n```\n```assembly [-gcc -O1]\n\t.intel_syntax noprefix\n\t.globl\tnumberOfPairs\nnumberOfPairs:\n\ttest\tsil, sil\n\tjle\t.L7\n\tpush\tr12\n\tpush\trbp\n\tpush\trbx\n\tmov\trbx, rdx\n\tmov\tebp, ecx\n\tmov\tr11, rdi\n\tmovsx\trsi, sil\n\tlea\tr12, [rdi+rsi*4]\n\tmovsx\trcx, cl\n\tlea\tr10, [rdx+rcx*4]\n\tmov\tr9d, 0\n\tmovsx\tr8d, r8b\n.L6:\n\tmovzx\tedi, BYTE PTR [r11]\n\ttest\tbpl, bpl\n\tjle\t.L3\n\tmov\trsi, rbx\n\tmovsx\tedi, dil\n.L5:\n\tmovsx\tecx, BYTE PTR [rsi]\n\timul\tecx, r8d\n\tmov\teax, edi\n\tcdq\n\tidiv\tecx\n\tcmp\tedx, 1\n\tadc\tr9w, 0\n\tadd\trsi, 4\n\tcmp\trsi, r10\n\tjne\t.L5\n.L3:\n\tadd\tr11, 4\n\tcmp\tr11, r12\n\tjne\t.L6\n\tmovzx\teax, r9w\n\tpop\trbx\n\tpop\trbp\n\tpop\tr12\n\tret\n.L7:\n\tmov\tr9d, 0\n\tmovzx\teax, r9w\n\tret\n```\n```assembly [-gcc -O2]\n\t.intel_syntax noprefix\n\t.globl\tnumberOfPairs\nnumberOfPairs:\n\ttest\tsil, sil\n\tjle\t.L9\n\tmov\teax, ecx\n\tmovsx\trsi, sil\n\tmovsx\trcx, cl\n\tpush\trbp\n\tmov\tr10, rdx\n\tpush\trbx\n\tlea\trbp, [rdi+rsi*4]\n\tmov\trbx, rdi\n\tlea\tr11, [rdx+rcx*4]\n.L8:\n\ttest\tal, al\n\tjg\t.L17\n\tadd\trbx, 4\n\tcmp\trbx, rbp\n\tjne\t.L8\n\txor\tr9d, r9d\n.L6:\n\tmovzx\teax, r9w\n\tpop\trbx\n\tpop\trbp\n\tret\n.L17:\n\tmovsx\tedi, BYTE PTR [rbx]\n\txor\tr9d, r9d\n\tmovsx\tr8d, r8b\n.L7:\n\tmov\trsi, r10\n.L5:\n\tmovsx\tecx, BYTE PTR [rsi]\n\tmov\teax, edi\n\tcdq\n\timul\tecx, r8d\n\tidiv\tecx\n\tcmp\tedx, 1\n\tadc\tr9w, 0\n\tadd\trsi, 4\n\tcmp\tr11, rsi\n\tjne\t.L5\n\tadd\trbx, 4\n\tcmp\trbp, rbx\n\tje\t.L6\n\tmovsx\tedi, BYTE PTR [rbx]\n\tjmp\t.L7\n.L9:\n\txor\teax, eax\n\tret\n```\n```assembly [-gcc -Os]\n\t.intel_syntax noprefix\n\t.globl\tnumberOfPairs\nnumberOfPairs:\n\tpush\tr12\n\tmov\tr10d, esi\n\tmov\tr11, rdi\n\txor\tesi, esi\n\tpush\trbp\n\tmovsx\tr8d, r8b\n\tmov\tebp, ecx\n\txor\tecx, ecx\n\tpush\trbx\n\tmov\trbx, rdx\n.L2:\n\tcmp\tr10b, sil\n\tjle\t.L9\n\tmovsx\tr12d, BYTE PTR [r11+rsi*4]\n\txor\tedi, edi\n.L3:\n\tcmp\tbpl, dil\n\tjle\t.L10\n\tmovsx\tr9d, BYTE PTR [rbx+rdi*4]\n\tmov\teax, r12d\n\tcdq\n\timul\tr9d, r8d\n\tidiv\tr9d\n\tcmp\tedx, 1\n\tadc\tcx, 0\n\tinc\trdi\n\tjmp\t.L3\n.L10:\n\tinc\trsi\n\tjmp\t.L2\n.L9:\n\tpop\trbx\n\tmovzx\teax, cx\n\tpop\trbp\n\tpop\tr12\n\tret\n```\n```assembly [-gcc -Oz]\n\t.intel_syntax noprefix\n\t.globl\tnumberOfPairs\nnumberOfPairs:\n\tpush\tr12\n\tmov\tr10d, esi\n\tmov\tr11, rdi\n\txor\tesi, esi\n\tpush\trbp\n\tmovsx\tr8d, r8b\n\tmov\tebp, ecx\n\txor\tecx, ecx\n\tpush\trbx\n\tmov\trbx, rdx\n.L2:\n\tcmp\tr10b, sil\n\tjle\t.L9\n\tmovsx\tr12d, BYTE PTR [r11+rsi*4]\n\txor\tedi, edi\n.L3:\n\tcmp\tbpl, dil\n\tjle\t.L10\n\tmovsx\tr9d, BYTE PTR [rbx+rdi*4]\n\tmov\teax, r12d\n\tcdq\n\timul\tr9d, r8d\n\tidiv\tr9d\n\tcmp\tedx, 1\n\tadc\tcx, 0\n\tinc\trdi\n\tjmp\t.L3\n.L10:\n\tinc\trsi\n\tjmp\t.L2\n.L9:\n\tpop\trbx\n\tmovzx\teax, cx\n\tpop\trbp\n\tpop\tr12\n\tret\n```\n```assembly [-my ASM]\n\t.intel_syntax noprefix\n\t.globl numberOfPairs\nnumberOfPairs:\n\txor r9, r9\n\txor r10, r10\n\tpush r12\n\tpush r13\n\txor r13, r13\n\tpush r14\n\tmov r14, rdx\nloop1:\n\tmov r11b, BYTE PTR[rdi+r9*4]\n\txor r10b, r10b\nloop2:\n\tmov al, BYTE PTR[r14+r10*4]\n\tmul r8b\n\tmov r12w, ax\n\tmovzx ax, r11b\n\txor dx, dx\n\tdiv r12w\n\ttest dx, dx\n\tjnz not_divisor\n\tinc r13w\nnot_divisor:\n\tinc r10b\n\tcmp r10b, cl\n\tjl loop2\n\tinc r9b\n\tcmp r9b, sil\n\tjl loop1\n\tmovzx eax, r13w\nepilog:\n\tpop r14\n\tpop r13\n\tpop r12\n\tret\n```\n\n\n# Run locally\n\nCompile locally the assembly code with GAS or the inline assembly with GCC.\n\n```bash\n$ as -o code.o assembly_code.s\n$ # or\n$ gcc -c -o code.o c_with_inline_assembly_code.c \n```\n\nThen, create a `main.c` file with the function prototype. Here you can include your tests.\n\n```c\n/* main.c */\n\nint numberOfPairs(int* nums1, int nums1Size, int* nums2, int nums2Size, int k);\n\nint main(int argc, const char *argv[]) {\n // Use numberOfPairs function\n return 0;\n}\n```\n\nThen, compile `main.c` and link to create the executable\n\n```bash\n$ gcc main.c code.o -o main\n```\n\nFinally, execute\n\n```bash\n$ ./main\n```\n\n | 6 | 0 | ['C'] | 2 |
find-the-number-of-good-pairs-i | Beats 100.00% of users with Python3 | beats-10000-of-users-with-python3-by-kaw-7l8d | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | KawinP | NORMAL | 2024-05-27T17:22:29.709857+00:00 | 2024-05-27T17:22:29.709880+00:00 | 328 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n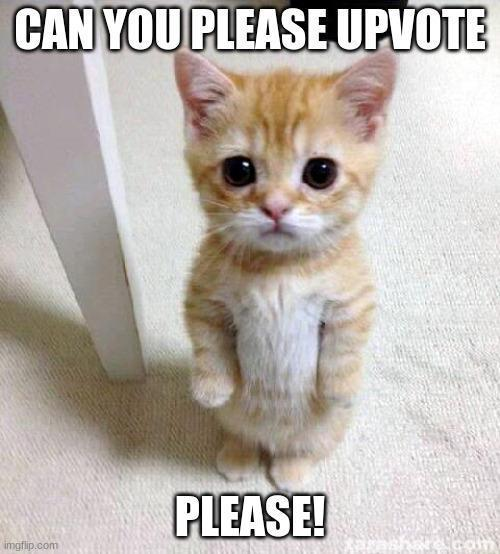\n\n\n# Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n c=0\n n1=len(nums1)\n n2=len(nums2)\n for i in range(n1):\n for j in range(n2):\n if nums1[i] % ( nums2[j] *k ) ==0:\n c = c+1\n return c \n\n``` | 6 | 0 | ['Python3'] | 1 |
find-the-number-of-good-pairs-i | Simple Brute-force & optimal solution | simple-brute-force-optimal-solution-by-k-rked | Here is the better solution then bruteforce approach : https://leetcode.com/problems/find-the-number-of-good-pairs-ii/solutions/5208919/check-all-factors-of-num | kreakEmp | NORMAL | 2024-05-26T04:03:15.533972+00:00 | 2024-05-26T04:17:12.070614+00:00 | 1,344 | false | Here is the better solution then bruteforce approach : https://leetcode.com/problems/find-the-number-of-good-pairs-ii/solutions/5208919/check-all-factors-of-nums1/\n\n# Code\n```\nint numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int ans = 0;\n for(int i = 0; i < nums1.size(); ++i){\n for(int j = 0; j < nums2.size(); ++j){\n int div = nums2[j]*k;\n if(nums1[i]%div == 0) ans++;\n }\n }\n return ans;\n}\n```\n\n\n\n---\n\n<b>Here is an article of my last interview experience - A Journey to FAANG Company, I recomand you to go through this to know which all resources I have used & how I cracked interview at Amazon:\nhttps://leetcode.com/discuss/interview-experience/3171859/Journey-to-a-FAANG-Company-Amazon-or-SDE2-(L5)-or-Bangalore-or-Oct-2022-Accepted\n\n--- | 6 | 1 | ['C++'] | 1 |
find-the-number-of-good-pairs-i | Python Elegant & Short | 1-Line | Pairwise | python-elegant-short-1-line-pairwise-by-apcsb | Complexity\n- Time complexity: O(n * m)\n- Space complexity: O(1)\n\n# Code\npython []\nclass Solution:\n def numberOfPairs(self, first: list[int], second: l | Kyrylo-Ktl | NORMAL | 2024-05-27T12:51:58.256153+00:00 | 2024-05-27T12:52:16.080487+00:00 | 347 | false | # Complexity\n- Time complexity: $$O(n * m)$$\n- Space complexity: $$O(1)$$\n\n# Code\n```python []\nclass Solution:\n def numberOfPairs(self, first: list[int], second: list[int], k: int) -> int:\n return sum(a % (b * k) == 0 for a, b in product(first, second))\n``` | 4 | 0 | ['Python3'] | 1 |
find-the-number-of-good-pairs-i | Beats 100% users... Dominate others with this code.. | beats-100-users-dominate-others-with-thi-cy66 | \n# Code\n\n\'\'\'class Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n\'\'\' \nclass Solution:\n def numberOfP | Aim_High_212 | NORMAL | 2024-05-27T02:08:08.077646+00:00 | 2024-05-27T02:08:08.077669+00:00 | 596 | false | \n# Code\n```\n\'\'\'class Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n\'\'\' \nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n c = 0\n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if nums1[i] % (nums2[j] * k) == 0:\n c += 1\n return c\n``` | 3 | 0 | ['C', 'Python', 'Java', 'Python3'] | 0 |
find-the-number-of-good-pairs-i | simple and easy C++ solution 😍❤️🔥 | simple-and-easy-c-solution-by-shishirrsi-en6e | if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n\n# Code\n\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k | shishirRsiam | NORMAL | 2024-05-26T05:56:05.647644+00:00 | 2024-05-26T05:56:05.647660+00:00 | 1,020 | false | # if it\'s help, please up \u2B06 vote! \u2764\uFE0F\n\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) \n {\n int ans = 0, div;\n for(auto val:nums1)\n {\n for(auto v:nums2)\n {\n div = v * k;\n if(val % div == 0) ans++;\n }\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['Array', 'Math', 'C++'] | 4 |
find-the-number-of-good-pairs-i | C++ || Easy || 5 Lines | c-easy-5-lines-by-dibendu07-gbha | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | dibendu07 | NORMAL | 2024-05-26T04:03:56.350063+00:00 | 2024-05-26T04:03:56.350088+00:00 | 528 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(N*M)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int n = nums1.size(),m = nums2.size(), cnt=0;\n for (int i=0; i<n; ++i) {\n for (int j=0; j<m; ++j) {\n int x=nums2[j]*k;\n if(nums1[i]%x == 0) cnt++;\n }\n }\n return cnt;\n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | MOST EASIEST PYTHON SOLUTION🔥🔥🔥 || BEGINNER FRIENDLY APPROACH | most-easiest-python-solution-beginner-fr-nmgq | Intuition\nThe problem likely involves finding pairs from two arrays nums1 and nums2 such that the elements in the pair satisfy a given condition. Specifically, | FAHAD_26 | NORMAL | 2024-09-24T19:18:52.351070+00:00 | 2024-09-24T19:18:52.351109+00:00 | 48 | false | # Intuition\nThe problem likely involves finding pairs from two arrays nums1 and nums2 such that the elements in the pair satisfy a given condition. Specifically, for each pair (nums1[i], nums2[j]), you\'re tasked with determining whether nums1[i] is divisible by nums2[j] * k (where k is an integer parameter provided). If the condition holds, the pair is considered valid, and you want to count how many such valid pairs exist.\n\n# Approach\nIterate through each element in nums1: For each element nums1[i], check its divisibility against all elements nums2[j] multiplied by k.\n\nNested loop structure: The outer loop will iterate over each element i in nums1.\nThe inner loop will iterate over each element j in nums2.\n\nCondition check: For every pair (i, j), check if nums1[i] % (nums2[j] * k) == 0.\nIf the condition is met, increase the count of valid pairs.\n\nReturn the count: After checking all possible pairs, return the total count of valid pairs.\n\n# Complexity\n- Time complexity:\n56 MS\n\n- Space complexity:\n11.67 MB\n\n# Code\n```python []\n# class Solution(object):\n\n# def __init__(self):\n# self.map = {}\n# self.c = 0\n\n# def checkcondition(nums1, nums2, i, j, k):\n# if( nums2[j] != 0 and nums1[i] % (nums2[j] * k) == 0):\n# self.map[i] = j\n# return True\n# return False\n \n# def numberOfPairs(self, nums1, nums2, k):\n# for i in range(len(nums1)):\n# for j in range(len(nums2)):\n# if (self.checkcondition(self, nums1, nums2, i, j, k)):\n# self.c = self.c + 1\n# return self.c\n\n# """\n# :type nums1: List[int]\n# :type nums2: List[int]\n# :type k: int\n# :rtype: int\n# """\nclass Solution(object):\n\n def __init__(self):\n self.map = {}\n self.c = 0\n\n def checkcondition(self, nums1, nums2, i, j, k): \n if nums2[j] != 0 and nums1[i] % (nums2[j] * k) == 0:\n self.map[i] = j \n return True\n return False\n \n def numberOfPairs(self, nums1, nums2, k):\n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if self.checkcondition(nums1, nums2, i, j, k): \n self.c += 1\n return self.c \n\n """\n :type nums1: List[int]\n :type nums2: List[int]\n :type k: int\n :rtype: int\n """\n\n``` | 2 | 0 | ['Python'] | 0 |
find-the-number-of-good-pairs-i | :D | d-by-yesyesem-xvu9 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | yesyesem | NORMAL | 2024-08-24T19:10:20.334856+00:00 | 2024-08-24T19:10:20.334880+00:00 | 169 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count=0;\n for(int i=0;i<nums1.size();i++)\n {\n for(int j=0;j<nums2.size();j++)\n {\n \n {\n if((nums1[i]%(nums2[j] * k ))==0)\n count++;\n }\n }\n }\n return count;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | 99.94% beats solution in java | 9994-beats-solution-in-java-by-mantosh_k-yjxe | \n\n# Code\n\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n \n int no_of_good_pair=0;\n for( int i=0; | mantosh_kumar04 | NORMAL | 2024-06-14T16:30:50.890593+00:00 | 2024-06-14T16:30:50.890632+00:00 | 296 | false | \n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n \n int no_of_good_pair=0;\n for( int i=0; i<nums1.length; i++)\n {\n for( int j=0; j<nums2.length; j++)\n {\n if(nums1[i]%(nums2[j]*k)==0){\n no_of_good_pair++;\n }\n }\n }\n return no_of_good_pair;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | Easiest Solution! | easiest-solution-by-saara208-txwy | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | saara208 | NORMAL | 2024-06-13T16:17:51.193851+00:00 | 2024-06-13T16:17:51.193881+00:00 | 119 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int c=0;\n for(int i=0;i<=nums1.length-1;i++){\n for(int j=0;j<=nums2.length-1;j++){\n if(nums1[i]%(nums2[j]*k)==0){\n c++;\n }\n }\n }\n return c;\n }\n}\n \n``` | 2 | 0 | ['Java'] | 1 |
find-the-number-of-good-pairs-i | Solution by Dare2Solve | Detail Explanation | O(n * sqrt(max(x))) | solution-by-dare2solve-detail-explanatio-lgwz | Explanation []\nauthorslog.vercel.app/blog/H8FBC3F9WU\n\n\n# Code\n\nC++ []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& n | Dare2Solve | NORMAL | 2024-06-08T11:49:16.699815+00:00 | 2024-06-08T11:56:35.411923+00:00 | 707 | false | ```Explanation []\nauthorslog.vercel.app/blog/H8FBC3F9WU\n```\n\n# Code\n\n```C++ []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n unordered_map<int, int> map1;\n unordered_map<int, int> map2;\n\n for (int x : nums1) {\n if (x % k == 0) {\n int f = x / k;\n map1[f]++;\n }\n }\n\n for (int x : nums2) {\n map2[x]++;\n }\n\n int res = 0;\n for (const auto& [x, y] : map1) {\n for (int i = 1; i <= sqrt(x); ++i) {\n if (x % i == 0) {\n int cur = x / i;\n if (map2.find(i) != map2.end()) {\n res += map2[i] * y;\n }\n if (cur != i && map2.find(cur) != map2.end()) {\n res += map2[cur] * y;\n }\n }\n }\n }\n\n return res;\n }\n};\n```\n\n```Python []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n map1 = defaultdict(int)\n map2 = defaultdict(int)\n\n for x in nums1:\n if x % k == 0:\n f = x // k\n map1[f] += 1\n\n for x in nums2:\n map2[x] += 1\n\n res = 0\n for x, y in map1.items():\n for i in range(1, int(math.sqrt(x)) + 1):\n if x % i == 0:\n cur = x // i\n if i in map2:\n res += map2[i] * y\n if cur != i and cur in map2:\n res += map2[cur] * y\n\n return res\n```\n\n```Java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n Map<Integer, Integer> map1 = new HashMap<>();\n Map<Integer, Integer> map2 = new HashMap<>();\n\n for (int x : nums1) {\n if (x % k == 0) {\n int f = x / k;\n map1.put(f, map1.getOrDefault(f, 0) + 1);\n }\n }\n\n for (int x : nums2) {\n map2.put(x, map2.getOrDefault(x, 0) + 1);\n }\n\n int res = 0;\n for (Map.Entry<Integer, Integer> entry : map1.entrySet()) {\n int x = entry.getKey();\n int y = entry.getValue();\n for (int i = 1; i <= Math.sqrt(x); ++i) {\n if (x % i == 0) {\n int cur = x / i;\n if (map2.containsKey(i)) {\n res += map2.get(i) * y;\n }\n if (cur != i && map2.containsKey(cur)) {\n res += map2.get(cur) * y;\n }\n }\n }\n }\n\n return res;\n }\n}\n```\n\n```JavaScript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @param {number} k\n * @return {number}\n */\nvar numberOfPairs = function (nums1, nums2, k) {\n var map1 = new Map();\n var map2 = new Map();\n\n nums1.forEach((x) => {\n if (x % k === 0) {\n var f = x / k;\n map1.set(f, (map1.get(f) || 0) + 1);\n }\n });\n\n nums2.forEach((x) => {\n map2.set(x, (map2.get(x) || 0) + 1);\n });\n\n var res = 0;\n for (var [x, y] of map1) {\n for (var i = 1; i <= Math.sqrt(x); ++i) {\n if (x % i === 0) {\n var cur = x / i;\n if (map2.has(i)) res += map2.get(i) * y;\n if (cur !== i && map2.has(cur)) res += map2.get(cur) * y;\n }\n }\n }\n return res;\n};\n```\n\n```Go []\nfunc numberOfPairs(nums1 []int, nums2 []int, k int) int {\n map1 := make(map[int]int)\n map2 := make(map[int]int)\n\n for _, x := range nums1 {\n if x % k == 0 {\n f := x / k\n map1[f] = map1[f] + 1\n }\n }\n\n for _, x := range nums2 {\n map2[x] = map2[x] + 1\n }\n\n var res int = 0\n for x, y := range map1 {\n for i := 1; i <= int(math.Sqrt(float64(x))); i++ {\n if x % i == 0 {\n cur := x / i\n if count, ok := map2[i]; ok {\n res += count * y\n }\n if cur != i {\n if count, ok := map2[cur]; ok {\n res += count * y\n }\n }\n }\n }\n }\n\n return res\n}\n```\n\n```C# []\npublic class Solution {\n public int NumberOfPairs(int[] nums1, int[] nums2, int k) {\n Dictionary<int, int> map1 = new Dictionary<int, int>();\n Dictionary<int, int> map2 = new Dictionary<int, int>();\n\n foreach (int x in nums1) {\n if (x % k == 0) {\n int f = x / k;\n if (map1.ContainsKey(f))\n map1[f]++;\n else\n map1[f] = 1;\n }\n }\n\n foreach (int x in nums2) {\n if (map2.ContainsKey(x))\n map2[x]++;\n else\n map2[x] = 1;\n }\n\n int res = 0;\n foreach (var entry in map1) {\n int x = entry.Key;\n int y = entry.Value;\n for (int i = 1; i <= Math.Sqrt(x); ++i) {\n if (x % i == 0) {\n int cur = x / i;\n if (map2.ContainsKey(i))\n res += map2[i] * y;\n if (cur != i && map2.ContainsKey(cur))\n res += map2[cur] * y;\n }\n }\n }\n\n return res;\n }\n}\n``` | 2 | 0 | ['Hash Table', 'Python', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'C#'] | 1 |
find-the-number-of-good-pairs-i | easy solution in all languages with their runtime (ms) - guess which is the fastest(this solution) | easy-solution-in-all-languages-with-thei-9mh1 | go - 0ms\n- java - 1ms\n- c++ - 6ms\n- python - 24ms\n- python3 - 53ms\n- javasript - 74ms\n- dart - 413ms\n\n\npython3 []\nclass Solution:\n def numberOfPai | Sajal_ | NORMAL | 2024-05-30T04:46:21.901080+00:00 | 2024-05-30T04:46:21.901100+00:00 | 691 | false | - go - 0ms\n- java - 1ms\n- c++ - 6ms\n- python - 24ms\n- python3 - 53ms\n- javasript - 74ms\n- dart - 413ms\n\n\n```python3 []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n for i in nums1:\n for j in nums2:\n if i % (j * k) == 0:\n count += 1\n return count\n```\n\n```javascript []\nvar numberOfPairs = function(nums1, nums2, k) {\n let count = 0\n for(let i = 0;i< nums1.length;i++){\n for(let j =0;j<nums2.length;j++){\n if (nums1[i] % (nums2[j]*k)==0){\n count ++\n }\n }\n }\n return count\n};\n```\n```c++ []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count = 0;\n for (int i = 0; i<nums1.size();i++){\n for (int j = 0;j<nums2.size();j++){\n if (nums1[i] % (nums2[j]*k) ==0){\n count ++ ;\n }\n }\n }\n return count;\n }\n};\n```\n```go []\nfunc numberOfPairs(nums1 []int, nums2 []int, k int) int {\n count := 0;\n for i := 0 ; i<len(nums1) ;i++{\n for j := 0 ;j<len(nums2);j++{\n if nums1[i] % (nums2[j]*k)==0{\n count ++;\n }\n }\n }\n return count;\n}\n```\n```java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count = 0;\n for (int i = 0; i<nums1.length;i++){\n if(nums1[i]%k!=0) continue;\n for (int j = 0;j<nums2.length;j++){\n if (nums1[i] % (nums2[j]*k) ==0){\n count ++ ;\n }\n }\n }\n return count;\n }\n}\n```\n```python []\nclass Solution(object):\n def numberOfPairs(self, nums1, nums2, k):\n """\n :type nums1: List[int]\n :type nums2: List[int]\n :type k: int\n :rtype: int\n """\n count = 0\n for i in nums1:\n for j in nums2:\n if i % (j * k) == 0:\n count += 1\n return count\n```\n```dart []\nclass Solution {\n int numberOfPairs(List<int> nums1, List<int> nums2, int k) {\n int count = 0;\n for (int i = 0; i<nums1.length;i++){\n if(nums1[i]%k!=0) continue;\n for (int j = 0;j<nums2.length;j++){\n if (nums1[i] % (nums2[j]*k) ==0){\n count ++ ;\n }\n }\n }\n return count;\n }\n}\n\n```\n\n | 2 | 0 | ['Array', 'C', 'Python', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'C#', 'Dart'] | 0 |
find-the-number-of-good-pairs-i | different language solutions | different-language-solutions-by-sayedadi-vicz | Intuition\njavascript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @param {number} k\n * @return {number}\n */\nvar numberOfPairs = funct | sayedadinan | NORMAL | 2024-05-30T04:39:06.847942+00:00 | 2024-05-30T04:40:33.614808+00:00 | 352 | false | # Intuition\n```javascript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @param {number} k\n * @return {number}\n */\nvar numberOfPairs = function(nums1, nums2, k) {\n let count=0;\n for(let i=0;i<nums1.length;i++){\n for(let j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0){\n count++;\n }\n }\n }\n return count;\n};\n```\n```python []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n result=0 \n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if nums1[i]%(nums2[j]*k)==0:\n result+=1\n return result \n\n```\n```java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int result=0;\n for(int i=0;i<nums1.length;i++){\n for(int j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0){\n result++;\n }\n }\n }\n return result;\n }\n}\n```\n```Go []\nfunc numberOfPairs(nums1 []int, nums2 []int, k int) int {\n count :=0\n for i := 0; i < len(nums1); i++{\n for j := 0; j < len(nums2); j++{\n if nums1[i] % (nums2[j] * k) == 0{\n count++;\n }\n } \n }\n return count;\n}\n```\n``` C []\n\nint numberOfPairs(int* nums1, int nums1Size, int* nums2, int nums2Size, int k) {\n int count=0;\n for(int i=0;i<nums1.Size;i++){\n for(int j=0;j<nums2.Size;j++){\n if(nums)\n }\n }\n}\n```\n``` Dart []\nclass Solution {\n int numberOfPairs(List<int> nums1, List<int> nums2, int k) {\n int result=0;\n for(int i=0;i<nums1.length;i++){\n for(int j=0;j<nums2.length;j++){\n if(nums1[i] % (nums2[j]*k)==0){\n result++;\n }\n }\n }\n return result;\n }\n} | 2 | 0 | ['Java', 'Go', 'Python3', 'JavaScript', 'C#', 'Dart'] | 0 |
find-the-number-of-good-pairs-i | Simple java solution | simple-java-solution-by-ankitbi1713-duil | \n# Code\n\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int goodpair=0;\n for(int i=0;i<nums1.length;i++){ | ANKITBI1713 | NORMAL | 2024-05-29T01:26:36.762464+00:00 | 2024-05-29T01:26:36.762483+00:00 | 249 | false | \n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int goodpair=0;\n for(int i=0;i<nums1.length;i++){\n for(int j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0){\n goodpair++;\n }\n }\n }\n return goodpair;\n }\n}\n``` | 2 | 0 | ['Array', 'Hash Table', 'C', 'C++', 'Java'] | 2 |
find-the-number-of-good-pairs-i | Easy Solution in C++ || Optimal Solution | easy-solution-in-c-optimal-solution-by-k-e1i2 | \n Describe your first thoughts on how to solve this problem. \n\n\n\n# Code\n\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int> | Kalyan1900 | NORMAL | 2024-05-26T08:46:15.938149+00:00 | 2024-05-26T08:46:15.938176+00:00 | 147 | false | \n<!-- Describe your first thoughts on how to solve this problem. -->\n\n\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count = 0;\n int i = 0, j = 0;\n while(i<nums1.size() && j<nums2.size()){\n if(nums1[i] % (nums2[j++]*k) == 0){\n count++;\n }\n if(j==nums2.size()){\n j = 0;\n i++;\n }\n }\n return count;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | C++ Multiple approaches | c-multiple-approaches-by-offline-keshav-rbza | To address the problem of counting pairs of integers (nums1[i], nums2[j]) where nums1[i] is divisible by nums2[j]*k, we explore two distinct approaches: a brute | offline-keshav | NORMAL | 2024-05-26T04:09:36.889874+00:00 | 2024-05-26T04:09:36.889897+00:00 | 99 | false | To address the problem of counting pairs of integers `(nums1[i], nums2[j])` where `nums1[i]` is divisible by `nums2[j]*k`, we explore two distinct approaches: a brute force method and an optimized strategy employing sorting and two pointers.\n\n# Brute Force Approach\n\n## Algorithm:\n1. **Initialize Counter**: Set `count` to 0.\n2. **Nested Loops**: Iterate through each element of `nums1` and `nums2` using nested loops.\n3. **Pair Validation**: For each pair `(nums1[i], nums2[j])`, increment `count` if `nums1[i]` is divisible by `nums2[j]*k`.\n4. **Return Count**: After all iterations, return `count`.\n\n## Complexity Analysis:\n- **Time Complexity**: O(n * m), where n and m are the sizes of `nums1` and `nums2` respectively, due to nested loops.\n- **Space Complexity**: O(1), minimal extra space apart from a few variables.\n\n## Code Implementation:\n```cpp\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count = 0;\n for (int i = 0; i < nums1.size(); ++i)\n for (int j = 0; j < nums2.size(); ++j)\n if (nums1[i] % (nums2[j] * k) == 0) ++count;\n return count;\n }\n};\n```\n\n# Optimized Approach\n\n## Algorithm:\n1. **Sort Arrays**: Sort `nums1` and `nums2`.\n2. **Two Pointers**: Initialize pointers `i` and `j` to iterate over `nums1` and `nums2`.\n3. **Pair Validation**: While `i` and `j` are within bounds, compare `nums1[i]` with `nums2[j]*k`:\n - If equal, increment `count` and move both pointers forward.\n - If `nums1[i]` is less than `nums2[j]*k`, increment `i`.\n - If greater, increment `j`.\n4. **Return Count**: Return `count`.\n\n## Complexity Analysis:\n- **Time Complexity**: O(n*log(n) + m*log(m) + n + m) due to sorting and traversal with two pointers.\n- **Space Complexity**: O(1), as sorting is in-place and minimal extra space is used.\n\n## Code Implementation:\n```cpp\n#include <vector>\n#include <algorithm>\n\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n sort(nums1.begin(), nums1.end());\n sort(nums2.begin(), nums2.end());\n \n int count = 0, i = 0, j = 0;\n while (i < nums1.size() && j < nums2.size()) {\n long long product = (long long)nums2[j] * k;\n if (nums1[i] == product) {\n ++count; ++i; ++j;\n } else if (nums1[i] < product) ++i;\n else ++j;\n }\n return count;\n }\n};\n```\n\n# Conclusion\n\nWhile the brute force approach offers a direct solution, its quadratic time complexity makes it less efficient for larger inputs. Conversely, the optimized method with sorting and two pointers provides a more efficient solution, significantly reducing time complexity. By understanding problem constraints and employing appropriate algorithms and data structures, we can effectively solve such counting problems.\n | 2 | 1 | ['Divide and Conquer', 'Ordered Map', 'C++'] | 2 |
find-the-number-of-good-pairs-i | ✅ Java Solution | java-solution-by-harsh__005-45s5 | CODE\nJava []\npublic int numberOfPairs(int[] nums1, int[] nums2, int k) {\n\tint n = nums1.length, m = nums2.length;\n\tint ct = 0;\n\tfor(int i=0; i<n; i++){\ | Harsh__005 | NORMAL | 2024-05-26T04:02:52.706905+00:00 | 2024-05-26T04:02:52.706933+00:00 | 555 | false | ## **CODE**\n```Java []\npublic int numberOfPairs(int[] nums1, int[] nums2, int k) {\n\tint n = nums1.length, m = nums2.length;\n\tint ct = 0;\n\tfor(int i=0; i<n; i++){\n\t\tfor(int j=0; j<m; j++) {\n\t\t\tif((nums1[i] % (nums2[j]*k)) == 0) ct++;\n\t\t}\n\t}\n\treturn ct;\n}\n``` | 2 | 0 | ['Java'] | 2 |
find-the-number-of-good-pairs-i | Hash Map || JavaScript || O(n*m) | hash-map-javascript-onm-by-poetryofcode-wrzp | Code | PoetryOfCode | NORMAL | 2025-03-31T00:17:31.663405+00:00 | 2025-03-31T00:17:31.663405+00:00 | 34 | false |
# Code
```javascript []
/**
* @param {number[]} nums1
* @param {number[]} nums2
* @param {number} k
* @return {number}
*/
var numberOfPairs = function(nums1, nums2, k) {
const freq = new Map();
for (const num of nums2) {
const key = num * k;
freq.set(key, (freq.get(key) || 0) + 1);
}
let count = 0;
for (const num of nums1) {
for (const [key, cnt] of freq) {
if (num % key === 0) {
count += cnt;
}
}
}
return count;
};
``` | 1 | 0 | ['JavaScript'] | 0 |
find-the-number-of-good-pairs-i | 0ms + 100% beats C++ | 0ms-100-beats-c-by-maaz_ali27-zohk | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Maaz_Ali27 | NORMAL | 2025-03-26T11:19:24.193230+00:00 | 2025-03-26T11:19:24.193230+00:00 | 38 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {
int cnt=0;
for(int i=0; i<nums1.size(); i++){
for(int j=0; j<nums2.size(); j++){
if(nums1[i] % (nums2[j]*k) == 0){
cnt++;
}
}
}
return cnt;
}
};
``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | EASY C# SOLUTION 100% | easy-c-solution-100-by-tequilasunset69-j0ws | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | TequilaSunset69 | NORMAL | 2025-03-16T20:33:15.858163+00:00 | 2025-03-16T20:33:15.858163+00:00 | 6 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```csharp []
public class Solution {
public int NumberOfPairs(int[] nums1, int[] nums2, int k) {
int result = 0;
for (int i = 0; i < nums1.Length; i++) {
for (int j = 0; j < nums2.Length; j++) {
if (nums1[i] % (nums2[j] * k) == 0) {
result++;
}
}
}
return result;
}
}
``` | 1 | 0 | ['C#'] | 0 |
find-the-number-of-good-pairs-i | Solution for Dart | solution-for-dart-by-bazil663-q974 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Bazil663 | NORMAL | 2025-03-06T07:12:15.511716+00:00 | 2025-03-06T07:12:15.511716+00:00 | 20 | false | # Intuition
<!-- The Simple way to do this. -->
# Approach
<!-- Straight forward and simple -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```dart []
class Solution {
int numberOfPairs(List<int> nums1, List<int> nums2, int k) {
int count=0;
for(int i=0;i<nums1.length;i++){
for(int j=0;j<nums2.length;j++){
if(nums1[i] % (nums2[j]*k)==0){
count++;
}
}
}
return count;
}
}
``` | 1 | 0 | ['Dart'] | 2 |
find-the-number-of-good-pairs-i | ⚡100.00% ⚡ || ✅SUPER SIMPLE CODE 💌 || Easy to understand 🍨 || C++ | 10000-super-simple-code-easy-to-understa-8rzt | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Thakur_Avaneesh | NORMAL | 2025-03-05T17:17:35.724547+00:00 | 2025-03-05T17:17:35.724547+00:00 | 70 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {
int count = 0;
for (int j = 0; j < nums2.size(); j++)
for (int i = 0; i < nums1.size(); i++)
if ((nums1[i] % (nums2[j] * k)) == 0)
count++;
return count;
}
};
``` | 1 | 0 | ['Array', 'Counting', 'C++'] | 0 |
find-the-number-of-good-pairs-i | beats 100% 🦅 | beats-100-by-asmit_kandwal-1aq3 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Asmit_Kandwal | NORMAL | 2025-02-06T15:32:25.661060+00:00 | 2025-02-06T15:32:25.661060+00:00 | 156 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {
int count = 0;
// Iterate through each element in nums1
for(int i = 0; i < nums1.size(); i++) {
// Iterate through each element in nums2
for(int j = 0; j < nums2.size(); j++) {
// Check if nums1[i] is divisible by (nums2[j] * k)
if(nums1[i] % (nums2[j] * k) == 0) {
count++; // Increase count if condition is met
}
}
}
return count; // Return the total count of valid pairs
}
};
``` | 1 | 0 | ['C++'] | 1 |
find-the-number-of-good-pairs-i | EASY GENERAL SOLUTION | easy-general-solution-by-nidhibaratam200-d846 | Code | nidhibaratam2005 | NORMAL | 2025-02-04T06:17:01.496602+00:00 | 2025-02-04T06:17:01.496602+00:00 | 138 | false |
# Code
```java []
class Solution {
public int numberOfPairs(int[] n1, int[] n2, int k)
{
int n=n1.length;
int m=n2.length;
int count=0;
for(int i=0;i<n;i++)
{
for(int j=0;j<m;j++)
{
if(n1[i]%(n2[j]*k)==0)
count++;
}
}
return count;
}
}
``` | 1 | 0 | ['Array', 'Hash Table', 'Java'] | 0 |
find-the-number-of-good-pairs-i | 1ms 100% beat in java very easiest code 😊. | 1ms-100-beat-in-java-very-easiest-code-b-jd6e | Code | Galani_jenis | NORMAL | 2025-01-09T09:22:24.926948+00:00 | 2025-01-09T09:22:24.926948+00:00 | 114 | false | 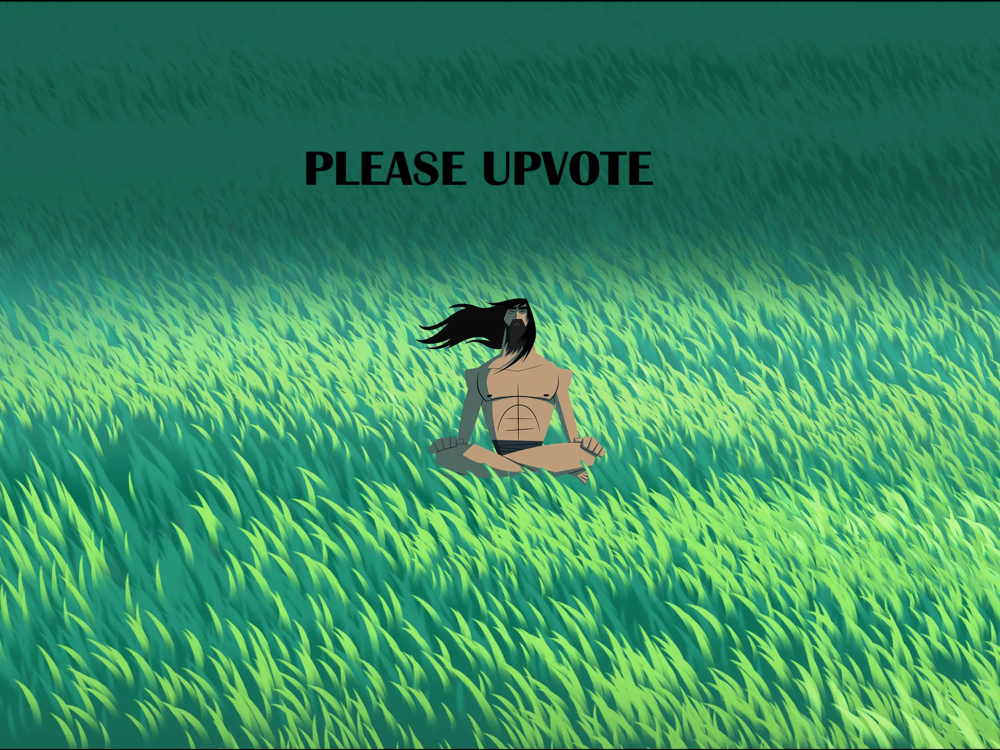
# Code
```java []
class Solution {
public int numberOfPairs(int[] nums1, int[] nums2, int k) {
int ans = 0;
for (int i = 0; i < nums1.length; i++) {
for (int j = 0; j < nums2.length; j++) {
if (nums1[i] % (nums2[j] * k) == 0) {
ans++;
}
}
}
return ans;
}
}
``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | Swift solution | swift-solution-by-shpwrckd-cj21 | Code | Shpwrckd | NORMAL | 2025-01-09T08:26:23.831005+00:00 | 2025-01-09T08:26:23.831005+00:00 | 26 | false |
# Code
```swift []
class Solution {
func numberOfPairs(_ nums1: [Int], _ nums2: [Int], _ k: Int) -> Int {
var c = 0
for i in 0..<nums1.count{
for j in 0..<nums2.count{
if nums1[i] % (nums2[j]*k) == 0{
c += 1
}
}
}
return c
}
}
``` | 1 | 0 | ['Swift'] | 0 |
find-the-number-of-good-pairs-i | Beginner Friendly | beginner-friendly-by-cs1a_2310397-91un | Easiest Approach100%Complexity
Time complexity:O(n^2)
Space complexity:O(1)
Code | cs1a_2310397 | NORMAL | 2025-01-06T09:56:19.103436+00:00 | 2025-01-06T09:56:19.103436+00:00 | 44 | false |
<!-- Describe your first thoughts on how to solve this problem. -->
# Easiest Approach
# 100%
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:O(n^2)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int numberOfPairs(int[] nums1, int[] nums2, int k) {
int count=0;
for(int i=0;i<nums1.length;i++){
for(int j=0;j<nums2.length;j++){
if(nums1[i]%(k*nums2[j])==0)
count++;
}
}
return count;
}
}
please UPVOTE
``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | Simple solution 100% beat | simple-solution-100-beat-by-cs_220164010-h7n0 | Complexity
Time complexity:O(n*n)
Space complexity:O(1)
Code | CS_2201640100153 | NORMAL | 2024-12-28T15:31:27.490677+00:00 | 2024-12-28T15:31:27.490677+00:00 | 136 | false |
# Complexity
- Time complexity:O(n*n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:
for i in range(len(nums2)):
nums2[i]=nums2[i]*k
cnt=0
for i in nums1:
for j in nums2:
if i%j==0:
cnt+=1
return cnt
``` | 1 | 0 | ['Array', 'Hash Table', 'Python3'] | 0 |
find-the-number-of-good-pairs-i | C++ || Precomputed divisors using hash map | c-precomputed-divisors-using-hash-map-by-3oti | ApproachThis optimized approach will be faster, especially for larger arrays with duplicate values in nums2.
Precomputation:
Precompute all possible divisors n | sajeeshpayolam | NORMAL | 2024-12-23T16:32:42.776793+00:00 | 2024-12-23T16:32:42.776793+00:00 | 160 | false | # Approach
This optimized approach will be faster, especially for larger arrays with duplicate values in `nums2`.
1. **Precomputation:**
Precompute all possible divisors `nums2[j] * k` and their frequencies.
2. **Efficient Divisibility Check:**
For each element in `nums1`, iterate through the precomputed divisors, checking divisibility and summing up counts.
# Complexity
- Time complexity:
`O(m + n x d)` where `d` is the unique `nums2[j] * k` values.
This is generally better than `O(mxn)`, especially if there are duplicate values in nums2.
- Space complexity:
`O(d)`, for storing the hashmap of divisors.
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k)
{
unordered_map<int,int> divisor;
for( const auto& n : nums2)
{
divisor[n*k]++;
}
int goodPair = 0;
for( int n : nums1)
{
for( const auto& [d ,c] : divisor )
{
if( n % d == 0)
goodPair += c;
}
}
return goodPair;
}
};
``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | C++ Solution 💻| Easy 😊| Explained🤔 | 100% Faster 🚀 | c-solution-easy-explained-100-faster-by-nnjic | Find the Number of Good Pairs\n\n\n## Intuition\nTo determine the number of "good pairs," the solution compares all possible pairs (i, j) of the arrays nums1 an | amols_15 | NORMAL | 2024-11-20T15:10:57.814731+00:00 | 2024-11-20T15:10:57.814793+00:00 | 78 | false | # Find the Number of Good Pairs\n\n\n## Intuition\nTo determine the number of "good pairs," the solution compares all possible pairs `(i, j)` of the arrays `nums1` and `nums2` and checks if the condition `nums1[i] % (nums2[j] * k) == 0` is satisfied.\n\n## Approach\n- Iterate through each element in `nums1` and `nums2` using nested loops.\n- For each pair `(i, j)`, check if the given condition holds.\n- Increment the count for each valid pair.\n- Return the count as the result.\n\n## Complexity\n- **Time complexity:** O(n * m) , where \\( n \\) and \\( m \\) are the sizes of `nums1` and `nums2`, respectively.\n- **Space complexity:** O(1) , as no extra space is used.\n\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count{0};\n for(int i=0;i<nums1.size();i++){\n for(int j=0;j<nums2.size();j++){\n if(nums1[i]%(nums2[j]*k)==0)\n count++;\n }\n }\n return count;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Simple Python Solution Beats 91.59% | simple-python-solution-beats-9159-by-eth-1k1j | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | ethan17225 | NORMAL | 2024-11-04T19:25:37.669085+00:00 | 2024-11-04T19:25:37.669128+00:00 | 18 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(mxn)\n- Space complexity:\nO(1)\n# Code\n```python3 []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n\n for num1 in nums1:\n for num2 in nums2:\n if num1 % (num2*k) == 0:\n count += 1\n\n return count\n``` | 1 | 0 | ['Python3'] | 0 |
find-the-number-of-good-pairs-i | Easy JS solution | easy-js-solution-by-joelll-533n | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Joelll | NORMAL | 2024-09-27T04:21:45.541985+00:00 | 2024-09-27T04:21:45.542009+00:00 | 36 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```javascript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @param {number} k\n * @return {number}\n */\nvar numberOfPairs = function(nums1, nums2, k) {\n let count = 0\n for(let i=0;i<nums1.length;i++){\n for(let j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0){\n count++\n }\n }\n }\n return count\n};\n``` | 1 | 0 | ['JavaScript'] | 0 |
find-the-number-of-good-pairs-i | ez soln. for beginners | beats 100% |please upvote | ez-soln-for-beginners-beats-100-please-u-m4xx | \n\n# Code\njava []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int cnt=0;\n for(int i=0;i<nums1.length;i | Lil_kidZ | NORMAL | 2024-09-26T17:23:51.248249+00:00 | 2024-09-26T17:23:51.248293+00:00 | 73 | false | \n\n# Code\n```java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int cnt=0;\n for(int i=0;i<nums1.length;i++){\n for(int j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0)cnt++;\n }\n }\n return cnt;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | EASY SOLUTION|| JAVA|| | easy-solution-java-by-robinrajput2869-pbem | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | robinrajput2869 | NORMAL | 2024-09-15T06:19:57.343993+00:00 | 2024-09-15T06:19:57.344022+00:00 | 17 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int good =0;\n for(int i =0; i<nums1.length; i++){\n for(int j =0; j<nums2.length; j++){\n if(nums1[i] % (nums2[j]*k) == 0){\n good++;\n }\n }\n }\n return good;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | Neat and Clean Solution in Java 🪄🪄 | neat-and-clean-solution-in-java-by-jasne-1qc0 | \uD83D\uDCBB Code\njava []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int n = nums1.length, m = nums2.length;\n | jasneet_aroraaa | NORMAL | 2024-08-21T16:03:38.866026+00:00 | 2024-08-21T16:03:38.866053+00:00 | 5 | false | # \uD83D\uDCBB Code\n```java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int n = nums1.length, m = nums2.length;\n int cnt = 0;\n\n for (int i = 0; i < n; i++) {\n for (int j = 0; j < m; j++) {\n if (nums1[i] % (nums2[j] * k) == 0) {\n cnt++;\n }\n }\n }\n\n return cnt;\n }\n}\n``` | 1 | 0 | ['Array', 'Java'] | 0 |
find-the-number-of-good-pairs-i | Python3 || Easy Solution😃😃 | python3-easy-solution-by-hanishb81-2rl2 | Code\n\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n for i in range(0, len(nums1 | hanishb81 | NORMAL | 2024-08-09T17:05:50.441172+00:00 | 2024-08-09T17:05:50.441199+00:00 | 95 | false | # Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n for i in range(0, len(nums1)):\n for j in range(0, len(nums2)):\n if nums1[i] % (nums2[j]*k) == 0:\n count += 1\n return count\n``` | 1 | 0 | ['Python3'] | 1 |
find-the-number-of-good-pairs-i | C# Simple and Easy to understand | c-simple-and-easy-to-understand-by-rhaze-qhml | \npublic class Solution {\n public int NumberOfPairs(int[] nums1, int[] nums2, int k) {\n int ans=0;\n for(int i=0;i<nums1.Length;i++){\n | rhazem13 | NORMAL | 2024-08-08T10:56:32.846491+00:00 | 2024-08-08T10:56:32.846510+00:00 | 40 | false | ```\npublic class Solution {\n public int NumberOfPairs(int[] nums1, int[] nums2, int k) {\n int ans=0;\n for(int i=0;i<nums1.Length;i++){\n for(int j=0;j<nums2.Length;j++){\n if(nums1[i]%(nums2[j]*k)==0)\n ans++;\n }\n }\n return ans;\n }\n}\n``` | 1 | 0 | [] | 0 |
find-the-number-of-good-pairs-i | Simple Approach || C++ Solution | simple-approach-c-solution-by-jeetgajera-h2jh | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Jeetgajera | NORMAL | 2024-08-08T03:31:29.520350+00:00 | 2024-08-08T03:31:29.520370+00:00 | 1 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count=0;\n for(int i=0;i<nums1.size();i++){\n for(int j=0;j<nums2.size();j++){\n if(nums1[i]%(nums2[j]*k)==0) count++;\n }\n }\n return count;\n }\n};\n``` | 1 | 0 | ['Array', 'C++'] | 0 |
find-the-number-of-good-pairs-i | ✔️✔️✔️very easy - brute force - PYTHON || C++ || JAVA⚡⚡⚡beats 99%⚡⚡⚡ | very-easy-brute-force-python-c-javabeats-s1lr | Code\nPython []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n for i in range(len | anish_sule | NORMAL | 2024-07-29T07:23:43.062782+00:00 | 2024-07-29T07:23:43.062812+00:00 | 434 | false | # Code\n```Python []\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n count = 0\n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if nums1[i] % (nums2[j] * k) == 0:\n count += 1\n return count\n```\n```C++ []\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count = 0;\n for (int i = 0; i < nums1.size(); i++){\n for (int j = 0; j < nums2.size(); j++){\n if (nums1[i] % (nums2[j] * k) == 0){\n count++;\n }\n }\n }\n return count;\n }\n};\n```\n```Java []\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count = 0;\n for (int i = 0; i < nums1.length; i++){\n for (int j = 0; j < nums2.length; j++){\n if (nums1[i] % (nums2[j] * k) == 0){\n count++;\n }\n }\n }\n return count;\n }\n}\n``` | 1 | 0 | ['Array', 'C++', 'Java', 'Python3'] | 0 |
find-the-number-of-good-pairs-i | Simple Approach! | simple-approach-by-ankit1317-veol | Code\n\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count=0;\n for(int i=0;i<nums1.length;i++){\n | Ankit1317 | NORMAL | 2024-07-13T07:50:58.715491+00:00 | 2024-07-13T07:50:58.715533+00:00 | 1,124 | false | # Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count=0;\n for(int i=0;i<nums1.length;i++){\n for(int j=0;j<nums2.length;j++){\n if(nums1[i]%(nums2[j]*k)==0)\n count++;\n }\n }\n return count;\n }\n}\n``` | 1 | 0 | ['Array', 'Hash Table', 'C++', 'Java'] | 1 |
find-the-number-of-good-pairs-i | Runtime 99.94% | runtime-9994-by-bekzodmirzayev-5l3a | Code\n\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int res=0; \n for(int i=0; i<nums1.length; i++){\n | bekzodmirzayev | NORMAL | 2024-06-15T13:32:44.977544+00:00 | 2024-06-15T13:32:44.977600+00:00 | 8 | false | # Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int res=0; \n for(int i=0; i<nums1.length; i++){\n for(int j=0; j<nums2.length; j++){\n if(nums1[i]%(nums2[j]*k)==0) res++;\n }\n }\n return res;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | easy swift solution | easy-swift-solution-by-sayedadinan-742a | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | sayedadinan | NORMAL | 2024-05-30T04:47:14.349400+00:00 | 2024-05-30T04:47:14.349429+00:00 | 142 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n func numberOfPairs(_ nums1: [Int], _ nums2: [Int], _ k: Int) -> Int {\n var count=0\n for i in 0..<nums1.count{\n for j in 0..<nums2.count{\n if nums1[i]%(nums2[j]*k)==0{\n count+=1\n }\n }\n }\n return count\n }\n}\n``` | 1 | 0 | ['Swift'] | 0 |
find-the-number-of-good-pairs-i | Simple Approach! | simple-approach-by-jasijasu959-xwoy | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | jasijasu959 | NORMAL | 2024-05-29T04:40:23.378570+00:00 | 2024-05-29T04:40:23.378592+00:00 | 102 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n int numberOfPairs(List<int> nums1, List<int> nums2, int k) {\n\n int count = 0;\n\n for(var num1 in nums1){\n for(var num2 in nums2){\n if(num1 % (num2 * k) == 0){\n count ++;\n }\n }\n }\n return count ;\n }\n}\n``` | 1 | 0 | ['Dart'] | 0 |
find-the-number-of-good-pairs-i | Time complexity 0(n) solution | time-complexity-0n-solution-by-ashita_ja-b6yn | Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem here is to reduce the time complexity from o(n2) to o(n) so taking this as | Ashita_java | NORMAL | 2024-05-28T09:28:13.445693+00:00 | 2024-05-28T09:28:13.445723+00:00 | 13 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem here is to reduce the time complexity from o(n2) to o(n) so taking this as the primary intution try to code with a single for loop\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nhere we have taken one pointer or we can say counter with respect to 2nd array and the basic operation to find the modulus is same .But the 2nd nums2 array is controlled by an if statement and any time the nums1 reaches the max index we set it to zero provided c1 the counter for the 2nd array is not out of length.we innitialize i=-1 because as the control reaches the top of the loop it starts with ++i.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n0(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int c1=0;int c2=0;\n for(int i=0;i<nums1.length;i++){\n if(nums1[i] % (nums2[c1]*k)==0){\n c2++;\n }\n if(i==nums1.length-1 && c1<nums2.length-1){\n i=-1;\n c1++;\n }\n }\n return c2;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | C# Two Pointers approach 65ms && 45.2MB | c-two-pointers-approach-65ms-452mb-by-de-yu45 | Sorting the arrays and moving the pointers around according to the combination of numbers\n\n# Code\n\npublic class Solution {\n public int NumberOfPairs(int | DeyanDiulgerov | NORMAL | 2024-05-27T10:00:38.555136+00:00 | 2024-05-27T10:00:38.555155+00:00 | 75 | false | Sorting the arrays and moving the pointers around according to the combination of numbers\n\n# Code\n```\npublic class Solution {\n public int NumberOfPairs(int[] nums1, int[] nums2, int k) {\n Array.Sort(nums1);\n Array.Sort(nums2);\n int n = nums1.Length, m = nums2.Length;\n int resCount = 0;\n int left = 0, right = 0;\n while (left < n)\n {\n if(right >= m || nums1[left] < nums2[right] * k)\n {\n left++;\n right = 0;\n }\n else if (nums1[left] % (nums2[right] * k) == 0)\n {\n resCount++;\n right++;\n }\n else if (nums1[left] > nums2[right] * k)\n right++;\n }\n return resCount;\n }\n}\n``` | 1 | 0 | ['C#'] | 0 |
find-the-number-of-good-pairs-i | Simple C++ | simple-c-by-deva766825_gupta-ig6m | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | deva766825_gupta | NORMAL | 2024-05-27T02:26:41.629948+00:00 | 2024-05-27T02:26:41.629965+00:00 | 1 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int n=nums1.size();\n int m=nums2.size();\n int count=0;\n\n for(int i=0;i<n;i++)\n {\n for(int j=0;j<m;j++)\n {\n int x =nums2[j]*k;\n if(nums1[i]%x==0)\n {\n count ++;\n\n }\n }\n\n }\n return count;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Simple Python3 with Speed Improvement | simple-python3-with-speed-improvement-by-mynn | Intuition\n Describe your first thoughts on how to solve this problem. \nIf the element X in nums1 is not devisable by k then we can avoid extra calculation for | maminnas | NORMAL | 2024-05-27T01:12:50.327521+00:00 | 2024-05-27T01:12:50.327549+00:00 | 100 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf the element X in nums1 is not devisable by k then we can avoid extra calculation for each member of nums2 for that element X.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nCheck divisibility by k and continue before getting into second loop.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(m*n) with m and n being length of nums1 and nums2 respectively, For worst case scenario that all elements are devisable by k and elements of nums2.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n ans = 0\n for m in nums1:\n if m%k!=0:\n continue\n for n in nums2:\n if m % (n*k)==0:\n ans+=1\n return ans\n``` | 1 | 0 | ['Python3'] | 0 |
find-the-number-of-good-pairs-i | Optimized | optimized-by-vipultawde000-ac6d | Intuition\n Describe your first thoughts on how to solve this problem. \nObvious solution of Brute Force is available.\n\nRemember - \nConstraints:\n\n1 <= n, m | vipultawde000 | NORMAL | 2024-05-26T16:14:59.407006+00:00 | 2024-05-26T16:14:59.407023+00:00 | 19 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nObvious solution of Brute Force is available.\n\nRemember - \nConstraints:\n\n1 <= n, m <= 50\n1 <= nums1[i], nums2[j] <= 50\n1 <= k <= 50\n\nBruteforce max iters = 50 x 50 = 2500\n\n# Approach\nAdding an extra check that will be executed at max m = 50 times\nEvery time the check fails it skips inner loop which itself can be a max of n = 50 iterations.\nLets say this check fails for 5% cases we should have upto \n\n+ 50 iter - 0.05 x 50 x 50 iters = 75 iter advantage on the worst case \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n) x O(m)$$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n ans=0\n for i in nums1:\n if i%k==0:\n for j in nums2: \n if i%(k*j)==0:\n ans+=1\n return ans\n \n``` | 1 | 0 | ['Python3'] | 0 |
find-the-number-of-good-pairs-i | CPP Solution Easy and Effective | cpp-solution-easy-and-effective-by-mriga-ck19 | Intuition\nSimple Brute force Used\n# Approach\n Describe your approach to solving the problem. \n1. Initialize a Counter : Start by initializing a counter cnt | Mrigaank | NORMAL | 2024-05-26T13:12:50.614093+00:00 | 2024-05-26T13:12:50.614112+00:00 | 41 | false | # Intuition\nSimple Brute force Used\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Initialize a Counter : Start by initializing a counter cnt to zero. This will keep track of the number of valid pairs.\n2. Nested Loop through Both Arrays : Use a nested loop to iterate through every possible pair of elements between nums1 and nums2.\nThe outer loop iterates over the elements of nums1 using an index i.\nThe inner loop iterates over the elements of nums2 using an index j.\n\n3. Check the Condition :\nFor each pair (nums1[i], nums2[j]), check if nums1[i] is divisible by nums2[j] * k.\nSpecifically, check if nums1[i] % (nums2[j] * k) == 0.\n\n4. Update the Counter : If the condition is satisfied, increment the counter cnt by 1. \n\n5. Return the Result : After completing the iterations, return the counter cnt as the result, which represents the number of valid pairs.\n\n\n# Complexity\n- Time complexity: O(n*m)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int cnt = 0;\n for(int i =0 ; i < nums1.size() ; i++){\n for(int j = 0 ; j < nums2.size() ; j++){\n if(nums1[i]%(nums2[j]*k)==0){\n cnt++;\n }\n }\n }\n return cnt; \n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Easy Beginner Solution || No HashMap | easy-beginner-solution-no-hashmap-by-shi-whnt | \n# Approach\n Describe your approach to solving the problem. \nUse 2 for loops to check every possible combination.\n\n# Complexity\n- Time complexity: 0(n^2)\ | ShivamKhator | NORMAL | 2024-05-26T12:55:47.382577+00:00 | 2024-05-26T12:55:47.382607+00:00 | 76 | false | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nUse 2 `for` loops to check every possible combination.\n\n# Complexity\n- Time complexity: 0(n^2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: 0(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int count = 0; // Initialize a counter to keep track of valid pairs\n\n // Iterate through each element in nums1\n for (int n1 : nums1)\n // For each element in nums2\n for (int n2 : nums2) \n // Check if n1 is divisible by (n2 * k)\n if (n1 % (n2 * k) == 0)\n count++;\n return count; // Return the total count of valid pairs\n }\n};\n\n```\n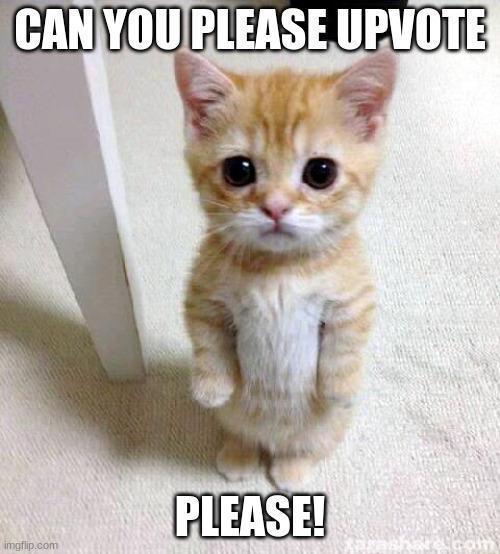\n | 1 | 0 | ['Array', 'C++'] | 2 |
find-the-number-of-good-pairs-i | Simple and very easy to understand solution | simple-and-very-easy-to-understand-solut-xev6 | Intuition\ncheck for every nnumber in nums1 how many nums2[i] divide it.\n\n# Approach\nsimple brute force\n\n# Complexity\n- Time complexity:\n O(n^2) \n\n- Sp | Saksham_Gulati | NORMAL | 2024-05-26T12:27:24.864838+00:00 | 2024-05-26T12:27:24.864866+00:00 | 280 | false | # Intuition\ncheck for every nnumber in nums1 how many nums2[i] divide it.\n\n# Approach\nsimple brute force\n\n# Complexity\n- Time complexity:\n $$O(n^2)$$ \n\n- Space complexity:\n$$O(1)$$ \n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int n=nums1.size();\n int m=nums2.size();\n int c=0;\n for(int i=0;i<m;i++)nums2[i]*=k;\n for(int i=0;i<n;i++)\n {\n for(int j=0;j<m;j++)\n {\n if(nums1[i]%nums2[j]==0)c++;\n }\n }\n return c;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Computing divisors (maths solution) | computing-divisors-maths-solution-by-alm-6bm0 | Intuition\n Describe your first thoughts on how to solve this problem. \nLet\'s find all divisors of an integer!\n\n# Approach\n Describe your approach to solvi | almostmonday | NORMAL | 2024-05-26T11:42:05.151141+00:00 | 2024-05-26T12:23:06.159203+00:00 | 273 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nLet\'s find all divisors of an integer!\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIf `nums1[i]` is divisible by `k`, you need to find all divisors of `nums1[i]` in `nums2`.\n\n# Complexity\n- Time complexity: $$(1 + x) \\cdot O(n) + O(m)$$, where $$x$$ is the sum of the unique integers in the array $$nums1$$ or `x = sum(set(nums1))`.\n- * It could be optimised if you\'re looking for divisors up to the square root of an integer.\n- * In the worst case, for $$nums1 = [1, 2, ..., 49, 50]$$, it\'s $$\\frac{50 \\cdot 51}{2} + 100=1375$$ operations.\n- * Due to constrains, $$1 <= n, m <= 50$$, it\'s around $$O(n \\cdot m) = 50 \\cdot 50 = 2500$$ operations.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n) + O(m)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n---\n* You could find some other extraordinary solutions in my [profile](https://leetcode.com/almostmonday/) on the Solutions tab (I don\'t post obvious or not interesting solutions at all.)\n* If this was helpful, please upvote so that others can see this solution too.\n---\n\n\n# Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n d, res = Counter(nums2), 0\n for x, cnt in Counter(nums1).items():\n if x % k == 0:\n x //= k\n for m in range(1, x + 1):\n if not x % m and m in d: res += d[m] * cnt\n\n return res\n``` | 1 | 0 | ['Math', 'Number Theory', 'Python', 'Python3'] | 0 |
find-the-number-of-good-pairs-i | EASY SOLUTION PYTHON | easy-solution-python-by-sakthivasan18-hhod | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Sakthivasan18 | NORMAL | 2024-05-26T07:25:40.560494+00:00 | 2024-05-26T07:25:40.560519+00:00 | 13 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n c=0\n for i in range(len(nums1)):\n for j in range(len(nums2)):\n if (nums1[i]%(nums2[j]*k)==0):\n c+=1\n return c\n \n``` | 1 | 0 | ['Python3'] | 0 |
find-the-number-of-good-pairs-i | Simplest Brute Force Solution Java || Count Number of Pairs | simplest-brute-force-solution-java-count-vsyx | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | tusharyadav11 | NORMAL | 2024-05-26T06:53:20.015948+00:00 | 2024-05-26T06:53:20.015966+00:00 | 218 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int goodPairs = 0;\n for (int i = 0; i < nums1.length; i++) {\n for (int j = 0; j < nums2.length; j++) {\n if (nums1[i] % (nums2[j] * k) == 0) {\n goodPairs++;\n }\n }\n }\n return goodPairs; \n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | ✔️✔️100% Faster JavaScript Solution || Optimized Approach ✌️✌️ | 100-faster-javascript-solution-optimized-icr9 | Intuition\n Describe your first thoughts on how to solve this problem. \nFor each number num in nums1 we just need to find how many factor of num/k is present i | Boolean_Autocrats | NORMAL | 2024-05-26T05:55:57.777010+00:00 | 2024-05-26T05:55:57.777027+00:00 | 232 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFor each number num in nums1 we just need to find how many factor of num/k is present in nums2 and sum of all these value will be our answer.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. First I will store frequency of each number of nums2 in map.\n2. Result = 0 \n2. Iterate over all element of nums1 and \n - If num % k == 0\n - find count of all divisors of num/k in nums1 and will sum all of these values.\n - Result += mp.get(divisor);\n3. Result will be the solution\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n1. $$O(m)$$ to store freq of each num of nums2\n2. $$O(n*sqrt(max(nums1)))$$ to find count of all such pairs \n- Space complexity:\n1. $$O(m)$$ to store freq of each num of nums2\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @param {number} k\n * @return {number}\n */\nvar numberOfPairs = function(nums1, nums2, k) {\n let ans = 0;\n let n = nums1.length , m = nums2.length;\n const mp = new Map();\n for(var num of nums2)\n {\n mp.set(num,mp.has(num) ? mp.get(num) + 1 : 1);\n }\n for(var v of nums1)\n {\n if(v%k ==0)\n {\n const val = v/k;\n for(let i = 1 ; i <= Math.sqrt(val) ; i++)\n {\n if(val % i == 0)\n {\n ans += mp.has(i) ? mp.get(i) : 0;\n if(val / i != i)\n {\n ans += mp.has(val / i) ? mp.get(val / i) : 0; \n }\n }\n }\n }\n }\n return ans;\n};\n``` | 1 | 0 | ['JavaScript'] | 0 |
find-the-number-of-good-pairs-i | ✅ Easy C++ Solution | easy-c-solution-by-moheat-wfqd | Code\n\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int n = nums1.size();\n int m = nums2. | moheat | NORMAL | 2024-05-26T05:36:56.381770+00:00 | 2024-05-26T05:36:56.381788+00:00 | 191 | false | # Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int n = nums1.size();\n int m = nums2.size();\n int ans = 0;\n\n for(int i=0;i<n;i++)\n {\n int temp = nums1[i];\n for(int j=0;j<m;j++)\n {\n if(temp % (nums2[j]*k) == 0)\n {\n ans++;\n }\n }\n }\n\n return ans;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | [ Python ] ✅✅ Simple Python Solution | 100 % Faster🥳✌👍 | python-simple-python-solution-100-faster-hsd7 | If You like the Solution, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Runtime: 54 ms, faster than 100.00% of Python3 online submissions for Find the Number of Go | ashok_kumar_meghvanshi | NORMAL | 2024-05-26T04:57:53.057388+00:00 | 2024-05-26T04:57:53.057412+00:00 | 232 | false | # If You like the Solution, Please UpVote! \uD83D\uDD3C\uD83D\uDE4F\n# Runtime: 54 ms, faster than 100.00% of Python3 online submissions for Find the Number of Good Pairs I.\n# Memory Usage: 16.6 MB, less than 100.00% of Python3 online submissions for Find the Number of Good Pairs I.\n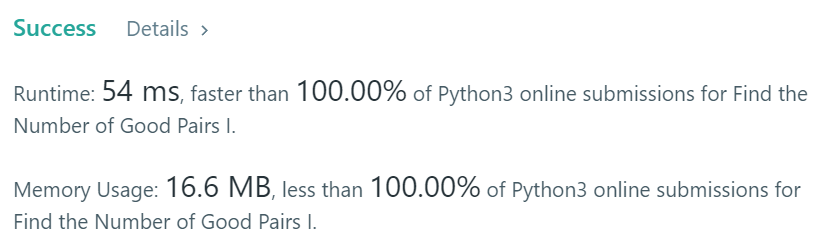\n\n\tclass Solution:\n\t\tdef numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:\n\n\t\t\tresult = 0\n\n\t\t\tfor num1 in nums1:\n\n\t\t\t\tfor num2 in nums2:\n\n\t\t\t\t\tif num1 % (num2 * k) == 0:\n\n\t\t\t\t\t\tresult = result + 1\n\n\t\t\treturn result\n\t\t\t\n\tTime Complexity : O(N ^ 2)\n\tSpace Complexity : O(1)\n# Thank You \uD83E\uDD73\u270C\uD83D\uDC4D | 1 | 0 | ['Python', 'Python3'] | 1 |
find-the-number-of-good-pairs-i | easy solution | beats 100% | easy-solution-beats-100-by-leet1101-3rwp | Intuition\nTo determine the number of good pairs (i, j) where nums1[i] is divisible by nums2[j]*k, we can use a straightforward nested loop approach. For each e | leet1101 | NORMAL | 2024-05-26T04:11:44.573127+00:00 | 2024-05-26T04:11:44.573145+00:00 | 219 | false | # Intuition\nTo determine the number of good pairs `(i, j)` where `nums1[i]` is divisible by `nums2[j]*k`, we can use a straightforward nested loop approach. For each element in `nums2`, calculate `nums2[j]*k` and then check all elements in `nums1` to see if they are divisible by this value.\n\n# Approach\n1. **Initialize Count**: Start with a counter set to 0 to keep track of the number of good pairs.\n2. **Nested Loop**: Use a nested loop where the outer loop iterates over each element in `nums2` and the inner loop iterates over each element in `nums1`.\n3. **Calculate and Check Divisibility**: For each combination of elements from `nums1` and `nums2`, compute the value `nums2[j]*k`. Check if `nums1[i]` is divisible by this computed value. If true, increment the counter.\n4. **Return Result**: After both loops complete, return the counter value.\n\n# Complexity\n- Time complexity: \n - The nested loop results in $$O(n*m)$$ operations, where n is the length of `nums1` and m is the length of `nums2`.\n\n- Space complexity: \n - $$O(1)$$, as we are using only a few extra variables that do not depend on the input size.\n\n# Code\n```cpp\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int ans = 0;\n for (int j = 0; j < nums2.size(); ++j) {\n long long num = nums2[j] * k;\n for (int i = 0; i < nums1.size(); ++i) {\n if (nums1[i] % num == 0) {\n ++ans;\n }\n }\n }\n return ans;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Java Simple Nive Solution | 26/5/2024 | java-simple-nive-solution-2652024-by-shr-63tf | Complexity\n- Time complexity:O(n^2)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity:O(1)\n Add your space complexity here, e.g. O(n) \n\n# Ot | Shree_Govind_Jee | NORMAL | 2024-05-26T04:11:35.677519+00:00 | 2024-05-26T04:11:35.677542+00:00 | 88 | false | # Complexity\n- Time complexity:$$O(n^2)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Other Version (SIMILAR QUESTION)\nhttps://leetcode.com/problems/find-the-number-of-good-pairs-ii/solutions/5208996/java-clean-solution-weekly-contest\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int count = 0;\n for (int i = 0; i < nums1.length; i++) {\n for (int j = 0; j < nums2.length; j++) {\n if (nums1[i] % (nums2[j] * k) == 0) {\n count++;\n }\n }\n }\n return count;\n }\n}\n```\n | 1 | 0 | ['Array', 'Math', 'Matrix', 'Java'] | 2 |
find-the-number-of-good-pairs-i | JAVA SOLUTION || 1MS SOLUTION || 100 % FASTER | java-solution-1ms-solution-100-faster-by-n8c8 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | viper_01 | NORMAL | 2024-05-26T04:08:29.057570+00:00 | 2024-05-26T04:08:29.057641+00:00 | 279 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N\xB2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public int numberOfPairs(int[] nums1, int[] nums2, int k) {\n int ans = 0;\n for(int i = 0; i < nums1.length; i++) {\n for(int j = 0; j < nums2.length; j++) {\n if(nums1[i] % (nums2[j] * k) == 0) ans++;\n }\n }\n \n return ans;\n }\n}\n``` | 1 | 0 | ['Java'] | 2 |
find-the-number-of-good-pairs-i | ✨⚡️✨ Fastest Solution ✨⚡️✨ | fastest-solution-by-crossmind-v920 | \n\n# Code\n\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int ans = 0;\n \n for (in | CrossMind | NORMAL | 2024-05-26T04:07:30.852706+00:00 | 2024-05-26T04:07:30.852735+00:00 | 197 | false | \n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n int ans = 0;\n \n for (int i = 0; i < nums1.size(); i++) {\n for (int j = 0; j < nums2.size(); j++) {\n if (nums1[i] % (nums2[j] * k) == 0) {\n ans++;\n }\n }\n }\n \n return ans;\n }\n};\n``` | 1 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | C++ || MAX LIMIT EASY BEATS 100% | c-max-limit-easy-beats-100-by-abhishek64-mds4 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Abhishek6487209 | NORMAL | 2024-05-26T04:05:54.537764+00:00 | 2024-05-26T04:06:11.912176+00:00 | 185 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {\n map<int,long long> a, b; \n for (int num : nums2) {\n b[num]++; \n } \n int mx=0;\n for (int num : nums1) {\n if(num%k!=0){ continue;}\n a[num]++;\n mx=max(mx,num);\n }\n vector<long long> kp;\n \n long long gp = 0;\n\n for(auto it:b){\n long long h=k*it.first;\n long long jp=0; \n int op=1;\n while(op*h<=mx){\n jp+=a[op*h];\n op++;\n }\n gp+=jp*it.second;\n }\n\n return gp;\n }\n};\n``` | 1 | 0 | ['C++'] | 2 |
find-the-number-of-good-pairs-i | Simple Python Solution | simple-python-solution-by-ascending-vo9j | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | ascending | NORMAL | 2025-04-05T15:47:29.556762+00:00 | 2025-04-05T15:47:29.556762+00:00 | 1 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```python3 []
class Solution:
def numberOfPairs(self, nums1: List[int], nums2: List[int], k: int) -> int:
nums2 = [k * num for num in nums2]
res = 0
for num1 in nums1:
for num2 in nums2:
if num1 % num2 == 0:
res += 1
return res
``` | 0 | 0 | ['Python3'] | 0 |
find-the-number-of-good-pairs-i | Simple Swift Solution | simple-swift-solution-by-felisviridis-7vcz | Code | Felisviridis | NORMAL | 2025-04-03T08:53:10.423394+00:00 | 2025-04-03T08:53:10.423394+00:00 | 3 | false | 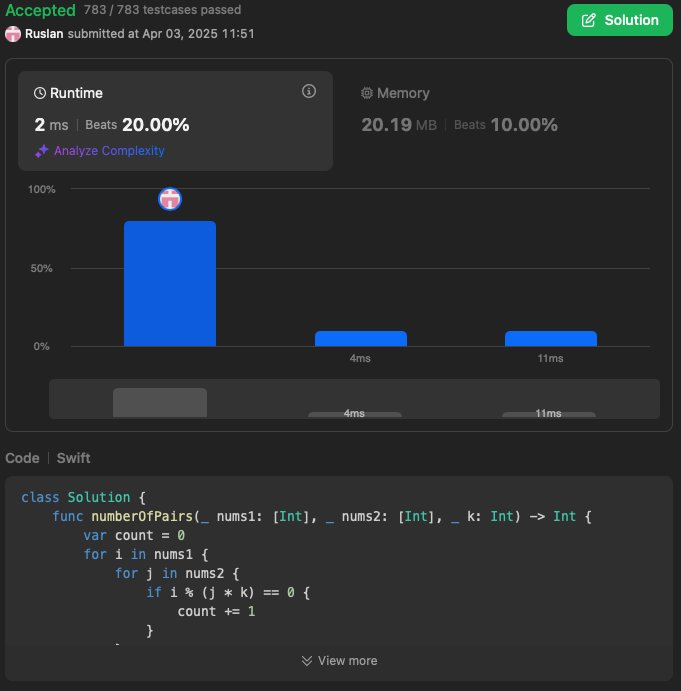
# Code
```swift []
class Solution {
func numberOfPairs(_ nums1: [Int], _ nums2: [Int], _ k: Int) -> Int {
var count = 0
for i in nums1 {
for j in nums2 {
if i % (j * k) == 0 {
count += 1
}
}
}
return count
}
}
``` | 0 | 0 | ['Array', 'Swift'] | 0 |
find-the-number-of-good-pairs-i | JAVA Solution | java-solution-by-yayjyvijaj-hevs | IntuitionApproachComplexity
Time complexity:
o(n2)
Space complexity:
o(1)Code | YaYJYVIJAj | NORMAL | 2025-04-02T10:48:43.998443+00:00 | 2025-04-02T10:48:43.998443+00:00 | 2 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
o(n2)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
o(1)
# Code
```java []
class Solution {
public int numberOfPairs(int[] nums1, int[] nums2, int k) {
int count=0;
for(int i=0;i<nums1.length;i++)
{
for(int j=0;j<nums2.length;j++)
{
if(nums1[i]%(nums2[j]*k)==0)
{
count++;
}
}
}
return count;
}
}
``` | 0 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | easy and best solution in c++ | easy-and-best-solution-in-c-by-ashish754-arox | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Ashish754937 | NORMAL | 2025-04-01T04:59:12.673871+00:00 | 2025-04-01T04:59:12.673871+00:00 | 3 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {
int ans=0;
for(int i=0;i<nums1.size();i++)
{
for(int j=0;j<nums2.size();j++)
{
if(nums1[i]%(k*nums2[j])==0) ans++;
}
}
return ans;
}
};
``` | 0 | 0 | ['C++'] | 0 |
find-the-number-of-good-pairs-i | Simple || Java ||easy to understand || Beats 100%✅ | simple-java-easy-to-understand-beats-100-ldk6 | Proof👇🏻https://leetcode.com/problems/find-the-number-of-good-pairs-i/submissions/1590830700Complexity
Time complexity:O(n)
Space complexity:O(1)
Code | SamirSyntax | NORMAL | 2025-03-30T07:05:12.374032+00:00 | 2025-03-30T07:05:12.374032+00:00 | 3 | false | # Proof👇🏻
https://leetcode.com/problems/find-the-number-of-good-pairs-i/submissions/1590830700
# Complexity
- Time complexity:O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public int numberOfPairs(int[] nums1, int[] nums2, int k) {
int gp =0;
for(int i=0; i<nums1.length; i++){
for(int j=0; j<nums2.length; j++){
if(nums1[i] % (nums2[j]*k)==0){
gp++;
}
}
}
return gp;
}
}
``` | 0 | 0 | ['Java'] | 0 |
find-the-number-of-good-pairs-i | basic and best code | basic-and-best-code-by-nikhilgupta932-3aoe | Code | Nikhilgupta932 | NORMAL | 2025-03-29T21:59:36.442796+00:00 | 2025-03-29T21:59:36.442796+00:00 | 1 | false |
# Code
```cpp []
class Solution {
public:
int numberOfPairs(vector<int>& nums1, vector<int>& nums2, int k) {
int n=nums1.size();
int m=nums2.size();
int count=0;
for(int i=0;i<n;i++){
for(int j=0;j<m;j++){
if(nums1[i]%(nums2[j]*k)==0)
count++;
}
}
return count;
}
};
``` | 0 | 0 | ['C++'] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.